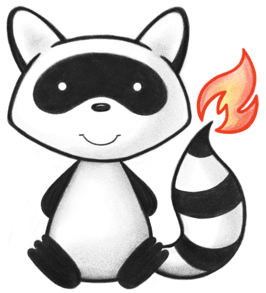
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The details of a healthcare service available at a location or in a catalog. In the case where there is a hierarchy of services (for example, Lab -> Pathology -> Wound Cultures), this can be represented using a set of linked HealthcareServices. 052 */ 053@ResourceDef(name="HealthcareService", profile="http://hl7.org/fhir/StructureDefinition/HealthcareService") 054public class HealthcareService extends DomainResource { 055 056 @Block() 057 public static class HealthcareServiceEligibilityComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Coded value for the eligibility. 060 */ 061 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="Coded value for the eligibility", formalDefinition="Coded value for the eligibility." ) 063 protected CodeableConcept code; 064 065 /** 066 * Describes the eligibility conditions for the service. 067 */ 068 @Child(name = "comment", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 069 @Description(shortDefinition="Describes the eligibility conditions for the service", formalDefinition="Describes the eligibility conditions for the service." ) 070 protected MarkdownType comment; 071 072 private static final long serialVersionUID = 1078065348L; 073 074 /** 075 * Constructor 076 */ 077 public HealthcareServiceEligibilityComponent() { 078 super(); 079 } 080 081 /** 082 * @return {@link #code} (Coded value for the eligibility.) 083 */ 084 public CodeableConcept getCode() { 085 if (this.code == null) 086 if (Configuration.errorOnAutoCreate()) 087 throw new Error("Attempt to auto-create HealthcareServiceEligibilityComponent.code"); 088 else if (Configuration.doAutoCreate()) 089 this.code = new CodeableConcept(); // cc 090 return this.code; 091 } 092 093 public boolean hasCode() { 094 return this.code != null && !this.code.isEmpty(); 095 } 096 097 /** 098 * @param value {@link #code} (Coded value for the eligibility.) 099 */ 100 public HealthcareServiceEligibilityComponent setCode(CodeableConcept value) { 101 this.code = value; 102 return this; 103 } 104 105 /** 106 * @return {@link #comment} (Describes the eligibility conditions for the service.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 107 */ 108 public MarkdownType getCommentElement() { 109 if (this.comment == null) 110 if (Configuration.errorOnAutoCreate()) 111 throw new Error("Attempt to auto-create HealthcareServiceEligibilityComponent.comment"); 112 else if (Configuration.doAutoCreate()) 113 this.comment = new MarkdownType(); // bb 114 return this.comment; 115 } 116 117 public boolean hasCommentElement() { 118 return this.comment != null && !this.comment.isEmpty(); 119 } 120 121 public boolean hasComment() { 122 return this.comment != null && !this.comment.isEmpty(); 123 } 124 125 /** 126 * @param value {@link #comment} (Describes the eligibility conditions for the service.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 127 */ 128 public HealthcareServiceEligibilityComponent setCommentElement(MarkdownType value) { 129 this.comment = value; 130 return this; 131 } 132 133 /** 134 * @return Describes the eligibility conditions for the service. 135 */ 136 public String getComment() { 137 return this.comment == null ? null : this.comment.getValue(); 138 } 139 140 /** 141 * @param value Describes the eligibility conditions for the service. 142 */ 143 public HealthcareServiceEligibilityComponent setComment(String value) { 144 if (Utilities.noString(value)) 145 this.comment = null; 146 else { 147 if (this.comment == null) 148 this.comment = new MarkdownType(); 149 this.comment.setValue(value); 150 } 151 return this; 152 } 153 154 protected void listChildren(List<Property> children) { 155 super.listChildren(children); 156 children.add(new Property("code", "CodeableConcept", "Coded value for the eligibility.", 0, 1, code)); 157 children.add(new Property("comment", "markdown", "Describes the eligibility conditions for the service.", 0, 1, comment)); 158 } 159 160 @Override 161 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 162 switch (_hash) { 163 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Coded value for the eligibility.", 0, 1, code); 164 case 950398559: /*comment*/ return new Property("comment", "markdown", "Describes the eligibility conditions for the service.", 0, 1, comment); 165 default: return super.getNamedProperty(_hash, _name, _checkValid); 166 } 167 168 } 169 170 @Override 171 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 172 switch (hash) { 173 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 174 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // MarkdownType 175 default: return super.getProperty(hash, name, checkValid); 176 } 177 178 } 179 180 @Override 181 public Base setProperty(int hash, String name, Base value) throws FHIRException { 182 switch (hash) { 183 case 3059181: // code 184 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 185 return value; 186 case 950398559: // comment 187 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 188 return value; 189 default: return super.setProperty(hash, name, value); 190 } 191 192 } 193 194 @Override 195 public Base setProperty(String name, Base value) throws FHIRException { 196 if (name.equals("code")) { 197 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 198 } else if (name.equals("comment")) { 199 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 200 } else 201 return super.setProperty(name, value); 202 return value; 203 } 204 205 @Override 206 public Base makeProperty(int hash, String name) throws FHIRException { 207 switch (hash) { 208 case 3059181: return getCode(); 209 case 950398559: return getCommentElement(); 210 default: return super.makeProperty(hash, name); 211 } 212 213 } 214 215 @Override 216 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 217 switch (hash) { 218 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 219 case 950398559: /*comment*/ return new String[] {"markdown"}; 220 default: return super.getTypesForProperty(hash, name); 221 } 222 223 } 224 225 @Override 226 public Base addChild(String name) throws FHIRException { 227 if (name.equals("code")) { 228 this.code = new CodeableConcept(); 229 return this.code; 230 } 231 else if (name.equals("comment")) { 232 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.eligibility.comment"); 233 } 234 else 235 return super.addChild(name); 236 } 237 238 public HealthcareServiceEligibilityComponent copy() { 239 HealthcareServiceEligibilityComponent dst = new HealthcareServiceEligibilityComponent(); 240 copyValues(dst); 241 return dst; 242 } 243 244 public void copyValues(HealthcareServiceEligibilityComponent dst) { 245 super.copyValues(dst); 246 dst.code = code == null ? null : code.copy(); 247 dst.comment = comment == null ? null : comment.copy(); 248 } 249 250 @Override 251 public boolean equalsDeep(Base other_) { 252 if (!super.equalsDeep(other_)) 253 return false; 254 if (!(other_ instanceof HealthcareServiceEligibilityComponent)) 255 return false; 256 HealthcareServiceEligibilityComponent o = (HealthcareServiceEligibilityComponent) other_; 257 return compareDeep(code, o.code, true) && compareDeep(comment, o.comment, true); 258 } 259 260 @Override 261 public boolean equalsShallow(Base other_) { 262 if (!super.equalsShallow(other_)) 263 return false; 264 if (!(other_ instanceof HealthcareServiceEligibilityComponent)) 265 return false; 266 HealthcareServiceEligibilityComponent o = (HealthcareServiceEligibilityComponent) other_; 267 return compareValues(comment, o.comment, true); 268 } 269 270 public boolean isEmpty() { 271 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, comment); 272 } 273 274 public String fhirType() { 275 return "HealthcareService.eligibility"; 276 277 } 278 279 } 280 281 /** 282 * External identifiers for this item. 283 */ 284 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 285 @Description(shortDefinition="External identifiers for this item", formalDefinition="External identifiers for this item." ) 286 protected List<Identifier> identifier; 287 288 /** 289 * This flag is used to mark the record to not be used. This is not used when a center is closed for maintenance, or for holidays, the notAvailable period is to be used for this. 290 */ 291 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=true, summary=true) 292 @Description(shortDefinition="Whether this HealthcareService record is in active use", formalDefinition="This flag is used to mark the record to not be used. This is not used when a center is closed for maintenance, or for holidays, the notAvailable period is to be used for this." ) 293 protected BooleanType active; 294 295 /** 296 * The organization that provides this healthcare service. 297 */ 298 @Child(name = "providedBy", type = {Organization.class}, order=2, min=0, max=1, modifier=false, summary=true) 299 @Description(shortDefinition="Organization that provides this service", formalDefinition="The organization that provides this healthcare service." ) 300 protected Reference providedBy; 301 302 /** 303 * When the HealthcareService is representing a specific, schedulable service, the availableIn property can refer to a generic service. 304 */ 305 @Child(name = "offeredIn", type = {HealthcareService.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 306 @Description(shortDefinition="The service within which this service is offered", formalDefinition="When the HealthcareService is representing a specific, schedulable service, the availableIn property can refer to a generic service." ) 307 protected List<Reference> offeredIn; 308 309 /** 310 * Identifies the broad category of service being performed or delivered. 311 */ 312 @Child(name = "category", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 313 @Description(shortDefinition="Broad category of service being performed or delivered", formalDefinition="Identifies the broad category of service being performed or delivered." ) 314 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-category") 315 protected List<CodeableConcept> category; 316 317 /** 318 * The specific type of service that may be delivered or performed. 319 */ 320 @Child(name = "type", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 321 @Description(shortDefinition="Type of service that may be delivered or performed", formalDefinition="The specific type of service that may be delivered or performed." ) 322 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-type") 323 protected List<CodeableConcept> type; 324 325 /** 326 * Collection of specialties handled by the Healthcare service. This is more of a medical term. 327 */ 328 @Child(name = "specialty", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 329 @Description(shortDefinition="Specialties handled by the HealthcareService", formalDefinition="Collection of specialties handled by the Healthcare service. This is more of a medical term." ) 330 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-practice-codes") 331 protected List<CodeableConcept> specialty; 332 333 /** 334 * The location(s) where this healthcare service may be provided. 335 */ 336 @Child(name = "location", type = {Location.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 337 @Description(shortDefinition="Location(s) where service may be provided", formalDefinition="The location(s) where this healthcare service may be provided." ) 338 protected List<Reference> location; 339 340 /** 341 * Further description of the service as it would be presented to a consumer while searching. 342 */ 343 @Child(name = "name", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=true) 344 @Description(shortDefinition="Description of service as presented to a consumer while searching", formalDefinition="Further description of the service as it would be presented to a consumer while searching." ) 345 protected StringType name; 346 347 /** 348 * Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName. 349 */ 350 @Child(name = "comment", type = {MarkdownType.class}, order=9, min=0, max=1, modifier=false, summary=true) 351 @Description(shortDefinition="Additional description and/or any specific issues not covered elsewhere", formalDefinition="Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName." ) 352 protected MarkdownType comment; 353 354 /** 355 * Extra details about the service that can't be placed in the other fields. 