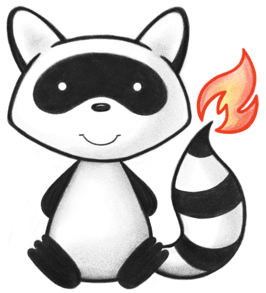
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.ChildOrder; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.Block; 046 047/** 048 * ExtendedContactDetail Type: Specifies contact information for a specific purpose over a period of time, might be handled/monitored by a specific named person or organization. 049 */ 050@DatatypeDef(name="ExtendedContactDetail") 051public class ExtendedContactDetail extends DataType implements ICompositeType { 052 053 /** 054 * The purpose/type of contact. 055 */ 056 @Child(name = "purpose", type = {CodeableConcept.class}, order=0, min=0, max=1, modifier=false, summary=true) 057 @Description(shortDefinition="The type of contact", formalDefinition="The purpose/type of contact." ) 058 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/contactentity-type") 059 protected CodeableConcept purpose; 060 061 /** 062 * The name of an individual to contact, some types of contact detail are usually blank. 063 */ 064 @Child(name = "name", type = {HumanName.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 065 @Description(shortDefinition="Name of an individual to contact", formalDefinition="The name of an individual to contact, some types of contact detail are usually blank." ) 066 protected List<HumanName> name; 067 068 /** 069 * The contact details application for the purpose defined. 070 */ 071 @Child(name = "telecom", type = {ContactPoint.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 072 @Description(shortDefinition="Contact details (e.g.phone/fax/url)", formalDefinition="The contact details application for the purpose defined." ) 073 protected List<ContactPoint> telecom; 074 075 /** 076 * Address for the contact. 077 */ 078 @Child(name = "address", type = {Address.class}, order=3, min=0, max=1, modifier=false, summary=true) 079 @Description(shortDefinition="Address for the contact", formalDefinition="Address for the contact." ) 080 protected Address address; 081 082 /** 083 * This contact detail is handled/monitored by a specific organization. If the name is provided in the contact, then it is referring to the named individual within this organization. 084 */ 085 @Child(name = "organization", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=true) 086 @Description(shortDefinition="This contact detail is handled/monitored by a specific organization", formalDefinition="This contact detail is handled/monitored by a specific organization. If the name is provided in the contact, then it is referring to the named individual within this organization." ) 087 protected Reference organization; 088 089 /** 090 * Period that this contact was valid for usage. 091 */ 092 @Child(name = "period", type = {Period.class}, order=5, min=0, max=1, modifier=false, summary=true) 093 @Description(shortDefinition="Period that this contact was valid for usage", formalDefinition="Period that this contact was valid for usage." ) 094 protected Period period; 095 096 private static final long serialVersionUID = 154672475L; 097 098 /** 099 * Constructor 100 */ 101 public ExtendedContactDetail() { 102 super(); 103 } 104 105 /** 106 * @return {@link #purpose} (The purpose/type of contact.) 107 */ 108 public CodeableConcept getPurpose() { 109 if (this.purpose == null) 110 if (Configuration.errorOnAutoCreate()) 111 throw new Error("Attempt to auto-create ExtendedContactDetail.purpose"); 112 else if (Configuration.doAutoCreate()) 113 this.purpose = new CodeableConcept(); // cc 114 return this.purpose; 115 } 116 117 public boolean hasPurpose() { 118 return this.purpose != null && !this.purpose.isEmpty(); 119 } 120 121 /** 122 * @param value {@link #purpose} (The purpose/type of contact.) 123 */ 124 public ExtendedContactDetail setPurpose(CodeableConcept value) { 125 this.purpose = value; 126 return this; 127 } 128 129 /** 130 * @return {@link #name} (The name of an individual to contact, some types of contact detail are usually blank.) 