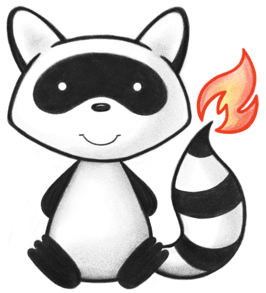
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc. 052 */ 053@ResourceDef(name="Goal", profile="http://hl7.org/fhir/StructureDefinition/Goal") 054public class Goal extends DomainResource { 055 056 public enum GoalLifecycleStatus { 057 /** 058 * A goal is proposed for this patient. 059 */ 060 PROPOSED, 061 /** 062 * A goal is planned for this patient. 063 */ 064 PLANNED, 065 /** 066 * A proposed goal was accepted or acknowledged. 067 */ 068 ACCEPTED, 069 /** 070 * The goal is being sought actively. 071 */ 072 ACTIVE, 073 /** 074 * The goal remains a long term objective but is no longer being actively pursued for a temporary period of time. 075 */ 076 ONHOLD, 077 /** 078 * The goal is no longer being sought. 079 */ 080 COMPLETED, 081 /** 082 * The goal has been abandoned. 083 */ 084 CANCELLED, 085 /** 086 * The goal was entered in error and voided. 087 */ 088 ENTEREDINERROR, 089 /** 090 * A proposed goal was rejected. 091 */ 092 REJECTED, 093 /** 094 * added to help the parsers with the generic types 095 */ 096 NULL; 097 public static GoalLifecycleStatus fromCode(String codeString) throws FHIRException { 098 if (codeString == null || "".equals(codeString)) 099 return null; 100 if ("proposed".equals(codeString)) 101 return PROPOSED; 102 if ("planned".equals(codeString)) 103 return PLANNED; 104 if ("accepted".equals(codeString)) 105 return ACCEPTED; 106 if ("active".equals(codeString)) 107 return ACTIVE; 108 if ("on-hold".equals(codeString)) 109 return ONHOLD; 110 if ("completed".equals(codeString)) 111 return COMPLETED; 112 if ("cancelled".equals(codeString)) 113 return CANCELLED; 114 if ("entered-in-error".equals(codeString)) 115 return ENTEREDINERROR; 116 if ("rejected".equals(codeString)) 117 return REJECTED; 118 if (Configuration.isAcceptInvalidEnums()) 119 return null; 120 else 121 throw new FHIRException("Unknown GoalLifecycleStatus code '"+codeString+"'"); 122 } 123 public String toCode() { 124 switch (this) { 125 case PROPOSED: return "proposed"; 126 case PLANNED: return "planned"; 127 case ACCEPTED: return "accepted"; 128 case ACTIVE: return "active"; 129 case ONHOLD: return "on-hold"; 130 case COMPLETED: return "completed"; 131 case CANCELLED: return "cancelled"; 132 case ENTEREDINERROR: return "entered-in-error"; 133 case REJECTED: return "rejected"; 134 case NULL: return null; 135 default: return "?"; 136 } 137 } 138 public String getSystem() { 139 switch (this) { 140 case PROPOSED: return "http://hl7.org/fhir/goal-status"; 141 case PLANNED: return "http://hl7.org/fhir/goal-status"; 142 case ACCEPTED: return "http://hl7.org/fhir/goal-status"; 143 case ACTIVE: return "http://hl7.org/fhir/goal-status"; 144 case ONHOLD: return "http://hl7.org/fhir/goal-status"; 145 case COMPLETED: return "http://hl7.org/fhir/goal-status"; 146 case CANCELLED: return "http://hl7.org/fhir/goal-status"; 147 case ENTEREDINERROR: return "http://hl7.org/fhir/goal-status"; 148 case REJECTED: return "http://hl7.org/fhir/goal-status"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 public String getDefinition() { 154 switch (this) { 155 case PROPOSED: return "A goal is proposed for this patient."; 156 case PLANNED: return "A goal is planned for this patient."; 157 case ACCEPTED: return "A proposed goal was accepted or acknowledged."; 158 case ACTIVE: return "The goal is being sought actively."; 159 case ONHOLD: return "The goal remains a long term objective but is no longer being actively pursued for a temporary period of time."; 160 case COMPLETED: return "The goal is no longer being sought."; 161 case CANCELLED: return "The goal has been abandoned."; 162 case ENTEREDINERROR: return "The goal was entered in error and voided."; 163 case REJECTED: return "A proposed goal was rejected."; 164 case NULL: return null; 165 default: return "?"; 166 } 167 } 168 public String getDisplay() { 169 switch (this) { 170 case PROPOSED: return "Proposed"; 171 case PLANNED: return "Planned"; 172 case ACCEPTED: return "Accepted"; 173 case ACTIVE: return "Active"; 174 case ONHOLD: return "On Hold"; 175 case COMPLETED: return "Completed"; 176 case CANCELLED: return "Cancelled"; 177 case ENTEREDINERROR: return "Entered in Error"; 178 case REJECTED: return "Rejected"; 179 case NULL: return null; 180 default: return "?"; 181 } 182 } 183 } 184 185 public static class GoalLifecycleStatusEnumFactory implements EnumFactory<GoalLifecycleStatus> { 186 public GoalLifecycleStatus fromCode(String codeString) throws IllegalArgumentException { 187 if (codeString == null || "".equals(codeString)) 188 if (codeString == null || "".equals(codeString)) 189 return null; 190 if ("proposed".equals(codeString)) 191 return GoalLifecycleStatus.PROPOSED; 192 if ("planned".equals(codeString)) 193 return GoalLifecycleStatus.PLANNED; 194 if ("accepted".equals(codeString)) 195 return GoalLifecycleStatus.ACCEPTED; 196 if ("active".equals(codeString)) 197 return GoalLifecycleStatus.ACTIVE; 198 if ("on-hold".equals(codeString)) 199 return GoalLifecycleStatus.ONHOLD; 200 if ("completed".equals(codeString)) 201 return GoalLifecycleStatus.COMPLETED; 202 if ("cancelled".equals(codeString)) 203 return GoalLifecycleStatus.CANCELLED; 204 if ("entered-in-error".equals(codeString)) 205 return GoalLifecycleStatus.ENTEREDINERROR; 206 if ("rejected".equals(codeString)) 207 return GoalLifecycleStatus.REJECTED; 208 throw new IllegalArgumentException("Unknown GoalLifecycleStatus code '"+codeString+"'"); 209 } 210 public Enumeration<GoalLifecycleStatus> fromType(PrimitiveType<?> code) throws FHIRException { 211 if (code == null) 212 return null; 213 if (code.isEmpty()) 214 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.NULL, code); 215 String codeString = ((PrimitiveType) code).asStringValue(); 216 if (codeString == null || "".equals(codeString)) 217 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.NULL, code); 218 if ("proposed".equals(codeString)) 219 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.PROPOSED, code); 220 if ("planned".equals(codeString)) 221 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.PLANNED, code); 222 if ("accepted".equals(codeString)) 223 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.ACCEPTED, code); 224 if ("active".equals(codeString)) 225 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.ACTIVE, code); 226 if ("on-hold".equals(codeString)) 227 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.ONHOLD, code); 228 if ("completed".equals(codeString)) 229 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.COMPLETED, code); 230 if ("cancelled".equals(codeString)) 231 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.CANCELLED, code); 232 if ("entered-in-error".equals(codeString)) 233 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.ENTEREDINERROR, code); 234 if ("rejected".equals(codeString)) 235 return new Enumeration<GoalLifecycleStatus>(this, GoalLifecycleStatus.REJECTED, code); 236 throw new FHIRException("Unknown GoalLifecycleStatus code '"+codeString+"'"); 237 } 238 public String toCode(GoalLifecycleStatus code) { 239 if (code == GoalLifecycleStatus.NULL) 240 return null; 241 if (code == GoalLifecycleStatus.PROPOSED) 242 return "proposed"; 243 if (code == GoalLifecycleStatus.PLANNED) 244 return "planned"; 245 if (code == GoalLifecycleStatus.ACCEPTED) 246 return "accepted"; 247 if (code == GoalLifecycleStatus.ACTIVE) 248 return "active"; 249 if (code == GoalLifecycleStatus.ONHOLD) 250 return "on-hold"; 251 if (code == GoalLifecycleStatus.COMPLETED) 252 return "completed"; 253 if (code == GoalLifecycleStatus.CANCELLED) 254 return "cancelled"; 255 if (code == GoalLifecycleStatus.ENTEREDINERROR) 256 return "entered-in-error"; 257 if (code == GoalLifecycleStatus.REJECTED) 258 return "rejected"; 259 return "?"; 260 } 261 public String toSystem(GoalLifecycleStatus code) { 262 return code.getSystem(); 263 } 264 } 265 266 @Block() 267 public static class GoalTargetComponent extends BackboneElement implements IBaseBackboneElement { 268 /** 269 * The parameter whose value is being tracked, e.g. body weight, blood pressure, or hemoglobin A1c level. 270 */ 271 @Child(name = "measure", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 272 @Description(shortDefinition="The parameter whose value is being tracked", formalDefinition="The parameter whose value is being tracked, e.g. body weight, blood pressure, or hemoglobin A1c level." ) 273 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 274 protected CodeableConcept measure; 275 276 /** 277 * The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value. 278 */ 279 @Child(name = "detail", type = {Quantity.class, Range.class, CodeableConcept.class, StringType.class, BooleanType.class, IntegerType.class, Ratio.class}, order=2, min=0, max=1, modifier=false, summary=true) 280 @Description(shortDefinition="The target value to be achieved", formalDefinition="The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value." ) 281 protected DataType detail; 282 283 /** 284 * Indicates either the date or the duration after start by which the goal should be met. 285 */ 286 @Child(name = "due", type = {DateType.class, Duration.class}, order=3, min=0, max=1, modifier=false, summary=true) 287 @Description(shortDefinition="Reach goal on or before", formalDefinition="Indicates either the date or the duration after start by which the goal should be met." ) 288 protected DataType due; 289 290 private static final long serialVersionUID = 1975697830L; 291 292 /** 293 * Constructor 294 */ 295 public GoalTargetComponent() { 296 super(); 297 } 298 299 /** 300 * @return {@link #measure} (The parameter whose value is being tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.) 301 */ 302 public CodeableConcept getMeasure() { 303 if (this.measure == null) 304 if (Configuration.errorOnAutoCreate()) 305 throw new Error("Attempt to auto-create GoalTargetComponent.measure"); 306 else if (Configuration.doAutoCreate()) 307 this.measure = new CodeableConcept(); // cc 308 return this.measure; 309 } 310 311 public boolean hasMeasure() { 312 return this.measure != null && !this.measure.isEmpty(); 313 } 314 315 /** 316 * @param value {@link #measure} (The parameter whose value is being tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.) 317 */ 318 public GoalTargetComponent setMeasure(CodeableConcept value) { 319 this.measure = value; 320 return this; 321 } 322 323 /** 324 * @return {@link #detail} (The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.) 325 */ 326 public DataType getDetail() { 327 return this.detail; 328 } 329 330 /** 331 * @return {@link #detail} (The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.) 332 */ 333 public Quantity getDetailQuantity() throws FHIRException { 334 if (this.detail == null) 335 this.detail = new Quantity(); 336 if (!(this.detail instanceof Quantity)) 337 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.detail.getClass().getName()+" was encountered"); 338 return (Quantity) this.detail; 339 } 340 341 public boolean hasDetailQuantity() { 342 return this != null && this.detail instanceof Quantity; 343 } 344 345 /** 346 * @return {@link #detail} (The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.) 347 */ 348 public Range getDetailRange() throws FHIRException { 349 if (this.detail == null) 350 this.detail = new Range(); 351 if (!(this.detail instanceof Range)) 352 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.detail.getClass().getName()+" was encountered"); 353 return (Range) this.detail; 354 } 355 356 public boolean hasDetailRange() { 357 return this != null && this.detail instanceof Range; 358 } 359 360 /** 361 * @return {@link #detail} (The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.) 362 */ 363 public CodeableConcept getDetailCodeableConcept() throws FHIRException { 364 if (this.detail == null) 365 this.detail = new CodeableConcept(); 366 if (!(this.detail instanceof CodeableConcept)) 367 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.detail.getClass().getName()+" was encountered"); 368 return (CodeableConcept) this.detail; 369 } 370 371 public boolean hasDetailCodeableConcept() { 372 return this != null && this.detail instanceof CodeableConcept; 373 } 374 375 /** 376 * @return {@link #detail} (The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.) 377 */ 378 public StringType getDetailStringType() throws FHIRException { 379 if (this.detail == null) 380 this.detail = new StringType(); 381 if (!(this.detail instanceof StringType)) 382 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.detail.getClass().getName()+" was encountered"); 383 return (StringType) this.detail; 384 } 385 386 public boolean hasDetailStringType() { 387 return this != null && this.detail instanceof StringType; 388 } 389 390 /** 391 * @return {@link #detail} (The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.) 