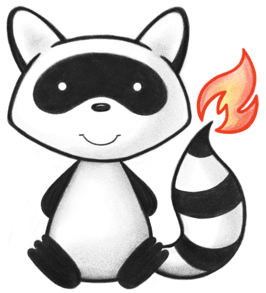
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048import org.hl7.fhir.instance.model.api.IBaseExtension; 049import org.hl7.fhir.instance.model.api.IBaseDatatype; 050import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 051/** 052 * Extension Type: Optional Extension Element - found in all resources. 053 */ 054@DatatypeDef(name="Extension") 055public class Extension extends BaseExtension implements IBaseExtension<Extension, DataType>, IBaseHasExtensions { 056 057 /** 058 * Source of the definition for the extension code - a logical name or a URL. 059 */ 060 @Child(name = "url", type = {UriType.class}, order=0, min=1, max=1, modifier=false, summary=false) 061 @Description(shortDefinition="identifies the meaning of the extension", formalDefinition="Source of the definition for the extension code - a logical name or a URL." ) 062 protected UriType url; 063 064 /** 065 * Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list). 066 */ 067 @Child(name = "value", type = {Base64BinaryType.class, BooleanType.class, CanonicalType.class, CodeType.class, DateType.class, DateTimeType.class, DecimalType.class, IdType.class, InstantType.class, IntegerType.class, Integer64Type.class, MarkdownType.class, OidType.class, PositiveIntType.class, StringType.class, TimeType.class, UnsignedIntType.class, UriType.class, UrlType.class, UuidType.class, Address.class, Age.class, Annotation.class, Attachment.class, CodeableConcept.class, CodeableReference.class, Coding.class, ContactPoint.class, Count.class, Distance.class, Duration.class, HumanName.class, Identifier.class, Money.class, Period.class, Quantity.class, Range.class, Ratio.class, RatioRange.class, Reference.class, SampledData.class, Signature.class, Timing.class, ContactDetail.class, DataRequirement.class, Expression.class, ParameterDefinition.class, RelatedArtifact.class, TriggerDefinition.class, UsageContext.class, Availability.class, ExtendedContactDetail.class, Dosage.class, Meta.class}, order=1, min=0, max=1, modifier=false, summary=false) 068 @Description(shortDefinition="Value of extension", formalDefinition="Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list)." ) 069 protected DataType value; 070 071 @Override 072 public String toString() { 073 return url + "=" + (value == null ? "null" : value.toString()); 074 } 075 076 private static final long serialVersionUID = 465890108L; 077 078 /** 079 * Constructor 080 */ 081 public Extension() { 082 super(); 083 } 084 085 /** 086 * Constructor 087 */ 088 public Extension(String url) { 089 super(); 090 this.setUrl(url); 091 } 092 093 /** 094 * Constructor 095 */ 096 public Extension(String theUrl, IBaseDatatype theValue) { 097 setUrl(theUrl); 098 setValue(theValue); 099 } 100 101 /** 102 * @return {@link #url} (Source of the definition for the extension code - a logical name or a URL.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 103 */ 104 public UriType getUrlElement() { 105 if (this.url == null) 106 if (Configuration.errorOnAutoCreate()) 107 throw new Error("Attempt to auto-create Extension.url"); 108 else if (Configuration.doAutoCreate()) 109 this.url = new UriType(); // bb 110 return this.url; 111 } 112 113 public boolean hasUrlElement() { 114 return this.url != null && !this.url.isEmpty(); 115 } 116 117 public boolean hasUrl() { 118 return this.url != null && !this.url.isEmpty(); 119 } 120 121 /** 122 * @param value {@link #url} (Source of the definition for the extension code - a logical name or a URL.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 123 */ 124 public Extension setUrlElement(UriType value) { 125 this.url = value; 126 return this; 127 } 128 129 /** 130 * @return Source of the definition for the extension code - a logical name or a URL. 131 */ 132 public String getUrl() { 133 return this.url == null ? null : this.url.getValue(); 134 } 135 136 /** 137 * @param value Source of the definition for the extension code - a logical name or a URL. 138 */ 139 public Extension setUrl(String value) { 140 if (this.url == null) 141 this.url = new UriType(); 142 this.url.setValue(value); 143 return this; 144 } 145 146 /** 147 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 148 */ 149 public DataType getValue() { 150 return this.value; 151 } 152 153 /** 154 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 155 */ 156 public Base64BinaryType getValueBase64BinaryType() throws FHIRException { 157 if (this.value == null) 158 this.value = new Base64BinaryType(); 159 if (!(this.value instanceof Base64BinaryType)) 160 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.value.getClass().getName()+" was encountered"); 161 return (Base64BinaryType) this.value; 162 } 163 164 public boolean hasValueBase64BinaryType() { 165 return this.value instanceof Base64BinaryType; 166 } 167 168 /** 169 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 170 */ 171 public BooleanType getValueBooleanType() throws FHIRException { 172 if (this.value == null) 173 this.value = new BooleanType(); 174 if (!(this.value instanceof BooleanType)) 175 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 176 return (BooleanType) this.value; 177 } 178 179 public boolean hasValueBooleanType() { 180 return this.value instanceof BooleanType; 181 } 182 183 /** 184 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 185 */ 186 public CanonicalType getValueCanonicalType() throws FHIRException { 187 if (this.value == null) 188 this.value = new CanonicalType(); 189 if (!(this.value instanceof CanonicalType)) 190 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.value.getClass().getName()+" was encountered"); 191 return (CanonicalType) this.value; 192 } 193 194 public boolean hasValueCanonicalType() { 195 return this.value instanceof CanonicalType; 196 } 197 198 /** 199 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 200 */ 201 public CodeType getValueCodeType() throws FHIRException { 202 if (this.value == null) 203 this.value = new CodeType(); 204 if (!(this.value instanceof CodeType)) 205 throw new FHIRException("Type mismatch: the type CodeType was expected, but "+this.value.getClass().getName()+" was encountered"); 206 return (CodeType) this.value; 207 } 208 209 public boolean hasValueCodeType() { 210 return this.value instanceof CodeType; 211 } 212 213 /** 214 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 215 */ 216 public DateType getValueDateType() throws FHIRException { 217 if (this.value == null) 218 this.value = new DateType(); 219 if (!