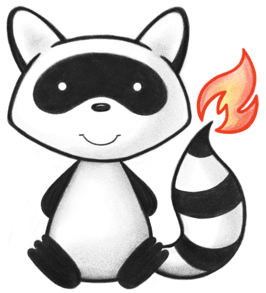
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051/** 052 * A selection of DICOM SOP instances and/or frames within a single Study and Series. This might include additional specifics such as an image region, an Observation UID or a Segmentation Number, allowing linkage to an Observation Resource or transferring this information along with the ImagingStudy Resource. 053 */ 054@ResourceDef(name="ImagingSelection", profile="http://hl7.org/fhir/StructureDefinition/ImagingSelection") 055public class ImagingSelection extends DomainResource { 056 057 public enum ImagingSelection2DGraphicType { 058 /** 059 * A single location denoted by a single (x,y) pair. 060 */ 061 POINT, 062 /** 063 * A series of connected line segments with ordered vertices denoted by (x,y) triplets; the points need not be coplanar. 064 */ 065 POLYLINE, 066 /** 067 * An n-tuple list of (x,y) pair end points between which some form of implementation dependent curved lines are to be drawn. The rendered line shall pass through all the specified points. 068 */ 069 INTERPOLATED, 070 /** 071 * Two points shall be present; the first point is to be interpreted as the center and the second point as a point on the circumference of a circle, some form of implementation dependent representation of which is to be drawn. 072 */ 073 CIRCLE, 074 /** 075 * An ellipse defined by four (x,y) pairs, the first two pairs specifying the endpoints of the major axis and the second two pairs specifying the endpoints of the minor axis. 076 */ 077 ELLIPSE, 078 /** 079 * added to help the parsers with the generic types 080 */ 081 NULL; 082 public static ImagingSelection2DGraphicType fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("point".equals(codeString)) 086 return POINT; 087 if ("polyline".equals(codeString)) 088 return POLYLINE; 089 if ("interpolated".equals(codeString)) 090 return INTERPOLATED; 091 if ("circle".equals(codeString)) 092 return CIRCLE; 093 if ("ellipse".equals(codeString)) 094 return ELLIPSE; 095 if (Configuration.isAcceptInvalidEnums()) 096 return null; 097 else 098 throw new FHIRException("Unknown ImagingSelection2DGraphicType code '"+codeString+"'"); 099 } 100 public String toCode() { 101 switch (this) { 102 case POINT: return "point"; 103 case POLYLINE: return "polyline"; 104 case INTERPOLATED: return "interpolated"; 105 case CIRCLE: return "circle"; 106 case ELLIPSE: return "ellipse"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getSystem() { 112 switch (this) { 113 case POINT: return "http://hl7.org/fhir/imagingselection-2dgraphictype"; 114 case POLYLINE: return "http://hl7.org/fhir/imagingselection-2dgraphictype"; 115 case INTERPOLATED: return "http://hl7.org/fhir/imagingselection-2dgraphictype"; 116 case CIRCLE: return "http://hl7.org/fhir/imagingselection-2dgraphictype"; 117 case ELLIPSE: return "http://hl7.org/fhir/imagingselection-2dgraphictype"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDefinition() { 123 switch (this) { 124 case POINT: return "A single location denoted by a single (x,y) pair."; 125 case POLYLINE: return "A series of connected line segments with ordered vertices denoted by (x,y) triplets; the points need not be coplanar."; 126 case INTERPOLATED: return "An n-tuple list of (x,y) pair end points between which some form of implementation dependent curved lines are to be drawn. The rendered line shall pass through all the specified points."; 127 case CIRCLE: return "Two points shall be present; the first point is to be interpreted as the center and the second point as a point on the circumference of a circle, some form of implementation dependent representation of which is to be drawn."; 128 case ELLIPSE: return "An ellipse defined by four (x,y) pairs, the first two pairs specifying the endpoints of the major axis and the second two pairs specifying the endpoints of the minor axis."; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 public String getDisplay() { 134 switch (this) { 135 case POINT: return "POINT"; 136 case POLYLINE: return "POLYLINE"; 137 case INTERPOLATED: return "INTERPOLATED"; 138 case CIRCLE: return "CIRCLE"; 139 case ELLIPSE: return "ELLIPSE"; 140 case NULL: return null; 141 default: return "?"; 142 } 143 } 144 } 145 146 public static class ImagingSelection2DGraphicTypeEnumFactory implements EnumFactory<ImagingSelection2DGraphicType> { 147 public ImagingSelection2DGraphicType fromCode(String codeString) throws IllegalArgumentException { 148 if (codeString == null || "".equals(codeString)) 149 if (codeString == null || "".equals(codeString)) 150 return null; 151 if ("point".equals(codeString)) 152 return ImagingSelection2DGraphicType.POINT; 153 if ("polyline".equals(codeString)) 154 return ImagingSelection2DGraphicType.POLYLINE; 155 if ("interpolated".equals(codeString)) 156 return ImagingSelection2DGraphicType.INTERPOLATED; 157 if ("circle".equals(codeString)) 158 return ImagingSelection2DGraphicType.CIRCLE; 159 if ("ellipse".equals(codeString)) 160 return ImagingSelection2DGraphicType.ELLIPSE; 161 throw new IllegalArgumentException("Unknown ImagingSelection2DGraphicType code '"+codeString+"'"); 162 } 163 public Enumeration<ImagingSelection2DGraphicType> fromType(PrimitiveType<?> code) throws FHIRException { 164 if (code == null) 165 return null; 166 if (code.isEmpty()) 167 return new Enumeration<ImagingSelection2DGraphicType>(this, ImagingSelection2DGraphicType.NULL, code); 168 String codeString = ((PrimitiveType) code).asStringValue(); 169 if (codeString == null || "".equals(codeString)) 170 return new Enumeration<ImagingSelection2DGraphicType>(this, ImagingSelection2DGraphicType.NULL, code); 171 if ("point".equals(codeString)) 172 return new Enumeration<ImagingSelection2DGraphicType>(this, ImagingSelection2DGraphicType.POINT, code); 173 if ("polyline".equals(codeString)) 174 return new Enumeration<ImagingSelection2DGraphicType>(this, ImagingSelection2DGraphicType.POLYLINE, code); 175 if ("interpolated".equals(codeString)) 176 return new Enumeration<ImagingSelection2DGraphicType>(this, ImagingSelection2DGraphicType.INTERPOLATED, code); 177 if ("circle".equals(codeString)) 178 return new Enumeration<ImagingSelection2DGraphicType>(this, ImagingSelection2DGraphicType.CIRCLE, code); 179 if ("ellipse".equals(codeString)) 180 return new Enumeration<ImagingSelection2DGraphicType>(this, ImagingSelection2DGraphicType.ELLIPSE, code); 181 throw new FHIRException("Unknown ImagingSelection2DGraphicType code '"+codeString+"'"); 182 } 183 public String toCode(ImagingSelection2DGraphicType code) { 184 if (code == ImagingSelection2DGraphicType.NULL) 185 return null; 186 if (code == ImagingSelection2DGraphicType.POINT) 187 return "point"; 188 if (code == ImagingSelection2DGraphicType.POLYLINE) 189 return "polyline"; 190 if (code == ImagingSelection2DGraphicType.INTERPOLATED) 191 return "interpolated"; 192 if (code == ImagingSelection2DGraphicType.CIRCLE) 193 return "circle"; 194 if (code == ImagingSelection2DGraphicType.ELLIPSE) 195 return "ellipse"; 196 return "?"; 197 } 198 public String toSystem(ImagingSelection2DGraphicType code) { 199 return code.getSystem(); 200 } 201 } 202 203 public enum ImagingSelection3DGraphicType { 204 /** 205 * A single location denoted by a single (x,y,z) triplet. 206 */ 207 POINT, 208 /** 209 * multiple locations each denoted by an (x,y,z) triplet; the points need not be coplanar. 210 */ 211 MULTIPOINT, 212 /** 213 * a series of connected line segments with ordered vertices denoted by (x,y,z) triplets; the points need not be coplanar. 214 */ 215 POLYLINE, 216 /** 217 * a series of connected line segments with ordered vertices denoted by (x,y,z) triplets, where the first and last vertices shall be the same forming a polygon; the points shall be coplanar. 218 */ 219 POLYGON, 220 /** 221 * an ellipse defined by four (x,y,z) triplets, the first two triplets specifying the endpoints of the major axis and the second two triplets specifying the endpoints of the minor axis. 222 */ 223 ELLIPSE, 224 /** 225 * a three-dimensional geometric surface whose plane sections are either ellipses or circles and contains three intersecting orthogonal axes, \"a\", \"b\", and \"c\"; the ellipsoid is defined by six (x,y,z) triplets, the first and second triplets specifying the endpoints of axis \"a\", the third and fourth triplets specifying the endpoints of axis \"b\", and the fifth and sixth triplets specifying the endpoints of axis \"c\". 226 */ 227 ELLIPSOID, 228 /** 229 * added to help the parsers with the generic types 230 */ 231 NULL; 232 public static ImagingSelection3DGraphicType fromCode(String codeString) throws FHIRException { 233 if (codeString == null || "".equals(codeString)) 234 return null; 235 if ("point".equals(codeString)) 236 return POINT; 237 if ("multipoint".equals(codeString)) 238 return MULTIPOINT; 239 if ("polyline".equals(codeString)) 240 return POLYLINE; 241 if ("polygon".equals(codeString)) 242 return POLYGON; 243 if ("ellipse".equals(codeString)) 244 return ELLIPSE; 245 if ("ellipsoid".equals(codeString)) 246 return ELLIPSOID; 247 if (Configuration.isAcceptInvalidEnums()) 248 return null; 249 else 250 throw new FHIRException("Unknown ImagingSelection3DGraphicType code '"+codeString+"'"); 251 } 252 public String toCode() { 253 switch (this) { 254 case POINT: return "point"; 255 case MULTIPOINT: return "multipoint"; 256 case POLYLINE: return "polyline"; 257 case POLYGON: return "polygon"; 258 case ELLIPSE: return "ellipse"; 259 case ELLIPSOID: return "ellipsoid"; 260 case NULL: return null; 261 default: return "?"; 262 } 263 } 264 public String getSystem() { 265 switch (this) { 266 case POINT: return "http://hl7.org/fhir/imagingselection-3dgraphictype"; 267 case MULTIPOINT: return "http://hl7.org/fhir/imagingselection-3dgraphictype"; 268 case POLYLINE: return "http://hl7.org/fhir/imagingselection-3dgraphictype"; 269 case POLYGON: return "http://hl7.org/fhir/imagingselection-3dgraphictype"; 270 case ELLIPSE: return "http://hl7.org/fhir/imagingselection-3dgraphictype"; 271 case ELLIPSOID: return "http://hl7.org/fhir/imagingselection-3dgraphictype"; 272 case NULL: return null; 273 default: return "?"; 274 } 275 } 276 public String getDefinition() { 277 switch (this) { 278 case POINT: return "A single location denoted by a single (x,y,z) triplet."; 279 case MULTIPOINT: return "multiple locations each denoted by an (x,y,z) triplet; the points need not be coplanar."; 280 case POLYLINE: return "a series of connected line segments with ordered vertices denoted by (x,y,z) triplets; the points need not be coplanar."; 281 case POLYGON: return "a series of connected line segments with ordered vertices denoted by (x,y,z) triplets, where the first and last vertices shall be the same forming a polygon; the points shall be coplanar."; 282 case ELLIPSE: return "an ellipse defined by four (x,y,z) triplets, the first two triplets specifying the endpoints of the major axis and the second two triplets specifying the endpoints of the minor axis."; 283 case ELLIPSOID: return "a three-dimensional geometric surface whose plane sections are either ellipses or circles and contains three intersecting orthogonal axes, \"a\", \"b\", and \"c\"; the ellipsoid is defined by six (x,y,z) triplets, the first and second triplets specifying the endpoints of axis \"a\", the third and fourth triplets specifying the endpoints of axis \"b\", and the fifth and sixth triplets specifying the endpoints of axis \"c\"."; 284 case NULL: return null; 285 default: return "?"; 286 } 287 } 288 public String getDisplay() { 289 switch (this) { 290 case POINT: return "POINT"; 291 case MULTIPOINT: return "MULTIPOINT"; 292 case POLYLINE: return "POLYLINE"; 293 case POLYGON: return "POLYGON"; 294 case ELLIPSE: return "ELLIPSE"; 295 case ELLIPSOID: return "ELLIPSOID"; 296 case NULL: return null; 297 default: return "?"; 298 } 299 } 300 } 301 302 public static class ImagingSelection3DGraphicTypeEnumFactory implements EnumFactory<ImagingSelection3DGraphicType> { 303 public ImagingSelection3DGraphicType fromCode(String codeString) throws IllegalArgumentException { 304 if (codeString == null || "".equals(codeString)) 305 if (codeString == null || "".equals(codeString)) 306 return null; 307 if ("point".equals(codeString)) 308 return ImagingSelection3DGraphicType.POINT; 309 if ("multipoint".equals(codeString)) 310 return ImagingSelection3DGraphicType.MULTIPOINT; 311 if ("polyline".equals(codeString)) 312 return ImagingSelection3DGraphicType.POLYLINE; 313 if ("polygon".equals(codeString)) 314 return ImagingSelection3DGraphicType.POLYGON; 315 if ("ellipse".equals(codeString)) 316 return ImagingSelection3DGraphicType.ELLIPSE; 317 if ("ellipsoid".equals(codeString)) 318 return ImagingSelection3DGraphicType.ELLIPSOID; 319 throw new IllegalArgumentException("Unknown ImagingSelection3DGraphicType code '"+codeString+"'"); 320 } 321 public Enumeration<ImagingSelection3DGraphicType> fromType(PrimitiveType<?> code) throws FHIRException { 322 if (code == null) 323 return null; 324 if (code.isEmpty()) 325 return new Enumeration<ImagingSelection3DGraphicType>(this, ImagingSelection3DGraphicType.NULL, code); 326 String codeString = ((PrimitiveType) code).asStringValue(); 327 if (codeString == null || "".equals(codeString)) 328 return new Enumeration<ImagingSelection3DGraphicType>(this, ImagingSelection3DGraphicType.NULL, code); 329 if ("point".equals(codeString)) 330 return new Enumeration<ImagingSelection3DGraphicType>(this, ImagingSelection3DGraphicType.POINT, code); 331 if ("multipoint".equals(codeString)) 332 return new Enumeration<ImagingSelection3DGraphicType>(this, ImagingSelection3DGraphicType.MULTIPOINT, code); 333 if ("polyline".equals(codeString)) 334 return new Enumeration<ImagingSelection3DGraphicType>(this, ImagingSelection3DGraphicType.POLYLINE, code); 335 if ("polygon".equals(codeString)) 336 return new Enumeration<ImagingSelection3DGraphicType>(this, ImagingSelection3DGraphicType.POLYGON, code); 337 if ("ellipse".equals(codeString)) 338 return new Enumeration<ImagingSelection3DGraphicType>(this, ImagingSelection3DGraphicType.ELLIPSE, code); 339 if ("ellipsoid".equals(codeString)) 340 return new Enumeration<ImagingSelection3DGraphicType>(this, ImagingSelection3DGraphicType.ELLIPSOID, code); 341 throw new FHIRException("Unknown ImagingSelection3DGraphicType code '"+codeString+"'"); 342 } 343 public String toCode(ImagingSelection3DGraphicType code) { 344 if (code == ImagingSelection3DGraphicType.NULL) 345 return null; 346 if (code == ImagingSelection3DGraphicType.POINT) 347 return "point"; 348 if (code == ImagingSelection3DGraphicType.MULTIPOINT) 349 return "multipoint"; 350 if (code == ImagingSelection3DGraphicType.POLYLINE) 351 return "polyline"; 352 if (code == ImagingSelection3DGraphicType.POLYGON) 353 return "polygon"; 354 if (code == ImagingSelection3DGraphicType.ELLIPSE) 355 return "ellipse"; 356 if (code == ImagingSelection3DGraphicType.ELLIPSOID) 357 return "ellipsoid"; 358 return "?"; 359 } 360 public String toSystem(ImagingSelection3DGraphicType code) { 361 return code.getSystem(); 362 } 363 } 364 365 public enum ImagingSelectionStatus { 366 /** 367 * The selected resources are available.. 368 */ 369 AVAILABLE, 370 /** 371 * The imaging selection has been withdrawn following a release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".). 372 */ 373 ENTEREDINERROR, 374 /** 375 * The system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one. 376 */ 377 UNKNOWN, 378 /** 379 * added to help the parsers with the generic types 380 */ 381 NULL; 382 public static ImagingSelectionStatus fromCode(String codeString) throws FHIRException { 383 if (codeString == null || "".equals(codeString)) 384 return null; 385 if ("available".equals(codeString)) 386 return AVAILABLE; 387 if ("entered-in-error".equals(codeString)) 388 return ENTEREDINERROR; 389 if ("unknown".equals(codeString)) 390 return UNKNOWN; 391 if (Configuration.isAcceptInvalidEnums()) 392 return null; 393 else 394 throw new FHIRException("Unknown ImagingSelectionStatus code '"+codeString+"'"); 395 } 396 public String toCode() { 397 switch (this) { 398 case AVAILABLE: return "available"; 399 case ENTEREDINERROR: return "entered-in-error"; 400 case UNKNOWN: return "unknown"; 401 case NULL: return null; 402 default: return "?"; 403 } 404 } 405 public String getSystem() { 406 switch (this) { 407 case AVAILABLE: return "http://hl7.org/fhir/imagingselection-status"; 408 case ENTEREDINERROR: return "http://hl7.org/fhir/imagingselection-status"; 409 case UNKNOWN: return "http://hl7.org/fhir/imagingselection-status"; 410 case NULL: return null; 411 default: return "?"; 412 } 413 } 414 public String getDefinition() { 415 switch (this) { 416 case AVAILABLE: return "The selected resources are available.."; 417 case ENTEREDINERROR: return "The imaging selection has been withdrawn following a release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 418 case UNKNOWN: return "The system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 419 case NULL: return null; 420 default: return "?"; 421 } 422 } 423 public String getDisplay() { 424 switch (this) { 425 case AVAILABLE: return "Available"; 426 case ENTEREDINERROR: return "Entered in Error"; 427 case UNKNOWN: return "Unknown"; 428 case NULL: return null; 429 default: return "?"; 430 } 431 } 432 } 433 434 public static class ImagingSelectionStatusEnumFactory implements EnumFactory<ImagingSelectionStatus> { 435 public ImagingSelectionStatus fromCode(String codeString) throws IllegalArgumentException { 436 if (codeString == null || "".equals(codeString)) 437 if (codeString == null || "".equals(codeString)) 438 return null; 439 if ("available".equals(codeString)) 440 return ImagingSelectionStatus.AVAILABLE; 441 if ("entered-in-error".equals(codeString)) 442 return ImagingSelectionStatus.ENTEREDINERROR; 443 if ("unknown".equals(codeString)) 444 return ImagingSelectionStatus.UNKNOWN; 445 throw new IllegalArgumentException("Unknown ImagingSelectionStatus code '"+codeString+"'"); 446 } 447 public Enumeration<ImagingSelectionStatus> fromType(PrimitiveType<?> code) throws FHIRException { 448 if (code == null) 449 return null; 450 if (code.isEmpty()) 451 return new Enumeration<ImagingSelectionStatus>(this, ImagingSelectionStatus.NULL, code); 452 String codeString = ((PrimitiveType) code).asStringValue(); 453 if (codeString == null || "".equals(codeString)) 454 return new Enumeration<ImagingSelectionStatus>(this, ImagingSelectionStatus.NULL, code); 455 if ("available".equals(codeString)) 456 return new Enumeration<ImagingSelectionStatus>(this, ImagingSelectionStatus.AVAILABLE, code); 457 if ("entered-in-error".equals(codeString)) 458 return new Enumeration<ImagingSelectionStatus>(this, ImagingSelectionStatus.ENTEREDINERROR, code); 459 if ("unknown".equals(codeString)) 460 return new Enumeration<ImagingSelectionStatus>(this, ImagingSelectionStatus.UNKNOWN, code); 461 throw new FHIRException("Unknown ImagingSelectionStatus code '"+codeString+"'"); 462 } 463 public String toCode(ImagingSelectionStatus code) { 464 if (code == ImagingSelectionStatus.NULL) 465 return null; 466 if (code == ImagingSelectionStatus.AVAILABLE) 467 return "available"; 468 if (code == ImagingSelectionStatus.ENTEREDINERROR) 469 return "entered-in-error"; 470 if (code == ImagingSelectionStatus.UNKNOWN) 471 return "unknown"; 472 return "?"; 473 } 474 public String toSystem(ImagingSelectionStatus code) { 475 return code.getSystem(); 476 } 477 } 478 479 @Block() 480 public static class ImagingSelectionPerformerComponent extends BackboneElement implements IBaseBackboneElement { 481 /** 482 * Distinguishes the type of involvement of the performer. 483 */ 484 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 485 @Description(shortDefinition="Type of performer", formalDefinition="Distinguishes the type of involvement of the performer." ) 486 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/series-performer-function") 487 protected CodeableConcept function; 488 489 /** 490 * Author ? human or machine. 491 */ 492 @Child(name = "actor", type = {Practitioner.class, PractitionerRole.class, Device.class, Organization.class, CareTeam.class, Patient.class, RelatedPerson.class, HealthcareService.class}, order=2, min=0, max=1, modifier=false, summary=true) 493 @Description(shortDefinition="Author (human or machine)", formalDefinition="Author ? human or machine." ) 494 protected Reference actor; 495 496 private static final long serialVersionUID = -576943815L; 497 498 /** 499 * Constructor 500 */ 501 public ImagingSelectionPerformerComponent() { 502 super(); 503 } 504 505 /** 506 * @return {@link #function} (Distinguishes the type of involvement of the performer.) 