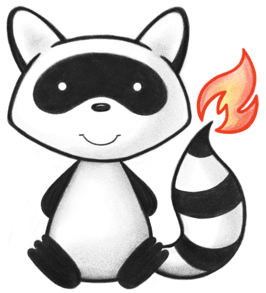
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048import ca.uhn.fhir.util.DatatypeUtil; 049import org.hl7.fhir.instance.model.api.IPrimitiveType; 050/** 051 * HumanName Type: A name, normally of a human, that can be used for other living entities (e.g. animals but not organizations) that have been assigned names by a human and may need the use of name parts or the need for usage information. 052 */ 053@DatatypeDef(name="HumanName") 054public class HumanName extends DataType implements ICompositeType { 055 056 public enum NameUse { 057 /** 058 * Known as/conventional/the one you normally use. 059 */ 060 USUAL, 061 /** 062 * The formal name as registered in an official (government) registry, but which name might not be commonly used. May be called \"legal name\". 063 */ 064 OFFICIAL, 065 /** 066 * A temporary name. Name.period can provide more detailed information. This may also be used for temporary names assigned at birth or in emergency situations. 067 */ 068 TEMP, 069 /** 070 * A name that is used to address the person in an informal manner, but is not part of their formal or usual name. 071 */ 072 NICKNAME, 073 /** 074 * Anonymous assigned name, alias, or pseudonym (used to protect a person's identity for privacy reasons). 075 */ 076 ANONYMOUS, 077 /** 078 * This name is no longer in use (or was never correct, but retained for records). 079 */ 080 OLD, 081 /** 082 * A name used prior to changing name because of marriage. This name use is for use by applications that collect and store names that were used prior to a marriage. Marriage naming customs vary greatly around the world, and are constantly changing. This term is not gender specific. The use of this term does not imply any particular history for a person's name. 083 */ 084 MAIDEN, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 public static NameUse fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("usual".equals(codeString)) 093 return USUAL; 094 if ("official".equals(codeString)) 095 return OFFICIAL; 096 if ("temp".equals(codeString)) 097 return TEMP; 098 if ("nickname".equals(codeString)) 099 return NICKNAME; 100 if ("anonymous".equals(codeString)) 101 return ANONYMOUS; 102 if ("old".equals(codeString)) 103 return OLD; 104 if ("maiden".equals(codeString)) 105 return MAIDEN; 106 if (Configuration.isAcceptInvalidEnums()) 107 return null; 108 else 109 throw new FHIRException("Unknown NameUse code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case USUAL: return "usual"; 114 case OFFICIAL: return "official"; 115 case TEMP: return "temp"; 116 case NICKNAME: return "nickname"; 117 case ANONYMOUS: return "anonymous"; 118 case OLD: return "old"; 119 case MAIDEN: return "maiden"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getSystem() { 125 switch (this) { 126 case USUAL: return "http://hl7.org/fhir/name-use"; 127 case OFFICIAL: return "http://hl7.org/fhir/name-use"; 128 case TEMP: return "http://hl7.org/fhir/name-use"; 129 case NICKNAME: return "http://hl7.org/fhir/name-use"; 130 case ANONYMOUS: return "http://hl7.org/fhir/name-use"; 131 case OLD: return "http://hl7.org/fhir/name-use"; 132 case MAIDEN: return "http://hl7.org/fhir/name-use"; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getDefinition() { 138 switch (this) { 139 case USUAL: return "Known as/conventional/the one you normally use."; 140 case OFFICIAL: return "The formal name as registered in an official (government) registry, but which name might not be commonly used. May be called \"legal name\"."; 141 case TEMP: return "A temporary name. Name.period can provide more detailed information. This may also be used for temporary names assigned at birth or in emergency situations."; 142 case NICKNAME: return "A name that is used to address the person in an informal manner, but is not part of their formal or usual name."; 143 case ANONYMOUS: return "Anonymous assigned name, alias, or pseudonym (used to protect a person's identity for privacy reasons)."; 144 case OLD: return "This name is no longer