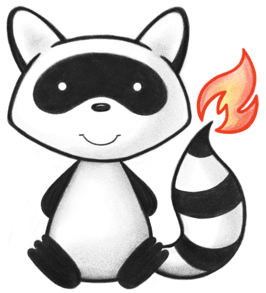
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * This resource describes a product or service that is available through a program and includes the conditions and constraints of availability. All of the information in this resource is specific to the inclusion of the item in the formulary and is not inherent to the item itself. 052 */ 053@ResourceDef(name="FormularyItem", profile="http://hl7.org/fhir/StructureDefinition/FormularyItem") 054public class FormularyItem extends DomainResource { 055 056 public enum FormularyItemStatusCodes { 057 /** 058 * The service or product referred to by this FormularyItem is in active use within the drug database or inventory system. 059 */ 060 ACTIVE, 061 /** 062 * The service or product referred to by this FormularyItem was entered in error within the drug database or inventory system. 063 */ 064 ENTEREDINERROR, 065 /** 066 * The service or product referred to by this FormularyItem is not in active use within the drug database or inventory system. 067 */ 068 INACTIVE, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static FormularyItemStatusCodes fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("entered-in-error".equals(codeString)) 079 return ENTEREDINERROR; 080 if ("inactive".equals(codeString)) 081 return INACTIVE; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown FormularyItemStatusCodes code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case ACTIVE: return "active"; 090 case ENTEREDINERROR: return "entered-in-error"; 091 case INACTIVE: return "inactive"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case ACTIVE: return "http://hl7.org/fhir/CodeSystem/formularyitem-status"; 099 case ENTEREDINERROR: return "http://hl7.org/fhir/CodeSystem/formularyitem-status"; 100 case INACTIVE: return "http://hl7.org/fhir/CodeSystem/formularyitem-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case ACTIVE: return "The service or product referred to by this FormularyItem is in active use within the drug database or inventory system."; 108 case ENTEREDINERROR: return "The service or product referred to by this FormularyItem was entered in error within the drug database or inventory system."; 109 case INACTIVE: return "The service or product referred to by this FormularyItem is not in active use within the drug database or inventory system."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case ACTIVE: return "Active"; 117 case ENTEREDINERROR: return "Entered in Error"; 118 case INACTIVE: return "Inactive"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class FormularyItemStatusCodesEnumFactory implements EnumFactory<FormularyItemStatusCodes> { 126 public FormularyItemStatusCodes fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("active".equals(codeString)) 131 return FormularyItemStatusCodes.ACTIVE; 132 if ("entered-in-error".equals(codeString)) 133 return FormularyItemStatusCodes.ENTEREDINERROR; 134 if ("inactive".equals(codeString)) 135 return FormularyItemStatusCodes.INACTIVE; 136 throw new IllegalArgumentException("Unknown FormularyItemStatusCodes code '"+codeString+"'"); 137 } 138 public Enumeration<FormularyItemStatusCodes> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<FormularyItemStatusCodes>(this, FormularyItemStatusCodes.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<FormularyItemStatusCodes>(this, FormularyItemStatusCodes.NULL, code); 146 if ("active".equals(codeString)) 147 return new Enumeration<FormularyItemStatusCodes>(this, FormularyItemStatusCodes.ACTIVE, code); 148 if ("entered-in-error".equals(codeString)) 149 return new Enumeration<FormularyItemStatusCodes>(this, FormularyItemStatusCodes.ENTEREDINERROR, code); 150 if ("inactive".equals(codeString)) 151 return new Enumeration<FormularyItemStatusCodes>(this, FormularyItemStatusCodes.INACTIVE, code); 152 throw new FHIRException("Unknown FormularyItemStatusCodes code '"+codeString+"'"); 153 } 154 public String toCode(FormularyItemStatusCodes code) { 155 if (code == FormularyItemStatusCodes.NULL) 156 return null; 157 if (code == FormularyItemStatusCodes.ACTIVE) 158 return "active"; 159 if (code == FormularyItemStatusCodes.ENTEREDINERROR) 160 return "entered-in-error"; 161 if (code == FormularyItemStatusCodes.INACTIVE) 162 return "inactive"; 163 return "?"; 164 } 165 public String toSystem(FormularyItemStatusCodes