356 */ 357 @Child(name = "extraDetails", type = {MarkdownType.class}, order=10, min=0, max=1, modifier=false, summary=false) 358 @Description(shortDefinition="Extra details about the service that can't be placed in the other fields", formalDefinition="Extra details about the service that can't be placed in the other fields." ) 359 protected MarkdownType extraDetails; 360 361 /** 362 * If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list. 363 */ 364 @Child(name = "photo", type = {Attachment.class}, order=11, min=0, max=1, modifier=false, summary=true) 365 @Description(shortDefinition="Facilitates quick identification of the service", formalDefinition="If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list." ) 366 protected Attachment photo; 367 368 /** 369 * The contact details of communication devices available relevant to the specific HealthcareService. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites. 370 */ 371 @Child(name = "contact", type = {ExtendedContactDetail.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 372 @Description(shortDefinition="Official contact details for the HealthcareService", formalDefinition="The contact details of communication devices available relevant to the specific HealthcareService. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites." ) 373 protected List<ExtendedContactDetail> contact; 374 375 /** 376 * The location(s) that this service is available to (not where the service is provided). 377 */ 378 @Child(name = "coverageArea", type = {Location.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 379 @Description(shortDefinition="Location(s) service is intended for/available to", formalDefinition="The location(s) that this service is available to (not where the service is provided)." ) 380 protected List<Reference> coverageArea; 381 382 /** 383 * The code(s) that detail the conditions under which the healthcare service is available/offered. 384 */ 385 @Child(name = "serviceProvisionCode", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 386 @Description(shortDefinition="Conditions under which service is available/offered", formalDefinition="The code(s) that detail the conditions under which the healthcare service is available/offered." ) 387 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-provision-conditions") 388 protected List<CodeableConcept> serviceProvisionCode; 389 390 /** 391 * Does this service have specific eligibility requirements that need to be met in order to use the service? 392 */ 393 @Child(name = "eligibility", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 394 @Description(shortDefinition="Specific eligibility requirements required to use the service", formalDefinition="Does this service have specific eligibility requirements that need to be met in order to use the service?" ) 395 protected List<HealthcareServiceEligibilityComponent> eligibility; 396 397 /** 398 * Programs that this service is applicable to. 399 */ 400 @Child(name = "program", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 401 @Description(shortDefinition="Programs that this service is applicable to", formalDefinition="Programs that this service is applicable to." ) 402 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/program") 403 protected List<CodeableConcept> program; 404 405 /** 406 * Collection of characteristics (attributes). 407 */ 408 @Child(name = "characteristic", type = {CodeableConcept.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 409 @Description(shortDefinition="Collection of characteristics (attributes)", formalDefinition="Collection of characteristics (attributes)." ) 410 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-mode") 411 protected List<CodeableConcept> characteristic; 412 413 /** 414 * Some services are specifically made available in multiple languages, this property permits a directory to declare the languages this is offered in. Typically this is only provided where a service operates in communities with mixed languages used. 415 */ 416 @Child(name = "communication", type = {CodeableConcept.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 417 @Description(shortDefinition="The language that this service is offered in", formalDefinition="Some services are specifically made available in multiple languages, this property permits a directory to declare the languages this is offered in. Typically this is only provided where a service operates in communities with mixed languages used." ) 418 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/all-languages") 419 protected List<CodeableConcept> communication; 420 421 /** 422 * Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required. 423 */ 424 @Child(name = "referralMethod", type = {CodeableConcept.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 425 @Description(shortDefinition="Ways that the service accepts referrals", formalDefinition="Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required." ) 426 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-referral-method") 427 protected List<CodeableConcept> referralMethod; 428 429 /** 430 * Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service. 431 */ 432 @Child(name = "appointmentRequired", type = {BooleanType.class}, order=20, min=0, max=1, modifier=false, summary=false) 433 @Description(shortDefinition="If an appointment is required for access to this service", formalDefinition="Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service." ) 434 protected BooleanType appointmentRequired; 435 436 /** 437 * A collection of times that the healthcare service is available. 438 */ 439 @Child(name = "availability", type = {Availability.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 440 @Description(shortDefinition="Times the healthcare service is available (including exceptions)", formalDefinition="A collection of times that the healthcare service is available." ) 441 protected List<Availability> availability; 442 443 /** 444 * Technical endpoints providing access to services operated for the specific healthcare services defined at this resource. 445 */ 446 @Child(name = "endpoint", type = {Endpoint.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 447 @Description(shortDefinition="Technical endpoints providing access to electronic services operated for the healthcare service", formalDefinition="Technical endpoints providing access to services operated for the specific healthcare services defined at this resource." ) 448 protected List<Reference> endpoint; 449 450 private static final long serialVersionUID = -438716159L; 451 452 /** 453 * Constructor 454 */ 455 public HealthcareService() { 456 super(); 457 } 458 459 /** 460 * @return {@link #identifier} (External identifiers for this item.) 461 */ 462 public List<Identifier> getIdentifier() { 463 if (this.identifier == null) 464 this.identifier = new ArrayList<Identifier>(); 465 return this.identifier; 466 } 467 468 /** 469 * @return Returns a reference to <code>this</code> for easy method chaining 470 */ 471 public HealthcareService setIdentifier(List<Identifier> theIdentifier) { 472 this.identifier = theIdentifier; 473 return this; 474 } 475 476 public boolean hasIdentifier() { 477 if (this.identifier == null) 478 return false; 479 for (Identifier item : this.identifier) 480 if (!item.isEmpty()) 481 return true; 482 return false; 483 } 484 485 public Identifier addIdentifier() { //3 486 Identifier t = new Identifier(); 487 if (this.identifier == null) 488 this.identifier = new ArrayList<Identifier>(); 489 this.identifier.add(t); 490 return t; 491 } 492 493 public HealthcareService addIdentifier(Identifier t) { //3 494 if (t == null) 495 return this; 496 if (this.identifier == null) 497 this.identifier = new ArrayList<Identifier>(); 498 this.identifier.add(t); 499 return this; 500 } 501 502 /** 503 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 504 */ 505 public Identifier getIdentifierFirstRep() { 506 if (getIdentifier().isEmpty()) { 507 addIdentifier(); 508 } 509 return getIdentifier().get(0); 510 } 511 512 /** 513 * @return {@link #active} (This flag is used to mark the record to not be used. This is not used when a center is closed for maintenance, or for holidays, the notAvailable period is to be used for this.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 514 */ 515 public BooleanType getActiveElement() { 516 if (this.active == null) 517 if (Configuration.errorOnAutoCreate()) 518 throw new Error("Attempt to auto-create HealthcareService.active"); 519 else if (Configuration.doAutoCreate()) 520 this.active = new BooleanType(); // bb 521 return this.active; 522 } 523 524 public boolean hasActiveElement() { 525 return this.active != null && !this.active.isEmpty(); 526 } 527 528 public boolean hasActive() { 529 return this.active != null && !this.active.isEmpty(); 530 } 531 532 /** 533 * @param value {@link #active} (This flag is used to mark the record to not be used. This is not used when a center is closed for maintenance, or for holidays, the notAvailable period is to be used for this.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 534 */ 535 public HealthcareService setActiveElement(BooleanType value) { 536 this.active = value; 537 return this; 538 } 539 540 /** 541 * @return This flag is used to mark the record to not be used. This is not used when a center is closed for maintenance, or for holidays, the notAvailable period is to be used for this. 542 */ 543 public boolean getActive() { 544 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 545 } 546 547 /** 548 * @param value This flag is used to mark the record to not be used. This is not used when a center is closed for maintenance, or for holidays, the notAvailable period is to be used for this. 549 */ 550 public HealthcareService setActive(boolean value) { 551 if (this.active == null) 552 this.active = new BooleanType(); 553 this.active.setValue(value); 554 return this; 555 } 556 557 /** 558 * @return {@link #providedBy} (The organization that provides this healthcare service.) 559 */ 560 public Reference getProvidedBy() { 561 if (this.providedBy == null) 562 if (Configuration.errorOnAutoCreate()) 563 throw new Error("Attempt to auto-create HealthcareService.providedBy"); 564 else if (Configuration.doAutoCreate()) 565 this.providedBy = new Reference(); // cc 566 return this.providedBy; 567 } 568 569 public boolean hasProvidedBy() { 570 return this.providedBy != null && !this.providedBy.isEmpty(); 571 } 572 573 /** 574 * @param value {@link #providedBy} (The organization that provides this healthcare service.) 575 */ 576 public HealthcareService setProvidedBy(Reference value) { 577 this.providedBy = value; 578 return this; 579 } 580 581 /** 582 * @return {@link #offeredIn} (When the HealthcareService is representing a specific, schedulable service, the availableIn property can refer to a generic service.) 583 */ 584 public List<Reference> getOfferedIn() { 585 if (this.offeredIn == null) 586 this.offeredIn = new ArrayList<Reference>(); 587 return this.offeredIn; 588 } 589 590 /** 591 * @return Returns a reference to <code>this</code> for easy method chaining 592 */ 593 public HealthcareService setOfferedIn(List<Reference> theOfferedIn) { 594 this.offeredIn = theOfferedIn; 595 return this; 596 } 597 598 public boolean hasOfferedIn() { 599 if (this.offeredIn == null) 600 return false; 601 for (Reference item : this.offeredIn) 602 if (!item.isEmpty()) 603 return true; 604 return false; 605 } 606 607 public Reference addOfferedIn() { //3 608 Reference t = new Reference(); 609 if (this.offeredIn == null) 610 this.offeredIn = new ArrayList<Reference>(); 611 this.offeredIn.add(t); 612 return t; 613 } 614 615 public HealthcareService addOfferedIn(Reference t) { //3 616 if (t == null) 617 return this; 618 if (this.offeredIn == null) 619 this.offeredIn = new ArrayList<Reference>(); 620 this.offeredIn.add(t); 621 return this; 622 } 623 624 /** 625 * @return The first repetition of repeating field {@link #offeredIn}, creating it if it does not already exist {3} 626 */ 627 public Reference getOfferedInFirstRep() { 628 if (getOfferedIn().isEmpty()) { 629 addOfferedIn(); 630 } 631 return getOfferedIn().get(0); 632 } 633 634 /** 635 * @return {@link #category} (Identifies the broad category of service being performed or delivered.) 