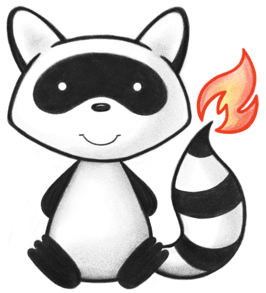
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * Identifier Type: An identifier - identifies some entity uniquely and unambiguously. Typically this is used for business identifiers. 050 */ 051@DatatypeDef(name="Identifier") 052public class Identifier extends DataType implements ICompositeType { 053 054 public enum IdentifierUse { 055 /** 056 * The identifier recommended for display and use in real-world interactions which should be used when such identifier is different from the \"official\" identifier. 057 */ 058 USUAL, 059 /** 060 * The identifier considered to be most trusted for the identification of this item. Sometimes also known as \"primary\" and \"main\". The determination of \"official\" is subjective and implementation guides often provide additional guidelines for use. 061 */ 062 OFFICIAL, 063 /** 064 * A temporary identifier. 065 */ 066 TEMP, 067 /** 068 * An identifier that was assigned in secondary use - it serves to identify the object in a relative context, but cannot be consistently assigned to the same object again in a different context. 069 */ 070 SECONDARY, 071 /** 072 * The identifier id no longer considered valid, but may be relevant for search purposes. E.g. Changes to identifier schemes, account merges, etc. 073 */ 074 OLD, 075 /** 076 * added to help the parsers with the generic types 077 */ 078 NULL; 079 public static IdentifierUse fromCode(String codeString) throws FHIRException { 080 if (codeString == null || "".equals(codeString)) 081 return null; 082 if ("usual".equals(codeString)) 083 return USUAL; 084 if ("official".equals(codeString)) 085 return OFFICIAL; 086 if ("temp".equals(codeString)) 087 return TEMP; 088 if ("secondary".equals(codeString)) 089 return SECONDARY; 090 if ("old".equals(codeString)) 091 return OLD; 092 if (Configuration.isAcceptInvalidEnums()) 093 return null; 094 else 095 throw new FHIRException("Unknown IdentifierUse code '"+codeString+"'"); 096 } 097 public String toCode() { 098 switch (this) { 099 case USUAL: return "usual"; 100 case OFFICIAL: return "official"; 101 case TEMP: return "temp"; 102 case SECONDARY: return "secondary"; 103 case OLD: return "old"; 104 case NULL: return null; 105 default: return "?"; 106 } 107 } 108 public String getSystem() { 109 switch (this) { 110 case USUAL: return "http://hl7.org/fhir/identifier-use"; 111 case OFFICIAL: return "http://hl7.org/fhir/identifier-use"; 112 case TEMP: return "http://hl7.org/fhir/identifier-use"; 113 case SECONDARY: return "http://hl7.org/fhir/identifier-use"; 114 case OLD: return "http://hl7.org/fhir/identifier-use"; 115 case NULL: return null; 116 default: return "?"; 117 } 118 } 119 public String getDefinition() { 120 switch (this) { 121 case USUAL: return "The identifier recommended for display and use in real-world interactions which should be used when such identifier is different from the \"official\" identifier."; 122 case OFFICIAL: return "The identifier considered to be most trusted for the identification of this item. Sometimes also known as \"primary\" and \"main\". The determination of \"official\" is subjective and implementation guides often provide additional guidelines for use."; 123 case TEMP: return "A temporary identifier."; 124 case SECONDARY: return "An identifier that was assigned in secondary use - it serves to identify the object in a relative context, but cannot be consistently assigned to the same object again in a different context."; 125 case OLD: return "The identifier id no longer considered valid, but may be relevant for search purposes. E.g. Changes to identifier schemes, account merges, etc."; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 public String getDisplay() { 131 switch (this) { 132 case USUAL: return "Usual"; 133 case OFFICIAL: return "Official"; 134 case TEMP: return "Temp"; 135 case SECONDARY: return "Secondary"; 136 case OLD: return "Old"; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 } 142 143 public static class IdentifierUseEnumFactory implements EnumFactory<IdentifierUse> { 144 public IdentifierUse fromCode(String codeString) throws IllegalArgumentException { 145 if (codeString == null || "".equals(codeString)) 146 if (codeString == null || "".equals(codeString)) 147 return null; 148 if ("usual".equals(codeString)) 149 return IdentifierUse.USUAL; 150 if ("official".equals(codeString)) 151 return IdentifierUse.OFFICIAL; 152 if ("temp".equals(codeString)) 153 return IdentifierUse.TEMP; 154 if ("secondary".equals(codeString)) 155 return IdentifierUse.SECONDARY; 156 if ("old".equals(codeString)) 157 return IdentifierUse.OLD; 158 throw new IllegalArgumentException("Unknown IdentifierUse code '"+codeString+"'"); 159 } 160 public Enumeration<IdentifierUse> fromType(PrimitiveType<?> code) throws FHIRException { 161 if (code == null) 162 return null; 163 if (code.isEmpty()) 164 return new Enumeration<IdentifierUse>(this, IdentifierUse.NULL, code); 165 String codeString = ((PrimitiveType) code).asStringValue(); 166 if (codeString == null || "".equals(codeString)) 167 return new Enumeration<IdentifierUse>(this, IdentifierUse.NULL, code); 168 if ("usual".equals(codeString)) 169 return new Enumeration<IdentifierUse>(this, IdentifierUse.USUAL, code); 170 if ("official".equals(codeString)) 171 return new Enumeration<IdentifierUse>(this, IdentifierUse.OFFICIAL, code); 172 if ("temp".equals(codeString)) 173 return new Enumeration<IdentifierUse>(this, IdentifierUse.TEMP, code); 174 if ("secondary".equals(codeString)) 175 return new Enumeration<IdentifierUse>(this, IdentifierUse.SECONDARY, code); 176 if ("old".equals(codeString)) 177 return new Enumeration<IdentifierUse>(this, IdentifierUse.OLD, code); 178 throw new FHIRException("Unknown IdentifierUse code '"+codeString+"'"); 179 } 180 public String toCode(IdentifierUse code) { 181 if (code == IdentifierUse.NULL) 182 return null; 183 if (code == IdentifierUse.USUAL) 184 return "usual"; 185 if (code == IdentifierUse.OFFICIAL) 186 return "official"; 187 if (code == IdentifierUse.TEMP) 188 return "temp"; 189 if (code == IdentifierUse.SECONDARY) 190 return "secondary"; 191 if (code == IdentifierUse.OLD) 192 return "old"; 193 return "?"; 194 } 195 public String toSystem(IdentifierUse code) { 196 return code.getSystem(); 197 } 198 } 199 200 /** 201 * The purpose of this identifier. 202 */ 203 @Child(name = "use", type = {CodeType.class}, order=0, min=0, max=1, modifier=true, summary=true) 204 @Description(shortDefinition="usual | official | temp | secondary | old (If known)", formalDefinition="The purpose of this identifier." ) 205 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/identifier-use") 206 protected Enumeration<IdentifierUse> use; 207 208 /** 209 * A coded type for the identifier that can be used to determine which identifier to use for a specific purpose. 210 */ 211 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 212 @Description(shortDefinition="Description of identifier", formalDefinition="A coded type for the identifier that can be used to determine which identifier to use for a specific purpose." ) 213 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/identifier-type") 214 protected CodeableConcept type; 215 216 /** 217 * Establishes the namespace for the value - that is, an absolute URL that describes a set values that are unique. 218 */ 219 @Child(name = "system", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 220 @Description(shortDefinition="The namespace for the identifier value", formalDefinition="Establishes the namespace for the value - that is, an absolute URL that describes a set values that are unique." ) 221 protected UriType system; 222 223 /** 224 * The portion of the identifier typically relevant to the user and which is unique within the context of the system. 225 */ 226 @Child(name = "value", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 227 @Description(shortDefinition="The value that is unique", formalDefinition="The portion of the identifier typically relevant to the user and which is unique within the context of the system." ) 228 protected StringType value; 229 230 /** 231 * Time period during which identifier is/was valid for use. 232 */ 233 @Child(name = "period", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=true) 234 @Description(shortDefinition="Time period when id is/was valid for use", formalDefinition="Time period during which identifier is/was valid for use." ) 235 protected Period period; 236 237 /** 238 * Organization that issued/manages the identifier. 