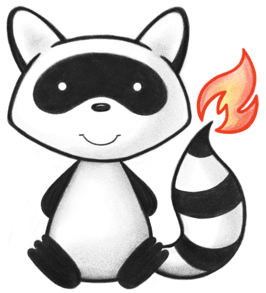
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set. 052 */ 053@ResourceDef(name="GraphDefinition", profile="http://hl7.org/fhir/StructureDefinition/GraphDefinition") 054public class GraphDefinition extends CanonicalResource { 055 056 public enum GraphCompartmentRule { 057 /** 058 * The compartment must be identical (the same literal reference). 059 */ 060 IDENTICAL, 061 /** 062 * The compartment must be the same - the record must be about the same patient, but the reference may be different. 063 */ 064 MATCHING, 065 /** 066 * The compartment must be different. 067 */ 068 DIFFERENT, 069 /** 070 * The compartment rule is defined in the accompanying FHIRPath expression. 071 */ 072 CUSTOM, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static GraphCompartmentRule fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("identical".equals(codeString)) 081 return IDENTICAL; 082 if ("matching".equals(codeString)) 083 return MATCHING; 084 if ("different".equals(codeString)) 085 return DIFFERENT; 086 if ("custom".equals(codeString)) 087 return CUSTOM; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown GraphCompartmentRule code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case IDENTICAL: return "identical"; 096 case MATCHING: return "matching"; 097 case DIFFERENT: return "different"; 098 case CUSTOM: return "custom"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case IDENTICAL: return "http://hl7.org/fhir/graph-compartment-rule"; 106 case MATCHING: return "http://hl7.org/fhir/graph-compartment-rule"; 107 case DIFFERENT: return "http://hl7.org/fhir/graph-compartment-rule"; 108 case CUSTOM: return "http://hl7.org/fhir/graph-compartment-rule"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case IDENTICAL: return "The compartment must be identical (the same literal reference)."; 116 case MATCHING: return "The compartment must be the same - the record must be about the same patient, but the reference may be different."; 117 case DIFFERENT: return "The compartment must be different."; 118 case CUSTOM: return "The compartment rule is defined in the accompanying FHIRPath expression."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case IDENTICAL: return "Identical"; 126 case MATCHING: return "Matching"; 127 case DIFFERENT: return "Different"; 128 case CUSTOM: return "Custom"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class GraphCompartmentRuleEnumFactory implements EnumFactory<GraphCompartmentRule> { 136 public GraphCompartmentRule fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("identical".equals(codeString)) 141 return GraphCompartmentRule.IDENTICAL; 142 if ("matching".equals(codeString)) 143 return GraphCompartmentRule.MATCHING; 144 if ("different".equals(codeString)) 145 return GraphCompartmentRule.DIFFERENT; 146 if ("custom".equals(codeString)) 147 return GraphCompartmentRule.CUSTOM; 148 throw new IllegalArgumentException("Unknown GraphCompartmentRule code '"+codeString+"'"); 149 } 150 public Enumeration<GraphCompartmentRule> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<GraphCompartmentRule>(this, GraphCompartmentRule.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<GraphCompartmentRule>(this, GraphCompartmentRule.NULL, code); 158 if ("identical".equals(codeString)) 159 return new Enumeration<GraphCompartmentRule>(this, GraphCompartmentRule.IDENTICAL, code); 160 if ("matching".equals(codeString)) 161 return new Enumeration<GraphCompartmentRule>(this, GraphCompartmentRule.MATCHING, code); 162 if ("different".equals(codeString)) 163 return new Enumeration<GraphCompartmentRule>(this, GraphCompartmentRule.DIFFERENT, code); 164 if ("custom".equals(codeString)) 165 return new Enumeration<GraphCompartmentRule>(this, GraphCompartmentRule.CUSTOM, code); 166 throw new FHIRException("Unknown GraphCompartmentRule code '"+codeString+"'"); 167 } 168 public String toCode(GraphCompartmentRule code) { 169 if (code == GraphCompartmentRule.NULL) 170 return null; 171 if (code == GraphCompartmentRule.IDENTICAL) 172 return "identical"; 173 if (code == GraphCompartmentRule.MATCHING) 174 return "matching"; 175 if (code == GraphCompartmentRule.DIFFERENT) 176 return "different"; 177 if (code == GraphCompartmentRule.CUSTOM) 178 return "custom"; 179 return "?"; 180 } 181 public String toSystem(GraphCompartmentRule code) { 182 return code.getSystem(); 183 } 184 } 185 186 public enum GraphCompartmentUse { 187 /** 188 * This compartment rule is a condition for whether the rule applies. 189 */ 190 WHERE, 191 /** 192 * This compartment rule is enforced on any relationships that meet the conditions. 193 */ 194 REQUIRES, 195 /** 196 * added to help the parsers with the generic types 197 */ 198 NULL; 199 public static GraphCompartmentUse fromCode(String codeString) throws FHIRException { 200 if (codeString == null || "".equals(codeString)) 201 return null; 202 if ("where".equals(codeString)) 203 return WHERE; 204 if ("requires".equals(codeString)) 205 return REQUIRES; 206 if (Configuration.isAcceptInvalidEnums()) 207 return null; 208 else 209 throw new FHIRException("Unknown GraphCompartmentUse code '"+codeString+"'"); 210 } 211 public String toCode() { 212 switch (this) { 213 case WHERE: return "where"; 214 case REQUIRES: return "requires"; 215 case NULL: return null; 216 default: return "?"; 217 } 218 } 219 public String getSystem() { 220 switch (this) { 221 case WHERE: return "http://hl7.org/fhir/graph-compartment-use"; 222 case REQUIRES: return "http://hl7.org/fhir/graph-compartment-use"; 223 case NULL: return null; 224 default: return "?"; 225 } 226 } 227 public String getDefinition() { 228 switch (this) { 229 case WHERE: return "This compartment rule is a condition for whether the rule applies."; 230 case REQUIRES: return "This compartment rule is enforced on any relationships that meet the conditions."; 231 case NULL: return null; 232 default: return "?"; 233 } 234 } 235 public String getDisplay() { 236 switch (this) { 237 case WHERE: return "Where"; 238 case REQUIRES: return "requires"; 239 case NULL: return null; 240 default: return "?"; 241 } 242 } 243 } 244 245 public static class GraphCompartmentUseEnumFactory implements EnumFactory<GraphCompartmentUse> { 246 public GraphCompartmentUse fromCode(String codeString) throws IllegalArgumentException { 247 if (codeString == null || "".equals(codeString)) 248 if (codeString == null || "".equals(codeString)) 249 return null; 250 if ("where".equals(codeString)) 251 return GraphCompartmentUse.WHERE; 252 if ("requires".equals(codeString)) 253 return GraphCompartmentUse.REQUIRES; 254 throw new IllegalArgumentException("Unknown GraphCompartmentUse code '"+codeString+"'"); 255 } 256 public Enumeration<GraphCompartmentUse> fromType(PrimitiveType<?> code) throws FHIRException { 257 if (code == null) 258 return null; 259 if (code.isEmpty()) 260 return new Enumeration<GraphCompartmentUse>(this, GraphCompartmentUse.NULL, code); 261 String codeString = ((PrimitiveType) code).asStringValue(); 262 if (codeString == null || "".equals(codeString)) 263 return new Enumeration<GraphCompartmentUse>(this, GraphCompartmentUse.NULL, code); 264 if ("where".equals(codeString)) 265 return new Enumeration<GraphCompartmentUse>(this, GraphCompartmentUse.WHERE, code); 266 if ("requires".equals(codeString)) 267 return new Enumeration<GraphCompartmentUse>(this, GraphCompartmentUse.REQUIRES, code); 268 throw new FHIRException("Unknown GraphCompartmentUse code '"+codeString+"'"); 269 } 270 public String toCode(GraphCompartmentUse code) { 271 if (code == GraphCompartmentUse.NULL) 272 return null; 273 if (code == GraphCompartmentUse.WHERE) 274 return "where"; 275 if (code == GraphCompartmentUse.REQUIRES) 276 return "requires"; 277 return "?"; 278 } 279 public String toSystem(GraphCompartmentUse code) { 280 return code.getSystem(); 281 } 282 } 283 284 @Block() 285 public static class GraphDefinitionNodeComponent extends BackboneElement implements IBaseBackboneElement { 286 /** 287 * Internal ID of node - target for link references. 288 */ 289 @Child(name = "nodeId", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 290 @Description(shortDefinition="Internal ID - target for link references", formalDefinition="Internal ID of node - target for link references." ) 291 protected IdType nodeId; 292 293 /** 294 * Information about why this node is of interest in this graph definition. 295 */ 296 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 297 @Description(shortDefinition="Why this node is specified", formalDefinition="Information about why this node is of interest in this graph definition." ) 298 protected StringType description; 299 300 /** 301 * Type of resource this link refers to. 302 */ 303 @Child(name = "type", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=false) 304 @Description(shortDefinition="Type of resource this link refers to", formalDefinition="Type of resource this link refers to." ) 305 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-independent-all-resource-types") 306 protected Enumeration<VersionIndependentResourceTypesAll> type; 307 308 /** 309 * Profile for the target resource. 310 */ 311 @Child(name = "profile", type = {CanonicalType.class}, order=4, min=0, max=1, modifier=false, summary=false) 312 @Description(shortDefinition="Profile for the target resource", formalDefinition="Profile for the target resource." ) 313 protected CanonicalType profile; 314 315 private static final long serialVersionUID = -1497429325L; 316 317 /** 318 * Constructor 319 */ 320 public GraphDefinitionNodeComponent() { 321 super(); 322 } 323 324 /** 325 * Constructor 326 */ 327 public GraphDefinitionNodeComponent(String nodeId, VersionIndependentResourceTypesAll type) { 328 super(); 329 this.setNodeId(nodeId); 330 this.setType(type); 331 } 332 333 /** 334 * @return {@link #nodeId} (Internal ID of node - target for link references.). This is the underlying object with id, value and extensions. The accessor "getNodeId" gives direct access to the value 335 */ 336 public IdType getNodeIdElement() { 337 if (this.nodeId == null) 338 if (Configuration.errorOnAutoCreate()) 339 throw new Error("Attempt to auto-create GraphDefinitionNodeComponent.nodeId"); 340 else if (Configuration.doAutoCreate()) 341 this.nodeId = new IdType(); // bb 342 return this.nodeId; 343 } 344 345 public boolean hasNodeIdElement() { 346 return this.nodeId != null && !this.nodeId.isEmpty(); 347 } 348 349 public boolean hasNodeId() { 350 return this.nodeId != null && !this.nodeId.isEmpty(); 351 } 352 353 /** 354 * @param value {@link #nodeId} (Internal ID of node - target for link references.). This is the underlying object with id, value and extensions. The accessor "getNodeId" gives direct access to the value 355 */ 356 public GraphDefinitionNodeComponent setNodeIdElement(IdType value) { 357 this.nodeId = value; 358 return this; 359 } 360 361 /** 362 * @return Internal ID of node - target for link references. 363 */ 364 public String getNodeId() { 365 return this.nodeId == null ? null : this.nodeId.getValue(); 366 } 367 368 /** 369 * @param value Internal ID of node - target for link references. 370 */ 371 public GraphDefinitionNodeComponent setNodeId(String value) { 372 if (this.nodeId == null) 373 this.nodeId = new IdType(); 374 this.nodeId.setValue(value); 375 return this; 376 } 377 378 /** 379 * @return {@link #description} (Information about why this node is of interest in this graph definition.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 380 */ 381 public StringType getDescriptionElement() { 382 if (this.description == null) 383 if (Configuration.errorOnAutoCreate()) 384 throw new Error("Attempt to auto-create GraphDefinitionNodeComponent.description"); 385 else if (Configuration.doAutoCreate()) 386 this.description = new StringType(); // bb 387 return this.description; 388 } 389 390 public boolean hasDescriptionElement() { 391 return this.description != null && !this.description.isEmpty(); 392 } 393 394 public boolean hasDescription() { 395 return this.description != null && !this.description.isEmpty(); 396 } 397 398 /** 399 * @param value {@link #description} (Information about why this node is of interest in this graph definition.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 400 */ 401 public GraphDefinitionNodeComponent setDescriptionElement(StringType value) { 402 this.description = value; 403 return this; 404 } 405 406 /** 407 * @return Information about why this node is of interest in this graph definition. 408 */ 409 public String getDescription() { 410 return this.description == null ? null : this.description.getValue(); 411 } 412 413 /** 414 * @param value Information about why this node is of interest in this graph definition. 415 */ 416 public GraphDefinitionNodeComponent setDescription(String value) { 417 if (Utilities.noString(value)) 418 this.description = null; 419 else { 420 if (this.description == null) 421 this.description = new StringType(); 422 this.description.setValue(value); 423 } 424 return this; 425 } 426 427 /** 428 * @return {@link #type} (Type of resource this link refers to.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 429 */ 430 public Enumeration<VersionIndependentResourceTypesAll> getTypeElement() { 431 if (this.type == null) 432 if (Configuration.errorOnAutoCreate()) 433 throw new Error("Attempt to auto-create GraphDefinitionNodeComponent.type"); 434 else if (Configuration.doAutoCreate()) 435 this.type = new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory()); // bb 436 return this.type; 437 } 438 439 public boolean hasTypeElement() { 440 return this.type != null && !this.type.isEmpty(); 441 } 442 443 public boolean hasType() { 444 return this.type != null && !this.type.isEmpty(); 445 } 446 447 /** 448 * @param value {@link #type} (Type of resource this link refers to.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 449 */ 450 public GraphDefinitionNodeComponent setTypeElement(Enumeration<VersionIndependentResourceTypesAll> value) { 451 this.type = value; 452 return this; 453 } 454 455 /** 456 * @return Type of resource this link refers to. 457 */ 458 public VersionIndependentResourceTypesAll getType() { 459 return this.type == null ? null : this.type.getValue(); 460 } 461 462 /** 463 * @param value Type of resource this link refers to. 464 */ 465 public GraphDefinitionNodeComponent setType(VersionIndependentResourceTypesAll value) { 466 if (this.type == null) 467 this.type = new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory()); 468 this.type.setValue(value); 469 return this; 470 } 471 472 /** 473 * @return {@link #profile} (Profile for the target resource.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 474 */ 475 public CanonicalType getProfileElement() { 476 if (this.profile == null) 477 if (Configuration.errorOnAutoCreate()) 478 throw new Error("Attempt to auto-create GraphDefinitionNodeComponent.profile"); 479 else if (Configuration.doAutoCreate()) 480 this.profile = new CanonicalType(); // bb 481 return this.profile; 482 } 483 484 public boolean hasProfileElement() { 485 return this.profile != null && !this.profile.isEmpty(); 486 } 487 488 public boolean hasProfile() { 489 return this.profile != null && !this.profile.isEmpty(); 490 } 491 492 /** 493 * @param value {@link #profile} (Profile for the target resource.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 494 */ 495 public GraphDefinitionNodeComponent setProfileElement(CanonicalType value) { 496 this.profile = value; 497 return this; 498 } 499 500 /** 501 * @return Profile for the target resource. 502 */ 503 public String getProfile() { 504 return this.profile == null ? null : this.profile.getValue(); 505 } 506 507 /** 508 * @param value Profile for the target resource. 509 */ 510 public GraphDefinitionNodeComponent setProfile(String value) { 511 if (Utilities.noString(value)) 512 this.profile = null; 513 else { 514 if (this.profile == null) 515 this.profile = new CanonicalType(); 516 this.profile.setValue(value); 517 } 518 return this; 519 } 520 521 protected void listChildren(List<Property> children) { 522 super.listChildren(children); 523 children.add(new Property("nodeId", "id", "Internal ID of node - target for link references.", 0, 1, nodeId)); 524 children.add(new Property("description", "string", "Information about why this node is of interest in this graph definition.", 0, 1, description)); 525 children.add(new Property("type", "code", "Type of resource this link refers to.", 0, 1, type)); 526 children.add(new Property("profile", "canonical(StructureDefinition)", "Profile for the target resource.", 0, 1, profile)); 527 } 528 529 @Override 530 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 531 switch (_hash) { 532 case -1040171331: /*nodeId*/ return new Property("nodeId", "id", "Internal ID of node - target for link references.", 0, 1, nodeId); 533 case -1724546052: /*description*/ return new Property("description", "string", "Information about why this node is of interest in this graph definition.", 0, 1, description); 534 case 3575610: /*type*/ return new Property("type", "code", "Type of resource this link refers to.", 0, 1, type); 535 case -309425751: /*profile*/ return new Property("profile", "canonical(StructureDefinition)", "Profile for the target resource.", 0, 1, profile); 536 default: return super.getNamedProperty(_hash, _name, _checkValid); 537 } 538 539 } 540 541 @Override 542 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 543 switch (hash) { 544 case -1040171331: /*nodeId*/ return this.nodeId == null ? new Base[0] : new Base[] {this.nodeId}; // IdType 545 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 546 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<VersionIndependentResourceTypesAll> 547 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // CanonicalType 548 default: return super.getProperty(hash, name, checkValid); 549 } 550 551 } 552 553 @Override 554 public Base setProperty(int hash, String name, Base value) throws FHIRException { 555 switch (hash) { 556 case -1040171331: // nodeId 557 this.nodeId = TypeConvertor.castToId(value); // IdType 558 return value; 559 case -1724546052: // description 560 this.description = TypeConvertor.castToString(value); // StringType 561 return value; 562 case 3575610: // type 563 value = new VersionIndependentResourceTypesAllEnumFactory().fromType(TypeConvertor.castToCode(value)); 564 this.type = (Enumeration) value; // Enumeration<VersionIndependentResourceTypesAll> 565 return value; 566 case -309425751: // profile 567 this.profile = TypeConvertor.castToCanonical(value); // CanonicalType 568 return value; 569 default: return super.setProperty(hash, name, value); 570 } 571 572 } 573 574 @Override 575 public Base setProperty(String name, Base value) throws FHIRException { 576 if (name.equals("nodeId")) { 577 this.nodeId = TypeConvertor.castToId(value); // IdType 578 } else if (name.equals("description")) { 579 this.description = TypeConvertor.castToString(value); // StringType 580 } else if (name.equals("type")) { 581 value = new VersionIndependentResourceTypesAllEnumFactory().fromType(TypeConvertor.castToCode(value)); 582 this.type = (Enumeration) value; // Enumeration<VersionIndependentResourceTypesAll> 583 } else if (name.equals("profile")) { 584 this.profile = TypeConvertor.castToCanonical(value); // CanonicalType 585 } else 586 return super.setProperty(name, value); 587 return value; 588 } 589 590 @Override 591 public void removeChild(String name, Base value) throws FHIRException { 592 if (name.equals("nodeId")) { 593 this.nodeId = null; 594 } else if (name.equals("description")) { 595 this.description = null; 596 } else if (name.equals("type")) { 597 value = new VersionIndependentResourceTypesAllEnumFactory().fromType(TypeConvertor.castToCode(value)); 598 this.type = (Enumeration) value; // Enumeration<VersionIndependentResourceTypesAll> 599 } else if (name.equals("profile")) { 600 this.profile = null; 601 } else 602 super.removeChild(name, value); 603 604 } 605 606 @Override 607 public Base makeProperty(int hash, String name) throws FHIRException { 608 switch (hash) { 609 case -1040171331: return getNodeIdElement(); 610 case -1724546052: return getDescriptionElement(); 611 case 3575610: return getTypeElement(); 612 case -309425751: return getProfileElement(); 613 default: return super.makeProperty(hash, name); 614 } 615 616 } 617 618 @Override 619 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 620 switch (hash) { 621 case -1040171331: /*nodeId*/ return new String[] {"id"}; 622 case -1724546052: /*description*/ return new String[] {"string"}; 623 case 3575610: /*type*/ return new String[] {"code"}; 624 case -309425751: /*profile*/ return new String[] {"canonical"}; 625 default: return super.getTypesForProperty(hash, name); 626 } 627 628 } 629 630 @Override 631 public Base addChild(String name) throws FHIRException { 632 if (name.equals("nodeId")) { 633 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.node.nodeId"); 634 } 635 else if (name.equals("description")) { 636 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.node.description"); 637 } 638 else if (name.equals("type")) { 639 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.node.type"); 640 } 641 else if (name.equals("profile")) { 642 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.node.profile"); 643 } 644 else 645 return super.addChild(name); 646 } 647 648 public GraphDefinitionNodeComponent copy() { 649 GraphDefinitionNodeComponent dst = new GraphDefinitionNodeComponent(); 650 copyValues(dst); 651 return dst; 652 } 653 654 public void copyValues(GraphDefinitionNodeComponent dst) { 655 super.copyValues(dst); 656 dst.nodeId = nodeId == null ? null : nodeId.copy(); 657 dst.description = description == null ? null : description.copy(); 658 dst.type = type == null ? null : type.copy(); 659 dst.profile = profile == null ? null : profile.copy(); 660 } 661 662 @Override 663 public boolean equalsDeep(Base other_) { 664 if (!super.equalsDeep(other_)) 665 return false; 666 if (!(other_ instanceof GraphDefinitionNodeComponent)) 667 return false; 668 GraphDefinitionNodeComponent o = (GraphDefinitionNodeComponent) other_; 669 return compareDeep(nodeId, o.nodeId, true) && compareDeep(description, o.description, true) && compareDeep(type, o.type, true) 670 && compareDeep(profile, o.profile, true); 671 } 672 673 @Override 674 public boolean equalsShallow(Base other_) { 675 if (!super.equalsShallow(other_)) 676 return false; 677 if (!(other_ instanceof GraphDefinitionNodeComponent)) 678 return false; 679 GraphDefinitionNodeComponent o = (GraphDefinitionNodeComponent) other_; 680 return compareValues(nodeId, o.nodeId, true) && compareValues(description, o.description, true) && compareValues(type, o.type, true) 681 && compareValues(profile, o.profile, true); 682 } 683 684 public boolean isEmpty() { 685 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(nodeId, description, type 686 , profile); 687 } 688 689 public String fhirType() { 690 return "GraphDefinition.node"; 691 692 } 693 694 } 695 696 @Block() 697 public static class GraphDefinitionLinkComponent extends BackboneElement implements IBaseBackboneElement { 698 /** 699 * Information about why this link is of interest in this graph definition. 700 */ 701 @Child(name = "description", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 702 @Description(shortDefinition="Why this link is specified", formalDefinition="Information about why this link is of interest in this graph definition." ) 703 protected StringType description; 704 705 /** 706 * Minimum occurrences for this link. 707 */ 708 @Child(name = "min", type = {IntegerType.class}, order=2, min=0, max=1, modifier=false, summary=false) 709 @Description(shortDefinition="Minimum occurrences for this link", formalDefinition="Minimum occurrences for this link." ) 710 protected IntegerType min; 711 712 /** 713 * Maximum occurrences for this link. 714 */ 715 @Child(name = "max", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 716 @Description(shortDefinition="Maximum occurrences for this link", formalDefinition="Maximum occurrences for this link." ) 717 protected StringType max; 718 719 /** 720 * The source node for this link. 721 */ 722 @Child(name = "sourceId", type = {IdType.class}, order=4, min=1, max=1, modifier=false, summary=false) 723 @Description(shortDefinition="Source Node for this link", formalDefinition="The source node for this link." ) 724 protected IdType sourceId; 725 726 /** 727 * A FHIRPath expression that identifies one of FHIR References to other resources. 728 */ 729 @Child(name = "path", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 730 @Description(shortDefinition="Path in the resource that contains the link", formalDefinition="A FHIRPath expression that identifies one of FHIR References to other resources." ) 731 protected StringType path; 732 733 /** 734 * Which slice (if profiled). 735 */ 736 @Child(name = "sliceName", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 737 @Description(shortDefinition="Which slice (if profiled)", formalDefinition="Which slice (if profiled)." ) 738 protected StringType sliceName; 739 740 /** 741 * The target node for this link. 742 */ 743 @Child(name = "targetId", type = {IdType.class}, order=7, min=1, max=1, modifier=false, summary=false) 744 @Description(shortDefinition="Target Node for this link", formalDefinition="The target node for this link." ) 745 protected IdType targetId; 746 747 /** 748 * A set of parameters to look up. 749 */ 750 @Child(name = "params", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=false) 751 @Description(shortDefinition="Criteria for reverse lookup", formalDefinition="A set of parameters to look up." ) 752 protected StringType params; 753 754 /** 755 * Compartment Consistency Rules. 