in use (or was never correct, but retained for records)."; 145 case MAIDEN: return "A name used prior to changing name because of marriage. This name use is for use by applications that collect and store names that were used prior to a marriage. Marriage naming customs vary greatly around the world, and are constantly changing. This term is not gender specific. The use of this term does not imply any particular history for a person's name."; 146 case NULL: return null; 147 default: return "?"; 148 } 149 } 150 public String getDisplay() { 151 switch (this) { 152 case USUAL: return "Usual"; 153 case OFFICIAL: return "Official"; 154 case TEMP: return "Temp"; 155 case NICKNAME: return "Nickname"; 156 case ANONYMOUS: return "Anonymous"; 157 case OLD: return "Old"; 158 case MAIDEN: return "Name changed for Marriage"; 159 case NULL: return null; 160 default: return "?"; 161 } 162 } 163 } 164 165 public static class NameUseEnumFactory implements EnumFactory<NameUse> { 166 public NameUse fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("usual".equals(codeString)) 171 return NameUse.USUAL; 172 if ("official".equals(codeString)) 173 return NameUse.OFFICIAL; 174 if ("temp".equals(codeString)) 175 return NameUse.TEMP; 176 if ("nickname".equals(codeString)) 177 return NameUse.NICKNAME; 178 if ("anonymous".equals(codeString)) 179 return NameUse.ANONYMOUS; 180 if ("old".equals(codeString)) 181 return NameUse.OLD; 182 if ("maiden".equals(codeString)) 183 return NameUse.MAIDEN; 184 throw new IllegalArgumentException("Unknown NameUse code '"+codeString+"'"); 185 } 186 public Enumeration<NameUse> fromType(PrimitiveType<?> code) throws FHIRException { 187 if (code == null) 188 return null; 189 if (code.isEmpty()) 190 return new Enumeration<NameUse>(this, NameUse.NULL, code); 191 String codeString = ((PrimitiveType) code).asStringValue(); 192 if (codeString == null || "".equals(codeString)) 193 return new Enumeration<NameUse>(this, NameUse.NULL, code); 194 if ("usual".equals(codeString)) 195 return new Enumeration<NameUse>(this, NameUse.USUAL, code); 196 if ("official".equals(codeString)) 197 return new Enumeration<NameUse>(this, NameUse.OFFICIAL, code); 198 if ("temp".equals(codeString)) 199 return new Enumeration<NameUse>(this, NameUse.TEMP, code); 200 if ("nickname".equals(codeString)) 201 return new Enumeration<NameUse>(this, NameUse.NICKNAME, code); 202 if ("anonymous".equals(codeString)) 203 return new Enumeration<NameUse>(this, NameUse.ANONYMOUS, code); 204 if ("old".equals(codeString)) 205 return new Enumeration<NameUse>(this, NameUse.OLD, code); 206 if ("maiden".equals(codeString)) 207 return new Enumeration<NameUse>(this, NameUse.MAIDEN, code); 208 throw new FHIRException("Unknown NameUse code '"+codeString+"'"); 209 } 210 public String toCode(NameUse code) { 211 if (code == NameUse.NULL) 212 return null; 213 if (code == NameUse.USUAL) 214 return "usual"; 215 if (code == NameUse.OFFICIAL) 216 return "official"; 217 if (code == NameUse.TEMP) 218 return "temp"; 219 if (code == NameUse.NICKNAME) 220 return "nickname"; 221 if (code == NameUse.ANONYMOUS) 222 return "anonymous"; 223 if (code == NameUse.OLD) 224 return "old"; 225 if (code == NameUse.MAIDEN) 226 return "maiden"; 227 return "?"; 228 } 229 public String toSystem(NameUse code) { 230 return code.getSystem(); 231 } 232 } 233 234 /** 235 * Identifies the purpose for this name. 236 */ 237 @Child(name = "use", type = {CodeType.class}, order=0, min=0, max=1, modifier=true, summary=true) 238 @Description(shortDefinition="usual | official | temp | nickname | anonymous | old | maiden", formalDefinition="Identifies the purpose for this name." ) 239 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/name-use") 240 protected Enumeration<NameUse> use; 241 242 /** 243 * Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts. 244 */ 245 @Child(name = "text", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 246 @Description(shortDefinition="Text representation of the full name", formalDefinition="Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts." ) 247 protected StringType text; 248 249 /** 250 * The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. 