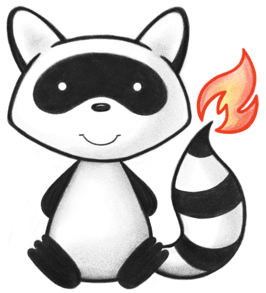
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A walkthrough of a workflow showing the interaction between systems and the instances shared, possibly including the evolution of instances over time. 052 */ 053@ResourceDef(name="ExampleScenario", profile="http://hl7.org/fhir/StructureDefinition/ExampleScenario") 054public class ExampleScenario extends CanonicalResource { 055 056 @Block() 057 public static class ExampleScenarioActorComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * A unique string within the scenario that is used to reference the actor. 060 */ 061 @Child(name = "key", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="ID or acronym of the actor", formalDefinition="A unique string within the scenario that is used to reference the actor." ) 063 protected StringType key; 064 065 /** 066 * The category of actor - person or system. 067 */ 068 @Child(name = "type", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 069 @Description(shortDefinition="person | system", formalDefinition="The category of actor - person or system." ) 070 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/examplescenario-actor-type") 071 protected Enumeration<ExampleScenarioActorType> type; 072 073 /** 074 * The human-readable name for the actor used when rendering the scenario. 075 */ 076 @Child(name = "title", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=false) 077 @Description(shortDefinition="Label for actor when rendering", formalDefinition="The human-readable name for the actor used when rendering the scenario." ) 078 protected StringType title; 079 080 /** 081 * An explanation of who/what the actor is and its role in the scenario. 082 */ 083 @Child(name = "description", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=false) 084 @Description(shortDefinition="Details about actor", formalDefinition="An explanation of who/what the actor is and its role in the scenario." ) 085 protected MarkdownType description; 086 087 private static final long serialVersionUID = 267911906L; 088 089 /** 090 * Constructor 091 */ 092 public ExampleScenarioActorComponent() { 093 super(); 094 } 095 096 /** 097 * Constructor 098 */ 099 public ExampleScenarioActorComponent(String key, ExampleScenarioActorType type, String title) { 100 super(); 101 this.setKey(key); 102 this.setType(type); 103 this.setTitle(title); 104 } 105 106 /** 107 * @return {@link #key} (A unique string within the scenario that is used to reference the actor.). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 108 */ 109 public StringType getKeyElement() { 110 if (this.key == null) 111 if (Configuration.errorOnAutoCreate()) 112 throw new Error("Attempt to auto-create ExampleScenarioActorComponent.key"); 113 else if (Configuration.doAutoCreate()) 114 this.key = new StringType(); // bb 115 return this.key; 116 } 117 118 public boolean hasKeyElement() { 119 return this.key != null && !this.key.isEmpty(); 120 } 121 122 public boolean hasKey() { 123 return this.key != null && !this.key.isEmpty(); 124 } 125 126 /** 127 * @param value {@link #key} (A unique string within the scenario that is used to reference the actor.). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 128 */ 129 public ExampleScenarioActorComponent setKeyElement(StringType value) { 130 this.key = value; 131 return this; 132 } 133 134 /** 135 * @return A unique string within the scenario that is used to reference the actor. 136 */ 137 public String getKey() { 138 return this.key == null ? null : this.key.getValue(); 139 } 140 141 /** 142 * @param value A unique string within the scenario that is used to reference the actor. 143 */ 144 public ExampleScenarioActorComponent setKey(String value) { 145 if (this.key == null) 146 this.key = new StringType(); 147 this.key.setValue(value); 148 return this; 149 } 150 151 /** 152 * @return {@link #type} (The category of actor - person or system.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 153 */ 154 public Enumeration<ExampleScenarioActorType> getTypeElement() { 155 if (this.type == null) 156 if (Configuration.errorOnAutoCreate()) 157 throw new Error("Attempt to auto-create ExampleScenarioActorComponent.type"); 158 else if (Configuration.doAutoCreate()) 159 this.type = new Enumeration<ExampleScenarioActorType>(new ExampleScenarioActorTypeEnumFactory()); // bb 160 return this.type; 161 } 162 163 public boolean hasTypeElement() { 164 return this.type != null && !this.type.isEmpty(); 165 } 166 167 public boolean hasType() { 168 return this.type != null && !this.type.isEmpty(); 169 } 170 171 /** 172 * @param value {@link #type} (The category of actor - person or system.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 173 */ 174 public ExampleScenarioActorComponent setTypeElement(Enumeration<ExampleScenarioActorType> value) { 175 this.type = value; 176 return this; 177 } 178 179 /** 180 * @return The category of actor - person or system. 181 */ 182 public ExampleScenarioActorType getType() { 183 return this.type == null ? null : this.type.getValue(); 184 } 185 186 /** 187 * @param value The category of actor - person or system. 188 */ 189 public ExampleScenarioActorComponent setType(ExampleScenarioActorType value) { 190 if (this.type == null) 191 this.type = new Enumeration<ExampleScenarioActorType>(new ExampleScenarioActorTypeEnumFactory()); 192 this.type.setValue(value); 193 return this; 194 } 195 196 /** 197 * @return {@link #title} (The human-readable name for the actor used when rendering the scenario.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 198 */ 199 public StringType getTitleElement() { 200 if (this.title == null) 201 if (Configuration.errorOnAutoCreate()) 202 throw new Error("Attempt to auto-create ExampleScenarioActorComponent.title"); 203 else if (Configuration.doAutoCreate()) 204 this.title = new StringType(); // bb 205 return this.title; 206 } 207 208 public boolean hasTitleElement() { 209 return this.title != null && !this.title.isEmpty(); 210 } 211 212 public boolean hasTitle() { 213 return this.title != null && !this.title.isEmpty(); 214 } 215 216 /** 217 * @param value {@link #title} (The human-readable name for the actor used when rendering the scenario.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 218 */ 219 public ExampleScenarioActorComponent setTitleElement(StringType value) { 220 this.title = value; 221 return this; 222 } 223 224 /** 225 * @return The human-readable name for the actor used when rendering the scenario. 226 */ 227 public String getTitle() { 228 return this.title == null ? null : this.title.getValue(); 229 } 230 231 /** 232 * @param value The human-readable name for the actor used when rendering the scenario. 233 */ 234 public ExampleScenarioActorComponent setTitle(String value) { 235 if (this.title == null) 236 this.title = new StringType(); 237 this.title.setValue(value); 238 return this; 239 } 240 241 /** 242 * @return {@link #description} (An explanation of who/what the actor is and its role in the scenario.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 243 */ 244 public MarkdownType getDescriptionElement() { 245 if (this.description == null) 246 if (Configuration.errorOnAutoCreate()) 247 throw new Error("Attempt to auto-create ExampleScenarioActorComponent.description"); 248 else if (Configuration.doAutoCreate()) 249 this.description = new MarkdownType(); // bb 250 return this.description; 251 } 252 253 public boolean hasDescriptionElement() { 254 return this.description != null && !this.description.isEmpty(); 255 } 256 257 public boolean hasDescription() { 258 return this.description != null && !this.description.isEmpty(); 259 } 260 261 /** 262 * @param value {@link #description} (An explanation of who/what the actor is and its role in the scenario.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 263 */ 264 public ExampleScenarioActorComponent setDescriptionElement(MarkdownType value) { 265 this.description = value; 266 return this; 267 } 268 269 /** 270 * @return An explanation of who/what the actor is and its role in the scenario. 271 */ 272 public String getDescription() { 273 return this.description == null ? null : this.description.getValue(); 274 } 275 276 /** 277 * @param value An explanation of who/what the actor is and its role in the scenario. 278 */ 279 public ExampleScenarioActorComponent setDescription(String value) { 280 if (Utilities.noString(value)) 281 this.description = null; 282 else { 283 if (this.description == null) 284 this.description = new MarkdownType(); 285 this.description.setValue(value); 286 } 287 return this; 288 } 289 290 protected void listChildren(List<Property> children) { 291 super.listChildren(children); 292 children.add(new Property("key", "string", "A unique string within the scenario that is used to reference the actor.", 0, 1, key)); 293 children.add(new Property("type", "code", "The category of actor - person or system.", 0, 1, type)); 294 children.add(new Property("title", "string", "The human-readable name for the actor used when rendering the scenario.", 0, 1, title)); 295 children.add(new Property("description", "markdown", "An explanation of who/what the actor is and its role in the scenario.", 0, 1, description)); 296 } 297 298 @Override 299 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 300 switch (_hash) { 301 case 106079: /*key*/ return new Property("key", "string", "A unique string within the scenario that is used to reference the actor.", 0, 1, key); 302 case 3575610: /*type*/ return new Property("type", "code", "The category of actor - person or system.", 0, 1, type); 303 case 110371416: /*title*/ return new Property("title", "string", "The human-readable name for the actor used when rendering the scenario.", 0, 1, title); 304 case -1724546052: /*description*/ return new Property("description", "markdown", "An explanation of who/what the actor is and its role in the scenario.", 0, 1, description); 305 default: return super.getNamedProperty(_hash, _name, _checkValid); 306 } 307 308 } 309 310 @Override 311 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 312 switch (hash) { 313 case 106079: /*key*/ return this.key == null ? new Base[0] : new Base[] {this.key}; // StringType 314 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<ExampleScenarioActorType> 315 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 316 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 317 default: return super.getProperty(hash, name, checkValid); 318 } 319 320 } 321 322 @Override 323 public Base setProperty(int hash, String name, Base value) throws FHIRException { 324 switch (hash) { 325 case 106079: // key 326 this.key = TypeConvertor.castToString(value); // StringType 327 return value; 328 case 3575610: // type 329 value = new ExampleScenarioActorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 330 this.type = (Enumeration) value; // Enumeration<ExampleScenarioActorType> 331 return value; 332 case 110371416: // title 333 this.title = TypeConvertor.castToString(value); // StringType 334 return value; 335 case -1724546052: // description 336 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 337 return value; 338 default: return super.setProperty(hash, name, value); 339 } 340 341 } 342 343 @Override 344 public Base setProperty(String name, Base value) throws FHIRException { 345 if (name.equals("key")) { 346 this.key = TypeConvertor.castToString(value); // StringType 347 } else if (name.equals("type")) { 348 value = new ExampleScenarioActorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 349 this.type = (Enumeration) value; // Enumeration<ExampleScenarioActorType> 350 } else if (name.equals("title")) { 351 this.title = TypeConvertor.castToString(value); // StringType 352 } else if (name.equals("description")) { 353 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 354 } else 355 return super.setProperty(name, value); 356 return value; 357 } 358 359 @Override 360 public void removeChild(String name, Base value) throws FHIRException { 361 if (name.equals("key")) { 362 this.key = null; 363 } else if (name.equals("type")) { 364 value = new ExampleScenarioActorTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 365 this.type = (Enumeration) value; // Enumeration<ExampleScenarioActorType> 366 } else if (name.equals("title")) { 367 this.title = null; 368 } else if (name.equals("description")) { 369 this.description = null; 370 } else 371 super.removeChild(name, value); 372 373 } 374 375 @Override 376 public Base makeProperty(int hash, String name) throws FHIRException { 377 switch (hash) { 378 case 106079: return getKeyElement(); 379 case 3575610: return getTypeElement(); 380 case 110371416: return getTitleElement(); 381 case -1724546052: return getDescriptionElement(); 382 default: return super.makeProperty(hash, name); 383 } 384 385 } 386 387 @Override 388 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 389 switch (hash) { 390 case 106079: /*key*/ return new String[] {"string"}; 391 case 3575610: /*type*/ return new String[] {"code"}; 392 case 110371416: /*title*/ return new String[] {"string"}; 393 case -1724546052: /*description*/ return new String[] {"markdown"}; 394 default: return super.getTypesForProperty(hash, name); 395 } 396 397 } 398 399 @Override 400 public Base addChild(String name) throws FHIRException { 401 if (name.equals("key")) { 402 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.actor.key"); 403 } 404 else if (name.equals("type")) { 405 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.actor.type"); 406 } 407 else if (name.equals("title")) { 408 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.actor.title"); 409 } 410 else if (name.equals("description")) { 411 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.actor.description"); 412 } 413 else 414 return super.addChild(name); 415 } 416 417 public ExampleScenarioActorComponent copy() { 418 ExampleScenarioActorComponent dst = new ExampleScenarioActorComponent(); 419 copyValues(dst); 420 return dst; 421 } 422 423 public void copyValues(ExampleScenarioActorComponent dst) { 424 super.copyValues(dst); 425 dst.key = key == null ? null : key.copy(); 426 dst.type = type == null ? null : type.copy(); 427 dst.title = title == null ? null : title.copy(); 428 dst.description = description == null ? null : description.copy(); 429 } 430 431 @Override 432 public boolean equalsDeep(Base other_) { 433 if (!super.equalsDeep(other_)) 434 return false; 435 if (!(other_ instanceof ExampleScenarioActorComponent)) 436 return false; 437 ExampleScenarioActorComponent o = (ExampleScenarioActorComponent) other_; 438 return compareDeep(key, o.key, true) && compareDeep(type, o.type, true) && compareDeep(title, o.title, true) 439 && compareDeep(description, o.description, true); 440 } 441 442 @Override 443 public boolean equalsShallow(Base other_) { 444 if (!super.equalsShallow(other_)) 445 return false; 446 if (!(other_ instanceof ExampleScenarioActorComponent)) 447 return false; 448 ExampleScenarioActorComponent o = (ExampleScenarioActorComponent) other_; 449 return compareValues(key, o.key, true) && compareValues(type, o.type, true) && compareValues(title, o.title, true) 450 && compareValues(description, o.description, true); 451 } 452 453 public boolean isEmpty() { 454 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(key, type, title, description 455 ); 456 } 457 458 public String fhirType() { 459 return "ExampleScenario.actor"; 460 461 } 462 463 } 464 465 @Block() 466 public static class ExampleScenarioInstanceComponent extends BackboneElement implements IBaseBackboneElement { 467 /** 468 * A unique string within the scenario that is used to reference the instance. 469 */ 470 @Child(name = "key", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 471 @Description(shortDefinition="ID or acronym of the instance", formalDefinition="A unique string within the scenario that is used to reference the instance." ) 472 protected StringType key; 473 474 /** 475 * A code indicating the kind of data structure (FHIR resource or some other standard) this is an instance of. 476 */ 477 @Child(name = "structureType", type = {Coding.class}, order=2, min=1, max=1, modifier=false, summary=false) 478 @Description(shortDefinition="Data structure for example", formalDefinition="A code indicating the kind of data structure (FHIR resource or some other standard) this is an instance of." ) 479 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/examplescenario-instance-type") 480 protected Coding structureType; 481 482 /** 483 * Conveys the version of the data structure instantiated. I.e. what release of FHIR, X12, OpenEHR, etc. is instance compliant with. 484 */ 485 @Child(name = "structureVersion", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 486 @Description(shortDefinition="E.g. 4.0.1", formalDefinition="Conveys the version of the data structure instantiated. I.e. what release of FHIR, X12, OpenEHR, etc. is instance compliant with." ) 487 protected StringType structureVersion; 488 489 /** 490 * Refers to a profile, template or other ruleset the instance adheres to. 491 */ 492 @Child(name = "structureProfile", type = {CanonicalType.class, UriType.class}, order=4, min=0, max=1, modifier=false, summary=false) 493 @Description(shortDefinition="Rules instance adheres to", formalDefinition="Refers to a profile, template or other ruleset the instance adheres to." ) 494 protected DataType structureProfile; 495 496 /** 497 * A short descriptive label the instance to be used in tables or diagrams. 498 */ 499 @Child(name = "title", type = {StringType.class}, order=5, min=1, max=1, modifier=false, summary=false) 500 @Description(shortDefinition="Label for instance", formalDefinition="A short descriptive label the instance to be used in tables or diagrams." ) 501 protected StringType title; 502 503 /** 504 * An explanation of what the instance contains and what it's for. 505 */ 506 @Child(name = "description", type = {MarkdownType.class}, order=6, min=0, max=1, modifier=false, summary=false) 507 @Description(shortDefinition="Human-friendly description of the instance", formalDefinition="An explanation of what the instance contains and what it's for." ) 508 protected MarkdownType description; 509 510 /** 511 * Points to an instance (typically an example) that shows the data that would corespond to this instance. 512 */ 513 @Child(name = "content", type = {Reference.class}, order=7, min=0, max=1, modifier=false, summary=false) 514 @Description(shortDefinition="Example instance data", formalDefinition="Points to an instance (typically an example) that shows the data that would corespond to this instance." ) 515 protected Reference content; 516 517 /** 518 * Represents the instance as it was at a specific time-point. 519 */ 520 @Child(name = "version", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 521 @Description(shortDefinition="Snapshot of instance that changes", formalDefinition="Represents the instance as it was at a specific time-point." ) 522 protected List<ExampleScenarioInstanceVersionComponent> version; 523 524 /** 525 * References to other instances that can be found within this instance (e.g. the observations contained in a bundle). 526 */ 527 @Child(name = "containedInstance", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 528 @Description(shortDefinition="Resources contained in the instance", formalDefinition="References to other instances that can be found within this instance (e.g. the observations contained in a bundle)." ) 529 protected List<ExampleScenarioInstanceContainedInstanceComponent> containedInstance; 530 531 private static final long serialVersionUID = -1366610733L; 532 533 /** 534 * Constructor 535 */ 536 public ExampleScenarioInstanceComponent() { 537 super(); 538 } 539 540 /** 541 * Constructor 542 */ 543 public ExampleScenarioInstanceComponent(String key, Coding structureType, String title) { 544 super(); 545 this.setKey(key); 546 this.setStructureType(structureType); 547 this.setTitle(title); 548 } 549 550 /** 551 * @return {@link #key} (A unique string within the scenario that is used to reference the instance.). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 552 */ 553 public StringType getKeyElement() { 554 if (this.key == null) 555 if (Configuration.errorOnAutoCreate()) 556 throw new Error("Attempt to auto-create ExampleScenarioInstanceComponent.key"); 557 else if (Configuration.doAutoCreate()) 558 this.key = new StringType(); // bb 559 return this.key; 560 } 561 562 public boolean hasKeyElement() { 563 return this.key != null && !this.key.isEmpty(); 564 } 565 566 public boolean hasKey() { 567 return this.key != null && !this.key.isEmpty(); 568 } 569 570 /** 571 * @param value {@link #key} (A unique string within the scenario that is used to reference the instance.). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 572 */ 573 public ExampleScenarioInstanceComponent setKeyElement(StringType value) { 574 this.key = value; 575 return this; 576 } 577 578 /** 579 * @return A unique string within the scenario that is used to reference the instance. 580 */ 581 public String getKey() { 582 return this.key == null ? null : this.key.getValue(); 583 } 584 585 /** 586 * @param value A unique string within the scenario that is used to reference the instance. 587 */ 588 public ExampleScenarioInstanceComponent setKey(String value) { 589 if (this.key == null) 590 this.key = new StringType(); 591 this.key.setValue(value); 592 return this; 593 } 594 595 /** 596 * @return {@link #structureType} (A code indicating the kind of data structure (FHIR resource or some other standard) this is an instance of.) 597 */ 598 public Coding getStructureType() { 599 if (this.structureType == null) 600 if (Configuration.errorOnAutoCreate()) 601 throw new Error("Attempt to auto-create ExampleScenarioInstanceComponent.structureType"); 602 else if (Configuration.doAutoCreate()) 603 this.structureType = new Coding(); // cc 604 return this.structureType; 605 } 606 607 public boolean hasStructureType() { 608 return this.structureType != null && !this.structureType.isEmpty(); 609 } 610 611 /** 612 * @param value {@link #structureType} (A code indicating the kind of data structure (FHIR resource or some other standard) this is an instance of.) 613 */ 614 public ExampleScenarioInstanceComponent setStructureType(Coding value) { 615 this.structureType = value; 616 return this; 617 } 618 619 /** 620 * @return {@link #structureVersion} (Conveys the version of the data structure instantiated. I.e. what release of FHIR, X12, OpenEHR, etc. is instance compliant with.). This is the underlying object with id, value and extensions. The accessor "getStructureVersion" gives direct access to the value 621 */ 622 public StringType getStructureVersionElement() { 623 if (this.structureVersion == null) 624 if (Configuration.errorOnAutoCreate()) 625 throw new Error("Attempt to auto-create ExampleScenarioInstanceComponent.structureVersion"); 626 else if (Configuration.doAutoCreate()) 627 this.structureVersion = new StringType(); // bb 628 return this.structureVersion; 629 } 630 631 public boolean hasStructureVersionElement() { 632 return this.structureVersion != null && !this.structureVersion.isEmpty(); 633 } 634 635 public boolean hasStructureVersion() { 636 return this.structureVersion != null && !this.structureVersion.isEmpty(); 637 } 638 639 /** 640 * @param value {@link #structureVersion} (Conveys the version of the data structure instantiated. I.e. what release of FHIR, X12, OpenEHR, etc. is instance compliant with.). This is the underlying object with id, value and extensions. The accessor "getStructureVersion" gives direct access to the value 641 */ 642 public ExampleScenarioInstanceComponent setStructureVersionElement(StringType value) { 643 this.structureVersion = value; 644 return this; 645 } 646 647 /** 648 * @return Conveys the version of the data structure instantiated. I.e. what release of FHIR, X12, OpenEHR, etc. is instance compliant with. 649 */ 650 public String getStructureVersion() { 651 return this.structureVersion == null ? null : this.structureVersion.getValue(); 652 } 653 654 /** 655 * @param value Conveys the version of the data structure instantiated. I.e. what release of FHIR, X12, OpenEHR, etc. is instance compliant with. 656 */ 657 public ExampleScenarioInstanceComponent setStructureVersion(String value) { 658 if (Utilities.noString(value)) 659 this.structureVersion = null; 660 else { 661 if (this.structureVersion == null) 662 this.structureVersion = new StringType(); 663 this.structureVersion.setValue(value); 664 } 665 return this; 666 } 667 668 /** 669 * @return {@link #structureProfile} (Refers to a profile, template or other ruleset the instance adheres to.) 670 */ 671 public DataType getStructureProfile() { 672 return this.structureProfile; 673 } 674 675 /** 676 * @return {@link #structureProfile} (Refers to a profile, template or other ruleset the instance adheres to.) 677 */ 678 public CanonicalType getStructureProfileCanonicalType() throws FHIRException { 679 if (this.structureProfile == null) 680 this.structureProfile = new CanonicalType(); 681 if (!(this.structureProfile instanceof CanonicalType)) 682 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.structureProfile.getClass().getName()+" was encountered"); 683 return (CanonicalType) this.structureProfile; 684 } 685 686 public boolean hasStructureProfileCanonicalType() { 687 return this != null && this.structureProfile instanceof CanonicalType; 688 } 689 690 /** 691 * @return {@link #structureProfile} (Refers to a profile, template or other ruleset the instance adheres to.) 692 */ 693 public UriType getStructureProfileUriType() throws FHIRException { 694 if (this.structureProfile == null) 695 this.structureProfile = new UriType(); 696 if (!(this.structureProfile instanceof UriType)) 697 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.structureProfile.getClass().getName()+" was encountered"); 698 return (UriType) this.structureProfile; 699 } 700 701 public boolean hasStructureProfileUriType() { 702 return this != null && this.structureProfile instanceof UriType; 703 } 704 705 public boolean hasStructureProfile() { 706 return this.structureProfile != null && !this.structureProfile.isEmpty(); 707 } 708 709 /** 710 * @param value {@link #structureProfile} (Refers to a profile, template or other ruleset the instance adheres to.) 711 */ 712 public ExampleScenarioInstanceComponent setStructureProfile(DataType value) { 713 if (value != null && !(value instanceof CanonicalType || value instanceof UriType)) 714 throw new FHIRException("Not the right type for ExampleScenario.instance.structureProfile[x]: "+value.fhirType()); 715 this.structureProfile = value; 716 return this; 717 } 718 719 /** 720 * @return {@link #title} (A short descriptive label the instance to be used in tables or diagrams.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 721 */ 722 public StringType getTitleElement() { 723 if (this.title == null) 724 if (Configuration.errorOnAutoCreate()) 725 throw new Error("Attempt to auto-create ExampleScenarioInstanceComponent.title"); 726 else if (Configuration.doAutoCreate()) 727 this.title = new StringType(); // bb 728 return this.title; 729 } 730 731 public boolean hasTitleElement() { 732 return this.title != null && !this.title.isEmpty(); 733 } 734 735 public boolean hasTitle() { 736 return this.title != null && !this.title.isEmpty(); 737 } 738 739 /** 740 * @param value {@link #title} (A short descriptive label the instance to be used in tables or diagrams.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 741 */ 742 public ExampleScenarioInstanceComponent setTitleElement(StringType value) { 743 this.title = value; 744 return this; 745 } 746 747 /** 748 * @return A short descriptive label the instance to be used in tables or diagrams. 749 */ 750 public String getTitle() { 751 return this.title == null ? null : this.title.getValue(); 752 } 753 754 /** 755 * @param value A short descriptive label the instance to be used in tables or diagrams. 756 */ 757 public ExampleScenarioInstanceComponent setTitle(String value) { 758 if (this.title == null) 759 this.title = new StringType(); 760 this.title.setValue(value); 761 return this; 762 } 763 764 /** 765 * @return {@link #description} (An explanation of what the instance contains and what it's for.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 766 */ 767 public MarkdownType getDescriptionElement() { 768 if (this.description == null) 769 if (Configuration.errorOnAutoCreate()) 770 throw new Error("Attempt to auto-create ExampleScenarioInstanceComponent.description"); 771 else if (Configuration.doAutoCreate()) 772 this.description = new MarkdownType(); // bb 773 return this.description; 774 } 775 776 public boolean hasDescriptionElement() { 777 return this.description != null && !this.description.isEmpty(); 778 } 779 780 public boolean hasDescription() { 781 return this.description != null && !this.description.isEmpty(); 782 } 783 784 /** 785 * @param value {@link #description} (An explanation of what the instance contains and what it's for.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 786 */ 787 public ExampleScenarioInstanceComponent setDescriptionElement(MarkdownType value) { 788 this.description = value; 789 return this; 790 } 791 792 /** 793 * @return An explanation of what the instance contains and what it's for. 794 */ 795 public String getDescription() { 796 return this.description == null ? null : this.description.getValue(); 797 } 798 799 /** 800 * @param value An explanation of what the instance contains and what it's for. 801 */ 802 public ExampleScenarioInstanceComponent setDescription(String value) { 803 if (Utilities.noString(value)) 804 this.description = null; 805 else { 806 if (this.description == null) 807 this.description = new MarkdownType(); 808 this.description.setValue(value); 809 } 810 return this; 811 } 812 813 /** 814 * @return {@link #content} (Points to an instance (typically an example) that shows the data that would corespond to this instance.) 815 */ 816 public Reference getContent() { 817 if (this.content == null) 818 if (Configuration.errorOnAutoCreate()) 819 throw new Error("Attempt to auto-create ExampleScenarioInstanceComponent.content"); 820 else if (Configuration.doAutoCreate()) 821 this.content = new Reference(); // cc 822 return this.content; 823 } 824 825 public boolean hasContent() { 826 return this.content != null && !this.content.isEmpty(); 827 } 828 829 /** 830 * @param value {@link #content} (Points to an instance (typically an example) that shows the data that would corespond to this instance.) 831 */ 832 public ExampleScenarioInstanceComponent setContent(Reference value) { 833 this.content = value; 834 return this; 835 } 836 837 /** 838 * @return {@link #version} (Represents the instance as it was at a specific time-point.) 839 */ 840 public List<ExampleScenarioInstanceVersionComponent> getVersion() { 841 if (this.version == null) 842 this.version = new ArrayList<ExampleScenarioInstanceVersionComponent>(); 843 return this.version; 844 } 845 846 /** 847 * @return Returns a reference to <code>this</code> for easy method chaining 848 */ 849 public ExampleScenarioInstanceComponent setVersion(List<ExampleScenarioInstanceVersionComponent> theVersion) { 850 this.version = theVersion; 851 return this; 852 } 853 854 public boolean hasVersion() { 855 if (this.version == null) 856 return false; 857 for (ExampleScenarioInstanceVersionComponent item : this.version) 858 if (!item.isEmpty()) 859 return true; 860 return false; 861 } 862 863 public ExampleScenarioInstanceVersionComponent addVersion() { //3 864 ExampleScenarioInstanceVersionComponent t = new ExampleScenarioInstanceVersionComponent(); 865 if (this.version == null) 866 this.version = new ArrayList<ExampleScenarioInstanceVersionComponent>(); 867 this.version.add(t); 868 return t; 869 } 870 871 public ExampleScenarioInstanceComponent addVersion(ExampleScenarioInstanceVersionComponent t) { //3 872 if (t == null) 873 return this; 874 if (this.version == null) 875 this.version = new ArrayList<ExampleScenarioInstanceVersionComponent>(); 876 this.version.add(t); 877 return this; 878 } 879 880 /** 881 * @return The first repetition of repeating field {@link #version}, creating it if it does not already exist {3} 882 */ 883 public ExampleScenarioInstanceVersionComponent getVersionFirstRep() { 884 if (getVersion().isEmpty()) { 885 addVersion(); 886 } 887 return getVersion().get(0); 888 } 889 890 /** 891 * @return {@link #containedInstance} (References to other instances that can be found within this instance (e.g. the observations contained in a bundle).) 