756 */ 757 @Child(name = "compartment", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 758 @Description(shortDefinition="Compartment Consistency Rules", formalDefinition="Compartment Consistency Rules." ) 759 protected List<GraphDefinitionLinkCompartmentComponent> compartment; 760 761 private static final long serialVersionUID = -433118895L; 762 763 /** 764 * Constructor 765 */ 766 public GraphDefinitionLinkComponent() { 767 super(); 768 } 769 770 /** 771 * Constructor 772 */ 773 public GraphDefinitionLinkComponent(String sourceId, String targetId) { 774 super(); 775 this.setSourceId(sourceId); 776 this.setTargetId(targetId); 777 } 778 779 /** 780 * @return {@link #description} (Information about why this link is of interest in this graph definition.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 781 */ 782 public StringType getDescriptionElement() { 783 if (this.description == null) 784 if (Configuration.errorOnAutoCreate()) 785 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.description"); 786 else if (Configuration.doAutoCreate()) 787 this.description = new StringType(); // bb 788 return this.description; 789 } 790 791 public boolean hasDescriptionElement() { 792 return this.description != null && !this.description.isEmpty(); 793 } 794 795 public boolean hasDescription() { 796 return this.description != null && !this.description.isEmpty(); 797 } 798 799 /** 800 * @param value {@link #description} (Information about why this link is of interest in this graph definition.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 801 */ 802 public GraphDefinitionLinkComponent setDescriptionElement(StringType value) { 803 this.description = value; 804 return this; 805 } 806 807 /** 808 * @return Information about why this link is of interest in this graph definition. 809 */ 810 public String getDescription() { 811 return this.description == null ? null : this.description.getValue(); 812 } 813 814 /** 815 * @param value Information about why this link is of interest in this graph definition. 816 */ 817 public GraphDefinitionLinkComponent setDescription(String value) { 818 if (Utilities.noString(value)) 819 this.description = null; 820 else { 821 if (this.description == null) 822 this.description = new StringType(); 823 this.description.setValue(value); 824 } 825 return this; 826 } 827 828 /** 829 * @return {@link #min} (Minimum occurrences for this link.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 830 */ 831 public IntegerType getMinElement() { 832 if (this.min == null) 833 if (Configuration.errorOnAutoCreate()) 834 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.min"); 835 else if (Configuration.doAutoCreate()) 836 this.min = new IntegerType(); // bb 837 return this.min; 838 } 839 840 public boolean hasMinElement() { 841 return this.min != null && !this.min.isEmpty(); 842 } 843 844 public boolean hasMin() { 845 return this.min != null && !this.min.isEmpty(); 846 } 847 848 /** 849 * @param value {@link #min} (Minimum occurrences for this link.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 850 */ 851 public GraphDefinitionLinkComponent setMinElement(IntegerType value) { 852 this.min = value; 853 return this; 854 } 855 856 /** 857 * @return Minimum occurrences for this link. 858 */ 859 public int getMin() { 860 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 861 } 862 863 /** 864 * @param value Minimum occurrences for this link. 865 */ 866 public GraphDefinitionLinkComponent setMin(int value) { 867 if (this.min == null) 868 this.min = new IntegerType(); 869 this.min.setValue(value); 870 return this; 871 } 872 873 /** 874 * @return {@link #max} (Maximum occurrences for this link.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 875 */ 876 public StringType getMaxElement() { 877 if (this.max == null) 878 if (Configuration.errorOnAutoCreate()) 879 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.max"); 880 else if (Configuration.doAutoCreate()) 881 this.max = new StringType(); // bb 882 return this.max; 883 } 884 885 public boolean hasMaxElement() { 886 return this.max != null && !this.max.isEmpty(); 887 } 888 889 public boolean hasMax() { 890 return this.max != null && !this.max.isEmpty(); 891 } 892 893 /** 894 * @param value {@link #max} (Maximum occurrences for this link.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 895 */ 896 public GraphDefinitionLinkComponent setMaxElement(StringType value) { 897 this.max = value; 898 return this; 899 } 900 901 /** 902 * @return Maximum occurrences for this link. 903 */ 904 public String getMax() { 905 return this.max == null ? null : this.max.getValue(); 906 } 907 908 /** 909 * @param value Maximum occurrences for this link. 910 */ 911 public GraphDefinitionLinkComponent setMax(String value) { 912 if (Utilities.noString(value)) 913 this.max = null; 914 else { 915 if (this.max == null) 916 this.max = new StringType(); 917 this.max.setValue(value); 918 } 919 return this; 920 } 921 922 /** 923 * @return {@link #sourceId} (The source node for this link.). This is the underlying object with id, value and extensions. The accessor "getSourceId" gives direct access to the value 924 */ 925 public IdType getSourceIdElement() { 926 if (this.sourceId == null) 927 if (Configuration.errorOnAutoCreate()) 928 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.sourceId"); 929 else if (Configuration.doAutoCreate()) 930 this.sourceId = new IdType(); // bb 931 return this.sourceId; 932 } 933 934 public boolean hasSourceIdElement() { 935 return this.sourceId != null && !this.sourceId.isEmpty(); 936 } 937 938 public boolean hasSourceId() { 939 return this.sourceId != null && !this.sourceId.isEmpty(); 940 } 941 942 /** 943 * @param value {@link #sourceId} (The source node for this link.). This is the underlying object with id, value and extensions. The accessor "getSourceId" gives direct access to the value 944 */ 945 public GraphDefinitionLinkComponent setSourceIdElement(IdType value) { 946 this.sourceId = value; 947 return this; 948 } 949 950 /** 951 * @return The source node for this link. 952 */ 953 public String getSourceId() { 954 return this.sourceId == null ? null : this.sourceId.getValue(); 955 } 956 957 /** 958 * @param value The source node for this link. 959 */ 960 public GraphDefinitionLinkComponent setSourceId(String value) { 961 if (this.sourceId == null) 962 this.sourceId = new IdType(); 963 this.sourceId.setValue(value); 964 return this; 965 } 966 967 /** 968 * @return {@link #path} (A FHIRPath expression that identifies one of FHIR References to other resources.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 969 */ 970 public StringType getPathElement() { 971 if (this.path == null) 972 if (Configuration.errorOnAutoCreate()) 973 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.path"); 974 else if (Configuration.doAutoCreate()) 975 this.path = new StringType(); // bb 976 return this.path; 977 } 978 979 public boolean hasPathElement() { 980 return this.path != null && !this.path.isEmpty(); 981 } 982 983 public boolean hasPath() { 984 return this.path != null && !this.path.isEmpty(); 985 } 986 987 /** 988 * @param value {@link #path} (A FHIRPath expression that identifies one of FHIR References to other resources.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 989 */ 990 public GraphDefinitionLinkComponent setPathElement(StringType value) { 991 this.path = value; 992 return this; 993 } 994 995 /** 996 * @return A FHIRPath expression that identifies one of FHIR References to other resources. 997 */ 998 public String getPath() { 999 return this.path == null ? null : this.path.getValue(); 1000 } 1001 1002 /** 1003 * @param value A FHIRPath expression that identifies one of FHIR References to other resources. 1004 */ 1005 public GraphDefinitionLinkComponent setPath(String value) { 1006 if (Utilities.noString(value)) 1007 this.path = null; 1008 else { 1009 if (this.path == null) 1010 this.path = new StringType(); 1011 this.path.setValue(value); 1012 } 1013 return this; 1014 } 1015 1016 /** 1017 * @return {@link #sliceName} (Which slice (if profiled).). This is the underlying object with id, value and extensions. The accessor "getSliceName" gives direct access to the value 1018 */ 1019 public StringType getSliceNameElement() { 1020 if (this.sliceName == null) 1021 if (Configuration.errorOnAutoCreate()) 1022 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.sliceName"); 1023 else if (Configuration.doAutoCreate()) 1024 this.sliceName = new StringType(); // bb 1025 return this.sliceName; 1026 } 1027 1028 public boolean hasSliceNameElement() { 1029 return this.sliceName != null && !this.sliceName.isEmpty(); 1030 } 1031 1032 public boolean hasSliceName() { 1033 return this.sliceName != null && !this.sliceName.isEmpty(); 1034 } 1035 1036 /** 1037 * @param value {@link #sliceName} (Which slice (if profiled).). This is the underlying object with id, value and extensions. The accessor "getSliceName" gives direct access to the value 1038 */ 1039 public GraphDefinitionLinkComponent setSliceNameElement(StringType value) { 1040 this.sliceName = value; 1041 return this; 1042 } 1043 1044 /** 1045 * @return Which slice (if profiled). 1046 */ 1047 public String getSliceName() { 1048 return this.sliceName == null ? null : this.sliceName.getValue(); 1049 } 1050 1051 /** 1052 * @param value Which slice (if profiled). 1053 */ 1054 public GraphDefinitionLinkComponent setSliceName(String value) { 1055 if (Utilities.noString(value)) 1056 this.sliceName = null; 1057 else { 1058 if (this.sliceName == null) 1059 this.sliceName = new StringType(); 1060 this.sliceName.setValue(value); 1061 } 1062 return this; 1063 } 1064 1065 /** 1066 * @return {@link #targetId} (The target node for this link.). This is the underlying object with id, value and extensions. The accessor "getTargetId" gives direct access to the value 1067 */ 1068 public IdType getTargetIdElement() { 1069 if (this.targetId == null) 1070 if (Configuration.errorOnAutoCreate()) 1071 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.targetId"); 1072 else if (Configuration.doAutoCreate()) 1073 this.targetId = new IdType(); // bb 1074 return this.targetId; 1075 } 1076 1077 public boolean hasTargetIdElement() { 1078 return this.targetId != null && !this.targetId.isEmpty(); 1079 } 1080 1081 public boolean hasTargetId() { 1082 return this.targetId != null && !this.targetId.isEmpty(); 1083 } 1084 1085 /** 1086 * @param value {@link #targetId} (The target node for this link.). This is the underlying object with id, value and extensions. The accessor "getTargetId" gives direct access to the value 1087 */ 1088 public GraphDefinitionLinkComponent setTargetIdElement(IdType value) { 1089 this.targetId = value; 1090 return this; 1091 } 1092 1093 /** 1094 * @return The target node for this link. 1095 */ 1096 public String getTargetId() { 1097 return this.targetId == null ? null : this.targetId.getValue(); 1098 } 1099 1100 /** 1101 * @param value The target node for this link. 1102 */ 1103 public GraphDefinitionLinkComponent setTargetId(String value) { 1104 if (this.targetId == null) 1105 this.targetId = new IdType(); 1106 this.targetId.setValue(value); 1107 return this; 1108 } 1109 1110 /** 1111 * @return {@link #params} (A set of parameters to look up.). This is the underlying object with id, value and extensions. The accessor "getParams" gives direct access to the value 1112 */ 1113 public StringType getParamsElement() { 1114 if (this.params == null) 1115 if (Configuration.errorOnAutoCreate()) 1116 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.params"); 1117 else if (Configuration.doAutoCreate()) 1118 this.params = new StringType(); // bb 1119 return this.params; 1120 } 1121 1122 public boolean hasParamsElement() { 1123 return this.params != null && !this.params.isEmpty(); 1124 } 1125 1126 public boolean hasParams() { 1127 return this.params != null && !this.params.isEmpty(); 1128 } 1129 1130 /** 1131 * @param value {@link #params} (A set of parameters to look up.). This is the underlying object with id, value and extensions. The accessor "getParams" gives direct access to the value 1132 */ 1133 public GraphDefinitionLinkComponent setParamsElement(StringType value) { 1134 this.params = value; 1135 return this; 1136 } 1137 1138 /** 1139 * @return A set of parameters to look up. 1140 */ 1141 public String getParams() { 1142 return this.params == null ? null : this.params.getValue(); 1143 } 1144 1145 /** 1146 * @param value A set of parameters to look up. 1147 */ 1148 public GraphDefinitionLinkComponent setParams(String value) { 1149 if (Utilities.noString(value)) 1150 this.params = null; 1151 else { 1152 if (this.params == null) 1153 this.params = new StringType(); 1154 this.params.setValue(value); 1155 } 1156 return this; 1157 } 1158 1159 /** 1160 * @return {@link #compartment} (Compartment Consistency Rules.) 1161 */ 1162 public List<GraphDefinitionLinkCompartmentComponent> getCompartment() { 1163 if (this.compartment == null) 1164 this.compartment = new ArrayList<GraphDefinitionLinkCompartmentComponent>(); 1165 return this.compartment; 1166 } 1167 1168 /** 1169 * @return Returns a reference to <code>this</code> for easy method chaining 1170 */ 1171 public GraphDefinitionLinkComponent setCompartment(List<GraphDefinitionLinkCompartmentComponent> theCompartment) { 1172 this.compartment = theCompartment; 1173 return this; 1174 } 1175 1176 public boolean hasCompartment() { 1177 if (this.compartment == null) 1178 return false; 1179 for (GraphDefinitionLinkCompartmentComponent item : this.compartment) 1180 if (!item.isEmpty()) 1181 return true; 1182 return false; 1183 } 1184 1185 public GraphDefinitionLinkCompartmentComponent addCompartment() { //3 1186 GraphDefinitionLinkCompartmentComponent t = new GraphDefinitionLinkCompartmentComponent(); 1187 if (this.compartment == null) 1188 this.compartment = new ArrayList<GraphDefinitionLinkCompartmentComponent>(); 1189 this.compartment.add(t); 1190 return t; 1191 } 1192 1193 public GraphDefinitionLinkComponent addCompartment(GraphDefinitionLinkCompartmentComponent t) { //3 1194 if (t == null) 1195 return this; 1196 if (this.compartment == null) 1197 this.compartment = new ArrayList<GraphDefinitionLinkCompartmentComponent>(); 1198 this.compartment.add(t); 1199 return this; 1200 } 1201 1202 /** 1203 * @return The first repetition of repeating field {@link #compartment}, creating it if it does not already exist {3} 1204 */ 1205 public GraphDefinitionLinkCompartmentComponent getCompartmentFirstRep() { 1206 if (getCompartment().isEmpty()) { 1207 addCompartment(); 1208 } 1209 return getCompartment().get(0); 1210 } 1211 1212 protected void listChildren(List<Property> children) { 1213 super.listChildren(children); 1214 children.add(new Property("description", "string", "Information about why this link is of interest in this graph definition.", 0, 1, description)); 1215 children.add(new Property("min", "integer", "Minimum occurrences for this link.", 0, 1, min)); 1216 children.add(new Property("max", "string", "Maximum occurrences for this link.", 0, 1, max)); 1217 children.add(new Property("sourceId", "id", "The source node for this link.", 0, 1, sourceId)); 1218 children.add(new Property("path", "string", "A FHIRPath expression that identifies one of FHIR References to other resources.", 0, 1, path)); 1219 children.add(new Property("sliceName", "string", "Which slice (if profiled).", 0, 1, sliceName)); 1220 children.add(new Property("targetId", "id", "The target node for this link.", 0, 1, targetId)); 1221 children.add(new Property("params", "string", "A set of parameters to look up.", 0, 1, params)); 1222 children.add(new Property("compartment", "", "Compartment Consistency Rules.", 0, java.lang.Integer.MAX_VALUE, compartment)); 1223 } 1224 1225 @Override 1226 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1227 switch (_hash) { 1228 case -1724546052: /*description*/ return new Property("description", "string", "Information about why this link is of interest in this graph definition.", 0, 1, description); 1229 case 108114: /*min*/ return new Property("min", "integer", "Minimum occurrences for this link.", 0, 1, min); 1230 case 107876: /*max*/ return new Property("max", "string", "Maximum occurrences for this link.", 0, 1, max); 1231 case 1746327190: /*sourceId*/ return new Property("sourceId", "id", "The source node for this link.", 0, 1, sourceId); 1232 case 3433509: /*path*/ return new Property("path", "string", "A FHIRPath expression that identifies one of FHIR References to other resources.", 0, 1, path); 1233 case -825289923: /*sliceName*/ return new Property("sliceName", "string", "Which slice (if profiled).", 0, 1, sliceName); 1234 case -441951604: /*targetId*/ return new Property("targetId", "id", "The target node for this link.", 0, 1, targetId); 1235 case -995427962: /*params*/ return new Property("params", "string", "A set of parameters to look up.", 0, 1, params); 1236 case -397756334: /*compartment*/ return new Property("compartment", "", "Compartment Consistency Rules.", 0, java.lang.Integer.MAX_VALUE, compartment); 1237 default: return super.getNamedProperty(_hash, _name, _checkValid); 1238 } 1239 1240 } 1241 1242 @Override 1243 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1244 switch (hash) { 1245 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1246 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // IntegerType 1247 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 1248 case 1746327190: /*sourceId*/ return this.sourceId == null ? new Base[0] : new Base[] {this.sourceId}; // IdType 1249 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 1250 case -825289923: /*sliceName*/ return this.sliceName == null ? new Base[0] : new Base[] {this.sliceName}; // StringType 1251 case -441951604: /*targetId*/ return this.targetId == null ? new Base[0] : new Base[] {this.targetId}; // IdType 1252 case -995427962: /*params*/ return this.params == null ? new Base[0] : new Base[] {this.params}; // StringType 1253 case -397756334: /*compartment*/ return this.compartment == null ? new Base[0] : this.compartment.toArray(new Base[this.compartment.size()]); // GraphDefinitionLinkCompartmentComponent 1254 default: return super.getProperty(hash, name, checkValid); 1255 } 1256 1257 } 1258 1259 @Override 1260 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1261 switch (hash) { 1262 case -1724546052: // description 1263 this.description = TypeConvertor.castToString(value); // StringType 1264 return value; 1265 case 108114: // min 1266 this.min = TypeConvertor.castToInteger(value); // IntegerType 1267 return value; 1268 case 107876: // max 1269 this.max = TypeConvertor.castToString(value); // StringType 1270 return value; 1271 case 1746327190: // sourceId 1272 this.sourceId = TypeConvertor.castToId(value); // IdType 1273 return value; 1274 case 3433509: // path 1275 this.path = TypeConvertor.castToString(value); // StringType 1276 return value; 1277 case -825289923: // sliceName 1278 this.sliceName = TypeConvertor.castToString(value); // StringType 1279 return value; 1280 case -441951604: // targetId 1281 this.targetId = TypeConvertor.castToId(value); // IdType 1282 return value; 1283 case -995427962: // params 1284 this.params = TypeConvertor.castToString(value); // StringType 1285 return value; 1286 case -397756334: // compartment 1287 this.getCompartment().add((GraphDefinitionLinkCompartmentComponent) value); // GraphDefinitionLinkCompartmentComponent 1288 return value; 1289 default: return super.setProperty(hash, name, value); 1290 } 1291 1292 } 1293 1294 @Override 1295 public Base setProperty(String name, Base value) throws FHIRException { 1296 if (name.equals("description")) { 1297 this.description = TypeConvertor.castToString(value); // StringType 1298 } else if (name.equals("min")) { 1299 this.min = TypeConvertor.castToInteger(value); // IntegerType 1300 } else if (name.equals("max")) { 1301 this.max = TypeConvertor.castToString(value); // StringType 1302 } else if (name.equals("sourceId")) { 1303 this.sourceId = TypeConvertor.castToId(value); // IdType 1304 } else if (name.equals("path")) { 1305 this.path = TypeConvertor.castToString(value); // StringType 1306 } else if (name.equals("sliceName")) { 1307 this.sliceName = TypeConvertor.castToString(value); // StringType 1308 } else if (name.equals("targetId")) { 1309 this.targetId = TypeConvertor.castToId(value); // IdType 1310 } else if (name.equals("params")) { 1311 this.params = TypeConvertor.castToString(value); // StringType 1312 } else if (name.equals("compartment")) { 1313 this.getCompartment().add((GraphDefinitionLinkCompartmentComponent) value); 1314 } else 1315 return super.setProperty(name, value); 1316 return value; 1317 } 1318 1319 @Override 1320 public void removeChild(String name, Base value) throws FHIRException { 1321 if (name.equals("description")) { 1322 this.description = null; 1323 } else if (name.equals("min")) { 1324 this.min = null; 1325 } else if (name.equals("max")) { 1326 this.max = null; 1327 } else if (name.equals("sourceId")) { 1328 this.sourceId = null; 1329 } else if (name.equals("path")) { 1330 this.path = null; 1331 } else if (name.equals("sliceName")) { 1332 this.sliceName = null; 1333 } else if (name.equals("targetId")) { 1334 this.targetId = null; 1335 } else if (name.equals("params")) { 1336 this.params = null; 1337 } else if (name.equals("compartment")) { 1338 this.getCompartment().remove((GraphDefinitionLinkCompartmentComponent) value); 1339 } else 1340 super.removeChild(name, value); 1341 1342 } 1343 1344 @Override 1345 public Base makeProperty(int hash, String name) throws FHIRException { 1346 switch (hash) { 1347 case -1724546052: return getDescriptionElement(); 1348 case 108114: return getMinElement(); 1349 case 107876: return getMaxElement(); 1350 case 1746327190: return getSourceIdElement(); 1351 case 3433509: return getPathElement(); 1352 case -825289923: return getSliceNameElement(); 1353 case -441951604: return getTargetIdElement(); 1354 case -995427962: return getParamsElement(); 1355 case -397756334: return addCompartment(); 1356 default: return super.makeProperty(hash, name); 1357 } 1358 1359 } 1360 1361 @Override 1362 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1363 switch (hash) { 1364 case -1724546052: /*description*/ return new String[] {"string"}; 1365 case 108114: /*min*/ return new String[] {"integer"}; 1366 case 107876: /*max*/ return new String[] {"string"}; 1367 case 1746327190: /*sourceId*/ return new String[] {"id"}; 1368 case 3433509: /*path*/ return new String[] {"string"}; 1369 case -825289923: /*sliceName*/ return new String[] {"string"}; 1370 case -441951604: /*targetId*/ return new String[] {"id"}; 1371 case -995427962: /*params*/ return new String[] {"string"}; 1372 case -397756334: /*compartment*/ return new String[] {}; 1373 default: return super.getTypesForProperty(hash, name); 1374 } 1375 1376 } 1377 1378 @Override 1379 public Base addChild(String name) throws FHIRException { 1380 if (name.equals("description")) { 1381 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.link.description"); 1382 } 1383 else if (name.equals("min")) { 1384 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.link.min"); 1385 } 1386 else if (name.equals("max")) { 1387 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.link.max"); 1388 } 1389 else if (name.equals("sourceId")) { 1390 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.link.sourceId"); 1391 } 1392 else if (name.equals("path")) { 1393 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.link.path"); 1394 } 1395 else if (name.equals("sliceName")) { 1396 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.link.sliceName"); 1397 } 1398 else if (name.equals("targetId")) { 1399 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.link.targetId"); 1400 } 1401 else if (name.equals("params")) { 1402 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.link.params"); 1403 } 1404 else if (name.equals("compartment")) { 1405 return addCompartment(); 1406 } 1407 else 1408 return super.addChild(name); 1409 } 1410 1411 public GraphDefinitionLinkComponent copy() { 1412 GraphDefinitionLinkComponent dst = new GraphDefinitionLinkComponent(); 1413 copyValues(dst); 1414 return dst; 1415 } 1416 1417 public void copyValues(GraphDefinitionLinkComponent dst) { 1418 super.copyValues(dst); 1419 dst.description = description == null ? null : description.copy(); 1420 dst.min = min == null ? null : min.copy(); 1421 dst.max = max == null ? null : max.copy(); 1422 dst.sourceId = sourceId == null ? null : sourceId.copy(); 1423 dst.path = path == null ? null : path.copy(); 1424 dst.sliceName = sliceName == null ? null : sliceName.copy(); 1425 dst.targetId = targetId == null ? null : targetId.copy(); 1426 dst.params = params == null ? null : params.copy(); 1427 if (compartment != null) { 1428 dst.compartment = new ArrayList<GraphDefinitionLinkCompartmentComponent>(); 1429 for (GraphDefinitionLinkCompartmentComponent i : compartment) 1430 dst.compartment.add(i.copy()); 1431 }; 1432 } 1433 1434 @Override 1435 public boolean equalsDeep(Base other_) { 1436 if (!super.equalsDeep(other_)) 1437 return false; 1438 if (!