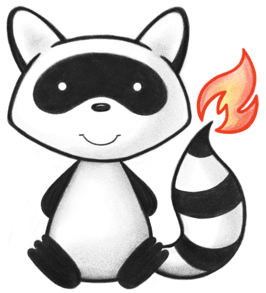
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A set of analyses performed to analyze and generate genomic data. 052 */ 053@ResourceDef(name="GenomicStudy", profile="http://hl7.org/fhir/StructureDefinition/GenomicStudy") 054public class GenomicStudy extends DomainResource { 055 056 public enum GenomicStudyStatus { 057 /** 058 * The existence of the genomic study is registered, but there is nothing yet available. 059 */ 060 REGISTERED, 061 /** 062 * At least one instance has been associated with this genomic study. 063 */ 064 AVAILABLE, 065 /** 066 * The genomic study is unavailable because the genomic study was not started or not completed (also sometimes called \"aborted\"). 067 */ 068 CANCELLED, 069 /** 070 * The genomic study has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".). 071 */ 072 ENTEREDINERROR, 073 /** 074 * The system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one. 075 */ 076 UNKNOWN, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static GenomicStudyStatus fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("registered".equals(codeString)) 085 return REGISTERED; 086 if ("available".equals(codeString)) 087 return AVAILABLE; 088 if ("cancelled".equals(codeString)) 089 return CANCELLED; 090 if ("entered-in-error".equals(codeString)) 091 return ENTEREDINERROR; 092 if ("unknown".equals(codeString)) 093 return UNKNOWN; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown GenomicStudyStatus code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case REGISTERED: return "registered"; 102 case AVAILABLE: return "available"; 103 case CANCELLED: return "cancelled"; 104 case ENTEREDINERROR: return "entered-in-error"; 105 case UNKNOWN: return "unknown"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case REGISTERED: return "http://hl7.org/fhir/genomicstudy-status"; 113 case AVAILABLE: return "http://hl7.org/fhir/genomicstudy-status"; 114 case CANCELLED: return "http://hl7.org/fhir/genomicstudy-status"; 115 case ENTEREDINERROR: return "http://hl7.org/fhir/genomicstudy-status"; 116 case UNKNOWN: return "http://hl7.org/fhir/genomicstudy-status"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case REGISTERED: return "The existence of the genomic study is registered, but there is nothing yet available."; 124 case AVAILABLE: return "At least one instance has been associated with this genomic study."; 125 case CANCELLED: return "The genomic study is unavailable because the genomic study was not started or not completed (also sometimes called \"aborted\")."; 126 case ENTEREDINERROR: return "The genomic study has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 127 case UNKNOWN: return "The system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case REGISTERED: return "Registered"; 135 case AVAILABLE: return "Available"; 136 case CANCELLED: return "Cancelled"; 137 case ENTEREDINERROR: return "Entered in Error"; 138 case UNKNOWN: return "Unknown"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class GenomicStudyStatusEnumFactory implements EnumFactory<GenomicStudyStatus> { 146 public GenomicStudyStatus fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("registered".equals(codeString)) 151 return GenomicStudyStatus.REGISTERED; 152 if ("available".equals(codeString)) 153 return GenomicStudyStatus.AVAILABLE; 154 if ("cancelled".equals(codeString)) 155 return GenomicStudyStatus.CANCELLED; 156 if ("entered-in-error".equals(codeString)) 157 return GenomicStudyStatus.ENTEREDINERROR; 158 if ("unknown".equals(codeString)) 159 return GenomicStudyStatus.UNKNOWN; 160 throw new IllegalArgumentException("Unknown GenomicStudyStatus code '"+codeString+"'"); 161 } 162 public Enumeration<GenomicStudyStatus> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<GenomicStudyStatus>(this, GenomicStudyStatus.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<GenomicStudyStatus>(this, GenomicStudyStatus.NULL, code); 170 if ("registered".equals(codeString)) 171 return new Enumeration<GenomicStudyStatus>(this, GenomicStudyStatus.REGISTERED, code); 172 if ("available".equals(codeString)) 173 return new Enumeration<GenomicStudyStatus>(this, GenomicStudyStatus.AVAILABLE, code); 174 if ("cancelled".equals(codeString)) 175 return new Enumeration<GenomicStudyStatus>(this, GenomicStudyStatus.CANCELLED, code); 176 if ("entered-in-error".equals(codeString)) 177 return new Enumeration<GenomicStudyStatus>(this, GenomicStudyStatus.ENTEREDINERROR, code); 178 if ("unknown".equals(codeString)) 179 return new Enumeration<GenomicStudyStatus>(this, GenomicStudyStatus.UNKNOWN, code); 180 throw new FHIRException("Unknown GenomicStudyStatus code '"+codeString+"'"); 181 } 182 public String toCode(GenomicStudyStatus code) { 183 if (code == GenomicStudyStatus.NULL) 184 return null; 185 if (code == GenomicStudyStatus.REGISTERED) 186 return "registered"; 187 if (code == GenomicStudyStatus.AVAILABLE) 188 return "available"; 189 if (code == GenomicStudyStatus.CANCELLED) 190 return "cancelled"; 191 if (code == GenomicStudyStatus.ENTEREDINERROR) 192 return "entered-in-error"; 193 if (code == GenomicStudyStatus.UNKNOWN) 194 return "unknown"; 195 return "?"; 196 } 197 public String toSystem(GenomicStudyStatus code) { 198 return code.getSystem(); 199 } 200 } 201 202 @Block() 203 public static class GenomicStudyAnalysisComponent extends BackboneElement implements IBaseBackboneElement { 204 /** 205 * Identifiers for the analysis event. 206 */ 207 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 208 @Description(shortDefinition="Identifiers for the analysis event", formalDefinition="Identifiers for the analysis event." ) 209 protected List<Identifier> identifier; 210 211 /** 212 * Type of the methods used in the analysis, e.g., Fluorescence in situ hybridization (FISH), Karyotyping, or Microsatellite instability testing (MSI). 213 */ 214 @Child(name = "methodType", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 215 @Description(shortDefinition="Type of the methods used in the analysis (e.g., FISH, Karyotyping, MSI)", formalDefinition="Type of the methods used in the analysis, e.g., Fluorescence in situ hybridization (FISH), Karyotyping, or Microsatellite instability testing (MSI)." ) 216 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/genomicstudy-methodtype") 217 protected List<CodeableConcept> methodType; 218 219 /** 220 * Type of the genomic changes studied in the analysis, e.g., DNA, RNA, or amino acid change. 221 */ 222 @Child(name = "changeType", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 223 @Description(shortDefinition="Type of the genomic changes studied in the analysis (e.g., DNA, RNA, or AA change)", formalDefinition="Type of the genomic changes studied in the analysis, e.g., DNA, RNA, or amino acid change." ) 224 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/genomicstudy-changetype") 225 protected List<CodeableConcept> changeType; 226 227 /** 228 * The reference genome build that is used in this analysis. 229 */ 230 @Child(name = "genomeBuild", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 231 @Description(shortDefinition="Genome build that is used in this analysis", formalDefinition="The reference genome build that is used in this analysis." ) 232 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://loinc.org/vs/LL1040-6") 233 protected CodeableConcept genomeBuild; 234 235 /** 236 * The defined protocol that describes the analysis. 237 */ 238 @Child(name = "instantiatesCanonical", type = {CanonicalType.class}, order=5, min=0, max=1, modifier=false, summary=false) 239 @Description(shortDefinition="The defined protocol that describes the analysis", formalDefinition="The defined protocol that describes the analysis." ) 240 protected CanonicalType instantiatesCanonical; 241 242 /** 243 * The URL pointing to an externally maintained protocol that describes the analysis. 244 */ 245 @Child(name = "instantiatesUri", type = {UriType.class}, order=6, min=0, max=1, modifier=false, summary=false) 246 @Description(shortDefinition="The URL pointing to an externally maintained protocol that describes the analysis", formalDefinition="The URL pointing to an externally maintained protocol that describes the analysis." ) 247 protected UriType instantiatesUri; 248 249 /** 250 * Name of the analysis event (human friendly). 251 */ 252 @Child(name = "title", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=true) 253 @Description(shortDefinition="Name of the analysis event (human friendly)", formalDefinition="Name of the analysis event (human friendly)." ) 254 protected StringType title; 255 256 /** 257 * The focus of a genomic analysis when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, child, or sibling. For example, in trio testing, the GenomicStudy.subject would be the child (proband) and the GenomicStudy.analysis.focus of a specific analysis would be the parent. 258 */ 259 @Child(name = "focus", type = {Reference.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 260 @Description(shortDefinition="What the genomic analysis is about, when it is not about the subject of record", formalDefinition="The focus of a genomic analysis when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, child, or sibling. For example, in trio testing, the GenomicStudy.subject would be the child (proband) and the GenomicStudy.analysis.focus of a specific analysis would be the parent." ) 261 protected List<Reference> focus; 262 263 /** 264 * The specimen used in the analysis event. 265 */ 266 @Child(name = "specimen", type = {Specimen.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 267 @Description(shortDefinition="The specimen used in the analysis event", formalDefinition="The specimen used in the analysis event." ) 268 protected List<Reference> specimen; 269 270 /** 271 * The date of the analysis event. 272 */ 273 @Child(name = "date", type = {DateTimeType.class}, order=10, min=0, max=1, modifier=false, summary=false) 274 @Description(shortDefinition="The date of the analysis event", formalDefinition="The date of the analysis event." ) 275 protected DateTimeType date; 276 277 /** 278 * Any notes capture with the analysis event. 279 */ 280 @Child(name = "note", type = {Annotation.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 281 @Description(shortDefinition="Any notes capture with the analysis event", formalDefinition="Any notes capture with the analysis event." ) 282 protected List<Annotation> note; 283 284 /** 285 * The protocol that was performed for the analysis event. 286 */ 287 @Child(name = "protocolPerformed", type = {Procedure.class, Task.class}, order=12, min=0, max=1, modifier=false, summary=false) 288 @Description(shortDefinition="The protocol that was performed for the analysis event", formalDefinition="The protocol that was performed for the analysis event." ) 289 protected Reference protocolPerformed; 290 291 /** 292 * The genomic regions to be studied in the analysis (BED file). 293 */ 294 @Child(name = "regionsStudied", type = {DocumentReference.class, Observation.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 295 @Description(shortDefinition="The genomic regions to be studied in the analysis (BED file)", formalDefinition="The genomic regions to be studied in the analysis (BED file)." ) 296 protected List<Reference> regionsStudied; 297 298 /** 299 * Genomic regions actually called in the analysis event (BED file). 300 */ 301 @Child(name = "regionsCalled", type = {DocumentReference.class, Observation.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 302 @Description(shortDefinition="Genomic regions actually called in the analysis event (BED file)", formalDefinition="Genomic regions actually called in the analysis event (BED file)." ) 303 protected List<Reference> regionsCalled; 304 305 /** 306 * Inputs for the analysis event. 307 */ 308 @Child(name = "input", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 309 @Description(shortDefinition="Inputs for the analysis event", formalDefinition="Inputs for the analysis event." ) 310 protected List<GenomicStudyAnalysisInputComponent> input; 311 312 /** 313 * Outputs for the analysis event. 314 */ 315 @Child(name = "output", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 316 @Description(shortDefinition="Outputs for the analysis event", formalDefinition="Outputs for the analysis event." ) 317 protected List<GenomicStudyAnalysisOutputComponent> output; 318 319 /** 320 * Performer for the analysis event. 321 */ 322 @Child(name = "performer", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 323 @Description(shortDefinition="Performer for the analysis event", formalDefinition="Performer for the analysis event." ) 324 protected List<GenomicStudyAnalysisPerformerComponent> performer; 325 326 /** 327 * Devices used for the analysis (e.g., instruments, software), with settings and parameters. 328 */ 329 @Child(name = "device", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 330 @Description(shortDefinition="Devices used for the analysis (e.g., instruments, software), with settings and parameters", formalDefinition="Devices used for the analysis (e.g., instruments, software), with settings and parameters." ) 331 protected List<GenomicStudyAnalysisDeviceComponent> device; 332 333 private static final long serialVersionUID = 467270310L; 334 335 /** 336 * Constructor 337 */ 338 public GenomicStudyAnalysisComponent() { 339 super(); 340 } 341 342 /** 343 * @return {@link #identifier} (Identifiers for the analysis event.) 344 */ 345 public List<Identifier> getIdentifier() { 346 if (this.identifier == null) 347 this.identifier = new ArrayList<Identifier>(); 348 return this.identifier; 349 } 350 351 /** 352 * @return Returns a reference to <code>this</code> for easy method chaining 353 */ 354 public GenomicStudyAnalysisComponent setIdentifier(List<Identifier> theIdentifier) { 355 this.identifier = theIdentifier; 356 return this; 357 } 358 359 public boolean hasIdentifier() { 360 if (this.identifier == null) 361 return false; 362 for (Identifier item : this.identifier) 363 if (!item.isEmpty()) 364 return true; 365 return false; 366 } 367 368 public Identifier addIdentifier() { //3 369 Identifier t = new Identifier(); 370 if (this.identifier == null) 371 this.identifier = new ArrayList<Identifier>(); 372 this.identifier.add(t); 373 return t; 374 } 375 376 public GenomicStudyAnalysisComponent addIdentifier(Identifier t) { //3 377 if (t == null) 378 return this; 379 if (this.identifier == null) 380 this.identifier = new ArrayList<Identifier>(); 381 this.identifier.add(t); 382 return this; 383 } 384 385 /** 386 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 387 */ 388 public Identifier getIdentifierFirstRep() { 389 if (getIdentifier().isEmpty()) { 390 addIdentifier(); 391 } 392 return getIdentifier().get(0); 393 } 394 395 /** 396 * @return {@link #methodType} (Type of the methods used in the analysis, e.g., Fluorescence in situ hybridization (FISH), Karyotyping, or Microsatellite instability testing (MSI).) 397 */ 398 public List<CodeableConcept> getMethodType() { 399 if (this.methodType == null) 400 this.methodType = new ArrayList<CodeableConcept>(); 401 return this.methodType; 402 } 403 404 /** 405 * @return Returns a reference to <code>this</code> for easy method chaining 406 */ 407 public GenomicStudyAnalysisComponent setMethodType(List<CodeableConcept> theMethodType) { 408 this.methodType = theMethodType; 409 return this; 410 } 411 412 public boolean hasMethodType() { 413 if (this.methodType == null) 414 return false; 415 for (CodeableConcept item : this.methodType) 416 if (!item.isEmpty()) 417 return true; 418 return false; 419 } 420 421 public CodeableConcept addMethodType() { //3 422 CodeableConcept t = new CodeableConcept(); 423 if (this.methodType == null) 424 this.methodType = new ArrayList<CodeableConcept>(); 425 this.methodType.add(t); 426 return t; 427 } 428 429 public GenomicStudyAnalysisComponent addMethodType(CodeableConcept t) { //3 430 if (t == null) 431 return this; 432 if (this.methodType == null) 433 this.methodType = new ArrayList<CodeableConcept>(); 434 this.methodType.add(t); 435 return this; 436 } 437 438 /** 439 * @return The first repetition of repeating field {@link #methodType}, creating it if it does not already exist {3} 440 */ 441 public CodeableConcept getMethodTypeFirstRep() { 442 if (getMethodType().isEmpty()) { 443 addMethodType(); 444 } 445 return getMethodType().get(0); 446 } 447 448 /** 449 * @return {@link #changeType} (Type of the genomic changes studied in the analysis, e.g., DNA, RNA, or amino acid change.) 450 */ 451 public List<CodeableConcept> getChangeType() { 452 if (this.changeType == null) 453 this.changeType = new ArrayList<CodeableConcept>(); 454 return this.changeType; 455 } 456 457 /** 458 * @return Returns a reference to <code>this</code> for easy method chaining 459 */ 460 public GenomicStudyAnalysisComponent setChangeType(List<CodeableConcept> theChangeType) { 461 this.changeType = theChangeType; 462 return this; 463 } 464 465 public boolean hasChangeType() { 466 if (this.changeType == null) 467 return false; 468 for (CodeableConcept item : this.changeType) 469 if (!item.isEmpty()) 470 return true; 471 return false; 472 } 473 474 public CodeableConcept addChangeType() { //3 475 CodeableConcept t = new CodeableConcept(); 476 if (this.changeType == null) 477 this.changeType = new ArrayList<CodeableConcept>(); 478 this.changeType.add(t); 479 return t; 480 } 481 482 public GenomicStudyAnalysisComponent addChangeType(CodeableConcept t) { //3 483 if (t == null) 484 return this; 485 if (this.changeType == null) 486 this.changeType = new ArrayList<CodeableConcept>(); 487 this.changeType.add(t); 488 return this; 489 } 490 491 /** 492 * @return The first repetition of repeating field {@link #changeType}, creating it if it does not already exist {3} 493 */ 494 public CodeableConcept getChangeTypeFirstRep() { 495 if (getChangeType().isEmpty()) { 496 addChangeType(); 497 } 498 return getChangeType().get(0); 499 } 500 501 /** 502 * @return {@link #genomeBuild} (The reference genome build that is used in this analysis.) 503 */ 504 public CodeableConcept getGenomeBuild() { 505 if (this.genomeBuild == null) 506 if (Configuration.errorOnAutoCreate()) 507 throw new Error("Attempt to auto-create GenomicStudyAnalysisComponent.genomeBuild"); 508 else if (Configuration.doAutoCreate()) 509 this.genomeBuild = new CodeableConcept(); // cc 510 return this.genomeBuild; 511 } 512 513 public boolean hasGenomeBuild() { 514 return this.genomeBuild != null && !this.genomeBuild.isEmpty(); 515 } 516 517 /** 518 * @param value {@link #genomeBuild} (The reference genome build that is used in this analysis.) 519 */ 520 public GenomicStudyAnalysisComponent setGenomeBuild(CodeableConcept value) { 521 this.genomeBuild = value; 522 return this; 523 } 524 525 /** 526 * @return {@link #instantiatesCanonical} (The defined protocol that describes the analysis.