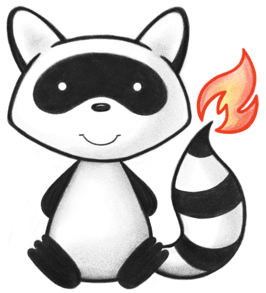
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051/** 052 * This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided. 053 */ 054@ResourceDef(name="ExplanationOfBenefit", profile="http://hl7.org/fhir/StructureDefinition/ExplanationOfBenefit") 055public class ExplanationOfBenefit extends DomainResource { 056 057 public enum ExplanationOfBenefitStatus { 058 /** 059 * The resource instance is currently in-force. 060 */ 061 ACTIVE, 062 /** 063 * The resource instance is withdrawn, rescinded or reversed. 064 */ 065 CANCELLED, 066 /** 067 * A new resource instance the contents of which is not complete. 068 */ 069 DRAFT, 070 /** 071 * The resource instance was entered in error. 072 */ 073 ENTEREDINERROR, 074 /** 075 * added to help the parsers with the generic types 076 */ 077 NULL; 078 public static ExplanationOfBenefitStatus fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("active".equals(codeString)) 082 return ACTIVE; 083 if ("cancelled".equals(codeString)) 084 return CANCELLED; 085 if ("draft".equals(codeString)) 086 return DRAFT; 087 if ("entered-in-error".equals(codeString)) 088 return ENTEREDINERROR; 089 if (Configuration.isAcceptInvalidEnums()) 090 return null; 091 else 092 throw new FHIRException("Unknown ExplanationOfBenefitStatus code '"+codeString+"'"); 093 } 094 public String toCode() { 095 switch (this) { 096 case ACTIVE: return "active"; 097 case CANCELLED: return "cancelled"; 098 case DRAFT: return "draft"; 099 case ENTEREDINERROR: return "entered-in-error"; 100 case NULL: return null; 101 default: return "?"; 102 } 103 } 104 public String getSystem() { 105 switch (this) { 106 case ACTIVE: return "http://hl7.org/fhir/explanationofbenefit-status"; 107 case CANCELLED: return "http://hl7.org/fhir/explanationofbenefit-status"; 108 case DRAFT: return "http://hl7.org/fhir/explanationofbenefit-status"; 109 case ENTEREDINERROR: return "http://hl7.org/fhir/explanationofbenefit-status"; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDefinition() { 115 switch (this) { 116 case ACTIVE: return "The resource instance is currently in-force."; 117 case CANCELLED: return "The resource instance is withdrawn, rescinded or reversed."; 118 case DRAFT: return "A new resource instance the contents of which is not complete."; 119 case ENTEREDINERROR: return "The resource instance was entered in error."; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getDisplay() { 125 switch (this) { 126 case ACTIVE: return "Active"; 127 case CANCELLED: return "Cancelled"; 128 case DRAFT: return "Draft"; 129 case ENTEREDINERROR: return "Entered In Error"; 130 case NULL: return null; 131 default: return "?"; 132 } 133 } 134 } 135 136 public static class ExplanationOfBenefitStatusEnumFactory implements EnumFactory<ExplanationOfBenefitStatus> { 137 public ExplanationOfBenefitStatus fromCode(String codeString) throws IllegalArgumentException { 138 if (codeString == null || "".equals(codeString)) 139 if (codeString == null || "".equals(codeString)) 140 return null; 141 if ("active".equals(codeString)) 142 return ExplanationOfBenefitStatus.ACTIVE; 143 if ("cancelled".equals(codeString)) 144 return ExplanationOfBenefitStatus.CANCELLED; 145 if ("draft".equals(codeString)) 146 return ExplanationOfBenefitStatus.DRAFT; 147 if ("entered-in-error".equals(codeString)) 148 return ExplanationOfBenefitStatus.ENTEREDINERROR; 149 throw new IllegalArgumentException("Unknown ExplanationOfBenefitStatus code '"+codeString+"'"); 150 } 151 public Enumeration<ExplanationOfBenefitStatus> fromType(PrimitiveType<?> code) throws FHIRException { 152 if (code == null) 153 return null; 154 if (code.isEmpty()) 155 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.NULL, code); 156 String codeString = ((PrimitiveType) code).asStringValue(); 157 if (codeString == null || "".equals(codeString)) 158 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.NULL, code); 159 if ("active".equals(codeString)) 160 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.ACTIVE, code); 161 if ("cancelled".equals(codeString)) 162 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.CANCELLED, code); 163 if ("draft".equals(codeString)) 164 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.DRAFT, code); 165 if ("entered-in-error".equals(codeString)) 166 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.ENTEREDINERROR, code); 167 throw new FHIRException("Unknown ExplanationOfBenefitStatus code '"+codeString+"'"); 168 } 169 public String toCode(ExplanationOfBenefitStatus code) { 170 if (code == ExplanationOfBenefitStatus.NULL) 171 return null; 172 if (code == ExplanationOfBenefitStatus.ACTIVE) 173 return "active"; 174 if (code == ExplanationOfBenefitStatus.CANCELLED) 175 return "cancelled"; 176 if (code == ExplanationOfBenefitStatus.DRAFT) 177 return "draft"; 178 if (code == ExplanationOfBenefitStatus.ENTEREDINERROR) 179 return "entered-in-error"; 180 return "?"; 181 } 182 public String toSystem(ExplanationOfBenefitStatus code) { 183 return code.getSystem(); 184 } 185 } 186 187 @Block() 188 public static class RelatedClaimComponent extends BackboneElement implements IBaseBackboneElement { 189 /** 190 * Reference to a related claim. 191 */ 192 @Child(name = "claim", type = {Claim.class}, order=1, min=0, max=1, modifier=false, summary=false) 193 @Description(shortDefinition="Reference to the related claim", formalDefinition="Reference to a related claim." ) 194 protected Reference claim; 195 196 /** 197 * A code to convey how the claims are related. 198 */ 199 @Child(name = "relationship", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 200 @Description(shortDefinition="How the reference claim is related", formalDefinition="A code to convey how the claims are related." ) 201 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/related-claim-relationship") 202 protected CodeableConcept relationship; 203 204 /** 205 * An alternate organizational reference to the case or file to which this particular claim pertains. 206 */ 207 @Child(name = "reference", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=false) 208 @Description(shortDefinition="File or case reference", formalDefinition="An alternate organizational reference to the case or file to which this particular claim pertains." ) 209 protected Identifier reference; 210 211 private static final long serialVersionUID = 1047077926L; 212 213 /** 214 * Constructor 215 */ 216 public RelatedClaimComponent() { 217 super(); 218 } 219 220 /** 221 * @return {@link #claim} (Reference to a related claim.) 222 */ 223 public Reference getClaim() { 224 if (this.claim == null) 225 if (Configuration.errorOnAutoCreate()) 226 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 227 else if (Configuration.doAutoCreate()) 228 this.claim = new Reference(); // cc 229 return this.claim; 230 } 231 232 public boolean hasClaim() { 233 return this.claim != null && !this.claim.isEmpty(); 234 } 235 236 /** 237 * @param value {@link #claim} (Reference to a related claim.) 238 */ 239 public RelatedClaimComponent setClaim(Reference value) { 240 this.claim = value; 241 return this; 242 } 243 244 /** 245 * @return {@link #relationship} (A code to convey how the claims are related.) 246 */ 247 public CodeableConcept getRelationship() { 248 if (this.relationship == null) 249 if (Configuration.errorOnAutoCreate()) 250 throw new Error("Attempt to auto-create RelatedClaimComponent.relationship"); 251 else if (Configuration.doAutoCreate()) 252 this.relationship = new CodeableConcept(); // cc 253 return this.relationship; 254 } 255 256 public boolean hasRelationship() { 257 return this.relationship != null && !this.relationship.isEmpty(); 258 } 259 260 /** 261 * @param value {@link #relationship} (A code to convey how the claims are related.) 262 */ 263 public RelatedClaimComponent setRelationship(CodeableConcept value) { 264 this.relationship = value; 265 return this; 266 } 267 268 /** 269 * @return {@link #reference} (An alternate organizational reference to the case or file to which this particular claim pertains.) 270 */ 271 public Identifier getReference() { 272 if (this.reference == null) 273 if (Configuration.errorOnAutoCreate()) 274 throw new Error("Attempt to auto-create RelatedClaimComponent.reference"); 275 else if (Configuration.doAutoCreate()) 276 this.reference = new Identifier(); // cc 277 return this.reference; 278 } 279 280 public boolean hasReference() { 281 return this.reference != null && !this.reference.isEmpty(); 282 } 283 284 /** 285 * @param value {@link #reference} (An alternate organizational reference to the case or file to which this particular claim pertains.) 286 */ 287 public RelatedClaimComponent setReference(Identifier value) { 288 this.reference = value; 289 return this; 290 } 291 292 protected void listChildren(List<Property> children) { 293 super.listChildren(children); 294 children.add(new Property("claim", "Reference(Claim)", "Reference to a related claim.", 0, 1, claim)); 295 children.add(new Property("relationship", "CodeableConcept", "A code to convey how the claims are related.", 0, 1, relationship)); 296 children.add(new Property("reference", "Identifier", "An alternate organizational reference to the case or file to which this particular claim pertains.", 0, 1, reference)); 297 } 298 299 @Override 300 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 301 switch (_hash) { 302 case 94742588: /*claim*/ return new Property("claim", "Reference(Claim)", "Reference to a related claim.", 0, 1, claim); 303 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "A code to convey how the claims are related.", 0, 1, relationship); 304 case -925155509: /*reference*/ return new Property("reference", "Identifier", "An alternate organizational reference to the case or file to which this particular claim pertains.", 0, 1, reference); 305 default: return super.getNamedProperty(_hash, _name, _checkValid); 306 } 307 308 } 309 310 @Override 311 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 312 switch (hash) { 313 case 94742588: /*claim*/ return this.claim == null ? new Base[0] : new Base[] {this.claim}; // Reference 314 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // CodeableConcept 315 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Identifier 316 default: return super.getProperty(hash, name, checkValid); 317 } 318 319 } 320 321 @Override 322 public Base setProperty(int hash, String name, Base value) throws FHIRException { 323 switch (hash) { 324 case 94742588: // claim 325 this.claim = TypeConvertor.castToReference(value); // Reference 326 return value; 327 case -261851592: // relationship 328 this.relationship = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 329 return value; 330 case -925155509: // reference 331 this.reference = TypeConvertor.castToIdentifier(value); // Identifier 332 return value; 333 default: return super.setProperty(hash, name, value); 334 } 335 336 } 337 338 @Override 339 public Base setProperty(String name, Base value) throws FHIRException { 340 if (name.equals("claim")) { 341 this.claim = TypeConvertor.castToReference(value); // Reference 342 } else if (name.equals("relationship")) { 343 this.relationship = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 344 } else if (name.equals("reference")) { 345 this.reference = TypeConvertor.castToIdentifier(value); // Identifier 346 } else 347 return super.setProperty(name, value); 348 return value; 349 } 350 351 @Override 352 public void removeChild(String name, Base value) throws FHIRException { 353 if (name.equals("claim")) { 354 this.claim = null; 355 } else if (name.equals("relationship")) { 356 this.relationship = null; 357 } else if (name.equals("reference")) { 358 this.reference = null; 359 } else 360 super.removeChild(name, value); 361 362 } 363 364 @Override 365 public Base makeProperty(int hash, String name) throws FHIRException { 366 switch (hash) { 367 case 94742588: return getClaim(); 368 case -261851592: return getRelationship(); 369 case -925155509: return getReference(); 370 default: return super.makeProperty(hash, name); 371 } 372 373 } 374 375 @Override 376 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 377 switch (hash) { 378 case 94742588: /*claim*/ return new String[] {"Reference"}; 379 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 380 case -925155509: /*reference*/ return new String[] {"Identifier"}; 381 default: return super.getTypesForProperty(hash, name); 382 } 383 384 } 385 386 @Override 387 public Base addChild(String name) throws FHIRException { 388 if (name.equals("claim")) { 389 this.claim = new Reference(); 390 return this.claim; 391 } 392 else if (name.equals("relationship")) { 393 this.relationship = new CodeableConcept(); 394 return this.relationship; 395 } 396 else if (name.equals("reference")) { 397 this.reference = new Identifier(); 398 return this.reference; 399 } 400 else 401 return super.addChild(name); 402 } 403 404 public RelatedClaimComponent copy() { 405 RelatedClaimComponent dst = new RelatedClaimComponent(); 406 copyValues(dst); 407 return dst; 408 } 409 410 public void copyValues(RelatedClaimComponent dst) { 411 super.copyValues(dst); 412 dst.claim = claim == null ? null : claim.copy(); 413 dst.relationship = relationship == null ? null : relationship.copy(); 414 dst.reference = reference == null ? null : reference.copy(); 415 } 416 417 @Override 418 public boolean equalsDeep(Base other_) { 419 if (!super.equalsDeep(other_)) 420 return false; 421 if (!(other_ instanceof RelatedClaimComponent)) 422 return false; 423 RelatedClaimComponent o = (RelatedClaimComponent) other_; 424 return compareDeep(claim, o.claim, true) && compareDeep(relationship, o.relationship, true) && compareDeep(reference, o.reference, true) 425 ; 426 } 427 428 @Override 429 public boolean equalsShallow(Base other_) { 430 if (!super.equalsShallow(other_)) 431 return false; 432 if (!(other_ instanceof RelatedClaimComponent)) 433 return false; 434 RelatedClaimComponent o = (RelatedClaimComponent) other_; 435 return true; 436 } 437 438 public boolean isEmpty() { 439 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(claim, relationship, reference 440 ); 441 } 442 443 public String fhirType() { 444 return "ExplanationOfBenefit.related"; 445 446 } 447 448 } 449 450 @Block() 451 public static class ExplanationOfBenefitEventComponent extends BackboneElement implements IBaseBackboneElement { 452 /** 453 * A coded event such as when a service is expected or a card printed. 454 */ 455 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 456 @Description(shortDefinition="Specific event", formalDefinition="A coded event such as when a service is expected or a card printed." ) 457 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/datestype") 458 protected CodeableConcept type; 459 460 /** 461 * A date or period in the past or future indicating when the event occurred or is expectd to occur. 462 */ 463 @Child(name = "when", type = {DateTimeType.class, Period.class}, order=2, min=1, max=1, modifier=false, summary=false) 464 @Description(shortDefinition="Occurance date or period", formalDefinition="A date or period in the past or future indicating when the event occurred or is expectd to occur." ) 465 protected DataType when; 466 467 private static final long serialVersionUID = -634897375L; 468 469 /** 470 * Constructor 471 */ 472 public ExplanationOfBenefitEventComponent() { 473 super(); 474 } 475 476 /** 477 * Constructor 478 */ 479 public ExplanationOfBenefitEventComponent(CodeableConcept type, DataType when) { 480 super(); 481 this.setType(type); 482 this.setWhen(when); 483 } 484 485 /** 486 * @return {@link #type} (A coded event such as when a service is expected or a card printed.) 487 */ 488 public CodeableConcept getType() { 489 if (this.type == null) 490 if (Configuration.errorOnAutoCreate()) 491 throw new Error("Attempt to auto-create ExplanationOfBenefitEventComponent.type"); 492 else if (Configuration.doAutoCreate()) 493 this.type = new CodeableConcept(); // cc 494 return this.type; 495 } 496 497 public boolean hasType() { 498 return this.type != null && !this.type.isEmpty(); 499 } 500 501 /** 502 * @param value {@link #type} (A coded event such as when a service is expected or a card printed.) 503 */ 504 public ExplanationOfBenefitEventComponent setType(CodeableConcept value) { 505 this.type = value; 506 return this; 507 } 508 509 /** 510 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 511 */ 512 public DataType getWhen() { 513 return this.when; 514 } 515 516 /** 517 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 518 */ 519 public DateTimeType getWhenDateTimeType() throws FHIRException { 520 if (this.when == null) 521 this.when = new DateTimeType(); 522 if (!(this.when instanceof DateTimeType)) 523 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.when.getClass().getName()+" was encountered"); 524 return (DateTimeType) this.when; 525 } 526 527 public boolean hasWhenDateTimeType() { 528 return this.when instanceof DateTimeType; 529 } 530 531 /** 532 * @return {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 533 */ 534 public Period getWhenPeriod() throws FHIRException { 535 if (this.when == null) 536 this.when = new Period(); 537 if (!(this.when instanceof Period)) 538 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.when.getClass().getName()+" was encountered"); 539 return (Period) this.when; 540 } 541 542 public boolean hasWhenPeriod() { 543 return this.when instanceof Period; 544 } 545 546 public boolean hasWhen() { 547 return this.when != null && !this.when.isEmpty(); 548 } 549 550 /** 551 * @param value {@link #when} (A date or period in the past or future indicating when the event occurred or is expectd to occur.) 552 */ 553 public ExplanationOfBenefitEventComponent setWhen(DataType value) { 554 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 555 throw new FHIRException("Not the right type for ExplanationOfBenefit.event.when[x]: "+value.fhirType()); 556 this.when = value; 557 return this; 558 } 559 560 protected void listChildren(List<Property> children) { 561 super.listChildren(children); 562 children.add(new Property("type", "CodeableConcept", "A coded event such as when a service is expected or a card printed.", 0, 1, type)); 563 children.add(new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when)); 564 } 565 566 @Override 567 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 568 switch (_hash) { 569 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A coded event such as when a service is expected or a card printed.", 0, 1, type); 570 case 1312831238: /*when[x]*/ return new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 571 case 3648314: /*when*/ return new Property("when[x]", "dateTime|Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 572 case -1785502475: /*whenDateTime*/ return new Property("when[x]", "dateTime", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 573 case 251476379: /*whenPeriod*/ return new Property("when[x]", "Period", "A date or period in the past or future indicating when the event occurred or is expectd to occur.", 0, 1, when); 574 default: return super.getNamedProperty(_hash, _name, _checkValid); 575 } 576 577 } 578 579 @Override 580 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 581 switch (hash) { 582 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 583 case 3648314: /*when*/ return this.when == null ? new Base[0] : new Base[] {this.when}; // DataType 584 default: return super.getProperty(hash, name, checkValid); 585 } 586 587 } 588 589 @Override 590 public Base setProperty(int hash, String name, Base value) throws FHIRException { 591 switch (hash) { 592 case 3575610: // type 593 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 594 return value; 595 case 3648314: // when 596 this.when = TypeConvertor.castToType(value); // DataType 597 return value; 598 default: return super.setProperty(hash, name, value); 599 } 600 601 } 602 603 @Override 604 public Base setProperty(String name, Base value) throws FHIRException { 605 if (name.equals("type")) { 606 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 607 } else if (name.equals("when[x]")) { 608 this.when = TypeConvertor.castToType(value); // DataType 609 } else 610 return super.setProperty(name, value); 611 return value; 612 } 613 614 @Override 615 public void removeChild(String name, Base value) throws FHIRException { 616 if (name.equals("type")) { 617 this.type = null; 618 } else if (name.equals("when[x]")) { 619 this.when = null; 620 } else 621 super.removeChild(name, value); 622 623 } 624 625 @Override 626 public Base makeProperty(int hash, String name) throws FHIRException { 627 switch (hash) { 628 case 3575610: return getType(); 629 case 1312831238: return getWhen(); 630 case 3648314: return getWhen(); 631 default: return super.makeProperty(hash, name); 632 } 633 634 } 635 636 @Override 637 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 638 switch (hash) { 639 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 640 case 3648314: /*when*/ return new String[] {"dateTime", "Period"}; 641 default: return super.getTypesForProperty(hash, name); 642 } 643 644 } 645 646 @Override 647 public Base addChild(String name) throws FHIRException { 648 if (name.equals("type")) { 649 this.type = new CodeableConcept(); 650 return this.type; 651 } 652 else if (name.equals("whenDateTime")) { 653 this.when = new DateTimeType(); 654 return this.when; 655 } 656 else if (name.equals("whenPeriod")) { 657 this.when = new Period(); 658 return this.when; 659 } 660 else 661 return super.addChild(name); 662 } 663 664 public ExplanationOfBenefitEventComponent copy() { 665 ExplanationOfBenefitEventComponent dst = new ExplanationOfBenefitEventComponent(); 666 copyValues(dst); 667 return dst; 668 } 669 670 public void copyValues(ExplanationOfBenefitEventComponent dst) { 671 super.copyValues(dst); 672 dst.type = type == null ? null : type.copy(); 673 dst.when = when == null ? null : when.copy(); 674 } 675 676 @Override 677 public boolean equalsDeep(Base other_) { 678 if (!super.equalsDeep(other_)) 679 return false; 680 if (!(other_ instanceof ExplanationOfBenefitEventComponent)) 681 return false; 682 ExplanationOfBenefitEventComponent o = (ExplanationOfBenefitEventComponent) other_; 683 return compareDeep(type, o.type, true) && compareDeep(when, o.when, true); 684 } 685 686 @Override 687 public boolean equalsShallow(Base other_) { 688 if (!super.equalsShallow(other_)) 689 return false; 690 if (!(other_ instanceof ExplanationOfBenefitEventComponent)) 691 return false; 692 ExplanationOfBenefitEventComponent o = (ExplanationOfBenefitEventComponent) other_; 693 return true; 694 } 695 696 public boolean isEmpty() { 697 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, when); 698 } 699 700 public String fhirType() { 701 return "ExplanationOfBenefit.event"; 702 703 } 704 705 } 706 707 @Block() 708 public static class PayeeComponent extends BackboneElement implements IBaseBackboneElement { 709 /** 710 * Type of Party to be reimbursed: Subscriber, provider, other. 711 */ 712 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 713 @Description(shortDefinition="Category of recipient", formalDefinition="Type of Party to be reimbursed: Subscriber, provider, other." ) 714 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payeetype") 715 protected CodeableConcept type; 716 717 /** 718 * Reference to the individual or organization to whom any payment will be made. 719 */ 720 @Child(name = "party", type = {Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, RelatedPerson.class}, order=2, min=0, max=1, modifier=false, summary=false) 721 @Description(shortDefinition="Recipient reference", formalDefinition="Reference to the individual or organization to whom any payment will be made." ) 722 protected Reference party; 723 724 private static final long serialVersionUID = -1948897146L; 725 726 /** 727 * Constructor 728 */ 729 public PayeeComponent() { 730 super(); 731 } 732 733 /** 734 * @return {@link #type} (Type of Party to be reimbursed: Subscriber, provider, other.) 735 */ 736 public CodeableConcept getType() { 737 if (this.type == null) 738 if (Configuration.errorOnAutoCreate()) 739 throw new Error("Attempt to auto-create PayeeComponent.type"); 740 else if (Configuration.doAutoCreate()) 741 this.type = new CodeableConcept(); // cc 742 return this.type; 743 } 744 745 public boolean hasType() { 746 return this.type != null && !this.type.isEmpty(); 747 } 748 749 /** 750 * @param value {@link #type} (Type of Party to be reimbursed: Subscriber, provider, other.) 751 */ 752 public PayeeComponent setType(CodeableConcept value) { 753 this.type = value; 754 return this; 755 } 756 757 /** 758 * @return {@link #party} (Reference to the individual or organization to whom any payment will be made.) 759 */ 760 public Reference getParty() { 761 if (this.party == null) 762 if (Configuration.errorOnAutoCreate()) 763 throw new Error("Attempt to auto-create PayeeComponent.party"); 764 else if (Configuration.doAutoCreate()) 765 this.party = new Reference(); // cc 766 return this.party; 767 } 768 769 public boolean hasParty() { 770 return this.party != null && !this.party.isEmpty(); 771 } 772 773 /** 774 * @param value {@link #party} (Reference to the individual or organization to whom any payment will be made.) 775 */ 776 public PayeeComponent setParty(Reference value) { 777 this.party = value; 778 return this; 779 } 780 781 protected void listChildren(List<Property> children) { 782 super.listChildren(children); 783 children.add(new Property("type", "CodeableConcept", "Type of Party to be reimbursed: Subscriber, provider, other.", 0, 1, type)); 784 children.add(new Property("party", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson)", "Reference to the individual or organization to whom any payment will be made.", 0, 1, party)); 785 } 786 787 @Override 788 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 789 switch (_hash) { 790 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of Party to be reimbursed: Subscriber, provider, other.", 0, 1, type); 791 case 106437350: /*party*/ return new Property("party", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson)", "Reference to the individual or organization to whom any payment will be made.", 0, 1, party); 792 default: return super.getNamedProperty(_hash, _name, _checkValid); 793 } 794 795 } 796 797 @Override 798 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 799 switch (hash) { 800 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 801 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 802 default: return super.getProperty(hash, name, checkValid); 803 } 804 805 } 806 807 @Override 808 public Base setProperty(int hash, String name, Base value) throws FHIRException { 809 switch (hash) { 810 case 3575610: // type 811 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 812 return value; 813 case 106437350: // party 814 this.party = TypeConvertor.castToReference(value); // Reference 815 return value; 816 default: return super.setProperty(hash, name, value); 817 } 818 819 } 820 821 @Override 822 public Base setProperty(String name, Base value) throws FHIRException { 823 if (name.equals("type")) { 824 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 825 } else if (name.equals("party")) { 826 this.party = TypeConvertor.castToReference(value); // Reference 827 } else 828 return super.setProperty(name, value); 829 return value; 830 } 831 832 @Override 833 public void removeChild(String name, Base value) throws FHIRException { 834 if (name.equals("type")) { 835 this.type = null; 836 } else if (name.equals("party")) { 837 this.party = null; 838 } else 839 super.removeChild(name, value); 840 841 } 842 843 @Override 844 public Base makeProperty(int hash, String name) throws FHIRException { 845 switch (hash) { 846 case 3575610: return getType(); 847 case 106437350: return getParty(); 848 default: return super.makeProperty(hash, name); 849 } 850 851 } 852 853 @Override 854 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 855 switch (hash) { 856 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 857 case 106437350: /*party*/ return new String[] {"Reference"}; 858 default: return super.getTypesForProperty(hash, name); 859 } 860 861 } 862 863 @Override 864 public Base addChild(String name) throws FHIRException { 865 if (name.equals("type")) { 866 this.type = new CodeableConcept(); 867 return this.type; 868 } 869 else if (name.equals("party")) { 870 this.party = new Reference(); 871 return this.party; 872 } 873 else 874 return super.addChild(name); 875 } 876 877 public PayeeComponent copy() { 878 PayeeComponent dst = new PayeeComponent(); 879 copyValues(dst); 880 return dst; 881 } 882 883 public void copyValues(PayeeComponent dst) { 884 super.copyValues(dst); 885 dst.type = type == null ? null : type.copy(); 886 dst.party = party == null ? null : party.copy(); 887 } 888 889 @Override 890 public boolean equalsDeep(Base other_) { 891 if (!super.equalsDeep(other_)) 892 return false; 893 if (!(other_ instanceof PayeeComponent)) 894 return false; 895 PayeeComponent o = (PayeeComponent) other_; 896 return compareDeep(type, o.type, true) && compareDeep(party, o.party, true); 897 } 898 899 @Override 900 public boolean equalsShallow(Base other_) { 901 if (!super.equalsShallow(other_)) 902 return false; 903 if (!(other_ instanceof PayeeComponent)) 904 return false; 905 PayeeComponent o = (PayeeComponent) other_; 906 return true; 907 } 908 909 public boolean isEmpty() { 910 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, party); 911 } 912 913 public String fhirType() { 914 return "ExplanationOfBenefit.payee"; 915 916 } 917 918 } 919 920 @Block() 921 public static class CareTeamComponent extends BackboneElement implements IBaseBackboneElement { 922 /** 923 * A number to uniquely identify care team entries. 924 */ 925 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 926 @Description(shortDefinition="Order of care team", formalDefinition="A number to uniquely identify care team entries." ) 927 protected PositiveIntType sequence; 928 929 /** 930 * Member of the team who provided the product or service. 931 */ 932 @Child(name = "provider", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=2, min=1, max=1, modifier=false, summary=false) 933 @Description(shortDefinition="Practitioner or organization", formalDefinition="Member of the team who provided the product or service." ) 934 protected Reference provider; 935 936 /** 937 * The party who is billing and/or responsible for the claimed products or services. 938 */ 939 @Child(name = "responsible", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 940 @Description(shortDefinition="Indicator of the lead practitioner", formalDefinition="The party who is billing and/or responsible for the claimed products or services." ) 941 protected BooleanType responsible; 942 943 /** 944 * The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team. 945 */ 946 @Child(name = "role", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 947 @Description(shortDefinition="Function within the team", formalDefinition="The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team." ) 948 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-careteamrole") 949 protected CodeableConcept role; 950 951 /** 952 * The specialization of the practitioner or provider which is applicable for this service. 953 */ 954 @Child(name = "specialty", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 955 @Description(shortDefinition="Practitioner or provider specialization", formalDefinition="The specialization of the practitioner or provider which is applicable for this service." ) 956 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/provider-qualification") 957 protected CodeableConcept specialty; 958 959 private static final long serialVersionUID = 1238813503L; 960 961 /** 962 * Constructor 963 */ 964 public CareTeamComponent() { 965 super(); 966 } 967 968 /** 969 * Constructor 970 */ 971 public CareTeamComponent(int sequence, Reference provider) { 972 super(); 973 this.setSequence(sequence); 974 this.setProvider(provider); 975 } 976 977 /** 978 * @return {@link #sequence} (A number to uniquely identify care team entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 979 */ 980 public PositiveIntType getSequenceElement() { 981 if (this.sequence == null) 982 if (Configuration.errorOnAutoCreate()) 983 throw new Error("Attempt to auto-create CareTeamComponent.sequence"); 984 else if (Configuration.doAutoCreate()) 985 this.sequence = new PositiveIntType(); // bb 986 return this.sequence; 987 } 988 989 public boolean hasSequenceElement() { 990 return this.sequence != null && !this.sequence.isEmpty(); 991 } 992 993 public boolean hasSequence() { 994 return this.sequence != null && !this.sequence.isEmpty(); 995 } 996 997 /** 998 * @param value {@link #sequence} (A number to uniquely identify care team entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 999 */ 1000 public CareTeamComponent setSequenceElement(PositiveIntType value) { 1001 this.sequence = value; 1002 return this; 1003 } 1004 1005 /** 1006 * @return A number to uniquely identify care team entries. 1007 */ 1008 public int getSequence() { 1009 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1010 } 1011 1012 /** 1013 * @param value A number to uniquely identify care team entries. 1014 */ 1015 public CareTeamComponent setSequence(int value) { 1016 if (this.sequence == null) 1017 this.sequence = new PositiveIntType(); 1018 this.sequence.setValue(value); 1019 return this; 1020 } 1021 1022 /** 1023 * @return {@link #provider} (Member of the team who provided the product or service.) 1024 */ 1025 public Reference getProvider() { 1026 if (this.provider == null) 1027 if (Configuration.errorOnAutoCreate()) 1028 throw new Error("Attempt to auto-create CareTeamComponent.provider"); 1029 else if (Configuration.doAutoCreate()) 1030 this.provider = new Reference(); // cc 1031 return this.provider; 1032 } 1033 1034 public boolean hasProvider() { 1035 return this.provider != null && !this.provider.isEmpty(); 1036 } 1037 1038 /** 1039 * @param value {@link #provider} (Member of the team who provided the product or service.) 1040 */ 1041 public CareTeamComponent setProvider(Reference value) { 1042 this.provider = value; 1043 return this; 1044 } 1045 1046 /** 1047 * @return {@link #responsible} (The party who is billing and/or responsible for the claimed products or services.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1048 */ 1049 public BooleanType getResponsibleElement() { 1050 if (this.responsible == null) 1051 if (Configuration.errorOnAutoCreate()) 1052 throw new Error("Attempt to auto-create CareTeamComponent.responsible"); 1053 else if (Configuration.doAutoCreate()) 1054 this.responsible = new BooleanType(); // bb 1055 return this.responsible; 1056 } 1057 1058 public boolean hasResponsibleElement() { 1059 return this.responsible != null && !this.responsible.isEmpty(); 1060 } 1061 1062 public boolean hasResponsible() { 1063 return this.responsible != null && !this.responsible.isEmpty(); 1064 } 1065 1066 /** 1067 * @param value {@link #responsible} (The party who is billing and/or responsible for the claimed products or services.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1068 */ 1069 public CareTeamComponent setResponsibleElement(BooleanType value) { 1070 this.responsible = value; 1071 return this; 1072 } 1073 1074 /** 1075 * @return The party who is billing and/or responsible for the claimed products or services. 1076 */ 1077 public boolean getResponsible() { 1078 return this.responsible == null || this.responsible.isEmpty() ? false : this.responsible.getValue(); 1079 } 1080 1081 /** 1082 * @param value The party who is billing and/or responsible for the claimed products or services. 1083 */ 1084 public CareTeamComponent setResponsible(boolean value) { 1085 if (this.responsible == null) 1086 this.responsible = new BooleanType(); 1087 this.responsible.setValue(value); 1088 return this; 1089 } 1090 1091 /** 1092 * @return {@link #role} (The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.) 1093 */ 1094 public CodeableConcept getRole() { 1095 if (this.role == null) 1096 if (Configuration.errorOnAutoCreate()) 1097 throw new Error("Attempt to auto-create CareTeamComponent.role"); 1098 else if (Configuration.doAutoCreate()) 1099 this.role = new CodeableConcept(); // cc 1100 return this.role; 1101 } 1102 1103 public boolean hasRole() { 1104 return this.role != null && !this.role.isEmpty(); 1105 } 1106 1107 /** 1108 * @param value {@link #role} (The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.) 1109 */ 1110 public CareTeamComponent setRole(CodeableConcept value) { 1111 this.role = value; 1112 return this; 1113 } 1114 1115 /** 1116 * @return {@link #specialty} (The specialization of the practitioner or provider which is applicable for this service.) 1117 */ 1118 public CodeableConcept getSpecialty() { 1119 if (this.specialty == null) 1120 if (Configuration.errorOnAutoCreate()) 1121 throw new Error("Attempt to auto-create CareTeamComponent.specialty"); 1122 else if (Configuration.doAutoCreate()) 1123 this.specialty = new CodeableConcept(); // cc 1124 return this.specialty; 1125 } 1126 1127 public boolean hasSpecialty() { 1128 return this.specialty != null && !this.specialty.isEmpty(); 1129 } 1130 1131 /** 1132 * @param value {@link #specialty} (The specialization of the practitioner or provider which is applicable for this service.) 1133 */ 1134 public CareTeamComponent setSpecialty(CodeableConcept value) { 1135 this.specialty = value; 1136 return this; 1137 } 1138 1139 protected void listChildren(List<Property> children) { 1140 super.listChildren(children); 1141 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify care team entries.", 0, 1, sequence)); 1142 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "Member of the team who provided the product or service.", 0, 1, provider)); 1143 children.add(new Property("responsible", "boolean", "The party who is billing and/or responsible for the claimed products or services.", 0, 1, responsible)); 1144 children.add(new Property("role", "CodeableConcept", "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.", 0, 1, role)); 1145 children.add(new Property("specialty", "CodeableConcept", "The specialization of the practitioner or provider which is applicable for this service.", 0, 1, specialty)); 1146 } 1147 1148 @Override 1149 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1150 switch (_hash) { 1151 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify care team entries.", 0, 1, sequence); 1152 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "Member of the team who provided the product or service.", 0, 1, provider); 1153 case 1847674614: /*responsible*/ return new Property("responsible", "boolean", "The party who is billing and/or responsible for the claimed products or services.", 0, 1, responsible); 1154 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The lead, assisting or supervising practitioner and their discipline if a multidisciplinary team.", 0, 1, role); 1155 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "The specialization of the practitioner or provider which is applicable for this service.", 0, 1, specialty); 1156 default: return super.getNamedProperty(_hash, _name, _checkValid); 1157 } 1158 1159 } 1160 1161 @Override 1162 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1163 switch (hash) { 1164 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1165 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 1166 case 1847674614: /*responsible*/ return this.responsible == null ? new Base[0] : new Base[] {this.responsible}; // BooleanType 1167 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 1168 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : new Base[] {this.specialty}; // CodeableConcept 1169 default: return super.getProperty(hash, name, checkValid); 1170 } 1171 1172 } 1173 1174 @Override 1175 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1176 switch (hash) { 1177 case 1349547969: // sequence 1178 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1179 return value; 1180 case -987494927: // provider 1181 this.provider = TypeConvertor.castToReference(value); // Reference 1182 return value; 1183 case 1847674614: // responsible 1184 this.responsible = TypeConvertor.castToBoolean(value); // BooleanType 1185 return value; 1186 case 3506294: // role 1187 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1188 return value; 1189 case -1694759682: // specialty 1190 this.specialty = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1191 return value; 1192 default: return super.setProperty(hash, name, value); 1193 } 1194 1195 } 1196 1197 @Override 1198 public Base setProperty(String name, Base value) throws FHIRException { 1199 if (name.equals("sequence")) { 1200 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1201 } else if (name.equals("provider")) { 1202 this.provider = TypeConvertor.castToReference(value); // Reference 1203 } else if (name.equals("responsible")) { 1204 this.responsible = TypeConvertor.castToBoolean(value); // BooleanType 1205 } else if (name.equals("role")) { 1206 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1207 } else if (name.equals("specialty")) { 1208 this.specialty = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1209 } else 1210 return super.setProperty(name, value); 1211 return value; 1212 } 1213 1214 @Override 1215 public void removeChild(String name, Base value) throws FHIRException { 1216 if (name.equals("sequence")) { 1217 this.sequence = null; 1218 } else if (name.equals("provider")) { 1219 this.provider = null; 1220 } else if (name.equals("responsible")) { 1221 this.responsible = null; 1222 } else if (name.equals("role")) { 1223 this.role = null; 1224 } else if (name.equals("specialty")) { 1225 this.specialty = null; 1226 } else 1227 super.removeChild(name, value); 1228 1229 } 1230 1231 @Override 1232 public Base makeProperty(int hash, String name) throws FHIRException { 1233 switch (hash) { 1234 case 1349547969: return getSequenceElement(); 1235 case -987494927: return getProvider(); 1236 case 1847674614: return getResponsibleElement(); 1237 case 3506294: return getRole(); 1238 case -1694759682: return getSpecialty(); 1239 default: return super.makeProperty(hash, name); 1240 } 1241 1242 } 1243 1244 @Override 1245 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1246 switch (hash) { 1247 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1248 case -987494927: /*provider*/ return new String[] {"Reference"}; 1249 case 1847674614: /*responsible*/ return new String[] {"boolean"}; 1250 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 1251 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 1252 default: return super.getTypesForProperty(hash, name); 1253 } 1254 1255 } 1256 1257 @Override 1258 public Base addChild(String name) throws FHIRException { 1259 if (name.equals("sequence")) { 1260 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.careTeam.sequence"); 1261 } 1262 else if (name.equals("provider")) { 1263 this.provider = new Reference(); 1264 return this.provider; 1265 } 1266 else if (name.equals("responsible")) { 1267 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.careTeam.responsible"); 1268 } 1269 else if (name.equals("role")) { 1270 this.role = new CodeableConcept(); 1271 return this.role; 1272 } 1273 else if (name.equals("specialty")) { 1274 this.specialty = new CodeableConcept(); 1275 return this.specialty; 1276 } 1277 else 1278 return super.addChild(name); 1279 } 1280 1281 public CareTeamComponent copy() { 1282 CareTeamComponent dst = new CareTeamComponent(); 1283 copyValues(dst); 1284 return dst; 1285 } 1286 1287 public void copyValues(CareTeamComponent dst) { 1288 super.copyValues(dst); 1289 dst.sequence = sequence == null ? null : sequence.copy(); 1290 dst.provider = provider == null ? null : provider.copy(); 1291 dst.responsible = responsible == null ? null : responsible.copy(); 1292 dst.role = role == null ? null : role.copy(); 1293 dst.specialty = specialty == null ? null : specialty.copy(); 1294 } 1295 1296 @Override 1297 public boolean equalsDeep(Base other_) { 1298 if (!super.equalsDeep(other_)) 1299 return false; 1300 if (!(other_ instanceof CareTeamComponent)) 1301 return false; 1302 CareTeamComponent o = (CareTeamComponent) other_; 1303 return compareDeep(sequence, o.sequence, true) && compareDeep(provider, o.provider, true) && compareDeep(responsible, o.responsible, true) 1304 && compareDeep(role, o.role, true) && compareDeep(specialty, o.specialty, true); 1305 } 1306 1307 @Override 1308 public boolean equalsShallow(Base other_) { 1309 if (!super.equalsShallow(other_)) 1310 return false; 1311 if (!(other_ instanceof CareTeamComponent)) 1312 return false; 1313 CareTeamComponent o = (CareTeamComponent) other_; 1314 return compareValues(sequence, o.sequence, true) && compareValues(responsible, o.responsible, true) 1315 ; 1316 } 1317 1318 public boolean isEmpty() { 1319 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, provider, responsible 1320 , role, specialty); 1321 } 1322 1323 public String fhirType() { 1324 return "ExplanationOfBenefit.careTeam"; 1325 1326 } 1327 1328 } 1329 1330 @Block() 1331 public static class SupportingInformationComponent extends BackboneElement implements IBaseBackboneElement { 1332 /** 1333 * A number to uniquely identify supporting information entries. 1334 */ 1335 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1336 @Description(shortDefinition="Information instance identifier", formalDefinition="A number to uniquely identify supporting information entries." ) 1337 protected PositiveIntType sequence; 1338 1339 /** 1340 * The general class of the information supplied: information; exception; accident, employment; onset, etc. 1341 */ 1342 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 1343 @Description(shortDefinition="Classification of the supplied information", formalDefinition="The general class of the information supplied: information; exception; accident, employment; onset, etc." ) 1344 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-informationcategory") 1345 protected CodeableConcept category; 1346 1347 /** 1348 * System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought. 1349 */ 1350 @Child(name = "code", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1351 @Description(shortDefinition="Type of information", formalDefinition="System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought." ) 1352 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-exception") 1353 protected CodeableConcept code; 1354 1355 /** 1356 * The date when or period to which this information refers. 1357 */ 1358 @Child(name = "timing", type = {DateType.class, Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 1359 @Description(shortDefinition="When it occurred", formalDefinition="The date when or period to which this information refers." ) 1360 protected DataType timing; 1361 1362 /** 1363 * Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data. 1364 */ 1365 @Child(name = "value", type = {BooleanType.class, StringType.class, Quantity.class, Attachment.class, Reference.class, Identifier.class}, order=5, min=0, max=1, modifier=false, summary=false) 1366 @Description(shortDefinition="Data to be provided", formalDefinition="Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data." ) 1367 protected DataType value; 1368 1369 /** 1370 * Provides the reason in the situation where a reason code is required in addition to the content. 1371 */ 1372 @Child(name = "reason", type = {Coding.class}, order=6, min=0, max=1, modifier=false, summary=false) 1373 @Description(shortDefinition="Explanation for the information", formalDefinition="Provides the reason in the situation where a reason code is required in addition to the content." ) 1374 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/missing-tooth-reason") 1375 protected Coding reason; 1376 1377 private static final long serialVersionUID = 1577205655L; 1378 1379 /** 1380 * Constructor 1381 */ 1382 public SupportingInformationComponent() { 1383 super(); 1384 } 1385 1386 /** 1387 * Constructor 1388 */ 1389 public SupportingInformationComponent(int sequence, CodeableConcept category) { 1390 super(); 1391 this.setSequence(sequence); 1392 this.setCategory(category); 1393 } 1394 1395 /** 1396 * @return {@link #sequence} (A number to uniquely identify supporting information entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1397 */ 1398 public PositiveIntType getSequenceElement() { 1399 if (this.sequence == null) 1400 if (Configuration.errorOnAutoCreate()) 1401 throw new Error("Attempt to auto-create SupportingInformationComponent.sequence"); 1402 else if (Configuration.doAutoCreate()) 1403 this.sequence = new PositiveIntType(); // bb 1404 return this.sequence; 1405 } 1406 1407 public boolean hasSequenceElement() { 1408 return this.sequence != null && !this.sequence.isEmpty(); 1409 } 1410 1411 public boolean hasSequence() { 1412 return this.sequence != null && !this.sequence.isEmpty(); 1413 } 1414 1415 /** 1416 * @param value {@link #sequence} (A number to uniquely identify supporting information entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1417 */ 1418 public SupportingInformationComponent setSequenceElement(PositiveIntType value) { 1419 this.sequence = value; 1420 return this; 1421 } 1422 1423 /** 1424 * @return A number to uniquely identify supporting information entries. 1425 */ 1426 public int getSequence() { 1427 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1428 } 1429 1430 /** 1431 * @param value A number to uniquely identify supporting information entries. 1432 */ 1433 public SupportingInformationComponent setSequence(int value) { 1434 if (this.sequence == null) 1435 this.sequence = new PositiveIntType(); 1436 this.sequence.setValue(value); 1437 return this; 1438 } 1439 1440 /** 1441 * @return {@link #category} (The general class of the information supplied: information; exception; accident, employment; onset, etc.) 1442 */ 1443 public CodeableConcept getCategory() { 1444 if (this.category == null) 1445 if (Configuration.errorOnAutoCreate()) 1446 throw new Error("Attempt to auto-create SupportingInformationComponent.category"); 1447 else if (Configuration.doAutoCreate()) 1448 this.category = new CodeableConcept(); // cc 1449 return this.category; 1450 } 1451 1452 public boolean hasCategory() { 1453 return this.category != null && !this.category.isEmpty(); 1454 } 1455 1456 /** 1457 * @param value {@link #category} (The general class of the information supplied: information; exception; accident, employment; onset, etc.) 1458 */ 1459 public SupportingInformationComponent setCategory(CodeableConcept value) { 1460 this.category = value; 1461 return this; 1462 } 1463 1464 /** 1465 * @return {@link #code} (System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.) 1466 */ 1467 public CodeableConcept getCode() { 1468 if (this.code == null) 1469 if (Configuration.errorOnAutoCreate()) 1470 throw new Error("Attempt to auto-create SupportingInformationComponent.code"); 1471 else if (Configuration.doAutoCreate()) 1472 this.code = new CodeableConcept(); // cc 1473 return this.code; 1474 } 1475 1476 public boolean hasCode() { 1477 return this.code != null && !this.code.isEmpty(); 1478 } 1479 1480 /** 1481 * @param value {@link #code} (System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.) 1482 */ 1483 public SupportingInformationComponent setCode(CodeableConcept value) { 1484 this.code = value; 1485 return this; 1486 } 1487 1488 /** 1489 * @return {@link #timing} (The date when or period to which this information refers.) 1490 */ 1491 public DataType getTiming() { 1492 return this.timing; 1493 } 1494 1495 /** 1496 * @return {@link #timing} (The date when or period to which this information refers.) 1497 */ 1498 public DateType getTimingDateType() throws FHIRException { 1499 if (this.timing == null) 1500 this.timing = new DateType(); 1501 if (!(this.timing instanceof DateType)) 1502 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.timing.getClass().getName()+" was encountered"); 1503 return (DateType) this.timing; 1504 } 1505 1506 public boolean hasTimingDateType() { 1507 return this.timing instanceof DateType; 1508 } 1509 1510 /** 1511 * @return {@link #timing} (The date when or period to which this information refers.) 1512 */ 1513 public Period getTimingPeriod() throws FHIRException { 1514 if (this.timing == null) 1515 this.timing = new Period(); 1516 if (!(this.timing instanceof Period)) 1517 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.timing.getClass().getName()+" was encountered"); 1518 return (Period) this.timing; 1519 } 1520 1521 public boolean hasTimingPeriod() { 1522 return this.timing instanceof Period; 1523 } 1524 1525 public boolean hasTiming() { 1526 return this.timing != null && !this.timing.isEmpty(); 1527 } 1528 1529 /** 1530 * @param value {@link #timing} (The date when or period to which this information refers.) 1531 */ 1532 public SupportingInformationComponent setTiming(DataType value) { 1533 if (value != null && !(value instanceof DateType || value instanceof Period)) 1534 throw new FHIRException("Not the right type for ExplanationOfBenefit.supportingInfo.timing[x]: "+value.fhirType()); 1535 this.timing = value; 1536 return this; 1537 } 1538 1539 /** 1540 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1541 */ 1542 public DataType getValue() { 1543 return this.value; 1544 } 1545 1546 /** 1547 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1548 */ 1549 public BooleanType getValueBooleanType() throws FHIRException { 1550 if (this.value == null) 1551 this.value = new BooleanType(); 1552 if (!(this.value instanceof BooleanType)) 1553 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 1554 return (BooleanType) this.value; 1555 } 1556 1557 public boolean hasValueBooleanType() { 1558 return this.value instanceof BooleanType; 1559 } 1560 1561 /** 1562 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1563 */ 1564 public StringType getValueStringType() throws FHIRException { 1565 if (this.value == null) 1566 this.value = new StringType(); 1567 if (!(this.value instanceof StringType)) 1568 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 1569 return (StringType) this.value; 1570 } 1571 1572 public boolean hasValueStringType() { 1573 return this.value instanceof StringType; 1574 } 1575 1576 /** 1577 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1578 */ 1579 public Quantity getValueQuantity() throws FHIRException { 1580 if (this.value == null) 1581 this.value = new Quantity(); 1582 if (!(this.value instanceof Quantity)) 1583 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1584 return (Quantity) this.value; 1585 } 1586 1587 public boolean hasValueQuantity() { 1588 return this.value instanceof Quantity; 1589 } 1590 1591 /** 1592 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1593 */ 1594 public Attachment getValueAttachment() throws FHIRException { 1595 if (this.value == null) 1596 this.value = new Attachment(); 1597 if (!(this.value instanceof Attachment)) 1598 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 1599 return (Attachment) this.value; 1600 } 1601 1602 public boolean hasValueAttachment() { 1603 return this.value instanceof Attachment; 1604 } 1605 1606 /** 1607 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1608 */ 1609 public Reference getValueReference() throws FHIRException { 1610 if (this.value == null) 1611 this.value = new Reference(); 1612 if (!(this.value instanceof Reference)) 1613 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 1614 return (Reference) this.value; 1615 } 1616 1617 public boolean hasValueReference() { 1618 return this.value instanceof Reference; 1619 } 1620 1621 /** 1622 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1623 */ 1624 public Identifier getValueIdentifier() throws FHIRException { 1625 if (this.value == null) 1626 this.value = new Identifier(); 1627 if (!(this.value instanceof Identifier)) 1628 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.value.getClass().getName()+" was encountered"); 1629 return (Identifier) this.value; 1630 } 1631 1632 public boolean hasValueIdentifier() { 1633 return this.value instanceof Identifier; 1634 } 1635 1636 public boolean hasValue() { 1637 return this.value != null && !this.value.isEmpty(); 1638 } 1639 1640 /** 1641 * @param value {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1642 */ 1643 public SupportingInformationComponent setValue(DataType value) { 1644 if (value != null && !(value instanceof BooleanType || value instanceof StringType || value instanceof Quantity || value instanceof Attachment || value instanceof Reference || value instanceof Identifier)) 1645 throw new FHIRException("Not the right type for ExplanationOfBenefit.supportingInfo.value[x]: "+value.fhirType()); 1646 this.value = value; 1647 return this; 1648 } 1649 1650 /** 1651 * @return {@link #reason} (Provides the reason in the situation where a reason code is required in addition to the content.) 1652 */ 1653 public Coding getReason() { 1654 if (this.reason == null) 1655 if (Configuration.errorOnAutoCreate()) 1656 throw new Error("Attempt to auto-create SupportingInformationComponent.reason"); 1657 else if (Configuration.doAutoCreate()) 1658 this.reason = new Coding(); // cc 1659 return this.reason; 1660 } 1661 1662 public boolean hasReason() { 1663 return this.reason != null && !this.reason.isEmpty(); 1664 } 1665 1666 /** 1667 * @param value {@link #reason} (Provides the reason in the situation where a reason code is required in addition to the content.) 1668 */ 1669 public SupportingInformationComponent setReason(Coding value) { 1670 this.reason = value; 1671 return this; 1672 } 1673 1674 protected void listChildren(List<Property> children) { 1675 super.listChildren(children); 1676 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify supporting information entries.", 0, 1, sequence)); 1677 children.add(new Property("category", "CodeableConcept", "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1, category)); 1678 children.add(new Property("code", "CodeableConcept", "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.", 0, 1, code)); 1679 children.add(new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing)); 1680 children.add(new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)|Identifier", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value)); 1681 children.add(new Property("reason", "Coding", "Provides the reason in the situation where a reason code is required in addition to the content.", 0, 1, reason)); 1682 } 1683 1684 @Override 1685 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1686 switch (_hash) { 1687 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify supporting information entries.", 0, 1, sequence); 1688 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1, category); 1689 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought.", 0, 1, code); 1690 case 164632566: /*timing[x]*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1691 case -873664438: /*timing*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1692 case 807935768: /*timingDate*/ return new Property("timing[x]", "date", "The date when or period to which this information refers.", 0, 1, timing); 1693 case -615615829: /*timingPeriod*/ return new Property("timing[x]", "Period", "The date when or period to which this information refers.", 0, 1, timing); 1694 case -1410166417: /*value[x]*/ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)|Identifier", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1695 case 111972721: /*value*/ return new Property("value[x]", "boolean|string|Quantity|Attachment|Reference(Any)|Identifier", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1696 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1697 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1698 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1699 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1700 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1701 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "Identifier", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1702 case -934964668: /*reason*/ return new Property("reason", "Coding", "Provides the reason in the situation where a reason code is required in addition to the content.", 0, 1, reason); 1703 default: return super.getNamedProperty(_hash, _name, _checkValid); 1704 } 1705 1706 } 1707 1708 @Override 1709 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1710 switch (hash) { 1711 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1712 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1713 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1714 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // DataType 1715 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1716 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // Coding 1717 default: return super.getProperty(hash, name, checkValid); 1718 } 1719 1720 } 1721 1722 @Override 1723 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1724 switch (hash) { 1725 case 1349547969: // sequence 1726 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1727 return value; 1728 case 50511102: // category 1729 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1730 return value; 1731 case 3059181: // code 1732 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1733 return value; 1734 case -873664438: // timing 1735 this.timing = TypeConvertor.castToType(value); // DataType 1736 return value; 1737 case 111972721: // value 1738 this.value = TypeConvertor.castToType(value); // DataType 1739 return value; 1740 case -934964668: // reason 1741 this.reason = TypeConvertor.castToCoding(value); // Coding 1742 return value; 1743 default: return super.setProperty(hash, name, value); 1744 } 1745 1746 } 1747 1748 @Override 1749 public Base setProperty(String name, Base value) throws FHIRException { 1750 if (name.equals("sequence")) { 1751 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1752 } else if (name.equals("category")) { 1753 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1754 } else if (name.equals("code")) { 1755 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1756 } else if (name.equals("timing[x]")) { 1757 this.timing = TypeConvertor.castToType(value); // DataType 1758 } else if (name.equals("value[x]")) { 1759 this.value = TypeConvertor.castToType(value); // DataType 1760 } else if (name.equals("reason")) { 1761 this.reason = TypeConvertor.castToCoding(value); // Coding 1762 } else 1763 return super.setProperty(name, value); 1764 return value; 1765 } 1766 1767 @Override 1768 public void removeChild(String name, Base value) throws FHIRException { 1769 if (name.equals("sequence")) { 1770 this.sequence = null; 1771 } else if (name.equals("category")) { 1772 this.category = null; 1773 } else if (name.equals("code")) { 1774 this.code = null; 1775 } else if (name.equals("timing[x]")) { 1776 this.timing = null; 1777 } else if (name.equals("value[x]")) { 1778 this.value = null; 1779 } else if (name.equals("reason")) { 1780 this.reason = null; 1781 } else 1782 super.removeChild(name, value); 1783 1784 } 1785 1786 @Override 1787 public Base makeProperty(int hash, String name) throws FHIRException { 1788 switch (hash) { 1789 case 1349547969: return getSequenceElement(); 1790 case 50511102: return getCategory(); 1791 case 3059181: return getCode(); 1792 case 164632566: return getTiming(); 1793 case -873664438: return getTiming(); 1794 case -1410166417: return getValue(); 1795 case 111972721: return getValue(); 1796 case -934964668: return getReason(); 1797 default: return super.makeProperty(hash, name); 1798 } 1799 1800 } 1801 1802 @Override 1803 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1804 switch (hash) { 1805 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1806 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1807 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1808 case -873664438: /*timing*/ return new String[] {"date", "Period"}; 1809 case 111972721: /*value*/ return new String[] {"boolean", "string", "Quantity", "Attachment", "Reference", "Identifier"}; 1810 case -934964668: /*reason*/ return new String[] {"Coding"}; 1811 default: return super.getTypesForProperty(hash, name); 1812 } 1813 1814 } 1815 1816 @Override 1817 public Base addChild(String name) throws FHIRException { 1818 if (name.equals("sequence")) { 1819 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.supportingInfo.sequence"); 1820 } 1821 else if (name.equals("category")) { 1822 this.category = new CodeableConcept(); 1823 return this.category; 1824 } 1825 else if (name.equals("code")) { 1826 this.code = new CodeableConcept(); 1827 return this.code; 1828 } 1829 else if (name.equals("timingDate")) { 1830 this.timing = new DateType(); 1831 return this.timing; 1832 } 1833 else if (name.equals("timingPeriod")) { 1834 this.timing = new Period(); 1835 return this.timing; 1836 } 1837 else if (name.equals("valueBoolean")) { 1838 this.value = new BooleanType(); 1839 return this.value; 1840 } 1841 else if (name.equals("valueString")) { 1842 this.value = new StringType(); 1843 return this.value; 1844 } 1845 else if (name.equals("valueQuantity")) { 1846 this.value = new Quantity(); 1847 return this.value; 1848 } 1849 else if (name.equals("valueAttachment")) { 1850 this.value = new Attachment(); 1851 return this.value; 1852 } 1853 else if (name.equals("valueReference")) { 1854 this.value = new Reference(); 1855 return this.value; 1856 } 1857 else if (name.equals("valueIdentifier")) { 1858 this.value = new Identifier(); 1859 return this.value; 1860 } 1861 else if (name.equals("reason")) { 1862 this.reason = new Coding(); 1863 return this.reason; 1864 } 1865 else 1866 return super.addChild(name); 1867 } 1868 1869 public SupportingInformationComponent copy() { 1870 SupportingInformationComponent dst = new SupportingInformationComponent(); 1871 copyValues(dst); 1872 return dst; 1873 } 1874 1875 public void copyValues(SupportingInformationComponent dst) { 1876 super.copyValues(dst); 1877 dst.sequence = sequence == null ? null : sequence.copy(); 1878 dst.category = category == null ? null : category.copy(); 1879 dst.code = code == null ? null : code.copy(); 1880 dst.timing = timing == null ? null : timing.copy(); 1881 dst.value = value == null ? null : value.copy(); 1882 dst.reason = reason == null ? null : reason.copy(); 1883 } 1884 1885 @Override 1886 public boolean equalsDeep(Base other_) { 1887 if (!super.equalsDeep(other_)) 1888 return false; 1889 if (!(other_ instanceof SupportingInformationComponent)) 1890 return false; 1891 SupportingInformationComponent o = (SupportingInformationComponent) other_; 1892 return compareDeep(sequence, o.sequence, true) && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 1893 && compareDeep(timing, o.timing, true) && compareDeep(value, o.value, true) && compareDeep(reason, o.reason, true) 1894 ; 1895 } 1896 1897 @Override 1898 public boolean equalsShallow(Base other_) { 1899 if (!super.equalsShallow(other_)) 1900 return false; 1901 if (!(other_ instanceof SupportingInformationComponent)) 1902 return false; 1903 SupportingInformationComponent o = (SupportingInformationComponent) other_; 1904 return compareValues(sequence, o.sequence, true); 1905 } 1906 1907 public boolean isEmpty() { 1908 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, category, code 1909 , timing, value, reason); 1910 } 1911 1912 public String fhirType() { 1913 return "ExplanationOfBenefit.supportingInfo"; 1914 1915 } 1916 1917 } 1918 1919 @Block() 1920 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 1921 /** 1922 * A number to uniquely identify diagnosis entries. 1923 */ 1924 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1925 @Description(shortDefinition="Diagnosis instance identifier", formalDefinition="A number to uniquely identify diagnosis entries." ) 1926 protected PositiveIntType sequence; 1927 1928 /** 1929 * The nature of illness or problem in a coded form or as a reference to an external defined Condition. 1930 */ 1931 @Child(name = "diagnosis", type = {CodeableConcept.class, Condition.class}, order=2, min=1, max=1, modifier=false, summary=false) 1932 @Description(shortDefinition="Nature of illness or problem", formalDefinition="The nature of illness or problem in a coded form or as a reference to an external defined Condition." ) 1933 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10") 1934 protected DataType diagnosis; 1935 1936 /** 1937 * When the condition was observed or the relative ranking. 1938 */ 1939 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1940 @Description(shortDefinition="Timing or nature of the diagnosis", formalDefinition="When the condition was observed or the relative ranking." ) 1941 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosistype") 1942 protected List<CodeableConcept> type; 1943 1944 /** 1945 * Indication of whether the diagnosis was present on admission to a facility. 1946 */ 1947 @Child(name = "onAdmission", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1948 @Description(shortDefinition="Present on admission", formalDefinition="Indication of whether the diagnosis was present on admission to a facility." ) 1949 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosis-on-admission") 1950 protected CodeableConcept onAdmission; 1951 1952 private static final long serialVersionUID = -320261526L; 1953 1954 /** 1955 * Constructor 1956 */ 1957 public DiagnosisComponent() { 1958 super(); 1959 } 1960 1961 /** 1962 * Constructor 1963 */ 1964 public DiagnosisComponent(int sequence, DataType diagnosis) { 1965 super(); 1966 this.setSequence(sequence); 1967 this.setDiagnosis(diagnosis); 1968 } 1969 1970 /** 1971 * @return {@link #sequence} (A number to uniquely identify diagnosis entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1972 */ 1973 public PositiveIntType getSequenceElement() { 1974 if (this.sequence == null) 1975 if (Configuration.errorOnAutoCreate()) 1976 throw new Error("Attempt to auto-create DiagnosisComponent.sequence"); 1977 else if (Configuration.doAutoCreate()) 1978 this.sequence = new PositiveIntType(); // bb 1979 return this.sequence; 1980 } 1981 1982 public boolean hasSequenceElement() { 1983 return this.sequence != null && !this.sequence.isEmpty(); 1984 } 1985 1986 public boolean hasSequence() { 1987 return this.sequence != null && !this.sequence.isEmpty(); 1988 } 1989 1990 /** 1991 * @param value {@link #sequence} (A number to uniquely identify diagnosis entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1992 */ 1993 public DiagnosisComponent setSequenceElement(PositiveIntType value) { 1994 this.sequence = value; 1995 return this; 1996 } 1997 1998 /** 1999 * @return A number to uniquely identify diagnosis entries. 2000 */ 2001 public int getSequence() { 2002 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2003 } 2004 2005 /** 2006 * @param value A number to uniquely identify diagnosis entries. 2007 */ 2008 public DiagnosisComponent setSequence(int value) { 2009 if (this.sequence == null) 2010 this.sequence = new PositiveIntType(); 2011 this.sequence.setValue(value); 2012 return this; 2013 } 2014 2015 /** 2016 * @return {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 2017 */ 2018 public DataType getDiagnosis() { 2019 return this.diagnosis; 2020 } 2021 2022 /** 2023 * @return {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 2024 */ 2025 public CodeableConcept getDiagnosisCodeableConcept() throws FHIRException { 2026 if (this.diagnosis == null) 2027 this.diagnosis = new CodeableConcept(); 2028 if (!(this.diagnosis instanceof CodeableConcept)) 2029 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 2030 return (CodeableConcept) this.diagnosis; 2031 } 2032 2033 public boolean hasDiagnosisCodeableConcept() { 2034 return this.diagnosis instanceof CodeableConcept; 2035 } 2036 2037 /** 2038 * @return {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 2039 */ 2040 public Reference getDiagnosisReference() throws FHIRException { 2041 if (this.diagnosis == null) 2042 this.diagnosis = new Reference(); 2043 if (!(this.diagnosis instanceof Reference)) 2044 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 2045 return (Reference) this.diagnosis; 2046 } 2047 2048 public boolean hasDiagnosisReference() { 2049 return this.diagnosis instanceof Reference; 2050 } 2051 2052 public boolean hasDiagnosis() { 2053 return this.diagnosis != null && !this.diagnosis.isEmpty(); 2054 } 2055 2056 /** 2057 * @param value {@link #diagnosis} (The nature of illness or problem in a coded form or as a reference to an external defined Condition.) 2058 */ 2059 public DiagnosisComponent setDiagnosis(DataType value) { 2060 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2061 throw new FHIRException("Not the right type for ExplanationOfBenefit.diagnosis.diagnosis[x]: "+value.fhirType()); 2062 this.diagnosis = value; 2063 return this; 2064 } 2065 2066 /** 2067 * @return {@link #type} (When the condition was observed or the relative ranking.) 2068 */ 2069 public List<CodeableConcept> getType() { 2070 if (this.type == null) 2071 this.type = new ArrayList<CodeableConcept>(); 2072 return this.type; 2073 } 2074 2075 /** 2076 * @return Returns a reference to <code>this</code> for easy method chaining 2077 */ 2078 public DiagnosisComponent setType(List<CodeableConcept> theType) { 2079 this.type = theType; 2080 return this; 2081 } 2082 2083 public boolean hasType() { 2084 if (this.type == null) 2085 return false; 2086 for (CodeableConcept item : this.type) 2087 if (!item.isEmpty()) 2088 return true; 2089 return false; 2090 } 2091 2092 public CodeableConcept addType() { //3 2093 CodeableConcept t = new CodeableConcept(); 2094 if (this.type == null) 2095 this.type = new ArrayList<CodeableConcept>(); 2096 this.type.add(t); 2097 return t; 2098 } 2099 2100 public DiagnosisComponent addType(CodeableConcept t) { //3 2101 if (t == null) 2102 return this; 2103 if (this.type == null) 2104 this.type = new ArrayList<CodeableConcept>(); 2105 this.type.add(t); 2106 return this; 2107 } 2108 2109 /** 2110 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 2111 */ 2112 public CodeableConcept getTypeFirstRep() { 2113 if (getType().isEmpty()) { 2114 addType(); 2115 } 2116 return getType().get(0); 2117 } 2118 2119 /** 2120 * @return {@link #onAdmission} (Indication of whether the diagnosis was present on admission to a facility.) 2121 */ 2122 public CodeableConcept getOnAdmission() { 2123 if (this.onAdmission == null) 2124 if (Configuration.errorOnAutoCreate()) 2125 throw new Error("Attempt to auto-create DiagnosisComponent.onAdmission"); 2126 else if (Configuration.doAutoCreate()) 2127 this.onAdmission = new CodeableConcept(); // cc 2128 return this.onAdmission; 2129 } 2130 2131 public boolean hasOnAdmission() { 2132 return this.onAdmission != null && !this.onAdmission.isEmpty(); 2133 } 2134 2135 /** 2136 * @param value {@link #onAdmission} (Indication of whether the diagnosis was present on admission to a facility.) 2137 */ 2138 public DiagnosisComponent setOnAdmission(CodeableConcept value) { 2139 this.onAdmission = value; 2140 return this; 2141 } 2142 2143 protected void listChildren(List<Property> children) { 2144 super.listChildren(children); 2145 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify diagnosis entries.", 0, 1, sequence)); 2146 children.add(new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis)); 2147 children.add(new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type)); 2148 children.add(new Property("onAdmission", "CodeableConcept", "Indication of whether the diagnosis was present on admission to a facility.", 0, 1, onAdmission)); 2149 } 2150 2151 @Override 2152 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2153 switch (_hash) { 2154 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify diagnosis entries.", 0, 1, sequence); 2155 case -1487009809: /*diagnosis[x]*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 2156 case 1196993265: /*diagnosis*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 2157 case 277781616: /*diagnosisCodeableConcept*/ return new Property("diagnosis[x]", "CodeableConcept", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 2158 case 2050454362: /*diagnosisReference*/ return new Property("diagnosis[x]", "Reference(Condition)", "The nature of illness or problem in a coded form or as a reference to an external defined Condition.", 0, 1, diagnosis); 2159 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type); 2160 case -3386134: /*onAdmission*/ return new Property("onAdmission", "CodeableConcept", "Indication of whether the diagnosis was present on admission to a facility.", 0, 1, onAdmission); 2161 default: return super.getNamedProperty(_hash, _name, _checkValid); 2162 } 2163 2164 } 2165 2166 @Override 2167 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2168 switch (hash) { 2169 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 2170 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : new Base[] {this.diagnosis}; // DataType 2171 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2172 case -3386134: /*onAdmission*/ return this.onAdmission == null ? new Base[0] : new Base[] {this.onAdmission}; // CodeableConcept 2173 default: return super.getProperty(hash, name, checkValid); 2174 } 2175 2176 } 2177 2178 @Override 2179 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2180 switch (hash) { 2181 case 1349547969: // sequence 2182 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2183 return value; 2184 case 1196993265: // diagnosis 2185 this.diagnosis = TypeConvertor.castToType(value); // DataType 2186 return value; 2187 case 3575610: // type 2188 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2189 return value; 2190 case -3386134: // onAdmission 2191 this.onAdmission = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2192 return value; 2193 default: return super.setProperty(hash, name, value); 2194 } 2195 2196 } 2197 2198 @Override 2199 public Base setProperty(String name, Base value) throws FHIRException { 2200 if (name.equals("sequence")) { 2201 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2202 } else if (name.equals("diagnosis[x]")) { 2203 this.diagnosis = TypeConvertor.castToType(value); // DataType 2204 } else if (name.equals("type")) { 2205 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 2206 } else if (name.equals("onAdmission")) { 2207 this.onAdmission = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2208 } else 2209 return super.setProperty(name, value); 2210 return value; 2211 } 2212 2213 @Override 2214 public void removeChild(String name, Base value) throws FHIRException { 2215 if (name.equals("sequence")) { 2216 this.sequence = null; 2217 } else if (name.equals("diagnosis[x]")) { 2218 this.diagnosis = null; 2219 } else if (name.equals("type")) { 2220 this.getType().remove(value); 2221 } else if (name.equals("onAdmission")) { 2222 this.onAdmission = null; 2223 } else 2224 super.removeChild(name, value); 2225 2226 } 2227 2228 @Override 2229 public Base makeProperty(int hash, String name) throws FHIRException { 2230 switch (hash) { 2231 case 1349547969: return getSequenceElement(); 2232 case -1487009809: return getDiagnosis(); 2233 case 1196993265: return getDiagnosis(); 2234 case 3575610: return addType(); 2235 case -3386134: return getOnAdmission(); 2236 default: return super.makeProperty(hash, name); 2237 } 2238 2239 } 2240 2241 @Override 2242 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2243 switch (hash) { 2244 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 2245 case 1196993265: /*diagnosis*/ return new String[] {"CodeableConcept", "Reference"}; 2246 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2247 case -3386134: /*onAdmission*/ return new String[] {"CodeableConcept"}; 2248 default: return super.getTypesForProperty(hash, name); 2249 } 2250 2251 } 2252 2253 @Override 2254 public Base addChild(String name) throws FHIRException { 2255 if (name.equals("sequence")) { 2256 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.diagnosis.sequence"); 2257 } 2258 else if (name.equals("diagnosisCodeableConcept")) { 2259 this.diagnosis = new CodeableConcept(); 2260 return this.diagnosis; 2261 } 2262 else if (name.equals("diagnosisReference")) { 2263 this.diagnosis = new Reference(); 2264 return this.diagnosis; 2265 } 2266 else if (name.equals("type")) { 2267 return addType(); 2268 } 2269 else if (name.equals("onAdmission")) { 2270 this.onAdmission = new CodeableConcept(); 2271 return this.onAdmission; 2272 } 2273 else 2274 return super.addChild(name); 2275 } 2276 2277 public DiagnosisComponent copy() { 2278 DiagnosisComponent dst = new DiagnosisComponent(); 2279 copyValues(dst); 2280 return dst; 2281 } 2282 2283 public void copyValues(DiagnosisComponent dst) { 2284 super.copyValues(dst); 2285 dst.sequence = sequence == null ? null : sequence.copy(); 2286 dst.diagnosis = diagnosis == null ? null : diagnosis.copy(); 2287 if (type != null) { 2288 dst.type = new ArrayList<CodeableConcept>(); 2289 for (CodeableConcept i : type) 2290 dst.type.add(i.copy()); 2291 }; 2292 dst.onAdmission = onAdmission == null ? null : onAdmission.copy(); 2293 } 2294 2295 @Override 2296 public boolean equalsDeep(Base other_) { 2297 if (!super.equalsDeep(other_)) 2298 return false; 2299 if (!(other_ instanceof DiagnosisComponent)) 2300 return false; 2301 DiagnosisComponent o = (DiagnosisComponent) other_; 2302 return compareDeep(sequence, o.sequence, true) && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(type, o.type, true) 2303 && compareDeep(onAdmission, o.onAdmission, true); 2304 } 2305 2306 @Override 2307 public boolean equalsShallow(Base other_) { 2308 if (!super.equalsShallow(other_)) 2309 return false; 2310 if (!(other_ instanceof DiagnosisComponent)) 2311 return false; 2312 DiagnosisComponent o = (DiagnosisComponent) other_; 2313 return compareValues(sequence, o.sequence, true); 2314 } 2315 2316 public boolean isEmpty() { 2317 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, diagnosis, type 2318 , onAdmission); 2319 } 2320 2321 public String fhirType() { 2322 return "ExplanationOfBenefit.diagnosis"; 2323 2324 } 2325 2326 } 2327 2328 @Block() 2329 public static class ProcedureComponent extends BackboneElement implements IBaseBackboneElement { 2330 /** 2331 * A number to uniquely identify procedure entries. 2332 */ 2333 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2334 @Description(shortDefinition="Procedure instance identifier", formalDefinition="A number to uniquely identify procedure entries." ) 2335 protected PositiveIntType sequence; 2336 2337 /** 2338 * When the condition was observed or the relative ranking. 2339 */ 2340 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2341 @Description(shortDefinition="Category of Procedure", formalDefinition="When the condition was observed or the relative ranking." ) 2342 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-procedure-type") 2343 protected List<CodeableConcept> type; 2344 2345 /** 2346 * Date and optionally time the procedure was performed. 2347 */ 2348 @Child(name = "date", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2349 @Description(shortDefinition="When the procedure was performed", formalDefinition="Date and optionally time the procedure was performed." ) 2350 protected DateTimeType date; 2351 2352 /** 2353 * The code or reference to a Procedure resource which identifies the clinical intervention performed. 2354 */ 2355 @Child(name = "procedure", type = {CodeableConcept.class, Procedure.class}, order=4, min=1, max=1, modifier=false, summary=false) 2356 @Description(shortDefinition="Specific clinical procedure", formalDefinition="The code or reference to a Procedure resource which identifies the clinical intervention performed." ) 2357 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10-procedures") 2358 protected DataType procedure; 2359 2360 /** 2361 * Unique Device Identifiers associated with this line item. 2362 */ 2363 @Child(name = "udi", type = {Device.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2364 @Description(shortDefinition="Unique device identifier", formalDefinition="Unique Device Identifiers associated with this line item." ) 2365 protected List<Reference> udi; 2366 2367 private static final long serialVersionUID = 1165684715L; 2368 2369 /** 2370 * Constructor 2371 */ 2372 public ProcedureComponent() { 2373 super(); 2374 } 2375 2376 /** 2377 * Constructor 2378 */ 2379 public ProcedureComponent(int sequence, DataType procedure) { 2380 super(); 2381 this.setSequence(sequence); 2382 this.setProcedure(procedure); 2383 } 2384 2385 /** 2386 * @return {@link #sequence} (A number to uniquely identify procedure entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2387 */ 2388 public PositiveIntType getSequenceElement() { 2389 if (this.sequence == null) 2390 if (Configuration.errorOnAutoCreate()) 2391 throw new Error("Attempt to auto-create ProcedureComponent.sequence"); 2392 else if (Configuration.doAutoCreate()) 2393 this.sequence = new PositiveIntType(); // bb 2394 return this.sequence; 2395 } 2396 2397 public boolean hasSequenceElement() { 2398 return this.sequence != null && !this.sequence.isEmpty(); 2399 } 2400 2401 public boolean hasSequence() { 2402 return this.sequence != null && !this.sequence.isEmpty(); 2403 } 2404 2405 /** 2406 * @param value {@link #sequence} (A number to uniquely identify procedure entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2407 */ 2408 public ProcedureComponent setSequenceElement(PositiveIntType value) { 2409 this.sequence = value; 2410 return this; 2411 } 2412 2413 /** 2414 * @return A number to uniquely identify procedure entries. 2415 */ 2416 public int getSequence() { 2417 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2418 } 2419 2420 /** 2421 * @param value A number to uniquely identify procedure entries. 2422 */ 2423 public ProcedureComponent setSequence(int value) { 2424 if (this.sequence == null) 2425 this.sequence = new PositiveIntType(); 2426 this.sequence.setValue(value); 2427 return this; 2428 } 2429 2430 /** 2431 * @return {@link #type} (When the condition was observed or the relative ranking.) 2432 */ 2433 public List<CodeableConcept> getType() { 2434 if (this.type == null) 2435 this.type = new ArrayList<CodeableConcept>(); 2436 return this.type; 2437 } 2438 2439 /** 2440 * @return Returns a reference to <code>this</code> for easy method chaining 2441 */ 2442 public ProcedureComponent setType(List<CodeableConcept> theType) { 2443 this.type = theType; 2444 return this; 2445 } 2446 2447 public boolean hasType() { 2448 if (this.type == null) 2449 return false; 2450 for (CodeableConcept item : this.type) 2451 if (!item.isEmpty()) 2452 return true; 2453 return false; 2454 } 2455 2456 public CodeableConcept addType() { //3 2457 CodeableConcept t = new CodeableConcept(); 2458 if (this.type == null) 2459 this.type = new ArrayList<CodeableConcept>(); 2460 this.type.add(t); 2461 return t; 2462 } 2463 2464 public ProcedureComponent addType(CodeableConcept t) { //3 2465 if (t == null) 2466 return this; 2467 if (this.type == null) 2468 this.type = new ArrayList<CodeableConcept>(); 2469 this.type.add(t); 2470 return this; 2471 } 2472 2473 /** 2474 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 2475 */ 2476 public CodeableConcept getTypeFirstRep() { 2477 if (getType().isEmpty()) { 2478 addType(); 2479 } 2480 return getType().get(0); 2481 } 2482 2483 /** 2484 * @return {@link #date} (Date and optionally time the procedure was performed.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2485 */ 2486 public DateTimeType getDateElement() { 2487 if (this.date == null) 2488 if (Configuration.errorOnAutoCreate()) 2489 throw new Error("Attempt to auto-create ProcedureComponent.date"); 2490 else if (Configuration.doAutoCreate()) 2491 this.date = new DateTimeType(); // bb 2492 return this.date; 2493 } 2494 2495 public boolean hasDateElement() { 2496 return this.date != null && !this.date.isEmpty(); 2497 } 2498 2499 public boolean hasDate() { 2500 return this.date != null && !this.date.isEmpty(); 2501 } 2502 2503 /** 2504 * @param value {@link #date} (Date and optionally time the procedure was performed.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2505 */ 2506 public ProcedureComponent setDateElement(DateTimeType value) { 2507 this.date = value; 2508 return this; 2509 } 2510 2511 /** 2512 * @return Date and optionally time the procedure was performed. 2513 */ 2514 public Date getDate() { 2515 return this.date == null ? null : this.date.getValue(); 2516 } 2517 2518 /** 2519 * @param value Date and optionally time the procedure was performed. 2520 */ 2521 public ProcedureComponent setDate(Date value) { 2522 if (value == null) 2523 this.date = null; 2524 else { 2525 if (this.date == null) 2526 this.date = new DateTimeType(); 2527 this.date.setValue(value); 2528 } 2529 return this; 2530 } 2531 2532 /** 2533 * @return {@link #procedure} (The code or reference to a Procedure resource which identifies the clinical intervention performed.) 2534 */ 2535 public DataType getProcedure() { 2536 return this.procedure; 2537 } 2538 2539 /** 2540 * @return {@link #procedure} (The code or reference to a Procedure resource which identifies the clinical intervention performed.) 2541 */ 2542 public CodeableConcept getProcedureCodeableConcept() throws FHIRException { 2543 if (this.procedure == null) 2544 this.procedure = new CodeableConcept(); 2545 if (!(this.procedure instanceof CodeableConcept)) 2546 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.procedure.getClass().getName()+" was encountered"); 2547 return (CodeableConcept) this.procedure; 2548 } 2549 2550 public boolean hasProcedureCodeableConcept() { 2551 return this.procedure instanceof CodeableConcept; 2552 } 2553 2554 /** 2555 * @return {@link #procedure} (The code or reference to a Procedure resource which identifies the clinical intervention performed.) 2556 */ 2557 public Reference getProcedureReference() throws FHIRException { 2558 if (this.procedure == null) 2559 this.procedure = new Reference(); 2560 if (!(this.procedure instanceof Reference)) 2561 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.procedure.getClass().getName()+" was encountered"); 2562 return (Reference) this.procedure; 2563 } 2564 2565 public boolean hasProcedureReference() { 2566 return this.procedure instanceof Reference; 2567 } 2568 2569 public boolean hasProcedure() { 2570 return this.procedure != null && !this.procedure.isEmpty(); 2571 } 2572 2573 /** 2574 * @param value {@link #procedure} (The code or reference to a Procedure resource which identifies the clinical intervention performed.) 