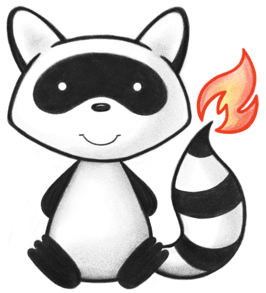
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Significant health conditions for a person related to the patient relevant in the context of care for the patient. 052 */ 053@ResourceDef(name="FamilyMemberHistory", profile="http://hl7.org/fhir/StructureDefinition/FamilyMemberHistory") 054public class FamilyMemberHistory extends DomainResource { 055 056 public enum FamilyHistoryStatus { 057 /** 058 * Some health information is known and captured, but not complete - see notes for details. 059 */ 060 PARTIAL, 061 /** 062 * All available related health information is captured as of the date (and possibly time) when the family member history was taken. 063 */ 064 COMPLETED, 065 /** 066 * This instance should not have been part of this patient's medical record. 067 */ 068 ENTEREDINERROR, 069 /** 070 * Health information for this family member is unavailable/unknown. 071 */ 072 HEALTHUNKNOWN, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static FamilyHistoryStatus fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("partial".equals(codeString)) 081 return PARTIAL; 082 if ("completed".equals(codeString)) 083 return COMPLETED; 084 if ("entered-in-error".equals(codeString)) 085 return ENTEREDINERROR; 086 if ("health-unknown".equals(codeString)) 087 return HEALTHUNKNOWN; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown FamilyHistoryStatus code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case PARTIAL: return "partial"; 096 case COMPLETED: return "completed"; 097 case ENTEREDINERROR: return "entered-in-error"; 098 case HEALTHUNKNOWN: return "health-unknown"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case PARTIAL: return "http://hl7.org/fhir/history-status"; 106 case COMPLETED: return "http://hl7.org/fhir/history-status"; 107 case ENTEREDINERROR: return "http://hl7.org/fhir/history-status"; 108 case HEALTHUNKNOWN: return "http://hl7.org/fhir/history-status"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case PARTIAL: return "Some health information is known and captured, but not complete - see notes for details."; 116 case COMPLETED: return "All available related health information is captured as of the date (and possibly time) when the family member history was taken."; 117 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 118 case HEALTHUNKNOWN: return "Health information for this family member is unavailable/unknown."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case PARTIAL: return "Partial"; 126 case COMPLETED: return "Completed"; 127 case ENTEREDINERROR: return "Entered in Error"; 128 case HEALTHUNKNOWN: return "Health Unknown"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class FamilyHistoryStatusEnumFactory implements EnumFactory<FamilyHistoryStatus> { 136 public FamilyHistoryStatus fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("partial".equals(codeString)) 141 return FamilyHistoryStatus.PARTIAL; 142 if ("completed".equals(codeString)) 143 return FamilyHistoryStatus.COMPLETED; 144 if ("entered-in-error".equals(codeString)) 145 return FamilyHistoryStatus.ENTEREDINERROR; 146 if ("health-unknown".equals(codeString)) 147 return FamilyHistoryStatus.HEALTHUNKNOWN; 148 throw new IllegalArgumentException("Unknown FamilyHistoryStatus code '"+codeString+"'"); 149 } 150 public Enumeration<FamilyHistoryStatus> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.NULL, code); 158 if ("partial".equals(codeString)) 159 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.PARTIAL, code); 160 if ("completed".equals(codeString)) 161 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.COMPLETED, code); 162 if ("entered-in-error".equals(codeString)) 163 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.ENTEREDINERROR, code); 164 if ("health-unknown".equals(codeString)) 165 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.HEALTHUNKNOWN, code); 166 throw new FHIRException("Unknown FamilyHistoryStatus code '"+codeString+"'"); 167 } 168 public String toCode(FamilyHistoryStatus code) { 169 if (code == FamilyHistoryStatus.NULL) 170 return null; 171 if (code == FamilyHistoryStatus.PARTIAL) 172 return "partial"; 173 if (code == FamilyHistoryStatus.COMPLETED) 174 return "completed"; 175 if (code == FamilyHistoryStatus.ENTEREDINERROR) 176 return "entered-in-error"; 177 if (code == FamilyHistoryStatus.HEALTHUNKNOWN) 178 return "health-unknown"; 179 return "?"; 180 } 181 public String toSystem(FamilyHistoryStatus code) { 182 return code.getSystem(); 183 } 184 } 185 186 @Block() 187 public static class FamilyMemberHistoryParticipantComponent extends BackboneElement implements IBaseBackboneElement { 188 /** 189 * Distinguishes the type of involvement of the actor in the activities related to the family member history. 190 */ 191 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 192 @Description(shortDefinition="Type of involvement", formalDefinition="Distinguishes the type of involvement of the actor in the activities related to the family member history." ) 193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participation-role-type") 194 protected CodeableConcept function; 195 196 /** 197 * Indicates who or what participated in the activities related to the family member history. 198 */ 199 @Child(name = "actor", type = {Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class, Device.class, Organization.class, CareTeam.class}, order=2, min=1, max=1, modifier=false, summary=true) 200 @Description(shortDefinition="Who or what participated in the activities related to the family member history", formalDefinition="Indicates who or what participated in the activities related to the family member history." ) 201 protected Reference actor; 202 203 private static final long serialVersionUID = -576943815L; 204 205 /** 206 * Constructor 207 */ 208 public FamilyMemberHistoryParticipantComponent() { 209 super(); 210 } 211 212 /** 213 * Constructor 214 */ 215 public FamilyMemberHistoryParticipantComponent(Reference actor) { 216 super(); 217 this.setActor(actor); 218 } 219 220 /** 221 * @return {@link #function} (Distinguishes the type of involvement of the actor in the activities related to the family member history.) 222 */ 223 public CodeableConcept getFunction() { 224 if (this.function == null) 225 if (Configuration.errorOnAutoCreate()) 226 throw new Error("Attempt to auto-create FamilyMemberHistoryParticipantComponent.function"); 227 else if (Configuration.doAutoCreate()) 228 this.function = new CodeableConcept(); // cc 229 return this.function; 230 } 231 232 public boolean hasFunction() { 233 return this.function != null && !this.function.isEmpty(); 234 } 235 236 /** 237 * @param value {@link #function} (Distinguishes the type of involvement of the actor in the activities related to the family member history.) 238 */ 239 public FamilyMemberHistoryParticipantComponent setFunction(CodeableConcept value) { 240 this.function = value; 241 return this; 242 } 243 244 /** 245 * @return {@link #actor} (Indicates who or what participated in the activities related to the family member history.) 246 */ 247 public Reference getActor() { 248 if (this.actor == null) 249 if (Configuration.errorOnAutoCreate()) 250 throw new Error("Attempt to auto-create FamilyMemberHistoryParticipantComponent.actor"); 251 else if (Configuration.doAutoCreate()) 252 this.actor = new Reference(); // cc 253 return this.actor; 254 } 255 256 public boolean hasActor() { 257 return this.actor != null && !this.actor.isEmpty(); 258 } 259 260 /** 261 * @param value {@link #actor} (Indicates who or what participated in the activities related to the family member history.) 262 */ 263 public FamilyMemberHistoryParticipantComponent setActor(Reference value) { 264 this.actor = value; 265 return this; 266 } 267 268 protected void listChildren(List<Property> children) { 269 super.listChildren(children); 270 children.add(new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the actor in the activities related to the family member history.", 0, 1, function)); 271 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Device|Organization|CareTeam)", "Indicates who or what participated in the activities related to the family member history.", 0, 1, actor)); 272 } 273 274 @Override 275 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 276 switch (_hash) { 277 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the actor in the activities related to the family member history.", 0, 1, function); 278 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Device|Organization|CareTeam)", "Indicates who or what participated in the activities related to the family member history.", 0, 1, actor); 279 default: return super.getNamedProperty(_hash, _name, _checkValid); 280 } 281 282 } 283 284 @Override 285 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 286 switch (hash) { 287 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 288 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 289 default: return super.getProperty(hash, name, checkValid); 290 } 291 292 } 293 294 @Override 295 public Base setProperty(int hash, String name, Base value) throws FHIRException { 296 switch (hash) { 297 case 1380938712: // function 298 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 299 return value; 300 case 92645877: // actor 301 this.actor = TypeConvertor.castToReference(value); // Reference 302 return value; 303 default: return super.setProperty(hash, name, value); 304 } 305 306 } 307 308 @Override 309 public Base setProperty(String name, Base value) throws FHIRException { 310 if (name.equals("function")) { 311 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 312 } else if (name.equals("actor")) { 313 this.actor = TypeConvertor.castToReference(value); // Reference 314 } else 315 return super.setProperty(name, value); 316 return value; 317 } 318 319 @Override 320 public void removeChild(String name, Base value) throws FHIRException { 321 if (name.equals("function")) { 322 this.function = null; 323 } else if (name.equals("actor")) { 324 this.actor = null; 325 } else 326 super.removeChild(name, value); 327 328 } 329 330 @Override 331 public Base makeProperty(int hash, String name) throws FHIRException { 332 switch (hash) { 333 case 1380938712: return getFunction(); 334 case 92645877: return getActor(); 335 default: return super.makeProperty(hash, name); 336 } 337 338 } 339 340 @Override 341 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 342 switch (hash) { 343 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 344 case 92645877: /*actor*/ return new String[] {"Reference"}; 345 default: return super.getTypesForProperty(hash, name); 346 } 347 348 } 349 350 @Override 351 public Base addChild(String name) throws FHIRException { 352 if (name.equals("function")) { 353 this.function = new CodeableConcept(); 354 return this.function; 355 } 356 else if (name.equals("actor")) { 357 this.actor = new Reference(); 358 return this.actor; 359 } 360 else 361 return super.addChild(name); 362 } 363 364 public FamilyMemberHistoryParticipantComponent copy() { 365 FamilyMemberHistoryParticipantComponent dst = new FamilyMemberHistoryParticipantComponent(); 366 copyValues(dst); 367 return dst; 368 } 369 370 public void copyValues(FamilyMemberHistoryParticipantComponent dst) { 371 super.copyValues(dst); 372 dst.function = function == null ? null : function.copy(); 373 dst.actor = actor == null ? null : actor.copy(); 374 } 375 376 @Override 377 public boolean equalsDeep(Base other_) { 378 if (!super.equalsDeep(other_)) 379 return false; 380 if (!(other_ instanceof FamilyMemberHistoryParticipantComponent)) 381 return false; 382 FamilyMemberHistoryParticipantComponent o = (FamilyMemberHistoryParticipantComponent) other_; 383 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 384 } 385 386 @Override 387 public boolean equalsShallow(Base other_) { 388 if (!super.equalsShallow(other_)) 389 return false; 390 if (!(other_ instanceof FamilyMemberHistoryParticipantComponent)) 391 return false; 392 FamilyMemberHistoryParticipantComponent o = (FamilyMemberHistoryParticipantComponent) other_; 393 return true; 394 } 395 396 public boolean isEmpty() { 397 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 398 } 399 400 public String fhirType() { 401 return "FamilyMemberHistory.participant"; 402 403 } 404 405 } 406 407 @Block() 408 public static class FamilyMemberHistoryConditionComponent extends BackboneElement implements IBaseBackboneElement { 409 /** 410 * The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system. 411 */ 412 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 413 @Description(shortDefinition="Condition suffered by relation", formalDefinition="The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system." ) 414 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 415 protected CodeableConcept code; 416 417 /** 418 * Indicates what happened following the condition. If the condition resulted in death, deceased date is captured on the relation. 419 */ 420 @Child(name = "outcome", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 421 @Description(shortDefinition="deceased | permanent disability | etc", formalDefinition="Indicates what happened following the condition. If the condition resulted in death, deceased date is captured on the relation." ) 422 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-outcome") 423 protected CodeableConcept outcome; 424 425 /** 426 * This condition contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown. 427 */ 428 @Child(name = "contributedToDeath", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 429 @Description(shortDefinition="Whether the condition contributed to the cause of death", formalDefinition="This condition contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown." ) 430 protected BooleanType contributedToDeath; 431 432 /** 433 * Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence. 434 */ 435 @Child(name = "onset", type = {Age.class, Range.class, Period.class, StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 436 @Description(shortDefinition="When condition first manifested", formalDefinition="Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence." ) 437 protected DataType onset; 438 439 /** 440 * An area where general notes can be placed about this specific condition. 441 */ 442 @Child(name = "note", type = {Annotation.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 443 @Description(shortDefinition="Extra information about condition", formalDefinition="An area where general notes can be placed about this specific condition." ) 444 protected List<Annotation> note; 445 446 private static final long serialVersionUID = -91335661L; 447 448 /** 449 * Constructor 450 */ 451 public FamilyMemberHistoryConditionComponent() { 452 super(); 453 } 454 455 /** 456 * Constructor 457 */ 458 public FamilyMemberHistoryConditionComponent(CodeableConcept code) { 459 super(); 460 this.setCode(code); 461 } 462 463 /** 464 * @return {@link #code} (The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system.) 465 */ 466 public CodeableConcept getCode() { 467 if (this.code == null) 468 if (Configuration.errorOnAutoCreate()) 469 throw new Error("Attempt to auto-create FamilyMemberHistoryConditionComponent.code"); 470 else if (Configuration.doAutoCreate()) 471 this.code = new CodeableConcept(); // cc 472 return this.code; 473 } 474 475 public boolean hasCode() { 476 return this.code != null && !this.code.isEmpty(); 477 } 478 479 /** 480 * @param value {@link #code} (The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system.) 481 */ 482 public FamilyMemberHistoryConditionComponent setCode(CodeableConcept value) { 483 this.code = value; 484 return this; 485 } 486 487 /** 488 * @return {@link #outcome} (Indicates what happened following the condition. If the condition resulted in death, deceased date is captured on the relation.) 489 */ 490 public CodeableConcept getOutcome() { 491 if (this.outcome == null) 492 if (Configuration.errorOnAutoCreate()) 493 throw new Error("Attempt to auto-create FamilyMemberHistoryConditionComponent.outcome"); 494 else if (Configuration.doAutoCreate()) 495 this.outcome = new CodeableConcept(); // cc 496 return this.outcome; 497 } 498 499 public boolean hasOutcome() { 500 return this.outcome != null && !this.outcome.isEmpty(); 501 } 502 503 /** 504 * @param value {@link #outcome} (Indicates what happened following the condition. If the condition resulted in death, deceased date is captured on the relation.) 505 */ 506 public FamilyMemberHistoryConditionComponent setOutcome(CodeableConcept value) { 507 this.outcome = value; 508 return this; 509 } 510 511 /** 512 * @return {@link #contributedToDeath} (This condition contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown.). This is the underlying object with id, value and extensions. The accessor "getContributedToDeath" gives direct access to the value 513 */ 514 public BooleanType getContributedToDeathElement() { 515 if (this.contributedToDeath == null) 516 if (Configuration.errorOnAutoCreate()) 517 throw new Error("Attempt to auto-create FamilyMemberHistoryConditionComponent.contributedToDeath"); 518 else if (Configuration.doAutoCreate()) 519 this.contributedToDeath = new BooleanType(); // bb 520 return this.contributedToDeath; 521 } 522 523 public boolean hasContributedToDeathElement() { 524 return this.contributedToDeath != null && !this.contributedToDeath.isEmpty(); 525 } 526 527 public boolean hasContributedToDeath() { 528 return this.contributedToDeath != null && !this.contributedToDeath.isEmpty(); 529 } 530 531 /** 532 * @param value {@link #contributedToDeath} (This condition contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown.). This is the underlying object with id, value and extensions. The accessor "getContributedToDeath" gives direct access to the value 533 */ 534 public FamilyMemberHistoryConditionComponent setContributedToDeathElement(BooleanType value) { 535 this.contributedToDeath = value; 536 return this; 537 } 538 539 /** 540 * @return This condition contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown. 541 */ 542 public boolean getContributedToDeath() { 543 return this.contributedToDeath == null || this.contributedToDeath.isEmpty() ? false : this.contributedToDeath.getValue(); 544 } 545 546 /** 547 * @param value This condition contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown. 548 */ 549 public FamilyMemberHistoryConditionComponent setContributedToDeath(boolean value) { 550 if (this.contributedToDeath == null) 551 this.contributedToDeath = new BooleanType(); 552 this.contributedToDeath.setValue(value); 553 return this; 554 } 555 556 /** 557 * @return {@link #onset} (Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.) 558 */ 559 public DataType getOnset() { 560 return this.onset; 561 } 562 563 /** 564 * @return {@link #onset} (Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.) 565 */ 566 public Age getOnsetAge() throws FHIRException { 567 if (this.onset == null) 568 this.onset = new Age(); 569 if (!(this.onset instanceof Age)) 570 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.onset.getClass().getName()+" was encountered"); 571 return (Age) this.onset; 572 } 573 574 public boolean hasOnsetAge() { 575 return this.onset instanceof Age; 576 } 577 578 /** 579 * @return {@link #onset} (Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.) 580 */ 581 public Range getOnsetRange() throws FHIRException { 582 if (this.onset == null) 583 this.onset = new Range(); 584 if (!