(this.value instanceof DateType)) 220 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 221 return (DateType) this.value; 222 } 223 224 public boolean hasValueDateType() { 225 return this.value instanceof DateType; 226 } 227 228 /** 229 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 230 */ 231 public DateTimeType getValueDateTimeType() throws FHIRException { 232 if (this.value == null) 233 this.value = new DateTimeType(); 234 if (!(this.value instanceof DateTimeType)) 235 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 236 return (DateTimeType) this.value; 237 } 238 239 public boolean hasValueDateTimeType() { 240 return this.value instanceof DateTimeType; 241 } 242 243 /** 244 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 245 */ 246 public DecimalType getValueDecimalType() throws FHIRException { 247 if (this.value == null) 248 this.value = new DecimalType(); 249 if (!(this.value instanceof DecimalType)) 250 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 251 return (DecimalType) this.value; 252 } 253 254 public boolean hasValueDecimalType() { 255 return this.value instanceof DecimalType; 256 } 257 258 /** 259 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 260 */ 261 public IdType getValueIdType() throws FHIRException { 262 if (this.value == null) 263 this.value = new IdType(); 264 if (!(this.value instanceof IdType)) 265 throw new FHIRException("Type mismatch: the type IdType was expected, but "+this.value.getClass().getName()+" was encountered"); 266 return (IdType) this.value; 267 } 268 269 public boolean hasValueIdType() { 270 return this.value instanceof IdType; 271 } 272 273 /** 274 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 275 */ 276 public InstantType getValueInstantType() throws FHIRException { 277 if (this.value == null) 278 this.value = new InstantType(); 279 if (!(this.value instanceof InstantType)) 280 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.value.getClass().getName()+" was encountered"); 281 return (InstantType) this.value; 282 } 283 284 public boolean hasValueInstantType() { 285 return this.value instanceof InstantType; 286 } 287 288 /** 289 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 290 */ 291 public IntegerType getValueIntegerType() throws FHIRException { 292 if (this.value == null) 293 this.value = new IntegerType(); 294 if (!(this.value instanceof IntegerType)) 295 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 296 return (IntegerType) this.value; 297 } 298 299 public boolean hasValueIntegerType() { 300 return this.value instanceof IntegerType; 301 } 302 303 /** 304 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 305 */ 306 public Integer64Type getValueInteger64Type() throws FHIRException { 307 if (this.value == null) 308 this.value = new Integer64Type(); 309 if (!(this.value instanceof Integer64Type)) 310 throw new FHIRException("Type mismatch: the type Integer64Type was expected, but "+this.value.getClass().getName()+" was encountered"); 311 return (Integer64Type) this.value; 312 } 313 314 public boolean hasValueInteger64Type() { 315 return this.value instanceof Integer64Type; 316 } 317 318 /** 319 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 320 */ 321 public MarkdownType getValueMarkdownType() throws FHIRException { 322 if (this.value == null) 323 this.value = new MarkdownType(); 324 if (!(this.value instanceof MarkdownType)) 325 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.value.getClass().getName()+" was encountered"); 326 return (MarkdownType) this.value; 327 } 328 329 public boolean hasValueMarkdownType() { 330 return this.value instanceof MarkdownType; 331 } 332 333 /** 334 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 335 */ 336 public OidType getValueOidType() throws FHIRException { 337 if (this.value == null) 338 this.value = new OidType(); 339 if (!(this.value instanceof OidType)) 340 throw new FHIRException("Type mismatch: the type OidType was expected, but "+this.value.getClass().getName()+" was encountered"); 341 return (OidType) this.value; 342 } 343 344 public boolean hasValueOidType() { 345 return this.value instanceof OidType; 346 } 347 348 /** 349 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 350 */ 351 public PositiveIntType getValuePositiveIntType() throws FHIRException { 352 if (this.value == null) 353 this.value = new PositiveIntType(); 354 if (!(this.value instanceof PositiveIntType)) 355 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.value.getClass().getName()+" was encountered"); 356 return (PositiveIntType) this.value; 357 } 358 359 public boolean hasValuePositiveIntType() { 360 return this.value instanceof PositiveIntType; 361 } 362 363 /** 364 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 365 */ 366 public StringType getValueStringType() throws FHIRException { 367 if (this.value == null) 368 this.value = new StringType(); 369 if (!(this.value instanceof StringType)) 370 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 371 return (StringType) this.value; 372 } 373 374 public boolean hasValueStringType() { 375 return this.value instanceof StringType; 376 } 377 378 /** 379 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 380 */ 381 public TimeType getValueTimeType() throws FHIRException { 382 if (this.value == null) 383 this.value = new TimeType(); 384 if (!(this.value instanceof TimeType)) 385 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 386 return (TimeType) this.value; 387 } 388 389 public boolean hasValueTimeType() { 390 return this.value instanceof TimeType; 391 } 392 393 /** 394 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 395 */ 396 public UnsignedIntType getValueUnsignedIntType() throws FHIRException { 397 if (this.value == null) 398 this.value = new UnsignedIntType(); 399 if (!(this.value instanceof UnsignedIntType)) 400 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.value.getClass().getName()+" was encountered"); 401 return (UnsignedIntType) this.value; 402 } 403 404 public boolean hasValueUnsignedIntType() { 405 return this.value instanceof UnsignedIntType; 406 } 407 408 /** 409 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 410 */ 411 public UriType getValueUriType() throws FHIRException { 412 if (this.value == null) 413 this.value = new UriType(); 414 if (!