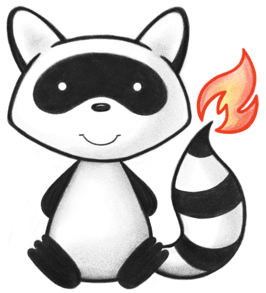
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051/** 052 * This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided. 053 */ 054@ResourceDef(name="ExplanationOfBenefit", profile="http://hl7.org/fhir/StructureDefinition/ExplanationOfBenefit") 055public class ExplanationOfBenefit extends DomainResource { 056 057 public enum ExplanationOfBenefitStatus { 058 /** 059 * The resource instance is currently in-force. 060 */ 061 ACTIVE, 062 /** 063 * The resource instance is withdrawn, rescinded or reversed. 064 */ 065 CANCELLED, 066 /** 067 * A new resource instance the contents of which is not complete. 068 */ 069 DRAFT, 070 /** 071 * The resource instance was entered in error. 072 */ 073 ENTEREDINERROR, 074 /** 075 * added to help the parsers with the generic types 076 */ 077 NULL; 078 public static ExplanationOfBenefitStatus fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("active".equals(codeString)) 082 return ACTIVE; 083 if ("cancelled".equals(codeString)) 084 return CANCELLED; 085 if ("draft".equals(codeString)) 086 return DRAFT; 087 if ("entered-in-error".equals(codeString)) 088 return ENTEREDINERROR; 089 if (Configuration.isAcceptInvalidEnums()) 090 return null; 091 else 092 throw new FHIRException("Unknown ExplanationOfBenefitStatus code '"+codeString+"'"); 093 } 094 public String toCode() { 095 switch (this) { 096 case ACTIVE: return "active"; 097 case CANCELLED: return "cancelled"; 098 case DRAFT: return "draft"; 099 case ENTEREDINERROR: return "entered-in-error"; 100 case NULL: return null; 101 default: return "?"; 102 } 103 } 104 public String getSystem() { 105 switch (this) { 106 case ACTIVE: return "http://hl7.org/fhir/explanationofbenefit-status"; 107 case CANCELLED: return "http://hl7.org/fhir/explanationofbenefit-status"; 108 case DRAFT: return "http://hl7.org/fhir/explanationofbenefit-status"; 109 case ENTEREDINERROR: return "http://hl7.org/fhir/explanationofbenefit-status"; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDefinition() { 115 switch (this) { 116 case ACTIVE: return "The resource instance is currently in-force."; 117 case CANCELLED: return "The resource instance is withdrawn, rescinded or reversed."; 118 case DRAFT: return "A new resource instance the contents of which is not complete."; 119 case ENTEREDINERROR: return "The resource instance was entered in error."; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getDisplay() { 125 switch (this) { 126 case ACTIVE: return "Active"; 127 case CANCELLED: return "Cancelled"; 128 case DRAFT: return "Draft"; 129 case ENTEREDINERROR: return "Entered In Error"; 130 case NULL: return null; 131 default: return "?"; 132 } 133 } 134 } 135 136 public static class ExplanationOfBenefitStatusEnumFactory implements EnumFactory<ExplanationOfBenefitStatus> { 137 public ExplanationOfBenefitStatus fromCode(String codeString) throws IllegalArgumentException { 138 if (codeString == null || "".equals(codeString)) 139 if (codeString == null || "".equals(codeString)) 140 return null; 141 if ("active".equals(codeString)) 142 return ExplanationOfBenefitStatus.ACTIVE; 143 if ("cancelled".equals(codeString)) 144 return ExplanationOfBenefitStatus.CANCELLED; 145 if ("draft".equals(codeString)) 146 return ExplanationOfBenefitStatus.DRAFT; 147 if ("entered-in-error".equals(codeString)) 148 return ExplanationOfBenefitStatus.ENTEREDINERROR; 149 throw new IllegalArgumentException("Unknown ExplanationOfBenefitStatus code '"+codeString+"'"); 150 } 151 public Enumeration<ExplanationOfBenefitStatus> fromType(PrimitiveType<?> code) throws FHIRException { 152 if (code == null) 153 return null; 154 if (code.isEmpty()) 155 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.NULL, code); 156 String codeString = ((PrimitiveType) code).asStringValue(); 157 if (codeString == null || "".equals(codeString)) 158 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.NULL, code); 159 if ("active".equals(codeString)) 160 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.ACTIVE, code); 161 if ("cancelled".equals(codeString)) 162 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.CANCELLED, code); 163 if ("draft".equals(codeString)) 164 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.DRAFT, code); 165 if ("entered-in-error".equals(codeString)) 166 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.ENTEREDINERROR, code); 167 throw new FHIRException("Unknown ExplanationOfBenefitStatus code '"+codeString+"'"); 168 } 169 public String toCode(ExplanationOfBenefitStatus code) { 170 if (code == ExplanationOfBenefitStatus.ACTIVE) 171 return "active"; 172 if (code == ExplanationOfBenefitStatus.CANCELLED) 173 return "cancelled"; 174 if (code == ExplanationOfBenefitStatus.DRAFT) 175 return "draft"; 176 if (code == ExplanationOfBenefitStatus.ENTEREDINERROR) 177 return "entered-in-error"; 178 return "?"; 179 } 180 public String toSystem(ExplanationOfBenefitStatus code) { 181 return code.getSystem(); 182 } 183 } 184 185 @Block() 186 public static class RelatedClaimComponent extends BackboneElement implements IBaseBackboneElement { 187 /** 188 * Reference to a related claim. 189 */ 190 @Child(name = "claim", type = {Claim.class}, order=1, min=0, max=1, modifier=false, summary=false) 191 @Description(shortDefinition="Reference to the related claim", formalDefinition="Reference to a related claim." ) 192 protected Reference claim; 193 194 /** 195 * A code to convey how the claims are related. 196 */ 197 @Child(name = "relationship", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 198 @Description(shortDefinition="How the reference claim is related", formalDefinition="A code to convey how the claims are related." ) 199 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/related-claim-relationship") 200 protected CodeableConcept relationship; 201 202 /** 203 * An alternate organizational reference to the case or file to which this particular claim pertains. 204 */ 205 @Child(name = "reference", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=false) 206 @Description(shortDefinition="File or case reference", formalDefinition="An alternate organizational reference to the case or file to which this particular claim pertains." ) 207 protected Identifier reference; 208 209 private static final long serialVersionUID = 1047077926L; 210 211 /** 212 * Constructor 213 */ 214 public RelatedClaimComponent() { 215 super(); 216 } 217 218 /** 219 * @return {@link #claim} (Reference to a related claim.) 220 */ 221 public Reference getClaim() { 222 if (this.claim == null) 223 if (Configuration.errorOnAutoCreate()) 224 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 225 else if (Configuration.doAutoCreate()) 226 this.claim = new Reference(); // cc 227 return this.claim; 228 } 229 230 public boolean hasClaim() { 231 return this.claim != null && !this.claim.isEmpty(); 232 } 233 234 /** 235 * @param value {@link #claim} (Reference to a related claim.) 236 */ 237 public RelatedClaimComponent setClaim(Reference value) { 238 this.claim = value; 239 return this; 240 } 241 242 /** 243 * @return {@link #relationship} (A code to convey how the claims are related.) 244 */ 245 public CodeableConcept getRelationship() { 246 if (this.relationship == null) 247 if (Configuration.errorOnAutoCreate()) 248 throw new Error("Attempt to auto-create RelatedClaimComponent.relationship"); 249 else if (Configuration.doAutoCreate()) 250 this.relationship = new CodeableConcept(); // cc 251 return this.relationship; 252 } 253 254 public boolean hasRelationship() { 255 return this.relationship != null && !this.relationship.isEmpty(); 256 } 257 258 /** 259 * @param value {@link #relationship} (A code to convey how the claims are related.) 260 */ 261 public RelatedClaimComponent setRelationship(CodeableConcept value) { 262 this.relationship = value; 263 return this; 264 } 265 266 /** 267 * @return {@link #reference} (An alternate organizational reference to the case or file to which this particular claim pertains.) 268 */ 269 public Identifier getReference() { 270 if (this.reference == null) 271 if (Configuration.errorOnAutoCreate()) 272 throw new Error("Attempt to auto-create RelatedClaimComponent.reference"); 273 else if (Configuration.doAutoCreate()) 274 this.reference = new Identifier(); // cc 275 return this.reference; 276 } 277 278 public boolean hasReference() { 279 return this.reference != null && !this.reference.isEmpty(); 280 } 281 282 /** 283 * @param value {@link #reference} (An alternate organizational reference to the case or file to which this particular claim pertains.) 284 */ 285 public RelatedClaimComponent setReference(Identifier value) { 286 this.reference = value; 287 return this; 288 } 289 290 protected void listChildren(List<Property> children) { 291 super.listChildren(children); 292 children.add(new Property("claim", "Reference(Claim)", "Reference to a related claim.", 0, 1, claim)); 293 children.add(new Property("relationship", "CodeableConcept", "A code to convey how the claims are related.", 0, 1, relationship)); 294 children.add(new Property("reference", "Identifier", "An alternate organizational reference to the case or file to which this particular claim pertains.", 0, 1, reference)); 295 } 296 297 @Override 298 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 299 switch (_hash) { 300 case 94742588: /*claim*/ return new Property("claim", "Reference(Claim)", "Reference to a related claim.", 0, 1, claim); 301 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "A code to convey how the claims are related.", 0, 1, relationship); 302 case -925155509: /*reference*/ return new Property("reference", "Identifier", "An alternate organizational reference to the case or file to which this particular claim pertains.", 0, 1, reference); 303 default: return super.getNamedProperty(_hash, _name, _checkValid); 304 } 305 306 } 307 308 @Override 309 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 310 switch (hash) { 311 case 94742588: /*claim*/ return this.claim == null ? new Base[0] : new Base[] {this.claim}; // Reference 312 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // CodeableConcept 313 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Identifier 314 default: return super.getProperty(hash, name, checkValid); 315 } 316 317 } 318 319 @Override 320 public Base setProperty(int hash, String name, Base value) throws FHIRException { 321 switch (hash) { 322 case 94742588: // claim 323 this.claim = TypeConvertor.castToReference(value); // Reference 324 return value; 325 case -261851592: // relationship 326 this.relationship = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 327 return value; 328 case -925155509: // reference 329 this.reference = TypeConvertor.castToIdentifier(value); // Identifier 330 return value; 331 default: return super.setProperty(hash, name, value); 332 } 333 334 } 335 336 @Override 337 public Base setProperty(String name, Base value) throws FHIRException { 338 if (name.equals("claim")) { 339 this.claim = TypeConvertor.castToReference(value); // Reference 340 } else if (name.equals("relationship")) { 341 this.relationship = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 342 } else if (name.equals("reference")) { 343 this.reference = TypeConvertor.castToIdentifier(value); // Identifier 344 } else 345 return super.setProperty(name, value); 346 return value; 347 } 348 349 @Override 350 public Base makeProperty(int hash, String name) throws FHIRException { 351 switch (hash) { 352 case 94742588: return getClaim(); 353 case -261851592: return getRelationship(); 354 case -925155509: return getReference(); 355 default: return super.makeProperty(hash, name); 356 } 357 358 } 359 360 @Override 361 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 362 switch (hash) { 363 case 94742588: /*claim*/ return new String[] {"Reference"}; 364 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 365 case -925155509: /*reference*/ return new String[] {"Identifier"}; 366 default: return super.getTypesForProperty(hash, name); 367 } 368 369 } 370 371 @Override 372 public Base addChild(String name) throws FHIRException { 373 if (name.equals("claim")) { 374 this.claim = new Reference(); 375 return this.claim; 376 } 377 else if (name.equals("relationship")) { 378 this.relationship = new CodeableConcept(); 379 return this.relationship; 380 } 381 else if (name.equals("reference")) { 382 this.reference = new Identifier(); 383 return this.reference; 384 } 385 else 386 return super.addChild(name); 387 } 388 389 public RelatedClaimComponent copy() { 390 RelatedClaimComponent dst = new RelatedClaimComponent(); 391 copyValues(dst); 392 return dst; 393 } 394 395 public void copyValues(RelatedClaimComponent dst) { 396 super.copyValues(dst); 397 dst.claim = claim == null ? null : claim.copy(); 398 dst.relationship = relationship == null ? null : relationship.copy(); 399 dst.reference = reference == null ? null : reference.copy(); 400 } 401 402 @Override 403 public boolean equalsDeep(Base other_) { 404 if (!super.equalsDeep(other_)) 405 return false; 406 if (!(other_ instanceof RelatedClaimComponent)) 407 return false; 408 RelatedClaimComponent o = (RelatedClaimComponent) other_; 409 return compareDeep(claim, o.claim, true) && compareDeep(relationship, o.relationship, true) && compareDeep(reference, o.reference, true) 410 ; 411 } 412 413 @Override 414 public boolean equalsShallow(Base other_) { 415 if (!super.equalsShallow(other_)) 416 return false; 417 if (!(other_ instanceof RelatedClaimComponent)) 418 return false; 419 RelatedClaimComponent o = (RelatedClaimComponent) other_; 420 return true; 421 } 422 423 public boolean isEmpty() { 424 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(claim, relationship, reference 425 ); 426 } 427 428 public String fhirType() { 429 return "ExplanationOfBenefit.related"; 430 431 } 432 433 } 434 435 @Block() 436 public static class ExplanationOfBenefitEventComponent extends BackboneElement implements IBaseBackboneElement { 437 /** 438 * A coded event such as when a service is expected or a card printed. 439 */ 440 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 441 @Description(shortDefinition="Specific event", formalDefinition="A coded event such as when a service is expected or a card printed." ) 442 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/datestype") 443 protected CodeableConcept type; 444 445 /** 446 * A date or period in the past or future indicating when the event occurred or is expectd to occur. 447 */ 448 @Child(name = "when", type = {DateTimeType.class, Period.class}, order=2, min=1, max=1, modifier=false, summary=false) 449 @Description(shortDefinition="Occurance date or period", formalDefinition="A date or period in the past or future indicating when the event occurred or is expectd to occur." ) 450 protected DataType when; 451 452 private static final long serialVersionUID = -634897375L; 453 454 /** 455 * Constructor 456 */ 457 public ExplanationOfBenefitEventComponent() { 458 super(); 459 } 460 461 /** 462 * Constructor 463 */ 464 public ExplanationOfBenefitEventComponent(CodeableConcept type, DataType when) { 465 super(); 466 this.setType(type); 467 this.setWhen(when); 468 } 469 470 /** 471 * @return {@link #type} (A coded event such as when a service is expected or a card printed.) 472 */ 473 public CodeableConcept getType() { 474 if (this.type == null) 475 if (Configuration.errorOnAutoCreate()) 476 throw new Error("Attempt to auto-create ExplanationOfBenefitEventComponent.type"); 477 else if (Configuration.doAutoCreate()) 478 this.type = new CodeableConcept(); // cc 479 return this.type; 480 } 481 482 public boolean hasType() { 483 return this.type != null && !this.type.isEmpty(); 484 } 485 486 /** 487 * @param value {@link #type} (A coded event such as when a service is expected or a card printed.) 488 */ 489 public ExplanationOfBenefitEventComponent setType(CodeableConcept value) { 490 this.type = value; 491 return this; 492 } 493 494 /** 495 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 496 */ 497 public DataType getWhen() { 498 return this.when; 499 } 500 501 /** 502 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 503 */ 504 public DateTimeType getWhenDateTimeType() throws FHIRException { 505 if (this.when == null) 506 this.when = new DateTimeType(); 507 if (!(this.when instanceof DateTimeType)) 508 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.when.getClass().getName()+" was encountered"); 509 return (DateTimeType) this.when; 510 } 511 512 public boolean hasWhenDateTimeType() { 513 return this != null && this.when instanceof DateTimeType; 514 } 515 516 /** 517 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 518 */ 519 public Period getWhenPeriod() throws FHIRException { 520 if (this.when == null) 521 this.when = new Period(); 522 if (!(this.when instanceof Period)) 523 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.when.getClass().getName()+" was encountered"); 524 return (Period) this.when; 525 } 526 527 public boolean hasWhenPeriod() { 528 return this != null && this.when instanceof Period; 529 } 530 531 public boolean hasWhen() { 532 return this.when != null && !this.when.isEmpty(); 533 } 534 535 /** 536 * @param value {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 537 */ 538 public ExplanationOfBenefitEventComponent setWhen(DataType value) { 539 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 540 throw new FHIRException("Not the right type for ExplanationOfBenefit.event.when[x]: "+value.fhirType()); 541 this.when = value; 542 return this; 543 } 544 545 protected void listChildren(List<Property> children) { 546 super.listChildren(children); 547 children.add(new Property("type", "CodeableConcept", "A coded event such as when a service is expected or a card printed.", 0, 1, type)); 548 children.add(new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when)); 549 } 550 551 @Override 552 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 553 switch (_hash) { 554 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A coded event such as when a service is expected or a card printed.", 0, 1, type); 555 case 1312831238: /*when[x]*/ return new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 556 case 3648314: /*when*/ return new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 557 case -1785502475: /*whenDateTime*/ return new Property("when[x]", "dateTime", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 558 case 251476379: /*whenPeriod*/ return new Property("when[x]", "Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 559 default: return super.getNamedProperty(_hash, _name, _checkValid); 560 } 561 562 } 563 564 @Override 565 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 566 switch (hash) { 567 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 568 case 3648314: /*when*/ return this.when == null ? new Base[0] : new Base[] {this.when}; // DataType 569 default: return super.getProperty(hash, name, checkValid); 570 } 571 572 } 573 574 @Override 575 public Base setProperty(int hash, String name, Base value) throws FHIRException { 576 switch (hash) { 577 case 3575610: // type 578 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 579 return value; 580 case 3648314: // when 581 this.when = TypeConvertor.castToType(value); // DataType 582 return value; 583 default: return super.setProperty(hash, name, value); 584 } 585 586 } 587 588 @Override 589 public Base setProperty(String name, Base value) throws FHIRException { 590 if (name.equals("type")) { 591 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 592 } else if (name.equals("when[x]")) { 593 this.when = TypeConvertor.castToType(value); // DataType 594 } else 595 return super.setProperty(name, value); 596 return value; 597 } 598 599 @Override 600 public Base makeProperty(int hash, String name) throws FHIRException { 601 switch (hash) { 602 case 3575610: return getType(); 603 case 1312831238: return getWhen(); 604 case 3648314: return getWhen(); 605 default: return super.makeProperty(hash, name); 606 } 607 608 } 609 610 @Override 611 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 612 switch (hash) { 613 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 614 case 3648314: /*when*/ return new String[] {"dateTime", "Period"}; 615 default: return super.getTypesForProperty(hash, name); 616 } 617 618 } 619 620 @Override 621 public Base addChild(String name) throws FHIRException { 622 if (name.equals("type")) { 623 this.type = new CodeableConcept(); 624 return this.type; 625 } 626 else if (name.equals("whenDateTime")) { 627 this.when = new DateTimeType(); 628 return this.when; 629 } 630 else if (name.equals("whenPeriod")) { 631 this.when = new Period(); 632 return this.when; 633 } 634 else 635 return super.addChild(name); 636 } 637 638 public ExplanationOfBenefitEventComponent copy() { 639 ExplanationOfBenefitEventComponent dst = new ExplanationOfBenefitEventComponent(); 640 copyValues(dst); 641 return dst; 642 } 643 644 public void copyValues(ExplanationOfBenefitEventComponent dst) { 645 super.copyValues(dst); 646 dst.type = type == null ? null : type.copy(); 647 dst.when = when == null ? null : when.copy(); 648 } 649 650 @Override 651 public boolean equalsDeep(Base other_) { 652 if (!super.equalsDeep(other_)) 653 return false; 654 if (!(other_ instanceof ExplanationOfBenefitEventComponent)) 655 return false; 656 ExplanationOfBenefitEventComponent o = (ExplanationOfBenefitEventComponent) other_; 657 return compareDeep(type, o.type, true) && compareDeep(when, o.when, true); 658 } 659 660 @Override 661 public boolean equalsShallow(Base other_) { 662 if (!super.equalsShallow(other_)) 663 return false; 664 if (!(other_ instanceof ExplanationOfBenefitEventComponent)) 665 return false; 666 ExplanationOfBenefitEventComponent o = (ExplanationOfBenefitEventComponent) other_; 667 return true; 668 } 669 670 public boolean isEmpty() { 671 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, when); 672 } 673 674 public String fhirType() { 675 return "ExplanationOfBenefit.event"; 676 677 } 678 679 } 680 681 @Block() 682 public static class PayeeComponent extends BackboneElement implements IBaseBackboneElement { 683 /** 684 * Type of Party to be reimbursed: Subscriber, provider, other. 685 */ 686 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 687 @Description(shortDefinition="Category of recipient", formalDefinition="Type of Party to be reimbursed: Subscriber, provider, other." ) 688 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payeetype") 689 protected CodeableConcept type; 690 691 /** 692 * Reference to the individual or organization to whom any payment will be made. 693 */ 694 @Child(name = "party", type = {Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, RelatedPerson.class}, order=2, min=0, max=1, modifier=false, summary=false) 695 @Description(shortDefinition="Recipient reference", formalDefinition="Reference to the individual or organization to whom any payment will be made." ) 696 protected Reference party; 697 698 private static final long serialVersionUID = -1948897146L; 699 700 /** 701 * Constructor 702 */ 703 public PayeeComponent() { 704 super(); 705 } 706 707 /** 708 * @return {@link #type} (Type of Party to be reimbursed: Subscriber, provider, other.) 709 */ 710 public CodeableConcept getType() { 711 if (this.type == null) 712 if (Configuration.errorOnAutoCreate()) 713 throw new Error("Attempt to auto-create PayeeComponent.type"); 714 else if (Configuration.doAutoCreate()) 715 this.type = new CodeableConcept(); // cc 716 return this.type; 717 } 718 719 public boolean hasType() { 720 return this.type != null && !this.type.isEmpty(); 721 } 722 723 /** 724 * @param value {@link #type} (Type of Party to be reimbursed: Subscriber, provider, other.) 725 */ 726 public PayeeComponent setType(CodeableConcept value) { 727 this.type = value; 728 return this; 729 } 730 731 /** 732 * @return {@link #party} (Reference to the individual or organization to whom any payment will be made.) 733 */ 734 public Reference getParty() { 735 if (this.party == null) 736 if (Configuration.errorOnAutoCreate()) 737 throw new Error("Attempt to auto-create PayeeComponent.party"); 738 else if (Configuration.doAutoCreate()) 739 this.party = new Reference(); // cc 740 return this.party; 741 } 742 743 public boolean hasParty() { 744 return this.party != null && !this.party.isEmpty(); 745 } 746 747 /** 748 * @param value {@link #party} (Reference to the individual or organization to whom any payment will be made.) 749 */ 750 public PayeeComponent setParty(Reference value) { 751 this.party = value; 752 return this; 753 } 754 755 protected void listChildren(List<Property> children) { 756 super.listChildren(children); 757 children.add(new Property("type", "CodeableConcept", "Type of Party to be reimbursed: Subscriber, provider, other.", 0, 1, type)); 758 children.add(new Property("party", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson)", "Reference to the individual or organization to whom any payment will be made.", 0, 1, party)); 759 } 760 761 @Override 762 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 763 switch (_hash) { 764 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of Party to be reimbursed: Subscriber, provider, other.", 0, 1, type); 765 case 106437350: /*party*/ return new Property("party", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson)", "Reference to the individual or organization to whom any payment will be made.", 0, 1, party); 766 default: return super.getNamedProperty(_hash, _name, _checkValid); 767 } 768 769 } 770 771 @Override 772 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 773 switch (hash) { 774 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 775 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 776 default: return super.getProperty(hash, name, checkValid); 777 } 778 779 } 780 781 @Override 782 public Base setProperty(int hash, String name, Base value) throws FHIRException { 783 switch (hash) { 784 case 3575610: // type 785 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 786 return value; 787 case 106437350: // party 788 this.party = TypeConvertor.castToReference(value); // Reference 789 return value; 790 default: return super.setProperty(hash, name, value); 791 } 792 793 } 794 795 @Override 796 public Base setProperty(String name, Base value) throws FHIRException { 797 if (name.equals("type")) { 798 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 799 } else if (name.equals("party")) { 800 this.party = TypeConvertor.castToReference(value); // Reference 801 } else 802 return super.setProperty(name, value); 803 return value; 804 } 805 806 @Override 807 public Base makeProperty(int hash, String name) throws FHIRException { 808 switch (hash) { 809 case 3575610: return getType(); 810 case 106437350: return getParty(); 811 default: return super.makeProperty(hash, name); 812 } 813 814 } 815 816 @Override 817 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 818 switch (hash) { 819 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 820 case 106437350: /*party*/ return new String[] {"Reference"}; 821 default: return super.getTypesForProperty(hash, name); 822 } 823 824 } 825 826 @Override 827 public Base addChild(String name) throws FHIRException { 828 if (name.equals("type")) { 829 this.type = new CodeableConcept(); 830 return this.type; 831 } 832 else if (name.equals("party")) { 833 this.party = new Reference(); 834 return this.party; 835 } 836 else 837 return super.addChild(name); 838 } 839 840 public PayeeComponent copy() { 841 PayeeComponent dst = new PayeeComponent(); 842 copyValues(dst); 843 return dst; 844 } 845 846 public void copyValues(PayeeComponent dst) { 847 super.copyValues(dst); 848 dst.type = type == null ? null : type.copy(); 849 dst.party = party == null ? null : party.copy(); 850 } 851 852 @Override 853 public boolean equalsDeep(Base other_) { 854 if (!super.equalsDeep(other_)) 855 return false; 856 if (!(other_ instanceof PayeeComponent)) 857 return false; 858 PayeeComponent o = (PayeeComponent) other_; 859 return compareDeep(type, o.type, true) && compareDeep(party, o.party, true); 860 } 861 862 @Override 863 public boolean equalsShallow(Base other_) { 864 if (!super.equalsShallow(other_)) 865 return false; 866 if (!(other_ instanceof PayeeComponent)) 867 return false; 868 PayeeComponent o = (PayeeComponent) other_; 869 return true; 870 } 871 872 public boolean isEmpty() { 873 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, party); 874 } 875 876 public String fhirType() { 877 return "ExplanationOfBenefit.payee"; 878 879 } 880 881 } 882 883 @Block() 884 public static class CareTeamComponent extends BackboneElement implements IBaseBackboneElement { 885 /** 886 * A number to uniquely identify care team entries. 887 */ 888 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 889 @Description(shortDefinition="Order of care team", formalDefinition="A number to uniquely identify care team entries." ) 890 protected PositiveIntType sequence; 891 892 /** 893 * Member of the team who provided the product or service. 894 */ 895 @Child(name = "provider", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=2, min=1, max=1, modifier=false, summary=false) 896 @Description(shortDefinition="Practitioner or organization", formalDefinition="Member of the team who provided the product or service." ) 897 protected Reference provider; 898 899 /** 900 * The party who is billing and/or responsible for the claimed products or services. 901 */ 902 @Child(name = "responsible", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 903 @Description(shortDefinition="Indicator of the lead practitioner", formalDefinition="The party who is billing and/or responsible for the claimed products or services." ) 904 protected BooleanType responsible; 905 906 /** 907 * The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team. 908 */ 909 @Child(name = "role", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 910 @Description(shortDefinition="Function within the team", formalDefinition="The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team." ) 911 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-careteamrole") 912 protected CodeableConcept role; 913 914 /** 915 * The specialization of the practitioner or provider which is applicable for this service. 916 */ 917 @Child(name = "specialty", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 918 @Description(shortDefinition="Practitioner or provider specialization", formalDefinition="The specialization of the practitioner or provider which is applicable for this service." ) 919 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/provider-qualification") 920 protected CodeableConcept specialty; 921 922 private static final long serialVersionUID = 1238813503L; 923 924 /** 925 * Constructor 926 */ 927 public CareTeamComponent() { 928 super(); 929 } 930 931 /** 932 * Constructor 933 */ 934 public CareTeamComponent(int sequence, Reference provider) { 935 super(); 936 this.setSequence(sequence); 937 this.setProvider(provider); 938 } 939 940 /** 941 * @return {@link #sequence} (A number to uniquely identify care team entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 942 */ 943 public PositiveIntType getSequenceElement() { 944 if (this.sequence == null) 945 if (Configuration.errorOnAutoCreate()) 946 throw new Error("Attempt to auto-create CareTeamComponent.sequence"); 947 else if (Configuration.doAutoCreate()) 948 this.sequence = new PositiveIntType(); // bb 949 return this.sequence; 950 } 951 952 public boolean hasSequenceElement() { 953 return this.sequence != null && !this.sequence.isEmpty(); 954 } 955 956 public boolean hasSequence() { 957 return this.sequence != null && !this.sequence.isEmpty(); 958 } 959 960 /** 961 * @param value {@link #sequence} (A number to uniquely identify care team entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 962 */ 963 public CareTeamComponent setSequenceElement(PositiveIntType value) { 964 this.sequence = value; 965 return this; 966 } 967 968 /** 969 * @return A number to uniquely identify care team entries. 970 */ 971 public int getSequence() { 972 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 973 } 974 975 /** 976 * @param value A number to uniquely identify care team entries. 977 */ 978 public CareTeamComponent setSequence(int value) { 979 if (this.sequence == null) 980 this.sequence = new PositiveIntType(); 981 this.sequence.setValue(value); 982 return this; 983 } 984 985 /** 986 * @return {@link #provider} (Member of the team who provided the product or service.) 987 */ 988 public Reference getProvider() { 989 if (this.provider == null) 990 if (Configuration.errorOnAutoCreate()) 991 throw new Error("Attempt to auto-create CareTeamComponent.provider"); 992 else if (Configuration.doAutoCreate()) 993 this.provider = new Reference(); // cc 994 return this.provider; 995 } 996 997 public boolean hasProvider() { 998 return this.provider != null && !this.provider.isEmpty(); 999 } 1000 1001 /** 1002 * @param value {@link #provider} (Member of the team who provided the product or service.) 1003 */ 1004 public CareTeamComponent setProvider(Reference value) { 1005 this.provider = value; 1006 return this; 1007 } 1008 1009 /** 1010 * @return {@link #responsible} (The party who is billing and/or responsible for the claimed products or services.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1011 */ 1012 public BooleanType getResponsibleElement() { 1013 if (this.responsible == null) 1014 if (Configuration.errorOnAutoCreate()) 1015 throw new Error("Attempt to auto-create CareTeamComponent.responsible"); 1016 else if (Configuration.doAutoCreate()) 1017 this.responsible = new BooleanType(); // bb 1018 return this.responsible; 1019 } 1020 1021 public boolean hasResponsibleElement() { 1022 return this.responsible != null && !this.responsible.isEmpty(); 1023 } 1024 1025 public boolean hasResponsible() { 1026 return this.responsible != null && !this.responsible.isEmpty(); 1027 } 1028 1029 /** 1030 * @param value {@link #responsible} (The party who is billing and/or responsible for the claimed products or services.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1031 */ 1032 public CareTeamComponent setResponsibleElement(BooleanType value) { 1033 this.responsible = value; 1034 return this; 1035 } 1036 1037 /** 1038 * @return The party who is billing and/or responsible for the claimed products or services. 1039 */ 1040 public boolean getResponsible() { 1041 return this.responsible == null || this.responsible.isEmpty() ? false : this.responsible.getValue(); 1042 } 1043 1044 /** 1045 * @param value The party who is billing and/or responsible for the claimed products or services. 1046 */ 1047 public CareTeamComponent setResponsible(boolean value) { 1048 if (this.responsible == null) 1049 this.responsible = new BooleanType(); 1050 this.responsible.setValue(value); 1051 return this; 1052 } 1053 1054 /** 1055 * @return {@link #role} (The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.) 1056 */ 1057 public CodeableConcept getRole() { 1058 if (this.role == null) 1059 if (Configuration.errorOnAutoCreate()) 1060 throw new Error("Attempt to auto-create CareTeamComponent.role"); 1061 else if (Configuration.doAutoCreate()) 1062 this.role = new CodeableConcept(); // cc 1063 return this.role; 1064 } 1065 1066 public boolean hasRole() { 1067 return this.role != null && !this.role.isEmpty(); 1068 } 1069 1070 /** 1071 * @param value {@link #role} (The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.) 1072 */ 1073 public CareTeamComponent setRole(CodeableConcept value) { 1074 this.role = value; 1075 return this; 1076 } 1077 1078 /** 1079 * @return {@link #specialty} (The specialization of the practitioner or provider which is applicable for this service.) 1080 */ 1081 public CodeableConcept getSpecialty() { 1082 if (this.specialty == null) 1083 if (Configuration.errorOnAutoCreate()) 1084 throw new Error("Attempt to auto-create CareTeamComponent.specialty"); 1085 else if (Configuration.doAutoCreate()) 1086 this.specialty = new CodeableConcept(); // cc 1087 return this.specialty; 1088 } 1089 1090 public boolean hasSpecialty() { 1091 return this.specialty != null && !this.specialty.isEmpty(); 1092 } 1093 1094 /** 1095 * @param value {@link #specialty} (The specialization of the practitioner or provider which is applicable for this service.) 1096 */ 1097 public CareTeamComponent setSpecialty(CodeableConcept value) { 1098 this.specialty = value; 1099 return this; 1100 } 1101 1102 protected void listChildren(List<Property> children) { 1103 super.listChildren(children); 1104 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify care team entries.", 0, 1, sequence)); 1105 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "Member of the team who provided the product or service.", 0, 1, provider)); 1106 children.add(new Property("responsible", "boolean", "The party who is billing and/or responsible for the claimed products or services.", 0, 1, responsible)); 1107 children.add(new Property("role", "CodeableConcept", "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.", 0, 1, role)); 1108 children.add(new Property("specialty", "CodeableConcept", "The specialization of the practitioner or provider which is applicable for this service.", 0, 1, specialty)); 1109 } 1110 1111 @Override 1112 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1113 switch (_hash) { 1114 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify care team entries.", 0, 1, sequence); 1115 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "Member of the team who provided the product or service.", 0, 1, provider); 1116 case 1847674614: /*responsible*/ return new Property("responsible", "boolean", "The party who is billing and/or responsible for the claimed products or services.", 0, 1, responsible); 1117 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.", 0, 1, role); 1118 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "The specialization of the practitioner or provider which is applicable for this service.", 0, 1, specialty); 1119 default: return super.getNamedProperty(_hash, _name, _checkValid); 1120 } 1121 1122 } 1123 1124 @Override 1125 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1126 switch (hash) { 1127 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1128 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 1129 case 1847674614: /*responsible*/ return this.responsible == null ? new Base[0] : new Base[] {this.responsible}; // BooleanType 1130 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 1131 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : new Base[] {this.specialty}; // CodeableConcept 1132 default: return super.getProperty(hash, name, checkValid); 1133 } 1134 1135 } 1136 1137 @Override 1138 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1139 switch (hash) { 1140 case 1349547969: // sequence 1141 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1142 return value; 1143 case -987494927: // provider 1144 this.provider = TypeConvertor.castToReference(value); // Reference 1145 return value; 1146 case 1847674614: // responsible 1147 this.responsible = TypeConvertor.castToBoolean(value); // BooleanType 1148 return value; 1149 case 3506294: // role 1150 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1151 return value; 1152 case -1694759682: // specialty 1153 this.specialty = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1154 return value; 1155 default: return super.setProperty(hash, name, value); 1156 } 1157 1158 } 1159 1160 @Override 1161 public Base setProperty(String name, Base value) throws FHIRException { 1162 if (name.equals("sequence")) { 1163 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1164 } else if (name.equals("provider")) { 1165 this.provider = TypeConvertor.castToReference(value); // Reference 1166 } else if (name.equals("responsible")) { 1167 this.responsible = TypeConvertor.castToBoolean(value); // BooleanType 1168 } else if (name.equals("role")) { 1169 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1170 } else if (name.equals("specialty")) { 1171 this.specialty = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1172 } else 1173 return super.setProperty(name, value); 1174 return value; 1175 } 1176 1177 @Override 1178 public Base makeProperty(int hash, String name) throws FHIRException { 1179 switch (hash) { 1180 case 1349547969: return getSequenceElement(); 1181 case -987494927: return getProvider(); 1182 case 1847674614: return getResponsibleElement(); 1183 case 3506294: return getRole(); 1184 case -1694759682: return getSpecialty(); 1185 default: return super.makeProperty(hash, name); 1186 } 1187 1188 } 1189 1190 @Override 1191 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1192 switch (hash) { 1193 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1194 case -987494927: /*provider*/ return new String[] {"Reference"}; 1195 case 1847674614: /*responsible*/ return new String[] {"boolean"}; 1196 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 1197 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 1198 default: return super.getTypesForProperty(hash, name); 1199 } 1200 1201 } 1202 1203 @Override 1204 public Base addChild(String name) throws FHIRException { 1205 if (name.equals("sequence")) { 1206 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.careTeam.sequence"); 1207 } 1208 else if (name.equals("provider")) { 1209 this.provider = new Reference(); 1210 return this.provider; 1211 } 1212 else if (name.equals("responsible")) { 1213 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.careTeam.responsible"); 1214 } 1215 else if (name.equals("role")) { 1216 this.role = new CodeableConcept(); 1217 return this.role; 1218 } 1219 else if (name.equals("specialty")) { 1220 this.specialty = new CodeableConcept(); 1221 return this.specialty; 1222 } 1223 else 1224 return super.addChild(name); 1225 } 1226 1227 public CareTeamComponent copy() { 1228 CareTeamComponent dst = new CareTeamComponent(); 1229 copyValues(dst); 1230 return dst; 1231 } 1232 1233 public void copyValues(CareTeamComponent dst) { 1234 super.copyValues(dst); 1235 dst.sequence = sequence == null ? null : sequence.copy(); 1236 dst.provider = provider == null ? null : provider.copy(); 1237 dst.responsible = responsible == null ? null : responsible.copy(); 1238 dst.role = role == null ? null : role.copy(); 1239 dst.specialty = specialty == null ? null : specialty.copy(); 1240 } 1241 1242 @Override 1243 public boolean equalsDeep(Base other_) { 1244 if (!super.equalsDeep(other_)) 1245 return false; 1246 if (!(other_ instanceof CareTeamComponent)) 1247 return false; 1248 CareTeamComponent o = (CareTeamComponent) other_; 1249 return compareDeep(sequence, o.sequence, true) && compareDeep(provider, o.provider, true) && compareDeep(responsible, o.responsible, true) 1250 && compareDeep(role, o.role, true) && compareDeep(specialty, o.specialty, true); 1251 } 1252 1253 @Override 1254 public boolean equalsShallow(Base other_) { 1255 if (!super.equalsShallow(other_)) 1256 return false; 1257 if (!(other_ instanceof CareTeamComponent)) 1258 return false; 1259 CareTeamComponent o = (CareTeamComponent) other_; 1260 return compareValues(sequence, o.sequence, true) && compareValues(responsible, o.responsible, true) 1261 ; 1262 } 1263 1264 public boolean isEmpty() { 1265 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, provider, responsible 1266 , role, specialty); 1267 } 1268 1269 public String fhirType() { 1270 return "ExplanationOfBenefit.careTeam"; 1271 1272 } 1273 1274 } 1275 1276 @Block() 1277 public static class SupportingInformationComponent extends BackboneElement implements IBaseBackboneElement { 1278 /** 1279 * A number to uniquely identify supporting information entries. 1280 */ 1281 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1282 @Description(shortDefinition="Information instance identifier", formalDefinition="A number to uniquely identify supporting information entries." ) 1283 protected PositiveIntType sequence; 1284 1285 /** 1286 * The general class of the information supplied: information; exception; accident, employment; onset, etc. 1287 */ 1288 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 1289 @Description(shortDefinition="Classification of the supplied information", formalDefinition="The general class of the information supplied: information; exception; accident, employment; onset, etc." ) 1290 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-informationcategory") 1291 protected CodeableConcept category; 1292 1293 /** 1294 * System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought. 1295 */ 1296 @Child(name = "code", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1297 @Description(shortDefinition="Type of information", formalDefinition="System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought." ) 1298 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-exception") 1299 protected CodeableConcept code; 1300 1301 /** 1302 * The date when or period to which this information refers. 1303 */ 1304 @Child(name = "timing", type = {DateType.class, Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 1305 @Description(shortDefinition="When it occurred", formalDefinition="The date when or period to which this information refers." ) 1306 protected DataType timing; 1307 1308 /** 1309 * Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data. 1310 */ 1311 @Child(name = "value", type = {BooleanType.class, StringType.class, Quantity.class, Attachment.class, Reference.class, Identifier.class}, order=5, min=0, max=1, modifier=false, summary=false) 1312 @Description(shortDefinition="Data to be provided", formalDefinition="Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data." ) 1313 protected DataType value; 1314 1315 /** 1316 * Provides the reason in the situation where a reason code is required in addition to the content. 1317 */ 1318 @Child(name = "reason", type = {Coding.class}, order=6, min=0, max=1, modifier=false, summary=false) 1319 @Description(shortDefinition="Explanation for the information", formalDefinition="Provides the reason in the situation where a reason code is required in addition to the content." ) 1320 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/missing-tooth-reason") 1321 protected Coding reason; 1322 1323 private static final long serialVersionUID = 1577205655L; 1324 1325 /** 1326 * Constructor 1327 */ 1328 public SupportingInformationComponent() { 1329 super(); 1330 } 1331 1332 /** 1333 * Constructor 1334 */ 1335 public SupportingInformationComponent(int sequence, CodeableConcept category) { 1336 super(); 1337 this.setSequence(sequence); 1338 this.setCategory(category); 1339 } 1340 1341 /** 1342 * @return {@link #sequence} (A number to uniquely identify supporting information entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1343 */ 1344 public PositiveIntType getSequenceElement() { 1345 if (this.sequence == null) 1346 if (Configuration.errorOnAutoCreate()) 1347 throw new Error("Attempt to auto-create SupportingInformationComponent.sequence"); 1348 else if (Configuration.doAutoCreate()) 1349 this.sequence = new PositiveIntType(); // bb 1350 return this.sequence; 1351 } 1352 1353 public boolean hasSequenceElement() { 1354 return this.sequence != null && !this.sequence.isEmpty(); 1355 } 1356 1357 public boolean hasSequence() { 1358 return this.sequence != null && !this.sequence.isEmpty(); 1359 } 1360 1361 /** 1362 * @param value {@link #sequence} (A number to uniquely identify supporting information entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1363 */ 1364 public SupportingInformationComponent setSequenceElement(PositiveIntType value) { 1365 this.sequence = value; 1366 return this; 1367 } 1368 1369 /** 1370 * @return A number to uniquely identify supporting information entries. 1371 */ 1372 public int getSequence() { 1373 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1374 } 1375 1376 /** 1377 * @param value A number to uniquely identify supporting information entries. 1378 */ 1379 public SupportingInformationComponent setSequence(int value) { 1380 if (this.sequence == null) 1381 this.sequence = new PositiveIntType(); 1382 this.sequence.setValue(value); 1383 return this; 1384 } 1385 1386 /** 1387 * @return {@link #category} (The general class of the information supplied: information; exception; accident, employment; onset, etc.) 1388 */ 1389 public CodeableConcept getCategory() { 1390 if (this.category == null) 1391 if (Configuration.errorOnAutoCreate()) 1392 throw new Error("Attempt to auto-create SupportingInformationComponent.category"); 1393 else if (Configuration.doAutoCreate()) 1394 this.category = new CodeableConcept(); // cc 1395 return this.category; 1396 } 1397 1398 public boolean hasCategory() { 1399 return this.category != null && !this.category.isEmpty(); 1400 } 1401 1402 /** 1403 * @param value {@link #category} (The general class of the information supplied: information; exception; accident, employment; onset, etc.) 1404 */ 1405 public SupportingInformationComponent setCategory(CodeableConcept value) { 1406 this.category = value; 1407 return this; 1408 } 1409 1410 /** 1411 * @return {@link #code} (System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.) 1412 */ 1413 public CodeableConcept getCode() { 1414 if (this.code == null) 1415 if (Configuration.errorOnAutoCreate()) 1416 throw new Error("Attempt to auto-create SupportingInformationComponent.code"); 1417 else if (Configuration.doAutoCreate()) 1418 this.code = new CodeableConcept(); // cc 1419 return this.code; 1420 } 1421 1422 public boolean hasCode() { 1423 return this.code != null && !this.code.isEmpty(); 1424 } 1425 1426 /** 1427 * @param value {@link #code} (System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.) 1428 */ 1429 public SupportingInformationComponent setCode(CodeableConcept value) { 1430 this.code = value; 1431 return this; 1432 } 1433 1434 /** 1435 * @return {@link #timing} (The date when or period to which this information refers.) 1436 */ 1437 public DataType getTiming() { 1438 return this.timing; 1439 } 1440 1441 /** 1442 * @return {@link #timing} (The date when or period to which this information refers.) 1443 */ 1444 public DateType getTimingDateType() throws FHIRException { 1445 if (this.timing == null) 1446 this.timing = new DateType(); 1447 if (!(this.timing instanceof DateType)) 1448 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.timing.getClass().getName()+" was encountered"); 1449 return (DateType) this.timing; 1450 } 1451 1452 public boolean hasTimingDateType() { 1453 return this != null && this.timing instanceof DateType; 1454 } 1455 1456 /** 1457 * @return {@link #timing} (The date when or period to which this information refers.) 1458 */ 1459 public Period getTimingPeriod() throws FHIRException { 1460 if (this.timing == null) 1461 this.timing = new Period(); 1462 if (!(this.timing instanceof Period)) 1463 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.timing.getClass().getName()+" was encountered"); 1464 return (Period) this.timing; 1465 } 1466 1467 public boolean hasTimingPeriod() { 1468 return this != null && this.timing instanceof Period; 1469 } 1470 1471 public boolean hasTiming() { 1472 return this.timing != null && !this.timing.isEmpty(); 1473 } 1474 1475 /** 1476 * @param value {@link #timing} (The date when or period to which this information refers.) 1477 */ 1478 public SupportingInformationComponent setTiming(DataType value) { 1479 if (value != null && !(value instanceof DateType || value instanceof Period)) 1480 throw new FHIRException("Not the right type for ExplanationOfBenefit.supportingInfo.timing[x]: "+value.fhirType()); 1481 this.timing = value; 1482 return this; 1483 } 1484 1485 /** 1486 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1487 */ 1488 public DataType getValue() { 1489 return this.value; 1490 } 1491 1492 /** 1493 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1494 */ 1495 public BooleanType getValueBooleanType() throws FHIRException { 1496 if (this.value == null) 1497 this.value = new BooleanType(); 1498 if (!(this.value instanceof BooleanType)) 1499 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 1500 return (BooleanType) this.value; 1501 } 1502 1503 public boolean hasValueBooleanType() { 1504 return this != null && this.value instanceof BooleanType; 1505 } 1506 1507 /** 1508 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1509 */ 1510 public StringType getValueStringType() throws FHIRException { 1511 if (this.value == null) 1512 this.value = new StringType(); 1513 if (!(this.value instanceof StringType)) 1514 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 1515 return (StringType) this.value; 1516 } 1517 1518 public boolean hasValueStringType() { 1519 return this != null && this.value instanceof StringType; 1520 } 1521 1522 /** 1523 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1524 */ 1525 public Quantity getValueQuantity() throws FHIRException { 1526 if (this.value == null) 1527 this.value = new Quantity(); 1528 if (!(this.value instanceof Quantity)) 1529 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1530 return (Quantity) this.value; 1531 } 1532 1533 public boolean hasValueQuantity() { 1534 return this != null && this.value instanceof Quantity; 1535 } 1536 1537 /** 1538 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1539 */ 1540 public Attachment getValueAttachment() throws FHIRException { 1541 if (this.value == null) 1542 this.value = new Attachment(); 1543 if (!(this.value instanceof Attachment)) 1544 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 1545 return (Attachment) this.value; 1546 } 1547 1548 public boolean hasValueAttachment() { 1549 return this != null && this.value instanceof Attachment; 1550 } 1551 1552 /** 1553 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1554 */ 1555 public Reference getValueReference() throws FHIRException { 1556 if (this.value == null) 1557 this.value = new Reference(); 1558 if (!(this.value instanceof Reference)) 1559 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 1560 return (Reference) this.value; 1561 } 1562 1563 public boolean hasValueReference() { 1564 return this != null && this.value instanceof Reference; 1565 } 1566 1567 /** 1568 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1569 */ 1570 public Identifier getValueIdentifier() throws FHIRException { 1571 if (this.value == null) 1572 this.value = new Identifier(); 1573 if (!(this.value instanceof Identifier)) 1574 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.value.getClass().getName()+" was encountered"); 1575 return (Identifier) this.value; 1576 } 1577 1578 public boolean hasValueIdentifier() { 1579 return this != null && this.value instanceof Identifier; 1580 } 1581 1582 public boolean hasValue() { 1583 return this.value != null && !this.value.isEmpty(); 1584 } 1585 1586 /** 1587 * @param value {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1588 */ 1589 public SupportingInformationComponent setValue(DataType value) { 1590 if (value != null && !(value instanceof BooleanType || value instanceof StringType || value instanceof Quantity || value instanceof Attachment || value instanceof Reference || value instanceof Identifier)) 1591 throw new FHIRException("Not the right type for ExplanationOfBenefit.supportingInfo.value[x]: "+value.fhirType()); 1592 this.value = value; 1593 return this; 1594 } 1595 1596 /** 1597 * @return {@link #reason} (Provides the reason in the situation where a reason code is required in addition to the content.) 1598 */ 1599 public Coding getReason() { 1600 if (this.reason == null) 1601 if (Configuration.errorOnAutoCreate()) 1602 throw new Error("Attempt to auto-create SupportingInformationComponent.reason"); 1603 else if (Configuration.doAutoCreate()) 1604 this.reason = new Coding(); // cc 1605 return this.reason; 1606 } 1607 1608 public boolean hasReason() { 1609 return this.reason != null && !this.reason.isEmpty(); 1610 } 1611 1612 /** 1613 * @param value {@link #reason} (Provides the reason in the situation where a reason code is required in addition to the content.) 1614 */ 1615 public SupportingInformationComponent setReason(Coding value) { 1616 this.reason = value; 1617 return this; 1618 } 1619 1620 protected void listChildren(List<Property> children) { 1621 super.listChildren(children); 1622 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify supporting information entries.", 0, 1, sequence)); 1623 children.add(new Property("category", "CodeableConcept", "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1, category)); 1624 children.add(new Property("code", "CodeableConcept", "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.", 0, 1, code)); 1625 children.add(new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing)); 1626 children.add(new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)|Identifier", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value)); 1627 children.add(new Property("reason", "Coding", "Provides the reason in the situation where a reason code is required in addition to the content.", 0, 1, reason)); 1628 } 1629 1630 @Override 1631 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1632 switch (_hash) { 1633 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify supporting information entries.", 0, 1, sequence); 1634 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1, category); 1635 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.", 0, 1, code); 1636 case 164632566: /*timing[x]*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1637 case -873664438: /*timing*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1638 case 807935768: /*timingDate*/ return new Property("timing[x]", "date", "The date when or period to which this information refers.", 0, 1, timing); 1639 case -615615829: /*timingPeriod*/ return new Property("timing[x]", "Period", "The date when or period to which this information refers.", 0, 1, timing); 1640 case -1410166417: /*value[x]*/ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)|Identifier", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1641 case 111972721: /*value*/ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)|Identifier", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1642 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1643 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1644 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1645 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1646 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1647 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "Identifier", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1648 case -934964668: /*reason*/ return new Property("reason", "Coding", "Provides the reason in the situation where a reason code is required in addition to the content.", 0, 1, reason); 1649 default: return super.getNamedProperty(_hash, _name, _checkValid); 1650 } 1651 1652 } 1653 1654 @Override 1655 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1656 switch (hash) { 1657 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1658 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1659 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1660 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // DataType 1661 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1662 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // Coding 1663 default: return super.getProperty(hash, name, checkValid); 1664 } 1665 1666 } 1667 1668 @Override 1669 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1670 switch (hash) { 1671 case 1349547969: // sequence 1672 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1673 return value; 1674 case 50511102: // category 1675 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1676 return value; 1677 case 3059181: // code 1678 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1679 return value; 1680 case -873664438: // timing 1681 this.timing = TypeConvertor.castToType(value); // DataType 1682 return value; 1683 case 111972721: // value 1684 this.value = TypeConvertor.castToType(value); // DataType 1685 return value; 1686 case -934964668: // reason 1687 this.reason = TypeConvertor.castToCoding(value); // Coding 1688 return value; 1689 default: return super.setProperty(hash, name, value); 1690 } 1691 1692 } 1693 1694 @Override 1695 public Base setProperty(String name, Base value) throws FHIRException { 1696 if (name.equals("sequence")) { 1697 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1698 } else if (name.equals("category")) { 1699 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1700 } else if (name.equals("code")) { 1701 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1702 } else if (name.equals("timing[x]")) { 1703 this.timing = TypeConvertor.castToType(value); // DataType 1704 } else if (name.equals("value[x]")) { 1705 this.value = TypeConvertor.castToType(value); // DataType 1706 } else if (name.equals("reason")) { 1707 this.reason = TypeConvertor.castToCoding(value); // Coding 1708 } else 1709 return super.setProperty(name, value); 1710 return value; 1711 } 1712 1713 @Override 1714 public Base makeProperty(int hash, String name) throws FHIRException { 1715 switch (hash) { 1716 case 1349547969: return getSequenceElement(); 1717 case 50511102: return getCategory(); 1718 case 3059181: return getCode(); 1719 case 164632566: return getTiming(); 1720 case -873664438: return getTiming(); 1721 case -1410166417: return getValue(); 1722 case 111972721: return getValue(); 1723 case -934964668: return getReason(); 1724 default: return super.makeProperty(hash, name); 1725 } 1726 1727 } 1728 1729 @Override 1730 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1731 switch (hash) { 1732 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1733 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1734 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1735 case -873664438: /*timing*/ return new String[] {"date", "Period"}; 1736 case 111972721: /*value*/ return new String[] {"boolean", "string", "Quantity", "Attachment", "Reference", "Identifier"}; 1737 case -934964668: /*reason*/ return new String[] {"Coding"}; 1738 default: return super.getTypesForProperty(hash, name); 1739 } 1740 1741 } 1742 1743 @Override 1744 public Base addChild(String name) throws FHIRException { 1745 if (name.equals("sequence")) { 1746 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.supportingInfo.sequence"); 1747 } 1748 else if (name.equals("category")) { 1749 this.category = new CodeableConcept(); 1750 return this.category; 1751 } 1752 else if (name.equals("code")) { 1753 this.code = new CodeableConcept(); 1754 return this.code; 1755 } 1756 else if (name.equals("timingDate")) { 1757 this.timing = new DateType(); 1758 return this.timing; 1759 } 1760 else if (name.equals("timingPeriod")) { 1761 this.timing = new Period(); 1762 return this.timing; 1763 } 1764 else if (name.equals("valueBoolean")) { 1765 this.value = new BooleanType(); 1766 return this.value; 1767 } 1768 else if (name.equals("valueString")) { 1769 this.value = new StringType(); 1770 return this.value; 1771 } 1772 else if (name.equals("valueQuantity")) { 1773 this.value = new Quantity(); 1774 return this.value; 1775 } 1776 else if (name.equals("valueAttachment")) { 1777 this.value = new Attachment(); 1778 return this.value; 1779 } 1780 else if (name.equals("valueReference")) { 1781 this.value = new Reference(); 1782 return this.value; 1783 } 1784 else if (name.equals("valueIdentifier")) { 1785 this.value = new Identifier(); 1786 return this.value; 1787 } 1788 else if (name.equals("reason")) { 1789 this.reason = new Coding(); 1790 return this.reason; 1791 } 1792 else 1793 return super.addChild(name); 1794 } 1795 1796 public SupportingInformationComponent copy() { 1797 SupportingInformationComponent dst = new SupportingInformationComponent(); 1798 copyValues(dst); 1799 return dst; 1800 } 1801 1802 public void copyValues(SupportingInformationComponent dst) { 1803 super.copyValues(dst); 1804 dst.sequence = sequence == null ? null : sequence.copy(); 1805 dst.category = category == null ? null : category.copy(); 1806 dst.code = code == null ? null : code.copy(); 1807 dst.timing = timing == null ? null : timing.copy(); 1808 dst.value = value == null ? null : value.copy(); 1809 dst.reason = reason == null ? null : reason.copy(); 1810 } 1811 1812 @Override 1813 public boolean equalsDeep(Base other_) { 1814 if (!super.equalsDeep(other_)) 1815 return false; 1816 if (!(other_ instanceof SupportingInformationComponent)) 1817 return false; 1818 SupportingInformationComponent o = (SupportingInformationComponent) other_; 1819 return compareDeep(sequence, o.sequence, true) && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 1820 && compareDeep(timing, o.timing, true) && compareDeep(value, o.value, true) && compareDeep(reason, o.reason, true) 1821 ; 1822 } 1823 1824 @Override 1825 public boolean equalsShallow(Base other_) { 1826 if (!super.equalsShallow(other_)) 1827 return false; 1828 if (!(other_ instanceof SupportingInformationComponent)) 1829 return false; 1830 SupportingInformationComponent o = (SupportingInformationComponent) other_; 1831 return compareValues(sequence, o.sequence, true); 1832 } 1833 1834 public boolean isEmpty() { 1835 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, category, code 1836 , timing, value, reason); 1837 } 1838 1839 public String fhirType() { 1840 return "ExplanationOfBenefit.supportingInfo"; 1841 1842 } 1843 1844 } 1845 1846 @Block() 1847 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 1848 /** 1849 * A number to uniquely identify diagnosis entries. 1850 */ 1851 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1852 @Description(shortDefinition="Diagnosis instance identifier", formalDefinition="A number to uniquely identify diagnosis entries." ) 1853 protected PositiveIntType sequence; 1854 1855 /** 1856 * The nature of illness or problem in a coded form or as a reference to an external defined Condition. 1857 */ 1858 @Child(name = "diagnosis", type = {CodeableConcept.class, Condition.class}, order=2, min=1, max=1, modifier=false, summary=false) 1859 @Description(shortDefinition="Nature of illness or problem", formalDefinition="The nature of illness or problem in a coded form or as a reference to an external defined Condition." ) 1860 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10") 1861 protected DataType diagnosis; 1862 1863 /** 1864 * When the condition was observed or the relative ranking. 1865 */ 1866 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1867 @Description(shortDefinition="Timing or nature of the diagnosis", formalDefinition="When the condition was observed or the relative ranking." ) 1868 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosistype") 1869 protected List<CodeableConcept> type; 1870 1871 /** 1872 * Indication of whether the diagnosis was present on admission to a facility. 1873 */ 1874 @Child(name = "onAdmission", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1875 @Description(shortDefinition="Present on admission", formalDefinition="Indication of whether the diagnosis was present on admission to a facility." ) 1876 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosis-on-admission") 1877 protected CodeableConcept onAdmission; 1878 1879 private static final long serialVersionUID = -320261526L; 1880 1881 /** 1882 * Constructor 1883 */ 1884 public DiagnosisComponent() { 1885 super(); 1886 } 1887 1888 /** 1889 * Constructor 1890 */ 1891 public DiagnosisComponent(int sequence, DataType diagnosis) { 1892 super(); 1893 this.setSequence(sequence); 1894 this.setDiagnosis(diagnosis); 1895 } 1896 1897 /** 1898 * @return {@link #sequence} (A number to uniquely identify diagnosis entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1899 */ 1900 public PositiveIntType getSequenceElement() { 1901 if (this.sequence == null) 1902 if (Configuration.errorOnAutoCreate()) 1903 throw new Error("Attempt to auto-create DiagnosisComponent.sequence"); 1904 else if (Configuration.doAutoCreate()) 1905 this.sequence = new PositiveIntType(); // bb 1906 return this.sequence; 1907 } 1908 1909 public boolean hasSequenceElement() { 1910 return this.sequence != null && !this.sequence.isEmpty(); 1911 } 1912 1913 public boolean hasSequence() { 1914 return this.sequence != null && !this.sequence.isEmpty(); 1915 } 1916 1917 /** 1918 * @param value {@link #sequence} (A number to uniquely identify diagnosis entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1919 */ 1920 public DiagnosisComponent setSequenceElement(PositiveIntType value) { 1921 this.sequence = value; 1922 return this; 1923 } 1924 1925 /** 1926 * @return A number to uniquely identify diagnosis entries. 1927 */ 1928 public int getSequence() { 1929 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1930 } 1931 1932 /** 1933 * @param value A number to uniquely identify diagnosis entries. 1934 */ 1935 public DiagnosisComponent setSequence(int value) { 1936 if (this.sequence == null) 1937 this.sequence = new PositiveIntType(); 1938 this.sequence.setValue(value); 1939 return this; 1940 } 1941 1942 /** 1943 * @return {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 1944 */ 1945 public DataType getDiagnosis() { 1946 return this.diagnosis; 1947 } 1948 1949 /** 1950 * @return {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 1951 */ 1952 public CodeableConcept getDiagnosisCodeableConcept() throws FHIRException { 1953 if (this.diagnosis == null) 1954 this.diagnosis = new CodeableConcept(); 1955 if (!(this.diagnosis instanceof CodeableConcept)) 1956 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 1957 return (CodeableConcept) this.diagnosis; 1958 } 1959 1960 public boolean hasDiagnosisCodeableConcept() { 1961 return this != null && this.diagnosis instanceof CodeableConcept; 1962 } 1963 1964 /** 1965 * @return {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 1966 */ 1967 public Reference getDiagnosisReference() throws FHIRException { 1968 if (this.diagnosis == null) 1969 this.diagnosis = new Reference(); 1970 if (!(this.diagnosis instanceof Reference)) 1971 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 1972 return (Reference) this.diagnosis; 1973 } 1974 1975 public boolean hasDiagnosisReference() { 1976 return this != null && this.diagnosis instanceof Reference; 1977 } 1978 1979 public boolean hasDiagnosis() { 1980 return this.diagnosis != null && !this.diagnosis.isEmpty(); 1981 } 1982 1983 /** 1984 * @param value {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 1985 */ 1986 public DiagnosisComponent setDiagnosis(DataType value) { 1987 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1988 throw new FHIRException("Not the right type for ExplanationOfBenefit.diagnosis.diagnosis[x]: "+value.fhirType()); 1989 this.diagnosis = value; 1990 return this; 1991 } 1992 1993 /** 1994 * @return {@link #type} (When the condition was observed or the relative ranking.) 1995 */ 1996 public List<CodeableConcept> getType() { 1997 if (this.type == null) 1998 this.type = new ArrayList<CodeableConcept>(); 1999 return this.type; 2000 } 2001 2002 /** 2003 * @return Returns a reference to <code>this</code> for easy method chaining 2004 */ 2005 public DiagnosisComponent setType(List<CodeableConcept> theType) { 2006 this.type = theType; 2007 return this; 2008 } 2009 2010 public boolean hasType() { 2011 if (this.type == null) 2012 return false; 2013 for (CodeableConcept item : this.type) 2014 if (!item.isEmpty()) 2015 return true; 2016 return false; 2017 } 2018 2019 public CodeableConcept addType() { //3 2020 CodeableConcept t = new CodeableConcept(); 2021 if (this.type == null) 2022 this.type = new ArrayList<CodeableConcept>(); 2023 this.type.add(t); 2024 return t; 2025 } 2026 2027 public DiagnosisComponent addType(CodeableConcept t) { //3 2028 if (t == null) 2029 return this; 2030 if (this.type == null) 2031 this.type = new ArrayList<CodeableConcept>(); 2032 this.type.add(t); 2033 return this; 2034 } 2035 2036 /** 2037 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 2038 */ 2039 public CodeableConcept getTypeFirstRep() { 2040 if (getType().isEmpty()) { 2041 addType(); 2042 } 2043 return getType().get(0); 2044 } 2045 2046 /** 2047 * @return {@link #onAdmission} (Indication of whether the diagnosis was present on admission to a facility.) 2048 */ 2049 public CodeableConcept getOnAdmission() { 2050 if (this.onAdmission == null) 2051 if (Configuration.errorOnAutoCreate()) 2052 throw new Error("Attempt to auto-create DiagnosisComponent.onAdmission"); 2053 else if (Configuration.doAutoCreate()) 2054 this.onAdmission = new CodeableConcept(); // cc 2055 return this.onAdmission; 2056 } 2057 2058 public boolean hasOnAdmission() { 2059 return this.onAdmission != null && !this.onAdmission.isEmpty(); 2060 } 2061 2062 /** 2063 * @param value {@link #onAdmission} (Indication of whether the diagnosis was present on admission to a facility.) 2064 */ 2065 public DiagnosisComponent setOnAdmission(CodeableConcept value) { 2066 this.onAdmission = value; 2067 return this; 2068 } 2069 2070 protected void listChildren(List<Property> children) { 2071 super.listChildren(children); 2072 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify diagnosis entries.", 0, 1, sequence)); 2073 children.add(new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis)); 2074 children.add(new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type)); 2075 children.add(new Property("onAdmission", "CodeableConcept", "Indication of whether the diagnosis was present on admission to a facility.", 0, 1, onAdmission)); 2076 } 2077 2078 @Override 2079 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2080 switch (_hash) { 2081 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify diagnosis entries.", 0, 1, sequence); 2082 case -1487009809: /*diagnosis[x]*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 2083 case 1196993265: /*diagnosis*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 2084 case 277781616: /*diagnosisCodeableConcept*/ return new Property("diagnosis[x]", "CodeableConcept", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 2085 case 2050454362: /*diagnosisReference*/ return new Property("diagnosis[x]", "Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 2086 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type); 2087 case -3386134: /*onAdmission*/ return new Property("onAdmission", "CodeableConcept", "Indication of whether the diagnosis was present on admission to a facility.", 0, 1, onAdmission); 2088 default: return super.getNamedProperty(_hash, _name, _checkValid); 2089 } 2090 2091 } 2092 2093 @Override 2094 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2095 switch (hash) { 2096 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 2097 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : new Base[] {this.diagnosis}; // DataType 2098 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2099 case -3386134: /*onAdmission*/ return this.onAdmission == null ? new Base[0] : new Base[] {this.onAdmission}; // CodeableConcept 2100 default: return super.getProperty(hash, name, checkValid); 2101 } 2102 2103 } 2104 2105 @Override 2106 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2107 switch (hash) { 2108 case 1349547969: // sequence 2109 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2110 return value; 2111 case 1196993265: // diagnosis 2112 this.diagnosis = TypeConvertor.castToType(value); // DataType 2113 return value; 2114 case 3575610: // type 2115 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2116 return value; 2117 case -3386134: // onAdmission 2118 this.onAdmission = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2119 return value; 2120 default: return super.setProperty(hash, name, value); 2121 } 2122 2123 } 2124 2125 @Override 2126 public Base setProperty(String name, Base value) throws FHIRException { 2127 if (name.equals("sequence")) { 2128 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2129 } else if (name.equals("diagnosis[x]")) { 2130 this.diagnosis = TypeConvertor.castToType(value); // DataType 2131 } else if (name.equals("type")) { 2132 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 2133 } else if (name.equals("onAdmission")) { 2134 this.onAdmission = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2135 } else 2136 return super.setProperty(name, value); 2137 return value; 2138 } 2139 2140 @Override 2141 public Base makeProperty(int hash, String name) throws FHIRException { 2142 switch (hash) { 2143 case 1349547969: return getSequenceElement(); 2144 case -1487009809: return getDiagnosis(); 2145 case 1196993265: return getDiagnosis(); 2146 case 3575610: return addType(); 2147 case -3386134: return getOnAdmission(); 2148 default: return super.makeProperty(hash, name); 2149 } 2150 2151 } 2152 2153 @Override 2154 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2155 switch (hash) { 2156 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 2157 case 1196993265: /*diagnosis*/ return new String[] {"CodeableConcept", "Reference"}; 2158 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2159 case -3386134: /*onAdmission*/ return new String[] {"CodeableConcept"}; 2160 default: return super.getTypesForProperty(hash, name); 2161 } 2162 2163 } 2164 2165 @Override 2166 public Base addChild(String name) throws FHIRException { 2167 if (name.equals("sequence")) { 2168 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.diagnosis.sequence"); 2169 } 2170 else if (name.equals("diagnosisCodeableConcept")) { 2171 this.diagnosis = new CodeableConcept(); 2172 return this.diagnosis; 2173 } 2174 else if (name.equals("diagnosisReference")) { 2175 this.diagnosis = new Reference(); 2176 return this.diagnosis; 2177 } 2178 else if (name.equals("type")) { 2179 return addType(); 2180 } 2181 else if (name.equals("onAdmission")) { 2182 this.onAdmission = new CodeableConcept(); 2183 return this.onAdmission; 2184 } 2185 else 2186 return super.addChild(name); 2187 } 2188 2189 public DiagnosisComponent copy() { 2190 DiagnosisComponent dst = new DiagnosisComponent(); 2191 copyValues(dst); 2192 return dst; 2193 } 2194 2195 public void copyValues(DiagnosisComponent dst) { 2196 super.copyValues(dst); 2197 dst.sequence = sequence == null ? null : sequence.copy(); 2198 dst.diagnosis = diagnosis == null ? null : diagnosis.copy(); 2199 if (type != null) { 2200 dst.type = new ArrayList<CodeableConcept>(); 2201 for (CodeableConcept i : type) 2202 dst.type.add(i.copy()); 2203 }; 2204 dst.onAdmission = onAdmission == null ? null : onAdmission.copy(); 2205 } 2206 2207 @Override 2208 public boolean equalsDeep(Base other_) { 2209 if (!super.equalsDeep(other_)) 2210 return false; 2211 if (!(other_ instanceof DiagnosisComponent)) 2212 return false; 2213 DiagnosisComponent o = (DiagnosisComponent) other_; 2214 return compareDeep(sequence, o.sequence, true) && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(type, o.type, true) 2215 && compareDeep(onAdmission, o.onAdmission, true); 2216 } 2217 2218 @Override 2219 public boolean equalsShallow(Base other_) { 2220 if (!super.equalsShallow(other_)) 2221 return false; 2222 if (!(other_ instanceof DiagnosisComponent)) 2223 return false; 2224 DiagnosisComponent o = (DiagnosisComponent) other_; 2225 return compareValues(sequence, o.sequence, true); 2226 } 2227 2228 public boolean isEmpty() { 2229 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, diagnosis, type 2230 , onAdmission); 2231 } 2232 2233 public String fhirType() { 2234 return "ExplanationOfBenefit.diagnosis"; 2235 2236 } 2237 2238 } 2239 2240 @Block() 2241 public static class ProcedureComponent extends BackboneElement implements IBaseBackboneElement { 2242 /** 2243 * A number to uniquely identify procedure entries. 2244 */ 2245 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2246 @Description(shortDefinition="Procedure instance identifier", formalDefinition="A number to uniquely identify procedure entries." ) 2247 protected PositiveIntType sequence; 2248 2249 /** 2250 * When the condition was observed or the relative ranking. 2251 */ 2252 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2253 @Description(shortDefinition="Category of Procedure", formalDefinition="When the condition was observed or the relative ranking." ) 2254 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-procedure-type") 2255 protected List<CodeableConcept> type; 2256 2257 /** 2258 * Date and optionally time the procedure was performed. 2259 */ 2260 @Child(name = "date", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2261 @Description(shortDefinition="When the procedure was performed", formalDefinition="Date and optionally time the procedure was performed." ) 2262 protected DateTimeType date; 2263 2264 /** 2265 * The code or reference to a Procedure resource which identifies the clinical intervention performed. 2266 */ 2267 @Child(name = "procedure", type = {CodeableConcept.class, Procedure.class}, order=4, min=1, max=1, modifier=false, summary=false) 2268 @Description(shortDefinition="Specific clinical procedure", formalDefinition="The code or reference to a Procedure resource which identifies the clinical intervention performed." ) 2269 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10-procedures") 2270 protected DataType procedure; 2271 2272 /** 2273 * Unique Device Identifiers associated with this line item. 2274 */ 2275 @Child(name = "udi", type = {Device.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2276 @Description(shortDefinition="Unique device identifier", formalDefinition="Unique Device Identifiers associated with this line item." ) 2277 protected List<Reference> udi; 2278 2279 private static final long serialVersionUID = 1165684715L; 2280 2281 /** 2282 * Constructor 2283 */ 2284 public ProcedureComponent() { 2285 super(); 2286 } 2287 2288 /** 2289 * Constructor 2290 */ 2291 public ProcedureComponent(int sequence, DataType procedure) { 2292 super(); 2293 this.setSequence(sequence); 2294 this.setProcedure(procedure); 2295 } 2296 2297 /** 2298 * @return {@link #sequence} (A number to uniquely identify procedure entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2299 */ 2300 public PositiveIntType getSequenceElement() { 2301 if (this.sequence == null) 2302 if (Configuration.errorOnAutoCreate()) 2303 throw new Error("Attempt to auto-create ProcedureComponent.sequence"); 2304 else if (Configuration.doAutoCreate()) 2305 this.sequence = new PositiveIntType(); // bb 2306 return this.sequence; 2307 } 2308 2309 public boolean hasSequenceElement() { 2310 return this.sequence != null && !this.sequence.isEmpty(); 2311 } 2312 2313 public boolean hasSequence() { 2314 return this.sequence != null && !this.sequence.isEmpty(); 2315 } 2316 2317 /** 2318 * @param value {@link #sequence} (A number to uniquely identify procedure entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2319 */ 2320 public ProcedureComponent setSequenceElement(PositiveIntType value) { 2321 this.sequence = value; 2322 return this; 2323 } 2324 2325 /** 2326 * @return A number to uniquely identify procedure entries. 2327 */ 2328 public int getSequence() { 2329 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2330 } 2331 2332 /** 2333 * @param value A number to uniquely identify procedure entries. 2334 */ 2335 public ProcedureComponent setSequence(int value) { 2336 if (this.sequence == null) 2337 this.sequence = new PositiveIntType(); 2338 this.sequence.setValue(value); 2339 return this; 2340 } 2341 2342 /** 2343 * @return {@link #type} (When the condition was observed or the relative ranking.) 2344 */ 2345 public List<CodeableConcept> getType() { 2346 if (this.type == null) 2347 this.type = new ArrayList<CodeableConcept>(); 2348 return this.type; 2349 } 2350 2351 /** 2352 * @return Returns a reference to <code>this</code> for easy method chaining 2353 */ 2354 public ProcedureComponent setType(List<CodeableConcept> theType) { 2355 this.type = theType; 2356 return this; 2357 } 2358 2359 public boolean hasType() { 2360 if (this.type == null) 2361 return false; 2362 for (CodeableConcept item : this.type) 2363 if (!item.isEmpty()) 2364 return true; 2365 return false; 2366 } 2367 2368 public CodeableConcept addType() { //3 2369 CodeableConcept t = new CodeableConcept(); 2370 if (this.type == null) 2371 this.type = new ArrayList<CodeableConcept>(); 2372 this.type.add(t); 2373 return t; 2374 } 2375 2376 public ProcedureComponent addType(CodeableConcept t) { //3 2377 if (t == null) 2378 return this; 2379 if (this.type == null) 2380 this.type = new ArrayList<CodeableConcept>(); 2381 this.type.add(t); 2382 return this; 2383 } 2384 2385 /** 2386 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 2387 */ 2388 public CodeableConcept getTypeFirstRep() { 2389 if (getType().isEmpty()) { 2390 addType(); 2391 } 2392 return getType().get(0); 2393 } 2394 2395 /** 2396 * @return {@link #date} (Date and optionally time the procedure was performed.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2397 */ 2398 public DateTimeType getDateElement() { 2399 if (this.date == null) 2400 if (Configuration.errorOnAutoCreate()) 2401 throw new Error("Attempt to auto-create ProcedureComponent.date"); 2402 else if (Configuration.doAutoCreate()) 2403 this.date = new DateTimeType(); // bb 2404 return this.date; 2405 } 2406 2407 public boolean hasDateElement() { 2408 return this.date != null && !this.date.isEmpty(); 2409 } 2410 2411 public boolean hasDate() { 2412 return this.date != null && !this.date.isEmpty(); 2413 } 2414 2415 /** 2416 * @param value {@link #date} (Date and optionally time the procedure was performed.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2417 */ 2418 public ProcedureComponent setDateElement(DateTimeType value) { 2419 this.date = value; 2420 return this; 2421 } 2422 2423 /** 2424 * @return Date and optionally time the procedure was performed. 2425 */ 2426 public Date getDate() { 2427 return this.date == null ? null : this.date.getValue(); 2428 } 2429 2430 /** 2431 * @param value Date and optionally time the procedure was performed. 2432 */ 2433 public ProcedureComponent setDate(Date value) { 2434 if (value == null) 2435 this.date = null; 2436 else { 2437 if (this.date == null) 2438 this.date = new DateTimeType(); 2439 this.date.setValue(value); 2440 } 2441 return this; 2442 } 2443 2444 /** 2445 * @return {@link #procedure} (The code or reference to a Procedure resource which identifies the clinical intervention performed.) 2446 */ 2447 public DataType getProcedure() { 2448 return this.procedure; 2449 } 2450 2451 /** 2452 * @return {@link #procedure} (The code or reference to a Procedure resource which identifies the clinical intervention performed.) 2453 */ 2454 public CodeableConcept getProcedureCodeableConcept() throws FHIRException { 2455 if (this.procedure == null) 2456 this.procedure = new CodeableConcept(); 2457 if (!(this.procedure instanceof CodeableConcept)) 2458 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.procedure.getClass().getName()+" was encountered"); 2459 return (CodeableConcept) this.procedure; 2460 } 2461 2462 public boolean hasProcedureCodeableConcept() { 2463 return this != null && this.procedure instanceof CodeableConcept; 2464 } 2465 2466 /** 2467 * @return {@link #procedure} (The code or reference to a Procedure resource which identifies the clinical intervention performed.) 2468 */ 2469 public Reference getProcedureReference() throws FHIRException { 2470 if (this.procedure == null) 2471 this.procedure = new Reference(); 2472 if (!(this.procedure instanceof Reference)) 2473 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.procedure.getClass().getName()+" was encountered"); 2474 return (Reference) this.procedure; 2475 } 2476 2477 public boolean hasProcedureReference() { 2478 return this != null && this.procedure instanceof Reference; 2479 } 2480 2481 public boolean hasProcedure() { 2482 return this.procedure != null && !this.procedure.isEmpty(); 2483 } 2484 2485 /** 2486 * @param value {@link #procedure} (The code or reference to a Procedure resource which identifies the clinical intervention performed.) 