(this.onset instanceof Range)) 585 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.onset.getClass().getName()+" was encountered"); 586 return (Range) this.onset; 587 } 588 589 public boolean hasOnsetRange() { 590 return this.onset instanceof Range; 591 } 592 593 /** 594 * @return {@link #onset} (Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.) 595 */ 596 public Period getOnsetPeriod() throws FHIRException { 597 if (this.onset == null) 598 this.onset = new Period(); 599 if (!(this.onset instanceof Period)) 600 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.onset.getClass().getName()+" was encountered"); 601 return (Period) this.onset; 602 } 603 604 public boolean hasOnsetPeriod() { 605 return this.onset instanceof Period; 606 } 607 608 /** 609 * @return {@link #onset} (Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.) 610 */ 611 public StringType getOnsetStringType() throws FHIRException { 612 if (this.onset == null) 613 this.onset = new StringType(); 614 if (!(this.onset instanceof StringType)) 615 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.onset.getClass().getName()+" was encountered"); 616 return (StringType) this.onset; 617 } 618 619 public boolean hasOnsetStringType() { 620 return this.onset instanceof StringType; 621 } 622 623 public boolean hasOnset() { 624 return this.onset != null && !this.onset.isEmpty(); 625 } 626 627 /** 628 * @param value {@link #onset} (Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.) 629 */ 630 public FamilyMemberHistoryConditionComponent setOnset(DataType value) { 631 if (value != null && !(value instanceof Age || value instanceof Range || value instanceof Period || value instanceof StringType)) 632 throw new FHIRException("Not the right type for FamilyMemberHistory.condition.onset[x]: "+value.fhirType()); 633 this.onset = value; 634 return this; 635 } 636 637 /** 638 * @return {@link #note} (An area where general notes can be placed about this specific condition.) 639 */ 640 public List<Annotation> getNote() { 641 if (this.note == null) 642 this.note = new ArrayList<Annotation>(); 643 return this.note; 644 } 645 646 /** 647 * @return Returns a reference to <code>this</code> for easy method chaining 648 */ 649 public FamilyMemberHistoryConditionComponent setNote(List<Annotation> theNote) { 650 this.note = theNote; 651 return this; 652 } 653 654 public boolean hasNote() { 655 if (this.note == null) 656 return false; 657 for (Annotation item : this.note) 658 if (!item.isEmpty()) 659 return true; 660 return false; 661 } 662 663 public Annotation addNote() { //3 664 Annotation t = new Annotation(); 665 if (this.note == null) 666 this.note = new ArrayList<Annotation>(); 667 this.note.add(t); 668 return t; 669 } 670 671 public FamilyMemberHistoryConditionComponent addNote(Annotation t) { //3 672 if (t == null) 673 return this; 674 if (this.note == null) 675 this.note = new ArrayList<Annotation>(); 676 this.note.add(t); 677 return this; 678 } 679 680 /** 681 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 682 */ 683 public Annotation getNoteFirstRep() { 684 if (getNote().isEmpty()) { 685 addNote(); 686 } 687 return getNote().get(0); 688 } 689 690 protected void listChildren(List<Property> children) { 691 super.listChildren(children); 692 children.add(new Property("code", "CodeableConcept", "The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system.", 0, 1, code)); 693 children.add(new Property("outcome", "CodeableConcept", "Indicates what happened following the condition. If the condition resulted in death, deceased date is captured on the relation.", 0, 1, outcome)); 694 children.add(new Property("contributedToDeath", "boolean", "This condition contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown.", 0, 1, contributedToDeath)); 695 children.add(new Property("onset[x]", "Age|Range|Period|string", "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 0, 1, onset)); 696 children.add(new Property("note", "Annotation", "An area where general notes can be placed about this specific condition.", 0, java.lang.Integer.MAX_VALUE, note)); 697 } 698 699 @Override 700 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 701 switch (_hash) { 702 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system.", 0, 1, code); 703 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "Indicates what happened following the condition. If the condition resulted in death, deceased date is captured on the relation.", 0, 1, outcome); 704 case -363644638: /*contributedToDeath*/ return new Property("contributedToDeath", "boolean", "This condition contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown.", 0, 1, contributedToDeath); 705 case -1886216323: /*onset[x]*/ return new Property("onset[x]", "Age|Range|Period|string", "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 0, 1, onset); 706 case 105901603: /*onset*/ return new Property("onset[x]", "Age|Range|Period|string", "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 0, 1, onset); 707 case -1886241828: /*onsetAge*/ return new Property("onset[x]", "Age", "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 0, 1, onset); 708 case -186664742: /*onsetRange*/ return new Property("onset[x]", "Range", "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 0, 1, onset); 709 case -1545082428: /*onsetPeriod*/ return new Property("onset[x]", "Period", "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 0, 1, onset); 710 case -1445342188: /*onsetString*/ return new Property("onset[x]", "string", "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 0, 1, onset); 711 case 3387378: /*note*/ return new Property("note", "Annotation", "An area where general notes can be placed about this specific condition.", 0, java.lang.Integer.MAX_VALUE, note); 712 default: return super.getNamedProperty(_hash, _name, _checkValid); 713 } 714 715 } 716 717 @Override 718 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 719 switch (hash) { 720 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 721 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // CodeableConcept 722 case -363644638: /*contributedToDeath*/ return this.contributedToDeath == null ? new Base[0] : new Base[] {this.contributedToDeath}; // BooleanType 723 case 105901603: /*onset*/ return this.onset == null ? new Base[0] : new Base[] {this.onset}; // DataType 724 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 725 default: return super.getProperty(hash, name, checkValid); 726 } 727 728 } 729 730 @Override 731 public Base setProperty(int hash, String name, Base value) throws FHIRException { 732 switch (hash) { 733 case 3059181: // code 734 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 735 return value; 736 case -1106507950: // outcome 737 this.outcome = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 738 return value; 739 case -363644638: // contributedToDeath 740 this.contributedToDeath = TypeConvertor.castToBoolean(value); // BooleanType 741 return value; 742 case 105901603: // onset 743 this.onset = TypeConvertor.castToType(value); // DataType 744 return value; 745 case 3387378: // note 746 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 747 return value; 748 default: return super.setProperty(hash, name, value); 749 } 750 751 } 752 753 @Override 754 public Base setProperty(String name, Base value) throws FHIRException { 755 if (name.equals("code")) { 756 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 757 } else if (name.equals("outcome")) { 758 this.outcome = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 759 } else if (name.equals("contributedToDeath")) { 760 this.contributedToDeath = TypeConvertor.castToBoolean(value); // BooleanType 761 } else if (name.equals("onset[x]")) { 762 this.onset = TypeConvertor.castToType(value); // DataType 763 } else if (name.equals("note")) { 764 this.getNote().add(TypeConvertor.castToAnnotation(value)); 765 } else 766 return super.setProperty(name, value); 767 return value; 768 } 769 770 @Override 771 public void removeChild(String name, Base value) throws FHIRException { 772 if (name.equals("code")) { 773 this.code = null; 774 } else if (name.equals("outcome")) { 775 this.outcome = null; 776 } else if (name.equals("contributedToDeath")) { 777 this.contributedToDeath = null; 778 } else if (name.equals("onset[x]")) { 779 this.onset = null; 780 } else if (name.equals("note")) { 781 this.getNote().remove(value); 782 } else 783 super.removeChild(name, value); 784 785 } 786 787 @Override 788 public Base makeProperty(int hash, String name) throws FHIRException { 789 switch (hash) { 790 case 3059181: return getCode(); 791 case -1106507950: return getOutcome(); 792 case -363644638: return getContributedToDeathElement(); 793 case -1886216323: return getOnset(); 794 case 105901603: return getOnset(); 795 case 3387378: return addNote(); 796 default: return super.makeProperty(hash, name); 797 } 798 799 } 800 801 @Override 802 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 803 switch (hash) { 804 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 805 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 806 case -363644638: /*contributedToDeath*/ return new String[] {"boolean"}; 807 case 105901603: /*onset*/ return new String[] {"Age", "Range", "Period", "string"}; 808 case 3387378: /*note*/ return new String[] {"Annotation"}; 809 default: return super.getTypesForProperty(hash, name); 810 } 811 812 } 813 814 @Override 815 public Base addChild(String name) throws FHIRException { 816 if (name.equals("code")) { 817 this.code = new CodeableConcept(); 818 return this.code; 819 } 820 else if (name.equals("outcome")) { 821 this.outcome = new CodeableConcept(); 822 return this.outcome; 823 } 824 else if (name.equals("contributedToDeath")) { 825 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.condition.contributedToDeath"); 826 } 827 else if (name.equals("onsetAge")) { 828 this.onset = new Age(); 829 return this.onset; 830 } 831 else if (name.equals("onsetRange")) { 832 this.onset = new Range(); 833 return this.onset; 834 } 835 else if (name.equals("onsetPeriod")) { 836 this.onset = new Period(); 837 return this.onset; 838 } 839 else if (name.equals("onsetString")) { 840 this.onset = new StringType(); 841 return this.onset; 842 } 843 else if (name.equals("note")) { 844 return addNote(); 845 } 846 else 847 return super.addChild(name); 848 } 849 850 public FamilyMemberHistoryConditionComponent copy() { 851 FamilyMemberHistoryConditionComponent dst = new FamilyMemberHistoryConditionComponent(); 852 copyValues(dst); 853 return dst; 854 } 855 856 public void copyValues(FamilyMemberHistoryConditionComponent dst) { 857 super.copyValues(dst); 858 dst.code = code == null ? null : code.copy(); 859 dst.outcome = outcome == null ? null : outcome.copy(); 860 dst.contributedToDeath = contributedToDeath == null ? null : contributedToDeath.copy(); 861 dst.onset = onset == null ? null : onset.copy(); 862 if (note != null) { 863 dst.note = new ArrayList<Annotation>(); 864 for (Annotation i : note) 865 dst.note.add(i.copy()); 866 }; 867 } 868 869 @Override 870 public boolean equalsDeep(Base other_) { 871 if (!super.equalsDeep(other_)) 872 return false; 873 if (!(other_ instanceof FamilyMemberHistoryConditionComponent)) 874 return false; 875 FamilyMemberHistoryConditionComponent o = (FamilyMemberHistoryConditionComponent) other_; 876 return compareDeep(code, o.code, true) && compareDeep(outcome, o.outcome, true) && compareDeep(contributedToDeath, o.contributedToDeath, true) 877 && compareDeep(onset, o.onset, true) && compareDeep(note, o.note, true); 878 } 879 880 @Override 881 public boolean equalsShallow(Base other_) { 882 if (!super.equalsShallow(other_)) 883 return false; 884 if (!(other_ instanceof FamilyMemberHistoryConditionComponent)) 885 return false; 886 FamilyMemberHistoryConditionComponent o = (FamilyMemberHistoryConditionComponent) other_; 887 return compareValues(contributedToDeath, o.contributedToDeath, true); 888 } 889 890 public boolean isEmpty() { 891 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, outcome, contributedToDeath 892 , onset, note); 893 } 894 895 public String fhirType() { 896 return "FamilyMemberHistory.condition"; 897 898 } 899 900 } 901 902 @Block() 903 public static class FamilyMemberHistoryProcedureComponent extends BackboneElement implements IBaseBackboneElement { 904 /** 905 * The actual procedure specified. Could be a coded procedure or a less specific string depending on how much is known about the procedure and the capabilities of the creating system. 906 */ 907 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 908 @Description(shortDefinition="Procedures performed on the related person", formalDefinition="The actual procedure specified. Could be a coded procedure or a less specific string depending on how much is known about the procedure and the capabilities of the creating system." ) 909 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-code") 910 protected CodeableConcept code; 911 912 /** 913 * Indicates what happened following the procedure. If the procedure resulted in death, deceased date is captured on the relation. 914 */ 915 @Child(name = "outcome", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 916 @Description(shortDefinition="What happened following the procedure", formalDefinition="Indicates what happened following the procedure. If the procedure resulted in death, deceased date is captured on the relation." ) 917 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 918 protected CodeableConcept outcome; 919 920 /** 921 * This procedure contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown. 922 */ 923 @Child(name = "contributedToDeath", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 924 @Description(shortDefinition="Whether the procedure contributed to the cause of death", formalDefinition="This procedure contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown." ) 925 protected BooleanType contributedToDeath; 926 927 /** 928 * Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured. 929 */ 930 @Child(name = "performed", type = {Age.class, Range.class, Period.class, StringType.class, DateTimeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 931 @Description(shortDefinition="When the procedure was performed", formalDefinition="Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured." ) 932 protected DataType performed; 933 934 /** 935 * An area where general notes can be placed about this specific procedure. 936 */ 937 @Child(name = "note", type = {Annotation.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 938 @Description(shortDefinition="Extra information about the procedure", formalDefinition="An area where general notes can be placed about this specific procedure." ) 939 protected List<Annotation> note; 940 941 private static final long serialVersionUID = 281271062L; 942 943 /** 944 * Constructor 945 */ 946 public FamilyMemberHistoryProcedureComponent() { 947 super(); 948 } 949 950 /** 951 * Constructor 952 */ 953 public FamilyMemberHistoryProcedureComponent(CodeableConcept code) { 954 super(); 955 this.setCode(code); 956 } 957 958 /** 959 * @return {@link #code} (The actual procedure specified. Could be a coded procedure or a less specific string depending on how much is known about the procedure and the capabilities of the creating system.) 960 */ 961 public CodeableConcept getCode() { 962 if (this.code == null) 963 if (Configuration.errorOnAutoCreate()) 964 throw new Error("Attempt to auto-create FamilyMemberHistoryProcedureComponent.code"); 965 else if (Configuration.doAutoCreate()) 966 this.code = new CodeableConcept(); // cc 967 return this.code; 968 } 969 970 public boolean hasCode() { 971 return this.code != null && !this.code.isEmpty(); 972 } 973 974 /** 975 * @param value {@link #code} (The actual procedure specified. Could be a coded procedure or a less specific string depending on how much is known about the procedure and the capabilities of the creating system.) 976 */ 977 public FamilyMemberHistoryProcedureComponent setCode(CodeableConcept value) { 978 this.code = value; 979 return this; 980 } 981 982 /** 983 * @return {@link #outcome} (Indicates what happened following the procedure. If the procedure resulted in death, deceased date is captured on the relation.) 984 */ 985 public CodeableConcept getOutcome() { 986 if (this.outcome == null) 987 if (Configuration.errorOnAutoCreate()) 988 throw new Error("Attempt to auto-create FamilyMemberHistoryProcedureComponent.outcome"); 989 else if (Configuration.doAutoCreate()) 990 this.outcome = new CodeableConcept(); // cc 991 return this.outcome; 992 } 993 994 public boolean hasOutcome() { 995 return this.outcome != null && !this.outcome.isEmpty(); 996 } 997 998 /** 999 * @param value {@link #outcome} (Indicates what happened following the procedure. If the procedure resulted in death, deceased date is captured on the relation.) 1000 */ 1001 public FamilyMemberHistoryProcedureComponent setOutcome(CodeableConcept value) { 1002 this.outcome = value; 1003 return this; 1004 } 1005 1006 /** 1007 * @return {@link #contributedToDeath} (This procedure contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown.). This is the underlying object with id, value and extensions. The accessor "getContributedToDeath" gives direct access to the value 1008 */ 1009 public BooleanType getContributedToDeathElement() { 1010 if (this.contributedToDeath == null) 1011 if (Configuration.errorOnAutoCreate()) 1012 throw new Error("Attempt to auto-create FamilyMemberHistoryProcedureComponent.contributedToDeath"); 1013 else if (Configuration.doAutoCreate()) 1014 this.contributedToDeath = new BooleanType(); // bb 1015 return this.contributedToDeath; 1016 } 1017 1018 public boolean hasContributedToDeathElement() { 1019 return this.contributedToDeath != null && !this.contributedToDeath.isEmpty(); 1020 } 1021 1022 public boolean hasContributedToDeath() { 1023 return this.contributedToDeath != null && !this.contributedToDeath.isEmpty(); 1024 } 1025 1026 /** 1027 * @param value {@link #contributedToDeath} (This procedure contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown.). This is the underlying object with id, value and extensions. The accessor "getContributedToDeath" gives direct access to the value 1028 */ 1029 public FamilyMemberHistoryProcedureComponent setContributedToDeathElement(BooleanType value) { 1030 this.contributedToDeath = value; 1031 return this; 1032 } 1033 1034 /** 1035 * @return This procedure contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown. 1036 */ 1037 public boolean getContributedToDeath() { 1038 return this.contributedToDeath == null || this.contributedToDeath.isEmpty() ? false : this.contributedToDeath.getValue(); 1039 } 1040 1041 /** 1042 * @param value This procedure contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown. 1043 */ 1044 public FamilyMemberHistoryProcedureComponent setContributedToDeath(boolean value) { 1045 if (this.contributedToDeath == null) 1046 this.contributedToDeath = new BooleanType(); 1047 this.contributedToDeath.setValue(value); 1048 return this; 1049 } 1050 1051 /** 1052 * @return {@link #performed} (Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1053 */ 1054 public DataType getPerformed() { 1055 return this.performed; 1056 } 1057 1058 /** 1059 * @return {@link #performed} (Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1060 */ 1061 public Age getPerformedAge() throws FHIRException { 1062 if (this.performed == null) 1063 this.performed = new Age(); 1064 if (!