(this.value instanceof UriType)) 415 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.value.getClass().getName()+" was encountered"); 416 return (UriType) this.value; 417 } 418 419 public boolean hasValueUriType() { 420 return this.value instanceof UriType; 421 } 422 423 /** 424 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 425 */ 426 public UrlType getValueUrlType() throws FHIRException { 427 if (this.value == null) 428 this.value = new UrlType(); 429 if (!(this.value instanceof UrlType)) 430 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.value.getClass().getName()+" was encountered"); 431 return (UrlType) this.value; 432 } 433 434 public boolean hasValueUrlType() { 435 return this.value instanceof UrlType; 436 } 437 438 /** 439 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 440 */ 441 public UuidType getValueUuidType() throws FHIRException { 442 if (this.value == null) 443 this.value = new UuidType(); 444 if (!(this.value instanceof UuidType)) 445 throw new FHIRException("Type mismatch: the type UuidType was expected, but "+this.value.getClass().getName()+" was encountered"); 446 return (UuidType) this.value; 447 } 448 449 public boolean hasValueUuidType() { 450 return this.value instanceof UuidType; 451 } 452 453 /** 454 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 455 */ 456 public Address getValueAddress() throws FHIRException { 457 if (this.value == null) 458 this.value = new Address(); 459 if (!(this.value instanceof Address)) 460 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.value.getClass().getName()+" was encountered"); 461 return (Address) this.value; 462 } 463 464 public boolean hasValueAddress() { 465 return this.value instanceof Address; 466 } 467 468 /** 469 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 470 */ 471 public Age getValueAge() throws FHIRException { 472 if (this.value == null) 473 this.value = new Age(); 474 if (!(this.value instanceof Age)) 475 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.value.getClass().getName()+" was encountered"); 476 return (Age) this.value; 477 } 478 479 public boolean hasValueAge() { 480 return this.value instanceof Age; 481 } 482 483 /** 484 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 485 */ 486 public Annotation getValueAnnotation() throws FHIRException { 487 if (this.value == null) 488 this.value = new Annotation(); 489 if (!(this.value instanceof Annotation)) 490 throw new FHIRException("Type mismatch: the type Annotation was expected, but "+this.value.getClass().getName()+" was encountered"); 491 return (Annotation) this.value; 492 } 493 494 public boolean hasValueAnnotation() { 495 return this.value instanceof Annotation; 496 } 497 498 /** 499 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 500 */ 501 public Attachment getValueAttachment() throws FHIRException { 502 if (this.value == null) 503 this.value = new Attachment(); 504 if (!(this.value instanceof Attachment)) 505 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 506 return (Attachment) this.value; 507 } 508 509 public boolean hasValueAttachment() { 510 return this.value instanceof Attachment; 511 } 512 513 /** 514 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 515 */ 516 public CodeableConcept getValueCodeableConcept() throws FHIRException { 517 if (this.value == null) 518 this.value = new CodeableConcept(); 519 if (!(this.value instanceof CodeableConcept)) 520 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 521 return (CodeableConcept) this.value; 522 } 523 524 public boolean hasValueCodeableConcept() { 525 return this.value instanceof CodeableConcept; 526 } 527 528 /** 529 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 530 */ 531 public CodeableReference getValueCodeableReference() throws FHIRException { 532 if (this.value == null) 533 this.value = new CodeableReference(); 534 if (!(this.value instanceof CodeableReference)) 535 throw new FHIRException("Type mismatch: the type CodeableReference was expected, but "+this.value.getClass().getName()+" was encountered"); 536 return (CodeableReference) this.value; 537 } 538 539 public boolean hasValueCodeableReference() { 540 return this.value instanceof CodeableReference; 541 } 542 543 /** 544 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 545 */ 546 public Coding getValueCoding() throws FHIRException { 547 if (this.value == null) 548 this.value = new Coding(); 549 if (!(this.value instanceof Coding)) 550 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.value.getClass().getName()+" was encountered"); 551 return (Coding) this.value; 552 } 553 554 public boolean hasValueCoding() { 555 return this.value instanceof Coding; 556 } 557 558 /** 559 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 560 */ 561 public ContactPoint getValueContactPoint() throws FHIRException { 562 if (this.value == null) 563 this.value = new ContactPoint(); 564 if (!(this.value instanceof ContactPoint)) 565 throw new FHIRException("Type mismatch: the type ContactPoint was expected, but "+this.value.getClass().getName()+" was encountered"); 566 return (ContactPoint) this.value; 567 } 568 569 public boolean hasValueContactPoint() { 570 return this.value instanceof ContactPoint; 571 } 572 573 /** 574 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 575 */ 576 public Count getValueCount() throws FHIRException { 577 if (this.value == null) 578 this.value = new Count(); 579 if (!(this.value instanceof Count)) 580 throw new FHIRException("Type mismatch: the type Count was expected, but "+this.value.getClass().getName()+" was encountered"); 581 return (Count) this.value; 582 } 583 584 public boolean hasValueCount() { 585 return this.value instanceof Count; 586 } 587 588 /** 589 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 590 */ 591 public Distance getValueDistance() throws FHIRException { 592 if (this.value == null) 593 this.value = new Distance(); 594 if (!(this.value instanceof Distance)) 595 throw new FHIRException("Type mismatch: the type Distance was expected, but "+this.value.getClass().getName()+" was encountered"); 596 return (Distance) this.value; 597 } 598 599 public boolean hasValueDistance() { 600 return this.value instanceof Distance; 601 } 602 603 /** 604 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 605 */ 606 public Duration getValueDuration() throws FHIRException { 607 if (this.value == null) 608 this.value = new Duration(); 609 if (!