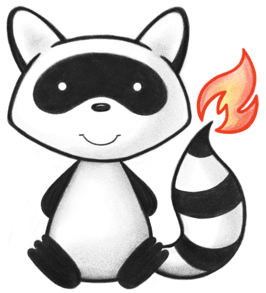
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048import ca.uhn.fhir.util.DatatypeUtil; 049import org.hl7.fhir.instance.model.api.IPrimitiveType; 050/** 051 * HumanName Type: A name, normally of a human, that can be used for other living entities (e.g. animals but not organizations) that have been assigned names by a human and may need the use of name parts or the need for usage information. 052 */ 053@DatatypeDef(name="HumanName") 054public class HumanName extends DataType implements ICompositeType { 055 056 public enum NameUse { 057 /** 058 * Known as/conventional/the one you normally use. 059 */ 060 USUAL, 061 /** 062 * The formal name as registered in an official (government) registry, but which name might not be commonly used. May be called \"legal name\". 063 */ 064 OFFICIAL, 065 /** 066 * A temporary name. Name.period can provide more detailed information. This may also be used for temporary names assigned at birth or in emergency situations. 067 */ 068 TEMP, 069 /** 070 * A name that is used to address the person in an informal manner, but is not part of their formal or usual name. 071 */ 072 NICKNAME, 073 /** 074 * Anonymous assigned name, alias, or pseudonym (used to protect a person's identity for privacy reasons). 075 */ 076 ANONYMOUS, 077 /** 078 * This name is no longer in use (or was never correct, but retained for records). 079 */ 080 OLD, 081 /** 082 * A name used prior to changing name because of marriage. This name use is for use by applications that collect and store names that were used prior to a marriage. Marriage naming customs vary greatly around the world, and are constantly changing. This term is not gender specific. The use of this term does not imply any particular history for a person's name. 083 */ 084 MAIDEN, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 public static NameUse fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("usual".equals(codeString)) 093 return USUAL; 094 if ("official".equals(codeString)) 095 return OFFICIAL; 096 if ("temp".equals(codeString)) 097 return TEMP; 098 if ("nickname".equals(codeString)) 099 return NICKNAME; 100 if ("anonymous".equals(codeString)) 101 return ANONYMOUS; 102 if ("old".equals(codeString)) 103 return OLD; 104 if ("maiden".equals(codeString)) 105 return MAIDEN; 106 if (Configuration.isAcceptInvalidEnums()) 107 return null; 108 else 109 throw new FHIRException("Unknown NameUse code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case USUAL: return "usual"; 114 case OFFICIAL: return "official"; 115 case TEMP: return "temp"; 116 case NICKNAME: return "nickname"; 117 case ANONYMOUS: return "anonymous"; 118 case OLD: return "old"; 119 case MAIDEN: return "maiden"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getSystem() { 125 switch (this) { 126 case USUAL: return "http://hl7.org/fhir/name-use"; 127 case OFFICIAL: return "http://hl7.org/fhir/name-use"; 128 case TEMP: return "http://hl7.org/fhir/name-use"; 129 case NICKNAME: return "http://hl7.org/fhir/name-use"; 130 case ANONYMOUS: return "http://hl7.org/fhir/name-use"; 131 case OLD: return "http://hl7.org/fhir/name-use"; 132 case MAIDEN: return "http://hl7.org/fhir/name-use"; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getDefinition() { 138 switch (this) { 139 case USUAL: return "Known as/conventional/the one you normally use."; 140 case OFFICIAL: return "The formal name as registered in an official (government) registry, but which name might not be commonly used. May be called \"legal name\"."; 141 case TEMP: return "A temporary name. Name.period can provide more detailed information. This may also be used for temporary names assigned at birth or in emergency situations."; 142 case NICKNAME: return "A name that is used to address the person in an informal manner, but is not part of their formal or usual name."; 143 case ANONYMOUS: return "Anonymous assigned name, alias, or pseudonym (used to protect a person's identity for privacy reasons)."; 144 case OLD: return "This name is no longer in use (or was never correct, but retained for records)."; 145 case MAIDEN: return "A name used prior to changing name because of marriage. This name use is for use by applications that collect and store names that were used prior to a marriage. Marriage naming customs vary greatly around the world, and are constantly changing. This term is not gender specific. The use of this term does not imply any particular history for a person's name."; 146 case NULL: return null; 147 default: return "?"; 148 } 149 } 150 public String getDisplay() { 151 switch (this) { 152 case USUAL: return "Usual"; 153 case OFFICIAL: return "Official"; 154 case TEMP: return "Temp"; 155 case NICKNAME: return "Nickname"; 156 case ANONYMOUS: return "Anonymous"; 157 case OLD: return "Old"; 158 case MAIDEN: return "Name changed for Marriage"; 159 case NULL: return null; 160 default: return "?"; 161 } 162 } 163 } 164 165 public static class NameUseEnumFactory implements EnumFactory<NameUse> { 166 public NameUse fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("usual".equals(codeString)) 171 return NameUse.USUAL; 172 if ("official".equals(codeString)) 173 return NameUse.OFFICIAL; 174 if ("temp".equals(codeString)) 175 return NameUse.TEMP; 176 if ("nickname".equals(codeString)) 177 return NameUse.NICKNAME; 178 if ("anonymous".equals(codeString)) 179 return NameUse.ANONYMOUS; 180 if ("old".equals(codeString)) 181 return NameUse.OLD; 182 if ("maiden".equals(codeString)) 183 return NameUse.MAIDEN; 184 throw new IllegalArgumentException("Unknown NameUse code '"+codeString+"'"); 185 } 186 public Enumeration<NameUse> fromType(PrimitiveType<?> code) throws FHIRException { 187 if (code == null) 188 return null; 189 if (code.isEmpty()) 190 return new Enumeration<NameUse>(this, NameUse.NULL, code); 191 String codeString = ((PrimitiveType) code).asStringValue(); 192 if (codeString == null || "".equals(codeString)) 193 return new Enumeration<NameUse>(this, NameUse.NULL, code); 194 if ("usual".equals(codeString)) 195 return new Enumeration<NameUse>(this, NameUse.USUAL, code); 196 if ("official".equals(codeString)) 197 return new Enumeration<NameUse>(this, NameUse.OFFICIAL, code); 198 if ("temp".equals(codeString)) 199 return new Enumeration<NameUse>(this, NameUse.TEMP, code); 200 if ("nickname".equals(codeString)) 201 return new Enumeration<NameUse>(this, NameUse.NICKNAME, code); 202 if ("anonymous".equals(codeString)) 203 return new Enumeration<NameUse>(this, NameUse.ANONYMOUS, code); 204 if ("old".equals(codeString)) 205 return new Enumeration<NameUse>(this, NameUse.OLD, code); 206 if ("maiden".equals(codeString)) 207 return new Enumeration<NameUse>(this, NameUse.MAIDEN, code); 208 throw new FHIRException("Unknown NameUse code '"+codeString+"'"); 209 } 210 public String toCode(NameUse code) { 211 if (code == NameUse.USUAL) 212 return "usual"; 213 if (code == NameUse.OFFICIAL) 214 return "official"; 215 if (code == NameUse.TEMP) 216 return "temp"; 217 if (code == NameUse.NICKNAME) 218 return "nickname"; 219 if (code == NameUse.ANONYMOUS) 220 return "anonymous"; 221 if (code == NameUse.OLD) 222 return "old"; 223 if (code == NameUse.MAIDEN) 224 return "maiden"; 225 return "?"; 226 } 227 public String toSystem(NameUse code) { 228 return code.getSystem(); 229 } 230 } 231 232 /** 233 * Identifies the purpose for this name. 234 */ 235 @Child(name = "use", type = {CodeType.class}, order=0, min=0, max=1, modifier=true, summary=true) 236 @Description(shortDefinition="usual | official | temp | nickname | anonymous | old | maiden", formalDefinition="Identifies the purpose for this name." ) 237 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/name-use") 238 protected Enumeration<NameUse> use; 239 240 /** 241 * Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts. 242 */ 243 @Child(name = "text", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 244 @Description(shortDefinition="Text representation of the full name", formalDefinition="Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts." ) 245 protected StringType text; 246 247 /** 248 * The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. 