code) { 166 return code.getSystem(); 167 } 168 } 169 170 /** 171 * Business identifier for this formulary item. 172 */ 173 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 174 @Description(shortDefinition="Business identifier for this formulary item", formalDefinition="Business identifier for this formulary item." ) 175 protected List<Identifier> identifier; 176 177 /** 178 * A code (or set of codes) that specify the product or service that is identified by this formulary item. 179 */ 180 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 181 @Description(shortDefinition="Codes that identify this formulary item", formalDefinition="A code (or set of codes) that specify the product or service that is identified by this formulary item." ) 182 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 183 protected CodeableConcept code; 184 185 /** 186 * The validity about the information of the formulary item and not of the underlying product or service itself. 187 */ 188 @Child(name = "status", type = {CodeType.class}, order=2, min=0, max=1, modifier=true, summary=true) 189 @Description(shortDefinition="active | entered-in-error | inactive", formalDefinition="The validity about the information of the formulary item and not of the underlying product or service itself." ) 190 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/formularyitem-status") 191 protected Enumeration<FormularyItemStatusCodes> status; 192 193 private static final long serialVersionUID = 601026823L; 194 195 /** 196 * Constructor 197 */ 198 public FormularyItem() { 199 super(); 200 } 201 202 /** 203 * @return {@link #identifier} (Business identifier for this formulary item.) 204 */ 205 public List<Identifier> getIdentifier() { 206 if (this.identifier == null) 207 this.identifier = new ArrayList<Identifier>(); 208 return this.identifier; 209 } 210 211 /** 212 * @return Returns a reference to <code>this</code> for easy method chaining 213 */ 214 public FormularyItem setIdentifier(List<Identifier> theIdentifier) { 215 this.identifier = theIdentifier; 216 return this; 217 } 218 219 public boolean hasIdentifier() { 220 if (this.identifier == null) 221 return false; 222 for (Identifier item : this.identifier) 223 if (!item.isEmpty()) 224 return true; 225 return false; 226 } 227 228 public Identifier addIdentifier() { //3 229 Identifier t = new Identifier(); 230 if (this.identifier == null) 231 this.identifier = new ArrayList<Identifier>(); 232 this.identifier.add(t); 233 return t; 234 } 235 236 public FormularyItem addIdentifier(Identifier t) { //3 237 if (t == null) 238 return this; 239 if (this.identifier == null) 240 this.identifier = new ArrayList<Identifier>(); 241 this.identifier.add(t); 242 return this; 243 } 244 245 /** 246 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 247 */ 248 public Identifier getIdentifierFirstRep() { 249 if (getIdentifier().isEmpty()) { 250 addIdentifier(); 251 } 252 return getIdentifier().get(0); 253 } 254 255 /** 256 * @return {@link #code} (A code (or set of codes) that specify the product or service that is identified by this formulary item.) 257 */ 258 public CodeableConcept getCode() { 259 if (this.code == null) 260 if (Configuration.errorOnAutoCreate()) 261 throw new Error("Attempt to auto-create FormularyItem.code"); 262 else if (Configuration.doAutoCreate()) 263 this.code = new CodeableConcept(); // cc 264 return this.code; 265 } 266 267 public boolean hasCode() { 268 return this.code != null && !this.code.isEmpty(); 269 } 270 271 /** 272 * @param value {@link #code} (A code (or set of codes) that specify the product or service that is identified by this formulary item.) 273 */ 274 public FormularyItem setCode(CodeableConcept value) { 275 this.code = value; 276 return this; 277 } 278 279 /** 280 * @return {@link #status} (The validity about the information of the formulary item and not of the underlying product or service itself.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 281 */ 282 public Enumeration<FormularyItemStatusCodes> getStatusElement() { 283 if (this.status == null) 284 if (Configuration.errorOnAutoCreate()) 285 throw new Error("Attempt to auto-create FormularyItem.status"); 286 else if (Configuration.doAutoCreate()) 287 this.status = new Enumeration<FormularyItemStatusCodes>(new FormularyItemStatusCodesEnumFactory()); // bb 288 return this.status; 289 } 290 291 public boolean hasStatusElement() { 292 return this.status != null && !this.status.isEmpty(); 293 } 294 295 public boolean hasStatus() { 296 return this.status != null && !this.status.isEmpty(); 297 } 298 299 /** 300 * @param value {@link #status} (The validity about the information of the formulary item and not of the underlying product or service itself.