892 */ 893 public List<ExampleScenarioInstanceContainedInstanceComponent> getContainedInstance() { 894 if (this.containedInstance == null) 895 this.containedInstance = new ArrayList<ExampleScenarioInstanceContainedInstanceComponent>(); 896 return this.containedInstance; 897 } 898 899 /** 900 * @return Returns a reference to <code>this</code> for easy method chaining 901 */ 902 public ExampleScenarioInstanceComponent setContainedInstance(List<ExampleScenarioInstanceContainedInstanceComponent> theContainedInstance) { 903 this.containedInstance = theContainedInstance; 904 return this; 905 } 906 907 public boolean hasContainedInstance() { 908 if (this.containedInstance == null) 909 return false; 910 for (ExampleScenarioInstanceContainedInstanceComponent item : this.containedInstance) 911 if (!item.isEmpty()) 912 return true; 913 return false; 914 } 915 916 public ExampleScenarioInstanceContainedInstanceComponent addContainedInstance() { //3 917 ExampleScenarioInstanceContainedInstanceComponent t = new ExampleScenarioInstanceContainedInstanceComponent(); 918 if (this.containedInstance == null) 919 this.containedInstance = new ArrayList<ExampleScenarioInstanceContainedInstanceComponent>(); 920 this.containedInstance.add(t); 921 return t; 922 } 923 924 public ExampleScenarioInstanceComponent addContainedInstance(ExampleScenarioInstanceContainedInstanceComponent t) { //3 925 if (t == null) 926 return this; 927 if (this.containedInstance == null) 928 this.containedInstance = new ArrayList<ExampleScenarioInstanceContainedInstanceComponent>(); 929 this.containedInstance.add(t); 930 return this; 931 } 932 933 /** 934 * @return The first repetition of repeating field {@link #containedInstance}, creating it if it does not already exist {3} 935 */ 936 public ExampleScenarioInstanceContainedInstanceComponent getContainedInstanceFirstRep() { 937 if (getContainedInstance().isEmpty()) { 938 addContainedInstance(); 939 } 940 return getContainedInstance().get(0); 941 } 942 943 protected void listChildren(List<Property> children) { 944 super.listChildren(children); 945 children.add(new Property("key", "string", "A unique string within the scenario that is used to reference the instance.", 0, 1, key)); 946 children.add(new Property("structureType", "Coding", "A code indicating the kind of data structure (FHIR resource or some other standard) this is an instance of.", 0, 1, structureType)); 947 children.add(new Property("structureVersion", "string", "Conveys the version of the data structure instantiated. I.e. what release of FHIR, X12, OpenEHR, etc. is instance compliant with.", 0, 1, structureVersion)); 948 children.add(new Property("structureProfile[x]", "canonical|uri", "Refers to a profile, template or other ruleset the instance adheres to.", 0, 1, structureProfile)); 949 children.add(new Property("title", "string", "A short descriptive label the instance to be used in tables or diagrams.", 0, 1, title)); 950 children.add(new Property("description", "markdown", "An explanation of what the instance contains and what it's for.", 0, 1, description)); 951 children.add(new Property("content", "Reference", "Points to an instance (typically an example) that shows the data that would corespond to this instance.", 0, 1, content)); 952 children.add(new Property("version", "", "Represents the instance as it was at a specific time-point.", 0, java.lang.Integer.MAX_VALUE, version)); 953 children.add(new Property("containedInstance", "", "References to other instances that can be found within this instance (e.g. the observations contained in a bundle).", 0, java.lang.Integer.MAX_VALUE, containedInstance)); 954 } 955 956 @Override 957 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 958 switch (_hash) { 959 case 106079: /*key*/ return new Property("key", "string", "A unique string within the scenario that is used to reference the instance.", 0, 1, key); 960 case -222609587: /*structureType*/ return new Property("structureType", "Coding", "A code indicating the kind of data structure (FHIR resource or some other standard) this is an instance of.", 0, 1, structureType); 961 case 872091621: /*structureVersion*/ return new Property("structureVersion", "string", "Conveys the version of the data structure instantiated. I.e. what release of FHIR, X12, OpenEHR, etc. is instance compliant with.", 0, 1, structureVersion); 962 case -207739894: /*structureProfile[x]*/ return new Property("structureProfile[x]", "canonical|uri", "Refers to a profile, template or other ruleset the instance adheres to.", 0, 1, structureProfile); 963 case 211057846: /*structureProfile*/ return new Property("structureProfile[x]", "canonical|uri", "Refers to a profile, template or other ruleset the instance adheres to.", 0, 1, structureProfile); 964 case -1044433698: /*structureProfileCanonical*/ return new Property("structureProfile[x]", "canonical", "Refers to a profile, template or other ruleset the instance adheres to.", 0, 1, structureProfile); 965 case -207745834: /*structureProfileUri*/ return new Property("structureProfile[x]", "uri", "Refers to a profile, template or other ruleset the instance adheres to.", 0, 1, structureProfile); 966 case 110371416: /*title*/ return new Property("title", "string", "A short descriptive label the instance to be used in tables or diagrams.", 0, 1, title); 967 case -1724546052: /*description*/ return new Property("description", "markdown", "An explanation of what the instance contains and what it's for.", 0, 1, description); 968 case 951530617: /*content*/ return new Property("content", "Reference", "Points to an instance (typically an example) that shows the data that would corespond to this instance.", 0, 1, content); 969 case 351608024: /*version*/ return new Property("version", "", "Represents the instance as it was at a specific time-point.", 0, java.lang.Integer.MAX_VALUE, version); 970 case -417062360: /*containedInstance*/ return new Property("containedInstance", "", "References to other instances that can be found within this instance (e.g. the observations contained in a bundle).", 0, java.lang.Integer.MAX_VALUE, containedInstance); 971 default: return super.getNamedProperty(_hash, _name, _checkValid); 972 } 973 974 } 975 976 @Override 977 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 978 switch (hash) { 979 case 106079: /*key*/ return this.key == null ? new Base[0] : new Base[] {this.key}; // StringType 980 case -222609587: /*structureType*/ return this.structureType == null ? new Base[0] : new Base[] {this.structureType}; // Coding 981 case 872091621: /*structureVersion*/ return this.structureVersion == null ? new Base[0] : new Base[] {this.structureVersion}; // StringType 982 case 211057846: /*structureProfile*/ return this.structureProfile == null ? new Base[0] : new Base[] {this.structureProfile}; // DataType 983 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 984 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 985 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // Reference 986 case 351608024: /*version*/ return this.version == null ? new Base[0] : this.version.toArray(new Base[this.version.size()]); // ExampleScenarioInstanceVersionComponent 987 case -417062360: /*containedInstance*/ return this.containedInstance == null ? new Base[0] : this.containedInstance.toArray(new Base[this.containedInstance.size()]); // ExampleScenarioInstanceContainedInstanceComponent 988 default: return super.getProperty(hash, name, checkValid); 989 } 990 991 } 992 993 @Override 994 public Base setProperty(int hash, String name, Base value) throws FHIRException { 995 switch (hash) { 996 case 106079: // key 997 this.key = TypeConvertor.castToString(value); // StringType 998 return value; 999 case -222609587: // structureType 1000 this.structureType = TypeConvertor.castToCoding(value); // Coding 1001 return value; 1002 case 872091621: // structureVersion 1003 this.structureVersion = TypeConvertor.castToString(value); // StringType 1004 return value; 1005 case 211057846: // structureProfile 1006 this.structureProfile = TypeConvertor.castToType(value); // DataType 1007 return value; 1008 case 110371416: // title 1009 this.title = TypeConvertor.castToString(value); // StringType 1010 return value; 1011 case -1724546052: // description 1012 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1013 return value; 1014 case 951530617: // content 1015 this.content = TypeConvertor.castToReference(value); // Reference 1016 return value; 1017 case 351608024: // version 1018 this.getVersion().add((ExampleScenarioInstanceVersionComponent) value); // ExampleScenarioInstanceVersionComponent 1019 return value; 1020 case -417062360: // containedInstance 1021 this.getContainedInstance().add((ExampleScenarioInstanceContainedInstanceComponent) value); // ExampleScenarioInstanceContainedInstanceComponent 1022 return value; 1023 default: return super.setProperty(hash, name, value); 1024 } 1025 1026 } 1027 1028 @Override 1029 public Base setProperty(String name, Base value) throws FHIRException { 1030 if (name.equals("key")) { 1031 this.key = TypeConvertor.castToString(value); // StringType 1032 } else if (name.equals("structureType")) { 1033 this.structureType = TypeConvertor.castToCoding(value); // Coding 1034 } else if (name.equals("structureVersion")) { 1035 this.structureVersion = TypeConvertor.castToString(value); // StringType 1036 } else if (name.equals("structureProfile[x]")) { 1037 this.structureProfile = TypeConvertor.castToType(value); // DataType 1038 } else if (name.equals("title")) { 1039 this.title = TypeConvertor.castToString(value); // StringType 1040 } else if (name.equals("description")) { 1041 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1042 } else if (name.equals("content")) { 1043 this.content = TypeConvertor.castToReference(value); // Reference 1044 } else if (name.equals("version")) { 1045 this.getVersion().add((ExampleScenarioInstanceVersionComponent) value); 1046 } else if (name.equals("containedInstance")) { 1047 this.getContainedInstance().add((ExampleScenarioInstanceContainedInstanceComponent) value); 1048 } else 1049 return super.setProperty(name, value); 1050 return value; 1051 } 1052 1053 @Override 1054 public void removeChild(String name, Base value) throws FHIRException { 1055 if (name.equals("key")) { 1056 this.key = null; 1057 } else if (name.equals("structureType")) { 1058 this.structureType = null; 1059 } else if (name.equals("structureVersion")) { 1060 this.structureVersion = null; 1061 } else if (name.equals("structureProfile[x]")) { 1062 this.structureProfile = null; 1063 } else if (name.equals("title")) { 1064 this.title = null; 1065 } else if (name.equals("description")) { 1066 this.description = null; 1067 } else if (name.equals("content")) { 1068 this.content = null; 1069 } else if (name.equals("version")) { 1070 this.getVersion().remove((ExampleScenarioInstanceVersionComponent) value); 1071 } else if (name.equals("containedInstance")) { 1072 this.getContainedInstance().remove((ExampleScenarioInstanceContainedInstanceComponent) value); 1073 } else 1074 super.removeChild(name, value); 1075 1076 } 1077 1078 @Override 1079 public Base makeProperty(int hash, String name) throws FHIRException { 1080 switch (hash) { 1081 case 106079: return getKeyElement(); 1082 case -222609587: return getStructureType(); 1083 case 872091621: return getStructureVersionElement(); 1084 case -207739894: return getStructureProfile(); 1085 case 211057846: return getStructureProfile(); 1086 case 110371416: return getTitleElement(); 1087 case -1724546052: return getDescriptionElement(); 1088 case 951530617: return getContent(); 1089 case 351608024: return addVersion(); 1090 case -417062360: return addContainedInstance(); 1091 default: return super.makeProperty(hash, name); 1092 } 1093 1094 } 1095 1096 @Override 1097 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1098 switch (hash) { 1099 case 106079: /*key*/ return new String[] {"string"}; 1100 case -222609587: /*structureType*/ return new String[] {"Coding"}; 1101 case 872091621: /*structureVersion*/ return new String[] {"string"}; 1102 case 211057846: /*structureProfile*/ return new String[] {"canonical", "uri"}; 1103 case 110371416: /*title*/ return new String[] {"string"}; 1104 case -1724546052: /*description*/ return new String[] {"markdown"}; 1105 case 951530617: /*content*/ return new String[] {"Reference"}; 1106 case 351608024: /*version*/ return new String[] {}; 1107 case -417062360: /*containedInstance*/ return new String[] {}; 1108 default: return super.getTypesForProperty(hash, name); 1109 } 1110 1111 } 1112 1113 @Override 1114 public Base addChild(String name) throws FHIRException { 1115 if (name.equals("key")) { 1116 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.instance.key"); 1117 } 1118 else if (name.equals("structureType")) { 1119 this.structureType = new Coding(); 1120 return this.structureType; 1121 } 1122 else if (name.equals("structureVersion")) { 1123 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.instance.structureVersion"); 1124 } 1125 else if (name.equals("structureProfileCanonical")) { 1126 this.structureProfile = new CanonicalType(); 1127 return this.structureProfile; 1128 } 1129 else if (name.equals("structureProfileUri")) { 1130 this.structureProfile = new UriType(); 1131 return this.structureProfile; 1132 } 1133 else if (name.equals("title")) { 1134 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.instance.title"); 1135 } 1136 else if (name.equals("description")) { 1137 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.instance.description"); 1138 } 1139 else if (name.equals("content")) { 1140 this.content = new Reference(); 1141 return this.content; 1142 } 1143 else if (name.equals("version")) { 1144 return addVersion(); 1145 } 1146 else if (name.equals("containedInstance")) { 1147 return addContainedInstance(); 1148 } 1149 else 1150 return super.addChild(name); 1151 } 1152 1153 public ExampleScenarioInstanceComponent copy() { 1154 ExampleScenarioInstanceComponent dst = new ExampleScenarioInstanceComponent(); 1155 copyValues(dst); 1156 return dst; 1157 } 1158 1159 public void copyValues(ExampleScenarioInstanceComponent dst) { 1160 super.copyValues(dst); 1161 dst.key = key == null ? null : key.copy(); 1162 dst.structureType = structureType == null ? null : structureType.copy(); 1163 dst.structureVersion = structureVersion == null ? null : structureVersion.copy(); 1164 dst.structureProfile = structureProfile == null ? null : structureProfile.copy(); 1165 dst.title = title == null ? null : title.copy(); 1166 dst.description = description == null ? null : description.copy(); 1167 dst.content = content == null ? null : content.copy(); 1168 if (version != null) { 1169 dst.version = new ArrayList<ExampleScenarioInstanceVersionComponent>(); 1170 for (ExampleScenarioInstanceVersionComponent i : version) 1171 dst.version.add(i.copy()); 1172 }; 1173 if (containedInstance != null) { 1174 dst.containedInstance = new ArrayList<ExampleScenarioInstanceContainedInstanceComponent>(); 1175 for (ExampleScenarioInstanceContainedInstanceComponent i : containedInstance) 1176 dst.containedInstance.add(i.copy()); 1177 }; 1178 } 1179 1180 @Override 1181 public boolean equalsDeep(Base other_) { 1182 if (!super.equalsDeep(other_)) 1183 return false; 1184 if (!(other_ instanceof ExampleScenarioInstanceComponent)) 1185 return false; 1186 ExampleScenarioInstanceComponent o = (ExampleScenarioInstanceComponent) other_; 1187 return compareDeep(key, o.key, true) && compareDeep(structureType, o.structureType, true) && compareDeep(structureVersion, o.structureVersion, true) 1188 && compareDeep(structureProfile, o.structureProfile, true) && compareDeep(title, o.title, true) 1189 && compareDeep(description, o.description, true) && compareDeep(content, o.content, true) && compareDeep(version, o.version, true) 1190 && compareDeep(containedInstance, o.containedInstance, true); 1191 } 1192 1193 @Override 1194 public boolean equalsShallow(Base other_) { 1195 if (!super.equalsShallow(other_)) 1196 return false; 1197 if (!(other_ instanceof ExampleScenarioInstanceComponent)) 1198 return false; 1199 ExampleScenarioInstanceComponent o = (ExampleScenarioInstanceComponent) other_; 1200 return compareValues(key, o.key, true) && compareValues(structureVersion, o.structureVersion, true) 1201 && compareValues(title, o.title, true) && compareValues(description, o.description, true); 1202 } 1203 1204 public boolean isEmpty() { 1205 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(key, structureType, structureVersion 1206 , structureProfile, title, description, content, version, containedInstance); 1207 } 1208 1209 public String fhirType() { 1210 return "ExampleScenario.instance"; 1211 1212 } 1213 1214 } 1215 1216 @Block() 1217 public static class ExampleScenarioInstanceVersionComponent extends BackboneElement implements IBaseBackboneElement { 1218 /** 1219 * A unique string within the instance that is used to reference the version of the instance. 1220 */ 1221 @Child(name = "key", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1222 @Description(shortDefinition="ID or acronym of the version", formalDefinition="A unique string within the instance that is used to reference the version of the instance." ) 1223 protected StringType key; 1224 1225 /** 1226 * A short descriptive label the version to be used in tables or diagrams. 1227 */ 1228 @Child(name = "title", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 1229 @Description(shortDefinition="Label for instance version", formalDefinition="A short descriptive label the version to be used in tables or diagrams." ) 1230 protected StringType title; 1231 1232 /** 1233 * An explanation of what this specific version of the instance contains and represents. 1234 */ 1235 @Child(name = "description", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1236 @Description(shortDefinition="Details about version", formalDefinition="An explanation of what this specific version of the instance contains and represents." ) 1237 protected MarkdownType description; 1238 1239 /** 1240 * Points to an instance (typically an example) that shows the data that would flow at this point in the scenario. 1241 */ 1242 @Child(name = "content", type = {Reference.class}, order=4, min=0, max=1, modifier=false, summary=false) 1243 @Description(shortDefinition="Example instance version data", formalDefinition="Points to an instance (typically an example) that shows the data that would flow at this point in the scenario." ) 1244 protected Reference content; 1245 1246 private static final long serialVersionUID = -1218548928L; 1247 1248 /** 1249 * Constructor 1250 */ 1251 public ExampleScenarioInstanceVersionComponent() { 1252 super(); 1253 } 1254 1255 /** 1256 * Constructor 1257 */ 1258 public ExampleScenarioInstanceVersionComponent(String key, String title) { 1259 super(); 1260 this.setKey(key); 1261 this.setTitle(title); 1262 } 1263 1264 /** 1265 * @return {@link #key} (A unique string within the instance that is used to reference the version of the instance.). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 1266 */ 1267 public StringType getKeyElement() { 1268 if (this.key == null) 1269 if (Configuration.errorOnAutoCreate()) 1270 throw new Error("Attempt to auto-create ExampleScenarioInstanceVersionComponent.key"); 1271 else if (Configuration.doAutoCreate()) 1272 this.key = new StringType(); // bb 1273 return this.key; 1274 } 1275 1276 public boolean hasKeyElement() { 1277 return this.key != null && !this.key.isEmpty(); 1278 } 1279 1280 public boolean hasKey() { 1281 return this.key != null && !this.key.isEmpty(); 1282 } 1283 1284 /** 1285 * @param value {@link #key} (A unique string within the instance that is used to reference the version of the instance.). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 1286 */ 1287 public ExampleScenarioInstanceVersionComponent setKeyElement(StringType value) { 1288 this.key = value; 1289 return this; 1290 } 1291 1292 /** 1293 * @return A unique string within the instance that is used to reference the version of the instance. 1294 */ 1295 public String getKey() { 1296 return this.key == null ? null : this.key.getValue(); 1297 } 1298 1299 /** 1300 * @param value A unique string within the instance that is used to reference the version of the instance. 1301 */ 1302 public ExampleScenarioInstanceVersionComponent setKey(String value) { 1303 if (this.key == null) 1304 this.key = new StringType(); 1305 this.key.setValue(value); 1306 return this; 1307 } 1308 1309 /** 1310 * @return {@link #title} (A short descriptive label the version to be used in tables or diagrams.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1311 */ 1312 public StringType getTitleElement() { 1313 if (this.title == null) 1314 if (Configuration.errorOnAutoCreate()) 1315 throw new Error("Attempt to auto-create ExampleScenarioInstanceVersionComponent.title"); 1316 else if (Configuration.doAutoCreate()) 1317 this.title = new StringType(); // bb 1318 return this.title; 1319 } 1320 1321 public boolean hasTitleElement() { 1322 return this.title != null && !this.title.isEmpty(); 1323 } 1324 1325 public boolean hasTitle() { 1326 return this.title != null && !this.title.isEmpty(); 1327 } 1328 1329 /** 1330 * @param value {@link #title} (A short descriptive label the version to be used in tables or diagrams.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1331 */ 1332 public ExampleScenarioInstanceVersionComponent setTitleElement(StringType value) { 1333 this.title = value; 1334 return this; 1335 } 1336 1337 /** 1338 * @return A short descriptive label the version to be used in tables or diagrams. 1339 */ 1340 public String getTitle() { 1341 return this.title == null ? null : this.title.getValue(); 1342 } 1343 1344 /** 1345 * @param value A short descriptive label the version to be used in tables or diagrams. 1346 */ 1347 public ExampleScenarioInstanceVersionComponent setTitle(String value) { 1348 if (this.title == null) 1349 this.title = new StringType(); 1350 this.title.setValue(value); 1351 return this; 1352 } 1353 1354 /** 1355 * @return {@link #description} (An explanation of what this specific version of the instance contains and represents.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1356 */ 1357 public MarkdownType getDescriptionElement() { 1358 if (this.description == null) 1359 if (Configuration.errorOnAutoCreate()) 1360 throw new Error("Attempt to auto-create ExampleScenarioInstanceVersionComponent.description"); 1361 else if (Configuration.doAutoCreate()) 1362 this.description = new MarkdownType(); // bb 1363 return this.description; 1364 } 1365 1366 public boolean hasDescriptionElement() { 1367 return this.description != null && !this.description.isEmpty(); 1368 } 1369 1370 public boolean hasDescription() { 1371 return this.description != null && !this.description.isEmpty(); 1372 } 1373 1374 /** 1375 * @param value {@link #description} (An explanation of what this specific version of the instance contains and represents.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1376 */ 1377 public ExampleScenarioInstanceVersionComponent setDescriptionElement(MarkdownType value) { 1378 this.description = value; 1379 return this; 1380 } 1381 1382 /** 1383 * @return An explanation of what this specific version of the instance contains and represents. 1384 */ 1385 public String getDescription() { 1386 return this.description == null ? null : this.description.getValue(); 1387 } 1388 1389 /** 1390 * @param value An explanation of what this specific version of the instance contains and represents. 1391 */ 1392 public ExampleScenarioInstanceVersionComponent setDescription(String value) { 1393 if (Utilities.noString(value)) 1394 this.description = null; 1395 else { 1396 if (this.description == null) 1397 this.description = new MarkdownType(); 1398 this.description.setValue(value); 1399 } 1400 return this; 1401 } 1402 1403 /** 1404 * @return {@link #content} (Points to an instance (typically an example) that shows the data that would flow at this point in the scenario.) 1405 */ 1406 public Reference getContent() { 1407 if (this.content == null) 1408 if (Configuration.errorOnAutoCreate()) 1409 throw new Error("Attempt to auto-create ExampleScenarioInstanceVersionComponent.content"); 1410 else if (Configuration.doAutoCreate()) 1411 this.content = new Reference(); // cc 1412 return this.content; 1413 } 1414 1415 public boolean hasContent() { 1416 return this.content != null && !this.content.isEmpty(); 1417 } 1418 1419 /** 1420 * @param value {@link #content} (Points to an instance (typically an example) that shows the data that would flow at this point in the scenario.) 1421 */ 1422 public ExampleScenarioInstanceVersionComponent setContent(Reference value) { 1423 this.content = value; 1424 return this; 1425 } 1426 1427 protected void listChildren(List<Property> children) { 1428 super.listChildren(children); 1429 children.add(new Property("key", "string", "A unique string within the instance that is used to reference the version of the instance.", 0, 1, key)); 1430 children.add(new Property("title", "string", "A short descriptive label the version to be used in tables or diagrams.", 0, 1, title)); 1431 children.add(new Property("description", "markdown", "An explanation of what this specific version of the instance contains and represents.", 0, 1, description)); 1432 children.add(new Property("content", "Reference", "Points to an instance (typically an example) that shows the data that would flow at this point in the scenario.", 0, 1, content)); 1433 } 1434 1435 @Override 1436 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1437 switch (_hash) { 1438 case 106079: /*key*/ return new Property("key", "string", "A unique string within the instance that is used to reference the version of the instance.", 0, 1, key); 1439 case 110371416: /*title*/ return new Property("title", "string", "A short descriptive label the version to be used in tables or diagrams.", 0, 1, title); 1440 case -1724546052: /*description*/ return new Property("description", "markdown", "An explanation of what this specific version of the instance contains and represents.", 0, 1, description); 1441 case 951530617: /*content*/ return new Property("content", "Reference", "Points to an instance (typically an example) that shows the data that would flow at this point in the scenario.", 0, 1, content); 1442 default: return super.getNamedProperty(_hash, _name, _checkValid); 1443 } 1444 1445 } 1446 1447 @Override 1448 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1449 switch (hash) { 1450 case 106079: /*key*/ return this.key == null ? new Base[0] : new Base[] {this.key}; // StringType 1451 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1452 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1453 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // Reference 1454 default: return super.getProperty(hash, name, checkValid); 1455 } 1456 1457 } 1458 1459 @Override 1460 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1461 switch (hash) { 1462 case 106079: // key 1463 this.key = TypeConvertor.castToString(value); // StringType 1464 return value; 1465 case 110371416: // title 1466 this.title = TypeConvertor.castToString(value); // StringType 1467 return value; 1468 case -1724546052: // description 1469 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1470 return value; 1471 case 951530617: // content 1472 this.content = TypeConvertor.castToReference(value); // Reference 1473 return value; 1474 default: return super.setProperty(hash, name, value); 1475 } 1476 1477 } 1478 1479 @Override 1480 public Base setProperty(String name, Base value) throws FHIRException { 1481 if (name.equals("key")) { 1482 this.key = TypeConvertor.castToString(value); // StringType 1483 } else if (name.equals("title")) { 1484 this.title = TypeConvertor.castToString(value); // StringType 1485 } else if (name.equals("description")) { 1486 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1487 } else if (name.equals("content")) { 1488 this.content = TypeConvertor.castToReference(value); // Reference 1489 } else 1490 return super.setProperty(name, value); 1491 return value; 1492 } 1493 1494 @Override 1495 public void removeChild(String name, Base value) throws FHIRException { 1496 if (name.equals("key")) { 1497 this.key = null; 1498 } else if (name.equals("title")) { 1499 this.title = null; 1500 } else if (name.equals("description")) { 1501 this.description = null; 1502 } else if (name.equals("content")) { 1503 this.content = null; 1504 } else 1505 super.removeChild(name, value); 1506 1507 } 1508 1509 @Override 1510 public Base makeProperty(int hash, String name) throws FHIRException { 1511 switch (hash) { 1512 case 106079: return getKeyElement(); 1513 case 110371416: return getTitleElement(); 1514 case -1724546052: return getDescriptionElement(); 1515 case 951530617: return getContent(); 1516 default: return super.makeProperty(hash, name); 1517 } 1518 1519 } 1520 1521 @Override 1522 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1523 switch (hash) { 1524 case 106079: /*key*/ return new String[] {"string"}; 1525 case 110371416: /*title*/ return new String[] {"string"}; 1526 case -1724546052: /*description*/ return new String[] {"markdown"}; 1527 case 951530617: /*content*/ return new String[] {"Reference"}; 1528 default: return super.getTypesForProperty(hash, name); 1529 } 1530 1531 } 1532 1533 @Override 1534 public Base addChild(String name) throws FHIRException { 1535 if (name.equals("key")) { 1536 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.instance.version.key"); 1537 } 1538 else if (name.equals("title")) { 1539 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.instance.version.title"); 1540 } 1541 else if (name.equals("description")) { 1542 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.instance.version.description"); 1543 } 1544 else if (name.equals("content")) { 1545 this.content = new Reference(); 1546 return this.content; 1547 } 1548 else 1549 return super.addChild(name); 1550 } 1551 1552 public ExampleScenarioInstanceVersionComponent copy() { 1553 ExampleScenarioInstanceVersionComponent dst = new ExampleScenarioInstanceVersionComponent(); 1554 copyValues(dst); 1555 return dst; 1556 } 1557 1558 public void copyValues(ExampleScenarioInstanceVersionComponent dst) { 1559 super.copyValues(dst); 1560 dst.key = key == null ? null : key.copy(); 1561 dst.title = title == null ? null : title.copy(); 1562 dst.description = description == null ? null : description.copy(); 1563 dst.content = content == null ? null : content.copy(); 1564 } 1565 1566 @Override 1567 public boolean equalsDeep(Base other_) { 1568 if (!super.equalsDeep(other_)) 1569 return false; 1570 if (!(other_ instanceof ExampleScenarioInstanceVersionComponent)) 1571 return false; 1572 ExampleScenarioInstanceVersionComponent o = (ExampleScenarioInstanceVersionComponent) other_; 1573 return compareDeep(key, o.key, true) && compareDeep(title, o.title, true) && compareDeep(description, o.description, true) 1574 && compareDeep(content, o.content, true); 1575 } 1576 1577 @Override 1578 public boolean equalsShallow(Base other_) { 1579 if (!super.equalsShallow(other_)) 1580 return false; 1581 if (!(other_ instanceof ExampleScenarioInstanceVersionComponent)) 1582 return false; 1583 ExampleScenarioInstanceVersionComponent o = (ExampleScenarioInstanceVersionComponent) other_; 1584 return compareValues(key, o.key, true) && compareValues(title, o.title, true) && compareValues(description, o.description, true) 1585 ; 1586 } 1587 1588 public boolean isEmpty() { 1589 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(key, title, description 1590 , content); 1591 } 1592 1593 public String fhirType() { 1594 return "ExampleScenario.instance.version"; 1595 1596 } 1597 1598 } 1599 1600 @Block() 1601 public static class ExampleScenarioInstanceContainedInstanceComponent extends BackboneElement implements IBaseBackboneElement { 1602 /** 1603 * A reference to the key of an instance found within this one. 1604 */ 1605 @Child(name = "instanceReference", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1606 @Description(shortDefinition="Key of contained instance", formalDefinition="A reference to the key of an instance found within this one." ) 1607 protected StringType instanceReference; 1608 1609 /** 1610 * A reference to the key of a specific version of an instance in this instance. 1611 */ 1612 @Child(name = "versionReference", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1613 @Description(shortDefinition="Key of contained instance version", formalDefinition="A reference to the key of a specific version of an instance in this instance." ) 1614 protected StringType versionReference; 1615 1616 private static final long serialVersionUID = 520704935L; 1617 1618 /** 1619 * Constructor 1620 */ 1621 public ExampleScenarioInstanceContainedInstanceComponent() { 1622 super(); 1623 } 1624 1625 /** 1626 * Constructor 1627 */ 1628 public ExampleScenarioInstanceContainedInstanceComponent(String instanceReference) { 1629 super(); 1630 this.setInstanceReference(instanceReference); 1631 } 1632 1633 /** 1634 * @return {@link #instanceReference} (A reference to the key of an instance found within this one.). This is the underlying object with id, value and extensions. The accessor "getInstanceReference" gives direct access to the value 1635 */ 1636 public StringType getInstanceReferenceElement() { 1637 if (this.instanceReference == null) 1638 if (Configuration.errorOnAutoCreate()) 1639 throw new Error("Attempt to auto-create ExampleScenarioInstanceContainedInstanceComponent.instanceReference"); 1640 else if (Configuration.doAutoCreate()) 1641 this.instanceReference = new StringType(); // bb 1642 return this.instanceReference; 1643 } 1644 1645 public boolean hasInstanceReferenceElement() { 1646 return this.instanceReference != null && !this.instanceReference.isEmpty(); 1647 } 1648 1649 public boolean hasInstanceReference() { 1650 return this.instanceReference != null && !this.instanceReference.isEmpty(); 1651 } 1652 1653 /** 1654 * @param value {@link #instanceReference} (A reference to the key of an instance found within this one.). This is the underlying object with id, value and extensions. The accessor "getInstanceReference" gives direct access to the value 1655 */ 1656 public ExampleScenarioInstanceContainedInstanceComponent setInstanceReferenceElement(StringType value) { 1657 this.instanceReference = value; 1658 return this; 1659 } 1660 1661 /** 1662 * @return A reference to the key of an instance found within this one. 1663 */ 1664 public String getInstanceReference() { 1665 return this.instanceReference == null ? null : this.instanceReference.getValue(); 1666 } 1667 1668 /** 1669 * @param value A reference to the key of an instance found within this one. 1670 */ 1671 public ExampleScenarioInstanceContainedInstanceComponent setInstanceReference(String value) { 1672 if (this.instanceReference == null) 1673 this.instanceReference = new StringType(); 1674 this.instanceReference.setValue(value); 1675 return this; 1676 } 1677 1678 /** 1679 * @return {@link #versionReference} (A reference to the key of a specific version of an instance in this instance.). This is the underlying object with id, value and extensions. The accessor "getVersionReference" gives direct access to the value 1680 */ 1681 public StringType getVersionReferenceElement() { 1682 if (this.versionReference == null) 1683 if (Configuration.errorOnAutoCreate()) 1684 throw new Error("Attempt to auto-create ExampleScenarioInstanceContainedInstanceComponent.versionReference"); 1685 else if (Configuration.doAutoCreate()) 1686 this.versionReference = new StringType(); // bb 1687 return this.versionReference; 1688 } 1689 1690 public boolean hasVersionReferenceElement() { 1691 return this.versionReference != null && !this.versionReference.isEmpty(); 1692 } 1693 1694 public boolean hasVersionReference() { 1695 return this.versionReference != null && !this.versionReference.isEmpty(); 1696 } 1697 1698 /** 1699 * @param value {@link #versionReference} (A reference to the key of a specific version of an instance in this instance.). This is the underlying object with id, value and extensions. The accessor "getVersionReference" gives direct access to the value 1700 */ 1701 public ExampleScenarioInstanceContainedInstanceComponent setVersionReferenceElement(StringType value) { 1702 this.versionReference = value; 1703 return this; 1704 } 1705 1706 /** 1707 * @return A reference to the key of a specific version of an instance in this instance. 1708 */ 1709 public String getVersionReference() { 1710 return this.versionReference == null ? null : this.versionReference.getValue(); 1711 } 1712 1713 /** 1714 * @param value A reference to the key of a specific version of an instance in this instance. 1715 */ 1716 public ExampleScenarioInstanceContainedInstanceComponent setVersionReference(String value) { 1717 if (Utilities.noString(value)) 1718 this.versionReference = null; 1719 else { 1720 if (this.versionReference == null) 1721 this.versionReference = new StringType(); 1722 this.versionReference.setValue(value); 1723 } 1724 return this; 1725 } 1726 1727 protected void listChildren(List<Property> children) { 1728 super.listChildren(children); 1729 children.add(new Property("instanceReference", "string", "A reference to the key of an instance found within this one.", 0, 1, instanceReference)); 1730 children.add(new Property("versionReference", "string", "A reference to the key of a specific version of an instance in this instance.", 0, 1, versionReference)); 1731 } 1732 1733 @Override 1734 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1735 switch (_hash) { 1736 case -1675877834: /*instanceReference*/ return new Property("instanceReference", "string", "A reference to the key of an instance found within this one.", 0, 1, instanceReference); 1737 case 357512531: /*versionReference*/ return new Property("versionReference", "string", "A reference to the key of a specific version of an instance in this instance.", 0, 1, versionReference); 1738 default: return super.getNamedProperty(_hash, _name, _checkValid); 1739 } 1740 1741 } 1742 1743 @Override 1744 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1745 switch (hash) { 1746 case -1675877834: /*instanceReference*/ return this.instanceReference == null ? new Base[0] : new Base[] {this.instanceReference}; // StringType 1747 case 357512531: /*versionReference*/ return this.versionReference == null ? new Base[0] : new Base[] {this.versionReference}; // StringType 1748 default: return super.getProperty(hash, name, checkValid); 1749 } 1750 1751 } 1752 1753 @Override 1754 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1755 switch (hash) { 1756 case -1675877834: // instanceReference 1757 this.instanceReference = TypeConvertor.castToString(value); // StringType 1758 return value; 1759 case 357512531: // versionReference 1760 this.versionReference = TypeConvertor.castToString(value); // StringType 1761 return value; 1762 default: return super.setProperty(hash, name, value); 1763 } 1764 1765 } 1766 1767 @Override 1768 public Base setProperty(String name, Base value) throws FHIRException { 1769 if (name.equals("instanceReference")) { 1770 this.instanceReference = TypeConvertor.castToString(value); // StringType 1771 } else if (name.equals("versionReference")) { 1772 this.versionReference = TypeConvertor.castToString(value); // StringType 1773 } else 1774 return super.setProperty(name, value); 1775 return value; 1776 } 1777 1778 @Override 1779 public void removeChild(String name, Base value) throws FHIRException { 1780 if (name.equals("instanceReference")) { 1781 this.instanceReference = null; 1782 } else if (name.equals("versionReference")) { 1783 this.versionReference = null; 1784 } else 1785 super.removeChild(name, value); 1786 1787 } 1788 1789 @Override 1790 public Base makeProperty(int hash, String name) throws FHIRException { 1791 switch (hash) { 1792 case -1675877834: return getInstanceReferenceElement(); 1793 case 357512531: return getVersionReferenceElement(); 1794 default: return super.makeProperty(hash, name); 1795 } 1796 1797 } 1798 1799 @Override 1800 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1801 switch (hash) { 1802 case -1675877834: /*instanceReference*/ return new String[] {"string"}; 1803 case 357512531: /*versionReference*/ return new String[] {"string"}; 1804 default: return super.getTypesForProperty(hash, name); 1805 } 1806 1807 } 1808 1809 @Override 1810 public Base addChild(String name) throws FHIRException { 1811 if (name.equals("instanceReference")) { 1812 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.instance.containedInstance.instanceReference"); 1813 } 1814 else if (name.equals("versionReference")) { 1815 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.instance.containedInstance.versionReference"); 1816 } 1817 else 1818 return super.addChild(name); 1819 } 1820 1821 public ExampleScenarioInstanceContainedInstanceComponent copy() { 1822 ExampleScenarioInstanceContainedInstanceComponent dst = new ExampleScenarioInstanceContainedInstanceComponent(); 1823 copyValues(dst); 1824 return dst; 1825 } 1826 1827 public void copyValues(ExampleScenarioInstanceContainedInstanceComponent dst) { 1828 super.copyValues(dst); 1829 dst.instanceReference = instanceReference == null ? null : instanceReference.copy(); 1830 dst.versionReference = versionReference == null ? null : versionReference.copy(); 1831 } 1832 1833 @Override 1834 public boolean equalsDeep(Base other_) { 1835 if (!super.equalsDeep(other_)) 1836 return false; 1837 if (!