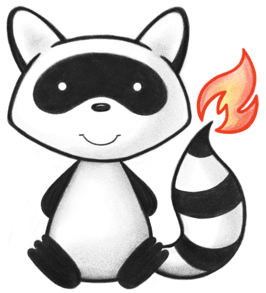
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively, and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization. 052 */ 053@ResourceDef(name="Group", profile="http://hl7.org/fhir/StructureDefinition/Group") 054public class Group extends DomainResource { 055 056 public enum GroupMembershipBasis { 057 /** 058 * The Group.characteristics specified are both necessary and sufficient to determine membership. All entities that meet the criteria are considered to be members of the group, whether referenced by the group or not. If members are present, they are individuals that happen to be known as meeting the Group.characteristics. The list cannot be presumed to be complete. 059 */ 060 DEFINITIONAL, 061 /** 062 * The Group.characteristics are necessary but not sufficient to determine membership. Membership is determined by being listed as one of the Group.member. 063 */ 064 ENUMERATED, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static GroupMembershipBasis fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("definitional".equals(codeString)) 073 return DEFINITIONAL; 074 if ("enumerated".equals(codeString)) 075 return ENUMERATED; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown GroupMembershipBasis code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case DEFINITIONAL: return "definitional"; 084 case ENUMERATED: return "enumerated"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 switch (this) { 091 case DEFINITIONAL: return "http://hl7.org/fhir/group-membership-basis"; 092 case ENUMERATED: return "http://hl7.org/fhir/group-membership-basis"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getDefinition() { 098 switch (this) { 099 case DEFINITIONAL: return "The Group.characteristics specified are both necessary and sufficient to determine membership. All entities that meet the criteria are considered to be members of the group, whether referenced by the group or not. If members are present, they are individuals that happen to be known as meeting the Group.characteristics. The list cannot be presumed to be complete."; 100 case ENUMERATED: return "The Group.characteristics are necessary but not sufficient to determine membership. Membership is determined by being listed as one of the Group.member."; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDisplay() { 106 switch (this) { 107 case DEFINITIONAL: return "Definitional"; 108 case ENUMERATED: return "Enumerated"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 } 114 115 public static class GroupMembershipBasisEnumFactory implements EnumFactory<GroupMembershipBasis> { 116 public GroupMembershipBasis fromCode(String codeString) throws IllegalArgumentException { 117 if (codeString == null || "".equals(codeString)) 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("definitional".equals(codeString)) 121 return GroupMembershipBasis.DEFINITIONAL; 122 if ("enumerated".equals(codeString)) 123 return GroupMembershipBasis.ENUMERATED; 124 throw new IllegalArgumentException("Unknown GroupMembershipBasis code '"+codeString+"'"); 125 } 126 public Enumeration<GroupMembershipBasis> fromType(PrimitiveType<?> code) throws FHIRException { 127 if (code == null) 128 return null; 129 if (code.isEmpty()) 130 return new Enumeration<GroupMembershipBasis>(this, GroupMembershipBasis.NULL, code); 131 String codeString = ((PrimitiveType) code).asStringValue(); 132 if (codeString == null || "".equals(codeString)) 133 return new Enumeration<GroupMembershipBasis>(this, GroupMembershipBasis.NULL, code); 134 if ("definitional".equals(codeString)) 135 return new Enumeration<GroupMembershipBasis>(this, GroupMembershipBasis.DEFINITIONAL, code); 136 if ("enumerated".equals(codeString)) 137 return new Enumeration<GroupMembershipBasis>(this, GroupMembershipBasis.ENUMERATED, code); 138 throw new FHIRException("Unknown GroupMembershipBasis code '"+codeString+"'"); 139 } 140 public String toCode(GroupMembershipBasis code) { 141 if (code == GroupMembershipBasis.NULL) 142 return null; 143 if (code == GroupMembershipBasis.DEFINITIONAL) 144 return "definitional"; 145 if (code == GroupMembershipBasis.ENUMERATED) 146 return "enumerated"; 147 return "?"; 148 } 149 public String toSystem(GroupMembershipBasis code) { 150 return code.getSystem(); 151 } 152 } 153 154 public enum GroupType { 155 /** 156 * Group contains \"person\" Patient resources. 157 */ 158 PERSON, 159 /** 160 * Group contains \"animal\" Patient resources. 161 */ 162 ANIMAL, 163 /** 164 * Group contains healthcare practitioner resources (Practitioner or PractitionerRole). 165 */ 166 PRACTITIONER, 167 /** 168 * Group contains Device resources. 169 */ 170 DEVICE, 171 /** 172 * Group contains CareTeam resources. 173 */ 174 CARETEAM, 175 /** 176 * Group contains HealthcareService resources. 177 */ 178 HEALTHCARESERVICE, 179 /** 180 * Group contains Location resources. 181 */ 182 LOCATION, 183 /** 184 * Group contains Organization resources. 185 */ 186 ORGANIZATION, 187 /** 188 * Group contains RelatedPerson resources. 189 */ 190 RELATEDPERSON, 191 /** 192 * Group contains Specimen resources. 193 */ 194 SPECIMEN, 195 /** 196 * added to help the parsers with the generic types 197 */ 198 NULL; 199 public static GroupType fromCode(String codeString) throws FHIRException { 200 if (codeString == null || "".equals(codeString)) 201 return null; 202 if ("person".equals(codeString)) 203 return PERSON; 204 if ("animal".equals(codeString)) 205 return ANIMAL; 206 if ("practitioner".equals(codeString)) 207 return PRACTITIONER; 208 if ("device".equals(codeString)) 209 return DEVICE; 210 if ("careteam".equals(codeString)) 211 return CARETEAM; 212 if ("healthcareservice".equals(codeString)) 213 return HEALTHCARESERVICE; 214 if ("location".equals(codeString)) 215 return LOCATION; 216 if ("organization".equals(codeString)) 217 return ORGANIZATION; 218 if ("relatedperson".equals(codeString)) 219 return RELATEDPERSON; 220 if ("specimen".equals(codeString)) 221 return SPECIMEN; 222 if (Configuration.isAcceptInvalidEnums()) 223 return null; 224 else 225 throw new FHIRException("Unknown GroupType code '"+codeString+"'"); 226 } 227 public String toCode() { 228 switch (this) { 229 case PERSON: return "person"; 230 case ANIMAL: return "animal"; 231 case PRACTITIONER: return "practitioner"; 232 case DEVICE: return "device"; 233 case CARETEAM: return "careteam"; 234 case HEALTHCARESERVICE: return "healthcareservice"; 235 case LOCATION: return "location"; 236 case ORGANIZATION: return "organization"; 237 case RELATEDPERSON: return "relatedperson"; 238 case SPECIMEN: return "specimen"; 239 case NULL: return null; 240 default: return "?"; 241 } 242 } 243 public String getSystem() { 244 switch (this) { 245 case PERSON: return "http://hl7.org/fhir/group-type"; 246 case ANIMAL: return "http://hl7.org/fhir/group-type"; 247 case PRACTITIONER: return "http://hl7.org/fhir/group-type"; 248 case DEVICE: return "http://hl7.org/fhir/group-type"; 249 case CARETEAM: return "http://hl7.org/fhir/group-type"; 250 case HEALTHCARESERVICE: return "http://hl7.org/fhir/group-type"; 251 case LOCATION: return "http://hl7.org/fhir/group-type"; 252 case ORGANIZATION: return "http://hl7.org/fhir/group-type"; 253 case RELATEDPERSON: return "http://hl7.org/fhir/group-type"; 254 case SPECIMEN: return "http://hl7.org/fhir/group-type"; 255 case NULL: return null; 256 default: return "?"; 257 } 258 } 259 public String getDefinition() { 260 switch (this) { 261 case PERSON: return "Group contains \"person\" Patient resources."; 262 case ANIMAL: return "Group contains \"animal\" Patient resources."; 263 case PRACTITIONER: return "Group contains healthcare practitioner resources (Practitioner or PractitionerRole)."; 264 case DEVICE: return "Group contains Device resources."; 265 case CARETEAM: return "Group contains CareTeam resources."; 266 case HEALTHCARESERVICE: return "Group contains HealthcareService resources."; 267 case LOCATION: return "Group contains Location resources."; 268 case ORGANIZATION: return "Group contains Organization resources."; 269 case RELATEDPERSON: return "Group contains RelatedPerson resources."; 270 case SPECIMEN: return "Group contains Specimen resources."; 271 case NULL: return null; 272 default: return "?"; 273 } 274 } 275 public String getDisplay() { 276 switch (this) { 277 case PERSON: return "Person"; 278 case ANIMAL: return "Animal"; 279 case PRACTITIONER: return "Practitioner"; 280 case DEVICE: return "Device"; 281 case CARETEAM: return "CareTeam"; 282 case HEALTHCARESERVICE: return "HealthcareService"; 283 case LOCATION: return "Location"; 284 case ORGANIZATION: return "Organization"; 285 case RELATEDPERSON: return "RelatedPerson"; 286 case SPECIMEN: return "Specimen"; 287 case NULL: return null; 288 default: return "?"; 289 } 290 } 291 } 292 293 public static class GroupTypeEnumFactory implements EnumFactory<GroupType> { 294 public GroupType fromCode(String codeString) throws IllegalArgumentException { 295 if (codeString == null || "".equals(codeString)) 296 if (codeString == null || "".equals(codeString)) 297 return null; 298 if ("person".equals(codeString)) 299 return GroupType.PERSON; 300 if ("animal".equals(codeString)) 301 return GroupType.ANIMAL; 302 if ("practitioner".equals(codeString)) 303 return GroupType.PRACTITIONER; 304 if ("device".equals(codeString)) 305 return GroupType.DEVICE; 306 if ("careteam".equals(codeString)) 307 return GroupType.CARETEAM; 308 if ("healthcareservice".equals(codeString)) 309 return GroupType.HEALTHCARESERVICE; 310 if ("location".equals(codeString)) 311 return GroupType.LOCATION; 312 if ("organization".equals(codeString)) 313 return GroupType.ORGANIZATION; 314 if ("relatedperson".equals(codeString)) 315 return GroupType.RELATEDPERSON; 316 if ("specimen".equals(codeString)) 317 return GroupType.SPECIMEN; 318 throw new IllegalArgumentException("Unknown GroupType code '"+codeString+"'"); 319 } 320 public Enumeration<GroupType> fromType(PrimitiveType<?> code) throws FHIRException { 321 if (code == null) 322 return null; 323 if (code.isEmpty()) 324 return new Enumeration<GroupType>(this, GroupType.NULL, code); 325 String codeString = ((PrimitiveType) code).asStringValue(); 326 if (codeString == null || "".equals(codeString)) 327 return new Enumeration<GroupType>(this, GroupType.NULL, code); 328 if ("person".equals(codeString)) 329 return new Enumeration<GroupType>(this, GroupType.PERSON, code); 330 if ("animal".equals(codeString)) 331 return new Enumeration<GroupType>(this, GroupType.ANIMAL, code); 332 if ("practitioner".equals(codeString)) 333 return new Enumeration<GroupType>(this, GroupType.PRACTITIONER, code); 334 if ("device".equals(codeString)) 335 return new Enumeration<GroupType>(this, GroupType.DEVICE, code); 336 if ("careteam".equals(codeString)) 337 return new Enumeration<GroupType>(this, GroupType.CARETEAM, code); 338 if ("healthcareservice".equals(codeString)) 339 return new Enumeration<GroupType>(this, GroupType.HEALTHCARESERVICE, code); 340 if ("location".equals(codeString)) 341 return new Enumeration<GroupType>(this, GroupType.LOCATION, code); 342 if ("organization".equals(codeString)) 343 return new Enumeration<GroupType>(this, GroupType.ORGANIZATION, code); 344 if ("relatedperson".equals(codeString)) 345 return new Enumeration<GroupType>(this, GroupType.RELATEDPERSON, code); 346 if ("specimen".equals(codeString)) 347 return new Enumeration<GroupType>(this, GroupType.SPECIMEN, code); 348 throw new FHIRException("Unknown GroupType code '"+codeString+"'"); 349 } 350 public String toCode(GroupType code) { 351 if (code == GroupType.NULL) 352 return null; 353 if (code == GroupType.PERSON) 354 return "person"; 355 if (code == GroupType.ANIMAL) 356 return "animal"; 357 if (code == GroupType.PRACTITIONER) 358 return "practitioner"; 359 if (code == GroupType.DEVICE) 360 return "device"; 361 if (code == GroupType.CARETEAM) 362 return "careteam"; 363 if (code == GroupType.HEALTHCARESERVICE) 364 return "healthcareservice"; 365 if (code == GroupType.LOCATION) 366 return "location"; 367 if (code == GroupType.ORGANIZATION) 368 return "organization"; 369 if (code == GroupType.RELATEDPERSON) 370 return "relatedperson"; 371 if (code == GroupType.SPECIMEN) 372 return "specimen"; 373 return "?"; 374 } 375 public String toSystem(GroupType code) { 376 return code.getSystem(); 377 } 378 } 379 380 @Block() 381 public static class GroupCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 382 /** 383 * A code that identifies the kind of trait being asserted. 