2487 */ 2488 public ProcedureComponent setProcedure(DataType value) { 2489 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2490 throw new FHIRException("Not the right type for ExplanationOfBenefit.procedure.procedure[x]: "+value.fhirType()); 2491 this.procedure = value; 2492 return this; 2493 } 2494 2495 /** 2496 * @return {@link #udi} (Unique Device Identifiers associated with this line item.) 2497 */ 2498 public List<Reference> getUdi() { 2499 if (this.udi == null) 2500 this.udi = new ArrayList<Reference>(); 2501 return this.udi; 2502 } 2503 2504 /** 2505 * @return Returns a reference to <code>this</code> for easy method chaining 2506 */ 2507 public ProcedureComponent setUdi(List<Reference> theUdi) { 2508 this.udi = theUdi; 2509 return this; 2510 } 2511 2512 public boolean hasUdi() { 2513 if (this.udi == null) 2514 return false; 2515 for (Reference item : this.udi) 2516 if (!item.isEmpty()) 2517 return true; 2518 return false; 2519 } 2520 2521 public Reference addUdi() { //3 2522 Reference t = new Reference(); 2523 if (this.udi == null) 2524 this.udi = new ArrayList<Reference>(); 2525 this.udi.add(t); 2526 return t; 2527 } 2528 2529 public ProcedureComponent addUdi(Reference t) { //3 2530 if (t == null) 2531 return this; 2532 if (this.udi == null) 2533 this.udi = new ArrayList<Reference>(); 2534 this.udi.add(t); 2535 return this; 2536 } 2537 2538 /** 2539 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist {3} 2540 */ 2541 public Reference getUdiFirstRep() { 2542 if (getUdi().isEmpty()) { 2543 addUdi(); 2544 } 2545 return getUdi().get(0); 2546 } 2547 2548 protected void listChildren(List<Property> children) { 2549 super.listChildren(children); 2550 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify procedure entries.", 0, 1, sequence)); 2551 children.add(new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type)); 2552 children.add(new Property("date", "dateTime", "Date and optionally time the procedure was performed.", 0, 1, date)); 2553 children.add(new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure)); 2554 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 2555 } 2556 2557 @Override 2558 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2559 switch (_hash) { 2560 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify procedure entries.", 0, 1, sequence); 2561 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type); 2562 case 3076014: /*date*/ return new Property("date", "dateTime", "Date and optionally time the procedure was performed.", 0, 1, date); 2563 case 1640074445: /*procedure[x]*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure); 2564 case -1095204141: /*procedure*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure); 2565 case -1284783026: /*procedureCodeableConcept*/ return new Property("procedure[x]", "CodeableConcept", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure); 2566 case 881809848: /*procedureReference*/ return new Property("procedure[x]", "Reference(Procedure)", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure); 2567 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 2568 default: return super.getNamedProperty(_hash, _name, _checkValid); 2569 } 2570 2571 } 2572 2573 @Override 2574 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2575 switch (hash) { 2576 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 2577 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2578 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2579 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : new Base[] {this.procedure}; // DataType 2580 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 2581 default: return super.getProperty(hash, name, checkValid); 2582 } 2583 2584 } 2585 2586 @Override 2587 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2588 switch (hash) { 2589 case 1349547969: // sequence 2590 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2591 return value; 2592 case 3575610: // type 2593 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2594 return value; 2595 case 3076014: // date 2596 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2597 return value; 2598 case -1095204141: // procedure 2599 this.procedure = TypeConvertor.castToType(value); // DataType 2600 return value; 2601 case 115642: // udi 2602 this.getUdi().add(TypeConvertor.castToReference(value)); // Reference 2603 return value; 2604 default: return super.setProperty(hash, name, value); 2605 } 2606 2607 } 2608 2609 @Override 2610 public Base setProperty(String name, Base value) throws FHIRException { 2611 if (name.equals("sequence")) { 2612 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2613 } else if (name.equals("type")) { 2614 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 2615 } else if (name.equals("date")) { 2616 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2617 } else if (name.equals("procedure[x]")) { 2618 this.procedure = TypeConvertor.castToType(value); // DataType 2619 } else if (name.equals("udi")) { 2620 this.getUdi().add(TypeConvertor.castToReference(value)); 2621 } else 2622 return super.setProperty(name, value); 2623 return value; 2624 } 2625 2626 @Override 2627 public Base makeProperty(int hash, String name) throws FHIRException { 2628 switch (hash) { 2629 case 1349547969: return getSequenceElement(); 2630 case 3575610: return addType(); 2631 case 3076014: return getDateElement(); 2632 case 1640074445: return getProcedure(); 2633 case -1095204141: return getProcedure(); 2634 case 115642: return addUdi(); 2635 default: return super.makeProperty(hash, name); 2636 } 2637 2638 } 2639 2640 @Override 2641 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2642 switch (hash) { 2643 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 2644 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2645 case 3076014: /*date*/ return new String[] {"dateTime"}; 2646 case -1095204141: /*procedure*/ return new String[] {"CodeableConcept", "Reference"}; 2647 case 115642: /*udi*/ return new String[] {"Reference"}; 2648 default: return super.getTypesForProperty(hash, name); 2649 } 2650 2651 } 2652 2653 @Override 2654 public Base addChild(String name) throws FHIRException { 2655 if (name.equals("sequence")) { 2656 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.procedure.sequence"); 2657 } 2658 else if (name.equals("type")) { 2659 return addType(); 2660 } 2661 else if (name.equals("date")) { 2662 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.procedure.date"); 2663 } 2664 else if (name.equals("procedureCodeableConcept")) { 2665 this.procedure = new CodeableConcept(); 2666 return this.procedure; 2667 } 2668 else if (name.equals("procedureReference")) { 2669 this.procedure = new Reference(); 2670 return this.procedure; 2671 } 2672 else if (name.equals("udi")) { 2673 return addUdi(); 2674 } 2675 else 2676 return super.addChild(name); 2677 } 2678 2679 public ProcedureComponent copy() { 2680 ProcedureComponent dst = new ProcedureComponent(); 2681 copyValues(dst); 2682 return dst; 2683 } 2684 2685 public void copyValues(ProcedureComponent dst) { 2686 super.copyValues(dst); 2687 dst.sequence = sequence == null ? null : sequence.copy(); 2688 if (type != null) { 2689 dst.type = new ArrayList<CodeableConcept>(); 2690 for (CodeableConcept i : type) 2691 dst.type.add(i.copy()); 2692 }; 2693 dst.date = date == null ? null : date.copy(); 2694 dst.procedure = procedure == null ? null : procedure.copy(); 2695 if (udi != null) { 2696 dst.udi = new ArrayList<Reference>(); 2697 for (Reference i : udi) 2698 dst.udi.add(i.copy()); 2699 }; 2700 } 2701 2702 @Override 2703 public boolean equalsDeep(Base other_) { 2704 if (!super.equalsDeep(other_)) 2705 return false; 2706 if (!(other_ instanceof ProcedureComponent)) 2707 return false; 2708 ProcedureComponent o = (ProcedureComponent) other_; 2709 return compareDeep(sequence, o.sequence, true) && compareDeep(type, o.type, true) && compareDeep(date, o.date, true) 2710 && compareDeep(procedure, o.procedure, true) && compareDeep(udi, o.udi, true); 2711 } 2712 2713 @Override 2714 public boolean equalsShallow(Base other_) { 2715 if (!super.equalsShallow(other_)) 2716 return false; 2717 if (!(other_ instanceof ProcedureComponent)) 2718 return false; 2719 ProcedureComponent o = (ProcedureComponent) other_; 2720 return compareValues(sequence, o.sequence, true) && compareValues(date, o.date, true); 2721 } 2722 2723 public boolean isEmpty() { 2724 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, type, date, procedure 2725 , udi); 2726 } 2727 2728 public String fhirType() { 2729 return "ExplanationOfBenefit.procedure"; 2730 2731 } 2732 2733 } 2734 2735 @Block() 2736 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 2737 /** 2738 * A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true. 2739 */ 2740 @Child(name = "focal", type = {BooleanType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2741 @Description(shortDefinition="Coverage to be used for adjudication", formalDefinition="A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true." ) 2742 protected BooleanType focal; 2743 2744 /** 2745 * Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system. 2746 */ 2747 @Child(name = "coverage", type = {Coverage.class}, order=2, min=1, max=1, modifier=false, summary=true) 2748 @Description(shortDefinition="Insurance information", formalDefinition="Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system." ) 2749 protected Reference coverage; 2750 2751 /** 2752 * Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization. 2753 */ 2754 @Child(name = "preAuthRef", type = {StringType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2755 @Description(shortDefinition="Prior authorization reference number", formalDefinition="Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization." ) 2756 protected List<StringType> preAuthRef; 2757 2758 private static final long serialVersionUID = 1519900285L; 2759 2760 /** 2761 * Constructor 2762 */ 2763 public InsuranceComponent() { 2764 super(); 2765 } 2766 2767 /** 2768 * Constructor 2769 */ 2770 public InsuranceComponent(boolean focal, Reference coverage) { 2771 super(); 2772 this.setFocal(focal); 2773 this.setCoverage(coverage); 2774 } 2775 2776 /** 2777 * @return {@link #focal} (A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 2778 */ 2779 public BooleanType getFocalElement() { 2780 if (this.focal == null) 2781 if (Configuration.errorOnAutoCreate()) 2782 throw new Error("Attempt to auto-create InsuranceComponent.focal"); 2783 else if (Configuration.doAutoCreate()) 2784 this.focal = new BooleanType(); // bb 2785 return this.focal; 2786 } 2787 2788 public boolean hasFocalElement() { 2789 return this.focal != null && !this.focal.isEmpty(); 2790 } 2791 2792 public boolean hasFocal() { 2793 return this.focal != null && !this.focal.isEmpty(); 2794 } 2795 2796 /** 2797 * @param value {@link #focal} (A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 2798 */ 2799 public InsuranceComponent setFocalElement(BooleanType value) { 2800 this.focal = value; 2801 return this; 2802 } 2803 2804 /** 2805 * @return A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true. 2806 */ 2807 public boolean getFocal() { 2808 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 2809 } 2810 2811 /** 2812 * @param value A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true. 2813 */ 2814 public InsuranceComponent setFocal(boolean value) { 2815 if (this.focal == null) 2816 this.focal = new BooleanType(); 2817 this.focal.setValue(value); 2818 return this; 2819 } 2820 2821 /** 2822 * @return {@link #coverage} (Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.) 2823 */ 2824 public Reference getCoverage() { 2825 if (this.coverage == null) 2826 if (Configuration.errorOnAutoCreate()) 2827 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 2828 else if (Configuration.doAutoCreate()) 2829 this.coverage = new Reference(); // cc 2830 return this.coverage; 2831 } 2832 2833 public boolean hasCoverage() { 2834 return this.coverage != null && !this.coverage.isEmpty(); 2835 } 2836 2837 /** 2838 * @param value {@link #coverage} (Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.) 2839 */ 2840 public InsuranceComponent setCoverage(Reference value) { 2841 this.coverage = value; 2842 return this; 2843 } 2844 2845 /** 2846 * @return {@link #preAuthRef} (Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.) 2847 */ 2848 public List<StringType> getPreAuthRef() { 2849 if (this.preAuthRef == null) 2850 this.preAuthRef = new ArrayList<StringType>(); 2851 return this.preAuthRef; 2852 } 2853 2854 /** 2855 * @return Returns a reference to <code>this</code> for easy method chaining 2856 */ 2857 public InsuranceComponent setPreAuthRef(List<StringType> thePreAuthRef) { 2858 this.preAuthRef = thePreAuthRef; 2859 return this; 2860 } 2861 2862 public boolean hasPreAuthRef() { 2863 if (this.preAuthRef == null) 2864 return false; 2865 for (StringType item : this.preAuthRef) 2866 if (!item.isEmpty()) 2867 return true; 2868 return false; 2869 } 2870 2871 /** 2872 * @return {@link #preAuthRef} (Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.) 2873 */ 2874 public StringType addPreAuthRefElement() {//2 2875 StringType t = new StringType(); 2876 if (this.preAuthRef == null) 2877 this.preAuthRef = new ArrayList<StringType>(); 2878 this.preAuthRef.add(t); 2879 return t; 2880 } 2881 2882 /** 2883 * @param value {@link #preAuthRef} (Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.) 2884 */ 2885 public InsuranceComponent addPreAuthRef(String value) { //1 2886 StringType t = new StringType(); 2887 t.setValue(value); 2888 if (this.preAuthRef == null) 2889 this.preAuthRef = new ArrayList<StringType>(); 2890 this.preAuthRef.add(t); 2891 return this; 2892 } 2893 2894 /** 2895 * @param value {@link #preAuthRef} (Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.) 2896 */ 2897 public boolean hasPreAuthRef(String value) { 2898 if (this.preAuthRef == null) 2899 return false; 2900 for (StringType v : this.preAuthRef) 2901 if (v.getValue().equals(value)) // string 2902 return true; 2903 return false; 2904 } 2905 2906 protected void listChildren(List<Property> children) { 2907 super.listChildren(children); 2908 children.add(new Property("focal", "boolean", "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 1, focal)); 2909 children.add(new Property("coverage", "Reference(Coverage)", "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 0, 1, coverage)); 2910 children.add(new Property("preAuthRef", "string", "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.", 0, java.lang.Integer.MAX_VALUE, preAuthRef)); 2911 } 2912 2913 @Override 2914 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2915 switch (_hash) { 2916 case 97604197: /*focal*/ return new Property("focal", "boolean", "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 1, focal); 2917 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 0, 1, coverage); 2918 case 522246568: /*preAuthRef*/ return new Property("preAuthRef", "string", "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.", 0, java.lang.Integer.MAX_VALUE, preAuthRef); 2919 default: return super.getNamedProperty(_hash, _name, _checkValid); 2920 } 2921 2922 } 2923 2924 @Override 2925 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2926 switch (hash) { 2927 case 97604197: /*focal*/ return this.focal == null ? new Base[0] : new Base[] {this.focal}; // BooleanType 2928 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 2929 case 522246568: /*preAuthRef*/ return this.preAuthRef == null ? new Base[0] : this.preAuthRef.toArray(new Base[this.preAuthRef.size()]); // StringType 2930 default: return super.getProperty(hash, name, checkValid); 2931 } 2932 2933 } 2934 2935 @Override 2936 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2937 switch (hash) { 2938 case 97604197: // focal 2939 this.focal = TypeConvertor.castToBoolean(value); // BooleanType 2940 return value; 2941 case -351767064: // coverage 2942 this.coverage = TypeConvertor.castToReference(value); // Reference 2943 return value; 2944 case 522246568: // preAuthRef 2945 this.getPreAuthRef().add(TypeConvertor.castToString(value)); // StringType 2946 return value; 2947 default: return super.setProperty(hash, name, value); 2948 } 2949 2950 } 2951 2952 @Override 2953 public Base setProperty(String name, Base value) throws FHIRException { 2954 if (name.equals("focal")) { 2955 this.focal = TypeConvertor.castToBoolean(value); // BooleanType 2956 } else if (name.equals("coverage")) { 2957 this.coverage = TypeConvertor.castToReference(value); // Reference 2958 } else if (name.equals("preAuthRef")) { 2959 this.getPreAuthRef().add(TypeConvertor.castToString(value)); 2960 } else 2961 return super.setProperty(name, value); 2962 return value; 2963 } 2964 2965 @Override 2966 public Base makeProperty(int hash, String name) throws FHIRException { 2967 switch (hash) { 2968 case 97604197: return getFocalElement(); 2969 case -351767064: return getCoverage(); 2970 case 522246568: return addPreAuthRefElement(); 2971 default: return super.makeProperty(hash, name); 2972 } 2973 2974 } 2975 2976 @Override 2977 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2978 switch (hash) { 2979 case 97604197: /*focal*/ return new String[] {"boolean"}; 2980 case -351767064: /*coverage*/ return new String[] {"Reference"}; 2981 case 522246568: /*preAuthRef*/ return new String[] {"string"}; 2982 default: return super.getTypesForProperty(hash, name); 2983 } 2984 2985 } 2986 2987 @Override 2988 public Base addChild(String name) throws FHIRException { 2989 if (name.equals("focal")) { 2990 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.insurance.focal"); 2991 } 2992 else if (name.equals("coverage")) { 2993 this.coverage = new Reference(); 2994 return this.coverage; 2995 } 2996 else if (name.equals("preAuthRef")) { 2997 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.insurance.preAuthRef"); 2998 } 2999 else 3000 return super.addChild(name); 3001 } 3002 3003 public InsuranceComponent copy() { 3004 InsuranceComponent dst = new InsuranceComponent(); 3005 copyValues(dst); 3006 return dst; 3007 } 3008 3009 public void copyValues(InsuranceComponent dst) { 3010 super.copyValues(dst); 3011 dst.focal = focal == null ? null : focal.copy(); 3012 dst.coverage = coverage == null ? null : coverage.copy(); 3013 if (preAuthRef != null) { 3014 dst.preAuthRef = new ArrayList<StringType>(); 3015 for (StringType i : preAuthRef) 3016 dst.preAuthRef.add(i.copy()); 3017 }; 3018 } 3019 3020 @Override 3021 public boolean equalsDeep(Base other_) { 3022 if (!super.equalsDeep(other_)) 3023 return false; 3024 if (!(other_ instanceof InsuranceComponent)) 3025 return false; 3026 InsuranceComponent o = (InsuranceComponent) other_; 3027 return compareDeep(focal, o.focal, true) && compareDeep(coverage, o.coverage, true) && compareDeep(preAuthRef, o.preAuthRef, true) 3028 ; 3029 } 3030 3031 @Override 3032 public boolean equalsShallow(Base other_) { 3033 if (!super.equalsShallow(other_)) 3034 return false; 3035 if (!(other_ instanceof InsuranceComponent)) 3036 return false; 3037 InsuranceComponent o = (InsuranceComponent) other_; 3038 return compareValues(focal, o.focal, true) && compareValues(preAuthRef, o.preAuthRef, true); 3039 } 3040 3041 public boolean isEmpty() { 3042 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(focal, coverage, preAuthRef 3043 ); 3044 } 3045 3046 public String fhirType() { 3047 return "ExplanationOfBenefit.insurance"; 3048 3049 } 3050 3051 } 3052 3053 @Block() 3054 public static class AccidentComponent extends BackboneElement implements IBaseBackboneElement { 3055 /** 3056 * Date of an accident event related to the products and services contained in the claim. 3057 */ 3058 @Child(name = "date", type = {DateType.class}, order=1, min=0, max=1, modifier=false, summary=false) 3059 @Description(shortDefinition="When the incident occurred", formalDefinition="Date of an accident event related to the products and services contained in the claim." ) 3060 protected DateType date; 3061 3062 /** 3063 * The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers. 3064 */ 3065 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 3066 @Description(shortDefinition="The nature of the accident", formalDefinition="The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers." ) 3067 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ActIncidentCode") 3068 protected CodeableConcept type; 3069 3070 /** 3071 * The physical location of the accident event. 3072 */ 3073 @Child(name = "location", type = {Address.class, Location.class}, order=3, min=0, max=1, modifier=false, summary=false) 3074 @Description(shortDefinition="Where the event occurred", formalDefinition="The physical location of the accident event." ) 3075 protected DataType location; 3076 3077 private static final long serialVersionUID = 11882722L; 3078 3079 /** 3080 * Constructor 3081 */ 3082 public AccidentComponent() { 3083 super(); 3084 } 3085 3086 /** 3087 * @return {@link #date} (Date of an accident event related to the products and services contained in the claim.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3088 */ 3089 public DateType getDateElement() { 3090 if (this.date == null) 3091 if (Configuration.errorOnAutoCreate()) 3092 throw new Error("Attempt to auto-create AccidentComponent.date"); 3093 else if (Configuration.doAutoCreate()) 3094 this.date = new DateType(); // bb 3095 return this.date; 3096 } 3097 3098 public boolean hasDateElement() { 3099 return this.date != null && !this.date.isEmpty(); 3100 } 3101 3102 public boolean hasDate() { 3103 return this.date != null && !this.date.isEmpty(); 3104 } 3105 3106 /** 3107 * @param value {@link #date} (Date of an accident event related to the products and services contained in the claim.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3108 */ 3109 public AccidentComponent setDateElement(DateType value) { 3110 this.date = value; 3111 return this; 3112 } 3113 3114 /** 3115 * @return Date of an accident event related to the products and services contained in the claim. 3116 */ 3117 public Date getDate() { 3118 return this.date == null ? null : this.date.getValue(); 3119 } 3120 3121 /** 3122 * @param value Date of an accident event related to the products and services contained in the claim. 3123 */ 3124 public AccidentComponent setDate(Date value) { 3125 if (value == null) 3126 this.date = null; 3127 else { 3128 if (this.date == null) 3129 this.date = new DateType(); 3130 this.date.setValue(value); 3131 } 3132 return this; 3133 } 3134 3135 /** 3136 * @return {@link #type} (The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.) 3137 */ 3138 public CodeableConcept getType() { 3139 if (this.type == null) 3140 if (Configuration.errorOnAutoCreate()) 3141 throw new Error("Attempt to auto-create AccidentComponent.type"); 3142 else if (Configuration.doAutoCreate()) 3143 this.type = new CodeableConcept(); // cc 3144 return this.type; 3145 } 3146 3147 public boolean hasType() { 3148 return this.type != null && !this.type.isEmpty(); 3149 } 3150 3151 /** 3152 * @param value {@link #type} (The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.) 3153 */ 3154 public AccidentComponent setType(CodeableConcept value) { 3155 this.type = value; 3156 return this; 3157 } 3158 3159 /** 3160 * @return {@link #location} (The physical location of the accident event.) 3161 */ 3162 public DataType getLocation() { 3163 return this.location; 3164 } 3165 3166 /** 3167 * @return {@link #location} (The physical location of the accident event.) 3168 */ 3169 public Address getLocationAddress() throws FHIRException { 3170 if (this.location == null) 3171 this.location = new Address(); 3172 if (!(this.location instanceof Address)) 3173 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 3174 return (Address) this.location; 3175 } 3176 3177 public boolean hasLocationAddress() { 3178 return this != null && this.location instanceof Address; 3179 } 3180 3181 /** 3182 * @return {@link #location} (The physical location of the accident event.) 3183 */ 3184 public Reference getLocationReference() throws FHIRException { 3185 if (this.location == null) 3186 this.location = new Reference(); 3187 if (!(this.location instanceof Reference)) 3188 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 3189 return (Reference) this.location; 3190 } 3191 3192 public boolean hasLocationReference() { 3193 return this != null && this.location instanceof Reference; 3194 } 3195 3196 public boolean hasLocation() { 3197 return this.location != null && !this.location.isEmpty(); 3198 } 3199 3200 /** 3201 * @param value {@link #location} (The physical location of the accident event.) 3202 */ 3203 public AccidentComponent setLocation(DataType value) { 3204 if (value != null && !(value instanceof Address || value instanceof Reference)) 3205 throw new FHIRException("Not the right type for ExplanationOfBenefit.accident.location[x]: "+value.fhirType()); 3206 this.location = value; 3207 return this; 3208 } 3209 3210 protected void listChildren(List<Property> children) { 3211 super.listChildren(children); 3212 children.add(new Property("date", "date", "Date of an accident event related to the products and services contained in the claim.", 0, 1, date)); 3213 children.add(new Property("type", "CodeableConcept", "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.", 0, 1, type)); 3214 children.add(new Property("location[x]", "Address|Reference(Location)", "The physical location of the accident event.", 0, 1, location)); 3215 } 3216 3217 @Override 3218 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3219 switch (_hash) { 3220 case 3076014: /*date*/ return new Property("date", "date", "Date of an accident event related to the products and services contained in the claim.", 0, 1, date); 3221 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.", 0, 1, type); 3222 case 552316075: /*location[x]*/ return new Property("location[x]", "Address|Reference(Location)", "The physical location of the accident event.", 0, 1, location); 3223 case 1901043637: /*location*/ return new Property("location[x]", "Address|Reference(Location)", "The physical location of the accident event.", 0, 1, location); 3224 case -1280020865: /*locationAddress*/ return new Property("location[x]", "Address", "The physical location of the accident event.", 0, 1, location); 3225 case 755866390: /*locationReference*/ return new Property("location[x]", "Reference(Location)", "The physical location of the accident event.", 0, 1, location); 3226 default: return super.getNamedProperty(_hash, _name, _checkValid); 3227 } 3228 3229 } 3230 3231 @Override 3232 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3233 switch (hash) { 3234 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 3235 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3236 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // DataType 3237 default: return super.getProperty(hash, name, checkValid); 3238 } 3239 3240 } 3241 3242 @Override 3243 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3244 switch (hash) { 3245 case 3076014: // date 3246 this.date = TypeConvertor.castToDate(value); // DateType 3247 return value; 3248 case 3575610: // type 3249 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3250 return value; 3251 case 1901043637: // location 3252 this.location = TypeConvertor.castToType(value); // DataType 3253 return value; 3254 default: return super.setProperty(hash, name, value); 3255 } 3256 3257 } 3258 3259 @Override 3260 public Base setProperty(String name, Base value) throws FHIRException { 3261 if (name.equals("date")) { 3262 this.date = TypeConvertor.castToDate(value); // DateType 3263 } else if (name.equals("type")) { 3264 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3265 } else if (name.equals("location[x]")) { 3266 this.location = TypeConvertor.castToType(value); // DataType 3267 } else 3268 return super.setProperty(name, value); 3269 return value; 3270 } 3271 3272 @Override 3273 public Base makeProperty(int hash, String name) throws FHIRException { 3274 switch (hash) { 3275 case 3076014: return getDateElement(); 3276 case 3575610: return getType(); 3277 case 552316075: return getLocation(); 3278 case 1901043637: return getLocation(); 3279 default: return super.makeProperty(hash, name); 3280 } 3281 3282 } 3283 3284 @Override 3285 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3286 switch (hash) { 3287 case 3076014: /*date*/ return new String[] {"date"}; 3288 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3289 case 1901043637: /*location*/ return new String[] {"Address", "Reference"}; 3290 default: return super.getTypesForProperty(hash, name); 3291 } 3292 3293 } 3294 3295 @Override 3296 public Base addChild(String name) throws FHIRException { 3297 if (name.equals("date")) { 3298 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.accident.date"); 3299 } 3300 else if (name.equals("type")) { 3301 this.type = new CodeableConcept(); 3302 return this.type; 3303 } 3304 else if (name.equals("locationAddress")) { 3305 this.location = new Address(); 3306 return this.location; 3307 } 3308 else if (name.equals("locationReference")) { 3309 this.location = new Reference(); 3310 return this.location; 3311 } 3312 else 3313 return super.addChild(name); 3314 } 3315 3316 public AccidentComponent copy() { 3317 AccidentComponent dst = new AccidentComponent(); 3318 copyValues(dst); 3319 return dst; 3320 } 3321 3322 public void copyValues(AccidentComponent dst) { 3323 super.copyValues(dst); 3324 dst.date = date == null ? null : date.copy(); 3325 dst.type = type == null ? null : type.copy(); 3326 dst.location = location == null ? null : location.copy(); 3327 } 3328 3329 @Override 3330 public boolean equalsDeep(Base other_) { 3331 if (!super.equalsDeep(other_)) 3332 return false; 3333 if (!(other_ instanceof AccidentComponent)) 3334 return false; 3335 AccidentComponent o = (AccidentComponent) other_; 3336 return compareDeep(date, o.date, true) && compareDeep(type, o.type, true) && compareDeep(location, o.location, true) 3337 ; 3338 } 3339 3340 @Override 3341 public boolean equalsShallow(Base other_) { 3342 if (!super.equalsShallow(other_)) 3343 return false; 3344 if (!(other_ instanceof AccidentComponent)) 3345 return false; 3346 AccidentComponent o = (AccidentComponent) other_; 3347 return compareValues(date, o.date, true); 3348 } 3349 3350 public boolean isEmpty() { 3351 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, type, location); 3352 } 3353 3354 public String fhirType() { 3355 return "ExplanationOfBenefit.accident"; 3356 3357 } 3358 3359 } 3360 3361 @Block() 3362 public static class ItemComponent extends BackboneElement implements IBaseBackboneElement { 3363 /** 3364 * A number to uniquely identify item entries. 3365 */ 3366 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3367 @Description(shortDefinition="Item instance identifier", formalDefinition="A number to uniquely identify item entries." ) 3368 protected PositiveIntType sequence; 3369 3370 /** 3371 * Care team members related to this service or product. 3372 */ 3373 @Child(name = "careTeamSequence", type = {PositiveIntType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3374 @Description(shortDefinition="Applicable care team members", formalDefinition="Care team members related to this service or product." ) 3375 protected List<PositiveIntType> careTeamSequence; 3376 3377 /** 3378 * Diagnoses applicable for this service or product. 3379 */ 3380 @Child(name = "diagnosisSequence", type = {PositiveIntType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3381 @Description(shortDefinition="Applicable diagnoses", formalDefinition="Diagnoses applicable for this service or product." ) 3382 protected List<PositiveIntType> diagnosisSequence; 3383 3384 /** 3385 * Procedures applicable for this service or product. 3386 */ 3387 @Child(name = "procedureSequence", type = {PositiveIntType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3388 @Description(shortDefinition="Applicable procedures", formalDefinition="Procedures applicable for this service or product." ) 3389 protected List<PositiveIntType> procedureSequence; 3390 3391 /** 3392 * Exceptions, special conditions and supporting information applicable for this service or product. 3393 */ 3394 @Child(name = "informationSequence", type = {PositiveIntType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3395 @Description(shortDefinition="Applicable exception and supporting information", formalDefinition="Exceptions, special conditions and supporting information applicable for this service or product." ) 3396 protected List<PositiveIntType> informationSequence; 3397 3398 /** 3399 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 3400 */ 3401 @Child(name = "traceNumber", type = {Identifier.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3402 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 3403 protected List<Identifier> traceNumber; 3404 3405 /** 3406 * The type of revenue or cost center providing the product and/or service. 3407 */ 3408 @Child(name = "revenue", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 3409 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 3410 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 3411 protected CodeableConcept revenue; 3412 3413 /** 3414 * Code to identify the general type of benefits under which products and services are provided. 3415 */ 3416 @Child(name = "category", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 3417 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 3418 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 3419 protected CodeableConcept category; 3420 3421 /** 3422 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 3423 */ 3424 @Child(name = "productOrService", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=false) 3425 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 3426 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 3427 protected CodeableConcept productOrService; 3428 3429 /** 3430 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 3431 */ 3432 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=false) 3433 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 3434 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 3435 protected CodeableConcept productOrServiceEnd; 3436 3437 /** 3438 * Request or Referral for Goods or Service to be rendered. 3439 */ 3440 @Child(name = "request", type = {DeviceRequest.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class, SupplyRequest.class, VisionPrescription.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3441 @Description(shortDefinition="Request or Referral for Service", formalDefinition="Request or Referral for Goods or Service to be rendered." ) 3442 protected List<Reference> request; 3443 3444 /** 3445 * Item typification or modifiers codes to convey additional context for the product or service. 3446 */ 3447 @Child(name = "modifier", type = {CodeableConcept.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3448 @Description(shortDefinition="Product or service billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 3449 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 3450 protected List<CodeableConcept> modifier; 3451 3452 /** 3453 * Identifies the program under which this may be recovered. 3454 */ 3455 @Child(name = "programCode", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3456 @Description(shortDefinition="Program the product or service is provided under", formalDefinition="Identifies the program under which this may be recovered." ) 3457 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 3458 protected List<CodeableConcept> programCode; 3459 3460 /** 3461 * The date or dates when the service or product was supplied, performed or completed. 3462 */ 3463 @Child(name = "serviced", type = {DateType.class, Period.class}, order=14, min=0, max=1, modifier=false, summary=false) 3464 @Description(shortDefinition="Date or dates of service or product delivery", formalDefinition="The date or dates when the service or product was supplied, performed or completed." ) 3465 protected DataType serviced; 3466 3467 /** 3468 * Where the product or service was provided. 3469 */ 3470 @Child(name = "location", type = {CodeableConcept.class, Address.class, Location.class}, order=15, min=0, max=1, modifier=false, summary=false) 3471 @Description(shortDefinition="Place of service or where product was supplied", formalDefinition="Where the product or service was provided." ) 3472 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-place") 3473 protected DataType location; 3474 3475 /** 3476 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 3477 */ 3478 @Child(name = "patientPaid", type = {Money.class}, order=16, min=0, max=1, modifier=false, summary=false) 3479 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 3480 protected Money patientPaid; 3481 3482 /** 3483 * The number of repetitions of a service or product. 3484 */ 3485 @Child(name = "quantity", type = {Quantity.class}, order=17, min=0, max=1, modifier=false, summary=false) 3486 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 3487 protected Quantity quantity; 3488 3489 /** 3490 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 3491 */ 3492 @Child(name = "unitPrice", type = {Money.class}, order=18, min=0, max=1, modifier=false, summary=false) 3493 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 3494 protected Money unitPrice; 3495 3496 /** 3497 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3498 */ 3499 @Child(name = "factor", type = {DecimalType.class}, order=19, min=0, max=1, modifier=false, summary=false) 3500 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 3501 protected DecimalType factor; 3502 3503 /** 3504 * The total of taxes applicable for this product or service. 3505 */ 3506 @Child(name = "tax", type = {Money.class}, order=20, min=0, max=1, modifier=false, summary=false) 3507 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 3508 protected Money tax; 3509 3510 /** 3511 * The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor. 3512 */ 3513 @Child(name = "net", type = {Money.class}, order=21, min=0, max=1, modifier=false, summary=false) 3514 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor." ) 3515 protected Money net; 3516 3517 /** 3518 * Unique Device Identifiers associated with this line item. 3519 */ 3520 @Child(name = "udi", type = {Device.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3521 @Description(shortDefinition="Unique device identifier", formalDefinition="Unique Device Identifiers associated with this line item." ) 3522 protected List<Reference> udi; 3523 3524 /** 3525 * Physical location where the service is performed or applies. 3526 */ 3527 @Child(name = "bodySite", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3528 @Description(shortDefinition="Anatomical location", formalDefinition="Physical location where the service is performed or applies." ) 3529 protected List<ItemBodySiteComponent> bodySite; 3530 3531 /** 3532 * Healthcare encounters related to this claim. 3533 */ 3534 @Child(name = "encounter", type = {Encounter.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3535 @Description(shortDefinition="Encounters associated with the listed treatments", formalDefinition="Healthcare encounters related to this claim." ) 3536 protected List<Reference> encounter; 3537 3538 /** 3539 * The numbers associated with notes below which apply to the adjudication of this item. 3540 */ 3541 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3542 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 3543 protected List<PositiveIntType> noteNumber; 3544 3545 /** 3546 * The high-level results of the adjudication if adjudication has been performed. 3547 */ 3548 @Child(name = "reviewOutcome", type = {}, order=26, min=0, max=1, modifier=false, summary=false) 3549 @Description(shortDefinition="Adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 3550 protected ItemReviewOutcomeComponent reviewOutcome; 3551 3552 /** 3553 * If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item. 3554 */ 3555 @Child(name = "adjudication", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3556 @Description(shortDefinition="Adjudication details", formalDefinition="If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item." ) 3557 protected List<AdjudicationComponent> adjudication; 3558 3559 /** 3560 * Second-tier of goods and services. 3561 */ 3562 @Child(name = "detail", type = {}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3563 @Description(shortDefinition="Additional items", formalDefinition="Second-tier of goods and services." ) 3564 protected List<DetailComponent> detail; 3565 3566 private static final long serialVersionUID = -1905277239L; 3567 3568 /** 3569 * Constructor 3570 */ 3571 public ItemComponent() { 3572 super(); 3573 } 3574 3575 /** 3576 * Constructor 3577 */ 3578 public ItemComponent(int sequence) { 3579 super(); 3580 this.setSequence(sequence); 3581 } 3582 3583 /** 3584 * @return {@link #sequence} (A number to uniquely identify item entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 3585 */ 3586 public PositiveIntType getSequenceElement() { 3587 if (this.sequence == null) 3588 if (Configuration.errorOnAutoCreate()) 3589 throw new Error("Attempt to auto-create ItemComponent.sequence"); 3590 else if (Configuration.doAutoCreate()) 3591 this.sequence = new PositiveIntType(); // bb 3592 return this.sequence; 3593 } 3594 3595 public boolean hasSequenceElement() { 3596 return this.sequence != null && !this.sequence.isEmpty(); 3597 } 3598 3599 public boolean hasSequence() { 3600 return this.sequence != null && !this.sequence.isEmpty(); 3601 } 3602 3603 /** 3604 * @param value {@link #sequence} (A number to uniquely identify item entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 3605 */ 3606 public ItemComponent setSequenceElement(PositiveIntType value) { 3607 this.sequence = value; 3608 return this; 3609 } 3610 3611 /** 3612 * @return A number to uniquely identify item entries. 3613 */ 3614 public int getSequence() { 3615 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 3616 } 3617 3618 /** 3619 * @param value A number to uniquely identify item entries. 3620 */ 3621 public ItemComponent setSequence(int value) { 3622 if (this.sequence == null) 3623 this.sequence = new PositiveIntType(); 3624 this.sequence.setValue(value); 3625 return this; 3626 } 3627 3628 /** 3629 * @return {@link #careTeamSequence} (Care team members related to this service or product.) 3630 */ 3631 public List<PositiveIntType> getCareTeamSequence() { 3632 if (this.careTeamSequence == null) 3633 this.careTeamSequence = new ArrayList<PositiveIntType>(); 3634 return this.careTeamSequence; 3635 } 3636 3637 /** 3638 * @return Returns a reference to <code>this</code> for easy method chaining 3639 */ 3640 public ItemComponent setCareTeamSequence(List<PositiveIntType> theCareTeamSequence) { 3641 this.careTeamSequence = theCareTeamSequence; 3642 return this; 3643 } 3644 3645 public boolean hasCareTeamSequence() { 3646 if (this.careTeamSequence == null) 3647 return false; 3648 for (PositiveIntType item : this.careTeamSequence) 3649 if (!item.isEmpty()) 3650 return true; 3651 return false; 3652 } 3653 3654 /** 3655 * @return {@link #careTeamSequence} (Care team members related to this service or product.) 3656 */ 3657 public PositiveIntType addCareTeamSequenceElement() {//2 3658 PositiveIntType t = new PositiveIntType(); 3659 if (this.careTeamSequence == null) 3660 this.careTeamSequence = new ArrayList<PositiveIntType>(); 3661 this.careTeamSequence.add(t); 3662 return t; 3663 } 3664 3665 /** 3666 * @param value {@link #careTeamSequence} (Care team members related to this service or product.) 3667 */ 3668 public ItemComponent addCareTeamSequence(int value) { //1 3669 PositiveIntType t = new PositiveIntType(); 3670 t.setValue(value); 3671 if (this.careTeamSequence == null) 3672 this.careTeamSequence = new ArrayList<PositiveIntType>(); 3673 this.careTeamSequence.add(t); 3674 return this; 3675 } 3676 3677 /** 3678 * @param value {@link #careTeamSequence} (Care team members related to this service or product.) 3679 */ 3680 public boolean hasCareTeamSequence(int value) { 3681 if (this.careTeamSequence == null) 3682 return false; 3683 for (PositiveIntType v : this.careTeamSequence) 3684 if (v.getValue().equals(value)) // positiveInt 3685 return true; 3686 return false; 3687 } 3688 3689 /** 3690 * @return {@link #diagnosisSequence} (Diagnoses applicable for this service or product.) 3691 */ 3692 public List<PositiveIntType> getDiagnosisSequence() { 3693 if (this.diagnosisSequence == null) 3694 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 3695 return this.diagnosisSequence; 3696 } 3697 3698 /** 3699 * @return Returns a reference to <code>this</code> for easy method chaining 3700 */ 3701 public ItemComponent setDiagnosisSequence(List<PositiveIntType> theDiagnosisSequence) { 3702 this.diagnosisSequence = theDiagnosisSequence; 3703 return this; 3704 } 3705 3706 public boolean hasDiagnosisSequence() { 3707 if (this.diagnosisSequence == null) 3708 return false; 3709 for (PositiveIntType item : this.diagnosisSequence) 3710 if (!item.isEmpty()) 3711 return true; 3712 return false; 3713 } 3714 3715 /** 3716 * @return {@link #diagnosisSequence} (Diagnoses applicable for this service or product.) 3717 */ 3718 public PositiveIntType addDiagnosisSequenceElement() {//2 3719 PositiveIntType t = new PositiveIntType(); 3720 if (this.diagnosisSequence == null) 3721 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 3722 this.diagnosisSequence.add(t); 3723 return t; 3724 } 3725 3726 /** 3727 * @param value {@link #diagnosisSequence} (Diagnoses applicable for this service or product.) 3728 */ 3729 public ItemComponent addDiagnosisSequence(int value) { //1 3730 PositiveIntType t = new PositiveIntType(); 3731 t.setValue(value); 3732 if (this.diagnosisSequence == null) 3733 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 3734 this.diagnosisSequence.add(t); 3735 return this; 3736 } 3737 3738 /** 3739 * @param value {@link #diagnosisSequence} (Diagnoses applicable for this service or product.) 3740 */ 3741 public boolean hasDiagnosisSequence(int value) { 3742 if (this.diagnosisSequence == null) 3743 return false; 3744 for (PositiveIntType v : this.diagnosisSequence) 3745 if (v.getValue().equals(value)) // positiveInt 3746 return true; 3747 return false; 3748 } 3749 3750 /** 3751 * @return {@link #procedureSequence} (Procedures applicable for this service or product.) 3752 */ 3753 public List<PositiveIntType> getProcedureSequence() { 3754 if (this.procedureSequence == null) 3755 this.procedureSequence = new ArrayList<PositiveIntType>(); 3756 return this.procedureSequence; 3757 } 3758 3759 /** 3760 * @return Returns a reference to <code>this</code> for easy method chaining 3761 */ 3762 public ItemComponent setProcedureSequence(List<PositiveIntType> theProcedureSequence) { 3763 this.procedureSequence = theProcedureSequence; 3764 return this; 3765 } 3766 3767 public boolean hasProcedureSequence() { 3768 if (this.procedureSequence == null) 3769 return false; 3770 for (PositiveIntType item : this.procedureSequence) 3771 if (!item.isEmpty()) 3772 return true; 3773 return false; 3774 } 3775 3776 /** 3777 * @return {@link #procedureSequence} (Procedures applicable for this service or product.) 3778 */ 3779 public PositiveIntType addProcedureSequenceElement() {//2 3780 PositiveIntType t = new PositiveIntType(); 3781 if (this.procedureSequence == null) 3782 this.procedureSequence = new ArrayList<PositiveIntType>(); 3783 this.procedureSequence.add(t); 3784 return t; 3785 } 3786 3787 /** 3788 * @param value {@link #procedureSequence} (Procedures applicable for this service or product.) 3789 */ 3790 public ItemComponent addProcedureSequence(int value) { //1 3791 PositiveIntType t = new PositiveIntType(); 3792 t.setValue(value); 3793 if (this.procedureSequence == null) 3794 this.procedureSequence = new ArrayList<PositiveIntType>(); 3795 this.procedureSequence.add(t); 3796 return this; 3797 } 3798 3799 /** 3800 * @param value {@link #procedureSequence} (Procedures applicable for this service or product.) 3801 */ 3802 public boolean hasProcedureSequence(int value) { 3803 if (this.procedureSequence == null) 3804 return false; 3805 for (PositiveIntType v : this.procedureSequence) 3806 if (v.getValue().equals(value)) // positiveInt 3807 return true; 3808 return false; 3809 } 3810 3811 /** 3812 * @return {@link #informationSequence} (Exceptions, special conditions and supporting information applicable for this service or product.) 3813 */ 3814 public List<PositiveIntType> getInformationSequence() { 3815 if (this.informationSequence == null) 3816 this.informationSequence = new ArrayList<PositiveIntType>(); 3817 return this.informationSequence; 3818 } 3819 3820 /** 3821 * @return Returns a reference to <code>this</code> for easy method chaining 3822 */ 3823 public ItemComponent setInformationSequence(List<PositiveIntType> theInformationSequence) { 3824 this.informationSequence = theInformationSequence; 3825 return this; 3826 } 3827 3828 public boolean hasInformationSequence() { 3829 if (this.informationSequence == null) 3830 return false; 3831 for (PositiveIntType item : this.informationSequence) 3832 if (!item.isEmpty()) 3833 return true; 3834 return false; 3835 } 3836 3837 /** 3838 * @return {@link #informationSequence} (Exceptions, special conditions and supporting information applicable for this service or product.) 3839 */ 3840 public PositiveIntType addInformationSequenceElement() {//2 3841 PositiveIntType t = new PositiveIntType(); 3842 if (this.informationSequence == null) 3843 this.informationSequence = new ArrayList<PositiveIntType>(); 3844 this.informationSequence.add(t); 3845 return t; 3846 } 3847 3848 /** 3849 * @param value {@link #informationSequence} (Exceptions, special conditions and supporting information applicable for this service or product.) 3850 */ 3851 public ItemComponent addInformationSequence(int value) { //1 3852 PositiveIntType t = new PositiveIntType(); 3853 t.setValue(value); 3854 if (this.informationSequence == null) 3855 this.informationSequence = new ArrayList<PositiveIntType>(); 3856 this.informationSequence.add(t); 3857 return this; 3858 } 3859 3860 /** 3861 * @param value {@link #informationSequence} (Exceptions, special conditions and supporting information applicable for this service or product.) 3862 */ 3863 public boolean hasInformationSequence(int value) { 3864 if (this.informationSequence == null) 3865 return false; 3866 for (PositiveIntType v : this.informationSequence) 3867 if (v.getValue().equals(value)) // positiveInt 3868 return true; 3869 return false; 3870 } 3871 3872 /** 3873 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 3874 */ 3875 public List<Identifier> getTraceNumber() { 3876 if (this.traceNumber == null) 3877 this.traceNumber = new ArrayList<Identifier>(); 3878 return this.traceNumber; 3879 } 3880 3881 /** 3882 * @return Returns a reference to <code>this</code> for easy method chaining 3883 */ 3884 public ItemComponent setTraceNumber(List<Identifier> theTraceNumber) { 3885 this.traceNumber = theTraceNumber; 3886 return this; 3887 } 3888 3889 public boolean hasTraceNumber() { 3890 if (this.traceNumber == null) 3891 return false; 3892 for (Identifier item : this.traceNumber) 3893 if (!item.isEmpty()) 3894 return true; 3895 return false; 3896 } 3897 3898 public Identifier addTraceNumber() { //3 3899 Identifier t = new Identifier(); 3900 if (this.traceNumber == null) 3901 this.traceNumber = new ArrayList<Identifier>(); 3902 this.traceNumber.add(t); 3903 return t; 3904 } 3905 3906 public ItemComponent addTraceNumber(Identifier t) { //3 3907 if (t == null) 3908 return this; 3909 if (this.traceNumber == null) 3910 this.traceNumber = new ArrayList<Identifier>(); 3911 this.traceNumber.add(t); 3912 return this; 3913 } 3914 3915 /** 3916 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 3917 */ 3918 public Identifier getTraceNumberFirstRep() { 3919 if (getTraceNumber().isEmpty()) { 3920 addTraceNumber(); 3921 } 3922 return getTraceNumber().get(0); 3923 } 3924 3925 /** 3926 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 3927 */ 3928 public CodeableConcept getRevenue() { 3929 if (this.revenue == null) 3930 if (Configuration.errorOnAutoCreate()) 3931 throw new Error("Attempt to auto-create ItemComponent.revenue"); 3932 else if (Configuration.doAutoCreate()) 3933 this.revenue = new CodeableConcept(); // cc 3934 return this.revenue; 3935 } 3936 3937 public boolean hasRevenue() { 3938 return this.revenue != null && !this.revenue.isEmpty(); 3939 } 3940 3941 /** 3942 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 3943 */ 3944 public ItemComponent setRevenue(CodeableConcept value) { 3945 this.revenue = value; 3946 return this; 3947 } 3948 3949 /** 3950 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 3951 */ 3952 public CodeableConcept getCategory() { 3953 if (this.category == null) 3954 if (Configuration.errorOnAutoCreate()) 3955 throw new Error("Attempt to auto-create ItemComponent.category"); 3956 else if (Configuration.doAutoCreate()) 3957 this.category = new CodeableConcept(); // cc 3958 return this.category; 3959 } 3960 3961 public boolean hasCategory() { 3962 return this.category != null && !this.category.isEmpty(); 3963 } 3964 3965 /** 3966 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 3967 */ 3968 public ItemComponent setCategory(CodeableConcept value) { 3969 this.category = value; 3970 return this; 3971 } 3972 3973 /** 3974 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 3975 */ 3976 public CodeableConcept getProductOrService() { 3977 if (this.productOrService == null) 3978 if (Configuration.errorOnAutoCreate()) 3979 throw new Error("Attempt to auto-create ItemComponent.productOrService"); 3980 else if (Configuration.doAutoCreate()) 3981 this.productOrService = new CodeableConcept(); // cc 3982 return this.productOrService; 3983 } 3984 3985 public boolean hasProductOrService() { 3986 return this.productOrService != null && !this.productOrService.isEmpty(); 3987 } 3988 3989 /** 3990 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 3991 */ 3992 public ItemComponent setProductOrService(CodeableConcept value) { 3993 this.productOrService = value; 3994 return this; 3995 } 3996 3997 /** 3998 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 3999 */ 4000 public CodeableConcept getProductOrServiceEnd() { 4001 if (this.productOrServiceEnd == null) 4002 if (Configuration.errorOnAutoCreate()) 4003 throw new Error("Attempt to auto-create ItemComponent.productOrServiceEnd"); 4004 else if (Configuration.doAutoCreate()) 4005 this.productOrServiceEnd = new CodeableConcept(); // cc 4006 return this.productOrServiceEnd; 4007 } 4008 4009 public boolean hasProductOrServiceEnd() { 4010 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 4011 } 4012 4013 /** 4014 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 4015 */ 4016 public ItemComponent setProductOrServiceEnd(CodeableConcept value) { 4017 this.productOrServiceEnd = value; 4018 return this; 4019 } 4020 4021 /** 4022 * @return {@link #request} (Request or Referral for Goods or Service to be rendered.) 4023 */ 4024 public List<Reference> getRequest() { 4025 if (this.request == null) 4026 this.request = new ArrayList<Reference>(); 4027 return this.request; 4028 } 4029 4030 /** 4031 * @return Returns a reference to <code>this</code> for easy method chaining 4032 */ 4033 public ItemComponent setRequest(List<Reference> theRequest) { 4034 this.request = theRequest; 4035 return this; 4036 } 4037 4038 public boolean hasRequest() { 4039 if (this.request == null) 4040 return false; 4041 for (Reference item : this.request) 4042 if (!item.isEmpty()) 4043 return true; 4044 return false; 4045 } 4046 4047 public Reference addRequest() { //3 4048 Reference t = new Reference(); 4049 if (this.request == null) 4050 this.request = new ArrayList<Reference>(); 4051 this.request.add(t); 4052 return t; 4053 } 4054 4055 public ItemComponent addRequest(Reference t) { //3 4056 if (t == null) 4057 return this; 4058 if (this.request == null) 4059 this.request = new ArrayList<Reference>(); 4060 this.request.add(t); 4061 return this; 4062 } 4063 4064 /** 4065 * @return The first repetition of repeating field {@link #request}, creating it if it does not already exist {3} 4066 */ 4067 public Reference getRequestFirstRep() { 4068 if (getRequest().isEmpty()) { 4069 addRequest(); 4070 } 4071 return getRequest().get(0); 4072 } 4073 4074 /** 4075 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 4076 */ 4077 public List<CodeableConcept> getModifier() { 4078 if (this.modifier == null) 4079 this.modifier = new ArrayList<CodeableConcept>(); 4080 return this.modifier; 4081 } 4082 4083 /** 4084 * @return Returns a reference to <code>this</code> for easy method chaining 4085 */ 4086 public ItemComponent setModifier(List<CodeableConcept> theModifier) { 4087 this.modifier = theModifier; 4088 return this; 4089 } 4090 4091 public boolean hasModifier() { 4092 if (this.modifier == null) 4093 return false; 4094 for (CodeableConcept item : this.modifier) 4095 if (!item.isEmpty()) 4096 return true; 4097 return false; 4098 } 4099 4100 public CodeableConcept addModifier() { //3 4101 CodeableConcept t = new CodeableConcept(); 4102 if (this.modifier == null) 4103 this.modifier = new ArrayList<CodeableConcept>(); 4104 this.modifier.add(t); 4105 return t; 4106 } 4107 4108 public ItemComponent addModifier(CodeableConcept t) { //3 4109 if (t == null) 4110 return this; 4111 if (this.modifier == null) 4112 this.modifier = new ArrayList<CodeableConcept>(); 4113 this.modifier.add(t); 4114 return this; 4115 } 4116 4117 /** 4118 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 4119 */ 4120 public CodeableConcept getModifierFirstRep() { 4121 if (getModifier().isEmpty()) { 4122 addModifier(); 4123 } 4124 return getModifier().get(0); 4125 } 4126 4127 /** 4128 * @return {@link #programCode} (Identifies the program under which this may be recovered.) 4129 */ 4130 public List<CodeableConcept> getProgramCode() { 4131 if (this.programCode == null) 4132 this.programCode = new ArrayList<CodeableConcept>(); 4133 return this.programCode; 4134 } 4135 4136 /** 4137 * @return Returns a reference to <code>this</code> for easy method chaining 4138 */ 4139 public ItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 4140 this.programCode = theProgramCode; 4141 return this; 4142 } 4143 4144 public boolean hasProgramCode() { 4145 if (this.programCode == null) 4146 return false; 4147 for (CodeableConcept item : this.programCode) 4148 if (!item.isEmpty()) 4149 return true; 4150 return false; 4151 } 4152 4153 public CodeableConcept addProgramCode() { //3 4154 CodeableConcept t = new CodeableConcept(); 4155 if (this.programCode == null) 4156 this.programCode = new ArrayList<CodeableConcept>(); 4157 this.programCode.add(t); 4158 return t; 4159 } 4160 4161 public ItemComponent addProgramCode(CodeableConcept t) { //3 4162 if (t == null) 4163 return this; 4164 if (this.programCode == null) 4165 this.programCode = new ArrayList<CodeableConcept>(); 4166 this.programCode.add(t); 4167 return this; 4168 } 4169 4170 /** 4171 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist {3} 4172 */ 4173 public CodeableConcept getProgramCodeFirstRep() { 4174 if (getProgramCode().isEmpty()) { 4175 addProgramCode(); 4176 } 4177 return getProgramCode().get(0); 4178 } 4179 4180 /** 4181 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 4182 */ 4183 public DataType getServiced() { 4184 return this.serviced; 4185 } 4186 4187 /** 4188 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 4189 */ 4190 public DateType getServicedDateType() throws FHIRException { 4191 if (this.serviced == null) 4192 this.serviced = new DateType(); 4193 if (!(this.serviced instanceof DateType)) 4194 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.serviced.getClass().getName()+" was encountered"); 4195 return (DateType) this.serviced; 4196 } 4197 4198 public boolean hasServicedDateType() { 4199 return this != null && this.serviced instanceof DateType; 4200 } 4201 4202 /** 4203 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 4204 */ 4205 public Period getServicedPeriod() throws FHIRException { 4206 if (this.serviced == null) 4207 this.serviced = new Period(); 4208 if (!(this.serviced instanceof Period)) 4209 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.serviced.getClass().getName()+" was encountered"); 4210 return (Period) this.serviced; 4211 } 4212 4213 public boolean hasServicedPeriod() { 4214 return this != null && this.serviced instanceof Period; 4215 } 4216 4217 public boolean hasServiced() { 4218 return this.serviced != null && !this.serviced.isEmpty(); 4219 } 4220 4221 /** 4222 * @param value {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 4223 */ 4224 public ItemComponent setServiced(DataType value) { 4225 if (value != null && !(value instanceof DateType || value instanceof Period)) 4226 throw new FHIRException("Not the right type for ExplanationOfBenefit.item.serviced[x]: "+value.fhirType()); 4227 this.serviced = value; 4228 return this; 4229 } 4230 4231 /** 4232 * @return {@link #location} (Where the product or service was provided.) 4233 */ 4234 public DataType getLocation() { 4235 return this.location; 4236 } 4237 4238 /** 4239 * @return {@link #location} (Where the product or service was provided.) 4240 */ 4241 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 4242 if (this.location == null) 4243 this.location = new CodeableConcept(); 4244 if (!(this.location instanceof CodeableConcept)) 4245 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.location.getClass().getName()+" was encountered"); 4246 return (CodeableConcept) this.location; 4247 } 4248 4249 public boolean hasLocationCodeableConcept() { 4250 return this != null && this.location instanceof CodeableConcept; 4251 } 4252 4253 /** 4254 * @return {@link #location} (Where the product or service was provided.) 4255 */ 4256 public Address getLocationAddress() throws FHIRException { 4257 if (this.location == null) 4258 this.location = new Address(); 4259 if (!(this.location instanceof Address)) 4260 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 4261 return (Address) this.location; 4262 } 4263 4264 public boolean hasLocationAddress() { 4265 return this != null && this.location instanceof Address; 4266 } 4267 4268 /** 4269 * @return {@link #location} (Where the product or service was provided.) 4270 */ 4271 public Reference getLocationReference() throws FHIRException { 4272 if (this.location == null) 4273 this.location = new Reference(); 4274 if (!(this.location instanceof Reference)) 4275 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 4276 return (Reference) this.location; 4277 } 4278 4279 public boolean hasLocationReference() { 4280 return this != null && this.location instanceof Reference; 4281 } 4282 4283 public boolean hasLocation() { 4284 return this.location != null && !this.location.isEmpty(); 4285 } 4286 4287 /** 4288 * @param value {@link #location} (Where the product or service was provided.) 4289 */ 4290 public ItemComponent setLocation(DataType value) { 4291 if (value != null && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 4292 throw new FHIRException("Not the right type for ExplanationOfBenefit.item.location[x]: "+value.fhirType()); 4293 this.location = value; 4294 return this; 4295 } 4296 4297 /** 4298 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 4299 */ 4300 public Money getPatientPaid() { 4301 if (this.patientPaid == null) 4302 if (Configuration.errorOnAutoCreate()) 4303 throw new Error("Attempt to auto-create ItemComponent.patientPaid"); 4304 else if (Configuration.doAutoCreate()) 4305 this.patientPaid = new Money(); // cc 4306 return this.patientPaid; 4307 } 4308 4309 public boolean hasPatientPaid() { 4310 return this.patientPaid != null && !this.patientPaid.isEmpty(); 4311 } 4312 4313 /** 4314 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 4315 */ 4316 public ItemComponent setPatientPaid(Money value) { 4317 this.patientPaid = value; 4318 return this; 4319 } 4320 4321 /** 4322 * @return {@link #quantity} (The number of repetitions of a service or product.) 4323 */ 4324 public Quantity getQuantity() { 4325 if (this.quantity == null) 4326 if (Configuration.errorOnAutoCreate()) 4327 throw new Error("Attempt to auto-create ItemComponent.quantity"); 4328 else if (Configuration.doAutoCreate()) 4329 this.quantity = new Quantity(); // cc 4330 return this.quantity; 4331 } 4332 4333 public boolean hasQuantity() { 4334 return this.quantity != null && !this.quantity.isEmpty(); 4335 } 4336 4337 /** 4338 * @param value {@link #quantity} (The number of repetitions of a service or product.) 4339 */ 4340 public ItemComponent setQuantity(Quantity value) { 4341 this.quantity = value; 4342 return this; 4343 } 4344 4345 /** 4346 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 4347 */ 4348 public Money getUnitPrice() { 4349 if (this.unitPrice == null) 4350 if (Configuration.errorOnAutoCreate()) 4351 throw new Error("Attempt to auto-create ItemComponent.unitPrice"); 4352 else if (Configuration.doAutoCreate()) 4353 this.unitPrice = new Money(); // cc 4354 return this.unitPrice; 4355 } 4356 4357 public boolean hasUnitPrice() { 4358 return this.unitPrice != null && !this.unitPrice.isEmpty(); 4359 } 4360 4361 /** 4362 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 4363 */ 4364 public ItemComponent setUnitPrice(Money value) { 4365 this.unitPrice = value; 4366 return this; 4367 } 4368 4369 /** 4370 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 4371 */ 4372 public DecimalType getFactorElement() { 4373 if (this.factor == null) 4374 if (Configuration.errorOnAutoCreate()) 4375 throw new Error("Attempt to auto-create ItemComponent.factor"); 4376 else if (Configuration.doAutoCreate()) 4377 this.factor = new DecimalType(); // bb 4378 return this.factor; 4379 } 4380 4381 public boolean hasFactorElement() { 4382 return this.factor != null && !this.factor.isEmpty(); 4383 } 4384 4385 public boolean hasFactor() { 4386 return this.factor != null && !this.factor.isEmpty(); 4387 } 4388 4389 /** 4390 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 4391 */ 4392 public ItemComponent setFactorElement(DecimalType value) { 4393 this.factor = value; 4394 return this; 4395 } 4396 4397 /** 4398 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4399 */ 4400 public BigDecimal getFactor() { 4401 return this.factor == null ? null : this.factor.getValue(); 4402 } 4403 4404 /** 4405 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4406 */ 4407 public ItemComponent setFactor(BigDecimal value) { 4408 if (value == null) 4409 this.factor = null; 4410 else { 4411 if (this.factor == null) 4412 this.factor = new DecimalType(); 4413 this.factor.setValue(value); 4414 } 4415 return this; 4416 } 4417 4418 /** 4419 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4420 */ 4421 public ItemComponent setFactor(long value) { 4422 this.factor = new DecimalType(); 4423 this.factor.setValue(value); 4424 return this; 4425 } 4426 4427 /** 4428 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4429 */ 4430 public ItemComponent setFactor(double value) { 4431 this.factor = new DecimalType(); 4432 this.factor.setValue(value); 4433 return this; 4434 } 4435 4436 /** 4437 * @return {@link #tax} (The total of taxes applicable for this product or service.) 4438 */ 4439 public Money getTax() { 4440 if (this.tax == null) 4441 if (Configuration.errorOnAutoCreate()) 4442 throw new Error("Attempt to auto-create ItemComponent.tax"); 4443 else if (Configuration.doAutoCreate()) 4444 this.tax = new Money(); // cc 4445 return this.tax; 4446 } 4447 4448 public boolean hasTax() { 4449 return this.tax != null && !this.tax.isEmpty(); 4450 } 4451 4452 /** 4453 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 4454 */ 4455 public ItemComponent setTax(Money value) { 4456 this.tax = value; 4457 return this; 4458 } 4459 4460 /** 4461 * @return {@link #net} (The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor.) 4462 */ 4463 public Money getNet() { 4464 if (this.net == null) 4465 if (Configuration.errorOnAutoCreate()) 4466 throw new Error("Attempt to auto-create ItemComponent.net"); 4467 else if (Configuration.doAutoCreate()) 4468 this.net = new Money(); // cc 4469 return this.net; 4470 } 4471 4472 public boolean hasNet() { 4473 return this.net != null && !this.net.isEmpty(); 4474 } 4475 4476 /** 4477 * @param value {@link #net} (The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor.) 4478 */ 4479 public ItemComponent setNet(Money value) { 4480 this.net = value; 4481 return this; 4482 } 4483 4484 /** 4485 * @return {@link #udi} (Unique Device Identifiers associated with this line item.) 4486 */ 4487 public List<Reference> getUdi() { 4488 if (this.udi == null) 4489 this.udi = new ArrayList<Reference>(); 4490 return this.udi; 4491 } 4492 4493 /** 4494 * @return Returns a reference to <code>this</code> for easy method chaining 4495 */ 4496 public ItemComponent setUdi(List<Reference> theUdi) { 4497 this.udi = theUdi; 4498 return this; 4499 } 4500 4501 public boolean hasUdi() { 4502 if (this.udi == null) 4503 return false; 4504 for (Reference item : this.udi) 4505 if (!item.isEmpty()) 4506 return true; 4507 return false; 4508 } 4509 4510 public Reference addUdi() { //3 4511 Reference t = new Reference(); 4512 if (this.udi == null) 4513 this.udi = new ArrayList<Reference>(); 4514 this.udi.add(t); 4515 return t; 4516 } 4517 4518 public ItemComponent addUdi(Reference t) { //3 4519 if (t == null) 4520 return this; 4521 if (this.udi == null) 4522 this.udi = new ArrayList<Reference>(); 4523 this.udi.add(t); 4524 return this; 4525 } 4526 4527 /** 4528 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist {3} 4529 */ 4530 public Reference getUdiFirstRep() { 4531 if (getUdi().isEmpty()) { 4532 addUdi(); 4533 } 4534 return getUdi().get(0); 4535 } 4536 4537 /** 4538 * @return {@link #bodySite} (Physical location where the service is performed or applies.) 4539 */ 4540 public List<ItemBodySiteComponent> getBodySite() { 4541 if (this.bodySite == null) 4542 this.bodySite = new ArrayList<ItemBodySiteComponent>(); 4543 return this.bodySite; 4544 } 4545 4546 /** 4547 * @return Returns a reference to <code>this</code> for easy method chaining 4548 */ 4549 public ItemComponent setBodySite(List<ItemBodySiteComponent> theBodySite) { 4550 this.bodySite = theBodySite; 4551 return this; 4552 } 4553 4554 public boolean hasBodySite() { 4555 if (this.bodySite == null) 4556 return false; 4557 for (ItemBodySiteComponent item : this.bodySite) 4558 if (!item.isEmpty()) 4559 return true; 4560 return false; 4561 } 4562 4563 public ItemBodySiteComponent addBodySite() { //3 4564 ItemBodySiteComponent t = new ItemBodySiteComponent(); 4565 if (this.bodySite == null) 4566 this.bodySite = new ArrayList<ItemBodySiteComponent>(); 4567 this.bodySite.add(t); 4568 return t; 4569 } 4570 4571 public ItemComponent addBodySite(ItemBodySiteComponent t) { //3 4572 if (t == null) 4573 return this; 4574 if (this.bodySite == null) 4575 this.bodySite = new ArrayList<ItemBodySiteComponent>(); 4576 this.bodySite.add(t); 4577 return this; 4578 } 4579 4580 /** 4581 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist {3} 4582 */ 4583 public ItemBodySiteComponent getBodySiteFirstRep() { 4584 if (getBodySite().isEmpty()) { 4585 addBodySite(); 4586 } 4587 return getBodySite().get(0); 4588 } 4589 4590 /** 4591 * @return {@link #encounter} (Healthcare encounters related to this claim.) 4592 */ 4593 public List<Reference> getEncounter() { 4594 if (this.encounter == null) 4595 this.encounter = new ArrayList<Reference>(); 4596 return this.encounter; 4597 } 4598 4599 /** 4600 * @return Returns a reference to <code>this</code> for easy method chaining 4601 */ 4602 public ItemComponent setEncounter(List<Reference> theEncounter) { 4603 this.encounter = theEncounter; 4604 return this; 4605 } 4606 4607 public boolean hasEncounter() { 4608 if (this.encounter == null) 4609 return false; 4610 for (Reference item : this.encounter) 4611 if (!item.isEmpty()) 4612 return true; 4613 return false; 4614 } 4615 4616 public Reference addEncounter() { //3 4617 Reference t = new Reference(); 4618 if (this.encounter == null) 4619 this.encounter = new ArrayList<Reference>(); 4620 this.encounter.add(t); 4621 return t; 4622 } 4623 4624 public ItemComponent addEncounter(Reference t) { //3 4625 if (t == null) 4626 return this; 4627 if (this.encounter == null) 4628 this.encounter = new ArrayList<Reference>(); 4629 this.encounter.add(t); 4630 return this; 4631 } 4632 4633 /** 4634 * @return The first repetition of repeating field {@link #encounter}, creating it if it does not already exist {3} 4635 */ 4636 public Reference getEncounterFirstRep() { 4637 if (getEncounter().isEmpty()) { 4638 addEncounter(); 4639 } 4640 return getEncounter().get(0); 4641 } 4642 4643 /** 4644 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 4645 */ 4646 public List<PositiveIntType> getNoteNumber() { 4647 if (this.noteNumber == null) 4648 this.noteNumber = new ArrayList<PositiveIntType>(); 4649 return this.noteNumber; 4650 } 4651 4652 /** 4653 * @return Returns a reference to <code>this</code> for easy method chaining 4654 */ 4655 public ItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 4656 this.noteNumber = theNoteNumber; 4657 return this; 4658 } 4659 4660 public boolean hasNoteNumber() { 4661 if (this.noteNumber == null) 4662 return false; 4663 for (PositiveIntType item : this.noteNumber) 4664 if (!item.isEmpty()) 4665 return true; 4666 return false; 4667 } 4668 4669 /** 4670 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 4671 */ 4672 public PositiveIntType addNoteNumberElement() {//2 4673 PositiveIntType t = new PositiveIntType(); 4674 if (this.noteNumber == null) 4675 this.noteNumber = new ArrayList<PositiveIntType>(); 4676 this.noteNumber.add(t); 4677 return t; 4678 } 4679 4680 /** 4681 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 4682 */ 4683 public ItemComponent addNoteNumber(int value) { //1 4684 PositiveIntType t = new PositiveIntType(); 4685 t.setValue(value); 4686 if (this.noteNumber == null) 4687 this.noteNumber = new ArrayList<PositiveIntType>(); 4688 this.noteNumber.add(t); 4689 return this; 4690 } 4691 4692 /** 4693 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 4694 */ 4695 public boolean hasNoteNumber(int value) { 4696 if (this.noteNumber == null) 4697 return false; 4698 for (PositiveIntType v : this.noteNumber) 4699 if (v.getValue().equals(value)) // positiveInt 4700 return true; 4701 return false; 4702 } 4703 4704 /** 4705 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 4706 */ 4707 public ItemReviewOutcomeComponent getReviewOutcome() { 4708 if (this.reviewOutcome == null) 4709 if (Configuration.errorOnAutoCreate()) 4710 throw new Error("Attempt to auto-create ItemComponent.reviewOutcome"); 4711 else if (Configuration.doAutoCreate()) 4712 this.reviewOutcome = new ItemReviewOutcomeComponent(); // cc 4713 return this.reviewOutcome; 4714 } 4715 4716 public boolean hasReviewOutcome() { 4717 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 4718 } 4719 4720 /** 4721 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 4722 */ 4723 public ItemComponent setReviewOutcome(ItemReviewOutcomeComponent value) { 4724 this.reviewOutcome = value; 4725 return this; 4726 } 4727 4728 /** 4729 * @return {@link #adjudication} (If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.) 4730 */ 4731 public List<AdjudicationComponent> getAdjudication() { 4732 if (this.adjudication == null) 4733 this.adjudication = new ArrayList<AdjudicationComponent>(); 4734 return this.adjudication; 4735 } 4736 4737 /** 4738 * @return Returns a reference to <code>this</code> for easy method chaining 4739 */ 4740 public ItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 4741 this.adjudication = theAdjudication; 4742 return this; 4743 } 4744 4745 public boolean hasAdjudication() { 4746 if (this.adjudication == null) 4747 return false; 4748 for (AdjudicationComponent item : this.adjudication) 4749 if (!item.isEmpty()) 4750 return true; 4751 return false; 4752 } 4753 4754 public AdjudicationComponent addAdjudication() { //3 4755 AdjudicationComponent t = new AdjudicationComponent(); 4756 if (this.adjudication == null) 4757 this.adjudication = new ArrayList<AdjudicationComponent>(); 4758 this.adjudication.add(t); 4759 return t; 4760 } 4761 4762 public ItemComponent addAdjudication(AdjudicationComponent t) { //3 4763 if (t == null) 4764 return this; 4765 if (this.adjudication == null) 4766 this.adjudication = new ArrayList<AdjudicationComponent>(); 4767 this.adjudication.add(t); 4768 return this; 4769 } 4770 4771 /** 4772 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 4773 */ 4774 public AdjudicationComponent getAdjudicationFirstRep() { 4775 if (getAdjudication().isEmpty()) { 4776 addAdjudication(); 4777 } 4778 return getAdjudication().get(0); 4779 } 4780 4781 /** 4782 * @return {@link #detail} (Second-tier of goods and services.) 4783 */ 4784 public List<DetailComponent> getDetail() { 4785 if (this.detail == null) 4786 this.detail = new ArrayList<DetailComponent>(); 4787 return this.detail; 4788 } 4789 4790 /** 4791 * @return Returns a reference to <code>this</code> for easy method chaining 4792 */ 4793 public ItemComponent setDetail(List<DetailComponent> theDetail) { 4794 this.detail = theDetail; 4795 return this; 4796 } 4797 4798 public boolean hasDetail() { 4799 if (this.detail == null) 4800 return false; 4801 for (DetailComponent item : this.detail) 4802 if (!item.isEmpty()) 4803 return true; 4804 return false; 4805 } 4806 4807 public DetailComponent addDetail() { //3 4808 DetailComponent t = new DetailComponent(); 4809 if (this.detail == null) 4810 this.detail = new ArrayList<DetailComponent>(); 4811 this.detail.add(t); 4812 return t; 4813 } 4814 4815 public ItemComponent addDetail(DetailComponent t) { //3 4816 if (t == null) 4817 return this; 4818 if (this.detail == null) 4819 this.detail = new ArrayList<DetailComponent>(); 4820 this.detail.add(t); 4821 return this; 4822 } 4823 4824 /** 4825 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist {3} 4826 */ 4827 public DetailComponent getDetailFirstRep() { 4828 if (getDetail().isEmpty()) { 4829 addDetail(); 4830 } 4831 return getDetail().get(0); 4832 } 4833 4834 protected void listChildren(List<Property> children) { 4835 super.listChildren(children); 4836 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence)); 4837 children.add(new Property("careTeamSequence", "positiveInt", "Care team members related to this service or product.", 0, java.lang.Integer.MAX_VALUE, careTeamSequence)); 4838 children.add(new Property("diagnosisSequence", "positiveInt", "Diagnoses applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, diagnosisSequence)); 4839 children.add(new Property("procedureSequence", "positiveInt", "Procedures applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, procedureSequence)); 4840 children.add(new Property("informationSequence", "positiveInt", "Exceptions, special conditions and supporting information applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, informationSequence)); 4841 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 4842 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 4843 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 4844 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 4845 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 4846 children.add(new Property("request", "Reference(DeviceRequest|MedicationRequest|NutritionOrder|ServiceRequest|SupplyRequest|VisionPrescription)", "Request or Referral for Goods or Service to be rendered.", 0, java.lang.Integer.MAX_VALUE, request)); 4847 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 4848 children.add(new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 4849 children.add(new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced)); 4850 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location)); 4851 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 4852 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 4853 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 4854 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 4855 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 4856 children.add(new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor.", 0, 1, net)); 4857 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 4858 children.add(new Property("bodySite", "", "Physical location where the service is performed or applies.