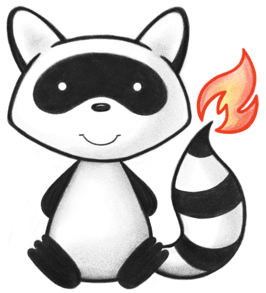
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities. 052 */ 053@ResourceDef(name="ImagingStudy", profile="http://hl7.org/fhir/StructureDefinition/ImagingStudy") 054public class ImagingStudy extends DomainResource { 055 056 public enum ImagingStudyStatus { 057 /** 058 * The existence of the imaging study is registered, but there is nothing yet available. 059 */ 060 REGISTERED, 061 /** 062 * At least one instance has been associated with this imaging study. 063 */ 064 AVAILABLE, 065 /** 066 * The imaging study is unavailable because the imaging study was not started or not completed (also sometimes called \"aborted\"). 067 */ 068 CANCELLED, 069 /** 070 * The imaging study has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".). 071 */ 072 ENTEREDINERROR, 073 /** 074 * The system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one. 075 */ 076 UNKNOWN, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static ImagingStudyStatus fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("registered".equals(codeString)) 085 return REGISTERED; 086 if ("available".equals(codeString)) 087 return AVAILABLE; 088 if ("cancelled".equals(codeString)) 089 return CANCELLED; 090 if ("entered-in-error".equals(codeString)) 091 return ENTEREDINERROR; 092 if ("unknown".equals(codeString)) 093 return UNKNOWN; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown ImagingStudyStatus code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case REGISTERED: return "registered"; 102 case AVAILABLE: return "available"; 103 case CANCELLED: return "cancelled"; 104 case ENTEREDINERROR: return "entered-in-error"; 105 case UNKNOWN: return "unknown"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case REGISTERED: return "http://hl7.org/fhir/imagingstudy-status"; 113 case AVAILABLE: return "http://hl7.org/fhir/imagingstudy-status"; 114 case CANCELLED: return "http://hl7.org/fhir/imagingstudy-status"; 115 case ENTEREDINERROR: return "http://hl7.org/fhir/imagingstudy-status"; 116 case UNKNOWN: return "http://hl7.org/fhir/imagingstudy-status"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case REGISTERED: return "The existence of the imaging study is registered, but there is nothing yet available."; 124 case AVAILABLE: return "At least one instance has been associated with this imaging study."; 125 case CANCELLED: return "The imaging study is unavailable because the imaging study was not started or not completed (also sometimes called \"aborted\")."; 126 case ENTEREDINERROR: return "The imaging study has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"cancelled\" rather than \"entered-in-error\".)."; 127 case UNKNOWN: return "The system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case REGISTERED: return "Registered"; 135 case AVAILABLE: return "Available"; 136 case CANCELLED: return "Cancelled"; 137 case ENTEREDINERROR: return "Entered in Error"; 138 case UNKNOWN: return "Unknown"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class ImagingStudyStatusEnumFactory implements EnumFactory<ImagingStudyStatus> { 146 public ImagingStudyStatus fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("registered".equals(codeString)) 151 return ImagingStudyStatus.REGISTERED; 152 if ("available".equals(codeString)) 153 return ImagingStudyStatus.AVAILABLE; 154 if ("cancelled".equals(codeString)) 155 return ImagingStudyStatus.CANCELLED; 156 if ("entered-in-error".equals(codeString)) 157 return ImagingStudyStatus.ENTEREDINERROR; 158 if ("unknown".equals(codeString)) 159 return ImagingStudyStatus.UNKNOWN; 160 throw new IllegalArgumentException("Unknown ImagingStudyStatus code '"+codeString+"'"); 161 } 162 public Enumeration<ImagingStudyStatus> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<ImagingStudyStatus>(this, ImagingStudyStatus.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<ImagingStudyStatus>(this, ImagingStudyStatus.NULL, code); 170 if ("registered".equals(codeString)) 171 return new Enumeration<ImagingStudyStatus>(this, ImagingStudyStatus.REGISTERED, code); 172 if ("available".equals(codeString)) 173 return new Enumeration<ImagingStudyStatus>(this, ImagingStudyStatus.AVAILABLE, code); 174 if ("cancelled".equals(codeString)) 175 return new Enumeration<ImagingStudyStatus>(this, ImagingStudyStatus.CANCELLED, code); 176 if ("entered-in-error".equals(codeString)) 177 return new Enumeration<ImagingStudyStatus>(this, ImagingStudyStatus.ENTEREDINERROR, code); 178 if ("unknown".equals(codeString)) 179 return new Enumeration<ImagingStudyStatus>(this, ImagingStudyStatus.UNKNOWN, code); 180 throw new FHIRException("Unknown ImagingStudyStatus code '"+codeString+"'"); 181 } 182 public String toCode(ImagingStudyStatus code) { 183 if (code == ImagingStudyStatus.NULL) 184 return null; 185 if (code == ImagingStudyStatus.REGISTERED) 186 return "registered"; 187 if (code == ImagingStudyStatus.AVAILABLE) 188 return "available"; 189 if (code == ImagingStudyStatus.CANCELLED) 190 return "cancelled"; 191 if (code == ImagingStudyStatus.ENTEREDINERROR) 192 return "entered-in-error"; 193 if (code == ImagingStudyStatus.UNKNOWN) 194 return "unknown"; 195 return "?"; 196 } 197 public String toSystem(ImagingStudyStatus code) { 198 return code.getSystem(); 199 } 200 } 201 202 @Block() 203 public static class ImagingStudySeriesComponent extends BackboneElement implements IBaseBackboneElement { 204 /** 205 * The DICOM Series Instance UID for the series. 206 */ 207 @Child(name = "uid", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 208 @Description(shortDefinition="DICOM Series Instance UID for the series", formalDefinition="The DICOM Series Instance UID for the series." ) 209 protected IdType uid; 210 211 /** 212 * The numeric identifier of this series in the study. 213 */ 214 @Child(name = "number", type = {UnsignedIntType.class}, order=2, min=0, max=1, modifier=false, summary=true) 215 @Description(shortDefinition="Numeric identifier of this series", formalDefinition="The numeric identifier of this series in the study." ) 216 protected UnsignedIntType number; 217 218 /** 219 * The distinct modality for this series. This may include both acquisition and non-acquisition modalities. 220 */ 221 @Child(name = "modality", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 222 @Description(shortDefinition="The modality used for this series", formalDefinition="The distinct modality for this series. This may include both acquisition and non-acquisition modalities." ) 223 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://dicom.nema.org/medical/dicom/current/output/chtml/part16/sect_CID_33.html") 224 protected CodeableConcept modality; 225 226 /** 227 * A description of the series. 228 */ 229 @Child(name = "description", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 230 @Description(shortDefinition="A short human readable summary of the series", formalDefinition="A description of the series." ) 231 protected StringType description; 232 233 /** 234 * Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present. 235 */ 236 @Child(name = "numberOfInstances", type = {UnsignedIntType.class}, order=5, min=0, max=1, modifier=false, summary=true) 237 @Description(shortDefinition="Number of Series Related Instances", formalDefinition="Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present." ) 238 protected UnsignedIntType numberOfInstances; 239 240 /** 241 * The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.connectionType. 242 */ 243 @Child(name = "endpoint", type = {Endpoint.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 244 @Description(shortDefinition="Series access endpoint", formalDefinition="The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.connectionType." ) 245 protected List<Reference> endpoint; 246 247 /** 248 * The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. The bodySite may indicate the laterality of body part imaged; if so, it shall be consistent with any content of ImagingStudy.series.laterality. 249 */ 250 @Child(name = "bodySite", type = {CodeableReference.class}, order=7, min=0, max=1, modifier=false, summary=true) 251 @Description(shortDefinition="Body part examined", formalDefinition="The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. The bodySite may indicate the laterality of body part imaged; if so, it shall be consistent with any content of ImagingStudy.series.laterality." ) 252 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 253 protected CodeableReference bodySite; 254 255 /** 256 * The laterality of the (possibly paired) anatomic structures examined. E.g., the left knee, both lungs, or unpaired abdomen. If present, shall be consistent with any laterality information indicated in ImagingStudy.series.bodySite. 257 */ 258 @Child(name = "laterality", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=true) 259 @Description(shortDefinition="Body part laterality", formalDefinition="The laterality of the (possibly paired) anatomic structures examined. E.g., the left knee, both lungs, or unpaired abdomen. If present, shall be consistent with any laterality information indicated in ImagingStudy.series.bodySite." ) 260 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://dicom.nema.org/medical/dicom/current/output/chtml/part16/sect_CID_244.html") 261 protected CodeableConcept laterality; 262 263 /** 264 * The specimen imaged, e.g., for whole slide imaging of a biopsy. 265 */ 266 @Child(name = "specimen", type = {Specimen.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 267 @Description(shortDefinition="Specimen imaged", formalDefinition="The specimen imaged, e.g., for whole slide imaging of a biopsy." ) 268 protected List<Reference> specimen; 269 270 /** 271 * The date and time the series was started. 272 */ 273 @Child(name = "started", type = {DateTimeType.class}, order=10, min=0, max=1, modifier=false, summary=true) 274 @Description(shortDefinition="When the series started", formalDefinition="The date and time the series was started." ) 275 protected DateTimeType started; 276 277 /** 278 * Indicates who or what performed the series and how they were involved. 279 */ 280 @Child(name = "performer", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 281 @Description(shortDefinition="Who performed the series", formalDefinition="Indicates who or what performed the series and how they were involved." ) 282 protected List<ImagingStudySeriesPerformerComponent> performer; 283 284 /** 285 * A single SOP instance within the series, e.g. an image, or presentation state. 286 */ 287 @Child(name = "instance", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 288 @Description(shortDefinition="A single SOP instance from the series", formalDefinition="A single SOP instance within the series, e.g. an image, or presentation state." ) 289 protected List<ImagingStudySeriesInstanceComponent> instance; 290 291 private static final long serialVersionUID = 755136591L; 292 293 /** 294 * Constructor 295 */ 296 public ImagingStudySeriesComponent() { 297 super(); 298 } 299 300 /** 301 * Constructor 302 */ 303 public ImagingStudySeriesComponent(String uid, CodeableConcept modality) { 304 super(); 305 this.setUid(uid); 306 this.setModality(modality); 307 } 308 309 /** 310 * @return {@link #uid} (The DICOM Series Instance UID for the series.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 311 */ 312 public IdType getUidElement() { 313 if (this.uid == null) 314 if (Configuration.errorOnAutoCreate()) 315 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.uid"); 316 else if (Configuration.doAutoCreate()) 317 this.uid = new IdType(); // bb 318 return this.uid; 319 } 320 321 public boolean hasUidElement() { 322 return this.uid != null && !this.uid.isEmpty(); 323 } 324 325 public boolean hasUid() { 326 return this.uid != null && !this.uid.isEmpty(); 327 } 328 329 /** 330 * @param value {@link #uid} (The DICOM Series Instance UID for the series.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 331 */ 332 public ImagingStudySeriesComponent setUidElement(IdType value) { 333 this.uid = value; 334 return this; 335 } 336 337 /** 338 * @return The DICOM Series Instance UID for the series. 339 */ 340 public String getUid() { 341 return this.uid == null ? null : this.uid.getValue(); 342 } 343 344 /** 345 * @param value The DICOM Series Instance UID for the series. 346 */ 347 public ImagingStudySeriesComponent setUid(String value) { 348 if (this.uid == null) 349 this.uid = new IdType(); 350 this.uid.setValue(value); 351 return this; 352 } 353 354 /** 355 * @return {@link #number} (The numeric identifier of this series in the study.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 356 */ 357 public UnsignedIntType getNumberElement() { 358 if (this.number == null) 359 if (Configuration.errorOnAutoCreate()) 360 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.number"); 361 else if (Configuration.doAutoCreate()) 362 this.number = new UnsignedIntType(); // bb 363 return this.number; 364 } 365 366 public boolean hasNumberElement() { 367 return this.number != null && !this.number.isEmpty(); 368 } 369 370 public boolean hasNumber() { 371 return this.number != null && !this.number.isEmpty(); 372 } 373 374 /** 375 * @param value {@link #number} (The numeric identifier of this series in the study.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 376 */ 377 public ImagingStudySeriesComponent setNumberElement(UnsignedIntType value) { 378 this.number = value; 379 return this; 380 } 381 382 /** 383 * @return The numeric identifier of this series in the study. 384 */ 385 public int getNumber() { 386 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 387 } 388 389 /** 390 * @param value The numeric identifier of this series in the study. 391 */ 392 public ImagingStudySeriesComponent setNumber(int value) { 393 if (this.number == null) 394 this.number = new UnsignedIntType(); 395 this.number.setValue(value); 396 return this; 397 } 398 399 /** 400 * @return {@link #modality} (The distinct modality for this series. This may include both acquisition and non-acquisition modalities.) 401 */ 402 public CodeableConcept getModality() { 403 if (this.modality == null) 404 if (Configuration.errorOnAutoCreate()) 405 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.modality"); 406 else if (Configuration.doAutoCreate()) 407 this.modality = new CodeableConcept(); // cc 408 return this.modality; 409 } 410 411 public boolean hasModality() { 412 return this.modality != null && !this.modality.isEmpty(); 413 } 414 415 /** 416 * @param value {@link #modality} (The distinct modality for this series. This may include both acquisition and non-acquisition modalities.) 417 */ 418 public ImagingStudySeriesComponent setModality(CodeableConcept value) { 419 this.modality = value; 420 return this; 421 } 422 423 /** 424 * @return {@link #description} (A description of the series.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 425 */ 426 public StringType getDescriptionElement() { 427 if (this.description == null) 428 if (Configuration.errorOnAutoCreate()) 429 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.description"); 430 else if (Configuration.doAutoCreate()) 431 this.description = new StringType(); // bb 432 return this.description; 433 } 434 435 public boolean hasDescriptionElement() { 436 return this.description != null && !this.description.isEmpty(); 437 } 438 439 public boolean hasDescription() { 440 return this.description != null && !this.description.isEmpty(); 441 } 442 443 /** 444 * @param value {@link #description} (A description of the series.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 445 */ 446 public ImagingStudySeriesComponent setDescriptionElement(StringType value) { 447 this.description = value; 448 return this; 449 } 450 451 /** 452 * @return A description of the series. 453 */ 454 public String getDescription() { 455 return this.description == null ? null : this.description.getValue(); 456 } 457 458 /** 459 * @param value A description of the series. 460 */ 461 public ImagingStudySeriesComponent setDescription(String value) { 462 if (Utilities.noString(value)) 463 this.description = null; 464 else { 465 if (this.description == null) 466 this.description = new StringType(); 467 this.description.setValue(value); 468 } 469 return this; 470 } 471 472 /** 473 * @return {@link #numberOfInstances} (Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.). This is the underlying object with id, value and extensions. The accessor "getNumberOfInstances" gives direct access to the value 474 */ 475 public UnsignedIntType getNumberOfInstancesElement() { 476 if (this.numberOfInstances == null) 477 if (Configuration.errorOnAutoCreate()) 478 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.numberOfInstances"); 479 else if (Configuration.doAutoCreate()) 480 this.numberOfInstances = new UnsignedIntType(); // bb 481 return this.numberOfInstances; 482 } 483 484 public boolean hasNumberOfInstancesElement() { 485 return this.numberOfInstances != null && !this.numberOfInstances.isEmpty(); 486 } 487 488 public boolean hasNumberOfInstances() { 489 return this.numberOfInstances != null && !this.numberOfInstances.isEmpty(); 490 } 491 492 /** 493 * @param value {@link #numberOfInstances} (Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.). This is the underlying object with id, value and extensions. The accessor "getNumberOfInstances" gives direct access to the value 494 */ 495 public ImagingStudySeriesComponent setNumberOfInstancesElement(UnsignedIntType value) { 496 this.numberOfInstances = value; 497 return this; 498 } 499 500 /** 501 * @return Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present. 502 */ 503 public int getNumberOfInstances() { 504 return this.numberOfInstances == null || this.numberOfInstances.isEmpty() ? 0 : this.numberOfInstances.getValue(); 505 } 506 507 /** 508 * @param value Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present. 509 */ 510 public ImagingStudySeriesComponent setNumberOfInstances(int value) { 511 if (this.numberOfInstances == null) 512 this.numberOfInstances = new UnsignedIntType(); 513 this.numberOfInstances.setValue(value); 514 return this; 515 } 516 517 /** 518 * @return {@link #endpoint} (The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.connectionType.) 519 */ 520 public List<Reference> getEndpoint() { 521 if (this.endpoint == null) 522 this.endpoint = new ArrayList<Reference>(); 523 return this.endpoint; 524 } 525 526 /** 527 * @return Returns a reference to <code>this</code> for easy method chaining 528 */ 529 public ImagingStudySeriesComponent setEndpoint(List<Reference> theEndpoint) { 530 this.endpoint = theEndpoint; 531 return this; 532 } 533 534 public boolean hasEndpoint() { 535 if (this.endpoint == null) 536 return false; 537 for (Reference item : this.endpoint) 538 if (!item.isEmpty()) 539 return true; 540 return false; 541 } 542 543 public Reference addEndpoint() { //3 544 Reference t = new Reference(); 545 if (this.endpoint == null) 546 this.endpoint = new ArrayList<Reference>(); 547 this.endpoint.add(t); 548 return t; 549 } 550 551 public ImagingStudySeriesComponent addEndpoint(Reference t) { //3 552 if (t == null) 553 return this; 554 if (this.endpoint == null) 555 this.endpoint = new ArrayList<Reference>(); 556 this.endpoint.add(t); 557 return this; 558 } 559 560 /** 561 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist {3} 562 */ 563 public Reference getEndpointFirstRep() { 564 if (getEndpoint().isEmpty()) { 565 addEndpoint(); 566 } 567 return getEndpoint().get(0); 568 } 569 570 /** 571 * @return {@link #bodySite} (The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. The bodySite may indicate the laterality of body part imaged; if so, it shall be consistent with any content of ImagingStudy.series.laterality.) 572 */ 573 public CodeableReference getBodySite() { 574 if (this.bodySite == null) 575 if (Configuration.errorOnAutoCreate()) 576 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.bodySite"); 577 else if (Configuration.doAutoCreate()) 578 this.bodySite = new CodeableReference(); // cc 579 return this.bodySite; 580 } 581 582 public boolean hasBodySite() { 583 return this.bodySite != null && !this.bodySite.isEmpty(); 584 } 585 586 /** 587 * @param value {@link #bodySite} (The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. The bodySite may indicate the laterality of body part imaged; if so, it shall be consistent with any content of ImagingStudy.series.laterality.) 