(this.performed instanceof Age)) 1065 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.performed.getClass().getName()+" was encountered"); 1066 return (Age) this.performed; 1067 } 1068 1069 public boolean hasPerformedAge() { 1070 return this.performed instanceof Age; 1071 } 1072 1073 /** 1074 * @return {@link #performed} (Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1075 */ 1076 public Range getPerformedRange() throws FHIRException { 1077 if (this.performed == null) 1078 this.performed = new Range(); 1079 if (!(this.performed instanceof Range)) 1080 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.performed.getClass().getName()+" was encountered"); 1081 return (Range) this.performed; 1082 } 1083 1084 public boolean hasPerformedRange() { 1085 return this.performed instanceof Range; 1086 } 1087 1088 /** 1089 * @return {@link #performed} (Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1090 */ 1091 public Period getPerformedPeriod() throws FHIRException { 1092 if (this.performed == null) 1093 this.performed = new Period(); 1094 if (!(this.performed instanceof Period)) 1095 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.performed.getClass().getName()+" was encountered"); 1096 return (Period) this.performed; 1097 } 1098 1099 public boolean hasPerformedPeriod() { 1100 return this.performed instanceof Period; 1101 } 1102 1103 /** 1104 * @return {@link #performed} (Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1105 */ 1106 public StringType getPerformedStringType() throws FHIRException { 1107 if (this.performed == null) 1108 this.performed = new StringType(); 1109 if (!(this.performed instanceof StringType)) 1110 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.performed.getClass().getName()+" was encountered"); 1111 return (StringType) this.performed; 1112 } 1113 1114 public boolean hasPerformedStringType() { 1115 return this.performed instanceof StringType; 1116 } 1117 1118 /** 1119 * @return {@link #performed} (Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1120 */ 1121 public DateTimeType getPerformedDateTimeType() throws FHIRException { 1122 if (this.performed == null) 1123 this.performed = new DateTimeType(); 1124 if (!(this.performed instanceof DateTimeType)) 1125 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.performed.getClass().getName()+" was encountered"); 1126 return (DateTimeType) this.performed; 1127 } 1128 1129 public boolean hasPerformedDateTimeType() { 1130 return this.performed instanceof DateTimeType; 1131 } 1132 1133 public boolean hasPerformed() { 1134 return this.performed != null && !this.performed.isEmpty(); 1135 } 1136 1137 /** 1138 * @param value {@link #performed} (Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.) 1139 */ 1140 public FamilyMemberHistoryProcedureComponent setPerformed(DataType value) { 1141 if (value != null && !(value instanceof Age || value instanceof Range || value instanceof Period || value instanceof StringType || value instanceof DateTimeType)) 1142 throw new FHIRException("Not the right type for FamilyMemberHistory.procedure.performed[x]: "+value.fhirType()); 1143 this.performed = value; 1144 return this; 1145 } 1146 1147 /** 1148 * @return {@link #note} (An area where general notes can be placed about this specific procedure.) 1149 */ 1150 public List<Annotation> getNote() { 1151 if (this.note == null) 1152 this.note = new ArrayList<Annotation>(); 1153 return this.note; 1154 } 1155 1156 /** 1157 * @return Returns a reference to <code>this</code> for easy method chaining 1158 */ 1159 public FamilyMemberHistoryProcedureComponent setNote(List<Annotation> theNote) { 1160 this.note = theNote; 1161 return this; 1162 } 1163 1164 public boolean hasNote() { 1165 if (this.note == null) 1166 return false; 1167 for (Annotation item : this.note) 1168 if (!item.isEmpty()) 1169 return true; 1170 return false; 1171 } 1172 1173 public Annotation addNote() { //3 1174 Annotation t = new Annotation(); 1175 if (this.note == null) 1176 this.note = new ArrayList<Annotation>(); 1177 this.note.add(t); 1178 return t; 1179 } 1180 1181 public FamilyMemberHistoryProcedureComponent addNote(Annotation t) { //3 1182 if (t == null) 1183 return this; 1184 if (this.note == null) 1185 this.note = new ArrayList<Annotation>(); 1186 this.note.add(t); 1187 return this; 1188 } 1189 1190 /** 1191 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1192 */ 1193 public Annotation getNoteFirstRep() { 1194 if (getNote().isEmpty()) { 1195 addNote(); 1196 } 1197 return getNote().get(0); 1198 } 1199 1200 protected void listChildren(List<Property> children) { 1201 super.listChildren(children); 1202 children.add(new Property("code", "CodeableConcept", "The actual procedure specified. Could be a coded procedure or a less specific string depending on how much is known about the procedure and the capabilities of the creating system.", 0, 1, code)); 1203 children.add(new Property("outcome", "CodeableConcept", "Indicates what happened following the procedure. If the procedure resulted in death, deceased date is captured on the relation.", 0, 1, outcome)); 1204 children.add(new Property("contributedToDeath", "boolean", "This procedure contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown.", 0, 1, contributedToDeath)); 1205 children.add(new Property("performed[x]", "Age|Range|Period|string|dateTime", "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, performed)); 1206 children.add(new Property("note", "Annotation", "An area where general notes can be placed about this specific procedure.", 0, java.lang.Integer.MAX_VALUE, note)); 1207 } 1208 1209 @Override 1210 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1211 switch (_hash) { 1212 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The actual procedure specified. Could be a coded procedure or a less specific string depending on how much is known about the procedure and the capabilities of the creating system.", 0, 1, code); 1213 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "Indicates what happened following the procedure. If the procedure resulted in death, deceased date is captured on the relation.", 0, 1, outcome); 1214 case -363644638: /*contributedToDeath*/ return new Property("contributedToDeath", "boolean", "This procedure contributed to the cause of death of the related person. If contributedToDeath is not populated, then it is unknown.", 0, 1, contributedToDeath); 1215 case 1355984064: /*performed[x]*/ return new Property("performed[x]", "Age|Range|Period|string|dateTime", "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, performed); 1216 case 481140672: /*performed*/ return new Property("performed[x]", "Age|Range|Period|string|dateTime", "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, performed); 1217 case 1355958559: /*performedAge*/ return new Property("performed[x]", "Age", "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, performed); 1218 case 1716617565: /*performedRange*/ return new Property("performed[x]", "Range", "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, performed); 1219 case 1622094241: /*performedPeriod*/ return new Property("performed[x]", "Period", "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, performed); 1220 case 1721834481: /*performedString*/ return new Property("performed[x]", "string", "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, performed); 1221 case 1118270331: /*performedDateTime*/ return new Property("performed[x]", "dateTime", "Estimated or actual date, date-time, period, or age when the procedure was performed. Allows a period to support complex procedures that span more than one date, and also allows for the length of the procedure to be captured.", 0, 1, performed); 1222 case 3387378: /*note*/ return new Property("note", "Annotation", "An area where general notes can be placed about this specific procedure.", 0, java.lang.Integer.MAX_VALUE, note); 1223 default: return super.getNamedProperty(_hash, _name, _checkValid); 1224 } 1225 1226 } 1227 1228 @Override 1229 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1230 switch (hash) { 1231 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1232 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // CodeableConcept 1233 case -363644638: /*contributedToDeath*/ return this.contributedToDeath == null ? new Base[0] : new Base[] {this.contributedToDeath}; // BooleanType 1234 case 481140672: /*performed*/ return this.performed == null ? new Base[0] : new Base[] {this.performed}; // DataType 1235 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1236 default: return super.getProperty(hash, name, checkValid); 1237 } 1238 1239 } 1240 1241 @Override 1242 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1243 switch (hash) { 1244 case 3059181: // code 1245 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1246 return value; 1247 case -1106507950: // outcome 1248 this.outcome = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1249 return value; 1250 case -363644638: // contributedToDeath 1251 this.contributedToDeath = TypeConvertor.castToBoolean(value); // BooleanType 1252 return value; 1253 case 481140672: // performed 1254 this.performed = TypeConvertor.castToType(value); // DataType 1255 return value; 1256 case 3387378: // note 1257 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1258 return value; 1259 default: return super.setProperty(hash, name, value); 1260 } 1261 1262 } 1263 1264 @Override 1265 public Base setProperty(String name, Base value) throws FHIRException { 1266 if (name.equals("code")) { 1267 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1268 } else if (name.equals("outcome")) { 1269 this.outcome = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1270 } else if (name.equals("contributedToDeath")) { 1271 this.contributedToDeath = TypeConvertor.castToBoolean(value); // BooleanType 1272 } else if (name.equals("performed[x]")) { 1273 this.performed = TypeConvertor.castToType(value); // DataType 1274 } else if (name.equals("note")) { 1275 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1276 } else 1277 return super.setProperty(name, value); 1278 return value; 1279 } 1280 1281 @Override 1282 public void removeChild(String name, Base value) throws FHIRException { 1283 if (name.equals("code")) { 1284 this.code = null; 1285 } else if (name.equals("outcome")) { 1286 this.outcome = null; 1287 } else if (name.equals("contributedToDeath")) { 1288 this.contributedToDeath = null; 1289 } else if (name.equals("performed[x]")) { 1290 this.performed = null; 1291 } else if (name.equals("note")) { 1292 this.getNote().remove(value); 1293 } else 1294 super.removeChild(name, value); 1295 1296 } 1297 1298 @Override 1299 public Base makeProperty(int hash, String name) throws FHIRException { 1300 switch (hash) { 1301 case 3059181: return getCode(); 1302 case -1106507950: return getOutcome(); 1303 case -363644638: return getContributedToDeathElement(); 1304 case 1355984064: return getPerformed(); 1305 case 481140672: return getPerformed(); 1306 case 3387378: return addNote(); 1307 default: return super.makeProperty(hash, name); 1308 } 1309 1310 } 1311 1312 @Override 1313 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1314 switch (hash) { 1315 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1316 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 1317 case -363644638: /*contributedToDeath*/ return new String[] {"boolean"}; 1318 case 481140672: /*performed*/ return new String[] {"Age", "Range", "Period", "string", "dateTime"}; 1319 case 3387378: /*note*/ return new String[] {"Annotation"}; 1320 default: return super.getTypesForProperty(hash, name); 1321 } 1322 1323 } 1324 1325 @Override 1326 public Base addChild(String name) throws FHIRException { 1327 if (name.equals("code")) { 1328 this.code = new CodeableConcept(); 1329 return this.code; 1330 } 1331 else if (name.equals("outcome")) { 1332 this.outcome = new CodeableConcept(); 1333 return this.outcome; 1334 } 1335 else if (name.equals("contributedToDeath")) { 1336 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.procedure.contributedToDeath"); 1337 } 1338 else if (name.equals("performedAge")) { 1339 this.performed = new Age(); 1340 return this.performed; 1341 } 1342 else if (name.equals("performedRange")) { 1343 this.performed = new Range(); 1344 return this.performed; 1345 } 1346 else if (name.equals("performedPeriod")) { 1347 this.performed = new Period(); 1348 return this.performed; 1349 } 1350 else if (name.equals("performedString")) { 1351 this.performed = new StringType(); 1352 return this.performed; 1353 } 1354 else if (name.equals("performedDateTime")) { 1355 this.performed = new DateTimeType(); 1356 return this.performed; 1357 } 1358 else if (name.equals("note")) { 1359 return addNote(); 1360 } 1361 else 1362 return super.addChild(name); 1363 } 1364 1365 public FamilyMemberHistoryProcedureComponent copy() { 1366 FamilyMemberHistoryProcedureComponent dst = new FamilyMemberHistoryProcedureComponent(); 1367 copyValues(dst); 1368 return dst; 1369 } 1370 1371 public void copyValues(FamilyMemberHistoryProcedureComponent dst) { 1372 super.copyValues(dst); 1373 dst.code = code == null ? null : code.copy(); 1374 dst.outcome = outcome == null ? null : outcome.copy(); 1375 dst.contributedToDeath = contributedToDeath == null ? null : contributedToDeath.copy(); 1376 dst.performed = performed == null ? null : performed.copy(); 1377 if (note != null) { 1378 dst.note = new ArrayList<Annotation>(); 1379 for (Annotation i : note) 1380 dst.note.add(i.copy()); 1381 }; 1382 } 1383 1384 @Override 1385 public boolean equalsDeep(Base other_) { 1386 if (!super.equalsDeep(other_)) 1387 return false; 1388 if (!(other_ instanceof FamilyMemberHistoryProcedureComponent)) 1389 return false; 1390 FamilyMemberHistoryProcedureComponent o = (FamilyMemberHistoryProcedureComponent) other_; 1391 return compareDeep(code, o.code, true) && compareDeep(outcome, o.outcome, true) && compareDeep(contributedToDeath, o.contributedToDeath, true) 1392 && compareDeep(performed, o.performed, true) && compareDeep(note, o.note, true); 1393 } 1394 1395 @Override 1396 public boolean equalsShallow(Base other_) { 1397 if (!super.equalsShallow(other_)) 1398 return false; 1399 if (!(other_ instanceof FamilyMemberHistoryProcedureComponent)) 1400 return false; 1401 FamilyMemberHistoryProcedureComponent o = (FamilyMemberHistoryProcedureComponent) other_; 1402 return compareValues(contributedToDeath, o.contributedToDeath, true); 1403 } 1404 1405 public boolean isEmpty() { 1406 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, outcome, contributedToDeath 1407 , performed, note); 1408 } 1409 1410 public String fhirType() { 1411 return "FamilyMemberHistory.procedure"; 1412 1413 } 1414 1415 } 1416 1417 /** 1418 * Business identifiers assigned to this family member history by the performer or other systems which remain constant as the resource is updated and propagates from server to server. 1419 */ 1420 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1421 @Description(shortDefinition="External Id(s) for this record", formalDefinition="Business identifiers assigned to this family member history by the performer or other systems which remain constant as the resource is updated and propagates from server to server." ) 1422 protected List<Identifier> identifier; 1423 1424 /** 1425 * The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory. 1426 */ 1427 @Child(name = "instantiatesCanonical", type = {CanonicalType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1428 @Description(shortDefinition="Instantiates FHIR protocol or definition", formalDefinition="The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory." ) 1429 protected List<CanonicalType> instantiatesCanonical; 1430 1431 /** 1432 * The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory. 1433 */ 1434 @Child(name = "instantiatesUri", type = {UriType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1435 @Description(shortDefinition="Instantiates external protocol or definition", formalDefinition="The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory." ) 1436 protected List<UriType> instantiatesUri; 1437 1438 /** 1439 * A code specifying the status of the record of the family history of a specific family member. 1440 */ 1441 @Child(name = "status", type = {CodeType.class}, order=3, min=1, max=1, modifier=true, summary=true) 1442 @Description(shortDefinition="partial | completed | entered-in-error | health-unknown", formalDefinition="A code specifying the status of the record of the family history of a specific family member." ) 1443 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/history-status") 1444 protected Enumeration<FamilyHistoryStatus> status; 1445 1446 /** 1447 * Describes why the family member's history is not available. 1448 */ 1449 @Child(name = "dataAbsentReason", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 1450 @Description(shortDefinition="subject-unknown | withheld | unable-to-obtain | deferred", formalDefinition="Describes why the family member's history is not available." ) 1451 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/history-absent-reason") 1452 protected CodeableConcept dataAbsentReason; 1453 1454 /** 1455 * The person who this history concerns. 1456 */ 1457 @Child(name = "patient", type = {Patient.class}, order=5, min=1, max=1, modifier=false, summary=true) 1458 @Description(shortDefinition="Patient history is about", formalDefinition="The person who this history concerns." ) 1459 protected Reference patient; 1460 1461 /** 1462 * The date (and possibly time) when the family member history was recorded or last updated. 1463 */ 1464 @Child(name = "date", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 1465 @Description(shortDefinition="When history was recorded or last updated", formalDefinition="The date (and possibly time) when the family member history was recorded or last updated." ) 1466 protected DateTimeType date; 1467 1468 /** 1469 * Indicates who or what participated in the activities related to the family member history and how they were involved. 1470 */ 1471 @Child(name = "participant", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1472 @Description(shortDefinition="Who or what participated in the activities related to the family member history and how they were involved", formalDefinition="Indicates who or what participated in the activities related to the family member history and how they were involved." ) 1473 protected List<FamilyMemberHistoryParticipantComponent> participant; 1474 1475 /** 1476 * This will either be a name or a description; e.g. "Aunt Susan", "my cousin with the red hair". 1477 */ 1478 @Child(name = "name", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1479 @Description(shortDefinition="The family member described", formalDefinition="This will either be a name or a description; e.g. \"Aunt Susan\", \"my cousin with the red hair\"." ) 1480 protected StringType name; 1481 1482 /** 1483 * The type of relationship this person has to the patient (father, mother, brother etc.). 1484 */ 1485 @Child(name = "relationship", type = {CodeableConcept.class}, order=9, min=1, max=1, modifier=false, summary=true) 1486 @Description(shortDefinition="Relationship to the subject", formalDefinition="The type of relationship this person has to the patient (father, mother, brother etc.)." ) 1487 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-FamilyMember") 1488 protected CodeableConcept relationship; 1489 1490 /** 1491 * The birth sex of the family member. 