2575 */ 2576 public ProcedureComponent setProcedure(DataType value) { 2577 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2578 throw new FHIRException("Not the right type for ExplanationOfBenefit.procedure.procedure[x]: "+value.fhirType()); 2579 this.procedure = value; 2580 return this; 2581 } 2582 2583 /** 2584 * @return {@link #udi} (Unique Device Identifiers associated with this line item.) 2585 */ 2586 public List<Reference> getUdi() { 2587 if (this.udi == null) 2588 this.udi = new ArrayList<Reference>(); 2589 return this.udi; 2590 } 2591 2592 /** 2593 * @return Returns a reference to <code>this</code> for easy method chaining 2594 */ 2595 public ProcedureComponent setUdi(List<Reference> theUdi) { 2596 this.udi = theUdi; 2597 return this; 2598 } 2599 2600 public boolean hasUdi() { 2601 if (this.udi == null) 2602 return false; 2603 for (Reference item : this.udi) 2604 if (!item.isEmpty()) 2605 return true; 2606 return false; 2607 } 2608 2609 public Reference addUdi() { //3 2610 Reference t = new Reference(); 2611 if (this.udi == null) 2612 this.udi = new ArrayList<Reference>(); 2613 this.udi.add(t); 2614 return t; 2615 } 2616 2617 public ProcedureComponent addUdi(Reference t) { //3 2618 if (t == null) 2619 return this; 2620 if (this.udi == null) 2621 this.udi = new ArrayList<Reference>(); 2622 this.udi.add(t); 2623 return this; 2624 } 2625 2626 /** 2627 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist {3} 2628 */ 2629 public Reference getUdiFirstRep() { 2630 if (getUdi().isEmpty()) { 2631 addUdi(); 2632 } 2633 return getUdi().get(0); 2634 } 2635 2636 protected void listChildren(List<Property> children) { 2637 super.listChildren(children); 2638 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify procedure entries.", 0, 1, sequence)); 2639 children.add(new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type)); 2640 children.add(new Property("date", "dateTime", "Date and optionally time the procedure was performed.", 0, 1, date)); 2641 children.add(new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure)); 2642 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 2643 } 2644 2645 @Override 2646 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2647 switch (_hash) { 2648 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify procedure entries.", 0, 1, sequence); 2649 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "When the condition was observed or the relative ranking.", 0, java.lang.Integer.MAX_VALUE, type); 2650 case 3076014: /*date*/ return new Property("date", "dateTime", "Date and optionally time the procedure was performed.", 0, 1, date); 2651 case 1640074445: /*procedure[x]*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure); 2652 case -1095204141: /*procedure*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure); 2653 case -1284783026: /*procedureCodeableConcept*/ return new Property("procedure[x]", "CodeableConcept", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure); 2654 case 881809848: /*procedureReference*/ return new Property("procedure[x]", "Reference(Procedure)", "The code or reference to a Procedure resource which identifies the clinical intervention performed.", 0, 1, procedure); 2655 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 2656 default: return super.getNamedProperty(_hash, _name, _checkValid); 2657 } 2658 2659 } 2660 2661 @Override 2662 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2663 switch (hash) { 2664 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 2665 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2666 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2667 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : new Base[] {this.procedure}; // DataType 2668 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 2669 default: return super.getProperty(hash, name, checkValid); 2670 } 2671 2672 } 2673 2674 @Override 2675 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2676 switch (hash) { 2677 case 1349547969: // sequence 2678 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2679 return value; 2680 case 3575610: // type 2681 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2682 return value; 2683 case 3076014: // date 2684 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2685 return value; 2686 case -1095204141: // procedure 2687 this.procedure = TypeConvertor.castToType(value); // DataType 2688 return value; 2689 case 115642: // udi 2690 this.getUdi().add(TypeConvertor.castToReference(value)); // Reference 2691 return value; 2692 default: return super.setProperty(hash, name, value); 2693 } 2694 2695 } 2696 2697 @Override 2698 public Base setProperty(String name, Base value) throws FHIRException { 2699 if (name.equals("sequence")) { 2700 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 2701 } else if (name.equals("type")) { 2702 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 2703 } else if (name.equals("date")) { 2704 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2705 } else if (name.equals("procedure[x]")) { 2706 this.procedure = TypeConvertor.castToType(value); // DataType 2707 } else if (name.equals("udi")) { 2708 this.getUdi().add(TypeConvertor.castToReference(value)); 2709 } else 2710 return super.setProperty(name, value); 2711 return value; 2712 } 2713 2714 @Override 2715 public void removeChild(String name, Base value) throws FHIRException { 2716 if (name.equals("sequence")) { 2717 this.sequence = null; 2718 } else if (name.equals("type")) { 2719 this.getType().remove(value); 2720 } else if (name.equals("date")) { 2721 this.date = null; 2722 } else if (name.equals("procedure[x]")) { 2723 this.procedure = null; 2724 } else if (name.equals("udi")) { 2725 this.getUdi().remove(value); 2726 } else 2727 super.removeChild(name, value); 2728 2729 } 2730 2731 @Override 2732 public Base makeProperty(int hash, String name) throws FHIRException { 2733 switch (hash) { 2734 case 1349547969: return getSequenceElement(); 2735 case 3575610: return addType(); 2736 case 3076014: return getDateElement(); 2737 case 1640074445: return getProcedure(); 2738 case -1095204141: return getProcedure(); 2739 case 115642: return addUdi(); 2740 default: return super.makeProperty(hash, name); 2741 } 2742 2743 } 2744 2745 @Override 2746 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2747 switch (hash) { 2748 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 2749 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2750 case 3076014: /*date*/ return new String[] {"dateTime"}; 2751 case -1095204141: /*procedure*/ return new String[] {"CodeableConcept", "Reference"}; 2752 case 115642: /*udi*/ return new String[] {"Reference"}; 2753 default: return super.getTypesForProperty(hash, name); 2754 } 2755 2756 } 2757 2758 @Override 2759 public Base addChild(String name) throws FHIRException { 2760 if (name.equals("sequence")) { 2761 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.procedure.sequence"); 2762 } 2763 else if (name.equals("type")) { 2764 return addType(); 2765 } 2766 else if (name.equals("date")) { 2767 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.procedure.date"); 2768 } 2769 else if (name.equals("procedureCodeableConcept")) { 2770 this.procedure = new CodeableConcept(); 2771 return this.procedure; 2772 } 2773 else if (name.equals("procedureReference")) { 2774 this.procedure = new Reference(); 2775 return this.procedure; 2776 } 2777 else if (name.equals("udi")) { 2778 return addUdi(); 2779 } 2780 else 2781 return super.addChild(name); 2782 } 2783 2784 public ProcedureComponent copy() { 2785 ProcedureComponent dst = new ProcedureComponent(); 2786 copyValues(dst); 2787 return dst; 2788 } 2789 2790 public void copyValues(ProcedureComponent dst) { 2791 super.copyValues(dst); 2792 dst.sequence = sequence == null ? null : sequence.copy(); 2793 if (type != null) { 2794 dst.type = new ArrayList<CodeableConcept>(); 2795 for (CodeableConcept i : type) 2796 dst.type.add(i.copy()); 2797 }; 2798 dst.date = date == null ? null : date.copy(); 2799 dst.procedure = procedure == null ? null : procedure.copy(); 2800 if (udi != null) { 2801 dst.udi = new ArrayList<Reference>(); 2802 for (Reference i : udi) 2803 dst.udi.add(i.copy()); 2804 }; 2805 } 2806 2807 @Override 2808 public boolean equalsDeep(Base other_) { 2809 if (!super.equalsDeep(other_)) 2810 return false; 2811 if (!(other_ instanceof ProcedureComponent)) 2812 return false; 2813 ProcedureComponent o = (ProcedureComponent) other_; 2814 return compareDeep(sequence, o.sequence, true) && compareDeep(type, o.type, true) && compareDeep(date, o.date, true) 2815 && compareDeep(procedure, o.procedure, true) && compareDeep(udi, o.udi, true); 2816 } 2817 2818 @Override 2819 public boolean equalsShallow(Base other_) { 2820 if (!super.equalsShallow(other_)) 2821 return false; 2822 if (!(other_ instanceof ProcedureComponent)) 2823 return false; 2824 ProcedureComponent o = (ProcedureComponent) other_; 2825 return compareValues(sequence, o.sequence, true) && compareValues(date, o.date, true); 2826 } 2827 2828 public boolean isEmpty() { 2829 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, type, date, procedure 2830 , udi); 2831 } 2832 2833 public String fhirType() { 2834 return "ExplanationOfBenefit.procedure"; 2835 2836 } 2837 2838 } 2839 2840 @Block() 2841 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 2842 /** 2843 * A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true. 2844 */ 2845 @Child(name = "focal", type = {BooleanType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2846 @Description(shortDefinition="Coverage to be used for adjudication", formalDefinition="A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true." ) 2847 protected BooleanType focal; 2848 2849 /** 2850 * Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system. 2851 */ 2852 @Child(name = "coverage", type = {Coverage.class}, order=2, min=1, max=1, modifier=false, summary=true) 2853 @Description(shortDefinition="Insurance information", formalDefinition="Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system." ) 2854 protected Reference coverage; 2855 2856 /** 2857 * Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization. 2858 */ 2859 @Child(name = "preAuthRef", type = {StringType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2860 @Description(shortDefinition="Prior authorization reference number", formalDefinition="Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization." ) 2861 protected List<StringType> preAuthRef; 2862 2863 private static final long serialVersionUID = 1519900285L; 2864 2865 /** 2866 * Constructor 2867 */ 2868 public InsuranceComponent() { 2869 super(); 2870 } 2871 2872 /** 2873 * Constructor 2874 */ 2875 public InsuranceComponent(boolean focal, Reference coverage) { 2876 super(); 2877 this.setFocal(focal); 2878 this.setCoverage(coverage); 2879 } 2880 2881 /** 2882 * @return {@link #focal} (A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 2883 */ 2884 public BooleanType getFocalElement() { 2885 if (this.focal == null) 2886 if (Configuration.errorOnAutoCreate()) 2887 throw new Error("Attempt to auto-create InsuranceComponent.focal"); 2888 else if (Configuration.doAutoCreate()) 2889 this.focal = new BooleanType(); // bb 2890 return this.focal; 2891 } 2892 2893 public boolean hasFocalElement() { 2894 return this.focal != null && !this.focal.isEmpty(); 2895 } 2896 2897 public boolean hasFocal() { 2898 return this.focal != null && !this.focal.isEmpty(); 2899 } 2900 2901 /** 2902 * @param value {@link #focal} (A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.). This is the underlying object with id, value and extensions. The accessor "getFocal" gives direct access to the value 2903 */ 2904 public InsuranceComponent setFocalElement(BooleanType value) { 2905 this.focal = value; 2906 return this; 2907 } 2908 2909 /** 2910 * @return A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true. 2911 */ 2912 public boolean getFocal() { 2913 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 2914 } 2915 2916 /** 2917 * @param value A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true. 2918 */ 2919 public InsuranceComponent setFocal(boolean value) { 2920 if (this.focal == null) 2921 this.focal = new BooleanType(); 2922 this.focal.setValue(value); 2923 return this; 2924 } 2925 2926 /** 2927 * @return {@link #coverage} (Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.) 2928 */ 2929 public Reference getCoverage() { 2930 if (this.coverage == null) 2931 if (Configuration.errorOnAutoCreate()) 2932 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 2933 else if (Configuration.doAutoCreate()) 2934 this.coverage = new Reference(); // cc 2935 return this.coverage; 2936 } 2937 2938 public boolean hasCoverage() { 2939 return this.coverage != null && !this.coverage.isEmpty(); 2940 } 2941 2942 /** 2943 * @param value {@link #coverage} (Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.) 2944 */ 2945 public InsuranceComponent setCoverage(Reference value) { 2946 this.coverage = value; 2947 return this; 2948 } 2949 2950 /** 2951 * @return {@link #preAuthRef} (Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.) 2952 */ 2953 public List<StringType> getPreAuthRef() { 2954 if (this.preAuthRef == null) 2955 this.preAuthRef = new ArrayList<StringType>(); 2956 return this.preAuthRef; 2957 } 2958 2959 /** 2960 * @return Returns a reference to <code>this</code> for easy method chaining 2961 */ 2962 public InsuranceComponent setPreAuthRef(List<StringType> thePreAuthRef) { 2963 this.preAuthRef = thePreAuthRef; 2964 return this; 2965 } 2966 2967 public boolean hasPreAuthRef() { 2968 if (this.preAuthRef == null) 2969 return false; 2970 for (StringType item : this.preAuthRef) 2971 if (!item.isEmpty()) 2972 return true; 2973 return false; 2974 } 2975 2976 /** 2977 * @return {@link #preAuthRef} (Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.) 2978 */ 2979 public StringType addPreAuthRefElement() {//2 2980 StringType t = new StringType(); 2981 if (this.preAuthRef == null) 2982 this.preAuthRef = new ArrayList<StringType>(); 2983 this.preAuthRef.add(t); 2984 return t; 2985 } 2986 2987 /** 2988 * @param value {@link #preAuthRef} (Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.) 2989 */ 2990 public InsuranceComponent addPreAuthRef(String value) { //1 2991 StringType t = new StringType(); 2992 t.setValue(value); 2993 if (this.preAuthRef == null) 2994 this.preAuthRef = new ArrayList<StringType>(); 2995 this.preAuthRef.add(t); 2996 return this; 2997 } 2998 2999 /** 3000 * @param value {@link #preAuthRef} (Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.) 3001 */ 3002 public boolean hasPreAuthRef(String value) { 3003 if (this.preAuthRef == null) 3004 return false; 3005 for (StringType v : this.preAuthRef) 3006 if (v.getValue().equals(value)) // string 3007 return true; 3008 return false; 3009 } 3010 3011 protected void listChildren(List<Property> children) { 3012 super.listChildren(children); 3013 children.add(new Property("focal", "boolean", "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 1, focal)); 3014 children.add(new Property("coverage", "Reference(Coverage)", "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 0, 1, coverage)); 3015 children.add(new Property("preAuthRef", "string", "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.", 0, java.lang.Integer.MAX_VALUE, preAuthRef)); 3016 } 3017 3018 @Override 3019 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3020 switch (_hash) { 3021 case 97604197: /*focal*/ return new Property("focal", "boolean", "A flag to indicate that this Coverage is to be used for adjudication of this claim when set to true.", 0, 1, focal); 3022 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "Reference to the insurance card level information contained in the Coverage resource. The coverage issuing insurer will use these details to locate the patient's actual coverage within the insurer's information system.", 0, 1, coverage); 3023 case 522246568: /*preAuthRef*/ return new Property("preAuthRef", "string", "Reference numbers previously provided by the insurer to the provider to be quoted on subsequent claims containing services or products related to the prior authorization.", 0, java.lang.Integer.MAX_VALUE, preAuthRef); 3024 default: return super.getNamedProperty(_hash, _name, _checkValid); 3025 } 3026 3027 } 3028 3029 @Override 3030 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3031 switch (hash) { 3032 case 97604197: /*focal*/ return this.focal == null ? new Base[0] : new Base[] {this.focal}; // BooleanType 3033 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 3034 case 522246568: /*preAuthRef*/ return this.preAuthRef == null ? new Base[0] : this.preAuthRef.toArray(new Base[this.preAuthRef.size()]); // StringType 3035 default: return super.getProperty(hash, name, checkValid); 3036 } 3037 3038 } 3039 3040 @Override 3041 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3042 switch (hash) { 3043 case 97604197: // focal 3044 this.focal = TypeConvertor.castToBoolean(value); // BooleanType 3045 return value; 3046 case -351767064: // coverage 3047 this.coverage = TypeConvertor.castToReference(value); // Reference 3048 return value; 3049 case 522246568: // preAuthRef 3050 this.getPreAuthRef().add(TypeConvertor.castToString(value)); // StringType 3051 return value; 3052 default: return super.setProperty(hash, name, value); 3053 } 3054 3055 } 3056 3057 @Override 3058 public Base setProperty(String name, Base value) throws FHIRException { 3059 if (name.equals("focal")) { 3060 this.focal = TypeConvertor.castToBoolean(value); // BooleanType 3061 } else if (name.equals("coverage")) { 3062 this.coverage = TypeConvertor.castToReference(value); // Reference 3063 } else if (name.equals("preAuthRef")) { 3064 this.getPreAuthRef().add(TypeConvertor.castToString(value)); 3065 } else 3066 return super.setProperty(name, value); 3067 return value; 3068 } 3069 3070 @Override 3071 public void removeChild(String name, Base value) throws FHIRException { 3072 if (name.equals("focal")) { 3073 this.focal = null; 3074 } else if (name.equals("coverage")) { 3075 this.coverage = null; 3076 } else if (name.equals("preAuthRef")) { 3077 this.getPreAuthRef().remove(value); 3078 } else 3079 super.removeChild(name, value); 3080 3081 } 3082 3083 @Override 3084 public Base makeProperty(int hash, String name) throws FHIRException { 3085 switch (hash) { 3086 case 97604197: return getFocalElement(); 3087 case -351767064: return getCoverage(); 3088 case 522246568: return addPreAuthRefElement(); 3089 default: return super.makeProperty(hash, name); 3090 } 3091 3092 } 3093 3094 @Override 3095 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3096 switch (hash) { 3097 case 97604197: /*focal*/ return new String[] {"boolean"}; 3098 case -351767064: /*coverage*/ return new String[] {"Reference"}; 3099 case 522246568: /*preAuthRef*/ return new String[] {"string"}; 3100 default: return super.getTypesForProperty(hash, name); 3101 } 3102 3103 } 3104 3105 @Override 3106 public Base addChild(String name) throws FHIRException { 3107 if (name.equals("focal")) { 3108 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.insurance.focal"); 3109 } 3110 else if (name.equals("coverage")) { 3111 this.coverage = new Reference(); 3112 return this.coverage; 3113 } 3114 else if (name.equals("preAuthRef")) { 3115 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.insurance.preAuthRef"); 3116 } 3117 else 3118 return super.addChild(name); 3119 } 3120 3121 public InsuranceComponent copy() { 3122 InsuranceComponent dst = new InsuranceComponent(); 3123 copyValues(dst); 3124 return dst; 3125 } 3126 3127 public void copyValues(InsuranceComponent dst) { 3128 super.copyValues(dst); 3129 dst.focal = focal == null ? null : focal.copy(); 3130 dst.coverage = coverage == null ? null : coverage.copy(); 3131 if (preAuthRef != null) { 3132 dst.preAuthRef = new ArrayList<StringType>(); 3133 for (StringType i : preAuthRef) 3134 dst.preAuthRef.add(i.copy()); 3135 }; 3136 } 3137 3138 @Override 3139 public boolean equalsDeep(Base other_) { 3140 if (!super.equalsDeep(other_)) 3141 return false; 3142 if (!(other_ instanceof InsuranceComponent)) 3143 return false; 3144 InsuranceComponent o = (InsuranceComponent) other_; 3145 return compareDeep(focal, o.focal, true) && compareDeep(coverage, o.coverage, true) && compareDeep(preAuthRef, o.preAuthRef, true) 3146 ; 3147 } 3148 3149 @Override 3150 public boolean equalsShallow(Base other_) { 3151 if (!super.equalsShallow(other_)) 3152 return false; 3153 if (!(other_ instanceof InsuranceComponent)) 3154 return false; 3155 InsuranceComponent o = (InsuranceComponent) other_; 3156 return compareValues(focal, o.focal, true) && compareValues(preAuthRef, o.preAuthRef, true); 3157 } 3158 3159 public boolean isEmpty() { 3160 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(focal, coverage, preAuthRef 3161 ); 3162 } 3163 3164 public String fhirType() { 3165 return "ExplanationOfBenefit.insurance"; 3166 3167 } 3168 3169 } 3170 3171 @Block() 3172 public static class AccidentComponent extends BackboneElement implements IBaseBackboneElement { 3173 /** 3174 * Date of an accident event related to the products and services contained in the claim. 3175 */ 3176 @Child(name = "date", type = {DateType.class}, order=1, min=0, max=1, modifier=false, summary=false) 3177 @Description(shortDefinition="When the incident occurred", formalDefinition="Date of an accident event related to the products and services contained in the claim." ) 3178 protected DateType date; 3179 3180 /** 3181 * The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers. 3182 */ 3183 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 3184 @Description(shortDefinition="The nature of the accident", formalDefinition="The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers." ) 3185 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ActIncidentCode") 3186 protected CodeableConcept type; 3187 3188 /** 3189 * The physical location of the accident event. 3190 */ 3191 @Child(name = "location", type = {Address.class, Location.class}, order=3, min=0, max=1, modifier=false, summary=false) 3192 @Description(shortDefinition="Where the event occurred", formalDefinition="The physical location of the accident event." ) 3193 protected DataType location; 3194 3195 private static final long serialVersionUID = 11882722L; 3196 3197 /** 3198 * Constructor 3199 */ 3200 public AccidentComponent() { 3201 super(); 3202 } 3203 3204 /** 3205 * @return {@link #date} (Date of an accident event related to the products and services contained in the claim.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3206 */ 3207 public DateType getDateElement() { 3208 if (this.date == null) 3209 if (Configuration.errorOnAutoCreate()) 3210 throw new Error("Attempt to auto-create AccidentComponent.date"); 3211 else if (Configuration.doAutoCreate()) 3212 this.date = new DateType(); // bb 3213 return this.date; 3214 } 3215 3216 public boolean hasDateElement() { 3217 return this.date != null && !this.date.isEmpty(); 3218 } 3219 3220 public boolean hasDate() { 3221 return this.date != null && !this.date.isEmpty(); 3222 } 3223 3224 /** 3225 * @param value {@link #date} (Date of an accident event related to the products and services contained in the claim.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3226 */ 3227 public AccidentComponent setDateElement(DateType value) { 3228 this.date = value; 3229 return this; 3230 } 3231 3232 /** 3233 * @return Date of an accident event related to the products and services contained in the claim. 3234 */ 3235 public Date getDate() { 3236 return this.date == null ? null : this.date.getValue(); 3237 } 3238 3239 /** 3240 * @param value Date of an accident event related to the products and services contained in the claim. 3241 */ 3242 public AccidentComponent setDate(Date value) { 3243 if (value == null) 3244 this.date = null; 3245 else { 3246 if (this.date == null) 3247 this.date = new DateType(); 3248 this.date.setValue(value); 3249 } 3250 return this; 3251 } 3252 3253 /** 3254 * @return {@link #type} (The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.) 3255 */ 3256 public CodeableConcept getType() { 3257 if (this.type == null) 3258 if (Configuration.errorOnAutoCreate()) 3259 throw new Error("Attempt to auto-create AccidentComponent.type"); 3260 else if (Configuration.doAutoCreate()) 3261 this.type = new CodeableConcept(); // cc 3262 return this.type; 3263 } 3264 3265 public boolean hasType() { 3266 return this.type != null && !this.type.isEmpty(); 3267 } 3268 3269 /** 3270 * @param value {@link #type} (The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.) 3271 */ 3272 public AccidentComponent setType(CodeableConcept value) { 3273 this.type = value; 3274 return this; 3275 } 3276 3277 /** 3278 * @return {@link #location} (The physical location of the accident event.) 3279 */ 3280 public DataType getLocation() { 3281 return this.location; 3282 } 3283 3284 /** 3285 * @return {@link #location} (The physical location of the accident event.) 3286 */ 3287 public Address getLocationAddress() throws FHIRException { 3288 if (this.location == null) 3289 this.location = new Address(); 3290 if (!(this.location instanceof Address)) 3291 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 3292 return (Address) this.location; 3293 } 3294 3295 public boolean hasLocationAddress() { 3296 return this.location instanceof Address; 3297 } 3298 3299 /** 3300 * @return {@link #location} (The physical location of the accident event.) 3301 */ 3302 public Reference getLocationReference() throws FHIRException { 3303 if (this.location == null) 3304 this.location = new Reference(); 3305 if (!(this.location instanceof Reference)) 3306 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 3307 return (Reference) this.location; 3308 } 3309 3310 public boolean hasLocationReference() { 3311 return this.location instanceof Reference; 3312 } 3313 3314 public boolean hasLocation() { 3315 return this.location != null && !this.location.isEmpty(); 3316 } 3317 3318 /** 3319 * @param value {@link #location} (The physical location of the accident event.) 3320 */ 3321 public AccidentComponent setLocation(DataType value) { 3322 if (value != null && !(value instanceof Address || value instanceof Reference)) 3323 throw new FHIRException("Not the right type for ExplanationOfBenefit.accident.location[x]: "+value.fhirType()); 3324 this.location = value; 3325 return this; 3326 } 3327 3328 protected void listChildren(List<Property> children) { 3329 super.listChildren(children); 3330 children.add(new Property("date", "date", "Date of an accident event related to the products and services contained in the claim.", 0, 1, date)); 3331 children.add(new Property("type", "CodeableConcept", "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.", 0, 1, type)); 3332 children.add(new Property("location[x]", "Address|Reference(Location)", "The physical location of the accident event.", 0, 1, location)); 3333 } 3334 3335 @Override 3336 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3337 switch (_hash) { 3338 case 3076014: /*date*/ return new Property("date", "date", "Date of an accident event related to the products and services contained in the claim.", 0, 1, date); 3339 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type or context of the accident event for the purposes of selection of potential insurance coverages and determination of coordination between insurers.", 0, 1, type); 3340 case 552316075: /*location[x]*/ return new Property("location[x]", "Address|Reference(Location)", "The physical location of the accident event.", 0, 1, location); 3341 case 1901043637: /*location*/ return new Property("location[x]", "Address|Reference(Location)", "The physical location of the accident event.", 0, 1, location); 3342 case -1280020865: /*locationAddress*/ return new Property("location[x]", "Address", "The physical location of the accident event.", 0, 1, location); 3343 case 755866390: /*locationReference*/ return new Property("location[x]", "Reference(Location)", "The physical location of the accident event.", 0, 1, location); 3344 default: return super.getNamedProperty(_hash, _name, _checkValid); 3345 } 3346 3347 } 3348 3349 @Override 3350 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3351 switch (hash) { 3352 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 3353 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3354 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // DataType 3355 default: return super.getProperty(hash, name, checkValid); 3356 } 3357 3358 } 3359 3360 @Override 3361 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3362 switch (hash) { 3363 case 3076014: // date 3364 this.date = TypeConvertor.castToDate(value); // DateType 3365 return value; 3366 case 3575610: // type 3367 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3368 return value; 3369 case 1901043637: // location 3370 this.location = TypeConvertor.castToType(value); // DataType 3371 return value; 3372 default: return super.setProperty(hash, name, value); 3373 } 3374 3375 } 3376 3377 @Override 3378 public Base setProperty(String name, Base value) throws FHIRException { 3379 if (name.equals("date")) { 3380 this.date = TypeConvertor.castToDate(value); // DateType 3381 } else if (name.equals("type")) { 3382 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3383 } else if (name.equals("location[x]")) { 3384 this.location = TypeConvertor.castToType(value); // DataType 3385 } else 3386 return super.setProperty(name, value); 3387 return value; 3388 } 3389 3390 @Override 3391 public void removeChild(String name, Base value) throws FHIRException { 3392 if (name.equals("date")) { 3393 this.date = null; 3394 } else if (name.equals("type")) { 3395 this.type = null; 3396 } else if (name.equals("location[x]")) { 3397 this.location = null; 3398 } else 3399 super.removeChild(name, value); 3400 3401 } 3402 3403 @Override 3404 public Base makeProperty(int hash, String name) throws FHIRException { 3405 switch (hash) { 3406 case 3076014: return getDateElement(); 3407 case 3575610: return getType(); 3408 case 552316075: return getLocation(); 3409 case 1901043637: return getLocation(); 3410 default: return super.makeProperty(hash, name); 3411 } 3412 3413 } 3414 3415 @Override 3416 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3417 switch (hash) { 3418 case 3076014: /*date*/ return new String[] {"date"}; 3419 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3420 case 1901043637: /*location*/ return new String[] {"Address", "Reference"}; 3421 default: return super.getTypesForProperty(hash, name); 3422 } 3423 3424 } 3425 3426 @Override 3427 public Base addChild(String name) throws FHIRException { 3428 if (name.equals("date")) { 3429 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.accident.date"); 3430 } 3431 else if (name.equals("type")) { 3432 this.type = new CodeableConcept(); 3433 return this.type; 3434 } 3435 else if (name.equals("locationAddress")) { 3436 this.location = new Address(); 3437 return this.location; 3438 } 3439 else if (name.equals("locationReference")) { 3440 this.location = new Reference(); 3441 return this.location; 3442 } 3443 else 3444 return super.addChild(name); 3445 } 3446 3447 public AccidentComponent copy() { 3448 AccidentComponent dst = new AccidentComponent(); 3449 copyValues(dst); 3450 return dst; 3451 } 3452 3453 public void copyValues(AccidentComponent dst) { 3454 super.copyValues(dst); 3455 dst.date = date == null ? null : date.copy(); 3456 dst.type = type == null ? null : type.copy(); 3457 dst.location = location == null ? null : location.copy(); 3458 } 3459 3460 @Override 3461 public boolean equalsDeep(Base other_) { 3462 if (!super.equalsDeep(other_)) 3463 return false; 3464 if (!(other_ instanceof AccidentComponent)) 3465 return false; 3466 AccidentComponent o = (AccidentComponent) other_; 3467 return compareDeep(date, o.date, true) && compareDeep(type, o.type, true) && compareDeep(location, o.location, true) 3468 ; 3469 } 3470 3471 @Override 3472 public boolean equalsShallow(Base other_) { 3473 if (!super.equalsShallow(other_)) 3474 return false; 3475 if (!(other_ instanceof AccidentComponent)) 3476 return false; 3477 AccidentComponent o = (AccidentComponent) other_; 3478 return compareValues(date, o.date, true); 3479 } 3480 3481 public boolean isEmpty() { 3482 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, type, location); 3483 } 3484 3485 public String fhirType() { 3486 return "ExplanationOfBenefit.accident"; 3487 3488 } 3489 3490 } 3491 3492 @Block() 3493 public static class ItemComponent extends BackboneElement implements IBaseBackboneElement { 3494 /** 3495 * A number to uniquely identify item entries. 3496 */ 3497 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 3498 @Description(shortDefinition="Item instance identifier", formalDefinition="A number to uniquely identify item entries." ) 3499 protected PositiveIntType sequence; 3500 3501 /** 3502 * Care team members related to this service or product. 3503 */ 3504 @Child(name = "careTeamSequence", type = {PositiveIntType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3505 @Description(shortDefinition="Applicable care team members", formalDefinition="Care team members related to this service or product." ) 3506 protected List<PositiveIntType> careTeamSequence; 3507 3508 /** 3509 * Diagnoses applicable for this service or product. 3510 */ 3511 @Child(name = "diagnosisSequence", type = {PositiveIntType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3512 @Description(shortDefinition="Applicable diagnoses", formalDefinition="Diagnoses applicable for this service or product." ) 3513 protected List<PositiveIntType> diagnosisSequence; 3514 3515 /** 3516 * Procedures applicable for this service or product. 3517 */ 3518 @Child(name = "procedureSequence", type = {PositiveIntType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3519 @Description(shortDefinition="Applicable procedures", formalDefinition="Procedures applicable for this service or product." ) 3520 protected List<PositiveIntType> procedureSequence; 3521 3522 /** 3523 * Exceptions, special conditions and supporting information applicable for this service or product. 3524 */ 3525 @Child(name = "informationSequence", type = {PositiveIntType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3526 @Description(shortDefinition="Applicable exception and supporting information", formalDefinition="Exceptions, special conditions and supporting information applicable for this service or product." ) 3527 protected List<PositiveIntType> informationSequence; 3528 3529 /** 3530 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 3531 */ 3532 @Child(name = "traceNumber", type = {Identifier.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3533 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 3534 protected List<Identifier> traceNumber; 3535 3536 /** 3537 * The type of revenue or cost center providing the product and/or service. 3538 */ 3539 @Child(name = "revenue", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 3540 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 3541 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 3542 protected CodeableConcept revenue; 3543 3544 /** 3545 * Code to identify the general type of benefits under which products and services are provided. 3546 */ 3547 @Child(name = "category", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 3548 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 3549 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 3550 protected CodeableConcept category; 3551 3552 /** 3553 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 3554 */ 3555 @Child(name = "productOrService", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=false) 3556 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 3557 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 3558 protected CodeableConcept productOrService; 3559 3560 /** 3561 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 3562 */ 3563 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=false) 3564 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 3565 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 3566 protected CodeableConcept productOrServiceEnd; 3567 3568 /** 3569 * Request or Referral for Goods or Service to be rendered. 3570 */ 3571 @Child(name = "request", type = {DeviceRequest.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class, SupplyRequest.class, VisionPrescription.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3572 @Description(shortDefinition="Request or Referral for Service", formalDefinition="Request or Referral for Goods or Service to be rendered." ) 3573 protected List<Reference> request; 3574 3575 /** 3576 * Item typification or modifiers codes to convey additional context for the product or service. 3577 */ 3578 @Child(name = "modifier", type = {CodeableConcept.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3579 @Description(shortDefinition="Product or service billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 3580 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 3581 protected List<CodeableConcept> modifier; 3582 3583 /** 3584 * Identifies the program under which this may be recovered. 3585 */ 3586 @Child(name = "programCode", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3587 @Description(shortDefinition="Program the product or service is provided under", formalDefinition="Identifies the program under which this may be recovered." ) 3588 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 3589 protected List<CodeableConcept> programCode; 3590 3591 /** 3592 * The date or dates when the service or product was supplied, performed or completed. 3593 */ 3594 @Child(name = "serviced", type = {DateType.class, Period.class}, order=14, min=0, max=1, modifier=false, summary=false) 3595 @Description(shortDefinition="Date or dates of service or product delivery", formalDefinition="The date or dates when the service or product was supplied, performed or completed." ) 3596 protected DataType serviced; 3597 3598 /** 3599 * Where the product or service was provided. 3600 */ 3601 @Child(name = "location", type = {CodeableConcept.class, Address.class, Location.class}, order=15, min=0, max=1, modifier=false, summary=false) 3602 @Description(shortDefinition="Place of service or where product was supplied", formalDefinition="Where the product or service was provided." ) 3603 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-place") 3604 protected DataType location; 3605 3606 /** 3607 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 3608 */ 3609 @Child(name = "patientPaid", type = {Money.class}, order=16, min=0, max=1, modifier=false, summary=false) 3610 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 3611 protected Money patientPaid; 3612 3613 /** 3614 * The number of repetitions of a service or product. 3615 */ 3616 @Child(name = "quantity", type = {Quantity.class}, order=17, min=0, max=1, modifier=false, summary=false) 3617 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 3618 protected Quantity quantity; 3619 3620 /** 3621 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 3622 */ 3623 @Child(name = "unitPrice", type = {Money.class}, order=18, min=0, max=1, modifier=false, summary=false) 3624 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 3625 protected Money unitPrice; 3626 3627 /** 3628 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3629 */ 3630 @Child(name = "factor", type = {DecimalType.class}, order=19, min=0, max=1, modifier=false, summary=false) 3631 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 3632 protected DecimalType factor; 3633 3634 /** 3635 * The total of taxes applicable for this product or service. 3636 */ 3637 @Child(name = "tax", type = {Money.class}, order=20, min=0, max=1, modifier=false, summary=false) 3638 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 3639 protected Money tax; 3640 3641 /** 3642 * The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor. 3643 */ 3644 @Child(name = "net", type = {Money.class}, order=21, min=0, max=1, modifier=false, summary=false) 3645 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor." ) 3646 protected Money net; 3647 3648 /** 3649 * Unique Device Identifiers associated with this line item. 3650 */ 3651 @Child(name = "udi", type = {Device.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3652 @Description(shortDefinition="Unique device identifier", formalDefinition="Unique Device Identifiers associated with this line item." ) 3653 protected List<Reference> udi; 3654 3655 /** 3656 * Physical location where the service is performed or applies. 3657 */ 3658 @Child(name = "bodySite", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3659 @Description(shortDefinition="Anatomical location", formalDefinition="Physical location where the service is performed or applies." ) 3660 protected List<ItemBodySiteComponent> bodySite; 3661 3662 /** 3663 * Healthcare encounters related to this claim. 3664 */ 3665 @Child(name = "encounter", type = {Encounter.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3666 @Description(shortDefinition="Encounters associated with the listed treatments", formalDefinition="Healthcare encounters related to this claim." ) 3667 protected List<Reference> encounter; 3668 3669 /** 3670 * The numbers associated with notes below which apply to the adjudication of this item. 3671 */ 3672 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3673 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 3674 protected List<PositiveIntType> noteNumber; 3675 3676 /** 3677 * The high-level results of the adjudication if adjudication has been performed. 3678 */ 3679 @Child(name = "reviewOutcome", type = {}, order=26, min=0, max=1, modifier=false, summary=false) 3680 @Description(shortDefinition="Adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 3681 protected ItemReviewOutcomeComponent reviewOutcome; 3682 3683 /** 3684 * If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item. 3685 */ 3686 @Child(name = "adjudication", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3687 @Description(shortDefinition="Adjudication details", formalDefinition="If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item." ) 3688 protected List<AdjudicationComponent> adjudication; 3689 3690 /** 3691 * Second-tier of goods and services. 3692 */ 3693 @Child(name = "detail", type = {}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3694 @Description(shortDefinition="Additional items", formalDefinition="Second-tier of goods and services." ) 3695 protected List<DetailComponent> detail; 3696 3697 private static final long serialVersionUID = -1905277239L; 3698 3699 /** 3700 * Constructor 3701 */ 3702 public ItemComponent() { 3703 super(); 3704 } 3705 3706 /** 3707 * Constructor 3708 */ 3709 public ItemComponent(int sequence) { 3710 super(); 3711 this.setSequence(sequence); 3712 } 3713 3714 /** 3715 * @return {@link #sequence} (A number to uniquely identify item entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 3716 */ 3717 public PositiveIntType getSequenceElement() { 3718 if (this.sequence == null) 3719 if (Configuration.errorOnAutoCreate()) 3720 throw new Error("Attempt to auto-create ItemComponent.sequence"); 3721 else if (Configuration.doAutoCreate()) 3722 this.sequence = new PositiveIntType(); // bb 3723 return this.sequence; 3724 } 3725 3726 public boolean hasSequenceElement() { 3727 return this.sequence != null && !this.sequence.isEmpty(); 3728 } 3729 3730 public boolean hasSequence() { 3731 return this.sequence != null && !this.sequence.isEmpty(); 3732 } 3733 3734 /** 3735 * @param value {@link #sequence} (A number to uniquely identify item entries.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 3736 */ 3737 public ItemComponent setSequenceElement(PositiveIntType value) { 3738 this.sequence = value; 3739 return this; 3740 } 3741 3742 /** 3743 * @return A number to uniquely identify item entries. 3744 */ 3745 public int getSequence() { 3746 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 3747 } 3748 3749 /** 3750 * @param value A number to uniquely identify item entries. 3751 */ 3752 public ItemComponent setSequence(int value) { 3753 if (this.sequence == null) 3754 this.sequence = new PositiveIntType(); 3755 this.sequence.setValue(value); 3756 return this; 3757 } 3758 3759 /** 3760 * @return {@link #careTeamSequence} (Care team members related to this service or product.) 3761 */ 3762 public List<PositiveIntType> getCareTeamSequence() { 3763 if (this.careTeamSequence == null) 3764 this.careTeamSequence = new ArrayList<PositiveIntType>(); 3765 return this.careTeamSequence; 3766 } 3767 3768 /** 3769 * @return Returns a reference to <code>this</code> for easy method chaining 3770 */ 3771 public ItemComponent setCareTeamSequence(List<PositiveIntType> theCareTeamSequence) { 3772 this.careTeamSequence = theCareTeamSequence; 3773 return this; 3774 } 3775 3776 public boolean hasCareTeamSequence() { 3777 if (this.careTeamSequence == null) 3778 return false; 3779 for (PositiveIntType item : this.careTeamSequence) 3780 if (!item.isEmpty()) 3781 return true; 3782 return false; 3783 } 3784 3785 /** 3786 * @return {@link #careTeamSequence} (Care team members related to this service or product.) 3787 */ 3788 public PositiveIntType addCareTeamSequenceElement() {//2 3789 PositiveIntType t = new PositiveIntType(); 3790 if (this.careTeamSequence == null) 3791 this.careTeamSequence = new ArrayList<PositiveIntType>(); 3792 this.careTeamSequence.add(t); 3793 return t; 3794 } 3795 3796 /** 3797 * @param value {@link #careTeamSequence} (Care team members related to this service or product.) 3798 */ 3799 public ItemComponent addCareTeamSequence(int value) { //1 3800 PositiveIntType t = new PositiveIntType(); 3801 t.setValue(value); 3802 if (this.careTeamSequence == null) 3803 this.careTeamSequence = new ArrayList<PositiveIntType>(); 3804 this.careTeamSequence.add(t); 3805 return this; 3806 } 3807 3808 /** 3809 * @param value {@link #careTeamSequence} (Care team members related to this service or product.) 3810 */ 3811 public boolean hasCareTeamSequence(int value) { 3812 if (this.careTeamSequence == null) 3813 return false; 3814 for (PositiveIntType v : this.careTeamSequence) 3815 if (v.getValue().equals(value)) // positiveInt 3816 return true; 3817 return false; 3818 } 3819 3820 /** 3821 * @return {@link #diagnosisSequence} (Diagnoses applicable for this service or product.) 3822 */ 3823 public List<PositiveIntType> getDiagnosisSequence() { 3824 if (this.diagnosisSequence == null) 3825 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 3826 return this.diagnosisSequence; 3827 } 3828 3829 /** 3830 * @return Returns a reference to <code>this</code> for easy method chaining 3831 */ 3832 public ItemComponent setDiagnosisSequence(List<PositiveIntType> theDiagnosisSequence) { 3833 this.diagnosisSequence = theDiagnosisSequence; 3834 return this; 3835 } 3836 3837 public boolean hasDiagnosisSequence() { 3838 if (this.diagnosisSequence == null) 3839 return false; 3840 for (PositiveIntType item : this.diagnosisSequence) 3841 if (!item.isEmpty()) 3842 return true; 3843 return false; 3844 } 3845 3846 /** 3847 * @return {@link #diagnosisSequence} (Diagnoses applicable for this service or product.) 3848 */ 3849 public PositiveIntType addDiagnosisSequenceElement() {//2 3850 PositiveIntType t = new PositiveIntType(); 3851 if (this.diagnosisSequence == null) 3852 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 3853 this.diagnosisSequence.add(t); 3854 return t; 3855 } 3856 3857 /** 3858 * @param value {@link #diagnosisSequence} (Diagnoses applicable for this service or product.) 3859 */ 3860 public ItemComponent addDiagnosisSequence(int value) { //1 3861 PositiveIntType t = new PositiveIntType(); 3862 t.setValue(value); 3863 if (this.diagnosisSequence == null) 3864 this.diagnosisSequence = new ArrayList<PositiveIntType>(); 3865 this.diagnosisSequence.add(t); 3866 return this; 3867 } 3868 3869 /** 3870 * @param value {@link #diagnosisSequence} (Diagnoses applicable for this service or product.) 3871 */ 3872 public boolean hasDiagnosisSequence(int value) { 3873 if (this.diagnosisSequence == null) 3874 return false; 3875 for (PositiveIntType v : this.diagnosisSequence) 3876 if (v.getValue().equals(value)) // positiveInt 3877 return true; 3878 return false; 3879 } 3880 3881 /** 3882 * @return {@link #procedureSequence} (Procedures applicable for this service or product.) 3883 */ 3884 public List<PositiveIntType> getProcedureSequence() { 3885 if (this.procedureSequence == null) 3886 this.procedureSequence = new ArrayList<PositiveIntType>(); 3887 return this.procedureSequence; 3888 } 3889 3890 /** 3891 * @return Returns a reference to <code>this</code> for easy method chaining 3892 */ 3893 public ItemComponent setProcedureSequence(List<PositiveIntType> theProcedureSequence) { 3894 this.procedureSequence = theProcedureSequence; 3895 return this; 3896 } 3897 3898 public boolean hasProcedureSequence() { 3899 if (this.procedureSequence == null) 3900 return false; 3901 for (PositiveIntType item : this.procedureSequence) 3902 if (!item.isEmpty()) 3903 return true; 3904 return false; 3905 } 3906 3907 /** 3908 * @return {@link #procedureSequence} (Procedures applicable for this service or product.) 3909 */ 3910 public PositiveIntType addProcedureSequenceElement() {//2 3911 PositiveIntType t = new PositiveIntType(); 3912 if (this.procedureSequence == null) 3913 this.procedureSequence = new ArrayList<PositiveIntType>(); 3914 this.procedureSequence.add(t); 3915 return t; 3916 } 3917 3918 /** 3919 * @param value {@link #procedureSequence} (Procedures applicable for this service or product.) 3920 */ 3921 public ItemComponent addProcedureSequence(int value) { //1 3922 PositiveIntType t = new PositiveIntType(); 3923 t.setValue(value); 3924 if (this.procedureSequence == null) 3925 this.procedureSequence = new ArrayList<PositiveIntType>(); 3926 this.procedureSequence.add(t); 3927 return this; 3928 } 3929 3930 /** 3931 * @param value {@link #procedureSequence} (Procedures applicable for this service or product.) 3932 */ 3933 public boolean hasProcedureSequence(int value) { 3934 if (this.procedureSequence == null) 3935 return false; 3936 for (PositiveIntType v : this.procedureSequence) 3937 if (v.getValue().equals(value)) // positiveInt 3938 return true; 3939 return false; 3940 } 3941 3942 /** 3943 * @return {@link #informationSequence} (Exceptions, special conditions and supporting information applicable for this service or product.) 3944 */ 3945 public List<PositiveIntType> getInformationSequence() { 3946 if (this.informationSequence == null) 3947 this.informationSequence = new ArrayList<PositiveIntType>(); 3948 return this.informationSequence; 3949 } 3950 3951 /** 3952 * @return Returns a reference to <code>this</code> for easy method chaining 3953 */ 3954 public ItemComponent setInformationSequence(List<PositiveIntType> theInformationSequence) { 3955 this.informationSequence = theInformationSequence; 3956 return this; 3957 } 3958 3959 public boolean hasInformationSequence() { 3960 if (this.informationSequence == null) 3961 return false; 3962 for (PositiveIntType item : this.informationSequence) 3963 if (!item.isEmpty()) 3964 return true; 3965 return false; 3966 } 3967 3968 /** 3969 * @return {@link #informationSequence} (Exceptions, special conditions and supporting information applicable for this service or product.) 3970 */ 3971 public PositiveIntType addInformationSequenceElement() {//2 3972 PositiveIntType t = new PositiveIntType(); 3973 if (this.informationSequence == null) 3974 this.informationSequence = new ArrayList<PositiveIntType>(); 3975 this.informationSequence.add(t); 3976 return t; 3977 } 3978 3979 /** 3980 * @param value {@link #informationSequence} (Exceptions, special conditions and supporting information applicable for this service or product.) 3981 */ 3982 public ItemComponent addInformationSequence(int value) { //1 3983 PositiveIntType t = new PositiveIntType(); 3984 t.setValue(value); 3985 if (this.informationSequence == null) 3986 this.informationSequence = new ArrayList<PositiveIntType>(); 3987 this.informationSequence.add(t); 3988 return this; 3989 } 3990 3991 /** 3992 * @param value {@link #informationSequence} (Exceptions, special conditions and supporting information applicable for this service or product.) 3993 */ 3994 public boolean hasInformationSequence(int value) { 3995 if (this.informationSequence == null) 3996 return false; 3997 for (PositiveIntType v : this.informationSequence) 3998 if (v.getValue().equals(value)) // positiveInt 3999 return true; 4000 return false; 4001 } 4002 4003 /** 4004 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 4005 */ 4006 public List<Identifier> getTraceNumber() { 4007 if (this.traceNumber == null) 4008 this.traceNumber = new ArrayList<Identifier>(); 4009 return this.traceNumber; 4010 } 4011 4012 /** 4013 * @return Returns a reference to <code>this</code> for easy method chaining 4014 */ 4015 public ItemComponent setTraceNumber(List<Identifier> theTraceNumber) { 4016 this.traceNumber = theTraceNumber; 4017 return this; 4018 } 4019 4020 public boolean hasTraceNumber() { 4021 if (this.traceNumber == null) 4022 return false; 4023 for (Identifier item : this.traceNumber) 4024 if (!item.isEmpty()) 4025 return true; 4026 return false; 4027 } 4028 4029 public Identifier addTraceNumber() { //3 4030 Identifier t = new Identifier(); 4031 if (this.traceNumber == null) 4032 this.traceNumber = new ArrayList<Identifier>(); 4033 this.traceNumber.add(t); 4034 return t; 4035 } 4036 4037 public ItemComponent addTraceNumber(Identifier t) { //3 4038 if (t == null) 4039 return this; 4040 if (this.traceNumber == null) 4041 this.traceNumber = new ArrayList<Identifier>(); 4042 this.traceNumber.add(t); 4043 return this; 4044 } 4045 4046 /** 4047 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 4048 */ 4049 public Identifier getTraceNumberFirstRep() { 4050 if (getTraceNumber().isEmpty()) { 4051 addTraceNumber(); 4052 } 4053 return getTraceNumber().get(0); 4054 } 4055 4056 /** 4057 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 4058 */ 4059 public CodeableConcept getRevenue() { 4060 if (this.revenue == null) 4061 if (Configuration.errorOnAutoCreate()) 4062 throw new Error("Attempt to auto-create ItemComponent.revenue"); 4063 else if (Configuration.doAutoCreate()) 4064 this.revenue = new CodeableConcept(); // cc 4065 return this.revenue; 4066 } 4067 4068 public boolean hasRevenue() { 4069 return this.revenue != null && !this.revenue.isEmpty(); 4070 } 4071 4072 /** 4073 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 4074 */ 4075 public ItemComponent setRevenue(CodeableConcept value) { 4076 this.revenue = value; 4077 return this; 4078 } 4079 4080 /** 4081 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 4082 */ 4083 public CodeableConcept getCategory() { 4084 if (this.category == null) 4085 if (Configuration.errorOnAutoCreate()) 4086 throw new Error("Attempt to auto-create ItemComponent.category"); 4087 else if (Configuration.doAutoCreate()) 4088 this.category = new CodeableConcept(); // cc 4089 return this.category; 4090 } 4091 4092 public boolean hasCategory() { 4093 return this.category != null && !this.category.isEmpty(); 4094 } 4095 4096 /** 4097 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 4098 */ 4099 public ItemComponent setCategory(CodeableConcept value) { 4100 this.category = value; 4101 return this; 4102 } 4103 4104 /** 4105 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 4106 */ 4107 public CodeableConcept getProductOrService() { 4108 if (this.productOrService == null) 4109 if (Configuration.errorOnAutoCreate()) 4110 throw new Error("Attempt to auto-create ItemComponent.productOrService"); 4111 else if (Configuration.doAutoCreate()) 4112 this.productOrService = new CodeableConcept(); // cc 4113 return this.productOrService; 4114 } 4115 4116 public boolean hasProductOrService() { 4117 return this.productOrService != null && !this.productOrService.isEmpty(); 4118 } 4119 4120 /** 4121 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 4122 */ 4123 public ItemComponent setProductOrService(CodeableConcept value) { 4124 this.productOrService = value; 4125 return this; 4126 } 4127 4128 /** 4129 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 4130 */ 4131 public CodeableConcept getProductOrServiceEnd() { 4132 if (this.productOrServiceEnd == null) 4133 if (Configuration.errorOnAutoCreate()) 4134 throw new Error("Attempt to auto-create ItemComponent.productOrServiceEnd"); 4135 else if (Configuration.doAutoCreate()) 4136 this.productOrServiceEnd = new CodeableConcept(); // cc 4137 return this.productOrServiceEnd; 4138 } 4139 4140 public boolean hasProductOrServiceEnd() { 4141 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 4142 } 4143 4144 /** 4145 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 4146 */ 4147 public ItemComponent setProductOrServiceEnd(CodeableConcept value) { 4148 this.productOrServiceEnd = value; 4149 return this; 4150 } 4151 4152 /** 4153 * @return {@link #request} (Request or Referral for Goods or Service to be rendered.) 4154 */ 4155 public List<Reference> getRequest() { 4156 if (this.request == null) 4157 this.request = new ArrayList<Reference>(); 4158 return this.request; 4159 } 4160 4161 /** 4162 * @return Returns a reference to <code>this</code> for easy method chaining 4163 */ 4164 public ItemComponent setRequest(List<Reference> theRequest) { 4165 this.request = theRequest; 4166 return this; 4167 } 4168 4169 public boolean hasRequest() { 4170 if (this.request == null) 4171 return false; 4172 for (Reference item : this.request) 4173 if (!item.isEmpty()) 4174 return true; 4175 return false; 4176 } 4177 4178 public Reference addRequest() { //3 4179 Reference t = new Reference(); 4180 if (this.request == null) 4181 this.request = new ArrayList<Reference>(); 4182 this.request.add(t); 4183 return t; 4184 } 4185 4186 public ItemComponent addRequest(Reference t) { //3 4187 if (t == null) 4188 return this; 4189 if (this.request == null) 4190 this.request = new ArrayList<Reference>(); 4191 this.request.add(t); 4192 return this; 4193 } 4194 4195 /** 4196 * @return The first repetition of repeating field {@link #request}, creating it if it does not already exist {3} 4197 */ 4198 public Reference getRequestFirstRep() { 4199 if (getRequest().isEmpty()) { 4200 addRequest(); 4201 } 4202 return getRequest().get(0); 4203 } 4204 4205 /** 4206 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 4207 */ 4208 public List<CodeableConcept> getModifier() { 4209 if (this.modifier == null) 4210 this.modifier = new ArrayList<CodeableConcept>(); 4211 return this.modifier; 4212 } 4213 4214 /** 4215 * @return Returns a reference to <code>this</code> for easy method chaining 4216 */ 4217 public ItemComponent setModifier(List<CodeableConcept> theModifier) { 4218 this.modifier = theModifier; 4219 return this; 4220 } 4221 4222 public boolean hasModifier() { 4223 if (this.modifier == null) 4224 return false; 4225 for (CodeableConcept item : this.modifier) 4226 if (!item.isEmpty()) 4227 return true; 4228 return false; 4229 } 4230 4231 public CodeableConcept addModifier() { //3 4232 CodeableConcept t = new CodeableConcept(); 4233 if (this.modifier == null) 4234 this.modifier = new ArrayList<CodeableConcept>(); 4235 this.modifier.add(t); 4236 return t; 4237 } 4238 4239 public ItemComponent addModifier(CodeableConcept t) { //3 4240 if (t == null) 4241 return this; 4242 if (this.modifier == null) 4243 this.modifier = new ArrayList<CodeableConcept>(); 4244 this.modifier.add(t); 4245 return this; 4246 } 4247 4248 /** 4249 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 4250 */ 4251 public CodeableConcept getModifierFirstRep() { 4252 if (getModifier().isEmpty()) { 4253 addModifier(); 4254 } 4255 return getModifier().get(0); 4256 } 4257 4258 /** 4259 * @return {@link #programCode} (Identifies the program under which this may be recovered.) 4260 */ 4261 public List<CodeableConcept> getProgramCode() { 4262 if (this.programCode == null) 4263 this.programCode = new ArrayList<CodeableConcept>(); 4264 return this.programCode; 4265 } 4266 4267 /** 4268 * @return Returns a reference to <code>this</code> for easy method chaining 4269 */ 4270 public ItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 4271 this.programCode = theProgramCode; 4272 return this; 4273 } 4274 4275 public boolean hasProgramCode() { 4276 if (this.programCode == null) 4277 return false; 4278 for (CodeableConcept item : this.programCode) 4279 if (!item.isEmpty()) 4280 return true; 4281 return false; 4282 } 4283 4284 public CodeableConcept addProgramCode() { //3 4285 CodeableConcept t = new CodeableConcept(); 4286 if (this.programCode == null) 4287 this.programCode = new ArrayList<CodeableConcept>(); 4288 this.programCode.add(t); 4289 return t; 4290 } 4291 4292 public ItemComponent addProgramCode(CodeableConcept t) { //3 4293 if (t == null) 4294 return this; 4295 if (this.programCode == null) 4296 this.programCode = new ArrayList<CodeableConcept>(); 4297 this.programCode.add(t); 4298 return this; 4299 } 4300 4301 /** 4302 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist {3} 4303 */ 4304 public CodeableConcept getProgramCodeFirstRep() { 4305 if (getProgramCode().isEmpty()) { 4306 addProgramCode(); 4307 } 4308 return getProgramCode().get(0); 4309 } 4310 4311 /** 4312 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 4313 */ 4314 public DataType getServiced() { 4315 return this.serviced; 4316 } 4317 4318 /** 4319 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 4320 */ 4321 public DateType getServicedDateType() throws FHIRException { 4322 if (this.serviced == null) 4323 this.serviced = new DateType(); 4324 if (!(this.serviced instanceof DateType)) 4325 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.serviced.getClass().getName()+" was encountered"); 4326 return (DateType) this.serviced; 4327 } 4328 4329 public boolean hasServicedDateType() { 4330 return this.serviced instanceof DateType; 4331 } 4332 4333 /** 4334 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 4335 */ 4336 public Period getServicedPeriod() throws FHIRException { 4337 if (this.serviced == null) 4338 this.serviced = new Period(); 4339 if (!(this.serviced instanceof Period)) 4340 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.serviced.getClass().getName()+" was encountered"); 4341 return (Period) this.serviced; 4342 } 4343 4344 public boolean hasServicedPeriod() { 4345 return this.serviced instanceof Period; 4346 } 4347 4348 public boolean hasServiced() { 4349 return this.serviced != null && !this.serviced.isEmpty(); 4350 } 4351 4352 /** 4353 * @param value {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 4354 */ 4355 public ItemComponent setServiced(DataType value) { 4356 if (value != null && !(value instanceof DateType || value instanceof Period)) 4357 throw new FHIRException("Not the right type for ExplanationOfBenefit.item.serviced[x]: "+value.fhirType()); 4358 this.serviced = value; 4359 return this; 4360 } 4361 4362 /** 4363 * @return {@link #location} (Where the product or service was provided.) 4364 */ 4365 public DataType getLocation() { 4366 return this.location; 4367 } 4368 4369 /** 4370 * @return {@link #location} (Where the product or service was provided.) 4371 */ 4372 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 4373 if (this.location == null) 4374 this.location = new CodeableConcept(); 4375 if (!(this.location instanceof CodeableConcept)) 4376 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.location.getClass().getName()+" was encountered"); 4377 return (CodeableConcept) this.location; 4378 } 4379 4380 public boolean hasLocationCodeableConcept() { 4381 return this.location instanceof CodeableConcept; 4382 } 4383 4384 /** 4385 * @return {@link #location} (Where the product or service was provided.) 4386 */ 4387 public Address getLocationAddress() throws FHIRException { 4388 if (this.location == null) 4389 this.location = new Address(); 4390 if (!(this.location instanceof Address)) 4391 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 4392 return (Address) this.location; 4393 } 4394 4395 public boolean hasLocationAddress() { 4396 return this.location instanceof Address; 4397 } 4398 4399 /** 4400 * @return {@link #location} (Where the product or service was provided.) 4401 */ 4402 public Reference getLocationReference() throws FHIRException { 4403 if (this.location == null) 4404 this.location = new Reference(); 4405 if (!(this.location instanceof Reference)) 4406 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 4407 return (Reference) this.location; 4408 } 4409 4410 public boolean hasLocationReference() { 4411 return this.location instanceof Reference; 4412 } 4413 4414 public boolean hasLocation() { 4415 return this.location != null && !this.location.isEmpty(); 4416 } 4417 4418 /** 4419 * @param value {@link #location} (Where the product or service was provided.) 4420 */ 4421 public ItemComponent setLocation(DataType value) { 4422 if (value != null && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 4423 throw new FHIRException("Not the right type for ExplanationOfBenefit.item.location[x]: "+value.fhirType()); 4424 this.location = value; 4425 return this; 4426 } 4427 4428 /** 4429 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 4430 */ 4431 public Money getPatientPaid() { 4432 if (this.patientPaid == null) 4433 if (Configuration.errorOnAutoCreate()) 4434 throw new Error("Attempt to auto-create ItemComponent.patientPaid"); 4435 else if (Configuration.doAutoCreate()) 4436 this.patientPaid = new Money(); // cc 4437 return this.patientPaid; 4438 } 4439 4440 public boolean hasPatientPaid() { 4441 return this.patientPaid != null && !this.patientPaid.isEmpty(); 4442 } 4443 4444 /** 4445 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 4446 */ 4447 public ItemComponent setPatientPaid(Money value) { 4448 this.patientPaid = value; 4449 return this; 4450 } 4451 4452 /** 4453 * @return {@link #quantity} (The number of repetitions of a service or product.) 4454 */ 4455 public Quantity getQuantity() { 4456 if (this.quantity == null) 4457 if (Configuration.errorOnAutoCreate()) 4458 throw new Error("Attempt to auto-create ItemComponent.quantity"); 4459 else if (Configuration.doAutoCreate()) 4460 this.quantity = new Quantity(); // cc 4461 return this.quantity; 4462 } 4463 4464 public boolean hasQuantity() { 4465 return this.quantity != null && !this.quantity.isEmpty(); 4466 } 4467 4468 /** 4469 * @param value {@link #quantity} (The number of repetitions of a service or product.) 4470 */ 4471 public ItemComponent setQuantity(Quantity value) { 4472 this.quantity = value; 4473 return this; 4474 } 4475 4476 /** 4477 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 4478 */ 4479 public Money getUnitPrice() { 4480 if (this.unitPrice == null) 4481 if (Configuration.errorOnAutoCreate()) 4482 throw new Error("Attempt to auto-create ItemComponent.unitPrice"); 4483 else if (Configuration.doAutoCreate()) 4484 this.unitPrice = new Money(); // cc 4485 return this.unitPrice; 4486 } 4487 4488 public boolean hasUnitPrice() { 4489 return this.unitPrice != null && !this.unitPrice.isEmpty(); 4490 } 4491 4492 /** 4493 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 4494 */ 4495 public ItemComponent setUnitPrice(Money value) { 4496 this.unitPrice = value; 4497 return this; 4498 } 4499 4500 /** 4501 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 4502 */ 4503 public DecimalType getFactorElement() { 4504 if (this.factor == null) 4505 if (Configuration.errorOnAutoCreate()) 4506 throw new Error("Attempt to auto-create ItemComponent.factor"); 4507 else if (Configuration.doAutoCreate()) 4508 this.factor = new DecimalType(); // bb 4509 return this.factor; 4510 } 4511 4512 public boolean hasFactorElement() { 4513 return this.factor != null && !this.factor.isEmpty(); 4514 } 4515 4516 public boolean hasFactor() { 4517 return this.factor != null && !this.factor.isEmpty(); 4518 } 4519 4520 /** 4521 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 4522 */ 4523 public ItemComponent setFactorElement(DecimalType value) { 4524 this.factor = value; 4525 return this; 4526 } 4527 4528 /** 4529 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4530 */ 4531 public BigDecimal getFactor() { 4532 return this.factor == null ? null : this.factor.getValue(); 4533 } 4534 4535 /** 4536 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4537 */ 4538 public ItemComponent setFactor(BigDecimal value) { 4539 if (value == null) 4540 this.factor = null; 4541 else { 4542 if (this.factor == null) 4543 this.factor = new DecimalType(); 4544 this.factor.setValue(value); 4545 } 4546 return this; 4547 } 4548 4549 /** 4550 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4551 */ 4552 public ItemComponent setFactor(long value) { 4553 this.factor = new DecimalType(); 4554 this.factor.setValue(value); 4555 return this; 4556 } 4557 4558 /** 4559 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 4560 */ 4561 public ItemComponent setFactor(double value) { 4562 this.factor = new DecimalType(); 4563 this.factor.setValue(value); 4564 return this; 4565 } 4566 4567 /** 4568 * @return {@link #tax} (The total of taxes applicable for this product or service.) 4569 */ 4570 public Money getTax() { 4571 if (this.tax == null) 4572 if (Configuration.errorOnAutoCreate()) 4573 throw new Error("Attempt to auto-create ItemComponent.tax"); 4574 else if (Configuration.doAutoCreate()) 4575 this.tax = new Money(); // cc 4576 return this.tax; 4577 } 4578 4579 public boolean hasTax() { 4580 return this.tax != null && !this.tax.isEmpty(); 4581 } 4582 4583 /** 4584 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 4585 */ 4586 public ItemComponent setTax(Money value) { 4587 this.tax = value; 4588 return this; 4589 } 4590 4591 /** 4592 * @return {@link #net} (The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor.) 4593 */ 4594 public Money getNet() { 4595 if (this.net == null) 4596 if (Configuration.errorOnAutoCreate()) 4597 throw new Error("Attempt to auto-create ItemComponent.net"); 4598 else if (Configuration.doAutoCreate()) 4599 this.net = new Money(); // cc 4600 return this.net; 4601 } 4602 4603 public boolean hasNet() { 4604 return this.net != null && !this.net.isEmpty(); 4605 } 4606 4607 /** 4608 * @param value {@link #net} (The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor.) 4609 */ 4610 public ItemComponent setNet(Money value) { 4611 this.net = value; 4612 return this; 4613 } 4614 4615 /** 4616 * @return {@link #udi} (Unique Device Identifiers associated with this line item.) 4617 */ 4618 public List<Reference> getUdi() { 4619 if (this.udi == null) 4620 this.udi = new ArrayList<Reference>(); 4621 return this.udi; 4622 } 4623 4624 /** 4625 * @return Returns a reference to <code>this</code> for easy method chaining 4626 */ 4627 public ItemComponent setUdi(List<Reference> theUdi) { 4628 this.udi = theUdi; 4629 return this; 4630 } 4631 4632 public boolean hasUdi() { 4633 if (this.udi == null) 4634 return false; 4635 for (Reference item : this.udi) 4636 if (!item.isEmpty()) 4637 return true; 4638 return false; 4639 } 4640 4641 public Reference addUdi() { //3 4642 Reference t = new Reference(); 4643 if (this.udi == null) 4644 this.udi = new ArrayList<Reference>(); 4645 this.udi.add(t); 4646 return t; 4647 } 4648 4649 public ItemComponent addUdi(Reference t) { //3 4650 if (t == null) 4651 return this; 4652 if (this.udi == null) 4653 this.udi = new ArrayList<Reference>(); 4654 this.udi.add(t); 4655 return this; 4656 } 4657 4658 /** 4659 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist {3} 4660 */ 4661 public Reference getUdiFirstRep() { 4662 if (getUdi().isEmpty()) { 4663 addUdi(); 4664 } 4665 return getUdi().get(0); 4666 } 4667 4668 /** 4669 * @return {@link #bodySite} (Physical location where the service is performed or applies.) 4670 */ 4671 public List<ItemBodySiteComponent> getBodySite() { 4672 if (this.bodySite == null) 4673 this.bodySite = new ArrayList<ItemBodySiteComponent>(); 4674 return this.bodySite; 4675 } 4676 4677 /** 4678 * @return Returns a reference to <code>this</code> for easy method chaining 4679 */ 4680 public ItemComponent setBodySite(List<ItemBodySiteComponent> theBodySite) { 4681 this.bodySite = theBodySite; 4682 return this; 4683 } 4684 4685 public boolean hasBodySite() { 4686 if (this.bodySite == null) 4687 return false; 4688 for (ItemBodySiteComponent item : this.bodySite) 4689 if (!item.isEmpty()) 4690 return true; 4691 return false; 4692 } 4693 4694 public ItemBodySiteComponent addBodySite() { //3 4695 ItemBodySiteComponent t = new ItemBodySiteComponent(); 4696 if (this.bodySite == null) 4697 this.bodySite = new ArrayList<ItemBodySiteComponent>(); 4698 this.bodySite.add(t); 4699 return t; 4700 } 4701 4702 public ItemComponent addBodySite(ItemBodySiteComponent t) { //3 4703 if (t == null) 4704 return this; 4705 if (this.bodySite == null) 4706 this.bodySite = new ArrayList<ItemBodySiteComponent>(); 4707 this.bodySite.add(t); 4708 return this; 4709 } 4710 4711 /** 4712 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist {3} 4713 */ 4714 public ItemBodySiteComponent getBodySiteFirstRep() { 4715 if (getBodySite().isEmpty()) { 4716 addBodySite(); 4717 } 4718 return getBodySite().get(0); 4719 } 4720 4721 /** 4722 * @return {@link #encounter} (Healthcare encounters related to this claim.) 4723 */ 4724 public List<Reference> getEncounter() { 4725 if (this.encounter == null) 4726 this.encounter = new ArrayList<Reference>(); 4727 return this.encounter; 4728 } 4729 4730 /** 4731 * @return Returns a reference to <code>this</code> for easy method chaining 4732 */ 4733 public ItemComponent setEncounter(List<Reference> theEncounter) { 4734 this.encounter = theEncounter; 4735 return this; 4736 } 4737 4738 public boolean hasEncounter() { 4739 if (this.encounter == null) 4740 return false; 4741 for (Reference item : this.encounter) 4742 if (!item.isEmpty()) 4743 return true; 4744 return false; 4745 } 4746 4747 public Reference addEncounter() { //3 4748 Reference t = new Reference(); 4749 if (this.encounter == null) 4750 this.encounter = new ArrayList<Reference>(); 4751 this.encounter.add(t); 4752 return t; 4753 } 4754 4755 public ItemComponent addEncounter(Reference t) { //3 4756 if (t == null) 4757 return this; 4758 if (this.encounter == null) 4759 this.encounter = new ArrayList<Reference>(); 4760 this.encounter.add(t); 4761 return this; 4762 } 4763 4764 /** 4765 * @return The first repetition of repeating field {@link #encounter}, creating it if it does not already exist {3} 4766 */ 4767 public Reference getEncounterFirstRep() { 4768 if (getEncounter().isEmpty()) { 4769 addEncounter(); 4770 } 4771 return getEncounter().get(0); 4772 } 4773 4774 /** 4775 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 4776 */ 4777 public List<PositiveIntType> getNoteNumber() { 4778 if (this.noteNumber == null) 4779 this.noteNumber = new ArrayList<PositiveIntType>(); 4780 return this.noteNumber; 4781 } 4782 4783 /** 4784 * @return Returns a reference to <code>this</code> for easy method chaining 4785 */ 4786 public ItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 4787 this.noteNumber = theNoteNumber; 4788 return this; 4789 } 4790 4791 public boolean hasNoteNumber() { 4792 if (this.noteNumber == null) 4793 return false; 4794 for (PositiveIntType item : this.noteNumber) 4795 if (!item.isEmpty()) 4796 return true; 4797 return false; 4798 } 4799 4800 /** 4801 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 4802 */ 4803 public PositiveIntType addNoteNumberElement() {//2 4804 PositiveIntType t = new PositiveIntType(); 4805 if (this.noteNumber == null) 4806 this.noteNumber = new ArrayList<PositiveIntType>(); 4807 this.noteNumber.add(t); 4808 return t; 4809 } 4810 4811 /** 4812 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 4813 */ 4814 public ItemComponent addNoteNumber(int value) { //1 4815 PositiveIntType t = new PositiveIntType(); 4816 t.setValue(value); 4817 if (this.noteNumber == null) 4818 this.noteNumber = new ArrayList<PositiveIntType>(); 4819 this.noteNumber.add(t); 4820 return this; 4821 } 4822 4823 /** 4824 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 4825 */ 4826 public boolean hasNoteNumber(int value) { 4827 if (this.noteNumber == null) 4828 return false; 4829 for (PositiveIntType v : this.noteNumber) 4830 if (v.getValue().equals(value)) // positiveInt 4831 return true; 4832 return false; 4833 } 4834 4835 /** 4836 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 4837 */ 4838 public ItemReviewOutcomeComponent getReviewOutcome() { 4839 if (this.reviewOutcome == null) 4840 if (Configuration.errorOnAutoCreate()) 4841 throw new Error("Attempt to auto-create ItemComponent.reviewOutcome"); 4842 else if (Configuration.doAutoCreate()) 4843 this.reviewOutcome = new ItemReviewOutcomeComponent(); // cc 4844 return this.reviewOutcome; 4845 } 4846 4847 public boolean hasReviewOutcome() { 4848 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 4849 } 4850 4851 /** 4852 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 4853 */ 4854 public ItemComponent setReviewOutcome(ItemReviewOutcomeComponent value) { 4855 this.reviewOutcome = value; 4856 return this; 4857 } 4858 4859 /** 4860 * @return {@link #adjudication} (If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.) 4861 */ 4862 public List<AdjudicationComponent> getAdjudication() { 4863 if (this.adjudication == null) 4864 this.adjudication = new ArrayList<AdjudicationComponent>(); 4865 return this.adjudication; 4866 } 4867 4868 /** 4869 * @return Returns a reference to <code>this</code> for easy method chaining 4870 */ 4871 public ItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 4872 this.adjudication = theAdjudication; 4873 return this; 4874 } 4875 4876 public boolean hasAdjudication() { 4877 if (this.adjudication == null) 4878 return false; 4879 for (AdjudicationComponent item : this.adjudication) 4880 if (!item.isEmpty()) 4881 return true; 4882 return false; 4883 } 4884 4885 public AdjudicationComponent addAdjudication() { //3 4886 AdjudicationComponent t = new AdjudicationComponent(); 4887 if (this.adjudication == null) 4888 this.adjudication = new ArrayList<AdjudicationComponent>(); 4889 this.adjudication.add(t); 4890 return t; 4891 } 4892 4893 public ItemComponent addAdjudication(AdjudicationComponent t) { //3 4894 if (t == null) 4895 return this; 4896 if (this.adjudication == null) 4897 this.adjudication = new ArrayList<AdjudicationComponent>(); 4898 this.adjudication.add(t); 4899 return this; 4900 } 4901 4902 /** 4903 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 4904 */ 4905 public AdjudicationComponent getAdjudicationFirstRep() { 4906 if (getAdjudication().isEmpty()) { 4907 addAdjudication(); 4908 } 4909 return getAdjudication().get(0); 4910 } 4911 4912 /** 4913 * @return {@link #detail} (Second-tier of goods and services.) 4914 */ 4915 public List<DetailComponent> getDetail() { 4916 if (this.detail == null) 4917 this.detail = new ArrayList<DetailComponent>(); 4918 return this.detail; 4919 } 4920 4921 /** 4922 * @return Returns a reference to <code>this</code> for easy method chaining 4923 */ 4924 public ItemComponent setDetail(List<DetailComponent> theDetail) { 4925 this.detail = theDetail; 4926 return this; 4927 } 4928 4929 public boolean hasDetail() { 4930 if (this.detail == null) 4931 return false; 4932 for (DetailComponent item : this.detail) 4933 if (!item.isEmpty()) 4934 return true; 4935 return false; 4936 } 4937 4938 public DetailComponent addDetail() { //3 4939 DetailComponent t = new DetailComponent(); 4940 if (this.detail == null) 4941 this.detail = new ArrayList<DetailComponent>(); 4942 this.detail.add(t); 4943 return t; 4944 } 4945 4946 public ItemComponent addDetail(DetailComponent t) { //3 4947 if (t == null) 4948 return this; 4949 if (this.detail == null) 4950 this.detail = new ArrayList<DetailComponent>(); 4951 this.detail.add(t); 4952 return this; 4953 } 4954 4955 /** 4956 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist {3} 4957 */ 4958 public DetailComponent getDetailFirstRep() { 4959 if (getDetail().isEmpty()) { 4960 addDetail(); 4961 } 4962 return getDetail().get(0); 4963 } 4964 4965 protected void listChildren(List<Property> children) { 4966 super.listChildren(children); 4967 children.add(new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence)); 4968 children.add(new Property("careTeamSequence", "positiveInt", "Care team members related to this service or product.", 0, java.lang.Integer.MAX_VALUE, careTeamSequence)); 4969 children.add(new Property("diagnosisSequence", "positiveInt", "Diagnoses applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, diagnosisSequence)); 4970 children.add(new Property("procedureSequence", "positiveInt", "Procedures applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, procedureSequence)); 4971 children.add(new Property("informationSequence", "positiveInt", "Exceptions, special conditions and supporting information applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, informationSequence)); 4972 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 4973 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 4974 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 4975 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 4976 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 4977 children.add(new Property("request", "Reference(DeviceRequest|MedicationRequest|NutritionOrder|ServiceRequest|SupplyRequest|VisionPrescription)", "Request or Referral for Goods or Service to be rendered.", 0, java.lang.Integer.MAX_VALUE, request)); 4978 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 4979 children.add(new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 4980 children.add(new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced)); 4981 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location)); 4982 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 4983 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 4984 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 4985 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 4986 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 4987 children.add(new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor.", 0, 1, net)); 4988 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 4989 children.add(new Property("bodySite", "", "Physical location where the service is performed or applies.