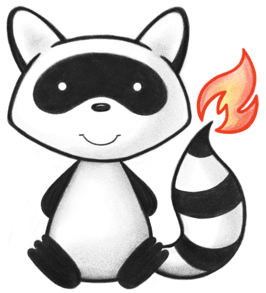
001package org.hl7.fhir.r5.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.io.IOException; 035import java.net.URISyntaxException; 036import java.text.ParseException; 037import java.util.UUID; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.r5.model.ContactPoint.ContactPointSystem; 041import org.hl7.fhir.r5.model.Narrative.NarrativeStatus; 042import org.hl7.fhir.utilities.Utilities; 043import org.hl7.fhir.utilities.xhtml.XhtmlParser; 044 045/* 046Copyright (c) 2011+, HL7, Inc 047All rights reserved. 048 049Redistribution and use in source and binary forms, with or without modification, 050are permitted provided that the following conditions are met: 051 052 * Redistributions of source code must retain the above copyright notice, this 053 list of conditions and the following disclaimer. 054 * Redistributions in binary form must reproduce the above copyright notice, 055 this list of conditions and the following disclaimer in the documentation 056 and/or other materials provided with the distribution. 057 * Neither the name of HL7 nor the names of its contributors may be used to 058 endorse or promote products derived from this software without specific 059 prior written permission. 060 061THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 062ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 063WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 064IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 065INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 066NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 067PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 068WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 069ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 070POSSIBILITY OF SUCH DAMAGE. 071 072*/ 073 074 075 076public class Factory { 077 078 public static IdType newId(String value) { 079 if (value == null) 080 return null; 081 IdType res = new IdType(); 082 res.setValue(value); 083 return res; 084 } 085 086 public static StringType newString_(String value) { 087 if (value == null) 088 return null; 089 StringType res = new StringType(); 090 res.setValue(value); 091 return res; 092 } 093 094 public static UriType newUri(String value) throws URISyntaxException { 095 if (value == null) 096 return null; 097 UriType res = new UriType(); 098 res.setValue(value); 099 return res; 100 } 101 102 public static UrlType newUrl(String value) throws URISyntaxException { 103 if (value == null) 104 return null; 105 UrlType res = new UrlType(); 106 res.setValue(value); 107 return res; 108 } 109 110 public static CanonicalType newCanonical(String value) throws URISyntaxException { 111 if (value == null) 112 return null; 113 CanonicalType res = new CanonicalType(); 114 res.setValue(value); 115 return res; 116 } 117 118 public static DateTimeType newDateTime(String value) throws ParseException { 119 if (value == null) 120 return null; 121 return new DateTimeType(value); 122 } 123 124 public static DateType newDate(String value) throws ParseException { 125 if (value == null) 126 return null; 127 return new DateType(value); 128 } 129 130 public static CodeType newCode(String value) { 131 if (value == null) 132 return null; 133 CodeType res = new CodeType(); 134 res.setValue(value); 135 return res; 136 } 137 138 public static IntegerType newInteger(int value) { 139 IntegerType res = new IntegerType(); 140 res.setValue(value); 141 return res; 142 } 143 144 public static IntegerType newInteger(java.lang.Integer value) { 145 if (value == null) 146 return null; 147 IntegerType res = new IntegerType(); 148 res.setValue(value); 149 return res; 150 } 151 152 public static BooleanType newBoolean(boolean value) { 153 BooleanType res = new BooleanType(); 154 res.setValue(value); 155 return res; 156 } 157 158 public static ContactPoint newContactPoint(ContactPointSystem system, String value) { 159 ContactPoint res = new ContactPoint(); 160 res.setSystem(system); 161 res.setValue(value); 162 return res; 163 } 164 165 public static Extension newExtension(String uri, DataType value, boolean evenIfNull) { 166 if (!evenIfNull && (value == null || value.isEmpty())) 167 return null; 168 Extension e = new Extension(); 169 