(this.value instanceof Duration)) 610 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.value.getClass().getName()+" was encountered"); 611 return (Duration) this.value; 612 } 613 614 public boolean hasValueDuration() { 615 return this.value instanceof Duration; 616 } 617 618 /** 619 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 620 */ 621 public HumanName getValueHumanName() throws FHIRException { 622 if (this.value == null) 623 this.value = new HumanName(); 624 if (!(this.value instanceof HumanName)) 625 throw new FHIRException("Type mismatch: the type HumanName was expected, but "+this.value.getClass().getName()+" was encountered"); 626 return (HumanName) this.value; 627 } 628 629 public boolean hasValueHumanName() { 630 return this.value instanceof HumanName; 631 } 632 633 /** 634 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 635 */ 636 public Identifier getValueIdentifier() throws FHIRException { 637 if (this.value == null) 638 this.value = new Identifier(); 639 if (!(this.value instanceof Identifier)) 640 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.value.getClass().getName()+" was encountered"); 641 return (Identifier) this.value; 642 } 643 644 public boolean hasValueIdentifier() { 645 return this.value instanceof Identifier; 646 } 647 648 /** 649 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 650 */ 651 public Money getValueMoney() throws FHIRException { 652 if (this.value == null) 653 this.value = new Money(); 654 if (!(this.value instanceof Money)) 655 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.value.getClass().getName()+" was encountered"); 656 return (Money) this.value; 657 } 658 659 public boolean hasValueMoney() { 660 return this.value instanceof Money; 661 } 662 663 /** 664 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 665 */ 666 public Period getValuePeriod() throws FHIRException { 667 if (this.value == null) 668 this.value = new Period(); 669 if (!(this.value instanceof Period)) 670 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.value.getClass().getName()+" was encountered"); 671 return (Period) this.value; 672 } 673 674 public boolean hasValuePeriod() { 675 return this.value instanceof Period; 676 } 677 678 /** 679 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 680 */ 681 public Quantity getValueQuantity() throws FHIRException { 682 if (this.value == null) 683 this.value = new Quantity(); 684 if (!(this.value instanceof Quantity)) 685 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 686 return (Quantity) this.value; 687 } 688 689 public boolean hasValueQuantity() { 690 return this.value instanceof Quantity; 691 } 692 693 /** 694 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 695 */ 696 public Range getValueRange() throws FHIRException { 697 if (this.value == null) 698 this.value = new Range(); 699 if (!(this.value instanceof Range)) 700 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 701 return (Range) this.value; 702 } 703 704 public boolean hasValueRange() { 705 return this.value instanceof Range; 706 } 707 708 /** 709 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 710 */ 711 public Ratio getValueRatio() throws FHIRException { 712 if (this.value == null) 713 this.value = new Ratio(); 714 if (!(this.value instanceof Ratio)) 715 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 716 return (Ratio) this.value; 717 } 718 719 public boolean hasValueRatio() { 720 return this.value instanceof Ratio; 721 } 722 723 /** 724 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 725 */ 726 public RatioRange getValueRatioRange() throws FHIRException { 727 if (this.value == null) 728 this.value = new RatioRange(); 729 if (!(this.value instanceof RatioRange)) 730 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.value.getClass().getName()+" was encountered"); 731 return (RatioRange) this.value; 732 } 733 734 public boolean hasValueRatioRange() { 735 return this.value instanceof RatioRange; 736 } 737 738 /** 739 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 740 */ 741 public Reference getValueReference() throws FHIRException { 742 if (this.value == null) 743 this.value = new Reference(); 744 if (!(this.value instanceof Reference)) 745 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 746 return (Reference) this.value; 747 } 748 749 public boolean hasValueReference() { 750 return this.value instanceof Reference; 751 } 752 753 /** 754 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 755 */ 756 public SampledData getValueSampledData() throws FHIRException { 757 if (this.value == null) 758 this.value = new SampledData(); 759 if (!(this.value instanceof SampledData)) 760 throw new FHIRException("Type mismatch: the type SampledData was expected, but "+this.value.getClass().getName()+" was encountered"); 761 return (SampledData) this.value; 762 } 763 764 public boolean hasValueSampledData() { 765 return this.value instanceof SampledData; 766 } 767 768 /** 769 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 770 */ 771 public Signature getValueSignature() throws FHIRException { 772 if (this.value == null) 773 this.value = new Signature(); 774 if (!(this.value instanceof Signature)) 775 throw new FHIRException("Type mismatch: the type Signature was expected, but "+this.value.getClass().getName()+" was encountered"); 776 return (Signature) this.value; 777 } 778 779 public boolean hasValueSignature() { 780 return this.value instanceof Signature; 781 } 782 783 /** 784 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 785 */ 786 public Timing getValueTiming() throws FHIRException { 787 if (this.value == null) 788 this.value = new Timing(); 789 if (!(this.value instanceof Timing)) 790 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.value.getClass().getName()+" was encountered"); 791 return (Timing) this.value; 792 } 793 794 public boolean hasValueTiming() { 795 return this.value instanceof Timing; 796 } 797 798 /** 799 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 800 */ 801 public ContactDetail getValueContactDetail() throws FHIRException { 802 if (this.value == null) 803 this.value = new ContactDetail(); 804 if (!(this.value instanceof ContactDetail)) 805 throw new FHIRException("Type mismatch: the type ContactDetail was expected, but "+this.value.getClass().getName()+" was encountered"); 806 return (ContactDetail) this.value; 807 } 808 809 public boolean hasValueContactDetail() { 810 return this.value instanceof ContactDetail; 811 } 812 813 /** 814 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 815 */ 816 public DataRequirement getValueDataRequirement() throws FHIRException { 817 if (this.value == null) 818 this.value = new DataRequirement(); 819 if (!