(other_ instanceof GraphDefinitionLinkComponent)) 1439 return false; 1440 GraphDefinitionLinkComponent o = (GraphDefinitionLinkComponent) other_; 1441 return compareDeep(description, o.description, true) && compareDeep(min, o.min, true) && compareDeep(max, o.max, true) 1442 && compareDeep(sourceId, o.sourceId, true) && compareDeep(path, o.path, true) && compareDeep(sliceName, o.sliceName, true) 1443 && compareDeep(targetId, o.targetId, true) && compareDeep(params, o.params, true) && compareDeep(compartment, o.compartment, true) 1444 ; 1445 } 1446 1447 @Override 1448 public boolean equalsShallow(Base other_) { 1449 if (!super.equalsShallow(other_)) 1450 return false; 1451 if (!(other_ instanceof GraphDefinitionLinkComponent)) 1452 return false; 1453 GraphDefinitionLinkComponent o = (GraphDefinitionLinkComponent) other_; 1454 return compareValues(description, o.description, true) && compareValues(min, o.min, true) && compareValues(max, o.max, true) 1455 && compareValues(sourceId, o.sourceId, true) && compareValues(path, o.path, true) && compareValues(sliceName, o.sliceName, true) 1456 && compareValues(targetId, o.targetId, true) && compareValues(params, o.params, true); 1457 } 1458 1459 public boolean isEmpty() { 1460 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, min, max, sourceId 1461 , path, sliceName, targetId, params, compartment); 1462 } 1463 1464 public String fhirType() { 1465 return "GraphDefinition.link"; 1466 1467 } 1468 1469 } 1470 1471 @Block() 1472 public static class GraphDefinitionLinkCompartmentComponent extends BackboneElement implements IBaseBackboneElement { 1473 /** 1474 * Defines how the compartment rule is used - whether it it is used to test whether resources are subject to the rule, or whether it is a rule that must be followed. 1475 */ 1476 @Child(name = "use", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1477 @Description(shortDefinition="where | requires", formalDefinition="Defines how the compartment rule is used - whether it it is used to test whether resources are subject to the rule, or whether it is a rule that must be followed." ) 1478 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/graph-compartment-use") 1479 protected Enumeration<GraphCompartmentUse> use; 1480 1481 /** 1482 * identical | matching | different | no-rule | custom. 1483 */ 1484 @Child(name = "rule", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 1485 @Description(shortDefinition="identical | matching | different | custom", formalDefinition="identical | matching | different | no-rule | custom." ) 1486 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/graph-compartment-rule") 1487 protected Enumeration<GraphCompartmentRule> rule; 1488 1489 /** 1490 * Identifies the compartment. 1491 */ 1492 @Child(name = "code", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=false) 1493 @Description(shortDefinition="Patient | Encounter | RelatedPerson | Practitioner | Device | EpisodeOfCare", formalDefinition="Identifies the compartment." ) 1494 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/compartment-type") 1495 protected Enumeration<CompartmentType> code; 1496 1497 /** 1498 * Custom rule, as a FHIRPath expression. 1499 */ 1500 @Child(name = "expression", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1501 @Description(shortDefinition="Custom rule, as a FHIRPath expression", formalDefinition="Custom rule, as a FHIRPath expression." ) 1502 protected StringType expression; 1503 1504 /** 1505 * Documentation for FHIRPath expression. 1506 */ 1507 @Child(name = "description", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 1508 @Description(shortDefinition="Documentation for FHIRPath expression", formalDefinition="Documentation for FHIRPath expression." ) 1509 protected StringType description; 1510 1511 private static final long serialVersionUID = 2140045786L; 1512 1513 /** 1514 * Constructor 1515 */ 1516 public GraphDefinitionLinkCompartmentComponent() { 1517 super(); 1518 } 1519 1520 /** 1521 * Constructor 1522 */ 1523 public GraphDefinitionLinkCompartmentComponent(GraphCompartmentUse use, GraphCompartmentRule rule, CompartmentType code) { 1524 super(); 1525 this.setUse(use); 1526 this.setRule(rule); 1527 this.setCode(code); 1528 } 1529 1530 /** 1531 * @return {@link #use} (Defines how the compartment rule is used - whether it it is used to test whether resources are subject to the rule, or whether it is a rule that must be followed.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 1532 */ 1533 public Enumeration<GraphCompartmentUse> getUseElement() { 1534 if (this.use == null) 1535 if (Configuration.errorOnAutoCreate()) 1536 throw new Error("Attempt to auto-create GraphDefinitionLinkCompartmentComponent.use"); 1537 else if (Configuration.doAutoCreate()) 1538 this.use = new Enumeration<GraphCompartmentUse>(new GraphCompartmentUseEnumFactory()); // bb 1539 return this.use; 1540 } 1541 1542 public boolean hasUseElement() { 1543 return this.use != null && !this.use.isEmpty(); 1544 } 1545 1546 public boolean hasUse() { 1547 return this.use != null && !this.use.isEmpty(); 1548 } 1549 1550 /** 1551 * @param value {@link #use} (Defines how the compartment rule is used - whether it it is used to test whether resources are subject to the rule, or whether it is a rule that must be followed.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 1552 */ 1553 public GraphDefinitionLinkCompartmentComponent setUseElement(Enumeration<GraphCompartmentUse> value) { 1554 this.use = value; 1555 return this; 1556 } 1557 1558 /** 1559 * @return Defines how the compartment rule is used - whether it it is used to test whether resources are subject to the rule, or whether it is a rule that must be followed. 1560 */ 1561 public GraphCompartmentUse getUse() { 1562 return this.use == null ? null : this.use.getValue(); 1563 } 1564 1565 /** 1566 * @param value Defines how the compartment rule is used - whether it it is used to test whether resources are subject to the rule, or whether it is a rule that must be followed. 1567 */ 1568 public GraphDefinitionLinkCompartmentComponent setUse(GraphCompartmentUse value) { 1569 if (this.use == null) 1570 this.use = new Enumeration<GraphCompartmentUse>(new GraphCompartmentUseEnumFactory()); 1571 this.use.setValue(value); 1572 return this; 1573 } 1574 1575 /** 1576 * @return {@link #rule} (identical | matching | different | no-rule | custom.). This is the underlying object with id, value and extensions. The accessor "getRule" gives direct access to the value 1577 */ 1578 public Enumeration<GraphCompartmentRule> getRuleElement() { 1579 if (this.rule == null) 1580 if (Configuration.errorOnAutoCreate()) 1581 throw new Error("Attempt to auto-create GraphDefinitionLinkCompartmentComponent.rule"); 1582 else if (Configuration.doAutoCreate()) 1583 this.rule = new Enumeration<GraphCompartmentRule>(new GraphCompartmentRuleEnumFactory()); // bb 1584 return this.rule; 1585 } 1586 1587 public boolean hasRuleElement() { 1588 return this.rule != null && !this.rule.isEmpty(); 1589 } 1590 1591 public boolean hasRule() { 1592 return this.rule != null && !this.rule.isEmpty(); 1593 } 1594 1595 /** 1596 * @param value {@link #rule} (identical | matching | different | no-rule | custom.). This is the underlying object with id, value and extensions. The accessor "getRule" gives direct access to the value 1597 */ 1598 public GraphDefinitionLinkCompartmentComponent setRuleElement(Enumeration<GraphCompartmentRule> value) { 1599 this.rule = value; 1600 return this; 1601 } 1602 1603 /** 1604 * @return identical | matching | different | no-rule | custom. 1605 */ 1606 public GraphCompartmentRule getRule() { 1607 return this.rule == null ? null : this.rule.getValue(); 1608 } 1609 1610 /** 1611 * @param value identical | matching | different | no-rule | custom. 1612 */ 1613 public GraphDefinitionLinkCompartmentComponent setRule(GraphCompartmentRule value) { 1614 if (this.rule == null) 1615 this.rule = new Enumeration<GraphCompartmentRule>(new GraphCompartmentRuleEnumFactory()); 1616 this.rule.setValue(value); 1617 return this; 1618 } 1619 1620 /** 1621 * @return {@link #code} (Identifies the compartment.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1622 */ 1623 public Enumeration<CompartmentType> getCodeElement() { 1624 if (this.code == null) 1625 if (Configuration.errorOnAutoCreate()) 1626 throw new Error("Attempt to auto-create GraphDefinitionLinkCompartmentComponent.code"); 1627 else if (Configuration.doAutoCreate()) 1628 this.code = new Enumeration<CompartmentType>(new CompartmentTypeEnumFactory()); // bb 1629 return this.code; 1630 } 1631 1632 public boolean hasCodeElement() { 1633 return this.code != null && !this.code.isEmpty(); 1634 } 1635 1636 public boolean hasCode() { 1637 return this.code != null && !this.code.isEmpty(); 1638 } 1639 1640 /** 1641 * @param value {@link #code} (Identifies the compartment.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1642 */ 1643 public GraphDefinitionLinkCompartmentComponent setCodeElement(Enumeration<CompartmentType> value) { 1644 this.code = value; 1645 return this; 1646 } 1647 1648 /** 1649 * @return Identifies the compartment. 1650 */ 1651 public CompartmentType getCode() { 1652 return this.code == null ? null : this.code.getValue(); 1653 } 1654 1655 /** 1656 * @param value Identifies the compartment. 1657 */ 1658 public GraphDefinitionLinkCompartmentComponent setCode(CompartmentType value) { 1659 if (this.code == null) 1660 this.code = new Enumeration<CompartmentType>(new CompartmentTypeEnumFactory()); 1661 this.code.setValue(value); 1662 return this; 1663 } 1664 1665 /** 1666 * @return {@link #expression} (Custom rule, as a FHIRPath expression.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 1667 */ 1668 public StringType getExpressionElement() { 1669 if (this.expression == null) 1670 if (Configuration.errorOnAutoCreate()) 1671 throw new Error("Attempt to auto-create GraphDefinitionLinkCompartmentComponent.expression"); 1672 else if (Configuration.doAutoCreate()) 1673 this.expression = new StringType(); // bb 1674 return this.expression; 1675 } 1676 1677 public boolean hasExpressionElement() { 1678 return this.expression != null && !this.expression.isEmpty(); 1679 } 1680 1681 public boolean hasExpression() { 1682 return this.expression != null && !this.expression.isEmpty(); 1683 } 1684 1685 /** 1686 * @param value {@link #expression} (Custom rule, as a FHIRPath expression.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 1687 */ 1688 public GraphDefinitionLinkCompartmentComponent setExpressionElement(StringType value) { 1689 this.expression = value; 1690 return this; 1691 } 1692 1693 /** 1694 * @return Custom rule, as a FHIRPath expression. 1695 */ 1696 public String getExpression() { 1697 return this.expression == null ? null : this.expression.getValue(); 1698 } 1699 1700 /** 1701 * @param value Custom rule, as a FHIRPath expression. 1702 */ 1703 public GraphDefinitionLinkCompartmentComponent setExpression(String value) { 1704 if (Utilities.noString(value)) 1705 this.expression = null; 1706 else { 1707 if (this.expression == null) 1708 this.expression = new StringType(); 1709 this.expression.setValue(value); 1710 } 1711 return this; 1712 } 1713 1714 /** 1715 * @return {@link #description} (Documentation for FHIRPath expression.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1716 */ 1717 public StringType getDescriptionElement() { 1718 if (this.description == null) 1719 if (Configuration.errorOnAutoCreate()) 1720 throw new Error("Attempt to auto-create GraphDefinitionLinkCompartmentComponent.description"); 1721 else if (Configuration.doAutoCreate()) 1722 this.description = new StringType(); // bb 1723 return this.description; 1724 } 1725 1726 public boolean hasDescriptionElement() { 1727 return this.description != null && !this.description.isEmpty(); 1728 } 1729 1730 public boolean hasDescription() { 1731 return this.description != null && !this.description.isEmpty(); 1732 } 1733 1734 /** 1735 * @param value {@link #description} (Documentation for FHIRPath expression.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1736 */ 1737 public GraphDefinitionLinkCompartmentComponent setDescriptionElement(StringType value) { 1738 this.description = value; 1739 return this; 1740 } 1741 1742 /** 1743 * @return Documentation for FHIRPath expression. 1744 */ 1745 public String getDescription() { 1746 return this.description == null ? null : this.description.getValue(); 1747 } 1748 1749 /** 1750 * @param value Documentation for FHIRPath expression. 1751 */ 1752 public GraphDefinitionLinkCompartmentComponent setDescription(String value) { 1753 if (Utilities.noString(value)) 1754 this.description = null; 1755 else { 1756 if (this.description == null) 1757 this.description = new StringType(); 1758 this.description.setValue(value); 1759 } 1760 return this; 1761 } 1762 1763 protected void listChildren(List<Property> children) { 1764 super.listChildren(children); 1765 children.add(new Property("use", "code", "Defines how the compartment rule is used - whether it it is used to test whether resources are subject to the rule, or whether it is a rule that must be followed.", 0, 1, use)); 1766 children.add(new Property("rule", "code", "identical | matching | different | no-rule | custom.", 0, 1, rule)); 1767 children.add(new Property("code", "code", "Identifies the compartment.", 0, 1, code)); 1768 children.add(new Property("expression", "string", "Custom rule, as a FHIRPath expression.", 0, 1, expression)); 1769 children.add(new Property("description", "string", "Documentation for FHIRPath expression.", 0, 1, description)); 1770 } 1771 1772 @Override 1773 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1774 switch (_hash) { 1775 case 116103: /*use*/ return new Property("use", "code", "Defines how the compartment rule is used - whether it it is used to test whether resources are subject to the rule, or whether it is a rule that must be followed.", 0, 1, use); 1776 case 3512060: /*rule*/ return new Property("rule", "code", "identical | matching | different | no-rule | custom.", 0, 1, rule); 1777 case 3059181: /*code*/ return new Property("code", "code", "Identifies the compartment.", 0, 1, code); 1778 case -1795452264: /*expression*/ return new Property("expression", "string", "Custom rule, as a FHIRPath expression.", 0, 1, expression); 1779 case -1724546052: /*description*/ return new Property("description", "string", "Documentation for FHIRPath expression.", 0, 1, description); 1780 default: return super.getNamedProperty(_hash, _name, _checkValid); 1781 } 1782 1783 } 1784 1785 @Override 1786 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1787 switch (hash) { 1788 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<GraphCompartmentUse> 1789 case 3512060: /*rule*/ return this.rule == null ? new Base[0] : new Base[] {this.rule}; // Enumeration<GraphCompartmentRule> 1790 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<CompartmentType> 1791 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 1792 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1793 default: return super.getProperty(hash, name, checkValid); 1794 } 1795 1796 } 1797 1798 @Override 1799 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1800 switch (hash) { 1801 case 116103: // use 1802 value = new GraphCompartmentUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 1803 this.use = (Enumeration) value; // Enumeration<GraphCompartmentUse> 1804 return value; 1805 case 3512060: // rule 1806 value = new GraphCompartmentRuleEnumFactory().fromType(TypeConvertor.castToCode(value)); 1807 this.rule = (Enumeration) value; // Enumeration<GraphCompartmentRule> 1808 return value; 1809 case 3059181: // code 1810 value = new CompartmentTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1811 this.code = (Enumeration) value; // Enumeration<CompartmentType> 1812 return value; 1813 case -1795452264: // expression 1814 this.expression = TypeConvertor.castToString(value); // StringType 1815 return value; 1816 case -1724546052: // description 1817 this.description = TypeConvertor.castToString(value); // StringType 1818 return value; 1819 default: return super.setProperty(hash, name, value); 1820 } 1821 1822 } 1823 1824 @Override 1825 public Base setProperty(String name, Base value) throws FHIRException { 1826 if (name.equals("use")) { 1827 value = new GraphCompartmentUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 1828 this.use = (Enumeration) value; // Enumeration<GraphCompartmentUse> 1829 } else if (name.equals("rule")) { 1830 value = new GraphCompartmentRuleEnumFactory().fromType(TypeConvertor.castToCode(value)); 1831 this.rule = (Enumeration) value; // Enumeration<GraphCompartmentRule> 1832 } else if (name.equals("code")) { 1833 value = new CompartmentTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1834 this.code = (Enumeration) value; // Enumeration<CompartmentType> 1835 } else if (name.equals("expression")) { 1836 this.expression = TypeConvertor.castToString(value); // StringType 1837 } else if (name.equals("description")) { 1838 this.description = TypeConvertor.castToString(value); // StringType 1839 } else 1840 return super.setProperty(name, value); 1841 return value; 1842 } 1843 1844 @Override 1845 public void removeChild(String name, Base value) throws FHIRException { 1846 if (name.equals("use")) { 1847 value = new GraphCompartmentUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 1848 this.use = (Enumeration) value; // Enumeration<GraphCompartmentUse> 1849 } else if (name.equals("rule")) { 1850 value = new GraphCompartmentRuleEnumFactory().fromType(TypeConvertor.castToCode(value)); 1851 this.rule = (Enumeration) value; // Enumeration<GraphCompartmentRule> 1852 } else if (name.equals("code")) { 1853 value = new CompartmentTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1854 this.code = (Enumeration) value; // Enumeration<CompartmentType> 1855 } else if (name.equals("expression")) { 1856 this.expression = null; 1857 } else if (name.equals("description")) { 1858 this.description = null; 1859 } else 1860 super.removeChild(name, value); 1861 1862 } 1863 1864 @Override 1865 public Base makeProperty(int hash, String name) throws FHIRException { 1866 switch (hash) { 1867 case 116103: return getUseElement(); 1868 case 3512060: return getRuleElement(); 1869 case 3059181: return getCodeElement(); 1870 case -1795452264: return getExpressionElement(); 1871 case -1724546052: return getDescriptionElement(); 1872 default: return super.makeProperty(hash, name); 1873 } 1874 1875 } 1876 1877 @Override 1878 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1879 switch (hash) { 1880 case 116103: /*use*/ return new String[] {"code"}; 1881 case 3512060: /*rule*/ return new String[] {"code"}; 1882 case 3059181: /*code*/ return new String[] {"code"}; 1883 case -1795452264: /*expression*/ return new String[] {"string"}; 1884 case -1724546052: /*description*/ return new String[] {"string"}; 1885 default: return super.getTypesForProperty(hash, name); 1886 } 1887 1888 } 1889 1890 @Override 1891 public Base addChild(String name) throws FHIRException { 1892 if (name.equals("use")) { 1893 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.link.compartment.use"); 1894 } 1895 else if (name.equals("rule")) { 1896 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.link.compartment.rule"); 1897 } 1898 else if (name.equals("code")) { 1899 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.link.compartment.code"); 1900 } 1901 else if (name.equals("expression")) { 1902 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.link.compartment.expression"); 1903 } 1904 else if (name.equals("description")) { 1905 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.link.compartment.description"); 1906 } 1907 else 1908 return super.addChild(name); 1909 } 1910 1911 public GraphDefinitionLinkCompartmentComponent copy() { 1912 GraphDefinitionLinkCompartmentComponent dst = new GraphDefinitionLinkCompartmentComponent(); 1913 copyValues(dst); 1914 return dst; 1915 } 1916 1917 public void copyValues(GraphDefinitionLinkCompartmentComponent dst) { 1918 super.copyValues(dst); 1919 dst.use = use == null ? null : use.copy(); 1920 dst.rule = rule == null ? null : rule.copy(); 1921 dst.code = code == null ? null : code.copy(); 1922 dst.expression = expression == null ? null : expression.copy(); 1923 dst.description = description == null ? null : description.copy(); 1924 } 1925 1926 @Override 1927 public boolean equalsDeep(Base other_) { 1928 if (!super.equalsDeep(other_)) 1929 return false; 1930 if (!(other_ instanceof GraphDefinitionLinkCompartmentComponent)) 1931 return false; 1932 GraphDefinitionLinkCompartmentComponent o = (GraphDefinitionLinkCompartmentComponent) other_; 1933 return compareDeep(use, o.use, true) && compareDeep(rule, o.rule, true) && compareDeep(code, o.code, true) 1934 && compareDeep(expression, o.expression, true) && compareDeep(description, o.description, true) 1935 ; 1936 } 1937 1938 @Override 1939 public boolean equalsShallow(Base other_) { 1940 if (!super.equalsShallow(other_)) 1941 return false; 1942 if (!(other_ instanceof GraphDefinitionLinkCompartmentComponent)) 1943 return false; 1944 GraphDefinitionLinkCompartmentComponent o = (GraphDefinitionLinkCompartmentComponent) other_; 1945 return compareValues(use, o.use, true) && compareValues(rule, o.rule, true) && compareValues(code, o.code, true) 1946 && compareValues(expression, o.expression, true) && compareValues(description, o.description, true) 1947 ; 1948 } 1949 1950 public boolean isEmpty() { 1951 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(use, rule, code, expression 1952 , description); 1953 } 1954 1955 public String fhirType() { 1956 return "GraphDefinition.link.compartment"; 1957 1958 } 1959 1960 } 1961 1962 /** 1963 * An absolute URI that is used to identify this graph definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this graph definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the graph definition is stored on different servers. 1964 */ 1965 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 1966 @Description(shortDefinition="Canonical identifier for this graph definition, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this graph definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this graph definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the graph definition is stored on different servers." ) 1967 protected UriType url; 1968 1969 /** 1970 * A formal identifier that is used to identify this GraphDefinition when it is represented in other formats, or referenced in a specification, model, design or an instance. 1971 */ 1972 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1973 @Description(shortDefinition="Additional identifier for the GraphDefinition (business identifier)", formalDefinition="A formal identifier that is used to identify this GraphDefinition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 1974 protected List<Identifier> identifier; 1975 1976 /** 1977 * The identifier that is used to identify this version of the graph definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the graph definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1978 */ 1979 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1980 @Description(shortDefinition="Business version of the graph definition", formalDefinition="The identifier that is used to identify this version of the graph definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the graph definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 1981 protected StringType version; 1982 1983 /** 1984 * Indicates the mechanism used to compare versions to determine which is more current. 1985 */ 1986 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 1987 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 1988 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 1989 protected DataType versionAlgorithm; 1990 1991 /** 1992 * A natural language name identifying the graph definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1993 */ 1994 @Child(name = "name", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=true) 1995 @Description(shortDefinition="Name for this graph definition (computer friendly)", formalDefinition="A natural language name identifying the graph definition. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 1996 protected StringType name; 1997 1998 /** 1999 * A short, descriptive, user-friendly title for the capability statement. 2000 */ 2001 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 2002 @Description(shortDefinition="Name for this graph definition (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the capability statement." ) 2003 protected StringType title; 2004 2005 /** 2006 * The status of this graph definition. Enables tracking the life-cycle of the content. 2007 */ 2008 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 2009 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this graph definition. Enables tracking the life-cycle of the content." ) 2010 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2011 protected Enumeration<PublicationStatus> status; 2012 2013 /** 2014 * A Boolean value to indicate that this graph definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2015 */ 2016 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 2017 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this graph definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 2018 protected BooleanType experimental; 2019 2020 /** 2021 * The date (and optionally time) when the graph definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the graph definition changes. 