239 */ 240 @Child(name = "assigner", type = {Organization.class}, order=5, min=0, max=1, modifier=false, summary=true) 241 @Description(shortDefinition="Organization that issued id (may be just text)", formalDefinition="Organization that issued/manages the identifier." ) 242 protected Reference assigner; 243 244 private static final long serialVersionUID = 2098433371L; 245 246 /** 247 * Constructor 248 */ 249 public Identifier() { 250 super(); 251 } 252 253 /** 254 * @return {@link #use} (The purpose of this identifier.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 255 */ 256 public Enumeration<IdentifierUse> getUseElement() { 257 if (this.use == null) 258 if (Configuration.errorOnAutoCreate()) 259 throw new Error("Attempt to auto-create Identifier.use"); 260 else if (Configuration.doAutoCreate()) 261 this.use = new Enumeration<IdentifierUse>(new IdentifierUseEnumFactory()); // bb 262 return this.use; 263 } 264 265 public boolean hasUseElement() { 266 return this.use != null && !this.use.isEmpty(); 267 } 268 269 public boolean hasUse() { 270 return this.use != null && !this.use.isEmpty(); 271 } 272 273 /** 274 * @param value {@link #use} (The purpose of this identifier.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 275 */ 276 public Identifier setUseElement(Enumeration<IdentifierUse> value) { 277 this.use = value; 278 return this; 279 } 280 281 /** 282 * @return The purpose of this identifier. 283 */ 284 public IdentifierUse getUse() { 285 return this.use == null ? null : this.use.getValue(); 286 } 287 288 /** 289 * @param value The purpose of this identifier. 290 */ 291 public Identifier setUse(IdentifierUse value) { 292 if (value == null) 293 this.use = null; 294 else { 295 if (this.use == null) 296 this.use = new Enumeration<IdentifierUse>(new IdentifierUseEnumFactory()); 297 this.use.setValue(value); 298 } 299 return this; 300 } 301 302 /** 303 * @return {@link #type} (A coded type for the identifier that can be used to determine which identifier to use for a specific purpose.) 304 */ 305 public CodeableConcept getType() { 306 if (this.type == null) 307 if (Configuration.errorOnAutoCreate()) 308 throw new Error("Attempt to auto-create Identifier.type"); 309 else if (Configuration.doAutoCreate()) 310 this.type = new CodeableConcept(); // cc 311 return this.type; 312 } 313 314 public boolean hasType() { 315 return this.type != null && !this.type.isEmpty(); 316 } 317 318 /** 319 * @param value {@link #type} (A coded type for the identifier that can be used to determine which identifier to use for a specific purpose.) 320 */ 321 public Identifier setType(CodeableConcept value) { 322 this.type = value; 323 return this; 324 } 325 326 /** 327 * @return {@link #system} (Establishes the namespace for the value - that is, an absolute URL that describes a set values that are unique.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 328 */ 329 public UriType getSystemElement() { 330 if (this.system == null) 331 if (Configuration.errorOnAutoCreate()) 332 throw new Error("Attempt to auto-create Identifier.system"); 333 else if (Configuration.doAutoCreate()) 334 this.system = new UriType(); // bb 335 return this.system; 336 } 337 338 public boolean hasSystemElement() { 339 return this.system != null && !this.system.isEmpty(); 340 } 341 342 public boolean hasSystem() { 343 return this.system != null && !this.system.isEmpty(); 344 } 345 346 /** 347 * @param value {@link #system} (Establishes the namespace for the value - that is, an absolute URL that describes a set values that are unique.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 348 */ 349 public Identifier setSystemElement(UriType value) { 350 this.system = value; 351 return this; 352 } 353 354 /** 355 * @return Establishes the namespace for the value - that is, an absolute URL that describes a set values that are unique. 