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 4859 children.add(new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter)); 4860 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 4861 children.add(new Property("reviewOutcome", "", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 4862 children.add(new Property("adjudication", "", "If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 4863 children.add(new Property("detail", "", "Second-tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, detail)); 4864 } 4865 4866 @Override 4867 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4868 switch (_hash) { 4869 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence); 4870 case 1070083823: /*careTeamSequence*/ return new Property("careTeamSequence", "positiveInt", "Care team members related to this service or product.", 0, java.lang.Integer.MAX_VALUE, careTeamSequence); 4871 case -909769262: /*diagnosisSequence*/ return new Property("diagnosisSequence", "positiveInt", "Diagnoses applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, diagnosisSequence); 4872 case -808920140: /*procedureSequence*/ return new Property("procedureSequence", "positiveInt", "Procedures applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, procedureSequence); 4873 case -702585587: /*informationSequence*/ return new Property("informationSequence", "positiveInt", "Exceptions, special conditions and supporting information applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, informationSequence); 4874 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 4875 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 4876 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 4877 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 4878 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 4879 case 1095692943: /*request*/ return new Property("request", "Reference(DeviceRequest|MedicationRequest|NutritionOrder|ServiceRequest|SupplyRequest|VisionPrescription)", "Request or Referral for Goods or Service to be rendered.", 0, java.lang.Integer.MAX_VALUE, request); 4880 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 4881 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 4882 case -1927922223: /*serviced[x]*/ return new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 4883 case 1379209295: /*serviced*/ return new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 4884 case 363246749: /*servicedDate*/ return new Property("serviced[x]", "date", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 4885 case 1534966512: /*servicedPeriod*/ return new Property("serviced[x]", "Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 4886 case 552316075: /*location[x]*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location); 4887 case 1901043637: /*location*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location); 4888 case -1224800468: /*locationCodeableConcept*/ return new Property("location[x]", "CodeableConcept", "Where the product or service was provided.", 0, 1, location); 4889 case -1280020865: /*locationAddress*/ return new Property("location[x]", "Address", "Where the product or service was provided.", 0, 1, location); 4890 case 755866390: /*locationReference*/ return new Property("location[x]", "Reference(Location)", "Where the product or service was provided.", 0, 1, location); 4891 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 4892 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 4893 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 4894 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 4895 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 4896 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor.", 0, 1, net); 4897 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 4898 case 1702620169: /*bodySite*/ return new Property("bodySite", "", "Physical location where the service is performed or applies.", 0, java.lang.Integer.MAX_VALUE, bodySite); 4899 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter); 4900 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 4901 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 4902 case -231349275: /*adjudication*/ return new Property("adjudication", "", "If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, adjudication); 4903 case -1335224239: /*detail*/ return new Property("detail", "", "Second-tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, detail); 4904 default: return super.getNamedProperty(_hash, _name, _checkValid); 4905 } 4906 4907 } 4908 4909 @Override 4910 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4911 switch (hash) { 4912 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 4913 case 1070083823: /*careTeamSequence*/ return this.careTeamSequence == null ? new Base[0] : this.careTeamSequence.toArray(new Base[this.careTeamSequence.size()]); // PositiveIntType 4914 case -909769262: /*diagnosisSequence*/ return this.diagnosisSequence == null ? new Base[0] : this.diagnosisSequence.toArray(new Base[this.diagnosisSequence.size()]); // PositiveIntType 4915 case -808920140: /*procedureSequence*/ return this.procedureSequence == null ? new Base[0] : this.procedureSequence.toArray(new Base[this.procedureSequence.size()]); // PositiveIntType 4916 case -702585587: /*informationSequence*/ return this.informationSequence == null ? new Base[0] : this.informationSequence.toArray(new Base[this.informationSequence.size()]); // PositiveIntType 4917 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 4918 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 4919 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 4920 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 4921 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 4922 case 1095692943: /*request*/ return this.request == null ? new Base[0] : this.request.toArray(new Base[this.request.size()]); // Reference 4923 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 4924 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 4925 case 1379209295: /*serviced*/ return this.serviced == null ? new Base[0] : new Base[] {this.serviced}; // DataType 4926 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // DataType 4927 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 4928 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 4929 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 4930 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 4931 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 4932 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 4933 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 4934 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // ItemBodySiteComponent 4935 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 4936 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 4937 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ItemReviewOutcomeComponent 4938 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 4939 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // DetailComponent 4940 default: return super.getProperty(hash, name, checkValid); 4941 } 4942 4943 } 4944 4945 @Override 4946 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4947 switch (hash) { 4948 case 1349547969: // sequence 4949 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 4950 return value; 4951 case 1070083823: // careTeamSequence 4952 this.getCareTeamSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 4953 return value; 4954 case -909769262: // diagnosisSequence 4955 this.getDiagnosisSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 4956 return value; 4957 case -808920140: // procedureSequence 4958 this.getProcedureSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 4959 return value; 4960 case -702585587: // informationSequence 4961 this.getInformationSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 4962 return value; 4963 case 82505966: // traceNumber 4964 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 4965 return value; 4966 case 1099842588: // revenue 4967 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4968 return value; 4969 case 50511102: // category 4970 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4971 return value; 4972 case 1957227299: // productOrService 4973 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4974 return value; 4975 case -717476168: // productOrServiceEnd 4976 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4977 return value; 4978 case 1095692943: // request 4979 this.getRequest().add(TypeConvertor.castToReference(value)); // Reference 4980 return value; 4981 case -615513385: // modifier 4982 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4983 return value; 4984 case 1010065041: // programCode 4985 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4986 return value; 4987 case 1379209295: // serviced 4988 this.serviced = TypeConvertor.castToType(value); // DataType 4989 return value; 4990 case 1901043637: // location 4991 this.location = TypeConvertor.castToType(value); // DataType 4992 return value; 4993 case 525514609: // patientPaid 4994 this.patientPaid = TypeConvertor.castToMoney(value); // Money 4995 return value; 4996 case -1285004149: // quantity 4997 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 4998 return value; 4999 case -486196699: // unitPrice 5000 this.unitPrice = TypeConvertor.castToMoney(value); // Money 5001 return value; 5002 case -1282148017: // factor 5003 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 5004 return value; 5005 case 114603: // tax 5006 this.tax = TypeConvertor.castToMoney(value); // Money 5007 return value; 5008 case 108957: // net 5009 this.net = TypeConvertor.castToMoney(value); // Money 5010 return value; 5011 case 115642: // udi 5012 this.getUdi().add(TypeConvertor.castToReference(value)); // Reference 5013 return value; 5014 case 1702620169: // bodySite 5015 this.getBodySite().add((ItemBodySiteComponent) value); // ItemBodySiteComponent 5016 return value; 5017 case 1524132147: // encounter 5018 this.getEncounter().add(TypeConvertor.castToReference(value)); // Reference 5019 return value; 5020 case -1110033957: // noteNumber 5021 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 5022 return value; 5023 case -51825446: // reviewOutcome 5024 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 5025 return value; 5026 case -231349275: // adjudication 5027 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 5028 return value; 5029 case -1335224239: // detail 5030 this.getDetail().add((DetailComponent) value); // DetailComponent 5031 return value; 5032 default: return super.setProperty(hash, name, value); 5033 } 5034 5035 } 5036 5037 @Override 5038 public Base setProperty(String name, Base value) throws FHIRException { 5039 if (name.equals("sequence")) { 5040 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 5041 } else if (name.equals("careTeamSequence")) { 5042 this.getCareTeamSequence().add(TypeConvertor.castToPositiveInt(value)); 5043 } else if (name.equals("diagnosisSequence")) { 5044 this.getDiagnosisSequence().add(TypeConvertor.castToPositiveInt(value)); 5045 } else if (name.equals("procedureSequence")) { 5046 this.getProcedureSequence().add(TypeConvertor.castToPositiveInt(value)); 5047 } else if (name.equals("informationSequence")) { 5048 this.getInformationSequence().add(TypeConvertor.castToPositiveInt(value)); 5049 } else if (name.equals("traceNumber")) { 5050 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 5051 } else if (name.equals("revenue")) { 5052 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5053 } else if (name.equals("category")) { 5054 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5055 } else if (name.equals("productOrService")) { 5056 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5057 } else if (name.equals("productOrServiceEnd")) { 5058 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5059 } else if (name.equals("request")) { 5060 this.getRequest().add(TypeConvertor.castToReference(value)); 5061 } else if (name.equals("modifier")) { 5062 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 5063 } else if (name.equals("programCode")) { 5064 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); 5065 } else if (name.equals("serviced[x]")) { 5066 this.serviced = TypeConvertor.castToType(value); // DataType 5067 } else if (name.equals("location[x]")) { 5068 this.location = TypeConvertor.castToType(value); // DataType 5069 } else if (name.equals("patientPaid")) { 5070 this.patientPaid = TypeConvertor.castToMoney(value); // Money 5071 } else if (name.equals("quantity")) { 5072 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 5073 } else if (name.equals("unitPrice")) { 5074 this.unitPrice = TypeConvertor.castToMoney(value); // Money 5075 } else if (name.equals("factor")) { 5076 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 5077 } else if (name.equals("tax")) { 5078 this.tax = TypeConvertor.castToMoney(value); // Money 5079 } else if (name.equals("net")) { 5080 this.net = TypeConvertor.castToMoney(value); // Money 5081 } else if (name.equals("udi")) { 5082 this.getUdi().add(TypeConvertor.castToReference(value)); 5083 } else if (name.equals("bodySite")) { 5084 this.getBodySite().add((ItemBodySiteComponent) value); 5085 } else if (name.equals("encounter")) { 5086 this.getEncounter().add(TypeConvertor.castToReference(value)); 5087 } else if (name.equals("noteNumber")) { 5088 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 5089 } else if (name.equals("reviewOutcome")) { 5090 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 5091 } else if (name.equals("adjudication")) { 5092 this.getAdjudication().add((AdjudicationComponent) value); 5093 } else if (name.equals("detail")) { 5094 this.getDetail().add((DetailComponent) value); 5095 } else 5096 return super.setProperty(name, value); 5097 return value; 5098 } 5099 5100 @Override 5101 public Base makeProperty(int hash, String name) throws FHIRException { 5102 switch (hash) { 5103 case 1349547969: return getSequenceElement(); 5104 case 1070083823: return addCareTeamSequenceElement(); 5105 case -909769262: return addDiagnosisSequenceElement(); 5106 case -808920140: return addProcedureSequenceElement(); 5107 case -702585587: return addInformationSequenceElement(); 5108 case 82505966: return addTraceNumber(); 5109 case 1099842588: return getRevenue(); 5110 case 50511102: return getCategory(); 5111 case 1957227299: return getProductOrService(); 5112 case -717476168: return getProductOrServiceEnd(); 5113 case 1095692943: return addRequest(); 5114 case -615513385: return addModifier(); 5115 case 1010065041: return addProgramCode(); 5116 case -1927922223: return getServiced(); 5117 case 1379209295: return getServiced(); 5118 case 552316075: return getLocation(); 5119 case 1901043637: return getLocation(); 5120 case 525514609: return getPatientPaid(); 5121 case -1285004149: return getQuantity(); 5122 case -486196699: return getUnitPrice(); 5123 case -1282148017: return getFactorElement(); 5124 case 114603: return getTax(); 5125 case 108957: return getNet(); 5126 case 115642: return addUdi(); 5127 case 1702620169: return addBodySite(); 5128 case 1524132147: return addEncounter(); 5129 case -1110033957: return addNoteNumberElement(); 5130 case -51825446: return getReviewOutcome(); 5131 case -231349275: return addAdjudication(); 5132 case -1335224239: return addDetail(); 5133 default: return super.makeProperty(hash, name); 5134 } 5135 5136 } 5137 5138 @Override 5139 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5140 switch (hash) { 5141 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 5142 case 1070083823: /*careTeamSequence*/ return new String[] {"positiveInt"}; 5143 case -909769262: /*diagnosisSequence*/ return new String[] {"positiveInt"}; 5144 case -808920140: /*procedureSequence*/ return new String[] {"positiveInt"}; 5145 case -702585587: /*informationSequence*/ return new String[] {"positiveInt"}; 5146 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 5147 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 5148 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 5149 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 5150 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 5151 case 1095692943: /*request*/ return new String[] {"Reference"}; 5152 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 5153 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 5154 case 1379209295: /*serviced*/ return new String[] {"date", "Period"}; 5155 case 1901043637: /*location*/ return new String[] {"CodeableConcept", "Address", "Reference"}; 5156 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 5157 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 5158 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 5159 case -1282148017: /*factor*/ return new String[] {"decimal"}; 5160 case 114603: /*tax*/ return new String[] {"Money"}; 5161 case 108957: /*net*/ return new String[] {"Money"}; 5162 case 115642: /*udi*/ return new String[] {"Reference"}; 5163 case 1702620169: /*bodySite*/ return new String[] {}; 5164 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 5165 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 5166 case -51825446: /*reviewOutcome*/ return new String[] {}; 5167 case -231349275: /*adjudication*/ return new String[] {}; 5168 case -1335224239: /*detail*/ return new String[] {}; 5169 default: return super.getTypesForProperty(hash, name); 5170 } 5171 5172 } 5173 5174 @Override 5175 public Base addChild(String name) throws FHIRException { 5176 if (name.equals("sequence")) { 5177 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.sequence"); 5178 } 5179 else if (name.equals("careTeamSequence")) { 5180 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.careTeamSequence"); 5181 } 5182 else if (name.equals("diagnosisSequence")) { 5183 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.diagnosisSequence"); 5184 } 5185 else if (name.equals("procedureSequence")) { 5186 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.procedureSequence"); 5187 } 5188 else if (name.equals("informationSequence")) { 5189 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.informationSequence"); 5190 } 5191 else if (name.equals("traceNumber")) { 5192 return addTraceNumber(); 5193 } 5194 else if (name.equals("revenue")) { 5195 this.revenue = new CodeableConcept(); 5196 return this.revenue; 5197 } 5198 else if (name.equals("category")) { 5199 this.category = new CodeableConcept(); 5200 return this.category; 5201 } 5202 else if (name.equals("productOrService")) { 5203 this.productOrService = new CodeableConcept(); 5204 return this.productOrService; 5205 } 5206 else if (name.equals("productOrServiceEnd")) { 5207 this.productOrServiceEnd = new CodeableConcept(); 5208 return this.productOrServiceEnd; 5209 } 5210 else if (name.equals("request")) { 5211 return addRequest(); 5212 } 5213 else if (name.equals("modifier")) { 5214 return addModifier(); 5215 } 5216 else if (name.equals("programCode")) { 5217 return addProgramCode(); 5218 } 5219 else if (name.equals("servicedDate")) { 5220 this.serviced = new DateType(); 5221 return this.serviced; 5222 } 5223 else if (name.equals("servicedPeriod")) { 5224 this.serviced = new Period(); 5225 return this.serviced; 5226 } 5227 else if (name.equals("locationCodeableConcept")) { 5228 this.location = new CodeableConcept(); 5229 return this.location; 5230 } 5231 else if (name.equals("locationAddress")) { 5232 this.location = new Address(); 5233 return this.location; 5234 } 5235 else if (name.equals("locationReference")) { 5236 this.location = new Reference(); 5237 return this.location; 5238 } 5239 else if (name.equals("patientPaid")) { 5240 this.patientPaid = new Money(); 5241 return this.patientPaid; 5242 } 5243 else if (name.equals("quantity")) { 5244 this.quantity = new Quantity(); 5245 return this.quantity; 5246 } 5247 else if (name.equals("unitPrice")) { 5248 this.unitPrice = new Money(); 5249 return this.unitPrice; 5250 } 5251 else if (name.equals("factor")) { 5252 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.factor"); 5253 } 5254 else if (name.equals("tax")) { 5255 this.tax = new Money(); 5256 return this.tax; 5257 } 5258 else if (name.equals("net")) { 5259 this.net = new Money(); 5260 return this.net; 5261 } 5262 else if (name.equals("udi")) { 5263 return addUdi(); 5264 } 5265 else if (name.equals("bodySite")) { 5266 return addBodySite(); 5267 } 5268 else if (name.equals("encounter")) { 5269 return addEncounter(); 5270 } 5271 else if (name.equals("noteNumber")) { 5272 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.noteNumber"); 5273 } 5274 else if (name.equals("reviewOutcome")) { 5275 this.reviewOutcome = new ItemReviewOutcomeComponent(); 5276 return this.reviewOutcome; 5277 } 5278 else if (name.equals("adjudication")) { 5279 return addAdjudication(); 5280 } 5281 else if (name.equals("detail")) { 5282 return addDetail(); 5283 } 5284 else 5285 return super.addChild(name); 5286 } 5287 5288 public ItemComponent copy() { 5289 ItemComponent dst = new ItemComponent(); 5290 copyValues(dst); 5291 return dst; 5292 } 5293 5294 public void copyValues(ItemComponent dst) { 5295 super.copyValues(dst); 5296 dst.sequence = sequence == null ? null : sequence.copy(); 5297 if (careTeamSequence != null) { 5298 dst.careTeamSequence = new ArrayList<PositiveIntType>(); 5299 for (PositiveIntType i : careTeamSequence) 5300 dst.careTeamSequence.add(i.copy()); 5301 }; 5302 if (diagnosisSequence != null) { 5303 dst.diagnosisSequence = new ArrayList<PositiveIntType>(); 5304 for (PositiveIntType i : diagnosisSequence) 5305 dst.diagnosisSequence.add(i.copy()); 5306 }; 5307 if (procedureSequence != null) { 5308 dst.procedureSequence = new ArrayList<PositiveIntType>(); 5309 for (PositiveIntType i : procedureSequence) 5310 dst.procedureSequence.add(i.copy()); 5311 }; 5312 if (informationSequence != null) { 5313 dst.informationSequence = new ArrayList<PositiveIntType>(); 5314 for (PositiveIntType i : informationSequence) 5315 dst.informationSequence.add(i.copy()); 5316 }; 5317 if (traceNumber != null) { 5318 dst.traceNumber = new ArrayList<Identifier>(); 5319 for (Identifier i : traceNumber) 5320 dst.traceNumber.add(i.copy()); 5321 }; 5322 dst.revenue = revenue == null ? null : revenue.copy(); 5323 dst.category = category == null ? null : category.copy(); 5324 dst.productOrService = productOrService == null ? null : productOrService.copy(); 5325 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 5326 if (request != null) { 5327 dst.request = new ArrayList<Reference>(); 5328 for (Reference i : request) 5329 dst.request.add(i.copy()); 5330 }; 5331 if (modifier != null) { 5332 dst.modifier = new ArrayList<CodeableConcept>(); 5333 for (CodeableConcept i : modifier) 5334 dst.modifier.add(i.copy()); 5335 }; 5336 if (programCode != null) { 5337 dst.programCode = new ArrayList<CodeableConcept>(); 5338 for (CodeableConcept i : programCode) 5339 dst.programCode.add(i.copy()); 5340 }; 5341 dst.serviced = serviced == null ? null : serviced.copy(); 5342 dst.location = location == null ? null : location.copy(); 5343 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 5344 dst.quantity = quantity == null ? null : quantity.copy(); 5345 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 5346 dst.factor = factor == null ? null : factor.copy(); 5347 dst.tax = tax == null ? null : tax.copy(); 5348 dst.net = net == null ? null : net.copy(); 5349 if (udi != null) { 5350 dst.udi = new ArrayList<Reference>(); 5351 for (Reference i : udi) 5352 dst.udi.add(i.copy()); 5353 }; 5354 if (bodySite != null) { 5355 dst.bodySite = new ArrayList<ItemBodySiteComponent>(); 5356 for (ItemBodySiteComponent i : bodySite) 5357 dst.bodySite.add(i.copy()); 5358 }; 5359 if (encounter != null) { 5360 dst.encounter = new ArrayList<Reference>(); 5361 for (Reference i : encounter) 5362 dst.encounter.add(i.copy()); 5363 }; 5364 if (noteNumber != null) { 5365 dst.noteNumber = new ArrayList<PositiveIntType>(); 5366 for (PositiveIntType i : noteNumber) 5367 dst.noteNumber.add(i.copy()); 5368 }; 5369 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 5370 if (adjudication != null) { 5371 dst.adjudication = new ArrayList<AdjudicationComponent>(); 5372 for (AdjudicationComponent i : adjudication) 5373 dst.adjudication.add(i.copy()); 5374 }; 5375 if (detail != null) { 5376 dst.detail = new ArrayList<DetailComponent>(); 5377 for (DetailComponent i : detail) 5378 dst.detail.add(i.copy()); 5379 }; 5380 } 5381 5382 @Override 5383 public boolean equalsDeep(Base other_) { 5384 if (!super.equalsDeep(other_)) 5385 return false; 5386 if (!(other_ instanceof ItemComponent)) 5387 return false; 5388 ItemComponent o = (ItemComponent) other_; 5389 return compareDeep(sequence, o.sequence, true) && compareDeep(careTeamSequence, o.careTeamSequence, true) 5390 && compareDeep(diagnosisSequence, o.diagnosisSequence, true) && compareDeep(procedureSequence, o.procedureSequence, true) 5391 && compareDeep(informationSequence, o.informationSequence, true) && compareDeep(traceNumber, o.traceNumber, true) 5392 && compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 5393 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(request, o.request, true) 5394 && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) && compareDeep(serviced, o.serviced, true) 5395 && compareDeep(location, o.location, true) && compareDeep(patientPaid, o.patientPaid, true) && compareDeep(quantity, o.quantity, true) 5396 && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) && compareDeep(tax, o.tax, true) 5397 && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) && compareDeep(bodySite, o.bodySite, true) 5398 && compareDeep(encounter, o.encounter, true) && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(reviewOutcome, o.reviewOutcome, true) 5399 && compareDeep(adjudication, o.adjudication, true) && compareDeep(detail, o.detail, true); 5400 } 5401 5402 @Override 5403 public boolean equalsShallow(Base other_) { 5404 if (!super.equalsShallow(other_)) 5405 return false; 5406 if (!(other_ instanceof ItemComponent)) 5407 return false; 5408 ItemComponent o = (ItemComponent) other_; 5409 return compareValues(sequence, o.sequence, true) && compareValues(careTeamSequence, o.careTeamSequence, true) 5410 && compareValues(diagnosisSequence, o.diagnosisSequence, true) && compareValues(procedureSequence, o.procedureSequence, true) 5411 && compareValues(informationSequence, o.informationSequence, true) && compareValues(factor, o.factor, true) 5412 && compareValues(noteNumber, o.noteNumber, true); 5413 } 5414 5415 public boolean isEmpty() { 5416 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, careTeamSequence 5417 , diagnosisSequence, procedureSequence, informationSequence, traceNumber, revenue 5418 , category, productOrService, productOrServiceEnd, request, modifier, programCode 5419 , serviced, location, patientPaid, quantity, unitPrice, factor, tax, net, udi 5420 , bodySite, encounter, noteNumber, reviewOutcome, adjudication, detail); 5421 } 5422 5423 public String fhirType() { 5424 return "ExplanationOfBenefit.item"; 5425 5426 } 5427 5428 } 5429 5430 @Block() 5431 public static class ItemBodySiteComponent extends BackboneElement implements IBaseBackboneElement { 5432 /** 5433 * Physical service site on the patient (limb, tooth, etc.). 5434 */ 5435 @Child(name = "site", type = {CodeableReference.class}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5436 @Description(shortDefinition="Location", formalDefinition="Physical service site on the patient (limb, tooth, etc.)." ) 5437 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/tooth") 5438 protected List<CodeableReference> site; 5439 5440 /** 5441 * A region or surface of the bodySite, e.g. limb region or tooth surface(s). 5442 */ 5443 @Child(name = "subSite", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5444 @Description(shortDefinition="Sub-location", formalDefinition="A region or surface of the bodySite, e.g. limb region or tooth surface(s)." ) 5445 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/surface") 5446 protected List<CodeableConcept> subSite; 5447 5448 private static final long serialVersionUID = 1190632415L; 5449 5450 /** 5451 * Constructor 5452 */ 5453 public ItemBodySiteComponent() { 5454 super(); 5455 } 5456 5457 /** 5458 * Constructor 5459 */ 5460 public ItemBodySiteComponent(CodeableReference site) { 5461 super(); 5462 this.addSite(site); 5463 } 5464 5465 /** 5466 * @return {@link #site} (Physical service site on the patient (limb, tooth, etc.).) 5467 */ 5468 public List<CodeableReference> getSite() { 5469 if (this.site == null) 5470 this.site = new ArrayList<CodeableReference>(); 5471 return this.site; 5472 } 5473 5474 /** 5475 * @return Returns a reference to <code>this</code> for easy method chaining 5476 */ 5477 public ItemBodySiteComponent setSite(List<CodeableReference> theSite) { 5478 this.site = theSite; 5479 return this; 5480 } 5481 5482 public boolean hasSite() { 5483 if (this.site == null) 5484 return false; 5485 for (CodeableReference item : this.site) 5486 if (!item.isEmpty()) 5487 return true; 5488 return false; 5489 } 5490 5491 public CodeableReference addSite() { //3 5492 CodeableReference t = new CodeableReference(); 5493 if (this.site == null) 5494 this.site = new ArrayList<CodeableReference>(); 5495 this.site.add(t); 5496 return t; 5497 } 5498 5499 public ItemBodySiteComponent addSite(CodeableReference t) { //3 5500 if (t == null) 5501 return this; 5502 if (this.site == null) 5503 this.site = new ArrayList<CodeableReference>(); 5504 this.site.add(t); 5505 return this; 5506 } 5507 5508 /** 5509 * @return The first repetition of repeating field {@link #site}, creating it if it does not already exist {3} 5510 */ 5511 public CodeableReference getSiteFirstRep() { 5512 if (getSite().isEmpty()) { 5513 addSite(); 5514 } 5515 return getSite().get(0); 5516 } 5517 5518 /** 5519 * @return {@link #subSite} (A region or surface of the bodySite, e.g. limb region or tooth surface(s).) 5520 */ 5521 public List<CodeableConcept> getSubSite() { 5522 if (this.subSite == null) 5523 this.subSite = new ArrayList<CodeableConcept>(); 5524 return this.subSite; 5525 } 5526 5527 /** 5528 * @return Returns a reference to <code>this</code> for easy method chaining 5529 */ 5530 public ItemBodySiteComponent setSubSite(List<CodeableConcept> theSubSite) { 5531 this.subSite = theSubSite; 5532 return this; 5533 } 5534 5535 public boolean hasSubSite() { 5536 if (this.subSite == null) 5537 return false; 5538 for (CodeableConcept item : this.subSite) 5539 if (!item.isEmpty()) 5540 return true; 5541 return false; 5542 } 5543 5544 public CodeableConcept addSubSite() { //3 5545 CodeableConcept t = new CodeableConcept(); 5546 if (this.subSite == null) 5547 this.subSite = new ArrayList<CodeableConcept>(); 5548 this.subSite.add(t); 5549 return t; 5550 } 5551 5552 public ItemBodySiteComponent addSubSite(CodeableConcept t) { //3 5553 if (t == null) 5554 return this; 5555 if (this.subSite == null) 5556 this.subSite = new ArrayList<CodeableConcept>(); 5557 this.subSite.add(t); 5558 return this; 5559 } 5560 5561 /** 5562 * @return The first repetition of repeating field {@link #subSite}, creating it if it does not already exist {3} 5563 */ 5564 public CodeableConcept getSubSiteFirstRep() { 5565 if (getSubSite().isEmpty()) { 5566 addSubSite(); 5567 } 5568 return getSubSite().get(0); 5569 } 5570 5571 protected void listChildren(List<Property> children) { 5572 super.listChildren(children); 5573 children.add(new Property("site", "CodeableReference(BodyStructure)", "Physical service site on the patient (limb, tooth, etc.).", 0, java.lang.Integer.MAX_VALUE, site)); 5574 children.add(new Property("subSite", "CodeableConcept", "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite)); 5575 } 5576 5577 @Override 5578 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5579 switch (_hash) { 5580 case 3530567: /*site*/ return new Property("site", "CodeableReference(BodyStructure)", "Physical service site on the patient (limb, tooth, etc.).", 0, java.lang.Integer.MAX_VALUE, site); 5581 case -1868566105: /*subSite*/ return new Property("subSite", "CodeableConcept", "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite); 5582 default: return super.getNamedProperty(_hash, _name, _checkValid); 5583 } 5584 5585 } 5586 5587 @Override 5588 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5589 switch (hash) { 5590 case 3530567: /*site*/ return this.site == null ? new Base[0] : this.site.toArray(new Base[this.site.size()]); // CodeableReference 5591 case -1868566105: /*subSite*/ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 5592 default: return super.getProperty(hash, name, checkValid); 5593 } 5594 5595 } 5596 5597 @Override 5598 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5599 switch (hash) { 5600 case 3530567: // site 5601 this.getSite().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 5602 return value; 5603 case -1868566105: // subSite 5604 this.getSubSite().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5605 return value; 5606 default: return super.setProperty(hash, name, value); 5607 } 5608 5609 } 5610 5611 @Override 5612 public Base setProperty(String name, Base value) throws FHIRException { 5613 if (name.equals("site")) { 5614 this.getSite().add(TypeConvertor.castToCodeableReference(value)); 5615 } else if (name.equals("subSite")) { 5616 this.getSubSite().add(TypeConvertor.castToCodeableConcept(value)); 5617 } else 5618 return super.setProperty(name, value); 5619 return value; 5620 } 5621 5622 @Override 5623 public Base makeProperty(int hash, String name) throws FHIRException { 5624 switch (hash) { 5625 case 3530567: return addSite(); 5626 case -1868566105: return addSubSite(); 5627 default: return super.makeProperty(hash, name); 5628 } 5629 5630 } 5631 5632 @Override 5633 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5634 switch (hash) { 5635 case 3530567: /*site*/ return new String[] {"CodeableReference"}; 5636 case -1868566105: /*subSite*/ return new String[] {"CodeableConcept"}; 5637 default: return super.getTypesForProperty(hash, name); 5638 } 5639 5640 } 5641 5642 @Override 5643 public Base addChild(String name) throws FHIRException { 5644 if (name.equals("site")) { 5645 return addSite(); 5646 } 5647 else if (name.equals("subSite")) { 5648 return addSubSite(); 5649 } 5650 else 5651 return super.addChild(name); 5652 } 5653 5654 public ItemBodySiteComponent copy() { 5655 ItemBodySiteComponent dst = new ItemBodySiteComponent(); 5656 copyValues(dst); 5657 return dst; 5658 } 5659 5660 public void copyValues(ItemBodySiteComponent dst) { 5661 super.copyValues(dst); 5662 if (site != null) { 5663 dst.site = new ArrayList<CodeableReference>(); 5664 for (CodeableReference i : site) 5665 dst.site.add(i.copy()); 5666 }; 5667 if (subSite != null) { 5668 dst.subSite = new ArrayList<CodeableConcept>(); 5669 for (CodeableConcept i : subSite) 5670 dst.subSite.add(i.copy()); 5671 }; 5672 } 5673 5674 @Override 5675 public boolean equalsDeep(Base other_) { 5676 if (!super.equalsDeep(other_)) 5677 return false; 5678 if (!(other_ instanceof ItemBodySiteComponent)) 5679 return false; 5680 ItemBodySiteComponent o = (ItemBodySiteComponent) other_; 5681 return compareDeep(site, o.site, true) && compareDeep(subSite, o.subSite, true); 5682 } 5683 5684 @Override 5685 public boolean equalsShallow(Base other_) { 5686 if (!super.equalsShallow(other_)) 5687 return false; 5688 if (!(other_ instanceof ItemBodySiteComponent)) 5689 return false; 5690 ItemBodySiteComponent o = (ItemBodySiteComponent) other_; 5691 return true; 5692 } 5693 5694 public boolean isEmpty() { 5695 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(site, subSite); 5696 } 5697 5698 public String fhirType() { 5699 return "ExplanationOfBenefit.item.bodySite"; 5700 5701 } 5702 5703 } 5704 5705 @Block() 5706 public static class ItemReviewOutcomeComponent extends BackboneElement implements IBaseBackboneElement { 5707 /** 5708 * The result of the claim, predetermination, or preauthorization adjudication. 5709 */ 5710 @Child(name = "decision", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 5711 @Description(shortDefinition="Result of the adjudication", formalDefinition="The result of the claim, predetermination, or preauthorization adjudication." ) 5712 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-decision") 5713 protected CodeableConcept decision; 5714 5715 /** 5716 * The reasons for the result of the claim, predetermination, or preauthorization adjudication. 5717 */ 5718 @Child(name = "reason", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5719 @Description(shortDefinition="Reason for result of the adjudication", formalDefinition="The reasons for the result of the claim, predetermination, or preauthorization adjudication." ) 5720 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-decision-reason") 5721 protected List<CodeableConcept> reason; 5722 5723 /** 5724 * Reference from the Insurer which is used in later communications which refers to this adjudication. 5725 */ 5726 @Child(name = "preAuthRef", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 5727 @Description(shortDefinition="Preauthorization reference", formalDefinition="Reference from the Insurer which is used in later communications which refers to this adjudication." ) 5728 protected StringType preAuthRef; 5729 5730 /** 5731 * The time frame during which this authorization is effective. 5732 */ 5733 @Child(name = "preAuthPeriod", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 5734 @Description(shortDefinition="Preauthorization reference effective period", formalDefinition="The time frame during which this authorization is effective." ) 5735 protected Period preAuthPeriod; 5736 5737 private static final long serialVersionUID = 2126097594L; 5738 5739 /** 5740 * Constructor 5741 */ 5742 public ItemReviewOutcomeComponent() { 5743 super(); 5744 } 5745 5746 /** 5747 * @return {@link #decision} (The result of the claim, predetermination, or preauthorization adjudication.) 5748 */ 5749 public CodeableConcept getDecision() { 5750 if (this.decision == null) 5751 if (Configuration.errorOnAutoCreate()) 5752 throw new Error("Attempt to auto-create ItemReviewOutcomeComponent.decision"); 5753 else if (Configuration.doAutoCreate()) 5754 this.decision = new CodeableConcept(); // cc 5755 return this.decision; 5756 } 5757 5758 public boolean hasDecision() { 5759 return this.decision != null && !this.decision.isEmpty(); 5760 } 5761 5762 /** 5763 * @param value {@link #decision} (The result of the claim, predetermination, or preauthorization adjudication.) 5764 */ 5765 public ItemReviewOutcomeComponent setDecision(CodeableConcept value) { 5766 this.decision = value; 5767 return this; 5768 } 5769 5770 /** 5771 * @return {@link #reason} (The reasons for the result of the claim, predetermination, or preauthorization adjudication.) 5772 */ 5773 public List<CodeableConcept> getReason() { 5774 if (this.reason == null) 5775 this.reason = new ArrayList<CodeableConcept>(); 5776 return this.reason; 5777 } 5778 5779 /** 5780 * @return Returns a reference to <code>this</code> for easy method chaining 5781 */ 5782 public ItemReviewOutcomeComponent setReason(List<CodeableConcept> theReason) { 5783 this.reason = theReason; 5784 return this; 5785 } 5786 5787 public boolean hasReason() { 5788 if (this.reason == null) 5789 return false; 5790 for (CodeableConcept item : this.reason) 5791 if (!item.isEmpty()) 5792 return true; 5793 return false; 5794 } 5795 5796 public CodeableConcept addReason() { //3 5797 CodeableConcept t = new CodeableConcept(); 5798 if (this.reason == null) 5799 this.reason = new ArrayList<CodeableConcept>(); 5800 this.reason.add(t); 5801 return t; 5802 } 5803 5804 public ItemReviewOutcomeComponent addReason(CodeableConcept t) { //3 5805 if (t == null) 5806 return this; 5807 if (this.reason == null) 5808 this.reason = new ArrayList<CodeableConcept>(); 5809 this.reason.add(t); 5810 return this; 5811 } 5812 5813 /** 5814 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 5815 */ 5816 public CodeableConcept getReasonFirstRep() { 5817 if (getReason().isEmpty()) { 5818 addReason(); 5819 } 5820 return getReason().get(0); 5821 } 5822 5823 /** 5824 * @return {@link #preAuthRef} (Reference from the Insurer which is used in later communications which refers to this adjudication.). This is the underlying object with id, value and extensions. The accessor "getPreAuthRef" gives direct access to the value 5825 */ 5826 public StringType getPreAuthRefElement() { 5827 if (this.preAuthRef == null) 5828 if (Configuration.errorOnAutoCreate()) 5829 throw new Error("Attempt to auto-create ItemReviewOutcomeComponent.preAuthRef"); 5830 else if (Configuration.doAutoCreate()) 5831 this.preAuthRef = new StringType(); // bb 5832 return this.preAuthRef; 5833 } 5834 5835 public boolean hasPreAuthRefElement() { 5836 return this.preAuthRef != null && !this.preAuthRef.isEmpty(); 5837 } 5838 5839 public boolean hasPreAuthRef() { 5840 return this.preAuthRef != null && !this.preAuthRef.isEmpty(); 5841 } 5842 5843 /** 5844 * @param value {@link #preAuthRef} (Reference from the Insurer which is used in later communications which refers to this adjudication.). This is the underlying object with id, value and extensions. The accessor "getPreAuthRef" gives direct access to the value 5845 */ 5846 public ItemReviewOutcomeComponent setPreAuthRefElement(StringType value) { 5847 this.preAuthRef = value; 5848 return this; 5849 } 5850 5851 /** 5852 * @return Reference from the Insurer which is used in later communications which refers to this adjudication. 5853 */ 5854 public String getPreAuthRef() { 5855 return this.preAuthRef == null ? null : this.preAuthRef.getValue(); 5856 } 5857 5858 /** 5859 * @param value Reference from the Insurer which is used in later communications which refers to this adjudication. 5860 */ 5861 public ItemReviewOutcomeComponent setPreAuthRef(String value) { 5862 if (Utilities.noString(value)) 5863 this.preAuthRef = null; 5864 else { 5865 if (this.preAuthRef == null) 5866 this.preAuthRef = new StringType(); 5867 this.preAuthRef.setValue(value); 5868 } 5869 return this; 5870 } 5871 5872 /** 5873 * @return {@link #preAuthPeriod} (The time frame during which this authorization is effective.) 5874 */ 5875 public Period getPreAuthPeriod() { 5876 if (this.preAuthPeriod == null) 5877 if (Configuration.errorOnAutoCreate()) 5878 throw new Error("Attempt to auto-create ItemReviewOutcomeComponent.preAuthPeriod"); 5879 else if (Configuration.doAutoCreate()) 5880 this.preAuthPeriod = new Period(); // cc 5881 return this.preAuthPeriod; 5882 } 5883 5884 public boolean hasPreAuthPeriod() { 5885 return this.preAuthPeriod != null && !this.preAuthPeriod.isEmpty(); 5886 } 5887 5888 /** 5889 * @param value {@link #preAuthPeriod} (The time frame during which this authorization is effective.) 5890 */ 5891 public ItemReviewOutcomeComponent setPreAuthPeriod(Period value) { 5892 this.preAuthPeriod = value; 5893 return this; 5894 } 5895 5896 protected void listChildren(List<Property> children) { 5897 super.listChildren(children); 5898 children.add(new Property("decision", "CodeableConcept", "The result of the claim, predetermination, or preauthorization adjudication.", 0, 1, decision)); 5899 children.add(new Property("reason", "CodeableConcept", "The reasons for the result of the claim, predetermination, or preauthorization adjudication.", 0, java.lang.Integer.MAX_VALUE, reason)); 5900 children.add(new Property("preAuthRef", "string", "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, 1, preAuthRef)); 5901 children.add(new Property("preAuthPeriod", "Period", "The time frame during which this authorization is effective.", 0, 1, preAuthPeriod)); 5902 } 5903 5904 @Override 5905 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5906 switch (_hash) { 5907 case 565719004: /*decision*/ return new Property("decision", "CodeableConcept", "The result of the claim, predetermination, or preauthorization adjudication.", 0, 1, decision); 5908 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "The reasons for the result of the claim, predetermination, or preauthorization adjudication.", 0, java.lang.Integer.MAX_VALUE, reason); 5909 case 522246568: /*preAuthRef*/ return new Property("preAuthRef", "string", "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, 1, preAuthRef); 5910 case 1819164812: /*preAuthPeriod*/ return new Property("preAuthPeriod", "Period", "The time frame during which this authorization is effective.", 0, 1, preAuthPeriod); 5911 default: return super.getNamedProperty(_hash, _name, _checkValid); 5912 } 5913 5914 } 5915 5916 @Override 5917 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5918 switch (hash) { 5919 case 565719004: /*decision*/ return this.decision == null ? new Base[0] : new Base[] {this.decision}; // CodeableConcept 5920 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 5921 case 522246568: /*preAuthRef*/ return this.preAuthRef == null ? new Base[0] : new Base[] {this.preAuthRef}; // StringType 5922 case 1819164812: /*preAuthPeriod*/ return this.preAuthPeriod == null ? new Base[0] : new Base[] {this.preAuthPeriod}; // Period 5923 default: return super.getProperty(hash, name, checkValid); 5924 } 5925 5926 } 5927 5928 @Override 5929 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5930 switch (hash) { 5931 case 565719004: // decision 5932 this.decision = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5933 return value; 5934 case -934964668: // reason 5935 this.getReason().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5936 return value; 5937 case 522246568: // preAuthRef 5938 this.preAuthRef = TypeConvertor.castToString(value); // StringType 5939 return value; 5940 case 1819164812: // preAuthPeriod 5941 this.preAuthPeriod = TypeConvertor.castToPeriod(value); // Period 5942 return value; 5943 default: return super.setProperty(hash, name, value); 5944 } 5945 5946 } 5947 5948 @Override 5949 public Base setProperty(String name, Base value) throws FHIRException { 5950 if (name.equals("decision")) { 5951 this.decision = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5952 } else if (name.equals("reason")) { 5953 this.getReason().add(TypeConvertor.castToCodeableConcept(value)); 5954 } else if (name.equals("preAuthRef")) { 5955 this.preAuthRef = TypeConvertor.castToString(value); // StringType 5956 } else if (name.equals("preAuthPeriod")) { 5957 this.preAuthPeriod = TypeConvertor.castToPeriod(value); // Period 5958 } else 5959 return super.setProperty(name, value); 5960 return value; 5961 } 5962 5963 @Override 5964 public Base makeProperty(int hash, String name) throws FHIRException { 5965 switch (hash) { 5966 case 565719004: return getDecision(); 5967 case -934964668: return addReason(); 5968 case 522246568: return getPreAuthRefElement(); 5969 case 1819164812: return getPreAuthPeriod(); 5970 default: return super.makeProperty(hash, name); 5971 } 5972 5973 } 5974 5975 @Override 5976 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5977 switch (hash) { 5978 case 565719004: /*decision*/ return new String[] {"CodeableConcept"}; 5979 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 5980 case 522246568: /*preAuthRef*/ return new String[] {"string"}; 5981 case 1819164812: /*preAuthPeriod*/ return new String[] {"Period"}; 5982 default: return super.getTypesForProperty(hash, name); 5983 } 5984 5985 } 5986 5987 @Override 5988 public Base addChild(String name) throws FHIRException { 5989 if (name.equals("decision")) { 5990 this.decision = new CodeableConcept(); 5991 return this.decision; 5992 } 5993 else if (name.equals("reason")) { 5994 return addReason(); 5995 } 5996 else if (name.equals("preAuthRef")) { 5997 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.reviewOutcome.preAuthRef"); 5998 } 5999 else if (name.equals("preAuthPeriod")) { 6000 this.preAuthPeriod = new Period(); 6001 return this.preAuthPeriod; 6002 } 6003 else 6004 return super.addChild(name); 6005 } 6006 6007 public ItemReviewOutcomeComponent copy() { 6008 ItemReviewOutcomeComponent dst = new ItemReviewOutcomeComponent(); 6009 copyValues(dst); 6010 return dst; 6011 } 6012 6013 public void copyValues(ItemReviewOutcomeComponent dst) { 6014 super.copyValues(dst); 6015 dst.decision = decision == null ? null : decision.copy(); 6016 if (reason != null) { 6017 dst.reason = new ArrayList<CodeableConcept>(); 6018 for (CodeableConcept i : reason) 6019 dst.reason.add(i.copy()); 6020 }; 6021 dst.preAuthRef = preAuthRef == null ? null : preAuthRef.copy(); 6022 dst.preAuthPeriod = preAuthPeriod == null ? null : preAuthPeriod.copy(); 6023 } 6024 6025 @Override 6026 public boolean equalsDeep(Base other_) { 6027 if (!super.equalsDeep(other_)) 6028 return false; 6029 if (!(other_ instanceof ItemReviewOutcomeComponent)) 6030 return false; 6031 ItemReviewOutcomeComponent o = (ItemReviewOutcomeComponent) other_; 6032 return compareDeep(decision, o.decision, true) && compareDeep(reason, o.reason, true) && compareDeep(preAuthRef, o.preAuthRef, true) 6033 && compareDeep(preAuthPeriod, o.preAuthPeriod, true); 6034 } 6035 6036 @Override 6037 public boolean equalsShallow(Base other_) { 6038 if (!super.equalsShallow(other_)) 6039 return false; 6040 if (!(other_ instanceof ItemReviewOutcomeComponent)) 6041 return false; 6042 ItemReviewOutcomeComponent o = (ItemReviewOutcomeComponent) other_; 6043 return compareValues(preAuthRef, o.preAuthRef, true); 6044 } 6045 6046 public boolean isEmpty() { 6047 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(decision, reason, preAuthRef 6048 , preAuthPeriod); 6049 } 6050 6051 public String fhirType() { 6052 return "ExplanationOfBenefit.item.reviewOutcome"; 6053 6054 } 6055 6056 } 6057 6058 @Block() 6059 public static class AdjudicationComponent extends BackboneElement implements IBaseBackboneElement { 6060 /** 6061 * A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in-aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item. 6062 */ 6063 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 6064 @Description(shortDefinition="Type of adjudication information", formalDefinition="A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in-aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item." ) 6065 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication") 6066 protected CodeableConcept category; 6067 6068 /** 6069 * A code supporting the understanding of the adjudication result and explaining variance from expected amount. 6070 */ 6071 @Child(name = "reason", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 6072 @Description(shortDefinition="Explanation of adjudication outcome", formalDefinition="A code supporting the understanding of the adjudication result and explaining variance from expected amount." ) 6073 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication-reason") 6074 protected CodeableConcept reason; 6075 6076 /** 6077 * Monetary amount associated with the category. 6078 */ 6079 @Child(name = "amount", type = {Money.class}, order=3, min=0, max=1, modifier=false, summary=false) 6080 @Description(shortDefinition="Monetary amount", formalDefinition="Monetary amount associated with the category." ) 6081 protected Money amount; 6082 6083 /** 6084 * A non-monetary value associated with the category. Mutually exclusive to the amount element above. 6085 */ 6086 @Child(name = "quantity", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=false) 6087 @Description(shortDefinition="Non-monitary value", formalDefinition="A non-monetary value associated with the category. Mutually exclusive to the amount element above." ) 6088 protected Quantity quantity; 6089 6090 private static final long serialVersionUID = 29312734L; 6091 6092 /** 6093 * Constructor 6094 */ 6095 public AdjudicationComponent() { 6096 super(); 6097 } 6098 6099 /** 6100 * Constructor 6101 */ 6102 public AdjudicationComponent(CodeableConcept category) { 6103 super(); 6104 this.setCategory(category); 6105 } 6106 6107 /** 6108 * @return {@link #category} (A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in-aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.) 6109 */ 6110 public CodeableConcept getCategory() { 6111 if (this.category == null) 6112 if (Configuration.errorOnAutoCreate()) 6113 throw new Error("Attempt to auto-create AdjudicationComponent.category"); 6114 else if (Configuration.doAutoCreate()) 6115 this.category = new CodeableConcept(); // cc 6116 return this.category; 6117 } 6118 6119 public boolean hasCategory() { 6120 return this.category != null && !this.category.isEmpty(); 6121 } 6122 6123 /** 6124 * @param value {@link #category} (A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in-aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.) 6125 */ 6126 public AdjudicationComponent setCategory(CodeableConcept value) { 6127 this.category = value; 6128 return this; 6129 } 6130 6131 /** 6132 * @return {@link #reason} (A code supporting the understanding of the adjudication result and explaining variance from expected amount.) 6133 */ 6134 public CodeableConcept getReason() { 6135 if (this.reason == null) 6136 if (Configuration.errorOnAutoCreate()) 6137 throw new Error("Attempt to auto-create AdjudicationComponent.reason"); 6138 else if (Configuration.doAutoCreate()) 6139 this.reason = new CodeableConcept(); // cc 6140 return this.reason; 6141 } 6142 6143 public boolean hasReason() { 6144 return this.reason != null && !this.reason.isEmpty(); 6145 } 6146 6147 /** 6148 * @param value {@link #reason} (A code supporting the understanding of the adjudication result and explaining variance from expected amount.) 6149 */ 6150 public AdjudicationComponent setReason(CodeableConcept value) { 6151 this.reason = value; 6152 return this; 6153 } 6154 6155 /** 6156 * @return {@link #amount} (Monetary amount associated with the category.) 6157 */ 6158 public Money getAmount() { 6159 if (this.amount == null) 6160 if (Configuration.errorOnAutoCreate()) 6161 throw new Error("Attempt to auto-create AdjudicationComponent.amount"); 6162 else if (Configuration.doAutoCreate()) 6163 this.amount = new Money(); // cc 6164 return this.amount; 6165 } 6166 6167 public boolean hasAmount() { 6168 return this.amount != null && !this.amount.isEmpty(); 6169 } 6170 6171 /** 6172 * @param value {@link #amount} (Monetary amount associated with the category.) 6173 */ 6174 public AdjudicationComponent setAmount(Money value) { 6175 this.amount = value; 6176 return this; 6177 } 6178 6179 /** 6180 * @return {@link #quantity} (A non-monetary value associated with the category. Mutually exclusive to the amount element above.) 6181 */ 6182 public Quantity getQuantity() { 6183 if (this.quantity == null) 6184 if (Configuration.errorOnAutoCreate()) 6185 throw new Error("Attempt to auto-create AdjudicationComponent.quantity"); 6186 else if (Configuration.doAutoCreate()) 6187 this.quantity = new Quantity(); // cc 6188 return this.quantity; 6189 } 6190 6191 public boolean hasQuantity() { 6192 return this.quantity != null && !this.quantity.isEmpty(); 6193 } 6194 6195 /** 6196 * @param value {@link #quantity} (A non-monetary value associated with the category. Mutually exclusive to the amount element above.) 6197 */ 6198 public AdjudicationComponent setQuantity(Quantity value) { 6199 this.quantity = value; 6200 return this; 6201 } 6202 6203 protected void listChildren(List<Property> children) { 6204 super.listChildren(children); 6205 children.add(new Property("category", "CodeableConcept", "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in-aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 0, 1, category)); 6206 children.add(new Property("reason", "CodeableConcept", "A code supporting the understanding of the adjudication result and explaining variance from expected amount.", 0, 1, reason)); 6207 children.add(new Property("amount", "Money", "Monetary amount associated with the category.", 0, 1, amount)); 6208 children.add(new Property("quantity", "Quantity", "A non-monetary value associated with the category. Mutually exclusive to the amount element above.", 0, 1, quantity)); 6209 } 6210 6211 @Override 6212 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6213 switch (_hash) { 6214 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in-aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 0, 1, category); 6215 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "A code supporting the understanding of the adjudication result and explaining variance from expected amount.", 0, 1, reason); 6216 case -1413853096: /*amount*/ return new Property("amount", "Money", "Monetary amount associated with the category.", 0, 1, amount); 6217 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "A non-monetary value associated with the category. Mutually exclusive to the amount element above.", 0, 1, quantity); 6218 default: return super.getNamedProperty(_hash, _name, _checkValid); 6219 } 6220 6221 } 6222 6223 @Override 6224 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6225 switch (hash) { 6226 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 6227 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 6228 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 6229 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 6230 default: return super.getProperty(hash, name, checkValid); 6231 } 6232 6233 } 6234 6235 @Override 6236 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6237 switch (hash) { 6238 case 50511102: // category 6239 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6240 return value; 6241 case -934964668: // reason 6242 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6243 return value; 6244 case -1413853096: // amount 6245 this.amount = TypeConvertor.castToMoney(value); // Money 6246 return value; 6247 case -1285004149: // quantity 6248 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 6249 return value; 6250 default: return super.setProperty(hash, name, value); 6251 } 6252 6253 } 6254 6255 @Override 6256 public Base setProperty(String name, Base value) throws FHIRException { 6257 if (name.equals("category")) { 6258 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6259 } else if (name.equals("reason")) { 6260 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6261 } else if (name.equals("amount")) { 6262 this.amount = TypeConvertor.castToMoney(value); // Money 6263 } else if (name.equals("quantity")) { 6264 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 6265 } else 6266 return super.setProperty(name, value); 6267 return value; 6268 } 6269 6270 @Override 6271 public Base makeProperty(int hash, String name) throws FHIRException { 6272 switch (hash) { 6273 case 50511102: return getCategory(); 6274 case -934964668: return getReason(); 6275 case -1413853096: return getAmount(); 6276 case -1285004149: return getQuantity(); 6277 default: return super.makeProperty(hash, name); 6278 } 6279 6280 } 6281 6282 @Override 6283 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6284 switch (hash) { 6285 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 6286 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 6287 case -1413853096: /*amount*/ return new String[] {"Money"}; 6288 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 6289 default: return super.getTypesForProperty(hash, name); 6290 } 6291 6292 } 6293 6294 @Override 6295 public Base addChild(String name) throws FHIRException { 6296 if (name.equals("category")) { 6297 this.category = new CodeableConcept(); 6298 return this.category; 6299 } 6300 else if (name.equals("reason")) { 6301 this.reason = new CodeableConcept(); 6302 return this.reason; 6303 } 6304 else if (name.equals("amount")) { 6305 this.amount = new Money(); 6306 return this.amount; 6307 } 6308 else if (name.equals("quantity")) { 6309 this.quantity = new Quantity(); 6310 return this.quantity; 6311 } 6312 else 6313 return super.addChild(name); 6314 } 6315 6316 public AdjudicationComponent copy() { 6317 AdjudicationComponent dst = new AdjudicationComponent(); 6318 copyValues(dst); 6319 return dst; 6320 } 6321 6322 public void copyValues(AdjudicationComponent dst) { 6323 super.copyValues(dst); 6324 dst.category = category == null ? null : category.copy(); 6325 dst.reason = reason == null ? null : reason.copy(); 6326 dst.amount = amount == null ? null : amount.copy(); 6327 dst.quantity = quantity == null ? null : quantity.copy(); 6328 } 6329 6330 @Override 6331 public boolean equalsDeep(Base other_) { 6332 if (!super.equalsDeep(other_)) 6333 return false; 6334 if (!(other_ instanceof AdjudicationComponent)) 6335 return false; 6336 AdjudicationComponent o = (AdjudicationComponent) other_; 6337 return compareDeep(category, o.category, true) && compareDeep(reason, o.reason, true) && compareDeep(amount, o.amount, true) 6338 && compareDeep(quantity, o.quantity, true); 6339 } 6340 6341 @Override 6342 public boolean equalsShallow(Base other_) { 6343 if (!super.equalsShallow(other_)) 6344 return false; 6345 if (!(other_ instanceof AdjudicationComponent)) 6346 return false; 6347 AdjudicationComponent o = (AdjudicationComponent) other_; 6348 return true; 6349 } 6350 6351 public boolean isEmpty() { 6352 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, reason, amount 6353 , quantity); 6354 } 6355 6356 public String fhirType() { 6357 return "ExplanationOfBenefit.item.adjudication"; 6358 6359 } 6360 6361 } 6362 6363 @Block() 6364 public static class DetailComponent extends BackboneElement implements IBaseBackboneElement { 6365 /** 6366 * A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 6367 */ 6368 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 6369 @Description(shortDefinition="Product or service provided", formalDefinition="A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items." ) 6370 protected PositiveIntType sequence; 6371 6372 /** 6373 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 6374 */ 6375 @Child(name = "traceNumber", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6376 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 6377 protected List<Identifier> traceNumber; 6378 6379 /** 6380 * The type of revenue or cost center providing the product and/or service. 6381 */ 6382 @Child(name = "revenue", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 6383 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 6384 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 6385 protected CodeableConcept revenue; 6386 6387 /** 6388 * Code to identify the general type of benefits under which products and services are provided. 6389 */ 6390 @Child(name = "category", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 6391 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 6392 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 6393 protected CodeableConcept category; 6394 6395 /** 6396 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 6397 */ 6398 @Child(name = "productOrService", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 6399 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 6400 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 6401 protected CodeableConcept productOrService; 6402 6403 /** 6404 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 6405 */ 6406 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 6407 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 6408 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 6409 protected CodeableConcept productOrServiceEnd; 6410 6411 /** 6412 * Item typification or modifiers codes to convey additional context for the product or service. 6413 */ 6414 @Child(name = "modifier", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6415 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 6416 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 6417 protected List<CodeableConcept> modifier; 6418 6419 /** 6420 * Identifies the program under which this may be recovered. 6421 */ 6422 @Child(name = "programCode", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6423 @Description(shortDefinition="Program the product or service is provided under", formalDefinition="Identifies the program under which this may be recovered." ) 6424 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 6425 protected List<CodeableConcept> programCode; 6426 6427 /** 6428 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 6429 */ 6430 @Child(name = "patientPaid", type = {Money.class}, order=9, min=0, max=1, modifier=false, summary=false) 6431 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 6432 protected Money patientPaid; 6433 6434 /** 6435 * The number of repetitions of a service or product. 6436 */ 6437 @Child(name = "quantity", type = {Quantity.class}, order=10, min=0, max=1, modifier=false, summary=false) 6438 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 6439 protected Quantity quantity; 6440 6441 /** 6442 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 6443 */ 6444 @Child(name = "unitPrice", type = {Money.class}, order=11, min=0, max=1, modifier=false, summary=false) 6445 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 6446 protected Money unitPrice; 6447 6448 /** 6449 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6450 */ 6451 @Child(name = "factor", type = {DecimalType.class}, order=12, min=0, max=1, modifier=false, summary=false) 6452 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 6453 protected DecimalType factor; 6454 6455 /** 6456 * The total of taxes applicable for this product or service. 6457 */ 6458 @Child(name = "tax", type = {Money.class}, order=13, min=0, max=1, modifier=false, summary=false) 6459 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 6460 protected Money tax; 6461 6462 /** 6463 * The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor. 6464 */ 6465 @Child(name = "net", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=false) 6466 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor." ) 6467 protected Money net; 6468 6469 /** 6470 * Unique Device Identifiers associated with this line item. 6471 */ 6472 @Child(name = "udi", type = {Device.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6473 @Description(shortDefinition="Unique device identifier", formalDefinition="Unique Device Identifiers associated with this line item." ) 6474 protected List<Reference> udi; 6475 6476 /** 6477 * The numbers associated with notes below which apply to the adjudication of this item. 6478 */ 6479 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6480 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 6481 protected List<PositiveIntType> noteNumber; 6482 6483 /** 6484 * The high-level results of the adjudication if adjudication has been performed. 6485 */ 6486 @Child(name = "reviewOutcome", type = {ItemReviewOutcomeComponent.class}, order=17, min=0, max=1, modifier=false, summary=false) 6487 @Description(shortDefinition="Detail level adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 6488 protected ItemReviewOutcomeComponent reviewOutcome; 6489 6490 /** 6491 * The adjudication results. 6492 */ 6493 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6494 @Description(shortDefinition="Detail level adjudication details", formalDefinition="The adjudication results." ) 6495 protected List<AdjudicationComponent> adjudication; 6496 6497 /** 6498 * Third-tier of goods and services. 6499 */ 6500 @Child(name = "subDetail", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6501 @Description(shortDefinition="Additional items", formalDefinition="Third-tier of goods and services." ) 6502 protected List<SubDetailComponent> subDetail; 6503 6504 private static final long serialVersionUID = -1951425443L; 6505 6506 /** 6507 * Constructor 6508 */ 6509 public DetailComponent() { 6510 super(); 6511 } 6512 6513 /** 6514 * Constructor 6515 */ 6516 public DetailComponent(int sequence) { 6517 super(); 6518 this.setSequence(sequence); 6519 } 6520 6521 /** 6522 * @return {@link #sequence} (A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 6523 */ 6524 public PositiveIntType getSequenceElement() { 6525 if (this.sequence == null) 6526 if (Configuration.errorOnAutoCreate()) 6527 throw new Error("Attempt to auto-create DetailComponent.sequence"); 6528 else if (Configuration.doAutoCreate()) 6529 this.sequence = new PositiveIntType(); // bb 6530 return this.sequence; 6531 } 6532 6533 public boolean hasSequenceElement() { 6534 return this.sequence != null && !this.sequence.isEmpty(); 6535 } 6536 6537 public boolean hasSequence() { 6538 return this.sequence != null && !this.sequence.isEmpty(); 6539 } 6540 6541 /** 6542 * @param value {@link #sequence} (A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 6543 */ 6544 public DetailComponent setSequenceElement(PositiveIntType value) { 6545 this.sequence = value; 6546 return this; 6547 } 6548 6549 /** 6550 * @return A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 6551 */ 6552 public int getSequence() { 6553 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 6554 } 6555 6556 /** 6557 * @param value A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 6558 */ 6559 public DetailComponent setSequence(int value) { 6560 if (this.sequence == null) 6561 this.sequence = new PositiveIntType(); 6562 this.sequence.setValue(value); 6563 return this; 6564 } 6565 6566 /** 6567 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 6568 */ 6569 public List<Identifier> getTraceNumber() { 6570 if (this.traceNumber == null) 6571 this.traceNumber = new ArrayList<Identifier>(); 6572 return this.traceNumber; 6573 } 6574 6575 /** 6576 * @return Returns a reference to <code>this</code> for easy method chaining 6577 */ 6578 public DetailComponent setTraceNumber(List<Identifier> theTraceNumber) { 6579 this.traceNumber = theTraceNumber; 6580 return this; 6581 } 6582 6583 public boolean hasTraceNumber() { 6584 if (this.traceNumber == null) 6585 return false; 6586 for (Identifier item : this.traceNumber) 6587 if (!item.isEmpty()) 6588 return true; 6589 return false; 6590 } 6591 6592 public Identifier addTraceNumber() { //3 6593 Identifier t = new Identifier(); 6594 if (this.traceNumber == null) 6595 this.traceNumber = new ArrayList<Identifier>(); 6596 this.traceNumber.add(t); 6597 return t; 6598 } 6599 6600 public DetailComponent addTraceNumber(Identifier t) { //3 6601 if (t == null) 6602 return this; 6603 if (this.traceNumber == null) 6604 this.traceNumber = new ArrayList<Identifier>(); 6605 this.traceNumber.add(t); 6606 return this; 6607 } 6608 6609 /** 6610 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 6611 */ 6612 public Identifier getTraceNumberFirstRep() { 6613 if (getTraceNumber().isEmpty()) { 6614 addTraceNumber(); 6615 } 6616 return getTraceNumber().get(0); 6617 } 6618 6619 /** 6620 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 6621 */ 6622 public CodeableConcept getRevenue() { 6623 if (this.revenue == null) 6624 if (Configuration.errorOnAutoCreate()) 6625 throw new Error("Attempt to auto-create DetailComponent.revenue"); 6626 else if (Configuration.doAutoCreate()) 6627 this.revenue = new CodeableConcept(); // cc 6628 return this.revenue; 6629 } 6630 6631 public boolean hasRevenue() { 6632 return this.revenue != null && !this.revenue.isEmpty(); 6633 } 6634 6635 /** 6636 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 6637 */ 6638 public DetailComponent setRevenue(CodeableConcept value) { 6639 this.revenue = value; 6640 return this; 6641 } 6642 6643 /** 6644 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 6645 */ 6646 public CodeableConcept getCategory() { 6647 if (this.category == null) 6648 if (Configuration.errorOnAutoCreate()) 6649 throw new Error("Attempt to auto-create DetailComponent.category"); 6650 else if (Configuration.doAutoCreate()) 6651 this.category = new CodeableConcept(); // cc 6652 return this.category; 6653 } 6654 6655 public boolean hasCategory() { 6656 return this.category != null && !this.category.isEmpty(); 6657 } 6658 6659 /** 6660 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 6661 */ 6662 public DetailComponent setCategory(CodeableConcept value) { 6663 this.category = value; 6664 return this; 6665 } 6666 6667 /** 6668 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 6669 */ 6670 public CodeableConcept getProductOrService() { 6671 if (this.productOrService == null) 6672 if (Configuration.errorOnAutoCreate()) 6673 throw new Error("Attempt to auto-create DetailComponent.productOrService"); 6674 else if (Configuration.doAutoCreate()) 6675 this.productOrService = new CodeableConcept(); // cc 6676 return this.productOrService; 6677 } 6678 6679 public boolean hasProductOrService() { 6680 return this.productOrService != null && !this.productOrService.isEmpty(); 6681 } 6682 6683 /** 6684 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 6685 */ 6686 public DetailComponent setProductOrService(CodeableConcept value) { 6687 this.productOrService = value; 6688 return this; 6689 } 6690 6691 /** 6692 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 6693 */ 6694 public CodeableConcept getProductOrServiceEnd() { 6695 if (this.productOrServiceEnd == null) 6696 if (Configuration.errorOnAutoCreate()) 6697 throw new Error("Attempt to auto-create DetailComponent.productOrServiceEnd"); 6698 else if (Configuration.doAutoCreate()) 6699 this.productOrServiceEnd = new CodeableConcept(); // cc 6700 return this.productOrServiceEnd; 6701 } 6702 6703 public boolean hasProductOrServiceEnd() { 6704 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 6705 } 6706 6707 /** 6708 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 6709 */ 6710 public DetailComponent setProductOrServiceEnd(CodeableConcept value) { 6711 this.productOrServiceEnd = value; 6712 return this; 6713 } 6714 6715 /** 6716 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 6717 */ 6718 public List<CodeableConcept> getModifier() { 6719 if (this.modifier == null) 6720 this.modifier = new ArrayList<CodeableConcept>(); 6721 return this.modifier; 6722 } 6723 6724 /** 6725 * @return Returns a reference to <code>this</code> for easy method chaining 6726 */ 6727 public DetailComponent setModifier(List<CodeableConcept> theModifier) { 6728 this.modifier = theModifier; 6729 return this; 6730 } 6731 6732 public boolean hasModifier() { 6733 if (this.modifier == null) 6734 return false; 6735 for (CodeableConcept item : this.modifier) 6736 if (!item.isEmpty()) 6737 return true; 6738 return false; 6739 } 6740 6741 public CodeableConcept addModifier() { //3 6742 CodeableConcept t = new CodeableConcept(); 6743 if (this.modifier == null) 6744 this.modifier = new ArrayList<CodeableConcept>(); 6745 this.modifier.add(t); 6746 return t; 6747 } 6748 6749 public DetailComponent addModifier(CodeableConcept t) { //3 6750 if (t == null) 6751 return this; 6752 if (this.modifier == null) 6753 this.modifier = new ArrayList<CodeableConcept>(); 6754 this.modifier.add(t); 6755 return this; 6756 } 6757 6758 /** 6759 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 6760 */ 6761 public CodeableConcept getModifierFirstRep() { 6762 if (getModifier().isEmpty()) { 6763 addModifier(); 6764 } 6765 return getModifier().get(0); 6766 } 6767 6768 /** 6769 * @return {@link #programCode} (Identifies the program under which this may be recovered.) 6770 */ 6771 public List<CodeableConcept> getProgramCode() { 6772 if (this.programCode == null) 6773 this.programCode = new ArrayList<CodeableConcept>(); 6774 return this.programCode; 6775 } 6776 6777 /** 6778 * @return Returns a reference to <code>this</code> for easy method chaining 6779 */ 6780 public DetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 6781 this.programCode = theProgramCode; 6782 return this; 6783 } 6784 6785 public boolean hasProgramCode() { 6786 if (this.programCode == null) 6787 return false; 6788 for (CodeableConcept item : this.programCode) 6789 if (!item.isEmpty()) 6790 return true; 6791 return false; 6792 } 6793 6794 public CodeableConcept addProgramCode() { //3 6795 CodeableConcept t = new CodeableConcept(); 6796 if (this.programCode == null) 6797 this.programCode = new ArrayList<CodeableConcept>(); 6798 this.programCode.add(t); 6799 return t; 6800 } 6801 6802 public DetailComponent addProgramCode(CodeableConcept t) { //3 6803 if (t == null) 6804 return this; 6805 if (this.programCode == null) 6806 this.programCode = new ArrayList<CodeableConcept>(); 6807 this.programCode.add(t); 6808 return this; 6809 } 6810 6811 /** 6812 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist {3} 6813 */ 6814 public CodeableConcept getProgramCodeFirstRep() { 6815 if (getProgramCode().isEmpty()) { 6816 addProgramCode(); 6817 } 6818 return getProgramCode().get(0); 6819 } 6820 6821 /** 6822 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 6823 */ 6824 public Money getPatientPaid() { 6825 if (this.patientPaid == null) 6826 if (Configuration.errorOnAutoCreate()) 6827 throw new Error("Attempt to auto-create DetailComponent.patientPaid"); 6828 else if (Configuration.doAutoCreate()) 6829 this.patientPaid = new Money(); // cc 6830 return this.patientPaid; 6831 } 6832 6833 public boolean hasPatientPaid() { 6834 return this.patientPaid != null && !this.patientPaid.isEmpty(); 6835 } 6836 6837 /** 6838 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 6839 */ 6840 public DetailComponent setPatientPaid(Money value) { 6841 this.patientPaid = value; 6842 return this; 6843 } 6844 6845 /** 6846 * @return {@link #quantity} (The number of repetitions of a service or product.) 6847 */ 6848 public Quantity getQuantity() { 6849 if (this.quantity == null) 6850 if (Configuration.errorOnAutoCreate()) 6851 throw new Error("Attempt to auto-create DetailComponent.quantity"); 6852 else if (Configuration.doAutoCreate()) 6853 this.quantity = new Quantity(); // cc 6854 return this.quantity; 6855 } 6856 6857 public boolean hasQuantity() { 6858 return this.quantity != null && !this.quantity.isEmpty(); 6859 } 6860 6861 /** 6862 * @param value {@link #quantity} (The number of repetitions of a service or product.) 6863 */ 6864 public DetailComponent setQuantity(Quantity value) { 6865 this.quantity = value; 6866 return this; 6867 } 6868 6869 /** 6870 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 6871 */ 6872 public Money getUnitPrice() { 6873 if (this.unitPrice == null) 6874 if (Configuration.errorOnAutoCreate()) 6875 throw new Error("Attempt to auto-create DetailComponent.unitPrice"); 6876 else if (Configuration.doAutoCreate()) 6877 this.unitPrice = new Money(); // cc 6878 return this.unitPrice; 6879 } 6880 6881 public boolean hasUnitPrice() { 6882 return this.unitPrice != null && !this.unitPrice.isEmpty(); 6883 } 6884 6885 /** 6886 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 6887 */ 6888 public DetailComponent setUnitPrice(Money value) { 6889 this.unitPrice = value; 6890 return this; 6891 } 6892 6893 /** 6894 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 6895 */ 6896 public DecimalType getFactorElement() { 6897 if (this.factor == null) 6898 if (Configuration.errorOnAutoCreate()) 6899 throw new Error("Attempt to auto-create DetailComponent.factor"); 6900 else if (Configuration.doAutoCreate()) 6901 this.factor = new DecimalType(); // bb 6902 return this.factor; 6903 } 6904 6905 public boolean hasFactorElement() { 6906 return this.factor != null && !this.factor.isEmpty(); 6907 } 6908 6909 public boolean hasFactor() { 6910 return this.factor != null && !this.factor.isEmpty(); 6911 } 6912 6913 /** 6914 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 6915 */ 6916 public DetailComponent setFactorElement(DecimalType value) { 6917 this.factor = value; 6918 return this; 6919 } 6920 6921 /** 6922 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6923 */ 6924 public BigDecimal getFactor() { 6925 return this.factor == null ? null : this.factor.getValue(); 6926 } 6927 6928 /** 6929 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6930 */ 6931 public DetailComponent setFactor(BigDecimal value) { 6932 if (value == null) 6933 this.factor = null; 6934 else { 6935 if (this.factor == null) 6936 this.factor = new DecimalType(); 6937 this.factor.setValue(value); 6938 } 6939 return this; 6940 } 6941 6942 /** 6943 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6944 */ 6945 public DetailComponent setFactor(long value) { 6946 this.factor = new DecimalType(); 6947 this.factor.setValue(value); 6948 return this; 6949 } 6950 6951 /** 6952 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6953 */ 6954 public DetailComponent setFactor(double value) { 6955 this.factor = new DecimalType(); 6956 this.factor.setValue(value); 6957 return this; 6958 } 6959 6960 /** 6961 * @return {@link #tax} (The total of taxes applicable for this product or service.) 6962 */ 6963 public Money getTax() { 6964 if (this.tax == null) 6965 if (Configuration.errorOnAutoCreate()) 6966 throw new Error("Attempt to auto-create DetailComponent.tax"); 6967 else if (Configuration.doAutoCreate()) 6968 this.tax = new Money(); // cc 6969 return this.tax; 6970 } 6971 6972 public boolean hasTax() { 6973 return this.tax != null && !this.tax.isEmpty(); 6974 } 6975 6976 /** 6977 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 6978 */ 6979 public DetailComponent setTax(Money value) { 6980 this.tax = value; 6981 return this; 6982 } 6983 6984 /** 6985 * @return {@link #net} (The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor.) 6986 */ 6987 public Money getNet() { 6988 if (this.net == null) 6989 if (Configuration.errorOnAutoCreate()) 6990 throw new Error("Attempt to auto-create DetailComponent.net"); 6991 else if (Configuration.doAutoCreate()) 6992 this.net = new Money(); // cc 6993 return this.net; 6994 } 6995 6996 public boolean hasNet() { 6997 return this.net != null && !this.net.isEmpty(); 6998 } 6999 7000 /** 7001 * @param value {@link #net} (The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor.) 7002 */ 7003 public DetailComponent setNet(Money value) { 7004 this.net = value; 7005 return this; 7006 } 7007 7008 /** 7009 * @return {@link #udi} (Unique Device Identifiers associated with this line item.) 7010 */ 7011 public List<Reference> getUdi() { 7012 if (this.udi == null) 7013 this.udi = new ArrayList<Reference>(); 7014 return this.udi; 7015 } 7016 7017 /** 7018 * @return Returns a reference to <code>this</code> for easy method chaining 7019 */ 7020 public DetailComponent setUdi(List<Reference> theUdi) { 7021 this.udi = theUdi; 7022 return this; 7023 } 7024 7025 public boolean hasUdi() { 7026 if (this.udi == null) 7027 return false; 7028 for (Reference item : this.udi) 7029 if (!item.isEmpty()) 7030 return true; 7031 return false; 7032 } 7033 7034 public Reference addUdi() { //3 7035 Reference t = new Reference(); 7036 if (this.udi == null) 7037 this.udi = new ArrayList<Reference>(); 7038 this.udi.add(t); 7039 return t; 7040 } 7041 7042 public DetailComponent addUdi(Reference t) { //3 7043 if (t == null) 7044 return this; 7045 if (this.udi == null) 7046 this.udi = new ArrayList<Reference>(); 7047 this.udi.add(t); 7048 return this; 7049 } 7050 7051 /** 7052 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist {3} 7053 */ 7054 public Reference getUdiFirstRep() { 7055 if (getUdi().isEmpty()) { 7056 addUdi(); 7057 } 7058 return getUdi().get(0); 7059 } 7060 7061 /** 7062 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 7063 */ 7064 public List<PositiveIntType> getNoteNumber() { 7065 if (this.noteNumber == null) 7066 this.noteNumber = new ArrayList<PositiveIntType>(); 7067 return this.noteNumber; 7068 } 7069 7070 /** 7071 * @return Returns a reference to <code>this</code> for easy method chaining 7072 */ 7073 public DetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 7074 this.noteNumber = theNoteNumber; 7075 return this; 7076 } 7077 7078 public boolean hasNoteNumber() { 7079 if (this.noteNumber == null) 7080 return false; 7081 for (PositiveIntType item : this.noteNumber) 7082 if (!item.isEmpty()) 7083 return true; 7084 return false; 7085 } 7086 7087 /** 7088 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 7089 */ 7090 public PositiveIntType addNoteNumberElement() {//2 7091 PositiveIntType t = new PositiveIntType(); 7092 if (this.noteNumber == null) 7093 this.noteNumber = new ArrayList<PositiveIntType>(); 7094 this.noteNumber.add(t); 7095 return t; 7096 } 7097 7098 /** 7099 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 7100 */ 7101 public DetailComponent addNoteNumber(int value) { //1 7102 PositiveIntType t = new PositiveIntType(); 7103 t.setValue(value); 7104 if (this.noteNumber == null) 7105 this.noteNumber = new ArrayList<PositiveIntType>(); 7106 this.noteNumber.add(t); 7107 return this; 7108 } 7109 7110 /** 7111 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 7112 */ 7113 public boolean hasNoteNumber(int value) { 7114 if (this.noteNumber == null) 7115 return false; 7116 for (PositiveIntType v : this.noteNumber) 7117 if (v.getValue().equals(value)) // positiveInt 7118 return true; 7119 return false; 7120 } 7121 7122 /** 7123 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 7124 */ 7125 public ItemReviewOutcomeComponent getReviewOutcome() { 7126 if (this.reviewOutcome == null) 7127 if (Configuration.errorOnAutoCreate()) 7128 throw new Error("Attempt to auto-create DetailComponent.reviewOutcome"); 7129 else if (Configuration.doAutoCreate()) 7130 this.reviewOutcome = new ItemReviewOutcomeComponent(); // cc 7131 return this.reviewOutcome; 7132 } 7133 7134 public boolean hasReviewOutcome() { 7135 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 7136 } 7137 7138 /** 7139 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 7140 */ 7141 public DetailComponent setReviewOutcome(ItemReviewOutcomeComponent value) { 7142 this.reviewOutcome = value; 7143 return this; 7144 } 7145 7146 /** 7147 * @return {@link #adjudication} (The adjudication results.) 7148 */ 7149 public List<AdjudicationComponent> getAdjudication() { 7150 if (this.adjudication == null) 7151 this.adjudication = new ArrayList<AdjudicationComponent>(); 7152 return this.adjudication; 7153 } 7154 7155 /** 7156 * @return Returns a reference to <code>this</code> for easy method chaining 7157 */ 7158 public DetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 7159 this.adjudication = theAdjudication; 7160 return this; 7161 } 7162 7163 public boolean hasAdjudication() { 7164 if (this.adjudication == null) 7165 return false; 7166 for (AdjudicationComponent item : this.adjudication) 7167 if (!item.isEmpty()) 7168 return true; 7169 return false; 7170 } 7171 7172 public AdjudicationComponent addAdjudication() { //3 7173 AdjudicationComponent t = new AdjudicationComponent(); 7174 if (this.adjudication == null) 7175 this.adjudication = new ArrayList<AdjudicationComponent>(); 7176 this.adjudication.add(t); 7177 return t; 7178 } 7179 7180 public DetailComponent addAdjudication(AdjudicationComponent t) { //3 7181 if (t == null) 7182 return this; 7183 if (this.adjudication == null) 7184 this.adjudication = new ArrayList<AdjudicationComponent>(); 7185 this.adjudication.add(t); 7186 return this; 7187 } 7188 7189 /** 7190 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 7191 */ 7192 public AdjudicationComponent getAdjudicationFirstRep() { 7193 if (getAdjudication().isEmpty()) { 7194 addAdjudication(); 7195 } 7196 return getAdjudication().get(0); 7197 } 7198 7199 /** 7200 * @return {@link #subDetail} (Third-tier of goods and services.) 7201 */ 7202 public List<SubDetailComponent> getSubDetail() { 7203 if (this.subDetail == null) 7204 this.subDetail = new ArrayList<SubDetailComponent>(); 7205 return this.subDetail; 7206 } 7207 7208 /** 7209 * @return Returns a reference to <code>this</code> for easy method chaining 7210 */ 7211 public DetailComponent setSubDetail(List<SubDetailComponent> theSubDetail) { 7212 this.subDetail = theSubDetail; 7213 return this; 7214 } 7215 7216 public boolean hasSubDetail() { 7217 if (this.subDetail == null) 7218 return false; 7219 for (SubDetailComponent item : this.subDetail) 7220 if (!item.isEmpty()) 7221 return true; 7222 return false; 7223 } 7224 7225 public SubDetailComponent addSubDetail() { //3 7226 SubDetailComponent t = new SubDetailComponent(); 7227 if (this.subDetail == null) 7228 this.subDetail = new ArrayList<SubDetailComponent>(); 7229 this.subDetail.add(t); 7230 return t; 7231 } 7232 7233 public DetailComponent addSubDetail(SubDetailComponent t) { //3 7234 if (t == null) 7235 return this; 7236 if (this.subDetail == null) 7237 this.subDetail = new ArrayList<SubDetailComponent>(); 7238 this.subDetail.add(t); 7239 return this; 7240 } 7241 7242 /** 7243 * @return The first repetition of repeating field {@link #subDetail}, creating it if it does not already exist {3} 7244 */ 7245 public SubDetailComponent getSubDetailFirstRep() { 7246 if (getSubDetail().isEmpty()) { 7247 addSubDetail(); 7248 } 7249 return getSubDetail().get(0); 7250 } 7251 7252 protected void listChildren(List<Property> children) { 7253 super.listChildren(children); 7254 children.add(new Property("sequence", "positiveInt", "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, 1, sequence)); 7255 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 7256 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 7257 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 7258 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 7259 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 7260 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 7261 children.add(new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 7262 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 7263 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 7264 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 7265 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 7266 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 7267 children.add(new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor.", 0, 1, net)); 7268 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 7269 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 7270 children.add(new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 7271 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 7272 children.add(new Property("subDetail", "", "Third-tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, subDetail)); 7273 } 7274 7275 @Override 7276 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7277 switch (_hash) { 7278 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, 1, sequence); 7279 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 7280 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 7281 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 7282 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 7283 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 7284 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 7285 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 7286 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 7287 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 7288 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 7289 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 7290 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 7291 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor.", 0, 1, net); 7292 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 7293 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 7294 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 7295 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 7296 case -828829007: /*subDetail*/ return new Property("subDetail", "", "Third-tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, subDetail); 7297 default: return super.getNamedProperty(_hash, _name, _checkValid); 7298 } 7299 7300 } 7301 7302 @Override 7303 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7304 switch (hash) { 7305 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 7306 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 7307 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 7308 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 7309 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 7310 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 7311 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 7312 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 7313 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 7314 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 7315 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 7316 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 7317 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 7318 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 7319 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 7320 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 7321 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ItemReviewOutcomeComponent 7322 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 7323 case -828829007: /*subDetail*/ return this.subDetail == null ? new Base[0] : this.subDetail.toArray(new Base[this.subDetail.size()]); // SubDetailComponent 7324 default: return super.getProperty(hash, name, checkValid); 7325 } 7326 7327 } 7328 7329 @Override 7330 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7331 switch (hash) { 7332 case 1349547969: // sequence 7333 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 7334 return value; 7335 case 82505966: // traceNumber 7336 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 7337 return value; 7338 case 1099842588: // revenue 7339 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7340 return value; 7341 case 50511102: // category 7342 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7343 return value; 7344 case 1957227299: // productOrService 7345 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7346 return value; 7347 case -717476168: // productOrServiceEnd 7348 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7349 return value; 7350 case -615513385: // modifier 7351 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 7352 return value; 7353 case 1010065041: // programCode 7354 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 7355 return value; 7356 case 525514609: // patientPaid 7357 this.patientPaid = TypeConvertor.castToMoney(value); // Money 7358 return value; 7359 case -1285004149: // quantity 7360 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 7361 return value; 7362 case -486196699: // unitPrice 7363 this.unitPrice = TypeConvertor.castToMoney(value); // Money 7364 return value; 7365 case -1282148017: // factor 7366 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 7367 return value; 7368 case 114603: // tax 7369 this.tax = TypeConvertor.castToMoney(value); // Money 7370 return value; 7371 case 108957: // net 7372 this.net = TypeConvertor.castToMoney(value); // Money 7373 return value; 7374 case 115642: // udi 7375 this.getUdi().add(TypeConvertor.castToReference(value)); // Reference 7376 return value; 7377 case -1110033957: // noteNumber 7378 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 7379 return value; 7380 case -51825446: // reviewOutcome 7381 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 7382 return value; 7383 case -231349275: // adjudication 7384 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 7385 return value; 7386 case -828829007: // subDetail 7387 this.getSubDetail().add((SubDetailComponent) value); // SubDetailComponent 7388 return value; 7389 default: return super.setProperty(hash, name, value); 7390 } 7391 7392 } 7393 7394 @Override 7395 public Base setProperty(String name, Base value) throws FHIRException { 7396 if (name.equals("sequence")) { 7397 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 7398 } else if (name.equals("traceNumber")) { 7399 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 7400 } else if (name.equals("revenue")) { 7401 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7402 } else if (name.equals("category")) { 7403 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7404 } else if (name.equals("productOrService")) { 7405 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7406 } else if (name.equals("productOrServiceEnd")) { 7407 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7408 } else if (name.equals("modifier")) { 7409 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 7410 } else if (name.equals("programCode")) { 7411 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); 7412 } else if (name.equals("patientPaid")) { 7413 this.patientPaid = TypeConvertor.castToMoney(value); // Money 7414 } else if (name.equals("quantity")) { 7415 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 7416 } else if (name.equals("unitPrice")) { 7417 this.unitPrice = TypeConvertor.castToMoney(value); // Money 7418 } else if (name.equals("factor")) { 7419 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 7420 } else if (name.equals("tax")) { 7421 this.tax = TypeConvertor.castToMoney(value); // Money 7422 } else if (name.equals("net")) { 7423 this.net = TypeConvertor.castToMoney(value); // Money 7424 } else if (name.equals("udi")) { 7425 this.getUdi().add(TypeConvertor.castToReference(value)); 7426 } else if (name.equals("noteNumber")) { 7427 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 7428 } else if (name.equals("reviewOutcome")) { 7429 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 7430 } else if (name.equals("adjudication")) { 7431 this.getAdjudication().add((AdjudicationComponent) value); 7432 } else if (name.equals("subDetail")) { 7433 this.getSubDetail().add((SubDetailComponent) value); 7434 } else 7435 return super.setProperty(name, value); 7436 return value; 7437 } 7438 7439 @Override 7440 public Base makeProperty(int hash, String name) throws FHIRException { 7441 switch (hash) { 7442 case 1349547969: return getSequenceElement(); 7443 case 82505966: return addTraceNumber(); 7444 case 1099842588: return getRevenue(); 7445 case 50511102: return getCategory(); 7446 case 1957227299: return getProductOrService(); 7447 case -717476168: return getProductOrServiceEnd(); 7448 case -615513385: return addModifier(); 7449 case 1010065041: return addProgramCode(); 7450 case 525514609: return getPatientPaid(); 7451 case -1285004149: return getQuantity(); 7452 case -486196699: return getUnitPrice(); 7453 case -1282148017: return getFactorElement(); 7454 case 114603: return getTax(); 7455 case 108957: return getNet(); 7456 case 115642: return addUdi(); 7457 case -1110033957: return addNoteNumberElement(); 7458 case -51825446: return getReviewOutcome(); 7459 case -231349275: return addAdjudication(); 7460 case -828829007: return addSubDetail(); 7461 default: return super.makeProperty(hash, name); 7462 } 7463 7464 } 7465 7466 @Override 7467 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7468 switch (hash) { 7469 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 7470 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 7471 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 7472 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 7473 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 7474 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 7475 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 7476 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 7477 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 7478 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 7479 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 7480 case -1282148017: /*factor*/ return new String[] {"decimal"}; 7481 case 114603: /*tax*/ return new String[] {"Money"}; 7482 case 108957: /*net*/ return new String[] {"Money"}; 7483 case 115642: /*udi*/ return new String[] {"Reference"}; 7484 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 7485 case -51825446: /*reviewOutcome*/ return new String[] {"@ExplanationOfBenefit.item.reviewOutcome"}; 7486 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 7487 case -828829007: /*subDetail*/ return new String[] {}; 7488 default: return super.getTypesForProperty(hash, name); 7489 } 7490 7491 } 7492 7493 @Override 7494 public Base addChild(String name) throws FHIRException { 7495 if (name.equals("sequence")) { 7496 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.detail.sequence"); 7497 } 7498 else if (name.equals("traceNumber")) { 7499 return addTraceNumber(); 7500 } 7501 else if (name.equals("revenue")) { 7502 this.revenue = new CodeableConcept(); 7503 return this.revenue; 7504 } 7505 else if (name.equals("category")) { 7506 this.category = new CodeableConcept(); 7507 return this.category; 7508 } 7509 else if (name.equals("productOrService")) { 7510 this.productOrService = new CodeableConcept(); 7511 return this.productOrService; 7512 } 7513 else if (name.equals("productOrServiceEnd")) { 7514 this.productOrServiceEnd = new CodeableConcept(); 7515 return this.productOrServiceEnd; 7516 } 7517 else if (name.equals("modifier")) { 7518 return addModifier(); 7519 } 7520 else if (name.equals("programCode")) { 7521 return addProgramCode(); 7522 } 7523 else if (name.equals("patientPaid")) { 7524 this.patientPaid = new Money(); 7525 return this.patientPaid; 7526 } 7527 else if (name.equals("quantity")) { 7528 this.quantity = new Quantity(); 7529 return this.quantity; 7530 } 7531 else if (name.equals("unitPrice")) { 7532 this.unitPrice = new Money(); 7533 return this.unitPrice; 7534 } 7535 else if (name.equals("factor")) { 7536 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.detail.factor"); 7537 } 7538 else if (name.equals("tax")) { 7539 this.tax = new Money(); 7540 return this.tax; 7541 } 7542 else if (name.equals("net")) { 7543 this.net = new Money(); 7544 return this.net; 7545 } 7546 else if (name.equals("udi")) { 7547 return addUdi(); 7548 } 7549 else if (name.equals("noteNumber")) { 7550 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.detail.noteNumber"); 7551 } 7552 else if (name.equals("reviewOutcome")) { 7553 this.reviewOutcome = new ItemReviewOutcomeComponent(); 7554 return this.reviewOutcome; 7555 } 7556 else if (name.equals("adjudication")) { 7557 return addAdjudication(); 7558 } 7559 else if (name.equals("subDetail")) { 7560 return addSubDetail(); 7561 } 7562 else 7563 return super.addChild(name); 7564 } 7565 7566 public DetailComponent copy() { 7567 DetailComponent dst = new DetailComponent(); 7568 copyValues(dst); 7569 return dst; 7570 } 7571 7572 public void copyValues(DetailComponent dst) { 7573 super.copyValues(dst); 7574 dst.sequence = sequence == null ? null : sequence.copy(); 7575 if (traceNumber != null) { 7576 dst.traceNumber = new ArrayList<Identifier>(); 7577 for (Identifier i : traceNumber) 7578 dst.traceNumber.add(i.copy()); 7579 }; 7580 dst.revenue = revenue == null ? null : revenue.copy(); 7581 dst.category = category == null ? null : category.copy(); 7582 dst.productOrService = productOrService == null ? null : productOrService.copy(); 7583 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 7584 if (modifier != null) { 7585 dst.modifier = new ArrayList<CodeableConcept>(); 7586 for (CodeableConcept i : modifier) 7587 dst.modifier.add(i.copy()); 7588 }; 7589 if (programCode != null) { 7590 dst.programCode = new ArrayList<CodeableConcept>(); 7591 for (CodeableConcept i : programCode) 7592 dst.programCode.add(i.copy()); 7593 }; 7594 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 7595 dst.quantity = quantity == null ? null : quantity.copy(); 7596 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 7597 dst.factor = factor == null ? null : factor.copy(); 7598 dst.tax = tax == null ? null : tax.copy(); 7599 dst.net = net == null ? null : net.copy(); 7600 if (udi != null) { 7601 dst.udi = new ArrayList<Reference>(); 7602 for (Reference i : udi) 7603 dst.udi.add(i.copy()); 7604 }; 7605 if (noteNumber != null) { 7606 dst.noteNumber = new ArrayList<PositiveIntType>(); 7607 for (PositiveIntType i : noteNumber) 7608 dst.noteNumber.add(i.copy()); 7609 }; 7610 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 7611 if (adjudication != null) { 7612 dst.adjudication = new ArrayList<AdjudicationComponent>(); 7613 for (AdjudicationComponent i : adjudication) 7614 dst.adjudication.add(i.copy()); 7615 }; 7616 if (subDetail != null) { 7617 dst.subDetail = new ArrayList<SubDetailComponent>(); 7618 for (SubDetailComponent i : subDetail) 7619 dst.subDetail.add(i.copy()); 7620 }; 7621 } 7622 7623 @Override 7624 public boolean equalsDeep(Base other_) { 7625 if (!super.equalsDeep(other_)) 7626 return false; 7627 if (!