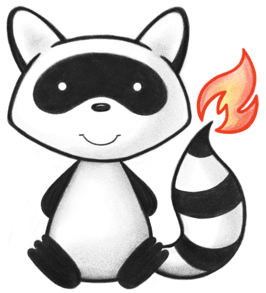
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051/** 052 * A selection of DICOM SOP instances and/or frames within a single Study and Series. This might include additional specifics such as an image region, an Observation UID or a Segmentation Number, allowing linkage to an Observation Resource or transferring this information along with the ImagingStudy Resource. 053 */ 054@ResourceDef(name="ImagingSelection", profile="http://hl7.org/fhir/StructureDefinition/ImagingSelection") 055public class ImagingSelection extends DomainResource { 056 057 public enum ImagingSelection2DGraphicType { 058 /** 059 * A single location denoted by a single (x,y) pair. 060 */ 061 POINT, 062 /** 063 * A series of connected line segments with ordered vertices denoted by (x,y) triplets; the points need not be coplanar. 064 */ 065 POLYLINE, 066 /** 067 * An n-tuple list of (x,y) pair end points between which some form of implementation dependent curved lines are to be drawn. The rendered line shall pass through all the specified points. 068 */ 069 INTERPOLATED, 070 /** 071 * Two points shall be present; the first point is to be interpreted as the center and the second point as a point on the circumference of a circle, some form of implementation dependent representation of which is to be drawn. 072 */ 073 CIRCLE, 074 /** 075 * An ellipse defined by four (x,y) pairs, the first two pairs specifying the endpoints of the major axis and the second two pairs specifying the endpoints of the minor axis. 076 */ 077 ELLIPSE, 078 /** 079 * added to help the parsers with the generic types 080 */ 081 NULL; 082 public static ImagingSelection2DGraphicType fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("point".equals(codeString)) 086 return POINT; 087 if ("polyline".equals(codeString)) 088 return POLYLINE; 089 if ("interpolated".equals(codeString)) 090 return INTERPOLATED; 091 if ("circle".equals(codeString)) 092 return CIRCLE; 093 if ("ellipse".equals(codeString)) 094 return ELLIPSE; 095 if (Configuration.isAcceptInvalidEnums()) 096 return null; 097 else 098 throw new FHIRException("Unknown ImagingSelection2DGraphicType code '"+codeString+"'"); 099 } 100 public String toCode() { 101 switch (this) { 102 case POINT: return "point"; 103 case POLYLINE: return "polyline"; 104 case INTERPOLATED: return "interpolated"; 105 case CIRCLE: return "circle"; 106 case ELLIPSE: return "ellipse"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getSystem() { 112 switch (this) { 113 case POINT: return "http://hl7.org/fhir/imagingselection-2dgraphictype"; 114 case POLYLINE: return "http://hl7.org/fhir/imagingselection-2dgraphictype"; 115 case INTERPOLATED: return "http://hl7.org/fhir/imagingselection-2dgraphictype"; 116 case CIRCLE: return "http://hl7.org/fhir/imagingselection-2dgraphictype"; 117 case ELLIPSE: return "http://hl7.org/fhir/imagingselection-2dgraphictype"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDefinition() { 123 switch (this) { 124 case POINT: return "A single location denoted by a single (x,y) pair."; 125 case POLYLINE: return "A series of connected line segments with ordered vertices denoted by (x,y) triplets; the points need not be coplanar."; 126 case INTERPOLATED: return "An n-tuple list of (x,y) pair end points between which some form of implementation dependent curved lines are to be drawn. The rendered line shall pass through all the specified points."; 127 case CIRCLE: return "Two points shall be present; the first point is to be interpreted as the center and the second point as a point on the circumference of a circle, some form of implementation dependent representation of which is to be drawn."; 128 case ELLIPSE: return "An ellipse defined by four (x,y) pairs, the first two pairs specifying the endpoints of the major axis and the second two pairs specifying the endpoints of the minor axis."; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 public String getDisplay() { 134 switch (this) { 135 case POINT: return "POINT"; 136 case POLYLINE: return "POLYLINE"; 137 case INTERPOLATED: return "INTERPOLATED"; 138 case CIRCLE: return "CIRCLE"; 139 case ELLIPSE: return "ELLIPSE"; 140 case NULL: return null; 141 default: return "?"; 142 } 143 } 144 } 145 146 public static class ImagingSelection2DGraphicTypeEnumFactory implements EnumFactory<ImagingSelection2DGraphicType> { 147 public ImagingSelection2DGraphicType fromCode(String codeString) throws IllegalArgumentException { 148 if (codeString == null || "".equals(codeString)) 149 if (codeString == null || "".equals(codeString)) 150 return null; 151 if ("point".equals(codeString)) 152 return ImagingSelection2DGraphicType.POINT; 153 if ("polyline".equals(codeString)) 154 return ImagingSelection2DGraphicType.POLYLINE; 155 if ("interpolated".equals(codeString)) 156 return ImagingSelection2DGraphicType.INTERPOLATED; 157 if ("circle".equals(codeString)) 158 return ImagingSelection2DGraphicType.CIRCLE; 159 if ("ellipse".equals(codeString)) 160 return ImagingSelection2DGraphicType.ELLIPSE; 161 throw new IllegalArgumentException("Unknown ImagingSelection2DGraphicType code '"+codeString+"'"); 162 } 163 public Enumeration<ImagingSelection2DGraphicType> fromType(PrimitiveType<?> code) throws FHIRException { 164 if (code == null) 165 return null; 166 if (code.isEmpty()) 167 return new Enumeration<ImagingSelection2DGraphicType>(this, ImagingSelection2DGraphicType.NULL, code); 168 String codeString = ((PrimitiveType) code).asStringValue(); 169 if (codeString == null || "".equals(codeString)) 170 return new Enumeration<ImagingSelection2DGraphicType>(this, ImagingSelection2DGraphicType.NULL, code); 171 if ("point".equals(codeString)) 172 return new Enumeration<ImagingSelection2DGraphicType>(this, ImagingSelection2DGraphicType.POINT, code); 173 if ("polyline".equals(codeString)) 174 return new Enumeration<ImagingSelection2DGraphicType>(this, ImagingSelection2DGraphicType.POLYLINE, code); 175 if ("interpolated".equals(codeString)) 176 return new Enumeration<ImagingSelection2DGraphicType>(this, ImagingSelection2DGraphicType.INTERPOLATED, code); 177 if ("circle".equals(codeString)) 178 return new Enumeration<ImagingSelection2DGraphicType>(this, ImagingSelection2DGraphicType.CIRCLE, code); 179 if ("ellipse".equals(codeString)) 180 return new Enumeration<ImagingSelection2DGraphicType>(this, ImagingSelection2DGraphicType.ELLIPSE, code); 181 throw new FHIRException("Unknown ImagingSelection2DGraphicType code '"+codeString+"'"); 182 } 183 public String toCode(ImagingSelection2DGraphicType code) { 184 if (code == ImagingSelection2DGraphicType.POINT) 185 return "point"; 186 if (code == ImagingSelection2DGraphicType.POLYLINE) 187 return "polyline"; 188 if (code == ImagingSelection2DGraphicType.INTERPOLATED) 189 return "interpolated"; 190 if (code == ImagingSelection2DGraphicType.CIRCLE) 191 return "circle"; 192 if (code == ImagingSelection2DGraphicType.ELLIPSE) 193 return "ellipse"; 194 return "?"; 195 } 196 public String toSystem(ImagingSelection2DGraphicType code) { 197 return code.getSystem(); 198 } 199 } 200 201 public enum ImagingSelection3DGraphicType { 202 /** 203 * A single location denoted by a single (x,y,z) triplet. 204 */ 205 POINT, 206 /** 207 * multiple locations each denoted by an (x,y,z) triplet; the points need not be coplanar. 208 */ 209 MULTIPOINT, 210 /** 211 * a series of connected line segments with ordered vertices denoted by (x,y,z) triplets; the points need not be coplanar. 212 */ 213 POLYLINE, 214 /** 215 * a series of connected line segments with ordered vertices denoted by (x,y,z) triplets, where the first and last vertices shall be the same forming a polygon; the points shall be coplanar. 216 */ 217 POLYGON, 218 /** 219 * an ellipse defined by four (x,y,z) triplets, the first two triplets specifying the endpoints of the major axis and the second two triplets specifying the endpoints of the minor axis. 220 */ 221 ELLIPSE, 222 /** 223 * a three-dimensional geometric surface whose plane sections are either ellipses or circles and contains three intersecting orthogonal axes, \"a\", \"b\", and \"c\"; the ellipsoid is defined by six (x,y,z) triplets, the first and second triplets specifying the endpoints of axis \"a\", the third and fourth triplets specifying the endpoints of axis \"b\", and the fifth and sixth triplets specifying the endpoints of axis \"c\". 224 */ 225 ELLIPSOID, 226 /** 227 * added to help the parsers with the generic types 228 */ 229 NULL; 230 public static ImagingSelection3DGraphicType fromCode(String codeString) throws FHIRException { 231 if (codeString == null || "".equals(codeString)) 232 return null; 233 if ("point".equals(codeString)) 234 return POINT; 235 if ("multipoint".equals(codeString)) 236 return MULTIPOINT; 237 if ("polyline".equals(codeString)) 238 return POLYLINE; 239 if ("polygon".equals(codeString)) 240 return POLYGON; 241 if ("ellipse".equals(codeString)) 242 return ELLIPSE; 243 if ("ellipsoid".equals(codeString)) 244 return ELLIPSOID; 245 if (Configuration.isAcceptInvalidEnums()) 246 return null; 247 else 248 throw new FHIRException("Unknown ImagingSelection3DGraphicType code '"+codeString+"'"); 249 } 250 public String toCode() { 251 switch (this) { 252 case POINT: return "point"; 253 case MULTIPOINT: return "multipoint"; 254 case POLYLINE: return "polyline"; 255 case POLYGON: return "polygon"; 256 case ELLIPSE: return "ellipse"; 257 case ELLIPSOID: return "ellipsoid"; 258 case NULL: return null; 259 default: return "?"; 260 } 261 } 262 public String getSystem() { 263 switch (this) { 264 case POINT: return "http://hl7.org/fhir/imagingselection-3dgraphictype"; 265 case MULTIPOINT: return "http://hl7.org/fhir/imagingselection-3dgraphictype"; 266 case POLYLINE: return "http://hl7.org/fhir/imagingselection-3dgraphictype"; 267 case POLYGON: return "http://hl7.org/fhir/imagingselection-3dgraphictype"; 268 case ELLIPSE: return "http://hl7.org/fhir/imagingselection-3dgraphictype"; 269 case ELLIPSOID: return "http://hl7.org/fhir/imagingselection-3dgraphictype"; 270 case NULL: return null; 271 default: return "?"; 272 } 273 } 274 public String getDefinition() { 275 switch (this) { 276 case POINT: return "A single location denoted by a single (x,y,z) triplet."; 277 case MULTIPOINT: return "multiple locations each denoted by an (x,y,z) triplet; the points need not be coplanar."; 278 case POLYLINE: return "a series of connected line segments with ordered vertices denoted by (x,y,z) triplets; the points need not be coplanar."; 279 case POLYGON: return "a series of connected line segments with ordered vertices denoted by (x,y,z) triplets, where the first and last vertices shall be the same forming a polygon; the points shall be coplanar."; 280 case ELLIPSE: return "an ellipse defined by four (x,y,z) triplets, the first two triplets specifying the endpoints of the major axis and the second two triplets specifying the endpoints of the minor axis."; 281 case ELLIPSOID: return "a three-dimensional geometric surface whose plane sections are either ellipses or circles and contains three intersecting orthogonal axes, \"a\", \"b\", and \"c\"; the ellipsoid is defined by six (x,y,z) triplets, the first and second triplets specifying the endpoints of axis \"a\", the third and fourth triplets specifying the endpoints of axis \"b\", and the fifth and sixth triplets specifying the endpoints of axis \"c\"."; 282 case NULL: return null; 283 default: return "?"; 284 } 285 } 286 public String getDisplay() { 287 switch (this) { 288 case POINT: return "POINT"; 289 case MULTIPOINT: return "MULTIPOINT"; 290 case POLYLINE: return "POLYLINE"; 291 case POLYGON: return "POLYGON"; 292 case ELLIPSE: return "ELLIPSE"; 293 case ELLIPSOID: return "ELLIPSOID"; 294 case NULL: return null; 295 default: return "?"; 296 } 297 } 298 } 299 300 public static class ImagingSelection3DGraphicTypeEnumFactory implements EnumFactory<ImagingSelection3DGraphicType> { 301 public ImagingSelection3DGraphicType fromCode(String codeString) throws IllegalArgumentException { 302 if (codeString == null || "".equals(codeString)) 303 if (codeString == null || "".equals(codeString)) 304 return null; 305 if ("point".equals(codeString)) 306 return ImagingSelection3DGraphicType.POINT; 307 if ("multipoint".equals(codeString)) 308 return ImagingSelection3DGraphicType.MULTIPOINT; 309 if ("polyline".equals(codeString)) 310 return ImagingSelection3DGraphicType.POLYLINE; 311 if ("polygon".equals(codeString)) 312 return ImagingSelection3DGraphicType.POLYGON; 313 if ("ellipse".equals(codeString)) 314 return ImagingSelection3DGraphicType.ELLIPSE; 315 if ("ellipsoid".equals(codeString)) 316 return ImagingSelection3DGraphicType.ELLIPSOID; 317 throw new IllegalArgumentException("Unknown ImagingSelection3DGraphicType code '"+codeString+"'"); 318 } 319 public Enumeration<ImagingSelection3DGraphicType> fromType(PrimitiveType<?> code) throws FHIRException { 320 if (code == null) 321 return null; 322 if (code.isEmpty()) 323 return new Enumeration<ImagingSelection3DGraphicType>(this, ImagingSelection3DGraphicType.NULL, code); 324 String codeString = ((PrimitiveType) code).asStringValue(); 325 if (codeString == null || "".equals(codeString)) 326 return new Enumeration<ImagingSelection3DGraphicType>(this, ImagingSelection3DGraphicType.NULL, code); 327 if ("point".equals(codeString)) 328 return new Enumeration<ImagingSelection3DGraphicType>(this, ImagingSelection3DGraphicType.POINT, code); 329 if ("multipoint".equals(codeString)) 330 return new Enumeration<ImagingSelection3DGraphicType>(this, ImagingSelection3DGraphicType.MULTIPOINT, code); 331 if ("polyline".equals(codeString)) 332 return new Enumeration<ImagingSelection3DGraphicType>(this, ImagingSelection3DGraphicType.POLYLINE, code); 333 if ("polygon".equals(codeString)) 334 return new Enumeration<ImagingSelection3DGraphicType>(this, ImagingSelection3DGraphicType.POLYGON, code); 335 if ("ellipse".equals(codeString)) 336 return new Enumeration<ImagingSelection3DGraphicType>(this, ImagingSelection3DGraphicType.ELLIPSE, code); 337 if ("ellipsoid".equals(codeString)) 338 return new Enumeration<ImagingSelection3DGraphicType>(this, ImagingSelection3DGraphicType.ELLIPSOID, code); 339 throw new FHIRException("Unknown ImagingSelection3DGraphicType code '"+codeString+"'"); 340 } 341 public String toCode(ImagingSelection3DGraphicType code) { 342 if (code == ImagingSelection3DGraphicType.POINT) 343 return "point"; 344 if (code == ImagingSelection3DGraphicType.MULTIPOINT) 345 return "multipoint"; 346 if (code == ImagingSelection3DGraphicType.POLYLINE) 347 return "polyline"; 348 if (code == ImagingSelection3DGraphicType.POLYGON) 349 return "polygon"; 350 if (code == ImagingSelection3DGraphicType.ELLIPSE) 351 return "ellipse"; 352 if (code == ImagingSelection3DGraphicType.ELLIPSOID) 353 return "ellipsoid"; 354 return "?"; 355 } 356 public String toSystem(ImagingSelection3DGraphicType code) { 357 return code.getSystem(); 358 } 359 } 360 361 public enum ImagingSelectionStatus { 362 /** 363 * The selected resources are available.. 364 */ 365 AVAILABLE, 366 /** 367 * The imaging selection has been withdrawn following a release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".). 368 */ 369 ENTEREDINERROR, 370 /** 371 * The system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one. 372 */ 373 UNKNOWN, 374 /** 375 * added to help the parsers with the generic types 376 */ 377 NULL; 378 public static ImagingSelectionStatus fromCode(String codeString) throws FHIRException { 379 if (codeString == null || "".equals(codeString)) 380 return null; 381 if ("available".equals(codeString)) 382 return AVAILABLE; 383 if ("entered-in-error".equals(codeString)) 384 return ENTEREDINERROR; 385 if ("unknown".equals(codeString)) 386 return UNKNOWN; 387 if (Configuration.isAcceptInvalidEnums()) 388 return null; 389 else 390 throw new FHIRException("Unknown ImagingSelectionStatus code '"+codeString+"'"); 391 } 392 public String toCode() { 393 switch (this) { 394 case AVAILABLE: return "available"; 395 case ENTEREDINERROR: return "entered-in-error"; 396 case UNKNOWN: return "unknown"; 397 case NULL: return null; 398 default: return "?"; 399 } 400 } 401 public String getSystem() { 402 switch (this) { 403 case AVAILABLE: return "http://hl7.org/fhir/imagingselection-status"; 404 case ENTEREDINERROR: return "http://hl7.org/fhir/imagingselection-status"; 405 case UNKNOWN: return "http://hl7.org/fhir/imagingselection-status"; 406 case NULL: return null; 407 default: return "?"; 408 } 409 } 410 public String getDefinition() { 411 switch (this) { 412 case AVAILABLE: return "The selected resources are available.."; 413 case ENTEREDINERROR: return "The imaging selection has been withdrawn following a release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 414 case UNKNOWN: return "The system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 415 case NULL: return null; 416 default: return "?"; 417 } 418 } 419 public String getDisplay() { 420 switch (this) { 421 case AVAILABLE: return "Available"; 422 case ENTEREDINERROR: return "Entered in Error"; 423 case UNKNOWN: return "Unknown"; 424 case NULL: return null; 425 default: return "?"; 426 } 427 } 428 } 429 430 public static class ImagingSelectionStatusEnumFactory implements EnumFactory<ImagingSelectionStatus> { 431 public ImagingSelectionStatus fromCode(String codeString) throws IllegalArgumentException { 432 if (codeString == null || "".equals(codeString)) 433 if (codeString == null || "".equals(codeString)) 434 return null; 435 if ("available".equals(codeString)) 436 return ImagingSelectionStatus.AVAILABLE; 437 if ("entered-in-error".equals(codeString)) 438 return ImagingSelectionStatus.ENTEREDINERROR; 439 if ("unknown".equals(codeString)) 440 return ImagingSelectionStatus.UNKNOWN; 441 throw new IllegalArgumentException("Unknown ImagingSelectionStatus code '"+codeString+"'"); 442 } 443 public Enumeration<ImagingSelectionStatus> fromType(PrimitiveType<?> code) throws FHIRException { 444 if (code == null) 445 return null; 446 if (code.isEmpty()) 447 return new Enumeration<ImagingSelectionStatus>(this, ImagingSelectionStatus.NULL, code); 448 String codeString = ((PrimitiveType) code).asStringValue(); 449 if (codeString == null || "".equals(codeString)) 450 return new Enumeration<ImagingSelectionStatus>(this, ImagingSelectionStatus.NULL, code); 451 if ("available".equals(codeString)) 452 return new Enumeration<ImagingSelectionStatus>(this, ImagingSelectionStatus.AVAILABLE, code); 453 if ("entered-in-error".equals(codeString)) 454 return new Enumeration<ImagingSelectionStatus>(this, ImagingSelectionStatus.ENTEREDINERROR, code); 455 if ("unknown".equals(codeString)) 456 return new Enumeration<ImagingSelectionStatus>(this, ImagingSelectionStatus.UNKNOWN, code); 457 throw new FHIRException("Unknown ImagingSelectionStatus code '"+codeString+"'"); 458 } 459 public String toCode(ImagingSelectionStatus code) { 460 if (code == ImagingSelectionStatus.AVAILABLE) 461 return "available"; 462 if (code == ImagingSelectionStatus.ENTEREDINERROR) 463 return "entered-in-error"; 464 if (code == ImagingSelectionStatus.UNKNOWN) 465 return "unknown"; 466 return "?"; 467 } 468 public String toSystem(ImagingSelectionStatus code) { 469 return code.getSystem(); 470 } 471 } 472 473 @Block() 474 public static class ImagingSelectionPerformerComponent extends BackboneElement implements IBaseBackboneElement { 475 /** 476 * Distinguishes the type of involvement of the performer. 477 */ 478 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 479 @Description(shortDefinition="Type of performer", formalDefinition="Distinguishes the type of involvement of the performer." ) 480 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/series-performer-function") 481 protected CodeableConcept function; 482 483 /** 484 * Author ? human or machine. 485 */ 486 @Child(name = "actor", type = {Practitioner.class, PractitionerRole.class, Device.class, Organization.class, CareTeam.class, Patient.class, RelatedPerson.class, HealthcareService.class}, order=2, min=0, max=1, modifier=false, summary=true) 487 @Description(shortDefinition="Author (human or machine)", formalDefinition="Author ? human or machine." ) 488 protected Reference actor; 489 490 private static final long serialVersionUID = -576943815L; 491 492 /** 493 * Constructor 494 */ 495 public ImagingSelectionPerformerComponent() { 496 super(); 497 } 498 499 /** 500 * @return {@link #function} (Distinguishes the type of involvement of the performer.) 501 */ 502 public CodeableConcept getFunction() { 503 if (this.function == null) 504 if (Configuration.errorOnAutoCreate()) 505 throw new Error("Attempt to auto-create ImagingSelectionPerformerComponent.function"); 506 else if (Configuration.doAutoCreate()) 507 this.function = new CodeableConcept(); // cc 508 return this.function; 509 } 510 511 public boolean hasFunction() { 512 return this.function != null && !this.function.isEmpty(); 513 } 514 515 /** 516 * @param value {@link #function} (Distinguishes the type of involvement of the performer.) 