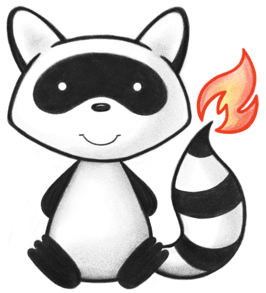
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Prospective warnings of potential issues when providing care to the patient. 052 */ 053@ResourceDef(name="Flag", profile="http://hl7.org/fhir/StructureDefinition/Flag") 054public class Flag extends DomainResource { 055 056 public enum FlagStatus { 057 /** 058 * A current flag that should be displayed to a user. A system may use the category to determine which user roles should view the flag. 059 */ 060 ACTIVE, 061 /** 062 * The flag no longer needs to be displayed. 063 */ 064 INACTIVE, 065 /** 066 * The flag was added in error and should no longer be displayed. 067 */ 068 ENTEREDINERROR, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static FlagStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("inactive".equals(codeString)) 079 return INACTIVE; 080 if ("entered-in-error".equals(codeString)) 081 return ENTEREDINERROR; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown FlagStatus code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case ACTIVE: return "active"; 090 case INACTIVE: return "inactive"; 091 case ENTEREDINERROR: return "entered-in-error"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case ACTIVE: return "http://hl7.org/fhir/flag-status"; 099 case INACTIVE: return "http://hl7.org/fhir/flag-status"; 100 case ENTEREDINERROR: return "http://hl7.org/fhir/flag-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case ACTIVE: return "A current flag that should be displayed to a user. A system may use the category to determine which user roles should view the flag."; 108 case INACTIVE: return "The flag no longer needs to be displayed."; 109 case ENTEREDINERROR: return "The flag was added in error and should no longer be displayed."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case ACTIVE: return "Active"; 117 case INACTIVE: return "Inactive"; 118 case ENTEREDINERROR: return "Entered in Error"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class FlagStatusEnumFactory implements EnumFactory<FlagStatus> { 126 public FlagStatus fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("active".equals(codeString)) 131 return FlagStatus.ACTIVE; 132 if ("inactive".equals(codeString)) 133 return FlagStatus.INACTIVE; 134 if ("entered-in-error".equals(codeString)) 135 return FlagStatus.ENTEREDINERROR; 136 throw new IllegalArgumentException("Unknown FlagStatus code '"+codeString+"'"); 137 } 138 public Enumeration<FlagStatus> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<FlagStatus>(this, FlagStatus.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<FlagStatus>(this, FlagStatus.NULL, code); 146 if ("active".equals(codeString)) 147 return new Enumeration<FlagStatus>(this, FlagStatus.ACTIVE, code); 148 if ("inactive".equals(codeString)) 149 return new Enumeration<FlagStatus>(this, FlagStatus.INACTIVE, code); 150 if ("entered-in-error".equals(codeString)) 151 return new Enumeration<FlagStatus>(this, FlagStatus.ENTEREDINERROR, code); 152 throw new FHIRException("Unknown FlagStatus code '"+codeString+"'"); 153 } 154 public String toCode(FlagStatus code) { 155 if (code == FlagStatus.NULL) 156 return null; 157 if (code == FlagStatus.ACTIVE) 158 return "active"; 159 if (code == FlagStatus.INACTIVE) 160 return "inactive"; 161 if (code == FlagStatus.ENTEREDINERROR) 162 return "entered-in-error"; 163 return "?"; 164 } 165 public String toSystem(FlagStatus code) { 166 return code.getSystem(); 167 } 168 } 169 170 /** 171 * Business identifiers assigned to this flag by the performer or other systems which remain constant as the resource is updated and propagates from server to server. 172 */ 173 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 174 @Description(shortDefinition="Business identifier", formalDefinition="Business identifiers assigned to this flag by the performer or other systems which remain constant as the resource is updated and propagates from server to server." ) 175 protected List<Identifier> identifier; 176 177 /** 178 * Supports basic workflow. 179 */ 180 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 181 @Description(shortDefinition="active | inactive | entered-in-error", formalDefinition="Supports basic workflow." ) 182 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/flag-status") 183 protected Enumeration<FlagStatus> status; 184 185 /** 186 * Allows a flag to be divided into different categories like clinical, administrative etc. Intended to be used as a means of filtering which flags are displayed to particular user or in a given context. 187 */ 188 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 189 @Description(shortDefinition="Clinical, administrative, etc", formalDefinition="Allows a flag to be divided into different categories like clinical, administrative etc. Intended to be used as a means of filtering which flags are displayed to particular user or in a given context." ) 190 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/flag-category") 191 protected List<CodeableConcept> category; 192 193 /** 194 * The coded value or textual component of the flag to display to the user. 195 */ 196 @Child(name = "code", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 197 @Description(shortDefinition="Coded or textual message to display to user", formalDefinition="The coded value or textual component of the flag to display to the user." ) 198 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/flag-code") 199 protected CodeableConcept code; 200 201 /** 202 * The patient, related person, location, group, organization, or practitioner etc. this is about record this flag is associated with. 203 */ 204 @Child(name = "subject", type = {Patient.class, RelatedPerson.class, Location.class, Group.class, Organization.class, Practitioner.class, PractitionerRole.class, PlanDefinition.class, Medication.class, Procedure.class}, order=4, min=1, max=1, modifier=false, summary=true) 205 @Description(shortDefinition="Who/What is flag about?", formalDefinition="The patient, related person, location, group, organization, or practitioner etc. this is about record this flag is associated with." ) 206 protected Reference subject; 207 208 /** 209 * The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified. 210 */ 211 @Child(name = "period", type = {Period.class}, order=5, min=0, max=1, modifier=false, summary=true) 212 @Description(shortDefinition="Time period when flag is active", formalDefinition="The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified." ) 213 protected Period period; 214 215 /** 216 * This alert is only relevant during the encounter. 217 */ 218 @Child(name = "encounter", type = {Encounter.class}, order=6, min=0, max=1, modifier=false, summary=true) 219 @Description(shortDefinition="Alert relevant during encounter", formalDefinition="This alert is only relevant during the encounter." ) 220 protected Reference encounter; 221 222 /** 223 * The person, organization or device that created the flag. 224 */ 225 @Child(name = "author", type = {Device.class, Organization.class, Patient.