392 */ 393 public BooleanType getDetailBooleanType() throws FHIRException { 394 if (this.detail == null) 395 this.detail = new BooleanType(); 396 if (!(this.detail instanceof BooleanType)) 397 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.detail.getClass().getName()+" was encountered"); 398 return (BooleanType) this.detail; 399 } 400 401 public boolean hasDetailBooleanType() { 402 return this != null && this.detail instanceof BooleanType; 403 } 404 405 /** 406 * @return {@link #detail} (The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.) 407 */ 408 public IntegerType getDetailIntegerType() throws FHIRException { 409 if (this.detail == null) 410 this.detail = new IntegerType(); 411 if (!(this.detail instanceof IntegerType)) 412 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.detail.getClass().getName()+" was encountered"); 413 return (IntegerType) this.detail; 414 } 415 416 public boolean hasDetailIntegerType() { 417 return this != null && this.detail instanceof IntegerType; 418 } 419 420 /** 421 * @return {@link #detail} (The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.) 422 */ 423 public Ratio getDetailRatio() throws FHIRException { 424 if (this.detail == null) 425 this.detail = new Ratio(); 426 if (!(this.detail instanceof Ratio)) 427 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.detail.getClass().getName()+" was encountered"); 428 return (Ratio) this.detail; 429 } 430 431 public boolean hasDetailRatio() { 432 return this != null && this.detail instanceof Ratio; 433 } 434 435 public boolean hasDetail() { 436 return this.detail != null && !this.detail.isEmpty(); 437 } 438 439 /** 440 * @param value {@link #detail} (The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.) 441 */ 442 public GoalTargetComponent setDetail(DataType value) { 443 if (value != null && !(value instanceof Quantity || value instanceof Range || value instanceof CodeableConcept || value instanceof StringType || value instanceof BooleanType || value instanceof IntegerType || value instanceof Ratio)) 444 throw new FHIRException("Not the right type for Goal.target.detail[x]: "+value.fhirType()); 445 this.detail = value; 446 return this; 447 } 448 449 /** 450 * @return {@link #due} (Indicates either the date or the duration after start by which the goal should be met.) 451 */ 452 public DataType getDue() { 453 return this.due; 454 } 455 456 /** 457 * @return {@link #due} (Indicates either the date or the duration after start by which the goal should be met.) 458 */ 459 public DateType getDueDateType() throws FHIRException { 460 if (this.due == null) 461 this.due = new DateType(); 462 if (!(this.due instanceof DateType)) 463 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.due.getClass().getName()+" was encountered"); 464 return (DateType) this.due; 465 } 466 467 public boolean hasDueDateType() { 468 return this != null && this.due instanceof DateType; 469 } 470 471 /** 472 * @return {@link #due} (Indicates either the date or the duration after start by which the goal should be met.) 473 */ 474 public Duration getDueDuration() throws FHIRException { 475 if (this.due == null) 476 this.due = new Duration(); 477 if (!(this.due instanceof Duration)) 478 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.due.getClass().getName()+" was encountered"); 479 return (Duration) this.due; 480 } 481 482 public boolean hasDueDuration() { 483 return this != null && this.due instanceof Duration; 484 } 485 486 public boolean hasDue() { 487 return this.due != null && !this.due.isEmpty(); 488 } 489 490 /** 491 * @param value {@link #due} (Indicates either the date or the duration after start by which the goal should be met.) 492 */ 493 public GoalTargetComponent setDue(DataType value) { 494 if (value != null && !(value instanceof DateType || value instanceof Duration)) 495 throw new FHIRException("Not the right type for Goal.target.due[x]: "+value.fhirType()); 496 this.due = value; 497 return this; 498 } 499 500 protected void listChildren(List<Property> children) { 501 super.listChildren(children); 502 children.add(new Property("measure", "CodeableConcept", "The parameter whose value is being tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.", 0, 1, measure)); 503 children.add(new Property("detail[x]", "Quantity|Range|CodeableConcept|string|boolean|integer|Ratio", "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 0, 1, detail)); 504 children.add(new Property("due[x]", "date|Duration", "Indicates either the date or the duration after start by which the goal should be met.", 0, 1, due)); 505 } 506 507 @Override 508 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 509 switch (_hash) { 510 case 938321246: /*measure*/ return new Property("measure", "CodeableConcept", "The parameter whose value is being tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.", 0, 1, measure); 511 case -1973084529: /*detail[x]*/ return new Property("detail[x]", "Quantity|Range|CodeableConcept|string|boolean|integer|Ratio", "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 0, 1, detail); 512 case -1335224239: /*detail*/ return new Property("detail[x]", "Quantity|Range|CodeableConcept|string|boolean|integer|Ratio", "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 0, 1, detail); 513 case -1313079300: /*detailQuantity*/ return new Property("detail[x]", "Quantity", "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 0, 1, detail); 514 case -2062632084: /*detailRange*/ return new Property("detail[x]", "Range", "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 0, 1, detail); 515 case -175586544: /*detailCodeableConcept*/ return new Property("detail[x]", "CodeableConcept", "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 0, 1, detail); 516 case 529212354: /*detailString*/ return new Property("detail[x]", "string", "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 0, 1, detail); 517 case 1172184727: /*detailBoolean*/ return new Property("detail[x]", "boolean", "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 0, 1, detail); 518 case -1229442131: /*detailInteger*/ return new Property("detail[x]", "integer", "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 0, 1, detail); 519 case -2062626246: /*detailRatio*/ return new Property("detail[x]", "Ratio", "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 0, 1, detail); 520 case -1320900084: /*due[x]*/ return new Property("due[x]", "date|Duration", "Indicates either the date or the duration after start by which the goal should be met.", 0, 1, due); 521 case 99828: /*due*/ return new Property("due[x]", "date|Duration", "Indicates either the date or the duration after start by which the goal should be met.", 0, 1, due); 522 case 2001063874: /*dueDate*/ return new Property("due[x]", "date", "Indicates either the date or the duration after start by which the goal should be met.", 0, 1, due); 523 case -620428376: /*dueDuration*/ return new Property("due[x]", "Duration", "Indicates either the date or the duration after start by which the goal should be met.", 0, 1, due); 524 default: return super.getNamedProperty(_hash, _name, _checkValid); 525 } 526 527 } 528 529 @Override 530 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 531 switch (hash) { 532 case 938321246: /*measure*/ return this.measure == null ? new Base[0] : new Base[] {this.measure}; // CodeableConcept 533 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // DataType 534 case 99828: /*due*/ return this.due == null ? new Base[0] : new Base[] {this.due}; // DataType 535 default: return super.getProperty(hash, name, checkValid); 536 } 537 538 } 539 540 @Override 541 public Base setProperty(int hash, String name, Base value) throws FHIRException { 542 switch (hash) { 543 case 938321246: // measure 544 this.measure = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 545 return value; 546 case -1335224239: // detail 547 this.detail = TypeConvertor.castToType(value); // DataType 548 return value; 549 case 99828: // due 550 this.due = TypeConvertor.castToType(value); // DataType 551 return value; 552 default: return super.setProperty(hash, name, value); 553 } 554 555 } 556 557 @Override 558 public Base setProperty(String name, Base value) throws FHIRException { 559 if (name.equals("measure")) { 560 this.measure = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 561 } else if (name.equals("detail[x]")) { 562 this.detail = TypeConvertor.castToType(value); // DataType 563 } else if (name.equals("due[x]")) { 564 this.due = TypeConvertor.castToType(value); // DataType 565 } else 566 return super.setProperty(name, value); 567 return value; 568 } 569 570 @Override 571 public void removeChild(String name, Base value) throws FHIRException { 572 if (name.equals("measure")) { 573 this.measure = null; 574 } else if (name.equals("detail[x]")) { 575 this.detail = null; 576 } else if (name.equals("due[x]")) { 577 this.due = null; 578 } else 579 super.removeChild(name, value); 580 581 } 582 583 @Override 584 public Base makeProperty(int hash, String name) throws FHIRException { 585 switch (hash) { 586 case 938321246: return getMeasure(); 587 case -1973084529: return getDetail(); 588 case -1335224239: return getDetail(); 589 case -1320900084: return getDue(); 590 case 99828: return getDue(); 591 default: return super.makeProperty(hash, name); 592 } 593 594 } 595 596 @Override 597 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 598 switch (hash) { 599 case 938321246: /*measure*/ return new String[] {"CodeableConcept"}; 600 case -1335224239: /*detail*/ return new String[] {"Quantity", "Range", "CodeableConcept", "string", "boolean", "integer", "Ratio"}; 601 case 99828: /*due*/ return new String[] {"date", "Duration"}; 602 default: return super.getTypesForProperty(hash, name); 603 } 604 605 } 606 607 @Override 608 public Base addChild(String name) throws FHIRException { 609 if (name.equals("measure")) { 610 this.measure = new CodeableConcept(); 611 return this.measure; 612 } 613 else if (name.equals("detailQuantity")) { 614 this.detail = new Quantity(); 615 return this.detail; 616 } 617 else if (name.equals("detailRange")) { 618 this.detail = new Range(); 619 return this.detail; 620 } 621 else if (name.equals("detailCodeableConcept")) { 622 this.detail = new CodeableConcept(); 623 return this.detail; 624 } 625 else if (name.equals("detailString")) { 626 this.detail = new StringType(); 627 return this.detail; 628 } 629 else if (name.equals("detailBoolean")) { 630 this.detail = new BooleanType(); 631 return this.detail; 632 } 633 else if (name.equals("detailInteger")) { 634 this.detail = new IntegerType(); 635 return this.detail; 636 } 637 else if (name.equals("detailRatio")) { 638 this.detail = new Ratio(); 639 return this.detail; 640 } 641 else if (name.equals("dueDate")) { 642 this.due = new DateType(); 643 return this.due; 644 } 645 else if (name.equals("dueDuration")) { 646 this.due = new Duration(); 647 return this.due; 648 } 649 else 650 return super.addChild(name); 651 } 652 653 public GoalTargetComponent copy() { 654 GoalTargetComponent dst = new GoalTargetComponent(); 655 copyValues(dst); 656 return dst; 657 } 658 659 public void copyValues(GoalTargetComponent dst) { 660 super.copyValues(dst); 661 dst.measure = measure == null ? null : measure.copy(); 662 dst.detail = detail == null ? null : detail.copy(); 663 dst.due = due == null ? null : due.copy(); 664 } 665 666 @Override 667 public boolean equalsDeep(Base other_) { 668 if (!super.equalsDeep(other_)) 669 return false; 670 if (!(other_ instanceof GoalTargetComponent)) 671 return false; 672 GoalTargetComponent o = (GoalTargetComponent) other_; 673 return compareDeep(measure, o.measure, true) && compareDeep(detail, o.detail, true) && compareDeep(due, o.due, true) 674 ; 675 } 676 677 @Override 678 public boolean equalsShallow(Base other_) { 679 if (!super.equalsShallow(other_)) 680 return false; 681 if (!(other_ instanceof GoalTargetComponent)) 682 return false; 683 GoalTargetComponent o = (GoalTargetComponent) other_; 684 return true; 685 } 686 687 public boolean isEmpty() { 688 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(measure, detail, due); 689 } 690 691 public String fhirType() { 692 return "Goal.target"; 693 694 } 695 696 } 697 698 /** 699 * Business identifiers assigned to this goal by the performer or other systems which remain constant as the resource is updated and propagates from server to server. 700 */ 701 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 702 @Description(shortDefinition="External Ids for this goal", formalDefinition="Business identifiers assigned to this goal by the performer or other systems which remain constant as the resource is updated and propagates from server to server." ) 703 protected List<Identifier> identifier; 704 705 /** 706 * The state of the goal throughout its lifecycle. 