517 */ 518 public ImagingSelectionPerformerComponent setFunction(CodeableConcept value) { 519 this.function = value; 520 return this; 521 } 522 523 /** 524 * @return {@link #actor} (Author ? human or machine.) 525 */ 526 public Reference getActor() { 527 if (this.actor == null) 528 if (Configuration.errorOnAutoCreate()) 529 throw new Error("Attempt to auto-create ImagingSelectionPerformerComponent.actor"); 530 else if (Configuration.doAutoCreate()) 531 this.actor = new Reference(); // cc 532 return this.actor; 533 } 534 535 public boolean hasActor() { 536 return this.actor != null && !this.actor.isEmpty(); 537 } 538 539 /** 540 * @param value {@link #actor} (Author ? human or machine.) 541 */ 542 public ImagingSelectionPerformerComponent setActor(Reference value) { 543 this.actor = value; 544 return this; 545 } 546 547 protected void listChildren(List<Property> children) { 548 super.listChildren(children); 549 children.add(new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the performer.", 0, 1, function)); 550 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Device|Organization|CareTeam|Patient|RelatedPerson|HealthcareService)", "Author ? human or machine.", 0, 1, actor)); 551 } 552 553 @Override 554 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 555 switch (_hash) { 556 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the performer.", 0, 1, function); 557 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|PractitionerRole|Device|Organization|CareTeam|Patient|RelatedPerson|HealthcareService)", "Author ? human or machine.", 0, 1, actor); 558 default: return super.getNamedProperty(_hash, _name, _checkValid); 559 } 560 561 } 562 563 @Override 564 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 565 switch (hash) { 566 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 567 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 568 default: return super.getProperty(hash, name, checkValid); 569 } 570 571 } 572 573 @Override 574 public Base setProperty(int hash, String name, Base value) throws FHIRException { 575 switch (hash) { 576 case 1380938712: // function 577 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 578 return value; 579 case 92645877: // actor 580 this.actor = TypeConvertor.castToReference(value); // Reference 581 return value; 582 default: return super.setProperty(hash, name, value); 583 } 584 585 } 586 587 @Override 588 public Base setProperty(String name, Base value) throws FHIRException { 589 if (name.equals("function")) { 590 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 591 } else if (name.equals("actor")) { 592 this.actor = TypeConvertor.castToReference(value); // Reference 593 } else 594 return super.setProperty(name, value); 595 return value; 596 } 597 598 @Override 599 public void removeChild(String name, Base value) throws FHIRException { 600 if (name.equals("function")) { 601 this.function = null; 602 } else if (name.equals("actor")) { 603 this.actor = null; 604 } else 605 super.removeChild(name, value); 606 607 } 608 609 @Override 610 public Base makeProperty(int hash, String name) throws FHIRException { 611 switch (hash) { 612 case 1380938712: return getFunction(); 613 case 92645877: return getActor(); 614 default: return super.makeProperty(hash, name); 615 } 616 617 } 618 619 @Override 620 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 621 switch (hash) { 622 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 623 case 92645877: /*actor*/ return new String[] {"Reference"}; 624 default: return super.getTypesForProperty(hash, name); 625 } 626 627 } 628 629 @Override 630 public Base addChild(String name) throws FHIRException { 631 if (name.equals("function")) { 632 this.function = new CodeableConcept(); 633 return this.function; 634 } 635 else if (name.equals("actor")) { 636 this.actor = new Reference(); 637 return this.actor; 638 } 639 else 640 return super.addChild(name); 641 } 642 643 public ImagingSelectionPerformerComponent copy() { 644 ImagingSelectionPerformerComponent dst = new ImagingSelectionPerformerComponent(); 645 copyValues(dst); 646 return dst; 647 } 648 649 public void copyValues(ImagingSelectionPerformerComponent dst) { 650 super.copyValues(dst); 651 dst.function = function == null ? null : function.copy(); 652 dst.actor = actor == null ? null : actor.copy(); 653 } 654 655 @Override 656 public boolean equalsDeep(Base other_) { 657 if (!super.equalsDeep(other_)) 658 return false; 659 if (!(other_ instanceof ImagingSelectionPerformerComponent)) 660 return false; 661 ImagingSelectionPerformerComponent o = (ImagingSelectionPerformerComponent) other_; 662 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 663 } 664 665 @Override 666 public boolean equalsShallow(Base other_) { 667 if (!super.equalsShallow(other_)) 668 return false; 669 if (!(other_ instanceof ImagingSelectionPerformerComponent)) 670 return false; 671 ImagingSelectionPerformerComponent o = (ImagingSelectionPerformerComponent) other_; 672 return true; 673 } 674 675 public boolean isEmpty() { 676 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 677 } 678 679 public String fhirType() { 680 return "ImagingSelection.performer"; 681 682 } 683 684 } 685 686 @Block() 687 public static class ImagingSelectionInstanceComponent extends BackboneElement implements IBaseBackboneElement { 688 /** 689 * The SOP Instance UID for the selected DICOM instance. 690 */ 691 @Child(name = "uid", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 692 @Description(shortDefinition="DICOM SOP Instance UID", formalDefinition="The SOP Instance UID for the selected DICOM instance." ) 693 protected IdType uid; 694 695 /** 696 * The Instance Number for the selected DICOM instance. 697 */ 698 @Child(name = "number", type = {UnsignedIntType.class}, order=2, min=0, max=1, modifier=false, summary=true) 699 @Description(shortDefinition="DICOM Instance Number", formalDefinition="The Instance Number for the selected DICOM instance." ) 700 protected UnsignedIntType number; 701 702 /** 703 * The SOP Class UID for the selected DICOM instance. 704 */ 705 @Child(name = "sopClass", type = {Coding.class}, order=3, min=0, max=1, modifier=false, summary=false) 706 @Description(shortDefinition="DICOM SOP Class UID", formalDefinition="The SOP Class UID for the selected DICOM instance." ) 707 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://dicom.nema.org/medical/dicom/current/output/chtml/part04/sect_B.5.html#table_B.5-1") 708 protected Coding sopClass; 709 710 /** 711 * Selected subset of the SOP Instance. The content and format of the subset item is determined by the SOP Class of the selected instance. 712 May be one of: 713 - A list of frame numbers selected from a multiframe SOP Instance. 714 - A list of Content Item Observation UID values selected from a DICOM SR or other structured document SOP Instance. 715 - A list of segment numbers selected from a segmentation SOP Instance. 716 - A list of Region of Interest (ROI) numbers selected from a radiotherapy structure set SOP Instance. 717 */ 718 @Child(name = "subset", type = {StringType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 719 @Description(shortDefinition="The selected subset of the SOP Instance", formalDefinition="Selected subset of the SOP Instance. The content and format of the subset item is determined by the SOP Class of the selected instance.\n May be one of:\n - A list of frame numbers selected from a multiframe SOP Instance.\n - A list of Content Item Observation UID values selected from a DICOM SR or other structured document SOP Instance.\n - A list of segment numbers selected from a segmentation SOP Instance.\n - A list of Region of Interest (ROI) numbers selected from a radiotherapy structure set SOP Instance." ) 720 protected List<StringType> subset; 721 722 /** 723 * Each imaging selection instance or frame list might includes an image region, specified by a region type and a set of 2D coordinates. 724 If the parent imagingSelection.instance contains a subset element of type frame, the image region applies to all frames in the subset list. 725 */ 726 @Child(name = "imageRegion2D", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 727 @Description(shortDefinition="A specific 2D region in a DICOM image / frame", formalDefinition="Each imaging selection instance or frame list might includes an image region, specified by a region type and a set of 2D coordinates.\n If the parent imagingSelection.instance contains a subset element of type frame, the image region applies to all frames in the subset list." ) 728 protected List<ImageRegion2DComponent> imageRegion2D; 729 730 /** 731 * Each imaging selection might includes a 3D image region, specified by a region type and a set of 3D coordinates. 732 */ 733 @Child(name = "imageRegion3D", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 734 @Description(shortDefinition="A specific 3D region in a DICOM frame of reference", formalDefinition="Each imaging selection might includes a 3D image region, specified by a region type and a set of 3D coordinates." ) 735 protected List<ImageRegion3DComponent> imageRegion3D; 736 737 private static final long serialVersionUID = 1783712351L; 738 739 /** 740 * Constructor 741 */ 742 public ImagingSelectionInstanceComponent() { 743 super(); 744 } 745 746 /** 747 * Constructor 748 */ 749 public ImagingSelectionInstanceComponent(String uid) { 750 super(); 751 this.setUid(uid); 752 } 753 754 /** 755 * @return {@link #uid} (The SOP Instance UID for the selected DICOM instance.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 756 */ 757 public IdType getUidElement() { 758 if (this.uid == null) 759 if (Configuration.errorOnAutoCreate()) 760 throw new Error("Attempt to auto-create ImagingSelectionInstanceComponent.uid"); 761 else if (Configuration.doAutoCreate()) 762 this.uid = new IdType(); // bb 763 return this.uid; 764 } 765 766 public boolean hasUidElement() { 767 return this.uid != null && !this.uid.isEmpty(); 768 } 769 770 public boolean hasUid() { 771 return this.uid != null && !this.uid.isEmpty(); 772 } 773 774 /** 775 * @param value {@link #uid} (The SOP Instance UID for the selected DICOM instance.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 776 */ 777 public ImagingSelectionInstanceComponent setUidElement(IdType value) { 778 this.uid = value; 779 return this; 780 } 781 782 /** 783 * @return The SOP Instance UID for the selected DICOM instance. 784 */ 785 public String getUid() { 786 return this.uid == null ? null : this.uid.getValue(); 787 } 788 789 /** 790 * @param value The SOP Instance UID for the selected DICOM instance. 791 */ 792 public ImagingSelectionInstanceComponent setUid(String value) { 793 if (this.uid == null) 794 this.uid = new IdType(); 795 this.uid.setValue(value); 796 return this; 797 } 798 799 /** 800 * @return {@link #number} (The Instance Number for the selected DICOM instance.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 801 */ 802 public UnsignedIntType getNumberElement() { 803 if (this.number == null) 804 if (Configuration.errorOnAutoCreate()) 805 throw new Error("Attempt to auto-create ImagingSelectionInstanceComponent.number"); 806 else if (Configuration.doAutoCreate()) 807 this.number = new UnsignedIntType(); // bb 808 return this.number; 809 } 810 811 public boolean hasNumberElement() { 812 return this.number != null && !this.number.isEmpty(); 813 } 814 815 public boolean hasNumber() { 816 return this.number != null && !this.number.isEmpty(); 817 } 818 819 /** 820 * @param value {@link #number} (The Instance Number for the selected DICOM instance.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 821 */ 822 public ImagingSelectionInstanceComponent setNumberElement(UnsignedIntType value) { 823 this.number = value; 824 return this; 825 } 826 827 /** 828 * @return The Instance Number for the selected DICOM instance. 829 */ 830 public int getNumber() { 831 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 832 } 833 834 /** 835 * @param value The Instance Number for the selected DICOM instance. 836 */ 837 public ImagingSelectionInstanceComponent setNumber(int value) { 838 if (this.number == null) 839 this.number = new UnsignedIntType(); 840 this.number.setValue(value); 841 return this; 842 } 843 844 /** 845 * @return {@link #sopClass} (The SOP Class UID for the selected DICOM instance.) 846 */ 847 public Coding getSopClass() { 848 if (this.sopClass == null) 849 if (Configuration.errorOnAutoCreate()) 850 throw new Error("Attempt to auto-create ImagingSelectionInstanceComponent.sopClass"); 851 else if (Configuration.doAutoCreate()) 852 this.sopClass = new Coding(); // cc 853 return this.sopClass; 854 } 855 856 public boolean hasSopClass() { 857 return this.sopClass != null && !this.sopClass.isEmpty(); 858 } 859 860 /** 861 * @param value {@link #sopClass} (The SOP Class UID for the selected DICOM instance.) 862 */ 863 public ImagingSelectionInstanceComponent setSopClass(Coding value) { 864 this.sopClass = value; 865 return this; 866 } 867 868 /** 869 * @return {@link #subset} (Selected subset of the SOP Instance. The content and format of the subset item is determined by the SOP Class of the selected instance. 870 May be one of: 871 - A list of frame numbers selected from a multiframe SOP Instance. 872 - A list of Content Item Observation UID values selected from a DICOM SR or other structured document SOP Instance. 873 - A list of segment numbers selected from a segmentation SOP Instance. 874 - A list of Region of Interest (ROI) numbers selected from a radiotherapy structure set SOP Instance.) 875 */ 876 public List<StringType> getSubset() { 877 if (this.subset == null) 878 this.subset = new ArrayList<StringType>(); 879 return this.subset; 880 } 881 882 /** 883 * @return Returns a reference to <code>this</code> for easy method chaining 884 */ 885 public ImagingSelectionInstanceComponent setSubset(List<StringType> theSubset) { 886 this.subset = theSubset; 887 return this; 888 } 889 890 public boolean hasSubset() { 891 if (this.subset == null) 892 return false; 893 for (StringType item : this.subset) 894 if (!item.isEmpty()) 895 return true; 896 return false; 897 } 898 899 /** 900 * @return {@link #subset} (Selected subset of the SOP Instance. The content and format of the subset item is determined by the SOP Class of the selected instance. 901 May be one of: 902 - A list of frame numbers selected from a multiframe SOP Instance. 903 - A list of Content Item Observation UID values selected from a DICOM SR or other structured document SOP Instance. 904 - A list of segment numbers selected from a segmentation SOP Instance. 905 - A list of Region of Interest (ROI) numbers selected from a radiotherapy structure set SOP Instance.) 906 */ 907 public StringType addSubsetElement() {//2 908 StringType t = new StringType(); 909 if (this.subset == null) 910 this.subset = new ArrayList<StringType>(); 911 this.subset.add(t); 912 return t; 913 } 914 915 /** 916 * @param value {@link #subset} (Selected subset of the SOP Instance. The content and format of the subset item is determined by the SOP Class of the selected instance. 917 May be one of: 918 - A list of frame numbers selected from a multiframe SOP Instance. 919 - A list of Content Item Observation UID values selected from a DICOM SR or other structured document SOP Instance. 920 - A list of segment numbers selected from a segmentation SOP Instance. 921 - A list of Region of Interest (ROI) numbers selected from a radiotherapy structure set SOP Instance.) 922 */ 923 public ImagingSelectionInstanceComponent addSubset(String value) { //1 924 StringType t = new StringType(); 925 t.setValue(value); 926 if (this.subset == null) 927 this.subset = new ArrayList<StringType>(); 928 this.subset.add(t); 929 return this; 930 } 931 932 /** 933 * @param value {@link #subset} (Selected subset of the SOP Instance. The content and format of the subset item is determined by the SOP Class of the selected instance. 934 May be one of: 935 - A list of frame numbers selected from a multiframe SOP Instance. 936 - A list of Content Item Observation UID values selected from a DICOM SR or other structured document SOP Instance. 937 - A list of segment numbers selected from a segmentation SOP Instance. 938 - A list of Region of Interest (ROI) numbers selected from a radiotherapy structure set SOP Instance.) 939 */ 940 public boolean hasSubset(String value) { 941 if (this.subset == null) 942 return false; 943 for (StringType v : this.subset) 944 if (v.getValue().equals(value)) // string 945 return true; 946 return false; 947 } 948 949 /** 950 * @return {@link #imageRegion2D} (Each imaging selection instance or frame list might includes an image region, specified by a region type and a set of 2D coordinates. 951 If the parent imagingSelection.instance contains a subset element of type frame, the image region applies to all frames in the subset list.) 952 */ 953 public List<ImageRegion2DComponent> getImageRegion2D() { 954 if (this.imageRegion2D == null) 955 this.imageRegion2D = new ArrayList<ImageRegion2DComponent>(); 956 return this.imageRegion2D; 957 } 958 959 /** 960 * @return Returns a reference to <code>this</code> for easy method chaining 961 */ 962 public ImagingSelectionInstanceComponent setImageRegion2D(List<ImageRegion2DComponent> theImageRegion2D) { 963 this.imageRegion2D = theImageRegion2D; 964 return this; 965 } 966 967 public boolean hasImageRegion2D() { 968 if (this.imageRegion2D == null) 969 return false; 970 for (ImageRegion2DComponent item : this.imageRegion2D) 971 if (!item.isEmpty()) 972 return true; 973 return false; 974 } 975 976 public ImageRegion2DComponent addImageRegion2D() { //3 977 ImageRegion2DComponent t = new ImageRegion2DComponent(); 978 if (this.imageRegion2D == null) 979 this.imageRegion2D = new ArrayList<ImageRegion2DComponent>(); 980 this.imageRegion2D.add(t); 981 return t; 982 } 983 984 public ImagingSelectionInstanceComponent addImageRegion2D(ImageRegion2DComponent t) { //3 985 if (t == null) 986 return this; 987 if (this.imageRegion2D == null) 988 this.imageRegion2D = new ArrayList<ImageRegion2DComponent>(); 989 this.imageRegion2D.add(t); 990 return this; 991 } 992 993 /** 994 * @return The first repetition of repeating field {@link #imageRegion2D}, creating it if it does not already exist {3} 995 */ 996 public ImageRegion2DComponent getImageRegion2DFirstRep() { 997 if (getImageRegion2D().isEmpty()) { 998 addImageRegion2D(); 999 } 1000 return getImageRegion2D().get(0); 1001 } 1002 1003 /** 1004 * @return {@link #imageRegion3D} (Each imaging selection might includes a 3D image region, specified by a region type and a set of 3D coordinates.) 1005 */ 1006 public List<ImageRegion3DComponent> getImageRegion3D() { 1007 if (this.imageRegion3D == null) 1008 this.imageRegion3D = new ArrayList<ImageRegion3DComponent>(); 1009 return this.imageRegion3D; 1010 } 1011 1012 /** 1013 * @return Returns a reference to <code>this</code> for easy method chaining 1014 */ 1015 public ImagingSelectionInstanceComponent setImageRegion3D(List<ImageRegion3DComponent> theImageRegion3D) { 1016 this.imageRegion3D = theImageRegion3D; 1017 return this; 1018 } 1019 1020 public boolean hasImageRegion3D() { 1021 if (this.imageRegion3D == null) 1022 return false; 1023 for (ImageRegion3DComponent item : this.imageRegion3D) 1024 if (!item.isEmpty()) 1025 return true; 1026 return false; 1027 } 1028 1029 public ImageRegion3DComponent addImageRegion3D() { //3 1030 ImageRegion3DComponent t = new ImageRegion3DComponent(); 1031 if (this.imageRegion3D == null) 1032 this.imageRegion3D = new ArrayList<ImageRegion3DComponent>(); 1033 this.imageRegion3D.add(t); 1034 return t; 1035 } 1036 1037 public ImagingSelectionInstanceComponent addImageRegion3D(ImageRegion3DComponent t) { //3 1038 if (t == null) 1039 return this; 1040 if (this.imageRegion3D == null) 1041 this.imageRegion3D = new ArrayList<ImageRegion3DComponent>(); 1042 this.imageRegion3D.add(t); 1043 return this; 1044 } 1045 1046 /** 1047 * @return The first repetition of repeating field {@link #imageRegion3D}, creating it if it does not already exist {3} 1048 */ 1049 public ImageRegion3DComponent getImageRegion3DFirstRep() { 1050 if (getImageRegion3D().isEmpty()) { 1051 addImageRegion3D(); 1052 } 1053 return getImageRegion3D().get(0); 1054 } 1055 1056 protected void listChildren(List<Property> children) { 1057 super.listChildren(children); 1058 children.add(new Property("uid", "id", "The SOP Instance UID for the selected DICOM instance.", 0, 1, uid)); 1059 children.add(new Property("number", "unsignedInt", "The Instance Number for the selected DICOM instance.", 0, 1, number)); 1060 children.add(new Property("sopClass", "Coding", "The SOP Class UID for the selected DICOM instance.", 0, 1, sopClass)); 1061 children.add(new Property("subset", "string", "Selected subset of the SOP Instance. The content and format of the subset item is determined by the SOP Class of the selected instance.