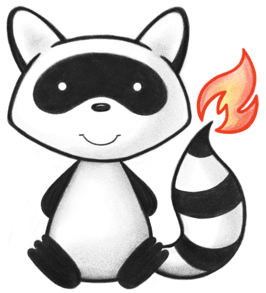
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Describes the event of a patient being administered a vaccine or a record of an immunization as reported by a patient, a clinician or another party. 052 */ 053@ResourceDef(name="Immunization", profile="http://hl7.org/fhir/StructureDefinition/Immunization") 054public class Immunization extends DomainResource { 055 056 public enum ImmunizationStatusCodes { 057 /** 058 * The event has now concluded. 059 */ 060 COMPLETED, 061 /** 062 * This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"stopped\" rather than \"entered-in-error\".). 063 */ 064 ENTEREDINERROR, 065 /** 066 * The event was terminated prior to any activity beyond preparation. I.e. The 'main' activity has not yet begun. The boundary between preparatory and the 'main' activity is context-specific. 067 */ 068 NOTDONE, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static ImmunizationStatusCodes fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("completed".equals(codeString)) 077 return COMPLETED; 078 if ("entered-in-error".equals(codeString)) 079 return ENTEREDINERROR; 080 if ("not-done".equals(codeString)) 081 return NOTDONE; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown ImmunizationStatusCodes code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case COMPLETED: return "completed"; 090 case ENTEREDINERROR: return "entered-in-error"; 091 case NOTDONE: return "not-done"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case COMPLETED: return "http://hl7.org/fhir/event-status"; 099 case ENTEREDINERROR: return "http://hl7.org/fhir/event-status"; 100 case NOTDONE: return "http://hl7.org/fhir/event-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case COMPLETED: return "The event has now concluded."; 108 case ENTEREDINERROR: return "This electronic record should never have existed, though it is possible that real-world decisions were based on it. (If real-world activity has occurred, the status should be \"stopped\" rather than \"entered-in-error\".)."; 109 case NOTDONE: return "The event was terminated prior to any activity beyond preparation. I.e. The 'main' activity has not yet begun. The boundary between preparatory and the 'main' activity is context-specific."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case COMPLETED: return "Completed"; 117 case ENTEREDINERROR: return "Entered in Error"; 118 case NOTDONE: return "Not Done"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class ImmunizationStatusCodesEnumFactory implements EnumFactory<ImmunizationStatusCodes> { 126 public ImmunizationStatusCodes fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("completed".equals(codeString)) 131 return ImmunizationStatusCodes.COMPLETED; 132 if ("entered-in-error".equals(codeString)) 133 return ImmunizationStatusCodes.ENTEREDINERROR; 134 if ("not-done".equals(codeString)) 135 return ImmunizationStatusCodes.NOTDONE; 136 throw new IllegalArgumentException("Unknown ImmunizationStatusCodes code '"+codeString+"'"); 137 } 138 public Enumeration<ImmunizationStatusCodes> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<ImmunizationStatusCodes>(this, ImmunizationStatusCodes.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<ImmunizationStatusCodes>(this, ImmunizationStatusCodes.NULL, code); 146 if ("completed".equals(codeString)) 147 return new Enumeration<ImmunizationStatusCodes>(this, ImmunizationStatusCodes.COMPLETED, code); 148 if ("entered-in-error".equals(codeString)) 149 return new Enumeration<ImmunizationStatusCodes>(this, ImmunizationStatusCodes.ENTEREDINERROR, code); 150 if ("not-done".equals(codeString)) 151 return new Enumeration<ImmunizationStatusCodes>(this, ImmunizationStatusCodes.NOTDONE, code); 152 throw new FHIRException("Unknown ImmunizationStatusCodes code '"+codeString+"'"); 153 } 154 public String toCode(ImmunizationStatusCodes code) { 155 if (code == ImmunizationStatusCodes.NULL) 156 return null; 157 if (code == ImmunizationStatusCodes.COMPLETED) 158 return "completed"; 159 if (code == ImmunizationStatusCodes.ENTEREDINERROR) 160 return "entered-in-error"; 161 if (code == ImmunizationStatusCodes.NOTDONE) 162 return "not-done"; 163 return "?"; 164 } 165 public String toSystem(ImmunizationStatusCodes code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class ImmunizationPerformerComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * Describes the type of performance (e.g. ordering provider, administering provider, etc.). 174 */ 175 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 176 @Description(shortDefinition="What type of performance was done", formalDefinition="Describes the type of performance (e.g. ordering provider, administering provider, etc.)." ) 177 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-function") 178 protected CodeableConcept function; 179 180 /** 181 * The practitioner or organization who performed the action. 