588 */ 589 public ImagingStudySeriesComponent setBodySite(CodeableReference value) { 590 this.bodySite = value; 591 return this; 592 } 593 594 /** 595 * @return {@link #laterality} (The laterality of the (possibly paired) anatomic structures examined. E.g., the left knee, both lungs, or unpaired abdomen. If present, shall be consistent with any laterality information indicated in ImagingStudy.series.bodySite.) 596 */ 597 public CodeableConcept getLaterality() { 598 if (this.laterality == null) 599 if (Configuration.errorOnAutoCreate()) 600 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.laterality"); 601 else if (Configuration.doAutoCreate()) 602 this.laterality = new CodeableConcept(); // cc 603 return this.laterality; 604 } 605 606 public boolean hasLaterality() { 607 return this.laterality != null && !this.laterality.isEmpty(); 608 } 609 610 /** 611 * @param value {@link #laterality} (The laterality of the (possibly paired) anatomic structures examined. E.g., the left knee, both lungs, or unpaired abdomen. If present, shall be consistent with any laterality information indicated in ImagingStudy.series.bodySite.) 612 */ 613 public ImagingStudySeriesComponent setLaterality(CodeableConcept value) { 614 this.laterality = value; 615 return this; 616 } 617 618 /** 619 * @return {@link #specimen} (The specimen imaged, e.g., for whole slide imaging of a biopsy.) 620 */ 621 public List<Reference> getSpecimen() { 622 if (this.specimen == null) 623 this.specimen = new ArrayList<Reference>(); 624 return this.specimen; 625 } 626 627 /** 628 * @return Returns a reference to <code>this</code> for easy method chaining 629 */ 630 public ImagingStudySeriesComponent setSpecimen(List<Reference> theSpecimen) { 631 this.specimen = theSpecimen; 632 return this; 633 } 634 635 public boolean hasSpecimen() { 636 if (this.specimen == null) 637 return false; 638 for (Reference item : this.specimen) 639 if (!item.isEmpty()) 640 return true; 641 return false; 642 } 643 644 public Reference addSpecimen() { //3 645 Reference t = new Reference(); 646 if (this.specimen == null) 647 this.specimen = new ArrayList<Reference>(); 648 this.specimen.add(t); 649 return t; 650 } 651 652 public ImagingStudySeriesComponent addSpecimen(Reference t) { //3 653 if (t == null) 654 return this; 655 if (this.specimen == null) 656 this.specimen = new ArrayList<Reference>(); 657 this.specimen.add(t); 658 return this; 659 } 660 661 /** 662 * @return The first repetition of repeating field {@link #specimen}, creating it if it does not already exist {3} 663 */ 664 public Reference getSpecimenFirstRep() { 665 if (getSpecimen().isEmpty()) { 666 addSpecimen(); 667 } 668 return getSpecimen().get(0); 669 } 670 671 /** 672 * @return {@link #started} (The date and time the series was started.). This is the underlying object with id, value and extensions. The accessor "getStarted" gives direct access to the value 673 */ 674 public DateTimeType getStartedElement() { 675 if (this.started == null) 676 if (Configuration.errorOnAutoCreate()) 677 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.started"); 678 else if (Configuration.doAutoCreate()) 679 this.started = new DateTimeType(); // bb 680 return this.started; 681 } 682 683 public boolean hasStartedElement() { 684 return this.started != null && !this.started.isEmpty(); 685 } 686 687 public boolean hasStarted() { 688 return this.started != null && !this.started.isEmpty(); 689 } 690 691 /** 692 * @param value {@link #started} (The date and time the series was started.). This is the underlying object with id, value and extensions. The accessor "getStarted" gives direct access to the value 693 */ 694 public ImagingStudySeriesComponent setStartedElement(DateTimeType value) { 695 this.started = value; 696 return this; 697 } 698 699 /** 700 * @return The date and time the series was started. 701 */ 702 public Date getStarted() { 703 return this.started == null ? null : this.started.getValue(); 704 } 705 706 /** 707 * @param value The date and time the series was started. 708 */ 709 public ImagingStudySeriesComponent setStarted(Date value) { 710 if (value == null) 711 this.started = null; 712 else { 713 if (this.started == null) 714 this.started = new DateTimeType(); 715 this.started.setValue(value); 716 } 717 return this; 718 } 719 720 /** 721 * @return {@link #performer} (Indicates who or what performed the series and how they were involved.) 722 */ 723 public List<ImagingStudySeriesPerformerComponent> getPerformer() { 724 if (this.performer == null) 725 this.performer = new ArrayList<ImagingStudySeriesPerformerComponent>(); 726 return this.performer; 727 } 728 729 /** 730 * @return Returns a reference to <code>this</code> for easy method chaining 731 */ 732 public ImagingStudySeriesComponent setPerformer(List<ImagingStudySeriesPerformerComponent> thePerformer) { 733 this.performer = thePerformer; 734 return this; 735 } 736 737 public boolean hasPerformer() { 738 if (this.performer == null) 739 return false; 740 for (ImagingStudySeriesPerformerComponent item : this.performer) 741 if (!item.isEmpty()) 742 return true; 743 return false; 744 } 745 746 public ImagingStudySeriesPerformerComponent addPerformer() { //3 747 ImagingStudySeriesPerformerComponent t = new ImagingStudySeriesPerformerComponent(); 748 if (this.performer == null) 749 this.performer = new ArrayList<ImagingStudySeriesPerformerComponent>(); 750 this.performer.add(t); 751 return t; 752 } 753 754 public ImagingStudySeriesComponent addPerformer(ImagingStudySeriesPerformerComponent t) { //3 755 if (t == null) 756 return this; 757 if (this.performer == null) 758 this.performer = new ArrayList<ImagingStudySeriesPerformerComponent>(); 759 this.performer.add(t); 760 return this; 761 } 762 763 /** 764 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 765 */ 766 public ImagingStudySeriesPerformerComponent getPerformerFirstRep() { 767 if (getPerformer().isEmpty()) { 768 addPerformer(); 769 } 770 return getPerformer().get(0); 771 } 772 773 /** 774 * @return {@link #instance} (A single SOP instance within the series, e.g. an image, or presentation state.) 775 */ 776 public List<ImagingStudySeriesInstanceComponent> getInstance() { 777 if (this.instance == null) 778 this.instance = new ArrayList<ImagingStudySeriesInstanceComponent>(); 779 return this.instance; 780 } 781 782 /** 783 * @return Returns a reference to <code>this</code> for easy method chaining 784 */ 785 public ImagingStudySeriesComponent setInstance(List<ImagingStudySeriesInstanceComponent> theInstance) { 786 this.instance = theInstance; 787 return this; 788 } 789 790 public boolean hasInstance() { 791 if (this.instance == null) 792 return false; 793 for (ImagingStudySeriesInstanceComponent item : this.instance) 794 if (!item.isEmpty()) 795 return true; 796 return false; 797 } 798 799 public ImagingStudySeriesInstanceComponent addInstance() { //3 800 ImagingStudySeriesInstanceComponent t = new ImagingStudySeriesInstanceComponent(); 801 if (this.instance == null) 802 this.instance = new ArrayList<ImagingStudySeriesInstanceComponent>(); 803 this.instance.add(t); 804 return t; 805 } 806 807 public ImagingStudySeriesComponent addInstance(ImagingStudySeriesInstanceComponent t) { //3 808 if (t == null) 809 return this; 810 if (this.instance == null) 811 this.instance = new ArrayList<ImagingStudySeriesInstanceComponent>(); 812 this.instance.add(t); 813 return this; 814 } 815 816 /** 817 * @return The first repetition of repeating field {@link #instance}, creating it if it does not already exist {3} 818 */ 819 public ImagingStudySeriesInstanceComponent getInstanceFirstRep() { 820 if (getInstance().isEmpty()) { 821 addInstance(); 822 } 823 return getInstance().get(0); 824 } 825 826 protected void listChildren(List<Property> children) { 827 super.listChildren(children); 828 children.add(new Property("uid", "id", "The DICOM Series Instance UID for the series.", 0, 1, uid)); 829 children.add(new Property("number", "unsignedInt", "The numeric identifier of this series in the study.", 0, 1, number)); 830 children.add(new Property("modality", "CodeableConcept", "The distinct modality for this series. This may include both acquisition and non-acquisition modalities.", 0, 1, modality)); 831 children.add(new Property("description", "string", "A description of the series.", 0, 1, description)); 832 children.add(new Property("numberOfInstances", "unsignedInt", "Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.", 0, 1, numberOfInstances)); 833 children.add(new Property("endpoint", "Reference(Endpoint)", "The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.connectionType.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 834 children.add(new Property("bodySite", "CodeableReference(BodyStructure)", "The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. The bodySite may indicate the laterality of body part imaged; if so, it shall be consistent with any content of ImagingStudy.series.laterality.", 0, 1, bodySite)); 835 children.add(new Property("laterality", "CodeableConcept", "The laterality of the (possibly paired) anatomic structures examined. E.g., the left knee, both lungs, or unpaired abdomen. If present, shall be consistent with any laterality information indicated in ImagingStudy.series.bodySite.", 0, 1, laterality)); 836 children.add(new Property("specimen", "Reference(Specimen)", "The specimen imaged, e.g., for whole slide imaging of a biopsy.", 0, java.lang.Integer.MAX_VALUE, specimen)); 837 children.add(new Property("started", "dateTime", "The date and time the series was started.", 0, 1, started)); 838 children.add(new Property("performer", "", "Indicates who or what performed the series and how they were involved.", 0, java.lang.Integer.MAX_VALUE, performer)); 839 children.add(new Property("instance", "", "A single SOP instance within the series, e.g. an image, or presentation state.", 0, java.lang.Integer.MAX_VALUE, instance)); 840 } 841 842 @Override 843 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 844 switch (_hash) { 845 case 115792: /*uid*/ return new Property("uid", "id", "The DICOM Series Instance UID for the series.", 0, 1, uid); 846 case -1034364087: /*number*/ return new Property("number", "unsignedInt", "The numeric identifier of this series in the study.", 0, 1, number); 847 case -622722335: /*modality*/ return new Property("modality", "CodeableConcept", "The distinct modality for this series. This may include both acquisition and non-acquisition modalities.", 0, 1, modality); 848 case -1724546052: /*description*/ return new Property("description", "string", "A description of the series.", 0, 1, description); 849 case -1043544226: /*numberOfInstances*/ return new Property("numberOfInstances", "unsignedInt", "Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.", 0, 1, numberOfInstances); 850 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.connectionType.", 0, java.lang.Integer.MAX_VALUE, endpoint); 851 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableReference(BodyStructure)", "The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. The bodySite may indicate the laterality of body part imaged; if so, it shall be consistent with any content of ImagingStudy.series.laterality.", 0, 1, bodySite); 852 case -170291817: /*laterality*/ return new Property("laterality", "CodeableConcept", "The laterality of the (possibly paired) anatomic structures examined. E.g., the left knee, both lungs, or unpaired abdomen. If present, shall be consistent with any laterality information indicated in ImagingStudy.series.bodySite.", 0, 1, laterality); 853 case -2132868344: /*specimen*/ return new Property("specimen", "Reference(Specimen)", "The specimen imaged, e.g., for whole slide imaging of a biopsy.", 0, java.lang.Integer.MAX_VALUE, specimen); 854 case -1897185151: /*started*/ return new Property("started", "dateTime", "The date and time the series was started.", 0, 1, started); 855 case 481140686: /*performer*/ return new Property("performer", "", "Indicates who or what performed the series and how they were involved.", 0, java.lang.Integer.MAX_VALUE, performer); 856 case 555127957: /*instance*/ return new Property("instance", "", "A single SOP instance within the series, e.g. an image, or presentation state.", 0, java.lang.Integer.MAX_VALUE, instance); 857 default: return super.getNamedProperty(_hash, _name, _checkValid); 858 } 859 860 } 861 862 @Override 863 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 864 switch (hash) { 865 case 115792: /*uid*/ return this.uid == null ? new Base[0] : new Base[] {this.uid}; // IdType 866 case -1034364087: /*number*/ return this.number == null ? new Base[0] : new Base[] {this.number}; // UnsignedIntType 867 case -622722335: /*modality*/ return this.modality == null ? new Base[0] : new Base[] {this.modality}; // CodeableConcept 868 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 869 case -1043544226: /*numberOfInstances*/ return this.numberOfInstances == null ? new Base[0] : new Base[] {this.numberOfInstances}; // UnsignedIntType 870 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 871 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableReference 872 case -170291817: /*laterality*/ return this.laterality == null ? new Base[0] : new Base[] {this.laterality}; // CodeableConcept 873 case -2132868344: /*specimen*/ return this.specimen == null ? new Base[0] : this.specimen.toArray(new Base[this.specimen.size()]); // Reference 874 case -1897185151: /*started*/ return this.started == null ? new Base[0] : new Base[] {this.started}; // DateTimeType 875 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // ImagingStudySeriesPerformerComponent 876 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : this.instance.toArray(new Base[this.instance.size()]); // ImagingStudySeriesInstanceComponent 877 default: return super.getProperty(hash, name, checkValid); 878 } 879 880 } 881 882 @Override 883 public Base setProperty(int hash, String name, Base value) throws FHIRException { 884 switch (hash) { 885 case 115792: // uid 886 this.uid = TypeConvertor.castToId(value); // IdType 887 return value; 888 case -1034364087: // number 889 this.number = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 890 return value; 891 case -622722335: // modality 892 this.modality = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 893 return value; 894 case -1724546052: // description 895 this.description = TypeConvertor.castToString(value); // StringType 896 return value; 897 case -1043544226: // numberOfInstances 898 this.numberOfInstances = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 899 return value; 900 case 1741102485: // endpoint 901 this.getEndpoint().add(TypeConvertor.castToReference(value)); // Reference 902 return value; 903 case 1702620169: // bodySite 904 this.bodySite = TypeConvertor.castToCodeableReference(value); // CodeableReference 905 return value; 906 case -170291817: // laterality 907 this.laterality = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 908 return value; 909 case -2132868344: // specimen 910 this.getSpecimen().add(TypeConvertor.castToReference(value)); // Reference 911 return value; 912 case -1897185151: // started 913 this.started = TypeConvertor.castToDateTime(value); // DateTimeType 914 return value; 915 case 481140686: // performer 916 this.getPerformer().add((ImagingStudySeriesPerformerComponent) value); // ImagingStudySeriesPerformerComponent 917 return value; 918 case 555127957: // instance 919 this.getInstance().add((ImagingStudySeriesInstanceComponent) value); // ImagingStudySeriesInstanceComponent 920 return value; 921 default: return super.setProperty(hash, name, value); 922 } 923 924 } 925 926 @Override 927 public Base setProperty(String name, Base value) throws FHIRException { 928 if (name.equals("uid")) { 929 this.uid = TypeConvertor.castToId(value); // IdType 930 } else if (name.equals("number")) { 931 this.number = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 932 } else if (name.equals("modality")) { 933 this.modality = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 934 } else if (name.equals("description")) { 935 this.description = TypeConvertor.castToString(value); // StringType 936 } else if (name.equals("numberOfInstances")) { 937 this.numberOfInstances = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 938 } else if (name.equals("endpoint")) { 939 this.getEndpoint().add(TypeConvertor.castToReference(value)); 940 } else if (name.equals("bodySite")) { 941 this.bodySite = TypeConvertor.castToCodeableReference(value); // CodeableReference 942 } else if (name.equals("laterality")) { 943 this.laterality = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 944 } else if (name.equals("specimen")) { 945 this.getSpecimen().add(TypeConvertor.castToReference(value)); 946 } else if (name.equals("started")) { 947 this.started = TypeConvertor.castToDateTime(value); // DateTimeType 948 } else if (name.equals("performer")) { 949 this.getPerformer().add((ImagingStudySeriesPerformerComponent) value); 950 } else if (name.equals("instance")) { 951 this.getInstance().add((ImagingStudySeriesInstanceComponent) value); 952 } else 953 return super.setProperty(name, value); 954 return value; 955 } 956 957 @Override 958 public void removeChild(String name, Base value) throws FHIRException { 959 if (name.equals("uid")) { 960 this.uid = null; 961 } else if (name.equals("number")) { 962 this.number = null; 963 } else if (name.equals("modality")) { 964 this.modality = null; 965 } else if (name.equals("description")) { 966 this.description = null; 967 } else if (name.equals("numberOfInstances")) { 968 this.numberOfInstances = null; 969 } else if (name.equals("endpoint")) { 970 this.getEndpoint().remove(value); 971 } else if (name.equals("bodySite")) { 972 this.bodySite = null; 973 } else if (name.equals("laterality")) { 974 this.laterality = null; 975 } else if (name.equals("specimen")) { 976 this.getSpecimen().remove(value); 977 } else if (name.equals("started")) { 978 this.started = null; 979 } else if (name.equals("performer")) { 980 this.getPerformer().remove((ImagingStudySeriesPerformerComponent) value); 981 } else if (name.equals("instance")) { 982 this.getInstance().remove((ImagingStudySeriesInstanceComponent) value); 983 } else 984 super.removeChild(name, value); 985 986 } 987 988 @Override 989 public Base makeProperty(int hash, String name) throws FHIRException { 990 switch (hash) { 991 case 115792: return getUidElement(); 992 case -1034364087: return getNumberElement(); 993 case -622722335: return getModality(); 994 case -1724546052: return getDescriptionElement(); 995 case -1043544226: return getNumberOfInstancesElement(); 996 case 1741102485: return addEndpoint(); 997 case 1702620169: return getBodySite(); 998 case -170291817: return getLaterality(); 999 case -2132868344: return addSpecimen(); 1000 case -1897185151: return getStartedElement(); 1001 case 481140686: return addPerformer(); 1002 case 555127957: return addInstance(); 1003 default: return super.makeProperty(hash, name); 1004 } 1005 1006 } 1007 1008 @Override 1009 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1010 switch (hash) { 1011 case 115792: /*uid*/ return new String[] {"id"}; 1012 case -1034364087: /*number*/ return new String[] {"unsignedInt"}; 1013 case -622722335: /*modality*/ return new String[] {"CodeableConcept"}; 1014 case -1724546052: /*description*/ return new String[] {"string"}; 1015 case -1043544226: /*numberOfInstances*/ return new String[] {"unsignedInt"}; 1016 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 1017 case 1702620169: /*bodySite*/ return new String[] {"CodeableReference"}; 1018 case -170291817: /*laterality*/ return new String[] {"CodeableConcept"}; 1019 case -2132868344: /*specimen*/ return new String[] {"Reference"}; 1020 case -1897185151: /*started*/ return new String[] {"dateTime"}; 1021 case 481140686: /*performer*/ return new String[] {}; 1022 case 555127957: /*instance*/ return new String[] {}; 1023 default: return