249 */ 250 @Child(name = "family", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 251 @Description(shortDefinition="Family name (often called 'Surname')", formalDefinition="The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father." ) 252 protected StringType family; 253 254 /** 255 * Given name. 256 */ 257 @Child(name = "given", type = {StringType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 258 @Description(shortDefinition="Given names (not always 'first'). Includes middle names", formalDefinition="Given name." ) 259 protected List<StringType> given; 260 261 /** 262 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name. 263 */ 264 @Child(name = "prefix", type = {StringType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 265 @Description(shortDefinition="Parts that come before the name", formalDefinition="Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name." ) 266 protected List<StringType> prefix; 267 268 /** 269 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name. 270 */ 271 @Child(name = "suffix", type = {StringType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 272 @Description(shortDefinition="Parts that come after the name", formalDefinition="Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name." ) 273 protected List<StringType> suffix; 274 275 /** 276 * Indicates the period of time when this name was valid for the named person. 277 */ 278 @Child(name = "period", type = {Period.class}, order=6, min=0, max=1, modifier=false, summary=true) 279 @Description(shortDefinition="Time period when name was/is in use", formalDefinition="Indicates the period of time when this name was valid for the named person." ) 280 protected Period period; 281 282 private static final long serialVersionUID = -507469160L; 283 284 /** 285 * Constructor 286 */ 287 public HumanName() { 288 super(); 289 } 290 291 /** 292 * @return {@link #use} (Identifies the purpose for this name.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 293 */ 294 public Enumeration<NameUse> getUseElement() { 295 if (this.use == null) 296 if (Configuration.errorOnAutoCreate()) 297 throw new Error("Attempt to auto-create HumanName.use"); 298 else if (Configuration.doAutoCreate()) 299 this.use = new Enumeration<NameUse>(new NameUseEnumFactory()); // bb 300 return this.use; 301 } 302 303 public boolean hasUseElement() { 304 return this.use != null && !this.use.isEmpty(); 305 } 306 307 public boolean hasUse() { 308 return this.use != null && !this.use.isEmpty(); 309 } 310 311 /** 312 * @param value {@link #use} (Identifies the purpose for this name.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 313 */ 314 public HumanName setUseElement(Enumeration<NameUse> value) { 315 this.use = value; 316 return this; 317 } 318 319 /** 320 * @return Identifies the purpose for this name. 321 */ 322 public NameUse getUse() { 323 return this.use == null ? null : this.use.getValue(); 324 } 325 326 /** 327 * @param value Identifies the purpose for this name. 328 */ 329 public HumanName setUse(NameUse value) { 330 if (value == null) 331 this.use = null; 332 else { 333 if (this.use == null) 334 this.use = new Enumeration<NameUse>(new NameUseEnumFactory()); 335 this.use.setValue(value); 336 } 337 return this; 338 } 339 340 /** 341 * @return {@link #text} (Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 342 */ 343 public StringType getTextElement() { 344 if (this.text == null) 345 if (Configuration.errorOnAutoCreate()) 346 throw new Error("Attempt to auto-create HumanName.text"); 347 else if (Configuration.doAutoCreate()) 348 this.text = new StringType(); // bb 349 return this.text; 350 } 351 