182 */ 183 @Child(name = "actor", type = {Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, RelatedPerson.class}, order=2, min=1, max=1, modifier=false, summary=true) 184 @Description(shortDefinition="Individual or organization who was performing", formalDefinition="The practitioner or organization who performed the action." ) 185 protected Reference actor; 186 187 private static final long serialVersionUID = -576943815L; 188 189 /** 190 * Constructor 191 */ 192 public ImmunizationPerformerComponent() { 193 super(); 194 } 195 196 /** 197 * Constructor 198 */ 199 public ImmunizationPerformerComponent(Reference actor) { 200 super(); 201 this.setActor(actor); 202 } 203 204 /** 205 * @return {@link #function} (Describes the type of performance (e.g. ordering provider, administering provider, etc.).) 206 */ 207 public CodeableConcept getFunction() { 208 if (this.function == null) 209 if (Configuration.errorOnAutoCreate()) 210 throw new Error("Attempt to auto-create ImmunizationPerformerComponent.function"); 211 else if (Configuration.doAutoCreate()) 212 this.function = new CodeableConcept(); // cc 213 return this.function; 214 } 215 216 public boolean hasFunction() { 217 return this.function != null && !this.function.isEmpty(); 218 } 219 220 /** 221 * @param value {@link #function} (Describes the type of performance (e.g. ordering provider, administering provider, etc.).) 222 */ 223 public ImmunizationPerformerComponent setFunction(CodeableConcept value) { 224 this.function = value; 225 return this; 226 } 227 228 /** 229 * @return {@link #actor} (The practitioner or organization who performed the action.) 230 */ 231 public Reference getActor() { 232 if (this.actor == null) 233 if (Configuration.errorOnAutoCreate()) 234 throw new Error("Attempt to auto-create ImmunizationPerformerComponent.actor"); 235 else if (Configuration.doAutoCreate()) 236 this.actor = new Reference(); // cc 237 return this.actor; 238 } 239 240 public boolean hasActor() { 241 return this.actor != null && !this.actor.isEmpty(); 242 } 243 244 /** 245 * @param value {@link #actor} (The practitioner or organization who performed the action.) 246 */ 247 public ImmunizationPerformerComponent setActor(Reference value) { 248 this.actor = value; 249 return this; 250 } 251 252 protected void listChildren(List<Property> children) { 253 super.listChildren(children); 254 children.add(new Property("function", "CodeableConcept", "Describes the type of performance (e.g. ordering provider, administering provider, etc.).", 0, 1, function)); 255 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson)", "The practitioner or organization who performed the action.", 0, 1, actor)); 256 } 257 258 @Override 259 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 260 switch (_hash) { 261 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Describes the type of performance (e.g. ordering provider, administering provider, etc.).", 0, 1, function); 262 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|Patient|RelatedPerson)", "The practitioner or organization who performed the action.", 0, 1, actor); 263 default: return super.getNamedProperty(_hash, _name, _checkValid); 264 } 265 266 } 267 268 @Override 269 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 270 switch (hash) { 271 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 272 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 273 default: return super.getProperty(hash, name, checkValid); 274 } 275 276 } 277 278 @Override 279 public Base setProperty(int hash, String name, Base value) throws FHIRException { 280 switch (hash) { 281 case 1380938712: // function 282 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 283 return value; 284 case 92645877: // actor 285 this.actor = TypeConvertor.castToReference(value); // Reference 286 return value; 287 default: return super.setProperty(hash, name, value); 288 } 289 290 } 291 292 @Override 293 public Base setProperty(String name, Base value) throws FHIRException { 294 if (name.equals("function")) { 295 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 296 } else if (name.equals("actor")) { 297 this.actor = TypeConvertor.castToReference(value); // Reference 298 } else 299 return super.setProperty(name, value); 300 return value; 301 } 302 303 @Override 304 public void removeChild(String name, Base value) throws FHIRException { 305 if (name.equals("function")) { 306 this.function = null; 307 } else if (name.equals("actor")) { 308 this.actor = null; 309 } else 310 super.removeChild(name, value); 311 312 } 313 314 @Override 315 public Base makeProperty(int hash, String name) throws FHIRException { 316 switch (hash) { 317 case 1380938712: return getFunction(); 318 case 92645877: return getActor(); 319 default: return super.makeProperty(hash, name); 320 } 321 322 } 323 324 @Override 325 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 326 switch (hash) { 327 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 328 case 92645877: /*actor*/ return new String[] {"Reference"}; 329 default: return super.getTypesForProperty(hash, name); 330 } 331 332 } 333 334 @Override 335 public Base addChild(String name) throws FHIRException { 336 if (name.equals("function")) { 337 this.function = new CodeableConcept(); 338 return this.function; 339 } 340 else if (name.equals("actor")) { 341 this.actor = new Reference(); 342 return this.actor; 343 } 344 else 345 return super.addChild(name); 346 } 347 348 public ImmunizationPerformerComponent copy() { 349 ImmunizationPerformerComponent dst = new ImmunizationPerformerComponent(); 350 copyValues(dst); 351 return dst; 352 } 353 354 public void copyValues(ImmunizationPerformerComponent dst) { 355 super.copyValues(dst); 356 dst.function = function == null ? null : function.copy(); 357 dst.actor = actor == null ? null : actor.copy(); 358 } 359 360 @Override 361 public boolean equalsDeep(Base other_) { 362 if (!super.equalsDeep(other_)) 363 return false; 364 if (!