507 */ 508 public CodeableConcept getFunction() { 509 if (this.function == null) 510 if (Configuration.errorOnAutoCreate()) 511 throw new Error("Attempt to auto-create ImagingSelectionPerformerComponent.function"); 512 else if (Configuration.doAutoCreate()) 513 this.function = new CodeableConcept(); // cc 514 return this.function; 515 } 516 517 public boolean hasFunction() { 518 return this.function != null && !this.function.isEmpty(); 519 } 520 521 /** 522 * @param value {@link #function} (Distinguishes the type of involvement of the performer.) 523 */ 524 public ImagingSelectionPerformerComponent setFunction(CodeableConcept value) { 525 this.function = value; 526 return this; 527 } 528 529 /** 530 * @return {@link #actor} (Author ? human or machine.) 531 */ 532 public Reference getActor() { 533 if (this.actor == null) 534 if (Configuration.errorOnAutoCreate()) 535 throw new Error("Attempt to auto-create ImagingSelectionPerformerComponent.actor"); 536 else if (Configuration.doAutoCreate()) 537 this.actor = new Reference(); // cc 538 return this.actor; 539 } 540 541 public boolean hasActor() { 542 return this.actor != null && !this.actor.isEmpty(); 543 } 544 545 /** 546 * @param value {@link #actor} (Author ? human or machine.) 547 */ 548 public ImagingSelectionPerformerComponent setActor(Reference value) { 549 this.actor = value; 550 return this; 551 } 552 553 protected void listChildren(List<Property> children) { 554 super.listChildren(children); 555 children.add(new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the performer.", 0, 1, function)); 556 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Device|Organization|CareTeam|Patient|RelatedPerson|HealthcareService)", "Author ? human or machine.", 0, 1, actor)); 557 } 558 559 @Override 560 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 561 switch (_hash) { 562 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the performer.", 0, 1, function); 563 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|PractitionerRole|Device|Organization|CareTeam|Patient|RelatedPerson|HealthcareService)", "Author ? human or machine.", 0, 1, actor); 564 default: return super.getNamedProperty(_hash, _name, _checkValid); 565 } 566 567 } 568 569 @Override 570 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 571 switch (hash) { 572 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 573 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 574 default: return super.getProperty(hash, name, checkValid); 575 } 576 577 } 578 579 @Override 580 public Base setProperty(int hash, String name, Base value) throws FHIRException { 581 switch (hash) { 582 case 1380938712: // function 583 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 584 return value; 585 case 92645877: // actor 586 this.actor = TypeConvertor.castToReference(value); // Reference 587 return value; 588 default: return super.setProperty(hash, name, value); 589 } 590 591 } 592 593 @Override 594 public Base setProperty(String name, Base value) throws FHIRException { 595 if (name.equals("function")) { 596 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 597 } else if (name.equals("actor")) { 598 this.actor = TypeConvertor.castToReference(value); // Reference 599 } else 600 return super.setProperty(name, value); 601 return value; 602 } 603 604 @Override 605 public void removeChild(String name, Base value) throws FHIRException { 606 if (name.equals("function")) { 607 this.function = null; 608 } else if (name.equals("actor")) { 609 this.actor = null; 610 } else 611 super.removeChild(name, value); 612 613 } 614 615 @Override 616 public Base makeProperty(int hash, String name) throws FHIRException { 617 switch (hash) { 618 case 1380938712: return getFunction(); 619 case 92645877: return getActor(); 620 default: return super.makeProperty(hash, name); 621 } 622 623 } 624 625 @Override 626 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 627 switch (hash) { 628 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 629 case 92645877: /*actor*/ return new String[] {"Reference"}; 630 default: return super.getTypesForProperty(hash, name); 631 } 632 633 } 634 635 @Override 636 public Base addChild(String name) throws FHIRException { 637 if (name.equals("function")) { 638 this.function = new CodeableConcept(); 639 return this.function; 640 } 641 else if (name.equals("actor")) { 642 this.actor = new Reference(); 643 return this.actor; 644 } 645 else 646 return super.addChild(name); 647 } 648 649 public ImagingSelectionPerformerComponent copy() { 650 ImagingSelectionPerformerComponent dst = new ImagingSelectionPerformerComponent(); 651 copyValues(dst); 652 return dst; 653 } 654 655 public void copyValues(ImagingSelectionPerformerComponent dst) { 656 super.copyValues(dst); 657 dst.function = function == null ? null : function.copy(); 658 dst.actor = actor == null ? null : actor.copy(); 659 } 660 661 @Override 662 public boolean equalsDeep(Base other_) { 663 if (!super.equalsDeep(other_)) 664 return false; 665 if (!(other_ instanceof ImagingSelectionPerformerComponent)) 666 return false; 667 ImagingSelectionPerformerComponent o = (ImagingSelectionPerformerComponent) other_; 668 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 669 } 670 671 @Override 672 public boolean equalsShallow(Base other_) { 673 if (!super.equalsShallow(other_)) 674 return false; 675 if (!(other_ instanceof ImagingSelectionPerformerComponent)) 676 return false; 677 ImagingSelectionPerformerComponent o = (ImagingSelectionPerformerComponent) other_; 678 return true; 679 } 680 681 public boolean isEmpty() { 682 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 683 } 684 685 public String fhirType() { 686 return "ImagingSelection.performer"; 687 688 } 689 690 } 691 692 @Block() 693 public static class ImagingSelectionInstanceComponent extends BackboneElement implements IBaseBackboneElement { 694 /** 695 * The SOP Instance UID for the selected DICOM instance. 696 */ 697 @Child(name = "uid", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 698 @Description(shortDefinition="DICOM SOP Instance UID", formalDefinition="The SOP Instance UID for the selected DICOM instance." ) 699 protected IdType uid; 700 701 /** 702 * The Instance Number for the selected DICOM instance. 703 */ 704 @Child(name = "number", type = {UnsignedIntType.class}, order=2, min=0, max=1, modifier=false, summary=true) 705 @Description(shortDefinition="DICOM Instance Number", formalDefinition="The Instance Number for the selected DICOM instance." ) 706 protected UnsignedIntType number; 707 708 /** 709 * The SOP Class UID for the selected DICOM instance. 710 */ 711 @Child(name = "sopClass", type = {Coding.class}, order=3, min=0, max=1, modifier=false, summary=false) 712 @Description(shortDefinition="DICOM SOP Class UID", formalDefinition="The SOP Class UID for the selected DICOM instance." ) 713 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://dicom.nema.org/medical/dicom/current/output/chtml/part04/sect_B.5.html#table_B.5-1") 714 protected Coding sopClass; 715 716 /** 717 * Selected subset of the SOP Instance. The content and format of the subset item is determined by the SOP Class of the selected instance. 718 May be one of: 719 - A list of frame numbers selected from a multiframe SOP Instance. 720 - A list of Content Item Observation UID values selected from a DICOM SR or other structured document SOP Instance. 721 - A list of segment numbers selected from a segmentation SOP Instance. 722 - A list of Region of Interest (ROI) numbers selected from a radiotherapy structure set SOP Instance. 723 */ 724 @Child(name = "subset", type = {StringType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 725 @Description(shortDefinition="The selected subset of the SOP Instance", formalDefinition="Selected subset of the SOP Instance. The content and format of the subset item is determined by the SOP Class of the selected instance.\n May be one of:\n - A list of frame numbers selected from a multiframe SOP Instance.\n - A list of Content Item Observation UID values selected from a DICOM SR or other structured document SOP Instance.\n - A list of segment numbers selected from a segmentation SOP Instance.\n - A list of Region of Interest (ROI) numbers selected from a radiotherapy structure set SOP Instance." ) 726 protected List<StringType> subset; 727 728 /** 729 * Each imaging selection instance or frame list might includes an image region, specified by a region type and a set of 2D coordinates. 730 If the parent imagingSelection.instance contains a subset element of type frame, the image region applies to all frames in the subset list. 731 */ 732 @Child(name = "imageRegion2D", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 733 @Description(shortDefinition="A specific 2D region in a DICOM image / frame", formalDefinition="Each imaging selection instance or frame list might includes an image region, specified by a region type and a set of 2D coordinates.\n If the parent imagingSelection.instance contains a subset element of type frame, the image region applies to all frames in the subset list." ) 734 protected List<ImageRegion2DComponent> imageRegion2D; 735 736 /** 737 * Each imaging selection might includes a 3D image region, specified by a region type and a set of 3D coordinates. 738 */ 739 @Child(name = "imageRegion3D", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 740 @Description(shortDefinition="A specific 3D region in a DICOM frame of reference", formalDefinition="Each imaging selection might includes a 3D image region, specified by a region type and a set of 3D coordinates." ) 741 protected List<ImageRegion3DComponent> imageRegion3D; 742 743 private static final long serialVersionUID = 1783712351L; 744 745 /** 746 * Constructor 747 */ 748 public ImagingSelectionInstanceComponent() { 749 super(); 750 } 751 752 /** 753 * Constructor 754 */ 755 public ImagingSelectionInstanceComponent(String uid) { 756 super(); 757 this.setUid(uid); 758 } 759 760 /** 761 * @return {@link #uid} (The SOP Instance UID for the selected DICOM instance.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 762 */ 763 public IdType getUidElement() { 764 if (this.uid == null) 765 if (Configuration.errorOnAutoCreate()) 766 throw new Error("Attempt to auto-create ImagingSelectionInstanceComponent.uid"); 767 else if (Configuration.doAutoCreate()) 768 this.uid = new IdType(); // bb 769 return this.uid; 770 } 771 772 public boolean hasUidElement() { 773 return this.uid != null && !this.uid.isEmpty(); 774 } 775 776 public boolean hasUid() { 777 return this.uid != null && !this.uid.isEmpty(); 778 } 779 780 /** 781 * @param value {@link #uid} (The SOP Instance UID for the selected DICOM instance.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 782 */ 783 public ImagingSelectionInstanceComponent setUidElement(IdType value) { 784 this.uid = value; 785 return this; 786 } 787 788 /** 789 * @return The SOP Instance UID for the selected DICOM instance. 790 */ 791 public String getUid() { 792 return this.uid == null ? null : this.uid.getValue(); 793 } 794 795 /** 796 * @param value The SOP Instance UID for the selected DICOM instance. 797 */ 798 public ImagingSelectionInstanceComponent setUid(String value) { 799 if (this.uid == null) 800 this.uid = new IdType(); 801 this.uid.setValue(value); 802 return this; 803 } 804 805 /** 806 * @return {@link #number} (The Instance Number for the selected DICOM instance.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 807 */ 808 public UnsignedIntType getNumberElement() { 809 if (this.number == null) 810 if (Configuration.errorOnAutoCreate()) 811 throw new Error("Attempt to auto-create ImagingSelectionInstanceComponent.number"); 812 else if (Configuration.doAutoCreate()) 813 this.number = new UnsignedIntType(); // bb 814 return this.number; 815 } 816 817 public boolean hasNumberElement() { 818 return this.number != null && !this.number.isEmpty(); 819 } 820 821 public boolean hasNumber() { 822 return this.number != null && !this.number.isEmpty(); 823 } 824 825 /** 826 * @param value {@link #number} (The Instance Number for the selected DICOM instance.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 827 */ 828 public ImagingSelectionInstanceComponent setNumberElement(UnsignedIntType value) { 829 this.number = value; 830 return this; 831 } 832 833 /** 834 * @return The Instance Number for the selected DICOM instance. 835 */ 836 public int getNumber() { 837 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 838 } 839 840 /** 841 * @param value The Instance Number for the selected DICOM instance. 842 */ 843 public ImagingSelectionInstanceComponent setNumber(int value) { 844 if (this.number == null) 845 this.number = new UnsignedIntType(); 846 this.number.setValue(value); 847 return this; 848 } 849 850 /** 851 * @return {@link #sopClass} (The SOP Class UID for the selected DICOM instance.) 852 */ 853 public Coding getSopClass() { 854 if (this.sopClass == null) 855 if (Configuration.errorOnAutoCreate()) 856 throw new Error("Attempt to auto-create ImagingSelectionInstanceComponent.sopClass"); 857 else if (Configuration.doAutoCreate()) 858 this.sopClass = new Coding(); // cc 859 return this.sopClass; 860 } 861 862 public boolean hasSopClass() { 863 return this.sopClass != null && !this.sopClass.isEmpty(); 864 } 865 866 /** 867 * @param value {@link #sopClass} (The SOP Class UID for the selected DICOM instance.) 868 */ 869 public ImagingSelectionInstanceComponent setSopClass(Coding value) { 870 this.sopClass = value; 871 return this; 872 } 873 874 /** 875 * @return {@link #subset} (Selected subset of the SOP Instance. The content and format of the subset item is determined by the SOP Class of the selected instance. 876 May be one of: 877 - A list of frame numbers selected from a multiframe SOP Instance. 878 - A list of Content Item Observation UID values selected from a DICOM SR or other structured document SOP Instance. 879 - A list of segment numbers selected from a segmentation SOP Instance. 880 - A list of Region of Interest (ROI) numbers selected from a radiotherapy structure set SOP Instance.) 881 */ 882 public List<StringType> getSubset() { 883 if (this.subset == null) 884 this.subset = new ArrayList<StringType>(); 885 return this.subset; 886 } 887 888 /** 889 * @return Returns a reference to <code>this</code> for easy method chaining 890 */ 891 public ImagingSelectionInstanceComponent setSubset(List<StringType> theSubset) { 892 this.subset = theSubset; 893 return this; 894 } 895 896 public boolean hasSubset() { 897 if (this.subset == null) 898 return false; 899 for (StringType item : this.subset) 900 if (!item.isEmpty()) 901 return true; 902 return false; 903 } 904 905 /** 906 * @return {@link #subset} (Selected subset of the SOP Instance. The content and format of the subset item is determined by the SOP Class of the selected instance. 907 May be one of: 908 - A list of frame numbers selected from a multiframe SOP Instance. 909 - A list of Content Item Observation UID values selected from a DICOM SR or other structured document SOP Instance. 910 - A list of segment numbers selected from a segmentation SOP Instance. 911 - A list of Region of Interest (ROI) numbers selected from a radiotherapy structure set SOP Instance.) 912 */ 913 public StringType addSubsetElement() {//2 914 StringType t = new StringType(); 915 if (this.subset == null) 916 this.subset = new ArrayList<StringType>(); 917 this.subset.add(t); 918 return t; 919 } 920 921 /** 922 * @param value {@link #subset} (Selected subset of the SOP Instance. The content and format of the subset item is determined by the SOP Class of the selected instance. 923 May be one of: 924 - A list of frame numbers selected from a multiframe SOP Instance. 925 - A list of Content Item Observation UID values selected from a DICOM SR or other structured document SOP Instance. 926 - A list of segment numbers selected from a segmentation SOP Instance. 927 - A list of Region of Interest (ROI) numbers selected from a radiotherapy structure set SOP Instance.) 928 */ 929 public ImagingSelectionInstanceComponent addSubset(String value) { //1 930 StringType t = new StringType(); 931 t.setValue(value); 932 if (this.subset == null) 933 this.subset = new ArrayList<StringType>(); 934 this.subset.add(t); 935 return this; 936 } 937 938 /** 939 * @param value {@link #subset} (Selected subset of the SOP Instance. The content and format of the subset item is determined by the SOP Class of the selected instance. 940 May be one of: 941 - A list of frame numbers selected from a multiframe SOP Instance. 942 - A list of Content Item Observation UID values selected from a DICOM SR or other structured document SOP Instance. 943 - A list of segment numbers selected from a segmentation SOP Instance. 944 - A list of Region of Interest (ROI) numbers selected from a radiotherapy structure set SOP Instance.) 945 */ 946 public boolean hasSubset(String value) { 947 if (this.subset == null) 948 return false; 949 for (StringType v : this.subset) 950 if (v.getValue().equals(value)) // string 951 return true; 952 return false; 953 } 954 955 /** 956 * @return {@link #imageRegion2D} (Each imaging selection instance or frame list might includes an image region, specified by a region type and a set of 2D coordinates. 957 If the parent imagingSelection.instance contains a subset element of type frame, the image region applies to all frames in the subset list.) 958 */ 959 public List<ImageRegion2DComponent> getImageRegion2D() { 960 if (this.imageRegion2D == null) 961 this.imageRegion2D = new ArrayList<ImageRegion2DComponent>(); 962 return this.imageRegion2D; 963 } 964 965 /** 966 * @return Returns a reference to <code>this</code> for easy method chaining 967 */ 968 public ImagingSelectionInstanceComponent setImageRegion2D(List<ImageRegion2DComponent> theImageRegion2D) { 969 this.imageRegion2D = theImageRegion2D; 970 return this; 971 } 972 973 public boolean hasImageRegion2D() { 974 if (this.imageRegion2D == null) 975 return false; 976 for (ImageRegion2DComponent item : this.imageRegion2D) 977 if (!item.isEmpty()) 978 return true; 979 return false; 980 } 981 982 public ImageRegion2DComponent addImageRegion2D() { //3 983 ImageRegion2DComponent t = new ImageRegion2DComponent(); 984 if (this.imageRegion2D == null) 985 this.imageRegion2D = new ArrayList<ImageRegion2DComponent>(); 986 this.imageRegion2D.add(t); 987 return t; 988 } 989 990 public ImagingSelectionInstanceComponent addImageRegion2D(ImageRegion2DComponent t) { //3 991 if (t == null) 992 return this; 993 if (this.imageRegion2D == null) 994 this.imageRegion2D = new ArrayList<ImageRegion2DComponent>(); 995 this.imageRegion2D.add(t); 996 return this; 997 } 998 999 /** 1000 * @return The first repetition of repeating field {@link #imageRegion2D}, creating it if it does not already exist {3} 1001 */ 1002 public ImageRegion2DComponent getImageRegion2DFirstRep() { 1003 if (getImageRegion2D().isEmpty()) { 1004 addImageRegion2D(); 1005 } 1006 return getImageRegion2D().get(0); 1007 } 1008 1009 /** 1010 * @return {@link #imageRegion3D} (Each imaging selection might includes a 3D image region, specified by a region type and a set of 3D coordinates.) 1011 */ 1012 public List<ImageRegion3DComponent> getImageRegion3D() { 1013 if (this.imageRegion3D == null) 1014 this.imageRegion3D = new ArrayList<ImageRegion3DComponent>(); 1015 return this.imageRegion3D; 1016 } 1017 1018 /** 1019 * @return Returns a reference to <code>this</code> for easy method chaining 1020 */ 1021 public ImagingSelectionInstanceComponent setImageRegion3D(List<ImageRegion3DComponent> theImageRegion3D) { 1022 this.imageRegion3D = theImageRegion3D; 1023 return this; 1024 } 1025 1026 public boolean hasImageRegion3D() { 1027 if (this.imageRegion3D == null) 1028 return false; 1029 for (ImageRegion3DComponent item : this.imageRegion3D) 1030 if (!item.isEmpty()) 1031 return true; 1032 return false; 1033 } 1034 1035 public ImageRegion3DComponent addImageRegion3D() { //3 1036 ImageRegion3DComponent t = new ImageRegion3DComponent(); 1037 if (this.imageRegion3D == null) 1038 this.imageRegion3D = new ArrayList<ImageRegion3DComponent>(); 1039 this.imageRegion3D.add(t); 1040 return t; 1041 } 1042 1043 public ImagingSelectionInstanceComponent addImageRegion3D(ImageRegion3DComponent t) { //3 1044 if (t == null) 1045 return this; 1046 if (this.imageRegion3D == null) 1047 this.imageRegion3D = new ArrayList<ImageRegion3DComponent>(); 1048 this.imageRegion3D.add(t); 1049 return this; 1050 } 1051 1052 /** 1053 * @return The first repetition of repeating field {@link #imageRegion3D}, creating it if it does not already exist {3} 1054 */ 1055 public ImageRegion3DComponent getImageRegion3DFirstRep() { 1056 if (getImageRegion3D().isEmpty()) { 1057 addImageRegion3D(); 1058 } 1059 return getImageRegion3D().get(0); 1060 } 1061 1062 protected void listChildren(List<Property> children) { 1063 super.listChildren(children); 1064 children.add(new Property("uid", "id", "The SOP Instance UID for the selected DICOM instance.", 0, 1, uid)); 1065 children.add(new Property("number", "unsignedInt", "The Instance Number for the selected DICOM instance.", 0, 1, number)); 1066 children.add(new Property("sopClass", "Coding", "The SOP Class UID for the selected DICOM instance.", 0, 1, sopClass)); 1067 children.add(new Property("subset", "string", "Selected subset of the SOP Instance. The content and format of the subset item is determined by the SOP Class of the selected instance.