(other_ instanceof DetailComponent)) 7628 return false; 7629 DetailComponent o = (DetailComponent) other_; 7630 return compareDeep(sequence, o.sequence, true) && compareDeep(traceNumber, o.traceNumber, true) 7631 && compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 7632 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(modifier, o.modifier, true) 7633 && compareDeep(programCode, o.programCode, true) && compareDeep(patientPaid, o.patientPaid, true) 7634 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 7635 && compareDeep(tax, o.tax, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 7636 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(reviewOutcome, o.reviewOutcome, true) 7637 && compareDeep(adjudication, o.adjudication, true) && compareDeep(subDetail, o.subDetail, true) 7638 ; 7639 } 7640 7641 @Override 7642 public boolean equalsShallow(Base other_) { 7643 if (!super.equalsShallow(other_)) 7644 return false; 7645 if (!(other_ instanceof DetailComponent)) 7646 return false; 7647 DetailComponent o = (DetailComponent) other_; 7648 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true) 7649 ; 7650 } 7651 7652 public boolean isEmpty() { 7653 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, traceNumber, revenue 7654 , category, productOrService, productOrServiceEnd, modifier, programCode, patientPaid 7655 , quantity, unitPrice, factor, tax, net, udi, noteNumber, reviewOutcome, adjudication 7656 , subDetail); 7657 } 7658 7659 public String fhirType() { 7660 return "ExplanationOfBenefit.item.detail"; 7661 7662 } 7663 7664 } 7665 7666 @Block() 7667 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 7668 /** 7669 * A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 7670 */ 7671 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 7672 @Description(shortDefinition="Product or service provided", formalDefinition="A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items." ) 7673 protected PositiveIntType sequence; 7674 7675 /** 7676 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 7677 */ 7678 @Child(name = "traceNumber", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7679 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 7680 protected List<Identifier> traceNumber; 7681 7682 /** 7683 * The type of revenue or cost center providing the product and/or service. 7684 */ 7685 @Child(name = "revenue", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 7686 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 7687 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 7688 protected CodeableConcept revenue; 7689 7690 /** 7691 * Code to identify the general type of benefits under which products and services are provided. 7692 */ 7693 @Child(name = "category", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 7694 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 7695 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 7696 protected CodeableConcept category; 7697 7698 /** 7699 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 7700 */ 7701 @Child(name = "productOrService", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 7702 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 7703 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 7704 protected CodeableConcept productOrService; 7705 7706 /** 7707 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 7708 */ 7709 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 7710 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 7711 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 7712 protected CodeableConcept productOrServiceEnd; 7713 7714 /** 7715 * Item typification or modifiers codes to convey additional context for the product or service. 7716 */ 7717 @Child(name = "modifier", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7718 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 7719 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 7720 protected List<CodeableConcept> modifier; 7721 7722 /** 7723 * Identifies the program under which this may be recovered. 7724 */ 7725 @Child(name = "programCode", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7726 @Description(shortDefinition="Program the product or service is provided under", formalDefinition="Identifies the program under which this may be recovered." ) 7727 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 7728 protected List<CodeableConcept> programCode; 7729 7730 /** 7731 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 7732 */ 7733 @Child(name = "patientPaid", type = {Money.class}, order=9, min=0, max=1, modifier=false, summary=false) 7734 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 7735 protected Money patientPaid; 7736 7737 /** 7738 * The number of repetitions of a service or product. 7739 */ 7740 @Child(name = "quantity", type = {Quantity.class}, order=10, min=0, max=1, modifier=false, summary=false) 7741 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 7742 protected Quantity quantity; 7743 7744 /** 7745 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 7746 */ 7747 @Child(name = "unitPrice", type = {Money.class}, order=11, min=0, max=1, modifier=false, summary=false) 7748 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 7749 protected Money unitPrice; 7750 7751 /** 7752 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 7753 */ 7754 @Child(name = "factor", type = {DecimalType.class}, order=12, min=0, max=1, modifier=false, summary=false) 7755 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 7756 protected DecimalType factor; 7757 7758 /** 7759 * The total of taxes applicable for this product or service. 7760 */ 7761 @Child(name = "tax", type = {Money.class}, order=13, min=0, max=1, modifier=false, summary=false) 7762 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 7763 protected Money tax; 7764 7765 /** 7766 * The total amount claimed for the line item.detail.subDetail. Net = unit price * quantity * factor. 7767 */ 7768 @Child(name = "net", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=false) 7769 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the line item.detail.subDetail. Net = unit price * quantity * factor." ) 7770 protected Money net; 7771 7772 /** 7773 * Unique Device Identifiers associated with this line item. 7774 */ 7775 @Child(name = "udi", type = {Device.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7776 @Description(shortDefinition="Unique device identifier", formalDefinition="Unique Device Identifiers associated with this line item." ) 7777 protected List<Reference> udi; 7778 7779 /** 7780 * The numbers associated with notes below which apply to the adjudication of this item. 7781 */ 7782 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7783 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 7784 protected List<PositiveIntType> noteNumber; 7785 7786 /** 7787 * The high-level results of the adjudication if adjudication has been performed. 7788 */ 7789 @Child(name = "reviewOutcome", type = {ItemReviewOutcomeComponent.class}, order=17, min=0, max=1, modifier=false, summary=false) 7790 @Description(shortDefinition="Subdetail level adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 7791 protected ItemReviewOutcomeComponent reviewOutcome; 7792 7793 /** 7794 * The adjudication results. 7795 */ 7796 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7797 @Description(shortDefinition="Subdetail level adjudication details", formalDefinition="The adjudication results." ) 7798 protected List<AdjudicationComponent> adjudication; 7799 7800 private static final long serialVersionUID = -560048316L; 7801 7802 /** 7803 * Constructor 7804 */ 7805 public SubDetailComponent() { 7806 super(); 7807 } 7808 7809 /** 7810 * Constructor 7811 */ 7812 public SubDetailComponent(int sequence) { 7813 super(); 7814 this.setSequence(sequence); 7815 } 7816 7817 /** 7818 * @return {@link #sequence} (A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 7819 */ 7820 public PositiveIntType getSequenceElement() { 7821 if (this.sequence == null) 7822 if (Configuration.errorOnAutoCreate()) 7823 throw new Error("Attempt to auto-create SubDetailComponent.sequence"); 7824 else if (Configuration.doAutoCreate()) 7825 this.sequence = new PositiveIntType(); // bb 7826 return this.sequence; 7827 } 7828 7829 public boolean hasSequenceElement() { 7830 return this.sequence != null && !this.sequence.isEmpty(); 7831 } 7832 7833 public boolean hasSequence() { 7834 return this.sequence != null && !this.sequence.isEmpty(); 7835 } 7836 7837 /** 7838 * @param value {@link #sequence} (A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 7839 */ 7840 public SubDetailComponent setSequenceElement(PositiveIntType value) { 7841 this.sequence = value; 7842 return this; 7843 } 7844 7845 /** 7846 * @return A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 7847 */ 7848 public int getSequence() { 7849 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 7850 } 7851 7852 /** 7853 * @param value A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 7854 */ 7855 public SubDetailComponent setSequence(int value) { 7856 if (this.sequence == null) 7857 this.sequence = new PositiveIntType(); 7858 this.sequence.setValue(value); 7859 return this; 7860 } 7861 7862 /** 7863 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 7864 */ 7865 public List<Identifier> getTraceNumber() { 7866 if (this.traceNumber == null) 7867 this.traceNumber = new ArrayList<Identifier>(); 7868 return this.traceNumber; 7869 } 7870 7871 /** 7872 * @return Returns a reference to <code>this</code> for easy method chaining 7873 */ 7874 public SubDetailComponent setTraceNumber(List<Identifier> theTraceNumber) { 7875 this.traceNumber = theTraceNumber; 7876 return this; 7877 } 7878 7879 public boolean hasTraceNumber() { 7880 if (this.traceNumber == null) 7881 return false; 7882 for (Identifier item : this.traceNumber) 7883 if (!item.isEmpty()) 7884 return true; 7885 return false; 7886 } 7887 7888 public Identifier addTraceNumber() { //3 7889 Identifier t = new Identifier(); 7890 if (this.traceNumber == null) 7891 this.traceNumber = new ArrayList<Identifier>(); 7892 this.traceNumber.add(t); 7893 return t; 7894 } 7895 7896 public SubDetailComponent addTraceNumber(Identifier t) { //3 7897 if (t == null) 7898 return this; 7899 if (this.traceNumber == null) 7900 this.traceNumber = new ArrayList<Identifier>(); 7901 this.traceNumber.add(t); 7902 return this; 7903 } 7904 7905 /** 7906 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 7907 */ 7908 public Identifier getTraceNumberFirstRep() { 7909 if (getTraceNumber().isEmpty()) { 7910 addTraceNumber(); 7911 } 7912 return getTraceNumber().get(0); 7913 } 7914 7915 /** 7916 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 7917 */ 7918 public CodeableConcept getRevenue() { 7919 if (this.revenue == null) 7920 if (Configuration.errorOnAutoCreate()) 7921 throw new Error("Attempt to auto-create SubDetailComponent.revenue"); 7922 else if (Configuration.doAutoCreate()) 7923 this.revenue = new CodeableConcept(); // cc 7924 return this.revenue; 7925 } 7926 7927 public boolean hasRevenue() { 7928 return this.revenue != null && !this.revenue.isEmpty(); 7929 } 7930 7931 /** 7932 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 7933 */ 7934 public SubDetailComponent setRevenue(CodeableConcept value) { 7935 this.revenue = value; 7936 return this; 7937 } 7938 7939 /** 7940 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 7941 */ 7942 public CodeableConcept getCategory() { 7943 if (this.category == null) 7944 if (Configuration.errorOnAutoCreate()) 7945 throw new Error("Attempt to auto-create SubDetailComponent.category"); 7946 else if (Configuration.doAutoCreate()) 7947 this.category = new CodeableConcept(); // cc 7948 return this.category; 7949 } 7950 7951 public boolean hasCategory() { 7952 return this.category != null && !this.category.isEmpty(); 7953 } 7954 7955 /** 7956 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 7957 */ 7958 public SubDetailComponent setCategory(CodeableConcept value) { 7959 this.category = value; 7960 return this; 7961 } 7962 7963 /** 7964 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 7965 */ 7966 public CodeableConcept getProductOrService() { 7967 if (this.productOrService == null) 7968 if (Configuration.errorOnAutoCreate()) 7969 throw new Error("Attempt to auto-create SubDetailComponent.productOrService"); 7970 else if (Configuration.doAutoCreate()) 7971 this.productOrService = new CodeableConcept(); // cc 7972 return this.productOrService; 7973 } 7974 7975 public boolean hasProductOrService() { 7976 return this.productOrService != null && !this.productOrService.isEmpty(); 7977 } 7978 7979 /** 7980 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 7981 */ 7982 public SubDetailComponent setProductOrService(CodeableConcept value) { 7983 this.productOrService = value; 7984 return this; 7985 } 7986 7987 /** 7988 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 7989 */ 7990 public CodeableConcept getProductOrServiceEnd() { 7991 if (this.productOrServiceEnd == null) 7992 if (Configuration.errorOnAutoCreate()) 7993 throw new Error("Attempt to auto-create SubDetailComponent.productOrServiceEnd"); 7994 else if (Configuration.doAutoCreate()) 7995 this.productOrServiceEnd = new CodeableConcept(); // cc 7996 return this.productOrServiceEnd; 7997 } 7998 7999 public boolean hasProductOrServiceEnd() { 8000 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 8001 } 8002 8003 /** 8004 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 8005 */ 8006 public SubDetailComponent setProductOrServiceEnd(CodeableConcept value) { 8007 this.productOrServiceEnd = value; 8008 return this; 8009 } 8010 8011 /** 8012 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 8013 */ 8014 public List<CodeableConcept> getModifier() { 8015 if (this.modifier == null) 8016 this.modifier = new ArrayList<CodeableConcept>(); 8017 return this.modifier; 8018 } 8019 8020 /** 8021 * @return Returns a reference to <code>this</code> for easy method chaining 8022 */ 8023 public SubDetailComponent setModifier(List<CodeableConcept> theModifier) { 8024 this.modifier = theModifier; 8025 return this; 8026 } 8027 8028 public boolean hasModifier() { 8029 if (this.modifier == null) 8030 return false; 8031 for (CodeableConcept item : this.modifier) 8032 if (!item.isEmpty()) 8033 return true; 8034 return false; 8035 } 8036 8037 public CodeableConcept addModifier() { //3 8038 CodeableConcept t = new CodeableConcept(); 8039 if (this.modifier == null) 8040 this.modifier = new ArrayList<CodeableConcept>(); 8041 this.modifier.add(t); 8042 return t; 8043 } 8044 8045 public SubDetailComponent addModifier(CodeableConcept t) { //3 8046 if (t == null) 8047 return this; 8048 if (this.modifier == null) 8049 this.modifier = new ArrayList<CodeableConcept>(); 8050 this.modifier.add(t); 8051 return this; 8052 } 8053 8054 /** 8055 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 8056 */ 8057 public CodeableConcept getModifierFirstRep() { 8058 if (getModifier().isEmpty()) { 8059 addModifier(); 8060 } 8061 return getModifier().get(0); 8062 } 8063 8064 /** 8065 * @return {@link #programCode} (Identifies the program under which this may be recovered.) 8066 */ 8067 public List<CodeableConcept> getProgramCode() { 8068 if (this.programCode == null) 8069 this.programCode = new ArrayList<CodeableConcept>(); 8070 return this.programCode; 8071 } 8072 8073 /** 8074 * @return Returns a reference to <code>this</code> for easy method chaining 8075 */ 8076 public SubDetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 8077 this.programCode = theProgramCode; 8078 return this; 8079 } 8080 8081 public boolean hasProgramCode() { 8082 if (this.programCode == null) 8083 return false; 8084 for (CodeableConcept item : this.programCode) 8085 if (!item.isEmpty()) 8086 return true; 8087 return false; 8088 } 8089 8090 public CodeableConcept addProgramCode() { //3 8091 CodeableConcept t = new CodeableConcept(); 8092 if (this.programCode == null) 8093 this.programCode = new ArrayList<CodeableConcept>(); 8094 this.programCode.add(t); 8095 return t; 8096 } 8097 8098 public SubDetailComponent addProgramCode(CodeableConcept t) { //3 8099 if (t == null) 8100 return this; 8101 if (this.programCode == null) 8102 this.programCode = new ArrayList<CodeableConcept>(); 8103 this.programCode.add(t); 8104 return this; 8105 } 8106 8107 /** 8108 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist {3} 8109 */ 8110 public CodeableConcept getProgramCodeFirstRep() { 8111 if (getProgramCode().isEmpty()) { 8112 addProgramCode(); 8113 } 8114 return getProgramCode().get(0); 8115 } 8116 8117 /** 8118 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 8119 */ 8120 public Money getPatientPaid() { 8121 if (this.patientPaid == null) 8122 if (Configuration.errorOnAutoCreate()) 8123 throw new Error("Attempt to auto-create SubDetailComponent.patientPaid"); 8124 else if (Configuration.doAutoCreate()) 8125 this.patientPaid = new Money(); // cc 8126 return this.patientPaid; 8127 } 8128 8129 public boolean hasPatientPaid() { 8130 return this.patientPaid != null && !this.patientPaid.isEmpty(); 8131 } 8132 8133 /** 8134 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 8135 */ 8136 public SubDetailComponent setPatientPaid(Money value) { 8137 this.patientPaid = value; 8138 return this; 8139 } 8140 8141 /** 8142 * @return {@link #quantity} (The number of repetitions of a service or product.) 8143 */ 8144 public Quantity getQuantity() { 8145 if (this.quantity == null) 8146 if (Configuration.errorOnAutoCreate()) 8147 throw new Error("Attempt to auto-create SubDetailComponent.quantity"); 8148 else if (Configuration.doAutoCreate()) 8149 this.quantity = new Quantity(); // cc 8150 return this.quantity; 8151 } 8152 8153 public boolean hasQuantity() { 8154 return this.quantity != null && !this.quantity.isEmpty(); 8155 } 8156 8157 /** 8158 * @param value {@link #quantity} (The number of repetitions of a service or product.) 8159 */ 8160 public SubDetailComponent setQuantity(Quantity value) { 8161 this.quantity = value; 8162 return this; 8163 } 8164 8165 /** 8166 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 8167 */ 8168 public Money getUnitPrice() { 8169 if (this.unitPrice == null) 8170 if (Configuration.errorOnAutoCreate()) 8171 throw new Error("Attempt to auto-create SubDetailComponent.unitPrice"); 8172 else if (Configuration.doAutoCreate()) 8173 this.unitPrice = new Money(); // cc 8174 return this.unitPrice; 8175 } 8176 8177 public boolean hasUnitPrice() { 8178 return this.unitPrice != null && !this.unitPrice.isEmpty(); 8179 } 8180 8181 /** 8182 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 8183 */ 8184 public SubDetailComponent setUnitPrice(Money value) { 8185 this.unitPrice = value; 8186 return this; 8187 } 8188 8189 /** 8190 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 8191 */ 8192 public DecimalType getFactorElement() { 8193 if (this.factor == null) 8194 if (Configuration.errorOnAutoCreate()) 8195 throw new Error("Attempt to auto-create SubDetailComponent.factor"); 8196 else if (Configuration.doAutoCreate()) 8197 this.factor = new DecimalType(); // bb 8198 return this.factor; 8199 } 8200 8201 public boolean hasFactorElement() { 8202 return this.factor != null && !this.factor.isEmpty(); 8203 } 8204 8205 public boolean hasFactor() { 8206 return this.factor != null && !this.factor.isEmpty(); 8207 } 8208 8209 /** 8210 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 8211 */ 8212 public SubDetailComponent setFactorElement(DecimalType value) { 8213 this.factor = value; 8214 return this; 8215 } 8216 8217 /** 8218 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 8219 */ 8220 public BigDecimal getFactor() { 8221 return this.factor == null ? null : this.factor.getValue(); 8222 } 8223 8224 /** 8225 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 8226 */ 8227 public SubDetailComponent setFactor(BigDecimal value) { 8228 if (value == null) 8229 this.factor = null; 8230 else { 8231 if (this.factor == null) 8232 this.factor = new DecimalType(); 8233 this.factor.setValue(value); 8234 } 8235 return this; 8236 } 8237 8238 /** 8239 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 8240 */ 8241 public SubDetailComponent setFactor(long value) { 8242 this.factor = new DecimalType(); 8243 this.factor.setValue(value); 8244 return this; 8245 } 8246 8247 /** 8248 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 8249 */ 8250 public SubDetailComponent setFactor(double value) { 8251 this.factor = new DecimalType(); 8252 this.factor.setValue(value); 8253 return this; 8254 } 8255 8256 /** 8257 * @return {@link #tax} (The total of taxes applicable for this product or service.) 8258 */ 8259 public Money getTax() { 8260 if (this.tax == null) 8261 if (Configuration.errorOnAutoCreate()) 8262 throw new Error("Attempt to auto-create SubDetailComponent.tax"); 8263 else if (Configuration.doAutoCreate()) 8264 this.tax = new Money(); // cc 8265 return this.tax; 8266 } 8267 8268 public boolean hasTax() { 8269 return this.tax != null && !this.tax.isEmpty(); 8270 } 8271 8272 /** 8273 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 8274 */ 8275 public SubDetailComponent setTax(Money value) { 8276 this.tax = value; 8277 return this; 8278 } 8279 8280 /** 8281 * @return {@link #net} (The total amount claimed for the line item.detail.subDetail. Net = unit price * quantity * factor.) 8282 */ 8283 public Money getNet() { 8284 if (this.net == null) 8285 if (Configuration.errorOnAutoCreate()) 8286 throw new Error("Attempt to auto-create SubDetailComponent.net"); 8287 else if (Configuration.doAutoCreate()) 8288 this.net = new Money(); // cc 8289 return this.net; 8290 } 8291 8292 public boolean hasNet() { 8293 return this.net != null && !this.net.isEmpty(); 8294 } 8295 8296 /** 8297 * @param value {@link #net} (The total amount claimed for the line item.detail.subDetail. Net = unit price * quantity * factor.) 8298 */ 8299 public SubDetailComponent setNet(Money value) { 8300 this.net = value; 8301 return this; 8302 } 8303 8304 /** 8305 * @return {@link #udi} (Unique Device Identifiers associated with this line item.) 8306 */ 8307 public List<Reference> getUdi() { 8308 if (this.udi == null) 8309 this.udi = new ArrayList<Reference>(); 8310 return this.udi; 8311 } 8312 8313 /** 8314 * @return Returns a reference to <code>this</code> for easy method chaining 8315 */ 8316 public SubDetailComponent setUdi(List<Reference> theUdi) { 8317 this.udi = theUdi; 8318 return this; 8319 } 8320 8321 public boolean hasUdi() { 8322 if (this.udi == null) 8323 return false; 8324 for (Reference item : this.udi) 8325 if (!item.isEmpty()) 8326 return true; 8327 return false; 8328 } 8329 8330 public Reference addUdi() { //3 8331 Reference t = new Reference(); 8332 if (this.udi == null) 8333 this.udi = new ArrayList<Reference>(); 8334 this.udi.add(t); 8335 return t; 8336 } 8337 8338 public SubDetailComponent addUdi(Reference t) { //3 8339 if (t == null) 8340 return this; 8341 if (this.udi == null) 8342 this.udi = new ArrayList<Reference>(); 8343 this.udi.add(t); 8344 return this; 8345 } 8346 8347 /** 8348 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist {3} 8349 */ 8350 public Reference getUdiFirstRep() { 8351 if (getUdi().isEmpty()) { 8352 addUdi(); 8353 } 8354 return getUdi().get(0); 8355 } 8356 8357 /** 8358 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 8359 */ 8360 public List<PositiveIntType> getNoteNumber() { 8361 if (this.noteNumber == null) 8362 this.noteNumber = new ArrayList<PositiveIntType>(); 8363 return this.noteNumber; 8364 } 8365 8366 /** 8367 * @return Returns a reference to <code>this</code> for easy method chaining 8368 */ 8369 public SubDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 8370 this.noteNumber = theNoteNumber; 8371 return this; 8372 } 8373 8374 public boolean hasNoteNumber() { 8375 if (this.noteNumber == null) 8376 return false; 8377 for (PositiveIntType item : this.noteNumber) 8378 if (!item.isEmpty()) 8379 return true; 8380 return false; 8381 } 8382 8383 /** 8384 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 8385 */ 8386 public PositiveIntType addNoteNumberElement() {//2 8387 PositiveIntType t = new PositiveIntType(); 8388 if (this.noteNumber == null) 8389 this.noteNumber = new ArrayList<PositiveIntType>(); 8390 this.noteNumber.add(t); 8391 return t; 8392 } 8393 8394 /** 8395 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 8396 */ 8397 public SubDetailComponent addNoteNumber(int value) { //1 8398 PositiveIntType t = new PositiveIntType(); 8399 t.setValue(value); 8400 if (this.noteNumber == null) 8401 this.noteNumber = new ArrayList<PositiveIntType>(); 8402 this.noteNumber.add(t); 8403 return this; 8404 } 8405 8406 /** 8407 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 8408 */ 8409 public boolean hasNoteNumber(int value) { 8410 if (this.noteNumber == null) 8411 return false; 8412 for (PositiveIntType v : this.noteNumber) 8413 if (v.getValue().equals(value)) // positiveInt 8414 return true; 8415 return false; 8416 } 8417 8418 /** 8419 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 8420 */ 8421 public ItemReviewOutcomeComponent getReviewOutcome() { 8422 if (this.reviewOutcome == null) 8423 if (Configuration.errorOnAutoCreate()) 8424 throw new Error("Attempt to auto-create SubDetailComponent.reviewOutcome"); 8425 else if (Configuration.doAutoCreate()) 8426 this.reviewOutcome = new ItemReviewOutcomeComponent(); // cc 8427 return this.reviewOutcome; 8428 } 8429 8430 public boolean hasReviewOutcome() { 8431 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 8432 } 8433 8434 /** 8435 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 8436 */ 8437 public SubDetailComponent setReviewOutcome(ItemReviewOutcomeComponent value) { 8438 this.reviewOutcome = value; 8439 return this; 8440 } 8441 8442 /** 8443 * @return {@link #adjudication} (The adjudication results.) 8444 */ 8445 public List<AdjudicationComponent> getAdjudication() { 8446 if (this.adjudication == null) 8447 this.adjudication = new ArrayList<AdjudicationComponent>(); 8448 return this.adjudication; 8449 } 8450 8451 /** 8452 * @return Returns a reference to <code>this</code> for easy method chaining 8453 */ 8454 public SubDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 8455 this.adjudication = theAdjudication; 8456 return this; 8457 } 8458 8459 public boolean hasAdjudication() { 8460 if (this.adjudication == null) 8461 return false; 8462 for (AdjudicationComponent item : this.adjudication) 8463 if (!item.isEmpty()) 8464 return true; 8465 return false; 8466 } 8467 8468 public AdjudicationComponent addAdjudication() { //3 8469 AdjudicationComponent t = new AdjudicationComponent(); 8470 if (this.adjudication == null) 8471 this.adjudication = new ArrayList<AdjudicationComponent>(); 8472 this.adjudication.add(t); 8473 return t; 8474 } 8475 8476 public SubDetailComponent addAdjudication(AdjudicationComponent t) { //3 8477 if (t == null) 8478 return this; 8479 if (this.adjudication == null) 8480 this.adjudication = new ArrayList<AdjudicationComponent>(); 8481 this.adjudication.add(t); 8482 return this; 8483 } 8484 8485 /** 8486 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 8487 */ 8488 public AdjudicationComponent getAdjudicationFirstRep() { 8489 if (getAdjudication().isEmpty()) { 8490 addAdjudication(); 8491 } 8492 return getAdjudication().get(0); 8493 } 8494 8495 protected void listChildren(List<Property> children) { 8496 super.listChildren(children); 8497 children.add(new Property("sequence", "positiveInt", "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, 1, sequence)); 8498 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 8499 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 8500 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 8501 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 8502 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 8503 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 8504 children.add(new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 8505 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 8506 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 8507 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 8508 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 8509 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 8510 children.add(new Property("net", "Money", "The total amount claimed for the line item.detail.subDetail. Net = unit price * quantity * factor.", 0, 1, net)); 8511 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 8512 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 8513 children.add(new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 8514 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 8515 } 8516 8517 @Override 8518 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8519 switch (_hash) { 8520 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, 1, sequence); 8521 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 8522 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 8523 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 8524 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 8525 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 8526 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 8527 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 8528 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 8529 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 8530 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 8531 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 8532 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 8533 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the line item.detail.subDetail. Net = unit price * quantity * factor.", 0, 1, net); 8534 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 8535 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 8536 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 8537 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 8538 default: return super.getNamedProperty(_hash, _name, _checkValid); 8539 } 8540 8541 } 8542 8543 @Override 8544 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8545 switch (hash) { 8546 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 8547 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 8548 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 8549 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 8550 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 8551 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 8552 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 8553 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 8554 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 8555 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 8556 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 8557 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 8558 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 8559 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 8560 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 8561 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 8562 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ItemReviewOutcomeComponent 8563 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 8564 default: return super.getProperty(hash, name, checkValid); 8565 } 8566 8567 } 8568 8569 @Override 8570 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8571 switch (hash) { 8572 case 1349547969: // sequence 8573 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 8574 return value; 8575 case 82505966: // traceNumber 8576 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 8577 return value; 8578 case 1099842588: // revenue 8579 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8580 return value; 8581 case 50511102: // category 8582 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8583 return value; 8584 case 1957227299: // productOrService 8585 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8586 return value; 8587 case -717476168: // productOrServiceEnd 8588 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8589 return value; 8590 case -615513385: // modifier 8591 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 8592 return value; 8593 case 1010065041: // programCode 8594 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 8595 return value; 8596 case 525514609: // patientPaid 8597 this.patientPaid = TypeConvertor.castToMoney(value); // Money 8598 return value; 8599 case -1285004149: // quantity 8600 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 8601 return value; 8602 case -486196699: // unitPrice 8603 this.unitPrice = TypeConvertor.castToMoney(value); // Money 8604 return value; 8605 case -1282148017: // factor 8606 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 8607 return value; 8608 case 114603: // tax 8609 this.tax = TypeConvertor.castToMoney(value); // Money 8610 return value; 8611 case 108957: // net 8612 this.net = TypeConvertor.castToMoney(value); // Money 8613 return value; 8614 case 115642: // udi 8615 this.getUdi().add(TypeConvertor.castToReference(value)); // Reference 8616 return value; 8617 case -1110033957: // noteNumber 8618 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 8619 return value; 8620 case -51825446: // reviewOutcome 8621 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 8622 return value; 8623 case -231349275: // adjudication 8624 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 8625 return value; 8626 default: return super.setProperty(hash, name, value); 8627 } 8628 8629 } 8630 8631 @Override 8632 public Base setProperty(String name, Base value) throws FHIRException { 8633 if (name.equals("sequence")) { 8634 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 8635 } else if (name.equals("traceNumber")) { 8636 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 8637 } else if (name.equals("revenue")) { 8638 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8639 } else if (name.equals("category")) { 8640 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8641 } else if (name.equals("productOrService")) { 8642 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8643 } else if (name.equals("productOrServiceEnd")) { 8644 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8645 } else if (name.equals("modifier")) { 8646 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 8647 } else if (name.equals("programCode")) { 8648 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); 8649 } else if (name.equals("patientPaid")) { 8650 this.patientPaid = TypeConvertor.castToMoney(value); // Money 8651 } else if (name.equals("quantity")) { 8652 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 8653 } else if (name.equals("unitPrice")) { 8654 this.unitPrice = TypeConvertor.castToMoney(value); // Money 8655 } else if (name.equals("factor")) { 8656 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 8657 } else if (name.equals("tax")) { 8658 this.tax = TypeConvertor.castToMoney(value); // Money 8659 } else if (name.equals("net")) { 8660 this.net = TypeConvertor.castToMoney(value); // Money 8661 } else if (name.equals("udi")) { 8662 this.getUdi().add(TypeConvertor.castToReference(value)); 8663 } else if (name.equals("noteNumber")) { 8664 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 8665 } else if (name.equals("reviewOutcome")) { 8666 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 8667 } else if (name.equals("adjudication")) { 8668 this.getAdjudication().add((AdjudicationComponent) value); 8669 } else 8670 return super.setProperty(name, value); 8671 return value; 8672 } 8673 8674 @Override 8675 public Base makeProperty(int hash, String name) throws FHIRException { 8676 switch (hash) { 8677 case 1349547969: return getSequenceElement(); 8678 case 82505966: return addTraceNumber(); 8679 case 1099842588: return getRevenue(); 8680 case 50511102: return getCategory(); 8681 case 1957227299: return getProductOrService(); 8682 case -717476168: return getProductOrServiceEnd(); 8683 case -615513385: return addModifier(); 8684 case 1010065041: return addProgramCode(); 8685 case 525514609: return getPatientPaid(); 8686 case -1285004149: return getQuantity(); 8687 case -486196699: return getUnitPrice(); 8688 case -1282148017: return getFactorElement(); 8689 case 114603: return getTax(); 8690 case 108957: return getNet(); 8691 case 115642: return addUdi(); 8692 case -1110033957: return addNoteNumberElement(); 8693 case -51825446: return getReviewOutcome(); 8694 case -231349275: return addAdjudication(); 8695 default: return super.makeProperty(hash, name); 8696 } 8697 8698 } 8699 8700 @Override 8701 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8702 switch (hash) { 8703 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 8704 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 8705 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 8706 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 8707 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 8708 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 8709 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 8710 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 8711 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 8712 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 8713 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 8714 case -1282148017: /*factor*/ return new String[] {"decimal"}; 8715 case 114603: /*tax*/ return new String[] {"Money"}; 8716 case 108957: /*net*/ return new String[] {"Money"}; 8717 case 115642: /*udi*/ return new String[] {"Reference"}; 8718 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 8719 case -51825446: /*reviewOutcome*/ return new String[] {"@ExplanationOfBenefit.item.reviewOutcome"}; 8720 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 8721 default: return super.getTypesForProperty(hash, name); 8722 } 8723 8724 } 8725 8726 @Override 8727 public Base addChild(String name) throws FHIRException { 8728 if (name.equals("sequence")) { 8729 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.detail.subDetail.sequence"); 8730 } 8731 else if (name.equals("traceNumber")) { 8732 return addTraceNumber(); 8733 } 8734 else if (name.equals("revenue")) { 8735 this.revenue = new CodeableConcept(); 8736 return this.revenue; 8737 } 8738 else if (name.equals("category")) { 8739 this.category = new CodeableConcept(); 8740 return this.category; 8741 } 8742 else if (name.equals("productOrService")) { 8743 this.productOrService = new CodeableConcept(); 8744 return this.productOrService; 8745 } 8746 else if (name.equals("productOrServiceEnd")) { 8747 this.productOrServiceEnd = new CodeableConcept(); 8748 return this.productOrServiceEnd; 8749 } 8750 else if (name.equals("modifier")) { 8751 return addModifier(); 8752 } 8753 else if (name.equals("programCode")) { 8754 return addProgramCode(); 8755 } 8756 else if (name.equals("patientPaid")) { 8757 this.patientPaid = new Money(); 8758 return this.patientPaid; 8759 } 8760 else if (name.equals("quantity")) { 8761 this.quantity = new Quantity(); 8762 return this.quantity; 8763 } 8764 else if (name.equals("unitPrice")) { 8765 this.unitPrice = new Money(); 8766 return this.unitPrice; 8767 } 8768 else if (name.equals("factor")) { 8769 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.detail.subDetail.factor"); 8770 } 8771 else if (name.equals("tax")) { 8772 this.tax = new Money(); 8773 return this.tax; 8774 } 8775 else if (name.equals("net")) { 8776 this.net = new Money(); 8777 return this.net; 8778 } 8779 else if (name.equals("udi")) { 8780 return addUdi(); 8781 } 8782 else if (name.equals("noteNumber")) { 8783 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.detail.subDetail.noteNumber"); 8784 } 8785 else if (name.equals("reviewOutcome")) { 8786 this.reviewOutcome = new ItemReviewOutcomeComponent(); 8787 return this.reviewOutcome; 8788 } 8789 else if (name.equals("adjudication")) { 8790 return addAdjudication(); 8791 } 8792 else 8793 return super.addChild(name); 8794 } 8795 8796 public SubDetailComponent copy() { 8797 SubDetailComponent dst = new SubDetailComponent(); 8798 copyValues(dst); 8799 return dst; 8800 } 8801 8802 public void copyValues(SubDetailComponent dst) { 8803 super.copyValues(dst); 8804 dst.sequence = sequence == null ? null : sequence.copy(); 8805 if (traceNumber != null) { 8806 dst.traceNumber = new ArrayList<Identifier>(); 8807 for (Identifier i : traceNumber) 8808 dst.traceNumber.add(i.copy()); 8809 }; 8810 dst.revenue = revenue == null ? null : revenue.copy(); 8811 dst.category = category == null ? null : category.copy(); 8812 dst.productOrService = productOrService == null ? null : productOrService.copy(); 8813 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 8814 if (modifier != null) { 8815 dst.modifier = new ArrayList<CodeableConcept>(); 8816 for (CodeableConcept i : modifier) 8817 dst.modifier.add(i.copy()); 8818 }; 8819 if (programCode != null) { 8820 dst.programCode = new ArrayList<CodeableConcept>(); 8821 for (CodeableConcept i : programCode) 8822 dst.programCode.add(i.copy()); 8823 }; 8824 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 8825 dst.quantity = quantity == null ? null : quantity.copy(); 8826 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 8827 dst.factor = factor == null ? null : factor.copy(); 8828 dst.tax = tax == null ? null : tax.copy(); 8829 dst.net = net == null ? null : net.copy(); 8830 if (udi != null) { 8831 dst.udi = new ArrayList<Reference>(); 8832 for (Reference i : udi) 8833 dst.udi.add(i.copy()); 8834 }; 8835 if (noteNumber != null) { 8836 dst.noteNumber = new ArrayList<PositiveIntType>(); 8837 for (PositiveIntType i : noteNumber) 8838 dst.noteNumber.add(i.copy()); 8839 }; 8840 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 8841 if (adjudication != null) { 8842 dst.adjudication = new ArrayList<AdjudicationComponent>(); 8843 for (AdjudicationComponent i : adjudication) 8844 dst.adjudication.add(i.copy()); 8845 }; 8846 } 8847 8848 @Override 8849 public boolean equalsDeep(Base other_) { 8850 if (!super.equalsDeep(other_)) 8851 return false; 8852 if (!(other_ instanceof SubDetailComponent)) 8853 return false; 8854 SubDetailComponent o = (SubDetailComponent) other_; 8855 return compareDeep(sequence, o.sequence, true) && compareDeep(traceNumber, o.traceNumber, true) 8856 && compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 8857 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(modifier, o.modifier, true) 8858 && compareDeep(programCode, o.programCode, true) && compareDeep(patientPaid, o.patientPaid, true) 8859 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 8860 && compareDeep(tax, o.tax, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 8861 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(reviewOutcome, o.reviewOutcome, true) 8862 && compareDeep(adjudication, o.adjudication, true); 8863 } 8864 8865 @Override 8866 public boolean equalsShallow(Base other_) { 8867 if (!super.equalsShallow(other_)) 8868 return false; 8869 if (!(other_ instanceof SubDetailComponent)) 8870 return false; 8871 SubDetailComponent o = (SubDetailComponent) other_; 8872 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true) 8873 ; 8874 } 8875 8876 public boolean isEmpty() { 8877 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, traceNumber, revenue 8878 , category, productOrService, productOrServiceEnd, modifier, programCode, patientPaid 8879 , quantity, unitPrice, factor, tax, net, udi, noteNumber, reviewOutcome, adjudication 8880 ); 8881 } 8882 8883 public String fhirType() { 8884 return "ExplanationOfBenefit.item.detail.subDetail"; 8885 8886 } 8887 8888 } 8889 8890 @Block() 8891 public static class AddedItemComponent extends BackboneElement implements IBaseBackboneElement { 8892 /** 8893 * Claim items which this service line is intended to replace. 8894 */ 8895 @Child(name = "itemSequence", type = {PositiveIntType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8896 @Description(shortDefinition="Item sequence number", formalDefinition="Claim items which this service line is intended to replace." ) 8897 protected List<PositiveIntType> itemSequence; 8898 8899 /** 8900 * The sequence number of the details within the claim item which this line is intended to replace. 8901 */ 8902 @Child(name = "detailSequence", type = {PositiveIntType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8903 @Description(shortDefinition="Detail sequence number", formalDefinition="The sequence number of the details within the claim item which this line is intended to replace." ) 8904 protected List<PositiveIntType> detailSequence; 8905 8906 /** 8907 * The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace. 8908 */ 8909 @Child(name = "subDetailSequence", type = {PositiveIntType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8910 @Description(shortDefinition="Subdetail sequence number", formalDefinition="The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace." ) 8911 protected List<PositiveIntType> subDetailSequence; 8912 8913 /** 8914 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 8915 */ 8916 @Child(name = "traceNumber", type = {Identifier.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8917 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 8918 protected List<Identifier> traceNumber; 8919 8920 /** 8921 * The providers who are authorized for the services rendered to the patient. 8922 */ 8923 @Child(name = "provider", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8924 @Description(shortDefinition="Authorized providers", formalDefinition="The providers who are authorized for the services rendered to the patient." ) 8925 protected List<Reference> provider; 8926 8927 /** 8928 * The type of revenue or cost center providing the product and/or service. 8929 */ 8930 @Child(name = "revenue", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 8931 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 8932 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 8933 protected CodeableConcept revenue; 8934 8935 /** 8936 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 8937 */ 8938 @Child(name = "productOrService", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 8939 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 8940 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 8941 protected CodeableConcept productOrService; 8942 8943 /** 8944 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 8945 */ 8946 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 8947 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 8948 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 8949 protected CodeableConcept productOrServiceEnd; 8950 8951 /** 8952 * Request or Referral for Goods or Service to be rendered. 8953 */ 8954 @Child(name = "request", type = {DeviceRequest.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class, SupplyRequest.class, VisionPrescription.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8955 @Description(shortDefinition="Request or Referral for Service", formalDefinition="Request or Referral for Goods or Service to be rendered." ) 8956 protected List<Reference> request; 8957 8958 /** 8959 * Item typification or modifiers codes to convey additional context for the product or service. 8960 */ 8961 @Child(name = "modifier", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8962 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 8963 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 8964 protected List<CodeableConcept> modifier; 8965 8966 /** 8967 * Identifies the program under which this may be recovered. 8968 */ 8969 @Child(name = "programCode", type = {CodeableConcept.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8970 @Description(shortDefinition="Program the product or service is provided under", formalDefinition="Identifies the program under which this may be recovered." ) 8971 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 8972 protected List<CodeableConcept> programCode; 8973 8974 /** 8975 * The date or dates when the service or product was supplied, performed or completed. 8976 */ 8977 @Child(name = "serviced", type = {DateType.class, Period.class}, order=12, min=0, max=1, modifier=false, summary=false) 8978 @Description(shortDefinition="Date or dates of service or product delivery", formalDefinition="The date or dates when the service or product was supplied, performed or completed." ) 8979 protected DataType serviced; 8980 8981 /** 8982 * Where the product or service was provided. 8983 */ 8984 @Child(name = "location", type = {CodeableConcept.class, Address.class, Location.class}, order=13, min=0, max=1, modifier=false, summary=false) 8985 @Description(shortDefinition="Place of service or where product was supplied", formalDefinition="Where the product or service was provided." ) 8986 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-place") 8987 protected DataType location; 8988 8989 /** 8990 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 8991 */ 8992 @Child(name = "patientPaid", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=false) 8993 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 8994 protected Money patientPaid; 8995 8996 /** 8997 * The number of repetitions of a service or product. 8998 */ 8999 @Child(name = "quantity", type = {Quantity.class}, order=15, min=0, max=1, modifier=false, summary=false) 9000 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 9001 protected Quantity quantity; 9002 9003 /** 9004 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 9005 */ 9006 @Child(name = "unitPrice", type = {Money.class}, order=16, min=0, max=1, modifier=false, summary=false) 9007 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 9008 protected Money unitPrice; 9009 9010 /** 9011 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 9012 */ 9013 @Child(name = "factor", type = {DecimalType.class}, order=17, min=0, max=1, modifier=false, summary=false) 9014 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 9015 protected DecimalType factor; 9016 9017 /** 9018 * The total of taxes applicable for this product or service. 9019 */ 9020 @Child(name = "tax", type = {Money.class}, order=18, min=0, max=1, modifier=false, summary=false) 9021 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 9022 protected Money tax; 9023 9024 /** 9025 * The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor. 9026 */ 9027 @Child(name = "net", type = {Money.class}, order=19, min=0, max=1, modifier=false, summary=false) 9028 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor." ) 9029 protected Money net; 9030 9031 /** 9032 * Physical location where the service is performed or applies. 9033 */ 9034 @Child(name = "bodySite", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9035 @Description(shortDefinition="Anatomical location", formalDefinition="Physical location where the service is performed or applies." ) 9036 protected List<AddedItemBodySiteComponent> bodySite; 9037 9038 /** 9039 * The numbers associated with notes below which apply to the adjudication of this item. 9040 */ 9041 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9042 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 9043 protected List<PositiveIntType> noteNumber; 9044 9045 /** 9046 * The high-level results of the adjudication if adjudication has been performed. 9047 */ 9048 @Child(name = "reviewOutcome", type = {ItemReviewOutcomeComponent.class}, order=22, min=0, max=1, modifier=false, summary=false) 9049 @Description(shortDefinition="Additem level adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 9050 protected ItemReviewOutcomeComponent reviewOutcome; 9051 9052 /** 9053 * The adjudication results. 9054 */ 9055 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9056 @Description(shortDefinition="Added items adjudication", formalDefinition="The adjudication results." ) 9057 protected List<AdjudicationComponent> adjudication; 9058 9059 /** 9060 * The second-tier service adjudications for payor added services. 9061 */ 9062 @Child(name = "detail", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9063 @Description(shortDefinition="Insurer added line items", formalDefinition="The second-tier service adjudications for payor added services." ) 9064 protected List<AddedItemDetailComponent> detail; 9065 9066 private static final long serialVersionUID = -1942324543L; 9067 9068 /** 9069 * Constructor 9070 */ 9071 public AddedItemComponent() { 9072 super(); 9073 } 9074 9075 /** 9076 * @return {@link #itemSequence} (Claim items which this service line is intended to replace.) 9077 */ 9078 public List<PositiveIntType> getItemSequence() { 9079 if (this.itemSequence == null) 9080 this.itemSequence = new ArrayList<PositiveIntType>(); 9081 return this.itemSequence; 9082 } 9083 9084 /** 9085 * @return Returns a reference to <code>this</code> for easy method chaining 9086 */ 9087 public AddedItemComponent setItemSequence(List<PositiveIntType> theItemSequence) { 9088 this.itemSequence = theItemSequence; 9089 return this; 9090 } 9091 9092 public boolean hasItemSequence() { 9093 if (this.itemSequence == null) 9094 return false; 9095 for (PositiveIntType item : this.itemSequence) 9096 if (!item.isEmpty()) 9097 return true; 9098 return false; 9099 } 9100 9101 /** 9102 * @return {@link #itemSequence} (Claim items which this service line is intended to replace.) 9103 */ 9104 public PositiveIntType addItemSequenceElement() {//2 9105 PositiveIntType t = new PositiveIntType(); 9106 if (this.itemSequence == null) 9107 this.itemSequence = new ArrayList<PositiveIntType>(); 9108 this.itemSequence.add(t); 9109 return t; 9110 } 9111 9112 /** 9113 * @param value {@link #itemSequence} (Claim items which this service line is intended to replace.) 9114 */ 9115 public AddedItemComponent addItemSequence(int value) { //1 9116 PositiveIntType t = new PositiveIntType(); 9117 t.setValue(value); 9118 if (this.itemSequence == null) 9119 this.itemSequence = new ArrayList<PositiveIntType>(); 9120 this.itemSequence.add(t); 9121 return this; 9122 } 9123 9124 /** 9125 * @param value {@link #itemSequence} (Claim items which this service line is intended to replace.) 9126 */ 9127 public boolean hasItemSequence(int value) { 9128 if (this.itemSequence == null) 9129 return false; 9130 for (PositiveIntType v : this.itemSequence) 9131 if (v.getValue().equals(value)) // positiveInt 9132 return true; 9133 return false; 9134 } 9135 9136 /** 9137 * @return {@link #detailSequence} (The sequence number of the details within the claim item which this line is intended to replace.) 9138 */ 9139 public List<PositiveIntType> getDetailSequence() { 9140 if (this.detailSequence == null) 9141 this.detailSequence = new ArrayList<PositiveIntType>(); 9142 return this.detailSequence; 9143 } 9144 9145 /** 9146 * @return Returns a reference to <code>this</code> for easy method chaining 9147 */ 9148 public AddedItemComponent setDetailSequence(List<PositiveIntType> theDetailSequence) { 9149 this.detailSequence = theDetailSequence; 9150 return this; 9151 } 9152 9153 public boolean hasDetailSequence() { 9154 if (this.detailSequence == null) 9155 return false; 9156 for (PositiveIntType item : this.detailSequence) 9157 if (!item.isEmpty()) 9158 return true; 9159 return false; 9160 } 9161 9162 /** 9163 * @return {@link #detailSequence} (The sequence number of the details within the claim item which this line is intended to replace.) 9164 */ 9165 public PositiveIntType addDetailSequenceElement() {//2 9166 PositiveIntType t = new PositiveIntType(); 9167 if (this.detailSequence == null) 9168 this.detailSequence = new ArrayList<PositiveIntType>(); 9169 this.detailSequence.add(t); 9170 return t; 9171 } 9172 9173 /** 9174 * @param value {@link #detailSequence} (The sequence number of the details within the claim item which this line is intended to replace.) 9175 */ 9176 public AddedItemComponent addDetailSequence(int value) { //1 9177 PositiveIntType t = new PositiveIntType(); 9178 t.setValue(value); 9179 if (this.detailSequence == null) 9180 this.detailSequence = new ArrayList<PositiveIntType>(); 9181 this.detailSequence.add(t); 9182 return this; 9183 } 9184 9185 /** 9186 * @param value {@link #detailSequence} (The sequence number of the details within the claim item which this line is intended to replace.) 9187 */ 9188 public boolean hasDetailSequence(int value) { 9189 if (this.detailSequence == null) 9190 return false; 9191 for (PositiveIntType v : this.detailSequence) 9192 if (v.getValue().equals(value)) // positiveInt 9193 return true; 9194 return false; 9195 } 9196 9197 /** 9198 * @return {@link #subDetailSequence} (The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace.) 9199 */ 9200 public List<PositiveIntType> getSubDetailSequence() { 9201 if (this.subDetailSequence == null) 9202 this.subDetailSequence = new ArrayList<PositiveIntType>(); 9203 return this.subDetailSequence; 9204 } 9205 9206 /** 9207 * @return Returns a reference to <code>this</code> for easy method chaining 9208 */ 9209 public AddedItemComponent setSubDetailSequence(List<PositiveIntType> theSubDetailSequence) { 9210 this.subDetailSequence = theSubDetailSequence; 9211 return this; 9212 } 9213 9214 public boolean hasSubDetailSequence() { 9215 if (this.subDetailSequence == null) 9216 return false; 9217 for (PositiveIntType item : this.subDetailSequence) 9218 if (!item.isEmpty()) 9219 return true; 9220 return false; 9221 } 9222 9223 /** 9224 * @return {@link #subDetailSequence} (The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace.) 9225 */ 9226 public PositiveIntType addSubDetailSequenceElement() {//2 9227 PositiveIntType t = new PositiveIntType(); 9228 if (this.subDetailSequence == null) 9229 this.subDetailSequence = new ArrayList<PositiveIntType>(); 9230 this.subDetailSequence.add(t); 9231 return t; 9232 } 9233 9234 /** 9235 * @param value {@link #subDetailSequence} (The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace.) 9236 */ 9237 public AddedItemComponent addSubDetailSequence(int value) { //1 9238 PositiveIntType t = new PositiveIntType(); 9239 t.setValue(value); 9240 if (this.subDetailSequence == null) 9241 this.subDetailSequence = new ArrayList<PositiveIntType>(); 9242 this.subDetailSequence.add(t); 9243 return this; 9244 } 9245 9246 /** 9247 * @param value {@link #subDetailSequence} (The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace.) 9248 */ 9249 public boolean hasSubDetailSequence(int value) { 9250 if (this.subDetailSequence == null) 9251 return false; 9252 for (PositiveIntType v : this.subDetailSequence) 9253 if (v.getValue().equals(value)) // positiveInt 9254 return true; 9255 return false; 9256 } 9257 9258 /** 9259 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 9260 */ 9261 public List<Identifier> getTraceNumber() { 9262 if (this.traceNumber == null) 9263 this.traceNumber = new ArrayList<Identifier>(); 9264 return this.traceNumber; 9265 } 9266 9267 /** 9268 * @return Returns a reference to <code>this</code> for easy method chaining 9269 */ 9270 public AddedItemComponent setTraceNumber(List<Identifier> theTraceNumber) { 9271 this.traceNumber = theTraceNumber; 9272 return this; 9273 } 9274 9275 public boolean hasTraceNumber() { 9276 if (this.traceNumber == null) 9277 return false; 9278 for (Identifier item : this.traceNumber) 9279 if (!item.isEmpty()) 9280 return true; 9281 return false; 9282 } 9283 9284 public Identifier addTraceNumber() { //3 9285 Identifier t = new Identifier(); 9286 if (this.traceNumber == null) 9287 this.traceNumber = new ArrayList<Identifier>(); 9288 this.traceNumber.add(t); 9289 return t; 9290 } 9291 9292 public AddedItemComponent addTraceNumber(Identifier t) { //3 9293 if (t == null) 9294 return this; 9295 if (this.traceNumber == null) 9296 this.traceNumber = new ArrayList<Identifier>(); 9297 this.traceNumber.add(t); 9298 return this; 9299 } 9300 9301 /** 9302 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 9303 */ 9304 public Identifier getTraceNumberFirstRep() { 9305 if (getTraceNumber().isEmpty()) { 9306 addTraceNumber(); 9307 } 9308 return getTraceNumber().get(0); 9309 } 9310 9311 /** 9312 * @return {@link #provider} (The providers who are authorized for the services rendered to the patient.) 9313 */ 9314 public List<Reference> getProvider() { 9315 if (this.provider == null) 9316 this.provider = new ArrayList<Reference>(); 9317 return this.provider; 9318 } 9319 9320 /** 9321 * @return Returns a reference to <code>this</code> for easy method chaining 9322 */ 9323 public AddedItemComponent setProvider(List<Reference> theProvider) { 9324 this.provider = theProvider; 9325 return this; 9326 } 9327 9328 public boolean hasProvider() { 9329 if (this.provider == null) 9330 return false; 9331 for (Reference item : this.provider) 9332 if (!item.isEmpty()) 9333 return true; 9334 return false; 9335 } 9336 9337 public Reference addProvider() { //3 9338 Reference t = new Reference(); 9339 if (this.provider == null) 9340 this.provider = new ArrayList<Reference>(); 9341 this.provider.add(t); 9342 return t; 9343 } 9344 9345 public AddedItemComponent addProvider(Reference t) { //3 9346 if (t == null) 9347 return this; 9348 if (this.provider == null) 9349 this.provider = new ArrayList<Reference>(); 9350 this.provider.add(t); 9351 return this; 9352 } 9353 9354 /** 9355 * @return The first repetition of repeating field {@link #provider}, creating it if it does not already exist {3} 9356 */ 9357 public Reference getProviderFirstRep() { 9358 if (getProvider().isEmpty()) { 9359 addProvider(); 9360 } 9361 return getProvider().get(0); 9362 } 9363 9364 /** 9365 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 9366 */ 9367 public CodeableConcept getRevenue() { 9368 if (this.revenue == null) 9369 if (Configuration.errorOnAutoCreate()) 9370 throw new Error("Attempt to auto-create AddedItemComponent.revenue"); 9371 else if (Configuration.doAutoCreate()) 9372 this.revenue = new CodeableConcept(); // cc 9373 return this.revenue; 9374 } 9375 9376 public boolean hasRevenue() { 9377 return this.revenue != null && !this.revenue.isEmpty(); 9378 } 9379 9380 /** 9381 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 9382 */ 9383 public AddedItemComponent setRevenue(CodeableConcept value) { 9384 this.revenue = value; 9385 return this; 9386 } 9387 9388 /** 9389 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 9390 */ 9391 public CodeableConcept getProductOrService() { 9392 if (this.productOrService == null) 9393 if (Configuration.errorOnAutoCreate()) 9394 throw new Error("Attempt to auto-create AddedItemComponent.productOrService"); 9395 else if (Configuration.doAutoCreate()) 9396 this.productOrService = new CodeableConcept(); // cc 9397 return this.productOrService; 9398 } 9399 9400 public boolean hasProductOrService() { 9401 return this.productOrService != null && !this.productOrService.isEmpty(); 9402 } 9403 9404 /** 9405 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 9406 */ 9407 public AddedItemComponent setProductOrService(CodeableConcept value) { 9408 this.productOrService = value; 9409 return this; 9410 } 9411 9412 /** 9413 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 9414 */ 9415 public CodeableConcept getProductOrServiceEnd() { 9416 if (this.productOrServiceEnd == null) 9417 if (Configuration.errorOnAutoCreate()) 9418 throw new Error("Attempt to auto-create AddedItemComponent.productOrServiceEnd"); 9419 else if (Configuration.doAutoCreate()) 9420 this.productOrServiceEnd = new CodeableConcept(); // cc 9421 return this.productOrServiceEnd; 9422 } 9423 9424 public boolean hasProductOrServiceEnd() { 9425 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 9426 } 9427 9428 /** 9429 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 9430 */ 9431 public AddedItemComponent setProductOrServiceEnd(CodeableConcept value) { 9432 this.productOrServiceEnd = value; 9433 return this; 9434 } 9435 9436 /** 9437 * @return {@link #request} (Request or Referral for Goods or Service to be rendered.) 9438 */ 9439 public List<Reference> getRequest() { 9440 if (this.request == null) 9441 this.request = new ArrayList<Reference>(); 9442 return this.request; 9443 } 9444 9445 /** 9446 * @return Returns a reference to <code>this</code> for easy method chaining 9447 */ 9448 public AddedItemComponent setRequest(List<Reference> theRequest) { 9449 this.request = theRequest; 9450 return this; 9451 } 9452 9453 public boolean hasRequest() { 9454 if (this.request == null) 9455 return false; 9456 for (Reference item : this.request) 9457 if (!item.isEmpty()) 9458 return true; 9459 return false; 9460 } 9461 9462 public Reference addRequest() { //3 9463 Reference t = new Reference(); 9464 if (this.request == null) 9465 this.request = new ArrayList<Reference>(); 9466 this.request.add(t); 9467 return t; 9468 } 9469 9470 public AddedItemComponent addRequest(Reference t) { //3 9471 if (t == null) 9472 return this; 9473 if (this.request == null) 9474 this.request = new ArrayList<Reference>(); 9475 this.request.add(t); 9476 return this; 9477 } 9478 9479 /** 9480 * @return The first repetition of repeating field {@link #request}, creating it if it does not already exist {3} 9481 */ 9482 public Reference getRequestFirstRep() { 9483 if (getRequest().isEmpty()) { 9484 addRequest(); 9485 } 9486 return getRequest().get(0); 9487 } 9488 9489 /** 9490 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 9491 */ 9492 public List<CodeableConcept> getModifier() { 9493 if (this.modifier == null) 9494 this.modifier = new ArrayList<CodeableConcept>(); 9495 return this.modifier; 9496 } 9497 9498 /** 9499 * @return Returns a reference to <code>this</code> for easy method chaining 9500 */ 9501 public AddedItemComponent setModifier(List<CodeableConcept> theModifier) { 9502 this.modifier = theModifier; 9503 return this; 9504 } 9505 9506 public boolean hasModifier() { 9507 if (this.modifier == null) 9508 return false; 9509 for (CodeableConcept item : this.modifier) 9510 if (!item.isEmpty()) 9511 return true; 9512 return false; 9513 } 9514 9515 public CodeableConcept addModifier() { //3 9516 CodeableConcept t = new CodeableConcept(); 9517 if (this.modifier == null) 9518 this.modifier = new ArrayList<CodeableConcept>(); 9519 this.modifier.add(t); 9520 return t; 9521 } 9522 9523 public AddedItemComponent addModifier(CodeableConcept t) { //3 9524 if (t == null) 9525 return this; 9526 if (this.modifier == null) 9527 this.modifier = new ArrayList<CodeableConcept>(); 9528 this.modifier.add(t); 9529 return this; 9530 } 9531 9532 /** 9533 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 9534 */ 9535 public CodeableConcept getModifierFirstRep() { 9536 if (getModifier().isEmpty()) { 9537 addModifier(); 9538 } 9539 return getModifier().get(0); 9540 } 9541 9542 /** 9543 * @return {@link #programCode} (Identifies the program under which this may be recovered.) 9544 */ 9545 public List<CodeableConcept> getProgramCode() { 9546 if (this.programCode == null) 9547 this.programCode = new ArrayList<CodeableConcept>(); 9548 return this.programCode; 9549 } 9550 9551 /** 9552 * @return Returns a reference to <code>this</code> for easy method chaining 9553 */ 9554 public AddedItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 9555 this.programCode = theProgramCode; 9556 return this; 9557 } 9558 9559 public boolean hasProgramCode() { 9560 if (this.programCode == null) 9561 return false; 9562 for (CodeableConcept item : this.programCode) 9563 if (!item.isEmpty()) 9564 return true; 9565 return false; 9566 } 9567 9568 public CodeableConcept addProgramCode() { //3 9569 CodeableConcept t = new CodeableConcept(); 9570 if (this.programCode == null) 9571 this.programCode = new ArrayList<CodeableConcept>(); 9572 this.programCode.add(t); 9573 return t; 9574 } 9575 9576 public AddedItemComponent addProgramCode(CodeableConcept t) { //3 9577 if (t == null) 9578 return this; 9579 if (this.programCode == null) 9580 this.programCode = new ArrayList<CodeableConcept>(); 9581 this.programCode.add(t); 9582 return this; 9583 } 9584 9585 /** 9586 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist {3} 9587 */ 9588 public CodeableConcept getProgramCodeFirstRep() { 9589 if (getProgramCode().isEmpty()) { 9590 addProgramCode(); 9591 } 9592 return getProgramCode().get(0); 9593 } 9594 9595 /** 9596 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 9597 */ 9598 public DataType getServiced() { 9599 return this.serviced; 9600 } 9601 9602 /** 9603 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 9604 */ 9605 public DateType getServicedDateType() throws FHIRException { 9606 if (this.serviced == null) 9607 this.serviced = new DateType(); 9608 if (!(this.serviced instanceof DateType)) 9609 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.serviced.getClass().getName()+" was encountered"); 9610 return (DateType) this.serviced; 9611 } 9612 9613 public boolean hasServicedDateType() { 9614 return this != null && this.serviced instanceof DateType; 9615 } 9616 9617 /** 9618 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 9619 */ 9620 public Period getServicedPeriod() throws FHIRException { 9621 if (this.serviced == null) 9622 this.serviced = new Period(); 9623 if (!(this.serviced instanceof Period)) 9624 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.serviced.getClass().getName()+" was encountered"); 9625 return (Period) this.serviced; 9626 } 9627 9628 public boolean hasServicedPeriod() { 9629 return this != null && this.serviced instanceof Period; 9630 } 9631 9632 public boolean hasServiced() { 9633 return this.serviced != null && !this.serviced.isEmpty(); 9634 } 9635 9636 /** 9637 * @param value {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 9638 */ 9639 public AddedItemComponent setServiced(DataType value) { 9640 if (value != null && !(value instanceof DateType || value instanceof Period)) 9641 throw new FHIRException("Not the right type for ExplanationOfBenefit.addItem.serviced[x]: "+value.fhirType()); 9642 this.serviced = value; 9643 return this; 9644 } 9645 9646 /** 9647 * @return {@link #location} (Where the product or service was provided.) 9648 */ 9649 public DataType getLocation() { 9650 return this.location; 9651 } 9652 9653 /** 9654 * @return {@link #location} (Where the product or service was provided.) 9655 */ 9656 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 9657 if (this.location == null) 9658 this.location = new CodeableConcept(); 9659 if (!(this.location instanceof CodeableConcept)) 9660 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.location.getClass().getName()+" was encountered"); 9661 return (CodeableConcept) this.location; 9662 } 9663 9664 public boolean hasLocationCodeableConcept() { 9665 return this != null && this.location instanceof CodeableConcept; 9666 } 9667 9668 /** 9669 * @return {@link #location} (Where the product or service was provided.) 9670 */ 9671 public Address getLocationAddress() throws FHIRException { 9672 if (this.location == null) 9673 this.location = new Address(); 9674 if (!(this.location instanceof Address)) 9675 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 9676 return (Address) this.location; 9677 } 9678 9679 public boolean hasLocationAddress() { 9680 return this != null && this.location instanceof Address; 9681 } 9682 9683 /** 9684 * @return {@link #location} (Where the product or service was provided.) 9685 */ 9686 public Reference getLocationReference() throws FHIRException { 9687 if (this.location == null) 9688 this.location = new Reference(); 9689 if (!(this.location instanceof Reference)) 9690 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 9691 return (Reference) this.location; 9692 } 9693 9694 public boolean hasLocationReference() { 9695 return this != null && this.location instanceof Reference; 9696 } 9697 9698 public boolean hasLocation() { 9699 return this.location != null && !this.location.isEmpty(); 9700 } 9701 9702 /** 9703 * @param value {@link #location} (Where the product or service was provided.) 9704 */ 9705 public AddedItemComponent setLocation(DataType value) { 9706 if (value != null && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 9707 throw new FHIRException("Not the right type for ExplanationOfBenefit.addItem.location[x]: "+value.fhirType()); 9708 this.location = value; 9709 return this; 9710 } 9711 9712 /** 9713 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 9714 */ 9715 public Money getPatientPaid() { 9716 if (this.patientPaid == null) 9717 if (Configuration.errorOnAutoCreate()) 9718 throw new Error("Attempt to auto-create AddedItemComponent.patientPaid"); 9719 else if (Configuration.doAutoCreate()) 9720 this.patientPaid = new Money(); // cc 9721 return this.patientPaid; 9722 } 9723 9724 public boolean hasPatientPaid() { 9725 return this.patientPaid != null && !this.patientPaid.isEmpty(); 9726 } 9727 9728 /** 9729 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 9730 */ 9731 public AddedItemComponent setPatientPaid(Money value) { 9732 this.patientPaid = value; 9733 return this; 9734 } 9735 9736 /** 9737 * @return {@link #quantity} (The number of repetitions of a service or product.) 9738 */ 9739 public Quantity getQuantity() { 9740 if (this.quantity == null) 9741 if (Configuration.errorOnAutoCreate()) 9742 throw new Error("Attempt to auto-create AddedItemComponent.quantity"); 9743 else if (Configuration.doAutoCreate()) 9744 this.quantity = new Quantity(); // cc 9745 return this.quantity; 9746 } 9747 9748 public boolean hasQuantity() { 9749 return this.quantity != null && !this.quantity.isEmpty(); 9750 } 9751 9752 /** 9753 * @param value {@link #quantity} (The number of repetitions of a service or product.) 9754 */ 9755 public AddedItemComponent setQuantity(Quantity value) { 9756 this.quantity = value; 9757 return this; 9758 } 9759 9760 /** 9761 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 9762 */ 9763 public Money getUnitPrice() { 9764 if (this.unitPrice == null) 9765 if (Configuration.errorOnAutoCreate()) 9766 throw new Error("Attempt to auto-create AddedItemComponent.unitPrice"); 9767 else if (Configuration.doAutoCreate()) 9768 this.unitPrice = new Money(); // cc 9769 return this.unitPrice; 9770 } 9771 9772 public boolean hasUnitPrice() { 9773 return this.unitPrice != null && !this.unitPrice.isEmpty(); 9774 } 9775 9776 /** 9777 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 9778 */ 9779 public AddedItemComponent setUnitPrice(Money value) { 9780 this.unitPrice = value; 9781 return this; 9782 } 9783 9784 /** 9785 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 9786 */ 9787 public DecimalType getFactorElement() { 9788 if (this.factor == null) 9789 if (Configuration.errorOnAutoCreate()) 9790 throw new Error("Attempt to auto-create AddedItemComponent.factor"); 9791 else if (Configuration.doAutoCreate()) 9792 this.factor = new DecimalType(); // bb 9793 return this.factor; 9794 } 9795 9796 public boolean hasFactorElement() { 9797 return this.factor != null && !this.factor.isEmpty(); 9798 } 9799 9800 public boolean hasFactor() { 9801 return this.factor != null && !this.factor.isEmpty(); 9802 } 9803 9804 /** 9805 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 9806 */ 9807 public AddedItemComponent setFactorElement(DecimalType value) { 9808 this.factor = value; 9809 return this; 9810 } 9811 9812 /** 9813 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 9814 */ 9815 public BigDecimal getFactor() { 9816 return this.factor == null ? null : this.factor.getValue(); 9817 } 9818 9819 /** 9820 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 9821 */ 9822 public AddedItemComponent setFactor(BigDecimal value) { 9823 if (value == null) 9824 this.factor = null; 9825 else { 9826 if (this.factor == null) 9827 this.factor = new DecimalType(); 9828 this.factor.setValue(value); 9829 } 9830 return this; 9831 } 9832 9833 /** 9834 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 9835 */ 9836 public AddedItemComponent setFactor(long value) { 9837 this.factor = new DecimalType(); 9838 this.factor.setValue(value); 9839 return this; 9840 } 9841 9842 /** 9843 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 9844 */ 9845 public AddedItemComponent setFactor(double value) { 9846 this.factor = new DecimalType(); 9847 this.factor.setValue(value); 9848 return this; 9849 } 9850 9851 /** 9852 * @return {@link #tax} (The total of taxes applicable for this product or service.) 9853 */ 9854 public Money getTax() { 9855 if (this.tax == null) 9856 if (Configuration.errorOnAutoCreate()) 9857 throw new Error("Attempt to auto-create AddedItemComponent.tax"); 9858 else if (Configuration.doAutoCreate()) 9859 this.tax = new Money(); // cc 9860 return this.tax; 9861 } 9862 9863 public boolean hasTax() { 9864 return this.tax != null && !this.tax.isEmpty(); 9865 } 9866 9867 /** 9868 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 9869 */ 9870 public AddedItemComponent setTax(Money value) { 9871 this.tax = value; 9872 return this; 9873 } 9874 9875 /** 9876 * @return {@link #net} (The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor.) 9877 */ 9878 public Money getNet() { 9879 if (this.net == null) 9880 if (Configuration.errorOnAutoCreate()) 9881 throw new Error("Attempt to auto-create AddedItemComponent.net"); 9882 else if (Configuration.doAutoCreate()) 9883 this.net = new Money(); // cc 9884 return this.net; 9885 } 9886 9887 public boolean hasNet() { 9888 return this.net != null && !this.net.isEmpty(); 9889 } 9890 9891 /** 9892 * @param value {@link #net} (The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor.) 9893 */ 9894 public AddedItemComponent setNet(Money value) { 9895 this.net = value; 9896 return this; 9897 } 9898 9899 /** 9900 * @return {@link #bodySite} (Physical location where the service is performed or applies.) 9901 */ 9902 public List<AddedItemBodySiteComponent> getBodySite() { 9903 if (this.bodySite == null) 9904 this.bodySite = new ArrayList<AddedItemBodySiteComponent>(); 9905 return this.bodySite; 9906 } 9907 9908 /** 9909 * @return Returns a reference to <code>this</code> for easy method chaining 9910 */ 9911 public AddedItemComponent setBodySite(List<AddedItemBodySiteComponent> theBodySite) { 9912 this.bodySite = theBodySite; 9913 return this; 9914 } 9915 9916 public boolean hasBodySite() { 9917 if (this.bodySite == null) 9918 return false; 9919 for (AddedItemBodySiteComponent item : this.bodySite) 9920 if (!item.isEmpty()) 9921 return true; 9922 return false; 9923 } 9924 9925 public AddedItemBodySiteComponent addBodySite() { //3 9926 AddedItemBodySiteComponent t = new AddedItemBodySiteComponent(); 9927 if (this.bodySite == null) 9928 this.bodySite = new ArrayList<AddedItemBodySiteComponent>(); 9929 this.bodySite.add(t); 9930 return t; 9931 } 9932 9933 public AddedItemComponent addBodySite(AddedItemBodySiteComponent t) { //3 9934 if (t == null) 9935 return this; 9936 if (this.bodySite == null) 9937 this.bodySite = new ArrayList<AddedItemBodySiteComponent>(); 9938 this.bodySite.add(t); 9939 return this; 9940 } 9941 9942 /** 9943 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist {3} 9944 */ 9945 public AddedItemBodySiteComponent getBodySiteFirstRep() { 9946 if (getBodySite().isEmpty()) { 9947 addBodySite(); 9948 } 9949 return getBodySite().get(0); 9950 } 9951 9952 /** 9953 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 9954 */ 9955 public List<PositiveIntType> getNoteNumber() { 9956 if (this.noteNumber == null) 9957 this.noteNumber = new ArrayList<PositiveIntType>(); 9958 return this.noteNumber; 9959 } 9960 9961 /** 9962 * @return Returns a reference to <code>this</code> for easy method chaining 9963 */ 9964 public AddedItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 9965 this.noteNumber = theNoteNumber; 9966 return this; 9967 } 9968 9969 public boolean hasNoteNumber() { 9970 if (this.noteNumber == null) 9971 return false; 9972 for (PositiveIntType item : this.noteNumber) 9973 if (!item.isEmpty()) 9974 return true; 9975 return false; 9976 } 9977 9978 /** 9979 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 9980 */ 9981 public PositiveIntType addNoteNumberElement() {//2 9982 PositiveIntType t = new PositiveIntType(); 9983 if (this.noteNumber == null) 9984 this.noteNumber = new ArrayList<PositiveIntType>(); 9985 this.noteNumber.add(t); 9986 return t; 9987 } 9988 9989 /** 9990 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 9991 */ 9992 public AddedItemComponent addNoteNumber(int value) { //1 9993 PositiveIntType t = new PositiveIntType(); 9994 t.setValue(value); 9995 if (this.noteNumber == null) 9996 this.noteNumber = new ArrayList<PositiveIntType>(); 9997 this.noteNumber.add(t); 9998 return this; 9999 } 10000 10001 /** 10002 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 10003 */ 10004 public boolean hasNoteNumber(int value) { 10005 if (this.noteNumber == null) 10006 return false; 10007 for (PositiveIntType v : this.noteNumber) 10008 if (v.getValue().equals(value)) // positiveInt 10009 return true; 10010 return false; 10011 } 10012 10013 /** 10014 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 10015 */ 10016 public ItemReviewOutcomeComponent getReviewOutcome() { 10017 if (this.reviewOutcome == null) 10018 if (Configuration.errorOnAutoCreate()) 10019 throw new Error("Attempt to auto-create AddedItemComponent.reviewOutcome"); 10020 else if (Configuration.doAutoCreate()) 10021 this.reviewOutcome = new ItemReviewOutcomeComponent(); // cc 10022 return this.reviewOutcome; 10023 } 10024 10025 public boolean hasReviewOutcome() { 10026 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 10027 } 10028 10029 /** 10030 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 10031 */ 10032 public AddedItemComponent setReviewOutcome(ItemReviewOutcomeComponent value) { 10033 this.reviewOutcome = value; 10034 return this; 10035 } 10036 10037 /** 10038 * @return {@link #adjudication} (The adjudication results.) 10039 */ 10040 public List<AdjudicationComponent> getAdjudication() { 10041 if (this.adjudication == null) 10042 this.adjudication = new ArrayList<AdjudicationComponent>(); 10043 return this.adjudication; 10044 } 10045 10046 /** 10047 * @return Returns a reference to <code>this</code> for easy method chaining 10048 */ 10049 public AddedItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 10050 this.adjudication = theAdjudication; 10051 return this; 10052 } 10053 10054 public boolean hasAdjudication() { 10055 if (this.adjudication == null) 10056 return false; 10057 for (AdjudicationComponent item : this.adjudication) 10058 if (!item.isEmpty()) 10059 return true; 10060 return false; 10061 } 10062 10063 public AdjudicationComponent addAdjudication() { //3 10064 AdjudicationComponent t = new AdjudicationComponent(); 10065 if (this.adjudication == null) 10066 this.adjudication = new ArrayList<AdjudicationComponent>(); 10067 this.adjudication.add(t); 10068 return t; 10069 } 10070 10071 public AddedItemComponent addAdjudication(AdjudicationComponent t) { //3 10072 if (t == null) 10073 return this; 10074 if (this.adjudication == null) 10075 this.adjudication = new ArrayList<AdjudicationComponent>(); 10076 this.adjudication.add(t); 10077 return this; 10078 } 10079 10080 /** 10081 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 10082 */ 10083 public AdjudicationComponent getAdjudicationFirstRep() { 10084 if (getAdjudication().isEmpty()) { 10085 addAdjudication(); 10086 } 10087 return getAdjudication().get(0); 10088 } 10089 10090 /** 10091 * @return {@link #detail} (The second-tier service adjudications for payor added services.) 