\n May be one of:\n - A list of frame numbers selected from a multiframe SOP Instance.\n - A list of Content Item Observation UID values selected from a DICOM SR or other structured document SOP Instance.\n - A list of segment numbers selected from a segmentation SOP Instance.\n - A list of Region of Interest (ROI) numbers selected from a radiotherapy structure set SOP Instance.", 0, java.lang.Integer.MAX_VALUE, subset)); 1062 children.add(new Property("imageRegion2D", "", "Each imaging selection instance or frame list might includes an image region, specified by a region type and a set of 2D coordinates.\n If the parent imagingSelection.instance contains a subset element of type frame, the image region applies to all frames in the subset list.", 0, java.lang.Integer.MAX_VALUE, imageRegion2D)); 1063 children.add(new Property("imageRegion3D", "", "Each imaging selection might includes a 3D image region, specified by a region type and a set of 3D coordinates.", 0, java.lang.Integer.MAX_VALUE, imageRegion3D)); 1064 } 1065 1066 @Override 1067 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1068 switch (_hash) { 1069 case 115792: /*uid*/ return new Property("uid", "id", "The SOP Instance UID for the selected DICOM instance.", 0, 1, uid); 1070 case -1034364087: /*number*/ return new Property("number", "unsignedInt", "The Instance Number for the selected DICOM instance.", 0, 1, number); 1071 case 1560041540: /*sopClass*/ return new Property("sopClass", "Coding", "The SOP Class UID for the selected DICOM instance.", 0, 1, sopClass); 1072 case -891529694: /*subset*/ return new Property("subset", "string", "Selected subset of the SOP Instance. The content and format of the subset item is determined by the SOP Class of the selected instance.\n May be one of:\n - A list of frame numbers selected from a multiframe SOP Instance.\n - A list of Content Item Observation UID values selected from a DICOM SR or other structured document SOP Instance.\n - A list of segment numbers selected from a segmentation SOP Instance.\n - A list of Region of Interest (ROI) numbers selected from a radiotherapy structure set SOP Instance.", 0, java.lang.Integer.MAX_VALUE, subset); 1073 case 675922625: /*imageRegion2D*/ return new Property("imageRegion2D", "", "Each imaging selection instance or frame list might includes an image region, specified by a region type and a set of 2D coordinates.\n If the parent imagingSelection.instance contains a subset element of type frame, the image region applies to all frames in the subset list.", 0, java.lang.Integer.MAX_VALUE, imageRegion2D); 1074 case 675922656: /*imageRegion3D*/ return new Property("imageRegion3D", "", "Each imaging selection might includes a 3D image region, specified by a region type and a set of 3D coordinates.", 0, java.lang.Integer.MAX_VALUE, imageRegion3D); 1075 default: return super.getNamedProperty(_hash, _name, _checkValid); 1076 } 1077 1078 } 1079 1080 @Override 1081 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1082 switch (hash) { 1083 case 115792: /*uid*/ return this.uid == null ? new Base[0] : new Base[] {this.uid}; // IdType 1084 case -1034364087: /*number*/ return this.number == null ? new Base[0] : new Base[] {this.number}; // UnsignedIntType 1085 case 1560041540: /*sopClass*/ return this.sopClass == null ? new Base[0] : new Base[] {this.sopClass}; // Coding 1086 case -891529694: /*subset*/ return this.subset == null ? new Base[0] : this.subset.toArray(new Base[this.subset.size()]); // StringType 1087 case 675922625: /*imageRegion2D*/ return this.imageRegion2D == null ? new Base[0] : this.imageRegion2D.toArray(new Base[this.imageRegion2D.size()]); // ImageRegion2DComponent 1088 case 675922656: /*imageRegion3D*/ return this.imageRegion3D == null ? new Base[0] : this.imageRegion3D.toArray(new Base[this.imageRegion3D.size()]); // ImageRegion3DComponent 1089 default: return super.getProperty(hash, name, checkValid); 1090 } 1091 1092 } 1093 1094 @Override 1095 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1096 switch (hash) { 1097 case 115792: // uid 1098 this.uid = TypeConvertor.castToId(value); // IdType 1099 return value; 1100 case -1034364087: // number 1101 this.number = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1102 return value; 1103 case 1560041540: // sopClass 1104 this.sopClass = TypeConvertor.castToCoding(value); // Coding 1105 return value; 1106 case -891529694: // subset 1107 this.getSubset().add(TypeConvertor.castToString(value)); // StringType 1108 return value; 1109 case 675922625: // imageRegion2D 1110 this.getImageRegion2D().add((ImageRegion2DComponent) value); // ImageRegion2DComponent 1111 return value; 1112 case 675922656: // imageRegion3D 1113 this.getImageRegion3D().add((ImageRegion3DComponent) value); // ImageRegion3DComponent 1114 return value; 1115 default: return super.setProperty(hash, name, value); 1116 } 1117 1118 } 1119 1120 @Override 1121 public Base setProperty(String name, Base value) throws FHIRException { 1122 if (name.equals("uid")) { 1123 this.uid = TypeConvertor.castToId(value); // IdType 1124 } else if (name.equals("number")) { 1125 this.number = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1126 } else if (name.equals("sopClass")) { 1127 this.sopClass = TypeConvertor.castToCoding(value); // Coding 1128 } else if (name.equals("subset")) { 1129 this.getSubset().add(TypeConvertor.castToString(value)); 1130 } else if (name.equals("imageRegion2D")) { 1131 this.getImageRegion2D().add((ImageRegion2DComponent) value); 1132 } else if (name.equals("imageRegion3D")) { 1133 this.getImageRegion3D().add((ImageRegion3DComponent) value); 1134 } else 1135 return super.setProperty(name, value); 1136 return value; 1137 } 1138 1139 @Override 1140 public void removeChild(String name, Base value) throws FHIRException { 1141 if (name.equals("uid")) { 1142 this.uid = null; 1143 } else if (name.equals("number")) { 1144 this.number = null; 1145 } else if (name.equals("sopClass")) { 1146 this.sopClass = null; 1147 } else if (name.equals("subset")) { 1148 this.getSubset().remove(value); 1149 } else if (name.equals("imageRegion2D")) { 1150 this.getImageRegion2D().remove((ImageRegion2DComponent) value); 1151 } else if (name.equals("imageRegion3D")) { 1152 this.getImageRegion3D().remove((ImageRegion3DComponent) value); 1153 } else 1154 super.removeChild(name, value); 1155 1156 } 1157 1158 @Override 1159 public Base makeProperty(int hash, String name) throws FHIRException { 1160 switch (hash) { 1161 case 115792: return getUidElement(); 1162 case -1034364087: return getNumberElement(); 1163 case 1560041540: return getSopClass(); 1164 case -891529694: return addSubsetElement(); 1165 case 675922625: return addImageRegion2D(); 1166 case 675922656: return addImageRegion3D(); 1167 default: return super.makeProperty(hash, name); 1168 } 1169 1170 } 1171 1172 @Override 1173 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1174 switch (hash) { 1175 case 115792: /*uid*/ return new String[] {"id"}; 1176 case -1034364087: /*number*/ return new String[] {"unsignedInt"}; 1177 case 1560041540: /*sopClass*/ return new String[] {"Coding"}; 1178 case -891529694: /*subset*/ return new String[] {"string"}; 1179 case 675922625: /*imageRegion2D*/ return new String[] {}; 1180 case 675922656: /*imageRegion3D*/ return new String[] {}; 1181 default: return super.getTypesForProperty(hash, name); 1182 } 1183 1184 } 1185 1186 @Override 1187 public Base addChild(String name) throws FHIRException { 1188 if (name.equals("uid")) { 1189 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.instance.uid"); 1190 } 1191 else if (name.equals("number")) { 1192 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.instance.number"); 1193 } 1194 else if (name.equals("sopClass")) { 1195 this.sopClass = new Coding(); 1196 return this.sopClass; 1197 } 1198 else if (name.equals("subset")) { 1199 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.instance.subset"); 1200 } 1201 else if (name.equals("imageRegion2D")) { 1202 return addImageRegion2D(); 1203 } 1204 else if (name.equals("imageRegion3D")) { 1205 return addImageRegion3D(); 1206 } 1207 else 1208 return super.addChild(name); 1209 } 1210 1211 public ImagingSelectionInstanceComponent copy() { 1212 ImagingSelectionInstanceComponent dst = new ImagingSelectionInstanceComponent(); 1213 copyValues(dst); 1214 return dst; 1215 } 1216 1217 public void copyValues(ImagingSelectionInstanceComponent dst) { 1218 super.copyValues(dst); 1219 dst.uid = uid == null ? null : uid.copy(); 1220 dst.number = number == null ? null : number.copy(); 1221 dst.sopClass = sopClass == null ? null : sopClass.copy(); 1222 if (subset != null) { 1223 dst.subset = new ArrayList<StringType>(); 1224 for (StringType i : subset) 1225 dst.subset.add(i.copy()); 1226 }; 1227 if (imageRegion2D != null) { 1228 dst.imageRegion2D = new ArrayList<ImageRegion2DComponent>(); 1229 for (ImageRegion2DComponent i : imageRegion2D) 1230 dst.imageRegion2D.add(i.copy()); 1231 }; 1232 if (imageRegion3D != null) { 1233 dst.imageRegion3D = new ArrayList<ImageRegion3DComponent>(); 1234 for (ImageRegion3DComponent i : imageRegion3D) 1235 dst.imageRegion3D.add(i.copy()); 1236 }; 1237 } 1238 1239 @Override 1240 public boolean equalsDeep(Base other_) { 1241 if (!super.equalsDeep(other_)) 1242 return false; 1243 if (!(other_ instanceof ImagingSelectionInstanceComponent)) 1244 return false; 1245 ImagingSelectionInstanceComponent o = (ImagingSelectionInstanceComponent) other_; 1246 return compareDeep(uid, o.uid, true) && compareDeep(number, o.number, true) && compareDeep(sopClass, o.sopClass, true) 1247 && compareDeep(subset, o.subset, true) && compareDeep(imageRegion2D, o.imageRegion2D, true) && compareDeep(imageRegion3D, o.imageRegion3D, true) 1248 ; 1249 } 1250 1251 @Override 1252 public boolean equalsShallow(Base other_) { 1253 if (!super.equalsShallow(other_)) 1254 return false; 1255 if (!(other_ instanceof ImagingSelectionInstanceComponent)) 1256 return false; 1257 ImagingSelectionInstanceComponent o = (ImagingSelectionInstanceComponent) other_; 1258 return compareValues(uid, o.uid, true) && compareValues(number, o.number, true) && compareValues(subset, o.subset, true) 1259 ; 1260 } 1261 1262 public boolean isEmpty() { 1263 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(uid, number, sopClass, subset 1264 , imageRegion2D, imageRegion3D); 1265 } 1266 1267 public String fhirType() { 1268 return "ImagingSelection.instance"; 1269 1270 } 1271 1272 } 1273 1274 @Block() 1275 public static class ImageRegion2DComponent extends BackboneElement implements IBaseBackboneElement { 1276 /** 1277 * Specifies the type of image region. 1278 */ 1279 @Child(name = "regionType", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1280 @Description(shortDefinition="point | polyline | interpolated | circle | ellipse", formalDefinition="Specifies the type of image region." ) 1281 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/imagingselection-2dgraphictype") 1282 protected Enumeration<ImagingSelection2DGraphicType> regionType; 1283 1284 /** 1285 * The coordinates describing the image region. Encoded as a set of (column, row) pairs that denote positions in the selected image / frames specified with sub-pixel resolution. 1286 The origin at the TLHC of the TLHC pixel is 0.0\0.0, the BRHC of the TLHC pixel is 1.0\1.0, and the BRHC of the BRHC pixel is the number of columns\rows in the image / frames. The values must be within the range 0\0 to the number of columns\rows in the image / frames. 1287 */ 1288 @Child(name = "coordinate", type = {DecimalType.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1289 @Description(shortDefinition="Specifies the coordinates that define the image region", formalDefinition="The coordinates describing the image region. Encoded as a set of (column, row) pairs that denote positions in the selected image / frames specified with sub-pixel resolution.\n The origin at the TLHC of the TLHC pixel is 0.0\\0.0, the BRHC of the TLHC pixel is 1.0\\1.0, and the BRHC of the BRHC pixel is the number of columns\\rows in the image / frames. The values must be within the range 0\\0 to the number of columns\\rows in the image / frames." ) 1290 protected List<DecimalType> coordinate; 1291 1292 private static final long serialVersionUID = 1518695052L; 1293 1294 /** 1295 * Constructor 1296 */ 1297 public ImageRegion2DComponent() { 1298 super(); 1299 } 1300 1301 /** 1302 * Constructor 1303 */ 1304 public ImageRegion2DComponent(ImagingSelection2DGraphicType regionType, BigDecimal coordinate) { 1305 super(); 1306 this.setRegionType(regionType); 1307 this.addCoordinate(coordinate); 1308 } 1309 1310 /** 1311 * @return {@link #regionType} (Specifies the type of image region.). This is the underlying object with id, value and extensions. The accessor "getRegionType" gives direct access to the value 1312 */ 1313 public Enumeration<ImagingSelection2DGraphicType> getRegionTypeElement() { 1314 if (this.regionType == null) 1315 if (Configuration.errorOnAutoCreate()) 1316 throw new Error("Attempt to auto-create ImageRegion2DComponent.regionType"); 1317 else if (Configuration.doAutoCreate()) 1318 this.regionType = new Enumeration<ImagingSelection2DGraphicType>(new ImagingSelection2DGraphicTypeEnumFactory()); // bb 1319 return this.regionType; 1320 } 1321 1322 public boolean hasRegionTypeElement() { 1323 return this.regionType != null && !this.regionType.isEmpty(); 1324 } 1325 1326 public boolean hasRegionType() { 1327 return this.regionType != null && !this.regionType.isEmpty(); 1328 } 1329 1330 /** 1331 * @param value {@link #regionType} (Specifies the type of image region.). This is the underlying object with id, value and extensions. The accessor "getRegionType" gives direct access to the value 1332 */ 1333 public ImageRegion2DComponent setRegionTypeElement(Enumeration<ImagingSelection2DGraphicType> value) { 1334 this.regionType = value; 1335 return this; 1336 } 1337 1338 /** 1339 * @return Specifies the type of image region. 1340 */ 1341 public ImagingSelection2DGraphicType getRegionType() { 1342 return this.regionType == null ? null : this.regionType.getValue(); 1343 } 1344 1345 /** 1346 * @param value Specifies the type of image region. 1347 */ 1348 public ImageRegion2DComponent setRegionType(ImagingSelection2DGraphicType value) { 1349 if (this.regionType == null) 1350 this.regionType = new Enumeration<ImagingSelection2DGraphicType>(new ImagingSelection2DGraphicTypeEnumFactory()); 1351 this.regionType.setValue(value); 1352 return this; 1353 } 1354 1355 /** 1356 * @return {@link #coordinate} (The coordinates describing the image region. Encoded as a set of (column, row) pairs that denote positions in the selected image / frames specified with sub-pixel resolution. 1357 The origin at the TLHC of the TLHC pixel is 0.0\0.0, the BRHC of the TLHC pixel is 1.0\1.0, and the BRHC of the BRHC pixel is the number of columns\rows in the image / frames. The values must be within the range 0\0 to the number of columns\rows in the image / frames.) 1358 */ 1359 public List<DecimalType> getCoordinate() { 1360 if (this.coordinate == null) 1361 this.coordinate = new ArrayList<DecimalType>(); 1362 return this.coordinate; 1363 } 1364 1365 /** 1366 * @return Returns a reference to <code>this</code> for easy method chaining 1367 */ 1368 public ImageRegion2DComponent setCoordinate(List<DecimalType> theCoordinate) { 1369 this.coordinate = theCoordinate; 1370 return this; 1371 } 1372 1373 public boolean hasCoordinate() { 1374 if (this.coordinate == null) 1375 return false; 1376 for (DecimalType item : this.coordinate) 1377 if (!item.isEmpty()) 1378 return true; 1379 return false; 1380 } 1381 1382 /** 1383 * @return {@link #coordinate} (The coordinates describing the image region. Encoded as a set of (column, row) pairs that denote positions in the selected image / frames specified with sub-pixel resolution. 1384 The origin at the TLHC of the TLHC pixel is 0.0\0.0, the BRHC of the TLHC pixel is 1.0\1.0, and the BRHC of the BRHC pixel is the number of columns\rows in the image / frames. The values must be within the range 0\0 to the number of columns\rows in the image / frames.) 1385 */ 1386 public DecimalType addCoordinateElement() {//2 1387 DecimalType t = new DecimalType(); 1388 if (this.coordinate == null) 1389 this.coordinate = new ArrayList<DecimalType>(); 1390 this.coordinate.add(t); 1391 return t; 1392 } 1393 1394 /** 1395 * @param value {@link #coordinate} (The coordinates describing the image region. Encoded as a set of (column, row) pairs that denote positions in the selected image / frames specified with sub-pixel resolution. 1396 The origin at the TLHC of the TLHC pixel is 0.0\0.0, the BRHC of the TLHC pixel is 1.0\1.0, and the BRHC of the BRHC pixel is the number of columns\rows in the image / frames. The values must be within the range 0\0 to the number of columns\rows in the image / frames.) 1397 */ 1398 public ImageRegion2DComponent addCoordinate(BigDecimal value) { //1 1399 DecimalType t = new DecimalType(); 1400 t.setValue(value); 1401 if (this.coordinate == null) 1402 this.coordinate = new ArrayList<DecimalType>(); 1403 this.coordinate.add(t); 1404 return this; 1405 } 1406 1407 /** 1408 * @param value {@link #coordinate} (The coordinates describing the image region. Encoded as a set of (column, row) pairs that denote positions in the selected image / frames specified with sub-pixel resolution. 1409 The origin at the TLHC of the TLHC pixel is 0.0\0.0, the BRHC of the TLHC pixel is 1.0\1.0, and the BRHC of the BRHC pixel is the number of columns\rows in the image / frames. The values must be within the range 0\0 to the number of columns\rows in the image / frames.) 1410 */ 1411 public boolean hasCoordinate(BigDecimal value) { 1412 if (this.coordinate == null) 1413 return false; 1414 for (DecimalType v : this.coordinate) 1415 if (v.getValue().equals(value)) // decimal 1416 return true; 1417 return false; 1418 } 1419 1420 protected void listChildren(List<Property> children) { 1421 super.listChildren(children); 1422 children.add(new Property("regionType", "code", "Specifies the type of image region.", 0, 1, regionType)); 1423 children.add(new Property("coordinate", "decimal", "The coordinates describing the image region. Encoded as a set of (column, row) pairs that denote positions in the selected image / frames specified with sub-pixel resolution.\n The origin at the TLHC of the TLHC pixel is 0.0\\0.0, the BRHC of the TLHC pixel is 1.0\\1.0, and the BRHC of the BRHC pixel is the number of columns\\rows in the image / frames. The values must be within the range 0\\0 to the number of columns\\rows in the image / frames.", 0, java.lang.Integer.MAX_VALUE, coordinate)); 1424 } 1425 1426 @Override 1427 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1428 switch (_hash) { 1429 case -1990487986: /*regionType*/ return new Property("regionType", "code", "Specifies the type of image region.", 0, 1, regionType); 1430 case 198931832: /*coordinate*/ return new Property("coordinate", "decimal", "The coordinates describing the image region. Encoded as a set of (column, row) pairs that denote positions in the selected image / frames specified with sub-pixel resolution.