2022 */ 2023 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 2024 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the graph definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the graph definition changes." ) 2025 protected DateTimeType date; 2026 2027 /** 2028 * The name of the organization or individual responsible for the release and ongoing maintenance of the graph definition. 2029 */ 2030 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 2031 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the graph definition." ) 2032 protected StringType publisher; 2033 2034 /** 2035 * Contact details to assist a user in finding and communicating with the publisher. 2036 */ 2037 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2038 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 2039 protected List<ContactDetail> contact; 2040 2041 /** 2042 * A free text natural language description of the graph definition from a consumer's perspective. 2043 */ 2044 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 2045 @Description(shortDefinition="Natural language description of the graph definition", formalDefinition="A free text natural language description of the graph definition from a consumer's perspective." ) 2046 protected MarkdownType description; 2047 2048 /** 2049 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate graph definition instances. 2050 */ 2051 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2052 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate graph definition instances." ) 2053 protected List<UsageContext> useContext; 2054 2055 /** 2056 * A legal or geographic region in which the graph definition is intended to be used. 2057 */ 2058 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2059 @Description(shortDefinition="Intended jurisdiction for graph definition (if applicable)", formalDefinition="A legal or geographic region in which the graph definition is intended to be used." ) 2060 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 2061 protected List<CodeableConcept> jurisdiction; 2062 2063 /** 2064 * Explanation of why this graph definition is needed and why it has been designed as it has. 2065 */ 2066 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 2067 @Description(shortDefinition="Why this graph definition is defined", formalDefinition="Explanation of why this graph definition is needed and why it has been designed as it has." ) 2068 protected MarkdownType purpose; 2069 2070 /** 2071 * A copyright statement relating to the graph definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the graph definition. 2072 */ 2073 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 2074 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the graph definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the graph definition." ) 2075 protected MarkdownType copyright; 2076 2077 /** 2078 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2079 */ 2080 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 2081 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 2082 protected StringType copyrightLabel; 2083 2084 /** 2085 * The Node at which instances of this graph start. If there is no nominated start, the graph can start at any of the nodes. 2086 */ 2087 @Child(name = "start", type = {IdType.class}, order=17, min=0, max=1, modifier=false, summary=false) 2088 @Description(shortDefinition="Starting Node", formalDefinition="The Node at which instances of this graph start. If there is no nominated start, the graph can start at any of the nodes." ) 2089 protected IdType start; 2090 2091 /** 2092 * Potential target for the link. 2093 */ 2094 @Child(name = "node", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2095 @Description(shortDefinition="Potential target for the link", formalDefinition="Potential target for the link." ) 2096 protected List<GraphDefinitionNodeComponent> node; 2097 2098 /** 2099 * Links this graph makes rules about. 2100 */ 2101 @Child(name = "link", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2102 @Description(shortDefinition="Links this graph makes rules about", formalDefinition="Links this graph makes rules about." ) 2103 protected List<GraphDefinitionLinkComponent> link; 2104 2105 private static final long serialVersionUID = 6161243L; 2106 2107 /** 2108 * Constructor 2109 */ 2110 public GraphDefinition() { 2111 super(); 2112 } 2113 2114 /** 2115 * Constructor 2116 */ 2117 public GraphDefinition(String name, PublicationStatus status) { 2118 super(); 2119 this.setName(name); 2120 this.setStatus(status); 2121 } 2122 2123 /** 2124 * @return {@link #url} (An absolute URI that is used to identify this graph definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this graph definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the graph definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2125 */ 2126 public UriType getUrlElement() { 2127 if (this.url == null) 2128 if (Configuration.errorOnAutoCreate()) 2129 throw new Error("Attempt to auto-create GraphDefinition.url"); 2130 else if (Configuration.doAutoCreate()) 2131 this.url = new UriType(); // bb 2132 return this.url; 2133 } 2134 2135 public boolean hasUrlElement() { 2136 return this.url != null && !this.url.isEmpty(); 2137 } 2138 2139 public boolean hasUrl() { 2140 return this.url != null && !this.url.isEmpty(); 2141 } 2142 2143 /** 2144 * @param value {@link #url} (An absolute URI that is used to identify this graph definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this graph definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the graph definition is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2145 */ 2146 public GraphDefinition setUrlElement(UriType value) { 2147 this.url = value; 2148 return this; 2149 } 2150 2151 /** 2152 * @return An absolute URI that is used to identify this graph definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this graph definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the graph definition is stored on different servers. 2153 */ 2154 public String getUrl() { 2155 return this.url == null ? null : this.url.getValue(); 2156 } 2157 2158 /** 2159 * @param value An absolute URI that is used to identify this graph definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this graph definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the graph definition is stored on different servers. 2160 */ 2161 public GraphDefinition setUrl(String value) { 2162 if (Utilities.noString(value)) 2163 this.url = null; 2164 else { 2165 if (this.url == null) 2166 this.url = new UriType(); 2167 this.url.setValue(value); 2168 } 2169 return this; 2170 } 2171 2172 /** 2173 * @return {@link #identifier} (A formal identifier that is used to identify this GraphDefinition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 2174 */ 2175 public List<Identifier> getIdentifier() { 2176 if (this.identifier == null) 2177 this.identifier = new ArrayList<Identifier>(); 2178 return this.identifier; 2179 } 2180 2181 /** 2182 * @return Returns a reference to <code>this</code> for easy method chaining 2183 */ 2184 public GraphDefinition setIdentifier(List<Identifier> theIdentifier) { 2185 this.identifier = theIdentifier; 2186 return this; 2187 } 2188 2189 public boolean hasIdentifier() { 2190 if (this.identifier == null) 2191 return false; 2192 for (Identifier item : this.identifier) 2193 if (!item.isEmpty()) 2194 return true; 2195 return false; 2196 } 2197 2198 public Identifier addIdentifier() { //3 2199 Identifier t = new Identifier(); 2200 if (this.identifier == null) 2201 this.identifier = new ArrayList<Identifier>(); 2202 this.identifier.add(t); 2203 return t; 2204 } 2205 2206 public GraphDefinition addIdentifier(Identifier t) { //3 2207 if (t == null) 2208 return this; 2209 if (this.identifier == null) 2210 this.identifier = new ArrayList<Identifier>(); 2211 this.identifier.add(t); 2212 return this; 2213 } 2214 2215 /** 2216 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2217 */ 2218 public Identifier getIdentifierFirstRep() { 2219 if (getIdentifier().isEmpty()) { 2220 addIdentifier(); 2221 } 2222 return getIdentifier().get(0); 2223 } 2224 2225 /** 2226 * @return {@link #version} (The identifier that is used to identify this version of the graph definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the graph definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2227 */ 2228 public StringType getVersionElement() { 2229 if (this.version == null) 2230 if (Configuration.errorOnAutoCreate()) 2231 throw new Error("Attempt to auto-create GraphDefinition.version"); 2232 else if (Configuration.doAutoCreate()) 2233 this.version = new StringType(); // bb 2234 return this.version; 2235 } 2236 2237 public boolean hasVersionElement() { 2238 return this.version != null && !this.version.isEmpty(); 2239 } 2240 2241 public boolean hasVersion() { 2242 return this.version != null && !this.version.isEmpty(); 2243 } 2244 2245 /** 2246 * @param value {@link #version} (The identifier that is used to identify this version of the graph definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the graph definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2247 */ 2248 public GraphDefinition setVersionElement(StringType value) { 2249 this.version = value; 2250 return this; 2251 } 2252 2253 /** 2254 * @return The identifier that is used to identify this version of the graph definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the graph definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 2255 */ 2256 public String getVersion() { 2257 return this.version == null ? null : this.version.getValue(); 2258 } 2259 2260 /** 2261 * @param value The identifier that is used to identify this version of the graph definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the graph definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 2262 */ 2263 public GraphDefinition setVersion(String value) { 2264 if (Utilities.noString(value)) 2265 this.version = null; 2266 else { 2267 if (this.version == null) 2268 this.version = new StringType(); 2269 this.version.setValue(value); 2270 } 2271 return this; 2272 } 2273 2274 /** 2275 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2276 */ 2277 public DataType getVersionAlgorithm() { 2278 return this.versionAlgorithm; 2279 } 2280 2281 /** 2282 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2283 */ 2284 public StringType getVersionAlgorithmStringType() throws FHIRException { 2285 if (this.versionAlgorithm == null) 2286 this.versionAlgorithm = new StringType(); 2287 if (!(this.versionAlgorithm instanceof StringType)) 2288 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 2289 return (StringType) this.versionAlgorithm; 2290 } 2291 2292 public boolean hasVersionAlgorithmStringType() { 2293 return this != null && this.versionAlgorithm instanceof StringType; 2294 } 2295 2296 /** 2297 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2298 */ 2299 public Coding getVersionAlgorithmCoding() throws FHIRException { 2300 if (this.versionAlgorithm == null) 2301 this.versionAlgorithm = new Coding(); 2302 if (!(this.versionAlgorithm instanceof Coding)) 2303 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 2304 return (Coding) this.versionAlgorithm; 2305 } 2306 2307 public boolean hasVersionAlgorithmCoding() { 2308 return this != null && this.versionAlgorithm instanceof Coding; 2309 } 2310 2311 public boolean hasVersionAlgorithm() { 2312 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 2313 } 2314 2315 /** 2316 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 2317 */ 2318 public GraphDefinition setVersionAlgorithm(DataType value) { 2319 if (value != null && !(value instanceof StringType || value instanceof Coding)) 2320 throw new FHIRException("Not the right type for GraphDefinition.versionAlgorithm[x]: "+value.fhirType()); 2321 this.versionAlgorithm = value; 2322 return this; 2323 } 2324 2325 /** 2326 * @return {@link #name} (A natural language name identifying the graph definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2327 */ 2328 public StringType getNameElement() { 2329 if (this.name == null) 2330 if (Configuration.errorOnAutoCreate()) 2331 throw new Error("Attempt to auto-create GraphDefinition.name"); 2332 else if (Configuration.doAutoCreate()) 2333 this.name = new StringType(); // bb 2334 return this.name; 2335 } 2336 2337 public boolean hasNameElement() { 2338 return this.name != null && !this.name.isEmpty(); 2339 } 2340 2341 public boolean hasName() { 2342 return this.name != null && !this.name.isEmpty(); 2343 } 2344 2345 /** 2346 * @param value {@link #name} (A natural language name identifying the graph definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2347 */ 2348 public GraphDefinition setNameElement(StringType value) { 2349 this.name = value; 2350 return this; 2351 } 2352 2353 /** 2354 * @return A natural language name identifying the graph definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2355 */ 2356 public String getName() { 2357 return this.name == null ? null : this.name.getValue(); 2358 } 2359 2360 /** 2361 * @param value A natural language name identifying the graph definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2362 */ 2363 public GraphDefinition setName(String value) { 2364 if (this.name == null) 2365 this.name = new StringType(); 2366 this.name.setValue(value); 2367 return this; 2368 } 2369 2370 /** 2371 * @return {@link #title} (A short, descriptive, user-friendly title for the capability statement.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2372 */ 2373 public StringType getTitleElement() { 2374 if (this.title == null) 2375 if (Configuration.errorOnAutoCreate()) 2376 throw new Error("Attempt to auto-create GraphDefinition.title"); 2377 else if (Configuration.doAutoCreate()) 2378 this.title = new StringType(); // bb 2379 return this.title; 2380 } 2381 2382 public boolean hasTitleElement() { 2383 return this.title != null && !this.title.isEmpty(); 2384 } 2385 2386 public boolean hasTitle() { 2387 return this.title != null && !this.title.isEmpty(); 2388 } 2389 2390 /** 2391 * @param value {@link #title} (A short, descriptive, user-friendly title for the capability statement.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2392 */ 2393 public GraphDefinition setTitleElement(StringType value) { 2394 this.title = value; 2395 return this; 2396 } 2397 2398 /** 2399 * @return A short, descriptive, user-friendly title for the capability statement. 2400 */ 2401 public String getTitle() { 2402 return this.title == null ? null : this.title.getValue(); 2403 } 2404 2405 /** 2406 * @param value A short, descriptive, user-friendly title for the capability statement. 2407 */ 2408 public GraphDefinition setTitle(String value) { 2409 if (Utilities.noString(value)) 2410 this.title = null; 2411 else { 2412 if (this.title == null) 2413 this.title = new StringType(); 2414 this.title.setValue(value); 2415 } 2416 return this; 2417 } 2418 2419 /** 2420 * @return {@link #status} (The status of this graph definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2421 */ 2422 public Enumeration<PublicationStatus> getStatusElement() { 2423 if (this.status == null) 2424 if (Configuration.errorOnAutoCreate()) 2425 throw new Error("Attempt to auto-create GraphDefinition.status"); 2426 else if (Configuration.doAutoCreate()) 2427 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2428 return this.status; 2429 } 2430 2431 public boolean hasStatusElement() { 2432 return this.status != null && !this.status.isEmpty(); 2433 } 2434 2435 public boolean hasStatus() { 2436 return this.status != null && !this.status.isEmpty(); 2437 } 2438 2439 /** 2440 * @param value {@link #status} (The status of this graph definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2441 */ 2442 public GraphDefinition setStatusElement(Enumeration<PublicationStatus> value) { 2443 this.status = value; 2444 return this; 2445 } 2446 2447 /** 2448 * @return The status of this graph definition. Enables tracking the life-cycle of the content. 2449 */ 2450 public PublicationStatus getStatus() { 2451 return this.status == null ? null : this.status.getValue(); 2452 } 2453 2454 /** 2455 * @param value The status of this graph definition. Enables tracking the life-cycle of the content. 2456 */ 2457 public GraphDefinition setStatus(PublicationStatus value) { 2458 if (this.status == null) 2459 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2460 this.status.setValue(value); 2461 return this; 2462 } 2463 2464 /** 2465 * @return {@link #experimental} (A Boolean value to indicate that this graph definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2466 */ 2467 public BooleanType getExperimentalElement() { 2468 if (this.experimental == null) 2469 if (Configuration.errorOnAutoCreate()) 2470 throw new Error("Attempt to auto-create GraphDefinition.experimental"); 2471 else if (Configuration.doAutoCreate()) 2472 this.experimental = new BooleanType(); // bb 2473 return this.experimental; 2474 } 2475 2476 public boolean hasExperimentalElement() { 2477 return this.experimental != null && !this.experimental.isEmpty(); 2478 } 2479 2480 public boolean hasExperimental() { 2481 return this.experimental != null && !this.experimental.isEmpty(); 2482 } 2483 2484 /** 2485 * @param value {@link #experimental} (A Boolean value to indicate that this graph definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2486 */ 2487 public GraphDefinition setExperimentalElement(BooleanType value) { 2488 this.experimental = value; 2489 return this; 2490 } 2491 2492 /** 2493 * @return A Boolean value to indicate that this graph definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2494 */ 2495 public boolean getExperimental() { 2496 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2497 } 2498 2499 /** 2500 * @param value A Boolean value to indicate that this graph definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 2501 */ 2502 public GraphDefinition setExperimental(boolean value) { 2503 if (this.experimental == null) 2504 this.experimental = new BooleanType(); 2505 this.experimental.setValue(value); 2506 return this; 2507 } 2508 2509 /** 2510 * @return {@link #date} (The date (and optionally time) when the graph definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the graph definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2511 */ 2512 public DateTimeType getDateElement() { 2513 if (this.date == null) 2514 if (Configuration.errorOnAutoCreate()) 2515 throw new Error("Attempt to auto-create GraphDefinition.date"); 2516 else if (Configuration.doAutoCreate()) 2517 this.date = new DateTimeType(); // bb 2518 return this.date; 2519 } 2520 2521 public boolean hasDateElement() { 2522 return this.date != null && !this.date.isEmpty(); 2523 } 2524 2525 public boolean hasDate() { 2526 return this.date != null && !this.date.isEmpty(); 2527 } 2528 2529 /** 2530 * @param value {@link #date} (The date (and optionally time) when the graph definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the graph definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2531 */ 2532 public GraphDefinition setDateElement(DateTimeType value) { 2533 this.date = value; 2534 return this; 2535 } 2536 2537 /** 2538 * @return The date (and optionally time) when the graph definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the graph definition changes. 2539 */ 2540 public Date getDate() { 2541 return this.date == null ? null : this.date.getValue(); 2542 } 2543 2544 /** 2545 * @param value The date (and optionally time) when the graph definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the graph definition changes. 2546 */ 2547 public GraphDefinition setDate(Date value) { 2548 if (value == null) 2549 this.date = null; 2550 else { 2551 if (this.date == null) 2552 this.date = new DateTimeType(); 2553 this.date.setValue(value); 2554 } 2555 return this; 2556 } 2557 2558 /** 2559 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the graph definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2560 */ 2561 public StringType getPublisherElement() { 2562 if (this.publisher == null) 2563 if (Configuration.errorOnAutoCreate()) 2564 throw new Error("Attempt to auto-create GraphDefinition.publisher"); 2565 else if (Configuration.doAutoCreate()) 2566 this.publisher = new StringType(); // bb 2567 return this.publisher; 2568 } 2569 2570 public boolean hasPublisherElement() { 2571 return this.publisher != null && !this.publisher.isEmpty(); 2572 } 2573 2574 public boolean hasPublisher() { 2575 return this.publisher != null && !this.publisher.isEmpty(); 2576 } 2577 2578 /** 2579 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the graph definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2580 */ 2581 public GraphDefinition setPublisherElement(StringType value) { 2582 this.publisher = value; 2583 return this; 2584 } 2585 2586 /** 2587 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the graph definition. 2588 */ 2589 public String getPublisher() { 2590 return this.publisher == null ? null : this.publisher.getValue(); 2591 } 2592 2593 /** 2594 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the graph definition. 2595 */ 2596 public GraphDefinition setPublisher(String value) { 2597 if (Utilities.noString(value)) 2598 this.publisher = null; 2599 else { 2600 if (this.publisher == null) 2601 this.publisher = new StringType(); 2602 this.publisher.setValue(value); 2603 } 2604 return this; 2605 } 2606 2607 /** 2608 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2609 */ 2610 public List<ContactDetail> getContact() { 2611 if (this.contact == null) 2612 this.contact = new ArrayList<ContactDetail>(); 2613 return this.contact; 2614 } 2615 2616 /** 2617 * @return Returns a reference to <code>this</code> for easy method chaining 2618 */ 2619 public GraphDefinition setContact(List<ContactDetail> theContact) { 2620 this.contact = theContact; 2621 return this; 2622 } 2623 2624 public boolean hasContact() { 2625 if (this.contact == null) 2626 return false; 2627 for (ContactDetail item : this.contact) 2628 if (!item.isEmpty()) 2629 return true; 2630 return false; 2631 } 2632 2633 public ContactDetail addContact() { //3 2634 ContactDetail t = new ContactDetail(); 2635 if (this.contact == null) 2636 this.contact = new ArrayList<ContactDetail>(); 2637 this.contact.add(t); 2638 return t; 2639 } 2640 2641 public GraphDefinition addContact(ContactDetail t) { //3 2642 if (t == null) 2643 return this; 2644 if (this.contact == null) 2645 this.contact = new ArrayList<ContactDetail>(); 2646 this.contact.add(t); 2647 return this; 2648 } 2649 2650 /** 2651 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 2652 */ 2653 public ContactDetail getContactFirstRep() { 2654 if (getContact().isEmpty()) { 2655 addContact(); 2656 } 2657 return getContact().get(0); 2658 } 2659 2660 /** 2661 * @return {@link #description} (A free text natural language description of the graph definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2662 */ 2663 public MarkdownType getDescriptionElement() { 2664 if (this.description == null) 2665 if (Configuration.errorOnAutoCreate()) 2666 throw new Error("Attempt to auto-create GraphDefinition.description"); 2667 else if (Configuration.doAutoCreate()) 2668 this.description = new MarkdownType(); // bb 2669 return this.description; 2670 } 2671 2672 public boolean hasDescriptionElement() { 2673 return this.description != null && !this.description.isEmpty(); 2674 } 2675 2676 public boolean hasDescription() { 2677 return this.description != null && !this.description.isEmpty(); 2678 } 2679 2680 /** 2681 * @param value {@link #description} (A free text natural language description of the graph definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2682 */ 2683 public GraphDefinition setDescriptionElement(MarkdownType value) { 2684 this.description = value; 2685 return this; 2686 } 2687 2688 /** 2689 * @return A free text natural language description of the graph definition from a consumer's perspective. 2690 */ 2691 public String getDescription() { 2692 return this.description == null ? null : this.description.getValue(); 2693 } 2694 2695 /** 2696 * @param value A free text natural language description of the graph definition from a consumer's perspective. 2697 */ 2698 public GraphDefinition setDescription(String value) { 2699 if (Utilities.noString(value)) 2700 this.description = null; 2701 else { 2702 if (this.description == null) 2703 this.description = new MarkdownType(); 2704 this.description.setValue(value); 2705 } 2706 return this; 2707 } 2708 2709 /** 2710 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate graph definition instances.) 