(other_ instanceof ExampleScenarioInstanceContainedInstanceComponent)) 1838 return false; 1839 ExampleScenarioInstanceContainedInstanceComponent o = (ExampleScenarioInstanceContainedInstanceComponent) other_; 1840 return compareDeep(instanceReference, o.instanceReference, true) && compareDeep(versionReference, o.versionReference, true) 1841 ; 1842 } 1843 1844 @Override 1845 public boolean equalsShallow(Base other_) { 1846 if (!super.equalsShallow(other_)) 1847 return false; 1848 if (!(other_ instanceof ExampleScenarioInstanceContainedInstanceComponent)) 1849 return false; 1850 ExampleScenarioInstanceContainedInstanceComponent o = (ExampleScenarioInstanceContainedInstanceComponent) other_; 1851 return compareValues(instanceReference, o.instanceReference, true) && compareValues(versionReference, o.versionReference, true) 1852 ; 1853 } 1854 1855 public boolean isEmpty() { 1856 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(instanceReference, versionReference 1857 ); 1858 } 1859 1860 public String fhirType() { 1861 return "ExampleScenario.instance.containedInstance"; 1862 1863 } 1864 1865 } 1866 1867 @Block() 1868 public static class ExampleScenarioProcessComponent extends BackboneElement implements IBaseBackboneElement { 1869 /** 1870 * A short descriptive label the process to be used in tables or diagrams. 1871 */ 1872 @Child(name = "title", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1873 @Description(shortDefinition="Label for procss", formalDefinition="A short descriptive label the process to be used in tables or diagrams." ) 1874 protected StringType title; 1875 1876 /** 1877 * An explanation of what the process represents and what it does. 1878 */ 1879 @Child(name = "description", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1880 @Description(shortDefinition="Human-friendly description of the process", formalDefinition="An explanation of what the process represents and what it does." ) 1881 protected MarkdownType description; 1882 1883 /** 1884 * Description of the initial state of the actors, environment and data before the process starts. 1885 */ 1886 @Child(name = "preConditions", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1887 @Description(shortDefinition="Status before process starts", formalDefinition="Description of the initial state of the actors, environment and data before the process starts." ) 1888 protected MarkdownType preConditions; 1889 1890 /** 1891 * Description of the final state of the actors, environment and data after the process has been successfully completed. 1892 */ 1893 @Child(name = "postConditions", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1894 @Description(shortDefinition="Status after successful completion", formalDefinition="Description of the final state of the actors, environment and data after the process has been successfully completed." ) 1895 protected MarkdownType postConditions; 1896 1897 /** 1898 * A significant action that occurs as part of the process. 1899 */ 1900 @Child(name = "step", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1901 @Description(shortDefinition="Event within of the process", formalDefinition="A significant action that occurs as part of the process." ) 1902 protected List<ExampleScenarioProcessStepComponent> step; 1903 1904 private static final long serialVersionUID = 325578043L; 1905 1906 /** 1907 * Constructor 1908 */ 1909 public ExampleScenarioProcessComponent() { 1910 super(); 1911 } 1912 1913 /** 1914 * Constructor 1915 */ 1916 public ExampleScenarioProcessComponent(String title) { 1917 super(); 1918 this.setTitle(title); 1919 } 1920 1921 /** 1922 * @return {@link #title} (A short descriptive label the process to be used in tables or diagrams.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1923 */ 1924 public StringType getTitleElement() { 1925 if (this.title == null) 1926 if (Configuration.errorOnAutoCreate()) 1927 throw new Error("Attempt to auto-create ExampleScenarioProcessComponent.title"); 1928 else if (Configuration.doAutoCreate()) 1929 this.title = new StringType(); // bb 1930 return this.title; 1931 } 1932 1933 public boolean hasTitleElement() { 1934 return this.title != null && !this.title.isEmpty(); 1935 } 1936 1937 public boolean hasTitle() { 1938 return this.title != null && !this.title.isEmpty(); 1939 } 1940 1941 /** 1942 * @param value {@link #title} (A short descriptive label the process to be used in tables or diagrams.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1943 */ 1944 public ExampleScenarioProcessComponent setTitleElement(StringType value) { 1945 this.title = value; 1946 return this; 1947 } 1948 1949 /** 1950 * @return A short descriptive label the process to be used in tables or diagrams. 1951 */ 1952 public String getTitle() { 1953 return this.title == null ? null : this.title.getValue(); 1954 } 1955 1956 /** 1957 * @param value A short descriptive label the process to be used in tables or diagrams. 1958 */ 1959 public ExampleScenarioProcessComponent setTitle(String value) { 1960 if (this.title == null) 1961 this.title = new StringType(); 1962 this.title.setValue(value); 1963 return this; 1964 } 1965 1966 /** 1967 * @return {@link #description} (An explanation of what the process represents and what it does.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1968 */ 1969 public MarkdownType getDescriptionElement() { 1970 if (this.description == null) 1971 if (Configuration.errorOnAutoCreate()) 1972 throw new Error("Attempt to auto-create ExampleScenarioProcessComponent.description"); 1973 else if (Configuration.doAutoCreate()) 1974 this.description = new MarkdownType(); // bb 1975 return this.description; 1976 } 1977 1978 public boolean hasDescriptionElement() { 1979 return this.description != null && !this.description.isEmpty(); 1980 } 1981 1982 public boolean hasDescription() { 1983 return this.description != null && !this.description.isEmpty(); 1984 } 1985 1986 /** 1987 * @param value {@link #description} (An explanation of what the process represents and what it does.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1988 */ 1989 public ExampleScenarioProcessComponent setDescriptionElement(MarkdownType value) { 1990 this.description = value; 1991 return this; 1992 } 1993 1994 /** 1995 * @return An explanation of what the process represents and what it does. 1996 */ 1997 public String getDescription() { 1998 return this.description == null ? null : this.description.getValue(); 1999 } 2000 2001 /** 2002 * @param value An explanation of what the process represents and what it does. 2003 */ 2004 public ExampleScenarioProcessComponent setDescription(String value) { 2005 if (Utilities.noString(value)) 2006 this.description = null; 2007 else { 2008 if (this.description == null) 2009 this.description = new MarkdownType(); 2010 this.description.setValue(value); 2011 } 2012 return this; 2013 } 2014 2015 /** 2016 * @return {@link #preConditions} (Description of the initial state of the actors, environment and data before the process starts.). This is the underlying object with id, value and extensions. The accessor "getPreConditions" gives direct access to the value 2017 */ 2018 public MarkdownType getPreConditionsElement() { 2019 if (this.preConditions == null) 2020 if (Configuration.errorOnAutoCreate()) 2021 throw new Error("Attempt to auto-create ExampleScenarioProcessComponent.preConditions"); 2022 else if (Configuration.doAutoCreate()) 2023 this.preConditions = new MarkdownType(); // bb 2024 return this.preConditions; 2025 } 2026 2027 public boolean hasPreConditionsElement() { 2028 return this.preConditions != null && !this.preConditions.isEmpty(); 2029 } 2030 2031 public boolean hasPreConditions() { 2032 return this.preConditions != null && !this.preConditions.isEmpty(); 2033 } 2034 2035 /** 2036 * @param value {@link #preConditions} (Description of the initial state of the actors, environment and data before the process starts.). This is the underlying object with id, value and extensions. The accessor "getPreConditions" gives direct access to the value 2037 */ 2038 public ExampleScenarioProcessComponent setPreConditionsElement(MarkdownType value) { 2039 this.preConditions = value; 2040 return this; 2041 } 2042 2043 /** 2044 * @return Description of the initial state of the actors, environment and data before the process starts. 2045 */ 2046 public String getPreConditions() { 2047 return this.preConditions == null ? null : this.preConditions.getValue(); 2048 } 2049 2050 /** 2051 * @param value Description of the initial state of the actors, environment and data before the process starts. 2052 */ 2053 public ExampleScenarioProcessComponent setPreConditions(String value) { 2054 if (Utilities.noString(value)) 2055 this.preConditions = null; 2056 else { 2057 if (this.preConditions == null) 2058 this.preConditions = new MarkdownType(); 2059 this.preConditions.setValue(value); 2060 } 2061 return this; 2062 } 2063 2064 /** 2065 * @return {@link #postConditions} (Description of the final state of the actors, environment and data after the process has been successfully completed.). This is the underlying object with id, value and extensions. The accessor "getPostConditions" gives direct access to the value 2066 */ 2067 public MarkdownType getPostConditionsElement() { 2068 if (this.postConditions == null) 2069 if (Configuration.errorOnAutoCreate()) 2070 throw new Error("Attempt to auto-create ExampleScenarioProcessComponent.postConditions"); 2071 else if (Configuration.doAutoCreate()) 2072 this.postConditions = new MarkdownType(); // bb 2073 return this.postConditions; 2074 } 2075 2076 public boolean hasPostConditionsElement() { 2077 return this.postConditions != null && !this.postConditions.isEmpty(); 2078 } 2079 2080 public boolean hasPostConditions() { 2081 return this.postConditions != null && !this.postConditions.isEmpty(); 2082 } 2083 2084 /** 2085 * @param value {@link #postConditions} (Description of the final state of the actors, environment and data after the process has been successfully completed.). This is the underlying object with id, value and extensions. The accessor "getPostConditions" gives direct access to the value 2086 */ 2087 public ExampleScenarioProcessComponent setPostConditionsElement(MarkdownType value) { 2088 this.postConditions = value; 2089 return this; 2090 } 2091 2092 /** 2093 * @return Description of the final state of the actors, environment and data after the process has been successfully completed. 2094 */ 2095 public String getPostConditions() { 2096 return this.postConditions == null ? null : this.postConditions.getValue(); 2097 } 2098 2099 /** 2100 * @param value Description of the final state of the actors, environment and data after the process has been successfully completed. 2101 */ 2102 public ExampleScenarioProcessComponent setPostConditions(String value) { 2103 if (Utilities.noString(value)) 2104 this.postConditions = null; 2105 else { 2106 if (this.postConditions == null) 2107 this.postConditions = new MarkdownType(); 2108 this.postConditions.setValue(value); 2109 } 2110 return this; 2111 } 2112 2113 /** 2114 * @return {@link #step} (A significant action that occurs as part of the process.) 2115 */ 2116 public List<ExampleScenarioProcessStepComponent> getStep() { 2117 if (this.step == null) 2118 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 2119 return this.step; 2120 } 2121 2122 /** 2123 * @return Returns a reference to <code>this</code> for easy method chaining 2124 */ 2125 public ExampleScenarioProcessComponent setStep(List<ExampleScenarioProcessStepComponent> theStep) { 2126 this.step = theStep; 2127 return this; 2128 } 2129 2130 public boolean hasStep() { 2131 if (this.step == null) 2132 return false; 2133 for (ExampleScenarioProcessStepComponent item : this.step) 2134 if (!item.isEmpty()) 2135 return true; 2136 return false; 2137 } 2138 2139 public ExampleScenarioProcessStepComponent addStep() { //3 2140 ExampleScenarioProcessStepComponent t = new ExampleScenarioProcessStepComponent(); 2141 if (this.step == null) 2142 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 2143 this.step.add(t); 2144 return t; 2145 } 2146 2147 public ExampleScenarioProcessComponent addStep(ExampleScenarioProcessStepComponent t) { //3 2148 if (t == null) 2149 return this; 2150 if (this.step == null) 2151 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 2152 this.step.add(t); 2153 return this; 2154 } 2155 2156 /** 2157 * @return The first repetition of repeating field {@link #step}, creating it if it does not already exist {3} 2158 */ 2159 public ExampleScenarioProcessStepComponent getStepFirstRep() { 2160 if (getStep().isEmpty()) { 2161 addStep(); 2162 } 2163 return getStep().get(0); 2164 } 2165 2166 protected void listChildren(List<Property> children) { 2167 super.listChildren(children); 2168 children.add(new Property("title", "string", "A short descriptive label the process to be used in tables or diagrams.", 0, 1, title)); 2169 children.add(new Property("description", "markdown", "An explanation of what the process represents and what it does.", 0, 1, description)); 2170 children.add(new Property("preConditions", "markdown", "Description of the initial state of the actors, environment and data before the process starts.", 0, 1, preConditions)); 2171 children.add(new Property("postConditions", "markdown", "Description of the final state of the actors, environment and data after the process has been successfully completed.", 0, 1, postConditions)); 2172 children.add(new Property("step", "", "A significant action that occurs as part of the process.", 0, java.lang.Integer.MAX_VALUE, step)); 2173 } 2174 2175 @Override 2176 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2177 switch (_hash) { 2178 case 110371416: /*title*/ return new Property("title", "string", "A short descriptive label the process to be used in tables or diagrams.", 0, 1, title); 2179 case -1724546052: /*description*/ return new Property("description", "markdown", "An explanation of what the process represents and what it does.", 0, 1, description); 2180 case -1006692933: /*preConditions*/ return new Property("preConditions", "markdown", "Description of the initial state of the actors, environment and data before the process starts.", 0, 1, preConditions); 2181 case 1738302328: /*postConditions*/ return new Property("postConditions", "markdown", "Description of the final state of the actors, environment and data after the process has been successfully completed.", 0, 1, postConditions); 2182 case 3540684: /*step*/ return new Property("step", "", "A significant action that occurs as part of the process.", 0, java.lang.Integer.MAX_VALUE, step); 2183 default: return super.getNamedProperty(_hash, _name, _checkValid); 2184 } 2185 2186 } 2187 2188 @Override 2189 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2190 switch (hash) { 2191 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2192 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2193 case -1006692933: /*preConditions*/ return this.preConditions == null ? new Base[0] : new Base[] {this.preConditions}; // MarkdownType 2194 case 1738302328: /*postConditions*/ return this.postConditions == null ? new Base[0] : new Base[] {this.postConditions}; // MarkdownType 2195 case 3540684: /*step*/ return this.step == null ? new Base[0] : this.step.toArray(new Base[this.step.size()]); // ExampleScenarioProcessStepComponent 2196 default: return super.getProperty(hash, name, checkValid); 2197 } 2198 2199 } 2200 2201 @Override 2202 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2203 switch (hash) { 2204 case 110371416: // title 2205 this.title = TypeConvertor.castToString(value); // StringType 2206 return value; 2207 case -1724546052: // description 2208 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2209 return value; 2210 case -1006692933: // preConditions 2211 this.preConditions = TypeConvertor.castToMarkdown(value); // MarkdownType 2212 return value; 2213 case 1738302328: // postConditions 2214 this.postConditions = TypeConvertor.castToMarkdown(value); // MarkdownType 2215 return value; 2216 case 3540684: // step 2217 this.getStep().add((ExampleScenarioProcessStepComponent) value); // ExampleScenarioProcessStepComponent 2218 return value; 2219 default: return super.setProperty(hash, name, value); 2220 } 2221 2222 } 2223 2224 @Override 2225 public Base setProperty(String name, Base value) throws FHIRException { 2226 if (name.equals("title")) { 2227 this.title = TypeConvertor.castToString(value); // StringType 2228 } else if (name.equals("description")) { 2229 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2230 } else if (name.equals("preConditions")) { 2231 this.preConditions = TypeConvertor.castToMarkdown(value); // MarkdownType 2232 } else if (name.equals("postConditions")) { 2233 this.postConditions = TypeConvertor.castToMarkdown(value); // MarkdownType 2234 } else if (name.equals("step")) { 2235 this.getStep().add((ExampleScenarioProcessStepComponent) value); 2236 } else 2237 return super.setProperty(name, value); 2238 return value; 2239 } 2240 2241 @Override 2242 public void removeChild(String name, Base value) throws FHIRException { 2243 if (name.equals("title")) { 2244 this.title = null; 2245 } else if (name.equals("description")) { 2246 this.description = null; 2247 } else if (name.equals("preConditions")) { 2248 this.preConditions = null; 2249 } else if (name.equals("postConditions")) { 2250 this.postConditions = null; 2251 } else if (name.equals("step")) { 2252 this.getStep().remove((ExampleScenarioProcessStepComponent) value); 2253 } else 2254 super.removeChild(name, value); 2255 2256 } 2257 2258 @Override 2259 public Base makeProperty(int hash, String name) throws FHIRException { 2260 switch (hash) { 2261 case 110371416: return getTitleElement(); 2262 case -1724546052: return getDescriptionElement(); 2263 case -1006692933: return getPreConditionsElement(); 2264 case 1738302328: return getPostConditionsElement(); 2265 case 3540684: return addStep(); 2266 default: return super.makeProperty(hash, name); 2267 } 2268 2269 } 2270 2271 @Override 2272 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2273 switch (hash) { 2274 case 110371416: /*title*/ return new String[] {"string"}; 2275 case -1724546052: /*description*/ return new String[] {"markdown"}; 2276 case -1006692933: /*preConditions*/ return new String[] {"markdown"}; 2277 case 1738302328: /*postConditions*/ return new String[] {"markdown"}; 2278 case 3540684: /*step*/ return new String[] {}; 2279 default: return super.getTypesForProperty(hash, name); 2280 } 2281 2282 } 2283 2284 @Override 2285 public Base addChild(String name) throws FHIRException { 2286 if (name.equals("title")) { 2287 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.process.title"); 2288 } 2289 else if (name.equals("description")) { 2290 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.process.description"); 2291 } 2292 else if (name.equals("preConditions")) { 2293 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.process.preConditions"); 2294 } 2295 else if (name.equals("postConditions")) { 2296 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.process.postConditions"); 2297 } 2298 else if (name.equals("step")) { 2299 return addStep(); 2300 } 2301 else 2302 return super.addChild(name); 2303 } 2304 2305 public ExampleScenarioProcessComponent copy() { 2306 ExampleScenarioProcessComponent dst = new ExampleScenarioProcessComponent(); 2307 copyValues(dst); 2308 return dst; 2309 } 2310 2311 public void copyValues(ExampleScenarioProcessComponent dst) { 2312 super.copyValues(dst); 2313 dst.title = title == null ? null : title.copy(); 2314 dst.description = description == null ? null : description.copy(); 2315 dst.preConditions = preConditions == null ? null : preConditions.copy(); 2316 dst.postConditions = postConditions == null ? null : postConditions.copy(); 2317 if (step != null) { 2318 dst.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 2319 for (ExampleScenarioProcessStepComponent i : step) 2320 dst.step.add(i.copy()); 2321 }; 2322 } 2323 2324 @Override 2325 public boolean equalsDeep(Base other_) { 2326 if (!super.equalsDeep(other_)) 2327 return false; 2328 if (!(other_ instanceof ExampleScenarioProcessComponent)) 2329 return false; 2330 ExampleScenarioProcessComponent o = (ExampleScenarioProcessComponent) other_; 2331 return compareDeep(title, o.title, true) && compareDeep(description, o.description, true) && compareDeep(preConditions, o.preConditions, true) 2332 && compareDeep(postConditions, o.postConditions, true) && compareDeep(step, o.step, true); 2333 } 2334 2335 @Override 2336 public boolean equalsShallow(Base other_) { 2337 if (!super.equalsShallow(other_)) 2338 return false; 2339 if (!(other_ instanceof ExampleScenarioProcessComponent)) 2340 return false; 2341 ExampleScenarioProcessComponent o = (ExampleScenarioProcessComponent) other_; 2342 return compareValues(title, o.title, true) && compareValues(description, o.description, true) && compareValues(preConditions, o.preConditions, true) 2343 && compareValues(postConditions, o.postConditions, true); 2344 } 2345 2346 public boolean isEmpty() { 2347 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(title, description, preConditions 2348 , postConditions, step); 2349 } 2350 2351 public String fhirType() { 2352 return "ExampleScenario.process"; 2353 2354 } 2355 2356 } 2357 2358 @Block() 2359 public static class ExampleScenarioProcessStepComponent extends BackboneElement implements IBaseBackboneElement { 2360 /** 2361 * The sequential number of the step, e.g. 1.2.5. 2362 */ 2363 @Child(name = "number", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2364 @Description(shortDefinition="Sequential number of the step", formalDefinition="The sequential number of the step, e.g. 1.2.5." ) 2365 protected StringType number; 2366 2367 /** 2368 * Indicates that the step is a complex sub-process with its own steps. 2369 */ 2370 @Child(name = "process", type = {ExampleScenarioProcessComponent.class}, order=2, min=0, max=1, modifier=false, summary=false) 2371 @Description(shortDefinition="Step is nested process", formalDefinition="Indicates that the step is a complex sub-process with its own steps." ) 2372 protected ExampleScenarioProcessComponent process; 2373 2374 /** 2375 * Indicates that the step is defined by a seaparate scenario instance. 2376 */ 2377 @Child(name = "workflow", type = {CanonicalType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2378 @Description(shortDefinition="Step is nested workflow", formalDefinition="Indicates that the step is defined by a seaparate scenario instance." ) 2379 protected CanonicalType workflow; 2380 2381 /** 2382 * The step represents a single operation invoked on receiver by sender. 2383 */ 2384 @Child(name = "operation", type = {}, order=4, min=0, max=1, modifier=false, summary=false) 2385 @Description(shortDefinition="Step is simple action", formalDefinition="The step represents a single operation invoked on receiver by sender." ) 2386 protected ExampleScenarioProcessStepOperationComponent operation; 2387 2388 /** 2389 * Indicates an alternative step that can be taken instead of the sub-process, scenario or operation. E.g. to represent non-happy-path/exceptional/atypical circumstances. 2390 */ 2391 @Child(name = "alternative", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2392 @Description(shortDefinition="Alternate non-typical step action", formalDefinition="Indicates an alternative step that can be taken instead of the sub-process, scenario or operation. E.g. to represent non-happy-path/exceptional/atypical circumstances." ) 2393 protected List<ExampleScenarioProcessStepAlternativeComponent> alternative; 2394 2395 /** 2396 * If true, indicates that, following this step, there is a pause in the flow and the subsequent step will occur at some later time (triggered by some event). 2397 */ 2398 @Child(name = "pause", type = {BooleanType.class}, order=6, min=0, max=1, modifier=false, summary=false) 2399 @Description(shortDefinition="Pause in the flow?", formalDefinition="If true, indicates that, following this step, there is a pause in the flow and the subsequent step will occur at some later time (triggered by some event)." ) 2400 protected BooleanType pause; 2401 2402 private static final long serialVersionUID = 674607242L; 2403 2404 /** 2405 * Constructor 2406 */ 2407 public ExampleScenarioProcessStepComponent() { 2408 super(); 2409 } 2410 2411 /** 2412 * @return {@link #number} (The sequential number of the step, e.g. 1.2.5.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 2413 */ 2414 public StringType getNumberElement() { 2415 if (this.number == null) 2416 if (Configuration.errorOnAutoCreate()) 2417 throw new Error("Attempt to auto-create ExampleScenarioProcessStepComponent.number"); 2418 else if (Configuration.doAutoCreate()) 2419 this.number = new StringType(); // bb 2420 return this.number; 2421 } 2422 2423 public boolean hasNumberElement() { 2424 return this.number != null && !this.number.isEmpty(); 2425 } 2426 2427 public boolean hasNumber() { 2428 return this.number != null && !this.number.isEmpty(); 2429 } 2430 2431 /** 2432 * @param value {@link #number} (The sequential number of the step, e.g. 1.2.5.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 2433 */ 2434 public ExampleScenarioProcessStepComponent setNumberElement(StringType value) { 2435 this.number = value; 2436 return this; 2437 } 2438 2439 /** 2440 * @return The sequential number of the step, e.g. 1.2.5. 2441 */ 2442 public String getNumber() { 2443 return this.number == null ? null : this.number.getValue(); 2444 } 2445 2446 /** 2447 * @param value The sequential number of the step, e.g. 1.2.5. 2448 */ 2449 public ExampleScenarioProcessStepComponent setNumber(String value) { 2450 if (Utilities.noString(value)) 2451 this.number = null; 2452 else { 2453 if (this.number == null) 2454 this.number = new StringType(); 2455 this.number.setValue(value); 2456 } 2457 return this; 2458 } 2459 2460 /** 2461 * @return {@link #process} (Indicates that the step is a complex sub-process with its own steps.) 2462 */ 2463 public ExampleScenarioProcessComponent getProcess() { 2464 if (this.process == null) 2465 if (Configuration.errorOnAutoCreate()) 2466 throw new Error("Attempt to auto-create ExampleScenarioProcessStepComponent.process"); 2467 else if (Configuration.doAutoCreate()) 2468 this.process = new ExampleScenarioProcessComponent(); // cc 2469 return this.process; 2470 } 2471 2472 public boolean hasProcess() { 2473 return this.process != null && !this.process.isEmpty(); 2474 } 2475 2476 /** 2477 * @param value {@link #process} (Indicates that the step is a complex sub-process with its own steps.) 2478 */ 2479 public ExampleScenarioProcessStepComponent setProcess(ExampleScenarioProcessComponent value) { 2480 this.process = value; 2481 return this; 2482 } 2483 2484 /** 2485 * @return {@link #workflow} (Indicates that the step is defined by a seaparate scenario instance.). This is the underlying object with id, value and extensions. The accessor "getWorkflow" gives direct access to the value 2486 */ 2487 public CanonicalType getWorkflowElement() { 2488 if (this.workflow == null) 2489 if (Configuration.errorOnAutoCreate()) 2490 throw new Error("Attempt to auto-create ExampleScenarioProcessStepComponent.workflow"); 2491 else if (Configuration.doAutoCreate()) 2492 this.workflow = new CanonicalType(); // bb 2493 return this.workflow; 2494 } 2495 2496 public boolean hasWorkflowElement() { 2497 return this.workflow != null && !this.workflow.isEmpty(); 2498 } 2499 2500 public boolean hasWorkflow() { 2501 return this.workflow != null && !this.workflow.isEmpty(); 2502 } 2503 2504 /** 2505 * @param value {@link #workflow} (Indicates that the step is defined by a seaparate scenario instance.). This is the underlying object with id, value and extensions. The accessor "getWorkflow" gives direct access to the value 2506 */ 2507 public ExampleScenarioProcessStepComponent setWorkflowElement(CanonicalType value) { 2508 this.workflow = value; 2509 return this; 2510 } 2511 2512 /** 2513 * @return Indicates that the step is defined by a seaparate scenario instance. 2514 */ 2515 public String getWorkflow() { 2516 return this.workflow == null ? null : this.workflow.getValue(); 2517 } 2518 2519 /** 2520 * @param value Indicates that the step is defined by a seaparate scenario instance. 2521 */ 2522 public ExampleScenarioProcessStepComponent setWorkflow(String value) { 2523 if (Utilities.noString(value)) 2524 this.workflow = null; 2525 else { 2526 if (this.workflow == null) 2527 this.workflow = new CanonicalType(); 2528 this.workflow.setValue(value); 2529 } 2530 return this; 2531 } 2532 2533 /** 2534 * @return {@link #operation} (The step represents a single operation invoked on receiver by sender.) 2535 */ 2536 public ExampleScenarioProcessStepOperationComponent getOperation() { 2537 if (this.operation == null) 2538 if (Configuration.errorOnAutoCreate()) 2539 throw new Error("Attempt to auto-create ExampleScenarioProcessStepComponent.operation"); 2540 else if (Configuration.doAutoCreate()) 2541 this.operation = new ExampleScenarioProcessStepOperationComponent(); // cc 2542 return this.operation; 2543 } 2544 2545 public boolean hasOperation() { 2546 return this.operation != null && !this.operation.isEmpty(); 2547 } 2548 2549 /** 2550 * @param value {@link #operation} (The step represents a single operation invoked on receiver by sender.) 2551 */ 2552 public ExampleScenarioProcessStepComponent setOperation(ExampleScenarioProcessStepOperationComponent value) { 2553 this.operation = value; 2554 return this; 2555 } 2556 2557 /** 2558 * @return {@link #alternative} (Indicates an alternative step that can be taken instead of the sub-process, scenario or operation. E.g. to represent non-happy-path/exceptional/atypical circumstances.) 2559 */ 2560 public List<ExampleScenarioProcessStepAlternativeComponent> getAlternative() { 2561 if (this.alternative == null) 2562 this.alternative = new ArrayList<ExampleScenarioProcessStepAlternativeComponent>(); 2563 return this.alternative; 2564 } 2565 2566 /** 2567 * @return Returns a reference to <code>this</code> for easy method chaining 2568 */ 2569 public ExampleScenarioProcessStepComponent setAlternative(List<ExampleScenarioProcessStepAlternativeComponent> theAlternative) { 2570 this.alternative = theAlternative; 2571 return this; 2572 } 2573 2574 public boolean hasAlternative() { 2575 if (this.alternative == null) 2576 return false; 2577 for (ExampleScenarioProcessStepAlternativeComponent item : this.alternative) 2578 if (!item.isEmpty()) 2579 return true; 2580 return false; 2581 } 2582 2583 public ExampleScenarioProcessStepAlternativeComponent addAlternative() { //3 2584 ExampleScenarioProcessStepAlternativeComponent t = new ExampleScenarioProcessStepAlternativeComponent(); 2585 if (this.alternative == null) 2586 this.alternative = new ArrayList<ExampleScenarioProcessStepAlternativeComponent>(); 2587 this.alternative.add(t); 2588 return t; 2589 } 2590 2591 public ExampleScenarioProcessStepComponent addAlternative(ExampleScenarioProcessStepAlternativeComponent t) { //3 2592 if (t == null) 2593 return this; 2594 if (this.alternative == null) 2595 this.alternative = new ArrayList<ExampleScenarioProcessStepAlternativeComponent>(); 2596 this.alternative.add(t); 2597 return this; 2598 } 2599 2600 /** 2601 * @return The first repetition of repeating field {@link #alternative}, creating it if it does not already exist {3} 2602 */ 2603 public ExampleScenarioProcessStepAlternativeComponent getAlternativeFirstRep() { 2604 if (getAlternative().isEmpty()) { 2605 addAlternative(); 2606 } 2607 return getAlternative().get(0); 2608 } 2609 2610 /** 2611 * @return {@link #pause} (If true, indicates that, following this step, there is a pause in the flow and the subsequent step will occur at some later time (triggered by some event).). This is the underlying object with id, value and extensions. The accessor "getPause" gives direct access to the value 2612 */ 2613 public BooleanType getPauseElement() { 2614 if (this.pause == null) 2615 if (Configuration.errorOnAutoCreate()) 2616 throw new Error("Attempt to auto-create ExampleScenarioProcessStepComponent.pause"); 2617 else if (Configuration.doAutoCreate()) 2618 this.pause = new BooleanType(); // bb 2619 return this.pause; 2620 } 2621 2622 public boolean hasPauseElement() { 2623 return this.pause != null && !this.pause.isEmpty(); 2624 } 2625 2626 public boolean hasPause() { 2627 return this.pause != null && !this.pause.isEmpty(); 2628 } 2629 2630 /** 2631 * @param value {@link #pause} (If true, indicates that, following this step, there is a pause in the flow and the subsequent step will occur at some later time (triggered by some event).). This is the underlying object with id, value and extensions. The accessor "getPause" gives direct access to the value 2632 */ 2633 public ExampleScenarioProcessStepComponent setPauseElement(BooleanType value) { 2634 this.pause = value; 2635 return this; 2636 } 2637 2638 /** 2639 * @return If true, indicates that, following this step, there is a pause in the flow and the subsequent step will occur at some later time (triggered by some event). 2640 */ 2641 public boolean getPause() { 2642 return this.pause == null || this.pause.isEmpty() ? false : this.pause.getValue(); 2643 } 2644 2645 /** 2646 * @param value If true, indicates that, following this step, there is a pause in the flow and the subsequent step will occur at some later time (triggered by some event). 