707 */ 708 @Child(name = "lifecycleStatus", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 709 @Description(shortDefinition="proposed | planned | accepted | active | on-hold | completed | cancelled | entered-in-error | rejected", formalDefinition="The state of the goal throughout its lifecycle." ) 710 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/goal-status") 711 protected Enumeration<GoalLifecycleStatus> lifecycleStatus; 712 713 /** 714 * Describes the progression, or lack thereof, towards the goal against the target. 715 */ 716 @Child(name = "achievementStatus", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 717 @Description(shortDefinition="in-progress | improving | worsening | no-change | achieved | sustaining | not-achieved | no-progress | not-attainable", formalDefinition="Describes the progression, or lack thereof, towards the goal against the target." ) 718 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/goal-achievement") 719 protected CodeableConcept achievementStatus; 720 721 /** 722 * Indicates a category the goal falls within. 723 */ 724 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 725 @Description(shortDefinition="E.g. Treatment, dietary, behavioral, etc", formalDefinition="Indicates a category the goal falls within." ) 726 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/goal-category") 727 protected List<CodeableConcept> category; 728 729 /** 730 * After meeting the goal, ongoing activity is needed to sustain the goal objective. 731 */ 732 @Child(name = "continuous", type = {BooleanType.class}, order=4, min=0, max=1, modifier=false, summary=false) 733 @Description(shortDefinition="After meeting the goal, ongoing activity is needed to sustain the goal objective", formalDefinition="After meeting the goal, ongoing activity is needed to sustain the goal objective." ) 734 protected BooleanType continuous; 735 736 /** 737 * Identifies the mutually agreed level of importance associated with reaching/sustaining the goal. 738 */ 739 @Child(name = "priority", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 740 @Description(shortDefinition="high-priority | medium-priority | low-priority", formalDefinition="Identifies the mutually agreed level of importance associated with reaching/sustaining the goal." ) 741 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/goal-priority") 742 protected CodeableConcept priority; 743 744 /** 745 * Human-readable and/or coded description of a specific desired objective of care, such as "control blood pressure" or "negotiate an obstacle course" or "dance with child at wedding". 746 */ 747 @Child(name = "description", type = {CodeableConcept.class}, order=6, min=1, max=1, modifier=false, summary=true) 748 @Description(shortDefinition="Code or text describing goal", formalDefinition="Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\"." ) 749 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 750 protected CodeableConcept description; 751 752 /** 753 * Identifies the patient, group or organization for whom the goal is being established. 754 */ 755 @Child(name = "subject", type = {Patient.class, Group.class, Organization.class}, order=7, min=1, max=1, modifier=false, summary=true) 756 @Description(shortDefinition="Who this goal is intended for", formalDefinition="Identifies the patient, group or organization for whom the goal is being established." ) 757 protected Reference subject; 758 759 /** 760 * The date or event after which the goal should begin being pursued. 761 */ 762 @Child(name = "start", type = {DateType.class, CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=true) 763 @Description(shortDefinition="When goal pursuit begins", formalDefinition="The date or event after which the goal should begin being pursued." ) 764 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/goal-start-event") 765 protected DataType start; 766 767 /** 768 * Indicates what should be done by when. 769 */ 770 @Child(name = "target", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 771 @Description(shortDefinition="Target outcome for the goal", formalDefinition="Indicates what should be done by when." ) 772 protected List<GoalTargetComponent> target; 773 774 /** 775 * Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc. 776 */ 777 @Child(name = "statusDate", type = {DateType.class}, order=10, min=0, max=1, modifier=false, summary=true) 778 @Description(shortDefinition="When goal status took effect", formalDefinition="Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc." ) 779 protected DateType statusDate; 780 781 /** 782 * Captures the reason for the current status. 783 */ 784 @Child(name = "statusReason", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=false) 785 @Description(shortDefinition="Reason for current status", formalDefinition="Captures the reason for the current status." ) 786 protected StringType statusReason; 787 788 /** 789 * Indicates whose goal this is - patient goal, practitioner goal, etc. 790 */ 791 @Child(name = "source", type = {Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, CareTeam.class}, order=12, min=0, max=1, modifier=false, summary=true) 792 @Description(shortDefinition="Who's responsible for creating Goal?", formalDefinition="Indicates whose goal this is - patient goal, practitioner goal, etc." ) 793 protected Reference source; 794 795 /** 796 * The identified conditions and other health record elements that are intended to be addressed by the goal. 797 */ 798 @Child(name = "addresses", type = {Condition.class, Observation.class, MedicationStatement.class, MedicationRequest.class, NutritionOrder.class, ServiceRequest.class, RiskAssessment.class, Procedure.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 799 @Description(shortDefinition="Issues addressed by this goal", formalDefinition="The identified conditions and other health record elements that are intended to be addressed by the goal." ) 800 protected List<Reference> addresses; 801 802 /** 803 * Any comments related to the goal. 804 */ 805 @Child(name = "note", type = {Annotation.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 806 @Description(shortDefinition="Comments about the goal", formalDefinition="Any comments related to the goal." ) 807 protected List<Annotation> note; 808 809 /** 810 * Identifies the change (or lack of change) at the point when the status of the goal is assessed. 811 */ 812 @Child(name = "outcome", type = {CodeableReference.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 813 @Description(shortDefinition="What result was achieved regarding the goal?", formalDefinition="Identifies the change (or lack of change) at the point when the status of the goal is assessed." ) 814 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 815 protected List<CodeableReference> outcome; 816 817 private static final long serialVersionUID = -884300976L; 818 819 /** 820 * Constructor 821 */ 822 public Goal() { 823 super(); 824 } 825 826 /** 827 * Constructor 828 */ 829 public Goal(GoalLifecycleStatus lifecycleStatus, CodeableConcept description, Reference subject) { 830 super(); 831 this.setLifecycleStatus(lifecycleStatus); 832 this.setDescription(description); 833 this.setSubject(subject); 834 } 835 836 /** 837 * @return {@link #identifier} (Business identifiers assigned to this goal by the performer or other systems which remain constant as the resource is updated and propagates from server to server.) 838 */ 839 public List<Identifier> getIdentifier() { 840 if (this.identifier == null) 841 this.identifier = new ArrayList<Identifier>(); 842 return this.identifier; 843 } 844 845 /** 846 * @return Returns a reference to <code>this</code> for easy method chaining 847 */ 848 public Goal setIdentifier(List<Identifier> theIdentifier) { 849 this.identifier = theIdentifier; 850 return this; 851 } 852 853 public boolean hasIdentifier() { 854 if (this.identifier == null) 855 return false; 856 for (Identifier item : this.identifier) 857 if (!item.isEmpty()) 858 return true; 859 return false; 860 } 861 862 public Identifier addIdentifier() { //3 863 Identifier t = new Identifier(); 864 if (this.identifier == null) 865 this.identifier = new ArrayList<Identifier>(); 866 this.identifier.add(t); 867 return t; 868 } 869 870 public Goal addIdentifier(Identifier t) { //3 871 if (t == null) 872 return this; 873 if (this.identifier == null) 874 this.identifier = new ArrayList<Identifier>(); 875 this.identifier.add(t); 876 return this; 877 } 878 879 /** 880 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 881 */ 882 public Identifier getIdentifierFirstRep() { 883 if (getIdentifier().isEmpty()) { 884 addIdentifier(); 885 } 886 return getIdentifier().get(0); 887 } 888 889 /** 890 * @return {@link #lifecycleStatus} (The state of the goal throughout its lifecycle.). This is the underlying object with id, value and extensions. The accessor "getLifecycleStatus" gives direct access to the value 891 */ 892 public Enumeration<GoalLifecycleStatus> getLifecycleStatusElement() { 893 if (this.lifecycleStatus == null) 894 if (Configuration.errorOnAutoCreate()) 895 throw new Error("Attempt to auto-create Goal.lifecycleStatus"); 896 else if (Configuration.doAutoCreate()) 897 this.lifecycleStatus = new Enumeration<GoalLifecycleStatus>(new GoalLifecycleStatusEnumFactory()); // bb 898 return this.lifecycleStatus; 899 } 900 901 public boolean hasLifecycleStatusElement() { 902 return this.lifecycleStatus != null && !this.lifecycleStatus.isEmpty(); 903 } 904 905 public boolean hasLifecycleStatus() { 906 return this.lifecycleStatus != null && !this.lifecycleStatus.isEmpty(); 907 } 908 909 /** 910 * @param value {@link #lifecycleStatus} (The state of the goal throughout its lifecycle.). This is the underlying object with id, value and extensions. The accessor "getLifecycleStatus" gives direct access to the value 911 */ 912 public Goal setLifecycleStatusElement(Enumeration<GoalLifecycleStatus> value) { 913 this.lifecycleStatus = value; 914 return this; 915 } 916 917 /** 918 * @return The state of the goal throughout its lifecycle. 919 */ 920 public GoalLifecycleStatus getLifecycleStatus() { 921 return this.lifecycleStatus == null ? null : this.lifecycleStatus.getValue(); 922 } 923 924 /** 925 * @param value The state of the goal throughout its lifecycle. 926 */ 927 public Goal setLifecycleStatus(GoalLifecycleStatus value) { 928 if (this.lifecycleStatus == null) 929 this.lifecycleStatus = new Enumeration<GoalLifecycleStatus>(new GoalLifecycleStatusEnumFactory()); 930 this.lifecycleStatus.setValue(value); 931 return this; 932 } 933 934 /** 935 * @return {@link #achievementStatus} (Describes the progression, or lack thereof, towards the goal against the target.) 936 */ 937 public CodeableConcept getAchievementStatus() { 938 if (this.achievementStatus == null) 939 if (Configuration.errorOnAutoCreate()) 940 throw new Error("Attempt to auto-create Goal.achievementStatus"); 941 else if (Configuration.doAutoCreate()) 942 this.achievementStatus = new CodeableConcept(); // cc 943 return this.achievementStatus; 944 } 945 946 public boolean hasAchievementStatus() { 947 return this.achievementStatus != null && !this.achievementStatus.isEmpty(); 948 } 949 950 /** 951 * @param value {@link #achievementStatus} (Describes the progression, or lack thereof, towards the goal against the target.) 952 */ 953 public Goal setAchievementStatus(CodeableConcept value) { 954 this.achievementStatus = value; 955 return this; 956 } 957 958 /** 959 * @return {@link #category} (Indicates a category the goal falls within.) 960 */ 961 public List<CodeableConcept> getCategory() { 962 if (this.category == null) 963 this.category = new ArrayList<CodeableConcept>(); 964 return this.category; 965 } 966 967 /** 968 * @return Returns a reference to <code>this</code> for easy method chaining 969 */ 970 public Goal setCategory(List<CodeableConcept> theCategory) { 971 this.category = theCategory; 972 return this; 973 } 974 975 public boolean hasCategory() { 976 if (this.category == null) 977 return false; 978 for (CodeableConcept item : this.category) 979 if (!item.isEmpty()) 980 return true; 981 return false; 982 } 983 984 public CodeableConcept addCategory() { //3 985 CodeableConcept t = new CodeableConcept(); 986 if (this.category == null) 987 this.category = new ArrayList<CodeableConcept>(); 988 this.category.add(t); 989 return t; 990 } 991 992 public Goal addCategory(CodeableConcept t) { //3 993 if (t == null) 994 return this; 995 if (this.category == null) 996 this.category = new ArrayList<CodeableConcept>(); 997 this.category.add(t); 998 return this; 999 } 1000 1001 /** 1002 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 1003 */ 1004 public CodeableConcept getCategoryFirstRep() { 1005 if (getCategory().isEmpty()) { 1006 addCategory(); 1007 } 1008 return getCategory().get(0); 1009 } 1010 1011 /** 1012 * @return {@link #continuous} (After meeting the goal, ongoing activity is needed to sustain the goal objective.). This is the underlying object with id, value and extensions. The accessor "getContinuous" gives direct access to the value 1013 */ 1014 public BooleanType getContinuousElement() { 1015 if (this.continuous == null) 1016 if (Configuration.errorOnAutoCreate()) 1017 throw new Error("Attempt to auto-create Goal.continuous"); 1018 else if (Configuration.doAutoCreate()) 1019 this.continuous = new BooleanType(); // bb 1020 return this.continuous; 1021 } 1022 1023 public boolean hasContinuousElement() { 1024 return this.continuous != null && !this.continuous.isEmpty(); 1025 } 1026 1027 public boolean hasContinuous() { 1028 return this.continuous != null && !this.continuous.isEmpty(); 1029 } 1030 1031 /** 1032 * @param value {@link #continuous} (After meeting the goal, ongoing activity is needed to sustain the goal objective.). This is the underlying object with id, value and extensions. The accessor "getContinuous" gives direct access to the value 1033 */ 1034 public Goal setContinuousElement(BooleanType value) { 1035 this.continuous = value; 1036 return this; 1037 } 1038 1039 /** 1040 * @return After meeting the goal, ongoing activity is needed to sustain the goal objective. 