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 4990 children.add(new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter)); 4991 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 4992 children.add(new Property("reviewOutcome", "", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 4993 children.add(new Property("adjudication", "", "If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 4994 children.add(new Property("detail", "", "Second-tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, detail)); 4995 } 4996 4997 @Override 4998 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4999 switch (_hash) { 5000 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A number to uniquely identify item entries.", 0, 1, sequence); 5001 case 1070083823: /*careTeamSequence*/ return new Property("careTeamSequence", "positiveInt", "Care team members related to this service or product.", 0, java.lang.Integer.MAX_VALUE, careTeamSequence); 5002 case -909769262: /*diagnosisSequence*/ return new Property("diagnosisSequence", "positiveInt", "Diagnoses applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, diagnosisSequence); 5003 case -808920140: /*procedureSequence*/ return new Property("procedureSequence", "positiveInt", "Procedures applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, procedureSequence); 5004 case -702585587: /*informationSequence*/ return new Property("informationSequence", "positiveInt", "Exceptions, special conditions and supporting information applicable for this service or product.", 0, java.lang.Integer.MAX_VALUE, informationSequence); 5005 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 5006 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 5007 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 5008 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 5009 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 5010 case 1095692943: /*request*/ return new Property("request", "Reference(DeviceRequest|MedicationRequest|NutritionOrder|ServiceRequest|SupplyRequest|VisionPrescription)", "Request or Referral for Goods or Service to be rendered.", 0, java.lang.Integer.MAX_VALUE, request); 5011 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 5012 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 5013 case -1927922223: /*serviced[x]*/ return new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 5014 case 1379209295: /*serviced*/ return new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 5015 case 363246749: /*servicedDate*/ return new Property("serviced[x]", "date", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 5016 case 1534966512: /*servicedPeriod*/ return new Property("serviced[x]", "Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 5017 case 552316075: /*location[x]*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location); 5018 case 1901043637: /*location*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location); 5019 case -1224800468: /*locationCodeableConcept*/ return new Property("location[x]", "CodeableConcept", "Where the product or service was provided.", 0, 1, location); 5020 case -1280020865: /*locationAddress*/ return new Property("location[x]", "Address", "Where the product or service was provided.", 0, 1, location); 5021 case 755866390: /*locationReference*/ return new Property("location[x]", "Reference(Location)", "Where the product or service was provided.", 0, 1, location); 5022 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 5023 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 5024 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 5025 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 5026 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 5027 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the line item. Net = unit price * quantity * factor.", 0, 1, net); 5028 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 5029 case 1702620169: /*bodySite*/ return new Property("bodySite", "", "Physical location where the service is performed or applies.", 0, java.lang.Integer.MAX_VALUE, bodySite); 5030 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter); 5031 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 5032 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 5033 case -231349275: /*adjudication*/ return new Property("adjudication", "", "If this item is a group then the values here are a summary of the adjudication of the detail items. If this item is a simple product or service then this is the result of the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, adjudication); 5034 case -1335224239: /*detail*/ return new Property("detail", "", "Second-tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, detail); 5035 default: return super.getNamedProperty(_hash, _name, _checkValid); 5036 } 5037 5038 } 5039 5040 @Override 5041 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5042 switch (hash) { 5043 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 5044 case 1070083823: /*careTeamSequence*/ return this.careTeamSequence == null ? new Base[0] : this.careTeamSequence.toArray(new Base[this.careTeamSequence.size()]); // PositiveIntType 5045 case -909769262: /*diagnosisSequence*/ return this.diagnosisSequence == null ? new Base[0] : this.diagnosisSequence.toArray(new Base[this.diagnosisSequence.size()]); // PositiveIntType 5046 case -808920140: /*procedureSequence*/ return this.procedureSequence == null ? new Base[0] : this.procedureSequence.toArray(new Base[this.procedureSequence.size()]); // PositiveIntType 5047 case -702585587: /*informationSequence*/ return this.informationSequence == null ? new Base[0] : this.informationSequence.toArray(new Base[this.informationSequence.size()]); // PositiveIntType 5048 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 5049 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 5050 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 5051 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 5052 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 5053 case 1095692943: /*request*/ return this.request == null ? new Base[0] : this.request.toArray(new Base[this.request.size()]); // Reference 5054 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 5055 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 5056 case 1379209295: /*serviced*/ return this.serviced == null ? new Base[0] : new Base[] {this.serviced}; // DataType 5057 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // DataType 5058 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 5059 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 5060 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 5061 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 5062 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 5063 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 5064 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 5065 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // ItemBodySiteComponent 5066 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 5067 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 5068 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ItemReviewOutcomeComponent 5069 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 5070 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // DetailComponent 5071 default: return super.getProperty(hash, name, checkValid); 5072 } 5073 5074 } 5075 5076 @Override 5077 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5078 switch (hash) { 5079 case 1349547969: // sequence 5080 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 5081 return value; 5082 case 1070083823: // careTeamSequence 5083 this.getCareTeamSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 5084 return value; 5085 case -909769262: // diagnosisSequence 5086 this.getDiagnosisSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 5087 return value; 5088 case -808920140: // procedureSequence 5089 this.getProcedureSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 5090 return value; 5091 case -702585587: // informationSequence 5092 this.getInformationSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 5093 return value; 5094 case 82505966: // traceNumber 5095 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 5096 return value; 5097 case 1099842588: // revenue 5098 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5099 return value; 5100 case 50511102: // category 5101 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5102 return value; 5103 case 1957227299: // productOrService 5104 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5105 return value; 5106 case -717476168: // productOrServiceEnd 5107 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5108 return value; 5109 case 1095692943: // request 5110 this.getRequest().add(TypeConvertor.castToReference(value)); // Reference 5111 return value; 5112 case -615513385: // modifier 5113 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5114 return value; 5115 case 1010065041: // programCode 5116 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5117 return value; 5118 case 1379209295: // serviced 5119 this.serviced = TypeConvertor.castToType(value); // DataType 5120 return value; 5121 case 1901043637: // location 5122 this.location = TypeConvertor.castToType(value); // DataType 5123 return value; 5124 case 525514609: // patientPaid 5125 this.patientPaid = TypeConvertor.castToMoney(value); // Money 5126 return value; 5127 case -1285004149: // quantity 5128 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 5129 return value; 5130 case -486196699: // unitPrice 5131 this.unitPrice = TypeConvertor.castToMoney(value); // Money 5132 return value; 5133 case -1282148017: // factor 5134 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 5135 return value; 5136 case 114603: // tax 5137 this.tax = TypeConvertor.castToMoney(value); // Money 5138 return value; 5139 case 108957: // net 5140 this.net = TypeConvertor.castToMoney(value); // Money 5141 return value; 5142 case 115642: // udi 5143 this.getUdi().add(TypeConvertor.castToReference(value)); // Reference 5144 return value; 5145 case 1702620169: // bodySite 5146 this.getBodySite().add((ItemBodySiteComponent) value); // ItemBodySiteComponent 5147 return value; 5148 case 1524132147: // encounter 5149 this.getEncounter().add(TypeConvertor.castToReference(value)); // Reference 5150 return value; 5151 case -1110033957: // noteNumber 5152 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 5153 return value; 5154 case -51825446: // reviewOutcome 5155 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 5156 return value; 5157 case -231349275: // adjudication 5158 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 5159 return value; 5160 case -1335224239: // detail 5161 this.getDetail().add((DetailComponent) value); // DetailComponent 5162 return value; 5163 default: return super.setProperty(hash, name, value); 5164 } 5165 5166 } 5167 5168 @Override 5169 public Base setProperty(String name, Base value) throws FHIRException { 5170 if (name.equals("sequence")) { 5171 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 5172 } else if (name.equals("careTeamSequence")) { 5173 this.getCareTeamSequence().add(TypeConvertor.castToPositiveInt(value)); 5174 } else if (name.equals("diagnosisSequence")) { 5175 this.getDiagnosisSequence().add(TypeConvertor.castToPositiveInt(value)); 5176 } else if (name.equals("procedureSequence")) { 5177 this.getProcedureSequence().add(TypeConvertor.castToPositiveInt(value)); 5178 } else if (name.equals("informationSequence")) { 5179 this.getInformationSequence().add(TypeConvertor.castToPositiveInt(value)); 5180 } else if (name.equals("traceNumber")) { 5181 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 5182 } else if (name.equals("revenue")) { 5183 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5184 } else if (name.equals("category")) { 5185 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5186 } else if (name.equals("productOrService")) { 5187 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5188 } else if (name.equals("productOrServiceEnd")) { 5189 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5190 } else if (name.equals("request")) { 5191 this.getRequest().add(TypeConvertor.castToReference(value)); 5192 } else if (name.equals("modifier")) { 5193 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 5194 } else if (name.equals("programCode")) { 5195 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); 5196 } else if (name.equals("serviced[x]")) { 5197 this.serviced = TypeConvertor.castToType(value); // DataType 5198 } else if (name.equals("location[x]")) { 5199 this.location = TypeConvertor.castToType(value); // DataType 5200 } else if (name.equals("patientPaid")) { 5201 this.patientPaid = TypeConvertor.castToMoney(value); // Money 5202 } else if (name.equals("quantity")) { 5203 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 5204 } else if (name.equals("unitPrice")) { 5205 this.unitPrice = TypeConvertor.castToMoney(value); // Money 5206 } else if (name.equals("factor")) { 5207 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 5208 } else if (name.equals("tax")) { 5209 this.tax = TypeConvertor.castToMoney(value); // Money 5210 } else if (name.equals("net")) { 5211 this.net = TypeConvertor.castToMoney(value); // Money 5212 } else if (name.equals("udi")) { 5213 this.getUdi().add(TypeConvertor.castToReference(value)); 5214 } else if (name.equals("bodySite")) { 5215 this.getBodySite().add((ItemBodySiteComponent) value); 5216 } else if (name.equals("encounter")) { 5217 this.getEncounter().add(TypeConvertor.castToReference(value)); 5218 } else if (name.equals("noteNumber")) { 5219 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 5220 } else if (name.equals("reviewOutcome")) { 5221 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 5222 } else if (name.equals("adjudication")) { 5223 this.getAdjudication().add((AdjudicationComponent) value); 5224 } else if (name.equals("detail")) { 5225 this.getDetail().add((DetailComponent) value); 5226 } else 5227 return super.setProperty(name, value); 5228 return value; 5229 } 5230 5231 @Override 5232 public void removeChild(String name, Base value) throws FHIRException { 5233 if (name.equals("sequence")) { 5234 this.sequence = null; 5235 } else if (name.equals("careTeamSequence")) { 5236 this.getCareTeamSequence().remove(value); 5237 } else if (name.equals("diagnosisSequence")) { 5238 this.getDiagnosisSequence().remove(value); 5239 } else if (name.equals("procedureSequence")) { 5240 this.getProcedureSequence().remove(value); 5241 } else if (name.equals("informationSequence")) { 5242 this.getInformationSequence().remove(value); 5243 } else if (name.equals("traceNumber")) { 5244 this.getTraceNumber().remove(value); 5245 } else if (name.equals("revenue")) { 5246 this.revenue = null; 5247 } else if (name.equals("category")) { 5248 this.category = null; 5249 } else if (name.equals("productOrService")) { 5250 this.productOrService = null; 5251 } else if (name.equals("productOrServiceEnd")) { 5252 this.productOrServiceEnd = null; 5253 } else if (name.equals("request")) { 5254 this.getRequest().remove(value); 5255 } else if (name.equals("modifier")) { 5256 this.getModifier().remove(value); 5257 } else if (name.equals("programCode")) { 5258 this.getProgramCode().remove(value); 5259 } else if (name.equals("serviced[x]")) { 5260 this.serviced = null; 5261 } else if (name.equals("location[x]")) { 5262 this.location = null; 5263 } else if (name.equals("patientPaid")) { 5264 this.patientPaid = null; 5265 } else if (name.equals("quantity")) { 5266 this.quantity = null; 5267 } else if (name.equals("unitPrice")) { 5268 this.unitPrice = null; 5269 } else if (name.equals("factor")) { 5270 this.factor = null; 5271 } else if (name.equals("tax")) { 5272 this.tax = null; 5273 } else if (name.equals("net")) { 5274 this.net = null; 5275 } else if (name.equals("udi")) { 5276 this.getUdi().remove(value); 5277 } else if (name.equals("bodySite")) { 5278 this.getBodySite().remove((ItemBodySiteComponent) value); 5279 } else if (name.equals("encounter")) { 5280 this.getEncounter().remove(value); 5281 } else if (name.equals("noteNumber")) { 5282 this.getNoteNumber().remove(value); 5283 } else if (name.equals("reviewOutcome")) { 5284 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 5285 } else if (name.equals("adjudication")) { 5286 this.getAdjudication().remove((AdjudicationComponent) value); 5287 } else if (name.equals("detail")) { 5288 this.getDetail().remove((DetailComponent) value); 5289 } else 5290 super.removeChild(name, value); 5291 5292 } 5293 5294 @Override 5295 public Base makeProperty(int hash, String name) throws FHIRException { 5296 switch (hash) { 5297 case 1349547969: return getSequenceElement(); 5298 case 1070083823: return addCareTeamSequenceElement(); 5299 case -909769262: return addDiagnosisSequenceElement(); 5300 case -808920140: return addProcedureSequenceElement(); 5301 case -702585587: return addInformationSequenceElement(); 5302 case 82505966: return addTraceNumber(); 5303 case 1099842588: return getRevenue(); 5304 case 50511102: return getCategory(); 5305 case 1957227299: return getProductOrService(); 5306 case -717476168: return getProductOrServiceEnd(); 5307 case 1095692943: return addRequest(); 5308 case -615513385: return addModifier(); 5309 case 1010065041: return addProgramCode(); 5310 case -1927922223: return getServiced(); 5311 case 1379209295: return getServiced(); 5312 case 552316075: return getLocation(); 5313 case 1901043637: return getLocation(); 5314 case 525514609: return getPatientPaid(); 5315 case -1285004149: return getQuantity(); 5316 case -486196699: return getUnitPrice(); 5317 case -1282148017: return getFactorElement(); 5318 case 114603: return getTax(); 5319 case 108957: return getNet(); 5320 case 115642: return addUdi(); 5321 case 1702620169: return addBodySite(); 5322 case 1524132147: return addEncounter(); 5323 case -1110033957: return addNoteNumberElement(); 5324 case -51825446: return getReviewOutcome(); 5325 case -231349275: return addAdjudication(); 5326 case -1335224239: return addDetail(); 5327 default: return super.makeProperty(hash, name); 5328 } 5329 5330 } 5331 5332 @Override 5333 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5334 switch (hash) { 5335 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 5336 case 1070083823: /*careTeamSequence*/ return new String[] {"positiveInt"}; 5337 case -909769262: /*diagnosisSequence*/ return new String[] {"positiveInt"}; 5338 case -808920140: /*procedureSequence*/ return new String[] {"positiveInt"}; 5339 case -702585587: /*informationSequence*/ return new String[] {"positiveInt"}; 5340 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 5341 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 5342 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 5343 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 5344 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 5345 case 1095692943: /*request*/ return new String[] {"Reference"}; 5346 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 5347 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 5348 case 1379209295: /*serviced*/ return new String[] {"date", "Period"}; 5349 case 1901043637: /*location*/ return new String[] {"CodeableConcept", "Address", "Reference"}; 5350 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 5351 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 5352 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 5353 case -1282148017: /*factor*/ return new String[] {"decimal"}; 5354 case 114603: /*tax*/ return new String[] {"Money"}; 5355 case 108957: /*net*/ return new String[] {"Money"}; 5356 case 115642: /*udi*/ return new String[] {"Reference"}; 5357 case 1702620169: /*bodySite*/ return new String[] {}; 5358 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 5359 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 5360 case -51825446: /*reviewOutcome*/ return new String[] {}; 5361 case -231349275: /*adjudication*/ return new String[] {}; 5362 case -1335224239: /*detail*/ return new String[] {}; 5363 default: return super.getTypesForProperty(hash, name); 5364 } 5365 5366 } 5367 5368 @Override 5369 public Base addChild(String name) throws FHIRException { 5370 if (name.equals("sequence")) { 5371 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.sequence"); 5372 } 5373 else if (name.equals("careTeamSequence")) { 5374 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.careTeamSequence"); 5375 } 5376 else if (name.equals("diagnosisSequence")) { 5377 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.diagnosisSequence"); 5378 } 5379 else if (name.equals("procedureSequence")) { 5380 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.procedureSequence"); 5381 } 5382 else if (name.equals("informationSequence")) { 5383 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.informationSequence"); 5384 } 5385 else if (name.equals("traceNumber")) { 5386 return addTraceNumber(); 5387 } 5388 else if (name.equals("revenue")) { 5389 this.revenue = new CodeableConcept(); 5390 return this.revenue; 5391 } 5392 else if (name.equals("category")) { 5393 this.category = new CodeableConcept(); 5394 return this.category; 5395 } 5396 else if (name.equals("productOrService")) { 5397 this.productOrService = new CodeableConcept(); 5398 return this.productOrService; 5399 } 5400 else if (name.equals("productOrServiceEnd")) { 5401 this.productOrServiceEnd = new CodeableConcept(); 5402 return this.productOrServiceEnd; 5403 } 5404 else if (name.equals("request")) { 5405 return addRequest(); 5406 } 5407 else if (name.equals("modifier")) { 5408 return addModifier(); 5409 } 5410 else if (name.equals("programCode")) { 5411 return addProgramCode(); 5412 } 5413 else if (name.equals("servicedDate")) { 5414 this.serviced = new DateType(); 5415 return this.serviced; 5416 } 5417 else if (name.equals("servicedPeriod")) { 5418 this.serviced = new Period(); 5419 return this.serviced; 5420 } 5421 else if (name.equals("locationCodeableConcept")) { 5422 this.location = new CodeableConcept(); 5423 return this.location; 5424 } 5425 else if (name.equals("locationAddress")) { 5426 this.location = new Address(); 5427 return this.location; 5428 } 5429 else if (name.equals("locationReference")) { 5430 this.location = new Reference(); 5431 return this.location; 5432 } 5433 else if (name.equals("patientPaid")) { 5434 this.patientPaid = new Money(); 5435 return this.patientPaid; 5436 } 5437 else if (name.equals("quantity")) { 5438 this.quantity = new Quantity(); 5439 return this.quantity; 5440 } 5441 else if (name.equals("unitPrice")) { 5442 this.unitPrice = new Money(); 5443 return this.unitPrice; 5444 } 5445 else if (name.equals("factor")) { 5446 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.factor"); 5447 } 5448 else if (name.equals("tax")) { 5449 this.tax = new Money(); 5450 return this.tax; 5451 } 5452 else if (name.equals("net")) { 5453 this.net = new Money(); 5454 return this.net; 5455 } 5456 else if (name.equals("udi")) { 5457 return addUdi(); 5458 } 5459 else if (name.equals("bodySite")) { 5460 return addBodySite(); 5461 } 5462 else if (name.equals("encounter")) { 5463 return addEncounter(); 5464 } 5465 else if (name.equals("noteNumber")) { 5466 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.noteNumber"); 5467 } 5468 else if (name.equals("reviewOutcome")) { 5469 this.reviewOutcome = new ItemReviewOutcomeComponent(); 5470 return this.reviewOutcome; 5471 } 5472 else if (name.equals("adjudication")) { 5473 return addAdjudication(); 5474 } 5475 else if (name.equals("detail")) { 5476 return addDetail(); 5477 } 5478 else 5479 return super.addChild(name); 5480 } 5481 5482 public ItemComponent copy() { 5483 ItemComponent dst = new ItemComponent(); 5484 copyValues(dst); 5485 return dst; 5486 } 5487 5488 public void copyValues(ItemComponent dst) { 5489 super.copyValues(dst); 5490 dst.sequence = sequence == null ? null : sequence.copy(); 5491 if (careTeamSequence != null) { 5492 dst.careTeamSequence = new ArrayList<PositiveIntType>(); 5493 for (PositiveIntType i : careTeamSequence) 5494 dst.careTeamSequence.add(i.copy()); 5495 }; 5496 if (diagnosisSequence != null) { 5497 dst.diagnosisSequence = new ArrayList<PositiveIntType>(); 5498 for (PositiveIntType i : diagnosisSequence) 5499 dst.diagnosisSequence.add(i.copy()); 5500 }; 5501 if (procedureSequence != null) { 5502 dst.procedureSequence = new ArrayList<PositiveIntType>(); 5503 for (PositiveIntType i : procedureSequence) 5504 dst.procedureSequence.add(i.copy()); 5505 }; 5506 if (informationSequence != null) { 5507 dst.informationSequence = new ArrayList<PositiveIntType>(); 5508 for (PositiveIntType i : informationSequence) 5509 dst.informationSequence.add(i.copy()); 5510 }; 5511 if (traceNumber != null) { 5512 dst.traceNumber = new ArrayList<Identifier>(); 5513 for (Identifier i : traceNumber) 5514 dst.traceNumber.add(i.copy()); 5515 }; 5516 dst.revenue = revenue == null ? null : revenue.copy(); 5517 dst.category = category == null ? null : category.copy(); 5518 dst.productOrService = productOrService == null ? null : productOrService.copy(); 5519 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 5520 if (request != null) { 5521 dst.request = new ArrayList<Reference>(); 5522 for (Reference i : request) 5523 dst.request.add(i.copy()); 5524 }; 5525 if (modifier != null) { 5526 dst.modifier = new ArrayList<CodeableConcept>(); 5527 for (CodeableConcept i : modifier) 5528 dst.modifier.add(i.copy()); 5529 }; 5530 if (programCode != null) { 5531 dst.programCode = new ArrayList<CodeableConcept>(); 5532 for (CodeableConcept i : programCode) 5533 dst.programCode.add(i.copy()); 5534 }; 5535 dst.serviced = serviced == null ? null : serviced.copy(); 5536 dst.location = location == null ? null : location.copy(); 5537 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 5538 dst.quantity = quantity == null ? null : quantity.copy(); 5539 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 5540 dst.factor = factor == null ? null : factor.copy(); 5541 dst.tax = tax == null ? null : tax.copy(); 5542 dst.net = net == null ? null : net.copy(); 5543 if (udi != null) { 5544 dst.udi = new ArrayList<Reference>(); 5545 for (Reference i : udi) 5546 dst.udi.add(i.copy()); 5547 }; 5548 if (bodySite != null) { 5549 dst.bodySite = new ArrayList<ItemBodySiteComponent>(); 5550 for (ItemBodySiteComponent i : bodySite) 5551 dst.bodySite.add(i.copy()); 5552 }; 5553 if (encounter != null) { 5554 dst.encounter = new ArrayList<Reference>(); 5555 for (Reference i : encounter) 5556 dst.encounter.add(i.copy()); 5557 }; 5558 if (noteNumber != null) { 5559 dst.noteNumber = new ArrayList<PositiveIntType>(); 5560 for (PositiveIntType i : noteNumber) 5561 dst.noteNumber.add(i.copy()); 5562 }; 5563 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 5564 if (adjudication != null) { 5565 dst.adjudication = new ArrayList<AdjudicationComponent>(); 5566 for (AdjudicationComponent i : adjudication) 5567 dst.adjudication.add(i.copy()); 5568 }; 5569 if (detail != null) { 5570 dst.detail = new ArrayList<DetailComponent>(); 5571 for (DetailComponent i : detail) 5572 dst.detail.add(i.copy()); 5573 }; 5574 } 5575 5576 @Override 5577 public boolean equalsDeep(Base other_) { 5578 if (!super.equalsDeep(other_)) 5579 return false; 5580 if (!(other_ instanceof ItemComponent)) 5581 return false; 5582 ItemComponent o = (ItemComponent) other_; 5583 return compareDeep(sequence, o.sequence, true) && compareDeep(careTeamSequence, o.careTeamSequence, true) 5584 && compareDeep(diagnosisSequence, o.diagnosisSequence, true) && compareDeep(procedureSequence, o.procedureSequence, true) 5585 && compareDeep(informationSequence, o.informationSequence, true) && compareDeep(traceNumber, o.traceNumber, true) 5586 && compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 5587 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(request, o.request, true) 5588 && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) && compareDeep(serviced, o.serviced, true) 5589 && compareDeep(location, o.location, true) && compareDeep(patientPaid, o.patientPaid, true) && compareDeep(quantity, o.quantity, true) 5590 && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) && compareDeep(tax, o.tax, true) 5591 && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) && compareDeep(bodySite, o.bodySite, true) 5592 && compareDeep(encounter, o.encounter, true) && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(reviewOutcome, o.reviewOutcome, true) 5593 && compareDeep(adjudication, o.adjudication, true) && compareDeep(detail, o.detail, true); 5594 } 5595 5596 @Override 5597 public boolean equalsShallow(Base other_) { 5598 if (!super.equalsShallow(other_)) 5599 return false; 5600 if (!(other_ instanceof ItemComponent)) 5601 return false; 5602 ItemComponent o = (ItemComponent) other_; 5603 return compareValues(sequence, o.sequence, true) && compareValues(careTeamSequence, o.careTeamSequence, true) 5604 && compareValues(diagnosisSequence, o.diagnosisSequence, true) && compareValues(procedureSequence, o.procedureSequence, true) 5605 && compareValues(informationSequence, o.informationSequence, true) && compareValues(factor, o.factor, true) 5606 && compareValues(noteNumber, o.noteNumber, true); 5607 } 5608 5609 public boolean isEmpty() { 5610 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, careTeamSequence 5611 , diagnosisSequence, procedureSequence, informationSequence, traceNumber, revenue 5612 , category, productOrService, productOrServiceEnd, request, modifier, programCode 5613 , serviced, location, patientPaid, quantity, unitPrice, factor, tax, net, udi 5614 , bodySite, encounter, noteNumber, reviewOutcome, adjudication, detail); 5615 } 5616 5617 public String fhirType() { 5618 return "ExplanationOfBenefit.item"; 5619 5620 } 5621 5622 } 5623 5624 @Block() 5625 public static class ItemBodySiteComponent extends BackboneElement implements IBaseBackboneElement { 5626 /** 5627 * Physical service site on the patient (limb, tooth, etc.). 5628 */ 5629 @Child(name = "site", type = {CodeableReference.class}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5630 @Description(shortDefinition="Location", formalDefinition="Physical service site on the patient (limb, tooth, etc.)." ) 5631 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/tooth") 5632 protected List<CodeableReference> site; 5633 5634 /** 5635 * A region or surface of the bodySite, e.g. limb region or tooth surface(s). 5636 */ 5637 @Child(name = "subSite", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5638 @Description(shortDefinition="Sub-location", formalDefinition="A region or surface of the bodySite, e.g. limb region or tooth surface(s)." ) 5639 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/surface") 5640 protected List<CodeableConcept> subSite; 5641 5642 private static final long serialVersionUID = 1190632415L; 5643 5644 /** 5645 * Constructor 5646 */ 5647 public ItemBodySiteComponent() { 5648 super(); 5649 } 5650 5651 /** 5652 * Constructor 5653 */ 5654 public ItemBodySiteComponent(CodeableReference site) { 5655 super(); 5656 this.addSite(site); 5657 } 5658 5659 /** 5660 * @return {@link #site} (Physical service site on the patient (limb, tooth, etc.).) 5661 */ 5662 public List<CodeableReference> getSite() { 5663 if (this.site == null) 5664 this.site = new ArrayList<CodeableReference>(); 5665 return this.site; 5666 } 5667 5668 /** 5669 * @return Returns a reference to <code>this</code> for easy method chaining 5670 */ 5671 public ItemBodySiteComponent setSite(List<CodeableReference> theSite) { 5672 this.site = theSite; 5673 return this; 5674 } 5675 5676 public boolean hasSite() { 5677 if (this.site == null) 5678 return false; 5679 for (CodeableReference item : this.site) 5680 if (!item.isEmpty()) 5681 return true; 5682 return false; 5683 } 5684 5685 public CodeableReference addSite() { //3 5686 CodeableReference t = new CodeableReference(); 5687 if (this.site == null) 5688 this.site = new ArrayList<CodeableReference>(); 5689 this.site.add(t); 5690 return t; 5691 } 5692 5693 public ItemBodySiteComponent addSite(CodeableReference t) { //3 5694 if (t == null) 5695 return this; 5696 if (this.site == null) 5697 this.site = new ArrayList<CodeableReference>(); 5698 this.site.add(t); 5699 return this; 5700 } 5701 5702 /** 5703 * @return The first repetition of repeating field {@link #site}, creating it if it does not already exist {3} 5704 */ 5705 public CodeableReference getSiteFirstRep() { 5706 if (getSite().isEmpty()) { 5707 addSite(); 5708 } 5709 return getSite().get(0); 5710 } 5711 5712 /** 5713 * @return {@link #subSite} (A region or surface of the bodySite, e.g. limb region or tooth surface(s).) 5714 */ 5715 public List<CodeableConcept> getSubSite() { 5716 if (this.subSite == null) 5717 this.subSite = new ArrayList<CodeableConcept>(); 5718 return this.subSite; 5719 } 5720 5721 /** 5722 * @return Returns a reference to <code>this</code> for easy method chaining 5723 */ 5724 public ItemBodySiteComponent setSubSite(List<CodeableConcept> theSubSite) { 5725 this.subSite = theSubSite; 5726 return this; 5727 } 5728 5729 public boolean hasSubSite() { 5730 if (this.subSite == null) 5731 return false; 5732 for (CodeableConcept item : this.subSite) 5733 if (!item.isEmpty()) 5734 return true; 5735 return false; 5736 } 5737 5738 public CodeableConcept addSubSite() { //3 5739 CodeableConcept t = new CodeableConcept(); 5740 if (this.subSite == null) 5741 this.subSite = new ArrayList<CodeableConcept>(); 5742 this.subSite.add(t); 5743 return t; 5744 } 5745 5746 public ItemBodySiteComponent addSubSite(CodeableConcept t) { //3 5747 if (t == null) 5748 return this; 5749 if (this.subSite == null) 5750 this.subSite = new ArrayList<CodeableConcept>(); 5751 this.subSite.add(t); 5752 return this; 5753 } 5754 5755 /** 5756 * @return The first repetition of repeating field {@link #subSite}, creating it if it does not already exist {3} 5757 */ 5758 public CodeableConcept getSubSiteFirstRep() { 5759 if (getSubSite().isEmpty()) { 5760 addSubSite(); 5761 } 5762 return getSubSite().get(0); 5763 } 5764 5765 protected void listChildren(List<Property> children) { 5766 super.listChildren(children); 5767 children.add(new Property("site", "CodeableReference(BodyStructure)", "Physical service site on the patient (limb, tooth, etc.).", 0, java.lang.Integer.MAX_VALUE, site)); 5768 children.add(new Property("subSite", "CodeableConcept", "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite)); 5769 } 5770 5771 @Override 5772 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5773 switch (_hash) { 5774 case 3530567: /*site*/ return new Property("site", "CodeableReference(BodyStructure)", "Physical service site on the patient (limb, tooth, etc.).", 0, java.lang.Integer.MAX_VALUE, site); 5775 case -1868566105: /*subSite*/ return new Property("subSite", "CodeableConcept", "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite); 5776 default: return super.getNamedProperty(_hash, _name, _checkValid); 5777 } 5778 5779 } 5780 5781 @Override 5782 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5783 switch (hash) { 5784 case 3530567: /*site*/ return this.site == null ? new Base[0] : this.site.toArray(new Base[this.site.size()]); // CodeableReference 5785 case -1868566105: /*subSite*/ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 5786 default: return super.getProperty(hash, name, checkValid); 5787 } 5788 5789 } 5790 5791 @Override 5792 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5793 switch (hash) { 5794 case 3530567: // site 5795 this.getSite().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 5796 return value; 5797 case -1868566105: // subSite 5798 this.getSubSite().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5799 return value; 5800 default: return super.setProperty(hash, name, value); 5801 } 5802 5803 } 5804 5805 @Override 5806 public Base setProperty(String name, Base value) throws FHIRException { 5807 if (name.equals("site")) { 5808 this.getSite().add(TypeConvertor.castToCodeableReference(value)); 5809 } else if (name.equals("subSite")) { 5810 this.getSubSite().add(TypeConvertor.castToCodeableConcept(value)); 5811 } else 5812 return super.setProperty(name, value); 5813 return value; 5814 } 5815 5816 @Override 5817 public void removeChild(String name, Base value) throws FHIRException { 5818 if (name.equals("site")) { 5819 this.getSite().remove(value); 5820 } else if (name.equals("subSite")) { 5821 this.getSubSite().remove(value); 5822 } else 5823 super.removeChild(name, value); 5824 5825 } 5826 5827 @Override 5828 public Base makeProperty(int hash, String name) throws FHIRException { 5829 switch (hash) { 5830 case 3530567: return addSite(); 5831 case -1868566105: return addSubSite(); 5832 default: return super.makeProperty(hash, name); 5833 } 5834 5835 } 5836 5837 @Override 5838 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5839 switch (hash) { 5840 case 3530567: /*site*/ return new String[] {"CodeableReference"}; 5841 case -1868566105: /*subSite*/ return new String[] {"CodeableConcept"}; 5842 default: return super.getTypesForProperty(hash, name); 5843 } 5844 5845 } 5846 5847 @Override 5848 public Base addChild(String name) throws FHIRException { 5849 if (name.equals("site")) { 5850 return addSite(); 5851 } 5852 else if (name.equals("subSite")) { 5853 return addSubSite(); 5854 } 5855 else 5856 return super.addChild(name); 5857 } 5858 5859 public ItemBodySiteComponent copy() { 5860 ItemBodySiteComponent dst = new ItemBodySiteComponent(); 5861 copyValues(dst); 5862 return dst; 5863 } 5864 5865 public void copyValues(ItemBodySiteComponent dst) { 5866 super.copyValues(dst); 5867 if (site != null) { 5868 dst.site = new ArrayList<CodeableReference>(); 5869 for (CodeableReference i : site) 5870 dst.site.add(i.copy()); 5871 }; 5872 if (subSite != null) { 5873 dst.subSite = new ArrayList<CodeableConcept>(); 5874 for (CodeableConcept i : subSite) 5875 dst.subSite.add(i.copy()); 5876 }; 5877 } 5878 5879 @Override 5880 public boolean equalsDeep(Base other_) { 5881 if (!super.equalsDeep(other_)) 5882 return false; 5883 if (!(other_ instanceof ItemBodySiteComponent)) 5884 return false; 5885 ItemBodySiteComponent o = (ItemBodySiteComponent) other_; 5886 return compareDeep(site, o.site, true) && compareDeep(subSite, o.subSite, true); 5887 } 5888 5889 @Override 5890 public boolean equalsShallow(Base other_) { 5891 if (!super.equalsShallow(other_)) 5892 return false; 5893 if (!(other_ instanceof ItemBodySiteComponent)) 5894 return false; 5895 ItemBodySiteComponent o = (ItemBodySiteComponent) other_; 5896 return true; 5897 } 5898 5899 public boolean isEmpty() { 5900 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(site, subSite); 5901 } 5902 5903 public String fhirType() { 5904 return "ExplanationOfBenefit.item.bodySite"; 5905 5906 } 5907 5908 } 5909 5910 @Block() 5911 public static class ItemReviewOutcomeComponent extends BackboneElement implements IBaseBackboneElement { 5912 /** 5913 * The result of the claim, predetermination, or preauthorization adjudication. 5914 */ 5915 @Child(name = "decision", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 5916 @Description(shortDefinition="Result of the adjudication", formalDefinition="The result of the claim, predetermination, or preauthorization adjudication." ) 5917 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-decision") 5918 protected CodeableConcept decision; 5919 5920 /** 5921 * The reasons for the result of the claim, predetermination, or preauthorization adjudication. 5922 */ 5923 @Child(name = "reason", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5924 @Description(shortDefinition="Reason for result of the adjudication", formalDefinition="The reasons for the result of the claim, predetermination, or preauthorization adjudication." ) 5925 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-decision-reason") 5926 protected List<CodeableConcept> reason; 5927 5928 /** 5929 * Reference from the Insurer which is used in later communications which refers to this adjudication. 5930 */ 5931 @Child(name = "preAuthRef", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 5932 @Description(shortDefinition="Preauthorization reference", formalDefinition="Reference from the Insurer which is used in later communications which refers to this adjudication." ) 5933 protected StringType preAuthRef; 5934 5935 /** 5936 * The time frame during which this authorization is effective. 5937 */ 5938 @Child(name = "preAuthPeriod", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 5939 @Description(shortDefinition="Preauthorization reference effective period", formalDefinition="The time frame during which this authorization is effective." ) 5940 protected Period preAuthPeriod; 5941 5942 private static final long serialVersionUID = 2126097594L; 5943 5944 /** 5945 * Constructor 5946 */ 5947 public ItemReviewOutcomeComponent() { 5948 super(); 5949 } 5950 5951 /** 5952 * @return {@link #decision} (The result of the claim, predetermination, or preauthorization adjudication.) 5953 */ 5954 public CodeableConcept getDecision() { 5955 if (this.decision == null) 5956 if (Configuration.errorOnAutoCreate()) 5957 throw new Error("Attempt to auto-create ItemReviewOutcomeComponent.decision"); 5958 else if (Configuration.doAutoCreate()) 5959 this.decision = new CodeableConcept(); // cc 5960 return this.decision; 5961 } 5962 5963 public boolean hasDecision() { 5964 return this.decision != null && !this.decision.isEmpty(); 5965 } 5966 5967 /** 5968 * @param value {@link #decision} (The result of the claim, predetermination, or preauthorization adjudication.) 5969 */ 5970 public ItemReviewOutcomeComponent setDecision(CodeableConcept value) { 5971 this.decision = value; 5972 return this; 5973 } 5974 5975 /** 5976 * @return {@link #reason} (The reasons for the result of the claim, predetermination, or preauthorization adjudication.) 5977 */ 5978 public List<CodeableConcept> getReason() { 5979 if (this.reason == null) 5980 this.reason = new ArrayList<CodeableConcept>(); 5981 return this.reason; 5982 } 5983 5984 /** 5985 * @return Returns a reference to <code>this</code> for easy method chaining 5986 */ 5987 public ItemReviewOutcomeComponent setReason(List<CodeableConcept> theReason) { 5988 this.reason = theReason; 5989 return this; 5990 } 5991 5992 public boolean hasReason() { 5993 if (this.reason == null) 5994 return false; 5995 for (CodeableConcept item : this.reason) 5996 if (!item.isEmpty()) 5997 return true; 5998 return false; 5999 } 6000 6001 public CodeableConcept addReason() { //3 6002 CodeableConcept t = new CodeableConcept(); 6003 if (this.reason == null) 6004 this.reason = new ArrayList<CodeableConcept>(); 6005 this.reason.add(t); 6006 return t; 6007 } 6008 6009 public ItemReviewOutcomeComponent addReason(CodeableConcept t) { //3 6010 if (t == null) 6011 return this; 6012 if (this.reason == null) 6013 this.reason = new ArrayList<CodeableConcept>(); 6014 this.reason.add(t); 6015 return this; 6016 } 6017 6018 /** 6019 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 6020 */ 6021 public CodeableConcept getReasonFirstRep() { 6022 if (getReason().isEmpty()) { 6023 addReason(); 6024 } 6025 return getReason().get(0); 6026 } 6027 6028 /** 6029 * @return {@link #preAuthRef} (Reference from the Insurer which is used in later communications which refers to this adjudication.). This is the underlying object with id, value and extensions. The accessor "getPreAuthRef" gives direct access to the value 6030 */ 6031 public StringType getPreAuthRefElement() { 6032 if (this.preAuthRef == null) 6033 if (Configuration.errorOnAutoCreate()) 6034 throw new Error("Attempt to auto-create ItemReviewOutcomeComponent.preAuthRef"); 6035 else if (Configuration.doAutoCreate()) 6036 this.preAuthRef = new StringType(); // bb 6037 return this.preAuthRef; 6038 } 6039 6040 public boolean hasPreAuthRefElement() { 6041 return this.preAuthRef != null && !this.preAuthRef.isEmpty(); 6042 } 6043 6044 public boolean hasPreAuthRef() { 6045 return this.preAuthRef != null && !this.preAuthRef.isEmpty(); 6046 } 6047 6048 /** 6049 * @param value {@link #preAuthRef} (Reference from the Insurer which is used in later communications which refers to this adjudication.). This is the underlying object with id, value and extensions. The accessor "getPreAuthRef" gives direct access to the value 6050 */ 6051 public ItemReviewOutcomeComponent setPreAuthRefElement(StringType value) { 6052 this.preAuthRef = value; 6053 return this; 6054 } 6055 6056 /** 6057 * @return Reference from the Insurer which is used in later communications which refers to this adjudication. 6058 */ 6059 public String getPreAuthRef() { 6060 return this.preAuthRef == null ? null : this.preAuthRef.getValue(); 6061 } 6062 6063 /** 6064 * @param value Reference from the Insurer which is used in later communications which refers to this adjudication. 6065 */ 6066 public ItemReviewOutcomeComponent setPreAuthRef(String value) { 6067 if (Utilities.noString(value)) 6068 this.preAuthRef = null; 6069 else { 6070 if (this.preAuthRef == null) 6071 this.preAuthRef = new StringType(); 6072 this.preAuthRef.setValue(value); 6073 } 6074 return this; 6075 } 6076 6077 /** 6078 * @return {@link #preAuthPeriod} (The time frame during which this authorization is effective.) 6079 */ 6080 public Period getPreAuthPeriod() { 6081 if (this.preAuthPeriod == null) 6082 if (Configuration.errorOnAutoCreate()) 6083 throw new Error("Attempt to auto-create ItemReviewOutcomeComponent.preAuthPeriod"); 6084 else if (Configuration.doAutoCreate()) 6085 this.preAuthPeriod = new Period(); // cc 6086 return this.preAuthPeriod; 6087 } 6088 6089 public boolean hasPreAuthPeriod() { 6090 return this.preAuthPeriod != null && !this.preAuthPeriod.isEmpty(); 6091 } 6092 6093 /** 6094 * @param value {@link #preAuthPeriod} (The time frame during which this authorization is effective.) 6095 */ 6096 public ItemReviewOutcomeComponent setPreAuthPeriod(Period value) { 6097 this.preAuthPeriod = value; 6098 return this; 6099 } 6100 6101 protected void listChildren(List<Property> children) { 6102 super.listChildren(children); 6103 children.add(new Property("decision", "CodeableConcept", "The result of the claim, predetermination, or preauthorization adjudication.", 0, 1, decision)); 6104 children.add(new Property("reason", "CodeableConcept", "The reasons for the result of the claim, predetermination, or preauthorization adjudication.", 0, java.lang.Integer.MAX_VALUE, reason)); 6105 children.add(new Property("preAuthRef", "string", "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, 1, preAuthRef)); 6106 children.add(new Property("preAuthPeriod", "Period", "The time frame during which this authorization is effective.", 0, 1, preAuthPeriod)); 6107 } 6108 6109 @Override 6110 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6111 switch (_hash) { 6112 case 565719004: /*decision*/ return new Property("decision", "CodeableConcept", "The result of the claim, predetermination, or preauthorization adjudication.", 0, 1, decision); 6113 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "The reasons for the result of the claim, predetermination, or preauthorization adjudication.", 0, java.lang.Integer.MAX_VALUE, reason); 6114 case 522246568: /*preAuthRef*/ return new Property("preAuthRef", "string", "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, 1, preAuthRef); 6115 case 1819164812: /*preAuthPeriod*/ return new Property("preAuthPeriod", "Period", "The time frame during which this authorization is effective.", 0, 1, preAuthPeriod); 6116 default: return super.getNamedProperty(_hash, _name, _checkValid); 6117 } 6118 6119 } 6120 6121 @Override 6122 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6123 switch (hash) { 6124 case 565719004: /*decision*/ return this.decision == null ? new Base[0] : new Base[] {this.decision}; // CodeableConcept 6125 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 6126 case 522246568: /*preAuthRef*/ return this.preAuthRef == null ? new Base[0] : new Base[] {this.preAuthRef}; // StringType 6127 case 1819164812: /*preAuthPeriod*/ return this.preAuthPeriod == null ? new Base[0] : new Base[] {this.preAuthPeriod}; // Period 6128 default: return super.getProperty(hash, name, checkValid); 6129 } 6130 6131 } 6132 6133 @Override 6134 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6135 switch (hash) { 6136 case 565719004: // decision 6137 this.decision = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6138 return value; 6139 case -934964668: // reason 6140 this.getReason().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6141 return value; 6142 case 522246568: // preAuthRef 6143 this.preAuthRef = TypeConvertor.castToString(value); // StringType 6144 return value; 6145 case 1819164812: // preAuthPeriod 6146 this.preAuthPeriod = TypeConvertor.castToPeriod(value); // Period 6147 return value; 6148 default: return super.setProperty(hash, name, value); 6149 } 6150 6151 } 6152 6153 @Override 6154 public Base setProperty(String name, Base value) throws FHIRException { 6155 if (name.equals("decision")) { 6156 this.decision = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6157 } else if (name.equals("reason")) { 6158 this.getReason().add(TypeConvertor.castToCodeableConcept(value)); 6159 } else if (name.equals("preAuthRef")) { 6160 this.preAuthRef = TypeConvertor.castToString(value); // StringType 6161 } else if (name.equals("preAuthPeriod")) { 6162 this.preAuthPeriod = TypeConvertor.castToPeriod(value); // Period 6163 } else 6164 return super.setProperty(name, value); 6165 return value; 6166 } 6167 6168 @Override 6169 public void removeChild(String name, Base value) throws FHIRException { 6170 if (name.equals("decision")) { 6171 this.decision = null; 6172 } else if (name.equals("reason")) { 6173 this.getReason().remove(value); 6174 } else if (name.equals("preAuthRef")) { 6175 this.preAuthRef = null; 6176 } else if (name.equals("preAuthPeriod")) { 6177 this.preAuthPeriod = null; 6178 } else 6179 super.removeChild(name, value); 6180 6181 } 6182 6183 @Override 6184 public Base makeProperty(int hash, String name) throws FHIRException { 6185 switch (hash) { 6186 case 565719004: return getDecision(); 6187 case -934964668: return addReason(); 6188 case 522246568: return getPreAuthRefElement(); 6189 case 1819164812: return getPreAuthPeriod(); 6190 default: return super.makeProperty(hash, name); 6191 } 6192 6193 } 6194 6195 @Override 6196 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6197 switch (hash) { 6198 case 565719004: /*decision*/ return new String[] {"CodeableConcept"}; 6199 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 6200 case 522246568: /*preAuthRef*/ return new String[] {"string"}; 6201 case 1819164812: /*preAuthPeriod*/ return new String[] {"Period"}; 6202 default: return super.getTypesForProperty(hash, name); 6203 } 6204 6205 } 6206 6207 @Override 6208 public Base addChild(String name) throws FHIRException { 6209 if (name.equals("decision")) { 6210 this.decision = new CodeableConcept(); 6211 return this.decision; 6212 } 6213 else if (name.equals("reason")) { 6214 return addReason(); 6215 } 6216 else if (name.equals("preAuthRef")) { 6217 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.reviewOutcome.preAuthRef"); 6218 } 6219 else if (name.equals("preAuthPeriod")) { 6220 this.preAuthPeriod = new Period(); 6221 return this.preAuthPeriod; 6222 } 6223 else 6224 return super.addChild(name); 6225 } 6226 6227 public ItemReviewOutcomeComponent copy() { 6228 ItemReviewOutcomeComponent dst = new ItemReviewOutcomeComponent(); 6229 copyValues(dst); 6230 return dst; 6231 } 6232 6233 public void copyValues(ItemReviewOutcomeComponent dst) { 6234 super.copyValues(dst); 6235 dst.decision = decision == null ? null : decision.copy(); 6236 if (reason != null) { 6237 dst.reason = new ArrayList<CodeableConcept>(); 6238 for (CodeableConcept i : reason) 6239 dst.reason.add(i.copy()); 6240 }; 6241 dst.preAuthRef = preAuthRef == null ? null : preAuthRef.copy(); 6242 dst.preAuthPeriod = preAuthPeriod == null ? null : preAuthPeriod.copy(); 6243 } 6244 6245 @Override 6246 public boolean equalsDeep(Base other_) { 6247 if (!super.equalsDeep(other_)) 6248 return false; 6249 if (!(other_ instanceof ItemReviewOutcomeComponent)) 6250 return false; 6251 ItemReviewOutcomeComponent o = (ItemReviewOutcomeComponent) other_; 6252 return compareDeep(decision, o.decision, true) && compareDeep(reason, o.reason, true) && compareDeep(preAuthRef, o.preAuthRef, true) 6253 && compareDeep(preAuthPeriod, o.preAuthPeriod, true); 6254 } 6255 6256 @Override 6257 public boolean equalsShallow(Base other_) { 6258 if (!super.equalsShallow(other_)) 6259 return false; 6260 if (!(other_ instanceof ItemReviewOutcomeComponent)) 6261 return false; 6262 ItemReviewOutcomeComponent o = (ItemReviewOutcomeComponent) other_; 6263 return compareValues(preAuthRef, o.preAuthRef, true); 6264 } 6265 6266 public boolean isEmpty() { 6267 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(decision, reason, preAuthRef 6268 , preAuthPeriod); 6269 } 6270 6271 public String fhirType() { 6272 return "ExplanationOfBenefit.item.reviewOutcome"; 6273 6274 } 6275 6276 } 6277 6278 @Block() 6279 public static class AdjudicationComponent extends BackboneElement implements IBaseBackboneElement { 6280 /** 6281 * A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in-aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item. 6282 */ 6283 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 6284 @Description(shortDefinition="Type of adjudication information", formalDefinition="A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in-aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item." ) 6285 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication") 6286 protected CodeableConcept category; 6287 6288 /** 6289 * A code supporting the understanding of the adjudication result and explaining variance from expected amount. 6290 */ 6291 @Child(name = "reason", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 6292 @Description(shortDefinition="Explanation of adjudication outcome", formalDefinition="A code supporting the understanding of the adjudication result and explaining variance from expected amount." ) 6293 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication-reason") 6294 protected CodeableConcept reason; 6295 6296 /** 6297 * Monetary amount associated with the category. 6298 */ 6299 @Child(name = "amount", type = {Money.class}, order=3, min=0, max=1, modifier=false, summary=false) 6300 @Description(shortDefinition="Monetary amount", formalDefinition="Monetary amount associated with the category." ) 6301 protected Money amount; 6302 6303 /** 6304 * A non-monetary value associated with the category. Mutually exclusive to the amount element above. 6305 */ 6306 @Child(name = "quantity", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=false) 6307 @Description(shortDefinition="Non-monitary value", formalDefinition="A non-monetary value associated with the category. Mutually exclusive to the amount element above." ) 6308 protected Quantity quantity; 6309 6310 private static final long serialVersionUID = 29312734L; 6311 6312 /** 6313 * Constructor 6314 */ 6315 public AdjudicationComponent() { 6316 super(); 6317 } 6318 6319 /** 6320 * Constructor 6321 */ 6322 public AdjudicationComponent(CodeableConcept category) { 6323 super(); 6324 this.setCategory(category); 6325 } 6326 6327 /** 6328 * @return {@link #category} (A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in-aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.) 6329 */ 6330 public CodeableConcept getCategory() { 6331 if (this.category == null) 6332 if (Configuration.errorOnAutoCreate()) 6333 throw new Error("Attempt to auto-create AdjudicationComponent.category"); 6334 else if (Configuration.doAutoCreate()) 6335 this.category = new CodeableConcept(); // cc 6336 return this.category; 6337 } 6338 6339 public boolean hasCategory() { 6340 return this.category != null && !this.category.isEmpty(); 6341 } 6342 6343 /** 6344 * @param value {@link #category} (A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in-aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.) 6345 */ 6346 public AdjudicationComponent setCategory(CodeableConcept value) { 6347 this.category = value; 6348 return this; 6349 } 6350 6351 /** 6352 * @return {@link #reason} (A code supporting the understanding of the adjudication result and explaining variance from expected amount.) 6353 */ 6354 public CodeableConcept getReason() { 6355 if (this.reason == null) 6356 if (Configuration.errorOnAutoCreate()) 6357 throw new Error("Attempt to auto-create AdjudicationComponent.reason"); 6358 else if (Configuration.doAutoCreate()) 6359 this.reason = new CodeableConcept(); // cc 6360 return this.reason; 6361 } 6362 6363 public boolean hasReason() { 6364 return this.reason != null && !this.reason.isEmpty(); 6365 } 6366 6367 /** 6368 * @param value {@link #reason} (A code supporting the understanding of the adjudication result and explaining variance from expected amount.) 6369 */ 6370 public AdjudicationComponent setReason(CodeableConcept value) { 6371 this.reason = value; 6372 return this; 6373 } 6374 6375 /** 6376 * @return {@link #amount} (Monetary amount associated with the category.) 6377 */ 6378 public Money getAmount() { 6379 if (this.amount == null) 6380 if (Configuration.errorOnAutoCreate()) 6381 throw new Error("Attempt to auto-create AdjudicationComponent.amount"); 6382 else if (Configuration.doAutoCreate()) 6383 this.amount = new Money(); // cc 6384 return this.amount; 6385 } 6386 6387 public boolean hasAmount() { 6388 return this.amount != null && !this.amount.isEmpty(); 6389 } 6390 6391 /** 6392 * @param value {@link #amount} (Monetary amount associated with the category.) 6393 */ 6394 public AdjudicationComponent setAmount(Money value) { 6395 this.amount = value; 6396 return this; 6397 } 6398 6399 /** 6400 * @return {@link #quantity} (A non-monetary value associated with the category. Mutually exclusive to the amount element above.) 6401 */ 6402 public Quantity getQuantity() { 6403 if (this.quantity == null) 6404 if (Configuration.errorOnAutoCreate()) 6405 throw new Error("Attempt to auto-create AdjudicationComponent.quantity"); 6406 else if (Configuration.doAutoCreate()) 6407 this.quantity = new Quantity(); // cc 6408 return this.quantity; 6409 } 6410 6411 public boolean hasQuantity() { 6412 return this.quantity != null && !this.quantity.isEmpty(); 6413 } 6414 6415 /** 6416 * @param value {@link #quantity} (A non-monetary value associated with the category. Mutually exclusive to the amount element above.) 6417 */ 6418 public AdjudicationComponent setQuantity(Quantity value) { 6419 this.quantity = value; 6420 return this; 6421 } 6422 6423 protected void listChildren(List<Property> children) { 6424 super.listChildren(children); 6425 children.add(new Property("category", "CodeableConcept", "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in-aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 0, 1, category)); 6426 children.add(new Property("reason", "CodeableConcept", "A code supporting the understanding of the adjudication result and explaining variance from expected amount.", 0, 1, reason)); 6427 children.add(new Property("amount", "Money", "Monetary amount associated with the category.", 0, 1, amount)); 6428 children.add(new Property("quantity", "Quantity", "A non-monetary value associated with the category. Mutually exclusive to the amount element above.", 0, 1, quantity)); 6429 } 6430 6431 @Override 6432 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6433 switch (_hash) { 6434 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in-aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 0, 1, category); 6435 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "A code supporting the understanding of the adjudication result and explaining variance from expected amount.", 0, 1, reason); 6436 case -1413853096: /*amount*/ return new Property("amount", "Money", "Monetary amount associated with the category.", 0, 1, amount); 6437 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "A non-monetary value associated with the category. Mutually exclusive to the amount element above.", 0, 1, quantity); 6438 default: return super.getNamedProperty(_hash, _name, _checkValid); 6439 } 6440 6441 } 6442 6443 @Override 6444 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6445 switch (hash) { 6446 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 6447 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 6448 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 6449 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 6450 default: return super.getProperty(hash, name, checkValid); 6451 } 6452 6453 } 6454 6455 @Override 6456 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6457 switch (hash) { 6458 case 50511102: // category 6459 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6460 return value; 6461 case -934964668: // reason 6462 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6463 return value; 6464 case -1413853096: // amount 6465 this.amount = TypeConvertor.castToMoney(value); // Money 6466 return value; 6467 case -1285004149: // quantity 6468 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 6469 return value; 6470 default: return super.setProperty(hash, name, value); 6471 } 6472 6473 } 6474 6475 @Override 6476 public Base setProperty(String name, Base value) throws FHIRException { 6477 if (name.equals("category")) { 6478 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6479 } else if (name.equals("reason")) { 6480 this.reason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6481 } else if (name.equals("amount")) { 6482 this.amount = TypeConvertor.castToMoney(value); // Money 6483 } else if (name.equals("quantity")) { 6484 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 6485 } else 6486 return super.setProperty(name, value); 6487 return value; 6488 } 6489 6490 @Override 6491 public void removeChild(String name, Base value) throws FHIRException { 6492 if (name.equals("category")) { 6493 this.category = null; 6494 } else if (name.equals("reason")) { 6495 this.reason = null; 6496 } else if (name.equals("amount")) { 6497 this.amount = null; 6498 } else if (name.equals("quantity")) { 6499 this.quantity = null; 6500 } else 6501 super.removeChild(name, value); 6502 6503 } 6504 6505 @Override 6506 public Base makeProperty(int hash, String name) throws FHIRException { 6507 switch (hash) { 6508 case 50511102: return getCategory(); 6509 case -934964668: return getReason(); 6510 case -1413853096: return getAmount(); 6511 case -1285004149: return getQuantity(); 6512 default: return super.makeProperty(hash, name); 6513 } 6514 6515 } 6516 6517 @Override 6518 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6519 switch (hash) { 6520 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 6521 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 6522 case -1413853096: /*amount*/ return new String[] {"Money"}; 6523 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 6524 default: return super.getTypesForProperty(hash, name); 6525 } 6526 6527 } 6528 6529 @Override 6530 public Base addChild(String name) throws FHIRException { 6531 if (name.equals("category")) { 6532 this.category = new CodeableConcept(); 6533 return this.category; 6534 } 6535 else if (name.equals("reason")) { 6536 this.reason = new CodeableConcept(); 6537 return this.reason; 6538 } 6539 else if (name.equals("amount")) { 6540 this.amount = new Money(); 6541 return this.amount; 6542 } 6543 else if (name.equals("quantity")) { 6544 this.quantity = new Quantity(); 6545 return this.quantity; 6546 } 6547 else 6548 return super.addChild(name); 6549 } 6550 6551 public AdjudicationComponent copy() { 6552 AdjudicationComponent dst = new AdjudicationComponent(); 6553 copyValues(dst); 6554 return dst; 6555 } 6556 6557 public void copyValues(AdjudicationComponent dst) { 6558 super.copyValues(dst); 6559 dst.category = category == null ? null : category.copy(); 6560 dst.reason = reason == null ? null : reason.copy(); 6561 dst.amount = amount == null ? null : amount.copy(); 6562 dst.quantity = quantity == null ? null : quantity.copy(); 6563 } 6564 6565 @Override 6566 public boolean equalsDeep(Base other_) { 6567 if (!super.equalsDeep(other_)) 6568 return false; 6569 if (!(other_ instanceof AdjudicationComponent)) 6570 return false; 6571 AdjudicationComponent o = (AdjudicationComponent) other_; 6572 return compareDeep(category, o.category, true) && compareDeep(reason, o.reason, true) && compareDeep(amount, o.amount, true) 6573 && compareDeep(quantity, o.quantity, true); 6574 } 6575 6576 @Override 6577 public boolean equalsShallow(Base other_) { 6578 if (!super.equalsShallow(other_)) 6579 return false; 6580 if (!(other_ instanceof AdjudicationComponent)) 6581 return false; 6582 AdjudicationComponent o = (AdjudicationComponent) other_; 6583 return true; 6584 } 6585 6586 public boolean isEmpty() { 6587 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, reason, amount 6588 , quantity); 6589 } 6590 6591 public String fhirType() { 6592 return "ExplanationOfBenefit.item.adjudication"; 6593 6594 } 6595 6596 } 6597 6598 @Block() 6599 public static class DetailComponent extends BackboneElement implements IBaseBackboneElement { 6600 /** 6601 * A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 6602 */ 6603 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 6604 @Description(shortDefinition="Product or service provided", formalDefinition="A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items." ) 6605 protected PositiveIntType sequence; 6606 6607 /** 6608 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 6609 */ 6610 @Child(name = "traceNumber", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6611 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 6612 protected List<Identifier> traceNumber; 6613 6614 /** 6615 * The type of revenue or cost center providing the product and/or service. 6616 */ 6617 @Child(name = "revenue", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 6618 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 6619 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 6620 protected CodeableConcept revenue; 6621 6622 /** 6623 * Code to identify the general type of benefits under which products and services are provided. 6624 */ 6625 @Child(name = "category", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 6626 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 6627 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 6628 protected CodeableConcept category; 6629 6630 /** 6631 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 6632 */ 6633 @Child(name = "productOrService", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 6634 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 6635 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 6636 protected CodeableConcept productOrService; 6637 6638 /** 6639 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 6640 */ 6641 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 6642 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 6643 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 6644 protected CodeableConcept productOrServiceEnd; 6645 6646 /** 6647 * Item typification or modifiers codes to convey additional context for the product or service. 6648 */ 6649 @Child(name = "modifier", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6650 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 6651 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 6652 protected List<CodeableConcept> modifier; 6653 6654 /** 6655 * Identifies the program under which this may be recovered. 6656 */ 6657 @Child(name = "programCode", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6658 @Description(shortDefinition="Program the product or service is provided under", formalDefinition="Identifies the program under which this may be recovered." ) 6659 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 6660 protected List<CodeableConcept> programCode; 6661 6662 /** 6663 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 6664 */ 6665 @Child(name = "patientPaid", type = {Money.class}, order=9, min=0, max=1, modifier=false, summary=false) 6666 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 6667 protected Money patientPaid; 6668 6669 /** 6670 * The number of repetitions of a service or product. 6671 */ 6672 @Child(name = "quantity", type = {Quantity.class}, order=10, min=0, max=1, modifier=false, summary=false) 6673 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 6674 protected Quantity quantity; 6675 6676 /** 6677 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 6678 */ 6679 @Child(name = "unitPrice", type = {Money.class}, order=11, min=0, max=1, modifier=false, summary=false) 6680 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 6681 protected Money unitPrice; 6682 6683 /** 6684 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6685 */ 6686 @Child(name = "factor", type = {DecimalType.class}, order=12, min=0, max=1, modifier=false, summary=false) 6687 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 6688 protected DecimalType factor; 6689 6690 /** 6691 * The total of taxes applicable for this product or service. 6692 */ 6693 @Child(name = "tax", type = {Money.class}, order=13, min=0, max=1, modifier=false, summary=false) 6694 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 6695 protected Money tax; 6696 6697 /** 6698 * The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor. 6699 */ 6700 @Child(name = "net", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=false) 6701 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor." ) 6702 protected Money net; 6703 6704 /** 6705 * Unique Device Identifiers associated with this line item. 6706 */ 6707 @Child(name = "udi", type = {Device.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6708 @Description(shortDefinition="Unique device identifier", formalDefinition="Unique Device Identifiers associated with this line item." ) 6709 protected List<Reference> udi; 6710 6711 /** 6712 * The numbers associated with notes below which apply to the adjudication of this item. 6713 */ 6714 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6715 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 6716 protected List<PositiveIntType> noteNumber; 6717 6718 /** 6719 * The high-level results of the adjudication if adjudication has been performed. 6720 */ 6721 @Child(name = "reviewOutcome", type = {ItemReviewOutcomeComponent.class}, order=17, min=0, max=1, modifier=false, summary=false) 6722 @Description(shortDefinition="Detail level adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 6723 protected ItemReviewOutcomeComponent reviewOutcome; 6724 6725 /** 6726 * The adjudication results. 6727 */ 6728 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6729 @Description(shortDefinition="Detail level adjudication details", formalDefinition="The adjudication results." ) 6730 protected List<AdjudicationComponent> adjudication; 6731 6732 /** 6733 * Third-tier of goods and services. 6734 */ 6735 @Child(name = "subDetail", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6736 @Description(shortDefinition="Additional items", formalDefinition="Third-tier of goods and services." ) 6737 protected List<SubDetailComponent> subDetail; 6738 6739 private static final long serialVersionUID = -1951425443L; 6740 6741 /** 6742 * Constructor 6743 */ 6744 public DetailComponent() { 6745 super(); 6746 } 6747 6748 /** 6749 * Constructor 6750 */ 6751 public DetailComponent(int sequence) { 6752 super(); 6753 this.setSequence(sequence); 6754 } 6755 6756 /** 6757 * @return {@link #sequence} (A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 6758 */ 6759 public PositiveIntType getSequenceElement() { 6760 if (this.sequence == null) 6761 if (Configuration.errorOnAutoCreate()) 6762 throw new Error("Attempt to auto-create DetailComponent.sequence"); 6763 else if (Configuration.doAutoCreate()) 6764 this.sequence = new PositiveIntType(); // bb 6765 return this.sequence; 6766 } 6767 6768 public boolean hasSequenceElement() { 6769 return this.sequence != null && !this.sequence.isEmpty(); 6770 } 6771 6772 public boolean hasSequence() { 6773 return this.sequence != null && !this.sequence.isEmpty(); 6774 } 6775 6776 /** 6777 * @param value {@link #sequence} (A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 6778 */ 6779 public DetailComponent setSequenceElement(PositiveIntType value) { 6780 this.sequence = value; 6781 return this; 6782 } 6783 6784 /** 6785 * @return A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 6786 */ 6787 public int getSequence() { 6788 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 6789 } 6790 6791 /** 6792 * @param value A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 6793 */ 6794 public DetailComponent setSequence(int value) { 6795 if (this.sequence == null) 6796 this.sequence = new PositiveIntType(); 6797 this.sequence.setValue(value); 6798 return this; 6799 } 6800 6801 /** 6802 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 6803 */ 6804 public List<Identifier> getTraceNumber() { 6805 if (this.traceNumber == null) 6806 this.traceNumber = new ArrayList<Identifier>(); 6807 return this.traceNumber; 6808 } 6809 6810 /** 6811 * @return Returns a reference to <code>this</code> for easy method chaining 6812 */ 6813 public DetailComponent setTraceNumber(List<Identifier> theTraceNumber) { 6814 this.traceNumber = theTraceNumber; 6815 return this; 6816 } 6817 6818 public boolean hasTraceNumber() { 6819 if (this.traceNumber == null) 6820 return false; 6821 for (Identifier item : this.traceNumber) 6822 if (!item.isEmpty()) 6823 return true; 6824 return false; 6825 } 6826 6827 public Identifier addTraceNumber() { //3 6828 Identifier t = new Identifier(); 6829 if (this.traceNumber == null) 6830 this.traceNumber = new ArrayList<Identifier>(); 6831 this.traceNumber.add(t); 6832 return t; 6833 } 6834 6835 public DetailComponent addTraceNumber(Identifier t) { //3 6836 if (t == null) 6837 return this; 6838 if (this.traceNumber == null) 6839 this.traceNumber = new ArrayList<Identifier>(); 6840 this.traceNumber.add(t); 6841 return this; 6842 } 6843 6844 /** 6845 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 6846 */ 6847 public Identifier getTraceNumberFirstRep() { 6848 if (getTraceNumber().isEmpty()) { 6849 addTraceNumber(); 6850 } 6851 return getTraceNumber().get(0); 6852 } 6853 6854 /** 6855 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 6856 */ 6857 public CodeableConcept getRevenue() { 6858 if (this.revenue == null) 6859 if (Configuration.errorOnAutoCreate()) 6860 throw new Error("Attempt to auto-create DetailComponent.revenue"); 6861 else if (Configuration.doAutoCreate()) 6862 this.revenue = new CodeableConcept(); // cc 6863 return this.revenue; 6864 } 6865 6866 public boolean hasRevenue() { 6867 return this.revenue != null && !this.revenue.isEmpty(); 6868 } 6869 6870 /** 6871 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 6872 */ 6873 public DetailComponent setRevenue(CodeableConcept value) { 6874 this.revenue = value; 6875 return this; 6876 } 6877 6878 /** 6879 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 6880 */ 6881 public CodeableConcept getCategory() { 6882 if (this.category == null) 6883 if (Configuration.errorOnAutoCreate()) 6884 throw new Error("Attempt to auto-create DetailComponent.category"); 6885 else if (Configuration.doAutoCreate()) 6886 this.category = new CodeableConcept(); // cc 6887 return this.category; 6888 } 6889 6890 public boolean hasCategory() { 6891 return this.category != null && !this.category.isEmpty(); 6892 } 6893 6894 /** 6895 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 6896 */ 6897 public DetailComponent setCategory(CodeableConcept value) { 6898 this.category = value; 6899 return this; 6900 } 6901 6902 /** 6903 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 6904 */ 6905 public CodeableConcept getProductOrService() { 6906 if (this.productOrService == null) 6907 if (Configuration.errorOnAutoCreate()) 6908 throw new Error("Attempt to auto-create DetailComponent.productOrService"); 6909 else if (Configuration.doAutoCreate()) 6910 this.productOrService = new CodeableConcept(); // cc 6911 return this.productOrService; 6912 } 6913 6914 public boolean hasProductOrService() { 6915 return this.productOrService != null && !this.productOrService.isEmpty(); 6916 } 6917 6918 /** 6919 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 6920 */ 6921 public DetailComponent setProductOrService(CodeableConcept value) { 6922 this.productOrService = value; 6923 return this; 6924 } 6925 6926 /** 6927 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 6928 */ 6929 public CodeableConcept getProductOrServiceEnd() { 6930 if (this.productOrServiceEnd == null) 6931 if (Configuration.errorOnAutoCreate()) 6932 throw new Error("Attempt to auto-create DetailComponent.productOrServiceEnd"); 6933 else if (Configuration.doAutoCreate()) 6934 this.productOrServiceEnd = new CodeableConcept(); // cc 6935 return this.productOrServiceEnd; 6936 } 6937 6938 public boolean hasProductOrServiceEnd() { 6939 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 6940 } 6941 6942 /** 6943 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 6944 */ 6945 public DetailComponent setProductOrServiceEnd(CodeableConcept value) { 6946 this.productOrServiceEnd = value; 6947 return this; 6948 } 6949 6950 /** 6951 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 6952 */ 6953 public List<CodeableConcept> getModifier() { 6954 if (this.modifier == null) 6955 this.modifier = new ArrayList<CodeableConcept>(); 6956 return this.modifier; 6957 } 6958 6959 /** 6960 * @return Returns a reference to <code>this</code> for easy method chaining 6961 */ 6962 public DetailComponent setModifier(List<CodeableConcept> theModifier) { 6963 this.modifier = theModifier; 6964 return this; 6965 } 6966 6967 public boolean hasModifier() { 6968 if (this.modifier == null) 6969 return false; 6970 for (CodeableConcept item : this.modifier) 6971 if (!item.isEmpty()) 6972 return true; 6973 return false; 6974 } 6975 6976 public CodeableConcept addModifier() { //3 6977 CodeableConcept t = new CodeableConcept(); 6978 if (this.modifier == null) 6979 this.modifier = new ArrayList<CodeableConcept>(); 6980 this.modifier.add(t); 6981 return t; 6982 } 6983 6984 public DetailComponent addModifier(CodeableConcept t) { //3 6985 if (t == null) 6986 return this; 6987 if (this.modifier == null) 6988 this.modifier = new ArrayList<CodeableConcept>(); 6989 this.modifier.add(t); 6990 return this; 6991 } 6992 6993 /** 6994 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 6995 */ 6996 public CodeableConcept getModifierFirstRep() { 6997 if (getModifier().isEmpty()) { 6998 addModifier(); 6999 } 7000 return getModifier().get(0); 7001 } 7002 7003 /** 7004 * @return {@link #programCode} (Identifies the program under which this may be recovered.) 7005 */ 7006 public List<CodeableConcept> getProgramCode() { 7007 if (this.programCode == null) 7008 this.programCode = new ArrayList<CodeableConcept>(); 7009 return this.programCode; 7010 } 7011 7012 /** 7013 * @return Returns a reference to <code>this</code> for easy method chaining 7014 */ 7015 public DetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 7016 this.programCode = theProgramCode; 7017 return this; 7018 } 7019 7020 public boolean hasProgramCode() { 7021 if (this.programCode == null) 7022 return false; 7023 for (CodeableConcept item : this.programCode) 7024 if (!item.isEmpty()) 7025 return true; 7026 return false; 7027 } 7028 7029 public CodeableConcept addProgramCode() { //3 7030 CodeableConcept t = new CodeableConcept(); 7031 if (this.programCode == null) 7032 this.programCode = new ArrayList<CodeableConcept>(); 7033 this.programCode.add(t); 7034 return t; 7035 } 7036 7037 public DetailComponent addProgramCode(CodeableConcept t) { //3 7038 if (t == null) 7039 return this; 7040 if (this.programCode == null) 7041 this.programCode = new ArrayList<CodeableConcept>(); 7042 this.programCode.add(t); 7043 return this; 7044 } 7045 7046 /** 7047 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist {3} 7048 */ 7049 public CodeableConcept getProgramCodeFirstRep() { 7050 if (getProgramCode().isEmpty()) { 7051 addProgramCode(); 7052 } 7053 return getProgramCode().get(0); 7054 } 7055 7056 /** 7057 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 7058 */ 7059 public Money getPatientPaid() { 7060 if (this.patientPaid == null) 7061 if (Configuration.errorOnAutoCreate()) 7062 throw new Error("Attempt to auto-create DetailComponent.patientPaid"); 7063 else if (Configuration.doAutoCreate()) 7064 this.patientPaid = new Money(); // cc 7065 return this.patientPaid; 7066 } 7067 7068 public boolean hasPatientPaid() { 7069 return this.patientPaid != null && !this.patientPaid.isEmpty(); 7070 } 7071 7072 /** 7073 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 7074 */ 7075 public DetailComponent setPatientPaid(Money value) { 7076 this.patientPaid = value; 7077 return this; 7078 } 7079 7080 /** 7081 * @return {@link #quantity} (The number of repetitions of a service or product.) 7082 */ 7083 public Quantity getQuantity() { 7084 if (this.quantity == null) 7085 if (Configuration.errorOnAutoCreate()) 7086 throw new Error("Attempt to auto-create DetailComponent.quantity"); 7087 else if (Configuration.doAutoCreate()) 7088 this.quantity = new Quantity(); // cc 7089 return this.quantity; 7090 } 7091 7092 public boolean hasQuantity() { 7093 return this.quantity != null && !this.quantity.isEmpty(); 7094 } 7095 7096 /** 7097 * @param value {@link #quantity} (The number of repetitions of a service or product.) 7098 */ 7099 public DetailComponent setQuantity(Quantity value) { 7100 this.quantity = value; 7101 return this; 7102 } 7103 7104 /** 7105 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 7106 */ 7107 public Money getUnitPrice() { 7108 if (this.unitPrice == null) 7109 if (Configuration.errorOnAutoCreate()) 7110 throw new Error("Attempt to auto-create DetailComponent.unitPrice"); 7111 else if (Configuration.doAutoCreate()) 7112 this.unitPrice = new Money(); // cc 7113 return this.unitPrice; 7114 } 7115 7116 public boolean hasUnitPrice() { 7117 return this.unitPrice != null && !this.unitPrice.isEmpty(); 7118 } 7119 7120 /** 7121 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 7122 */ 7123 public DetailComponent setUnitPrice(Money value) { 7124 this.unitPrice = value; 7125 return this; 7126 } 7127 7128 /** 7129 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 7130 */ 7131 public DecimalType getFactorElement() { 7132 if (this.factor == null) 7133 if (Configuration.errorOnAutoCreate()) 7134 throw new Error("Attempt to auto-create DetailComponent.factor"); 7135 else if (Configuration.doAutoCreate()) 7136 this.factor = new DecimalType(); // bb 7137 return this.factor; 7138 } 7139 7140 public boolean hasFactorElement() { 7141 return this.factor != null && !this.factor.isEmpty(); 7142 } 7143 7144 public boolean hasFactor() { 7145 return this.factor != null && !this.factor.isEmpty(); 7146 } 7147 7148 /** 7149 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 7150 */ 7151 public DetailComponent setFactorElement(DecimalType value) { 7152 this.factor = value; 7153 return this; 7154 } 7155 7156 /** 7157 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 7158 */ 7159 public BigDecimal getFactor() { 7160 return this.factor == null ? null : this.factor.getValue(); 7161 } 7162 7163 /** 7164 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 7165 */ 7166 public DetailComponent setFactor(BigDecimal value) { 7167 if (value == null) 7168 this.factor = null; 7169 else { 7170 if (this.factor == null) 7171 this.factor = new DecimalType(); 7172 this.factor.setValue(value); 7173 } 7174 return this; 7175 } 7176 7177 /** 7178 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 7179 */ 7180 public DetailComponent setFactor(long value) { 7181 this.factor = new DecimalType(); 7182 this.factor.setValue(value); 7183 return this; 7184 } 7185 7186 /** 7187 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 7188 */ 7189 public DetailComponent setFactor(double value) { 7190 this.factor = new DecimalType(); 7191 this.factor.setValue(value); 7192 return this; 7193 } 7194 7195 /** 7196 * @return {@link #tax} (The total of taxes applicable for this product or service.) 7197 */ 7198 public Money getTax() { 7199 if (this.tax == null) 7200 if (Configuration.errorOnAutoCreate()) 7201 throw new Error("Attempt to auto-create DetailComponent.tax"); 7202 else if (Configuration.doAutoCreate()) 7203 this.tax = new Money(); // cc 7204 return this.tax; 7205 } 7206 7207 public boolean hasTax() { 7208 return this.tax != null && !this.tax.isEmpty(); 7209 } 7210 7211 /** 7212 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 7213 */ 7214 public DetailComponent setTax(Money value) { 7215 this.tax = value; 7216 return this; 7217 } 7218 7219 /** 7220 * @return {@link #net} (The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor.) 7221 */ 7222 public Money getNet() { 7223 if (this.net == null) 7224 if (Configuration.errorOnAutoCreate()) 7225 throw new Error("Attempt to auto-create DetailComponent.net"); 7226 else if (Configuration.doAutoCreate()) 7227 this.net = new Money(); // cc 7228 return this.net; 7229 } 7230 7231 public boolean hasNet() { 7232 return this.net != null && !this.net.isEmpty(); 7233 } 7234 7235 /** 7236 * @param value {@link #net} (The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor.) 7237 */ 7238 public DetailComponent setNet(Money value) { 7239 this.net = value; 7240 return this; 7241 } 7242 7243 /** 7244 * @return {@link #udi} (Unique Device Identifiers associated with this line item.) 7245 */ 7246 public List<Reference> getUdi() { 7247 if (this.udi == null) 7248 this.udi = new ArrayList<Reference>(); 7249 return this.udi; 7250 } 7251 7252 /** 7253 * @return Returns a reference to <code>this</code> for easy method chaining 7254 */ 7255 public DetailComponent setUdi(List<Reference> theUdi) { 7256 this.udi = theUdi; 7257 return this; 7258 } 7259 7260 public boolean hasUdi() { 7261 if (this.udi == null) 7262 return false; 7263 for (Reference item : this.udi) 7264 if (!item.isEmpty()) 7265 return true; 7266 return false; 7267 } 7268 7269 public Reference addUdi() { //3 7270 Reference t = new Reference(); 7271 if (this.udi == null) 7272 this.udi = new ArrayList<Reference>(); 7273 this.udi.add(t); 7274 return t; 7275 } 7276 7277 public DetailComponent addUdi(Reference t) { //3 7278 if (t == null) 7279 return this; 7280 if (this.udi == null) 7281 this.udi = new ArrayList<Reference>(); 7282 this.udi.add(t); 7283 return this; 7284 } 7285 7286 /** 7287 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist {3} 7288 */ 7289 public Reference getUdiFirstRep() { 7290 if (getUdi().isEmpty()) { 7291 addUdi(); 7292 } 7293 return getUdi().get(0); 7294 } 7295 7296 /** 7297 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 7298 */ 7299 public List<PositiveIntType> getNoteNumber() { 7300 if (this.noteNumber == null) 7301 this.noteNumber = new ArrayList<PositiveIntType>(); 7302 return this.noteNumber; 7303 } 7304 7305 /** 7306 * @return Returns a reference to <code>this</code> for easy method chaining 7307 */ 7308 public DetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 7309 this.noteNumber = theNoteNumber; 7310 return this; 7311 } 7312 7313 public boolean hasNoteNumber() { 7314 if (this.noteNumber == null) 7315 return false; 7316 for (PositiveIntType item : this.noteNumber) 7317 if (!item.isEmpty()) 7318 return true; 7319 return false; 7320 } 7321 7322 /** 7323 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 7324 */ 7325 public PositiveIntType addNoteNumberElement() {//2 7326 PositiveIntType t = new PositiveIntType(); 7327 if (this.noteNumber == null) 7328 this.noteNumber = new ArrayList<PositiveIntType>(); 7329 this.noteNumber.add(t); 7330 return t; 7331 } 7332 7333 /** 7334 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 7335 */ 7336 public DetailComponent addNoteNumber(int value) { //1 7337 PositiveIntType t = new PositiveIntType(); 7338 t.setValue(value); 7339 if (this.noteNumber == null) 7340 this.noteNumber = new ArrayList<PositiveIntType>(); 7341 this.noteNumber.add(t); 7342 return this; 7343 } 7344 7345 /** 7346 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 7347 */ 7348 public boolean hasNoteNumber(int value) { 7349 if (this.noteNumber == null) 7350 return false; 7351 for (PositiveIntType v : this.noteNumber) 7352 if (v.getValue().equals(value)) // positiveInt 7353 return true; 7354 return false; 7355 } 7356 7357 /** 7358 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 7359 */ 7360 public ItemReviewOutcomeComponent getReviewOutcome() { 7361 if (this.reviewOutcome == null) 7362 if (Configuration.errorOnAutoCreate()) 7363 throw new Error("Attempt to auto-create DetailComponent.reviewOutcome"); 7364 else if (Configuration.doAutoCreate()) 7365 this.reviewOutcome = new ItemReviewOutcomeComponent(); // cc 7366 return this.reviewOutcome; 7367 } 7368 7369 public boolean hasReviewOutcome() { 7370 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 7371 } 7372 7373 /** 7374 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 7375 */ 7376 public DetailComponent setReviewOutcome(ItemReviewOutcomeComponent value) { 7377 this.reviewOutcome = value; 7378 return this; 7379 } 7380 7381 /** 7382 * @return {@link #adjudication} (The adjudication results.) 7383 */ 7384 public List<AdjudicationComponent> getAdjudication() { 7385 if (this.adjudication == null) 7386 this.adjudication = new ArrayList<AdjudicationComponent>(); 7387 return this.adjudication; 7388 } 7389 7390 /** 7391 * @return Returns a reference to <code>this</code> for easy method chaining 7392 */ 7393 public DetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 7394 this.adjudication = theAdjudication; 7395 return this; 7396 } 7397 7398 public boolean hasAdjudication() { 7399 if (this.adjudication == null) 7400 return false; 7401 for (AdjudicationComponent item : this.adjudication) 7402 if (!item.isEmpty()) 7403 return true; 7404 return false; 7405 } 7406 7407 public AdjudicationComponent addAdjudication() { //3 7408 AdjudicationComponent t = new AdjudicationComponent(); 7409 if (this.adjudication == null) 7410 this.adjudication = new ArrayList<AdjudicationComponent>(); 7411 this.adjudication.add(t); 7412 return t; 7413 } 7414 7415 public DetailComponent addAdjudication(AdjudicationComponent t) { //3 7416 if (t == null) 7417 return this; 7418 if (this.adjudication == null) 7419 this.adjudication = new ArrayList<AdjudicationComponent>(); 7420 this.adjudication.add(t); 7421 return this; 7422 } 7423 7424 /** 7425 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 7426 */ 7427 public AdjudicationComponent getAdjudicationFirstRep() { 7428 if (getAdjudication().isEmpty()) { 7429 addAdjudication(); 7430 } 7431 return getAdjudication().get(0); 7432 } 7433 7434 /** 7435 * @return {@link #subDetail} (Third-tier of goods and services.) 7436 */ 7437 public List<SubDetailComponent> getSubDetail() { 7438 if (this.subDetail == null) 7439 this.subDetail = new ArrayList<SubDetailComponent>(); 7440 return this.subDetail; 7441 } 7442 7443 /** 7444 * @return Returns a reference to <code>this</code> for easy method chaining 7445 */ 7446 public DetailComponent setSubDetail(List<SubDetailComponent> theSubDetail) { 7447 this.subDetail = theSubDetail; 7448 return this; 7449 } 7450 7451 public boolean hasSubDetail() { 7452 if (this.subDetail == null) 7453 return false; 7454 for (SubDetailComponent item : this.subDetail) 7455 if (!item.isEmpty()) 7456 return true; 7457 return false; 7458 } 7459 7460 public SubDetailComponent addSubDetail() { //3 7461 SubDetailComponent t = new SubDetailComponent(); 7462 if (this.subDetail == null) 7463 this.subDetail = new ArrayList<SubDetailComponent>(); 7464 this.subDetail.add(t); 7465 return t; 7466 } 7467 7468 public DetailComponent addSubDetail(SubDetailComponent t) { //3 7469 if (t == null) 7470 return this; 7471 if (this.subDetail == null) 7472 this.subDetail = new ArrayList<SubDetailComponent>(); 7473 this.subDetail.add(t); 7474 return this; 7475 } 7476 7477 /** 7478 * @return The first repetition of repeating field {@link #subDetail}, creating it if it does not already exist {3} 7479 */ 7480 public SubDetailComponent getSubDetailFirstRep() { 7481 if (getSubDetail().isEmpty()) { 7482 addSubDetail(); 7483 } 7484 return getSubDetail().get(0); 7485 } 7486 7487 protected void listChildren(List<Property> children) { 7488 super.listChildren(children); 7489 children.add(new Property("sequence", "positiveInt", "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, 1, sequence)); 7490 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 7491 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 7492 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 7493 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 7494 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 7495 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 7496 children.add(new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 7497 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 7498 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 7499 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 7500 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 7501 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 7502 children.add(new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor.", 0, 1, net)); 7503 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 7504 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 7505 children.add(new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 7506 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 7507 children.add(new Property("subDetail", "", "Third-tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, subDetail)); 7508 } 7509 7510 @Override 7511 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7512 switch (_hash) { 7513 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, 1, sequence); 7514 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 7515 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 7516 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 7517 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 7518 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 7519 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 7520 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 7521 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 7522 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 7523 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 7524 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 7525 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 7526 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the line item.detail. Net = unit price * quantity * factor.", 0, 1, net); 7527 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 7528 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 7529 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 7530 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 7531 case -828829007: /*subDetail*/ return new Property("subDetail", "", "Third-tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, subDetail); 7532 default: return super.getNamedProperty(_hash, _name, _checkValid); 7533 } 7534 7535 } 7536 7537 @Override 7538 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7539 switch (hash) { 7540 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 7541 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 7542 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 7543 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 7544 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 7545 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 7546 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 7547 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 7548 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 7549 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 7550 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 7551 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 7552 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 7553 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 7554 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 7555 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 7556 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ItemReviewOutcomeComponent 7557 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 7558 case -828829007: /*subDetail*/ return this.subDetail == null ? new Base[0] : this.subDetail.toArray(new Base[this.subDetail.size()]); // SubDetailComponent 7559 default: return super.getProperty(hash, name, checkValid); 7560 } 7561 7562 } 7563 7564 @Override 7565 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7566 switch (hash) { 7567 case 1349547969: // sequence 7568 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 7569 return value; 7570 case 82505966: // traceNumber 7571 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 7572 return value; 7573 case 1099842588: // revenue 7574 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7575 return value; 7576 case 50511102: // category 7577 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7578 return value; 7579 case 1957227299: // productOrService 7580 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7581 return value; 7582 case -717476168: // productOrServiceEnd 7583 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7584 return value; 7585 case -615513385: // modifier 7586 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 7587 return value; 7588 case 1010065041: // programCode 7589 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 7590 return value; 7591 case 525514609: // patientPaid 7592 this.patientPaid = TypeConvertor.castToMoney(value); // Money 7593 return value; 7594 case -1285004149: // quantity 7595 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 7596 return value; 7597 case -486196699: // unitPrice 7598 this.unitPrice = TypeConvertor.castToMoney(value); // Money 7599 return value; 7600 case -1282148017: // factor 7601 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 7602 return value; 7603 case 114603: // tax 7604 this.tax = TypeConvertor.castToMoney(value); // Money 7605 return value; 7606 case 108957: // net 7607 this.net = TypeConvertor.castToMoney(value); // Money 7608 return value; 7609 case 115642: // udi 7610 this.getUdi().add(TypeConvertor.castToReference(value)); // Reference 7611 return value; 7612 case -1110033957: // noteNumber 7613 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 7614 return value; 7615 case -51825446: // reviewOutcome 7616 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 7617 return value; 7618 case -231349275: // adjudication 7619 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 7620 return value; 7621 case -828829007: // subDetail 7622 this.getSubDetail().add((SubDetailComponent) value); // SubDetailComponent 7623 return value; 7624 default: return super.setProperty(hash, name, value); 7625 } 7626 7627 } 7628 7629 @Override 7630 public Base setProperty(String name, Base value) throws FHIRException { 7631 if (name.equals("sequence")) { 7632 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 7633 } else if (name.equals("traceNumber")) { 7634 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 7635 } else if (name.equals("revenue")) { 7636 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7637 } else if (name.equals("category")) { 7638 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7639 } else if (name.equals("productOrService")) { 7640 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7641 } else if (name.equals("productOrServiceEnd")) { 7642 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 7643 } else if (name.equals("modifier")) { 7644 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 7645 } else if (name.equals("programCode")) { 7646 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); 7647 } else if (name.equals("patientPaid")) { 7648 this.patientPaid = TypeConvertor.castToMoney(value); // Money 7649 } else if (name.equals("quantity")) { 7650 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 7651 } else if (name.equals("unitPrice")) { 7652 this.unitPrice = TypeConvertor.castToMoney(value); // Money 7653 } else if (name.equals("factor")) { 7654 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 7655 } else if (name.equals("tax")) { 7656 this.tax = TypeConvertor.castToMoney(value); // Money 7657 } else if (name.equals("net")) { 7658 this.net = TypeConvertor.castToMoney(value); // Money 7659 } else if (name.equals("udi")) { 7660 this.getUdi().add(TypeConvertor.castToReference(value)); 7661 } else if (name.equals("noteNumber")) { 7662 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 7663 } else if (name.equals("reviewOutcome")) { 7664 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 7665 } else if (name.equals("adjudication")) { 7666 this.getAdjudication().add((AdjudicationComponent) value); 7667 } else if (name.equals("subDetail")) { 7668 this.getSubDetail().add((SubDetailComponent) value); 7669 } else 7670 return super.setProperty(name, value); 7671 return value; 7672 } 7673 7674 @Override 7675 public void removeChild(String name, Base value) throws FHIRException { 7676 if (name.equals("sequence")) { 7677 this.sequence = null; 7678 } else if (name.equals("traceNumber")) { 7679 this.getTraceNumber().remove(value); 7680 } else if (name.equals("revenue")) { 7681 this.revenue = null; 7682 } else if (name.equals("category")) { 7683 this.category = null; 7684 } else if (name.equals("productOrService")) { 7685 this.productOrService = null; 7686 } else if (name.equals("productOrServiceEnd")) { 7687 this.productOrServiceEnd = null; 7688 } else if (name.equals("modifier")) { 7689 this.getModifier().remove(value); 7690 } else if (name.equals("programCode")) { 7691 this.getProgramCode().remove(value); 7692 } else if (name.equals("patientPaid")) { 7693 this.patientPaid = null; 7694 } else if (name.equals("quantity")) { 7695 this.quantity = null; 7696 } else if (name.equals("unitPrice")) { 7697 this.unitPrice = null; 7698 } else if (name.equals("factor")) { 7699 this.factor = null; 7700 } else if (name.equals("tax")) { 7701 this.tax = null; 7702 } else if (name.equals("net")) { 7703 this.net = null; 7704 } else if (name.equals("udi")) { 7705 this.getUdi().remove(value); 7706 } else if (name.equals("noteNumber")) { 7707 this.getNoteNumber().remove(value); 7708 } else if (name.equals("reviewOutcome")) { 7709 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 7710 } else if (name.equals("adjudication")) { 7711 this.getAdjudication().remove((AdjudicationComponent) value); 7712 } else if (name.equals("subDetail")) { 7713 this.getSubDetail().remove((SubDetailComponent) value); 7714 } else 7715 super.removeChild(name, value); 7716 7717 } 7718 7719 @Override 7720 public Base makeProperty(int hash, String name) throws FHIRException { 7721 switch (hash) { 7722 case 1349547969: return getSequenceElement(); 7723 case 82505966: return addTraceNumber(); 7724 case 1099842588: return getRevenue(); 7725 case 50511102: return getCategory(); 7726 case 1957227299: return getProductOrService(); 7727 case -717476168: return getProductOrServiceEnd(); 7728 case -615513385: return addModifier(); 7729 case 1010065041: return addProgramCode(); 7730 case 525514609: return getPatientPaid(); 7731 case -1285004149: return getQuantity(); 7732 case -486196699: return getUnitPrice(); 7733 case -1282148017: return getFactorElement(); 7734 case 114603: return getTax(); 7735 case 108957: return getNet(); 7736 case 115642: return addUdi(); 7737 case -1110033957: return addNoteNumberElement(); 7738 case -51825446: return getReviewOutcome(); 7739 case -231349275: return addAdjudication(); 7740 case -828829007: return addSubDetail(); 7741 default: return super.makeProperty(hash, name); 7742 } 7743 7744 } 7745 7746 @Override 7747 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7748 switch (hash) { 7749 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 7750 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 7751 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 7752 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 7753 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 7754 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 7755 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 7756 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 7757 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 7758 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 7759 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 7760 case -1282148017: /*factor*/ return new String[] {"decimal"}; 7761 case 114603: /*tax*/ return new String[] {"Money"}; 7762 case 108957: /*net*/ return new String[] {"Money"}; 7763 case 115642: /*udi*/ return new String[] {"Reference"}; 7764 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 7765 case -51825446: /*reviewOutcome*/ return new String[] {"@ExplanationOfBenefit.item.reviewOutcome"}; 7766 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 7767 case -828829007: /*subDetail*/ return new String[] {}; 7768 default: return super.getTypesForProperty(hash, name); 7769 } 7770 7771 } 7772 7773 @Override 7774 public Base addChild(String name) throws FHIRException { 7775 if (name.equals("sequence")) { 7776 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.detail.sequence"); 7777 } 7778 else if (name.equals("traceNumber")) { 7779 return addTraceNumber(); 7780 } 7781 else if (name.equals("revenue")) { 7782 this.revenue = new CodeableConcept(); 7783 return this.revenue; 7784 } 7785 else if (name.equals("category")) { 7786 this.category = new CodeableConcept(); 7787 return this.category; 7788 } 7789 else if (name.equals("productOrService")) { 7790 this.productOrService = new CodeableConcept(); 7791 return this.productOrService; 7792 } 7793 else if (name.equals("productOrServiceEnd")) { 7794 this.productOrServiceEnd = new CodeableConcept(); 7795 return this.productOrServiceEnd; 7796 } 7797 else if (name.equals("modifier")) { 7798 return addModifier(); 7799 } 7800 else if (name.equals("programCode")) { 7801 return addProgramCode(); 7802 } 7803 else if (name.equals("patientPaid")) { 7804 this.patientPaid = new Money(); 7805 return this.patientPaid; 7806 } 7807 else if (name.equals("quantity")) { 7808 this.quantity = new Quantity(); 7809 return this.quantity; 7810 } 7811 else if (name.equals("unitPrice")) { 7812 this.unitPrice = new Money(); 7813 return this.unitPrice; 7814 } 7815 else if (name.equals("factor")) { 7816 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.detail.factor"); 7817 } 7818 else if (name.equals("tax")) { 7819 this.tax = new Money(); 7820 return this.tax; 7821 } 7822 else if (name.equals("net")) { 7823 this.net = new Money(); 7824 return this.net; 7825 } 7826 else if (name.equals("udi")) { 7827 return addUdi(); 7828 } 7829 else if (name.equals("noteNumber")) { 7830 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.detail.noteNumber"); 7831 } 7832 else if (name.equals("reviewOutcome")) { 7833 this.reviewOutcome = new ItemReviewOutcomeComponent(); 7834 return this.reviewOutcome; 7835 } 7836 else if (name.equals("adjudication")) { 7837 return addAdjudication(); 7838 } 7839 else if (name.equals("subDetail")) { 7840 return addSubDetail(); 7841 } 7842 else 7843 return super.addChild(name); 7844 } 7845 7846 public DetailComponent copy() { 7847 DetailComponent dst = new DetailComponent(); 7848 copyValues(dst); 7849 return dst; 7850 } 7851 7852 public void copyValues(DetailComponent dst) { 7853 super.copyValues(dst); 7854 dst.sequence = sequence == null ? null : sequence.copy(); 7855 if (traceNumber != null) { 7856 dst.traceNumber = new ArrayList<Identifier>(); 7857 for (Identifier i : traceNumber) 7858 dst.traceNumber.add(i.copy()); 7859 }; 7860 dst.revenue = revenue == null ? null : revenue.copy(); 7861 dst.category = category == null ? null : category.copy(); 7862 dst.productOrService = productOrService == null ? null : productOrService.copy(); 7863 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 7864 if (modifier != null) { 7865 dst.modifier = new ArrayList<CodeableConcept>(); 7866 for (CodeableConcept i : modifier) 7867 dst.modifier.add(i.copy()); 7868 }; 7869 if (programCode != null) { 7870 dst.programCode = new ArrayList<CodeableConcept>(); 7871 for (CodeableConcept i : programCode) 7872 dst.programCode.add(i.copy()); 7873 }; 7874 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 7875 dst.quantity = quantity == null ? null : quantity.copy(); 7876 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 7877 dst.factor = factor == null ? null : factor.copy(); 7878 dst.tax = tax == null ? null : tax.copy(); 7879 dst.net = net == null ? null : net.copy(); 7880 if (udi != null) { 7881 dst.udi = new ArrayList<Reference>(); 7882 for (Reference i : udi) 7883 dst.udi.add(i.copy()); 7884 }; 7885 if (noteNumber != null) { 7886 dst.noteNumber = new ArrayList<PositiveIntType>(); 7887 for (PositiveIntType i : noteNumber) 7888 dst.noteNumber.add(i.copy()); 7889 }; 7890 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 7891 if (adjudication != null) { 7892 dst.adjudication = new ArrayList<AdjudicationComponent>(); 7893 for (AdjudicationComponent i : adjudication) 7894 dst.adjudication.add(i.copy()); 7895 }; 7896 if (subDetail != null) { 7897 dst.subDetail = new ArrayList<SubDetailComponent>(); 7898 for (SubDetailComponent i : subDetail) 7899 dst.subDetail.add(i.copy()); 7900 }; 7901 } 7902 7903 @Override 7904 public boolean equalsDeep(Base other_) { 7905 if (!super.equalsDeep(other_)) 7906 return false; 7907 if (!