1492 */ 1493 @Child(name = "sex", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=true) 1494 @Description(shortDefinition="male | female | other | unknown", formalDefinition="The birth sex of the family member." ) 1495 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administrative-gender") 1496 protected CodeableConcept sex; 1497 1498 /** 1499 * The actual or approximate date of birth of the relative. 1500 */ 1501 @Child(name = "born", type = {Period.class, DateType.class, StringType.class}, order=11, min=0, max=1, modifier=false, summary=false) 1502 @Description(shortDefinition="(approximate) date of birth", formalDefinition="The actual or approximate date of birth of the relative." ) 1503 protected DataType born; 1504 1505 /** 1506 * The age of the relative at the time the family member history is recorded. 1507 */ 1508 @Child(name = "age", type = {Age.class, Range.class, StringType.class}, order=12, min=0, max=1, modifier=false, summary=true) 1509 @Description(shortDefinition="(approximate) age", formalDefinition="The age of the relative at the time the family member history is recorded." ) 1510 protected DataType age; 1511 1512 /** 1513 * If true, indicates that the age value specified is an estimated value. 1514 */ 1515 @Child(name = "estimatedAge", type = {BooleanType.class}, order=13, min=0, max=1, modifier=false, summary=true) 1516 @Description(shortDefinition="Age is estimated?", formalDefinition="If true, indicates that the age value specified is an estimated value." ) 1517 protected BooleanType estimatedAge; 1518 1519 /** 1520 * Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record. 1521 */ 1522 @Child(name = "deceased", type = {BooleanType.class, Age.class, Range.class, DateType.class, StringType.class}, order=14, min=0, max=1, modifier=false, summary=true) 1523 @Description(shortDefinition="Dead? How old/when?", formalDefinition="Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record." ) 1524 protected DataType deceased; 1525 1526 /** 1527 * Describes why the family member history occurred in coded or textual form, or Indicates a Condition, Observation, AllergyIntolerance, or QuestionnaireResponse that justifies this family member history event. 1528 */ 1529 @Child(name = "reason", type = {CodeableReference.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1530 @Description(shortDefinition="Why was family member history performed?", formalDefinition="Describes why the family member history occurred in coded or textual form, or Indicates a Condition, Observation, AllergyIntolerance, or QuestionnaireResponse that justifies this family member history event." ) 1531 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 1532 protected List<CodeableReference> reason; 1533 1534 /** 1535 * This property allows a non condition-specific note to the made about the related person. Ideally, the note would be in the condition property, but this is not always possible. 1536 */ 1537 @Child(name = "note", type = {Annotation.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1538 @Description(shortDefinition="General note about related person", formalDefinition="This property allows a non condition-specific note to the made about the related person. Ideally, the note would be in the condition property, but this is not always possible." ) 1539 protected List<Annotation> note; 1540 1541 /** 1542 * The significant Conditions (or condition) that the family member had. This is a repeating section to allow a system to represent more than one condition per resource, though there is nothing stopping multiple resources - one per condition. 1543 */ 1544 @Child(name = "condition", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1545 @Description(shortDefinition="Condition that the related person had", formalDefinition="The significant Conditions (or condition) that the family member had. This is a repeating section to allow a system to represent more than one condition per resource, though there is nothing stopping multiple resources - one per condition." ) 1546 protected List<FamilyMemberHistoryConditionComponent> condition; 1547 1548 /** 1549 * The significant Procedures (or procedure) that the family member had. This is a repeating section to allow a system to represent more than one procedure per resource, though there is nothing stopping multiple resources - one per procedure. 1550 */ 1551 @Child(name = "procedure", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1552 @Description(shortDefinition="Procedures that the related person had", formalDefinition="The significant Procedures (or procedure) that the family member had. This is a repeating section to allow a system to represent more than one procedure per resource, though there is nothing stopping multiple resources - one per procedure." ) 1553 protected List<FamilyMemberHistoryProcedureComponent> procedure; 1554 1555 private static final long serialVersionUID = -1025621277L; 1556 1557 /** 1558 * Constructor 1559 */ 1560 public FamilyMemberHistory() { 1561 super(); 1562 } 1563 1564 /** 1565 * Constructor 1566 */ 1567 public FamilyMemberHistory(FamilyHistoryStatus status, Reference patient, CodeableConcept relationship) { 1568 super(); 1569 this.setStatus(status); 1570 this.setPatient(patient); 1571 this.setRelationship(relationship); 1572 } 1573 1574 /** 1575 * @return {@link #identifier} (Business identifiers assigned to this family member history by the performer or other systems which remain constant as the resource is updated and propagates from server to server.) 1576 */ 1577 public List<Identifier> getIdentifier() { 1578 if (this.identifier == null) 1579 this.identifier = new ArrayList<Identifier>(); 1580 return this.identifier; 1581 } 1582 1583 /** 1584 * @return Returns a reference to <code>this</code> for easy method chaining 1585 */ 1586 public FamilyMemberHistory setIdentifier(List<Identifier> theIdentifier) { 1587 this.identifier = theIdentifier; 1588 return this; 1589 } 1590 1591 public boolean hasIdentifier() { 1592 if (this.identifier == null) 1593 return false; 1594 for (Identifier item : this.identifier) 1595 if (!item.isEmpty()) 1596 return true; 1597 return false; 1598 } 1599 1600 public Identifier addIdentifier() { //3 1601 Identifier t = new Identifier(); 1602 if (this.identifier == null) 1603 this.identifier = new ArrayList<Identifier>(); 1604 this.identifier.add(t); 1605 return t; 1606 } 1607 1608 public FamilyMemberHistory addIdentifier(Identifier t) { //3 1609 if (t == null) 1610 return this; 1611 if (this.identifier == null) 1612 this.identifier = new ArrayList<Identifier>(); 1613 this.identifier.add(t); 1614 return this; 1615 } 1616 1617 /** 1618 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1619 */ 1620 public Identifier getIdentifierFirstRep() { 1621 if (getIdentifier().isEmpty()) { 1622 addIdentifier(); 1623 } 1624 return getIdentifier().get(0); 1625 } 1626 1627 /** 1628 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.) 1629 */ 1630 public List<CanonicalType> getInstantiatesCanonical() { 1631 if (this.instantiatesCanonical == null) 1632 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1633 return this.instantiatesCanonical; 1634 } 1635 1636 /** 1637 * @return Returns a reference to <code>this</code> for easy method chaining 1638 */ 1639 public FamilyMemberHistory setInstantiatesCanonical(List<CanonicalType> theInstantiatesCanonical) { 1640 this.instantiatesCanonical = theInstantiatesCanonical; 1641 return this; 1642 } 1643 1644 public boolean hasInstantiatesCanonical() { 1645 if (this.instantiatesCanonical == null) 1646 return false; 1647 for (CanonicalType item : this.instantiatesCanonical) 1648 if (!item.isEmpty()) 1649 return true; 1650 return false; 1651 } 1652 1653 /** 1654 * @return {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.) 1655 */ 1656 public CanonicalType addInstantiatesCanonicalElement() {//2 1657 CanonicalType t = new CanonicalType(); 1658 if (this.instantiatesCanonical == null) 1659 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1660 this.instantiatesCanonical.add(t); 1661 return t; 1662 } 1663 1664 /** 1665 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.) 1666 */ 1667 public FamilyMemberHistory addInstantiatesCanonical(String value) { //1 1668 CanonicalType t = new CanonicalType(); 1669 t.setValue(value); 1670 if (this.instantiatesCanonical == null) 1671 this.instantiatesCanonical = new ArrayList<CanonicalType>(); 1672 this.instantiatesCanonical.add(t); 1673 return this; 1674 } 1675 1676 /** 1677 * @param value {@link #instantiatesCanonical} (The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.) 1678 */ 1679 public boolean hasInstantiatesCanonical(String value) { 1680 if (this.instantiatesCanonical == null) 1681 return false; 1682 for (CanonicalType v : this.instantiatesCanonical) 1683 if (v.getValue().equals(value)) // canonical 1684 return true; 1685 return false; 1686 } 1687 1688 /** 1689 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.) 1690 */ 1691 public List<UriType> getInstantiatesUri() { 1692 if (this.instantiatesUri == null) 1693 this.instantiatesUri = new ArrayList<UriType>(); 1694 return this.instantiatesUri; 1695 } 1696 1697 /** 1698 * @return Returns a reference to <code>this</code> for easy method chaining 1699 */ 1700 public FamilyMemberHistory setInstantiatesUri(List<UriType> theInstantiatesUri) { 1701 this.instantiatesUri = theInstantiatesUri; 1702 return this; 1703 } 1704 1705 public boolean hasInstantiatesUri() { 1706 if (this.instantiatesUri == null) 1707 return false; 1708 for (UriType item : this.instantiatesUri) 1709 if (!item.isEmpty()) 1710 return true; 1711 return false; 1712 } 1713 1714 /** 1715 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.) 1716 */ 1717 public UriType addInstantiatesUriElement() {//2 1718 UriType t = new UriType(); 1719 if (this.instantiatesUri == null) 1720 this.instantiatesUri = new ArrayList<UriType>(); 1721 this.instantiatesUri.add(t); 1722 return t; 1723 } 1724 1725 /** 1726 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.) 1727 */ 1728 public FamilyMemberHistory addInstantiatesUri(String value) { //1 1729 UriType t = new UriType(); 1730 t.setValue(value); 1731 if (this.instantiatesUri == null) 1732 this.instantiatesUri = new ArrayList<UriType>(); 1733 this.instantiatesUri.add(t); 1734 return this; 1735 } 1736 1737 /** 1738 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.) 1739 */ 1740 public boolean hasInstantiatesUri(String value) { 1741 if (this.instantiatesUri == null) 1742 return false; 1743 for (UriType v : this.instantiatesUri) 1744 if (v.getValue().equals(value)) // uri 1745 return true; 1746 return false; 1747 } 1748 1749 /** 1750 * @return {@link #status} (A code specifying the status of the record of the family history of a specific family member.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1751 */ 1752 public Enumeration<FamilyHistoryStatus> getStatusElement() { 1753 if (this.status == null) 1754 if (Configuration.errorOnAutoCreate()) 1755 throw new Error("Attempt to auto-create FamilyMemberHistory.status"); 1756 else if (Configuration.doAutoCreate()) 1757 this.status = new Enumeration<FamilyHistoryStatus>(new FamilyHistoryStatusEnumFactory()); // bb 1758 return this.status; 1759 } 1760 1761 public boolean hasStatusElement() { 1762 return this.status != null && !this.status.isEmpty(); 1763 } 1764 1765 public boolean hasStatus() { 1766 return this.status != null && !this.status.isEmpty(); 1767 } 1768 1769 /** 1770 * @param value {@link #status} (A code specifying the status of the record of the family history of a specific family member.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1771 */ 1772 public FamilyMemberHistory setStatusElement(Enumeration<FamilyHistoryStatus> value) { 1773 this.status = value; 1774 return this; 1775 } 1776 1777 /** 1778 * @return A code specifying the status of the record of the family history of a specific family member. 1779 */ 1780 public FamilyHistoryStatus getStatus() { 1781 return this.status == null ? null : this.status.getValue(); 1782 } 1783 1784 /** 1785 * @param value A code specifying the status of the record of the family history of a specific family member. 1786 */ 1787 public FamilyMemberHistory setStatus(FamilyHistoryStatus value) { 1788 if (this.status == null) 1789 this.status = new Enumeration<FamilyHistoryStatus>(new FamilyHistoryStatusEnumFactory()); 1790 this.status.setValue(value); 1791 return this; 1792 } 1793 1794 /** 1795 * @return {@link #dataAbsentReason} (Describes why the family member's history is not available.) 1796 */ 1797 public CodeableConcept getDataAbsentReason() { 1798 if (this.dataAbsentReason == null) 1799 if (Configuration.errorOnAutoCreate()) 1800 throw new Error("Attempt to auto-create FamilyMemberHistory.dataAbsentReason"); 1801 else if (Configuration.doAutoCreate()) 1802 this.dataAbsentReason = new CodeableConcept(); // cc 1803 return this.dataAbsentReason; 1804 } 1805 1806 public boolean hasDataAbsentReason() { 1807 return this.dataAbsentReason != null && !this.dataAbsentReason.isEmpty(); 1808 } 1809 1810 /** 1811 * @param value {@link #dataAbsentReason} (Describes why the family member's history is not available.) 1812 */ 1813 public FamilyMemberHistory setDataAbsentReason(CodeableConcept value) { 1814 this.dataAbsentReason = value; 1815 return this; 1816 } 1817 1818 /** 1819 * @return {@link #patient} (The person who this history concerns.) 1820 */ 1821 public Reference getPatient() { 1822 if (this.patient == null) 1823 if (Configuration.errorOnAutoCreate()) 1824 throw new Error("Attempt to auto-create FamilyMemberHistory.patient"); 1825 else if (Configuration.doAutoCreate()) 1826 this.patient = new Reference(); // cc 1827 return this.patient; 1828 } 1829 1830 public boolean hasPatient() { 1831 return this.patient != null && !this.patient.isEmpty(); 1832 } 1833 1834 /** 1835 * @param value {@link #patient} (The person who this history concerns.) 1836 */ 1837 public FamilyMemberHistory setPatient(Reference value) { 1838 this.patient = value; 1839 return this; 1840 } 1841 1842 /** 1843 * @return {@link #date} (The date (and possibly time) when the family member history was recorded or last updated.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1844 */ 1845 public DateTimeType getDateElement() { 1846 if (this.date == null) 1847 if (Configuration.errorOnAutoCreate()) 1848 throw new Error("Attempt to auto-create FamilyMemberHistory.date"); 1849 else if (Configuration.doAutoCreate()) 1850 this.date = new DateTimeType(); // bb 1851 return this.date; 1852 } 1853 1854 public boolean hasDateElement() { 1855 return this.date != null && !this.date.isEmpty(); 1856 } 1857 1858 public boolean hasDate() { 1859 return this.date != null && !this.date.isEmpty(); 1860 } 1861 1862 /** 1863 * @param value {@link #date} (The date (and possibly time) when the family member history was recorded or last updated.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1864 */ 1865 public FamilyMemberHistory setDateElement(DateTimeType value) { 1866 this.date = value; 1867 return this; 1868 } 1869 1870 /** 1871 * @return The date (and possibly time) when the family member history was recorded or last updated. 1872 */ 1873 public Date getDate() { 1874 return this.date == null ? null : this.date.getValue(); 1875 } 1876 1877 /** 1878 * @param value The date (and possibly time) when the family member history was recorded or last updated. 1879 */ 1880 public FamilyMemberHistory setDate(Date value) { 1881 if (value == null) 1882 this.date = null; 1883 else { 1884 if (this.date == null) 1885 this.date = new DateTimeType(); 1886 this.date.setValue(value); 1887 } 1888 return this; 1889 } 1890 1891 /** 1892 * @return {@link #participant} (Indicates who or what participated in the activities related to the family member history and how they were involved.) 1893 */ 1894 public List<FamilyMemberHistoryParticipantComponent> getParticipant() { 1895 if (this.participant == null) 1896 this.participant = new ArrayList<FamilyMemberHistoryParticipantComponent>(); 1897 return this.participant; 1898 } 1899 1900 /** 1901 * @return Returns a reference to <code>this</code> for easy method chaining 1902 */ 1903 public FamilyMemberHistory setParticipant(List<FamilyMemberHistoryParticipantComponent> theParticipant) { 1904 this.participant = theParticipant; 1905 return this; 1906 } 1907 1908 public boolean hasParticipant() { 1909 if (this.participant == null) 1910 return false; 1911 for (FamilyMemberHistoryParticipantComponent item : this.participant) 1912 if (!item.isEmpty()) 1913 return true; 1914 return false; 1915 } 1916 1917 public FamilyMemberHistoryParticipantComponent addParticipant() { //3 1918 FamilyMemberHistoryParticipantComponent t = new FamilyMemberHistoryParticipantComponent(); 1919 if (this.participant == null) 1920 this.participant = new ArrayList<FamilyMemberHistoryParticipantComponent>(); 1921 this.participant.add(t); 1922 return t; 1923 } 1924 1925 public FamilyMemberHistory addParticipant(FamilyMemberHistoryParticipantComponent t) { //3 1926 if (t == null) 1927 return this; 1928 if (this.participant == null) 1929 this.participant = new ArrayList<FamilyMemberHistoryParticipantComponent>(); 1930 this.participant.add(t); 1931 return this; 1932 } 1933 1934 /** 1935 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist {3} 1936 */ 1937 public FamilyMemberHistoryParticipantComponent getParticipantFirstRep() { 1938 if (getParticipant().isEmpty()) { 1939 addParticipant(); 1940 } 1941 return getParticipant().get(0); 1942 } 1943 1944 /** 1945 * @return {@link #name} (This will either be a name or a description; e.g. "Aunt Susan", "my cousin with the red hair".). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1946 */ 1947 public StringType getNameElement() { 1948 if (this.name == null) 1949 if (Configuration.errorOnAutoCreate()) 1950 throw new Error("Attempt to auto-create FamilyMemberHistory.name"); 1951 else if (Configuration.doAutoCreate()) 1952 this.name = new StringType(); // bb 1953 return this.name; 1954 } 1955 1956 public boolean hasNameElement() { 1957 return this.name != null && !this.name.isEmpty(); 1958 } 1959 1960 public boolean hasName() { 1961 return this.name != null && !this.name.isEmpty(); 1962 } 1963 1964 /** 1965 * @param value {@link #name} (This will either be a name or a description; e.g. "Aunt Susan", "my cousin with the red hair".). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1966 */ 1967 public FamilyMemberHistory setNameElement(StringType value) { 1968 this.name = value; 1969 return this; 1970 } 1971 1972 /** 1973 * @return This will either be a name or a description; e.g. "Aunt Susan", "my cousin with the red hair". 1974 */ 1975 public String getName() { 1976 return this.name == null ? null : this.name.getValue(); 1977 } 1978 1979 /** 1980 * @param value This will either be a name or a description; e.g. "Aunt Susan", "my cousin with the red hair". 1981 */ 1982 public FamilyMemberHistory setName(String value) { 1983 if (Utilities.noString(value)) 1984 this.name = null; 1985 else { 1986 if (this.name == null) 1987 this.name = new StringType(); 1988 this.name.setValue(value); 1989 } 1990 return this; 1991 } 1992 1993 /** 1994 * @return {@link #relationship} (The type of relationship this person has to the patient (father, mother, brother etc.).) 1995 */ 1996 public CodeableConcept getRelationship() { 1997 if (this.relationship == null) 1998 if (Configuration.errorOnAutoCreate()) 1999 throw new Error("Attempt to auto-create FamilyMemberHistory.relationship"); 2000 else if (Configuration.doAutoCreate()) 2001 this.relationship = new CodeableConcept(); // cc 2002 return this.relationship; 2003 } 2004 2005 public boolean hasRelationship() { 2006 return this.relationship != null && !this.relationship.isEmpty(); 2007 } 2008 2009 /** 2010 * @param value {@link #relationship} (The type of relationship this person has to the patient (father, mother, brother etc.).) 