1041 */ 1042 public boolean getContinuous() { 1043 return this.continuous == null || this.continuous.isEmpty() ? false : this.continuous.getValue(); 1044 } 1045 1046 /** 1047 * @param value After meeting the goal, ongoing activity is needed to sustain the goal objective. 1048 */ 1049 public Goal setContinuous(boolean value) { 1050 if (this.continuous == null) 1051 this.continuous = new BooleanType(); 1052 this.continuous.setValue(value); 1053 return this; 1054 } 1055 1056 /** 1057 * @return {@link #priority} (Identifies the mutually agreed level of importance associated with reaching/sustaining the goal.) 1058 */ 1059 public CodeableConcept getPriority() { 1060 if (this.priority == null) 1061 if (Configuration.errorOnAutoCreate()) 1062 throw new Error("Attempt to auto-create Goal.priority"); 1063 else if (Configuration.doAutoCreate()) 1064 this.priority = new CodeableConcept(); // cc 1065 return this.priority; 1066 } 1067 1068 public boolean hasPriority() { 1069 return this.priority != null && !this.priority.isEmpty(); 1070 } 1071 1072 /** 1073 * @param value {@link #priority} (Identifies the mutually agreed level of importance associated with reaching/sustaining the goal.) 1074 */ 1075 public Goal setPriority(CodeableConcept value) { 1076 this.priority = value; 1077 return this; 1078 } 1079 1080 /** 1081 * @return {@link #description} (Human-readable and/or coded description of a specific desired objective of care, such as "control blood pressure" or "negotiate an obstacle course" or "dance with child at wedding".) 1082 */ 1083 public CodeableConcept getDescription() { 1084 if (this.description == null) 1085 if (Configuration.errorOnAutoCreate()) 1086 throw new Error("Attempt to auto-create Goal.description"); 1087 else if (Configuration.doAutoCreate()) 1088 this.description = new CodeableConcept(); // cc 1089 return this.description; 1090 } 1091 1092 public boolean hasDescription() { 1093 return this.description != null && !this.description.isEmpty(); 1094 } 1095 1096 /** 1097 * @param value {@link #description} (Human-readable and/or coded description of a specific desired objective of care, such as "control blood pressure" or "negotiate an obstacle course" or "dance with child at wedding".) 1098 */ 1099 public Goal setDescription(CodeableConcept value) { 1100 this.description = value; 1101 return this; 1102 } 1103 1104 /** 1105 * @return {@link #subject} (Identifies the patient, group or organization for whom the goal is being established.) 1106 */ 1107 public Reference getSubject() { 1108 if (this.subject == null) 1109 if (Configuration.errorOnAutoCreate()) 1110 throw new Error("Attempt to auto-create Goal.subject"); 1111 else if (Configuration.doAutoCreate()) 1112 this.subject = new Reference(); // cc 1113 return this.subject; 1114 } 1115 1116 public boolean hasSubject() { 1117 return this.subject != null && !this.subject.isEmpty(); 1118 } 1119 1120 /** 1121 * @param value {@link #subject} (Identifies the patient, group or organization for whom the goal is being established.) 1122 */ 1123 public Goal setSubject(Reference value) { 1124 this.subject = value; 1125 return this; 1126 } 1127 1128 /** 1129 * @return {@link #start} (The date or event after which the goal should begin being pursued.) 1130 */ 1131 public DataType getStart() { 1132 return this.start; 1133 } 1134 1135 /** 1136 * @return {@link #start} (The date or event after which the goal should begin being pursued.) 1137 */ 1138 public DateType getStartDateType() throws FHIRException { 1139 if (this.start == null) 1140 this.start = new DateType(); 1141 if (!(this.start instanceof DateType)) 1142 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.start.getClass().getName()+" was encountered"); 1143 return (DateType) this.start; 1144 } 1145 1146 public boolean hasStartDateType() { 1147 return this != null && this.start instanceof DateType; 1148 } 1149 1150 /** 1151 * @return {@link #start} (The date or event after which the goal should begin being pursued.) 1152 */ 1153 public CodeableConcept getStartCodeableConcept() throws FHIRException { 1154 if (this.start == null) 1155 this.start = new CodeableConcept(); 1156 if (!(this.start instanceof CodeableConcept)) 1157 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.start.getClass().getName()+" was encountered"); 1158 return (CodeableConcept) this.start; 1159 } 1160 1161 public boolean hasStartCodeableConcept() { 1162 return this != null && this.start instanceof CodeableConcept; 1163 } 1164 1165 public boolean hasStart() { 1166 return this.start != null && !this.start.isEmpty(); 1167 } 1168 1169 /** 1170 * @param value {@link #start} (The date or event after which the goal should begin being pursued.) 1171 */ 1172 public Goal setStart(DataType value) { 1173 if (value != null && !(value instanceof DateType || value instanceof CodeableConcept)) 1174 throw new FHIRException("Not the right type for Goal.start[x]: "+value.fhirType()); 1175 this.start = value; 1176 return this; 1177 } 1178 1179 /** 1180 * @return {@link #target} (Indicates what should be done by when.) 1181 */ 1182 public List<GoalTargetComponent> getTarget() { 1183 if (this.target == null) 1184 this.target = new ArrayList<GoalTargetComponent>(); 1185 return this.target; 1186 } 1187 1188 /** 1189 * @return Returns a reference to <code>this</code> for easy method chaining 1190 */ 1191 public Goal setTarget(List<GoalTargetComponent> theTarget) { 1192 this.target = theTarget; 1193 return this; 1194 } 1195 1196 public boolean hasTarget() { 1197 if (this.target == null) 1198 return false; 1199 for (GoalTargetComponent item : this.target) 1200 if (!item.isEmpty()) 1201 return true; 1202 return false; 1203 } 1204 1205 public GoalTargetComponent addTarget() { //3 1206 GoalTargetComponent t = new GoalTargetComponent(); 1207 if (this.target == null) 1208 this.target = new ArrayList<GoalTargetComponent>(); 1209 this.target.add(t); 1210 return t; 1211 } 1212 1213 public Goal addTarget(GoalTargetComponent t) { //3 1214 if (t == null) 1215 return this; 1216 if (this.target == null) 1217 this.target = new ArrayList<GoalTargetComponent>(); 1218 this.target.add(t); 1219 return this; 1220 } 1221 1222 /** 1223 * @return The first repetition of repeating field {@link #target}, creating it if it does not already exist {3} 1224 */ 1225 public GoalTargetComponent getTargetFirstRep() { 1226 if (getTarget().isEmpty()) { 1227 addTarget(); 1228 } 1229 return getTarget().get(0); 1230 } 1231 1232 /** 1233 * @return {@link #statusDate} (Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 1234 */ 1235 public DateType getStatusDateElement() { 1236 if (this.statusDate == null) 1237 if (Configuration.errorOnAutoCreate()) 1238 throw new Error("Attempt to auto-create Goal.statusDate"); 1239 else if (Configuration.doAutoCreate()) 1240 this.statusDate = new DateType(); // bb 1241 return this.statusDate; 1242 } 1243 1244 public boolean hasStatusDateElement() { 1245 return this.statusDate != null && !this.statusDate.isEmpty(); 1246 } 1247 1248 public boolean hasStatusDate() { 1249 return this.statusDate != null && !this.statusDate.isEmpty(); 1250 } 1251 1252 /** 1253 * @param value {@link #statusDate} (Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 1254 */ 1255 public Goal setStatusDateElement(DateType value) { 1256 this.statusDate = value; 1257 return this; 1258 } 1259 1260 /** 1261 * @return Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc. 1262 */ 1263 public Date getStatusDate() { 1264 return this.statusDate == null ? null : this.statusDate.getValue(); 1265 } 1266 1267 /** 1268 * @param value Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc. 1269 */ 1270 public Goal setStatusDate(Date value) { 1271 if (value == null) 1272 this.statusDate = null; 1273 else { 1274 if (this.statusDate == null) 1275 this.statusDate = new DateType(); 1276 this.statusDate.setValue(value); 1277 } 1278 return this; 1279 } 1280 1281 /** 1282 * @return {@link #statusReason} (Captures the reason for the current status.). This is the underlying object with id, value and extensions. The accessor "getStatusReason" gives direct access to the value 1283 */ 1284 public StringType getStatusReasonElement() { 1285 if (this.statusReason == null) 1286 if (Configuration.errorOnAutoCreate()) 1287 throw new Error("Attempt to auto-create Goal.statusReason"); 1288 else if (Configuration.doAutoCreate()) 1289 this.statusReason = new StringType(); // bb 1290 return this.statusReason; 1291 } 1292 1293 public boolean hasStatusReasonElement() { 1294 return this.statusReason != null && !this.statusReason.isEmpty(); 1295 } 1296 1297 public boolean hasStatusReason() { 1298 return this.statusReason != null && !this.statusReason.isEmpty(); 1299 } 1300 1301 /** 1302 * @param value {@link #statusReason} (Captures the reason for the current status.). This is the underlying object with id, value and extensions. The accessor "getStatusReason" gives direct access to the value 1303 */ 1304 public Goal setStatusReasonElement(StringType value) { 1305 this.statusReason = value; 1306 return this; 1307 } 1308 1309 /** 1310 * @return Captures the reason for the current status. 1311 */ 1312 public String getStatusReason() { 1313 return this.statusReason == null ? null : this.statusReason.getValue(); 1314 } 1315 1316 /** 1317 * @param value Captures the reason for the current status. 1318 */ 1319 public Goal setStatusReason(String value) { 1320 if (Utilities.noString(value)) 1321 this.statusReason = null; 1322 else { 1323 if (this.statusReason == null) 1324 this.statusReason = new StringType(); 1325 this.statusReason.setValue(value); 1326 } 1327 return this; 1328 } 1329 1330 /** 1331 * @return {@link #source} (Indicates whose goal this is - patient goal, practitioner goal, etc.) 1332 */ 1333 public Reference getSource() { 1334 if (this.source == null) 1335 if (Configuration.errorOnAutoCreate()) 1336 throw new Error("Attempt to auto-create Goal.source"); 1337 else if (Configuration.doAutoCreate()) 1338 this.source = new Reference(); // cc 1339 return this.source; 1340 } 1341 1342 public boolean hasSource() { 1343 return this.source != null && !this.source.isEmpty(); 1344 } 1345 1346 /** 1347 * @param value {@link #source} (Indicates whose goal this is - patient goal, practitioner goal, etc.) 1348 */ 1349 public Goal setSource(Reference value) { 1350 this.source = value; 1351 return this; 1352 } 1353 1354 /** 1355 * @return {@link #addresses} (The identified conditions and other health record elements that are intended to be addressed by the goal.) 1356 */ 1357 public List<Reference> getAddresses() { 1358 if (this.addresses == null) 1359 this.addresses = new ArrayList<Reference>(); 1360 return this.addresses; 1361 } 1362 1363 /** 1364 * @return Returns a reference to <code>this</code> for easy method chaining 1365 */ 1366 public Goal setAddresses(List<Reference> theAddresses) { 1367 this.addresses = theAddresses; 1368 return this; 1369 } 1370 1371 public boolean hasAddresses() { 1372 if (this.addresses == null) 1373 return false; 1374 for (Reference item : this.addresses) 1375 if (!item.isEmpty()) 1376 return true; 1377 return false; 1378 } 1379 1380 public Reference addAddresses() { //3 1381 Reference t = new Reference(); 1382 if (this.addresses == null) 1383 this.addresses = new ArrayList<Reference>(); 1384 this.addresses.add(t); 1385 return t; 1386 } 1387 1388 public Goal addAddresses(Reference t) { //3 1389 if (t == null) 1390 return this; 1391 if (this.addresses == null) 1392 this.addresses = new ArrayList<Reference>(); 1393 this.addresses.add(t); 1394 return this; 1395 } 1396 1397 /** 1398 * @return The first repetition of repeating field {@link #addresses}, creating it if it does not already exist {3} 1399 */ 1400 public Reference getAddressesFirstRep() { 1401 if (getAddresses().isEmpty()) { 1402 addAddresses(); 1403 } 1404 return getAddresses().get(0); 1405 } 1406 1407 /** 1408 * @return {@link #note} (Any comments related to the goal.) 1409 */ 1410 public List<Annotation> getNote() { 1411 if (this.note == null) 1412 this.note = new ArrayList<Annotation>(); 1413 return this.note; 1414 } 1415 1416 /** 1417 * @return Returns a reference to <code>this</code> for easy method chaining 1418 */ 1419 public Goal setNote(List<Annotation> theNote) { 1420 this.note = theNote; 1421 return this; 1422 } 1423 1424 public boolean hasNote() { 1425 if (this.note == null) 1426 return false; 1427 for (Annotation item : this.note) 1428 if (!item.isEmpty()) 1429 return true; 1430 return false; 1431 } 1432 1433 public Annotation addNote() { //3 1434 Annotation t = new Annotation(); 1435 if (this.note == null) 1436 this.note = new ArrayList<Annotation>(); 1437 this.note.add(t); 1438 return t; 1439 } 1440 1441 public Goal addNote(Annotation t) { //3 1442 if (t == null) 1443 return this; 1444 if (this.note == null) 1445 this.note = new ArrayList<Annotation>(); 1446 this.note.add(t); 1447 return this; 1448 } 1449 1450 /** 1451 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1452 */ 1453 public Annotation getNoteFirstRep() { 1454 if (getNote().isEmpty()) { 1455 addNote(); 1456 } 1457 return getNote().get(0); 1458 } 1459 1460 /** 1461 * @return {@link #outcome} (Identifies the change (or lack of change) at the point when the status of the goal is assessed.) 