\n The origin at the TLHC of the TLHC pixel is 0.0\\0.0, the BRHC of the TLHC pixel is 1.0\\1.0, and the BRHC of the BRHC pixel is the number of columns\\rows in the image / frames. The values must be within the range 0\\0 to the number of columns\\rows in the image / frames.", 0, java.lang.Integer.MAX_VALUE, coordinate); 1431 default: return super.getNamedProperty(_hash, _name, _checkValid); 1432 } 1433 1434 } 1435 1436 @Override 1437 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1438 switch (hash) { 1439 case -1990487986: /*regionType*/ return this.regionType == null ? new Base[0] : new Base[] {this.regionType}; // Enumeration<ImagingSelection2DGraphicType> 1440 case 198931832: /*coordinate*/ return this.coordinate == null ? new Base[0] : this.coordinate.toArray(new Base[this.coordinate.size()]); // DecimalType 1441 default: return super.getProperty(hash, name, checkValid); 1442 } 1443 1444 } 1445 1446 @Override 1447 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1448 switch (hash) { 1449 case -1990487986: // regionType 1450 value = new ImagingSelection2DGraphicTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1451 this.regionType = (Enumeration) value; // Enumeration<ImagingSelection2DGraphicType> 1452 return value; 1453 case 198931832: // coordinate 1454 this.getCoordinate().add(TypeConvertor.castToDecimal(value)); // DecimalType 1455 return value; 1456 default: return super.setProperty(hash, name, value); 1457 } 1458 1459 } 1460 1461 @Override 1462 public Base setProperty(String name, Base value) throws FHIRException { 1463 if (name.equals("regionType")) { 1464 value = new ImagingSelection2DGraphicTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1465 this.regionType = (Enumeration) value; // Enumeration<ImagingSelection2DGraphicType> 1466 } else if (name.equals("coordinate")) { 1467 this.getCoordinate().add(TypeConvertor.castToDecimal(value)); 1468 } else 1469 return super.setProperty(name, value); 1470 return value; 1471 } 1472 1473 @Override 1474 public void removeChild(String name, Base value) throws FHIRException { 1475 if (name.equals("regionType")) { 1476 value = new ImagingSelection2DGraphicTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1477 this.regionType = (Enumeration) value; // Enumeration<ImagingSelection2DGraphicType> 1478 } else if (name.equals("coordinate")) { 1479 this.getCoordinate().remove(value); 1480 } else 1481 super.removeChild(name, value); 1482 1483 } 1484 1485 @Override 1486 public Base makeProperty(int hash, String name) throws FHIRException { 1487 switch (hash) { 1488 case -1990487986: return getRegionTypeElement(); 1489 case 198931832: return addCoordinateElement(); 1490 default: return super.makeProperty(hash, name); 1491 } 1492 1493 } 1494 1495 @Override 1496 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1497 switch (hash) { 1498 case -1990487986: /*regionType*/ return new String[] {"code"}; 1499 case 198931832: /*coordinate*/ return new String[] {"decimal"}; 1500 default: return super.getTypesForProperty(hash, name); 1501 } 1502 1503 } 1504 1505 @Override 1506 public Base addChild(String name) throws FHIRException { 1507 if (name.equals("regionType")) { 1508 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.instance.imageRegion2D.regionType"); 1509 } 1510 else if (name.equals("coordinate")) { 1511 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.instance.imageRegion2D.coordinate"); 1512 } 1513 else 1514 return super.addChild(name); 1515 } 1516 1517 public ImageRegion2DComponent copy() { 1518 ImageRegion2DComponent dst = new ImageRegion2DComponent(); 1519 copyValues(dst); 1520 return dst; 1521 } 1522 1523 public void copyValues(ImageRegion2DComponent dst) { 1524 super.copyValues(dst); 1525 dst.regionType = regionType == null ? null : regionType.copy(); 1526 if (coordinate != null) { 1527 dst.coordinate = new ArrayList<DecimalType>(); 1528 for (DecimalType i : coordinate) 1529 dst.coordinate.add(i.copy()); 1530 }; 1531 } 1532 1533 @Override 1534 public boolean equalsDeep(Base other_) { 1535 if (!super.equalsDeep(other_)) 1536 return false; 1537 if (!(other_ instanceof ImageRegion2DComponent)) 1538 return false; 1539 ImageRegion2DComponent o = (ImageRegion2DComponent) other_; 1540 return compareDeep(regionType, o.regionType, true) && compareDeep(coordinate, o.coordinate, true) 1541 ; 1542 } 1543 1544 @Override 1545 public boolean equalsShallow(Base other_) { 1546 if (!super.equalsShallow(other_)) 1547 return false; 1548 if (!(other_ instanceof ImageRegion2DComponent)) 1549 return false; 1550 ImageRegion2DComponent o = (ImageRegion2DComponent) other_; 1551 return compareValues(regionType, o.regionType, true) && compareValues(coordinate, o.coordinate, true) 1552 ; 1553 } 1554 1555 public boolean isEmpty() { 1556 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(regionType, coordinate); 1557 } 1558 1559 public String fhirType() { 1560 return "ImagingSelection.instance.imageRegion2D"; 1561 1562 } 1563 1564 } 1565 1566 @Block() 1567 public static class ImageRegion3DComponent extends BackboneElement implements IBaseBackboneElement { 1568 /** 1569 * Specifies the type of image region. 1570 */ 1571 @Child(name = "regionType", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1572 @Description(shortDefinition="point | multipoint | polyline | polygon | ellipse | ellipsoid", formalDefinition="Specifies the type of image region." ) 1573 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/imagingselection-3dgraphictype") 1574 protected Enumeration<ImagingSelection3DGraphicType> regionType; 1575 1576 /** 1577 * The coordinates describing the image region. Encoded as an ordered set of (x,y,z) triplets (in mm and may be negative) that define a region of interest in the patient-relative Reference Coordinate System defined by ImagingSelection.frameOfReferenceUid element. 1578 */ 1579 @Child(name = "coordinate", type = {DecimalType.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1580 @Description(shortDefinition="Specifies the coordinates that define the image region", formalDefinition="The coordinates describing the image region. Encoded as an ordered set of (x,y,z) triplets (in mm and may be negative) that define a region of interest in the patient-relative Reference Coordinate System defined by ImagingSelection.frameOfReferenceUid element." ) 1581 protected List<DecimalType> coordinate; 1582 1583 private static final long serialVersionUID = 1532227853L; 1584 1585 /** 1586 * Constructor 1587 */ 1588 public ImageRegion3DComponent() { 1589 super(); 1590 } 1591 1592 /** 1593 * Constructor 1594 */ 1595 public ImageRegion3DComponent(ImagingSelection3DGraphicType regionType, BigDecimal coordinate) { 1596 super(); 1597 this.setRegionType(regionType); 1598 this.addCoordinate(coordinate); 1599 } 1600 1601 /** 1602 * @return {@link #regionType} (Specifies the type of image region.). This is the underlying object with id, value and extensions. The accessor "getRegionType" gives direct access to the value 1603 */ 1604 public Enumeration<ImagingSelection3DGraphicType> getRegionTypeElement() { 1605 if (this.regionType == null) 1606 if (Configuration.errorOnAutoCreate()) 1607 throw new Error("Attempt to auto-create ImageRegion3DComponent.regionType"); 1608 else if (Configuration.doAutoCreate()) 1609 this.regionType = new Enumeration<ImagingSelection3DGraphicType>(new ImagingSelection3DGraphicTypeEnumFactory()); // bb 1610 return this.regionType; 1611 } 1612 1613 public boolean hasRegionTypeElement() { 1614 return this.regionType != null && !this.regionType.isEmpty(); 1615 } 1616 1617 public boolean hasRegionType() { 1618 return this.regionType != null && !this.regionType.isEmpty(); 1619 } 1620 1621 /** 1622 * @param value {@link #regionType} (Specifies the type of image region.). This is the underlying object with id, value and extensions. The accessor "getRegionType" gives direct access to the value 1623 */ 1624 public ImageRegion3DComponent setRegionTypeElement(Enumeration<ImagingSelection3DGraphicType> value) { 1625 this.regionType = value; 1626 return this; 1627 } 1628 1629 /** 1630 * @return Specifies the type of image region. 1631 */ 1632 public ImagingSelection3DGraphicType getRegionType() { 1633 return this.regionType == null ? null : this.regionType.getValue(); 1634 } 1635 1636 /** 1637 * @param value Specifies the type of image region. 1638 */ 1639 public ImageRegion3DComponent setRegionType(ImagingSelection3DGraphicType value) { 1640 if (this.regionType == null) 1641 this.regionType = new Enumeration<ImagingSelection3DGraphicType>(new ImagingSelection3DGraphicTypeEnumFactory()); 1642 this.regionType.setValue(value); 1643 return this; 1644 } 1645 1646 /** 1647 * @return {@link #coordinate} (The coordinates describing the image region. Encoded as an ordered set of (x,y,z) triplets (in mm and may be negative) that define a region of interest in the patient-relative Reference Coordinate System defined by ImagingSelection.frameOfReferenceUid element.) 1648 */ 1649 public List<DecimalType> getCoordinate() { 1650 if (this.coordinate == null) 1651 this.coordinate = new ArrayList<DecimalType>(); 1652 return this.coordinate; 1653 } 1654 1655 /** 1656 * @return Returns a reference to <code>this</code> for easy method chaining 1657 */ 1658 public ImageRegion3DComponent setCoordinate(List<DecimalType> theCoordinate) { 1659 this.coordinate = theCoordinate; 1660 return this; 1661 } 1662 1663 public boolean hasCoordinate() { 1664 if (this.coordinate == null) 1665 return false; 1666 for (DecimalType item : this.coordinate) 1667 if (!item.isEmpty()) 1668 return true; 1669 return false; 1670 } 1671 1672 /** 1673 * @return {@link #coordinate} (The coordinates describing the image region. Encoded as an ordered set of (x,y,z) triplets (in mm and may be negative) that define a region of interest in the patient-relative Reference Coordinate System defined by ImagingSelection.frameOfReferenceUid element.) 1674 */ 1675 public DecimalType addCoordinateElement() {//2 1676 DecimalType t = new DecimalType(); 1677 if (this.coordinate == null) 1678 this.coordinate = new ArrayList<DecimalType>(); 1679 this.coordinate.add(t); 1680 return t; 1681 } 1682 1683 /** 1684 * @param value {@link #coordinate} (The coordinates describing the image region. Encoded as an ordered set of (x,y,z) triplets (in mm and may be negative) that define a region of interest in the patient-relative Reference Coordinate System defined by ImagingSelection.frameOfReferenceUid element.) 1685 */ 1686 public ImageRegion3DComponent addCoordinate(BigDecimal value) { //1 1687 DecimalType t = new DecimalType(); 1688 t.setValue(value); 1689 if (this.coordinate == null) 1690 this.coordinate = new ArrayList<DecimalType>(); 1691 this.coordinate.add(t); 1692 return this; 1693 } 1694 1695 /** 1696 * @param value {@link #coordinate} (The coordinates describing the image region. Encoded as an ordered set of (x,y,z) triplets (in mm and may be negative) that define a region of interest in the patient-relative Reference Coordinate System defined by ImagingSelection.frameOfReferenceUid element.) 1697 */ 1698 public boolean hasCoordinate(BigDecimal value) { 1699 if (this.coordinate == null) 1700 return false; 1701 for (DecimalType v : this.coordinate) 1702 if (v.getValue().equals(value)) // decimal 1703 return true; 1704 return false; 1705 } 1706 1707 protected void listChildren(List<Property> children) { 1708 super.listChildren(children); 1709 children.add(new Property("regionType", "code", "Specifies the type of image region.", 0, 1, regionType)); 1710 children.add(new Property("coordinate", "decimal", "The coordinates describing the image region. Encoded as an ordered set of (x,y,z) triplets (in mm and may be negative) that define a region of interest in the patient-relative Reference Coordinate System defined by ImagingSelection.frameOfReferenceUid element.", 0, java.lang.Integer.MAX_VALUE, coordinate)); 1711 } 1712 1713 @Override 1714 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1715 switch (_hash) { 1716 case -1990487986: /*regionType*/ return new Property("regionType", "code", "Specifies the type of image region.", 0, 1, regionType); 1717 case 198931832: /*coordinate*/ return new Property("coordinate", "decimal", "The coordinates describing the image region. Encoded as an ordered set of (x,y,z) triplets (in mm and may be negative) that define a region of interest in the patient-relative Reference Coordinate System defined by ImagingSelection.frameOfReferenceUid element.", 0, java.lang.Integer.MAX_VALUE, coordinate); 1718 default: return super.getNamedProperty(_hash, _name, _checkValid); 1719 } 1720 1721 } 1722 1723 @Override 1724 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1725 switch (hash) { 1726 case -1990487986: /*regionType*/ return this.regionType == null ? new Base[0] : new Base[] {this.regionType}; // Enumeration<ImagingSelection3DGraphicType> 1727 case 198931832: /*coordinate*/ return this.coordinate == null ? new Base[0] : this.coordinate.toArray(new Base[this.coordinate.size()]); // DecimalType 1728 default: return super.getProperty(hash, name, checkValid); 1729 } 1730 1731 } 1732 1733 @Override 1734 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1735 switch (hash) { 1736 case -1990487986: // regionType 1737 value = new ImagingSelection3DGraphicTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1738 this.regionType = (Enumeration) value; // Enumeration<ImagingSelection3DGraphicType> 1739 return value; 1740 case 198931832: // coordinate 1741 this.getCoordinate().add(TypeConvertor.castToDecimal(value)); // DecimalType 1742 return value; 1743 default: return super.setProperty(hash, name, value); 1744 } 1745 1746 } 1747 1748 @Override 1749 public Base setProperty(String name, Base value) throws FHIRException { 1750 if (name.equals("regionType")) { 1751 value = new ImagingSelection3DGraphicTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1752 this.regionType = (Enumeration) value; // Enumeration<ImagingSelection3DGraphicType> 1753 } else if (name.equals("coordinate")) { 1754 this.getCoordinate().add(TypeConvertor.castToDecimal(value)); 1755 } else 1756 return super.setProperty(name, value); 1757 return value; 1758 } 1759 1760 @Override 1761 public void removeChild(String name, Base value) throws FHIRException { 1762 if (name.equals("regionType")) { 1763 value = new ImagingSelection3DGraphicTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1764 this.regionType = (Enumeration) value; // Enumeration<ImagingSelection3DGraphicType> 1765 } else if (name.equals("coordinate")) { 1766 this.getCoordinate().remove(value); 1767 } else 1768 super.removeChild(name, value); 1769 1770 } 1771 1772 @Override 1773 public Base makeProperty(int hash, String name) throws FHIRException { 1774 switch (hash) { 1775 case -1990487986: return getRegionTypeElement(); 1776 case 198931832: return addCoordinateElement(); 1777 default: return super.makeProperty(hash, name); 1778 } 1779 1780 } 1781 1782 @Override 1783 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1784 switch (hash) { 1785 case -1990487986: /*regionType*/ return new String[] {"code"}; 1786 case 198931832: /*coordinate*/ return new String[] {"decimal"}; 1787 default: return super.getTypesForProperty(hash, name); 1788 } 1789 1790 } 1791 1792 @Override 1793 public Base addChild(String name) throws FHIRException { 1794 if (name.equals("regionType")) { 1795 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.instance.imageRegion3D.regionType"); 1796 } 1797 else if (name.equals("coordinate")) { 1798 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.instance.imageRegion3D.coordinate"); 1799 } 1800 else 1801 return super.addChild(name); 1802 } 1803 1804 public ImageRegion3DComponent copy() { 1805 ImageRegion3DComponent dst = new ImageRegion3DComponent(); 1806 copyValues(dst); 1807 return dst; 1808 } 1809 1810 public void copyValues(ImageRegion3DComponent dst) { 1811 super.copyValues(dst); 1812 dst.regionType = regionType == null ? null : regionType.copy(); 1813 if (coordinate != null) { 1814 dst.coordinate = new ArrayList<DecimalType>(); 1815 for (DecimalType i : coordinate) 1816 dst.coordinate.add(i.copy()); 1817 }; 1818 } 1819 1820 @Override 1821 public boolean equalsDeep(Base other_) { 1822 if (!super.equalsDeep(other_)) 1823 return false; 1824 if (!(other_ instanceof ImageRegion3DComponent)) 1825 return false; 1826 ImageRegion3DComponent o = (ImageRegion3DComponent) other_; 1827 return compareDeep(regionType, o.regionType, true) && compareDeep(coordinate, o.coordinate, true) 1828 ; 1829 } 1830 1831 @Override 1832 public boolean equalsShallow(Base other_) { 1833 if (!super.equalsShallow(other_)) 1834 return false; 1835 if (!(other_ instanceof ImageRegion3DComponent)) 1836 return false; 1837 ImageRegion3DComponent o = (ImageRegion3DComponent) other_; 1838 return compareValues(regionType, o.regionType, true) && compareValues(coordinate, o.coordinate, true) 1839 ; 1840 } 1841 1842 public boolean isEmpty() { 1843 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(regionType, coordinate); 1844 } 1845 1846 public String fhirType() { 1847 return "ImagingSelection.instance.imageRegion3D"; 1848 1849 } 1850 1851 } 1852 1853 /** 1854 * A unique identifier assigned to this imaging selection. 1855 */ 1856 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1857 @Description(shortDefinition="Business Identifier for Imaging Selection", formalDefinition="A unique identifier assigned to this imaging selection." ) 1858 protected List<Identifier> identifier; 1859 1860 /** 1861 * The current state of the ImagingSelection resource. This is not the status of any ImagingStudy, ServiceRequest, or Task resources associated with the ImagingSelection. 1862 */ 1863 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 1864 @Description(shortDefinition="available | entered-in-error | unknown", formalDefinition="The current state of the ImagingSelection resource. This is not the status of any ImagingStudy, ServiceRequest, or Task resources associated with the ImagingSelection." ) 1865 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/imagingselection-status") 1866 protected Enumeration<ImagingSelectionStatus> status; 1867 1868 /** 1869 * The patient, or group of patients, location, device, organization, procedure or practitioner this imaging selection is about and into whose or what record the imaging selection is placed. 1870 */ 1871 @Child(name = "subject", type = {Patient.class, Group.class, Device.class, Location.class, Organization.class, Procedure.class, Practitioner.class, Medication.class, Substance.class, Specimen.class}, order=2, min=0, max=1, modifier=false, summary=true) 1872 @Description(shortDefinition="Subject of the selected instances", formalDefinition="The patient, or group of patients, location, device, organization, procedure or practitioner this imaging selection is about and into whose or what record the imaging selection is placed." ) 1873 protected Reference subject; 1874 1875 /** 1876 * The date and time this imaging selection was created. 1877 */ 1878 @Child(name = "issued", type = {InstantType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1879 @Description(shortDefinition="Date / Time when this imaging selection was created", formalDefinition="The date and time this imaging selection was created." ) 1880 protected InstantType issued; 1881 1882 /** 1883 * Selector of the instances ? human or machine. 1884 */ 1885 @Child(name = "performer", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1886 @Description(shortDefinition="Selector of the instances (human or machine)", formalDefinition="Selector of the instances ? human or machine." ) 1887 protected List<ImagingSelectionPerformerComponent> performer; 1888 1889 /** 1890 * A list of the diagnostic requests that resulted in this imaging selection being performed. 1891 */ 1892 @Child(name = "basedOn", type = {CarePlan.class, ServiceRequest.class, Appointment.class, AppointmentResponse.class, Task.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1893 @Description(shortDefinition="Associated request", formalDefinition="A list of the diagnostic requests that resulted in this imaging selection being performed." ) 1894 protected List<Reference> basedOn; 1895 1896 /** 1897 * Classifies the imaging selection. 1898 */ 1899 @Child(name = "category", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1900 @Description(shortDefinition="Classifies the imaging selection", formalDefinition="Classifies the imaging selection." ) 1901 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://dicom.nema.org/medical/dicom/current/output/chtml/part16/sect_CID_7010.html") 1902 protected List<CodeableConcept> category; 1903 1904 /** 1905 * Reason for referencing the selected content. 1906 */ 1907 @Child(name = "code", type = {CodeableConcept.class}, order=7, min=1, max=1, modifier=false, summary=true) 1908 @Description(shortDefinition="Imaging Selection purpose text or code", formalDefinition="Reason for referencing the selected content." ) 1909 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://dicom.nema.org/medical/dicom/current/output/chtml/part16/sect_CID_7010.html") 1910 protected CodeableConcept code; 1911 1912 /** 1913 * The Study Instance UID for the DICOM Study from which the images were selected. 1914 */ 1915 @Child(name = "studyUid", type = {IdType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1916 @Description(shortDefinition="DICOM Study Instance UID", formalDefinition="The Study Instance UID for the DICOM Study from which the images were selected." ) 1917 protected IdType studyUid; 1918 1919 /** 1920 * The imaging study from which the imaging selection is made. 1921 */ 1922 @Child(name = "derivedFrom", type = {ImagingStudy.class, DocumentReference.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1923 @Description(shortDefinition="The imaging study from which the imaging selection is derived", formalDefinition="The imaging study from which the imaging selection is made." ) 1924 protected List<Reference> derivedFrom; 1925 1926 /** 1927 * The network service providing retrieval access to the selected images, frames, etc. See implementation notes for information about using DICOM endpoints. 