(other_ instanceof ImmunizationPerformerComponent)) 365 return false; 366 ImmunizationPerformerComponent o = (ImmunizationPerformerComponent) other_; 367 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 368 } 369 370 @Override 371 public boolean equalsShallow(Base other_) { 372 if (!super.equalsShallow(other_)) 373 return false; 374 if (!(other_ instanceof ImmunizationPerformerComponent)) 375 return false; 376 ImmunizationPerformerComponent o = (ImmunizationPerformerComponent) other_; 377 return true; 378 } 379 380 public boolean isEmpty() { 381 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 382 } 383 384 public String fhirType() { 385 return "Immunization.performer"; 386 387 } 388 389 } 390 391 @Block() 392 public static class ImmunizationProgramEligibilityComponent extends BackboneElement implements IBaseBackboneElement { 393 /** 394 * Indicates which program the patient had their eligility evaluated for. 395 */ 396 @Child(name = "program", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 397 @Description(shortDefinition="The program that eligibility is declared for", formalDefinition="Indicates which program the patient had their eligility evaluated for." ) 398 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-vaccine-funding-program") 399 protected CodeableConcept program; 400 401 /** 402 * Indicates the patient's eligility status for for a specific payment program. 403 */ 404 @Child(name = "programStatus", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 405 @Description(shortDefinition="The patient's eligibility status for the program", formalDefinition="Indicates the patient's eligility status for for a specific payment program." ) 406 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-program-eligibility") 407 protected CodeableConcept programStatus; 408 409 private static final long serialVersionUID = -442289864L; 410 411 /** 412 * Constructor 413 */ 414 public ImmunizationProgramEligibilityComponent() { 415 super(); 416 } 417 418 /** 419 * Constructor 420 */ 421 public ImmunizationProgramEligibilityComponent(CodeableConcept program, CodeableConcept programStatus) { 422 super(); 423 this.setProgram(program); 424 this.setProgramStatus(programStatus); 425 } 426 427 /** 428 * @return {@link #program} (Indicates which program the patient had their eligility evaluated for.) 429 */ 430 public CodeableConcept getProgram() { 431 if (this.program == null) 432 if (Configuration.errorOnAutoCreate()) 433 throw new Error("Attempt to auto-create ImmunizationProgramEligibilityComponent.program"); 434 else if (Configuration.doAutoCreate()) 435 this.program = new CodeableConcept(); // cc 436 return this.program; 437 } 438 439 public boolean hasProgram() { 440 return this.program != null && !this.program.isEmpty(); 441 } 442 443 /** 444 * @param value {@link #program} (Indicates which program the patient had their eligility evaluated for.) 445 */ 446 public ImmunizationProgramEligibilityComponent setProgram(CodeableConcept value) { 447 this.program = value; 448 return this; 449 } 450 451 /** 452 * @return {@link #programStatus} (Indicates the patient's eligility status for for a specific payment program.) 453 */ 454 public CodeableConcept getProgramStatus() { 455 if (this.programStatus == null) 456 if (Configuration.errorOnAutoCreate()) 457 throw new Error("Attempt to auto-create ImmunizationProgramEligibilityComponent.programStatus"); 458 else if (Configuration.doAutoCreate()) 459 this.programStatus = new CodeableConcept(); // cc 460 return this.programStatus; 461 } 462 463 public boolean hasProgramStatus() { 464 return this.programStatus != null && !this.programStatus.isEmpty(); 465 } 466 467 /** 468 * @param value {@link #programStatus} (Indicates the patient's eligility status for for a specific payment program.) 