352 public boolean hasTextElement() { 353 return this.text != null && !this.text.isEmpty(); 354 } 355 356 public boolean hasText() { 357 return this.text != null && !this.text.isEmpty(); 358 } 359 360 /** 361 * @param value {@link #text} (Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 362 */ 363 public HumanName setTextElement(StringType value) { 364 this.text = value; 365 return this; 366 } 367 368 /** 369 * @return Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts. 370 */ 371 public String getText() { 372 return this.text == null ? null : this.text.getValue(); 373 } 374 375 /** 376 * @param value Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts. 377 */ 378 public HumanName setText(String value) { 379 if (Utilities.noString(value)) 380 this.text = null; 381 else { 382 if (this.text == null) 383 this.text = new StringType(); 384 this.text.setValue(value); 385 } 386 return this; 387 } 388 389 /** 390 * @return {@link #family} (The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.). This is the underlying object with id, value and extensions. The accessor "getFamily" gives direct access to the value 391 */ 392 public StringType getFamilyElement() { 393 if (this.family == null) 394 if (Configuration.errorOnAutoCreate()) 395 throw new Error("Attempt to auto-create HumanName.family"); 396 else if (Configuration.doAutoCreate()) 397 this.family = new StringType(); // bb 398 return this.family; 399 } 400 401 public boolean hasFamilyElement() { 402 return this.family != null && !this.family.isEmpty(); 403 } 404 405 public boolean hasFamily() { 406 return this.family != null && !this.family.isEmpty(); 407 } 408 409 /** 410 * @param value {@link #family} (The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.). This is the underlying object with id, value and extensions. The accessor "getFamily" gives direct access to the value 411 */ 412 public HumanName setFamilyElement(StringType value) { 413 this.family = value; 414 return this; 415 } 416 417 /** 418 * @return The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. 419 */ 420 public String getFamily() { 421 return this.family == null ? null : this.family.getValue(); 422 } 423 424 /** 425 * @param value The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. 426 */ 427 public HumanName setFamily(String value) { 428 if (Utilities.noString(value)) 429 this.family = null; 430 else { 431 if (this.family == null) 432 this.family = new StringType(); 433 this.family.setValue(value); 434 } 435 return this; 436 } 437 438 /** 439 * @return {@link #given} (Given name.) 440 */ 441 public List<StringType> getGiven() { 442 if (this.given == null) 443 this.given = new ArrayList<StringType>(); 444 return this.given; 445 } 446 447 /** 448 * @return Returns a reference to <code>this</code> for easy method chaining 449 */ 450 public HumanName setGiven(List<StringType> theGiven) { 451 this.given = theGiven; 452 return this; 453 } 454 455 public boolean hasGiven() { 456 if (this.given == null) 457 return false; 458 for (StringType item : this.given) 459 if (!item.isEmpty()) 460 return true; 461 return false; 462 } 463 464 /** 465 * @return {@link #given} (Given name.) 466 */ 467 public StringType addGivenElement() {//2 468 StringType t = new StringType(); 469 if (this.given == null) 470 this.given = new ArrayList<StringType>(); 471 this.given.add(t); 472 return t; 473 } 474 475 /** 476 * @param value {@link #given} (Given name.) 477 */ 478 public HumanName addGiven(String value) { //1 479 StringType t = new StringType(); 480 t.setValue(value); 481 if (this.given == null) 482 this.given = new ArrayList<StringType>(); 483 this.given.add(t); 484 return this; 485 } 486 487 /** 488 * @param value {@link #given} (Given name.) 489 */ 490 public boolean hasGiven(String value) { 491 if (this.given == null) 492 return false; 493 for (StringType v : this.given) 494 if (v.getValue().equals(value)) // string 495 return true; 496 return false; 497 } 498 499 /** 500 * @return {@link #prefix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.) 