super.getTypesForProperty(hash, name); 1024 } 1025 1026 } 1027 1028 @Override 1029 public Base addChild(String name) throws FHIRException { 1030 if (name.equals("uid")) { 1031 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.series.uid"); 1032 } 1033 else if (name.equals("number")) { 1034 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.series.number"); 1035 } 1036 else if (name.equals("modality")) { 1037 this.modality = new CodeableConcept(); 1038 return this.modality; 1039 } 1040 else if (name.equals("description")) { 1041 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.series.description"); 1042 } 1043 else if (name.equals("numberOfInstances")) { 1044 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.series.numberOfInstances"); 1045 } 1046 else if (name.equals("endpoint")) { 1047 return addEndpoint(); 1048 } 1049 else if (name.equals("bodySite")) { 1050 this.bodySite = new CodeableReference(); 1051 return this.bodySite; 1052 } 1053 else if (name.equals("laterality")) { 1054 this.laterality = new CodeableConcept(); 1055 return this.laterality; 1056 } 1057 else if (name.equals("specimen")) { 1058 return addSpecimen(); 1059 } 1060 else if (name.equals("started")) { 1061 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.series.started"); 1062 } 1063 else if (name.equals("performer")) { 1064 return addPerformer(); 1065 } 1066 else if (name.equals("instance")) { 1067 return addInstance(); 1068 } 1069 else 1070 return super.addChild(name); 1071 } 1072 1073 public ImagingStudySeriesComponent copy() { 1074 ImagingStudySeriesComponent dst = new ImagingStudySeriesComponent(); 1075 copyValues(dst); 1076 return dst; 1077 } 1078 1079 public void copyValues(ImagingStudySeriesComponent dst) { 1080 super.copyValues(dst); 1081 dst.uid = uid == null ? null : uid.copy(); 1082 dst.number = number == null ? null : number.copy(); 1083 dst.modality = modality == null ? null : modality.copy(); 1084 dst.description = description == null ? null : description.copy(); 1085 dst.numberOfInstances = numberOfInstances == null ? null : numberOfInstances.copy(); 1086 if (endpoint != null) { 1087 dst.endpoint = new ArrayList<Reference>(); 1088 for (Reference i : endpoint) 1089 dst.endpoint.add(i.copy()); 1090 }; 1091 dst.bodySite = bodySite == null ? null : bodySite.copy(); 1092 dst.laterality = laterality == null ? null : laterality.copy(); 1093 if (specimen != null) { 1094 dst.specimen = new ArrayList<Reference>(); 1095 for (Reference i : specimen) 1096 dst.specimen.add(i.copy()); 1097 }; 1098 dst.started = started == null ? null : started.copy(); 1099 if (performer != null) { 1100 dst.performer = new ArrayList<ImagingStudySeriesPerformerComponent>(); 1101 for (ImagingStudySeriesPerformerComponent i : performer) 1102 dst.performer.add(i.copy()); 1103 }; 1104 if (instance != null) { 1105 dst.instance = new ArrayList<ImagingStudySeriesInstanceComponent>(); 1106 for (ImagingStudySeriesInstanceComponent i : instance) 1107 dst.instance.add(i.copy()); 1108 }; 1109 } 1110 1111 @Override 1112 public boolean equalsDeep(Base other_) { 1113 if (!super.equalsDeep(other_)) 1114 return false; 1115 if (!(other_ instanceof ImagingStudySeriesComponent)) 1116 return false; 1117 ImagingStudySeriesComponent o = (ImagingStudySeriesComponent) other_; 1118 return compareDeep(uid, o.uid, true) && compareDeep(number, o.number, true) && compareDeep(modality, o.modality, true) 1119 && compareDeep(description, o.description, true) && compareDeep(numberOfInstances, o.numberOfInstances, true) 1120 && compareDeep(endpoint, o.endpoint, true) && compareDeep(bodySite, o.bodySite, true) && compareDeep(laterality, o.laterality, true) 1121 && compareDeep(specimen, o.specimen, true) && compareDeep(started, o.started, true) && compareDeep(performer, o.performer, true) 1122 && compareDeep(instance, o.instance, true); 1123 } 1124 1125 @Override 1126 public boolean equalsShallow(Base other_) { 1127 if (!super.equalsShallow(other_)) 1128 return false; 1129 if (!(other_ instanceof ImagingStudySeriesComponent)) 1130 return false; 1131 ImagingStudySeriesComponent o = (ImagingStudySeriesComponent) other_; 1132 return compareValues(uid, o.uid, true) && compareValues(number, o.number, true) && compareValues(description, o.description, true) 1133 && compareValues(numberOfInstances, o.numberOfInstances, true) && compareValues(started, o.started, true) 1134 ; 1135 } 1136 1137 public boolean isEmpty() { 1138 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(uid, number, modality, description 1139 , numberOfInstances, endpoint, bodySite, laterality, specimen, started, performer 1140 , instance); 1141 } 1142 1143 public String fhirType() { 1144 return "ImagingStudy.series"; 1145 1146 } 1147 1148 } 1149 1150 @Block() 1151 public static class ImagingStudySeriesPerformerComponent extends BackboneElement implements IBaseBackboneElement { 1152 /** 1153 * Distinguishes the type of involvement of the performer in the series. 1154 */ 1155 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 1156 @Description(shortDefinition="Type of performance", formalDefinition="Distinguishes the type of involvement of the performer in the series." ) 1157 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/series-performer-function") 1158 protected CodeableConcept function; 1159 1160 /** 1161 * Indicates who or what performed the series. 1162 */ 1163 @Child(name = "actor", type = {Practitioner.class, PractitionerRole.class, Organization.class, CareTeam.class, Patient.class, Device.class, RelatedPerson.class, HealthcareService.class}, order=2, min=1, max=1, modifier=false, summary=true) 1164 @Description(shortDefinition="Who performed the series", formalDefinition="Indicates who or what performed the series." ) 1165 protected Reference actor; 1166 1167 private static final long serialVersionUID = -576943815L; 1168 1169 /** 1170 * Constructor 1171 */ 1172 public ImagingStudySeriesPerformerComponent() { 1173 super(); 1174 } 1175 1176 /** 1177 * Constructor 1178 */ 1179 public ImagingStudySeriesPerformerComponent(Reference actor) { 1180 super(); 1181 this.setActor(actor); 1182 } 1183 1184 /** 1185 * @return {@link #function} (Distinguishes the type of involvement of the performer in the series.) 1186 */ 1187 public CodeableConcept getFunction() { 1188 if (this.function == null) 1189 if (Configuration.errorOnAutoCreate()) 1190 throw new Error("Attempt to auto-create ImagingStudySeriesPerformerComponent.function"); 1191 else if (Configuration.doAutoCreate()) 1192 this.function = new CodeableConcept(); // cc 1193 return this.function; 1194 } 1195 1196 public boolean hasFunction() { 1197 return this.function != null && !this.function.isEmpty(); 1198 } 1199 1200 /** 1201 * @param value {@link #function} (Distinguishes the type of involvement of the performer in the series.) 1202 */ 1203 public ImagingStudySeriesPerformerComponent setFunction(CodeableConcept value) { 1204 this.function = value; 1205 return this; 1206 } 1207 1208 /** 1209 * @return {@link #actor} (Indicates who or what performed the series.) 1210 */ 1211 public Reference getActor() { 1212 if (this.actor == null) 1213 if (Configuration.errorOnAutoCreate()) 1214 throw new Error("Attempt to auto-create ImagingStudySeriesPerformerComponent.actor"); 1215 else if (Configuration.doAutoCreate()) 1216 this.actor = new Reference(); // cc 1217 return this.actor; 1218 } 1219 1220 public boolean hasActor() { 1221 return this.actor != null && !this.actor.isEmpty(); 1222 } 1223 1224 /** 1225 * @param value {@link #actor} (Indicates who or what performed the series.) 1226 */ 1227 public ImagingStudySeriesPerformerComponent setActor(Reference value) { 1228 this.actor = value; 1229 return this; 1230 } 1231 1232 protected void listChildren(List<Property> children) { 1233 super.listChildren(children); 1234 children.add(new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the performer in the series.", 0, 1, function)); 1235 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson|HealthcareService)", "Indicates who or what performed the series.", 0, 1, actor)); 1236 } 1237 1238 @Override 1239 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1240 switch (_hash) { 1241 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the performer in the series.", 0, 1, function); 1242 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|CareTeam|Patient|Device|RelatedPerson|HealthcareService)", "Indicates who or what performed the series.", 0, 1, actor); 1243 default: return super.getNamedProperty(_hash, _name, _checkValid); 1244 } 1245 1246 } 1247 1248 @Override 1249 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1250 switch (hash) { 1251 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 1252 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 1253 default: return super.getProperty(hash, name, checkValid); 1254 } 1255 1256 } 1257 1258 @Override 1259 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1260 switch (hash) { 1261 case 1380938712: // function 1262 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1263 return value; 1264 case 92645877: // actor 1265 this.actor = TypeConvertor.castToReference(value); // Reference 1266 return value; 1267 default: return super.setProperty(hash, name, value); 1268 } 1269 1270 } 1271 1272 @Override 1273 public Base setProperty(String name, Base value) throws FHIRException { 1274 if (name.equals("function")) { 1275 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1276 } else if (name.equals("actor")) { 1277 this.actor = TypeConvertor.castToReference(value); // Reference 1278 } else 1279 return super.setProperty(name, value); 1280 return value; 1281 } 1282 1283 @Override 1284 public void removeChild(String name, Base value) throws FHIRException { 1285 if (name.equals("function")) { 1286 this.function = null; 1287 } else if (name.equals("actor")) { 1288 this.actor = null; 1289 } else 1290 super.removeChild(name, value); 1291 1292 } 1293 1294 @Override 1295 public Base makeProperty(int hash, String name) throws FHIRException { 1296 switch (hash) { 1297 case 1380938712: return getFunction(); 1298 case 92645877: return getActor(); 1299 default: return super.makeProperty(hash, name); 1300 } 1301 1302 } 1303 1304 @Override 1305 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1306 switch (hash) { 1307 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 1308 case 92645877: /*actor*/ return new String[] {"Reference"}; 1309 default: return super.getTypesForProperty(hash, name); 1310 } 1311 1312 } 1313 1314 @Override 1315 public Base addChild(String name) throws FHIRException { 1316 if (name.equals("function")) { 1317 this.function = new CodeableConcept(); 1318 return this.function; 1319 } 1320 else if (name.equals("actor")) { 1321 this.actor = new Reference(); 1322 return this.actor; 1323 } 1324 else 1325 return super.addChild(name); 1326 } 1327 1328 public ImagingStudySeriesPerformerComponent copy() { 1329 ImagingStudySeriesPerformerComponent dst = new ImagingStudySeriesPerformerComponent(); 1330 copyValues(dst); 1331 return dst; 1332 } 1333 1334 public void copyValues(ImagingStudySeriesPerformerComponent dst) { 1335 super.copyValues(dst); 1336 dst.function = function == null ? null : function.copy(); 1337 dst.actor = actor == null ? null : actor.copy(); 1338 } 1339 1340 @Override 1341 public boolean equalsDeep(Base other_) { 1342 if (!super.equalsDeep(other_)) 1343 return false; 1344 if (!(other_ instanceof ImagingStudySeriesPerformerComponent)) 1345 return false; 1346 ImagingStudySeriesPerformerComponent o = (ImagingStudySeriesPerformerComponent) other_; 1347 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 1348 } 1349 1350 @Override 1351 public boolean equalsShallow(Base other_) { 1352 if (!super.equalsShallow(other_)) 1353 return false; 1354 if (!(other_ instanceof ImagingStudySeriesPerformerComponent)) 1355 return false; 1356 ImagingStudySeriesPerformerComponent o = (ImagingStudySeriesPerformerComponent) other_; 1357 return true; 1358 } 1359 1360 public boolean isEmpty() { 1361 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 1362 } 1363 1364 public String fhirType() { 1365 return "ImagingStudy.series.performer"; 1366 1367 } 1368 1369 } 1370 1371 @Block() 1372 public static class ImagingStudySeriesInstanceComponent extends BackboneElement implements IBaseBackboneElement { 1373 /** 1374 * The DICOM SOP Instance UID for this image or other DICOM content. 1375 */ 1376 @Child(name = "uid", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1377 @Description(shortDefinition="DICOM SOP Instance UID", formalDefinition="The DICOM SOP Instance UID for this image or other DICOM content." ) 1378 protected IdType uid; 1379 1380 /** 1381 * DICOM instance type. 1382 */ 1383 @Child(name = "sopClass", type = {Coding.class}, order=2, min=1, max=1, modifier=false, summary=false) 1384 @Description(shortDefinition="DICOM class type", formalDefinition="DICOM instance type." ) 1385 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://dicom.nema.org/medical/dicom/current/output/chtml/part04/sect_B.5.html#table_B.5-1") 1386 protected Coding sopClass; 1387 1388 /** 1389 * The number of instance in the series. 1390 */ 1391 @Child(name = "number", type = {UnsignedIntType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1392 @Description(shortDefinition="The number of this instance in the series", formalDefinition="The number of instance in the series." ) 1393 protected UnsignedIntType number; 1394 1395 /** 1396 * The description of the instance. 1397 */ 1398 @Child(name = "title", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1399 @Description(shortDefinition="Description of instance", formalDefinition="The description of the instance." ) 1400 protected StringType title; 1401 1402 private static final long serialVersionUID = -888152445L; 1403 1404 /** 1405 * Constructor 1406 */ 1407 public ImagingStudySeriesInstanceComponent() { 1408 super(); 1409 } 1410 1411 /** 1412 * Constructor 1413 */ 1414 public ImagingStudySeriesInstanceComponent(String uid, Coding sopClass) { 1415 super(); 1416 this.setUid(uid); 1417 this.setSopClass(sopClass); 1418 } 1419 1420 /** 1421 * @return {@link #uid} (The DICOM SOP Instance UID for this image or other DICOM content.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 1422 */ 1423 public IdType getUidElement() { 1424 if (this.uid == null) 1425 if (Configuration.errorOnAutoCreate()) 1426 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.uid"); 1427 else if (Configuration.doAutoCreate()) 1428 this.uid = new IdType(); // bb 1429 return this.uid; 1430 } 1431 1432 public boolean hasUidElement() { 1433 return this.uid != null && !this.uid.isEmpty(); 1434 } 1435 1436 public boolean hasUid() { 1437 return this.uid != null && !this.uid.isEmpty(); 1438 } 1439 1440 /** 1441 * @param value {@link #uid} (The DICOM SOP Instance UID for this image or other DICOM content.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 1442 */ 1443 public ImagingStudySeriesInstanceComponent setUidElement(IdType value) { 1444 this.uid = value; 1445 return this; 1446 } 1447 1448 /** 1449 * @return The DICOM SOP Instance UID for this image or other DICOM content. 1450 */ 1451 public String getUid() { 1452 return this.uid == null ? null : this.uid.getValue(); 1453 } 1454 1455 /** 1456 * @param value The DICOM SOP Instance UID for this image or other DICOM content. 1457 */ 1458 public ImagingStudySeriesInstanceComponent setUid(String value) { 1459 if (this.uid == null) 1460 this.uid = new IdType(); 1461 this.uid.setValue(value); 1462 return this; 1463 } 1464 1465 /** 1466 * @return {@link #sopClass} (DICOM instance type.) 1467 */ 1468 public Coding getSopClass() { 1469 if (this.sopClass == null) 1470 if (Configuration.errorOnAutoCreate()) 1471 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.sopClass"); 1472 else if (Configuration.doAutoCreate()) 1473 this.sopClass = new Coding(); // cc 1474 return this.sopClass; 1475 } 1476 1477 public boolean hasSopClass() { 1478 return this.sopClass != null && !this.sopClass.isEmpty(); 1479 } 1480 1481 /** 1482 * @param value {@link #sopClass} (DICOM instance type.) 1483 */ 1484 public ImagingStudySeriesInstanceComponent setSopClass(Coding value) { 1485 this.sopClass = value; 1486 return this; 1487 } 1488 1489 /** 1490 * @return {@link #number} (The number of instance in the series.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 1491 */ 1492 public UnsignedIntType getNumberElement() { 1493 if (this.number == null) 1494 if (Configuration.errorOnAutoCreate()) 1495 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.number"); 1496 else if (Configuration.doAutoCreate()) 1497 this.number = new UnsignedIntType(); // bb 1498 return this.number; 1499 } 1500 1501 public boolean hasNumberElement() { 1502 return this.number != null && !this.number.isEmpty(); 1503 } 1504 1505 public boolean hasNumber() { 1506 return this.number != null && !this.number.isEmpty(); 1507 } 1508 1509 /** 1510 * @param value {@link #number} (The number of instance in the series.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 1511 */ 1512 public ImagingStudySeriesInstanceComponent setNumberElement(UnsignedIntType value) { 1513 this.number = value; 1514 return this; 1515 } 1516 1517 /** 1518 * @return The number of instance in the series. 1519 */ 1520 public int getNumber() { 1521 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 1522 } 1523 1524 /** 1525 * @param value The number of instance in the series. 1526 */ 1527 public ImagingStudySeriesInstanceComponent setNumber(int value) { 1528 if (this.number == null) 1529 this.number = new UnsignedIntType(); 1530 this.number.setValue(value); 1531 return this; 1532 } 1533 1534 /** 1535 * @return {@link #title} (The description of the instance.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1536 */ 1537 public StringType getTitleElement() { 1538 if (this.title == null) 1539 if (Configuration.errorOnAutoCreate()) 1540 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.title"); 1541 else if (Configuration.doAutoCreate()) 1542 this.title = new StringType(); // bb 1543 return this.title; 1544 } 1545 1546 public boolean hasTitleElement() { 1547 return this.title != null && !this.title.isEmpty(); 1548 } 1549 1550 public boolean hasTitle() { 1551 return this.title != null && !this.title.isEmpty(); 1552 } 1553 1554 /** 1555 * @param value {@link #title} (The description of the instance.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1556 */ 1557 public ImagingStudySeriesInstanceComponent setTitleElement(StringType value) { 1558 this.title = value; 1559 return this; 1560 } 1561 1562 /** 1563 * @return The description of the instance. 1564 */ 1565 public String getTitle() { 1566 return this.title == null ? null : this.title.getValue(); 1567 } 1568 1569 /** 1570 * @param value The description of the instance. 