2647 */ 2648 public ExampleScenarioProcessStepComponent setPause(boolean value) { 2649 if (this.pause == null) 2650 this.pause = new BooleanType(); 2651 this.pause.setValue(value); 2652 return this; 2653 } 2654 2655 protected void listChildren(List<Property> children) { 2656 super.listChildren(children); 2657 children.add(new Property("number", "string", "The sequential number of the step, e.g. 1.2.5.", 0, 1, number)); 2658 children.add(new Property("process", "@ExampleScenario.process", "Indicates that the step is a complex sub-process with its own steps.", 0, 1, process)); 2659 children.add(new Property("workflow", "canonical(ExampleScenario)", "Indicates that the step is defined by a seaparate scenario instance.", 0, 1, workflow)); 2660 children.add(new Property("operation", "", "The step represents a single operation invoked on receiver by sender.", 0, 1, operation)); 2661 children.add(new Property("alternative", "", "Indicates an alternative step that can be taken instead of the sub-process, scenario or operation. E.g. to represent non-happy-path/exceptional/atypical circumstances.", 0, java.lang.Integer.MAX_VALUE, alternative)); 2662 children.add(new Property("pause", "boolean", "If true, indicates that, following this step, there is a pause in the flow and the subsequent step will occur at some later time (triggered by some event).", 0, 1, pause)); 2663 } 2664 2665 @Override 2666 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2667 switch (_hash) { 2668 case -1034364087: /*number*/ return new Property("number", "string", "The sequential number of the step, e.g. 1.2.5.", 0, 1, number); 2669 case -309518737: /*process*/ return new Property("process", "@ExampleScenario.process", "Indicates that the step is a complex sub-process with its own steps.", 0, 1, process); 2670 case 35379135: /*workflow*/ return new Property("workflow", "canonical(ExampleScenario)", "Indicates that the step is defined by a seaparate scenario instance.", 0, 1, workflow); 2671 case 1662702951: /*operation*/ return new Property("operation", "", "The step represents a single operation invoked on receiver by sender.", 0, 1, operation); 2672 case -196794451: /*alternative*/ return new Property("alternative", "", "Indicates an alternative step that can be taken instead of the sub-process, scenario or operation. E.g. to represent non-happy-path/exceptional/atypical circumstances.", 0, java.lang.Integer.MAX_VALUE, alternative); 2673 case 106440182: /*pause*/ return new Property("pause", "boolean", "If true, indicates that, following this step, there is a pause in the flow and the subsequent step will occur at some later time (triggered by some event).", 0, 1, pause); 2674 default: return super.getNamedProperty(_hash, _name, _checkValid); 2675 } 2676 2677 } 2678 2679 @Override 2680 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2681 switch (hash) { 2682 case -1034364087: /*number*/ return this.number == null ? new Base[0] : new Base[] {this.number}; // StringType 2683 case -309518737: /*process*/ return this.process == null ? new Base[0] : new Base[] {this.process}; // ExampleScenarioProcessComponent 2684 case 35379135: /*workflow*/ return this.workflow == null ? new Base[0] : new Base[] {this.workflow}; // CanonicalType 2685 case 1662702951: /*operation*/ return this.operation == null ? new Base[0] : new Base[] {this.operation}; // ExampleScenarioProcessStepOperationComponent 2686 case -196794451: /*alternative*/ return this.alternative == null ? new Base[0] : this.alternative.toArray(new Base[this.alternative.size()]); // ExampleScenarioProcessStepAlternativeComponent 2687 case 106440182: /*pause*/ return this.pause == null ? new Base[0] : new Base[] {this.pause}; // BooleanType 2688 default: return super.getProperty(hash, name, checkValid); 2689 } 2690 2691 } 2692 2693 @Override 2694 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2695 switch (hash) { 2696 case -1034364087: // number 2697 this.number = TypeConvertor.castToString(value); // StringType 2698 return value; 2699 case -309518737: // process 2700 this.process = (ExampleScenarioProcessComponent) value; // ExampleScenarioProcessComponent 2701 return value; 2702 case 35379135: // workflow 2703 this.workflow = TypeConvertor.castToCanonical(value); // CanonicalType 2704 return value; 2705 case 1662702951: // operation 2706 this.operation = (ExampleScenarioProcessStepOperationComponent) value; // ExampleScenarioProcessStepOperationComponent 2707 return value; 2708 case -196794451: // alternative 2709 this.getAlternative().add((ExampleScenarioProcessStepAlternativeComponent) value); // ExampleScenarioProcessStepAlternativeComponent 2710 return value; 2711 case 106440182: // pause 2712 this.pause = TypeConvertor.castToBoolean(value); // BooleanType 2713 return value; 2714 default: return super.setProperty(hash, name, value); 2715 } 2716 2717 } 2718 2719 @Override 2720 public Base setProperty(String name, Base value) throws FHIRException { 2721 if (name.equals("number")) { 2722 this.number = TypeConvertor.castToString(value); // StringType 2723 } else if (name.equals("process")) { 2724 this.process = (ExampleScenarioProcessComponent) value; // ExampleScenarioProcessComponent 2725 } else if (name.equals("workflow")) { 2726 this.workflow = TypeConvertor.castToCanonical(value); // CanonicalType 2727 } else if (name.equals("operation")) { 2728 this.operation = (ExampleScenarioProcessStepOperationComponent) value; // ExampleScenarioProcessStepOperationComponent 2729 } else if (name.equals("alternative")) { 2730 this.getAlternative().add((ExampleScenarioProcessStepAlternativeComponent) value); 2731 } else if (name.equals("pause")) { 2732 this.pause = TypeConvertor.castToBoolean(value); // BooleanType 2733 } else 2734 return super.setProperty(name, value); 2735 return value; 2736 } 2737 2738 @Override 2739 public void removeChild(String name, Base value) throws FHIRException { 2740 if (name.equals("number")) { 2741 this.number = null; 2742 } else if (name.equals("process")) { 2743 this.process = (ExampleScenarioProcessComponent) value; // ExampleScenarioProcessComponent 2744 } else if (name.equals("workflow")) { 2745 this.workflow = null; 2746 } else if (name.equals("operation")) { 2747 this.operation = (ExampleScenarioProcessStepOperationComponent) value; // ExampleScenarioProcessStepOperationComponent 2748 } else if (name.equals("alternative")) { 2749 this.getAlternative().remove((ExampleScenarioProcessStepAlternativeComponent) value); 2750 } else if (name.equals("pause")) { 2751 this.pause = null; 2752 } else 2753 super.removeChild(name, value); 2754 2755 } 2756 2757 @Override 2758 public Base makeProperty(int hash, String name) throws FHIRException { 2759 switch (hash) { 2760 case -1034364087: return getNumberElement(); 2761 case -309518737: return getProcess(); 2762 case 35379135: return getWorkflowElement(); 2763 case 1662702951: return getOperation(); 2764 case -196794451: return addAlternative(); 2765 case 106440182: return getPauseElement(); 2766 default: return super.makeProperty(hash, name); 2767 } 2768 2769 } 2770 2771 @Override 2772 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2773 switch (hash) { 2774 case -1034364087: /*number*/ return new String[] {"string"}; 2775 case -309518737: /*process*/ return new String[] {"@ExampleScenario.process"}; 2776 case 35379135: /*workflow*/ return new String[] {"canonical"}; 2777 case 1662702951: /*operation*/ return new String[] {}; 2778 case -196794451: /*alternative*/ return new String[] {}; 2779 case 106440182: /*pause*/ return new String[] {"boolean"}; 2780 default: return super.getTypesForProperty(hash, name); 2781 } 2782 2783 } 2784 2785 @Override 2786 public Base addChild(String name) throws FHIRException { 2787 if (name.equals("number")) { 2788 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.process.step.number"); 2789 } 2790 else if (name.equals("process")) { 2791 this.process = new ExampleScenarioProcessComponent(); 2792 return this.process; 2793 } 2794 else if (name.equals("workflow")) { 2795 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.process.step.workflow"); 2796 } 2797 else if (name.equals("operation")) { 2798 this.operation = new ExampleScenarioProcessStepOperationComponent(); 2799 return this.operation; 2800 } 2801 else if (name.equals("alternative")) { 2802 return addAlternative(); 2803 } 2804 else if (name.equals("pause")) { 2805 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.process.step.pause"); 2806 } 2807 else 2808 return super.addChild(name); 2809 } 2810 2811 public ExampleScenarioProcessStepComponent copy() { 2812 ExampleScenarioProcessStepComponent dst = new ExampleScenarioProcessStepComponent(); 2813 copyValues(dst); 2814 return dst; 2815 } 2816 2817 public void copyValues(ExampleScenarioProcessStepComponent dst) { 2818 super.copyValues(dst); 2819 dst.number = number == null ? null : number.copy(); 2820 dst.process = process == null ? null : process.copy(); 2821 dst.workflow = workflow == null ? null : workflow.copy(); 2822 dst.operation = operation == null ? null : operation.copy(); 2823 if (alternative != null) { 2824 dst.alternative = new ArrayList<ExampleScenarioProcessStepAlternativeComponent>(); 2825 for (ExampleScenarioProcessStepAlternativeComponent i : alternative) 2826 dst.alternative.add(i.copy()); 2827 }; 2828 dst.pause = pause == null ? null : pause.copy(); 2829 } 2830 2831 @Override 2832 public boolean equalsDeep(Base other_) { 2833 if (!super.equalsDeep(other_)) 2834 return false; 2835 if (!(other_ instanceof ExampleScenarioProcessStepComponent)) 2836 return false; 2837 ExampleScenarioProcessStepComponent o = (ExampleScenarioProcessStepComponent) other_; 2838 return compareDeep(number, o.number, true) && compareDeep(process, o.process, true) && compareDeep(workflow, o.workflow, true) 2839 && compareDeep(operation, o.operation, true) && compareDeep(alternative, o.alternative, true) && compareDeep(pause, o.pause, true) 2840 ; 2841 } 2842 2843 @Override 2844 public boolean equalsShallow(Base other_) { 2845 if (!super.equalsShallow(other_)) 2846 return false; 2847 if (!(other_ instanceof ExampleScenarioProcessStepComponent)) 2848 return false; 2849 ExampleScenarioProcessStepComponent o = (ExampleScenarioProcessStepComponent) other_; 2850 return compareValues(number, o.number, true) && compareValues(workflow, o.workflow, true) && compareValues(pause, o.pause, true) 2851 ; 2852 } 2853 2854 public boolean isEmpty() { 2855 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(number, process, workflow 2856 , operation, alternative, pause); 2857 } 2858 2859 public String fhirType() { 2860 return "ExampleScenario.process.step"; 2861 2862 } 2863 2864 } 2865 2866 @Block() 2867 public static class ExampleScenarioProcessStepOperationComponent extends BackboneElement implements IBaseBackboneElement { 2868 /** 2869 * The standardized type of action (FHIR or otherwise). 2870 */ 2871 @Child(name = "type", type = {Coding.class}, order=1, min=0, max=1, modifier=false, summary=false) 2872 @Description(shortDefinition="Kind of action", formalDefinition="The standardized type of action (FHIR or otherwise)." ) 2873 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/testscript-operation-codes") 2874 protected Coding type; 2875 2876 /** 2877 * A short descriptive label the step to be used in tables or diagrams. 2878 */ 2879 @Child(name = "title", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 2880 @Description(shortDefinition="Label for step", formalDefinition="A short descriptive label the step to be used in tables or diagrams." ) 2881 protected StringType title; 2882 2883 /** 2884 * The system that invokes the action/transmits the data. 2885 */ 2886 @Child(name = "initiator", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2887 @Description(shortDefinition="Who starts the operation", formalDefinition="The system that invokes the action/transmits the data." ) 2888 protected StringType initiator; 2889 2890 /** 2891 * The system on which the action is invoked/receives the data. 2892 */ 2893 @Child(name = "receiver", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2894 @Description(shortDefinition="Who receives the operation", formalDefinition="The system on which the action is invoked/receives the data." ) 2895 protected StringType receiver; 2896 2897 /** 2898 * An explanation of what the operation represents and what it does. 2899 */ 2900 @Child(name = "description", type = {MarkdownType.class}, order=5, min=0, max=1, modifier=false, summary=false) 2901 @Description(shortDefinition="Human-friendly description of the operation", formalDefinition="An explanation of what the operation represents and what it does." ) 2902 protected MarkdownType description; 2903 2904 /** 2905 * If false, the initiator is deactivated right after the operation. 2906 */ 2907 @Child(name = "initiatorActive", type = {BooleanType.class}, order=6, min=0, max=1, modifier=false, summary=false) 2908 @Description(shortDefinition="Initiator stays active?", formalDefinition="If false, the initiator is deactivated right after the operation." ) 2909 protected BooleanType initiatorActive; 2910 2911 /** 2912 * If false, the receiver is deactivated right after the operation. 2913 */ 2914 @Child(name = "receiverActive", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=false) 2915 @Description(shortDefinition="Receiver stays active?", formalDefinition="If false, the receiver is deactivated right after the operation." ) 2916 protected BooleanType receiverActive; 2917 2918 /** 2919 * A reference to the instance that is transmitted from requester to receiver as part of the invocation of the operation. 2920 */ 2921 @Child(name = "request", type = {ExampleScenarioInstanceContainedInstanceComponent.class}, order=8, min=0, max=1, modifier=false, summary=false) 2922 @Description(shortDefinition="Instance transmitted on invocation", formalDefinition="A reference to the instance that is transmitted from requester to receiver as part of the invocation of the operation." ) 2923 protected ExampleScenarioInstanceContainedInstanceComponent request; 2924 2925 /** 2926 * A reference to the instance that is transmitted from receiver to requester as part of the operation's synchronous response (if any). 2927 */ 2928 @Child(name = "response", type = {ExampleScenarioInstanceContainedInstanceComponent.class}, order=9, min=0, max=1, modifier=false, summary=false) 2929 @Description(shortDefinition="Instance transmitted on invocation response", formalDefinition="A reference to the instance that is transmitted from receiver to requester as part of the operation's synchronous response (if any)." ) 2930 protected ExampleScenarioInstanceContainedInstanceComponent response; 2931 2932 private static final long serialVersionUID = -252586646L; 2933 2934 /** 2935 * Constructor 2936 */ 2937 public ExampleScenarioProcessStepOperationComponent() { 2938 super(); 2939 } 2940 2941 /** 2942 * Constructor 2943 */ 2944 public ExampleScenarioProcessStepOperationComponent(String title) { 2945 super(); 2946 this.setTitle(title); 2947 } 2948 2949 /** 2950 * @return {@link #type} (The standardized type of action (FHIR or otherwise).) 2951 */ 2952 public Coding getType() { 2953 if (this.type == null) 2954 if (Configuration.errorOnAutoCreate()) 2955 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.type"); 2956 else if (Configuration.doAutoCreate()) 2957 this.type = new Coding(); // cc 2958 return this.type; 2959 } 2960 2961 public boolean hasType() { 2962 return this.type != null && !this.type.isEmpty(); 2963 } 2964 2965 /** 2966 * @param value {@link #type} (The standardized type of action (FHIR or otherwise).) 2967 */ 2968 public ExampleScenarioProcessStepOperationComponent setType(Coding value) { 2969 this.type = value; 2970 return this; 2971 } 2972 2973 /** 2974 * @return {@link #title} (A short descriptive label the step to be used in tables or diagrams.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2975 */ 2976 public StringType getTitleElement() { 2977 if (this.title == null) 2978 if (Configuration.errorOnAutoCreate()) 2979 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.title"); 2980 else if (Configuration.doAutoCreate()) 2981 this.title = new StringType(); // bb 2982 return this.title; 2983 } 2984 2985 public boolean hasTitleElement() { 2986 return this.title != null && !this.title.isEmpty(); 2987 } 2988 2989 public boolean hasTitle() { 2990 return this.title != null && !this.title.isEmpty(); 2991 } 2992 2993 /** 2994 * @param value {@link #title} (A short descriptive label the step to be used in tables or diagrams.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2995 */ 2996 public ExampleScenarioProcessStepOperationComponent setTitleElement(StringType value) { 2997 this.title = value; 2998 return this; 2999 } 3000 3001 /** 3002 * @return A short descriptive label the step to be used in tables or diagrams. 3003 */ 3004 public String getTitle() { 3005 return this.title == null ? null : this.title.getValue(); 3006 } 3007 3008 /** 3009 * @param value A short descriptive label the step to be used in tables or diagrams. 3010 */ 3011 public ExampleScenarioProcessStepOperationComponent setTitle(String value) { 3012 if (this.title == null) 3013 this.title = new StringType(); 3014 this.title.setValue(value); 3015 return this; 3016 } 3017 3018 /** 3019 * @return {@link #initiator} (The system that invokes the action/transmits the data.). This is the underlying object with id, value and extensions. The accessor "getInitiator" gives direct access to the value 3020 */ 3021 public StringType getInitiatorElement() { 3022 if (this.initiator == null) 3023 if (Configuration.errorOnAutoCreate()) 3024 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.initiator"); 3025 else if (Configuration.doAutoCreate()) 3026 this.initiator = new StringType(); // bb 3027 return this.initiator; 3028 } 3029 3030 public boolean hasInitiatorElement() { 3031 return this.initiator != null && !this.initiator.isEmpty(); 3032 } 3033 3034 public boolean hasInitiator() { 3035 return this.initiator != null && !this.initiator.isEmpty(); 3036 } 3037 3038 /** 3039 * @param value {@link #initiator} (The system that invokes the action/transmits the data.). This is the underlying object with id, value and extensions. The accessor "getInitiator" gives direct access to the value 3040 */ 3041 public ExampleScenarioProcessStepOperationComponent setInitiatorElement(StringType value) { 3042 this.initiator = value; 3043 return this; 3044 } 3045 3046 /** 3047 * @return The system that invokes the action/transmits the data. 3048 */ 3049 public String getInitiator() { 3050 return this.initiator == null ? null : this.initiator.getValue(); 3051 } 3052 3053 /** 3054 * @param value The system that invokes the action/transmits the data. 3055 */ 3056 public ExampleScenarioProcessStepOperationComponent setInitiator(String value) { 3057 if (Utilities.noString(value)) 3058 this.initiator = null; 3059 else { 3060 if (this.initiator == null) 3061 this.initiator = new StringType(); 3062 this.initiator.setValue(value); 3063 } 3064 return this; 3065 } 3066 3067 /** 3068 * @return {@link #receiver} (The system on which the action is invoked/receives the data.). This is the underlying object with id, value and extensions. The accessor "getReceiver" gives direct access to the value 3069 */ 3070 public StringType getReceiverElement() { 3071 if (this.receiver == null) 3072 if (Configuration.errorOnAutoCreate()) 3073 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.receiver"); 3074 else if (Configuration.doAutoCreate()) 3075 this.receiver = new StringType(); // bb 3076 return this.receiver; 3077 } 3078 3079 public boolean hasReceiverElement() { 3080 return this.receiver != null && !this.receiver.isEmpty(); 3081 } 3082 3083 public boolean hasReceiver() { 3084 return this.receiver != null && !this.receiver.isEmpty(); 3085 } 3086 3087 /** 3088 * @param value {@link #receiver} (The system on which the action is invoked/receives the data.). This is the underlying object with id, value and extensions. The accessor "getReceiver" gives direct access to the value 3089 */ 3090 public ExampleScenarioProcessStepOperationComponent setReceiverElement(StringType value) { 3091 this.receiver = value; 3092 return this; 3093 } 3094 3095 /** 3096 * @return The system on which the action is invoked/receives the data. 3097 */ 3098 public String getReceiver() { 3099 return this.receiver == null ? null : this.receiver.getValue(); 3100 } 3101 3102 /** 3103 * @param value The system on which the action is invoked/receives the data. 3104 */ 3105 public ExampleScenarioProcessStepOperationComponent setReceiver(String value) { 3106 if (Utilities.noString(value)) 3107 this.receiver = null; 3108 else { 3109 if (this.receiver == null) 3110 this.receiver = new StringType(); 3111 this.receiver.setValue(value); 3112 } 3113 return this; 3114 } 3115 3116 /** 3117 * @return {@link #description} (An explanation of what the operation represents and what it does.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3118 */ 3119 public MarkdownType getDescriptionElement() { 3120 if (this.description == null) 3121 if (Configuration.errorOnAutoCreate()) 3122 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.description"); 3123 else if (Configuration.doAutoCreate()) 3124 this.description = new MarkdownType(); // bb 3125 return this.description; 3126 } 3127 3128 public boolean hasDescriptionElement() { 3129 return this.description != null && !this.description.isEmpty(); 3130 } 3131 3132 public boolean hasDescription() { 3133 return this.description != null && !this.description.isEmpty(); 3134 } 3135 3136 /** 3137 * @param value {@link #description} (An explanation of what the operation represents and what it does.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3138 */ 3139 public ExampleScenarioProcessStepOperationComponent setDescriptionElement(MarkdownType value) { 3140 this.description = value; 3141 return this; 3142 } 3143 3144 /** 3145 * @return An explanation of what the operation represents and what it does. 3146 */ 3147 public String getDescription() { 3148 return this.description == null ? null : this.description.getValue(); 3149 } 3150 3151 /** 3152 * @param value An explanation of what the operation represents and what it does. 3153 */ 3154 public ExampleScenarioProcessStepOperationComponent setDescription(String value) { 3155 if (Utilities.noString(value)) 3156 this.description = null; 3157 else { 3158 if (this.description == null) 3159 this.description = new MarkdownType(); 3160 this.description.setValue(value); 3161 } 3162 return this; 3163 } 3164 3165 /** 3166 * @return {@link #initiatorActive} (If false, the initiator is deactivated right after the operation.). This is the underlying object with id, value and extensions. The accessor "getInitiatorActive" gives direct access to the value 3167 */ 3168 public BooleanType getInitiatorActiveElement() { 3169 if (this.initiatorActive == null) 3170 if (Configuration.errorOnAutoCreate()) 3171 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.initiatorActive"); 3172 else if (Configuration.doAutoCreate()) 3173 this.initiatorActive = new BooleanType(); // bb 3174 return this.initiatorActive; 3175 } 3176 3177 public boolean hasInitiatorActiveElement() { 3178 return this.initiatorActive != null && !this.initiatorActive.isEmpty(); 3179 } 3180 3181 public boolean hasInitiatorActive() { 3182 return this.initiatorActive != null && !this.initiatorActive.isEmpty(); 3183 } 3184 3185 /** 3186 * @param value {@link #initiatorActive} (If false, the initiator is deactivated right after the operation.). This is the underlying object with id, value and extensions. The accessor "getInitiatorActive" gives direct access to the value 3187 */ 3188 public ExampleScenarioProcessStepOperationComponent setInitiatorActiveElement(BooleanType value) { 3189 this.initiatorActive = value; 3190 return this; 3191 } 3192 3193 /** 3194 * @return If false, the initiator is deactivated right after the operation. 3195 */ 3196 public boolean getInitiatorActive() { 3197 return this.initiatorActive == null || this.initiatorActive.isEmpty() ? false : this.initiatorActive.getValue(); 3198 } 3199 3200 /** 3201 * @param value If false, the initiator is deactivated right after the operation. 3202 */ 3203 public ExampleScenarioProcessStepOperationComponent setInitiatorActive(boolean value) { 3204 if (this.initiatorActive == null) 3205 this.initiatorActive = new BooleanType(); 3206 this.initiatorActive.setValue(value); 3207 return this; 3208 } 3209 3210 /** 3211 * @return {@link #receiverActive} (If false, the receiver is deactivated right after the operation.). This is the underlying object with id, value and extensions. The accessor "getReceiverActive" gives direct access to the value 3212 */ 3213 public BooleanType getReceiverActiveElement() { 3214 if (this.receiverActive == null) 3215 if (Configuration.errorOnAutoCreate()) 3216 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.receiverActive"); 3217 else if (Configuration.doAutoCreate()) 3218 this.receiverActive = new BooleanType(); // bb 3219 return this.receiverActive; 3220 } 3221 3222 public boolean hasReceiverActiveElement() { 3223 return this.receiverActive != null && !this.receiverActive.isEmpty(); 3224 } 3225 3226 public boolean hasReceiverActive() { 3227 return this.receiverActive != null && !this.receiverActive.isEmpty(); 3228 } 3229 3230 /** 3231 * @param value {@link #receiverActive} (If false, the receiver is deactivated right after the operation.). This is the underlying object with id, value and extensions. The accessor "getReceiverActive" gives direct access to the value 3232 */ 3233 public ExampleScenarioProcessStepOperationComponent setReceiverActiveElement(BooleanType value) { 3234 this.receiverActive = value; 3235 return this; 3236 } 3237 3238 /** 3239 * @return If false, the receiver is deactivated right after the operation. 3240 */ 3241 public boolean getReceiverActive() { 3242 return this.receiverActive == null || this.receiverActive.isEmpty() ? false : this.receiverActive.getValue(); 3243 } 3244 3245 /** 3246 * @param value If false, the receiver is deactivated right after the operation. 3247 */ 3248 public ExampleScenarioProcessStepOperationComponent setReceiverActive(boolean value) { 3249 if (this.receiverActive == null) 3250 this.receiverActive = new BooleanType(); 3251 this.receiverActive.setValue(value); 3252 return this; 3253 } 3254 3255 /** 3256 * @return {@link #request} (A reference to the instance that is transmitted from requester to receiver as part of the invocation of the operation.) 3257 */ 3258 public ExampleScenarioInstanceContainedInstanceComponent getRequest() { 3259 if (this.request == null) 3260 if (Configuration.errorOnAutoCreate()) 3261 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.request"); 3262 else if (Configuration.doAutoCreate()) 3263 this.request = new ExampleScenarioInstanceContainedInstanceComponent(); // cc 3264 return this.request; 3265 } 3266 3267 public boolean hasRequest() { 3268 return this.request != null && !this.request.isEmpty(); 3269 } 3270 3271 /** 3272 * @param value {@link #request} (A reference to the instance that is transmitted from requester to receiver as part of the invocation of the operation.) 3273 */ 3274 public ExampleScenarioProcessStepOperationComponent setRequest(ExampleScenarioInstanceContainedInstanceComponent value) { 3275 this.request = value; 3276 return this; 3277 } 3278 3279 /** 3280 * @return {@link #response} (A reference to the instance that is transmitted from receiver to requester as part of the operation's synchronous response (if any).) 3281 */ 3282 public ExampleScenarioInstanceContainedInstanceComponent getResponse() { 3283 if (this.response == null) 3284 if (Configuration.errorOnAutoCreate()) 3285 throw new Error("Attempt to auto-create ExampleScenarioProcessStepOperationComponent.response"); 3286 else if (Configuration.doAutoCreate()) 3287 this.response = new ExampleScenarioInstanceContainedInstanceComponent(); // cc 3288 return this.response; 3289 } 3290 3291 public boolean hasResponse() { 3292 return this.response != null && !this.response.isEmpty(); 3293 } 3294 3295 /** 3296 * @param value {@link #response} (A reference to the instance that is transmitted from receiver to requester as part of the operation's synchronous response (if any).) 3297 */ 3298 public ExampleScenarioProcessStepOperationComponent setResponse(ExampleScenarioInstanceContainedInstanceComponent value) { 3299 this.response = value; 3300 return this; 3301 } 3302 3303 protected void listChildren(List<Property> children) { 3304 super.listChildren(children); 3305 children.add(new Property("type", "Coding", "The standardized type of action (FHIR or otherwise).", 0, 1, type)); 3306 children.add(new Property("title", "string", "A short descriptive label the step to be used in tables or diagrams.", 0, 1, title)); 3307 children.add(new Property("initiator", "string", "The system that invokes the action/transmits the data.", 0, 1, initiator)); 3308 children.add(new Property("receiver", "string", "The system on which the action is invoked/receives the data.", 0, 1, receiver)); 3309 children.add(new Property("description", "markdown", "An explanation of what the operation represents and what it does.", 0, 1, description)); 3310 children.add(new Property("initiatorActive", "boolean", "If false, the initiator is deactivated right after the operation.", 0, 1, initiatorActive)); 3311 children.add(new Property("receiverActive", "boolean", "If false, the receiver is deactivated right after the operation.", 0, 1, receiverActive)); 3312 children.add(new Property("request", "@ExampleScenario.instance.containedInstance", "A reference to the instance that is transmitted from requester to receiver as part of the invocation of the operation.", 0, 1, request)); 3313 children.add(new Property("response", "@ExampleScenario.instance.containedInstance", "A reference to the instance that is transmitted from receiver to requester as part of the operation's synchronous response (if any).", 0, 1, response)); 3314 } 3315 3316 @Override 3317 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3318 switch (_hash) { 3319 case 3575610: /*type*/ return new Property("type", "Coding", "The standardized type of action (FHIR or otherwise).", 0, 1, type); 3320 case 110371416: /*title*/ return new Property("title", "string", "A short descriptive label the step to be used in tables or diagrams.", 0, 1, title); 3321 case -248987089: /*initiator*/ return new Property("initiator", "string", "The system that invokes the action/transmits the data.", 0, 1, initiator); 3322 case -808719889: /*receiver*/ return new Property("receiver", "string", "The system on which the action is invoked/receives the data.", 0, 1, receiver); 3323 case -1724546052: /*description*/ return new Property("description", "markdown", "An explanation of what the operation represents and what it does.", 0, 1, description); 3324 case 384339477: /*initiatorActive*/ return new Property("initiatorActive", "boolean", "If false, the initiator is deactivated right after the operation.", 0, 1, initiatorActive); 3325 case -285284907: /*receiverActive*/ return new Property("receiverActive", "boolean", "If false, the receiver is deactivated right after the operation.", 0, 1, receiverActive); 3326 case 1095692943: /*request*/ return new Property("request", "@ExampleScenario.instance.containedInstance", "A reference to the instance that is transmitted from requester to receiver as part of the invocation of the operation.", 0, 1, request); 3327 case -340323263: /*response*/ return new Property("response", "@ExampleScenario.instance.containedInstance", "A reference to the instance that is transmitted from receiver to requester as part of the operation's synchronous response (if any).", 0, 1, response); 3328 default: return super.getNamedProperty(_hash, _name, _checkValid); 3329 } 3330 3331 } 3332 3333 @Override 3334 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3335 switch (hash) { 3336 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Coding 3337 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3338 case -248987089: /*initiator*/ return this.initiator == null ? new Base[0] : new Base[] {this.initiator}; // StringType 3339 case -808719889: /*receiver*/ return this.receiver == null ? new Base[0] : new Base[] {this.receiver}; // StringType 3340 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3341 case 384339477: /*initiatorActive*/ return this.initiatorActive == null ? new Base[0] : new Base[] {this.initiatorActive}; // BooleanType 3342 case -285284907: /*receiverActive*/ return this.receiverActive == null ? new Base[0] : new Base[] {this.receiverActive}; // BooleanType 3343 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // ExampleScenarioInstanceContainedInstanceComponent 3344 case -340323263: /*response*/ return this.response == null ? new Base[0] : new Base[] {this.response}; // ExampleScenarioInstanceContainedInstanceComponent 3345 default: return super.getProperty(hash, name, checkValid); 3346 } 3347 3348 } 3349 3350 @Override 3351 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3352 switch (hash) { 3353 case 3575610: // type 3354 this.type = TypeConvertor.castToCoding(value); // Coding 3355 return value; 3356 case 110371416: // title 3357 this.title = TypeConvertor.castToString(value); // StringType 3358 return value; 3359 case -248987089: // initiator 3360 this.initiator = TypeConvertor.castToString(value); // StringType 3361 return value; 3362 case -808719889: // receiver 3363 this.receiver = TypeConvertor.castToString(value); // StringType 3364 return value; 3365 case -1724546052: // description 3366 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3367 return value; 3368 case 384339477: // initiatorActive 3369 this.initiatorActive = TypeConvertor.castToBoolean(value); // BooleanType 3370 return value; 3371 case -285284907: // receiverActive 3372 this.receiverActive = TypeConvertor.castToBoolean(value); // BooleanType 3373 return value; 3374 case 1095692943: // request 3375 this.request = (ExampleScenarioInstanceContainedInstanceComponent) value; // ExampleScenarioInstanceContainedInstanceComponent 3376 return value; 3377 case -340323263: // response 3378 this.response = (ExampleScenarioInstanceContainedInstanceComponent) value; // ExampleScenarioInstanceContainedInstanceComponent 3379 return value; 3380 default: return super.setProperty(hash, name, value); 3381 } 3382 3383 } 3384 3385 @Override 3386 public Base setProperty(String name, Base value) throws FHIRException { 3387 if (name.equals("type")) { 3388 this.type = TypeConvertor.castToCoding(value); // Coding 3389 } else if (name.equals("title")) { 3390 this.title = TypeConvertor.castToString(value); // StringType 3391 } else if (name.equals("initiator")) { 3392 this.initiator = TypeConvertor.castToString(value); // StringType 3393 } else if (name.equals("receiver")) { 3394 this.receiver = TypeConvertor.castToString(value); // StringType 3395 } else if (name.equals("description")) { 3396 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3397 } else if (name.equals("initiatorActive")) { 3398 this.initiatorActive = TypeConvertor.castToBoolean(value); // BooleanType 3399 } else if (name.equals("receiverActive")) { 3400 this.receiverActive = TypeConvertor.castToBoolean(value); // BooleanType 3401 } else if (name.equals("request")) { 3402 this.request = (ExampleScenarioInstanceContainedInstanceComponent) value; // ExampleScenarioInstanceContainedInstanceComponent 3403 } else if (name.equals("response")) { 3404 this.response = (ExampleScenarioInstanceContainedInstanceComponent) value; // ExampleScenarioInstanceContainedInstanceComponent 3405 } else 3406 return super.setProperty(name, value); 3407 return value; 3408 } 3409 3410 @Override 3411 public void removeChild(String name, Base value) throws FHIRException { 3412 if (name.equals("type")) { 3413 this.type = null; 3414 } else if (name.equals("title")) { 3415 this.title = null; 3416 } else if (name.equals("initiator")) { 3417 this.initiator = null; 3418 } else if (name.equals("receiver")) { 3419 this.receiver = null; 3420 } else if (name.equals("description")) { 3421 this.description = null; 3422 } else if (name.equals("initiatorActive")) { 3423 this.initiatorActive = null; 3424 } else if (name.equals("receiverActive")) { 3425 this.receiverActive = null; 3426 } else if (name.equals("request")) { 3427 this.request = (ExampleScenarioInstanceContainedInstanceComponent) value; // ExampleScenarioInstanceContainedInstanceComponent 3428 } else if (name.equals("response")) { 3429 this.response = (ExampleScenarioInstanceContainedInstanceComponent) value; // ExampleScenarioInstanceContainedInstanceComponent 3430 } else 3431 super.removeChild(name, value); 3432 3433 } 3434 3435 @Override 3436 public Base makeProperty(int hash, String name) throws FHIRException { 3437 switch (hash) { 3438 case 3575610: return getType(); 3439 case 110371416: return getTitleElement(); 3440 case -248987089: return getInitiatorElement(); 3441 case -808719889: return getReceiverElement(); 3442 case -1724546052: return getDescriptionElement(); 3443 case 384339477: return getInitiatorActiveElement(); 3444 case -285284907: return getReceiverActiveElement(); 3445 case 1095692943: return getRequest(); 3446 case -340323263: return getResponse(); 3447 default: return super.makeProperty(hash, name); 3448 } 3449 3450 } 3451 3452 @Override 3453 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3454 switch (hash) { 3455 case 3575610: /*type*/ return new String[] {"Coding"}; 3456 case 110371416: /*title*/ return new String[] {"string"}; 3457 case -248987089: /*initiator*/ return new String[] {"string"}; 3458 case -808719889: /*receiver*/ return new String[] {"string"}; 3459 case -1724546052: /*description*/ return new String[] {"markdown"}; 3460 case 384339477: /*initiatorActive*/ return new String[] {"boolean"}; 3461 case -285284907: /*receiverActive*/ return new String[] {"boolean"}; 3462 case 1095692943: /*request*/ return new String[] {"@ExampleScenario.instance.containedInstance"}; 3463 case -340323263: /*response*/ return new String[] {"@ExampleScenario.instance.containedInstance"}; 3464 default: return super.getTypesForProperty(hash, name); 3465 } 3466 3467 } 3468 3469 @Override 3470 public Base addChild(String name) throws FHIRException { 3471 if (name.equals("type")) { 3472 this.type = new Coding(); 3473 return this.type; 3474 } 3475 else if (name.equals("title")) { 3476 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.process.step.operation.title"); 3477 } 3478 else if (name.equals("initiator")) { 3479 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.process.step.operation.initiator"); 3480 } 3481 else if (name.equals("receiver")) { 3482 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.process.step.operation.receiver"); 3483 } 3484 else if (name.equals("description")) { 3485 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.process.step.operation.description"); 3486 } 3487 else if (name.equals("initiatorActive")) { 3488 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.process.step.operation.initiatorActive"); 3489 } 3490 else if (name.equals("receiverActive")) { 3491 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.process.step.operation.receiverActive"); 3492 } 3493 else if (name.equals("request")) { 3494 this.request = new ExampleScenarioInstanceContainedInstanceComponent(); 3495 return this.request; 3496 } 3497 else if (name.equals("response")) { 3498 this.response = new ExampleScenarioInstanceContainedInstanceComponent(); 3499 return this.response; 3500 } 3501 else 3502 return super.addChild(name); 3503 } 3504 3505 public ExampleScenarioProcessStepOperationComponent copy() { 3506 ExampleScenarioProcessStepOperationComponent dst = new ExampleScenarioProcessStepOperationComponent(); 3507 copyValues(dst); 3508 return dst; 3509 } 3510 3511 public void copyValues(ExampleScenarioProcessStepOperationComponent dst) { 3512 super.copyValues(dst); 3513 dst.type = type == null ? null : type.copy(); 3514 dst.title = title == null ? null : title.copy(); 3515 dst.initiator = initiator == null ? null : initiator.copy(); 3516 dst.receiver = receiver == null ? null : receiver.copy(); 3517 dst.description = description == null ? null : description.copy(); 3518 dst.initiatorActive = initiatorActive == null ? null : initiatorActive.copy(); 3519 dst.receiverActive = receiverActive == null ? null : receiverActive.copy(); 3520 dst.request = request == null ? null : request.copy(); 3521 dst.response = response == null ? null : response.copy(); 3522 } 3523 3524 @Override 3525 public boolean equalsDeep(Base other_) { 3526 if (!super.equalsDeep(other_)) 3527 return false; 3528 if (!