384 */ 385 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 386 @Description(shortDefinition="Kind of characteristic", formalDefinition="A code that identifies the kind of trait being asserted." ) 387 protected CodeableConcept code; 388 389 /** 390 * The value of the trait that holds (or does not hold - see 'exclude') for members of the group. 391 */ 392 @Child(name = "value", type = {CodeableConcept.class, BooleanType.class, Quantity.class, Range.class, Reference.class}, order=2, min=1, max=1, modifier=false, summary=true) 393 @Description(shortDefinition="Value held by characteristic", formalDefinition="The value of the trait that holds (or does not hold - see 'exclude') for members of the group." ) 394 protected DataType value; 395 396 /** 397 * If true, indicates the characteristic is one that is NOT held by members of the group. 398 */ 399 @Child(name = "exclude", type = {BooleanType.class}, order=3, min=1, max=1, modifier=false, summary=true) 400 @Description(shortDefinition="Group includes or excludes", formalDefinition="If true, indicates the characteristic is one that is NOT held by members of the group." ) 401 protected BooleanType exclude; 402 403 /** 404 * The period over which the characteristic is tested; e.g. the patient had an operation during the month of June. 405 */ 406 @Child(name = "period", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 407 @Description(shortDefinition="Period over which characteristic is tested", formalDefinition="The period over which the characteristic is tested; e.g. the patient had an operation during the month of June." ) 408 protected Period period; 409 410 private static final long serialVersionUID = 279867823L; 411 412 /** 413 * Constructor 414 */ 415 public GroupCharacteristicComponent() { 416 super(); 417 } 418 419 /** 420 * Constructor 421 */ 422 public GroupCharacteristicComponent(CodeableConcept code, DataType value, boolean exclude) { 423 super(); 424 this.setCode(code); 425 this.setValue(value); 426 this.setExclude(exclude); 427 } 428 429 /** 430 * @return {@link #code} (A code that identifies the kind of trait being asserted.) 431 */ 432 public CodeableConcept getCode() { 433 if (this.code == null) 434 if (Configuration.errorOnAutoCreate()) 435 throw new Error("Attempt to auto-create GroupCharacteristicComponent.code"); 436 else if (Configuration.doAutoCreate()) 437 this.code = new CodeableConcept(); // cc 438 return this.code; 439 } 440 441 public boolean hasCode() { 442 return this.code != null && !this.code.isEmpty(); 443 } 444 445 /** 446 * @param value {@link #code} (A code that identifies the kind of trait being asserted.) 447 */ 448 public GroupCharacteristicComponent setCode(CodeableConcept value) { 449 this.code = value; 450 return this; 451 } 452 453 /** 454 * @return {@link #value} (The value of the trait that holds (or does not hold - see 'exclude') for members of the group.) 455 */ 456 public DataType getValue() { 457 return this.value; 458 } 459 460 /** 461 * @return {@link #value} (The value of the trait that holds (or does not hold - see 'exclude') for members of the group.) 462 */ 463 public CodeableConcept getValueCodeableConcept() throws FHIRException { 464 if (this.value == null) 465 this.value = new CodeableConcept(); 466 if (!(this.value instanceof CodeableConcept)) 467 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 468 return (CodeableConcept) this.value; 469 } 470 471 public boolean hasValueCodeableConcept() { 472 return this != null && this.value instanceof CodeableConcept; 473 } 474 475 /** 476 * @return {@link #value} (The value of the trait that holds (or does not hold - see 'exclude') for members of the group.) 477 */ 478 public BooleanType getValueBooleanType() throws FHIRException { 479 if (this.value == null) 480 this.value = new BooleanType(); 481 if (!(this.value instanceof BooleanType)) 482 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 483 return (BooleanType) this.value; 484 } 485 486 public boolean hasValueBooleanType() { 487 return this != null && this.value instanceof BooleanType; 488 } 489 490 /** 491 * @return {@link #value} (The value of the trait that holds (or does not hold - see 'exclude') for members of the group.) 492 */ 493 public Quantity getValueQuantity() throws FHIRException { 494 if (this.value == null) 495 this.value = new Quantity(); 496 if (!(this.value instanceof Quantity)) 497 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 498 return (Quantity) this.value; 499 } 500 501 public boolean hasValueQuantity() { 502 return this != null && this.value instanceof Quantity; 503 } 504 505 /** 506 * @return {@link #value} (The value of the trait that holds (or does not hold - see 'exclude') for members of the group.) 507 */ 508 public Range getValueRange() throws FHIRException { 509 if (this.value == null) 510 this.value = new Range(); 511 if (!(this.value instanceof Range)) 512 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 513 return (Range) this.value; 514 } 515 516 public boolean hasValueRange() { 517 return this != null && this.value instanceof Range; 518 } 519 520 /** 521 * @return {@link #value} (The value of the trait that holds (or does not hold - see 'exclude') for members of the group.) 522 */ 523 public Reference getValueReference() throws FHIRException { 524 if (this.value == null) 525 this.value = new Reference(); 526 if (!(this.value instanceof Reference)) 527 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 528 return (Reference) this.value; 529 } 530 531 public boolean hasValueReference() { 532 return this != null && this.value instanceof Reference; 533 } 534 535 public boolean hasValue() { 536 return this.value != null && !this.value.isEmpty(); 537 } 538 539 /** 540 * @param value {@link #value} (The value of the trait that holds (or does not hold - see 'exclude') for members of the group.) 541 */ 542 public GroupCharacteristicComponent setValue(DataType value) { 543 if (value != null && !(value instanceof CodeableConcept || value instanceof BooleanType || value instanceof Quantity || value instanceof Range || value instanceof Reference)) 544 throw new FHIRException("Not the right type for Group.characteristic.value[x]: "+value.fhirType()); 545 this.value = value; 546 return this; 547 } 548 549 /** 550 * @return {@link #exclude} (If true, indicates the characteristic is one that is NOT held by members of the group.). This is the underlying object with id, value and extensions. The accessor "getExclude" gives direct access to the value 551 */ 552 public BooleanType getExcludeElement() { 553 if (this.exclude == null) 554 if (Configuration.errorOnAutoCreate()) 555 throw new Error("Attempt to auto-create GroupCharacteristicComponent.exclude"); 556 else if (Configuration.doAutoCreate()) 557 this.exclude = new BooleanType(); // bb 558 return this.exclude; 559 } 560 561 public boolean hasExcludeElement() { 562 return this.exclude != null && !this.exclude.isEmpty(); 563 } 564 565 public boolean hasExclude() { 566 return this.exclude != null && !this.exclude.isEmpty(); 567 } 568 569 /** 570 * @param value {@link #exclude} (If true, indicates the characteristic is one that is NOT held by members of the group.). This is the underlying object with id, value and extensions. The accessor "getExclude" gives direct access to the value 571 */ 572 public GroupCharacteristicComponent setExcludeElement(BooleanType value) { 573 this.exclude = value; 574 return this; 575 } 576 577 /** 578 * @return If true, indicates the characteristic is one that is NOT held by members of the group. 579 */ 580 public boolean getExclude() { 581 return this.exclude == null || this.exclude.isEmpty() ? false : this.exclude.getValue(); 582 } 583 584 /** 585 * @param value If true, indicates the characteristic is one that is NOT held by members of the group. 586 */ 587 public GroupCharacteristicComponent setExclude(boolean value) { 588 if (this.exclude == null) 589 this.exclude = new BooleanType(); 590 this.exclude.setValue(value); 591 return this; 592 } 593 594 /** 595 * @return {@link #period} (The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.) 596 */ 597 public Period getPeriod() { 598 if (this.period == null) 599 if (Configuration.errorOnAutoCreate()) 600 throw new Error("Attempt to auto-create GroupCharacteristicComponent.period"); 601 else if (Configuration.doAutoCreate()) 602 this.period = new Period(); // cc 603 return this.period; 604 } 605 606 public boolean hasPeriod() { 607 return this.period != null && !this.period.isEmpty(); 608 } 609 610 /** 611 * @param value {@link #period} (The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.) 612 */ 613 public GroupCharacteristicComponent setPeriod(Period value) { 614 this.period = value; 615 return this; 616 } 617 618 protected void listChildren(List<Property> children) { 619 super.listChildren(children); 620 children.add(new Property("code", "CodeableConcept", "A code that identifies the kind of trait being asserted.", 0, 1, code)); 621 children.add(new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, value)); 622 children.add(new Property("exclude", "boolean", "If true, indicates the characteristic is one that is NOT held by members of the group.", 0, 1, exclude)); 623 children.add(new Property("period", "Period", "The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.", 0, 1, period)); 624 } 625 626 @Override 627 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 628 switch (_hash) { 629 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code that identifies the kind of trait being asserted.", 0, 1, code); 630 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, value); 631 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, value); 632 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, value); 633 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, value); 634 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, value); 635 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, value); 636 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference", "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, value); 637 case -1321148966: /*exclude*/ return new Property("exclude", "boolean", "If true, indicates the characteristic is one that is NOT held by members of the group.", 0, 1, exclude); 638 case -991726143: /*period*/ return new Property("period", "Period", "The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.", 0, 1, period); 639 default: return super.getNamedProperty(_hash, _name, _checkValid); 640 } 641 642 } 643 644 @Override 645 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 