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 301 */ 302 public FormularyItem setStatusElement(Enumeration<FormularyItemStatusCodes> value) { 303 this.status = value; 304 return this; 305 } 306 307 /** 308 * @return The validity about the information of the formulary item and not of the underlying product or service itself. 309 */ 310 public FormularyItemStatusCodes getStatus() { 311 return this.status == null ? null : this.status.getValue(); 312 } 313 314 /** 315 * @param value The validity about the information of the formulary item and not of the underlying product or service itself. 316 */ 317 public FormularyItem setStatus(FormularyItemStatusCodes value) { 318 if (value == null) 319 this.status = null; 320 else { 321 if (this.status == null) 322 this.status = new Enumeration<FormularyItemStatusCodes>(new FormularyItemStatusCodesEnumFactory()); 323 this.status.setValue(value); 324 } 325 return this; 326 } 327 328 protected void listChildren(List<Property> children) { 329 super.listChildren(children); 330 children.add(new Property("identifier", "Identifier", "Business identifier for this formulary item.", 0, java.lang.Integer.MAX_VALUE, identifier)); 331 children.add(new Property("code", "CodeableConcept", "A code (or set of codes) that specify the product or service that is identified by this formulary item.", 0, 1, code)); 332 children.add(new Property("status", "code", "The validity about the information of the formulary item and not of the underlying product or service itself.", 0, 1, status)); 333 } 334 335 @Override 336 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 337 switch (_hash) { 338 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier for this formulary item.", 0, java.lang.Integer.MAX_VALUE, identifier); 339 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code (or set of codes) that specify the product or service that is identified by this formulary item.", 0, 1, code); 340 case -892481550: /*status*/ return new Property("status", "code", "The validity about the information of the formulary item and not of the underlying product or service itself.", 0, 1, status); 341 default: return super.getNamedProperty(_hash, _name, _checkValid); 342 } 343 344 } 345 346 @Override 347 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 348 switch (hash) { 349 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 350 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 351 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FormularyItemStatusCodes> 352 default: return super.getProperty(hash, name, checkValid); 353 } 354 355 } 356 357 @Override 358 public Base setProperty(int hash, String name, Base value) throws FHIRException { 359 switch (hash) { 360 case -1618432855: // identifier 361 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 362 return value; 363 case 3059181: // code 364 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 365 return value; 366 case -892481550: // status 367 value = new FormularyItemStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 368 this.status = (Enumeration) value; // Enumeration<FormularyItemStatusCodes> 369 return value; 370 default: return super.setProperty(hash, name, value); 371 } 372 373 } 374 375 @Override 376 public Base setProperty(String name, Base value) throws FHIRException { 377 if (name.equals("identifier")) { 378 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 379 } else if (name.equals("code")) { 380 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 381 } else if (name.equals("status")) { 382 value = new FormularyItemStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 383 this.status = (Enumeration) value; // Enumeration<FormularyItemStatusCodes> 384 } else 385 return super.setProperty(name, value); 386 return value; 387 } 388 389 @Override 390 public void removeChild(String name, Base value) throws FHIRException { 391 if (name.equals("identifier")) { 392 this.getIdentifier().remove(value); 393 } else if (name.equals("code")) { 