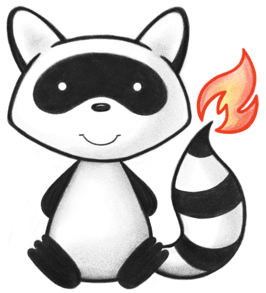
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken. 052 */ 053@ResourceDef(name="GuidanceResponse", profile="http://hl7.org/fhir/StructureDefinition/GuidanceResponse") 054public class GuidanceResponse extends DomainResource { 055 056 public enum GuidanceResponseStatus { 057 /** 058 * The request was processed successfully. 059 */ 060 SUCCESS, 061 /** 062 * The request was processed successfully, but more data may result in a more complete evaluation. 063 */ 064 DATAREQUESTED, 065 /** 066 * The request was processed, but more data is required to complete the evaluation. 067 */ 068 DATAREQUIRED, 069 /** 070 * The request is currently being processed. 071 */ 072 INPROGRESS, 073 /** 074 * The request was not processed successfully. 075 */ 076 FAILURE, 077 /** 078 * The response was entered in error. 079 */ 080 ENTEREDINERROR, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 public static GuidanceResponseStatus fromCode(String codeString) throws FHIRException { 086 if (codeString == null || "".equals(codeString)) 087 return null; 088 if ("success".equals(codeString)) 089 return SUCCESS; 090 if ("data-requested".equals(codeString)) 091 return DATAREQUESTED; 092 if ("data-required".equals(codeString)) 093 return DATAREQUIRED; 094 if ("in-progress".equals(codeString)) 095 return INPROGRESS; 096 if ("failure".equals(codeString)) 097 return FAILURE; 098 if ("entered-in-error".equals(codeString)) 099 return ENTEREDINERROR; 100 if (Configuration.isAcceptInvalidEnums()) 101 return null; 102 else 103 throw new FHIRException("Unknown GuidanceResponseStatus code '"+codeString+"'"); 104 } 105 public String toCode() { 106 switch (this) { 107 case SUCCESS: return "success"; 108 case DATAREQUESTED: return "data-requested"; 109 case DATAREQUIRED: return "data-required"; 110 case INPROGRESS: return "in-progress"; 111 case FAILURE: return "failure"; 112 case ENTEREDINERROR: return "entered-in-error"; 113 case NULL: return null; 114 default: return "?"; 115 } 116 } 117 public String getSystem() { 118 switch (this) { 119 case SUCCESS: return "http://hl7.org/fhir/guidance-response-status"; 120 case DATAREQUESTED: return "http://hl7.org/fhir/guidance-response-status"; 121 case DATAREQUIRED: return "http://hl7.org/fhir/guidance-response-status"; 122 case INPROGRESS: return "http://hl7.org/fhir/guidance-response-status"; 123 case FAILURE: return "http://hl7.org/fhir/guidance-response-status"; 124 case ENTEREDINERROR: return "http://hl7.org/fhir/guidance-response-status"; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 public String getDefinition() { 130 switch (this) { 131 case SUCCESS: return "The request was processed successfully."; 132 case DATAREQUESTED: return "The request was processed successfully, but more data may result in a more complete evaluation."; 133 case DATAREQUIRED: return "The request was processed, but more data is required to complete the evaluation."; 134 case INPROGRESS: return "The request is currently being processed."; 135 case FAILURE: return "The request was not processed successfully."; 136 case ENTEREDINERROR: return "The response was entered in error."; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 public String getDisplay() { 142 switch (this) { 143 case SUCCESS: return "Success"; 144 case DATAREQUESTED: return "Data Requested"; 145 case DATAREQUIRED: return "Data Required"; 146 case INPROGRESS: return "In Progress"; 147 case FAILURE: return "Failure"; 148 case ENTEREDINERROR: return "Entered In Error"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 } 154 155 public static class GuidanceResponseStatusEnumFactory implements EnumFactory<GuidanceResponseStatus> { 156 public GuidanceResponseStatus fromCode(String codeString) throws IllegalArgumentException { 157 if (codeString == null || "".equals(codeString)) 158 if (codeString == null || "".equals(codeString)) 159 return null; 160 if ("success".equals(codeString)) 161 return GuidanceResponseStatus.SUCCESS; 162 if ("data-requested".equals(codeString)) 163 return GuidanceResponseStatus.DATAREQUESTED; 164 if ("data-required".equals(codeString)) 165 return GuidanceResponseStatus.DATAREQUIRED; 166 if ("in-progress".equals(codeString)) 167 return GuidanceResponseStatus.INPROGRESS; 168 if ("failure".equals(codeString)) 169 return GuidanceResponseStatus.FAILURE; 170 if ("entered-in-error".equals(codeString)) 171 return GuidanceResponseStatus.ENTEREDINERROR; 172 throw new IllegalArgumentException("Unknown GuidanceResponseStatus code '"+codeString+"'"); 173 } 174 public Enumeration<GuidanceResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 175 if (code == null) 176 return null; 177 if (code.isEmpty()) 178 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.NULL, code); 179 String codeString = ((PrimitiveType) code).asStringValue(); 180 if (codeString == null || "".equals(codeString)) 181 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.NULL, code); 182 if ("success".equals(codeString)) 183 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.SUCCESS, code); 184 if ("data-requested".equals(codeString)) 185 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.DATAREQUESTED, code); 186 if ("data-required".equals(codeString)) 187 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.DATAREQUIRED, code); 188 if ("in-progress".equals(codeString)) 189 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.INPROGRESS, code); 190 if ("failure".equals(codeString)) 191 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.FAILURE, code); 192 if ("entered-in-error".equals(codeString)) 193 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.ENTEREDINERROR, code); 194 throw new FHIRException("Unknown GuidanceResponseStatus code '"+codeString+"'"); 195 } 196 public String toCode(GuidanceResponseStatus code) { 197 if (code == GuidanceResponseStatus.NULL) 198 return null; 199 if (code == GuidanceResponseStatus.SUCCESS) 200 return "success"; 201 if (code == GuidanceResponseStatus.DATAREQUESTED) 202 return "data-requested"; 203 if (code == GuidanceResponseStatus.DATAREQUIRED) 204 return "data-required"; 205 if (code == GuidanceResponseStatus.INPROGRESS) 206 return "in-progress"; 207 if (code == GuidanceResponseStatus.FAILURE) 208 return "failure"; 209 if (code == GuidanceResponseStatus.ENTEREDINERROR) 210 return "entered-in-error"; 211 return "?"; 212 } 213 public String toSystem(GuidanceResponseStatus code) { 214 return code.getSystem(); 215 } 216 } 217 218 /** 219 * The identifier of the request associated with this response. If an identifier was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario. 220 */ 221 @Child(name = "requestIdentifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 222 @Description(shortDefinition="The identifier of the request associated with this response, if any", formalDefinition="The identifier of the request associated with this response. If an identifier was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario." ) 223 protected Identifier requestIdentifier; 224 225 /** 226 * Allows a service to provide unique, business identifiers for the response. 227 */ 228 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 229 @Description(shortDefinition="Business identifier", formalDefinition="Allows a service to provide unique, business identifiers for the response." ) 230 protected List<Identifier> identifier; 231 232 /** 233 * An identifier, CodeableConcept or canonical reference to the guidance that was requested. 234 */ 235 @Child(name = "module", type = {UriType.class, CanonicalType.class, CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=true) 236 @Description(shortDefinition="What guidance was requested", formalDefinition="An identifier, CodeableConcept or canonical reference to the guidance that was requested." ) 237 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/guidance-module-code") 238 protected DataType module; 239 240 /** 241 * The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information. 242 */ 243 @Child(name = "status", type = {CodeType.class}, order=3, min=1, max=1, modifier=true, summary=true) 244 @Description(shortDefinition="success | data-requested | data-required | in-progress | failure | entered-in-error", formalDefinition="The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information." ) 245 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/guidance-response-status") 246 protected Enumeration<GuidanceResponseStatus> status; 247 248 /** 249 * The patient for which the request was processed. 250 */ 251 @Child(name = "subject", type = {Patient.class, Group.class}, order=4, min=0, max=1, modifier=false, summary=false) 252 @Description(shortDefinition="Patient the request was performed for", formalDefinition="The patient for which the request was processed." ) 253 protected Reference subject; 254 255 /** 256 * The encounter during which this response was created or to which the creation of this record is tightly associated. 257 */ 258 @Child(name = "encounter", type = {Encounter.class}, order=5, min=0, max=1, modifier=false, summary=false) 259 @Description(shortDefinition="Encounter during which the response was returned", formalDefinition="The encounter during which this response was created or to which the creation of this record is tightly associated." ) 260 protected Reference encounter; 261 262 /** 263 * Indicates when the guidance response was processed. 