501 */ 502 public List<StringType> getPrefix() { 503 if (this.prefix == null) 504 this.prefix = new ArrayList<StringType>(); 505 return this.prefix; 506 } 507 508 /** 509 * @return Returns a reference to <code>this</code> for easy method chaining 510 */ 511 public HumanName setPrefix(List<StringType> thePrefix) { 512 this.prefix = thePrefix; 513 return this; 514 } 515 516 public boolean hasPrefix() { 517 if (this.prefix == null) 518 return false; 519 for (StringType item : this.prefix) 520 if (!item.isEmpty()) 521 return true; 522 return false; 523 } 524 525 /** 526 * @return {@link #prefix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.) 527 */ 528 public StringType addPrefixElement() {//2 529 StringType t = new StringType(); 530 if (this.prefix == null) 531 this.prefix = new ArrayList<StringType>(); 532 this.prefix.add(t); 533 return t; 534 } 535 536 /** 537 * @param value {@link #prefix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.) 538 */ 539 public HumanName addPrefix(String value) { //1 540 StringType t = new StringType(); 541 t.setValue(value); 542 if (this.prefix == null) 543 this.prefix = new ArrayList<StringType>(); 544 this.prefix.add(t); 545 return this; 546 } 547 548 /** 549 * @param value {@link #prefix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.) 550 */ 551 public boolean hasPrefix(String value) { 552 if (this.prefix == null) 553 return false; 554 for (StringType v : this.prefix) 555 if (v.getValue().equals(value)) // string 556 return true; 557 return false; 558 } 559 560 /** 561 * @return {@link #suffix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.) 562 */ 563 public List<StringType> getSuffix() { 564 if (this.suffix == null) 565 this.suffix = new ArrayList<StringType>(); 566 return this.suffix; 567 } 568 569 /** 570 * @return Returns a reference to <code>this</code> for easy method chaining 571 */ 572 public HumanName setSuffix(List<StringType> theSuffix) { 573 this.suffix = theSuffix; 574 return this; 575 } 576 577 public boolean hasSuffix() { 578 if (this.suffix == null) 579 return false; 580 for (StringType item : this.suffix) 581 if (!item.isEmpty()) 582 return true; 583 return false; 584 } 585 586 /** 587 * @return {@link #suffix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.) 588 */ 589 public StringType addSuffixElement() {//2 590 StringType t = new StringType(); 591 if (this.suffix == null) 592 this.suffix = new ArrayList<StringType>(); 593 this.suffix.add(t); 594 return t; 595 } 596 597 /** 598 * @param value {@link #suffix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.) 599 */ 600 public HumanName addSuffix(String value) { //1 601 StringType t = new StringType(); 602 t.setValue(value); 603 if (this.suffix == null) 604 this.suffix = new ArrayList<StringType>(); 605 this.suffix.add(t); 606 return this; 607 } 608 609 /** 610 * @param value {@link #suffix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.) 611 */ 612 public boolean hasSuffix(String value) { 613 if (this.suffix == null) 614 return false; 615 for (StringType v : this.suffix) 616 if (v.getValue().equals(value)) // string 617 return true; 618 return false; 619 } 620 621 /** 622 * @return {@link #period} (Indicates the period of time when this name was valid for the named person.) 623 */ 624 public Period getPeriod() { 625 if (this.period == null) 626 if (Configuration.errorOnAutoCreate()) 627 throw new Error("Attempt to auto-create HumanName.period"); 628 else if (Configuration.doAutoCreate()) 629 this.period = new Period(); // cc 630 return this.period; 631 } 632 633 public boolean hasPeriod() { 634 return this.period != null && !this.period.isEmpty(); 635 } 636 637 /** 638 * @param value {@link #period} (Indicates the period of time when this name was valid for the named person.) 