251 */ 252 @Child(name = "family", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 253 @Description(shortDefinition="Family name (often called 'Surname')", formalDefinition="The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father." ) 254 protected StringType family; 255 256 /** 257 * Given name. 258 */ 259 @Child(name = "given", type = {StringType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 260 @Description(shortDefinition="Given names (not always 'first'). Includes middle names", formalDefinition="Given name." ) 261 protected List<StringType> given; 262 263 /** 264 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name. 265 */ 266 @Child(name = "prefix", type = {StringType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 267 @Description(shortDefinition="Parts that come before the name", formalDefinition="Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name." ) 268 protected List<StringType> prefix; 269 270 /** 271 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name. 272 */ 273 @Child(name = "suffix", type = {StringType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 274 @Description(shortDefinition="Parts that come after the name", formalDefinition="Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name." ) 275 protected List<StringType> suffix; 276 277 /** 278 * Indicates the period of time when this name was valid for the named person. 279 */ 280 @Child(name = "period", type = {Period.class}, order=6, min=0, max=1, modifier=false, summary=true) 281 @Description(shortDefinition="Time period when name was/is in use", formalDefinition="Indicates the period of time when this name was valid for the named person." ) 282 protected Period period; 283 284 private static final long serialVersionUID = -507469160L; 285 286 /** 287 * Constructor 288 */ 289 public HumanName() { 290 super(); 291 } 292 293 /** 294 * @return {@link #use} (Identifies the purpose for this name.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 295 */ 296 public Enumeration<NameUse> getUseElement() { 297 if (this.use == null) 298 if (Configuration.errorOnAutoCreate()) 299 throw new Error("Attempt to auto-create HumanName.use"); 300 else if (Configuration.doAutoCreate()) 301 this.use = new Enumeration<NameUse>(new NameUseEnumFactory()); // bb 302 return this.use; 303 } 304 305 public boolean hasUseElement() { 306 return this.use != null && !this.use.isEmpty(); 307 } 308 309 public boolean hasUse() { 310 return this.use != null && !this.use.isEmpty(); 311 } 312 313 /** 314 * @param value {@link #use} (Identifies the purpose for this name.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 315 */ 316 public HumanName setUseElement(Enumeration<NameUse> value) { 317 this.use = value; 318 return this; 319 } 320 321 /** 322 * @return Identifies the purpose for this name. 323 */ 324 public NameUse getUse() { 325 return this.use == null ? null : this.use.getValue(); 326 } 327 328 /** 329 * @param value Identifies the purpose for this name. 330 */ 331 public HumanName setUse(NameUse value) { 332 if (value == null) 333 this.use = null; 334 else { 335 if (this.use == null) 336 this.use = new Enumeration<NameUse>(new NameUseEnumFactory()); 337 this.use.setValue(value); 338 } 339 return this; 340 } 341 342 /** 343 * @return {@link #text} (Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 344 */ 345 public StringType getTextElement() { 346 if (this.text == null) 347 if (Configuration.errorOnAutoCreate()) 348 throw new Error("Attempt to auto-create HumanName.text"); 349 else if (Configuration.doAutoCreate()) 350 this.text = new StringType(); // bb 351 return this.text; 352 } 353 354 public boolean hasTextElement() { 355 return this.text != null && !this.text.isEmpty(); 356 } 357 358 public boolean hasText() { 359 return this.text != null && !this.text.isEmpty(); 360 } 361 362 /** 363 * @param value {@link #text} (Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 