636 */ 637 public List<CodeableConcept> getCategory() { 638 if (this.category == null) 639 this.category = new ArrayList<CodeableConcept>(); 640 return this.category; 641 } 642 643 /** 644 * @return Returns a reference to <code>this</code> for easy method chaining 645 */ 646 public HealthcareService setCategory(List<CodeableConcept> theCategory) { 647 this.category = theCategory; 648 return this; 649 } 650 651 public boolean hasCategory() { 652 if (this.category == null) 653 return false; 654 for (CodeableConcept item : this.category) 655 if (!item.isEmpty()) 656 return true; 657 return false; 658 } 659 660 public CodeableConcept addCategory() { //3 661 CodeableConcept t = new CodeableConcept(); 662 if (this.category == null) 663 this.category = new ArrayList<CodeableConcept>(); 664 this.category.add(t); 665 return t; 666 } 667 668 public HealthcareService addCategory(CodeableConcept t) { //3 669 if (t == null) 670 return this; 671 if (this.category == null) 672 this.category = new ArrayList<CodeableConcept>(); 673 this.category.add(t); 674 return this; 675 } 676 677 /** 678 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 679 */ 680 public CodeableConcept getCategoryFirstRep() { 681 if (getCategory().isEmpty()) { 682 addCategory(); 683 } 684 return getCategory().get(0); 685 } 686 687 /** 688 * @return {@link #type} (The specific type of service that may be delivered or performed.) 689 */ 690 public List<CodeableConcept> getType() { 691 if (this.type == null) 692 this.type = new ArrayList<CodeableConcept>(); 693 return this.type; 694 } 695 696 /** 697 * @return Returns a reference to <code>this</code> for easy method chaining 698 */ 699 public HealthcareService setType(List<CodeableConcept> theType) { 700 this.type = theType; 701 return this; 702 } 703 704 public boolean hasType() { 705 if (this.type == null) 706 return false; 707 for (CodeableConcept item : this.type) 708 if (!item.isEmpty()) 709 return true; 710 return false; 711 } 712 713 public CodeableConcept addType() { //3 714 CodeableConcept t = new CodeableConcept(); 715 if (this.type == null) 716 this.type = new ArrayList<CodeableConcept>(); 717 this.type.add(t); 718 return t; 719 } 720 721 public HealthcareService addType(CodeableConcept t) { //3 722 if (t == null) 723 return this; 724 if (this.type == null) 725 this.type = new ArrayList<CodeableConcept>(); 726 this.type.add(t); 727 return this; 728 } 729 730 /** 731 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 732 */ 733 public CodeableConcept getTypeFirstRep() { 734 if (getType().isEmpty()) { 735 addType(); 736 } 737 return getType().get(0); 738 } 739 740 /** 741 * @return {@link #specialty} (Collection of specialties handled by the Healthcare service. This is more of a medical term.) 742 */ 743 public List<CodeableConcept> getSpecialty() { 744 if (this.specialty == null) 745 this.specialty = new ArrayList<CodeableConcept>(); 746 return this.specialty; 747 } 748 749 /** 750 * @return Returns a reference to <code>this</code> for easy method chaining 751 */ 752 public HealthcareService setSpecialty(List<CodeableConcept> theSpecialty) { 753 this.specialty = theSpecialty; 754 return this; 755 } 756 757 public boolean hasSpecialty() { 758 if (this.specialty == null) 759 return false; 760 for (CodeableConcept item : this.specialty) 761 if (!item.isEmpty()) 762 return true; 763 return false; 764 } 765 766 public CodeableConcept addSpecialty() { //3 767 CodeableConcept t = new CodeableConcept(); 768 if (this.specialty == null) 769 this.specialty = new ArrayList<CodeableConcept>(); 770 this.specialty.add(t); 771 return t; 772 } 773 774 public HealthcareService addSpecialty(CodeableConcept t) { //3 775 if (t == null) 776 return this; 777 if (this.specialty == null) 778 this.specialty = new ArrayList<CodeableConcept>(); 779 this.specialty.add(t); 780 return this; 781 } 782 783 /** 784 * @return The first repetition of repeating field {@link #specialty}, creating it if it does not already exist {3} 785 */ 786 public CodeableConcept getSpecialtyFirstRep() { 787 if (getSpecialty().isEmpty()) { 788 addSpecialty(); 789 } 790 return getSpecialty().get(0); 791 } 792 793 /** 794 * @return {@link #location} (The location(s) where this healthcare service may be provided.) 795 */ 796 public List<Reference> getLocation() { 797 if (this.location == null) 798 this.location = new ArrayList<Reference>(); 799 return this.location; 800 } 801 802 /** 803 * @return Returns a reference to <code>this</code> for easy method chaining 804 */ 805 public HealthcareService setLocation(List<Reference> theLocation) { 806 this.location = theLocation; 807 return this; 808 } 809 810 public boolean hasLocation() { 811 if (this.location == null) 812 return false; 813 for (Reference item : this.location) 814 if (!item.isEmpty()) 815 return true; 816 return false; 817 } 818 819 public Reference addLocation() { //3 820 Reference t = new Reference(); 821 if (this.location == null) 822 this.location = new ArrayList<Reference>(); 823 this.location.add(t); 824 return t; 825 } 826 827 public HealthcareService addLocation(Reference t) { //3 828 if (t == null) 829 return this; 830 if (this.location == null) 831 this.location = new ArrayList<Reference>(); 832 this.location.add(t); 833 return this; 834 } 835 836 /** 837 * @return The first repetition of repeating field {@link #location}, creating it if it does not already exist {3} 838 */ 839 public Reference getLocationFirstRep() { 840 if (getLocation().isEmpty()) { 841 addLocation(); 842 } 843 return getLocation().get(0); 844 } 845 846 /** 847 * @return {@link #name} (Further description of the service as it would be presented to a consumer while searching.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 848 */ 849 public StringType getNameElement() { 850 if (this.name == null) 851 if (Configuration.errorOnAutoCreate()) 852 throw new Error("Attempt to auto-create HealthcareService.name"); 853 else if (Configuration.doAutoCreate()) 854 this.name = new StringType(); // bb 855 return this.name; 856 } 857 858 public boolean hasNameElement() { 859 return this.name != null && !this.name.isEmpty(); 860 } 861 862 public boolean hasName() { 863 return this.name != null && !this.name.isEmpty(); 864 } 865 866 /** 867 * @param value {@link #name} (Further description of the service as it would be presented to a consumer while searching.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 868 */ 869 public HealthcareService setNameElement(StringType value) { 870 this.name = value; 871 return this; 872 } 873 874 /** 875 * @return Further description of the service as it would be presented to a consumer while searching. 876 */ 877 public String getName() { 878 return this.name == null ? null : this.name.getValue(); 879 } 880 881 /** 882 * @param value Further description of the service as it would be presented to a consumer while searching. 883 */ 884 public HealthcareService setName(String value) { 885 if (Utilities.noString(value)) 886 this.name = null; 887 else { 888 if (this.name == null) 889 this.name = new StringType(); 890 this.name.setValue(value); 891 } 892 return this; 893 } 894 895 /** 896 * @return {@link #comment} (Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 897 */ 898 public MarkdownType getCommentElement() { 899 if (this.comment == null) 900 if (Configuration.errorOnAutoCreate()) 901 throw new Error("Attempt to auto-create HealthcareService.comment"); 902 else if (Configuration.doAutoCreate()) 903 this.comment = new MarkdownType(); // bb 904 return this.comment; 905 } 906 907 public boolean hasCommentElement() { 908 return this.comment != null && !this.comment.isEmpty(); 909 } 910 911 public boolean hasComment() { 912 return this.comment != null && !this.comment.isEmpty(); 913 } 914 915 /** 916 * @param value {@link #comment} (Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 917 */ 918 public HealthcareService setCommentElement(MarkdownType value) { 919 this.comment = value; 920 return this; 921 } 922 923 /** 924 * @return Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName. 925 */ 926 public String getComment() { 927 return this.comment == null ? null : this.comment.getValue(); 928 } 929 930 /** 931 * @param value Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName. 932 */ 933 public HealthcareService setComment(String value) { 934 if (Utilities.noString(value)) 935 this.comment = null; 936 else { 937 if (this.comment == null) 938 this.comment = new MarkdownType(); 939 this.comment.setValue(value); 940 } 941 return this; 942 } 943 944 /** 945 * @return {@link #extraDetails} (Extra details about the service that can't be placed in the other fields.). This is the underlying object with id, value and extensions. The accessor "getExtraDetails" gives direct access to the value 946 */ 947 public MarkdownType getExtraDetailsElement() { 948 if (this.extraDetails == null) 949 if (Configuration.errorOnAutoCreate()) 950 throw new Error("Attempt to auto-create HealthcareService.extraDetails"); 951 else if (Configuration.doAutoCreate()) 952 this.extraDetails = new MarkdownType(); // bb 953 return this.extraDetails; 954 } 955 956 public boolean hasExtraDetailsElement() { 957 return this.extraDetails != null && !this.extraDetails.isEmpty(); 958 } 959 960 public boolean hasExtraDetails() { 961 return this.extraDetails != null && !this.extraDetails.isEmpty(); 962 } 963 964 /** 965 * @param value {@link #extraDetails} (Extra details about the service that can't be placed in the other fields.). This is the underlying object with id, value and extensions. The accessor "getExtraDetails" gives direct access to the value 966 */ 967 public HealthcareService setExtraDetailsElement(MarkdownType value) { 968 this.extraDetails = value; 969 return this; 970 } 971 972 /** 973 * @return Extra details about the service that can't be placed in the other fields. 974 */ 975 public String getExtraDetails() { 976 return this.extraDetails == null ? null : this.extraDetails.getValue(); 977 } 978 979 /** 980 * @param value Extra details about the service that can't be placed in the other fields. 981 */ 982 public HealthcareService setExtraDetails(String value) { 983 if (Utilities.noString(value)) 984 this.extraDetails = null; 985 else { 986 if (this.extraDetails == null) 987 this.extraDetails = new MarkdownType(); 988 this.extraDetails.setValue(value); 989 } 990 return this; 991 } 992 993 /** 994 * @return {@link #photo} (If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list.) 995 */ 996 public Attachment getPhoto() { 997 if (this.photo == null) 998 if (Configuration.errorOnAutoCreate()) 999 throw new Error("Attempt to auto-create HealthcareService.photo"); 1000 else if (Configuration.doAutoCreate()) 1001 this.photo = new Attachment(); // cc 1002 return this.photo; 1003 } 1004 1005 public boolean hasPhoto() { 1006 return this.photo != null && !this.photo.isEmpty(); 1007 } 1008 1009 /** 1010 * @param value {@link #photo} (If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list.) 1011 */ 1012 public HealthcareService setPhoto(Attachment value) { 1013 this.photo = value; 1014 return this; 1015 } 1016 1017 /** 1018 * @return {@link #contact} (The contact details of communication devices available relevant to the specific HealthcareService. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites.) 1019 */ 1020 public List<ExtendedContactDetail> getContact() { 1021 if (this.contact == null) 1022 this.contact = new ArrayList<ExtendedContactDetail>(); 1023 return this.contact; 1024 } 1025 1026 /** 1027 * @return Returns a reference to <code>this</code> for easy method chaining 1028 */ 1029 public HealthcareService setContact(List<ExtendedContactDetail> theContact) { 1030 this.contact = theContact; 1031 return this; 1032 } 1033 1034 public boolean hasContact() { 1035 if (this.contact == null) 1036 return false; 1037 for (ExtendedContactDetail item : this.contact) 1038 if (!item.isEmpty()) 1039 return true; 1040 return false; 1041 } 1042 1043 public ExtendedContactDetail addContact() { //3 1044 ExtendedContactDetail t = new ExtendedContactDetail(); 1045 if (this.contact == null) 1046 this.contact = new ArrayList<ExtendedContactDetail>(); 1047 this.contact.add(t); 1048 return t; 1049 } 1050 1051 public HealthcareService addContact(ExtendedContactDetail t) { //3 1052 if (t == null) 1053 return this; 1054 if (this.contact == null) 1055 this.contact = new ArrayList<ExtendedContactDetail>(); 1056 this.contact.add(t); 1057 return this; 1058 } 1059 1060 /** 1061 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 1062 */ 1063 public ExtendedContactDetail getContactFirstRep() { 1064 if (getContact().isEmpty()) { 1065 addContact(); 1066 } 1067 return getContact().get(0); 1068 } 1069 1070 /** 1071 * @return {@link #coverageArea} (The location(s) that this service is available to (not where the service is provided).) 