2011 */ 2012 public FamilyMemberHistory setRelationship(CodeableConcept value) { 2013 this.relationship = value; 2014 return this; 2015 } 2016 2017 /** 2018 * @return {@link #sex} (The birth sex of the family member.) 2019 */ 2020 public CodeableConcept getSex() { 2021 if (this.sex == null) 2022 if (Configuration.errorOnAutoCreate()) 2023 throw new Error("Attempt to auto-create FamilyMemberHistory.sex"); 2024 else if (Configuration.doAutoCreate()) 2025 this.sex = new CodeableConcept(); // cc 2026 return this.sex; 2027 } 2028 2029 public boolean hasSex() { 2030 return this.sex != null && !this.sex.isEmpty(); 2031 } 2032 2033 /** 2034 * @param value {@link #sex} (The birth sex of the family member.) 2035 */ 2036 public FamilyMemberHistory setSex(CodeableConcept value) { 2037 this.sex = value; 2038 return this; 2039 } 2040 2041 /** 2042 * @return {@link #born} (The actual or approximate date of birth of the relative.) 2043 */ 2044 public DataType getBorn() { 2045 return this.born; 2046 } 2047 2048 /** 2049 * @return {@link #born} (The actual or approximate date of birth of the relative.) 2050 */ 2051 public Period getBornPeriod() throws FHIRException { 2052 if (this.born == null) 2053 this.born = new Period(); 2054 if (!(this.born instanceof Period)) 2055 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.born.getClass().getName()+" was encountered"); 2056 return (Period) this.born; 2057 } 2058 2059 public boolean hasBornPeriod() { 2060 return this.born instanceof Period; 2061 } 2062 2063 /** 2064 * @return {@link #born} (The actual or approximate date of birth of the relative.) 2065 */ 2066 public DateType getBornDateType() throws FHIRException { 2067 if (this.born == null) 2068 this.born = new DateType(); 2069 if (!(this.born instanceof DateType)) 2070 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.born.getClass().getName()+" was encountered"); 2071 return (DateType) this.born; 2072 } 2073 2074 public boolean hasBornDateType() { 2075 return this.born instanceof DateType; 2076 } 2077 2078 /** 2079 * @return {@link #born} (The actual or approximate date of birth of the relative.) 2080 */ 2081 public StringType getBornStringType() throws FHIRException { 2082 if (this.born == null) 2083 this.born = new StringType(); 2084 if (!(this.born instanceof StringType)) 2085 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.born.getClass().getName()+" was encountered"); 2086 return (StringType) this.born; 2087 } 2088 2089 public boolean hasBornStringType() { 2090 return this.born instanceof StringType; 2091 } 2092 2093 public boolean hasBorn() { 2094 return this.born != null && !this.born.isEmpty(); 2095 } 2096 2097 /** 2098 * @param value {@link #born} (The actual or approximate date of birth of the relative.) 2099 */ 2100 public FamilyMemberHistory setBorn(DataType value) { 2101 if (value != null && !(value instanceof Period || value instanceof DateType || value instanceof StringType)) 2102 throw new FHIRException("Not the right type for FamilyMemberHistory.born[x]: "+value.fhirType()); 2103 this.born = value; 2104 return this; 2105 } 2106 2107 /** 2108 * @return {@link #age} (The age of the relative at the time the family member history is recorded.) 2109 */ 2110 public DataType getAge() { 2111 return this.age; 2112 } 2113 2114 /** 2115 * @return {@link #age} (The age of the relative at the time the family member history is recorded.) 2116 */ 2117 public Age getAgeAge() throws FHIRException { 2118 if (this.age == null) 2119 this.age = new Age(); 2120 if (!(this.age instanceof Age)) 2121 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.age.getClass().getName()+" was encountered"); 2122 return (Age) this.age; 2123 } 2124 2125 public boolean hasAgeAge() { 2126 return this.age instanceof Age; 2127 } 2128 2129 /** 2130 * @return {@link #age} (The age of the relative at the time the family member history is recorded.) 2131 */ 2132 public Range getAgeRange() throws FHIRException { 2133 if (this.age == null) 2134 this.age = new Range(); 2135 if (!(this.age instanceof Range)) 2136 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.age.getClass().getName()+" was encountered"); 2137 return (Range) this.age; 2138 } 2139 2140 public boolean hasAgeRange() { 2141 return this.age instanceof Range; 2142 } 2143 2144 /** 2145 * @return {@link #age} (The age of the relative at the time the family member history is recorded.) 2146 */ 2147 public StringType getAgeStringType() throws FHIRException { 2148 if (this.age == null) 2149 this.age = new StringType(); 2150 if (!(this.age instanceof StringType)) 2151 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.age.getClass().getName()+" was encountered"); 2152 return (StringType) this.age; 2153 } 2154 2155 public boolean hasAgeStringType() { 2156 return this.age instanceof StringType; 2157 } 2158 2159 public boolean hasAge() { 2160 return this.age != null && !this.age.isEmpty(); 2161 } 2162 2163 /** 2164 * @param value {@link #age} (The age of the relative at the time the family member history is recorded.) 2165 */ 2166 public FamilyMemberHistory setAge(DataType value) { 2167 if (value != null && !(value instanceof Age || value instanceof Range || value instanceof StringType)) 2168 throw new FHIRException("Not the right type for FamilyMemberHistory.age[x]: "+value.fhirType()); 2169 this.age = value; 2170 return this; 2171 } 2172 2173 /** 2174 * @return {@link #estimatedAge} (If true, indicates that the age value specified is an estimated value.). This is the underlying object with id, value and extensions. The accessor "getEstimatedAge" gives direct access to the value 2175 */ 2176 public BooleanType getEstimatedAgeElement() { 2177 if (this.estimatedAge == null) 2178 if (Configuration.errorOnAutoCreate()) 2179 throw new Error("Attempt to auto-create FamilyMemberHistory.estimatedAge"); 2180 else if (Configuration.doAutoCreate()) 2181 this.estimatedAge = new BooleanType(); // bb 2182 return this.estimatedAge; 2183 } 2184 2185 public boolean hasEstimatedAgeElement() { 2186 return this.estimatedAge != null && !this.estimatedAge.isEmpty(); 2187 } 2188 2189 public boolean hasEstimatedAge() { 2190 return this.estimatedAge != null && !this.estimatedAge.isEmpty(); 2191 } 2192 2193 /** 2194 * @param value {@link #estimatedAge} (If true, indicates that the age value specified is an estimated value.). This is the underlying object with id, value and extensions. The accessor "getEstimatedAge" gives direct access to the value 2195 */ 2196 public FamilyMemberHistory setEstimatedAgeElement(BooleanType value) { 2197 this.estimatedAge = value; 2198 return this; 2199 } 2200 2201 /** 2202 * @return If true, indicates that the age value specified is an estimated value. 2203 */ 2204 public boolean getEstimatedAge() { 2205 return this.estimatedAge == null || this.estimatedAge.isEmpty() ? false : this.estimatedAge.getValue(); 2206 } 2207 2208 /** 2209 * @param value If true, indicates that the age value specified is an estimated value. 2210 */ 2211 public FamilyMemberHistory setEstimatedAge(boolean value) { 2212 if (this.estimatedAge == null) 2213 this.estimatedAge = new BooleanType(); 2214 this.estimatedAge.setValue(value); 2215 return this; 2216 } 2217 2218 /** 2219 * @return {@link #deceased} (Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.) 2220 */ 2221 public DataType getDeceased() { 2222 return this.deceased; 2223 } 2224 2225 /** 2226 * @return {@link #deceased} (Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.) 2227 */ 2228 public BooleanType getDeceasedBooleanType() throws FHIRException { 2229 if (this.deceased == null) 2230 this.deceased = new BooleanType(); 2231 if (!(this.deceased instanceof BooleanType)) 2232 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.deceased.getClass().getName()+" was encountered"); 2233 return (BooleanType) this.deceased; 2234 } 2235 2236 public boolean hasDeceasedBooleanType() { 2237 return this.deceased instanceof BooleanType; 2238 } 2239 2240 /** 2241 * @return {@link #deceased} (Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.) 2242 */ 2243 public Age getDeceasedAge() throws FHIRException { 2244 if (this.deceased == null) 2245 this.deceased = new Age(); 2246 if (!(this.deceased instanceof Age)) 2247 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.deceased.getClass().getName()+" was encountered"); 2248 return (Age) this.deceased; 2249 } 2250 2251 public boolean hasDeceasedAge() { 2252 return this.deceased instanceof Age; 2253 } 2254 2255 /** 2256 * @return {@link #deceased} (Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.) 2257 */ 2258 public Range getDeceasedRange() throws FHIRException { 2259 if (this.deceased == null) 2260 this.deceased = new Range(); 2261 if (!(this.deceased instanceof Range)) 2262 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.deceased.getClass().getName()+" was encountered"); 2263 return (Range) this.deceased; 2264 } 2265 2266 public boolean hasDeceasedRange() { 2267 return this.deceased instanceof Range; 2268 } 2269 2270 /** 2271 * @return {@link #deceased} (Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.) 2272 */ 2273 public DateType getDeceasedDateType() throws FHIRException { 2274 if (this.deceased == null) 2275 this.deceased = new DateType(); 2276 if (!(this.deceased instanceof DateType)) 2277 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.deceased.getClass().getName()+" was encountered"); 2278 return (DateType) this.deceased; 2279 } 2280 2281 public boolean hasDeceasedDateType() { 2282 return this.deceased instanceof DateType; 2283 } 2284 2285 /** 2286 * @return {@link #deceased} (Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.) 2287 */ 2288 public StringType getDeceasedStringType() throws FHIRException { 2289 if (this.deceased == null) 2290 this.deceased = new StringType(); 2291 if (!(this.deceased instanceof StringType)) 2292 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.deceased.getClass().getName()+" was encountered"); 2293 return (StringType) this.deceased; 2294 } 2295 2296 public boolean hasDeceasedStringType() { 2297 return this.deceased instanceof StringType; 2298 } 2299 2300 public boolean hasDeceased() { 2301 return this.deceased != null && !this.deceased.isEmpty(); 2302 } 2303 2304 /** 2305 * @param value {@link #deceased} (Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.) 2306 */ 2307 public FamilyMemberHistory setDeceased(DataType value) { 2308 if (value != null && !(value instanceof BooleanType || value instanceof Age || value instanceof Range || value instanceof DateType || value instanceof StringType)) 2309 throw new FHIRException("Not the right type for FamilyMemberHistory.deceased[x]: "+value.fhirType()); 2310 this.deceased = value; 2311 return this; 2312 } 2313 2314 /** 2315 * @return {@link #reason} (Describes why the family member history occurred in coded or textual form, or Indicates a Condition, Observation, AllergyIntolerance, or QuestionnaireResponse that justifies this family member history event.) 2316 */ 2317 public List<CodeableReference> getReason() { 2318 if (this.reason == null) 2319 this.reason = new ArrayList<CodeableReference>(); 2320 return this.reason; 2321 } 2322 2323 /** 2324 * @return Returns a reference to <code>this</code> for easy method chaining 2325 */ 2326 public FamilyMemberHistory setReason(List<CodeableReference> theReason) { 2327 this.reason = theReason; 2328 return this; 2329 } 2330 2331 public boolean hasReason() { 2332 if (this.reason == null) 2333 return false; 2334 for (CodeableReference item : this.reason) 2335 if (!item.isEmpty()) 2336 return true; 2337 return false; 2338 } 2339 2340 public CodeableReference addReason() { //3 2341 CodeableReference t = new CodeableReference(); 2342 if (this.reason == null) 2343 this.reason = new ArrayList<CodeableReference>(); 2344 this.reason.add(t); 2345 return t; 2346 } 2347 2348 public FamilyMemberHistory addReason(CodeableReference t) { //3 2349 if (t == null) 2350 return this; 2351 if (this.reason == null) 2352 this.reason = new ArrayList<CodeableReference>(); 2353 this.reason.add(t); 2354 return this; 2355 } 2356 2357 /** 2358 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 2359 */ 2360 public CodeableReference getReasonFirstRep() { 2361 if (getReason().isEmpty()) { 2362 addReason(); 2363 } 2364 return getReason().get(0); 2365 } 2366 2367 /** 2368 * @return {@link #note} (This property allows a non condition-specific note to the made about the related person. Ideally, the note would be in the condition property, but this is not always possible.) 2369 */ 2370 public List<Annotation> getNote() { 2371 if (this.note == null) 2372 this.note = new ArrayList<Annotation>(); 2373 return this.note; 2374 } 2375 2376 /** 2377 * @return Returns a reference to <code>this</code> for easy method chaining 2378 */ 2379 public FamilyMemberHistory setNote(List<Annotation> theNote) { 2380 this.note = theNote; 2381 return this; 2382 } 2383 2384 public boolean hasNote() { 2385 if (this.note == null) 2386 return false; 2387 for (Annotation item : this.note) 2388 if (!item.isEmpty()) 2389 return true; 2390 return false; 2391 } 2392 2393 public Annotation addNote() { //3 2394 Annotation t = new Annotation(); 2395 if (this.note == null) 2396 this.note = new ArrayList<Annotation>(); 2397 this.note.add(t); 2398 return t; 2399 } 2400 2401 public FamilyMemberHistory addNote(Annotation t) { //3 2402 if (t == null) 2403 return this; 2404 if (this.note == null) 2405 this.note = new ArrayList<Annotation>(); 2406 this.note.add(t); 2407 return this; 2408 } 2409 2410 /** 2411 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2412 */ 2413 public Annotation getNoteFirstRep() { 2414 if (getNote().isEmpty()) { 2415 addNote(); 2416 } 2417 return getNote().get(0); 2418 } 2419 2420 /** 2421 * @return {@link #condition} (The significant Conditions (or condition) that the family member had. This is a repeating section to allow a system to represent more than one condition per resource, though there is nothing stopping multiple resources - one per condition.) 2422 */ 2423 public List<FamilyMemberHistoryConditionComponent> getCondition() { 2424 if (this.condition == null) 2425 this.condition = new ArrayList<FamilyMemberHistoryConditionComponent>(); 2426 return this.condition; 2427 } 2428 2429 /** 2430 * @return Returns a reference to <code>this</code> for easy method chaining 2431 */ 2432 public FamilyMemberHistory setCondition(List<FamilyMemberHistoryConditionComponent> theCondition) { 2433 this.condition = theCondition; 2434 return this; 2435 } 2436 2437 public boolean hasCondition() { 2438 if (this.condition == null) 2439 return false; 2440 for (FamilyMemberHistoryConditionComponent item : this.condition) 2441 if (!item.isEmpty()) 2442 return true; 2443 return false; 2444 } 2445 2446 public FamilyMemberHistoryConditionComponent addCondition() { //3 2447 FamilyMemberHistoryConditionComponent t = new FamilyMemberHistoryConditionComponent(); 2448 if (this.condition == null) 2449 this.condition = new ArrayList<FamilyMemberHistoryConditionComponent>(); 2450 this.condition.add(t); 2451 return t; 2452 } 2453 2454 public FamilyMemberHistory addCondition(FamilyMemberHistoryConditionComponent t) { //3 2455 if (t == null) 2456 return this; 2457 if (this.condition == null) 2458 this.condition = new ArrayList<FamilyMemberHistoryConditionComponent>(); 2459 this.condition.add(t); 2460 return this; 2461 } 2462 2463 /** 2464 * @return The first repetition of repeating field {@link #condition}, creating it if it does not already exist {3} 2465 */ 2466 public FamilyMemberHistoryConditionComponent getConditionFirstRep() { 2467 if (getCondition().isEmpty()) { 2468 addCondition(); 2469 } 2470 return getCondition().get(0); 2471 } 2472 2473 /** 2474 * @return {@link #procedure} (The significant Procedures (or procedure) that the family member had. This is a repeating section to allow a system to represent more than one procedure per resource, though there is nothing stopping multiple resources - one per procedure.) 2475 */ 2476 public List<FamilyMemberHistoryProcedureComponent> getProcedure() { 2477 if (this.procedure == null) 2478 this.procedure = new ArrayList<FamilyMemberHistoryProcedureComponent>(); 2479 return this.procedure; 2480 } 2481 2482 /** 2483 * @return Returns a reference to <code>this</code> for easy method chaining 2484 */ 2485 public FamilyMemberHistory setProcedure(List<FamilyMemberHistoryProcedureComponent> theProcedure) { 2486 this.procedure = theProcedure; 2487 return this; 2488 } 2489 2490 public boolean hasProcedure() { 2491 if (this.procedure == null) 2492 return false; 2493 for (FamilyMemberHistoryProcedureComponent item : this.procedure) 2494 if (!item.isEmpty()) 2495 return true; 2496 return false; 2497 } 2498 2499 public FamilyMemberHistoryProcedureComponent addProcedure() { //3 2500 FamilyMemberHistoryProcedureComponent t = new FamilyMemberHistoryProcedureComponent(); 2501 if (this.procedure == null) 2502 this.procedure = new ArrayList<FamilyMemberHistoryProcedureComponent>(); 2503 this.procedure.add(t); 2504 return t; 2505 } 2506 2507 public FamilyMemberHistory addProcedure(FamilyMemberHistoryProcedureComponent t) { //3 2508 if (t == null) 2509 return this; 2510 if (this.procedure == null) 2511 this.procedure = new ArrayList<FamilyMemberHistoryProcedureComponent>(); 2512 this.procedure.add(t); 2513 return this; 2514 } 2515 2516 /** 2517 * @return The first repetition of repeating field {@link #procedure}, creating it if it does not already exist {3} 2518 */ 2519 public FamilyMemberHistoryProcedureComponent getProcedureFirstRep() { 2520 if (getProcedure().isEmpty()) { 2521 addProcedure(); 2522 } 2523 return getProcedure().get(0); 2524 } 2525 2526 protected void listChildren(List<Property> children) { 2527 super.listChildren(children); 2528 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this family member history by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2529 children.add(new Property("instantiatesCanonical", "canonical(PlanDefinition|Questionnaire|ActivityDefinition|Measure|OperationDefinition)", "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical)); 2530 children.add(new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri)); 2531 children.add(new Property("status", "code", "A code specifying the status of the record of the family history of a specific family member.", 0, 1, status)); 2532 children.add(new Property("dataAbsentReason", "CodeableConcept", "Describes why the family member's history is not available.", 0, 1, dataAbsentReason)); 2533 children.add(new Property("patient", "Reference(Patient)", "The person who this history concerns.", 0, 1, patient)); 2534 children.add(new Property("date", "dateTime", "The date (and possibly time) when the family member history was recorded or last updated.", 0, 1, date)); 2535 children.add(new Property("participant", "", "Indicates who or what participated in the activities related to the family member history and how they were involved.", 0, java.lang.Integer.MAX_VALUE, participant)); 2536 children.add(new Property("name", "string", "This will either be a name or a description; e.g. \"Aunt Susan\", \"my cousin with the red hair\".", 0, 1, name)); 2537 children.add(new Property("relationship", "CodeableConcept", "The type of relationship this person has to the patient (father, mother, brother etc.).", 0, 1, relationship)); 