646 switch (hash) { 647 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 648 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 649 case -1321148966: /*exclude*/ return this.exclude == null ? new Base[0] : new Base[] {this.exclude}; // BooleanType 650 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 651 default: return super.getProperty(hash, name, checkValid); 652 } 653 654 } 655 656 @Override 657 public Base setProperty(int hash, String name, Base value) throws FHIRException { 658 switch (hash) { 659 case 3059181: // code 660 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 661 return value; 662 case 111972721: // value 663 this.value = TypeConvertor.castToType(value); // DataType 664 return value; 665 case -1321148966: // exclude 666 this.exclude = TypeConvertor.castToBoolean(value); // BooleanType 667 return value; 668 case -991726143: // period 669 this.period = TypeConvertor.castToPeriod(value); // Period 670 return value; 671 default: return super.setProperty(hash, name, value); 672 } 673 674 } 675 676 @Override 677 public Base setProperty(String name, Base value) throws FHIRException { 678 if (name.equals("code")) { 679 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 680 } else if (name.equals("value[x]")) { 681 this.value = TypeConvertor.castToType(value); // DataType 682 } else if (name.equals("exclude")) { 683 this.exclude = TypeConvertor.castToBoolean(value); // BooleanType 684 } else if (name.equals("period")) { 685 this.period = TypeConvertor.castToPeriod(value); // Period 686 } else 687 return super.setProperty(name, value); 688 return value; 689 } 690 691 @Override 692 public void removeChild(String name, Base value) throws FHIRException { 693 if (name.equals("code")) { 694 this.code = null; 695 } else if (name.equals("value[x]")) { 696 this.value = null; 697 } else if (name.equals("exclude")) { 698 this.exclude = null; 699 } else if (name.equals("period")) { 700 this.period = null; 701 } else 702 super.removeChild(name, value); 703 704 } 705 706 @Override 707 public Base makeProperty(int hash, String name) throws FHIRException { 708 switch (hash) { 709 case 3059181: return getCode(); 710 case -1410166417: return getValue(); 711 case 111972721: return getValue(); 712 case -1321148966: return getExcludeElement(); 713 case -991726143: return getPeriod(); 714 default: return super.makeProperty(hash, name); 715 } 716 717 } 718 719 @Override 720 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 721 switch (hash) { 722 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 723 case 111972721: /*value*/ return new String[] {"CodeableConcept", "boolean", "Quantity", "Range", "Reference"}; 724 case -1321148966: /*exclude*/ return new String[] {"boolean"}; 725 case -991726143: /*period*/ return new String[] {"Period"}; 726 default: return super.getTypesForProperty(hash, name); 727 } 728 729 } 730 731 @Override 732 public Base addChild(String name) throws FHIRException { 733 if (name.equals("code")) { 734 this.code = new CodeableConcept(); 735 return this.code; 736 } 737 else if (name.equals("valueCodeableConcept")) { 738 this.value = new CodeableConcept(); 739 return this.value; 740 } 741 else if (name.equals("valueBoolean")) { 742 this.value = new BooleanType(); 743 return this.value; 744 } 745 else if (name.equals("valueQuantity")) { 746 this.value = new Quantity(); 747 return this.value; 748 } 749 else if (name.equals("valueRange")) { 750 this.value = new Range(); 751 return this.value; 752 } 753 else if (name.equals("valueReference")) { 754 this.value = new Reference(); 755 return this.value; 756 } 757 else if (name.equals("exclude")) { 758 throw new FHIRException("Cannot call addChild on a singleton property Group.characteristic.exclude"); 759 } 760 else if (name.equals("period")) { 761 this.period = new Period(); 762 return this.period; 763 } 764 else 765 return super.addChild(name); 766 } 767 768 public GroupCharacteristicComponent copy() { 769 GroupCharacteristicComponent dst = new GroupCharacteristicComponent(); 770 copyValues(dst); 771 return dst; 772 } 773 774 public void copyValues(GroupCharacteristicComponent dst) { 775 super.copyValues(dst); 776 dst.code = code == null ? null : code.copy(); 777 dst.value = value == null ? null : value.copy(); 778 dst.exclude = exclude == null ? null : exclude.copy(); 779 dst.period = period == null ? null : period.copy(); 780 } 781 782 @Override 783 public boolean equalsDeep(Base other_) { 784 if (!super.equalsDeep(other_)) 785 return false; 786 if (!(other_ instanceof GroupCharacteristicComponent)) 787 return false; 788 GroupCharacteristicComponent o = (GroupCharacteristicComponent) other_; 789 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) && compareDeep(exclude, o.exclude, true) 790 && compareDeep(period, o.period, true); 791 } 792 793 @Override 794 public boolean equalsShallow(Base other_) { 795 if (!super.equalsShallow(other_)) 796 return false; 797 if (!(other_ instanceof GroupCharacteristicComponent)) 798 return false; 799 GroupCharacteristicComponent o = (GroupCharacteristicComponent) other_; 800 return compareValues(exclude, o.exclude, true); 801 } 802 803 public boolean isEmpty() { 804 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value, exclude, period 805 ); 806 } 807 808 public String fhirType() { 809 return "Group.characteristic"; 810 811 } 812 813 } 814 815 @Block() 816 public static class GroupMemberComponent extends BackboneElement implements IBaseBackboneElement { 817 /** 818 * A reference to the entity that is a member of the group. Must be consistent with Group.type. If the entity is another group, then the type must be the same. 819 */ 820 @Child(name = "entity", type = {CareTeam.class, Device.class, Group.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, Specimen.class}, order=1, min=1, max=1, modifier=false, summary=false) 821 @Description(shortDefinition="Reference to the group member", formalDefinition="A reference to the entity that is a member of the group. Must be consistent with Group.type. If the entity is another group, then the type must be the same." ) 822 protected Reference entity; 823 824 /** 825 * The period that the member was in the group, if known. 826 */ 827 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=false) 828 @Description(shortDefinition="Period member belonged to the group", formalDefinition="The period that the member was in the group, if known." ) 829 protected Period period; 830 831 /** 832 * A flag to indicate that the member is no longer in the group, but previously may have been a member. 833 */ 834 @Child(name = "inactive", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 835 @Description(shortDefinition="If member is no longer in group", formalDefinition="A flag to indicate that the member is no longer in the group, but previously may have been a member." ) 836 protected BooleanType inactive; 837 838 private static final long serialVersionUID = -1206153083L; 839 840 /** 841 * Constructor 842 */ 843 public GroupMemberComponent() { 844 super(); 845 } 846 847 /** 848 * Constructor 849 */ 850 public GroupMemberComponent(Reference entity) { 851 super(); 852 this.setEntity(entity); 853 } 854 855 /** 856 * @return {@link #entity} (A reference to the entity that is a member of the group. Must be consistent with Group.type. If the entity is another group, then the type must be the same.) 857 */ 858 public Reference getEntity() { 859 if (this.entity == null) 860 if (Configuration.errorOnAutoCreate()) 861 throw new Error("Attempt to auto-create GroupMemberComponent.entity"); 862 else if (Configuration.doAutoCreate()) 863 this.entity = new Reference(); // cc 864 return this.entity; 865 } 866 867 public boolean hasEntity() { 868 return this.entity != null && !this.entity.isEmpty(); 869 } 870 871 /** 872 * @param value {@link #entity} (A reference to the entity that is a member of the group. Must be consistent with Group.type. If the entity is another group, then the type must be the same.) 873 */ 874 public GroupMemberComponent setEntity(Reference value) { 875 this.entity = value; 876 return this; 877 } 878 879 /** 880 * @return {@link #period} (The period that the member was in the group, if known.) 881 */ 882 public Period getPeriod() { 883 if (this.period == null) 884 if (Configuration.errorOnAutoCreate()) 885 throw new Error("Attempt to auto-create GroupMemberComponent.period"); 886 else if (Configuration.doAutoCreate()) 887 this.period = new Period(); // cc 888 return this.period; 889 } 890 891 public boolean hasPeriod() { 892 return this.period != null && !this.period.isEmpty(); 893 } 894 895 /** 896 * @param value {@link #period} (The period that the member was in the group, if known.) 897 */ 898 public GroupMemberComponent setPeriod(Period value) { 899 this.period = value; 900 return this; 901 } 902 903 /** 904 * @return {@link #inactive} (A flag to indicate that the member is no longer in the group, but previously may have been a member.). This is the underlying object with id, value and extensions. The accessor "getInactive" gives direct access to the value 905 */ 906 public BooleanType getInactiveElement() { 907 if (this.inactive == null) 908 if (Configuration.errorOnAutoCreate()) 909 throw new Error("Attempt to auto-create GroupMemberComponent.inactive"); 910 else if (Configuration.doAutoCreate()) 911 this.inactive = new BooleanType(); // bb 912 return this.inactive; 913 } 914 915 public boolean hasInactiveElement() { 916 return this.inactive != null && !this.inactive.isEmpty(); 917 } 918 919 public boolean hasInactive() { 920 return this.inactive != null && !this.inactive.isEmpty(); 921 } 922 923 /** 924 * @param value {@link #inactive} (A flag to indicate that the member is no longer in the group, but previously may have been a member.). This is the underlying object with id, value and extensions. The accessor "getInactive" gives direct access to the value 925 */ 926 public GroupMemberComponent setInactiveElement(BooleanType value) { 927 this.inactive = value; 928 return this; 929 } 930 931 /** 932 * @return A flag to indicate that the member is no longer in the group, but previously may have been a member. 933 */ 934 public boolean getInactive() { 935 return this.inactive == null || this.inactive.isEmpty() ? false : this.inactive.getValue(); 936 } 937 938 /** 939 * @param value A flag to indicate that the member is no longer in the group, but previously may have been a member. 