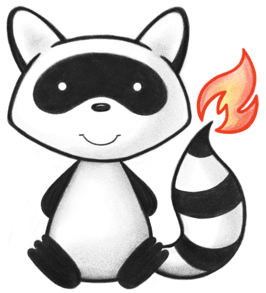
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * Expression Type: A expression that is evaluated in a specified context and returns a value. The context of use of the expression must specify the context in which the expression is evaluated, and how the result of the expression is used. 050 */ 051@DatatypeDef(name="Expression") 052public class Expression extends DataType implements ICompositeType { 053 054 /** 055 * A brief, natural language description of the condition that effectively communicates the intended semantics. 056 */ 057 @Child(name = "description", type = {StringType.class}, order=0, min=0, max=1, modifier=false, summary=true) 058 @Description(shortDefinition="Natural language description of the condition", formalDefinition="A brief, natural language description of the condition that effectively communicates the intended semantics." ) 059 protected StringType description; 060 061 /** 062 * A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined. 063 */ 064 @Child(name = "name", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 065 @Description(shortDefinition="Short name assigned to expression for reuse", formalDefinition="A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined." ) 066 protected CodeType name; 067 068 /** 069 * The media type of the language for the expression. 070 */ 071 @Child(name = "language", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 072 @Description(shortDefinition="text/cql | text/fhirpath | application/x-fhir-query | etc.", formalDefinition="The media type of the language for the expression." ) 073 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/expression-language") 074 protected CodeType language; 075 076 /** 077 * An expression in the specified language that returns a value. 078 */ 079 @Child(name = "expression", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 080 @Description(shortDefinition="Expression in specified language", formalDefinition="An expression in the specified language that returns a value." ) 081 protected StringType expression; 082 083 /** 084 * A URI that defines where the expression is found. 085 */ 086 @Child(name = "reference", type = {UriType.class}, order=4, min=0, max=1, modifier=false, summary=true) 087 @Description(shortDefinition="Where the expression is found", formalDefinition="A URI that defines where the expression is found." ) 088 protected UriType reference; 089 090 private static final long serialVersionUID = -1266682572L; 091 092 /** 093 * Constructor 094 */ 095 public Expression() { 096 super(); 097 } 098 099 /** 100 * @return {@link #description} (A brief, natural language description of the condition that effectively communicates the intended semantics.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 101 */ 102 public StringType getDescriptionElement() { 103 if (this.description == null) 104 if (Configuration.errorOnAutoCreate()) 105 throw new Error("Attempt to auto-create Expression.description"); 106 else if (Configuration.doAutoCreate()) 107 this.description = new StringType(); // bb 108 return this.description; 109 } 110 111 public boolean hasDescriptionElement() { 112 return this.description != null && !this.description.isEmpty(); 113 } 114 115 public boolean hasDescription() { 116 return this.description != null && !this.description.isEmpty(); 117 } 118 119 /** 120 * @param value {@link #description} (A brief, natural language description of the condition that effectively communicates the intended semantics.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 121 */ 122 public Expression setDescriptionElement(StringType value) { 123 this.description = value; 124 return this; 125 } 126 127 /** 128 * @return A brief, natural language description of the condition that effectively communicates the intended semantics. 129 */ 130 public String getDescription() { 131 return this.description == null ? null : this.description.getValue(); 132 } 133 134 /** 135 * @param value A brief, natural language description of the condition that effectively communicates the intended semantics. 