(other_ instanceof ExampleScenarioProcessStepOperationComponent)) 3529 return false; 3530 ExampleScenarioProcessStepOperationComponent o = (ExampleScenarioProcessStepOperationComponent) other_; 3531 return compareDeep(type, o.type, true) && compareDeep(title, o.title, true) && compareDeep(initiator, o.initiator, true) 3532 && compareDeep(receiver, o.receiver, true) && compareDeep(description, o.description, true) && compareDeep(initiatorActive, o.initiatorActive, true) 3533 && compareDeep(receiverActive, o.receiverActive, true) && compareDeep(request, o.request, true) 3534 && compareDeep(response, o.response, true); 3535 } 3536 3537 @Override 3538 public boolean equalsShallow(Base other_) { 3539 if (!super.equalsShallow(other_)) 3540 return false; 3541 if (!(other_ instanceof ExampleScenarioProcessStepOperationComponent)) 3542 return false; 3543 ExampleScenarioProcessStepOperationComponent o = (ExampleScenarioProcessStepOperationComponent) other_; 3544 return compareValues(title, o.title, true) && compareValues(initiator, o.initiator, true) && compareValues(receiver, o.receiver, true) 3545 && compareValues(description, o.description, true) && compareValues(initiatorActive, o.initiatorActive, true) 3546 && compareValues(receiverActive, o.receiverActive, true); 3547 } 3548 3549 public boolean isEmpty() { 3550 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, title, initiator, receiver 3551 , description, initiatorActive, receiverActive, request, response); 3552 } 3553 3554 public String fhirType() { 3555 return "ExampleScenario.process.step.operation"; 3556 3557 } 3558 3559 } 3560 3561 @Block() 3562 public static class ExampleScenarioProcessStepAlternativeComponent extends BackboneElement implements IBaseBackboneElement { 3563 /** 3564 * The label to display for the alternative that gives a sense of the circumstance in which the alternative should be invoked. 3565 */ 3566 @Child(name = "title", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3567 @Description(shortDefinition="Label for alternative", formalDefinition="The label to display for the alternative that gives a sense of the circumstance in which the alternative should be invoked." ) 3568 protected StringType title; 3569 3570 /** 3571 * A human-readable description of the alternative explaining when the alternative should occur rather than the base step. 3572 */ 3573 @Child(name = "description", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 3574 @Description(shortDefinition="Human-readable description of option", formalDefinition="A human-readable description of the alternative explaining when the alternative should occur rather than the base step." ) 3575 protected MarkdownType description; 3576 3577 /** 3578 * Indicates the operation, sub-process or scenario that happens if the alternative option is selected. 3579 */ 3580 @Child(name = "step", type = {ExampleScenarioProcessStepComponent.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3581 @Description(shortDefinition="Alternative action(s)", formalDefinition="Indicates the operation, sub-process or scenario that happens if the alternative option is selected." ) 3582 protected List<ExampleScenarioProcessStepComponent> step; 3583 3584 private static final long serialVersionUID = -254687460L; 3585 3586 /** 3587 * Constructor 3588 */ 3589 public ExampleScenarioProcessStepAlternativeComponent() { 3590 super(); 3591 } 3592 3593 /** 3594 * Constructor 3595 */ 3596 public ExampleScenarioProcessStepAlternativeComponent(String title) { 3597 super(); 3598 this.setTitle(title); 3599 } 3600 3601 /** 3602 * @return {@link #title} (The label to display for the alternative that gives a sense of the circumstance in which the alternative should be invoked.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3603 */ 3604 public StringType getTitleElement() { 3605 if (this.title == null) 3606 if (Configuration.errorOnAutoCreate()) 3607 throw new Error("Attempt to auto-create ExampleScenarioProcessStepAlternativeComponent.title"); 3608 else if (Configuration.doAutoCreate()) 3609 this.title = new StringType(); // bb 3610 return this.title; 3611 } 3612 3613 public boolean hasTitleElement() { 3614 return this.title != null && !this.title.isEmpty(); 3615 } 3616 3617 public boolean hasTitle() { 3618 return this.title != null && !this.title.isEmpty(); 3619 } 3620 3621 /** 3622 * @param value {@link #title} (The label to display for the alternative that gives a sense of the circumstance in which the alternative should be invoked.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3623 */ 3624 public ExampleScenarioProcessStepAlternativeComponent setTitleElement(StringType value) { 3625 this.title = value; 3626 return this; 3627 } 3628 3629 /** 3630 * @return The label to display for the alternative that gives a sense of the circumstance in which the alternative should be invoked. 3631 */ 3632 public String getTitle() { 3633 return this.title == null ? null : this.title.getValue(); 3634 } 3635 3636 /** 3637 * @param value The label to display for the alternative that gives a sense of the circumstance in which the alternative should be invoked. 3638 */ 3639 public ExampleScenarioProcessStepAlternativeComponent setTitle(String value) { 3640 if (this.title == null) 3641 this.title = new StringType(); 3642 this.title.setValue(value); 3643 return this; 3644 } 3645 3646 /** 3647 * @return {@link #description} (A human-readable description of the alternative explaining when the alternative should occur rather than the base step.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3648 */ 3649 public MarkdownType getDescriptionElement() { 3650 if (this.description == null) 3651 if (Configuration.errorOnAutoCreate()) 3652 throw new Error("Attempt to auto-create ExampleScenarioProcessStepAlternativeComponent.description"); 3653 else if (Configuration.doAutoCreate()) 3654 this.description = new MarkdownType(); // bb 3655 return this.description; 3656 } 3657 3658 public boolean hasDescriptionElement() { 3659 return this.description != null && !this.description.isEmpty(); 3660 } 3661 3662 public boolean hasDescription() { 3663 return this.description != null && !this.description.isEmpty(); 3664 } 3665 3666 /** 3667 * @param value {@link #description} (A human-readable description of the alternative explaining when the alternative should occur rather than the base step.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3668 */ 3669 public ExampleScenarioProcessStepAlternativeComponent setDescriptionElement(MarkdownType value) { 3670 this.description = value; 3671 return this; 3672 } 3673 3674 /** 3675 * @return A human-readable description of the alternative explaining when the alternative should occur rather than the base step. 3676 */ 3677 public String getDescription() { 3678 return this.description == null ? null : this.description.getValue(); 3679 } 3680 3681 /** 3682 * @param value A human-readable description of the alternative explaining when the alternative should occur rather than the base step. 3683 */ 3684 public ExampleScenarioProcessStepAlternativeComponent setDescription(String value) { 3685 if (Utilities.noString(value)) 3686 this.description = null; 3687 else { 3688 if (this.description == null) 3689 this.description = new MarkdownType(); 3690 this.description.setValue(value); 3691 } 3692 return this; 3693 } 3694 3695 /** 3696 * @return {@link #step} (Indicates the operation, sub-process or scenario that happens if the alternative option is selected.) 3697 */ 3698 public List<ExampleScenarioProcessStepComponent> getStep() { 3699 if (this.step == null) 3700 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 3701 return this.step; 3702 } 3703 3704 /** 3705 * @return Returns a reference to <code>this</code> for easy method chaining 3706 */ 3707 public ExampleScenarioProcessStepAlternativeComponent setStep(List<ExampleScenarioProcessStepComponent> theStep) { 3708 this.step = theStep; 3709 return this; 3710 } 3711 3712 public boolean hasStep() { 3713 if (this.step == null) 3714 return false; 3715 for (ExampleScenarioProcessStepComponent item : this.step) 3716 if (!item.isEmpty()) 3717 return true; 3718 return false; 3719 } 3720 3721 public ExampleScenarioProcessStepComponent addStep() { //3 3722 ExampleScenarioProcessStepComponent t = new ExampleScenarioProcessStepComponent(); 3723 if (this.step == null) 3724 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 3725 this.step.add(t); 3726 return t; 3727 } 3728 3729 public ExampleScenarioProcessStepAlternativeComponent addStep(ExampleScenarioProcessStepComponent t) { //3 3730 if (t == null) 3731 return this; 3732 if (this.step == null) 3733 this.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 3734 this.step.add(t); 3735 return this; 3736 } 3737 3738 /** 3739 * @return The first repetition of repeating field {@link #step}, creating it if it does not already exist {3} 3740 */ 3741 public ExampleScenarioProcessStepComponent getStepFirstRep() { 3742 if (getStep().isEmpty()) { 3743 addStep(); 3744 } 3745 return getStep().get(0); 3746 } 3747 3748 protected void listChildren(List<Property> children) { 3749 super.listChildren(children); 3750 children.add(new Property("title", "string", "The label to display for the alternative that gives a sense of the circumstance in which the alternative should be invoked.", 0, 1, title)); 3751 children.add(new Property("description", "markdown", "A human-readable description of the alternative explaining when the alternative should occur rather than the base step.", 0, 1, description)); 3752 children.add(new Property("step", "@ExampleScenario.process.step", "Indicates the operation, sub-process or scenario that happens if the alternative option is selected.", 0, java.lang.Integer.MAX_VALUE, step)); 3753 } 3754 3755 @Override 3756 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3757 switch (_hash) { 3758 case 110371416: /*title*/ return new Property("title", "string", "The label to display for the alternative that gives a sense of the circumstance in which the alternative should be invoked.", 0, 1, title); 3759 case -1724546052: /*description*/ return new Property("description", "markdown", "A human-readable description of the alternative explaining when the alternative should occur rather than the base step.", 0, 1, description); 3760 case 3540684: /*step*/ return new Property("step", "@ExampleScenario.process.step", "Indicates the operation, sub-process or scenario that happens if the alternative option is selected.", 0, java.lang.Integer.MAX_VALUE, step); 3761 default: return super.getNamedProperty(_hash, _name, _checkValid); 3762 } 3763 3764 } 3765 3766 @Override 3767 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3768 switch (hash) { 3769 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3770 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3771 case 3540684: /*step*/ return this.step == null ? new Base[0] : this.step.toArray(new Base[this.step.size()]); // ExampleScenarioProcessStepComponent 3772 default: return super.getProperty(hash, name, checkValid); 3773 } 3774 3775 } 3776 3777 @Override 3778 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3779 switch (hash) { 3780 case 110371416: // title 3781 this.title = TypeConvertor.castToString(value); // StringType 3782 return value; 3783 case -1724546052: // description 3784 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3785 return value; 3786 case 3540684: // step 3787 this.getStep().add((ExampleScenarioProcessStepComponent) value); // ExampleScenarioProcessStepComponent 3788 return value; 3789 default: return super.setProperty(hash, name, value); 3790 } 3791 3792 } 3793 3794 @Override 3795 public Base setProperty(String name, Base value) throws FHIRException { 3796 if (name.equals("title")) { 3797 this.title = TypeConvertor.castToString(value); // StringType 3798 } else if (name.equals("description")) { 3799 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3800 } else if (name.equals("step")) { 3801 this.getStep().add((ExampleScenarioProcessStepComponent) value); 3802 } else 3803 return super.setProperty(name, value); 3804 return value; 3805 } 3806 3807 @Override 3808 public void removeChild(String name, Base value) throws FHIRException { 3809 if (name.equals("title")) { 3810 this.title = null; 3811 } else if (name.equals("description")) { 3812 this.description = null; 3813 } else if (name.equals("step")) { 3814 this.getStep().remove((ExampleScenarioProcessStepComponent) value); 3815 } else 3816 super.removeChild(name, value); 3817 3818 } 3819 3820 @Override 3821 public Base makeProperty(int hash, String name) throws FHIRException { 3822 switch (hash) { 3823 case 110371416: return getTitleElement(); 3824 case -1724546052: return getDescriptionElement(); 3825 case 3540684: return addStep(); 3826 default: return super.makeProperty(hash, name); 3827 } 3828 3829 } 3830 3831 @Override 3832 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3833 switch (hash) { 3834 case 110371416: /*title*/ return new String[] {"string"}; 3835 case -1724546052: /*description*/ return new String[] {"markdown"}; 3836 case 3540684: /*step*/ return new String[] {"@ExampleScenario.process.step"}; 3837 default: return super.getTypesForProperty(hash, name); 3838 } 3839 3840 } 3841 3842 @Override 3843 public Base addChild(String name) throws FHIRException { 3844 if (name.equals("title")) { 3845 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.process.step.alternative.title"); 3846 } 3847 else if (name.equals("description")) { 3848 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.process.step.alternative.description"); 3849 } 3850 else if (name.equals("step")) { 3851 return addStep(); 3852 } 3853 else 3854 return super.addChild(name); 3855 } 3856 3857 public ExampleScenarioProcessStepAlternativeComponent copy() { 3858 ExampleScenarioProcessStepAlternativeComponent dst = new ExampleScenarioProcessStepAlternativeComponent(); 3859 copyValues(dst); 3860 return dst; 3861 } 3862 3863 public void copyValues(ExampleScenarioProcessStepAlternativeComponent dst) { 3864 super.copyValues(dst); 3865 dst.title = title == null ? null : title.copy(); 3866 dst.description = description == null ? null : description.copy(); 3867 if (step != null) { 3868 dst.step = new ArrayList<ExampleScenarioProcessStepComponent>(); 3869 for (ExampleScenarioProcessStepComponent i : step) 3870 dst.step.add(i.copy()); 3871 }; 3872 } 3873 3874 @Override 3875 public boolean equalsDeep(Base other_) { 3876 if (!super.equalsDeep(other_)) 3877 return false; 3878 if (!(other_ instanceof ExampleScenarioProcessStepAlternativeComponent)) 3879 return false; 3880 ExampleScenarioProcessStepAlternativeComponent o = (ExampleScenarioProcessStepAlternativeComponent) other_; 3881 return compareDeep(title, o.title, true) && compareDeep(description, o.description, true) && compareDeep(step, o.step, true) 3882 ; 3883 } 3884 3885 @Override 3886 public boolean equalsShallow(Base other_) { 3887 if (!super.equalsShallow(other_)) 3888 return false; 3889 if (!(other_ instanceof ExampleScenarioProcessStepAlternativeComponent)) 3890 return false; 3891 ExampleScenarioProcessStepAlternativeComponent o = (ExampleScenarioProcessStepAlternativeComponent) other_; 3892 return compareValues(title, o.title, true) && compareValues(description, o.description, true); 3893 } 3894 3895 public boolean isEmpty() { 3896 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(title, description, step 3897 ); 3898 } 3899 3900 public String fhirType() { 3901 return "ExampleScenario.process.step.alternative"; 3902 3903 } 3904 3905 } 3906 3907 /** 3908 * An absolute URI that is used to identify this example scenario when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this example scenario is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the example scenario is stored on different servers. 3909 */ 3910 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 3911 @Description(shortDefinition="Canonical identifier for this example scenario, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this example scenario when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this example scenario is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the example scenario is stored on different servers." ) 3912 protected UriType url; 3913 3914 /** 3915 * A formal identifier that is used to identify this example scenario when it is represented in other formats, or referenced in a specification, model, design or an instance. 3916 */ 3917 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3918 @Description(shortDefinition="Additional identifier for the example scenario", formalDefinition="A formal identifier that is used to identify this example scenario when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 3919 protected List<Identifier> identifier; 3920 3921 /** 3922 * The identifier that is used to identify this version of the example scenario when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the example scenario author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 3923 */ 3924 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 3925 @Description(shortDefinition="Business version of the example scenario", formalDefinition="The identifier that is used to identify this version of the example scenario when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the example scenario author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 3926 protected StringType version; 3927 3928 /** 3929 * Indicates the mechanism used to compare versions to determine which is more current. 3930 */ 3931 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 3932 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 3933 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 3934 protected DataType versionAlgorithm; 3935 3936 /** 3937 * Temporarily retained for tooling purposes. 3938 */ 3939 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 3940 @Description(shortDefinition="To be removed?", formalDefinition="Temporarily retained for tooling purposes." ) 3941 protected StringType name; 3942 3943 /** 3944 * A short, descriptive, user-friendly title for the ExampleScenario. 3945 */ 3946 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 3947 @Description(shortDefinition="Name for this example scenario (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the ExampleScenario." ) 3948 protected StringType title; 3949 3950 /** 3951 * The status of this example scenario. Enables tracking the life-cycle of the content. 3952 */ 3953 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 3954 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this example scenario. Enables tracking the life-cycle of the content." ) 3955 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 3956 protected Enumeration<PublicationStatus> status; 3957 3958 /** 3959 * A Boolean value to indicate that this example scenario is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 3960 */ 3961 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 3962 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this example scenario is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 3963 protected BooleanType experimental; 3964 3965 /** 3966 * The date (and optionally time) when the example scenario was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the example scenario changes. (e.g. the 'content logical definition'). 3967 */ 3968 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 3969 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the example scenario was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the example scenario changes. (e.g. the 'content logical definition')." ) 3970 protected DateTimeType date; 3971 3972 /** 3973 * The name of the organization or individual responsible for the release and ongoing maintenance of the example scenario. 3974 */ 3975 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 3976 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the example scenario." ) 3977 protected StringType publisher; 3978 3979 /** 3980 * Contact details to assist a user in finding and communicating with the publisher. 3981 */ 3982 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3983 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 3984 protected List<ContactDetail> contact; 3985 3986 /** 3987 * A free text natural language description of the ExampleScenario from a consumer's perspective. 3988 */ 3989 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=true) 3990 @Description(shortDefinition="Natural language description of the ExampleScenario", formalDefinition="A free text natural language description of the ExampleScenario from a consumer's perspective." ) 3991 protected MarkdownType description; 3992 3993 /** 3994 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate example scenario instances. 3995 */ 3996 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3997 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate example scenario instances." ) 3998 protected List<UsageContext> useContext; 3999 4000 /** 4001 * A legal or geographic region in which the example scenario is intended to be used. 4002 */ 4003 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4004 @Description(shortDefinition="Intended jurisdiction for example scenario (if applicable)", formalDefinition="A legal or geographic region in which the example scenario is intended to be used." ) 4005 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 4006 protected List<CodeableConcept> jurisdiction; 4007 4008 /** 4009 * What the example scenario resource is created for. This should not be used to show the business purpose of the scenario itself, but the purpose of documenting a scenario. 4010 */ 4011 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 4012 @Description(shortDefinition="The purpose of the example, e.g. to illustrate a scenario", formalDefinition="What the example scenario resource is created for. This should not be used to show the business purpose of the scenario itself, but the purpose of documenting a scenario." ) 4013 protected MarkdownType purpose; 4014 4015 /** 4016 * A copyright statement relating to the example scenario and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the example scenario. 4017 */ 4018 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 4019 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the example scenario and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the example scenario." ) 4020 protected MarkdownType copyright; 4021 4022 /** 4023 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 4024 */ 4025 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 4026 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 4027 protected StringType copyrightLabel; 4028 4029 /** 4030 * A system or person who shares or receives an instance within the scenario. 4031 */ 4032 @Child(name = "actor", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4033 @Description(shortDefinition="Individual involved in exchange", formalDefinition="A system or person who shares or receives an instance within the scenario." ) 4034 protected List<ExampleScenarioActorComponent> actor; 4035 4036 /** 4037 * A single data collection that is shared as part of the scenario. 4038 */ 4039 @Child(name = "instance", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4040 @Description(shortDefinition="Data used in the scenario", formalDefinition="A single data collection that is shared as part of the scenario." ) 4041 protected List<ExampleScenarioInstanceComponent> instance; 4042 4043 /** 4044 * A group of operations that represents a significant step within a scenario. 4045 */ 4046 @Child(name = "process", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4047 @Description(shortDefinition="Major process within scenario", formalDefinition="A group of operations that represents a significant step within a scenario." ) 4048 protected List<ExampleScenarioProcessComponent> process; 4049 4050 private static final long serialVersionUID = 292494233L; 4051 4052 /** 4053 * Constructor 4054 */ 4055 public ExampleScenario() { 4056 super(); 4057 } 4058 4059 /** 4060 * Constructor 4061 */ 4062 public ExampleScenario(PublicationStatus status) { 4063 super(); 4064 this.setStatus(status); 4065 } 4066 4067 /** 4068 * @return {@link #url} (An absolute URI that is used to identify this example scenario when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this example scenario is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the example scenario is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 4069 */ 4070 public UriType getUrlElement() { 4071 if (this.url == null) 4072 if (Configuration.errorOnAutoCreate()) 4073 throw new Error("Attempt to auto-create ExampleScenario.url"); 4074 else if (Configuration.doAutoCreate()) 4075 this.url = new UriType(); // bb 4076 return this.url; 4077 } 4078 4079 public boolean hasUrlElement() { 4080 return this.url != null && !this.url.isEmpty(); 4081 } 4082 4083 public boolean hasUrl() { 4084 return this.url != null && !this.url.isEmpty(); 4085 } 4086 4087 /** 4088 * @param value {@link #url} (An absolute URI that is used to identify this example scenario when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this example scenario is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the example scenario is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 4089 */ 4090 public ExampleScenario setUrlElement(UriType value) { 4091 this.url = value; 4092 return this; 4093 } 4094 4095 /** 4096 * @return An absolute URI that is used to identify this example scenario when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this example scenario is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the example scenario is stored on different servers. 4097 */ 4098 public String getUrl() { 4099 return this.url == null ? null : this.url.getValue(); 4100 } 4101 4102 /** 4103 * @param value An absolute URI that is used to identify this example scenario when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this example scenario is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the example scenario is stored on different servers. 4104 */ 4105 public ExampleScenario setUrl(String value) { 4106 if (Utilities.noString(value)) 4107 this.url = null; 4108 else { 4109 if (this.url == null) 4110 this.url = new UriType(); 4111 this.url.setValue(value); 4112 } 4113 return this; 4114 } 4115 4116 /** 4117 * @return {@link #identifier} (A formal identifier that is used to identify this example scenario when it is represented in other formats, or referenced in a specification, model, design or an instance.) 4118 */ 4119 public List<Identifier> getIdentifier() { 4120 if (this.identifier == null) 4121 this.identifier = new ArrayList<Identifier>(); 4122 return this.identifier; 4123 } 4124 4125 /** 4126 * @return Returns a reference to <code>this</code> for easy method chaining 4127 */ 4128 public ExampleScenario setIdentifier(List<Identifier> theIdentifier) { 4129 this.identifier = theIdentifier; 4130 return this; 4131 } 4132 4133 public boolean hasIdentifier() { 4134 if (this.identifier == null) 4135 return false; 4136 for (Identifier item : this.identifier) 4137 if (!item.isEmpty()) 4138 return true; 4139 return false; 4140 } 4141 4142 public Identifier addIdentifier() { //3 4143 Identifier t = new Identifier(); 4144 if (this.identifier == null) 4145 this.identifier = new ArrayList<Identifier>(); 4146 this.identifier.add(t); 4147 return t; 4148 } 4149 4150 public ExampleScenario addIdentifier(Identifier t) { //3 4151 if (t == null) 4152 return this; 4153 if (this.identifier == null) 4154 this.identifier = new ArrayList<Identifier>(); 4155 this.identifier.add(t); 4156 return this; 4157 } 4158 4159 /** 4160 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 4161 */ 4162 public Identifier getIdentifierFirstRep() { 4163 if (getIdentifier().isEmpty()) { 4164 addIdentifier(); 4165 } 4166 return getIdentifier().get(0); 4167 } 4168 4169 /** 4170 * @return {@link #version} (The identifier that is used to identify this version of the example scenario when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the example scenario author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 4171 */ 4172 public StringType getVersionElement() { 4173 if (this.version == null) 4174 if (Configuration.errorOnAutoCreate()) 4175 throw new Error("Attempt to auto-create ExampleScenario.version"); 4176 else if (Configuration.doAutoCreate()) 4177 this.version = new StringType(); // bb 4178 return this.version; 4179 } 4180 4181 public boolean hasVersionElement() { 4182 return this.version != null && !this.version.isEmpty(); 4183 } 4184 4185 public boolean hasVersion() { 4186 return this.version != null && !this.version.isEmpty(); 4187 } 4188 4189 /** 4190 * @param value {@link #version} (The identifier that is used to identify this version of the example scenario when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the example scenario author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 4191 */ 4192 public ExampleScenario setVersionElement(StringType value) { 4193 this.version = value; 4194 return this; 4195 } 4196 4197 /** 4198 * @return The identifier that is used to identify this version of the example scenario when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the example scenario author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 4199 */ 4200 public String getVersion() { 4201 return this.version == null ? null : this.version.getValue(); 4202 } 4203 4204 /** 4205 * @param value The identifier that is used to identify this version of the example scenario when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the example scenario author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 4206 */ 4207 public ExampleScenario setVersion(String value) { 4208 if (Utilities.noString(value)) 4209 this.version = null; 4210 else { 4211 if (this.version == null) 4212 this.version = new StringType(); 4213 this.version.setValue(value); 4214 } 4215 return this; 4216 } 4217 4218 /** 4219 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 4220 */ 4221 public DataType getVersionAlgorithm() { 4222 return this.versionAlgorithm; 4223 } 4224 4225 /** 4226 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 4227 */ 4228 public StringType getVersionAlgorithmStringType() throws FHIRException { 4229 if (this.versionAlgorithm == null) 4230 this.versionAlgorithm = new StringType(); 4231 if (!(this.versionAlgorithm instanceof StringType)) 4232 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 4233 return (StringType) this.versionAlgorithm; 4234 } 4235 4236 public boolean hasVersionAlgorithmStringType() { 4237 return this != null && this.versionAlgorithm instanceof StringType; 4238 } 4239 4240 /** 4241 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 4242 */ 4243 public Coding getVersionAlgorithmCoding() throws FHIRException { 4244 if (this.versionAlgorithm == null) 4245 this.versionAlgorithm = new Coding(); 4246 if (!(this.versionAlgorithm instanceof Coding)) 4247 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 4248 return (Coding) this.versionAlgorithm; 4249 } 4250 4251 public boolean hasVersionAlgorithmCoding() { 4252 return this != null && this.versionAlgorithm instanceof Coding; 4253 } 4254 4255 public boolean hasVersionAlgorithm() { 4256 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 4257 } 4258 4259 /** 4260 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 4261 */ 4262 public ExampleScenario setVersionAlgorithm(DataType value) { 4263 if (value != null && !(value instanceof StringType || value instanceof Coding)) 4264 throw new FHIRException("Not the right type for ExampleScenario.versionAlgorithm[x]: "+value.fhirType()); 4265 this.versionAlgorithm = value; 4266 return this; 4267 } 4268 4269 /** 4270 * @return {@link #name} (Temporarily retained for tooling purposes.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 4271 */ 4272 public StringType getNameElement() { 4273 if (this.name == null) 4274 if (Configuration.errorOnAutoCreate()) 4275 throw new Error("Attempt to auto-create ExampleScenario.name"); 4276 else if (Configuration.doAutoCreate()) 4277 this.name = new StringType(); // bb 4278 return this.name; 4279 } 4280 4281 public boolean hasNameElement() { 4282 return this.name != null && !this.name.isEmpty(); 4283 } 4284 4285 public boolean hasName() { 4286 return this.name != null && !this.name.isEmpty(); 4287 } 4288 4289 /** 4290 * @param value {@link #name} (Temporarily retained for tooling purposes.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 4291 */ 4292 public ExampleScenario setNameElement(StringType value) { 4293 this.name = value; 4294 return this; 4295 } 4296 4297 /** 4298 * @return Temporarily retained for tooling purposes. 4299 */ 4300 public String getName() { 4301 return this.name == null ? null : this.name.getValue(); 4302 } 4303 4304 /** 4305 * @param value Temporarily retained for tooling purposes. 4306 */ 4307 public ExampleScenario setName(String value) { 4308 if (Utilities.noString(value)) 4309 this.name = null; 4310 else { 4311 if (this.name == null) 4312 this.name = new StringType(); 4313 this.name.setValue(value); 4314 } 4315 return this; 4316 } 4317 4318 /** 4319 * @return {@link #title} (A short, descriptive, user-friendly title for the ExampleScenario.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 4320 */ 4321 public StringType getTitleElement() { 4322 if (this.title == null) 4323 if (Configuration.errorOnAutoCreate()) 4324 throw new Error("Attempt to auto-create ExampleScenario.title"); 4325 else if (Configuration.doAutoCreate()) 4326 this.title = new StringType(); // bb 4327 return this.title; 4328 } 4329 4330 public boolean hasTitleElement() { 4331 return this.title != null && !this.title.isEmpty(); 4332 } 4333 4334 public boolean hasTitle() { 4335 return this.title != null && !this.title.isEmpty(); 4336 } 4337 4338 /** 4339 * @param value {@link #title} (A short, descriptive, user-friendly title for the ExampleScenario.