940 */ 941 public GroupMemberComponent setInactive(boolean value) { 942 if (this.inactive == null) 943 this.inactive = new BooleanType(); 944 this.inactive.setValue(value); 945 return this; 946 } 947 948 protected void listChildren(List<Property> children) { 949 super.listChildren(children); 950 children.add(new Property("entity", "Reference(CareTeam|Device|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|Specimen)", "A reference to the entity that is a member of the group. Must be consistent with Group.type. If the entity is another group, then the type must be the same.", 0, 1, entity)); 951 children.add(new Property("period", "Period", "The period that the member was in the group, if known.", 0, 1, period)); 952 children.add(new Property("inactive", "boolean", "A flag to indicate that the member is no longer in the group, but previously may have been a member.", 0, 1, inactive)); 953 } 954 955 @Override 956 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 957 switch (_hash) { 958 case -1298275357: /*entity*/ return new Property("entity", "Reference(CareTeam|Device|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson|Specimen)", "A reference to the entity that is a member of the group. Must be consistent with Group.type. If the entity is another group, then the type must be the same.", 0, 1, entity); 959 case -991726143: /*period*/ return new Property("period", "Period", "The period that the member was in the group, if known.", 0, 1, period); 960 case 24665195: /*inactive*/ return new Property("inactive", "boolean", "A flag to indicate that the member is no longer in the group, but previously may have been a member.", 0, 1, inactive); 961 default: return super.getNamedProperty(_hash, _name, _checkValid); 962 } 963 964 } 965 966 @Override 967 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 968 switch (hash) { 969 case -1298275357: /*entity*/ return this.entity == null ? new Base[0] : new Base[] {this.entity}; // Reference 970 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 971 case 24665195: /*inactive*/ return this.inactive == null ? new Base[0] : new Base[] {this.inactive}; // BooleanType 972 default: return super.getProperty(hash, name, checkValid); 973 } 974 975 } 976 977 @Override 978 public Base setProperty(int hash, String name, Base value) throws FHIRException { 979 switch (hash) { 980 case -1298275357: // entity 981 this.entity = TypeConvertor.castToReference(value); // Reference 982 return value; 983 case -991726143: // period 984 this.period = TypeConvertor.castToPeriod(value); // Period 985 return value; 986 case 24665195: // inactive 987 this.inactive = TypeConvertor.castToBoolean(value); // BooleanType 988 return value; 989 default: return super.setProperty(hash, name, value); 990 } 991 992 } 993 994 @Override 995 public Base setProperty(String name, Base value) throws FHIRException { 996 if (name.equals("entity")) { 997 this.entity = TypeConvertor.castToReference(value); // Reference 998 } else if (name.equals("period")) { 999 this.period = TypeConvertor.castToPeriod(value); // Period 1000 } else if (name.equals("inactive")) { 1001 this.inactive = TypeConvertor.castToBoolean(value); // BooleanType 1002 } else 1003 return super.setProperty(name, value); 1004 return value; 1005 } 1006 1007 @Override 1008 public void removeChild(String name, Base value) throws FHIRException { 1009 if (name.equals("entity")) { 1010 this.entity = null; 1011 } else if (name.equals("period")) { 1012 this.period = null; 1013 } else if (name.equals("inactive")) { 1014 this.inactive = null; 1015 } else 1016 super.removeChild(name, value); 1017 1018 } 1019 1020 @Override 1021 public Base makeProperty(int hash, String name) throws FHIRException { 1022 switch (hash) { 1023 case -1298275357: return getEntity(); 1024 case -991726143: return getPeriod(); 1025 case 24665195: return getInactiveElement(); 1026 default: return super.makeProperty(hash, name); 1027 } 1028 1029 } 1030 1031 @Override 1032 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1033 switch (hash) { 1034 case -1298275357: /*entity*/ return new String[] {"Reference"}; 1035 case -991726143: /*period*/ return new String[] {"Period"}; 1036 case 24665195: /*inactive*/ return new String[] {"boolean"}; 1037 default: return super.getTypesForProperty(hash, name); 1038 } 1039 1040 } 1041 1042 @Override 1043 public Base addChild(String name) throws FHIRException { 1044 if (name.equals("entity")) { 1045 this.entity = new Reference(); 1046 return this.entity; 1047 } 1048 else if (name.equals("period")) { 1049 this.period = new Period(); 1050 return this.period; 1051 } 1052 else if (name.equals("inactive")) { 1053 throw new FHIRException("Cannot call addChild on a singleton property Group.member.inactive"); 1054 } 1055 else 1056 return super.addChild(name); 1057 } 1058 1059 public GroupMemberComponent copy() { 1060 GroupMemberComponent dst = new GroupMemberComponent(); 1061 copyValues(dst); 1062 return dst; 1063 } 1064 1065 public void copyValues(GroupMemberComponent dst) { 1066 super.copyValues(dst); 1067 dst.entity = entity == null ? null : entity.copy(); 1068 dst.period = period == null ? null : period.copy(); 1069 dst.inactive = inactive == null ? null : inactive.copy(); 1070 } 1071 1072 @Override 1073 public boolean equalsDeep(Base other_) { 1074 if (!super.equalsDeep(other_)) 1075 return false; 1076 if (!(other_ instanceof GroupMemberComponent)) 1077 return false; 1078 GroupMemberComponent o = (GroupMemberComponent) other_; 1079 return compareDeep(entity, o.entity, true) && compareDeep(period, o.period, true) && compareDeep(inactive, o.inactive, true) 1080 ; 1081 } 1082 1083 @Override 1084 public boolean equalsShallow(Base other_) { 1085 if (!super.equalsShallow(other_)) 1086 return false; 1087 if (!(other_ instanceof GroupMemberComponent)) 1088 return false; 1089 GroupMemberComponent o = (GroupMemberComponent) other_; 1090 return compareValues(inactive, o.inactive, true); 1091 } 1092 1093 public boolean isEmpty() { 1094 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(entity, period, inactive 1095 ); 1096 } 1097 1098 public String fhirType() { 1099 return "Group.member"; 1100 1101 } 1102 1103 } 1104 1105 /** 1106 * Business identifiers assigned to this participant by one of the applications involved. These identifiers remain constant as the resource is updated and propagates from server to server. 1107 */ 1108 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1109 @Description(shortDefinition="Business Identifier for this Group", formalDefinition="Business identifiers assigned to this participant by one of the applications involved. These identifiers remain constant as the resource is updated and propagates from server to server." ) 1110 protected List<Identifier> identifier; 1111 1112 /** 1113 * Indicates whether the record for the group is available for use or is merely being retained for historical purposes. 1114 */ 1115 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=true, summary=true) 1116 @Description(shortDefinition="Whether this group's record is in active use", formalDefinition="Indicates whether the record for the group is available for use or is merely being retained for historical purposes." ) 1117 protected BooleanType active; 1118 1119 /** 1120 * Identifies the broad classification of the kind of resources the group includes. 1121 */ 1122 @Child(name = "type", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1123 @Description(shortDefinition="person | animal | practitioner | device | careteam | healthcareservice | location | organization | relatedperson | specimen", formalDefinition="Identifies the broad classification of the kind of resources the group includes." ) 1124 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/group-type") 1125 protected Enumeration<GroupType> type; 1126 1127 /** 1128 * Basis for membership in the Group: 1129 1130* 'definitional': The Group.characteristics specified are both necessary and sufficient to determine membership. All entities that meet the criteria are considered to be members of the group, whether referenced by the group or not. If members are present, they are individuals that happen to be known as meeting the Group.characteristics. The list cannot be presumed to be complete. 1131* 'enumerated': The Group.characteristics are necessary but not sufficient to determine membership. Membership is determined by being listed as one of the Group.member. 1132 */ 1133 @Child(name = "membership", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 1134 @Description(shortDefinition="definitional | enumerated", formalDefinition="Basis for membership in the Group:\n\n* 'definitional': The Group.characteristics specified are both necessary and sufficient to determine membership. All entities that meet the criteria are considered to be members of the group, whether referenced by the group or not. If members are present, they are individuals that happen to be known as meeting the Group.characteristics. The list cannot be presumed to be complete.\n* 'enumerated': The Group.characteristics are necessary but not sufficient to determine membership. Membership is determined by being listed as one of the Group.member." ) 1135 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/group-membership-basis") 1136 protected Enumeration<GroupMembershipBasis> membership; 1137 1138 /** 1139 * Provides a specific type of resource the group includes; e.g. "cow", "syringe", etc. 1140 */ 1141 @Child(name = "code", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 1142 @Description(shortDefinition="Kind of Group members", formalDefinition="Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc." ) 1143 protected CodeableConcept code; 1144 1145 /** 1146 * A label assigned to the group for human identification and communication. 1147 */ 1148 @Child(name = "name", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1149 @Description(shortDefinition="Label for Group", formalDefinition="A label assigned to the group for human identification and communication." ) 1150 protected StringType name; 1151 1152 /** 1153 * Explanation of what the group represents and how it is intended to be used. 1154 */ 1155 @Child(name = "description", type = {MarkdownType.class}, order=6, min=0, max=1, modifier=false, summary=false) 1156 @Description(shortDefinition="Natural language description of the group", formalDefinition="Explanation of what the group represents and how it is intended to be used." ) 1157 protected MarkdownType description; 1158 1159 /** 1160 * A count of the number of resource instances that are part of the group. 1161 */ 1162 @Child(name = "quantity", type = {UnsignedIntType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1163 @Description(shortDefinition="Number of members", formalDefinition="A count of the number of resource instances that are part of the group." ) 1164 protected UnsignedIntType quantity; 1165 1166 /** 1167 * Entity responsible for defining and maintaining Group characteristics and/or registered members. 1168 */ 1169 @Child(name = "managingEntity", type = {Organization.class, RelatedPerson.class, Practitioner.class, PractitionerRole.class}, order=8, min=0, max=1, modifier=false, summary=true) 1170 @Description(shortDefinition="Entity that is the custodian of the Group's definition", formalDefinition="Entity responsible for defining and maintaining Group characteristics and/or registered members." ) 1171 protected Reference managingEntity; 1172 1173 /** 1174 * Identifies traits whose presence r absence is shared by members of the group. 1175 */ 1176 @Child(name = "characteristic", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1177 @Description(shortDefinition="Include / Exclude group members by Trait", formalDefinition="Identifies traits whose presence r absence is shared by members of the group." ) 1178 protected List<GroupCharacteristicComponent> characteristic; 1179 1180 /** 1181 * Identifies the resource instances that are members of the group. 1182 */ 1183 @Child(name = "member", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1184 @Description(shortDefinition="Who or what is in group", formalDefinition="Identifies the resource instances that are members of the group." ) 1185 protected List<GroupMemberComponent> member; 1186 1187 private static final long serialVersionUID = -39542514L; 1188 1189 /** 1190 * Constructor 1191 */ 1192 public Group() { 1193 super(); 1194 } 1195 1196 /** 1197 * Constructor 1198 */ 1199 public Group(GroupType type, GroupMembershipBasis membership) { 1200 super(); 1201 this.setType(type); 1202 this.setMembership(membership); 1203 } 1204 1205 /** 1206 * @return {@link #identifier} (Business identifiers assigned to this participant by one of the applications involved. These identifiers remain constant as the resource is updated and propagates from server to server.) 