264 */ 265 @Child(name = "occurrenceDateTime", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 266 @Description(shortDefinition="When the guidance response was processed", formalDefinition="Indicates when the guidance response was processed." ) 267 protected DateTimeType occurrenceDateTime; 268 269 /** 270 * Provides a reference to the device that performed the guidance. 271 */ 272 @Child(name = "performer", type = {Device.class}, order=7, min=0, max=1, modifier=false, summary=false) 273 @Description(shortDefinition="Device returning the guidance", formalDefinition="Provides a reference to the device that performed the guidance." ) 274 protected Reference performer; 275 276 /** 277 * Describes the reason for the guidance response in coded or textual form, or Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response. 278 */ 279 @Child(name = "reason", type = {CodeableReference.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 280 @Description(shortDefinition="Why guidance is needed", formalDefinition="Describes the reason for the guidance response in coded or textual form, or Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response." ) 281 protected List<CodeableReference> reason; 282 283 /** 284 * Provides a mechanism to communicate additional information about the response. 285 */ 286 @Child(name = "note", type = {Annotation.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 287 @Description(shortDefinition="Additional notes about the response", formalDefinition="Provides a mechanism to communicate additional information about the response." ) 288 protected List<Annotation> note; 289 290 /** 291 * Messages resulting from the evaluation of the artifact or artifacts. As part of evaluating the request, the engine may produce informational or warning messages. These messages will be provided by this element. 292 */ 293 @Child(name = "evaluationMessage", type = {OperationOutcome.class}, order=10, min=0, max=1, modifier=false, summary=false) 294 @Description(shortDefinition="Messages resulting from the evaluation of the artifact or artifacts", formalDefinition="Messages resulting from the evaluation of the artifact or artifacts. As part of evaluating the request, the engine may produce informational or warning messages. These messages will be provided by this element." ) 295 protected Reference evaluationMessage; 296 297 /** 298 * The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element. 299 */ 300 @Child(name = "outputParameters", type = {Parameters.class}, order=11, min=0, max=1, modifier=false, summary=false) 301 @Description(shortDefinition="The output parameters of the evaluation, if any", formalDefinition="The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element." ) 302 protected Reference outputParameters; 303 304 /** 305 * The actions, if any, produced by the evaluation of the artifact. 306 */ 307 @Child(name = "result", type = {Appointment.class, AppointmentResponse.class, CarePlan.class, Claim.class, CommunicationRequest.class, Contract.class, CoverageEligibilityRequest.class, DeviceRequest.class, EnrollmentRequest.class, ImmunizationRecommendation.class, MedicationRequest.class, NutritionOrder.class, RequestOrchestration.class, ServiceRequest.class, SupplyRequest.class, Task.class, VisionPrescription.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 308 @Description(shortDefinition="Proposed actions, if any", formalDefinition="The actions, if any, produced by the evaluation of the artifact." ) 309 protected List<Reference> result; 310 311 /** 312 * If the evaluation could not be completed due to lack of information, or additional information would potentially result in a more accurate response, this element will a description of the data required in order to proceed with the evaluation. A subsequent request to the service should include this data. 313 */ 314 @Child(name = "dataRequirement", type = {DataRequirement.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 315 @Description(shortDefinition="Additional required data", formalDefinition="If the evaluation could not be completed due to lack of information, or additional information would potentially result in a more accurate response, this element will a description of the data required in order to proceed with the evaluation. A subsequent request to the service should include this data." ) 316 protected List<DataRequirement> dataRequirement; 317 318 private static final long serialVersionUID = 1439061923L; 319 320 /** 321 * Constructor 322 */ 323 public GuidanceResponse() { 324 super(); 325 } 326 327 /** 328 * Constructor 329 */ 330 public GuidanceResponse(DataType module, GuidanceResponseStatus status) { 331 super(); 332 this.setModule(module); 333 this.setStatus(status); 334 } 335 336 /** 337 * @return {@link #requestIdentifier} (The identifier of the request associated with this response. If an identifier was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario.) 338 */ 339 public Identifier getRequestIdentifier() { 340 if (this.requestIdentifier == null) 341 if (Configuration.errorOnAutoCreate()) 342 throw new Error("Attempt to auto-create GuidanceResponse.requestIdentifier"); 343 else if (Configuration.doAutoCreate()) 344 this.requestIdentifier = new Identifier(); // cc 345 return this.requestIdentifier; 346 } 347 348 public boolean hasRequestIdentifier() { 349 return this.requestIdentifier != null && !this.requestIdentifier.isEmpty(); 350 } 351 352 /** 353 * @param value {@link #requestIdentifier} (The identifier of the request associated with this response. If an identifier was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario.) 354 */ 355 public GuidanceResponse setRequestIdentifier(Identifier value) { 356 this.requestIdentifier = value; 357 return this; 358 } 359 360 /** 361 * @return {@link #identifier} (Allows a service to provide unique, business identifiers for the response.) 362 */ 363 public List<Identifier> getIdentifier() { 364 if (this.identifier == null) 365 this.identifier = new ArrayList<Identifier>(); 366 return this.identifier; 367 } 368 369 /** 370 * @return Returns a reference to <code>this</code> for easy method chaining 371 */ 372 public GuidanceResponse setIdentifier(List<Identifier> theIdentifier) { 373 this.identifier = theIdentifier; 374 return this; 375 } 376 377 public boolean hasIdentifier() { 378 if (this.identifier == null) 379 return false; 380 for (Identifier item : this.identifier) 381 if (!item.isEmpty()) 382 return true; 383 return false; 384 } 385 386 public Identifier addIdentifier() { //3 387 Identifier t = new Identifier(); 388 if (this.identifier == null) 389 this.identifier = new ArrayList<Identifier>(); 390 this.identifier.add(t); 391 return t; 392 } 393 394 public GuidanceResponse addIdentifier(Identifier t) { //3 395 if (t == null) 396 return this; 397 if (this.identifier == null) 398 this.identifier = new ArrayList<Identifier>(); 399 this.identifier.add(t); 400 return this; 401 } 402 403 /** 404 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 405 */ 406 public Identifier getIdentifierFirstRep() { 407 if (getIdentifier().isEmpty()) { 408 addIdentifier(); 409 } 410 return getIdentifier().get(0); 411 } 412 413 /** 414 * @return {@link #module} (An identifier, CodeableConcept or canonical reference to the guidance that was requested.) 415 */ 416 public DataType getModule() { 417 return this.module; 418 } 419 420 /** 421 * @return {@link #module} (An identifier, CodeableConcept or canonical reference to the guidance that was requested.) 422 */ 423 public UriType getModuleUriType() throws FHIRException { 424 if (this.module == null) 425 this.module = new UriType(); 426 if (!(this.module instanceof UriType)) 427 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.module.getClass().getName()+" was encountered"); 428 return (UriType) this.module; 429 } 430 431 public boolean hasModuleUriType() { 432 return this != null && this.module instanceof UriType; 433 } 434 435 /** 436 * @return {@link #module} (An identifier, CodeableConcept or canonical reference to the guidance that was requested.) 437 */ 438 public CanonicalType getModuleCanonicalType() throws FHIRException { 439 if (this.module == null) 440 this.module = new CanonicalType(); 441 if (!(this.module instanceof CanonicalType)) 442 throw new FHIRException("Type mismatch: the type CanonicalType was expected, but "+this.module.getClass().getName()+" was encountered"); 443 return (CanonicalType) this.module; 444 } 445 446 public boolean hasModuleCanonicalType() { 447 return this != null && this.module instanceof CanonicalType; 448 } 449 450 /** 451 * @return {@link #module} (An identifier, CodeableConcept or canonical reference to the guidance that was requested.) 452 */ 453 public CodeableConcept getModuleCodeableConcept() throws FHIRException { 454 if (this.module == null) 455 this.module = new CodeableConcept(); 456 if (!(this.module instanceof CodeableConcept)) 457 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.module.getClass().getName()+" was encountered"); 458 return (CodeableConcept) this.module; 459 } 460 461 public boolean hasModuleCodeableConcept() { 462 return this != null && this.module instanceof CodeableConcept; 463 } 464 465 public boolean hasModule() { 466 return this.module != null && !this.module.isEmpty(); 467 } 468 469 /** 470 * @param value {@link #module} (An identifier, CodeableConcept or canonical reference to the guidance that was requested.) 471 */ 472 public GuidanceResponse setModule(DataType value) { 473 if (value != null && !