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesCanonical" gives direct access to the value 527 */ 528 public CanonicalType getInstantiatesCanonicalElement() { 529 if (this.instantiatesCanonical == null) 530 if (Configuration.errorOnAutoCreate()) 531 throw new Error("Attempt to auto-create GenomicStudyAnalysisComponent.instantiatesCanonical"); 532 else if (Configuration.doAutoCreate()) 533 this.instantiatesCanonical = new CanonicalType(); // bb 534 return this.instantiatesCanonical; 535 } 536 537 public boolean hasInstantiatesCanonicalElement() { 538 return this.instantiatesCanonical != null && !this.instantiatesCanonical.isEmpty(); 539 } 540 541 public boolean hasInstantiatesCanonical() { 542 return this.instantiatesCanonical != null && !this.instantiatesCanonical.isEmpty(); 543 } 544 545 /** 546 * @param value {@link #instantiatesCanonical} (The defined protocol that describes the analysis.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesCanonical" gives direct access to the value 547 */ 548 public GenomicStudyAnalysisComponent setInstantiatesCanonicalElement(CanonicalType value) { 549 this.instantiatesCanonical = value; 550 return this; 551 } 552 553 /** 554 * @return The defined protocol that describes the analysis. 555 */ 556 public String getInstantiatesCanonical() { 557 return this.instantiatesCanonical == null ? null : this.instantiatesCanonical.getValue(); 558 } 559 560 /** 561 * @param value The defined protocol that describes the analysis. 562 */ 563 public GenomicStudyAnalysisComponent setInstantiatesCanonical(String value) { 564 if (Utilities.noString(value)) 565 this.instantiatesCanonical = null; 566 else { 567 if (this.instantiatesCanonical == null) 568 this.instantiatesCanonical = new CanonicalType(); 569 this.instantiatesCanonical.setValue(value); 570 } 571 return this; 572 } 573 574 /** 575 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol that describes the analysis.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesUri" gives direct access to the value 576 */ 577 public UriType getInstantiatesUriElement() { 578 if (this.instantiatesUri == null) 579 if (Configuration.errorOnAutoCreate()) 580 throw new Error("Attempt to auto-create GenomicStudyAnalysisComponent.instantiatesUri"); 581 else if (Configuration.doAutoCreate()) 582 this.instantiatesUri = new UriType(); // bb 583 return this.instantiatesUri; 584 } 585 586 public boolean hasInstantiatesUriElement() { 587 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 588 } 589 590 public boolean hasInstantiatesUri() { 591 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 592 } 593 594 /** 595 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol that describes the analysis.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesUri" gives direct access to the value 596 */ 597 public GenomicStudyAnalysisComponent setInstantiatesUriElement(UriType value) { 598 this.instantiatesUri = value; 599 return this; 600 } 601 602 /** 603 * @return The URL pointing to an externally maintained protocol that describes the analysis. 604 */ 605 public String getInstantiatesUri() { 606 return this.instantiatesUri == null ? null : this.instantiatesUri.getValue(); 607 } 608 609 /** 610 * @param value The URL pointing to an externally maintained protocol that describes the analysis. 611 */ 612 public GenomicStudyAnalysisComponent setInstantiatesUri(String value) { 613 if (Utilities.noString(value)) 614 this.instantiatesUri = null; 615 else { 616 if (this.instantiatesUri == null) 617 this.instantiatesUri = new UriType(); 618 this.instantiatesUri.setValue(value); 619 } 620 return this; 621 } 622 623 /** 624 * @return {@link #title} (Name of the analysis event (human friendly).). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 625 */ 626 public StringType getTitleElement() { 627 if (this.title == null) 628 if (Configuration.errorOnAutoCreate()) 629 throw new Error("Attempt to auto-create GenomicStudyAnalysisComponent.title"); 630 else if (Configuration.doAutoCreate()) 631 this.title = new StringType(); // bb 632 return this.title; 633 } 634 635 public boolean hasTitleElement() { 636 return this.title != null && !this.title.isEmpty(); 637 } 638 639 public boolean hasTitle() { 640 return this.title != null && !this.title.isEmpty(); 641 } 642 643 /** 644 * @param value {@link #title} (Name of the analysis event (human friendly).). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 645 */ 646 public GenomicStudyAnalysisComponent setTitleElement(StringType value) { 647 this.title = value; 648 return this; 649 } 650 651 /** 652 * @return Name of the analysis event (human friendly). 653 */ 654 public String getTitle() { 655 return this.title == null ? null : this.title.getValue(); 656 } 657 658 /** 659 * @param value Name of the analysis event (human friendly). 660 */ 661 public GenomicStudyAnalysisComponent setTitle(String value) { 662 if (Utilities.noString(value)) 663 this.title = null; 664 else { 665 if (this.title == null) 666 this.title = new StringType(); 667 this.title.setValue(value); 668 } 669 return this; 670 } 671 672 /** 673 * @return {@link #focus} (The focus of a genomic analysis when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, child, or sibling. For example, in trio testing, the GenomicStudy.subject would be the child (proband) and the GenomicStudy.analysis.focus of a specific analysis would be the parent.) 674 */ 675 public List<Reference> getFocus() { 676 if (this.focus == null) 677 this.focus = new ArrayList<Reference>(); 678 return this.focus; 679 } 680 681 /** 682 * @return Returns a reference to <code>this</code> for easy method chaining 683 */ 684 public GenomicStudyAnalysisComponent setFocus(List<Reference> theFocus) { 685 this.focus = theFocus; 686 return this; 687 } 688 689 public boolean hasFocus() { 690 if (this.focus == null) 691 return false; 692 for (Reference item : this.focus) 693 if (!item.isEmpty()) 694 return true; 695 return false; 696 } 697 698 public Reference addFocus() { //3 699 Reference t = new Reference(); 700 if (this.focus == null) 701 this.focus = new ArrayList<Reference>(); 702 this.focus.add(t); 703 return t; 704 } 705 706 public GenomicStudyAnalysisComponent addFocus(Reference t) { //3 707 if (t == null) 708 return this; 709 if (this.focus == null) 710 this.focus = new ArrayList<Reference>(); 711 this.focus.add(t); 712 return this; 713 } 714 715 /** 716 * @return The first repetition of repeating field {@link #focus}, creating it if it does not already exist {3} 717 */ 718 public Reference getFocusFirstRep() { 719 if (getFocus().isEmpty()) { 720 addFocus(); 721 } 722 return getFocus().get(0); 723 } 724 725 /** 726 * @return {@link #specimen} (The specimen used in the analysis event.) 727 */ 728 public List<Reference> getSpecimen() { 729 if (this.specimen == null) 730 this.specimen = new ArrayList<Reference>(); 731 return this.specimen; 732 } 733 734 /** 735 * @return Returns a reference to <code>this</code> for easy method chaining 736 */ 737 public GenomicStudyAnalysisComponent setSpecimen(List<Reference> theSpecimen) { 738 this.specimen = theSpecimen; 739 return this; 740 } 741 742 public boolean hasSpecimen() { 743 if (this.specimen == null) 744 return false; 745 for (Reference item : this.specimen) 746 if (!item.isEmpty()) 747 return true; 748 return false; 749 } 750 751 public Reference addSpecimen() { //3 752 Reference t = new Reference(); 753 if (this.specimen == null) 754 this.specimen = new ArrayList<Reference>(); 755 this.specimen.add(t); 756 return t; 757 } 758 759 public GenomicStudyAnalysisComponent addSpecimen(Reference t) { //3 760 if (t == null) 761 return this; 762 if (this.specimen == null) 763 this.specimen = new ArrayList<Reference>(); 764 this.specimen.add(t); 765 return this; 766 } 767 768 /** 769 * @return The first repetition of repeating field {@link #specimen}, creating it if it does not already exist {3} 770 */ 771 public Reference getSpecimenFirstRep() { 772 if (getSpecimen().isEmpty()) { 773 addSpecimen(); 774 } 775 return getSpecimen().get(0); 776 } 777 778 /** 779 * @return {@link #date} (The date of the analysis event.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 780 */ 781 public DateTimeType getDateElement() { 782 if (this.date == null) 783 if (Configuration.errorOnAutoCreate()) 784 throw new Error("Attempt to auto-create GenomicStudyAnalysisComponent.date"); 785 else if (Configuration.doAutoCreate()) 786 this.date = new DateTimeType(); // bb 787 return this.date; 788 } 789 790 public boolean hasDateElement() { 791 return this.date != null && !this.date.isEmpty(); 792 } 793 794 public boolean hasDate() { 795 return this.date != null && !this.date.isEmpty(); 796 } 797 798 /** 799 * @param value {@link #date} (The date of the analysis event.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 800 */ 801 public GenomicStudyAnalysisComponent setDateElement(DateTimeType value) { 802 this.date = value; 803 return this; 804 } 805 806 /** 807 * @return The date of the analysis event. 808 */ 809 public Date getDate() { 810 return this.date == null ? null : this.date.getValue(); 811 } 812 813 /** 814 * @param value The date of the analysis event. 815 */ 816 public GenomicStudyAnalysisComponent setDate(Date value) { 817 if (value == null) 818 this.date = null; 819 else { 820 if (this.date == null) 821 this.date = new DateTimeType(); 822 this.date.setValue(value); 823 } 824 return this; 825 } 826 827 /** 828 * @return {@link #note} (Any notes capture with the analysis event.) 829 */ 830 public List<Annotation> getNote() { 831 if (this.note == null) 832 this.note = new ArrayList<Annotation>(); 833 return this.note; 834 } 835 836 /** 837 * @return Returns a reference to <code>this</code> for easy method chaining 838 */ 839 public GenomicStudyAnalysisComponent setNote(List<Annotation> theNote) { 840 this.note = theNote; 841 return this; 842 } 843 844 public boolean hasNote() { 845 if (this.note == null) 846 return false; 847 for (Annotation item : this.note) 848 if (!item.isEmpty()) 849 return true; 850 return false; 851 } 852 853 public Annotation addNote() { //3 854 Annotation t = new Annotation(); 855 if (this.note == null) 856 this.note = new ArrayList<Annotation>(); 857 this.note.add(t); 858 return t; 859 } 860 861 public GenomicStudyAnalysisComponent addNote(Annotation t) { //3 862 if (t == null) 863 return this; 864 if (this.note == null) 865 this.note = new ArrayList<Annotation>(); 866 this.note.add(t); 867 return this; 868 } 869 870 /** 871 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 872 */ 873 public Annotation getNoteFirstRep() { 874 if (getNote().isEmpty()) { 875 addNote(); 876 } 877 return getNote().get(0); 878 } 879 880 /** 881 * @return {@link #protocolPerformed} (The protocol that was performed for the analysis event.) 882 */ 883 public Reference getProtocolPerformed() { 884 if (this.protocolPerformed == null) 885 if (Configuration.errorOnAutoCreate()) 886 throw new Error("Attempt to auto-create GenomicStudyAnalysisComponent.protocolPerformed"); 887 else if (Configuration.doAutoCreate()) 888 this.protocolPerformed = new Reference(); // cc 889 return this.protocolPerformed; 890 } 891 892 public boolean hasProtocolPerformed() { 893 return this.protocolPerformed != null && !this.protocolPerformed.isEmpty(); 894 } 895 896 /** 897 * @param value {@link #protocolPerformed} (The protocol that was performed for the analysis event.) 898 */ 899 public GenomicStudyAnalysisComponent setProtocolPerformed(Reference value) { 900 this.protocolPerformed = value; 901 return this; 902 } 903 904 /** 905 * @return {@link #regionsStudied} (The genomic regions to be studied in the analysis (BED file).) 906 */ 907 public List<Reference> getRegionsStudied() { 908 if (this.regionsStudied == null) 909 this.regionsStudied = new ArrayList<Reference>(); 910 return this.regionsStudied; 911 } 912 913 /** 914 * @return Returns a reference to <code>this</code> for easy method chaining 915 */ 916 public GenomicStudyAnalysisComponent setRegionsStudied(List<Reference> theRegionsStudied) { 917 this.regionsStudied = theRegionsStudied; 918 return this; 919 } 920 921 public boolean hasRegionsStudied() { 922 if (this.regionsStudied == null) 923 return false; 924 for (Reference item : this.regionsStudied) 925 if (!item.isEmpty()) 926 return true; 927 return false; 928 } 929 930 public Reference addRegionsStudied() { //3 931 Reference t = new Reference(); 932 if (this.regionsStudied == null) 933 this.regionsStudied = new ArrayList<Reference>(); 934 this.regionsStudied.add(t); 935 return t; 936 } 937 938 public GenomicStudyAnalysisComponent addRegionsStudied(Reference t) { //3 939 if (t == null) 940 return this; 941 if (this.regionsStudied == null) 942 this.regionsStudied = new ArrayList<Reference>(); 943 this.regionsStudied.add(t); 944 return this; 945 } 946 947 /** 948 * @return The first repetition of repeating field {@link #regionsStudied}, creating it if it does not already exist {3} 949 */ 950 public Reference getRegionsStudiedFirstRep() { 951 if (getRegionsStudied().isEmpty()) { 952 addRegionsStudied(); 953 } 954 return getRegionsStudied().get(0); 955 } 956 957 /** 958 * @return {@link #regionsCalled} (Genomic regions actually called in the analysis event (BED file).) 959 */ 960 public List<Reference> getRegionsCalled() { 961 if (this.regionsCalled == null) 962 this.regionsCalled = new ArrayList<Reference>(); 963 return this.regionsCalled; 964 } 965 966 /** 967 * @return Returns a reference to <code>this</code> for easy method chaining 968 */ 969 public GenomicStudyAnalysisComponent setRegionsCalled(List<Reference> theRegionsCalled) { 970 this.regionsCalled = theRegionsCalled; 971 return this; 972 } 973 974 public boolean hasRegionsCalled() { 975 if (this.regionsCalled == null) 976 return false; 977 for (Reference item : this.regionsCalled) 978 if (!item.isEmpty()) 979 return true; 980 return false; 981 } 982 983 public Reference addRegionsCalled() { //3 984 Reference t = new Reference(); 985 if (this.regionsCalled == null) 986 this.regionsCalled = new ArrayList<Reference>(); 987 this.regionsCalled.add(t); 988 return t; 989 } 990 991 public GenomicStudyAnalysisComponent addRegionsCalled(Reference t) { //3 992 if (t == null) 993 return this; 994 if (this.regionsCalled == null) 995 this.regionsCalled = new ArrayList<Reference>(); 996 this.regionsCalled.add(t); 997 return this; 998 } 999 1000 /** 1001 * @return The first repetition of repeating field {@link #regionsCalled}, creating it if it does not already exist {3} 1002 */ 1003 public Reference getRegionsCalledFirstRep() { 1004 if (getRegionsCalled().isEmpty()) { 1005 addRegionsCalled(); 1006 } 1007 return getRegionsCalled().get(0); 1008 } 1009 1010 /** 1011 * @return {@link #input} (Inputs for the analysis event.) 1012 */ 1013 public List<GenomicStudyAnalysisInputComponent> getInput() { 1014 if (this.input == null) 1015 this.input = new ArrayList<GenomicStudyAnalysisInputComponent>(); 1016 return this.input; 1017 } 1018 1019 /** 1020 * @return Returns a reference to <code>this</code> for easy method chaining 1021 */ 1022 public GenomicStudyAnalysisComponent setInput(List<GenomicStudyAnalysisInputComponent> theInput) { 1023 this.input = theInput; 1024 return this; 1025 } 1026 1027 public boolean hasInput() { 1028 if (this.input == null) 1029 return false; 1030 for (GenomicStudyAnalysisInputComponent item : this.input) 1031 if (!item.isEmpty()) 1032 return true; 1033 return false; 1034 } 1035 1036 public GenomicStudyAnalysisInputComponent addInput() { //3 1037 GenomicStudyAnalysisInputComponent t = new GenomicStudyAnalysisInputComponent(); 1038 if (this.input == null) 1039 this.input = new ArrayList<GenomicStudyAnalysisInputComponent>(); 1040 this.input.add(t); 1041 return t; 1042 } 1043 1044 public GenomicStudyAnalysisComponent addInput(GenomicStudyAnalysisInputComponent t) { //3 1045 if (t == null) 1046 return this; 1047 if (this.input == null) 1048 this.input = new ArrayList<GenomicStudyAnalysisInputComponent>(); 1049 this.input.add(t); 1050 return this; 1051 } 1052 1053 /** 1054 * @return The first repetition of repeating field {@link #input}, creating it if it does not already exist {3} 1055 */ 1056 public GenomicStudyAnalysisInputComponent getInputFirstRep() { 1057 if (getInput().isEmpty()) { 1058 addInput(); 1059 } 1060 return getInput().get(0); 1061 } 1062 1063 /** 1064 * @return {@link #output} (Outputs for the analysis event.) 1065 */ 1066 public List<GenomicStudyAnalysisOutputComponent> getOutput() { 1067 if (this.output == null) 1068 this.output = new ArrayList<GenomicStudyAnalysisOutputComponent>(); 1069 return this.output; 1070 } 1071 1072 /** 1073 * @return Returns a reference to <code>this</code> for easy method chaining 1074 */ 1075 public GenomicStudyAnalysisComponent setOutput(List<GenomicStudyAnalysisOutputComponent> theOutput) { 1076 this.output = theOutput; 1077 return this; 1078 } 1079 1080 public boolean hasOutput() { 1081 if (this.output == null) 1082 return false; 1083 for (GenomicStudyAnalysisOutputComponent item : this.output) 1084 if (!item.isEmpty()) 1085 return true; 1086 return false; 1087 } 1088 1089 public GenomicStudyAnalysisOutputComponent addOutput() { //3 1090 GenomicStudyAnalysisOutputComponent t = new GenomicStudyAnalysisOutputComponent(); 1091 if (this.output == null) 1092 this.output = new ArrayList<GenomicStudyAnalysisOutputComponent>(); 1093 this.output.add(t); 1094 return t; 1095 } 1096 1097 public GenomicStudyAnalysisComponent addOutput(GenomicStudyAnalysisOutputComponent t) { //3 1098 if (t == null) 1099 return this; 1100 if (this.output == null) 1101 this.output = new ArrayList<GenomicStudyAnalysisOutputComponent>(); 1102 this.output.add(t); 1103 return this; 1104 } 1105 1106 /** 1107 * @return The first repetition of repeating field {@link #output}, creating it if it does not already exist {3} 1108 */ 1109 public GenomicStudyAnalysisOutputComponent getOutputFirstRep() { 1110 if (getOutput().isEmpty()) { 1111 addOutput(); 1112 } 1113 return getOutput().get(0); 1114 } 1115 1116 /** 1117 * @return {@link #performer} (Performer for the analysis event.) 