131 */ 132 public List<HumanName> getName() { 133 if (this.name == null) 134 this.name = new ArrayList<HumanName>(); 135 return this.name; 136 } 137 138 /** 139 * @return Returns a reference to <code>this</code> for easy method chaining 140 */ 141 public ExtendedContactDetail setName(List<HumanName> theName) { 142 this.name = theName; 143 return this; 144 } 145 146 public boolean hasName() { 147 if (this.name == null) 148 return false; 149 for (HumanName item : this.name) 150 if (!item.isEmpty()) 151 return true; 152 return false; 153 } 154 155 public HumanName addName() { //3 156 HumanName t = new HumanName(); 157 if (this.name == null) 158 this.name = new ArrayList<HumanName>(); 159 this.name.add(t); 160 return t; 161 } 162 163 public ExtendedContactDetail addName(HumanName t) { //3 164 if (t == null) 165 return this; 166 if (this.name == null) 167 this.name = new ArrayList<HumanName>(); 168 this.name.add(t); 169 return this; 170 } 171 172 /** 173 * @return The first repetition of repeating field {@link #name}, creating it if it does not already exist {3} 174 */ 175 public HumanName getNameFirstRep() { 176 if (getName().isEmpty()) { 177 addName(); 178 } 179 return getName().get(0); 180 } 181 182 /** 183 * @return {@link #telecom} (The contact details application for the purpose defined.) 184 */ 185 public List<ContactPoint> getTelecom() { 186 if (this.telecom == null) 187 this.telecom = new ArrayList<ContactPoint>(); 188 return this.telecom; 189 } 190 191 /** 192 * @return Returns a reference to <code>this</code> for easy method chaining 193 */ 194 public ExtendedContactDetail setTelecom(List<ContactPoint> theTelecom) { 195 this.telecom = theTelecom; 196 return this; 197 } 198 199 public boolean hasTelecom() { 200 if (this.telecom == null) 201 return false; 202 for (ContactPoint item : this.telecom) 203 if (!item.isEmpty()) 204 return true; 205 return false; 206 } 207 208 public ContactPoint addTelecom() { //3 209 ContactPoint t = new ContactPoint(); 210 if (this.telecom == null) 211 this.telecom = new ArrayList<ContactPoint>(); 212 this.telecom.add(t); 213 return t; 214 } 215 216 public ExtendedContactDetail addTelecom(ContactPoint t) { //3 217 if (t == null) 218 return this; 219 if (this.telecom == null) 220 this.telecom = new ArrayList<ContactPoint>(); 221 this.telecom.add(t); 222 return this; 223 } 224 225 /** 226 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist {3} 227 */ 228 public ContactPoint getTelecomFirstRep() { 229 if (getTelecom().isEmpty()) { 230 addTelecom(); 231 } 232 return getTelecom().get(0); 233 } 234 235 /** 236 * @return {@link #address} (Address for the contact.) 237 */ 238 public Address getAddress() { 239 if (this.address == null) 240 if (Configuration.errorOnAutoCreate()) 241 throw new Error("Attempt to auto-create ExtendedContactDetail.address"); 242 else if (Configuration.doAutoCreate()) 243 this.address = new Address(); // cc 244 return this.address; 245 } 246 247 public boolean hasAddress() { 248 return this.address != null && !this.address.isEmpty(); 249 } 250 251 /** 252 * @param value {@link #address} (Address for the contact.) 253 */ 254 public ExtendedContactDetail setAddress(Address value) { 255 this.address = value; 256 return this; 257 } 258 259 /** 260 * @return {@link #organization} (This contact detail is handled/monitored by a specific organization. If the name is provided in the contact, then it is referring to the named individual within this organization.) 261 */ 262 public Reference getOrganization() { 263 if (this.organization == null) 264 if (Configuration.errorOnAutoCreate()) 265 throw new Error("Attempt to auto-create ExtendedContactDetail.organization"); 266 else if (Configuration.doAutoCreate()) 267 this.organization = new Reference(); // cc 268 return this.organization; 269 } 270 271 public boolean hasOrganization() { 272 return this.organization != null && !this.organization.isEmpty(); 273 } 274 275 /** 276 * @param value {@link #organization} (This contact detail is handled/monitored by a specific organization. If the name is provided in the contact, then it is referring to the named individual within this organization.) 277 */ 278 public ExtendedContactDetail setOrganization(Reference value) { 279 this.organization = value; 280 return this; 281 } 282 283 /** 284 * @return {@link #period} (Period that this contact was valid for usage.) 285 */ 286 public Period getPeriod() { 287 if (this.period == null) 288 if (Configuration.errorOnAutoCreate()) 289 throw new Error("Attempt to auto-create ExtendedContactDetail.period"); 290 else if (Configuration.doAutoCreate()) 291 this.period = new Period(); // cc 292 return this.period; 293 } 294 295 public boolean hasPeriod() { 296 return this.period != null && !this.period.isEmpty(); 297 } 298 299 /** 300 * @param value {@link #period} (Period that this contact was valid for usage.) 