\n May be one of:\n - A list of frame numbers selected from a multiframe SOP Instance.\n - A list of Content Item Observation UID values selected from a DICOM SR or other structured document SOP Instance.\n - A list of segment numbers selected from a segmentation SOP Instance.\n - A list of Region of Interest (ROI) numbers selected from a radiotherapy structure set SOP Instance.", 0, java.lang.Integer.MAX_VALUE, subset)); 1068 children.add(new Property("imageRegion2D", "", "Each imaging selection instance or frame list might includes an image region, specified by a region type and a set of 2D coordinates.\n If the parent imagingSelection.instance contains a subset element of type frame, the image region applies to all frames in the subset list.", 0, java.lang.Integer.MAX_VALUE, imageRegion2D)); 1069 children.add(new Property("imageRegion3D", "", "Each imaging selection might includes a 3D image region, specified by a region type and a set of 3D coordinates.", 0, java.lang.Integer.MAX_VALUE, imageRegion3D)); 1070 } 1071 1072 @Override 1073 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1074 switch (_hash) { 1075 case 115792: /*uid*/ return new Property("uid", "id", "The SOP Instance UID for the selected DICOM instance.", 0, 1, uid); 1076 case -1034364087: /*number*/ return new Property("number", "unsignedInt", "The Instance Number for the selected DICOM instance.", 0, 1, number); 1077 case 1560041540: /*sopClass*/ return new Property("sopClass", "Coding", "The SOP Class UID for the selected DICOM instance.", 0, 1, sopClass); 1078 case -891529694: /*subset*/ return new Property("subset", "string", "Selected subset of the SOP Instance. The content and format of the subset item is determined by the SOP Class of the selected instance.\n May be one of:\n - A list of frame numbers selected from a multiframe SOP Instance.\n - A list of Content Item Observation UID values selected from a DICOM SR or other structured document SOP Instance.\n - A list of segment numbers selected from a segmentation SOP Instance.\n - A list of Region of Interest (ROI) numbers selected from a radiotherapy structure set SOP Instance.", 0, java.lang.Integer.MAX_VALUE, subset); 1079 case 675922625: /*imageRegion2D*/ return new Property("imageRegion2D", "", "Each imaging selection instance or frame list might includes an image region, specified by a region type and a set of 2D coordinates.\n If the parent imagingSelection.instance contains a subset element of type frame, the image region applies to all frames in the subset list.", 0, java.lang.Integer.MAX_VALUE, imageRegion2D); 1080 case 675922656: /*imageRegion3D*/ return new Property("imageRegion3D", "", "Each imaging selection might includes a 3D image region, specified by a region type and a set of 3D coordinates.", 0, java.lang.Integer.MAX_VALUE, imageRegion3D); 1081 default: return super.getNamedProperty(_hash, _name, _checkValid); 1082 } 1083 1084 } 1085 1086 @Override 1087 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1088 switch (hash) { 1089 case 115792: /*uid*/ return this.uid == null ? new Base[0] : new Base[] {this.uid}; // IdType 1090 case -1034364087: /*number*/ return this.number == null ? new Base[0] : new Base[] {this.number}; // UnsignedIntType 1091 case 1560041540: /*sopClass*/ return this.sopClass == null ? new Base[0] : new Base[] {this.sopClass}; // Coding 1092 case -891529694: /*subset*/ return this.subset == null ? new Base[0] : this.subset.toArray(new Base[this.subset.size()]); // StringType 1093 case 675922625: /*imageRegion2D*/ return this.imageRegion2D == null ? new Base[0] : this.imageRegion2D.toArray(new Base[this.imageRegion2D.size()]); // ImageRegion2DComponent 1094 case 675922656: /*imageRegion3D*/ return this.imageRegion3D == null ? new Base[0] : this.imageRegion3D.toArray(new Base[this.imageRegion3D.size()]); // ImageRegion3DComponent 1095 default: return super.getProperty(hash, name, checkValid); 1096 } 1097 1098 } 1099 1100 @Override 1101 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1102 switch (hash) { 1103 case 115792: // uid 1104 this.uid = TypeConvertor.castToId(value); // IdType 1105 return value; 1106 case -1034364087: // number 1107 this.number = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1108 return value; 1109 case 1560041540: // sopClass 1110 this.sopClass = TypeConvertor.castToCoding(value); // Coding 1111 return value; 1112 case -891529694: // subset 1113 this.getSubset().add(TypeConvertor.castToString(value)); // StringType 1114 return value; 1115 case 675922625: // imageRegion2D 1116 this.getImageRegion2D().add((ImageRegion2DComponent) value); // ImageRegion2DComponent 1117 return value; 1118 case 675922656: // imageRegion3D 1119 this.getImageRegion3D().add((ImageRegion3DComponent) value); // ImageRegion3DComponent 1120 return value; 1121 default: return super.setProperty(hash, name, value); 1122 } 1123 1124 } 1125 1126 @Override 1127 public Base setProperty(String name, Base value) throws FHIRException { 1128 if (name.equals("uid")) { 1129 this.uid = TypeConvertor.castToId(value); // IdType 1130 } else if (name.equals("number")) { 1131 this.number = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1132 } else if (name.equals("sopClass")) { 1133 this.sopClass = TypeConvertor.castToCoding(value); // Coding 1134 } else if (name.equals("subset")) { 1135 this.getSubset().add(TypeConvertor.castToString(value)); 1136 } else if (name.equals("imageRegion2D")) { 1137 this.getImageRegion2D().add((ImageRegion2DComponent) value); 1138 } else if (name.equals("imageRegion3D")) { 1139 this.getImageRegion3D().add((ImageRegion3DComponent) value); 1140 } else 1141 return super.setProperty(name, value); 1142 return value; 1143 } 1144 1145 @Override 1146 public void removeChild(String name, Base value) throws FHIRException { 1147 if (name.equals("uid")) { 1148 this.uid = null; 1149 } else if (name.equals("number")) { 1150 this.number = null; 1151 } else if (name.equals("sopClass")) { 1152 this.sopClass = null; 1153 } else if (name.equals("subset")) { 1154 this.getSubset().remove(value); 1155 } else if (name.equals("imageRegion2D")) { 1156 this.getImageRegion2D().remove((ImageRegion2DComponent) value); 1157 } else if (name.equals("imageRegion3D")) { 1158 this.getImageRegion3D().remove((ImageRegion3DComponent) value); 1159 } else 1160 super.removeChild(name, value); 1161 1162 } 1163 1164 @Override 1165 public Base makeProperty(int hash, String name) throws FHIRException { 1166 switch (hash) { 1167 case 115792: return getUidElement(); 1168 case -1034364087: return getNumberElement(); 1169 case 1560041540: return getSopClass(); 1170 case -891529694: return addSubsetElement(); 1171 case 675922625: return addImageRegion2D(); 1172 case 675922656: return addImageRegion3D(); 1173 default: return super.makeProperty(hash, name); 1174 } 1175 1176 } 1177 1178 @Override 1179 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1180 switch (hash) { 1181 case 115792: /*uid*/ return new String[] {"id"}; 1182 case -1034364087: /*number*/ return new String[] {"unsignedInt"}; 1183 case 1560041540: /*sopClass*/ return new String[] {"Coding"}; 1184 case -891529694: /*subset*/ return new String[] {"string"}; 1185 case 675922625: /*imageRegion2D*/ return new String[] {}; 1186 case 675922656: /*imageRegion3D*/ return new String[] {}; 1187 default: return super.getTypesForProperty(hash, name); 1188 } 1189 1190 } 1191 1192 @Override 1193 public Base addChild(String name) throws FHIRException { 1194 if (name.equals("uid")) { 1195 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.instance.uid"); 1196 } 1197 else if (name.equals("number")) { 1198 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.instance.number"); 1199 } 1200 else if (name.equals("sopClass")) { 1201 this.sopClass = new Coding(); 1202 return this.sopClass; 1203 } 1204 else if (name.equals("subset")) { 1205 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.instance.subset"); 1206 } 1207 else if (name.equals("imageRegion2D")) { 1208 return addImageRegion2D(); 1209 } 1210 else if (name.equals("imageRegion3D")) { 1211 return addImageRegion3D(); 1212 } 1213 else 1214 return super.addChild(name); 1215 } 1216 1217 public ImagingSelectionInstanceComponent copy() { 1218 ImagingSelectionInstanceComponent dst = new ImagingSelectionInstanceComponent(); 1219 copyValues(dst); 1220 return dst; 1221 } 1222 1223 public void copyValues(ImagingSelectionInstanceComponent dst) { 1224 super.copyValues(dst); 1225 dst.uid = uid == null ? null : uid.copy(); 1226 dst.number = number == null ? null : number.copy(); 1227 dst.sopClass = sopClass == null ? null : sopClass.copy(); 1228 if (subset != null) { 1229 dst.subset = new ArrayList<StringType>(); 1230 for (StringType i : subset) 1231 dst.subset.add(i.copy()); 1232 }; 1233 if (imageRegion2D != null) { 1234 dst.imageRegion2D = new ArrayList<ImageRegion2DComponent>(); 1235 for (ImageRegion2DComponent i : imageRegion2D) 1236 dst.imageRegion2D.add(i.copy()); 1237 }; 1238 if (imageRegion3D != null) { 1239 dst.imageRegion3D = new ArrayList<ImageRegion3DComponent>(); 1240 for (ImageRegion3DComponent i : imageRegion3D) 1241 dst.imageRegion3D.add(i.copy()); 1242 }; 1243 } 1244 1245 @Override 1246 public boolean equalsDeep(Base other_) { 1247 if (!super.equalsDeep(other_)) 1248 return false; 1249 if (!(other_ instanceof ImagingSelectionInstanceComponent)) 1250 return false; 1251 ImagingSelectionInstanceComponent o = (ImagingSelectionInstanceComponent) other_; 1252 return compareDeep(uid, o.uid, true) && compareDeep(number, o.number, true) && compareDeep(sopClass, o.sopClass, true) 1253 && compareDeep(subset, o.subset, true) && compareDeep(imageRegion2D, o.imageRegion2D, true) && compareDeep(imageRegion3D, o.imageRegion3D, true) 1254 ; 1255 } 1256 1257 @Override 1258 public boolean equalsShallow(Base other_) { 1259 if (!super.equalsShallow(other_)) 1260 return false; 1261 if (!(other_ instanceof ImagingSelectionInstanceComponent)) 1262 return false; 1263 ImagingSelectionInstanceComponent o = (ImagingSelectionInstanceComponent) other_; 1264 return compareValues(uid, o.uid, true) && compareValues(number, o.number, true) && compareValues(subset, o.subset, true) 1265 ; 1266 } 1267 1268 public boolean isEmpty() { 1269 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(uid, number, sopClass, subset 1270 , imageRegion2D, imageRegion3D); 1271 } 1272 1273 public String fhirType() { 1274 return "ImagingSelection.instance"; 1275 1276 } 1277 1278 } 1279 1280 @Block() 1281 public static class ImageRegion2DComponent extends BackboneElement implements IBaseBackboneElement { 1282 /** 1283 * Specifies the type of image region. 1284 */ 1285 @Child(name = "regionType", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1286 @Description(shortDefinition="point | polyline | interpolated | circle | ellipse", formalDefinition="Specifies the type of image region." ) 1287 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/imagingselection-2dgraphictype") 1288 protected Enumeration<ImagingSelection2DGraphicType> regionType; 1289 1290 /** 1291 * The coordinates describing the image region. Encoded as a set of (column, row) pairs that denote positions in the selected image / frames specified with sub-pixel resolution. 1292 The origin at the TLHC of the TLHC pixel is 0.0\0.0, the BRHC of the TLHC pixel is 1.0\1.0, and the BRHC of the BRHC pixel is the number of columns\rows in the image / frames. The values must be within the range 0\0 to the number of columns\rows in the image / frames. 1293 */ 1294 @Child(name = "coordinate", type = {DecimalType.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1295 @Description(shortDefinition="Specifies the coordinates that define the image region", formalDefinition="The coordinates describing the image region. Encoded as a set of (column, row) pairs that denote positions in the selected image / frames specified with sub-pixel resolution.\n The origin at the TLHC of the TLHC pixel is 0.0\\0.0, the BRHC of the TLHC pixel is 1.0\\1.0, and the BRHC of the BRHC pixel is the number of columns\\rows in the image / frames. The values must be within the range 0\\0 to the number of columns\\rows in the image / frames." ) 1296 protected List<DecimalType> coordinate; 1297 1298 private static final long serialVersionUID = 1518695052L; 1299 1300 /** 1301 * Constructor 1302 */ 1303 public ImageRegion2DComponent() { 1304 super(); 1305 } 1306 1307 /** 1308 * Constructor 1309 */ 1310 public ImageRegion2DComponent(ImagingSelection2DGraphicType regionType, BigDecimal coordinate) { 1311 super(); 1312 this.setRegionType(regionType); 1313 this.addCoordinate(coordinate); 1314 } 1315 1316 /** 1317 * @return {@link #regionType} (Specifies the type of image region.). This is the underlying object with id, value and extensions. The accessor "getRegionType" gives direct access to the value 1318 */ 1319 public Enumeration<ImagingSelection2DGraphicType> getRegionTypeElement() { 1320 if (this.regionType == null) 1321 if (Configuration.errorOnAutoCreate()) 1322 throw new Error("Attempt to auto-create ImageRegion2DComponent.regionType"); 1323 else if (Configuration.doAutoCreate()) 1324 this.regionType = new Enumeration<ImagingSelection2DGraphicType>(new ImagingSelection2DGraphicTypeEnumFactory()); // bb 1325 return this.regionType; 1326 } 1327 1328 public boolean hasRegionTypeElement() { 1329 return this.regionType != null && !this.regionType.isEmpty(); 1330 } 1331 1332 public boolean hasRegionType() { 1333 return this.regionType != null && !this.regionType.isEmpty(); 1334 } 1335 1336 /** 1337 * @param value {@link #regionType} (Specifies the type of image region.). This is the underlying object with id, value and extensions. The accessor "getRegionType" gives direct access to the value 1338 */ 1339 public ImageRegion2DComponent setRegionTypeElement(Enumeration<ImagingSelection2DGraphicType> value) { 1340 this.regionType = value; 1341 return this; 1342 } 1343 1344 /** 1345 * @return Specifies the type of image region. 1346 */ 1347 public ImagingSelection2DGraphicType getRegionType() { 1348 return this.regionType == null ? null : this.regionType.getValue(); 1349 } 1350 1351 /** 1352 * @param value Specifies the type of image region. 1353 */ 1354 public ImageRegion2DComponent setRegionType(ImagingSelection2DGraphicType value) { 1355 if (this.regionType == null) 1356 this.regionType = new Enumeration<ImagingSelection2DGraphicType>(new ImagingSelection2DGraphicTypeEnumFactory()); 1357 this.regionType.setValue(value); 1358 return this; 1359 } 1360 1361 /** 1362 * @return {@link #coordinate} (The coordinates describing the image region. Encoded as a set of (column, row) pairs that denote positions in the selected image / frames specified with sub-pixel resolution. 1363 The origin at the TLHC of the TLHC pixel is 0.0\0.0, the BRHC of the TLHC pixel is 1.0\1.0, and the BRHC of the BRHC pixel is the number of columns\rows in the image / frames. The values must be within the range 0\0 to the number of columns\rows in the image / frames.) 1364 */ 1365 public List<DecimalType> getCoordinate() { 1366 if (this.coordinate == null) 1367 this.coordinate = new ArrayList<DecimalType>(); 1368 return this.coordinate; 1369 } 1370 1371 /** 1372 * @return Returns a reference to <code>this</code> for easy method chaining 1373 */ 1374 public ImageRegion2DComponent setCoordinate(List<DecimalType> theCoordinate) { 1375 this.coordinate = theCoordinate; 1376 return this; 1377 } 1378 1379 public boolean hasCoordinate() { 1380 if (this.coordinate == null) 1381 return false; 1382 for (DecimalType item : this.coordinate) 1383 if (!item.isEmpty()) 1384 return true; 1385 return false; 1386 } 1387 1388 /** 1389 * @return {@link #coordinate} (The coordinates describing the image region. Encoded as a set of (column, row) pairs that denote positions in the selected image / frames specified with sub-pixel resolution. 1390 The origin at the TLHC of the TLHC pixel is 0.0\0.0, the BRHC of the TLHC pixel is 1.0\1.0, and the BRHC of the BRHC pixel is the number of columns\rows in the image / frames. The values must be within the range 0\0 to the number of columns\rows in the image / frames.) 1391 */ 1392 public DecimalType addCoordinateElement() {//2 1393 DecimalType t = new DecimalType(); 1394 if (this.coordinate == null) 1395 this.coordinate = new ArrayList<DecimalType>(); 1396 this.coordinate.add(t); 1397 return t; 1398 } 1399 1400 /** 1401 * @param value {@link #coordinate} (The coordinates describing the image region. Encoded as a set of (column, row) pairs that denote positions in the selected image / frames specified with sub-pixel resolution. 1402 The origin at the TLHC of the TLHC pixel is 0.0\0.0, the BRHC of the TLHC pixel is 1.0\1.0, and the BRHC of the BRHC pixel is the number of columns\rows in the image / frames. The values must be within the range 0\0 to the number of columns\rows in the image / frames.) 1403 */ 1404 public ImageRegion2DComponent addCoordinate(BigDecimal value) { //1 1405 DecimalType t = new DecimalType(); 1406 t.setValue(value); 1407 if (this.coordinate == null) 1408 this.coordinate = new ArrayList<DecimalType>(); 1409 this.coordinate.add(t); 1410 return this; 1411 } 1412 1413 /** 1414 * @param value {@link #coordinate} (The coordinates describing the image region. Encoded as a set of (column, row) pairs that denote positions in the selected image / frames specified with sub-pixel resolution. 1415 The origin at the TLHC of the TLHC pixel is 0.0\0.0, the BRHC of the TLHC pixel is 1.0\1.0, and the BRHC of the BRHC pixel is the number of columns\rows in the image / frames. The values must be within the range 0\0 to the number of columns\rows in the image / frames.) 1416 */ 1417 public boolean hasCoordinate(BigDecimal value) { 1418 if (this.coordinate == null) 1419 return false; 1420 for (DecimalType v : this.coordinate) 1421 if (v.getValue().equals(value)) // decimal 1422 return true; 1423 return false; 1424 } 1425 1426 protected void listChildren(List<Property> children) { 1427 super.listChildren(children); 1428 children.add(new Property("regionType", "code", "Specifies the type of image region.", 0, 1, regionType)); 1429 children.add(new Property("coordinate", "decimal", "The coordinates describing the image region. Encoded as a set of (column, row) pairs that denote positions in the selected image / frames specified with sub-pixel resolution.\n The origin at the TLHC of the TLHC pixel is 0.0\\0.0, the BRHC of the TLHC pixel is 1.0\\1.0, and the BRHC of the BRHC pixel is the number of columns\\rows in the image / frames. The values must be within the range 0\\0 to the number of columns\\rows in the image / frames.", 0, java.lang.Integer.MAX_VALUE, coordinate)); 1430 } 1431 1432 @Override 1433 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1434 switch (_hash) { 1435 case -1990487986: /*regionType*/ return new Property("regionType", "code", "Specifies the type of image region.", 0, 1, regionType); 1436 case 198931832: /*coordinate*/ return new Property("coordinate", "decimal", "The coordinates describing the image region. Encoded as a set of (column, row) pairs that denote positions in the selected image / frames specified with sub-pixel resolution.