1571 */ 1572 public ImagingStudySeriesInstanceComponent setTitle(String value) { 1573 if (Utilities.noString(value)) 1574 this.title = null; 1575 else { 1576 if (this.title == null) 1577 this.title = new StringType(); 1578 this.title.setValue(value); 1579 } 1580 return this; 1581 } 1582 1583 protected void listChildren(List<Property> children) { 1584 super.listChildren(children); 1585 children.add(new Property("uid", "id", "The DICOM SOP Instance UID for this image or other DICOM content.", 0, 1, uid)); 1586 children.add(new Property("sopClass", "Coding", "DICOM instance type.", 0, 1, sopClass)); 1587 children.add(new Property("number", "unsignedInt", "The number of instance in the series.", 0, 1, number)); 1588 children.add(new Property("title", "string", "The description of the instance.", 0, 1, title)); 1589 } 1590 1591 @Override 1592 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1593 switch (_hash) { 1594 case 115792: /*uid*/ return new Property("uid", "id", "The DICOM SOP Instance UID for this image or other DICOM content.", 0, 1, uid); 1595 case 1560041540: /*sopClass*/ return new Property("sopClass", "Coding", "DICOM instance type.", 0, 1, sopClass); 1596 case -1034364087: /*number*/ return new Property("number", "unsignedInt", "The number of instance in the series.", 0, 1, number); 1597 case 110371416: /*title*/ return new Property("title", "string", "The description of the instance.", 0, 1, title); 1598 default: return super.getNamedProperty(_hash, _name, _checkValid); 1599 } 1600 1601 } 1602 1603 @Override 1604 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1605 switch (hash) { 1606 case 115792: /*uid*/ return this.uid == null ? new Base[0] : new Base[] {this.uid}; // IdType 1607 case 1560041540: /*sopClass*/ return this.sopClass == null ? new Base[0] : new Base[] {this.sopClass}; // Coding 1608 case -1034364087: /*number*/ return this.number == null ? new Base[0] : new Base[] {this.number}; // UnsignedIntType 1609 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1610 default: return super.getProperty(hash, name, checkValid); 1611 } 1612 1613 } 1614 1615 @Override 1616 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1617 switch (hash) { 1618 case 115792: // uid 1619 this.uid = TypeConvertor.castToId(value); // IdType 1620 return value; 1621 case 1560041540: // sopClass 1622 this.sopClass = TypeConvertor.castToCoding(value); // Coding 1623 return value; 1624 case -1034364087: // number 1625 this.number = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1626 return value; 1627 case 110371416: // title 1628 this.title = TypeConvertor.castToString(value); // StringType 1629 return value; 1630 default: return super.setProperty(hash, name, value); 1631 } 1632 1633 } 1634 1635 @Override 1636 public Base setProperty(String name, Base value) throws FHIRException { 1637 if (name.equals("uid")) { 1638 this.uid = TypeConvertor.castToId(value); // IdType 1639 } else if (name.equals("sopClass")) { 1640 this.sopClass = TypeConvertor.castToCoding(value); // Coding 1641 } else if (name.equals("number")) { 1642 this.number = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 1643 } else if (name.equals("title")) { 1644 this.title = TypeConvertor.castToString(value); // StringType 1645 } else 1646 return super.setProperty(name, value); 1647 return value; 1648 } 1649 1650 @Override 1651 public void removeChild(String name, Base value) throws FHIRException { 1652 if (name.equals("uid")) { 1653 this.uid = null; 1654 } else if (name.equals("sopClass")) { 1655 this.sopClass = null; 1656 } else if (name.equals("number")) { 1657 this.number = null; 1658 } else if (name.equals("title")) { 1659 this.title = null; 1660 } else 1661 super.removeChild(name, value); 1662 1663 } 1664 1665 @Override 1666 public Base makeProperty(int hash, String name) throws FHIRException { 1667 switch (hash) { 1668 case 115792: return getUidElement(); 1669 case 1560041540: return getSopClass(); 1670 case -1034364087: return getNumberElement(); 1671 case 110371416: return getTitleElement(); 1672 default: return super.makeProperty(hash, name); 1673 } 1674 1675 } 1676 1677 @Override 1678 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1679 switch (hash) { 1680 case 115792: /*uid*/ return new String[] {"id"}; 1681 case 1560041540: /*sopClass*/ return new String[] {"Coding"}; 1682 case -1034364087: /*number*/ return new String[] {"unsignedInt"}; 1683 case 110371416: /*title*/ return new String[] {"string"}; 1684 default: return super.getTypesForProperty(hash, name); 1685 } 1686 1687 } 1688 1689 @Override 1690 public Base addChild(String name) throws FHIRException { 1691 if (name.equals("uid")) { 1692 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.series.instance.uid"); 1693 } 1694 else if (name.equals("sopClass")) { 1695 this.sopClass = new Coding(); 1696 return this.sopClass; 1697 } 1698 else if (name.equals("number")) { 1699 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.series.instance.number"); 1700 } 1701 else if (name.equals("title")) { 1702 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.series.instance.title"); 1703 } 1704 else 1705 return super.addChild(name); 1706 } 1707 1708 public ImagingStudySeriesInstanceComponent copy() { 1709 ImagingStudySeriesInstanceComponent dst = new ImagingStudySeriesInstanceComponent(); 1710 copyValues(dst); 1711 return dst; 1712 } 1713 1714 public void copyValues(ImagingStudySeriesInstanceComponent dst) { 1715 super.copyValues(dst); 1716 dst.uid = uid == null ? null : uid.copy(); 1717 dst.sopClass = sopClass == null ? null : sopClass.copy(); 1718 dst.number = number == null ? null : number.copy(); 1719 dst.title = title == null ? null : title.copy(); 1720 } 1721 1722 @Override 1723 public boolean equalsDeep(Base other_) { 1724 if (!super.equalsDeep(other_)) 1725 return false; 1726 if (!(other_ instanceof ImagingStudySeriesInstanceComponent)) 1727 return false; 1728 ImagingStudySeriesInstanceComponent o = (ImagingStudySeriesInstanceComponent) other_; 1729 return compareDeep(uid, o.uid, true) && compareDeep(sopClass, o.sopClass, true) && compareDeep(number, o.number, true) 1730 && compareDeep(title, o.title, true); 1731 } 1732 1733 @Override 1734 public boolean equalsShallow(Base other_) { 1735 if (!super.equalsShallow(other_)) 1736 return false; 1737 if (!(other_ instanceof ImagingStudySeriesInstanceComponent)) 1738 return false; 1739 ImagingStudySeriesInstanceComponent o = (ImagingStudySeriesInstanceComponent) other_; 1740 return compareValues(uid, o.uid, true) && compareValues(number, o.number, true) && compareValues(title, o.title, true) 1741 ; 1742 } 1743 1744 public boolean isEmpty() { 1745 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(uid, sopClass, number, title 1746 ); 1747 } 1748 1749 public String fhirType() { 1750 return "ImagingStudy.series.instance"; 1751 1752 } 1753 1754 } 1755 1756 /** 1757 * Identifiers for the ImagingStudy such as DICOM Study Instance UID. 1758 */ 1759 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1760 @Description(shortDefinition="Identifiers for the whole study", formalDefinition="Identifiers for the ImagingStudy such as DICOM Study Instance UID." ) 1761 protected List<Identifier> identifier; 1762 1763 /** 1764 * The current state of the ImagingStudy resource. This is not the status of any ServiceRequest or Task resources associated with the ImagingStudy. 1765 */ 1766 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 1767 @Description(shortDefinition="registered | available | cancelled | entered-in-error | unknown", formalDefinition="The current state of the ImagingStudy resource. This is not the status of any ServiceRequest or Task resources associated with the ImagingStudy." ) 1768 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/imagingstudy-status") 1769 protected Enumeration<ImagingStudyStatus> status; 1770 1771 /** 1772 * A list of all the distinct values of series.modality. This may include both acquisition and non-acquisition modalities. 1773 */ 1774 @Child(name = "modality", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1775 @Description(shortDefinition="All of the distinct values for series' modalities", formalDefinition="A list of all the distinct values of series.modality. This may include both acquisition and non-acquisition modalities." ) 1776 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://dicom.nema.org/medical/dicom/current/output/chtml/part16/sect_CID_33.html") 1777 protected List<CodeableConcept> modality; 1778 1779 /** 1780 * The subject, typically a patient, of the imaging study. 1781 */ 1782 @Child(name = "subject", type = {Patient.class, Device.class, Group.class}, order=3, min=1, max=1, modifier=false, summary=true) 1783 @Description(shortDefinition="Who or what is the subject of the study", formalDefinition="The subject, typically a patient, of the imaging study." ) 1784 protected Reference subject; 1785 1786 /** 1787 * The healthcare event (e.g. a patient and healthcare provider interaction) during which this ImagingStudy is made. 1788 */ 1789 @Child(name = "encounter", type = {Encounter.class}, order=4, min=0, max=1, modifier=false, summary=true) 1790 @Description(shortDefinition="Encounter with which this imaging study is associated", formalDefinition="The healthcare event (e.g. a patient and healthcare provider interaction) during which this ImagingStudy is made." ) 1791 protected Reference encounter; 1792 1793 /** 1794 * Date and time the study started. 1795 */ 1796 @Child(name = "started", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1797 @Description(shortDefinition="When the study was started", formalDefinition="Date and time the study started." ) 1798 protected DateTimeType started; 1799 1800 /** 1801 * A list of the diagnostic requests that resulted in this imaging study being performed. 1802 */ 1803 @Child(name = "basedOn", type = {CarePlan.class, ServiceRequest.class, Appointment.class, AppointmentResponse.class, Task.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1804 @Description(shortDefinition="Request fulfilled", formalDefinition="A list of the diagnostic requests that resulted in this imaging study being performed." ) 1805 protected List<Reference> basedOn; 1806 1807 /** 1808 * A larger event of which this particular ImagingStudy is a component or step. For example, an ImagingStudy as part of a procedure. 1809 */ 1810 @Child(name = "partOf", type = {Procedure.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1811 @Description(shortDefinition="Part of referenced event", formalDefinition="A larger event of which this particular ImagingStudy is a component or step. For example, an ImagingStudy as part of a procedure." ) 1812 protected List<Reference> partOf; 1813 1814 /** 1815 * The requesting/referring physician. 1816 */ 1817 @Child(name = "referrer", type = {Practitioner.class, PractitionerRole.class}, order=8, min=0, max=1, modifier=false, summary=true) 1818 @Description(shortDefinition="Referring physician", formalDefinition="The requesting/referring physician." ) 1819 protected Reference referrer; 1820 1821 /** 1822 * The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.connectionType. 1823 */ 1824 @Child(name = "endpoint", type = {Endpoint.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1825 @Description(shortDefinition="Study access endpoint", formalDefinition="The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.connectionType." ) 1826 protected List<Reference> endpoint; 1827 1828 /** 1829 * Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present. 1830 */ 1831 @Child(name = "numberOfSeries", type = {UnsignedIntType.class}, order=10, min=0, max=1, modifier=false, summary=true) 1832 @Description(shortDefinition="Number of Study Related Series", formalDefinition="Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present." ) 1833 protected UnsignedIntType numberOfSeries; 1834 1835 /** 1836 * Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present. 1837 */ 1838 @Child(name = "numberOfInstances", type = {UnsignedIntType.class}, order=11, min=0, max=1, modifier=false, summary=true) 1839 @Description(shortDefinition="Number of Study Related Instances", formalDefinition="Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present." ) 1840 protected UnsignedIntType numberOfInstances; 1841 1842 /** 1843 * This field corresponds to the DICOM Procedure Code Sequence (0008,1032). This is different from the FHIR Procedure resource that may include the ImagingStudy. 1844 */ 1845 @Child(name = "procedure", type = {CodeableReference.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1846 @Description(shortDefinition="The performed procedure or code", formalDefinition="This field corresponds to the DICOM Procedure Code Sequence (0008,1032). This is different from the FHIR Procedure resource that may include the ImagingStudy." ) 1847 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://loinc.org/vs/loinc-rsna-radiology-playbook") 1848 protected List<CodeableReference> procedure; 1849 1850 /** 1851 * The principal physical location where the ImagingStudy was performed. 1852 */ 1853 @Child(name = "location", type = {Location.class}, order=13, min=0, max=1, modifier=false, summary=true) 1854 @Description(shortDefinition="Where ImagingStudy occurred", formalDefinition="The principal physical location where the ImagingStudy was performed." ) 1855 protected Reference location; 1856 1857 /** 1858 * Description of clinical condition indicating why the ImagingStudy was requested, and/or Indicates another resource whose existence justifies this Study. 1859 */ 1860 @Child(name = "reason", type = {CodeableReference.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1861 @Description(shortDefinition="Why the study was requested / performed", formalDefinition="Description of clinical condition indicating why the ImagingStudy was requested, and/or Indicates another resource whose existence justifies this Study." ) 1862 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-reason") 1863 protected List<CodeableReference> reason; 1864 1865 /** 1866 * Per the recommended DICOM mapping, this element is derived from the Study Description attribute (0008,1030). Observations or findings about the imaging study should be recorded in another resource, e.g. Observation, and not in this element. 1867 */ 1868 @Child(name = "note", type = {Annotation.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1869 @Description(shortDefinition="User-defined comments", formalDefinition="Per the recommended DICOM mapping, this element is derived from the Study Description attribute (0008,1030). Observations or findings about the imaging study should be recorded in another resource, e.g. Observation, and not in this element." ) 1870 protected List<Annotation> note; 1871 1872 /** 1873 * The Imaging Manager description of the study. Institution-generated description or classification of the Study (component) performed. 1874 */ 1875 @Child(name = "description", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=true) 1876 @Description(shortDefinition="Institution-generated description", formalDefinition="The Imaging Manager description of the study. Institution-generated description or classification of the Study (component) performed." ) 1877 protected StringType description; 1878 1879 /** 1880 * Each study has one or more series of images or other content. 1881 */ 1882 @Child(name = "series", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1883 @Description(shortDefinition="Each study has one or more series of instances", formalDefinition="Each study has one or more series of images or other content." ) 1884 protected List<ImagingStudySeriesComponent> series; 1885 1886 private static final long serialVersionUID = -1519153867L; 1887 1888 /** 1889 * Constructor 1890 */ 1891 public ImagingStudy() { 1892 super(); 1893 } 1894 1895 /** 1896 * Constructor 1897 */ 1898 public ImagingStudy(ImagingStudyStatus status, Reference subject) { 1899 super(); 1900 this.setStatus(status); 1901 this.setSubject(subject); 1902 } 1903 1904 /** 1905 * @return {@link #identifier} (Identifiers for the ImagingStudy such as DICOM Study Instance UID.) 1906 */ 1907 public List<Identifier> getIdentifier() { 1908 if (this.identifier == null) 1909 this.identifier = new ArrayList<Identifier>(); 1910 return this.identifier; 1911 } 1912 1913 /** 1914 * @return Returns a reference to <code>this</code> for easy method chaining 1915 */ 1916 public ImagingStudy setIdentifier(List<Identifier> theIdentifier) { 1917 this.identifier = theIdentifier; 1918 return this; 1919 } 1920 1921 public boolean hasIdentifier() { 1922 if (this.identifier == null) 1923 return false; 1924 for (Identifier item : this.identifier) 1925 if (!item.isEmpty()) 1926 return true; 1927 return false; 1928 } 1929 1930 public Identifier addIdentifier() { //3 1931 Identifier t = new Identifier(); 1932 if (this.identifier == null) 1933 this.identifier = new ArrayList<Identifier>(); 1934 this.identifier.add(t); 1935 return t; 1936 } 1937 1938 public ImagingStudy addIdentifier(Identifier t) { //3 1939 if (t == null) 1940 return this; 1941 if (this.identifier == null) 1942 this.identifier = new ArrayList<Identifier>(); 1943 this.identifier.add(t); 1944 return this; 1945 } 1946 1947 /** 1948 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1949 */ 1950 public Identifier getIdentifierFirstRep() { 1951 if (getIdentifier().isEmpty()) { 1952 addIdentifier(); 1953 } 1954 return getIdentifier().get(0); 1955 } 1956 1957 /** 1958 * @return {@link #status} (The current state of the ImagingStudy resource. This is not the status of any ServiceRequest or Task resources associated with the ImagingStudy.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1959 */ 1960 public Enumeration<ImagingStudyStatus> getStatusElement() { 1961 if (this.status == null) 1962 if (Configuration.errorOnAutoCreate()) 1963 throw new Error("Attempt to auto-create ImagingStudy.status"); 1964 else if (Configuration.doAutoCreate()) 1965 this.status = new Enumeration<ImagingStudyStatus>(new ImagingStudyStatusEnumFactory()); // bb 1966 return this.status; 1967 } 1968 1969 public boolean hasStatusElement() { 1970 return this.status != null && !this.status.isEmpty(); 1971 } 1972 1973 public boolean hasStatus() { 1974 return this.status != null && !this.status.isEmpty(); 1975 } 1976 1977 /** 1978 * @param value {@link #status} (The current state of the ImagingStudy resource. This is not the status of any ServiceRequest or Task resources associated with the ImagingStudy.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1979 */ 1980 public ImagingStudy setStatusElement(Enumeration<ImagingStudyStatus> value) { 1981 this.status = value; 1982 return this; 1983 } 1984 1985 /** 1986 * @return The current state of the ImagingStudy resource. This is not the status of any ServiceRequest or Task resources associated with the ImagingStudy. 1987 */ 1988 public ImagingStudyStatus getStatus() { 1989 return this.status == null ? null : this.status.getValue(); 1990 } 1991 1992 /** 1993 * @param value The current state of the ImagingStudy resource. This is not the status of any ServiceRequest or Task resources associated with the ImagingStudy. 1994 */ 1995 public ImagingStudy setStatus(ImagingStudyStatus value) { 1996 if (this.status == null) 1997 this.status = new Enumeration<ImagingStudyStatus>(new ImagingStudyStatusEnumFactory()); 1998 this.status.setValue(value); 1999 return this; 2000 } 2001 2002 /** 2003 * @return {@link #modality} (A list of all the distinct values of series.modality. This may include both acquisition and non-acquisition modalities.) 2004 */ 2005 public List<CodeableConcept> getModality() { 2006 if (this.modality == null) 2007 this.modality = new ArrayList<CodeableConcept>(); 2008 return this.modality; 2009 } 2010 2011 /** 2012 * @return Returns a reference to <code>this</code> for easy method chaining 2013 */ 2014 public ImagingStudy setModality(List<CodeableConcept> theModality) { 2015 this.modality = theModality; 2016 return this; 2017 } 2018 2019 public boolean hasModality() { 2020 if (this.modality == null) 2021 return false; 2022 for (CodeableConcept item : this.modality) 2023 if (!item.isEmpty()) 2024 return true; 2025 return false; 2026 } 2027 2028 public CodeableConcept addModality() { //3 2029 CodeableConcept t = new CodeableConcept(); 2030 if (this.modality == null) 2031 this.modality = new ArrayList<CodeableConcept>(); 2032 this.modality.add(t); 2033 return t; 2034 } 2035 2036 public ImagingStudy addModality(CodeableConcept t) { //3 2037 if (t == null) 2038 return this; 2039 if (this.modality == null) 2040 this.modality = new ArrayList<CodeableConcept>(); 2041 this.modality.add(t); 2042 return this; 2043 } 2044 2045 /** 2046 * @return The first repetition of repeating field {@link #modality}, creating it if it does not already exist {3} 2047 */ 2048 public CodeableConcept getModalityFirstRep() { 2049 if (getModality().isEmpty()) { 2050 addModality(); 2051 } 2052 return getModality().get(0); 2053 } 2054 2055 /** 2056 * @return {@link #subject} (The subject, typically a patient, of the imaging study.) 2057 */ 2058 public Reference getSubject() { 2059 if (this.subject == null) 2060 if (Configuration.errorOnAutoCreate()) 2061 throw new Error("Attempt to auto-create ImagingStudy.subject"); 2062 else if (Configuration.doAutoCreate()) 2063 this.subject = new Reference(); // cc 2064 return this.subject; 2065 } 2066 2067 public boolean hasSubject() { 2068 return this.subject != null && !this.subject.isEmpty(); 2069 } 2070 2071 /** 2072 * @param value {@link #subject} (The subject, typically a patient, of the imaging study.) 