1928 */ 1929 @Child(name = "endpoint", type = {Endpoint.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1930 @Description(shortDefinition="The network service providing retrieval for the images referenced in the imaging selection", formalDefinition="The network service providing retrieval access to the selected images, frames, etc. See implementation notes for information about using DICOM endpoints." ) 1931 protected List<Reference> endpoint; 1932 1933 /** 1934 * The Series Instance UID for the DICOM Series from which the images were selected. 1935 */ 1936 @Child(name = "seriesUid", type = {IdType.class}, order=11, min=0, max=1, modifier=false, summary=true) 1937 @Description(shortDefinition="DICOM Series Instance UID", formalDefinition="The Series Instance UID for the DICOM Series from which the images were selected." ) 1938 protected IdType seriesUid; 1939 1940 /** 1941 * The Series Number for the DICOM Series from which the images were selected. 1942 */ 1943 @Child(name = "seriesNumber", type = {UnsignedIntType.class}, order=12, min=0, max=1, modifier=false, summary=true) 1944 @Description(shortDefinition="DICOM Series Number", formalDefinition="The Series Number for the DICOM Series from which the images were selected." ) 1945 protected UnsignedIntType seriesNumber; 1946 1947 /** 1948 * The Frame of Reference UID identifying the coordinate system that conveys spatial and/or temporal information for the selected images or frames. 1949 */ 1950 @Child(name = "frameOfReferenceUid", type = {IdType.class}, order=13, min=0, max=1, modifier=false, summary=true) 1951 @Description(shortDefinition="The Frame of Reference UID for the selected images", formalDefinition="The Frame of Reference UID identifying the coordinate system that conveys spatial and/or temporal information for the selected images or frames." ) 1952 protected IdType frameOfReferenceUid; 1953 1954 /** 1955 * The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. 1956 */ 1957 @Child(name = "bodySite", type = {CodeableReference.class}, order=14, min=0, max=1, modifier=false, summary=true) 1958 @Description(shortDefinition="Body part examined", formalDefinition="The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings." ) 1959 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 1960 protected CodeableReference bodySite; 1961 1962 /** 1963 * The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus. 1964 */ 1965 @Child(name = "focus", type = {ImagingSelection.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1966 @Description(shortDefinition="Related resource that is the focus for the imaging selection", formalDefinition="The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus." ) 1967 protected List<Reference> focus; 1968 1969 /** 1970 * Each imaging selection includes one or more selected DICOM SOP instances. 1971 */ 1972 @Child(name = "instance", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1973 @Description(shortDefinition="The selected instances", formalDefinition="Each imaging selection includes one or more selected DICOM SOP instances." ) 1974 protected List<ImagingSelectionInstanceComponent> instance; 1975 1976 private static final long serialVersionUID = -1733487270L; 1977 1978 /** 1979 * Constructor 1980 */ 1981 public ImagingSelection() { 1982 super(); 1983 } 1984 1985 /** 1986 * Constructor 1987 */ 1988 public ImagingSelection(ImagingSelectionStatus status, CodeableConcept code) { 1989 super(); 1990 this.setStatus(status); 1991 this.setCode(code); 1992 } 1993 1994 /** 1995 * @return {@link #identifier} (A unique identifier assigned to this imaging selection.) 1996 */ 1997 public List<Identifier> getIdentifier() { 1998 if (this.identifier == null) 1999 this.identifier = new ArrayList<Identifier>(); 2000 return this.identifier; 2001 } 2002 2003 /** 2004 * @return Returns a reference to <code>this</code> for easy method chaining 2005 */ 2006 public ImagingSelection setIdentifier(List<Identifier> theIdentifier) { 2007 this.identifier = theIdentifier; 2008 return this; 2009 } 2010 2011 public boolean hasIdentifier() { 2012 if (this.identifier == null) 2013 return false; 2014 for (Identifier item : this.identifier) 2015 if (!item.isEmpty()) 2016 return true; 2017 return false; 2018 } 2019 2020 public Identifier addIdentifier() { //3 2021 Identifier t = new Identifier(); 2022 if (this.identifier == null) 2023 this.identifier = new ArrayList<Identifier>(); 2024 this.identifier.add(t); 2025 return t; 2026 } 2027 2028 public ImagingSelection addIdentifier(Identifier t) { //3 2029 if (t == null) 2030 return this; 2031 if (this.identifier == null) 2032 this.identifier = new ArrayList<Identifier>(); 2033 this.identifier.add(t); 2034 return this; 2035 } 2036 2037 /** 2038 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2039 */ 2040 public Identifier getIdentifierFirstRep() { 2041 if (getIdentifier().isEmpty()) { 2042 addIdentifier(); 2043 } 2044 return getIdentifier().get(0); 2045 } 2046 2047 /** 2048 * @return {@link #status} (The current state of the ImagingSelection resource. This is not the status of any ImagingStudy, ServiceRequest, or Task resources associated with the ImagingSelection.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2049 */ 2050 public Enumeration<ImagingSelectionStatus> getStatusElement() { 2051 if (this.status == null) 2052 if (Configuration.errorOnAutoCreate()) 2053 throw new Error("Attempt to auto-create ImagingSelection.status"); 2054 else if (Configuration.doAutoCreate()) 2055 this.status = new Enumeration<ImagingSelectionStatus>(new ImagingSelectionStatusEnumFactory()); // bb 2056 return this.status; 2057 } 2058 2059 public boolean hasStatusElement() { 2060 return this.status != null && !this.status.isEmpty(); 2061 } 2062 2063 public boolean hasStatus() { 2064 return this.status != null && !this.status.isEmpty(); 2065 } 2066 2067 /** 2068 * @param value {@link #status} (The current state of the ImagingSelection resource. This is not the status of any ImagingStudy, ServiceRequest, or Task resources associated with the ImagingSelection.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2069 */ 2070 public ImagingSelection setStatusElement(Enumeration<ImagingSelectionStatus> value) { 2071 this.status = value; 2072 return this; 2073 } 2074 2075 /** 2076 * @return The current state of the ImagingSelection resource. This is not the status of any ImagingStudy, ServiceRequest, or Task resources associated with the ImagingSelection. 2077 */ 2078 public ImagingSelectionStatus getStatus() { 2079 return this.status == null ? null : this.status.getValue(); 2080 } 2081 2082 /** 2083 * @param value The current state of the ImagingSelection resource. This is not the status of any ImagingStudy, ServiceRequest, or Task resources associated with the ImagingSelection. 2084 */ 2085 public ImagingSelection setStatus(ImagingSelectionStatus value) { 2086 if (this.status == null) 2087 this.status = new Enumeration<ImagingSelectionStatus>(new ImagingSelectionStatusEnumFactory()); 2088 this.status.setValue(value); 2089 return this; 2090 } 2091 2092 /** 2093 * @return {@link #subject} (The patient, or group of patients, location, device, organization, procedure or practitioner this imaging selection is about and into whose or what record the imaging selection is placed.) 2094 */ 2095 public Reference getSubject() { 2096 if (this.subject == null) 2097 if (Configuration.errorOnAutoCreate()) 2098 throw new Error("Attempt to auto-create ImagingSelection.subject"); 2099 else if (Configuration.doAutoCreate()) 2100 this.subject = new Reference(); // cc 2101 return this.subject; 2102 } 2103 2104 public boolean hasSubject() { 2105 return this.subject != null && !this.subject.isEmpty(); 2106 } 2107 2108 /** 2109 * @param value {@link #subject} (The patient, or group of patients, location, device, organization, procedure or practitioner this imaging selection is about and into whose or what record the imaging selection is placed.) 2110 */ 2111 public ImagingSelection setSubject(Reference value) { 2112 this.subject = value; 2113 return this; 2114 } 2115 2116 /** 2117 * @return {@link #issued} (The date and time this imaging selection was created.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 2118 */ 2119 public InstantType getIssuedElement() { 2120 if (this.issued == null) 2121 if (Configuration.errorOnAutoCreate()) 2122 throw new Error("Attempt to auto-create ImagingSelection.issued"); 2123 else if (Configuration.doAutoCreate()) 2124 this.issued = new InstantType(); // bb 2125 return this.issued; 2126 } 2127 2128 public boolean hasIssuedElement() { 2129 return this.issued != null && !this.issued.isEmpty(); 2130 } 2131 2132 public boolean hasIssued() { 2133 return this.issued != null && !this.issued.isEmpty(); 2134 } 2135 2136 /** 2137 * @param value {@link #issued} (The date and time this imaging selection was created.). This is the underlying object with id, value and extensions. The accessor "getIssued" gives direct access to the value 2138 */ 2139 public ImagingSelection setIssuedElement(InstantType value) { 2140 this.issued = value; 2141 return this; 2142 } 2143 2144 /** 2145 * @return The date and time this imaging selection was created. 2146 */ 2147 public Date getIssued() { 2148 return this.issued == null ? null : this.issued.getValue(); 2149 } 2150 2151 /** 2152 * @param value The date and time this imaging selection was created. 2153 */ 2154 public ImagingSelection setIssued(Date value) { 2155 if (value == null) 2156 this.issued = null; 2157 else { 2158 if (this.issued == null) 2159 this.issued = new InstantType(); 2160 this.issued.setValue(value); 2161 } 2162 return this; 2163 } 2164 2165 /** 2166 * @return {@link #performer} (Selector of the instances ? human or machine.) 2167 */ 2168 public List<ImagingSelectionPerformerComponent> getPerformer() { 2169 if (this.performer == null) 2170 this.performer = new ArrayList<ImagingSelectionPerformerComponent>(); 2171 return this.performer; 2172 } 2173 2174 /** 2175 * @return Returns a reference to <code>this</code> for easy method chaining 2176 */ 2177 public ImagingSelection setPerformer(List<ImagingSelectionPerformerComponent> thePerformer) { 2178 this.performer = thePerformer; 2179 return this; 2180 } 2181 2182 public boolean hasPerformer() { 2183 if (this.performer == null) 2184 return false; 2185 for (ImagingSelectionPerformerComponent item : this.performer) 2186 if (!item.isEmpty()) 2187 return true; 2188 return false; 2189 } 2190 2191 public ImagingSelectionPerformerComponent addPerformer() { //3 2192 ImagingSelectionPerformerComponent t = new ImagingSelectionPerformerComponent(); 2193 if (this.performer == null) 2194 this.performer = new ArrayList<ImagingSelectionPerformerComponent>(); 2195 this.performer.add(t); 2196 return t; 2197 } 2198 2199 public ImagingSelection addPerformer(ImagingSelectionPerformerComponent t) { //3 2200 if (t == null) 2201 return this; 2202 if (this.performer == null) 2203 this.performer = new ArrayList<ImagingSelectionPerformerComponent>(); 2204 this.performer.add(t); 2205 return this; 2206 } 2207 2208 /** 2209 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 2210 */ 2211 public ImagingSelectionPerformerComponent getPerformerFirstRep() { 2212 if (getPerformer().isEmpty()) { 2213 addPerformer(); 2214 } 2215 return getPerformer().get(0); 2216 } 2217 2218 /** 2219 * @return {@link #basedOn} (A list of the diagnostic requests that resulted in this imaging selection being performed.) 2220 */ 2221 public List<Reference> getBasedOn() { 2222 if (this.basedOn == null) 2223 this.basedOn = new ArrayList<Reference>(); 2224 return this.basedOn; 2225 } 2226 2227 /** 2228 * @return Returns a reference to <code>this</code> for easy method chaining 2229 */ 2230 public ImagingSelection setBasedOn(List<Reference> theBasedOn) { 2231 this.basedOn = theBasedOn; 2232 return this; 2233 } 2234 2235 public boolean hasBasedOn() { 2236 if (this.basedOn == null) 2237 return false; 2238 for (Reference item : this.basedOn) 2239 if (!item.isEmpty()) 2240 return true; 2241 return false; 2242 } 2243 2244 public Reference addBasedOn() { //3 2245 Reference t = new Reference(); 2246 if (this.basedOn == null) 2247 this.basedOn = new ArrayList<Reference>(); 2248 this.basedOn.add(t); 2249 return t; 2250 } 2251 2252 public ImagingSelection addBasedOn(Reference t) { //3 2253 if (t == null) 2254 return this; 2255 if (this.basedOn == null) 2256 this.basedOn = new ArrayList<Reference>(); 2257 this.basedOn.add(t); 2258 return this; 2259 } 2260 2261 /** 2262 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 2263 */ 2264 public Reference getBasedOnFirstRep() { 2265 if (getBasedOn().isEmpty()) { 2266 addBasedOn(); 2267 } 2268 return getBasedOn().get(0); 2269 } 2270 2271 /** 2272 * @return {@link #category} (Classifies the imaging selection.) 2273 */ 2274 public List<CodeableConcept> getCategory() { 2275 if (this.category == null) 2276 this.category = new ArrayList<CodeableConcept>(); 2277 return this.category; 2278 } 2279 2280 /** 2281 * @return Returns a reference to <code>this</code> for easy method chaining 2282 */ 2283 public ImagingSelection setCategory(List<CodeableConcept> theCategory) { 2284 this.category = theCategory; 2285 return this; 2286 } 2287 2288 public boolean hasCategory() { 2289 if (this.category == null) 2290 return false; 2291 for (CodeableConcept item : this.category) 2292 if (!item.isEmpty()) 2293 return true; 2294 return false; 2295 } 2296 2297 public CodeableConcept addCategory() { //3 2298 CodeableConcept t = new CodeableConcept(); 2299 if (this.category == null) 2300 this.category = new ArrayList<CodeableConcept>(); 2301 this.category.add(t); 2302 return t; 2303 } 2304 2305 public ImagingSelection addCategory(CodeableConcept t) { //3 2306 if (t == null) 2307 return this; 2308 if (this.category == null) 2309 this.category = new ArrayList<CodeableConcept>(); 2310 this.category.add(t); 2311 return this; 2312 } 2313 2314 /** 2315 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 2316 */ 2317 public CodeableConcept getCategoryFirstRep() { 2318 if (getCategory().isEmpty()) { 2319 addCategory(); 2320 } 2321 return getCategory().get(0); 2322 } 2323 2324 /** 2325 * @return {@link #code} (Reason for referencing the selected content.) 2326 */ 2327 public CodeableConcept getCode() { 2328 if (this.code == null) 2329 if (Configuration.errorOnAutoCreate()) 2330 throw new Error("Attempt to auto-create ImagingSelection.code"); 2331 else if (Configuration.doAutoCreate()) 2332 this.code = new CodeableConcept(); // cc 2333 return this.code; 2334 } 2335 2336 public boolean hasCode() { 2337 return this.code != null && !this.code.isEmpty(); 2338 } 2339 2340 /** 2341 * @param value {@link #code} (Reason for referencing the selected content.) 2342 */ 2343 public ImagingSelection setCode(CodeableConcept value) { 2344 this.code = value; 2345 return this; 2346 } 2347 2348 /** 2349 * @return {@link #studyUid} (The Study Instance UID for the DICOM Study from which the images were selected.). This is the underlying object with id, value and extensions. The accessor "getStudyUid" gives direct access to the value 2350 */ 2351 public IdType getStudyUidElement() { 2352 if (this.studyUid == null) 2353 if (Configuration.errorOnAutoCreate()) 2354 throw new Error("Attempt to auto-create ImagingSelection.studyUid"); 2355 else if (Configuration.doAutoCreate()) 2356 this.studyUid = new IdType(); // bb 2357 return this.studyUid; 2358 } 2359 2360 public boolean hasStudyUidElement() { 2361 return this.studyUid != null && !this.studyUid.isEmpty(); 2362 } 2363 2364 public boolean hasStudyUid() { 2365 return this.studyUid != null && !this.studyUid.isEmpty(); 2366 } 2367 2368 /** 2369 * @param value {@link #studyUid} (The Study Instance UID for the DICOM Study from which the images were selected.). This is the underlying object with id, value and extensions. The accessor "getStudyUid" gives direct access to the value 2370 */ 2371 public ImagingSelection setStudyUidElement(IdType value) { 2372 this.studyUid = value; 2373 return this; 2374 } 2375 2376 /** 2377 * @return The Study Instance UID for the DICOM Study from which the images were selected. 2378 */ 2379 public String getStudyUid() { 2380 return this.studyUid == null ? null : this.studyUid.getValue(); 2381 } 2382 2383 /** 2384 * @param value The Study Instance UID for the DICOM Study from which the images were selected. 2385 */ 2386 public ImagingSelection setStudyUid(String value) { 2387 if (Utilities.noString(value)) 2388 this.studyUid = null; 2389 else { 2390 if (this.studyUid == null) 2391 this.studyUid = new IdType(); 2392 this.studyUid.setValue(value); 2393 } 2394 return this; 2395 } 2396 2397 /** 2398 * @return {@link #derivedFrom} (The imaging study from which the imaging selection is made.) 2399 */ 2400 public List<Reference> getDerivedFrom() { 2401 if (this.derivedFrom == null) 2402 this.derivedFrom = new ArrayList<Reference>(); 2403 return this.derivedFrom; 2404 } 2405 2406 /** 2407 * @return Returns a reference to <code>this</code> for easy method chaining 2408 */ 2409 public ImagingSelection setDerivedFrom(List<Reference> theDerivedFrom) { 2410 this.derivedFrom = theDerivedFrom; 2411 return this; 2412 } 2413 2414 public boolean hasDerivedFrom() { 2415 if (this.derivedFrom == null) 2416 return false; 2417 for (Reference item : this.derivedFrom) 2418 if (!item.isEmpty()) 2419 return true; 2420 return false; 2421 } 2422 2423 public Reference addDerivedFrom() { //3 2424 Reference t = new Reference(); 2425 if (this.derivedFrom == null) 2426 this.derivedFrom = new ArrayList<Reference>(); 2427 this.derivedFrom.add(t); 2428 return t; 2429 } 2430 2431 public ImagingSelection addDerivedFrom(Reference t) { //3 2432 if (t == null) 2433 return this; 2434 if (this.derivedFrom == null) 2435 this.derivedFrom = new ArrayList<Reference>(); 2436 this.derivedFrom.add(t); 2437 return this; 2438 } 2439 2440 /** 2441 * @return The first repetition of repeating field {@link #derivedFrom}, creating it if it does not already exist {3} 2442 */ 2443 public Reference getDerivedFromFirstRep() { 2444 if (getDerivedFrom().isEmpty()) { 2445 addDerivedFrom(); 2446 } 2447 return getDerivedFrom().get(0); 2448 } 2449 2450 /** 2451 * @return {@link #endpoint} (The network service providing retrieval access to the selected images, frames, etc. See implementation notes for information about using DICOM endpoints.) 2452 */ 2453 public List<Reference> getEndpoint() { 2454 if (this.endpoint == null) 2455 this.endpoint = new ArrayList<Reference>(); 2456 return this.endpoint; 2457 } 2458 2459 /** 2460 * @return Returns a reference to <code>this</code> for easy method chaining 2461 */ 2462 public ImagingSelection setEndpoint(List<Reference> theEndpoint) { 2463 this.endpoint = theEndpoint; 2464 return this; 2465 } 2466 2467 public boolean hasEndpoint() { 2468 if (this.endpoint == null) 2469 return false; 2470 for (Reference item : this.endpoint) 2471 if (!item.isEmpty()) 2472 return true; 2473 return false; 2474 } 2475 2476 public Reference addEndpoint() { //3 2477 Reference t = new Reference(); 2478 if (this.endpoint == null) 2479 this.endpoint = new ArrayList<Reference>(); 2480 this.endpoint.add(t); 2481 return t; 2482 } 2483 2484 public ImagingSelection addEndpoint(Reference t) { //3 2485 if (t == null) 2486 return this; 2487 if (this.endpoint == null) 2488 this.endpoint = new ArrayList<Reference>(); 2489 this.endpoint.add(t); 2490 return this; 2491 } 2492 2493 /** 2494 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist {3} 2495 */ 2496 public Reference getEndpointFirstRep() { 2497 if (getEndpoint().isEmpty()) { 2498 addEndpoint(); 2499 } 2500 return getEndpoint().get(0); 2501 } 2502 2503 /** 2504 * @return {@link #seriesUid} (The Series Instance UID for the DICOM Series from which the images were selected.). This is the underlying object with id, value and extensions. The accessor "getSeriesUid" gives direct access to the value 2505 */ 2506 public IdType getSeriesUidElement() { 2507 if (this.seriesUid == null) 2508 if (Configuration.errorOnAutoCreate()) 2509 throw new Error("Attempt to auto-create ImagingSelection.seriesUid"); 2510 else if (Configuration.doAutoCreate()) 2511 this.seriesUid = new IdType(); // bb 2512 return this.seriesUid; 2513 } 2514 2515 public boolean hasSeriesUidElement() { 2516 return this.seriesUid != null && !this.seriesUid.isEmpty(); 2517 } 2518 2519 public boolean hasSeriesUid() { 2520 return this.seriesUid != null && !this.seriesUid.isEmpty(); 2521 } 2522 2523 /** 2524 * @param value {@link #seriesUid} (The Series Instance UID for the DICOM Series from which the images were selected.). This is the underlying object with id, value and extensions. The accessor "getSeriesUid" gives direct access to the value 2525 */ 2526 public ImagingSelection setSeriesUidElement(IdType value) { 2527 this.seriesUid = value; 2528 return this; 2529 } 2530 2531 /** 2532 * @return The Series Instance UID for the DICOM Series from which the images were selected. 