\n The origin at the TLHC of the TLHC pixel is 0.0\\0.0, the BRHC of the TLHC pixel is 1.0\\1.0, and the BRHC of the BRHC pixel is the number of columns\\rows in the image / frames. The values must be within the range 0\\0 to the number of columns\\rows in the image / frames.", 0, java.lang.Integer.MAX_VALUE, coordinate); 1437 default: return super.getNamedProperty(_hash, _name, _checkValid); 1438 } 1439 1440 } 1441 1442 @Override 1443 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1444 switch (hash) { 1445 case -1990487986: /*regionType*/ return this.regionType == null ? new Base[0] : new Base[] {this.regionType}; // Enumeration<ImagingSelection2DGraphicType> 1446 case 198931832: /*coordinate*/ return this.coordinate == null ? new Base[0] : this.coordinate.toArray(new Base[this.coordinate.size()]); // DecimalType 1447 default: return super.getProperty(hash, name, checkValid); 1448 } 1449 1450 } 1451 1452 @Override 1453 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1454 switch (hash) { 1455 case -1990487986: // regionType 1456 value = new ImagingSelection2DGraphicTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1457 this.regionType = (Enumeration) value; // Enumeration<ImagingSelection2DGraphicType> 1458 return value; 1459 case 198931832: // coordinate 1460 this.getCoordinate().add(TypeConvertor.castToDecimal(value)); // DecimalType 1461 return value; 1462 default: return super.setProperty(hash, name, value); 1463 } 1464 1465 } 1466 1467 @Override 1468 public Base setProperty(String name, Base value) throws FHIRException { 1469 if (name.equals("regionType")) { 1470 value = new ImagingSelection2DGraphicTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1471 this.regionType = (Enumeration) value; // Enumeration<ImagingSelection2DGraphicType> 1472 } else if (name.equals("coordinate")) { 1473 this.getCoordinate().add(TypeConvertor.castToDecimal(value)); 1474 } else 1475 return super.setProperty(name, value); 1476 return value; 1477 } 1478 1479 @Override 1480 public void removeChild(String name, Base value) throws FHIRException { 1481 if (name.equals("regionType")) { 1482 value = new ImagingSelection2DGraphicTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1483 this.regionType = (Enumeration) value; // Enumeration<ImagingSelection2DGraphicType> 1484 } else if (name.equals("coordinate")) { 1485 this.getCoordinate().remove(value); 1486 } else 1487 super.removeChild(name, value); 1488 1489 } 1490 1491 @Override 1492 public Base makeProperty(int hash, String name) throws FHIRException { 1493 switch (hash) { 1494 case -1990487986: return getRegionTypeElement(); 1495 case 198931832: return addCoordinateElement(); 1496 default: return super.makeProperty(hash, name); 1497 } 1498 1499 } 1500 1501 @Override 1502 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1503 switch (hash) { 1504 case -1990487986: /*regionType*/ return new String[] {"code"}; 1505 case 198931832: /*coordinate*/ return new String[] {"decimal"}; 1506 default: return super.getTypesForProperty(hash, name); 1507 } 1508 1509 } 1510 1511 @Override 1512 public Base addChild(String name) throws FHIRException { 1513 if (name.equals("regionType")) { 1514 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.instance.imageRegion2D.regionType"); 1515 } 1516 else if (name.equals("coordinate")) { 1517 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.instance.imageRegion2D.coordinate"); 1518 } 1519 else 1520 return super.addChild(name); 1521 } 1522 1523 public ImageRegion2DComponent copy() { 1524 ImageRegion2DComponent dst = new ImageRegion2DComponent(); 1525 copyValues(dst); 1526 return dst; 1527 } 1528 1529 public void copyValues(ImageRegion2DComponent dst) { 1530 super.copyValues(dst); 1531 dst.regionType = regionType == null ? null : regionType.copy(); 1532 if (coordinate != null) { 1533 dst.coordinate = new ArrayList<DecimalType>(); 1534 for (DecimalType i : coordinate) 1535 dst.coordinate.add(i.copy()); 1536 }; 1537 } 1538 1539 @Override 1540 public boolean equalsDeep(Base other_) { 1541 if (!super.equalsDeep(other_)) 1542 return false; 1543 if (!(other_ instanceof ImageRegion2DComponent)) 1544 return false; 1545 ImageRegion2DComponent o = (ImageRegion2DComponent) other_; 1546 return compareDeep(regionType, o.regionType, true) && compareDeep(coordinate, o.coordinate, true) 1547 ; 1548 } 1549 1550 @Override 1551 public boolean equalsShallow(Base other_) { 1552 if (!super.equalsShallow(other_)) 1553 return false; 1554 if (!(other_ instanceof ImageRegion2DComponent)) 1555 return false; 1556 ImageRegion2DComponent o = (ImageRegion2DComponent) other_; 1557 return compareValues(regionType, o.regionType, true) && compareValues(coordinate, o.coordinate, true) 1558 ; 1559 } 1560 1561 public boolean isEmpty() { 1562 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(regionType, coordinate); 1563 } 1564 1565 public String fhirType() { 1566 return "ImagingSelection.instance.imageRegion2D"; 1567 1568 } 1569 1570 } 1571 1572 @Block() 1573 public static class ImageRegion3DComponent extends BackboneElement implements IBaseBackboneElement { 1574 /** 1575 * Specifies the type of image region. 1576 */ 1577 @Child(name = "regionType", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1578 @Description(shortDefinition="point | multipoint | polyline | polygon | ellipse | ellipsoid", formalDefinition="Specifies the type of image region." ) 1579 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/imagingselection-3dgraphictype") 1580 protected Enumeration<ImagingSelection3DGraphicType> regionType; 1581 1582 /** 1583 * The coordinates describing the image region. Encoded as an ordered set of (x,y,z) triplets (in mm and may be negative) that define a region of interest in the patient-relative Reference Coordinate System defined by ImagingSelection.frameOfReferenceUid element. 1584 */ 1585 @Child(name = "coordinate", type = {DecimalType.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1586 @Description(shortDefinition="Specifies the coordinates that define the image region", formalDefinition="The coordinates describing the image region. Encoded as an ordered set of (x,y,z) triplets (in mm and may be negative) that define a region of interest in the patient-relative Reference Coordinate System defined by ImagingSelection.frameOfReferenceUid element." ) 1587 protected List<DecimalType> coordinate; 1588 1589 private static final long serialVersionUID = 1532227853L; 1590 1591 /** 1592 * Constructor 1593 */ 1594 public ImageRegion3DComponent() { 1595 super(); 1596 } 1597 1598 /** 1599 * Constructor 1600 */ 1601 public ImageRegion3DComponent(ImagingSelection3DGraphicType regionType, BigDecimal coordinate) { 1602 super(); 1603 this.setRegionType(regionType); 1604 this.addCoordinate(coordinate); 1605 } 1606 1607 /** 1608 * @return {@link #regionType} (Specifies the type of image region.). This is the underlying object with id, value and extensions. The accessor "getRegionType" gives direct access to the value 1609 */ 1610 public Enumeration<ImagingSelection3DGraphicType> getRegionTypeElement() { 1611 if (this.regionType == null) 1612 if (Configuration.errorOnAutoCreate()) 1613 throw new Error("Attempt to auto-create ImageRegion3DComponent.regionType"); 1614 else if (Configuration.doAutoCreate()) 1615 this.regionType = new Enumeration<ImagingSelection3DGraphicType>(new ImagingSelection3DGraphicTypeEnumFactory()); // bb 1616 return this.regionType; 1617 } 1618 1619 public boolean hasRegionTypeElement() { 1620 return this.regionType != null && !this.regionType.isEmpty(); 1621 } 1622 1623 public boolean hasRegionType() { 1624 return this.regionType != null && !this.regionType.isEmpty(); 1625 } 1626 1627 /** 1628 * @param value {@link #regionType} (Specifies the type of image region.). This is the underlying object with id, value and extensions. The accessor "getRegionType" gives direct access to the value 1629 */ 1630 public ImageRegion3DComponent setRegionTypeElement(Enumeration<ImagingSelection3DGraphicType> value) { 1631 this.regionType = value; 1632 return this; 1633 } 1634 1635 /** 1636 * @return Specifies the type of image region. 1637 */ 1638 public ImagingSelection3DGraphicType getRegionType() { 1639 return this.regionType == null ? null : this.regionType.getValue(); 1640 } 1641 1642 /** 1643 * @param value Specifies the type of image region. 1644 */ 1645 public ImageRegion3DComponent setRegionType(ImagingSelection3DGraphicType value) { 1646 if (this.regionType == null) 1647 this.regionType = new Enumeration<ImagingSelection3DGraphicType>(new ImagingSelection3DGraphicTypeEnumFactory()); 1648 this.regionType.setValue(value); 1649 return this; 1650 } 1651 1652 /** 1653 * @return {@link #coordinate} (The coordinates describing the image region. Encoded as an ordered set of (x,y,z) triplets (in mm and may be negative) that define a region of interest in the patient-relative Reference Coordinate System defined by ImagingSelection.frameOfReferenceUid element.) 1654 */ 1655 public List<DecimalType> getCoordinate() { 1656 if (this.coordinate == null) 1657 this.coordinate = new ArrayList<DecimalType>(); 1658 return this.coordinate; 1659 } 1660 1661 /** 1662 * @return Returns a reference to <code>this</code> for easy method chaining 1663 */ 1664 public ImageRegion3DComponent setCoordinate(List<DecimalType> theCoordinate) { 1665 this.coordinate = theCoordinate; 1666 return this; 1667 } 1668 1669 public boolean hasCoordinate() { 1670 if (this.coordinate == null) 1671 return false; 1672 for (DecimalType item : this.coordinate) 1673 if (!item.isEmpty()) 1674 return true; 1675 return false; 1676 } 1677 1678 /** 1679 * @return {@link #coordinate} (The coordinates describing the image region. Encoded as an ordered set of (x,y,z) triplets (in mm and may be negative) that define a region of interest in the patient-relative Reference Coordinate System defined by ImagingSelection.frameOfReferenceUid element.) 1680 */ 1681 public DecimalType addCoordinateElement() {//2 1682 DecimalType t = new DecimalType(); 1683 if (this.coordinate == null) 1684 this.coordinate = new ArrayList<DecimalType>(); 1685 this.coordinate.add(t); 1686 return t; 1687 } 1688 1689 /** 1690 * @param value {@link #coordinate} (The coordinates describing the image region. Encoded as an ordered set of (x,y,z) triplets (in mm and may be negative) that define a region of interest in the patient-relative Reference Coordinate System defined by ImagingSelection.frameOfReferenceUid element.) 1691 */ 1692 public ImageRegion3DComponent addCoordinate(BigDecimal value) { //1 1693 DecimalType t = new DecimalType(); 1694 t.setValue(value); 1695 if (this.coordinate == null) 1696 this.coordinate = new ArrayList<DecimalType>(); 1697 this.coordinate.add(t); 1698 return this; 1699 } 1700 1701 /** 1702 * @param value {@link #coordinate} (The coordinates describing the image region. Encoded as an ordered set of (x,y,z) triplets (in mm and may be negative) that define a region of interest in the patient-relative Reference Coordinate System defined by ImagingSelection.frameOfReferenceUid element.) 1703 */ 1704 public boolean hasCoordinate(BigDecimal value) { 1705 if (this.coordinate == null) 1706 return false; 1707 for (DecimalType v : this.coordinate) 1708 if (v.getValue().equals(value)) // decimal 1709 return true; 1710 return false; 1711 } 1712 1713 protected void listChildren(List<Property> children) { 1714 super.listChildren(children); 1715 children.add(new Property("regionType", "code", "Specifies the type of image region.", 0, 1, regionType)); 1716 children.add(new Property("coordinate", "decimal", "The coordinates describing the image region. Encoded as an ordered set of (x,y,z) triplets (in mm and may be negative) that define a region of interest in the patient-relative Reference Coordinate System defined by ImagingSelection.frameOfReferenceUid element.", 0, java.lang.Integer.MAX_VALUE, coordinate)); 1717 } 1718 1719 @Override 1720 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1721 switch (_hash) { 1722 case -1990487986: /*regionType*/ return new Property("regionType", "code", "Specifies the type of image region.", 0, 1, regionType); 1723 case 198931832: /*coordinate*/ return new Property("coordinate", "decimal", "The coordinates describing the image region. Encoded as an ordered set of (x,y,z) triplets (in mm and may be negative) that define a region of interest in the patient-relative Reference Coordinate System defined by ImagingSelection.frameOfReferenceUid element.", 0, java.lang.Integer.MAX_VALUE, coordinate); 1724 default: return super.getNamedProperty(_hash, _name, _checkValid); 1725 } 1726 1727 } 1728 1729 @Override 1730 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1731 switch (hash) { 1732 case -1990487986: /*regionType*/ return this.regionType == null ? new Base[0] : new Base[] {this.regionType}; // Enumeration<ImagingSelection3DGraphicType> 1733 case 198931832: /*coordinate*/ return this.coordinate == null ? new Base[0] : this.coordinate.toArray(new Base[this.coordinate.size()]); // DecimalType 1734 default: return super.getProperty(hash, name, checkValid); 1735 } 1736 1737 } 1738 1739 @Override 1740 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1741 switch (hash) { 1742 case -1990487986: // regionType 1743 value = new ImagingSelection3DGraphicTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1744 this.regionType = (Enumeration) value; // Enumeration<ImagingSelection3DGraphicType> 1745 return value; 1746 case 198931832: // coordinate 1747 this.getCoordinate().add(TypeConvertor.castToDecimal(value)); // DecimalType 1748 return value; 1749 default: return super.setProperty(hash, name, value); 1750 } 1751 1752 } 1753 1754 @Override 1755 public Base setProperty(String name, Base value) throws FHIRException { 1756 if (name.equals("regionType")) { 1757 value = new ImagingSelection3DGraphicTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1758 this.regionType = (Enumeration) value; // Enumeration<ImagingSelection3DGraphicType> 1759 } else if (name.equals("coordinate")) { 1760 this.getCoordinate().add(TypeConvertor.castToDecimal(value)); 1761 } else 1762 return super.setProperty(name, value); 1763 return value; 1764 } 1765 1766 @Override 1767 public void removeChild(String name, Base value) throws FHIRException { 1768 if (name.equals("regionType")) { 1769 value = new ImagingSelection3DGraphicTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1770 this.regionType = (Enumeration) value; // Enumeration<ImagingSelection3DGraphicType> 1771 } else if (name.equals("coordinate")) { 1772 this.getCoordinate().remove(value); 1773 } else 1774 super.removeChild(name, value); 1775 1776 } 1777 1778 @Override 1779 public Base makeProperty(int hash, String name) throws FHIRException { 1780 switch (hash) { 1781 case -1990487986: return getRegionTypeElement(); 1782 case 198931832: return addCoordinateElement(); 1783 default: return super.makeProperty(hash, name); 1784 } 1785 1786 } 1787 1788 @Override 1789 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1790 switch (hash) { 1791 case -1990487986: /*regionType*/ return new String[] {"code"}; 1792 case 198931832: /*coordinate*/ return new String[] {"decimal"}; 1793 default: return super.getTypesForProperty(hash, name); 1794 } 1795 1796 } 1797 1798 @Override 1799 public Base addChild(String name) throws FHIRException { 1800 if (name.equals("regionType")) { 1801 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.instance.imageRegion3D.regionType"); 1802 } 1803 else if (name.equals("coordinate")) { 1804 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.instance.imageRegion3D.coordinate"); 1805 } 1806 else 1807 return super.addChild(name); 1808 } 1809 1810 public ImageRegion3DComponent copy() { 1811 ImageRegion3DComponent dst = new ImageRegion3DComponent(); 1812 copyValues(dst); 1813 return dst; 1814 } 1815 1816 public void copyValues(ImageRegion3DComponent dst) { 1817 super.copyValues(dst); 1818 dst.regionType = regionType == null ? null : regionType.copy(); 1819 if (coordinate != null) { 1820 dst.coordinate = new ArrayList<DecimalType>(); 1821 for (DecimalType i : coordinate) 1822 dst.coordinate.add(i.copy()); 1823 }; 1824 } 1825 1826 @Override 1827 public boolean equalsDeep(Base other_) { 1828 if (!super.equalsDeep(other_)) 1829 return false; 1830 if (!(other_ instanceof ImageRegion3DComponent)) 1831 return false; 1832 ImageRegion3DComponent o = (ImageRegion3DComponent) other_; 1833 return compareDeep(regionType, o.regionType, true) && compareDeep(coordinate, o.coordinate, true) 1834 ; 1835 } 1836 1837 @Override 1838 public boolean equalsShallow(Base other_) { 1839 if (!super.equalsShallow(other_)) 1840 return false; 1841 if (!(other_ instanceof ImageRegion3DComponent)) 1842 return false; 1843 ImageRegion3DComponent o = (ImageRegion3DComponent) other_; 1844 return compareValues(regionType, o.regionType, true) && compareValues(coordinate, o.coordinate, true) 1845 ; 1846 } 1847 1848 public boolean isEmpty() { 1849 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(regionType, coordinate); 1850 } 1851 1852 public String fhirType() { 1853 return "ImagingSelection.instance.imageRegion3D"; 1854 1855 } 1856 1857 } 1858 1859 /** 1860 * A unique identifier assigned to this imaging selection. 1861 */ 1862 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1863 @Description(shortDefinition="Business Identifier for Imaging Selection", formalDefinition="A unique identifier assigned to this imaging selection." ) 1864 protected List<Identifier> identifier; 1865 1866 /** 1867 * The current state of the ImagingSelection resource. This is not the status of any ImagingStudy, ServiceRequest, or Task resources associated with the ImagingSelection. 1868 */ 1869 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 1870 @Description(shortDefinition="available | entered-in-error | unknown", formalDefinition="The current state of the ImagingSelection resource. This is not the status of any ImagingStudy, ServiceRequest, or Task resources associated with the ImagingSelection." ) 1871 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/imagingselection-status") 1872 protected Enumeration<ImagingSelectionStatus> status; 1873 1874 /** 1875 * The patient, or group of patients, location, device, organization, procedure or practitioner this imaging selection is about and into whose or what record the imaging selection is placed. 1876 */ 1877 @Child(name = "subject", type = {Patient.class, Group.class, Device.class, Location.class, Organization.class, Procedure.class, Practitioner.class, Medication.class, Substance.class, Specimen.class}, order=2, min=0, max=1, modifier=false, summary=true) 1878 @Description(shortDefinition="Subject of the selected instances", formalDefinition="The patient, or group of patients, location, device, organization, procedure or practitioner this imaging selection is about and into whose or what record the imaging selection is placed." ) 1879 protected Reference subject; 1880 1881 /** 1882 * The date and time this imaging selection was created. 1883 */ 1884 @Child(name = "issued", type = {InstantType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1885 @Description(shortDefinition="Date / Time when this imaging selection was created", formalDefinition="The date and time this imaging selection was created." ) 1886 protected InstantType issued; 1887 1888 /** 1889 * Selector of the instances ? human or machine. 1890 */ 1891 @Child(name = "performer", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1892 @Description(shortDefinition="Selector of the instances (human or machine)", formalDefinition="Selector of the instances ? human or machine." ) 1893 protected List<ImagingSelectionPerformerComponent> performer; 1894 1895 /** 1896 * A list of the diagnostic requests that resulted in this imaging selection being performed. 1897 */ 1898 @Child(name = "basedOn", type = {CarePlan.class, ServiceRequest.class, Appointment.class, AppointmentResponse.class, Task.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1899 @Description(shortDefinition="Associated request", formalDefinition="A list of the diagnostic requests that resulted in this imaging selection being performed." ) 1900 protected List<Reference> basedOn; 1901 1902 /** 1903 * Classifies the imaging selection. 1904 */ 1905 @Child(name = "category", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1906 @Description(shortDefinition="Classifies the imaging selection", formalDefinition="Classifies the imaging selection." ) 1907 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://dicom.nema.org/medical/dicom/current/output/chtml/part16/sect_CID_7010.html") 1908 protected List<CodeableConcept> category; 1909 1910 /** 1911 * Reason for referencing the selected content. 1912 */ 1913 @Child(name = "code", type = {CodeableConcept.class}, order=7, min=1, max=1, modifier=false, summary=true) 1914 @Description(shortDefinition="Imaging Selection purpose text or code", formalDefinition="Reason for referencing the selected content." ) 1915 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://dicom.nema.org/medical/dicom/current/output/chtml/part16/sect_CID_7010.html") 1916 protected CodeableConcept code; 1917 1918 /** 1919 * The Study Instance UID for the DICOM Study from which the images were selected. 1920 */ 1921 @Child(name = "studyUid", type = {IdType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1922 @Description(shortDefinition="DICOM Study Instance UID", formalDefinition="The Study Instance UID for the DICOM Study from which the images were selected." ) 1923 protected IdType studyUid; 1924 1925 /** 1926 * The imaging study from which the imaging selection is made. 1927 */ 1928 @Child(name = "derivedFrom", type = {ImagingStudy.class, DocumentReference.