1118 */ 1119 public List<GenomicStudyAnalysisPerformerComponent> getPerformer() { 1120 if (this.performer == null) 1121 this.performer = new ArrayList<GenomicStudyAnalysisPerformerComponent>(); 1122 return this.performer; 1123 } 1124 1125 /** 1126 * @return Returns a reference to <code>this</code> for easy method chaining 1127 */ 1128 public GenomicStudyAnalysisComponent setPerformer(List<GenomicStudyAnalysisPerformerComponent> thePerformer) { 1129 this.performer = thePerformer; 1130 return this; 1131 } 1132 1133 public boolean hasPerformer() { 1134 if (this.performer == null) 1135 return false; 1136 for (GenomicStudyAnalysisPerformerComponent item : this.performer) 1137 if (!item.isEmpty()) 1138 return true; 1139 return false; 1140 } 1141 1142 public GenomicStudyAnalysisPerformerComponent addPerformer() { //3 1143 GenomicStudyAnalysisPerformerComponent t = new GenomicStudyAnalysisPerformerComponent(); 1144 if (this.performer == null) 1145 this.performer = new ArrayList<GenomicStudyAnalysisPerformerComponent>(); 1146 this.performer.add(t); 1147 return t; 1148 } 1149 1150 public GenomicStudyAnalysisComponent addPerformer(GenomicStudyAnalysisPerformerComponent t) { //3 1151 if (t == null) 1152 return this; 1153 if (this.performer == null) 1154 this.performer = new ArrayList<GenomicStudyAnalysisPerformerComponent>(); 1155 this.performer.add(t); 1156 return this; 1157 } 1158 1159 /** 1160 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 1161 */ 1162 public GenomicStudyAnalysisPerformerComponent getPerformerFirstRep() { 1163 if (getPerformer().isEmpty()) { 1164 addPerformer(); 1165 } 1166 return getPerformer().get(0); 1167 } 1168 1169 /** 1170 * @return {@link #device} (Devices used for the analysis (e.g., instruments, software), with settings and parameters.) 1171 */ 1172 public List<GenomicStudyAnalysisDeviceComponent> getDevice() { 1173 if (this.device == null) 1174 this.device = new ArrayList<GenomicStudyAnalysisDeviceComponent>(); 1175 return this.device; 1176 } 1177 1178 /** 1179 * @return Returns a reference to <code>this</code> for easy method chaining 1180 */ 1181 public GenomicStudyAnalysisComponent setDevice(List<GenomicStudyAnalysisDeviceComponent> theDevice) { 1182 this.device = theDevice; 1183 return this; 1184 } 1185 1186 public boolean hasDevice() { 1187 if (this.device == null) 1188 return false; 1189 for (GenomicStudyAnalysisDeviceComponent item : this.device) 1190 if (!item.isEmpty()) 1191 return true; 1192 return false; 1193 } 1194 1195 public GenomicStudyAnalysisDeviceComponent addDevice() { //3 1196 GenomicStudyAnalysisDeviceComponent t = new GenomicStudyAnalysisDeviceComponent(); 1197 if (this.device == null) 1198 this.device = new ArrayList<GenomicStudyAnalysisDeviceComponent>(); 1199 this.device.add(t); 1200 return t; 1201 } 1202 1203 public GenomicStudyAnalysisComponent addDevice(GenomicStudyAnalysisDeviceComponent t) { //3 1204 if (t == null) 1205 return this; 1206 if (this.device == null) 1207 this.device = new ArrayList<GenomicStudyAnalysisDeviceComponent>(); 1208 this.device.add(t); 1209 return this; 1210 } 1211 1212 /** 1213 * @return The first repetition of repeating field {@link #device}, creating it if it does not already exist {3} 1214 */ 1215 public GenomicStudyAnalysisDeviceComponent getDeviceFirstRep() { 1216 if (getDevice().isEmpty()) { 1217 addDevice(); 1218 } 1219 return getDevice().get(0); 1220 } 1221 1222 protected void listChildren(List<Property> children) { 1223 super.listChildren(children); 1224 children.add(new Property("identifier", "Identifier", "Identifiers for the analysis event.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1225 children.add(new Property("methodType", "CodeableConcept", "Type of the methods used in the analysis, e.g., Fluorescence in situ hybridization (FISH), Karyotyping, or Microsatellite instability testing (MSI).", 0, java.lang.Integer.MAX_VALUE, methodType)); 1226 children.add(new Property("changeType", "CodeableConcept", "Type of the genomic changes studied in the analysis, e.g., DNA, RNA, or amino acid change.", 0, java.lang.Integer.MAX_VALUE, changeType)); 1227 children.add(new Property("genomeBuild", "CodeableConcept", "The reference genome build that is used in this analysis.", 0, 1, genomeBuild)); 1228 children.add(new Property("instantiatesCanonical", "canonical(PlanDefinition|ActivityDefinition)", "The defined protocol that describes the analysis.", 0, 1, instantiatesCanonical)); 1229 children.add(new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol that describes the analysis.", 0, 1, instantiatesUri)); 1230 children.add(new Property("title", "string", "Name of the analysis event (human friendly).", 0, 1, title)); 1231 children.add(new Property("focus", "Reference(Any)", "The focus of a genomic analysis when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, child, or sibling. For example, in trio testing, the GenomicStudy.subject would be the child (proband) and the GenomicStudy.analysis.focus of a specific analysis would be the parent.", 0, java.lang.Integer.MAX_VALUE, focus)); 1232 children.add(new Property("specimen", "Reference(Specimen)", "The specimen used in the analysis event.", 0, java.lang.Integer.MAX_VALUE, specimen)); 1233 children.add(new Property("date", "dateTime", "The date of the analysis event.", 0, 1, date)); 1234 children.add(new Property("note", "Annotation", "Any notes capture with the analysis event.", 0, java.lang.Integer.MAX_VALUE, note)); 1235 children.add(new Property("protocolPerformed", "Reference(Procedure|Task)", "The protocol that was performed for the analysis event.", 0, 1, protocolPerformed)); 1236 children.add(new Property("regionsStudied", "Reference(DocumentReference|Observation)", "The genomic regions to be studied in the analysis (BED file).", 0, java.lang.Integer.MAX_VALUE, regionsStudied)); 1237 children.add(new Property("regionsCalled", "Reference(DocumentReference|Observation)", "Genomic regions actually called in the analysis event (BED file).", 0, java.lang.Integer.MAX_VALUE, regionsCalled)); 1238 children.add(new Property("input", "", "Inputs for the analysis event.", 0, java.lang.Integer.MAX_VALUE, input)); 1239 children.add(new Property("output", "", "Outputs for the analysis event.", 0, java.lang.Integer.MAX_VALUE, output)); 1240 children.add(new Property("performer", "", "Performer for the analysis event.", 0, java.lang.Integer.MAX_VALUE, performer)); 1241 children.add(new Property("device", "", "Devices used for the analysis (e.g., instruments, software), with settings and parameters.", 0, java.lang.Integer.MAX_VALUE, device)); 1242 } 1243 1244 @Override 1245 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1246 switch (_hash) { 1247 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers for the analysis event.", 0, java.lang.Integer.MAX_VALUE, identifier); 1248 case -722961477: /*methodType*/ return new Property("methodType", "CodeableConcept", "Type of the methods used in the analysis, e.g., Fluorescence in situ hybridization (FISH), Karyotyping, or Microsatellite instability testing (MSI).", 0, java.lang.Integer.MAX_VALUE, methodType); 1249 case -2131902710: /*changeType*/ return new Property("changeType", "CodeableConcept", "Type of the genomic changes studied in the analysis, e.g., DNA, RNA, or amino acid change.", 0, java.lang.Integer.MAX_VALUE, changeType); 1250 case 1061239735: /*genomeBuild*/ return new Property("genomeBuild", "CodeableConcept", "The reference genome build that is used in this analysis.", 0, 1, genomeBuild); 1251 case 8911915: /*instantiatesCanonical*/ return new Property("instantiatesCanonical", "canonical(PlanDefinition|ActivityDefinition)", "The defined protocol that describes the analysis.", 0, 1, instantiatesCanonical); 1252 case -1926393373: /*instantiatesUri*/ return new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol that describes the analysis.", 0, 1, instantiatesUri); 1253 case 110371416: /*title*/ return new Property("title", "string", "Name of the analysis event (human friendly).", 0, 1, title); 1254 case 97604824: /*focus*/ return new Property("focus", "Reference(Any)", "The focus of a genomic analysis when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, child, or sibling. For example, in trio testing, the GenomicStudy.subject would be the child (proband) and the GenomicStudy.analysis.focus of a specific analysis would be the parent.", 0, java.lang.Integer.MAX_VALUE, focus); 1255 case -2132868344: /*specimen*/ return new Property("specimen", "Reference(Specimen)", "The specimen used in the analysis event.", 0, java.lang.Integer.MAX_VALUE, specimen); 1256 case 3076014: /*date*/ return new Property("date", "dateTime", "The date of the analysis event.", 0, 1, date); 1257 case 3387378: /*note*/ return new Property("note", "Annotation", "Any notes capture with the analysis event.", 0, java.lang.Integer.MAX_VALUE, note); 1258 case -1565516792: /*protocolPerformed*/ return new Property("protocolPerformed", "Reference(Procedure|Task)", "The protocol that was performed for the analysis event.", 0, 1, protocolPerformed); 1259 case 391791385: /*regionsStudied*/ return new Property("regionsStudied", "Reference(DocumentReference|Observation)", "The genomic regions to be studied in the analysis (BED file).", 0, java.lang.Integer.MAX_VALUE, regionsStudied); 1260 case -2125803428: /*regionsCalled*/ return new Property("regionsCalled", "Reference(DocumentReference|Observation)", "Genomic regions actually called in the analysis event (BED file).", 0, java.lang.Integer.MAX_VALUE, regionsCalled); 1261 case 100358090: /*input*/ return new Property("input", "", "Inputs for the analysis event.", 0, java.lang.Integer.MAX_VALUE, input); 1262 case -1005512447: /*output*/ return new Property("output", "", "Outputs for the analysis event.", 0, java.lang.Integer.MAX_VALUE, output); 1263 case 481140686: /*performer*/ return new Property("performer", "", "Performer for the analysis event.", 0, java.lang.Integer.MAX_VALUE, performer); 1264 case -1335157162: /*device*/ return new Property("device", "", "Devices used for the analysis (e.g., instruments, software), with settings and parameters.", 0, java.lang.Integer.MAX_VALUE, device); 1265 default: return super.getNamedProperty(_hash, _name, _checkValid); 1266 } 1267 1268 } 1269 1270 @Override 1271 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1272 switch (hash) { 1273 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1274 case -722961477: /*methodType*/ return this.methodType == null ? new Base[0] : this.methodType.toArray(new Base[this.methodType.size()]); // CodeableConcept 1275 case -2131902710: /*changeType*/ return this.changeType == null ? new Base[0] : this.changeType.toArray(new Base[this.changeType.size()]); // CodeableConcept 1276 case 1061239735: /*genomeBuild*/ return this.genomeBuild == null ? new Base[0] : new Base[] {this.genomeBuild}; // CodeableConcept 1277 case 8911915: /*instantiatesCanonical*/ return this.instantiatesCanonical == null ? new Base[0] : new Base[] {this.instantiatesCanonical}; // CanonicalType 1278 case -1926393373: /*instantiatesUri*/ return this.instantiatesUri == null ? new Base[0] : new Base[] {this.instantiatesUri}; // UriType 1279 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1280 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // Reference 1281 case -2132868344: /*specimen*/ return this.specimen == null ? new Base[0] : this.specimen.toArray(new Base[this.specimen.size()]); // Reference 1282 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1283 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1284 case -1565516792: /*protocolPerformed*/ return this.protocolPerformed == null ? new Base[0] : new Base[] {this.protocolPerformed}; // Reference 1285 case 391791385: /*regionsStudied*/ return this.regionsStudied == null ? new Base[0] : this.regionsStudied.toArray(new Base[this.regionsStudied.size()]); // Reference 1286 case -2125803428: /*regionsCalled*/ return this.regionsCalled == null ? new Base[0] : this.regionsCalled.toArray(new Base[this.regionsCalled.size()]); // Reference 1287 case 100358090: /*input*/ return this.input == null ? new Base[0] : this.input.toArray(new Base[this.input.size()]); // GenomicStudyAnalysisInputComponent 1288 case -1005512447: /*output*/ return this.output == null ? new Base[0] : this.output.toArray(new Base[this.output.size()]); // GenomicStudyAnalysisOutputComponent 1289 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // GenomicStudyAnalysisPerformerComponent 1290 case -1335157162: /*device*/ return this.device == null ? new Base[0] : this.device.toArray(new Base[this.device.size()]); // GenomicStudyAnalysisDeviceComponent 1291 default: return super.getProperty(hash, name, checkValid); 1292 } 1293 1294 } 1295 1296 @Override 1297 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1298 switch (hash) { 1299 case -1618432855: // identifier 1300 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1301 return value; 1302 case -722961477: // methodType 1303 this.getMethodType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1304 return value; 1305 case -2131902710: // changeType 1306 this.getChangeType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1307 return value; 1308 case 1061239735: // genomeBuild 1309 this.genomeBuild = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1310 return value; 1311 case 8911915: // instantiatesCanonical 1312 this.instantiatesCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 1313 return value; 1314 case -1926393373: // instantiatesUri 1315 this.instantiatesUri = TypeConvertor.castToUri(value); // UriType 1316 return value; 1317 case 110371416: // title 1318 this.title = TypeConvertor.castToString(value); // StringType 1319 return value; 1320 case 97604824: // focus 1321 this.getFocus().add(TypeConvertor.castToReference(value)); // Reference 1322 return value; 1323 case -2132868344: // specimen 1324 this.getSpecimen().add(TypeConvertor.castToReference(value)); // Reference 1325 return value; 1326 case 3076014: // date 1327 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1328 return value; 1329 case 3387378: // note 1330 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1331 return value; 1332 case -1565516792: // protocolPerformed 1333 this.protocolPerformed = TypeConvertor.castToReference(value); // Reference 1334 return value; 1335 case 391791385: // regionsStudied 1336 this.getRegionsStudied().add(TypeConvertor.castToReference(value)); // Reference 1337 return value; 1338 case -2125803428: // regionsCalled 1339 this.getRegionsCalled().add(TypeConvertor.castToReference(value)); // Reference 1340 return value; 1341 case 100358090: // input 1342 this.getInput().add((GenomicStudyAnalysisInputComponent) value); // GenomicStudyAnalysisInputComponent 1343 return value; 1344 case -1005512447: // output 1345 this.getOutput().add((GenomicStudyAnalysisOutputComponent) value); // GenomicStudyAnalysisOutputComponent 1346 return value; 1347 case 481140686: // performer 1348 this.getPerformer().add((GenomicStudyAnalysisPerformerComponent) value); // GenomicStudyAnalysisPerformerComponent 1349 return value; 1350 case -1335157162: // device 1351 this.getDevice().add((GenomicStudyAnalysisDeviceComponent) value); // GenomicStudyAnalysisDeviceComponent 1352 return value; 1353 default: return super.setProperty(hash, name, value); 1354 } 1355 1356 } 1357 1358 @Override 1359 public Base setProperty(String name, Base value) throws FHIRException { 1360 if (name.equals("identifier")) { 1361 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1362 } else if (name.equals("methodType")) { 1363 this.getMethodType().add(TypeConvertor.castToCodeableConcept(value)); 1364 } else if (name.equals("changeType")) { 1365 this.getChangeType().add(TypeConvertor.castToCodeableConcept(value)); 1366 } else if (name.equals("genomeBuild")) { 1367 this.genomeBuild = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1368 } else if (name.equals("instantiatesCanonical")) { 1369 this.instantiatesCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 1370 } else if (name.equals("instantiatesUri")) { 1371 this.instantiatesUri = TypeConvertor.castToUri(value); // UriType 1372 } else if (name.equals("title")) { 1373 this.title = TypeConvertor.castToString(value); // StringType 1374 } else if (name.equals("focus")) { 1375 this.getFocus().add(TypeConvertor.castToReference(value)); 1376 } else if (name.equals("specimen")) { 1377 this.getSpecimen().add(TypeConvertor.castToReference(value)); 1378 } else if (name.equals("date")) { 1379 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1380 } else if (name.equals("note")) { 1381 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1382 } else if (name.equals("protocolPerformed")) { 1383 this.protocolPerformed = TypeConvertor.castToReference(value); // Reference 1384 } else if (name.equals("regionsStudied")) { 1385 this.getRegionsStudied().add(TypeConvertor.castToReference(value)); 1386 } else if (name.equals("regionsCalled")) { 1387 this.getRegionsCalled().add(TypeConvertor.castToReference(value)); 1388 } else if (name.equals("input")) { 1389 this.getInput().add((GenomicStudyAnalysisInputComponent) value); 1390 } else if (name.equals("output")) { 1391 this.getOutput().add((GenomicStudyAnalysisOutputComponent) value); 1392 } else if (name.equals("performer")) { 1393 this.getPerformer().add((GenomicStudyAnalysisPerformerComponent) value); 1394 } else if (name.equals("device")) { 1395 this.getDevice().add((GenomicStudyAnalysisDeviceComponent) value); 1396 } else 1397 return super.setProperty(name, value); 1398 return value; 1399 } 1400 1401 @Override 1402 public void removeChild(String name, Base value) throws FHIRException { 1403 if (name.equals("identifier")) { 1404 this.getIdentifier().remove(value); 1405 } else if (name.equals("methodType")) { 1406 this.getMethodType().remove(value); 1407 } else if (name.equals("changeType")) { 1408 this.getChangeType().remove(value); 1409 } else if (name.equals("genomeBuild")) { 1410 this.genomeBuild = null; 1411 } else if (name.equals("instantiatesCanonical")) { 1412 this.instantiatesCanonical = null; 1413 } else if (name.equals("instantiatesUri")) { 1414 this.instantiatesUri = null; 1415 } else if (name.equals("title")) { 1416 this.title = null; 1417 } else if (name.equals("focus")) { 1418 this.getFocus().remove(value); 1419 } else if (name.equals("specimen")) { 1420 this.getSpecimen().remove(value); 1421 } else if (name.equals("date")) { 1422 this.date = null; 1423 } else if (name.equals("note")) { 1424 this.getNote().remove(value); 1425 } else if (name.equals("protocolPerformed")) { 1426 this.protocolPerformed = null; 1427 } else if (name.equals("regionsStudied")) { 1428 this.getRegionsStudied().remove(value); 1429 } else if (name.equals("regionsCalled")) { 1430 this.getRegionsCalled().remove(value); 1431 } else if (name.equals("input")) { 1432 this.getInput().remove((GenomicStudyAnalysisInputComponent) value); 1433 } else if (name.equals("output")) { 1434 this.getOutput().remove((GenomicStudyAnalysisOutputComponent) value); 1435 } else if (name.equals("performer")) { 1436 this.getPerformer().remove((GenomicStudyAnalysisPerformerComponent) value); 1437 } else if (name.equals("device")) { 1438 this.getDevice().remove((GenomicStudyAnalysisDeviceComponent) value); 1439 } else 1440 super.removeChild(name, value); 1441 1442 } 1443 1444 @Override 1445 public Base makeProperty(int hash, String