1207 */ 1208 public List<Identifier> getIdentifier() { 1209 if (this.identifier == null) 1210 this.identifier = new ArrayList<Identifier>(); 1211 return this.identifier; 1212 } 1213 1214 /** 1215 * @return Returns a reference to <code>this</code> for easy method chaining 1216 */ 1217 public Group setIdentifier(List<Identifier> theIdentifier) { 1218 this.identifier = theIdentifier; 1219 return this; 1220 } 1221 1222 public boolean hasIdentifier() { 1223 if (this.identifier == null) 1224 return false; 1225 for (Identifier item : this.identifier) 1226 if (!item.isEmpty()) 1227 return true; 1228 return false; 1229 } 1230 1231 public Identifier addIdentifier() { //3 1232 Identifier t = new Identifier(); 1233 if (this.identifier == null) 1234 this.identifier = new ArrayList<Identifier>(); 1235 this.identifier.add(t); 1236 return t; 1237 } 1238 1239 public Group addIdentifier(Identifier t) { //3 1240 if (t == null) 1241 return this; 1242 if (this.identifier == null) 1243 this.identifier = new ArrayList<Identifier>(); 1244 this.identifier.add(t); 1245 return this; 1246 } 1247 1248 /** 1249 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1250 */ 1251 public Identifier getIdentifierFirstRep() { 1252 if (getIdentifier().isEmpty()) { 1253 addIdentifier(); 1254 } 1255 return getIdentifier().get(0); 1256 } 1257 1258 /** 1259 * @return {@link #active} (Indicates whether the record for the group is available for use or is merely being retained for historical purposes.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 1260 */ 1261 public BooleanType getActiveElement() { 1262 if (this.active == null) 1263 if (Configuration.errorOnAutoCreate()) 1264 throw new Error("Attempt to auto-create Group.active"); 1265 else if (Configuration.doAutoCreate()) 1266 this.active = new BooleanType(); // bb 1267 return this.active; 1268 } 1269 1270 public boolean hasActiveElement() { 1271 return this.active != null && !this.active.isEmpty(); 1272 } 1273 1274 public boolean hasActive() { 1275 return this.active != null && !this.active.isEmpty(); 1276 } 1277 1278 /** 1279 * @param value {@link #active} (Indicates whether the record for the group is available for use or is merely being retained for historical purposes.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 1280 */ 1281 public Group setActiveElement(BooleanType value) { 1282 this.active = value; 1283 return this; 1284 } 1285 1286 /** 1287 * @return Indicates whether the record for the group is available for use or is merely being retained for historical purposes. 1288 */ 1289 public boolean getActive() { 1290 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1291 } 1292 1293 /** 1294 * @param value Indicates whether the record for the group is available for use or is merely being retained for historical purposes. 1295 */ 1296 public Group setActive(boolean value) { 1297 if (this.active == null) 1298 this.active = new BooleanType(); 1299 this.active.setValue(value); 1300 return this; 1301 } 1302 1303 /** 1304 * @return {@link #type} (Identifies the broad classification of the kind of resources the group includes.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1305 */ 1306 public Enumeration<GroupType> getTypeElement() { 1307 if (this.type == null) 1308 if (Configuration.errorOnAutoCreate()) 1309 throw new Error("Attempt to auto-create Group.type"); 1310 else if (Configuration.doAutoCreate()) 1311 this.type = new Enumeration<GroupType>(new GroupTypeEnumFactory()); // bb 1312 return this.type; 1313 } 1314 1315 public boolean hasTypeElement() { 1316 return this.type != null && !this.type.isEmpty(); 1317 } 1318 1319 public boolean hasType() { 1320 return this.type != null && !this.type.isEmpty(); 1321 } 1322 1323 /** 1324 * @param value {@link #type} (Identifies the broad classification of the kind of resources the group includes.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1325 */ 1326 public Group setTypeElement(Enumeration<GroupType> value) { 1327 this.type = value; 1328 return this; 1329 } 1330 1331 /** 1332 * @return Identifies the broad classification of the kind of resources the group includes. 1333 */ 1334 public GroupType getType() { 1335 return this.type == null ? null : this.type.getValue(); 1336 } 1337 1338 /** 1339 * @param value Identifies the broad classification of the kind of resources the group includes. 1340 */ 1341 public Group setType(GroupType value) { 1342 if (this.type == null) 1343 this.type = new Enumeration<GroupType>(new GroupTypeEnumFactory()); 1344 this.type.setValue(value); 1345 return this; 1346 } 1347 1348 /** 1349 * @return {@link #membership} (Basis for membership in the Group: 1350 1351* 'definitional': The Group.characteristics specified are both necessary and sufficient to determine membership. All entities that meet the criteria are considered to be members of the group, whether referenced by the group or not. If members are present, they are individuals that happen to be known as meeting the Group.characteristics. The list cannot be presumed to be complete. 1352* 'enumerated': The Group.characteristics are necessary but not sufficient to determine membership. Membership is determined by being listed as one of the Group.member.). This is the underlying object with id, value and extensions. The accessor "getMembership" gives direct access to the value 1353 */ 1354 public Enumeration<GroupMembershipBasis> getMembershipElement() { 1355 if (this.membership == null) 1356 if (Configuration.errorOnAutoCreate()) 1357 throw new Error("Attempt to auto-create Group.membership"); 1358 else if (Configuration.doAutoCreate()) 1359 this.membership = new Enumeration<GroupMembershipBasis>(new GroupMembershipBasisEnumFactory()); // bb 1360 return this.membership; 1361 } 1362 1363 public boolean hasMembershipElement() { 1364 return this.membership != null && !this.membership.isEmpty(); 1365 } 1366 1367 public boolean hasMembership() { 1368 return this.membership != null && !this.membership.isEmpty(); 1369 } 1370 1371 /** 1372 * @param value {@link #membership} (Basis for membership in the Group: 1373 1374* 'definitional': The Group.characteristics specified are both necessary and sufficient to determine membership. All entities that meet the criteria are considered to be members of the group, whether referenced by the group or not. If members are present, they are individuals that happen to be known as meeting the Group.characteristics. The list cannot be presumed to be complete. 1375* 'enumerated': The Group.characteristics are necessary but not sufficient to determine membership. Membership is determined by being listed as one of the Group.member.). This is the underlying object with id, value and extensions. The accessor "getMembership" gives direct access to the value 1376 */ 1377 public Group setMembershipElement(Enumeration<GroupMembershipBasis> value) { 1378 this.membership = value; 1379 return this; 1380 } 1381 1382 /** 1383 * @return Basis for membership in the Group: 1384 1385* 'definitional': The Group.characteristics specified are both necessary and sufficient to determine membership. All entities that meet the criteria are considered to be members of the group, whether referenced by the group or not. If members are present, they are individuals that happen to be known as meeting the Group.characteristics. The list cannot be presumed to be complete. 1386* 'enumerated': The Group.characteristics are necessary but not sufficient to determine membership. Membership is determined by being listed as one of the Group.member. 1387 */ 1388 public GroupMembershipBasis getMembership() { 1389 return this.membership == null ? null : this.membership.getValue(); 1390 } 1391 1392 /** 1393 * @param value Basis for membership in the Group: 1394 1395* 'definitional': The Group.characteristics specified are both necessary and sufficient to determine membership. All entities that meet the criteria are considered to be members of the group, whether referenced by the group or not. If members are present, they are individuals that happen to be known as meeting the Group.characteristics. The list cannot be presumed to be complete. 1396* 'enumerated': The Group.characteristics are necessary but not sufficient to determine membership. Membership is determined by being listed as one of the Group.member. 1397 */ 1398 public Group setMembership(GroupMembershipBasis value) { 1399 if (this.membership == null) 1400 this.membership = new Enumeration<GroupMembershipBasis>(new GroupMembershipBasisEnumFactory()); 1401 this.membership.setValue(value); 1402 return this; 1403 } 1404 1405 /** 1406 * @return {@link #code} (Provides a specific type of resource the group includes; e.g. "cow", "syringe", etc.) 1407 */ 1408 public CodeableConcept getCode() { 1409 if (this.code == null) 1410 if (Configuration.errorOnAutoCreate()) 1411 throw new Error("Attempt to auto-create Group.code"); 1412 else if (Configuration.doAutoCreate()) 1413 this.code = new CodeableConcept(); // cc 1414 return this.code; 1415 } 1416 1417 public boolean hasCode() { 1418 return this.code != null && !this.code.isEmpty(); 1419 } 1420 1421 /** 1422 * @param value {@link #code} (Provides a specific type of resource the group includes; e.g. "cow", "syringe", etc.) 1423 */ 1424 public Group setCode(CodeableConcept value) { 1425 this.code = value; 1426 return this; 1427 } 1428 1429 /** 1430 * @return {@link #name} (A label assigned to the group for human identification and communication.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1431 */ 1432 public StringType getNameElement() { 1433 if (this.name == null) 1434 if (Configuration.errorOnAutoCreate()) 1435 throw new Error("Attempt to auto-create Group.name"); 1436 else if (Configuration.doAutoCreate()) 1437 this.name = new StringType(); // bb 1438 return this.name; 1439 } 1440 1441 public boolean hasNameElement() { 1442 return this.name != null && !this.name.isEmpty(); 1443 } 1444 1445 public boolean hasName() { 1446 return this.name != null && !this.name.isEmpty(); 1447 } 1448 1449 /** 1450 * @param value {@link #name} (A label assigned to the group for human identification and communication.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1451 */ 1452 public Group setNameElement(StringType value) { 1453 this.name = value; 1454 return this; 1455 } 1456 1457 /** 1458 * @return A label assigned to the group for human identification and communication. 1459 */ 1460 public String getName() { 1461 return this.name == null ? null : this.name.getValue(); 1462 } 1463 1464 /** 1465 * @param value A label assigned to the group for human identification and communication. 