(value instanceof UriType || value instanceof CanonicalType || value instanceof CodeableConcept)) 474 throw new FHIRException("Not the right type for GuidanceResponse.module[x]: "+value.fhirType()); 475 this.module = value; 476 return this; 477 } 478 479 /** 480 * @return {@link #status} (The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 481 */ 482 public Enumeration<GuidanceResponseStatus> getStatusElement() { 483 if (this.status == null) 484 if (Configuration.errorOnAutoCreate()) 485 throw new Error("Attempt to auto-create GuidanceResponse.status"); 486 else if (Configuration.doAutoCreate()) 487 this.status = new Enumeration<GuidanceResponseStatus>(new GuidanceResponseStatusEnumFactory()); // bb 488 return this.status; 489 } 490 491 public boolean hasStatusElement() { 492 return this.status != null && !this.status.isEmpty(); 493 } 494 495 public boolean hasStatus() { 496 return this.status != null && !this.status.isEmpty(); 497 } 498 499 /** 500 * @param value {@link #status} (The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 501 */ 502 public GuidanceResponse setStatusElement(Enumeration<GuidanceResponseStatus> value) { 503 this.status = value; 504 return this; 505 } 506 507 /** 508 * @return The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information. 509 */ 510 public GuidanceResponseStatus getStatus() { 511 return this.status == null ? null : this.status.getValue(); 512 } 513 514 /** 515 * @param value The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information. 516 */ 517 public GuidanceResponse setStatus(GuidanceResponseStatus value) { 518 if (this.status == null) 519 this.status = new Enumeration<GuidanceResponseStatus>(new GuidanceResponseStatusEnumFactory()); 520 this.status.setValue(value); 521 return this; 522 } 523 524 /** 525 * @return {@link #subject} (The patient for which the request was processed.) 526 */ 527 public Reference getSubject() { 528 if (this.subject == null) 529 if (Configuration.errorOnAutoCreate()) 530 throw new Error("Attempt to auto-create GuidanceResponse.subject"); 531 else if (Configuration.doAutoCreate()) 532 this.subject = new Reference(); // cc 533 return this.subject; 534 } 535 536 public boolean hasSubject() { 537 return this.subject != null && !this.subject.isEmpty(); 538 } 539 540 /** 541 * @param value {@link #subject} (The patient for which the request was processed.) 542 */ 543 public GuidanceResponse setSubject(Reference value) { 544 this.subject = value; 545 return this; 546 } 547 548 /** 549 * @return {@link #encounter} (The encounter during which this response was created or to which the creation of this record is tightly associated.) 550 */ 551 public Reference getEncounter() { 552 if (this.encounter == null) 553 if (Configuration.errorOnAutoCreate()) 554 throw new Error("Attempt to auto-create GuidanceResponse.encounter"); 555 else if (Configuration.doAutoCreate()) 556 this.encounter = new Reference(); // cc 557 return this.encounter; 558 } 559 560 public boolean hasEncounter() { 561 return this.encounter != null && !this.encounter.isEmpty(); 562 } 563 564 /** 565 * @param value {@link #encounter} (The encounter during which this response was created or to which the creation of this record is tightly associated.) 566 */ 567 public GuidanceResponse setEncounter(Reference value) { 568 this.encounter = value; 569 return this; 570 } 571 572 /** 573 * @return {@link #occurrenceDateTime} (Indicates when the guidance response was processed.). This is the underlying object with id, value and extensions. The accessor "getOccurrenceDateTime" gives direct access to the value 574 */ 575 public DateTimeType getOccurrenceDateTimeElement() { 576 if (this.occurrenceDateTime == null) 577 if (Configuration.errorOnAutoCreate()) 578 throw new Error("Attempt to auto-create GuidanceResponse.occurrenceDateTime"); 579 else if (Configuration.doAutoCreate()) 580 this.occurrenceDateTime = new DateTimeType(); // bb 581 return this.occurrenceDateTime; 582 } 583 584 public boolean hasOccurrenceDateTimeElement() { 585 return this.occurrenceDateTime != null && !this.occurrenceDateTime.isEmpty(); 586 } 587 588 public boolean hasOccurrenceDateTime() { 589 return this.occurrenceDateTime != null && !this.occurrenceDateTime.isEmpty(); 590 } 591 592 /** 593 * @param value {@link #occurrenceDateTime} (Indicates when the guidance response was processed.). This is the underlying object with id, value and extensions. The accessor "getOccurrenceDateTime" gives direct access to the value 594 */ 595 public GuidanceResponse setOccurrenceDateTimeElement(DateTimeType value) { 596 this.occurrenceDateTime = value; 597 return this; 598 } 599 600 /** 601 * @return Indicates when the guidance response was processed. 602 */ 603 public Date getOccurrenceDateTime() { 604 return this.occurrenceDateTime == null ? null : this.occurrenceDateTime.getValue(); 605 } 606 607 /** 608 * @param value Indicates when the guidance response was processed. 609 */ 610 public GuidanceResponse setOccurrenceDateTime(Date value) { 611 if (value == null) 612 this.occurrenceDateTime = null; 613 else { 614 if (this.occurrenceDateTime == null) 615 this.occurrenceDateTime = new DateTimeType(); 616 this.occurrenceDateTime.setValue(value); 617 } 618 return this; 619 } 620 621 /** 622 * @return {@link #performer} (Provides a reference to the device that performed the guidance.) 623 */ 624 public Reference getPerformer() { 625 if (this.performer == null) 626 if (Configuration.errorOnAutoCreate()) 627 throw new Error("Attempt to auto-create GuidanceResponse.performer"); 628 else if (Configuration.doAutoCreate()) 629 this.performer = new Reference(); // cc 630 return this.performer; 631 } 632 633 public boolean hasPerformer() { 634 return this.performer != null && !this.performer.isEmpty(); 635 } 636 637 /** 638 * @param value {@link #performer} (Provides a reference to the device that performed the guidance.) 639 */ 640 public GuidanceResponse setPerformer(Reference value) { 641 this.performer = value; 642 return this; 643 } 644 645 /** 646 * @return {@link #reason} (Describes the reason for the guidance response in coded or textual form, or Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.) 647 */ 648 public List<CodeableReference> getReason() { 649 if (this.reason == null) 650 this.reason = new ArrayList<CodeableReference>(); 651 return this.reason; 652 } 653 654 /** 655 * @return Returns a reference to <code>this</code> for easy method chaining 656 */ 657 public GuidanceResponse setReason(List<CodeableReference> theReason) { 658 this.reason = theReason; 659 return this; 660 } 661 662 public boolean hasReason() { 663 if (this.reason == null) 664 return false; 665 for (CodeableReference item : this.reason) 666 if (!item.isEmpty()) 667 return true; 668 return false; 669 } 670 671 public CodeableReference addReason() { //3 672 CodeableReference t = new CodeableReference(); 673 if (this.reason == null) 674 this.reason = new ArrayList<CodeableReference>(); 675 this.reason.add(t); 676 return t; 677 } 678 679 public GuidanceResponse addReason(CodeableReference t) { //3 680 if (t == null) 681 return this; 682 if (this.reason == null) 683 this.reason = new ArrayList<CodeableReference>(); 684 this.reason.add(t); 685 return this; 686 } 687 688 /** 689 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 690 */ 691 public CodeableReference getReasonFirstRep() { 692 if (getReason().isEmpty()) { 693 addReason(); 694 } 695 return getReason().get(0); 696 } 697 698 /** 699 * @return {@link #note} (Provides a mechanism to communicate additional information about the response.) 700 */ 701 public List<Annotation> getNote() { 702 if (this.note == null) 703 this.note = new ArrayList<Annotation>(); 704 return this.note; 705 } 706 707 /** 708 * @return Returns a reference to <code>this</code> for easy method chaining 709 */ 710 public GuidanceResponse setNote(List<Annotation> theNote) { 711 this.note = theNote; 712 return this; 713 } 714 715 public boolean hasNote() { 716 if (this.note == null) 717 return false; 718 for (Annotation item : this.note) 719 if (!item.isEmpty()) 720 return true; 721 return false; 722 } 723 724 public Annotation addNote() { //3 725 Annotation t = new Annotation(); 726 if (this.note == null) 727 this.note = new ArrayList<Annotation>(); 728 this.note.add(t); 729 return t; 730 } 731 732 public GuidanceResponse addNote(Annotation t) { //3 733 if (t == null) 734 return this; 735 if (this.note == null) 736 this.note = new ArrayList<Annotation>(); 737 this.note.add(t); 738 return this; 739 } 740 741 /** 742 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 743 */ 744 public Annotation getNoteFirstRep() { 745 if (getNote().isEmpty()) { 746 addNote(); 747 } 748 return getNote().get(0); 749 } 750 751 /** 752 * @return {@link #evaluationMessage} (Messages resulting from the evaluation of the artifact or artifacts. As part of evaluating the request, the engine may produce informational or warning messages. These messages will be provided by this element.) 753 */ 754 public Reference getEvaluationMessage() { 755 if (this.evaluationMessage == null) 756 if (Configuration.errorOnAutoCreate()) 757 throw new Error("Attempt to auto-create GuidanceResponse.evaluationMessage"); 758 else if (Configuration.doAutoCreate()) 759 this.evaluationMessage = new Reference(); // cc 760 return this.evaluationMessage; 761 } 762 763 public boolean hasEvaluationMessage() { 764 return this.evaluationMessage != null && !this.evaluationMessage.isEmpty(); 765 } 766 767 /** 768 * @param value {@link #evaluationMessage} (Messages resulting from the evaluation of the artifact or artifacts. As part of evaluating the request, the engine may produce informational or warning messages. These messages will be provided by this element.) 