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class}, order=7, min=0, max=1, modifier=false, summary=true) 226 @Description(shortDefinition="Flag creator", formalDefinition="The person, organization or device that created the flag." ) 227 protected Reference author; 228 229 private static final long serialVersionUID = -901823137L; 230 231 /** 232 * Constructor 233 */ 234 public Flag() { 235 super(); 236 } 237 238 /** 239 * Constructor 240 */ 241 public Flag(FlagStatus status, CodeableConcept code, Reference subject) { 242 super(); 243 this.setStatus(status); 244 this.setCode(code); 245 this.setSubject(subject); 246 } 247 248 /** 249 * @return {@link #identifier} (Business identifiers assigned to this flag by the performer or other systems which remain constant as the resource is updated and propagates from server to server.) 250 */ 251 public List<Identifier> getIdentifier() { 252 if (this.identifier == null) 253 this.identifier = new ArrayList<Identifier>(); 254 return this.identifier; 255 } 256 257 /** 258 * @return Returns a reference to <code>this</code> for easy method chaining 259 */ 260 public Flag setIdentifier(List<Identifier> theIdentifier) { 261 this.identifier = theIdentifier; 262 return this; 263 } 264 265 public boolean hasIdentifier() { 266 if (this.identifier == null) 267 return false; 268 for (Identifier item : this.identifier) 269 if (!item.isEmpty()) 270 return true; 271 return false; 272 } 273 274 public Identifier addIdentifier() { //3 275 Identifier t = new Identifier(); 276 if (this.identifier == null) 277 this.identifier = new ArrayList<Identifier>(); 278 this.identifier.add(t); 279 return t; 280 } 281 282 public Flag addIdentifier(Identifier t) { //3 283 if (t == null) 284 return this; 285 if (this.identifier == null) 286 this.identifier = new ArrayList<Identifier>(); 287 this.identifier.add(t); 288 return this; 289 } 290 291 /** 292 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 293 */ 294 public Identifier getIdentifierFirstRep() { 295 if (getIdentifier().isEmpty()) { 296 addIdentifier(); 297 } 298 return getIdentifier().get(0); 299 } 300 301 /** 302 * @return {@link #status} (Supports basic workflow.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 303 */ 304 public Enumeration<FlagStatus> getStatusElement() { 305 if (this.status == null) 306 if (Configuration.errorOnAutoCreate()) 307 throw new Error("Attempt to auto-create Flag.status"); 308 else if (Configuration.doAutoCreate()) 309 this.status = new Enumeration<FlagStatus>(new FlagStatusEnumFactory()); // bb 310 return this.status; 311 } 312 313 public boolean hasStatusElement() { 314 return this.status != null && !this.status.isEmpty(); 315 } 316 317 public boolean hasStatus() { 318 return this.status != null && !this.status.isEmpty(); 319 } 320 321 /** 322 * @param value {@link #status} (Supports basic workflow.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 323 */ 324 public Flag setStatusElement(Enumeration<FlagStatus> value) { 325 this.status = value; 326 return this; 327 } 328 329 /** 330 * @return Supports basic workflow. 331 */ 332 public FlagStatus getStatus() { 333 return this.status == null ? null : this.status.getValue(); 334 } 335 336 /** 337 * @param value Supports basic workflow. 338 */ 339 public Flag setStatus(FlagStatus value) { 340 if (this.status == null) 341 this.status = new Enumeration<FlagStatus>(new FlagStatusEnumFactory()); 342 this.status.setValue(value); 343 return this; 344 } 345 346 /** 347 * @return {@link #category} (Allows a flag to be divided into different categories like clinical, administrative etc. Intended to be used as a means of filtering which flags are displayed to particular user or in a given context.) 348 */ 349 public List<CodeableConcept> getCategory() { 350 if (this.category == null) 351 this.category = new ArrayList<CodeableConcept>(); 352 return this.category; 353 } 354 355 /** 356 * @return Returns a reference to <code>this</code> for easy method chaining 357 */ 358 public Flag setCategory(List<CodeableConcept> theCategory) { 359 this.category = theCategory; 360 return this; 361 } 362 363 public boolean hasCategory() { 364 if (this.category == null) 365 return false; 366 for (CodeableConcept item : this.category) 367 if (!item.isEmpty()) 368 return true; 369 return false; 370 } 371 372 public CodeableConcept addCategory() { //3 373 CodeableConcept t = new CodeableConcept(); 374 if (this.category == null) 375 this.category = new ArrayList<CodeableConcept>(); 376 this.category.add(t); 377 return t; 378 } 379 380 public Flag addCategory(CodeableConcept t) { //3 381 if (t == null) 382 return this; 383 if (this.category == null) 384 this.category = new ArrayList<CodeableConcept>(); 385 this.category.add(t); 386 return this; 387 } 388 389 /** 390 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 391 */ 392 public CodeableConcept getCategoryFirstRep() { 393 if (getCategory().isEmpty()) { 394 addCategory(); 395 } 396 return getCategory().get(0); 397 } 398 399 /** 400 * @return {@link #code} (The coded value or textual component of the flag to display to the user.) 401 */ 402 public CodeableConcept getCode() { 403 if (this.code == null) 404 if (Configuration.errorOnAutoCreate()) 405 throw new Error("Attempt to auto-create Flag.code"); 406 else if (Configuration.doAutoCreate()) 407 this.code = new CodeableConcept(); // cc 408 return this.code; 409 } 410 411 public boolean hasCode() { 412 return this.code != null && !this.code.isEmpty(); 413 } 414 415 /** 416 * @param value {@link #code} (The coded value or textual component of the flag to display to the user.) 417 */ 418 public Flag setCode(CodeableConcept value) { 419 this.code = value; 420 return this; 421 } 422 423 /** 424 * @return {@link #subject} (The patient, related person, location, group, organization, or practitioner etc. this is about record this flag is associated with.) 425 */ 426 public Reference getSubject() { 427 if (this.subject == null) 428 if (Configuration.errorOnAutoCreate()) 429 throw new Error("Attempt to auto-create Flag.subject"); 430 else if (Configuration.doAutoCreate()) 431 this.subject = new Reference(); // cc 432 return this.subject; 433 } 434 435 public boolean hasSubject() { 436 return this.subject != null && !this.subject.isEmpty(); 437 } 438 439 /** 440 * @param value {@link #subject} (The patient, related person, location, group, organization, or practitioner etc. this is about record this flag is associated with.) 441 */ 442 public Flag setSubject(Reference value) { 443 this.subject = value; 444 return this; 445 } 446 447 /** 448 * @return {@link #period} (The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified.) 449 */ 450 public Period getPeriod() { 451 if (this.period == null) 452 if (Configuration.errorOnAutoCreate()) 453 throw new Error("Attempt to auto-create Flag.period"); 454 else if (Configuration.doAutoCreate()) 455 this.period = new Period(); // cc 456 return this.period; 457 } 458 459 public boolean hasPeriod() { 460 return this.period != null && !this.period.isEmpty(); 461 } 462 463 /** 464 * @param value {@link #period} (The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified.) 