name) throws FHIRException { 1446 switch (hash) { 1447 case -1618432855: return addIdentifier(); 1448 case -722961477: return addMethodType(); 1449 case -2131902710: return addChangeType(); 1450 case 1061239735: return getGenomeBuild(); 1451 case 8911915: return getInstantiatesCanonicalElement(); 1452 case -1926393373: return getInstantiatesUriElement(); 1453 case 110371416: return getTitleElement(); 1454 case 97604824: return addFocus(); 1455 case -2132868344: return addSpecimen(); 1456 case 3076014: return getDateElement(); 1457 case 3387378: return addNote(); 1458 case -1565516792: return getProtocolPerformed(); 1459 case 391791385: return addRegionsStudied(); 1460 case -2125803428: return addRegionsCalled(); 1461 case 100358090: return addInput(); 1462 case -1005512447: return addOutput(); 1463 case 481140686: return addPerformer(); 1464 case -1335157162: return addDevice(); 1465 default: return super.makeProperty(hash, name); 1466 } 1467 1468 } 1469 1470 @Override 1471 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1472 switch (hash) { 1473 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1474 case -722961477: /*methodType*/ return new String[] {"CodeableConcept"}; 1475 case -2131902710: /*changeType*/ return new String[] {"CodeableConcept"}; 1476 case 1061239735: /*genomeBuild*/ return new String[] {"CodeableConcept"}; 1477 case 8911915: /*instantiatesCanonical*/ return new String[] {"canonical"}; 1478 case -1926393373: /*instantiatesUri*/ return new String[] {"uri"}; 1479 case 110371416: /*title*/ return new String[] {"string"}; 1480 case 97604824: /*focus*/ return new String[] {"Reference"}; 1481 case -2132868344: /*specimen*/ return new String[] {"Reference"}; 1482 case 3076014: /*date*/ return new String[] {"dateTime"}; 1483 case 3387378: /*note*/ return new String[] {"Annotation"}; 1484 case -1565516792: /*protocolPerformed*/ return new String[] {"Reference"}; 1485 case 391791385: /*regionsStudied*/ return new String[] {"Reference"}; 1486 case -2125803428: /*regionsCalled*/ return new String[] {"Reference"}; 1487 case 100358090: /*input*/ return new String[] {}; 1488 case -1005512447: /*output*/ return new String[] {}; 1489 case 481140686: /*performer*/ return new String[] {}; 1490 case -1335157162: /*device*/ return new String[] {}; 1491 default: return super.getTypesForProperty(hash, name); 1492 } 1493 1494 } 1495 1496 @Override 1497 public Base addChild(String name) throws FHIRException { 1498 if (name.equals("identifier")) { 1499 return addIdentifier(); 1500 } 1501 else if (name.equals("methodType")) { 1502 return addMethodType(); 1503 } 1504 else if (name.equals("changeType")) { 1505 return addChangeType(); 1506 } 1507 else if (name.equals("genomeBuild")) { 1508 this.genomeBuild = new CodeableConcept(); 1509 return this.genomeBuild; 1510 } 1511 else if (name.equals("instantiatesCanonical")) { 1512 throw new FHIRException("Cannot call addChild on a singleton property GenomicStudy.analysis.instantiatesCanonical"); 1513 } 1514 else if (name.equals("instantiatesUri")) { 1515 throw new FHIRException("Cannot call addChild on a singleton property GenomicStudy.analysis.instantiatesUri"); 1516 } 1517 else if (name.equals("title")) { 1518 throw new FHIRException("Cannot call addChild on a singleton property GenomicStudy.analysis.title"); 1519 } 1520 else if (name.equals("focus")) { 1521 return addFocus(); 1522 } 1523 else if (name.equals("specimen")) { 1524 return addSpecimen(); 1525 } 1526 else if (name.equals("date")) { 1527 throw new FHIRException("Cannot call addChild on a singleton property GenomicStudy.analysis.date"); 1528 } 1529 else if (name.equals("note")) { 1530 return addNote(); 1531 } 1532 else if (name.equals("protocolPerformed")) { 1533 this.protocolPerformed = new Reference(); 1534 return this.protocolPerformed; 1535 } 1536 else if (name.equals("regionsStudied")) { 1537 return addRegionsStudied(); 1538 } 1539 else if (name.equals("regionsCalled")) { 1540 return addRegionsCalled(); 1541 } 1542 else if (name.equals("input")) { 1543 return addInput(); 1544 } 1545 else if (name.equals("output")) { 1546 return addOutput(); 1547 } 1548 else if (name.equals("performer")) { 1549 return addPerformer(); 1550 } 1551 else if (name.equals("device")) { 1552 return addDevice(); 1553 } 1554 else 1555 return super.addChild(name); 1556 } 1557 1558 public GenomicStudyAnalysisComponent copy() { 1559 GenomicStudyAnalysisComponent dst = new GenomicStudyAnalysisComponent(); 1560 copyValues(dst); 1561 return dst; 1562 } 1563 1564 public void copyValues(GenomicStudyAnalysisComponent dst) { 1565 super.copyValues(dst); 1566 if (identifier != null) { 1567 dst.identifier = new ArrayList<Identifier>(); 1568 for (Identifier i : identifier) 1569 dst.identifier.add(i.copy()); 1570 }; 1571 if (methodType != null) { 1572 dst.methodType = new ArrayList<CodeableConcept>(); 1573 for (CodeableConcept i : methodType) 1574 dst.methodType.add(i.copy()); 1575 }; 1576 if (changeType != null) { 1577 dst.changeType = new ArrayList<CodeableConcept>(); 1578 for (CodeableConcept i : changeType) 1579 dst.changeType.add(i.copy()); 1580 }; 1581 dst.genomeBuild = genomeBuild == null ? null : genomeBuild.copy(); 1582 dst.instantiatesCanonical = instantiatesCanonical == null ? null : instantiatesCanonical.copy(); 1583 dst.instantiatesUri = instantiatesUri == null ? null : instantiatesUri.copy(); 1584 dst.title = title == null ? null : title.copy(); 1585 if (focus != null) { 1586 dst.focus = new ArrayList<Reference>(); 1587 for (Reference i : focus) 1588 dst.focus.add(i.copy()); 1589 }; 1590 if (specimen != null) { 1591 dst.specimen = new ArrayList<Reference>(); 1592 for (Reference i : specimen) 1593 dst.specimen.add(i.copy()); 1594 }; 1595 dst.date = date == null ? null : date.copy(); 1596 if (note != null) { 1597 dst.note = new ArrayList<Annotation>(); 1598 for (Annotation i : note) 1599 dst.note.add(i.copy()); 1600 }; 1601 dst.protocolPerformed = protocolPerformed == null ? null : protocolPerformed.copy(); 1602 if (regionsStudied != null) { 1603 dst.regionsStudied = new ArrayList<Reference>(); 1604 for (Reference i : regionsStudied) 1605 dst.regionsStudied.add(i.copy()); 1606 }; 1607 if (regionsCalled != null) { 1608 dst.regionsCalled = new ArrayList<Reference>(); 1609 for (Reference i : regionsCalled) 1610 dst.regionsCalled.add(i.copy()); 1611 }; 1612 if (input != null) { 1613 dst.input = new ArrayList<GenomicStudyAnalysisInputComponent>(); 1614 for (GenomicStudyAnalysisInputComponent i : input) 1615 dst.input.add(i.copy()); 1616 }; 1617 if (output != null) { 1618 dst.output = new ArrayList<GenomicStudyAnalysisOutputComponent>(); 1619 for (GenomicStudyAnalysisOutputComponent i : output) 1620 dst.output.add(i.copy()); 1621 }; 1622 if (performer != null) { 1623 dst.performer = new ArrayList<GenomicStudyAnalysisPerformerComponent>(); 1624 for (GenomicStudyAnalysisPerformerComponent i : performer) 1625 dst.performer.add(i.copy()); 1626 }; 1627 if (device != null) { 1628 dst.device = new ArrayList<GenomicStudyAnalysisDeviceComponent>(); 1629 for (GenomicStudyAnalysisDeviceComponent i : device) 1630 dst.device.add(i.copy()); 1631 }; 1632 } 1633 1634 @Override 1635 public boolean equalsDeep(Base other_) { 1636 if (!super.equalsDeep(other_)) 1637 return false; 1638 if (!(other_ instanceof GenomicStudyAnalysisComponent)) 1639 return false; 1640 GenomicStudyAnalysisComponent o = (GenomicStudyAnalysisComponent) other_; 1641 return compareDeep(identifier, o.identifier, true) && compareDeep(methodType, o.methodType, true) 1642 && compareDeep(changeType, o.changeType, true) && compareDeep(genomeBuild, o.genomeBuild, true) 1643 && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) && compareDeep(instantiatesUri, o.instantiatesUri, true) 1644 && compareDeep(title, o.title, true) && compareDeep(focus, o.focus, true) && compareDeep(specimen, o.specimen, true) 1645 && compareDeep(date, o.date, true) && compareDeep(note, o.note, true) && compareDeep(protocolPerformed, o.protocolPerformed, true) 1646 && compareDeep(regionsStudied, o.regionsStudied, true) && compareDeep(regionsCalled, o.regionsCalled, true) 1647 && compareDeep(input, o.input, true) && compareDeep(output, o.output, true) && compareDeep(performer, o.performer, true) 1648 && compareDeep(device, o.device, true); 1649 } 1650 1651 @Override 1652 public boolean equalsShallow(Base other_) { 1653 if (!super.equalsShallow(other_)) 1654 return false; 1655 if (!(other_ instanceof GenomicStudyAnalysisComponent)) 1656 return false; 1657 GenomicStudyAnalysisComponent o = (GenomicStudyAnalysisComponent) other_; 1658 return compareValues(instantiatesCanonical, o.instantiatesCanonical, true) && compareValues(instantiatesUri, o.instantiatesUri, true) 1659 && compareValues(title, o.title, true) && compareValues(date, o.date, true); 1660 } 1661 1662 public boolean isEmpty() { 1663 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, methodType, changeType 1664 , genomeBuild, instantiatesCanonical, instantiatesUri, title, focus, specimen, date 1665 , note, protocolPerformed, regionsStudied, regionsCalled, input, output, performer 1666 , device); 1667 } 1668 1669 public String fhirType() { 1670 return "GenomicStudy.analysis"; 1671 1672 } 1673 1674 } 1675 1676 @Block() 1677 public static class GenomicStudyAnalysisInputComponent extends BackboneElement implements IBaseBackboneElement { 1678 /** 1679 * File containing input data. 1680 */ 1681 @Child(name = "file", type = {DocumentReference.class}, order=1, min=0, max=1, modifier=false, summary=true) 1682 @Description(shortDefinition="File containing input data", formalDefinition="File containing input data." ) 1683 protected Reference file; 1684 1685 /** 1686 * Type of input data, e.g., BAM, CRAM, or FASTA. 1687 */ 1688 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1689 @Description(shortDefinition="Type of input data (e.g., BAM, CRAM, or FASTA)", formalDefinition="Type of input data, e.g., BAM, CRAM, or FASTA." ) 1690 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/genomicstudy-dataformat") 1691 protected CodeableConcept type; 1692 1693 /** 1694 * The analysis event or other GenomicStudy that generated this input file. 1695 */ 1696 @Child(name = "generatedBy", type = {Identifier.class, GenomicStudy.class}, order=3, min=0, max=1, modifier=false, summary=false) 1697 @Description(shortDefinition="The analysis event or other GenomicStudy that generated this input file", formalDefinition="The analysis event or other GenomicStudy that generated this input file." ) 1698 protected DataType generatedBy; 1699 1700 private static final long serialVersionUID = -650883036L; 1701 1702 /** 1703 * Constructor 1704 */ 1705 public GenomicStudyAnalysisInputComponent() { 1706 super(); 1707 } 1708 1709 /** 1710 * @return {@link #file} (File containing input data.) 1711 */ 1712 public Reference getFile() { 1713 if (this.file == null) 1714 if (Configuration.errorOnAutoCreate()) 1715 throw new Error("Attempt to auto-create GenomicStudyAnalysisInputComponent.file"); 1716 else if (Configuration.doAutoCreate()) 1717 this.file = new Reference(); // cc 1718 return this.file; 1719 } 1720 1721 public boolean hasFile() { 1722 return this.file != null && !this.file.isEmpty(); 1723 } 1724 1725 /** 1726 * @param value {@link #file} (File containing input data.) 1727 */ 1728 public GenomicStudyAnalysisInputComponent setFile(Reference value) { 1729 this.file = value; 1730 return this; 1731 } 1732 1733 /** 1734 * @return {@link #type} (Type of input data, e.g., BAM, CRAM, or FASTA.) 1735 */ 1736 public CodeableConcept getType() { 1737 if (this.type == null) 1738 if (Configuration.errorOnAutoCreate()) 1739 throw new Error("Attempt to auto-create GenomicStudyAnalysisInputComponent.type"); 1740 else if (Configuration.doAutoCreate()) 1741 this.type = new CodeableConcept(); // cc 1742 return this.type; 1743 } 1744 1745 public boolean hasType() { 1746 return this.type != null && !this.type.isEmpty(); 1747 } 1748 1749 /** 1750 * @param value {@link #type} (Type of input data, e.g., BAM, CRAM, or FASTA.) 1751 */ 1752 public GenomicStudyAnalysisInputComponent setType(CodeableConcept value) { 1753 this.type = value; 1754 return this; 1755 } 1756 1757 /** 1758 * @return {@link #generatedBy} (The analysis event or other GenomicStudy that generated this input file.) 1759 */ 1760 public DataType getGeneratedBy() { 1761 return this.generatedBy; 1762 } 1763 1764 /** 1765 * @return {@link #generatedBy} (The analysis event or other GenomicStudy that generated this input file.) 1766 */ 1767 public Identifier getGeneratedByIdentifier() throws FHIRException { 1768 if (this.generatedBy == null) 1769 this.generatedBy = new Identifier(); 1770 if (!(this.generatedBy instanceof Identifier)) 1771 throw new FHIRException("Type mismatch: the type Identifier was expected, but "+this.generatedBy.getClass().getName()+" was encountered"); 1772 return (Identifier) this.generatedBy; 1773 } 1774 1775 public boolean hasGeneratedByIdentifier() { 1776 return this != null && this.generatedBy instanceof Identifier; 1777 } 1778 1779 /** 1780 * @return {@link #generatedBy} (The analysis event or other GenomicStudy that generated this input file.) 1781 */ 1782 public Reference getGeneratedByReference() throws FHIRException { 1783 if (this.generatedBy == null) 1784 this.generatedBy = new Reference(); 1785 if (!(this.generatedBy instanceof Reference)) 1786 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.generatedBy.getClass().getName()+" was encountered"); 1787 return (Reference) this.generatedBy; 1788 } 1789 1790 public boolean hasGeneratedByReference() { 1791 return this != null && this.generatedBy instanceof Reference; 1792 } 1793 1794 public boolean hasGeneratedBy() { 1795 return this.generatedBy != null && !this.generatedBy.isEmpty(); 1796 } 1797 1798 /** 1799 * @param value {@link #generatedBy} (The analysis event or other GenomicStudy that generated this input file.) 1800 */ 1801 public GenomicStudyAnalysisInputComponent setGeneratedBy(DataType value) { 1802 if (value != null && !(value instanceof Identifier || value instanceof Reference)) 1803 throw new FHIRException("Not the right type for GenomicStudy.analysis.input.generatedBy[x]: "+value.fhirType()); 1804 this.generatedBy = value; 1805 return this; 1806 } 1807 1808 protected void listChildren(List<Property> children) { 1809 super.listChildren(children); 1810 children.add(new Property("file", "Reference(DocumentReference)", "File containing input data.", 0, 1, file)); 1811 children.add(new Property("type", "CodeableConcept", "Type of input data, e.g., BAM, CRAM, or FASTA.", 0, 1, type)); 1812 children.add(new Property("generatedBy[x]", "Identifier|Reference(GenomicStudy)", "The analysis event or other GenomicStudy that generated this input file.", 0, 1, generatedBy)); 1813 } 1814 1815 @Override 1816 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1817 switch (_hash) { 1818 case 3143036: /*file*/ return new Property("file", "Reference(DocumentReference)", "File containing input data.", 0, 1, file); 1819 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of input data, e.g., BAM, CRAM, or FASTA.", 0, 1, type); 1820 case -1669563270: /*generatedBy[x]*/ return new Property("generatedBy[x]", "Identifier|Reference(GenomicStudy)", "The analysis event or other GenomicStudy that generated this input file.", 0, 1, generatedBy); 1821 case 886733382: /*generatedBy*/ return new Property("generatedBy[x]", "Identifier|Reference(GenomicStudy)", "The analysis event or other GenomicStudy that generated this input file.", 0, 1, generatedBy); 1822 case 913138575: /*generatedByIdentifier*/ return new Property("generatedBy[x]", "Identifier", "The analysis event or other GenomicStudy that generated this input file.", 0, 1, generatedBy); 1823 case -1397681243: /*generatedByReference*/ return new Property("generatedBy[x]", "Reference(GenomicStudy)", "The analysis event or other GenomicStudy that generated this input file.", 0, 1, generatedBy); 1824 default: return super.getNamedProperty(_hash, _name, _checkValid); 1825 } 1826 1827 } 1828 1829 @Override 1830 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1831 switch (hash) { 1832 case 3143036: /*file*/ return this.file == null ? new Base[0] : new Base[] {this.file}; // Reference 1833 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1834 case 886733382: /*generatedBy*/ return this.generatedBy == null ? new Base[0] : new Base[] {this.generatedBy}; // DataType 1835 default: return super.getProperty(hash, name, checkValid); 1836 } 1837 1838 } 1839 1840 @Override 1841 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1842 switch (hash) { 1843 case 3143036: // file 1844 this.file = TypeConvertor.castToReference(value); // Reference 1845 return value; 1846 case 3575610: // type 1847 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1848 return value; 1849 case 886733382: // generatedBy 1850 this.generatedBy = TypeConvertor.castToType(value); // DataType 1851 return value; 1852 default: return super.setProperty(hash, name, value); 1853 } 1854 1855 } 1856 1857 @Override 1858 public Base setProperty(String name, Base value) throws FHIRException { 1859 if (name.equals("file")) { 1860 this.file = TypeConvertor.castToReference(value); // Reference 1861 } else if (name.equals("type")) { 1862 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1863 } else if (name.equals("generatedBy[x]")) { 1864 this.generatedBy = TypeConvertor.castToType(value); // DataType 1865 } else 1866 return super.setProperty(name, value); 1867 return value; 1868 } 1869 1870 @Override 1871 public void removeChild(String name, Base value) throws FHIRException { 1872 if (name.equals("file")) { 1873 this.file = null; 1874 } else if (name.equals("type")) { 1875 this.type = null; 1876 } else if (name.equals("generatedBy[x]")) { 1877 this.generatedBy = null; 1878 } else 1879 super.removeChild(name, value); 1880 1881 } 1882 1883 @Override 1884 public Base makeProperty(int hash, String name) throws FHIRException { 1885 switch (hash) { 1886 case 3143036: return getFile(); 1887 case 3575610: return getType(); 1888 case -1669563270: return getGeneratedBy(); 1889 case 886733382: return getGeneratedBy(); 1890 default: return super.makeProperty(hash, name); 1891 } 1892 1893 } 1894 1895 @Override 1896 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1897 switch (hash) { 1898 case 3143036: /*file*/ return new String[] {"Reference"}; 1899 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1900 case 886733382: /*generatedBy*/ return new String[] {"Identifier", "Reference"}; 1901 default: return super.getTypesForProperty(hash, name); 1902 } 1903 1904 } 1905 1906 @Override 1907 public Base addChild(String name) throws FHIRException { 1908 if (name.equals("file")) { 1909 this.file = new Reference(); 1910 return this.file; 1911 } 1912 else if (name.equals("type")) { 1913 this.type = new CodeableConcept(); 1914 return this.type; 1915 } 1916 else if (name.equals("generatedByIdentifier")) { 1917 this.generatedBy = new Identifier(); 1918 return this.generatedBy; 1919 } 1920 else if (name.equals("generatedByReference")) { 1921 this.generatedBy = new Reference(); 1922 return this.generatedBy; 1923 } 1924 else 1925 return super.addChild(name); 1926 } 1927 1928 public GenomicStudyAnalysisInputComponent copy() { 1929 GenomicStudyAnalysisInputComponent dst = new GenomicStudyAnalysisInputComponent(); 1930 copyValues(dst); 1931 return dst; 1932 } 1933 1934 public void copyValues(GenomicStudyAnalysisInputComponent dst) { 1935 super.copyValues(dst); 1936 dst.file = file == null ? null : file.copy(); 1937 dst.type = type == null ? null : type.copy(); 1938 dst.generatedBy = generatedBy == null ? null : generatedBy.copy(); 1939 } 1940 1941 @Override 1942 public boolean equalsDeep(Base other_) { 1943 if (!super.equalsDeep(other_)) 1944 return false; 1945 if (!