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1929 @Description(shortDefinition="The imaging study from which the imaging selection is derived", formalDefinition="The imaging study from which the imaging selection is made." ) 1930 protected List<Reference> derivedFrom; 1931 1932 /** 1933 * The network service providing retrieval access to the selected images, frames, etc. See implementation notes for information about using DICOM endpoints. 1934 */ 1935 @Child(name = "endpoint", type = {Endpoint.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1936 @Description(shortDefinition="The network service providing retrieval for the images referenced in the imaging selection", formalDefinition="The network service providing retrieval access to the selected images, frames, etc. See implementation notes for information about using DICOM endpoints." ) 1937 protected List<Reference> endpoint; 1938 1939 /** 1940 * The Series Instance UID for the DICOM Series from which the images were selected. 1941 */ 1942 @Child(name = "seriesUid", type = {IdType.class}, order=11, min=0, max=1, modifier=false, summary=true) 1943 @Description(shortDefinition="DICOM Series Instance UID", formalDefinition="The Series Instance UID for the DICOM Series from which the images were selected." ) 1944 protected IdType seriesUid; 1945 1946 /** 1947 * The Series Number for the DICOM Series from which the images were selected. 1948 */ 1949 @Child(name = "seriesNumber", type = {UnsignedIntType.class}, order=12, min=0, max=1, modifier=false, summary=true) 1950 @Description(shortDefinition="DICOM Series Number", formalDefinition="The Series Number for the DICOM Series from which the images were selected." ) 1951 protected UnsignedIntType seriesNumber; 1952 1953 /** 1954 * The Frame of Reference UID identifying the coordinate system that conveys spatial and/or temporal information for the selected images or frames. 1955 */ 1956 @Child(name = "frameOfReferenceUid", type = {IdType.class}, order=13, min=0, max=1, modifier=false, summary=true) 1957 @Description(shortDefinition="The Frame of Reference UID for the selected images", formalDefinition="The Frame of Reference UID identifying the coordinate system that conveys spatial and/or temporal information for the selected images or frames." ) 1958 protected IdType frameOfReferenceUid; 1959 1960 /** 1961 * The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. 1962 */ 1963 @Child(name = "bodySite", type = {CodeableReference.class}, order=14, min=0, max=1, modifier=false, summary=true) 1964 @Description(shortDefinition="Body part examined", formalDefinition="The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings." ) 1965 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 1966 protected CodeableReference bodySite; 1967 1968 /** 1969 * The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus. 1970 */ 1971 @Child(name = "focus", type = {ImagingSelection.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1972 @Description(shortDefinition="Related resource that is the focus for the imaging selection", formalDefinition="The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus." ) 1973 protected List<Reference> focus; 1974 1975 /** 1976 * Each imaging selection includes one or more selected DICOM SOP instances. 1977 */ 1978 @Child(name = "instance", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1979 @Description(shortDefinition="The selected instances", formalDefinition="Each imaging selection includes one or more selected DICOM SOP instances." ) 1980 protected List<ImagingSelectionInstanceComponent> instance; 1981 1982 private static final long serialVersionUID = -1733487270L; 1983 1984 /** 1985 * Constructor 1986 */ 1987 public ImagingSelection() { 1988 super(); 1989 } 1990 1991 /** 1992 * Constructor 1993 */ 1994 public ImagingSelection(ImagingSelectionStatus status, CodeableConcept code) { 1995 super(); 1996 this.setStatus(status); 1997 this.setCode(code); 1998 } 1999 2000 /** 2001 * @return {@link #identifier} (A unique identifier assigned to this imaging selection.) 2002 */ 2003 public List<Identifier> getIdentifier() { 2004 if (this.identifier == null) 2005 this.identifier = new ArrayList<Identifier>(); 2006 return this.identifier; 2007 } 2008 2009 /** 2010 * @return Returns a reference to <code>this</code> for easy method chaining 2011 */ 2012 public ImagingSelection setIdentifier(List<Identifier> theIdentifier) { 2013 this.identifier = theIdentifier; 2014 return this; 2015 } 2016 2017 public boolean hasIdentifier() { 2018 if (this.identifier == null) 2019 return false; 2020 for (Identifier item : this.identifier) 2021 if (!item.isEmpty()) 2022 return true; 2023 return false; 2024 } 2025 2026 public Identifier addIdentifier() { //3 2027 Identifier t = new Identifier(); 2028 if (this.identifier == null) 2029 this.identifier = new ArrayList<Identifier>(); 2030 this.identifier.add(t); 2031 return t; 2032 } 2033 2034 public ImagingSelection addIdentifier(Identifier t) { //3 2035 if (t == null) 2036 return this; 2037 if (this.identifier == null) 2038 this.identifier = new ArrayList<Identifier>(); 2039 this.identifier.add(t); 2040 return this; 2041 } 2042 2043 /** 2044 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2045 */ 2046 public Identifier getIdentifierFirstRep() { 2047 if (getIdentifier().isEmpty()) { 2048 addIdentifier(); 2049 } 2050 return getIdentifier().get(0); 2051 } 2052 2053 /** 2054 * @return {@link #status} (The current state of the ImagingSelection resource. This is not the status of any ImagingStudy, ServiceRequest, or Task resources associated with the ImagingSelection.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2055 */ 2056 public Enumeration<ImagingSelectionStatus> getStatusElement() { 2057 if (this.status == null) 2058 if (Configuration.errorOnAutoCreate()) 2059 throw new Error("Attempt to auto-create ImagingSelection.status"); 2060 else if (Configuration.doAutoCreate()) 2061 this.status = new Enumeration<ImagingSelectionStatus>(new ImagingSelectionStatusEnumFactory()); // bb 2062 return this.status; 2063 } 2064 2065 public boolean hasStatusElement() { 2066 return this.status != null && !this.status.isEmpty(); 2067 } 2068 2069 public boolean hasStatus() { 2070 return this.status != null && !this.status.isEmpty(); 2071 } 2072 2073 /** 2074 * @param value {@link #status} (The current state of the ImagingSelection resource. This is not the status of any ImagingStudy, ServiceRequest, or Task resources associated with the ImagingSelection.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2075 */ 2076 public ImagingSelection setStatusElement(Enumeration<ImagingSelectionStatus> value) { 2077 this.status = value; 2078 return this; 2079 } 2080 2081 /** 2082 * @return The current state of the ImagingSelection resource. This is not the status of any ImagingStudy, ServiceRequest, or Task resources associated with the ImagingSelection. 2083 */ 2084 public ImagingSelectionStatus getStatus() { 2085 return this.status == null ? null : this.status.getValue(); 2086 } 2087 2088 /** 2089 * @param value The current state of the ImagingSelection resource. This is not the status of any ImagingStudy, ServiceRequest, or Task resources associated with the ImagingSelection. 2090 */ 2091 public ImagingSelection setStatus(ImagingSelectionStatus value) { 2092 if (this.status == null) 2093 this.status = new Enumeration<ImagingSelectionStatus>(new ImagingSelectionStatusEnumFactory()); 2094 this.status.setValue(value); 2095 return this; 2096 } 2097 2098 /** 2099 * @return {@link #subject} (The patient, or group of patients, location, device, organization, procedure or practitioner this imaging selection is about and into whose or what record the imaging selection is placed.) 2100 */ 2101 public Reference getSubject() { 2102 if (this.subject == null) 2103 if (Configuration.errorOnAutoCreate()) 2104 throw new Error("Attempt to auto-create ImagingSelection.subject"); 2105 else if (Configuration.doAutoCreate()) 2106 this.subject = new Reference(); // cc 2107 return this.subject; 2108 } 2109 2110 public boolean hasSubject() { 2111 return this.subject != null && !this.subject.isEmpty(); 2112 } 2113 2114 /** 2115 * @param value {@link #subject} (The patient, or group of patients, location, device, organization, procedure or practitioner this imaging selection is about and into whose or what record the imaging selection is placed.) 2116 */ 2117 public ImagingSelection setSubject(Reference value) { 2118 this.subject = value; 2119 return this; 2120 } 2121 2122 /** 2123 * @return {@link #issued} (The date and time this imaging selection was created.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 2124 */ 2125 public InstantType getIssuedElement() { 2126 if (this.issued == null) 2127 if (Configuration.errorOnAutoCreate()) 2128 throw new Error("Attempt to auto-create ImagingSelection.issued"); 2129 else if (Configuration.doAutoCreate()) 2130 this.issued = new InstantType(); // bb 2131 return this.issued; 2132 } 2133 2134 public boolean hasIssuedElement() { 2135 return this.issued != null && !this.issued.isEmpty(); 2136 } 2137 2138 public boolean hasIssued() { 2139 return this.issued != null && !this.issued.isEmpty(); 2140 } 2141 2142 /** 2143 * @param value {@link #issued} (The date and time this imaging selection was created.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 2144 */ 2145 public ImagingSelection setIssuedElement(InstantType value) { 2146 this.issued = value; 2147 return this; 2148 } 2149 2150 /** 2151 * @return The date and time this imaging selection was created. 2152 */ 2153 public Date getIssued() { 2154 return this.issued == null ? null : this.issued.getValue(); 2155 } 2156 2157 /** 2158 * @param value The date and time this imaging selection was created. 2159 */ 2160 public ImagingSelection setIssued(Date value) { 2161 if (value == null) 2162 this.issued = null; 2163 else { 2164 if (this.issued == null) 2165 this.issued = new InstantType(); 2166 this.issued.setValue(value); 2167 } 2168 return this; 2169 } 2170 2171 /** 2172 * @return {@link #performer} (Selector of the instances ? human or machine.) 2173 */ 2174 public List<ImagingSelectionPerformerComponent> getPerformer() { 2175 if (this.performer == null) 2176 this.performer = new ArrayList<ImagingSelectionPerformerComponent>(); 2177 return this.performer; 2178 } 2179 2180 /** 2181 * @return Returns a reference to <code>this</code> for easy method chaining 2182 */ 2183 public ImagingSelection setPerformer(List<ImagingSelectionPerformerComponent> thePerformer) { 2184 this.performer = thePerformer; 2185 return this; 2186 } 2187 2188 public boolean hasPerformer() { 2189 if (this.performer == null) 2190 return false; 2191 for (ImagingSelectionPerformerComponent item : this.performer) 2192 if (!item.isEmpty()) 2193 return true; 2194 return false; 2195 } 2196 2197 public ImagingSelectionPerformerComponent addPerformer() { //3 2198 ImagingSelectionPerformerComponent t = new ImagingSelectionPerformerComponent(); 2199 if (this.performer == null) 2200 this.performer = new ArrayList<ImagingSelectionPerformerComponent>(); 2201 this.performer.add(t); 2202 return t; 2203 } 2204 2205 public ImagingSelection addPerformer(ImagingSelectionPerformerComponent t) { //3 2206 if (t == null) 2207 return this; 2208 if (this.performer == null) 2209 this.performer = new ArrayList<ImagingSelectionPerformerComponent>(); 2210 this.performer.add(t); 2211 return this; 2212 } 2213 2214 /** 2215 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 2216 */ 2217 public ImagingSelectionPerformerComponent getPerformerFirstRep() { 2218 if (getPerformer().isEmpty()) { 2219 addPerformer(); 2220 } 2221 return getPerformer().get(0); 2222 } 2223 2224 /** 2225 * @return {@link #basedOn} (A list of the diagnostic requests that resulted in this imaging selection being performed.) 2226 */ 2227 public List<Reference> getBasedOn() { 2228 if (this.basedOn == null) 2229 this.basedOn = new ArrayList<Reference>(); 2230 return this.basedOn; 2231 } 2232 2233 /** 2234 * @return Returns a reference to <code>this</code> for easy method chaining 2235 */ 2236 public ImagingSelection setBasedOn(List<Reference> theBasedOn) { 2237 this.basedOn = theBasedOn; 2238 return this; 2239 } 2240 2241 public boolean hasBasedOn() { 2242 if (this.basedOn == null) 2243 return false; 2244 for (Reference item : this.basedOn) 2245 if (!item.isEmpty()) 2246 return true; 2247 return false; 2248 } 2249 2250 public Reference addBasedOn() { //3 2251 Reference t = new Reference(); 2252 if (this.basedOn == null) 2253 this.basedOn = new ArrayList<Reference>(); 2254 this.basedOn.add(t); 2255 return t; 2256 } 2257 2258 public ImagingSelection addBasedOn(Reference t) { //3 2259 if (t == null) 2260 return this; 2261 if (this.basedOn == null) 2262 this.basedOn = new ArrayList<Reference>(); 2263 this.basedOn.add(t); 2264 return this; 2265 } 2266 2267 /** 2268 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 2269 */ 2270 public Reference getBasedOnFirstRep() { 2271 if (getBasedOn().isEmpty()) { 2272 addBasedOn(); 2273 } 2274 return getBasedOn().get(0); 2275 } 2276 2277 /** 2278 * @return {@link #category} (Classifies the imaging selection.) 2279 */ 2280 public List<CodeableConcept> getCategory() { 2281 if (this.category == null) 2282 this.category = new ArrayList<CodeableConcept>(); 2283 return this.category; 2284 } 2285 2286 /** 2287 * @return Returns a reference to <code>this</code> for easy method chaining 2288 */ 2289 public ImagingSelection setCategory(List<CodeableConcept> theCategory) { 2290 this.category = theCategory; 2291 return this; 2292 } 2293 2294 public boolean hasCategory() { 2295 if (this.category == null) 2296 return false; 2297 for (CodeableConcept item : this.category) 2298 if (!item.isEmpty()) 2299 return true; 2300 return false; 2301 } 2302 2303 public CodeableConcept addCategory() { //3 2304 CodeableConcept t = new CodeableConcept(); 2305 if (this.category == null) 2306 this.category = new ArrayList<CodeableConcept>(); 2307 this.category.add(t); 2308 return t; 2309 } 2310 2311 public ImagingSelection addCategory(CodeableConcept t) { //3 2312 if (t == null) 2313 return this; 2314 if (this.category == null) 2315 this.category = new ArrayList<CodeableConcept>(); 2316 this.category.add(t); 2317 return this; 2318 } 2319 2320 /** 2321 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 2322 */ 2323 public CodeableConcept getCategoryFirstRep() { 2324 if (getCategory().isEmpty()) { 2325 addCategory(); 2326 } 2327 return getCategory().get(0); 2328 } 2329 2330 /** 2331 * @return {@link #code} (Reason for referencing the selected content.) 2332 */ 2333 public CodeableConcept getCode() { 2334 if (this.code == null) 2335 if (Configuration.errorOnAutoCreate()) 2336 throw new Error("Attempt to auto-create ImagingSelection.code"); 2337 else if (Configuration.doAutoCreate()) 2338 this.code = new CodeableConcept(); // cc 2339 return this.code; 2340 } 2341 2342 public boolean hasCode() { 2343 return this.code != null && !this.code.isEmpty(); 2344 } 2345 2346 /** 2347 * @param value {@link #code} (Reason for referencing the selected content.) 2348 */ 2349 public ImagingSelection setCode(CodeableConcept value) { 2350 this.code = value; 2351 return this; 2352 } 2353 2354 /** 2355 * @return {@link #studyUid} (The Study Instance UID for the DICOM Study from which the images were selected.). This is the underlying object with id, value and extensions. The accessor "getStudyUid" gives direct access to the value 2356 */ 2357 public IdType getStudyUidElement() { 2358 if (this.studyUid == null) 2359 if (Configuration.errorOnAutoCreate()) 2360 throw new Error("Attempt to auto-create ImagingSelection.studyUid"); 2361 else if (Configuration.doAutoCreate()) 2362 this.studyUid = new IdType(); // bb 2363 return this.studyUid; 2364 } 2365 2366 public boolean hasStudyUidElement() { 2367 return this.studyUid != null && !this.studyUid.isEmpty(); 2368 } 2369 2370 public boolean hasStudyUid() { 2371 return this.studyUid != null && !this.studyUid.isEmpty(); 2372 } 2373 2374 /** 2375 * @param value {@link #studyUid} (The Study Instance UID for the DICOM Study from which the images were selected.). This is the underlying object with id, value and extensions. The accessor "getStudyUid" gives direct access to the value 2376 */ 2377 public ImagingSelection setStudyUidElement(IdType value) { 2378 this.studyUid = value; 2379 return this; 2380 } 2381 2382 /** 2383 * @return The Study Instance UID for the DICOM Study from which the images were selected. 2384 */ 2385 public String getStudyUid() { 2386 return this.studyUid == null ? null : this.studyUid.getValue(); 2387 } 2388 2389 /** 2390 * @param value The Study Instance UID for the DICOM Study from which the images were selected. 2391 */ 2392 public ImagingSelection setStudyUid(String value) { 2393 if (Utilities.noString(value)) 2394 this.studyUid = null; 2395 else { 2396 if (this.studyUid == null) 2397 this.studyUid = new IdType(); 2398 this.studyUid.setValue(value); 2399 } 2400 return this; 2401 } 2402 2403 /** 2404 * @return {@link #derivedFrom} (The imaging study from which the imaging selection is made.) 2405 */ 2406 public List<Reference> getDerivedFrom() { 2407 if (this.derivedFrom == null) 2408 this.derivedFrom = new ArrayList<Reference>(); 2409 return this.derivedFrom; 2410 } 2411 2412 /** 2413 * @return Returns a reference to <code>this</code> for easy method chaining 2414 */ 2415 public ImagingSelection setDerivedFrom(List<Reference> theDerivedFrom) { 2416 this.derivedFrom = theDerivedFrom; 2417 return this; 2418 } 2419 2420 public boolean hasDerivedFrom() { 2421 if (this.derivedFrom == null) 2422 return false; 2423 for (Reference item : this.derivedFrom) 2424 if (!item.isEmpty()) 2425 return true; 2426 return false; 2427 } 2428 2429 public Reference addDerivedFrom() { //3 2430 Reference t = new Reference(); 2431 if (this.derivedFrom == null) 2432 this.derivedFrom = new ArrayList<Reference>(); 2433 this.derivedFrom.add(t); 2434 return t; 2435 } 2436 2437 public ImagingSelection addDerivedFrom(Reference t) { //3 2438 if (t == null) 2439 return this; 2440 if (this.derivedFrom == null) 2441 this.derivedFrom = new ArrayList<Reference>(); 2442 this.derivedFrom.add(t); 2443 return this; 2444 } 2445 2446 /** 2447 * @return The first repetition of repeating field {@link #derivedFrom}, creating it if it does not already exist {3} 2448 */ 2449 public Reference getDerivedFromFirstRep() { 2450 if (getDerivedFrom().isEmpty()) { 2451 addDerivedFrom(); 2452 } 2453 return getDerivedFrom().get(0); 2454 } 2455 2456 /** 2457 * @return {@link #endpoint} (The network service providing retrieval access to the selected images, frames, etc. See implementation notes for information about using DICOM endpoints.) 2458 */ 2459 public List<Reference> getEndpoint() { 2460 if (this.endpoint == null) 2461 this.endpoint = new ArrayList<Reference>(); 2462 return this.endpoint; 2463 } 2464 2465 /** 2466 * @return Returns a reference to <code>this</code> for easy method chaining 2467 */ 2468 public ImagingSelection setEndpoint(List<Reference> theEndpoint) { 2469 this.endpoint = theEndpoint; 2470 return this; 2471 } 2472 2473 public boolean hasEndpoint() { 2474 if (this.endpoint == null) 2475 return false; 2476 for (Reference item : this.endpoint) 2477 if (!item.isEmpty()) 2478 return true; 2479 return false; 2480 } 2481 2482 public Reference addEndpoint() { //3 2483 Reference t = new Reference(); 2484 if (this.endpoint == null) 2485 this.endpoint = new ArrayList<Reference>(); 2486 this.endpoint.add(t); 2487 return t; 2488 } 2489 2490 public ImagingSelection addEndpoint(Reference t) { //3 2491 if (t == null) 2492 return this; 2493 if (this.endpoint == null) 2494 this.endpoint = new ArrayList<Reference>(); 2495 this.endpoint.add(t); 2496 return this; 2497 } 2498 2499 /** 2500 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist {3} 2501 */ 2502 public Reference getEndpointFirstRep() { 2503 if (getEndpoint().isEmpty()) { 2504 addEndpoint(); 2505 } 2506 return getEndpoint().get(0); 2507 } 2508 2509 /** 2510 * @return {@link #seriesUid} (The Series Instance UID for the DICOM Series from which the images were selected.). This is the underlying object with id, value and extensions. The accessor "getSeriesUid" gives direct access to the value 2511 */ 2512 public IdType getSeriesUidElement() { 2513 if (this.seriesUid == null) 2514 if (Configuration.errorOnAutoCreate()) 2515 throw new Error("Attempt to auto-create ImagingSelection.seriesUid"); 2516 else if (Configuration.doAutoCreate()) 2517 this.seriesUid = new IdType(); // bb 2518 return this.seriesUid; 2519 } 2520 2521 public boolean hasSeriesUidElement() { 2522 return this.seriesUid != null && !this.seriesUid.isEmpty(); 2523 } 2524 2525 public boolean hasSeriesUid() { 2526 return this.seriesUid != null && !this.seriesUid.isEmpty(); 2527 } 2528 2529 /** 2530 * @param value {@link #seriesUid} (The Series Instance UID for the DICOM Series from which the images were selected.). This is the underlying object with id, value and extensions. The accessor "getSeriesUid" gives direct access to the value 2531 */ 2532 public ImagingSelection setSeriesUidElement(IdType value) { 2533 this.seriesUid = value; 2534 return this; 2535 } 2536 2537 /** 2538 * @return The Series Instance UID for the DICOM Series from which the images were selected. 