469 */ 470 public ImmunizationProgramEligibilityComponent setProgramStatus(CodeableConcept value) { 471 this.programStatus = value; 472 return this; 473 } 474 475 protected void listChildren(List<Property> children) { 476 super.listChildren(children); 477 children.add(new Property("program", "CodeableConcept", "Indicates which program the patient had their eligility evaluated for.", 0, 1, program)); 478 children.add(new Property("programStatus", "CodeableConcept", "Indicates the patient's eligility status for for a specific payment program.", 0, 1, programStatus)); 479 } 480 481 @Override 482 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 483 switch (_hash) { 484 case -309387644: /*program*/ return new Property("program", "CodeableConcept", "Indicates which program the patient had their eligility evaluated for.", 0, 1, program); 485 case 472508310: /*programStatus*/ return new Property("programStatus", "CodeableConcept", "Indicates the patient's eligility status for for a specific payment program.", 0, 1, programStatus); 486 default: return super.getNamedProperty(_hash, _name, _checkValid); 487 } 488 489 } 490 491 @Override 492 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 493 switch (hash) { 494 case -309387644: /*program*/ return this.program == null ? new Base[0] : new Base[] {this.program}; // CodeableConcept 495 case 472508310: /*programStatus*/ return this.programStatus == null ? new Base[0] : new Base[] {this.programStatus}; // CodeableConcept 496 default: return super.getProperty(hash, name, checkValid); 497 } 498 499 } 500 501 @Override 502 public Base setProperty(int hash, String name, Base value) throws FHIRException { 503 switch (hash) { 504 case -309387644: // program 505 this.program = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 506 return value; 507 case 472508310: // programStatus 508 this.programStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 509 return value; 510 default: return super.setProperty(hash, name, value); 511 } 512 513 } 514 515 @Override 516 public Base setProperty(String name, Base value) throws FHIRException { 517 if (name.equals("program")) { 518 this.program = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 519 } else if (name.equals("programStatus")) { 520 this.programStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 521 } else 522 return super.setProperty(name, value); 523 return value; 524 } 525 526 @Override 527 public void removeChild(String name, Base value) throws FHIRException { 528 if (name.equals("program")) { 529 this.program = null; 530 } else if (name.equals("programStatus")) { 531 this.programStatus = null; 532 } else 533 super.removeChild(name, value); 534 535 } 536 537 @Override 538 public Base makeProperty(int hash, String name) throws FHIRException { 539 switch (hash) { 540 case -309387644: return getProgram(); 541 case 472508310: return getProgramStatus(); 542 default: return super.makeProperty(hash, name); 543 } 544 545 } 546 547 @Override 548 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 549 switch (hash) { 550 case -309387644: /*program*/ return new String[] {"CodeableConcept"}; 551 case 472508310: /*programStatus*/ return new String[] {"CodeableConcept"}; 552 default: return super.getTypesForProperty(hash, name); 553 } 554 555 } 556 557 @Override 558 public Base addChild(String name) throws FHIRException { 559 if (name.equals("program")) { 560 this.program = new CodeableConcept(); 561 return this.program; 562 } 563 else if (name.equals("programStatus")) { 564 this.programStatus = new CodeableConcept(); 565 return this.programStatus; 566 } 567 else 568 return super.addChild(name); 569 } 570 571 public ImmunizationProgramEligibilityComponent copy() { 572 ImmunizationProgramEligibilityComponent dst = new ImmunizationProgramEligibilityComponent(); 573 copyValues(dst); 574 return dst; 575 } 576 577 public void copyValues(ImmunizationProgramEligibilityComponent dst) { 578 super.copyValues(dst); 579 dst.program = program == null ? null : program.copy(); 580 dst.programStatus = programStatus == null ? null : programStatus.copy(); 581 } 582 583 @Override 584 public boolean equalsDeep(Base other_) { 585 if (!super.equalsDeep(other_)) 586 return false; 587 if (!(other_ instanceof ImmunizationProgramEligibilityComponent)) 588 return false; 589 ImmunizationProgramEligibilityComponent o = (ImmunizationProgramEligibilityComponent) other_; 590 return compareDeep(program, o.program, true) && compareDeep(programStatus, o.programStatus, true) 591 ; 592 } 593 594 @Override 595 public boolean equalsShallow(Base other_) { 596 if (!super.equalsShallow(other_)) 597 return false; 598 if (!(other_ instanceof ImmunizationProgramEligibilityComponent)) 599 return false; 600 ImmunizationProgramEligibilityComponent o = (ImmunizationProgramEligibilityComponent) other_; 601 return true; 602 } 603 604 public boolean isEmpty() { 605 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(program, programStatus); 606 } 607 608 public String fhirType() { 609 return "Immunization.programEligibility"; 610 611 } 612 613 } 614 615 @Block() 616 public static class ImmunizationReactionComponent extends BackboneElement implements IBaseBackboneElement { 617 /** 618 * Date of reaction to the immunization. 619 */ 620 @Child(name = "date", type = {DateTimeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 621 @Description(shortDefinition="When reaction started", formalDefinition="Date of reaction to the immunization." ) 622 protected DateTimeType date; 623 624 /** 625 * Details of the reaction. 