1072 */ 1073 public List<Reference> getCoverageArea() { 1074 if (this.coverageArea == null) 1075 this.coverageArea = new ArrayList<Reference>(); 1076 return this.coverageArea; 1077 } 1078 1079 /** 1080 * @return Returns a reference to <code>this</code> for easy method chaining 1081 */ 1082 public HealthcareService setCoverageArea(List<Reference> theCoverageArea) { 1083 this.coverageArea = theCoverageArea; 1084 return this; 1085 } 1086 1087 public boolean hasCoverageArea() { 1088 if (this.coverageArea == null) 1089 return false; 1090 for (Reference item : this.coverageArea) 1091 if (!item.isEmpty()) 1092 return true; 1093 return false; 1094 } 1095 1096 public Reference addCoverageArea() { //3 1097 Reference t = new Reference(); 1098 if (this.coverageArea == null) 1099 this.coverageArea = new ArrayList<Reference>(); 1100 this.coverageArea.add(t); 1101 return t; 1102 } 1103 1104 public HealthcareService addCoverageArea(Reference t) { //3 1105 if (t == null) 1106 return this; 1107 if (this.coverageArea == null) 1108 this.coverageArea = new ArrayList<Reference>(); 1109 this.coverageArea.add(t); 1110 return this; 1111 } 1112 1113 /** 1114 * @return The first repetition of repeating field {@link #coverageArea}, creating it if it does not already exist {3} 1115 */ 1116 public Reference getCoverageAreaFirstRep() { 1117 if (getCoverageArea().isEmpty()) { 1118 addCoverageArea(); 1119 } 1120 return getCoverageArea().get(0); 1121 } 1122 1123 /** 1124 * @return {@link #serviceProvisionCode} (The code(s) that detail the conditions under which the healthcare service is available/offered.) 1125 */ 1126 public List<CodeableConcept> getServiceProvisionCode() { 1127 if (this.serviceProvisionCode == null) 1128 this.serviceProvisionCode = new ArrayList<CodeableConcept>(); 1129 return this.serviceProvisionCode; 1130 } 1131 1132 /** 1133 * @return Returns a reference to <code>this</code> for easy method chaining 1134 */ 1135 public HealthcareService setServiceProvisionCode(List<CodeableConcept> theServiceProvisionCode) { 1136 this.serviceProvisionCode = theServiceProvisionCode; 1137 return this; 1138 } 1139 1140 public boolean hasServiceProvisionCode() { 1141 if (this.serviceProvisionCode == null) 1142 return false; 1143 for (CodeableConcept item : this.serviceProvisionCode) 1144 if (!item.isEmpty()) 1145 return true; 1146 return false; 1147 } 1148 1149 public CodeableConcept addServiceProvisionCode() { //3 1150 CodeableConcept t = new CodeableConcept(); 1151 if (this.serviceProvisionCode == null) 1152 this.serviceProvisionCode = new ArrayList<CodeableConcept>(); 1153 this.serviceProvisionCode.add(t); 1154 return t; 1155 } 1156 1157 public HealthcareService addServiceProvisionCode(CodeableConcept t) { //3 1158 if (t == null) 1159 return this; 1160 if (this.serviceProvisionCode == null) 1161 this.serviceProvisionCode = new ArrayList<CodeableConcept>(); 1162 this.serviceProvisionCode.add(t); 1163 return this; 1164 } 1165 1166 /** 1167 * @return The first repetition of repeating field {@link #serviceProvisionCode}, creating it if it does not already exist {3} 1168 */ 1169 public CodeableConcept getServiceProvisionCodeFirstRep() { 1170 if (getServiceProvisionCode().isEmpty()) { 1171 addServiceProvisionCode(); 1172 } 1173 return getServiceProvisionCode().get(0); 1174 } 1175 1176 /** 1177 * @return {@link #eligibility} (Does this service have specific eligibility requirements that need to be met in order to use the service?) 1178 */ 1179 public List<HealthcareServiceEligibilityComponent> getEligibility() { 1180 if (this.eligibility == null) 1181 this.eligibility = new ArrayList<HealthcareServiceEligibilityComponent>(); 1182 return this.eligibility; 1183 } 1184 1185 /** 1186 * @return Returns a reference to <code>this</code> for easy method chaining 1187 */ 1188 public HealthcareService setEligibility(List<HealthcareServiceEligibilityComponent> theEligibility) { 1189 this.eligibility = theEligibility; 1190 return this; 1191 } 1192 1193 public boolean hasEligibility() { 1194 if (this.eligibility == null) 1195 return false; 1196 for (HealthcareServiceEligibilityComponent item : this.eligibility) 1197 if (!item.isEmpty()) 1198 return true; 1199 return false; 1200 } 1201 1202 public HealthcareServiceEligibilityComponent addEligibility() { //3 1203 HealthcareServiceEligibilityComponent t = new HealthcareServiceEligibilityComponent(); 1204 if (this.eligibility == null) 1205 this.eligibility = new ArrayList<HealthcareServiceEligibilityComponent>(); 1206 this.eligibility.add(t); 1207 return t; 1208 } 1209 1210 public HealthcareService addEligibility(HealthcareServiceEligibilityComponent t) { //3 1211 if (t == null) 1212 return this; 1213 if (this.eligibility == null) 1214 this.eligibility = new ArrayList<HealthcareServiceEligibilityComponent>(); 1215 this.eligibility.add(t); 1216 return this; 1217 } 1218 1219 /** 1220 * @return The first repetition of repeating field {@link #eligibility}, creating it if it does not already exist {3} 1221 */ 1222 public HealthcareServiceEligibilityComponent getEligibilityFirstRep() { 1223 if (getEligibility().isEmpty()) { 1224 addEligibility(); 1225 } 1226 return getEligibility().get(0); 1227 } 1228 1229 /** 1230 * @return {@link #program} (Programs that this service is applicable to.) 1231 */ 1232 public List<CodeableConcept> getProgram() { 1233 if (this.program == null) 1234 this.program = new ArrayList<CodeableConcept>(); 1235 return this.program; 1236 } 1237 1238 /** 1239 * @return Returns a reference to <code>this</code> for easy method chaining 1240 */ 1241 public HealthcareService setProgram(List<CodeableConcept> theProgram) { 1242 this.program = theProgram; 1243 return this; 1244 } 1245 1246 public boolean hasProgram() { 1247 if (this.program == null) 1248 return false; 1249 for (CodeableConcept item : this.program) 1250 if (!item.isEmpty()) 1251 return true; 1252 return false; 1253 } 1254 1255 public CodeableConcept addProgram() { //3 1256 CodeableConcept t = new CodeableConcept(); 1257 if (this.program == null) 1258 this.program = new ArrayList<CodeableConcept>(); 1259 this.program.add(t); 1260 return t; 1261 } 1262 1263 public HealthcareService addProgram(CodeableConcept t) { //3 1264 if (t == null) 1265 return this; 1266 if (this.program == null) 1267 this.program = new ArrayList<CodeableConcept>(); 1268 this.program.add(t); 1269 return this; 1270 } 1271 1272 /** 1273 * @return The first repetition of repeating field {@link #program}, creating it if it does not already exist {3} 1274 */ 1275 public CodeableConcept getProgramFirstRep() { 1276 if (getProgram().isEmpty()) { 1277 addProgram(); 1278 } 1279 return getProgram().get(0); 1280 } 1281 1282 /** 1283 * @return {@link #characteristic} (Collection of characteristics (attributes).) 1284 */ 1285 public List<CodeableConcept> getCharacteristic() { 1286 if (this.characteristic == null) 1287 this.characteristic = new ArrayList<CodeableConcept>(); 1288 return this.characteristic; 1289 } 1290 1291 /** 1292 * @return Returns a reference to <code>this</code> for easy method chaining 1293 */ 1294 public HealthcareService setCharacteristic(List<CodeableConcept> theCharacteristic) { 1295 this.characteristic = theCharacteristic; 1296 return this; 1297 } 1298 1299 public boolean hasCharacteristic() { 1300 if (this.characteristic == null) 1301 return false; 1302 for (CodeableConcept item : this.characteristic) 1303 if (!item.isEmpty()) 1304 return true; 1305 return false; 1306 } 1307 1308 public CodeableConcept addCharacteristic() { //3 1309 CodeableConcept t = new CodeableConcept(); 1310 if (this.characteristic == null) 1311 this.characteristic = new ArrayList<CodeableConcept>(); 1312 this.characteristic.add(t); 1313 return t; 1314 } 1315 1316 public HealthcareService addCharacteristic(CodeableConcept t) { //3 1317 if (t == null) 1318 return this; 1319 if (this.characteristic == null) 1320 this.characteristic = new ArrayList<CodeableConcept>(); 1321 this.characteristic.add(t); 1322 return this; 1323 } 1324 1325 /** 1326 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist {3} 1327 */ 1328 public CodeableConcept getCharacteristicFirstRep() { 1329 if (getCharacteristic().isEmpty()) { 1330 addCharacteristic(); 1331 } 1332 return getCharacteristic().get(0); 1333 } 1334 1335 /** 1336 * @return {@link #communication} (Some services are specifically made available in multiple languages, this property permits a directory to declare the languages this is offered in. Typically this is only provided where a service operates in communities with mixed languages used.) 1337 */ 1338 public List<CodeableConcept> getCommunication() { 1339 if (this.communication == null) 1340 this.communication = new ArrayList<CodeableConcept>(); 1341 return this.communication; 1342 } 1343 1344 /** 1345 * @return Returns a reference to <code>this</code> for easy method chaining 1346 */ 1347 public HealthcareService setCommunication(List<CodeableConcept> theCommunication) { 1348 this.communication = theCommunication; 1349 return this; 1350 } 1351 1352 public boolean hasCommunication() { 1353 if (this.communication == null) 1354 return false; 1355 for (CodeableConcept item : this.communication) 1356 if (!item.isEmpty()) 1357 return true; 1358 return false; 1359 } 1360 1361 public CodeableConcept addCommunication() { //3 1362 CodeableConcept t = new CodeableConcept(); 1363 if (this.communication == null) 1364 this.communication = new ArrayList<CodeableConcept>(); 1365 this.communication.add(t); 1366 return t; 1367 } 1368 1369 public HealthcareService addCommunication(CodeableConcept t) { //3 1370 if (t == null) 1371 return this; 1372 if (this.communication == null) 1373 this.communication = new ArrayList<CodeableConcept>(); 1374 this.communication.add(t); 1375 return this; 1376 } 1377 1378 /** 1379 * @return The first repetition of repeating field {@link #communication}, creating it if it does not already exist {3} 1380 */ 1381 public CodeableConcept getCommunicationFirstRep() { 1382 if (getCommunication().isEmpty()) { 1383 addCommunication(); 1384 } 1385 return getCommunication().get(0); 1386 } 1387 1388 /** 1389 * @return {@link #referralMethod} (Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required.) 