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 4340 */ 4341 public ExampleScenario setTitleElement(StringType value) { 4342 this.title = value; 4343 return this; 4344 } 4345 4346 /** 4347 * @return A short, descriptive, user-friendly title for the ExampleScenario. 4348 */ 4349 public String getTitle() { 4350 return this.title == null ? null : this.title.getValue(); 4351 } 4352 4353 /** 4354 * @param value A short, descriptive, user-friendly title for the ExampleScenario. 4355 */ 4356 public ExampleScenario setTitle(String value) { 4357 if (Utilities.noString(value)) 4358 this.title = null; 4359 else { 4360 if (this.title == null) 4361 this.title = new StringType(); 4362 this.title.setValue(value); 4363 } 4364 return this; 4365 } 4366 4367 /** 4368 * @return {@link #status} (The status of this example scenario. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4369 */ 4370 public Enumeration<PublicationStatus> getStatusElement() { 4371 if (this.status == null) 4372 if (Configuration.errorOnAutoCreate()) 4373 throw new Error("Attempt to auto-create ExampleScenario.status"); 4374 else if (Configuration.doAutoCreate()) 4375 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 4376 return this.status; 4377 } 4378 4379 public boolean hasStatusElement() { 4380 return this.status != null && !this.status.isEmpty(); 4381 } 4382 4383 public boolean hasStatus() { 4384 return this.status != null && !this.status.isEmpty(); 4385 } 4386 4387 /** 4388 * @param value {@link #status} (The status of this example scenario. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 4389 */ 4390 public ExampleScenario setStatusElement(Enumeration<PublicationStatus> value) { 4391 this.status = value; 4392 return this; 4393 } 4394 4395 /** 4396 * @return The status of this example scenario. Enables tracking the life-cycle of the content. 4397 */ 4398 public PublicationStatus getStatus() { 4399 return this.status == null ? null : this.status.getValue(); 4400 } 4401 4402 /** 4403 * @param value The status of this example scenario. Enables tracking the life-cycle of the content. 4404 */ 4405 public ExampleScenario setStatus(PublicationStatus value) { 4406 if (this.status == null) 4407 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 4408 this.status.setValue(value); 4409 return this; 4410 } 4411 4412 /** 4413 * @return {@link #experimental} (A Boolean value to indicate that this example scenario is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 4414 */ 4415 public BooleanType getExperimentalElement() { 4416 if (this.experimental == null) 4417 if (Configuration.errorOnAutoCreate()) 4418 throw new Error("Attempt to auto-create ExampleScenario.experimental"); 4419 else if (Configuration.doAutoCreate()) 4420 this.experimental = new BooleanType(); // bb 4421 return this.experimental; 4422 } 4423 4424 public boolean hasExperimentalElement() { 4425 return this.experimental != null && !this.experimental.isEmpty(); 4426 } 4427 4428 public boolean hasExperimental() { 4429 return this.experimental != null && !this.experimental.isEmpty(); 4430 } 4431 4432 /** 4433 * @param value {@link #experimental} (A Boolean value to indicate that this example scenario is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 4434 */ 4435 public ExampleScenario setExperimentalElement(BooleanType value) { 4436 this.experimental = value; 4437 return this; 4438 } 4439 4440 /** 4441 * @return A Boolean value to indicate that this example scenario is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 4442 */ 4443 public boolean getExperimental() { 4444 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 4445 } 4446 4447 /** 4448 * @param value A Boolean value to indicate that this example scenario is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 4449 */ 4450 public ExampleScenario setExperimental(boolean value) { 4451 if (this.experimental == null) 4452 this.experimental = new BooleanType(); 4453 this.experimental.setValue(value); 4454 return this; 4455 } 4456 4457 /** 4458 * @return {@link #date} (The date (and optionally time) when the example scenario was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the example scenario changes. (e.g. the 'content logical definition').). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 4459 */ 4460 public DateTimeType getDateElement() { 4461 if (this.date == null) 4462 if (Configuration.errorOnAutoCreate()) 4463 throw new Error("Attempt to auto-create ExampleScenario.date"); 4464 else if (Configuration.doAutoCreate()) 4465 this.date = new DateTimeType(); // bb 4466 return this.date; 4467 } 4468 4469 public boolean hasDateElement() { 4470 return this.date != null && !this.date.isEmpty(); 4471 } 4472 4473 public boolean hasDate() { 4474 return this.date != null && !this.date.isEmpty(); 4475 } 4476 4477 /** 4478 * @param value {@link #date} (The date (and optionally time) when the example scenario was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the example scenario changes. (e.g. the 'content logical definition').). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 4479 */ 4480 public ExampleScenario setDateElement(DateTimeType value) { 4481 this.date = value; 4482 return this; 4483 } 4484 4485 /** 4486 * @return The date (and optionally time) when the example scenario was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the example scenario changes. (e.g. the 'content logical definition'). 4487 */ 4488 public Date getDate() { 4489 return this.date == null ? null : this.date.getValue(); 4490 } 4491 4492 /** 4493 * @param value The date (and optionally time) when the example scenario was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the example scenario changes. (e.g. the 'content logical definition'). 4494 */ 4495 public ExampleScenario setDate(Date value) { 4496 if (value == null) 4497 this.date = null; 4498 else { 4499 if (this.date == null) 4500 this.date = new DateTimeType(); 4501 this.date.setValue(value); 4502 } 4503 return this; 4504 } 4505 4506 /** 4507 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the example scenario.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 4508 */ 4509 public StringType getPublisherElement() { 4510 if (this.publisher == null) 4511 if (Configuration.errorOnAutoCreate()) 4512 throw new Error("Attempt to auto-create ExampleScenario.publisher"); 4513 else if (Configuration.doAutoCreate()) 4514 this.publisher = new StringType(); // bb 4515 return this.publisher; 4516 } 4517 4518 public boolean hasPublisherElement() { 4519 return this.publisher != null && !this.publisher.isEmpty(); 4520 } 4521 4522 public boolean hasPublisher() { 4523 return this.publisher != null && !this.publisher.isEmpty(); 4524 } 4525 4526 /** 4527 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the example scenario.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 4528 */ 4529 public ExampleScenario setPublisherElement(StringType value) { 4530 this.publisher = value; 4531 return this; 4532 } 4533 4534 /** 4535 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the example scenario. 4536 */ 4537 public String getPublisher() { 4538 return this.publisher == null ? null : this.publisher.getValue(); 4539 } 4540 4541 /** 4542 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the example scenario. 4543 */ 4544 public ExampleScenario setPublisher(String value) { 4545 if (Utilities.noString(value)) 4546 this.publisher = null; 4547 else { 4548 if (this.publisher == null) 4549 this.publisher = new StringType(); 4550 this.publisher.setValue(value); 4551 } 4552 return this; 4553 } 4554 4555 /** 4556 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 4557 */ 4558 public List<ContactDetail> getContact() { 4559 if (this.contact == null) 4560 this.contact = new ArrayList<ContactDetail>(); 4561 return this.contact; 4562 } 4563 4564 /** 4565 * @return Returns a reference to <code>this</code> for easy method chaining 4566 */ 4567 public ExampleScenario setContact(List<ContactDetail> theContact) { 4568 this.contact = theContact; 4569 return this; 4570 } 4571 4572 public boolean hasContact() { 4573 if (this.contact == null) 4574 return false; 4575 for (ContactDetail item : this.contact) 4576 if (!item.isEmpty()) 4577 return true; 4578 return false; 4579 } 4580 4581 public ContactDetail addContact() { //3 4582 ContactDetail t = new ContactDetail(); 4583 if (this.contact == null) 4584 this.contact = new ArrayList<ContactDetail>(); 4585 this.contact.add(t); 4586 return t; 4587 } 4588 4589 public ExampleScenario addContact(ContactDetail t) { //3 4590 if (t == null) 4591 return this; 4592 if (this.contact == null) 4593 this.contact = new ArrayList<ContactDetail>(); 4594 this.contact.add(t); 4595 return this; 4596 } 4597 4598 /** 4599 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 4600 */ 4601 public ContactDetail getContactFirstRep() { 4602 if (getContact().isEmpty()) { 4603 addContact(); 4604 } 4605 return getContact().get(0); 4606 } 4607 4608 /** 4609 * @return {@link #description} (A free text natural language description of the ExampleScenario from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4610 */ 4611 public MarkdownType getDescriptionElement() { 4612 if (this.description == null) 4613 if (Configuration.errorOnAutoCreate()) 4614 throw new Error("Attempt to auto-create ExampleScenario.description"); 4615 else if (Configuration.doAutoCreate()) 4616 this.description = new MarkdownType(); // bb 4617 return this.description; 4618 } 4619 4620 public boolean hasDescriptionElement() { 4621 return this.description != null && !this.description.isEmpty(); 4622 } 4623 4624 public boolean hasDescription() { 4625 return this.description != null && !this.description.isEmpty(); 4626 } 4627 4628 /** 4629 * @param value {@link #description} (A free text natural language description of the ExampleScenario from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4630 */ 4631 public ExampleScenario setDescriptionElement(MarkdownType value) { 4632 this.description = value; 4633 return this; 4634 } 4635 4636 /** 4637 * @return A free text natural language description of the ExampleScenario from a consumer's perspective. 4638 */ 4639 public String getDescription() { 4640 return this.description == null ? null : this.description.getValue(); 4641 } 4642 4643 /** 4644 * @param value A free text natural language description of the ExampleScenario from a consumer's perspective. 4645 */ 4646 public ExampleScenario setDescription(String value) { 4647 if (Utilities.noString(value)) 4648 this.description = null; 4649 else { 4650 if (this.description == null) 4651 this.description = new MarkdownType(); 4652 this.description.setValue(value); 4653 } 4654 return this; 4655 } 4656 4657 /** 4658 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate example scenario instances.) 4659 */ 4660 public List<UsageContext> getUseContext() { 4661 if (this.useContext == null) 4662 this.useContext = new ArrayList<UsageContext>(); 4663 return this.useContext; 4664 } 4665 4666 /** 4667 * @return Returns a reference to <code>this</code> for easy method chaining 4668 */ 4669 public ExampleScenario setUseContext(List<UsageContext> theUseContext) { 4670 this.useContext = theUseContext; 4671 return this; 4672 } 4673 4674 public boolean hasUseContext() { 4675 if (this.useContext == null) 4676 return false; 4677 for (UsageContext item : this.useContext) 4678 if (!item.isEmpty()) 4679 return true; 4680 return false; 4681 } 4682 4683 public UsageContext addUseContext() { //3 4684 UsageContext t = new UsageContext(); 4685 if (this.useContext == null) 4686 this.useContext = new ArrayList<UsageContext>(); 4687 this.useContext.add(t); 4688 return t; 4689 } 4690 4691 public ExampleScenario addUseContext(UsageContext t) { //3 4692 if (t == null) 4693 return this; 4694 if (this.useContext == null) 4695 this.useContext = new ArrayList<UsageContext>(); 4696 this.useContext.add(t); 4697 return this; 4698 } 4699 4700 /** 4701 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 4702 */ 4703 public UsageContext getUseContextFirstRep() { 4704 if (getUseContext().isEmpty()) { 4705 addUseContext(); 4706 } 4707 return getUseContext().get(0); 4708 } 4709 4710 /** 4711 * @return {@link #jurisdiction} (A legal or geographic region in which the example scenario is intended to be used.) 4712 */ 4713 public List<CodeableConcept> getJurisdiction() { 4714 if (this.jurisdiction == null) 4715 this.jurisdiction = new ArrayList<CodeableConcept>(); 4716 return this.jurisdiction; 4717 } 4718 4719 /** 4720 * @return Returns a reference to <code>this</code> for easy method chaining 4721 */ 4722 public ExampleScenario setJurisdiction(List<CodeableConcept> theJurisdiction) { 4723 this.jurisdiction = theJurisdiction; 4724 return this; 4725 } 4726 4727 public boolean hasJurisdiction() { 4728 if (this.jurisdiction == null) 4729 return false; 4730 for (CodeableConcept item : this.jurisdiction) 4731 if (!item.isEmpty()) 4732 return true; 4733 return false; 4734 } 4735 4736 public CodeableConcept addJurisdiction() { //3 4737 CodeableConcept t = new CodeableConcept(); 4738 if (this.jurisdiction == null) 4739 this.jurisdiction = new ArrayList<CodeableConcept>(); 4740 this.jurisdiction.add(t); 4741 return t; 4742 } 4743 4744 public ExampleScenario addJurisdiction(CodeableConcept t) { //3 4745 if (t == null) 4746 return this; 4747 if (this.jurisdiction == null) 4748 this.jurisdiction = new ArrayList<CodeableConcept>(); 4749 this.jurisdiction.add(t); 4750 return this; 4751 } 4752 4753 /** 4754 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 4755 */ 4756 public CodeableConcept getJurisdictionFirstRep() { 4757 if (getJurisdiction().isEmpty()) { 4758 addJurisdiction(); 4759 } 4760 return getJurisdiction().get(0); 4761 } 4762 4763 /** 4764 * @return {@link #purpose} (What the example scenario resource is created for. This should not be used to show the business purpose of the scenario itself, but the purpose of documenting a scenario.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 4765 */ 4766 public MarkdownType getPurposeElement() { 4767 if (this.purpose == null) 4768 if (Configuration.errorOnAutoCreate()) 4769 throw new Error("Attempt to auto-create ExampleScenario.purpose"); 4770 else if (Configuration.doAutoCreate()) 4771 this.purpose = new MarkdownType(); // bb 4772 return this.purpose; 4773 } 4774 4775 public boolean hasPurposeElement() { 4776 return this.purpose != null && !this.purpose.isEmpty(); 4777 } 4778 4779 public boolean hasPurpose() { 4780 return this.purpose != null && !this.purpose.isEmpty(); 4781 } 4782 4783 /** 4784 * @param value {@link #purpose} (What the example scenario resource is created for. This should not be used to show the business purpose of the scenario itself, but the purpose of documenting a scenario.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 4785 */ 4786 public ExampleScenario setPurposeElement(MarkdownType value) { 4787 this.purpose = value; 4788 return this; 4789 } 4790 4791 /** 4792 * @return What the example scenario resource is created for. This should not be used to show the business purpose of the scenario itself, but the purpose of documenting a scenario. 4793 */ 4794 public String getPurpose() { 4795 return this.purpose == null ? null : this.purpose.getValue(); 4796 } 4797 4798 /** 4799 * @param value What the example scenario resource is created for. This should not be used to show the business purpose of the scenario itself, but the purpose of documenting a scenario. 4800 */ 4801 public ExampleScenario setPurpose(String value) { 4802 if (Utilities.noString(value)) 4803 this.purpose = null; 4804 else { 4805 if (this.purpose == null) 4806 this.purpose = new MarkdownType(); 4807 this.purpose.setValue(value); 4808 } 4809 return this; 4810 } 4811 4812 /** 4813 * @return {@link #copyright} (A copyright statement relating to the example scenario and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the example scenario.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 4814 */ 4815 public MarkdownType getCopyrightElement() { 4816 if (this.copyright == null) 4817 if (Configuration.errorOnAutoCreate()) 4818 throw new Error("Attempt to auto-create ExampleScenario.copyright"); 4819 else if (Configuration.doAutoCreate()) 4820 this.copyright = new MarkdownType(); // bb 4821 return this.copyright; 4822 } 4823 4824 public boolean hasCopyrightElement() { 4825 return this.copyright != null && !this.copyright.isEmpty(); 4826 } 4827 4828 public boolean hasCopyright() { 4829 return this.copyright != null && !this.copyright.isEmpty(); 4830 } 4831 4832 /** 4833 * @param value {@link #copyright} (A copyright statement relating to the example scenario and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the example scenario.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 4834 */ 4835 public ExampleScenario setCopyrightElement(MarkdownType value) { 4836 this.copyright = value; 4837 return this; 4838 } 4839 4840 /** 4841 * @return A copyright statement relating to the example scenario and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the example scenario. 4842 */ 4843 public String getCopyright() { 4844 return this.copyright == null ? null : this.copyright.getValue(); 4845 } 4846 4847 /** 4848 * @param value A copyright statement relating to the example scenario and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the example scenario. 4849 */ 4850 public ExampleScenario setCopyright(String value) { 4851 if (Utilities.noString(value)) 4852 this.copyright = null; 4853 else { 4854 if (this.copyright == null) 4855 this.copyright = new MarkdownType(); 4856 this.copyright.setValue(value); 4857 } 4858 return this; 4859 } 4860 4861 /** 4862 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 4863 */ 4864 public StringType getCopyrightLabelElement() { 4865 if (this.copyrightLabel == null) 4866 if (Configuration.errorOnAutoCreate()) 4867 throw new Error("Attempt to auto-create ExampleScenario.copyrightLabel"); 4868 else if (Configuration.doAutoCreate()) 4869 this.copyrightLabel = new StringType(); // bb 4870 return this.copyrightLabel; 4871 } 4872 4873 public boolean hasCopyrightLabelElement() { 4874 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 4875 } 4876 4877 public boolean hasCopyrightLabel() { 4878 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 4879 } 4880 4881 /** 4882 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 4883 */ 4884 public ExampleScenario setCopyrightLabelElement(StringType value) { 4885 this.copyrightLabel = value; 4886 return this; 4887 } 4888 4889 /** 4890 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 4891 */ 4892 public String getCopyrightLabel() { 4893 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 4894 } 4895 4896 /** 4897 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 4898 */ 4899 public ExampleScenario setCopyrightLabel(String value) { 4900 if (Utilities.noString(value)) 4901 this.copyrightLabel = null; 4902 else { 4903 if (this.copyrightLabel == null) 4904 this.copyrightLabel = new StringType(); 4905 this.copyrightLabel.setValue(value); 4906 } 4907 return this; 4908 } 4909 4910 /** 4911 * @return {@link #actor} (A system or person who shares or receives an instance within the scenario.) 4912 */ 4913 public List<ExampleScenarioActorComponent> getActor() { 4914 if (this.actor == null) 4915 this.actor = new ArrayList<ExampleScenarioActorComponent>(); 4916 return this.actor; 4917 } 4918 4919 /** 4920 * @return Returns a reference to <code>this</code> for easy method chaining 4921 */ 4922 public ExampleScenario setActor(List<ExampleScenarioActorComponent> theActor) { 4923 this.actor = theActor; 4924 return this; 4925 } 4926 4927 public boolean hasActor() { 4928 if (this.actor == null) 4929 return false; 4930 for (ExampleScenarioActorComponent item : this.actor) 4931 if (!item.isEmpty()) 4932 return true; 4933 return false; 4934 } 4935 4936 public ExampleScenarioActorComponent addActor() { //3 4937 ExampleScenarioActorComponent t = new ExampleScenarioActorComponent(); 4938 if (this.actor == null) 4939 this.actor = new ArrayList<ExampleScenarioActorComponent>(); 4940 this.actor.add(t); 4941 return t; 4942 } 4943 4944 public ExampleScenario addActor(ExampleScenarioActorComponent t) { //3 4945 if (t == null) 4946 return this; 4947 if (this.actor == null) 4948 this.actor = new ArrayList<ExampleScenarioActorComponent>(); 4949 this.actor.add(t); 4950 return this; 4951 } 4952 4953 /** 4954 * @return The first repetition of repeating field {@link #actor}, creating it if it does not already exist {3} 4955 */ 4956 public ExampleScenarioActorComponent getActorFirstRep() { 4957 if (getActor().isEmpty()) { 4958 addActor(); 4959 } 4960 return getActor().get(0); 4961 } 4962 4963 /** 4964 * @return {@link #instance} (A single data collection that is shared as part of the scenario.) 4965 */ 4966 public List<ExampleScenarioInstanceComponent> getInstance() { 4967 if (this.instance == null) 4968 this.instance = new ArrayList<ExampleScenarioInstanceComponent>(); 4969 return this.instance; 4970 } 4971 4972 /** 4973 * @return Returns a reference to <code>this</code> for easy method chaining 4974 */ 4975 public ExampleScenario setInstance(List<ExampleScenarioInstanceComponent> theInstance) { 4976 this.instance = theInstance; 4977 return this; 4978 } 4979 4980 public boolean hasInstance() { 4981 if (this.instance == null) 4982 return false; 4983 for (ExampleScenarioInstanceComponent item : this.instance) 4984 if (!item.isEmpty()) 4985 return true; 4986 return false; 4987 } 4988 4989 public ExampleScenarioInstanceComponent addInstance() { //3 4990 ExampleScenarioInstanceComponent t = new ExampleScenarioInstanceComponent(); 4991 if (this.instance == null) 4992 this.instance = new ArrayList<ExampleScenarioInstanceComponent>(); 4993 this.instance.add(t); 4994 return t; 4995 } 4996 4997 public ExampleScenario addInstance(ExampleScenarioInstanceComponent t) { //3 4998 if (t == null) 4999 return this; 5000 if (this.instance == null) 5001 this.instance = new ArrayList<ExampleScenarioInstanceComponent>(); 5002 this.instance.add(t); 5003 return this; 5004 } 5005 5006 /** 5007 * @return The first repetition of repeating field {@link #instance}, creating it if it does not already exist {3} 5008 */ 5009 public ExampleScenarioInstanceComponent getInstanceFirstRep() { 5010 if (getInstance().isEmpty()) { 5011 addInstance(); 5012 } 5013 return getInstance().get(0); 5014 } 5015 5016 /** 5017 * @return {@link #process} (A group of operations that represents a significant step within a scenario.) 5018 */ 5019 public List<ExampleScenarioProcessComponent> getProcess() { 5020 if (this.process == null) 5021 this.process = new ArrayList<ExampleScenarioProcessComponent>(); 5022 return this.process; 5023 } 5024 5025 /** 5026 * @return Returns a reference to <code>this</code> for easy method chaining 5027 */ 5028 public ExampleScenario setProcess(List<ExampleScenarioProcessComponent> theProcess) { 5029 this.process = theProcess; 5030 return this; 5031 } 5032 5033 public boolean hasProcess() { 5034 if (this.process == null) 5035 return false; 5036 for (ExampleScenarioProcessComponent item : this.process) 5037 if (!item.isEmpty()) 5038 return true; 5039 return false; 5040 } 5041 5042 public ExampleScenarioProcessComponent addProcess() { //3 5043 ExampleScenarioProcessComponent t = new ExampleScenarioProcessComponent(); 5044 if (this.process == null) 5045 this.process = new ArrayList<ExampleScenarioProcessComponent>(); 5046 this.process.add(t); 5047 return t; 5048 } 5049 5050 public ExampleScenario addProcess(ExampleScenarioProcessComponent t) { //3 5051 if (t == null) 5052 return this; 5053 if (this.process == null) 5054 this.process = new ArrayList<ExampleScenarioProcessComponent>(); 5055 this.process.add(t); 5056 return this; 5057 } 5058 5059 /** 5060 * @return The first repetition of repeating field {@link #process}, creating it if it does not already exist {3} 5061 */ 5062 public ExampleScenarioProcessComponent getProcessFirstRep() { 5063 if (getProcess().isEmpty()) { 5064 addProcess(); 5065 } 5066 return getProcess().get(0); 5067 } 5068 5069 protected void listChildren(List<Property> children) { 5070 super.listChildren(children); 5071 children.add(new Property("url", "uri", "An absolute URI that is used to identify this example scenario when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this example scenario is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the example scenario is stored on different servers.", 0, 1, url)); 5072 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this example scenario when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 5073 children.add(new Property("version", "string", "The identifier that is used to identify this version of the example scenario when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the example scenario author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 5074 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 5075 children.add(new Property("name", "string", "Temporarily retained for tooling purposes.", 0, 1, name)); 5076 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the ExampleScenario.", 0, 1, title)); 5077 children.add(new Property("status", "code", "The status of this example scenario. Enables tracking the life-cycle of the content.", 0, 1, status)); 5078 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this example scenario is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 5079 children.add(new Property("date", "dateTime", "The date (and optionally time) when the example scenario was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the example scenario changes. (e.g. the 'content logical definition').", 0, 1, date)); 5080 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the example scenario.", 0, 1, publisher)); 5081 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 5082 children.add(new Property("description", "markdown", "A free text natural language description of the ExampleScenario from a consumer's perspective.", 0, 1, description)); 5083 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate example scenario instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 5084 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the example scenario is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 5085 children.add(new Property("purpose", "markdown", "What the example scenario resource is created for. This should not be used to show the business purpose of the scenario itself, but the purpose of documenting a scenario.", 0, 1, purpose)); 5086 children.add(new Property("copyright", "markdown", "A copyright statement relating to the example scenario and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the example scenario.", 0, 1, copyright)); 5087 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 5088 children.add(new Property("actor", "", "A system or person who shares or receives an instance within the scenario.", 0, java.lang.Integer.MAX_VALUE, actor)); 5089 children.add(new Property("instance", "", "A single data collection that is shared as part of the scenario.", 0, java.lang.Integer.MAX_VALUE, instance)); 5090 children.add(new Property("process", "", "A group of operations that represents a significant step within a scenario.", 0, java.lang.Integer.MAX_VALUE, process)); 5091 } 5092 5093 @Override 5094 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5095 switch (_hash) { 5096 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this example scenario when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this example scenario is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the example scenario is stored on different servers.", 0, 1, url); 5097 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this example scenario when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 5098 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the example scenario when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the example scenario author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 5099 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 5100 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 5101 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 5102 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 5103 case 3373707: /*name*/ return new Property("name", "string", "Temporarily retained for tooling purposes.", 0, 1, name); 5104 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the ExampleScenario.", 0, 1, title); 5105 case -892481550: /*status*/ return new Property("status", "code", "The status of this example scenario. Enables tracking the life-cycle of the content.", 0, 1, status); 5106 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this example scenario is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 5107 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the example scenario was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the example scenario changes. (e.g. the 'content logical definition').", 0, 1, date); 5108 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the example scenario.", 0, 1, publisher); 5109 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 5110 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the ExampleScenario from a consumer's perspective.", 0, 1, description); 5111 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate example scenario instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 5112 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the example scenario is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 5113 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "What the example scenario resource is created for. This should not be used to show the business purpose of the scenario itself, but the purpose of documenting a scenario.", 0, 1, purpose); 5114 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the example scenario and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the example scenario.", 0, 1, copyright); 5115 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 5116 case 92645877: /*actor*/ return new Property("actor", "", "A system or person who shares or receives an instance within the scenario.", 0, java.lang.Integer.MAX_VALUE, actor); 5117 case 555127957: /*instance*/ return new Property("instance", "", "A single data collection that is shared as part of the scenario.", 0, java.lang.Integer.MAX_VALUE, instance); 5118 case -309518737: /*process*/ return new Property("process", "", "A group of operations that represents a significant step within a scenario.", 0, java.lang.Integer.MAX_VALUE, process); 5119 default: return super.getNamedProperty(_hash, _name, _checkValid); 5120 } 5121 5122 } 5123 5124 @Override 5125 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5126 switch (hash) { 5127 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 5128 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5129 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 5130 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 5131 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 5132 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 5133 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 5134 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 5135 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 5136 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 5137 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 5138 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 5139 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 5140 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 5141 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 5142 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 5143 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 5144 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // ExampleScenarioActorComponent 5145 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : this.instance.toArray(new Base[this.instance.size()]); // ExampleScenarioInstanceComponent 5146 case -309518737: /*process*/ return this.process == null ? new Base[0] : this.process.toArray(new Base[this.process.size()]); // ExampleScenarioProcessComponent 5147 default: return super.getProperty(hash, name, checkValid); 5148 } 5149 5150 } 5151 5152 @Override 5153 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5154 switch (hash) { 5155 case 116079: // url 5156 this.url = TypeConvertor.castToUri(value); // UriType 5157 return value; 5158 case -1618432855: // identifier 5159 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 5160 return value; 5161 case 351608024: // version 5162 this.version = TypeConvertor.castToString(value); // StringType 5163 return value; 5164 case 1508158071: // versionAlgorithm 5165 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 5166 return value; 5167 case 3373707: // name 5168 this.name = TypeConvertor.castToString(value); // StringType 5169 return value; 5170 case 110371416: // title 5171 this.title = TypeConvertor.castToString(value); // StringType 5172 return value; 5173 case -892481550: // status 5174 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5175 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5176 return value; 5177 case -404562712: // experimental 5178 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 5179 return value; 5180 case 3076014: // date 5181 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 5182 return value; 5183 case 1447404028: // publisher 5184 this.publisher = TypeConvertor.castToString(value); // StringType 5185 return value; 5186 case 951526432: // contact 5187 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 5188 return value; 5189 case -1724546052: // description 5190 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 5191 return value; 5192 case -669707736: // useContext 5193 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 5194 return value; 5195 case -507075711: // jurisdiction 5196 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5197 return value; 5198 case -220463842: // purpose 5199 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 5200 return value; 5201 case 1522889671: // copyright 5202 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 5203 return value; 5204 case 765157229: // copyrightLabel 5205 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 5206 return value; 5207 case 92645877: // actor 5208 this.getActor().add((ExampleScenarioActorComponent) value); // ExampleScenarioActorComponent 5209 return value; 5210 case 555127957: // instance 5211 this.getInstance().add((ExampleScenarioInstanceComponent) value); // ExampleScenarioInstanceComponent 5212 return value; 5213 case -309518737: // process 5214 this.getProcess().add((ExampleScenarioProcessComponent) value); // ExampleScenarioProcessComponent 5215 return value; 5216 default: return super.setProperty(hash, name, value); 5217 } 5218 5219 } 5220 5221 @Override 5222 public Base setProperty(String name, Base value) throws FHIRException { 5223 if (name.equals("url")) { 5224 this.url = TypeConvertor.castToUri(value); // UriType 5225 } else if (name.equals("identifier")) { 5226 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 5227 } else if (name.equals("version")) { 5228 this.version = TypeConvertor.castToString(value); // StringType 5229 } else if (name.equals("versionAlgorithm[x]")) { 5230 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 5231 } else if (name.equals("name")) { 5232 this.name = TypeConvertor.castToString(value); // StringType 5233 } else if (name.equals("title")) { 5234 this.title = TypeConvertor.castToString(value); // StringType 5235 } else if (name.equals("status")) { 5236 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5237 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5238 } else if (name.equals("experimental")) { 5239 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 5240 } else if (name.equals("date")) { 5241 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 5242 } else if (name.equals("publisher")) { 5243 this.publisher = TypeConvertor.castToString(value); // StringType 5244 } else if (name.equals("contact")) { 5245 this.getContact().add(TypeConvertor.castToContactDetail(value)); 5246 } else if (name.equals("description")) { 5247 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 5248 } else if (name.equals("useContext")) { 5249 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 