356 */ 357 public String getSystem() { 358 return this.system == null ? null : this.system.getValue(); 359 } 360 361 /** 362 * @param value Establishes the namespace for the value - that is, an absolute URL that describes a set values that are unique. 363 */ 364 public Identifier setSystem(String value) { 365 if (Utilities.noString(value)) 366 this.system = null; 367 else { 368 if (this.system == null) 369 this.system = new UriType(); 370 this.system.setValue(value); 371 } 372 return this; 373 } 374 375 /** 376 * @return {@link #value} (The portion of the identifier typically relevant to the user and which is unique within the context of the system.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 377 */ 378 public StringType getValueElement() { 379 if (this.value == null) 380 if (Configuration.errorOnAutoCreate()) 381 throw new Error("Attempt to auto-create Identifier.value"); 382 else if (Configuration.doAutoCreate()) 383 this.value = new StringType(); // bb 384 return this.value; 385 } 386 387 public boolean hasValueElement() { 388 return this.value != null && !this.value.isEmpty(); 389 } 390 391 public boolean hasValue() { 392 return this.value != null && !this.value.isEmpty(); 393 } 394 395 /** 396 * @param value {@link #value} (The portion of the identifier typically relevant to the user and which is unique within the context of the system.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 397 */ 398 public Identifier setValueElement(StringType value) { 399 this.value = value; 400 return this; 401 } 402 403 /** 404 * @return The portion of the identifier typically relevant to the user and which is unique within the context of the system. 405 */ 406 public String getValue() { 407 return this.value == null ? null : this.value.getValue(); 408 } 409 410 /** 411 * @param value The portion of the identifier typically relevant to the user and which is unique within the context of the system. 412 */ 413 public Identifier setValue(String value) { 414 if (Utilities.noString(value)) 415 this.value = null; 416 else { 417 if (this.value == null) 418 this.value = new StringType(); 419 this.value.setValue(value); 420 } 421 return this; 422 } 423 424 /** 425 * @return {@link #period} (Time period during which identifier is/was valid for use.) 426 */ 427 public Period getPeriod() { 428 if (this.period == null) 429 if (Configuration.errorOnAutoCreate()) 430 throw new Error("Attempt to auto-create Identifier.period"); 431 else if (Configuration.doAutoCreate()) 432 this.period = new Period(); // cc 433 return this.period; 434 } 435 436 public boolean hasPeriod() { 437 return this.period != null && !this.period.isEmpty(); 438 } 439 440 /** 441 * @param value {@link #period} (Time period during which identifier is/was valid for use.) 442 */ 443 public Identifier setPeriod(Period value) { 444 this.period = value; 445 return this; 446 } 447 448 /** 449 * @return {@link #assigner} (Organization that issued/manages the identifier.) 450 */ 451 public Reference getAssigner() { 452 if (this.assigner == null) 453 if (Configuration.errorOnAutoCreate()) 454 throw new Error("Attempt to auto-create Identifier.assigner"); 455 else if (Configuration.doAutoCreate()) 456 this.assigner = new Reference(); // cc 457 return this.assigner; 458 } 459 460 public boolean hasAssigner() { 461 return this.assigner != null && !this.assigner.isEmpty(); 462 } 463 464 /** 465 * @param value {@link #assigner} (Organization that issued/manages the identifier.) 466 */ 467 public Identifier setAssigner(Reference value) { 468 this.assigner = value; 469 return this; 470 } 471 472 protected void listChildren(List<Property> children) { 473 super.listChildren(children); 474 children.add(new Property("use", "code", "The purpose of this identifier.", 0, 1, use)); 475 children.add(new Property("type", "CodeableConcept", "A coded type for the identifier that can be used to determine which identifier to use for a specific purpose.", 0, 1, type)); 476 children.add(new Property("system", "uri", "Establishes the namespace for the value - that is, an absolute URL that describes a set values that are unique.", 0, 1, system)); 477 children.add(new Property("value", "string", "The