2711 */ 2712 public List<UsageContext> getUseContext() { 2713 if (this.useContext == null) 2714 this.useContext = new ArrayList<UsageContext>(); 2715 return this.useContext; 2716 } 2717 2718 /** 2719 * @return Returns a reference to <code>this</code> for easy method chaining 2720 */ 2721 public GraphDefinition setUseContext(List<UsageContext> theUseContext) { 2722 this.useContext = theUseContext; 2723 return this; 2724 } 2725 2726 public boolean hasUseContext() { 2727 if (this.useContext == null) 2728 return false; 2729 for (UsageContext item : this.useContext) 2730 if (!item.isEmpty()) 2731 return true; 2732 return false; 2733 } 2734 2735 public UsageContext addUseContext() { //3 2736 UsageContext t = new UsageContext(); 2737 if (this.useContext == null) 2738 this.useContext = new ArrayList<UsageContext>(); 2739 this.useContext.add(t); 2740 return t; 2741 } 2742 2743 public GraphDefinition addUseContext(UsageContext t) { //3 2744 if (t == null) 2745 return this; 2746 if (this.useContext == null) 2747 this.useContext = new ArrayList<UsageContext>(); 2748 this.useContext.add(t); 2749 return this; 2750 } 2751 2752 /** 2753 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 2754 */ 2755 public UsageContext getUseContextFirstRep() { 2756 if (getUseContext().isEmpty()) { 2757 addUseContext(); 2758 } 2759 return getUseContext().get(0); 2760 } 2761 2762 /** 2763 * @return {@link #jurisdiction} (A legal or geographic region in which the graph definition is intended to be used.) 2764 */ 2765 public List<CodeableConcept> getJurisdiction() { 2766 if (this.jurisdiction == null) 2767 this.jurisdiction = new ArrayList<CodeableConcept>(); 2768 return this.jurisdiction; 2769 } 2770 2771 /** 2772 * @return Returns a reference to <code>this</code> for easy method chaining 2773 */ 2774 public GraphDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2775 this.jurisdiction = theJurisdiction; 2776 return this; 2777 } 2778 2779 public boolean hasJurisdiction() { 2780 if (this.jurisdiction == null) 2781 return false; 2782 for (CodeableConcept item : this.jurisdiction) 2783 if (!item.isEmpty()) 2784 return true; 2785 return false; 2786 } 2787 2788 public CodeableConcept addJurisdiction() { //3 2789 CodeableConcept t = new CodeableConcept(); 2790 if (this.jurisdiction == null) 2791 this.jurisdiction = new ArrayList<CodeableConcept>(); 2792 this.jurisdiction.add(t); 2793 return t; 2794 } 2795 2796 public GraphDefinition addJurisdiction(CodeableConcept t) { //3 2797 if (t == null) 2798 return this; 2799 if (this.jurisdiction == null) 2800 this.jurisdiction = new ArrayList<CodeableConcept>(); 2801 this.jurisdiction.add(t); 2802 return this; 2803 } 2804 2805 /** 2806 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 2807 */ 2808 public CodeableConcept getJurisdictionFirstRep() { 2809 if (getJurisdiction().isEmpty()) { 2810 addJurisdiction(); 2811 } 2812 return getJurisdiction().get(0); 2813 } 2814 2815 /** 2816 * @return {@link #purpose} (Explanation of why this graph definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2817 */ 2818 public MarkdownType getPurposeElement() { 2819 if (this.purpose == null) 2820 if (Configuration.errorOnAutoCreate()) 2821 throw new Error("Attempt to auto-create GraphDefinition.purpose"); 2822 else if (Configuration.doAutoCreate()) 2823 this.purpose = new MarkdownType(); // bb 2824 return this.purpose; 2825 } 2826 2827 public boolean hasPurposeElement() { 2828 return this.purpose != null && !this.purpose.isEmpty(); 2829 } 2830 2831 public boolean hasPurpose() { 2832 return this.purpose != null && !this.purpose.isEmpty(); 2833 } 2834 2835 /** 2836 * @param value {@link #purpose} (Explanation of why this graph definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2837 */ 2838 public GraphDefinition setPurposeElement(MarkdownType value) { 2839 this.purpose = value; 2840 return this; 2841 } 2842 2843 /** 2844 * @return Explanation of why this graph definition is needed and why it has been designed as it has. 2845 */ 2846 public String getPurpose() { 2847 return this.purpose == null ? null : this.purpose.getValue(); 2848 } 2849 2850 /** 2851 * @param value Explanation of why this graph definition is needed and why it has been designed as it has. 2852 */ 2853 public GraphDefinition setPurpose(String value) { 2854 if (Utilities.noString(value)) 2855 this.purpose = null; 2856 else { 2857 if (this.purpose == null) 2858 this.purpose = new MarkdownType(); 2859 this.purpose.setValue(value); 2860 } 2861 return this; 2862 } 2863 2864 /** 2865 * @return {@link #copyright} (A copyright statement relating to the graph definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the graph definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2866 */ 2867 public MarkdownType getCopyrightElement() { 2868 if (this.copyright == null) 2869 if (Configuration.errorOnAutoCreate()) 2870 throw new Error("Attempt to auto-create GraphDefinition.copyright"); 2871 else if (Configuration.doAutoCreate()) 2872 this.copyright = new MarkdownType(); // bb 2873 return this.copyright; 2874 } 2875 2876 public boolean hasCopyrightElement() { 2877 return this.copyright != null && !this.copyright.isEmpty(); 2878 } 2879 2880 public boolean hasCopyright() { 2881 return this.copyright != null && !this.copyright.isEmpty(); 2882 } 2883 2884 /** 2885 * @param value {@link #copyright} (A copyright statement relating to the graph definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the graph definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2886 */ 2887 public GraphDefinition setCopyrightElement(MarkdownType value) { 2888 this.copyright = value; 2889 return this; 2890 } 2891 2892 /** 2893 * @return A copyright statement relating to the graph definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the graph definition. 2894 */ 2895 public String getCopyright() { 2896 return this.copyright == null ? null : this.copyright.getValue(); 2897 } 2898 2899 /** 2900 * @param value A copyright statement relating to the graph definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the graph definition. 2901 */ 2902 public GraphDefinition setCopyright(String value) { 2903 if (Utilities.noString(value)) 2904 this.copyright = null; 2905 else { 2906 if (this.copyright == null) 2907 this.copyright = new MarkdownType(); 2908 this.copyright.setValue(value); 2909 } 2910 return this; 2911 } 2912 2913 /** 2914 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2915 */ 2916 public StringType getCopyrightLabelElement() { 2917 if (this.copyrightLabel == null) 2918 if (Configuration.errorOnAutoCreate()) 2919 throw new Error("Attempt to auto-create GraphDefinition.copyrightLabel"); 2920 else if (Configuration.doAutoCreate()) 2921 this.copyrightLabel = new StringType(); // bb 2922 return this.copyrightLabel; 2923 } 2924 2925 public boolean hasCopyrightLabelElement() { 2926 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2927 } 2928 2929 public boolean hasCopyrightLabel() { 2930 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 2931 } 2932 2933 /** 2934 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 2935 */ 2936 public GraphDefinition setCopyrightLabelElement(StringType value) { 2937 this.copyrightLabel = value; 2938 return this; 2939 } 2940 2941 /** 2942 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2943 */ 2944 public String getCopyrightLabel() { 2945 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 2946 } 2947 2948 /** 2949 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 2950 */ 2951 public GraphDefinition setCopyrightLabel(String value) { 2952 if (Utilities.noString(value)) 2953 this.copyrightLabel = null; 2954 else { 2955 if (this.copyrightLabel == null) 2956 this.copyrightLabel = new StringType(); 2957 this.copyrightLabel.setValue(value); 2958 } 2959 return this; 2960 } 2961 2962 /** 2963 * @return {@link #start} (The Node at which instances of this graph start. If there is no nominated start, the graph can start at any of the nodes.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 2964 */ 2965 public IdType getStartElement() { 2966 if (this.start == null) 2967 if (Configuration.errorOnAutoCreate()) 2968 throw new Error("Attempt to auto-create GraphDefinition.start"); 2969 else if (Configuration.doAutoCreate()) 2970 this.start = new IdType(); // bb 2971 return this.start; 2972 } 2973 2974 public boolean hasStartElement() { 2975 return this.start != null && !this.start.isEmpty(); 2976 } 2977 2978 public boolean hasStart() { 2979 return this.start != null && !this.start.isEmpty(); 2980 } 2981 2982 /** 2983 * @param value {@link #start} (The Node at which instances of this graph start. If there is no nominated start, the graph can start at any of the nodes.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 2984 */ 2985 public GraphDefinition setStartElement(IdType value) { 2986 this.start = value; 2987 return this; 2988 } 2989 2990 /** 2991 * @return The Node at which instances of this graph start. If there is no nominated start, the graph can start at any of the nodes. 2992 */ 2993 public String getStart() { 2994 return this.start == null ? null : this.start.getValue(); 2995 } 2996 2997 /** 2998 * @param value The Node at which instances of this graph start. If there is no nominated start, the graph can start at any of the nodes. 2999 */ 3000 public GraphDefinition setStart(String value) { 3001 if (Utilities.noString(value)) 3002 this.start = null; 3003 else { 3004 if (this.start == null) 3005 this.start = new IdType(); 3006 this.start.setValue(value); 3007 } 3008 return this; 3009 } 3010 3011 /** 3012 * @return {@link #node} (Potential target for the link.) 3013 */ 3014 public List<GraphDefinitionNodeComponent> getNode() { 3015 if (this.node == null) 3016 this.node = new ArrayList<GraphDefinitionNodeComponent>(); 3017 return this.node; 3018 } 3019 3020 /** 3021 * @return Returns a reference to <code>this</code> for easy method chaining 3022 */ 3023 public GraphDefinition setNode(List<GraphDefinitionNodeComponent> theNode) { 3024 this.node = theNode; 3025 return this; 3026 } 3027 3028 public boolean hasNode() { 3029 if (this.node == null) 3030 return false; 3031 for (GraphDefinitionNodeComponent item : this.node) 3032 if (!item.isEmpty()) 3033 return true; 3034 return false; 3035 } 3036 3037 public GraphDefinitionNodeComponent addNode() { //3 3038 GraphDefinitionNodeComponent t = new GraphDefinitionNodeComponent(); 3039 if (this.node == null) 3040 this.node = new ArrayList<GraphDefinitionNodeComponent>(); 3041 this.node.add(t); 3042 return t; 3043 } 3044 3045 public GraphDefinition addNode(GraphDefinitionNodeComponent t) { //3 3046 if (t == null) 3047 return this; 3048 if (this.node == null) 3049 this.node = new ArrayList<GraphDefinitionNodeComponent>(); 3050 this.node.add(t); 3051 return this; 3052 } 3053 3054 /** 3055 * @return The first repetition of repeating field {@link #node}, creating it if it does not already exist {3} 3056 */ 3057 public GraphDefinitionNodeComponent getNodeFirstRep() { 3058 if (getNode().isEmpty()) { 3059 addNode(); 3060 } 3061 return getNode().get(0); 3062 } 3063 3064 /** 3065 * @return {@link #link} (Links this graph makes rules about.) 3066 */ 3067 public List<GraphDefinitionLinkComponent> getLink() { 3068 if (this.link == null) 3069 this.link = new ArrayList<GraphDefinitionLinkComponent>(); 3070 return this.link; 3071 } 3072 3073 /** 3074 * @return Returns a reference to <code>this</code> for easy method chaining 3075 */ 3076 public GraphDefinition setLink(List<GraphDefinitionLinkComponent> theLink) { 3077 this.link = theLink; 3078 return this; 3079 } 3080 3081 public boolean hasLink() { 3082 if (this.link == null) 3083 return false; 3084 for (GraphDefinitionLinkComponent item : this.link) 3085 if (!item.isEmpty()) 3086 return true; 3087 return false; 3088 } 3089 3090 public GraphDefinitionLinkComponent addLink() { //3 3091 GraphDefinitionLinkComponent t = new GraphDefinitionLinkComponent(); 3092 if (this.link == null) 3093 this.link = new ArrayList<GraphDefinitionLinkComponent>(); 3094 this.link.add(t); 3095 return t; 3096 } 3097 3098 public GraphDefinition addLink(GraphDefinitionLinkComponent t) { //3 3099 if (t == null) 3100 return this; 3101 if (this.link == null) 3102 this.link = new ArrayList<GraphDefinitionLinkComponent>(); 3103 this.link.add(t); 3104 return this; 3105 } 3106 3107 /** 3108 * @return The first repetition of repeating field {@link #link}, creating it if it does not already exist {3} 3109 */ 3110 public GraphDefinitionLinkComponent getLinkFirstRep() { 3111 if (getLink().isEmpty()) { 3112 addLink(); 3113 } 3114 return getLink().get(0); 3115 } 3116 3117 protected void listChildren(List<Property> children) { 3118 super.listChildren(children); 3119 children.add(new Property("url", "uri", "An absolute URI that is used to identify this graph definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this graph definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the graph definition is stored on different servers.", 0, 1, url)); 3120 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this GraphDefinition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3121 children.add(new Property("version", "string", "The identifier that is used to identify this version of the graph definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the graph definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 3122 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 3123 children.add(new Property("name", "string", "A natural language name identifying the graph definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3124 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the capability statement.", 0, 1, title)); 3125 children.add(new Property("status", "code", "The status of this graph definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 3126 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this graph definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 3127 children.add(new Property("date", "dateTime", "The date (and optionally time) when the graph definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the graph definition changes.", 0, 1, date)); 3128 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the graph definition.", 0, 1, publisher)); 3129 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3130 children.add(new Property("description", "markdown", "A free text natural language description of the graph definition from a consumer's perspective.", 0, 1, description)); 3131 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate graph definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3132 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the graph definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3133 children.add(new Property("purpose", "markdown", "Explanation of why this graph definition is needed and why it has been designed as it has.", 0, 1, purpose)); 3134 children.add(new Property("copyright", "markdown", "A copyright statement relating to the graph definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the graph definition.", 0, 1, copyright)); 3135 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 3136 children.add(new Property("start", "id", "The Node at which instances of this graph start. If there is no nominated start, the graph can start at any of the nodes.", 0, 1, start)); 3137 children.add(new Property("node", "", "Potential target for the link.", 0, java.lang.Integer.MAX_VALUE, node)); 3138 children.add(new Property("link", "", "Links this graph makes rules about.", 0, java.lang.Integer.MAX_VALUE, link)); 3139 } 3140 3141 @Override 3142 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3143 switch (_hash) { 3144 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this graph definition when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this graph definition is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the graph definition is stored on different servers.", 0, 1, url); 3145 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this GraphDefinition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 3146 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the graph definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the graph definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 3147 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3148 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3149 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3150 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 3151 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the graph definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3152 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the capability statement.", 0, 1, title); 3153 case -892481550: /*status*/ return new Property("status", "code", "The status of this graph definition. Enables tracking the life-cycle of the content.", 0, 1, status); 3154 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this graph definition is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 3155 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the graph definition was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the graph definition changes.", 0, 1, date); 3156 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the graph definition.", 0, 1, publisher); 3157 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 3158 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the graph definition from a consumer's perspective.", 0, 1, description); 3159 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate graph definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 3160 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the graph definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3161 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this graph definition is needed and why it has been designed as it has.", 0, 1, purpose); 3162 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the graph definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the graph definition.", 0, 1, copyright); 3163 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 3164 case 109757538: /*start*/ return new Property("start", "id", "The Node at which instances of this graph start. If there is no nominated start, the graph can start at any of the nodes.", 0, 1, start); 3165 case 3386882: /*node*/ return new Property("node", "", "Potential target for the link.", 0, java.lang.Integer.MAX_VALUE, node); 3166 case 3321850: /*link*/ return new Property("link", "", "Links this graph makes rules about.", 0, java.lang.Integer.MAX_VALUE, link); 3167 default: return super.getNamedProperty(_hash, _name, _checkValid); 3168 } 3169 3170 } 3171 3172 @Override 3173 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3174 switch (hash) { 3175 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3176 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3177 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 3178 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 3179 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3180 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3181 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 3182 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 3183 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 3184 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 3185 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3186 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3187 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3188 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3189 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 3190 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 3191 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 3192 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // IdType 3193 case 3386882: /*node*/ return this.node == null ? new Base[0] : this.node.toArray(new Base[this.node.size()]); // GraphDefinitionNodeComponent 3194 case 3321850: /*link*/ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // GraphDefinitionLinkComponent 3195 default: return super.getProperty(hash, name, checkValid); 3196 } 3197 3198 } 3199 3200 @Override 3201 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3202 switch (hash) { 3203 case 116079: // url 3204 this.url = TypeConvertor.castToUri(value); // UriType 3205 return value; 3206 case -1618432855: // identifier 3207 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3208 return value; 3209 case 351608024: // version 3210 this.version = TypeConvertor.castToString(value); // StringType 3211 return value; 3212 case 1508158071: // versionAlgorithm 3213 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3214 return value; 3215 case 3373707: // name 3216 this.name = TypeConvertor.castToString(value); // StringType 3217 return value; 3218 case 110371416: // title 3219 this.title = TypeConvertor.castToString(value); // StringType 3220 return value; 3221 case -892481550: // status 3222 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3223 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3224 return value; 3225 case -404562712: // experimental 3226 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3227 return value; 3228 case 3076014: // date 3229 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3230 return value; 3231 case 1447404028: // publisher 3232 this.publisher = TypeConvertor.castToString(value); // StringType 3233 return value; 3234 case 951526432: // contact 3235 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 3236 return value; 3237 case -1724546052: // description 3238 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3239 return value; 3240 case -669707736: // useContext 3241 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 3242 return value; 3243 case -507075711: // jurisdiction 3244 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3245 return value; 3246 case -220463842: // purpose 3247 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 3248 return value; 3249 case 1522889671: // copyright 3250 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 3251 return value; 3252 case 765157229: // copyrightLabel 3253 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 3254 return value; 3255 case 109757538: // start 3256 this.start = TypeConvertor.castToId(value); // IdType 3257 return value; 3258 case 3386882: // node 3259 this.getNode().add((GraphDefinitionNodeComponent) value); // GraphDefinitionNodeComponent 3260 return value; 3261 case 3321850: // link 3262 this.getLink().add((GraphDefinitionLinkComponent) value); // GraphDefinitionLinkComponent 3263 return value; 3264 default: return super.setProperty(hash, name, value); 3265 } 3266 3267 } 3268 3269 @Override 3270 public Base setProperty(String name, Base value) throws FHIRException { 3271 if (name.equals("url")) { 3272 this.url = TypeConvertor.castToUri(value); // UriType 3273 } else if (name.equals("identifier")) { 3274 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3275 } else if (name.equals("version")) { 3276 this.version = TypeConvertor.castToString(value); // StringType 3277 } else if (name.equals("versionAlgorithm[x]")) { 3278 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 3279 } else if (name.equals("name")) { 3280 this.name = TypeConvertor.castToString(value); // StringType 3281 } else if (name.equals("title")) { 3282 this.title = TypeConvertor.castToString(value); // StringType 3283 } else if (name.equals("status")) { 3284 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3285 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3286 } else if (name.equals("experimental")) { 3287 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 3288 } else if (name.equals("date")) { 3289 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 3290 } else if (name.equals("publisher")) { 3291 this.publisher = TypeConvertor.castToString(value); // StringType 3292 } else if (name.equals("contact")) { 3293 this.getContact().add(TypeConvertor.castToContactDetail(value)); 3294 } else if (name.equals("description")) { 3295 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3296 } else if (name.equals("useContext")) { 3297 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 3298 } else if (name.equals("jurisdiction")) { 3299 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 3300 } else if (name.equals("purpose")) { 3301 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 3302 } else if (name.equals("copyright")) { 3303 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 3304 } else if (name.equals("copyrightLabel")) { 3305 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 3306 } else if (name.equals("start")) { 3307 this.start = TypeConvertor.castToId(value); // IdType 3308 } else if (name.equals("node")) { 3309 this.getNode().add((GraphDefinitionNodeComponent) value); 3310 } else if (name.equals("link")) { 3311 this.getLink().add((GraphDefinitionLinkComponent) value); 3312 } else 3313 return super.setProperty(name, value); 3314 return value; 3315 } 3316 3317 @Override 3318 public void removeChild(String name, Base value) throws FHIRException { 3319 if (name.equals("url")) { 3320 this.url = null; 3321 } else if (name.equals("identifier")) { 3322 this.getIdentifier().remove(value); 3323 } else if (name.equals("version")) { 3324 this.version = null; 3325 } else if (name.equals("versionAlgorithm[x]")) { 3326 this.versionAlgorithm = null; 3327 } else if (name.equals("name")) { 3328 this.name = null; 3329 } else if (name.equals("title")) { 3330 this.title = null; 3331 } else if (name.equals("status")) { 3332 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3333 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3334 } else if (name.equals("experimental")) { 3335 