465 */ 466 public Flag setPeriod(Period value) { 467 this.period = value; 468 return this; 469 } 470 471 /** 472 * @return {@link #encounter} (This alert is only relevant during the encounter.) 473 */ 474 public Reference getEncounter() { 475 if (this.encounter == null) 476 if (Configuration.errorOnAutoCreate()) 477 throw new Error("Attempt to auto-create Flag.encounter"); 478 else if (Configuration.doAutoCreate()) 479 this.encounter = new Reference(); // cc 480 return this.encounter; 481 } 482 483 public boolean hasEncounter() { 484 return this.encounter != null && !this.encounter.isEmpty(); 485 } 486 487 /** 488 * @param value {@link #encounter} (This alert is only relevant during the encounter.) 489 */ 490 public Flag setEncounter(Reference value) { 491 this.encounter = value; 492 return this; 493 } 494 495 /** 496 * @return {@link #author} (The person, organization or device that created the flag.) 497 */ 498 public Reference getAuthor() { 499 if (this.author == null) 500 if (Configuration.errorOnAutoCreate()) 501 throw new Error("Attempt to auto-create Flag.author"); 502 else if (Configuration.doAutoCreate()) 503 this.author = new Reference(); // cc 504 return this.author; 505 } 506 507 public boolean hasAuthor() { 508 return this.author != null && !this.author.isEmpty(); 509 } 510 511 /** 512 * @param value {@link #author} (The person, organization or device that created the flag.) 513 */ 514 public Flag setAuthor(Reference value) { 515 this.author = value; 516 return this; 517 } 518 519 protected void listChildren(List<Property> children) { 520 super.listChildren(children); 521 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this flag by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 522 children.add(new Property("status", "code", "Supports basic workflow.", 0, 1, status)); 523 children.add(new Property("category", "CodeableConcept", "Allows a flag to be divided into different categories like clinical, administrative etc. Intended to be used as a means of filtering which flags are displayed to particular user or in a given context.", 0, java.lang.Integer.MAX_VALUE, category)); 524 children.add(new Property("code", "CodeableConcept", "The coded value or textual component of the flag to display to the user.", 0, 1, code)); 525 children.add(new Property("subject", "Reference(Patient|RelatedPerson|Location|Group|Organization|Practitioner|PractitionerRole|PlanDefinition|Medication|Procedure)", "The patient, related person, location, group, organization, or practitioner etc. this is about record this flag is associated with.", 0, 1, subject)); 526 children.add(new Property("period", "Period", "The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified.", 0, 1, period)); 527 children.add(new Property("encounter", "Reference(Encounter)", "This alert is only relevant during the encounter.", 0, 1, encounter)); 528 children.add(new Property("author", "Reference(Device|Organization|Patient|RelatedPerson|Practitioner|PractitionerRole)", "The person, organization or device that created the flag.", 0, 1, author)); 529 } 530 531 @Override 532 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 533 switch (_hash) { 534 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this flag by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 535 case -892481550: /*status*/ return new Property("status", "code", "Supports basic workflow.", 0, 1, status); 536 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Allows a flag to be divided into different categories like clinical, administrative etc. Intended to be used as a means of filtering which flags are displayed to particular user or in a given context.", 0, java.lang.Integer.MAX_VALUE, category); 537 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The coded value or textual component of the flag to display to the user.", 0, 1, code); 538 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|RelatedPerson|Location|Group|Organization|Practitioner|PractitionerRole|PlanDefinition|Medication|Procedure)", "The patient, related person, location, group, organization, or practitioner etc. this is about record this flag is associated with.", 0, 1, subject); 539 case -991726143: /*period*/ return new Property("period", "Period", "The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified.", 0, 1, period); 540 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "This alert is only relevant during the encounter.", 0, 1, encounter); 541 case -1406328437: /*author*/ return new Property("author", "Reference(Device|Organization|Patient|RelatedPerson|Practitioner|PractitionerRole)", "The person, organization or device that created the flag.", 0, 1, author); 542 default: return super.getNamedProperty(_hash, _name, _checkValid); 543 } 544 545 } 546 547 @Override 548 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 549 switch (hash) { 550 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 551 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FlagStatus> 552 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 553 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 554 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 555 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 556 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 557 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 558 default: return super.getProperty(hash, name, checkValid); 559 } 560 561 } 562 563 @Override 564 public Base setProperty(int hash, String name, Base value) throws FHIRException { 565 switch (hash) { 566 case -1618432855: // identifier 567 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 568 return value; 569 case -892481550: // status 570 value = new FlagStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 571 this.status = (Enumeration) value; // Enumeration<FlagStatus> 572 return value; 573 case 50511102: // category 574 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 575 return value; 576 case 3059181: // code 577 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 578 return value; 579 case -1867885268: // subject 580 this.subject = TypeConvertor.castToReference(value); // Reference 581 return value; 582 case -991726143: // period 583 this.period = TypeConvertor.castToPeriod(value); // Period 584 return value; 585 case 1524132147: // encounter 586 this.encounter = TypeConvertor.castToReference(value); // Reference 587 return value; 588 case -1406328437: // author 589 this.author = TypeConvertor.castToReference(value); // Reference 590 return value; 591 default: return super.setProperty(hash, name, value); 592 } 593 594 } 595 596 @Override 597 public Base setProperty(String name, Base value) throws FHIRException { 598 if (name.equals("identifier")) { 599 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 600 } else if (name.equals("status")) { 601 value = new FlagStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 602 this.status = (Enumeration) value; // Enumeration<FlagStatus> 603 } else if (name.equals("category")) { 604 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 605 } else if (name.equals("code")) { 606 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 607 } else if (name.equals("subject")) { 608 this.subject = TypeConvertor.castToReference(value); // Reference 609 } else if (name.equals("period")) { 610 this.period = TypeConvertor.castToPeriod(value); // Period 611 } else if (name.equals("encounter")) { 612 this.encounter = TypeConvertor.castToReference(value); // Reference 613 } else if (name.equals("author")) { 614 this.author = TypeConvertor.castToReference(value); // Reference 615 } else 616 return super.setProperty(name, value); 617 return value; 618 } 619 620 @Override 621 public void removeChild(String name, Base value) throws FHIRException { 622 if (name.equals("identifier")) { 623 this.getIdentifier().remove(value); 624 } else if (name.equals("status")) { 625 value = new FlagStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 626 this.status = (Enumeration) value; // Enumeration<FlagStatus> 627 } else if (name.equals("category")) { 628 this.getCategory().remove(value); 629 } else if (name.equals("code")) { 630 this.code = null; 631 } else if (name.equals("subject")) { 632 this.subject = null; 633 } else if (name.equals("period")) { 634 this.period = null; 635 } else if (name.equals("encounter")) { 636 this.encounter = null; 637 } else if (name.equals("author")) { 638 this.author = null; 639 } else 640 super.removeChild(name, value); 641 642 } 643 644 @Override 645 public Base makeProperty(int hash, String name) throws FHIRException { 646 switch (hash) { 647 case -1618432855: return addIdentifier(); 648 case -892481550: return getStatusElement(); 649 case 50511102: return addCategory(); 650 case 3059181: return getCode(); 651 case -1867885268: return getSubject(); 652 case -991726143: return getPeriod(); 653 case 1524132147: return getEncounter(); 654 case -1406328437: return getAuthor(); 655 default: return super.makeProperty(hash, name); 656 } 657 658 } 659 660 @Override 661 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 662 switch (hash) { 663 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 664 case -892481550: /*status*/ return new String[] {"code"}; 665 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 666 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 667 case -1867885268: /*subject*/ return new String[] {"Reference"}; 668 case -991726143: /*period*/ return new String[] {"Period"}; 669 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 670 case -1406328437: /*author*/ return new String[] {"Reference"}; 671 default: return super.getTypesForProperty(hash, name); 672 } 673 674 } 675 676 @Override 677 public Base addChild(String name) throws FHIRException { 678 if (name.equals("identifier")) { 679 return addIdentifier(); 680 } 681 else if (name.equals("status")) { 682 throw new FHIRException("Cannot call addChild on a singleton property Flag.status"); 683 } 684 else if (name.equals("category")) { 685 return addCategory(); 686 } 687 else if (name.equals("code")) { 688 this.code = new CodeableConcept(); 689 return this.code; 690 } 691 else if (name.equals("subject")) { 692 this.subject = new Reference(); 693 return this.subject; 694 } 695 else if (name.equals("period")) { 696 this.period = new Period(); 697 return this.period; 698 } 699 else if (name.equals("encounter")) { 700 this.encounter = new Reference(); 701 return this.encounter; 702 } 703 else if (name.equals("author")) { 704 this.author = new Reference(); 705 return this.author; 706 } 707 else 708 return super.addChild(name); 709 } 710 711 public String fhirType() { 712 return "Flag"; 713 714 } 715 716 public Flag copy() { 717 Flag dst = new Flag(); 718 copyValues(dst); 719 return dst; 720 } 721 722 public void copyValues(Flag dst) { 723 super.copyValues(dst); 724 if (identifier != null) { 725 dst.identifier = new ArrayList<Identifier>(); 726 for (Identifier i : identifier) 727 dst.identifier.add(i.copy()); 728 }; 729 dst.status = status == null ? null : status.copy(); 730 if (category != null) { 731 dst.category = new ArrayList<CodeableConcept>(); 732 for (CodeableConcept i : category) 733 dst.category.add(i.copy()); 734 }; 735 dst.code = code == null ? null : code.copy(); 736 dst.subject = subject == null ? null : subject.copy(); 737 dst.period = period == null ? null : period.copy(); 738 dst.encounter = encounter == null ? null : encounter.copy(); 739 dst.author = author == null ? null : author.copy(); 740 } 741 742 protected Flag typedCopy() { 743 return copy(); 744 } 745 746 @Override 747 public boolean equalsDeep(Base other_) { 748 if (!super.equalsDeep(other_)) 749 return false; 750 if (!(other_ instanceof Flag)) 751 return false; 752 Flag o = (Flag) other_; 753 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 754 && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) && compareDeep(period, o.period, true) 755 && compareDeep(encounter, o.encounter, true) && compareDeep(author, o.author, true); 756 } 757 758 @Override 759 public boolean equalsShallow(Base other_) { 760 if (!super.equalsShallow(other_)) 761 return false; 762 if (!(other_ instanceof Flag)) 763 return false; 764 Flag o = (Flag) other_; 765 return compareValues(status, o.status, true); 766 } 767 768 public boolean isEmpty() { 769 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 770 , code, subject, period, encounter, author); 771 } 772 773 @Override 774 public ResourceType getResourceType() { 775 return ResourceType.Flag; 776 } 777 778 /** 779 * Search parameter: <b>author</b> 780 * <p> 781 * Description: <b>Flag creator</b><br> 782 * Type: <b>reference</b><br> 783 * Path: <b>Flag.author</b><br> 784 * </p> 785 */ 786 @SearchParamDefinition(name="author", path="Flag.author", description="Flag creator", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 787 public static final String SP_AUTHOR = "author"; 788 /** 789 * <b>Fluent Client</b> search parameter constant for <b>author</b> 790 * <p> 791 * Description: <b>Flag creator</b><br> 792 * Type: <b>reference</b><br> 793 * Path: <b>Flag.author</b><br> 794 * </p> 795 */ 796 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 797 798/** 799 * Constant for fluent queries to be used to add include statements. Specifies 800 * the path value of "<b>Flag:author</b>". 801 */ 802 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("Flag:author").toLocked(); 803 804 /** 805 * Search parameter: <b>category</b> 806 * <p> 807 * Description: <b>The category of the flag, such as clinical, administrative, etc.</b><br> 808 * Type: <b>token</b><br> 809 * Path: <b>Flag.category</b><br> 810 * </p> 811 */ 812 @SearchParamDefinition(name="category", path="Flag.category", description="The category of the flag, such as clinical, administrative, etc.", type="token" ) 813 public static final String SP_CATEGORY = "category"; 814 /** 815 * <b>Fluent Client</b> search parameter constant for <b>category</b> 816 * <p> 817 * Description: <b>The category of the flag, such as clinical, administrative, etc.