136 */ 137 public Expression setDescription(String value) { 138 if (Utilities.noString(value)) 139 this.description = null; 140 else { 141 if (this.description == null) 142 this.description = new StringType(); 143 this.description.setValue(value); 144 } 145 return this; 146 } 147 148 /** 149 * @return {@link #name} (A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 150 */ 151 public CodeType getNameElement() { 152 if (this.name == null) 153 if (Configuration.errorOnAutoCreate()) 154 throw new Error("Attempt to auto-create Expression.name"); 155 else if (Configuration.doAutoCreate()) 156 this.name = new CodeType(); // bb 157 return this.name; 158 } 159 160 public boolean hasNameElement() { 161 return this.name != null && !this.name.isEmpty(); 162 } 163 164 public boolean hasName() { 165 return this.name != null && !this.name.isEmpty(); 166 } 167 168 /** 169 * @param value {@link #name} (A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 170 */ 171 public Expression setNameElement(CodeType value) { 172 this.name = value; 173 return this; 174 } 175 176 /** 177 * @return A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined. 178 */ 179 public String getName() { 180 return this.name == null ? null : this.name.getValue(); 181 } 182 183 /** 184 * @param value A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined. 185 */ 186 public Expression setName(String value) { 187 if (Utilities.noString(value)) 188 this.name = null; 189 else { 190 if (this.name == null) 191 this.name = new CodeType(); 192 this.name.setValue(value); 193 } 194 return this; 195 } 196 197 /** 198 * @return {@link #language} (The media type of the language for the expression.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 199 */ 200 public CodeType getLanguageElement() { 201 if (this.language == null) 202 if (Configuration.errorOnAutoCreate()) 203 throw new Error("Attempt to auto-create Expression.language"); 204 else if (Configuration.doAutoCreate()) 205 this.language = new CodeType(); // bb 206 return this.language; 207 } 208 209 public boolean hasLanguageElement() { 210 return this.language != null && !this.language.isEmpty(); 211 } 212 213 public boolean hasLanguage() { 214 return this.language != null && !this.language.isEmpty(); 215 } 216 217 /** 218 * @param value {@link #language} (The media type of the language for the expression.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 219 */ 220 public Expression setLanguageElement(CodeType value) { 221 this.language = value; 222 return this; 223 } 224 225 /** 226 * @return The media type of the language for the expression. 227 */ 228 public String getLanguage() { 229 return this.language == null ? null : this.language.getValue(); 230 } 231 232 /** 233 * @param value The media type of the language for the expression. 234 */ 235 public Expression setLanguage(String value) { 236 if (Utilities.noString(value)) 237 this.language = null; 238 else { 239 if (this.language == null) 240 this.language = new CodeType(); 241 this.language.setValue(value); 242 } 243 return this; 244 } 245 246 /** 247 * @return {@link #expression} (An expression in the specified language that returns a value.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 248 */ 249 public StringType getExpressionElement() { 250 if (this.expression == null) 251 if (Configuration.errorOnAutoCreate()) 252 throw new Error("Attempt to auto-create Expression.expression"); 253 else if (Configuration.doAutoCreate()) 254 this.expression = new StringType(); // bb 255 return this.expression; 256 } 257 258 public boolean hasExpressionElement() { 259 return this.expression != null && !this.expression.isEmpty(); 260 } 261 262 public boolean hasExpression() { 263 return this.expression != null && !this.expression.isEmpty(); 264 } 265 266 /** 267 * @param value {@link #expression} (An expression in the specified language that returns a value.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 268 */ 269 public Expression setExpressionElement(StringType value) { 270 this.expression = value; 271 return this; 272 } 273 274 /** 275 * @return An expression in the specified language that returns a value. 276 */ 277 public String getExpression() { 278 return this.expression == null ? null : this.expression.getValue(); 279 } 280 281 /** 282 * @param value An expression in the specified language that returns a value. 283 */ 284 public Expression setExpression(String value) { 285 if (Utilities.noString(value)) 286 this.expression = null; 287 else { 288 if (this.expression == null) 289 this.expression = new StringType(); 290 this.expression.setValue(value); 291 } 292 return this; 293 } 294 295 /** 296 * @return {@link #reference} (A URI that defines where the expression is found.