1466 */ 1467 public Group setName(String value) { 1468 if (Utilities.noString(value)) 1469 this.name = null; 1470 else { 1471 if (this.name == null) 1472 this.name = new StringType(); 1473 this.name.setValue(value); 1474 } 1475 return this; 1476 } 1477 1478 /** 1479 * @return {@link #description} (Explanation of what the group represents and how it is intended to be used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1480 */ 1481 public MarkdownType getDescriptionElement() { 1482 if (this.description == null) 1483 if (Configuration.errorOnAutoCreate()) 1484 throw new Error("Attempt to auto-create Group.description"); 1485 else if (Configuration.doAutoCreate()) 1486 this.description = new MarkdownType(); // bb 1487 return this.description; 1488 } 1489 1490 public boolean hasDescriptionElement() { 1491 return this.description != null && !this.description.isEmpty(); 1492 } 1493 1494 public boolean hasDescription() { 1495 return this.description != null && !this.description.isEmpty(); 1496 } 1497 1498 /** 1499 * @param value {@link #description} (Explanation of what the group represents and how it is intended to be used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1500 */ 1501 public Group setDescriptionElement(MarkdownType value) { 1502 this.description = value; 1503 return this; 1504 } 1505 1506 /** 1507 * @return Explanation of what the group represents and how it is intended to be used. 1508 */ 1509 public String getDescription() { 1510 return this.description == null ? null : this.description.getValue(); 1511 } 1512 1513 /** 1514 * @param value Explanation of what the group represents and how it is intended to be used. 1515 */ 1516 public Group setDescription(String value) { 1517 if (Utilities.noString(value)) 1518 this.description = null; 1519 else { 1520 if (this.description == null) 1521 this.description = new MarkdownType(); 1522 this.description.setValue(value); 1523 } 1524 return this; 1525 } 1526 1527 /** 1528 * @return {@link #quantity} (A count of the number of resource instances that are part of the group.). This is the underlying object with id, value and extensions. The accessor "getQuantity" gives direct access to the value 1529 */ 1530 public UnsignedIntType getQuantityElement() { 1531 if (this.quantity == null) 1532 if (Configuration.errorOnAutoCreate()) 1533 throw new Error("Attempt to auto-create Group.quantity"); 1534 else if (Configuration.doAutoCreate()) 1535 this.quantity = new UnsignedIntType(); // bb 1536 return this.quantity; 1537 } 1538 1539 public boolean hasQuantityElement() { 1540 return this.quantity != null && !this.quantity.isEmpty(); 1541 } 1542 1543 public boolean hasQuantity() { 1544 return this.quantity != null && !this.quantity.isEmpty(); 1545 } 1546 1547 /** 1548 * @param value {@link #quantity} (A count of the number of resource instances that are part of the group.). This is the underlying object with id, value and extensions. The accessor "getQuantity" gives direct access to the value 1549 */ 1550 public Group setQuantityElement(UnsignedIntType value) { 1551 this.quantity = value; 1552 return this; 1553 } 1554 1555 /** 1556 * @return A count of the number of resource instances that are part of the group. 1557 */ 1558 public int getQuantity() { 1559 return this.quantity == null || this.quantity.isEmpty() ? 0 : this.quantity.getValue(); 1560 } 1561 1562 /** 1563 * @param value A count of the number of resource instances that are part of the group. 1564 */ 1565 public Group setQuantity(int value) { 1566 if (this.quantity == null) 1567 this.quantity = new UnsignedIntType(); 1568 this.quantity.setValue(value); 1569 return this; 1570 } 1571 1572 /** 1573 * @return {@link #managingEntity} (Entity responsible for defining and maintaining Group characteristics and/or registered members.) 1574 */ 1575 public Reference getManagingEntity() { 1576 if (this.managingEntity == null) 1577 if (Configuration.errorOnAutoCreate()) 1578 throw new Error("Attempt to auto-create Group.managingEntity"); 1579 else if (Configuration.doAutoCreate()) 1580 this.managingEntity = new Reference(); // cc 1581 return this.managingEntity; 1582 } 1583 1584 public boolean hasManagingEntity() { 1585 return this.managingEntity != null && !this.managingEntity.isEmpty(); 1586 } 1587 1588 /** 1589 * @param value {@link #managingEntity} (Entity responsible for defining and maintaining Group characteristics and/or registered members.) 1590 */ 1591 public Group setManagingEntity(Reference value) { 1592 this.managingEntity = value; 1593 return this; 1594 } 1595 1596 /** 1597 * @return {@link #characteristic} (Identifies traits whose presence r absence is shared by members of the group.) 1598 */ 1599 public List<GroupCharacteristicComponent> getCharacteristic() { 1600 if (this.characteristic == null) 1601 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1602 return this.characteristic; 1603 } 1604 1605 /** 1606 * @return Returns a reference to <code>this</code> for easy method chaining 1607 */ 1608 public Group setCharacteristic(List<GroupCharacteristicComponent> theCharacteristic) { 1609 this.characteristic = theCharacteristic; 1610 return this; 1611 } 1612 1613 public boolean hasCharacteristic() { 1614 if (this.characteristic == null) 1615 return false; 1616 for (GroupCharacteristicComponent item : this.characteristic) 1617 if (!item.isEmpty()) 1618 return true; 1619 return false; 1620 } 1621 1622 public GroupCharacteristicComponent addCharacteristic() { //3 1623 GroupCharacteristicComponent t = new GroupCharacteristicComponent(); 1624 if (this.characteristic == null) 1625 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1626 this.characteristic.add(t); 1627 return t; 1628 } 1629 1630 public Group addCharacteristic(GroupCharacteristicComponent t) { //3 1631 if (t == null) 1632 return this; 1633 if (this.characteristic == null) 1634 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1635 this.characteristic.add(t); 1636 return this; 1637 } 1638 1639 /** 1640 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist {3} 1641 */ 1642 public GroupCharacteristicComponent getCharacteristicFirstRep() { 1643 if (getCharacteristic().isEmpty()) { 1644 addCharacteristic(); 1645 } 1646 return getCharacteristic().get(0); 1647 } 1648 1649 /** 1650 * @return {@link #member} (Identifies the resource instances that are members of the group.) 1651 */ 1652 public List<GroupMemberComponent> getMember() { 1653 if (this.member == null) 1654 this.member = new ArrayList<GroupMemberComponent>(); 1655 return this.member; 1656 } 1657 1658 /** 1659 * @return Returns a reference to <code>this</code> for easy method chaining 1660 */ 1661 public Group setMember(List<GroupMemberComponent> theMember) { 1662 this.member = theMember; 1663 return this; 1664 } 1665 1666 public boolean hasMember() { 1667 if (this.member == null) 1668 return false; 1669 for (GroupMemberComponent item : this.member) 1670 if (!item.isEmpty()) 1671 return true; 1672 return false; 1673 } 1674 1675 public GroupMemberComponent addMember() { //3 1676 GroupMemberComponent t = new GroupMemberComponent(); 1677 if (this.member == null) 1678 this.member = new ArrayList<GroupMemberComponent>(); 1679 this.member.add(t); 1680 return t; 1681 } 1682 1683 public Group addMember(GroupMemberComponent t) { //3 1684 if (t == null) 1685 return this; 1686 if (this.member == null) 1687 this.member = new ArrayList<GroupMemberComponent>(); 1688 this.member.add(t); 1689 return this; 1690 } 1691 1692 /** 1693 * @return The first repetition of repeating field {@link #member}, creating it if it does not already exist {3} 1694 */ 1695 public GroupMemberComponent getMemberFirstRep() { 1696 if (getMember().isEmpty()) { 1697 addMember(); 1698 } 1699 return getMember().get(0); 1700 } 1701 1702 protected void listChildren(List<Property> children) { 1703 super.listChildren(children); 1704 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this participant by one of the applications involved. These identifiers remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1705 children.add(new Property("active", "boolean", "Indicates whether the record for the group is available for use or is merely being retained for historical purposes.", 0, 1, active)); 1706 children.add(new Property("type", "code", "Identifies the broad classification of the kind of resources the group includes.", 0, 1, type)); 1707 children.add(new Property("membership", "code", "Basis for membership in the Group:\n\n* 'definitional': The Group.characteristics specified are both necessary and sufficient to determine membership. All entities that meet the criteria are considered to be members of the group, whether referenced by the group or not. If members are present, they are individuals that happen to be known as meeting the Group.characteristics. The list cannot be presumed to be complete.\n* 'enumerated': The Group.characteristics are necessary but not sufficient to determine membership. Membership is determined by being listed as one of the Group.member.", 0, 1, membership)); 1708 children.add(new Property("code", "CodeableConcept", "Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc.", 0, 1, code)); 1709 children.add(new Property("name", "string", "A label assigned to the group for human identification and communication.", 0, 1, name)); 1710 children.add(new Property("description", "markdown", "Explanation of what the group represents and how it is intended to be used.", 0, 1, description)); 1711 children.add(new Property("quantity", "unsignedInt", "A count of the number of resource instances that are part of the group.", 0, 1, quantity)); 1712 children.add(new Property("managingEntity", "Reference(Organization|RelatedPerson|Practitioner|PractitionerRole)", "Entity responsible for defining and maintaining Group characteristics and/or registered members.", 0, 1, managingEntity)); 1713 children.add(new Property("characteristic", "", "Identifies traits whose presence r absence is shared by members of the group.", 0, java.lang.Integer.MAX_VALUE, characteristic)); 1714 children.add(new Property("member", "", "Identifies the resource instances that are members of the group.", 0, java.lang.Integer.MAX_VALUE, member)); 1715 } 1716 1717 @Override 1718 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1719 switch (_hash) { 1720 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this participant by one of the applications involved. These identifiers remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 1721 case -1422950650: /*active*/ return new Property("active", "boolean", "Indicates whether the record for the group is available for use or is merely being retained for historical purposes.", 0, 1, active); 1722 case 3575610: /*type*/ return new Property("type", "code", "Identifies the broad classification of the kind of resources the group includes.", 0, 1, type); 1723 case -1340241962: /*membership*/ return new Property("membership", "code", "Basis for membership in the Group:\n\n* 'definitional': The Group.characteristics specified are both necessary and sufficient to determine membership. All entities that meet the criteria are considered to be members of the group, whether referenced by the group or not. If members are present, they are individuals that happen to be known as meeting the Group.characteristics. The list cannot be presumed to be complete.