394 this.code = null; 395 } else if (name.equals("status")) { 396 value = new FormularyItemStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 397 this.status = (Enumeration) value; // Enumeration<FormularyItemStatusCodes> 398 } else 399 super.removeChild(name, value); 400 401 } 402 403 @Override 404 public Base makeProperty(int hash, String name) throws FHIRException { 405 switch (hash) { 406 case -1618432855: return addIdentifier(); 407 case 3059181: return getCode(); 408 case -892481550: return getStatusElement(); 409 default: return super.makeProperty(hash, name); 410 } 411 412 } 413 414 @Override 415 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 416 switch (hash) { 417 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 418 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 419 case -892481550: /*status*/ return new String[] {"code"}; 420 default: return super.getTypesForProperty(hash, name); 421 } 422 423 } 424 425 @Override 426 public Base addChild(String name) throws FHIRException { 427 if (name.equals("identifier")) { 428 return addIdentifier(); 429 } 430 else if (name.equals("code")) { 431 this.code = new CodeableConcept(); 432 return this.code; 433 } 434 else if (name.equals("status")) { 435 throw new FHIRException("Cannot call addChild on a singleton property FormularyItem.status"); 436 } 437 else 438 return super.addChild(name); 439 } 440 441 public String fhirType() { 442 return "FormularyItem"; 443 444 } 445 446 public FormularyItem copy() { 447 FormularyItem dst = new FormularyItem(); 448 copyValues(dst); 449 return dst; 450 } 451 452 public void copyValues(FormularyItem dst) { 453 super.copyValues(dst); 454 if (identifier != null) { 455 dst.identifier = new ArrayList<Identifier>(); 456 for (Identifier i : identifier) 457 dst.identifier.add(i.copy()); 458 }; 459 dst.code = code == null ? null : code.copy(); 460 dst.status = status == null ? null : status.copy(); 461 } 462 463 protected FormularyItem typedCopy() { 464 return copy(); 465 } 466 467 @Override 468 public boolean equalsDeep(Base other_) { 469 if (!super.equalsDeep(other_)) 470 return false; 471 if (!(other_ instanceof FormularyItem)) 472 return false; 473 FormularyItem o = (FormularyItem) other_; 474 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) && compareDeep(status, o.status, true) 475 ; 476 } 477 478 @Override 479 public boolean equalsShallow(Base other_) { 480 if (!super.equalsShallow(other_)) 481 return false; 482 if (!(other_ instanceof FormularyItem)) 483 return false; 484 FormularyItem o = (FormularyItem) other_; 485 return compareValues(status, o.status, true); 486 } 487 488 public boolean isEmpty() { 489 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, status 490 ); 491 } 492 493 @Override 494 public ResourceType getResourceType() { 495 return ResourceType.FormularyItem; 496 } 497 498 /** 499 * Search parameter: <b>code</b> 500 * <p> 501 * Description: <b>Returns formulary items for a specific code</b><br> 502 * Type: <b>token</b><br> 503 * Path: <b>FormularyItem.code</b><br> 504 * </p> 505 */ 506 @SearchParamDefinition(name="code", path="FormularyItem.code", description="Returns formulary items for a specific code", type="token" ) 507 public static final String SP_CODE = "code"; 508 /** 509 * <b>Fluent Client</b> search parameter constant for <b>code</b> 510 * <p> 511 * Description: <b>Returns formulary items for a specific code</b><br> 512 * Type: <b>token</b><br> 513 * Path: <b>FormularyItem.code</b><br> 514 * </p> 515 */ 516 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 517 518 /** 519 * Search parameter: <b>identifier</b> 520 * <p> 521 * Description: <b>Returns formulary items with this external identifier</b><br> 522 * Type: <b>token</b><br> 523 * Path: <b>FormularyItem.identifier</b><br> 524 * </p> 525 */ 526 @SearchParamDefinition(name="identifier", path="FormularyItem.identifier", description="Returns formulary items with this external identifier", type="token" ) 527 public static final String SP_IDENTIFIER = "identifier"; 528 /** 529 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 530 * <p> 531 * Description: <b>Returns formulary items with this external identifier</b><br> 532 * Type: <b>token</b><br> 533 * Path: <b>FormularyItem.identifier</b><br> 534 * </p> 535 */ 536 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 537 538 539} 540