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 297 */ 298 public UriType getReferenceElement() { 299 if (this.reference == null) 300 if (Configuration.errorOnAutoCreate()) 301 throw new Error("Attempt to auto-create Expression.reference"); 302 else if (Configuration.doAutoCreate()) 303 this.reference = new UriType(); // bb 304 return this.reference; 305 } 306 307 public boolean hasReferenceElement() { 308 return this.reference != null && !this.reference.isEmpty(); 309 } 310 311 public boolean hasReference() { 312 return this.reference != null && !this.reference.isEmpty(); 313 } 314 315 /** 316 * @param value {@link #reference} (A URI that defines where the expression is found.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 317 */ 318 public Expression setReferenceElement(UriType value) { 319 this.reference = value; 320 return this; 321 } 322 323 /** 324 * @return A URI that defines where the expression is found. 325 */ 326 public String getReference() { 327 return this.reference == null ? null : this.reference.getValue(); 328 } 329 330 /** 331 * @param value A URI that defines where the expression is found. 332 */ 333 public Expression setReference(String value) { 334 if (Utilities.noString(value)) 335 this.reference = null; 336 else { 337 if (this.reference == null) 338 this.reference = new UriType(); 339 this.reference.setValue(value); 340 } 341 return this; 342 } 343 344 protected void listChildren(List<Property> children) { 345 super.listChildren(children); 346 children.add(new Property("description", "string", "A brief, natural language description of the condition that effectively communicates the intended semantics.", 0, 1, description)); 347 children.add(new Property("name", "code", "A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined.", 0, 1, name)); 348 children.add(new Property("language", "code", "The media type of the language for the expression.", 0, 1, language)); 349 children.add(new Property("expression", "string", "An expression in the specified language that returns a value.", 0, 1, expression)); 350 children.add(new Property("reference", "uri", "A URI that defines where the expression is found.", 0, 1, reference)); 351 } 352 353 @Override 354 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 355 switch (_hash) { 356 case -1724546052: /*description*/ return new Property("description", "string", "A brief, natural language description of the condition that effectively communicates the intended semantics.", 0, 1, description); 357 case 3373707: /*name*/ return new Property("name", "code", "A short name assigned to the expression to allow for multiple reuse of the expression in the context where it is defined.", 0, 1, name); 358 case -1613589672: /*language*/ return new Property("language", "code", "The media type of the language for the expression.", 0, 1, language); 359 case -1795452264: /*expression*/ return new Property("expression", "string", "An expression in the specified language that returns a value.", 0, 1, expression); 360 case -925155509: /*reference*/ return new Property("reference", "uri", "A URI that defines where the expression is found.", 0, 1, reference); 361 default: return super.getNamedProperty(_hash, _name, _checkValid); 362 } 363 364 } 365 366 @Override 367 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 368 switch (hash) { 369 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 370 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // CodeType 371 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeType 372 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 373 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // UriType 374 default: return super.getProperty(hash, name, checkValid); 375 } 376 377 } 378 379 @Override 380 public Base setProperty(int hash, String name, Base value) throws FHIRException { 381 switch (hash) { 382 case -1724546052: // description 383 this.description = TypeConvertor.castToString(value); // StringType 384 return value; 385 case 3373707: // name 386 this.name = TypeConvertor.castToCode(value); // CodeType 387 return value; 388 case -1613589672: // language 389 this.language = TypeConvertor.castToCode(value); // CodeType 390 return value; 391 case -1795452264: // expression 392 this.expression = TypeConvertor.castToString(value); // StringType 393 return value; 394 case -925155509: // reference 395 this.reference = TypeConvertor.castToUri(value); // UriType 396 return value; 397 default: return super.setProperty(hash, name, value); 398 } 399 400 } 401 402 @Override 403 public Base setProperty(String name, Base value) throws FHIRException { 404 if (name.equals("description")) { 405 this.description = TypeConvertor.castToString(value); // StringType 