(other_ instanceof DetailComponent)) 7908 return false; 7909 DetailComponent o = (DetailComponent) other_; 7910 return compareDeep(sequence, o.sequence, true) && compareDeep(traceNumber, o.traceNumber, true) 7911 && compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 7912 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(modifier, o.modifier, true) 7913 && compareDeep(programCode, o.programCode, true) && compareDeep(patientPaid, o.patientPaid, true) 7914 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 7915 && compareDeep(tax, o.tax, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 7916 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(reviewOutcome, o.reviewOutcome, true) 7917 && compareDeep(adjudication, o.adjudication, true) && compareDeep(subDetail, o.subDetail, true) 7918 ; 7919 } 7920 7921 @Override 7922 public boolean equalsShallow(Base other_) { 7923 if (!super.equalsShallow(other_)) 7924 return false; 7925 if (!(other_ instanceof DetailComponent)) 7926 return false; 7927 DetailComponent o = (DetailComponent) other_; 7928 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true) 7929 ; 7930 } 7931 7932 public boolean isEmpty() { 7933 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, traceNumber, revenue 7934 , category, productOrService, productOrServiceEnd, modifier, programCode, patientPaid 7935 , quantity, unitPrice, factor, tax, net, udi, noteNumber, reviewOutcome, adjudication 7936 , subDetail); 7937 } 7938 7939 public String fhirType() { 7940 return "ExplanationOfBenefit.item.detail"; 7941 7942 } 7943 7944 } 7945 7946 @Block() 7947 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 7948 /** 7949 * A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 7950 */ 7951 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 7952 @Description(shortDefinition="Product or service provided", formalDefinition="A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items." ) 7953 protected PositiveIntType sequence; 7954 7955 /** 7956 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 7957 */ 7958 @Child(name = "traceNumber", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7959 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 7960 protected List<Identifier> traceNumber; 7961 7962 /** 7963 * The type of revenue or cost center providing the product and/or service. 7964 */ 7965 @Child(name = "revenue", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 7966 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 7967 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 7968 protected CodeableConcept revenue; 7969 7970 /** 7971 * Code to identify the general type of benefits under which products and services are provided. 7972 */ 7973 @Child(name = "category", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 7974 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 7975 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 7976 protected CodeableConcept category; 7977 7978 /** 7979 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 7980 */ 7981 @Child(name = "productOrService", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 7982 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 7983 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 7984 protected CodeableConcept productOrService; 7985 7986 /** 7987 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 7988 */ 7989 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 7990 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 7991 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 7992 protected CodeableConcept productOrServiceEnd; 7993 7994 /** 7995 * Item typification or modifiers codes to convey additional context for the product or service. 7996 */ 7997 @Child(name = "modifier", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7998 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 7999 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 8000 protected List<CodeableConcept> modifier; 8001 8002 /** 8003 * Identifies the program under which this may be recovered. 8004 */ 8005 @Child(name = "programCode", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8006 @Description(shortDefinition="Program the product or service is provided under", formalDefinition="Identifies the program under which this may be recovered." ) 8007 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 8008 protected List<CodeableConcept> programCode; 8009 8010 /** 8011 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 8012 */ 8013 @Child(name = "patientPaid", type = {Money.class}, order=9, min=0, max=1, modifier=false, summary=false) 8014 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 8015 protected Money patientPaid; 8016 8017 /** 8018 * The number of repetitions of a service or product. 8019 */ 8020 @Child(name = "quantity", type = {Quantity.class}, order=10, min=0, max=1, modifier=false, summary=false) 8021 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 8022 protected Quantity quantity; 8023 8024 /** 8025 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 8026 */ 8027 @Child(name = "unitPrice", type = {Money.class}, order=11, min=0, max=1, modifier=false, summary=false) 8028 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 8029 protected Money unitPrice; 8030 8031 /** 8032 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 8033 */ 8034 @Child(name = "factor", type = {DecimalType.class}, order=12, min=0, max=1, modifier=false, summary=false) 8035 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 8036 protected DecimalType factor; 8037 8038 /** 8039 * The total of taxes applicable for this product or service. 8040 */ 8041 @Child(name = "tax", type = {Money.class}, order=13, min=0, max=1, modifier=false, summary=false) 8042 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 8043 protected Money tax; 8044 8045 /** 8046 * The total amount claimed for the line item.detail.subDetail. Net = unit price * quantity * factor. 8047 */ 8048 @Child(name = "net", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=false) 8049 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the line item.detail.subDetail. Net = unit price * quantity * factor." ) 8050 protected Money net; 8051 8052 /** 8053 * Unique Device Identifiers associated with this line item. 8054 */ 8055 @Child(name = "udi", type = {Device.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8056 @Description(shortDefinition="Unique device identifier", formalDefinition="Unique Device Identifiers associated with this line item." ) 8057 protected List<Reference> udi; 8058 8059 /** 8060 * The numbers associated with notes below which apply to the adjudication of this item. 8061 */ 8062 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8063 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 8064 protected List<PositiveIntType> noteNumber; 8065 8066 /** 8067 * The high-level results of the adjudication if adjudication has been performed. 8068 */ 8069 @Child(name = "reviewOutcome", type = {ItemReviewOutcomeComponent.class}, order=17, min=0, max=1, modifier=false, summary=false) 8070 @Description(shortDefinition="Subdetail level adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 8071 protected ItemReviewOutcomeComponent reviewOutcome; 8072 8073 /** 8074 * The adjudication results. 8075 */ 8076 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8077 @Description(shortDefinition="Subdetail level adjudication details", formalDefinition="The adjudication results." ) 8078 protected List<AdjudicationComponent> adjudication; 8079 8080 private static final long serialVersionUID = -560048316L; 8081 8082 /** 8083 * Constructor 8084 */ 8085 public SubDetailComponent() { 8086 super(); 8087 } 8088 8089 /** 8090 * Constructor 8091 */ 8092 public SubDetailComponent(int sequence) { 8093 super(); 8094 this.setSequence(sequence); 8095 } 8096 8097 /** 8098 * @return {@link #sequence} (A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 8099 */ 8100 public PositiveIntType getSequenceElement() { 8101 if (this.sequence == null) 8102 if (Configuration.errorOnAutoCreate()) 8103 throw new Error("Attempt to auto-create SubDetailComponent.sequence"); 8104 else if (Configuration.doAutoCreate()) 8105 this.sequence = new PositiveIntType(); // bb 8106 return this.sequence; 8107 } 8108 8109 public boolean hasSequenceElement() { 8110 return this.sequence != null && !this.sequence.isEmpty(); 8111 } 8112 8113 public boolean hasSequence() { 8114 return this.sequence != null && !this.sequence.isEmpty(); 8115 } 8116 8117 /** 8118 * @param value {@link #sequence} (A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 8119 */ 8120 public SubDetailComponent setSequenceElement(PositiveIntType value) { 8121 this.sequence = value; 8122 return this; 8123 } 8124 8125 /** 8126 * @return A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 8127 */ 8128 public int getSequence() { 8129 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 8130 } 8131 8132 /** 8133 * @param value A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items. 8134 */ 8135 public SubDetailComponent setSequence(int value) { 8136 if (this.sequence == null) 8137 this.sequence = new PositiveIntType(); 8138 this.sequence.setValue(value); 8139 return this; 8140 } 8141 8142 /** 8143 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 8144 */ 8145 public List<Identifier> getTraceNumber() { 8146 if (this.traceNumber == null) 8147 this.traceNumber = new ArrayList<Identifier>(); 8148 return this.traceNumber; 8149 } 8150 8151 /** 8152 * @return Returns a reference to <code>this</code> for easy method chaining 8153 */ 8154 public SubDetailComponent setTraceNumber(List<Identifier> theTraceNumber) { 8155 this.traceNumber = theTraceNumber; 8156 return this; 8157 } 8158 8159 public boolean hasTraceNumber() { 8160 if (this.traceNumber == null) 8161 return false; 8162 for (Identifier item : this.traceNumber) 8163 if (!item.isEmpty()) 8164 return true; 8165 return false; 8166 } 8167 8168 public Identifier addTraceNumber() { //3 8169 Identifier t = new Identifier(); 8170 if (this.traceNumber == null) 8171 this.traceNumber = new ArrayList<Identifier>(); 8172 this.traceNumber.add(t); 8173 return t; 8174 } 8175 8176 public SubDetailComponent addTraceNumber(Identifier t) { //3 8177 if (t == null) 8178 return this; 8179 if (this.traceNumber == null) 8180 this.traceNumber = new ArrayList<Identifier>(); 8181 this.traceNumber.add(t); 8182 return this; 8183 } 8184 8185 /** 8186 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 8187 */ 8188 public Identifier getTraceNumberFirstRep() { 8189 if (getTraceNumber().isEmpty()) { 8190 addTraceNumber(); 8191 } 8192 return getTraceNumber().get(0); 8193 } 8194 8195 /** 8196 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 8197 */ 8198 public CodeableConcept getRevenue() { 8199 if (this.revenue == null) 8200 if (Configuration.errorOnAutoCreate()) 8201 throw new Error("Attempt to auto-create SubDetailComponent.revenue"); 8202 else if (Configuration.doAutoCreate()) 8203 this.revenue = new CodeableConcept(); // cc 8204 return this.revenue; 8205 } 8206 8207 public boolean hasRevenue() { 8208 return this.revenue != null && !this.revenue.isEmpty(); 8209 } 8210 8211 /** 8212 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 8213 */ 8214 public SubDetailComponent setRevenue(CodeableConcept value) { 8215 this.revenue = value; 8216 return this; 8217 } 8218 8219 /** 8220 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 8221 */ 8222 public CodeableConcept getCategory() { 8223 if (this.category == null) 8224 if (Configuration.errorOnAutoCreate()) 8225 throw new Error("Attempt to auto-create SubDetailComponent.category"); 8226 else if (Configuration.doAutoCreate()) 8227 this.category = new CodeableConcept(); // cc 8228 return this.category; 8229 } 8230 8231 public boolean hasCategory() { 8232 return this.category != null && !this.category.isEmpty(); 8233 } 8234 8235 /** 8236 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 8237 */ 8238 public SubDetailComponent setCategory(CodeableConcept value) { 8239 this.category = value; 8240 return this; 8241 } 8242 8243 /** 8244 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 8245 */ 8246 public CodeableConcept getProductOrService() { 8247 if (this.productOrService == null) 8248 if (Configuration.errorOnAutoCreate()) 8249 throw new Error("Attempt to auto-create SubDetailComponent.productOrService"); 8250 else if (Configuration.doAutoCreate()) 8251 this.productOrService = new CodeableConcept(); // cc 8252 return this.productOrService; 8253 } 8254 8255 public boolean hasProductOrService() { 8256 return this.productOrService != null && !this.productOrService.isEmpty(); 8257 } 8258 8259 /** 8260 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 8261 */ 8262 public SubDetailComponent setProductOrService(CodeableConcept value) { 8263 this.productOrService = value; 8264 return this; 8265 } 8266 8267 /** 8268 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 8269 */ 8270 public CodeableConcept getProductOrServiceEnd() { 8271 if (this.productOrServiceEnd == null) 8272 if (Configuration.errorOnAutoCreate()) 8273 throw new Error("Attempt to auto-create SubDetailComponent.productOrServiceEnd"); 8274 else if (Configuration.doAutoCreate()) 8275 this.productOrServiceEnd = new CodeableConcept(); // cc 8276 return this.productOrServiceEnd; 8277 } 8278 8279 public boolean hasProductOrServiceEnd() { 8280 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 8281 } 8282 8283 /** 8284 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 8285 */ 8286 public SubDetailComponent setProductOrServiceEnd(CodeableConcept value) { 8287 this.productOrServiceEnd = value; 8288 return this; 8289 } 8290 8291 /** 8292 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 8293 */ 8294 public List<CodeableConcept> getModifier() { 8295 if (this.modifier == null) 8296 this.modifier = new ArrayList<CodeableConcept>(); 8297 return this.modifier; 8298 } 8299 8300 /** 8301 * @return Returns a reference to <code>this</code> for easy method chaining 8302 */ 8303 public SubDetailComponent setModifier(List<CodeableConcept> theModifier) { 8304 this.modifier = theModifier; 8305 return this; 8306 } 8307 8308 public boolean hasModifier() { 8309 if (this.modifier == null) 8310 return false; 8311 for (CodeableConcept item : this.modifier) 8312 if (!item.isEmpty()) 8313 return true; 8314 return false; 8315 } 8316 8317 public CodeableConcept addModifier() { //3 8318 CodeableConcept t = new CodeableConcept(); 8319 if (this.modifier == null) 8320 this.modifier = new ArrayList<CodeableConcept>(); 8321 this.modifier.add(t); 8322 return t; 8323 } 8324 8325 public SubDetailComponent addModifier(CodeableConcept t) { //3 8326 if (t == null) 8327 return this; 8328 if (this.modifier == null) 8329 this.modifier = new ArrayList<CodeableConcept>(); 8330 this.modifier.add(t); 8331 return this; 8332 } 8333 8334 /** 8335 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 8336 */ 8337 public CodeableConcept getModifierFirstRep() { 8338 if (getModifier().isEmpty()) { 8339 addModifier(); 8340 } 8341 return getModifier().get(0); 8342 } 8343 8344 /** 8345 * @return {@link #programCode} (Identifies the program under which this may be recovered.) 8346 */ 8347 public List<CodeableConcept> getProgramCode() { 8348 if (this.programCode == null) 8349 this.programCode = new ArrayList<CodeableConcept>(); 8350 return this.programCode; 8351 } 8352 8353 /** 8354 * @return Returns a reference to <code>this</code> for easy method chaining 8355 */ 8356 public SubDetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 8357 this.programCode = theProgramCode; 8358 return this; 8359 } 8360 8361 public boolean hasProgramCode() { 8362 if (this.programCode == null) 8363 return false; 8364 for (CodeableConcept item : this.programCode) 8365 if (!item.isEmpty()) 8366 return true; 8367 return false; 8368 } 8369 8370 public CodeableConcept addProgramCode() { //3 8371 CodeableConcept t = new CodeableConcept(); 8372 if (this.programCode == null) 8373 this.programCode = new ArrayList<CodeableConcept>(); 8374 this.programCode.add(t); 8375 return t; 8376 } 8377 8378 public SubDetailComponent addProgramCode(CodeableConcept t) { //3 8379 if (t == null) 8380 return this; 8381 if (this.programCode == null) 8382 this.programCode = new ArrayList<CodeableConcept>(); 8383 this.programCode.add(t); 8384 return this; 8385 } 8386 8387 /** 8388 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist {3} 8389 */ 8390 public CodeableConcept getProgramCodeFirstRep() { 8391 if (getProgramCode().isEmpty()) { 8392 addProgramCode(); 8393 } 8394 return getProgramCode().get(0); 8395 } 8396 8397 /** 8398 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 8399 */ 8400 public Money getPatientPaid() { 8401 if (this.patientPaid == null) 8402 if (Configuration.errorOnAutoCreate()) 8403 throw new Error("Attempt to auto-create SubDetailComponent.patientPaid"); 8404 else if (Configuration.doAutoCreate()) 8405 this.patientPaid = new Money(); // cc 8406 return this.patientPaid; 8407 } 8408 8409 public boolean hasPatientPaid() { 8410 return this.patientPaid != null && !this.patientPaid.isEmpty(); 8411 } 8412 8413 /** 8414 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 8415 */ 8416 public SubDetailComponent setPatientPaid(Money value) { 8417 this.patientPaid = value; 8418 return this; 8419 } 8420 8421 /** 8422 * @return {@link #quantity} (The number of repetitions of a service or product.) 8423 */ 8424 public Quantity getQuantity() { 8425 if (this.quantity == null) 8426 if (Configuration.errorOnAutoCreate()) 8427 throw new Error("Attempt to auto-create SubDetailComponent.quantity"); 8428 else if (Configuration.doAutoCreate()) 8429 this.quantity = new Quantity(); // cc 8430 return this.quantity; 8431 } 8432 8433 public boolean hasQuantity() { 8434 return this.quantity != null && !this.quantity.isEmpty(); 8435 } 8436 8437 /** 8438 * @param value {@link #quantity} (The number of repetitions of a service or product.) 8439 */ 8440 public SubDetailComponent setQuantity(Quantity value) { 8441 this.quantity = value; 8442 return this; 8443 } 8444 8445 /** 8446 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 8447 */ 8448 public Money getUnitPrice() { 8449 if (this.unitPrice == null) 8450 if (Configuration.errorOnAutoCreate()) 8451 throw new Error("Attempt to auto-create SubDetailComponent.unitPrice"); 8452 else if (Configuration.doAutoCreate()) 8453 this.unitPrice = new Money(); // cc 8454 return this.unitPrice; 8455 } 8456 8457 public boolean hasUnitPrice() { 8458 return this.unitPrice != null && !this.unitPrice.isEmpty(); 8459 } 8460 8461 /** 8462 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 8463 */ 8464 public SubDetailComponent setUnitPrice(Money value) { 8465 this.unitPrice = value; 8466 return this; 8467 } 8468 8469 /** 8470 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 8471 */ 8472 public DecimalType getFactorElement() { 8473 if (this.factor == null) 8474 if (Configuration.errorOnAutoCreate()) 8475 throw new Error("Attempt to auto-create SubDetailComponent.factor"); 8476 else if (Configuration.doAutoCreate()) 8477 this.factor = new DecimalType(); // bb 8478 return this.factor; 8479 } 8480 8481 public boolean hasFactorElement() { 8482 return this.factor != null && !this.factor.isEmpty(); 8483 } 8484 8485 public boolean hasFactor() { 8486 return this.factor != null && !this.factor.isEmpty(); 8487 } 8488 8489 /** 8490 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 8491 */ 8492 public SubDetailComponent setFactorElement(DecimalType value) { 8493 this.factor = value; 8494 return this; 8495 } 8496 8497 /** 8498 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 8499 */ 8500 public BigDecimal getFactor() { 8501 return this.factor == null ? null : this.factor.getValue(); 8502 } 8503 8504 /** 8505 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 8506 */ 8507 public SubDetailComponent setFactor(BigDecimal value) { 8508 if (value == null) 8509 this.factor = null; 8510 else { 8511 if (this.factor == null) 8512 this.factor = new DecimalType(); 8513 this.factor.setValue(value); 8514 } 8515 return this; 8516 } 8517 8518 /** 8519 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 8520 */ 8521 public SubDetailComponent setFactor(long value) { 8522 this.factor = new DecimalType(); 8523 this.factor.setValue(value); 8524 return this; 8525 } 8526 8527 /** 8528 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 8529 */ 8530 public SubDetailComponent setFactor(double value) { 8531 this.factor = new DecimalType(); 8532 this.factor.setValue(value); 8533 return this; 8534 } 8535 8536 /** 8537 * @return {@link #tax} (The total of taxes applicable for this product or service.) 8538 */ 8539 public Money getTax() { 8540 if (this.tax == null) 8541 if (Configuration.errorOnAutoCreate()) 8542 throw new Error("Attempt to auto-create SubDetailComponent.tax"); 8543 else if (Configuration.doAutoCreate()) 8544 this.tax = new Money(); // cc 8545 return this.tax; 8546 } 8547 8548 public boolean hasTax() { 8549 return this.tax != null && !this.tax.isEmpty(); 8550 } 8551 8552 /** 8553 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 8554 */ 8555 public SubDetailComponent setTax(Money value) { 8556 this.tax = value; 8557 return this; 8558 } 8559 8560 /** 8561 * @return {@link #net} (The total amount claimed for the line item.detail.subDetail. Net = unit price * quantity * factor.) 8562 */ 8563 public Money getNet() { 8564 if (this.net == null) 8565 if (Configuration.errorOnAutoCreate()) 8566 throw new Error("Attempt to auto-create SubDetailComponent.net"); 8567 else if (Configuration.doAutoCreate()) 8568 this.net = new Money(); // cc 8569 return this.net; 8570 } 8571 8572 public boolean hasNet() { 8573 return this.net != null && !this.net.isEmpty(); 8574 } 8575 8576 /** 8577 * @param value {@link #net} (The total amount claimed for the line item.detail.subDetail. Net = unit price * quantity * factor.) 8578 */ 8579 public SubDetailComponent setNet(Money value) { 8580 this.net = value; 8581 return this; 8582 } 8583 8584 /** 8585 * @return {@link #udi} (Unique Device Identifiers associated with this line item.) 8586 */ 8587 public List<Reference> getUdi() { 8588 if (this.udi == null) 8589 this.udi = new ArrayList<Reference>(); 8590 return this.udi; 8591 } 8592 8593 /** 8594 * @return Returns a reference to <code>this</code> for easy method chaining 8595 */ 8596 public SubDetailComponent setUdi(List<Reference> theUdi) { 8597 this.udi = theUdi; 8598 return this; 8599 } 8600 8601 public boolean hasUdi() { 8602 if (this.udi == null) 8603 return false; 8604 for (Reference item : this.udi) 8605 if (!item.isEmpty()) 8606 return true; 8607 return false; 8608 } 8609 8610 public Reference addUdi() { //3 8611 Reference t = new Reference(); 8612 if (this.udi == null) 8613 this.udi = new ArrayList<Reference>(); 8614 this.udi.add(t); 8615 return t; 8616 } 8617 8618 public SubDetailComponent addUdi(Reference t) { //3 8619 if (t == null) 8620 return this; 8621 if (this.udi == null) 8622 this.udi = new ArrayList<Reference>(); 8623 this.udi.add(t); 8624 return this; 8625 } 8626 8627 /** 8628 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist {3} 8629 */ 8630 public Reference getUdiFirstRep() { 8631 if (getUdi().isEmpty()) { 8632 addUdi(); 8633 } 8634 return getUdi().get(0); 8635 } 8636 8637 /** 8638 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 8639 */ 8640 public List<PositiveIntType> getNoteNumber() { 8641 if (this.noteNumber == null) 8642 this.noteNumber = new ArrayList<PositiveIntType>(); 8643 return this.noteNumber; 8644 } 8645 8646 /** 8647 * @return Returns a reference to <code>this</code> for easy method chaining 8648 */ 8649 public SubDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 8650 this.noteNumber = theNoteNumber; 8651 return this; 8652 } 8653 8654 public boolean hasNoteNumber() { 8655 if (this.noteNumber == null) 8656 return false; 8657 for (PositiveIntType item : this.noteNumber) 8658 if (!item.isEmpty()) 8659 return true; 8660 return false; 8661 } 8662 8663 /** 8664 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 8665 */ 8666 public PositiveIntType addNoteNumberElement() {//2 8667 PositiveIntType t = new PositiveIntType(); 8668 if (this.noteNumber == null) 8669 this.noteNumber = new ArrayList<PositiveIntType>(); 8670 this.noteNumber.add(t); 8671 return t; 8672 } 8673 8674 /** 8675 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 8676 */ 8677 public SubDetailComponent addNoteNumber(int value) { //1 8678 PositiveIntType t = new PositiveIntType(); 8679 t.setValue(value); 8680 if (this.noteNumber == null) 8681 this.noteNumber = new ArrayList<PositiveIntType>(); 8682 this.noteNumber.add(t); 8683 return this; 8684 } 8685 8686 /** 8687 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 8688 */ 8689 public boolean hasNoteNumber(int value) { 8690 if (this.noteNumber == null) 8691 return false; 8692 for (PositiveIntType v : this.noteNumber) 8693 if (v.getValue().equals(value)) // positiveInt 8694 return true; 8695 return false; 8696 } 8697 8698 /** 8699 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 8700 */ 8701 public ItemReviewOutcomeComponent getReviewOutcome() { 8702 if (this.reviewOutcome == null) 8703 if (Configuration.errorOnAutoCreate()) 8704 throw new Error("Attempt to auto-create SubDetailComponent.reviewOutcome"); 8705 else if (Configuration.doAutoCreate()) 8706 this.reviewOutcome = new ItemReviewOutcomeComponent(); // cc 8707 return this.reviewOutcome; 8708 } 8709 8710 public boolean hasReviewOutcome() { 8711 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 8712 } 8713 8714 /** 8715 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 8716 */ 8717 public SubDetailComponent setReviewOutcome(ItemReviewOutcomeComponent value) { 8718 this.reviewOutcome = value; 8719 return this; 8720 } 8721 8722 /** 8723 * @return {@link #adjudication} (The adjudication results.) 8724 */ 8725 public List<AdjudicationComponent> getAdjudication() { 8726 if (this.adjudication == null) 8727 this.adjudication = new ArrayList<AdjudicationComponent>(); 8728 return this.adjudication; 8729 } 8730 8731 /** 8732 * @return Returns a reference to <code>this</code> for easy method chaining 8733 */ 8734 public SubDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 8735 this.adjudication = theAdjudication; 8736 return this; 8737 } 8738 8739 public boolean hasAdjudication() { 8740 if (this.adjudication == null) 8741 return false; 8742 for (AdjudicationComponent item : this.adjudication) 8743 if (!item.isEmpty()) 8744 return true; 8745 return false; 8746 } 8747 8748 public AdjudicationComponent addAdjudication() { //3 8749 AdjudicationComponent t = new AdjudicationComponent(); 8750 if (this.adjudication == null) 8751 this.adjudication = new ArrayList<AdjudicationComponent>(); 8752 this.adjudication.add(t); 8753 return t; 8754 } 8755 8756 public SubDetailComponent addAdjudication(AdjudicationComponent t) { //3 8757 if (t == null) 8758 return this; 8759 if (this.adjudication == null) 8760 this.adjudication = new ArrayList<AdjudicationComponent>(); 8761 this.adjudication.add(t); 8762 return this; 8763 } 8764 8765 /** 8766 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 8767 */ 8768 public AdjudicationComponent getAdjudicationFirstRep() { 8769 if (getAdjudication().isEmpty()) { 8770 addAdjudication(); 8771 } 8772 return getAdjudication().get(0); 8773 } 8774 8775 protected void listChildren(List<Property> children) { 8776 super.listChildren(children); 8777 children.add(new Property("sequence", "positiveInt", "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, 1, sequence)); 8778 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 8779 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 8780 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 8781 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 8782 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 8783 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 8784 children.add(new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 8785 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 8786 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 8787 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 8788 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 8789 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 8790 children.add(new Property("net", "Money", "The total amount claimed for the line item.detail.subDetail. Net = unit price * quantity * factor.", 0, 1, net)); 8791 children.add(new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 8792 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 8793 children.add(new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 8794 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 8795 } 8796 8797 @Override 8798 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8799 switch (_hash) { 8800 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A claim detail line. Either a simple (a product or service) or a 'group' of sub-details which are simple items.", 0, 1, sequence); 8801 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 8802 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 8803 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 8804 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 8805 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 8806 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 8807 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 8808 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 8809 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 8810 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 8811 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 8812 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 8813 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the line item.detail.subDetail. Net = unit price * quantity * factor.", 0, 1, net); 8814 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 8815 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 8816 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 8817 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 8818 default: return super.getNamedProperty(_hash, _name, _checkValid); 8819 } 8820 8821 } 8822 8823 @Override 8824 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8825 switch (hash) { 8826 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 8827 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 8828 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 8829 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 8830 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 8831 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 8832 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 8833 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 8834 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 8835 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 8836 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 8837 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 8838 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 8839 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 8840 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 8841 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 8842 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ItemReviewOutcomeComponent 8843 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 8844 default: return super.getProperty(hash, name, checkValid); 8845 } 8846 8847 } 8848 8849 @Override 8850 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8851 switch (hash) { 8852 case 1349547969: // sequence 8853 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 8854 return value; 8855 case 82505966: // traceNumber 8856 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 8857 return value; 8858 case 1099842588: // revenue 8859 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8860 return value; 8861 case 50511102: // category 8862 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8863 return value; 8864 case 1957227299: // productOrService 8865 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8866 return value; 8867 case -717476168: // productOrServiceEnd 8868 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8869 return value; 8870 case -615513385: // modifier 8871 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 8872 return value; 8873 case 1010065041: // programCode 8874 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 8875 return value; 8876 case 525514609: // patientPaid 8877 this.patientPaid = TypeConvertor.castToMoney(value); // Money 8878 return value; 8879 case -1285004149: // quantity 8880 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 8881 return value; 8882 case -486196699: // unitPrice 8883 this.unitPrice = TypeConvertor.castToMoney(value); // Money 8884 return value; 8885 case -1282148017: // factor 8886 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 8887 return value; 8888 case 114603: // tax 8889 this.tax = TypeConvertor.castToMoney(value); // Money 8890 return value; 8891 case 108957: // net 8892 this.net = TypeConvertor.castToMoney(value); // Money 8893 return value; 8894 case 115642: // udi 8895 this.getUdi().add(TypeConvertor.castToReference(value)); // Reference 8896 return value; 8897 case -1110033957: // noteNumber 8898 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 8899 return value; 8900 case -51825446: // reviewOutcome 8901 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 8902 return value; 8903 case -231349275: // adjudication 8904 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 8905 return value; 8906 default: return super.setProperty(hash, name, value); 8907 } 8908 8909 } 8910 8911 @Override 8912 public Base setProperty(String name, Base value) throws FHIRException { 8913 if (name.equals("sequence")) { 8914 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 8915 } else if (name.equals("traceNumber")) { 8916 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 8917 } else if (name.equals("revenue")) { 8918 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8919 } else if (name.equals("category")) { 8920 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8921 } else if (name.equals("productOrService")) { 8922 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8923 } else if (name.equals("productOrServiceEnd")) { 8924 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 8925 } else if (name.equals("modifier")) { 8926 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 8927 } else if (name.equals("programCode")) { 8928 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); 8929 } else if (name.equals("patientPaid")) { 8930 this.patientPaid = TypeConvertor.castToMoney(value); // Money 8931 } else if (name.equals("quantity")) { 8932 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 8933 } else if (name.equals("unitPrice")) { 8934 this.unitPrice = TypeConvertor.castToMoney(value); // Money 8935 } else if (name.equals("factor")) { 8936 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 8937 } else if (name.equals("tax")) { 8938 this.tax = TypeConvertor.castToMoney(value); // Money 8939 } else if (name.equals("net")) { 8940 this.net = TypeConvertor.castToMoney(value); // Money 8941 } else if (name.equals("udi")) { 8942 this.getUdi().add(TypeConvertor.castToReference(value)); 8943 } else if (name.equals("noteNumber")) { 8944 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 8945 } else if (name.equals("reviewOutcome")) { 8946 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 8947 } else if (name.equals("adjudication")) { 8948 this.getAdjudication().add((AdjudicationComponent) value); 8949 } else 8950 return super.setProperty(name, value); 8951 return value; 8952 } 8953 8954 @Override 8955 public void removeChild(String name, Base value) throws FHIRException { 8956 if (name.equals("sequence")) { 8957 this.sequence = null; 8958 } else if (name.equals("traceNumber")) { 8959 this.getTraceNumber().remove(value); 8960 } else if (name.equals("revenue")) { 8961 this.revenue = null; 8962 } else if (name.equals("category")) { 8963 this.category = null; 8964 } else if (name.equals("productOrService")) { 8965 this.productOrService = null; 8966 } else if (name.equals("productOrServiceEnd")) { 8967 this.productOrServiceEnd = null; 8968 } else if (name.equals("modifier")) { 8969 this.getModifier().remove(value); 8970 } else if (name.equals("programCode")) { 8971 this.getProgramCode().remove(value); 8972 } else if (name.equals("patientPaid")) { 8973 this.patientPaid = null; 8974 } else if (name.equals("quantity")) { 8975 this.quantity = null; 8976 } else if (name.equals("unitPrice")) { 8977 this.unitPrice = null; 8978 } else if (name.equals("factor")) { 8979 this.factor = null; 8980 } else if (name.equals("tax")) { 8981 this.tax = null; 8982 } else if (name.equals("net")) { 8983 this.net = null; 8984 } else if (name.equals("udi")) { 8985 this.getUdi().remove(value); 8986 } else if (name.equals("noteNumber")) { 8987 this.getNoteNumber().remove(value); 8988 } else if (name.equals("reviewOutcome")) { 8989 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 8990 } else if (name.equals("adjudication")) { 8991 this.getAdjudication().remove((AdjudicationComponent) value); 8992 } else 8993 super.removeChild(name, value); 8994 8995 } 8996 8997 @Override 8998 public Base makeProperty(int hash, String name) throws FHIRException { 8999 switch (hash) { 9000 case 1349547969: return getSequenceElement(); 9001 case 82505966: return addTraceNumber(); 9002 case 1099842588: return getRevenue(); 9003 case 50511102: return getCategory(); 9004 case 1957227299: return getProductOrService(); 9005 case -717476168: return getProductOrServiceEnd(); 9006 case -615513385: return addModifier(); 9007 case 1010065041: return addProgramCode(); 9008 case 525514609: return getPatientPaid(); 9009 case -1285004149: return getQuantity(); 9010 case -486196699: return getUnitPrice(); 9011 case -1282148017: return getFactorElement(); 9012 case 114603: return getTax(); 9013 case 108957: return getNet(); 9014 case 115642: return addUdi(); 9015 case -1110033957: return addNoteNumberElement(); 9016 case -51825446: return getReviewOutcome(); 9017 case -231349275: return addAdjudication(); 9018 default: return super.makeProperty(hash, name); 9019 } 9020 9021 } 9022 9023 @Override 9024 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9025 switch (hash) { 9026 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 9027 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 9028 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 9029 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 9030 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 9031 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 9032 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 9033 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 9034 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 9035 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 9036 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 9037 case -1282148017: /*factor*/ return new String[] {"decimal"}; 9038 case 114603: /*tax*/ return new String[] {"Money"}; 9039 case 108957: /*net*/ return new String[] {"Money"}; 9040 case 115642: /*udi*/ return new String[] {"Reference"}; 9041 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 9042 case -51825446: /*reviewOutcome*/ return new String[] {"@ExplanationOfBenefit.item.reviewOutcome"}; 9043 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 9044 default: return super.getTypesForProperty(hash, name); 9045 } 9046 9047 } 9048 9049 @Override 9050 public Base addChild(String name) throws FHIRException { 9051 if (name.equals("sequence")) { 9052 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.detail.subDetail.sequence"); 9053 } 9054 else if (name.equals("traceNumber")) { 9055 return addTraceNumber(); 9056 } 9057 else if (name.equals("revenue")) { 9058 this.revenue = new CodeableConcept(); 9059 return this.revenue; 9060 } 9061 else if (name.equals("category")) { 9062 this.category = new CodeableConcept(); 9063 return this.category; 9064 } 9065 else if (name.equals("productOrService")) { 9066 this.productOrService = new CodeableConcept(); 9067 return this.productOrService; 9068 } 9069 else if (name.equals("productOrServiceEnd")) { 9070 this.productOrServiceEnd = new CodeableConcept(); 9071 return this.productOrServiceEnd; 9072 } 9073 else if (name.equals("modifier")) { 9074 return addModifier(); 9075 } 9076 else if (name.equals("programCode")) { 9077 return addProgramCode(); 9078 } 9079 else if (name.equals("patientPaid")) { 9080 this.patientPaid = new Money(); 9081 return this.patientPaid; 9082 } 9083 else if (name.equals("quantity")) { 9084 this.quantity = new Quantity(); 9085 return this.quantity; 9086 } 9087 else if (name.equals("unitPrice")) { 9088 this.unitPrice = new Money(); 9089 return this.unitPrice; 9090 } 9091 else if (name.equals("factor")) { 9092 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.detail.subDetail.factor"); 9093 } 9094 else if (name.equals("tax")) { 9095 this.tax = new Money(); 9096 return this.tax; 9097 } 9098 else if (name.equals("net")) { 9099 this.net = new Money(); 9100 return this.net; 9101 } 9102 else if (name.equals("udi")) { 9103 return addUdi(); 9104 } 9105 else if (name.equals("noteNumber")) { 9106 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.item.detail.subDetail.noteNumber"); 9107 } 9108 else if (name.equals("reviewOutcome")) { 9109 this.reviewOutcome = new ItemReviewOutcomeComponent(); 9110 return this.reviewOutcome; 9111 } 9112 else if (name.equals("adjudication")) { 9113 return addAdjudication(); 9114 } 9115 else 9116 return super.addChild(name); 9117 } 9118 9119 public SubDetailComponent copy() { 9120 SubDetailComponent dst = new SubDetailComponent(); 9121 copyValues(dst); 9122 return dst; 9123 } 9124 9125 public void copyValues(SubDetailComponent dst) { 9126 super.copyValues(dst); 9127 dst.sequence = sequence == null ? null : sequence.copy(); 9128 if (traceNumber != null) { 9129 dst.traceNumber = new ArrayList<Identifier>(); 9130 for (Identifier i : traceNumber) 9131 dst.traceNumber.add(i.copy()); 9132 }; 9133 dst.revenue = revenue == null ? null : revenue.copy(); 9134 dst.category = category == null ? null : category.copy(); 9135 dst.productOrService = productOrService == null ? null : productOrService.copy(); 9136 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 9137 if (modifier != null) { 9138 dst.modifier = new ArrayList<CodeableConcept>(); 9139 for (CodeableConcept i : modifier) 9140 dst.modifier.add(i.copy()); 9141 }; 9142 if (programCode != null) { 9143 dst.programCode = new ArrayList<CodeableConcept>(); 9144 for (CodeableConcept i : programCode) 9145 dst.programCode.add(i.copy()); 9146 }; 9147 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 9148 dst.quantity = quantity == null ? null : quantity.copy(); 9149 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 9150 dst.factor = factor == null ? null : factor.copy(); 9151 dst.tax = tax == null ? null : tax.copy(); 9152 dst.net = net == null ? null : net.copy(); 9153 if (udi != null) { 9154 dst.udi = new ArrayList<Reference>(); 9155 for (Reference i : udi) 9156 dst.udi.add(i.copy()); 9157 }; 9158 if (noteNumber != null) { 9159 dst.noteNumber = new ArrayList<PositiveIntType>(); 9160 for (PositiveIntType i : noteNumber) 9161 dst.noteNumber.add(i.copy()); 9162 }; 9163 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 9164 if (adjudication != null) { 9165 dst.adjudication = new ArrayList<AdjudicationComponent>(); 9166 for (AdjudicationComponent i : adjudication) 9167 dst.adjudication.add(i.copy()); 9168 }; 9169 } 9170 9171 @Override 9172 public boolean equalsDeep(Base other_) { 9173 if (!super.equalsDeep(other_)) 9174 return false; 9175 if (!(other_ instanceof SubDetailComponent)) 9176 return false; 9177 SubDetailComponent o = (SubDetailComponent) other_; 9178 return compareDeep(sequence, o.sequence, true) && compareDeep(traceNumber, o.traceNumber, true) 9179 && compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) && compareDeep(productOrService, o.productOrService, true) 9180 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(modifier, o.modifier, true) 9181 && compareDeep(programCode, o.programCode, true) && compareDeep(patientPaid, o.patientPaid, true) 9182 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 9183 && compareDeep(tax, o.tax, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 9184 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(reviewOutcome, o.reviewOutcome, true) 9185 && compareDeep(adjudication, o.adjudication, true); 9186 } 9187 9188 @Override 9189 public boolean equalsShallow(Base other_) { 9190 if (!super.equalsShallow(other_)) 9191 return false; 9192 if (!(other_ instanceof SubDetailComponent)) 9193 return false; 9194 SubDetailComponent o = (SubDetailComponent) other_; 9195 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true) 9196 ; 9197 } 9198 9199 public boolean isEmpty() { 9200 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, traceNumber, revenue 9201 , category, productOrService, productOrServiceEnd, modifier, programCode, patientPaid 9202 , quantity, unitPrice, factor, tax, net, udi, noteNumber, reviewOutcome, adjudication 9203 ); 9204 } 9205 9206 public String fhirType() { 9207 return "ExplanationOfBenefit.item.detail.subDetail"; 9208 9209 } 9210 9211 } 9212 9213 @Block() 9214 public static class AddedItemComponent extends BackboneElement implements IBaseBackboneElement { 9215 /** 9216 * Claim items which this service line is intended to replace. 9217 */ 9218 @Child(name = "itemSequence", type = {PositiveIntType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9219 @Description(shortDefinition="Item sequence number", formalDefinition="Claim items which this service line is intended to replace." ) 9220 protected List<PositiveIntType> itemSequence; 9221 9222 /** 9223 * The sequence number of the details within the claim item which this line is intended to replace. 9224 */ 9225 @Child(name = "detailSequence", type = {PositiveIntType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9226 @Description(shortDefinition="Detail sequence number", formalDefinition="The sequence number of the details within the claim item which this line is intended to replace." ) 9227 protected List<PositiveIntType> detailSequence; 9228 9229 /** 9230 * The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace. 9231 */ 9232 @Child(name = "subDetailSequence", type = {PositiveIntType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9233 @Description(shortDefinition="Subdetail sequence number", formalDefinition="The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace." ) 9234 protected List<PositiveIntType> subDetailSequence; 9235 9236 /** 9237 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 9238 */ 9239 @Child(name = "traceNumber", type = {Identifier.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9240 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 9241 protected List<Identifier> traceNumber; 9242 9243 /** 9244 * The providers who are authorized for the services rendered to the patient. 9245 */ 9246 @Child(name = "provider", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9247 @Description(shortDefinition="Authorized providers", formalDefinition="The providers who are authorized for the services rendered to the patient." ) 9248 protected List<Reference> provider; 9249 9250 /** 9251 * The type of revenue or cost center providing the product and/or service. 9252 */ 9253 @Child(name = "revenue", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 9254 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 9255 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 9256 protected CodeableConcept revenue; 9257 9258 /** 9259 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 9260 */ 9261 @Child(name = "productOrService", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 9262 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 9263 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 9264 protected CodeableConcept productOrService; 9265 9266 /** 9267 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 9268 */ 9269 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 9270 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 9271 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 9272 protected CodeableConcept productOrServiceEnd; 9273 9274 /** 9275 * Request or Referral for Goods or Service to be rendered. 9276 */ 9277 @Child(name = "request", type = {DeviceRequest.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class, SupplyRequest.class, VisionPrescription.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9278 @Description(shortDefinition="Request or Referral for Service", formalDefinition="Request or Referral for Goods or Service to be rendered." ) 9279 protected List<Reference> request; 9280 9281 /** 9282 * Item typification or modifiers codes to convey additional context for the product or service. 9283 */ 9284 @Child(name = "modifier", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9285 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 9286 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 9287 protected List<CodeableConcept> modifier; 9288 9289 /** 9290 * Identifies the program under which this may be recovered. 9291 */ 9292 @Child(name = "programCode", type = {CodeableConcept.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9293 @Description(shortDefinition="Program the product or service is provided under", formalDefinition="Identifies the program under which this may be recovered." ) 9294 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 9295 protected List<CodeableConcept> programCode; 9296 9297 /** 9298 * The date or dates when the service or product was supplied, performed or completed. 9299 */ 9300 @Child(name = "serviced", type = {DateType.class, Period.class}, order=12, min=0, max=1, modifier=false, summary=false) 9301 @Description(shortDefinition="Date or dates of service or product delivery", formalDefinition="The date or dates when the service or product was supplied, performed or completed." ) 9302 protected DataType serviced; 9303 9304 /** 9305 * Where the product or service was provided. 9306 */ 9307 @Child(name = "location", type = {CodeableConcept.class, Address.class, Location.class}, order=13, min=0, max=1, modifier=false, summary=false) 9308 @Description(shortDefinition="Place of service or where product was supplied", formalDefinition="Where the product or service was provided." ) 9309 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-place") 9310 protected DataType location; 9311 9312 /** 9313 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 9314 */ 9315 @Child(name = "patientPaid", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=false) 9316 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 9317 protected Money patientPaid; 9318 9319 /** 9320 * The number of repetitions of a service or product. 9321 */ 9322 @Child(name = "quantity", type = {Quantity.class}, order=15, min=0, max=1, modifier=false, summary=false) 9323 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 9324 protected Quantity quantity; 9325 9326 /** 9327 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 9328 */ 9329 @Child(name = "unitPrice", type = {Money.class}, order=16, min=0, max=1, modifier=false, summary=false) 9330 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 9331 protected Money unitPrice; 9332 9333 /** 9334 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 9335 */ 9336 @Child(name = "factor", type = {DecimalType.class}, order=17, min=0, max=1, modifier=false, summary=false) 9337 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 9338 protected DecimalType factor; 9339 9340 /** 9341 * The total of taxes applicable for this product or service. 9342 */ 9343 @Child(name = "tax", type = {Money.class}, order=18, min=0, max=1, modifier=false, summary=false) 9344 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 9345 protected Money tax; 9346 9347 /** 9348 * The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor. 9349 */ 9350 @Child(name = "net", type = {Money.class}, order=19, min=0, max=1, modifier=false, summary=false) 9351 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor." ) 9352 protected Money net; 9353 9354 /** 9355 * Physical location where the service is performed or applies. 9356 */ 9357 @Child(name = "bodySite", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9358 @Description(shortDefinition="Anatomical location", formalDefinition="Physical location where the service is performed or applies." ) 9359 protected List<AddedItemBodySiteComponent> bodySite; 9360 9361 /** 9362 * The numbers associated with notes below which apply to the adjudication of this item. 9363 */ 9364 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9365 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 9366 protected List<PositiveIntType> noteNumber; 9367 9368 /** 9369 * The high-level results of the adjudication if adjudication has been performed. 9370 */ 9371 @Child(name = "reviewOutcome", type = {ItemReviewOutcomeComponent.class}, order=22, min=0, max=1, modifier=false, summary=false) 9372 @Description(shortDefinition="Additem level adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 9373 protected ItemReviewOutcomeComponent reviewOutcome; 9374 9375 /** 9376 * The adjudication results. 9377 */ 9378 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9379 @Description(shortDefinition="Added items adjudication", formalDefinition="The adjudication results." ) 9380 protected List<AdjudicationComponent> adjudication; 9381 9382 /** 9383 * The second-tier service adjudications for payor added services. 9384 */ 9385 @Child(name = "detail", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9386 @Description(shortDefinition="Insurer added line items", formalDefinition="The second-tier service adjudications for payor added services." ) 9387 protected List<AddedItemDetailComponent> detail; 9388 9389 private static final long serialVersionUID = -1942324543L; 9390 9391 /** 9392 * Constructor 9393 */ 9394 public AddedItemComponent() { 9395 super(); 9396 } 9397 9398 /** 9399 * @return {@link #itemSequence} (Claim items which this service line is intended to replace.) 9400 */ 9401 public List<PositiveIntType> getItemSequence() { 9402 if (this.itemSequence == null) 9403 this.itemSequence = new ArrayList<PositiveIntType>(); 9404 return this.itemSequence; 9405 } 9406 9407 /** 9408 * @return Returns a reference to <code>this</code> for easy method chaining 9409 */ 9410 public AddedItemComponent setItemSequence(List<PositiveIntType> theItemSequence) { 9411 this.itemSequence = theItemSequence; 9412 return this; 9413 } 9414 9415 public boolean hasItemSequence() { 9416 if (this.itemSequence == null) 9417 return false; 9418 for (PositiveIntType item : this.itemSequence) 9419 if (!item.isEmpty()) 9420 return true; 9421 return false; 9422 } 9423 9424 /** 9425 * @return {@link #itemSequence} (Claim items which this service line is intended to replace.) 9426 */ 9427 public PositiveIntType addItemSequenceElement() {//2 9428 PositiveIntType t = new PositiveIntType(); 9429 if (this.itemSequence == null) 9430 this.itemSequence = new ArrayList<PositiveIntType>(); 9431 this.itemSequence.add(t); 9432 return t; 9433 } 9434 9435 /** 9436 * @param value {@link #itemSequence} (Claim items which this service line is intended to replace.) 9437 */ 9438 public AddedItemComponent addItemSequence(int value) { //1 9439 PositiveIntType t = new PositiveIntType(); 9440 t.setValue(value); 9441 if (this.itemSequence == null) 9442 this.itemSequence = new ArrayList<PositiveIntType>(); 9443 this.itemSequence.add(t); 9444 return this; 9445 } 9446 9447 /** 9448 * @param value {@link #itemSequence} (Claim items which this service line is intended to replace.) 9449 */ 9450 public boolean hasItemSequence(int value) { 9451 if (this.itemSequence == null) 9452 return false; 9453 for (PositiveIntType v : this.itemSequence) 9454 if (v.getValue().equals(value)) // positiveInt 9455 return true; 9456 return false; 9457 } 9458 9459 /** 9460 * @return {@link #detailSequence} (The sequence number of the details within the claim item which this line is intended to replace.) 9461 */ 9462 public List<PositiveIntType> getDetailSequence() { 9463 if (this.detailSequence == null) 9464 this.detailSequence = new ArrayList<PositiveIntType>(); 9465 return this.detailSequence; 9466 } 9467 9468 /** 9469 * @return Returns a reference to <code>this</code> for easy method chaining 9470 */ 9471 public AddedItemComponent setDetailSequence(List<PositiveIntType> theDetailSequence) { 9472 this.detailSequence = theDetailSequence; 9473 return this; 9474 } 9475 9476 public boolean hasDetailSequence() { 9477 if (this.detailSequence == null) 9478 return false; 9479 for (PositiveIntType item : this.detailSequence) 9480 if (!item.isEmpty()) 9481 return true; 9482 return false; 9483 } 9484 9485 /** 9486 * @return {@link #detailSequence} (The sequence number of the details within the claim item which this line is intended to replace.) 9487 */ 9488 public PositiveIntType addDetailSequenceElement() {//2 9489 PositiveIntType t = new PositiveIntType(); 9490 if (this.detailSequence == null) 9491 this.detailSequence = new ArrayList<PositiveIntType>(); 9492 this.detailSequence.add(t); 9493 return t; 9494 } 9495 9496 /** 9497 * @param value {@link #detailSequence} (The sequence number of the details within the claim item which this line is intended to replace.) 9498 */ 9499 public AddedItemComponent addDetailSequence(int value) { //1 9500 PositiveIntType t = new PositiveIntType(); 9501 t.setValue(value); 9502 if (this.detailSequence == null) 9503 this.detailSequence = new ArrayList<PositiveIntType>(); 9504 this.detailSequence.add(t); 9505 return this; 9506 } 9507 9508 /** 9509 * @param value {@link #detailSequence} (The sequence number of the details within the claim item which this line is intended to replace.) 9510 */ 9511 public boolean hasDetailSequence(int value) { 9512 if (this.detailSequence == null) 9513 return false; 9514 for (PositiveIntType v : this.detailSequence) 9515 if (v.getValue().equals(value)) // positiveInt 9516 return true; 9517 return false; 9518 } 9519 9520 /** 9521 * @return {@link #subDetailSequence} (The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace.) 9522 */ 9523 public List<PositiveIntType> getSubDetailSequence() { 9524 if (this.subDetailSequence == null) 9525 this.subDetailSequence = new ArrayList<PositiveIntType>(); 9526 return this.subDetailSequence; 9527 } 9528 9529 /** 9530 * @return Returns a reference to <code>this</code> for easy method chaining 9531 */ 9532 public AddedItemComponent setSubDetailSequence(List<PositiveIntType> theSubDetailSequence) { 9533 this.subDetailSequence = theSubDetailSequence; 9534 return this; 9535 } 9536 9537 public boolean hasSubDetailSequence() { 9538 if (this.subDetailSequence == null) 9539 return false; 9540 for (PositiveIntType item : this.subDetailSequence) 9541 if (!item.isEmpty()) 9542 return true; 9543 return false; 9544 } 9545 9546 /** 9547 * @return {@link #subDetailSequence} (The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace.) 9548 */ 9549 public PositiveIntType addSubDetailSequenceElement() {//2 9550 PositiveIntType t = new PositiveIntType(); 9551 if (this.subDetailSequence == null) 9552 this.subDetailSequence = new ArrayList<PositiveIntType>(); 9553 this.subDetailSequence.add(t); 9554 return t; 9555 } 9556 9557 /** 9558 * @param value {@link #subDetailSequence} (The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace.) 9559 */ 9560 public AddedItemComponent addSubDetailSequence(int value) { //1 9561 PositiveIntType t = new PositiveIntType(); 9562 t.setValue(value); 9563 if (this.subDetailSequence == null) 9564 this.subDetailSequence = new ArrayList<PositiveIntType>(); 9565 this.subDetailSequence.add(t); 9566 return this; 9567 } 9568 9569 /** 9570 * @param value {@link #subDetailSequence} (The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace.) 9571 */ 9572 public boolean hasSubDetailSequence(int value) { 9573 if (this.subDetailSequence == null) 9574 return false; 9575 for (PositiveIntType v : this.subDetailSequence) 9576 if (v.getValue().equals(value)) // positiveInt 9577 return true; 9578 return false; 9579 } 9580 9581 /** 9582 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 9583 */ 9584 public List<Identifier> getTraceNumber() { 9585 if (this.traceNumber == null) 9586 this.traceNumber = new ArrayList<Identifier>(); 9587 return this.traceNumber; 9588 } 9589 9590 /** 9591 * @return Returns a reference to <code>this</code> for easy method chaining 9592 */ 9593 public AddedItemComponent setTraceNumber(List<Identifier> theTraceNumber) { 9594 this.traceNumber = theTraceNumber; 9595 return this; 9596 } 9597 9598 public boolean hasTraceNumber() { 9599 if (this.traceNumber == null) 9600 return false; 9601 for (Identifier item : this.traceNumber) 9602 if (!item.isEmpty()) 9603 return true; 9604 return false; 9605 } 9606 9607 public Identifier addTraceNumber() { //3 9608 Identifier t = new Identifier(); 9609 if (this.traceNumber == null) 9610 this.traceNumber = new ArrayList<Identifier>(); 9611 this.traceNumber.add(t); 9612 return t; 9613 } 9614 9615 public AddedItemComponent addTraceNumber(Identifier t) { //3 9616 if (t == null) 9617 return this; 9618 if (this.traceNumber == null) 9619 this.traceNumber = new ArrayList<Identifier>(); 9620 this.traceNumber.add(t); 9621 return this; 9622 } 9623 9624 /** 9625 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 9626 */ 9627 public Identifier getTraceNumberFirstRep() { 9628 if (getTraceNumber().isEmpty()) { 9629 addTraceNumber(); 9630 } 9631 return getTraceNumber().get(0); 9632 } 9633 9634 /** 9635 * @return {@link #provider} (The providers who are authorized for the services rendered to the patient.) 9636 */ 9637 public List<Reference> getProvider() { 9638 if (this.provider == null) 9639 this.provider = new ArrayList<Reference>(); 9640 return this.provider; 9641 } 9642 9643 /** 9644 * @return Returns a reference to <code>this</code> for easy method chaining 9645 */ 9646 public AddedItemComponent setProvider(List<Reference> theProvider) { 9647 this.provider = theProvider; 9648 return this; 9649 } 9650 9651 public boolean hasProvider() { 9652 if (this.provider == null) 9653 return false; 9654 for (Reference item : this.provider) 9655 if (!item.isEmpty()) 9656 return true; 9657 return false; 9658 } 9659 9660 public Reference addProvider() { //3 9661 Reference t = new Reference(); 9662 if (this.provider == null) 9663 this.provider = new ArrayList<Reference>(); 9664 this.provider.add(t); 9665 return t; 9666 } 9667 9668 public AddedItemComponent addProvider(Reference t) { //3 9669 if (t == null) 9670 return this; 9671 if (this.provider == null) 9672 this.provider = new ArrayList<Reference>(); 9673 this.provider.add(t); 9674 return this; 9675 } 9676 9677 /** 9678 * @return The first repetition of repeating field {@link #provider}, creating it if it does not already exist {3} 9679 */ 9680 public Reference getProviderFirstRep() { 9681 if (getProvider().isEmpty()) { 9682 addProvider(); 9683 } 9684 return getProvider().get(0); 9685 } 9686 9687 /** 9688 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 9689 */ 9690 public CodeableConcept getRevenue() { 9691 if (this.revenue == null) 9692 if (Configuration.errorOnAutoCreate()) 9693 throw new Error("Attempt to auto-create AddedItemComponent.revenue"); 9694 else if (Configuration.doAutoCreate()) 9695 this.revenue = new CodeableConcept(); // cc 9696 return this.revenue; 9697 } 9698 9699 public boolean hasRevenue() { 9700 return this.revenue != null && !this.revenue.isEmpty(); 9701 } 9702 9703 /** 9704 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 9705 */ 9706 public AddedItemComponent setRevenue(CodeableConcept value) { 9707 this.revenue = value; 9708 return this; 9709 } 9710 9711 /** 9712 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 9713 */ 9714 public CodeableConcept getProductOrService() { 9715 if (this.productOrService == null) 9716 if (Configuration.errorOnAutoCreate()) 9717 throw new Error("Attempt to auto-create AddedItemComponent.productOrService"); 9718 else if (Configuration.doAutoCreate()) 9719 this.productOrService = new CodeableConcept(); // cc 9720 return this.productOrService; 9721 } 9722 9723 public boolean hasProductOrService() { 9724 return this.productOrService != null && !this.productOrService.isEmpty(); 9725 } 9726 9727 /** 9728 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 9729 */ 9730 public AddedItemComponent setProductOrService(CodeableConcept value) { 9731 this.productOrService = value; 9732 return this; 9733 } 9734 9735 /** 9736 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 9737 */ 9738 public CodeableConcept getProductOrServiceEnd() { 9739 if (this.productOrServiceEnd == null) 9740 if (Configuration.errorOnAutoCreate()) 9741 throw new Error("Attempt to auto-create AddedItemComponent.productOrServiceEnd"); 9742 else if (Configuration.doAutoCreate()) 9743 this.productOrServiceEnd = new CodeableConcept(); // cc 9744 return this.productOrServiceEnd; 9745 } 9746 9747 public boolean hasProductOrServiceEnd() { 9748 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 9749 } 9750 9751 /** 9752 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 9753 */ 9754 public AddedItemComponent setProductOrServiceEnd(CodeableConcept value) { 9755 this.productOrServiceEnd = value; 9756 return this; 9757 } 9758 9759 /** 9760 * @return {@link #request} (Request or Referral for Goods or Service to be rendered.) 9761 */ 9762 public List<Reference> getRequest() { 9763 if (this.request == null) 9764 this.request = new ArrayList<Reference>(); 9765 return this.request; 9766 } 9767 9768 /** 9769 * @return Returns a reference to <code>this</code> for easy method chaining 9770 */ 9771 public AddedItemComponent setRequest(List<Reference> theRequest) { 9772 this.request = theRequest; 9773 return this; 9774 } 9775 9776 public boolean hasRequest() { 9777 if (this.request == null) 9778 return false; 9779 for (Reference item : this.request) 9780 if (!item.isEmpty()) 9781 return true; 9782 return false; 9783 } 9784 9785 public Reference addRequest() { //3 9786 Reference t = new Reference(); 9787 if (this.request == null) 9788 this.request = new ArrayList<Reference>(); 9789 this.request.add(t); 9790 return t; 9791 } 9792 9793 public AddedItemComponent addRequest(Reference t) { //3 9794 if (t == null) 9795 return this; 9796 if (this.request == null) 9797 this.request = new ArrayList<Reference>(); 9798 this.request.add(t); 9799 return this; 9800 } 9801 9802 /** 9803 * @return The first repetition of repeating field {@link #request}, creating it if it does not already exist {3} 9804 */ 9805 public Reference getRequestFirstRep() { 9806 if (getRequest().isEmpty()) { 9807 addRequest(); 9808 } 9809 return getRequest().get(0); 9810 } 9811 9812 /** 9813 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 9814 */ 9815 public List<CodeableConcept> getModifier() { 9816 if (this.modifier == null) 9817 this.modifier = new ArrayList<CodeableConcept>(); 9818 return this.modifier; 9819 } 9820 9821 /** 9822 * @return Returns a reference to <code>this</code> for easy method chaining 9823 */ 9824 public AddedItemComponent setModifier(List<CodeableConcept> theModifier) { 9825 this.modifier = theModifier; 9826 return this; 9827 } 9828 9829 public boolean hasModifier() { 9830 if (this.modifier == null) 9831 return false; 9832 for (CodeableConcept item : this.modifier) 9833 if (!item.isEmpty()) 9834 return true; 9835 return false; 9836 } 9837 9838 public CodeableConcept addModifier() { //3 9839 CodeableConcept t = new CodeableConcept(); 9840 if (this.modifier == null) 9841 this.modifier = new ArrayList<CodeableConcept>(); 9842 this.modifier.add(t); 9843 return t; 9844 } 9845 9846 public AddedItemComponent addModifier(CodeableConcept t) { //3 9847 if (t == null) 9848 return this; 9849 if (this.modifier == null) 9850 this.modifier = new ArrayList<CodeableConcept>(); 9851 this.modifier.add(t); 9852 return this; 9853 } 9854 9855 /** 9856 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 9857 */ 9858 public CodeableConcept getModifierFirstRep() { 9859 if (getModifier().isEmpty()) { 9860 addModifier(); 9861 } 9862 return getModifier().get(0); 9863 } 9864 9865 /** 9866 * @return {@link #programCode} (Identifies the program under which this may be recovered.) 9867 */ 9868 public List<CodeableConcept> getProgramCode() { 9869 if (this.programCode == null) 9870 this.programCode = new ArrayList<CodeableConcept>(); 9871 return this.programCode; 9872 } 9873 9874 /** 9875 * @return Returns a reference to <code>this</code> for easy method chaining 9876 */ 9877 public AddedItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 9878 this.programCode = theProgramCode; 9879 return this; 9880 } 9881 9882 public boolean hasProgramCode() { 9883 if (this.programCode == null) 9884 return false; 9885 for (CodeableConcept item : this.programCode) 9886 if (!item.isEmpty()) 9887 return true; 9888 return false; 9889 } 9890 9891 public CodeableConcept addProgramCode() { //3 9892 CodeableConcept t = new CodeableConcept(); 9893 if (this.programCode == null) 9894 this.programCode = new ArrayList<CodeableConcept>(); 9895 this.programCode.add(t); 9896 return t; 9897 } 9898 9899 public AddedItemComponent addProgramCode(CodeableConcept t) { //3 9900 if (t == null) 9901 return this; 9902 if (this.programCode == null) 9903 this.programCode = new ArrayList<CodeableConcept>(); 9904 this.programCode.add(t); 9905 return this; 9906 } 9907 9908 /** 9909 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist {3} 9910 */ 9911 public CodeableConcept getProgramCodeFirstRep() { 9912 if (getProgramCode().isEmpty()) { 9913 addProgramCode(); 9914 } 9915 return getProgramCode().get(0); 9916 } 9917 9918 /** 9919 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 9920 */ 9921 public DataType getServiced() { 9922 return this.serviced; 9923 } 9924 9925 /** 9926 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 9927 */ 9928 public DateType getServicedDateType() throws FHIRException { 9929 if (this.serviced == null) 9930 this.serviced = new DateType(); 9931 if (!(this.serviced instanceof DateType)) 9932 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.serviced.getClass().getName()+" was encountered"); 9933 return (DateType) this.serviced; 9934 } 9935 9936 public boolean hasServicedDateType() { 9937 return this.serviced instanceof DateType; 9938 } 9939 9940 /** 9941 * @return {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 9942 */ 9943 public Period getServicedPeriod() throws FHIRException { 9944 if (this.serviced == null) 9945 this.serviced = new Period(); 9946 if (!(this.serviced instanceof Period)) 9947 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.serviced.getClass().getName()+" was encountered"); 9948 return (Period) this.serviced; 9949 } 9950 9951 public boolean hasServicedPeriod() { 9952 return this.serviced instanceof Period; 9953 } 9954 9955 public boolean hasServiced() { 9956 return this.serviced != null && !this.serviced.isEmpty(); 9957 } 9958 9959 /** 9960 * @param value {@link #serviced} (The date or dates when the service or product was supplied, performed or completed.) 9961 */ 9962 public AddedItemComponent setServiced(DataType value) { 9963 if (value != null && !(value instanceof DateType || value instanceof Period)) 9964 throw new FHIRException("Not the right type for ExplanationOfBenefit.addItem.serviced[x]: "+value.fhirType()); 9965 this.serviced = value; 9966 return this; 9967 } 9968 9969 /** 9970 * @return {@link #location} (Where the product or service was provided.) 9971 */ 9972 public DataType getLocation() { 9973 return this.location; 9974 } 9975 9976 /** 9977 * @return {@link #location} (Where the product or service was provided.) 9978 */ 9979 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 9980 if (this.location == null) 9981 this.location = new CodeableConcept(); 9982 if (!(this.location instanceof CodeableConcept)) 9983 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.location.getClass().getName()+" was encountered"); 9984 return (CodeableConcept) this.location; 9985 } 9986 9987 public boolean hasLocationCodeableConcept() { 9988 return this.location instanceof CodeableConcept; 9989 } 9990 9991 /** 9992 * @return {@link #location} (Where the product or service was provided.) 9993 */ 9994 public Address getLocationAddress() throws FHIRException { 9995 if (this.location == null) 9996 this.location = new Address(); 9997 if (!(this.location instanceof Address)) 9998 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 9999 return (Address) this.location; 10000 } 10001 10002 public boolean hasLocationAddress() { 10003 return this.location instanceof Address; 10004 } 10005 10006 /** 10007 * @return {@link #location} (Where the product or service was provided.) 10008 */ 10009 public Reference getLocationReference() throws FHIRException { 10010 if (this.location == null) 10011 this.location = new Reference(); 10012 if (!(this.location instanceof Reference)) 10013 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 10014 return (Reference) this.location; 10015 } 10016 10017 public boolean hasLocationReference() { 10018 return this.location instanceof Reference; 10019 } 10020 10021 public boolean hasLocation() { 10022 return this.location != null && !this.location.isEmpty(); 10023 } 10024 10025 /** 10026 * @param value {@link #location} (Where the product or service was provided.) 10027 */ 10028 public AddedItemComponent setLocation(DataType value) { 10029 if (value != null && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 10030 throw new FHIRException("Not the right type for ExplanationOfBenefit.addItem.location[x]: "+value.fhirType()); 10031 this.location = value; 10032 return this; 10033 } 10034 10035 /** 10036 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 10037 */ 10038 public Money getPatientPaid() { 10039 if (this.patientPaid == null) 10040 if (Configuration.errorOnAutoCreate()) 10041 throw new Error("Attempt to auto-create AddedItemComponent.patientPaid"); 10042 else if (Configuration.doAutoCreate()) 10043 this.patientPaid = new Money(); // cc 10044 return this.patientPaid; 10045 } 10046 10047 public boolean hasPatientPaid() { 10048 return this.patientPaid != null && !this.patientPaid.isEmpty(); 10049 } 10050 10051 /** 10052 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 10053 */ 10054 public AddedItemComponent setPatientPaid(Money value) { 10055 this.patientPaid = value; 10056 return this; 10057 } 10058 10059 /** 10060 * @return {@link #quantity} (The number of repetitions of a service or product.) 10061 */ 10062 public Quantity getQuantity() { 10063 if (this.quantity == null) 10064 if (Configuration.errorOnAutoCreate()) 10065 throw new Error("Attempt to auto-create AddedItemComponent.quantity"); 10066 else if (Configuration.doAutoCreate()) 10067 this.quantity = new Quantity(); // cc 10068 return this.quantity; 10069 } 10070 10071 public boolean hasQuantity() { 10072 return this.quantity != null && !this.quantity.isEmpty(); 10073 } 10074 10075 /** 10076 * @param value {@link #quantity} (The number of repetitions of a service or product.) 10077 */ 10078 public AddedItemComponent setQuantity(Quantity value) { 10079 this.quantity = value; 10080 return this; 10081 } 10082 10083 /** 10084 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 10085 */ 10086 public Money getUnitPrice() { 10087 if (this.unitPrice == null) 10088 if (Configuration.errorOnAutoCreate()) 10089 throw new Error("Attempt to auto-create AddedItemComponent.unitPrice"); 10090 else if (Configuration.doAutoCreate()) 10091 this.unitPrice = new Money(); // cc 10092 return this.unitPrice; 10093 } 10094 10095 public boolean hasUnitPrice() { 10096 return this.unitPrice != null && !this.unitPrice.isEmpty(); 10097 } 10098 10099 /** 10100 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 10101 */ 10102 public AddedItemComponent setUnitPrice(Money value) { 10103 this.unitPrice = value; 10104 return this; 10105 } 10106 10107 /** 10108 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 10109 */ 10110 public DecimalType getFactorElement() { 10111 if (this.factor == null) 10112 if (Configuration.errorOnAutoCreate()) 10113 throw new Error("Attempt to auto-create AddedItemComponent.factor"); 10114 else if (Configuration.doAutoCreate()) 10115 this.factor = new DecimalType(); // bb 10116 return this.factor; 10117 } 10118 10119 public boolean hasFactorElement() { 10120 return this.factor != null && !this.factor.isEmpty(); 10121 } 10122 10123 public boolean hasFactor() { 10124 return this.factor != null && !this.factor.isEmpty(); 10125 } 10126 10127 /** 10128 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 10129 */ 10130 public AddedItemComponent setFactorElement(DecimalType value) { 10131 this.factor = value; 10132 return this; 10133 } 10134 10135 /** 10136 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 10137 */ 10138 public BigDecimal getFactor() { 10139 return this.factor == null ? null : this.factor.getValue(); 10140 } 10141 10142 /** 10143 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 10144 */ 10145 public AddedItemComponent setFactor(BigDecimal value) { 10146 if (value == null) 10147 this.factor = null; 10148 else { 10149 if (this.factor == null) 10150 this.factor = new DecimalType(); 10151 this.factor.setValue(value); 10152 } 10153 return this; 10154 } 10155 10156 /** 10157 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 10158 */ 10159 public AddedItemComponent setFactor(long value) { 10160 this.factor = new DecimalType(); 10161 this.factor.setValue(value); 10162 return this; 10163 } 10164 10165 /** 10166 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 10167 */ 10168 public AddedItemComponent setFactor(double value) { 10169 this.factor = new DecimalType(); 10170 this.factor.setValue(value); 10171 return this; 10172 } 10173 10174 /** 10175 * @return {@link #tax} (The total of taxes applicable for this product or service.) 10176 */ 10177 public Money getTax() { 10178 if (this.tax == null) 10179 if (Configuration.errorOnAutoCreate()) 10180 throw new Error("Attempt to auto-create AddedItemComponent.tax"); 10181 else if (Configuration.doAutoCreate()) 10182 this.tax = new Money(); // cc 10183 return this.tax; 10184 } 10185 10186 public boolean hasTax() { 10187 return this.tax != null && !this.tax.isEmpty(); 10188 } 10189 10190 /** 10191 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 10192 */ 10193 public AddedItemComponent setTax(Money value) { 10194 this.tax = value; 10195 return this; 10196 } 10197 10198 /** 10199 * @return {@link #net} (The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor.) 10200 */ 10201 public Money getNet() { 10202 if (this.net == null) 10203 if (Configuration.errorOnAutoCreate()) 10204 throw new Error("Attempt to auto-create AddedItemComponent.net"); 10205 else if (Configuration.doAutoCreate()) 10206 this.net = new Money(); // cc 10207 return this.net; 10208 } 10209 10210 public boolean hasNet() { 10211 return this.net != null && !this.net.isEmpty(); 10212 } 10213 10214 /** 10215 * @param value {@link #net} (The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor.) 10216 */ 10217 public AddedItemComponent setNet(Money value) { 10218 this.net = value; 10219 return this; 10220 } 10221 10222 /** 10223 * @return {@link #bodySite} (Physical location where the service is performed or applies.) 10224 */ 10225 public List<AddedItemBodySiteComponent> getBodySite() { 10226 if (this.bodySite == null) 10227 this.bodySite = new ArrayList<AddedItemBodySiteComponent>(); 10228 return this.bodySite; 10229 } 10230 10231 /** 10232 * @return Returns a reference to <code>this</code> for easy method chaining 10233 */ 10234 public AddedItemComponent setBodySite(List<AddedItemBodySiteComponent> theBodySite) { 10235 this.bodySite = theBodySite; 10236 return this; 10237 } 10238 10239 public boolean hasBodySite() { 10240 if (this.bodySite == null) 10241 return false; 10242 for (AddedItemBodySiteComponent item : this.bodySite) 10243 if (!item.isEmpty()) 10244 return true; 10245 return false; 10246 } 10247 10248 public AddedItemBodySiteComponent addBodySite() { //3 10249 AddedItemBodySiteComponent t = new AddedItemBodySiteComponent(); 10250 if (this.bodySite == null) 10251 this.bodySite = new ArrayList<AddedItemBodySiteComponent>(); 10252 this.bodySite.add(t); 10253 return t; 10254 } 10255 10256 public AddedItemComponent addBodySite(AddedItemBodySiteComponent t) { //3 10257 if (t == null) 10258 return this; 10259 if (this.bodySite == null) 10260 this.bodySite = new ArrayList<AddedItemBodySiteComponent>(); 10261 this.bodySite.add(t); 10262 return this; 10263 } 10264 10265 /** 10266 * @return The first repetition of repeating field {@link #bodySite}, creating it if it does not already exist {3} 10267 */ 10268 public AddedItemBodySiteComponent getBodySiteFirstRep() { 10269 if (getBodySite().isEmpty()) { 10270 addBodySite(); 10271 } 10272 return getBodySite().get(0); 10273 } 10274 10275 /** 10276 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 10277 */ 10278 public List<PositiveIntType> getNoteNumber() { 10279 if (this.noteNumber == null) 10280 this.noteNumber = new ArrayList<PositiveIntType>(); 10281 return this.noteNumber; 10282 } 10283 10284 /** 10285 * @return Returns a reference to <code>this</code> for easy method chaining 10286 */ 10287 public AddedItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 10288 this.noteNumber = theNoteNumber; 10289 return this; 10290 } 10291 10292 public boolean hasNoteNumber() { 10293 if (this.noteNumber == null) 10294 return false; 10295 for (PositiveIntType item : this.noteNumber) 10296 if (!item.isEmpty()) 10297 return true; 10298 return false; 10299 } 10300 10301 /** 10302 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 10303 */ 10304 public PositiveIntType addNoteNumberElement() {//2 10305 PositiveIntType t = new PositiveIntType(); 10306 if (this.noteNumber == null) 10307 this.noteNumber = new ArrayList<PositiveIntType>(); 10308 this.noteNumber.add(t); 10309 return t; 10310 } 10311 10312 /** 10313 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 10314 */ 10315 public AddedItemComponent addNoteNumber(int value) { //1 10316 PositiveIntType t = new PositiveIntType(); 10317 t.setValue(value); 10318 if (this.noteNumber == null) 10319 this.noteNumber = new ArrayList<PositiveIntType>(); 10320 this.noteNumber.add(t); 10321 return this; 10322 } 10323 10324 /** 10325 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 10326 */ 10327 public boolean hasNoteNumber(int value) { 10328 if (this.noteNumber == null) 10329 return false; 10330 for (PositiveIntType v : this.noteNumber) 10331 if (v.getValue().equals(value)) // positiveInt 10332 return true; 10333 return false; 10334 } 10335 10336 /** 10337 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 10338 */ 10339 public ItemReviewOutcomeComponent getReviewOutcome() { 10340 if (this.reviewOutcome == null) 10341 if (Configuration.errorOnAutoCreate()) 10342 throw new Error("Attempt to auto-create AddedItemComponent.reviewOutcome"); 10343 else if (Configuration.doAutoCreate()) 10344 this.reviewOutcome = new ItemReviewOutcomeComponent(); // cc 10345 return this.reviewOutcome; 10346 } 10347 10348 public boolean hasReviewOutcome() { 10349 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 10350 } 10351 10352 /** 10353 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 10354 */ 10355 public AddedItemComponent setReviewOutcome(ItemReviewOutcomeComponent value) { 10356 this.reviewOutcome = value; 10357 return this; 10358 } 10359 10360 /** 10361 * @return {@link #adjudication} (The adjudication results.) 10362 */ 10363 public List<AdjudicationComponent> getAdjudication() { 10364 if (this.adjudication == null) 10365 this.adjudication = new ArrayList<AdjudicationComponent>(); 10366 return this.adjudication; 10367 } 10368 10369 /** 10370 * @return Returns a reference to <code>this</code> for easy method chaining 10371 */ 10372 public AddedItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 10373 this.adjudication = theAdjudication; 10374 return this; 10375 } 10376 10377 public boolean hasAdjudication() { 10378 if (this.adjudication == null) 10379 return false; 10380 for (AdjudicationComponent item : this.adjudication) 10381 if (!item.isEmpty()) 10382 return true; 10383 return false; 10384 } 10385 10386 public AdjudicationComponent addAdjudication() { //3 10387 AdjudicationComponent t = new AdjudicationComponent(); 10388 if (this.adjudication == null) 10389 this.adjudication = new ArrayList<AdjudicationComponent>(); 10390 this.adjudication.add(t); 10391 return t; 10392 } 10393 10394 public AddedItemComponent addAdjudication(AdjudicationComponent t) { //3 10395 if (t == null) 10396 return this; 10397 if (this.adjudication == null) 10398 this.adjudication = new ArrayList<AdjudicationComponent>(); 10399 this.adjudication.add(t); 10400 return this; 10401 } 10402 10403 /** 10404 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 10405 */ 10406 public AdjudicationComponent getAdjudicationFirstRep() { 10407 if (getAdjudication().isEmpty()) { 10408 addAdjudication(); 10409 } 10410 return getAdjudication().get(0); 10411 } 10412 10413 /** 10414 * @return {@link #detail} (The second-tier service adjudications for payor added services.) 