364 */ 365 public HumanName setTextElement(StringType value) { 366 this.text = value; 367 return this; 368 } 369 370 /** 371 * @return Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts. 372 */ 373 public String getText() { 374 return this.text == null ? null : this.text.getValue(); 375 } 376 377 /** 378 * @param value Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts. 379 */ 380 public HumanName setText(String value) { 381 if (Utilities.noString(value)) 382 this.text = null; 383 else { 384 if (this.text == null) 385 this.text = new StringType(); 386 this.text.setValue(value); 387 } 388 return this; 389 } 390 391 /** 392 * @return {@link #family} (The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.). This is the underlying object with id, value and extensions. The accessor "getFamily" gives direct access to the value 393 */ 394 public StringType getFamilyElement() { 395 if (this.family == null) 396 if (Configuration.errorOnAutoCreate()) 397 throw new Error("Attempt to auto-create HumanName.family"); 398 else if (Configuration.doAutoCreate()) 399 this.family = new StringType(); // bb 400 return this.family; 401 } 402 403 public boolean hasFamilyElement() { 404 return this.family != null && !this.family.isEmpty(); 405 } 406 407 public boolean hasFamily() { 408 return this.family != null && !this.family.isEmpty(); 409 } 410 411 /** 412 * @param value {@link #family} (The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.). This is the underlying object with id, value and extensions. The accessor "getFamily" gives direct access to the value 413 */ 414 public HumanName setFamilyElement(StringType value) { 415 this.family = value; 416 return this; 417 } 418 419 /** 420 * @return The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. 421 */ 422 public String getFamily() { 423 return this.family == null ? null : this.family.getValue(); 424 } 425 426 /** 427 * @param value The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. 428 */ 429 public HumanName setFamily(String value) { 430 if (Utilities.noString(value)) 431 this.family = null; 432 else { 433 if (this.family == null) 434 this.family = new StringType(); 435 this.family.setValue(value); 436 } 437 return this; 438 } 439 440 /** 441 * @return {@link #given} (Given name.) 442 */ 443 public List<StringType> getGiven() { 444 if (this.given == null) 445 this.given = new ArrayList<StringType>(); 446 return this.given; 447 } 448 449 /** 450 * @return Returns a reference to <code>this</code> for easy method chaining 451 */ 452 public HumanName setGiven(List<StringType> theGiven) { 453 this.given = theGiven; 454 return this; 455 } 456 457 public boolean hasGiven() { 458 if (this.given == null) 459 return false; 460 for (StringType item : this.given) 461 if (!item.isEmpty()) 462 return true; 463 return false; 464 } 465 466 /** 467 * @return {@link #given} (Given name.) 468 */ 469 public StringType addGivenElement() {//2 470 StringType t = new StringType(); 471 if (this.given == null) 472 this.given = new ArrayList<StringType>(); 473 this.given.add(t); 474 return t; 475 } 476 477 /** 478 * @param value {@link #given} (Given name.) 479 */ 480 public HumanName addGiven(String value) { //1 481 StringType t = new StringType(); 482 t.setValue(value); 483 if (this.given == null) 484 this.given = new ArrayList<StringType>(); 485 this.given.add(t); 486 return this; 487 } 488 489 /** 490 * @param value {@link #given} (Given name.) 491 */ 492 public boolean hasGiven(String value) { 493 if (this.given == null) 494 return false; 495 for (StringType v : this.given) 496 if (v.getValue().equals(value)) // string 497 return true; 498 return false; 499 } 500 501 /** 502 * @return {@link #prefix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.) 