2533 */ 2534 public String getSeriesUid() { 2535 return this.seriesUid == null ? null : this.seriesUid.getValue(); 2536 } 2537 2538 /** 2539 * @param value The Series Instance UID for the DICOM Series from which the images were selected. 2540 */ 2541 public ImagingSelection setSeriesUid(String value) { 2542 if (Utilities.noString(value)) 2543 this.seriesUid = null; 2544 else { 2545 if (this.seriesUid == null) 2546 this.seriesUid = new IdType(); 2547 this.seriesUid.setValue(value); 2548 } 2549 return this; 2550 } 2551 2552 /** 2553 * @return {@link #seriesNumber} (The Series Number for the DICOM Series from which the images were selected.). This is the underlying object with id, value and extensions. The accessor "getSeriesNumber" gives direct access to the value 2554 */ 2555 public UnsignedIntType getSeriesNumberElement() { 2556 if (this.seriesNumber == null) 2557 if (Configuration.errorOnAutoCreate()) 2558 throw new Error("Attempt to auto-create ImagingSelection.seriesNumber"); 2559 else if (Configuration.doAutoCreate()) 2560 this.seriesNumber = new UnsignedIntType(); // bb 2561 return this.seriesNumber; 2562 } 2563 2564 public boolean hasSeriesNumberElement() { 2565 return this.seriesNumber != null && !this.seriesNumber.isEmpty(); 2566 } 2567 2568 public boolean hasSeriesNumber() { 2569 return this.seriesNumber != null && !this.seriesNumber.isEmpty(); 2570 } 2571 2572 /** 2573 * @param value {@link #seriesNumber} (The Series Number for the DICOM Series from which the images were selected.). This is the underlying object with id, value and extensions. The accessor "getSeriesNumber" gives direct access to the value 2574 */ 2575 public ImagingSelection setSeriesNumberElement(UnsignedIntType value) { 2576 this.seriesNumber = value; 2577 return this; 2578 } 2579 2580 /** 2581 * @return The Series Number for the DICOM Series from which the images were selected. 2582 */ 2583 public int getSeriesNumber() { 2584 return this.seriesNumber == null || this.seriesNumber.isEmpty() ? 0 : this.seriesNumber.getValue(); 2585 } 2586 2587 /** 2588 * @param value The Series Number for the DICOM Series from which the images were selected. 2589 */ 2590 public ImagingSelection setSeriesNumber(int value) { 2591 if (this.seriesNumber == null) 2592 this.seriesNumber = new UnsignedIntType(); 2593 this.seriesNumber.setValue(value); 2594 return this; 2595 } 2596 2597 /** 2598 * @return {@link #frameOfReferenceUid} (The Frame of Reference UID identifying the coordinate system that conveys spatial and/or temporal information for the selected images or frames.). This is the underlying object with id, value and extensions. The accessor "getFrameOfReferenceUid" gives direct access to the value 2599 */ 2600 public IdType getFrameOfReferenceUidElement() { 2601 if (this.frameOfReferenceUid == null) 2602 if (Configuration.errorOnAutoCreate()) 2603 throw new Error("Attempt to auto-create ImagingSelection.frameOfReferenceUid"); 2604 else if (Configuration.doAutoCreate()) 2605 this.frameOfReferenceUid = new IdType(); // bb 2606 return this.frameOfReferenceUid; 2607 } 2608 2609 public boolean hasFrameOfReferenceUidElement() { 2610 return this.frameOfReferenceUid != null && !this.frameOfReferenceUid.isEmpty(); 2611 } 2612 2613 public boolean hasFrameOfReferenceUid() { 2614 return this.frameOfReferenceUid != null && !this.frameOfReferenceUid.isEmpty(); 2615 } 2616 2617 /** 2618 * @param value {@link #frameOfReferenceUid} (The Frame of Reference UID identifying the coordinate system that conveys spatial and/or temporal information for the selected images or frames.). This is the underlying object with id, value and extensions. The accessor "getFrameOfReferenceUid" gives direct access to the value 2619 */ 2620 public ImagingSelection setFrameOfReferenceUidElement(IdType value) { 2621 this.frameOfReferenceUid = value; 2622 return this; 2623 } 2624 2625 /** 2626 * @return The Frame of Reference UID identifying the coordinate system that conveys spatial and/or temporal information for the selected images or frames. 2627 */ 2628 public String getFrameOfReferenceUid() { 2629 return this.frameOfReferenceUid == null ? null : this.frameOfReferenceUid.getValue(); 2630 } 2631 2632 /** 2633 * @param value The Frame of Reference UID identifying the coordinate system that conveys spatial and/or temporal information for the selected images or frames. 2634 */ 2635 public ImagingSelection setFrameOfReferenceUid(String value) { 2636 if (Utilities.noString(value)) 2637 this.frameOfReferenceUid = null; 2638 else { 2639 if (this.frameOfReferenceUid == null) 2640 this.frameOfReferenceUid = new IdType(); 2641 this.frameOfReferenceUid.setValue(value); 2642 } 2643 return this; 2644 } 2645 2646 /** 2647 * @return {@link #bodySite} (The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings.) 2648 */ 2649 public CodeableReference getBodySite() { 2650 if (this.bodySite == null) 2651 if (Configuration.errorOnAutoCreate()) 2652 throw new Error("Attempt to auto-create ImagingSelection.bodySite"); 2653 else if (Configuration.doAutoCreate()) 2654 this.bodySite = new CodeableReference(); // cc 2655 return this.bodySite; 2656 } 2657 2658 public boolean hasBodySite() { 2659 return this.bodySite != null && !this.bodySite.isEmpty(); 2660 } 2661 2662 /** 2663 * @param value {@link #bodySite} (The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings.) 2664 */ 2665 public ImagingSelection setBodySite(CodeableReference value) { 2666 this.bodySite = value; 2667 return this; 2668 } 2669 2670 /** 2671 * @return {@link #focus} (The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.) 2672 */ 2673 public List<Reference> getFocus() { 2674 if (this.focus == null) 2675 this.focus = new ArrayList<Reference>(); 2676 return this.focus; 2677 } 2678 2679 /** 2680 * @return Returns a reference to <code>this</code> for easy method chaining 2681 */ 2682 public ImagingSelection setFocus(List<Reference> theFocus) { 2683 this.focus = theFocus; 2684 return this; 2685 } 2686 2687 public boolean hasFocus() { 2688 if (this.focus == null) 2689 return false; 2690 for (Reference item : this.focus) 2691 if (!item.isEmpty()) 2692 return true; 2693 return false; 2694 } 2695 2696 public Reference addFocus() { //3 2697 Reference t = new Reference(); 2698 if (this.focus == null) 2699 this.focus = new ArrayList<Reference>(); 2700 this.focus.add(t); 2701 return t; 2702 } 2703 2704 public ImagingSelection addFocus(Reference t) { //3 2705 if (t == null) 2706 return this; 2707 if (this.focus == null) 2708 this.focus = new ArrayList<Reference>(); 2709 this.focus.add(t); 2710 return this; 2711 } 2712 2713 /** 2714 * @return The first repetition of repeating field {@link #focus}, creating it if it does not already exist {3} 2715 */ 2716 public Reference getFocusFirstRep() { 2717 if (getFocus().isEmpty()) { 2718 addFocus(); 2719 } 2720 return getFocus().get(0); 2721 } 2722 2723 /** 2724 * @return {@link #instance} (Each imaging selection includes one or more selected DICOM SOP instances.) 2725 */ 2726 public List<ImagingSelectionInstanceComponent> getInstance() { 2727 if (this.instance == null) 2728 this.instance = new ArrayList<ImagingSelectionInstanceComponent>(); 2729 return this.instance; 2730 } 2731 2732 /** 2733 * @return Returns a reference to <code>this</code> for easy method chaining 2734 */ 2735 public ImagingSelection setInstance(List<ImagingSelectionInstanceComponent> theInstance) { 2736 this.instance = theInstance; 2737 return this; 2738 } 2739 2740 public boolean hasInstance() { 2741 if (this.instance == null) 2742 return false; 2743 for (ImagingSelectionInstanceComponent item : this.instance) 2744 if (!item.isEmpty()) 2745 return true; 2746 return false; 2747 } 2748 2749 public ImagingSelectionInstanceComponent addInstance() { //3 2750 ImagingSelectionInstanceComponent t = new ImagingSelectionInstanceComponent(); 2751 if (this.instance == null) 2752 this.instance = new ArrayList<ImagingSelectionInstanceComponent>(); 2753 this.instance.add(t); 2754 return t; 2755 } 2756 2757 public ImagingSelection addInstance(ImagingSelectionInstanceComponent t) { //3 2758 if (t == null) 2759 return this; 2760 if (this.instance == null) 2761 this.instance = new ArrayList<ImagingSelectionInstanceComponent>(); 2762 this.instance.add(t); 2763 return this; 2764 } 2765 2766 /** 2767 * @return The first repetition of repeating field {@link #instance}, creating it if it does not already exist {3} 2768 */ 2769 public ImagingSelectionInstanceComponent getInstanceFirstRep() { 2770 if (getInstance().isEmpty()) { 2771 addInstance(); 2772 } 2773 return getInstance().get(0); 2774 } 2775 2776 protected void listChildren(List<Property> children) { 2777 super.listChildren(children); 2778 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this imaging selection.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2779 children.add(new Property("status", "code", "The current state of the ImagingSelection resource. This is not the status of any ImagingStudy, ServiceRequest, or Task resources associated with the ImagingSelection.", 0, 1, status)); 2780 children.add(new Property("subject", "Reference(Patient|Group|Device|Location|Organization|Procedure|Practitioner|Medication|Substance|Specimen)", "The patient, or group of patients, location, device, organization, procedure or practitioner this imaging selection is about and into whose or what record the imaging selection is placed.", 0, 1, subject)); 2781 children.add(new Property("issued", "instant", "The date and time this imaging selection was created.", 0, 1, issued)); 2782 children.add(new Property("performer", "", "Selector of the instances ? human or machine.", 0, java.lang.Integer.MAX_VALUE, performer)); 2783 children.add(new Property("basedOn", "Reference(CarePlan|ServiceRequest|Appointment|AppointmentResponse|Task)", "A list of the diagnostic requests that resulted in this imaging selection being performed.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2784 children.add(new Property("category", "CodeableConcept", "Classifies the imaging selection.", 0, java.lang.Integer.MAX_VALUE, category)); 2785 children.add(new Property("code", "CodeableConcept", "Reason for referencing the selected content.", 0, 1, code)); 2786 children.add(new Property("studyUid", "id", "The Study Instance UID for the DICOM Study from which the images were selected.", 0, 1, studyUid)); 2787 children.add(new Property("derivedFrom", "Reference(ImagingStudy|DocumentReference)", "The imaging study from which the imaging selection is made.", 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 2788 children.add(new Property("endpoint", "Reference(Endpoint)", "The network service providing retrieval access to the selected images, frames, etc. See implementation notes for information about using DICOM endpoints.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 2789 children.add(new Property("seriesUid", "id", "The Series Instance UID for the DICOM Series from which the images were selected.", 0, 1, seriesUid)); 2790 children.add(new Property("seriesNumber", "unsignedInt", "The Series Number for the DICOM Series from which the images were selected.", 0, 1, seriesNumber)); 2791 children.add(new Property("frameOfReferenceUid", "id", "The Frame of Reference UID identifying the coordinate system that conveys spatial and/or temporal information for the selected images or frames.", 0, 1, frameOfReferenceUid)); 2792 children.add(new Property("bodySite", "CodeableReference(BodyStructure)", "The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings.", 0, 1, bodySite)); 2793 children.add(new Property("focus", "Reference(ImagingSelection)", "The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.", 0, java.lang.Integer.MAX_VALUE, focus)); 2794 children.add(new Property("instance", "", "Each imaging selection includes one or more selected DICOM SOP instances.", 0, java.lang.Integer.MAX_VALUE, instance)); 2795 } 2796 2797 @Override 2798 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2799 switch (_hash) { 2800 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this imaging selection.", 0, java.lang.Integer.MAX_VALUE, identifier); 2801 case -892481550: /*status*/ return new Property("status", "code", "The current state of the ImagingSelection resource. This is not the status of any ImagingStudy, ServiceRequest, or Task resources associated with the ImagingSelection.", 0, 1, status); 2802 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Device|Location|Organization|Procedure|Practitioner|Medication|Substance|Specimen)", "The patient, or group of patients, location, device, organization, procedure or practitioner this imaging selection is about and into whose or what record the imaging selection is placed.", 0, 1, subject); 2803 case -1179159893: /*issued*/ return new Property("issued", "instant", "The date and time this imaging selection was created.", 0, 1, issued); 2804 case 481140686: /*performer*/ return new Property("performer", "", "Selector of the instances ? human or machine.", 0, java.lang.Integer.MAX_VALUE, performer); 2805 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|ServiceRequest|Appointment|AppointmentResponse|Task)", "A list of the diagnostic requests that resulted in this imaging selection being performed.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2806 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Classifies the imaging selection.", 0, java.lang.Integer.MAX_VALUE, category); 2807 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Reason for referencing the selected content.", 0, 1, code); 2808 case 1876590023: /*studyUid*/ return new Property("studyUid", "id", "The Study Instance UID for the DICOM Study from which the images were selected.", 0, 1, studyUid); 2809 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "Reference(ImagingStudy|DocumentReference)", "The imaging study from which the imaging selection is made.", 0, java.lang.Integer.MAX_VALUE, derivedFrom); 2810 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "The network service providing retrieval access to the selected images, frames, etc. See implementation notes for information about using DICOM endpoints.", 0, java.lang.Integer.MAX_VALUE, endpoint); 2811 case -569596327: /*seriesUid*/ return new Property("seriesUid", "id", "The Series Instance UID for the DICOM Series from which the images were selected.", 0, 1, seriesUid); 2812 case 382652576: /*seriesNumber*/ return new Property("seriesNumber", "unsignedInt", "The Series Number for the DICOM Series from which the images were selected.", 0, 1, seriesNumber); 2813 case 828378953: /*frameOfReferenceUid*/ return new Property("frameOfReferenceUid", "id", "The Frame of Reference UID identifying the coordinate system that conveys spatial and/or temporal information for the selected images or frames.", 0, 1, frameOfReferenceUid); 2814 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableReference(BodyStructure)", "The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings.", 0, 1, bodySite); 2815 case 97604824: /*focus*/ return new Property("focus", "Reference(ImagingSelection)", "The actual focus of an observation when it is not the patient of record representing something or someone associated with the patient such as a spouse, parent, fetus, or donor. For example, fetus observations in a mother's record. The focus of an observation could also be an existing condition, an intervention, the subject's diet, another observation of the subject, or a body structure such as tumor or implanted device. An example use case would be using the Observation resource to capture whether the mother is trained to change her child's tracheostomy tube. In this example, the child is the patient of record and the mother is the focus.", 0, java.lang.Integer.MAX_VALUE, focus); 2816 case 555127957: /*instance*/ return new Property("instance", "", "Each imaging selection includes one or more selected DICOM SOP instances.", 0, java.lang.Integer.MAX_VALUE, instance); 2817 default: return super.getNamedProperty(_hash, _name, _checkValid); 2818 } 2819 2820 } 2821 2822 @Override 2823 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2824 switch (hash) { 2825 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2826 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ImagingSelectionStatus> 2827 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2828 case -1179159893: /*issued*/ return this.issued == null ? new Base[0] : new Base[] {this.issued}; // InstantType 2829 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // ImagingSelectionPerformerComponent 2830 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2831 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2832 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2833 case 1876590023: /*studyUid*/ return this.studyUid == null ? new Base[0] : new Base[] {this.studyUid}; // IdType 2834 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // Reference 2835 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 2836 case -569596327: /*seriesUid*/ return this.seriesUid == null ? new Base[0] : new Base[] {this.seriesUid}; // IdType 2837 case 382652576: /*seriesNumber*/ return this.seriesNumber == null ? new Base[0] : new Base[] {this.seriesNumber}; // UnsignedIntType 2838 case 828378953: /*frameOfReferenceUid*/ return this.frameOfReferenceUid == null ? new Base[0] : new Base[] {this.frameOfReferenceUid}; // IdType 2839 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableReference 2840 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // Reference 2841 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : this.instance.toArray(new Base[this.instance.size()]); // ImagingSelectionInstanceComponent 2842 default: return super.getProperty(hash, name, checkValid); 2843 } 2844 2845 } 2846 2847 @Override 2848 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2849 switch (hash) { 2850 case -1618432855: // identifier 2851 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2852 return value; 2853 case -892481550: // status 2854 value = new ImagingSelectionStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2855 this.status = (Enumeration) value; // Enumeration<ImagingSelectionStatus> 2856 return value; 2857 case -1867885268: // subject 2858 this.subject = TypeConvertor.castToReference(value); // Reference 2859 return value; 2860 case -1179159893: // issued 2861 this.issued = TypeConvertor.castToInstant(value); // InstantType 2862 return value; 2863 case 481140686: // performer 2864 this.getPerformer().add((ImagingSelectionPerformerComponent) value); // ImagingSelectionPerformerComponent 2865 return value; 2866 case -332612366: // basedOn 2867 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 2868 return value; 2869 case 50511102: // category 2870 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2871 return value; 2872 case 3059181: // code 2873 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2874 return value; 2875 case 1876590023: // studyUid 2876 this.studyUid = TypeConvertor.castToId(value); // IdType 2877 return value; 2878 case 1077922663: // derivedFrom 2879 this.getDerivedFrom().add(TypeConvertor.castToReference(value)); // Reference 2880 return value; 2881 case 1741102485: // endpoint 2882 this.getEndpoint().add(TypeConvertor.castToReference(value)); // Reference 2883 return value; 2884 case -569596327: // seriesUid 2885 this.seriesUid = TypeConvertor.castToId(value); // IdType 2886 return value; 2887 case 382652576: // seriesNumber 2888 this.seriesNumber = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 2889 return value; 2890 case 828378953: // frameOfReferenceUid 2891 this.frameOfReferenceUid = TypeConvertor.castToId(value); // IdType 2892 return value; 2893 case 1702620169: // bodySite 2894 this.bodySite = TypeConvertor.castToCodeableReference(value); // CodeableReference 2895 return value; 2896 case 97604824: // focus 2897 this.getFocus().add(TypeConvertor.castToReference(value)); // Reference 2898 return value; 2899 case 555127957: // instance 2900 this.getInstance().add((ImagingSelectionInstanceComponent) value); // ImagingSelectionInstanceComponent 2901 return value; 2902 default: return super.setProperty(hash, name, value); 2903 } 2904 2905 } 2906 2907 @Override 2908 public Base setProperty(String name, Base value) throws FHIRException { 2909 if (name.equals("identifier")) { 2910 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2911 } else if (name.equals("status")) { 2912 value = new ImagingSelectionStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2913 this.status = (Enumeration) value; // Enumeration<ImagingSelectionStatus> 2914 } else if (name.equals("subject")) { 2915 this.subject = TypeConvertor.castToReference(value); // Reference 2916 } else if (name.equals("issued")) { 2917 this.issued = TypeConvertor.castToInstant(value); // InstantType 2918 } else if (name.equals("performer")) { 2919 this.getPerformer().add((ImagingSelectionPerformerComponent) value); 2920 } else if (name.equals("basedOn")) { 2921 this.getBasedOn().add(TypeConvertor.castToReference(value)); 2922 } else if (name.equals("category")) { 2923 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 2924 } else if (name.equals("code")) { 2925 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2926 } else if (name.equals("studyUid")) { 2927 this.studyUid = TypeConvertor.castToId(value); // IdType 2928 } else if (name.equals("derivedFrom")) { 2929 this.getDerivedFrom().add(TypeConvertor.castToReference(value)); 2930 } else if (name.equals("endpoint")) { 2931 this.getEndpoint().add(TypeConvertor.castToReference(value)); 2932 } else if (name.equals("seriesUid")) { 2933 this.seriesUid = TypeConvertor.castToId(value); // IdType 2934 } else if (name.equals("seriesNumber")) { 2935 this.seriesNumber = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 2936 } else if (name.equals("frameOfReferenceUid")) { 2937 this.frameOfReferenceUid = TypeConvertor.castToId(value); // IdType 2938 } else if (name.equals("bodySite")) { 2939 this.bodySite = TypeConvertor.castToCodeableReference(value); // CodeableReference 2940 } else if (name.equals("focus")) { 2941 this.getFocus().add(TypeConvertor.castToReference(value)); 2942 } else if (name.equals("instance")) { 2943 this.getInstance().add((ImagingSelectionInstanceComponent) value); 2944 } else 2945 return super.setProperty(name, value); 2946 return value; 2947 } 2948 2949 @Override 2950 public void removeChild(String name, Base value) throws FHIRException { 2951 if (name.equals("identifier")) { 2952 this.getIdentifier().remove(value); 2953 } else if (name.equals("status")) { 2954 value = new ImagingSelectionStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2955 this.status = (Enumeration) value; // Enumeration<ImagingSelectionStatus> 2956 } else if (name.equals("subject")) { 2957 this.subject = null; 2958 } else if (name.equals("issued")) { 2959 this.issued = null; 2960 } else if (name.equals("performer")) { 2961 this.getPerformer().remove((ImagingSelectionPerformerComponent) value); 2962 } else if (name.equals("basedOn")) { 2963 this.getBasedOn().remove(value); 2964 } else if (name.equals("category")) { 2965 this.getCategory().remove(value); 2966 } else if (name.equals("code")) { 2967 this.code = null; 2968 } else if (name.equals("studyUid")) { 2969 this.studyUid = null; 2970 } else if (name.equals("derivedFrom")) { 2971 this.getDerivedFrom().remove(value); 2972 } else if (name.equals("endpoint")) { 2973 this.getEndpoint().remove(value); 2974 } else if (name.equals("seriesUid")) { 2975 this.seriesUid = null; 2976 } else if (name.equals("seriesNumber")) { 2977 this.seriesNumber = null; 2978 } else if (name.equals("frameOfReferenceUid")) { 2979 this.frameOfReferenceUid = null; 2980 } else if (name.equals("bodySite")) { 2981 this.bodySite = null; 2982 } else if (name.equals("focus")) { 2983 this.getFocus().remove(value); 2984 } else if (name.equals("instance")) { 2985 this.getInstance().remove((ImagingSelectionInstanceComponent) value); 2986 } else 2987 super.removeChild(name, value); 2988 2989 } 2990 2991 @Override 2992 public Base makeProperty(int hash, String name) throws FHIRException { 2993 switch (hash) { 2994 case -1618432855: return addIdentifier(); 2995 case -892481550: return getStatusElement(); 2996 case -1867885268: return getSubject(); 2997 case -1179159893: return getIssuedElement(); 2998 case 481140686: return addPerformer(); 2999 case -332612366: return addBasedOn(); 3000 case 50511102: return addCategory(); 3001 case 3059181: return getCode(); 3002 case 1876590023: return getStudyUidElement(); 3003 case 1077922663: return addDerivedFrom(); 3004 case 1741102485: return addEndpoint(); 3005 case -569596327: return getSeriesUidElement(); 3006 case 382652576: return getSeriesNumberElement(); 3007 case 828378953: return getFrameOfReferenceUidElement(); 3008 case 1702620169: return getBodySite(); 3009 case 97604824: return addFocus(); 3010 case 555127957: return addInstance(); 3011 default: return super.makeProperty(hash, name); 3012 } 3013 3014 } 3015 3016 @Override 3017 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3018 switch (hash) { 3019 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3020 case -892481550: /*status*/ return new String[] {"code"}; 3021 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3022 case -1179159893: /*issued*/ return new String[] {"instant"}; 3023 case 481140686: /*performer*/ return new String[] {}; 3024 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 3025 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3026 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3027 case 1876590023: /*studyUid*/ return new String[] {"id"}; 3028 case 1077922663: /*derivedFrom*/ return new String[] {"Reference"}; 3029 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 3030 case -569596327: /*seriesUid*/ return new String[] {"id"}; 3031 case 382652576: /*seriesNumber*/ return new String[] {"unsignedInt"}; 3032 case 828378953: /*frameOfReferenceUid*/ return new String[] {"id"}; 3033 case 1702620169: /*bodySite*/ return new String[] {"CodeableReference"}; 3034 case 97604824: /*focus*/ return new String[] {"Reference"}; 3035 case 555127957: /*instance*/ return new String[] {}; 3036 default: return super.getTypesForProperty(hash, name); 3037 } 3038 3039 } 3040 3041 @Override 3042 public Base addChild(String name) throws FHIRException { 3043 if (name.equals("identifier")) { 3044 return addIdentifier(); 3045 } 3046 else if (name.equals("status")) { 3047 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.status"); 3048 } 3049 else if (name.equals("subject")) { 3050 this.subject = new Reference(); 3051 return this.subject; 3052 } 3053 else if (name.equals("issued")) { 3054 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.issued"); 3055 } 3056 else if (name.equals("performer")) { 3057 return addPerformer(); 3058 } 3059 else if (name.equals("basedOn")) { 3060 return addBasedOn(); 3061 } 3062 else if (name.equals("category")) { 3063 return addCategory(); 3064 } 3065 else if (name.equals("code")) { 3066 this.code = new CodeableConcept(); 3067 return this.code; 3068 } 3069 else if (name.equals("studyUid")) { 3070 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.studyUid"); 3071 } 3072 else if (name.equals("derivedFrom")) { 3073 return addDerivedFrom(); 3074 } 3075 else if (name.equals("endpoint")) { 3076 return addEndpoint(); 3077 } 3078 else if (name.equals("seriesUid")) { 3079 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.seriesUid"); 3080 } 3081 else if (name.equals("seriesNumber")) { 3082 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.seriesNumber"); 3083 } 3084 else if (name.equals("frameOfReferenceUid")) { 3085 throw new FHIRException("Cannot call addChild on a singleton property ImagingSelection.frameOfReferenceUid"); 3086 } 3087 else if (name.equals("bodySite")) { 3088 this.bodySite = new CodeableReference(); 3089 return this.bodySite; 3090 } 3091 else if (name.equals("focus")) { 3092 return addFocus(); 3093 } 3094 else if (name.equals("instance")) { 3095 return addInstance(); 3096 } 3097 else 3098 return super.addChild(name); 3099 } 3100 3101 public String fhirType() { 3102 return "ImagingSelection"; 3103 3104 } 3105 3106 public ImagingSelection copy() { 3107 ImagingSelection dst = new ImagingSelection(); 3108 copyValues(dst); 3109 return dst; 3110 } 3111 3112 public void copyValues(ImagingSelection dst) { 3113 super.copyValues(dst); 3114 if (identifier != null) { 3115 dst.identifier = new ArrayList<Identifier>(); 3116 for (Identifier i : identifier) 3117 dst.identifier.add(i.copy()); 3118 }; 3119 dst.status = status == null ? null : status.copy(); 3120 dst.subject = subject == null ? null : subject.copy(); 3121 dst.issued = issued == null ? null : issued.copy(); 3122 if (performer != null) { 3123 dst.performer = new ArrayList<ImagingSelectionPerformerComponent>(); 3124 for (ImagingSelectionPerformerComponent i : performer) 3125 dst.performer.add(i.copy()); 3126 }; 3127 if (basedOn != null) { 3128 dst.basedOn = new ArrayList<Reference>(); 3129 for (Reference i : basedOn) 3130 dst.basedOn.add(i.copy()); 3131 }; 3132 if (category != null) { 3133 dst.category = new ArrayList<CodeableConcept>(); 3134 for (CodeableConcept i : category) 3135 dst.category.add(i.copy()); 3136 }; 3137 dst.code = code == null ? null : code.copy(); 3138 dst.studyUid = studyUid == null ? null : studyUid.copy(); 3139 if (derivedFrom != null) { 3140 dst.derivedFrom = new ArrayList<Reference>(); 3141 for (Reference i : derivedFrom) 3142 dst.derivedFrom.add(i.copy()); 3143 }; 3144 if (endpoint != null) { 3145 dst.endpoint = new ArrayList<Reference>(); 3146 for (Reference i : endpoint) 3147 dst.endpoint.add(i.copy()); 3148 }; 3149 dst.seriesUid = seriesUid == null ? null : seriesUid.copy(); 3150 dst.seriesNumber = seriesNumber == null ? null : seriesNumber.copy(); 3151 dst.frameOfReferenceUid = frameOfReferenceUid == null ? null : frameOfReferenceUid.copy(); 3152 dst.bodySite = bodySite == null ? null : bodySite.copy(); 3153 if (focus != null) { 3154 dst.focus = new ArrayList<Reference>(); 3155 for (Reference i : focus) 3156 dst.focus.add(i.copy()); 3157 }; 3158 if (instance != null) { 3159 dst.instance = new ArrayList<ImagingSelectionInstanceComponent>(); 3160 for (ImagingSelectionInstanceComponent i : instance) 3161 dst.instance.add(i.copy()); 3162 }; 3163 } 3164 3165 protected ImagingSelection typedCopy() { 3166 return copy(); 3167 } 3168 3169 @Override 3170 public boolean equalsDeep(Base other_) { 3171 if (!super.equalsDeep(other_)) 3172 return false; 3173 if (!(other_ instanceof ImagingSelection)) 3174 return false; 3175 ImagingSelection o = (ImagingSelection) other_; 3176 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(subject, o.subject, true) 3177 && compareDeep(issued, o.issued, true) && compareDeep(performer, o.performer, true) && compareDeep(basedOn, o.basedOn, true) 3178 && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) && compareDeep(studyUid, o.studyUid, true) 3179 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(endpoint, o.endpoint, true) && compareDeep(seriesUid, o.seriesUid, true) 3180 && compareDeep(seriesNumber, o.seriesNumber, true) && compareDeep(frameOfReferenceUid, o.frameOfReferenceUid, true) 3181 && compareDeep(bodySite, o.bodySite, true) && compareDeep(focus, o.focus, true) && compareDeep(instance, o.instance, true) 3182 ; 3183 } 3184 3185 @Override 3186 public boolean equalsShallow(Base other_) { 3187 if (!super.equalsShallow(other_)) 3188 return false; 3189 if (!(other_ instanceof ImagingSelection)) 3190 return false; 3191 ImagingSelection o = (ImagingSelection) other_; 3192 return compareValues(status, o.status, true) && compareValues(issued, o.issued, true) && compareValues(studyUid, o.studyUid, true) 3193 && compareValues(seriesUid, o.seriesUid, true) && compareValues(seriesNumber, o.seriesNumber, true) 3194 && compareValues(frameOfReferenceUid, o.frameOfReferenceUid, true); 3195 } 3196 3197 public boolean isEmpty() { 3198 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, subject 3199 , issued, performer, basedOn, category, code, studyUid, derivedFrom, endpoint 3200 , seriesUid, seriesNumber, frameOfReferenceUid, bodySite, focus, instance); 3201 } 3202 3203 @Override 3204 public ResourceType getResourceType() { 3205 return ResourceType.ImagingSelection; 3206 } 3207 3208 /** 3209 * Search parameter: <b>based-on</b> 3210 * <p> 3211 * Description: <b>The request associated with an imaging selection</b><br> 3212 * Type: <b>reference</b><br> 3213 * Path: <b>ImagingSelection.basedOn</b><br> 3214 * </p> 3215 */ 3216 @SearchParamDefinition(name="based-on", path="ImagingSelection.basedOn", description="The request associated with an imaging selection", type="reference", target={Appointment.class, AppointmentResponse.class, CarePlan.class, ServiceRequest.class, Task.class } ) 3217 public static final String SP_BASED_ON = "based-on"; 3218 /** 3219 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3220 * <p> 3221 * Description: <b>The request associated with an imaging selection</b><br> 3222 * Type: <b>reference</b><br> 3223 * Path: <b>ImagingSelection.basedOn</b><br> 3224 * </p> 3225 */ 3226 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 3227 3228/** 3229 * Constant for fluent queries to be used to add include statements. Specifies 3230 * the path value of "<b>ImagingSelection:based-on</b>". 3231 */ 3232 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("ImagingSelection:based-on").toLocked(); 3233 3234 /** 3235 * Search parameter: <b>body-site</b> 3236 * <p> 3237 * Description: <b>The body site code associated with the imaging selection</b><br> 3238 * Type: <b>token</b><br> 3239 * Path: <b>ImagingSelection.bodySite.concept</b><br> 3240 * </p> 3241 */ 3242 @SearchParamDefinition(name="body-site", path="ImagingSelection.bodySite.concept", description="The body site code associated with the imaging selection", type="token" ) 3243 public static final String SP_BODY_SITE = "body-site"; 3244 /** 3245 * <b>Fluent Client</b> search parameter constant for <b>body-site</b> 3246 * <p> 3247 * Description: <b>The body site code associated with the imaging selection</b><br> 3248 * Type: <b>token</b><br> 3249 * Path: <b>ImagingSelection.bodySite.concept</b><br> 3250 * </p> 3251 */ 3252 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BODY_SITE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BODY_SITE); 3253 3254 /** 3255 * Search parameter: <b>body-structure</b> 3256 * <p> 3257 * Description: <b>The body structure associated with the imaging selection</b><br> 3258 * Type: <b>reference</b><br> 3259 * Path: <b>ImagingSelection.bodySite.reference</b><br> 3260 * </p> 3261 */ 3262 @SearchParamDefinition(name="body-structure", path="ImagingSelection.bodySite.reference", description="The body structure associated with the imaging selection", type="reference", target={BodyStructure.class } ) 3263 public static final String SP_BODY_STRUCTURE = "body-structure"; 3264 /** 3265 * <b>Fluent Client</b> search parameter constant for <b>body-structure</b> 3266 * <p> 3267 * Description: <b>The body structure associated with the imaging selection</b><br> 3268 * Type: <b>reference</b><br> 3269 * Path: <b>ImagingSelection.bodySite.reference</b><br> 3270 * </p> 3271 */ 3272 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BODY_STRUCTURE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BODY_STRUCTURE); 3273 3274/** 3275 * Constant for fluent queries to be used to add include statements. Specifies 3276 * the path value of "<b>ImagingSelection:body-structure</b>". 3277 */ 3278 public static final ca.uhn.fhir.model.api.Include INCLUDE_BODY_STRUCTURE = new ca.uhn.fhir.model.api.Include("ImagingSelection:body-structure").toLocked(); 3279 3280 /** 3281 * Search parameter: <b>derived-from</b> 3282 * <p> 3283 * Description: <b>The imaging study from which the imaging selection was derived</b><br> 3284 * Type: <b>reference</b><br> 3285 * Path: <b>ImagingSelection.derivedFrom</b><br> 3286 * </p> 3287 */ 3288 @SearchParamDefinition(name="derived-from", path="ImagingSelection.derivedFrom", description="The imaging study from which the imaging selection was derived", type="reference", target={DocumentReference.class, ImagingStudy.class } ) 3289 public static final String SP_DERIVED_FROM = "derived-from"; 3290 /** 3291 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 3292 * <p> 3293 * Description: <b>The imaging study from which the imaging selection was derived</b><br> 3294 * Type: <b>reference</b><br> 3295 * Path: <b>ImagingSelection.derivedFrom</b><br> 3296 * </p> 3297 */ 3298 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 3299 3300/** 3301 * Constant for fluent queries to be used to add include statements. Specifies 3302 * the path value of "<b>ImagingSelection:derived-from</b>". 3303 */ 3304 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("ImagingSelection:derived-from").toLocked(); 3305 3306 /** 3307 * Search parameter: <b>issued</b> 3308 * <p> 3309 * Description: <b>The date / time the imaging selection was created</b><br> 3310 * Type: <b>date</b><br> 3311 * Path: <b>ImagingSelection.issued</b><br> 3312 * </p> 3313 */ 3314 @SearchParamDefinition(name="issued", path="ImagingSelection.issued", description="The date / time the imaging selection was created", type="date" ) 3315 public static final String SP_ISSUED = "issued"; 3316 /** 3317 * <b>Fluent Client</b> search parameter constant for <b>issued</b> 3318 * <p> 3319 * Description: <b>The date / time the imaging selection was created</b><br> 3320 * Type: <b>date</b><br> 3321 * Path: <b>ImagingSelection.issued</b><br> 3322 * </p> 3323 */ 3324 public static final ca.uhn.fhir.rest.gclient.DateClientParam ISSUED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_ISSUED); 3325 3326 /** 3327 * Search parameter: <b>status</b> 3328 * <p> 3329 * Description: <b>The status of the imaging selection</b><br> 3330 * Type: <b>token</b><br> 3331 * Path: <b>ImagingSelection.status</b><br> 3332 * </p> 3333 */ 3334 @SearchParamDefinition(name="status", path="ImagingSelection.status", description="The status of the imaging selection", type="token" ) 3335 public static final String SP_STATUS = "status"; 3336 /** 3337 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3338 * <p> 3339 * Description: <b>The status of the imaging selection</b><br> 3340 * Type: <b>token</b><br> 3341 * Path: <b>ImagingSelection.status</b><br> 3342 * </p> 3343 */ 3344 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3345 3346 /** 3347 * Search parameter: <b>study-uid</b> 3348 * <p> 3349 * Description: <b>The DICOM Study Instance UID from which the images were selected</b><br> 3350 * Type: <b>token</b><br> 3351 * Path: <b>ImagingSelection.studyUid</b><br> 3352 * </p> 3353 */ 3354 @SearchParamDefinition(name="study-uid", path="ImagingSelection.studyUid", description="The DICOM Study Instance UID from which the images were selected", type="token" ) 3355 public static final String SP_STUDY_UID = "study-uid"; 3356 /** 3357 * <b>Fluent Client</b> search parameter constant for <b>study-uid</b> 3358 * <p> 3359 * Description: <b>The DICOM Study Instance UID from which the images were selected</b><br> 3360 * Type: <b>token</b><br> 3361 * Path: <b>ImagingSelection.studyUid</b><br> 3362 * </p> 3363 */ 3364 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STUDY_UID = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STUDY_UID); 3365 3366 /** 3367 * Search parameter: <b>subject</b> 3368 * <p> 3369 * Description: <b>The subject of the Imaging Selection, such as the associated Patient</b><br> 3370 * Type: <b>reference</b><br> 3371 * Path: <b>ImagingSelection.subject</b><br> 3372 * </p> 3373 */ 3374 @SearchParamDefinition(name="subject", path="ImagingSelection.subject", description="The subject of the Imaging Selection, such as the associated Patient", type="reference", target={Device.class, Group.class, Location.class, Medication.class, Organization.class, Patient.class, Practitioner.class, Procedure.class, Specimen.class, Substance.class } ) 3375 public static final String SP_SUBJECT = "subject"; 3376 /** 3377 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3378 * <p> 3379 * Description: <b>The subject of the Imaging Selection, such as the associated Patient</b><br> 3380 * Type: <b>reference</b><br> 3381 * Path: <b>ImagingSelection.subject</b><br> 3382 * </p> 3383 */ 3384 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3385 3386/** 3387 * Constant for fluent queries to be used to add include statements. Specifies 3388 * the path value of "<b>ImagingSelection:subject</b>". 