2539 */ 2540 public String getSeriesUid() { 2541 return this.seriesUid == null ? null : this.seriesUid.getValue(); 2542 } 2543 2544 /** 2545 * @param value The Series Instance UID for the DICOM Series from which the images were selected. 2546 */ 2547 public ImagingSelection setSeriesUid(String value) { 2548 if (Utilities.noString(value)) 2549 this.seriesUid = null; 2550 else { 2551 if (this.seriesUid == null) 2552 this.seriesUid = new IdType(); 2553 this.seriesUid.setValue(value); 2554 } 2555 return this; 2556 } 2557 2558 /** 2559 * @return {@link #seriesNumber} (The Series Number for the DICOM Series from which the images were selected.). This is the underlying object with id, value and extensions. The accessor "getSeriesNumber" gives direct access to the value 2560 */ 2561 public UnsignedIntType getSeriesNumberElement() { 2562 if (this.seriesNumber == null) 2563 if (Configuration.errorOnAutoCreate()) 2564 throw new Error("Attempt to auto-create ImagingSelection.seriesNumber"); 2565 else if (Configuration.doAutoCreate()) 2566 this.seriesNumber = new UnsignedIntType(); // bb 2567 return this.seriesNumber; 2568 } 2569 2570 public boolean hasSeriesNumberElement() { 2571 return this.seriesNumber != null && !this.seriesNumber.isEmpty(); 2572 } 2573 2574 public boolean hasSeriesNumber() { 2575 return this.seriesNumber != null && !this.seriesNumber.isEmpty(); 2576 } 2577 2578 /** 2579 * @param value {@link #seriesNumber} (The Series Number for the DICOM Series from which the images were selected.). This is the underlying object with id, value and extensions. The accessor "getSeriesNumber" gives direct access to the value 2580 */ 2581 public ImagingSelection setSeriesNumberElement(UnsignedIntType value) { 2582 this.seriesNumber = value; 2583 return this; 2584 } 2585 2586 /** 2587 * @return The Series Number for the DICOM Series from which the images were selected. 2588 */ 2589 public int getSeriesNumber() { 2590 return this.seriesNumber == null || this.seriesNumber.isEmpty() ? 0 : this.seriesNumber.getValue(); 2591 } 2592 2593 /** 2594 * @param value The Series Number for the DICOM Series from which the images were selected. 2595 */ 2596 public ImagingSelection setSeriesNumber(int value) { 2597 if (this.seriesNumber == null) 2598 this.seriesNumber = new UnsignedIntType(); 2599 this.seriesNumber.setValue(value); 2600 return this; 2601 } 2602 2603 /** 2604 * @return {@link #frameOfReferenceUid} (The Frame of Reference UID identifying the coordinate system that conveys spatial and/or temporal information for the selected images or frames.). This is the underlying object with id, value and extensions. The accessor "getFrameOfReferenceUid" gives direct access to the value 2605 */ 2606 public IdType getFrameOfReferenceUidElement() { 2607 if (this.frameOfReferenceUid == null) 2608 if (Configuration.errorOnAutoCreate()) 2609 throw new Error("Attempt to auto-create ImagingSelection.frameOfReferenceUid"); 2610 else if (Configuration.doAutoCreate()) 2611 this.frameOfReferenceUid = new IdType(); // bb 2612 return this.frameOfReferenceUid; 2613 } 2614 2615 public boolean hasFrameOfReferenceUidElement() { 2616 return this.frameOfReferenceUid != null && !this.frameOfReferenceUid.isEmpty(); 2617 } 2618 2619 public boolean hasFrameOfReferenceUid() { 2620 return this.frameOfReferenceUid != null && !this.frameOfReferenceUid.isEmpty(); 2621 } 2622 2623 /** 2624 * @param value {@link #frameOfReferenceUid} (The Frame of Reference UID identifying the coordinate system that conveys spatial and/or temporal information for the selected images or frames.). This is the underlying object with id, value and extensions. The accessor "getFrameOfReferenceUid" gives direct access to the value 2625 */ 2626 public ImagingSelection setFrameOfReferenceUidElement(IdType value) { 2627 this.frameOfReferenceUid = value; 2628 return this; 2629 } 2630 2631 /** 2632 * @return The Frame of Reference UID identifying the coordinate system that conveys spatial and/or temporal information for the selected images or frames. 2633 */ 2634 public String getFrameOfReferenceUid() { 2635 return this.frameOfReferenceUid == null ? null : this.frameOfReferenceUid.getValue(); 2636 } 2637 2638 /** 2639 * @param value The Frame of Reference UID identifying the coordinate system that conveys spatial and/or temporal information for the selected images or frames. 2640 */ 2641 public ImagingSelection setFrameOfReferenceUid(String value) { 2642 if (Utilities.noString(value)) 2643 this.frameOfReferenceUid = null; 2644 else { 2645 if (this.frameOfReferenceUid == null) 2646 this.frameOfReferenceUid = new IdType(); 2647 this.frameOfReferenceUid.setValue(value); 2648 } 2649 return this; 2650 } 2651 2652 /** 2653 * @return {@link #bodySite} (The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings.) 2654 */ 2655 public CodeableReference getBodySite() { 2656 if (this.bodySite == null) 2657 if (Configuration.errorOnAutoCreate()) 2658 throw new Error("Attempt to auto-create ImagingSelection.bodySite"); 2659 else if (Configuration.doAutoCreate()) 2660 this.bodySite = new CodeableReference(); // cc 2661 return this.bodySite; 2662 } 2663 2664 public boolean hasBodySite() { 2665 return this.bodySite != null && !this.bodySite.isEmpty(); 2666 } 2667 2668 /** 2669 * @param value {@link #bodySite} (The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings.) 2670 */ 2671 public ImagingSelection setBodySite(CodeableReference value) { 2672 this.bodySite = value; 2673 return this; 2674 } 2675 2676 /** 2677 * @return {@link #focus} (The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.) 2678 */ 2679 public List<Reference> getFocus() { 2680 if (this.focus == null) 2681 this.focus = new ArrayList<Reference>(); 2682 return this.focus; 2683 } 2684 2685 /** 2686 * @return Returns a reference to <code>this</code> for easy method chaining 2687 */ 2688 public ImagingSelection setFocus(List<Reference> theFocus) { 2689 this.focus = theFocus; 2690 return this; 2691 } 2692 2693 public boolean hasFocus() { 2694 if (this.focus == null) 2695 return false; 2696 for (Reference item : this.focus) 2697 if (!item.isEmpty()) 2698 return true; 2699 return false; 2700 } 2701 2702 public Reference addFocus() { //3 2703 Reference t = new Reference(); 2704 if (this.focus == null) 2705 this.focus = new ArrayList<Reference>(); 2706 this.focus.add(t); 2707 return t; 2708 } 2709 2710 public ImagingSelection addFocus(Reference t) { //3 2711 if (t == null) 2712 return this; 2713 if (this.focus == null) 2714 this.focus = new ArrayList<Reference>(); 2715 this.focus.add(t); 2716 return this; 2717 } 2718 2719 /** 2720 * @return The first repetition of repeating field {@link #focus}, creating it if it does not already exist {3} 2721 */ 2722 public Reference getFocusFirstRep() { 2723 if (getFocus().isEmpty()) { 2724 addFocus(); 2725 } 2726 return getFocus().get(0); 2727 } 2728 2729 /** 2730 * @return {@link #instance} (Each imaging selection includes one or more selected DICOM SOP instances.) 2731 */ 2732 public List<ImagingSelectionInstanceComponent> getInstance() { 2733 if (this.instance == null) 2734 this.instance = new ArrayList<ImagingSelectionInstanceComponent>(); 2735 return this.instance; 2736 } 2737 2738 /** 2739 * @return Returns a reference to <code>this</code> for easy method chaining 2740 */ 2741 public ImagingSelection setInstance(List<ImagingSelectionInstanceComponent> theInstance) { 2742 this.instance = theInstance; 2743 return this; 2744 } 2745 2746 public boolean hasInstance() { 2747 if (this.instance == null) 2748 return false; 2749 for (ImagingSelectionInstanceComponent item : this.instance) 2750 if (!item.isEmpty()) 2751 return true; 2752 return false; 2753 } 2754 2755 public ImagingSelectionInstanceComponent addInstance() { //3 2756 ImagingSelectionInstanceComponent t = new ImagingSelectionInstanceComponent(); 2757 if (this.instance == null) 2758 this.instance = new ArrayList<ImagingSelectionInstanceComponent>(); 2759 this.instance.add(t); 2760 return t; 2761 } 2762 2763 public ImagingSelection addInstance(ImagingSelectionInstanceComponent t) { //3 2764 if (t == null) 2765 return this; 2766 if (this.instance == null) 2767 this.instance = new ArrayList<ImagingSelectionInstanceComponent>(); 2768 this.instance.add(t); 2769 return this; 2770 } 2771 2772 /** 2773 * @return The first repetition of repeating field {@link #instance}, creating it if it does not already exist {3} 2774 */ 2775 public ImagingSelectionInstanceComponent getInstanceFirstRep() { 2776 if (getInstance().isEmpty()) { 2777 addInstance(); 2778 } 2779 return getInstance().get(0); 2780 } 2781 2782 protected void listChildren(List<Property> children) { 2783 super.listChildren(children); 2784 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this imaging selection.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2785 children.add(new Property("status", "code", "The current state of the ImagingSelection resource. This is not the status of any ImagingStudy, ServiceRequest, or Task resources associated with the ImagingSelection.", 0, 1, status)); 2786 children.add(new Property("subject", "Reference(Patient|Group|Device|Location|Organization|Procedure|Practitioner|Medication|Substance|Specimen)", "The patient, or group of patients, location, device, organization, procedure or practitioner this imaging selection is about and into whose or what record the imaging selection is placed.", 0, 1, subject)); 2787 children.add(new Property("issued", "instant", "The date and time this imaging selection was created.", 0, 1, issued)); 2788 children.add(new Property("performer", "", "Selector of the instances ? human or machine.", 0, java.lang.Integer.MAX_VALUE, performer)); 2789 children.add(new Property("basedOn", "Reference(CarePlan|ServiceRequest|Appointment|AppointmentResponse|Task)", "A list of the diagnostic requests that resulted in this imaging selection being performed.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2790 children.add(new Property("category", "CodeableConcept", "Classifies the imaging selection.", 0, java.lang.Integer.MAX_VALUE, category)); 2791 children.add(new Property("code", "CodeableConcept", "Reason for referencing the selected content.", 0, 1, code)); 2792 children.add(new Property("studyUid", "id", "The Study Instance UID for the DICOM Study from which the images were selected.", 0, 1, studyUid)); 2793 children.add(new Property("derivedFrom", "Reference(ImagingStudy|DocumentReference)", "The imaging study from which the imaging selection is made.", 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 2794 children.add(new Property("endpoint", "Reference(Endpoint)", "The network service providing retrieval access to the selected images, frames, etc. See implementation notes for information about using DICOM endpoints.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 2795 children.add(new Property("seriesUid", "id", "The Series Instance UID for the DICOM Series from which the images were selected.", 0, 1, seriesUid)); 2796 children.add(new Property("seriesNumber", "unsignedInt", "The Series Number for the DICOM Series from which the images were selected.", 0, 1, seriesNumber)); 2797 children.add(new Property("frameOfReferenceUid", "id", "The Frame of Reference UID identifying the coordinate system that conveys spatial and/or temporal information for the selected images or frames.", 0, 1, frameOfReferenceUid)); 2798 children.add(new Property("bodySite", "CodeableReference(BodyStructure)", "The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings.", 0, 1, bodySite)); 2799 children.add(new Property("focus", "Reference(ImagingSelection)", "The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.", 0, java.lang.Integer.MAX_VALUE, focus)); 2800 children.add(new Property("instance", "", "Each imaging selection includes one or more selected DICOM SOP instances.", 0, java.lang.Integer.MAX_VALUE, instance)); 2801 } 2802 2803 @Override 2804 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2805 switch (_hash) { 2806 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this imaging selection.", 0, java.lang.Integer.MAX_VALUE, identifier); 2807 case -892481550: /*status*/ return new Property("status", "code", "The current state of the ImagingSelection resource. This is not the status of any ImagingStudy, ServiceRequest, or Task resources associated with the ImagingSelection.", 0, 1, status); 2808 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Device|Location|Organization|Procedure|Practitioner|Medication|Substance|Specimen)", "The patient, or group of patients, location, device, organization, procedure or practitioner this imaging selection is about and into whose or what record the imaging selection is placed.", 0, 1, subject); 2809 case -1179159893: /*issued*/ return new Property("issued", "instant", "The date and time this imaging selection was created.", 0, 1, issued); 2810 case 481140686: /*performer*/ return new Property("performer", "", "Selector of the instances ? human or machine.", 0, java.lang.Integer.MAX_VALUE, performer); 2811 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|ServiceRequest|Appointment|AppointmentResponse|Task)", "A list of the diagnostic requests that resulted in this imaging selection being performed.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2812 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Classifies the imaging selection.", 0, java.lang.Integer.MAX_VALUE, category); 2813 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Reason for referencing the selected content.", 0, 1, code); 2814 case 1876590023: /*studyUid*/ return new Property("studyUid", "id", "The Study Instance UID for the DICOM Study from which the images were selected.", 0, 1, studyUid); 2815 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "Reference(ImagingStudy|DocumentReference)", "The imaging study from which the imaging selection is made.", 0, java.lang.Integer.MAX_VALUE, derivedFrom); 2816 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "The network service providing retrieval access to the selected images, frames, etc. See implementation notes for information about using DICOM endpoints.", 0, java.lang.Integer.MAX_VALUE, endpoint); 2817 case -569596327: /*seriesUid*/ return new Property("seriesUid", "id", "The Series Instance UID for the DICOM Series from which the images were selected.", 0, 1, seriesUid); 2818 case 382652576: /*seriesNumber*/ return new Property("seriesNumber", "unsignedInt", "The Series Number for the DICOM Series from which the images were selected.", 0, 1, seriesNumber); 2819 case 828378953: /*frameOfReferenceUid*/ return new Property("frameOfReferenceUid", "id", "The Frame of Reference UID identifying the coordinate system that conveys spatial and/or temporal information for the selected images or frames.", 0, 1, frameOfReferenceUid); 2820 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableReference(BodyStructure)", "The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings.", 0, 1, bodySite); 2821 case 97604824: /*focus*/ return new Property("focus", "Reference(ImagingSelection)", "The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.", 0, java.lang.Integer.MAX_VALUE, focus); 2822 case 555127957: /*instance*/ return new Property("instance", "", "Each imaging selection includes one or more selected DICOM SOP instances.", 0, java.lang.Integer.MAX_VALUE, instance); 2823 default: return super.getNamedProperty(_hash, _name, _checkValid); 2824 } 2825 2826 } 2827 2828 @Override 2829 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2830 switch (hash) { 2831 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2832 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ImagingSelectionStatus> 2833 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2834 case -1179159893: /*issued*/ return this.issued == null ? new Base[0] : new Base[] {this.issued}; // InstantType 2835 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // ImagingSelectionPerformerComponent 2836 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2837 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2838 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2839 case 1876590023: /*studyUid*/ return this.studyUid == null ? new Base[0] : new Base[] {this.studyUid}; // IdType 2840 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // Reference 2841 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 2842 case -569596327: /*seriesUid*/ return this.seriesUid == null ? new Base[0] : new Base[] {this.seriesUid}; // IdType 2843 case 382652576: /*seriesNumber*/ return this.seriesNumber == null ? new Base[0] : new Base[] {this.seriesNumber}; // UnsignedIntType 2844 case 828378953: /*frameOfReferenceUid*/ return this.frameOfReferenceUid == null ? new Base[0] : new Base[] {this.frameOfReferenceUid}; // IdType 2845 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableReference 2846 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // Reference 2847 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : this.instance.toArray(new Base[this.instance.size()]); // ImagingSelectionInstanceComponent 2848 default: return super.getProperty(hash, name, checkValid); 2849 } 2850 2851 } 2852 2853 @Override 2854 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2855 switch (hash) { 2856 case -1618432855: // identifier 2857 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2858 return value; 2859 case -892481550: // status 2860 value = new ImagingSelectionStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2861 this.status = (Enumeration) value; // Enumeration<ImagingSelectionStatus> 2862 return value; 2863 case -1867885268: // subject 2864 this.subject = TypeConvertor.castToReference(value); // Reference 2865 return value; 2866 case -1179159893: // issued 2867 this.issued = TypeConvertor.castToInstant(value); // InstantType 2868 return value; 2869 case 481140686: // performer 2870 this.getPerformer().add((ImagingSelectionPerformerComponent) value); // ImagingSelectionPerformerComponent 2871 return value; 2872 case -332612366: // basedOn 2873 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 2874 return value; 2875 case 50511102: // category 2876 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2877 return value; 2878 case 3059181: // code 2879 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2880 return value; 2881 case 1876590023: // studyUid 2882 this.studyUid = TypeConvertor.castToId(value); // IdType 2883 return value; 2884 case 1077922663: // derivedFrom 2885 this.getDerivedFrom().add(TypeConvertor.castToReference(value)); // Reference 2886 return value; 2887 case 1741102485: // endpoint 2888 this.getEndpoint().add(TypeConvertor.castToReference(value)); // Reference 2889 return value; 2890 case -569596327: // seriesUid 2891 this.seriesUid = TypeConvertor.castToId(value); // IdType 2892 return value; 2893 case 382652576: // seriesNumber 2894 this.seriesNumber = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 2895 return value; 2896 case 828378953: // frameOfReferenceUid 2897 this.frameOfReferenceUid = TypeConvertor.castToId(value); // IdType 2898 return value; 2899 case 1702620169: // bodySite 2900 this.bodySite = TypeConvertor.castToCodeableReference(value); // CodeableReference 2901 return value; 2902 case 97604824: // focus 2903 this.getFocus().add(TypeConvertor.castToReference(value)); // Reference 2904 return value; 2905 case 555127957: // instance 2906 this.getInstance().add((ImagingSelectionInstanceComponent) value); // ImagingSelectionInstanceComponent 2907 return value; 2908 default: return super.setProperty(hash, name, value); 2909 } 2910 2911 } 2912 2913 @Override 2914 public Base setProperty(String name, Base value) throws FHIRException { 2915 if (name.equals("identifier")) { 2916 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2917 } else if (name.equals("status")) { 2918 value = new ImagingSelectionStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2919 this.status = (Enumeration) value; // Enumeration<ImagingSelectionStatus> 2920 } else if (name.equals("subject")) { 2921 this.subject = TypeConvertor.castToReference(value); // Reference 2922 } else if (name.equals("issued")) { 2923 this.issued = TypeConvertor.castToInstant(value); // InstantType 2924 } else if (name.equals("performer")) { 2925 this.getPerformer().add((ImagingSelectionPerformerComponent) value); 2926 } else if (name.equals("basedOn")) { 2927 this.getBasedOn().add(TypeConvertor.castToReference(value)); 2928 } else if (name.equals("category")) { 2929 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 2930 } else if (name.equals("code")) { 2931 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2932 } else if (name.equals("studyUid")) { 2933 this.studyUid = TypeConvertor.castToId(value); // IdType 2934 } else if (name.equals("derivedFrom")) { 2935 this.getDerivedFrom().add(TypeConvertor.castToReference(value)); 2936 } else if (name.equals("endpoint")) { 2937 this.getEndpoint().add(TypeConvertor.castToReference(value)); 2938 } else if (name.equals("seriesUid")) { 2939 this.seriesUid = TypeConvertor.castToId(value); // IdType 2940 } else if (name.equals("seriesNumber")) { 2941 this.seriesNumber = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 2942 } else if (name.equals("frameOfReferenceUid")) { 2943 this.frameOfReferenceUid = TypeConvertor.castToId(value); // IdType 2944 } else if (name.equals("bodySite")) { 2945 this.bodySite = TypeConvertor.castToCodeableReference(value); // CodeableReference 2946 } else if (name.equals("focus")) { 2947 this.getFocus().add(TypeConvertor.castToReference(value)); 2948 } else if (name.equals("instance")) { 2949 this.getInstance().add((ImagingSelectionInstanceComponent) value); 2950 } else 2951 return super.setProperty(name, value); 2952 return value; 2953 } 2954 2955 @Override 2956 public void removeChild(String name, Base value) throws FHIRException { 2957 if (name.equals("identifier")) { 2958 this.getIdentifier().remove(value); 2959 } else if (name.equals("status")) { 2960 value = new ImagingSelectionStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2961 this.status = (Enumeration) value; // Enumeration<ImagingSelectionStatus> 2962 } else if (name.equals("subject")) { 2963 this.subject = null; 2964 } else if (name.equals("issued")) { 2965 this.issued = null; 2966 } else if (name.equals("performer")) { 2967 this.getPerformer().remove((ImagingSelectionPerformerComponent) value); 2968 } else if (name.equals("basedOn")) { 2969 this.getBasedOn().remove(value); 2970 } else if (name.equals("category")) { 2971 this.getCategory().remove(value); 2972 } else if (name.equals("code")) { 2973 this.code = null; 2974 } else if (name.equals("studyUid")) { 2975 this.studyUid = null; 2976 } else if (name.equals("derivedFrom")) { 2977 this.getDerivedFrom().remove(value); 2978 } else if (name.equals("endpoint")) { 2979 this.getEndpoint().remove(value); 2980 } else if (name.equals("seriesUid")) { 2981 this.seriesUid = null; 2982 } else if (name.equals("seriesNumber")) { 2983 this.seriesNumber = null; 2984 } else if (name.equals("frameOfReferenceUid")) { 2985 this.frameOfReferenceUid = null; 2986 } else if (name.equals("bodySite")) { 2987 this.bodySite = null; 2988 } else if (name.equals("focus")) { 2989 this.getFocus().remove(value); 2990 } else if (name.equals("instance")) { 2991 this.getInstance().remove((ImagingSelectionInstanceComponent) value); 2992 } else 2993 super.removeChild(name, value); 2994 2995 } 2996 2997 @Override 2998 public Base makeProperty(int hash, String name) throws FHIRException { 2999 switch (hash) { 3000 case -1618432855: return addIdentifier(); 3001 case -892481550: return getStatusElement(); 3002 case -1867885268: return getSubject(); 3003 case -1179159893: return getIssuedElement(); 3004 case 481140686: return addPerformer(); 3005 case -332612366: return addBasedOn(); 3006 case 50511102: return addCategory(); 3007 case 3059181: return getCode(); 3008 case 1876590023: return getStudyUidElement(); 3009 case 1077922663: return addDerivedFrom(); 3010 case 1741102485: return addEndpoint(); 3011 case -569596327: return getSeriesUidElement(); 3012 case 382652576: return getSeriesNumberElement(); 3013 case 828378953: return getFrameOfReferenceUidElement(); 3014 case 1702620169: return getBodySite(); 3015 case 97604824: return addFocus(); 3016 case 555127957: return addInstance(); 3017 default: return super.makeProperty(hash, name); 3018 } 3019 3020 } 3021 3022 @Override 3023 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3024 switch (hash) { 3025 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3026 case -892481550: /*status*/ return new String[] {"code"}; 3027 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3028 case -1179159893: /*issued*/ return new String[] {"instant"}; 3029 case 481140686: /*performer*/ return new String[] {}; 3030 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 3031 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3032 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3033 case 1876590023: /*studyUid*/ return new String[] {"id"}; 3034 case 1077922663: /*derivedFrom*/ return new String[] {"Reference"}; 3035 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 3036 case -569596327: /*seriesUid*/ return new String[] {"id"}; 3037 case 382652576: /*seriesNumber*/ return new String[] {"unsignedInt"}; 3038 case 828378953: /*frameOfReferenceUid*/ return new String[] {"id"}; 3039 case 1702620169: /*bodySite*/ return new String[] {"CodeableReference"}; 3040 case 97604824: /*focus*/ return new String[] {"Reference"}; 3041 case 555127957: /*instance*/ return new String[] {}; 3042 default: return super.getTypesForProperty(hash, name); 3043 } 3044 3045 } 3046 3047 @Override 3048 public Base addChild(String name) throws FHIRException { 3049 if (name.equals("identifier")) { 3050 return addIdentifier(); 3051 } 3052 else if (name.equals("status")) { 3053 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.status"); 3054 } 3055 else if (name.equals("subject")) { 3056 this.subject = new Reference(); 3057 return this.subject; 3058 } 3059 else if (name.equals("issued")) { 3060 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.issued"); 3061 } 3062 else if (name.equals("performer")) { 3063 return addPerformer(); 3064 } 3065 else if (name.equals("basedOn")) { 3066 return addBasedOn(); 3067 } 3068 else if (name.equals("category")) { 3069 return addCategory(); 3070 } 3071 else if (name.equals("code")) { 3072 this.code = new CodeableConcept(); 3073 return this.code; 3074 } 3075 else if (name.equals("studyUid")) { 3076 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.studyUid"); 3077 } 3078 else if (name.equals("derivedFrom")) { 3079 return addDerivedFrom(); 3080 } 3081 else if (name.equals("endpoint")) { 3082 return addEndpoint(); 3083 } 3084 else if (name.equals("seriesUid")) { 3085 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.seriesUid"); 3086 } 3087 else if (name.equals("seriesNumber")) { 3088 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.seriesNumber"); 3089 } 3090 else if (name.equals("frameOfReferenceUid")) { 3091 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.frameOfReferenceUid"); 3092 } 3093 else if (name.equals("bodySite")) { 3094 this.bodySite = new CodeableReference(); 3095 return this.bodySite; 3096 } 3097 else if (name.equals("focus")) { 3098 return addFocus(); 3099 } 3100 else if (name.equals("instance")) { 3101 return addInstance(); 3102 } 3103 else 3104 return super.addChild(name); 3105 } 3106 3107 public String fhirType() { 3108 return "ImagingSelection"; 3109 3110 } 3111 3112 public ImagingSelection copy() { 3113 ImagingSelection dst = new ImagingSelection(); 3114 copyValues(dst); 3115 return dst; 3116 } 3117 3118 public void copyValues(ImagingSelection dst) { 3119 super.copyValues(dst); 3120 if (identifier != null) { 3121 dst.identifier = new ArrayList<Identifier>(); 3122 for (Identifier i : identifier) 3123 dst.identifier.add(i.copy()); 3124 }; 3125 dst.status = status == null ? null : status.copy(); 3126 dst.subject = subject == null ? null : subject.copy(); 3127 dst.issued = issued == null ? null : issued.copy(); 3128 if (performer != null) { 3129 dst.performer = new ArrayList<ImagingSelectionPerformerComponent>(); 3130 for (ImagingSelectionPerformerComponent i : performer) 3131 dst.performer.add(i.copy()); 3132 }; 3133 if (basedOn != null) { 3134 dst.basedOn = new ArrayList<Reference>(); 3135 for (Reference i : basedOn) 3136 dst.basedOn.add(i.copy()); 3137 }; 3138 if (category != null) { 3139 dst.category = new ArrayList<CodeableConcept>(); 3140 for (CodeableConcept i : category) 3141 dst.category.add(i.copy()); 3142 }; 3143 dst.code = code == null ? null : code.copy(); 3144 dst.studyUid = studyUid == null ? null : studyUid.copy(); 3145 if (derivedFrom != null) { 3146 dst.derivedFrom = new ArrayList<Reference>(); 3147 for (Reference i : derivedFrom) 3148 dst.derivedFrom.add(i.copy()); 3149 }; 3150 if (endpoint != null) { 3151 dst.endpoint = new ArrayList<Reference>(); 3152 for (Reference i : endpoint) 3153 dst.endpoint.add(i.copy()); 3154 }; 3155 dst.seriesUid = seriesUid == null ? null : seriesUid.copy(); 3156 dst.seriesNumber = seriesNumber == null ? null : seriesNumber.copy(); 3157 dst.frameOfReferenceUid = frameOfReferenceUid == null ? null : frameOfReferenceUid.copy(); 3158 dst.bodySite = bodySite == null ? null : bodySite.copy(); 3159 if (focus != null) { 3160 dst.focus = new ArrayList<Reference>(); 3161 for (Reference i : focus) 3162 dst.focus.add(i.copy()); 3163 }; 3164 if (instance != null) { 3165 dst.instance = new ArrayList<ImagingSelectionInstanceComponent>(); 3166 for (ImagingSelectionInstanceComponent i : instance) 3167 dst.instance.add(i.copy()); 3168 }; 3169 } 3170 3171 protected ImagingSelection typedCopy() { 3172 return copy(); 3173 } 3174 3175 @Override 3176 public boolean equalsDeep(Base other_) { 3177 if (!super.equalsDeep(other_)) 3178 return false; 3179 if (!(other_ instanceof ImagingSelection)) 3180 return false; 3181 ImagingSelection o = (ImagingSelection) other_; 3182 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(subject, o.subject, true) 3183 && compareDeep(issued, o.issued, true) && compareDeep(performer, o.performer, true) && compareDeep(basedOn, o.basedOn, true) 3184 && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) && compareDeep(studyUid, o.studyUid, true) 3185 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(endpoint, o.endpoint, true) && compareDeep(seriesUid, o.seriesUid, true) 3186 && compareDeep(seriesNumber, o.seriesNumber, true) && compareDeep(frameOfReferenceUid, o.frameOfReferenceUid, true) 3187 && compareDeep(bodySite, o.bodySite, true) && compareDeep(focus, o.focus, true) && compareDeep(instance, o.instance, true) 3188 ; 3189 } 3190 3191 @Override 3192 public boolean equalsShallow(Base other_) { 3193 if (!super.equalsShallow(other_)) 3194 return false; 3195 if (!(other_ instanceof ImagingSelection)) 3196 return false; 3197 ImagingSelection o = (ImagingSelection) other_; 3198 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true) && compareValues(studyUid, o.studyUid, true) 3199 && compareValues(seriesUid, o.seriesUid, true) && compareValues(seriesNumber, o.seriesNumber, true) 3200 && compareValues(frameOfReferenceUid, o.frameOfReferenceUid, true); 3201 } 3202 3203 public boolean isEmpty() { 3204 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, subject 3205 , issued, performer, basedOn, category, code, studyUid, derivedFrom, endpoint 3206 , seriesUid, seriesNumber, frameOfReferenceUid, bodySite, focus, instance); 3207 } 3208 3209 @Override 3210 public ResourceType getResourceType() { 3211 return ResourceType.ImagingSelection; 3212 } 3213 3214 /** 3215 * Search parameter: <b>based-on</b> 3216 * <p> 3217 * Description: <b>The request associated with an imaging selection</b><br> 3218 * Type: <b>reference</b><br> 3219 * Path: <b>ImagingSelection.basedOn</b><br> 3220 * </p> 3221 */ 3222 @SearchParamDefinition(name="based-on", path="ImagingSelection.basedOn", description="The request associated with an imaging selection", type="reference", target={Appointment.class, AppointmentResponse.class, CarePlan.class, ServiceRequest.class, Task.class } ) 3223 public static final String SP_BASED_ON = "based-on"; 3224 /** 3225 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3226 * <p> 3227 * Description: <b>The request associated with an imaging selection</b><br> 3228 * Type: <b>reference</b><br> 3229 * Path: <b>ImagingSelection.basedOn</b><br> 3230 * </p> 3231 */ 3232 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 3233 3234/** 3235 * Constant for fluent queries to be used to add include statements. Specifies 3236 * the path value of "<b>ImagingSelection:based-on</b>". 3237 */ 3238 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("ImagingSelection:based-on").toLocked(); 3239 3240 /** 3241 * Search parameter: <b>body-site</b> 3242 * <p> 3243 * Description: <b>The body site code associated with the imaging selection</b><br> 3244 * Type: <b>token</b><br> 3245 * Path: <b>ImagingSelection.bodySite.concept</b><br> 3246 * </p> 3247 */ 3248 @SearchParamDefinition(name="body-site", path="ImagingSelection.bodySite.concept", description="The body site code associated with the imaging selection", type="token" ) 3249 public static final String SP_BODY_SITE = "body-site"; 3250 /** 3251 * <b>Fluent Client</b> search parameter constant for <b>body-site</b> 3252 * <p> 3253 * Description: <b>The body site code associated with the imaging selection</b><br> 3254 * Type: <b>token</b><br> 3255 * Path: <b>ImagingSelection.bodySite.concept</b><br> 3256 * </p> 3257 */ 3258 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BODY_SITE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BODY_SITE); 3259 3260 /** 3261 * Search parameter: <b>body-structure</b> 3262 * <p> 3263 * Description: <b>The body structure associated with the imaging selection</b><br> 3264 * Type: <b>reference</b><br> 3265 * Path: <b>ImagingSelection.bodySite.reference</b><br> 3266 * </p> 3267 */ 3268 @SearchParamDefinition(name="body-structure", path="ImagingSelection.bodySite.reference", description="The body structure associated with the imaging selection", type="reference", target={BodyStructure.class } ) 3269 public static final String SP_BODY_STRUCTURE = "body-structure"; 3270 /** 3271 * <b>Fluent Client</b> search parameter constant for <b>body-structure</b> 3272 * <p> 3273 * Description: <b>The body structure associated with the imaging selection</b><br> 3274 * Type: <b>reference</b><br> 3275 * Path: <b>ImagingSelection.bodySite.reference</b><br> 3276 * </p> 3277 */ 3278 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BODY_STRUCTURE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BODY_STRUCTURE); 3279 3280/** 3281 * Constant for fluent queries to be used to add include statements. Specifies 3282 * the path value of "<b>ImagingSelection:body-structure</b>". 3283 */ 3284 public static final ca.uhn.fhir.model.api.Include INCLUDE_BODY_STRUCTURE = new ca.uhn.fhir.model.api.Include("ImagingSelection:body-structure").toLocked(); 3285 3286 /** 3287 * Search parameter: <b>derived-from</b> 3288 * <p> 3289 * Description: <b>The imaging study from which the imaging selection was derived</b><br> 3290 * Type: <b>reference</b><br> 3291 * Path: <b>ImagingSelection.derivedFrom</b><br> 3292 * </p> 3293 */ 3294 @SearchParamDefinition(name="derived-from", path="ImagingSelection.derivedFrom", description="The imaging study from which the imaging selection was derived", type="reference", target={DocumentReference.class, ImagingStudy.class } ) 3295 public static final String SP_DERIVED_FROM = "derived-from"; 3296 /** 3297 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 3298 * <p> 3299 * Description: <b>The imaging study from which the imaging selection was derived</b><br> 3300 * Type: <b>reference</b><br> 3301 * Path: <b>ImagingSelection.derivedFrom</b><br> 3302 * </p> 3303 */ 3304 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 3305 3306/** 3307 * Constant for fluent queries to be used to add include statements. Specifies 3308 * the path value of "<b>ImagingSelection:derived-from</b>". 3309 */ 3310 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("ImagingSelection:derived-from").toLocked(); 3311 3312 /** 3313 * Search parameter: <b>issued</b> 3314 * <p> 3315 * Description: <b>The date / time the imaging selection was created</b><br> 3316 * Type: <b>date</b><br> 3317 * Path: <b>ImagingSelection.issued</b><br> 3318 * </p> 3319 */ 3320 @SearchParamDefinition(name="issued", path="ImagingSelection.issued", description="The date / time the imaging selection was created", type="date" ) 3321 public static final String SP_ISSUED = "issued"; 3322 /** 3323 * <b>Fluent Client</b> search parameter constant for <b>issued</b> 3324 * <p> 3325 * Description: <b>The date / time the imaging selection was created</b><br> 3326 * Type: <b>date</b><br> 3327 * Path: <b>ImagingSelection.issued</b><br> 3328 * </p> 3329 */ 3330 public static final ca.uhn.fhir.rest.gclient.DateClientParam ISSUED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ISSUED); 3331 3332 /** 3333 * Search parameter: <b>status</b> 3334 * <p> 3335 * Description: <b>The status of the imaging selection</b><br> 3336 * Type: <b>token</b><br> 3337 * Path: <b>ImagingSelection.status</b><br> 3338 * </p> 3339 */ 3340 @SearchParamDefinition(name="status", path="ImagingSelection.status", description="The status of the imaging selection", type="token" ) 3341 public static final String SP_STATUS = "status"; 3342 /** 3343 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3344 * <p> 3345 * Description: <b>The status of the imaging selection</b><br> 3346 * Type: <b>token</b><br> 3347 * Path: <b>ImagingSelection.status</b><br> 3348 * </p> 3349 */ 3350 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3351 3352 /** 3353 * Search parameter: <b>study-uid</b> 3354 * <p> 3355 * Description: <b>The DICOM Study Instance UID from which the images were selected</b><br> 3356 * Type: <b>token</b><br> 3357 * Path: <b>ImagingSelection.studyUid</b><br> 3358 * </p> 3359 */ 3360 @SearchParamDefinition(name="study-uid", path="ImagingSelection.studyUid", description="The DICOM Study Instance UID from which the images were selected", type="token" ) 3361 public static final String SP_STUDY_UID = "study-uid"; 3362 /** 3363 * <b>Fluent Client</b> search parameter constant for <b>study-uid</b> 3364 * <p> 3365 * Description: <b>The DICOM Study Instance UID from which the images were selected</b><br> 3366 * Type: <b>token</b><br> 3367 * Path: <b>ImagingSelection.studyUid</b><br> 3368 * </p> 3369 */ 3370 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STUDY_UID = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STUDY_UID); 3371 3372 /** 3373 * Search parameter: <b>subject</b> 3374 * <p> 3375 * Description: <b>The subject of the Imaging Selection, such as the associated Patient</b><br> 3376 * Type: <b>reference</b><br> 3377 * Path: <b>ImagingSelection.subject</b><br> 3378 * </p> 3379 */ 3380 @SearchParamDefinition(name="subject", path="ImagingSelection.subject", description="The subject of the Imaging Selection, such as the associated Patient", type="reference", target={Device.class, Group.class, Location.class, Medication.class, Organization.class, Patient.class, Practitioner.class, Procedure.class, Specimen.class, Substance.class } ) 3381 public static final String SP_SUBJECT = "subject"; 3382 /** 3383 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3384 * <p> 3385 * Description: <b>The subject of the Imaging Selection, such as the associated Patient</b><br> 3386 * Type: <b>reference</b><br> 3387 * Path: <b>ImagingSelection.subject</b><br> 3388 * </p> 3389 */ 3390 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3391 3392/** 3393 * Constant for fluent queries to be used to add include statements. Specifies 3394 * the path value of "<b>ImagingSelection:subject</b>". 