(other_ instanceof GenomicStudyAnalysisInputComponent)) 1946 return false; 1947 GenomicStudyAnalysisInputComponent o = (GenomicStudyAnalysisInputComponent) other_; 1948 return compareDeep(file, o.file, true) && compareDeep(type, o.type, true) && compareDeep(generatedBy, o.generatedBy, true) 1949 ; 1950 } 1951 1952 @Override 1953 public boolean equalsShallow(Base other_) { 1954 if (!super.equalsShallow(other_)) 1955 return false; 1956 if (!(other_ instanceof GenomicStudyAnalysisInputComponent)) 1957 return false; 1958 GenomicStudyAnalysisInputComponent o = (GenomicStudyAnalysisInputComponent) other_; 1959 return true; 1960 } 1961 1962 public boolean isEmpty() { 1963 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(file, type, generatedBy 1964 ); 1965 } 1966 1967 public String fhirType() { 1968 return "GenomicStudy.analysis.input"; 1969 1970 } 1971 1972 } 1973 1974 @Block() 1975 public static class GenomicStudyAnalysisOutputComponent extends BackboneElement implements IBaseBackboneElement { 1976 /** 1977 * File containing output data. 1978 */ 1979 @Child(name = "file", type = {DocumentReference.class}, order=1, min=0, max=1, modifier=false, summary=true) 1980 @Description(shortDefinition="File containing output data", formalDefinition="File containing output data." ) 1981 protected Reference file; 1982 1983 /** 1984 * Type of output data, e.g., VCF, MAF, or BAM. 1985 */ 1986 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1987 @Description(shortDefinition="Type of output data (e.g., VCF, MAF, or BAM)", formalDefinition="Type of output data, e.g., VCF, MAF, or BAM." ) 1988 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/genomicstudy-dataformat") 1989 protected CodeableConcept type; 1990 1991 private static final long serialVersionUID = -2075265800L; 1992 1993 /** 1994 * Constructor 1995 */ 1996 public GenomicStudyAnalysisOutputComponent() { 1997 super(); 1998 } 1999 2000 /** 2001 * @return {@link #file} (File containing output data.) 2002 */ 2003 public Reference getFile() { 2004 if (this.file == null) 2005 if (Configuration.errorOnAutoCreate()) 2006 throw new Error("Attempt to auto-create GenomicStudyAnalysisOutputComponent.file"); 2007 else if (Configuration.doAutoCreate()) 2008 this.file = new Reference(); // cc 2009 return this.file; 2010 } 2011 2012 public boolean hasFile() { 2013 return this.file != null && !this.file.isEmpty(); 2014 } 2015 2016 /** 2017 * @param value {@link #file} (File containing output data.) 2018 */ 2019 public GenomicStudyAnalysisOutputComponent setFile(Reference value) { 2020 this.file = value; 2021 return this; 2022 } 2023 2024 /** 2025 * @return {@link #type} (Type of output data, e.g., VCF, MAF, or BAM.) 2026 */ 2027 public CodeableConcept getType() { 2028 if (this.type == null) 2029 if (Configuration.errorOnAutoCreate()) 2030 throw new Error("Attempt to auto-create GenomicStudyAnalysisOutputComponent.type"); 2031 else if (Configuration.doAutoCreate()) 2032 this.type = new CodeableConcept(); // cc 2033 return this.type; 2034 } 2035 2036 public boolean hasType() { 2037 return this.type != null && !this.type.isEmpty(); 2038 } 2039 2040 /** 2041 * @param value {@link #type} (Type of output data, e.g., VCF, MAF, or BAM.) 2042 */ 2043 public GenomicStudyAnalysisOutputComponent setType(CodeableConcept value) { 2044 this.type = value; 2045 return this; 2046 } 2047 2048 protected void listChildren(List<Property> children) { 2049 super.listChildren(children); 2050 children.add(new Property("file", "Reference(DocumentReference)", "File containing output data.", 0, 1, file)); 2051 children.add(new Property("type", "CodeableConcept", "Type of output data, e.g., VCF, MAF, or BAM.", 0, 1, type)); 2052 } 2053 2054 @Override 2055 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2056 switch (_hash) { 2057 case 3143036: /*file*/ return new Property("file", "Reference(DocumentReference)", "File containing output data.", 0, 1, file); 2058 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of output data, e.g., VCF, MAF, or BAM.", 0, 1, type); 2059 default: return super.getNamedProperty(_hash, _name, _checkValid); 2060 } 2061 2062 } 2063 2064 @Override 2065 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2066 switch (hash) { 2067 case 3143036: /*file*/ return this.file == null ? new Base[0] : new Base[] {this.file}; // Reference 2068 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2069 default: return super.getProperty(hash, name, checkValid); 2070 } 2071 2072 } 2073 2074 @Override 2075 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2076 switch (hash) { 2077 case 3143036: // file 2078 this.file = TypeConvertor.castToReference(value); // Reference 2079 return value; 2080 case 3575610: // type 2081 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2082 return value; 2083 default: return super.setProperty(hash, name, value); 2084 } 2085 2086 } 2087 2088 @Override 2089 public Base setProperty(String name, Base value) throws FHIRException { 2090 if (name.equals("file")) { 2091 this.file = TypeConvertor.castToReference(value); // Reference 2092 } else if (name.equals("type")) { 2093 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2094 } else 2095 return super.setProperty(name, value); 2096 return value; 2097 } 2098 2099 @Override 2100 public void removeChild(String name, Base value) throws FHIRException { 2101 if (name.equals("file")) { 2102 this.file = null; 2103 } else if (name.equals("type")) { 2104 this.type = null; 2105 } else 2106 super.removeChild(name, value); 2107 2108 } 2109 2110 @Override 2111 public Base makeProperty(int hash, String name) throws FHIRException { 2112 switch (hash) { 2113 case 3143036: return getFile(); 2114 case 3575610: return getType(); 2115 default: return super.makeProperty(hash, name); 2116 } 2117 2118 } 2119 2120 @Override 2121 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2122 switch (hash) { 2123 case 3143036: /*file*/ return new String[] {"Reference"}; 2124 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2125 default: return super.getTypesForProperty(hash, name); 2126 } 2127 2128 } 2129 2130 @Override 2131 public Base addChild(String name) throws FHIRException { 2132 if (name.equals("file")) { 2133 this.file = new Reference(); 2134 return this.file; 2135 } 2136 else if (name.equals("type")) { 2137 this.type = new CodeableConcept(); 2138 return this.type; 2139 } 2140 else 2141 return super.addChild(name); 2142 } 2143 2144 public GenomicStudyAnalysisOutputComponent copy() { 2145 GenomicStudyAnalysisOutputComponent dst = new GenomicStudyAnalysisOutputComponent(); 2146 copyValues(dst); 2147 return dst; 2148 } 2149 2150 public void copyValues(GenomicStudyAnalysisOutputComponent dst) { 2151 super.copyValues(dst); 2152 dst.file = file == null ? null : file.copy(); 2153 dst.type = type == null ? null : type.copy(); 2154 } 2155 2156 @Override 2157 public boolean equalsDeep(Base other_) { 2158 if (!super.equalsDeep(other_)) 2159 return false; 2160 if (!(other_ instanceof GenomicStudyAnalysisOutputComponent)) 2161 return false; 2162 GenomicStudyAnalysisOutputComponent o = (GenomicStudyAnalysisOutputComponent) other_; 2163 return compareDeep(file, o.file, true) && compareDeep(type, o.type, true); 2164 } 2165 2166 @Override 2167 public boolean equalsShallow(Base other_) { 2168 if (!super.equalsShallow(other_)) 2169 return false; 2170 if (!(other_ instanceof GenomicStudyAnalysisOutputComponent)) 2171 return false; 2172 GenomicStudyAnalysisOutputComponent o = (GenomicStudyAnalysisOutputComponent) other_; 2173 return true; 2174 } 2175 2176 public boolean isEmpty() { 2177 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(file, type); 2178 } 2179 2180 public String fhirType() { 2181 return "GenomicStudy.analysis.output"; 2182 2183 } 2184 2185 } 2186 2187 @Block() 2188 public static class GenomicStudyAnalysisPerformerComponent extends BackboneElement implements IBaseBackboneElement { 2189 /** 2190 * The organization, healthcare professional, or others who participated in performing this analysis. 2191 */ 2192 @Child(name = "actor", type = {Practitioner.class, PractitionerRole.class, Organization.class, Device.class}, order=1, min=0, max=1, modifier=false, summary=false) 2193 @Description(shortDefinition="The organization, healthcare professional, or others who participated in performing this analysis", formalDefinition="The organization, healthcare professional, or others who participated in performing this analysis." ) 2194 protected Reference actor; 2195 2196 /** 2197 * Role of the actor for this analysis. 2198 */ 2199 @Child(name = "role", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 2200 @Description(shortDefinition="Role of the actor for this analysis", formalDefinition="Role of the actor for this analysis." ) 2201 protected CodeableConcept role; 2202 2203 private static final long serialVersionUID = 827444743L; 2204 2205 /** 2206 * Constructor 2207 */ 2208 public GenomicStudyAnalysisPerformerComponent() { 2209 super(); 2210 } 2211 2212 /** 2213 * @return {@link #actor} (The organization, healthcare professional, or others who participated in performing this analysis.) 2214 */ 2215 public Reference getActor() { 2216 if (this.actor == null) 2217 if (Configuration.errorOnAutoCreate()) 2218 throw new Error("Attempt to auto-create GenomicStudyAnalysisPerformerComponent.actor"); 2219 else if (Configuration.doAutoCreate()) 2220 this.actor = new Reference(); // cc 2221 return this.actor; 2222 } 2223 2224 public boolean hasActor() { 2225 return this.actor != null && !this.actor.isEmpty(); 2226 } 2227 2228 /** 2229 * @param value {@link #actor} (The organization, healthcare professional, or others who participated in performing this analysis.) 2230 */ 2231 public GenomicStudyAnalysisPerformerComponent setActor(Reference value) { 2232 this.actor = value; 2233 return this; 2234 } 2235 2236 /** 2237 * @return {@link #role} (Role of the actor for this analysis.) 2238 */ 2239 public CodeableConcept getRole() { 2240 if (this.role == null) 2241 if (Configuration.errorOnAutoCreate()) 2242 throw new Error("Attempt to auto-create GenomicStudyAnalysisPerformerComponent.role"); 2243 else if (Configuration.doAutoCreate()) 2244 this.role = new CodeableConcept(); // cc 2245 return this.role; 2246 } 2247 2248 public boolean hasRole() { 2249 return this.role != null && !this.role.isEmpty(); 2250 } 2251 2252 /** 2253 * @param value {@link #role} (Role of the actor for this analysis.) 2254 */ 2255 public GenomicStudyAnalysisPerformerComponent setRole(CodeableConcept value) { 2256 this.role = value; 2257 return this; 2258 } 2259 2260 protected void listChildren(List<Property> children) { 2261 super.listChildren(children); 2262 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|Device)", "The organization, healthcare professional, or others who participated in performing this analysis.", 0, 1, actor)); 2263 children.add(new Property("role", "CodeableConcept", "Role of the actor for this analysis.", 0, 1, role)); 2264 } 2265 2266 @Override 2267 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2268 switch (_hash) { 2269 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|Device)", "The organization, healthcare professional, or others who participated in performing this analysis.", 0, 1, actor); 2270 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Role of the actor for this analysis.", 0, 1, role); 2271 default: return super.getNamedProperty(_hash, _name, _checkValid); 2272 } 2273 2274 } 2275 2276 @Override 2277 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2278 switch (hash) { 2279 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 2280 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 2281 default: return super.getProperty(hash, name, checkValid); 2282 } 2283 2284 } 2285 2286 @Override 2287 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2288 switch (hash) { 2289 case 92645877: // actor 2290 this.actor = TypeConvertor.castToReference(value); // Reference 2291 return value; 2292 case 3506294: // role 2293 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2294 return value; 2295 default: return super.setProperty(hash, name, value); 2296 } 2297 2298 } 2299 2300 @Override 2301 public Base setProperty(String name, Base value) throws FHIRException { 2302 if (name.equals("actor")) { 2303 this.actor = TypeConvertor.castToReference(value); // Reference 2304 } else if (name.equals("role")) { 2305 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2306 } else 2307 return super.setProperty(name, value); 2308 return value; 2309 } 2310 2311 @Override 2312 public void removeChild(String name, Base value) throws FHIRException { 2313 if (name.equals("actor")) { 2314 this.actor = null; 2315 } else if (name.equals("role")) { 2316 this.role = null; 2317 } else 2318 super.removeChild(name, value); 2319 2320 } 2321 2322 @Override 2323 public Base makeProperty(int hash, String name) throws FHIRException { 2324 switch (hash) { 2325 case 92645877: return getActor(); 2326 case 3506294: return getRole(); 2327 default: return super.makeProperty(hash, name); 2328 } 2329 2330 } 2331 2332 @Override 2333 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2334 switch (hash) { 2335 case 92645877: /*actor*/ return new String[] {"Reference"}; 2336 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 2337 default: return super.getTypesForProperty(hash, name); 2338 } 2339 2340 } 2341 2342 @Override 2343 public Base addChild(String name) throws FHIRException { 2344 if (name.equals("actor")) { 2345 this.actor = new Reference(); 2346 return this.actor; 2347 } 2348 else if (name.equals("role")) { 2349 this.role = new CodeableConcept(); 2350 return this.role; 2351 } 2352 else 2353 return super.addChild(name); 2354 } 2355 2356 public GenomicStudyAnalysisPerformerComponent copy() { 2357 GenomicStudyAnalysisPerformerComponent dst = new GenomicStudyAnalysisPerformerComponent(); 2358 copyValues(dst); 2359 return dst; 2360 } 2361 2362 public void copyValues(GenomicStudyAnalysisPerformerComponent dst) { 2363 super.copyValues(dst); 2364 dst.actor = actor == null ? null : actor.copy(); 2365 dst.role = role == null ? null : role.copy(); 2366 } 2367 2368 @Override 2369 public boolean equalsDeep(Base other_) { 2370 if (!super.equalsDeep(other_)) 2371 return false; 2372 if (!(other_ instanceof GenomicStudyAnalysisPerformerComponent)) 2373 return false; 2374 GenomicStudyAnalysisPerformerComponent o = (GenomicStudyAnalysisPerformerComponent) other_; 2375 return compareDeep(actor, o.actor, true) && compareDeep(role, o.role, true); 2376 } 2377 2378 @Override 2379 public boolean equalsShallow(Base other_) { 2380 if (!super.equalsShallow(other_)) 2381 return false; 2382 if (!(other_ instanceof GenomicStudyAnalysisPerformerComponent)) 2383 return false; 2384 GenomicStudyAnalysisPerformerComponent o = (GenomicStudyAnalysisPerformerComponent) other_; 2385 return true; 2386 } 2387 2388 public boolean isEmpty() { 2389 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(actor, role); 2390 } 2391 2392 public String fhirType() { 2393 return "GenomicStudy.analysis.performer"; 2394 2395 } 2396 2397 } 2398 2399 @Block() 2400 public static class GenomicStudyAnalysisDeviceComponent extends BackboneElement implements IBaseBackboneElement { 2401 /** 2402 * Device used for the analysis. 2403 */ 2404 @Child(name = "device", type = {Device.class}, order=1, min=0, max=1, modifier=false, summary=false) 2405 @Description(shortDefinition="Device used for the analysis", formalDefinition="Device used for the analysis." ) 2406 protected Reference device; 2407 2408 /** 2409 * Specific function for the device used for the analysis. 2410 */ 2411 @Child(name = "function", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 2412 @Description(shortDefinition="Specific function for the device used for the analysis", formalDefinition="Specific function for the device used for the analysis." ) 2413 protected CodeableConcept function; 2414 2415 private static final long serialVersionUID = 1643933108L; 2416 2417 /** 2418 * Constructor 2419 */ 2420 public GenomicStudyAnalysisDeviceComponent() { 2421 super(); 2422 } 2423 2424 /** 2425 * @return {@link #device} (Device used for the analysis.) 2426 */ 2427 public Reference getDevice() { 2428 if (this.device == null) 2429 if (Configuration.errorOnAutoCreate()) 2430 throw new Error("Attempt to auto-create GenomicStudyAnalysisDeviceComponent.device"); 2431 else if (Configuration.doAutoCreate()) 2432 this.device = new Reference(); // cc 2433 return this.device; 2434 } 2435 2436 public boolean hasDevice() { 2437 return this.device != null && !this.device.isEmpty(); 2438 } 2439 2440 /** 2441 * @param value {@link #device} (Device used for the analysis.) 2442 */ 2443 public GenomicStudyAnalysisDeviceComponent setDevice(Reference value) { 2444 this.device = value; 2445 return this; 2446 } 2447 2448 /** 2449 * @return {@link #function} (Specific function for the device used for the analysis.) 2450 */ 2451 public CodeableConcept getFunction() { 2452 if (this.function == null) 2453 if (Configuration.errorOnAutoCreate()) 2454 throw new Error("Attempt to auto-create GenomicStudyAnalysisDeviceComponent.function"); 2455 else if (Configuration.doAutoCreate()) 2456 this.function = new CodeableConcept(); // cc 2457 return this.function; 2458 } 2459 2460 public boolean hasFunction() { 2461 return this.function != null && !this.function.isEmpty(); 2462 } 2463 2464 /** 2465 * @param value {@link #function} (Specific function for the device used for the analysis.) 