2538 children.add(new Property("sex", "CodeableConcept", "The birth sex of the family member.", 0, 1, sex)); 2539 children.add(new Property("born[x]", "Period|date|string", "The actual or approximate date of birth of the relative.", 0, 1, born)); 2540 children.add(new Property("age[x]", "Age|Range|string", "The age of the relative at the time the family member history is recorded.", 0, 1, age)); 2541 children.add(new Property("estimatedAge", "boolean", "If true, indicates that the age value specified is an estimated value.", 0, 1, estimatedAge)); 2542 children.add(new Property("deceased[x]", "boolean|Age|Range|date|string", "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 0, 1, deceased)); 2543 children.add(new Property("reason", "CodeableReference(Condition|Observation|AllergyIntolerance|QuestionnaireResponse|DiagnosticReport|DocumentReference)", "Describes why the family member history occurred in coded or textual form, or Indicates a Condition, Observation, AllergyIntolerance, or QuestionnaireResponse that justifies this family member history event.", 0, java.lang.Integer.MAX_VALUE, reason)); 2544 children.add(new Property("note", "Annotation", "This property allows a non condition-specific note to the made about the related person. Ideally, the note would be in the condition property, but this is not always possible.", 0, java.lang.Integer.MAX_VALUE, note)); 2545 children.add(new Property("condition", "", "The significant Conditions (or condition) that the family member had. This is a repeating section to allow a system to represent more than one condition per resource, though there is nothing stopping multiple resources - one per condition.", 0, java.lang.Integer.MAX_VALUE, condition)); 2546 children.add(new Property("procedure", "", "The significant Procedures (or procedure) that the family member had. This is a repeating section to allow a system to represent more than one procedure per resource, though there is nothing stopping multiple resources - one per procedure.", 0, java.lang.Integer.MAX_VALUE, procedure)); 2547 } 2548 2549 @Override 2550 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2551 switch (_hash) { 2552 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this family member history by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 2553 case 8911915: /*instantiatesCanonical*/ return new Property("instantiatesCanonical", "canonical(PlanDefinition|Questionnaire|ActivityDefinition|Measure|OperationDefinition)", "The URL pointing to a FHIR-defined protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.", 0, java.lang.Integer.MAX_VALUE, instantiatesCanonical); 2554 case -1926393373: /*instantiatesUri*/ return new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol, guideline, orderset or other definition that is adhered to in whole or in part by this FamilyMemberHistory.", 0, java.lang.Integer.MAX_VALUE, instantiatesUri); 2555 case -892481550: /*status*/ return new Property("status", "code", "A code specifying the status of the record of the family history of a specific family member.", 0, 1, status); 2556 case 1034315687: /*dataAbsentReason*/ return new Property("dataAbsentReason", "CodeableConcept", "Describes why the family member's history is not available.", 0, 1, dataAbsentReason); 2557 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The person who this history concerns.", 0, 1, patient); 2558 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and possibly time) when the family member history was recorded or last updated.", 0, 1, date); 2559 case 767422259: /*participant*/ return new Property("participant", "", "Indicates who or what participated in the activities related to the family member history and how they were involved.", 0, java.lang.Integer.MAX_VALUE, participant); 2560 case 3373707: /*name*/ return new Property("name", "string", "This will either be a name or a description; e.g. \"Aunt Susan\", \"my cousin with the red hair\".", 0, 1, name); 2561 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "The type of relationship this person has to the patient (father, mother, brother etc.).", 0, 1, relationship); 2562 case 113766: /*sex*/ return new Property("sex", "CodeableConcept", "The birth sex of the family member.", 0, 1, sex); 2563 case 67532951: /*born[x]*/ return new Property("born[x]", "Period|date|string", "The actual or approximate date of birth of the relative.", 0, 1, born); 2564 case 3029833: /*born*/ return new Property("born[x]", "Period|date|string", "The actual or approximate date of birth of the relative.", 0, 1, born); 2565 case 1497711210: /*bornPeriod*/ return new Property("born[x]", "Period", "The actual or approximate date of birth of the relative.", 0, 1, born); 2566 case 2092814999: /*bornDate*/ return new Property("born[x]", "date", "The actual or approximate date of birth of the relative.", 0, 1, born); 2567 case 1597451450: /*bornString*/ return new Property("born[x]", "string", "The actual or approximate date of birth of the relative.", 0, 1, born); 2568 case -1419716831: /*age[x]*/ return new Property("age[x]", "Age|Range|string", "The age of the relative at the time the family member history is recorded.", 0, 1, age); 2569 case 96511: /*age*/ return new Property("age[x]", "Age|Range|string", "The age of the relative at the time the family member history is recorded.", 0, 1, age); 2570 case -1419742336: /*ageAge*/ return new Property("age[x]", "Age", "The age of the relative at the time the family member history is recorded.", 0, 1, age); 2571 case 1442748286: /*ageRange*/ return new Property("age[x]", "Range", "The age of the relative at the time the family member history is recorded.", 0, 1, age); 2572 case 1821821424: /*ageString*/ return new Property("age[x]", "string", "The age of the relative at the time the family member history is recorded.", 0, 1, age); 2573 case 2130167587: /*estimatedAge*/ return new Property("estimatedAge", "boolean", "If true, indicates that the age value specified is an estimated value.", 0, 1, estimatedAge); 2574 case -1311442804: /*deceased[x]*/ return new Property("deceased[x]", "boolean|Age|Range|date|string", "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 0, 1, deceased); 2575 case 561497972: /*deceased*/ return new Property("deceased[x]", "boolean|Age|Range|date|string", "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 0, 1, deceased); 2576 case 497463828: /*deceasedBoolean*/ return new Property("deceased[x]", "boolean", "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 0, 1, deceased); 2577 case -1311468309: /*deceasedAge*/ return new Property("deceased[x]", "Age", "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 0, 1, deceased); 2578 case -1880094167: /*deceasedRange*/ return new Property("deceased[x]", "Range", "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 0, 1, deceased); 2579 case -2000727742: /*deceasedDate*/ return new Property("deceased[x]", "date", "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 0, 1, deceased); 2580 case 1892920485: /*deceasedString*/ return new Property("deceased[x]", "string", "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 0, 1, deceased); 2581 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation|AllergyIntolerance|QuestionnaireResponse|DiagnosticReport|DocumentReference)", "Describes why the family member history occurred in coded or textual form, or Indicates a Condition, Observation, AllergyIntolerance, or QuestionnaireResponse that justifies this family member history event.", 0, java.lang.Integer.MAX_VALUE, reason); 2582 case 3387378: /*note*/ return new Property("note", "Annotation", "This property allows a non condition-specific note to the made about the related person. Ideally, the note would be in the condition property, but this is not always possible.", 0, java.lang.Integer.MAX_VALUE, note); 2583 case -861311717: /*condition*/ return new Property("condition", "", "The significant Conditions (or condition) that the family member had. This is a repeating section to allow a system to represent more than one condition per resource, though there is nothing stopping multiple resources - one per condition.", 0, java.lang.Integer.MAX_VALUE, condition); 2584 case -1095204141: /*procedure*/ return new Property("procedure", "", "The significant Procedures (or procedure) that the family member had. This is a repeating section to allow a system to represent more than one procedure per resource, though there is nothing stopping multiple resources - one per procedure.", 0, java.lang.Integer.MAX_VALUE, procedure); 2585 default: return super.getNamedProperty(_hash, _name, _checkValid); 2586 } 2587 2588 } 2589 2590 @Override 2591 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2592 switch (hash) { 2593 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2594 case 8911915: /*instantiatesCanonical*/ return this.instantiatesCanonical == null ? new Base[0] : this.instantiatesCanonical.toArray(new Base[this.instantiatesCanonical.size()]); // CanonicalType 2595 case -1926393373: /*instantiatesUri*/ return this.instantiatesUri == null ? new Base[0] : this.instantiatesUri.toArray(new Base[this.instantiatesUri.size()]); // UriType 2596 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FamilyHistoryStatus> 2597 case 1034315687: /*dataAbsentReason*/ return this.dataAbsentReason == null ? new Base[0] : new Base[] {this.dataAbsentReason}; // CodeableConcept 2598 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 2599 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2600 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // FamilyMemberHistoryParticipantComponent 2601 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2602 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // CodeableConcept 2603 case 113766: /*sex*/ return this.sex == null ? new Base[0] : new Base[] {this.sex}; // CodeableConcept 2604 case 3029833: /*born*/ return this.born == null ? new Base[0] : new Base[] {this.born}; // DataType 2605 case 96511: /*age*/ return this.age == null ? new Base[0] : new Base[] {this.age}; // DataType 2606 case 2130167587: /*estimatedAge*/ return this.estimatedAge == null ? new Base[0] : new Base[] {this.estimatedAge}; // BooleanType 2607 case 561497972: /*deceased*/ return this.deceased == null ? new Base[0] : new Base[] {this.deceased}; // DataType 2608 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 2609 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2610 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : this.condition.toArray(new Base[this.condition.size()]); // FamilyMemberHistoryConditionComponent 2611 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : this.procedure.toArray(new Base[this.procedure.size()]); // FamilyMemberHistoryProcedureComponent 2612 default: return super.getProperty(hash, name, checkValid); 2613 } 2614 2615 } 2616 2617 @Override 2618 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2619 switch (hash) { 2620 case -1618432855: // identifier 2621 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2622 return value; 2623 case 8911915: // instantiatesCanonical 2624 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); // CanonicalType 2625 return value; 2626 case -1926393373: // instantiatesUri 2627 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); // UriType 2628 return value; 2629 case -892481550: // status 2630 value = new FamilyHistoryStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2631 this.status = (Enumeration) value; // Enumeration<FamilyHistoryStatus> 2632 return value; 2633 case 1034315687: // dataAbsentReason 2634 this.dataAbsentReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2635 return value; 2636 case -791418107: // patient 2637 this.patient = TypeConvertor.castToReference(value); // Reference 2638 return value; 2639 case 3076014: // date 2640 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2641 return value; 2642 case 767422259: // participant 2643 this.getParticipant().add((FamilyMemberHistoryParticipantComponent) value); // FamilyMemberHistoryParticipantComponent 2644 return value; 2645 case 3373707: // name 2646 this.name = TypeConvertor.castToString(value); // StringType 2647 return value; 2648 case -261851592: // relationship 2649 this.relationship = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2650 return value; 2651 case 113766: // sex 2652 this.sex = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2653 return value; 2654 case 3029833: // born 2655 this.born = TypeConvertor.castToType(value); // DataType 2656 return value; 2657 case 96511: // age 2658 this.age = TypeConvertor.castToType(value); // DataType 2659 return value; 2660 case 2130167587: // estimatedAge 2661 this.estimatedAge = TypeConvertor.castToBoolean(value); // BooleanType 2662 return value; 2663 case 561497972: // deceased 2664 this.deceased = TypeConvertor.castToType(value); // DataType 2665 return value; 2666 case -934964668: // reason 2667 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2668 return value; 2669 case 3387378: // note 2670 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2671 return value; 2672 case -861311717: // condition 2673 this.getCondition().add((FamilyMemberHistoryConditionComponent) value); // FamilyMemberHistoryConditionComponent 2674 return value; 2675 case -1095204141: // procedure 2676 this.getProcedure().add((FamilyMemberHistoryProcedureComponent) value); // FamilyMemberHistoryProcedureComponent 2677 return value; 2678 default: return super.setProperty(hash, name, value); 2679 } 2680 2681 } 2682 2683 @Override 2684 public Base setProperty(String name, Base value) throws FHIRException { 2685 if (name.equals("identifier")) { 2686 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2687 } else if (name.equals("instantiatesCanonical")) { 2688 this.getInstantiatesCanonical().add(TypeConvertor.castToCanonical(value)); 2689 } else if (name.equals("instantiatesUri")) { 2690 this.getInstantiatesUri().add(TypeConvertor.castToUri(value)); 2691 } else if (name.equals("status")) { 2692 value = new FamilyHistoryStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2693 this.status = (Enumeration) value; // Enumeration<FamilyHistoryStatus> 2694 } else if (name.equals("dataAbsentReason")) { 2695 this.dataAbsentReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2696 } else if (name.equals("patient")) { 2697 this.patient = TypeConvertor.castToReference(value); // Reference 2698 } else if (name.equals("date")) { 2699 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2700 } else if (name.equals("participant")) { 2701 this.getParticipant().add((FamilyMemberHistoryParticipantComponent) value); 2702 } else if (name.equals("name")) { 2703 this.name = TypeConvertor.castToString(value); // StringType 2704 } else if (name.equals("relationship")) { 2705 this.relationship = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2706 } else if (name.equals("sex")) { 2707 this.sex = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2708 } else if (name.equals("born[x]")) { 2709 this.born = TypeConvertor.castToType(value); // DataType 2710 } else if (name.equals("age[x]")) { 2711 this.age = TypeConvertor.castToType(value); // DataType 2712 } else if (name.equals("estimatedAge")) { 2713 this.estimatedAge = TypeConvertor.castToBoolean(value); // BooleanType 2714 } else if (name.equals("deceased[x]")) { 2715 this.deceased = TypeConvertor.castToType(value); // DataType 2716 } else if (name.equals("reason")) { 2717 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 2718 } else if (name.equals("note")) { 2719 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2720 } else if (name.equals("condition")) { 2721 this.getCondition().add((FamilyMemberHistoryConditionComponent) value); 2722 } else if (name.equals("procedure")) { 2723 this.getProcedure().add((FamilyMemberHistoryProcedureComponent) value); 2724 } else 2725 return super.setProperty(name, value); 2726 return value; 2727 } 2728 2729 @Override 2730 public void removeChild(String name, Base value) throws FHIRException { 2731 if (name.equals("identifier")) { 2732 this.getIdentifier().remove(value); 2733 } else if (name.equals("instantiatesCanonical")) { 2734 this.getInstantiatesCanonical().remove(value); 2735 } else if (name.equals("instantiatesUri")) { 2736 this.getInstantiatesUri().remove(value); 2737 } else if (name.equals("status")) { 2738 value = new FamilyHistoryStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2739 this.status = (Enumeration) value; // Enumeration<FamilyHistoryStatus> 2740 } else if (name.equals("dataAbsentReason")) { 2741 this.dataAbsentReason = null; 2742 } else if (name.equals("patient")) { 2743 this.patient = null; 2744 } else if (name.equals("date")) { 2745 this.date = null; 2746 } else if (name.equals("participant")) { 2747 this.getParticipant().remove((FamilyMemberHistoryParticipantComponent) value); 2748 } else if (name.equals("name")) { 2749 this.name = null; 2750 } else if (name.equals("relationship")) { 2751 this.relationship = null; 2752 } else if (name.equals("sex")) { 2753 this.sex = null; 2754 } else if (name.equals("born[x]")) { 2755 this.born = null; 2756 } else if (name.equals("age[x]")) { 2757 this.age = null; 2758 } else if (name.equals("estimatedAge")) { 2759 this.estimatedAge = null; 2760 } else if (name.equals("deceased[x]")) { 2761 this.deceased = null; 2762 } else if (name.equals("reason")) { 2763 this.getReason().remove(value); 2764 } else if (name.equals("note")) { 2765 this.getNote().remove(value); 2766 } else if (name.equals("condition")) { 2767 this.getCondition().remove((FamilyMemberHistoryConditionComponent) value); 2768 } else if (name.equals("procedure")) { 2769 this.getProcedure().remove((FamilyMemberHistoryProcedureComponent) value); 2770 } else 2771 super.removeChild(name, value); 2772 2773 } 2774 2775 @Override 2776 public Base makeProperty(int hash, String name) throws FHIRException { 2777 switch (hash) { 2778 case -1618432855: return addIdentifier(); 2779 case 8911915: return addInstantiatesCanonicalElement(); 2780 case -1926393373: return addInstantiatesUriElement(); 2781 case -892481550: return getStatusElement(); 2782 case 1034315687: return getDataAbsentReason(); 2783 case -791418107: return getPatient(); 2784 case 3076014: return getDateElement(); 2785 case 767422259: return addParticipant(); 2786 case 3373707: return getNameElement(); 2787 case -261851592: return getRelationship(); 2788 case 113766: return getSex(); 2789 case 67532951: return getBorn(); 2790 case 3029833: return getBorn(); 2791 case -1419716831: return getAge(); 2792 case 96511: return getAge(); 2793 case 2130167587: return getEstimatedAgeElement(); 2794 case -1311442804: return getDeceased(); 2795 case 561497972: return getDeceased(); 2796 case -934964668: return addReason(); 2797 case 3387378: return addNote(); 2798 case -861311717: return addCondition(); 2799 case -1095204141: return addProcedure(); 2800 default: return super.makeProperty(hash, name); 2801 } 2802 2803 } 2804 2805 @Override 2806 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2807 switch (hash) { 2808 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2809 case 8911915: /*instantiatesCanonical*/ return new String[] {"canonical"}; 2810 case -1926393373: /*instantiatesUri*/ return new String[] {"uri"}; 2811 case -892481550: /*status*/ return new String[] {"code"}; 2812 case 1034315687: /*dataAbsentReason*/ return new String[] {"CodeableConcept"}; 2813 case -791418107: /*patient*/ return new String[] {"Reference"}; 2814 case 3076014: /*date*/ return new String[] {"dateTime"}; 