503 */ 504 public List<StringType> getPrefix() { 505 if (this.prefix == null) 506 this.prefix = new ArrayList<StringType>(); 507 return this.prefix; 508 } 509 510 /** 511 * @return Returns a reference to <code>this</code> for easy method chaining 512 */ 513 public HumanName setPrefix(List<StringType> thePrefix) { 514 this.prefix = thePrefix; 515 return this; 516 } 517 518 public boolean hasPrefix() { 519 if (this.prefix == null) 520 return false; 521 for (StringType item : this.prefix) 522 if (!item.isEmpty()) 523 return true; 524 return false; 525 } 526 527 /** 528 * @return {@link #prefix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.) 529 */ 530 public StringType addPrefixElement() {//2 531 StringType t = new StringType(); 532 if (this.prefix == null) 533 this.prefix = new ArrayList<StringType>(); 534 this.prefix.add(t); 535 return t; 536 } 537 538 /** 539 * @param value {@link #prefix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.) 540 */ 541 public HumanName addPrefix(String value) { //1 542 StringType t = new StringType(); 543 t.setValue(value); 544 if (this.prefix == null) 545 this.prefix = new ArrayList<StringType>(); 546 this.prefix.add(t); 547 return this; 548 } 549 550 /** 551 * @param value {@link #prefix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.) 552 */ 553 public boolean hasPrefix(String value) { 554 if (this.prefix == null) 555 return false; 556 for (StringType v : this.prefix) 557 if (v.getValue().equals(value)) // string 558 return true; 559 return false; 560 } 561 562 /** 563 * @return {@link #suffix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.) 564 */ 565 public List<StringType> getSuffix() { 566 if (this.suffix == null) 567 this.suffix = new ArrayList<StringType>(); 568 return this.suffix; 569 } 570 571 /** 572 * @return Returns a reference to <code>this</code> for easy method chaining 573 */ 574 public HumanName setSuffix(List<StringType> theSuffix) { 575 this.suffix = theSuffix; 576 return this; 577 } 578 579 public boolean hasSuffix() { 580 if (this.suffix == null) 581 return false; 582 for (StringType item : this.suffix) 583 if (!item.isEmpty()) 584 return true; 585 return false; 586 } 587 588 /** 589 * @return {@link #suffix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.) 590 */ 591 public StringType addSuffixElement() {//2 592 StringType t = new StringType(); 593 if (this.suffix == null) 594 this.suffix = new ArrayList<StringType>(); 595 this.suffix.add(t); 596 return t; 597 } 598 599 /** 600 * @param value {@link #suffix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.) 601 */ 602 public HumanName addSuffix(String value) { //1 603 StringType t = new StringType(); 604 t.setValue(value); 605 if (this.suffix == null) 606 this.suffix = new ArrayList<StringType>(); 607 this.suffix.add(t); 608 return this; 609 } 610 611 /** 612 * @param value {@link #suffix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.) 613 */ 614 public boolean hasSuffix(String value) { 615 if (this.suffix == null) 616 return false; 617 for (StringType v : this.suffix) 618 if (v.getValue().equals(value)) // string 619 return true; 620 return false; 621 } 622 623 /** 624 * @return {@link #period} (Indicates the period of time when this name was valid for the named person.) 625 */ 626 public Period getPeriod() { 627 if (this.period == null) 628 if (Configuration.errorOnAutoCreate()) 629 throw new Error("Attempt to auto-create HumanName.period"); 630 else if (Configuration.doAutoCreate()) 631 this.period = new Period(); // cc 632 return this.period; 633 } 634 635 public boolean hasPeriod() { 636 return this.period != null && !this.period.isEmpty(); 637 } 638 639 /** 640 * @param value {@link #period} (Indicates the period of time when this name was valid for the named person.) 