10092 */ 10093 public List<AddedItemDetailComponent> getDetail() { 10094 if (this.detail == null) 10095 this.detail = new ArrayList<AddedItemDetailComponent>(); 10096 return this.detail; 10097 } 10098 10099 /** 10100 * @return Returns a reference to <code>this</code> for easy method chaining 10101 */ 10102 public AddedItemComponent setDetail(List<AddedItemDetailComponent> theDetail) { 10103 this.detail = theDetail; 10104 return this; 10105 } 10106 10107 public boolean hasDetail() { 10108 if (this.detail == null) 10109 return false; 10110 for (AddedItemDetailComponent item : this.detail) 10111 if (!item.isEmpty()) 10112 return true; 10113 return false; 10114 } 10115 10116 public AddedItemDetailComponent addDetail() { //3 10117 AddedItemDetailComponent t = new AddedItemDetailComponent(); 10118 if (this.detail == null) 10119 this.detail = new ArrayList<AddedItemDetailComponent>(); 10120 this.detail.add(t); 10121 return t; 10122 } 10123 10124 public AddedItemComponent addDetail(AddedItemDetailComponent t) { //3 10125 if (t == null) 10126 return this; 10127 if (this.detail == null) 10128 this.detail = new ArrayList<AddedItemDetailComponent>(); 10129 this.detail.add(t); 10130 return this; 10131 } 10132 10133 /** 10134 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist {3} 10135 */ 10136 public AddedItemDetailComponent getDetailFirstRep() { 10137 if (getDetail().isEmpty()) { 10138 addDetail(); 10139 } 10140 return getDetail().get(0); 10141 } 10142 10143 protected void listChildren(List<Property> children) { 10144 super.listChildren(children); 10145 children.add(new Property("itemSequence", "positiveInt", "Claim items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, itemSequence)); 10146 children.add(new Property("detailSequence", "positiveInt", "The sequence number of the details within the claim item which this line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, detailSequence)); 10147 children.add(new Property("subDetailSequence", "positiveInt", "The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, subDetailSequence)); 10148 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 10149 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The providers who are authorized for the services rendered to the patient.", 0, java.lang.Integer.MAX_VALUE, provider)); 10150 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 10151 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 10152 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 10153 children.add(new Property("request", "Reference(DeviceRequest|MedicationRequest|NutritionOrder|ServiceRequest|SupplyRequest|VisionPrescription)", "Request or Referral for Goods or Service to be rendered.", 0, java.lang.Integer.MAX_VALUE, request)); 10154 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 10155 children.add(new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 10156 children.add(new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced)); 10157 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location)); 10158 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 10159 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 10160 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 10161 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 10162 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 10163 children.add(new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor.", 0, 1, net)); 10164 children.add(new Property("bodySite", "", "Physical location where the service is performed or applies.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 10165 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 10166 children.add(new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 10167 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 10168 children.add(new Property("detail", "", "The second-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, detail)); 10169 } 10170 10171 @Override 10172 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10173 switch (_hash) { 10174 case 1977979892: /*itemSequence*/ return new Property("itemSequence", "positiveInt", "Claim items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, itemSequence); 10175 case 1321472818: /*detailSequence*/ return new Property("detailSequence", "positiveInt", "The sequence number of the details within the claim item which this line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, detailSequence); 10176 case -855462510: /*subDetailSequence*/ return new Property("subDetailSequence", "positiveInt", "The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, subDetailSequence); 10177 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 10178 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The providers who are authorized for the services rendered to the patient.", 0, java.lang.Integer.MAX_VALUE, provider); 10179 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 10180 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 10181 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 10182 case 1095692943: /*request*/ return new Property("request", "Reference(DeviceRequest|MedicationRequest|NutritionOrder|ServiceRequest|SupplyRequest|VisionPrescription)", "Request or Referral for Goods or Service to be rendered.", 0, java.lang.Integer.MAX_VALUE, request); 10183 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 10184 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 10185 case -1927922223: /*serviced[x]*/ return new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 10186 case 1379209295: /*serviced*/ return new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 10187 case 363246749: /*servicedDate*/ return new Property("serviced[x]", "date", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 10188 case 1534966512: /*servicedPeriod*/ return new Property("serviced[x]", "Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 10189 case 552316075: /*location[x]*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location); 10190 case 1901043637: /*location*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location); 10191 case -1224800468: /*locationCodeableConcept*/ return new Property("location[x]", "CodeableConcept", "Where the product or service was provided.", 0, 1, location); 10192 case -1280020865: /*locationAddress*/ return new Property("location[x]", "Address", "Where the product or service was provided.", 0, 1, location); 10193 case 755866390: /*locationReference*/ return new Property("location[x]", "Reference(Location)", "Where the product or service was provided.", 0, 1, location); 10194 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 10195 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 10196 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 10197 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 10198 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 10199 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor.", 0, 1, net); 10200 case 1702620169: /*bodySite*/ return new Property("bodySite", "", "Physical location where the service is performed or applies.", 0, java.lang.Integer.MAX_VALUE, bodySite); 10201 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 10202 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 10203 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 10204 case -1335224239: /*detail*/ return new Property("detail", "", "The second-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, detail); 10205 default: return super.getNamedProperty(_hash, _name, _checkValid); 10206 } 10207 10208 } 10209 10210 @Override 10211 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10212 switch (hash) { 10213 case 1977979892: /*itemSequence*/ return this.itemSequence == null ? new Base[0] : this.itemSequence.toArray(new Base[this.itemSequence.size()]); // PositiveIntType 10214 case 1321472818: /*detailSequence*/ return this.detailSequence == null ? new Base[0] : this.detailSequence.toArray(new Base[this.detailSequence.size()]); // PositiveIntType 10215 case -855462510: /*subDetailSequence*/ return this.subDetailSequence == null ? new Base[0] : this.subDetailSequence.toArray(new Base[this.subDetailSequence.size()]); // PositiveIntType 10216 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 10217 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : this.provider.toArray(new Base[this.provider.size()]); // Reference 10218 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 10219 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 10220 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 10221 case 1095692943: /*request*/ return this.request == null ? new Base[0] : this.request.toArray(new Base[this.request.size()]); // Reference 10222 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 10223 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 10224 case 1379209295: /*serviced*/ return this.serviced == null ? new Base[0] : new Base[] {this.serviced}; // DataType 10225 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // DataType 10226 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 10227 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 10228 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 10229 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 10230 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 10231 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 10232 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // AddedItemBodySiteComponent 10233 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 10234 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ItemReviewOutcomeComponent 10235 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 10236 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // AddedItemDetailComponent 10237 default: return super.getProperty(hash, name, checkValid); 10238 } 10239 10240 } 10241 10242 @Override 10243 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10244 switch (hash) { 10245 case 1977979892: // itemSequence 10246 this.getItemSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 10247 return value; 10248 case 1321472818: // detailSequence 10249 this.getDetailSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 10250 return value; 10251 case -855462510: // subDetailSequence 10252 this.getSubDetailSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 10253 return value; 10254 case 82505966: // traceNumber 10255 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 10256 return value; 10257 case -987494927: // provider 10258 this.getProvider().add(TypeConvertor.castToReference(value)); // Reference 10259 return value; 10260 case 1099842588: // revenue 10261 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10262 return value; 10263 case 1957227299: // productOrService 10264 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10265 return value; 10266 case -717476168: // productOrServiceEnd 10267 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10268 return value; 10269 case 1095692943: // request 10270 this.getRequest().add(TypeConvertor.castToReference(value)); // Reference 10271 return value; 10272 case -615513385: // modifier 10273 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 10274 return value; 10275 case 1010065041: // programCode 10276 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 10277 return value; 10278 case 1379209295: // serviced 10279 this.serviced = TypeConvertor.castToType(value); // DataType 10280 return value; 10281 case 1901043637: // location 10282 this.location = TypeConvertor.castToType(value); // DataType 10283 return value; 10284 case 525514609: // patientPaid 10285 this.patientPaid = TypeConvertor.castToMoney(value); // Money 10286 return value; 10287 case -1285004149: // quantity 10288 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 10289 return value; 10290 case -486196699: // unitPrice 10291 this.unitPrice = TypeConvertor.castToMoney(value); // Money 10292 return value; 10293 case -1282148017: // factor 10294 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 10295 return value; 10296 case 114603: // tax 10297 this.tax = TypeConvertor.castToMoney(value); // Money 10298 return value; 10299 case 108957: // net 10300 this.net = TypeConvertor.castToMoney(value); // Money 10301 return value; 10302 case 1702620169: // bodySite 10303 this.getBodySite().add((AddedItemBodySiteComponent) value); // AddedItemBodySiteComponent 10304 return value; 10305 case -1110033957: // noteNumber 10306 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 10307 return value; 10308 case -51825446: // reviewOutcome 10309 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 10310 return value; 10311 case -231349275: // adjudication 10312 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 10313 return value; 10314 case -1335224239: // detail 10315 this.getDetail().add((AddedItemDetailComponent) value); // AddedItemDetailComponent 10316 return value; 10317 default: return super.setProperty(hash, name, value); 10318 } 10319 10320 } 10321 10322 @Override 10323 public Base setProperty(String name, Base value) throws FHIRException { 10324 if (name.equals("itemSequence")) { 10325 this.getItemSequence().add(TypeConvertor.castToPositiveInt(value)); 10326 } else if (name.equals("detailSequence")) { 10327 this.getDetailSequence().add(TypeConvertor.castToPositiveInt(value)); 10328 } else if (name.equals("subDetailSequence")) { 10329 this.getSubDetailSequence().add(TypeConvertor.castToPositiveInt(value)); 10330 } else if (name.equals("traceNumber")) { 10331 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 10332 } else if (name.equals("provider")) { 10333 this.getProvider().add(TypeConvertor.castToReference(value)); 10334 } else if (name.equals("revenue")) { 10335 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10336 } else if (name.equals("productOrService")) { 10337 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10338 } else if (name.equals("productOrServiceEnd")) { 10339 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10340 } else if (name.equals("request")) { 10341 this.getRequest().add(TypeConvertor.castToReference(value)); 10342 } else if (name.equals("modifier")) { 10343 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 10344 } else if (name.equals("programCode")) { 10345 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); 10346 } else if (name.equals("serviced[x]")) { 10347 this.serviced = TypeConvertor.castToType(value); // DataType 10348 } else if (name.equals("location[x]")) { 10349 this.location = TypeConvertor.castToType(value); // DataType 10350 } else if (name.equals("patientPaid")) { 10351 this.patientPaid = TypeConvertor.castToMoney(value); // Money 10352 } else if (name.equals("quantity")) { 10353 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 10354 } else if (name.equals("unitPrice")) { 10355 this.unitPrice = TypeConvertor.castToMoney(value); // Money 10356 } else if (name.equals("factor")) { 10357 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 10358 } else if (name.equals("tax")) { 10359 this.tax = TypeConvertor.castToMoney(value); // Money 10360 } else if (name.equals("net")) { 10361 this.net = TypeConvertor.castToMoney(value); // Money 10362 } else if (name.equals("bodySite")) { 10363 this.getBodySite().add((AddedItemBodySiteComponent) value); 10364 } else if (name.equals("noteNumber")) { 10365 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 10366 } else if (name.equals("reviewOutcome")) { 10367 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 10368 } else if (name.equals("adjudication")) { 10369 this.getAdjudication().add((AdjudicationComponent) value); 10370 } else if (name.equals("detail")) { 10371 this.getDetail().add((AddedItemDetailComponent) value); 10372 } else 10373 return super.setProperty(name, value); 10374 return value; 10375 } 10376 10377 @Override 10378 public Base makeProperty(int hash, String name) throws FHIRException { 10379 switch (hash) { 10380 case 1977979892: return addItemSequenceElement(); 10381 case 1321472818: return addDetailSequenceElement(); 10382 case -855462510: return addSubDetailSequenceElement(); 10383 case 82505966: return addTraceNumber(); 10384 case -987494927: return addProvider(); 10385 case 1099842588: return getRevenue(); 10386 case 1957227299: return getProductOrService(); 10387 case -717476168: return getProductOrServiceEnd(); 10388 case 1095692943: return addRequest(); 10389 case -615513385: return addModifier(); 10390 case 1010065041: return addProgramCode(); 10391 case -1927922223: return getServiced(); 10392 case 1379209295: return getServiced(); 10393 case 552316075: return getLocation(); 10394 case 1901043637: return getLocation(); 10395 case 525514609: return getPatientPaid(); 10396 case -1285004149: return getQuantity(); 10397 case -486196699: return getUnitPrice(); 10398 case -1282148017: return getFactorElement(); 10399 case 114603: return getTax(); 10400 case 108957: return getNet(); 10401 case 1702620169: return addBodySite(); 10402 case -1110033957: return addNoteNumberElement(); 10403 case -51825446: return getReviewOutcome(); 10404 case -231349275: return addAdjudication(); 10405 case -1335224239: return addDetail(); 10406 default: return super.makeProperty(hash, name); 10407 } 10408 10409 } 10410 10411 @Override 10412 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10413 switch (hash) { 10414 case 1977979892: /*itemSequence*/ return new String[] {"positiveInt"}; 10415 case 1321472818: /*detailSequence*/ return new String[] {"positiveInt"}; 10416 case -855462510: /*subDetailSequence*/ return new String[] {"positiveInt"}; 10417 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 10418 case -987494927: /*provider*/ return new String[] {"Reference"}; 10419 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 10420 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 10421 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 10422 case 1095692943: /*request*/ return new String[] {"Reference"}; 10423 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 10424 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 10425 case 1379209295: /*serviced*/ return new String[] {"date", "Period"}; 10426 case 1901043637: /*location*/ return new String[] {"CodeableConcept", "Address", "Reference"}; 10427 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 10428 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 10429 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 10430 case -1282148017: /*factor*/ return new String[] {"decimal"}; 10431 case 114603: /*tax*/ return new String[] {"Money"}; 10432 case 108957: /*net*/ return new String[] {"Money"}; 10433 case 1702620169: /*bodySite*/ return new String[] {}; 10434 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 10435 case -51825446: /*reviewOutcome*/ return new String[] {"@ExplanationOfBenefit.item.reviewOutcome"}; 10436 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 10437 case -1335224239: /*detail*/ return new String[] {}; 10438 default: return super.getTypesForProperty(hash, name); 10439 } 10440 10441 } 10442 10443 @Override 10444 public Base addChild(String name) throws FHIRException { 10445 if (name.equals("itemSequence")) { 10446 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.itemSequence"); 10447 } 10448 else if (name.equals("detailSequence")) { 10449 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.detailSequence"); 10450 } 10451 else if (name.equals("subDetailSequence")) { 10452 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.subDetailSequence"); 10453 } 10454 else if (name.equals("traceNumber")) { 10455 return addTraceNumber(); 10456 } 10457 else if (name.equals("provider")) { 10458 return addProvider(); 10459 } 10460 else if (name.equals("revenue")) { 10461 this.revenue = new CodeableConcept(); 10462 return this.revenue; 10463 } 10464 else if (name.equals("productOrService")) { 10465 this.productOrService = new CodeableConcept(); 10466 return this.productOrService; 10467 } 10468 else if (name.equals("productOrServiceEnd")) { 10469 this.productOrServiceEnd = new CodeableConcept(); 10470 return this.productOrServiceEnd; 10471 } 10472 else if (name.equals("request")) { 10473 return addRequest(); 10474 } 10475 else if (name.equals("modifier")) { 10476 return addModifier(); 10477 } 10478 else if (name.equals("programCode")) { 10479 return addProgramCode(); 10480 } 10481 else if (name.equals("servicedDate")) { 10482 this.serviced = new DateType(); 10483 return this.serviced; 10484 } 10485 else if (name.equals("servicedPeriod")) { 10486 this.serviced = new Period(); 10487 return this.serviced; 10488 } 10489 else if (name.equals("locationCodeableConcept")) { 10490 this.location = new CodeableConcept(); 10491 return this.location; 10492 } 10493 else if (name.equals("locationAddress")) { 10494 this.location = new Address(); 10495 return this.location; 10496 } 10497 else if (name.equals("locationReference")) { 10498 this.location = new Reference(); 10499 return this.location; 10500 } 10501 else if (name.equals("patientPaid")) { 10502 this.patientPaid = new Money(); 10503 return this.patientPaid; 10504 } 10505 else if (name.equals("quantity")) { 10506 this.quantity = new Quantity(); 10507 return this.quantity; 10508 } 10509 else if (name.equals("unitPrice")) { 10510 this.unitPrice = new Money(); 10511 return this.unitPrice; 10512 } 10513 else if (name.equals("factor")) { 10514 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.factor"); 10515 } 10516 else if (name.equals("tax")) { 10517 this.tax = new Money(); 10518 return this.tax; 10519 } 10520 else if (name.equals("net")) { 10521 this.net = new Money(); 10522 return this.net; 10523 } 10524 else if (name.equals("bodySite")) { 10525 return addBodySite(); 10526 } 10527 else if (name.equals("noteNumber")) { 10528 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.noteNumber"); 10529 } 10530 else if (name.equals("reviewOutcome")) { 10531 this.reviewOutcome = new ItemReviewOutcomeComponent(); 10532 return this.reviewOutcome; 10533 } 10534 else if (name.equals("adjudication")) { 10535 return addAdjudication(); 10536 } 10537 else if (name.equals("detail")) { 10538 return addDetail(); 10539 } 10540 else 10541 return super.addChild(name); 10542 } 10543 10544 public AddedItemComponent copy() { 10545 AddedItemComponent dst = new AddedItemComponent(); 10546 copyValues(dst); 10547 return dst; 10548 } 10549 10550 public void copyValues(AddedItemComponent dst) { 10551 super.copyValues(dst); 10552 if (itemSequence != null) { 10553 dst.itemSequence = new ArrayList<PositiveIntType>(); 10554 for (PositiveIntType i : itemSequence) 10555 dst.itemSequence.add(i.copy()); 10556 }; 10557 if (detailSequence != null) { 10558 dst.detailSequence = new ArrayList<PositiveIntType>(); 10559 for (PositiveIntType i : detailSequence) 10560 dst.detailSequence.add(i.copy()); 10561 }; 10562 if (subDetailSequence != null) { 10563 dst.subDetailSequence = new ArrayList<PositiveIntType>(); 10564 for (PositiveIntType i : subDetailSequence) 10565 dst.subDetailSequence.add(i.copy()); 10566 }; 10567 if (traceNumber != null) { 10568 dst.traceNumber = new ArrayList<Identifier>(); 10569 for (Identifier i : traceNumber) 10570 dst.traceNumber.add(i.copy()); 10571 }; 10572 if (provider != null) { 10573 dst.provider = new ArrayList<Reference>(); 10574 for (Reference i : provider) 10575 dst.provider.add(i.copy()); 10576 }; 10577 dst.revenue = revenue == null ? null : revenue.copy(); 10578 dst.productOrService = productOrService == null ? null : productOrService.copy(); 10579 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 10580 if (request != null) { 10581 dst.request = new ArrayList<Reference>(); 10582 for (Reference i : request) 10583 dst.request.add(i.copy()); 10584 }; 10585 if (modifier != null) { 10586 dst.modifier = new ArrayList<CodeableConcept>(); 10587 for (CodeableConcept i : modifier) 10588 dst.modifier.add(i.copy()); 10589 }; 10590 if (programCode != null) { 10591 dst.programCode = new ArrayList<CodeableConcept>(); 10592 for (CodeableConcept i : programCode) 10593 dst.programCode.add(i.copy()); 10594 }; 10595 dst.serviced = serviced == null ? null : serviced.copy(); 10596 dst.location = location == null ? null : location.copy(); 10597 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 10598 dst.quantity = quantity == null ? null : quantity.copy(); 10599 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 10600 dst.factor = factor == null ? null : factor.copy(); 10601 dst.tax = tax == null ? null : tax.copy(); 10602 dst.net = net == null ? null : net.copy(); 10603 if (bodySite != null) { 10604 dst.bodySite = new ArrayList<AddedItemBodySiteComponent>(); 10605 for (AddedItemBodySiteComponent i : bodySite) 10606 dst.bodySite.add(i.copy()); 10607 }; 10608 if (noteNumber != null) { 10609 dst.noteNumber = new ArrayList<PositiveIntType>(); 10610 for (PositiveIntType i : noteNumber) 10611 dst.noteNumber.add(i.copy()); 10612 }; 10613 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 10614 if (adjudication != null) { 10615 dst.adjudication = new ArrayList<AdjudicationComponent>(); 10616 for (AdjudicationComponent i : adjudication) 10617 dst.adjudication.add(i.copy()); 10618 }; 10619 if (detail != null) { 10620 dst.detail = new ArrayList<AddedItemDetailComponent>(); 10621 for (AddedItemDetailComponent i : detail) 10622 dst.detail.add(i.copy()); 10623 }; 10624 } 10625 10626 @Override 10627 public boolean equalsDeep(Base other_) { 10628 if (!super.equalsDeep(other_)) 10629 return false; 10630 if (!(other_ instanceof AddedItemComponent)) 10631 return false; 10632 AddedItemComponent o = (AddedItemComponent) other_; 10633 return compareDeep(itemSequence, o.itemSequence, true) && compareDeep(detailSequence, o.detailSequence, true) 10634 && compareDeep(subDetailSequence, o.subDetailSequence, true) && compareDeep(traceNumber, o.traceNumber, true) 10635 && compareDeep(provider, o.provider, true) && compareDeep(revenue, o.revenue, true) && compareDeep(productOrService, o.productOrService, true) 10636 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(request, o.request, true) 10637 && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) && compareDeep(serviced, o.serviced, true) 10638 && compareDeep(location, o.location, true) && compareDeep(patientPaid, o.patientPaid, true) && compareDeep(quantity, o.quantity, true) 10639 && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) && compareDeep(tax, o.tax, true) 10640 && compareDeep(net, o.net, true) && compareDeep(bodySite, o.bodySite, true) && compareDeep(noteNumber, o.noteNumber, true) 10641 && compareDeep(reviewOutcome, o.reviewOutcome, true) && compareDeep(adjudication, o.adjudication, true) 10642 && compareDeep(detail, o.detail, true); 10643 } 10644 10645 @Override 10646 public boolean equalsShallow(Base other_) { 10647 if (!super.equalsShallow(other_)) 10648 return false; 10649 if (!(other_ instanceof AddedItemComponent)) 10650 return false; 10651 AddedItemComponent o = (AddedItemComponent) other_; 10652 return compareValues(itemSequence, o.itemSequence, true) && compareValues(detailSequence, o.detailSequence, true) 10653 && compareValues(subDetailSequence, o.subDetailSequence, true) && compareValues(factor, o.factor, true) 10654 && compareValues(noteNumber, o.noteNumber, true); 10655 } 10656 10657 public boolean isEmpty() { 10658 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(itemSequence, detailSequence 10659 , subDetailSequence, traceNumber, provider, revenue, productOrService, productOrServiceEnd 10660 , request, modifier, programCode, serviced, location, patientPaid, quantity, unitPrice 10661 , factor, tax, net, bodySite, noteNumber, reviewOutcome, adjudication, detail 10662 ); 10663 } 10664 10665 public String fhirType() { 10666 return "ExplanationOfBenefit.addItem"; 10667 10668 } 10669 10670 } 10671 10672 @Block() 10673 public static class AddedItemBodySiteComponent extends BackboneElement implements IBaseBackboneElement { 10674 /** 10675 * Physical service site on the patient (limb, tooth, etc.). 10676 */ 10677 @Child(name = "site", type = {CodeableReference.class}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10678 @Description(shortDefinition="Location", formalDefinition="Physical service site on the patient (limb, tooth, etc.)." ) 10679 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/tooth") 10680 protected List<CodeableReference> site; 10681 10682 /** 10683 * A region or surface of the bodySite, e.g. limb region or tooth surface(s). 10684 */ 10685 @Child(name = "subSite", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10686 @Description(shortDefinition="Sub-location", formalDefinition="A region or surface of the bodySite, e.g. limb region or tooth surface(s)." ) 10687 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/surface") 10688 protected List<CodeableConcept> subSite; 10689 10690 private static final long serialVersionUID = 1190632415L; 10691 10692 /** 10693 * Constructor 10694 */ 10695 public AddedItemBodySiteComponent() { 10696 super(); 10697 } 10698 10699 /** 10700 * Constructor 10701 */ 10702 public AddedItemBodySiteComponent(CodeableReference site) { 10703 super(); 10704 this.addSite(site); 10705 } 10706 10707 /** 10708 * @return {@link #site} (Physical service site on the patient (limb, tooth, etc.).) 10709 */ 10710 public List<CodeableReference> getSite() { 10711 if (this.site == null) 10712 this.site = new ArrayList<CodeableReference>(); 10713 return this.site; 10714 } 10715 10716 /** 10717 * @return Returns a reference to <code>this</code> for easy method chaining 10718 */ 10719 public AddedItemBodySiteComponent setSite(List<CodeableReference> theSite) { 10720 this.site = theSite; 10721 return this; 10722 } 10723 10724 public boolean hasSite() { 10725 if (this.site == null) 10726 return false; 10727 for (CodeableReference item : this.site) 10728 if (!item.isEmpty()) 10729 return true; 10730 return false; 10731 } 10732 10733 public CodeableReference addSite() { //3 10734 CodeableReference t = new CodeableReference(); 10735 if (this.site == null) 10736 this.site = new ArrayList<CodeableReference>(); 10737 this.site.add(t); 10738 return t; 10739 } 10740 10741 public AddedItemBodySiteComponent addSite(CodeableReference t) { //3 10742 if (t == null) 10743 return this; 10744 if (this.site == null) 10745 this.site = new ArrayList<CodeableReference>(); 10746 this.site.add(t); 10747 return this; 10748 } 10749 10750 /** 10751 * @return The first repetition of repeating field {@link #site}, creating it if it does not already exist {3} 10752 */ 10753 public CodeableReference getSiteFirstRep() { 10754 if (getSite().isEmpty()) { 10755 addSite(); 10756 } 10757 return getSite().get(0); 10758 } 10759 10760 /** 10761 * @return {@link #subSite} (A region or surface of the bodySite, e.g. limb region or tooth surface(s).) 10762 */ 10763 public List<CodeableConcept> getSubSite() { 10764 if (this.subSite == null) 10765 this.subSite = new ArrayList<CodeableConcept>(); 10766 return this.subSite; 10767 } 10768 10769 /** 10770 * @return Returns a reference to <code>this</code> for easy method chaining 10771 */ 10772 public AddedItemBodySiteComponent setSubSite(List<CodeableConcept> theSubSite) { 10773 this.subSite = theSubSite; 10774 return this; 10775 } 10776 10777 public boolean hasSubSite() { 10778 if (this.subSite == null) 10779 return false; 10780 for (CodeableConcept item : this.subSite) 10781 if (!item.isEmpty()) 10782 return true; 10783 return false; 10784 } 10785 10786 public CodeableConcept addSubSite() { //3 10787 CodeableConcept t = new CodeableConcept(); 10788 if (this.subSite == null) 10789 this.subSite = new ArrayList<CodeableConcept>(); 10790 this.subSite.add(t); 10791 return t; 10792 } 10793 10794 public AddedItemBodySiteComponent addSubSite(CodeableConcept t) { //3 10795 if (t == null) 10796 return this; 10797 if (this.subSite == null) 10798 this.subSite = new ArrayList<CodeableConcept>(); 10799 this.subSite.add(t); 10800 return this; 10801 } 10802 10803 /** 10804 * @return The first repetition of repeating field {@link #subSite}, creating it if it does not already exist {3} 10805 */ 10806 public CodeableConcept getSubSiteFirstRep() { 10807 if (getSubSite().isEmpty()) { 10808 addSubSite(); 10809 } 10810 return getSubSite().get(0); 10811 } 10812 10813 protected void listChildren(List<Property> children) { 10814 super.listChildren(children); 10815 children.add(new Property("site", "CodeableReference(BodyStructure)", "Physical service site on the patient (limb, tooth, etc.).", 0, java.lang.Integer.MAX_VALUE, site)); 10816 children.add(new Property("subSite", "CodeableConcept", "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite)); 10817 } 10818 10819 @Override 10820 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10821 switch (_hash) { 10822 case 3530567: /*site*/ return new Property("site", "CodeableReference(BodyStructure)", "Physical service site on the patient (limb, tooth, etc.).", 0, java.lang.Integer.MAX_VALUE, site); 10823 case -1868566105: /*subSite*/ return new Property("subSite", "CodeableConcept", "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite); 10824 default: return super.getNamedProperty(_hash, _name, _checkValid); 10825 } 10826 10827 } 10828 10829 @Override 10830 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10831 switch (hash) { 10832 case 3530567: /*site*/ return this.site == null ? new Base[0] : this.site.toArray(new Base[this.site.size()]); // CodeableReference 10833 case -1868566105: /*subSite*/ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 10834 default: return super.getProperty(hash, name, checkValid); 10835 } 10836 10837 } 10838 10839 @Override 10840 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10841 switch (hash) { 10842 case 3530567: // site 10843 this.getSite().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 10844 return value; 10845 case -1868566105: // subSite 10846 this.getSubSite().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 10847 return value; 10848 default: return super.setProperty(hash, name, value); 10849 } 10850 10851 } 10852 10853 @Override 10854 public Base setProperty(String name, Base value) throws FHIRException { 10855 if (name.equals("site")) { 10856 this.getSite().add(TypeConvertor.castToCodeableReference(value)); 10857 } else if (name.equals("subSite")) { 10858 this.getSubSite().add(TypeConvertor.castToCodeableConcept(value)); 10859 } else 10860 return super.setProperty(name, value); 10861 return value; 10862 } 10863 10864 @Override 10865 public Base makeProperty(int hash, String name) throws FHIRException { 10866 switch (hash) { 10867 case 3530567: return addSite(); 10868 case -1868566105: return addSubSite(); 10869 default: return super.makeProperty(hash, name); 10870 } 10871 10872 } 10873 10874 @Override 10875 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10876 switch (hash) { 10877 case 3530567: /*site*/ return new String[] {"CodeableReference"}; 10878 case -1868566105: /*subSite*/ return new String[] {"CodeableConcept"}; 10879 default: return super.getTypesForProperty(hash, name); 10880 } 10881 10882 } 10883 10884 @Override 10885 public Base addChild(String name) throws FHIRException { 10886 if (name.equals("site")) { 10887 return addSite(); 10888 } 10889 else if (name.equals("subSite")) { 10890 return addSubSite(); 10891 } 10892 else 10893 return super.addChild(name); 10894 } 10895 10896 public AddedItemBodySiteComponent copy() { 10897 AddedItemBodySiteComponent dst = new AddedItemBodySiteComponent(); 10898 copyValues(dst); 10899 return dst; 10900 } 10901 10902 public void copyValues(AddedItemBodySiteComponent dst) { 10903 super.copyValues(dst); 10904 if (site != null) { 10905 dst.site = new ArrayList<CodeableReference>(); 10906 for (CodeableReference i : site) 10907 dst.site.add(i.copy()); 10908 }; 10909 if (subSite != null) { 10910 dst.subSite = new ArrayList<CodeableConcept>(); 10911 for (CodeableConcept i : subSite) 10912 dst.subSite.add(i.copy()); 10913 }; 10914 } 10915 10916 @Override 10917 public boolean equalsDeep(Base other_) { 10918 if (!super.equalsDeep(other_)) 10919 return false; 10920 if (!(other_ instanceof AddedItemBodySiteComponent)) 10921 return false; 10922 AddedItemBodySiteComponent o = (AddedItemBodySiteComponent) other_; 10923 return compareDeep(site, o.site, true) && compareDeep(subSite, o.subSite, true); 10924 } 10925 10926 @Override 10927 public boolean equalsShallow(Base other_) { 10928 if (!super.equalsShallow(other_)) 10929 return false; 10930 if (!(other_ instanceof AddedItemBodySiteComponent)) 10931 return false; 10932 AddedItemBodySiteComponent o = (AddedItemBodySiteComponent) other_; 10933 return true; 10934 } 10935 10936 public boolean isEmpty() { 10937 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(site, subSite); 10938 } 10939 10940 public String fhirType() { 10941 return "ExplanationOfBenefit.addItem.bodySite"; 10942 10943 } 10944 10945 } 10946 10947 @Block() 10948 public static class AddedItemDetailComponent extends BackboneElement implements IBaseBackboneElement { 10949 /** 10950 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 10951 */ 10952 @Child(name = "traceNumber", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10953 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 10954 protected List<Identifier> traceNumber; 10955 10956 /** 10957 * The type of revenue or cost center providing the product and/or service. 10958 */ 10959 @Child(name = "revenue", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 10960 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 10961 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 10962 protected CodeableConcept revenue; 10963 10964 /** 10965 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 10966 */ 10967 @Child(name = "productOrService", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 10968 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 10969 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 10970 protected CodeableConcept productOrService; 10971 10972 /** 10973 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 10974 */ 10975 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 10976 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 10977 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 10978 protected CodeableConcept productOrServiceEnd; 10979 10980 /** 10981 * Item typification or modifiers codes to convey additional context for the product or service. 10982 */ 10983 @Child(name = "modifier", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10984 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 10985 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 10986 protected List<CodeableConcept> modifier; 10987 10988 /** 10989 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 10990 */ 10991 @Child(name = "patientPaid", type = {Money.class}, order=6, min=0, max=1, modifier=false, summary=false) 10992 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 10993 protected Money patientPaid; 10994 10995 /** 10996 * The number of repetitions of a service or product. 10997 */ 10998 @Child(name = "quantity", type = {Quantity.class}, order=7, min=0, max=1, modifier=false, summary=false) 10999 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 11000 protected Quantity quantity; 11001 11002 /** 11003 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 11004 */ 11005 @Child(name = "unitPrice", type = {Money.class}, order=8, min=0, max=1, modifier=false, summary=false) 11006 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 11007 protected Money unitPrice; 11008 11009 /** 11010 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 11011 */ 11012 @Child(name = "factor", type = {DecimalType.class}, order=9, min=0, max=1, modifier=false, summary=false) 11013 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 11014 protected DecimalType factor; 11015 11016 /** 11017 * The total of taxes applicable for this product or service. 11018 */ 11019 @Child(name = "tax", type = {Money.class}, order=10, min=0, max=1, modifier=false, summary=false) 11020 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 11021 protected Money tax; 11022 11023 /** 11024 * The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor. 11025 */ 11026 @Child(name = "net", type = {Money.class}, order=11, min=0, max=1, modifier=false, summary=false) 11027 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor." ) 11028 protected Money net; 11029 11030 /** 11031 * The numbers associated with notes below which apply to the adjudication of this item. 11032 */ 11033 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 11034 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 11035 protected List<PositiveIntType> noteNumber; 11036 11037 /** 11038 * The high-level results of the adjudication if adjudication has been performed. 11039 */ 11040 @Child(name = "reviewOutcome", type = {ItemReviewOutcomeComponent.class}, order=13, min=0, max=1, modifier=false, summary=false) 11041 @Description(shortDefinition="Additem detail level adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 11042 protected ItemReviewOutcomeComponent reviewOutcome; 11043 11044 /** 11045 * The adjudication results. 11046 */ 11047 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 11048 @Description(shortDefinition="Added items adjudication", formalDefinition="The adjudication results." ) 11049 protected List<AdjudicationComponent> adjudication; 11050 11051 /** 11052 * The third-tier service adjudications for payor added services. 11053 */ 11054 @Child(name = "subDetail", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 11055 @Description(shortDefinition="Insurer added line items", formalDefinition="The third-tier service adjudications for payor added services." ) 11056 protected List<AddedItemDetailSubDetailComponent> subDetail; 11057 11058 private static final long serialVersionUID = 1088072336L; 11059 11060 /** 11061 * Constructor 11062 */ 11063 public AddedItemDetailComponent() { 11064 super(); 11065 } 11066 11067 /** 11068 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 11069 */ 11070 public List<Identifier> getTraceNumber() { 11071 if (this.traceNumber == null) 11072 this.traceNumber = new ArrayList<Identifier>(); 11073 return this.traceNumber; 11074 } 11075 11076 /** 11077 * @return Returns a reference to <code>this</code> for easy method chaining 11078 */ 11079 public AddedItemDetailComponent setTraceNumber(List<Identifier> theTraceNumber) { 11080 this.traceNumber = theTraceNumber; 11081 return this; 11082 } 11083 11084 public boolean hasTraceNumber() { 11085 if (this.traceNumber == null) 11086 return false; 11087 for (Identifier item : this.traceNumber) 11088 if (!item.isEmpty()) 11089 return true; 11090 return false; 11091 } 11092 11093 public Identifier addTraceNumber() { //3 11094 Identifier t = new Identifier(); 11095 if (this.traceNumber == null) 11096 this.traceNumber = new ArrayList<Identifier>(); 11097 this.traceNumber.add(t); 11098 return t; 11099 } 11100 11101 public AddedItemDetailComponent addTraceNumber(Identifier t) { //3 11102 if (t == null) 11103 return this; 11104 if (this.traceNumber == null) 11105 this.traceNumber = new ArrayList<Identifier>(); 11106 this.traceNumber.add(t); 11107 return this; 11108 } 11109 11110 /** 11111 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 11112 */ 11113 public Identifier getTraceNumberFirstRep() { 11114 if (getTraceNumber().isEmpty()) { 11115 addTraceNumber(); 11116 } 11117 return getTraceNumber().get(0); 11118 } 11119 11120 /** 11121 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 11122 */ 11123 public CodeableConcept getRevenue() { 11124 if (this.revenue == null) 11125 if (Configuration.errorOnAutoCreate()) 11126 throw new Error("Attempt to auto-create AddedItemDetailComponent.revenue"); 11127 else if (Configuration.doAutoCreate()) 11128 this.revenue = new CodeableConcept(); // cc 11129 return this.revenue; 11130 } 11131 11132 public boolean hasRevenue() { 11133 return this.revenue != null && !this.revenue.isEmpty(); 11134 } 11135 11136 /** 11137 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 11138 */ 11139 public AddedItemDetailComponent setRevenue(CodeableConcept value) { 11140 this.revenue = value; 11141 return this; 11142 } 11143 11144 /** 11145 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 11146 */ 11147 public CodeableConcept getProductOrService() { 11148 if (this.productOrService == null) 11149 if (Configuration.errorOnAutoCreate()) 11150 throw new Error("Attempt to auto-create AddedItemDetailComponent.productOrService"); 11151 else if (Configuration.doAutoCreate()) 11152 this.productOrService = new CodeableConcept(); // cc 11153 return this.productOrService; 11154 } 11155 11156 public boolean hasProductOrService() { 11157 return this.productOrService != null && !this.productOrService.isEmpty(); 11158 } 11159 11160 /** 11161 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 11162 */ 11163 public AddedItemDetailComponent setProductOrService(CodeableConcept value) { 11164 this.productOrService = value; 11165 return this; 11166 } 11167 11168 /** 11169 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 11170 */ 11171 public CodeableConcept getProductOrServiceEnd() { 11172 if (this.productOrServiceEnd == null) 11173 if (Configuration.errorOnAutoCreate()) 11174 throw new Error("Attempt to auto-create AddedItemDetailComponent.productOrServiceEnd"); 11175 else if (Configuration.doAutoCreate()) 11176 this.productOrServiceEnd = new CodeableConcept(); // cc 11177 return this.productOrServiceEnd; 11178 } 11179 11180 public boolean hasProductOrServiceEnd() { 11181 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 11182 } 11183 11184 /** 11185 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 11186 */ 11187 public AddedItemDetailComponent setProductOrServiceEnd(CodeableConcept value) { 11188 this.productOrServiceEnd = value; 11189 return this; 11190 } 11191 11192 /** 11193 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 11194 */ 11195 public List<CodeableConcept> getModifier() { 11196 if (this.modifier == null) 11197 this.modifier = new ArrayList<CodeableConcept>(); 11198 return this.modifier; 11199 } 11200 11201 /** 11202 * @return Returns a reference to <code>this</code> for easy method chaining 11203 */ 11204 public AddedItemDetailComponent setModifier(List<CodeableConcept> theModifier) { 11205 this.modifier = theModifier; 11206 return this; 11207 } 11208 11209 public boolean hasModifier() { 11210 if (this.modifier == null) 11211 return false; 11212 for (CodeableConcept item : this.modifier) 11213 if (!item.isEmpty()) 11214 return true; 11215 return false; 11216 } 11217 11218 public CodeableConcept addModifier() { //3 11219 CodeableConcept t = new CodeableConcept(); 11220 if (this.modifier == null) 11221 this.modifier = new ArrayList<CodeableConcept>(); 11222 this.modifier.add(t); 11223 return t; 11224 } 11225 11226 public AddedItemDetailComponent addModifier(CodeableConcept t) { //3 11227 if (t == null) 11228 return this; 11229 if (this.modifier == null) 11230 this.modifier = new ArrayList<CodeableConcept>(); 11231 this.modifier.add(t); 11232 return this; 11233 } 11234 11235 /** 11236 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 11237 */ 11238 public CodeableConcept getModifierFirstRep() { 11239 if (getModifier().isEmpty()) { 11240 addModifier(); 11241 } 11242 return getModifier().get(0); 11243 } 11244 11245 /** 11246 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 11247 */ 11248 public Money getPatientPaid() { 11249 if (this.patientPaid == null) 11250 if (Configuration.errorOnAutoCreate()) 11251 throw new Error("Attempt to auto-create AddedItemDetailComponent.patientPaid"); 11252 else if (Configuration.doAutoCreate()) 11253 this.patientPaid = new Money(); // cc 11254 return this.patientPaid; 11255 } 11256 11257 public boolean hasPatientPaid() { 11258 return this.patientPaid != null && !this.patientPaid.isEmpty(); 11259 } 11260 11261 /** 11262 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 11263 */ 11264 public AddedItemDetailComponent setPatientPaid(Money value) { 11265 this.patientPaid = value; 11266 return this; 11267 } 11268 11269 /** 11270 * @return {@link #quantity} (The number of repetitions of a service or product.) 11271 */ 11272 public Quantity getQuantity() { 11273 if (this.quantity == null) 11274 if (Configuration.errorOnAutoCreate()) 11275 throw new Error("Attempt to auto-create AddedItemDetailComponent.quantity"); 11276 else if (Configuration.doAutoCreate()) 11277 this.quantity = new Quantity(); // cc 11278 return this.quantity; 11279 } 11280 11281 public boolean hasQuantity() { 11282 return this.quantity != null && !this.quantity.isEmpty(); 11283 } 11284 11285 /** 11286 * @param value {@link #quantity} (The number of repetitions of a service or product.) 11287 */ 11288 public AddedItemDetailComponent setQuantity(Quantity value) { 11289 this.quantity = value; 11290 return this; 11291 } 11292 11293 /** 11294 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 11295 */ 11296 public Money getUnitPrice() { 11297 if (this.unitPrice == null) 11298 if (Configuration.errorOnAutoCreate()) 11299 throw new Error("Attempt to auto-create AddedItemDetailComponent.unitPrice"); 11300 else if (Configuration.doAutoCreate()) 11301 this.unitPrice = new Money(); // cc 11302 return this.unitPrice; 11303 } 11304 11305 public boolean hasUnitPrice() { 11306 return this.unitPrice != null && !this.unitPrice.isEmpty(); 11307 } 11308 11309 /** 11310 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 11311 */ 11312 public AddedItemDetailComponent setUnitPrice(Money value) { 11313 this.unitPrice = value; 11314 return this; 11315 } 11316 11317 /** 11318 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 11319 */ 11320 public DecimalType getFactorElement() { 11321 if (this.factor == null) 11322 if (Configuration.errorOnAutoCreate()) 11323 throw new Error("Attempt to auto-create AddedItemDetailComponent.factor"); 11324 else if (Configuration.doAutoCreate()) 11325 this.factor = new DecimalType(); // bb 11326 return this.factor; 11327 } 11328 11329 public boolean hasFactorElement() { 11330 return this.factor != null && !this.factor.isEmpty(); 11331 } 11332 11333 public boolean hasFactor() { 11334 return this.factor != null && !this.factor.isEmpty(); 11335 } 11336 11337 /** 11338 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 11339 */ 11340 public AddedItemDetailComponent setFactorElement(DecimalType value) { 11341 this.factor = value; 11342 return this; 11343 } 11344 11345 /** 11346 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 11347 */ 11348 public BigDecimal getFactor() { 11349 return this.factor == null ? null : this.factor.getValue(); 11350 } 11351 11352 /** 11353 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 11354 */ 11355 public AddedItemDetailComponent setFactor(BigDecimal value) { 11356 if (value == null) 11357 this.factor = null; 11358 else { 11359 if (this.factor == null) 11360 this.factor = new DecimalType(); 11361 this.factor.setValue(value); 11362 } 11363 return this; 11364 } 11365 11366 /** 11367 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 11368 */ 11369 public AddedItemDetailComponent setFactor(long value) { 11370 this.factor = new DecimalType(); 11371 this.factor.setValue(value); 11372 return this; 11373 } 11374 11375 /** 11376 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 11377 */ 11378 public AddedItemDetailComponent setFactor(double value) { 11379 this.factor = new DecimalType(); 11380 this.factor.setValue(value); 11381 return this; 11382 } 11383 11384 /** 11385 * @return {@link #tax} (The total of taxes applicable for this product or service.) 11386 */ 11387 public Money getTax() { 11388 if (this.tax == null) 11389 if (Configuration.errorOnAutoCreate()) 11390 throw new Error("Attempt to auto-create AddedItemDetailComponent.tax"); 11391 else if (Configuration.doAutoCreate()) 11392 this.tax = new Money(); // cc 11393 return this.tax; 11394 } 11395 11396 public boolean hasTax() { 11397 return this.tax != null && !this.tax.isEmpty(); 11398 } 11399 11400 /** 11401 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 11402 */ 11403 public AddedItemDetailComponent setTax(Money value) { 11404 this.tax = value; 11405 return this; 11406 } 11407 11408 /** 11409 * @return {@link #net} (The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor.) 11410 */ 11411 public Money getNet() { 11412 if (this.net == null) 11413 if (Configuration.errorOnAutoCreate()) 11414 throw new Error("Attempt to auto-create AddedItemDetailComponent.net"); 11415 else if (Configuration.doAutoCreate()) 11416 this.net = new Money(); // cc 11417 return this.net; 11418 } 11419 11420 public boolean hasNet() { 11421 return this.net != null && !this.net.isEmpty(); 11422 } 11423 11424 /** 11425 * @param value {@link #net} (The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor.) 11426 */ 11427 public AddedItemDetailComponent setNet(Money value) { 11428 this.net = value; 11429 return this; 11430 } 11431 11432 /** 11433 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 11434 */ 11435 public List<PositiveIntType> getNoteNumber() { 11436 if (this.noteNumber == null) 11437 this.noteNumber = new ArrayList<PositiveIntType>(); 11438 return this.noteNumber; 11439 } 11440 11441 /** 11442 * @return Returns a reference to <code>this</code> for easy method chaining 11443 */ 11444 public AddedItemDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 11445 this.noteNumber = theNoteNumber; 11446 return this; 11447 } 11448 11449 public boolean hasNoteNumber() { 11450 if (this.noteNumber == null) 11451 return false; 11452 for (PositiveIntType item : this.noteNumber) 11453 if (!item.isEmpty()) 11454 return true; 11455 return false; 11456 } 11457 11458 /** 11459 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 11460 */ 11461 public PositiveIntType addNoteNumberElement() {//2 11462 PositiveIntType t = new PositiveIntType(); 11463 if (this.noteNumber == null) 11464 this.noteNumber = new ArrayList<PositiveIntType>(); 11465 this.noteNumber.add(t); 11466 return t; 11467 } 11468 11469 /** 11470 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 11471 */ 11472 public AddedItemDetailComponent addNoteNumber(int value) { //1 11473 PositiveIntType t = new PositiveIntType(); 11474 t.setValue(value); 11475 if (this.noteNumber == null) 11476 this.noteNumber = new ArrayList<PositiveIntType>(); 11477 this.noteNumber.add(t); 11478 return this; 11479 } 11480 11481 /** 11482 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 11483 */ 11484 public boolean hasNoteNumber(int value) { 11485 if (this.noteNumber == null) 11486 return false; 11487 for (PositiveIntType v : this.noteNumber) 11488 if (v.getValue().equals(value)) // positiveInt 11489 return true; 11490 return false; 11491 } 11492 11493 /** 11494 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 11495 */ 11496 public ItemReviewOutcomeComponent getReviewOutcome() { 11497 if (this.reviewOutcome == null) 11498 if (Configuration.errorOnAutoCreate()) 11499 throw new Error("Attempt to auto-create AddedItemDetailComponent.reviewOutcome"); 11500 else if (Configuration.doAutoCreate()) 11501 this.reviewOutcome = new ItemReviewOutcomeComponent(); // cc 11502 return this.reviewOutcome; 11503 } 11504 11505 public boolean hasReviewOutcome() { 11506 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 11507 } 11508 11509 /** 11510 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 11511 */ 11512 public AddedItemDetailComponent setReviewOutcome(ItemReviewOutcomeComponent value) { 11513 this.reviewOutcome = value; 11514 return this; 11515 } 11516 11517 /** 11518 * @return {@link #adjudication} (The adjudication results.) 11519 */ 11520 public List<AdjudicationComponent> getAdjudication() { 11521 if (this.adjudication == null) 11522 this.adjudication = new ArrayList<AdjudicationComponent>(); 11523 return this.adjudication; 11524 } 11525 11526 /** 11527 * @return Returns a reference to <code>this</code> for easy method chaining 11528 */ 11529 public AddedItemDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 11530 this.adjudication = theAdjudication; 11531 return this; 11532 } 11533 11534 public boolean hasAdjudication() { 11535 if (this.adjudication == null) 11536 return false; 11537 for (AdjudicationComponent item : this.adjudication) 11538 if (!item.isEmpty()) 11539 return true; 11540 return false; 11541 } 11542 11543 public AdjudicationComponent addAdjudication() { //3 11544 AdjudicationComponent t = new AdjudicationComponent(); 11545 if (this.adjudication == null) 11546 this.adjudication = new ArrayList<AdjudicationComponent>(); 11547 this.adjudication.add(t); 11548 return t; 11549 } 11550 11551 public AddedItemDetailComponent addAdjudication(AdjudicationComponent t) { //3 11552 if (t == null) 11553 return this; 11554 if (this.adjudication == null) 11555 this.adjudication = new ArrayList<AdjudicationComponent>(); 11556 this.adjudication.add(t); 11557 return this; 11558 } 11559 11560 /** 11561 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 11562 */ 11563 public AdjudicationComponent getAdjudicationFirstRep() { 11564 if (getAdjudication().isEmpty()) { 11565 addAdjudication(); 11566 } 11567 return getAdjudication().get(0); 11568 } 11569 11570 /** 11571 * @return {@link #subDetail} (The third-tier service adjudications for payor added services.) 11572 */ 11573 public List<AddedItemDetailSubDetailComponent> getSubDetail() { 11574 if (this.subDetail == null) 11575 this.subDetail = new ArrayList<AddedItemDetailSubDetailComponent>(); 11576 return this.subDetail; 11577 } 11578 11579 /** 11580 * @return Returns a reference to <code>this</code> for easy method chaining 11581 */ 11582 public AddedItemDetailComponent setSubDetail(List<AddedItemDetailSubDetailComponent> theSubDetail) { 11583 this.subDetail = theSubDetail; 11584 return this; 11585 } 11586 11587 public boolean hasSubDetail() { 11588 if (this.subDetail == null) 11589 return false; 11590 for (AddedItemDetailSubDetailComponent item : this.subDetail) 11591 if (!item.isEmpty()) 11592 return true; 11593 return false; 11594 } 11595 11596 public AddedItemDetailSubDetailComponent addSubDetail() { //3 11597 AddedItemDetailSubDetailComponent t = new AddedItemDetailSubDetailComponent(); 11598 if (this.subDetail == null) 11599 this.subDetail = new ArrayList<AddedItemDetailSubDetailComponent>(); 11600 this.subDetail.add(t); 11601 return t; 11602 } 11603 11604 public AddedItemDetailComponent addSubDetail(AddedItemDetailSubDetailComponent t) { //3 11605 if (t == null) 11606 return this; 11607 if (this.subDetail == null) 11608 this.subDetail = new ArrayList<AddedItemDetailSubDetailComponent>(); 11609 this.subDetail.add(t); 11610 return this; 11611 } 11612 11613 /** 11614 * @return The first repetition of repeating field {@link #subDetail}, creating it if it does not already exist {3} 11615 */ 11616 public AddedItemDetailSubDetailComponent getSubDetailFirstRep() { 11617 if (getSubDetail().isEmpty()) { 11618 addSubDetail(); 11619 } 11620 return getSubDetail().get(0); 11621 } 11622 11623 protected void listChildren(List<Property> children) { 11624 super.listChildren(children); 11625 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 11626 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 11627 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 11628 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 11629 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 11630 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 11631 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 11632 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 11633 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 11634 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 11635 children.add(new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor.", 0, 1, net)); 11636 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 11637 children.add(new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 11638 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 11639 children.add(new Property("subDetail", "", "The third-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, subDetail)); 11640 } 11641 11642 @Override 11643 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 11644 switch (_hash) { 11645 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 11646 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 11647 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 11648 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 11649 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 11650 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 11651 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 11652 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 11653 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 11654 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 11655 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor.", 0, 1, net); 11656 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 11657 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 11658 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 11659 case -828829007: /*subDetail*/ return new Property("subDetail", "", "The third-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, subDetail); 11660 default: return super.getNamedProperty(_hash, _name, _checkValid); 11661 } 11662 11663 } 11664 11665 @Override 11666 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 11667 switch (hash) { 11668 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 11669 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 11670 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 11671 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 11672 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 11673 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 11674 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 11675 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 11676 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 11677 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 11678 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 11679 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 11680 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ItemReviewOutcomeComponent 11681 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 11682 case -828829007: /*subDetail*/ return this.subDetail == null ? new Base[0] : this.subDetail.toArray(new Base[this.subDetail.size()]); // AddedItemDetailSubDetailComponent 11683 default: return super.getProperty(hash, name, checkValid); 11684 } 11685 11686 } 11687 11688 @Override 11689 public Base setProperty(int hash, String name, Base value) throws FHIRException { 11690 switch (hash) { 11691 case 82505966: // traceNumber 11692 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 11693 return value; 11694 case 1099842588: // revenue 11695 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 11696 return value; 11697 case 1957227299: // productOrService 11698 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 11699 return value; 11700 case -717476168: // productOrServiceEnd 11701 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 11702 return value; 11703 case -615513385: // modifier 11704 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 11705 return value; 11706 case 525514609: // patientPaid 11707 this.patientPaid = TypeConvertor.castToMoney(value); // Money 11708 return value; 11709 case -1285004149: // quantity 11710 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 11711 return value; 11712 case -486196699: // unitPrice 11713 this.unitPrice = TypeConvertor.castToMoney(value); // Money 11714 return value; 11715 case -1282148017: // factor 11716 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 11717 return value; 11718 case 114603: // tax 11719 this.tax = TypeConvertor.castToMoney(value); // Money 11720 return value; 11721 case 108957: // net 11722 this.net = TypeConvertor.castToMoney(value); // Money 11723 return value; 11724 case -1110033957: // noteNumber 11725 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 11726 return value; 11727 case -51825446: // reviewOutcome 11728 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 11729 return value; 11730 case -231349275: // adjudication 11731 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 11732 return value; 11733 case -828829007: // subDetail 11734 this.getSubDetail().add((AddedItemDetailSubDetailComponent) value); // AddedItemDetailSubDetailComponent 11735 return value; 11736 default: return super.setProperty(hash, name, value); 11737 } 11738 11739 } 11740 11741 @Override 11742 public Base setProperty(String name, Base value) throws FHIRException { 11743 if (name.equals("traceNumber")) { 11744 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 11745 } else if (name.equals("revenue")) { 11746 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 11747 } else if (name.equals("productOrService")) { 11748 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 11749 } else if (name.equals("productOrServiceEnd")) { 11750 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 11751 } else if (name.equals("modifier")) { 11752 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 11753 } else if (name.equals("patientPaid")) { 11754 this.patientPaid = TypeConvertor.castToMoney(value); // Money 11755 } else if (name.equals("quantity")) { 11756 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 11757 } else if (name.equals("unitPrice")) { 11758 this.unitPrice = TypeConvertor.castToMoney(value); // Money 11759 } else if (name.equals("factor")) { 11760 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 11761 } else if (name.equals("tax")) { 11762 this.tax = TypeConvertor.castToMoney(value); // Money 11763 } else if (name.equals("net")) { 11764 this.net = TypeConvertor.castToMoney(value); // Money 11765 } else if (name.equals("noteNumber")) { 11766 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 11767 } else if (name.equals("reviewOutcome")) { 11768 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 11769 } else if (name.equals("adjudication")) { 11770 this.getAdjudication().add((AdjudicationComponent) value); 11771 } else if (name.equals("subDetail")) { 11772 this.getSubDetail().add((AddedItemDetailSubDetailComponent) value); 11773 } else 11774 return super.setProperty(name, value); 11775 return value; 11776 } 11777 11778 @Override 11779 public Base makeProperty(int hash, String name) throws FHIRException { 11780 switch (hash) { 11781 case 82505966: return addTraceNumber(); 11782 case 1099842588: return getRevenue(); 11783 case 1957227299: return getProductOrService(); 11784 case -717476168: return getProductOrServiceEnd(); 11785 case -615513385: return addModifier(); 11786 case 525514609: return getPatientPaid(); 11787 case -1285004149: return getQuantity(); 11788 case -486196699: return getUnitPrice(); 11789 case -1282148017: return getFactorElement(); 11790 case 114603: return getTax(); 11791 case 108957: return getNet(); 11792 case -1110033957: return addNoteNumberElement(); 11793 case -51825446: return getReviewOutcome(); 11794 case -231349275: return addAdjudication(); 11795 case -828829007: return addSubDetail(); 11796 default: return super.makeProperty(hash, name); 11797 } 11798 11799 } 11800 11801 @Override 11802 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 11803 switch (hash) { 11804 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 11805 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 11806 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 11807 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 11808 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 11809 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 11810 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 11811 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 11812 case -1282148017: /*factor*/ return new String[] {"decimal"}; 11813 case 114603: /*tax*/ return new String[] {"Money"}; 11814 case 108957: /*net*/ return new String[] {"Money"}; 11815 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 11816 case -51825446: /*reviewOutcome*/ return new String[] {"@ExplanationOfBenefit.item.reviewOutcome"}; 11817 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 11818 case -828829007: /*subDetail*/ return new String[] {}; 11819 default: return super.getTypesForProperty(hash, name); 11820 } 11821 11822 } 11823 11824 @Override 11825 public Base addChild(String name) throws FHIRException { 11826 if (name.equals("traceNumber")) { 11827 return addTraceNumber(); 11828 } 11829 else if (name.equals("revenue")) { 11830 this.revenue = new CodeableConcept(); 11831 return this.revenue; 11832 } 11833 else if (name.equals("productOrService")) { 11834 this.productOrService = new CodeableConcept(); 11835 return this.productOrService; 11836 } 11837 else if (name.equals("productOrServiceEnd")) { 11838 this.productOrServiceEnd = new CodeableConcept(); 11839 return this.productOrServiceEnd; 11840 } 11841 else if (name.equals("modifier")) { 11842 return addModifier(); 11843 } 11844 else if (name.equals("patientPaid")) { 11845 this.patientPaid = new Money(); 11846 return this.patientPaid; 11847 } 11848 else if (name.equals("quantity")) { 11849 this.quantity = new Quantity(); 11850 return this.quantity; 11851 } 11852 else if (name.equals("unitPrice")) { 11853 this.unitPrice = new Money(); 11854 return this.unitPrice; 11855 } 11856 else if (name.equals("factor")) { 11857 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.detail.factor"); 11858 } 11859 else if (name.equals("tax")) { 11860 this.tax = new Money(); 11861 return this.tax; 11862 } 11863 else if (name.equals("net")) { 11864 this.net = new Money(); 11865 return this.net; 11866 } 11867 else if (name.equals("noteNumber")) { 11868 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.detail.noteNumber"); 11869 } 11870 else if (name.equals("reviewOutcome")) { 11871 this.reviewOutcome = new ItemReviewOutcomeComponent(); 11872 return this.reviewOutcome; 11873 } 11874 else if (name.equals("adjudication")) { 11875 return addAdjudication(); 11876 } 11877 else if (name.equals("subDetail")) { 11878 return addSubDetail(); 11879 } 11880 else 11881 return super.addChild(name); 11882 } 11883 11884 public AddedItemDetailComponent copy() { 11885 AddedItemDetailComponent dst = new AddedItemDetailComponent(); 11886 copyValues(dst); 11887 return dst; 11888 } 11889 11890 public void copyValues(AddedItemDetailComponent dst) { 11891 super.copyValues(dst); 11892 if (traceNumber != null) { 11893 dst.traceNumber = new ArrayList<Identifier>(); 11894 for (Identifier i : traceNumber) 11895 dst.traceNumber.add(i.copy()); 11896 }; 11897 dst.revenue = revenue == null ? null : revenue.copy(); 11898 dst.productOrService = productOrService == null ? null : productOrService.copy(); 11899 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 11900 if (modifier != null) { 11901 dst.modifier = new ArrayList<CodeableConcept>(); 11902 for (CodeableConcept i : modifier) 11903 dst.modifier.add(i.copy()); 11904 }; 11905 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 11906 dst.quantity = quantity == null ? null : quantity.copy(); 11907 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 11908 dst.factor = factor == null ? null : factor.copy(); 11909 dst.tax = tax == null ? null : tax.copy(); 11910 dst.net = net == null ? null : net.copy(); 11911 if (noteNumber != null) { 11912 dst.noteNumber = new ArrayList<PositiveIntType>(); 11913 for (PositiveIntType i : noteNumber) 11914 dst.noteNumber.add(i.copy()); 11915 }; 11916 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 11917 if (adjudication != null) { 11918 dst.adjudication = new ArrayList<AdjudicationComponent>(); 11919 for (AdjudicationComponent i : adjudication) 11920 dst.adjudication.add(i.copy()); 11921 }; 11922 if (subDetail != null) { 11923 dst.subDetail = new ArrayList<AddedItemDetailSubDetailComponent>(); 11924 for (AddedItemDetailSubDetailComponent i : subDetail) 11925 dst.subDetail.add(i.copy()); 11926 }; 11927 } 11928 11929 @Override 11930 public boolean equalsDeep(Base other_) { 11931 if (!super.equalsDeep(other_)) 11932 return false; 11933 if (!(other_ instanceof AddedItemDetailComponent)) 11934 return false; 11935 AddedItemDetailComponent o = (AddedItemDetailComponent) other_; 11936 return compareDeep(traceNumber, o.traceNumber, true) && compareDeep(revenue, o.revenue, true) && compareDeep(productOrService, o.productOrService, true) 11937 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(modifier, o.modifier, true) 11938 && compareDeep(patientPaid, o.patientPaid, true) && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 11939 && compareDeep(factor, o.factor, true) && compareDeep(tax, o.tax, true) && compareDeep(net, o.net, true) 11940 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(reviewOutcome, o.reviewOutcome, true) 11941 && compareDeep(adjudication, o.adjudication, true) && compareDeep(subDetail, o.subDetail, true) 11942 ; 11943 } 11944 11945 @Override 11946 public boolean equalsShallow(Base other_) { 11947 if (!super.equalsShallow(other_)) 11948 return false; 11949 if (!(other_ instanceof AddedItemDetailComponent)) 11950 return false; 11951 AddedItemDetailComponent o = (AddedItemDetailComponent) other_; 11952 return compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true); 11953 } 11954 11955 public boolean isEmpty() { 11956 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(traceNumber, revenue, productOrService 11957 , productOrServiceEnd, modifier, patientPaid, quantity, unitPrice, factor, tax 11958 , net, noteNumber, reviewOutcome, adjudication, subDetail); 11959 } 11960 11961 public String fhirType() { 11962 return "ExplanationOfBenefit.addItem.detail"; 11963 11964 } 11965 11966 } 11967 11968 @Block() 11969 public static class AddedItemDetailSubDetailComponent extends BackboneElement implements IBaseBackboneElement { 11970 /** 11971 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 11972 */ 11973 @Child(name = "traceNumber", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 11974 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 11975 protected List<Identifier> traceNumber; 11976 11977 /** 11978 * The type of revenue or cost center providing the product and/or service. 11979 */ 11980 @Child(name = "revenue", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 11981 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 11982 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 11983 protected CodeableConcept revenue; 11984 11985 /** 11986 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 11987 */ 11988 @Child(name = "productOrService", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 11989 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 11990 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 11991 protected CodeableConcept productOrService; 11992 11993 /** 11994 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 11995 */ 11996 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 11997 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 11998 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 11999 protected CodeableConcept productOrServiceEnd; 12000 12001 /** 12002 * Item typification or modifiers codes to convey additional context for the product or service. 12003 */ 12004 @Child(name = "modifier", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 12005 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 12006 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 12007 protected List<CodeableConcept> modifier; 12008 12009 /** 12010 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 12011 */ 12012 @Child(name = "patientPaid", type = {Money.class}, order=6, min=0, max=1, modifier=false, summary=false) 12013 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 12014 protected Money patientPaid; 12015 12016 /** 12017 * The number of repetitions of a service or product. 12018 */ 12019 @Child(name = "quantity", type = {Quantity.class}, order=7, min=0, max=1, modifier=false, summary=false) 12020 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 12021 protected Quantity quantity; 12022 12023 /** 12024 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 12025 */ 12026 @Child(name = "unitPrice", type = {Money.class}, order=8, min=0, max=1, modifier=false, summary=false) 12027 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 12028 protected Money unitPrice; 12029 12030 /** 12031 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 12032 */ 12033 @Child(name = "factor", type = {DecimalType.class}, order=9, min=0, max=1, modifier=false, summary=false) 12034 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 12035 protected DecimalType factor; 12036 12037 /** 12038 * The total of taxes applicable for this product or service. 12039 */ 12040 @Child(name = "tax", type = {Money.class}, order=10, min=0, max=1, modifier=false, summary=false) 12041 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 12042 protected Money tax; 12043 12044 /** 12045 * The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor. 12046 */ 12047 @Child(name = "net", type = {Money.class}, order=11, min=0, max=1, modifier=false, summary=false) 12048 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor." ) 12049 protected Money net; 12050 12051 /** 12052 * The numbers associated with notes below which apply to the adjudication of this item. 12053 */ 12054 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 12055 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 12056 protected List<PositiveIntType> noteNumber; 12057 12058 /** 12059 * The high-level results of the adjudication if adjudication has been performed. 12060 */ 12061 @Child(name = "reviewOutcome", type = {ItemReviewOutcomeComponent.class}, order=13, min=0, max=1, modifier=false, summary=false) 12062 @Description(shortDefinition="Additem subdetail level adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 12063 protected ItemReviewOutcomeComponent reviewOutcome; 12064 12065 /** 12066 * The adjudication results. 12067 */ 12068 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 12069 @Description(shortDefinition="Added items adjudication", formalDefinition="The adjudication results." ) 12070 protected List<AdjudicationComponent> adjudication; 12071 12072 private static final long serialVersionUID = -71243645L; 12073 12074 /** 12075 * Constructor 12076 */ 12077 public AddedItemDetailSubDetailComponent() { 12078 super(); 12079 } 12080 12081 /** 12082 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 12083 */ 12084 public List<Identifier> getTraceNumber() { 12085 if (this.traceNumber == null) 12086 this.traceNumber = new ArrayList<Identifier>(); 12087 return this.traceNumber; 12088 } 12089 12090 /** 12091 * @return Returns a reference to <code>this</code> for easy method chaining 12092 */ 12093 public AddedItemDetailSubDetailComponent setTraceNumber(List<Identifier> theTraceNumber) { 12094 this.traceNumber = theTraceNumber; 12095 return this; 12096 } 12097 12098 public boolean hasTraceNumber() { 12099 if (this.traceNumber == null) 12100 return false; 12101 for (Identifier item : this.traceNumber) 12102 if (!item.isEmpty()) 12103 return true; 12104 return false; 12105 } 12106 12107 public Identifier addTraceNumber() { //3 12108 Identifier t = new Identifier(); 12109 if (this.traceNumber == null) 12110 this.traceNumber = new ArrayList<Identifier>(); 12111 this.traceNumber.add(t); 12112 return t; 12113 } 12114 12115 public AddedItemDetailSubDetailComponent addTraceNumber(Identifier t) { //3 12116 if (t == null) 12117 return this; 12118 if (this.traceNumber == null) 12119 this.traceNumber = new ArrayList<Identifier>(); 12120 this.traceNumber.add(t); 12121 return this; 12122 } 12123 12124 /** 12125 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 12126 */ 12127 public Identifier getTraceNumberFirstRep() { 12128 if (getTraceNumber().isEmpty()) { 12129 addTraceNumber(); 12130 } 12131 return getTraceNumber().get(0); 12132 } 12133 12134 /** 12135 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 12136 */ 12137 public CodeableConcept getRevenue() { 12138 if (this.revenue == null) 12139 if (Configuration.errorOnAutoCreate()) 12140 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.revenue"); 12141 else if (Configuration.doAutoCreate()) 12142 this.revenue = new CodeableConcept(); // cc 12143 return this.revenue; 12144 } 12145 12146 public boolean hasRevenue() { 12147 return this.revenue != null && !this.revenue.isEmpty(); 12148 } 12149 12150 /** 12151 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 12152 */ 12153 public AddedItemDetailSubDetailComponent setRevenue(CodeableConcept value) { 12154 this.revenue = value; 12155 return this; 12156 } 12157 12158 /** 12159 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 12160 */ 12161 public CodeableConcept getProductOrService() { 12162 if (this.productOrService == null) 12163 if (Configuration.errorOnAutoCreate()) 12164 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.productOrService"); 12165 else if (Configuration.doAutoCreate()) 12166 this.productOrService = new CodeableConcept(); // cc 12167 return this.productOrService; 12168 } 12169 12170 public boolean hasProductOrService() { 12171 return this.productOrService != null && !this.productOrService.isEmpty(); 12172 } 12173 12174 /** 12175 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 12176 */ 12177 public AddedItemDetailSubDetailComponent setProductOrService(CodeableConcept value) { 12178 this.productOrService = value; 12179 return this; 12180 } 12181 12182 /** 12183 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 12184 */ 12185 public CodeableConcept getProductOrServiceEnd() { 12186 if (this.productOrServiceEnd == null) 12187 if (Configuration.errorOnAutoCreate()) 12188 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.productOrServiceEnd"); 12189 else if (Configuration.doAutoCreate()) 12190 this.productOrServiceEnd = new CodeableConcept(); // cc 12191 return this.productOrServiceEnd; 12192 } 12193 12194 public boolean hasProductOrServiceEnd() { 12195 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 12196 } 12197 12198 /** 12199 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 12200 */ 12201 public AddedItemDetailSubDetailComponent setProductOrServiceEnd(CodeableConcept value) { 12202 this.productOrServiceEnd = value; 12203 return this; 12204 } 12205 12206 /** 12207 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 12208 */ 12209 public List<CodeableConcept> getModifier() { 12210 if (this.modifier == null) 12211 this.modifier = new ArrayList<CodeableConcept>(); 12212 return this.modifier; 12213 } 12214 12215 /** 12216 * @return Returns a reference to <code>this</code> for easy method chaining 12217 */ 12218 public AddedItemDetailSubDetailComponent setModifier(List<CodeableConcept> theModifier) { 12219 this.modifier = theModifier; 12220 return this; 12221 } 12222 12223 public boolean hasModifier() { 12224 if (this.modifier == null) 12225 return false; 12226 for (CodeableConcept item : this.modifier) 12227 if (!item.isEmpty()) 12228 return true; 12229 return false; 12230 } 12231 12232 public CodeableConcept addModifier() { //3 12233 CodeableConcept t = new CodeableConcept(); 12234 if (this.modifier == null) 12235 this.modifier = new ArrayList<CodeableConcept>(); 12236 this.modifier.add(t); 12237 return t; 12238 } 12239 12240 public AddedItemDetailSubDetailComponent addModifier(CodeableConcept t) { //3 12241 if (t == null) 12242 return this; 12243 if (this.modifier == null) 12244 this.modifier = new ArrayList<CodeableConcept>(); 12245 this.modifier.add(t); 12246 return this; 12247 } 12248 12249 /** 12250 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 12251 */ 12252 public CodeableConcept getModifierFirstRep() { 12253 if (getModifier().isEmpty()) { 12254 addModifier(); 12255 } 12256 return getModifier().get(0); 12257 } 12258 12259 /** 12260 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 12261 */ 12262 public Money getPatientPaid() { 12263 if (this.patientPaid == null) 12264 if (Configuration.errorOnAutoCreate()) 12265 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.patientPaid"); 12266 else if (Configuration.doAutoCreate()) 12267 this.patientPaid = new Money(); // cc 12268 return this.patientPaid; 12269 } 12270 12271 public boolean hasPatientPaid() { 12272 return this.patientPaid != null && !this.patientPaid.isEmpty(); 12273 } 12274 12275 /** 12276 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 12277 */ 12278 public AddedItemDetailSubDetailComponent setPatientPaid(Money value) { 12279 this.patientPaid = value; 12280 return this; 12281 } 12282 12283 /** 12284 * @return {@link #quantity} (The number of repetitions of a service or product.) 12285 */ 12286 public Quantity getQuantity() { 12287 if (this.quantity == null) 12288 if (Configuration.errorOnAutoCreate()) 12289 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.quantity"); 12290 else if (Configuration.doAutoCreate()) 12291 this.quantity = new Quantity(); // cc 12292 return this.quantity; 12293 } 12294 12295 public boolean hasQuantity() { 12296 return this.quantity != null && !this.quantity.isEmpty(); 12297 } 12298 12299 /** 12300 * @param value {@link #quantity} (The number of repetitions of a service or product.) 12301 */ 12302 public AddedItemDetailSubDetailComponent setQuantity(Quantity value) { 12303 this.quantity = value; 12304 return this; 12305 } 12306 12307 /** 12308 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 12309 */ 12310 public Money getUnitPrice() { 12311 if (this.unitPrice == null) 12312 if (Configuration.errorOnAutoCreate()) 12313 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.unitPrice"); 12314 else if (Configuration.doAutoCreate()) 12315 this.unitPrice = new Money(); // cc 12316 return this.unitPrice; 12317 } 12318 12319 public boolean hasUnitPrice() { 12320 return this.unitPrice != null && !this.unitPrice.isEmpty(); 12321 } 12322 12323 /** 12324 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 12325 */ 12326 public AddedItemDetailSubDetailComponent setUnitPrice(Money value) { 12327 this.unitPrice = value; 12328 return this; 12329 } 12330 12331 /** 12332 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 12333 */ 12334 public DecimalType getFactorElement() { 12335 if (this.factor == null) 12336 if (Configuration.errorOnAutoCreate()) 12337 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.factor"); 12338 else if (Configuration.doAutoCreate()) 12339 this.factor = new DecimalType(); // bb 12340 return this.factor; 12341 } 12342 12343 public boolean hasFactorElement() { 12344 return this.factor != null && !this.factor.isEmpty(); 12345 } 12346 12347 public boolean hasFactor() { 12348 return this.factor != null && !this.factor.isEmpty(); 12349 } 12350 12351 /** 12352 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 12353 */ 12354 public AddedItemDetailSubDetailComponent setFactorElement(DecimalType value) { 12355 this.factor = value; 12356 return this; 12357 } 12358 12359 /** 12360 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 12361 */ 12362 public BigDecimal getFactor() { 12363 return this.factor == null ? null : this.factor.getValue(); 12364 } 12365 12366 /** 12367 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 12368 */ 12369 public AddedItemDetailSubDetailComponent setFactor(BigDecimal value) { 12370 if (value == null) 12371 this.factor = null; 12372 else { 12373 if (this.factor == null) 12374 this.factor = new DecimalType(); 12375 this.factor.setValue(value); 12376 } 12377 return this; 12378 } 12379 12380 /** 12381 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 12382 */ 12383 public AddedItemDetailSubDetailComponent setFactor(long value) { 12384 this.factor = new DecimalType(); 12385 this.factor.setValue(value); 12386 return this; 12387 } 12388 12389 /** 12390 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 12391 */ 12392 public AddedItemDetailSubDetailComponent setFactor(double value) { 12393 this.factor = new DecimalType(); 12394 this.factor.setValue(value); 12395 return this; 12396 } 12397 12398 /** 12399 * @return {@link #tax} (The total of taxes applicable for this product or service.) 