10415 */ 10416 public List<AddedItemDetailComponent> getDetail() { 10417 if (this.detail == null) 10418 this.detail = new ArrayList<AddedItemDetailComponent>(); 10419 return this.detail; 10420 } 10421 10422 /** 10423 * @return Returns a reference to <code>this</code> for easy method chaining 10424 */ 10425 public AddedItemComponent setDetail(List<AddedItemDetailComponent> theDetail) { 10426 this.detail = theDetail; 10427 return this; 10428 } 10429 10430 public boolean hasDetail() { 10431 if (this.detail == null) 10432 return false; 10433 for (AddedItemDetailComponent item : this.detail) 10434 if (!item.isEmpty()) 10435 return true; 10436 return false; 10437 } 10438 10439 public AddedItemDetailComponent addDetail() { //3 10440 AddedItemDetailComponent t = new AddedItemDetailComponent(); 10441 if (this.detail == null) 10442 this.detail = new ArrayList<AddedItemDetailComponent>(); 10443 this.detail.add(t); 10444 return t; 10445 } 10446 10447 public AddedItemComponent addDetail(AddedItemDetailComponent t) { //3 10448 if (t == null) 10449 return this; 10450 if (this.detail == null) 10451 this.detail = new ArrayList<AddedItemDetailComponent>(); 10452 this.detail.add(t); 10453 return this; 10454 } 10455 10456 /** 10457 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist {3} 10458 */ 10459 public AddedItemDetailComponent getDetailFirstRep() { 10460 if (getDetail().isEmpty()) { 10461 addDetail(); 10462 } 10463 return getDetail().get(0); 10464 } 10465 10466 protected void listChildren(List<Property> children) { 10467 super.listChildren(children); 10468 children.add(new Property("itemSequence", "positiveInt", "Claim items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, itemSequence)); 10469 children.add(new Property("detailSequence", "positiveInt", "The sequence number of the details within the claim item which this line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, detailSequence)); 10470 children.add(new Property("subDetailSequence", "positiveInt", "The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, subDetailSequence)); 10471 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 10472 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The providers who are authorized for the services rendered to the patient.", 0, java.lang.Integer.MAX_VALUE, provider)); 10473 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 10474 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 10475 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 10476 children.add(new Property("request", "Reference(DeviceRequest|MedicationRequest|NutritionOrder|ServiceRequest|SupplyRequest|VisionPrescription)", "Request or Referral for Goods or Service to be rendered.", 0, java.lang.Integer.MAX_VALUE, request)); 10477 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 10478 children.add(new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode)); 10479 children.add(new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced)); 10480 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location)); 10481 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 10482 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 10483 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 10484 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 10485 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 10486 children.add(new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor.", 0, 1, net)); 10487 children.add(new Property("bodySite", "", "Physical location where the service is performed or applies.", 0, java.lang.Integer.MAX_VALUE, bodySite)); 10488 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 10489 children.add(new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 10490 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 10491 children.add(new Property("detail", "", "The second-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, detail)); 10492 } 10493 10494 @Override 10495 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10496 switch (_hash) { 10497 case 1977979892: /*itemSequence*/ return new Property("itemSequence", "positiveInt", "Claim items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, itemSequence); 10498 case 1321472818: /*detailSequence*/ return new Property("detailSequence", "positiveInt", "The sequence number of the details within the claim item which this line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, detailSequence); 10499 case -855462510: /*subDetailSequence*/ return new Property("subDetailSequence", "positiveInt", "The sequence number of the sub-details woithin the details within the claim item which this line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, subDetailSequence); 10500 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 10501 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The providers who are authorized for the services rendered to the patient.", 0, java.lang.Integer.MAX_VALUE, provider); 10502 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 10503 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 10504 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 10505 case 1095692943: /*request*/ return new Property("request", "Reference(DeviceRequest|MedicationRequest|NutritionOrder|ServiceRequest|SupplyRequest|VisionPrescription)", "Request or Referral for Goods or Service to be rendered.", 0, java.lang.Integer.MAX_VALUE, request); 10506 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 10507 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "Identifies the program under which this may be recovered.", 0, java.lang.Integer.MAX_VALUE, programCode); 10508 case -1927922223: /*serviced[x]*/ return new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 10509 case 1379209295: /*serviced*/ return new Property("serviced[x]", "date|Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 10510 case 363246749: /*servicedDate*/ return new Property("serviced[x]", "date", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 10511 case 1534966512: /*servicedPeriod*/ return new Property("serviced[x]", "Period", "The date or dates when the service or product was supplied, performed or completed.", 0, 1, serviced); 10512 case 552316075: /*location[x]*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location); 10513 case 1901043637: /*location*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the product or service was provided.", 0, 1, location); 10514 case -1224800468: /*locationCodeableConcept*/ return new Property("location[x]", "CodeableConcept", "Where the product or service was provided.", 0, 1, location); 10515 case -1280020865: /*locationAddress*/ return new Property("location[x]", "Address", "Where the product or service was provided.", 0, 1, location); 10516 case 755866390: /*locationReference*/ return new Property("location[x]", "Reference(Location)", "Where the product or service was provided.", 0, 1, location); 10517 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 10518 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 10519 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 10520 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 10521 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 10522 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the addItem. Net = unit price * quantity * factor.", 0, 1, net); 10523 case 1702620169: /*bodySite*/ return new Property("bodySite", "", "Physical location where the service is performed or applies.", 0, java.lang.Integer.MAX_VALUE, bodySite); 10524 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 10525 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 10526 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 10527 case -1335224239: /*detail*/ return new Property("detail", "", "The second-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, detail); 10528 default: return super.getNamedProperty(_hash, _name, _checkValid); 10529 } 10530 10531 } 10532 10533 @Override 10534 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10535 switch (hash) { 10536 case 1977979892: /*itemSequence*/ return this.itemSequence == null ? new Base[0] : this.itemSequence.toArray(new Base[this.itemSequence.size()]); // PositiveIntType 10537 case 1321472818: /*detailSequence*/ return this.detailSequence == null ? new Base[0] : this.detailSequence.toArray(new Base[this.detailSequence.size()]); // PositiveIntType 10538 case -855462510: /*subDetailSequence*/ return this.subDetailSequence == null ? new Base[0] : this.subDetailSequence.toArray(new Base[this.subDetailSequence.size()]); // PositiveIntType 10539 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 10540 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : this.provider.toArray(new Base[this.provider.size()]); // Reference 10541 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 10542 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 10543 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 10544 case 1095692943: /*request*/ return this.request == null ? new Base[0] : this.request.toArray(new Base[this.request.size()]); // Reference 10545 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 10546 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 10547 case 1379209295: /*serviced*/ return this.serviced == null ? new Base[0] : new Base[] {this.serviced}; // DataType 10548 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // DataType 10549 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 10550 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 10551 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 10552 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 10553 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 10554 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 10555 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : this.bodySite.toArray(new Base[this.bodySite.size()]); // AddedItemBodySiteComponent 10556 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 10557 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ItemReviewOutcomeComponent 10558 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 10559 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // AddedItemDetailComponent 10560 default: return super.getProperty(hash, name, checkValid); 10561 } 10562 10563 } 10564 10565 @Override 10566 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10567 switch (hash) { 10568 case 1977979892: // itemSequence 10569 this.getItemSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 10570 return value; 10571 case 1321472818: // detailSequence 10572 this.getDetailSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 10573 return value; 10574 case -855462510: // subDetailSequence 10575 this.getSubDetailSequence().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 10576 return value; 10577 case 82505966: // traceNumber 10578 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 10579 return value; 10580 case -987494927: // provider 10581 this.getProvider().add(TypeConvertor.castToReference(value)); // Reference 10582 return value; 10583 case 1099842588: // revenue 10584 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10585 return value; 10586 case 1957227299: // productOrService 10587 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10588 return value; 10589 case -717476168: // productOrServiceEnd 10590 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10591 return value; 10592 case 1095692943: // request 10593 this.getRequest().add(TypeConvertor.castToReference(value)); // Reference 10594 return value; 10595 case -615513385: // modifier 10596 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 10597 return value; 10598 case 1010065041: // programCode 10599 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 10600 return value; 10601 case 1379209295: // serviced 10602 this.serviced = TypeConvertor.castToType(value); // DataType 10603 return value; 10604 case 1901043637: // location 10605 this.location = TypeConvertor.castToType(value); // DataType 10606 return value; 10607 case 525514609: // patientPaid 10608 this.patientPaid = TypeConvertor.castToMoney(value); // Money 10609 return value; 10610 case -1285004149: // quantity 10611 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 10612 return value; 10613 case -486196699: // unitPrice 10614 this.unitPrice = TypeConvertor.castToMoney(value); // Money 10615 return value; 10616 case -1282148017: // factor 10617 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 10618 return value; 10619 case 114603: // tax 10620 this.tax = TypeConvertor.castToMoney(value); // Money 10621 return value; 10622 case 108957: // net 10623 this.net = TypeConvertor.castToMoney(value); // Money 10624 return value; 10625 case 1702620169: // bodySite 10626 this.getBodySite().add((AddedItemBodySiteComponent) value); // AddedItemBodySiteComponent 10627 return value; 10628 case -1110033957: // noteNumber 10629 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 10630 return value; 10631 case -51825446: // reviewOutcome 10632 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 10633 return value; 10634 case -231349275: // adjudication 10635 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 10636 return value; 10637 case -1335224239: // detail 10638 this.getDetail().add((AddedItemDetailComponent) value); // AddedItemDetailComponent 10639 return value; 10640 default: return super.setProperty(hash, name, value); 10641 } 10642 10643 } 10644 10645 @Override 10646 public Base setProperty(String name, Base value) throws FHIRException { 10647 if (name.equals("itemSequence")) { 10648 this.getItemSequence().add(TypeConvertor.castToPositiveInt(value)); 10649 } else if (name.equals("detailSequence")) { 10650 this.getDetailSequence().add(TypeConvertor.castToPositiveInt(value)); 10651 } else if (name.equals("subDetailSequence")) { 10652 this.getSubDetailSequence().add(TypeConvertor.castToPositiveInt(value)); 10653 } else if (name.equals("traceNumber")) { 10654 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 10655 } else if (name.equals("provider")) { 10656 this.getProvider().add(TypeConvertor.castToReference(value)); 10657 } else if (name.equals("revenue")) { 10658 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10659 } else if (name.equals("productOrService")) { 10660 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10661 } else if (name.equals("productOrServiceEnd")) { 10662 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 10663 } else if (name.equals("request")) { 10664 this.getRequest().add(TypeConvertor.castToReference(value)); 10665 } else if (name.equals("modifier")) { 10666 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 10667 } else if (name.equals("programCode")) { 10668 this.getProgramCode().add(TypeConvertor.castToCodeableConcept(value)); 10669 } else if (name.equals("serviced[x]")) { 10670 this.serviced = TypeConvertor.castToType(value); // DataType 10671 } else if (name.equals("location[x]")) { 10672 this.location = TypeConvertor.castToType(value); // DataType 10673 } else if (name.equals("patientPaid")) { 10674 this.patientPaid = TypeConvertor.castToMoney(value); // Money 10675 } else if (name.equals("quantity")) { 10676 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 10677 } else if (name.equals("unitPrice")) { 10678 this.unitPrice = TypeConvertor.castToMoney(value); // Money 10679 } else if (name.equals("factor")) { 10680 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 10681 } else if (name.equals("tax")) { 10682 this.tax = TypeConvertor.castToMoney(value); // Money 10683 } else if (name.equals("net")) { 10684 this.net = TypeConvertor.castToMoney(value); // Money 10685 } else if (name.equals("bodySite")) { 10686 this.getBodySite().add((AddedItemBodySiteComponent) value); 10687 } else if (name.equals("noteNumber")) { 10688 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 10689 } else if (name.equals("reviewOutcome")) { 10690 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 10691 } else if (name.equals("adjudication")) { 10692 this.getAdjudication().add((AdjudicationComponent) value); 10693 } else if (name.equals("detail")) { 10694 this.getDetail().add((AddedItemDetailComponent) value); 10695 } else 10696 return super.setProperty(name, value); 10697 return value; 10698 } 10699 10700 @Override 10701 public void removeChild(String name, Base value) throws FHIRException { 10702 if (name.equals("itemSequence")) { 10703 this.getItemSequence().remove(value); 10704 } else if (name.equals("detailSequence")) { 10705 this.getDetailSequence().remove(value); 10706 } else if (name.equals("subDetailSequence")) { 10707 this.getSubDetailSequence().remove(value); 10708 } else if (name.equals("traceNumber")) { 10709 this.getTraceNumber().remove(value); 10710 } else if (name.equals("provider")) { 10711 this.getProvider().remove(value); 10712 } else if (name.equals("revenue")) { 10713 this.revenue = null; 10714 } else if (name.equals("productOrService")) { 10715 this.productOrService = null; 10716 } else if (name.equals("productOrServiceEnd")) { 10717 this.productOrServiceEnd = null; 10718 } else if (name.equals("request")) { 10719 this.getRequest().remove(value); 10720 } else if (name.equals("modifier")) { 10721 this.getModifier().remove(value); 10722 } else if (name.equals("programCode")) { 10723 this.getProgramCode().remove(value); 10724 } else if (name.equals("serviced[x]")) { 10725 this.serviced = null; 10726 } else if (name.equals("location[x]")) { 10727 this.location = null; 10728 } else if (name.equals("patientPaid")) { 10729 this.patientPaid = null; 10730 } else if (name.equals("quantity")) { 10731 this.quantity = null; 10732 } else if (name.equals("unitPrice")) { 10733 this.unitPrice = null; 10734 } else if (name.equals("factor")) { 10735 this.factor = null; 10736 } else if (name.equals("tax")) { 10737 this.tax = null; 10738 } else if (name.equals("net")) { 10739 this.net = null; 10740 } else if (name.equals("bodySite")) { 10741 this.getBodySite().remove((AddedItemBodySiteComponent) value); 10742 } else if (name.equals("noteNumber")) { 10743 this.getNoteNumber().remove(value); 10744 } else if (name.equals("reviewOutcome")) { 10745 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 10746 } else if (name.equals("adjudication")) { 10747 this.getAdjudication().remove((AdjudicationComponent) value); 10748 } else if (name.equals("detail")) { 10749 this.getDetail().remove((AddedItemDetailComponent) value); 10750 } else 10751 super.removeChild(name, value); 10752 10753 } 10754 10755 @Override 10756 public Base makeProperty(int hash, String name) throws FHIRException { 10757 switch (hash) { 10758 case 1977979892: return addItemSequenceElement(); 10759 case 1321472818: return addDetailSequenceElement(); 10760 case -855462510: return addSubDetailSequenceElement(); 10761 case 82505966: return addTraceNumber(); 10762 case -987494927: return addProvider(); 10763 case 1099842588: return getRevenue(); 10764 case 1957227299: return getProductOrService(); 10765 case -717476168: return getProductOrServiceEnd(); 10766 case 1095692943: return addRequest(); 10767 case -615513385: return addModifier(); 10768 case 1010065041: return addProgramCode(); 10769 case -1927922223: return getServiced(); 10770 case 1379209295: return getServiced(); 10771 case 552316075: return getLocation(); 10772 case 1901043637: return getLocation(); 10773 case 525514609: return getPatientPaid(); 10774 case -1285004149: return getQuantity(); 10775 case -486196699: return getUnitPrice(); 10776 case -1282148017: return getFactorElement(); 10777 case 114603: return getTax(); 10778 case 108957: return getNet(); 10779 case 1702620169: return addBodySite(); 10780 case -1110033957: return addNoteNumberElement(); 10781 case -51825446: return getReviewOutcome(); 10782 case -231349275: return addAdjudication(); 10783 case -1335224239: return addDetail(); 10784 default: return super.makeProperty(hash, name); 10785 } 10786 10787 } 10788 10789 @Override 10790 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10791 switch (hash) { 10792 case 1977979892: /*itemSequence*/ return new String[] {"positiveInt"}; 10793 case 1321472818: /*detailSequence*/ return new String[] {"positiveInt"}; 10794 case -855462510: /*subDetailSequence*/ return new String[] {"positiveInt"}; 10795 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 10796 case -987494927: /*provider*/ return new String[] {"Reference"}; 10797 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 10798 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 10799 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 10800 case 1095692943: /*request*/ return new String[] {"Reference"}; 10801 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 10802 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 10803 case 1379209295: /*serviced*/ return new String[] {"date", "Period"}; 10804 case 1901043637: /*location*/ return new String[] {"CodeableConcept", "Address", "Reference"}; 10805 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 10806 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 10807 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 10808 case -1282148017: /*factor*/ return new String[] {"decimal"}; 10809 case 114603: /*tax*/ return new String[] {"Money"}; 10810 case 108957: /*net*/ return new String[] {"Money"}; 10811 case 1702620169: /*bodySite*/ return new String[] {}; 10812 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 10813 case -51825446: /*reviewOutcome*/ return new String[] {"@ExplanationOfBenefit.item.reviewOutcome"}; 10814 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 10815 case -1335224239: /*detail*/ return new String[] {}; 10816 default: return super.getTypesForProperty(hash, name); 10817 } 10818 10819 } 10820 10821 @Override 10822 public Base addChild(String name) throws FHIRException { 10823 if (name.equals("itemSequence")) { 10824 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.itemSequence"); 10825 } 10826 else if (name.equals("detailSequence")) { 10827 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.detailSequence"); 10828 } 10829 else if (name.equals("subDetailSequence")) { 10830 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.subDetailSequence"); 10831 } 10832 else if (name.equals("traceNumber")) { 10833 return addTraceNumber(); 10834 } 10835 else if (name.equals("provider")) { 10836 return addProvider(); 10837 } 10838 else if (name.equals("revenue")) { 10839 this.revenue = new CodeableConcept(); 10840 return this.revenue; 10841 } 10842 else if (name.equals("productOrService")) { 10843 this.productOrService = new CodeableConcept(); 10844 return this.productOrService; 10845 } 10846 else if (name.equals("productOrServiceEnd")) { 10847 this.productOrServiceEnd = new CodeableConcept(); 10848 return this.productOrServiceEnd; 10849 } 10850 else if (name.equals("request")) { 10851 return addRequest(); 10852 } 10853 else if (name.equals("modifier")) { 10854 return addModifier(); 10855 } 10856 else if (name.equals("programCode")) { 10857 return addProgramCode(); 10858 } 10859 else if (name.equals("servicedDate")) { 10860 this.serviced = new DateType(); 10861 return this.serviced; 10862 } 10863 else if (name.equals("servicedPeriod")) { 10864 this.serviced = new Period(); 10865 return this.serviced; 10866 } 10867 else if (name.equals("locationCodeableConcept")) { 10868 this.location = new CodeableConcept(); 10869 return this.location; 10870 } 10871 else if (name.equals("locationAddress")) { 10872 this.location = new Address(); 10873 return this.location; 10874 } 10875 else if (name.equals("locationReference")) { 10876 this.location = new Reference(); 10877 return this.location; 10878 } 10879 else if (name.equals("patientPaid")) { 10880 this.patientPaid = new Money(); 10881 return this.patientPaid; 10882 } 10883 else if (name.equals("quantity")) { 10884 this.quantity = new Quantity(); 10885 return this.quantity; 10886 } 10887 else if (name.equals("unitPrice")) { 10888 this.unitPrice = new Money(); 10889 return this.unitPrice; 10890 } 10891 else if (name.equals("factor")) { 10892 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.factor"); 10893 } 10894 else if (name.equals("tax")) { 10895 this.tax = new Money(); 10896 return this.tax; 10897 } 10898 else if (name.equals("net")) { 10899 this.net = new Money(); 10900 return this.net; 10901 } 10902 else if (name.equals("bodySite")) { 10903 return addBodySite(); 10904 } 10905 else if (name.equals("noteNumber")) { 10906 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.noteNumber"); 10907 } 10908 else if (name.equals("reviewOutcome")) { 10909 this.reviewOutcome = new ItemReviewOutcomeComponent(); 10910 return this.reviewOutcome; 10911 } 10912 else if (name.equals("adjudication")) { 10913 return addAdjudication(); 10914 } 10915 else if (name.equals("detail")) { 10916 return addDetail(); 10917 } 10918 else 10919 return super.addChild(name); 10920 } 10921 10922 public AddedItemComponent copy() { 10923 AddedItemComponent dst = new AddedItemComponent(); 10924 copyValues(dst); 10925 return dst; 10926 } 10927 10928 public void copyValues(AddedItemComponent dst) { 10929 super.copyValues(dst); 10930 if (itemSequence != null) { 10931 dst.itemSequence = new ArrayList<PositiveIntType>(); 10932 for (PositiveIntType i : itemSequence) 10933 dst.itemSequence.add(i.copy()); 10934 }; 10935 if (detailSequence != null) { 10936 dst.detailSequence = new ArrayList<PositiveIntType>(); 10937 for (PositiveIntType i : detailSequence) 10938 dst.detailSequence.add(i.copy()); 10939 }; 10940 if (subDetailSequence != null) { 10941 dst.subDetailSequence = new ArrayList<PositiveIntType>(); 10942 for (PositiveIntType i : subDetailSequence) 10943 dst.subDetailSequence.add(i.copy()); 10944 }; 10945 if (traceNumber != null) { 10946 dst.traceNumber = new ArrayList<Identifier>(); 10947 for (Identifier i : traceNumber) 10948 dst.traceNumber.add(i.copy()); 10949 }; 10950 if (provider != null) { 10951 dst.provider = new ArrayList<Reference>(); 10952 for (Reference i : provider) 10953 dst.provider.add(i.copy()); 10954 }; 10955 dst.revenue = revenue == null ? null : revenue.copy(); 10956 dst.productOrService = productOrService == null ? null : productOrService.copy(); 10957 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 10958 if (request != null) { 10959 dst.request = new ArrayList<Reference>(); 10960 for (Reference i : request) 10961 dst.request.add(i.copy()); 10962 }; 10963 if (modifier != null) { 10964 dst.modifier = new ArrayList<CodeableConcept>(); 10965 for (CodeableConcept i : modifier) 10966 dst.modifier.add(i.copy()); 10967 }; 10968 if (programCode != null) { 10969 dst.programCode = new ArrayList<CodeableConcept>(); 10970 for (CodeableConcept i : programCode) 10971 dst.programCode.add(i.copy()); 10972 }; 10973 dst.serviced = serviced == null ? null : serviced.copy(); 10974 dst.location = location == null ? null : location.copy(); 10975 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 10976 dst.quantity = quantity == null ? null : quantity.copy(); 10977 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 10978 dst.factor = factor == null ? null : factor.copy(); 10979 dst.tax = tax == null ? null : tax.copy(); 10980 dst.net = net == null ? null : net.copy(); 10981 if (bodySite != null) { 10982 dst.bodySite = new ArrayList<AddedItemBodySiteComponent>(); 10983 for (AddedItemBodySiteComponent i : bodySite) 10984 dst.bodySite.add(i.copy()); 10985 }; 10986 if (noteNumber != null) { 10987 dst.noteNumber = new ArrayList<PositiveIntType>(); 10988 for (PositiveIntType i : noteNumber) 10989 dst.noteNumber.add(i.copy()); 10990 }; 10991 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 10992 if (adjudication != null) { 10993 dst.adjudication = new ArrayList<AdjudicationComponent>(); 10994 for (AdjudicationComponent i : adjudication) 10995 dst.adjudication.add(i.copy()); 10996 }; 10997 if (detail != null) { 10998 dst.detail = new ArrayList<AddedItemDetailComponent>(); 10999 for (AddedItemDetailComponent i : detail) 11000 dst.detail.add(i.copy()); 11001 }; 11002 } 11003 11004 @Override 11005 public boolean equalsDeep(Base other_) { 11006 if (!super.equalsDeep(other_)) 11007 return false; 11008 if (!(other_ instanceof AddedItemComponent)) 11009 return false; 11010 AddedItemComponent o = (AddedItemComponent) other_; 11011 return compareDeep(itemSequence, o.itemSequence, true) && compareDeep(detailSequence, o.detailSequence, true) 11012 && compareDeep(subDetailSequence, o.subDetailSequence, true) && compareDeep(traceNumber, o.traceNumber, true) 11013 && compareDeep(provider, o.provider, true) && compareDeep(revenue, o.revenue, true) && compareDeep(productOrService, o.productOrService, true) 11014 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(request, o.request, true) 11015 && compareDeep(modifier, o.modifier, true) && compareDeep(programCode, o.programCode, true) && compareDeep(serviced, o.serviced, true) 11016 && compareDeep(location, o.location, true) && compareDeep(patientPaid, o.patientPaid, true) && compareDeep(quantity, o.quantity, true) 11017 && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) && compareDeep(tax, o.tax, true) 11018 && compareDeep(net, o.net, true) && compareDeep(bodySite, o.bodySite, true) && compareDeep(noteNumber, o.noteNumber, true) 11019 && compareDeep(reviewOutcome, o.reviewOutcome, true) && compareDeep(adjudication, o.adjudication, true) 11020 && compareDeep(detail, o.detail, true); 11021 } 11022 11023 @Override 11024 public boolean equalsShallow(Base other_) { 11025 if (!super.equalsShallow(other_)) 11026 return false; 11027 if (!(other_ instanceof AddedItemComponent)) 11028 return false; 11029 AddedItemComponent o = (AddedItemComponent) other_; 11030 return compareValues(itemSequence, o.itemSequence, true) && compareValues(detailSequence, o.detailSequence, true) 11031 && compareValues(subDetailSequence, o.subDetailSequence, true) && compareValues(factor, o.factor, true) 11032 && compareValues(noteNumber, o.noteNumber, true); 11033 } 11034 11035 public boolean isEmpty() { 11036 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(itemSequence, detailSequence 11037 , subDetailSequence, traceNumber, provider, revenue, productOrService, productOrServiceEnd 11038 , request, modifier, programCode, serviced, location, patientPaid, quantity, unitPrice 11039 , factor, tax, net, bodySite, noteNumber, reviewOutcome, adjudication, detail 11040 ); 11041 } 11042 11043 public String fhirType() { 11044 return "ExplanationOfBenefit.addItem"; 11045 11046 } 11047 11048 } 11049 11050 @Block() 11051 public static class AddedItemBodySiteComponent extends BackboneElement implements IBaseBackboneElement { 11052 /** 11053 * Physical service site on the patient (limb, tooth, etc.). 11054 */ 11055 @Child(name = "site", type = {CodeableReference.class}, order=1, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 11056 @Description(shortDefinition="Location", formalDefinition="Physical service site on the patient (limb, tooth, etc.)." ) 11057 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/tooth") 11058 protected List<CodeableReference> site; 11059 11060 /** 11061 * A region or surface of the bodySite, e.g. limb region or tooth surface(s). 11062 */ 11063 @Child(name = "subSite", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 11064 @Description(shortDefinition="Sub-location", formalDefinition="A region or surface of the bodySite, e.g. limb region or tooth surface(s)." ) 11065 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/surface") 11066 protected List<CodeableConcept> subSite; 11067 11068 private static final long serialVersionUID = 1190632415L; 11069 11070 /** 11071 * Constructor 11072 */ 11073 public AddedItemBodySiteComponent() { 11074 super(); 11075 } 11076 11077 /** 11078 * Constructor 11079 */ 11080 public AddedItemBodySiteComponent(CodeableReference site) { 11081 super(); 11082 this.addSite(site); 11083 } 11084 11085 /** 11086 * @return {@link #site} (Physical service site on the patient (limb, tooth, etc.).) 11087 */ 11088 public List<CodeableReference> getSite() { 11089 if (this.site == null) 11090 this.site = new ArrayList<CodeableReference>(); 11091 return this.site; 11092 } 11093 11094 /** 11095 * @return Returns a reference to <code>this</code> for easy method chaining 11096 */ 11097 public AddedItemBodySiteComponent setSite(List<CodeableReference> theSite) { 11098 this.site = theSite; 11099 return this; 11100 } 11101 11102 public boolean hasSite() { 11103 if (this.site == null) 11104 return false; 11105 for (CodeableReference item : this.site) 11106 if (!item.isEmpty()) 11107 return true; 11108 return false; 11109 } 11110 11111 public CodeableReference addSite() { //3 11112 CodeableReference t = new CodeableReference(); 11113 if (this.site == null) 11114 this.site = new ArrayList<CodeableReference>(); 11115 this.site.add(t); 11116 return t; 11117 } 11118 11119 public AddedItemBodySiteComponent addSite(CodeableReference t) { //3 11120 if (t == null) 11121 return this; 11122 if (this.site == null) 11123 this.site = new ArrayList<CodeableReference>(); 11124 this.site.add(t); 11125 return this; 11126 } 11127 11128 /** 11129 * @return The first repetition of repeating field {@link #site}, creating it if it does not already exist {3} 11130 */ 11131 public CodeableReference getSiteFirstRep() { 11132 if (getSite().isEmpty()) { 11133 addSite(); 11134 } 11135 return getSite().get(0); 11136 } 11137 11138 /** 11139 * @return {@link #subSite} (A region or surface of the bodySite, e.g. limb region or tooth surface(s).) 11140 */ 11141 public List<CodeableConcept> getSubSite() { 11142 if (this.subSite == null) 11143 this.subSite = new ArrayList<CodeableConcept>(); 11144 return this.subSite; 11145 } 11146 11147 /** 11148 * @return Returns a reference to <code>this</code> for easy method chaining 11149 */ 11150 public AddedItemBodySiteComponent setSubSite(List<CodeableConcept> theSubSite) { 11151 this.subSite = theSubSite; 11152 return this; 11153 } 11154 11155 public boolean hasSubSite() { 11156 if (this.subSite == null) 11157 return false; 11158 for (CodeableConcept item : this.subSite) 11159 if (!item.isEmpty()) 11160 return true; 11161 return false; 11162 } 11163 11164 public CodeableConcept addSubSite() { //3 11165 CodeableConcept t = new CodeableConcept(); 11166 if (this.subSite == null) 11167 this.subSite = new ArrayList<CodeableConcept>(); 11168 this.subSite.add(t); 11169 return t; 11170 } 11171 11172 public AddedItemBodySiteComponent addSubSite(CodeableConcept t) { //3 11173 if (t == null) 11174 return this; 11175 if (this.subSite == null) 11176 this.subSite = new ArrayList<CodeableConcept>(); 11177 this.subSite.add(t); 11178 return this; 11179 } 11180 11181 /** 11182 * @return The first repetition of repeating field {@link #subSite}, creating it if it does not already exist {3} 11183 */ 11184 public CodeableConcept getSubSiteFirstRep() { 11185 if (getSubSite().isEmpty()) { 11186 addSubSite(); 11187 } 11188 return getSubSite().get(0); 11189 } 11190 11191 protected void listChildren(List<Property> children) { 11192 super.listChildren(children); 11193 children.add(new Property("site", "CodeableReference(BodyStructure)", "Physical service site on the patient (limb, tooth, etc.).", 0, java.lang.Integer.MAX_VALUE, site)); 11194 children.add(new Property("subSite", "CodeableConcept", "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite)); 11195 } 11196 11197 @Override 11198 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 11199 switch (_hash) { 11200 case 3530567: /*site*/ return new Property("site", "CodeableReference(BodyStructure)", "Physical service site on the patient (limb, tooth, etc.).", 0, java.lang.Integer.MAX_VALUE, site); 11201 case -1868566105: /*subSite*/ return new Property("subSite", "CodeableConcept", "A region or surface of the bodySite, e.g. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite); 11202 default: return super.getNamedProperty(_hash, _name, _checkValid); 11203 } 11204 11205 } 11206 11207 @Override 11208 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 11209 switch (hash) { 11210 case 3530567: /*site*/ return this.site == null ? new Base[0] : this.site.toArray(new Base[this.site.size()]); // CodeableReference 11211 case -1868566105: /*subSite*/ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 11212 default: return super.getProperty(hash, name, checkValid); 11213 } 11214 11215 } 11216 11217 @Override 11218 public Base setProperty(int hash, String name, Base value) throws FHIRException { 11219 switch (hash) { 11220 case 3530567: // site 11221 this.getSite().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 11222 return value; 11223 case -1868566105: // subSite 11224 this.getSubSite().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 11225 return value; 11226 default: return super.setProperty(hash, name, value); 11227 } 11228 11229 } 11230 11231 @Override 11232 public Base setProperty(String name, Base value) throws FHIRException { 11233 if (name.equals("site")) { 11234 this.getSite().add(TypeConvertor.castToCodeableReference(value)); 11235 } else if (name.equals("subSite")) { 11236 this.getSubSite().add(TypeConvertor.castToCodeableConcept(value)); 11237 } else 11238 return super.setProperty(name, value); 11239 return value; 11240 } 11241 11242 @Override 11243 public void removeChild(String name, Base value) throws FHIRException { 11244 if (name.equals("site")) { 11245 this.getSite().remove(value); 11246 } else if (name.equals("subSite")) { 11247 this.getSubSite().remove(value); 11248 } else 11249 super.removeChild(name, value); 11250 11251 } 11252 11253 @Override 11254 public Base makeProperty(int hash, String name) throws FHIRException { 11255 switch (hash) { 11256 case 3530567: return addSite(); 11257 case -1868566105: return addSubSite(); 11258 default: return super.makeProperty(hash, name); 11259 } 11260 11261 } 11262 11263 @Override 11264 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 11265 switch (hash) { 11266 case 3530567: /*site*/ return new String[] {"CodeableReference"}; 11267 case -1868566105: /*subSite*/ return new String[] {"CodeableConcept"}; 11268 default: return super.getTypesForProperty(hash, name); 11269 } 11270 11271 } 11272 11273 @Override 11274 public Base addChild(String name) throws FHIRException { 11275 if (name.equals("site")) { 11276 return addSite(); 11277 } 11278 else if (name.equals("subSite")) { 11279 return addSubSite(); 11280 } 11281 else 11282 return super.addChild(name); 11283 } 11284 11285 public AddedItemBodySiteComponent copy() { 11286 AddedItemBodySiteComponent dst = new AddedItemBodySiteComponent(); 11287 copyValues(dst); 11288 return dst; 11289 } 11290 11291 public void copyValues(AddedItemBodySiteComponent dst) { 11292 super.copyValues(dst); 11293 if (site != null) { 11294 dst.site = new ArrayList<CodeableReference>(); 11295 for (CodeableReference i : site) 11296 dst.site.add(i.copy()); 11297 }; 11298 if (subSite != null) { 11299 dst.subSite = new ArrayList<CodeableConcept>(); 11300 for (CodeableConcept i : subSite) 11301 dst.subSite.add(i.copy()); 11302 }; 11303 } 11304 11305 @Override 11306 public boolean equalsDeep(Base other_) { 11307 if (!super.equalsDeep(other_)) 11308 return false; 11309 if (!(other_ instanceof AddedItemBodySiteComponent)) 11310 return false; 11311 AddedItemBodySiteComponent o = (AddedItemBodySiteComponent) other_; 11312 return compareDeep(site, o.site, true) && compareDeep(subSite, o.subSite, true); 11313 } 11314 11315 @Override 11316 public boolean equalsShallow(Base other_) { 11317 if (!super.equalsShallow(other_)) 11318 return false; 11319 if (!(other_ instanceof AddedItemBodySiteComponent)) 11320 return false; 11321 AddedItemBodySiteComponent o = (AddedItemBodySiteComponent) other_; 11322 return true; 11323 } 11324 11325 public boolean isEmpty() { 11326 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(site, subSite); 11327 } 11328 11329 public String fhirType() { 11330 return "ExplanationOfBenefit.addItem.bodySite"; 11331 11332 } 11333 11334 } 11335 11336 @Block() 11337 public static class AddedItemDetailComponent extends BackboneElement implements IBaseBackboneElement { 11338 /** 11339 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 11340 */ 11341 @Child(name = "traceNumber", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 11342 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 11343 protected List<Identifier> traceNumber; 11344 11345 /** 11346 * The type of revenue or cost center providing the product and/or service. 11347 */ 11348 @Child(name = "revenue", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 11349 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 11350 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 11351 protected CodeableConcept revenue; 11352 11353 /** 11354 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 11355 */ 11356 @Child(name = "productOrService", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 11357 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 11358 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 11359 protected CodeableConcept productOrService; 11360 11361 /** 11362 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 11363 */ 11364 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 11365 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 11366 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 11367 protected CodeableConcept productOrServiceEnd; 11368 11369 /** 11370 * Item typification or modifiers codes to convey additional context for the product or service. 11371 */ 11372 @Child(name = "modifier", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 11373 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 11374 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 11375 protected List<CodeableConcept> modifier; 11376 11377 /** 11378 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 11379 */ 11380 @Child(name = "patientPaid", type = {Money.class}, order=6, min=0, max=1, modifier=false, summary=false) 11381 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 11382 protected Money patientPaid; 11383 11384 /** 11385 * The number of repetitions of a service or product. 11386 */ 11387 @Child(name = "quantity", type = {Quantity.class}, order=7, min=0, max=1, modifier=false, summary=false) 11388 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 11389 protected Quantity quantity; 11390 11391 /** 11392 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 11393 */ 11394 @Child(name = "unitPrice", type = {Money.class}, order=8, min=0, max=1, modifier=false, summary=false) 11395 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 11396 protected Money unitPrice; 11397 11398 /** 11399 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 11400 */ 11401 @Child(name = "factor", type = {DecimalType.class}, order=9, min=0, max=1, modifier=false, summary=false) 11402 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 11403 protected DecimalType factor; 11404 11405 /** 11406 * The total of taxes applicable for this product or service. 11407 */ 11408 @Child(name = "tax", type = {Money.class}, order=10, min=0, max=1, modifier=false, summary=false) 11409 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 11410 protected Money tax; 11411 11412 /** 11413 * The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor. 11414 */ 11415 @Child(name = "net", type = {Money.class}, order=11, min=0, max=1, modifier=false, summary=false) 11416 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor." ) 11417 protected Money net; 11418 11419 /** 11420 * The numbers associated with notes below which apply to the adjudication of this item. 11421 */ 11422 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 11423 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 11424 protected List<PositiveIntType> noteNumber; 11425 11426 /** 11427 * The high-level results of the adjudication if adjudication has been performed. 11428 */ 11429 @Child(name = "reviewOutcome", type = {ItemReviewOutcomeComponent.class}, order=13, min=0, max=1, modifier=false, summary=false) 11430 @Description(shortDefinition="Additem detail level adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 11431 protected ItemReviewOutcomeComponent reviewOutcome; 11432 11433 /** 11434 * The adjudication results. 11435 */ 11436 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 11437 @Description(shortDefinition="Added items adjudication", formalDefinition="The adjudication results." ) 11438 protected List<AdjudicationComponent> adjudication; 11439 11440 /** 11441 * The third-tier service adjudications for payor added services. 11442 */ 11443 @Child(name = "subDetail", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 11444 @Description(shortDefinition="Insurer added line items", formalDefinition="The third-tier service adjudications for payor added services." ) 11445 protected List<AddedItemDetailSubDetailComponent> subDetail; 11446 11447 private static final long serialVersionUID = 1088072336L; 11448 11449 /** 11450 * Constructor 11451 */ 11452 public AddedItemDetailComponent() { 11453 super(); 11454 } 11455 11456 /** 11457 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 11458 */ 11459 public List<Identifier> getTraceNumber() { 11460 if (this.traceNumber == null) 11461 this.traceNumber = new ArrayList<Identifier>(); 11462 return this.traceNumber; 11463 } 11464 11465 /** 11466 * @return Returns a reference to <code>this</code> for easy method chaining 11467 */ 11468 public AddedItemDetailComponent setTraceNumber(List<Identifier> theTraceNumber) { 11469 this.traceNumber = theTraceNumber; 11470 return this; 11471 } 11472 11473 public boolean hasTraceNumber() { 11474 if (this.traceNumber == null) 11475 return false; 11476 for (Identifier item : this.traceNumber) 11477 if (!item.isEmpty()) 11478 return true; 11479 return false; 11480 } 11481 11482 public Identifier addTraceNumber() { //3 11483 Identifier t = new Identifier(); 11484 if (this.traceNumber == null) 11485 this.traceNumber = new ArrayList<Identifier>(); 11486 this.traceNumber.add(t); 11487 return t; 11488 } 11489 11490 public AddedItemDetailComponent addTraceNumber(Identifier t) { //3 11491 if (t == null) 11492 return this; 11493 if (this.traceNumber == null) 11494 this.traceNumber = new ArrayList<Identifier>(); 11495 this.traceNumber.add(t); 11496 return this; 11497 } 11498 11499 /** 11500 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 11501 */ 11502 public Identifier getTraceNumberFirstRep() { 11503 if (getTraceNumber().isEmpty()) { 11504 addTraceNumber(); 11505 } 11506 return getTraceNumber().get(0); 11507 } 11508 11509 /** 11510 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 11511 */ 11512 public CodeableConcept getRevenue() { 11513 if (this.revenue == null) 11514 if (Configuration.errorOnAutoCreate()) 11515 throw new Error("Attempt to auto-create AddedItemDetailComponent.revenue"); 11516 else if (Configuration.doAutoCreate()) 11517 this.revenue = new CodeableConcept(); // cc 11518 return this.revenue; 11519 } 11520 11521 public boolean hasRevenue() { 11522 return this.revenue != null && !this.revenue.isEmpty(); 11523 } 11524 11525 /** 11526 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 11527 */ 11528 public AddedItemDetailComponent setRevenue(CodeableConcept value) { 11529 this.revenue = value; 11530 return this; 11531 } 11532 11533 /** 11534 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 11535 */ 11536 public CodeableConcept getProductOrService() { 11537 if (this.productOrService == null) 11538 if (Configuration.errorOnAutoCreate()) 11539 throw new Error("Attempt to auto-create AddedItemDetailComponent.productOrService"); 11540 else if (Configuration.doAutoCreate()) 11541 this.productOrService = new CodeableConcept(); // cc 11542 return this.productOrService; 11543 } 11544 11545 public boolean hasProductOrService() { 11546 return this.productOrService != null && !this.productOrService.isEmpty(); 11547 } 11548 11549 /** 11550 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 11551 */ 11552 public AddedItemDetailComponent setProductOrService(CodeableConcept value) { 11553 this.productOrService = value; 11554 return this; 11555 } 11556 11557 /** 11558 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 11559 */ 11560 public CodeableConcept getProductOrServiceEnd() { 11561 if (this.productOrServiceEnd == null) 11562 if (Configuration.errorOnAutoCreate()) 11563 throw new Error("Attempt to auto-create AddedItemDetailComponent.productOrServiceEnd"); 11564 else if (Configuration.doAutoCreate()) 11565 this.productOrServiceEnd = new CodeableConcept(); // cc 11566 return this.productOrServiceEnd; 11567 } 11568 11569 public boolean hasProductOrServiceEnd() { 11570 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 11571 } 11572 11573 /** 11574 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 11575 */ 11576 public AddedItemDetailComponent setProductOrServiceEnd(CodeableConcept value) { 11577 this.productOrServiceEnd = value; 11578 return this; 11579 } 11580 11581 /** 11582 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 11583 */ 11584 public List<CodeableConcept> getModifier() { 11585 if (this.modifier == null) 11586 this.modifier = new ArrayList<CodeableConcept>(); 11587 return this.modifier; 11588 } 11589 11590 /** 11591 * @return Returns a reference to <code>this</code> for easy method chaining 11592 */ 11593 public AddedItemDetailComponent setModifier(List<CodeableConcept> theModifier) { 11594 this.modifier = theModifier; 11595 return this; 11596 } 11597 11598 public boolean hasModifier() { 11599 if (this.modifier == null) 11600 return false; 11601 for (CodeableConcept item : this.modifier) 11602 if (!item.isEmpty()) 11603 return true; 11604 return false; 11605 } 11606 11607 public CodeableConcept addModifier() { //3 11608 CodeableConcept t = new CodeableConcept(); 11609 if (this.modifier == null) 11610 this.modifier = new ArrayList<CodeableConcept>(); 11611 this.modifier.add(t); 11612 return t; 11613 } 11614 11615 public AddedItemDetailComponent addModifier(CodeableConcept t) { //3 11616 if (t == null) 11617 return this; 11618 if (this.modifier == null) 11619 this.modifier = new ArrayList<CodeableConcept>(); 11620 this.modifier.add(t); 11621 return this; 11622 } 11623 11624 /** 11625 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 11626 */ 11627 public CodeableConcept getModifierFirstRep() { 11628 if (getModifier().isEmpty()) { 11629 addModifier(); 11630 } 11631 return getModifier().get(0); 11632 } 11633 11634 /** 11635 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 11636 */ 11637 public Money getPatientPaid() { 11638 if (this.patientPaid == null) 11639 if (Configuration.errorOnAutoCreate()) 11640 throw new Error("Attempt to auto-create AddedItemDetailComponent.patientPaid"); 11641 else if (Configuration.doAutoCreate()) 11642 this.patientPaid = new Money(); // cc 11643 return this.patientPaid; 11644 } 11645 11646 public boolean hasPatientPaid() { 11647 return this.patientPaid != null && !this.patientPaid.isEmpty(); 11648 } 11649 11650 /** 11651 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 11652 */ 11653 public AddedItemDetailComponent setPatientPaid(Money value) { 11654 this.patientPaid = value; 11655 return this; 11656 } 11657 11658 /** 11659 * @return {@link #quantity} (The number of repetitions of a service or product.) 11660 */ 11661 public Quantity getQuantity() { 11662 if (this.quantity == null) 11663 if (Configuration.errorOnAutoCreate()) 11664 throw new Error("Attempt to auto-create AddedItemDetailComponent.quantity"); 11665 else if (Configuration.doAutoCreate()) 11666 this.quantity = new Quantity(); // cc 11667 return this.quantity; 11668 } 11669 11670 public boolean hasQuantity() { 11671 return this.quantity != null && !this.quantity.isEmpty(); 11672 } 11673 11674 /** 11675 * @param value {@link #quantity} (The number of repetitions of a service or product.) 11676 */ 11677 public AddedItemDetailComponent setQuantity(Quantity value) { 11678 this.quantity = value; 11679 return this; 11680 } 11681 11682 /** 11683 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 11684 */ 11685 public Money getUnitPrice() { 11686 if (this.unitPrice == null) 11687 if (Configuration.errorOnAutoCreate()) 11688 throw new Error("Attempt to auto-create AddedItemDetailComponent.unitPrice"); 11689 else if (Configuration.doAutoCreate()) 11690 this.unitPrice = new Money(); // cc 11691 return this.unitPrice; 11692 } 11693 11694 public boolean hasUnitPrice() { 11695 return this.unitPrice != null && !this.unitPrice.isEmpty(); 11696 } 11697 11698 /** 11699 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 11700 */ 11701 public AddedItemDetailComponent setUnitPrice(Money value) { 11702 this.unitPrice = value; 11703 return this; 11704 } 11705 11706 /** 11707 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 11708 */ 11709 public DecimalType getFactorElement() { 11710 if (this.factor == null) 11711 if (Configuration.errorOnAutoCreate()) 11712 throw new Error("Attempt to auto-create AddedItemDetailComponent.factor"); 11713 else if (Configuration.doAutoCreate()) 11714 this.factor = new DecimalType(); // bb 11715 return this.factor; 11716 } 11717 11718 public boolean hasFactorElement() { 11719 return this.factor != null && !this.factor.isEmpty(); 11720 } 11721 11722 public boolean hasFactor() { 11723 return this.factor != null && !this.factor.isEmpty(); 11724 } 11725 11726 /** 11727 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 11728 */ 11729 public AddedItemDetailComponent setFactorElement(DecimalType value) { 11730 this.factor = value; 11731 return this; 11732 } 11733 11734 /** 11735 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 11736 */ 11737 public BigDecimal getFactor() { 11738 return this.factor == null ? null : this.factor.getValue(); 11739 } 11740 11741 /** 11742 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 11743 */ 11744 public AddedItemDetailComponent setFactor(BigDecimal value) { 11745 if (value == null) 11746 this.factor = null; 11747 else { 11748 if (this.factor == null) 11749 this.factor = new DecimalType(); 11750 this.factor.setValue(value); 11751 } 11752 return this; 11753 } 11754 11755 /** 11756 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 11757 */ 11758 public AddedItemDetailComponent setFactor(long value) { 11759 this.factor = new DecimalType(); 11760 this.factor.setValue(value); 11761 return this; 11762 } 11763 11764 /** 11765 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 11766 */ 11767 public AddedItemDetailComponent setFactor(double value) { 11768 this.factor = new DecimalType(); 11769 this.factor.setValue(value); 11770 return this; 11771 } 11772 11773 /** 11774 * @return {@link #tax} (The total of taxes applicable for this product or service.) 11775 */ 11776 public Money getTax() { 11777 if (this.tax == null) 11778 if (Configuration.errorOnAutoCreate()) 11779 throw new Error("Attempt to auto-create AddedItemDetailComponent.tax"); 11780 else if (Configuration.doAutoCreate()) 11781 this.tax = new Money(); // cc 11782 return this.tax; 11783 } 11784 11785 public boolean hasTax() { 11786 return this.tax != null && !this.tax.isEmpty(); 11787 } 11788 11789 /** 11790 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 11791 */ 11792 public AddedItemDetailComponent setTax(Money value) { 11793 this.tax = value; 11794 return this; 11795 } 11796 11797 /** 11798 * @return {@link #net} (The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor.) 11799 */ 11800 public Money getNet() { 11801 if (this.net == null) 11802 if (Configuration.errorOnAutoCreate()) 11803 throw new Error("Attempt to auto-create AddedItemDetailComponent.net"); 11804 else if (Configuration.doAutoCreate()) 11805 this.net = new Money(); // cc 11806 return this.net; 11807 } 11808 11809 public boolean hasNet() { 11810 return this.net != null && !this.net.isEmpty(); 11811 } 11812 11813 /** 11814 * @param value {@link #net} (The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor.) 11815 */ 11816 public AddedItemDetailComponent setNet(Money value) { 11817 this.net = value; 11818 return this; 11819 } 11820 11821 /** 11822 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 11823 */ 11824 public List<PositiveIntType> getNoteNumber() { 11825 if (this.noteNumber == null) 11826 this.noteNumber = new ArrayList<PositiveIntType>(); 11827 return this.noteNumber; 11828 } 11829 11830 /** 11831 * @return Returns a reference to <code>this</code> for easy method chaining 11832 */ 11833 public AddedItemDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 11834 this.noteNumber = theNoteNumber; 11835 return this; 11836 } 11837 11838 public boolean hasNoteNumber() { 11839 if (this.noteNumber == null) 11840 return false; 11841 for (PositiveIntType item : this.noteNumber) 11842 if (!item.isEmpty()) 11843 return true; 11844 return false; 11845 } 11846 11847 /** 11848 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 11849 */ 11850 public PositiveIntType addNoteNumberElement() {//2 11851 PositiveIntType t = new PositiveIntType(); 11852 if (this.noteNumber == null) 11853 this.noteNumber = new ArrayList<PositiveIntType>(); 11854 this.noteNumber.add(t); 11855 return t; 11856 } 11857 11858 /** 11859 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 11860 */ 11861 public AddedItemDetailComponent addNoteNumber(int value) { //1 11862 PositiveIntType t = new PositiveIntType(); 11863 t.setValue(value); 11864 if (this.noteNumber == null) 11865 this.noteNumber = new ArrayList<PositiveIntType>(); 11866 this.noteNumber.add(t); 11867 return this; 11868 } 11869 11870 /** 11871 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 11872 */ 11873 public boolean hasNoteNumber(int value) { 11874 if (this.noteNumber == null) 11875 return false; 11876 for (PositiveIntType v : this.noteNumber) 11877 if (v.getValue().equals(value)) // positiveInt 11878 return true; 11879 return false; 11880 } 11881 11882 /** 11883 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 11884 */ 11885 public ItemReviewOutcomeComponent getReviewOutcome() { 11886 if (this.reviewOutcome == null) 11887 if (Configuration.errorOnAutoCreate()) 11888 throw new Error("Attempt to auto-create AddedItemDetailComponent.reviewOutcome"); 11889 else if (Configuration.doAutoCreate()) 11890 this.reviewOutcome = new ItemReviewOutcomeComponent(); // cc 11891 return this.reviewOutcome; 11892 } 11893 11894 public boolean hasReviewOutcome() { 11895 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 11896 } 11897 11898 /** 11899 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 11900 */ 11901 public AddedItemDetailComponent setReviewOutcome(ItemReviewOutcomeComponent value) { 11902 this.reviewOutcome = value; 11903 return this; 11904 } 11905 11906 /** 11907 * @return {@link #adjudication} (The adjudication results.) 11908 */ 11909 public List<AdjudicationComponent> getAdjudication() { 11910 if (this.adjudication == null) 11911 this.adjudication = new ArrayList<AdjudicationComponent>(); 11912 return this.adjudication; 11913 } 11914 11915 /** 11916 * @return Returns a reference to <code>this</code> for easy method chaining 11917 */ 11918 public AddedItemDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 11919 this.adjudication = theAdjudication; 11920 return this; 11921 } 11922 11923 public boolean hasAdjudication() { 11924 if (this.adjudication == null) 11925 return false; 11926 for (AdjudicationComponent item : this.adjudication) 11927 if (!item.isEmpty()) 11928 return true; 11929 return false; 11930 } 11931 11932 public AdjudicationComponent addAdjudication() { //3 11933 AdjudicationComponent t = new AdjudicationComponent(); 11934 if (this.adjudication == null) 11935 this.adjudication = new ArrayList<AdjudicationComponent>(); 11936 this.adjudication.add(t); 11937 return t; 11938 } 11939 11940 public AddedItemDetailComponent addAdjudication(AdjudicationComponent t) { //3 11941 if (t == null) 11942 return this; 11943 if (this.adjudication == null) 11944 this.adjudication = new ArrayList<AdjudicationComponent>(); 11945 this.adjudication.add(t); 11946 return this; 11947 } 11948 11949 /** 11950 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 11951 */ 11952 public AdjudicationComponent getAdjudicationFirstRep() { 11953 if (getAdjudication().isEmpty()) { 11954 addAdjudication(); 11955 } 11956 return getAdjudication().get(0); 11957 } 11958 11959 /** 11960 * @return {@link #subDetail} (The third-tier service adjudications for payor added services.) 11961 */ 11962 public List<AddedItemDetailSubDetailComponent> getSubDetail() { 11963 if (this.subDetail == null) 11964 this.subDetail = new ArrayList<AddedItemDetailSubDetailComponent>(); 11965 return this.subDetail; 11966 } 11967 11968 /** 11969 * @return Returns a reference to <code>this</code> for easy method chaining 11970 */ 11971 public AddedItemDetailComponent setSubDetail(List<AddedItemDetailSubDetailComponent> theSubDetail) { 11972 this.subDetail = theSubDetail; 11973 return this; 11974 } 11975 11976 public boolean hasSubDetail() { 11977 if (this.subDetail == null) 11978 return false; 11979 for (AddedItemDetailSubDetailComponent item : this.subDetail) 11980 if (!item.isEmpty()) 11981 return true; 11982 return false; 11983 } 11984 11985 public AddedItemDetailSubDetailComponent addSubDetail() { //3 11986 AddedItemDetailSubDetailComponent t = new AddedItemDetailSubDetailComponent(); 11987 if (this.subDetail == null) 11988 this.subDetail = new ArrayList<AddedItemDetailSubDetailComponent>(); 11989 this.subDetail.add(t); 11990 return t; 11991 } 11992 11993 public AddedItemDetailComponent addSubDetail(AddedItemDetailSubDetailComponent t) { //3 11994 if (t == null) 11995 return this; 11996 if (this.subDetail == null) 11997 this.subDetail = new ArrayList<AddedItemDetailSubDetailComponent>(); 11998 this.subDetail.add(t); 11999 return this; 12000 } 12001 12002 /** 12003 * @return The first repetition of repeating field {@link #subDetail}, creating it if it does not already exist {3} 12004 */ 12005 public AddedItemDetailSubDetailComponent getSubDetailFirstRep() { 12006 if (getSubDetail().isEmpty()) { 12007 addSubDetail(); 12008 } 12009 return getSubDetail().get(0); 12010 } 12011 12012 protected void listChildren(List<Property> children) { 12013 super.listChildren(children); 12014 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 12015 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 12016 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 12017 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 12018 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 12019 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 12020 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 12021 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 12022 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 12023 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 12024 children.add(new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor.", 0, 1, net)); 12025 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 12026 children.add(new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 12027 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 12028 children.add(new Property("subDetail", "", "The third-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, subDetail)); 12029 } 12030 12031 @Override 12032 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 12033 switch (_hash) { 12034 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 12035 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 12036 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 12037 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 12038 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 12039 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 12040 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 12041 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 12042 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 12043 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 12044 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the group (if a grouper) or the addItem.detail. Net = unit price * quantity * factor.", 0, 1, net); 12045 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 12046 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 12047 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 12048 case -828829007: /*subDetail*/ return new Property("subDetail", "", "The third-tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, subDetail); 12049 default: return super.getNamedProperty(_hash, _name, _checkValid); 12050 } 12051 12052 } 12053 12054 @Override 12055 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 12056 switch (hash) { 12057 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 12058 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 12059 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 12060 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 12061 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 12062 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 12063 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 12064 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 12065 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 12066 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 12067 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 12068 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 12069 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ItemReviewOutcomeComponent 12070 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 12071 case -828829007: /*subDetail*/ return this.subDetail == null ? new Base[0] : this.subDetail.toArray(new Base[this.subDetail.size()]); // AddedItemDetailSubDetailComponent 12072 default: return super.getProperty(hash, name, checkValid); 12073 } 12074 12075 } 12076 12077 @Override 12078 public Base setProperty(int hash, String name, Base value) throws FHIRException { 12079 switch (hash) { 12080 case 82505966: // traceNumber 12081 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 12082 return value; 12083 case 1099842588: // revenue 12084 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 12085 return value; 12086 case 1957227299: // productOrService 12087 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 12088 return value; 12089 case -717476168: // productOrServiceEnd 12090 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 12091 return value; 12092 case -615513385: // modifier 12093 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 12094 return value; 12095 case 525514609: // patientPaid 12096 this.patientPaid = TypeConvertor.castToMoney(value); // Money 12097 return value; 12098 case -1285004149: // quantity 12099 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 12100 return value; 12101 case -486196699: // unitPrice 12102 this.unitPrice = TypeConvertor.castToMoney(value); // Money 12103 return value; 12104 case -1282148017: // factor 12105 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 12106 return value; 12107 case 114603: // tax 12108 this.tax = TypeConvertor.castToMoney(value); // Money 12109 return value; 12110 case 108957: // net 12111 this.net = TypeConvertor.castToMoney(value); // Money 12112 return value; 12113 case -1110033957: // noteNumber 12114 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 12115 return value; 12116 case -51825446: // reviewOutcome 12117 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 12118 return value; 12119 case -231349275: // adjudication 12120 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 12121 return value; 12122 case -828829007: // subDetail 12123 this.getSubDetail().add((AddedItemDetailSubDetailComponent) value); // AddedItemDetailSubDetailComponent 12124 return value; 12125 default: return super.setProperty(hash, name, value); 12126 } 12127 12128 } 12129 12130 @Override 12131 public Base setProperty(String name, Base value) throws FHIRException { 12132 if (name.equals("traceNumber")) { 12133 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 12134 } else if (name.equals("revenue")) { 12135 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 12136 } else if (name.equals("productOrService")) { 12137 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 12138 } else if (name.equals("productOrServiceEnd")) { 12139 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 12140 } else if (name.equals("modifier")) { 12141 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 12142 } else if (name.equals("patientPaid")) { 12143 this.patientPaid = TypeConvertor.castToMoney(value); // Money 12144 } else if (name.equals("quantity")) { 12145 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 12146 } else if (name.equals("unitPrice")) { 12147 this.unitPrice = TypeConvertor.castToMoney(value); // Money 12148 } else if (name.equals("factor")) { 12149 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 12150 } else if (name.equals("tax")) { 12151 this.tax = TypeConvertor.castToMoney(value); // Money 12152 } else if (name.equals("net")) { 12153 this.net = TypeConvertor.castToMoney(value); // Money 12154 } else if (name.equals("noteNumber")) { 12155 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 12156 } else if (name.equals("reviewOutcome")) { 12157 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 12158 } else if (name.equals("adjudication")) { 12159 this.getAdjudication().add((AdjudicationComponent) value); 12160 } else if (name.equals("subDetail")) { 12161 this.getSubDetail().add((AddedItemDetailSubDetailComponent) value); 12162 } else 12163 return super.setProperty(name, value); 12164 return value; 12165 } 12166 12167 @Override 12168 public void removeChild(String name, Base value) throws FHIRException { 12169 if (name.equals("traceNumber")) { 12170 this.getTraceNumber().remove(value); 12171 } else if (name.equals("revenue")) { 12172 this.revenue = null; 12173 } else if (name.equals("productOrService")) { 12174 this.productOrService = null; 12175 } else if (name.equals("productOrServiceEnd")) { 12176 this.productOrServiceEnd = null; 12177 } else if (name.equals("modifier")) { 12178 this.getModifier().remove(value); 12179 } else if (name.equals("patientPaid")) { 12180 this.patientPaid = null; 12181 } else if (name.equals("quantity")) { 12182 this.quantity = null; 12183 } else if (name.equals("unitPrice")) { 12184 this.unitPrice = null; 12185 } else if (name.equals("factor")) { 12186 this.factor = null; 12187 } else if (name.equals("tax")) { 12188 this.tax = null; 12189 } else if (name.equals("net")) { 12190 this.net = null; 12191 } else if (name.equals("noteNumber")) { 12192 this.getNoteNumber().remove(value); 12193 } else if (name.equals("reviewOutcome")) { 12194 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 12195 } else if (name.equals("adjudication")) { 12196 this.getAdjudication().remove((AdjudicationComponent) value); 12197 } else if (name.equals("subDetail")) { 12198 this.getSubDetail().remove((AddedItemDetailSubDetailComponent) value); 12199 } else 12200 super.removeChild(name, value); 12201 12202 } 12203 12204 @Override 12205 public Base makeProperty(int hash, String name) throws FHIRException { 12206 switch (hash) { 12207 case 82505966: return addTraceNumber(); 12208 case 1099842588: return getRevenue(); 12209 case 1957227299: return getProductOrService(); 12210 case -717476168: return getProductOrServiceEnd(); 12211 case -615513385: return addModifier(); 12212 case 525514609: return getPatientPaid(); 12213 case -1285004149: return getQuantity(); 12214 case -486196699: return getUnitPrice(); 12215 case -1282148017: return getFactorElement(); 12216 case 114603: return getTax(); 12217 case 108957: return getNet(); 12218 case -1110033957: return addNoteNumberElement(); 12219 case -51825446: return getReviewOutcome(); 12220 case -231349275: return addAdjudication(); 12221 case -828829007: return addSubDetail(); 12222 default: return super.makeProperty(hash, name); 12223 } 12224 12225 } 12226 12227 @Override 12228 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 12229 switch (hash) { 12230 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 12231 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 12232 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 12233 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 12234 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 12235 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 12236 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 12237 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 12238 case -1282148017: /*factor*/ return new String[] {"decimal"}; 12239 case 114603: /*tax*/ return new String[] {"Money"}; 12240 case 108957: /*net*/ return new String[] {"Money"}; 12241 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 12242 case -51825446: /*reviewOutcome*/ return new String[] {"@ExplanationOfBenefit.item.reviewOutcome"}; 12243 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 12244 case -828829007: /*subDetail*/ return new String[] {}; 12245 default: return super.getTypesForProperty(hash, name); 12246 } 12247 12248 } 12249 12250 @Override 12251 public Base addChild(String name) throws FHIRException { 12252 if (name.equals("traceNumber")) { 12253 return addTraceNumber(); 12254 } 12255 else if (name.equals("revenue")) { 12256 this.revenue = new CodeableConcept(); 12257 return this.revenue; 12258 } 12259 else if (name.equals("productOrService")) { 12260 this.productOrService = new CodeableConcept(); 12261 return this.productOrService; 12262 } 12263 else if (name.equals("productOrServiceEnd")) { 12264 this.productOrServiceEnd = new CodeableConcept(); 12265 return this.productOrServiceEnd; 12266 } 12267 else if (name.equals("modifier")) { 12268 return addModifier(); 12269 } 12270 else if (name.equals("patientPaid")) { 12271 this.patientPaid = new Money(); 12272 return this.patientPaid; 12273 } 12274 else if (name.equals("quantity")) { 12275 this.quantity = new Quantity(); 12276 return this.quantity; 12277 } 12278 else if (name.equals("unitPrice")) { 12279 this.unitPrice = new Money(); 12280 return this.unitPrice; 12281 } 12282 else if (name.equals("factor")) { 12283 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.detail.factor"); 12284 } 12285 else if (name.equals("tax")) { 12286 this.tax = new Money(); 12287 return this.tax; 12288 } 12289 else if (name.equals("net")) { 12290 this.net = new Money(); 12291 return this.net; 12292 } 12293 else if (name.equals("noteNumber")) { 12294 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.detail.noteNumber"); 12295 } 12296 else if (name.equals("reviewOutcome")) { 12297 this.reviewOutcome = new ItemReviewOutcomeComponent(); 12298 return this.reviewOutcome; 12299 } 12300 else if (name.equals("adjudication")) { 12301 return addAdjudication(); 12302 } 12303 else if (name.equals("subDetail")) { 12304 return addSubDetail(); 12305 } 12306 else 12307 return super.addChild(name); 12308 } 12309 12310 public AddedItemDetailComponent copy() { 12311 AddedItemDetailComponent dst = new AddedItemDetailComponent(); 12312 copyValues(dst); 12313 return dst; 12314 } 12315 12316 public void copyValues(AddedItemDetailComponent dst) { 12317 super.copyValues(dst); 12318 if (traceNumber != null) { 12319 dst.traceNumber = new ArrayList<Identifier>(); 12320 for (Identifier i : traceNumber) 12321 dst.traceNumber.add(i.copy()); 12322 }; 12323 dst.revenue = revenue == null ? null : revenue.copy(); 12324 dst.productOrService = productOrService == null ? null : productOrService.copy(); 12325 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 12326 if (modifier != null) { 12327 dst.modifier = new ArrayList<CodeableConcept>(); 12328 for (CodeableConcept i : modifier) 12329 dst.modifier.add(i.copy()); 12330 }; 12331 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 12332 dst.quantity = quantity == null ? null : quantity.copy(); 12333 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 12334 dst.factor = factor == null ? null : factor.copy(); 12335 dst.tax = tax == null ? null : tax.copy(); 12336 dst.net = net == null ? null : net.copy(); 12337 if (noteNumber != null) { 12338 dst.noteNumber = new ArrayList<PositiveIntType>(); 12339 for (PositiveIntType i : noteNumber) 12340 dst.noteNumber.add(i.copy()); 12341 }; 12342 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 12343 if (adjudication != null) { 12344 dst.adjudication = new ArrayList<AdjudicationComponent>(); 12345 for (AdjudicationComponent i : adjudication) 12346 dst.adjudication.add(i.copy()); 12347 }; 12348 if (subDetail != null) { 12349 dst.subDetail = new ArrayList<AddedItemDetailSubDetailComponent>(); 12350 for (AddedItemDetailSubDetailComponent i : subDetail) 12351 dst.subDetail.add(i.copy()); 12352 }; 12353 } 12354 12355 @Override 12356 public boolean equalsDeep(Base other_) { 12357 if (!super.equalsDeep(other_)) 12358 return false; 12359 if (!(other_ instanceof AddedItemDetailComponent)) 12360 return false; 12361 AddedItemDetailComponent o = (AddedItemDetailComponent) other_; 12362 return compareDeep(traceNumber, o.traceNumber, true) && compareDeep(revenue, o.revenue, true) && compareDeep(productOrService, o.productOrService, true) 12363 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(modifier, o.modifier, true) 12364 && compareDeep(patientPaid, o.patientPaid, true) && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 12365 && compareDeep(factor, o.factor, true) && compareDeep(tax, o.tax, true) && compareDeep(net, o.net, true) 12366 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(reviewOutcome, o.reviewOutcome, true) 12367 && compareDeep(adjudication, o.adjudication, true) && compareDeep(subDetail, o.subDetail, true) 12368 ; 12369 } 12370 12371 @Override 12372 public boolean equalsShallow(Base other_) { 12373 if (!super.equalsShallow(other_)) 12374 return false; 12375 if (!(other_ instanceof AddedItemDetailComponent)) 12376 return false; 12377 AddedItemDetailComponent o = (AddedItemDetailComponent) other_; 12378 return compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true); 12379 } 12380 12381 public boolean isEmpty() { 12382 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(traceNumber, revenue, productOrService 12383 , productOrServiceEnd, modifier, patientPaid, quantity, unitPrice, factor, tax 12384 , net, noteNumber, reviewOutcome, adjudication, subDetail); 12385 } 12386 12387 public String fhirType() { 12388 return "ExplanationOfBenefit.addItem.detail"; 12389 12390 } 12391 12392 } 12393 12394 @Block() 12395 public static class AddedItemDetailSubDetailComponent extends BackboneElement implements IBaseBackboneElement { 12396 /** 12397 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 12398 */ 12399 @Child(name = "traceNumber", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 12400 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 12401 protected List<Identifier> traceNumber; 12402 12403 /** 12404 * The type of revenue or cost center providing the product and/or service. 12405 */ 12406 @Child(name = "revenue", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 12407 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of revenue or cost center providing the product and/or service." ) 12408 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 12409 protected CodeableConcept revenue; 12410 12411 /** 12412 * When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used. 12413 */ 12414 @Child(name = "productOrService", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 12415 @Description(shortDefinition="Billing, service, product, or drug code", formalDefinition="When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used." ) 12416 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 12417 protected CodeableConcept productOrService; 12418 12419 /** 12420 * This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims. 12421 */ 12422 @Child(name = "productOrServiceEnd", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 12423 @Description(shortDefinition="End of a range of codes", formalDefinition="This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims." ) 12424 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 12425 protected CodeableConcept productOrServiceEnd; 12426 12427 /** 12428 * Item typification or modifiers codes to convey additional context for the product or service. 12429 */ 12430 @Child(name = "modifier", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 12431 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes to convey additional context for the product or service." ) 12432 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 12433 protected List<CodeableConcept> modifier; 12434 12435 /** 12436 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 12437 */ 12438 @Child(name = "patientPaid", type = {Money.class}, order=6, min=0, max=1, modifier=false, summary=false) 12439 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 12440 protected Money patientPaid; 12441 12442 /** 12443 * The number of repetitions of a service or product. 12444 */ 12445 @Child(name = "quantity", type = {Quantity.class}, order=7, min=0, max=1, modifier=false, summary=false) 12446 @Description(shortDefinition="Count of products or services", formalDefinition="The number of repetitions of a service or product." ) 12447 protected Quantity quantity; 12448 12449 /** 12450 * If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group. 12451 */ 12452 @Child(name = "unitPrice", type = {Money.class}, order=8, min=0, max=1, modifier=false, summary=false) 12453 @Description(shortDefinition="Fee, charge or cost per item", formalDefinition="If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group." ) 12454 protected Money unitPrice; 12455 12456 /** 12457 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 12458 */ 12459 @Child(name = "factor", type = {DecimalType.class}, order=9, min=0, max=1, modifier=false, summary=false) 12460 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 12461 protected DecimalType factor; 12462 12463 /** 12464 * The total of taxes applicable for this product or service. 12465 */ 12466 @Child(name = "tax", type = {Money.class}, order=10, min=0, max=1, modifier=false, summary=false) 12467 @Description(shortDefinition="Total tax", formalDefinition="The total of taxes applicable for this product or service." ) 12468 protected Money tax; 12469 12470 /** 12471 * The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor. 12472 */ 12473 @Child(name = "net", type = {Money.class}, order=11, min=0, max=1, modifier=false, summary=false) 12474 @Description(shortDefinition="Total item cost", formalDefinition="The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor." ) 12475 protected Money net; 12476 12477 /** 12478 * The numbers associated with notes below which apply to the adjudication of this item. 12479 */ 12480 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 12481 @Description(shortDefinition="Applicable note numbers", formalDefinition="The numbers associated with notes below which apply to the adjudication of this item." ) 12482 protected List<PositiveIntType> noteNumber; 12483 12484 /** 12485 * The high-level results of the adjudication if adjudication has been performed. 12486 */ 12487 @Child(name = "reviewOutcome", type = {ItemReviewOutcomeComponent.class}, order=13, min=0, max=1, modifier=false, summary=false) 12488 @Description(shortDefinition="Additem subdetail level adjudication results", formalDefinition="The high-level results of the adjudication if adjudication has been performed." ) 12489 protected ItemReviewOutcomeComponent reviewOutcome; 12490 12491 /** 12492 * The adjudication results. 12493 */ 12494 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 12495 @Description(shortDefinition="Added items adjudication", formalDefinition="The adjudication results." ) 12496 protected List<AdjudicationComponent> adjudication; 12497 12498 private static final long serialVersionUID = -71243645L; 12499 12500 /** 12501 * Constructor 12502 */ 12503 public AddedItemDetailSubDetailComponent() { 12504 super(); 12505 } 12506 12507 /** 12508 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 12509 */ 12510 public List<Identifier> getTraceNumber() { 12511 if (this.traceNumber == null) 12512 this.traceNumber = new ArrayList<Identifier>(); 12513 return this.traceNumber; 12514 } 12515 12516 /** 12517 * @return Returns a reference to <code>this</code> for easy method chaining 12518 */ 12519 public AddedItemDetailSubDetailComponent setTraceNumber(List<Identifier> theTraceNumber) { 12520 this.traceNumber = theTraceNumber; 12521 return this; 12522 } 12523 12524 public boolean hasTraceNumber() { 12525 if (this.traceNumber == null) 12526 return false; 12527 for (Identifier item : this.traceNumber) 12528 if (!item.isEmpty()) 12529 return true; 12530 return false; 12531 } 12532 12533 public Identifier addTraceNumber() { //3 12534 Identifier t = new Identifier(); 12535 if (this.traceNumber == null) 12536 this.traceNumber = new ArrayList<Identifier>(); 12537 this.traceNumber.add(t); 12538 return t; 12539 } 12540 12541 public AddedItemDetailSubDetailComponent addTraceNumber(Identifier t) { //3 12542 if (t == null) 12543 return this; 12544 if (this.traceNumber == null) 12545 this.traceNumber = new ArrayList<Identifier>(); 12546 this.traceNumber.add(t); 12547 return this; 12548 } 12549 12550 /** 12551 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 12552 */ 12553 public Identifier getTraceNumberFirstRep() { 12554 if (getTraceNumber().isEmpty()) { 12555 addTraceNumber(); 12556 } 12557 return getTraceNumber().get(0); 12558 } 12559 12560 /** 12561 * @return {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 12562 */ 12563 public CodeableConcept getRevenue() { 12564 if (this.revenue == null) 12565 if (Configuration.errorOnAutoCreate()) 12566 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.revenue"); 12567 else if (Configuration.doAutoCreate()) 12568 this.revenue = new CodeableConcept(); // cc 12569 return this.revenue; 12570 } 12571 12572 public boolean hasRevenue() { 12573 return this.revenue != null && !this.revenue.isEmpty(); 12574 } 12575 12576 /** 12577 * @param value {@link #revenue} (The type of revenue or cost center providing the product and/or service.) 12578 */ 12579 public AddedItemDetailSubDetailComponent setRevenue(CodeableConcept value) { 12580 this.revenue = value; 12581 return this; 12582 } 12583 12584 /** 12585 * @return {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 12586 */ 12587 public CodeableConcept getProductOrService() { 12588 if (this.productOrService == null) 12589 if (Configuration.errorOnAutoCreate()) 12590 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.productOrService"); 12591 else if (Configuration.doAutoCreate()) 12592 this.productOrService = new CodeableConcept(); // cc 12593 return this.productOrService; 12594 } 12595 12596 public boolean hasProductOrService() { 12597 return this.productOrService != null && !this.productOrService.isEmpty(); 12598 } 12599 12600 /** 12601 * @param value {@link #productOrService} (When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.) 12602 */ 12603 public AddedItemDetailSubDetailComponent setProductOrService(CodeableConcept value) { 12604 this.productOrService = value; 12605 return this; 12606 } 12607 12608 /** 12609 * @return {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 12610 */ 12611 public CodeableConcept getProductOrServiceEnd() { 12612 if (this.productOrServiceEnd == null) 12613 if (Configuration.errorOnAutoCreate()) 12614 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.productOrServiceEnd"); 12615 else if (Configuration.doAutoCreate()) 12616 this.productOrServiceEnd = new CodeableConcept(); // cc 12617 return this.productOrServiceEnd; 12618 } 12619 12620 public boolean hasProductOrServiceEnd() { 12621 return this.productOrServiceEnd != null && !this.productOrServiceEnd.isEmpty(); 12622 } 12623 12624 /** 12625 * @param value {@link #productOrServiceEnd} (This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.) 12626 */ 12627 public AddedItemDetailSubDetailComponent setProductOrServiceEnd(CodeableConcept value) { 12628 this.productOrServiceEnd = value; 12629 return this; 12630 } 12631 12632 /** 12633 * @return {@link #modifier} (Item typification or modifiers codes to convey additional context for the product or service.) 12634 */ 12635 public List<CodeableConcept> getModifier() { 12636 if (this.modifier == null) 12637 this.modifier = new ArrayList<CodeableConcept>(); 12638 return this.modifier; 12639 } 12640 12641 /** 12642 * @return Returns a reference to <code>this</code> for easy method chaining 12643 */ 12644 public AddedItemDetailSubDetailComponent setModifier(List<CodeableConcept> theModifier) { 12645 this.modifier = theModifier; 12646 return this; 12647 } 12648 12649 public boolean hasModifier() { 12650 if (this.modifier == null) 12651 return false; 12652 for (CodeableConcept item : this.modifier) 12653 if (!item.isEmpty()) 12654 return true; 12655 return false; 12656 } 12657 12658 public CodeableConcept addModifier() { //3 12659 CodeableConcept t = new CodeableConcept(); 12660 if (this.modifier == null) 12661 this.modifier = new ArrayList<CodeableConcept>(); 12662 this.modifier.add(t); 12663 return t; 12664 } 12665 12666 public AddedItemDetailSubDetailComponent addModifier(CodeableConcept t) { //3 12667 if (t == null) 12668 return this; 12669 if (this.modifier == null) 12670 this.modifier = new ArrayList<CodeableConcept>(); 12671 this.modifier.add(t); 12672 return this; 12673 } 12674 12675 /** 12676 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist {3} 12677 */ 12678 public CodeableConcept getModifierFirstRep() { 12679 if (getModifier().isEmpty()) { 12680 addModifier(); 12681 } 12682 return getModifier().get(0); 12683 } 12684 12685 /** 12686 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 12687 */ 12688 public Money getPatientPaid() { 12689 if (this.patientPaid == null) 12690 if (Configuration.errorOnAutoCreate()) 12691 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.patientPaid"); 12692 else if (Configuration.doAutoCreate()) 12693 this.patientPaid = new Money(); // cc 12694 return this.patientPaid; 12695 } 12696 12697 public boolean hasPatientPaid() { 12698 return this.patientPaid != null && !this.patientPaid.isEmpty(); 12699 } 12700 12701 /** 12702 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 12703 */ 12704 public AddedItemDetailSubDetailComponent setPatientPaid(Money value) { 12705 this.patientPaid = value; 12706 return this; 12707 } 12708 12709 /** 12710 * @return {@link #quantity} (The number of repetitions of a service or product.) 12711 */ 12712 public Quantity getQuantity() { 12713 if (this.quantity == null) 12714 if (Configuration.errorOnAutoCreate()) 12715 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.quantity"); 12716 else if (Configuration.doAutoCreate()) 12717 this.quantity = new Quantity(); // cc 12718 return this.quantity; 12719 } 12720 12721 public boolean hasQuantity() { 12722 return this.quantity != null && !this.quantity.isEmpty(); 12723 } 12724 12725 /** 12726 * @param value {@link #quantity} (The number of repetitions of a service or product.) 12727 */ 12728 public AddedItemDetailSubDetailComponent setQuantity(Quantity value) { 12729 this.quantity = value; 12730 return this; 12731 } 12732 12733 /** 12734 * @return {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 12735 */ 12736 public Money getUnitPrice() { 12737 if (this.unitPrice == null) 12738 if (Configuration.errorOnAutoCreate()) 12739 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.unitPrice"); 12740 else if (Configuration.doAutoCreate()) 12741 this.unitPrice = new Money(); // cc 12742 return this.unitPrice; 12743 } 12744 12745 public boolean hasUnitPrice() { 12746 return this.unitPrice != null && !this.unitPrice.isEmpty(); 12747 } 12748 12749 /** 12750 * @param value {@link #unitPrice} (If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.) 12751 */ 12752 public AddedItemDetailSubDetailComponent setUnitPrice(Money value) { 12753 this.unitPrice = value; 12754 return this; 12755 } 12756 12757 /** 12758 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 12759 */ 12760 public DecimalType getFactorElement() { 12761 if (this.factor == null) 12762 if (Configuration.errorOnAutoCreate()) 12763 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.factor"); 12764 else if (Configuration.doAutoCreate()) 12765 this.factor = new DecimalType(); // bb 12766 return this.factor; 12767 } 12768 12769 public boolean hasFactorElement() { 12770 return this.factor != null && !this.factor.isEmpty(); 12771 } 12772 12773 public boolean hasFactor() { 12774 return this.factor != null && !this.factor.isEmpty(); 12775 } 12776 12777 /** 12778 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 12779 */ 12780 public AddedItemDetailSubDetailComponent setFactorElement(DecimalType value) { 12781 this.factor = value; 12782 return this; 12783 } 12784 12785 /** 12786 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 12787 */ 12788 public BigDecimal getFactor() { 12789 return this.factor == null ? null : this.factor.getValue(); 12790 } 12791 12792 /** 12793 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 12794 */ 12795 public AddedItemDetailSubDetailComponent setFactor(BigDecimal value) { 12796 if (value == null) 12797 this.factor = null; 12798 else { 12799 if (this.factor == null) 12800 this.factor = new DecimalType(); 12801 this.factor.setValue(value); 12802 } 12803 return this; 12804 } 12805 12806 /** 12807 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 12808 */ 12809 public AddedItemDetailSubDetailComponent setFactor(long value) { 12810 this.factor = new DecimalType(); 12811 this.factor.setValue(value); 12812 return this; 12813 } 12814 12815 /** 12816 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 12817 */ 12818 public AddedItemDetailSubDetailComponent setFactor(double value) { 12819 this.factor = new DecimalType(); 12820 this.factor.setValue(value); 12821 return this; 12822 } 12823 12824 /** 12825 * @return {@link #tax} (The total of taxes applicable for this product or service.) 12826 */ 12827 public Money getTax() { 12828 if (this.tax == null) 12829 if (Configuration.errorOnAutoCreate()) 12830 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.tax"); 12831 else if (Configuration.doAutoCreate()) 12832 this.tax = new Money(); // cc 12833 return this.tax; 12834 } 12835 12836 public boolean hasTax() { 12837 return this.tax != null && !this.tax.isEmpty(); 12838 } 12839 12840 /** 12841 * @param value {@link #tax} (The total of taxes applicable for this product or service.) 12842 */ 12843 public AddedItemDetailSubDetailComponent setTax(Money value) { 12844 this.tax = value; 12845 return this; 12846 } 12847 12848 /** 12849 * @return {@link #net} (The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor.) 12850 */ 12851 public Money getNet() { 12852 if (this.net == null) 12853 if (Configuration.errorOnAutoCreate()) 12854 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.net"); 12855 else if (Configuration.doAutoCreate()) 12856 this.net = new Money(); // cc 12857 return this.net; 12858 } 12859 12860 public boolean hasNet() { 12861 return this.net != null && !this.net.isEmpty(); 12862 } 12863 12864 /** 12865 * @param value {@link #net} (The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor.) 12866 */ 12867 public AddedItemDetailSubDetailComponent setNet(Money value) { 12868 this.net = value; 12869 return this; 12870 } 12871 12872 /** 12873 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 12874 */ 12875 public List<PositiveIntType> getNoteNumber() { 12876 if (this.noteNumber == null) 12877 this.noteNumber = new ArrayList<PositiveIntType>(); 12878 return this.noteNumber; 12879 } 12880 12881 /** 12882 * @return Returns a reference to <code>this</code> for easy method chaining 12883 */ 12884 public AddedItemDetailSubDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 12885 this.noteNumber = theNoteNumber; 12886 return this; 12887 } 12888 12889 public boolean hasNoteNumber() { 12890 if (this.noteNumber == null) 12891 return false; 12892 for (PositiveIntType item : this.noteNumber) 12893 if (!item.isEmpty()) 12894 return true; 12895 return false; 12896 } 12897 12898 /** 12899 * @return {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 12900 */ 12901 public PositiveIntType addNoteNumberElement() {//2 12902 PositiveIntType t = new PositiveIntType(); 12903 if (this.noteNumber == null) 12904 this.noteNumber = new ArrayList<PositiveIntType>(); 12905 this.noteNumber.add(t); 12906 return t; 12907 } 12908 12909 /** 12910 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 12911 */ 12912 public AddedItemDetailSubDetailComponent addNoteNumber(int value) { //1 12913 PositiveIntType t = new PositiveIntType(); 12914 t.setValue(value); 12915 if (this.noteNumber == null) 12916 this.noteNumber = new ArrayList<PositiveIntType>(); 12917 this.noteNumber.add(t); 12918 return this; 12919 } 12920 12921 /** 12922 * @param value {@link #noteNumber} (The numbers associated with notes below which apply to the adjudication of this item.) 12923 */ 12924 public boolean hasNoteNumber(int value) { 12925 if (this.noteNumber == null) 12926 return false; 12927 for (PositiveIntType v : this.noteNumber) 12928 if (v.getValue().equals(value)) // positiveInt 12929 return true; 12930 return false; 12931 } 12932 12933 /** 12934 * @return {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 12935 */ 12936 public ItemReviewOutcomeComponent getReviewOutcome() { 12937 if (this.reviewOutcome == null) 12938 if (Configuration.errorOnAutoCreate()) 12939 throw new Error("Attempt to auto-create AddedItemDetailSubDetailComponent.reviewOutcome"); 12940 else if (Configuration.doAutoCreate()) 12941 this.reviewOutcome = new ItemReviewOutcomeComponent(); // cc 12942 return this.reviewOutcome; 12943 } 12944 12945 public boolean hasReviewOutcome() { 12946 return this.reviewOutcome != null && !this.reviewOutcome.isEmpty(); 12947 } 12948 12949 /** 12950 * @param value {@link #reviewOutcome} (The high-level results of the adjudication if adjudication has been performed.) 12951 */ 12952 public AddedItemDetailSubDetailComponent setReviewOutcome(ItemReviewOutcomeComponent value) { 12953 this.reviewOutcome = value; 12954 return this; 12955 } 12956 12957 /** 12958 * @return {@link #adjudication} (The adjudication results.) 