</b><br> 818 * Type: <b>token</b><br> 819 * Path: <b>Flag.category</b><br> 820 * </p> 821 */ 822 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 823 824 /** 825 * Search parameter: <b>status</b> 826 * <p> 827 * Description: <b>active | inactive | entered-in-error</b><br> 828 * Type: <b>token</b><br> 829 * Path: <b>Flag.status</b><br> 830 * </p> 831 */ 832 @SearchParamDefinition(name="status", path="Flag.status", description="active | inactive | entered-in-error", type="token" ) 833 public static final String SP_STATUS = "status"; 834 /** 835 * <b>Fluent Client</b> search parameter constant for <b>status</b> 836 * <p> 837 * Description: <b>active | inactive | entered-in-error</b><br> 838 * Type: <b>token</b><br> 839 * Path: <b>Flag.status</b><br> 840 * </p> 841 */ 842 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 843 844 /** 845 * Search parameter: <b>subject</b> 846 * <p> 847 * Description: <b>The identity of a subject to list flags for</b><br> 848 * Type: <b>reference</b><br> 849 * Path: <b>Flag.subject</b><br> 850 * </p> 851 */ 852 @SearchParamDefinition(name="subject", path="Flag.subject", description="The identity of a subject to list flags for", type="reference", target={Group.class, Location.class, Medication.class, Organization.class, Patient.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, RelatedPerson.class } ) 853 public static final String SP_SUBJECT = "subject"; 854 /** 855 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 856 * <p> 857 * Description: <b>The identity of a subject to list flags for</b><br> 858 * Type: <b>reference</b><br> 859 * Path: <b>Flag.subject</b><br> 860 * </p> 861 */ 862 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 863 864/** 865 * Constant for fluent queries to be used to add include statements. Specifies 866 * the path value of "<b>Flag:subject</b>". 867 */ 868 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Flag:subject").toLocked(); 869 870 /** 871 * Search parameter: <b>date</b> 872 * <p> 873 * Description: <b>Multiple Resources: 874 875* [AdverseEvent](adverseevent.html): When the event occurred 876* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 877* [Appointment](appointment.html): Appointment date/time. 878* [AuditEvent](auditevent.html): Time when the event was recorded 879* [CarePlan](careplan.html): Time period plan covers 880* [CareTeam](careteam.html): A date within the coverage time period. 881* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 882* [Composition](composition.html): Composition editing time 883* [Consent](consent.html): When consent was agreed to 884* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 885* [DocumentReference](documentreference.html): When this document reference was created 886* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 887* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 888* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 889* [Flag](flag.html): Time period when flag is active 890* [Immunization](immunization.html): Vaccination (non)-Administration Date 891* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 892* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 893* [Invoice](invoice.html): Invoice date / posting date 894* [List](list.html): When the list was prepared 895* [MeasureReport](measurereport.html): The date of the measure report 896* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 897* [Observation](observation.html): Clinically relevant time/time-period for observation 898* [Procedure](procedure.html): When the procedure occurred or is occurring 899* [ResearchSubject](researchsubject.html): Start and end of participation 900* [RiskAssessment](riskassessment.html): When was assessment made? 901* [SupplyRequest](supplyrequest.html): When the request was made 902</b><br> 903 * Type: <b>date</b><br> 904 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 905 * </p> 906 */ 907 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 908 public static final String SP_DATE = "date"; 909 /** 910 * <b>Fluent Client</b> search parameter constant for <b>date</b> 911 * <p> 912 * Description: <b>Multiple Resources: 913 914* [AdverseEvent](adverseevent.html): When the event occurred 915* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 916* [Appointment](appointment.html): Appointment date/time. 917* [AuditEvent](auditevent.html): Time when the event was recorded 918* [CarePlan](careplan.html): Time period plan covers 919* [CareTeam](careteam.html): A date within the coverage time period. 920* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 921* [Composition](composition.html): Composition editing time 922* [Consent](consent.html): When consent was agreed to 923* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 924* [DocumentReference](documentreference.html): When this document reference was created 925* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 926* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 927* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 928* [Flag](flag.html): Time period when flag is active 929* [Immunization](immunization.html): Vaccination (non)-Administration Date 930* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 931* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 932* [Invoice](invoice.html): Invoice date / posting date 933* [List](list.html): When the list was prepared 934* [MeasureReport](measurereport.html): The date of the measure report 935* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 936* [Observation](observation.html): Clinically relevant time/time-period for observation 937* [Procedure](procedure.html): When the procedure occurred or is occurring 938* [ResearchSubject](researchsubject.html): Start and end of participation 939* [RiskAssessment](riskassessment.html): When was assessment made? 940* [SupplyRequest](supplyrequest.html): When the request was made 941</b><br> 942 * Type: <b>date</b><br> 943 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 944 * </p> 945 */ 946 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 947 948 /** 949 * Search parameter: <b>encounter</b> 950 * <p> 951 * Description: <b>Multiple Resources: 952 953* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 954* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 955* [ChargeItem](chargeitem.html): Encounter associated with event 956* [Claim](claim.html): Encounters associated with a billed line item 957* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 958* [Communication](communication.html): The Encounter during which this Communication was created 959* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 960* [Composition](composition.html): Context of the Composition 961* [Condition](condition.html): The Encounter during which this Condition was created 962* [DeviceRequest](devicerequest.html): Encounter during which request was created 963* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 964* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 965* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 966* [Flag](flag.html): Alert relevant during encounter 967* [ImagingStudy](imagingstudy.html): The context of the study 968* [List](list.html): Context in which list created 969* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 970* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 971* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 972* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 973* [Observation](observation.html): Encounter related to the observation 974* [Procedure](procedure.html): The Encounter during which this Procedure was created 975* [Provenance](provenance.html): Encounter related to the Provenance 976* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 977* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 978* [RiskAssessment](riskassessment.html): Where was assessment performed? 