this.experimental = null; 3336 } else if (name.equals("date")) { 3337 this.date = null; 3338 } else if (name.equals("publisher")) { 3339 this.publisher = null; 3340 } else if (name.equals("contact")) { 3341 this.getContact().remove(value); 3342 } else if (name.equals("description")) { 3343 this.description = null; 3344 } else if (name.equals("useContext")) { 3345 this.getUseContext().remove(value); 3346 } else if (name.equals("jurisdiction")) { 3347 this.getJurisdiction().remove(value); 3348 } else if (name.equals("purpose")) { 3349 this.purpose = null; 3350 } else if (name.equals("copyright")) { 3351 this.copyright = null; 3352 } else if (name.equals("copyrightLabel")) { 3353 this.copyrightLabel = null; 3354 } else if (name.equals("start")) { 3355 this.start = null; 3356 } else if (name.equals("node")) { 3357 this.getNode().remove((GraphDefinitionNodeComponent) value); 3358 } else if (name.equals("link")) { 3359 this.getLink().remove((GraphDefinitionLinkComponent) value); 3360 } else 3361 super.removeChild(name, value); 3362 3363 } 3364 3365 @Override 3366 public Base makeProperty(int hash, String name) throws FHIRException { 3367 switch (hash) { 3368 case 116079: return getUrlElement(); 3369 case -1618432855: return addIdentifier(); 3370 case 351608024: return getVersionElement(); 3371 case -115699031: return getVersionAlgorithm(); 3372 case 1508158071: return getVersionAlgorithm(); 3373 case 3373707: return getNameElement(); 3374 case 110371416: return getTitleElement(); 3375 case -892481550: return getStatusElement(); 3376 case -404562712: return getExperimentalElement(); 3377 case 3076014: return getDateElement(); 3378 case 1447404028: return getPublisherElement(); 3379 case 951526432: return addContact(); 3380 case -1724546052: return getDescriptionElement(); 3381 case -669707736: return addUseContext(); 3382 case -507075711: return addJurisdiction(); 3383 case -220463842: return getPurposeElement(); 3384 case 1522889671: return getCopyrightElement(); 3385 case 765157229: return getCopyrightLabelElement(); 3386 case 109757538: return getStartElement(); 3387 case 3386882: return addNode(); 3388 case 3321850: return addLink(); 3389 default: return super.makeProperty(hash, name); 3390 } 3391 3392 } 3393 3394 @Override 3395 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3396 switch (hash) { 3397 case 116079: /*url*/ return new String[] {"uri"}; 3398 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3399 case 351608024: /*version*/ return new String[] {"string"}; 3400 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 3401 case 3373707: /*name*/ return new String[] {"string"}; 3402 case 110371416: /*title*/ return new String[] {"string"}; 3403 case -892481550: /*status*/ return new String[] {"code"}; 3404 case -404562712: /*experimental*/ return new String[] {"boolean"}; 3405 case 3076014: /*date*/ return new String[] {"dateTime"}; 3406 case 1447404028: /*publisher*/ return new String[] {"string"}; 3407 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 3408 case -1724546052: /*description*/ return new String[] {"markdown"}; 3409 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 3410 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 3411 case -220463842: /*purpose*/ return new String[] {"markdown"}; 3412 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 3413 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 3414 case 109757538: /*start*/ return new String[] {"id"}; 3415 case 3386882: /*node*/ return new String[] {}; 3416 case 3321850: /*link*/ return new String[] {}; 3417 default: return super.getTypesForProperty(hash, name); 3418 } 3419 3420 } 3421 3422 @Override 3423 public Base addChild(String name) throws FHIRException { 3424 if (name.equals("url")) { 3425 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.url"); 3426 } 3427 else if (name.equals("identifier")) { 3428 return addIdentifier(); 3429 } 3430 else if (name.equals("version")) { 3431 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.version"); 3432 } 3433 else if (name.equals("versionAlgorithmString")) { 3434 this.versionAlgorithm = new StringType(); 3435 return this.versionAlgorithm; 3436 } 3437 else if (name.equals("versionAlgorithmCoding")) { 3438 this.versionAlgorithm = new Coding(); 3439 return this.versionAlgorithm; 3440 } 3441 else if (name.equals("name")) { 3442 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.name"); 3443 } 3444 else if (name.equals("title")) { 3445 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.title"); 3446 } 3447 else if (name.equals("status")) { 3448 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.status"); 3449 } 3450 else if (name.equals("experimental")) { 3451 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.experimental"); 3452 } 3453 else if (name.equals("date")) { 3454 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.date"); 3455 } 3456 else if (name.equals("publisher")) { 3457 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.publisher"); 3458 } 3459 else if (name.equals("contact")) { 3460 return addContact(); 3461 } 3462 else if (name.equals("description")) { 3463 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.description"); 3464 } 3465 else if (name.equals("useContext")) { 3466 return addUseContext(); 3467 } 3468 else if (name.equals("jurisdiction")) { 3469 return addJurisdiction(); 3470 } 3471 else if (name.equals("purpose")) { 3472 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.purpose"); 3473 } 3474 else if (name.equals("copyright")) { 3475 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.copyright"); 3476 } 3477 else if (name.equals("copyrightLabel")) { 3478 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.copyrightLabel"); 3479 } 3480 else if (name.equals("start")) { 3481 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.start"); 3482 } 3483 else if (name.equals("node")) { 3484 return addNode(); 3485 } 3486 else if (name.equals("link")) { 3487 return addLink(); 3488 } 3489 else 3490 return super.addChild(name); 3491 } 3492 3493 public String fhirType() { 3494 return "GraphDefinition"; 3495 3496 } 3497 3498 public GraphDefinition copy() { 3499 GraphDefinition dst = new GraphDefinition(); 3500 copyValues(dst); 3501 return dst; 3502 } 3503 3504 public void copyValues(GraphDefinition dst) { 3505 super.copyValues(dst); 3506 dst.url = url == null ? null : url.copy(); 3507 if (identifier != null) { 3508 dst.identifier = new ArrayList<Identifier>(); 3509 for (Identifier i : identifier) 3510 dst.identifier.add(i.copy()); 3511 }; 3512 dst.version = version == null ? null : version.copy(); 3513 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 3514 dst.name = name == null ? null : name.copy(); 3515 dst.title = title == null ? null : title.copy(); 3516 dst.status = status == null ? null : status.copy(); 3517 dst.experimental = experimental == null ? null : experimental.copy(); 3518 dst.date = date == null ? null : date.copy(); 3519 dst.publisher = publisher == null ? null : publisher.copy(); 3520 if (contact != null) { 3521 dst.contact = new ArrayList<ContactDetail>(); 3522 for (ContactDetail i : contact) 3523 dst.contact.add(i.copy()); 3524 }; 3525 dst.description = description == null ? null : description.copy(); 3526 if (useContext != null) { 3527 dst.useContext = new ArrayList<UsageContext>(); 3528 for (UsageContext i : useContext) 3529 dst.useContext.add(i.copy()); 3530 }; 3531 if (jurisdiction != null) { 3532 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3533 for (CodeableConcept i : jurisdiction) 3534 dst.jurisdiction.add(i.copy()); 3535 }; 3536 dst.purpose = purpose == null ? null : purpose.copy(); 3537 dst.copyright = copyright == null ? null : copyright.copy(); 3538 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 3539 dst.start = start == null ? null : start.copy(); 3540 if (node != null) { 3541 dst.node = new ArrayList<GraphDefinitionNodeComponent>(); 3542 for (GraphDefinitionNodeComponent i : node) 3543 dst.node.add(i.copy()); 3544 }; 3545 if (link != null) { 3546 dst.link = new ArrayList<GraphDefinitionLinkComponent>(); 3547 for (GraphDefinitionLinkComponent i : link) 3548 dst.link.add(i.copy()); 3549 }; 3550 } 3551 3552 protected GraphDefinition typedCopy() { 3553 return copy(); 3554 } 3555 3556 @Override 3557 public boolean equalsDeep(Base other_) { 3558 if (!super.equalsDeep(other_)) 3559 return false; 3560 if (!(other_ instanceof GraphDefinition)) 3561 return false; 3562 GraphDefinition o = (GraphDefinition) other_; 3563 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 3564 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 3565 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 3566 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 3567 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 3568 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 3569 && compareDeep(start, o.start, true) && compareDeep(node, o.node, true) && compareDeep(link, o.link, true) 3570 ; 3571 } 3572 3573 @Override 3574 public boolean equalsShallow(Base other_) { 3575 if (!super.equalsShallow(other_)) 3576 return false; 3577 if (!(other_ instanceof GraphDefinition)) 3578 return false; 3579 GraphDefinition o = (GraphDefinition) other_; 3580 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 3581 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 3582 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 3583 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 3584 && compareValues(start, o.start, true); 3585 } 3586 3587 public boolean isEmpty() { 3588 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 3589 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 3590 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, start 3591 , node, link); 3592 } 3593 3594 @Override 3595 public ResourceType getResourceType() { 3596 return ResourceType.GraphDefinition; 3597 } 3598 3599 /** 3600 * Search parameter: <b>context-quantity</b> 3601 * <p> 3602 * Description: <b>Multiple Resources: 3603 3604* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 3605* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 3606* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 3607* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 3608* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 3609* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 3610* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 3611* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 3612* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 3613* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 3614* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 3615* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 3616* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 3617* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 3618* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 3619* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 3620* [Library](library.html): A quantity- or range-valued use context assigned to the library 3621* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 3622* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 3623* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 3624* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 3625* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 3626* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 3627* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 3628* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 3629* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 3630* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 3631* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 3632* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 3633* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 3634</b><br> 3635 * Type: <b>quantity</b><br> 3636 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 3637 * </p> 3638 */ 3639 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 3640 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3641 /** 3642 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3643 * <p> 3644 * Description: <b>Multiple Resources: 3645 3646* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 3647* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 3648* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 3649* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 3650* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 3651* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 3652* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 3653* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 3654* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 3655* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 3656* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 3657* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 3658* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 3659* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 3660* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 3661* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 3662* [Library](library.html): A quantity- or range-valued use context assigned to the library 3663* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 3664* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 3665* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 3666* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 3667* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 3668* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 3669* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 3670* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 3671* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 3672* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 3673* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 3674* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 3675* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 3676</b><br> 3677 * Type: <b>quantity</b><br> 3678 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 3679 * </p> 3680 */ 3681 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 3682 3683 /** 3684 * Search parameter: <b>context-type-quantity</b> 3685 * <p> 3686 * Description: <b>Multiple Resources: 3687 3688* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3689* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3690* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3691* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3692* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3693* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3694* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3695* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3696* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3697* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3698* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3699* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3700* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3701* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3702* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3703* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3704* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3705* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3706* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3707* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3708* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3709* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3710* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3711* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3712* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3713* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3714* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3715* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3716* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3717* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3718</b><br> 3719 * Type: <b>composite</b><br> 3720 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3721 * </p> 3722 */ 3723 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 3724 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3725 /** 3726 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 3727 * <p> 3728 * Description: <b>Multiple Resources: 3729 3730* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 3731* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 3732* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 3733* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 3734* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 3735* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 3736* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 3737* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 3738* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 3739* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 3740* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 3741* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 3742* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 3743* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 3744* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 3745* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 3746* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 3747* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 3748* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 3749* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 3750* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 3751* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 3752* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 3753* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 3754* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 3755* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 3756* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 3757* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 3758* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 3759* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 3760</b><br> 3761 * Type: <b>composite</b><br> 3762 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3763 * </p> 3764 */ 3765 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 3766 3767 /** 3768 * Search parameter: <b>context-type-value</b> 3769 * <p> 3770 * Description: <b>Multiple Resources: 3771 3772* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3773* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3774* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3775* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3776* [Citation](citation.html): A use context type and value assigned to the citation 3777* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3778* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3779* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3780* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3781* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3782* [Evidence](evidence.html): A use context type and value assigned to the evidence 3783* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3784* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3785* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3786* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3787* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3788* [Library](library.html): A use context type and value assigned to the library 3789* [Measure](measure.html): A use context type and value assigned to the measure 3790* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3791* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3792* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3793* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3794* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3795* [Requirements](requirements.html): A use context type and value assigned to the requirements 3796* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3797* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3798* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3799* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3800* [TestScript](testscript.html): A use context type and value assigned to the test script 3801* [ValueSet](valueset.html): A use context type and value assigned to the value set 3802</b><br> 3803 * Type: <b>composite</b><br> 3804 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3805 * </p> 3806 */ 3807 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 3808 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 3809 /** 3810 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 3811 * <p> 3812 * Description: <b>Multiple Resources: 3813 3814* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 3815* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 3816* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 3817* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 3818* [Citation](citation.html): A use context type and value assigned to the citation 3819* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 3820* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 3821* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 3822* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 3823* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 3824* [Evidence](evidence.html): A use context type and value assigned to the evidence 3825* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 3826* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 3827* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 3828* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 3829* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 3830* [Library](library.html): A use context type and value assigned to the library 3831* [Measure](measure.html): A use context type and value assigned to the measure 3832* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 3833* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 3834* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 3835* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 3836* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 3837* [Requirements](requirements.html): A use context type and value assigned to the requirements 3838* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 3839* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 3840* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 3841* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 3842* [TestScript](testscript.html): A use context type and value assigned to the test script 3843* [ValueSet](valueset.html): A use context type and value assigned to the value set 3844</b><br> 3845 * Type: <b>composite</b><br> 3846 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 3847 * </p> 3848 */ 3849 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 3850 3851 /** 3852 * Search parameter: <b>context-type</b> 3853 * <p> 3854 * Description: <b>Multiple Resources: 3855 3856* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3857* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3858* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3859* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3860* [Citation](citation.html): A type of use context assigned to the citation 3861* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3862* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3863* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3864* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3865* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3866* [Evidence](evidence.html): A type of use context assigned to the evidence 3867* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3868* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3869* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3870* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3871* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3872* [Library](library.html): A type of use context assigned to the library 3873* [Measure](measure.html): A type of use context assigned to the measure 3874* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3875* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3876* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3877* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3878* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3879* [Requirements](requirements.html): A type of use context assigned to the requirements 3880* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3881* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3882* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3883* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3884* [TestScript](testscript.html): A type of use context assigned to the test script 3885* [ValueSet](valueset.html): A type of use context assigned to the value set 3886</b><br> 3887 * Type: <b>token</b><br> 3888 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3889 * </p> 3890 */ 3891 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 3892 public static final String SP_CONTEXT_TYPE = "context-type"; 3893 /** 3894 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 3895 * <p> 3896 * Description: <b>Multiple Resources: 3897 3898* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 3899* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 