2815 case 767422259: /*participant*/ return new String[] {}; 2816 case 3373707: /*name*/ return new String[] {"string"}; 2817 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 2818 case 113766: /*sex*/ return new String[] {"CodeableConcept"}; 2819 case 3029833: /*born*/ return new String[] {"Period", "date", "string"}; 2820 case 96511: /*age*/ return new String[] {"Age", "Range", "string"}; 2821 case 2130167587: /*estimatedAge*/ return new String[] {"boolean"}; 2822 case 561497972: /*deceased*/ return new String[] {"boolean", "Age", "Range", "date", "string"}; 2823 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 2824 case 3387378: /*note*/ return new String[] {"Annotation"}; 2825 case -861311717: /*condition*/ return new String[] {}; 2826 case -1095204141: /*procedure*/ return new String[] {}; 2827 default: return super.getTypesForProperty(hash, name); 2828 } 2829 2830 } 2831 2832 @Override 2833 public Base addChild(String name) throws FHIRException { 2834 if (name.equals("identifier")) { 2835 return addIdentifier(); 2836 } 2837 else if (name.equals("instantiatesCanonical")) { 2838 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.instantiatesCanonical"); 2839 } 2840 else if (name.equals("instantiatesUri")) { 2841 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.instantiatesUri"); 2842 } 2843 else if (name.equals("status")) { 2844 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.status"); 2845 } 2846 else if (name.equals("dataAbsentReason")) { 2847 this.dataAbsentReason = new CodeableConcept(); 2848 return this.dataAbsentReason; 2849 } 2850 else if (name.equals("patient")) { 2851 this.patient = new Reference(); 2852 return this.patient; 2853 } 2854 else if (name.equals("date")) { 2855 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.date"); 2856 } 2857 else if (name.equals("participant")) { 2858 return addParticipant(); 2859 } 2860 else if (name.equals("name")) { 2861 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.name"); 2862 } 2863 else if (name.equals("relationship")) { 2864 this.relationship = new CodeableConcept(); 2865 return this.relationship; 2866 } 2867 else if (name.equals("sex")) { 2868 this.sex = new CodeableConcept(); 2869 return this.sex; 2870 } 2871 else if (name.equals("bornPeriod")) { 2872 this.born = new Period(); 2873 return this.born; 2874 } 2875 else if (name.equals("bornDate")) { 2876 this.born = new DateType(); 2877 return this.born; 2878 } 2879 else if (name.equals("bornString")) { 2880 this.born = new StringType(); 2881 return this.born; 2882 } 2883 else if (name.equals("ageAge")) { 2884 this.age = new Age(); 2885 return this.age; 2886 } 2887 else if (name.equals("ageRange")) { 2888 this.age = new Range(); 2889 return this.age; 2890 } 2891 else if (name.equals("ageString")) { 2892 this.age = new StringType(); 2893 return this.age; 2894 } 2895 else if (name.equals("estimatedAge")) { 2896 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.estimatedAge"); 2897 } 2898 else if (name.equals("deceasedBoolean")) { 2899 this.deceased = new BooleanType(); 2900 return this.deceased; 2901 } 2902 else if (name.equals("deceasedAge")) { 2903 this.deceased = new Age(); 2904 return this.deceased; 2905 } 2906 else if (name.equals("deceasedRange")) { 2907 this.deceased = new Range(); 2908 return this.deceased; 2909 } 2910 else if (name.equals("deceasedDate")) { 2911 this.deceased = new DateType(); 2912 return this.deceased; 2913 } 2914 else if (name.equals("deceasedString")) { 2915 this.deceased = new StringType(); 2916 return this.deceased; 2917 } 2918 else if (name.equals("reason")) { 2919 return addReason(); 2920 } 2921 else if (name.equals("note")) { 2922 return addNote(); 2923 } 2924 else if (name.equals("condition")) { 2925 return addCondition(); 2926 } 2927 else if (name.equals("procedure")) { 2928 return addProcedure(); 2929 } 2930 else 2931 return super.addChild(name); 2932 } 2933 2934 public String fhirType() { 2935 return "FamilyMemberHistory"; 2936 2937 } 2938 2939 public FamilyMemberHistory copy() { 2940 FamilyMemberHistory dst = new FamilyMemberHistory(); 2941 copyValues(dst); 2942 return dst; 2943 } 2944 2945 public void copyValues(FamilyMemberHistory dst) { 2946 super.copyValues(dst); 2947 if (identifier != null) { 2948 dst.identifier = new ArrayList<Identifier>(); 2949 for (Identifier i : identifier) 2950 dst.identifier.add(i.copy()); 2951 }; 2952 if (instantiatesCanonical != null) { 2953 dst.instantiatesCanonical = new ArrayList<CanonicalType>(); 2954 for (CanonicalType i : instantiatesCanonical) 2955 dst.instantiatesCanonical.add(i.copy()); 2956 }; 2957 if (instantiatesUri != null) { 2958 dst.instantiatesUri = new ArrayList<UriType>(); 2959 for (UriType i : instantiatesUri) 2960 dst.instantiatesUri.add(i.copy()); 2961 }; 2962 dst.status = status == null ? null : status.copy(); 2963 dst.dataAbsentReason = dataAbsentReason == null ? null : dataAbsentReason.copy(); 2964 dst.patient = patient == null ? null : patient.copy(); 2965 dst.date = date == null ? null : date.copy(); 2966 if (participant != null) { 2967 dst.participant = new ArrayList<FamilyMemberHistoryParticipantComponent>(); 2968 for (FamilyMemberHistoryParticipantComponent i : participant) 2969 dst.participant.add(i.copy()); 2970 }; 2971 dst.name = name == null ? null : name.copy(); 2972 dst.relationship = relationship == null ? null : relationship.copy(); 2973 dst.sex = sex == null ? null : sex.copy(); 2974 dst.born = born == null ? null : born.copy(); 2975 dst.age = age == null ? null : age.copy(); 2976 dst.estimatedAge = estimatedAge == null ? null : estimatedAge.copy(); 2977 dst.deceased = deceased == null ? null : deceased.copy(); 2978 if (reason != null) { 2979 dst.reason = new ArrayList<CodeableReference>(); 2980 for (CodeableReference i : reason) 2981 dst.reason.add(i.copy()); 2982 }; 2983 if (note != null) { 2984 dst.note = new ArrayList<Annotation>(); 2985 for (Annotation i : note) 2986 dst.note.add(i.copy()); 2987 }; 2988 if (condition != null) { 2989 dst.condition = new ArrayList<FamilyMemberHistoryConditionComponent>(); 2990 for (FamilyMemberHistoryConditionComponent i : condition) 2991 dst.condition.add(i.copy()); 2992 }; 2993 if (procedure != null) { 2994 dst.procedure = new ArrayList<FamilyMemberHistoryProcedureComponent>(); 2995 for (FamilyMemberHistoryProcedureComponent i : procedure) 2996 dst.procedure.add(i.copy()); 2997 }; 2998 } 2999 3000 protected FamilyMemberHistory typedCopy() { 3001 return copy(); 3002 } 3003 3004 @Override 3005 public boolean equalsDeep(Base other_) { 3006 if (!super.equalsDeep(other_)) 3007 return false; 3008 if (!(other_ instanceof FamilyMemberHistory)) 3009 return false; 3010 FamilyMemberHistory o = (FamilyMemberHistory) other_; 3011 return compareDeep(identifier, o.identifier, true) && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 3012 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(status, o.status, true) 3013 && compareDeep(dataAbsentReason, o.dataAbsentReason, true) && compareDeep(patient, o.patient, true) 3014 && compareDeep(date, o.date, true) && compareDeep(participant, o.participant, true) && compareDeep(name, o.name, true) 3015 && compareDeep(relationship, o.relationship, true) && compareDeep(sex, o.sex, true) && compareDeep(born, o.born, true) 3016 && compareDeep(age, o.age, true) && compareDeep(estimatedAge, o.estimatedAge, true) && compareDeep(deceased, o.deceased, true) 3017 && compareDeep(reason, o.reason, true) && compareDeep(note, o.note, true) && compareDeep(condition, o.condition, true) 3018 && compareDeep(procedure, o.procedure, true); 3019 } 3020 3021 @Override 3022 public boolean equalsShallow(Base other_) { 3023 if (!super.equalsShallow(other_)) 3024 return false; 3025 if (!(other_ instanceof FamilyMemberHistory)) 3026 return false; 3027 FamilyMemberHistory o = (FamilyMemberHistory) other_; 3028 return compareValues(instantiatesCanonical, o.instantiatesCanonical, true) && compareValues(instantiatesUri, o.instantiatesUri, true) 3029 && compareValues(status, o.status, true) && compareValues(date, o.date, true) && compareValues(name, o.name, true) 3030 && compareValues(estimatedAge, o.estimatedAge, true); 3031 } 3032 3033 public boolean isEmpty() { 3034 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, instantiatesCanonical 3035 , instantiatesUri, status, dataAbsentReason, patient, date, participant, name 3036 , relationship, sex, born, age, estimatedAge, deceased, reason, note, condition 3037 , procedure); 3038 } 3039 3040 @Override 3041 public ResourceType getResourceType() { 3042 return ResourceType.FamilyMemberHistory; 3043 } 3044 3045 /** 3046 * Search parameter: <b>instantiates-canonical</b> 3047 * <p> 3048 * Description: <b>Instantiates FHIR protocol or definition</b><br> 3049 * Type: <b>reference</b><br> 3050 * Path: <b>FamilyMemberHistory.instantiatesCanonical</b><br> 3051 * </p> 3052 */ 3053 @SearchParamDefinition(name="instantiates-canonical", path="FamilyMemberHistory.instantiatesCanonical", description="Instantiates FHIR protocol or definition", type="reference", target={ActivityDefinition.class, Measure.class, OperationDefinition.class, PlanDefinition.class, Questionnaire.class } ) 3054 public static final String SP_INSTANTIATES_CANONICAL = "instantiates-canonical"; 3055 /** 3056 * <b>Fluent Client</b> search parameter constant for <b>instantiates-canonical</b> 3057 * <p> 3058 * Description: <b>Instantiates FHIR protocol or definition</b><br> 3059 * Type: <b>reference</b><br> 3060 * Path: <b>FamilyMemberHistory.instantiatesCanonical</b><br> 3061 * </p> 3062 */ 3063 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSTANTIATES_CANONICAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSTANTIATES_CANONICAL); 3064 3065/** 3066 * Constant for fluent queries to be used to add include statements. Specifies 3067 * the path value of "<b>FamilyMemberHistory:instantiates-canonical</b>". 3068 */ 3069 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSTANTIATES_CANONICAL = new ca.uhn.fhir.model.api.Include("FamilyMemberHistory:instantiates-canonical").toLocked(); 3070 3071 /** 3072 * Search parameter: <b>instantiates-uri</b> 3073 * <p> 3074 * Description: <b>Instantiates external protocol or definition</b><br> 3075 * Type: <b>uri</b><br> 3076 * Path: <b>FamilyMemberHistory.instantiatesUri</b><br> 3077 * </p> 3078 */ 3079 @SearchParamDefinition(name="instantiates-uri", path="FamilyMemberHistory.instantiatesUri", description="Instantiates external protocol or definition", type="uri" ) 3080 public static final String SP_INSTANTIATES_URI = "instantiates-uri"; 3081 /** 3082 * <b>Fluent Client</b> search parameter constant for <b>instantiates-uri</b> 3083 * <p> 3084 * Description: <b>Instantiates external protocol or definition</b><br> 3085 * Type: <b>uri</b><br> 3086 * Path: <b>FamilyMemberHistory.instantiatesUri</b><br> 3087 * </p> 3088 */ 3089 public static final ca.uhn.fhir.rest.gclient.UriClientParam INSTANTIATES_URI = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_INSTANTIATES_URI); 3090 3091 /** 3092 * Search parameter: <b>relationship</b> 3093 * <p> 3094 * Description: <b>A search by a relationship type</b><br> 3095 * Type: <b>token</b><br> 3096 * Path: <b>FamilyMemberHistory.relationship</b><br> 3097 * </p> 3098 */ 3099 @SearchParamDefinition(name="relationship", path="FamilyMemberHistory.relationship", description="A search by a relationship type", type="token" ) 3100 public static final String SP_RELATIONSHIP = "relationship"; 3101 /** 3102 * <b>Fluent Client</b> search parameter constant for <b>relationship</b> 3103 * <p> 3104 * Description: <b>A search by a relationship type</b><br> 3105 * Type: <b>token</b><br> 3106 * Path: <b>FamilyMemberHistory.relationship</b><br> 3107 * </p> 3108 */ 3109 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATIONSHIP = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RELATIONSHIP); 3110 3111 /** 3112 * Search parameter: <b>sex</b> 3113 * <p> 3114 * Description: <b>A search by a sex code of a family member</b><br> 3115 * Type: <b>token</b><br> 3116 * Path: <b>FamilyMemberHistory.sex</b><br> 3117 * </p> 3118 */ 3119 @SearchParamDefinition(name="sex", path="FamilyMemberHistory.sex", description="A search by a sex code of a family member", type="token" ) 3120 public static final String SP_SEX = "sex"; 3121 /** 3122 * <b>Fluent Client</b> search parameter constant for <b>sex</b> 3123 * <p> 3124 * Description: <b>A search by a sex code of a family member</b><br> 3125 * Type: <b>token</b><br> 3126 * Path: <b>FamilyMemberHistory.sex</b><br> 3127 * </p> 3128 */ 3129 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SEX = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SEX); 3130 3131 /** 3132 * Search parameter: <b>status</b> 3133 * <p> 3134 * Description: <b>partial | completed | entered-in-error | health-unknown</b><br> 3135 * Type: <b>token</b><br> 3136 * Path: <b>FamilyMemberHistory.status</b><br> 3137 * </p> 3138 */ 3139 @SearchParamDefinition(name="status", path="FamilyMemberHistory.status", description="partial | completed | entered-in-error | health-unknown", type="token" ) 3140 public static final String SP_STATUS = "status"; 3141 /** 3142 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3143 * <p> 3144 * Description: <b>partial | completed | entered-in-error | health-unknown</b><br> 3145 * Type: <b>token</b><br> 3146 * Path: <b>FamilyMemberHistory.status</b><br> 3147 * </p> 3148 */ 3149 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3150 3151 /** 3152 * Search parameter: <b>code</b> 3153 * <p> 3154 * Description: <b>Multiple Resources: 3155 3156* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 3157* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 3158* [AuditEvent](auditevent.html): More specific code for the event 3159* [Basic](basic.html): Kind of Resource 3160* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 3161* [Condition](condition.html): Code for the condition 3162* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 3163* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 3164* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 3165* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 3166* [ImagingSelection](imagingselection.html): The imaging selection status 3167* [List](list.html): What the purpose of this list is 3168* [Medication](medication.html): Returns medications for a specific code 3169* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 3170* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 3171* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 3172* [MedicationStatement](medicationstatement.html): Return statements of this medication code 3173* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 3174* [Observation](observation.html): The code of the observation type 3175* [Procedure](procedure.html): A code to identify a procedure 3176* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 3177* [Task](task.html): Search by task code 3178</b><br> 3179 * Type: <b>token</b><br> 3180 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 3181 * </p> 3182 */ 3183 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 3184 public static final String SP_CODE = "code"; 3185 /** 3186 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3187 * <p> 3188 * Description: <b>Multiple Resources: 3189 3190* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 3191* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 3192* [AuditEvent](auditevent.html): More specific code for the event 3193* [Basic](basic.html): Kind of Resource 3194* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 3195* [Condition](condition.html): Code for the condition 3196* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 3197* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 3198* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 3199* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 3200* [ImagingSelection](imagingselection.html): The imaging selection status 3201* [List](list.html): What the purpose of this list is 3202* [Medication](medication.html): Returns medications for a specific code 3203* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 3204* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 3205* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 3206* [MedicationStatement](medicationstatement.html): Return statements of this medication code 3207* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 3208* [Observation](observation.html): The code of the observation type 3209* [Procedure](procedure.html): A code to identify a procedure 3210* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 3211* [Task](task.html): Search by task code 3212</b><br> 3213 * Type: <b>token</b><br> 3214 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 3215 * </p> 3216 */ 3217 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 3218 3219 /** 3220 * Search parameter: <b>date</b> 3221 * <p> 3222 * Description: <b>Multiple Resources: 3223 3224* [AdverseEvent](adverseevent.html): When the event occurred 3225* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 3226* [Appointment](appointment.html): Appointment date/time. 3227* [AuditEvent](auditevent.html): Time when the event was recorded 3228* [CarePlan](careplan.html): Time period plan covers 3229* [CareTeam](careteam.html): A date within the coverage time period. 3230* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 3231* [Composition](composition.html): Composition editing time 3232* [Consent](consent.html): When consent was agreed to 3233* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 3234* [DocumentReference](documentreference.html): When this document reference was created 3235* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 3236* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 3237* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 3238* [Flag](flag.html): Time period when flag is active 3239* [Immunization](immunization.html): Vaccination (non)-Administration Date 3240* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 3241* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 3242* [Invoice](invoice.html): Invoice date / posting date 3243* [List](list.html): When the list was prepared 3244* [MeasureReport](measurereport.html): The date of the measure report 3245* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 3246* [Observation](observation.html): Clinically relevant time/time-period for observation 3247* [Procedure](procedure.html): When the procedure occurred or is occurring 3248* [ResearchSubject](researchsubject.html): Start and end of participation 3249* [RiskAssessment](riskassessment.html): When was assessment made? 3250* [SupplyRequest](supplyrequest.html): When the request was made 3251</b><br> 3252 * Type: <b>date</b><br> 3253 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 3254 * </p> 3255 */ 3256 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 3257 public static final String SP_DATE = "date"; 3258 /** 3259 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3260 * <p> 3261 * Description: <b>Multiple Resources: 3262 3263* [AdverseEvent](adverseevent.html): When the event occurred 3264* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 3265* [Appointment](appointment.html): Appointment date/time. 3266* [AuditEvent](auditevent.html): Time when the event was recorded 3267* [CarePlan](careplan.html): Time period plan covers 3268* [CareTeam](careteam.html): A date within the coverage time period. 