e.setUrl(uri); 170 e.setValue(value); 171 return e; 172 } 173 174 public static CodeableConcept newCodeableConcept(String code, String system, String display) { 175 CodeableConcept cc = new CodeableConcept(); 176 Coding c = new Coding(); 177 c.setCode(code); 178 c.setSystem(system); 179 c.setDisplay(display); 180 cc.getCoding().add(c); 181 return cc; 182 } 183 184 public static Reference makeReference(String url) { 185 Reference rr = new Reference(); 186 rr.setReference(url); 187 return rr; 188 } 189 190 public static Narrative newNarrative(NarrativeStatus status, String html) throws IOException, FHIRException { 191 Narrative n = new Narrative(); 192 n.setStatus(status); 193 try { 194 n.setDiv(new XhtmlParser().parseFragment("<div>"+Utilities.escapeXml(html)+"</div>")); 195 } catch (org.hl7.fhir.exceptions.FHIRException e) { 196 throw new FHIRException(e.getMessage(), e); 197 } 198 return n; 199 } 200 201 public static Coding makeCoding(String code) throws FHIRException { 202 String[] parts = code.split("\\|"); 203 Coding c = new Coding(); 204 if (parts.length == 2) { 205 c.setSystem(parts[0]); 206 c.setCode(parts[1]); 207 } else if (parts.length == 3) { 208 c.setSystem(parts[0]); 209 c.setCode(parts[1]); 210 c.setDisplay(parts[2]); 211 } else 212 throw new FHIRException("Unable to understand the code '"+code+"'. Use the format system|code(|display)"); 213 return c; 214 } 215 216 public static Reference makeReference(String url, String text) { 217 Reference rr = new Reference(); 218 rr.setReference(url); 219 if (!Utilities.noString(text)) 220 rr.setDisplay(text); 221 return rr; 222 } 223 224 public static String createUUID() { 225 return "urn:uuid:"+UUID.randomUUID().toString().toLowerCase(); 226 } 227 228 public DataType create(String name) throws FHIRException { 229 if (name.equals("boolean")) 230 return new BooleanType(); 231 else if (name.equals("integer")) 232 return new IntegerType(); 233 else if (name.equals("integer64")) 234 return new Integer64Type(); 235 else if (name.equals("decimal")) 236 return new DecimalType(); 237 else if (name.equals("base64Binary")) 238 return new Base64BinaryType(); 239 else if (name.equals("instant")) 240 return new InstantType(); 241 else if (name.equals("string")) 242 return new StringType(); 243 else if (name.equals("uri")) 244 return new UriType(); 245 else if (name.equals("url")) 246 return new UrlType(); 247 else if (name.equals("canonical")) 248 return new CanonicalType(); 249 else if (name.equals("date")) 250 return new DateType(); 251 else if (name.equals("dateTime")) 252 return new DateTimeType(); 253 else if (name.equals("time")) 254 return new TimeType(); 255 else if (name.equals("code")) 256 return new CodeType(); 257 else if (name.equals("oid")) 258 return new OidType(); 259 else if (name.equals("id")) 260 return new IdType(); 261 else if (name.equals("unsignedInt")) 262 return new UnsignedIntType(); 263 else if (name.equals("positiveInt")) 264 return new PositiveIntType(); 265 else if (name.equals("markdown")) 266 return new MarkdownType(); 267 else if (name.equals("Annotation")) 268 return new Annotation(); 269 else if (name.equals("Attachment")) 270 return new Attachment(); 271 else if (name.equals("Identifier")) 272 return new Identifier(); 273 else if (name.equals("CodeableConcept")) 274 return new CodeableConcept(); 275 else if (name.equals("Coding")) 276 return new Coding(); 277 else if (name.equals("Quantity")) 278 return new Quantity(); 279 else if (name.equals("Range")) 280 return new Range(); 281 else if (name.equals("Period")) 282 return new Period(); 283 else if (name.equals("Ratio")) 284 return new Ratio(); 285 else if (name.equals("SampledData")) 286 return new SampledData(); 287 else if (name.equals("Signature")) 288 return new Signature(); 289 else if (name.equals("HumanName")) 290 return new HumanName(); 291 else if (name.equals("Address")) 292 return new Address(); 293 else if (name.equals("ContactPoint")) 294 return new ContactPoint(); 295 else if (name.equals("Timing")) 296 return new Timing(); 297 else if (name.equals("Reference")) 298 return new Reference(); 299 else if (name.equals("Meta")) 300 return new Meta(); 301 else 302 throw new FHIRException("Unknown data type name "+name); 303 } 304}