1462 */ 1463 public List<CodeableReference> getOutcome() { 1464 if (this.outcome == null) 1465 this.outcome = new ArrayList<CodeableReference>(); 1466 return this.outcome; 1467 } 1468 1469 /** 1470 * @return Returns a reference to <code>this</code> for easy method chaining 1471 */ 1472 public Goal setOutcome(List<CodeableReference> theOutcome) { 1473 this.outcome = theOutcome; 1474 return this; 1475 } 1476 1477 public boolean hasOutcome() { 1478 if (this.outcome == null) 1479 return false; 1480 for (CodeableReference item : this.outcome) 1481 if (!item.isEmpty()) 1482 return true; 1483 return false; 1484 } 1485 1486 public CodeableReference addOutcome() { //3 1487 CodeableReference t = new CodeableReference(); 1488 if (this.outcome == null) 1489 this.outcome = new ArrayList<CodeableReference>(); 1490 this.outcome.add(t); 1491 return t; 1492 } 1493 1494 public Goal addOutcome(CodeableReference t) { //3 1495 if (t == null) 1496 return this; 1497 if (this.outcome == null) 1498 this.outcome = new ArrayList<CodeableReference>(); 1499 this.outcome.add(t); 1500 return this; 1501 } 1502 1503 /** 1504 * @return The first repetition of repeating field {@link #outcome}, creating it if it does not already exist {3} 1505 */ 1506 public CodeableReference getOutcomeFirstRep() { 1507 if (getOutcome().isEmpty()) { 1508 addOutcome(); 1509 } 1510 return getOutcome().get(0); 1511 } 1512 1513 protected void listChildren(List<Property> children) { 1514 super.listChildren(children); 1515 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this goal by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1516 children.add(new Property("lifecycleStatus", "code", "The state of the goal throughout its lifecycle.", 0, 1, lifecycleStatus)); 1517 children.add(new Property("achievementStatus", "CodeableConcept", "Describes the progression, or lack thereof, towards the goal against the target.", 0, 1, achievementStatus)); 1518 children.add(new Property("category", "CodeableConcept", "Indicates a category the goal falls within.", 0, java.lang.Integer.MAX_VALUE, category)); 1519 children.add(new Property("continuous", "boolean", "After meeting the goal, ongoing activity is needed to sustain the goal objective.", 0, 1, continuous)); 1520 children.add(new Property("priority", "CodeableConcept", "Identifies the mutually agreed level of importance associated with reaching/sustaining the goal.", 0, 1, priority)); 1521 children.add(new Property("description", "CodeableConcept", "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".", 0, 1, description)); 1522 children.add(new Property("subject", "Reference(Patient|Group|Organization)", "Identifies the patient, group or organization for whom the goal is being established.", 0, 1, subject)); 1523 children.add(new Property("start[x]", "date|CodeableConcept", "The date or event after which the goal should begin being pursued.", 0, 1, start)); 1524 children.add(new Property("target", "", "Indicates what should be done by when.", 0, java.lang.Integer.MAX_VALUE, target)); 1525 children.add(new Property("statusDate", "date", "Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc.", 0, 1, statusDate)); 1526 children.add(new Property("statusReason", "string", "Captures the reason for the current status.", 0, 1, statusReason)); 1527 children.add(new Property("source", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|CareTeam)", "Indicates whose goal this is - patient goal, practitioner goal, etc.", 0, 1, source)); 1528 children.add(new Property("addresses", "Reference(Condition|Observation|MedicationStatement|MedicationRequest|NutritionOrder|ServiceRequest|RiskAssessment|Procedure)", "The identified conditions and other health record elements that are intended to be addressed by the goal.", 0, java.lang.Integer.MAX_VALUE, addresses)); 1529 children.add(new Property("note", "Annotation", "Any comments related to the goal.", 0, java.lang.Integer.MAX_VALUE, note)); 1530 children.add(new Property("outcome", "CodeableReference(Observation)", "Identifies the change (or lack of change) at the point when the status of the goal is assessed.", 0, java.lang.Integer.MAX_VALUE, outcome)); 1531 } 1532 1533 @Override 1534 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1535 switch (_hash) { 1536 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this goal by the performer or other systems which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 1537 case 1165552636: /*lifecycleStatus*/ return new Property("lifecycleStatus", "code", "The state of the goal throughout its lifecycle.", 0, 1, lifecycleStatus); 1538 case 104524801: /*achievementStatus*/ return new Property("achievementStatus", "CodeableConcept", "Describes the progression, or lack thereof, towards the goal against the target.", 0, 1, achievementStatus); 1539 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Indicates a category the goal falls within.", 0, java.lang.Integer.MAX_VALUE, category); 1540 case 379114255: /*continuous*/ return new Property("continuous", "boolean", "After meeting the goal, ongoing activity is needed to sustain the goal objective.", 0, 1, continuous); 1541 case -1165461084: /*priority*/ return new Property("priority", "CodeableConcept", "Identifies the mutually agreed level of importance associated with reaching/sustaining the goal.", 0, 1, priority); 1542 case -1724546052: /*description*/ return new Property("description", "CodeableConcept", "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".", 0, 1, description); 1543 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Organization)", "Identifies the patient, group or organization for whom the goal is being established.", 0, 1, subject); 1544 case 1316793566: /*start[x]*/ return new Property("start[x]", "date|CodeableConcept", "The date or event after which the goal should begin being pursued.", 0, 1, start); 1545 case 109757538: /*start*/ return new Property("start[x]", "date|CodeableConcept", "The date or event after which the goal should begin being pursued.", 0, 1, start); 1546 case -2129778896: /*startDate*/ return new Property("start[x]", "date", "The date or event after which the goal should begin being pursued.", 0, 1, start); 1547 case -1758833953: /*startCodeableConcept*/ return new Property("start[x]", "CodeableConcept", "The date or event after which the goal should begin being pursued.", 0, 1, start); 1548 case -880905839: /*target*/ return new Property("target", "", "Indicates what should be done by when.", 0, java.lang.Integer.MAX_VALUE, target); 1549 case 247524032: /*statusDate*/ return new Property("statusDate", "date", "Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc.", 0, 1, statusDate); 1550 case 2051346646: /*statusReason*/ return new Property("statusReason", "string", "Captures the reason for the current status.", 0, 1, statusReason); 1551 case -896505829: /*source*/ return new Property("source", "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|CareTeam)", "Indicates whose goal this is - patient goal, practitioner goal, etc.", 0, 1, source); 1552 case 874544034: /*addresses*/ return new Property("addresses", "Reference(Condition|Observation|MedicationStatement|MedicationRequest|NutritionOrder|ServiceRequest|RiskAssessment|Procedure)", "The identified conditions and other health record elements that are intended to be addressed by the goal.", 0, java.lang.Integer.MAX_VALUE, addresses); 1553 case 3387378: /*note*/ return new Property("note", "Annotation", "Any comments related to the goal.", 0, java.lang.Integer.MAX_VALUE, note); 1554 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableReference(Observation)", "Identifies the change (or lack of change) at the point when the status of the goal is assessed.", 0, java.lang.Integer.MAX_VALUE, outcome); 1555 default: return super.getNamedProperty(_hash, _name, _checkValid); 1556 } 1557 1558 } 1559 1560 @Override 1561 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1562 switch (hash) { 1563 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1564 case 1165552636: /*lifecycleStatus*/ return this.lifecycleStatus == null ? new Base[0] : new Base[] {this.lifecycleStatus}; // Enumeration<GoalLifecycleStatus> 1565 case 104524801: /*achievementStatus*/ return this.achievementStatus == null ? new Base[0] : new Base[] {this.achievementStatus}; // CodeableConcept 1566 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1567 case 379114255: /*continuous*/ return this.continuous == null ? new Base[0] : new Base[] {this.continuous}; // BooleanType 1568 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // CodeableConcept 1569 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // CodeableConcept 1570 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1571 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // DataType 1572 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // GoalTargetComponent 1573 case 247524032: /*statusDate*/ return this.statusDate == null ? new Base[0] : new Base[] {this.statusDate}; // DateType 1574 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : new Base[] {this.statusReason}; // StringType 1575 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // Reference 1576 case 874544034: /*addresses*/ return this.addresses == null ? new Base[0] : this.addresses.toArray(new Base[this.addresses.size()]); // Reference 1577 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1578 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : this.outcome.toArray(new Base[this.outcome.size()]); // CodeableReference 1579 default: return super.getProperty(hash, name, checkValid); 1580 } 1581 1582 } 1583 1584 @Override 1585 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1586 switch (hash) { 1587 case -1618432855: // identifier 1588 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1589 return value; 1590 case 1165552636: // lifecycleStatus 1591 value = new GoalLifecycleStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1592 this.lifecycleStatus = (Enumeration) value; // Enumeration<GoalLifecycleStatus> 1593 return value; 1594 case 104524801: // achievementStatus 1595 this.achievementStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1596 return value; 1597 case 50511102: // category 1598 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1599 return value; 1600 case 379114255: // continuous 1601 this.continuous = TypeConvertor.castToBoolean(value); // BooleanType 1602 return value; 1603 case -1165461084: // priority 1604 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1605 return value; 1606 case -1724546052: // description 1607 this.description = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1608 return value; 1609 case -1867885268: // subject 1610 this.subject = TypeConvertor.castToReference(value); // Reference 1611 return value; 1612 case 109757538: // start 1613 this.start = TypeConvertor.castToType(value); // DataType 1614 return value; 1615 case -880905839: // target 1616 this.getTarget().add((GoalTargetComponent) value); // GoalTargetComponent 1617 return value; 1618 case 247524032: // statusDate 1619 this.statusDate = TypeConvertor.castToDate(value); // DateType 1620 return value; 1621 case 2051346646: // statusReason 1622 this.statusReason = TypeConvertor.castToString(value); // StringType 1623 return value; 1624 case -896505829: // source 1625 this.source = TypeConvertor.castToReference(value); // Reference 1626 return value; 1627 case 874544034: // addresses 1628 this.getAddresses().add(TypeConvertor.castToReference(value)); // Reference 1629 return value; 1630 case 3387378: // note 1631 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1632 return value; 1633 case -1106507950: // outcome 1634 this.getOutcome().