301 */ 302 public ExtendedContactDetail setPeriod(Period value) { 303 this.period = value; 304 return this; 305 } 306 307 protected void listChildren(List<Property> children) { 308 super.listChildren(children); 309 children.add(new Property("purpose", "CodeableConcept", "The purpose/type of contact.", 0, 1, purpose)); 310 children.add(new Property("name", "HumanName", "The name of an individual to contact, some types of contact detail are usually blank.", 0, java.lang.Integer.MAX_VALUE, name)); 311 children.add(new Property("telecom", "ContactPoint", "The contact details application for the purpose defined.", 0, java.lang.Integer.MAX_VALUE, telecom)); 312 children.add(new Property("address", "Address", "Address for the contact.", 0, 1, address)); 313 children.add(new Property("organization", "Reference(Organization)", "This contact detail is handled/monitored by a specific organization. If the name is provided in the contact, then it is referring to the named individual within this organization.", 0, 1, organization)); 314 children.add(new Property("period", "Period", "Period that this contact was valid for usage.", 0, 1, period)); 315 } 316 317 @Override 318 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 319 switch (_hash) { 320 case -220463842: /*purpose*/ return new Property("purpose", "CodeableConcept", "The purpose/type of contact.", 0, 1, purpose); 321 case 3373707: /*name*/ return new Property("name", "HumanName", "The name of an individual to contact, some types of contact detail are usually blank.", 0, java.lang.Integer.MAX_VALUE, name); 322 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "The contact details application for the purpose defined.", 0, java.lang.Integer.MAX_VALUE, telecom); 323 case -1147692044: /*address*/ return new Property("address", "Address", "Address for the contact.", 0, 1, address); 324 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "This contact detail is handled/monitored by a specific organization. If the name is provided in the contact, then it is referring to the named individual within this organization.", 0, 1, organization); 325 case -991726143: /*period*/ return new Property("period", "Period", "Period that this contact was valid for usage.", 0, 1, period); 326 default: return super.getNamedProperty(_hash, _name, _checkValid); 327 } 328 329 } 330 331 @Override 332 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 333 switch (hash) { 334 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // CodeableConcept 335 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // HumanName 336 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 337 case -1147692044: /*address*/ return this.address == null ? new Base[0] : new Base[] {this.address}; // Address 338 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 339 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 340 default: return super.getProperty(hash, name, checkValid); 341 } 342 343 } 344 345 @Override 346 public Base setProperty(int hash, String name, Base value) throws FHIRException { 347 switch (hash) { 348 case -220463842: // purpose 349 this.purpose = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 350 return value; 351 case 3373707: // name 352 this.getName().add(TypeConvertor.castToHumanName(value)); // HumanName 353 return value; 354 case -1429363305: // telecom 355 this.getTelecom().add(TypeConvertor.castToContactPoint(value)); // ContactPoint 356 return value; 357 case -1147692044: // address 358 this.address = TypeConvertor.castToAddress(value); // Address 359 return value; 360 case 1178922291: // organization 361 this.organization = TypeConvertor.castToReference(value); // Reference 362 return value; 363 case -991726143: // period 364 this.period = TypeConvertor.castToPeriod(value); // Period 365 return value; 366 default: return super.setProperty(hash, name, value); 367 } 368 369 } 370 371 @Override 372 public Base setProperty(String name, Base value) throws FHIRException { 373 if (name.equals("purpose")) { 374 this.purpose = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 375 } else if (name.equals("name")) { 376 this.getName().add(TypeConvertor.castToHumanName(value)); 377 } else if (name.equals("telecom")) { 378 this.getTelecom().add(TypeConvertor.castToContactPoint(value)); 379 } else if (name.equals("address")) { 380 this.address = TypeConvertor.castToAddress(value); // Address 381 } else if (name.equals("organization")) { 382 this.organization = TypeConvertor.castToReference(value); // Reference 383 } else if (name.equals("period")) { 384 this.period = TypeConvertor.castToPeriod(value); // Period 385 } else 386 return super.setProperty(name, value); 387 return value; 388 } 389 390 @Override 391 public void removeChild(String name, Base value) throws FHIRException { 392 if (name.equals("purpose")) { 393 this.purpose = null; 394 } else if (name.equals("name")) { 395 this.getName().remove(value); 396 } else if (name.equals("telecom")) { 397 this.getTelecom().remove(value); 398 } else if (name.equals("address")) { 399 this.address = null; 400 } else if (name.equals("organization")) { 401 this.organization = null; 402 } else if (name.equals("period")) { 403 this.period = null; 404 } else 405 super.removeChild(name, value); 406 407 } 408 409 @Override 410 public Base makeProperty(int hash, String name) throws FHIRException { 411 switch (hash) { 412 case -220463842: return getPurpose(); 413 case 3373707: return addName(); 414 case -1429363305: return addTelecom(); 415 case -1147692044: return getAddress(); 416 case 1178922291: return getOrganization(); 417 case -991726143: return getPeriod(); 418 default: return super.makeProperty(hash, name); 419 } 420 421 } 422 423 @Override 424 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 425 switch (hash) { 426 case -220463842: /*purpose*/ return new String[] {"CodeableConcept"}; 427 case 3373707: /*name*/ return new String[] {"HumanName"}; 428 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 429 case -1147692044: /*address*/ return new String[] {"Address"}; 430 case 1178922291: /*organization*/ return new String[] {"Reference"}; 431 case -991726143: /*period*/ return new String[] {"Period"}; 432 default: return super.getTypesForProperty(hash, name); 433 } 434 435 } 436 437 @Override 438 public Base addChild(String name) throws FHIRException { 439 if (name.equals("purpose")) { 440 this.purpose = new CodeableConcept(); 441 return this.purpose; 442 } 443 else if (name.equals("name")) { 444 return addName(); 445 } 446 else if (name.equals("telecom")) { 447 return addTelecom(); 448 } 449 else if (name.equals("address")) { 450 this.address = new Address(); 451 return this.address; 452 } 453 else if (name.equals("organization")) { 454 this.organization = new Reference(); 455 return this.organization; 456 } 457 else if (name.equals("period")) { 458 this.period = new Period(); 459 return this.period; 460 } 461 else 462 return super.addChild(name); 463 } 464 465 public String fhirType() { 466 return "ExtendedContactDetail"; 467 468 } 469 470 public ExtendedContactDetail copy() { 471 ExtendedContactDetail dst = new ExtendedContactDetail(); 472 copyValues(dst); 473 return dst; 474 } 475 476 public void copyValues(ExtendedContactDetail dst) { 477 super.copyValues(dst); 478 dst.purpose = purpose == null ? null : purpose.copy(); 479 if (name != null) { 480 dst.name = new ArrayList<HumanName>(); 481 for (HumanName i : name) 482 dst.name.add(i.copy()); 483 }; 484 if (telecom != null) { 485 dst.telecom = new ArrayList<ContactPoint>(); 486 for (ContactPoint i : telecom) 487 dst.telecom.add(i.copy()); 488 }; 489 dst.address = address == null ? null : address.copy(); 490 dst.organization = organization == null ? null : organization.copy(); 491 dst.period = period == null ? null : period.copy(); 492 } 493 494 protected ExtendedContactDetail typedCopy() { 495 return copy(); 496 } 497 498 @Override 499 public boolean equalsDeep(Base other_) { 500 if (!super.equalsDeep(other_)) 501 return false; 502 if (!(other_ instanceof ExtendedContactDetail)) 503 return false; 504 ExtendedContactDetail o = (ExtendedContactDetail) other_; 505 return compareDeep(purpose, o.purpose, true) && compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true) 506 && compareDeep(address, o.address, true) && compareDeep(organization, o.organization, true) && compareDeep(period, o.period, true) 507 ; 508 } 509 510 @Override 511 public boolean equalsShallow(Base other_) { 512 if (!super.equalsShallow(other_)) 513 return false; 514 if (!(other_ instanceof ExtendedContactDetail)) 515 return false; 516 ExtendedContactDetail o = (ExtendedContactDetail) other_; 517 return true; 518 } 519 520 public boolean isEmpty() { 521 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, name, telecom, address 522 , organization, period); 523 } 524 525 526} 527