12959 */ 12960 public List<AdjudicationComponent> getAdjudication() { 12961 if (this.adjudication == null) 12962 this.adjudication = new ArrayList<AdjudicationComponent>(); 12963 return this.adjudication; 12964 } 12965 12966 /** 12967 * @return Returns a reference to <code>this</code> for easy method chaining 12968 */ 12969 public AddedItemDetailSubDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 12970 this.adjudication = theAdjudication; 12971 return this; 12972 } 12973 12974 public boolean hasAdjudication() { 12975 if (this.adjudication == null) 12976 return false; 12977 for (AdjudicationComponent item : this.adjudication) 12978 if (!item.isEmpty()) 12979 return true; 12980 return false; 12981 } 12982 12983 public AdjudicationComponent addAdjudication() { //3 12984 AdjudicationComponent t = new AdjudicationComponent(); 12985 if (this.adjudication == null) 12986 this.adjudication = new ArrayList<AdjudicationComponent>(); 12987 this.adjudication.add(t); 12988 return t; 12989 } 12990 12991 public AddedItemDetailSubDetailComponent addAdjudication(AdjudicationComponent t) { //3 12992 if (t == null) 12993 return this; 12994 if (this.adjudication == null) 12995 this.adjudication = new ArrayList<AdjudicationComponent>(); 12996 this.adjudication.add(t); 12997 return this; 12998 } 12999 13000 /** 13001 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 13002 */ 13003 public AdjudicationComponent getAdjudicationFirstRep() { 13004 if (getAdjudication().isEmpty()) { 13005 addAdjudication(); 13006 } 13007 return getAdjudication().get(0); 13008 } 13009 13010 protected void listChildren(List<Property> children) { 13011 super.listChildren(children); 13012 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 13013 children.add(new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue)); 13014 children.add(new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService)); 13015 children.add(new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd)); 13016 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier)); 13017 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 13018 children.add(new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 13019 children.add(new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice)); 13020 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 13021 children.add(new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax)); 13022 children.add(new Property("net", "Money", "The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor.", 0, 1, net)); 13023 children.add(new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 13024 children.add(new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome)); 13025 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 13026 } 13027 13028 @Override 13029 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 13030 switch (_hash) { 13031 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 13032 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of revenue or cost center providing the product and/or service.", 0, 1, revenue); 13033 case 1957227299: /*productOrService*/ return new Property("productOrService", "CodeableConcept", "When the value is a group code then this item collects a set of related item details, otherwise this contains the product, service, drug or other billing code for the item. This element may be the start of a range of .productOrService codes used in conjunction with .productOrServiceEnd or it may be a solo element where .productOrServiceEnd is not used.", 0, 1, productOrService); 13034 case -717476168: /*productOrServiceEnd*/ return new Property("productOrServiceEnd", "CodeableConcept", "This contains the end of a range of product, service, drug or other billing codes for the item. This element is not used when the .productOrService is a group code. This value may only be present when a .productOfService code has been provided to convey the start of the range. Typically this value may be used only with preauthorizations and not with claims.", 0, 1, productOrServiceEnd); 13035 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes to convey additional context for the product or service.", 0, java.lang.Integer.MAX_VALUE, modifier); 13036 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 13037 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The number of repetitions of a service or product.", 0, 1, quantity); 13038 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is not a group then this is the fee for the product or service, otherwise this is the total of the fees for the details of the group.", 0, 1, unitPrice); 13039 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 13040 case 114603: /*tax*/ return new Property("tax", "Money", "The total of taxes applicable for this product or service.", 0, 1, tax); 13041 case 108957: /*net*/ return new Property("net", "Money", "The total amount claimed for the addItem.detail.subDetail. Net = unit price * quantity * factor.", 0, 1, net); 13042 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "The numbers associated with notes below which apply to the adjudication of this item.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 13043 case -51825446: /*reviewOutcome*/ return new Property("reviewOutcome", "@ExplanationOfBenefit.item.reviewOutcome", "The high-level results of the adjudication if adjudication has been performed.", 0, 1, reviewOutcome); 13044 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 13045 default: return super.getNamedProperty(_hash, _name, _checkValid); 13046 } 13047 13048 } 13049 13050 @Override 13051 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 13052 switch (hash) { 13053 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 13054 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 13055 case 1957227299: /*productOrService*/ return this.productOrService == null ? new Base[0] : new Base[] {this.productOrService}; // CodeableConcept 13056 case -717476168: /*productOrServiceEnd*/ return this.productOrServiceEnd == null ? new Base[0] : new Base[] {this.productOrServiceEnd}; // CodeableConcept 13057 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 13058 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 13059 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 13060 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 13061 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 13062 case 114603: /*tax*/ return this.tax == null ? new Base[0] : new Base[] {this.tax}; // Money 13063 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 13064 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 13065 case -51825446: /*reviewOutcome*/ return this.reviewOutcome == null ? new Base[0] : new Base[] {this.reviewOutcome}; // ItemReviewOutcomeComponent 13066 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 13067 default: return super.getProperty(hash, name, checkValid); 13068 } 13069 13070 } 13071 13072 @Override 13073 public Base setProperty(int hash, String name, Base value) throws FHIRException { 13074 switch (hash) { 13075 case 82505966: // traceNumber 13076 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 13077 return value; 13078 case 1099842588: // revenue 13079 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13080 return value; 13081 case 1957227299: // productOrService 13082 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13083 return value; 13084 case -717476168: // productOrServiceEnd 13085 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13086 return value; 13087 case -615513385: // modifier 13088 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 13089 return value; 13090 case 525514609: // patientPaid 13091 this.patientPaid = TypeConvertor.castToMoney(value); // Money 13092 return value; 13093 case -1285004149: // quantity 13094 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 13095 return value; 13096 case -486196699: // unitPrice 13097 this.unitPrice = TypeConvertor.castToMoney(value); // Money 13098 return value; 13099 case -1282148017: // factor 13100 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 13101 return value; 13102 case 114603: // tax 13103 this.tax = TypeConvertor.castToMoney(value); // Money 13104 return value; 13105 case 108957: // net 13106 this.net = TypeConvertor.castToMoney(value); // Money 13107 return value; 13108 case -1110033957: // noteNumber 13109 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); // PositiveIntType 13110 return value; 13111 case -51825446: // reviewOutcome 13112 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 13113 return value; 13114 case -231349275: // adjudication 13115 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 13116 return value; 13117 default: return super.setProperty(hash, name, value); 13118 } 13119 13120 } 13121 13122 @Override 13123 public Base setProperty(String name, Base value) throws FHIRException { 13124 if (name.equals("traceNumber")) { 13125 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 13126 } else if (name.equals("revenue")) { 13127 this.revenue = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13128 } else if (name.equals("productOrService")) { 13129 this.productOrService = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13130 } else if (name.equals("productOrServiceEnd")) { 13131 this.productOrServiceEnd = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13132 } else if (name.equals("modifier")) { 13133 this.getModifier().add(TypeConvertor.castToCodeableConcept(value)); 13134 } else if (name.equals("patientPaid")) { 13135 this.patientPaid = TypeConvertor.castToMoney(value); // Money 13136 } else if (name.equals("quantity")) { 13137 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 13138 } else if (name.equals("unitPrice")) { 13139 this.unitPrice = TypeConvertor.castToMoney(value); // Money 13140 } else if (name.equals("factor")) { 13141 this.factor = TypeConvertor.castToDecimal(value); // DecimalType 13142 } else if (name.equals("tax")) { 13143 this.tax = TypeConvertor.castToMoney(value); // Money 13144 } else if (name.equals("net")) { 13145 this.net = TypeConvertor.castToMoney(value); // Money 13146 } else if (name.equals("noteNumber")) { 13147 this.getNoteNumber().add(TypeConvertor.castToPositiveInt(value)); 13148 } else if (name.equals("reviewOutcome")) { 13149 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 13150 } else if (name.equals("adjudication")) { 13151 this.getAdjudication().add((AdjudicationComponent) value); 13152 } else 13153 return super.setProperty(name, value); 13154 return value; 13155 } 13156 13157 @Override 13158 public void removeChild(String name, Base value) throws FHIRException { 13159 if (name.equals("traceNumber")) { 13160 this.getTraceNumber().remove(value); 13161 } else if (name.equals("revenue")) { 13162 this.revenue = null; 13163 } else if (name.equals("productOrService")) { 13164 this.productOrService = null; 13165 } else if (name.equals("productOrServiceEnd")) { 13166 this.productOrServiceEnd = null; 13167 } else if (name.equals("modifier")) { 13168 this.getModifier().remove(value); 13169 } else if (name.equals("patientPaid")) { 13170 this.patientPaid = null; 13171 } else if (name.equals("quantity")) { 13172 this.quantity = null; 13173 } else if (name.equals("unitPrice")) { 13174 this.unitPrice = null; 13175 } else if (name.equals("factor")) { 13176 this.factor = null; 13177 } else if (name.equals("tax")) { 13178 this.tax = null; 13179 } else if (name.equals("net")) { 13180 this.net = null; 13181 } else if (name.equals("noteNumber")) { 13182 this.getNoteNumber().remove(value); 13183 } else if (name.equals("reviewOutcome")) { 13184 this.reviewOutcome = (ItemReviewOutcomeComponent) value; // ItemReviewOutcomeComponent 13185 } else if (name.equals("adjudication")) { 13186 this.getAdjudication().remove((AdjudicationComponent) value); 13187 } else 13188 super.removeChild(name, value); 13189 13190 } 13191 13192 @Override 13193 public Base makeProperty(int hash, String name) throws FHIRException { 13194 switch (hash) { 13195 case 82505966: return addTraceNumber(); 13196 case 1099842588: return getRevenue(); 13197 case 1957227299: return getProductOrService(); 13198 case -717476168: return getProductOrServiceEnd(); 13199 case -615513385: return addModifier(); 13200 case 525514609: return getPatientPaid(); 13201 case -1285004149: return getQuantity(); 13202 case -486196699: return getUnitPrice(); 13203 case -1282148017: return getFactorElement(); 13204 case 114603: return getTax(); 13205 case 108957: return getNet(); 13206 case -1110033957: return addNoteNumberElement(); 13207 case -51825446: return getReviewOutcome(); 13208 case -231349275: return addAdjudication(); 13209 default: return super.makeProperty(hash, name); 13210 } 13211 13212 } 13213 13214 @Override 13215 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 13216 switch (hash) { 13217 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 13218 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 13219 case 1957227299: /*productOrService*/ return new String[] {"CodeableConcept"}; 13220 case -717476168: /*productOrServiceEnd*/ return new String[] {"CodeableConcept"}; 13221 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 13222 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 13223 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 13224 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 13225 case -1282148017: /*factor*/ return new String[] {"decimal"}; 13226 case 114603: /*tax*/ return new String[] {"Money"}; 13227 case 108957: /*net*/ return new String[] {"Money"}; 13228 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 13229 case -51825446: /*reviewOutcome*/ return new String[] {"@ExplanationOfBenefit.item.reviewOutcome"}; 13230 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 13231 default: return super.getTypesForProperty(hash, name); 13232 } 13233 13234 } 13235 13236 @Override 13237 public Base addChild(String name) throws FHIRException { 13238 if (name.equals("traceNumber")) { 13239 return addTraceNumber(); 13240 } 13241 else if (name.equals("revenue")) { 13242 this.revenue = new CodeableConcept(); 13243 return this.revenue; 13244 } 13245 else if (name.equals("productOrService")) { 13246 this.productOrService = new CodeableConcept(); 13247 return this.productOrService; 13248 } 13249 else if (name.equals("productOrServiceEnd")) { 13250 this.productOrServiceEnd = new CodeableConcept(); 13251 return this.productOrServiceEnd; 13252 } 13253 else if (name.equals("modifier")) { 13254 return addModifier(); 13255 } 13256 else if (name.equals("patientPaid")) { 13257 this.patientPaid = new Money(); 13258 return this.patientPaid; 13259 } 13260 else if (name.equals("quantity")) { 13261 this.quantity = new Quantity(); 13262 return this.quantity; 13263 } 13264 else if (name.equals("unitPrice")) { 13265 this.unitPrice = new Money(); 13266 return this.unitPrice; 13267 } 13268 else if (name.equals("factor")) { 13269 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.detail.subDetail.factor"); 13270 } 13271 else if (name.equals("tax")) { 13272 this.tax = new Money(); 13273 return this.tax; 13274 } 13275 else if (name.equals("net")) { 13276 this.net = new Money(); 13277 return this.net; 13278 } 13279 else if (name.equals("noteNumber")) { 13280 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.addItem.detail.subDetail.noteNumber"); 13281 } 13282 else if (name.equals("reviewOutcome")) { 13283 this.reviewOutcome = new ItemReviewOutcomeComponent(); 13284 return this.reviewOutcome; 13285 } 13286 else if (name.equals("adjudication")) { 13287 return addAdjudication(); 13288 } 13289 else 13290 return super.addChild(name); 13291 } 13292 13293 public AddedItemDetailSubDetailComponent copy() { 13294 AddedItemDetailSubDetailComponent dst = new AddedItemDetailSubDetailComponent(); 13295 copyValues(dst); 13296 return dst; 13297 } 13298 13299 public void copyValues(AddedItemDetailSubDetailComponent dst) { 13300 super.copyValues(dst); 13301 if (traceNumber != null) { 13302 dst.traceNumber = new ArrayList<Identifier>(); 13303 for (Identifier i : traceNumber) 13304 dst.traceNumber.add(i.copy()); 13305 }; 13306 dst.revenue = revenue == null ? null : revenue.copy(); 13307 dst.productOrService = productOrService == null ? null : productOrService.copy(); 13308 dst.productOrServiceEnd = productOrServiceEnd == null ? null : productOrServiceEnd.copy(); 13309 if (modifier != null) { 13310 dst.modifier = new ArrayList<CodeableConcept>(); 13311 for (CodeableConcept i : modifier) 13312 dst.modifier.add(i.copy()); 13313 }; 13314 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 13315 dst.quantity = quantity == null ? null : quantity.copy(); 13316 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 13317 dst.factor = factor == null ? null : factor.copy(); 13318 dst.tax = tax == null ? null : tax.copy(); 13319 dst.net = net == null ? null : net.copy(); 13320 if (noteNumber != null) { 13321 dst.noteNumber = new ArrayList<PositiveIntType>(); 13322 for (PositiveIntType i : noteNumber) 13323 dst.noteNumber.add(i.copy()); 13324 }; 13325 dst.reviewOutcome = reviewOutcome == null ? null : reviewOutcome.copy(); 13326 if (adjudication != null) { 13327 dst.adjudication = new ArrayList<AdjudicationComponent>(); 13328 for (AdjudicationComponent i : adjudication) 13329 dst.adjudication.add(i.copy()); 13330 }; 13331 } 13332 13333 @Override 13334 public boolean equalsDeep(Base other_) { 13335 if (!super.equalsDeep(other_)) 13336 return false; 13337 if (!(other_ instanceof AddedItemDetailSubDetailComponent)) 13338 return false; 13339 AddedItemDetailSubDetailComponent o = (AddedItemDetailSubDetailComponent) other_; 13340 return compareDeep(traceNumber, o.traceNumber, true) && compareDeep(revenue, o.revenue, true) && compareDeep(productOrService, o.productOrService, true) 13341 && compareDeep(productOrServiceEnd, o.productOrServiceEnd, true) && compareDeep(modifier, o.modifier, true) 13342 && compareDeep(patientPaid, o.patientPaid, true) && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 13343 && compareDeep(factor, o.factor, true) && compareDeep(tax, o.tax, true) && compareDeep(net, o.net, true) 13344 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(reviewOutcome, o.reviewOutcome, true) 13345 && compareDeep(adjudication, o.adjudication, true); 13346 } 13347 13348 @Override 13349 public boolean equalsShallow(Base other_) { 13350 if (!super.equalsShallow(other_)) 13351 return false; 13352 if (!(other_ instanceof AddedItemDetailSubDetailComponent)) 13353 return false; 13354 AddedItemDetailSubDetailComponent o = (AddedItemDetailSubDetailComponent) other_; 13355 return compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true); 13356 } 13357 13358 public boolean isEmpty() { 13359 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(traceNumber, revenue, productOrService 13360 , productOrServiceEnd, modifier, patientPaid, quantity, unitPrice, factor, tax 13361 , net, noteNumber, reviewOutcome, adjudication); 13362 } 13363 13364 public String fhirType() { 13365 return "ExplanationOfBenefit.addItem.detail.subDetail"; 13366 13367 } 13368 13369 } 13370 13371 @Block() 13372 public static class TotalComponent extends BackboneElement implements IBaseBackboneElement { 13373 /** 13374 * A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item. 13375 */ 13376 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 13377 @Description(shortDefinition="Type of adjudication information", formalDefinition="A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item." ) 13378 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication") 13379 protected CodeableConcept category; 13380 13381 /** 13382 * Monetary total amount associated with the category. 13383 */ 13384 @Child(name = "amount", type = {Money.class}, order=2, min=1, max=1, modifier=false, summary=true) 13385 @Description(shortDefinition="Financial total for the category", formalDefinition="Monetary total amount associated with the category." ) 13386 protected Money amount; 13387 13388 private static final long serialVersionUID = 2012310309L; 13389 13390 /** 13391 * Constructor 13392 */ 13393 public TotalComponent() { 13394 super(); 13395 } 13396 13397 /** 13398 * Constructor 13399 */ 13400 public TotalComponent(CodeableConcept category, Money amount) { 13401 super(); 13402 this.setCategory(category); 13403 this.setAmount(amount); 13404 } 13405 13406 /** 13407 * @return {@link #category} (A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.) 13408 */ 13409 public CodeableConcept getCategory() { 13410 if (this.category == null) 13411 if (Configuration.errorOnAutoCreate()) 13412 throw new Error("Attempt to auto-create TotalComponent.category"); 13413 else if (Configuration.doAutoCreate()) 13414 this.category = new CodeableConcept(); // cc 13415 return this.category; 13416 } 13417 13418 public boolean hasCategory() { 13419 return this.category != null && !this.category.isEmpty(); 13420 } 13421 13422 /** 13423 * @param value {@link #category} (A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.) 13424 */ 13425 public TotalComponent setCategory(CodeableConcept value) { 13426 this.category = value; 13427 return this; 13428 } 13429 13430 /** 13431 * @return {@link #amount} (Monetary total amount associated with the category.) 13432 */ 13433 public Money getAmount() { 13434 if (this.amount == null) 13435 if (Configuration.errorOnAutoCreate()) 13436 throw new Error("Attempt to auto-create TotalComponent.amount"); 13437 else if (Configuration.doAutoCreate()) 13438 this.amount = new Money(); // cc 13439 return this.amount; 13440 } 13441 13442 public boolean hasAmount() { 13443 return this.amount != null && !this.amount.isEmpty(); 13444 } 13445 13446 /** 13447 * @param value {@link #amount} (Monetary total amount associated with the category.) 13448 */ 13449 public TotalComponent setAmount(Money value) { 13450 this.amount = value; 13451 return this; 13452 } 13453 13454 protected void listChildren(List<Property> children) { 13455 super.listChildren(children); 13456 children.add(new Property("category", "CodeableConcept", "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 0, 1, category)); 13457 children.add(new Property("amount", "Money", "Monetary total amount associated with the category.", 0, 1, amount)); 13458 } 13459 13460 @Override 13461 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 13462 switch (_hash) { 13463 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "A code to indicate the information type of this adjudication record. Information types may include: the value submitted, maximum values or percentages allowed or payable under the plan, amounts that the patient is responsible for in aggregate or pertaining to this item, amounts paid by other coverages, and the benefit payable for this item.", 0, 1, category); 13464 case -1413853096: /*amount*/ return new Property("amount", "Money", "Monetary total amount associated with the category.", 0, 1, amount); 13465 default: return super.getNamedProperty(_hash, _name, _checkValid); 13466 } 13467 13468 } 13469 13470 @Override 13471 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 13472 switch (hash) { 13473 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 13474 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 13475 default: return super.getProperty(hash, name, checkValid); 13476 } 13477 13478 } 13479 13480 @Override 13481 public Base setProperty(int hash, String name, Base value) throws FHIRException { 13482 switch (hash) { 13483 case 50511102: // category 13484 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13485 return value; 13486 case -1413853096: // amount 13487 this.amount = TypeConvertor.castToMoney(value); // Money 13488 return value; 13489 default: return super.setProperty(hash, name, value); 13490 } 13491 13492 } 13493 13494 @Override 13495 public Base setProperty(String name, Base value) throws FHIRException { 13496 if (name.equals("category")) { 13497 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13498 } else if (name.equals("amount")) { 13499 this.amount = TypeConvertor.castToMoney(value); // Money 13500 } else 13501 return super.setProperty(name, value); 13502 return value; 13503 } 13504 13505 @Override 13506 public void removeChild(String name, Base value) throws FHIRException { 13507 if (name.equals("category")) { 13508 this.category = null; 13509 } else if (name.equals("amount")) { 13510 this.amount = null; 13511 } else 13512 super.removeChild(name, value); 13513 13514 } 13515 13516 @Override 13517 public Base makeProperty(int hash, String name) throws FHIRException { 13518 switch (hash) { 13519 case 50511102: return getCategory(); 13520 case -1413853096: return getAmount(); 13521 default: return super.makeProperty(hash, name); 13522 } 13523 13524 } 13525 13526 @Override 13527 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 13528 switch (hash) { 13529 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 13530 case -1413853096: /*amount*/ return new String[] {"Money"}; 13531 default: return super.getTypesForProperty(hash, name); 13532 } 13533 13534 } 13535 13536 @Override 13537 public Base addChild(String name) throws FHIRException { 13538 if (name.equals("category")) { 13539 this.category = new CodeableConcept(); 13540 return this.category; 13541 } 13542 else if (name.equals("amount")) { 13543 this.amount = new Money(); 13544 return this.amount; 13545 } 13546 else 13547 return super.addChild(name); 13548 } 13549 13550 public TotalComponent copy() { 13551 TotalComponent dst = new TotalComponent(); 13552 copyValues(dst); 13553 return dst; 13554 } 13555 13556 public void copyValues(TotalComponent dst) { 13557 super.copyValues(dst); 13558 dst.category = category == null ? null : category.copy(); 13559 dst.amount = amount == null ? null : amount.copy(); 13560 } 13561 13562 @Override 13563 public boolean equalsDeep(Base other_) { 13564 if (!super.equalsDeep(other_)) 13565 return false; 13566 if (!(other_ instanceof TotalComponent)) 13567 return false; 13568 TotalComponent o = (TotalComponent) other_; 13569 return compareDeep(category, o.category, true) && compareDeep(amount, o.amount, true); 13570 } 13571 13572 @Override 13573 public boolean equalsShallow(Base other_) { 13574 if (!super.equalsShallow(other_)) 13575 return false; 13576 if (!(other_ instanceof TotalComponent)) 13577 return false; 13578 TotalComponent o = (TotalComponent) other_; 13579 return true; 13580 } 13581 13582 public boolean isEmpty() { 13583 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, amount); 13584 } 13585 13586 public String fhirType() { 13587 return "ExplanationOfBenefit.total"; 13588 13589 } 13590 13591 } 13592 13593 @Block() 13594 public static class PaymentComponent extends BackboneElement implements IBaseBackboneElement { 13595 /** 13596 * Whether this represents partial or complete payment of the benefits payable. 13597 */ 13598 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 13599 @Description(shortDefinition="Partial or complete payment", formalDefinition="Whether this represents partial or complete payment of the benefits payable." ) 13600 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-paymenttype") 13601 protected CodeableConcept type; 13602 13603 /** 13604 * Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication. 13605 */ 13606 @Child(name = "adjustment", type = {Money.class}, order=2, min=0, max=1, modifier=false, summary=false) 13607 @Description(shortDefinition="Payment adjustment for non-claim issues", formalDefinition="Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication." ) 13608 protected Money adjustment; 13609 13610 /** 13611 * Reason for the payment adjustment. 13612 */ 13613 @Child(name = "adjustmentReason", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 13614 @Description(shortDefinition="Explanation for the variance", formalDefinition="Reason for the payment adjustment." ) 13615 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payment-adjustment-reason") 13616 protected CodeableConcept adjustmentReason; 13617 13618 /** 13619 * Estimated date the payment will be issued or the actual issue date of payment. 13620 */ 13621 @Child(name = "date", type = {DateType.class}, order=4, min=0, max=1, modifier=false, summary=false) 13622 @Description(shortDefinition="Expected date of payment", formalDefinition="Estimated date the payment will be issued or the actual issue date of payment." ) 13623 protected DateType date; 13624 13625 /** 13626 * Benefits payable less any payment adjustment. 13627 */ 13628 @Child(name = "amount", type = {Money.class}, order=5, min=0, max=1, modifier=false, summary=false) 13629 @Description(shortDefinition="Payable amount after adjustment", formalDefinition="Benefits payable less any payment adjustment." ) 13630 protected Money amount; 13631 13632 /** 13633 * Issuer's unique identifier for the payment instrument. 13634 */ 13635 @Child(name = "identifier", type = {Identifier.class}, order=6, min=0, max=1, modifier=false, summary=false) 13636 @Description(shortDefinition="Business identifier for the payment", formalDefinition="Issuer's unique identifier for the payment instrument." ) 13637 protected Identifier identifier; 13638 13639 private static final long serialVersionUID = 1539906026L; 13640 13641 /** 13642 * Constructor 13643 */ 13644 public PaymentComponent() { 13645 super(); 13646 } 13647 13648 /** 13649 * @return {@link #type} (Whether this represents partial or complete payment of the benefits payable.) 13650 */ 13651 public CodeableConcept getType() { 13652 if (this.type == null) 13653 if (Configuration.errorOnAutoCreate()) 13654 throw new Error("Attempt to auto-create PaymentComponent.type"); 13655 else if (Configuration.doAutoCreate()) 13656 this.type = new CodeableConcept(); // cc 13657 return this.type; 13658 } 13659 13660 public boolean hasType() { 13661 return this.type != null && !this.type.isEmpty(); 13662 } 13663 13664 /** 13665 * @param value {@link #type} (Whether this represents partial or complete payment of the benefits payable.) 13666 */ 13667 public PaymentComponent setType(CodeableConcept value) { 13668 this.type = value; 13669 return this; 13670 } 13671 13672 /** 13673 * @return {@link #adjustment} (Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.) 13674 */ 13675 public Money getAdjustment() { 13676 if (this.adjustment == null) 13677 if (Configuration.errorOnAutoCreate()) 13678 throw new Error("Attempt to auto-create PaymentComponent.adjustment"); 13679 else if (Configuration.doAutoCreate()) 13680 this.adjustment = new Money(); // cc 13681 return this.adjustment; 13682 } 13683 13684 public boolean hasAdjustment() { 13685 return this.adjustment != null && !this.adjustment.isEmpty(); 13686 } 13687 13688 /** 13689 * @param value {@link #adjustment} (Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.) 13690 */ 13691 public PaymentComponent setAdjustment(Money value) { 13692 this.adjustment = value; 13693 return this; 13694 } 13695 13696 /** 13697 * @return {@link #adjustmentReason} (Reason for the payment adjustment.) 13698 */ 13699 public CodeableConcept getAdjustmentReason() { 13700 if (this.adjustmentReason == null) 13701 if (Configuration.errorOnAutoCreate()) 13702 throw new Error("Attempt to auto-create PaymentComponent.adjustmentReason"); 13703 else if (Configuration.doAutoCreate()) 13704 this.adjustmentReason = new CodeableConcept(); // cc 13705 return this.adjustmentReason; 13706 } 13707 13708 public boolean hasAdjustmentReason() { 13709 return this.adjustmentReason != null && !this.adjustmentReason.isEmpty(); 13710 } 13711 13712 /** 13713 * @param value {@link #adjustmentReason} (Reason for the payment adjustment.) 13714 */ 13715 public PaymentComponent setAdjustmentReason(CodeableConcept value) { 13716 this.adjustmentReason = value; 13717 return this; 13718 } 13719 13720 /** 13721 * @return {@link #date} (Estimated date the payment will be issued or the actual issue date of payment.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 13722 */ 13723 public DateType getDateElement() { 13724 if (this.date == null) 13725 if (Configuration.errorOnAutoCreate()) 13726 throw new Error("Attempt to auto-create PaymentComponent.date"); 13727 else if (Configuration.doAutoCreate()) 13728 this.date = new DateType(); // bb 13729 return this.date; 13730 } 13731 13732 public boolean hasDateElement() { 13733 return this.date != null && !this.date.isEmpty(); 13734 } 13735 13736 public boolean hasDate() { 13737 return this.date != null && !this.date.isEmpty(); 13738 } 13739 13740 /** 13741 * @param value {@link #date} (Estimated date the payment will be issued or the actual issue date of payment.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 13742 */ 13743 public PaymentComponent setDateElement(DateType value) { 13744 this.date = value; 13745 return this; 13746 } 13747 13748 /** 13749 * @return Estimated date the payment will be issued or the actual issue date of payment. 13750 */ 13751 public Date getDate() { 13752 return this.date == null ? null : this.date.getValue(); 13753 } 13754 13755 /** 13756 * @param value Estimated date the payment will be issued or the actual issue date of payment. 13757 */ 13758 public PaymentComponent setDate(Date value) { 13759 if (value == null) 13760 this.date = null; 13761 else { 13762 if (this.date == null) 13763 this.date = new DateType(); 13764 this.date.setValue(value); 13765 } 13766 return this; 13767 } 13768 13769 /** 13770 * @return {@link #amount} (Benefits payable less any payment adjustment.) 13771 */ 13772 public Money getAmount() { 13773 if (this.amount == null) 13774 if (Configuration.errorOnAutoCreate()) 13775 throw new Error("Attempt to auto-create PaymentComponent.amount"); 13776 else if (Configuration.doAutoCreate()) 13777 this.amount = new Money(); // cc 13778 return this.amount; 13779 } 13780 13781 public boolean hasAmount() { 13782 return this.amount != null && !this.amount.isEmpty(); 13783 } 13784 13785 /** 13786 * @param value {@link #amount} (Benefits payable less any payment adjustment.) 13787 */ 13788 public PaymentComponent setAmount(Money value) { 13789 this.amount = value; 13790 return this; 13791 } 13792 13793 /** 13794 * @return {@link #identifier} (Issuer's unique identifier for the payment instrument.) 13795 */ 13796 public Identifier getIdentifier() { 13797 if (this.identifier == null) 13798 if (Configuration.errorOnAutoCreate()) 13799 throw new Error("Attempt to auto-create PaymentComponent.identifier"); 13800 else if (Configuration.doAutoCreate()) 13801 this.identifier = new Identifier(); // cc 13802 return this.identifier; 13803 } 13804 13805 public boolean hasIdentifier() { 13806 return this.identifier != null && !this.identifier.isEmpty(); 13807 } 13808 13809 /** 13810 * @param value {@link #identifier} (Issuer's unique identifier for the payment instrument.) 13811 */ 13812 public PaymentComponent setIdentifier(Identifier value) { 13813 this.identifier = value; 13814 return this; 13815 } 13816 13817 protected void listChildren(List<Property> children) { 13818 super.listChildren(children); 13819 children.add(new Property("type", "CodeableConcept", "Whether this represents partial or complete payment of the benefits payable.", 0, 1, type)); 13820 children.add(new Property("adjustment", "Money", "Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.", 0, 1, adjustment)); 13821 children.add(new Property("adjustmentReason", "CodeableConcept", "Reason for the payment adjustment.", 0, 1, adjustmentReason)); 13822 children.add(new Property("date", "date", "Estimated date the payment will be issued or the actual issue date of payment.", 0, 1, date)); 13823 children.add(new Property("amount", "Money", "Benefits payable less any payment adjustment.", 0, 1, amount)); 13824 children.add(new Property("identifier", "Identifier", "Issuer's unique identifier for the payment instrument.", 0, 1, identifier)); 13825 } 13826 13827 @Override 13828 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 13829 switch (_hash) { 13830 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Whether this represents partial or complete payment of the benefits payable.", 0, 1, type); 13831 case 1977085293: /*adjustment*/ return new Property("adjustment", "Money", "Total amount of all adjustments to this payment included in this transaction which are not related to this claim's adjudication.", 0, 1, adjustment); 13832 case -1255938543: /*adjustmentReason*/ return new Property("adjustmentReason", "CodeableConcept", "Reason for the payment adjustment.", 0, 1, adjustmentReason); 13833 case 3076014: /*date*/ return new Property("date", "date", "Estimated date the payment will be issued or the actual issue date of payment.", 0, 1, date); 13834 case -1413853096: /*amount*/ return new Property("amount", "Money", "Benefits payable less any payment adjustment.", 0, 1, amount); 13835 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Issuer's unique identifier for the payment instrument.", 0, 1, identifier); 13836 default: return super.getNamedProperty(_hash, _name, _checkValid); 13837 } 13838 13839 } 13840 13841 @Override 13842 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 13843 switch (hash) { 13844 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 13845 case 1977085293: /*adjustment*/ return this.adjustment == null ? new Base[0] : new Base[] {this.adjustment}; // Money 13846 case -1255938543: /*adjustmentReason*/ return this.adjustmentReason == null ? new Base[0] : new Base[] {this.adjustmentReason}; // CodeableConcept 13847 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 13848 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 13849 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 13850 default: return super.getProperty(hash, name, checkValid); 13851 } 13852 13853 } 13854 13855 @Override 13856 public Base setProperty(int hash, String name, Base value) throws FHIRException { 13857 switch (hash) { 13858 case 3575610: // type 13859 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13860 return value; 13861 case 1977085293: // adjustment 13862 this.adjustment = TypeConvertor.castToMoney(value); // Money 13863 return value; 13864 case -1255938543: // adjustmentReason 13865 this.adjustmentReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13866 return value; 13867 case 3076014: // date 13868 this.date = TypeConvertor.castToDate(value); // DateType 13869 return value; 13870 case -1413853096: // amount 13871 this.amount = TypeConvertor.castToMoney(value); // Money 13872 return value; 13873 case -1618432855: // identifier 13874 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 13875 return value; 13876 default: return super.setProperty(hash, name, value); 13877 } 13878 13879 } 13880 13881 @Override 13882 public Base setProperty(String name, Base value) throws FHIRException { 13883 if (name.equals("type")) { 13884 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13885 } else if (name.equals("adjustment")) { 13886 this.adjustment = TypeConvertor.castToMoney(value); // Money 13887 } else if (name.equals("adjustmentReason")) { 13888 this.adjustmentReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 13889 } else if (name.equals("date")) { 13890 this.date = TypeConvertor.castToDate(value); // DateType 13891 } else if (name.equals("amount")) { 13892 this.amount = TypeConvertor.castToMoney(value); // Money 13893 } else if (name.equals("identifier")) { 13894 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 13895 } else 13896 return super.setProperty(name, value); 13897 return value; 13898 } 13899 13900 @Override 13901 public void removeChild(String name, Base value) throws FHIRException { 13902 if (name.equals("type")) { 13903 this.type = null; 13904 } else if (name.equals("adjustment")) { 13905 this.adjustment = null; 13906 } else if (name.equals("adjustmentReason")) { 13907 this.adjustmentReason = null; 13908 } else if (name.equals("date")) { 13909 this.date = null; 13910 } else if (name.equals("amount")) { 13911 this.amount = null; 13912 } else if (name.equals("identifier")) { 13913 this.identifier = null; 13914 } else 13915 super.removeChild(name, value); 13916 13917 } 13918 13919 @Override 13920 public Base makeProperty(int hash, String name) throws FHIRException { 13921 switch (hash) { 13922 case 3575610: return getType(); 13923 case 1977085293: return getAdjustment(); 13924 case -1255938543: return getAdjustmentReason(); 13925 case 3076014: return getDateElement(); 13926 case -1413853096: return getAmount(); 13927 case -1618432855: return getIdentifier(); 13928 default: return super.makeProperty(hash, name); 13929 } 13930 13931 } 13932 13933 @Override 13934 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 13935 switch (hash) { 13936 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 13937 case 1977085293: /*adjustment*/ return new String[] {"Money"}; 13938 case -1255938543: /*adjustmentReason*/ return new String[] {"CodeableConcept"}; 13939 case 3076014: /*date*/ return new String[] {"date"}; 13940 case -1413853096: /*amount*/ return new String[] {"Money"}; 13941 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 13942 default: return super.getTypesForProperty(hash, name); 13943 } 13944 13945 } 13946 13947 @Override 13948 public Base addChild(String name) throws FHIRException { 13949 if (name.equals("type")) { 13950 this.type = new CodeableConcept(); 13951 return this.type; 13952 } 13953 else if (name.equals("adjustment")) { 13954 this.adjustment = new Money(); 13955 return this.adjustment; 13956 } 13957 else if (name.equals("adjustmentReason")) { 13958 this.adjustmentReason = new CodeableConcept(); 13959 return this.adjustmentReason; 13960 } 13961 else if (name.equals("date")) { 13962 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.payment.date"); 13963 } 13964 else if (name.equals("amount")) { 13965 this.amount = new Money(); 13966 return this.amount; 13967 } 13968 else if (name.equals("identifier")) { 13969 this.identifier = new Identifier(); 13970 return this.identifier; 13971 } 13972 else 13973 return super.addChild(name); 13974 } 13975 13976 public PaymentComponent copy() { 13977 PaymentComponent dst = new PaymentComponent(); 13978 copyValues(dst); 13979 return dst; 13980 } 13981 13982 public void copyValues(PaymentComponent dst) { 13983 super.copyValues(dst); 13984 dst.type = type == null ? null : type.copy(); 13985 dst.adjustment = adjustment == null ? null : adjustment.copy(); 13986 dst.adjustmentReason = adjustmentReason == null ? null : adjustmentReason.copy(); 13987 dst.date = date == null ? null : date.copy(); 13988 dst.amount = amount == null ? null : amount.copy(); 13989 dst.identifier = identifier == null ? null : identifier.copy(); 13990 } 13991 13992 @Override 13993 public boolean equalsDeep(Base other_) { 13994 if (!super.equalsDeep(other_)) 13995 return false; 13996 if (!(other_ instanceof PaymentComponent)) 13997 return false; 13998 PaymentComponent o = (PaymentComponent) other_; 13999 return compareDeep(type, o.type, true) && compareDeep(adjustment, o.adjustment, true) && compareDeep(adjustmentReason, o.adjustmentReason, true) 14000 && compareDeep(date, o.date, true) && compareDeep(amount, o.amount, true) && compareDeep(identifier, o.identifier, true) 14001 ; 14002 } 14003 14004 @Override 14005 public boolean equalsShallow(Base other_) { 14006 if (!super.equalsShallow(other_)) 14007 return false; 14008 if (!(other_ instanceof PaymentComponent)) 14009 return false; 14010 PaymentComponent o = (PaymentComponent) other_; 14011 return compareValues(date, o.date, true); 14012 } 14013 14014 public boolean isEmpty() { 14015 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, adjustment, adjustmentReason 14016 , date, amount, identifier); 14017 } 14018 14019 public String fhirType() { 14020 return "ExplanationOfBenefit.payment"; 14021 14022 } 14023 14024 } 14025 14026 @Block() 14027 public static class NoteComponent extends BackboneElement implements IBaseBackboneElement { 14028 /** 14029 * A number to uniquely identify a note entry. 14030 */ 14031 @Child(name = "number", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 14032 @Description(shortDefinition="Note instance identifier", formalDefinition="A number to uniquely identify a note entry." ) 14033 protected PositiveIntType number; 14034 14035 /** 14036 * The business purpose of the note text. 14037 */ 14038 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 14039 @Description(shortDefinition="Note purpose", formalDefinition="The business purpose of the note text." ) 14040 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/note-type") 14041 protected CodeableConcept type; 14042 14043 /** 14044 * The explanation or description associated with the processing. 14045 */ 14046 @Child(name = "text", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 14047 @Description(shortDefinition="Note explanatory text", formalDefinition="The explanation or description associated with the processing." ) 14048 protected StringType text; 14049 14050 /** 14051 * A code to define the language used in the text of the note. 14052 */ 14053 @Child(name = "language", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 14054 @Description(shortDefinition="Language of the text", formalDefinition="A code to define the language used in the text of the note." ) 14055 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-languages") 14056 protected CodeableConcept language; 14057 14058 private static final long serialVersionUID = -944255449L; 14059 14060 /** 14061 * Constructor 14062 */ 14063 public NoteComponent() { 14064 super(); 14065 } 14066 14067 /** 14068 * @return {@link #number} (A number to uniquely identify a note entry.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 14069 */ 14070 public PositiveIntType getNumberElement() { 14071 if (this.number == null) 14072 if (Configuration.errorOnAutoCreate()) 14073 throw new Error("Attempt to auto-create NoteComponent.number"); 14074 else if (Configuration.doAutoCreate()) 14075 this.number = new PositiveIntType(); // bb 14076 return this.number; 14077 } 14078 14079 public boolean hasNumberElement() { 14080 return this.number != null && !this.number.isEmpty(); 14081 } 14082 14083 public boolean hasNumber() { 14084 return this.number != null && !this.number.isEmpty(); 14085 } 14086 14087 /** 14088 * @param value {@link #number} (A number to uniquely identify a note entry.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 14089 */ 14090 public NoteComponent setNumberElement(PositiveIntType value) { 14091 this.number = value; 14092 return this; 14093 } 14094 14095 /** 14096 * @return A number to uniquely identify a note entry. 14097 */ 14098 public int getNumber() { 14099 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 14100 } 14101 14102 /** 14103 * @param value A number to uniquely identify a note entry. 14104 */ 14105 public NoteComponent setNumber(int value) { 14106 if (this.number == null) 14107 this.number = new PositiveIntType(); 14108 this.number.setValue(value); 14109 return this; 14110 } 14111 14112 /** 14113 * @return {@link #type} (The business purpose of the note text.) 14114 */ 14115 public CodeableConcept getType() { 14116 if (this.type == null) 14117 if (Configuration.errorOnAutoCreate()) 14118 throw new Error("Attempt to auto-create NoteComponent.type"); 14119 else if (Configuration.doAutoCreate()) 14120 this.type = new CodeableConcept(); // cc 14121 return this.type; 14122 } 14123 14124 public boolean hasType() { 14125 return this.type != null && !this.type.isEmpty(); 14126 } 14127 14128 /** 14129 * @param value {@link #type} (The business purpose of the note text.) 14130 */ 14131 public NoteComponent setType(CodeableConcept value) { 14132 this.type = value; 14133 return this; 14134 } 14135 14136 /** 14137 * @return {@link #text} (The explanation or description associated with the processing.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 14138 */ 14139 public StringType getTextElement() { 14140 if (this.text == null) 14141 if (Configuration.errorOnAutoCreate()) 14142 throw new Error("Attempt to auto-create NoteComponent.text"); 14143 else if (Configuration.doAutoCreate()) 14144 this.text = new StringType(); // bb 14145 return this.text; 14146 } 14147 14148 public boolean hasTextElement() { 14149 return this.text != null && !this.text.isEmpty(); 14150 } 14151 14152 public boolean hasText() { 14153 return this.text != null && !this.text.isEmpty(); 14154 } 14155 14156 /** 14157 * @param value {@link #text} (The explanation or description associated with the processing.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 14158 */ 14159 public NoteComponent setTextElement(StringType value) { 14160 this.text = value; 14161 return this; 14162 } 14163 14164 /** 14165 * @return The explanation or description associated with the processing. 14166 */ 14167 public String getText() { 14168 return this.text == null ? null : this.text.getValue(); 14169 } 14170 14171 /** 14172 * @param value The explanation or description associated with the processing. 14173 */ 14174 public NoteComponent setText(String value) { 14175 if (Utilities.noString(value)) 14176 this.text = null; 14177 else { 14178 if (this.text == null) 14179 this.text = new StringType(); 14180 this.text.setValue(value); 14181 } 14182 return this; 14183 } 14184 14185 /** 14186 * @return {@link #language} (A code to define the language used in the text of the note.) 14187 */ 14188 public CodeableConcept getLanguage() { 14189 if (this.language == null) 14190 if (Configuration.errorOnAutoCreate()) 14191 throw new Error("Attempt to auto-create NoteComponent.language"); 14192 else if (Configuration.doAutoCreate()) 14193 this.language = new CodeableConcept(); // cc 14194 return this.language; 14195 } 14196 14197 public boolean hasLanguage() { 14198 return this.language != null && !this.language.isEmpty(); 14199 } 14200 14201 /** 14202 * @param value {@link #language} (A code to define the language used in the text of the note.) 14203 */ 14204 public NoteComponent setLanguage(CodeableConcept value) { 14205 this.language = value; 14206 return this; 14207 } 14208 14209 protected void listChildren(List<Property> children) { 14210 super.listChildren(children); 14211 children.add(new Property("number", "positiveInt", "A number to uniquely identify a note entry.", 0, 1, number)); 14212 children.add(new Property("type", "CodeableConcept", "The business purpose of the note text.", 0, 1, type)); 14213 children.add(new Property("text", "string", "The explanation or description associated with the processing.", 0, 1, text)); 14214 children.add(new Property("language", "CodeableConcept", "A code to define the language used in the text of the note.", 0, 1, language)); 14215 } 14216 14217 @Override 14218 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 14219 switch (_hash) { 14220 case -1034364087: /*number*/ return new Property("number", "positiveInt", "A number to uniquely identify a note entry.", 0, 1, number); 14221 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The business purpose of the note text.", 0, 1, type); 14222 case 3556653: /*text*/ return new Property("text", "string", "The explanation or description associated with the processing.", 0, 1, text); 14223 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "A code to define the language used in the text of the note.", 0, 1, language); 14224 default: return super.getNamedProperty(_hash, _name, _checkValid); 14225 } 14226 14227 } 14228 14229 @Override 14230 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 14231 switch (hash) { 14232 case -1034364087: /*number*/ return this.number == null ? new Base[0] : new Base[] {this.number}; // PositiveIntType 14233 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 14234 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 14235 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeableConcept 14236 default: return super.getProperty(hash, name, checkValid); 14237 } 14238 14239 } 14240 14241 @Override 14242 public Base setProperty(int hash, String name, Base value) throws FHIRException { 14243 switch (hash) { 14244 case -1034364087: // number 14245 this.number = TypeConvertor.castToPositiveInt(value); // PositiveIntType 14246 return value; 14247 case 3575610: // type 14248 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14249 return value; 14250 case 3556653: // text 14251 this.text = TypeConvertor.castToString(value); // StringType 14252 return value; 14253 case -1613589672: // language 14254 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14255 return value; 14256 default: return super.setProperty(hash, name, value); 14257 } 14258 14259 } 14260 14261 @Override 14262 public Base setProperty(String name, Base value) throws FHIRException { 14263 if (name.equals("number")) { 14264 this.number = TypeConvertor.castToPositiveInt(value); // PositiveIntType 14265 } else if (name.equals("type")) { 14266 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14267 } else if (name.equals("text")) { 14268 this.text = TypeConvertor.castToString(value); // StringType 14269 } else if (name.equals("language")) { 14270 this.language = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14271 } else 14272 return super.setProperty(name, value); 14273 return value; 14274 } 14275 14276 @Override 14277 public void removeChild(String name, Base value) throws FHIRException { 14278 if (name.equals("number")) { 14279 this.number = null; 14280 } else if (name.equals("type")) { 14281 this.type = null; 14282 } else if (name.equals("text")) { 14283 this.text = null; 14284 } else if (name.equals("language")) { 14285 this.language = null; 14286 } else 14287 super.removeChild(name, value); 14288 14289 } 14290 14291 @Override 14292 public Base makeProperty(int hash, String name) throws FHIRException { 14293 switch (hash) { 14294 case -1034364087: return getNumberElement(); 14295 case 3575610: return getType(); 14296 case 3556653: return getTextElement(); 14297 case -1613589672: return getLanguage(); 14298 default: return super.makeProperty(hash, name); 14299 } 14300 14301 } 14302 14303 @Override 14304 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 14305 switch (hash) { 14306 case -1034364087: /*number*/ return new String[] {"positiveInt"}; 14307 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 14308 case 3556653: /*text*/ return new String[] {"string"}; 14309 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 14310 default: return super.getTypesForProperty(hash, name); 14311 } 14312 14313 } 14314 14315 @Override 14316 public Base addChild(String name) throws FHIRException { 14317 if (name.equals("number")) { 14318 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.processNote.number"); 14319 } 14320 else if (name.equals("type")) { 14321 this.type = new CodeableConcept(); 14322 return this.type; 14323 } 14324 else if (name.equals("text")) { 14325 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.processNote.text"); 14326 } 14327 else if (name.equals("language")) { 14328 this.language = new CodeableConcept(); 14329 return this.language; 14330 } 14331 else 14332 return super.addChild(name); 14333 } 14334 14335 public NoteComponent copy() { 14336 NoteComponent dst = new NoteComponent(); 14337 copyValues(dst); 14338 return dst; 14339 } 14340 14341 public void copyValues(NoteComponent dst) { 14342 super.copyValues(dst); 14343 dst.number = number == null ? null : number.copy(); 14344 dst.type = type == null ? null : type.copy(); 14345 dst.text = text == null ? null : text.copy(); 14346 dst.language = language == null ? null : language.copy(); 14347 } 14348 14349 @Override 14350 public boolean equalsDeep(Base other_) { 14351 if (!super.equalsDeep(other_)) 14352 return false; 14353 if (!(other_ instanceof NoteComponent)) 14354 return false; 14355 NoteComponent o = (NoteComponent) other_; 14356 return compareDeep(number, o.number, true) && compareDeep(type, o.type, true) && compareDeep(text, o.text, true) 14357 && compareDeep(language, o.language, true); 14358 } 14359 14360 @Override 14361 public boolean equalsShallow(Base other_) { 14362 if (!super.equalsShallow(other_)) 14363 return false; 14364 if (!(other_ instanceof NoteComponent)) 14365 return false; 14366 NoteComponent o = (NoteComponent) other_; 14367 return compareValues(number, o.number, true) && compareValues(text, o.text, true); 14368 } 14369 14370 public boolean isEmpty() { 14371 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(number, type, text, language 14372 ); 14373 } 14374 14375 public String fhirType() { 14376 return "ExplanationOfBenefit.processNote"; 14377 14378 } 14379 14380 } 14381 14382 @Block() 14383 public static class BenefitBalanceComponent extends BackboneElement implements IBaseBackboneElement { 14384 /** 14385 * Code to identify the general type of benefits under which products and services are provided. 14386 */ 14387 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 14388 @Description(shortDefinition="Benefit classification", formalDefinition="Code to identify the general type of benefits under which products and services are provided." ) 14389 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-benefitcategory") 14390 protected CodeableConcept category; 14391 14392 /** 14393 * True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage. 14394 */ 14395 @Child(name = "excluded", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 14396 @Description(shortDefinition="Excluded from the plan", formalDefinition="True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage." ) 14397 protected BooleanType excluded; 14398 14399 /** 14400 * A short name or tag for the benefit. 14401 */ 14402 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 14403 @Description(shortDefinition="Short name for the benefit", formalDefinition="A short name or tag for the benefit." ) 14404 protected StringType name; 14405 14406 /** 14407 * A richer description of the benefit or services covered. 14408 */ 14409 @Child(name = "description", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 14410 @Description(shortDefinition="Description of the benefit or services covered", formalDefinition="A richer description of the benefit or services covered." ) 14411 protected StringType description; 14412 14413 /** 14414 * Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers. 14415 */ 14416 @Child(name = "network", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 14417 @Description(shortDefinition="In or out of network", formalDefinition="Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers." ) 14418 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-network") 14419 protected CodeableConcept network; 14420 14421 /** 14422 * Indicates if the benefits apply to an individual or to the family. 14423 */ 14424 @Child(name = "unit", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 14425 @Description(shortDefinition="Individual or family", formalDefinition="Indicates if the benefits apply to an individual or to the family." ) 14426 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-unit") 14427 protected CodeableConcept unit; 14428 14429 /** 14430 * The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'. 14431 */ 14432 @Child(name = "term", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 14433 @Description(shortDefinition="Annual or lifetime", formalDefinition="The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'." ) 14434 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-term") 14435 protected CodeableConcept term; 14436 14437 /** 14438 * Benefits Used to date. 14439 */ 14440 @Child(name = "financial", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 14441 @Description(shortDefinition="Benefit Summary", formalDefinition="Benefits Used to date." ) 14442 protected List<BenefitComponent> financial; 14443 14444 private static final long serialVersionUID = -1889655824L; 14445 14446 /** 14447 * Constructor 14448 */ 14449 public BenefitBalanceComponent() { 14450 super(); 14451 } 14452 14453 /** 14454 * Constructor 14455 */ 14456 public BenefitBalanceComponent(CodeableConcept category) { 14457 super(); 14458 this.setCategory(category); 14459 } 14460 14461 /** 14462 * @return {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 14463 */ 14464 public CodeableConcept getCategory() { 14465 if (this.category == null) 14466 if (Configuration.errorOnAutoCreate()) 14467 throw new Error("Attempt to auto-create BenefitBalanceComponent.category"); 14468 else if (Configuration.doAutoCreate()) 14469 this.category = new CodeableConcept(); // cc 14470 return this.category; 14471 } 14472 14473 public boolean hasCategory() { 14474 return this.category != null && !this.category.isEmpty(); 14475 } 14476 14477 /** 14478 * @param value {@link #category} (Code to identify the general type of benefits under which products and services are provided.) 14479 */ 14480 public BenefitBalanceComponent setCategory(CodeableConcept value) { 14481 this.category = value; 14482 return this; 14483 } 14484 14485 /** 14486 * @return {@link #excluded} (True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.). This is the underlying object with id, value and extensions. The accessor "getExcluded" gives direct access to the value 14487 */ 14488 public BooleanType getExcludedElement() { 14489 if (this.excluded == null) 14490 if (Configuration.errorOnAutoCreate()) 14491 throw new Error("Attempt to auto-create BenefitBalanceComponent.excluded"); 14492 else if (Configuration.doAutoCreate()) 14493 this.excluded = new BooleanType(); // bb 14494 return this.excluded; 14495 } 14496 14497 public boolean hasExcludedElement() { 14498 return this.excluded != null && !this.excluded.isEmpty(); 14499 } 14500 14501 public boolean hasExcluded() { 14502 return this.excluded != null && !this.excluded.isEmpty(); 14503 } 14504 14505 /** 14506 * @param value {@link #excluded} (True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.). This is the underlying object with id, value and extensions. The accessor "getExcluded" gives direct access to the value 14507 */ 14508 public BenefitBalanceComponent setExcludedElement(BooleanType value) { 14509 this.excluded = value; 14510 return this; 14511 } 14512 14513 /** 14514 * @return True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage. 14515 */ 14516 public boolean getExcluded() { 14517 return this.excluded == null || this.excluded.isEmpty() ? false : this.excluded.getValue(); 14518 } 14519 14520 /** 14521 * @param value True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage. 14522 */ 14523 public BenefitBalanceComponent setExcluded(boolean value) { 14524 if (this.excluded == null) 14525 this.excluded = new BooleanType(); 14526 this.excluded.setValue(value); 14527 return this; 14528 } 14529 14530 /** 14531 * @return {@link #name} (A short name or tag for the benefit.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 14532 */ 14533 public StringType getNameElement() { 14534 if (this.name == null) 14535 if (Configuration.errorOnAutoCreate()) 14536 throw new Error("Attempt to auto-create BenefitBalanceComponent.name"); 14537 else if (Configuration.doAutoCreate()) 14538 this.name = new StringType(); // bb 14539 return this.name; 14540 } 14541 14542 public boolean hasNameElement() { 14543 return this.name != null && !this.name.isEmpty(); 14544 } 14545 14546 public boolean hasName() { 14547 return this.name != null && !this.name.isEmpty(); 14548 } 14549 14550 /** 14551 * @param value {@link #name} (A short name or tag for the benefit.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 14552 */ 14553 public BenefitBalanceComponent setNameElement(StringType value) { 14554 this.name = value; 14555 return this; 14556 } 14557 14558 /** 14559 * @return A short name or tag for the benefit. 14560 */ 14561 public String getName() { 14562 return this.name == null ? null : this.name.getValue(); 14563 } 14564 14565 /** 14566 * @param value A short name or tag for the benefit. 14567 */ 14568 public BenefitBalanceComponent setName(String value) { 14569 if (Utilities.noString(value)) 14570 this.name = null; 14571 else { 14572 if (this.name == null) 14573 this.name = new StringType(); 14574 this.name.setValue(value); 14575 } 14576 return this; 14577 } 14578 14579 /** 14580 * @return {@link #description} (A richer description of the benefit or services covered.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 14581 */ 14582 public StringType getDescriptionElement() { 14583 if (this.description == null) 14584 if (Configuration.errorOnAutoCreate()) 14585 throw new Error("Attempt to auto-create BenefitBalanceComponent.description"); 14586 else if (Configuration.doAutoCreate()) 14587 this.description = new StringType(); // bb 14588 return this.description; 14589 } 14590 14591 public boolean hasDescriptionElement() { 14592 return this.description != null && !this.description.isEmpty(); 14593 } 14594 14595 public boolean hasDescription() { 14596 return this.description != null && !this.description.isEmpty(); 14597 } 14598 14599 /** 14600 * @param value {@link #description} (A richer description of the benefit or services covered.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 14601 */ 14602 public BenefitBalanceComponent setDescriptionElement(StringType value) { 14603 this.description = value; 14604 return this; 14605 } 14606 14607 /** 14608 * @return A richer description of the benefit or services covered. 14609 */ 14610 public String getDescription() { 14611 return this.description == null ? null : this.description.getValue(); 14612 } 14613 14614 /** 14615 * @param value A richer description of the benefit or services covered. 14616 */ 14617 public BenefitBalanceComponent setDescription(String value) { 14618 if (Utilities.noString(value)) 14619 this.description = null; 14620 else { 14621 if (this.description == null) 14622 this.description = new StringType(); 14623 this.description.setValue(value); 14624 } 14625 return this; 14626 } 14627 14628 /** 14629 * @return {@link #network} (Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.) 14630 */ 14631 public CodeableConcept getNetwork() { 14632 if (this.network == null) 14633 if (Configuration.errorOnAutoCreate()) 14634 throw new Error("Attempt to auto-create BenefitBalanceComponent.network"); 14635 else if (Configuration.doAutoCreate()) 14636 this.network = new CodeableConcept(); // cc 14637 return this.network; 14638 } 14639 14640 public boolean hasNetwork() { 14641 return this.network != null && !this.network.isEmpty(); 14642 } 14643 14644 /** 14645 * @param value {@link #network} (Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.) 14646 */ 14647 public BenefitBalanceComponent setNetwork(CodeableConcept value) { 14648 this.network = value; 14649 return this; 14650 } 14651 14652 /** 14653 * @return {@link #unit} (Indicates if the benefits apply to an individual or to the family.) 14654 */ 14655 public CodeableConcept getUnit() { 14656 if (this.unit == null) 14657 if (Configuration.errorOnAutoCreate()) 14658 throw new Error("Attempt to auto-create BenefitBalanceComponent.unit"); 14659 else if (Configuration.doAutoCreate()) 14660 this.unit = new CodeableConcept(); // cc 14661 return this.unit; 14662 } 14663 14664 public boolean hasUnit() { 14665 return this.unit != null && !this.unit.isEmpty(); 14666 } 14667 14668 /** 14669 * @param value {@link #unit} (Indicates if the benefits apply to an individual or to the family.) 14670 */ 14671 public BenefitBalanceComponent setUnit(CodeableConcept value) { 14672 this.unit = value; 14673 return this; 14674 } 14675 14676 /** 14677 * @return {@link #term} (The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.) 14678 */ 14679 public CodeableConcept getTerm() { 14680 if (this.term == null) 14681 if (Configuration.errorOnAutoCreate()) 14682 throw new Error("Attempt to auto-create BenefitBalanceComponent.term"); 14683 else if (Configuration.doAutoCreate()) 14684 this.term = new CodeableConcept(); // cc 14685 return this.term; 14686 } 14687 14688 public boolean hasTerm() { 14689 return this.term != null && !this.term.isEmpty(); 14690 } 14691 14692 /** 14693 * @param value {@link #term} (The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.) 14694 */ 14695 public BenefitBalanceComponent setTerm(CodeableConcept value) { 14696 this.term = value; 14697 return this; 14698 } 14699 14700 /** 14701 * @return {@link #financial} (Benefits Used to date.) 14702 */ 14703 public List<BenefitComponent> getFinancial() { 14704 if (this.financial == null) 14705 this.financial = new ArrayList<BenefitComponent>(); 14706 return this.financial; 14707 } 14708 14709 /** 14710 * @return Returns a reference to <code>this</code> for easy method chaining 14711 */ 14712 public BenefitBalanceComponent setFinancial(List<BenefitComponent> theFinancial) { 14713 this.financial = theFinancial; 14714 return this; 14715 } 14716 14717 public boolean hasFinancial() { 14718 if (this.financial == null) 14719 return false; 14720 for (BenefitComponent item : this.financial) 14721 if (!item.isEmpty()) 14722 return true; 14723 return false; 14724 } 14725 14726 public BenefitComponent addFinancial() { //3 14727 BenefitComponent t = new BenefitComponent(); 14728 if (this.financial == null) 14729 this.financial = new ArrayList<BenefitComponent>(); 14730 this.financial.add(t); 14731 return t; 14732 } 14733 14734 public BenefitBalanceComponent addFinancial(BenefitComponent t) { //3 14735 if (t == null) 14736 return this; 14737 if (this.financial == null) 14738 this.financial = new ArrayList<BenefitComponent>(); 14739 this.financial.add(t); 14740 return this; 14741 } 14742 14743 /** 14744 * @return The first repetition of repeating field {@link #financial}, creating it if it does not already exist {3} 14745 */ 14746 public BenefitComponent getFinancialFirstRep() { 14747 if (getFinancial().isEmpty()) { 14748 addFinancial(); 14749 } 14750 return getFinancial().get(0); 14751 } 14752 14753 protected void listChildren(List<Property> children) { 14754 super.listChildren(children); 14755 children.add(new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category)); 14756 children.add(new Property("excluded", "boolean", "True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.", 0, 1, excluded)); 14757 children.add(new Property("name", "string", "A short name or tag for the benefit.", 0, 1, name)); 14758 children.add(new Property("description", "string", "A richer description of the benefit or services covered.", 0, 1, description)); 14759 children.add(new Property("network", "CodeableConcept", "Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.", 0, 1, network)); 14760 children.add(new Property("unit", "CodeableConcept", "Indicates if the benefits apply to an individual or to the family.", 0, 1, unit)); 14761 children.add(new Property("term", "CodeableConcept", "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.", 0, 1, term)); 14762 children.add(new Property("financial", "", "Benefits Used to date.", 0, java.lang.Integer.MAX_VALUE, financial)); 14763 } 14764 14765 @Override 14766 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 14767 switch (_hash) { 14768 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code to identify the general type of benefits under which products and services are provided.", 0, 1, category); 14769 case 1994055114: /*excluded*/ return new Property("excluded", "boolean", "True if the indicated class of service is excluded from the plan, missing or False indicates the product or service is included in the coverage.", 0, 1, excluded); 14770 case 3373707: /*name*/ return new Property("name", "string", "A short name or tag for the benefit.", 0, 1, name); 14771 case -1724546052: /*description*/ return new Property("description", "string", "A richer description of the benefit or services covered.", 0, 1, description); 14772 case 1843485230: /*network*/ return new Property("network", "CodeableConcept", "Is a flag to indicate whether the benefits refer to in-network providers or out-of-network providers.", 0, 1, network); 14773 case 3594628: /*unit*/ return new Property("unit", "CodeableConcept", "Indicates if the benefits apply to an individual or to the family.", 0, 1, unit); 14774 case 3556460: /*term*/ return new Property("term", "CodeableConcept", "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual visits'.", 0, 1, term); 14775 case 357555337: /*financial*/ return new Property("financial", "", "Benefits Used to date.", 0, java.lang.Integer.MAX_VALUE, financial); 14776 default: return super.getNamedProperty(_hash, _name, _checkValid); 14777 } 14778 14779 } 14780 14781 @Override 14782 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 14783 switch (hash) { 14784 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 14785 case 1994055114: /*excluded*/ return this.excluded == null ? new Base[0] : new Base[] {this.excluded}; // BooleanType 14786 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 14787 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 14788 case 1843485230: /*network*/ return this.network == null ? new Base[0] : new Base[] {this.network}; // CodeableConcept 14789 case 3594628: /*unit*/ return this.unit == null ? new Base[0] : new Base[] {this.unit}; // CodeableConcept 14790 case 3556460: /*term*/ return this.term == null ? new Base[0] : new Base[] {this.term}; // CodeableConcept 14791 case 357555337: /*financial*/ return this.financial == null ? new Base[0] : this.financial.toArray(new Base[this.financial.size()]); // BenefitComponent 14792 default: return super.getProperty(hash, name, checkValid); 14793 } 14794 14795 } 14796 14797 @Override 14798 public Base setProperty(int hash, String name, Base value) throws FHIRException { 14799 switch (hash) { 14800 case 50511102: // category 14801 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14802 return value; 14803 case 1994055114: // excluded 14804 this.excluded = TypeConvertor.castToBoolean(value); // BooleanType 14805 return value; 14806 case 3373707: // name 14807 this.name = TypeConvertor.castToString(value); // StringType 14808 return value; 14809 case -1724546052: // description 14810 this.description = TypeConvertor.castToString(value); // StringType 14811 return value; 14812 case 1843485230: // network 14813 this.network = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14814 return value; 14815 case 3594628: // unit 14816 this.unit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14817 return value; 14818 case 3556460: // term 14819 this.term = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14820 return value; 14821 case 357555337: // financial 14822 this.getFinancial().add((BenefitComponent) value); // BenefitComponent 14823 return value; 14824 default: return super.setProperty(hash, name, value); 14825 } 14826 14827 } 14828 14829 @Override 14830 public Base setProperty(String name, Base value) throws FHIRException { 14831 if (name.equals("category")) { 14832 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14833 } else if (name.equals("excluded")) { 14834 this.excluded = TypeConvertor.castToBoolean(value); // BooleanType 14835 } else if (name.equals("name")) { 14836 this.name = TypeConvertor.castToString(value); // StringType 14837 } else if (name.equals("description")) { 14838 this.description = TypeConvertor.castToString(value); // StringType 14839 } else if (name.equals("network")) { 14840 this.network = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14841 } else if (name.equals("unit")) { 14842 this.unit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14843 } else if (name.equals("term")) { 14844 this.term = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 14845 } else if (name.equals("financial")) { 14846 this.getFinancial().add((BenefitComponent) value); 14847 } else 14848 return super.setProperty(name, value); 14849 return value; 14850 } 14851 14852 @Override 14853 public void removeChild(String name, Base value) throws FHIRException { 14854 if (name.equals("category")) { 14855 this.category = null; 14856 } else if (name.equals("excluded")) { 14857 this.excluded = null; 14858 } else if (name.equals("name")) { 14859 this.name = null; 14860 } else if (name.equals("description")) { 14861 this.description = null; 14862 } else if (name.equals("network")) { 14863 this.network = null; 14864 } else if (name.equals("unit")) { 14865 this.unit = null; 14866 } else if (name.equals("term")) { 14867 this.term = null; 14868 } else if (name.equals("financial")) { 14869 this.getFinancial().remove((BenefitComponent) value); 14870 } else 14871 super.removeChild(name, value); 14872 14873 } 14874 14875 @Override 14876 public Base makeProperty(int hash, String name) throws FHIRException { 14877 switch (hash) { 14878 case 50511102: return getCategory(); 14879 case 1994055114: return getExcludedElement(); 14880 case 3373707: return getNameElement(); 14881 case -1724546052: return getDescriptionElement(); 14882 case 1843485230: return getNetwork(); 14883 case 3594628: return getUnit(); 14884 case 3556460: return getTerm(); 14885 case 357555337: return addFinancial(); 14886 default: return super.makeProperty(hash, name); 14887 } 14888 14889 } 14890 14891 @Override 14892 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 14893 switch (hash) { 14894 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 14895 case 1994055114: /*excluded*/ return new String[] {"boolean"}; 14896 case 3373707: /*name*/ return new String[] {"string"}; 14897 case -1724546052: /*description*/ return new String[] {"string"}; 14898 case 1843485230: /*network*/ return new String[] {"CodeableConcept"}; 14899 case 3594628: /*unit*/ return new String[] {"CodeableConcept"}; 14900 case 3556460: /*term*/ return new String[] {"CodeableConcept"}; 14901 case 357555337: /*financial*/ return new String[] {}; 14902 default: return super.getTypesForProperty(hash, name); 14903 } 14904 14905 } 14906 14907 @Override 14908 public Base addChild(String name) throws FHIRException { 14909 if (name.equals("category")) { 14910 this.category = new CodeableConcept(); 14911 return this.category; 14912 } 14913 else if (name.equals("excluded")) { 14914 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.benefitBalance.excluded"); 14915 } 14916 else if (name.equals("name")) { 14917 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.benefitBalance.name"); 14918 } 14919 else if (name.equals("description")) { 14920 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.benefitBalance.description"); 14921 } 14922 else if (name.equals("network")) { 14923 this.network = new CodeableConcept(); 14924 return this.network; 14925 } 14926 else if (name.equals("unit")) { 14927 this.unit = new CodeableConcept(); 14928 return this.unit; 14929 } 14930 else if (name.equals("term")) { 14931 this.term = new CodeableConcept(); 14932 return this.term; 14933 } 14934 else if (name.equals("financial")) { 14935 return addFinancial(); 14936 } 14937 else 14938 return super.addChild(name); 14939 } 14940 14941 public BenefitBalanceComponent copy() { 14942 BenefitBalanceComponent dst = new BenefitBalanceComponent(); 14943 copyValues(dst); 14944 return dst; 14945 } 14946 14947 public void copyValues(BenefitBalanceComponent dst) { 14948 super.copyValues(dst); 14949 dst.category = category == null ? null : category.copy(); 14950 dst.excluded = excluded == null ? null : excluded.copy(); 14951 dst.name = name == null ? null : name.copy(); 14952 dst.description = description == null ? null : description.copy(); 14953 dst.network = network == null ? null : network.copy(); 14954 dst.unit = unit == null ? null : unit.copy(); 14955 dst.term = term == null ? null : term.copy(); 14956 if (financial != null) { 14957 dst.financial = new ArrayList<BenefitComponent>(); 14958 for (BenefitComponent i : financial) 14959 dst.financial.add(i.copy()); 14960 }; 14961 } 14962 14963 @Override 14964 public boolean equalsDeep(Base other_) { 14965 if (!super.equalsDeep(other_)) 14966 return false; 14967 if (!(other_ instanceof BenefitBalanceComponent)) 14968 return false; 14969 BenefitBalanceComponent o = (BenefitBalanceComponent) other_; 14970 return compareDeep(category, o.category, true) && compareDeep(excluded, o.excluded, true) && compareDeep(name, o.name, true) 14971 && compareDeep(description, o.description, true) && compareDeep(network, o.network, true) && compareDeep(unit, o.unit, true) 14972 && compareDeep(term, o.term, true) && compareDeep(financial, o.financial, true); 14973 } 14974 14975 @Override 14976 public boolean equalsShallow(Base other_) { 14977 if (!super.equalsShallow(other_)) 14978 return false; 14979 if (!(other_ instanceof BenefitBalanceComponent)) 14980 return false; 14981 BenefitBalanceComponent o = (BenefitBalanceComponent) other_; 14982 return compareValues(excluded, o.excluded, true) && compareValues(name, o.name, true) && compareValues(description, o.description, true) 14983 ; 14984 } 14985 14986 public boolean isEmpty() { 14987 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, excluded, name 14988 , description, network, unit, term, financial); 14989 } 14990 14991 public String fhirType() { 14992 return "ExplanationOfBenefit.benefitBalance"; 14993 14994 } 14995 14996 } 14997 14998 @Block() 14999 public static class BenefitComponent extends BackboneElement implements IBaseBackboneElement { 15000 /** 15001 * Classification of benefit being provided. 15002 */ 15003 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 15004 @Description(shortDefinition="Benefit classification", formalDefinition="Classification of benefit being provided." ) 15005 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-type") 15006 protected CodeableConcept type; 15007 15008 /** 15009 * The quantity of the benefit which is permitted under the coverage. 15010 */ 15011 @Child(name = "allowed", type = {UnsignedIntType.class, StringType.class, Money.class}, order=2, min=0, max=1, modifier=false, summary=false) 15012 @Description(shortDefinition="Benefits allowed", formalDefinition="The quantity of the benefit which is permitted under the coverage." ) 15013 protected DataType allowed; 15014 15015 /** 15016 * The quantity of the benefit which have been consumed to date. 15017 */ 15018 @Child(name = "used", type = {UnsignedIntType.class, Money.class}, order=3, min=0, max=1, modifier=false, summary=false) 15019 @Description(shortDefinition="Benefits used", formalDefinition="The quantity of the benefit which have been consumed to date." ) 15020 protected DataType used; 15021 15022 private static final long serialVersionUID = 1900247614L; 15023 15024 /** 15025 * Constructor 15026 */ 15027 public BenefitComponent() { 15028 super(); 15029 } 15030 15031 /** 15032 * Constructor 15033 */ 15034 public BenefitComponent(CodeableConcept type) { 15035 super(); 15036 this.setType(type); 15037 } 15038 15039 /** 15040 * @return {@link #type} (Classification of benefit being provided.) 15041 */ 15042 public CodeableConcept getType() { 15043 if (this.type == null) 15044 if (Configuration.errorOnAutoCreate()) 15045 throw new Error("Attempt to auto-create BenefitComponent.type"); 15046 else if (Configuration.doAutoCreate()) 15047 this.type = new CodeableConcept(); // cc 15048 return this.type; 15049 } 15050 15051 public boolean hasType() { 15052 return this.type != null && !this.type.isEmpty(); 15053 } 15054 15055 /** 15056 * @param value {@link #type} (Classification of benefit being provided.) 15057 */ 15058 public BenefitComponent setType(CodeableConcept value) { 15059 this.type = value; 15060 return this; 15061 } 15062 15063 /** 15064 * @return {@link #allowed} (The quantity of the benefit which is permitted under the coverage.) 15065 */ 15066 public DataType getAllowed() { 15067 return this.allowed; 15068 } 15069 15070 /** 15071 * @return {@link #allowed} (The quantity of the benefit which is permitted under the coverage.) 15072 */ 15073 public UnsignedIntType getAllowedUnsignedIntType() throws FHIRException { 15074 if (this.allowed == null) 15075 this.allowed = new UnsignedIntType(); 15076 if (!(this.allowed instanceof UnsignedIntType)) 15077 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.allowed.getClass().getName()+" was encountered"); 15078 return (UnsignedIntType) this.allowed; 15079 } 15080 15081 public boolean hasAllowedUnsignedIntType() { 15082 return this.allowed instanceof UnsignedIntType; 15083 } 15084 15085 /** 15086 * @return {@link #allowed} (The quantity of the benefit which is permitted under the coverage.) 15087 */ 15088 public StringType getAllowedStringType() throws FHIRException { 15089 if (this.allowed == null) 15090 this.allowed = new StringType(); 15091 if (!(this.allowed instanceof StringType)) 15092 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.allowed.getClass().getName()+" was encountered"); 15093 return (StringType) this.allowed; 15094 } 15095 15096 public boolean hasAllowedStringType() { 15097 return this.allowed instanceof StringType; 15098 } 15099 15100 /** 15101 * @return {@link #allowed} (The quantity of the benefit which is permitted under the coverage.) 15102 */ 15103 public Money getAllowedMoney() throws FHIRException { 15104 if (this.allowed == null) 15105 this.allowed = new Money(); 15106 if (!(this.allowed instanceof Money)) 15107 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.allowed.getClass().getName()+" was encountered"); 15108 return (Money) this.allowed; 15109 } 15110 15111 public boolean hasAllowedMoney() { 15112 return this.allowed instanceof Money; 15113 } 15114 15115 public boolean hasAllowed() { 15116 return this.allowed != null && !this.allowed.isEmpty(); 15117 } 15118 15119 /** 15120 * @param value {@link #allowed} (The quantity of the benefit which is permitted under the coverage.) 15121 */ 15122 public BenefitComponent setAllowed(DataType value) { 15123 if (value != null && !(value instanceof UnsignedIntType || value instanceof StringType || value instanceof Money)) 15124 throw new FHIRException("Not the right type for ExplanationOfBenefit.benefitBalance.financial.allowed[x]: "+value.fhirType()); 15125 this.allowed = value; 15126 return this; 15127 } 15128 15129 /** 15130 * @return {@link #used} (The quantity of the benefit which have been consumed to date.) 15131 */ 15132 public DataType getUsed() { 15133 return this.used; 15134 } 15135 15136 /** 15137 * @return {@link #used} (The quantity of the benefit which have been consumed to date.) 15138 */ 15139 public UnsignedIntType getUsedUnsignedIntType() throws FHIRException { 15140 if (this.used == null) 15141 this.used = new UnsignedIntType(); 15142 if (!(this.used instanceof UnsignedIntType)) 15143 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.used.getClass().getName()+" was encountered"); 15144 return (UnsignedIntType) this.used; 15145 } 15146 15147 public boolean hasUsedUnsignedIntType() { 15148 return this.used instanceof UnsignedIntType; 15149 } 15150 15151 /** 15152 * @return {@link #used} (The quantity of the benefit which have been consumed to date.) 15153 */ 15154 public Money getUsedMoney() throws FHIRException { 15155 if (this.used == null) 15156 this.used = new Money(); 15157 if (!(this.used instanceof Money)) 15158 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.used.getClass().getName()+" was encountered"); 15159 return (Money) this.used; 15160 } 15161 15162 public boolean hasUsedMoney() { 15163 return this.used instanceof Money; 15164 } 15165 15166 public boolean hasUsed() { 15167 return this.used != null && !this.used.isEmpty(); 15168 } 15169 15170 /** 15171 * @param value {@link #used} (The quantity of the benefit which have been consumed to date.) 15172 */ 15173 public BenefitComponent setUsed(DataType value) { 15174 if (value != null && !(value instanceof UnsignedIntType || value instanceof Money)) 15175 throw new FHIRException("Not the right type for ExplanationOfBenefit.benefitBalance.financial.used[x]: "+value.fhirType()); 15176 this.used = value; 15177 return this; 15178 } 15179 15180 protected void listChildren(List<Property> children) { 15181 super.listChildren(children); 15182 children.add(new Property("type", "CodeableConcept", "Classification of benefit being provided.", 0, 1, type)); 15183 children.add(new Property("allowed[x]", "unsignedInt|string|Money", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed)); 15184 children.add(new Property("used[x]", "unsignedInt|Money", "The quantity of the benefit which have been consumed to date.", 0, 1, used)); 15185 } 15186 15187 @Override 15188 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 15189 switch (_hash) { 15190 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Classification of benefit being provided.", 0, 1, type); 15191 case -1336663592: /*allowed[x]*/ return new Property("allowed[x]", "unsignedInt|string|Money", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 15192 case -911343192: /*allowed*/ return new Property("allowed[x]", "unsignedInt|string|Money", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 15193 case 1668802034: /*allowedUnsignedInt*/ return new Property("allowed[x]", "unsignedInt", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 15194 case -2135265319: /*allowedString*/ return new Property("allowed[x]", "string", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 15195 case -351668232: /*allowedMoney*/ return new Property("allowed[x]", "Money", "The quantity of the benefit which is permitted under the coverage.", 0, 1, allowed); 15196 case -147553373: /*used[x]*/ return new Property("used[x]", "unsignedInt|Money", "The quantity of the benefit which have been consumed to date.", 0, 1, used); 15197 case 3599293: /*used*/ return new Property("used[x]", "unsignedInt|Money", "The quantity of the benefit which have been consumed to date.", 0, 1, used); 15198 case 1252740285: /*usedUnsignedInt*/ return new Property("used[x]", "unsignedInt", "The quantity of the benefit which have been consumed to date.", 0, 1, used); 15199 case -78048509: /*usedMoney*/ return new Property("used[x]", "Money", "The quantity of the benefit which have been consumed to date.", 0, 1, used); 15200 default: return super.getNamedProperty(_hash, _name, _checkValid); 15201 } 15202 15203 } 15204 15205 @Override 15206 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 15207 switch (hash) { 15208 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 15209 case -911343192: /*allowed*/ return this.allowed == null ? new Base[0] : new Base[] {this.allowed}; // DataType 15210 case 3599293: /*used*/ return this.used == null ? new Base[0] : new Base[] {this.used}; // DataType 15211 default: return super.getProperty(hash, name, checkValid); 15212 } 15213 15214 } 15215 15216 @Override 15217 public Base setProperty(int hash, String name, Base value) throws FHIRException { 15218 switch (hash) { 15219 case 3575610: // type 15220 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 15221 return value; 15222 case -911343192: // allowed 15223 this.allowed = TypeConvertor.castToType(value); // DataType 15224 return value; 15225 case 3599293: // used 15226 this.used = TypeConvertor.castToType(value); // DataType 15227 return value; 15228 default: return super.setProperty(hash, name, value); 15229 } 15230 15231 } 15232 15233 @Override 15234 public Base setProperty(String name, Base value) throws FHIRException { 15235 if (name.equals("type")) { 15236 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 15237 } else if (name.equals("allowed[x]")) { 15238 this.allowed = TypeConvertor.castToType(value); // DataType 15239 } else if (name.equals("used[x]")) { 15240 this.used = TypeConvertor.castToType(value); // DataType 15241 } else 15242 return super.setProperty(name, value); 15243 return value; 15244 } 15245 15246 @Override 15247 public void removeChild(String name, Base value) throws FHIRException { 15248 if (name.equals("type")) { 15249 this.type = null; 15250 } else if (name.equals("allowed[x]")) { 15251 this.allowed = null; 15252 } else if (name.equals("used[x]")) { 15253 this.used = null; 15254 } else 15255 super.removeChild(name, value); 15256 15257 } 15258 15259 @Override 15260 public Base makeProperty(int hash, String name) throws FHIRException { 15261 switch (hash) { 15262 case 3575610: return getType(); 15263 case -1336663592: return getAllowed(); 15264 case -911343192: return getAllowed(); 15265 case -147553373: return getUsed(); 15266 case 3599293: return getUsed(); 15267 default: return super.makeProperty(hash, name); 15268 } 15269 15270 } 15271 15272 @Override 15273 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 15274 switch (hash) { 15275 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 15276 case -911343192: /*allowed*/ return new String[] {"unsignedInt", "string", "Money"}; 15277 case 3599293: /*used*/ return new String[] {"unsignedInt", "Money"}; 15278 default: return super.getTypesForProperty(hash, name); 15279 } 15280 15281 } 15282 15283 @Override 15284 public Base addChild(String name) throws FHIRException { 15285 if (name.equals("type")) { 15286 this.type = new CodeableConcept(); 15287 return this.type; 15288 } 15289 else if (name.equals("allowedUnsignedInt")) { 15290 this.allowed = new UnsignedIntType(); 15291 return this.allowed; 15292 } 15293 else if (name.equals("allowedString")) { 15294 this.allowed = new StringType(); 15295 return this.allowed; 15296 } 15297 else if (name.equals("allowedMoney")) { 15298 this.allowed = new Money(); 15299 return this.allowed; 15300 } 15301 else if (name.equals("usedUnsignedInt")) { 15302 this.used = new UnsignedIntType(); 15303 return this.used; 15304 } 15305 else if (name.equals("usedMoney")) { 15306 this.used = new Money(); 15307 return this.used; 15308 } 15309 else 15310 return super.addChild(name); 15311 } 15312 15313 public BenefitComponent copy() { 15314 BenefitComponent dst = new BenefitComponent(); 15315 copyValues(dst); 15316 return dst; 15317 } 15318 15319 public void copyValues(BenefitComponent dst) { 15320 super.copyValues(dst); 15321 dst.type = type == null ? null : type.copy(); 15322 dst.allowed = allowed == null ? null : allowed.copy(); 15323 dst.used = used == null ? null : used.copy(); 15324 } 15325 15326 @Override 15327 public boolean equalsDeep(Base other_) { 15328 if (!super.equalsDeep(other_)) 15329 return false; 15330 if (!