639 */ 640 public HumanName setPeriod(Period value) { 641 this.period = value; 642 return this; 643 } 644 645 protected void listChildren(List<Property> children) { 646 super.listChildren(children); 647 children.add(new Property("use", "code", "Identifies the purpose for this name.", 0, 1, use)); 648 children.add(new Property("text", "string", "Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts.", 0, 1, text)); 649 children.add(new Property("family", "string", "The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.", 0, 1, family)); 650 children.add(new Property("given", "string", "Given name.", 0, java.lang.Integer.MAX_VALUE, given)); 651 children.add(new Property("prefix", "string", "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.", 0, java.lang.Integer.MAX_VALUE, prefix)); 652 children.add(new Property("suffix", "string", "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.", 0, java.lang.Integer.MAX_VALUE, suffix)); 653 children.add(new Property("period", "Period", "Indicates the period of time when this name was valid for the named person.", 0, 1, period)); 654 } 655 656 @Override 657 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 658 switch (_hash) { 659 case 116103: /*use*/ return new Property("use", "code", "Identifies the purpose for this name.", 0, 1, use); 660 case 3556653: /*text*/ return new Property("text", "string", "Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts.", 0, 1, text); 661 case -1281860764: /*family*/ return new Property("family", "string", "The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.", 0, 1, family); 662 case 98367357: /*given*/ return new Property("given", "string", "Given name.", 0, java.lang.Integer.MAX_VALUE, given); 663 case -980110702: /*prefix*/ return new Property("prefix", "string", "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.", 0, java.lang.Integer.MAX_VALUE, prefix); 664 case -891422895: /*suffix*/ return new Property("suffix", "string", "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.", 0, java.lang.Integer.MAX_VALUE, suffix); 665 case -991726143: /*period*/ return new Property("period", "Period", "Indicates the period of time when this name was valid for the named person.", 0, 1, period); 666 default: return super.getNamedProperty(_hash, _name, _checkValid); 667 } 668 669 } 670 671 @Override 672 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 673 switch (hash) { 674 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<NameUse> 675 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 676 case -1281860764: /*family*/ return this.family == null ? new Base[0] : new Base[] {this.family}; // StringType 677 case 98367357: /*given*/ return this.given == null ? new Base[0] : this.given.toArray(new Base[this.given.size()]); // StringType 678 case -980110702: /*prefix*/ return this.prefix == null ? new Base[0] : this.prefix.toArray(new Base[this.prefix.size()]); // StringType 679 case -891422895: /*suffix*/ return this.suffix == null ? new Base[0] : this.suffix.toArray(new Base[this.suffix.size()]); // StringType 680 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 681 default: return super.getProperty(hash, name, checkValid); 682 } 683 684 } 685 686 @Override 687 public Base setProperty(int hash, String name, Base value) throws FHIRException { 688 switch (hash) { 689 case 116103: // use 690 value = new NameUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 691 this.use = (Enumeration) value; // Enumeration<NameUse> 692 return value; 693 case 3556653: // text 694 this.text = TypeConvertor.castToString(value); // StringType 695 return value; 696 case -1281860764: // family 697 this.family = TypeConvertor.castToString(value); // StringType 698 return value; 699 case 98367357: // given 700 this.getGiven().add(TypeConvertor.castToString(value)); // StringType 701 return value; 702 case -980110702: // prefix 703 this.getPrefix().add(TypeConvertor.castToString(value)); // StringType 704 return value; 705 case -891422895: // suffix 706 this.getSuffix().add(TypeConvertor.castToString(value)); // StringType 707 return value; 708 case -991726143: // period 709 this.period = TypeConvertor.castToPeriod(value); // Period 710 return value; 711 default: return super.setProperty(hash, name, value); 712 } 713 714 } 715 716 @Override 717 public Base setProperty(String name, Base value) throws FHIRException { 718 if (name.equals("use")) { 719 value = new NameUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 720 this.use = (Enumeration) value; // Enumeration<NameUse> 721 } else if (name.equals("text")) { 722 this.text = TypeConvertor.castToString(value); // StringType 723 } else if (name.equals("family")) { 724 this.family = TypeConvertor.castToString(value); // StringType 725 } else if (name.equals("given")) { 726 this.getGiven().add(TypeConvertor.castToString(value)); 727 } else if (name.equals("prefix")) { 728 this.getPrefix().add(TypeConvertor.castToString(value)); 729 } else if (name.equals("suffix")) { 730 this.getSuffix().add(TypeConvertor.castToString(value)); 731 } else if (name.equals("period")) { 732 this.period = TypeConvertor.castToPeriod(value); // Period 733 } else 734 return super.setProperty(name, value); 735 return value; 736 } 737 738 @Override 739 public Base makeProperty(int hash, String name) throws FHIRException { 740 switch (hash) { 741 case 116103: return getUseElement(); 742 case 3556653: return getTextElement(); 743 case -1281860764: return getFamilyElement(); 744 case 98367357: return addGivenElement(); 745 case -980110702: return addPrefixElement(); 746 case -891422895: return addSuffixElement(); 747 case -991726143: return getPeriod(); 748 default: return super.makeProperty(hash, name); 749 } 750 751 } 752 753 @Override 754 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 755 switch (hash) { 756 case 116103: /*use*/ return new String[] {"code"}; 757 case 3556653: /*text*/ return new String[] {"string"}; 758 case -1281860764: /*family*/ return new String[] {"string"}; 759 case 98367357: /*given*/ return new String[] {"string"}; 760 case -980110702: /*prefix*/ return new String[] {"string"}; 761 case -891422895: /*suffix*/ return new String[] {"string"}; 762 case -991726143: /*period*/ return new String[] {"Period"}; 763 default: return super.getTypesForProperty(hash, name); 764 } 765 766 } 767 768 @Override 769 public Base addChild(String name) throws FHIRException { 770 if (name.equals("use")) { 771 throw new FHIRException("Cannot call addChild on a singleton property HumanName.use"); 772 } 773 else if (name.equals("text")) { 774 throw new FHIRException("Cannot call addChild on a singleton property HumanName.text"); 775 } 776 else if (name.equals("family")) { 777 throw new FHIRException("Cannot call addChild on a singleton property HumanName.family"); 778 } 779 else if (name.equals("given")) { 780 throw new FHIRException("Cannot call addChild on a singleton property HumanName.given"); 781 } 782 else if (name.equals("prefix")) { 783 throw new FHIRException("Cannot call addChild on a singleton property HumanName.prefix"); 784 } 785 else if (name.equals("suffix")) { 786 throw new FHIRException("Cannot call addChild on a singleton property HumanName.suffix"); 787 } 788 else if (name.equals("period")) { 789 this.period = new Period(); 790 return this.period; 791 } 792 else 793 return super.addChild(name); 794 } 795 796 public String fhirType() { 797 return "HumanName"; 798 799 } 800 801 public HumanName copy() { 802 HumanName dst = new HumanName(); 803 copyValues(dst); 804 return dst; 805 } 806 807 public void copyValues(HumanName dst) { 808 super.copyValues(dst); 809 dst.use = use == null ? null : use.copy(); 810 dst.text = text == null ? null : text.copy(); 811 dst.family = family == null ? null : family.copy(); 812 if (given != null) { 813 dst.given = new ArrayList<StringType>(); 814 for (StringType i : given) 815 dst.given.add(i.copy()); 816 }; 817 if (prefix != null) { 818 dst.prefix = new ArrayList<StringType>(); 819 for (StringType i : prefix) 820 dst.prefix.add(i.copy()); 821 }; 822 if (suffix != null) { 823 dst.suffix = new ArrayList<StringType>(); 824 for (StringType i : suffix) 825 dst.suffix.add(i.copy()); 826 }; 827 dst.period = period == null ? null : period.copy(); 828 } 829 830 protected HumanName typedCopy() { 831 return copy(); 832 } 833 834 @Override 835 public boolean equalsDeep(Base other_) { 836 if (!super.equalsDeep(other_)) 837 return false; 838 if (!