979* [ServiceRequest](servicerequest.html): An encounter in which this request is made 980* [Task](task.html): Search by encounter 981* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 982</b><br> 983 * Type: <b>reference</b><br> 984 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 985 * </p> 986 */ 987 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", target={Encounter.class } ) 988 public static final String SP_ENCOUNTER = "encounter"; 989 /** 990 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 991 * <p> 992 * Description: <b>Multiple Resources: 993 994* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 995* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 996* [ChargeItem](chargeitem.html): Encounter associated with event 997* [Claim](claim.html): Encounters associated with a billed line item 998* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 999* [Communication](communication.html): The Encounter during which this Communication was created 1000* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 1001* [Composition](composition.html): Context of the Composition 1002* [Condition](condition.html): The Encounter during which this Condition was created 1003* [DeviceRequest](devicerequest.html): Encounter during which request was created 1004* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 1005* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 1006* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 1007* [Flag](flag.html): Alert relevant during encounter 1008* [ImagingStudy](imagingstudy.html): The context of the study 1009* [List](list.html): Context in which list created 1010* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 1011* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 1012* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 1013* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 1014* [Observation](observation.html): Encounter related to the observation 1015* [Procedure](procedure.html): The Encounter during which this Procedure was created 1016* [Provenance](provenance.html): Encounter related to the Provenance 1017* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 1018* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 1019* [RiskAssessment](riskassessment.html): Where was assessment performed? 1020* [ServiceRequest](servicerequest.html): An encounter in which this request is made 1021* [Task](task.html): Search by encounter 1022* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 1023</b><br> 1024 * Type: <b>reference</b><br> 1025 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 1026 * </p> 1027 */ 1028 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 1029 1030/** 1031 * Constant for fluent queries to be used to add include statements. Specifies 1032 * the path value of "<b>Flag:encounter</b>". 1033 */ 1034 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Flag:encounter").toLocked(); 1035 1036 /** 1037 * Search parameter: <b>identifier</b> 1038 * <p> 1039 * Description: <b>Multiple Resources: 1040 1041* [Account](account.html): Account number 1042* [AdverseEvent](adverseevent.html): Business identifier for the event 1043* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1044* [Appointment](appointment.html): An Identifier of the Appointment 1045* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1046* [Basic](basic.html): Business identifier 1047* [BodyStructure](bodystructure.html): Bodystructure identifier 1048* [CarePlan](careplan.html): External Ids for this plan 1049* [CareTeam](careteam.html): External Ids for this team 1050* [ChargeItem](chargeitem.html): Business Identifier for item 1051* [Claim](claim.html): The primary identifier of the financial resource 1052* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1053* [ClinicalImpression](clinicalimpression.html): Business identifier 1054* [Communication](communication.html): Unique identifier 1055* [CommunicationRequest](communicationrequest.html): Unique identifier 1056* [Composition](composition.html): Version-independent identifier for the Composition 1057* [Condition](condition.html): A unique identifier of the condition record 1058* [Consent](consent.html): Identifier for this record (external references) 1059* [Contract](contract.html): The identity of the contract 1060* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1061* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1062* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1063* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1064* [DeviceRequest](devicerequest.html): Business identifier for request/order 1065* [DeviceUsage](deviceusage.html): Search by identifier 1066* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1067* [DocumentReference](documentreference.html): Identifier of the attachment binary 1068* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1069* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1070* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1071* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1072* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1073* [Flag](flag.html): Business identifier 1074* [Goal](goal.html): External Ids for this goal 1075* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1076* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1077* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1078* [Immunization](immunization.html): Business identifier 1079* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1080* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1081* [Invoice](invoice.html): Business Identifier for item 1082* [List](list.html): Business identifier 1083* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1084* [Medication](medication.html): Returns medications with this external identifier 1085* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1086* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1087* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1088* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1089* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1090* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1091* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1092* [Observation](observation.html): The unique id for a particular observation 1093* [Person](person.html): A person Identifier 1094* [Procedure](procedure.html): A unique identifier for a procedure 1095* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1096* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1097* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1098* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1099* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1100* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1101* [Specimen](specimen.html): The