3269* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 3270* [Composition](composition.html): Composition editing time 3271* [Consent](consent.html): When consent was agreed to 3272* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 3273* [DocumentReference](documentreference.html): When this document reference was created 3274* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 3275* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 3276* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 3277* [Flag](flag.html): Time period when flag is active 3278* [Immunization](immunization.html): Vaccination (non)-Administration Date 3279* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 3280* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 3281* [Invoice](invoice.html): Invoice date / posting date 3282* [List](list.html): When the list was prepared 3283* [MeasureReport](measurereport.html): The date of the measure report 3284* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 3285* [Observation](observation.html): Clinically relevant time/time-period for observation 3286* [Procedure](procedure.html): When the procedure occurred or is occurring 3287* [ResearchSubject](researchsubject.html): Start and end of participation 3288* [RiskAssessment](riskassessment.html): When was assessment made? 3289* [SupplyRequest](supplyrequest.html): When the request was made 3290</b><br> 3291 * Type: <b>date</b><br> 3292 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 3293 * </p> 3294 */ 3295 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3296 3297 /** 3298 * Search parameter: <b>identifier</b> 3299 * <p> 3300 * Description: <b>Multiple Resources: 3301 3302* [Account](account.html): Account number 3303* [AdverseEvent](adverseevent.html): Business identifier for the event 3304* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3305* [Appointment](appointment.html): An Identifier of the Appointment 3306* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3307* [Basic](basic.html): Business identifier 3308* [BodyStructure](bodystructure.html): Bodystructure identifier 3309* [CarePlan](careplan.html): External Ids for this plan 3310* [CareTeam](careteam.html): External Ids for this team 3311* [ChargeItem](chargeitem.html): Business Identifier for item 3312* [Claim](claim.html): The primary identifier of the financial resource 3313* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3314* [ClinicalImpression](clinicalimpression.html): Business identifier 3315* [Communication](communication.html): Unique identifier 3316* [CommunicationRequest](communicationrequest.html): Unique identifier 3317* [Composition](composition.html): Version-independent identifier for the Composition 3318* [Condition](condition.html): A unique identifier of the condition record 3319* [Consent](consent.html): Identifier for this record (external references) 3320* [Contract](contract.html): The identity of the contract 3321* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3322* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3323* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3324* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3325* [DeviceRequest](devicerequest.html): Business identifier for request/order 3326* [DeviceUsage](deviceusage.html): Search by identifier 3327* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3328* [DocumentReference](documentreference.html): Identifier of the attachment binary 3329* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3330* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3331* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3332* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3333* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3334* [Flag](flag.html): Business identifier 3335* [Goal](goal.html): External Ids for this goal 3336* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3337* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3338* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3339* [Immunization](immunization.html): Business identifier 3340* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3341* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3342* [Invoice](invoice.html): Business Identifier for item 3343* [List](list.html): Business identifier 3344* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3345* [Medication](medication.html): Returns medications with this external identifier 3346* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3347* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3348* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3349* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3350* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3351* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3352* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3353* [Observation](observation.html): The unique id for a particular observation 3354* [Person](person.html): A person Identifier 3355* [Procedure](procedure.html): A unique identifier for a procedure 3356* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3357* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3358* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3359* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3360* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3361* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3362* [Specimen](specimen.html): The unique identifier associated with the specimen 3363* [SupplyDelivery](supplydelivery.html): External identifier 3364* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3365* [Task](task.html): Search for a task instance by its business identifier 3366* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3367</b><br> 3368 * Type: <b>token</b><br> 3369 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3370 * </p> 3371 */ 3372 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 3373 public static final String SP_IDENTIFIER = "identifier"; 3374 /** 3375 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3376 * <p> 3377 * Description: <b>Multiple Resources: 3378 3379* [Account](account.html): Account number 3380* [AdverseEvent](adverseevent.html): Business identifier for the event 3381* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3382* [Appointment](appointment.html): An Identifier of the Appointment 3383* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3384* [Basic](basic.html): Business identifier 3385* [BodyStructure](bodystructure.html): Bodystructure identifier 3386* [CarePlan](careplan.html): External Ids for this plan 3387* [CareTeam](careteam.html): External Ids for this team 3388* [ChargeItem](chargeitem.html): Business Identifier for item 3389* [Claim](claim.html): The primary identifier of the financial resource 3390* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3391* [ClinicalImpression](clinicalimpression.html): Business identifier 3392* [Communication](communication.html): Unique identifier 3393* [CommunicationRequest](communicationrequest.html): Unique identifier 3394* [Composition](composition.html): Version-independent identifier for the Composition 3395* [Condition](condition.html): A unique identifier of the condition record 3396* [Consent](consent.html): Identifier for this record (external references) 3397* [Contract](contract.html): The identity of the contract 3398* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3399* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3400* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3401* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3402* [DeviceRequest](devicerequest.html): Business identifier for request/order 3403* [DeviceUsage](deviceusage.html): Search by identifier 3404* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3405* [DocumentReference](documentreference.html): Identifier of the attachment binary 3406* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3407* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3408* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3409* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3410* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3411* [Flag](flag.html): Business identifier 3412* [Goal](goal.html): External Ids for this goal 3413* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3414* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3415* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3416* [Immunization](immunization.html): Business identifier 3417* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3418* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3419* [Invoice](invoice.html): Business Identifier for item 3420* [List](list.html): Business identifier 3421* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3422* [Medication](medication.html): Returns medications with this external identifier 3423* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3424* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3425* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3426* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3427* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3428* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3429* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3430* [Observation](observation.html): The unique id for a particular observation 3431* [Person](person.html): A person Identifier 3432* [Procedure](procedure.html): A unique identifier for a procedure 3433* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3434* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3435* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3436* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3437* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3438* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3439* [Specimen](specimen.html): The unique identifier associated with the specimen 3440* [SupplyDelivery](supplydelivery.html): External identifier 3441* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3442* [Task](task.html): Search for a task instance by its business identifier 3443* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3444</b><br> 3445 * Type: <b>token</b><br> 3446 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3447 * </p> 3448 */ 3449 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3450 3451 /** 3452 * Search parameter: <b>patient</b> 3453 * <p> 3454 * Description: <b>Multiple Resources: 3455 3456* [Account](account.html): The entity that caused the expenses 3457* [AdverseEvent](adverseevent.html): Subject impacted by event 3458* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3459* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3460* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3461* [AuditEvent](auditevent.html): Where the activity involved patient data 3462* [Basic](basic.html): Identifies the focus of this resource 3463* [BodyStructure](bodystructure.html): Who this is about 3464* [CarePlan](careplan.html): Who the care plan is for 3465* [CareTeam](careteam.html): Who care team is for 3466* [ChargeItem](chargeitem.html): Individual service was done for/to 3467* [Claim](claim.html): Patient receiving the products or services 3468* [ClaimResponse](claimresponse.html): The subject of care 3469* [ClinicalImpression](clinicalimpression.html): Patient assessed 3470* [Communication](communication.html): Focus of message 3471* [CommunicationRequest](communicationrequest.html): Focus of message 3472* [Composition](composition.html): Who and/or what the composition is about 3473* [Condition](condition.html): Who has the condition? 3474* [Consent](consent.html): Who the consent applies to 3475* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3476* [Coverage](coverage.html): Retrieve coverages for a patient 3477* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3478* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3479* [DetectedIssue](detectedissue.html): Associated patient 3480* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3481* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3482* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3483* [DocumentReference](documentreference.html): Who/what is the subject of the document 3484* [Encounter](encounter.html): The patient present at the encounter 3485* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3486* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3487* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3488* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3489* [Flag](flag.html): The identity of a subject to list flags for 3490* [Goal](goal.html): Who this goal is intended for 3491* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3492* [ImagingSelection](imagingselection.html): Who the study is about 3493* [ImagingStudy](imagingstudy.html): Who the study is about 3494* [Immunization](immunization.html): The patient for the vaccination record 3495* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3496* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3497* [Invoice](invoice.html): Recipient(s) of goods and services 3498* [List](list.html): If all resources have the same subject 3499* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3500* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3501* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3502* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3503* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3504* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3505* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3506* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3507* [Observation](observation.html): The subject that the observation is about (if patient) 3508* [Person](person.html): The Person links to this Patient 3509* [Procedure](procedure.html): Search by subject - a patient 3510* [Provenance](provenance.html): Where the activity involved patient data 3511* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3512* [RelatedPerson](relatedperson.html): The patient this related person is related to 3513* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3514* [ResearchSubject](researchsubject.html): Who or what is part of study 3515* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3516* [ServiceRequest](servicerequest.html): Search by subject - a patient 3517* [Specimen](specimen.html): The patient the specimen comes from 3518* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3519* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3520* [Task](task.html): Search by patient 3521* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3522</b><br> 3523 * Type: <b>reference</b><br> 3524 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3525 * </p> 3526 */ 3527 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 3528 public static final String SP_PATIENT = "patient"; 3529 /** 3530 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3531 * <p> 3532 * Description: <b>Multiple Resources: 3533 3534* [Account](account.html): The entity that caused the expenses 3535* [AdverseEvent](adverseevent.html): Subject impacted by event 3536* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3537* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3538* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3539* [AuditEvent](auditevent.html): Where the activity involved patient data 3540* [Basic](basic.html): Identifies the focus of this resource 3541* [BodyStructure](bodystructure.html): Who this is about 3542* [CarePlan](careplan.html): Who the care plan is for 3543* [CareTeam](careteam.html): Who care team is for 3544* [ChargeItem](chargeitem.html): Individual service was done for/to 3545* [Claim](claim.html): Patient receiving the products or services 3546* [ClaimResponse](claimresponse.html): The subject of care 3547* [ClinicalImpression](clinicalimpression.html): Patient assessed 3548* [Communication](communication.html): Focus of message 3549* [CommunicationRequest](communicationrequest.html): Focus of message 3550* [Composition](composition.html): Who and/or what the composition is about 3551* [Condition](condition.html): Who has the condition? 3552* [Consent](consent.html): Who the consent applies to 3553* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3554* [Coverage](coverage.html): Retrieve coverages for a patient 3555* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3556* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3557* [DetectedIssue](detectedissue.html): Associated patient 3558* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3559* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3560* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3561* [DocumentReference](documentreference.html): Who/what is the subject of the document 3562* [Encounter](encounter.html): The patient present at the encounter 3563* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3564* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3565* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3566* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3567* [Flag](flag.html): The identity of a subject to list flags for 3568* [Goal](goal.html): Who this goal is intended for 3569* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3570* [ImagingSelection](imagingselection.html): Who the study is about 3571* [ImagingStudy](imagingstudy.html): Who the study is about 3572* [Immunization](immunization.html): The patient for the vaccination record 3573* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3574* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3575* [Invoice](invoice.html): Recipient(s) of goods and services 3576* [List](list.html): If all resources have the same subject 3577* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3578* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3579* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3580* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3581* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3582* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3583* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3584* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3585* [Observation](observation.html): The subject that the observation is about (if patient) 3586* [Person](person.html): The Person links to this Patient 3587* [Procedure](procedure.html): Search by subject - a patient 3588* [Provenance](provenance.html): Where the activity involved patient data 3589* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3590* [RelatedPerson](relatedperson.html): The patient this related person is related to 3591* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3592* [ResearchSubject](researchsubject.html): Who or what is part of study 3593* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3594* [ServiceRequest](servicerequest.html): Search by subject - a patient 3595* [Specimen](specimen.html): The patient the specimen comes from 3596* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3597* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3598* [Task](task.html): Search by patient 3599* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3600</b><br> 3601 * Type: <b>reference</b><br> 3602 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3603 * </p> 3604 */ 3605 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3606 3607/** 3608 * Constant for fluent queries to be used to add include statements. Specifies 3609 * the path value of "<b>FamilyMemberHistory:patient</b>". 3610 */ 3611 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("FamilyMemberHistory:patient").toLocked(); 3612 3613 3614} 3615