(this.value instanceof DataRequirement)) 820 throw new FHIRException("Type mismatch: the type DataRequirement was expected, but "+this.value.getClass().getName()+" was encountered"); 821 return (DataRequirement) this.value; 822 } 823 824 public boolean hasValueDataRequirement() { 825 return this.value instanceof DataRequirement; 826 } 827 828 /** 829 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 830 */ 831 public Expression getValueExpression() throws FHIRException { 832 if (this.value == null) 833 this.value = new Expression(); 834 if (!(this.value instanceof Expression)) 835 throw new FHIRException("Type mismatch: the type Expression was expected, but "+this.value.getClass().getName()+" was encountered"); 836 return (Expression) this.value; 837 } 838 839 public boolean hasValueExpression() { 840 return this.value instanceof Expression; 841 } 842 843 /** 844 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 845 */ 846 public ParameterDefinition getValueParameterDefinition() throws FHIRException { 847 if (this.value == null) 848 this.value = new ParameterDefinition(); 849 if (!(this.value instanceof ParameterDefinition)) 850 throw new FHIRException("Type mismatch: the type ParameterDefinition was expected, but "+this.value.getClass().getName()+" was encountered"); 851 return (ParameterDefinition) this.value; 852 } 853 854 public boolean hasValueParameterDefinition() { 855 return this.value instanceof ParameterDefinition; 856 } 857 858 /** 859 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 860 */ 861 public RelatedArtifact getValueRelatedArtifact() throws FHIRException { 862 if (this.value == null) 863 this.value = new RelatedArtifact(); 864 if (!(this.value instanceof RelatedArtifact)) 865 throw new FHIRException("Type mismatch: the type RelatedArtifact was expected, but "+this.value.getClass().getName()+" was encountered"); 866 return (RelatedArtifact) this.value; 867 } 868 869 public boolean hasValueRelatedArtifact() { 870 return this.value instanceof RelatedArtifact; 871 } 872 873 /** 874 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 875 */ 876 public TriggerDefinition getValueTriggerDefinition() throws FHIRException { 877 if (this.value == null) 878 this.value = new TriggerDefinition(); 879 if (!(this.value instanceof TriggerDefinition)) 880 throw new FHIRException("Type mismatch: the type TriggerDefinition was expected, but "+this.value.getClass().getName()+" was encountered"); 881 return (TriggerDefinition) this.value; 882 } 883 884 public boolean hasValueTriggerDefinition() { 885 return this.value instanceof TriggerDefinition; 886 } 887 888 /** 889 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 890 */ 891 public UsageContext getValueUsageContext() throws FHIRException { 892 if (this.value == null) 893 this.value = new UsageContext(); 894 if (!(this.value instanceof UsageContext)) 895 throw new FHIRException("Type mismatch: the type UsageContext was expected, but "+this.value.getClass().getName()+" was encountered"); 896 return (UsageContext) this.value; 897 } 898 899 public boolean hasValueUsageContext() { 900 return this.value instanceof UsageContext; 901 } 902 903 /** 904 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 905 */ 906 public Availability getValueAvailability() throws FHIRException { 907 if (this.value == null) 908 this.value = new Availability(); 909 if (!(this.value instanceof Availability)) 910 throw new FHIRException("Type mismatch: the type Availability was expected, but "+this.value.getClass().getName()+" was encountered"); 911 return (Availability) this.value; 912 } 913 914 public boolean hasValueAvailability() { 915 return this.value instanceof Availability; 916 } 917 918 /** 919 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 920 */ 921 public ExtendedContactDetail getValueExtendedContactDetail() throws FHIRException { 922 if (this.value == null) 923 this.value = new ExtendedContactDetail(); 924 if (!(this.value instanceof ExtendedContactDetail)) 925 throw new FHIRException("Type mismatch: the type ExtendedContactDetail was expected, but "+this.value.getClass().getName()+" was encountered"); 926 return (ExtendedContactDetail) this.value; 927 } 928 929 public boolean hasValueExtendedContactDetail() { 930 return this.value instanceof ExtendedContactDetail; 931 } 932 933 /** 934 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 935 */ 936 public Dosage getValueDosage() throws FHIRException { 937 if (this.value == null) 938 this.value = new Dosage(); 939 if (!(this.value instanceof Dosage)) 940 throw new FHIRException("Type mismatch: the type Dosage was expected, but "+this.value.getClass().getName()+" was encountered"); 941 return (Dosage) this.value; 942 } 943 944 public boolean hasValueDosage() { 945 return this.value instanceof Dosage; 946 } 947 948 /** 949 * @return {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 950 */ 951 public Meta getValueMeta() throws FHIRException { 952 if (this.value == null) 953 this.value = new Meta(); 954 if (!(this.value instanceof Meta)) 955 throw new FHIRException("Type mismatch: the type Meta was expected, but "+this.value.getClass().getName()+" was encountered"); 956 return (Meta) this.value; 957 } 958 959 public boolean hasValueMeta() { 960 return this.value instanceof Meta; 961 } 962 963 public boolean hasValue() { 964 return this.value != null && !this.value.isEmpty(); 965 } 966 967 /** 968 * @param value {@link #value} (Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).) 969 */ 970 public Extension setValue(DataType value) { 971 if (value != null && !