3900* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 3901* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 3902* [Citation](citation.html): A type of use context assigned to the citation 3903* [CodeSystem](codesystem.html): A type of use context assigned to the code system 3904* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 3905* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 3906* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 3907* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 3908* [Evidence](evidence.html): A type of use context assigned to the evidence 3909* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 3910* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 3911* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 3912* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 3913* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 3914* [Library](library.html): A type of use context assigned to the library 3915* [Measure](measure.html): A type of use context assigned to the measure 3916* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 3917* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 3918* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 3919* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 3920* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 3921* [Requirements](requirements.html): A type of use context assigned to the requirements 3922* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 3923* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 3924* [StructureMap](structuremap.html): A type of use context assigned to the structure map 3925* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 3926* [TestScript](testscript.html): A type of use context assigned to the test script 3927* [ValueSet](valueset.html): A type of use context assigned to the value set 3928</b><br> 3929 * Type: <b>token</b><br> 3930 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 3931 * </p> 3932 */ 3933 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 3934 3935 /** 3936 * Search parameter: <b>context</b> 3937 * <p> 3938 * Description: <b>Multiple Resources: 3939 3940* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3941* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3942* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3943* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3944* [Citation](citation.html): A use context assigned to the citation 3945* [CodeSystem](codesystem.html): A use context assigned to the code system 3946* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3947* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3948* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3949* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3950* [Evidence](evidence.html): A use context assigned to the evidence 3951* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3952* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3953* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3954* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3955* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3956* [Library](library.html): A use context assigned to the library 3957* [Measure](measure.html): A use context assigned to the measure 3958* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3959* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3960* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3961* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3962* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3963* [Requirements](requirements.html): A use context assigned to the requirements 3964* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3965* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3966* [StructureMap](structuremap.html): A use context assigned to the structure map 3967* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3968* [TestScript](testscript.html): A use context assigned to the test script 3969* [ValueSet](valueset.html): A use context assigned to the value set 3970</b><br> 3971 * Type: <b>token</b><br> 3972 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3973 * </p> 3974 */ 3975 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 3976 public static final String SP_CONTEXT = "context"; 3977 /** 3978 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3979 * <p> 3980 * Description: <b>Multiple Resources: 3981 3982* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3983* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3984* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3985* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3986* [Citation](citation.html): A use context assigned to the citation 3987* [CodeSystem](codesystem.html): A use context assigned to the code system 3988* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3989* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3990* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3991* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3992* [Evidence](evidence.html): A use context assigned to the evidence 3993* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3994* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3995* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3996* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3997* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3998* [Library](library.html): A use context assigned to the library 3999* [Measure](measure.html): A use context assigned to the measure 4000* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 4001* [NamingSystem](namingsystem.html): A use context assigned to the naming system 4002* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 4003* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 4004* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 4005* [Requirements](requirements.html): A use context assigned to the requirements 4006* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 4007* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 4008* [StructureMap](structuremap.html): A use context assigned to the structure map 4009* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 4010* [TestScript](testscript.html): A use context assigned to the test script 4011* [ValueSet](valueset.html): A use context assigned to the value set 4012</b><br> 4013 * Type: <b>token</b><br> 4014 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 4015 * </p> 4016 */ 4017 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 4018 4019 /** 4020 * Search parameter: <b>date</b> 4021 * <p> 4022 * Description: <b>Multiple Resources: 4023 4024* [ActivityDefinition](activitydefinition.html): The activity definition publication date 4025* [ActorDefinition](actordefinition.html): The Actor Definition publication date 4026* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 4027* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 4028* [Citation](citation.html): The citation publication date 4029* [CodeSystem](codesystem.html): The code system publication date 4030* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 4031* [ConceptMap](conceptmap.html): The concept map publication date 4032* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 4033* [EventDefinition](eventdefinition.html): The event definition publication date 4034* [Evidence](evidence.html): The evidence publication date 4035* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 4036* [ExampleScenario](examplescenario.html): The example scenario publication date 4037* [GraphDefinition](graphdefinition.html): The graph definition publication date 4038* [ImplementationGuide](implementationguide.html): The implementation guide publication date 4039* [Library](library.html): The library publication date 4040* [Measure](measure.html): The measure publication date 4041* [MessageDefinition](messagedefinition.html): The message definition publication date 4042* [NamingSystem](namingsystem.html): The naming system publication date 4043* [OperationDefinition](operationdefinition.html): The operation definition publication date 4044* [PlanDefinition](plandefinition.html): The plan definition publication date 4045* [Questionnaire](questionnaire.html): The questionnaire publication date 4046* [Requirements](requirements.html): The requirements publication date 4047* [SearchParameter](searchparameter.html): The search parameter publication date 4048* [StructureDefinition](structuredefinition.html): The structure definition publication date 4049* [StructureMap](structuremap.html): The structure map publication date 4050* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 4051* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 4052* [TestScript](testscript.html): The test script publication date 4053* [ValueSet](valueset.html): The value set publication date 4054</b><br> 4055 * Type: <b>date</b><br> 4056 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 4057 * </p> 4058 */ 4059 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 4060 public static final String SP_DATE = "date"; 4061 /** 4062 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4063 * <p> 4064 * Description: <b>Multiple Resources: 4065 4066* [ActivityDefinition](activitydefinition.html): The activity definition publication date 4067* [ActorDefinition](actordefinition.html): The Actor Definition publication date 4068* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 4069* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 4070* [Citation](citation.html): The citation publication date 4071* [CodeSystem](codesystem.html): The code system publication date 4072* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 4073* [ConceptMap](conceptmap.html): The concept map publication date 4074* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 4075* [EventDefinition](eventdefinition.html): The event definition publication date 4076* [Evidence](evidence.html): The evidence publication date 4077* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 4078* [ExampleScenario](examplescenario.html): The example scenario publication date 4079* [GraphDefinition](graphdefinition.html): The graph definition publication date 4080* [ImplementationGuide](implementationguide.html): The implementation guide publication date 4081* [Library](library.html): The library publication date 4082* [Measure](measure.html): The measure publication date 4083* [MessageDefinition](messagedefinition.html): The message definition publication date 4084* [NamingSystem](namingsystem.html): The naming system publication date 4085* [OperationDefinition](operationdefinition.html): The operation definition publication date 4086* [PlanDefinition](plandefinition.html): The plan definition publication date 4087* [Questionnaire](questionnaire.html): The questionnaire publication date 4088* [Requirements](requirements.html): The requirements publication date 4089* [SearchParameter](searchparameter.html): The search parameter publication date 4090* [StructureDefinition](structuredefinition.html): The structure definition publication date 4091* [StructureMap](structuremap.html): The structure map publication date 4092* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 4093* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 4094* [TestScript](testscript.html): The test script publication date 4095* [ValueSet](valueset.html): The value set publication date 4096</b><br> 4097 * Type: <b>date</b><br> 4098 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 4099 * </p> 4100 */ 4101 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4102 4103 /** 4104 * Search parameter: <b>description</b> 4105 * <p> 4106 * Description: <b>Multiple Resources: 4107 4108* [ActivityDefinition](activitydefinition.html): The description of the activity definition 4109* [ActorDefinition](actordefinition.html): The description of the Actor Definition 4110* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 4111* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 4112* [Citation](citation.html): The description of the citation 4113* [CodeSystem](codesystem.html): The description of the code system 4114* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 4115* [ConceptMap](conceptmap.html): The description of the concept map 4116* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 4117* [EventDefinition](eventdefinition.html): The description of the event definition 4118* [Evidence](evidence.html): The description of the evidence 4119* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 4120* [GraphDefinition](graphdefinition.html): The description of the graph definition 4121* [ImplementationGuide](implementationguide.html): The description of the implementation guide 4122* [Library](library.html): The description of the library 4123* [Measure](measure.html): The description of the measure 4124* [MessageDefinition](messagedefinition.html): The description of the message definition 4125* [NamingSystem](namingsystem.html): The description of the naming system 4126* [OperationDefinition](operationdefinition.html): The description of the operation definition 4127* [PlanDefinition](plandefinition.html): The description of the plan definition 4128* [Questionnaire](questionnaire.html): The description of the questionnaire 4129* [Requirements](requirements.html): The description of the requirements 4130* [SearchParameter](searchparameter.html): The description of the search parameter 4131* [StructureDefinition](structuredefinition.html): The description of the structure definition 4132* [StructureMap](structuremap.html): The description of the structure map 4133* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 4134* [TestScript](testscript.html): The description of the test script 4135* [ValueSet](valueset.html): The description of the value set 4136</b><br> 4137 * Type: <b>string</b><br> 4138 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 4139 * </p> 4140 */ 4141 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 4142 public static final String SP_DESCRIPTION = "description"; 4143 /** 4144 * <b>Fluent Client</b> search parameter constant for <b>description</b> 4145 * <p> 4146 * Description: <b>Multiple Resources: 4147 4148* [ActivityDefinition](activitydefinition.html): The description of the activity definition 4149* [ActorDefinition](actordefinition.html): The description of the Actor Definition 4150* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 4151* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 4152* [Citation](citation.html): The description of the citation 4153* [CodeSystem](codesystem.html): The description of the code system 4154* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 4155* [ConceptMap](conceptmap.html): The description of the concept map 4156* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 4157* [EventDefinition](eventdefinition.html): The description of the event definition 4158* [Evidence](evidence.html): The description of the evidence 4159* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 4160* [GraphDefinition](graphdefinition.html): The description of the graph definition 4161* [ImplementationGuide](implementationguide.html): The description of the implementation guide 4162* [Library](library.html): The description of the library 4163* [Measure](measure.html): The description of the measure 4164* [MessageDefinition](messagedefinition.html): The description of the message definition 4165* [NamingSystem](namingsystem.html): The description of the naming system 4166* [OperationDefinition](operationdefinition.html): The description of the operation definition 4167* [PlanDefinition](plandefinition.html): The description of the plan definition 4168* [Questionnaire](questionnaire.html): The description of the questionnaire 4169* [Requirements](requirements.html): The description of the requirements 4170* [SearchParameter](searchparameter.html): The description of the search parameter 4171* [StructureDefinition](structuredefinition.html): The description of the structure definition 4172* [StructureMap](structuremap.html): The description of the structure map 4173* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 4174* [TestScript](testscript.html): The description of the test script 4175* [ValueSet](valueset.html): The description of the value set 4176</b><br> 4177 * Type: <b>string</b><br> 4178 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 4179 * </p> 4180 */ 4181 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 4182 4183 /** 4184 * Search parameter: <b>identifier</b> 4185 * <p> 4186 * Description: <b>Multiple Resources: 4187 4188* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4189* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4190* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4191* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4192* [Citation](citation.html): External identifier for the citation 4193* [CodeSystem](codesystem.html): External identifier for the code system 4194* [ConceptMap](conceptmap.html): External identifier for the concept map 4195* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4196* [EventDefinition](eventdefinition.html): External identifier for the event definition 4197* [Evidence](evidence.html): External identifier for the evidence 4198* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4199* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4200* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4201* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4202* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4203* [Library](library.html): External identifier for the library 4204* [Measure](measure.html): External identifier for the measure 4205* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4206* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4207* [NamingSystem](namingsystem.html): External identifier for the naming system 4208* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4209* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4210* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4211* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4212* [Requirements](requirements.html): External identifier for the requirements 4213* [SearchParameter](searchparameter.html): External identifier for the search parameter 4214* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4215* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4216* [StructureMap](structuremap.html): External identifier for the structure map 4217* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4218* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4219* [TestPlan](testplan.html): An identifier for the test plan 4220* [TestScript](testscript.html): External identifier for the test script 4221* [ValueSet](valueset.html): External identifier for the value set 4222</b><br> 4223 * Type: <b>token</b><br> 4224 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4225 * </p> 4226 */ 4227 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 4228 public static final String SP_IDENTIFIER = "identifier"; 4229 /** 4230 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4231 * <p> 4232 * Description: <b>Multiple Resources: 4233 4234* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 4235* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 4236* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 4237* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 4238* [Citation](citation.html): External identifier for the citation 4239* [CodeSystem](codesystem.html): External identifier for the code system 4240* [ConceptMap](conceptmap.html): External identifier for the concept map 4241* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 4242* [EventDefinition](eventdefinition.html): External identifier for the event definition 4243* [Evidence](evidence.html): External identifier for the evidence 4244* [EvidenceReport](evidencereport.html): External identifier for the evidence report 4245* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 4246* [ExampleScenario](examplescenario.html): External identifier for the example scenario 4247* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 4248* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 4249* [Library](library.html): External identifier for the library 4250* [Measure](measure.html): External identifier for the measure 4251* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 4252* [MessageDefinition](messagedefinition.html): External identifier for the message definition 4253* [NamingSystem](namingsystem.html): External identifier for the naming system 4254* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 4255* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 4256* [PlanDefinition](plandefinition.html): External identifier for the plan definition 4257* [Questionnaire](questionnaire.html): External identifier for the questionnaire 4258* [Requirements](requirements.html): External identifier for the requirements 4259* [SearchParameter](searchparameter.html): External identifier for the search parameter 4260* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 4261* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 4262* [StructureMap](structuremap.html): External identifier for the structure map 4263* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 4264* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 4265* [TestPlan](testplan.html): An identifier for the test plan 4266* [TestScript](testscript.html): External identifier for the test script 4267* [ValueSet](valueset.html): External identifier for the value set 4268</b><br> 4269 * Type: <b>token</b><br> 4270 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 4271 * </p> 4272 */ 4273 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4274 4275 /** 4276 * Search parameter: <b>jurisdiction</b> 4277 * <p> 4278 * Description: <b>Multiple Resources: 4279 4280* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 4281* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 4282* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 4283* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 4284* [Citation](citation.html): Intended jurisdiction for the citation 4285* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 4286* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 4287* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 4288* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 4289* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 4290* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 4291* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 4292* [Library](library.html): Intended jurisdiction for the library 4293* [Measure](measure.html): Intended jurisdiction for the measure 4294* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 4295* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 4296* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 4297* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 4298* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 4299* [Requirements](requirements.html): Intended jurisdiction for the requirements 4300* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 4301* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 4302* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 4303* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 4304* [TestScript](testscript.html): Intended jurisdiction for the test script 4305* [ValueSet](valueset.html): Intended jurisdiction for the value set 4306</b><br> 4307 * Type: <b>token</b><br> 4308 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 4309 * </p> 4310 */ 4311 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 4312 public static final String SP_JURISDICTION = "jurisdiction"; 4313 /** 4314 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4315 * <p> 4316 * Description: <b>Multiple Resources: 4317 4318* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 4319* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 4320* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 4321* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 4322* [Citation](citation.html): Intended jurisdiction for the citation 4323* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 4324* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 4325* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 4326* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 4327* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 4328* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 4329* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 4330* [Library](library.html): Intended jurisdiction for the library 4331* [Measure](measure.html): Intended jurisdiction for the measure 4332* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 4333* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 4334* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 4335* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 4336* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 4337* [Requirements](requirements.html): Intended jurisdiction for the requirements 4338* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 4339* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 4340* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 4341* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 4342* [TestScript](testscript.html): Intended