626 */ 627 @Child(name = "manifestation", type = {CodeableReference.class}, order=2, min=0, max=1, modifier=false, summary=false) 628 @Description(shortDefinition="Additional information on reaction", formalDefinition="Details of the reaction." ) 629 protected CodeableReference manifestation; 630 631 /** 632 * Self-reported indicator. 633 */ 634 @Child(name = "reported", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 635 @Description(shortDefinition="Indicates self-reported reaction", formalDefinition="Self-reported indicator." ) 636 protected BooleanType reported; 637 638 private static final long serialVersionUID = 1181151012L; 639 640 /** 641 * Constructor 642 */ 643 public ImmunizationReactionComponent() { 644 super(); 645 } 646 647 /** 648 * @return {@link #date} (Date of reaction to the immunization.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 649 */ 650 public DateTimeType getDateElement() { 651 if (this.date == null) 652 if (Configuration.errorOnAutoCreate()) 653 throw new Error("Attempt to auto-create ImmunizationReactionComponent.date"); 654 else if (Configuration.doAutoCreate()) 655 this.date = new DateTimeType(); // bb 656 return this.date; 657 } 658 659 public boolean hasDateElement() { 660 return this.date != null && !this.date.isEmpty(); 661 } 662 663 public boolean hasDate() { 664 return this.date != null && !this.date.isEmpty(); 665 } 666 667 /** 668 * @param value {@link #date} (Date of reaction to the immunization.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 669 */ 670 public ImmunizationReactionComponent setDateElement(DateTimeType value) { 671 this.date = value; 672 return this; 673 } 674 675 /** 676 * @return Date of reaction to the immunization. 677 */ 678 public Date getDate() { 679 return this.date == null ? null : this.date.getValue(); 680 } 681 682 /** 683 * @param value Date of reaction to the immunization. 684 */ 685 public ImmunizationReactionComponent setDate(Date value) { 686 if (value == null) 687 this.date = null; 688 else { 689 if (this.date == null) 690 this.date = new DateTimeType(); 691 this.date.setValue(value); 692 } 693 return this; 694 } 695 696 /** 697 * @return {@link #manifestation} (Details of the reaction.) 698 */ 699 public CodeableReference getManifestation() { 700 if (this.manifestation == null) 701 if (Configuration.errorOnAutoCreate()) 702 throw new Error("Attempt to auto-create ImmunizationReactionComponent.manifestation"); 703 else if (Configuration.doAutoCreate()) 704 this.manifestation = new CodeableReference(); // cc 705 return this.manifestation; 706 } 707 708 public boolean hasManifestation() { 709 return this.manifestation != null && !this.manifestation.isEmpty(); 710 } 711 712 /** 713 * @param value {@link #manifestation} (Details of the reaction.) 714 */ 715 public ImmunizationReactionComponent setManifestation(CodeableReference value) { 716 this.manifestation = value; 717 return this; 718 } 719 720 /** 721 * @return {@link #reported} (Self-reported indicator.). This is the underlying object with id, value and extensions. The accessor "getReported" gives direct access to the value 722 */ 723 public BooleanType getReportedElement() { 724 if (this.reported == null) 725 if (Configuration.errorOnAutoCreate()) 726 throw new Error("Attempt to auto-create ImmunizationReactionComponent.reported"); 727 else if (Configuration.doAutoCreate()) 728 this.reported = new BooleanType(); // bb 729 return this.reported; 730 } 731 732 public boolean hasReportedElement() { 733 return this.reported != null && !this.reported.isEmpty(); 734 } 735 736 public boolean hasReported() { 737 return this.reported != null && !this.reported.isEmpty(); 738 } 739 740 /** 741 * @param value {@link #reported} (Self-reported indicator.). This is the underlying object with id, value and extensions. The accessor "getReported" gives direct access to the value 742 */ 743 public ImmunizationReactionComponent setReportedElement(BooleanType value) { 744 this.reported = value; 745 return this; 746 } 747 748 /** 749 * @return Self-reported indicator. 750 */ 751 public boolean getReported() { 752 return this.reported == null || this.reported.isEmpty() ? false : this.reported.getValue(); 753 } 754 755 /** 756 * @param value Self-reported indicator. 757 */ 758 public ImmunizationReactionComponent setReported(boolean value) { 759 if (this.reported == null) 760 this.reported = new BooleanType(); 761 this.reported.setValue(value); 762 return this; 763 } 764 765 protected void listChildren(List<Property> children) { 766 super.listChildren(children); 767 children.add(new Property("date", "dateTime", "Date of reaction to the immunization.", 0, 1, date)); 768 children.add(new Property("manifestation", "CodeableReference(Observation)", "Details of the reaction.", 0, 1, manifestation)); 769 children.add(new Property("reported", "boolean", "Self-reported indicator.", 0, 1, reported)); 770 } 771 772 @Override 773 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 774 switch (_hash) { 775 case 3076014: /*date*/ return new Property("date", "dateTime", "Date of reaction to the immunization.", 0, 1, date); 776 case 1115984422: /*manifestation*/ return new Property("manifestation", "CodeableReference(Observation)", "Details of the reaction.", 0, 1, manifestation); 777 case -427039533: /*reported*/ return new Property("reported", "boolean", "Self-reported indicator.", 0, 1, reported); 778 default: return super.getNamedProperty(_hash, _name, _checkValid); 779 } 780 781 } 782 783 @Override 784 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 785 switch (hash) { 786 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 787 case 1115984422: /*manifestation*/ return this.manifestation == null ? new Base[0] : new Base[] {this.manifestation}; // CodeableReference 788 case -427039533: /*reported*/ return this.reported == null ? new Base[0] : new Base[] {this.reported}; // BooleanType 789 default: return super.getProperty(hash, name, checkValid); 790 } 791 792 } 793 794 @Override 795 public Base setProperty(int hash, String name, Base value) throws FHIRException { 796 switch (hash) { 797 case 3076014: // date 798 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 799 return value; 800 case 1115984422: // manifestation 801 this.manifestation = TypeConvertor.castToCodeableReference(value); // CodeableReference 802 return value; 803 case -427039533: // reported 804 this.reported = TypeConvertor.castToBoolean(value); // BooleanType 805 return value; 806 default: return super.setProperty(hash, name, value); 807 } 808 809 } 810 811 @Override 812 public Base setProperty(String name, Base value) throws FHIRException { 813 if (name.equals("date")) { 814 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 815 } else if (name.equals("manifestation")) { 816 this.manifestation = TypeConvertor.castToCodeableReference(value); // CodeableReference 817 } else if (name.equals("reported")) { 818 this.reported = TypeConvertor.castToBoolean(value); // BooleanType 819 } else 820 return super.setProperty(name, value); 821 return value; 822 } 823 824 @Override 825 public void removeChild(String name, Base value) throws FHIRException { 826 if (name.equals("date")) { 827 this.date = null; 828 } else if (name.equals("manifestation")) { 829 this.manifestation = null; 830 } else if (name.equals("reported")) { 831 this.reported = null; 832 } else 833 super.removeChild(name, value); 834 835 } 836 837 @Override 838 public Base makeProperty(int hash, String name) throws FHIRException { 839 switch (hash) { 840 case 3076014: return getDateElement(); 841 case 1115984422: return getManifestation(); 842 case -427039533: return getReportedElement(); 843 default: return super.makeProperty(hash, name); 844 } 845 846 } 847 848 @Override 849 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 850 switch (hash) { 851 case 3076014: /*date*/ return new String[] {"dateTime"}; 852 case 1115984422: /*manifestation*/ return new String[] {"CodeableReference"}; 853 case -427039533: /*reported*/ return new String[] {"boolean"}; 854 default: return super.getTypesForProperty(hash, name); 855 } 856 857 } 858 859 @Override 860 public Base addChild(String name) throws FHIRException { 861 if (name.equals("date")) { 862 throw new FHIRException("Cannot call addChild on a singleton property Immunization.reaction.date"); 863 } 864 else if (name.equals("manifestation")) { 865 this.manifestation = new CodeableReference(); 866 return this.manifestation; 867 } 868 else if (name.equals("reported")) { 869 throw new FHIRException("Cannot call addChild on a singleton property Immunization.reaction.reported"); 870 } 871 else 872 return super.addChild(name); 873 } 874 875 public ImmunizationReactionComponent copy() { 876 ImmunizationReactionComponent dst = new ImmunizationReactionComponent(); 877 copyValues(dst); 878 return dst; 879 } 880 881 public void copyValues(ImmunizationReactionComponent dst) { 882 super.copyValues(dst); 883 dst.date = date == null ? null : date.copy(); 884 dst.manifestation = manifestation == null ? null : manifestation.copy(); 885 dst.reported = reported == null ? null : reported.copy(); 886 } 887 888 @Override 889 public boolean equalsDeep(Base other_) { 890 if (!super.equalsDeep(other_)) 891 return false; 892 if (!(other_ instanceof ImmunizationReactionComponent)) 893 return false; 894 ImmunizationReactionComponent o = (ImmunizationReactionComponent) other_; 895 return compareDeep(date, o.date, true) && compareDeep(manifestation, o.manifestation, true) && compareDeep(reported, o.reported, true) 896 ; 897 } 898 899 @Override 900 public boolean equalsShallow(Base other_) { 901 if (!super.equalsShallow(other_)) 902 return false; 903 if (!(other_ instanceof ImmunizationReactionComponent)) 904 return false; 905 ImmunizationReactionComponent o = (ImmunizationReactionComponent) other_; 906 return compareValues(date, o.date, true) && compareValues(reported, o.reported, true); 907 } 908 909 public boolean isEmpty() { 910 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, manifestation, reported 911 ); 912 } 913 914 public String fhirType() { 915 return "Immunization.reaction"; 916 917 } 918 919 } 920 921 @Block() 922 public static class ImmunizationProtocolAppliedComponent extends BackboneElement implements IBaseBackboneElement { 923 /** 924 * One possible path to achieve presumed immunity against a disease - within the context of an authority. 