641 */ 642 public HumanName setPeriod(Period value) { 643 this.period = value; 644 return this; 645 } 646 647 protected void listChildren(List<Property> children) { 648 super.listChildren(children); 649 children.add(new Property("use", "code", "Identifies the purpose for this name.", 0, 1, use)); 650 children.add(new Property("text", "string", "Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts.", 0, 1, text)); 651 children.add(new Property("family", "string", "The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.", 0, 1, family)); 652 children.add(new Property("given", "string", "Given name.", 0, java.lang.Integer.MAX_VALUE, given)); 653 children.add(new Property("prefix", "string", "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.", 0, java.lang.Integer.MAX_VALUE, prefix)); 654 children.add(new Property("suffix", "string", "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.", 0, java.lang.Integer.MAX_VALUE, suffix)); 655 children.add(new Property("period", "Period", "Indicates the period of time when this name was valid for the named person.", 0, 1, period)); 656 } 657 658 @Override 659 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 660 switch (_hash) { 661 case 116103: /*use*/ return new Property("use", "code", "Identifies the purpose for this name.", 0, 1, use); 662 case 3556653: /*text*/ return new Property("text", "string", "Specifies the entire name as it should be displayed e.g. on an application UI. This may be provided instead of or as well as the specific parts.", 0, 1, text); 663 case -1281860764: /*family*/ return new Property("family", "string", "The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.", 0, 1, family); 664 case 98367357: /*given*/ return new Property("given", "string", "Given name.", 0, java.lang.Integer.MAX_VALUE, given); 665 case -980110702: /*prefix*/ return new Property("prefix", "string", "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.", 0, java.lang.Integer.MAX_VALUE, prefix); 666 case -891422895: /*suffix*/ return new Property("suffix", "string", "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.", 0, java.lang.Integer.MAX_VALUE, suffix); 667 case -991726143: /*period*/ return new Property("period", "Period", "Indicates the period of time when this name was valid for the named person.", 0, 1, period); 668 default: return super.getNamedProperty(_hash, _name, _checkValid); 669 } 670 671 } 672 673 @Override 674 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 675 switch (hash) { 676 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<NameUse> 677 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 678 case -1281860764: /*family*/ return this.family == null ? new Base[0] : new Base[] {this.family}; // StringType 679 case 98367357: /*given*/ return this.given == null ? new Base[0] : this.given.toArray(new Base[this.given.size()]); // StringType 680 case -980110702: /*prefix*/ return this.prefix == null ? new Base[0] : this.prefix.toArray(new Base[this.prefix.size()]); // StringType 681 case -891422895: /*suffix*/ return this.suffix == null ? new Base[0] : this.suffix.toArray(new Base[this.suffix.size()]); // StringType 682 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 683 default: return super.getProperty(hash, name, checkValid); 684 } 685 686 } 687 688 @Override 689 public Base setProperty(int hash, String name, Base value) throws FHIRException { 690 switch (hash) { 691 case 116103: // use 692 value = new NameUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 693 this.use = (Enumeration) value; // Enumeration<NameUse> 694 return value; 695 case 3556653: // text 696 this.text = TypeConvertor.castToString(value); // StringType 697 return value; 698 case -1281860764: // family 699 this.family = TypeConvertor.castToString(value); // StringType 700 return value; 701 case 98367357: // given 702 this.getGiven().add(TypeConvertor.castToString(value)); // StringType 703 return value; 704 case -980110702: // prefix 705 this.getPrefix().add(TypeConvertor.castToString(value)); // StringType 706 return value; 707 case -891422895: // suffix 708 this.getSuffix().add(TypeConvertor.castToString(value)); // StringType 709 return value; 710 case -991726143: // period 711 this.period = TypeConvertor.castToPeriod(value); // Period 712 return value; 713 default: return super.setProperty(hash, name, value); 714 } 715 716 } 717 718 @Override 719 public Base setProperty(String name, Base value) throws FHIRException { 720 if (name.equals("use")) { 721 value = new NameUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 722 this.use = (Enumeration) value; // Enumeration<NameUse> 723 } else if (name.equals("text")) { 724 this.text = TypeConvertor.castToString(value); // StringType 725 } else if (name.equals("family")) { 726 this.family = TypeConvertor.castToString(value); // StringType 727 } else if (name.equals("given")) { 728 this.getGiven().add(TypeConvertor.castToString(value)); 729 } else if (name.equals("prefix")) { 730 this.getPrefix().add(TypeConvertor.castToString(value)); 731 } else if (name.equals("suffix")) { 732 this.getSuffix().add(TypeConvertor.castToString(value)); 733 } else if (name.equals("period")) { 734 this.period = TypeConvertor.castToPeriod(value); // Period 735 } else 736 return super.setProperty(name, value); 737 return value; 738 } 739 740 @Override 741 public void removeChild(String name, Base value) throws FHIRException { 742 if (name.equals("use")) { 743 value = new NameUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 744 this.use = (Enumeration) value; // Enumeration<NameUse> 745 } else if (name.equals("text")) { 746 this.text = null; 747 } else if (name.equals("family")) { 748 this.family = null; 749 } else if (name.equals("given")) { 750 this.getGiven().remove(value); 751 } else if (name.equals("prefix")) { 752 this.getPrefix().remove(value); 753 } else if (name.equals("suffix")) { 754 this.getSuffix().remove(value); 755 } else if (name.equals("period")) { 756 this.period = null; 757 } else 758 super.removeChild(name, value); 759 760 } 761 762 @Override 763 public Base makeProperty(int hash, String name) throws FHIRException { 764 switch (hash) { 765 case 116103: return getUseElement(); 766 case 3556653: return getTextElement(); 767 case -1281860764: return getFamilyElement(); 768 case 98367357: return addGivenElement(); 769 case -980110702: return addPrefixElement(); 770 case -891422895: return addSuffixElement(); 771 case -991726143: return getPeriod(); 772 default: return super.makeProperty(hash, name); 773 } 774 775 } 776 777 @Override 778 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 779 switch (hash) { 780 case 116103: /*use*/ return new String[] {"code"}; 781 case 3556653: /*text*/ return new String[] {"string"}; 782 case -1281860764: /*family*/ return new String[] {"string"}; 783 case 98367357: /*given*/ return new String[] {"string"}; 784 case -980110702: /*prefix*/ return new String[] {"string"}; 785 case -891422895: /*suffix*/ return new String[] {"string"}; 786 case -991726143: /*period*/ return new String[] {"Period"}; 787 default: return super.getTypesForProperty(hash, name); 788 } 789 790 } 791 792 @Override 793 public Base addChild(String name) throws FHIRException { 794 if (name.equals("use")) { 795 throw new FHIRException("Cannot call addChild on a singleton property HumanName.use"); 796 } 797 else if (name.equals("text")) { 798 throw new FHIRException("Cannot call addChild on a singleton property HumanName.text"); 799 } 800 else if (name.equals("family")) { 801 throw new FHIRException("Cannot call addChild on a singleton property HumanName.family"); 802 } 803 else if (name.equals("given")) { 804 throw new FHIRException("Cannot call addChild on a singleton property HumanName.given"); 805 } 806 else if (name.equals("prefix")) { 807 throw new FHIRException("Cannot call addChild on a singleton property HumanName.prefix"); 808 } 809 else if (name.equals("suffix")) { 810 throw new FHIRException("Cannot call addChild on a singleton property HumanName.suffix"); 811 } 812 else if (name.equals("period")) { 813 this.period = new Period(); 814 return this.period; 815 } 816 else 817 return super.addChild(name); 818 } 819 820 public String fhirType() { 821 return "HumanName"; 822 823 } 824 825 public HumanName copy() { 826 HumanName dst = new HumanName(); 827 copyValues(dst); 828 return dst; 829 } 830 831 public void copyValues(HumanName dst) { 832 super.copyValues(dst); 833 dst.use = use == null ? null : use.copy(); 834 dst.text = text == null ? null : text.copy(); 835 dst.family = family == null ? null : family.copy(); 836 if (given != null) { 837 dst.given = new