portion of the identifier typically relevant to the user and which is unique within the context of the system.", 0, 1, value)); 478 children.add(new Property("period", "Period", "Time period during which identifier is/was valid for use.", 0, 1, period)); 479 children.add(new Property("assigner", "Reference(Organization)", "Organization that issued/manages the identifier.", 0, 1, assigner)); 480 } 481 482 @Override 483 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 484 switch (_hash) { 485 case 116103: /*use*/ return new Property("use", "code", "The purpose of this identifier.", 0, 1, use); 486 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A coded type for the identifier that can be used to determine which identifier to use for a specific purpose.", 0, 1, type); 487 case -887328209: /*system*/ return new Property("system", "uri", "Establishes the namespace for the value - that is, an absolute URL that describes a set values that are unique.", 0, 1, system); 488 case 111972721: /*value*/ return new Property("value", "string", "The portion of the identifier typically relevant to the user and which is unique within the context of the system.", 0, 1, value); 489 case -991726143: /*period*/ return new Property("period", "Period", "Time period during which identifier is/was valid for use.", 0, 1, period); 490 case -369881636: /*assigner*/ return new Property("assigner", "Reference(Organization)", "Organization that issued/manages the identifier.", 0, 1, assigner); 491 default: return super.getNamedProperty(_hash, _name, _checkValid); 492 } 493 494 } 495 496 @Override 497 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 498 switch (hash) { 499 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<IdentifierUse> 500 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 501 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // UriType 502 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 503 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 504 case -369881636: /*assigner*/ return this.assigner == null ? new Base[0] : new Base[] {this.assigner}; // Reference 505 default: return super.getProperty(hash, name, checkValid); 506 } 507 508 } 509 510 @Override 511 public Base setProperty(int hash, String name, Base value) throws FHIRException { 512 switch (hash) { 513 case 116103: // use 514 value = new IdentifierUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 515 this.use = (Enumeration) value; // Enumeration<IdentifierUse> 516 return value; 517 case 3575610: // type 518 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 519 return value; 520 case -887328209: // system 521 this.system = TypeConvertor.castToUri(value); // UriType 522 return value; 523 case 111972721: // value 524 this.value = TypeConvertor.castToString(value); // StringType 525 return value; 526 case -991726143: // period 527 this.period = TypeConvertor.castToPeriod(value); // Period 528 return value; 529 case -369881636: // assigner 530 this.assigner = TypeConvertor.castToReference(value); // Reference 531 return value; 532 default: return super.setProperty(hash, name, value); 533 } 534 535 } 536 537 @Override 538 public Base setProperty(String name, Base value) throws FHIRException { 539 if (name.equals("use")) { 540 value = new IdentifierUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 541 this.use = (Enumeration) value; // Enumeration<IdentifierUse> 542 } else if (name.equals("type")) { 543 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 544 } else if (name.equals("system")) { 545 this.system = TypeConvertor.castToUri(value); // UriType 546 } else if (name.equals("value")) { 547 this.value = TypeConvertor.castToString(value); // StringType 548 } else if (name.equals("period")) { 549 this.period = TypeConvertor.castToPeriod(value); // Period 550 } else if (name.equals("assigner")) { 551 this.assigner = TypeConvertor.castToReference(value); // Reference 552 } else 553 return super.setProperty(name, value); 554 return value; 555 } 556 557 @Override 558 public void removeChild(String name, Base value) throws FHIRException { 559 if (name.equals("use")) { 560 value = new