(other_ instanceof HumanName)) 839 return false; 840 HumanName o = (HumanName) other_; 841 return compareDeep(use, o.use, true) && compareDeep(text, o.text, true) && compareDeep(family, o.family, true) 842 && compareDeep(given, o.given, true) && compareDeep(prefix, o.prefix, true) && compareDeep(suffix, o.suffix, true) 843 && compareDeep(period, o.period, true); 844 } 845 846 @Override 847 public boolean equalsShallow(Base other_) { 848 if (!super.equalsShallow(other_)) 849 return false; 850 if (!(other_ instanceof HumanName)) 851 return false; 852 HumanName o = (HumanName) other_; 853 return compareValues(use, o.use, true) && compareValues(text, o.text, true) && compareValues(family, o.family, true) 854 && compareValues(given, o.given, true) && compareValues(prefix, o.prefix, true) && compareValues(suffix, o.suffix, true) 855 ; 856 } 857 858 public boolean isEmpty() { 859 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(use, text, family, given 860 , prefix, suffix, period); 861 } 862 863// Manual code (from Configuration.txt): 864/** 865 /** 866 * Returns all repetitions of {@link #getGiven() given name} as a space separated string 867 * 868 * @see DatatypeUtil#joinStringsSpaceSeparated(List) 869 */ 870 public String getGivenAsSingleString() { 871 return joinStringsSpaceSeparated(getGiven()); 872 } 873 874 /** 875 * Returns all repetitions of {@link #getPrefix() prefix name} as a space separated string 876 * 877 * @see DatatypeUtil#joinStringsSpaceSeparated(List) 878 */ 879 public String getPrefixAsSingleString() { 880 return joinStringsSpaceSeparated(getPrefix()); 881 } 882 883 /** 884 * Returns all repetitions of {@link #getSuffix() suffix} as a space separated string 885 * 886 * @see DatatypeUtil#joinStringsSpaceSeparated(List) 887 */ 888 public String getSuffixAsSingleString() { 889 return joinStringsSpaceSeparated(getSuffix()); 890 } 891 892 /** 893 * <p>Returns the {@link #getTextElement() text} element value if it is not null.</p> 894 895 * <p>If the {@link #getTextElement() text} element value is null, returns all the components of the name (prefix, 896 * given, family, suffix) as a single string with a single spaced string separating each part. </p> 897 * 898 * @return the human name as a single string 899 */ 900 public String getNameAsSingleString() { 901 if (hasText()) { 902 return getText().toString(); 903 } 904 905 List<StringType> nameParts = new ArrayList<StringType>(); 906 nameParts.addAll(getPrefix()); 907 nameParts.addAll(getGiven()); 908 if (hasFamilyElement()) { 909 nameParts.add(getFamilyElement()); 910 } 911 nameParts.addAll(getSuffix()); 912 if (nameParts.size() > 0) { 913 return joinStringsSpaceSeparated(nameParts); 914 } else { 915 return getTextElement().getValue(); 916 } 917 } 918 919 /** 920 * Joins a list of strings with a single space (' ') between each string 921 * 922 * TODO: replace with call to ca.uhn.fhir.util.DatatypeUtil.joinStringsSpaceSeparated when HAPI upgrades to 1.4 923 */ 924 private static String joinStringsSpaceSeparated(List<? extends IPrimitiveType<String>> theStrings) { 925 StringBuilder stringBuilder = new StringBuilder(); 926 for (IPrimitiveType<String> string : theStrings) { 927 if (string.isEmpty()) { 928 continue; 929 } 930 if (stringBuilder.length() > 0) { 931 stringBuilder.append(' '); 932 } 933 stringBuilder.append(string.getValue()); 934 } 935 return stringBuilder.toString(); 936 } 937// end addition 938 939} 940