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1635 return value; 1636 default: return super.setProperty(hash, name, value); 1637 } 1638 1639 } 1640 1641 @Override 1642 public Base setProperty(String name, Base value) throws FHIRException { 1643 if (name.equals("identifier")) { 1644 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1645 } else if (name.equals("lifecycleStatus")) { 1646 value = new GoalLifecycleStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1647 this.lifecycleStatus = (Enumeration) value; // Enumeration<GoalLifecycleStatus> 1648 } else if (name.equals("achievementStatus")) { 1649 this.achievementStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1650 } else if (name.equals("category")) { 1651 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 1652 } else if (name.equals("continuous")) { 1653 this.continuous = TypeConvertor.castToBoolean(value); // BooleanType 1654 } else if (name.equals("priority")) { 1655 this.priority = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1656 } else if (name.equals("description")) { 1657 this.description = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1658 } else if (name.equals("subject")) { 1659 this.subject = TypeConvertor.castToReference(value); // Reference 1660 } else if (name.equals("start[x]")) { 1661 this.start = TypeConvertor.castToType(value); // DataType 1662 } else if (name.equals("target")) { 1663 this.getTarget().add((GoalTargetComponent) value); 1664 } else if (name.equals("statusDate")) { 1665 this.statusDate = TypeConvertor.castToDate(value); // DateType 1666 } else if (name.equals("statusReason")) { 1667 this.statusReason = TypeConvertor.castToString(value); // StringType 1668 } else if (name.equals("source")) { 1669 this.source = TypeConvertor.castToReference(value); // Reference 1670 } else if (name.equals("addresses")) { 1671 this.getAddresses().add(TypeConvertor.castToReference(value)); 1672 } else if (name.equals("note")) { 1673 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1674 } else if (name.equals("outcome")) { 1675 this.getOutcome().add(TypeConvertor.castToCodeableReference(value)); 1676 } else 1677 return super.setProperty(name, value); 1678 return value; 1679 } 1680 1681 @Override 1682 public void removeChild(String name, Base value) throws FHIRException { 1683 if (name.equals("identifier")) { 1684 this.getIdentifier().remove(value); 1685 } else if (name.equals("lifecycleStatus")) { 1686 value = new GoalLifecycleStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1687 this.lifecycleStatus = (Enumeration) value; // Enumeration<GoalLifecycleStatus> 1688 } else if (name.equals("achievementStatus")) { 1689 this.achievementStatus = null; 1690 } else if (name.equals("category")) { 1691 this.getCategory().remove(value); 1692 } else if (name.equals("continuous")) { 1693 this.continuous = null; 1694 } else if (name.equals("priority")) { 1695 this.priority = null; 1696 } else if (name.equals("description")) { 1697 this.description = null; 1698 } else if (name.equals("subject")) { 1699 this.subject = null; 1700 } else if (name.equals("start[x]")) { 1701 this.start = null; 1702 } else if (name.equals("target")) { 1703 this.getTarget().remove((GoalTargetComponent) value); 1704 } else if (name.equals("statusDate")) { 1705 this.statusDate = null; 1706 } else if (name.equals("statusReason")) { 1707 this.statusReason = null; 1708 } else if (name.equals("source")) { 1709 this.source = null; 1710 } else if (name.equals("addresses")) { 1711 this.getAddresses().remove(value); 1712 } else if (name.equals("note")) { 1713 this.getNote().remove(value); 1714 } else if (name.equals("outcome")) { 1715 this.getOutcome().remove(value); 1716 } else 1717 super.removeChild(name, value); 1718 1719 } 1720 1721 @Override 1722 public Base makeProperty(int hash, String name) throws FHIRException { 1723 switch (hash) { 1724 case -1618432855: return addIdentifier(); 1725 case 1165552636: return getLifecycleStatusElement(); 1726 case 104524801: return getAchievementStatus(); 1727 case 50511102: return addCategory(); 1728 case 379114255: return getContinuousElement(); 1729 case -1165461084: return getPriority(); 1730 case -1724546052: return getDescription(); 1731 case -1867885268: return getSubject(); 1732 case 1316793566: return getStart(); 1733 case 109757538: return getStart(); 1734 case -880905839: return addTarget(); 1735 case 247524032: return getStatusDateElement(); 1736 case 2051346646: return getStatusReasonElement(); 1737 case -896505829: return getSource(); 1738 case 874544034: return addAddresses(); 1739 case 3387378: return addNote(); 1740 case -1106507950: return addOutcome(); 1741 default: return super.makeProperty(hash, name); 1742 } 1743 1744 } 1745 1746 @Override 1747 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1748 switch (hash) { 1749 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1750 case 1165552636: /*lifecycleStatus*/ return new String[] {"code"}; 1751 case 104524801: /*achievementStatus*/ return new String[] {"CodeableConcept"}; 1752 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1753 case 379114255: /*continuous*/ return new String[] {"boolean"}; 1754 case -1165461084: /*priority*/ return new String[] {"CodeableConcept"}; 1755 case -1724546052: /*description*/ return new String[] {"CodeableConcept"}; 1756 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1757 case 109757538: /*start*/ return new String[] {"date", "CodeableConcept"}; 1758 case -880905839: /*target*/ return new String[] {}; 1759 case 247524032: /*statusDate*/ return new String[] {"date"}; 1760 case 2051346646: /*statusReason*/ return new String[] {"string"}; 1761 case -896505829: /*source*/ return new String[] {"Reference"}; 1762 case 874544034: /*addresses*/ return new String[] {"Reference"}; 1763 case 3387378: /*note*/ return new String[] {"Annotation"}; 1764 case -1106507950: /*outcome*/ return new String[] {"CodeableReference"}; 1765 default: return super.getTypesForProperty(hash, name); 1766 } 1767 1768 } 1769 1770 @Override 1771 public Base addChild(String name) throws FHIRException { 1772 if (name.equals("identifier")) { 1773 return addIdentifier(); 1774 } 1775 else if (name.equals("lifecycleStatus")) { 1776 throw new FHIRException("Cannot call addChild on a singleton property Goal.lifecycleStatus"); 1777 } 1778 else if (name.equals("achievementStatus")) { 1779 this.achievementStatus = new CodeableConcept(); 1780 return this.achievementStatus; 1781 } 1782 else if (name.equals("category")) { 1783 return addCategory(); 1784 } 1785 else if (name.equals("continuous")) { 1786 throw new FHIRException("Cannot call addChild on a singleton property Goal.continuous"); 1787 } 1788 else if (name.equals("priority")) { 1789 this.priority = new CodeableConcept(); 1790 return this.priority; 1791 } 1792 else if (name.equals("description")) { 1793 this.description = new CodeableConcept(); 1794 return this.description; 1795 } 1796 else if (name.equals("subject")) { 1797 this.subject = new Reference(); 1798 return this.subject; 1799 } 1800 else if (name.equals("startDate")) { 1801 this.start = new DateType(); 1802 return this.start; 1803 } 1804 else if (name.equals("startCodeableConcept")) { 1805 this.start = new CodeableConcept(); 1806 return this.start; 1807 } 1808 else if (name.equals("target")) { 1809 return addTarget(); 1810 } 1811 else if (name.equals("statusDate")) { 1812 throw new FHIRException("Cannot call addChild on a singleton property Goal.statusDate"); 1813 } 1814 else if (name.equals("statusReason")) { 1815 throw new FHIRException("Cannot call addChild on a singleton property Goal.statusReason"); 1816 } 1817 else if (name.equals("source")) { 1818 this.source = new Reference(); 1819 return this.source; 1820 } 1821 else if (name.equals("addresses")) { 1822 return addAddresses(); 1823 } 1824 else if (name.equals("note")) { 1825 return addNote(); 1826 } 1827 else if (name.equals("outcome")) { 1828 return addOutcome(); 1829 } 1830 else 1831 return super.addChild(name); 1832 } 1833 1834 public String fhirType() { 1835 return "Goal"; 1836 1837 } 1838 1839 public Goal copy() { 1840 Goal dst = new Goal(); 1841 copyValues(dst); 1842 return dst; 1843 } 1844 1845 public void copyValues(Goal dst) { 1846 super.copyValues(dst); 1847 if (identifier != null) { 1848 dst.identifier = new ArrayList<Identifier>(); 1849 for (Identifier i : identifier) 1850 dst.identifier.add(i.copy()); 1851 }; 1852 dst.lifecycleStatus = lifecycleStatus == null ? null : lifecycleStatus.copy(); 1853 dst.achievementStatus = achievementStatus == null ? null : achievementStatus.copy(); 1854 if (category != null) { 1855 dst.category = new ArrayList<CodeableConcept>(); 1856 for (CodeableConcept i : category) 1857 dst.category.add(i.copy()); 1858 }; 1859 dst.continuous = continuous == null ? null : continuous.copy(); 1860 dst.priority = priority == null ? null : priority.copy(); 1861 dst.description = description == null ? null : description.copy(); 1862 dst.subject = subject == null ? null : subject.copy(); 1863 dst.start = start == null ? null : start.copy(); 1864 if (target != null) { 1865 dst.target = new ArrayList<GoalTargetComponent>(); 1866 for (GoalTargetComponent i : target) 1867 dst.target.add(i.copy()); 1868 }; 1869 dst.statusDate = statusDate == null ? null : statusDate.copy(); 1870 dst.statusReason = statusReason == null ? null : statusReason.copy(); 1871 dst.source = source == null ? null : source.copy(); 1872 if (addresses != null) { 1873 dst.addresses = new ArrayList<Reference>(); 1874 for (Reference i : addresses) 1875 dst.addresses.add(i.copy()); 1876 }; 1877 if (note != null) { 1878 dst.note = new ArrayList<Annotation>(); 1879 for (Annotation i : note) 1880 dst.note.add(i.copy()); 1881 }; 1882 if (outcome != null) { 1883 dst.outcome = new ArrayList<CodeableReference>(); 1884 for (CodeableReference i : outcome) 1885 dst.outcome.add(i.copy()); 1886 }; 1887 } 1888 1889 protected Goal typedCopy() { 1890 return copy(); 1891 } 1892 1893 @Override 1894 public boolean equalsDeep(Base other_) { 1895 if (!super.equalsDeep(other_)) 1896 return false; 1897 if (!(other_ instanceof Goal)) 1898 return false; 1899 Goal o = (Goal) other_; 1900 return compareDeep(identifier, o.identifier, true) && compareDeep(lifecycleStatus, o.lifecycleStatus, true) 1901 && compareDeep(achievementStatus, o.achievementStatus, true) && compareDeep(category, o.category, true) 1902 && compareDeep(continuous, o.continuous, true) && compareDeep(priority, o.priority, true) && compareDeep(description, o.description, true) 1903 && compareDeep(subject, o.subject, true) && compareDeep(start, o.start, true) && compareDeep(target, o.target, true) 1904 && compareDeep(statusDate, o.statusDate, true) && compareDeep(statusReason, o.statusReason, true) 1905 && compareDeep(source, o.source, true) && compareDeep(addresses, o.addresses, true) && compareDeep(note, o.note, true) 1906 && compareDeep(outcome, o.outcome, true); 1907 } 1908 1909 @Override 1910 public boolean equalsShallow(Base other_) { 1911 if (!super.equalsShallow(other_)) 1912 return false; 1913 if (!