ArrayList<StringType>(); 838 for (StringType i : given) 839 dst.given.add(i.copy()); 840 }; 841 if (prefix != null) { 842 dst.prefix = new ArrayList<StringType>(); 843 for (StringType i : prefix) 844 dst.prefix.add(i.copy()); 845 }; 846 if (suffix != null) { 847 dst.suffix = new ArrayList<StringType>(); 848 for (StringType i : suffix) 849 dst.suffix.add(i.copy()); 850 }; 851 dst.period = period == null ? null : period.copy(); 852 } 853 854 protected HumanName typedCopy() { 855 return copy(); 856 } 857 858 @Override 859 public boolean equalsDeep(Base other_) { 860 if (!super.equalsDeep(other_)) 861 return false; 862 if (!(other_ instanceof HumanName)) 863 return false; 864 HumanName o = (HumanName) other_; 865 return compareDeep(use, o.use, true) && compareDeep(text, o.text, true) && compareDeep(family, o.family, true) 866 && compareDeep(given, o.given, true) && compareDeep(prefix, o.prefix, true) && compareDeep(suffix, o.suffix, true) 867 && compareDeep(period, o.period, true); 868 } 869 870 @Override 871 public boolean equalsShallow(Base other_) { 872 if (!super.equalsShallow(other_)) 873 return false; 874 if (!(other_ instanceof HumanName)) 875 return false; 876 HumanName o = (HumanName) other_; 877 return compareValues(use, o.use, true) && compareValues(text, o.text, true) && compareValues(family, o.family, true) 878 && compareValues(given, o.given, true) && compareValues(prefix, o.prefix, true) && compareValues(suffix, o.suffix, true) 879 ; 880 } 881 882 public boolean isEmpty() { 883 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(use, text, family, given 884 , prefix, suffix, period); 885 } 886 887// Manual code (from Configuration.txt): 888/** 889 /** 890 * Returns all repetitions of {@link #getGiven() given name} as a space separated string 891 * 892 * @see DatatypeUtil#joinStringsSpaceSeparated(List) 893 */ 894 public String getGivenAsSingleString() { 895 return joinStringsSpaceSeparated(getGiven()); 896 } 897 898 /** 899 * Returns all repetitions of {@link #getPrefix() prefix name} as a space separated string 900 * 901 * @see DatatypeUtil#joinStringsSpaceSeparated(List) 902 */ 903 public String getPrefixAsSingleString() { 904 return joinStringsSpaceSeparated(getPrefix()); 905 } 906 907 /** 908 * Returns all repetitions of {@link #getSuffix() suffix} as a space separated string 909 * 910 * @see DatatypeUtil#joinStringsSpaceSeparated(List) 911 */ 912 public String getSuffixAsSingleString() { 913 return joinStringsSpaceSeparated(getSuffix()); 914 } 915 916 /** 917 * <p>Returns the {@link #getTextElement() text} element value if it is not null.</p> 918 919 * <p>If the {@link #getTextElement() text} element value is null, returns all the components of the name (prefix, 920 * given, family, suffix) as a single string with a single spaced string separating each part. </p> 921 * 922 * @return the human name as a single string 923 */ 924 public String getNameAsSingleString() { 925 if (hasText()) { 926 return getText().toString(); 927 } 928 929 List<StringType> nameParts = new ArrayList<StringType>(); 930 nameParts.addAll(getPrefix()); 931 nameParts.addAll(getGiven()); 932 if (hasFamilyElement()) { 933 nameParts.add(getFamilyElement()); 934 } 935 nameParts.addAll(getSuffix()); 936 if (nameParts.size() > 0) { 937 return joinStringsSpaceSeparated(nameParts); 938 } else { 939 return getTextElement().getValue(); 940 } 941 } 942 943 /** 944 * Joins a list of strings with a single space (' ') between each string 945 * 946 * TODO: replace with call to ca.uhn.fhir.util.DatatypeUtil.joinStringsSpaceSeparated when HAPI upgrades to 1.4 947 */ 948 private static String joinStringsSpaceSeparated(List<? extends IPrimitiveType<String>> theStrings) { 949 StringBuilder stringBuilder = new StringBuilder(); 950 for (IPrimitiveType<String> string : theStrings) { 951 if (string.isEmpty()) { 952 continue; 953 } 954 if (stringBuilder.length() > 0) { 955 stringBuilder.append(' '); 956 } 957 stringBuilder.append(string.getValue()); 958 } 959 return stringBuilder.toString(); 960 } 961// end addition 962 963} 964