5250 } else if (name.equals("jurisdiction")) { 5251 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 5252 } else if (name.equals("purpose")) { 5253 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 5254 } else if (name.equals("copyright")) { 5255 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 5256 } else if (name.equals("copyrightLabel")) { 5257 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 5258 } else if (name.equals("actor")) { 5259 this.getActor().add((ExampleScenarioActorComponent) value); 5260 } else if (name.equals("instance")) { 5261 this.getInstance().add((ExampleScenarioInstanceComponent) value); 5262 } else if (name.equals("process")) { 5263 this.getProcess().add((ExampleScenarioProcessComponent) value); 5264 } else 5265 return super.setProperty(name, value); 5266 return value; 5267 } 5268 5269 @Override 5270 public void removeChild(String name, Base value) throws FHIRException { 5271 if (name.equals("url")) { 5272 this.url = null; 5273 } else if (name.equals("identifier")) { 5274 this.getIdentifier().remove(value); 5275 } else if (name.equals("version")) { 5276 this.version = null; 5277 } else if (name.equals("versionAlgorithm[x]")) { 5278 this.versionAlgorithm = null; 5279 } else if (name.equals("name")) { 5280 this.name = null; 5281 } else if (name.equals("title")) { 5282 this.title = null; 5283 } else if (name.equals("status")) { 5284 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5285 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5286 } else if (name.equals("experimental")) { 5287 this.experimental = null; 5288 } else if (name.equals("date")) { 5289 this.date = null; 5290 } else if (name.equals("publisher")) { 5291 this.publisher = null; 5292 } else if (name.equals("contact")) { 5293 this.getContact().remove(value); 5294 } else if (name.equals("description")) { 5295 this.description = null; 5296 } else if (name.equals("useContext")) { 5297 this.getUseContext().remove(value); 5298 } else if (name.equals("jurisdiction")) { 5299 this.getJurisdiction().remove(value); 5300 } else if (name.equals("purpose")) { 5301 this.purpose = null; 5302 } else if (name.equals("copyright")) { 5303 this.copyright = null; 5304 } else if (name.equals("copyrightLabel")) { 5305 this.copyrightLabel = null; 5306 } else if (name.equals("actor")) { 5307 this.getActor().remove((ExampleScenarioActorComponent) value); 5308 } else if (name.equals("instance")) { 5309 this.getInstance().remove((ExampleScenarioInstanceComponent) value); 5310 } else if (name.equals("process")) { 5311 this.getProcess().remove((ExampleScenarioProcessComponent) value); 5312 } else 5313 super.removeChild(name, value); 5314 5315 } 5316 5317 @Override 5318 public Base makeProperty(int hash, String name) throws FHIRException { 5319 switch (hash) { 5320 case 116079: return getUrlElement(); 5321 case -1618432855: return addIdentifier(); 5322 case 351608024: return getVersionElement(); 5323 case -115699031: return getVersionAlgorithm(); 5324 case 1508158071: return getVersionAlgorithm(); 5325 case 3373707: return getNameElement(); 5326 case 110371416: return getTitleElement(); 5327 case -892481550: return getStatusElement(); 5328 case -404562712: return getExperimentalElement(); 5329 case 3076014: return getDateElement(); 5330 case 1447404028: return getPublisherElement(); 5331 case 951526432: return addContact(); 5332 case -1724546052: return getDescriptionElement(); 5333 case -669707736: return addUseContext(); 5334 case -507075711: return addJurisdiction(); 5335 case -220463842: return getPurposeElement(); 5336 case 1522889671: return getCopyrightElement(); 5337 case 765157229: return getCopyrightLabelElement(); 5338 case 92645877: return addActor(); 5339 case 555127957: return addInstance(); 5340 case -309518737: return addProcess(); 5341 default: return super.makeProperty(hash, name); 5342 } 5343 5344 } 5345 5346 @Override 5347 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5348 switch (hash) { 5349 case 116079: /*url*/ return new String[] {"uri"}; 5350 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 5351 case 351608024: /*version*/ return new String[] {"string"}; 5352 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 5353 case 3373707: /*name*/ return new String[] {"string"}; 5354 case 110371416: /*title*/ return new String[] {"string"}; 5355 case -892481550: /*status*/ return new String[] {"code"}; 5356 case -404562712: /*experimental*/ return new String[] {"boolean"}; 5357 case 3076014: /*date*/ return new String[] {"dateTime"}; 5358 case 1447404028: /*publisher*/ return new String[] {"string"}; 5359 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 5360 case -1724546052: /*description*/ return new String[] {"markdown"}; 5361 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 5362 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 5363 case -220463842: /*purpose*/ return new String[] {"markdown"}; 5364 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 5365 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 5366 case 92645877: /*actor*/ return new String[] {}; 5367 case 555127957: /*instance*/ return new String[] {}; 5368 case -309518737: /*process*/ return new String[] {}; 5369 default: return super.getTypesForProperty(hash, name); 5370 } 5371 5372 } 5373 5374 @Override 5375 public Base addChild(String name) throws FHIRException { 5376 if (name.equals("url")) { 5377 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.url"); 5378 } 5379 else if (name.equals("identifier")) { 5380 return addIdentifier(); 5381 } 5382 else if (name.equals("version")) { 5383 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.version"); 5384 } 5385 else if (name.equals("versionAlgorithmString")) { 5386 this.versionAlgorithm = new StringType(); 5387 return this.versionAlgorithm; 5388 } 5389 else if (name.equals("versionAlgorithmCoding")) { 5390 this.versionAlgorithm = new Coding(); 5391 return this.versionAlgorithm; 5392 } 5393 else if (name.equals("name")) { 5394 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.name"); 5395 } 5396 else if (name.equals("title")) { 5397 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.title"); 5398 } 5399 else if (name.equals("status")) { 5400 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.status"); 5401 } 5402 else if (name.equals("experimental")) { 5403 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.experimental"); 5404 } 5405 else if (name.equals("date")) { 5406 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.date"); 5407 } 5408 else if (name.equals("publisher")) { 5409 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.publisher"); 5410 } 5411 else if (name.equals("contact")) { 5412 return addContact(); 5413 } 5414 else if (name.equals("description")) { 5415 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.description"); 5416 } 5417 else if (name.equals("useContext")) { 5418 return addUseContext(); 5419 } 5420 else if (name.equals("jurisdiction")) { 5421 return addJurisdiction(); 5422 } 5423 else if (name.equals("purpose")) { 5424 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.purpose"); 5425 } 5426 else if (name.equals("copyright")) { 5427 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.copyright"); 5428 } 5429 else if (name.equals("copyrightLabel")) { 5430 throw new FHIRException("Cannot call addChild on a singleton property ExampleScenario.copyrightLabel"); 5431 } 5432 else if (name.equals("actor")) { 5433 return addActor(); 5434 } 5435 else if (name.equals("instance")) { 5436 return addInstance(); 5437 } 5438 else if (name.equals("process")) { 5439 return addProcess(); 5440 } 5441 else 5442 return super.addChild(name); 5443 } 5444 5445 public String fhirType() { 5446 return "ExampleScenario"; 5447 5448 } 5449 5450 public ExampleScenario copy() { 5451 ExampleScenario dst = new ExampleScenario(); 5452 copyValues(dst); 5453 return dst; 5454 } 5455 5456 public void copyValues(ExampleScenario dst) { 5457 super.copyValues(dst); 5458 dst.url = url == null ? null : url.copy(); 5459 if (identifier != null) { 5460 dst.identifier = new ArrayList<Identifier>(); 5461 for (Identifier i : identifier) 5462 dst.identifier.add(i.copy()); 5463 }; 5464 dst.version = version == null ? null : version.copy(); 5465 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 5466 dst.name = name == null ? null : name.copy(); 5467 dst.title = title == null ? null : title.copy(); 5468 dst.status = status == null ? null : status.copy(); 5469 dst.experimental = experimental == null ? null : experimental.copy(); 5470 dst.date = date == null ? null : date.copy(); 5471 dst.publisher = publisher == null ? null : publisher.copy(); 5472 if (contact != null) { 5473 dst.contact = new ArrayList<ContactDetail>(); 5474 for (ContactDetail i : contact) 5475 dst.contact.add(i.copy()); 5476 }; 5477 dst.description = description == null ? null : description.copy(); 5478 if (useContext != null) { 5479 dst.useContext = new ArrayList<UsageContext>(); 5480 for (UsageContext i : useContext) 5481 dst.useContext.add(i.copy()); 5482 }; 5483 if (jurisdiction != null) { 5484 dst.jurisdiction = new ArrayList<CodeableConcept>(); 5485 for (CodeableConcept i : jurisdiction) 5486 dst.jurisdiction.add(i.copy()); 5487 }; 5488 dst.purpose = purpose == null ? null : purpose.copy(); 5489 dst.copyright = copyright == null ? null : copyright.copy(); 5490 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 5491 if (actor != null) { 5492 dst.actor = new ArrayList<ExampleScenarioActorComponent>(); 5493 for (ExampleScenarioActorComponent i : actor) 5494 dst.actor.add(i.copy()); 5495 }; 5496 if (instance != null) { 5497 dst.instance = new ArrayList<ExampleScenarioInstanceComponent>(); 5498 for (ExampleScenarioInstanceComponent i : instance) 5499 dst.instance.add(i.copy()); 5500 }; 5501 if (process != null) { 5502 dst.process = new ArrayList<ExampleScenarioProcessComponent>(); 5503 for (ExampleScenarioProcessComponent i : process) 5504 dst.process.add(i.copy()); 5505 }; 5506 } 5507 5508 protected ExampleScenario typedCopy() { 5509 return copy(); 5510 } 5511 5512 @Override 5513 public boolean equalsDeep(Base other_) { 5514 if (!super.equalsDeep(other_)) 5515 return false; 5516 if (!(other_ instanceof ExampleScenario)) 5517 return false; 5518 ExampleScenario o = (ExampleScenario) other_; 5519 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 5520 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 5521 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 5522 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 5523 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 5524 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 5525 && compareDeep(actor, o.actor, true) && compareDeep(instance, o.instance, true) && compareDeep(process, o.process, true) 5526 ; 5527 } 5528 5529 @Override 5530 public boolean equalsShallow(Base other_) { 5531 if (!super.equalsShallow(other_)) 5532 return false; 5533 if (!(other_ instanceof ExampleScenario)) 5534 return false; 5535 ExampleScenario o = (ExampleScenario) other_; 5536 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 5537 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 5538 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 5539 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 5540 ; 5541 } 5542 5543 public boolean isEmpty() { 5544 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 5545 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 5546 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, actor 5547 , instance, process); 5548 } 5549 5550 @Override 5551 public ResourceType getResourceType() { 5552 return ResourceType.ExampleScenario; 5553 } 5554 5555 /** 5556 * Search parameter: <b>context-quantity</b> 5557 * <p> 5558 * Description: <b>Multiple Resources: 5559 5560* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 5561* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 5562* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 5563* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 5564* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 5565* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 5566* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 5567* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 5568* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 5569* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 5570* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 5571* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 5572* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 5573* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 5574* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 5575* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 5576* [Library](library.html): A quantity- or range-valued use context assigned to the library 5577* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 5578* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 5579* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 5580* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 5581* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 5582* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 5583* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 5584* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 5585* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 5586* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 5587* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 5588* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 5589* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 5590</b><br> 5591 * Type: <b>quantity</b><br> 5592 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 5593 * </p> 5594 */ 5595 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 5596 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 5597 /** 5598 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 5599 * <p> 5600 * Description: <b>Multiple Resources: 5601 5602* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 5603* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 5604* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 5605* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 5606* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 5607* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 5608* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 5609* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 5610* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 5611* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 5612* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 5613* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 5614* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 5615* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 5616* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 5617* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 5618* [Library](library.html): A quantity- or range-valued use context assigned to the library 5619* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 5620* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 5621* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 5622* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 5623* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 5624* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 5625* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 5626* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 5627* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 5628* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 5629* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 5630* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 5631* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 5632</b><br> 5633 * Type: <b>quantity</b><br> 5634 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 5635 * </p> 5636 */ 5637 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 5638 5639 /** 5640 * Search parameter: <b>context-type-quantity</b> 5641 * <p> 5642 * Description: <b>Multiple Resources: 5643 5644* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 5645* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 5646* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 5647* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 5648* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 5649* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 5650* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 5651* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 5652* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 5653* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 5654* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 5655* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 5656* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 5657* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 5658* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 5659* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 5660* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 5661* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 5662* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 5663* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 5664* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 5665* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 5666* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 5667* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 5668* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 5669* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 5670* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 5671* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 5672* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 5673* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 5674</b><br> 5675 * Type: <b>composite</b><br> 5676 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5677 * </p> 5678 */ 5679 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 5680 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 5681 /** 5682 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 5683 * <p> 5684 * Description: <b>Multiple Resources: 5685 5686* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 5687* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 5688* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 5689* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 5690* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 5691* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 5692* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 5693* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 5694* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 5695* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 5696* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 5697* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 5698* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 5699* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 5700* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 5701* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 5702* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 5703* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 5704* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 5705* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 5706* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 5707* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 5708* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 5709* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 5710* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 5711* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 5712* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 5713* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 5714* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 5715* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 5716</b><br> 5717 * Type: <b>composite</b><br> 5718 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5719 * </p> 5720 */ 5721 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 5722 5723 /** 5724 * Search parameter: <b>context-type-value</b> 5725 * <p> 5726 * Description: <b>Multiple Resources: 5727 5728* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 5729* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 5730* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 5731* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 5732* [Citation](citation.html): A use context type and value assigned to the citation 5733* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 5734* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 5735* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 5736* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 5737* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 5738* [Evidence](evidence.html): A use context type and value assigned to the evidence 5739* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 5740* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 5741* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 5742* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 5743* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 5744* [Library](library.html): A use context type and value assigned to the library 5745* [Measure](measure.html): A use context type and value assigned to the measure 5746* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 5747* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 5748* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 5749* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 5750* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 5751* [Requirements](requirements.html): A use context type and value assigned to the requirements 5752* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 5753* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 5754* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 5755* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 5756* [TestScript](testscript.html): A use context type and value assigned to the test script 5757* [ValueSet](valueset.html): A use context type and value assigned to the value set 5758</b><br> 5759 * Type: <b>composite</b><br> 5760 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5761 * </p> 5762 */ 5763 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 5764 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 5765 /** 5766 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 5767 * <p> 5768 * Description: <b>Multiple Resources: 5769 5770* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 5771* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 5772* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 5773* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 5774* [Citation](citation.html): A use context type and value assigned to the citation 5775* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 5776* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 5777* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 5778* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 5779* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 5780* [Evidence](evidence.html): A use context type and value assigned to the evidence 5781* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 5782* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 5783* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 5784* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 5785* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 5786* [Library](library.html): A use context type and value assigned to the library 5787* [Measure](measure.html): A use context type and value assigned to the measure 5788* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 5789* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 5790* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 5791* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 5792* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 5793* [Requirements](requirements.html): A use context type and value assigned to the requirements 5794* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 5795* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 5796* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 5797* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 5798* [TestScript](testscript.html): A use context type and value assigned to the test script 5799* [ValueSet](valueset.html): A use context type and value assigned to the value set 5800</b><br> 5801 * Type: <b>composite</b><br> 5802 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 5803 * </p> 5804 */ 5805 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 5806 5807 /** 5808 * Search parameter: <b>context-type</b> 5809 * <p> 5810 * Description: <b>Multiple Resources: 5811 5812* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 5813* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 5814* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 5815* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 5816* [Citation](citation.html): A type of use context assigned to the citation 5817* [CodeSystem](codesystem.html): A type of use context assigned to the code system 5818* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 5819* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 5820* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 5821* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 5822* [Evidence](evidence.html): A type of use context assigned to the evidence 5823* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 5824* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 5825* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 5826* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 5827* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 5828* [Library](library.html): A type of use context assigned to the library 5829* [Measure](measure.html): A type of use context assigned to the measure 5830* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 5831* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 5832* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 5833* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 5834* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 5835* [Requirements](requirements.html): A type of use context assigned to the requirements 5836* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 5837* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 5838* [StructureMap](structuremap.html): A type of use context assigned to the structure map 5839* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 5840* [TestScript](testscript.html): A type of use context assigned to the test script 5841* [ValueSet](valueset.html): A type of use context assigned to the value set 5842</b><br> 5843 * Type: <b>token</b><br> 5844 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 5845 * </p> 5846 */ 5847 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 5848 public static final String SP_CONTEXT_TYPE = "context-type"; 5849 /** 5850 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 5851 * <p> 5852 * Description: <b>Multiple Resources: 5853 5854* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 5855* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 5856* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 5857* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 5858* [Citation](citation.html): A type of use context assigned to the citation 5859* [CodeSystem](codesystem.html): A type of use context assigned to the code system 5860* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 5861* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 5862* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 5863* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 5864* [Evidence](evidence.html): A type of use context assigned to the evidence 5865* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 5866* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 5867* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 5868* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 5869* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 5870* [Library](library.html): A type of use context assigned to the library 5871* [Measure](measure.html): A type of use context assigned to the measure 5872* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 5873* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 5874* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 5875* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 5876* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 5877* [Requirements](requirements.html): A type of use context assigned to the requirements 5878* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 5879* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 5880* [StructureMap](structuremap.html): A type of use context assigned to the structure map 5881* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 5882* [TestScript](testscript.html): A type of use context assigned to the test script 5883* [ValueSet](valueset.html): A type of use context assigned to the value set 5884</b><br> 5885 * Type: <b>token</b><br> 5886 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 5887 * </p> 5888 */ 5889 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 5890 5891 /** 5892 * Search parameter: <b>context</b> 5893 * <p> 5894 * Description: <b>Multiple Resources: 5895 5896* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 5897* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 5898* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 5899* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 5900* [Citation](citation.html): A use context assigned to the citation 5901* [CodeSystem](codesystem.html): A use context assigned to the code system 5902* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 5903* [ConceptMap](conceptmap.html): A use context assigned to the concept map 5904* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 5905* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 5906* [Evidence](evidence.html): A use context assigned to the evidence 5907* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 5908* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 5909* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 5910* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 5911* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 5912* [Library](library.html): A use context assigned to the library 5913* [Measure](measure.html): A use context assigned to the measure 5914* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 5915* [NamingSystem](namingsystem.html): A use context assigned to the naming system 5916* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 5917* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 5918* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 5919* [Requirements](requirements.html): A use context assigned to the requirements 5920* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 5921* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 5922* [StructureMap](structuremap.html): A use context assigned to the structure map 5923* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 5924* [TestScript](testscript.html): A use context assigned to the test script 5925* [ValueSet](valueset.html): A use context assigned to the value set 5926</b><br> 5927 * Type: <b>token</b><br> 5928 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 5929 * </p> 5930 */ 5931 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 5932 public static final String SP_CONTEXT = "context"; 5933 /** 5934 * <b>Fluent Client</b> search parameter constant for <b>context</b> 5935 * <p> 5936 * Description: <b>Multiple Resources: 5937 5938* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 5939* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 5940* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 5941* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 5942* [Citation](citation.html): A use context assigned to the citation 5943* [CodeSystem](codesystem.html): A use context assigned to the code system 5944* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 5945* [ConceptMap](conceptmap.html): A use context assigned to the concept map 5946* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 5947* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 5948* [Evidence](evidence.html): A use context assigned to the evidence 5949* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 5950* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 5951* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 5952* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 5953* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 5954* [Library](library.html): A use context assigned to the library 5955* [Measure](measure.html): A use context assigned to the measure 5956* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 5957* [NamingSystem](namingsystem.html): A use context assigned to the naming system 5958* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 5959* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 5960* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 5961* [Requirements](requirements.html): A use context assigned to the requirements 5962* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 5963* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 5964* [StructureMap](structuremap.html): A use context assigned to the structure map 5965* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 5966* [TestScript](testscript.html): A use context assigned to the test script 5967* [ValueSet](valueset.html): A use context assigned to the value set 5968</b><br> 5969 * Type: <b>token</b><br> 5970 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 5971 * </p> 5972 */ 5973 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 5974 5975 /** 5976 * Search parameter: <b>date</b> 5977 * <p> 5978 * Description: <b>Multiple Resources: 5979 5980* [ActivityDefinition](activitydefinition.html): The activity definition publication date 5981* [ActorDefinition](actordefinition.html): The Actor Definition publication date 5982* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 5983* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 5984* [Citation](citation.html): The citation publication date 5985* [CodeSystem](codesystem.html): The code system publication date 5986* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 5987* [ConceptMap](conceptmap.html): The concept map publication date 5988* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 5989* [EventDefinition](eventdefinition.html): The event definition publication date 5990* [Evidence](evidence.html): The evidence publication date 5991* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 5992* [ExampleScenario](examplescenario.html): The example scenario publication date 5993* [GraphDefinition](graphdefinition.html): The graph definition publication date 5994* [ImplementationGuide](implementationguide.html): The implementation guide publication date 5995* [Library](library.html): The library publication date 5996* [Measure](measure.html): The measure publication date 5997* [MessageDefinition](messagedefinition.html): The message definition publication date 5998* [NamingSystem](namingsystem.html): The naming system publication date 5999* [OperationDefinition](operationdefinition.html): The operation definition publication date 6000* [PlanDefinition](plandefinition.html): The plan definition publication date 6001* [Questionnaire](questionnaire.html): The questionnaire publication date 6002* [Requirements](requirements.html): The requirements publication date 6003* [SearchParameter](searchparameter.html): The search parameter publication date 6004* [StructureDefinition](structuredefinition.html): The structure definition publication date 6005* [StructureMap](structuremap.html): The structure map publication date 6006* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 6007* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 6008* [TestScript](testscript.html): The test script publication date 6009* [ValueSet](valueset.html): The value set publication date 6010</b><br> 6011 * Type: <b>date</b><br> 6012 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 6013 * </p> 6014 */ 6015 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 6016 public static