(other_ instanceof Goal)) 1914 return false; 1915 Goal o = (Goal) other_; 1916 return compareValues(lifecycleStatus, o.lifecycleStatus, true) && compareValues(continuous, o.continuous, true) 1917 && compareValues(statusDate, o.statusDate, true) && compareValues(statusReason, o.statusReason, true) 1918 ; 1919 } 1920 1921 public boolean isEmpty() { 1922 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, lifecycleStatus 1923 , achievementStatus, category, continuous, priority, description, subject, start 1924 , target, statusDate, statusReason, source, addresses, note, outcome); 1925 } 1926 1927 @Override 1928 public ResourceType getResourceType() { 1929 return ResourceType.Goal; 1930 } 1931 1932 /** 1933 * Search parameter: <b>achievement-status</b> 1934 * <p> 1935 * Description: <b>in-progress | improving | worsening | no-change | achieved | sustaining | not-achieved | no-progress | not-attainable</b><br> 1936 * Type: <b>token</b><br> 1937 * Path: <b>Goal.achievementStatus</b><br> 1938 * </p> 1939 */ 1940 @SearchParamDefinition(name="achievement-status", path="Goal.achievementStatus", description="in-progress | improving | worsening | no-change | achieved | sustaining | not-achieved | no-progress | not-attainable", type="token" ) 1941 public static final String SP_ACHIEVEMENT_STATUS = "achievement-status"; 1942 /** 1943 * <b>Fluent Client</b> search parameter constant for <b>achievement-status</b> 1944 * <p> 1945 * Description: <b>in-progress | improving | worsening | no-change | achieved | sustaining | not-achieved | no-progress | not-attainable</b><br> 1946 * Type: <b>token</b><br> 1947 * Path: <b>Goal.achievementStatus</b><br> 1948 * </p> 1949 */ 1950 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACHIEVEMENT_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACHIEVEMENT_STATUS); 1951 1952 /** 1953 * Search parameter: <b>addresses</b> 1954 * <p> 1955 * Description: <b>Issues addressed by this goal</b><br> 1956 * Type: <b>reference</b><br> 1957 * Path: <b>Goal.addresses</b><br> 1958 * </p> 1959 */ 1960 @SearchParamDefinition(name="addresses", path="Goal.addresses", description="Issues addressed by this goal", type="reference", target={Condition.class, MedicationRequest.class, MedicationStatement.class, NutritionOrder.class, Observation.class, Procedure.class, RiskAssessment.class, ServiceRequest.class } ) 1961 public static final String SP_ADDRESSES = "addresses"; 1962 /** 1963 * <b>Fluent Client</b> search parameter constant for <b>addresses</b> 1964 * <p> 1965 * Description: <b>Issues addressed by this goal</b><br> 1966 * Type: <b>reference</b><br> 1967 * Path: <b>Goal.addresses</b><br> 1968 * </p> 1969 */ 1970 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ADDRESSES = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ADDRESSES); 1971 1972/** 1973 * Constant for fluent queries to be used to add include statements. Specifies 1974 * the path value of "<b>Goal:addresses</b>". 1975 */ 1976 public static final ca.uhn.fhir.model.api.Include INCLUDE_ADDRESSES = new ca.uhn.fhir.model.api.Include("Goal:addresses").toLocked(); 1977 1978 /** 1979 * Search parameter: <b>category</b> 1980 * <p> 1981 * Description: <b>E.g. Treatment, dietary, behavioral, etc.</b><br> 1982 * Type: <b>token</b><br> 1983 * Path: <b>Goal.category</b><br> 1984 * </p> 1985 */ 1986 @SearchParamDefinition(name="category", path="Goal.category", description="E.g. Treatment, dietary, behavioral, etc.", type="token" ) 1987 public static final String SP_CATEGORY = "category"; 1988 /** 1989 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1990 * <p> 1991 * Description: <b>E.g. Treatment, dietary, behavioral, etc.</b><br> 1992 * Type: <b>token</b><br> 1993 * Path: <b>Goal.category</b><br> 1994 * </p> 1995 */ 1996 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1997 1998 /** 1999 * Search parameter: <b>description</b> 2000 * <p> 2001 * Description: <b>Code or text describing goal</b><br> 2002 * Type: <b>token</b><br> 2003 * Path: <b>Goal.description</b><br> 2004 * </p> 2005 */ 2006 @SearchParamDefinition(name="description", path="Goal.description", description="Code or text describing goal", type="token" ) 2007 public static final String SP_DESCRIPTION = "description"; 2008 /** 2009 * <b>Fluent Client</b> search parameter constant for <b>description</b> 2010 * <p> 2011 * Description: <b>Code or text describing goal</b><br> 2012 * Type: <b>token</b><br> 2013 * Path: <b>Goal.description</b><br> 2014 * </p> 2015 */ 2016 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DESCRIPTION); 2017 2018 /** 2019 * Search parameter: <b>lifecycle-status</b> 2020 * <p> 2021 * Description: <b>proposed | planned | accepted | active | on-hold | completed | cancelled | entered-in-error | rejected</b><br> 2022 * Type: <b>token</b><br> 2023 * Path: <b>Goal.lifecycleStatus</b><br> 2024 * </p> 2025 */ 2026 @SearchParamDefinition(name="lifecycle-status", path="Goal.lifecycleStatus", description="proposed | planned | accepted | active | on-hold | completed | cancelled | entered-in-error | rejected", type="token" ) 2027 public static final String SP_LIFECYCLE_STATUS = "lifecycle-status"; 2028 /** 2029 * <b>Fluent Client</b> search parameter constant for <b>lifecycle-status</b> 2030 * <p> 2031 * Description: <b>proposed | planned | accepted | active | on-hold | completed | cancelled | entered-in-error | rejected</b><br> 2032 * Type: <b>token</b><br> 2033 * Path: <b>Goal.lifecycleStatus</b><br> 2034 * </p> 2035 */ 2036 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LIFECYCLE_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_LIFECYCLE_STATUS); 2037 2038 /** 2039 * Search parameter: <b>start-date</b> 2040 * <p> 2041 * Description: <b>When goal pursuit begins</b><br> 2042 * Type: <b>date</b><br> 2043 * Path: <b>(Goal.start.ofType(date))</b><br> 2044 * </p> 2045 */ 2046 @SearchParamDefinition(name="start-date", path="(Goal.start.ofType(date))", description="When goal pursuit begins", type="date" ) 2047 public static final String SP_START_DATE = "start-date"; 2048 /** 2049 * <b>Fluent Client</b> search parameter constant for <b>start-date</b> 2050 * <p> 2051 * Description: <b>When goal pursuit begins</b><br> 2052 * Type: <b>date</b><br> 2053 * Path: <b>(Goal.start.ofType(date))</b><br> 2054 * </p> 2055 */ 2056 public static final ca.uhn.fhir.rest.gclient.DateClientParam START_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_START_DATE); 2057 2058 /** 2059 * Search parameter: <b>subject</b> 2060 * <p> 2061 * Description: <b>Who this goal is intended for</b><br> 2062 * Type: <b>reference</b><br> 2063 * Path: <b>Goal.subject</b><br> 2064 * </p> 2065 */ 2066 @SearchParamDefinition(name="subject", path="Goal.subject", description="Who this goal is intended for", type="reference", target={Group.class, Organization.class, Patient.class } ) 2067 public static final String SP_SUBJECT = "subject"; 2068 /** 2069 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2070 * <p> 2071 * Description: <b>Who this goal is intended for</b><br> 2072 * Type: <b>reference</b><br> 2073 * Path: <b>Goal.subject</b><br> 2074 * </p> 2075 */ 2076 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2077 2078/** 2079 * Constant for fluent queries to be used to add include statements. Specifies 2080 * the path value of "<b>Goal:subject</b>". 2081 */ 2082 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Goal:subject").toLocked(); 2083 2084 /** 2085 * Search parameter: <b>target-date</b> 2086 * <p> 2087 * Description: <b>Reach goal on or before</b><br> 2088 * Type: <b>date</b><br> 2089 * Path: <b>(Goal.target.due.ofType(date))</b><br> 2090 * </p> 2091 */ 2092 @SearchParamDefinition(name="target-date", path="(Goal.target.due.ofType(date))", description="Reach goal on or before", type="date" ) 2093 public static final String SP_TARGET_DATE = "target-date"; 2094 /** 2095 * <b>Fluent Client</b> search parameter constant for <b>target-date</b> 2096 * <p> 2097 * Description: <b>Reach goal on or before</b><br> 2098 * Type: <b>date</b><br> 2099 * Path: <b>(Goal.target.due.ofType(date))</b><br> 2100 * </p> 2101 */ 2102 public static final ca.uhn.fhir.rest.gclient.DateClientParam TARGET_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_TARGET_DATE); 2103 2104 /** 2105 * Search parameter: <b>target-measure</b> 2106 * <p> 2107 * Description: <b>The parameter whose value is being tracked</b><br> 2108 * Type: <b>token</b><br> 2109 * Path: <b>Goal.target.measure</b><br> 2110 * </p> 2111 */ 2112 @SearchParamDefinition(name="target-measure", path="Goal.target.measure", description="The parameter whose value is being tracked", type="token" ) 2113 public static final String SP_TARGET_MEASURE = "target-measure"; 2114 /** 2115 * <b>Fluent Client</b> search parameter constant for <b>target-measure</b> 2116 * <p> 2117 * Description: <b>The parameter whose value is being tracked</b><br> 2118 * Type: <b>token</b><br> 2119 * Path: <b>Goal.target.measure</b><br> 2120 * </p> 2121 */ 2122 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_MEASURE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TARGET_MEASURE); 2123 2124 /** 2125 * Search parameter: <b>identifier</b> 2126 * <p> 2127 * Description: <b>Multiple Resources: 2128 2129* [Account](account.html): Account number 2130* [AdverseEvent](adverseevent.html): Business identifier for the event 2131* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2132* [Appointment](appointment.html): An Identifier of the Appointment 2133* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2134* [Basic](basic.html): Business identifier 2135* [BodyStructure](bodystructure.html): Bodystructure identifier 2136* [CarePlan](careplan.html): External Ids for this plan 2137* [CareTeam](careteam.html): External Ids for this team 2138* [ChargeItem](chargeitem.html): Business Identifier for item 2139* [Claim](claim.html): The primary identifier of the financial resource 2140* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2141* [ClinicalImpression](clinicalimpression.html): Business identifier 2142* [Communication](communication.html): Unique identifier 2143* [CommunicationRequest](communicationrequest.html): Unique identifier 2144* [Composition](composition.html): Version-independent identifier for the Composition 2145* [Condition](condition.html): A unique identifier of the condition record 2146* [Consent](consent.html): Identifier for this record (external references) 2147* [Contract](contract.html): The identity of the contract 2148* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2149* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2150* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2151* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2152* [DeviceRequest](devicerequest.html): Business identifier for request/order 2153* [DeviceUsage](deviceusage.html): Search by identifier 2154* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2155* [DocumentReference](documentreference.html): Identifier of the attachment binary 2156* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2157* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2158* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2159* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2160* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2161* [Flag](flag.html): Business identifier 2162* [Goal](goal.html): External Ids for this goal 2163* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2164* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2165* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2166* [Immunization](immunization.html): Business identifier 2167* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2168* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2169* [Invoice](invoice.html): Business Identifier for item 2170* [List](list.html): Business identifier 2171* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2172* [Medication](medication.html): Returns medications with this external identifier 2173* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2174* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2175* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2176* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2177* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2178* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2179* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2180* [Observation](observation.html): The unique id for a particular observation 2181* [Person](person.html): A person Identifier 2182* [Procedure](procedure.html): A unique identifier for a procedure 2183* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2184* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2185* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2186* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2187* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2188* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2189* [Specimen](specimen.html): The unique identifier associated with the specimen 2190* [SupplyDelivery](supplydelivery.html): External identifier 2191* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2192* [Task](task.html): Search for a task instance by its business identifier 2193* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2194</b><br> 2195 * Type: <b>token</b><br> 2196 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2197 * </p> 2198 */ 2199 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2200 public static final String SP_IDENTIFIER = "identifier"; 2201 /** 2202 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2203 * <p> 2204 * Description: <b>Multiple Resources: 2205 2206* [Account](account.html): Account number 2207* [AdverseEvent](adverseevent.html): Business identifier for the event 2208* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2209* [Appointment](appointment.html): An Identifier of the Appointment 2210* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2211* [Basic](basic.html): Business identifier 2212* [BodyStructure](bodystructure.html): Bodystructure identifier 2213* [CarePlan](careplan.html): External Ids for this plan 2214* [CareTeam](careteam.html): External Ids for this team 2215* [ChargeItem](chargeitem.html): Business Identifier for item 2216* [Claim](claim.html): The primary identifier of the financial