925 */ 926 @Child(name = "series", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 927 @Description(shortDefinition="Name of vaccine series", formalDefinition="One possible path to achieve presumed immunity against a disease - within the context of an authority." ) 928 protected StringType series; 929 930 /** 931 * Indicates the authority who published the protocol (e.g. ACIP) that is being followed. 932 */ 933 @Child(name = "authority", type = {Organization.class}, order=2, min=0, max=1, modifier=false, summary=false) 934 @Description(shortDefinition="Who is responsible for publishing the recommendations", formalDefinition="Indicates the authority who published the protocol (e.g. ACIP) that is being followed." ) 935 protected Reference authority; 936 937 /** 938 * The vaccine preventable disease the dose is being administered against. 939 */ 940 @Child(name = "targetDisease", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 941 @Description(shortDefinition="Vaccine preventatable disease being targeted", formalDefinition="The vaccine preventable disease the dose is being administered against." ) 942 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-target-disease") 943 protected List<CodeableConcept> targetDisease; 944 945 /** 946 * Nominal position in a series as intended by the practitioner administering the dose. 947 */ 948 @Child(name = "doseNumber", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=false) 949 @Description(shortDefinition="Dose number within series", formalDefinition="Nominal position in a series as intended by the practitioner administering the dose." ) 950 protected StringType doseNumber; 951 952 /** 953 * The recommended number of doses to achieve immunity as intended by the practitioner administering the dose. 954 */ 955 @Child(name = "seriesDoses", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 956 @Description(shortDefinition="Recommended number of doses for immunity", formalDefinition="The recommended number of doses to achieve immunity as intended by the practitioner administering the dose." ) 957 protected StringType seriesDoses; 958 959 private static final long serialVersionUID = 660613103L; 960 961 /** 962 * Constructor 963 */ 964 public ImmunizationProtocolAppliedComponent() { 965 super(); 966 } 967 968 /** 969 * Constructor 970 */ 971 public ImmunizationProtocolAppliedComponent(String doseNumber) { 972 super(); 973 this.setDoseNumber(doseNumber); 974 } 975 976 /** 977 * @return {@link #series} (One possible path to achieve presumed immunity against a disease - within the context of an authority.). This is the underlying object with id, value and extensions. The accessor "getSeries" gives direct access to the value 978 */ 979 public StringType getSeriesElement() { 980 if (this.series == null) 981 if (Configuration.errorOnAutoCreate()) 982 throw new Error("Attempt to auto-create ImmunizationProtocolAppliedComponent.series"); 983 else if (Configuration.doAutoCreate()) 984 this.series = new StringType(); // bb 985 return this.series; 986 } 987 988 public boolean hasSeriesElement() { 989 return this.series != null && !this.series.isEmpty(); 990 } 991 992 public boolean hasSeries() { 993 return this.series != null && !this.series.isEmpty(); 994 } 995 996 /** 997 * @param value {@link #series} (One possible path to achieve presumed immunity against a disease - within the context of an authority.). This is the underlying object with id, value and extensions. The accessor "getSeries" gives direct access to the value 998 */ 999 public ImmunizationProtocolAppliedComponent setSeriesElement(StringType value) { 1000 this.series = value; 1001 return this; 1002 } 1003 1004 /** 1005 * @return One possible path to achieve presumed immunity against a disease - within the context of an authority. 1006 */ 1007 public String getSeries() { 1008 return this.series == null ? null : this.series.getValue(); 1009 } 1010 1011 /** 1012 * @param value One possible path to achieve presumed immunity against a disease - within the context of an authority. 1013 */ 1014 public ImmunizationProtocolAppliedComponent setSeries(String value) { 1015 if (Utilities.noString(value)) 1016 this.series = null; 1017 else { 1018 if (this.series == null) 1019 this.series = new StringType(); 1020 this.series.setValue(value); 1021 } 1022 return this; 1023 } 1024 1025 /** 1026 * @return {@link #authority} (Indicates the authority who published the protocol (e.g. ACIP) that is being followed.) 1027 */ 1028 public Reference getAuthority() { 1029 if (this.authority == null) 1030 if (Configuration.errorOnAutoCreate()) 1031 throw new Error("Attempt to auto-create ImmunizationProtocolAppliedComponent.authority"); 1032 else if (Configuration.doAutoCreate()) 1033 this.authority = new Reference(); // cc 1034 return this.authority; 1035 } 1036 1037 public boolean hasAuthority() { 1038 return this.authority != null && !this.authority.isEmpty(); 1039 } 1040 1041 /** 1042 * @param value {@link #authority} (Indicates the authority who published the protocol (e.g. ACIP) that is being followed.) 