(other_ instanceof BenefitComponent)) 15331 return false; 15332 BenefitComponent o = (BenefitComponent) other_; 15333 return compareDeep(type, o.type, true) && compareDeep(allowed, o.allowed, true) && compareDeep(used, o.used, true) 15334 ; 15335 } 15336 15337 @Override 15338 public boolean equalsShallow(Base other_) { 15339 if (!super.equalsShallow(other_)) 15340 return false; 15341 if (!(other_ instanceof BenefitComponent)) 15342 return false; 15343 BenefitComponent o = (BenefitComponent) other_; 15344 return true; 15345 } 15346 15347 public boolean isEmpty() { 15348 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, allowed, used); 15349 } 15350 15351 public String fhirType() { 15352 return "ExplanationOfBenefit.benefitBalance.financial"; 15353 15354 } 15355 15356 } 15357 15358 /** 15359 * A unique identifier assigned to this explanation of benefit. 15360 */ 15361 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15362 @Description(shortDefinition="Business Identifier for the resource", formalDefinition="A unique identifier assigned to this explanation of benefit." ) 15363 protected List<Identifier> identifier; 15364 15365 /** 15366 * Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners. 15367 */ 15368 @Child(name = "traceNumber", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15369 @Description(shortDefinition="Number for tracking", formalDefinition="Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners." ) 15370 protected List<Identifier> traceNumber; 15371 15372 /** 15373 * The status of the resource instance. 15374 */ 15375 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 15376 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 15377 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/explanationofbenefit-status") 15378 protected Enumeration<ExplanationOfBenefitStatus> status; 15379 15380 /** 15381 * The category of claim, e.g. oral, pharmacy, vision, institutional, professional. 15382 */ 15383 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 15384 @Description(shortDefinition="Category or discipline", formalDefinition="The category of claim, e.g. oral, pharmacy, vision, institutional, professional." ) 15385 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-type") 15386 protected CodeableConcept type; 15387 15388 /** 15389 * A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service. 15390 */ 15391 @Child(name = "subType", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 15392 @Description(shortDefinition="More granular claim type", formalDefinition="A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service." ) 15393 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-subtype") 15394 protected CodeableConcept subType; 15395 15396 /** 15397 * A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided. 15398 */ 15399 @Child(name = "use", type = {CodeType.class}, order=5, min=1, max=1, modifier=false, summary=true) 15400 @Description(shortDefinition="claim | preauthorization | predetermination", formalDefinition="A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided." ) 15401 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-use") 15402 protected Enumeration<Use> use; 15403 15404 /** 15405 * The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for forecast reimbursement is sought. 15406 */ 15407 @Child(name = "patient", type = {Patient.class}, order=6, min=1, max=1, modifier=false, summary=true) 15408 @Description(shortDefinition="The recipient of the products and services", formalDefinition="The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for forecast reimbursement is sought." ) 15409 protected Reference patient; 15410 15411 /** 15412 * The period for which charges are being submitted. 15413 */ 15414 @Child(name = "billablePeriod", type = {Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 15415 @Description(shortDefinition="Relevant time frame for the claim", formalDefinition="The period for which charges are being submitted." ) 15416 protected Period billablePeriod; 15417 15418 /** 15419 * The date this resource was created. 15420 */ 15421 @Child(name = "created", type = {DateTimeType.class}, order=8, min=1, max=1, modifier=false, summary=true) 15422 @Description(shortDefinition="Response creation date", formalDefinition="The date this resource was created." ) 15423 protected DateTimeType created; 15424 15425 /** 15426 * Individual who created the claim, predetermination or preauthorization. 15427 */ 15428 @Child(name = "enterer", type = {Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class}, order=9, min=0, max=1, modifier=false, summary=false) 15429 @Description(shortDefinition="Author of the claim", formalDefinition="Individual who created the claim, predetermination or preauthorization." ) 15430 protected Reference enterer; 15431 15432 /** 15433 * The party responsible for authorization, adjudication and reimbursement. 15434 */ 15435 @Child(name = "insurer", type = {Organization.class}, order=10, min=0, max=1, modifier=false, summary=true) 15436 @Description(shortDefinition="Party responsible for reimbursement", formalDefinition="The party responsible for authorization, adjudication and reimbursement." ) 15437 protected Reference insurer; 15438 15439 /** 15440 * The provider which is responsible for the claim, predetermination or preauthorization. 15441 */ 15442 @Child(name = "provider", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=11, min=0, max=1, modifier=false, summary=true) 15443 @Description(shortDefinition="Party responsible for the claim", formalDefinition="The provider which is responsible for the claim, predetermination or preauthorization." ) 15444 protected Reference provider; 15445 15446 /** 15447 * The provider-required urgency of processing the request. Typical values include: stat, normal deferred. 15448 */ 15449 @Child(name = "priority", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=false) 15450 @Description(shortDefinition="Desired processing urgency", formalDefinition="The provider-required urgency of processing the request. Typical values include: stat, normal deferred." ) 15451 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/process-priority") 15452 protected CodeableConcept priority; 15453 15454 /** 15455 * A code to indicate whether and for whom funds are to be reserved for future claims. 15456 */ 15457 @Child(name = "fundsReserveRequested", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=false) 15458 @Description(shortDefinition="For whom to reserve funds", formalDefinition="A code to indicate whether and for whom funds are to be reserved for future claims." ) 15459 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fundsreserve") 15460 protected CodeableConcept fundsReserveRequested; 15461 15462 /** 15463 * A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom. 15464 */ 15465 @Child(name = "fundsReserve", type = {CodeableConcept.class}, order=14, min=0, max=1, modifier=false, summary=false) 15466 @Description(shortDefinition="Funds reserved status", formalDefinition="A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom." ) 15467 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fundsreserve") 15468 protected CodeableConcept fundsReserve; 15469 15470 /** 15471 * Other claims which are related to this claim such as prior submissions or claims for related services or for the same event. 15472 */ 15473 @Child(name = "related", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15474 @Description(shortDefinition="Prior or corollary claims", formalDefinition="Other claims which are related to this claim such as prior submissions or claims for related services or for the same event." ) 15475 protected List<RelatedClaimComponent> related; 15476 15477 /** 15478 * Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments. 15479 */ 15480 @Child(name = "prescription", type = {MedicationRequest.class, VisionPrescription.class}, order=16, min=0, max=1, modifier=false, summary=false) 15481 @Description(shortDefinition="Prescription authorizing services or products", formalDefinition="Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments." ) 15482 protected Reference prescription; 15483 15484 /** 15485 * Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products. 15486 */ 15487 @Child(name = "originalPrescription", type = {MedicationRequest.class}, order=17, min=0, max=1, modifier=false, summary=false) 15488 @Description(shortDefinition="Original prescription if superceded by fulfiller", formalDefinition="Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products." ) 15489 protected Reference originalPrescription; 15490 15491 /** 15492 * Information code for an event with a corresponding date or period. 15493 */ 15494 @Child(name = "event", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15495 @Description(shortDefinition="Event information", formalDefinition="Information code for an event with a corresponding date or period." ) 15496 protected List<ExplanationOfBenefitEventComponent> event; 15497 15498 /** 15499 * The party to be reimbursed for cost of the products and services according to the terms of the policy. 15500 */ 15501 @Child(name = "payee", type = {}, order=19, min=0, max=1, modifier=false, summary=false) 15502 @Description(shortDefinition="Recipient of benefits payable", formalDefinition="The party to be reimbursed for cost of the products and services according to the terms of the policy." ) 15503 protected PayeeComponent payee; 15504 15505 /** 15506 * The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services. 15507 */ 15508 @Child(name = "referral", type = {ServiceRequest.class}, order=20, min=0, max=1, modifier=false, summary=false) 15509 @Description(shortDefinition="Treatment Referral", formalDefinition="The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services." ) 15510 protected Reference referral; 15511 15512 /** 15513 * Healthcare encounters related to this claim. 15514 */ 15515 @Child(name = "encounter", type = {Encounter.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15516 @Description(shortDefinition="Encounters associated with the listed treatments", formalDefinition="Healthcare encounters related to this claim." ) 15517 protected List<Reference> encounter; 15518 15519 /** 15520 * Facility where the services were provided. 15521 */ 15522 @Child(name = "facility", type = {Location.class, Organization.class}, order=22, min=0, max=1, modifier=false, summary=false) 15523 @Description(shortDefinition="Servicing Facility", formalDefinition="Facility where the services were provided." ) 15524 protected Reference facility; 15525 15526 /** 15527 * The business identifier for the instance of the adjudication request: claim predetermination or preauthorization. 15528 */ 15529 @Child(name = "claim", type = {Claim.class}, order=23, min=0, max=1, modifier=false, summary=false) 15530 @Description(shortDefinition="Claim reference", formalDefinition="The business identifier for the instance of the adjudication request: claim predetermination or preauthorization." ) 15531 protected Reference claim; 15532 15533 /** 15534 * The business identifier for the instance of the adjudication response: claim, predetermination or preauthorization response. 15535 */ 15536 @Child(name = "claimResponse", type = {ClaimResponse.class}, order=24, min=0, max=1, modifier=false, summary=false) 15537 @Description(shortDefinition="Claim response reference", formalDefinition="The business identifier for the instance of the adjudication response: claim, predetermination or preauthorization response." ) 15538 protected Reference claimResponse; 15539 15540 /** 15541 * The outcome of the claim, predetermination, or preauthorization processing. 15542 */ 15543 @Child(name = "outcome", type = {CodeType.class}, order=25, min=1, max=1, modifier=false, summary=true) 15544 @Description(shortDefinition="queued | complete | error | partial", formalDefinition="The outcome of the claim, predetermination, or preauthorization processing." ) 15545 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-outcome") 15546 protected Enumeration<ClaimProcessingCodes> outcome; 15547 15548 /** 15549 * The result of the claim, predetermination, or preauthorization adjudication. 15550 */ 15551 @Child(name = "decision", type = {CodeableConcept.class}, order=26, min=0, max=1, modifier=false, summary=true) 15552 @Description(shortDefinition="Result of the adjudication", formalDefinition="The result of the claim, predetermination, or preauthorization adjudication." ) 15553 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-decision") 15554 protected CodeableConcept decision; 15555 15556 /** 15557 * A human readable description of the status of the adjudication. 15558 */ 15559 @Child(name = "disposition", type = {StringType.class}, order=27, min=0, max=1, modifier=false, summary=false) 15560 @Description(shortDefinition="Disposition Message", formalDefinition="A human readable description of the status of the adjudication." ) 15561 protected StringType disposition; 15562 15563 /** 15564 * Reference from the Insurer which is used in later communications which refers to this adjudication. 15565 */ 15566 @Child(name = "preAuthRef", type = {StringType.class}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15567 @Description(shortDefinition="Preauthorization reference", formalDefinition="Reference from the Insurer which is used in later communications which refers to this adjudication." ) 15568 protected List<StringType> preAuthRef; 15569 15570 /** 15571 * The timeframe during which the supplied preauthorization reference may be quoted on claims to obtain the adjudication as provided. 15572 */ 15573 @Child(name = "preAuthRefPeriod", type = {Period.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15574 @Description(shortDefinition="Preauthorization in-effect period", formalDefinition="The timeframe during which the supplied preauthorization reference may be quoted on claims to obtain the adjudication as provided." ) 15575 protected List<Period> preAuthRefPeriod; 15576 15577 /** 15578 * A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system. 15579 */ 15580 @Child(name = "diagnosisRelatedGroup", type = {CodeableConcept.class}, order=30, min=0, max=1, modifier=false, summary=false) 15581 @Description(shortDefinition="Package billing code", formalDefinition="A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system." ) 15582 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosisrelatedgroup") 15583 protected CodeableConcept diagnosisRelatedGroup; 15584 15585 /** 15586 * The members of the team who provided the products and services. 15587 */ 15588 @Child(name = "careTeam", type = {}, order=31, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15589 @Description(shortDefinition="Care Team members", formalDefinition="The members of the team who provided the products and services." ) 15590 protected List<CareTeamComponent> careTeam; 15591 15592 /** 15593 * Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. 15594 */ 15595 @Child(name = "supportingInfo", type = {}, order=32, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15596 @Description(shortDefinition="Supporting information", formalDefinition="Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues." ) 15597 protected List<SupportingInformationComponent> supportingInfo; 15598 15599 /** 15600 * Information about diagnoses relevant to the claim items. 15601 */ 15602 @Child(name = "diagnosis", type = {}, order=33, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15603 @Description(shortDefinition="Pertinent diagnosis information", formalDefinition="Information about diagnoses relevant to the claim items." ) 15604 protected List<DiagnosisComponent> diagnosis; 15605 15606 /** 15607 * Procedures performed on the patient relevant to the billing items with the claim. 15608 */ 15609 @Child(name = "procedure", type = {}, order=34, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15610 @Description(shortDefinition="Clinical procedures performed", formalDefinition="Procedures performed on the patient relevant to the billing items with the claim." ) 15611 protected List<ProcedureComponent> procedure; 15612 15613 /** 15614 * This indicates the relative order of a series of EOBs related to different coverages for the same suite of services. 15615 */ 15616 @Child(name = "precedence", type = {PositiveIntType.class}, order=35, min=0, max=1, modifier=false, summary=false) 15617 @Description(shortDefinition="Precedence (primary, secondary, etc.)", formalDefinition="This indicates the relative order of a series of EOBs related to different coverages for the same suite of services." ) 15618 protected PositiveIntType precedence; 15619 15620 /** 15621 * Financial instruments for reimbursement for the health care products and services specified on the claim. 15622 */ 15623 @Child(name = "insurance", type = {}, order=36, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 15624 @Description(shortDefinition="Patient insurance information", formalDefinition="Financial instruments for reimbursement for the health care products and services specified on the claim." ) 15625 protected List<InsuranceComponent> insurance; 15626 15627 /** 15628 * Details of a accident which resulted in injuries which required the products and services listed in the claim. 15629 */ 15630 @Child(name = "accident", type = {}, order=37, min=0, max=1, modifier=false, summary=false) 15631 @Description(shortDefinition="Details of the event", formalDefinition="Details of a accident which resulted in injuries which required the products and services listed in the claim." ) 15632 protected AccidentComponent accident; 15633 15634 /** 15635 * The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services. 15636 */ 15637 @Child(name = "patientPaid", type = {Money.class}, order=38, min=0, max=1, modifier=false, summary=false) 15638 @Description(shortDefinition="Paid by the patient", formalDefinition="The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services." ) 15639 protected Money patientPaid; 15640 15641 /** 15642 * A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details. 15643 */ 15644 @Child(name = "item", type = {}, order=39, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15645 @Description(shortDefinition="Product or service provided", formalDefinition="A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details." ) 15646 protected List<ItemComponent> item; 15647 15648 /** 15649 * The first-tier service adjudications for payor added product or service lines. 15650 */ 15651 @Child(name = "addItem", type = {}, order=40, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15652 @Description(shortDefinition="Insurer added line items", formalDefinition="The first-tier service adjudications for payor added product or service lines." ) 15653 protected List<AddedItemComponent> addItem; 15654 15655 /** 15656 * The adjudication results which are presented at the header level rather than at the line-item or add-item levels. 15657 */ 15658 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=41, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15659 @Description(shortDefinition="Header-level adjudication", formalDefinition="The adjudication results which are presented at the header level rather than at the line-item or add-item levels." ) 15660 protected List<AdjudicationComponent> adjudication; 15661 15662 /** 15663 * Categorized monetary totals for the adjudication. 15664 */ 15665 @Child(name = "total", type = {}, order=42, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 15666 @Description(shortDefinition="Adjudication totals", formalDefinition="Categorized monetary totals for the adjudication." ) 15667 protected List<TotalComponent> total; 15668 15669 /** 15670 * Payment details for the adjudication of the claim. 15671 */ 15672 @Child(name = "payment", type = {}, order=43, min=0, max=1, modifier=false, summary=false) 15673 @Description(shortDefinition="Payment Details", formalDefinition="Payment details for the adjudication of the claim." ) 15674 protected PaymentComponent payment; 15675 15676 /** 15677 * A code for the form to be used for printing the content. 15678 */ 15679 @Child(name = "formCode", type = {CodeableConcept.class}, order=44, min=0, max=1, modifier=false, summary=false) 15680 @Description(shortDefinition="Printed form identifier", formalDefinition="A code for the form to be used for printing the content." ) 15681 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/forms") 15682 protected CodeableConcept formCode; 15683 15684 /** 15685 * The actual form, by reference or inclusion, for printing the content or an EOB. 15686 */ 15687 @Child(name = "form", type = {Attachment.class}, order=45, min=0, max=1, modifier=false, summary=false) 15688 @Description(shortDefinition="Printed reference or actual form", formalDefinition="The actual form, by reference or inclusion, for printing the content or an EOB." ) 15689 protected Attachment form; 15690 15691 /** 15692 * A note that describes or explains adjudication results in a human readable form. 15693 */ 15694 @Child(name = "processNote", type = {}, order=46, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15695 @Description(shortDefinition="Note concerning adjudication", formalDefinition="A note that describes or explains adjudication results in a human readable form." ) 15696 protected List<NoteComponent> processNote; 15697 15698 /** 15699 * The term of the benefits documented in this response. 15700 */ 15701 @Child(name = "benefitPeriod", type = {Period.class}, order=47, min=0, max=1, modifier=false, summary=false) 15702 @Description(shortDefinition="When the benefits are applicable", formalDefinition="The term of the benefits documented in this response." ) 15703 protected Period benefitPeriod; 15704 15705 /** 15706 * Balance by Benefit Category. 15707 */ 15708 @Child(name = "benefitBalance", type = {}, order=48, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 15709 @Description(shortDefinition="Balance by Benefit Category", formalDefinition="Balance by Benefit Category." ) 15710 protected List<BenefitBalanceComponent> benefitBalance; 15711 15712 private static final long serialVersionUID = 106661060L; 15713 15714 /** 15715 * Constructor 15716 */ 15717 public ExplanationOfBenefit() { 15718 super(); 15719 } 15720 15721 /** 15722 * Constructor 15723 */ 15724 public ExplanationOfBenefit(ExplanationOfBenefitStatus status, CodeableConcept type, Use use, Reference patient, Date created, ClaimProcessingCodes outcome) { 15725 super(); 15726 this.setStatus(status); 15727 this.setType(type); 15728 this.setUse(use); 15729 this.setPatient(patient); 15730 this.setCreated(created); 15731 this.setOutcome(outcome); 15732 } 15733 15734 /** 15735 * @return {@link #identifier} (A unique identifier assigned to this explanation of benefit.) 15736 */ 15737 public List<Identifier> getIdentifier() { 15738 if (this.identifier == null) 15739 this.identifier = new ArrayList<Identifier>(); 15740 return this.identifier; 15741 } 15742 15743 /** 15744 * @return Returns a reference to <code>this</code> for easy method chaining 15745 */ 15746 public ExplanationOfBenefit setIdentifier(List<Identifier> theIdentifier) { 15747 this.identifier = theIdentifier; 15748 return this; 15749 } 15750 15751 public boolean hasIdentifier() { 15752 if (this.identifier == null) 15753 return false; 15754 for (Identifier item : this.identifier) 15755 if (!item.isEmpty()) 15756 return true; 15757 return false; 15758 } 15759 15760 public Identifier addIdentifier() { //3 15761 Identifier t = new Identifier(); 15762 if (this.identifier == null) 15763 this.identifier = new ArrayList<Identifier>(); 15764 this.identifier.add(t); 15765 return t; 15766 } 15767 15768 public ExplanationOfBenefit addIdentifier(Identifier t) { //3 15769 if (t == null) 15770 return this; 15771 if (this.identifier == null) 15772 this.identifier = new ArrayList<Identifier>(); 15773 this.identifier.add(t); 15774 return this; 15775 } 15776 15777 /** 15778 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 15779 */ 15780 public Identifier getIdentifierFirstRep() { 15781 if (getIdentifier().isEmpty()) { 15782 addIdentifier(); 15783 } 15784 return getIdentifier().get(0); 15785 } 15786 15787 /** 15788 * @return {@link #traceNumber} (Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.) 15789 */ 15790 public List<Identifier> getTraceNumber() { 15791 if (this.traceNumber == null) 15792 this.traceNumber = new ArrayList<Identifier>(); 15793 return this.traceNumber; 15794 } 15795 15796 /** 15797 * @return Returns a reference to <code>this</code> for easy method chaining 15798 */ 15799 public ExplanationOfBenefit setTraceNumber(List<Identifier> theTraceNumber) { 15800 this.traceNumber = theTraceNumber; 15801 return this; 15802 } 15803 15804 public boolean hasTraceNumber() { 15805 if (this.traceNumber == null) 15806 return false; 15807 for (Identifier item : this.traceNumber) 15808 if (!item.isEmpty()) 15809 return true; 15810 return false; 15811 } 15812 15813 public Identifier addTraceNumber() { //3 15814 Identifier t = new Identifier(); 15815 if (this.traceNumber == null) 15816 this.traceNumber = new ArrayList<Identifier>(); 15817 this.traceNumber.add(t); 15818 return t; 15819 } 15820 15821 public ExplanationOfBenefit addTraceNumber(Identifier t) { //3 15822 if (t == null) 15823 return this; 15824 if (this.traceNumber == null) 15825 this.traceNumber = new ArrayList<Identifier>(); 15826 this.traceNumber.add(t); 15827 return this; 15828 } 15829 15830 /** 15831 * @return The first repetition of repeating field {@link #traceNumber}, creating it if it does not already exist {3} 15832 */ 15833 public Identifier getTraceNumberFirstRep() { 15834 if (getTraceNumber().isEmpty()) { 15835 addTraceNumber(); 15836 } 15837 return getTraceNumber().get(0); 15838 } 15839 15840 /** 15841 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 15842 */ 15843 public Enumeration<ExplanationOfBenefitStatus> getStatusElement() { 15844 if (this.status == null) 15845 if (Configuration.errorOnAutoCreate()) 15846 throw new Error("Attempt to auto-create ExplanationOfBenefit.status"); 15847 else if (Configuration.doAutoCreate()) 15848 this.status = new Enumeration<ExplanationOfBenefitStatus>(new ExplanationOfBenefitStatusEnumFactory()); // bb 15849 return this.status; 15850 } 15851 15852 public boolean hasStatusElement() { 15853 return this.status != null && !this.status.isEmpty(); 15854 } 15855 15856 public boolean hasStatus() { 15857 return this.status != null && !this.status.isEmpty(); 15858 } 15859 15860 /** 15861 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 15862 */ 15863 public ExplanationOfBenefit setStatusElement(Enumeration<ExplanationOfBenefitStatus> value) { 15864 this.status = value; 15865 return this; 15866 } 15867 15868 /** 15869 * @return The status of the resource instance. 15870 */ 15871 public ExplanationOfBenefitStatus getStatus() { 15872 return this.status == null ? null : this.status.getValue(); 15873 } 15874 15875 /** 15876 * @param value The status of the resource instance. 15877 */ 15878 public ExplanationOfBenefit setStatus(ExplanationOfBenefitStatus value) { 15879 if (this.status == null) 15880 this.status = new Enumeration<ExplanationOfBenefitStatus>(new ExplanationOfBenefitStatusEnumFactory()); 15881 this.status.setValue(value); 15882 return this; 15883 } 15884 15885 /** 15886 * @return {@link #type} (The category of claim, e.g. oral, pharmacy, vision, institutional, professional.) 15887 */ 15888 public CodeableConcept getType() { 15889 if (this.type == null) 15890 if (Configuration.errorOnAutoCreate()) 15891 throw new Error("Attempt to auto-create ExplanationOfBenefit.type"); 15892 else if (Configuration.doAutoCreate()) 15893 this.type = new CodeableConcept(); // cc 15894 return this.type; 15895 } 15896 15897 public boolean hasType() { 15898 return this.type != null && !this.type.isEmpty(); 15899 } 15900 15901 /** 15902 * @param value {@link #type} (The category of claim, e.g. oral, pharmacy, vision, institutional, professional.) 15903 */ 15904 public ExplanationOfBenefit setType(CodeableConcept value) { 15905 this.type = value; 15906 return this; 15907 } 15908 15909 /** 15910 * @return {@link #subType} (A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.) 15911 */ 15912 public CodeableConcept getSubType() { 15913 if (this.subType == null) 15914 if (Configuration.errorOnAutoCreate()) 15915 throw new Error("Attempt to auto-create ExplanationOfBenefit.subType"); 15916 else if (Configuration.doAutoCreate()) 15917 this.subType = new CodeableConcept(); // cc 15918 return this.subType; 15919 } 15920 15921 public boolean hasSubType() { 15922 return this.subType != null && !this.subType.isEmpty(); 15923 } 15924 15925 /** 15926 * @param value {@link #subType} (A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.) 15927 */ 15928 public ExplanationOfBenefit setSubType(CodeableConcept value) { 15929 this.subType = value; 15930 return this; 15931 } 15932 15933 /** 15934 * @return {@link #use} (A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 15935 */ 15936 public Enumeration<Use> getUseElement() { 15937 if (this.use == null) 15938 if (Configuration.errorOnAutoCreate()) 15939 throw new Error("Attempt to auto-create ExplanationOfBenefit.use"); 15940 else if (Configuration.doAutoCreate()) 15941 this.use = new Enumeration<Use>(new UseEnumFactory()); // bb 15942 return this.use; 15943 } 15944 15945 public boolean hasUseElement() { 15946 return this.use != null && !this.use.isEmpty(); 15947 } 15948 15949 public boolean hasUse() { 15950 return this.use != null && !this.use.isEmpty(); 15951 } 15952 15953 /** 15954 * @param value {@link #use} (A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 15955 */ 15956 public ExplanationOfBenefit setUseElement(Enumeration<Use> value) { 15957 this.use = value; 15958 return this; 15959 } 15960 15961 /** 15962 * @return A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided. 15963 */ 15964 public Use getUse() { 15965 return this.use == null ? null : this.use.getValue(); 15966 } 15967 15968 /** 15969 * @param value A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided. 15970 */ 15971 public ExplanationOfBenefit setUse(Use value) { 15972 if (this.use == null) 15973 this.use = new Enumeration<Use>(new UseEnumFactory()); 15974 this.use.setValue(value); 15975 return this; 15976 } 15977 15978 /** 15979 * @return {@link #patient} (The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for forecast reimbursement is sought.) 15980 */ 15981 public Reference getPatient() { 15982 if (this.patient == null) 15983 if (Configuration.errorOnAutoCreate()) 15984 throw new Error("Attempt to auto-create ExplanationOfBenefit.patient"); 15985 else if (Configuration.doAutoCreate()) 15986 this.patient = new Reference(); // cc 15987 return this.patient; 15988 } 15989 15990 public boolean hasPatient() { 15991 return this.patient != null && !this.patient.isEmpty(); 15992 } 15993 15994 /** 15995 * @param value {@link #patient} (The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for forecast reimbursement is sought.) 15996 */ 15997 public ExplanationOfBenefit setPatient(Reference value) { 15998 this.patient = value; 15999 return this; 16000 } 16001 16002 /** 16003 * @return {@link #billablePeriod} (The period for which charges are being submitted.) 16004 */ 16005 public Period getBillablePeriod() { 16006 if (this.billablePeriod == null) 16007 if (Configuration.errorOnAutoCreate()) 16008 throw new Error("Attempt to auto-create ExplanationOfBenefit.billablePeriod"); 16009 else if (Configuration.doAutoCreate()) 16010 this.billablePeriod = new Period(); // cc 16011 return this.billablePeriod; 16012 } 16013 16014 public boolean hasBillablePeriod() { 16015 return this.billablePeriod != null && !this.billablePeriod.isEmpty(); 16016 } 16017 16018 /** 16019 * @param value {@link #billablePeriod} (The period for which charges are being submitted.) 16020 */ 16021 public ExplanationOfBenefit setBillablePeriod(Period value) { 16022 this.billablePeriod = value; 16023 return this; 16024 } 16025 16026 /** 16027 * @return {@link #created} (The date this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 16028 */ 16029 public DateTimeType getCreatedElement() { 16030 if (this.created == null) 16031 if (Configuration.errorOnAutoCreate()) 16032 throw new Error("Attempt to auto-create ExplanationOfBenefit.created"); 16033 else if (Configuration.doAutoCreate()) 16034 this.created = new DateTimeType(); // bb 16035 return this.created; 16036 } 16037 16038 public boolean hasCreatedElement() { 16039 return this.created != null && !this.created.isEmpty(); 16040 } 16041 16042 public boolean hasCreated() { 16043 return this.created != null && !this.created.isEmpty(); 16044 } 16045 16046 /** 16047 * @param value {@link #created} (The date this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 16048 */ 16049 public ExplanationOfBenefit setCreatedElement(DateTimeType value) { 16050 this.created = value; 16051 return this; 16052 } 16053 16054 /** 16055 * @return The date this resource was created. 16056 */ 16057 public Date getCreated() { 16058 return this.created == null ? null : this.created.getValue(); 16059 } 16060 16061 /** 16062 * @param value The date this resource was created. 16063 */ 16064 public ExplanationOfBenefit setCreated(Date value) { 16065 if (this.created == null) 16066 this.created = new DateTimeType(); 16067 this.created.setValue(value); 16068 return this; 16069 } 16070 16071 /** 16072 * @return {@link #enterer} (Individual who created the claim, predetermination or preauthorization.) 16073 */ 16074 public Reference getEnterer() { 16075 if (this.enterer == null) 16076 if (Configuration.errorOnAutoCreate()) 16077 throw new Error("Attempt to auto-create ExplanationOfBenefit.enterer"); 16078 else if (Configuration.doAutoCreate()) 16079 this.enterer = new Reference(); // cc 16080 return this.enterer; 16081 } 16082 16083 public boolean hasEnterer() { 16084 return this.enterer != null && !this.enterer.isEmpty(); 16085 } 16086 16087 /** 16088 * @param value {@link #enterer} (Individual who created the claim, predetermination or preauthorization.) 16089 */ 16090 public ExplanationOfBenefit setEnterer(Reference value) { 16091 this.enterer = value; 16092 return this; 16093 } 16094 16095 /** 16096 * @return {@link #insurer} (The party responsible for authorization, adjudication and reimbursement.) 16097 */ 16098 public Reference getInsurer() { 16099 if (this.insurer == null) 16100 if (Configuration.errorOnAutoCreate()) 16101 throw new Error("Attempt to auto-create ExplanationOfBenefit.insurer"); 16102 else if (Configuration.doAutoCreate()) 16103 this.insurer = new Reference(); // cc 16104 return this.insurer; 16105 } 16106 16107 public boolean hasInsurer() { 16108 return this.insurer != null && !this.insurer.isEmpty(); 16109 } 16110 16111 /** 16112 * @param value {@link #insurer} (The party responsible for authorization, adjudication and reimbursement.) 16113 */ 16114 public ExplanationOfBenefit setInsurer(Reference value) { 16115 this.insurer = value; 16116 return this; 16117 } 16118 16119 /** 16120 * @return {@link #provider} (The provider which is responsible for the claim, predetermination or preauthorization.) 16121 */ 16122 public Reference getProvider() { 16123 if (this.provider == null) 16124 if (Configuration.errorOnAutoCreate()) 16125 throw new Error("Attempt to auto-create ExplanationOfBenefit.provider"); 16126 else if (Configuration.doAutoCreate()) 16127 this.provider = new Reference(); // cc 16128 return this.provider; 16129 } 16130 16131 public boolean hasProvider() { 16132 return this.provider != null && !this.provider.isEmpty(); 16133 } 16134 16135 /** 16136 * @param value {@link #provider} (The provider which is responsible for the claim, predetermination or preauthorization.) 16137 */ 16138 public ExplanationOfBenefit setProvider(Reference value) { 16139 this.provider = value; 16140 return this; 16141 } 16142 16143 /** 16144 * @return {@link #priority} (The provider-required urgency of processing the request. Typical values include: stat, normal deferred.) 16145 */ 16146 public CodeableConcept getPriority() { 16147 if (this.priority == null) 16148 if (Configuration.errorOnAutoCreate()) 16149 throw new Error("Attempt to auto-create ExplanationOfBenefit.priority"); 16150 else if (Configuration.doAutoCreate()) 16151 this.priority = new CodeableConcept(); // cc 16152 return this.priority; 16153 } 16154 16155 public boolean hasPriority() { 16156 return this.priority != null && !this.priority.isEmpty(); 16157 } 16158 16159 /** 16160 * @param value {@link #priority} (The provider-required urgency of processing the request. Typical values include: stat, normal deferred.) 16161 */ 16162 public ExplanationOfBenefit setPriority(CodeableConcept value) { 16163 this.priority = value; 16164 return this; 16165 } 16166 16167 /** 16168 * @return {@link #fundsReserveRequested} (A code to indicate whether and for whom funds are to be reserved for future claims.) 16169 */ 16170 public CodeableConcept getFundsReserveRequested() { 16171 if (this.fundsReserveRequested == null) 16172 if (Configuration.errorOnAutoCreate()) 16173 throw new Error("Attempt to auto-create ExplanationOfBenefit.fundsReserveRequested"); 16174 else if (Configuration.doAutoCreate()) 16175 this.fundsReserveRequested = new CodeableConcept(); // cc 16176 return this.fundsReserveRequested; 16177 } 16178 16179 public boolean hasFundsReserveRequested() { 16180 return this.fundsReserveRequested != null && !this.fundsReserveRequested.isEmpty(); 16181 } 16182 16183 /** 16184 * @param value {@link #fundsReserveRequested} (A code to indicate whether and for whom funds are to be reserved for future claims.) 16185 */ 16186 public ExplanationOfBenefit setFundsReserveRequested(CodeableConcept value) { 16187 this.fundsReserveRequested = value; 16188 return this; 16189 } 16190 16191 /** 16192 * @return {@link #fundsReserve} (A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.) 16193 */ 16194 public CodeableConcept getFundsReserve() { 16195 if (this.fundsReserve == null) 16196 if (Configuration.errorOnAutoCreate()) 16197 throw new Error("Attempt to auto-create ExplanationOfBenefit.fundsReserve"); 16198 else if (Configuration.doAutoCreate()) 16199 this.fundsReserve = new CodeableConcept(); // cc 16200 return this.fundsReserve; 16201 } 16202 16203 public boolean hasFundsReserve() { 16204 return this.fundsReserve != null && !this.fundsReserve.isEmpty(); 16205 } 16206 16207 /** 16208 * @param value {@link #fundsReserve} (A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.) 16209 */ 16210 public ExplanationOfBenefit setFundsReserve(CodeableConcept value) { 16211 this.fundsReserve = value; 16212 return this; 16213 } 16214 16215 /** 16216 * @return {@link #related} (Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.) 16217 */ 16218 public List<RelatedClaimComponent> getRelated() { 16219 if (this.related == null) 16220 this.related = new ArrayList<RelatedClaimComponent>(); 16221 return this.related; 16222 } 16223 16224 /** 16225 * @return Returns a reference to <code>this</code> for easy method chaining 16226 */ 16227 public ExplanationOfBenefit setRelated(List<RelatedClaimComponent> theRelated) { 16228 this.related = theRelated; 16229 return this; 16230 } 16231 16232 public boolean hasRelated() { 16233 if (this.related == null) 16234 return false; 16235 for (RelatedClaimComponent item : this.related) 16236 if (!item.isEmpty()) 16237 return true; 16238 return false; 16239 } 16240 16241 public RelatedClaimComponent addRelated() { //3 16242 RelatedClaimComponent t = new RelatedClaimComponent(); 16243 if (this.related == null) 16244 this.related = new ArrayList<RelatedClaimComponent>(); 16245 this.related.add(t); 16246 return t; 16247 } 16248 16249 public ExplanationOfBenefit addRelated(RelatedClaimComponent t) { //3 16250 if (t == null) 16251 return this; 16252 if (this.related == null) 16253 this.related = new ArrayList<RelatedClaimComponent>(); 16254 this.related.add(t); 16255 return this; 16256 } 16257 16258 /** 16259 * @return The first repetition of repeating field {@link #related}, creating it if it does not already exist {3} 16260 */ 16261 public RelatedClaimComponent getRelatedFirstRep() { 16262 if (getRelated().isEmpty()) { 16263 addRelated(); 16264 } 16265 return getRelated().get(0); 16266 } 16267 16268 /** 16269 * @return {@link #prescription} (Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments.) 16270 */ 16271 public Reference getPrescription() { 16272 if (this.prescription == null) 16273 if (Configuration.errorOnAutoCreate()) 16274 throw new Error("Attempt to auto-create ExplanationOfBenefit.prescription"); 16275 else if (Configuration.doAutoCreate()) 16276 this.prescription = new Reference(); // cc 16277 return this.prescription; 16278 } 16279 16280 public boolean hasPrescription() { 16281 return this.prescription != null && !this.prescription.isEmpty(); 16282 } 16283 16284 /** 16285 * @param value {@link #prescription} (Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments.) 16286 */ 16287 public ExplanationOfBenefit setPrescription(Reference value) { 16288 this.prescription = value; 16289 return this; 16290 } 16291 16292 /** 16293 * @return {@link #originalPrescription} (Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.) 16294 */ 16295 public Reference getOriginalPrescription() { 16296 if (this.originalPrescription == null) 16297 if (Configuration.errorOnAutoCreate()) 16298 throw new Error("Attempt to auto-create ExplanationOfBenefit.originalPrescription"); 16299 else if (Configuration.doAutoCreate()) 16300 this.originalPrescription = new Reference(); // cc 16301 return this.originalPrescription; 16302 } 16303 16304 public boolean hasOriginalPrescription() { 16305 return this.originalPrescription != null && !this.originalPrescription.isEmpty(); 16306 } 16307 16308 /** 16309 * @param value {@link #originalPrescription} (Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.) 16310 */ 16311 public ExplanationOfBenefit setOriginalPrescription(Reference value) { 16312 this.originalPrescription = value; 16313 return this; 16314 } 16315 16316 /** 16317 * @return {@link #event} (Information code for an event with a corresponding date or period.) 16318 */ 16319 public List<ExplanationOfBenefitEventComponent> getEvent() { 16320 if (this.event == null) 16321 this.event = new ArrayList<ExplanationOfBenefitEventComponent>(); 16322 return this.event; 16323 } 16324 16325 /** 16326 * @return Returns a reference to <code>this</code> for easy method chaining 16327 */ 16328 public ExplanationOfBenefit setEvent(List<ExplanationOfBenefitEventComponent> theEvent) { 16329 this.event = theEvent; 16330 return this; 16331 } 16332 16333 public boolean hasEvent() { 16334 if (this.event == null) 16335 return false; 16336 for (ExplanationOfBenefitEventComponent item : this.event) 16337 if (!item.isEmpty()) 16338 return true; 16339 return false; 16340 } 16341 16342 public ExplanationOfBenefitEventComponent addEvent() { //3 16343 ExplanationOfBenefitEventComponent t = new ExplanationOfBenefitEventComponent(); 16344 if (this.event == null) 16345 this.event = new ArrayList<ExplanationOfBenefitEventComponent>(); 16346 this.event.add(t); 16347 return t; 16348 } 16349 16350 public ExplanationOfBenefit addEvent(ExplanationOfBenefitEventComponent t) { //3 16351 if (t == null) 16352 return this; 16353 if (this.event == null) 16354 this.event = new ArrayList<ExplanationOfBenefitEventComponent>(); 16355 this.event.add(t); 16356 return this; 16357 } 16358 16359 /** 16360 * @return The first repetition of repeating field {@link #event}, creating it if it does not already exist {3} 16361 */ 16362 public ExplanationOfBenefitEventComponent getEventFirstRep() { 16363 if (getEvent().isEmpty()) { 16364 addEvent(); 16365 } 16366 return getEvent().get(0); 16367 } 16368 16369 /** 16370 * @return {@link #payee} (The party to be reimbursed for cost of the products and services according to the terms of the policy.) 16371 */ 16372 public PayeeComponent getPayee() { 16373 if (this.payee == null) 16374 if (Configuration.errorOnAutoCreate()) 16375 throw new Error("Attempt to auto-create ExplanationOfBenefit.payee"); 16376 else if (Configuration.doAutoCreate()) 16377 this.payee = new PayeeComponent(); // cc 16378 return this.payee; 16379 } 16380 16381 public boolean hasPayee() { 16382 return this.payee != null && !this.payee.isEmpty(); 16383 } 16384 16385 /** 16386 * @param value {@link #payee} (The party to be reimbursed for cost of the products and services according to the terms of the policy.) 16387 */ 16388 public ExplanationOfBenefit setPayee(PayeeComponent value) { 16389 this.payee = value; 16390 return this; 16391 } 16392 16393 /** 16394 * @return {@link #referral} (The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services.) 16395 */ 16396 public Reference getReferral() { 16397 if (this.referral == null) 16398 if (Configuration.errorOnAutoCreate()) 16399 throw new Error("Attempt to auto-create ExplanationOfBenefit.referral"); 16400 else if (Configuration.doAutoCreate()) 16401 this.referral = new Reference(); // cc 16402 return this.referral; 16403 } 16404 16405 public boolean hasReferral() { 16406 return this.referral != null && !this.referral.isEmpty(); 16407 } 16408 16409 /** 16410 * @param value {@link #referral} (The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services.) 16411 */ 16412 public ExplanationOfBenefit setReferral(Reference value) { 16413 this.referral = value; 16414 return this; 16415 } 16416 16417 /** 16418 * @return {@link #encounter} (Healthcare encounters related to this claim.) 16419 */ 16420 public List<Reference> getEncounter() { 16421 if (this.encounter == null) 16422 this.encounter = new ArrayList<Reference>(); 16423 return this.encounter; 16424 } 16425 16426 /** 16427 * @return Returns a reference to <code>this</code> for easy method chaining 16428 */ 16429 public ExplanationOfBenefit setEncounter(List<Reference> theEncounter) { 16430 this.encounter = theEncounter; 16431 return this; 16432 } 16433 16434 public boolean hasEncounter() { 16435 if (this.encounter == null) 16436 return false; 16437 for (Reference item : this.encounter) 16438 if (!item.isEmpty()) 16439 return true; 16440 return false; 16441 } 16442 16443 public Reference addEncounter() { //3 16444 Reference t = new Reference(); 16445 if (this.encounter == null) 16446 this.encounter = new ArrayList<Reference>(); 16447 this.encounter.add(t); 16448 return t; 16449 } 16450 16451 public ExplanationOfBenefit addEncounter(Reference t) { //3 16452 if (t == null) 16453 return this; 16454 if (this.encounter == null) 16455 this.encounter = new ArrayList<Reference>(); 16456 this.encounter.add(t); 16457 return this; 16458 } 16459 16460 /** 16461 * @return The first repetition of repeating field {@link #encounter}, creating it if it does not already exist {3} 16462 */ 16463 public Reference getEncounterFirstRep() { 16464 if (getEncounter().isEmpty()) { 16465 addEncounter(); 16466 } 16467 return getEncounter().get(0); 16468 } 16469 16470 /** 16471 * @return {@link #facility} (Facility where the services were provided.) 16472 */ 16473 public Reference getFacility() { 16474 if (this.facility == null) 16475 if (Configuration.errorOnAutoCreate()) 16476 throw new Error("Attempt to auto-create ExplanationOfBenefit.facility"); 16477 else if (Configuration.doAutoCreate()) 16478 this.facility = new Reference(); // cc 16479 return this.facility; 16480 } 16481 16482 public boolean hasFacility() { 16483 return this.facility != null && !this.facility.isEmpty(); 16484 } 16485 16486 /** 16487 * @param value {@link #facility} (Facility where the services were provided.) 16488 */ 16489 public ExplanationOfBenefit setFacility(Reference value) { 16490 this.facility = value; 16491 return this; 16492 } 16493 16494 /** 16495 * @return {@link #claim} (The business identifier for the instance of the adjudication request: claim predetermination or preauthorization.) 16496 */ 16497 public Reference getClaim() { 16498 if (this.claim == null) 16499 if (Configuration.errorOnAutoCreate()) 16500 throw new Error("Attempt to auto-create ExplanationOfBenefit.claim"); 16501 else if (Configuration.doAutoCreate()) 16502 this.claim = new Reference(); // cc 16503 return this.claim; 16504 } 16505 16506 public boolean hasClaim() { 16507 return this.claim != null && !this.claim.isEmpty(); 16508 } 16509 16510 /** 16511 * @param value {@link #claim} (The business identifier for the instance of the adjudication request: claim predetermination or preauthorization.) 16512 */ 16513 public ExplanationOfBenefit setClaim(Reference value) { 16514 this.claim = value; 16515 return this; 16516 } 16517 16518 /** 16519 * @return {@link #claimResponse} (The business identifier for the instance of the adjudication response: claim, predetermination or preauthorization response.) 16520 */ 16521 public Reference getClaimResponse() { 16522 if (this.claimResponse == null) 16523 if (Configuration.errorOnAutoCreate()) 16524 throw new Error("Attempt to auto-create ExplanationOfBenefit.claimResponse"); 16525 else if (Configuration.doAutoCreate()) 16526 this.claimResponse = new Reference(); // cc 16527 return this.claimResponse; 16528 } 16529 16530 public boolean hasClaimResponse() { 16531 return this.claimResponse != null && !this.claimResponse.isEmpty(); 16532 } 16533 16534 /** 16535 * @param value {@link #claimResponse} (The business identifier for the instance of the adjudication response: claim, predetermination or preauthorization response.) 16536 */ 16537 public ExplanationOfBenefit setClaimResponse(Reference value) { 16538 this.claimResponse = value; 16539 return this; 16540 } 16541 16542 /** 16543 * @return {@link #outcome} (The outcome of the claim, predetermination, or preauthorization processing.). This is the underlying object with id, value and extensions. The accessor "getOutcome" gives direct access to the value 16544 */ 16545 public Enumeration<ClaimProcessingCodes> getOutcomeElement() { 16546 if (this.outcome == null) 16547 if (Configuration.errorOnAutoCreate()) 16548 throw new Error("Attempt to auto-create ExplanationOfBenefit.outcome"); 16549 else if (Configuration.doAutoCreate()) 16550 this.outcome = new Enumeration<ClaimProcessingCodes>(new ClaimProcessingCodesEnumFactory()); // bb 16551 return this.outcome; 16552 } 16553 16554 public boolean hasOutcomeElement() { 16555 return this.outcome != null && !this.outcome.isEmpty(); 16556 } 16557 16558 public boolean hasOutcome() { 16559 return this.outcome != null && !this.outcome.isEmpty(); 16560 } 16561 16562 /** 16563 * @param value {@link #outcome} (The outcome of the claim, predetermination, or preauthorization processing.). This is the underlying object with id, value and extensions. The accessor "getOutcome" gives direct access to the value 16564 */ 16565 public ExplanationOfBenefit setOutcomeElement(Enumeration<ClaimProcessingCodes> value) { 16566 this.outcome = value; 16567 return this; 16568 } 16569 16570 /** 16571 * @return The outcome of the claim, predetermination, or preauthorization processing. 16572 */ 16573 public ClaimProcessingCodes getOutcome() { 16574 return this.outcome == null ? null : this.outcome.getValue(); 16575 } 16576 16577 /** 16578 * @param value The outcome of the claim, predetermination, or preauthorization processing. 16579 */ 16580 public ExplanationOfBenefit setOutcome(ClaimProcessingCodes value) { 16581 if (this.outcome == null) 16582 this.outcome = new Enumeration<ClaimProcessingCodes>(new ClaimProcessingCodesEnumFactory()); 16583 this.outcome.setValue(value); 16584 return this; 16585 } 16586 16587 /** 16588 * @return {@link #decision} (The result of the claim, predetermination, or preauthorization adjudication.) 16589 */ 16590 public CodeableConcept getDecision() { 16591 if (this.decision == null) 16592 if (Configuration.errorOnAutoCreate()) 16593 throw new Error("Attempt to auto-create ExplanationOfBenefit.decision"); 16594 else if (Configuration.doAutoCreate()) 16595 this.decision = new CodeableConcept(); // cc 16596 return this.decision; 16597 } 16598 16599 public boolean hasDecision() { 16600 return this.decision != null && !this.decision.isEmpty(); 16601 } 16602 16603 /** 16604 * @param value {@link #decision} (The result of the claim, predetermination, or preauthorization adjudication.) 16605 */ 16606 public ExplanationOfBenefit setDecision(CodeableConcept value) { 16607 this.decision = value; 16608 return this; 16609 } 16610 16611 /** 16612 * @return {@link #disposition} (A human readable description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 16613 */ 16614 public StringType getDispositionElement() { 16615 if (this.disposition == null) 16616 if (Configuration.errorOnAutoCreate()) 16617 throw new Error("Attempt to auto-create ExplanationOfBenefit.disposition"); 16618 else if (Configuration.doAutoCreate()) 16619 this.disposition = new StringType(); // bb 16620 return this.disposition; 16621 } 16622 16623 public boolean hasDispositionElement() { 16624 return this.disposition != null && !this.disposition.isEmpty(); 16625 } 16626 16627 public boolean hasDisposition() { 16628 return this.disposition != null && !this.disposition.isEmpty(); 16629 } 16630 16631 /** 16632 * @param value {@link #disposition} (A human readable description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 16633 */ 16634 public ExplanationOfBenefit setDispositionElement(StringType value) { 16635 this.disposition = value; 16636 return this; 16637 } 16638 16639 /** 16640 * @return A human readable description of the status of the adjudication. 16641 */ 16642 public String getDisposition() { 16643 return this.disposition == null ? null : this.disposition.getValue(); 16644 } 16645 16646 /** 16647 * @param value A human readable description of the status of the adjudication. 16648 */ 16649 public ExplanationOfBenefit setDisposition(String value) { 16650 if (Utilities.noString(value)) 16651 this.disposition = null; 16652 else { 16653 if (this.disposition == null) 16654 this.disposition = new StringType(); 16655 this.disposition.setValue(value); 16656 } 16657 return this; 16658 } 16659 16660 /** 16661 * @return {@link #preAuthRef} (Reference from the Insurer which is used in later communications which refers to this adjudication.) 16662 */ 16663 public List<StringType> getPreAuthRef() { 16664 if (this.preAuthRef == null) 16665 this.preAuthRef = new ArrayList<StringType>(); 16666 return this.preAuthRef; 16667 } 16668 16669 /** 16670 * @return Returns a reference to <code>this</code> for easy method chaining 16671 */ 16672 public ExplanationOfBenefit setPreAuthRef(List<StringType> thePreAuthRef) { 16673 this.preAuthRef = thePreAuthRef; 16674 return this; 16675 } 16676 16677 public boolean hasPreAuthRef() { 16678 if (this.preAuthRef == null) 16679 return false; 16680 for (StringType item : this.preAuthRef) 16681 if (!item.isEmpty()) 16682 return true; 16683 return false; 16684 } 16685 16686 /** 16687 * @return {@link #preAuthRef} (Reference from the Insurer which is used in later communications which refers to this adjudication.) 16688 */ 16689 public StringType addPreAuthRefElement() {//2 16690 StringType t = new StringType(); 16691 if (this.preAuthRef == null) 16692 this.preAuthRef = new ArrayList<StringType>(); 16693 this.preAuthRef.add(t); 16694 return t; 16695 } 16696 16697 /** 16698 * @param value {@link #preAuthRef} (Reference from the Insurer which is used in later communications which refers to this adjudication.) 16699 */ 16700 public ExplanationOfBenefit addPreAuthRef(String value) { //1 16701 StringType t = new StringType(); 16702 t.setValue(value); 16703 if (this.preAuthRef == null) 16704 this.preAuthRef = new ArrayList<StringType>(); 16705 this.preAuthRef.add(t); 16706 return this; 16707 } 16708 16709 /** 16710 * @param value {@link #preAuthRef} (Reference from the Insurer which is used in later communications which refers to this adjudication.) 16711 */ 16712 public boolean hasPreAuthRef(String value) { 16713 if (this.preAuthRef == null) 16714 return false; 16715 for (StringType v : this.preAuthRef) 16716 if (v.getValue().equals(value)) // string 16717 return true; 16718 return false; 16719 } 16720 16721 /** 16722 * @return {@link #preAuthRefPeriod} (The timeframe during which the supplied preauthorization reference may be quoted on claims to obtain the adjudication as provided.) 16723 */ 16724 public List<Period> getPreAuthRefPeriod() { 16725 if (this.preAuthRefPeriod == null) 16726 this.preAuthRefPeriod = new ArrayList<Period>(); 16727 return this.preAuthRefPeriod; 16728 } 16729 16730 /** 16731 * @return Returns a reference to <code>this</code> for easy method chaining 16732 */ 16733 public ExplanationOfBenefit setPreAuthRefPeriod(List<Period> thePreAuthRefPeriod) { 16734 this.preAuthRefPeriod = thePreAuthRefPeriod; 16735 return this; 16736 } 16737 16738 public boolean hasPreAuthRefPeriod() { 16739 if (this.preAuthRefPeriod == null) 16740 return false; 16741 for (Period item : this.preAuthRefPeriod) 16742 if (!item.isEmpty()) 16743 return true; 16744 return false; 16745 } 16746 16747 public Period addPreAuthRefPeriod() { //3 16748 Period t = new Period(); 16749 if (this.preAuthRefPeriod == null) 16750 this.preAuthRefPeriod = new ArrayList<Period>(); 16751 this.preAuthRefPeriod.add(t); 16752 return t; 16753 } 16754 16755 public ExplanationOfBenefit addPreAuthRefPeriod(Period t) { //3 16756 if (t == null) 16757 return this; 16758 if (this.preAuthRefPeriod == null) 16759 this.preAuthRefPeriod = new ArrayList<Period>(); 16760 this.preAuthRefPeriod.add(t); 16761 return this; 16762 } 16763 16764 /** 16765 * @return The first repetition of repeating field {@link #preAuthRefPeriod}, creating it if it does not already exist {3} 16766 */ 16767 public Period getPreAuthRefPeriodFirstRep() { 16768 if (getPreAuthRefPeriod().isEmpty()) { 16769 addPreAuthRefPeriod(); 16770 } 16771 return getPreAuthRefPeriod().get(0); 16772 } 16773 16774 /** 16775 * @return {@link #diagnosisRelatedGroup} (A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.) 16776 */ 16777 public CodeableConcept getDiagnosisRelatedGroup() { 16778 if (this.diagnosisRelatedGroup == null) 16779 if (Configuration.errorOnAutoCreate()) 16780 throw new Error("Attempt to auto-create ExplanationOfBenefit.diagnosisRelatedGroup"); 16781 else if (Configuration.doAutoCreate()) 16782 this.diagnosisRelatedGroup = new CodeableConcept(); // cc 16783 return this.diagnosisRelatedGroup; 16784 } 16785 16786 public boolean hasDiagnosisRelatedGroup() { 16787 return this.diagnosisRelatedGroup != null && !this.diagnosisRelatedGroup.isEmpty(); 16788 } 16789 16790 /** 16791 * @param value {@link #diagnosisRelatedGroup} (A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.) 16792 */ 16793 public ExplanationOfBenefit setDiagnosisRelatedGroup(CodeableConcept value) { 16794 this.diagnosisRelatedGroup = value; 16795 return this; 16796 } 16797 16798 /** 16799 * @return {@link #careTeam} (The members of the team who provided the products and services.) 16800 */ 16801 public List<CareTeamComponent> getCareTeam() { 16802 if (this.careTeam == null) 16803 this.careTeam = new ArrayList<CareTeamComponent>(); 16804 return this.careTeam; 16805 } 16806 16807 /** 16808 * @return Returns a reference to <code>this</code> for easy method chaining 16809 */ 16810 public ExplanationOfBenefit setCareTeam(List<CareTeamComponent> theCareTeam) { 16811 this.careTeam = theCareTeam; 16812 return this; 16813 } 16814 16815 public boolean hasCareTeam() { 16816 if (this.careTeam == null) 16817 return false; 16818 for (CareTeamComponent item : this.careTeam) 16819 if (!item.isEmpty()) 16820 return true; 16821 return false; 16822 } 16823 16824 public CareTeamComponent addCareTeam() { //3 16825 CareTeamComponent t = new CareTeamComponent(); 16826 if (this.careTeam == null) 16827 this.careTeam = new ArrayList<CareTeamComponent>(); 16828 this.careTeam.add(t); 16829 return t; 16830 } 16831 16832 public ExplanationOfBenefit addCareTeam(CareTeamComponent t) { //3 16833 if (t == null) 16834 return this; 16835 if (this.careTeam == null) 16836 this.careTeam = new ArrayList<CareTeamComponent>(); 16837 this.careTeam.add(t); 16838 return this; 16839 } 16840 16841 /** 16842 * @return The first repetition of repeating field {@link #careTeam}, creating it if it does not already exist {3} 16843 */ 16844 public CareTeamComponent getCareTeamFirstRep() { 16845 if (getCareTeam().isEmpty()) { 16846 addCareTeam(); 16847 } 16848 return getCareTeam().get(0); 16849 } 16850 16851 /** 16852 * @return {@link #supportingInfo} (Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.) 16853 */ 16854 public List<SupportingInformationComponent> getSupportingInfo() { 16855 if (this.supportingInfo == null) 16856 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 16857 return this.supportingInfo; 16858 } 16859 16860 /** 16861 * @return Returns a reference to <code>this</code> for easy method chaining 16862 */ 16863 public ExplanationOfBenefit setSupportingInfo(List<SupportingInformationComponent> theSupportingInfo) { 16864 this.supportingInfo = theSupportingInfo; 16865 return this; 16866 } 16867 16868 public boolean hasSupportingInfo() { 16869 if (this.supportingInfo == null) 16870 return false; 16871 for (SupportingInformationComponent item : this.supportingInfo) 16872 if (!item.isEmpty()) 16873 return true; 16874 return false; 16875 } 16876 16877 public SupportingInformationComponent addSupportingInfo() { //3 16878 SupportingInformationComponent t = new SupportingInformationComponent(); 16879 if (this.supportingInfo == null) 16880 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 16881 this.supportingInfo.add(t); 16882 return t; 16883 } 16884 16885 public ExplanationOfBenefit addSupportingInfo(SupportingInformationComponent t) { //3 16886 if (t == null) 16887 return this; 16888 if (this.supportingInfo == null) 16889 this.supportingInfo = new ArrayList<SupportingInformationComponent>(); 16890 this.supportingInfo.add(t); 16891 return this; 16892 } 16893 16894 /** 16895 * @return The first repetition of repeating field {@link #supportingInfo}, creating it if it does not already exist {3} 16896 */ 16897 public SupportingInformationComponent getSupportingInfoFirstRep() { 16898 if (getSupportingInfo().isEmpty()) { 16899 addSupportingInfo(); 16900 } 16901 return getSupportingInfo().get(0); 16902 } 16903 16904 /** 16905 * @return {@link #diagnosis} (Information about diagnoses relevant to the claim items.) 16906 */ 16907 public List<DiagnosisComponent> getDiagnosis() { 16908 if (this.diagnosis == null) 16909 this.diagnosis = new ArrayList<DiagnosisComponent>(); 16910 return this.diagnosis; 16911 } 16912 16913 /** 16914 * @return Returns a reference to <code>this</code> for easy method chaining 16915 */ 16916 public ExplanationOfBenefit setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 16917 this.diagnosis = theDiagnosis; 16918 return this; 16919 } 16920 16921 public boolean hasDiagnosis() { 16922 if (this.diagnosis == null) 16923 return false; 16924 for (DiagnosisComponent item : this.diagnosis) 16925 if (!item.isEmpty()) 16926 return true; 16927 return false; 16928 } 16929 16930 public DiagnosisComponent addDiagnosis() { //3 16931 DiagnosisComponent t = new DiagnosisComponent(); 16932 if (this.diagnosis == null) 16933 this.diagnosis = new ArrayList<DiagnosisComponent>(); 16934 this.diagnosis.add(t); 16935 return t; 16936 } 16937 16938 public ExplanationOfBenefit addDiagnosis(DiagnosisComponent t) { //3 16939 if (t == null) 16940 return this; 16941 if (this.diagnosis == null) 16942 this.diagnosis = new ArrayList<DiagnosisComponent>(); 16943 this.diagnosis.add(t); 16944 return this; 16945 } 16946 16947 /** 16948 * @return The first repetition of repeating field {@link #diagnosis}, creating it if it does not already exist {3} 16949 */ 16950 public DiagnosisComponent getDiagnosisFirstRep() { 16951 if (getDiagnosis().isEmpty()) { 16952 addDiagnosis(); 16953 } 16954 return getDiagnosis().get(0); 16955 } 16956 16957 /** 16958 * @return {@link #procedure} (Procedures performed on the patient relevant to the billing items with the claim.) 16959 */ 16960 public List<ProcedureComponent> getProcedure() { 16961 if (this.procedure == null) 16962 this.procedure = new ArrayList<ProcedureComponent>(); 16963 return this.procedure; 16964 } 16965 16966 /** 16967 * @return Returns a reference to <code>this</code> for easy method chaining 16968 */ 16969 public ExplanationOfBenefit setProcedure(List<ProcedureComponent> theProcedure) { 16970 this.procedure = theProcedure; 16971 return this; 16972 } 16973 16974 public boolean hasProcedure() { 16975 if (this.procedure == null) 16976 return false; 16977 for (ProcedureComponent item : this.procedure) 16978 if (!item.isEmpty()) 16979 return true; 16980 return false; 16981 } 16982 16983 public ProcedureComponent addProcedure() { //3 16984 ProcedureComponent t = new ProcedureComponent(); 16985 if (this.procedure == null) 16986 this.procedure = new ArrayList<ProcedureComponent>(); 16987 this.procedure.add(t); 16988 return t; 16989 } 16990 16991 public ExplanationOfBenefit addProcedure(ProcedureComponent t) { //3 16992 if (t == null) 16993 return this; 16994 if (this.procedure == null) 16995 this.procedure = new ArrayList<ProcedureComponent>(); 16996 this.procedure.add(t); 16997 return this; 16998 } 16999 17000 /** 17001 * @return The first repetition of repeating field {@link #procedure}, creating it if it does not already exist {3} 17002 */ 17003 public ProcedureComponent getProcedureFirstRep() { 17004 if (getProcedure().isEmpty()) { 17005 addProcedure(); 17006 } 17007 return getProcedure().get(0); 17008 } 17009 17010 /** 17011 * @return {@link #precedence} (This indicates the relative order of a series of EOBs related to different coverages for the same suite of services.). This is the underlying object with id, value and extensions. The accessor "getPrecedence" gives direct access to the value 17012 */ 17013 public PositiveIntType getPrecedenceElement() { 17014 if (this.precedence == null) 17015 if (Configuration.errorOnAutoCreate()) 17016 throw new Error("Attempt to auto-create ExplanationOfBenefit.precedence"); 17017 else if (Configuration.doAutoCreate()) 17018 this.precedence = new PositiveIntType(); // bb 17019 return this.precedence; 17020 } 17021 17022 public boolean hasPrecedenceElement() { 17023 return this.precedence != null && !this.precedence.isEmpty(); 17024 } 17025 17026 public boolean hasPrecedence() { 17027 return this.precedence != null && !this.precedence.isEmpty(); 17028 } 17029 17030 /** 17031 * @param value {@link #precedence} (This indicates the relative order of a series of EOBs related to different coverages for the same suite of services.). This is the underlying object with id, value and extensions. The accessor "getPrecedence" gives direct access to the value 17032 */ 17033 public ExplanationOfBenefit setPrecedenceElement(PositiveIntType value) { 17034 this.precedence = value; 17035 return this; 17036 } 17037 17038 /** 17039 * @return This indicates the relative order of a series of EOBs related to different coverages for the same suite of services. 17040 */ 17041 public int getPrecedence() { 17042 return this.precedence == null || this.precedence.isEmpty() ? 0 : this.precedence.getValue(); 17043 } 17044 17045 /** 17046 * @param value This indicates the relative order of a series of EOBs related to different coverages for the same suite of services. 17047 */ 17048 public ExplanationOfBenefit setPrecedence(int value) { 17049 if (this.precedence == null) 17050 this.precedence = new PositiveIntType(); 17051 this.precedence.setValue(value); 17052 return this; 17053 } 17054 17055 /** 17056 * @return {@link #insurance} (Financial instruments for reimbursement for the health care products and services specified on the claim.) 17057 */ 17058 public List<InsuranceComponent> getInsurance() { 17059 if (this.insurance == null) 17060 this.insurance = new ArrayList<InsuranceComponent>(); 17061 return this.insurance; 17062 } 17063 17064 /** 17065 * @return Returns a reference to <code>this</code> for easy method chaining 17066 */ 17067 public ExplanationOfBenefit setInsurance(List<InsuranceComponent> theInsurance) { 17068 this.insurance = theInsurance; 17069 return this; 17070 } 17071 17072 public boolean hasInsurance() { 17073 if (this.insurance == null) 17074 return false; 17075 for (InsuranceComponent item : this.insurance) 17076 if (!item.isEmpty()) 17077 return true; 17078 return false; 17079 } 17080 17081 public InsuranceComponent addInsurance() { //3 17082 InsuranceComponent t = new InsuranceComponent(); 17083 if (this.insurance == null) 17084 this.insurance = new ArrayList<InsuranceComponent>(); 17085 this.insurance.add(t); 17086 return t; 17087 } 17088 17089 public ExplanationOfBenefit addInsurance(InsuranceComponent t) { //3 17090 if (t == null) 17091 return this; 17092 if (this.insurance == null) 17093 this.insurance = new ArrayList<InsuranceComponent>(); 17094 this.insurance.add(t); 17095 return this; 17096 } 17097 17098 /** 17099 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist {3} 17100 */ 17101 public InsuranceComponent getInsuranceFirstRep() { 17102 if (getInsurance().isEmpty()) { 17103 addInsurance(); 17104 } 17105 return getInsurance().get(0); 17106 } 17107 17108 /** 17109 * @return {@link #accident} (Details of a accident which resulted in injuries which required the products and services listed in the claim.) 17110 */ 17111 public AccidentComponent getAccident() { 17112 if (this.accident == null) 17113 if (Configuration.errorOnAutoCreate()) 17114 throw new Error("Attempt to auto-create ExplanationOfBenefit.accident"); 17115 else if (Configuration.doAutoCreate()) 17116 this.accident = new AccidentComponent(); // cc 17117 return this.accident; 17118 } 17119 17120 public boolean hasAccident() { 17121 return this.accident != null && !this.accident.isEmpty(); 17122 } 17123 17124 /** 17125 * @param value {@link #accident} (Details of a accident which resulted in injuries which required the products and services listed in the claim.) 17126 */ 17127 public ExplanationOfBenefit setAccident(AccidentComponent value) { 17128 this.accident = value; 17129 return this; 17130 } 17131 17132 /** 17133 * @return {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 17134 */ 17135 public Money getPatientPaid() { 17136 if (this.patientPaid == null) 17137 if (Configuration.errorOnAutoCreate()) 17138 throw new Error("Attempt to auto-create ExplanationOfBenefit.patientPaid"); 17139 else if (Configuration.doAutoCreate()) 17140 this.patientPaid = new Money(); // cc 17141 return this.patientPaid; 17142 } 17143 17144 public boolean hasPatientPaid() { 17145 return this.patientPaid != null && !this.patientPaid.isEmpty(); 17146 } 17147 17148 /** 17149 * @param value {@link #patientPaid} (The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.) 17150 */ 17151 public ExplanationOfBenefit setPatientPaid(Money value) { 17152 this.patientPaid = value; 17153 return this; 17154 } 17155 17156 /** 17157 * @return {@link #item} (A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.) 17158 */ 17159 public List<ItemComponent> getItem() { 17160 if (this.item == null) 17161 this.item = new ArrayList<ItemComponent>(); 17162 return this.item; 17163 } 17164 17165 /** 17166 * @return Returns a reference to <code>this</code> for easy method chaining 17167 */ 17168 public ExplanationOfBenefit setItem(List<ItemComponent> theItem) { 17169 this.item = theItem; 17170 return this; 17171 } 17172 17173 public boolean hasItem() { 17174 if (this.item == null) 17175 return false; 17176 for (ItemComponent item : this.item) 17177 if (!item.isEmpty()) 17178 return true; 17179 return false; 17180 } 17181 17182 public ItemComponent addItem() { //3 17183 ItemComponent t = new ItemComponent(); 17184 if (this.item == null) 17185 this.item = new ArrayList<ItemComponent>(); 17186 this.item.add(t); 17187 return t; 17188 } 17189 17190 public ExplanationOfBenefit addItem(ItemComponent t) { //3 17191 if (t == null) 17192 return this; 17193 if (this.item == null) 17194 this.item = new ArrayList<ItemComponent>(); 17195 this.item.add(t); 17196 return this; 17197 } 17198 17199 /** 17200 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 17201 */ 17202 public ItemComponent getItemFirstRep() { 17203 if (getItem().isEmpty()) { 17204 addItem(); 17205 } 17206 return getItem().get(0); 17207 } 17208 17209 /** 17210 * @return {@link #addItem} (The first-tier service adjudications for payor added product or service lines.) 17211 */ 17212 public List<AddedItemComponent> getAddItem() { 17213 if (this.addItem == null) 17214 this.addItem = new ArrayList<AddedItemComponent>(); 17215 return this.addItem; 17216 } 17217 17218 /** 17219 * @return Returns a reference to <code>this</code> for easy method chaining 17220 */ 17221 public ExplanationOfBenefit setAddItem(List<AddedItemComponent> theAddItem) { 17222 this.addItem = theAddItem; 17223 return this; 17224 } 17225 17226 public boolean hasAddItem() { 17227 if (this.addItem == null) 17228 return false; 17229 for (AddedItemComponent item : this.addItem) 17230 if (!item.isEmpty()) 17231 return true; 17232 return false; 17233 } 17234 17235 public AddedItemComponent addAddItem() { //3 17236 AddedItemComponent t = new AddedItemComponent(); 17237 if (this.addItem == null) 17238 this.addItem = new ArrayList<AddedItemComponent>(); 17239 this.addItem.add(t); 17240 return t; 17241 } 17242 17243 public ExplanationOfBenefit addAddItem(AddedItemComponent t) { //3 17244 if (t == null) 17245 return this; 17246 if (this.addItem == null) 17247 this.addItem = new ArrayList<AddedItemComponent>(); 17248 this.addItem.add(t); 17249 return this; 17250 } 17251 17252 /** 17253 * @return The first repetition of repeating field {@link #addItem}, creating it if it does not already exist {3} 17254 */ 17255 public AddedItemComponent getAddItemFirstRep() { 17256 if (getAddItem().isEmpty()) { 17257 addAddItem(); 17258 } 17259 return getAddItem().get(0); 17260 } 17261 17262 /** 17263 * @return {@link #adjudication} (The adjudication results which are presented at the header level rather than at the line-item or add-item levels.) 17264 */ 17265 public List<AdjudicationComponent> getAdjudication() { 17266 if (this.adjudication == null) 17267 this.adjudication = new ArrayList<AdjudicationComponent>(); 17268 return this.adjudication; 17269 } 17270 17271 /** 17272 * @return Returns a reference to <code>this</code> for easy method chaining 17273 */ 17274 public ExplanationOfBenefit setAdjudication(List<AdjudicationComponent> theAdjudication) { 17275 this.adjudication = theAdjudication; 17276 return this; 17277 } 17278 17279 public boolean hasAdjudication() { 17280 if (this.adjudication == null) 17281 return false; 17282 for (AdjudicationComponent item : this.adjudication) 17283 if (!item.isEmpty()) 17284 return true; 17285 return false; 17286 } 17287 17288 public AdjudicationComponent addAdjudication() { //3 17289 AdjudicationComponent t = new AdjudicationComponent(); 17290 if (this.adjudication == null) 17291 this.adjudication = new ArrayList<AdjudicationComponent>(); 17292 this.adjudication.add(t); 17293 return t; 17294 } 17295 17296 public ExplanationOfBenefit addAdjudication(AdjudicationComponent t) { //3 17297 if (t == null) 17298 return this; 17299 if (this.adjudication == null) 17300 this.adjudication = new ArrayList<AdjudicationComponent>(); 17301 this.adjudication.add(t); 17302 return this; 17303 } 17304 17305 /** 17306 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist {3} 17307 */ 17308 public AdjudicationComponent getAdjudicationFirstRep() { 17309 if (getAdjudication().isEmpty()) { 17310 addAdjudication(); 17311 } 17312 return getAdjudication().get(0); 17313 } 17314 17315 /** 17316 * @return {@link #total} (Categorized monetary totals for the adjudication.) 17317 */ 17318 public List<TotalComponent> getTotal() { 17319 if (this.total == null) 17320 this.total = new ArrayList<TotalComponent>(); 17321 return this.total; 17322 } 17323 17324 /** 17325 * @return Returns a reference to <code>this</code> for easy method chaining 17326 */ 17327 public ExplanationOfBenefit setTotal(List<TotalComponent> theTotal) { 17328 this.total = theTotal; 17329 return this; 17330 } 17331 17332 public boolean hasTotal() { 17333 if (this.total == null) 17334 return false; 17335 for (TotalComponent item : this.total) 17336 if (!item.isEmpty()) 17337 return true; 17338 return false; 17339 } 17340 17341 public TotalComponent addTotal() { //3 17342 TotalComponent t = new TotalComponent(); 17343 if (this.total == null) 17344 this.total = new ArrayList<TotalComponent>(); 17345 this.total.add(t); 17346 return t; 17347 } 17348 17349 public ExplanationOfBenefit addTotal(TotalComponent t) { //3 17350 if (t == null) 17351 return this; 17352 if (this.total == null) 17353 this.total = new ArrayList<TotalComponent>(); 17354 this.total.add(t); 17355 return this; 17356 } 17357 17358 /** 17359 * @return The first repetition of repeating field {@link #total}, creating it if it does not already exist {3} 17360 */ 17361 public TotalComponent getTotalFirstRep() { 17362 if (getTotal().isEmpty()) { 17363 addTotal(); 17364 } 17365 return getTotal().get(0); 17366 } 17367 17368 /** 17369 * @return {@link #payment} (Payment details for the adjudication of the claim.) 17370 */ 17371 public PaymentComponent getPayment() { 17372 if (this.payment == null) 17373 if (Configuration.errorOnAutoCreate()) 17374 throw new Error("Attempt to auto-create ExplanationOfBenefit.payment"); 17375 else if (Configuration.doAutoCreate()) 17376 this.payment = new PaymentComponent(); // cc 17377 return this.payment; 17378 } 17379 17380 public boolean hasPayment() { 17381 return this.payment != null && !this.payment.isEmpty(); 17382 } 17383 17384 /** 17385 * @param value {@link #payment} (Payment details for the adjudication of the claim.) 17386 */ 17387 public ExplanationOfBenefit setPayment(PaymentComponent value) { 17388 this.payment = value; 17389 return this; 17390 } 17391 17392 /** 17393 * @return {@link #formCode} (A code for the form to be used for printing the content.) 17394 */ 17395 public CodeableConcept getFormCode() { 17396 if (this.formCode == null) 17397 if (Configuration.errorOnAutoCreate()) 17398 throw new Error("Attempt to auto-create ExplanationOfBenefit.formCode"); 17399 else if (Configuration.doAutoCreate()) 17400 this.formCode = new CodeableConcept(); // cc 17401 return this.formCode; 17402 } 17403 17404 public boolean hasFormCode() { 17405 return this.formCode != null && !this.formCode.isEmpty(); 17406 } 17407 17408 /** 17409 * @param value {@link #formCode} (A code for the form to be used for printing the content.) 17410 */ 17411 public ExplanationOfBenefit setFormCode(CodeableConcept value) { 17412 this.formCode = value; 17413 return this; 17414 } 17415 17416 /** 17417 * @return {@link #form} (The actual form, by reference or inclusion, for printing the content or an EOB.) 17418 */ 17419 public Attachment getForm() { 17420 if (this.form == null) 17421 if (Configuration.errorOnAutoCreate()) 17422 throw new Error("Attempt to auto-create ExplanationOfBenefit.form"); 17423 else if (Configuration.doAutoCreate()) 17424 this.form = new Attachment(); // cc 17425 return this.form; 17426 } 17427 17428 public boolean hasForm() { 17429 return this.form != null && !this.form.isEmpty(); 17430 } 17431 17432 /** 17433 * @param value {@link #form} (The actual form, by reference or inclusion, for printing the content or an EOB.) 17434 */ 17435 public ExplanationOfBenefit setForm(Attachment value) { 17436 this.form = value; 17437 return this; 17438 } 17439 17440 /** 17441 * @return {@link #processNote} (A note that describes or explains adjudication results in a human readable form.) 17442 */ 17443 public List<NoteComponent> getProcessNote() { 17444 if (this.processNote == null) 17445 this.processNote = new ArrayList<NoteComponent>(); 17446 return this.processNote; 17447 } 17448 17449 /** 17450 * @return Returns a reference to <code>this</code> for easy method chaining 17451 */ 17452 public ExplanationOfBenefit setProcessNote(List<NoteComponent> theProcessNote) { 17453 this.processNote = theProcessNote; 17454 return this; 17455 } 17456 17457 public boolean hasProcessNote() { 17458 if (this.processNote == null) 17459 return false; 17460 for (NoteComponent item : this.processNote) 17461 if (!item.isEmpty()) 17462 return true; 17463 return false; 17464 } 17465 17466 public NoteComponent addProcessNote() { //3 17467 NoteComponent t = new NoteComponent(); 17468 if (this.processNote == null) 17469 this.processNote = new ArrayList<NoteComponent>(); 17470 this.processNote.add(t); 17471 return t; 17472 } 17473 17474 public ExplanationOfBenefit addProcessNote(NoteComponent t) { //3 17475 if (t == null) 17476 return this; 17477 if (this.processNote == null) 17478 this.processNote = new ArrayList<NoteComponent>(); 17479 this.processNote.add(t); 17480 return this; 17481 } 17482 17483 /** 17484 * @return The first repetition of repeating field {@link #processNote}, creating it if it does not already exist {3} 17485 */ 17486 public NoteComponent getProcessNoteFirstRep() { 17487 if (getProcessNote().isEmpty()) { 17488 addProcessNote(); 17489 } 17490 return getProcessNote().get(0); 17491 } 17492 17493 /** 17494 * @return {@link #benefitPeriod} (The term of the benefits documented in this response.) 17495 */ 17496 public Period getBenefitPeriod() { 17497 if (this.benefitPeriod == null) 17498 if (Configuration.errorOnAutoCreate()) 17499 throw new Error("Attempt to auto-create ExplanationOfBenefit.benefitPeriod"); 17500 else if (Configuration.doAutoCreate()) 17501 this.benefitPeriod = new Period(); // cc 17502 return this.benefitPeriod; 17503 } 17504 17505 public boolean hasBenefitPeriod() { 17506 return this.benefitPeriod != null && !this.benefitPeriod.isEmpty(); 17507 } 17508 17509 /** 17510 * @param value {@link #benefitPeriod} (The term of the benefits documented in this response.) 17511 */ 17512 public ExplanationOfBenefit setBenefitPeriod(Period value) { 17513 this.benefitPeriod = value; 17514 return this; 17515 } 17516 17517 /** 17518 * @return {@link #benefitBalance} (Balance by Benefit Category.) 17519 */ 17520 public List<BenefitBalanceComponent> getBenefitBalance() { 17521 if (this.benefitBalance == null) 17522 this.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 17523 return this.benefitBalance; 17524 } 17525 17526 /** 17527 * @return Returns a reference to <code>this</code> for easy method chaining 17528 */ 17529 public ExplanationOfBenefit setBenefitBalance(List<BenefitBalanceComponent> theBenefitBalance) { 17530 this.benefitBalance = theBenefitBalance; 17531 return this; 17532 } 17533 17534 public boolean hasBenefitBalance() { 17535 if (this.benefitBalance == null) 17536 return false; 17537 for (BenefitBalanceComponent item : this.benefitBalance) 17538 if (!item.isEmpty()) 17539 return true; 17540 return false; 17541 } 17542 17543 public BenefitBalanceComponent addBenefitBalance() { //3 17544 BenefitBalanceComponent t = new BenefitBalanceComponent(); 17545 if (this.benefitBalance == null) 17546 this.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 17547 this.benefitBalance.add(t); 17548 return t; 17549 } 17550 17551 public ExplanationOfBenefit addBenefitBalance(BenefitBalanceComponent t) { //3 17552 if (t == null) 17553 return this; 17554 if (this.benefitBalance == null) 17555 this.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 17556 this.benefitBalance.add(t); 17557 return this; 17558 } 17559 17560 /** 17561 * @return The first repetition of repeating field {@link #benefitBalance}, creating it if it does not already exist {3} 17562 */ 17563 public BenefitBalanceComponent getBenefitBalanceFirstRep() { 17564 if (getBenefitBalance().isEmpty()) { 17565 addBenefitBalance(); 17566 } 17567 return getBenefitBalance().get(0); 17568 } 17569 17570 protected void listChildren(List<Property> children) { 17571 super.listChildren(children); 17572 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this explanation of benefit.", 0, java.lang.Integer.MAX_VALUE, identifier)); 17573 children.add(new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber)); 17574 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 17575 children.add(new Property("type", "CodeableConcept", "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.", 0, 1, type)); 17576 children.add(new Property("subType", "CodeableConcept", "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 0, 1, subType)); 17577 children.add(new Property("use", "code", "A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.", 0, 1, use)); 17578 children.add(new Property("patient", "Reference(Patient)", "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for forecast reimbursement is sought.", 0, 1, patient)); 17579 children.add(new Property("billablePeriod", "Period", "The period for which charges are being submitted.", 0, 1, billablePeriod)); 17580 children.add(new Property("created", "dateTime", "The date this resource was created.", 0, 1, created)); 17581 children.add(new Property("enterer", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", "Individual who created the claim, predetermination or preauthorization.", 0, 1, enterer)); 17582 children.add(new Property("insurer", "Reference(Organization)", "The party responsible for authorization, adjudication and reimbursement.", 0, 1, insurer)); 17583 children.add(new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, provider)); 17584 children.add(new Property("priority", "CodeableConcept", "The provider-required urgency of processing the request. Typical values include: stat, normal deferred.", 0, 1, priority)); 17585 children.add(new Property("fundsReserveRequested", "CodeableConcept", "A code to indicate whether and for whom funds are to be reserved for future claims.", 0, 1, fundsReserveRequested)); 17586 children.add(new Property("fundsReserve", "CodeableConcept", "A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.", 0, 1, fundsReserve)); 17587 children.add(new Property("related", "", "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.", 0, java.lang.Integer.MAX_VALUE, related)); 17588 children.add(new Property("prescription", "Reference(MedicationRequest|VisionPrescription)", "Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments.", 0, 1, prescription)); 17589 children.add(new Property("originalPrescription", "Reference(MedicationRequest)", "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.", 0, 1, originalPrescription)); 17590 children.add(new Property("event", "", "Information code for an event with a corresponding date or period.", 0, java.lang.Integer.MAX_VALUE, event)); 17591 children.add(new Property("payee", "", "The party to be reimbursed for cost of the products and services according to the terms of the policy.", 0, 1, payee)); 17592 children.add(new Property("referral", "Reference(ServiceRequest)", "The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services.", 0, 1, referral)); 17593 children.add(new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter)); 17594 children.add(new Property("facility", "Reference(Location|Organization)", "Facility where the services were provided.", 0, 1, facility)); 17595 children.add(new Property("claim", "Reference(Claim)", "The business identifier for the instance of the adjudication request: claim predetermination or preauthorization.", 0, 1, claim)); 17596 children.add(new Property("claimResponse", "Reference(ClaimResponse)", "The business identifier for the instance of the adjudication response: claim, predetermination or preauthorization response.", 0, 1, claimResponse)); 17597 children.add(new Property("outcome", "code", "The outcome of the claim, predetermination, or preauthorization processing.", 0, 1, outcome)); 17598 children.add(new Property("decision", "CodeableConcept", "The result of the claim, predetermination, or preauthorization adjudication.", 0, 1, decision)); 17599 children.add(new Property("disposition", "string", "A human readable description of the status of the adjudication.", 0, 1, disposition)); 17600 children.add(new Property("preAuthRef", "string", "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, java.lang.Integer.MAX_VALUE, preAuthRef)); 17601 children.add(new Property("preAuthRefPeriod", "Period", "The timeframe during which the supplied preauthorization reference may be quoted on claims to obtain the adjudication as provided.", 0, java.lang.Integer.MAX_VALUE, preAuthRefPeriod)); 17602 children.add(new Property("diagnosisRelatedGroup", "CodeableConcept", "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.", 0, 1, diagnosisRelatedGroup)); 17603 children.add(new Property("careTeam", "", "The members of the team who provided the products and services.", 0, java.lang.Integer.MAX_VALUE, careTeam)); 17604 children.add(new Property("supportingInfo", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 0, java.lang.Integer.MAX_VALUE, supportingInfo)); 17605 children.add(new Property("diagnosis", "", "Information about diagnoses relevant to the claim items.", 0, java.lang.Integer.MAX_VALUE, diagnosis)); 17606 children.add(new Property("procedure", "", "Procedures performed on the patient relevant to the billing items with the claim.", 0, java.lang.Integer.MAX_VALUE, procedure)); 17607 children.add(new Property("precedence", "positiveInt", "This indicates the relative order of a series of EOBs related to different coverages for the same suite of services.", 0, 1, precedence)); 17608 children.add(new Property("insurance", "", "Financial instruments for reimbursement for the health care products and services specified on the claim.", 0, java.lang.Integer.MAX_VALUE, insurance)); 17609 children.add(new Property("accident", "", "Details of a accident which resulted in injuries which required the products and services listed in the claim.", 0, 1, accident)); 17610 children.add(new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid)); 17611 children.add(new Property("item", "", "A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.", 0, java.lang.Integer.MAX_VALUE, item)); 17612 children.add(new Property("addItem", "", "The first-tier service adjudications for payor added product or service lines.", 0, java.lang.Integer.MAX_VALUE, addItem)); 17613 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results which are presented at the header level rather than at the line-item or add-item levels.