resource 2217* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2218* [ClinicalImpression](clinicalimpression.html): Business identifier 2219* [Communication](communication.html): Unique identifier 2220* [CommunicationRequest](communicationrequest.html): Unique identifier 2221* [Composition](composition.html): Version-independent identifier for the Composition 2222* [Condition](condition.html): A unique identifier of the condition record 2223* [Consent](consent.html): Identifier for this record (external references) 2224* [Contract](contract.html): The identity of the contract 2225* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2226* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2227* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2228* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2229* [DeviceRequest](devicerequest.html): Business identifier for request/order 2230* [DeviceUsage](deviceusage.html): Search by identifier 2231* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2232* [DocumentReference](documentreference.html): Identifier of the attachment binary 2233* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2234* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2235* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2236* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2237* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2238* [Flag](flag.html): Business identifier 2239* [Goal](goal.html): External Ids for this goal 2240* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2241* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2242* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2243* [Immunization](immunization.html): Business identifier 2244* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2245* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2246* [Invoice](invoice.html): Business Identifier for item 2247* [List](list.html): Business identifier 2248* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2249* [Medication](medication.html): Returns medications with this external identifier 2250* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2251* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2252* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2253* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2254* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2255* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2256* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2257* [Observation](observation.html): The unique id for a particular observation 2258* [Person](person.html): A person Identifier 2259* [Procedure](procedure.html): A unique identifier for a procedure 2260* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2261* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2262* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2263* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2264* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2265* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2266* [Specimen](specimen.html): The unique identifier associated with the specimen 2267* [SupplyDelivery](supplydelivery.html): External identifier 2268* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2269* [Task](task.html): Search for a task instance by its business identifier 2270* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2271</b><br> 2272 * Type: <b>token</b><br> 2273 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2274 * </p> 2275 */ 2276 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2277 2278 /** 2279 * Search parameter: <b>patient</b> 2280 * <p> 2281 * Description: <b>Multiple Resources: 2282 2283* [Account](account.html): The entity that caused the expenses 2284* [AdverseEvent](adverseevent.html): Subject impacted by event 2285* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2286* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2287* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2288* [AuditEvent](auditevent.html): Where the activity involved patient data 2289* [Basic](basic.html): Identifies the focus of this resource 2290* [BodyStructure](bodystructure.html): Who this is about 2291* [CarePlan](careplan.html): Who the care plan is for 2292* [CareTeam](careteam.html): Who care team is for 2293* [ChargeItem](chargeitem.html): Individual service was done for/to 2294* [Claim](claim.html): Patient receiving the products or services 2295* [ClaimResponse](claimresponse.html): The subject of care 2296* [ClinicalImpression](clinicalimpression.html): Patient assessed 2297* [Communication](communication.html): Focus of message 2298* [CommunicationRequest](communicationrequest.html): Focus of message 2299* [Composition](composition.html): Who and/or what the composition is about 2300* [Condition](condition.html): Who has the condition? 2301* [Consent](consent.html): Who the consent applies to 2302* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2303* [Coverage](coverage.html): Retrieve coverages for a patient 2304* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2305* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2306* [DetectedIssue](detectedissue.html): Associated patient 2307* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2308* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2309* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2310* [DocumentReference](documentreference.html): Who/what is the subject of the document 2311* [Encounter](encounter.html): The patient present at the encounter 2312* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2313* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2314* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2315* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2316* [Flag](flag.html): The identity of a subject to list flags for 2317* [Goal](goal.html): Who this goal is intended for 2318* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2319* [ImagingSelection](imagingselection.html): Who the study is about 2320* [ImagingStudy](imagingstudy.html): Who the study is about 2321* [Immunization](immunization.html): The patient for the vaccination record 2322* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2323* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2324* [Invoice](invoice.html): Recipient(s) of goods and services 2325* [List](list.html): If all resources have the same subject 2326* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2327* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2328* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2329* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2330* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2331* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2332* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2333* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2334* [Observation](observation.html): The subject that the observation is about (if patient) 2335* [Person](person.html): The Person links to this Patient 2336* [Procedure](procedure.html): Search by subject - a patient 2337* [Provenance](provenance.html): Where the activity involved patient data 2338* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2339* [RelatedPerson](relatedperson.html): The patient this related person is related to 2340* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2341* [ResearchSubject](researchsubject.html): Who or what is part of study 2342* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2343* [ServiceRequest](servicerequest.html): Search by subject - a patient 2344* [Specimen](specimen.html): The patient the specimen comes from 2345* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2346* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2347* [Task](task.html): Search by patient 2348* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2349</b><br> 2350 * Type: <b>reference</b><br> 2351 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2352 * </p> 2353 */ 2354 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 2355 public static final String SP_PATIENT = "patient"; 2356 /** 2357 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2358 * <p> 2359 * Description: <b>Multiple Resources: 2360 2361* [Account](account.html): The entity that caused the expenses 2362* [AdverseEvent](adverseevent.html): Subject impacted by event 2363* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2364* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2365* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2366* [AuditEvent](auditevent.html): Where the activity involved patient data 2367* [Basic](basic.html): Identifies the focus of this resource 2368* [BodyStructure](bodystructure.html): Who this is about 2369* [CarePlan](careplan.html): Who the care plan is for 2370* [CareTeam](careteam.html): Who care team is for 2371* [ChargeItem](chargeitem.html): Individual service was done for/to 2372* [Claim](claim.html): Patient receiving the products or services 2373* [ClaimResponse](claimresponse.html): The subject of care 2374* [ClinicalImpression](clinicalimpression.html): Patient assessed 2375* [Communication](communication.html): Focus of message 2376* [CommunicationRequest](communicationrequest.html): Focus of message 2377* [Composition](composition.html): Who and/or what the composition is about 2378* [Condition](condition.html): Who has the condition? 2379* [Consent](consent.html): Who the consent applies to 2380* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2381* [Coverage](coverage.html): Retrieve coverages for a patient 2382* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2383* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2384* [DetectedIssue](detectedissue.html): Associated patient 2385* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2386* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2387* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2388* [DocumentReference](documentreference.html): Who/what is the subject of the document 2389* [Encounter](encounter.html): The patient present at the encounter 2390* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2391* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2392* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2393* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2394* [Flag](flag.html): The identity of a subject to list flags for 2395* [Goal](goal.html): Who this goal is intended for 2396* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2397* [ImagingSelection](imagingselection.html): Who the study is about 2398* [ImagingStudy](imagingstudy.html): Who the study is about 2399* [Immunization](immunization.html): The patient for the vaccination record 2400* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2401* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2402* [Invoice](invoice.html): Recipient(s) of goods and services 2403* [List](list.html): If all resources have the same subject 2404* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2405* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2406* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2407* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2408* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2409* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2410* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2411* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2412* [Observation](observation.html): The subject that the observation is about (if patient) 2413* [Person](person.html): The Person links to this Patient 2414* [Procedure](procedure.html): Search by subject - a patient 2415* [Provenance](provenance.html): Where the activity involved patient data 2416* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2417* [RelatedPerson](relatedperson.html): The patient this related person is related to 2418* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2419* [ResearchSubject](researchsubject.html): Who or what is part of study 2420* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2421* [ServiceRequest](servicerequest.html): Search by subject - a patient 2422* [Specimen](specimen.html): The patient the specimen comes from 2423* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2424* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2425* [Task](task.html): Search by patient 2426* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2427</b><br> 2428 * Type: <b>reference</b><br> 2429 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2430 * </p> 2431 */ 2432 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2433 2434/** 2435 * Constant for fluent queries to be used to add include statements. Specifies 2436 * the path value of "<b>Goal:patient</b>". 2437 */ 2438 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Goal:patient").toLocked(); 2439 2440 2441} 2442