(value instanceof Base64BinaryType || value instanceof BooleanType || value instanceof CanonicalType || value instanceof CodeType || value instanceof DateType || value instanceof DateTimeType || value instanceof DecimalType || value instanceof IdType || value instanceof InstantType || value instanceof IntegerType || value instanceof Integer64Type || value instanceof MarkdownType || value instanceof OidType || value instanceof PositiveIntType || value instanceof StringType || value instanceof TimeType || value instanceof UnsignedIntType || value instanceof UriType || value instanceof UrlType || value instanceof UuidType || value instanceof Address || value instanceof Age || value instanceof Annotation || value instanceof Attachment || value instanceof CodeableConcept || value instanceof CodeableReference || value instanceof Coding || value instanceof ContactPoint || value instanceof Count || value instanceof Distance || value instanceof Duration || value instanceof HumanName || value instanceof Identifier || value instanceof Money || value instanceof Period || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof RatioRange || value instanceof Reference || value instanceof SampledData || value instanceof Signature || value instanceof Timing || value instanceof ContactDetail || value instanceof DataRequirement || value instanceof Expression || value instanceof ParameterDefinition || value instanceof RelatedArtifact || value instanceof TriggerDefinition || value instanceof UsageContext || value instanceof Availability || value instanceof ExtendedContactDetail || value instanceof Dosage || value instanceof Meta)) 972 throw new FHIRException("Not the right type for Extension.value[x]: "+value.fhirType()); 973 this.value = value; 974 return this; 975 } 976 977 protected void listChildren(List<Property> children) { 978 super.listChildren(children); 979 children.add(new Property("url", "uri", "Source of the definition for the extension code - a logical name or a URL.", 0, 1, url)); 980 children.add(new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value)); 981 } 982 983 @Override 984 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 985 switch (_hash) { 986 case 116079: /*url*/ return new Property("url", "uri", "Source of the definition for the extension code - a logical name or a URL.", 0, 1, url); 987 case -1410166417: /*value[x]*/ return new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 988 case 111972721: /*value*/ return new Property("value[x]", "base64Binary|boolean|canonical|code|date|dateTime|decimal|id|instant|integer|integer64|markdown|oid|positiveInt|string|time|unsignedInt|uri|url|uuid|Address|Age|Annotation|Attachment|CodeableConcept|CodeableReference|Coding|ContactPoint|Count|Distance|Duration|HumanName|Identifier|Money|Period|Quantity|Range|Ratio|RatioRange|Reference|SampledData|Signature|Timing|ContactDetail|DataRequirement|Expression|ParameterDefinition|RelatedArtifact|TriggerDefinition|UsageContext|Availability|ExtendedContactDetail|Dosage|Meta", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 989 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "base64Binary", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 990 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 991 case -786218365: /*valueCanonical*/ return new Property("value[x]", "canonical", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 992 case -766209282: /*valueCode*/ return new Property("value[x]", "code", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 993 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 994 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 995 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 996 case 231604844: /*valueId*/ return new Property("value[x]", "id", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 997 case -1668687056: /*valueInstant*/ return new Property("value[x]", "instant", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 998 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 999 case -1122120181: /*valueInteger64*/ return new Property("value[x]", "integer64", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1000 case -497880704: /*valueMarkdown*/ return new Property("value[x]", "markdown", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1001 case -1410178407: /*valueOid*/ return new Property("value[x]", "oid", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1002 case -1249932027: /*valuePositiveInt*/ return new Property("value[x]", "positiveInt", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1003 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1004 case -765708322: /*valueTime*/ return new Property("value[x]", "time", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1005 case 26529417: /*valueUnsignedInt*/ return new Property("value[x]", "unsignedInt", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1006 case -1410172357: /*valueUri*/ return new Property("value[x]", "uri", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1007 case -1410172354: /*valueUrl*/ return new Property("value[x]", "url", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1008 case -765667124: /*valueUuid*/ return new Property("value[x]", "uuid", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1009 case -478981821: /*valueAddress*/ return new Property("value[x]", "Address", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1010 case -1410191922: /*valueAge*/ return new Property("value[x]", "Age", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1011 case -67108992: /*valueAnnotation*/ return new Property("value[x]", "Annotation", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1012 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1013 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1014 case -257955629: /*valueCodeableReference*/ return new Property("value[x]", "CodeableReference", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1015 case -1887705029: /*valueCoding*/ return new Property("value[x]", "Coding", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1016 case 944904545: /*valueContactPoint*/ return new Property("value[x]", "ContactPoint", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1017 case 2017332766: /*valueCount*/ return new Property("value[x]", "Count", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1018 case -456359802: /*valueDistance*/ return new Property("value[x]", "Distance", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1019 case 1558135333: /*valueDuration*/ return new Property("value[x]", "Duration", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1020 case -2026205465: /*valueHumanName*/ return new Property("value[x]", "HumanName", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1021 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "Identifier", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1022 case 2026560975: /*valueMoney*/ return new Property("value[x]", "Money", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1023 