unique identifier associated with the specimen 1102* [SupplyDelivery](supplydelivery.html): External identifier 1103* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1104* [Task](task.html): Search for a task instance by its business identifier 1105* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1106</b><br> 1107 * Type: <b>token</b><br> 1108 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1109 * </p> 1110 */ 1111 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 1112 public static final String SP_IDENTIFIER = "identifier"; 1113 /** 1114 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1115 * <p> 1116 * Description: <b>Multiple Resources: 1117 1118* [Account](account.html): Account number 1119* [AdverseEvent](adverseevent.html): Business identifier for the event 1120* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1121* [Appointment](appointment.html): An Identifier of the Appointment 1122* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1123* [Basic](basic.html): Business identifier 1124* [BodyStructure](bodystructure.html): Bodystructure identifier 1125* [CarePlan](careplan.html): External Ids for this plan 1126* [CareTeam](careteam.html): External Ids for this team 1127* [ChargeItem](chargeitem.html): Business Identifier for item 1128* [Claim](claim.html): The primary identifier of the financial resource 1129* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1130* [ClinicalImpression](clinicalimpression.html): Business identifier 1131* [Communication](communication.html): Unique identifier 1132* [CommunicationRequest](communicationrequest.html): Unique identifier 1133* [Composition](composition.html): Version-independent identifier for the Composition 1134* [Condition](condition.html): A unique identifier of the condition record 1135* [Consent](consent.html): Identifier for this record (external references) 1136* [Contract](contract.html): The identity of the contract 1137* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1138* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1139* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1140* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1141* [DeviceRequest](devicerequest.html): Business identifier for request/order 1142* [DeviceUsage](deviceusage.html): Search by identifier 1143* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1144* [DocumentReference](documentreference.html): Identifier of the attachment binary 1145* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1146* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1147* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1148* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1149* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1150* [Flag](flag.html): Business identifier 1151* [Goal](goal.html): External Ids for this goal 1152* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1153* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1154* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1155* [Immunization](immunization.html): Business identifier 1156* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1157* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1158* [Invoice](invoice.html): Business Identifier for item 1159* [List](list.html): Business identifier 1160* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1161* [Medication](medication.html): Returns medications with this external identifier 1162* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1163* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1164* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1165* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1166* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1167* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1168* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1169* [Observation](observation.html): The unique id for a particular observation 1170* [Person](person.html): A person Identifier 1171* [Procedure](procedure.html): A unique identifier for a procedure 1172* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1173* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1174* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1175* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1176* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1177* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1178* [Specimen](specimen.html): The unique identifier associated with the specimen 1179* [SupplyDelivery](supplydelivery.html): External identifier 1180* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1181* [Task](task.html): Search for a task instance by its business identifier 1182* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1183</b><br> 1184 * Type: <b>token</b><br> 1185 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1186 * </p> 1187 */ 1188 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1189 1190 /** 1191 * Search parameter: <b>patient</b> 1192 * <p> 1193 * Description: <b>Multiple Resources: 1194 1195* [Account](account.html): The entity that caused the expenses 1196* [AdverseEvent](adverseevent.html): Subject impacted by event 1197* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1198* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1199* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1200* [AuditEvent](auditevent.html): Where the activity involved patient data 1201* [Basic](basic.html): Identifies the focus of this resource 1202* [BodyStructure](bodystructure.html): Who this is about 1203* [CarePlan](careplan.html): Who the care plan is for 1204* [CareTeam](careteam.html): Who care team is for 1205* [ChargeItem](chargeitem.html): Individual service was done for/to 1206* [Claim](claim.html): Patient receiving the products or services 1207* [ClaimResponse](claimresponse.html): The subject of care 1208* [ClinicalImpression](clinicalimpression.html): Patient assessed 1209* [Communication](communication.html): Focus of message 1210* [CommunicationRequest](communicationrequest.html): Focus of message 1211* [Composition](composition.html): Who and/or what the composition is about 1212* [Condition](condition.html): Who has the condition? 