769 */ 770 public GuidanceResponse setEvaluationMessage(Reference value) { 771 this.evaluationMessage = value; 772 return this; 773 } 774 775 /** 776 * @return {@link #outputParameters} (The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element.) 777 */ 778 public Reference getOutputParameters() { 779 if (this.outputParameters == null) 780 if (Configuration.errorOnAutoCreate()) 781 throw new Error("Attempt to auto-create GuidanceResponse.outputParameters"); 782 else if (Configuration.doAutoCreate()) 783 this.outputParameters = new Reference(); // cc 784 return this.outputParameters; 785 } 786 787 public boolean hasOutputParameters() { 788 return this.outputParameters != null && !this.outputParameters.isEmpty(); 789 } 790 791 /** 792 * @param value {@link #outputParameters} (The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element.) 793 */ 794 public GuidanceResponse setOutputParameters(Reference value) { 795 this.outputParameters = value; 796 return this; 797 } 798 799 /** 800 * @return {@link #result} (The actions, if any, produced by the evaluation of the artifact.) 801 */ 802 public List<Reference> getResult() { 803 if (this.result == null) 804 this.result = new ArrayList<Reference>(); 805 return this.result; 806 } 807 808 /** 809 * @return Returns a reference to <code>this</code> for easy method chaining 810 */ 811 public GuidanceResponse setResult(List<Reference> theResult) { 812 this.result = theResult; 813 return this; 814 } 815 816 public boolean hasResult() { 817 if (this.result == null) 818 return false; 819 for (Reference item : this.result) 820 if (!item.isEmpty()) 821 return true; 822 return false; 823 } 824 825 public Reference addResult() { //3 826 Reference t = new Reference(); 827 if (this.result == null) 828 this.result = new ArrayList<Reference>(); 829 this.result.add(t); 830 return t; 831 } 832 833 public GuidanceResponse addResult(Reference t) { //3 834 if (t == null) 835 return this; 836 if (this.result == null) 837 this.result = new ArrayList<Reference>(); 838 this.result.add(t); 839 return this; 840 } 841 842 /** 843 * @return The first repetition of repeating field {@link #result}, creating it if it does not already exist {3} 844 */ 845 public Reference getResultFirstRep() { 846 if (getResult().isEmpty()) { 847 addResult(); 848 } 849 return getResult().get(0); 850 } 851 852 /** 853 * @return {@link #dataRequirement} (If the evaluation could not be completed due to lack of information, or additional information would potentially result in a more accurate response, this element will a description of the data required in order to proceed with the evaluation. A subsequent request to the service should include this data.) 854 */ 855 public List<DataRequirement> getDataRequirement() { 856 if (this.dataRequirement == null) 857 this.dataRequirement = new ArrayList<DataRequirement>(); 858 return this.dataRequirement; 859 } 860 861 /** 862 * @return Returns a reference to <code>this</code> for easy method chaining 863 */ 864 public GuidanceResponse setDataRequirement(List<DataRequirement> theDataRequirement) { 865 this.dataRequirement = theDataRequirement; 866 return this; 867 } 868 869 public boolean hasDataRequirement() { 870 if (this.dataRequirement == null) 871 return false; 872 for (DataRequirement item : this.dataRequirement) 873 if (!item.isEmpty()) 874 return true; 875 return false; 876 } 877 878 public DataRequirement addDataRequirement() { //3 879 DataRequirement t = new DataRequirement(); 880 if (this.dataRequirement == null) 881 this.dataRequirement = new ArrayList<DataRequirement>(); 882 this.dataRequirement.add(t); 883 return t; 884 } 885 886 public GuidanceResponse addDataRequirement(DataRequirement t) { //3 887 if (t == null) 888 return this; 889 if (this.dataRequirement == null) 890 this.dataRequirement = new ArrayList<DataRequirement>(); 891 this.dataRequirement.add(t); 892 return this; 893 } 894 895 /** 896 * @return The first repetition of repeating field {@link #dataRequirement}, creating it if it does not already exist {3} 897 */ 898 public DataRequirement getDataRequirementFirstRep() { 899 if (getDataRequirement().isEmpty()) { 900 addDataRequirement(); 901 } 902 return getDataRequirement().get(0); 903 } 904 905 protected void listChildren(List<Property> children) { 906 super.listChildren(children); 907 children.add(new Property("requestIdentifier", "Identifier", "The identifier of the request associated with this response. If an identifier was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario.", 0, 1, requestIdentifier)); 908 children.add(new Property("identifier", "Identifier", "Allows a service to provide unique, business identifiers for the response.", 0, java.lang.Integer.MAX_VALUE, identifier)); 909 children.add(new Property("module[x]", "uri|canonical|CodeableConcept", "An identifier, CodeableConcept or canonical reference to the guidance that was requested.", 0, 1, module)); 910 children.add(new Property("status", "code", "The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information.", 0, 1, status)); 911 children.add(new Property("subject", "Reference(Patient|Group)", "The patient for which the request was processed.", 0, 1, subject)); 912 children.add(new Property("encounter", "Reference(Encounter)", "The encounter during which this response was created or to which the creation of this record is tightly associated.", 0, 1, encounter)); 913 children.add(new Property("occurrenceDateTime", "dateTime", "Indicates when the guidance response was processed.", 0, 1, occurrenceDateTime)); 914 children.add(new Property("performer", "Reference(Device)", "Provides a reference to the device that performed the guidance.", 0, 1, performer)); 915 children.add(new Property("reason", "CodeableReference", "Describes the reason for the guidance response in coded or textual form, or Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, java.lang.Integer.MAX_VALUE, reason)); 916 children.add(new Property("note", "Annotation", "Provides a mechanism to communicate additional information about the response.", 0, java.lang.Integer.MAX_VALUE, note)); 917 children.add(new Property("evaluationMessage", "Reference(OperationOutcome)", "Messages resulting from the evaluation of the artifact or artifacts. As part of evaluating the request, the engine may produce informational or warning messages. These messages will be provided by this element.", 0, 1, evaluationMessage)); 918 children.add(new Property("outputParameters", "Reference(Parameters)", "The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element.", 0, 1, outputParameters)); 919 children.add(new Property("result", "Reference(Appointment|AppointmentResponse|CarePlan|Claim|CommunicationRequest|Contract|CoverageEligibilityRequest|DeviceRequest|EnrollmentRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|RequestOrchestration|ServiceRequest|SupplyRequest|Task|VisionPrescription)", "The actions, if any, produced by the evaluation of the artifact.", 0, java.lang.Integer.MAX_VALUE, result)); 920 children.add(new Property("dataRequirement", "DataRequirement", "If the evaluation could not be completed due to lack of information, or additional information would potentially result in a more accurate response, this element will a description of the data required in order to proceed with the evaluation. A subsequent request to the service should include this data.", 0, java.lang.Integer.MAX_VALUE, dataRequirement)); 921 } 922 923 @Override 924 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 925 switch (_hash) { 926 case -354233192: /*requestIdentifier*/ return new Property("requestIdentifier", "Identifier", "The identifier of the request associated with this response. If an identifier was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario.", 0, 1, requestIdentifier); 927 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Allows a service to provide unique, business identifiers for the response.", 0, java.lang.Integer.MAX_VALUE, identifier); 928 case -1552083308: /*module[x]*/ return new Property("module[x]", "uri|canonical|CodeableConcept", "An identifier, CodeableConcept or canonical reference to the guidance that was requested.", 0, 1, module); 929 case -1068784020: /*module*/ return new Property("module[x]", "uri|canonical|CodeableConcept", "An identifier, CodeableConcept or canonical reference to the guidance that was requested.", 0, 1, module); 930 case -1552089248: /*moduleUri*/ return new Property("module[x]", "uri", "An identifier, CodeableConcept or canonical reference to the guidance that was requested.", 0, 1, module); 931 case -1153656856: /*moduleCanonical*/ return new Property("module[x]", "canonical", "An identifier, CodeableConcept or canonical reference to the guidance that was requested.", 0, 1, module); 932 case -1157899371: /*moduleCodeableConcept*/ return new Property("module[x]", "CodeableConcept", "An identifier, CodeableConcept or canonical reference to the guidance that was requested.", 0, 1, module); 933 case -892481550: /*status*/ return new Property("status", "code", "The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information.", 0, 1, status); 934 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient for which the request was processed.", 0, 1, subject); 935 