\n* 'enumerated': The Group.characteristics are necessary but not sufficient to determine membership. Membership is determined by being listed as one of the Group.member.", 0, 1, membership); 1724 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc.", 0, 1, code); 1725 case 3373707: /*name*/ return new Property("name", "string", "A label assigned to the group for human identification and communication.", 0, 1, name); 1726 case -1724546052: /*description*/ return new Property("description", "markdown", "Explanation of what the group represents and how it is intended to be used.", 0, 1, description); 1727 case -1285004149: /*quantity*/ return new Property("quantity", "unsignedInt", "A count of the number of resource instances that are part of the group.", 0, 1, quantity); 1728 case -988474523: /*managingEntity*/ return new Property("managingEntity", "Reference(Organization|RelatedPerson|Practitioner|PractitionerRole)", "Entity responsible for defining and maintaining Group characteristics and/or registered members.", 0, 1, managingEntity); 1729 case 366313883: /*characteristic*/ return new Property("characteristic", "", "Identifies traits whose presence r absence is shared by members of the group.", 0, java.lang.Integer.MAX_VALUE, characteristic); 1730 case -1077769574: /*member*/ return new Property("member", "", "Identifies the resource instances that are members of the group.", 0, java.lang.Integer.MAX_VALUE, member); 1731 default: return super.getNamedProperty(_hash, _name, _checkValid); 1732 } 1733 1734 } 1735 1736 @Override 1737 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1738 switch (hash) { 1739 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1740 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 1741 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<GroupType> 1742 case -1340241962: /*membership*/ return this.membership == null ? new Base[0] : new Base[] {this.membership}; // Enumeration<GroupMembershipBasis> 1743 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1744 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1745 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1746 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // UnsignedIntType 1747 case -988474523: /*managingEntity*/ return this.managingEntity == null ? new Base[0] : new Base[] {this.managingEntity}; // Reference 1748 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // GroupCharacteristicComponent 1749 case -1077769574: /*member*/ return this.member == null ? new Base[0] : this.member.toArray(new Base[this.member.size()]); // GroupMemberComponent 1750 default: return super.getProperty(hash, name, checkValid); 1751 } 1752 1753 } 1754 1755 @Override 1756 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1757 switch (hash) { 1758 case -1618432855: // identifier 1759 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1760 return value; 1761 case -1422950650: // active 1762 this.active = TypeConvertor.castToBoolean(value); // BooleanType 1763 return value; 1764 case 3575610: // type 1765 value = new GroupTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1766 this.type = (Enumeration) value; // Enumeration<GroupType> 1767 return value; 1768 case -1340241962: // membership 1769 value = new GroupMembershipBasisEnumFactory().fromType(TypeConvertor.castToCode(value)); 1770 this.membership = (Enumeration) value; // Enumeration<GroupMembershipBasis> 1771 return value; 1772 case 3059181: // code 1773 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1774 return value; 1775 case 3373707: // name 1776 this.name = TypeConvertor.castToString(value); // StringType 1777 return value; 1778 case -1724546052: // description 1779 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1780 return value; 1781 case -1285004149: // quantity 1782 this.quantity = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1783 return value; 1784 case -988474523: // managingEntity 1785 this.managingEntity = TypeConvertor.castToReference(value); // Reference 1786 return value; 1787 case 366313883: // characteristic 1788 this.getCharacteristic().add((GroupCharacteristicComponent) value); // GroupCharacteristicComponent 1789 return value; 1790 case -1077769574: // member 1791 this.getMember().add((GroupMemberComponent) value); // GroupMemberComponent 1792 return value; 1793 default: return super.setProperty(hash, name, value); 1794 } 1795 1796 } 1797 1798 @Override 1799 public Base setProperty(String name, Base value) throws FHIRException { 1800 if (name.equals("identifier")) { 1801 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1802 } else if (name.equals("active")) { 1803 this.active = TypeConvertor.castToBoolean(value); // BooleanType 1804 } else if (name.equals("type")) { 1805 value = new GroupTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1806 this.type = (Enumeration) value; // Enumeration<GroupType> 1807 } else if (name.equals("membership")) { 1808 value = new GroupMembershipBasisEnumFactory().fromType(TypeConvertor.castToCode(value)); 1809 this.membership = (Enumeration) value; // Enumeration<GroupMembershipBasis> 1810 } else if (name.equals("code")) { 1811 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1812 } else if (name.equals("name")) { 1813 this.name = TypeConvertor.castToString(value); // StringType 1814 } else if (name.equals("description")) { 1815 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1816 } else if (name.equals("quantity")) { 1817 this.quantity = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1818 } else if (name.equals("managingEntity")) { 1819 this.managingEntity = TypeConvertor.castToReference(value); // Reference 1820 } else if (name.equals("characteristic")) { 1821 this.getCharacteristic().add((GroupCharacteristicComponent) value); 1822 } else if (name.equals("member")) { 1823 this.getMember().add((GroupMemberComponent) value); 1824 } else 1825 return super.setProperty(name, value); 1826 return value; 1827 } 1828 1829 @Override 1830 public void removeChild(String name, Base value) throws FHIRException { 1831 if (name.equals("identifier")) { 1832 this.getIdentifier().remove(value); 1833 } else if (name.equals("active")) { 1834 this.active = null; 1835 } else if (name.equals("type")) { 1836 value = new GroupTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1837 this.type = (Enumeration) value; // Enumeration<GroupType> 1838 } else if (name.equals("membership")) { 1839 value = new GroupMembershipBasisEnumFactory().fromType(TypeConvertor.castToCode(value)); 1840 this.membership = (Enumeration) value; // Enumeration<GroupMembershipBasis> 1841 } else if (name.equals("code")) { 1842 this.code = null; 1843 } else if (name.equals("name")) { 1844 this.name = null; 1845 } else if (name.equals("description")) { 1846 this.description = null; 1847 } else if (name.equals("quantity")) { 1848 this.quantity = null; 1849 } else if (name.equals("managingEntity")) { 1850 this.managingEntity = null; 1851 } else if (name.equals("characteristic")) { 1852 this.getCharacteristic().remove((GroupCharacteristicComponent) value); 1853 } else if (name.equals("member")) { 1854 this.getMember().remove((GroupMemberComponent) value); 1855 } else 1856 super.removeChild(name, value); 1857 1858 } 1859 1860 @Override 1861 public Base makeProperty(int hash, String name) throws FHIRException { 1862 switch (hash) { 1863 case -1618432855: return addIdentifier(); 1864 case -1422950650: return getActiveElement(); 1865 case 3575610: return getTypeElement(); 1866 case -1340241962: return getMembershipElement(); 1867 case 3059181: return getCode(); 1868 case 3373707: return getNameElement(); 1869 case -1724546052: return getDescriptionElement(); 1870 case -1285004149: return getQuantityElement(); 1871 case -988474523: return getManagingEntity(); 1872 case 366313883: return addCharacteristic(); 1873 case -1077769574: return addMember(); 1874 default: return super.makeProperty(hash, name); 1875 } 1876 1877 } 1878 1879 @Override 1880 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1881 switch (hash) { 1882 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1883 case -1422950650: /*active*/ return new String[] {"boolean"}; 1884 case 3575610: /*type*/ return new String[] {"code"}; 1885 case -1340241962: /*membership*/ return new String[] {"code"}; 1886 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1887 case 3373707: /*name*/ return new String[] {"string"}; 1888 case -1724546052: /*description*/ return new String[] {"markdown"}; 1889 case -1285004149: /*quantity*/ return new String[] {"unsignedInt"}; 1890 case -988474523: /*managingEntity*/ return new String[] {"Reference"}; 1891 case 366313883: /*characteristic*/ return new String[] {}; 1892 case -1077769574: /*member*/ return new String[] {}; 1893 default: return super.getTypesForProperty(hash, name); 1894 } 1895 1896 } 1897 1898 @Override 1899 public Base addChild(String name) throws FHIRException { 1900 if (name.equals("identifier")) { 1901 return addIdentifier(); 1902 } 1903 else if (name.equals("active")) { 1904 throw new FHIRException("Cannot call addChild on a singleton property Group.active"); 1905 } 1906 else if (name.equals("type")) { 1907 throw new FHIRException("Cannot call addChild on a singleton property Group.type"); 1908 } 1909 else if (name.equals("membership")) { 1910 throw new FHIRException("Cannot call addChild on a singleton property Group.membership"); 1911 } 1912 else if (name.equals("code")) { 1913 this.code = new CodeableConcept(); 1914 return this.code; 1915 } 1916 else if (name.equals("name")) { 1917 throw new FHIRException("Cannot call addChild on a singleton property Group.name"); 1918 } 1919 else if (name.equals("description")) { 1920 throw new FHIRException("Cannot call addChild on a singleton property Group.description"); 1921 } 1922 else if (name.equals("quantity")) { 1923 throw new FHIRException("Cannot call addChild on a singleton property Group.quantity"); 1924 } 1925 else if (name.equals("managingEntity")) { 1926 this.managingEntity = new Reference(); 1927 return this.managingEntity; 1928 } 1929 else if (name.equals("characteristic")) { 1930 return addCharacteristic(); 1931 } 1932 else if (name.equals("member")) { 1933 return addMember(); 1934 } 1935 else 1936 return super.addChild(name); 1937 } 1938 1939 public String fhirType() { 1940 return "Group"; 1941 1942 } 1943 1944 public Group copy() { 1945 Group dst = new Group(); 1946 copyValues(dst); 1947 return dst; 1948 } 1949 1950 public void copyValues(Group dst) { 1951 super.copyValues(dst); 1952 if (identifier != null) { 1953 dst.identifier = new ArrayList<Identifier>(); 1954 for (Identifier i : identifier) 1955 dst.identifier.add(i.copy()); 1956 }; 1957 dst.active = active == null ? null : active.copy(); 1958 dst.type = type == null ? null : type.copy(); 1959 dst.membership = membership == null ? null : membership.copy(); 1960 dst.code = code == null ? null : code.copy(); 1961 dst.name = name == null ? null : name.copy(); 1962 dst.description = description == null ? null : description.copy(); 1963 dst.quantity = quantity == null ? null : quantity.copy(); 1964 dst.managingEntity = managingEntity == null ? null : managingEntity.copy(); 1965 if (characteristic != null) { 1966 dst.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1967 for (GroupCharacteristicComponent i : characteristic) 1968 dst.characteristic.add(i.copy()); 1969 }; 1970 if (member != null) { 1971 dst.member = new ArrayList<GroupMemberComponent>(); 1972 for (GroupMemberComponent i : member) 1973 dst.member.add(i.copy()); 1974 }; 1975 } 1976 1977 protected Group typedCopy() { 1978 return copy(); 1979 } 1980 1981 @Override 1982 public boolean equalsDeep(Base other_) { 1983 if (!super.equalsDeep(other_)) 1984 return false; 1985 if (!(other_ instanceof Group)) 1986 return false; 1987 Group o = (Group) other_; 1988 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(type, o.type, true) 1989 && compareDeep(membership, o.membership, true) && compareDeep(code, o.code, true) && compareDeep(name, o.name, true) 1990 && compareDeep(description, o.description, true) && compareDeep(quantity, o.quantity, true) && compareDeep(managingEntity, o.managingEntity, true) 1991 && compareDeep(characteristic, o.characteristic, true) && compareDeep(member, o.member, true); 1992 } 1993 1994 @Override 1995 public boolean equalsShallow(Base other_) { 1996 if (!super.equalsShallow(other_)) 1997 return false; 1998 if (!