2073 */ 2074 public ImagingStudy setSubject(Reference value) { 2075 this.subject = value; 2076 return this; 2077 } 2078 2079 /** 2080 * @return {@link #encounter} (The healthcare event (e.g. a patient and healthcare provider interaction) during which this ImagingStudy is made.) 2081 */ 2082 public Reference getEncounter() { 2083 if (this.encounter == null) 2084 if (Configuration.errorOnAutoCreate()) 2085 throw new Error("Attempt to auto-create ImagingStudy.encounter"); 2086 else if (Configuration.doAutoCreate()) 2087 this.encounter = new Reference(); // cc 2088 return this.encounter; 2089 } 2090 2091 public boolean hasEncounter() { 2092 return this.encounter != null && !this.encounter.isEmpty(); 2093 } 2094 2095 /** 2096 * @param value {@link #encounter} (The healthcare event (e.g. a patient and healthcare provider interaction) during which this ImagingStudy is made.) 2097 */ 2098 public ImagingStudy setEncounter(Reference value) { 2099 this.encounter = value; 2100 return this; 2101 } 2102 2103 /** 2104 * @return {@link #started} (Date and time the study started.). This is the underlying object with id, value and extensions. The accessor "getStarted" gives direct access to the value 2105 */ 2106 public DateTimeType getStartedElement() { 2107 if (this.started == null) 2108 if (Configuration.errorOnAutoCreate()) 2109 throw new Error("Attempt to auto-create ImagingStudy.started"); 2110 else if (Configuration.doAutoCreate()) 2111 this.started = new DateTimeType(); // bb 2112 return this.started; 2113 } 2114 2115 public boolean hasStartedElement() { 2116 return this.started != null && !this.started.isEmpty(); 2117 } 2118 2119 public boolean hasStarted() { 2120 return this.started != null && !this.started.isEmpty(); 2121 } 2122 2123 /** 2124 * @param value {@link #started} (Date and time the study started.). This is the underlying object with id, value and extensions. The accessor "getStarted" gives direct access to the value 2125 */ 2126 public ImagingStudy setStartedElement(DateTimeType value) { 2127 this.started = value; 2128 return this; 2129 } 2130 2131 /** 2132 * @return Date and time the study started. 2133 */ 2134 public Date getStarted() { 2135 return this.started == null ? null : this.started.getValue(); 2136 } 2137 2138 /** 2139 * @param value Date and time the study started. 2140 */ 2141 public ImagingStudy setStarted(Date value) { 2142 if (value == null) 2143 this.started = null; 2144 else { 2145 if (this.started == null) 2146 this.started = new DateTimeType(); 2147 this.started.setValue(value); 2148 } 2149 return this; 2150 } 2151 2152 /** 2153 * @return {@link #basedOn} (A list of the diagnostic requests that resulted in this imaging study being performed.) 2154 */ 2155 public List<Reference> getBasedOn() { 2156 if (this.basedOn == null) 2157 this.basedOn = new ArrayList<Reference>(); 2158 return this.basedOn; 2159 } 2160 2161 /** 2162 * @return Returns a reference to <code>this</code> for easy method chaining 2163 */ 2164 public ImagingStudy setBasedOn(List<Reference> theBasedOn) { 2165 this.basedOn = theBasedOn; 2166 return this; 2167 } 2168 2169 public boolean hasBasedOn() { 2170 if (this.basedOn == null) 2171 return false; 2172 for (Reference item : this.basedOn) 2173 if (!item.isEmpty()) 2174 return true; 2175 return false; 2176 } 2177 2178 public Reference addBasedOn() { //3 2179 Reference t = new Reference(); 2180 if (this.basedOn == null) 2181 this.basedOn = new ArrayList<Reference>(); 2182 this.basedOn.add(t); 2183 return t; 2184 } 2185 2186 public ImagingStudy addBasedOn(Reference t) { //3 2187 if (t == null) 2188 return this; 2189 if (this.basedOn == null) 2190 this.basedOn = new ArrayList<Reference>(); 2191 this.basedOn.add(t); 2192 return this; 2193 } 2194 2195 /** 2196 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 2197 */ 2198 public Reference getBasedOnFirstRep() { 2199 if (getBasedOn().isEmpty()) { 2200 addBasedOn(); 2201 } 2202 return getBasedOn().get(0); 2203 } 2204 2205 /** 2206 * @return {@link #partOf} (A larger event of which this particular ImagingStudy is a component or step. For example, an ImagingStudy as part of a procedure.) 2207 */ 2208 public List<Reference> getPartOf() { 2209 if (this.partOf == null) 2210 this.partOf = new ArrayList<Reference>(); 2211 return this.partOf; 2212 } 2213 2214 /** 2215 * @return Returns a reference to <code>this</code> for easy method chaining 2216 */ 2217 public ImagingStudy setPartOf(List<Reference> thePartOf) { 2218 this.partOf = thePartOf; 2219 return this; 2220 } 2221 2222 public boolean hasPartOf() { 2223 if (this.partOf == null) 2224 return false; 2225 for (Reference item : this.partOf) 2226 if (!item.isEmpty()) 2227 return true; 2228 return false; 2229 } 2230 2231 public Reference addPartOf() { //3 2232 Reference t = new Reference(); 2233 if (this.partOf == null) 2234 this.partOf = new ArrayList<Reference>(); 2235 this.partOf.add(t); 2236 return t; 2237 } 2238 2239 public ImagingStudy addPartOf(Reference t) { //3 2240 if (t == null) 2241 return this; 2242 if (this.partOf == null) 2243 this.partOf = new ArrayList<Reference>(); 2244 this.partOf.add(t); 2245 return this; 2246 } 2247 2248 /** 2249 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 2250 */ 2251 public Reference getPartOfFirstRep() { 2252 if (getPartOf().isEmpty()) { 2253 addPartOf(); 2254 } 2255 return getPartOf().get(0); 2256 } 2257 2258 /** 2259 * @return {@link #referrer} (The requesting/referring physician.) 2260 */ 2261 public Reference getReferrer() { 2262 if (this.referrer == null) 2263 if (Configuration.errorOnAutoCreate()) 2264 throw new Error("Attempt to auto-create ImagingStudy.referrer"); 2265 else if (Configuration.doAutoCreate()) 2266 this.referrer = new Reference(); // cc 2267 return this.referrer; 2268 } 2269 2270 public boolean hasReferrer() { 2271 return this.referrer != null && !this.referrer.isEmpty(); 2272 } 2273 2274 /** 2275 * @param value {@link #referrer} (The requesting/referring physician.) 2276 */ 2277 public ImagingStudy setReferrer(Reference value) { 2278 this.referrer = value; 2279 return this; 2280 } 2281 2282 /** 2283 * @return {@link #endpoint} (The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.connectionType.) 2284 */ 2285 public List<Reference> getEndpoint() { 2286 if (this.endpoint == null) 2287 this.endpoint = new ArrayList<Reference>(); 2288 return this.endpoint; 2289 } 2290 2291 /** 2292 * @return Returns a reference to <code>this</code> for easy method chaining 2293 */ 2294 public ImagingStudy setEndpoint(List<Reference> theEndpoint) { 2295 this.endpoint = theEndpoint; 2296 return this; 2297 } 2298 2299 public boolean hasEndpoint() { 2300 if (this.endpoint == null) 2301 return false; 2302 for (Reference item : this.endpoint) 2303 if (!item.isEmpty()) 2304 return true; 2305 return false; 2306 } 2307 2308 public Reference addEndpoint() { //3 2309 Reference t = new Reference(); 2310 if (this.endpoint == null) 2311 this.endpoint = new ArrayList<Reference>(); 2312 this.endpoint.add(t); 2313 return t; 2314 } 2315 2316 public ImagingStudy addEndpoint(Reference t) { //3 2317 if (t == null) 2318 return this; 2319 if (this.endpoint == null) 2320 this.endpoint = new ArrayList<Reference>(); 2321 this.endpoint.add(t); 2322 return this; 2323 } 2324 2325 /** 2326 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist {3} 2327 */ 2328 public Reference getEndpointFirstRep() { 2329 if (getEndpoint().isEmpty()) { 2330 addEndpoint(); 2331 } 2332 return getEndpoint().get(0); 2333 } 2334 2335 /** 2336 * @return {@link #numberOfSeries} (Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present.). This is the underlying object with id, value and extensions. The accessor "getNumberOfSeries" gives direct access to the value 2337 */ 2338 public UnsignedIntType getNumberOfSeriesElement() { 2339 if (this.numberOfSeries == null) 2340 if (Configuration.errorOnAutoCreate()) 2341 throw new Error("Attempt to auto-create ImagingStudy.numberOfSeries"); 2342 else if (Configuration.doAutoCreate()) 2343 this.numberOfSeries = new UnsignedIntType(); // bb 2344 return this.numberOfSeries; 2345 } 2346 2347 public boolean hasNumberOfSeriesElement() { 2348 return this.numberOfSeries != null && !this.numberOfSeries.isEmpty(); 2349 } 2350 2351 public boolean hasNumberOfSeries() { 2352 return this.numberOfSeries != null && !this.numberOfSeries.isEmpty(); 2353 } 2354 2355 /** 2356 * @param value {@link #numberOfSeries} (Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present.). This is the underlying object with id, value and extensions. The accessor "getNumberOfSeries" gives direct access to the value 2357 */ 2358 public ImagingStudy setNumberOfSeriesElement(UnsignedIntType value) { 2359 this.numberOfSeries = value; 2360 return this; 2361 } 2362 2363 /** 2364 * @return Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present. 2365 */ 2366 public int getNumberOfSeries() { 2367 return this.numberOfSeries == null || this.numberOfSeries.isEmpty() ? 0 : this.numberOfSeries.getValue(); 2368 } 2369 2370 /** 2371 * @param value Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present. 2372 */ 2373 public ImagingStudy setNumberOfSeries(int value) { 2374 if (this.numberOfSeries == null) 2375 this.numberOfSeries = new UnsignedIntType(); 2376 this.numberOfSeries.setValue(value); 2377 return this; 2378 } 2379 2380 /** 2381 * @return {@link #numberOfInstances} (Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.). This is the underlying object with id, value and extensions. The accessor "getNumberOfInstances" gives direct access to the value 2382 */ 2383 public UnsignedIntType getNumberOfInstancesElement() { 2384 if (this.numberOfInstances == null) 2385 if (Configuration.errorOnAutoCreate()) 2386 throw new Error("Attempt to auto-create ImagingStudy.numberOfInstances"); 2387 else if (Configuration.doAutoCreate()) 2388 this.numberOfInstances = new UnsignedIntType(); // bb 2389 return this.numberOfInstances; 2390 } 2391 2392 public boolean hasNumberOfInstancesElement() { 2393 return this.numberOfInstances != null && !this.numberOfInstances.isEmpty(); 2394 } 2395 2396 public boolean hasNumberOfInstances() { 2397 return this.numberOfInstances != null && !this.numberOfInstances.isEmpty(); 2398 } 2399 2400 /** 2401 * @param value {@link #numberOfInstances} (Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.). This is the underlying object with id, value and extensions. The accessor "getNumberOfInstances" gives direct access to the value 2402 */ 2403 public ImagingStudy setNumberOfInstancesElement(UnsignedIntType value) { 2404 this.numberOfInstances = value; 2405 return this; 2406 } 2407 2408 /** 2409 * @return Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present. 2410 */ 2411 public int getNumberOfInstances() { 2412 return this.numberOfInstances == null || this.numberOfInstances.isEmpty() ? 0 : this.numberOfInstances.getValue(); 2413 } 2414 2415 /** 2416 * @param value Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present. 2417 */ 2418 public ImagingStudy setNumberOfInstances(int value) { 2419 if (this.numberOfInstances == null) 2420 this.numberOfInstances = new UnsignedIntType(); 2421 this.numberOfInstances.setValue(value); 2422 return this; 2423 } 2424 2425 /** 2426 * @return {@link #procedure} (This field corresponds to the DICOM Procedure Code Sequence (0008,1032). This is different from the FHIR Procedure resource that may include the ImagingStudy.) 2427 */ 2428 public List<CodeableReference> getProcedure() { 2429 if (this.procedure == null) 2430 this.procedure = new ArrayList<CodeableReference>(); 2431 return this.procedure; 2432 } 2433 2434 /** 2435 * @return Returns a reference to <code>this</code> for easy method chaining 2436 */ 2437 public ImagingStudy setProcedure(List<CodeableReference> theProcedure) { 2438 this.procedure = theProcedure; 2439 return this; 2440 } 2441 2442 public boolean hasProcedure() { 2443 if (this.procedure == null) 2444 return false; 2445 for (CodeableReference item : this.procedure) 2446 if (!item.isEmpty()) 2447 return true; 2448 return false; 2449 } 2450 2451 public CodeableReference addProcedure() { //3 2452 CodeableReference t = new CodeableReference(); 2453 if (this.procedure == null) 2454 this.procedure = new ArrayList<CodeableReference>(); 2455 this.procedure.add(t); 2456 return t; 2457 } 2458 2459 public ImagingStudy addProcedure(CodeableReference t) { //3 2460 if (t == null) 2461 return this; 2462 if (this.procedure == null) 2463 this.procedure = new ArrayList<CodeableReference>(); 2464 this.procedure.add(t); 2465 return this; 2466 } 2467 2468 /** 2469 * @return The first repetition of repeating field {@link #procedure}, creating it if it does not already exist {3} 2470 */ 2471 public CodeableReference getProcedureFirstRep() { 2472 if (getProcedure().isEmpty()) { 2473 addProcedure(); 2474 } 2475 return getProcedure().get(0); 2476 } 2477 2478 /** 2479 * @return {@link #location} (The principal physical location where the ImagingStudy was performed.) 2480 */ 2481 public Reference getLocation() { 2482 if (this.location == null) 2483 if (Configuration.errorOnAutoCreate()) 2484 throw new Error("Attempt to auto-create ImagingStudy.location"); 2485 else if (Configuration.doAutoCreate()) 2486 this.location = new Reference(); // cc 2487 return this.location; 2488 } 2489 2490 public boolean hasLocation() { 2491 return this.location != null && !this.location.isEmpty(); 2492 } 2493 2494 /** 2495 * @param value {@link #location} (The principal physical location where the ImagingStudy was performed.) 2496 */ 2497 public ImagingStudy setLocation(Reference value) { 2498 this.location = value; 2499 return this; 2500 } 2501 2502 /** 2503 * @return {@link #reason} (Description of clinical condition indicating why the ImagingStudy was requested, and/or Indicates another resource whose existence justifies this Study.) 2504 */ 2505 public List<CodeableReference> getReason() { 2506 if (this.reason == null) 2507 this.reason = new ArrayList<CodeableReference>(); 2508 return this.reason; 2509 } 2510 2511 /** 2512 * @return Returns a reference to <code>this</code> for easy method chaining 2513 */ 2514 public ImagingStudy setReason(List<CodeableReference> theReason) { 2515 this.reason = theReason; 2516 return this; 2517 } 2518 2519 public boolean hasReason() { 2520 if (this.reason == null) 2521 return false; 2522 for (CodeableReference item : this.reason) 2523 if (!item.isEmpty()) 2524 return true; 2525 return false; 2526 } 2527 2528 public CodeableReference addReason() { //3 2529 CodeableReference t = new CodeableReference(); 2530 if (this.reason == null) 2531 this.reason = new ArrayList<CodeableReference>(); 2532 this.reason.add(t); 2533 return t; 2534 } 2535 2536 public ImagingStudy addReason(CodeableReference t) { //3 2537 if (t == null) 2538 return this; 2539 if (this.reason == null) 2540 this.reason = new ArrayList<CodeableReference>(); 2541 this.reason.add(t); 2542 return this; 2543 } 2544 2545 /** 2546 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 2547 */ 2548 public CodeableReference getReasonFirstRep() { 2549 if (getReason().isEmpty()) { 2550 addReason(); 2551 } 2552 return getReason().get(0); 2553 } 2554 2555 /** 2556 * @return {@link #note} (Per the recommended DICOM mapping, this element is derived from the Study Description attribute (0008,1030). Observations or findings about the imaging study should be recorded in another resource, e.g. Observation, and not in this element.) 2557 */ 2558 public List<Annotation> getNote() { 2559 if (this.note == null) 2560 this.note = new ArrayList<Annotation>(); 2561 return this.note; 2562 } 2563 2564 /** 2565 * @return Returns a reference to <code>this</code> for easy method chaining 2566 */ 2567 public ImagingStudy setNote(List<Annotation> theNote) { 2568 this.note = theNote; 2569 return this; 2570 } 2571 2572 public boolean hasNote() { 2573 if (this.note == null) 2574 return false; 2575 for (Annotation item : this.note) 2576 if (!item.isEmpty()) 2577 return true; 2578 return false; 2579 } 2580 2581 public Annotation addNote() { //3 2582 Annotation t = new Annotation(); 2583 if (this.note == null) 2584 this.note = new ArrayList<Annotation>(); 2585 this.note.add(t); 2586 return t; 2587 } 2588 2589 public ImagingStudy addNote(Annotation t) { //3 2590 if (t == null) 2591 return this; 2592 if (this.note == null) 2593 this.note = new ArrayList<Annotation>(); 2594 this.note.add(t); 2595 return this; 2596 } 2597 2598 /** 2599 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2600 */ 2601 public Annotation getNoteFirstRep() { 2602 if (getNote().isEmpty()) { 2603 addNote(); 2604 } 2605 return getNote().get(0); 2606 } 2607 2608 /** 2609 * @return {@link #description} (The Imaging Manager description of the study. Institution-generated description or classification of the Study (component) performed.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2610 */ 2611 public StringType getDescriptionElement() { 2612 if (this.description == null) 2613 if (Configuration.errorOnAutoCreate()) 2614 throw new Error("Attempt to auto-create ImagingStudy.description"); 2615 else if (Configuration.doAutoCreate()) 2616 this.description = new StringType(); // bb 2617 return this.description; 2618 } 2619 2620 public boolean hasDescriptionElement() { 2621 return this.description != null && !this.description.isEmpty(); 2622 } 2623 2624 public boolean hasDescription() { 2625 return this.description != null && !this.description.isEmpty(); 2626 } 2627 2628 /** 2629 * @param value {@link #description} (The Imaging Manager description of the study. Institution-generated description or classification of the Study (component) performed.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2630 */ 2631 public ImagingStudy setDescriptionElement(StringType value) { 2632 this.description = value; 2633 return this; 2634 } 2635 2636 /** 2637 * @return The Imaging Manager description of the study. Institution-generated description or classification of the Study (component) performed. 2638 */ 2639 public String getDescription() { 2640 return this.description == null ? null : this.description.getValue(); 2641 } 2642 2643 /** 2644 * @param value The Imaging Manager description of the study. Institution-generated description or classification of the Study (component) performed. 2645 */ 2646 public ImagingStudy setDescription(String value) { 2647 if (Utilities.noString(value)) 2648 this.description = null; 2649 else { 2650 if (this.description == null) 2651 this.description = new StringType(); 2652 this.description.setValue(value); 2653 } 2654 return this; 2655 } 2656 2657 /** 2658 * @return {@link #series} (Each study has one or more series of images or other content.) 2659 */ 2660 public List<ImagingStudySeriesComponent> getSeries() { 2661 if (this.series == null) 2662 this.series = new ArrayList<ImagingStudySeriesComponent>(); 2663 return this.series; 2664 } 2665 2666 /** 2667 * @return Returns a reference to <code>this</code> for easy method chaining 2668 */ 2669 public ImagingStudy setSeries(List<ImagingStudySeriesComponent> theSeries) { 2670 this.series = theSeries; 2671 return this; 2672 } 2673 2674 public boolean hasSeries() { 2675 if (this.series == null) 2676 return false; 2677 for (ImagingStudySeriesComponent item : this.series) 2678 if (!item.isEmpty()) 2679 return true; 2680 return false; 2681 } 2682 2683 public ImagingStudySeriesComponent addSeries() { //3 2684 ImagingStudySeriesComponent t = new ImagingStudySeriesComponent(); 2685 if (this.series == null) 2686 this.series = new ArrayList<ImagingStudySeriesComponent>(); 2687 this.series.add(t); 2688 return t; 2689 } 2690 2691 public ImagingStudy addSeries(ImagingStudySeriesComponent t) { //3 2692 if (t == null) 2693 return this; 2694 if (this.series == null) 2695 this.series = new ArrayList<ImagingStudySeriesComponent>(); 2696 this.series.add(t); 2697 return this; 2698 } 2699 2700 /** 2701 * @return The first repetition of repeating field {@link #series}, creating it if it does not already exist {3} 2702 */ 2703 public ImagingStudySeriesComponent getSeriesFirstRep() { 2704 if (getSeries().isEmpty()) { 2705 addSeries(); 2706 } 2707 return getSeries().get(0); 2708 } 2709 2710 protected void listChildren(List<Property> children) { 2711 super.listChildren(children); 2712 children.add(new Property("identifier", "Identifier", "Identifiers for the ImagingStudy such as DICOM Study Instance UID.