2466 */ 2467 public GenomicStudyAnalysisDeviceComponent setFunction(CodeableConcept value) { 2468 this.function = value; 2469 return this; 2470 } 2471 2472 protected void listChildren(List<Property> children) { 2473 super.listChildren(children); 2474 children.add(new Property("device", "Reference(Device)", "Device used for the analysis.", 0, 1, device)); 2475 children.add(new Property("function", "CodeableConcept", "Specific function for the device used for the analysis.", 0, 1, function)); 2476 } 2477 2478 @Override 2479 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2480 switch (_hash) { 2481 case -1335157162: /*device*/ return new Property("device", "Reference(Device)", "Device used for the analysis.", 0, 1, device); 2482 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Specific function for the device used for the analysis.", 0, 1, function); 2483 default: return super.getNamedProperty(_hash, _name, _checkValid); 2484 } 2485 2486 } 2487 2488 @Override 2489 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2490 switch (hash) { 2491 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 2492 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 2493 default: return super.getProperty(hash, name, checkValid); 2494 } 2495 2496 } 2497 2498 @Override 2499 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2500 switch (hash) { 2501 case -1335157162: // device 2502 this.device = TypeConvertor.castToReference(value); // Reference 2503 return value; 2504 case 1380938712: // function 2505 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2506 return value; 2507 default: return super.setProperty(hash, name, value); 2508 } 2509 2510 } 2511 2512 @Override 2513 public Base setProperty(String name, Base value) throws FHIRException { 2514 if (name.equals("device")) { 2515 this.device = TypeConvertor.castToReference(value); // Reference 2516 } else if (name.equals("function")) { 2517 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2518 } else 2519 return super.setProperty(name, value); 2520 return value; 2521 } 2522 2523 @Override 2524 public void removeChild(String name, Base value) throws FHIRException { 2525 if (name.equals("device")) { 2526 this.device = null; 2527 } else if (name.equals("function")) { 2528 this.function = null; 2529 } else 2530 super.removeChild(name, value); 2531 2532 } 2533 2534 @Override 2535 public Base makeProperty(int hash, String name) throws FHIRException { 2536 switch (hash) { 2537 case -1335157162: return getDevice(); 2538 case 1380938712: return getFunction(); 2539 default: return super.makeProperty(hash, name); 2540 } 2541 2542 } 2543 2544 @Override 2545 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2546 switch (hash) { 2547 case -1335157162: /*device*/ return new String[] {"Reference"}; 2548 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 2549 default: return super.getTypesForProperty(hash, name); 2550 } 2551 2552 } 2553 2554 @Override 2555 public Base addChild(String name) throws FHIRException { 2556 if (name.equals("device")) { 2557 this.device = new Reference(); 2558 return this.device; 2559 } 2560 else if (name.equals("function")) { 2561 this.function = new CodeableConcept(); 2562 return this.function; 2563 } 2564 else 2565 return super.addChild(name); 2566 } 2567 2568 public GenomicStudyAnalysisDeviceComponent copy() { 2569 GenomicStudyAnalysisDeviceComponent dst = new GenomicStudyAnalysisDeviceComponent(); 2570 copyValues(dst); 2571 return dst; 2572 } 2573 2574 public void copyValues(GenomicStudyAnalysisDeviceComponent dst) { 2575 super.copyValues(dst); 2576 dst.device = device == null ? null : device.copy(); 2577 dst.function = function == null ? null : function.copy(); 2578 } 2579 2580 @Override 2581 public boolean equalsDeep(Base other_) { 2582 if (!super.equalsDeep(other_)) 2583 return false; 2584 if (!(other_ instanceof GenomicStudyAnalysisDeviceComponent)) 2585 return false; 2586 GenomicStudyAnalysisDeviceComponent o = (GenomicStudyAnalysisDeviceComponent) other_; 2587 return compareDeep(device, o.device, true) && compareDeep(function, o.function, true); 2588 } 2589 2590 @Override 2591 public boolean equalsShallow(Base other_) { 2592 if (!super.equalsShallow(other_)) 2593 return false; 2594 if (!(other_ instanceof GenomicStudyAnalysisDeviceComponent)) 2595 return false; 2596 GenomicStudyAnalysisDeviceComponent o = (GenomicStudyAnalysisDeviceComponent) other_; 2597 return true; 2598 } 2599 2600 public boolean isEmpty() { 2601 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(device, function); 2602 } 2603 2604 public String fhirType() { 2605 return "GenomicStudy.analysis.device"; 2606 2607 } 2608 2609 } 2610 2611 /** 2612 * Identifiers for this genomic study. 2613 */ 2614 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2615 @Description(shortDefinition="Identifiers for this genomic study", formalDefinition="Identifiers for this genomic study." ) 2616 protected List<Identifier> identifier; 2617 2618 /** 2619 * The status of the genomic study. 2620 */ 2621 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 2622 @Description(shortDefinition="registered | available | cancelled | entered-in-error | unknown", formalDefinition="The status of the genomic study." ) 2623 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/genomicstudy-status") 2624 protected Enumeration<GenomicStudyStatus> status; 2625 2626 /** 2627 * The type of the study, e.g., Familial variant segregation, Functional variation detection, or Gene expression profiling. 2628 */ 2629 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2630 @Description(shortDefinition="The type of the study (e.g., Familial variant segregation, Functional variation detection, or Gene expression profiling)", formalDefinition="The type of the study, e.g., Familial variant segregation, Functional variation detection, or Gene expression profiling." ) 2631 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/genomicstudy-type") 2632 protected List<CodeableConcept> type; 2633 2634 /** 2635 * The primary subject of the genomic study. 2636 */ 2637 @Child(name = "subject", type = {Patient.class, Group.class, Substance.class, BiologicallyDerivedProduct.class, NutritionProduct.class}, order=3, min=1, max=1, modifier=false, summary=true) 2638 @Description(shortDefinition="The primary subject of the genomic study", formalDefinition="The primary subject of the genomic study." ) 2639 protected Reference subject; 2640 2641 /** 2642 * The healthcare event with which this genomics study is associated. 2643 */ 2644 @Child(name = "encounter", type = {Encounter.class}, order=4, min=0, max=1, modifier=false, summary=true) 2645 @Description(shortDefinition="The healthcare event with which this genomics study is associated", formalDefinition="The healthcare event with which this genomics study is associated." ) 2646 protected Reference encounter; 2647 2648 /** 2649 * When the genomic study was started. 2650 */ 2651 @Child(name = "startDate", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 2652 @Description(shortDefinition="When the genomic study was started", formalDefinition="When the genomic study was started." ) 2653 protected DateTimeType startDate; 2654 2655 /** 2656 * Event resources that the genomic study is based on. 2657 */ 2658 @Child(name = "basedOn", type = {ServiceRequest.class, Task.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2659 @Description(shortDefinition="Event resources that the genomic study is based on", formalDefinition="Event resources that the genomic study is based on." ) 2660 protected List<Reference> basedOn; 2661 2662 /** 2663 * Healthcare professional who requested or referred the genomic study. 2664 */ 2665 @Child(name = "referrer", type = {Practitioner.class, PractitionerRole.class}, order=7, min=0, max=1, modifier=false, summary=false) 2666 @Description(shortDefinition="Healthcare professional who requested or referred the genomic study", formalDefinition="Healthcare professional who requested or referred the genomic study." ) 2667 protected Reference referrer; 2668 2669 /** 2670 * Healthcare professionals who interpreted the genomic study. 2671 */ 2672 @Child(name = "interpreter", type = {Practitioner.class, PractitionerRole.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2673 @Description(shortDefinition="Healthcare professionals who interpreted the genomic study", formalDefinition="Healthcare professionals who interpreted the genomic study." ) 2674 protected List<Reference> interpreter; 2675 2676 /** 2677 * Why the genomic study was performed. 2678 */ 2679 @Child(name = "reason", type = {CodeableReference.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2680 @Description(shortDefinition="Why the genomic study was performed", formalDefinition="Why the genomic study was performed." ) 2681 protected List<CodeableReference> reason; 2682 2683 /** 2684 * The defined protocol that describes the study. 2685 */ 2686 @Child(name = "instantiatesCanonical", type = {CanonicalType.class}, order=10, min=0, max=1, modifier=false, summary=false) 2687 @Description(shortDefinition="The defined protocol that describes the study", formalDefinition="The defined protocol that describes the study." ) 2688 protected CanonicalType instantiatesCanonical; 2689 2690 /** 2691 * The URL pointing to an externally maintained protocol that describes the study. 2692 */ 2693 @Child(name = "instantiatesUri", type = {UriType.class}, order=11, min=0, max=1, modifier=false, summary=false) 2694 @Description(shortDefinition="The URL pointing to an externally maintained protocol that describes the study", formalDefinition="The URL pointing to an externally maintained protocol that describes the study." ) 2695 protected UriType instantiatesUri; 2696 2697 /** 2698 * Comments related to the genomic study. 2699 */ 2700 @Child(name = "note", type = {Annotation.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2701 @Description(shortDefinition="Comments related to the genomic study", formalDefinition="Comments related to the genomic study." ) 2702 protected List<Annotation> note; 2703 2704 /** 2705 * Description of the genomic study. 2706 */ 2707 @Child(name = "description", type = {MarkdownType.class}, order=13, min=0, max=1, modifier=false, summary=false) 2708 @Description(shortDefinition="Description of the genomic study", formalDefinition="Description of the genomic study." ) 2709 protected MarkdownType description; 2710 2711 /** 2712 * The details about a specific analysis that was performed in this GenomicStudy. 2713 */ 2714 @Child(name = "analysis", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2715 @Description(shortDefinition="Genomic Analysis Event", formalDefinition="The details about a specific analysis that was performed in this GenomicStudy." ) 2716 protected List<GenomicStudyAnalysisComponent> analysis; 2717 2718 private static final long serialVersionUID = 644053021L; 2719 2720 /** 2721 * Constructor 2722 */ 2723 public GenomicStudy() { 2724 super(); 2725 } 2726 2727 /** 2728 * Constructor 2729 */ 2730 public GenomicStudy(GenomicStudyStatus status, Reference subject) { 2731 super(); 2732 this.setStatus(status); 2733 this.setSubject(subject); 2734 } 2735 2736 /** 2737 * @return {@link #identifier} (Identifiers for this genomic study.) 2738 */ 2739 public List<Identifier> getIdentifier() { 2740 if (this.identifier == null) 2741 this.identifier = new ArrayList<Identifier>(); 2742 return this.identifier; 2743 } 2744 2745 /** 2746 * @return Returns a reference to <code>this</code> for easy method chaining 2747 */ 2748 public GenomicStudy setIdentifier(List<Identifier> theIdentifier) { 2749 this.identifier = theIdentifier; 2750 return this; 2751 } 2752 2753 public boolean hasIdentifier() { 2754 if (this.identifier == null) 2755 return false; 2756 for (Identifier item : this.identifier) 2757 if (!item.isEmpty()) 2758 return true; 2759 return false; 2760 } 2761 2762 public Identifier addIdentifier() { //3 2763 Identifier t = new Identifier(); 2764 if (this.identifier == null) 2765 this.identifier = new ArrayList<Identifier>(); 2766 this.identifier.add(t); 2767 return t; 2768 } 2769 2770 public GenomicStudy addIdentifier(Identifier t) { //3 2771 if (t == null) 2772 return this; 2773 if (this.identifier == null) 2774 this.identifier = new ArrayList<Identifier>(); 2775 this.identifier.add(t); 2776 return this; 2777 } 2778 2779 /** 2780 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2781 */ 2782 public Identifier getIdentifierFirstRep() { 2783 if (getIdentifier().isEmpty()) { 2784 addIdentifier(); 2785 } 2786 return getIdentifier().get(0); 2787 } 2788 2789 /** 2790 * @return {@link #status} (The status of the genomic study.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2791 */ 2792 public Enumeration<GenomicStudyStatus> getStatusElement() { 2793 if (this.status == null) 2794 if (Configuration.errorOnAutoCreate()) 2795 throw new Error("Attempt to auto-create GenomicStudy.status"); 2796 else if (Configuration.doAutoCreate()) 2797 this.status = new Enumeration<GenomicStudyStatus>(new GenomicStudyStatusEnumFactory()); // bb 2798 return this.status; 2799 } 2800 2801 public boolean hasStatusElement() { 2802 return this.status != null && !this.status.isEmpty(); 2803 } 2804 2805 public boolean hasStatus() { 2806 return this.status != null && !this.status.isEmpty(); 2807 } 2808 2809 /** 2810 * @param value {@link #status} (The status of the genomic study.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2811 */ 2812 public GenomicStudy setStatusElement(Enumeration<GenomicStudyStatus> value) { 2813 this.status = value; 2814 return this; 2815 } 2816 2817 /** 2818 * @return The status of the genomic study. 2819 */ 2820 public GenomicStudyStatus getStatus() { 2821 return this.status == null ? null : this.status.getValue(); 2822 } 2823 2824 /** 2825 * @param value The status of the genomic study. 2826 */ 2827 public GenomicStudy setStatus(GenomicStudyStatus value) { 2828 if (this.status == null) 2829 this.status = new Enumeration<GenomicStudyStatus>(new GenomicStudyStatusEnumFactory()); 2830 this.status.setValue(value); 2831 return this; 2832 } 2833 2834 /** 2835 * @return {@link #type} (The type of the study, e.g., Familial variant segregation, Functional variation detection, or Gene expression profiling.) 2836 */ 2837 public List<CodeableConcept> getType() { 2838 if (this.type == null) 2839 this.type = new ArrayList<CodeableConcept>(); 2840 return this.type; 2841 } 2842 2843 /** 2844 * @return Returns a reference to <code>this</code> for easy method chaining 2845 */ 2846 public GenomicStudy setType(List<CodeableConcept> theType) { 2847 this.type = theType; 2848 return this; 2849 } 2850 2851 public boolean hasType() { 2852 if (this.type == null) 2853 return false; 2854 for (CodeableConcept item : this.type) 2855 if (!item.isEmpty()) 2856 return true; 2857 return false; 2858 } 2859 2860 public CodeableConcept addType() { //3 2861 CodeableConcept t = new CodeableConcept(); 2862 if (this.type == null) 2863 this.type = new ArrayList<CodeableConcept>(); 2864 this.type.add(t); 2865 return t; 2866 } 2867 2868 public GenomicStudy addType(CodeableConcept t) { //3 2869 if (t == null) 2870 return this; 2871 if (this.type == null) 2872 this.type = new ArrayList<CodeableConcept>(); 2873 this.type.add(t); 2874 return this; 2875 } 2876 2877 /** 2878 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 2879 */ 2880 public CodeableConcept getTypeFirstRep() { 2881 if (getType().isEmpty()) { 2882 addType(); 2883 } 2884 return getType().get(0); 2885 } 2886 2887 /** 2888 * @return {@link #subject} (The primary subject of the genomic study.) 2889 */ 2890 public Reference getSubject() { 2891 if (this.subject == null) 2892 if (Configuration.errorOnAutoCreate()) 2893 throw new Error("Attempt to auto-create GenomicStudy.subject"); 2894 else if (Configuration.doAutoCreate()) 2895 this.subject = new Reference(); // cc 2896 return this.subject; 2897 } 2898 2899 public boolean hasSubject() { 2900 return this.subject != null && !this.subject.isEmpty(); 2901 } 2902 2903 /** 2904 * @param value {@link #subject} (The primary subject of the genomic study.) 2905 */ 2906 public GenomicStudy setSubject(Reference value) { 2907 this.subject = value; 2908 return this; 2909 } 2910 2911 /** 2912 * @return {@link #encounter} (The healthcare event with which this genomics study is associated.) 2913 */ 2914 public Reference getEncounter() { 2915 if (this.encounter == null) 2916 if (Configuration.errorOnAutoCreate()) 2917 throw new Error("Attempt to auto-create GenomicStudy.encounter"); 2918 else if (Configuration.doAutoCreate()) 2919 this.encounter = new Reference(); // cc 2920 return this.encounter; 2921 } 2922 2923 public boolean hasEncounter() { 2924 return this.encounter != null && !this.encounter.isEmpty(); 2925 } 2926 2927 /** 2928 * @param value {@link #encounter} (The healthcare event with which this genomics study is associated.) 2929 */ 2930 public GenomicStudy setEncounter(Reference value) { 2931 this.encounter = value; 2932 return this; 2933 } 2934 2935 /** 2936 * @return {@link #startDate} (When the genomic study was started.). This is the underlying object with id, value and extensions. The accessor "getStartDate" gives direct access to the value 2937 */ 2938 public DateTimeType getStartDateElement() { 2939 if (this.startDate == null) 2940 if (Configuration.errorOnAutoCreate()) 2941 throw new Error("Attempt to auto-create GenomicStudy.startDate"); 2942 else if (Configuration.doAutoCreate()) 2943 this.startDate = new DateTimeType(); // bb 2944 return this.startDate; 2945 } 2946 2947 public boolean hasStartDateElement() { 2948 return this.startDate != null && !this.startDate.isEmpty(); 2949 } 2950 2951 public boolean hasStartDate() { 2952 return this.startDate != null && !this.startDate.isEmpty(); 2953 } 2954 2955 /** 2956 * @param value {@link #startDate} (When the genomic study was started.). This is the underlying object with id, value and extensions. The accessor "getStartDate" gives direct access to the value 2957 */ 2958 public GenomicStudy setStartDateElement(DateTimeType value) { 2959 this.startDate = value; 2960 return this; 2961 } 2962 2963 /** 2964 * @return When the genomic study was started. 2965 */ 2966 public Date getStartDate() { 2967 return this.startDate == null ? null : this.startDate.getValue(); 2968 } 2969 2970 /** 2971 * @param value When the genomic study was started. 