1390 */ 1391 public List<CodeableConcept> getReferralMethod() { 1392 if (this.referralMethod == null) 1393 this.referralMethod = new ArrayList<CodeableConcept>(); 1394 return this.referralMethod; 1395 } 1396 1397 /** 1398 * @return Returns a reference to <code>this</code> for easy method chaining 1399 */ 1400 public HealthcareService setReferralMethod(List<CodeableConcept> theReferralMethod) { 1401 this.referralMethod = theReferralMethod; 1402 return this; 1403 } 1404 1405 public boolean hasReferralMethod() { 1406 if (this.referralMethod == null) 1407 return false; 1408 for (CodeableConcept item : this.referralMethod) 1409 if (!item.isEmpty()) 1410 return true; 1411 return false; 1412 } 1413 1414 public CodeableConcept addReferralMethod() { //3 1415 CodeableConcept t = new CodeableConcept(); 1416 if (this.referralMethod == null) 1417 this.referralMethod = new ArrayList<CodeableConcept>(); 1418 this.referralMethod.add(t); 1419 return t; 1420 } 1421 1422 public HealthcareService addReferralMethod(CodeableConcept t) { //3 1423 if (t == null) 1424 return this; 1425 if (this.referralMethod == null) 1426 this.referralMethod = new ArrayList<CodeableConcept>(); 1427 this.referralMethod.add(t); 1428 return this; 1429 } 1430 1431 /** 1432 * @return The first repetition of repeating field {@link #referralMethod}, creating it if it does not already exist {3} 1433 */ 1434 public CodeableConcept getReferralMethodFirstRep() { 1435 if (getReferralMethod().isEmpty()) { 1436 addReferralMethod(); 1437 } 1438 return getReferralMethod().get(0); 1439 } 1440 1441 /** 1442 * @return {@link #appointmentRequired} (Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service.). This is the underlying object with id, value and extensions. The accessor "getAppointmentRequired" gives direct access to the value 1443 */ 1444 public BooleanType getAppointmentRequiredElement() { 1445 if (this.appointmentRequired == null) 1446 if (Configuration.errorOnAutoCreate()) 1447 throw new Error("Attempt to auto-create HealthcareService.appointmentRequired"); 1448 else if (Configuration.doAutoCreate()) 1449 this.appointmentRequired = new BooleanType(); // bb 1450 return this.appointmentRequired; 1451 } 1452 1453 public boolean hasAppointmentRequiredElement() { 1454 return this.appointmentRequired != null && !this.appointmentRequired.isEmpty(); 1455 } 1456 1457 public boolean hasAppointmentRequired() { 1458 return this.appointmentRequired != null && !this.appointmentRequired.isEmpty(); 1459 } 1460 1461 /** 1462 * @param value {@link #appointmentRequired} (Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service.). This is the underlying object with id, value and extensions. The accessor "getAppointmentRequired" gives direct access to the value 1463 */ 1464 public HealthcareService setAppointmentRequiredElement(BooleanType value) { 1465 this.appointmentRequired = value; 1466 return this; 1467 } 1468 1469 /** 1470 * @return Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service. 1471 */ 1472 public boolean getAppointmentRequired() { 1473 return this.appointmentRequired == null || this.appointmentRequired.isEmpty() ? false : this.appointmentRequired.getValue(); 1474 } 1475 1476 /** 1477 * @param value Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service. 1478 */ 1479 public HealthcareService setAppointmentRequired(boolean value) { 1480 if (this.appointmentRequired == null) 1481 this.appointmentRequired = new BooleanType(); 1482 this.appointmentRequired.setValue(value); 1483 return this; 1484 } 1485 1486 /** 1487 * @return {@link #availability} (A collection of times that the healthcare service is available.) 1488 */ 1489 public List<Availability> getAvailability() { 1490 if (this.availability == null) 1491 this.availability = new ArrayList<Availability>(); 1492 return this.availability; 1493 } 1494 1495 /** 1496 * @return Returns a reference to <code>this</code> for easy method chaining 1497 */ 1498 public HealthcareService setAvailability(List<Availability> theAvailability) { 1499 this.availability = theAvailability; 1500 return this; 1501 } 1502 1503 public boolean hasAvailability() { 1504 if (this.availability == null) 1505 return false; 1506 for (Availability item : this.availability) 1507 if (!item.isEmpty()) 1508 return true; 1509 return false; 1510 } 1511 1512 public Availability addAvailability() { //3 1513 Availability t = new Availability(); 1514 if (this.availability == null) 1515 this.availability = new ArrayList<Availability>(); 1516 this.availability.add(t); 1517 return t; 1518 } 1519 1520 public HealthcareService addAvailability(Availability t) { //3 1521 if (t == null) 1522 return this; 1523 if (this.availability == null) 1524 this.availability = new ArrayList<Availability>(); 1525 this.availability.add(t); 1526 return this; 1527 } 1528 1529 /** 1530 * @return The first repetition of repeating field {@link #availability}, creating it if it does not already exist {3} 1531 */ 1532 public Availability getAvailabilityFirstRep() { 1533 if (getAvailability().isEmpty()) { 1534 addAvailability(); 1535 } 1536 return getAvailability().get(0); 1537 } 1538 1539 /** 1540 * @return {@link #endpoint} (Technical endpoints providing access to services operated for the specific healthcare services defined at this resource.) 1541 */ 1542 public List<Reference> getEndpoint() { 1543 if (this.endpoint == null) 1544 this.endpoint = new ArrayList<Reference>(); 1545 return this.endpoint; 1546 } 1547 1548 /** 1549 * @return Returns a reference to <code>this</code> for easy method chaining 1550 */ 1551 public HealthcareService setEndpoint(List<Reference> theEndpoint) { 1552 this.endpoint = theEndpoint; 1553 return this; 1554 } 1555 1556 public boolean hasEndpoint() { 1557 if (this.endpoint == null) 1558 return false; 1559 for (Reference item : this.endpoint) 1560 if (!item.isEmpty()) 1561 return true; 1562 return false; 1563 } 1564 1565 public Reference addEndpoint() { //3 1566 Reference t = new Reference(); 1567 if (this.endpoint == null) 1568 this.endpoint = new ArrayList<Reference>(); 1569 this.endpoint.add(t); 1570 return t; 1571 } 1572 1573 public HealthcareService addEndpoint(Reference t) { //3 1574 if (t == null) 1575 return this; 1576 if (this.endpoint == null) 1577 this.endpoint = new ArrayList<Reference>(); 1578 this.endpoint.add(t); 1579 return this; 1580 } 1581 1582 /** 1583 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist {3} 1584 */ 1585 public Reference getEndpointFirstRep() { 1586 if (getEndpoint().isEmpty()) { 1587 addEndpoint(); 1588 } 1589 return getEndpoint().get(0); 1590 } 1591 1592 protected void listChildren(List<Property> children) { 1593 super.listChildren(children); 1594 children.add(new Property("identifier", "Identifier", "External identifiers for this item.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1595 children.add(new Property("active", "boolean", "This flag is used to mark the record to not be used. This is not used when a center is closed for maintenance, or for holidays, the notAvailable period is to be used for this.", 0, 1, active)); 1596 children.add(new Property("providedBy", "Reference(Organization)", "The organization that provides this healthcare service.", 0, 1, providedBy)); 1597 children.add(new Property("offeredIn", "Reference(HealthcareService)", "When the HealthcareService is representing a specific, schedulable service, the availableIn property can refer to a generic service.", 0, java.lang.Integer.MAX_VALUE, offeredIn)); 1598 children.add(new Property("category", "CodeableConcept", "Identifies the broad category of service being performed or delivered.", 0, java.lang.Integer.MAX_VALUE, category)); 1599 children.add(new Property("type", "CodeableConcept", "The specific type of service that may be delivered or performed.", 0, java.lang.Integer.MAX_VALUE, type)); 1600 children.add(new Property("specialty", "CodeableConcept", "Collection of specialties handled by the Healthcare service. This is more of a medical term.", 0, java.lang.Integer.MAX_VALUE, specialty)); 1601 children.add(new Property("location", "Reference(Location)", "The location(s) where this healthcare service may be provided.", 0, java.lang.Integer.MAX_VALUE, location)); 1602 children.add(new Property("name", "string", "Further description of the service as it would be presented to a consumer while searching.", 0, 1, name)); 1603 children.add(new Property("comment", "markdown", "Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName.", 0, 1, comment)); 1604 children.add(new Property("extraDetails", "markdown", "Extra details about the service that can't be placed in the other fields.", 0, 1, extraDetails)); 1605 children.add(new Property("photo", "Attachment", "If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list.", 0, 1, photo)); 1606 children.add(new Property("contact", "ExtendedContactDetail", "The contact details of communication devices available relevant to the specific HealthcareService. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 0, java.lang.Integer.MAX_VALUE, contact)); 1607 children.add(new Property("coverageArea", "Reference(Location)", "The location(s) that this service is available to (not where the service is provided).", 0, java.lang.Integer.MAX_VALUE, coverageArea)); 1608 children.add(new Property("serviceProvisionCode", "CodeableConcept", "The code(s) that detail the conditions under which the healthcare service is available/offered.", 0, java.lang.Integer.MAX_VALUE, serviceProvisionCode)); 1609 children.add(new Property("eligibility", "", "Does this service have specific eligibility requirements that need to be met in order to use the service?", 0, java.lang.Integer.MAX_VALUE, eligibility)); 1610 children.add(new Property("program", "CodeableConcept", "Programs that this service is applicable to.", 0, java.lang.Integer.MAX_VALUE, program)); 1611 children.add(new Property("characteristic", "CodeableConcept", "Collection of characteristics (attributes).", 0, java.lang.Integer.MAX_VALUE, characteristic)); 1612 children.add(new Property("communication", "CodeableConcept", "Some services are specifically made available in multiple languages, this property permits a directory to declare the languages this is offered in. Typically this is only provided where a service operates in communities with mixed languages used.", 0, java.lang.Integer.MAX_VALUE, communication)); 1613 children.add(new Property("referralMethod", "CodeableConcept", "Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required.", 0, java.lang.Integer.MAX_VALUE, referralMethod)); 1614 children.add(new Property("appointmentRequired", "boolean", "Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service.", 0, 1, appointmentRequired)); 1615 children.add(new Property("availability", "Availability", "A collection of times that the healthcare service is available.", 0, java.lang.Integer.MAX_VALUE, availability)); 1616 children.add(new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for the specific healthcare services defined at this resource.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 1617 } 1618 1619 @Override 1620 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1621 switch (_hash) { 1622 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "External identifiers for this item.", 0, java.lang.Integer.MAX_VALUE, identifier); 1623 case -1422950650: /*active*/ return new Property("active", "boolean", "This flag is used to mark the record to not be used. This is not used when a center is closed for maintenance, or for holidays, the notAvailable period is to be used for this.", 0, 1, active); 1624 case 205136282: /*providedBy*/ return new Property("providedBy", "Reference(Organization)", "The organization that provides this healthcare service.", 0, 1, providedBy); 1625 case 1945040512: /*offeredIn*/ return new Property("offeredIn", "Reference(HealthcareService)", "When the HealthcareService is representing a specific, schedulable service, the availableIn property can refer to a generic service.", 0, java.lang.Integer.MAX_VALUE, offeredIn); 1626 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Identifies the broad category of service being performed or delivered.", 0, java.lang.Integer.MAX_VALUE, category); 1627 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The specific type of service that may be delivered or performed.", 0, java.lang.Integer.MAX_VALUE, type); 1628 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "Collection of specialties handled by the Healthcare service. This is more of a medical term.", 0, java.lang.Integer.MAX_VALUE, specialty); 1629 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The location(s) where this healthcare service may be provided.", 0, java.lang.Integer.MAX_VALUE, location); 1630 case 3373707: /*name*/ return new Property("name", "string", "Further description of the service as it would be presented to a consumer while searching.", 0, 1, name); 1631 case 950398559: /*comment*/ return new Property("comment", "markdown", "Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName.", 0, 1, comment); 1632 case -1469168622: /*extraDetails*/ return new Property("extraDetails", "markdown", "Extra details about the service that can't be placed in the other fields.", 0, 1, extraDetails); 1633 case 106642994: /*photo*/ return new Property("photo", "Attachment", "If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list.", 0, 1, photo); 1634 case 951526432: /*contact*/ return new Property("contact", "ExtendedContactDetail", "The contact details of communication devices available relevant to the specific HealthcareService. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 0, java.lang.Integer.MAX_VALUE, contact); 1635 case -1532328299: /*coverageArea*/ return new Property("coverageArea", "Reference(Location)", "The location(s) that this service is available to (not where the service is provided).", 0, java.lang.Integer.MAX_VALUE, coverageArea); 1636 case 1504575405: /*serviceProvisionCode*/ return new Property("serviceProvisionCode", "CodeableConcept", "The code(s) that detail the conditions under which the healthcare service is available/offered.", 0, java.lang.Integer.MAX_VALUE, serviceProvisionCode); 1637 case -930847859: /*eligibility*/ return new Property("eligibility", "", "Does this service have specific eligibility requirements that need to be met in order to use the service?", 0, java.lang.Integer.MAX_VALUE, eligibility); 1638 case -309387644: /*program*/ return new Property("program", "CodeableConcept", "Programs that this service is applicable to.", 0, java.lang.Integer.MAX_VALUE, program); 1639 case 366313883: /*characteristic*/ return new Property("characteristic", "CodeableConcept", "Collection of characteristics (attributes).", 0, java.lang.Integer.MAX_VALUE, characteristic); 1640 case -1035284522: /*communication*/ return new Property("communication", "CodeableConcept", "Some services are specifically made available in multiple languages, this property permits a directory to declare the languages this is offered in. Typically this is only provided where a service operates in communities with mixed languages used.", 0, java.lang.Integer.MAX_VALUE, communication); 1641 case -2092740898: /*referralMethod*/ return new Property("referralMethod", "CodeableConcept", "Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required.", 0, java.lang.Integer.MAX_VALUE, referralMethod); 1642 case 427220062: /*appointmentRequired*/ return new Property("appointmentRequired", "boolean", "Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service.", 0, 1, appointmentRequired); 1643 case 1997542747: /*availability*/ return new Property("availability", "Availability", "A collection of times that the healthcare service is available.", 0, java.lang.Integer.MAX_VALUE, availability); 1644 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for the specific healthcare services defined at this resource.", 0, java.lang.Integer.MAX_VALUE, endpoint); 1645 default: return super.getNamedProperty(_hash, _name, _checkValid); 1646 } 1647 1648 } 1649 1650 @Override 1651 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1652 switch (hash) { 1653 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1654 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 1655 case 205136282: /*providedBy*/ return this.providedBy == null ? new Base[0] : new Base[] {this.providedBy}; // Reference 1656 case 1945040512: /*offeredIn*/ return this.offeredIn == null ? new Base[0] : this.offeredIn.toArray(new Base[this.offeredIn.size()]); // Reference 1657 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1658 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1659 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 1660 case 1901043637: /*location*/ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // Reference 1661 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1662 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // MarkdownType 1663 case -1469168622: /*extraDetails*/ return this.extraDetails == null ? new Base[0] : new Base[] {this.extraDetails}; // MarkdownType 1664 case 106642994: /*photo*/ return this.photo == null ? new Base[0] : new Base[] {this.photo}; // Attachment 1665 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ExtendedContactDetail 1666 case -1532328299: /*coverageArea*/ return this.coverageArea == null ? new Base[0] : this.coverageArea.toArray(new Base[this.coverageArea.size()]); // Reference 1667 case 1504575405: /*serviceProvisionCode*/ return this.serviceProvisionCode == null ? new Base[0] : this.serviceProvisionCode.toArray(new Base[this.serviceProvisionCode.size()]); // CodeableConcept 1668 case -930847859: /*eligibility*/ return this.eligibility == null ? new Base[0] : this.eligibility.toArray(new Base[this.eligibility.size()]); // HealthcareServiceEligibilityComponent 1669 case -309387644: /*program*/ return this.program == null ? new Base[0] : this.program.toArray(new Base[this.program.size()]); // CodeableConcept 1670 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // CodeableConcept 1671 case -1035284522: /*communication*/ return this.communication == null ? new Base[0] : this.communication.toArray(new Base[this.communication.size()]); // CodeableConcept 1672 case -2092740898: /*referralMethod*/ return this.referralMethod == null ? new Base[0] : this.referralMethod.toArray(new Base[this.referralMethod.size()]); // CodeableConcept 1673 case 427220062: /*appointmentRequired*/ return this.appointmentRequired == null ? new Base[0] : new Base[] {this.appointmentRequired}; // BooleanType 1674 case 1997542747: /*availability*/ return this.availability == null ? new Base[0] : this.availability.toArray(new Base[this.availability.size()]); // Availability 1675 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 1676 default: return super.getProperty(hash, name, checkValid); 1677 } 1678 1679 } 1680 1681 @Override 1682 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1683 switch (hash) { 1684 case -1618432855: // identifier 1685 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1686 return value; 1687 case -1422950650: // active 1688 this.active = TypeConvertor.castToBoolean(value); // BooleanType 1689 return value; 1690 case 205136282: // providedBy 1691 this.providedBy = TypeConvertor.castToReference(value); // Reference 1692 return value; 1693 case 1945040512: // offeredIn 1694 this.getOfferedIn().add(TypeConvertor.castToReference(value)); // Reference 1695 return value; 1696 case 50511102: // category 1697 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1698 return value; 1699 case 3575610: // type 1700 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1701 return value; 1702 case -1694759682: // specialty 1703 this.getSpecialty().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1704 return value; 1705 case 1901043637: // location 1706 this.getLocation().add(TypeConvertor.castToReference(value)); // Reference 1707 return value; 1708 case 3373707: // name 1709 this.name = TypeConvertor.castToString(value); // StringType 1710 return value; 1711 case 950398559: // comment 1712 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 1713 return value; 1714 case -1469168622: // extraDetails 1715 this.extraDetails = TypeConvertor.castToMarkdown(value); // MarkdownType 1716 return value; 1717 case 106642994: // photo 1718 this.photo = TypeConvertor.castToAttachment(value); // Attachment 1719 return value; 1720 case 951526432: // contact 1721 this.getContact().add(TypeConvertor.castToExtendedContactDetail(value)); // ExtendedContactDetail 1722 return value; 1723 case -1532328299: // coverageArea 1724 this.getCoverageArea().add(TypeConvertor.castToReference(value)); // Reference 1725 return value; 1726 case 1504575405: // serviceProvisionCode 1727 this.getServiceProvisionCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1728 return value; 1729 case -930847859: // eligibility 1730 this.getEligibility().add((HealthcareServiceEligibilityComponent) value); // HealthcareServiceEligibilityComponent 1731 return value; 1732 case -309387644: // program 1733 this.getProgram().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1734 return value; 1735 case 366313883: // characteristic 1736 this.getCharacteristic().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1737 return value; 1738 case -1035284522: // communication 1739 this.getCommunication().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1740 return value; 1741 case -2092740898: // referralMethod 1742 this.getReferralMethod().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1743 return value; 1744 case 427220062: // appointmentRequired 1745 this.appointmentRequired = TypeConvertor.castToBoolean(value); // BooleanType 1746 return value; 1747 case 1997542747: // availability 1748 this.getAvailability().add(TypeConvertor.castToAvailability(value)); // Availability 1749 return value; 1750 case 1741102485: // endpoint 1751 this.getEndpoint().add(TypeConvertor.castToReference(value)); // Reference 1752 return value; 1753 default: return super.setProperty(hash, name, value); 1754 } 1755 1756 } 1757 1758 @Override 1759 public Base setProperty(String name, Base value) throws FHIRException { 1760 if (name.equals("identifier")) { 1761 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1762 } else if (name.equals("active")) { 1763 this.active = TypeConvertor.castToBoolean(value); // BooleanType 1764 } else if (name.equals("providedBy")) { 1765 this.providedBy = TypeConvertor.castToReference(value); // Reference 1766 } else if (name.equals("offeredIn")) { 1767 this.getOfferedIn().add(TypeConvertor.castToReference(value)); 1768 } else if (name.equals("category")) { 1769 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 1770 } else if (name.equals("type")) { 1771 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 1772 } else if (name.equals("specialty")) { 1773 this.getSpecialty().add(TypeConvertor.castToCodeableConcept(value)); 1774 } else if (name.equals("location")) { 1775 this.getLocation().add(TypeConvertor.castToReference(value)); 1776 } else if (name.equals("name")) { 1777 this.name = TypeConvertor.castToString(value); // StringType 1778 } else if (name.equals("comment")) { 1779 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 1780 } else if (name.equals("extraDetails")) { 1781 this.extraDetails = TypeConvertor.castToMarkdown(value); // MarkdownType 1782 } else if (name.equals("photo")) { 1783 this.photo = TypeConvertor.castToAttachment(value); // Attachment 1784 } else if (name.equals("contact")) { 1785 this.getContact().add(TypeConvertor.castToExtendedContactDetail(value)); 1786 } else if (name.equals("coverageArea")) { 1787 this.getCoverageArea().add(TypeConvertor.castToReference(value)); 1788 } else if (name.equals("serviceProvisionCode")) { 1789 this.getServiceProvisionCode().add(TypeConvertor.castToCodeableConcept(value)); 1790 } else if (name.equals("eligibility")) { 1791 this.getEligibility().add((HealthcareServiceEligibilityComponent) value); 1792 } else if (name.equals("program")) { 1793 this.getProgram().add(TypeConvertor.castToCodeableConcept(value)); 1794 } else if (name.equals("characteristic")) { 1795 this.getCharacteristic().add(TypeConvertor.castToCodeableConcept(value)); 1796 } else if (name.equals("communication")) { 1797 this.getCommunication().add(TypeConvertor.castToCodeableConcept(value)); 1798 } else if (name.equals("referralMethod")) { 1799 this.getReferralMethod().add(TypeConvertor.castToCodeableConcept(value)); 1800 } else if (name.equals("appointmentRequired")) { 1801 this.appointmentRequired = TypeConvertor.castToBoolean(value); // BooleanType 1802 } else if (name.equals("availability")) { 1803 this.getAvailability().add(TypeConvertor.castToAvailability(value)); 1804 } else if (name.equals("endpoint")) { 1805 this.getEndpoint().add(TypeConvertor.castToReference(value)); 1806 } else 1807 return super.setProperty(name, value); 1808 return value; 1809 } 1810 1811 @Override 1812 public Base makeProperty(int hash, String name) throws FHIRException { 1813 switch (hash) { 1814 case -1618432855: return addIdentifier(); 1815 case -1422950650: return getActiveElement(); 1816 case 205136282: return getProvidedBy(); 1817 case 1945040512: return addOfferedIn(); 1818 case 50511102: return addCategory(); 1819 case 3575610: return addType(); 1820 case -1694759682: return addSpecialty(); 1821 case 1901043637: return addLocation(); 1822 case 3373707: return getNameElement(); 1823 case 950398559: return getCommentElement(); 1824 case -1469168622: return getExtraDetailsElement(); 1825 case 106642994: return getPhoto(); 1826 case 951526432: return addContact(); 1827 case -1532328299: return addCoverageArea(); 1828 case 1504575405: return addServiceProvisionCode(); 1829 case -930847859: return addEligibility(); 1830 case -309387644: return addProgram(); 1831 case 366313883: return addCharacteristic(); 1832 case -1035284522: return addCommunication(); 1833 case -2092740898: return addReferralMethod(); 1834 case 427220062: return getAppointmentRequiredElement(); 