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 17614 children.add(new Property("total", "", "Categorized monetary totals for the adjudication.", 0, java.lang.Integer.MAX_VALUE, total)); 17615 children.add(new Property("payment", "", "Payment details for the adjudication of the claim.", 0, 1, payment)); 17616 children.add(new Property("formCode", "CodeableConcept", "A code for the form to be used for printing the content.", 0, 1, formCode)); 17617 children.add(new Property("form", "Attachment", "The actual form, by reference or inclusion, for printing the content or an EOB.", 0, 1, form)); 17618 children.add(new Property("processNote", "", "A note that describes or explains adjudication results in a human readable form.", 0, java.lang.Integer.MAX_VALUE, processNote)); 17619 children.add(new Property("benefitPeriod", "Period", "The term of the benefits documented in this response.", 0, 1, benefitPeriod)); 17620 children.add(new Property("benefitBalance", "", "Balance by Benefit Category.", 0, java.lang.Integer.MAX_VALUE, benefitBalance)); 17621 } 17622 17623 @Override 17624 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 17625 switch (_hash) { 17626 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this explanation of benefit.", 0, java.lang.Integer.MAX_VALUE, identifier); 17627 case 82505966: /*traceNumber*/ return new Property("traceNumber", "Identifier", "Trace number for tracking purposes. May be defined at the jurisdiction level or between trading partners.", 0, java.lang.Integer.MAX_VALUE, traceNumber); 17628 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 17629 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The category of claim, e.g. oral, pharmacy, vision, institutional, professional.", 0, 1, type); 17630 case -1868521062: /*subType*/ return new Property("subType", "CodeableConcept", "A finer grained suite of claim type codes which may convey additional information such as Inpatient vs Outpatient and/or a specialty service.", 0, 1, subType); 17631 case 116103: /*use*/ return new Property("use", "code", "A code to indicate whether the nature of the request is: Claim - A request to an Insurer to adjudicate the supplied charges for health care goods and services under the identified policy and to pay the determined Benefit amount, if any; Preauthorization - A request to an Insurer to adjudicate the supplied proposed future charges for health care goods and services under the identified policy and to approve the services and provide the expected benefit amounts and potentially to reserve funds to pay the benefits when Claims for the indicated services are later submitted; or, Pre-determination - A request to an Insurer to adjudicate the supplied 'what if' charges for health care goods and services under the identified policy and report back what the Benefit payable would be had the services actually been provided.", 0, 1, use); 17632 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The party to whom the professional services and/or products have been supplied or are being considered and for whom actual for forecast reimbursement is sought.", 0, 1, patient); 17633 case -332066046: /*billablePeriod*/ return new Property("billablePeriod", "Period", "The period for which charges are being submitted.", 0, 1, billablePeriod); 17634 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date this resource was created.", 0, 1, created); 17635 case -1591951995: /*enterer*/ return new Property("enterer", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson)", "Individual who created the claim, predetermination or preauthorization.", 0, 1, enterer); 17636 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The party responsible for authorization, adjudication and reimbursement.", 0, 1, insurer); 17637 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|PractitionerRole|Organization)", "The provider which is responsible for the claim, predetermination or preauthorization.", 0, 1, provider); 17638 case -1165461084: /*priority*/ return new Property("priority", "CodeableConcept", "The provider-required urgency of processing the request. Typical values include: stat, normal deferred.", 0, 1, priority); 17639 case -1688904576: /*fundsReserveRequested*/ return new Property("fundsReserveRequested", "CodeableConcept", "A code to indicate whether and for whom funds are to be reserved for future claims.", 0, 1, fundsReserveRequested); 17640 case 1314609806: /*fundsReserve*/ return new Property("fundsReserve", "CodeableConcept", "A code, used only on a response to a preauthorization, to indicate whether the benefits payable have been reserved and for whom.", 0, 1, fundsReserve); 17641 case 1090493483: /*related*/ return new Property("related", "", "Other claims which are related to this claim such as prior submissions or claims for related services or for the same event.", 0, java.lang.Integer.MAX_VALUE, related); 17642 case 460301338: /*prescription*/ return new Property("prescription", "Reference(MedicationRequest|VisionPrescription)", "Prescription is the document/authorization given to the claim author for them to provide products and services for which consideration (reimbursement) is sought. Could be a RX for medications, an 'order' for oxygen or wheelchair or physiotherapy treatments.", 0, 1, prescription); 17643 case -1814015861: /*originalPrescription*/ return new Property("originalPrescription", "Reference(MedicationRequest)", "Original prescription which has been superseded by this prescription to support the dispensing of pharmacy services, medications or products.", 0, 1, originalPrescription); 17644 case 96891546: /*event*/ return new Property("event", "", "Information code for an event with a corresponding date or period.", 0, java.lang.Integer.MAX_VALUE, event); 17645 case 106443592: /*payee*/ return new Property("payee", "", "The party to be reimbursed for cost of the products and services according to the terms of the policy.", 0, 1, payee); 17646 case -722568291: /*referral*/ return new Property("referral", "Reference(ServiceRequest)", "The referral information received by the claim author, it is not to be used when the author generates a referral for a patient. A copy of that referral may be provided as supporting information. Some insurers require proof of referral to pay for services or to pay specialist rates for services.", 0, 1, referral); 17647 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "Healthcare encounters related to this claim.", 0, java.lang.Integer.MAX_VALUE, encounter); 17648 case 501116579: /*facility*/ return new Property("facility", "Reference(Location|Organization)", "Facility where the services were provided.", 0, 1, facility); 17649 case 94742588: /*claim*/ return new Property("claim", "Reference(Claim)", "The business identifier for the instance of the adjudication request: claim predetermination or preauthorization.", 0, 1, claim); 17650 case 689513629: /*claimResponse*/ return new Property("claimResponse", "Reference(ClaimResponse)", "The business identifier for the instance of the adjudication response: claim, predetermination or preauthorization response.", 0, 1, claimResponse); 17651 case -1106507950: /*outcome*/ return new Property("outcome", "code", "The outcome of the claim, predetermination, or preauthorization processing.", 0, 1, outcome); 17652 case 565719004: /*decision*/ return new Property("decision", "CodeableConcept", "The result of the claim, predetermination, or preauthorization adjudication.", 0, 1, decision); 17653 case 583380919: /*disposition*/ return new Property("disposition", "string", "A human readable description of the status of the adjudication.", 0, 1, disposition); 17654 case 522246568: /*preAuthRef*/ return new Property("preAuthRef", "string", "Reference from the Insurer which is used in later communications which refers to this adjudication.", 0, java.lang.Integer.MAX_VALUE, preAuthRef); 17655 case -1262920311: /*preAuthRefPeriod*/ return new Property("preAuthRefPeriod", "Period", "The timeframe during which the supplied preauthorization reference may be quoted on claims to obtain the adjudication as provided.", 0, java.lang.Integer.MAX_VALUE, preAuthRefPeriod); 17656 case -1599182171: /*diagnosisRelatedGroup*/ return new Property("diagnosisRelatedGroup", "CodeableConcept", "A package billing code or bundle code used to group products and services to a particular health condition (such as heart attack) which is based on a predetermined grouping code system.", 0, 1, diagnosisRelatedGroup); 17657 case -7323378: /*careTeam*/ return new Property("careTeam", "", "The members of the team who provided the products and services.", 0, java.lang.Integer.MAX_VALUE, careTeam); 17658 case 1922406657: /*supportingInfo*/ return new Property("supportingInfo", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues.", 0, java.lang.Integer.MAX_VALUE, supportingInfo); 17659 case 1196993265: /*diagnosis*/ return new Property("diagnosis", "", "Information about diagnoses relevant to the claim items.", 0, java.lang.Integer.MAX_VALUE, diagnosis); 17660 case -1095204141: /*procedure*/ return new Property("procedure", "", "Procedures performed on the patient relevant to the billing items with the claim.", 0, java.lang.Integer.MAX_VALUE, procedure); 17661 case 159695370: /*precedence*/ return new Property("precedence", "positiveInt", "This indicates the relative order of a series of EOBs related to different coverages for the same suite of services.", 0, 1, precedence); 17662 case 73049818: /*insurance*/ return new Property("insurance", "", "Financial instruments for reimbursement for the health care products and services specified on the claim.", 0, java.lang.Integer.MAX_VALUE, insurance); 17663 case -2143202801: /*accident*/ return new Property("accident", "", "Details of a accident which resulted in injuries which required the products and services listed in the claim.", 0, 1, accident); 17664 case 525514609: /*patientPaid*/ return new Property("patientPaid", "Money", "The amount paid by the patient, in total at the claim claim level or specifically for the item and detail level, to the provider for goods and services.", 0, 1, patientPaid); 17665 case 3242771: /*item*/ return new Property("item", "", "A claim line. Either a simple (a product or service) or a 'group' of details which can also be a simple items or groups of sub-details.", 0, java.lang.Integer.MAX_VALUE, item); 17666 case -1148899500: /*addItem*/ return new Property("addItem", "", "The first-tier service adjudications for payor added product or service lines.", 0, java.lang.Integer.MAX_VALUE, addItem); 17667 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudication results which are presented at the header level rather than at the line-item or add-item levels.", 0, java.lang.Integer.MAX_VALUE, adjudication); 17668 case 110549828: /*total*/ return new Property("total", "", "Categorized monetary totals for the adjudication.", 0, java.lang.Integer.MAX_VALUE, total); 17669 case -786681338: /*payment*/ return new Property("payment", "", "Payment details for the adjudication of the claim.", 0, 1, payment); 17670 case 473181393: /*formCode*/ return new Property("formCode", "CodeableConcept", "A code for the form to be used for printing the content.", 0, 1, formCode); 17671 case 3148996: /*form*/ return new Property("form", "Attachment", "The actual form, by reference or inclusion, for printing the content or an EOB.", 0, 1, form); 17672 case 202339073: /*processNote*/ return new Property("processNote", "", "A note that describes or explains adjudication results in a human readable form.", 0, java.lang.Integer.MAX_VALUE, processNote); 17673 case -407369416: /*benefitPeriod*/ return new Property("benefitPeriod", "Period", "The term of the benefits documented in this response.", 0, 1, benefitPeriod); 17674 case 596003397: /*benefitBalance*/ return new Property("benefitBalance", "", "Balance by Benefit Category.", 0, java.lang.Integer.MAX_VALUE, benefitBalance); 17675 default: return super.getNamedProperty(_hash, _name, _checkValid); 17676 } 17677 17678 } 17679 17680 @Override 17681 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 17682 switch (hash) { 17683 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 17684 case 82505966: /*traceNumber*/ return this.traceNumber == null ? new Base[0] : this.traceNumber.toArray(new Base[this.traceNumber.size()]); // Identifier 17685 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ExplanationOfBenefitStatus> 17686 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 17687 case -1868521062: /*subType*/ return this.subType == null ? new Base[0] : new Base[] {this.subType}; // CodeableConcept 17688 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<Use> 17689 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 17690 case -332066046: /*billablePeriod*/ return this.billablePeriod == null ? new Base[0] : new Base[] {this.billablePeriod}; // Period 17691 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 17692 case -1591951995: /*enterer*/ return this.enterer == null ? new Base[0] : new Base[] {this.enterer}; // Reference 17693 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 17694 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 17695 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // CodeableConcept 17696 case -1688904576: /*fundsReserveRequested*/ return this.fundsReserveRequested == null ? new Base[0] : new Base[] {this.fundsReserveRequested}; // CodeableConcept 17697 case 1314609806: /*fundsReserve*/ return this.fundsReserve == null ? new Base[0] : new Base[] {this.fundsReserve}; // CodeableConcept 17698 case 1090493483: /*related*/ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // RelatedClaimComponent 17699 case 460301338: /*prescription*/ return this.prescription == null ? new Base[0] : new Base[] {this.prescription}; // Reference 17700 case -1814015861: /*originalPrescription*/ return this.originalPrescription == null ? new Base[0] : new Base[] {this.originalPrescription}; // Reference 17701 case 96891546: /*event*/ return this.event == null ? new Base[0] : this.event.toArray(new Base[this.event.size()]); // ExplanationOfBenefitEventComponent 17702 case 106443592: /*payee*/ return this.payee == null ? new Base[0] : new Base[] {this.payee}; // PayeeComponent 17703 case -722568291: /*referral*/ return this.referral == null ? new Base[0] : new Base[] {this.referral}; // Reference 17704 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 17705 case 501116579: /*facility*/ return this.facility == null ? new Base[0] : new Base[] {this.facility}; // Reference 17706 case 94742588: /*claim*/ return this.claim == null ? new Base[0] : new Base[] {this.claim}; // Reference 17707 case 689513629: /*claimResponse*/ return this.claimResponse == null ? new Base[0] : new Base[] {this.claimResponse}; // Reference 17708 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // Enumeration<ClaimProcessingCodes> 17709 case 565719004: /*decision*/ return this.decision == null ? new Base[0] : new Base[] {this.decision}; // CodeableConcept 17710 case 583380919: /*disposition*/ return this.disposition == null ? new Base[0] : new Base[] {this.disposition}; // StringType 17711 case 522246568: /*preAuthRef*/ return this.preAuthRef == null ? new Base[0] : this.preAuthRef.toArray(new Base[this.preAuthRef.size()]); // StringType 17712 case -1262920311: /*preAuthRefPeriod*/ return this.preAuthRefPeriod == null ? new Base[0] : this.preAuthRefPeriod.toArray(new Base[this.preAuthRefPeriod.size()]); // Period 17713 case -1599182171: /*diagnosisRelatedGroup*/ return this.diagnosisRelatedGroup == null ? new Base[0] : new Base[] {this.diagnosisRelatedGroup}; // CodeableConcept 17714 case -7323378: /*careTeam*/ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // CareTeamComponent 17715 case 1922406657: /*supportingInfo*/ return this.supportingInfo == null ? new Base[0] : this.supportingInfo.toArray(new Base[this.supportingInfo.size()]); // SupportingInformationComponent 17716 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 17717 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : this.procedure.toArray(new Base[this.procedure.size()]); // ProcedureComponent 17718 case 159695370: /*precedence*/ return this.precedence == null ? new Base[0] : new Base[] {this.precedence}; // PositiveIntType 17719 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 17720 case -2143202801: /*accident*/ return this.accident == null ? new Base[0] : new Base[] {this.accident}; // AccidentComponent 17721 case 525514609: /*patientPaid*/ return this.patientPaid == null ? new Base[0] : new Base[] {this.patientPaid}; // Money 17722 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemComponent 17723 case -1148899500: /*addItem*/ return this.addItem == null ? new Base[0] : this.addItem.toArray(new Base[this.addItem.size()]); // AddedItemComponent 17724 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 17725 case 110549828: /*total*/ return this.total == null ? new Base[0] : this.total.toArray(new Base[this.total.size()]); // TotalComponent 17726 case -786681338: /*payment*/ return this.payment == null ? new Base[0] : new Base[] {this.payment}; // PaymentComponent 17727 case 473181393: /*formCode*/ return this.formCode == null ? new Base[0] : new Base[] {this.formCode}; // CodeableConcept 17728 case 3148996: /*form*/ return this.form == null ? new Base[0] : new Base[] {this.form}; // Attachment 17729 case 202339073: /*processNote*/ return this.processNote == null ? new Base[0] : this.processNote.toArray(new Base[this.processNote.size()]); // NoteComponent 17730 case -407369416: /*benefitPeriod*/ return this.benefitPeriod == null ? new Base[0] : new Base[] {this.benefitPeriod}; // Period 17731 case 596003397: /*benefitBalance*/ return this.benefitBalance == null ? new Base[0] : this.benefitBalance.toArray(new Base[this.benefitBalance.size()]); // BenefitBalanceComponent 17732 default: return super.getProperty(hash, name, checkValid); 17733 } 17734 17735 } 17736 17737 @Override 17738 public Base setProperty(int hash, String name, Base value) throws FHIRException { 17739 switch (hash) { 17740 case -1618432855: // identifier 17741 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 17742 return value; 17743 case 82505966: // traceNumber 17744 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); // Identifier 17745 return value; 17746 case -892481550: // status 17747 value = new ExplanationOfBenefitStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 17748 this.status = (Enumeration) value; // Enumeration<ExplanationOfBenefitStatus> 17749 return value; 17750 case 3575610: // type 17751 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17752 return value; 17753 case -1868521062: // subType 17754 this.subType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17755 return value; 17756 case 116103: // use 17757 value = new UseEnumFactory().fromType(TypeConvertor.castToCode(value)); 17758 this.use = (Enumeration) value; // Enumeration<Use> 17759 return value; 17760 case -791418107: // patient 17761 this.patient = TypeConvertor.castToReference(value); // Reference 17762 return value; 17763 case -332066046: // billablePeriod 17764 this.billablePeriod = TypeConvertor.castToPeriod(value); // Period 17765 return value; 17766 case 1028554472: // created 17767 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 17768 return value; 17769 case -1591951995: // enterer 17770 this.enterer = TypeConvertor.castToReference(value); // Reference 17771 return value; 17772 case 1957615864: // insurer 17773 this.insurer = TypeConvertor.castToReference(value); // Reference 17774 return value; 17775 case -987494927: // provider 17776 this.provider = TypeConvertor.castToReference(value); // Reference 17777 return value; 17778 case -1165461084: // priority 17779 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17780 return value; 17781 case -1688904576: // fundsReserveRequested 17782 this.fundsReserveRequested = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17783 return value; 17784 case 1314609806: // fundsReserve 17785 this.fundsReserve = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17786 return value; 17787 case 1090493483: // related 17788 this.getRelated().add((RelatedClaimComponent) value); // RelatedClaimComponent 17789 return value; 17790 case 460301338: // prescription 17791 this.prescription = TypeConvertor.castToReference(value); // Reference 17792 return value; 17793 case -1814015861: // originalPrescription 17794 this.originalPrescription = TypeConvertor.castToReference(value); // Reference 17795 return value; 17796 case 96891546: // event 17797 this.getEvent().add((ExplanationOfBenefitEventComponent) value); // ExplanationOfBenefitEventComponent 17798 return value; 17799 case 106443592: // payee 17800 this.payee = (PayeeComponent) value; // PayeeComponent 17801 return value; 17802 case -722568291: // referral 17803 this.referral = TypeConvertor.castToReference(value); // Reference 17804 return value; 17805 case 1524132147: // encounter 17806 this.getEncounter().add(TypeConvertor.castToReference(value)); // Reference 17807 return value; 17808 case 501116579: // facility 17809 this.facility = TypeConvertor.castToReference(value); // Reference 17810 return value; 17811 case 94742588: // claim 17812 this.claim = TypeConvertor.castToReference(value); // Reference 17813 return value; 17814 case 689513629: // claimResponse 17815 this.claimResponse = TypeConvertor.castToReference(value); // Reference 17816 return value; 17817 case -1106507950: // outcome 17818 value = new ClaimProcessingCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 17819 this.outcome = (Enumeration) value; // Enumeration<ClaimProcessingCodes> 17820 return value; 17821 case 565719004: // decision 17822 this.decision = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17823 return value; 17824 case 583380919: // disposition 17825 this.disposition = TypeConvertor.castToString(value); // StringType 17826 return value; 17827 case 522246568: // preAuthRef 17828 this.getPreAuthRef().add(TypeConvertor.castToString(value)); // StringType 17829 return value; 17830 case -1262920311: // preAuthRefPeriod 17831 this.getPreAuthRefPeriod().add(TypeConvertor.castToPeriod(value)); // Period 17832 return value; 17833 case -1599182171: // diagnosisRelatedGroup 17834 this.diagnosisRelatedGroup = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17835 return value; 17836 case -7323378: // careTeam 17837 this.getCareTeam().add((CareTeamComponent) value); // CareTeamComponent 17838 return value; 17839 case 1922406657: // supportingInfo 17840 this.getSupportingInfo().add((SupportingInformationComponent) value); // SupportingInformationComponent 17841 return value; 17842 case 1196993265: // diagnosis 17843 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 17844 return value; 17845 case -1095204141: // procedure 17846 this.getProcedure().add((ProcedureComponent) value); // ProcedureComponent 17847 return value; 17848 case 159695370: // precedence 17849 this.precedence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 17850 return value; 17851 case 73049818: // insurance 17852 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 17853 return value; 17854 case -2143202801: // accident 17855 this.accident = (AccidentComponent) value; // AccidentComponent 17856 return value; 17857 case 525514609: // patientPaid 17858 this.patientPaid = TypeConvertor.castToMoney(value); // Money 17859 return value; 17860 case 3242771: // item 17861 this.getItem().add((ItemComponent) value); // ItemComponent 17862 return value; 17863 case -1148899500: // addItem 17864 this.getAddItem().add((AddedItemComponent) value); // AddedItemComponent 17865 return value; 17866 case -231349275: // adjudication 17867 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 17868 return value; 17869 case 110549828: // total 17870 this.getTotal().add((TotalComponent) value); // TotalComponent 17871 return value; 17872 case -786681338: // payment 17873 this.payment = (PaymentComponent) value; // PaymentComponent 17874 return value; 17875 case 473181393: // formCode 17876 this.formCode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17877 return value; 17878 case 3148996: // form 17879 this.form = TypeConvertor.castToAttachment(value); // Attachment 17880 return value; 17881 case 202339073: // processNote 17882 this.getProcessNote().add((NoteComponent) value); // NoteComponent 17883 return value; 17884 case -407369416: // benefitPeriod 17885 this.benefitPeriod = TypeConvertor.castToPeriod(value); // Period 17886 return value; 17887 case 596003397: // benefitBalance 17888 this.getBenefitBalance().add((BenefitBalanceComponent) value); // BenefitBalanceComponent 17889 return value; 17890 default: return super.setProperty(hash, name, value); 17891 } 17892 17893 } 17894 17895 @Override 17896 public Base setProperty(String name, Base value) throws FHIRException { 17897 if (name.equals("identifier")) { 17898 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 17899 } else if (name.equals("traceNumber")) { 17900 this.getTraceNumber().add(TypeConvertor.castToIdentifier(value)); 17901 } else if (name.equals("status")) { 17902 value = new ExplanationOfBenefitStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 17903 this.status = (Enumeration) value; // Enumeration<ExplanationOfBenefitStatus> 17904 } else if (name.equals("type")) { 17905 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17906 } else if (name.equals("subType")) { 17907 this.subType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17908 } else if (name.equals("use")) { 17909 value = new UseEnumFactory().fromType(TypeConvertor.castToCode(value)); 17910 this.use = (Enumeration) value; // Enumeration<Use> 17911 } else if (name.equals("patient")) { 17912 this.patient = TypeConvertor.castToReference(value); // Reference 17913 } else if (name.equals("billablePeriod")) { 17914 this.billablePeriod = TypeConvertor.castToPeriod(value); // Period 17915 } else if (name.equals("created")) { 17916 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 17917 } else if (name.equals("enterer")) { 17918 this.enterer = TypeConvertor.castToReference(value); // Reference 17919 } else if (name.equals("insurer")) { 17920 this.insurer = TypeConvertor.castToReference(value); // Reference 17921 } else if (name.equals("provider")) { 17922 this.provider = TypeConvertor.castToReference(value); // Reference 17923 } else if (name.equals("priority")) { 17924 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17925 } else if (name.equals("fundsReserveRequested")) { 17926 this.fundsReserveRequested = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17927 } else if (name.equals("fundsReserve")) { 17928 this.fundsReserve = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17929 } else if (name.equals("related")) { 17930 this.getRelated().add((RelatedClaimComponent) value); 17931 } else if (name.equals("prescription")) { 17932 this.prescription = TypeConvertor.castToReference(value); // Reference 17933 } else if (name.equals("originalPrescription")) { 17934 this.originalPrescription = TypeConvertor.castToReference(value); // Reference 17935 } else if (name.equals("event")) { 17936 this.getEvent().add((ExplanationOfBenefitEventComponent) value); 17937 } else if (name.equals("payee")) { 17938 this.payee = (PayeeComponent) value; // PayeeComponent 17939 } else if (name.equals("referral")) { 17940 this.referral = TypeConvertor.castToReference(value); // Reference 17941 } else if (name.equals("encounter")) { 17942 this.getEncounter().add(TypeConvertor.castToReference(value)); 17943 } else if (name.equals("facility")) { 17944 this.facility = TypeConvertor.castToReference(value); // Reference 17945 } else if (name.equals("claim")) { 17946 this.claim = TypeConvertor.castToReference(value); // Reference 17947 } else if (name.equals("claimResponse")) { 17948 this.claimResponse = TypeConvertor.castToReference(value); // Reference 17949 } else if (name.equals("outcome")) { 17950 value = new ClaimProcessingCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 17951 this.outcome = (Enumeration) value; // Enumeration<ClaimProcessingCodes> 17952 } else if (name.equals("decision")) { 17953 this.decision = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17954 } else if (name.equals("disposition")) { 17955 this.disposition = TypeConvertor.castToString(value); // StringType 17956 } else if (name.equals("preAuthRef")) { 17957 this.getPreAuthRef().add(TypeConvertor.castToString(value)); 17958 } else if (name.equals("preAuthRefPeriod")) { 17959 this.getPreAuthRefPeriod().add(TypeConvertor.castToPeriod(value)); 17960 } else if (name.equals("diagnosisRelatedGroup")) { 17961 this.diagnosisRelatedGroup = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17962 } else if (name.equals("careTeam")) { 17963 this.getCareTeam().add((CareTeamComponent) value); 17964 } else if (name.equals("supportingInfo")) { 17965 this.getSupportingInfo().add((SupportingInformationComponent) value); 17966 } else if (name.equals("diagnosis")) { 17967 this.getDiagnosis().add((DiagnosisComponent) value); 17968 } else if (name.equals("procedure")) { 17969 this.getProcedure().add((ProcedureComponent) value); 17970 } else if (name.equals("precedence")) { 17971 this.precedence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 17972 } else if (name.equals("insurance")) { 17973 this.getInsurance().add((InsuranceComponent) value); 17974 } else if (name.equals("accident")) { 17975 this.accident = (AccidentComponent) value; // AccidentComponent 17976 } else if (name.equals("patientPaid")) { 17977 this.patientPaid = TypeConvertor.castToMoney(value); // Money 17978 } else if (name.equals("item")) { 17979 this.getItem().add((ItemComponent) value); 17980 } else if (name.equals("addItem")) { 17981 this.getAddItem().add((AddedItemComponent) value); 17982 } else if (name.equals("adjudication")) { 17983 this.getAdjudication().add((AdjudicationComponent) value); 17984 } else if (name.equals("total")) { 17985 this.getTotal().add((TotalComponent) value); 17986 } else if (name.equals("payment")) { 17987 this.payment = (PaymentComponent) value; // PaymentComponent 17988 } else if (name.equals("formCode")) { 17989 this.formCode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 17990 } else if (name.equals("form")) { 17991 this.form = TypeConvertor.castToAttachment(value); // Attachment 17992 } else if (name.equals("processNote")) { 17993 this.getProcessNote().add((NoteComponent) value); 17994 } else if (name.equals("benefitPeriod")) { 17995 this.benefitPeriod = TypeConvertor.castToPeriod(value); // Period 17996 } else if (name.equals("benefitBalance")) { 17997 this.getBenefitBalance().add((BenefitBalanceComponent) value); 17998 } else 17999 return super.setProperty(name, value); 18000 return value; 18001 } 18002 18003 @Override 18004 public void removeChild(String name, Base value) throws FHIRException { 18005 if (name.equals("identifier")) { 18006 this.getIdentifier().remove(value); 18007 } else if (name.equals("traceNumber")) { 18008 this.getTraceNumber().remove(value); 18009 } else if (name.equals("status")) { 18010 value = new ExplanationOfBenefitStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 18011 this.status = (Enumeration) value; // Enumeration<ExplanationOfBenefitStatus> 18012 } else if (name.equals("type")) { 18013 this.type = null; 18014 } else if (name.equals("subType")) { 18015 this.subType = null; 18016 } else if (name.equals("use")) { 18017 value = new UseEnumFactory().fromType(TypeConvertor.castToCode(value)); 18018 this.use = (Enumeration) value; // Enumeration<Use> 18019 } else if (name.equals("patient")) { 18020 this.patient = null; 18021 } else if (name.equals("billablePeriod")) { 18022 this.billablePeriod = null; 18023 } else if (name.equals("created")) { 18024 this.created = null; 18025 } else if (name.equals("enterer")) { 18026 this.enterer = null; 18027 } else if (name.equals("insurer")) { 18028 this.insurer = null; 18029 } else if (name.equals("provider")) { 18030 this.provider = null; 18031 } else if (name.equals("priority")) { 18032 this.priority = null; 18033 } else if (name.equals("fundsReserveRequested")) { 18034 this.fundsReserveRequested = null; 18035 } else if (name.equals("fundsReserve")) { 18036 this.fundsReserve = null; 18037 } else if (name.equals("related")) { 18038 this.getRelated().remove((RelatedClaimComponent) value); 18039 } else if (name.equals("prescription")) { 18040 this.prescription = null; 18041 } else if (name.equals("originalPrescription")) { 18042 this.originalPrescription = null; 18043 } else if (name.equals("event")) { 18044 this.getEvent().remove((ExplanationOfBenefitEventComponent) value); 18045 } else if (name.equals("payee")) { 18046 this.payee = (PayeeComponent) value; // PayeeComponent 18047 } else if (name.equals("referral")) { 18048 this.referral = null; 18049 } else if (name.equals("encounter")) { 18050 this.getEncounter().remove(value); 18051 } else if (name.equals("facility")) { 18052 this.facility = null; 18053 } else if (name.equals("claim")) { 18054 this.claim = null; 18055 } else if (name.equals("claimResponse")) { 18056 this.claimResponse = null; 18057 } else if (name.equals("outcome")) { 18058 value = new ClaimProcessingCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 18059 this.outcome = (Enumeration) value; // Enumeration<ClaimProcessingCodes> 18060 } else if (name.equals("decision")) { 18061 this.decision = null; 18062 } else if (name.equals("disposition")) { 18063 this.disposition = null; 18064 } else if (name.equals("preAuthRef")) { 18065 this.getPreAuthRef().remove(value); 18066 } else if (name.equals("preAuthRefPeriod")) { 18067 this.getPreAuthRefPeriod().remove(value); 18068 } else if (name.equals("diagnosisRelatedGroup")) { 18069 this.diagnosisRelatedGroup = null; 18070 } else if (name.equals("careTeam")) { 18071 this.getCareTeam().remove((CareTeamComponent) value); 18072 } else if (name.equals("supportingInfo")) { 18073 this.getSupportingInfo().remove((SupportingInformationComponent) value); 18074 } else if (name.equals("diagnosis")) { 18075 this.getDiagnosis().remove((DiagnosisComponent) value); 18076 } else if (name.equals("procedure")) { 18077 this.getProcedure().remove((ProcedureComponent) value); 18078 } else if (name.equals("precedence")) { 18079 this.precedence = null; 18080 } else if (name.equals("insurance")) { 18081 this.getInsurance().remove((InsuranceComponent) value); 18082 } else if (name.equals("accident")) { 18083 this.accident = (AccidentComponent) value; // AccidentComponent 18084 } else if (name.equals("patientPaid")) { 18085 this.patientPaid = null; 18086 } else if (name.equals("item")) { 18087 this.getItem().remove((ItemComponent) value); 18088 } else if (name.equals("addItem")) { 18089 this.getAddItem().remove((AddedItemComponent) value); 18090 } else if (name.equals("adjudication")) { 18091 this.getAdjudication().remove((AdjudicationComponent) value); 18092 } else if (name.equals("total")) { 18093 this.getTotal().remove((TotalComponent) value); 18094 } else if (name.equals("payment")) { 18095 this.payment = (PaymentComponent) value; // PaymentComponent 18096 } else if (name.equals("formCode")) { 18097 this.formCode = null; 18098 } else if (name.equals("form")) { 18099 this.form = null; 18100 } else if (name.equals("processNote")) { 18101 this.getProcessNote().remove((NoteComponent) value); 18102 } else if (name.equals("benefitPeriod")) { 18103 this.benefitPeriod = null; 18104 } else if (name.equals("benefitBalance")) { 18105 this.getBenefitBalance().remove((BenefitBalanceComponent) value); 18106 } else 18107 super.removeChild(name, value); 18108 18109 } 18110 18111 @Override 18112 public Base makeProperty(int hash, String name) throws FHIRException { 18113 switch (hash) { 18114 case -1618432855: return addIdentifier(); 18115 case 82505966: return addTraceNumber(); 18116 case -892481550: return getStatusElement(); 18117 case 3575610: return getType(); 18118 case -1868521062: return getSubType(); 18119 case 116103: return getUseElement(); 18120 case -791418107: return getPatient(); 18121 case -332066046: return getBillablePeriod(); 18122 case 1028554472: return getCreatedElement(); 18123 case -1591951995: return getEnterer(); 18124 case 1957615864: return getInsurer(); 18125 case -987494927: return getProvider(); 18126 case -1165461084: return getPriority(); 18127 case -1688904576: return getFundsReserveRequested(); 18128 case 1314609806: return getFundsReserve(); 18129 case 1090493483: return addRelated(); 18130 case 460301338: return getPrescription(); 18131 case -1814015861: return getOriginalPrescription(); 18132 case 96891546: return addEvent(); 18133 case 106443592: return getPayee(); 18134 case -722568291: return getReferral(); 18135 case 1524132147: return addEncounter(); 18136 case 501116579: return getFacility(); 18137 case 94742588: return getClaim(); 18138 case 689513629: return getClaimResponse(); 18139 case -1106507950: return getOutcomeElement(); 18140 case 565719004: return getDecision(); 18141 case 583380919: return getDispositionElement(); 18142 case 522246568: return addPreAuthRefElement(); 18143 case -1262920311: return addPreAuthRefPeriod(); 18144 case -1599182171: return getDiagnosisRelatedGroup(); 18145 case -7323378: return addCareTeam(); 18146 case 1922406657: return addSupportingInfo(); 18147 case 1196993265: return addDiagnosis(); 18148 case -1095204141: return addProcedure(); 18149 case 159695370: return getPrecedenceElement(); 18150 case 73049818: return addInsurance(); 18151 case -2143202801: return getAccident(); 18152 case 525514609: return getPatientPaid(); 18153 case 3242771: return addItem(); 18154 case -1148899500: return addAddItem(); 18155 case -231349275: return addAdjudication(); 18156 case 110549828: return addTotal(); 18157 case -786681338: return getPayment(); 18158 case 473181393: return getFormCode(); 18159 case 3148996: return getForm(); 18160 case 202339073: return addProcessNote(); 18161 case -407369416: return getBenefitPeriod(); 18162 case 596003397: return addBenefitBalance(); 18163 default: return super.makeProperty(hash, name); 18164 } 18165 18166 } 18167 18168 @Override 18169 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 18170 switch (hash) { 18171 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 18172 case 82505966: /*traceNumber*/ return new String[] {"Identifier"}; 18173 case -892481550: /*status*/ return new String[] {"code"}; 18174 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 18175 case -1868521062: /*subType*/ return new String[] {"CodeableConcept"}; 18176 case 116103: /*use*/ return new String[] {"code"}; 18177 case -791418107: /*patient*/ return new String[] {"Reference"}; 18178 case -332066046: /*billablePeriod*/ return new String[] {"Period"}; 18179 case 1028554472: /*created*/ return new String[] {"dateTime"}; 18180 case -1591951995: /*enterer*/ return new String[] {"Reference"}; 18181 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 18182 case -987494927: /*provider*/ return new String[] {"Reference"}; 18183 case -1165461084: /*priority*/ return new String[] {"CodeableConcept"}; 18184 case -1688904576: /*fundsReserveRequested*/ return new String[] {"CodeableConcept"}; 18185 case 1314609806: /*fundsReserve*/ return new String[] {"CodeableConcept"}; 18186 case 1090493483: /*related*/ return new String[] {}; 18187 case 460301338: /*prescription*/ return new String[] {"Reference"}; 18188 case -1814015861: /*originalPrescription*/ return new String[] {"Reference"}; 18189 case 96891546: /*event*/ return new String[] {}; 18190 case 106443592: /*payee*/ return new String[] {}; 18191 case -722568291: /*referral*/ return new String[] {"Reference"}; 18192 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 18193 case 501116579: /*facility*/ return new String[] {"Reference"}; 18194 case 94742588: /*claim*/ return new String[] {"Reference"}; 18195 case 689513629: /*claimResponse*/ return new String[] {"Reference"}; 18196 case -1106507950: /*outcome*/ return new String[] {"code"}; 18197 case 565719004: /*decision*/ return new String[] {"CodeableConcept"}; 18198 case 583380919: /*disposition*/ return new String[] {"string"}; 18199 case 522246568: /*preAuthRef*/ return new String[] {"string"}; 18200 case -1262920311: /*preAuthRefPeriod*/ return new String[] {"Period"}; 18201 case -1599182171: /*diagnosisRelatedGroup*/ return new String[] {"CodeableConcept"}; 18202 case -7323378: /*careTeam*/ return new String[] {}; 18203 case 1922406657: /*supportingInfo*/ return new String[] {}; 18204 case 1196993265: /*diagnosis*/ return new String[] {}; 18205 case -1095204141: /*procedure*/ return new String[] {}; 18206 case 159695370: /*precedence*/ return new String[] {"positiveInt"}; 18207 case 73049818: /*insurance*/ return new String[] {}; 18208 case -2143202801: /*accident*/ return new String[] {}; 18209 case 525514609: /*patientPaid*/ return new String[] {"Money"}; 18210 case 3242771: /*item*/ return new String[] {}; 18211 case -1148899500: /*addItem*/ return new String[] {}; 18212 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 18213 case 110549828: /*total*/ return new String[] {}; 18214 case -786681338: /*payment*/ return new String[] {}; 18215 case 473181393: /*formCode*/ return new String[] {"CodeableConcept"}; 18216 case 3148996: /*form*/ return new String[] {"Attachment"}; 18217 case 202339073: /*processNote*/ return new String[] {}; 18218 case -407369416: /*benefitPeriod*/ return new String[] {"Period"}; 18219 case 596003397: /*benefitBalance*/ return new String[] {}; 18220 default: return super.getTypesForProperty(hash, name); 18221 } 18222 18223 } 18224 18225 @Override 18226 public Base addChild(String name) throws FHIRException { 18227 if (name.equals("identifier")) { 18228 return addIdentifier(); 18229 } 18230 else if (name.equals("traceNumber")) { 18231 return addTraceNumber(); 18232 } 18233 else if (name.equals("status")) { 18234 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.status"); 18235 } 18236 else if (name.equals("type")) { 18237 this.type = new CodeableConcept(); 18238 return this.type; 18239 } 18240 else if (name.equals("subType")) { 18241 this.subType = new CodeableConcept(); 18242 return this.subType; 18243 } 18244 else if (name.equals("use")) { 18245 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.use"); 18246 } 18247 else if (name.equals("patient")) { 18248 this.patient = new Reference(); 18249 return this.patient; 18250 } 18251 else if (name.equals("billablePeriod")) { 18252 this.billablePeriod = new Period(); 18253 return this.billablePeriod; 18254 } 18255 else if (name.equals("created")) { 18256 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.created"); 18257 } 18258 else if (name.equals("enterer")) { 18259 this.enterer = new Reference(); 18260 return this.enterer; 18261 } 18262 else if (name.equals("insurer")) { 18263 this.insurer = new Reference(); 18264 return this.insurer; 18265 } 18266 else if (name.equals("provider")) { 18267 this.provider = new Reference(); 18268 return this.provider; 18269 } 18270 else if (name.equals("priority")) { 18271 this.priority = new CodeableConcept(); 18272 return this.priority; 18273 } 18274 else if (name.equals("fundsReserveRequested")) { 18275 this.fundsReserveRequested = new CodeableConcept(); 18276 return this.fundsReserveRequested; 18277 } 18278 else if (name.equals("fundsReserve")) { 18279 this.fundsReserve = new CodeableConcept(); 18280 return this.fundsReserve; 18281 } 18282 else if (name.equals("related")) { 18283 return addRelated(); 18284 } 18285 else if (name.equals("prescription")) { 18286 this.prescription = new Reference(); 18287 return this.prescription; 18288 } 18289 else if (name.equals("originalPrescription")) { 18290 this.originalPrescription = new Reference(); 18291 return this.originalPrescription; 18292 } 18293 else if (name.equals("event")) { 18294 return addEvent(); 18295 } 18296 else if (name.equals("payee")) { 18297 this.payee = new PayeeComponent(); 18298 return this.payee; 18299 } 18300 else if (name.equals("referral")) { 18301 this.referral = new Reference(); 18302 return this.referral; 18303 } 18304 else if (name.equals("encounter")) { 18305 return addEncounter(); 18306 } 18307 else if (name.equals("facility")) { 18308 this.facility = new Reference(); 18309 return this.facility; 18310 } 18311 else if (name.equals("claim")) { 18312 this.claim = new Reference(); 18313 return this.claim; 18314 } 18315 else if (name.equals("claimResponse")) { 18316 this.claimResponse = new Reference(); 18317 return this.claimResponse; 18318 } 18319 else if (name.equals("outcome")) { 18320 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.outcome"); 18321 } 18322 else if (name.equals("decision")) { 18323 this.decision = new CodeableConcept(); 18324 return this.decision; 18325 } 18326 else if (name.equals("disposition")) { 18327 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.disposition"); 18328 } 18329 else if (name.equals("preAuthRef")) { 18330 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.preAuthRef"); 18331 } 18332 else if (name.equals("preAuthRefPeriod")) { 18333 return addPreAuthRefPeriod(); 18334 } 18335 else if (name.equals("diagnosisRelatedGroup")) { 18336 this.diagnosisRelatedGroup = new CodeableConcept(); 18337 return this.diagnosisRelatedGroup; 18338 } 18339 else if (name.equals("careTeam")) { 18340 return addCareTeam(); 18341 } 18342 else if (name.equals("supportingInfo")) { 18343 return addSupportingInfo(); 18344 } 18345 else if (name.equals("diagnosis")) { 18346 return addDiagnosis(); 18347 } 18348 else if (name.equals("procedure")) { 18349 return addProcedure(); 18350 } 18351 else if (name.equals("precedence")) { 18352 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.precedence"); 18353 } 18354 else if (name.equals("insurance")) { 18355 return addInsurance(); 18356 } 18357 else if (name.equals("accident")) { 18358 this.accident = new AccidentComponent(); 18359 return this.accident; 18360 } 18361 else if (name.equals("patientPaid")) { 18362 this.patientPaid = new Money(); 18363 return this.patientPaid; 18364 } 18365 else if (name.equals("item")) { 18366 return addItem(); 18367 } 18368 else if (name.equals("addItem")) { 18369 return addAddItem(); 18370 } 18371 else if (name.equals("adjudication")) { 18372 return addAdjudication(); 18373 } 18374 else if (name.equals("total")) { 18375 return addTotal(); 18376 } 18377 else if (name.equals("payment")) { 18378 this.payment = new PaymentComponent(); 18379 return this.payment; 18380 } 18381 else if (name.equals("formCode")) { 18382 this.formCode = new CodeableConcept(); 18383 return this.formCode; 18384 } 18385 else if (name.equals("form")) { 18386 this.form = new Attachment(); 18387 return this.form; 18388 } 18389 else if (name.equals("processNote")) { 18390 return addProcessNote(); 18391 } 18392 else if (name.equals("benefitPeriod")) { 18393 this.benefitPeriod = new Period(); 18394 return this.benefitPeriod; 18395 } 18396 else if (name.equals("benefitBalance")) { 18397 return addBenefitBalance(); 18398 } 18399 else 18400 return super.addChild(name); 18401 } 18402 18403 public String fhirType() { 18404 return "ExplanationOfBenefit"; 18405 18406 } 18407 18408 public ExplanationOfBenefit copy() { 18409 ExplanationOfBenefit dst = new ExplanationOfBenefit(); 18410 copyValues(dst); 18411 return dst; 18412 } 18413 18414 public void copyValues(ExplanationOfBenefit dst) { 18415 super.copyValues(dst); 18416 if (identifier != null) { 18417 dst.identifier = new ArrayList<Identifier>(); 18418 for (Identifier i : identifier) 18419 dst.identifier.add(i.copy()); 18420 }; 18421 if (traceNumber != null) { 18422 dst.traceNumber = new ArrayList<Identifier>(); 18423 for (Identifier i : traceNumber) 18424 dst.traceNumber.add(i.copy()); 18425 }; 18426 dst.status = status == null ? null : status.copy(); 18427 dst.type = type == null ? null : type.copy(); 18428 dst.subType = subType == null ? null : subType.copy(); 18429 dst.use = use == null ? null : use.copy(); 18430 dst.patient = patient == null ? null : patient.copy(); 18431 dst.billablePeriod = billablePeriod == null ? null : billablePeriod.copy(); 18432 dst.created = created == null ? null : created.copy(); 18433 dst.enterer = enterer == null ? null : enterer.copy(); 18434 dst.insurer = insurer == null ? null : insurer.copy(); 18435 dst.provider = provider == null ? null : provider.copy(); 18436 dst.priority = priority == null ? null : priority.copy(); 18437 dst.fundsReserveRequested = fundsReserveRequested == null ? null : fundsReserveRequested.copy(); 18438 dst.fundsReserve = fundsReserve == null ? null : fundsReserve.copy(); 18439 if (related != null) { 18440 dst.related = new ArrayList<RelatedClaimComponent>(); 18441 for (RelatedClaimComponent i : related) 18442 dst.related.add(i.copy()); 18443 }; 18444 dst.prescription = prescription == null ? null : prescription.copy(); 18445 dst.originalPrescription = originalPrescription == null ? null : originalPrescription.copy(); 18446 if (event != null) { 18447 dst.event = new ArrayList<ExplanationOfBenefitEventComponent>(); 18448 for (ExplanationOfBenefitEventComponent i : event) 18449 dst.event.add(i.copy()); 18450 }; 18451 dst.payee = payee == null ? null : payee.copy(); 18452 dst.referral = referral == null ? null : referral.copy(); 18453 if (encounter != null) { 18454 dst.encounter = new ArrayList<Reference>(); 18455 for (Reference i : encounter) 18456 dst.encounter.add(i.copy()); 18457 }; 18458 dst.facility = facility == null ? null : facility.copy(); 18459 dst.claim = claim == null ? null : claim.copy(); 18460 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 18461 dst.outcome = outcome == null ? null : outcome.copy(); 18462 dst.decision = decision == null ? null : decision.copy(); 18463 dst.disposition = disposition == null ? null : disposition.copy(); 18464 if (preAuthRef != null) { 18465 dst.preAuthRef = new ArrayList<StringType>(); 18466 for (StringType i : preAuthRef) 18467 dst.preAuthRef.add(i.copy()); 18468 }; 18469 if (preAuthRefPeriod != null) { 18470 dst.preAuthRefPeriod = new ArrayList<Period>(); 18471 for (Period i : preAuthRefPeriod) 18472 dst.preAuthRefPeriod.add(i.copy()); 18473 }; 18474 dst.diagnosisRelatedGroup = diagnosisRelatedGroup == null ? null : diagnosisRelatedGroup.copy(); 18475 if (careTeam != null) { 18476 dst.careTeam = new ArrayList<CareTeamComponent>(); 18477 for (CareTeamComponent i : careTeam) 18478 dst.careTeam.add(i.copy()); 18479 }; 18480 if (supportingInfo != null) { 18481 dst.supportingInfo = new ArrayList<SupportingInformationComponent>(); 18482 for (SupportingInformationComponent i : supportingInfo) 18483 dst.supportingInfo.add(i.copy()); 18484 }; 18485 if (diagnosis != null) { 18486 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 18487 for (DiagnosisComponent i : diagnosis) 18488 dst.diagnosis.add(i.copy()); 18489 }; 18490 if (procedure != null) { 18491 dst.procedure = new ArrayList<ProcedureComponent>(); 18492 for (ProcedureComponent i : procedure) 18493 dst.procedure.add(i.copy()); 18494 }; 18495 dst.precedence = precedence == null ? null : precedence.copy(); 18496 if (insurance != null) { 18497 dst.insurance = new ArrayList<InsuranceComponent>(); 18498 for (InsuranceComponent i : insurance) 18499 dst.insurance.add(i.copy()); 18500 }; 18501 dst.accident = accident == null ? null : accident.copy(); 18502 dst.patientPaid = patientPaid == null ? null : patientPaid.copy(); 18503 if (item != null) { 18504 dst.item = new ArrayList<ItemComponent>(); 18505 for (ItemComponent i : item) 18506 dst.item.add(i.copy()); 18507 }; 18508 if (addItem != null) { 18509 dst.addItem = new ArrayList<AddedItemComponent>(); 18510 for (AddedItemComponent i : addItem) 18511 dst.addItem.add(i.copy()); 18512 }; 18513 if (adjudication != null) { 18514 dst.adjudication = new ArrayList<AdjudicationComponent>(); 18515 for (AdjudicationComponent i : adjudication) 18516 dst.adjudication.add(i.copy()); 18517 }; 18518 if (total != null) { 18519 dst.total = new ArrayList<TotalComponent>(); 18520 for (TotalComponent i : total) 18521 dst.total.add(i.copy()); 18522 }; 18523 dst.payment = payment == null ? null : payment.copy(); 18524 dst.formCode = formCode == null ? null : formCode.copy(); 18525 dst.form = form == null ? null : form.copy(); 18526 if (processNote != null) { 18527 dst.processNote = new ArrayList<NoteComponent>(); 18528 for (NoteComponent i : processNote) 18529 dst.processNote.add(i.copy()); 18530 }; 18531 dst.benefitPeriod = benefitPeriod == null ? null : benefitPeriod.copy(); 18532 if (benefitBalance != null) { 18533 dst.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 18534 for (BenefitBalanceComponent i : benefitBalance) 18535 dst.benefitBalance.add(i.copy()); 18536 }; 18537 } 18538 18539 protected ExplanationOfBenefit typedCopy() { 18540 return copy(); 18541 } 18542 18543 @Override 18544 public boolean equalsDeep(Base other_) { 18545 if (!super.equalsDeep(other_)) 18546 return false; 18547 if (!(other_ instanceof ExplanationOfBenefit)) 18548 return false; 18549 ExplanationOfBenefit o = (ExplanationOfBenefit) other_; 18550 return compareDeep(identifier, o.identifier, true) && compareDeep(traceNumber, o.traceNumber, true) 18551 && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) && compareDeep(subType, o.subType, true) 18552 && compareDeep(use, o.use, true) && compareDeep(patient, o.patient, true) && compareDeep(billablePeriod, o.billablePeriod, true) 18553 && compareDeep(created, o.created, true) && compareDeep(enterer, o.enterer, true) && compareDeep(insurer, o.insurer, true) 18554 && compareDeep(provider, o.provider, true) && compareDeep(priority, o.priority, true) && compareDeep(fundsReserveRequested, o.fundsReserveRequested, true) 18555 && compareDeep(fundsReserve, o.fundsReserve, true) && compareDeep(related, o.related, true) && compareDeep(prescription, o.prescription, true) 18556 && compareDeep(originalPrescription, o.originalPrescription, true) && compareDeep(event, o.event, true) 18557 && compareDeep(payee, o.payee, true) && compareDeep(referral, o.referral, true) && compareDeep(encounter, o.encounter, true) 18558 && compareDeep(facility, o.facility, true) && compareDeep(claim, o.claim, true) && compareDeep(claimResponse, o.claimResponse, true) 18559 && compareDeep(outcome, o.outcome, true) && compareDeep(decision, o.decision, true) && compareDeep(disposition, o.disposition, true) 18560 && compareDeep(preAuthRef, o.preAuthRef, true) && compareDeep(preAuthRefPeriod, o.preAuthRefPeriod, true) 18561 && compareDeep(diagnosisRelatedGroup, o.diagnosisRelatedGroup, true) && compareDeep(careTeam, o.careTeam, true) 18562 && compareDeep(supportingInfo, o.supportingInfo, true) && compareDeep(diagnosis, o.diagnosis, true) 18563 && compareDeep(procedure, o.procedure, true) && compareDeep(precedence, o.precedence, true) && compareDeep(insurance, o.insurance, true) 18564 && compareDeep(accident, o.accident, true) && compareDeep(patientPaid, o.patientPaid, true) && compareDeep(item, o.item, true) 18565 && compareDeep(addItem, o.addItem, true) && compareDeep(adjudication, o.adjudication, true) && compareDeep(total, o.total, true) 18566 && compareDeep(payment, o.payment, true) && compareDeep(formCode, o.formCode, true) && compareDeep(form, o.form, true) 18567 && compareDeep(processNote, o.processNote, true) && compareDeep(benefitPeriod, o.benefitPeriod, true) 18568 && compareDeep(benefitBalance, o.benefitBalance, true); 18569 } 18570 18571 @Override 18572 public boolean equalsShallow(Base other_) { 18573 if (!super.equalsShallow(other_)) 18574 return false; 18575 if (!(other_ instanceof ExplanationOfBenefit)) 18576 return false; 18577 ExplanationOfBenefit o = (ExplanationOfBenefit) other_; 18578 return compareValues(status, o.status, true) && compareValues(use, o.use, true) && compareValues(created, o.created, true) 18579 && compareValues(outcome, o.outcome, true) && compareValues(disposition, o.disposition, true) && compareValues(preAuthRef, o.preAuthRef, true) 18580 && compareValues(precedence, o.precedence, true); 18581 } 18582 18583 public boolean isEmpty() { 18584 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, traceNumber, status 18585 , type, subType, use, patient, billablePeriod, created, enterer, insurer, provider 18586 , priority, fundsReserveRequested, fundsReserve, related, prescription, originalPrescription 18587 , event, payee, referral, encounter, facility, claim, claimResponse, outcome 18588 , decision, disposition, preAuthRef, preAuthRefPeriod, diagnosisRelatedGroup, careTeam 18589 , supportingInfo, diagnosis, procedure, precedence, insurance, accident, patientPaid 18590 , item, addItem, adjudication, total, payment, formCode, form, processNote, benefitPeriod 18591 , benefitBalance); 18592 } 18593 18594 @Override 18595 public ResourceType getResourceType() { 18596 return ResourceType.ExplanationOfBenefit; 18597 } 18598 18599 /** 18600 * Search parameter: <b>care-team</b> 18601 * <p> 18602 * Description: <b>Member of the CareTeam</b><br> 18603 * Type: <b>reference</b><br> 18604 * Path: <b>ExplanationOfBenefit.careTeam.provider</b><br> 18605 * </p> 18606 */ 18607 @SearchParamDefinition(name="care-team", path="ExplanationOfBenefit.careTeam.provider", description="Member of the CareTeam", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 18608 public static final String SP_CARE_TEAM = "care-team"; 18609 /** 18610 * <b>Fluent Client</b> search parameter constant for <b>care-team</b> 18611 * <p> 18612 * Description: <b>Member of the CareTeam</b><br> 18613 * Type: <b>reference</b><br> 18614 * Path: <b>ExplanationOfBenefit.careTeam.provider</b><br> 18615 * </p> 18616 */ 18617 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_TEAM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CARE_TEAM); 18618 18619/** 18620 * Constant for fluent queries to be used to add include statements. Specifies 18621 * the path value of "<b>ExplanationOfBenefit:care-team</b>". 18622 */ 18623 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_TEAM = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:care-team").toLocked(); 18624 18625 /** 18626 * Search parameter: <b>claim</b> 18627 * <p> 18628 * Description: <b>The reference to the claim</b><br> 18629 * Type: <b>reference</b><br> 18630 * Path: <b>ExplanationOfBenefit.claim</b><br> 18631 * </p> 18632 */ 18633 @SearchParamDefinition(name="claim", path="ExplanationOfBenefit.claim", description="The reference to the claim", type="reference", target={Claim.class } ) 18634 public static final String SP_CLAIM = "claim"; 18635 /** 18636 * <b>Fluent Client</b> search parameter constant for <b>claim</b> 18637 * <p> 18638 * Description: <b>The reference to the claim</b><br> 18639 * Type: <b>reference</b><br> 18640 * Path: <b>ExplanationOfBenefit.claim</b><br> 18641 * </p> 18642 */ 18643 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CLAIM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CLAIM); 18644 18645/** 18646 * Constant for fluent queries to be used to add include statements. Specifies 18647 * the path value of "<b>ExplanationOfBenefit:claim</b>". 18648 */ 18649 public static final ca.uhn.fhir.model.api.Include INCLUDE_CLAIM = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:claim").toLocked(); 18650 18651 /** 18652 * Search parameter: <b>coverage</b> 18653 * <p> 18654 * Description: <b>The plan under which the claim was adjudicated</b><br> 18655 * Type: <b>reference</b><br> 18656 * Path: <b>ExplanationOfBenefit.insurance.coverage</b><br> 18657 * </p> 18658 */ 18659 @SearchParamDefinition(name="coverage", path="ExplanationOfBenefit.insurance.coverage", description="The plan under which the claim was adjudicated", type="reference", target={Coverage.class } ) 18660 public static final String SP_COVERAGE = "coverage"; 18661 /** 18662 * <b>Fluent Client</b> search parameter constant for <b>coverage</b> 18663 * <p> 18664 * Description: <b>The plan under which the claim was adjudicated</b><br> 18665 * Type: <b>reference</b><br> 18666 * Path: <b>ExplanationOfBenefit.insurance.coverage</b><br> 18667 * </p> 18668 */ 18669 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COVERAGE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COVERAGE); 18670 18671/** 18672 * Constant for fluent queries to be used to add include statements. Specifies 18673 * the path value of "<b>ExplanationOfBenefit:coverage</b>". 18674 */ 18675 public static final ca.uhn.fhir.model.api.Include INCLUDE_COVERAGE = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:coverage").toLocked(); 18676 18677 /** 18678 * Search parameter: <b>created</b> 18679 * <p> 18680 * Description: <b>The creation date for the EOB</b><br> 18681 * Type: <b>date</b><br> 18682 * Path: <b>ExplanationOfBenefit.created</b><br> 18683 * </p> 18684 */ 18685 @SearchParamDefinition(name="created", path="ExplanationOfBenefit.created", description="The creation date for the EOB", type="date" ) 18686 public static final String SP_CREATED = "created"; 18687 /** 18688 * <b>Fluent Client</b> search parameter constant for <b>created</b> 18689 * <p> 18690 * Description: <b>The creation date for the EOB</b><br> 18691 * Type: <b>date</b><br> 18692 * Path: <b>ExplanationOfBenefit.created</b><br> 18693 * </p> 18694 */ 18695 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 18696 18697 /** 18698 * Search parameter: <b>detail-udi</b> 18699 * <p> 18700 * Description: <b>UDI associated with a line item detail product or service</b><br> 18701 * Type: <b>reference</b><br> 18702 * Path: <b>ExplanationOfBenefit.item.detail.udi</b><br> 18703 * </p> 18704 */ 18705 @SearchParamDefinition(name="detail-udi", path="ExplanationOfBenefit.item.detail.udi", description="UDI associated with a line item detail product or service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 18706 public static final String SP_DETAIL_UDI = "detail-udi"; 18707 /** 18708 * <b>Fluent Client</b> search parameter constant for <b>detail-udi</b> 18709 * <p> 18710 * Description: <b>UDI associated with a line item detail product or service</b><br> 18711 * Type: <b>reference</b><br> 18712 * Path: <b>ExplanationOfBenefit.item.detail.udi</b><br> 18713 * </p> 18714 */ 18715 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DETAIL_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DETAIL_UDI); 18716 18717/** 18718 * Constant for fluent queries to be used to add include statements. Specifies 18719 * the path value of "<b>ExplanationOfBenefit:detail-udi</b>". 18720 */ 18721 public static final ca.uhn.fhir.model.api.Include INCLUDE_DETAIL_UDI = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:detail-udi").toLocked(); 18722 18723 /** 18724 * Search parameter: <b>disposition</b> 18725 * <p> 18726 * Description: <b>The contents of the disposition message</b><br> 18727 * Type: <b>string</b><br> 18728 * Path: <b>ExplanationOfBenefit.disposition</b><br> 18729 * </p> 18730 */ 18731 @SearchParamDefinition(name="disposition", path="ExplanationOfBenefit.disposition", description="The contents of the disposition message", type="string" ) 18732 public static final String SP_DISPOSITION = "disposition"; 18733 /** 18734 * <b>Fluent Client</b> search parameter constant for <b>disposition</b> 18735 * <p> 18736 * Description: <b>The contents of the disposition message</b><br> 18737 * Type: <b>string</b><br> 18738 * Path: <b>ExplanationOfBenefit.disposition</b><br> 18739 * </p> 18740 */ 18741 public static final ca.uhn.fhir.rest.gclient.StringClientParam DISPOSITION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DISPOSITION); 18742 18743 /** 18744 * Search parameter: <b>enterer</b> 18745 * <p> 18746 * Description: <b>The party responsible for the entry of the Claim</b><br> 18747 * Type: <b>reference</b><br> 18748 * Path: <b>ExplanationOfBenefit.enterer</b><br> 18749 * </p> 18750 */ 18751 @SearchParamDefinition(name="enterer", path="ExplanationOfBenefit.enterer", description="The party responsible for the entry of the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 18752 public static final String SP_ENTERER = "enterer"; 18753 /** 18754 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 18755 * <p> 18756 * Description: <b>The party responsible for the entry of the Claim</b><br> 18757 * Type: <b>reference</b><br> 18758 * Path: <b>ExplanationOfBenefit.enterer</b><br> 18759 * </p> 18760 */ 18761 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTERER); 18762 18763/** 18764 * Constant for fluent queries to be used to add include statements. Specifies 18765 * the path value of "<b>ExplanationOfBenefit:enterer</b>". 18766 */ 18767 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:enterer").toLocked(); 18768 18769 /** 18770 * Search parameter: <b>facility</b> 18771 * <p> 18772 * Description: <b>Facility responsible for the goods and services</b><br> 18773 * Type: <b>reference</b><br> 18774 * Path: <b>ExplanationOfBenefit.facility</b><br> 18775 * </p> 18776 */ 18777 @SearchParamDefinition(name="facility", path="ExplanationOfBenefit.facility", description="Facility responsible for the goods and services", type="reference", target={Location.class, Organization.class } ) 18778 public static final String SP_FACILITY = "facility"; 18779 /** 18780 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 18781 * <p> 18782 * Description: <b>Facility responsible for the goods and services</b><br> 18783 * Type: <b>reference</b><br> 18784 * Path: <b>ExplanationOfBenefit.facility</b><br> 18785 * </p> 18786 */ 18787 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FACILITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FACILITY); 18788 18789/** 18790 * Constant for fluent queries to be used to add include statements. Specifies 18791 * the path value of "<b>ExplanationOfBenefit:facility</b>". 18792 */ 18793 public static final ca.uhn.fhir.model.api.Include INCLUDE_FACILITY = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:facility").toLocked(); 18794 18795 /** 18796 * Search parameter: <b>item-udi</b> 18797 * <p> 18798 * Description: <b>UDI associated with a line item product or service</b><br> 18799 * Type: <b>reference</b><br> 18800 * Path: <b>ExplanationOfBenefit.item.udi</b><br> 18801 * </p> 18802 */ 18803 @SearchParamDefinition(name="item-udi", path="ExplanationOfBenefit.item.udi", description="UDI associated with a line item product or service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 18804 public static final String SP_ITEM_UDI = "item-udi"; 18805 /** 18806 * <b>Fluent Client</b> search parameter constant for <b>item-udi</b> 18807 * <p> 18808 * Description: <b>UDI associated with a line item product or service</b><br> 18809 * Type: <b>reference</b><br> 18810 * Path: <b>ExplanationOfBenefit.item.udi</b><br> 18811 * </p> 18812 */ 18813 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ITEM_UDI); 18814 18815/** 18816 * Constant for fluent queries to be used to add include statements. Specifies 18817 * the path value of "<b>ExplanationOfBenefit:item-udi</b>". 18818 */ 18819 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM_UDI = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:item-udi").toLocked(); 18820 18821 /** 18822 * Search parameter: <b>payee</b> 18823 * <p> 18824 * Description: <b>The party receiving any payment for the Claim</b><br> 18825 * Type: <b>reference</b><br> 18826 * Path: <b>ExplanationOfBenefit.payee.party</b><br> 18827 * </p> 18828 */ 18829 @SearchParamDefinition(name="payee", path="ExplanationOfBenefit.payee.party", description="The party receiving any payment for the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 18830 public static final String SP_PAYEE = "payee"; 18831 /** 18832 * <b>Fluent Client</b> search parameter constant for <b>payee</b> 18833 * <p> 18834 * Description: <b>The party receiving any payment for the Claim</b><br> 18835 * Type: <b>reference</b><br> 18836 * Path: <b>ExplanationOfBenefit.payee.party</b><br> 18837 * </p> 18838 */ 18839 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYEE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PAYEE); 18840 18841/** 18842 * Constant for fluent queries to be used to add include statements. Specifies 18843 * the path value of "<b>ExplanationOfBenefit:payee</b>". 18844 */ 18845 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYEE = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:payee").toLocked(); 18846 18847 /** 18848 * Search parameter: <b>procedure-udi</b> 18849 * <p> 18850 * Description: <b>UDI associated with a procedure</b><br> 18851 * Type: <b>reference</b><br> 18852 * Path: <b>ExplanationOfBenefit.procedure.udi</b><br> 18853 * </p> 18854 */ 18855 @SearchParamDefinition(name="procedure-udi", path="ExplanationOfBenefit.procedure.udi", description="UDI associated with a procedure", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 18856 public static final String SP_PROCEDURE_UDI = "procedure-udi"; 18857 /** 18858 * <b>Fluent Client</b> search parameter constant for <b>procedure-udi</b> 18859 * <p> 18860 * Description: <b>UDI associated with a procedure</b><br> 18861 * Type: <b>reference</b><br> 18862 * Path: <b>ExplanationOfBenefit.procedure.udi</b><br> 18863 * </p> 18864 */ 18865 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROCEDURE_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROCEDURE_UDI); 18866 18867/** 18868 * Constant for fluent queries to be used to add include statements. Specifies 18869 * the path value of "<b>ExplanationOfBenefit:procedure-udi</b>". 18870 */ 18871 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROCEDURE_UDI = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:procedure-udi").toLocked(); 18872 18873 /** 18874 * Search parameter: <b>provider</b> 18875 * <p> 18876 * Description: <b>The reference to the provider</b><br> 18877 * Type: <b>reference</b><br> 18878 * Path: <b>ExplanationOfBenefit.provider</b><br> 18879 * </p> 18880 */ 18881 @SearchParamDefinition(name="provider", path="ExplanationOfBenefit.provider", description="The reference to the provider", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 18882 public static final String SP_PROVIDER = "provider"; 18883 /** 18884 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 18885 * <p> 18886 * Description: <b>The reference to the provider</b><br> 18887 * Type: <b>reference</b><br> 18888 * Path: <b>ExplanationOfBenefit.provider</b><br> 18889 * </p> 18890 */ 18891 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROVIDER); 18892 18893/** 18894 * Constant for fluent queries to be used to add include statements. Specifies 18895 * the path value of "<b>ExplanationOfBenefit:provider</b>". 18896 */ 18897 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:provider").toLocked(); 18898 18899 /** 18900 * Search parameter: <b>status</b> 18901 * <p> 18902 * Description: <b>Status of the instance</b><br> 18903 * Type: <b>token</b><br> 18904 * Path: <b>ExplanationOfBenefit.status</b><br> 18905 * </p> 18906 */ 18907 @SearchParamDefinition(name="status", path="ExplanationOfBenefit.status", description="Status of the instance", type="token" ) 18908 public static final String SP_STATUS = "status"; 18909 /** 18910 * <b>Fluent Client</b> search parameter constant for <b>status</b> 18911 * <p> 18912 * Description: <b>Status of the instance</b><br> 18913 * Type: <b>token</b><br> 18914 * Path: <b>ExplanationOfBenefit.status</b><br> 18915 * </p> 18916 */ 18917 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 18918 18919 /** 18920 * Search parameter: <b>subdetail-udi</b> 18921 * <p> 18922 * Description: <b>UDI associated with a line item detail subdetail product or service</b><br> 18923 * Type: <b>reference</b><br> 18924 * Path: <b>ExplanationOfBenefit.item.detail.subDetail.udi</b><br> 18925 * </p> 18926 */ 18927 @SearchParamDefinition(name="subdetail-udi", path="ExplanationOfBenefit.item.detail.subDetail.udi", description="UDI associated with a line item detail subdetail product or service", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device") }, target={Device.class } ) 18928 public static final String SP_SUBDETAIL_UDI = "subdetail-udi"; 18929 /** 18930 * <b>Fluent Client</b> search parameter constant for <b>subdetail-udi</b> 18931 * <p> 18932 * Description: <b>UDI associated with a line item detail subdetail product or service</b><br> 18933 * Type: <b>reference</b><br> 18934 * Path: <b>ExplanationOfBenefit.item.detail.subDetail.udi</b><br> 18935 * </p> 18936 */ 18937 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBDETAIL_UDI = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBDETAIL_UDI); 18938 18939/** 18940 * Constant for fluent queries to be used to add include statements. Specifies 18941 * the path value of "<b>ExplanationOfBenefit:subdetail-udi</b>". 18942 */ 18943 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBDETAIL_UDI = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:subdetail-udi").toLocked(); 18944 18945 /** 18946 * Search parameter: <b>encounter</b> 18947 * <p> 18948 * Description: <b>Multiple Resources: 18949 18950* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 18951* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 18952* [ChargeItem](chargeitem.html): Encounter associated with event 18953* [Claim](claim.html): Encounters associated with a billed line item 18954* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 18955* [Communication](communication.html): The Encounter during which this Communication was created 18956* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 18957* [Composition](composition.html): Context of the Composition 18958* [Condition](condition.html): The Encounter during which this Condition was created 18959* [DeviceRequest](devicerequest.html): Encounter during which request was created 18960* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 18961* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 18962* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 18963* [Flag](flag.html): Alert relevant during encounter 18964* [ImagingStudy](imagingstudy.html): The context of the study 18965* [List](list.html): Context in which list created 18966* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 18967* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 18968* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 18969* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 18970* [Observation](observation.html): Encounter related to the observation 18971* [Procedure](procedure.html): The Encounter during which this Procedure was created 18972* [Provenance](provenance.html): Encounter related to the Provenance 18973* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 18974* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 18975* [RiskAssessment](riskassessment.html): Where was assessment performed? 18976* [ServiceRequest](servicerequest.html): An encounter in which this request is made 18977* [Task](task.html): Search by encounter 18978* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 18979</b><br> 18980 * Type: <b>reference</b><br> 18981 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 18982 * </p> 18983 */ 18984 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 18985 public static final String SP_ENCOUNTER = "encounter"; 18986 /** 18987 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 18988 * <p> 18989 * Description: <b>Multiple Resources: 18990 18991* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 18992* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 18993* [ChargeItem](chargeitem.html): Encounter associated with event 18994* [Claim](claim.html): Encounters associated with a billed line item 18995* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 18996* [Communication](communication.html): The Encounter during which this Communication was created 18997* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 18998* [Composition](composition.html): Context of the Composition 18999* [Condition](condition.html): The Encounter during which this Condition was created 19000* [DeviceRequest](devicerequest.html): Encounter during which request was created 19001* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 19002* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 19003* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 19004* [Flag](flag.html): Alert relevant during encounter 19005* [ImagingStudy](imagingstudy.html): The context of the study 19006* [List](list.html): Context in which list created 19007* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 19008* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 19009* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 19010* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 19011* [Observation](observation.html): Encounter related to the observation 19012* [Procedure](procedure.html): The Encounter during which this Procedure was created 19013* [Provenance](provenance.html): Encounter related to the Provenance 19014* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 19015* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 19016* [RiskAssessment](riskassessment.html): Where was assessment performed? 19017* [ServiceRequest](servicerequest.html): An encounter in which this request is made 19018* [Task](task.html): Search by encounter 19019* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 19020</b><br> 19021 * Type: <b>reference</b><br> 19022 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 19023 * </p> 19024 */ 19025 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 19026 19027/** 19028 * Constant for fluent queries to be used to add include statements. Specifies 19029 * the path value of "<b>ExplanationOfBenefit:encounter</b>". 19030 */ 19031 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:encounter").toLocked(); 19032 19033 /** 19034 * Search parameter: <b>identifier</b> 19035 * <p> 19036 * Description: <b>Multiple Resources: 19037 19038* [Account](account.html): Account number 19039* [AdverseEvent](adverseevent.html): Business identifier for the event 19040* [AllergyIntolerance](allergyintolerance.html): External ids for this item 19041* [Appointment](appointment.html): An Identifier of the Appointment 19042* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 19043* [Basic](basic.html): Business identifier 19044* [BodyStructure](bodystructure.html): Bodystructure identifier 19045* [CarePlan](careplan.html): External Ids for this plan 19046* [CareTeam](careteam.html): External Ids for this team 19047* [ChargeItem](chargeitem.html): Business Identifier for item 19048* [Claim](claim.html): The primary identifier of the financial resource 19049* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 19050* [ClinicalImpression](clinicalimpression.html): Business identifier 19051* [Communication](communication.html): Unique identifier 19052* [CommunicationRequest](communicationrequest.html): Unique identifier 19053* [Composition](composition.html): Version-independent identifier for the Composition 19054* [Condition](condition.html): A unique identifier of the condition record 19055* [Consent](consent.html): Identifier for this record (external references) 19056* [Contract](contract.html): The identity of the contract 19057* [Coverage](coverage.html): The primary identifier of the insured and the coverage 19058* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 19059* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 19060* [DetectedIssue](detectedissue.html): Unique id for the detected issue 19061* [DeviceRequest](devicerequest.html): Business identifier for request/order 19062* [DeviceUsage](deviceusage.html): Search by identifier 19063* [DiagnosticReport](diagnosticreport.html): An identifier for the report 19064* [DocumentReference](documentreference.html): Identifier of the attachment binary 19065* [Encounter](encounter.html): Identifier(s) by which this encounter is known 19066* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 19067* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 19068* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 19069* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 19070* [Flag](flag.html): Business identifier 19071* [Goal](goal.html): External Ids for this goal 19072* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 19073* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 19074* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 19075* [Immunization](immunization.html): Business identifier 19076* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 19077* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 19078* [Invoice](invoice.html): Business Identifier for item 19079* [List](list.html): Business identifier 19080* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 19081* [Medication](medication.html): Returns medications with this external identifier 19082* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 19083* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 19084* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 19085* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 19086* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 19087* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 19088* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 19089* [Observation](observation.html): The unique id for a particular observation 19090* [Person](person.html): A person Identifier 19091* [Procedure](procedure.html): A unique identifier for a procedure 19092* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 19093* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 19094* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 19095* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 19096* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 19097* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 19098* [Specimen](specimen.html): The unique identifier associated with the specimen 19099* [SupplyDelivery](supplydelivery.html): External identifier 19100* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 19101* [Task](task.html): Search for a task instance by its business identifier 19102* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 19103</b><br> 19104 * Type: <b>token</b><br> 19105 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 19106 * </p> 19107 */ 19108 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 19109 public static final String SP_IDENTIFIER = "identifier"; 19110 /** 19111 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 19112 * <p> 19113 * Description: <b>Multiple Resources: 19114 19115* [Account](account.html): Account number 19116* [AdverseEvent](adverseevent.html): Business identifier for the event 19117* [AllergyIntolerance](allergyintolerance.html): External ids for this item 19118* [Appointment](appointment.html): An Identifier of the Appointment 19119* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 19120* [Basic](basic.html): Business identifier 19121* [BodyStructure](bodystructure.html): Bodystructure identifier 19122* [CarePlan](careplan.html): External Ids for this plan 19123* [CareTeam](careteam.html): External Ids for this team 19124* [ChargeItem](chargeitem.html): Business Identifier for item 19125* [Claim](claim.html): The primary identifier of the financial resource 19126* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 19127* [ClinicalImpression](clinicalimpression.html): Business identifier 19128* [Communication](communication.html): Unique identifier 19129* [CommunicationRequest](communicationrequest.html): Unique identifier 19130* [Composition](composition.html): Version-independent identifier for the Composition 19131* [Condition](condition.html): A unique identifier of the condition record 19132* [Consent](consent.html): Identifier for this record (external references) 19133* [Contract](contract.html): The identity of the contract 19134* [Coverage](coverage.html): The primary identifier of the insured and the coverage 19135* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 19136* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 19137* [DetectedIssue](detectedissue.html): Unique id for the detected issue 19138* [DeviceRequest](devicerequest.html): Business identifier for request/order 19139* [DeviceUsage](deviceusage.html): Search by identifier 19140* [DiagnosticReport](diagnosticreport.html): An identifier for the report 19141* [DocumentReference](documentreference.html): Identifier of the attachment binary 19142* [Encounter](encounter.html): Identifier(s) by which this encounter is known 19143* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 19144* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 19145* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 19146* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 19147* [Flag](flag.html): Business identifier 19148* [Goal](goal.html): External Ids for this goal 19149* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 19150* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 19151* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 19152* [Immunization](immunization.html): Business identifier 19153* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 19154* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 19155* [Invoice](invoice.html): Business Identifier for item 19156* [List](list.html): Business identifier 19157* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 19158* [Medication](medication.html): Returns medications with this external identifier 19159* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 19160* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 19161* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 19162* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 19163* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 19164* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 19165* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 19166* [Observation](observation.html): The unique id for a particular observation 19167* [Person](person.html): A person Identifier 19168* [Procedure](procedure.html): A unique identifier for a procedure 19169* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 19170* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 19171* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 19172* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 19173* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 19174* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 19175* [Specimen](specimen.html): The unique identifier associated with the specimen 19176* [SupplyDelivery](supplydelivery.html): External identifier 19177* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 19178* [Task](task.html): Search for a task instance by its business identifier 19179* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 19180</b><br> 19181 * Type: <b>token</b><br> 19182 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 19183 * </p> 19184 */ 19185 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 19186 19187 /** 19188 * Search parameter: <b>patient</b> 19189 * <p> 19190 * Description: <b>Multiple Resources: 19191 19192* [Account](account.html): The entity that caused the expenses 19193* [AdverseEvent](adverseevent.html): Subject impacted by event 19194* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 19195* [Appointment](appointment.html): One of the individuals of the appointment is this patient 19196* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 19197* [AuditEvent](auditevent.html): Where the activity involved patient data 19198* [Basic](basic.html): Identifies the focus of this resource 19199* [BodyStructure](bodystructure.html): Who this is about 19200* [CarePlan](careplan.html): Who the care plan is for 19201* [CareTeam](careteam.html): Who care team is for 19202* [ChargeItem](chargeitem.html): Individual service was done for/to 19203* [Claim](claim.html): Patient receiving the products or services 19204* [ClaimResponse](claimresponse.html): The subject of care 19205* [ClinicalImpression](clinicalimpression.html): Patient assessed 19206* [Communication](communication.html): Focus of message 19207* [CommunicationRequest](communicationrequest.html): Focus of message 19208* [Composition](composition.html): Who and/or what the composition is about 19209* [Condition](condition.html): Who has the condition? 19210* [Consent](consent.html): Who the consent applies to 19211* [Contract](contract.html): The identity of the subject of the contract (if a patient) 19212* [Coverage](coverage.html): Retrieve coverages for a patient 19213* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 19214* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 19215* [DetectedIssue](detectedissue.html): Associated patient 19216* [DeviceRequest](devicerequest.html): Individual the service is ordered for 19217* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 19218* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 19219* [DocumentReference](documentreference.html): Who/what is the subject of the document 19220* [Encounter](encounter.html): The patient present at the encounter 19221* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 19222* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 19223* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 19224* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 19225* [Flag](flag.html): The identity of a subject to list flags for 19226* [Goal](goal.html): Who this goal is intended for 19227* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 19228* [ImagingSelection](imagingselection.html): Who the study is about 19229* [ImagingStudy](imagingstudy.html): Who the study is about 19230* [Immunization](immunization.html): The patient for the vaccination record 19231* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 19232* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 19233* [Invoice](invoice.html): Recipient(s) of goods and services 19234* [List](list.html): If all resources have the same subject 19235* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 19236* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 19237* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 19238* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 19239* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 19240* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 19241* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 19242* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 19243* [Observation](observation.html): The subject that the observation is about (if patient) 19244* [Person](person.html): The Person links to this Patient 19245* [Procedure](procedure.html): Search by subject - a patient 19246* [Provenance](provenance.html): Where the activity involved patient data 19247* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 19248* [RelatedPerson](relatedperson.html): The patient this related person is related to 19249* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 19250* [ResearchSubject](researchsubject.html): Who or what is part of study 19251* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 19252* [ServiceRequest](servicerequest.html): Search by subject - a patient 19253* [Specimen](specimen.html): The patient the specimen comes from 19254* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 19255* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 19256* [Task](task.html): Search by patient 19257* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 19258</b><br> 19259 * Type: <b>reference</b><br> 19260 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 19261 * </p> 19262 */ 19263 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 19264 public static final String SP_PATIENT = "patient"; 19265 /** 19266 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 19267 * <p> 19268 * Description: <b>Multiple Resources: 19269 19270* [Account](account.html): The entity that caused the expenses 19271* [AdverseEvent](adverseevent.html): Subject impacted by event 19272* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 19273* [Appointment](appointment.html): One of the individuals of the appointment is this patient 19274* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 19275* [AuditEvent](auditevent.html): Where the activity involved patient data 19276* [Basic](basic.html): Identifies the focus of this resource 19277* [BodyStructure](bodystructure.html): Who this is about 19278* [CarePlan](careplan.html): Who the care plan is for 19279* [CareTeam](careteam.html): Who care team is for 19280* [ChargeItem](chargeitem.html): Individual service was done for/to 19281* [Claim](claim.html): Patient receiving the products or services 19282* [ClaimResponse](claimresponse.html): The subject of care 19283* [ClinicalImpression](clinicalimpression.html): Patient assessed 19284* [Communication](communication.html): Focus of message 19285* [CommunicationRequest](communicationrequest.html): Focus of message 19286* [Composition](composition.html): Who and/or what the composition is about 19287* [Condition](condition.html): Who has the condition? 19288* [Consent](consent.html): Who the consent applies to 19289* [Contract](contract.html): The identity of the subject of the contract (if a patient) 19290* [Coverage](coverage.html): Retrieve coverages for a patient 19291* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 19292* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 19293* [DetectedIssue](detectedissue.html): Associated patient 19294* [DeviceRequest](devicerequest.html): Individual the service is ordered for 19295* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 19296* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 19297* [DocumentReference](documentreference.html): Who/what is the subject of the document 19298* [Encounter](encounter.html): The patient present at the encounter 19299* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 19300* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 19301* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 19302* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 19303* [Flag](flag.html): The identity of a subject to list flags for 19304* [Goal](goal.html): Who this goal is intended for 19305* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 19306* [ImagingSelection](imagingselection.html): Who the study is about 19307* [ImagingStudy](imagingstudy.html): Who the study is about 19308* [Immunization](immunization.html): The patient for the vaccination record 19309* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 19310* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 19311* [Invoice](invoice.html): Recipient(s) of goods and services 19312* [List](list.html): If all resources have the same subject 19313* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 19314* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 19315* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 19316* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 19317* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 19318* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 19319* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 19320* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 19321* [Observation](observation.html): The subject that the observation is about (if patient) 19322* [Person](person.html): The Person links to this Patient 19323* [Procedure](procedure.html): Search by subject - a patient 19324* [Provenance](provenance.html): Where the activity involved patient data 19325* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 19326* [RelatedPerson](relatedperson.html): The patient this related person is related to 19327* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 19328* [ResearchSubject](researchsubject.html): Who or what is part of study 19329* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 19330* [ServiceRequest](servicerequest.html): Search by subject - a patient 19331* [Specimen](specimen.html): The patient the specimen comes from 19332* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 19333* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 19334* [Task](task.html): Search by patient 19335* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 19336</b><br> 19337 * Type: <b>reference</b><br> 19338 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 19339 * </p> 19340 */ 19341 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 19342 19343/** 19344 * Constant for fluent queries to be used to add include statements. Specifies 19345 * the path value of "<b>ExplanationOfBenefit:patient</b>". 19346 */ 19347 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:patient").toLocked(); 19348 19349 19350} 19351