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "Period", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1024 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1025 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1026 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1027 case -706454461: /*valueRatioRange*/ return new Property("value[x]", "RatioRange", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1028 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1029 case -962229101: /*valueSampledData*/ return new Property("value[x]", "SampledData", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1030 case -540985785: /*valueSignature*/ return new Property("value[x]", "Signature", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1031 case -1406282469: /*valueTiming*/ return new Property("value[x]", "Timing", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1032 case -1125200224: /*valueContactDetail*/ return new Property("value[x]", "ContactDetail", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1033 case 1710554248: /*valueDataRequirement*/ return new Property("value[x]", "DataRequirement", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1034 case -307517719: /*valueExpression*/ return new Property("value[x]", "Expression", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1035 case 1387478187: /*valueParameterDefinition*/ return new Property("value[x]", "ParameterDefinition", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1036 case 1748214124: /*valueRelatedArtifact*/ return new Property("value[x]", "RelatedArtifact", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1037 case 976830394: /*valueTriggerDefinition*/ return new Property("value[x]", "TriggerDefinition", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1038 case 588000479: /*valueUsageContext*/ return new Property("value[x]", "UsageContext", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1039 case 1678530924: /*valueAvailability*/ return new Property("value[x]", "Availability", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1040 case -1567222041: /*valueExtendedContactDetail*/ return new Property("value[x]", "ExtendedContactDetail", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1041 case -1858636920: /*valueDosage*/ return new Property("value[x]", "Dosage", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1042 case -765920490: /*valueMeta*/ return new Property("value[x]", "Meta", "Value of extension - must be one of a constrained set of the data types (see [Extensibility](extensibility.html) for a list).", 0, 1, value); 1043 default: return super.getNamedProperty(_hash, _name, _checkValid); 1044 } 1045 1046 } 1047 1048 @Override 1049 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1050 switch (hash) { 1051 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 1052 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1053 default: return super.getProperty(hash, name, checkValid); 1054 } 1055 1056 } 1057 1058 @Override 1059 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1060 switch (hash) { 1061 case 116079: // url 1062 this.url = TypeConvertor.castToUri(value); // UriType 1063 return value; 1064 case 111972721: // value 1065 this.value = TypeConvertor.castToType(value); // DataType 1066 return value; 1067 default: return super.setProperty(hash, name, value); 1068 } 1069 1070 } 1071 1072 @Override 1073 public Base setProperty(String name, Base value) throws FHIRException { 1074 if (name.equals("url")) { 1075 this.url = TypeConvertor.castToUri(value); // UriType 1076 } else if (name.equals("value[x]")) { 1077 this.value = TypeConvertor.castToType(value); // DataType 1078 } else 1079 return super.setProperty(name, value); 1080 return value; 1081 } 1082 1083 @Override 1084 public void removeChild(String name, Base value) throws FHIRException { 1085 if (name.equals("url")) { 1086 this.url = null; 1087 } else if (name.equals("value[x]")) { 1088 this.value = null; 1089 } else 1090 super.removeChild(name, value); 1091 1092 } 1093 1094 @Override 1095 public Base makeProperty(int hash, String name) throws FHIRException { 1096 switch (hash) { 1097 case 116079: return getUrlElement(); 1098 case -1410166417: return getValue(); 1099 case 111972721: return getValue(); 1100 default: return super.makeProperty(hash, name); 1101 } 1102 1103 } 1104 1105 @Override 1106 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1107 switch (hash) { 1108 case 116079: /*url*/ return new String[] {"uri"}; 1109 case 111972721: /*value*/ return new String[] {"base64Binary", "boolean", "canonical", "code", "date", "dateTime", "decimal", "id", "instant", "integer", "integer64", "markdown", "oid", "positiveInt", "string", "time", "unsignedInt", "uri", "url", "uuid", "Address", "Age", "Annotation", "Attachment", "CodeableConcept", "CodeableReference", "Coding", "ContactPoint", "Count", "Distance", "Duration", "HumanName", "Identifier", "Money", "Period", "Quantity", "Range", "Ratio", "RatioRange", "Reference", "SampledData", "Signature", "Timing", "ContactDetail", "DataRequirement", "Expression", "ParameterDefinition", "RelatedArtifact", "TriggerDefinition", "UsageContext", "Availability", "ExtendedContactDetail", "Dosage", "Meta"}; 1110 default: return super.getTypesForProperty(hash, name); 1111 } 1112 1113 } 1114 1115 @Override 1116 public Base addChild(String name) throws FHIRException { 1117 if (name.equals("url")) { 1118 throw new FHIRException("Cannot call addChild on a singleton property Extension.url"); 1119 } 1120 else if (name.equals("valueBase64Binary")) { 1121 this.value = new Base64BinaryType(); 1122 return this.value; 1123 } 1124 else if (name.equals("valueBoolean")) { 1125 this.value = new BooleanType(); 1126 return this.value; 1127 } 1128 else if (name.equals("valueCanonical")) { 1129 this.value = new CanonicalType(); 1130 return this.value; 1131 } 1132 else if (name.equals("valueCode")) { 1133 this.value = new CodeType(); 1134 return this.value; 1135 } 1136 else if (name.equals("valueDate")) { 1137 this.value = new DateType(); 1138 return this.value; 1139 } 1140 else if (name.equals("valueDateTime")) { 1141 this.value = new DateTimeType(); 1142 return this.value; 1143 } 1144 else if (name.equals("valueDecimal")) { 1145 this.value = new DecimalType(); 1146 return this.value; 1147 } 1148 else if (name.equals("valueId")) { 1149 this.value = new IdType(); 1150 return this.value; 1151 } 1152 else if (name.equals("valueInstant")) { 