3389 */ 3390 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("ImagingSelection:subject").toLocked(); 3391 3392 /** 3393 * Search parameter: <b>code</b> 3394 * <p> 3395 * Description: <b>Multiple Resources: 3396 3397* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 3398* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 3399* [AuditEvent](auditevent.html): More specific code for the event 3400* [Basic](basic.html): Kind of Resource 3401* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 3402* [Condition](condition.html): Code for the condition 3403* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 3404* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 3405* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 3406* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 3407* [ImagingSelection](imagingselection.html): The imaging selection status 3408* [List](list.html): What the purpose of this list is 3409* [Medication](medication.html): Returns medications for a specific code 3410* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 3411* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 3412* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 3413* [MedicationStatement](medicationstatement.html): Return statements of this medication code 3414* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 3415* [Observation](observation.html): The code of the observation type 3416* [Procedure](procedure.html): A code to identify a procedure 3417* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 3418* [Task](task.html): Search by task code 3419</b><br> 3420 * Type: <b>token</b><br> 3421 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 3422 * </p> 3423 */ 3424 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 3425 public static final String SP_CODE = "code"; 3426 /** 3427 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3428 * <p> 3429 * Description: <b>Multiple Resources: 3430 3431* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 3432* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 3433* [AuditEvent](auditevent.html): More specific code for the event 3434* [Basic](basic.html): Kind of Resource 3435* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 3436* [Condition](condition.html): Code for the condition 3437* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 3438* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 3439* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 3440* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 3441* [ImagingSelection](imagingselection.html): The imaging selection status 3442* [List](list.html): What the purpose of this list is 3443* [Medication](medication.html): Returns medications for a specific code 3444* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 3445* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 3446* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 3447* [MedicationStatement](medicationstatement.html): Return statements of this medication code 3448* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 3449* [Observation](observation.html): The code of the observation type 3450* [Procedure](procedure.html): A code to identify a procedure 3451* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 3452* [Task](task.html): Search by task code 3453</b><br> 3454 * Type: <b>token</b><br> 3455 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 3456 * </p> 3457 */ 3458 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 3459 3460 /** 3461 * Search parameter: <b>identifier</b> 3462 * <p> 3463 * Description: <b>Multiple Resources: 3464 3465* [Account](account.html): Account number 3466* [AdverseEvent](adverseevent.html): Business identifier for the event 3467* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3468* [Appointment](appointment.html): An Identifier of the Appointment 3469* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3470* [Basic](basic.html): Business identifier 3471* [BodyStructure](bodystructure.html): Bodystructure identifier 3472* [CarePlan](careplan.html): External Ids for this plan 3473* [CareTeam](careteam.html): External Ids for this team 3474* [ChargeItem](chargeitem.html): Business Identifier for item 3475* [Claim](claim.html): The primary identifier of the financial resource 3476* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3477* [ClinicalImpression](clinicalimpression.html): Business identifier 3478* [Communication](communication.html): Unique identifier 3479* [CommunicationRequest](communicationrequest.html): Unique identifier 3480* [Composition](composition.html): Version-independent identifier for the Composition 3481* [Condition](condition.html): A unique identifier of the condition record 3482* [Consent](consent.html): Identifier for this record (external references) 3483* [Contract](contract.html): The identity of the contract 3484* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3485* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3486* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3487* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3488* [DeviceRequest](devicerequest.html): Business identifier for request/order 3489* [DeviceUsage](deviceusage.html): Search by identifier 3490* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3491* [DocumentReference](documentreference.html): Identifier of the attachment binary 3492* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3493* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3494* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3495* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3496* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3497* [Flag](flag.html): Business identifier 3498* [Goal](goal.html): External Ids for this goal 3499* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3500* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3501* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3502* [Immunization](immunization.html): Business identifier 3503* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3504* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3505* [Invoice](invoice.html): Business Identifier for item 3506* [List](list.html): Business identifier 3507* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3508* [Medication](medication.html): Returns medications with this external identifier 3509* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3510* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3511* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3512* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3513* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3514* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3515* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3516* [Observation](observation.html): The unique id for a particular observation 3517* [Person](person.html): A person Identifier 3518* [Procedure](procedure.html): A unique identifier for a procedure 3519* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3520* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3521* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3522* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3523* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3524* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3525* [Specimen](specimen.html): The unique identifier associated with the specimen 3526* [SupplyDelivery](supplydelivery.html): External identifier 3527* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3528* [Task](task.html): Search for a task instance by its business identifier 3529* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3530</b><br> 3531 * Type: <b>token</b><br> 3532 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3533 * </p> 3534 */ 3535 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 3536 public static final String SP_IDENTIFIER = "identifier"; 3537 /** 3538 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3539 * <p> 3540 * Description: <b>Multiple Resources: 3541 3542* [Account](account.html): Account number 3543* [AdverseEvent](adverseevent.html): Business identifier for the event 3544* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3545* [Appointment](appointment.html): An Identifier of the Appointment 3546* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3547* [Basic](basic.html): Business identifier 3548* [BodyStructure](bodystructure.html): Bodystructure identifier 3549* [CarePlan](careplan.html): External Ids for this plan 3550* [CareTeam](careteam.html): External Ids for this team 3551* [ChargeItem](chargeitem.html): Business Identifier for item 3552* [Claim](claim.html): The primary identifier of the financial resource 3553* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3554* [ClinicalImpression](clinicalimpression.html): Business identifier 3555* [Communication](communication.html): Unique identifier 3556* [CommunicationRequest](communicationrequest.html): Unique identifier 3557* [Composition](composition.html): Version-independent identifier for the Composition 3558* [Condition](condition.html): A unique identifier of the condition record 3559* [Consent](consent.html): Identifier for this record (external references) 3560* [Contract](contract.html): The identity of the contract 3561* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3562* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3563* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3564* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3565* [DeviceRequest](devicerequest.html): Business identifier for request/order 3566* [DeviceUsage](deviceusage.html): Search by identifier 3567* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3568* [DocumentReference](documentreference.html): Identifier of the attachment binary 3569* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3570* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3571* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3572* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3573* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3574* [Flag](flag.html): Business identifier 3575* [Goal](goal.html): External Ids for this goal 3576* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3577* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3578* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3579* [Immunization](immunization.html): Business identifier 3580* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3581* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3582* [Invoice](invoice.html): Business Identifier for item 3583* [List](list.html): Business identifier 3584* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3585* [Medication](medication.html): Returns medications with this external identifier 3586* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3587* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3588* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3589* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3590* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3591* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3592* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3593* [Observation](observation.html): The unique id for a particular observation 3594* [Person](person.html): A person Identifier 3595* [Procedure](procedure.html): A unique identifier for a procedure 3596* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3597* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3598* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3599* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3600* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3601* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3602* [Specimen](specimen.html): The unique identifier associated with the specimen 3603* [SupplyDelivery](supplydelivery.html): External identifier 3604* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3605* [Task](task.html): Search for a task instance by its business identifier 3606* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3607</b><br> 3608 * Type: <b>token</b><br> 3609 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3610 * </p> 3611 */ 3612 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3613 3614 /** 3615 * Search parameter: <b>patient</b> 3616 * <p> 3617 * Description: <b>Multiple Resources: 3618 3619* [Account](account.html): The entity that caused the expenses 3620* [AdverseEvent](adverseevent.html): Subject impacted by event 3621* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3622* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3623* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3624* [AuditEvent](auditevent.html): Where the activity involved patient data 3625* [Basic](basic.html): Identifies the focus of this resource 3626* [BodyStructure](bodystructure.html): Who this is about 3627* [CarePlan](careplan.html): Who the care plan is for 3628* [CareTeam](careteam.html): Who care team is for 3629* [ChargeItem](chargeitem.html): Individual service was done for/to 3630* [Claim](claim.html): Patient receiving the products or services 3631* [ClaimResponse](claimresponse.html): The subject of care 3632* [ClinicalImpression](clinicalimpression.html): Patient assessed 3633* [Communication](communication.html): Focus of message 3634* [CommunicationRequest](communicationrequest.html): Focus of message 3635* [Composition](composition.html): Who and/or what the composition is about 3636* [Condition](condition.html): Who has the condition? 3637* [Consent](consent.html): Who the consent applies to 3638* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3639* [Coverage](coverage.html): Retrieve coverages for a patient 3640* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3641* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3642* [DetectedIssue](detectedissue.html): Associated patient 3643* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3644* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3645* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3646* [DocumentReference](documentreference.html): Who/what is the subject of the document 3647* [Encounter](encounter.html): The patient present at the encounter 3648* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3649* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3650* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3651* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3652* [Flag](flag.html): The identity of a subject to list flags for 3653* [Goal](goal.html): Who this goal is intended for 3654* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3655* [ImagingSelection](imagingselection.html): Who the study is about 3656* [ImagingStudy](imagingstudy.html): Who the study is about 3657* [Immunization](immunization.html): The patient for the vaccination record 3658* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3659* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3660* [Invoice](invoice.html): Recipient(s) of goods and services 3661* [List](list.html): If all resources have the same subject 3662* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3663* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3664* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3665* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3666* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3667* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3668* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3669* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3670* [Observation](observation.html): The subject that the observation is about (if patient) 3671* [Person](person.html): The Person links to this Patient 3672* [Procedure](procedure.html): Search by subject - a patient 3673* [Provenance](provenance.html): Where the activity involved patient data 3674* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3675* [RelatedPerson](relatedperson.html): The patient this related person is related to 3676* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3677* [ResearchSubject](researchsubject.html): Who or what is part of study 3678* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3679* [ServiceRequest](servicerequest.html): Search by subject - a patient 3680* [Specimen](specimen.html): The patient the specimen comes from 3681* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3682* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3683* [Task](task.html): Search by patient 3684* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3685</b><br> 3686 * Type: <b>reference</b><br> 3687 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3688 * </p> 3689 */ 3690 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 3691 public static final String SP_PATIENT = "patient"; 3692 /** 3693 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3694 * <p> 3695 * Description: <b>Multiple Resources: 3696 3697* [Account](account.html): The entity that caused the expenses 3698* [AdverseEvent](adverseevent.html): Subject impacted by event 3699* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3700* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3701* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3702* [AuditEvent](auditevent.html): Where the activity involved patient data 3703* [Basic](basic.html): Identifies the focus of this resource 3704* [BodyStructure](bodystructure.html): Who this is about 3705* [CarePlan](careplan.html): Who the care plan is for 3706* [CareTeam](careteam.html): Who care team is for 3707* [ChargeItem](chargeitem.html): Individual service was done for/to 3708* [Claim](claim.html): Patient receiving the products or services 3709* [ClaimResponse](claimresponse.html): The subject of care 3710* [ClinicalImpression](clinicalimpression.html): Patient assessed 3711* [Communication](communication.html): Focus of message 3712* [CommunicationRequest](communicationrequest.html): Focus of message 3713* [Composition](composition.html): Who and/or what the composition is about 3714* [Condition](condition.html): Who has the condition? 3715* [Consent](consent.html): Who the consent applies to 3716* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3717* [Coverage](coverage.html): Retrieve coverages for a patient 3718* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3719* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3720* [DetectedIssue](detectedissue.html): Associated patient 3721* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3722* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3723* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3724* [DocumentReference](documentreference.html): Who/what is the subject of the document 3725* [Encounter](encounter.html): The patient present at the encounter 3726* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3727* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3728* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3729* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3730* [Flag](flag.html): The identity of a subject to list flags for 3731* [Goal](goal.html): Who this goal is intended for 3732* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3733* [ImagingSelection](imagingselection.html): Who the study is about 3734* [ImagingStudy](imagingstudy.html): Who the study is about 3735* [Immunization](immunization.html): The patient for the vaccination record 3736* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3737* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3738* [Invoice](invoice.html): Recipient(s) of goods and services 3739* [List](list.html): If all resources have the same subject 3740* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3741* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3742* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3743* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3744* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3745* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3746* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3747* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3748* [Observation](observation.html): The subject that the observation is about (if patient) 3749* [Person](person.html): The Person links to this Patient 3750* [Procedure](procedure.html): Search by subject - a patient 3751* [Provenance](provenance.html): Where the activity involved patient data 3752* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3753* [RelatedPerson](relatedperson.html): The patient this related person is related to 3754* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3755* [ResearchSubject](researchsubject.html): Who or what is part of study 3756* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3757* [ServiceRequest](servicerequest.html): Search by subject - a patient 3758* [Specimen](specimen.html): The patient the specimen comes from 3759* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3760* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3761* [Task](task.html): Search by patient 3762* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3763</b><br> 3764 * Type: <b>reference</b><br> 3765 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3766 * </p> 3767 */ 3768 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3769 3770/** 3771 * Constant for fluent queries to be used to add include statements. Specifies 3772 * the path value of "<b>ImagingSelection:patient</b>". 3773 */ 3774 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ImagingSelection:patient").toLocked(); 3775 3776 3777} 3778