12400 */ 12401 public Money getTax() { 12402 if (this.tax == null) 12403 if (Configuration.errorOnAutoCreate()) 12404 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.tax"); 12405 else if (Configuration.doAutoCreate()) 12406 this.tax = new Money(); // cc 12407 return this.tax; 12408 } 12409 12410 public boolean hasTax() { 12411 return this.tax != null && !this.tax.isEmpty(); 12412 } 12413 12414 /** 12415 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 12416 */ 12417 public AddedItemDetailSubDetailComponent setTax(Money value) { 12418 this.tax = value; 12419 return this; 12420 } 12421 12422 /** 12423 * @return {@link #net} (The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor.) 12424 */ 12425 public Money getNet() { 12426 if (this.net == null) 12427 if (Configuration.errorOnAutoCreate()) 12428 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.net"); 12429 else if (Configuration.doAutoCreate()) 12430 this.net = new Money(); // cc 12431 return this.net; 12432 } 12433 12434 public boolean hasNet() { 12435 return this.net != null && !this.net.isEmpty(); 12436 } 12437 12438 /** 12439 * @param value {@link #net} (The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor.) 12440 */ 12441 public AddedItemDetailSubDetailComponent setNet(Money value) { 12442 this.net = value; 12443 return this; 12444 } 12445 12446 /** 12447 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 12448 */ 12449 public List<PositiveIntType> getNoteNumber() { 12450 if (this.noteNumber == null) 12451 this.noteNumber = new ArrayList<PositiveIntType>(); 12452 return this.noteNumber; 12453 } 12454 12455 /** 12456 * @return Returns a reference to <code>this</code> for easy method chaining 12457 */ 12458 public AddedItemDetailSubDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 12459 this.noteNumber = theNoteNumber; 12460 return this; 12461 } 12462 12463 public boolean hasNoteNumber() { 12464 if (this.noteNumber == null) 12465 return false; 12466 for (PositiveIntType item : this.noteNumber) 12467 if (!item.isEmpty()) 12468 return true; 12469 return false; 12470 } 12471 12472 /** 12473 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 12474 */ 12475 public PositiveIntType addNoteNumberElement() {//2 12476 PositiveIntType t = new PositiveIntType(); 12477 if (this.noteNumber == null) 12478 this.noteNumber = new ArrayList<PositiveIntType>(); 12479 this.noteNumber.add(t); 12480 return t; 12481 } 12482 12483 /** 12484 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 12485 */ 12486 public AddedItemDetailSubDetailComponent addNoteNumber(int value) { //1 12487 PositiveIntType t = new PositiveIntType(); 12488 t.setValue(value); 12489 if (this.noteNumber == null) 12490 this.noteNumber = new ArrayList<PositiveIntType>(); 12491 this.noteNumber.add(t); 12492 return this; 12493 } 12494 12495 /** 12496 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 12497 */ 12498 public boolean hasNoteNumber(int value) { 12499 if (this.noteNumber == null) 12500 return false; 12501 for (PositiveIntType v : this.noteNumber) 12502 if (v.getValue().equals(value)) // positiveInt 12503 return true; 12504 return false; 12505 } 12506 12507 /** 12508 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 12509 */ 12510 public ItemReviewOutcomeComponent getReviewOutcome() { 12511 if (this.reviewOutcome == null) 12512 if (Configuration.errorOnAutoCreate()) 12513 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.reviewOutcome"); 12514 else if (Configuration.doAutoCreate()) 12515 this.reviewOutcome = new ItemReviewOutcomeComponent(); // cc 12516 return this.reviewOutcome; 12517 } 12518 12519 public boolean hasReviewOutcome() { 12520 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 12521 } 12522 12523 /** 12524 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 12525 */ 12526 public AddedItemDetailSubDetailComponent setReviewOutcome(ItemReviewOutcomeComponent value) { 12527 this.reviewOutcome = value; 12528 return this; 12529 } 12530 12531 /** 12532 * @return {@link #adjudication} (The adjudication results.) 12533 */ 12534 public List<AdjudicationComponent> getAdjudication() { 12535 if (this.adjudication == null) 12536 this.adjudication = new ArrayList<AdjudicationComponent>(); 12537 return this.adjudication; 12538 } 12539 12540 /** 12541 * @return Returns a reference to <code>this</code> for easy method chaining 12542 */ 12543 public AddedItemDetailSubDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 12544 this.adjudication = theAdjudication; 12545 return this; 12546 } 12547 12548 public boolean hasAdjudication() { 12549 if (this.adjudication == null) 12550 return false; 12551 for (AdjudicationComponent item : this.adjudication) 12552 if (!item.isEmpty()) 12553 return true; 12554 return false; 12555 } 12556 12557 public AdjudicationComponent addAdjudication() { //3 12558 AdjudicationComponent t = new AdjudicationComponent(); 12559 if (this.adjudication == null) 12560 this.adjudication = new ArrayList<AdjudicationComponent>(); 12561 this.adjudication.add(t); 12562 return t; 12563 } 12564 12565 public AddedItemDetailSubDetailComponent addAdjudication(AdjudicationComponent t) { //3 12566 if (t == null) 12567 return this; 12568 if (this.adjudication == null) 12569 this.adjudication = new ArrayList<AdjudicationComponent>(); 12570 this.adjudication.add(t); 12571 return this; 12572 } 12573 12574 /** 12575 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 12576 */ 12577 public AdjudicationComponent getAdjudicationFirstRep() { 12578 if (getAdjudication().isEmpty()) { 12579 addAdjudication(); 12580 } 12581 return getAdjudication().get(0); 12582 } 12583 12584 protected void listChildren(List<Property> children) { 12585 super.listChildren(children); 12586 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 12587 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 12588 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 12589 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 12590 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 12591 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 12592 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 12593 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 12594 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 12595 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 12596 children.add(new Property("net", "Money", "The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor.", 0, 1, net)); 12597 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 12598 children.add(new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 12599 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 12600 } 12601 12602 @Override 12603 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 12604 switch (_hash) { 12605 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 12606 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 12607 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 12608 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 12609 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 12610 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 12611 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 12612 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 12613 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 12614 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 12615 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor.", 0, 1, net); 12616 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 12617 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 12618 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 12619 default: return super.getNamedProperty(_hash, _name, _checkValid); 12620 } 12621 12622 } 12623 12624 @Override 12625 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 12626 switch (hash) { 12627 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 12628 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 12629 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 12630 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 12631 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 12632 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 12633 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 12634 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 12635 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 12636 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 12637 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 12638 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 12639 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ItemReviewOutcomeComponent 12640 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 12641 default: return super.getProperty(hash, name, checkValid); 12642 } 12643 12644 } 12645 12646 @Override 12647 public Base setProperty(int hash, String name, Base value) throws FHIRException { 12648 switch (hash) { 12649 case 82505966: // traceNumber 12650 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 12651 return value; 12652 case 1099842588: // revenue 12653 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 12654 return value; 12655 case 1957227299: // productOrService 12656 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 12657 return value; 12658 case -717476168: // productOrServiceEnd 12659 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 12660 return value; 12661 case -615513385: // modifier 12662 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 12663 return value; 12664 case 525514609: // patientPaid 12665 this.patientPaid = TypeConvertor.castToMoney(value); // Money 12666 return value; 12667 case -1285004149: // quantity 12668 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 12669 return value; 12670 case -486196699: // unitPrice 12671 this.unitPrice = TypeConvertor.castToMoney(value); // Money 12672 return value; 12673 case -1282148017: // factor 12674 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 12675 return value; 12676 case 114603: // tax 12677 this.tax = TypeConvertor.castToMoney(value); // Money 12678 return value; 12679 case 108957: // net 12680 this.net = TypeConvertor.castToMoney(value); // Money 12681 return value; 12682 case -1110033957: // noteNumber 12683 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 12684 return value; 12685 case -51825446: // reviewOutcome 12686 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 12687 return value; 12688 case -231349275: // adjudication 12689 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 12690 return value; 12691 default: return super.setProperty(hash, name, value); 12692 } 12693 12694 } 12695 12696 @Override 12697 public Base setProperty(String name, Base value) throws FHIRException { 12698 if (name.equals("traceNumber")) { 12699 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 12700 } else if (name.equals("revenue")) { 12701 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 12702 } else if (name.equals("productOrService")) { 12703 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 12704 } else if (name.equals("productOrServiceEnd")) { 12705 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 12706 } else if (name.equals("modifier")) { 12707 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 12708 } else if (name.equals("patientPaid")) { 12709 this.patientPaid = TypeConvertor.castToMoney(value); // Money 12710 } else if (name.equals("quantity")) { 12711 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 12712 } else if (name.equals("unitPrice")) { 12713 this.unitPrice = TypeConvertor.castToMoney(value); // Money 12714 } else if (name.equals("factor")) { 12715 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 12716 } else if (name.equals("tax")) { 12717 this.tax = TypeConvertor.castToMoney(value); // Money 12718 } else if (name.equals("net")) { 12719 this.net = TypeConvertor.castToMoney(value); // Money 12720 } else if (name.equals("noteNumber")) { 12721 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 12722 } else if (name.equals("reviewOutcome")) { 12723 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 12724 } else if (name.equals("adjudication")) { 12725 this.getAdjudication().add((AdjudicationComponent) value); 12726 } else 12727 return super.setProperty(name, value); 12728 return value; 12729 } 12730 12731 @Override 12732 public Base makeProperty(int hash, String name) throws FHIRException { 12733 switch (hash) { 12734 case 82505966: return addTraceNumber(); 12735 case 1099842588: return getRevenue(); 12736 case 1957227299: return getProductOrService(); 12737 case -717476168: return getProductOrServiceEnd(); 12738 case -615513385: return addModifier(); 12739 case 525514609: return getPatientPaid(); 12740 case -1285004149: return getQuantity(); 12741 case -486196699: return getUnitPrice(); 12742 case -1282148017: return getFactorElement(); 12743 case 114603: return getTax(); 12744 case 108957: return getNet(); 12745 case -1110033957: return addNoteNumberElement(); 12746 case -51825446: return getReviewOutcome(); 12747 case -231349275: return addAdjudication(); 12748 default: return super.makeProperty(hash, name); 12749 } 12750 12751 } 12752 12753 @Override 12754 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 12755 switch (hash) { 12756 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 12757 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 12758 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 12759 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 12760 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 12761 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 12762 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 12763 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 12764 case -1282148017: /*factor*/ return new String[] {"decimal"}; 12765 case 114603: /*tax*/ return new String[] {"Money"}; 12766 case 108957: /*net*/ return new String[] {"Money"}; 12767 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 12768 case -51825446: /*reviewOutcome*/ return new String[] {"@ExplanationOfBenefit.item.reviewOutcome"}; 12769 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 12770 default: return super.getTypesForProperty(hash, name); 12771 } 12772 12773 } 12774 12775 @Override 12776 public Base addChild(String name) throws FHIRException { 12777 if (name.equals("traceNumber")) { 12778 return addTraceNumber(); 12779 } 12780 else if (name.equals("revenue")) { 12781 this.revenue = new CodeableConcept(); 12782 return this.revenue; 12783 } 12784 else if (name.equals("productOrService")) { 12785 this.productOrService = new CodeableConcept(); 12786 return this.productOrService; 12787 } 12788 else if (name.equals("productOrServiceEnd")) { 12789 this.productOrServiceEnd = new CodeableConcept(); 12790 return this.productOrServiceEnd; 12791 } 12792 else if (name.equals("modifier")) { 12793 return addModifier(); 12794 } 12795 else if (name.equals("patientPaid")) { 12796 this.patientPaid = new Money(); 12797 return this.patientPaid; 12798 } 12799 else if (name.equals("quantity")) { 12800 this.quantity = new Quantity(); 12801 return this.quantity; 12802 } 12803 else if (name.equals("unitPrice")) { 12804 this.unitPrice = new Money(); 12805 return this.unitPrice; 12806 } 12807 else if (name.equals("factor")) { 12808 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.detail.subDetail.factor"); 12809 } 12810 else if (name.equals("tax")) { 12811 this.tax = new Money(); 12812 return this.tax; 12813 } 12814 else if (name.equals("net")) { 12815 this.net = new Money(); 12816 return this.net; 12817 } 12818 else if (name.equals("noteNumber")) { 12819 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.detail.subDetail.noteNumber"); 12820 } 12821 else if (name.equals("reviewOutcome")) { 12822 this.reviewOutcome = new ItemReviewOutcomeComponent(); 12823 return this.reviewOutcome; 12824 } 12825 else if (name.equals("adjudication")) { 12826 return addAdjudication(); 12827 } 12828 else 12829 return super.addChild(name); 12830 } 12831 12832 public AddedItemDetailSubDetailComponent copy() { 12833 AddedItemDetailSubDetailComponent dst = new AddedItemDetailSubDetailComponent(); 12834 copyValues(dst); 12835 return dst; 12836 } 12837 12838 public void copyValues(AddedItemDetailSubDetailComponent dst) { 12839 super.copyValues(dst); 12840 if (traceNumber != null) { 12841 dst.traceNumber = new ArrayList<Identifier>(); 12842 for (Identifier i : traceNumber) 12843 dst.traceNumber.add(i.copy()); 12844 }; 12845 dst.revenue = revenue == null ? null : revenue.copy(); 12846 dst.productOrService = productOrService == null ? null : productOrService.copy(); 12847 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 12848 if (modifier != null) { 12849 dst.modifier = new ArrayList<CodeableConcept>(); 12850 for (CodeableConcept i : modifier) 12851 dst.modifier.add(i.copy()); 12852 }; 12853 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 12854 dst.quantity = quantity == null ? null : quantity.copy(); 12855 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 12856 dst.factor = factor == null ? null : factor.copy(); 12857 dst.tax = tax == null ? null : tax.copy(); 12858 dst.net = net == null ? null : net.copy(); 12859 if (noteNumber != null) { 12860 dst.noteNumber = new ArrayList<PositiveIntType>(); 12861 for (PositiveIntType i : noteNumber) 12862 dst.noteNumber.add(i.copy()); 12863 }; 12864 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 12865 if (adjudication != null) { 12866 dst.adjudication = new ArrayList<AdjudicationComponent>(); 12867 for (AdjudicationComponent i : adjudication) 12868 dst.adjudication.add(i.copy()); 12869 }; 12870 } 12871 12872 @Override 12873 public boolean equalsDeep(Base other_) { 12874 if (!super.equalsDeep(other_)) 12875 return false; 12876 if (!(other_ instanceof AddedItemDetailSubDetailComponent)) 12877 return false; 12878 AddedItemDetailSubDetailComponent o = (AddedItemDetailSubDetailComponent) other_; 12879 return compareDeep(traceNumber, o.traceNumber, true) && compareDeep(revenue, o.revenue, true) && compareDeep(productOrService, o.productOrService, true) 12880 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(modifier, o.modifier, true) 12881 && compareDeep(patientPaid, o.patientPaid, true) && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 12882 && compareDeep(factor, o.factor, true) && compareDeep(tax, o.tax, true) && compareDeep(net, o.net, true) 12883 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(reviewOutcome, o.reviewOutcome, true) 12884 && compareDeep(adjudication, o.adjudication, true); 12885 } 12886 12887 @Override 12888 public boolean equalsShallow(Base other_) { 12889 if (!super.equalsShallow(other_)) 12890 return false; 12891 if (!(other_ instanceof AddedItemDetailSubDetailComponent)) 12892 return false; 12893 AddedItemDetailSubDetailComponent o = (AddedItemDetailSubDetailComponent) other_; 12894 return compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true); 12895 } 12896 12897 public boolean isEmpty() { 12898 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(traceNumber, revenue, productOrService 12899 , productOrServiceEnd, modifier, patientPaid, quantity, unitPrice, factor, tax 12900 , net, noteNumber, reviewOutcome, adjudication); 12901 } 12902 12903 public String fhirType() { 12904 return "ExplanationOfBenefit.addItem.detail.subDetail"; 12905 12906 } 12907 12908 } 12909 12910 @Block() 12911 public static class TotalComponent extends BackboneElement implements IBaseBackboneElement { 12912 /** 12913 * A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item. 12914 */ 12915 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 12916 @Description(shortDefinition="Type of adjudication information", formalDefinition="A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item." ) 12917 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication") 12918 protected CodeableConcept category; 12919 12920 /** 12921 * Monetary total amount associated with the category. 12922 */ 12923 @Child(name = "amount", type = {Money.class}, order=2, min=1, max=1, modifier=false, summary=true) 12924 @Description(shortDefinition="Financial total for the category", formalDefinition="Monetary total amount associated with the category." ) 12925 protected Money amount; 12926 12927 private static final long serialVersionUID = 2012310309L; 12928 12929 /** 12930 * Constructor 12931 */ 12932 public TotalComponent() { 12933 super(); 12934 } 12935 12936 /** 12937 * Constructor 12938 */ 12939 public TotalComponent(CodeableConcept category, Money amount) { 12940 super(); 12941 this.setCategory(category); 12942 this.setAmount(amount); 12943 } 12944 12945 /** 12946 * @return {@link #category} (A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.) 12947 */ 12948 public CodeableConcept getCategory() { 12949 if (this.category == null) 12950 if (Configuration.errorOnAutoCreate()) 12951 throw new Error("Attempt to auto-create TotalComponent.category"); 12952 else if (Configuration.doAutoCreate()) 12953 this.category = new CodeableConcept(); // cc 12954 return this.category; 12955 } 12956 12957 public boolean hasCategory() { 12958 return this.category != null && !this.category.isEmpty(); 12959 } 12960 12961 /** 12962 * @param value {@link #category} (A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.) 12963 */ 12964 public TotalComponent setCategory(CodeableConcept value) { 12965 this.category = value; 12966 return this; 12967 } 12968 12969 /** 12970 * @return {@link #amount} (Monetary total amount associated with the category.) 12971 */ 12972 public Money getAmount() { 12973 if (this.amount == null) 12974 if (Configuration.errorOnAutoCreate()) 12975 throw new Error("Attempt to auto-create TotalComponent.amount"); 12976 else if (Configuration.doAutoCreate()) 12977 this.amount = new Money(); // cc 12978 return this.amount; 12979 } 12980 12981 public boolean hasAmount() { 12982 return this.amount != null && !this.amount.isEmpty(); 12983 } 12984 12985 /** 12986 * @param value {@link #amount} (Monetary total amount associated with the category.) 12987 */ 12988 public TotalComponent setAmount(Money value) { 12989 this.amount = value; 12990 return this; 12991 } 12992 12993 protected void listChildren(List<Property> children) { 12994 super.listChildren(children); 12995 children.add(new Property("category", "CodeableConcept", "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 0, 1, category)); 12996 children.add(new Property("amount", "Money", "Monetary total amount associated with the category.", 0, 1, amount)); 12997 } 12998 12999 @Override 13000 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 13001 switch (_hash) { 13002 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 0, 1, category); 13003 case -1413853096: /*amount*/ return new Property("amount", "Money", "Monetary total amount associated with the category.", 0, 1, amount); 13004 default: return super.getNamedProperty(_hash, _name, _checkValid); 13005 } 13006 13007 } 13008 13009 @Override 13010 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 13011 switch (hash) { 13012 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 13013 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 13014 default: return super.getProperty(hash, name, checkValid); 13015 } 13016 13017 } 13018 13019 @Override 13020 public Base setProperty(int hash, String name, Base value) throws FHIRException { 13021 switch (hash) { 13022 case 50511102: // category 13023 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13024 return value; 13025 case -1413853096: // amount 13026 this.amount = TypeConvertor.castToMoney(value); // Money 13027 return value; 13028 default: return super.setProperty(hash, name, value); 13029 } 13030 13031 } 13032 13033 @Override 13034 public Base setProperty(String name, Base value) throws FHIRException { 13035 if (name.equals("category")) { 13036 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13037 } else if (name.equals("amount")) { 13038 this.amount = TypeConvertor.castToMoney(value); // Money 13039 } else 13040 return super.setProperty(name, value); 13041 return value; 13042 } 13043 13044 @Override 13045 public Base makeProperty(int hash, String name) throws FHIRException { 13046 switch (hash) { 13047 case 50511102: return getCategory(); 13048 case -1413853096: return getAmount(); 13049 default: return super.makeProperty(hash, name); 13050 } 13051 13052 } 13053 13054 @Override 13055 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 13056 switch (hash) { 13057 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 13058 case -1413853096: /*amount*/ return new String[] {"Money"}; 13059 default: return super.getTypesForProperty(hash, name); 13060 } 13061 13062 } 13063 13064 @Override 13065 public Base addChild(String name) throws FHIRException { 13066 if (name.equals("category")) { 13067 this.category = new CodeableConcept(); 13068 return this.category; 13069 } 13070 else if (name.equals("amount")) { 13071 this.amount = new Money(); 13072 return this.amount; 13073 } 13074 else 13075 return super.addChild(name); 13076 } 13077 13078 public TotalComponent copy() { 13079 TotalComponent dst = new TotalComponent(); 13080 copyValues(dst); 13081 return dst; 13082 } 13083 13084 public void copyValues(TotalComponent dst) { 13085 super.copyValues(dst); 13086 dst.category = category == null ? null : category.copy(); 13087 dst.amount = amount == null ? null : amount.copy(); 13088 } 13089 13090 @Override 13091 public boolean equalsDeep(Base other_) { 13092 if (!super.equalsDeep(other_)) 13093 return false; 13094 if (!(other_ instanceof TotalComponent)) 13095 return false; 13096 TotalComponent o = (TotalComponent) other_; 13097 return compareDeep(category, o.category, true) && compareDeep(amount, o.amount, true); 13098 } 13099 13100 @Override 13101 public boolean equalsShallow(Base other_) { 13102 if (!super.equalsShallow(other_)) 13103 return false; 13104 if (!(other_ instanceof TotalComponent)) 13105 return false; 13106 TotalComponent o = (TotalComponent) other_; 13107 return true; 13108 } 13109 13110 public boolean isEmpty() { 13111 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, amount); 13112 } 13113 13114 public String fhirType() { 13115 return "ExplanationOfBenefit.total"; 13116 13117 } 13118 13119 } 13120 13121 @Block() 13122 public static class PaymentComponent extends BackboneElement implements IBaseBackboneElement { 13123 /** 13124 * Whether this represents partial or complete payment of the benefits payable. 13125 */ 13126 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 13127 @Description(shortDefinition="Partial or complete payment", formalDefinition="Whether this represents partial or complete payment of the benefits payable." ) 13128 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-paymenttype") 13129 protected CodeableConcept type; 13130 13131 /** 13132 * Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication. 13133 */ 13134 @Child(name = "adjustment", type = {Money.class}, order=2, min=0, max=1, modifier=false, summary=false) 13135 @Description(shortDefinition="Payment adjustment for non-claim issues", formalDefinition="Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication." ) 13136 protected Money adjustment; 13137 13138 /** 13139 * Reason for the payment adjustment. 13140 */ 13141 @Child(name = "adjustmentReason", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 13142 @Description(shortDefinition="Explanation for the variance", formalDefinition="Reason for the payment adjustment." ) 13143 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payment-adjustment-reason") 13144 protected CodeableConcept adjustmentReason; 13145 13146 /** 13147 * Estimated date the payment will be issued or the actual issue date of payment. 13148 */ 13149 @Child(name = "date", type = {DateType.class}, order=4, min=0, max=1, modifier=false, summary=false) 13150 @Description(shortDefinition="Expected date of payment", formalDefinition="Estimated date the payment will be issued or the actual issue date of payment." ) 13151 protected DateType date; 13152 13153 /** 13154 * Benefits payable less any payment adjustment. 13155 */ 13156 @Child(name = "amount", type = {Money.class}, order=5, min=0, max=1, modifier=false, summary=false) 13157 @Description(shortDefinition="Payable amount after adjustment", formalDefinition="Benefits payable less any payment adjustment." ) 13158 protected Money amount; 13159 13160 /** 13161 * Issuer's unique identifier for the payment instrument. 13162 */ 13163 @Child(name = "identifier", type = {Identifier.class}, order=6, min=0, max=1, modifier=false, summary=false) 13164 @Description(shortDefinition="Business identifier for the payment", formalDefinition="Issuer's unique identifier for the payment instrument." ) 13165 protected Identifier identifier; 13166 13167 private static final long serialVersionUID = 1539906026L; 13168 13169 /** 13170 * Constructor 13171 */ 13172 public PaymentComponent() { 13173 super(); 13174 } 13175 13176 /** 13177 * @return {@link #type} (Whether this represents partial or complete payment of the benefits payable.) 13178 */ 13179 public CodeableConcept getType() { 13180 if (this.type == null) 13181 if (Configuration.errorOnAutoCreate()) 13182 throw new Error("Attempt to auto-create PaymentComponent.type"); 13183 else if (Configuration.doAutoCreate()) 13184 this.type = new CodeableConcept(); // cc 13185 return this.type; 13186 } 13187 13188 public boolean hasType() { 13189 return this.type != null && !this.type.isEmpty(); 13190 } 13191 13192 /** 13193 * @param value {@link #type} (Whether this represents partial or complete payment of the benefits payable.) 13194 */ 13195 public PaymentComponent setType(CodeableConcept value) { 13196 this.type = value; 13197 return this; 13198 } 13199 13200 /** 13201 * @return {@link #adjustment} (Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.) 13202 */ 13203 public Money getAdjustment() { 13204 if (this.adjustment == null) 13205 if (Configuration.errorOnAutoCreate()) 13206 throw new Error("Attempt to auto-create PaymentComponent.adjustment"); 13207 else if (Configuration.doAutoCreate()) 13208 this.adjustment = new Money(); // cc 13209 return this.adjustment; 13210 } 13211 13212 public boolean hasAdjustment() { 13213 return this.adjustment != null && !this.adjustment.isEmpty(); 13214 } 13215 13216 /** 13217 * @param value {@link #adjustment} (Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.) 13218 */ 13219 public PaymentComponent setAdjustment(Money value) { 13220 this.adjustment = value; 13221 return this; 13222 } 13223 13224 /** 13225 * @return {@link #adjustmentReason} (Reason for the payment adjustment.) 13226 */ 13227 public CodeableConcept getAdjustmentReason() { 13228 if (this.adjustmentReason == null) 13229 if (Configuration.errorOnAutoCreate()) 13230 throw new Error("Attempt to auto-create PaymentComponent.adjustmentReason"); 13231 else if (Configuration.doAutoCreate()) 13232 this.adjustmentReason = new CodeableConcept(); // cc 13233 return this.adjustmentReason; 13234 } 13235 13236 public boolean hasAdjustmentReason() { 13237 return this.adjustmentReason != null && !this.adjustmentReason.isEmpty(); 13238 } 13239 13240 /** 13241 * @param value {@link #adjustmentReason} (Reason for the payment adjustment.) 13242 */ 13243 public PaymentComponent setAdjustmentReason(CodeableConcept value) { 13244 this.adjustmentReason = value; 13245 return this; 13246 } 13247 13248 /** 13249 * @return {@link #date} (Estimated date the payment will be issued or the actual issue date of payment.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 13250 */ 13251 public DateType getDateElement() { 13252 if (this.date == null) 13253 if (Configuration.errorOnAutoCreate()) 13254 throw new Error("Attempt to auto-create PaymentComponent.date"); 13255 else if (Configuration.doAutoCreate()) 13256 this.date = new DateType(); // bb 13257 return this.date; 13258 } 13259 13260 public boolean hasDateElement() { 13261 return this.date != null && !this.date.isEmpty(); 13262 } 13263 13264 public boolean hasDate() { 13265 return this.date != null && !this.date.isEmpty(); 13266 } 13267 13268 /** 13269 * @param value {@link #date} (Estimated date the payment will be issued or the actual issue date of payment.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 13270 */ 13271 public PaymentComponent setDateElement(DateType value) { 13272 this.date = value; 13273 return this; 13274 } 13275 13276 /** 13277 * @return Estimated date the payment will be issued or the actual issue date of payment. 13278 */ 13279 public Date getDate() { 13280 return this.date == null ? null : this.date.getValue(); 13281 } 13282 13283 /** 13284 * @param value Estimated date the payment will be issued or the actual issue date of payment. 13285 */ 13286 public PaymentComponent setDate(Date value) { 13287 if (value == null) 13288 this.date = null; 13289 else { 13290 if (this.date == null) 13291 this.date = new DateType(); 13292 this.date.setValue(value); 13293 } 13294 return this; 13295 } 13296 13297 /** 13298 * @return {@link #amount} (Benefits payable less any payment adjustment.) 13299 */ 13300 public Money getAmount() { 13301 if (this.amount == null) 13302 if (Configuration.errorOnAutoCreate()) 13303 throw new Error("Attempt to auto-create PaymentComponent.amount"); 13304 else if (Configuration.doAutoCreate()) 13305 this.amount = new Money(); // cc 13306 return this.amount; 13307 } 13308 13309 public boolean hasAmount() { 13310 return this.amount != null && !this.amount.isEmpty(); 13311 } 13312 13313 /** 13314 * @param value {@link #amount} (Benefits payable less any payment adjustment.) 13315 */ 13316 public PaymentComponent setAmount(Money value) { 13317 this.amount = value; 13318 return this; 13319 } 13320 13321 /** 13322 * @return {@link #identifier} (Issuer's unique identifier for the payment instrument.) 13323 */ 13324 public Identifier getIdentifier() { 13325 if (this.identifier == null) 13326 if (Configuration.errorOnAutoCreate()) 13327 throw new Error("Attempt to auto-create PaymentComponent.identifier"); 13328 else if (Configuration.doAutoCreate()) 13329 this.identifier = new Identifier(); // cc 13330 return this.identifier; 13331 } 13332 13333 public boolean hasIdentifier() { 13334 return this.identifier != null && !this.identifier.isEmpty(); 13335 } 13336 13337 /** 13338 * @param value {@link #identifier} (Issuer's unique identifier for the payment instrument.) 13339 */ 13340 public PaymentComponent setIdentifier(Identifier value) { 13341 this.identifier = value; 13342 return this; 13343 } 13344 13345 protected void listChildren(List<Property> children) { 13346 super.listChildren(children); 13347 children.add(new Property("type", "CodeableConcept", "Whether this represents partial or complete payment of the benefits payable.", 0, 1, type)); 13348 children.add(new Property("adjustment", "Money", "Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.", 0, 1, adjustment)); 13349 children.add(new Property("adjustmentReason", "CodeableConcept", "Reason for the payment adjustment.", 0, 1, adjustmentReason)); 13350 children.add(new Property("date", "date", "Estimated date the payment will be issued or the actual issue date of payment.", 0, 1, date)); 13351 children.add(new Property("amount", "Money", "Benefits payable less any payment adjustment.", 0, 1, amount)); 13352 children.add(new Property("identifier", "Identifier", "Issuer's unique identifier for the payment instrument.", 0, 1, identifier)); 13353 } 13354 13355 @Override 13356 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 13357 switch (_hash) { 13358 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Whether this represents partial or complete payment of the benefits payable.", 0, 1, type); 13359 case 1977085293: /*adjustment*/ return new Property("adjustment", "Money", "Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.", 0, 1, adjustment); 13360 case -1255938543: /*adjustmentReason*/ return new Property("adjustmentReason", "CodeableConcept", "Reason for the payment adjustment.", 0, 1, adjustmentReason); 13361 case 3076014: /*date*/ return new Property("date", "date", "Estimated date the payment will be issued or the actual issue date of payment.", 0, 1, date); 13362 case -1413853096: /*amount*/ return new Property("amount", "Money", "Benefits payable less any payment adjustment.", 0, 1, amount); 13363 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Issuer's unique identifier for the payment instrument.", 0, 1, identifier); 13364 default: return super.getNamedProperty(_hash, _name, _checkValid); 13365 } 13366 13367 } 13368 13369 @Override 13370 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 13371 switch (hash) { 13372 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 13373 case 1977085293: /*adjustment*/ return this.adjustment == null ? new Base[0] : new Base[] {this.adjustment}; // Money 13374 case -1255938543: /*adjustmentReason*/ return this.adjustmentReason == null ? new Base[0] : new Base[] {this.adjustmentReason}; // CodeableConcept 13375 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 13376 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 13377 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 13378 default: return super.getProperty(hash, name, checkValid); 13379 } 13380 13381 } 13382 13383 @Override 13384 public Base setProperty(int hash, String name, Base value) throws FHIRException { 13385 switch (hash) { 13386 case 3575610: // type 13387 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13388 return value; 13389 case 1977085293: // adjustment 13390 this.adjustment = TypeConvertor.castToMoney(value); // Money 13391 return value; 13392 case -1255938543: // adjustmentReason 13393 this.adjustmentReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13394 return value; 13395 case 3076014: // date 13396 this.date = TypeConvertor.castToDate(value); // DateType 13397 return value; 13398 case -1413853096: // amount 13399 this.amount = TypeConvertor.castToMoney(value); // Money 13400 return value; 13401 case -1618432855: // identifier 13402 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 13403 return value; 13404 default: return super.setProperty(hash, name, value); 13405 } 13406 13407 } 13408 13409 @Override 13410 public Base setProperty(String name, Base value) throws FHIRException { 13411 if (name.equals("type")) { 13412 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13413 } else if (name.equals("adjustment")) { 13414 this.adjustment = TypeConvertor.castToMoney(value); // Money 13415 } else if (name.equals("adjustmentReason")) { 13416 this.adjustmentReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13417 } else if (name.equals("date")) { 13418 this.date = TypeConvertor.castToDate(value); // DateType 13419 } else if (name.equals("amount")) { 13420 this.amount = TypeConvertor.castToMoney(value); // Money 13421 } else if (name.equals("identifier")) { 13422 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 13423 } else 13424 return super.setProperty(name, value); 13425 return value; 13426 } 13427 13428 @Override 13429 public Base makeProperty(int hash, String name) throws FHIRException { 13430 switch (hash) { 13431 case 3575610: return getType(); 13432 case 1977085293: return getAdjustment(); 13433 case -1255938543: return getAdjustmentReason(); 13434 case 3076014: return getDateElement(); 13435 case -1413853096: return getAmount(); 13436 case -1618432855: return getIdentifier(); 13437 default: return super.makeProperty(hash, name); 13438 } 13439 13440 } 13441 13442 @Override 13443 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 13444 switch (hash) { 13445 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 13446 case 1977085293: /*adjustment*/ return new String[] {"Money"}; 13447 case -1255938543: /*adjustmentReason*/ return new String[] {"CodeableConcept"}; 13448 case 3076014: /*date*/ return new String[] {"date"}; 13449 case -1413853096: /*amount*/ return new String[] {"Money"}; 13450 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 13451 default: return super.getTypesForProperty(hash, name); 13452 } 13453 13454 } 13455 13456 @Override 13457 public Base addChild(String name) throws FHIRException { 13458 if (name.equals("type")) { 13459 this.type = new CodeableConcept(); 13460 return this.type; 13461 } 13462 else if (name.equals("adjustment")) { 13463 this.adjustment = new Money(); 13464 return this.adjustment; 13465 } 13466 else if (name.equals("adjustmentReason")) { 13467 this.adjustmentReason = new CodeableConcept(); 13468 return this.adjustmentReason; 13469 } 13470 else if (name.equals("date")) { 13471 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.payment.date"); 13472 } 13473 else if (name.equals("amount")) { 13474 this.amount = new Money(); 13475 return this.amount; 13476 } 13477 else if (name.equals("identifier")) { 13478 this.identifier = new Identifier(); 13479 return this.identifier; 13480 } 13481 else 13482 return super.addChild(name); 13483 } 13484 13485 public PaymentComponent copy() { 13486 PaymentComponent dst = new PaymentComponent(); 13487 copyValues(dst); 13488 return dst; 13489 } 13490 13491 public void copyValues(PaymentComponent dst) { 13492 super.copyValues(dst); 13493 dst.type = type == null ? null : type.copy(); 13494 dst.adjustment = adjustment == null ? null : adjustment.copy(); 13495 dst.adjustmentReason = adjustmentReason == null ? null : adjustmentReason.copy(); 13496 dst.date = date == null ? null : date.copy(); 13497 dst.amount = amount == null ? null : amount.copy(); 13498 dst.identifier = identifier == null ? null : identifier.copy(); 13499 } 13500 13501 @Override 13502 public boolean equalsDeep(Base other_) { 13503 if (!super.equalsDeep(other_)) 13504 return false; 13505 if (!(other_ instanceof PaymentComponent)) 13506 return false; 13507 PaymentComponent o = (PaymentComponent) other_; 13508 return compareDeep(type, o.type, true) && compareDeep(adjustment, o.adjustment, true) && compareDeep(adjustmentReason, o.adjustmentReason, true) 13509 && compareDeep(date, o.date, true) && compareDeep(amount, o.amount, true) && compareDeep(identifier, o.identifier, true) 13510 ; 13511 } 13512 13513 @Override 13514 public boolean equalsShallow(Base other_) { 13515 if (!super.equalsShallow(other_)) 13516 return false; 13517 if (!(other_ instanceof PaymentComponent)) 13518 return false; 13519 PaymentComponent o = (PaymentComponent) other_; 13520 return compareValues(date, o.date, true); 13521 } 13522 13523 public boolean isEmpty() { 13524 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, adjustment, adjustmentReason 13525 , date, amount, identifier); 13526 } 13527 13528 public String fhirType() { 13529 return "ExplanationOfBenefit.payment"; 13530 13531 } 13532 13533 } 13534 13535 @Block() 13536 public static class NoteComponent extends BackboneElement implements IBaseBackboneElement { 13537 /** 13538 * A number to uniquely identify a note entry. 13539 */ 13540 @Child(name = "number", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 13541 @Description(shortDefinition="Note instance identifier", formalDefinition="A number to uniquely identify a note entry." ) 13542 protected PositiveIntType number; 13543 13544 /** 13545 * The business purpose of the note text. 13546 */ 13547 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 13548 @Description(shortDefinition="Note purpose", formalDefinition="The business purpose of the note text." ) 13549 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/note-type") 13550 protected CodeableConcept type; 13551 13552 /** 13553 * The explanation or description associated with the processing. 13554 */ 13555 @Child(name = "text", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 13556 @Description(shortDefinition="Note explanatory text", formalDefinition="The explanation or description associated with the processing." ) 13557 protected StringType text; 13558 13559 /** 13560 * A code to define the language used in the text of the note. 13561 */ 13562 @Child(name = "language", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 13563 @Description(shortDefinition="Language of the text", formalDefinition="A code to define the language used in the text of the note." ) 13564 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-languages") 13565 protected CodeableConcept language; 13566 13567 private static final long serialVersionUID = -944255449L; 13568 13569 /** 13570 * Constructor 13571 */ 13572 public NoteComponent() { 13573 super(); 13574 } 13575 13576 /** 13577 * @return {@link #number} (A number to uniquely identify a note entry.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 13578 */ 13579 public PositiveIntType getNumberElement() { 13580 if (this.number == null) 13581 if (Configuration.errorOnAutoCreate()) 13582 throw new Error("Attempt to auto-create NoteComponent.number"); 13583 else if (Configuration.doAutoCreate()) 13584 this.number = new PositiveIntType(); // bb 13585 return this.number; 13586 } 13587 13588 public boolean hasNumberElement() { 13589 return this.number != null && !this.number.isEmpty(); 13590 } 13591 13592 public boolean hasNumber() { 13593 return this.number != null && !this.number.isEmpty(); 13594 } 13595 13596 /** 13597 * @param value {@link #number} (A number to uniquely identify a note entry.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 13598 */ 13599 public NoteComponent setNumberElement(PositiveIntType value) { 13600 this.number = value; 13601 return this; 13602 } 13603 13604 /** 13605 * @return A number to uniquely identify a note entry. 13606 */ 13607 public int getNumber() { 13608 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 13609 } 13610 13611 /** 13612 * @param value A number to uniquely identify a note entry. 13613 */ 13614 public NoteComponent setNumber(int value) { 13615 if (this.number == null) 13616 this.number = new PositiveIntType(); 13617 this.number.setValue(value); 13618 return this; 13619 } 13620 13621 /** 13622 * @return {@link #type} (The business purpose of the note text.) 13623 */ 13624 public CodeableConcept getType() { 13625 if (this.type == null) 13626 if (Configuration.errorOnAutoCreate()) 13627 throw new Error("Attempt to auto-create NoteComponent.type"); 13628 else if (Configuration.doAutoCreate()) 13629 this.type = new CodeableConcept(); // cc 13630 return this.type; 13631 } 13632 13633 public boolean hasType() { 13634 return this.type != null && !this.type.isEmpty(); 13635 } 13636 13637 /** 13638 * @param value {@link #type} (The business purpose of the note text.) 13639 */ 13640 public NoteComponent setType(CodeableConcept value) { 13641 this.type = value; 13642 return this; 13643 } 13644 13645 /** 13646 * @return {@link #text} (The explanation or description associated with the processing.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 13647 */ 13648 public StringType getTextElement() { 13649 if (this.text == null) 13650 if (Configuration.errorOnAutoCreate()) 13651 throw new Error("Attempt to auto-create NoteComponent.text"); 13652 else if (Configuration.doAutoCreate()) 13653 this.text = new StringType(); // bb 13654 return this.text; 13655 } 13656 13657 public boolean hasTextElement() { 13658 return this.text != null && !this.text.isEmpty(); 13659 } 13660 13661 public boolean hasText() { 13662 return this.text != null && !this.text.isEmpty(); 13663 } 13664 13665 /** 13666 * @param value {@link #text} (The explanation or description associated with the processing.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 13667 */ 13668 public NoteComponent setTextElement(StringType value) { 13669 this.text = value; 13670 return this; 13671 } 13672 13673 /** 13674 * @return The explanation or description associated with the processing. 13675 */ 13676 public String getText() { 13677 return this.text == null ? null : this.text.getValue(); 13678 } 13679 13680 /** 13681 * @param value The explanation or description associated with the processing. 13682 */ 13683 public NoteComponent setText(String value) { 13684 if (Utilities.noString(value)) 13685 this.text = null; 13686 else { 13687 if (this.text == null) 13688 this.text = new StringType(); 13689 this.text.setValue(value); 13690 } 13691 return this; 13692 } 13693 13694 /** 13695 * @return {@link #language} (A code to define the language used in the text of the note.) 13696 */ 13697 public CodeableConcept getLanguage() { 13698 if (this.language == null) 13699 if (Configuration.errorOnAutoCreate()) 13700 throw new Error("Attempt to auto-create NoteComponent.language"); 13701 else if (Configuration.doAutoCreate()) 13702 this.language = new CodeableConcept(); // cc 13703 return this.language; 13704 } 13705 13706 public boolean hasLanguage() { 13707 return this.language != null && !this.language.isEmpty(); 13708 } 13709 13710 /** 13711 * @param value {@link #language} (A code to define the language used in the text of the note.) 13712 */ 13713 public NoteComponent setLanguage(CodeableConcept value) { 13714 this.language = value; 13715 return this; 13716 } 13717 13718 protected void listChildren(List<Property> children) { 13719 super.listChildren(children); 13720 children.add(new Property("number", "positiveInt", "A number to uniquely identify a note entry.", 0, 1, number)); 13721 children.add(new Property("type", "CodeableConcept", "The business purpose of the note text.", 0, 1, type)); 13722 children.add(new Property("text", "string", "The explanation or description associated with the processing.", 0, 1, text)); 13723 children.add(new Property("language", "CodeableConcept", "A code to define the language used in the text of the note.", 0, 1, language)); 13724 } 13725 13726 @Override 13727 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 13728 switch (_hash) { 13729 case -1034364087: /*number*/ return new Property("number", "positiveInt", "A number to uniquely identify a note entry.", 0, 1, number); 13730 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The business purpose of the note text.", 0, 1, type); 13731 case 3556653: /*text*/ return new Property("text", "string", "The explanation or description associated with the processing.", 0, 1, text); 13732 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "A code to define the language used in the text of the note.", 0, 1, language); 13733 default: return super.getNamedProperty(_hash, _name, _checkValid); 13734 } 13735 13736 } 13737 13738 @Override 13739 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 13740 switch (hash) { 13741 case -1034364087: /*number*/ return this.number == null ? new Base[0] : new Base[] {this.number}; // PositiveIntType 13742 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 13743 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 13744 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeableConcept 13745 default: return super.getProperty(hash, name, checkValid); 13746 } 13747 13748 } 13749 13750 @Override 13751 public Base setProperty(int hash, String name, Base value) throws FHIRException { 13752 switch (hash) { 13753 case -1034364087: // number 13754 this.number = TypeConvertor.castToPositiveInt(value); // PositiveIntType 13755 return value; 13756 case 3575610: // type 13757 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13758 return value; 13759 case 3556653: // text 13760 this.text = TypeConvertor.castToString(value); // StringType 13761 return value; 13762 case -1613589672: // language 13763 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13764 return value; 13765 default: return super.setProperty(hash, name, value); 13766 } 13767 13768 } 13769 13770 @Override 13771 public Base setProperty(String name, Base value) throws FHIRException { 13772 if (name.equals("number")) { 13773 this.number = TypeConvertor.castToPositiveInt(value); // PositiveIntType 13774 } else if (name.equals("type")) { 13775 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13776 } else if (name.equals("text")) { 13777 this.text = TypeConvertor.castToString(value); // StringType 13778 } else if (name.equals("language")) { 13779 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13780 } else 13781 return super.setProperty(name, value); 13782 return value; 13783 } 13784 13785 @Override 13786 public Base makeProperty(int hash, String name) throws FHIRException { 13787 switch (hash) { 13788 case -1034364087: return getNumberElement(); 13789 case 3575610: return getType(); 13790 case 3556653: return getTextElement(); 13791 case -1613589672: return getLanguage(); 13792 default: return super.makeProperty(hash, name); 13793 } 13794 13795 } 13796 13797 @Override 13798 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 13799 switch (hash) { 13800 case -1034364087: /*number*/ return new String[] {"positiveInt"}; 13801 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 13802 case 3556653: /*text*/ return new String[] {"string"}; 13803 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 13804 default: return super.getTypesForProperty(hash, name); 13805 } 13806 13807 } 13808 13809 @Override 13810 public Base addChild(String name) throws FHIRException { 13811 if (name.equals("number")) { 13812 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.processNote.number"); 13813 } 13814 else if (name.equals("type")) { 13815 this.type = new CodeableConcept(); 13816 return this.type; 13817 } 13818 else if (name.equals("text")) { 13819 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.processNote.text"); 13820 } 13821 else if (name.equals("language")) { 13822 this.language = new CodeableConcept(); 13823 return this.language; 13824 } 13825 else 13826 return super.addChild(name); 13827 } 13828 13829 public NoteComponent copy() { 13830 NoteComponent dst = new NoteComponent(); 13831 copyValues(dst); 13832 return dst; 13833 } 13834 13835 public void copyValues(NoteComponent dst) { 13836 super.copyValues(dst); 13837 dst.number = number == null ? null : number.copy(); 13838 dst.type = type == null ? null : type.copy(); 13839 dst.text = text == null ? null : text.copy(); 13840 dst.language = language == null ? null : language.copy(); 13841 } 13842 13843 @Override 13844 public boolean equalsDeep(Base other_) { 13845 if (!super.equalsDeep(other_)) 13846 return false; 13847 if (!(other_ instanceof NoteComponent)) 13848 return false; 13849 NoteComponent o = (NoteComponent) other_; 13850 return compareDeep(number, o.number, true) && compareDeep(type, o.type, true) && compareDeep(text, o.text, true) 13851 && compareDeep(language, o.language, true); 13852 } 13853 13854 @Override 13855 public boolean equalsShallow(Base other_) { 13856 if (!super.equalsShallow(other_)) 13857 return false; 13858 if (!(other_ instanceof NoteComponent)) 13859 return false; 13860 NoteComponent o = (NoteComponent) other_; 13861 return compareValues(number, o.number, true) && compareValues(text, o.text, true); 13862 } 13863 13864 public boolean isEmpty() { 13865 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(number, type, text, language 13866 ); 13867 } 13868 13869 public String fhirType() { 13870 return "ExplanationOfBenefit.processNote"; 13871 13872 } 13873 13874 } 13875 13876 @Block() 13877 public static class BenefitBalanceComponent extends BackboneElement implements IBaseBackboneElement { 13878 /** 13879 * Code to identify the general type of benefits under which products and services are provided. 13880 */ 13881 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 13882 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 13883 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 13884 protected CodeableConcept category; 13885 13886 /** 13887 * True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage. 13888 */ 13889 @Child(name = "excluded", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 13890 @Description(shortDefinition="Excluded from the plan", formalDefinition="True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage." ) 13891 protected BooleanType excluded; 13892 13893 /** 13894 * A short name or tag for the benefit. 13895 */ 13896 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 13897 @Description(shortDefinition="Short name for the benefit", formalDefinition="A short name or tag for the benefit." ) 13898 protected StringType name; 13899 13900 /** 13901 * A richer description of the benefit or services covered. 13902 */ 13903 @Child(name = "description", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 13904 @Description(shortDefinition="Description of the benefit or services covered", formalDefinition="A richer description of the benefit or services covered." ) 13905 protected StringType description; 13906 13907 /** 13908 * Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers. 13909 */ 13910 @Child(name = "network", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 13911 @Description(shortDefinition="In or out of network", formalDefinition="Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers." ) 13912 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-network") 13913 protected CodeableConcept network; 13914 13915 /** 13916 * Indicates if the benefits apply to an individual or to the family. 13917 */ 13918 @Child(name = "unit", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 13919 @Description(shortDefinition="Individual or family", formalDefinition="Indicates if the benefits apply to an individual or to the family." ) 13920 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-unit") 13921 protected CodeableConcept unit; 13922 13923 /** 13924 * The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'. 13925 */ 13926 @Child(name = "term", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 13927 @Description(shortDefinition="Annual or lifetime", formalDefinition="The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'." ) 13928 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-term") 13929 protected CodeableConcept term; 13930 13931 /** 13932 * Benefits Used to date. 13933 */ 13934 @Child(name = "financial", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 13935 @Description(shortDefinition="Benefit Summary", formalDefinition="Benefits Used to date." ) 13936 protected List<BenefitComponent> financial; 13937 13938 private static final long serialVersionUID = -1889655824L; 13939 13940 /** 13941 * Constructor 13942 */ 13943 public BenefitBalanceComponent() { 13944 super(); 13945 } 13946 13947 /** 13948 * Constructor 13949 */ 13950 public BenefitBalanceComponent(CodeableConcept category) { 13951 super(); 13952 this.setCategory(category); 13953 } 13954 13955 /** 13956 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 13957 */ 13958 public CodeableConcept getCategory() { 13959 if (this.category == null) 13960 if (Configuration.errorOnAutoCreate()) 13961 throw new Error("Attempt to auto-create BenefitBalanceComponent.category"); 13962 else if (Configuration.doAutoCreate()) 13963 this.category = new CodeableConcept(); // cc 13964 return this.category; 13965 } 13966 13967 public boolean hasCategory() { 13968 return this.category != null && !this.category.isEmpty(); 13969 } 13970 13971 /** 13972 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 13973 */ 13974 public BenefitBalanceComponent setCategory(CodeableConcept value) { 13975 this.category = value; 13976 return this; 13977 } 13978 13979 /** 13980 * @return {@link #excluded} (True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.). This is the underlying object with id, value and extensions. The accessor "getExcluded" gives direct access to the value 13981 */ 13982 public BooleanType getExcludedElement() { 13983 if (this.excluded == null) 13984 if (Configuration.errorOnAutoCreate()) 13985 throw new Error("Attempt to auto-create BenefitBalanceComponent.excluded"); 13986 else if (Configuration.doAutoCreate()) 13987 this.excluded = new BooleanType(); // bb 13988 return this.excluded; 13989 } 13990 13991 public boolean hasExcludedElement() { 13992 return this.excluded != null && !this.excluded.isEmpty(); 13993 } 13994 13995 public boolean hasExcluded() { 13996 return this.excluded != null && !this.excluded.isEmpty(); 13997 } 13998 13999 /** 14000 * @param value {@link #excluded} (True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.). This is the underlying object with id, value and extensions. The accessor "getExcluded" gives direct access to the value 14001 */ 14002 public BenefitBalanceComponent setExcludedElement(BooleanType value) { 14003 this.excluded = value; 14004 return this; 14005 } 14006 14007 /** 14008 * @return True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage. 14009 */ 14010 public boolean getExcluded() { 14011 return this.excluded == null || this.excluded.isEmpty() ? false : this.excluded.getValue(); 14012 } 14013 14014 /** 14015 * @param value True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage. 14016 */ 14017 public BenefitBalanceComponent setExcluded(boolean value) { 14018 if (this.excluded == null) 14019 this.excluded = new BooleanType(); 14020 this.excluded.setValue(value); 14021 return this; 14022 } 14023 14024 /** 14025 * @return {@link #name} (A short name or tag for the benefit.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 14026 */ 14027 public StringType getNameElement() { 14028 if (this.name == null) 14029 if (Configuration.errorOnAutoCreate()) 14030 throw new Error("Attempt to auto-create BenefitBalanceComponent.name"); 14031 else if (Configuration.doAutoCreate()) 14032 this.name = new StringType(); // bb 14033 return this.name; 14034 } 14035 14036 public boolean hasNameElement() { 14037 return this.name != null && !this.name.isEmpty(); 14038 } 14039 14040 public boolean hasName() { 14041 return this.name != null && !this.name.isEmpty(); 14042 } 14043 14044 /** 14045 * @param value {@link #name} (A short name or tag for the benefit.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 14046 */ 14047 public BenefitBalanceComponent setNameElement(StringType value) { 14048 this.name = value; 14049 return this; 14050 } 14051 14052 /** 14053 * @return A short name or tag for the benefit. 14054 */ 14055 public String getName() { 14056 return this.name == null ? null : this.name.getValue(); 14057 } 14058 14059 /** 14060 * @param value A short name or tag for the benefit. 14061 */ 14062 public BenefitBalanceComponent setName(String value) { 14063 if (Utilities.noString(value)) 14064 this.name = null; 14065 else { 14066 if (this.name == null) 14067 this.name = new StringType(); 14068 this.name.setValue(value); 14069 } 14070 return this; 14071 } 14072 14073 /** 14074 * @return {@link #description} (A richer description of the benefit or services covered.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 14075 */ 14076 public StringType getDescriptionElement() { 14077 if (this.description == null) 14078 if (Configuration.errorOnAutoCreate()) 14079 throw new Error("Attempt to auto-create BenefitBalanceComponent.description"); 14080 else if (Configuration.doAutoCreate()) 14081 this.description = new StringType(); // bb 14082 return this.description; 14083 } 14084 14085 public boolean hasDescriptionElement() { 14086 return this.description != null && !this.description.isEmpty(); 14087 } 14088 14089 public boolean hasDescription() { 14090 return this.description != null && !this.description.isEmpty(); 14091 } 14092 14093 /** 14094 * @param value {@link #description} (A richer description of the benefit or services covered.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 14095 */ 14096 public BenefitBalanceComponent setDescriptionElement(StringType value) { 14097 this.description = value; 14098 return this; 14099 } 14100 14101 /** 14102 * @return A richer description of the benefit or services covered. 14103 */ 14104 public String getDescription() { 14105 return this.description == null ? null : this.description.getValue(); 14106 } 14107 14108 /** 14109 * @param value A richer description of the benefit or services covered. 14110 */ 14111 public BenefitBalanceComponent setDescription(String value) { 14112 if (Utilities.noString(value)) 14113 this.description = null; 14114 else { 14115 if (this.description == null) 14116 this.description = new StringType(); 14117 this.description.setValue(value); 14118 } 14119 return this; 14120 } 14121 14122 /** 14123 * @return {@link #network} (Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.) 14124 */ 14125 public CodeableConcept getNetwork() { 14126 if (this.network == null) 14127 if (Configuration.errorOnAutoCreate()) 14128 throw new Error("Attempt to auto-create BenefitBalanceComponent.network"); 14129 else if (Configuration.doAutoCreate()) 14130 this.network = new CodeableConcept(); // cc 14131 return this.network; 14132 } 14133 14134 public boolean hasNetwork() { 14135 return this.network != null && !this.network.isEmpty(); 14136 } 14137 14138 /** 14139 * @param value {@link #network} (Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.) 14140 */ 14141 public BenefitBalanceComponent setNetwork(CodeableConcept value) { 14142 this.network = value; 14143 return this; 14144 } 14145 14146 /** 14147 * @return {@link #unit} (Indicates if the benefits apply to an individual or to the family.) 14148 */ 14149 public CodeableConcept getUnit() { 14150 if (this.unit == null) 14151 if (Configuration.errorOnAutoCreate()) 14152 throw new Error("Attempt to auto-create BenefitBalanceComponent.unit"); 14153 else if (Configuration.doAutoCreate()) 14154 this.unit = new CodeableConcept(); // cc 14155 return this.unit; 14156 } 14157 14158 public boolean hasUnit() { 14159 return this.unit != null && !this.unit.isEmpty(); 14160 } 14161 14162 /** 14163 * @param value {@link #unit} (Indicates if the benefits apply to an individual or to the family.) 14164 */ 14165 public BenefitBalanceComponent setUnit(CodeableConcept value) { 14166 this.unit = value; 14167 return this; 14168 } 14169 14170 /** 14171 * @return {@link #term} (The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.) 14172 */ 14173 public CodeableConcept getTerm() { 14174 if (this.term == null) 14175 if (Configuration.errorOnAutoCreate()) 14176 throw new Error("Attempt to auto-create BenefitBalanceComponent.term"); 14177 else if (Configuration.doAutoCreate()) 14178 this.term = new CodeableConcept(); // cc 14179 return this.term; 14180 } 14181 14182 public boolean hasTerm() { 14183 return this.term != null && !this.term.isEmpty(); 14184 } 14185 14186 /** 14187 * @param value {@link #term} (The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.) 14188 */ 14189 public BenefitBalanceComponent setTerm(CodeableConcept value) { 14190 this.term = value; 14191 return this; 14192 } 14193 14194 /** 14195 * @return {@link #financial} (Benefits Used to date.) 14196 */ 14197 public List<BenefitComponent> getFinancial() { 14198 if (this.financial == null) 14199 this.financial = new ArrayList<BenefitComponent>(); 14200 return this.financial; 14201 } 14202 14203 /** 14204 * @return Returns a reference to <code>this</code> for easy method chaining 14205 */ 14206 public BenefitBalanceComponent setFinancial(List<BenefitComponent> theFinancial) { 14207 this.financial = theFinancial; 14208 return this; 14209 } 14210 14211 public boolean hasFinancial() { 14212 if (this.financial == null) 14213 return false; 14214 for (BenefitComponent item : this.financial) 14215 if (!item.isEmpty()) 14216 return true; 14217 return false; 14218 } 14219 14220 public BenefitComponent addFinancial() { //3 14221 BenefitComponent t = new BenefitComponent(); 14222 if (this.financial == null) 14223 this.financial = new ArrayList<BenefitComponent>(); 14224 this.financial.add(t); 14225 return t; 14226 } 14227 14228 public BenefitBalanceComponent addFinancial(BenefitComponent t) { //3 14229 if (t == null) 14230 return this; 14231 if (this.financial == null) 14232 this.financial = new ArrayList<BenefitComponent>(); 14233 this.financial.add(t); 14234 return this; 14235 } 14236 14237 /** 14238 * @return The first repetition of repeating field {@link #financial}, creating it if it does not already exist {3} 14239 */ 14240 public BenefitComponent getFinancialFirstRep() { 14241 if (getFinancial().isEmpty()) { 14242 addFinancial(); 14243 } 14244 return getFinancial().get(0); 14245 } 14246 14247 protected void listChildren(List<Property> children) { 14248 super.listChildren(children); 14249 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 14250 children.add(new Property("excluded", "boolean", "True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.", 0, 1, excluded)); 14251 children.add(new Property("name", "string", "A short name or tag for the benefit.", 0, 1, name)); 14252 children.add(new Property("description", "string", "A richer description of the benefit or services covered.", 0, 1, description)); 14253 children.add(new Property("network", "CodeableConcept", "Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.", 0, 1, network)); 14254 children.add(new Property("unit", "CodeableConcept", "Indicates if the benefits apply to an individual or to the family.", 0, 1, unit)); 14255 children.add(new Property("term", "CodeableConcept", "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.", 0, 1, term)); 14256 children.add(new Property("financial", "", "Benefits Used to date.", 0, java.lang.Integer.MAX_VALUE, financial)); 14257 } 14258 14259 @Override 14260 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 14261 switch (_hash) { 14262 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 14263 case 1994055114: /*excluded*/ return new Property("excluded", "boolean", "True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.", 0, 1, excluded); 14264 case 3373707: /*name*/ return new Property("name", "string", "A short name or tag for the benefit.", 0, 1, name); 14265 case -1724546052: /*description*/ return new Property("description", "string", "A richer description of the benefit or services covered.", 0, 1, description); 14266 case 1843485230: /*network*/ return new Property("network", "CodeableConcept", "Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.", 0, 1, network); 14267 case 3594628: /*unit*/ return new Property("unit", "CodeableConcept", "Indicates if the benefits apply to an individual or to the family.", 0, 1, unit); 14268 case 3556460: /*term*/ return new Property("term", "CodeableConcept", "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.", 0, 1, term); 14269 case 357555337: /*financial*/ return new Property("financial", "", "Benefits Used to date.", 0, java.lang.Integer.MAX_VALUE, financial); 14270 default: return super.getNamedProperty(_hash, _name, _checkValid); 14271 } 14272 14273 } 14274 14275 @Override 14276 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 14277 switch (hash) { 14278 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 14279 case 1994055114: /*excluded*/ return this.excluded == null ? new Base[0] : new Base[] {this.excluded}; // BooleanType 14280 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 14281 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 14282 case 1843485230: /*network*/ return this.network == null ? new Base[0] : new Base[] {this.network}; // CodeableConcept 14283 case 3594628: /*unit*/ return this.unit == null ? new Base[0] : new Base[] {this.unit}; // CodeableConcept 14284 case 3556460: /*term*/ return this.term == null ? new Base[0] : new Base[] {this.term}; // CodeableConcept 14285 case 357555337: /*financial*/ return this.financial == null ? new Base[0] : this.financial.toArray(new Base[this.financial.size()]); // BenefitComponent 14286 default: return super.getProperty(hash, name, checkValid); 14287 } 14288 14289 } 14290 14291 @Override 14292 public Base setProperty(int hash, String name, Base value) throws FHIRException { 14293 switch (hash) { 14294 case 50511102: // category 14295 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14296 return value; 14297 case 1994055114: // excluded 14298 this.excluded = TypeConvertor.castToBoolean(value); // BooleanType 14299 return value; 14300 case 3373707: // name 14301 this.name = TypeConvertor.castToString(value); // StringType 14302 return value; 14303 case -1724546052: // description 14304 this.description = TypeConvertor.castToString(value); // StringType 14305 return value; 14306 case 1843485230: // network 14307 this.network = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14308 return value; 14309 case 3594628: // unit 14310 this.unit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14311 return value; 14312 case 3556460: // term 14313 this.term = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14314 return value; 14315 case 357555337: // financial 14316 this.getFinancial().add((BenefitComponent) value); // BenefitComponent 14317 return value; 14318 default: return super.setProperty(hash, name, value); 14319 } 14320 14321 } 14322 14323 @Override 14324 public Base setProperty(String name, Base value) throws FHIRException { 14325 if (name.equals("category")) { 14326 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14327 } else if (name.equals("excluded")) { 14328 this.excluded = TypeConvertor.castToBoolean(value); // BooleanType 14329 } else if (name.equals("name")) { 14330 this.name = TypeConvertor.castToString(value); // StringType 14331 } else if (name.equals("description")) { 14332 this.description = TypeConvertor.castToString(value); // StringType 14333 } else if (name.equals("network")) { 14334 this.network = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14335 } else if (name.equals("unit")) { 14336 this.unit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14337 } else if (name.equals("term")) { 14338 this.term = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14339 } else if (name.equals("financial")) { 14340 this.getFinancial().add((BenefitComponent) value); 14341 } else 14342 return super.setProperty(name, value); 14343 return value; 14344 } 14345 14346 @Override 14347 public Base makeProperty(int hash, String name) throws FHIRException { 14348 switch (hash) { 14349 case 50511102: return getCategory(); 14350 case 1994055114: return getExcludedElement(); 14351 case 3373707: return getNameElement(); 14352 case -1724546052: return getDescriptionElement(); 14353 case 1843485230: return getNetwork(); 14354 case 3594628: return getUnit(); 14355 case 3556460: return getTerm(); 14356 case 357555337: return addFinancial(); 14357 default: return super.makeProperty(hash, name); 14358 } 14359 14360 } 14361 14362 @Override 14363 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 14364 switch (hash) { 14365 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 14366 case 1994055114: /*excluded*/ return new String[] {"boolean"}; 14367 case 3373707: /*name*/ return new String[] {"string"}; 14368 case -1724546052: /*description*/ return new String[] {"string"}; 14369 case 1843485230: /*network*/ return new String[] {"CodeableConcept"}; 14370 case 3594628: /*unit*/ return new String[] {"CodeableConcept"}; 14371 case 3556460: /*term*/ return new String[] {"CodeableConcept"}; 14372 case 357555337: /*financial*/ return new String[] {}; 14373 default: return super.getTypesForProperty(hash, name); 14374 } 14375 14376 } 14377 14378 @Override 14379 public Base addChild(String name) throws FHIRException { 14380 if (name.equals("category")) { 14381 this.category = new CodeableConcept(); 14382 return this.category; 14383 } 14384 else if (name.equals("excluded")) { 14385 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.benefitBalance.excluded"); 14386 } 14387 else if (name.equals("name")) { 14388 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.benefitBalance.name"); 14389 } 14390 else if (name.equals("description")) { 14391 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.benefitBalance.description"); 14392 } 14393 else if (name.equals("network")) { 14394 this.network = new CodeableConcept(); 14395 return this.network; 14396 } 14397 else if (name.equals("unit")) { 14398 this.unit = new CodeableConcept(); 14399 return this.unit; 14400 } 14401 else if (name.equals("term")) { 14402 this.term = new CodeableConcept(); 14403 return this.term; 14404 } 14405 else if (name.equals("financial")) { 14406 return addFinancial(); 14407 } 14408 else 14409 return super.addChild(name); 14410 } 14411 14412 public BenefitBalanceComponent copy() { 14413 BenefitBalanceComponent dst = new BenefitBalanceComponent(); 14414 copyValues(dst); 14415 return dst; 14416 } 14417 14418 public void copyValues(BenefitBalanceComponent dst) { 14419 super.copyValues(dst); 14420 dst.category = category == null ? null : category.copy(); 14421 dst.excluded = excluded == null ? null : excluded.copy(); 14422 dst.name = name == null ? null : name.copy(); 14423 dst.description = description == null ? null : description.copy(); 14424 dst.network = network == null ? null : network.copy(); 14425 dst.unit = unit == null ? null : unit.copy(); 14426 dst.term = term == null ? null : term.copy(); 14427 if (financial != null) { 14428 dst.financial = new ArrayList<BenefitComponent>(); 14429 for (BenefitComponent i : financial) 14430 dst.financial.add(i.copy()); 14431 }; 14432 } 14433 14434 @Override 14435 public boolean equalsDeep(Base other_) { 14436 if (!super.equalsDeep(other_)) 14437 return false; 14438 if (!(other_ instanceof BenefitBalanceComponent)) 14439 return false; 14440 BenefitBalanceComponent o = (BenefitBalanceComponent) other_; 14441 return compareDeep(category, o.category, true) && compareDeep(excluded, o.excluded, true) && compareDeep(name, o.name, true) 14442 && compareDeep(description, o.description, true) && compareDeep(network, o.network, true) && compareDeep(unit, o.unit, true) 14443 && compareDeep(term, o.term, true) && compareDeep(financial, o.financial, true); 14444 } 14445 14446 @Override 14447 public boolean equalsShallow(Base other_) { 14448 if (!super.equalsShallow(other_)) 14449 return false; 14450 if (!(other_ instanceof BenefitBalanceComponent)) 14451 return false; 14452 BenefitBalanceComponent o = (BenefitBalanceComponent) other_; 14453 return compareValues(excluded, o.excluded, true) && compareValues(name, o.name, true) && compareValues(description, o.description, true) 14454 ; 14455 } 14456 14457 public boolean isEmpty() { 14458 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, excluded, name 14459 , description, network, unit, term, financial); 14460 } 14461 14462 public String fhirType() { 14463 return "ExplanationOfBenefit.benefitBalance"; 14464 14465 } 14466 14467 } 14468 14469 @Block() 14470 public static class BenefitComponent extends BackboneElement implements IBaseBackboneElement { 14471 /** 14472 * Classification of benefit being provided. 14473 */ 14474 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 14475 @Description(shortDefinition="Benefit classification", formalDefinition="Classification of benefit being provided." ) 14476 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-type") 14477 protected CodeableConcept type; 14478 14479 /** 14480 * The quantity of the benefit which is permitted under the coverage. 14481 */ 14482 @Child(name = "allowed", type = {UnsignedIntType.class, StringType.class, Money.class}, order=2, min=0, max=1, modifier=false, summary=false) 14483 @Description(shortDefinition="Benefits allowed", formalDefinition="The quantity of the benefit which is permitted under the coverage." ) 14484 protected DataType allowed; 14485 14486 /** 14487 * The quantity of the benefit which have been consumed to date. 14488 */ 14489 @Child(name = "used", type = {UnsignedIntType.class, Money.class}, order=3, min=0, max=1, modifier=false, summary=false) 14490 @Description(shortDefinition="Benefits used", formalDefinition="The quantity of the benefit which have been consumed to date." ) 14491 protected DataType used; 14492 14493 private static final long serialVersionUID = 1900247614L; 14494 14495 /** 14496 * Constructor 14497 */ 14498 public BenefitComponent() { 14499 super(); 14500 } 14501 14502 /** 14503 * Constructor 14504 */ 14505 public BenefitComponent(CodeableConcept type) { 14506 super(); 14507 this.setType(type); 14508 } 14509 14510 /** 14511 * @return {@link #type} (Classification of benefit being provided.) 14512 */ 14513 public CodeableConcept getType() { 14514 if (this.type == null) 14515 if (Configuration.errorOnAutoCreate()) 14516 throw new Error("Attempt to auto-create BenefitComponent.type"); 14517 else if (Configuration.doAutoCreate()) 14518 this.type = new CodeableConcept(); // cc 14519 return this.type; 14520 } 14521 14522 public boolean hasType() { 14523 return this.type != null && !this.type.isEmpty(); 14524 } 14525 14526 /** 14527 * @param value {@link #type} (Classification of benefit being provided.) 14528 */ 14529 public BenefitComponent setType(CodeableConcept value) { 14530 this.type = value; 14531 return this; 14532 } 14533 14534 /** 14535 * @return {@link #allowed} (The quantity of the benefit which is permitted under the coverage.) 14536 */ 14537 public DataType getAllowed() { 14538 return this.allowed; 14539 } 14540 14541 /** 14542 * @return {@link #allowed} (The quantity of the benefit which is permitted under the coverage.) 14543 */ 14544 public UnsignedIntType getAllowedUnsignedIntType() throws FHIRException { 14545 if (this.allowed == null) 14546 this.allowed = new UnsignedIntType(); 14547 if (!(this.allowed instanceof UnsignedIntType)) 14548 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.allowed.getClass().getName()+" was encountered"); 14549 return (UnsignedIntType) this.allowed; 14550 } 14551 14552 public boolean hasAllowedUnsignedIntType() { 14553 return this != null && this.allowed instanceof UnsignedIntType; 14554 } 14555 14556 /** 14557 * @return {@link #allowed} (The quantity of the benefit which is permitted under the coverage.) 14558 */ 14559 public StringType getAllowedStringType() throws FHIRException { 14560 if (this.allowed == null) 14561 this.allowed = new StringType(); 14562 if (!(this.allowed instanceof StringType)) 14563 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.allowed.getClass().getName()+" was encountered"); 14564 return (StringType) this.allowed; 14565 } 14566 14567 public boolean hasAllowedStringType() { 14568 return this != null && this.allowed instanceof StringType; 14569 } 14570 14571 /** 14572 * @return {@link #allowed} (The quantity of the benefit which is permitted under the coverage.) 14573 */ 14574 public Money getAllowedMoney() throws FHIRException { 14575 if (this.allowed == null) 14576 this.allowed = new Money(); 14577 if (!(this.allowed instanceof Money)) 14578 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.allowed.getClass().getName()+" was encountered"); 14579 return (Money) this.allowed; 14580 } 14581 14582 public boolean hasAllowedMoney() { 14583 return this != null && this.allowed instanceof Money; 14584 } 14585 14586 public boolean hasAllowed() { 14587 return this.allowed != null && !this.allowed.isEmpty(); 14588 } 14589 14590 /** 14591 * @param value {@link #allowed} (The quantity of the benefit which is permitted under the coverage.) 14592 */ 14593 public BenefitComponent setAllowed(DataType value) { 14594 if (value != null && !(value instanceof UnsignedIntType || value instanceof StringType || value instanceof Money)) 14595 throw new FHIRException("Not the right type for ExplanationOfBenefit.benefitBalance.financial.allowed[x]: "+value.fhirType()); 14596 this.allowed = value; 14597 return this; 14598 } 14599 14600 /** 14601 * @return {@link #used} (The quantity of the benefit which have been consumed to date.) 14602 */ 14603 public DataType getUsed() { 14604 return this.used; 14605 } 14606 14607 /** 14608 * @return {@link #used} (The quantity of the benefit which have been consumed to date.) 14609 */ 14610 public UnsignedIntType getUsedUnsignedIntType() throws FHIRException { 14611 if (this.used == null) 14612 this.used = new UnsignedIntType(); 14613 if (!(this.used instanceof UnsignedIntType)) 14614 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.used.getClass().getName()+" was encountered"); 14615 return (UnsignedIntType) this.used; 14616 } 14617 14618 public boolean hasUsedUnsignedIntType() { 14619 return this != null && this.used instanceof UnsignedIntType; 14620 } 14621 14622 /** 14623 * @return {@link #used} (The quantity of the benefit which have been consumed to date.) 14624 */ 14625 public Money getUsedMoney() throws FHIRException { 14626 if (this.used == null) 14627 this.used = new Money(); 14628 if (!(this.used instanceof Money)) 14629 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.used.getClass().getName()+" was encountered"); 14630 return (Money) this.used; 14631 } 14632 14633 public boolean hasUsedMoney() { 14634 return this != null && this.used instanceof Money; 14635 } 14636 14637 public boolean hasUsed() { 14638 return this.used != null && !this.used.isEmpty(); 14639 } 14640 14641 /** 14642 * @param value {@link #used} (The quantity of the benefit which have been consumed to date.) 14643 */ 14644 public BenefitComponent setUsed(DataType value) { 14645 if (value != null && !(value instanceof UnsignedIntType || value instanceof Money)) 14646 throw new FHIRException("Not the right type for ExplanationOfBenefit.benefitBalance.financial.used[x]: "+value.fhirType()); 14647 this.used = value; 14648 return this; 14649 } 14650 14651 protected void listChildren(List<Property> children) { 14652 super.listChildren(children); 14653 children.add(new Property("type", "CodeableConcept", "Classification of benefit being provided.", 0, 1, type)); 14654 children.add(new Property("allowed[x]", "unsignedInt|string|Money", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed)); 14655 children.add(new Property("used[x]", "unsignedInt|Money", "The quantity of the benefit which have been consumed to date.", 0, 1, used)); 14656 } 14657 14658 @Override 14659 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 14660 switch (_hash) { 14661 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Classification of benefit being provided.", 0, 1, type); 14662 case -1336663592: /*allowed[x]*/ return new Property("allowed[x]", "unsignedInt|string|Money", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 14663 case -911343192: /*allowed*/ return new Property("allowed[x]", "unsignedInt|string|Money", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 14664 case 1668802034: /*allowedUnsignedInt*/ return new Property("allowed[x]", "unsignedInt", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 14665 case -2135265319: /*allowedString*/ return new Property("allowed[x]", "string", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 14666 case -351668232: /*allowedMoney*/ return new Property("allowed[x]", "Money", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 14667 case -147553373: /*used[x]*/ return new Property("used[x]", "unsignedInt|Money", "The quantity of the benefit which have been consumed to date.", 0, 1, used); 14668 case 3599293: /*used*/ return new Property("used[x]", "unsignedInt|Money", "The quantity of the benefit which have been consumed to date.", 0, 1, used); 14669 case 1252740285: /*usedUnsignedInt*/ return new Property("used[x]", "unsignedInt", "The quantity of the benefit which have been consumed to date.", 0, 1, used); 14670 case -78048509: /*usedMoney*/ return new Property("used[x]", "Money", "The quantity of the benefit which have been consumed to date.", 0, 1, used); 14671 default: return super.getNamedProperty(_hash, _name, _checkValid); 14672 } 14673 14674 } 14675 14676 @Override 14677 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 14678 switch (hash) { 14679 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 14680 case -911343192: /*allowed*/ return this.allowed == null ? new Base[0] : new Base[] {this.allowed}; // DataType 14681 case 3599293: /*used*/ return this.used == null ? new Base[0] : new Base[] {this.used}; // DataType 14682 default: return super.getProperty(hash, name, checkValid); 14683 } 14684 14685 } 14686 14687 @Override 14688 public Base setProperty(int hash, String name, Base value) throws FHIRException { 14689 switch (hash) { 14690 case 3575610: // type 14691 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14692 return value; 14693 case -911343192: // allowed 14694 this.allowed = TypeConvertor.castToType(value); // DataType 14695 return value; 14696 case 3599293: // used 14697 this.used = TypeConvertor.castToType(value); // DataType 14698 return value; 14699 default: return super.setProperty(hash, name, value); 14700 } 14701 14702 } 14703 14704 @Override 14705 public Base setProperty(String name, Base value) throws FHIRException { 14706 if (name.equals("type")) { 14707 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14708 } else if (name.equals("allowed[x]")) { 14709 this.allowed = TypeConvertor.castToType(value); // DataType 14710 } else if (name.equals("used[x]")) { 14711 this.used = TypeConvertor.castToType(value); // DataType 14712 } else 14713 return super.setProperty(name, value); 14714 return value; 14715 } 14716 14717 @Override 14718 public Base makeProperty(int hash, String name) throws FHIRException { 14719 switch (hash) { 14720 case 3575610: return getType(); 14721 case -1336663592: return getAllowed(); 14722 case -911343192: return getAllowed(); 14723 case -147553373: return getUsed(); 14724 case 3599293: return getUsed(); 14725 default: return super.makeProperty(hash, name); 14726 } 14727 14728 } 14729 14730 @Override 14731 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 14732 switch (hash) { 14733 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 14734 case -911343192: /*allowed*/ return new String[] {"unsignedInt", "string", "Money"}; 14735 case 3599293: /*used*/ return new String[] {"unsignedInt", "Money"}; 14736 default: return super.getTypesForProperty(hash, name); 14737 } 14738 14739 } 14740 14741 @Override 14742 public Base addChild(String name) throws FHIRException { 14743 if (name.equals("type")) { 14744 this.type = new CodeableConcept(); 14745 return this.type; 14746 } 14747 else if (name.equals("allowedUnsignedInt")) { 14748 this.allowed = new UnsignedIntType(); 14749 return this.allowed; 14750 } 14751 else if (name.equals("allowedString")) { 14752 this.allowed = new StringType(); 14753 return this.allowed; 14754 } 14755 else if (name.equals("allowedMoney")) { 14756 this.allowed = new Money(); 14757 return this.allowed; 14758 } 14759 else if (name.equals("usedUnsignedInt")) { 14760 this.used = new UnsignedIntType(); 14761 return this.used; 14762 } 14763 else if (name.equals("usedMoney")) { 14764 this.used = new Money(); 14765 return this.used; 14766 } 14767 else 14768 return super.addChild(name); 14769 } 14770 14771 public BenefitComponent copy() { 14772 BenefitComponent dst = new BenefitComponent(); 14773 copyValues(dst); 14774 return dst; 14775 } 14776 14777 public void copyValues(BenefitComponent dst) { 14778 super.copyValues(dst); 14779 dst.type = type == null ? null : type.copy(); 14780 dst.allowed = allowed == null ? null : allowed.copy(); 14781 dst.used = used == null ? null : used.copy(); 14782 } 14783 14784 @Override 14785 public boolean equalsDeep(Base other_) { 14786 if (!super.equalsDeep(other_)) 14787 return false; 14788 if (!