1835 case 1997542747: return addAvailability(); 1836 case 1741102485: return addEndpoint(); 1837 default: return super.makeProperty(hash, name); 1838 } 1839 1840 } 1841 1842 @Override 1843 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1844 switch (hash) { 1845 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1846 case -1422950650: /*active*/ return new String[] {"boolean"}; 1847 case 205136282: /*providedBy*/ return new String[] {"Reference"}; 1848 case 1945040512: /*offeredIn*/ return new String[] {"Reference"}; 1849 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1850 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1851 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 1852 case 1901043637: /*location*/ return new String[] {"Reference"}; 1853 case 3373707: /*name*/ return new String[] {"string"}; 1854 case 950398559: /*comment*/ return new String[] {"markdown"}; 1855 case -1469168622: /*extraDetails*/ return new String[] {"markdown"}; 1856 case 106642994: /*photo*/ return new String[] {"Attachment"}; 1857 case 951526432: /*contact*/ return new String[] {"ExtendedContactDetail"}; 1858 case -1532328299: /*coverageArea*/ return new String[] {"Reference"}; 1859 case 1504575405: /*serviceProvisionCode*/ return new String[] {"CodeableConcept"}; 1860 case -930847859: /*eligibility*/ return new String[] {}; 1861 case -309387644: /*program*/ return new String[] {"CodeableConcept"}; 1862 case 366313883: /*characteristic*/ return new String[] {"CodeableConcept"}; 1863 case -1035284522: /*communication*/ return new String[] {"CodeableConcept"}; 1864 case -2092740898: /*referralMethod*/ return new String[] {"CodeableConcept"}; 1865 case 427220062: /*appointmentRequired*/ return new String[] {"boolean"}; 1866 case 1997542747: /*availability*/ return new String[] {"Availability"}; 1867 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 1868 default: return super.getTypesForProperty(hash, name); 1869 } 1870 1871 } 1872 1873 @Override 1874 public Base addChild(String name) throws FHIRException { 1875 if (name.equals("identifier")) { 1876 return addIdentifier(); 1877 } 1878 else if (name.equals("active")) { 1879 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.active"); 1880 } 1881 else if (name.equals("providedBy")) { 1882 this.providedBy = new Reference(); 1883 return this.providedBy; 1884 } 1885 else if (name.equals("offeredIn")) { 1886 return addOfferedIn(); 1887 } 1888 else if (name.equals("category")) { 1889 return addCategory(); 1890 } 1891 else if (name.equals("type")) { 1892 return addType(); 1893 } 1894 else if (name.equals("specialty")) { 1895 return addSpecialty(); 1896 } 1897 else if (name.equals("location")) { 1898 return addLocation(); 1899 } 1900 else if (name.equals("name")) { 1901 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.name"); 1902 } 1903 else if (name.equals("comment")) { 1904 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.comment"); 1905 } 1906 else if (name.equals("extraDetails")) { 1907 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.extraDetails"); 1908 } 1909 else if (name.equals("photo")) { 1910 this.photo = new Attachment(); 1911 return this.photo; 1912 } 1913 else if (name.equals("contact")) { 1914 return addContact(); 1915 } 1916 else if (name.equals("coverageArea")) { 1917 return addCoverageArea(); 1918 } 1919 else if (name.equals("serviceProvisionCode")) { 1920 return addServiceProvisionCode(); 1921 } 1922 else if (name.equals("eligibility")) { 1923 return addEligibility(); 1924 } 1925 else if (name.equals("program")) { 1926 return addProgram(); 1927 } 1928 else if (name.equals("characteristic")) { 1929 return addCharacteristic(); 1930 } 1931 else if (name.equals("communication")) { 1932 return addCommunication(); 1933 } 1934 else if (name.equals("referralMethod")) { 1935 return addReferralMethod(); 1936 } 1937 else if (name.equals("appointmentRequired")) { 1938 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.appointmentRequired"); 1939 } 1940 else if (name.equals("availability")) { 1941 return addAvailability(); 1942 } 1943 else if (name.equals("endpoint")) { 1944 return addEndpoint(); 1945 } 1946 else 1947 return super.addChild(name); 1948 } 1949 1950 public String fhirType() { 1951 return "HealthcareService"; 1952 1953 } 1954 1955 public HealthcareService copy() { 1956 HealthcareService dst = new HealthcareService(); 1957 copyValues(dst); 1958 return dst; 1959 } 1960 1961 public void copyValues(HealthcareService dst) { 1962 super.copyValues(dst); 1963 if (identifier != null) { 1964 dst.identifier = new ArrayList<Identifier>(); 1965 for (Identifier i : identifier) 1966 dst.identifier.add(i.copy()); 1967 }; 1968 dst.active = active == null ? null : active.copy(); 1969 dst.providedBy = providedBy == null ? null : providedBy.copy(); 1970 if (offeredIn != null) { 1971 dst.offeredIn = new ArrayList<Reference>(); 1972 for (Reference i : offeredIn) 1973 dst.offeredIn.add(i.copy()); 1974 }; 1975 if (category != null) { 1976 dst.category = new ArrayList<CodeableConcept>(); 1977 for (CodeableConcept i : category) 1978 dst.category.add(i.copy()); 1979 }; 1980 if (type != null) { 1981 dst.type = new ArrayList<CodeableConcept>(); 1982 for (CodeableConcept i : type) 1983 dst.type.add(i.copy()); 1984 }; 1985 if (specialty != null) { 1986 dst.specialty = new ArrayList<CodeableConcept>(); 1987 for (CodeableConcept i : specialty) 1988 dst.specialty.add(i.copy()); 1989 }; 1990 if (location != null) { 1991 dst.location = new ArrayList<Reference>(); 1992 for (Reference i : location) 1993 dst.location.add(i.copy()); 1994 }; 1995 dst.name = name == null ? null : name.copy(); 1996 dst.comment = comment == null ? null : comment.copy(); 1997 dst.extraDetails = extraDetails == null ? null : extraDetails.copy(); 1998 dst.photo = photo == null ? null : photo.copy(); 1999 if (contact != null) { 2000 dst.contact = new ArrayList<ExtendedContactDetail>(); 2001 for (ExtendedContactDetail i : contact) 2002 dst.contact.add(i.copy()); 2003 }; 2004 if (coverageArea != null) { 2005 dst.coverageArea = new ArrayList<Reference>(); 2006 for (Reference i : coverageArea) 2007 dst.coverageArea.add(i.copy()); 2008 }; 2009 if (serviceProvisionCode != null) { 2010 dst.serviceProvisionCode = new ArrayList<CodeableConcept>(); 2011 for (CodeableConcept i : serviceProvisionCode) 2012 dst.serviceProvisionCode.add(i.copy()); 2013 }; 2014 if (eligibility != null) { 2015 dst.eligibility = new ArrayList<HealthcareServiceEligibilityComponent>(); 2016 for (HealthcareServiceEligibilityComponent i : eligibility) 2017 dst.eligibility.add(i.copy()); 2018 }; 2019 if (program != null) { 2020 dst.program = new ArrayList<CodeableConcept>(); 2021 for (CodeableConcept i : program) 2022 dst.program.add(i.copy()); 2023 }; 2024 if (characteristic != null) { 2025 dst.characteristic = new ArrayList<CodeableConcept>(); 2026 for (CodeableConcept i : characteristic) 2027 dst.characteristic.add(i.copy()); 2028 }; 2029 if (communication != null) { 2030 dst.communication = new ArrayList<CodeableConcept>(); 2031 for (CodeableConcept i : communication) 2032 dst.communication.add(i.copy()); 2033 }; 2034 if (referralMethod != null) { 2035 dst.referralMethod = new ArrayList<CodeableConcept>(); 2036 for (CodeableConcept i : referralMethod) 2037 dst.referralMethod.add(i.copy()); 2038 }; 2039 dst.appointmentRequired = appointmentRequired == null ? null : appointmentRequired.copy(); 2040 if (availability != null) { 2041 dst.availability = new ArrayList<Availability>(); 2042 for (Availability i : availability) 2043 dst.availability.add(i.copy()); 2044 }; 2045 if (endpoint != null) { 2046 dst.endpoint = new ArrayList<Reference>(); 2047 for (Reference i : endpoint) 2048 dst.endpoint.add(i.copy()); 2049 }; 2050 } 2051 2052 protected HealthcareService typedCopy() { 2053 return copy(); 2054 } 2055 2056 @Override 2057 public boolean equalsDeep(Base other_) { 2058 if (!super.equalsDeep(other_)) 2059 return false; 2060 if (!(other_ instanceof HealthcareService)) 2061 return false; 2062 HealthcareService o = (HealthcareService) other_; 2063 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(providedBy, o.providedBy, true) 2064 && compareDeep(offeredIn, o.offeredIn, true) && compareDeep(category, o.category, true) && compareDeep(type, o.type, true) 2065 && compareDeep(specialty, o.specialty, true) && compareDeep(location, o.location, true) && compareDeep(name, o.name, true) 2066 && compareDeep(comment, o.comment, true) && compareDeep(extraDetails, o.extraDetails, true) && compareDeep(photo, o.photo, true) 2067 && compareDeep(contact, o.contact, true) && compareDeep(coverageArea, o.coverageArea, true) && compareDeep(serviceProvisionCode, o.serviceProvisionCode, true) 2068 && compareDeep(eligibility, o.eligibility, true) && compareDeep(program, o.program, true) && compareDeep(characteristic, o.characteristic, true) 2069 && compareDeep(communication, o.communication, true) && compareDeep(referralMethod, o.referralMethod, true) 2070 && compareDeep(appointmentRequired, o.appointmentRequired, true) && compareDeep(availability, o.availability, true) 2071 && compareDeep(endpoint, o.endpoint, true); 2072 } 2073 2074 @Override 2075 public boolean equalsShallow(Base other_) { 2076 if (!super.equalsShallow(other_)) 2077 return false; 2078 if (!(other_ instanceof HealthcareService)) 2079 return false; 2080 HealthcareService o = (HealthcareService) other_; 2081 return compareValues(active, o.active, true) && compareValues(name, o.name, true) && compareValues(comment, o.comment, true) 2082 && compareValues(extraDetails, o.extraDetails, true) && compareValues(appointmentRequired, o.appointmentRequired, true) 2083 ; 2084 } 2085 2086 public boolean isEmpty() { 2087 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, providedBy 2088 , offeredIn, category, type, specialty, location, name, comment, extraDetails 2089 , photo, contact, coverageArea, serviceProvisionCode, eligibility, program, characteristic 2090 , communication, referralMethod, appointmentRequired, availability, endpoint); 2091 } 2092 2093 @Override 2094 public ResourceType getResourceType() { 2095 return ResourceType.HealthcareService; 2096 } 2097 2098 /** 2099 * Search parameter: <b>active</b> 2100 * <p> 2101 * Description: <b>The Healthcare Service is currently marked as active</b><br> 2102 * Type: <b>token</b><br> 2103 * Path: <b>HealthcareService.active</b><br> 2104 * </p> 2105 */ 2106 @SearchParamDefinition(name="active", path="HealthcareService.active", description="The Healthcare Service is currently marked as active", type="token" ) 2107 public static final String SP_ACTIVE = "active"; 2108 /** 2109 * <b>Fluent Client</b> search parameter constant for <b>active</b> 2110 * <p> 2111 * Description: <b>The Healthcare Service is currently marked as active</b><br> 2112 * Type: <b>token</b><br> 2113 * Path: <b>HealthcareService.active</b><br> 2114 * </p> 2115 */ 2116 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVE); 2117 2118 /** 2119 * Search parameter: <b>characteristic</b> 2120 * <p> 2121 * Description: <b>One of the HealthcareService's characteristics</b><br> 2122 * Type: <b>token</b><br> 2123 * Path: <b>HealthcareService.characteristic</b><br> 2124 * </p> 2125 */ 2126 @SearchParamDefinition(name="characteristic", path="HealthcareService.characteristic", description="One of the HealthcareService's characteristics", type="token" ) 2127 public static final String SP_CHARACTERISTIC = "characteristic"; 2128 /** 2129 * <b>Fluent Client</b> search parameter constant for <b>characteristic</b> 2130 * <p> 2131 * Description: <b>One of the HealthcareService's characteristics</b><br> 2132 * Type: <b>token</b><br> 2133 * Path: <b>HealthcareService.characteristic</b><br> 2134 * </p> 2135 */ 2136 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHARACTERISTIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CHARACTERISTIC); 2137 2138 /** 2139 * Search parameter: <b>communication</b> 2140 * <p> 2141 * Description: <b>Languages that are available at this service</b><br> 2142 * Type: <b>token</b><br> 2143 * Path: <b>HealthcareService.communication</b><br> 2144 * </p> 2145 */ 2146 @SearchParamDefinition(name="communication", path="HealthcareService.communication", description="Languages that are available at this service", type="token" ) 2147 public static final String SP_COMMUNICATION = "communication"; 2148 /** 2149 * <b>Fluent Client</b> search parameter constant for <b>communication</b> 2150 * <p> 2151 * Description: <b>Languages that are available at this service</b><br> 2152 * Type: <b>token</b><br> 2153 * Path: <b>HealthcareService.communication</b><br> 2154 * </p> 2155 */ 2156 public static final ca.uhn.fhir.rest.gclient.TokenClientParam COMMUNICATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_COMMUNICATION); 2157 2158 /** 2159 * Search parameter: <b>coverage-area</b> 2160 * <p> 2161 * Description: <b>Location(s) service is intended for/available to</b><br> 2162 * Type: <b>reference</b><br> 2163 * Path: <b>HealthcareService.coverageArea</b><br> 2164 * </p> 2165 */ 2166 @SearchParamDefinition(name="coverage-area", path="HealthcareService.coverageArea", description="Location(s) service is intended for/available to", type="reference", target={Location.class } ) 2167 public static final String SP_COVERAGE_AREA = "coverage-area"; 2168 /** 2169 * <b>Fluent Client</b> search parameter constant for <b>coverage-area</b> 2170 * <p> 2171 * Description: <b>Location(s) service is intended for/available to</b><br> 2172 * Type: <b>reference</b><br> 2173 * Path: <b>HealthcareService.coverageArea</b><br> 2174 * </p> 2175 */ 2176 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COVERAGE_AREA = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COVERAGE_AREA); 2177 2178/** 2179 * Constant for fluent queries to be used to add include statements. Specifies 2180 * the path value of "<b>HealthcareService:coverage-area</b>". 