1153 this.value = new InstantType(); 1154 return this.value; 1155 } 1156 else if (name.equals("valueInteger")) { 1157 this.value = new IntegerType(); 1158 return this.value; 1159 } 1160 else if (name.equals("valueInteger64")) { 1161 this.value = new Integer64Type(); 1162 return this.value; 1163 } 1164 else if (name.equals("valueMarkdown")) { 1165 this.value = new MarkdownType(); 1166 return this.value; 1167 } 1168 else if (name.equals("valueOid")) { 1169 this.value = new OidType(); 1170 return this.value; 1171 } 1172 else if (name.equals("valuePositiveInt")) { 1173 this.value = new PositiveIntType(); 1174 return this.value; 1175 } 1176 else if (name.equals("valueString")) { 1177 this.value = new StringType(); 1178 return this.value; 1179 } 1180 else if (name.equals("valueTime")) { 1181 this.value = new TimeType(); 1182 return this.value; 1183 } 1184 else if (name.equals("valueUnsignedInt")) { 1185 this.value = new UnsignedIntType(); 1186 return this.value; 1187 } 1188 else if (name.equals("valueUri")) { 1189 this.value = new UriType(); 1190 return this.value; 1191 } 1192 else if (name.equals("valueUrl")) { 1193 this.value = new UrlType(); 1194 return this.value; 1195 } 1196 else if (name.equals("valueUuid")) { 1197 this.value = new UuidType(); 1198 return this.value; 1199 } 1200 else if (name.equals("valueAddress")) { 1201 this.value = new Address(); 1202 return this.value; 1203 } 1204 else if (name.equals("valueAge")) { 1205 this.value = new Age(); 1206 return this.value; 1207 } 1208 else if (name.equals("valueAnnotation")) { 1209 this.value = new Annotation(); 1210 return this.value; 1211 } 1212 else if (name.equals("valueAttachment")) { 1213 this.value = new Attachment(); 1214 return this.value; 1215 } 1216 else if (name.equals("valueCodeableConcept")) { 1217 this.value = new CodeableConcept(); 1218 return this.value; 1219 } 1220 else if (name.equals("valueCodeableReference")) { 1221 this.value = new CodeableReference(); 1222 return this.value; 1223 } 1224 else if (name.equals("valueCoding")) { 1225 this.value = new Coding(); 1226 return this.value; 1227 } 1228 else if (name.equals("valueContactPoint")) { 1229 this.value = new ContactPoint(); 1230 return this.value; 1231 } 1232 else if (name.equals("valueCount")) { 1233 this.value = new Count(); 1234 return this.value; 1235 } 1236 else if (name.equals("valueDistance")) { 1237 this.value = new Distance(); 1238 return this.value; 1239 } 1240 else if (name.equals("valueDuration")) { 1241 this.value = new Duration(); 1242 return this.value; 1243 } 1244 else if (name.equals("valueHumanName")) { 1245 this.value = new HumanName(); 1246 return this.value; 1247 } 1248 else if (name.equals("valueIdentifier")) { 1249 this.value = new Identifier(); 1250 return this.value; 1251 } 1252 else if (name.equals("valueMoney")) { 1253 this.value = new Money(); 1254 return this.value; 1255 } 1256 else if (name.equals("valuePeriod")) { 1257 this.value = new Period(); 1258 return this.value; 1259 } 1260 else if (name.equals("valueQuantity")) { 1261 this.value = new Quantity(); 1262 return this.value; 1263 } 1264 else if (name.equals("valueRange")) { 1265 this.value = new Range(); 1266 return this.value; 1267 } 1268 else if (name.equals("valueRatio")) { 1269 this.value = new Ratio(); 1270 return this.value; 1271 } 1272 else if (name.equals("valueRatioRange")) { 1273 this.value = new RatioRange(); 1274 return this.value; 1275 } 1276 else if (name.equals("valueReference")) { 1277 this.value = new Reference(); 1278 return this.value; 1279 } 1280 else if (name.equals("valueSampledData")) { 1281 this.value = new SampledData(); 1282 return this.value; 1283 } 1284 else if (name.equals("valueSignature")) { 1285 this.value = new Signature(); 1286 return this.value; 1287 } 1288 else if (name.equals("valueTiming")) { 1289 this.value = new Timing(); 1290 return this.value; 1291 } 1292 else if (name.equals("valueContactDetail")) { 1293 this.value = new ContactDetail(); 1294 return this.value; 1295 } 1296 else if (name.equals("valueDataRequirement")) { 1297 this.value = new DataRequirement(); 1298 return this.value; 1299 } 1300 else if (name.equals("valueExpression")) { 1301 this.value = new Expression(); 1302 return this.value; 1303 } 1304 else if (name.equals("valueParameterDefinition")) { 1305 this.value = new ParameterDefinition(); 1306 return this.value; 1307 } 1308 else if (name.equals("valueRelatedArtifact")) { 1309 this.value = new RelatedArtifact(); 1310 return this.value; 1311 } 1312 else if (name.equals("valueTriggerDefinition")) { 1313 this.value = new TriggerDefinition(); 1314 return this.value; 1315 } 1316 else if (name.equals("valueUsageContext")) { 1317 this.value = new UsageContext(); 1318 return this.value; 1319 } 1320 else if (name.equals("valueAvailability")) { 1321 this.value = new Availability(); 1322 return this.value; 1323 } 1324 else if (name.equals("valueExtendedContactDetail")) { 1325 this.value = new ExtendedContactDetail(); 1326 return this.value; 1327 } 1328 else if (name.equals("valueDosage")) { 1329 this.value = new Dosage(); 1330 return this.value; 1331 } 1332 else if (name.equals("valueMeta")) { 1333 this.value = new Meta(); 1334 return this.value; 1335 } 1336 else 1337 return super.addChild(name); 1338 } 1339 1340 public String fhirType() { 1341 return "Extension"; 1342 1343 } 1344 1345 public Extension copy() { 1346 Extension dst = new Extension(); 1347 copyValues(dst); 1348 return dst; 1349 } 1350 1351 public void copyValues(Extension dst) { 1352 super.copyValues(dst); 1353 dst.url = url == null ? null : url.copy(); 1354 dst.value = value == null ? null : value.copy(); 1355 } 1356 1357 protected Extension typedCopy() { 1358 return copy(); 1359 } 1360 1361 @Override 1362 public boolean equalsDeep(Base other_) { 1363 if (!super.equalsDeep(other_)) 1364 return false; 1365 if (!(other_ instanceof Extension)) 1366 return false; 1367 Extension o = (Extension) other_; 1368 return compareDeep(url, o.url, true) && compareDeep(value, o.value, true); 1369 } 1370 1371 @Override 1372 public boolean equalsShallow(Base other_) { 1373 if (!super.equalsShallow(other_)) 1374 return false; 1375 if (!(other_ instanceof Extension)) 1376 return false; 1377 Extension o = (Extension) other_; 1378 return compareValues(url, o.url, true); 1379 } 1380 1381 public boolean isEmpty() { 1382 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, value); 1383 } 1384 1385 1386} 1387