(other_ instanceof BenefitComponent)) 14789 return false; 14790 BenefitComponent o = (BenefitComponent) other_; 14791 return compareDeep(type, o.type, true) && compareDeep(allowed, o.allowed, true) && compareDeep(used, o.used, true) 14792 ; 14793 } 14794 14795 @Override 14796 public boolean equalsShallow(Base other_) { 14797 if (!super.equalsShallow(other_)) 14798 return false; 14799 if (!(other_ instanceof BenefitComponent)) 14800 return false; 14801 BenefitComponent o = (BenefitComponent) other_; 14802 return true; 14803 } 14804 14805 public boolean isEmpty() { 14806 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, allowed, used); 14807 } 14808 14809 public String fhirType() { 14810 return "ExplanationOfBenefit.benefitBalance.financial"; 14811 14812 } 14813 14814 } 14815 14816 /** 14817 * A unique identifier assigned to this explanation of benefit. 14818 */ 14819 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 14820 @Description(shortDefinition="Business Identifier for the resource", formalDefinition="A unique identifier assigned to this explanation of benefit." ) 14821 protected List<Identifier> identifier; 14822 14823 /** 14824 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 14825 */ 14826 @Child(name = "traceNumber", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 14827 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 14828 protected List<Identifier> traceNumber; 14829 14830 /** 14831 * The status of the resource instance. 14832 */ 14833 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 14834 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 14835 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/explanationofbenefit-status") 14836 protected Enumeration<ExplanationOfBenefitStatus> status; 14837 14838 /** 14839 * The category of claim, e.g. oral, pharmacy, vision, institutional, professional. 14840 */ 14841 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 14842 @Description(shortDefinition="Category or discipline", formalDefinition="The category of claim, e.g. oral, pharmacy, vision, institutional, professional." ) 14843 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-type") 14844 protected CodeableConcept type; 14845 14846 /** 14847 * A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service. 14848 */ 14849 @Child(name = "subType", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 14850 @Description(shortDefinition="More granular claim type", formalDefinition="A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service." ) 14851 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-subtype") 14852 protected CodeableConcept subType; 14853 14854 /** 14855 * A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided. 14856 */ 14857 @Child(name = "use", type = {CodeType.class}, order=5, min=1, max=1, modifier=false, summary=true) 14858 @Description(shortDefinition="claim | preauthorization | predetermination", formalDefinition="A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided." ) 14859 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-use") 14860 protected Enumeration<Use> use; 14861 14862 /** 14863 * The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for forecast reimbursement is sought. 14864 */ 14865 @Child(name = "patient", type = {Patient.class}, order=6, min=1, max=1, modifier=false, summary=true) 14866 @Description(shortDefinition="The recipient of the products and services", formalDefinition="The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for forecast reimbursement is sought." ) 14867 protected Reference patient; 14868 14869 /** 14870 * The period for which charges are being submitted. 14871 */ 14872 @Child(name = "billablePeriod", type = {Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 14873 @Description(shortDefinition="Relevant time frame for the claim", formalDefinition="The period for which charges are being submitted." ) 14874 protected Period billablePeriod; 14875 14876 /** 14877 * The date this resource was created. 14878 */ 14879 @Child(name = "created", type = {DateTimeType.class}, order=8, min=1, max=1, modifier=false, summary=true) 14880 @Description(shortDefinition="Response creation date", formalDefinition="The date this resource was created." ) 14881 protected DateTimeType created; 14882 14883 /** 14884 * Individual who created the claim, predetermination or preauthorization. 14885 */ 14886 @Child(name = "enterer", type = {Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class}, order=9, min=0, max=1, modifier=false, summary=false) 14887 @Description(shortDefinition="Author of the claim", formalDefinition="Individual who created the claim, predetermination or preauthorization." ) 14888 protected Reference enterer; 14889 14890 /** 14891 * The party responsible for authorization, adjudication and reimbursement. 14892 */ 14893 @Child(name = "insurer", type = {Organization.class}, order=10, min=0, max=1, modifier=false, summary=true) 14894 @Description(shortDefinition="Party responsible for reimbursement", formalDefinition="The party responsible for authorization, adjudication and reimbursement." ) 14895 protected Reference insurer; 14896 14897 /** 14898 * The provider which is responsible for the claim, predetermination or preauthorization. 14899 */ 14900 @Child(name = "provider", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=11, min=0, max=1, modifier=false, summary=true) 14901 @Description(shortDefinition="Party responsible for the claim", formalDefinition="The provider which is responsible for the claim, predetermination or preauthorization." ) 14902 protected Reference provider; 14903 14904 /** 14905 * The provider-required urgency of processing the request. Typical values include: stat, normal deferred. 14906 */ 14907 @Child(name = "priority", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=false) 14908 @Description(shortDefinition="Desired processing urgency", formalDefinition="The provider-required urgency of processing the request. Typical values include: stat, normal deferred." ) 14909 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/process-priority") 14910 protected CodeableConcept priority; 14911 14912 /** 14913 * A code to indicate whether and for whom funds are to be reserved for future claims. 14914 */ 14915 @Child(name = "fundsReserveRequested", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=false) 14916 @Description(shortDefinition="For whom to reserve funds", formalDefinition="A code to indicate whether and for whom funds are to be reserved for future claims." ) 14917 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fundsreserve") 14918 protected CodeableConcept fundsReserveRequested; 14919 14920 /** 14921 * A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom. 14922 */ 14923 @Child(name = "fundsReserve", type = {CodeableConcept.class}, order=14, min=0, max=1, modifier=false, summary=false) 14924 @Description(shortDefinition="Funds reserved status", formalDefinition="A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom." ) 14925 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fundsreserve") 14926 protected CodeableConcept fundsReserve; 14927 14928 /** 14929 * Other claims which are related to this claim such as prior submissions or claims for related services or for the same event. 14930 */ 14931 @Child(name = "related", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 14932 @Description(shortDefinition="Prior or corollary claims", formalDefinition="Other claims which are related to this claim such as prior submissions or claims for related services or for the same event." ) 14933 protected List<RelatedClaimComponent> related; 14934 14935 /** 14936 * Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments. 14937 */ 14938 @Child(name = "prescription", type = {MedicationRequest.class, VisionPrescription.class}, order=16, min=0, max=1, modifier=false, summary=false) 14939 @Description(shortDefinition="Prescription authorizing services or products", formalDefinition="Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments." ) 14940 protected Reference prescription; 14941 14942 /** 14943 * Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products. 14944 */ 14945 @Child(name = "originalPrescription", type = {MedicationRequest.class}, order=17, min=0, max=1, modifier=false, summary=false) 14946 @Description(shortDefinition="Original prescription if superceded by fulfiller", formalDefinition="Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products." ) 14947 protected Reference originalPrescription; 14948 14949 /** 14950 * Information code for an event with a corresponding date or period. 14951 */ 14952 @Child(name = "event", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 14953 @Description(shortDefinition="Event information", formalDefinition="Information code for an event with a corresponding date or period." ) 14954 protected List<ExplanationOfBenefitEventComponent> event; 14955 14956 /** 14957 * The party to be reimbursed for cost of the products and services according to the terms of the policy. 14958 */ 14959 @Child(name = "payee", type = {}, order=19, min=0, max=1, modifier=false, summary=false) 14960 @Description(shortDefinition="Recipient of benefits payable", formalDefinition="The party to be reimbursed for cost of the products and services according to the terms of the policy." ) 14961 protected PayeeComponent payee; 14962 14963 /** 14964 * The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services. 14965 */ 14966 @Child(name = "referral", type = {ServiceRequest.class}, order=20, min=0, max=1, modifier=false, summary=false) 14967 @Description(shortDefinition="Treatment Referral", formalDefinition="The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services." ) 14968 protected Reference referral; 14969 14970 /** 14971 * Healthcare encounters related to this claim. 14972 */ 14973 @Child(name = "encounter", type = {Encounter.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 14974 @Description(shortDefinition="Encounters associated with the listed treatments", formalDefinition="Healthcare encounters related to this claim." ) 14975 protected List<Reference> encounter; 14976 14977 /** 14978 * Facility where the services were provided. 14979 */ 14980 @Child(name = "facility", type = {Location.class, Organization.class}, order=22, min=0, max=1, modifier=false, summary=false) 14981 @Description(shortDefinition="Servicing Facility", formalDefinition="Facility where the services were provided." ) 14982 protected Reference facility; 14983 14984 /** 14985 * The business identifier for the instance of the adjudication request: claim predetermination or preauthorization. 14986 */ 14987 @Child(name = "claim", type = {Claim.class}, order=23, min=0, max=1, modifier=false, summary=false) 14988 @Description(shortDefinition="Claim reference", formalDefinition="The business identifier for the instance of the adjudication request: claim predetermination or preauthorization." ) 14989 protected Reference claim; 14990 14991 /** 14992 * The business identifier for the instance of the adjudication response: claim, predetermination or preauthorization response. 14993 */ 14994 @Child(name = "claimResponse", type = {ClaimResponse.class}, order=24, min=0, max=1, modifier=false, summary=false) 14995 @Description(shortDefinition="Claim response reference", formalDefinition="The business identifier for the instance of the adjudication response: claim, predetermination or preauthorization response." ) 14996 protected Reference claimResponse; 14997 14998 /** 14999 * The outcome of the claim, predetermination, or preauthorization processing. 15000 */ 15001 @Child(name = "outcome", type = {CodeType.class}, order=25, min=1, max=1, modifier=false, summary=true) 15002 @Description(shortDefinition="queued | complete | error | partial", formalDefinition="The outcome of the claim, predetermination, or preauthorization processing." ) 15003 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-outcome") 15004 protected Enumeration<ClaimProcessingCodes> outcome; 15005 15006 /** 15007 * The result of the claim, predetermination, or preauthorization adjudication. 15008 */ 15009 @Child(name = "decision", type = {CodeableConcept.class}, order=26, min=0, max=1, modifier=false, summary=true) 15010 @Description(shortDefinition="Result of the adjudication", formalDefinition="The result of the claim, predetermination, or preauthorization adjudication." ) 15011 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-decision") 15012 protected CodeableConcept decision; 15013 15014 /** 15015 * A human readable description of the status of the adjudication. 15016 */ 15017 @Child(name = "disposition", type = {StringType.class}, order=27, min=0, max=1, modifier=false, summary=false) 15018 @Description(shortDefinition="Disposition Message", formalDefinition="A human readable description of the status of the adjudication." ) 15019 protected StringType disposition; 15020 15021 /** 15022 * Reference from the Insurer which is used in later communications which refers to this adjudication. 15023 */ 15024 @Child(name = "preAuthRef", type = {StringType.class}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15025 @Description(shortDefinition="Preauthorization reference", formalDefinition="Reference from the Insurer which is used in later communications which refers to this adjudication." ) 15026 protected List<StringType> preAuthRef; 15027 15028 /** 15029 * The timeframe during which the supplied preauthorization reference may be quoted on claims to obtain the adjudication as provided. 15030 */ 15031 @Child(name = "preAuthRefPeriod", type = {Period.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15032 @Description(shortDefinition="Preauthorization in-effect period", formalDefinition="The timeframe during which the supplied preauthorization reference may be quoted on claims to obtain the adjudication as provided." ) 15033 protected List<Period> preAuthRefPeriod; 15034 15035 /** 15036 * A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system. 15037 */ 15038 @Child(name = "diagnosisRelatedGroup", type = {CodeableConcept.class}, order=30, min=0, max=1, modifier=false, summary=false) 15039 @Description(shortDefinition="Package billing code", formalDefinition="A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system." ) 15040 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosisrelatedgroup") 15041 protected CodeableConcept diagnosisRelatedGroup; 15042 15043 /** 15044 * The members of the team who provided the products and services. 15045 */ 15046 @Child(name = "careTeam", type = {}, order=31, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15047 @Description(shortDefinition="Care Team members", formalDefinition="The members of the team who provided the products and services." ) 15048 protected List<CareTeamComponent> careTeam; 15049 15050 /** 15051 * Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. 15052 */ 15053 @Child(name = "supportingInfo", type = {}, order=32, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15054 @Description(shortDefinition="Supporting information", formalDefinition="Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues." ) 15055 protected List<SupportingInformationComponent> supportingInfo; 15056 15057 /** 15058 * Information about diagnoses relevant to the claim items. 15059 */ 15060 @Child(name = "diagnosis", type = {}, order=33, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15061 @Description(shortDefinition="Pertinent diagnosis information", formalDefinition="Information about diagnoses relevant to the claim items." ) 15062 protected List<DiagnosisComponent> diagnosis; 15063 15064 /** 15065 * Procedures performed on the patient relevant to the billing items with the claim. 15066 */ 15067 @Child(name = "procedure", type = {}, order=34, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15068 @Description(shortDefinition="Clinical procedures performed", formalDefinition="Procedures performed on the patient relevant to the billing items with the claim." ) 15069 protected List<ProcedureComponent> procedure; 15070 15071 /** 15072 * This indicates the relative order of a series of EOBs related to different coverages for the same suite of services. 15073 */ 15074 @Child(name = "precedence", type = {PositiveIntType.class}, order=35, min=0, max=1, modifier=false, summary=false) 15075 @Description(shortDefinition="Precedence (primary, secondary, etc.)", formalDefinition="This indicates the relative order of a series of EOBs related to different coverages for the same suite of services." ) 15076 protected PositiveIntType precedence; 15077 15078 /** 15079 * Financial instruments for reimbursement for the health care products and services specified on the claim. 15080 */ 15081 @Child(name = "insurance", type = {}, order=36, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 15082 @Description(shortDefinition="Patient insurance information", formalDefinition="Financial instruments for reimbursement for the health care products and services specified on the claim." ) 15083 protected List<InsuranceComponent> insurance; 15084 15085 /** 15086 * Details of a accident which resulted in injuries which required the products and services listed in the claim. 15087 */ 15088 @Child(name = "accident", type = {}, order=37, min=0, max=1, modifier=false, summary=false) 15089 @Description(shortDefinition="Details of the event", formalDefinition="Details of a accident which resulted in injuries which required the products and services listed in the claim." ) 15090 protected AccidentComponent accident; 15091 15092 /** 15093 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 15094 */ 15095 @Child(name = "patientPaid", type = {Money.class}, order=38, min=0, max=1, modifier=false, summary=false) 15096 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 15097 protected Money patientPaid; 15098 15099 /** 15100 * A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details. 15101 */ 15102 @Child(name = "item", type = {}, order=39, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15103 @Description(shortDefinition="Product or service provided", formalDefinition="A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details." ) 15104 protected List<ItemComponent> item; 15105 15106 /** 15107 * The first-tier service adjudications for payor added product or service lines. 15108 */ 15109 @Child(name = "addItem", type = {}, order=40, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15110 @Description(shortDefinition="Insurer added line items", formalDefinition="The first-tier service adjudications for payor added product or service lines." ) 15111 protected List<AddedItemComponent> addItem; 15112 15113 /** 15114 * The adjudication results which are presented at the header level rather than at the line-item or add-item levels. 15115 */ 15116 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=41, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15117 @Description(shortDefinition="Header-level adjudication", formalDefinition="The adjudication results which are presented at the header level rather than at the line-item or add-item levels." ) 15118 protected List<AdjudicationComponent> adjudication; 15119 15120 /** 15121 * Categorized monetary totals for the adjudication. 15122 */ 15123 @Child(name = "total", type = {}, order=42, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 15124 @Description(shortDefinition="Adjudication totals", formalDefinition="Categorized monetary totals for the adjudication." ) 15125 protected List<TotalComponent> total; 15126 15127 /** 15128 * Payment details for the adjudication of the claim. 15129 */ 15130 @Child(name = "payment", type = {}, order=43, min=0, max=1, modifier=false, summary=false) 15131 @Description(shortDefinition="Payment Details", formalDefinition="Payment details for the adjudication of the claim." ) 15132 protected PaymentComponent payment; 15133 15134 /** 15135 * A code for the form to be used for printing the content. 15136 */ 15137 @Child(name = "formCode", type = {CodeableConcept.class}, order=44, min=0, max=1, modifier=false, summary=false) 15138 @Description(shortDefinition="Printed form identifier", formalDefinition="A code for the form to be used for printing the content." ) 15139 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/forms") 15140 protected CodeableConcept formCode; 15141 15142 /** 15143 * The actual form, by reference or inclusion, for printing the content or an EOB. 15144 */ 15145 @Child(name = "form", type = {Attachment.class}, order=45, min=0, max=1, modifier=false, summary=false) 15146 @Description(shortDefinition="Printed reference or actual form", formalDefinition="The actual form, by reference or inclusion, for printing the content or an EOB." ) 15147 protected Attachment form; 15148 15149 /** 15150 * A note that describes or explains adjudication results in a human readable form. 15151 */ 15152 @Child(name = "processNote", type = {}, order=46, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15153 @Description(shortDefinition="Note concerning adjudication", formalDefinition="A note that describes or explains adjudication results in a human readable form." ) 15154 protected List<NoteComponent> processNote; 15155 15156 /** 15157 * The term of the benefits documented in this response. 15158 */ 15159 @Child(name = "benefitPeriod", type = {Period.class}, order=47, min=0, max=1, modifier=false, summary=false) 15160 @Description(shortDefinition="When the benefits are applicable", formalDefinition="The term of the benefits documented in this response." ) 15161 protected Period benefitPeriod; 15162 15163 /** 15164 * Balance by Benefit Category. 15165 */ 15166 @Child(name = "benefitBalance", type = {}, order=48, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15167 @Description(shortDefinition="Balance by Benefit Category", formalDefinition="Balance by Benefit Category." ) 15168 protected List<BenefitBalanceComponent> benefitBalance; 15169 15170 private static final long serialVersionUID = 106661060L; 15171 15172 /** 15173 * Constructor 15174 */ 15175 public ExplanationOfBenefit() { 15176 super(); 15177 } 15178 15179 /** 15180 * Constructor 15181 */ 15182 public ExplanationOfBenefit(ExplanationOfBenefitStatus status, CodeableConcept type, Use use, Reference patient, Date created, ClaimProcessingCodes outcome) { 15183 super(); 15184 this.setStatus(status); 15185 this.setType(type); 15186 this.setUse(use); 15187 this.setPatient(patient); 15188 this.setCreated(created); 15189 this.setOutcome(outcome); 15190 } 15191 15192 /** 15193 * @return {@link #identifier} (A unique identifier assigned to this explanation of benefit.) 15194 */ 15195 public List<Identifier> getIdentifier() { 15196 if (this.identifier == null) 15197 this.identifier = new ArrayList<Identifier>(); 15198 return this.identifier; 15199 } 15200 15201 /** 15202 * @return Returns a reference to <code>this</code> for easy method chaining 15203 */ 15204 public ExplanationOfBenefit setIdentifier(List<Identifier> theIdentifier) { 15205 this.identifier = theIdentifier; 15206 return this; 15207 } 15208 15209 public boolean hasIdentifier() { 15210 if (this.identifier == null) 15211 return false; 15212 for (Identifier item : this.identifier) 15213 if (!item.isEmpty()) 15214 return true; 15215 return false; 15216 } 15217 15218 public Identifier addIdentifier() { //3 15219 Identifier t = new Identifier(); 15220 if (this.identifier == null) 15221 this.identifier = new ArrayList<Identifier>(); 15222 this.identifier.add(t); 15223 return t; 15224 } 15225 15226 public ExplanationOfBenefit addIdentifier(Identifier t) { //3 15227 if (t == null) 15228 return this; 15229 if (this.identifier == null) 15230 this.identifier = new ArrayList<Identifier>(); 15231 this.identifier.add(t); 15232 return this; 15233 } 15234 15235 /** 15236 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 15237 */ 15238 public Identifier getIdentifierFirstRep() { 15239 if (getIdentifier().isEmpty()) { 15240 addIdentifier(); 15241 } 15242 return getIdentifier().get(0); 15243 } 15244 15245 /** 15246 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 15247 */ 15248 public List<Identifier> getTraceNumber() { 15249 if (this.traceNumber == null) 15250 this.traceNumber = new ArrayList<Identifier>(); 15251 return this.traceNumber; 15252 } 15253 15254 /** 15255 * @return Returns a reference to <code>this</code> for easy method chaining 15256 */ 15257 public ExplanationOfBenefit setTraceNumber(List<Identifier> theTraceNumber) { 15258 this.traceNumber = theTraceNumber; 15259 return this; 15260 } 15261 15262 public boolean hasTraceNumber() { 15263 if (this.traceNumber == null) 15264 return false; 15265 for (Identifier item : this.traceNumber) 15266 if (!item.isEmpty()) 15267 return true; 15268 return false; 15269 } 15270 15271 public Identifier addTraceNumber() { //3 15272 Identifier t = new Identifier(); 15273 if (this.traceNumber == null) 15274 this.traceNumber = new ArrayList<Identifier>(); 15275 this.traceNumber.add(t); 15276 return t; 15277 } 15278 15279 public ExplanationOfBenefit addTraceNumber(Identifier t) { //3 15280 if (t == null) 15281 return this; 15282 if (this.traceNumber == null) 15283 this.traceNumber = new ArrayList<Identifier>(); 15284 this.traceNumber.add(t); 15285 return this; 15286 } 15287 15288 /** 15289 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 15290 */ 15291 public Identifier getTraceNumberFirstRep() { 15292 if (getTraceNumber().isEmpty()) { 15293 addTraceNumber(); 15294 } 15295 return getTraceNumber().get(0); 15296 } 15297 15298 /** 15299 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 15300 */ 15301 public Enumeration<ExplanationOfBenefitStatus> getStatusElement() { 15302 if (this.status == null) 15303 if (Configuration.errorOnAutoCreate()) 15304 throw new Error("Attempt to auto-create ExplanationOfBenefit.status"); 15305 else if (Configuration.doAutoCreate()) 15306 this.status = new Enumeration<ExplanationOfBenefitStatus>(new ExplanationOfBenefitStatusEnumFactory()); // bb 15307 return this.status; 15308 } 15309 15310 public boolean hasStatusElement() { 15311 return this.status != null && !this.status.isEmpty(); 15312 } 15313 15314 public boolean hasStatus() { 15315 return this.status != null && !this.status.isEmpty(); 15316 } 15317 15318 /** 15319 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 15320 */ 15321 public ExplanationOfBenefit setStatusElement(Enumeration<ExplanationOfBenefitStatus> value) { 15322 this.status = value; 15323 return this; 15324 } 15325 15326 /** 15327 * @return The status of the resource instance. 15328 */ 15329 public ExplanationOfBenefitStatus getStatus() { 15330 return this.status == null ? null : this.status.getValue(); 15331 } 15332 15333 /** 15334 * @param value The status of the resource instance. 15335 */ 15336 public ExplanationOfBenefit setStatus(ExplanationOfBenefitStatus value) { 15337 if (this.status == null) 15338 this.status = new Enumeration<ExplanationOfBenefitStatus>(new ExplanationOfBenefitStatusEnumFactory()); 15339 this.status.setValue(value); 15340 return this; 15341 } 15342 15343 /** 15344 * @return {@link #type} (The category of claim, e.g. oral, pharmacy, vision, institutional, professional.) 15345 */ 15346 public CodeableConcept getType() { 15347 if (this.type == null) 15348 if (Configuration.errorOnAutoCreate()) 15349 throw new Error("Attempt to auto-create ExplanationOfBenefit.type"); 15350 else if (Configuration.doAutoCreate()) 15351 this.type = new CodeableConcept(); // cc 15352 return this.type; 15353 } 15354 15355 public boolean hasType() { 15356 return this.type != null && !this.type.isEmpty(); 15357 } 15358 15359 /** 15360 * @param value {@link #type} (The category of claim, e.g. oral, pharmacy, vision, institutional, professional.) 15361 */ 15362 public ExplanationOfBenefit setType(CodeableConcept value) { 15363 this.type = value; 15364 return this; 15365 } 15366 15367 /** 15368 * @return {@link #subType} (A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.) 15369 */ 15370 public CodeableConcept getSubType() { 15371 if (this.subType == null) 15372 if (Configuration.errorOnAutoCreate()) 15373 throw new Error("Attempt to auto-create ExplanationOfBenefit.subType"); 15374 else if (Configuration.doAutoCreate()) 15375 this.subType = new CodeableConcept(); // cc 15376 return this.subType; 15377 } 15378 15379 public boolean hasSubType() { 15380 return this.subType != null && !this.subType.isEmpty(); 15381 } 15382 15383 /** 15384 * @param value {@link #subType} (A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.) 15385 */ 15386 public ExplanationOfBenefit setSubType(CodeableConcept value) { 15387 this.subType = value; 15388 return this; 15389 } 15390 15391 /** 15392 * @return {@link #use} (A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 15393 */ 15394 public Enumeration<Use> getUseElement() { 15395 if (this.use == null) 15396 if (Configuration.errorOnAutoCreate()) 15397 throw new Error("Attempt to auto-create ExplanationOfBenefit.use"); 15398 else if (Configuration.doAutoCreate()) 15399 this.use = new Enumeration<Use>(new UseEnumFactory()); // bb 15400 return this.use; 15401 } 15402 15403 public boolean hasUseElement() { 15404 return this.use != null && !this.use.isEmpty(); 15405 } 15406 15407 public boolean hasUse() { 15408 return this.use != null && !this.use.isEmpty(); 15409 } 15410 15411 /** 15412 * @param value {@link #use} (A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 15413 */ 15414 public ExplanationOfBenefit setUseElement(Enumeration<Use> value) { 15415 this.use = value; 15416 return this; 15417 } 15418 15419 /** 15420 * @return A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided. 15421 */ 15422 public Use getUse() { 15423 return this.use == null ? null : this.use.getValue(); 15424 } 15425 15426 /** 15427 * @param value A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided. 15428 */ 15429 public ExplanationOfBenefit setUse(Use value) { 15430 if (this.use == null) 15431 this.use = new Enumeration<Use>(new UseEnumFactory()); 15432 this.use.setValue(value); 15433 return this; 15434 } 15435 15436 /** 15437 * @return {@link #patient} (The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for forecast reimbursement is sought.) 15438 */ 15439 public Reference getPatient() { 15440 if (this.patient == null) 15441 if (Configuration.errorOnAutoCreate()) 15442 throw new Error("Attempt to auto-create ExplanationOfBenefit.patient"); 15443 else if (Configuration.doAutoCreate()) 15444 this.patient = new Reference(); // cc 15445 return this.patient; 15446 } 15447 15448 public boolean hasPatient() { 15449 return this.patient != null && !this.patient.isEmpty(); 15450 } 15451 15452 /** 15453 * @param value {@link #patient} (The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for forecast reimbursement is sought.) 15454 */ 15455 public ExplanationOfBenefit setPatient(Reference value) { 15456 this.patient = value; 15457 return this; 15458 } 15459 15460 /** 15461 * @return {@link #billablePeriod} (The period for which charges are being submitted.) 15462 */ 15463 public Period getBillablePeriod() { 15464 if (this.billablePeriod == null) 15465 if (Configuration.errorOnAutoCreate()) 15466 throw new Error("Attempt to auto-create ExplanationOfBenefit.billablePeriod"); 15467 else if (Configuration.doAutoCreate()) 15468 this.billablePeriod = new Period(); // cc 15469 return this.billablePeriod; 15470 } 15471 15472 public boolean hasBillablePeriod() { 15473 return this.billablePeriod != null && !this.billablePeriod.isEmpty(); 15474 } 15475 15476 /** 15477 * @param value {@link #billablePeriod} (The period for which charges are being submitted.) 15478 */ 15479 public ExplanationOfBenefit setBillablePeriod(Period value) { 15480 this.billablePeriod = value; 15481 return this; 15482 } 15483 15484 /** 15485 * @return {@link #created} (The date this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 15486 */ 15487 public DateTimeType getCreatedElement() { 15488 if (this.created == null) 15489 if (Configuration.errorOnAutoCreate()) 15490 throw new Error("Attempt to auto-create ExplanationOfBenefit.created"); 15491 else if (Configuration.doAutoCreate()) 15492 this.created = new DateTimeType(); // bb 15493 return this.created; 15494 } 15495 15496 public boolean hasCreatedElement() { 15497 return this.created != null && !this.created.isEmpty(); 15498 } 15499 15500 public boolean hasCreated() { 15501 return this.created != null && !this.created.isEmpty(); 15502 } 15503 15504 /** 15505 * @param value {@link #created} (The date this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 15506 */ 15507 public ExplanationOfBenefit setCreatedElement(DateTimeType value) { 15508 this.created = value; 15509 return this; 15510 } 15511 15512 /** 15513 * @return The date this resource was created. 15514 */ 15515 public Date getCreated() { 15516 return this.created == null ? null : this.created.getValue(); 15517 } 15518 15519 /** 15520 * @param value The date this resource was created. 15521 */ 15522 public ExplanationOfBenefit setCreated(Date value) { 15523 if (this.created == null) 15524 this.created = new DateTimeType(); 15525 this.created.setValue(value); 15526 return this; 15527 } 15528 15529 /** 15530 * @return {@link #enterer} (Individual who created the claim, predetermination or preauthorization.) 15531 */ 15532 public Reference getEnterer() { 15533 if (this.enterer == null) 15534 if (Configuration.errorOnAutoCreate()) 15535 throw new Error("Attempt to auto-create ExplanationOfBenefit.enterer"); 15536 else if (Configuration.doAutoCreate()) 15537 this.enterer = new Reference(); // cc 15538 return this.enterer; 15539 } 15540 15541 public boolean hasEnterer() { 15542 return this.enterer != null && !this.enterer.isEmpty(); 15543 } 15544 15545 /** 15546 * @param value {@link #enterer} (Individual who created the claim, predetermination or preauthorization.) 15547 */ 15548 public ExplanationOfBenefit setEnterer(Reference value) { 15549 this.enterer = value; 15550 return this; 15551 } 15552 15553 /** 15554 * @return {@link #insurer} (The party responsible for authorization, adjudication and reimbursement.) 15555 */ 15556 public Reference getInsurer() { 15557 if (this.insurer == null) 15558 if (Configuration.errorOnAutoCreate()) 15559 throw new Error("Attempt to auto-create ExplanationOfBenefit.insurer"); 15560 else if (Configuration.doAutoCreate()) 15561 this.insurer = new Reference(); // cc 15562 return this.insurer; 15563 } 15564 15565 public boolean hasInsurer() { 15566 return this.insurer != null && !this.insurer.isEmpty(); 15567 } 15568 15569 /** 15570 * @param value {@link #insurer} (The party responsible for authorization, adjudication and reimbursement.) 15571 */ 15572 public ExplanationOfBenefit setInsurer(Reference value) { 15573 this.insurer = value; 15574 return this; 15575 } 15576 15577 /** 15578 * @return {@link #provider} (The provider which is responsible for the claim, predetermination or preauthorization.) 15579 */ 15580 public Reference getProvider() { 15581 if (this.provider == null) 15582 if (Configuration.errorOnAutoCreate()) 15583 throw new Error("Attempt to auto-create ExplanationOfBenefit.provider"); 15584 else if (Configuration.doAutoCreate()) 15585 this.provider = new Reference(); // cc 15586 return this.provider; 15587 } 15588 15589 public boolean hasProvider() { 15590 return this.provider != null && !this.provider.isEmpty(); 15591 } 15592 15593 /** 15594 * @param value {@link #provider} (The provider which is responsible for the claim, predetermination or preauthorization.) 15595 */ 15596 public ExplanationOfBenefit setProvider(Reference value) { 15597 this.provider = value; 15598 return this; 15599 } 15600 15601 /** 15602 * @return {@link #priority} (The provider-required urgency of processing the request. Typical values include: stat, normal deferred.) 15603 */ 15604 public CodeableConcept getPriority() { 15605 if (this.priority == null) 15606 if (Configuration.errorOnAutoCreate()) 15607 throw new Error("Attempt to auto-create ExplanationOfBenefit.priority"); 15608 else if (Configuration.doAutoCreate()) 15609 this.priority = new CodeableConcept(); // cc 15610 return this.priority; 15611 } 15612 15613 public boolean hasPriority() { 15614 return this.priority != null && !this.priority.isEmpty(); 15615 } 15616 15617 /** 15618 * @param value {@link #priority} (The provider-required urgency of processing the request. Typical values include: stat, normal deferred.) 15619 */ 15620 public ExplanationOfBenefit setPriority(CodeableConcept value) { 15621 this.priority = value; 15622 return this; 15623 } 15624 15625 /** 15626 * @return {@link #fundsReserveRequested} (A code to indicate whether and for whom funds are to be reserved for future claims.) 15627 */ 15628 public CodeableConcept getFundsReserveRequested() { 15629 if (this.fundsReserveRequested == null) 15630 if (Configuration.errorOnAutoCreate()) 15631 throw new Error("Attempt to auto-create ExplanationOfBenefit.fundsReserveRequested"); 15632 else if (Configuration.doAutoCreate()) 15633 this.fundsReserveRequested = new CodeableConcept(); // cc 15634 return this.fundsReserveRequested; 15635 } 15636 15637 public boolean hasFundsReserveRequested() { 15638 return this.fundsReserveRequested != null && !this.fundsReserveRequested.isEmpty(); 15639 } 15640 15641 /** 15642 * @param value {@link #fundsReserveRequested} (A code to indicate whether and for whom funds are to be reserved for future claims.) 15643 */ 15644 public ExplanationOfBenefit setFundsReserveRequested(CodeableConcept value) { 15645 this.fundsReserveRequested = value; 15646 return this; 15647 } 15648 15649 /** 15650 * @return {@link #fundsReserve} (A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.) 15651 */ 15652 public CodeableConcept getFundsReserve() { 15653 if (this.fundsReserve == null) 15654 if (Configuration.errorOnAutoCreate()) 15655 throw new Error("Attempt to auto-create ExplanationOfBenefit.fundsReserve"); 15656 else if (Configuration.doAutoCreate()) 15657 this.fundsReserve = new CodeableConcept(); // cc 15658 return this.fundsReserve; 15659 } 15660 15661 public boolean hasFundsReserve() { 15662 return this.fundsReserve != null && !this.fundsReserve.isEmpty(); 15663 } 15664 15665 /** 15666 * @param value {@link #fundsReserve} (A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.) 15667 */ 15668 public ExplanationOfBenefit setFundsReserve(CodeableConcept value) { 15669 this.fundsReserve = value; 15670 return this; 15671 } 15672 15673 /** 15674 * @return {@link #related} (Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.) 15675 */ 15676 public List<RelatedClaimComponent> getRelated() { 15677 if (this.related == null) 15678 this.related = new ArrayList<RelatedClaimComponent>(); 15679 return this.related; 15680 } 15681 15682 /** 15683 * @return Returns a reference to <code>this</code> for easy method chaining 15684 */ 15685 public ExplanationOfBenefit setRelated(List<RelatedClaimComponent> theRelated) { 15686 this.related = theRelated; 15687 return this; 15688 } 15689 15690 public boolean hasRelated() { 15691 if (this.related == null) 15692 return false; 15693 for (RelatedClaimComponent item : this.related) 15694 if (!item.isEmpty()) 15695 return true; 15696 return false; 15697 } 15698 15699 public RelatedClaimComponent addRelated() { //3 15700 RelatedClaimComponent t = new RelatedClaimComponent(); 15701 if (this.related == null) 15702 this.related = new ArrayList<RelatedClaimComponent>(); 15703 this.related.add(t); 15704 return t; 15705 } 15706 15707 public ExplanationOfBenefit addRelated(RelatedClaimComponent t) { //3 15708 if (t == null) 15709 return this; 15710 if (this.related == null) 15711 this.related = new ArrayList<RelatedClaimComponent>(); 15712 this.related.add(t); 15713 return this; 15714 } 15715 15716 /** 15717 * @return The first repetition of repeating field {@link #related}, creating it if it does not already exist {3} 15718 */ 15719 public RelatedClaimComponent getRelatedFirstRep() { 15720 if (getRelated().isEmpty()) { 15721 addRelated(); 15722 } 15723 return getRelated().get(0); 15724 } 15725 15726 /** 15727 * @return {@link #prescription} (Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments.) 15728 */ 15729 public Reference getPrescription() { 15730 if (this.prescription == null) 15731 if (Configuration.errorOnAutoCreate()) 15732 throw new Error("Attempt to auto-create ExplanationOfBenefit.prescription"); 15733 else if (Configuration.doAutoCreate()) 15734 this.prescription = new Reference(); // cc 15735 return this.prescription; 15736 } 15737 15738 public boolean hasPrescription() { 15739 return this.prescription != null && !this.prescription.isEmpty(); 15740 } 15741 15742 /** 15743 * @param value {@link #prescription} (Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments.) 15744 */ 15745 public ExplanationOfBenefit setPrescription(Reference value) { 15746 this.prescription = value; 15747 return this; 15748 } 15749 15750 /** 15751 * @return {@link #originalPrescription} (Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.) 15752 */ 15753 public Reference getOriginalPrescription() { 15754 if (this.originalPrescription == null) 15755 if (Configuration.errorOnAutoCreate()) 15756 throw new Error("Attempt to auto-create ExplanationOfBenefit.originalPrescription"); 15757 else if (Configuration.doAutoCreate()) 15758 this.originalPrescription = new Reference(); // cc 15759 return this.originalPrescription; 15760 } 15761 15762 public boolean hasOriginalPrescription() { 15763 return this.originalPrescription != null && !this.originalPrescription.isEmpty(); 15764 } 15765 15766 /** 15767 * @param value {@link #originalPrescription} (Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.) 15768 */ 15769 public ExplanationOfBenefit setOriginalPrescription(Reference value) { 15770 this.originalPrescription = value; 15771 return this; 15772 } 15773 15774 /** 15775 * @return {@link #event} (Information code for an event with a corresponding date or period.) 15776 */ 15777 public List<ExplanationOfBenefitEventComponent> getEvent() { 15778 if (this.event == null) 15779 this.event = new ArrayList<ExplanationOfBenefitEventComponent>(); 15780 return this.event; 15781 } 15782 15783 /** 15784 * @return Returns a reference to <code>this</code> for easy method chaining 15785 */ 15786 public ExplanationOfBenefit setEvent(List<ExplanationOfBenefitEventComponent> theEvent) { 15787 this.event = theEvent; 15788 return this; 15789 } 15790 15791 public boolean hasEvent() { 15792 if (this.event == null) 15793 return false; 15794 for (ExplanationOfBenefitEventComponent item : this.event) 15795 if (!item.isEmpty()) 15796 return true; 15797 return false; 15798 } 15799 15800 public ExplanationOfBenefitEventComponent addEvent() { //3 15801 ExplanationOfBenefitEventComponent t = new ExplanationOfBenefitEventComponent(); 15802 if (this.event == null) 15803 this.event = new ArrayList<ExplanationOfBenefitEventComponent>(); 15804 this.event.add(t); 15805 return t; 15806 } 15807 15808 public ExplanationOfBenefit addEvent(ExplanationOfBenefitEventComponent t) { //3 15809 if (t == null) 15810 return this; 15811 if (this.event == null) 15812 this.event = new ArrayList<ExplanationOfBenefitEventComponent>(); 15813 this.event.add(t); 15814 return this; 15815 } 15816 15817 /** 15818 * @return The first repetition of repeating field {@link #event}, creating it if it does not already exist {3} 15819 */ 15820 public ExplanationOfBenefitEventComponent getEventFirstRep() { 15821 if (getEvent().isEmpty()) { 15822 addEvent(); 15823 } 15824 return getEvent().get(0); 15825 } 15826 15827 /** 15828 * @return {@link #payee} (The party to be reimbursed for cost of the products and services according to the terms of the policy.) 15829 */ 15830 public PayeeComponent getPayee() { 15831 if (this.payee == null) 15832 if (Configuration.errorOnAutoCreate()) 15833 throw new Error("Attempt to auto-create ExplanationOfBenefit.payee"); 15834 else if (Configuration.doAutoCreate()) 15835 this.payee = new PayeeComponent(); // cc 15836 return this.payee; 15837 } 15838 15839 public boolean hasPayee() { 15840 return this.payee != null && !this.payee.isEmpty(); 15841 } 15842 15843 /** 15844 * @param value {@link #payee} (The party to be reimbursed for cost of the products and services according to the terms of the policy.) 15845 */ 15846 public ExplanationOfBenefit setPayee(PayeeComponent value) { 15847 this.payee = value; 15848 return this; 15849 } 15850 15851 /** 15852 * @return {@link #referral} (The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services.) 15853 */ 15854 public Reference getReferral() { 15855 if (this.referral == null) 15856 if (Configuration.errorOnAutoCreate()) 15857 throw new Error("Attempt to auto-create ExplanationOfBenefit.referral"); 15858 else if (Configuration.doAutoCreate()) 15859 this.referral = new Reference(); // cc 15860 return this.referral; 15861 } 15862 15863 public boolean hasReferral() { 15864 return this.referral != null && !this.referral.isEmpty(); 15865 } 15866 15867 /** 15868 * @param value {@link #referral} (The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services.) 15869 */ 15870 public ExplanationOfBenefit setReferral(Reference value) { 15871 this.referral = value; 15872 return this; 15873 } 15874 15875 /** 15876 * @return {@link #encounter} (Healthcare encounters related to this claim.) 15877 */ 15878 public List<Reference> getEncounter() { 15879 if (this.encounter == null) 15880 this.encounter = new ArrayList<Reference>(); 15881 return this.encounter; 15882 } 15883 15884 /** 15885 * @return Returns a reference to <code>this</code> for easy method chaining 15886 */ 15887 public ExplanationOfBenefit setEncounter(List<Reference> theEncounter) { 15888 this.encounter = theEncounter; 15889 return this; 15890 } 15891 15892 public boolean hasEncounter() { 15893 if (this.encounter == null) 15894 return false; 15895 for (Reference item : this.encounter) 15896 if (!item.isEmpty()) 15897 return true; 15898 return false; 15899 } 15900 15901 public Reference addEncounter() { //3 15902 Reference t = new Reference(); 15903 if (this.encounter == null) 15904 this.encounter = new ArrayList<Reference>(); 15905 this.encounter.add(t); 15906 return t; 15907 } 15908 15909 public ExplanationOfBenefit addEncounter(Reference t) { //3 15910 if (t == null) 15911 return this; 15912 if (this.encounter == null) 15913 this.encounter = new ArrayList<Reference>(); 15914 this.encounter.add(t); 15915 return this; 15916 } 15917 15918 /** 15919 * @return The first repetition of repeating field {@link #encounter}, creating it if it does not already exist {3} 15920 */ 15921 public Reference getEncounterFirstRep() { 15922 if (getEncounter().isEmpty()) { 15923 addEncounter(); 15924 } 15925 return getEncounter().get(0); 15926 } 15927 15928 /** 15929 * @return {@link #facility} (Facility where the services were provided.) 15930 */ 15931 public Reference getFacility() { 15932 if (this.facility == null) 15933 if (Configuration.errorOnAutoCreate()) 15934 throw new Error("Attempt to auto-create ExplanationOfBenefit.facility"); 15935 else if (Configuration.doAutoCreate()) 15936 this.facility = new Reference(); // cc 15937 return this.facility; 15938 } 15939 15940 public boolean hasFacility() { 15941 return this.facility != null && !this.facility.isEmpty(); 15942 } 15943 15944 /** 15945 * @param value {@link #facility} (Facility where the services were provided.) 15946 */ 15947 public ExplanationOfBenefit setFacility(Reference value) { 15948 this.facility = value; 15949 return this; 15950 } 15951 15952 /** 15953 * @return {@link #claim} (The business identifier for the instance of the adjudication request: claim predetermination or preauthorization.) 15954 */ 15955 public Reference getClaim() { 15956 if (this.claim == null) 15957 if (Configuration.errorOnAutoCreate()) 15958 throw new Error("Attempt to auto-create ExplanationOfBenefit.claim"); 15959 else if (Configuration.doAutoCreate()) 15960 this.claim = new Reference(); // cc 15961 return this.claim; 15962 } 15963 15964 public boolean hasClaim() { 15965 return this.claim != null && !this.claim.isEmpty(); 15966 } 15967 15968 /** 15969 * @param value {@link #claim} (The business identifier for the instance of the adjudication request: claim predetermination or preauthorization.) 15970 */ 15971 public ExplanationOfBenefit setClaim(Reference value) { 15972 this.claim = value; 15973 return this; 15974 } 15975 15976 /** 15977 * @return {@link #claimResponse} (The business identifier for the instance of the adjudication response: claim, predetermination or preauthorization response.) 15978 */ 15979 public Reference getClaimResponse() { 15980 if (this.claimResponse == null) 15981 if (Configuration.errorOnAutoCreate()) 15982 throw new Error("Attempt to auto-create ExplanationOfBenefit.claimResponse"); 15983 else if (Configuration.doAutoCreate()) 15984 this.claimResponse = new Reference(); // cc 15985 return this.claimResponse; 15986 } 15987 15988 public boolean hasClaimResponse() { 15989 return this.claimResponse != null && !this.claimResponse.isEmpty(); 15990 } 15991 15992 /** 15993 * @param value {@link #claimResponse} (The business identifier for the instance of the adjudication response: claim, predetermination or preauthorization response.) 15994 */ 15995 public ExplanationOfBenefit setClaimResponse(Reference value) { 15996 this.claimResponse = value; 15997 return this; 15998 } 15999 16000 /** 16001 * @return {@link #outcome} (The outcome of the claim, predetermination, or preauthorization processing.). This is the underlying object with id, value and extensions. The accessor "getOutcome" gives direct access to the value 16002 */ 16003 public Enumeration<ClaimProcessingCodes> getOutcomeElement() { 16004 if (this.outcome == null) 16005 if (Configuration.errorOnAutoCreate()) 16006 throw new Error("Attempt to auto-create ExplanationOfBenefit.outcome"); 16007 else if (Configuration.doAutoCreate()) 16008 this.outcome = new Enumeration<ClaimProcessingCodes>(new ClaimProcessingCodesEnumFactory()); // bb 16009 return this.outcome; 16010 } 16011 16012 public boolean hasOutcomeElement() { 16013 return this.outcome != null && !this.outcome.isEmpty(); 16014 } 16015 16016 public boolean hasOutcome() { 16017 return this.outcome != null && !this.outcome.isEmpty(); 16018 } 16019 16020 /** 16021 * @param value {@link #outcome} (The outcome of the claim, predetermination, or preauthorization processing.). This is the underlying object with id, value and extensions. The accessor "getOutcome" gives direct access to the value 16022 */ 16023 public ExplanationOfBenefit setOutcomeElement(Enumeration<ClaimProcessingCodes> value) { 16024 this.outcome = value; 16025 return this; 16026 } 16027 16028 /** 16029 * @return The outcome of the claim, predetermination, or preauthorization processing. 16030 */ 16031 public ClaimProcessingCodes getOutcome() { 16032 return this.outcome == null ? null : this.outcome.getValue(); 16033 } 16034 16035 /** 16036 * @param value The outcome of the claim, predetermination, or preauthorization processing. 16037 */ 16038 public ExplanationOfBenefit setOutcome(ClaimProcessingCodes value) { 16039 if (this.outcome == null) 16040 this.outcome = new Enumeration<ClaimProcessingCodes>(new ClaimProcessingCodesEnumFactory()); 16041 this.outcome.setValue(value); 16042 return this; 16043 } 16044 16045 /** 16046 * @return {@link #decision} (The result of the claim, predetermination, or preauthorization adjudication.) 16047 */ 16048 public CodeableConcept getDecision() { 16049 if (this.decision == null) 16050 if (Configuration.errorOnAutoCreate()) 16051 throw new Error("Attempt to auto-create ExplanationOfBenefit.decision"); 16052 else if (Configuration.doAutoCreate()) 16053 this.decision = new CodeableConcept(); // cc 16054 return this.decision; 16055 } 16056 16057 public boolean hasDecision() { 16058 return this.decision != null && !this.decision.isEmpty(); 16059 } 16060 16061 /** 16062 * @param value {@link #decision} (The result of the claim, predetermination, or preauthorization adjudication.) 16063 */ 16064 public ExplanationOfBenefit setDecision(CodeableConcept value) { 16065 this.decision = value; 16066 return this; 16067 } 16068 16069 /** 16070 * @return {@link #disposition} (A human readable description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 16071 */ 16072 public StringType getDispositionElement() { 16073 if (this.disposition == null) 16074 if (Configuration.errorOnAutoCreate()) 16075 throw new Error("Attempt to auto-create ExplanationOfBenefit.disposition"); 16076 else if (Configuration.doAutoCreate()) 16077 this.disposition = new StringType(); // bb 16078 return this.disposition; 16079 } 16080 16081 public boolean hasDispositionElement() { 16082 return this.disposition != null && !this.disposition.isEmpty(); 16083 } 16084 16085 public boolean hasDisposition() { 16086 return this.disposition != null && !this.disposition.isEmpty(); 16087 } 16088 16089 /** 16090 * @param value {@link #disposition} (A human readable description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 16091 */ 16092 public ExplanationOfBenefit setDispositionElement(StringType value) { 16093 this.disposition = value; 16094 return this; 16095 } 16096 16097 /** 16098 * @return A human readable description of the status of the adjudication. 16099 */ 16100 public String getDisposition() { 16101 return this.disposition == null ? null : this.disposition.getValue(); 16102 } 16103 16104 /** 16105 * @param value A human readable description of the status of the adjudication. 16106 */ 16107 public ExplanationOfBenefit setDisposition(String value) { 16108 if (Utilities.noString(value)) 16109 this.disposition = null; 16110 else { 16111 if (this.disposition == null) 16112 this.disposition = new StringType(); 16113 this.disposition.setValue(value); 16114 } 16115 return this; 16116 } 16117 16118 /** 16119 * @return {@link #preAuthRef} (Reference from the Insurer which is used in later communications which refers to this adjudication.) 16120 */ 16121 public List<StringType> getPreAuthRef() { 16122 if (this.preAuthRef == null) 16123 this.preAuthRef = new ArrayList<StringType>(); 16124 return this.preAuthRef; 16125 } 16126 16127 /** 16128 * @return Returns a reference to <code>this</code> for easy method chaining 16129 */ 16130 public ExplanationOfBenefit setPreAuthRef(List<StringType> thePreAuthRef) { 16131 this.preAuthRef = thePreAuthRef; 16132 return this; 16133 } 16134 16135 public boolean hasPreAuthRef() { 16136 if (this.preAuthRef == null) 16137 return false; 16138 for (StringType item : this.preAuthRef) 16139 if (!item.isEmpty()) 16140 return true; 16141 return false; 16142 } 16143 16144 /** 16145 * @return {@link #preAuthRef} (Reference from the Insurer which is used in later communications which refers to this adjudication.) 16146 */ 16147 public StringType addPreAuthRefElement() {//2 16148 StringType t = new StringType(); 16149 if (this.preAuthRef == null) 16150 this.preAuthRef = new ArrayList<StringType>(); 16151 this.preAuthRef.add(t); 16152 return t; 16153 } 16154 16155 /** 16156 * @param value {@link #preAuthRef} (Reference from the Insurer which is used in later communications which refers to this adjudication.) 16157 */ 16158 public ExplanationOfBenefit addPreAuthRef(String value) { //1 16159 StringType t = new StringType(); 16160 t.setValue(value); 16161 if (this.preAuthRef == null) 16162 this.preAuthRef = new ArrayList<StringType>(); 16163 this.preAuthRef.add(t); 16164 return this; 16165 } 16166 16167 /** 16168 * @param value {@link #preAuthRef} (Reference from the Insurer which is used in later communications which refers to this adjudication.) 16169 */ 16170 public boolean hasPreAuthRef(String value) { 16171 if (this.preAuthRef == null) 16172 return false; 16173 for (StringType v : this.preAuthRef) 16174 if (v.getValue().equals(value)) // string 16175 return true; 16176 return false; 16177 } 16178 16179 /** 16180 * @return {@link #preAuthRefPeriod} (The timeframe during which the supplied preauthorization reference may be quoted on claims to obtain the adjudication as provided.) 16181 */ 16182 public List<Period> getPreAuthRefPeriod() { 16183 if (this.preAuthRefPeriod == null) 16184 this.preAuthRefPeriod = new ArrayList<Period>(); 16185 return this.preAuthRefPeriod; 16186 } 16187 16188 /** 16189 * @return Returns a reference to <code>this</code> for easy method chaining 16190 */ 16191 public ExplanationOfBenefit setPreAuthRefPeriod(List<Period> thePreAuthRefPeriod) { 16192 this.preAuthRefPeriod = thePreAuthRefPeriod; 16193 return this; 16194 } 16195 16196 public boolean hasPreAuthRefPeriod() { 16197 if (this.preAuthRefPeriod == null) 16198 return false; 16199 for (Period item : this.preAuthRefPeriod) 16200 if (!item.isEmpty()) 16201 return true; 16202 return false; 16203 } 16204 16205 public Period addPreAuthRefPeriod() { //3 16206 Period t = new Period(); 16207 if (this.preAuthRefPeriod == null) 16208 this.preAuthRefPeriod = new ArrayList<Period>(); 16209 this.preAuthRefPeriod.add(t); 16210 return t; 16211 } 16212 16213 public ExplanationOfBenefit addPreAuthRefPeriod(Period t) { //3 16214 if (t == null) 16215 return this; 16216 if (this.preAuthRefPeriod == null) 16217 this.preAuthRefPeriod = new ArrayList<Period>(); 16218 this.preAuthRefPeriod.add(t); 16219 return this; 16220 } 16221 16222 /** 16223 * @return The first repetition of repeating field {@link #preAuthRefPeriod}, creating it if it does not already exist {3} 16224 */ 16225 public Period getPreAuthRefPeriodFirstRep() { 16226 if (getPreAuthRefPeriod().isEmpty()) { 16227 addPreAuthRefPeriod(); 16228 } 16229 return getPreAuthRefPeriod().get(0); 16230 } 16231 16232 /** 16233 * @return {@link #diagnosisRelatedGroup} (A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.) 16234 */ 16235 public CodeableConcept getDiagnosisRelatedGroup() { 16236 if (this.diagnosisRelatedGroup == null) 16237 if (Configuration.errorOnAutoCreate()) 16238 throw new Error("Attempt to auto-create ExplanationOfBenefit.diagnosisRelatedGroup"); 16239 else if (Configuration.doAutoCreate()) 16240 this.diagnosisRelatedGroup = new CodeableConcept(); // cc 16241 return this.diagnosisRelatedGroup; 16242 } 16243 16244 public boolean hasDiagnosisRelatedGroup() { 16245 return this.diagnosisRelatedGroup != null && !this.diagnosisRelatedGroup.isEmpty(); 16246 } 16247 16248 /** 16249 * @param value {@link #diagnosisRelatedGroup} (A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.) 16250 */ 16251 public ExplanationOfBenefit setDiagnosisRelatedGroup(CodeableConcept value) { 16252 this.diagnosisRelatedGroup = value; 16253 return this; 16254 } 16255 16256 /** 16257 * @return {@link #careTeam} (The members of the team who provided the products and services.) 16258 */ 16259 public List<CareTeamComponent> getCareTeam() { 16260 if (this.careTeam == null) 16261 this.careTeam = new ArrayList<CareTeamComponent>(); 16262 return this.careTeam; 16263 } 16264 16265 /** 16266 * @return Returns a reference to <code>this</code> for easy method chaining 16267 */ 16268 public ExplanationOfBenefit setCareTeam(List<CareTeamComponent> theCareTeam) { 16269 this.careTeam = theCareTeam; 16270 return this; 16271 } 16272 16273 public boolean hasCareTeam() { 16274 if (this.careTeam == null) 16275 return false; 16276 for (CareTeamComponent item : this.careTeam) 16277 if (!item.isEmpty()) 16278 return true; 16279 return false; 16280 } 16281 16282 public CareTeamComponent addCareTeam() { //3 16283 CareTeamComponent t = new CareTeamComponent(); 16284 if (this.careTeam == null) 16285 this.careTeam = new ArrayList<CareTeamComponent>(); 16286 this.careTeam.add(t); 16287 return t; 16288 } 16289 16290 public ExplanationOfBenefit addCareTeam(CareTeamComponent t) { //3 16291 if (t == null) 16292 return this; 16293 if (this.careTeam == null) 16294 this.careTeam = new ArrayList<CareTeamComponent>(); 16295 this.careTeam.add(t); 16296 return this; 16297 } 16298 16299 /** 16300 * @return The first repetition of repeating field {@link #careTeam}, creating it if it does not already exist {3} 16301 */ 16302 public CareTeamComponent getCareTeamFirstRep() { 16303 if (getCareTeam().isEmpty()) { 16304 addCareTeam(); 16305 } 16306 return getCareTeam().get(0); 16307 } 16308 16309 /** 16310 * @return {@link #supportingInfo} (Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.) 16311 */ 16312 public List<SupportingInformationComponent> getSupportingInfo() { 16313 if (this.supportingInfo == null) 16314 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 16315 return this.supportingInfo; 16316 } 16317 16318 /** 16319 * @return Returns a reference to <code>this</code> for easy method chaining 16320 */ 16321 public ExplanationOfBenefit setSupportingInfo(List<SupportingInformationComponent> theSupportingInfo) { 16322 this.supportingInfo = theSupportingInfo; 16323 return this; 16324 } 16325 16326 public boolean hasSupportingInfo() { 16327 if (this.supportingInfo == null) 16328 return false; 16329 for (SupportingInformationComponent item : this.supportingInfo) 16330 if (!item.isEmpty()) 16331 return true; 16332 return false; 16333 } 16334 16335 public SupportingInformationComponent addSupportingInfo() { //3 16336 SupportingInformationComponent t = new SupportingInformationComponent(); 16337 if (this.supportingInfo == null) 16338 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 16339 this.supportingInfo.add(t); 16340 return t; 16341 } 16342 16343 public ExplanationOfBenefit addSupportingInfo(SupportingInformationComponent t) { //3 16344 if (t == null) 16345 return this; 16346 if (this.supportingInfo == null) 16347 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 16348 this.supportingInfo.add(t); 16349 return this; 16350 } 16351 16352 /** 16353 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist {3} 16354 */ 16355 public SupportingInformationComponent getSupportingInfoFirstRep() { 16356 if (getSupportingInfo().isEmpty()) { 16357 addSupportingInfo(); 16358 } 16359 return getSupportingInfo().get(0); 16360 } 16361 16362 /** 16363 * @return {@link #diagnosis} (Information about diagnoses relevant to the claim items.) 16364 */ 16365 public List<DiagnosisComponent> getDiagnosis() { 16366 if (this.diagnosis == null) 16367 this.diagnosis = new ArrayList<DiagnosisComponent>(); 16368 return this.diagnosis; 16369 } 16370 16371 /** 16372 * @return Returns a reference to <code>this</code> for easy method chaining 16373 */ 16374 public ExplanationOfBenefit setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 16375 this.diagnosis = theDiagnosis; 16376 return this; 16377 } 16378 16379 public boolean hasDiagnosis() { 16380 if (this.diagnosis == null) 16381 return false; 16382 for (DiagnosisComponent item : this.diagnosis) 16383 if (!item.isEmpty()) 16384 return true; 16385 return false; 16386 } 16387 16388 public DiagnosisComponent addDiagnosis() { //3 16389 DiagnosisComponent t = new DiagnosisComponent(); 16390 if (this.diagnosis == null) 16391 this.diagnosis = new ArrayList<DiagnosisComponent>(); 16392 this.diagnosis.add(t); 16393 return t; 16394 } 16395 16396 public ExplanationOfBenefit addDiagnosis(DiagnosisComponent t) { //3 16397 if (t == null) 16398 return this; 16399 if (this.diagnosis == null) 16400 this.diagnosis = new ArrayList<DiagnosisComponent>(); 16401 this.diagnosis.add(t); 16402 return this; 16403 } 16404 16405 /** 16406 * @return The first repetition of repeating field {@link #diagnosis}, creating it if it does not already exist {3} 16407 */ 16408 public DiagnosisComponent getDiagnosisFirstRep() { 16409 if (getDiagnosis().isEmpty()) { 16410 addDiagnosis(); 16411 } 16412 return getDiagnosis().get(0); 16413 } 16414 16415 /** 16416 * @return {@link #procedure} (Procedures performed on the patient relevant to the billing items with the claim.) 16417 */ 16418 public List<ProcedureComponent> getProcedure() { 16419 if (this.procedure == null) 16420 this.procedure = new ArrayList<ProcedureComponent>(); 16421 return this.procedure; 16422 } 16423 16424 /** 16425 * @return Returns a reference to <code>this</code> for easy method chaining 16426 */ 16427 public ExplanationOfBenefit setProcedure(List<ProcedureComponent> theProcedure) { 16428 this.procedure = theProcedure; 16429 return this; 16430 } 16431 16432 public boolean hasProcedure() { 16433 if (this.procedure == null) 16434 return false; 16435 for (ProcedureComponent item : this.procedure) 16436 if (!item.isEmpty()) 16437 return true; 16438 return false; 16439 } 16440 16441 public ProcedureComponent addProcedure() { //3 16442 ProcedureComponent t = new ProcedureComponent(); 16443 if (this.procedure == null) 16444 this.procedure = new ArrayList<ProcedureComponent>(); 16445 this.procedure.add(t); 16446 return t; 16447 } 16448 16449 public ExplanationOfBenefit addProcedure(ProcedureComponent t) { //3 16450 if (t == null) 16451 return this; 16452 if (this.procedure == null) 16453 this.procedure = new ArrayList<ProcedureComponent>(); 16454 this.procedure.add(t); 16455 return this; 16456 } 16457 16458 /** 16459 * @return The first repetition of repeating field {@link #procedure}, creating it if it does not already exist {3} 16460 */ 16461 public ProcedureComponent getProcedureFirstRep() { 16462 if (getProcedure().isEmpty()) { 16463 addProcedure(); 16464 } 16465 return getProcedure().get(0); 16466 } 16467 16468 /** 16469 * @return {@link #precedence} (This indicates the relative order of a series of EOBs related to different coverages for the same suite of services.). This is the underlying object with id, value and extensions. The accessor "getPrecedence" gives direct access to the value 16470 */ 16471 public PositiveIntType getPrecedenceElement() { 16472 if (this.precedence == null) 16473 if (Configuration.errorOnAutoCreate()) 16474 throw new Error("Attempt to auto-create ExplanationOfBenefit.precedence"); 16475 else if (Configuration.doAutoCreate()) 16476 this.precedence = new PositiveIntType(); // bb 16477 return this.precedence; 16478 } 16479 16480 public boolean hasPrecedenceElement() { 16481 return this.precedence != null && !this.precedence.isEmpty(); 16482 } 16483 16484 public boolean hasPrecedence() { 16485 return this.precedence != null && !this.precedence.isEmpty(); 16486 } 16487 16488 /** 16489 * @param value {@link #precedence} (This indicates the relative order of a series of EOBs related to different coverages for the same suite of services.). This is the underlying object with id, value and extensions. The accessor "getPrecedence" gives direct access to the value 16490 */ 16491 public ExplanationOfBenefit setPrecedenceElement(PositiveIntType value) { 16492 this.precedence = value; 16493 return this; 16494 } 16495 16496 /** 16497 * @return This indicates the relative order of a series of EOBs related to different coverages for the same suite of services. 16498 */ 16499 public int getPrecedence() { 16500 return this.precedence == null || this.precedence.isEmpty() ? 0 : this.precedence.getValue(); 16501 } 16502 16503 /** 16504 * @param value This indicates the relative order of a series of EOBs related to different coverages for the same suite of services. 16505 */ 16506 public ExplanationOfBenefit setPrecedence(int value) { 16507 if (this.precedence == null) 16508 this.precedence = new PositiveIntType(); 16509 this.precedence.setValue(value); 16510 return this; 16511 } 16512 16513 /** 16514 * @return {@link #insurance} (Financial instruments for reimbursement for the health care products and services specified on the claim.) 16515 */ 16516 public List<InsuranceComponent> getInsurance() { 16517 if (this.insurance == null) 16518 this.insurance = new ArrayList<InsuranceComponent>(); 16519 return this.insurance; 16520 } 16521 16522 /** 16523 * @return Returns a reference to <code>this</code> for easy method chaining 16524 */ 16525 public ExplanationOfBenefit setInsurance(List<InsuranceComponent> theInsurance) { 16526 this.insurance = theInsurance; 16527 return this; 16528 } 16529 16530 public boolean hasInsurance() { 16531 if (this.insurance == null) 16532 return false; 16533 for (InsuranceComponent item : this.insurance) 16534 if (!item.isEmpty()) 16535 return true; 16536 return false; 16537 } 16538 16539 public InsuranceComponent addInsurance() { //3 16540 InsuranceComponent t = new InsuranceComponent(); 16541 if (this.insurance == null) 16542 this.insurance = new ArrayList<InsuranceComponent>(); 16543 this.insurance.add(t); 16544 return t; 16545 } 16546 16547 public ExplanationOfBenefit addInsurance(InsuranceComponent t) { //3 16548 if (t == null) 16549 return this; 16550 if (this.insurance == null) 16551 this.insurance = new ArrayList<InsuranceComponent>(); 16552 this.insurance.add(t); 16553 return this; 16554 } 16555 16556 /** 16557 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist {3} 16558 */ 16559 public InsuranceComponent getInsuranceFirstRep() { 16560 if (getInsurance().isEmpty()) { 16561 addInsurance(); 16562 } 16563 return getInsurance().get(0); 16564 } 16565 16566 /** 16567 * @return {@link #accident} (Details of a accident which resulted in injuries which required the products and services listed in the claim.) 16568 */ 16569 public AccidentComponent getAccident() { 16570 if (this.accident == null) 16571 if (Configuration.errorOnAutoCreate()) 16572 throw new Error("Attempt to auto-create ExplanationOfBenefit.accident"); 16573 else if (Configuration.doAutoCreate()) 16574 this.accident = new AccidentComponent(); // cc 16575 return this.accident; 16576 } 16577 16578 public boolean hasAccident() { 16579 return this.accident != null && !this.accident.isEmpty(); 16580 } 16581 16582 /** 16583 * @param value {@link #accident} (Details of a accident which resulted in injuries which required the products and services listed in the claim.) 16584 */ 16585 public ExplanationOfBenefit setAccident(AccidentComponent value) { 16586 this.accident = value; 16587 return this; 16588 } 16589 16590 /** 16591 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 16592 */ 16593 public Money getPatientPaid() { 16594 if (this.patientPaid == null) 16595 if (Configuration.errorOnAutoCreate()) 16596 throw new Error("Attempt to auto-create ExplanationOfBenefit.patientPaid"); 16597 else if (Configuration.doAutoCreate()) 16598 this.patientPaid = new Money(); // cc 16599 return this.patientPaid; 16600 } 16601 16602 public boolean hasPatientPaid() { 16603 return this.patientPaid != null && !this.patientPaid.isEmpty(); 16604 } 16605 16606 /** 16607 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 16608 */ 16609 public ExplanationOfBenefit setPatientPaid(Money value) { 16610 this.patientPaid = value; 16611 return this; 16612 } 16613 16614 /** 16615 * @return {@link #item} (A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.) 16616 */ 16617 public List<ItemComponent> getItem() { 16618 if (this.item == null) 16619 this.item = new ArrayList<ItemComponent>(); 16620 return this.item; 16621 } 16622 16623 /** 16624 * @return Returns a reference to <code>this</code> for easy method chaining 16625 */ 16626 public ExplanationOfBenefit setItem(List<ItemComponent> theItem) { 16627 this.item = theItem; 16628 return this; 16629 } 16630 16631 public boolean hasItem() { 16632 if (this.item == null) 16633 return false; 16634 for (ItemComponent item : this.item) 16635 if (!item.isEmpty()) 16636 return true; 16637 return false; 16638 } 16639 16640 public ItemComponent addItem() { //3 16641 ItemComponent t = new ItemComponent(); 16642 if (this.item == null) 16643 this.item = new ArrayList<ItemComponent>(); 16644 this.item.add(t); 16645 return t; 16646 } 16647 16648 public ExplanationOfBenefit addItem(ItemComponent t) { //3 16649 if (t == null) 16650 return this; 16651 if (this.item == null) 16652 this.item = new ArrayList<ItemComponent>(); 16653 this.item.add(t); 16654 return this; 16655 } 16656 16657 /** 16658 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 16659 */ 16660 public ItemComponent getItemFirstRep() { 16661 if (getItem().isEmpty()) { 16662 addItem(); 16663 } 16664 return getItem().get(0); 16665 } 16666 16667 /** 16668 * @return {@link #addItem} (The first-tier service adjudications for payor added product or service lines.) 16669 */ 16670 public List<AddedItemComponent> getAddItem() { 16671 if (this.addItem == null) 16672 this.addItem = new ArrayList<AddedItemComponent>(); 16673 return this.addItem; 16674 } 16675 16676 /** 16677 * @return Returns a reference to <code>this</code> for easy method chaining 16678 */ 16679 public ExplanationOfBenefit setAddItem(List<AddedItemComponent> theAddItem) { 16680 this.addItem = theAddItem; 16681 return this; 16682 } 16683 16684 public boolean hasAddItem() { 16685 if (this.addItem == null) 16686 return false; 16687 for (AddedItemComponent item : this.addItem) 16688 if (!item.isEmpty()) 16689 return true; 16690 return false; 16691 } 16692 16693 public AddedItemComponent addAddItem() { //3 16694 AddedItemComponent t = new AddedItemComponent(); 16695 if (this.addItem == null) 16696 this.addItem = new ArrayList<AddedItemComponent>(); 16697 this.addItem.add(t); 16698 return t; 16699 } 16700 16701 public ExplanationOfBenefit addAddItem(AddedItemComponent t) { //3 16702 if (t == null) 16703 return this; 16704 if (this.addItem == null) 16705 this.addItem = new ArrayList<AddedItemComponent>(); 16706 this.addItem.add(t); 16707 return this; 16708 } 16709 16710 /** 16711 * @return The first repetition of repeating field {@link #addItem}, creating it if it does not already exist {3} 16712 */ 16713 public AddedItemComponent getAddItemFirstRep() { 16714 if (getAddItem().isEmpty()) { 16715 addAddItem(); 16716 } 16717 return getAddItem().get(0); 16718 } 16719 16720 /** 16721 * @return {@link #adjudication} (The adjudication results which are presented at the header level rather than at the line-item or add-item levels.) 16722 */ 16723 public List<AdjudicationComponent> getAdjudication() { 16724 if (this.adjudication == null) 16725 this.adjudication = new ArrayList<AdjudicationComponent>(); 16726 return this.adjudication; 16727 } 16728 16729 /** 16730 * @return Returns a reference to <code>this</code> for easy method chaining 16731 */ 16732 public ExplanationOfBenefit setAdjudication(List<AdjudicationComponent> theAdjudication) { 16733 this.adjudication = theAdjudication; 16734 return this; 16735 } 16736 16737 public boolean hasAdjudication() { 16738 if (this.adjudication == null) 16739 return false; 16740 for (AdjudicationComponent item : this.adjudication) 16741 if (!item.isEmpty()) 16742 return true; 16743 return false; 16744 } 16745 16746 public AdjudicationComponent addAdjudication() { //3 16747 AdjudicationComponent t = new AdjudicationComponent(); 16748 if (this.adjudication == null) 16749 this.adjudication = new ArrayList<AdjudicationComponent>(); 16750 this.adjudication.add(t); 16751 return t; 16752 } 16753 16754 public ExplanationOfBenefit addAdjudication(AdjudicationComponent t) { //3 16755 if (t == null) 16756 return this; 16757 if (this.adjudication == null) 16758 this.adjudication = new ArrayList<AdjudicationComponent>(); 16759 this.adjudication.add(t); 16760 return this; 16761 } 16762 16763 /** 16764 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 16765 */ 16766 public AdjudicationComponent getAdjudicationFirstRep() { 16767 if (getAdjudication().isEmpty()) { 16768 addAdjudication(); 16769 } 16770 return getAdjudication().get(0); 16771 } 16772 16773 /** 16774 * @return {@link #total} (Categorized monetary totals for the adjudication.) 16775 */ 16776 public List<TotalComponent> getTotal() { 16777 if (this.total == null) 16778 this.total = new ArrayList<TotalComponent>(); 16779 return this.total; 16780 } 16781 16782 /** 16783 * @return Returns a reference to <code>this</code> for easy method chaining 16784 */ 16785 public ExplanationOfBenefit setTotal(List<TotalComponent> theTotal) { 16786 this.total = theTotal; 16787 return this; 16788 } 16789 16790 public boolean hasTotal() { 16791 if (this.total == null) 16792 return false; 16793 for (TotalComponent item : this.total) 16794 if (!item.isEmpty()) 16795 return true; 16796 return false; 16797 } 16798 16799 public TotalComponent addTotal() { //3 16800 TotalComponent t = new TotalComponent(); 16801 if (this.total == null) 16802 this.total = new ArrayList<TotalComponent>(); 16803 this.total.add(t); 16804 return t; 16805 } 16806 16807 public ExplanationOfBenefit addTotal(TotalComponent t) { //3 16808 if (t == null) 16809 return this; 16810 if (this.total == null) 16811 this.total = new ArrayList<TotalComponent>(); 16812 this.total.add(t); 16813 return this; 16814 } 16815 16816 /** 16817 * @return The first repetition of repeating field {@link #total}, creating it if it does not already exist {3} 16818 */ 16819 public TotalComponent getTotalFirstRep() { 16820 if (getTotal().isEmpty()) { 16821 addTotal(); 16822 } 16823 return getTotal().get(0); 16824 } 16825 16826 /** 16827 * @return {@link #payment} (Payment details for the adjudication of the claim.) 16828 */ 16829 public PaymentComponent getPayment() { 16830 if (this.payment == null) 16831 if (Configuration.errorOnAutoCreate()) 16832 throw new Error("Attempt to auto-create ExplanationOfBenefit.payment"); 16833 else if (Configuration.doAutoCreate()) 16834 this.payment = new PaymentComponent(); // cc 16835 return this.payment; 16836 } 16837 16838 public boolean hasPayment() { 16839 return this.payment != null && !this.payment.isEmpty(); 16840 } 16841 16842 /** 16843 * @param value {@link #payment} (Payment details for the adjudication of the claim.) 16844 */ 16845 public ExplanationOfBenefit setPayment(PaymentComponent value) { 16846 this.payment = value; 16847 return this; 16848 } 16849 16850 /** 16851 * @return {@link #formCode} (A code for the form to be used for printing the content.) 16852 */ 16853 public CodeableConcept getFormCode() { 16854 if (this.formCode == null) 16855 if (Configuration.errorOnAutoCreate()) 16856 throw new Error("Attempt to auto-create ExplanationOfBenefit.formCode"); 16857 else if (Configuration.doAutoCreate()) 16858 this.formCode = new CodeableConcept(); // cc 16859 return this.formCode; 16860 } 16861 16862 public boolean hasFormCode() { 16863 return this.formCode != null && !this.formCode.isEmpty(); 16864 } 16865 16866 /** 16867 * @param value {@link #formCode} (A code for the form to be used for printing the content.) 16868 */ 16869 public ExplanationOfBenefit setFormCode(CodeableConcept value) { 16870 this.formCode = value; 16871 return this; 16872 } 16873 16874 /** 16875 * @return {@link #form} (The actual form, by reference or inclusion, for printing the content or an EOB.) 16876 */ 16877 public Attachment getForm() { 16878 if (this.form == null) 16879 if (Configuration.errorOnAutoCreate()) 16880 throw new Error("Attempt to auto-create ExplanationOfBenefit.form"); 16881 else if (Configuration.doAutoCreate()) 16882 this.form = new Attachment(); // cc 16883 return this.form; 16884 } 16885 16886 public boolean hasForm() { 16887 return this.form != null && !this.form.isEmpty(); 16888 } 16889 16890 /** 16891 * @param value {@link #form} (The actual form, by reference or inclusion, for printing the content or an EOB.) 16892 */ 16893 public ExplanationOfBenefit setForm(Attachment value) { 16894 this.form = value; 16895 return this; 16896 } 16897 16898 /** 16899 * @return {@link #processNote} (A note that describes or explains adjudication results in a human readable form.) 16900 */ 16901 public List<NoteComponent> getProcessNote() { 16902 if (this.processNote == null) 16903 this.processNote = new ArrayList<NoteComponent>(); 16904 return this.processNote; 16905 } 16906 16907 /** 16908 * @return Returns a reference to <code>this</code> for easy method chaining 16909 */ 16910 public ExplanationOfBenefit setProcessNote(List<NoteComponent> theProcessNote) { 16911 this.processNote = theProcessNote; 16912 return this; 16913 } 16914 16915 public boolean hasProcessNote() { 16916 if (this.processNote == null) 16917 return false; 16918 for (NoteComponent item : this.processNote) 16919 if (!item.isEmpty()) 16920 return true; 16921 return false; 16922 } 16923 16924 public NoteComponent addProcessNote() { //3 16925 NoteComponent t = new NoteComponent(); 16926 if (this.processNote == null) 16927 this.processNote = new ArrayList<NoteComponent>(); 16928 this.processNote.add(t); 16929 return t; 16930 } 16931 16932 public ExplanationOfBenefit addProcessNote(NoteComponent t) { //3 16933 if (t == null) 16934 return this; 16935 if (this.processNote == null) 16936 this.processNote = new ArrayList<NoteComponent>(); 16937 this.processNote.add(t); 16938 return this; 16939 } 16940 16941 /** 16942 * @return The first repetition of repeating field {@link #processNote}, creating it if it does not already exist {3} 16943 */ 16944 public NoteComponent getProcessNoteFirstRep() { 16945 if (getProcessNote().isEmpty()) { 16946 addProcessNote(); 16947 } 16948 return getProcessNote().get(0); 16949 } 16950 16951 /** 16952 * @return {@link #benefitPeriod} (The term of the benefits documented in this response.) 16953 */ 16954 public Period getBenefitPeriod() { 16955 if (this.benefitPeriod == null) 16956 if (Configuration.errorOnAutoCreate()) 16957 throw new Error("Attempt to auto-create ExplanationOfBenefit.benefitPeriod"); 16958 else if (Configuration.doAutoCreate()) 16959 this.benefitPeriod = new Period(); // cc 16960 return this.benefitPeriod; 16961 } 16962 16963 public boolean hasBenefitPeriod() { 16964 return this.benefitPeriod != null && !this.benefitPeriod.isEmpty(); 16965 } 16966 16967 /** 16968 * @param value {@link #benefitPeriod} (The term of the benefits documented in this response.) 16969 */ 16970 public ExplanationOfBenefit setBenefitPeriod(Period value) { 16971 this.benefitPeriod = value; 16972 return this; 16973 } 16974 16975 /** 16976 * @return {@link #benefitBalance} (Balance by Benefit Category.) 16977 */ 16978 public List<BenefitBalanceComponent> getBenefitBalance() { 16979 if (this.benefitBalance == null) 16980 this.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 16981 return this.benefitBalance; 16982 } 16983 16984 /** 16985 * @return Returns a reference to <code>this</code> for easy method chaining 16986 */ 16987 public ExplanationOfBenefit setBenefitBalance(List<BenefitBalanceComponent> theBenefitBalance) { 16988 this.benefitBalance = theBenefitBalance; 16989 return this; 16990 } 16991 16992 public boolean hasBenefitBalance() { 16993 if (this.benefitBalance == null) 16994 return false; 16995 for (BenefitBalanceComponent item : this.benefitBalance) 16996 if (!item.isEmpty()) 16997 return true; 16998 return false; 16999 } 17000 17001 public BenefitBalanceComponent addBenefitBalance() { //3 17002 BenefitBalanceComponent t = new BenefitBalanceComponent(); 17003 if (this.benefitBalance == null) 17004 this.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 17005 this.benefitBalance.add(t); 17006 return t; 17007 } 17008 17009 public ExplanationOfBenefit addBenefitBalance(BenefitBalanceComponent t) { //3 17010 if (t == null) 17011 return this; 17012 if (this.benefitBalance == null) 17013 this.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 17014 this.benefitBalance.add(t); 17015 return this; 17016 } 17017 17018 /** 17019 * @return The first repetition of repeating field {@link #benefitBalance}, creating it if it does not already exist {3} 17020 */ 17021 public BenefitBalanceComponent getBenefitBalanceFirstRep() { 17022 if (getBenefitBalance().isEmpty()) { 17023 addBenefitBalance(); 17024 } 17025 return getBenefitBalance().get(0); 17026 } 17027 17028 protected void listChildren(List<Property> children) { 17029 super.listChildren(children); 17030 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this explanation of benefit.", 0, java.lang.Integer.MAX_VALUE, identifier)); 17031 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 17032 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 17033 children.add(new Property("type", "CodeableConcept", "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.", 0, 1, type)); 17034 children.add(new Property("subType", "CodeableConcept", "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 0, 1, subType)); 17035 children.add(new Property("use", "code", "A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.", 0, 1, use)); 17036 children.add(new Property("patient", "Reference(Patient)", "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for forecast reimbursement is sought.", 0, 1, patient)); 17037 children.add(new Property("billablePeriod", "Period", "The period for which charges are being submitted.", 0, 1, billablePeriod)); 17038 children.add(new Property("created", "dateTime", "The date this resource was created.", 0, 1, created)); 17039 children.add(new Property("enterer", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", "Individual who created the claim, predetermination or preauthorization.", 0, 1, enterer)); 17040 children.add(new Property("insurer", "Reference(Organization)", "The party responsible for authorization, adjudication and reimbursement.", 0, 1, insurer)); 17041 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, provider)); 17042 children.add(new Property("priority", "CodeableConcept", "The provider-required urgency of processing the request. Typical values include: stat, normal deferred.", 0, 1, priority)); 17043 children.add(new Property("fundsReserveRequested", "CodeableConcept", "A code to indicate whether and for whom funds are to be reserved for future claims.", 0, 1, fundsReserveRequested)); 17044 children.add(new Property("fundsReserve", "CodeableConcept", "A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.", 0, 1, fundsReserve)); 17045 children.add(new Property("related", "", "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.", 0, java.lang.Integer.MAX_VALUE, related)); 17046 children.add(new Property("prescription", "Reference(MedicationRequest|VisionPrescription)", "Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments.", 0, 1, prescription)); 17047 children.add(new Property("originalPrescription", "Reference(MedicationRequest)", "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.", 0, 1, originalPrescription)); 17048 children.add(new Property("event", "", "Information code for an event with a corresponding date or period.", 0, java.lang.Integer.MAX_VALUE, event)); 17049 children.add(new Property("payee", "", "The party to be reimbursed for cost of the products and services according to the terms of the policy.", 0, 1, payee)); 17050 children.add(new Property("referral", "Reference(ServiceRequest)", "The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services.", 0, 1, referral)); 17051 children.add(new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter)); 17052 children.add(new Property("facility", "Reference(Location|Organization)", "Facility where the services were provided.", 0, 1, facility)); 17053 children.add(new Property("claim", "Reference(Claim)", "The business identifier for the instance of the adjudication request: claim predetermination or preauthorization.", 0, 1, claim)); 17054 children.add(new Property("claimResponse", "Reference(ClaimResponse)", "The business identifier for the instance of the adjudication response: claim, predetermination or preauthorization response.", 0, 1, claimResponse)); 17055 children.add(new Property("outcome", "code", "The outcome of the claim, predetermination, or preauthorization processing.", 0, 1, outcome)); 17056 children.add(new Property("decision", "CodeableConcept", "The result of the claim, predetermination, or preauthorization adjudication.", 0, 1, decision)); 17057 children.add(new Property("disposition", "string", "A human readable description of the status of the adjudication.", 0, 1, disposition)); 17058 children.add(new Property("preAuthRef", "string", "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, java.lang.Integer.MAX_VALUE, preAuthRef)); 17059 children.add(new Property("preAuthRefPeriod", "Period", "The timeframe during which the supplied preauthorization reference may be quoted on claims to obtain the adjudication as provided.", 0, java.lang.Integer.MAX_VALUE, preAuthRefPeriod)); 17060 children.add(new Property("diagnosisRelatedGroup", "CodeableConcept", "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.", 0, 1, diagnosisRelatedGroup)); 17061 children.add(new Property("careTeam", "", "The members of the team who provided the products and services.", 0, java.lang.Integer.MAX_VALUE, careTeam)); 17062 children.add(new Property("supportingInfo", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 17063 children.add(new Property("diagnosis", "", "Information about diagnoses relevant to the claim items.", 0, java.lang.Integer.MAX_VALUE, diagnosis)); 17064 children.add(new Property("procedure", "", "Procedures performed on the patient relevant to the billing items with the claim.", 0, java.lang.Integer.MAX_VALUE, procedure)); 17065 children.add(new Property("precedence", "positiveInt", "This indicates the relative order of a series of EOBs related to different coverages for the same suite of services.", 0, 1, precedence)); 17066 children.add(new Property("insurance", "", "Financial instruments for reimbursement for the health care products and services specified on the claim.", 0, java.lang.Integer.MAX_VALUE, insurance)); 17067 children.add(new Property("accident", "", "Details of a accident which resulted in injuries which required the products and services listed in the claim.", 0, 1, accident)); 17068 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 17069 children.add(new Property("item", "", "A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.", 0, java.lang.Integer.MAX_VALUE, item)); 17070 children.add(new Property("addItem", "", "The first-tier service adjudications for payor added product or service lines.", 0, java.lang.Integer.MAX_VALUE, addItem)); 17071 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results which are presented at the header level rather than at the line-item or add-item levels.