jurisdiction for the test script 4343* [ValueSet](valueset.html): Intended jurisdiction for the value set 4344</b><br> 4345 * Type: <b>token</b><br> 4346 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 4347 * </p> 4348 */ 4349 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 4350 4351 /** 4352 * Search parameter: <b>name</b> 4353 * <p> 4354 * Description: <b>Multiple Resources: 4355 4356* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 4357* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 4358* [Citation](citation.html): Computationally friendly name of the citation 4359* [CodeSystem](codesystem.html): Computationally friendly name of the code system 4360* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 4361* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 4362* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 4363* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 4364* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 4365* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 4366* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 4367* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 4368* [Library](library.html): Computationally friendly name of the library 4369* [Measure](measure.html): Computationally friendly name of the measure 4370* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 4371* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 4372* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 4373* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 4374* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 4375* [Requirements](requirements.html): Computationally friendly name of the requirements 4376* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 4377* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 4378* [StructureMap](structuremap.html): Computationally friendly name of the structure map 4379* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 4380* [TestScript](testscript.html): Computationally friendly name of the test script 4381* [ValueSet](valueset.html): Computationally friendly name of the value set 4382</b><br> 4383 * Type: <b>string</b><br> 4384 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 4385 * </p> 4386 */ 4387 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 4388 public static final String SP_NAME = "name"; 4389 /** 4390 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4391 * <p> 4392 * Description: <b>Multiple Resources: 4393 4394* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 4395* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 4396* [Citation](citation.html): Computationally friendly name of the citation 4397* [CodeSystem](codesystem.html): Computationally friendly name of the code system 4398* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 4399* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 4400* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 4401* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 4402* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 4403* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 4404* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 4405* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 4406* [Library](library.html): Computationally friendly name of the library 4407* [Measure](measure.html): Computationally friendly name of the measure 4408* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 4409* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 4410* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 4411* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 4412* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 4413* [Requirements](requirements.html): Computationally friendly name of the requirements 4414* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 4415* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 4416* [StructureMap](structuremap.html): Computationally friendly name of the structure map 4417* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 4418* [TestScript](testscript.html): Computationally friendly name of the test script 4419* [ValueSet](valueset.html): Computationally friendly name of the value set 4420</b><br> 4421 * Type: <b>string</b><br> 4422 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 4423 * </p> 4424 */ 4425 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 4426 4427 /** 4428 * Search parameter: <b>publisher</b> 4429 * <p> 4430 * Description: <b>Multiple Resources: 4431 4432* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 4433* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 4434* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 4435* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 4436* [Citation](citation.html): Name of the publisher of the citation 4437* [CodeSystem](codesystem.html): Name of the publisher of the code system 4438* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 4439* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 4440* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 4441* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 4442* [Evidence](evidence.html): Name of the publisher of the evidence 4443* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 4444* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 4445* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 4446* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 4447* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 4448* [Library](library.html): Name of the publisher of the library 4449* [Measure](measure.html): Name of the publisher of the measure 4450* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 4451* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 4452* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 4453* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 4454* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 4455* [Requirements](requirements.html): Name of the publisher of the requirements 4456* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 4457* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 4458* [StructureMap](structuremap.html): Name of the publisher of the structure map 4459* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 4460* [TestScript](testscript.html): Name of the publisher of the test script 4461* [ValueSet](valueset.html): Name of the publisher of the value set 4462</b><br> 4463 * Type: <b>string</b><br> 4464 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 4465 * </p> 4466 */ 4467 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 4468 public static final String SP_PUBLISHER = "publisher"; 4469 /** 4470 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 4471 * <p> 4472 * Description: <b>Multiple Resources: 4473 4474* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 4475* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 4476* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 4477* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 4478* [Citation](citation.html): Name of the publisher of the citation 4479* [CodeSystem](codesystem.html): Name of the publisher of the code system 4480* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 4481* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 4482* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 4483* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 4484* [Evidence](evidence.html): Name of the publisher of the evidence 4485* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 4486* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 4487* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 4488* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 4489* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 4490* [Library](library.html): Name of the publisher of the library 4491* [Measure](measure.html): Name of the publisher of the measure 4492* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 4493* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 4494* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 4495* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 4496* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 4497* [Requirements](requirements.html): Name of the publisher of the requirements 4498* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 4499* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 4500* [StructureMap](structuremap.html): Name of the publisher of the structure map 4501* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 4502* [TestScript](testscript.html): Name of the publisher of the test script 4503* [ValueSet](valueset.html): Name of the publisher of the value set 4504</b><br> 4505 * Type: <b>string</b><br> 4506 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 4507 * </p> 4508 */ 4509 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 4510 4511 /** 4512 * Search parameter: <b>status</b> 4513 * <p> 4514 * Description: <b>Multiple Resources: 4515 4516* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4517* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4518* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4519* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4520* [Citation](citation.html): The current status of the citation 4521* [CodeSystem](codesystem.html): The current status of the code system 4522* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4523* [ConceptMap](conceptmap.html): The current status of the concept map 4524* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4525* [EventDefinition](eventdefinition.html): The current status of the event definition 4526* [Evidence](evidence.html): The current status of the evidence 4527* [EvidenceReport](evidencereport.html): The current status of the evidence report 4528* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4529* [ExampleScenario](examplescenario.html): The current status of the example scenario 4530* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4531* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4532* [Library](library.html): The current status of the library 4533* [Measure](measure.html): The current status of the measure 4534* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4535* [MessageDefinition](messagedefinition.html): The current status of the message definition 4536* [NamingSystem](namingsystem.html): The current status of the naming system 4537* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4538* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4539* [PlanDefinition](plandefinition.html): The current status of the plan definition 4540* [Questionnaire](questionnaire.html): The current status of the questionnaire 4541* [Requirements](requirements.html): The current status of the requirements 4542* [SearchParameter](searchparameter.html): The current status of the search parameter 4543* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4544* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4545* [StructureMap](structuremap.html): The current status of the structure map 4546* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4547* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4548* [TestPlan](testplan.html): The current status of the test plan 4549* [TestScript](testscript.html): The current status of the test script 4550* [ValueSet](valueset.html): The current status of the value set 4551</b><br> 4552 * Type: <b>token</b><br> 4553 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4554 * </p> 4555 */ 4556 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 4557 public static final String SP_STATUS = "status"; 4558 /** 4559 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4560 * <p> 4561 * Description: <b>Multiple Resources: 4562 4563* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 4564* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 4565* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 4566* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 4567* [Citation](citation.html): The current status of the citation 4568* [CodeSystem](codesystem.html): The current status of the code system 4569* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 4570* [ConceptMap](conceptmap.html): The current status of the concept map 4571* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 4572* [EventDefinition](eventdefinition.html): The current status of the event definition 4573* [Evidence](evidence.html): The current status of the evidence 4574* [EvidenceReport](evidencereport.html): The current status of the evidence report 4575* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 4576* [ExampleScenario](examplescenario.html): The current status of the example scenario 4577* [GraphDefinition](graphdefinition.html): The current status of the graph definition 4578* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 4579* [Library](library.html): The current status of the library 4580* [Measure](measure.html): The current status of the measure 4581* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 4582* [MessageDefinition](messagedefinition.html): The current status of the message definition 4583* [NamingSystem](namingsystem.html): The current status of the naming system 4584* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 4585* [OperationDefinition](operationdefinition.html): The current status of the operation definition 4586* [PlanDefinition](plandefinition.html): The current status of the plan definition 4587* [Questionnaire](questionnaire.html): The current status of the questionnaire 4588* [Requirements](requirements.html): The current status of the requirements 4589* [SearchParameter](searchparameter.html): The current status of the search parameter 4590* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 4591* [StructureDefinition](structuredefinition.html): The current status of the structure definition 4592* [StructureMap](structuremap.html): The current status of the structure map 4593* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 4594* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 4595* [TestPlan](testplan.html): The current status of the test plan 4596* [TestScript](testscript.html): The current status of the test script 4597* [ValueSet](valueset.html): The current status of the value set 4598</b><br> 4599 * Type: <b>token</b><br> 4600 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 4601 * </p> 4602 */ 4603 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4604 4605 /** 4606 * Search parameter: <b>url</b> 4607 * <p> 4608 * Description: <b>Multiple Resources: 4609 4610* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4611* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4612* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4613* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4614* [Citation](citation.html): The uri that identifies the citation 4615* [CodeSystem](codesystem.html): The uri that identifies the code system 4616* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4617* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4618* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4619* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4620* [Evidence](evidence.html): The uri that identifies the evidence 4621* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4622* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4623* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4624* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4625* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4626* [Library](library.html): The uri that identifies the library 4627* [Measure](measure.html): The uri that identifies the measure 4628* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4629* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4630* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4631* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4632* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4633* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4634* [Requirements](requirements.html): The uri that identifies the requirements 4635* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4636* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4637* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4638* [StructureMap](structuremap.html): The uri that identifies the structure map 4639* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4640* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4641* [TestPlan](testplan.html): The uri that identifies the test plan 4642* [TestScript](testscript.html): The uri that identifies the test script 4643* [ValueSet](valueset.html): The uri that identifies the value set 4644</b><br> 4645 * Type: <b>uri</b><br> 4646 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4647 * </p> 4648 */ 4649 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 4650 public static final String SP_URL = "url"; 4651 /** 4652 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4653 * <p> 4654 * Description: <b>Multiple Resources: 4655 4656* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 4657* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 4658* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 4659* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 4660* [Citation](citation.html): The uri that identifies the citation 4661* [CodeSystem](codesystem.html): The uri that identifies the code system 4662* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 4663* [ConceptMap](conceptmap.html): The URI that identifies the concept map 4664* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 4665* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 4666* [Evidence](evidence.html): The uri that identifies the evidence 4667* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 4668* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 4669* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 4670* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 4671* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 4672* [Library](library.html): The uri that identifies the library 4673* [Measure](measure.html): The uri that identifies the measure 4674* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 4675* [NamingSystem](namingsystem.html): The uri that identifies the naming system 4676* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 4677* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 4678* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 4679* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 4680* [Requirements](requirements.html): The uri that identifies the requirements 4681* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 4682* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 4683* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 4684* [StructureMap](structuremap.html): The uri that identifies the structure map 4685* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 4686* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 4687* [TestPlan](testplan.html): The uri that identifies the test plan 4688* [TestScript](testscript.html): The uri that identifies the test script 4689* [ValueSet](valueset.html): The uri that identifies the value set 4690</b><br> 4691 * Type: <b>uri</b><br> 4692 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 4693 * </p> 4694 */ 4695 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4696 4697 /** 4698 * Search parameter: <b>version</b> 4699 * <p> 4700 * Description: <b>Multiple Resources: 4701 4702* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4703* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4704* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4705* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4706* [Citation](citation.html): The business version of the citation 4707* [CodeSystem](codesystem.html): The business version of the code system 4708* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4709* [ConceptMap](conceptmap.html): The business version of the concept map 4710* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4711* [EventDefinition](eventdefinition.html): The business version of the event definition 4712* [Evidence](evidence.html): The business version of the evidence 4713* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4714* [ExampleScenario](examplescenario.html): The business version of the example scenario 4715* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4716* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4717* [Library](library.html): The business version of the library 4718* [Measure](measure.html): The business version of the measure 4719* [MessageDefinition](messagedefinition.html): The business version of the message definition 4720* [NamingSystem](namingsystem.html): The business version of the naming system 4721* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4722* [PlanDefinition](plandefinition.html): The business version of the plan definition 4723* [Questionnaire](questionnaire.html): The business version of the questionnaire 4724* [Requirements](requirements.html): The business version of the requirements 4725* [SearchParameter](searchparameter.html): The business version of the search parameter 4726* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4727* [StructureMap](structuremap.html): The business version of the structure map 4728* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4729* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4730* [TestScript](testscript.html): The business version of the test script 4731* [ValueSet](valueset.html): The business version of the value set 4732</b><br> 4733 * Type: <b>token</b><br> 4734 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4735 * </p> 4736 */ 4737 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 4738 public static final String SP_VERSION = "version"; 4739 /** 4740 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4741 * <p> 4742 * Description: <b>Multiple Resources: 4743 4744* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 4745* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 4746* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 4747* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 4748* [Citation](citation.html): The business version of the citation 4749* [CodeSystem](codesystem.html): The business version of the code system 4750* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 4751* [ConceptMap](conceptmap.html): The business version of the concept map 4752* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 4753* [EventDefinition](eventdefinition.html): The business version of the event definition 4754* [Evidence](evidence.html): The business version of the evidence 4755* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 4756* [ExampleScenario](examplescenario.html): The business version of the example scenario 4757* [GraphDefinition](graphdefinition.html): The business version of the graph definition 4758* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 4759* [Library](library.html): The business version of the library 4760* [Measure](measure.html): The business version of the measure 4761* [MessageDefinition](messagedefinition.html): The business version of the message definition 4762* [NamingSystem](namingsystem.html): The business version of the naming system 4763* [OperationDefinition](operationdefinition.html): The business version of the operation definition 4764* [PlanDefinition](plandefinition.html): The business version of the plan definition 4765* [Questionnaire](questionnaire.html): The business version of the questionnaire 4766* [Requirements](requirements.html): The business version of the requirements 4767* [SearchParameter](searchparameter.html): The business version of the search parameter 4768* [StructureDefinition](structuredefinition.html): The business version of the structure definition 4769* [StructureMap](structuremap.html): The business version of the structure map 4770* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 4771* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 4772* [TestScript](testscript.html): The business version of the test script 4773* [ValueSet](valueset.html): The business version of the value set 4774</b><br> 4775 * Type: <b>token</b><br> 4776 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 4777 * </p> 4778 */ 4779 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 4780 4781 /** 4782 * Search parameter: <b>start</b> 4783 * <p> 4784 * Description: <b>Type of resource at which the graph starts</b><br> 4785 * Type: <b>token</b><br> 4786 * Path: <b>GraphDefinition.start</b><br> 4787 * </p> 4788 */ 4789 @SearchParamDefinition(name="start", path="GraphDefinition.start", description="Type of resource at which the graph starts", type="token" ) 4790 public static final String SP_START = "start"; 4791 /** 4792 * <b>Fluent Client</b> search parameter constant for <b>start</b> 4793 * <p> 4794 * Description: <b>Type of resource at which the graph starts</b><br> 4795 * Type: <b>token</b><br> 4796 * Path: <b>GraphDefinition.start</b><br> 4797 * </p> 4798 */ 4799 public static final ca.uhn.fhir.rest.gclient.TokenClientParam START = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_START); 4800 4801// Manual code (from Configuration.txt): 4802 public boolean supportsCopyright() { 4803 return true; 4804 } 4805 4806// end addition 4807 4808} 4809