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The encounter during which this response was created or to which the creation of this record is tightly associated.", 0, 1, encounter); 936 case -298443636: /*occurrenceDateTime*/ return new Property("occurrenceDateTime", "dateTime", "Indicates when the guidance response was processed.", 0, 1, occurrenceDateTime); 937 case 481140686: /*performer*/ return new Property("performer", "Reference(Device)", "Provides a reference to the device that performed the guidance.", 0, 1, performer); 938 case -934964668: /*reason*/ return new Property("reason", "CodeableReference", "Describes the reason for the guidance response in coded or textual form, or Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, java.lang.Integer.MAX_VALUE, reason); 939 case 3387378: /*note*/ return new Property("note", "Annotation", "Provides a mechanism to communicate additional information about the response.", 0, java.lang.Integer.MAX_VALUE, note); 940 case 1081619755: /*evaluationMessage*/ return new Property("evaluationMessage", "Reference(OperationOutcome)", "Messages resulting from the evaluation of the artifact or artifacts. As part of evaluating the request, the engine may produce informational or warning messages. These messages will be provided by this element.", 0, 1, evaluationMessage); 941 case 525609419: /*outputParameters*/ return new Property("outputParameters", "Reference(Parameters)", "The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element.", 0, 1, outputParameters); 942 case -934426595: /*result*/ return new Property("result", "Reference(Appointment|AppointmentResponse|CarePlan|Claim|CommunicationRequest|Contract|CoverageEligibilityRequest|DeviceRequest|EnrollmentRequest|ImmunizationRecommendation|MedicationRequest|NutritionOrder|RequestOrchestration|ServiceRequest|SupplyRequest|Task|VisionPrescription)", "The actions, if any, produced by the evaluation of the artifact.", 0, java.lang.Integer.MAX_VALUE, result); 943 case 629147193: /*dataRequirement*/ return new Property("dataRequirement", "DataRequirement", "If the evaluation could not be completed due to lack of information, or additional information would potentially result in a more accurate response, this element will a description of the data required in order to proceed with the evaluation. A subsequent request to the service should include this data.", 0, java.lang.Integer.MAX_VALUE, dataRequirement); 944 default: return super.getNamedProperty(_hash, _name, _checkValid); 945 } 946 947 } 948 949 @Override 950 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 951 switch (hash) { 952 case -354233192: /*requestIdentifier*/ return this.requestIdentifier == null ? new Base[0] : new Base[] {this.requestIdentifier}; // Identifier 953 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 954 case -1068784020: /*module*/ return this.module == null ? new Base[0] : new Base[] {this.module}; // DataType 955 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<GuidanceResponseStatus> 956 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 957 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 958 case -298443636: /*occurrenceDateTime*/ return this.occurrenceDateTime == null ? new Base[0] : new Base[] {this.occurrenceDateTime}; // DateTimeType 959 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : new Base[] {this.performer}; // Reference 960 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 961 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 962 case 1081619755: /*evaluationMessage*/ return this.evaluationMessage == null ? new Base[0] : new Base[] {this.evaluationMessage}; // Reference 963 case 525609419: /*outputParameters*/ return this.outputParameters == null ? new Base[0] : new Base[] {this.outputParameters}; // Reference 964 case -934426595: /*result*/ return this.result == null ? new Base[0] : this.result.toArray(new Base[this.result.size()]); // Reference 965 case 629147193: /*dataRequirement*/ return this.dataRequirement == null ? new Base[0] : this.dataRequirement.toArray(new Base[this.dataRequirement.size()]); // DataRequirement 966 default: return super.getProperty(hash, name, checkValid); 967 } 968 969 } 970 971 @Override 972 public Base setProperty(int hash, String name, Base value) throws FHIRException { 973 switch (hash) { 974 case -354233192: // requestIdentifier 975 this.requestIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 976 return value; 977 case -1618432855: // identifier 978 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 979 return value; 980 case -1068784020: // module 981 this.module = TypeConvertor.castToType(value); // DataType 982 return value; 983 case -892481550: // status 984 value = new GuidanceResponseStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 985 this.status = (Enumeration) value; // Enumeration<GuidanceResponseStatus> 986 return value; 987 case -1867885268: // subject 988 this.subject = TypeConvertor.castToReference(value); // Reference 989 return value; 990 case 1524132147: // encounter 991 this.encounter = TypeConvertor.castToReference(value); // Reference 992 return value; 993 case -298443636: // occurrenceDateTime 994 this.occurrenceDateTime = TypeConvertor.castToDateTime(value); // DateTimeType 995 return value; 996 case 481140686: // performer 997 this.performer = TypeConvertor.castToReference(value); // Reference 998 return value; 999 case -934964668: // reason 1000 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1001 return value; 1002 case 3387378: // note 1003 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1004 return value; 1005 case 1081619755: // evaluationMessage 1006 this.evaluationMessage = TypeConvertor.castToReference(value); // Reference 1007 return value; 1008 case 525609419: // outputParameters 1009 this.outputParameters = TypeConvertor.castToReference(value); // Reference 1010 return value; 1011 case -934426595: // result 1012 this.getResult().add(TypeConvertor.castToReference(value)); // Reference 1013 return value; 1014 case 629147193: // dataRequirement 1015 this.getDataRequirement().add(TypeConvertor.castToDataRequirement(value)); // DataRequirement 1016 return value; 1017 default: return super.setProperty(hash, name, value); 1018 } 1019 1020 } 1021 1022 @Override 1023 public Base setProperty(String name, Base value) throws FHIRException { 1024 if (name.equals("requestIdentifier")) { 1025 this.requestIdentifier = TypeConvertor.castToIdentifier(value); // Identifier 1026 } else if (name.equals("identifier")) { 1027 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1028 } else if (name.equals("module[x]")) { 1029 this.module = TypeConvertor.castToType(value); // DataType 1030 } else if (name.equals("status")) { 1031 value = new GuidanceResponseStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1032 this.status = (Enumeration) value; // Enumeration<GuidanceResponseStatus> 1033 } else if (name.equals("subject")) { 1034 this.subject = TypeConvertor.castToReference(value); // Reference 1035 } else if (name.equals("encounter")) { 1036 this.encounter = TypeConvertor.castToReference(value); // Reference 1037 } else if (name.equals("occurrenceDateTime")) { 1038 this.occurrenceDateTime = TypeConvertor.castToDateTime(value); // DateTimeType 1039 } else if (name.equals("performer")) { 1040 this.performer = TypeConvertor.castToReference(value); // Reference 1041 } else if (name.equals("reason")) { 1042 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 1043 } else if (name.equals("note")) { 1044 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1045 } else if (name.equals("evaluationMessage")) { 1046 this.evaluationMessage = TypeConvertor.castToReference(value); // Reference 1047 } else if (name.equals("outputParameters")) { 1048 this.outputParameters = TypeConvertor.castToReference(value); // Reference 1049 } else if (name.equals("result")) { 1050 this.getResult().add(TypeConvertor.castToReference(value)); 1051 } else if (name.equals("dataRequirement")) { 1052 this.getDataRequirement().add(TypeConvertor.castToDataRequirement(value)); 1053 } else 1054 return super.setProperty(name, value); 1055 return value; 1056 } 1057 1058 @Override 1059 public void removeChild(String name, Base value) throws FHIRException { 1060 if (name.equals("requestIdentifier")) { 1061 this.requestIdentifier = null; 1062 } else if (name.equals("identifier")) { 1063 this.getIdentifier().remove(value); 1064 } else if (name.equals("module[x]")) { 1065 this.module = null; 1066 } else if (name.equals("status")) { 1067 value = new GuidanceResponseStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1068 this.status = (Enumeration) value; // Enumeration<GuidanceResponseStatus> 1069 } else if (name.equals("subject")) { 1070 this.subject = null; 1071 } else if (name.equals("encounter")) { 1072 this.encounter = null; 1073 } else if (name.equals("occurrenceDateTime")) { 1074 this.occurrenceDateTime = null; 1075 } else if (name.equals("performer")) { 1076 this.performer = null; 1077 } else if (name.equals("reason")) { 1078 this.getReason().remove(value); 1079 } else if (name.equals("note")) { 1080 this.getNote().remove(value); 1081 } else if (name.equals("evaluationMessage")) { 1082 this.evaluationMessage = null; 1083 } else if (name.equals("outputParameters")) { 1084 this.outputParameters = null; 1085 } else if (name.equals("result")) { 1086 this.getResult().remove(value); 1087 } else if (name.equals("dataRequirement")) { 1088 this.getDataRequirement().remove(value); 