3395 */ 3396 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("ImagingSelection:subject").toLocked(); 3397 3398 /** 3399 * Search parameter: <b>code</b> 3400 * <p> 3401 * Description: <b>Multiple Resources: 3402 3403* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 3404* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 3405* [AuditEvent](auditevent.html): More specific code for the event 3406* [Basic](basic.html): Kind of Resource 3407* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 3408* [Condition](condition.html): Code for the condition 3409* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 3410* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 3411* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 3412* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 3413* [ImagingSelection](imagingselection.html): The imaging selection status 3414* [List](list.html): What the purpose of this list is 3415* [Medication](medication.html): Returns medications for a specific code 3416* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 3417* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 3418* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 3419* [MedicationStatement](medicationstatement.html): Return statements of this medication code 3420* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 3421* [Observation](observation.html): The code of the observation type 3422* [Procedure](procedure.html): A code to identify a procedure 3423* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 3424* [Task](task.html): Search by task code 3425</b><br> 3426 * Type: <b>token</b><br> 3427 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 3428 * </p> 3429 */ 3430 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 3431 public static final String SP_CODE = "code"; 3432 /** 3433 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3434 * <p> 3435 * Description: <b>Multiple Resources: 3436 3437* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 3438* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 3439* [AuditEvent](auditevent.html): More specific code for the event 3440* [Basic](basic.html): Kind of Resource 3441* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 3442* [Condition](condition.html): Code for the condition 3443* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 3444* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 3445* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 3446* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 3447* [ImagingSelection](imagingselection.html): The imaging selection status 3448* [List](list.html): What the purpose of this list is 3449* [Medication](medication.html): Returns medications for a specific code 3450* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 3451* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 3452* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 3453* [MedicationStatement](medicationstatement.html): Return statements of this medication code 3454* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 3455* [Observation](observation.html): The code of the observation type 3456* [Procedure](procedure.html): A code to identify a procedure 3457* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 3458* [Task](task.html): Search by task code 3459</b><br> 3460 * Type: <b>token</b><br> 3461 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 3462 * </p> 3463 */ 3464 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 3465 3466 /** 3467 * Search parameter: <b>identifier</b> 3468 * <p> 3469 * Description: <b>Multiple Resources: 3470 3471* [Account](account.html): Account number 3472* [AdverseEvent](adverseevent.html): Business identifier for the event 3473* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3474* [Appointment](appointment.html): An Identifier of the Appointment 3475* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3476* [Basic](basic.html): Business identifier 3477* [BodyStructure](bodystructure.html): Bodystructure identifier 3478* [CarePlan](careplan.html): External Ids for this plan 3479* [CareTeam](careteam.html): External Ids for this team 3480* [ChargeItem](chargeitem.html): Business Identifier for item 3481* [Claim](claim.html): The primary identifier of the financial resource 3482* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3483* [ClinicalImpression](clinicalimpression.html): Business identifier 3484* [Communication](communication.html): Unique identifier 3485* [CommunicationRequest](communicationrequest.html): Unique identifier 3486* [Composition](composition.html): Version-independent identifier for the Composition 3487* [Condition](condition.html): A unique identifier of the condition record 3488* [Consent](consent.html): Identifier for this record (external references) 3489* [Contract](contract.html): The identity of the contract 3490* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3491* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3492* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3493* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3494* [DeviceRequest](devicerequest.html): Business identifier for request/order 3495* [DeviceUsage](deviceusage.html): Search by identifier 3496* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3497* [DocumentReference](documentreference.html): Identifier of the attachment binary 3498* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3499* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3500* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3501* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3502* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3503* [Flag](flag.html): Business identifier 3504* [Goal](goal.html): External Ids for this goal 3505* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3506* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3507* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3508* [Immunization](immunization.html): Business identifier 3509* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3510* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3511* [Invoice](invoice.html): Business Identifier for item 3512* [List](list.html): Business identifier 3513* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3514* [Medication](medication.html): Returns medications with this external identifier 3515* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3516* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3517* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3518* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3519* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3520* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3521* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3522* [Observation](observation.html): The unique id for a particular observation 3523* [Person](person.html): A person Identifier 3524* [Procedure](procedure.html): A unique identifier for a procedure 3525* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3526* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3527* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3528* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3529* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3530* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3531* [Specimen](specimen.html): The unique identifier associated with the specimen 3532* [SupplyDelivery](supplydelivery.html): External identifier 3533* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3534* [Task](task.html): Search for a task instance by its business identifier 3535* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3536</b><br> 3537 * Type: <b>token</b><br> 3538 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3539 * </p> 3540 */ 3541 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 3542 public static final String SP_IDENTIFIER = "identifier"; 3543 /** 3544 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3545 * <p> 3546 * Description: <b>Multiple Resources: 3547 3548* [Account](account.html): Account number 3549* [AdverseEvent](adverseevent.html): Business identifier for the event 3550* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3551* [Appointment](appointment.html): An Identifier of the Appointment 3552* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3553* [Basic](basic.html): Business identifier 3554* [BodyStructure](bodystructure.html): Bodystructure identifier 3555* [CarePlan](careplan.html): External Ids for this plan 3556* [CareTeam](careteam.html): External Ids for this team 3557* [ChargeItem](chargeitem.html): Business Identifier for item 3558* [Claim](claim.html): The primary identifier of the financial resource 3559* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3560* [ClinicalImpression](clinicalimpression.html): Business identifier 3561* [Communication](communication.html): Unique identifier 3562* [CommunicationRequest](communicationrequest.html): Unique identifier 3563* [Composition](composition.html): Version-independent identifier for the Composition 3564* [Condition](condition.html): A unique identifier of the condition record 3565* [Consent](consent.html): Identifier for this record (external references) 3566* [Contract](contract.html): The identity of the contract 3567* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3568* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3569* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3570* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3571* [DeviceRequest](devicerequest.html): Business identifier for request/order 3572* [DeviceUsage](deviceusage.html): Search by identifier 3573* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3574* [DocumentReference](documentreference.html): Identifier of the attachment binary 3575* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3576* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3577* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3578* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3579* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3580* [Flag](flag.html): Business identifier 3581* [Goal](goal.html): External Ids for this goal 3582* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3583* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3584* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3585* [Immunization](immunization.html): Business identifier 3586* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3587* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3588* [Invoice](invoice.html): Business Identifier for item 3589* [List](list.html): Business identifier 3590* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3591* [Medication](medication.html): Returns medications with this external identifier 3592* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3593* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3594* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3595* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3596* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3597* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3598* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3599* [Observation](observation.html): The unique id for a particular observation 3600* [Person](person.html): A person Identifier 3601* [Procedure](procedure.html): A unique identifier for a procedure 3602* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3603* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3604* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3605* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3606* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3607* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3608* [Specimen](specimen.html): The unique identifier associated with the specimen 3609* [SupplyDelivery](supplydelivery.html): External identifier 3610* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3611* [Task](task.html): Search for a task instance by its business identifier 3612* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3613</b><br> 3614 * Type: <b>token</b><br> 3615 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3616 * </p> 3617 */ 3618 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3619 3620 /** 3621 * Search parameter: <b>patient</b> 3622 * <p> 3623 * Description: <b>Multiple Resources: 3624 3625* [Account](account.html): The entity that caused the expenses 3626* [AdverseEvent](adverseevent.html): Subject impacted by event 3627* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3628* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3629* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3630* [AuditEvent](auditevent.html): Where the activity involved patient data 3631* [Basic](basic.html): Identifies the focus of this resource 3632* [BodyStructure](bodystructure.html): Who this is about 3633* [CarePlan](careplan.html): Who the care plan is for 3634* [CareTeam](careteam.html): Who care team is for 3635* [ChargeItem](chargeitem.html): Individual service was done for/to 3636* [Claim](claim.html): Patient receiving the products or services 3637* [ClaimResponse](claimresponse.html): The subject of care 3638* [ClinicalImpression](clinicalimpression.html): Patient assessed 3639* [Communication](communication.html): Focus of message 3640* [CommunicationRequest](communicationrequest.html): Focus of message 3641* [Composition](composition.html): Who and/or what the composition is about 3642* [Condition](condition.html): Who has the condition? 3643* [Consent](consent.html): Who the consent applies to 3644* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3645* [Coverage](coverage.html): Retrieve coverages for a patient 3646* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3647* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3648* [DetectedIssue](detectedissue.html): Associated patient 3649* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3650* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3651* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3652* [DocumentReference](documentreference.html): Who/what is the subject of the document 3653* [Encounter](encounter.html): The patient present at the encounter 3654* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3655* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3656* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3657* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3658* [Flag](flag.html): The identity of a subject to list flags for 3659* [Goal](goal.html): Who this goal is intended for 3660* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3661* [ImagingSelection](imagingselection.html): Who the study is about 3662* [ImagingStudy](imagingstudy.html): Who the study is about 3663* [Immunization](immunization.html): The patient for the vaccination record 3664* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3665* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3666* [Invoice](invoice.html): Recipient(s) of goods and services 3667* [List](list.html): If all resources have the same subject 3668* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3669* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3670* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3671* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3672* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3673* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3674* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3675* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3676* [Observation](observation.html): The subject that the observation is about (if patient) 3677* [Person](person.html): The Person links to this Patient 3678* [Procedure](procedure.html): Search by subject - a patient 3679* [Provenance](provenance.html): Where the activity involved patient data 3680* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3681* [RelatedPerson](relatedperson.html): The patient this related person is related to 3682* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3683* [ResearchSubject](researchsubject.html): Who or what is part of study 3684* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3685* [ServiceRequest](servicerequest.html): Search by subject - a patient 3686* [Specimen](specimen.html): The patient the specimen comes from 3687* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3688* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3689* [Task](task.html): Search by patient 3690* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3691</b><br> 3692 * Type: <b>reference</b><br> 3693 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3694 * </p> 3695 */ 3696 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 3697 public static final String SP_PATIENT = "patient"; 3698 /** 3699 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3700 * <p> 3701 * Description: <b>Multiple Resources: 3702 3703* [Account](account.html): The entity that caused the expenses 3704* [AdverseEvent](adverseevent.html): Subject impacted by event 3705* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3706* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3707* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3708* [AuditEvent](auditevent.html): Where the activity involved patient data 3709* [Basic](basic.html): Identifies the focus of this resource 3710* [BodyStructure](bodystructure.html): Who this is about 3711* [CarePlan](careplan.html): Who the care plan is for 3712* [CareTeam](careteam.html): Who care team is for 3713* [ChargeItem](chargeitem.html): Individual service was done for/to 3714* [Claim](claim.html): Patient receiving the products or services 3715* [ClaimResponse](claimresponse.html): The subject of care 3716* [ClinicalImpression](clinicalimpression.html): Patient assessed 3717* [Communication](communication.html): Focus of message 3718* [CommunicationRequest](communicationrequest.html): Focus of message 3719* [Composition](composition.html): Who and/or what the composition is about 3720* [Condition](condition.html): Who has the condition? 3721* [Consent](consent.html): Who the consent applies to 3722* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3723* [Coverage](coverage.html): Retrieve coverages for a patient 3724* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3725* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3726* [DetectedIssue](detectedissue.html): Associated patient 3727* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3728* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3729* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3730* [DocumentReference](documentreference.html): Who/what is the subject of the document 3731* [Encounter](encounter.html): The patient present at the encounter 3732* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3733* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3734* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3735* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3736* [Flag](flag.html): The identity of a subject to list flags for 3737* [Goal](goal.html): Who this goal is intended for 3738* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3739* [ImagingSelection](imagingselection.html): Who the study is about 3740* [ImagingStudy](imagingstudy.html): Who the study is about 3741* [Immunization](immunization.html): The patient for the vaccination record 3742* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3743* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3744* [Invoice](invoice.html): Recipient(s) of goods and services 3745* [List](list.html): If all resources have the same subject 3746* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3747* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3748* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3749* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3750* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3751* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3752* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3753* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3754* [Observation](observation.html): The subject that the observation is about (if patient) 3755* [Person](person.html): The Person links to this Patient 3756* [Procedure](procedure.html): Search by subject - a patient 3757* [Provenance](provenance.html): Where the activity involved patient data 3758* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3759* [RelatedPerson](relatedperson.html): The patient this related person is related to 3760* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3761* [ResearchSubject](researchsubject.html): Who or what is part of study 3762* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3763* [ServiceRequest](servicerequest.html): Search by subject - a patient 3764* [Specimen](specimen.html): The patient the specimen comes from 3765* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3766* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3767* [Task](task.html): Search by patient 3768* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3769</b><br> 3770 * Type: <b>reference</b><br> 3771 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3772 * </p> 3773 */ 3774 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3775 3776/** 3777 * Constant for fluent queries to be used to add include statements. Specifies 3778 * the path value of "<b>ImagingSelection:patient</b>". 3779 */ 3780 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ImagingSelection:patient").toLocked(); 3781 3782 3783} 3784