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2713 children.add(new Property("status", "code", "The current state of the ImagingStudy resource. This is not the status of any ServiceRequest or Task resources associated with the ImagingStudy.", 0, 1, status)); 2714 children.add(new Property("modality", "CodeableConcept", "A list of all the distinct values of series.modality. This may include both acquisition and non-acquisition modalities.", 0, java.lang.Integer.MAX_VALUE, modality)); 2715 children.add(new Property("subject", "Reference(Patient|Device|Group)", "The subject, typically a patient, of the imaging study.", 0, 1, subject)); 2716 children.add(new Property("encounter", "Reference(Encounter)", "The healthcare event (e.g. a patient and healthcare provider interaction) during which this ImagingStudy is made.", 0, 1, encounter)); 2717 children.add(new Property("started", "dateTime", "Date and time the study started.", 0, 1, started)); 2718 children.add(new Property("basedOn", "Reference(CarePlan|ServiceRequest|Appointment|AppointmentResponse|Task)", "A list of the diagnostic requests that resulted in this imaging study being performed.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2719 children.add(new Property("partOf", "Reference(Procedure)", "A larger event of which this particular ImagingStudy is a component or step. For example, an ImagingStudy as part of a procedure.", 0, java.lang.Integer.MAX_VALUE, partOf)); 2720 children.add(new Property("referrer", "Reference(Practitioner|PractitionerRole)", "The requesting/referring physician.", 0, 1, referrer)); 2721 children.add(new Property("endpoint", "Reference(Endpoint)", "The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.connectionType.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 2722 children.add(new Property("numberOfSeries", "unsignedInt", "Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present.", 0, 1, numberOfSeries)); 2723 children.add(new Property("numberOfInstances", "unsignedInt", "Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.", 0, 1, numberOfInstances)); 2724 children.add(new Property("procedure", "CodeableReference(PlanDefinition|ActivityDefinition)", "This field corresponds to the DICOM Procedure Code Sequence (0008,1032). This is different from the FHIR Procedure resource that may include the ImagingStudy.", 0, java.lang.Integer.MAX_VALUE, procedure)); 2725 children.add(new Property("location", "Reference(Location)", "The principal physical location where the ImagingStudy was performed.", 0, 1, location)); 2726 children.add(new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference)", "Description of clinical condition indicating why the ImagingStudy was requested, and/or Indicates another resource whose existence justifies this Study.", 0, java.lang.Integer.MAX_VALUE, reason)); 2727 children.add(new Property("note", "Annotation", "Per the recommended DICOM mapping, this element is derived from the Study Description attribute (0008,1030). Observations or findings about the imaging study should be recorded in another resource, e.g. Observation, and not in this element.", 0, java.lang.Integer.MAX_VALUE, note)); 2728 children.add(new Property("description", "string", "The Imaging Manager description of the study. Institution-generated description or classification of the Study (component) performed.", 0, 1, description)); 2729 children.add(new Property("series", "", "Each study has one or more series of images or other content.", 0, java.lang.Integer.MAX_VALUE, series)); 2730 } 2731 2732 @Override 2733 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2734 switch (_hash) { 2735 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers for the ImagingStudy such as DICOM Study Instance UID.", 0, java.lang.Integer.MAX_VALUE, identifier); 2736 case -892481550: /*status*/ return new Property("status", "code", "The current state of the ImagingStudy resource. This is not the status of any ServiceRequest or Task resources associated with the ImagingStudy.", 0, 1, status); 2737 case -622722335: /*modality*/ return new Property("modality", "CodeableConcept", "A list of all the distinct values of series.modality. This may include both acquisition and non-acquisition modalities.", 0, java.lang.Integer.MAX_VALUE, modality); 2738 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Device|Group)", "The subject, typically a patient, of the imaging study.", 0, 1, subject); 2739 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The healthcare event (e.g. a patient and healthcare provider interaction) during which this ImagingStudy is made.", 0, 1, encounter); 2740 case -1897185151: /*started*/ return new Property("started", "dateTime", "Date and time the study started.", 0, 1, started); 2741 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|ServiceRequest|Appointment|AppointmentResponse|Task)", "A list of the diagnostic requests that resulted in this imaging study being performed.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2742 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Procedure)", "A larger event of which this particular ImagingStudy is a component or step. For example, an ImagingStudy as part of a procedure.", 0, java.lang.Integer.MAX_VALUE, partOf); 2743 case -722568161: /*referrer*/ return new Property("referrer", "Reference(Practitioner|PractitionerRole)", "The requesting/referring physician.", 0, 1, referrer); 2744 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.connectionType.", 0, java.lang.Integer.MAX_VALUE, endpoint); 2745 case 1920000407: /*numberOfSeries*/ return new Property("numberOfSeries", "unsignedInt", "Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present.", 0, 1, numberOfSeries); 2746 case -1043544226: /*numberOfInstances*/ return new Property("numberOfInstances", "unsignedInt", "Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.", 0, 1, numberOfInstances); 2747 case -1095204141: /*procedure*/ return new Property("procedure", "CodeableReference(PlanDefinition|ActivityDefinition)", "This field corresponds to the DICOM Procedure Code Sequence (0008,1032). This is different from the FHIR Procedure resource that may include the ImagingStudy.", 0, java.lang.Integer.MAX_VALUE, procedure); 2748 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The principal physical location where the ImagingStudy was performed.", 0, 1, location); 2749 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport|DocumentReference)", "Description of clinical condition indicating why the ImagingStudy was requested, and/or Indicates another resource whose existence justifies this Study.", 0, java.lang.Integer.MAX_VALUE, reason); 2750 case 3387378: /*note*/ return new Property("note", "Annotation", "Per the recommended DICOM mapping, this element is derived from the Study Description attribute (0008,1030). Observations or findings about the imaging study should be recorded in another resource, e.g. Observation, and not in this element.", 0, java.lang.Integer.MAX_VALUE, note); 2751 case -1724546052: /*description*/ return new Property("description", "string", "The Imaging Manager description of the study. Institution-generated description or classification of the Study (component) performed.", 0, 1, description); 2752 case -905838985: /*series*/ return new Property("series", "", "Each study has one or more series of images or other content.", 0, java.lang.Integer.MAX_VALUE, series); 2753 default: return super.getNamedProperty(_hash, _name, _checkValid); 2754 } 2755 2756 } 2757 2758 @Override 2759 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2760 switch (hash) { 2761 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2762 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ImagingStudyStatus> 2763 case -622722335: /*modality*/ return this.modality == null ? new Base[0] : this.modality.toArray(new Base[this.modality.size()]); // CodeableConcept 2764 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2765 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 2766 case -1897185151: /*started*/ return this.started == null ? new Base[0] : new Base[] {this.started}; // DateTimeType 2767 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2768 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2769 case -722568161: /*referrer*/ return this.referrer == null ? new Base[0] : new Base[] {this.referrer}; // Reference 2770 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 2771 case 1920000407: /*numberOfSeries*/ return this.numberOfSeries == null ? new Base[0] : new Base[] {this.numberOfSeries}; // UnsignedIntType 2772 case -1043544226: /*numberOfInstances*/ return this.numberOfInstances == null ? new Base[0] : new Base[] {this.numberOfInstances}; // UnsignedIntType 2773 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : this.procedure.toArray(new Base[this.procedure.size()]); // CodeableReference 2774 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 2775 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 2776 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2777 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2778 case -905838985: /*series*/ return this.series == null ? new Base[0] : this.series.toArray(new Base[this.series.size()]); // ImagingStudySeriesComponent 2779 default: return super.getProperty(hash, name, checkValid); 2780 } 2781 2782 } 2783 2784 @Override 2785 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2786 switch (hash) { 2787 case -1618432855: // identifier 2788 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2789 return value; 2790 case -892481550: // status 2791 value = new ImagingStudyStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2792 this.status = (Enumeration) value; // Enumeration<ImagingStudyStatus> 2793 return value; 2794 case -622722335: // modality 2795 this.getModality().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2796 return value; 2797 case -1867885268: // subject 2798 this.subject = TypeConvertor.castToReference(value); // Reference 2799 return value; 2800 case 1524132147: // encounter 2801 this.encounter = TypeConvertor.castToReference(value); // Reference 2802 return value; 2803 case -1897185151: // started 2804 this.started = TypeConvertor.castToDateTime(value); // DateTimeType 2805 return value; 2806 case -332612366: // basedOn 2807 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 2808 return value; 2809 case -995410646: // partOf 2810 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 2811 return value; 2812 case -722568161: // referrer 2813 this.referrer = TypeConvertor.castToReference(value); // Reference 2814 return value; 2815 case 1741102485: // endpoint 2816 this.getEndpoint().add(TypeConvertor.castToReference(value)); // Reference 2817 return value; 2818 case 1920000407: // numberOfSeries 2819 this.numberOfSeries = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 2820 return value; 2821 case -1043544226: // numberOfInstances 2822 this.numberOfInstances = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 2823 return value; 2824 case -1095204141: // procedure 2825 this.getProcedure().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2826 return value; 2827 case 1901043637: // location 2828 this.location = TypeConvertor.castToReference(value); // Reference 2829 return value; 2830 case -934964668: // reason 2831 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2832 return value; 2833 case 3387378: // note 2834 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2835 return value; 2836 case -1724546052: // description 2837 this.description = TypeConvertor.castToString(value); // StringType 2838 return value; 2839 case -905838985: // series 2840 this.getSeries().add((ImagingStudySeriesComponent) value); // ImagingStudySeriesComponent 2841 return value; 2842 default: return super.setProperty(hash, name, value); 2843 } 2844 2845 } 2846 2847 @Override 2848 public Base setProperty(String name, Base value) throws FHIRException { 2849 if (name.equals("identifier")) { 2850 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2851 } else if (name.equals("status")) { 2852 value = new ImagingStudyStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2853 this.status = (Enumeration) value; // Enumeration<ImagingStudyStatus> 2854 } else if (name.equals("modality")) { 2855 this.getModality().add(TypeConvertor.castToCodeableConcept(value)); 2856 } else if (name.equals("subject")) { 2857 this.subject = TypeConvertor.castToReference(value); // Reference 2858 } else if (name.equals("encounter")) { 2859 this.encounter = TypeConvertor.castToReference(value); // Reference 2860 } else if (name.equals("started")) { 2861 this.started = TypeConvertor.castToDateTime(value); // DateTimeType 2862 } else if (name.equals("basedOn")) { 2863 this.getBasedOn().add(TypeConvertor.castToReference(value)); 2864 } else if (name.equals("partOf")) { 2865 this.getPartOf().add(TypeConvertor.castToReference(value)); 2866 } else if (name.equals("referrer")) { 2867 this.referrer = TypeConvertor.castToReference(value); // Reference 2868 } else if (name.equals("endpoint")) { 2869 this.getEndpoint().add(TypeConvertor.castToReference(value)); 2870 } else if (name.equals("numberOfSeries")) { 2871 this.numberOfSeries = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 2872 } else if (name.equals("numberOfInstances")) { 2873 this.numberOfInstances = TypeConvertor.castToUnsignedInt(value); // UnsignedIntType 2874 } else if (name.equals("procedure")) { 2875 this.getProcedure().add(TypeConvertor.castToCodeableReference(value)); 2876 } else if (name.equals("location")) { 2877 this.location = TypeConvertor.castToReference(value); // Reference 2878 } else if (name.equals("reason")) { 2879 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 2880 } else if (name.equals("note")) { 2881 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2882 } else if (name.equals("description")) { 2883 this.description = TypeConvertor.castToString(value); // StringType 2884 } else if (name.equals("series")) { 2885 this.getSeries().add((ImagingStudySeriesComponent) value); 2886 } else 2887 return super.setProperty(name, value); 2888 return value; 2889 } 2890 2891 @Override 2892 public void removeChild(String name, Base value) throws FHIRException { 2893 if (name.equals("identifier")) { 2894 this.getIdentifier().remove(value); 2895 } else if (name.equals("status")) { 2896 value = new ImagingStudyStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2897 this.status = (Enumeration) value; // Enumeration<ImagingStudyStatus> 2898 } else if (name.equals("modality")) { 2899 this.getModality().remove(value); 2900 } else if (name.equals("subject")) { 2901 this.subject = null; 2902 } else if (name.equals("encounter")) { 2903 this.encounter = null; 2904 } else if (name.equals("started")) { 2905 this.started = null; 2906 } else if (name.equals("basedOn")) { 2907 this.getBasedOn().remove(value); 2908 } else if (name.equals("partOf")) { 2909 this.getPartOf().remove(value); 2910 } else if (name.equals("referrer")) { 2911 this.referrer = null; 2912 } else if (name.equals("endpoint")) { 2913 this.getEndpoint().remove(value); 2914 } else if (name.equals("numberOfSeries")) { 2915 this.numberOfSeries = null; 2916 } else if (name.equals("numberOfInstances")) { 2917 this.numberOfInstances = null; 2918 } else if (name.equals("procedure")) { 2919 this.getProcedure().remove(value); 2920 } else if (name.equals("location")) { 2921 this.location = null; 2922 } else if (name.equals("reason")) { 2923 this.getReason().remove(value); 2924 } else if (name.equals("note")) { 2925 this.getNote().remove(value); 2926 } else if (name.equals("description")) { 2927 this.description = null; 2928 } else if (name.equals("series")) { 2929 this.getSeries().remove((ImagingStudySeriesComponent) value); 2930 } else 2931 super.removeChild(name, value); 2932 2933 } 2934 2935 @Override 2936 public Base makeProperty(int hash, String name) throws FHIRException { 2937 switch (hash) { 2938 case -1618432855: return addIdentifier(); 2939 case -892481550: return getStatusElement(); 2940 case -622722335: return addModality(); 2941 case -1867885268: return getSubject(); 2942 case 1524132147: return getEncounter(); 2943 case -1897185151: return getStartedElement(); 2944 case -332612366: return addBasedOn(); 2945 case -995410646: return addPartOf(); 2946 case -722568161: return getReferrer(); 2947 case 1741102485: return addEndpoint(); 2948 case 1920000407: return getNumberOfSeriesElement(); 2949 case -1043544226: return getNumberOfInstancesElement(); 2950 case -1095204141: return addProcedure(); 2951 case 1901043637: return getLocation(); 2952 case -934964668: return addReason(); 2953 case 3387378: return addNote(); 2954 case -1724546052: return getDescriptionElement(); 2955 case -905838985: return addSeries(); 2956 default: return super.makeProperty(hash, name); 2957 } 2958 2959 } 2960 2961 @Override 2962 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2963 switch (hash) { 2964 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2965 case -892481550: /*status*/ return new String[] {"code"}; 2966 case -622722335: /*modality*/ return new String[] {"CodeableConcept"}; 2967 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2968 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 2969 case -1897185151: /*started*/ return new String[] {"dateTime"}; 2970 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2971 case -995410646: /*partOf*/ return new String[] {"Reference"}; 2972 case -722568161: /*referrer*/ return new String[] {"Reference"}; 2973 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 2974 case 1920000407: /*numberOfSeries*/ return new String[] {"unsignedInt"}; 2975 case -1043544226: /*numberOfInstances*/ return new String[] {"unsignedInt"}; 2976 case -1095204141: /*procedure*/ return new String[] {"CodeableReference"}; 2977 case 1901043637: /*location*/ return new String[] {"Reference"}; 2978 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 2979 case 3387378: /*note*/ return new String[] {"Annotation"}; 2980 case -1724546052: /*description*/ return new String[] {"string"}; 2981 case -905838985: /*series*/ return new String[] {}; 2982 default: return super.getTypesForProperty(hash, name); 2983 } 2984 2985 } 2986 2987 @Override 2988 public Base addChild(String name) throws FHIRException { 2989 if (name.equals("identifier")) { 2990 return addIdentifier(); 2991 } 2992 else if (name.equals("status")) { 2993 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.status"); 2994 } 2995 else if (name.equals("modality")) { 2996 return addModality(); 2997 } 2998 else if (name.equals("subject")) { 2999 this.subject = new Reference(); 3000 return this.subject; 3001 } 3002 else if (name.equals("encounter")) { 3003 this.encounter = new Reference(); 3004 return this.encounter; 3005 } 3006 else if (name.equals("started")) { 3007 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.started"); 3008 } 3009 else if (name.equals("basedOn")) { 3010 return addBasedOn(); 3011 } 3012 else if (name.equals("partOf")) { 3013 return addPartOf(); 3014 } 3015 else if (name.equals("referrer")) { 3016 this.referrer = new Reference(); 3017 return this.referrer; 3018 } 3019 else if (name.equals("endpoint")) { 3020 return addEndpoint(); 3021 } 3022 else if (name.equals("numberOfSeries")) { 3023 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.numberOfSeries"); 3024 } 3025 else if (name.equals("numberOfInstances")) { 3026 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.numberOfInstances"); 3027 } 3028 else if (name.equals("procedure")) { 3029 return addProcedure(); 3030 } 3031 else if (name.equals("location")) { 3032 this.location = new Reference(); 3033 return this.location; 3034 } 3035 else if (name.equals("reason")) { 3036 return addReason(); 3037 } 3038 else if (name.equals("note")) { 3039 return addNote(); 3040 } 3041 else if (name.equals("description")) { 3042 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.description"); 3043 } 3044 else if (name.equals("series")) { 3045 return addSeries(); 3046 } 3047 else 3048 return super.addChild(name); 3049 } 3050 3051 public String fhirType() { 3052 return "ImagingStudy"; 3053 3054 } 3055 3056 public ImagingStudy copy() { 3057 ImagingStudy dst = new ImagingStudy(); 3058 copyValues(dst); 3059 return dst; 3060 } 3061 3062 public void copyValues(ImagingStudy dst) { 3063 super.copyValues(dst); 3064 if (identifier != null) { 3065 dst.identifier = new ArrayList<Identifier>(); 3066 for (Identifier i : identifier) 3067 dst.identifier.add(i.copy()); 3068 }; 3069 dst.status = status == null ? null : status.copy(); 3070 if (modality != null) { 3071 dst.modality = new ArrayList<CodeableConcept>(); 3072 for (CodeableConcept i : modality) 3073 dst.modality.add(i.copy()); 3074 }; 3075 dst.subject = subject == null ? null : subject.copy(); 3076 dst.encounter = encounter == null ? null : encounter.copy(); 3077 dst.started = started == null ? null : started.copy(); 3078 if (basedOn != null) { 3079 dst.basedOn = new ArrayList<Reference>(); 3080 for (Reference i : basedOn) 3081 dst.basedOn.add(i.copy()); 3082 }; 3083 if (partOf != null) { 3084 dst.partOf = new ArrayList<Reference>(); 3085 for (Reference i : partOf) 3086 dst.partOf.add(i.copy()); 3087 }; 3088 dst.referrer = referrer == null ? null : referrer.copy(); 3089 if (endpoint != null) { 3090 dst.endpoint = new ArrayList<Reference>(); 3091 for (Reference i : endpoint) 3092 dst.endpoint.add(i.copy()); 3093 }; 3094 dst.numberOfSeries = numberOfSeries == null ? null : numberOfSeries.copy(); 3095 dst.numberOfInstances = numberOfInstances == null ? null : numberOfInstances.copy(); 3096 if (procedure != null) { 3097 dst.procedure = new ArrayList<CodeableReference>(); 3098 for (CodeableReference i : procedure) 3099 dst.procedure.add(i.copy()); 3100 }; 3101 dst.location = location == null ? null : location.copy(); 3102 if (reason != null) { 3103 dst.reason = new ArrayList<CodeableReference>(); 3104 for (CodeableReference i : reason) 3105 dst.reason.add(i.copy()); 3106 }; 3107 if (note != null) { 3108 dst.note = new ArrayList<Annotation>(); 3109 for (Annotation i : note) 3110 dst.note.add(i.copy()); 3111 }; 3112 dst.description = description == null ? null : description.copy(); 3113 if (series != null) { 3114 dst.series = new ArrayList<ImagingStudySeriesComponent>(); 3115 for (ImagingStudySeriesComponent i : series) 3116 dst.series.add(i.copy()); 3117 }; 3118 } 3119 3120 protected ImagingStudy typedCopy() { 3121 return copy(); 3122 } 3123 3124 @Override 3125 public boolean equalsDeep(Base other_) { 3126 if (!super.equalsDeep(other_)) 3127 return false; 3128 if (!