1089 } else 1090 super.removeChild(name, value); 1091 1092 } 1093 1094 @Override 1095 public Base makeProperty(int hash, String name) throws FHIRException { 1096 switch (hash) { 1097 case -354233192: return getRequestIdentifier(); 1098 case -1618432855: return addIdentifier(); 1099 case -1552083308: return getModule(); 1100 case -1068784020: return getModule(); 1101 case -892481550: return getStatusElement(); 1102 case -1867885268: return getSubject(); 1103 case 1524132147: return getEncounter(); 1104 case -298443636: return getOccurrenceDateTimeElement(); 1105 case 481140686: return getPerformer(); 1106 case -934964668: return addReason(); 1107 case 3387378: return addNote(); 1108 case 1081619755: return getEvaluationMessage(); 1109 case 525609419: return getOutputParameters(); 1110 case -934426595: return addResult(); 1111 case 629147193: return addDataRequirement(); 1112 default: return super.makeProperty(hash, name); 1113 } 1114 1115 } 1116 1117 @Override 1118 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1119 switch (hash) { 1120 case -354233192: /*requestIdentifier*/ return new String[] {"Identifier"}; 1121 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1122 case -1068784020: /*module*/ return new String[] {"uri", "canonical", "CodeableConcept"}; 1123 case -892481550: /*status*/ return new String[] {"code"}; 1124 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1125 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1126 case -298443636: /*occurrenceDateTime*/ return new String[] {"dateTime"}; 1127 case 481140686: /*performer*/ return new String[] {"Reference"}; 1128 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 1129 case 3387378: /*note*/ return new String[] {"Annotation"}; 1130 case 1081619755: /*evaluationMessage*/ return new String[] {"Reference"}; 1131 case 525609419: /*outputParameters*/ return new String[] {"Reference"}; 1132 case -934426595: /*result*/ return new String[] {"Reference"}; 1133 case 629147193: /*dataRequirement*/ return new String[] {"DataRequirement"}; 1134 default: return super.getTypesForProperty(hash, name); 1135 } 1136 1137 } 1138 1139 @Override 1140 public Base addChild(String name) throws FHIRException { 1141 if (name.equals("requestIdentifier")) { 1142 this.requestIdentifier = new Identifier(); 1143 return this.requestIdentifier; 1144 } 1145 else if (name.equals("identifier")) { 1146 return addIdentifier(); 1147 } 1148 else if (name.equals("moduleUri")) { 1149 this.module = new UriType(); 1150 return this.module; 1151 } 1152 else if (name.equals("moduleCanonical")) { 1153 this.module = new CanonicalType(); 1154 return this.module; 1155 } 1156 else if (name.equals("moduleCodeableConcept")) { 1157 this.module = new CodeableConcept(); 1158 return this.module; 1159 } 1160 else if (name.equals("status")) { 1161 throw new FHIRException("Cannot call addChild on a singleton property GuidanceResponse.status"); 1162 } 1163 else if (name.equals("subject")) { 1164 this.subject = new Reference(); 1165 return this.subject; 1166 } 1167 else if (name.equals("encounter")) { 1168 this.encounter = new Reference(); 1169 return this.encounter; 1170 } 1171 else if (name.equals("occurrenceDateTime")) { 1172 throw new FHIRException("Cannot call addChild on a singleton property GuidanceResponse.occurrenceDateTime"); 1173 } 1174 else if (name.equals("performer")) { 1175 this.performer = new Reference(); 1176 return this.performer; 1177 } 1178 else if (name.equals("reason")) { 1179 return addReason(); 1180 } 1181 else if (name.equals("note")) { 1182 return addNote(); 1183 } 1184 else if (name.equals("evaluationMessage")) { 1185 this.evaluationMessage = new Reference(); 1186 return this.evaluationMessage; 1187 } 1188 else if (name.equals("outputParameters")) { 1189 this.outputParameters = new Reference(); 1190 return this.outputParameters; 1191 } 1192 else if (name.equals("result")) { 1193 return addResult(); 1194 } 1195 else if (name.equals("dataRequirement")) { 1196 return addDataRequirement(); 1197 } 1198 else 1199 return super.addChild(name); 1200 } 1201 1202 public String fhirType() { 1203 return "GuidanceResponse"; 1204 1205 } 1206 1207 public GuidanceResponse copy() { 1208 GuidanceResponse dst = new GuidanceResponse(); 1209 copyValues(dst); 1210 return dst; 1211 } 1212 1213 public void copyValues(GuidanceResponse dst) { 1214 super.copyValues(dst); 1215 dst.requestIdentifier = requestIdentifier == null ? null : requestIdentifier.copy(); 1216 if (identifier != null) { 1217 dst.identifier = new ArrayList<Identifier>(); 1218 for (Identifier i : identifier) 1219 dst.identifier.add(i.copy()); 1220 }; 1221 dst.module = module == null ? null : module.copy(); 1222 dst.status = status == null ? null : status.copy(); 1223 dst.subject = subject == null ? null : subject.copy(); 1224 dst.encounter = encounter == null ? null : encounter.copy(); 1225 dst.occurrenceDateTime = occurrenceDateTime == null ? null : occurrenceDateTime.copy(); 1226 dst.performer = performer == null ? null : performer.copy(); 1227 if (reason != null) { 1228 dst.reason = new ArrayList<CodeableReference>(); 1229 for (CodeableReference i : reason) 1230 dst.reason.add(i.copy()); 1231 }; 1232 if (note != null) { 1233 dst.note = new ArrayList<Annotation>(); 1234 for (Annotation i : note) 1235 dst.note.add(i.copy()); 1236 }; 1237 dst.evaluationMessage = evaluationMessage == null ? null : evaluationMessage.copy(); 1238 dst.outputParameters = outputParameters == null ? null : outputParameters.copy(); 1239 if (result != null) { 1240 dst.result = new ArrayList<Reference>(); 1241 for (Reference i : result) 1242 dst.result.add(i.copy()); 1243 }; 1244 if (dataRequirement != null) { 1245 dst.dataRequirement = new ArrayList<DataRequirement>(); 1246 for (DataRequirement i : dataRequirement) 1247 dst.dataRequirement.add(i.copy()); 1248 }; 1249 } 1250 1251 protected GuidanceResponse typedCopy() { 1252 return copy(); 1253 } 1254 1255 @Override 1256 public boolean equalsDeep(Base other_) { 1257 if (!super.equalsDeep(other_)) 1258 return false; 1259 if (!(other_ instanceof GuidanceResponse)) 1260 return false; 1261 GuidanceResponse o = (GuidanceResponse) other_; 1262 return compareDeep(requestIdentifier, o.requestIdentifier, true) && compareDeep(identifier, o.identifier, true) 1263 && compareDeep(module, o.module, true) && compareDeep(status, o.status, true) && compareDeep(subject, o.subject, true) 1264 && compareDeep(encounter, o.encounter, true) && compareDeep(occurrenceDateTime, o.occurrenceDateTime, true) 1265 && compareDeep(performer, o.performer, true) && compareDeep(reason, o.reason, true) && compareDeep(note, o.note, true) 1266 && compareDeep(evaluationMessage, o.evaluationMessage, true) && compareDeep(outputParameters, o.outputParameters, true) 1267 && compareDeep(result, o.result, true) && compareDeep(dataRequirement, o.dataRequirement, true) 1268 ; 1269 } 1270 1271 @Override 1272 public boolean equalsShallow(Base other_) { 1273 if (!super.equalsShallow(other_)) 1274 return false; 1275 if (!(other_ instanceof GuidanceResponse)) 1276 return false; 1277 GuidanceResponse o = (GuidanceResponse) other_; 1278 return compareValues(status, o.status, true) && compareValues(occurrenceDateTime, o.occurrenceDateTime, true) 1279 ; 1280 } 1281 1282 public boolean isEmpty() { 1283 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(requestIdentifier, identifier 1284 , module, status, subject, encounter, occurrenceDateTime, performer, reason, note 1285 , evaluationMessage, outputParameters, result, dataRequirement); 1286 } 1287 1288 @Override 1289 public ResourceType getResourceType() { 1290 return ResourceType.GuidanceResponse; 1291 } 1292 1293 /** 1294 * Search parameter: <b>request</b> 1295 * <p> 1296 * Description: <b>The identifier of the request associated with the response</b><br> 1297 * Type: <b>token</b><br> 1298 * Path: <b>GuidanceResponse.requestIdentifier</b><br> 1299 * </p> 1300 */ 1301 @SearchParamDefinition(name="request", path="GuidanceResponse.requestIdentifier", description="The identifier of the request associated with the response", type="token" ) 1302 public static final String SP_REQUEST = "request"; 1303 /** 1304 * <b>Fluent Client</b> search parameter constant for <b>request</b> 1305 * <p> 1306 * Description: <b>The identifier of the request associated with the response</b><br> 1307 * Type: <b>token</b><br> 1308 * Path: <b>GuidanceResponse.requestIdentifier</b><br> 1309 * </p> 1310 */ 1311 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REQUEST = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REQUEST); 1312 1313 /** 1314 * Search parameter: <b>status</b> 1315 * <p> 1316 * Description: <b>The status of the guidance response</b><br> 1317 * Type: <b>token</b><br> 1318 * Path: <b>GuidanceResponse.status</b><br> 1319 * </p> 1320 */ 1321 @SearchParamDefinition(name="status", path="GuidanceResponse.status", description="The status of the guidance response", type="token" ) 1322 public static final String SP_STATUS = "status"; 1323 /** 1324 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1325 * <p> 1326 * Description: <b>The status of the guidance response</b><br> 1327 * Type: <b>token</b><br> 1328 * Path: <b>GuidanceResponse.status</b><br> 1329 * </p> 1330 */ 1331 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1332 1333 /** 1334 * Search parameter: <b>subject</b> 1335 * <p> 1336 * Description: <b>The subject that the guidance response is about</b><br> 1337 * Type: <b>reference</b><br> 1338 * Path: <b>GuidanceResponse.subject</b><br> 1339 * </p> 1340 */ 1341 @SearchParamDefinition(name="subject", path="GuidanceResponse.subject", description="The subject that the guidance response is about", type="reference", target={Group.class, Patient.class } ) 1342 public static final String SP_SUBJECT = "subject"; 1343 /** 1344 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1345 * <p> 1346 * Description: <b>The subject that the guidance response is about</b><br> 1347 * Type: <b>reference</b><br> 1348 * Path: <b>GuidanceResponse.subject</b><br> 1349 * </p> 1350 */ 1351 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1352 1353/** 1354 * Constant for fluent queries to be used to add include statements. Specifies 1355 * the path value of "<b>GuidanceResponse:subject</b>". 