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 17072 children.add(new Property("total", "", "Categorized monetary totals for the adjudication.", 0, java.lang.Integer.MAX_VALUE, total)); 17073 children.add(new Property("payment", "", "Payment details for the adjudication of the claim.", 0, 1, payment)); 17074 children.add(new Property("formCode", "CodeableConcept", "A code for the form to be used for printing the content.", 0, 1, formCode)); 17075 children.add(new Property("form", "Attachment", "The actual form, by reference or inclusion, for printing the content or an EOB.", 0, 1, form)); 17076 children.add(new Property("processNote", "", "A note that describes or explains adjudication results in a human readable form.", 0, java.lang.Integer.MAX_VALUE, processNote)); 17077 children.add(new Property("benefitPeriod", "Period", "The term of the benefits documented in this response.", 0, 1, benefitPeriod)); 17078 children.add(new Property("benefitBalance", "", "Balance by Benefit Category.", 0, java.lang.Integer.MAX_VALUE, benefitBalance)); 17079 } 17080 17081 @Override 17082 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 17083 switch (_hash) { 17084 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this explanation of benefit.", 0, java.lang.Integer.MAX_VALUE, identifier); 17085 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 17086 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 17087 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.", 0, 1, type); 17088 case -1868521062: /*subType*/ return new Property("subType", "CodeableConcept", "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 0, 1, subType); 17089 case 116103: /*use*/ return new Property("use", "code", "A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.", 0, 1, use); 17090 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for forecast reimbursement is sought.", 0, 1, patient); 17091 case -332066046: /*billablePeriod*/ return new Property("billablePeriod", "Period", "The period for which charges are being submitted.", 0, 1, billablePeriod); 17092 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date this resource was created.", 0, 1, created); 17093 case -1591951995: /*enterer*/ return new Property("enterer", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", "Individual who created the claim, predetermination or preauthorization.", 0, 1, enterer); 17094 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The party responsible for authorization, adjudication and reimbursement.", 0, 1, insurer); 17095 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, provider); 17096 case -1165461084: /*priority*/ return new Property("priority", "CodeableConcept", "The provider-required urgency of processing the request. Typical values include: stat, normal deferred.", 0, 1, priority); 17097 case -1688904576: /*fundsReserveRequested*/ return new Property("fundsReserveRequested", "CodeableConcept", "A code to indicate whether and for whom funds are to be reserved for future claims.", 0, 1, fundsReserveRequested); 17098 case 1314609806: /*fundsReserve*/ return new Property("fundsReserve", "CodeableConcept", "A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.", 0, 1, fundsReserve); 17099 case 1090493483: /*related*/ return new Property("related", "", "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.", 0, java.lang.Integer.MAX_VALUE, related); 17100 case 460301338: /*prescription*/ return new Property("prescription", "Reference(MedicationRequest|VisionPrescription)", "Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments.", 0, 1, prescription); 17101 case -1814015861: /*originalPrescription*/ return new Property("originalPrescription", "Reference(MedicationRequest)", "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.", 0, 1, originalPrescription); 17102 case 96891546: /*event*/ return new Property("event", "", "Information code for an event with a corresponding date or period.", 0, java.lang.Integer.MAX_VALUE, event); 17103 case 106443592: /*payee*/ return new Property("payee", "", "The party to be reimbursed for cost of the products and services according to the terms of the policy.", 0, 1, payee); 17104 case -722568291: /*referral*/ return new Property("referral", "Reference(ServiceRequest)", "The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services.", 0, 1, referral); 17105 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter); 17106 case 501116579: /*facility*/ return new Property("facility", "Reference(Location|Organization)", "Facility where the services were provided.", 0, 1, facility); 17107 case 94742588: /*claim*/ return new Property("claim", "Reference(Claim)", "The business identifier for the instance of the adjudication request: claim predetermination or preauthorization.", 0, 1, claim); 17108 case 689513629: /*claimResponse*/ return new Property("claimResponse", "Reference(ClaimResponse)", "The business identifier for the instance of the adjudication response: claim, predetermination or preauthorization response.", 0, 1, claimResponse); 17109 case -1106507950: /*outcome*/ return new Property("outcome", "code", "The outcome of the claim, predetermination, or preauthorization processing.", 0, 1, outcome); 17110 case 565719004: /*decision*/ return new Property("decision", "CodeableConcept", "The result of the claim, predetermination, or preauthorization adjudication.", 0, 1, decision); 17111 case 583380919: /*disposition*/ return new Property("disposition", "string", "A human readable description of the status of the adjudication.", 0, 1, disposition); 17112 case 522246568: /*preAuthRef*/ return new Property("preAuthRef", "string", "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, java.lang.Integer.MAX_VALUE, preAuthRef); 17113 case -1262920311: /*preAuthRefPeriod*/ return new Property("preAuthRefPeriod", "Period", "The timeframe during which the supplied preauthorization reference may be quoted on claims to obtain the adjudication as provided.", 0, java.lang.Integer.MAX_VALUE, preAuthRefPeriod); 17114 case -1599182171: /*diagnosisRelatedGroup*/ return new Property("diagnosisRelatedGroup", "CodeableConcept", "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.", 0, 1, diagnosisRelatedGroup); 17115 case -7323378: /*careTeam*/ return new Property("careTeam", "", "The members of the team who provided the products and services.", 0, java.lang.Integer.MAX_VALUE, careTeam); 17116 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 17117 case 1196993265: /*diagnosis*/ return new Property("diagnosis", "", "Information about diagnoses relevant to the claim items.", 0, java.lang.Integer.MAX_VALUE, diagnosis); 17118 case -1095204141: /*procedure*/ return new Property("procedure", "", "Procedures performed on the patient relevant to the billing items with the claim.", 0, java.lang.Integer.MAX_VALUE, procedure); 17119 case 159695370: /*precedence*/ return new Property("precedence", "positiveInt", "This indicates the relative order of a series of EOBs related to different coverages for the same suite of services.", 0, 1, precedence); 17120 case 73049818: /*insurance*/ return new Property("insurance", "", "Financial instruments for reimbursement for the health care products and services specified on the claim.", 0, java.lang.Integer.MAX_VALUE, insurance); 17121 case -2143202801: /*accident*/ return new Property("accident", "", "Details of a accident which resulted in injuries which required the products and services listed in the claim.", 0, 1, accident); 17122 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 17123 case 3242771: /*item*/ return new Property("item", "", "A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.", 0, java.lang.Integer.MAX_VALUE, item); 17124 case -1148899500: /*addItem*/ return new Property("addItem", "", "The first-tier service adjudications for payor added product or service lines.", 0, java.lang.Integer.MAX_VALUE, addItem); 17125 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results which are presented at the header level rather than at the line-item or add-item levels.", 0, java.lang.Integer.MAX_VALUE, adjudication); 17126 case 110549828: /*total*/ return new Property("total", "", "Categorized monetary totals for the adjudication.", 0, java.lang.Integer.MAX_VALUE, total); 17127 case -786681338: /*payment*/ return new Property("payment", "", "Payment details for the adjudication of the claim.", 0, 1, payment); 17128 case 473181393: /*formCode*/ return new Property("formCode", "CodeableConcept", "A code for the form to be used for printing the content.", 0, 1, formCode); 17129 case 3148996: /*form*/ return new Property("form", "Attachment", "The actual form, by reference or inclusion, for printing the content or an EOB.", 0, 1, form); 17130 case 202339073: /*processNote*/ return new Property("processNote", "", "A note that describes or explains adjudication results in a human readable form.", 0, java.lang.Integer.MAX_VALUE, processNote); 17131 case -407369416: /*benefitPeriod*/ return new Property("benefitPeriod", "Period", "The term of the benefits documented in this response.", 0, 1, benefitPeriod); 17132 case 596003397: /*benefitBalance*/ return new Property("benefitBalance", "", "Balance by Benefit Category.", 0, java.lang.Integer.MAX_VALUE, benefitBalance); 17133 default: return super.getNamedProperty(_hash, _name, _checkValid); 17134 } 17135 17136 } 17137 17138 @Override 17139 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 17140 switch (hash) { 17141 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 17142 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 17143 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ExplanationOfBenefitStatus> 17144 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 17145 case -1868521062: /*subType*/ return this.subType == null ? new Base[0] : new Base[] {this.subType}; // CodeableConcept 17146 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<Use> 17147 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 17148 case -332066046: /*billablePeriod*/ return this.billablePeriod == null ? new Base[0] : new Base[] {this.billablePeriod}; // Period 17149 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 17150 case -1591951995: /*enterer*/ return this.enterer == null ? new Base[0] : new Base[] {this.enterer}; // Reference 17151 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 17152 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 17153 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // CodeableConcept 17154 case -1688904576: /*fundsReserveRequested*/ return this.fundsReserveRequested == null ? new Base[0] : new Base[] {this.fundsReserveRequested}; // CodeableConcept 17155 case 1314609806: /*fundsReserve*/ return this.fundsReserve == null ? new Base[0] : new Base[] {this.fundsReserve}; // CodeableConcept 17156 case 1090493483: /*related*/ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // RelatedClaimComponent 17157 case 460301338: /*prescription*/ return this.prescription == null ? new Base[0] : new Base[] {this.prescription}; // Reference 17158 case -1814015861: /*originalPrescription*/ return this.originalPrescription == null ? new Base[0] : new Base[] {this.originalPrescription}; // Reference 17159 case 96891546: /*event*/ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // ExplanationOfBenefitEventComponent 17160 case 106443592: /*payee*/ return this.payee == null ? new Base[0] : new Base[] {this.payee}; // PayeeComponent 17161 case -722568291: /*referral*/ return this.referral == null ? new Base[0] : new Base[] {this.referral}; // Reference 17162 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 17163 case 501116579: /*facility*/ return this.facility == null ? new Base[0] : new Base[] {this.facility}; // Reference 17164 case 94742588: /*claim*/ return this.claim == null ? new Base[0] : new Base[] {this.claim}; // Reference 17165 case 689513629: /*claimResponse*/ return this.claimResponse == null ? new Base[0] : new Base[] {this.claimResponse}; // Reference 17166 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // Enumeration<ClaimProcessingCodes> 17167 case 565719004: /*decision*/ return this.decision == null ? new Base[0] : new Base[] {this.decision}; // CodeableConcept 17168 case 583380919: /*disposition*/ return this.disposition == null ? new Base[0] : new Base[] {this.disposition}; // StringType 17169 case 522246568: /*preAuthRef*/ return this.preAuthRef == null ? new Base[0] : this.preAuthRef.toArray(new Base[this.preAuthRef.size()]); // StringType 17170 case -1262920311: /*preAuthRefPeriod*/ return this.preAuthRefPeriod == null ? new Base[0] : this.preAuthRefPeriod.toArray(new Base[this.preAuthRefPeriod.size()]); // Period 17171 case -1599182171: /*diagnosisRelatedGroup*/ return this.diagnosisRelatedGroup == null ? new Base[0] : new Base[] {this.diagnosisRelatedGroup}; // CodeableConcept 17172 case -7323378: /*careTeam*/ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // CareTeamComponent 17173 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // SupportingInformationComponent 17174 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 17175 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : this.procedure.toArray(new Base[this.procedure.size()]); // ProcedureComponent 17176 case 159695370: /*precedence*/ return this.precedence == null ? new Base[0] : new Base[] {this.precedence}; // PositiveIntType 17177 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 17178 case -2143202801: /*accident*/ return this.accident == null ? new Base[0] : new Base[] {this.accident}; // AccidentComponent 17179 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 17180 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemComponent 17181 case -1148899500: /*addItem*/ return this.addItem == null ? new Base[0] : this.addItem.toArray(new Base[this.addItem.size()]); // AddedItemComponent 17182 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 17183 case 110549828: /*total*/ return this.total == null ? new Base[0] : this.total.toArray(new Base[this.total.size()]); // TotalComponent 17184 case -786681338: /*payment*/ return this.payment == null ? new Base[0] : new Base[] {this.payment}; // PaymentComponent 17185 case 473181393: /*formCode*/ return this.formCode == null ? new Base[0] : new Base[] {this.formCode}; // CodeableConcept 17186 case 3148996: /*form*/ return this.form == null ? new Base[0] : new Base[] {this.form}; // Attachment 17187 case 202339073: /*processNote*/ return this.processNote == null ? new Base[0] : this.processNote.toArray(new Base[this.processNote.size()]); // NoteComponent 17188 case -407369416: /*benefitPeriod*/ return this.benefitPeriod == null ? new Base[0] : new Base[] {this.benefitPeriod}; // Period 17189 case 596003397: /*benefitBalance*/ return this.benefitBalance == null ? new Base[0] : this.benefitBalance.toArray(new Base[this.benefitBalance.size()]); // BenefitBalanceComponent 17190 default: return super.getProperty(hash, name, checkValid); 17191 } 17192 17193 } 17194 17195 @Override 17196 public Base setProperty(int hash, String name, Base value) throws FHIRException { 17197 switch (hash) { 17198 case -1618432855: // identifier 17199 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 17200 return value; 17201 case 82505966: // traceNumber 17202 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 17203 return value; 17204 case -892481550: // status 17205 value = new ExplanationOfBenefitStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 17206 this.status = (Enumeration) value; // Enumeration<ExplanationOfBenefitStatus> 17207 return value; 17208 case 3575610: // type 17209 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17210 return value; 17211 case -1868521062: // subType 17212 this.subType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17213 return value; 17214 case 116103: // use 17215 value = new UseEnumFactory().fromType(TypeConvertor.castToCode(value)); 17216 this.use = (Enumeration) value; // Enumeration<Use> 17217 return value; 17218 case -791418107: // patient 17219 this.patient = TypeConvertor.castToReference(value); // Reference 17220 return value; 17221 case -332066046: // billablePeriod 17222 this.billablePeriod = TypeConvertor.castToPeriod(value); // Period 17223 return value; 17224 case 1028554472: // created 17225 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 17226 return value; 17227 case -1591951995: // enterer 17228 this.enterer = TypeConvertor.castToReference(value); // Reference 17229 return value; 17230 case 1957615864: // insurer 17231 this.insurer = TypeConvertor.castToReference(value); // Reference 17232 return value; 17233 case -987494927: // provider 17234 this.provider = TypeConvertor.castToReference(value); // Reference 17235 return value; 17236 case -1165461084: // priority 17237 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17238 return value; 17239 case -1688904576: // fundsReserveRequested 17240 this.fundsReserveRequested = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17241 return value; 17242 case 1314609806: // fundsReserve 17243 this.fundsReserve = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17244 return value; 17245 case 1090493483: // related 17246 this.getRelated().add((RelatedClaimComponent) value); // RelatedClaimComponent 17247 return value; 17248 case 460301338: // prescription 17249 this.prescription = TypeConvertor.castToReference(value); // Reference 17250 return value; 17251 case -1814015861: // originalPrescription 17252 this.originalPrescription = TypeConvertor.castToReference(value); // Reference 17253 return value; 17254 case 96891546: // event 17255 this.getEvent().add((ExplanationOfBenefitEventComponent) value); // ExplanationOfBenefitEventComponent 17256 return value; 17257 case 106443592: // payee 17258 this.payee = (PayeeComponent) value; // PayeeComponent 17259 return value; 17260 case -722568291: // referral 17261 this.referral = TypeConvertor.castToReference(value); // Reference 17262 return value; 17263 case 1524132147: // encounter 17264 this.getEncounter().add(TypeConvertor.castToReference(value)); // Reference 17265 return value; 17266 case 501116579: // facility 17267 this.facility = TypeConvertor.castToReference(value); // Reference 17268 return value; 17269 case 94742588: // claim 17270 this.claim = TypeConvertor.castToReference(value); // Reference 17271 return value; 17272 case 689513629: // claimResponse 17273 this.claimResponse = TypeConvertor.castToReference(value); // Reference 17274 return value; 17275 case -1106507950: // outcome 17276 value = new ClaimProcessingCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 17277 this.outcome = (Enumeration) value; // Enumeration<ClaimProcessingCodes> 17278 return value; 17279 case 565719004: // decision 17280 this.decision = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17281 return value; 17282 case 583380919: // disposition 17283 this.disposition = TypeConvertor.castToString(value); // StringType 17284 return value; 17285 case 522246568: // preAuthRef 17286 this.getPreAuthRef().add(TypeConvertor.castToString(value)); // StringType 17287 return value; 17288 case -1262920311: // preAuthRefPeriod 17289 this.getPreAuthRefPeriod().add(TypeConvertor.castToPeriod(value)); // Period 17290 return value; 17291 case -1599182171: // diagnosisRelatedGroup 17292 this.diagnosisRelatedGroup = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17293 return value; 17294 case -7323378: // careTeam 17295 this.getCareTeam().add((CareTeamComponent) value); // CareTeamComponent 17296 return value; 17297 case 1922406657: // supportingInfo 17298 this.getSupportingInfo().add((SupportingInformationComponent) value); // SupportingInformationComponent 17299 return value; 17300 case 1196993265: // diagnosis 17301 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 17302 return value; 17303 case -1095204141: // procedure 17304 this.getProcedure().add((ProcedureComponent) value); // ProcedureComponent 17305 return value; 17306 case 159695370: // precedence 17307 this.precedence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 17308 return value; 17309 case 73049818: // insurance 17310 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 17311 return value; 17312 case -2143202801: // accident 17313 this.accident = (AccidentComponent) value; // AccidentComponent 17314 return value; 17315 case 525514609: // patientPaid 17316 this.patientPaid = TypeConvertor.castToMoney(value); // Money 17317 return value; 17318 case 3242771: // item 17319 this.getItem().add((ItemComponent) value); // ItemComponent 17320 return value; 17321 case -1148899500: // addItem 17322 this.getAddItem().add((AddedItemComponent) value); // AddedItemComponent 17323 return value; 17324 case -231349275: // adjudication 17325 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 17326 return value; 17327 case 110549828: // total 17328 this.getTotal().add((TotalComponent) value); // TotalComponent 17329 return value; 17330 case -786681338: // payment 17331 this.payment = (PaymentComponent) value; // PaymentComponent 17332 return value; 17333 case 473181393: // formCode 17334 this.formCode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17335 return value; 17336 case 3148996: // form 17337 this.form = TypeConvertor.castToAttachment(value); // Attachment 17338 return value; 17339 case 202339073: // processNote 17340 this.getProcessNote().add((NoteComponent) value); // NoteComponent 17341 return value; 17342 case -407369416: // benefitPeriod 17343 this.benefitPeriod = TypeConvertor.castToPeriod(value); // Period 17344 return value; 17345 case 596003397: // benefitBalance 17346 this.getBenefitBalance().add((BenefitBalanceComponent) value); // BenefitBalanceComponent 17347 return value; 17348 default: return super.setProperty(hash, name, value); 17349 } 17350 17351 } 17352 17353 @Override 17354 public Base setProperty(String name, Base value) throws FHIRException { 17355 if (name.equals("identifier")) { 17356 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 17357 } else if (name.equals("traceNumber")) { 17358 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 17359 } else if (name.equals("status")) { 17360 value = new ExplanationOfBenefitStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 17361 this.status = (Enumeration) value; // Enumeration<ExplanationOfBenefitStatus> 17362 } else if (name.equals("type")) { 17363 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17364 } else if (name.equals("subType")) { 17365 this.subType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17366 } else if (name.equals("use")) { 17367 value = new UseEnumFactory().fromType(TypeConvertor.castToCode(value)); 17368 this.use = (Enumeration) value; // Enumeration<Use> 17369 } else if (name.equals("patient")) { 17370 this.patient = TypeConvertor.castToReference(value); // Reference 17371 } else if (name.equals("billablePeriod")) { 17372 this.billablePeriod = TypeConvertor.castToPeriod(value); // Period 17373 } else if (name.equals("created")) { 17374 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 17375 } else if (name.equals("enterer")) { 17376 this.enterer = TypeConvertor.castToReference(value); // Reference 17377 } else if (name.equals("insurer")) { 17378 this.insurer = TypeConvertor.castToReference(value); // Reference 17379 } else if (name.equals("provider")) { 17380 this.provider = TypeConvertor.castToReference(value); // Reference 17381 } else if (name.equals("priority")) { 17382 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17383 } else if (name.equals("fundsReserveRequested")) { 17384 this.fundsReserveRequested = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17385 } else if (name.equals("fundsReserve")) { 17386 this.fundsReserve = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17387 } else if (name.equals("related")) { 17388 this.getRelated().add((RelatedClaimComponent) value); 17389 } else if (name.equals("prescription")) { 17390 this.prescription = TypeConvertor.castToReference(value); // Reference 17391 } else if (name.equals("originalPrescription")) { 17392 this.originalPrescription = TypeConvertor.castToReference(value); // Reference 17393 } else if (name.equals("event")) { 17394 this.getEvent().add((ExplanationOfBenefitEventComponent) value); 17395 } else if (name.equals("payee")) { 17396 this.payee = (PayeeComponent) value; // PayeeComponent 17397 } else if (name.equals("referral")) { 17398 this.referral = TypeConvertor.castToReference(value); // Reference 17399 } else if (name.equals("encounter")) { 17400 this.getEncounter().add(TypeConvertor.castToReference(value)); 17401 } else if (name.equals("facility")) { 17402 this.facility = TypeConvertor.castToReference(value); // Reference 17403 } else if (name.equals("claim")) { 17404 this.claim = TypeConvertor.castToReference(value); // Reference 17405 } else if (name.equals("claimResponse")) { 17406 this.claimResponse = TypeConvertor.castToReference(value); // Reference 17407 } else if (name.equals("outcome")) { 17408 value = new ClaimProcessingCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 17409 this.outcome = (Enumeration) value; // Enumeration<ClaimProcessingCodes> 17410 } else if (name.equals("decision")) { 17411 this.decision = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17412 } else if (name.equals("disposition")) { 17413 this.disposition = TypeConvertor.castToString(value); // StringType 17414 } else if (name.equals("preAuthRef")) { 17415 this.getPreAuthRef().add(TypeConvertor.castToString(value)); 17416 } else if (name.equals("preAuthRefPeriod")) { 17417 this.getPreAuthRefPeriod().add(TypeConvertor.castToPeriod(value)); 17418 } else if (name.equals("diagnosisRelatedGroup")) { 17419 this.diagnosisRelatedGroup = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17420 } else if (name.equals("careTeam")) { 17421 this.getCareTeam().add((CareTeamComponent) value); 17422 } else if (name.equals("supportingInfo")) { 17423 this.getSupportingInfo().add((SupportingInformationComponent) value); 17424 } else if (name.equals("diagnosis")) { 17425 this.getDiagnosis().add((DiagnosisComponent) value); 17426 } else if (name.equals("procedure")) { 17427 this.getProcedure().add((ProcedureComponent) value); 17428 } else if (name.equals("precedence")) { 17429 this.precedence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 17430 } else if (name.equals("insurance")) { 17431 this.getInsurance().add((InsuranceComponent) value); 17432 } else if (name.equals("accident")) { 17433 this.accident = (AccidentComponent) value; // AccidentComponent 17434 } else if (name.equals("patientPaid")) { 17435 this.patientPaid = TypeConvertor.castToMoney(value); // Money 17436 } else if (name.equals("item")) { 17437 this.getItem().add((ItemComponent) value); 17438 } else if (name.equals("addItem")) { 17439 this.getAddItem().add((AddedItemComponent) value); 17440 } else if (name.equals("adjudication")) { 17441 this.getAdjudication().add((AdjudicationComponent) value); 17442 } else if (name.equals("total")) { 17443 this.getTotal().add((TotalComponent) value); 17444 } else if (name.equals("payment")) { 17445 this.payment = (PaymentComponent) value; // PaymentComponent 17446 } else if (name.equals("formCode")) { 17447 this.formCode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17448 } else if (name.equals("form")) { 17449 this.form = TypeConvertor.castToAttachment(value); // Attachment 17450 } else if (name.equals("processNote")) { 17451 this.getProcessNote().add((NoteComponent) value); 17452 } else if (name.equals("benefitPeriod")) { 17453 this.benefitPeriod = TypeConvertor.castToPeriod(value); // Period 17454 } else if (name.equals("benefitBalance")) { 17455 this.getBenefitBalance().add((BenefitBalanceComponent) value); 17456 } else 17457 return super.setProperty(name, value); 17458 return value; 17459 } 17460 17461 @Override 17462 public Base makeProperty(int hash, String name) throws FHIRException { 17463 switch (hash) { 17464 case -1618432855: return addIdentifier(); 17465 case 82505966: return addTraceNumber(); 17466 case -892481550: return getStatusElement(); 17467 case 3575610: return getType(); 17468 case -1868521062: return getSubType(); 17469 case 116103: return getUseElement(); 17470 case -791418107: return getPatient(); 17471 case -332066046: return getBillablePeriod(); 17472 case 1028554472: return getCreatedElement(); 17473 case -1591951995: return getEnterer(); 17474 case 1957615864: return getInsurer(); 17475 case -987494927: return getProvider(); 17476 case -1165461084: return getPriority(); 17477 case -1688904576: return getFundsReserveRequested(); 17478 case 1314609806: return getFundsReserve(); 17479 case 1090493483: return addRelated(); 17480 case 460301338: return getPrescription(); 17481 case -1814015861: return getOriginalPrescription(); 17482 case 96891546: return addEvent(); 17483 case 106443592: return getPayee(); 17484 case -722568291: return getReferral(); 17485 case 1524132147: return addEncounter(); 17486 case 501116579: return getFacility(); 17487 case 94742588: return getClaim(); 17488 case 689513629: return getClaimResponse(); 17489 case -1106507950: return getOutcomeElement(); 17490 case 565719004: return getDecision(); 17491 case 583380919: return getDispositionElement(); 17492 case 522246568: return addPreAuthRefElement(); 17493 case -1262920311: return addPreAuthRefPeriod(); 17494 case -1599182171: return getDiagnosisRelatedGroup(); 17495 case -7323378: return addCareTeam(); 17496 case 1922406657: return addSupportingInfo(); 17497 case 1196993265: return addDiagnosis(); 17498 case -1095204141: return addProcedure(); 17499 case 159695370: return getPrecedenceElement(); 17500 case 73049818: return addInsurance(); 17501 case -2143202801: return getAccident(); 17502 case 525514609: return getPatientPaid(); 17503 case 3242771: return addItem(); 17504 case -1148899500: return addAddItem(); 17505 case -231349275: return addAdjudication(); 17506 case 110549828: return addTotal(); 17507 case -786681338: return getPayment(); 17508 case 473181393: return getFormCode(); 17509 case 3148996: return getForm(); 17510 case 202339073: return addProcessNote(); 17511 case -407369416: return getBenefitPeriod(); 17512 case 596003397: return addBenefitBalance(); 17513 default: return super.makeProperty(hash, name); 17514 } 17515 17516 } 17517 17518 @Override 17519 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 17520 switch (hash) { 17521 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 17522 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 17523 case -892481550: /*status*/ return new String[] {"code"}; 17524 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 17525 case -1868521062: /*subType*/ return new String[] {"CodeableConcept"}; 17526 case 116103: /*use*/ return new String[] {"code"}; 17527 case -791418107: /*patient*/ return new String[] {"Reference"}; 17528 case -332066046: /*billablePeriod*/ return new String[] {"Period"}; 17529 case 1028554472: /*created*/ return new String[] {"dateTime"}; 17530 case -1591951995: /*enterer*/ return new String[] {"Reference"}; 17531 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 17532 case -987494927: /*provider*/ return new String[] {"Reference"}; 17533 case -1165461084: /*priority*/ return new String[] {"CodeableConcept"}; 17534 case -1688904576: /*fundsReserveRequested*/ return new String[] {"CodeableConcept"}; 17535 case 1314609806: /*fundsReserve*/ return new String[] {"CodeableConcept"}; 17536 case 1090493483: /*related*/ return new String[] {}; 17537 case 460301338: /*prescription*/ return new String[] {"Reference"}; 17538 case -1814015861: /*originalPrescription*/ return new String[] {"Reference"}; 17539 case 96891546: /*event*/ return new String[] {}; 17540 case 106443592: /*payee*/ return new String[] {}; 17541 case -722568291: /*referral*/ return new String[] {"Reference"}; 17542 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 17543 case 501116579: /*facility*/ return new String[] {"Reference"}; 17544 case 94742588: /*claim*/ return new String[] {"Reference"}; 17545 case 689513629: /*claimResponse*/ return new String[] {"Reference"}; 17546 case -1106507950: /*outcome*/ return new String[] {"code"}; 17547 case 565719004: /*decision*/ return new String[] {"CodeableConcept"}; 17548 case 583380919: /*disposition*/ return new String[] {"string"}; 17549 case 522246568: /*preAuthRef*/ return new String[] {"string"}; 17550 case -1262920311: /*preAuthRefPeriod*/ return new String[] {"Period"}; 17551 case -1599182171: /*diagnosisRelatedGroup*/ return new String[] {"CodeableConcept"}; 17552 case -7323378: /*careTeam*/ return new String[] {}; 17553 case 1922406657: /*supportingInfo*/ return new String[] {}; 17554 case 1196993265: /*diagnosis*/ return new String[] {}; 17555 case -1095204141: /*procedure*/ return new String[] {}; 17556 case 159695370: /*precedence*/ return new String[] {"positiveInt"}; 17557 case 73049818: /*insurance*/ return new String[] {}; 17558 case -2143202801: /*accident*/ return new String[] {}; 17559 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 17560 case 3242771: /*item*/ return new String[] {}; 17561 case -1148899500: /*addItem*/ return new String[] {}; 17562 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 17563 case 110549828: /*total*/ return new String[] {}; 17564 case -786681338: /*payment*/ return new String[] {}; 17565 case 473181393: /*formCode*/ return new String[] {"CodeableConcept"}; 17566 case 3148996: /*form*/ return new String[] {"Attachment"}; 17567 case 202339073: /*processNote*/ return new String[] {}; 17568 case -407369416: /*benefitPeriod*/ return new String[] {"Period"}; 17569 case 596003397: /*benefitBalance*/ return new String[] {}; 17570 default: return super.getTypesForProperty(hash, name); 17571 } 17572 17573 } 17574 17575 @Override 17576 public Base addChild(String name) throws FHIRException { 17577 if (name.equals("identifier")) { 17578 return addIdentifier(); 17579 } 17580 else if (name.equals("traceNumber")) { 17581 return addTraceNumber(); 17582 } 17583 else if (name.equals("status")) { 17584 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.status"); 17585 } 17586 else if (name.equals("type")) { 17587 this.type = new CodeableConcept(); 17588 return this.type; 17589 } 17590 else if (name.equals("subType")) { 17591 this.subType = new CodeableConcept(); 17592 return this.subType; 17593 } 17594 else if (name.equals("use")) { 17595 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.use"); 17596 } 17597 else if (name.equals("patient")) { 17598 this.patient = new Reference(); 17599 return this.patient; 17600 } 17601 else if (name.equals("billablePeriod")) { 17602 this.billablePeriod = new Period(); 17603 return this.billablePeriod; 17604 } 17605 else if (name.equals("created")) { 17606 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.created"); 17607 } 17608 else if (name.equals("enterer")) { 17609 this.enterer = new Reference(); 17610 return this.enterer; 17611 } 17612 else if (name.equals("insurer")) { 17613 this.insurer = new Reference(); 17614 return this.insurer; 17615 } 17616 else if (name.equals("provider")) { 17617 this.provider = new Reference(); 17618 return this.provider; 17619 } 17620 else if (name.equals("priority")) { 17621 this.priority = new CodeableConcept(); 17622 return this.priority; 17623 } 17624 else if (name.equals("fundsReserveRequested")) { 17625 this.fundsReserveRequested = new CodeableConcept(); 17626 return this.fundsReserveRequested; 17627 } 17628 else if (name.equals("fundsReserve")) { 17629 this.fundsReserve = new CodeableConcept(); 17630 return this.fundsReserve; 17631 } 17632 else if (name.equals("related")) { 17633 return addRelated(); 17634 } 17635 else if (name.equals("prescription")) { 17636 this.prescription = new Reference(); 17637 return this.prescription; 17638 } 17639 else if (name.equals("originalPrescription")) { 17640 this.originalPrescription = new Reference(); 17641 return this.originalPrescription; 17642 } 17643 else if (name.equals("event")) { 17644 return addEvent(); 17645 } 17646 else if (name.equals("payee")) { 17647 this.payee = new PayeeComponent(); 17648 return this.payee; 17649 } 17650 else if (name.equals("referral")) { 17651 this.referral = new Reference(); 17652 return this.referral; 17653 } 17654 else if (name.equals("encounter")) { 17655 return addEncounter(); 17656 } 17657 else if (name.equals("facility")) { 17658 this.facility = new Reference(); 17659 return this.facility; 17660 } 17661 else if (name.equals("claim")) { 17662 this.claim = new Reference(); 17663 return this.claim; 17664 } 17665 else if (name.equals("claimResponse")) { 17666 this.claimResponse = new Reference(); 17667 return this.claimResponse; 17668 } 17669 else if (name.equals("outcome")) { 17670 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.outcome"); 17671 } 17672 else if (name.equals("decision")) { 17673 this.decision = new CodeableConcept(); 17674 return this.decision; 17675 } 17676 else if (name.equals("disposition")) { 17677 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.disposition"); 17678 } 17679 else if (name.equals("preAuthRef")) { 17680 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.preAuthRef"); 17681 } 17682 else if (name.equals("preAuthRefPeriod")) { 17683 return addPreAuthRefPeriod(); 17684 } 17685 else if (name.equals("diagnosisRelatedGroup")) { 17686 this.diagnosisRelatedGroup = new CodeableConcept(); 17687 return this.diagnosisRelatedGroup; 17688 } 17689 else if (name.equals("careTeam")) { 17690 return addCareTeam(); 17691 } 17692 else if (name.equals("supportingInfo")) { 17693 return addSupportingInfo(); 17694 } 17695 else if (name.equals("diagnosis")) { 17696 return addDiagnosis(); 17697 } 17698 else if (name.equals("procedure")) { 17699 return addProcedure(); 17700 } 17701 else if (name.equals("precedence")) { 17702 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.precedence"); 17703 } 17704 else if (name.equals("insurance")) { 17705 return addInsurance(); 17706 } 17707 else if (name.equals("accident")) { 17708 this.accident = new AccidentComponent(); 17709 return this.accident; 17710 } 17711 else if (name.equals("patientPaid")) { 17712 this.patientPaid = new Money(); 17713 return this.patientPaid; 17714 } 17715 else if (name.equals("item")) { 17716 return addItem(); 17717 } 17718 else if (name.equals("addItem")) { 17719 return addAddItem(); 17720 } 17721 else if (name.equals("adjudication")) { 17722 return addAdjudication(); 17723 } 17724 else if (name.equals("total")) { 17725 return addTotal(); 17726 } 17727 else if (name.equals("payment")) { 17728 this.payment = new PaymentComponent(); 17729 return this.payment; 17730 } 17731 else if (name.equals("formCode")) { 17732 this.formCode = new CodeableConcept(); 17733 return this.formCode; 17734 } 17735 else if (name.equals("form")) { 17736 this.form = new Attachment(); 17737 return this.form; 17738 } 17739 else if (name.equals("processNote")) { 17740 return addProcessNote(); 17741 } 17742 else if (name.equals("benefitPeriod")) { 17743 this.benefitPeriod = new Period(); 17744 return this.benefitPeriod; 17745 } 17746 else if (name.equals("benefitBalance")) { 17747 return addBenefitBalance(); 17748 } 17749 else 17750 return super.addChild(name); 17751 } 17752 17753 public String fhirType() { 17754 return "ExplanationOfBenefit"; 17755 17756 } 17757 17758 public ExplanationOfBenefit copy() { 17759 ExplanationOfBenefit dst = new ExplanationOfBenefit(); 17760 copyValues(dst); 17761 return dst; 17762 } 17763 17764 public void copyValues(ExplanationOfBenefit dst) { 17765 super.copyValues(dst); 17766 if (identifier != null) { 17767 dst.identifier = new ArrayList<Identifier>(); 17768 for (Identifier i : identifier) 17769 dst.identifier.add(i.copy()); 17770 }; 17771 if (traceNumber != null) { 17772 dst.traceNumber = new ArrayList<Identifier>(); 17773 for (Identifier i : traceNumber) 17774 dst.traceNumber.add(i.copy()); 17775 }; 17776 dst.status = status == null ? null : status.copy(); 17777 dst.type = type == null ? null : type.copy(); 17778 dst.subType = subType == null ? null : subType.copy(); 17779 dst.use = use == null ? null : use.copy(); 17780 dst.patient = patient == null ? null : patient.copy(); 17781 dst.billablePeriod = billablePeriod == null ? null : billablePeriod.copy(); 17782 dst.created = created == null ? null : created.copy(); 17783 dst.enterer = enterer == null ? null : enterer.copy(); 17784 dst.insurer = insurer == null ? null : insurer.copy(); 17785 dst.provider = provider == null ? null : provider.copy(); 17786 dst.priority = priority == null ? null : priority.copy(); 17787 dst.fundsReserveRequested = fundsReserveRequested == null ? null : fundsReserveRequested.copy(); 17788 dst.fundsReserve = fundsReserve == null ? null : fundsReserve.copy(); 17789 if (related != null) { 17790 dst.related = new ArrayList<RelatedClaimComponent>(); 17791 for (RelatedClaimComponent i : related) 17792 dst.related.add(i.copy()); 17793 }; 17794 dst.prescription = prescription == null ? null : prescription.copy(); 17795 dst.originalPrescription = originalPrescription == null ? null : originalPrescription.copy(); 17796 if (event != null) { 17797 dst.event = new ArrayList<ExplanationOfBenefitEventComponent>(); 17798 for (ExplanationOfBenefitEventComponent i : event) 17799 dst.event.add(i.copy()); 17800 }; 17801 dst.payee = payee == null ? null : payee.copy(); 17802 dst.referral = referral == null ? null : referral.copy(); 17803 if (encounter != null) { 17804 dst.encounter = new ArrayList<Reference>(); 17805 for (Reference i : encounter) 17806 dst.encounter.add(i.copy()); 17807 }; 17808 dst.facility = facility == null ? null : facility.copy(); 17809 dst.claim = claim == null ? null : claim.copy(); 17810 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 17811 dst.outcome = outcome == null ? null : outcome.copy(); 17812 dst.decision = decision == null ? null : decision.copy(); 17813 dst.disposition = disposition == null ? null : disposition.copy(); 17814 if (preAuthRef != null) { 17815 dst.preAuthRef = new ArrayList<StringType>(); 17816 for (StringType i : preAuthRef) 17817 dst.preAuthRef.add(i.copy()); 17818 }; 17819 if (preAuthRefPeriod != null) { 17820 dst.preAuthRefPeriod = new ArrayList<Period>(); 17821 for (Period i : preAuthRefPeriod) 17822 dst.preAuthRefPeriod.add(i.copy()); 17823 }; 17824 dst.diagnosisRelatedGroup = diagnosisRelatedGroup == null ? null : diagnosisRelatedGroup.copy(); 17825 if (careTeam != null) { 17826 dst.careTeam = new ArrayList<CareTeamComponent>(); 17827 for (CareTeamComponent i : careTeam) 17828 dst.careTeam.add(i.copy()); 17829 }; 17830 if (supportingInfo != null) { 17831 dst.supportingInfo = new ArrayList<SupportingInformationComponent>(); 17832 for (SupportingInformationComponent i : supportingInfo) 17833 dst.supportingInfo.add(i.copy()); 17834 }; 17835 if (diagnosis != null) { 17836 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 17837 for (DiagnosisComponent i : diagnosis) 17838 dst.diagnosis.add(i.copy()); 17839 }; 17840 if (procedure != null) { 17841 dst.procedure = new ArrayList<ProcedureComponent>(); 17842 for (ProcedureComponent i : procedure) 17843 dst.procedure.add(i.copy()); 17844 }; 17845 dst.precedence = precedence == null ? null : precedence.copy(); 17846 if (insurance != null) { 17847 dst.insurance = new ArrayList<InsuranceComponent>(); 17848 for (InsuranceComponent i : insurance) 17849 dst.insurance.add(i.copy()); 17850 }; 17851 dst.accident = accident == null ? null : accident.copy(); 17852 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 17853 if (item != null) { 17854 dst.item = new ArrayList<ItemComponent>(); 17855 for (ItemComponent i : item) 17856 dst.item.add(i.copy()); 17857 }; 17858 if (addItem != null) { 17859 dst.addItem = new ArrayList<AddedItemComponent>(); 17860 for (AddedItemComponent i : addItem) 17861 dst.addItem.add(i.copy()); 17862 }; 17863 if (adjudication != null) { 17864 dst.adjudication = new ArrayList<AdjudicationComponent>(); 17865 for (AdjudicationComponent i : adjudication) 17866 dst.adjudication.add(i.copy()); 17867 }; 17868 if (total != null) { 17869 dst.total = new ArrayList<TotalComponent>(); 17870 for (TotalComponent i : total) 17871 dst.total.add(i.copy()); 17872 }; 17873 dst.payment = payment == null ? null : payment.copy(); 17874 dst.formCode = formCode == null ? null : formCode.copy(); 17875 dst.form = form == null ? null : form.copy(); 17876 if (processNote != null) { 17877 dst.processNote = new ArrayList<NoteComponent>(); 17878 for (NoteComponent i : processNote) 17879 dst.processNote.add(i.copy()); 17880 }; 17881 dst.benefitPeriod = benefitPeriod == null ? null : benefitPeriod.copy(); 17882 if (benefitBalance != null) { 17883 dst.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 17884 for (BenefitBalanceComponent i : benefitBalance) 17885 dst.benefitBalance.add(i.copy()); 17886 }; 17887 } 17888 17889 protected ExplanationOfBenefit typedCopy() { 17890 return copy(); 17891 } 17892 17893 @Override 17894 public boolean equalsDeep(Base other_) { 17895 if (!super.equalsDeep(other_)) 17896 return false; 17897 if (!(other_ instanceof ExplanationOfBenefit)) 17898 return false; 17899 ExplanationOfBenefit o = (ExplanationOfBenefit) other_; 17900 return compareDeep(identifier, o.identifier, true) && compareDeep(traceNumber, o.traceNumber, true) 17901 && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) 17902 && compareDeep(use, o.use, true) && compareDeep(patient, o.patient, true) && compareDeep(billablePeriod, o.billablePeriod, true) 17903 && compareDeep(created, o.created, true) && compareDeep(enterer, o.enterer, true) && compareDeep(insurer, o.insurer, true) 17904 && compareDeep(provider, o.provider, true) && compareDeep(priority, o.priority, true) && compareDeep(fundsReserveRequested, o.fundsReserveRequested, true) 17905 && compareDeep(fundsReserve, o.fundsReserve, true) && compareDeep(related, o.related, true) && compareDeep(prescription, o.prescription, true) 17906 && compareDeep(originalPrescription, o.originalPrescription, true) && compareDeep(event, o.event, true) 17907 && compareDeep(payee, o.payee, true) && compareDeep(referral, o.referral, true) && compareDeep(encounter, o.encounter, true) 17908 && compareDeep(facility, o.facility, true) && compareDeep(claim, o.claim, true) && compareDeep(claimResponse, o.claimResponse, true) 17909 && compareDeep(outcome, o.outcome, true) && compareDeep(decision, o.decision, true) && compareDeep(disposition, o.disposition, true) 17910 && compareDeep(preAuthRef, o.preAuthRef, true) && compareDeep(preAuthRefPeriod, o.preAuthRefPeriod, true) 17911 && compareDeep(diagnosisRelatedGroup, o.diagnosisRelatedGroup, true) && compareDeep(careTeam, o.careTeam, true) 17912 && compareDeep(supportingInfo, o.supportingInfo, true) && compareDeep(diagnosis, o.diagnosis, true) 17913 && compareDeep(procedure, o.procedure, true) && compareDeep(precedence, o.precedence, true) && compareDeep(insurance, o.insurance, true) 17914 && compareDeep(accident, o.accident, true) && compareDeep(patientPaid, o.patientPaid, true) && compareDeep(item, o.item, true) 17915 && compareDeep(addItem, o.addItem, true) && compareDeep(adjudication, o.adjudication, true) && compareDeep(total, o.total, true) 17916 && compareDeep(payment, o.payment, true) && compareDeep(formCode, o.formCode, true) && compareDeep(form, o.form, true) 17917 && compareDeep(processNote, o.processNote, true) && compareDeep(benefitPeriod, o.benefitPeriod, true) 17918 && compareDeep(benefitBalance, o.benefitBalance, true); 17919 } 17920 17921 @Override 17922 public boolean equalsShallow(Base other_) { 17923 if (!super.equalsShallow(other_)) 17924 return false; 17925 if (!(other_ instanceof ExplanationOfBenefit)) 17926 return false; 17927 ExplanationOfBenefit o = (ExplanationOfBenefit) other_; 17928 return compareValues(status, o.status, true) && compareValues(use, o.use, true) && compareValues(created, o.created, true) 17929 && compareValues(outcome, o.outcome, true) && compareValues(disposition, o.disposition, true) && compareValues(preAuthRef, o.preAuthRef, true) 17930 && compareValues(precedence, o.precedence, true); 17931 } 17932 17933 public boolean isEmpty() { 17934 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, traceNumber, status 17935 , type, subType, use, patient, billablePeriod, created, enterer, insurer, provider 17936 , priority, fundsReserveRequested, fundsReserve, related, prescription, originalPrescription 17937 , event, payee, referral, encounter, facility, claim, claimResponse, outcome 17938 , decision, disposition, preAuthRef, preAuthRefPeriod, diagnosisRelatedGroup, careTeam 17939 , supportingInfo, diagnosis, procedure, precedence, insurance, accident, patientPaid 17940 , item, addItem, adjudication, total, payment, formCode, form, processNote, benefitPeriod 17941 , benefitBalance); 17942 } 17943 17944 @Override 17945 public ResourceType getResourceType() { 17946 return ResourceType.ExplanationOfBenefit; 17947 } 17948 17949 /** 17950 * Search parameter: <b>care-team</b> 17951 * <p> 17952 * Description: <b>Member of the CareTeam</b><br> 17953 * Type: <b>reference</b><br> 17954 * Path: <b>ExplanationOfBenefit.careTeam.provider</b><br> 17955 * </p> 17956 */ 17957 @SearchParamDefinition(name="care-team", path="ExplanationOfBenefit.careTeam.provider", description="Member of the CareTeam", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 17958 public static final String SP_CARE_TEAM = "care-team"; 17959 /** 17960 * <b>Fluent Client</b> search parameter constant for <b>care-team</b> 17961 * <p> 17962 * Description: <b>Member of the CareTeam</b><br> 17963 * Type: <b>reference</b><br> 17964 * Path: <b>ExplanationOfBenefit.careTeam.provider</b><br> 17965 * </p> 17966 */ 17967 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_TEAM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CARE_TEAM); 17968 17969/** 17970 * Constant for fluent queries to be used to add include statements. Specifies 17971 * the path value of "<b>ExplanationOfBenefit:care-team</b>". 17972 */ 17973 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_TEAM = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:care-team").toLocked(); 17974 17975 /** 17976 * Search parameter: <b>claim</b> 17977 * <p> 17978 * Description: <b>The reference to the claim</b><br> 17979 * Type: <b>reference</b><br> 17980 * Path: <b>ExplanationOfBenefit.claim</b><br> 17981 * </p> 17982 */ 17983 @SearchParamDefinition(name="claim", path="ExplanationOfBenefit.claim", description="The reference to the claim", type="reference", target={Claim.class } ) 17984 public static final String SP_CLAIM = "claim"; 17985 /** 17986 * <b>Fluent Client</b> search parameter constant for <b>claim</b> 17987 * <p> 17988 * Description: <b>The reference to the claim</b><br> 17989 * Type: <b>reference</b><br> 17990 * Path: <b>ExplanationOfBenefit.claim</b><br> 17991 * </p> 17992 */ 17993 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CLAIM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CLAIM); 17994 17995/** 17996 * Constant for fluent queries to be used to add include statements. Specifies 17997 * the path value of "<b>ExplanationOfBenefit:claim</b>". 17998 */ 17999 public static final ca.uhn.fhir.model.api.Include INCLUDE_CLAIM = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:claim").toLocked(); 18000 18001 /** 18002 * Search parameter: <b>coverage</b> 18003 * <p> 18004 * Description: <b>The plan under which the claim was adjudicated</b><br> 18005 * Type: <b>reference</b><br> 18006 * Path: <b>ExplanationOfBenefit.insurance.coverage</b><br> 18007 * </p> 18008 */ 18009 @SearchParamDefinition(name="coverage", path="ExplanationOfBenefit.insurance.coverage", description="The plan under which the claim was adjudicated", type="reference", target={Coverage.class } ) 18010 public static final String SP_COVERAGE = "coverage"; 18011 /** 18012 * <b>Fluent Client</b> search parameter constant for <b>coverage</b> 18013 * <p> 18014 * Description: <b>The plan under which the claim was adjudicated</b><br> 18015 * Type: <b>reference</b><br> 18016 * Path: <b>ExplanationOfBenefit.insurance.coverage</b><br> 18017 * </p> 18018 */ 18019 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COVERAGE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COVERAGE); 18020 18021/** 18022 * Constant for fluent queries to be used to add include statements. Specifies 18023 * the path value of "<b>ExplanationOfBenefit:coverage</b>". 18024 */ 18025 public static final ca.uhn.fhir.model.api.Include INCLUDE_COVERAGE = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:coverage").toLocked(); 18026 18027 /** 18028 * Search parameter: <b>created</b> 18029 * <p> 18030 * Description: <b>The creation date for the EOB</b><br> 18031 * Type: <b>date</b><br> 18032 * Path: <b>ExplanationOfBenefit.created</b><br> 18033 * </p> 18034 */ 18035 @SearchParamDefinition(name="created", path="ExplanationOfBenefit.created", description="The creation date for the EOB", type="date" ) 18036 public static final String SP_CREATED = "created"; 18037 /** 18038 * <b>Fluent Client</b> search parameter constant for <b>created</b> 18039 * <p> 18040 * Description: <b>The creation date for the EOB</b><br> 18041 * Type: <b>date</b><br> 18042 * Path: <b>ExplanationOfBenefit.created</b><br> 18043 * </p> 18044 */ 18045 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 18046 18047 /** 18048 * Search parameter: <b>detail-udi</b> 18049 * <p> 18050 * Description: <b>UDI associated with a line item detail product or service</b><br> 18051 * Type: <b>reference</b><br> 18052 * Path: <b>ExplanationOfBenefit.item.detail.udi</b><br> 18053 * </p> 18054 */ 18055 @SearchParamDefinition(name="detail-udi", path="ExplanationOfBenefit.item.detail.udi", description="UDI associated with a line item detail product or service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 18056 public static final String SP_DETAIL_UDI = "detail-udi"; 18057 /** 18058 * <b>Fluent Client</b> search parameter constant for <b>detail-udi</b> 18059 * <p> 18060 * Description: <b>UDI associated with a line item detail product or service</b><br> 18061 * Type: <b>reference</b><br> 18062 * Path: <b>ExplanationOfBenefit.item.detail.udi</b><br> 18063 * </p> 18064 */ 18065 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DETAIL_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DETAIL_UDI); 18066 18067/** 18068 * Constant for fluent queries to be used to add include statements. Specifies 18069 * the path value of "<b>ExplanationOfBenefit:detail-udi</b>". 18070 */ 18071 public static final ca.uhn.fhir.model.api.Include INCLUDE_DETAIL_UDI = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:detail-udi").toLocked(); 18072 18073 /** 18074 * Search parameter: <b>disposition</b> 18075 * <p> 18076 * Description: <b>The contents of the disposition message</b><br> 18077 * Type: <b>string</b><br> 18078 * Path: <b>ExplanationOfBenefit.disposition</b><br> 18079 * </p> 18080 */ 18081 @SearchParamDefinition(name="disposition", path="ExplanationOfBenefit.disposition", description="The contents of the disposition message", type="string" ) 18082 public static final String SP_DISPOSITION = "disposition"; 18083 /** 18084 * <b>Fluent Client</b> search parameter constant for <b>disposition</b> 18085 * <p> 18086 * Description: <b>The contents of the disposition message</b><br> 18087 * Type: <b>string</b><br> 18088 * Path: <b>ExplanationOfBenefit.disposition</b><br> 18089 * </p> 18090 */ 18091 public static final ca.uhn.fhir.rest.gclient.StringClientParam DISPOSITION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DISPOSITION); 18092 18093 /** 18094 * Search parameter: <b>enterer</b> 18095 * <p> 18096 * Description: <b>The party responsible for the entry of the Claim</b><br> 18097 * Type: <b>reference</b><br> 18098 * Path: <b>ExplanationOfBenefit.enterer</b><br> 18099 * </p> 18100 */ 18101 @SearchParamDefinition(name="enterer", path="ExplanationOfBenefit.enterer", description="The party responsible for the entry of the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 18102 public static final String SP_ENTERER = "enterer"; 18103 /** 18104 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 18105 * <p> 18106 * Description: <b>The party responsible for the entry of the Claim</b><br> 18107 * Type: <b>reference</b><br> 18108 * Path: <b>ExplanationOfBenefit.enterer</b><br> 18109 * </p> 18110 */ 18111 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTERER); 18112 18113/** 18114 * Constant for fluent queries to be used to add include statements. Specifies 18115 * the path value of "<b>ExplanationOfBenefit:enterer</b>". 18116 */ 18117 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:enterer").toLocked(); 18118 18119 /** 18120 * Search parameter: <b>facility</b> 18121 * <p> 18122 * Description: <b>Facility responsible for the goods and services</b><br> 18123 * Type: <b>reference</b><br> 18124 * Path: <b>ExplanationOfBenefit.facility</b><br> 18125 * </p> 18126 */ 18127 @SearchParamDefinition(name="facility", path="ExplanationOfBenefit.facility", description="Facility responsible for the goods and services", type="reference", target={Location.class, Organization.class } ) 18128 public static final String SP_FACILITY = "facility"; 18129 /** 18130 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 18131 * <p> 18132 * Description: <b>Facility responsible for the goods and services</b><br> 18133 * Type: <b>reference</b><br> 18134 * Path: <b>ExplanationOfBenefit.facility</b><br> 18135 * </p> 18136 */ 18137 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FACILITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FACILITY); 18138 18139/** 18140 * Constant for fluent queries to be used to add include statements. Specifies 18141 * the path value of "<b>ExplanationOfBenefit:facility</b>". 18142 */ 18143 public static final ca.uhn.fhir.model.api.Include INCLUDE_FACILITY = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:facility").toLocked(); 18144 18145 /** 18146 * Search parameter: <b>item-udi</b> 18147 * <p> 18148 * Description: <b>UDI associated with a line item product or service</b><br> 18149 * Type: <b>reference</b><br> 18150 * Path: <b>ExplanationOfBenefit.item.udi</b><br> 18151 * </p> 18152 */ 18153 @SearchParamDefinition(name="item-udi", path="ExplanationOfBenefit.item.udi", description="UDI associated with a line item product or service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 18154 public static final String SP_ITEM_UDI = "item-udi"; 18155 /** 18156 * <b>Fluent Client</b> search parameter constant for <b>item-udi</b> 18157 * <p> 18158 * Description: <b>UDI associated with a line item product or service</b><br> 18159 * Type: <b>reference</b><br> 18160 * Path: <b>ExplanationOfBenefit.item.udi</b><br> 18161 * </p> 18162 */ 18163 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ITEM_UDI); 18164 18165/** 18166 * Constant for fluent queries to be used to add include statements. Specifies 18167 * the path value of "<b>ExplanationOfBenefit:item-udi</b>". 18168 */ 18169 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM_UDI = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:item-udi").toLocked(); 18170 18171 /** 18172 * Search parameter: <b>payee</b> 18173 * <p> 18174 * Description: <b>The party receiving any payment for the Claim</b><br> 18175 * Type: <b>reference</b><br> 18176 * Path: <b>ExplanationOfBenefit.payee.party</b><br> 18177 * </p> 18178 */ 18179 @SearchParamDefinition(name="payee", path="ExplanationOfBenefit.payee.party", description="The party receiving any payment for the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 18180 public static final String SP_PAYEE = "payee"; 18181 /** 18182 * <b>Fluent Client</b> search parameter constant for <b>payee</b> 18183 * <p> 18184 * Description: <b>The party receiving any payment for the Claim</b><br> 18185 * Type: <b>reference</b><br> 18186 * Path: <b>ExplanationOfBenefit.payee.party</b><br> 18187 * </p> 18188 */ 18189 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYEE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PAYEE); 18190 18191/** 18192 * Constant for fluent queries to be used to add include statements. Specifies 18193 * the path value of "<b>ExplanationOfBenefit:payee</b>". 18194 */ 18195 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYEE = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:payee").toLocked(); 18196 18197 /** 18198 * Search parameter: <b>procedure-udi</b> 18199 * <p> 18200 * Description: <b>UDI associated with a procedure</b><br> 18201 * Type: <b>reference</b><br> 18202 * Path: <b>ExplanationOfBenefit.procedure.udi</b><br> 18203 * </p> 18204 */ 18205 @SearchParamDefinition(name="procedure-udi", path="ExplanationOfBenefit.procedure.udi", description="UDI associated with a procedure", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 18206 public static final String SP_PROCEDURE_UDI = "procedure-udi"; 18207 /** 18208 * <b>Fluent Client</b> search parameter constant for <b>procedure-udi</b> 18209 * <p> 18210 * Description: <b>UDI associated with a procedure</b><br> 18211 * Type: <b>reference</b><br> 18212 * Path: <b>ExplanationOfBenefit.procedure.udi</b><br> 18213 * </p> 18214 */ 18215 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROCEDURE_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROCEDURE_UDI); 18216 18217/** 18218 * Constant for fluent queries to be used to add include statements. Specifies 18219 * the path value of "<b>ExplanationOfBenefit:procedure-udi</b>". 18220 */ 18221 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROCEDURE_UDI = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:procedure-udi").toLocked(); 18222 18223 /** 18224 * Search parameter: <b>provider</b> 18225 * <p> 18226 * Description: <b>The reference to the provider</b><br> 18227 * Type: <b>reference</b><br> 18228 * Path: <b>ExplanationOfBenefit.provider</b><br> 18229 * </p> 18230 */ 18231 @SearchParamDefinition(name="provider", path="ExplanationOfBenefit.provider", description="The reference to the provider", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 18232 public static final String SP_PROVIDER = "provider"; 18233 /** 18234 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 18235 * <p> 18236 * Description: <b>The reference to the provider</b><br> 18237 * Type: <b>reference</b><br> 18238 * Path: <b>ExplanationOfBenefit.provider</b><br> 18239 * </p> 18240 */ 18241 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROVIDER); 18242 18243/** 18244 * Constant for fluent queries to be used to add include statements. Specifies 18245 * the path value of "<b>ExplanationOfBenefit:provider</b>". 18246 */ 18247 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:provider").toLocked(); 18248 18249 /** 18250 * Search parameter: <b>status</b> 18251 * <p> 18252 * Description: <b>Status of the instance</b><br> 18253 * Type: <b>token</b><br> 18254 * Path: <b>ExplanationOfBenefit.status</b><br> 18255 * </p> 18256 */ 18257 @SearchParamDefinition(name="status", path="ExplanationOfBenefit.status", description="Status of the instance", type="token" ) 18258 public static final String SP_STATUS = "status"; 18259 /** 18260 * <b>Fluent Client</b> search parameter constant for <b>status</b> 18261 * <p> 18262 * Description: <b>Status of the instance</b><br> 18263 * Type: <b>token</b><br> 18264 * Path: <b>ExplanationOfBenefit.status</b><br> 18265 * </p> 18266 */ 18267 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 18268 18269 /** 18270 * Search parameter: <b>subdetail-udi</b> 18271 * <p> 18272 * Description: <b>UDI associated with a line item detail subdetail product or service</b><br> 18273 * Type: <b>reference</b><br> 18274 * Path: <b>ExplanationOfBenefit.item.detail.subDetail.udi</b><br> 18275 * </p> 18276 */ 18277 @SearchParamDefinition(name="subdetail-udi", path="ExplanationOfBenefit.item.detail.subDetail.udi", description="UDI associated with a line item detail subdetail product or service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 18278 public static final String SP_SUBDETAIL_UDI = "subdetail-udi"; 18279 /** 18280 * <b>Fluent Client</b> search parameter constant for <b>subdetail-udi</b> 18281 * <p> 18282 * Description: <b>UDI associated with a line item detail subdetail product or service</b><br> 18283 * Type: <b>reference</b><br> 18284 * Path: <b>ExplanationOfBenefit.item.detail.subDetail.udi</b><br> 18285 * </p> 18286 */ 18287 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBDETAIL_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBDETAIL_UDI); 18288 18289/** 18290 * Constant for fluent queries to be used to add include statements. Specifies 18291 * the path value of "<b>ExplanationOfBenefit:subdetail-udi</b>". 18292 */ 18293 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBDETAIL_UDI = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:subdetail-udi").toLocked(); 18294 18295 /** 18296 * Search parameter: <b>encounter</b> 18297 * <p> 18298 * Description: <b>Multiple Resources: 18299 18300* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 18301* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 18302* [ChargeItem](chargeitem.html): Encounter associated with event 18303* [Claim](claim.html): Encounters associated with a billed line item 18304* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 18305* [Communication](communication.html): The Encounter during which this Communication was created 18306* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 18307* [Composition](composition.html): Context of the Composition 18308* [Condition](condition.html): The Encounter during which this Condition was created 18309* [DeviceRequest](devicerequest.html): Encounter during which request was created 18310* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 18311* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 18312* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 18313* [Flag](flag.html): Alert relevant during encounter 18314* [ImagingStudy](imagingstudy.html): The context of the study 18315* [List](list.html): Context in which list created 18316* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 18317* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 18318* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 18319* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 18320* [Observation](observation.html): Encounter related to the observation 18321* [Procedure](procedure.html): The Encounter during which this Procedure was created 18322* [Provenance](provenance.html): Encounter related to the Provenance 18323* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 18324* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 18325* [RiskAssessment](riskassessment.html): Where was assessment performed? 18326* [ServiceRequest](servicerequest.html): An encounter in which this request is made 18327* [Task](task.html): Search by encounter 18328* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 18329</b><br> 18330 * Type: <b>reference</b><br> 18331 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 18332 * </p> 18333 */ 18334 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 18335 public static final String SP_ENCOUNTER = "encounter"; 18336 /** 18337 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 18338 * <p> 18339 * Description: <b>Multiple Resources: 18340 18341* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 18342* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 18343* [ChargeItem](chargeitem.html): Encounter associated with event 18344* [Claim](claim.html): Encounters associated with a billed line item 18345* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 18346* [Communication](communication.html): The Encounter during which this Communication was created 18347* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 18348* [Composition](composition.html): Context of the Composition 18349* [Condition](condition.html): The Encounter during which this Condition was created 18350* [DeviceRequest](devicerequest.html): Encounter during which request was created 18351* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 18352* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 18353* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 18354* [Flag](flag.html): Alert relevant during encounter 18355* [ImagingStudy](imagingstudy.html): The context of the study 18356* [List](list.html): Context in which list created 18357* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 18358* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 18359* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 18360* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 18361* [Observation](observation.html): Encounter related to the observation 18362* [Procedure](procedure.html): The Encounter during which this Procedure was created 18363* [Provenance](provenance.html): Encounter related to the Provenance 18364* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 18365* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 18366* [RiskAssessment](riskassessment.html): Where was assessment performed? 18367* [ServiceRequest](servicerequest.html): An encounter in which this request is made 18368* [Task](task.html): Search by encounter 18369* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 18370</b><br> 18371 * Type: <b>reference</b><br> 18372 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 18373 * </p> 18374 */ 18375 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 18376 18377/** 18378 * Constant for fluent queries to be used to add include statements. Specifies 18379 * the path value of "<b>ExplanationOfBenefit:encounter</b>". 18380 */ 18381 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:encounter").toLocked(); 18382 18383 /** 18384 * Search parameter: <b>identifier</b> 18385 * <p> 18386 * Description: <b>Multiple Resources: 18387 18388* [Account](account.html): Account number 18389* [AdverseEvent](adverseevent.html): Business identifier for the event 18390* [AllergyIntolerance](allergyintolerance.html): External ids for this item 18391* [Appointment](appointment.html): An Identifier of the Appointment 18392* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 18393* [Basic](basic.html): Business identifier 18394* [BodyStructure](bodystructure.html): Bodystructure identifier 18395* [CarePlan](careplan.html): External Ids for this plan 18396* [CareTeam](careteam.html): External Ids for this team 18397* [ChargeItem](chargeitem.html): Business Identifier for item 18398* [Claim](claim.html): The primary identifier of the financial resource 18399* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 18400* [ClinicalImpression](clinicalimpression.html): Business identifier 18401* [Communication](communication.html): Unique identifier 18402* [CommunicationRequest](communicationrequest.html): Unique identifier 18403* [Composition](composition.html): Version-independent identifier for the Composition 18404* [Condition](condition.html): A unique identifier of the condition record 18405* [Consent](consent.html): Identifier for this record (external references) 18406* [Contract](contract.html): The identity of the contract 18407* [Coverage](coverage.html): The primary identifier of the insured and the coverage 18408* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 18409* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 18410* [DetectedIssue](detectedissue.html): Unique id for the detected issue 18411* [DeviceRequest](devicerequest.html): Business identifier for request/order 18412* [DeviceUsage](deviceusage.html): Search by identifier 18413* [DiagnosticReport](diagnosticreport.html): An identifier for the report 18414* [DocumentReference](documentreference.html): Identifier of the attachment binary 18415* [Encounter](encounter.html): Identifier(s) by which this encounter is known 18416* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 18417* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 18418* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 18419* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 18420* [Flag](flag.html): Business identifier 18421* [Goal](goal.html): External Ids for this goal 18422* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 18423* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 18424* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 18425* [Immunization](immunization.html): Business identifier 18426* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 18427* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 18428* [Invoice](invoice.html): Business Identifier for item 18429* [List](list.html): Business identifier 18430* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 18431* [Medication](medication.html): Returns medications with this external identifier 18432* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 18433* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 18434* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 18435* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 18436* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 18437* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 18438* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 18439* [Observation](observation.html): The unique id for a particular observation 18440* [Person](person.html): A person Identifier 18441* [Procedure](procedure.html): A unique identifier for a procedure 18442* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 18443* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 18444* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 18445* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 18446* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 18447* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 18448* [Specimen](specimen.html): The unique identifier associated with the specimen 18449* [SupplyDelivery](supplydelivery.html): External identifier 18450* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 18451* [Task](task.html): Search for a task instance by its business identifier 18452* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 18453</b><br> 18454 * Type: <b>token</b><br> 18455 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 18456 * </p> 18457 */ 18458 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 18459 public static final String SP_IDENTIFIER = "identifier"; 18460 /** 18461 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 18462 * <p> 18463 * Description: <b>Multiple Resources: 18464 18465* [Account](account.html): Account number 18466* [AdverseEvent](adverseevent.html): Business identifier for the event 18467* [AllergyIntolerance](allergyintolerance.html): External ids for this item 18468* [Appointment](appointment.html): An Identifier of the Appointment 18469* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 18470* [Basic](basic.html): Business identifier 18471* [BodyStructure](bodystructure.html): Bodystructure identifier 18472* [CarePlan](careplan.html): External Ids for this plan 18473* [CareTeam](careteam.html): External Ids for this team 18474* [ChargeItem](chargeitem.html): Business Identifier for item 18475* [Claim](claim.html): The primary identifier of the financial resource 18476* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 18477* [ClinicalImpression](clinicalimpression.html): Business identifier 18478* [Communication](communication.html): Unique identifier 18479* [CommunicationRequest](communicationrequest.html): Unique identifier 18480* [Composition](composition.html): Version-independent identifier for the Composition 18481* [Condition](condition.html): A unique identifier of the condition record 18482* [Consent](consent.html): Identifier for this record (external references) 18483* [Contract](contract.html): The identity of the contract 18484* [Coverage](coverage.html): The primary identifier of the insured and the coverage 18485* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 18486* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 18487* [DetectedIssue](detectedissue.html): Unique id for the detected issue 18488* [DeviceRequest](devicerequest.html): Business identifier for request/order 18489* [DeviceUsage](deviceusage.html): Search by identifier 18490* [DiagnosticReport](diagnosticreport.html): An identifier for the report 18491* [DocumentReference](documentreference.html): Identifier of the attachment binary 18492* [Encounter](encounter.html): Identifier(s) by which this encounter is known 18493* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 18494* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 18495* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 18496* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 18497* [Flag](flag.html): Business identifier 18498* [Goal](goal.html): External Ids for this goal 18499* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 18500* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 18501* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 18502* [Immunization](immunization.html): Business identifier 18503* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 18504* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 18505* [Invoice](invoice.html): Business Identifier for item 18506* [List](list.html): Business identifier 18507* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 18508* [Medication](medication.html): Returns medications with this external identifier 18509* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 18510* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 18511* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 18512* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 18513* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 18514* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 18515* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 18516* [Observation](observation.html): The unique id for a particular observation 18517* [Person](person.html): A person Identifier 18518* [Procedure](procedure.html): A unique identifier for a procedure 18519* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 18520* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 18521* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 18522* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 18523* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 18524* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 18525* [Specimen](specimen.html): The unique identifier associated with the specimen 18526* [SupplyDelivery](supplydelivery.html): External identifier 18527* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 18528* [Task](task.html): Search for a task instance by its business identifier 18529* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 18530</b><br> 18531 * Type: <b>token</b><br> 18532 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 18533 * </p> 18534 */ 18535 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 18536 18537 /** 18538 * Search parameter: <b>patient</b> 18539 * <p> 18540 * Description: <b>Multiple Resources: 18541 18542* [Account](account.html): The entity that caused the expenses 18543* [AdverseEvent](adverseevent.html): Subject impacted by event 18544* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 18545* [Appointment](appointment.html): One of the individuals of the appointment is this patient 18546* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 18547* [AuditEvent](auditevent.html): Where the activity involved patient data 18548* [Basic](basic.html): Identifies the focus of this resource 18549* [BodyStructure](bodystructure.html): Who this is about 18550* [CarePlan](careplan.html): Who the care plan is for 18551* [CareTeam](careteam.html): Who care team is for 18552* [ChargeItem](chargeitem.html): Individual service was done for/to 18553* [Claim](claim.html): Patient receiving the products or services 18554* [ClaimResponse](claimresponse.html): The subject of care 18555* [ClinicalImpression](clinicalimpression.html): Patient assessed 18556* [Communication](communication.html): Focus of message 18557* [CommunicationRequest](communicationrequest.html): Focus of message 18558* [Composition](composition.html): Who and/or what the composition is about 18559* [Condition](condition.html): Who has the condition? 18560* [Consent](consent.html): Who the consent applies to 18561* [Contract](contract.html): The identity of the subject of the contract (if a patient) 18562* [Coverage](coverage.html): Retrieve coverages for a patient 18563* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 18564* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 18565* [DetectedIssue](detectedissue.html): Associated patient 18566* [DeviceRequest](devicerequest.html): Individual the service is ordered for 18567* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 18568* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 18569* [DocumentReference](documentreference.html): Who/what is the subject of the document 18570* [Encounter](encounter.html): The patient present at the encounter 18571* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 18572* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 18573* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 18574* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 18575* [Flag](flag.html): The identity of a subject to list flags for 18576* [Goal](goal.html): Who this goal is intended for 18577* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 18578* [ImagingSelection](imagingselection.html): Who the study is about 18579* [ImagingStudy](imagingstudy.html): Who the study is about 18580* [Immunization](immunization.html): The patient for the vaccination record 18581* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 18582* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 18583* [Invoice](invoice.html): Recipient(s) of goods and services 18584* [List](list.html): If all resources have the same subject 18585* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 18586* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 18587* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 18588* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 18589* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 18590* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 18591* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 18592* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 18593* [Observation](observation.html): The subject that the observation is about (if patient) 18594* [Person](person.html): The Person links to this Patient 18595* [Procedure](procedure.html): Search by subject - a patient 18596* [Provenance](provenance.html): Where the activity involved patient data 18597* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 18598* [RelatedPerson](relatedperson.html): The patient this related person is related to 18599* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 18600* [ResearchSubject](researchsubject.html): Who or what is part of study 18601* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 18602* [ServiceRequest](servicerequest.html): Search by subject - a patient 18603* [Specimen](specimen.html): The patient the specimen comes from 18604* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 18605* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 18606* [Task](task.html): Search by patient 18607* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 18608</b><br> 18609 * Type: <b>reference</b><br> 18610 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 18611 * </p> 18612 */ 18613 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 18614 public static final String SP_PATIENT = "patient"; 18615 /** 18616 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 18617 * <p> 18618 * Description: <b>Multiple Resources: 18619 18620* [Account](account.html): The entity that caused the expenses 18621* [AdverseEvent](adverseevent.html): Subject impacted by event 18622* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 18623* [Appointment](appointment.html): One of the individuals of the appointment is this patient 18624* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 18625* [AuditEvent](auditevent.html): Where the activity involved patient data 18626* [Basic](basic.html): Identifies the focus of this resource 18627* [BodyStructure](bodystructure.html): Who this is about 18628* [CarePlan](careplan.html): Who the care plan is for 18629* [CareTeam](careteam.html): Who care team is for 18630* [ChargeItem](chargeitem.html): Individual service was done for/to 18631* [Claim](claim.html): Patient receiving the products or services 18632* [ClaimResponse](claimresponse.html): The subject of care 18633* [ClinicalImpression](clinicalimpression.html): Patient assessed 18634* [Communication](communication.html): Focus of message 18635* [CommunicationRequest](communicationrequest.html): Focus of message 18636* [Composition](composition.html): Who and/or what the composition is about 18637* [Condition](condition.html): Who has the condition? 18638* [Consent](consent.html): Who the consent applies to 18639* [Contract](contract.html): The identity of the subject of the contract (if a patient) 18640* [Coverage](coverage.html): Retrieve coverages for a patient 18641* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 18642* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 18643* [DetectedIssue](detectedissue.html): Associated patient 18644* [DeviceRequest](devicerequest.html): Individual the service is ordered for 18645* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 18646* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 18647* [DocumentReference](documentreference.html): Who/what is the subject of the document 18648* [Encounter](encounter.html): The patient present at the encounter 18649* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 18650* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 18651* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 18652* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 18653* [Flag](flag.html): The identity of a subject to list flags for 18654* [Goal](goal.html): Who this goal is intended for 18655* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 18656* [ImagingSelection](imagingselection.html): Who the study is about 18657* [ImagingStudy](imagingstudy.html): Who the study is about 18658* [Immunization](immunization.html): The patient for the vaccination record 18659* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 18660* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 18661* [Invoice](invoice.html): Recipient(s) of goods and services 18662* [List](list.html): If all resources have the same subject 18663* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 18664* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 18665* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 18666* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 18667* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 18668* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 18669* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 18670* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 18671* [Observation](observation.html): The subject that the observation is about (if patient) 18672* [Person](person.html): The Person links to this Patient 18673* [Procedure](procedure.html): Search by subject - a patient 18674* [Provenance](provenance.html): Where the activity involved patient data 18675* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 18676* [RelatedPerson](relatedperson.html): The patient this related person is related to 18677* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 18678* [ResearchSubject](researchsubject.html): Who or what is part of study 18679* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 18680* [ServiceRequest](servicerequest.html): Search by subject - a patient 18681* [Specimen](specimen.html): The patient the specimen comes from 18682* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 18683* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 18684* [Task](task.html): Search by patient 18685* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 18686</b><br> 18687 * Type: <b>reference</b><br> 18688 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 18689 * </p> 18690 */ 18691 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 18692 18693/** 18694 * Constant for fluent queries to be used to add include statements. Specifies 18695 * the path value of "<b>ExplanationOfBenefit:patient</b>". 18696 */ 18697 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:patient").toLocked(); 18698 18699 18700} 18701