(other_ instanceof ImagingStudy)) 3129 return false; 3130 ImagingStudy o = (ImagingStudy) other_; 3131 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(modality, o.modality, true) 3132 && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) && compareDeep(started, o.started, true) 3133 && compareDeep(basedOn, o.basedOn, true) && compareDeep(partOf, o.partOf, true) && compareDeep(referrer, o.referrer, true) 3134 && compareDeep(endpoint, o.endpoint, true) && compareDeep(numberOfSeries, o.numberOfSeries, true) 3135 && compareDeep(numberOfInstances, o.numberOfInstances, true) && compareDeep(procedure, o.procedure, true) 3136 && compareDeep(location, o.location, true) && compareDeep(reason, o.reason, true) && compareDeep(note, o.note, true) 3137 && compareDeep(description, o.description, true) && compareDeep(series, o.series, true); 3138 } 3139 3140 @Override 3141 public boolean equalsShallow(Base other_) { 3142 if (!super.equalsShallow(other_)) 3143 return false; 3144 if (!(other_ instanceof ImagingStudy)) 3145 return false; 3146 ImagingStudy o = (ImagingStudy) other_; 3147 return compareValues(status, o.status, true) && compareValues(started, o.started, true) && compareValues(numberOfSeries, o.numberOfSeries, true) 3148 && compareValues(numberOfInstances, o.numberOfInstances, true) && compareValues(description, o.description, true) 3149 ; 3150 } 3151 3152 public boolean isEmpty() { 3153 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, modality 3154 , subject, encounter, started, basedOn, partOf, referrer, endpoint, numberOfSeries 3155 , numberOfInstances, procedure, location, reason, note, description, series); 3156 } 3157 3158 @Override 3159 public ResourceType getResourceType() { 3160 return ResourceType.ImagingStudy; 3161 } 3162 3163 /** 3164 * Search parameter: <b>based-on</b> 3165 * <p> 3166 * Description: <b>The order for the image, such as Accession Number associated with a ServiceRequest</b><br> 3167 * Type: <b>reference</b><br> 3168 * Path: <b>ImagingStudy.basedOn</b><br> 3169 * </p> 3170 */ 3171 @SearchParamDefinition(name="based-on", path="ImagingStudy.basedOn", description="The order for the image, such as Accession Number associated with a ServiceRequest", type="reference", target={Appointment.class, AppointmentResponse.class, CarePlan.class, ServiceRequest.class, Task.class } ) 3172 public static final String SP_BASED_ON = "based-on"; 3173 /** 3174 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 3175 * <p> 3176 * Description: <b>The order for the image, such as Accession Number associated with a ServiceRequest</b><br> 3177 * Type: <b>reference</b><br> 3178 * Path: <b>ImagingStudy.basedOn</b><br> 3179 * </p> 3180 */ 3181 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 3182 3183/** 3184 * Constant for fluent queries to be used to add include statements. Specifies 3185 * the path value of "<b>ImagingStudy:based-on</b>". 3186 */ 3187 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("ImagingStudy:based-on").toLocked(); 3188 3189 /** 3190 * Search parameter: <b>body-site</b> 3191 * <p> 3192 * Description: <b>The body site code studied</b><br> 3193 * Type: <b>token</b><br> 3194 * Path: <b>ImagingStudy.series.bodySite.concept</b><br> 3195 * </p> 3196 */ 3197 @SearchParamDefinition(name="body-site", path="ImagingStudy.series.bodySite.concept", description="The body site code studied", type="token" ) 3198 public static final String SP_BODY_SITE = "body-site"; 3199 /** 3200 * <b>Fluent Client</b> search parameter constant for <b>body-site</b> 3201 * <p> 3202 * Description: <b>The body site code studied</b><br> 3203 * Type: <b>token</b><br> 3204 * Path: <b>ImagingStudy.series.bodySite.concept</b><br> 3205 * </p> 3206 */ 3207 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BODY_SITE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BODY_SITE); 3208 3209 /** 3210 * Search parameter: <b>body-structure</b> 3211 * <p> 3212 * Description: <b>The body structure resource associated with the ImagingStudy</b><br> 3213 * Type: <b>reference</b><br> 3214 * Path: <b>ImagingStudy.series.bodySite.reference</b><br> 3215 * </p> 3216 */ 3217 @SearchParamDefinition(name="body-structure", path="ImagingStudy.series.bodySite.reference", description="The body structure resource associated with the ImagingStudy", type="reference", target={BodyStructure.class } ) 3218 public static final String SP_BODY_STRUCTURE = "body-structure"; 3219 /** 3220 * <b>Fluent Client</b> search parameter constant for <b>body-structure</b> 3221 * <p> 3222 * Description: <b>The body structure resource associated with the ImagingStudy</b><br> 3223 * Type: <b>reference</b><br> 3224 * Path: <b>ImagingStudy.series.bodySite.reference</b><br> 3225 * </p> 3226 */ 3227 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BODY_STRUCTURE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BODY_STRUCTURE); 3228 3229/** 3230 * Constant for fluent queries to be used to add include statements. Specifies 3231 * the path value of "<b>ImagingStudy:body-structure</b>". 3232 */ 3233 public static final ca.uhn.fhir.model.api.Include INCLUDE_BODY_STRUCTURE = new ca.uhn.fhir.model.api.Include("ImagingStudy:body-structure").toLocked(); 3234 3235 /** 3236 * Search parameter: <b>dicom-class</b> 3237 * <p> 3238 * Description: <b>The type of the instance</b><br> 3239 * Type: <b>token</b><br> 3240 * Path: <b>ImagingStudy.series.instance.sopClass</b><br> 3241 * </p> 3242 */ 3243 @SearchParamDefinition(name="dicom-class", path="ImagingStudy.series.instance.sopClass", description="The type of the instance", type="token" ) 3244 public static final String SP_DICOM_CLASS = "dicom-class"; 3245 /** 3246 * <b>Fluent Client</b> search parameter constant for <b>dicom-class</b> 3247 * <p> 3248 * Description: <b>The type of the instance</b><br> 3249 * Type: <b>token</b><br> 3250 * Path: <b>ImagingStudy.series.instance.sopClass</b><br> 3251 * </p> 3252 */ 3253 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DICOM_CLASS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DICOM_CLASS); 3254 3255 /** 3256 * Search parameter: <b>endpoint</b> 3257 * <p> 3258 * Description: <b>The endpoint for the study or series</b><br> 3259 * Type: <b>reference</b><br> 3260 * Path: <b>ImagingStudy.endpoint | ImagingStudy.series.endpoint</b><br> 3261 * </p> 3262 */ 3263 @SearchParamDefinition(name="endpoint", path="ImagingStudy.endpoint | ImagingStudy.series.endpoint", description="The endpoint for the study or series", type="reference", target={Endpoint.class } ) 3264 public static final String SP_ENDPOINT = "endpoint"; 3265 /** 3266 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 3267 * <p> 3268 * Description: <b>The endpoint for the study or series</b><br> 3269 * Type: <b>reference</b><br> 3270 * Path: <b>ImagingStudy.endpoint | ImagingStudy.series.endpoint</b><br> 3271 * </p> 3272 */ 3273 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENDPOINT); 3274 3275/** 3276 * Constant for fluent queries to be used to add include statements. Specifies 3277 * the path value of "<b>ImagingStudy:endpoint</b>". 3278 */ 3279 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include("ImagingStudy:endpoint").toLocked(); 3280 3281 /** 3282 * Search parameter: <b>instance</b> 3283 * <p> 3284 * Description: <b>SOP Instance UID for an instance</b><br> 3285 * Type: <b>token</b><br> 3286 * Path: <b>ImagingStudy.series.instance.uid</b><br> 3287 * </p> 3288 */ 3289 @SearchParamDefinition(name="instance", path="ImagingStudy.series.instance.uid", description="SOP Instance UID for an instance", type="token" ) 3290 public static final String SP_INSTANCE = "instance"; 3291 /** 3292 * <b>Fluent Client</b> search parameter constant for <b>instance</b> 3293 * <p> 3294 * Description: <b>SOP Instance UID for an instance</b><br> 3295 * Type: <b>token</b><br> 3296 * Path: <b>ImagingStudy.series.instance.uid</b><br> 3297 * </p> 3298 */ 3299 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INSTANCE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INSTANCE); 3300 3301 /** 3302 * Search parameter: <b>modality</b> 3303 * <p> 3304 * Description: <b>The modality of the series</b><br> 3305 * Type: <b>token</b><br> 3306 * Path: <b>ImagingStudy.series.modality</b><br> 3307 * </p> 3308 */ 3309 @SearchParamDefinition(name="modality", path="ImagingStudy.series.modality", description="The modality of the series", type="token" ) 3310 public static final String SP_MODALITY = "modality"; 3311 /** 3312 * <b>Fluent Client</b> search parameter constant for <b>modality</b> 3313 * <p> 3314 * Description: <b>The modality of the series</b><br> 3315 * Type: <b>token</b><br> 3316 * Path: <b>ImagingStudy.series.modality</b><br> 3317 * </p> 3318 */ 3319 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MODALITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MODALITY); 3320 3321 /** 3322 * Search parameter: <b>performer</b> 3323 * <p> 3324 * Description: <b>The person who performed the study</b><br> 3325 * Type: <b>reference</b><br> 3326 * Path: <b>ImagingStudy.series.performer.actor</b><br> 3327 * </p> 3328 */ 3329 @SearchParamDefinition(name="performer", path="ImagingStudy.series.performer.actor", description="The person who performed the study", type="reference", target={CareTeam.class, Device.class, HealthcareService.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 3330 public static final String SP_PERFORMER = "performer"; 3331 /** 3332 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 3333 * <p> 3334 * Description: <b>The person who performed the study</b><br> 3335 * Type: <b>reference</b><br> 3336 * Path: <b>ImagingStudy.series.performer.actor</b><br> 3337 * </p> 3338 */ 3339 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 3340 3341/** 3342 * Constant for fluent queries to be used to add include statements. Specifies 3343 * the path value of "<b>ImagingStudy:performer</b>". 3344 */ 3345 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("ImagingStudy:performer").toLocked(); 3346 3347 /** 3348 * Search parameter: <b>reason</b> 3349 * <p> 3350 * Description: <b>The reason for the study</b><br> 3351 * Type: <b>token</b><br> 3352 * Path: <b>null</b><br> 3353 * </p> 3354 */ 3355 @SearchParamDefinition(name="reason", path="", description="The reason for the study", type="token" ) 3356 public static final String SP_REASON = "reason"; 3357 /** 3358 * <b>Fluent Client</b> search parameter constant for <b>reason</b> 3359 * <p> 3360 * Description: <b>The reason for the study</b><br> 3361 * Type: <b>token</b><br> 3362 * Path: <b>null</b><br> 3363 * </p> 3364 */ 3365 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REASON); 3366 3367 /** 3368 * Search parameter: <b>referrer</b> 3369 * <p> 3370 * Description: <b>The referring physician</b><br> 3371 * Type: <b>reference</b><br> 3372 * Path: <b>ImagingStudy.referrer</b><br> 3373 * </p> 3374 */ 3375 @SearchParamDefinition(name="referrer", path="ImagingStudy.referrer", description="The referring physician", type="reference", target={Practitioner.class, PractitionerRole.class } ) 3376 public static final String SP_REFERRER = "referrer"; 3377 /** 3378 * <b>Fluent Client</b> search parameter constant for <b>referrer</b> 3379 * <p> 3380 * Description: <b>The referring physician</b><br> 3381 * Type: <b>reference</b><br> 3382 * Path: <b>ImagingStudy.referrer</b><br> 3383 * </p> 3384 */ 3385 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REFERRER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REFERRER); 3386 3387/** 3388 * Constant for fluent queries to be used to add include statements. Specifies 3389 * the path value of "<b>ImagingStudy:referrer</b>". 3390 */ 3391 public static final ca.uhn.fhir.model.api.Include INCLUDE_REFERRER = new ca.uhn.fhir.model.api.Include("ImagingStudy:referrer").toLocked(); 3392 3393 /** 3394 * Search parameter: <b>series</b> 3395 * <p> 3396 * Description: <b>DICOM Series Instance UID for a series</b><br> 3397 * Type: <b>token</b><br> 3398 * Path: <b>ImagingStudy.series.uid</b><br> 3399 * </p> 3400 */ 3401 @SearchParamDefinition(name="series", path="ImagingStudy.series.uid", description="DICOM Series Instance UID for a series", type="token" ) 3402 public static final String SP_SERIES = "series"; 3403 /** 3404 * <b>Fluent Client</b> search parameter constant for <b>series</b> 3405 * <p> 3406 * Description: <b>DICOM Series Instance UID for a series</b><br> 3407 * Type: <b>token</b><br> 3408 * Path: <b>ImagingStudy.series.uid</b><br> 3409 * </p> 3410 */ 3411 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERIES = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERIES); 3412 3413 /** 3414 * Search parameter: <b>started</b> 3415 * <p> 3416 * Description: <b>When the study was started</b><br> 3417 * Type: <b>date</b><br> 3418 * Path: <b>ImagingStudy.started</b><br> 3419 * </p> 3420 */ 3421 @SearchParamDefinition(name="started", path="ImagingStudy.started", description="When the study was started", type="date" ) 3422 public static final String SP_STARTED = "started"; 3423 /** 3424 * <b>Fluent Client</b> search parameter constant for <b>started</b> 3425 * <p> 3426 * Description: <b>When the study was started</b><br> 3427 * Type: <b>date</b><br> 3428 * Path: <b>ImagingStudy.started</b><br> 3429 * </p> 3430 */ 3431 public static final ca.uhn.fhir.rest.gclient.DateClientParam STARTED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_STARTED); 3432 3433 /** 3434 * Search parameter: <b>status</b> 3435 * <p> 3436 * Description: <b>The status of the study</b><br> 3437 * Type: <b>token</b><br> 3438 * Path: <b>ImagingStudy.status</b><br> 3439 * </p> 3440 */ 3441 @SearchParamDefinition(name="status", path="ImagingStudy.status", description="The status of the study", type="token" ) 3442 public static final String SP_STATUS = "status"; 3443 /** 3444 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3445 * <p> 3446 * Description: <b>The status of the study</b><br> 3447 * Type: <b>token</b><br> 3448 * Path: <b>ImagingStudy.status</b><br> 3449 * </p> 3450 */ 3451 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3452 3453 /** 3454 * Search parameter: <b>subject</b> 3455 * <p> 3456 * Description: <b>Who the study is about</b><br> 3457 * Type: <b>reference</b><br> 3458 * Path: <b>ImagingStudy.subject</b><br> 3459 * </p> 3460 */ 3461 @SearchParamDefinition(name="subject", path="ImagingStudy.subject", description="Who the study is about", type="reference", target={Device.class, Group.class, Patient.class } ) 3462 public static final String SP_SUBJECT = "subject"; 3463 /** 3464 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3465 * <p> 3466 * Description: <b>Who the study is about</b><br> 3467 * Type: <b>reference</b><br> 3468 * Path: <b>ImagingStudy.subject</b><br> 3469 * </p> 3470 */ 3471 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3472 3473/** 3474 * Constant for fluent queries to be used to add include statements. Specifies 3475 * the path value of "<b>ImagingStudy:subject</b>". 3476 */ 3477 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("ImagingStudy:subject").toLocked(); 3478 3479 /** 3480 * Search parameter: <b>encounter</b> 3481 * <p> 3482 * Description: <b>Multiple Resources: 3483 3484* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 3485* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 3486* [ChargeItem](chargeitem.html): Encounter associated with event 3487* [Claim](claim.html): Encounters associated with a billed line item 3488* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 3489* [Communication](communication.html): The Encounter during which this Communication was created 3490* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 3491* [Composition](composition.html): Context of the Composition 3492* [Condition](condition.html): The Encounter during which this Condition was created 3493* [DeviceRequest](devicerequest.html): Encounter during which request was created 3494* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 3495* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 3496* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 3497* [Flag](flag.html): Alert relevant during encounter 3498* [ImagingStudy](imagingstudy.html): The context of the study 3499* [List](list.html): Context in which list created 3500* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 3501* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 3502* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 3503* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 3504* [Observation](observation.html): Encounter related to the observation 3505* [Procedure](procedure.html): The Encounter during which this Procedure was created 3506* [Provenance](provenance.html): Encounter related to the Provenance 3507* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 3508* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 3509* [RiskAssessment](riskassessment.html): Where was assessment performed? 