406 } else if (name.equals("name")) { 407 this.name = TypeConvertor.castToCode(value); // CodeType 408 } else if (name.equals("language")) { 409 this.language = TypeConvertor.castToCode(value); // CodeType 410 } else if (name.equals("expression")) { 411 this.expression = TypeConvertor.castToString(value); // StringType 412 } else if (name.equals("reference")) { 413 this.reference = TypeConvertor.castToUri(value); // UriType 414 } else 415 return super.setProperty(name, value); 416 return value; 417 } 418 419 @Override 420 public void removeChild(String name, Base value) throws FHIRException { 421 if (name.equals("description")) { 422 this.description = null; 423 } else if (name.equals("name")) { 424 this.name = null; 425 } else if (name.equals("language")) { 426 this.language = null; 427 } else if (name.equals("expression")) { 428 this.expression = null; 429 } else if (name.equals("reference")) { 430 this.reference = null; 431 } else 432 super.removeChild(name, value); 433 434 } 435 436 @Override 437 public Base makeProperty(int hash, String name) throws FHIRException { 438 switch (hash) { 439 case -1724546052: return getDescriptionElement(); 440 case 3373707: return getNameElement(); 441 case -1613589672: return getLanguageElement(); 442 case -1795452264: return getExpressionElement(); 443 case -925155509: return getReferenceElement(); 444 default: return super.makeProperty(hash, name); 445 } 446 447 } 448 449 @Override 450 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 451 switch (hash) { 452 case -1724546052: /*description*/ return new String[] {"string"}; 453 case 3373707: /*name*/ return new String[] {"code"}; 454 case -1613589672: /*language*/ return new String[] {"code"}; 455 case -1795452264: /*expression*/ return new String[] {"string"}; 456 case -925155509: /*reference*/ return new String[] {"uri"}; 457 default: return super.getTypesForProperty(hash, name); 458 } 459 460 } 461 462 @Override 463 public Base addChild(String name) throws FHIRException { 464 if (name.equals("description")) { 465 throw new FHIRException("Cannot call addChild on a singleton property Expression.description"); 466 } 467 else if (name.equals("name")) { 468 throw new FHIRException("Cannot call addChild on a singleton property Expression.name"); 469 } 470 else if (name.equals("language")) { 471 throw new FHIRException("Cannot call addChild on a singleton property Expression.language"); 472 } 473 else if (name.equals("expression")) { 474 throw new FHIRException("Cannot call addChild on a singleton property Expression.expression"); 475 } 476 else if (name.equals("reference")) { 477 throw new FHIRException("Cannot call addChild on a singleton property Expression.reference"); 478 } 479 else 480 return super.addChild(name); 481 } 482 483 public String fhirType() { 484 return "Expression"; 485 486 } 487 488 public Expression copy() { 489 Expression dst = new Expression(); 490 copyValues(dst); 491 return dst; 492 } 493 494 public void copyValues(Expression dst) { 495 super.copyValues(dst); 496 dst.description = description == null ? null : description.copy(); 497 dst.name = name == null ? null : name.copy(); 498 dst.language = language == null ? null : language.copy(); 499 dst.expression = expression == null ? null : expression.copy(); 500 dst.reference = reference == null ? null : reference.copy(); 501 } 502 503 protected Expression typedCopy() { 504 return copy(); 505 } 506 507 @Override 508 public boolean equalsDeep(Base other_) { 509 if (!super.equalsDeep(other_)) 510 return false; 511 if (!(other_ instanceof Expression)) 512 return false; 513 Expression o = (Expression) other_; 514 return compareDeep(description, o.description, true) && compareDeep(name, o.name, true) && compareDeep(language, o.language, true) 515 && compareDeep(expression, o.expression, true) && compareDeep(reference, o.reference, true); 516 } 517 518 @Override 519 public boolean equalsShallow(Base other_) { 520 if (!super.equalsShallow(other_)) 521 return false; 522 if (!(other_ instanceof Expression)) 523 return false; 524 Expression o = (Expression) other_; 525 return compareValues(description, o.description, true) && compareValues(name, o.name, true) && compareValues(language, o.language, true) 526 && compareValues(expression, o.expression, true) && compareValues(reference, o.reference, true); 527 } 528 529 public boolean isEmpty() { 530 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, name, language 531 , expression, reference); 532 } 533 534 535} 536