1356 */ 1357 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("GuidanceResponse:subject").toLocked(); 1358 1359 /** 1360 * Search parameter: <b>identifier</b> 1361 * <p> 1362 * Description: <b>Multiple Resources: 1363 1364* [Account](account.html): Account number 1365* [AdverseEvent](adverseevent.html): Business identifier for the event 1366* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1367* [Appointment](appointment.html): An Identifier of the Appointment 1368* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1369* [Basic](basic.html): Business identifier 1370* [BodyStructure](bodystructure.html): Bodystructure identifier 1371* [CarePlan](careplan.html): External Ids for this plan 1372* [CareTeam](careteam.html): External Ids for this team 1373* [ChargeItem](chargeitem.html): Business Identifier for item 1374* [Claim](claim.html): The primary identifier of the financial resource 1375* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1376* [ClinicalImpression](clinicalimpression.html): Business identifier 1377* [Communication](communication.html): Unique identifier 1378* [CommunicationRequest](communicationrequest.html): Unique identifier 1379* [Composition](composition.html): Version-independent identifier for the Composition 1380* [Condition](condition.html): A unique identifier of the condition record 1381* [Consent](consent.html): Identifier for this record (external references) 1382* [Contract](contract.html): The identity of the contract 1383* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1384* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1385* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1386* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1387* [DeviceRequest](devicerequest.html): Business identifier for request/order 1388* [DeviceUsage](deviceusage.html): Search by identifier 1389* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1390* [DocumentReference](documentreference.html): Identifier of the attachment binary 1391* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1392* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1393* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1394* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1395* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1396* [Flag](flag.html): Business identifier 1397* [Goal](goal.html): External Ids for this goal 1398* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1399* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1400* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1401* [Immunization](immunization.html): Business identifier 1402* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1403* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1404* [Invoice](invoice.html): Business Identifier for item 1405* [List](list.html): Business identifier 1406* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1407* [Medication](medication.html): Returns medications with this external identifier 1408* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1409* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1410* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1411* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1412* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1413* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1414* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1415* [Observation](observation.html): The unique id for a particular observation 1416* [Person](person.html): A person Identifier 1417* [Procedure](procedure.html): A unique identifier for a procedure 1418* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1419* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1420* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1421* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1422* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1423* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1424* [Specimen](specimen.html): The unique identifier associated with the specimen 1425* [SupplyDelivery](supplydelivery.html): External identifier 1426* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1427* [Task](task.html): Search for a task instance by its business identifier 1428* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1429</b><br> 1430 * Type: <b>token</b><br> 1431 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1432 * </p> 1433 */ 1434 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 1435 public static final String SP_IDENTIFIER = "identifier"; 1436 /** 1437 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1438 * <p> 1439 * Description: <b>Multiple Resources: 1440 1441* [Account](account.html): Account number 1442* [AdverseEvent](adverseevent.html): Business identifier for the event 1443* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1444* [Appointment](appointment.html): An Identifier of the Appointment 1445* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1446* [Basic](basic.html): Business identifier 1447* [BodyStructure](bodystructure.html): Bodystructure identifier 1448* [CarePlan](careplan.html): External Ids for this plan 1449* [CareTeam](careteam.html): External Ids for this team 1450* [ChargeItem](chargeitem.html): Business Identifier for item 1451* [Claim](claim.html): The primary identifier of the financial resource 1452* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1453* [ClinicalImpression](clinicalimpression.html): Business identifier 1454* [Communication](communication.html): Unique identifier 1455* [CommunicationRequest](communicationrequest.html): Unique identifier 1456* [Composition](composition.html): Version-independent identifier for the Composition 1457* [Condition](condition.html): A unique identifier of the condition record 1458* [Consent](consent.html): Identifier for this record (external references) 1459* [Contract](contract.html): The identity of the contract 1460* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1461* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1462* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1463* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1464* [DeviceRequest](devicerequest.html): Business identifier for request/order 1465* [DeviceUsage](deviceusage.html): Search by identifier 1466* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1467* [DocumentReference](documentreference.html): Identifier of the attachment binary 1468* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1469* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1470* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1471* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1472* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1473* [Flag](flag.html): Business identifier 1474* [Goal](goal.html): External Ids for this goal 1475* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1476* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1477* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1478* [Immunization](immunization.html): Business identifier 1479* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1480* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1481* [Invoice](invoice.html): Business Identifier for item 1482* [List](list.html): Business identifier 1483* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1484* [Medication](medication.html): Returns medications with this external identifier 1485* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1486* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1487* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1488* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1489* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1490* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1491* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1492* [Observation](observation.html): The unique id for a particular observation 1493* [Person](person.html): A person Identifier 1494* [Procedure](procedure.html): A unique identifier for a procedure 1495* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1496* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1497* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1498* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1499* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1500* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1501* [Specimen](specimen.html): The unique identifier associated with the specimen 1502* [SupplyDelivery](supplydelivery.html): External identifier 1503* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1504* [Task](task.html): Search for a task instance by its business identifier 1505* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1506</b><br> 1507 * Type: <b>token</b><br> 1508 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1509 * </p> 1510 */ 1511 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1512 1513 /** 1514 * Search parameter: <b>patient</b> 1515 * <p> 1516 * Description: <b>Multiple Resources: 1517 1518* [Account](account.html): The entity that caused the expenses 1519* [AdverseEvent](adverseevent.html): Subject impacted by event 1520* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1521* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1522* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1523* [AuditEvent](auditevent.html): Where the activity involved patient data 1524* [Basic](basic.html): Identifies the focus of this resource 1525* [BodyStructure](bodystructure.html): Who this is about 1526* [CarePlan](careplan.html): Who the care plan is for 1527* [CareTeam](careteam.html): Who care team is for 1528* [ChargeItem](chargeitem.html): Individual service was done for/to 1529* [Claim](claim.html): Patient receiving the products or services 1530* [ClaimResponse](claimresponse.html): The subject of care 1531* [ClinicalImpression](clinicalimpression.html): Patient assessed 1532* [Communication](communication.html): Focus of message 1533* [CommunicationRequest](communicationrequest.html): Focus of message 1534* [Composition](composition.html): Who and/or what the composition is about 1535* [Condition](condition.html): Who has the condition? 1536* [Consent](consent.html): Who the consent applies to 1537* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1538* [Coverage](coverage.html): Retrieve coverages for a patient 1539* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1540* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1541* [DetectedIssue](detectedissue.html): Associated patient 1542* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1543* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1544* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1545* [DocumentReference](documentreference.html): Who/what is the subject of the document 1546* [Encounter](encounter.html): The patient present at the encounter 1547* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1548* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1549* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1550* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1551* [Flag](flag.html): The identity of a subject to list flags for 1552* [Goal](goal.html): Who this goal is intended for 1553* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1554* [ImagingSelection](imagingselection.html): Who the study is about 1555* [ImagingStudy](imagingstudy.html): Who the study is about 1556* [Immunization](immunization.html): The patient for the vaccination record 1557* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1558* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1559* [Invoice](invoice.html): Recipient(s) of goods and services 1560* [List](list.html): If all resources have the same subject 1561* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1562* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1563* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1564* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1565* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1566* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1567* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1568* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1569* [Observation](observation.html): The subject that the observation is about (if patient) 1570* [Person](person.html): The Person links to this Patient 1571* [Procedure](procedure.html): Search by subject - a patient 1572* [Provenance](provenance.html): Where the activity involved patient data 1573* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1574* [RelatedPerson](relatedperson.html): The patient this related person is related to 1575* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1576* [ResearchSubject](researchsubject.html): Who or what is part of study 1577* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1578* [ServiceRequest](servicerequest.html): Search by subject - a patient 1579* [Specimen](specimen.html): The patient the specimen comes from 1580* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1581* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1582* [Task](task.html): Search by patient 1583* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1584</b><br> 1585 * Type: <b>reference</b><br> 1586 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1587 * </p> 1588 */ 1589 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 1590 public static final String SP_PATIENT = "patient"; 1591 /** 1592 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1593 * <p> 1594 * Description: <b>Multiple Resources: 1595 1596* [Account](account.html): The entity that caused the expenses 1597* [AdverseEvent](adverseevent.html): Subject impacted by event 1598* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1599* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1600* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1601* [AuditEvent](auditevent.html): Where the activity involved patient data 1602* [Basic](basic.html): Identifies the focus of this resource 1603* [BodyStructure](bodystructure.html): Who this is about 1604* [CarePlan](careplan.html): Who the care plan is for 1605* [CareTeam](careteam.html): Who care team is for 1606* [ChargeItem](chargeitem.html): Individual service was done for/to 1607* [Claim](claim.html): Patient receiving the products or services 1608* [ClaimResponse](claimresponse.html): The subject of care 1609* [ClinicalImpression](clinicalimpression.html): Patient assessed 1610* [Communication](communication.html): Focus of message 1611* [CommunicationRequest](communicationrequest.html): Focus of message 1612* [Composition](composition.html): Who and/or what the composition is about 1613* [Condition](condition.html): Who has the condition? 1614* [Consent](consent.html): Who the consent applies to 1615* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1616* [Coverage](coverage.html): Retrieve coverages for a patient 1617* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1618* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1619* [DetectedIssue](detectedissue.html): Associated patient 1620* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1621* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1622* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1623* [DocumentReference](documentreference.html): Who/what is the subject of the document 1624* [Encounter](encounter.html): The patient present at the encounter 1625* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1626* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1627* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1628* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1629* [Flag](flag.html): The identity of a subject to list flags for 1630* [Goal](goal.html): Who this goal is intended for 1631* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1632* [ImagingSelection](imagingselection.html): Who the study is about 1633* [ImagingStudy](imagingstudy.html): Who the study is about 1634* [Immunization](immunization.html): The patient for the vaccination record 1635* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1636* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1637* [Invoice](invoice.html): Recipient(s) of goods and services 1638* [List](list.html): If all resources have the same subject 1639* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1640* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1641* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1642* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1643* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1644* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1645* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1646* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1647* [Observation](observation.html): The subject that the observation is about (if patient) 1648* [Person](person.html): The Person links to this Patient 1649* [Procedure](procedure.html): Search by subject - a patient 1650* [Provenance](provenance.html): Where the activity involved patient data 1651* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1652* [RelatedPerson](relatedperson.html): The patient this related person is related to 1653* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1654* [ResearchSubject](researchsubject.html): Who or what is part of study 1655* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1656* [ServiceRequest](servicerequest.html): Search by subject - a patient 1657* [Specimen](specimen.html): The patient the specimen comes from 1658* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1659* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1660* [Task](task.html): Search by patient 1661* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1662</b><br> 1663 * Type: <b>reference</b><br> 1664 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1665 * </p> 1666 */ 1667 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1668 1669/** 1670 * Constant for fluent queries to be used to add include statements. Specifies 1671 * the path value of "<b>GuidanceResponse:patient</b>". 1672 */ 1673 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("GuidanceResponse:patient").toLocked(); 1674 1675 1676} 1677