3510* [ServiceRequest](servicerequest.html): An encounter in which this request is made 3511* [Task](task.html): Search by encounter 3512* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 3513</b><br> 3514 * Type: <b>reference</b><br> 3515 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 3516 * </p> 3517 */ 3518 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", target={Encounter.class } ) 3519 public static final String SP_ENCOUNTER = "encounter"; 3520 /** 3521 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3522 * <p> 3523 * Description: <b>Multiple Resources: 3524 3525* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 3526* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 3527* [ChargeItem](chargeitem.html): Encounter associated with event 3528* [Claim](claim.html): Encounters associated with a billed line item 3529* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 3530* [Communication](communication.html): The Encounter during which this Communication was created 3531* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 3532* [Composition](composition.html): Context of the Composition 3533* [Condition](condition.html): The Encounter during which this Condition was created 3534* [DeviceRequest](devicerequest.html): Encounter during which request was created 3535* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 3536* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 3537* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 3538* [Flag](flag.html): Alert relevant during encounter 3539* [ImagingStudy](imagingstudy.html): The context of the study 3540* [List](list.html): Context in which list created 3541* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 3542* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 3543* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 3544* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 3545* [Observation](observation.html): Encounter related to the observation 3546* [Procedure](procedure.html): The Encounter during which this Procedure was created 3547* [Provenance](provenance.html): Encounter related to the Provenance 3548* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 3549* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 3550* [RiskAssessment](riskassessment.html): Where was assessment performed? 3551* [ServiceRequest](servicerequest.html): An encounter in which this request is made 3552* [Task](task.html): Search by encounter 3553* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 3554</b><br> 3555 * Type: <b>reference</b><br> 3556 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 3557 * </p> 3558 */ 3559 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 3560 3561/** 3562 * Constant for fluent queries to be used to add include statements. Specifies 3563 * the path value of "<b>ImagingStudy:encounter</b>". 3564 */ 3565 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("ImagingStudy:encounter").toLocked(); 3566 3567 /** 3568 * Search parameter: <b>identifier</b> 3569 * <p> 3570 * Description: <b>Multiple Resources: 3571 3572* [Account](account.html): Account number 3573* [AdverseEvent](adverseevent.html): Business identifier for the event 3574* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3575* [Appointment](appointment.html): An Identifier of the Appointment 3576* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3577* [Basic](basic.html): Business identifier 3578* [BodyStructure](bodystructure.html): Bodystructure identifier 3579* [CarePlan](careplan.html): External Ids for this plan 3580* [CareTeam](careteam.html): External Ids for this team 3581* [ChargeItem](chargeitem.html): Business Identifier for item 3582* [Claim](claim.html): The primary identifier of the financial resource 3583* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3584* [ClinicalImpression](clinicalimpression.html): Business identifier 3585* [Communication](communication.html): Unique identifier 3586* [CommunicationRequest](communicationrequest.html): Unique identifier 3587* [Composition](composition.html): Version-independent identifier for the Composition 3588* [Condition](condition.html): A unique identifier of the condition record 3589* [Consent](consent.html): Identifier for this record (external references) 3590* [Contract](contract.html): The identity of the contract 3591* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3592* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3593* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3594* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3595* [DeviceRequest](devicerequest.html): Business identifier for request/order 3596* [DeviceUsage](deviceusage.html): Search by identifier 3597* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3598* [DocumentReference](documentreference.html): Identifier of the attachment binary 3599* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3600* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3601* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3602* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3603* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3604* [Flag](flag.html): Business identifier 3605* [Goal](goal.html): External Ids for this goal 3606* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3607* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3608* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3609* [Immunization](immunization.html): Business identifier 3610* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3611* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3612* [Invoice](invoice.html): Business Identifier for item 3613* [List](list.html): Business identifier 3614* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3615* [Medication](medication.html): Returns medications with this external identifier 3616* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3617* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3618* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3619* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3620* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3621* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3622* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3623* [Observation](observation.html): The unique id for a particular observation 3624* [Person](person.html): A person Identifier 3625* [Procedure](procedure.html): A unique identifier for a procedure 3626* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3627* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3628* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3629* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3630* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3631* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3632* [Specimen](specimen.html): The unique identifier associated with the specimen 3633* [SupplyDelivery](supplydelivery.html): External identifier 3634* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3635* [Task](task.html): Search for a task instance by its business identifier 3636* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3637</b><br> 3638 * Type: <b>token</b><br> 3639 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3640 * </p> 3641 */ 3642 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 3643 public static final String SP_IDENTIFIER = "identifier"; 3644 /** 3645 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3646 * <p> 3647 * Description: <b>Multiple Resources: 3648 3649* [Account](account.html): Account number 3650* [AdverseEvent](adverseevent.html): Business identifier for the event 3651* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3652* [Appointment](appointment.html): An Identifier of the Appointment 3653* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3654* [Basic](basic.html): Business identifier 3655* [BodyStructure](bodystructure.html): Bodystructure identifier 3656* [CarePlan](careplan.html): External Ids for this plan 3657* [CareTeam](careteam.html): External Ids for this team 3658* [ChargeItem](chargeitem.html): Business Identifier for item 3659* [Claim](claim.html): The primary identifier of the financial resource 3660* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3661* [ClinicalImpression](clinicalimpression.html): Business identifier 3662* [Communication](communication.html): Unique identifier 3663* [CommunicationRequest](communicationrequest.html): Unique identifier 3664* [Composition](composition.html): Version-independent identifier for the Composition 3665* [Condition](condition.html): A unique identifier of the condition record 3666* [Consent](consent.html): Identifier for this record (external references) 3667* [Contract](contract.html): The identity of the contract 3668* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3669* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3670* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3671* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3672* [DeviceRequest](devicerequest.html): Business identifier for request/order 3673* [DeviceUsage](deviceusage.html): Search by identifier 3674* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3675* [DocumentReference](documentreference.html): Identifier of the attachment binary 3676* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3677* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3678* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3679* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3680* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3681* [Flag](flag.html): Business identifier 3682* [Goal](goal.html): External Ids for this goal 3683* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3684* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3685* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3686* [Immunization](immunization.html): Business identifier 3687* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3688* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3689* [Invoice](invoice.html): Business Identifier for item 3690* [List](list.html): Business identifier 3691* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3692* [Medication](medication.html): Returns medications with this external identifier 3693* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3694* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3695* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3696* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3697* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3698* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3699* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3700* [Observation](observation.html): The unique id for a particular observation 3701* [Person](person.html): A person Identifier 3702* [Procedure](procedure.html): A unique identifier for a procedure 3703* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3704* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3705* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3706* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3707* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3708* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3709* [Specimen](specimen.html): The unique identifier associated with the specimen 3710* [SupplyDelivery](supplydelivery.html): External identifier 3711* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3712* [Task](task.html): Search for a task instance by its business identifier 3713* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3714</b><br> 3715 * Type: <b>token</b><br> 3716 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3717 * </p> 3718 */ 3719 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3720 3721 /** 3722 * Search parameter: <b>patient</b> 3723 * <p> 3724 * Description: <b>Multiple Resources: 3725 3726* [Account](account.html): The entity that caused the expenses 3727* [AdverseEvent](adverseevent.html): Subject impacted by event 3728* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3729* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3730* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3731* [AuditEvent](auditevent.html): Where the activity involved patient data 3732* [Basic](basic.html): Identifies the focus of this resource 3733* [BodyStructure](bodystructure.html): Who this is about 3734* [CarePlan](careplan.html): Who the care plan is for 3735* [CareTeam](careteam.html): Who care team is for 3736* [ChargeItem](chargeitem.html): Individual service was done for/to 3737* [Claim](claim.html): Patient receiving the products or services 3738* [ClaimResponse](claimresponse.html): The subject of care 3739* [ClinicalImpression](clinicalimpression.html): Patient assessed 3740* [Communication](communication.html): Focus of message 3741* [CommunicationRequest](communicationrequest.html): Focus of message 3742* [Composition](composition.html): Who and/or what the composition is about 3743* [Condition](condition.html): Who has the condition? 3744* [Consent](consent.html): Who the consent applies to 3745* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3746* [Coverage](coverage.html): Retrieve coverages for a patient 3747* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3748* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3749* [DetectedIssue](detectedissue.html): Associated patient 3750* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3751* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3752* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3753* [DocumentReference](documentreference.html): Who/what is the subject of the document 3754* [Encounter](encounter.html): The patient present at the encounter 3755* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3756* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3757* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3758* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3759* [Flag](flag.html): The identity of a subject to list flags for 3760* [Goal](goal.html): Who this goal is intended for 3761* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3762* [ImagingSelection](imagingselection.html): Who the study is about 3763* [ImagingStudy](imagingstudy.html): Who the study is about 3764* [Immunization](immunization.html): The patient for the vaccination record 3765* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3766* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3767* [Invoice](invoice.html): Recipient(s) of goods and services 3768* [List](list.html): If all resources have the same subject 3769* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3770* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3771* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3772* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3773* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3774* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3775* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3776* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3777* [Observation](observation.html): The subject that the observation is about (if patient) 3778* [Person](person.html): The Person links to this Patient 3779* [Procedure](procedure.html): Search by subject - a patient 3780* [Provenance](provenance.html): Where the activity involved patient data 3781* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3782* [RelatedPerson](relatedperson.html): The patient this related person is related to 3783* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3784* [ResearchSubject](researchsubject.html): Who or what is part of study 3785* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3786* [ServiceRequest](servicerequest.html): Search by subject - a patient 3787* [Specimen](specimen.html): The patient the specimen comes from 3788* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3789* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3790* [Task](task.html): Search by patient 3791* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3792</b><br> 3793 * Type: <b>reference</b><br> 3794 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3795 * </p> 3796 */ 3797 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 3798 public static final String SP_PATIENT = "patient"; 3799 /** 3800 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3801 * <p> 3802 * Description: <b>Multiple Resources: 3803 3804* [Account](account.html): The entity that caused the expenses 3805* [AdverseEvent](adverseevent.html): Subject impacted by event 3806* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3807* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3808* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3809* [AuditEvent](auditevent.html): Where the activity involved patient data 3810* [Basic](basic.html): Identifies the focus of this resource 3811* [BodyStructure](bodystructure.html): Who this is about 3812* [CarePlan](careplan.html): Who the care plan is for 3813* [CareTeam](careteam.html): Who care team is for 3814* [ChargeItem](chargeitem.html): Individual service was done for/to 3815* [Claim](claim.html): Patient receiving the products or services 3816* [ClaimResponse](claimresponse.html): The subject of care 3817* [ClinicalImpression](clinicalimpression.html): Patient assessed 3818* [Communication](communication.html): Focus of message 3819* [CommunicationRequest](communicationrequest.html): Focus of message 3820* [Composition](composition.html): Who and/or what the composition is about 3821* [Condition](condition.html): Who has the condition? 3822* [Consent](consent.html): Who the consent applies to 3823* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3824* [Coverage](coverage.html): Retrieve coverages for a patient 3825* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3826* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3827* [DetectedIssue](detectedissue.html): Associated patient 3828* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3829* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3830* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3831* [DocumentReference](documentreference.html): Who/what is the subject of the document 3832* [Encounter](encounter.html): The patient present at the encounter 3833* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3834* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3835* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3836* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3837* [Flag](flag.html): The identity of a subject to list flags for 3838* [Goal](goal.html): Who this goal is intended for 3839* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3840* [ImagingSelection](imagingselection.html): Who the study is about 3841* [ImagingStudy](imagingstudy.html): Who the study is about 3842* [Immunization](immunization.html): The patient for the vaccination record 3843* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3844* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3845* [Invoice](invoice.html): Recipient(s) of goods and services 3846* [List](list.html): If all resources have the same subject 3847* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3848* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3849* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3850* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3851* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3852* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3853* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3854* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3855* [Observation](observation.html): The subject that the observation is about (if patient) 3856* [Person](person.html): The Person links to this Patient 3857* [Procedure](procedure.html): Search by subject - a patient 3858* [Provenance](provenance.html): Where the activity involved patient data 3859* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3860* [RelatedPerson](relatedperson.html): The patient this related person is related to 3861* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3862* [ResearchSubject](researchsubject.html): Who or what is part of study 3863* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3864* [ServiceRequest](servicerequest.html): Search by subject - a patient 3865* [Specimen](specimen.html): The patient the specimen comes from 3866* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3867* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3868* [Task](task.html): Search by patient 3869* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3870</b><br> 3871 * Type: <b>reference</b><br> 3872 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3873 * </p> 3874 */ 3875 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3876 3877/** 3878 * Constant for fluent queries to be used to add include statements. Specifies 3879 * the path value of "<b>ImagingStudy:patient</b>". 3880 */ 3881 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ImagingStudy:patient").toLocked(); 3882 3883 3884} 3885