(other_ instanceof Group)) 1999 return false; 2000 Group o = (Group) other_; 2001 return compareValues(active, o.active, true) && compareValues(type, o.type, true) && compareValues(membership, o.membership, true) 2002 && compareValues(name, o.name, true) && compareValues(description, o.description, true) && compareValues(quantity, o.quantity, true) 2003 ; 2004 } 2005 2006 public boolean isEmpty() { 2007 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, type 2008 , membership, code, name, description, quantity, managingEntity, characteristic 2009 , member); 2010 } 2011 2012 @Override 2013 public ResourceType getResourceType() { 2014 return ResourceType.Group; 2015 } 2016 2017 /** 2018 * Search parameter: <b>characteristic-reference</b> 2019 * <p> 2020 * Description: <b>An entity referenced in a characteristic</b><br> 2021 * Type: <b>reference</b><br> 2022 * Path: <b>(Group.characteristic.value.ofType(Reference))</b><br> 2023 * </p> 2024 */ 2025 @SearchParamDefinition(name="characteristic-reference", path="(Group.characteristic.value.ofType(Reference))", description="An entity referenced in a characteristic", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 2026 public static final String SP_CHARACTERISTIC_REFERENCE = "characteristic-reference"; 2027 /** 2028 * <b>Fluent Client</b> search parameter constant for <b>characteristic-reference</b> 2029 * <p> 2030 * Description: <b>An entity referenced in a characteristic</b><br> 2031 * Type: <b>reference</b><br> 2032 * Path: <b>(Group.characteristic.value.ofType(Reference))</b><br> 2033 * </p> 2034 */ 2035 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CHARACTERISTIC_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CHARACTERISTIC_REFERENCE); 2036 2037/** 2038 * Constant for fluent queries to be used to add include statements. Specifies 2039 * the path value of "<b>Group:characteristic-reference</b>". 2040 */ 2041 public static final ca.uhn.fhir.model.api.Include INCLUDE_CHARACTERISTIC_REFERENCE = new ca.uhn.fhir.model.api.Include("Group:characteristic-reference").toLocked(); 2042 2043 /** 2044 * Search parameter: <b>characteristic-value</b> 2045 * <p> 2046 * Description: <b>A composite of both characteristic and value</b><br> 2047 * Type: <b>composite</b><br> 2048 * Path: <b>Group.characteristic</b><br> 2049 * </p> 2050 */ 2051 @SearchParamDefinition(name="characteristic-value", path="Group.characteristic", description="A composite of both characteristic and value", type="composite", compositeOf={"characteristic", "value"} ) 2052 public static final String SP_CHARACTERISTIC_VALUE = "characteristic-value"; 2053 /** 2054 * <b>Fluent Client</b> search parameter constant for <b>characteristic-value</b> 2055 * <p> 2056 * Description: <b>A composite of both characteristic and value</b><br> 2057 * Type: <b>composite</b><br> 2058 * Path: <b>Group.characteristic</b><br> 2059 * </p> 2060 */ 2061 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CHARACTERISTIC_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CHARACTERISTIC_VALUE); 2062 2063 /** 2064 * Search parameter: <b>characteristic</b> 2065 * <p> 2066 * Description: <b>Kind of characteristic</b><br> 2067 * Type: <b>token</b><br> 2068 * Path: <b>Group.characteristic.code</b><br> 2069 * </p> 2070 */ 2071 @SearchParamDefinition(name="characteristic", path="Group.characteristic.code", description="Kind of characteristic", type="token" ) 2072 public static final String SP_CHARACTERISTIC = "characteristic"; 2073 /** 2074 * <b>Fluent Client</b> search parameter constant for <b>characteristic</b> 2075 * <p> 2076 * Description: <b>Kind of characteristic</b><br> 2077 * Type: <b>token</b><br> 2078 * Path: <b>Group.characteristic.code</b><br> 2079 * </p> 2080 */ 2081 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHARACTERISTIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CHARACTERISTIC); 2082 2083 /** 2084 * Search parameter: <b>code</b> 2085 * <p> 2086 * Description: <b>The kind of resources contained</b><br> 2087 * Type: <b>token</b><br> 2088 * Path: <b>Group.code</b><br> 2089 * </p> 2090 */ 2091 @SearchParamDefinition(name="code", path="Group.code", description="The kind of resources contained", type="token" ) 2092 public static final String SP_CODE = "code"; 2093 /** 2094 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2095 * <p> 2096 * Description: <b>The kind of resources contained</b><br> 2097 * Type: <b>token</b><br> 2098 * Path: <b>Group.code</b><br> 2099 * </p> 2100 */ 2101 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2102 2103 /** 2104 * Search parameter: <b>exclude</b> 2105 * <p> 2106 * Description: <b>Group includes or excludes</b><br> 2107 * Type: <b>token</b><br> 2108 * Path: <b>Group.characteristic.exclude</b><br> 2109 * </p> 2110 */ 2111 @SearchParamDefinition(name="exclude", path="Group.characteristic.exclude", description="Group includes or excludes", type="token" ) 2112 public static final String SP_EXCLUDE = "exclude"; 2113 /** 2114 * <b>Fluent Client</b> search parameter constant for <b>exclude</b> 2115 * <p> 2116 * Description: <b>Group includes or excludes</b><br> 2117 * Type: <b>token</b><br> 2118 * Path: <b>Group.characteristic.exclude</b><br> 2119 * </p> 2120 */ 2121 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXCLUDE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EXCLUDE); 2122 2123 /** 2124 * Search parameter: <b>identifier</b> 2125 * <p> 2126 * Description: <b>Unique id</b><br> 2127 * Type: <b>token</b><br> 2128 * Path: <b>Group.identifier</b><br> 2129 * </p> 2130 */ 2131 @SearchParamDefinition(name="identifier", path="Group.identifier", description="Unique id", type="token" ) 2132 public static final String SP_IDENTIFIER = "identifier"; 2133 /** 2134 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2135 * <p> 2136 * Description: <b>Unique id</b><br> 2137 * Type: <b>token</b><br> 2138 * Path: <b>Group.identifier</b><br> 2139 * </p> 2140 */ 2141 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2142 2143 /** 2144 * Search parameter: <b>managing-entity</b> 2145 * <p> 2146 * Description: <b>Entity that is the custodian of the Group's definition</b><br> 2147 * Type: <b>reference</b><br> 2148 * Path: <b>Group.managingEntity</b><br> 2149 * </p> 2150 */ 2151 @SearchParamDefinition(name="managing-entity", path="Group.managingEntity", description="Entity that is the custodian of the Group's definition", type="reference", target={Organization.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2152 public static final String SP_MANAGING_ENTITY = "managing-entity"; 2153 /** 2154 * <b>Fluent Client</b> search parameter constant for <b>managing-entity</b> 2155 * <p> 2156 * Description: <b>Entity that is the custodian of the Group's definition</b><br> 2157 * Type: <b>reference</b><br> 2158 * Path: <b>Group.managingEntity</b><br> 2159 * </p> 2160 */ 2161 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANAGING_ENTITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MANAGING_ENTITY); 2162 2163/** 2164 * Constant for fluent queries to be used to add include statements. Specifies 2165 * the path value of "<b>Group:managing-entity</b>". 2166 */ 2167 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANAGING_ENTITY = new ca.uhn.fhir.model.api.Include("Group:managing-entity").toLocked(); 2168 2169 /** 2170 * Search parameter: <b>member</b> 2171 * <p> 2172 * Description: <b>Reference to the group member</b><br> 2173 * Type: <b>reference</b><br> 2174 * Path: <b>Group.member.entity</b><br> 2175 * </p> 2176 */ 2177 @SearchParamDefinition(name="member", path="Group.member.entity", description="Reference to the group member", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={CareTeam.class, Device.class, Group.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, Specimen.class } ) 2178 public static final String SP_MEMBER = "member"; 2179 /** 2180 * <b>Fluent Client</b> search parameter constant for <b>member</b> 2181 * <p> 2182 * Description: <b>Reference to the group member</b><br> 2183 * Type: <b>reference</b><br> 2184 * Path: <b>Group.member.entity</b><br> 2185 * </p> 2186 */ 2187 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEMBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MEMBER); 2188 2189/** 2190 * Constant for fluent queries to be used to add include statements. Specifies 2191 * the path value of "<b>Group:member</b>". 2192 */ 2193 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEMBER = new ca.uhn.fhir.model.api.Include("Group:member").toLocked(); 2194 2195 /** 2196 * Search parameter: <b>membership</b> 2197 * <p> 2198 * Description: <b>Definitional or enumerated group</b><br> 2199 * Type: <b>token</b><br> 2200 * Path: <b>Group.membership</b><br> 2201 * </p> 2202 */ 2203 @SearchParamDefinition(name="membership", path="Group.membership", description="Definitional or enumerated group", type="token" ) 2204 public static final String SP_MEMBERSHIP = "membership"; 2205 /** 2206 * <b>Fluent Client</b> search parameter constant for <b>membership</b> 2207 * <p> 2208 * Description: <b>Definitional or enumerated group</b><br> 2209 * Type: <b>token</b><br> 2210 * Path: <b>Group.membership</b><br> 2211 * </p> 2212 */ 2213 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MEMBERSHIP = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MEMBERSHIP); 2214 2215 /** 2216 * Search parameter: <b>name</b> 2217 * <p> 2218 * Description: <b>A portion of the Group's name</b><br> 2219 * Type: <b>string</b><br> 2220 * Path: <b>Group.name</b><br> 2221 * </p> 2222 */ 2223 @SearchParamDefinition(name="name", path="Group.name", description="A portion of the Group's name", type="string" ) 2224 public static final String SP_NAME = "name"; 2225 /** 2226 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2227 * <p> 2228 * Description: <b>A portion of the Group's name</b><br> 2229 * Type: <b>string</b><br> 2230 * Path: <b>Group.name</b><br> 2231 * </p> 2232 */ 2233 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 2234 2235 /** 2236 * Search parameter: <b>type</b> 2237 * <p> 2238 * Description: <b>The type of resources the group contains</b><br> 2239 * Type: <b>token</b><br> 2240 * Path: <b>Group.type</b><br> 2241 * </p> 2242 */ 2243 @SearchParamDefinition(name="type", path="Group.type", description="The type of resources the group contains", type="token" ) 2244 public static final String SP_TYPE = "type"; 2245 /** 2246 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2247 * <p> 2248 * Description: <b>The type of resources the group contains</b><br> 2249 * Type: <b>token</b><br> 2250 * Path: <b>Group.type</b><br> 2251 * </p> 2252 */ 2253 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2254 2255 /** 2256 * Search parameter: <b>value</b> 2257 * <p> 2258 * Description: <b>Value held by characteristic</b><br> 2259 * Type: <b>token</b><br> 2260 * Path: <b>(Group.characteristic.value.ofType(CodeableConcept)) | (Group.characteristic.value.ofType(boolean))</b><br> 2261 * </p> 2262 */ 2263 @SearchParamDefinition(name="value", path="(Group.characteristic.value.ofType(CodeableConcept)) | (Group.characteristic.value.ofType(boolean))", description="Value held by characteristic", type="token" ) 2264 public static final String SP_VALUE = "value"; 2265 /** 2266 * <b>Fluent Client</b> search parameter constant for <b>value</b> 2267 * <p> 2268 * Description: <b>Value held by characteristic</b><br> 2269 * Type: <b>token</b><br> 2270 * Path: <b>(Group.characteristic.value.ofType(CodeableConcept)) | (Group.characteristic.value.ofType(boolean))</b><br> 2271 * </p> 2272 */ 2273 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VALUE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VALUE); 2274 2275 2276} 2277