IdentifierUseEnumFactory().fromType(TypeConvertor.castToCode(value)); 561 this.use = (Enumeration) value; // Enumeration<IdentifierUse> 562 } else if (name.equals("type")) { 563 this.type = null; 564 } else if (name.equals("system")) { 565 this.system = null; 566 } else if (name.equals("value")) { 567 this.value = null; 568 } else if (name.equals("period")) { 569 this.period = null; 570 } else if (name.equals("assigner")) { 571 this.assigner = null; 572 } else 573 super.removeChild(name, value); 574 575 } 576 577 @Override 578 public Base makeProperty(int hash, String name) throws FHIRException { 579 switch (hash) { 580 case 116103: return getUseElement(); 581 case 3575610: return getType(); 582 case -887328209: return getSystemElement(); 583 case 111972721: return getValueElement(); 584 case -991726143: return getPeriod(); 585 case -369881636: return getAssigner(); 586 default: return super.makeProperty(hash, name); 587 } 588 589 } 590 591 @Override 592 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 593 switch (hash) { 594 case 116103: /*use*/ return new String[] {"code"}; 595 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 596 case -887328209: /*system*/ return new String[] {"uri"}; 597 case 111972721: /*value*/ return new String[] {"string"}; 598 case -991726143: /*period*/ return new String[] {"Period"}; 599 case -369881636: /*assigner*/ return new String[] {"Reference"}; 600 default: return super.getTypesForProperty(hash, name); 601 } 602 603 } 604 605 @Override 606 public Base addChild(String name) throws FHIRException { 607 if (name.equals("use")) { 608 throw new FHIRException("Cannot call addChild on a singleton property Identifier.use"); 609 } 610 else if (name.equals("type")) { 611 this.type = new CodeableConcept(); 612 return this.type; 613 } 614 else if (name.equals("system")) { 615 throw new FHIRException("Cannot call addChild on a singleton property Identifier.system"); 616 } 617 else if (name.equals("value")) { 618 throw new FHIRException("Cannot call addChild on a singleton property Identifier.value"); 619 } 620 else if (name.equals("period")) { 621 this.period = new Period(); 622 return this.period; 623 } 624 else if (name.equals("assigner")) { 625 this.assigner = new Reference(); 626 return this.assigner; 627 } 628 else 629 return super.addChild(name); 630 } 631 632 public String fhirType() { 633 return "Identifier"; 634 635 } 636 637 public Identifier copy() { 638 Identifier dst = new Identifier(); 639 copyValues(dst); 640 return dst; 641 } 642 643 public void copyValues(Identifier dst) { 644 super.copyValues(dst); 645 dst.use = use == null ? null : use.copy(); 646 dst.type = type == null ? null : type.copy(); 647 dst.system = system == null ? null : system.copy(); 648 dst.value = value == null ? null : value.copy(); 649 dst.period = period == null ? null : period.copy(); 650 dst.assigner = assigner == null ? null : assigner.copy(); 651 } 652 653 protected Identifier typedCopy() { 654 return copy(); 655 } 656 657 @Override 658 public boolean equalsDeep(Base other_) { 659 if (!super.equalsDeep(other_)) 660 return false; 661 if (!(other_ instanceof Identifier)) 662 return false; 663 Identifier o = (Identifier) other_; 664 return compareDeep(use, o.use, true) && compareDeep(type, o.type, true) && compareDeep(system, o.system, true) 665 && compareDeep(value, o.value, true) && compareDeep(period, o.period, true) && compareDeep(assigner, o.assigner, true) 666 ; 667 } 668 669 @Override 670 public boolean equalsShallow(Base other_) { 671 if (!super.equalsShallow(other_)) 672 return false; 673 if (!(other_ instanceof Identifier)) 674 return false; 675 Identifier o = (Identifier) other_; 676 return compareValues(use, o.use, true) && compareValues(system, o.system, true) && compareValues(value, o.value, true) 677 ; 678 } 679 680 public boolean isEmpty() { 681 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(use, type, system, value 682 , period, assigner); 683 } 684 685 public boolean matches(Identifier other) { 686 return hasSystem() && hasValue() && getSystem().matches(other.getSystem()) && getValue().matches(other.getValue()); 687 } 688 689 690} 691