1213* [Consent](consent.html): Who the consent applies to 1214* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1215* [Coverage](coverage.html): Retrieve coverages for a patient 1216* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1217* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1218* [DetectedIssue](detectedissue.html): Associated patient 1219* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1220* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1221* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1222* [DocumentReference](documentreference.html): Who/what is the subject of the document 1223* [Encounter](encounter.html): The patient present at the encounter 1224* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1225* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1226* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1227* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1228* [Flag](flag.html): The identity of a subject to list flags for 1229* [Goal](goal.html): Who this goal is intended for 1230* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1231* [ImagingSelection](imagingselection.html): Who the study is about 1232* [ImagingStudy](imagingstudy.html): Who the study is about 1233* [Immunization](immunization.html): The patient for the vaccination record 1234* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1235* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1236* [Invoice](invoice.html): Recipient(s) of goods and services 1237* [List](list.html): If all resources have the same subject 1238* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1239* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1240* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1241* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1242* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1243* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1244* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1245* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1246* [Observation](observation.html): The subject that the observation is about (if patient) 1247* [Person](person.html): The Person links to this Patient 1248* [Procedure](procedure.html): Search by subject - a patient 1249* [Provenance](provenance.html): Where the activity involved patient data 1250* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1251* [RelatedPerson](relatedperson.html): The patient this related person is related to 1252* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1253* [ResearchSubject](researchsubject.html): Who or what is part of study 1254* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1255* [ServiceRequest](servicerequest.html): Search by subject - a patient 1256* [Specimen](specimen.html): The patient the specimen comes from 1257* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1258* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1259* [Task](task.html): Search by patient 1260* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1261</b><br> 1262 * Type: <b>reference</b><br> 1263 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1264 * </p> 1265 */ 1266 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 1267 public static final String SP_PATIENT = "patient"; 1268 /** 1269 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1270 * <p> 1271 * Description: <b>Multiple Resources: 1272 1273* [Account](account.html): The entity that caused the expenses 1274* [AdverseEvent](adverseevent.html): Subject impacted by event 1275* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1276* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1277* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1278* [AuditEvent](auditevent.html): Where the activity involved patient data 1279* [Basic](basic.html): Identifies the focus of this resource 1280* [BodyStructure](bodystructure.html): Who this is about 1281* [CarePlan](careplan.html): Who the care plan is for 1282* [CareTeam](careteam.html): Who care team is for 1283* [ChargeItem](chargeitem.html): Individual service was done for/to 1284* [Claim](claim.html): Patient receiving the products or services 1285* [ClaimResponse](claimresponse.html): The subject of care 1286* [ClinicalImpression](clinicalimpression.html): Patient assessed 1287* [Communication](communication.html): Focus of message 1288* [CommunicationRequest](communicationrequest.html): Focus of message 1289* [Composition](composition.html): Who and/or what the composition is about 1290* [Condition](condition.html): Who has the condition? 1291* [Consent](consent.html): Who the consent applies to 1292* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1293* [Coverage](coverage.html): Retrieve coverages for a patient 1294* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1295* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1296* [DetectedIssue](detectedissue.html): Associated patient 1297* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1298* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1299* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1300* [DocumentReference](documentreference.html): Who/what is the subject of the document 1301* [Encounter](encounter.html): The patient present at the encounter 1302* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1303* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1304* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1305* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1306* [Flag](flag.html): The identity of a subject to list flags for 1307* [Goal](goal.html): Who this goal is intended for 1308* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1309* [ImagingSelection](imagingselection.html): Who the study is about 1310* [ImagingStudy](imagingstudy.html): Who the study is about 1311* [Immunization](immunization.html): The patient for the vaccination record 1312* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1313* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1314* [Invoice](invoice.html): Recipient(s) of goods and services 1315* [List](list.html): If all resources have the same subject 1316* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1317* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1318* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1319* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1320* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1321* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1322* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1323* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1324* [Observation](observation.html): The subject that the observation is about (if patient) 1325* [Person](person.html): The Person links to this Patient 1326* [Procedure](procedure.html): Search by subject - a patient 1327* [Provenance](provenance.html): Where the activity involved patient data 1328* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1329* [RelatedPerson](relatedperson.html): The patient this related person is related to 1330* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1331* [ResearchSubject](researchsubject.html): Who or what is part of study 1332* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1333* [ServiceRequest](servicerequest.html): Search by subject - a patient 1334* [Specimen](specimen.html): The patient the specimen comes from 1335* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1336* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1337* [Task](task.html): Search by patient 1338* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1339</b><br> 1340 * Type: <b>reference</b><br> 1341 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1342 * </p> 1343 */ 1344 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1345 1346/** 1347 * Constant for fluent queries to be used to add include statements. Specifies 1348 * the path value of "<b>Flag:patient</b>". 1349 */ 1350 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Flag:patient").toLocked(); 1351 1352 1353} 1354