final String SP_DATE = "date"; 6017 /** 6018 * <b>Fluent Client</b> search parameter constant for <b>date</b> 6019 * <p> 6020 * Description: <b>Multiple Resources: 6021 6022* [ActivityDefinition](activitydefinition.html): The activity definition publication date 6023* [ActorDefinition](actordefinition.html): The Actor Definition publication date 6024* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 6025* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 6026* [Citation](citation.html): The citation publication date 6027* [CodeSystem](codesystem.html): The code system publication date 6028* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 6029* [ConceptMap](conceptmap.html): The concept map publication date 6030* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 6031* [EventDefinition](eventdefinition.html): The event definition publication date 6032* [Evidence](evidence.html): The evidence publication date 6033* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 6034* [ExampleScenario](examplescenario.html): The example scenario publication date 6035* [GraphDefinition](graphdefinition.html): The graph definition publication date 6036* [ImplementationGuide](implementationguide.html): The implementation guide publication date 6037* [Library](library.html): The library publication date 6038* [Measure](measure.html): The measure publication date 6039* [MessageDefinition](messagedefinition.html): The message definition publication date 6040* [NamingSystem](namingsystem.html): The naming system publication date 6041* [OperationDefinition](operationdefinition.html): The operation definition publication date 6042* [PlanDefinition](plandefinition.html): The plan definition publication date 6043* [Questionnaire](questionnaire.html): The questionnaire publication date 6044* [Requirements](requirements.html): The requirements publication date 6045* [SearchParameter](searchparameter.html): The search parameter publication date 6046* [StructureDefinition](structuredefinition.html): The structure definition publication date 6047* [StructureMap](structuremap.html): The structure map publication date 6048* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 6049* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 6050* [TestScript](testscript.html): The test script publication date 6051* [ValueSet](valueset.html): The value set publication date 6052</b><br> 6053 * Type: <b>date</b><br> 6054 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 6055 * </p> 6056 */ 6057 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 6058 6059 /** 6060 * Search parameter: <b>identifier</b> 6061 * <p> 6062 * Description: <b>Multiple Resources: 6063 6064* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 6065* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 6066* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 6067* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 6068* [Citation](citation.html): External identifier for the citation 6069* [CodeSystem](codesystem.html): External identifier for the code system 6070* [ConceptMap](conceptmap.html): External identifier for the concept map 6071* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 6072* [EventDefinition](eventdefinition.html): External identifier for the event definition 6073* [Evidence](evidence.html): External identifier for the evidence 6074* [EvidenceReport](evidencereport.html): External identifier for the evidence report 6075* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 6076* [ExampleScenario](examplescenario.html): External identifier for the example scenario 6077* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 6078* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 6079* [Library](library.html): External identifier for the library 6080* [Measure](measure.html): External identifier for the measure 6081* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 6082* [MessageDefinition](messagedefinition.html): External identifier for the message definition 6083* [NamingSystem](namingsystem.html): External identifier for the naming system 6084* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 6085* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 6086* [PlanDefinition](plandefinition.html): External identifier for the plan definition 6087* [Questionnaire](questionnaire.html): External identifier for the questionnaire 6088* [Requirements](requirements.html): External identifier for the requirements 6089* [SearchParameter](searchparameter.html): External identifier for the search parameter 6090* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 6091* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 6092* [StructureMap](structuremap.html): External identifier for the structure map 6093* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 6094* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 6095* [TestPlan](testplan.html): An identifier for the test plan 6096* [TestScript](testscript.html): External identifier for the test script 6097* [ValueSet](valueset.html): External identifier for the value set 6098</b><br> 6099 * Type: <b>token</b><br> 6100 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 6101 * </p> 6102 */ 6103 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 6104 public static final String SP_IDENTIFIER = "identifier"; 6105 /** 6106 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 6107 * <p> 6108 * Description: <b>Multiple Resources: 6109 6110* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 6111* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 6112* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 6113* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 6114* [Citation](citation.html): External identifier for the citation 6115* [CodeSystem](codesystem.html): External identifier for the code system 6116* [ConceptMap](conceptmap.html): External identifier for the concept map 6117* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 6118* [EventDefinition](eventdefinition.html): External identifier for the event definition 6119* [Evidence](evidence.html): External identifier for the evidence 6120* [EvidenceReport](evidencereport.html): External identifier for the evidence report 6121* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 6122* [ExampleScenario](examplescenario.html): External identifier for the example scenario 6123* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 6124* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 6125* [Library](library.html): External identifier for the library 6126* [Measure](measure.html): External identifier for the measure 6127* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 6128* [MessageDefinition](messagedefinition.html): External identifier for the message definition 6129* [NamingSystem](namingsystem.html): External identifier for the naming system 6130* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 6131* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 6132* [PlanDefinition](plandefinition.html): External identifier for the plan definition 6133* [Questionnaire](questionnaire.html): External identifier for the questionnaire 6134* [Requirements](requirements.html): External identifier for the requirements 6135* [SearchParameter](searchparameter.html): External identifier for the search parameter 6136* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 6137* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 6138* [StructureMap](structuremap.html): External identifier for the structure map 6139* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 6140* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 6141* [TestPlan](testplan.html): An identifier for the test plan 6142* [TestScript](testscript.html): External identifier for the test script 6143* [ValueSet](valueset.html): External identifier for the value set 6144</b><br> 6145 * Type: <b>token</b><br> 6146 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 6147 * </p> 6148 */ 6149 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 6150 6151 /** 6152 * Search parameter: <b>jurisdiction</b> 6153 * <p> 6154 * Description: <b>Multiple Resources: 6155 6156* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 6157* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 6158* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 6159* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 6160* [Citation](citation.html): Intended jurisdiction for the citation 6161* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 6162* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 6163* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 6164* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 6165* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 6166* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 6167* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 6168* [Library](library.html): Intended jurisdiction for the library 6169* [Measure](measure.html): Intended jurisdiction for the measure 6170* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 6171* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 6172* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 6173* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 6174* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 6175* [Requirements](requirements.html): Intended jurisdiction for the requirements 6176* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 6177* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 6178* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 6179* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 6180* [TestScript](testscript.html): Intended jurisdiction for the test script 6181* [ValueSet](valueset.html): Intended jurisdiction for the value set 6182</b><br> 6183 * Type: <b>token</b><br> 6184 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 6185 * </p> 6186 */ 6187 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 6188 public static final String SP_JURISDICTION = "jurisdiction"; 6189 /** 6190 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 6191 * <p> 6192 * Description: <b>Multiple Resources: 6193 6194* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 6195* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 6196* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 6197* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 6198* [Citation](citation.html): Intended jurisdiction for the citation 6199* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 6200* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 6201* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 6202* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 6203* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 6204* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 6205* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 6206* [Library](library.html): Intended jurisdiction for the library 6207* [Measure](measure.html): Intended jurisdiction for the measure 6208* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 6209* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 6210* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 6211* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 6212* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 6213* [Requirements](requirements.html): Intended jurisdiction for the requirements 6214* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 6215* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 6216* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 6217* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 6218* [TestScript](testscript.html): Intended jurisdiction for the test script 6219* [ValueSet](valueset.html): Intended jurisdiction for the value set 6220</b><br> 6221 * Type: <b>token</b><br> 6222 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 6223 * </p> 6224 */ 6225 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 6226 6227 /** 6228 * Search parameter: <b>name</b> 6229 * <p> 6230 * Description: <b>Multiple Resources: 6231 6232* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 6233* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 6234* [Citation](citation.html): Computationally friendly name of the citation 6235* [CodeSystem](codesystem.html): Computationally friendly name of the code system 6236* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 6237* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 6238* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 6239* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 6240* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 6241* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 6242* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 6243* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 6244* [Library](library.html): Computationally friendly name of the library 6245* [Measure](measure.html): Computationally friendly name of the measure 6246* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 6247* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 6248* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 6249* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 6250* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 6251* [Requirements](requirements.html): Computationally friendly name of the requirements 6252* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 6253* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 6254* [StructureMap](structuremap.html): Computationally friendly name of the structure map 6255* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 6256* [TestScript](testscript.html): Computationally friendly name of the test script 6257* [ValueSet](valueset.html): Computationally friendly name of the value set 6258</b><br> 6259 * Type: <b>string</b><br> 6260 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 6261 * </p> 6262 */ 6263 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 6264 public static final String SP_NAME = "name"; 6265 /** 6266 * <b>Fluent Client</b> search parameter constant for <b>name</b> 6267 * <p> 6268 * Description: <b>Multiple Resources: 6269 6270* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 6271* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 6272* [Citation](citation.html): Computationally friendly name of the citation 6273* [CodeSystem](codesystem.html): Computationally friendly name of the code system 6274* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 6275* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 6276* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 6277* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 6278* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 6279* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 6280* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 6281* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 6282* [Library](library.html): Computationally friendly name of the library 6283* [Measure](measure.html): Computationally friendly name of the measure 6284* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 6285* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 6286* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 6287* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 6288* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 6289* [Requirements](requirements.html): Computationally friendly name of the requirements 6290* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 6291* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 6292* [StructureMap](structuremap.html): Computationally friendly name of the structure map 6293* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 6294* [TestScript](testscript.html): Computationally friendly name of the test script 6295* [ValueSet](valueset.html): Computationally friendly name of the value set 6296</b><br> 6297 * Type: <b>string</b><br> 6298 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 6299 * </p> 6300 */ 6301 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 6302 6303 /** 6304 * Search parameter: <b>publisher</b> 6305 * <p> 6306 * Description: <b>Multiple Resources: 6307 6308* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 6309* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 6310* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 6311* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 6312* [Citation](citation.html): Name of the publisher of the citation 6313* [CodeSystem](codesystem.html): Name of the publisher of the code system 6314* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 6315* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 6316* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 6317* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 6318* [Evidence](evidence.html): Name of the publisher of the evidence 6319* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 6320* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 6321* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 6322* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 6323* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 6324* [Library](library.html): Name of the publisher of the library 6325* [Measure](measure.html): Name of the publisher of the measure 6326* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 6327* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 6328* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 6329* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 6330* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 6331* [Requirements](requirements.html): Name of the publisher of the requirements 6332* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 6333* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 6334* [StructureMap](structuremap.html): Name of the publisher of the structure map 6335* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 6336* [TestScript](testscript.html): Name of the publisher of the test script 6337* [ValueSet](valueset.html): Name of the publisher of the value set 6338</b><br> 6339 * Type: <b>string</b><br> 6340 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 6341 * </p> 6342 */ 6343 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 6344 public static final String SP_PUBLISHER = "publisher"; 6345 /** 6346 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 6347 * <p> 6348 * Description: <b>Multiple Resources: 6349 6350* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 6351* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 6352* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 6353* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 6354* [Citation](citation.html): Name of the publisher of the citation 6355* [CodeSystem](codesystem.html): Name of the publisher of the code system 6356* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 6357* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 6358* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 6359* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 6360* [Evidence](evidence.html): Name of the publisher of the evidence 6361* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 6362* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 6363* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 6364* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 6365* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 6366* [Library](library.html): Name of the publisher of the library 6367* [Measure](measure.html): Name of the publisher of the measure 6368* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 6369* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 6370* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 6371* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 6372* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 6373* [Requirements](requirements.html): Name of the publisher of the requirements 6374* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 6375* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 6376* [StructureMap](structuremap.html): Name of the publisher of the structure map 6377* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 6378* [TestScript](testscript.html): Name of the publisher of the test script 6379* [ValueSet](valueset.html): Name of the publisher of the value set 6380</b><br> 6381 * Type: <b>string</b><br> 6382 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 6383 * </p> 6384 */ 6385 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 6386 6387 /** 6388 * Search parameter: <b>status</b> 6389 * <p> 6390 * Description: <b>Multiple Resources: 6391 6392* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 6393* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 6394* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 6395* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 6396* [Citation](citation.html): The current status of the citation 6397* [CodeSystem](codesystem.html): The current status of the code system 6398* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 6399* [ConceptMap](conceptmap.html): The current status of the concept map 6400* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 6401* [EventDefinition](eventdefinition.html): The current status of the event definition 6402* [Evidence](evidence.html): The current status of the evidence 6403* [EvidenceReport](evidencereport.html): The current status of the evidence report 6404* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 6405* [ExampleScenario](examplescenario.html): The current status of the example scenario 6406* [GraphDefinition](graphdefinition.html): The current status of the graph definition 6407* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 6408* [Library](library.html): The current status of the library 6409* [Measure](measure.html): The current status of the measure 6410* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 6411* [MessageDefinition](messagedefinition.html): The current status of the message definition 6412* [NamingSystem](namingsystem.html): The current status of the naming system 6413* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 6414* [OperationDefinition](operationdefinition.html): The current status of the operation definition 6415* [PlanDefinition](plandefinition.html): The current status of the plan definition 6416* [Questionnaire](questionnaire.html): The current status of the questionnaire 6417* [Requirements](requirements.html): The current status of the requirements 6418* [SearchParameter](searchparameter.html): The current status of the search parameter 6419* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 6420* [StructureDefinition](structuredefinition.html): The current status of the structure definition 6421* [StructureMap](structuremap.html): The current status of the structure map 6422* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 6423* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 6424* [TestPlan](testplan.html): The current status of the test plan 6425* [TestScript](testscript.html): The current status of the test script 6426* [ValueSet](valueset.html): The current status of the value set 6427</b><br> 6428 * Type: <b>token</b><br> 6429 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 6430 * </p> 6431 */ 6432 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 6433 public static final String SP_STATUS = "status"; 6434 /** 6435 * <b>Fluent Client</b> search parameter constant for <b>status</b> 6436 * <p> 6437 * Description: <b>Multiple Resources: 6438 6439* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 6440* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 6441* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 6442* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 6443* [Citation](citation.html): The current status of the citation 6444* [CodeSystem](codesystem.html): The current status of the code system 6445* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 6446* [ConceptMap](conceptmap.html): The current status of the concept map 6447* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 6448* [EventDefinition](eventdefinition.html): The current status of the event definition 6449* [Evidence](evidence.html): The current status of the evidence 6450* [EvidenceReport](evidencereport.html): The current status of the evidence report 6451* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 6452* [ExampleScenario](examplescenario.html): The current status of the example scenario 6453* [GraphDefinition](graphdefinition.html): The current status of the graph definition 6454* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 6455* [Library](library.html): The current status of the library 6456* [Measure](measure.html): The current status of the measure 6457* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 6458* [MessageDefinition](messagedefinition.html): The current status of the message definition 6459* [NamingSystem](namingsystem.html): The current status of the naming system 6460* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 6461* [OperationDefinition](operationdefinition.html): The current status of the operation definition 6462* [PlanDefinition](plandefinition.html): The current status of the plan definition 6463* [Questionnaire](questionnaire.html): The current status of the questionnaire 6464* [Requirements](requirements.html): The current status of the requirements 6465* [SearchParameter](searchparameter.html): The current status of the search parameter 6466* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 6467* [StructureDefinition](structuredefinition.html): The current status of the structure definition 6468* [StructureMap](structuremap.html): The current status of the structure map 6469* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 6470* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 6471* [TestPlan](testplan.html): The current status of the test plan 6472* [TestScript](testscript.html): The current status of the test script 6473* [ValueSet](valueset.html): The current status of the value set 6474</b><br> 6475 * Type: <b>token</b><br> 6476 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 6477 * </p> 6478 */ 6479 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 6480 6481 /** 6482 * Search parameter: <b>url</b> 6483 * <p> 6484 * Description: <b>Multiple Resources: 6485 6486* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 6487* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 6488* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 6489* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 6490* [Citation](citation.html): The uri that identifies the citation 6491* [CodeSystem](codesystem.html): The uri that identifies the code system 6492* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 6493* [ConceptMap](conceptmap.html): The URI that identifies the concept map 6494* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 6495* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 6496* [Evidence](evidence.html): The uri that identifies the evidence 6497* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 6498* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 6499* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 6500* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 6501* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 6502* [Library](library.html): The uri that identifies the library 6503* [Measure](measure.html): The uri that identifies the measure 6504* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 6505* [NamingSystem](namingsystem.html): The uri that identifies the naming system 6506* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 6507* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 6508* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 6509* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 6510* [Requirements](requirements.html): The uri that identifies the requirements 6511* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 6512* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 6513* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 6514* [StructureMap](structuremap.html): The uri that identifies the structure map 6515* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 6516* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 6517* [TestPlan](testplan.html): The uri that identifies the test plan 6518* [TestScript](testscript.html): The uri that identifies the test script 6519* [ValueSet](valueset.html): The uri that identifies the value set 6520</b><br> 6521 * Type: <b>uri</b><br> 6522 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 6523 * </p> 6524 */ 6525 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 6526 public static final String SP_URL = "url"; 6527 /** 6528 * <b>Fluent Client</b> search parameter constant for <b>url</b> 6529 * <p> 6530 * Description: <b>Multiple Resources: 6531 6532* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 6533* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 6534* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 6535* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 6536* [Citation](citation.html): The uri that identifies the citation 6537* [CodeSystem](codesystem.html): The uri that identifies the code system 6538* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 6539* [ConceptMap](conceptmap.html): The URI that identifies the concept map 6540* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 6541* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 6542* [Evidence](evidence.html): The uri that identifies the evidence 6543* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 6544* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 6545* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 6546* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 6547* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 6548* [Library](library.html): The uri that identifies the library 6549* [Measure](measure.html): The uri that identifies the measure 6550* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 6551* [NamingSystem](namingsystem.html): The uri that identifies the naming system 6552* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 6553* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 6554* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 6555* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 6556* [Requirements](requirements.html): The uri that identifies the requirements 6557* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 6558* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 6559* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 6560* [StructureMap](structuremap.html): The uri that identifies the structure map 6561* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 6562* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 6563* [TestPlan](testplan.html): The uri that identifies the test plan 6564* [TestScript](testscript.html): The uri that identifies the test script 6565* [ValueSet](valueset.html): The uri that identifies the value set 6566</b><br> 6567 * Type: <b>uri</b><br> 6568 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 6569 * </p> 6570 */ 6571 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 6572 6573 /** 6574 * Search parameter: <b>version</b> 6575 * <p> 6576 * Description: <b>Multiple Resources: 6577 6578* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 6579* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 6580* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 6581* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 6582* [Citation](citation.html): The business version of the citation 6583* [CodeSystem](codesystem.html): The business version of the code system 6584* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 6585* [ConceptMap](conceptmap.html): The business version of the concept map 6586* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 6587* [EventDefinition](eventdefinition.html): The business version of the event definition 6588* [Evidence](evidence.html): The business version of the evidence 6589* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 6590* [ExampleScenario](examplescenario.html): The business version of the example scenario 6591* [GraphDefinition](graphdefinition.html): The business version of the graph definition 6592* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 6593* [Library](library.html): The business version of the library 6594* [Measure](measure.html): The business version of the measure 6595* [MessageDefinition](messagedefinition.html): The business version of the message definition 6596* [NamingSystem](namingsystem.html): The business version of the naming system 6597* [OperationDefinition](operationdefinition.html): The business version of the operation definition 6598* [PlanDefinition](plandefinition.html): The business version of the plan definition 6599* [Questionnaire](questionnaire.html): The business version of the questionnaire 6600* [Requirements](requirements.html): The business version of the requirements 6601* [SearchParameter](searchparameter.html): The business version of the search parameter 6602* [StructureDefinition](structuredefinition.html): The business version of the structure definition 6603* [StructureMap](structuremap.html): The business version of the structure map 6604* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 6605* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 6606* [TestScript](testscript.html): The business version of the test script 6607* [ValueSet](valueset.html): The business version of the value set 6608</b><br> 6609 * Type: <b>token</b><br> 6610 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 6611 * </p> 6612 */ 6613 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 6614 public static final String SP_VERSION = "version"; 6615 /** 6616 * <b>Fluent Client</b> search parameter constant for <b>version</b> 6617 * <p> 6618 * Description: <b>Multiple Resources: 6619 6620* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 6621* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 6622* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 6623* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 6624* [Citation](citation.html): The business version of the citation 6625* [CodeSystem](codesystem.html): The business version of the code system 6626* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 6627* [ConceptMap](conceptmap.html): The business version of the concept map 6628* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 6629* [EventDefinition](eventdefinition.html): The business version of the event definition 6630* [Evidence](evidence.html): The business version of the evidence 6631* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 6632* [ExampleScenario](examplescenario.html): The business version of the example scenario 6633* [GraphDefinition](graphdefinition.html): The business version of the graph definition 6634* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 6635* [Library](library.html): The business version of the library 6636* [Measure](measure.html): The business version of the measure 6637* [MessageDefinition](messagedefinition.html): The business version of the message definition 6638* [NamingSystem](namingsystem.html): The business version of the naming system 6639* [OperationDefinition](operationdefinition.html): The business version of the operation definition 6640* [PlanDefinition](plandefinition.html): The business version of the plan definition 6641* [Questionnaire](questionnaire.html): The business version of the questionnaire 6642* [Requirements](requirements.html): The business version of the requirements 6643* [SearchParameter](searchparameter.html): The business version of the search parameter 6644* [StructureDefinition](structuredefinition.html): The business version of the structure definition 6645* [StructureMap](structuremap.html): The business version of the structure map 6646* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 6647* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 6648* [TestScript](testscript.html): The business version of the test script 6649* [ValueSet](valueset.html): The business version of the value set 6650</b><br> 6651 * Type: <b>token</b><br> 6652 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 6653 * </p> 6654 */ 6655 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 6656 6657 6658} 6659