2181 */ 2182 public static final ca.uhn.fhir.model.api.Include INCLUDE_COVERAGE_AREA = new ca.uhn.fhir.model.api.Include("HealthcareService:coverage-area").toLocked(); 2183 2184 /** 2185 * Search parameter: <b>eligibility</b> 2186 * <p> 2187 * Description: <b>One of the HealthcareService's eligibility requirements</b><br> 2188 * Type: <b>token</b><br> 2189 * Path: <b>HealthcareService.eligibility.code</b><br> 2190 * </p> 2191 */ 2192 @SearchParamDefinition(name="eligibility", path="HealthcareService.eligibility.code", description="One of the HealthcareService's eligibility requirements", type="token" ) 2193 public static final String SP_ELIGIBILITY = "eligibility"; 2194 /** 2195 * <b>Fluent Client</b> search parameter constant for <b>eligibility</b> 2196 * <p> 2197 * Description: <b>One of the HealthcareService's eligibility requirements</b><br> 2198 * Type: <b>token</b><br> 2199 * Path: <b>HealthcareService.eligibility.code</b><br> 2200 * </p> 2201 */ 2202 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ELIGIBILITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ELIGIBILITY); 2203 2204 /** 2205 * Search parameter: <b>endpoint</b> 2206 * <p> 2207 * Description: <b>Technical endpoints providing access to electronic services operated for the healthcare service</b><br> 2208 * Type: <b>reference</b><br> 2209 * Path: <b>HealthcareService.endpoint</b><br> 2210 * </p> 2211 */ 2212 @SearchParamDefinition(name="endpoint", path="HealthcareService.endpoint", description="Technical endpoints providing access to electronic services operated for the healthcare service", type="reference", target={Endpoint.class } ) 2213 public static final String SP_ENDPOINT = "endpoint"; 2214 /** 2215 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 2216 * <p> 2217 * Description: <b>Technical endpoints providing access to electronic services operated for the healthcare service</b><br> 2218 * Type: <b>reference</b><br> 2219 * Path: <b>HealthcareService.endpoint</b><br> 2220 * </p> 2221 */ 2222 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENDPOINT); 2223 2224/** 2225 * Constant for fluent queries to be used to add include statements. Specifies 2226 * the path value of "<b>HealthcareService:endpoint</b>". 2227 */ 2228 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include("HealthcareService:endpoint").toLocked(); 2229 2230 /** 2231 * Search parameter: <b>identifier</b> 2232 * <p> 2233 * Description: <b>External identifiers for this item</b><br> 2234 * Type: <b>token</b><br> 2235 * Path: <b>HealthcareService.identifier</b><br> 2236 * </p> 2237 */ 2238 @SearchParamDefinition(name="identifier", path="HealthcareService.identifier", description="External identifiers for this item", type="token" ) 2239 public static final String SP_IDENTIFIER = "identifier"; 2240 /** 2241 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2242 * <p> 2243 * Description: <b>External identifiers for this item</b><br> 2244 * Type: <b>token</b><br> 2245 * Path: <b>HealthcareService.identifier</b><br> 2246 * </p> 2247 */ 2248 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2249 2250 /** 2251 * Search parameter: <b>location</b> 2252 * <p> 2253 * Description: <b>The location of the Healthcare Service</b><br> 2254 * Type: <b>reference</b><br> 2255 * Path: <b>HealthcareService.location</b><br> 2256 * </p> 2257 */ 2258 @SearchParamDefinition(name="location", path="HealthcareService.location", description="The location of the Healthcare Service", type="reference", target={Location.class } ) 2259 public static final String SP_LOCATION = "location"; 2260 /** 2261 * <b>Fluent Client</b> search parameter constant for <b>location</b> 2262 * <p> 2263 * Description: <b>The location of the Healthcare Service</b><br> 2264 * Type: <b>reference</b><br> 2265 * Path: <b>HealthcareService.location</b><br> 2266 * </p> 2267 */ 2268 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 2269 2270/** 2271 * Constant for fluent queries to be used to add include statements. Specifies 2272 * the path value of "<b>HealthcareService:location</b>". 2273 */ 2274 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("HealthcareService:location").toLocked(); 2275 2276 /** 2277 * Search parameter: <b>name</b> 2278 * <p> 2279 * Description: <b>A portion of the Healthcare service name</b><br> 2280 * Type: <b>string</b><br> 2281 * Path: <b>HealthcareService.name</b><br> 2282 * </p> 2283 */ 2284 @SearchParamDefinition(name="name", path="HealthcareService.name", description="A portion of the Healthcare service name", type="string" ) 2285 public static final String SP_NAME = "name"; 2286 /** 2287 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2288 * <p> 2289 * Description: <b>A portion of the Healthcare service name</b><br> 2290 * Type: <b>string</b><br> 2291 * Path: <b>HealthcareService.name</b><br> 2292 * </p> 2293 */ 2294 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 2295 2296 /** 2297 * Search parameter: <b>offered-in</b> 2298 * <p> 2299 * Description: <b>The service within which this service is offered</b><br> 2300 * Type: <b>reference</b><br> 2301 * Path: <b>HealthcareService.offeredIn</b><br> 2302 * </p> 2303 */ 2304 @SearchParamDefinition(name="offered-in", path="HealthcareService.offeredIn", description="The service within which this service is offered", type="reference", target={HealthcareService.class } ) 2305 public static final String SP_OFFERED_IN = "offered-in"; 2306 /** 2307 * <b>Fluent Client</b> search parameter constant for <b>offered-in</b> 2308 * <p> 2309 * Description: <b>The service within which this service is offered</b><br> 2310 * Type: <b>reference</b><br> 2311 * Path: <b>HealthcareService.offeredIn</b><br> 2312 * </p> 2313 */ 2314 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OFFERED_IN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_OFFERED_IN); 2315 2316/** 2317 * Constant for fluent queries to be used to add include statements. Specifies 2318 * the path value of "<b>HealthcareService:offered-in</b>". 2319 */ 2320 public static final ca.uhn.fhir.model.api.Include INCLUDE_OFFERED_IN = new ca.uhn.fhir.model.api.Include("HealthcareService:offered-in").toLocked(); 2321 2322 /** 2323 * Search parameter: <b>organization</b> 2324 * <p> 2325 * Description: <b>The organization that provides this Healthcare Service</b><br> 2326 * Type: <b>reference</b><br> 2327 * Path: <b>HealthcareService.providedBy</b><br> 2328 * </p> 2329 */ 2330 @SearchParamDefinition(name="organization", path="HealthcareService.providedBy", description="The organization that provides this Healthcare Service", type="reference", target={Organization.class } ) 2331 public static final String SP_ORGANIZATION = "organization"; 2332 /** 2333 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 2334 * <p> 2335 * Description: <b>The organization that provides this Healthcare Service</b><br> 2336 * Type: <b>reference</b><br> 2337 * Path: <b>HealthcareService.providedBy</b><br> 2338 * </p> 2339 */ 2340 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 2341 2342/** 2343 * Constant for fluent queries to be used to add include statements. Specifies 2344 * the path value of "<b>HealthcareService:organization</b>". 2345 */ 2346 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("HealthcareService:organization").toLocked(); 2347 2348 /** 2349 * Search parameter: <b>program</b> 2350 * <p> 2351 * Description: <b>One of the Programs supported by this HealthcareService</b><br> 2352 * Type: <b>token</b><br> 2353 * Path: <b>HealthcareService.program</b><br> 2354 * </p> 2355 */ 2356 @SearchParamDefinition(name="program", path="HealthcareService.program", description="One of the Programs supported by this HealthcareService", type="token" ) 2357 public static final String SP_PROGRAM = "program"; 2358 /** 2359 * <b>Fluent Client</b> search parameter constant for <b>program</b> 2360 * <p> 2361 * Description: <b>One of the Programs supported by this HealthcareService</b><br> 2362 * Type: <b>token</b><br> 2363 * Path: <b>HealthcareService.program</b><br> 2364 * </p> 2365 */ 2366 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PROGRAM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PROGRAM); 2367 2368 /** 2369 * Search parameter: <b>service-category</b> 2370 * <p> 2371 * Description: <b>Service Category of the Healthcare Service</b><br> 2372 * Type: <b>token</b><br> 2373 * Path: <b>HealthcareService.category</b><br> 2374 * </p> 2375 */ 2376 @SearchParamDefinition(name="service-category", path="HealthcareService.category", description="Service Category of the Healthcare Service", type="token" ) 2377 public static final String SP_SERVICE_CATEGORY = "service-category"; 2378 /** 2379 * <b>Fluent Client</b> search parameter constant for <b>service-category</b> 2380 * <p> 2381 * Description: <b>Service Category of the Healthcare Service</b><br> 2382 * Type: <b>token</b><br> 2383 * Path: <b>HealthcareService.category</b><br> 2384 * </p> 2385 */ 2386 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERVICE_CATEGORY); 2387 2388 /** 2389 * Search parameter: <b>service-type</b> 2390 * <p> 2391 * Description: <b>The type of service provided by this healthcare service</b><br> 2392 * Type: <b>token</b><br> 2393 * Path: <b>HealthcareService.type</b><br> 2394 * </p> 2395 */ 2396 @SearchParamDefinition(name="service-type", path="HealthcareService.type", description="The type of service provided by this healthcare service", type="token" ) 2397 public static final String SP_SERVICE_TYPE = "service-type"; 2398 /** 2399 * <b>Fluent Client</b> search parameter constant for <b>service-type</b> 2400 * <p> 2401 * Description: <b>The type of service provided by this healthcare service</b><br> 2402 * Type: <b>token</b><br> 2403 * Path: <b>HealthcareService.type</b><br> 2404 * </p> 2405 */ 2406 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERVICE_TYPE); 2407 2408 /** 2409 * Search parameter: <b>specialty</b> 2410 * <p> 2411 * Description: <b>The specialty of the service provided by this healthcare service</b><br> 2412 * Type: <b>token</b><br> 2413 * Path: <b>HealthcareService.specialty</b><br> 2414 * </p> 2415 */ 2416 @SearchParamDefinition(name="specialty", path="HealthcareService.specialty", description="The specialty of the service provided by this healthcare service", type="token" ) 2417 public static final String SP_SPECIALTY = "specialty"; 2418 /** 2419 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 2420 * <p> 2421 * Description: <b>The specialty of the service provided by this healthcare service</b><br> 2422 * Type: <b>token</b><br> 2423 * Path: <b>HealthcareService.specialty</b><br> 2424 * </p> 2425 */ 2426 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SPECIALTY); 2427 2428 2429} 2430