2972 */ 2973 public GenomicStudy setStartDate(Date value) { 2974 if (value == null) 2975 this.startDate = null; 2976 else { 2977 if (this.startDate == null) 2978 this.startDate = new DateTimeType(); 2979 this.startDate.setValue(value); 2980 } 2981 return this; 2982 } 2983 2984 /** 2985 * @return {@link #basedOn} (Event resources that the genomic study is based on.) 2986 */ 2987 public List<Reference> getBasedOn() { 2988 if (this.basedOn == null) 2989 this.basedOn = new ArrayList<Reference>(); 2990 return this.basedOn; 2991 } 2992 2993 /** 2994 * @return Returns a reference to <code>this</code> for easy method chaining 2995 */ 2996 public GenomicStudy setBasedOn(List<Reference> theBasedOn) { 2997 this.basedOn = theBasedOn; 2998 return this; 2999 } 3000 3001 public boolean hasBasedOn() { 3002 if (this.basedOn == null) 3003 return false; 3004 for (Reference item : this.basedOn) 3005 if (!item.isEmpty()) 3006 return true; 3007 return false; 3008 } 3009 3010 public Reference addBasedOn() { //3 3011 Reference t = new Reference(); 3012 if (this.basedOn == null) 3013 this.basedOn = new ArrayList<Reference>(); 3014 this.basedOn.add(t); 3015 return t; 3016 } 3017 3018 public GenomicStudy addBasedOn(Reference t) { //3 3019 if (t == null) 3020 return this; 3021 if (this.basedOn == null) 3022 this.basedOn = new ArrayList<Reference>(); 3023 this.basedOn.add(t); 3024 return this; 3025 } 3026 3027 /** 3028 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 3029 */ 3030 public Reference getBasedOnFirstRep() { 3031 if (getBasedOn().isEmpty()) { 3032 addBasedOn(); 3033 } 3034 return getBasedOn().get(0); 3035 } 3036 3037 /** 3038 * @return {@link #referrer} (Healthcare professional who requested or referred the genomic study.) 3039 */ 3040 public Reference getReferrer() { 3041 if (this.referrer == null) 3042 if (Configuration.errorOnAutoCreate()) 3043 throw new Error("Attempt to auto-create GenomicStudy.referrer"); 3044 else if (Configuration.doAutoCreate()) 3045 this.referrer = new Reference(); // cc 3046 return this.referrer; 3047 } 3048 3049 public boolean hasReferrer() { 3050 return this.referrer != null && !this.referrer.isEmpty(); 3051 } 3052 3053 /** 3054 * @param value {@link #referrer} (Healthcare professional who requested or referred the genomic study.) 3055 */ 3056 public GenomicStudy setReferrer(Reference value) { 3057 this.referrer = value; 3058 return this; 3059 } 3060 3061 /** 3062 * @return {@link #interpreter} (Healthcare professionals who interpreted the genomic study.) 3063 */ 3064 public List<Reference> getInterpreter() { 3065 if (this.interpreter == null) 3066 this.interpreter = new ArrayList<Reference>(); 3067 return this.interpreter; 3068 } 3069 3070 /** 3071 * @return Returns a reference to <code>this</code> for easy method chaining 3072 */ 3073 public GenomicStudy setInterpreter(List<Reference> theInterpreter) { 3074 this.interpreter = theInterpreter; 3075 return this; 3076 } 3077 3078 public boolean hasInterpreter() { 3079 if (this.interpreter == null) 3080 return false; 3081 for (Reference item : this.interpreter) 3082 if (!item.isEmpty()) 3083 return true; 3084 return false; 3085 } 3086 3087 public Reference addInterpreter() { //3 3088 Reference t = new Reference(); 3089 if (this.interpreter == null) 3090 this.interpreter = new ArrayList<Reference>(); 3091 this.interpreter.add(t); 3092 return t; 3093 } 3094 3095 public GenomicStudy addInterpreter(Reference t) { //3 3096 if (t == null) 3097 return this; 3098 if (this.interpreter == null) 3099 this.interpreter = new ArrayList<Reference>(); 3100 this.interpreter.add(t); 3101 return this; 3102 } 3103 3104 /** 3105 * @return The first repetition of repeating field {@link #interpreter}, creating it if it does not already exist {3} 3106 */ 3107 public Reference getInterpreterFirstRep() { 3108 if (getInterpreter().isEmpty()) { 3109 addInterpreter(); 3110 } 3111 return getInterpreter().get(0); 3112 } 3113 3114 /** 3115 * @return {@link #reason} (Why the genomic study was performed.) 3116 */ 3117 public List<CodeableReference> getReason() { 3118 if (this.reason == null) 3119 this.reason = new ArrayList<CodeableReference>(); 3120 return this.reason; 3121 } 3122 3123 /** 3124 * @return Returns a reference to <code>this</code> for easy method chaining 3125 */ 3126 public GenomicStudy setReason(List<CodeableReference> theReason) { 3127 this.reason = theReason; 3128 return this; 3129 } 3130 3131 public boolean hasReason() { 3132 if (this.reason == null) 3133 return false; 3134 for (CodeableReference item : this.reason) 3135 if (!item.isEmpty()) 3136 return true; 3137 return false; 3138 } 3139 3140 public CodeableReference addReason() { //3 3141 CodeableReference t = new CodeableReference(); 3142 if (this.reason == null) 3143 this.reason = new ArrayList<CodeableReference>(); 3144 this.reason.add(t); 3145 return t; 3146 } 3147 3148 public GenomicStudy addReason(CodeableReference t) { //3 3149 if (t == null) 3150 return this; 3151 if (this.reason == null) 3152 this.reason = new ArrayList<CodeableReference>(); 3153 this.reason.add(t); 3154 return this; 3155 } 3156 3157 /** 3158 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 3159 */ 3160 public CodeableReference getReasonFirstRep() { 3161 if (getReason().isEmpty()) { 3162 addReason(); 3163 } 3164 return getReason().get(0); 3165 } 3166 3167 /** 3168 * @return {@link #instantiatesCanonical} (The defined protocol that describes the study.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesCanonical" gives direct access to the value 3169 */ 3170 public CanonicalType getInstantiatesCanonicalElement() { 3171 if (this.instantiatesCanonical == null) 3172 if (Configuration.errorOnAutoCreate()) 3173 throw new Error("Attempt to auto-create GenomicStudy.instantiatesCanonical"); 3174 else if (Configuration.doAutoCreate()) 3175 this.instantiatesCanonical = new CanonicalType(); // bb 3176 return this.instantiatesCanonical; 3177 } 3178 3179 public boolean hasInstantiatesCanonicalElement() { 3180 return this.instantiatesCanonical != null && !this.instantiatesCanonical.isEmpty(); 3181 } 3182 3183 public boolean hasInstantiatesCanonical() { 3184 return this.instantiatesCanonical != null && !this.instantiatesCanonical.isEmpty(); 3185 } 3186 3187 /** 3188 * @param value {@link #instantiatesCanonical} (The defined protocol that describes the study.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesCanonical" gives direct access to the value 3189 */ 3190 public GenomicStudy setInstantiatesCanonicalElement(CanonicalType value) { 3191 this.instantiatesCanonical = value; 3192 return this; 3193 } 3194 3195 /** 3196 * @return The defined protocol that describes the study. 3197 */ 3198 public String getInstantiatesCanonical() { 3199 return this.instantiatesCanonical == null ? null : this.instantiatesCanonical.getValue(); 3200 } 3201 3202 /** 3203 * @param value The defined protocol that describes the study. 3204 */ 3205 public GenomicStudy setInstantiatesCanonical(String value) { 3206 if (Utilities.noString(value)) 3207 this.instantiatesCanonical = null; 3208 else { 3209 if (this.instantiatesCanonical == null) 3210 this.instantiatesCanonical = new CanonicalType(); 3211 this.instantiatesCanonical.setValue(value); 3212 } 3213 return this; 3214 } 3215 3216 /** 3217 * @return {@link #instantiatesUri} (The URL pointing to an externally maintained protocol that describes the study.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesUri" gives direct access to the value 3218 */ 3219 public UriType getInstantiatesUriElement() { 3220 if (this.instantiatesUri == null) 3221 if (Configuration.errorOnAutoCreate()) 3222 throw new Error("Attempt to auto-create GenomicStudy.instantiatesUri"); 3223 else if (Configuration.doAutoCreate()) 3224 this.instantiatesUri = new UriType(); // bb 3225 return this.instantiatesUri; 3226 } 3227 3228 public boolean hasInstantiatesUriElement() { 3229 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 3230 } 3231 3232 public boolean hasInstantiatesUri() { 3233 return this.instantiatesUri != null && !this.instantiatesUri.isEmpty(); 3234 } 3235 3236 /** 3237 * @param value {@link #instantiatesUri} (The URL pointing to an externally maintained protocol that describes the study.). This is the underlying object with id, value and extensions. The accessor "getInstantiatesUri" gives direct access to the value 3238 */ 3239 public GenomicStudy setInstantiatesUriElement(UriType value) { 3240 this.instantiatesUri = value; 3241 return this; 3242 } 3243 3244 /** 3245 * @return The URL pointing to an externally maintained protocol that describes the study. 3246 */ 3247 public String getInstantiatesUri() { 3248 return this.instantiatesUri == null ? null : this.instantiatesUri.getValue(); 3249 } 3250 3251 /** 3252 * @param value The URL pointing to an externally maintained protocol that describes the study. 3253 */ 3254 public GenomicStudy setInstantiatesUri(String value) { 3255 if (Utilities.noString(value)) 3256 this.instantiatesUri = null; 3257 else { 3258 if (this.instantiatesUri == null) 3259 this.instantiatesUri = new UriType(); 3260 this.instantiatesUri.setValue(value); 3261 } 3262 return this; 3263 } 3264 3265 /** 3266 * @return {@link #note} (Comments related to the genomic study.) 3267 */ 3268 public List<Annotation> getNote() { 3269 if (this.note == null) 3270 this.note = new ArrayList<Annotation>(); 3271 return this.note; 3272 } 3273 3274 /** 3275 * @return Returns a reference to <code>this</code> for easy method chaining 3276 */ 3277 public GenomicStudy setNote(List<Annotation> theNote) { 3278 this.note = theNote; 3279 return this; 3280 } 3281 3282 public boolean hasNote() { 3283 if (this.note == null) 3284 return false; 3285 for (Annotation item : this.note) 3286 if (!item.isEmpty()) 3287 return true; 3288 return false; 3289 } 3290 3291 public Annotation addNote() { //3 3292 Annotation t = new Annotation(); 3293 if (this.note == null) 3294 this.note = new ArrayList<Annotation>(); 3295 this.note.add(t); 3296 return t; 3297 } 3298 3299 public GenomicStudy addNote(Annotation t) { //3 3300 if (t == null) 3301 return this; 3302 if (this.note == null) 3303 this.note = new ArrayList<Annotation>(); 3304 this.note.add(t); 3305 return this; 3306 } 3307 3308 /** 3309 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 3310 */ 3311 public Annotation getNoteFirstRep() { 3312 if (getNote().isEmpty()) { 3313 addNote(); 3314 } 3315 return getNote().get(0); 3316 } 3317 3318 /** 3319 * @return {@link #description} (Description of the genomic study.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3320 */ 3321 public MarkdownType getDescriptionElement() { 3322 if (this.description == null) 3323 if (Configuration.errorOnAutoCreate()) 3324 throw new Error("Attempt to auto-create GenomicStudy.description"); 3325 else if (Configuration.doAutoCreate()) 3326 this.description = new MarkdownType(); // bb 3327 return this.description; 3328 } 3329 3330 public boolean hasDescriptionElement() { 3331 return this.description != null && !this.description.isEmpty(); 3332 } 3333 3334 public boolean hasDescription() { 3335 return this.description != null && !this.description.isEmpty(); 3336 } 3337 3338 /** 3339 * @param value {@link #description} (Description of the genomic study.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3340 */ 3341 public GenomicStudy setDescriptionElement(MarkdownType value) { 3342 this.description = value; 3343 return this; 3344 } 3345 3346 /** 3347 * @return Description of the genomic study. 3348 */ 3349 public String getDescription() { 3350 return this.description == null ? null : this.description.getValue(); 3351 } 3352 3353 /** 3354 * @param value Description of the genomic study. 3355 */ 3356 public GenomicStudy setDescription(String value) { 3357 if (Utilities.noString(value)) 3358 this.description = null; 3359 else { 3360 if (this.description == null) 3361 this.description = new MarkdownType(); 3362 this.description.setValue(value); 3363 } 3364 return this; 3365 } 3366 3367 /** 3368 * @return {@link #analysis} (The details about a specific analysis that was performed in this GenomicStudy.) 3369 */ 3370 public List<GenomicStudyAnalysisComponent> getAnalysis() { 3371 if (this.analysis == null) 3372 this.analysis = new ArrayList<GenomicStudyAnalysisComponent>(); 3373 return this.analysis; 3374 } 3375 3376 /** 3377 * @return Returns a reference to <code>this</code> for easy method chaining 3378 */ 3379 public GenomicStudy setAnalysis(List<GenomicStudyAnalysisComponent> theAnalysis) { 3380 this.analysis = theAnalysis; 3381 return this; 3382 } 3383 3384 public boolean hasAnalysis() { 3385 if (this.analysis == null) 3386 return false; 3387 for (GenomicStudyAnalysisComponent item : this.analysis) 3388 if (!item.isEmpty()) 3389 return true; 3390 return false; 3391 } 3392 3393 public GenomicStudyAnalysisComponent addAnalysis() { //3 3394 GenomicStudyAnalysisComponent t = new GenomicStudyAnalysisComponent(); 3395 if (this.analysis == null) 3396 this.analysis = new ArrayList<GenomicStudyAnalysisComponent>(); 3397 this.analysis.add(t); 3398 return t; 3399 } 3400 3401 public GenomicStudy addAnalysis(GenomicStudyAnalysisComponent t) { //3 3402 if (t == null) 3403 return this; 3404 if (this.analysis == null) 3405 this.analysis = new ArrayList<GenomicStudyAnalysisComponent>(); 3406 this.analysis.add(t); 3407 return this; 3408 } 3409 3410 /** 3411 * @return The first repetition of repeating field {@link #analysis}, creating it if it does not already exist {3} 3412 */ 3413 public GenomicStudyAnalysisComponent getAnalysisFirstRep() { 3414 if (getAnalysis().isEmpty()) { 3415 addAnalysis(); 3416 } 3417 return getAnalysis().get(0); 3418 } 3419 3420 protected void listChildren(List<Property> children) { 3421 super.listChildren(children); 3422 children.add(new Property("identifier", "Identifier", "Identifiers for this genomic study.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3423 children.add(new Property("status", "code", "The status of the genomic study.", 0, 1, status)); 3424 children.add(new Property("type", "CodeableConcept", "The type of the study, e.g., Familial variant segregation, Functional variation detection, or Gene expression profiling.", 0, java.lang.Integer.MAX_VALUE, type)); 3425 children.add(new Property("subject", "Reference(Patient|Group|Substance|BiologicallyDerivedProduct|NutritionProduct)", "The primary subject of the genomic study.", 0, 1, subject)); 3426 children.add(new Property("encounter", "Reference(Encounter)", "The healthcare event with which this genomics study is associated.", 0, 1, encounter)); 3427 children.add(new Property("startDate", "dateTime", "When the genomic study was started.", 0, 1, startDate)); 3428 children.add(new Property("basedOn", "Reference(ServiceRequest|Task)", "Event resources that the genomic study is based on.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 3429 children.add(new Property("referrer", "Reference(Practitioner|PractitionerRole)", "Healthcare professional who requested or referred the genomic study.", 0, 1, referrer)); 3430 children.add(new Property("interpreter", "Reference(Practitioner|PractitionerRole)", "Healthcare professionals who interpreted the genomic study.", 0, java.lang.Integer.MAX_VALUE, interpreter)); 3431 children.add(new Property("reason", "CodeableReference(Condition|Observation)", "Why the genomic study was performed.", 0, java.lang.Integer.MAX_VALUE, reason)); 3432 children.add(new Property("instantiatesCanonical", "canonical(PlanDefinition)", "The defined protocol that describes the study.", 0, 1, instantiatesCanonical)); 3433 children.add(new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol that describes the study.", 0, 1, instantiatesUri)); 3434 children.add(new Property("note", "Annotation", "Comments related to the genomic study.", 0, java.lang.Integer.MAX_VALUE, note)); 3435 children.add(new Property("description", "markdown", "Description of the genomic study.", 0, 1, description)); 3436 children.add(new Property("analysis", "", "The details about a specific analysis that was performed in this GenomicStudy.", 0, java.lang.Integer.MAX_VALUE, analysis)); 3437 } 3438 3439 @Override 3440 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3441 switch (_hash) { 3442 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers for this genomic study.", 0, java.lang.Integer.MAX_VALUE, identifier); 3443 case -892481550: /*status*/ return new Property("status", "code", "The status of the genomic study.", 0, 1, status); 3444 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of the study, e.g., Familial variant segregation, Functional variation detection, or Gene expression profiling.", 0, java.lang.Integer.MAX_VALUE, type); 3445 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Substance|BiologicallyDerivedProduct|NutritionProduct)", "The primary subject of the genomic study.", 0, 1, subject); 3446 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The healthcare event with which this genomics study is associated.", 0, 1, encounter); 3447 case -2129778896: /*startDate*/ return new Property("startDate", "dateTime", "When the genomic study was started.", 0, 1, startDate); 3448 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(ServiceRequest|Task)", "Event resources that the genomic study is based on.", 0, java.lang.Integer.MAX_VALUE, basedOn); 3449 case -722568161: /*referrer*/ return new Property("referrer", "Reference(Practitioner|PractitionerRole)", "Healthcare professional who requested or referred the genomic study.", 0, 1, referrer); 3450 case -2008009094: /*interpreter*/ return new Property("interpreter", "Reference(Practitioner|PractitionerRole)", "Healthcare professionals who interpreted the genomic study.", 0, java.lang.Integer.MAX_VALUE, interpreter); 3451 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation)", "Why the genomic study was performed.", 0, java.lang.Integer.MAX_VALUE, reason); 3452 case 8911915: /*instantiatesCanonical*/ return new Property("instantiatesCanonical", "canonical(PlanDefinition)", "The defined protocol that describes the study.", 0, 1, instantiatesCanonical); 3453 case -1926393373: /*instantiatesUri*/ return new Property("instantiatesUri", "uri", "The URL pointing to an externally maintained protocol that describes the study.", 0, 1, instantiatesUri); 3454 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments related to the genomic study.", 0, java.lang.Integer.MAX_VALUE, note); 3455 case -1724546052: /*description*/ return new Property("description", "markdown", "Description of the genomic study.", 0, 1, description); 3456 case -1024445732: /*analysis*/ return new Property("analysis", "", "The details about a specific analysis that was performed in this GenomicStudy.", 0, java.lang.Integer.MAX_VALUE, analysis); 3457 default: return super.getNamedProperty(_hash, _name, _checkValid); 3458 } 3459 3460 } 3461 3462 @Override 3463 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3464 switch (hash) { 3465 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3466 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<GenomicStudyStatus> 3467 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 3468 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 3469 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 3470 case -2129778896: /*startDate*/ return this.startDate == null ? new Base[0] : new Base[] {this.startDate}; // DateTimeType 3471 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 3472 case -722568161: /*referrer*/ return this.referrer == null ? new Base[0] : new Base[] {this.referrer}; // Reference 3473 case -2008009094: /*interpreter*/ return this.interpreter == null ? new Base[0] : this.interpreter.toArray(new Base[this.interpreter.size()]); // Reference 3474 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 3475 case 8911915: /*instantiatesCanonical*/ return this.instantiatesCanonical == null ? new Base[0] : new Base[] {this.instantiatesCanonical}; // CanonicalType 3476 case -1926393373: /*instantiatesUri*/ return this.instantiatesUri == null ? new Base[0] : new Base[] {this.instantiatesUri}; // UriType 3477 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3478 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3479 case -1024445732: /*analysis*/ return this.analysis == null ? new Base[0] : this.analysis.toArray(new Base[this.analysis.size()]); // GenomicStudyAnalysisComponent 3480 default: return super.getProperty(hash, name, checkValid); 3481 } 3482 3483 } 3484 3485 @Override 3486 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3487 switch (hash) { 3488 case -1618432855: // identifier 3489 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3490 return value; 3491 case -892481550: // status 3492 value = new GenomicStudyStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3493 this.status = (Enumeration) value; // Enumeration<GenomicStudyStatus> 3494 return value; 3495 case 3575610: // type 3496 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3497 return value; 3498 case -1867885268: // subject 3499 this.subject = TypeConvertor.castToReference(value); // Reference 3500 return value; 3501 case 1524132147: // encounter 3502 this.encounter = TypeConvertor.castToReference(value); // Reference 3503 return value; 3504 case -2129778896: // startDate 3505 this.startDate = TypeConvertor.castToDateTime(value); // DateTimeType 3506 return value; 3507 case -332612366: // basedOn 3508 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 3509 return value; 3510 case -722568161: // referrer 3511 this.referrer = TypeConvertor.castToReference(value); // Reference 3512 return value; 3513 case -2008009094: // interpreter 3514 this.getInterpreter().add(TypeConvertor.castToReference(value)); // Reference 3515 return value; 3516 case -934964668: // reason 3517 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 3518 return value; 3519 case 8911915: // instantiatesCanonical 3520 this.instantiatesCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 3521 return value; 3522 case -1926393373: // instantiatesUri 3523 this.instantiatesUri = TypeConvertor.castToUri(value); // UriType 3524 return value; 3525 case 3387378: // note 3526 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 3527 return value; 3528 case -1724546052: // description 3529 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3530 return value; 3531 case -1024445732: // analysis 3532 this.getAnalysis().add((GenomicStudyAnalysisComponent) value); // GenomicStudyAnalysisComponent 3533 return value; 3534 default: return super.setProperty(hash, name, value); 3535 } 3536 3537 } 3538 3539 @Override 3540 public Base setProperty(String name, Base value) throws FHIRException { 3541 if (name.equals("identifier")) { 3542 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3543 } else if (name.equals("status")) { 3544 value = new GenomicStudyStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3545 this.status = (Enumeration) value; // Enumeration<GenomicStudyStatus> 3546 } else if (name.equals("type")) { 3547 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 3548 } else if (name.equals("subject")) { 3549 this.subject = TypeConvertor.castToReference(value); // Reference 3550 } else if (name.equals("encounter")) { 3551 this.encounter = TypeConvertor.castToReference(value); // Reference 3552 } else if (name.equals("startDate")) { 3553 this.startDate = TypeConvertor.castToDateTime(value); // DateTimeType 3554 } else if (name.equals("basedOn")) { 3555 this.getBasedOn().add(TypeConvertor.castToReference(value)); 3556 } else if (name.equals("referrer")) { 3557 this.referrer = TypeConvertor.castToReference(value); // Reference 3558 } else if (name.equals("interpreter")) { 3559 this.getInterpreter().add(TypeConvertor.castToReference(value)); 3560 } else if (name.equals("reason")) { 3561 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 3562 } else if (name.equals("instantiatesCanonical")) { 3563 this.instantiatesCanonical = TypeConvertor.castToCanonical(value); // CanonicalType 3564 } else if (name.equals("instantiatesUri")) { 3565 this.instantiatesUri = TypeConvertor.castToUri(value); // UriType 3566 } else if (name.equals("note")) { 3567 this.getNote().add(TypeConvertor.castToAnnotation(value)); 3568 } else if (name.equals("description")) { 3569 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3570 } else if (name.equals("analysis")) { 3571 this.getAnalysis().add((GenomicStudyAnalysisComponent) value); 3572 } else 3573 return super.setProperty(name, value); 3574 return value; 3575 } 3576 3577 @Override 3578 public void removeChild(String name, Base value) throws FHIRException { 3579 if (name.equals("identifier")) { 3580 this.getIdentifier().remove(value); 3581 } else if (name.equals("status")) { 3582 value = new GenomicStudyStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3583 this.status = (Enumeration) value; // Enumeration<GenomicStudyStatus> 3584 } else if (name.equals("type")) { 3585 this.getType().remove(value); 3586 } else if (name.equals("subject")) { 3587 this.subject = null; 3588 } else if (name.equals("encounter")) { 3589 this.encounter = null; 3590 } else if (name.equals("startDate")) { 3591 this.startDate = null; 3592 } else if (name.equals("basedOn")) { 3593 this.getBasedOn().remove(value); 3594 } else if (name.equals("referrer")) { 3595 this.referrer = null; 3596 } else if (name.equals("interpreter")) { 3597 this.getInterpreter().remove(value); 3598 } else if (name.equals("reason")) { 3599 this.getReason().remove(value); 3600 } else if (name.equals("instantiatesCanonical")) { 3601 this.instantiatesCanonical = null; 3602 } else if (name.equals("instantiatesUri")) { 3603 this.instantiatesUri = null; 3604 } else if (name.equals("note")) { 3605 this.getNote().remove(value); 3606 } else if (name.equals("description")) { 3607 this.description = null; 3608 } else if (name.equals("analysis")) { 3609 this.getAnalysis().remove((GenomicStudyAnalysisComponent) value); 3610 } else 3611 super.removeChild(name, value); 3612 3613 } 3614 3615 @Override 3616 public Base makeProperty(int hash, String name) throws FHIRException { 3617 switch (hash) { 3618 case -1618432855: return addIdentifier(); 3619 case -892481550: return getStatusElement(); 3620 case 3575610: return addType(); 3621 case -1867885268: return getSubject(); 3622 case 1524132147: return getEncounter(); 3623 case -2129778896: return getStartDateElement(); 3624 case -332612366: return addBasedOn(); 3625 case -722568161: return getReferrer(); 3626 case -2008009094: return addInterpreter(); 3627 case -934964668: return addReason(); 3628 case 8911915: return getInstantiatesCanonicalElement(); 3629 case -1926393373: return getInstantiatesUriElement(); 3630 case 3387378: return addNote(); 3631 case -1724546052: return getDescriptionElement(); 3632 case -1024445732: return addAnalysis(); 3633 default: return super.makeProperty(hash, name); 3634 } 3635 3636 } 3637 3638 @Override 3639 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3640 switch (hash) { 3641 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3642 case -892481550: /*status*/ return new String[] {"code"}; 3643 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3644 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3645 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 3646 case -2129778896: /*startDate*/ return new String[] {"dateTime"}; 3647 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 3648 case -722568161: /*referrer*/ return new String[] {"Reference"}; 3649 case -2008009094: /*interpreter*/ return new String[] {"Reference"}; 3650 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 3651 case 8911915: /*instantiatesCanonical*/ return new String[] {"canonical"}; 3652 case -1926393373: /*instantiatesUri*/ return new String[] {"uri"}; 3653 case 3387378: /*note*/ return new String[] {"Annotation"}; 3654 case -1724546052: /*description*/ return new String[] {"markdown"}; 3655 case -1024445732: /*analysis*/ return new String[] {}; 3656 default: return super.getTypesForProperty(hash, name); 3657 } 3658 3659 } 3660 3661 @Override 3662 public Base addChild(String name) throws FHIRException { 3663 if (name.equals("identifier")) { 3664 return addIdentifier(); 3665 } 3666 else if (name.equals("status")) { 3667 throw new FHIRException("Cannot call addChild on a singleton property GenomicStudy.status"); 3668 } 3669 else if (name.equals("type")) { 3670 return addType(); 3671 } 3672 else if (name.equals("subject")) { 3673 this.subject = new Reference(); 3674 return this.subject; 3675 } 3676 else if (name.equals("encounter")) { 3677 this.encounter = new Reference(); 3678 return this.encounter; 3679 } 3680 else if (name.equals("startDate")) { 3681 throw new FHIRException("Cannot call addChild on a singleton property GenomicStudy.startDate"); 3682 } 3683 else if (name.equals("basedOn")) { 3684 return addBasedOn(); 3685 } 3686 else if (name.equals("referrer")) { 3687 this.referrer = new Reference(); 3688 return this.referrer; 3689 } 3690 else if (name.equals("interpreter")) { 3691 return addInterpreter(); 3692 } 3693 else if (name.equals("reason")) { 3694 return addReason(); 3695 } 3696 else if (name.equals("instantiatesCanonical")) { 3697 throw new FHIRException("Cannot call addChild on a singleton property GenomicStudy.instantiatesCanonical"); 3698 } 3699 else if (name.equals("instantiatesUri")) { 3700 throw new FHIRException("Cannot call addChild on a singleton property GenomicStudy.instantiatesUri"); 3701 } 3702 else if (name.equals("note")) { 3703 return addNote(); 3704 } 3705 else if (name.equals("description")) { 3706 throw new FHIRException("Cannot call addChild on a singleton property GenomicStudy.description"); 3707 } 3708 else if (name.equals("analysis")) { 3709 return addAnalysis(); 3710 } 3711 else 3712 return super.addChild(name); 3713 } 3714 3715 public String fhirType() { 3716 return "GenomicStudy"; 3717 3718 } 3719 3720 public GenomicStudy copy() { 3721 GenomicStudy dst = new GenomicStudy(); 3722 copyValues(dst); 3723 return dst; 3724 } 3725 3726 public void copyValues(GenomicStudy dst) { 3727 super.copyValues(dst); 3728 if (identifier != null) { 3729 dst.identifier = new ArrayList<Identifier>(); 3730 for (Identifier i : identifier) 3731 dst.identifier.add(i.copy()); 3732 }; 3733 dst.status = status == null ? null : status.copy(); 3734 if (type != null) { 3735 dst.type = new ArrayList<CodeableConcept>(); 3736 for (CodeableConcept i : type) 3737 dst.type.add(i.copy()); 3738 }; 3739 dst.subject = subject == null ? null : subject.copy(); 3740 dst.encounter = encounter == null ? null : encounter.copy(); 3741 dst.startDate = startDate == null ? null : startDate.copy(); 3742 if (basedOn != null) { 3743 dst.basedOn = new ArrayList<Reference>(); 3744 for (Reference i : basedOn) 3745 dst.basedOn.add(i.copy()); 3746 }; 3747 dst.referrer = referrer == null ? null : referrer.copy(); 3748 if (interpreter != null) { 3749 dst.interpreter = new ArrayList<Reference>(); 3750 for (Reference i : interpreter) 3751 dst.interpreter.add(i.copy()); 3752 }; 3753 if (reason != null) { 3754 dst.reason = new ArrayList<CodeableReference>(); 3755 for (CodeableReference i : reason) 3756 dst.reason.add(i.copy()); 3757 }; 3758 dst.instantiatesCanonical = instantiatesCanonical == null ? null : instantiatesCanonical.copy(); 3759 dst.instantiatesUri = instantiatesUri == null ? null : instantiatesUri.copy(); 3760 if (note != null) { 3761 dst.note = new ArrayList<Annotation>(); 3762 for (Annotation i : note) 3763 dst.note.add(i.copy()); 3764 }; 3765 dst.description = description == null ? null : description.copy(); 3766 if (analysis != null) { 3767 dst.analysis = new ArrayList<GenomicStudyAnalysisComponent>(); 3768 for (GenomicStudyAnalysisComponent i : analysis) 3769 dst.analysis.add(i.copy()); 3770 }; 3771 } 3772 3773 protected GenomicStudy typedCopy() { 3774 return copy(); 3775 } 3776 3777 @Override 3778 public boolean equalsDeep(Base other_) { 3779 if (!super.equalsDeep(other_)) 3780 return false; 3781 if (!(other_ instanceof GenomicStudy)) 3782 return false; 3783 GenomicStudy o = (GenomicStudy) other_; 3784 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 3785 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) && compareDeep(startDate, o.startDate, true) 3786 && compareDeep(basedOn, o.basedOn, true) && compareDeep(referrer, o.referrer, true) && compareDeep(interpreter, o.interpreter, true) 3787 && compareDeep(reason, o.reason, true) && compareDeep(instantiatesCanonical, o.instantiatesCanonical, true) 3788 && compareDeep(instantiatesUri, o.instantiatesUri, true) && compareDeep(note, o.note, true) && compareDeep(description, o.description, true) 3789 && compareDeep(analysis, o.analysis, true); 3790 } 3791 3792 @Override 3793 public boolean equalsShallow(Base other_) { 3794 if (!super.equalsShallow(other_)) 3795 return false; 3796 if (!(other_ instanceof GenomicStudy)) 3797 return false; 3798 GenomicStudy o = (GenomicStudy) other_; 3799 return compareValues(status, o.status, true) && compareValues(startDate, o.startDate, true) && compareValues(instantiatesCanonical, o.instantiatesCanonical, true) 3800 && compareValues(instantiatesUri, o.instantiatesUri, true) && compareValues(description, o.description, true) 3801 ; 3802 } 3803 3804 public boolean isEmpty() { 3805 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type 3806 , subject, encounter, startDate, basedOn, referrer, interpreter, reason, instantiatesCanonical 3807 , instantiatesUri, note, description, analysis); 3808 } 3809 3810 @Override 3811 public ResourceType getResourceType() { 3812 return ResourceType.GenomicStudy; 3813 } 3814 3815 /** 3816 * Search parameter: <b>focus</b> 3817 * <p> 3818 * Description: <b>What the genomic study analysis is about, when it is not about the subject of record</b><br> 3819 * Type: <b>reference</b><br> 3820 * Path: <b>GenomicStudy.analysis.focus</b><br> 3821 * </p> 3822 */ 3823 @SearchParamDefinition(name="focus", path="GenomicStudy.analysis.focus", description="What the genomic study analysis is about, when it is not about the subject of record", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 3824 public static final String SP_FOCUS = "focus"; 3825 /** 3826 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 3827 * <p> 3828 * Description: <b>What the genomic study analysis is about, when it is not about the subject of record</b><br> 3829 * Type: <b>reference</b><br> 3830 * Path: <b>GenomicStudy.analysis.focus</b><br> 3831 * </p> 3832 */ 3833 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FOCUS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FOCUS); 3834 3835/** 3836 * Constant for fluent queries to be used to add include statements. Specifies 3837 * the path value of "<b>GenomicStudy:focus</b>". 3838 */ 3839 public static final ca.uhn.fhir.model.api.Include INCLUDE_FOCUS = new ca.uhn.fhir.model.api.Include("GenomicStudy:focus").toLocked(); 3840 3841 /** 3842 * Search parameter: <b>identifier</b> 3843 * <p> 3844 * Description: <b>Identifiers for the Study</b><br> 3845 * Type: <b>token</b><br> 3846 * Path: <b>GenomicStudy.identifier</b><br> 3847 * </p> 3848 */ 3849 @SearchParamDefinition(name="identifier", path="GenomicStudy.identifier", description="Identifiers for the Study", type="token" ) 3850 public static final String SP_IDENTIFIER = "identifier"; 3851 /** 3852 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3853 * <p> 3854 * Description: <b>Identifiers for the Study</b><br> 3855 * Type: <b>token</b><br> 3856 * Path: <b>GenomicStudy.identifier</b><br> 3857 * </p> 3858 */ 3859 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3860 3861 /** 3862 * Search parameter: <b>patient</b> 3863 * <p> 3864 * Description: <b>Who the study is about</b><br> 3865 * Type: <b>reference</b><br> 3866 * Path: <b>GenomicStudy.subject.where(resolve() is Patient)</b><br> 3867 * </p> 3868 */ 3869 @SearchParamDefinition(name="patient", path="GenomicStudy.subject.where(resolve() is Patient)", description="Who the study is about", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 3870 public static final String SP_PATIENT = "patient"; 3871 /** 3872 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3873 * <p> 3874 * Description: <b>Who the study is about</b><br> 3875 * Type: <b>reference</b><br> 3876 * Path: <b>GenomicStudy.subject.where(resolve() is Patient)</b><br> 3877 * </p> 3878 */ 3879 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3880 3881/** 3882 * Constant for fluent queries to be used to add include statements. Specifies 3883 * the path value of "<b>GenomicStudy:patient</b>". 3884 */ 3885 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("GenomicStudy:patient").toLocked(); 3886 3887 /** 3888 * Search parameter: <b>status</b> 3889 * <p> 3890 * Description: <b>The status of the study</b><br> 3891 * Type: <b>token</b><br> 3892 * Path: <b>GenomicStudy.status</b><br> 3893 * </p> 3894 */ 3895 @SearchParamDefinition(name="status", path="GenomicStudy.status", description="The status of the study", type="token" ) 3896 public static final String SP_STATUS = "status"; 3897 /** 3898 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3899 * <p> 3900 * Description: <b>The status of the study</b><br> 3901 * Type: <b>token</b><br> 3902 * Path: <b>GenomicStudy.status</b><br> 3903 * </p> 3904 */ 3905 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3906 3907 /** 3908 * Search parameter: <b>subject</b> 3909 * <p> 3910 * Description: <b>Who the study is about</b><br> 3911 * Type: <b>reference</b><br> 3912 * Path: <b>GenomicStudy.subject</b><br> 3913 * </p> 3914 */ 3915 @SearchParamDefinition(name="subject", path="GenomicStudy.subject", description="Who the study is about", type="reference", target={BiologicallyDerivedProduct.class, Group.class, NutritionProduct.class, Patient.class, Substance.class } ) 3916 public static final String SP_SUBJECT = "subject"; 3917 /** 3918 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3919 * <p> 3920 * Description: <b>Who the study is about</b><br> 3921 * Type: <b>reference</b><br> 3922 * Path: <b>GenomicStudy.subject</b><br> 3923 * </p> 3924 */ 3925 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3926 3927/** 3928 * Constant for fluent queries to be used to add include statements. Specifies 3929 * the path value of "<b>GenomicStudy:subject</b>". 3930 */ 3931 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("GenomicStudy:subject").toLocked(); 3932 3933 3934} 3935