1043 */ 1044 public ImmunizationProtocolAppliedComponent setAuthority(Reference value) { 1045 this.authority = value; 1046 return this; 1047 } 1048 1049 /** 1050 * @return {@link #targetDisease} (The vaccine preventable disease the dose is being administered against.) 1051 */ 1052 public List<CodeableConcept> getTargetDisease() { 1053 if (this.targetDisease == null) 1054 this.targetDisease = new ArrayList<CodeableConcept>(); 1055 return this.targetDisease; 1056 } 1057 1058 /** 1059 * @return Returns a reference to <code>this</code> for easy method chaining 1060 */ 1061 public ImmunizationProtocolAppliedComponent setTargetDisease(List<CodeableConcept> theTargetDisease) { 1062 this.targetDisease = theTargetDisease; 1063 return this; 1064 } 1065 1066 public boolean hasTargetDisease() { 1067 if (this.targetDisease == null) 1068 return false; 1069 for (CodeableConcept item : this.targetDisease) 1070 if (!item.isEmpty()) 1071 return true; 1072 return false; 1073 } 1074 1075 public CodeableConcept addTargetDisease() { //3 1076 CodeableConcept t = new CodeableConcept(); 1077 if (this.targetDisease == null) 1078 this.targetDisease = new ArrayList<CodeableConcept>(); 1079 this.targetDisease.add(t); 1080 return t; 1081 } 1082 1083 public ImmunizationProtocolAppliedComponent addTargetDisease(CodeableConcept t) { //3 1084 if (t == null) 1085 return this; 1086 if (this.targetDisease == null) 1087 this.targetDisease = new ArrayList<CodeableConcept>(); 1088 this.targetDisease.add(t); 1089 return this; 1090 } 1091 1092 /** 1093 * @return The first repetition of repeating field {@link #targetDisease}, creating it if it does not already exist {3} 1094 */ 1095 public CodeableConcept getTargetDiseaseFirstRep() { 1096 if (getTargetDisease().isEmpty()) { 1097 addTargetDisease(); 1098 } 1099 return getTargetDisease().get(0); 1100 } 1101 1102 /** 1103 * @return {@link #doseNumber} (Nominal position in a series as intended by the practitioner administering the dose.). This is the underlying object with id, value and extensions. The accessor "getDoseNumber" gives direct access to the value 1104 */ 1105 public StringType getDoseNumberElement() { 1106 if (this.doseNumber == null) 1107 if (Configuration.errorOnAutoCreate()) 1108 throw new Error("Attempt to auto-create ImmunizationProtocolAppliedComponent.doseNumber"); 1109 else if (Configuration.doAutoCreate()) 1110 this.doseNumber = new StringType(); // bb 1111 return this.doseNumber; 1112 } 1113 1114 public boolean hasDoseNumberElement() { 1115 return this.doseNumber != null && !this.doseNumber.isEmpty(); 1116 } 1117 1118 public boolean hasDoseNumber() { 1119 return this.doseNumber != null && !this.doseNumber.isEmpty(); 1120 } 1121 1122 /** 1123 * @param value {@link #doseNumber} (Nominal position in a series as intended by the practitioner administering the dose.). This is the underlying object with id, value and extensions. The accessor "getDoseNumber" gives direct access to the value 1124 */ 1125 public ImmunizationProtocolAppliedComponent setDoseNumberElement(StringType value) { 1126 this.doseNumber = value; 1127 return this; 1128 } 1129 1130 /** 1131 * @return Nominal position in a series as intended by the practitioner administering the dose. 1132 */ 1133 public String getDoseNumber() { 1134 return this.doseNumber == null ? null : this.doseNumber.getValue(); 1135 } 1136 1137 /** 1138 * @param value Nominal position in a series as intended by the practitioner administering the dose. 1139 */ 1140 public ImmunizationProtocolAppliedComponent setDoseNumber(String value) { 1141 if (this.doseNumber == null) 1142 this.doseNumber = new StringType(); 1143 this.doseNumber.setValue(value); 1144 return this; 1145 } 1146 1147 /** 1148 * @return {@link #seriesDoses} (The recommended number of doses to achieve immunity as intended by the practitioner administering the dose.). This is the underlying object with id, value and extensions. The accessor "getSeriesDoses" gives direct access to the value 1149 */ 1150 public StringType getSeriesDosesElement() { 1151 if (this.seriesDoses == null) 1152 if (Configuration.errorOnAutoCreate()) 1153 throw new Error("Attempt to auto-create ImmunizationProtocolAppliedComponent.seriesDoses"); 1154 else if (Configuration.doAutoCreate()) 1155 this.seriesDoses = new StringType(); // bb 1156 return this.seriesDoses; 1157 } 1158 1159 public boolean hasSeriesDosesElement() { 1160 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 1161 } 1162 1163 public boolean hasSeriesDoses() { 1164 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 1165 } 1166 1167 /** 1168 * @param value {@link #seriesDoses} (The recommended number of doses to achieve immunity as intended by the practitioner administering the dose.). This is the underlying object with id, value and extensions. The accessor "getSeriesDoses" gives direct access to the value 1169 */ 1170 public ImmunizationProtocolAppliedComponent setSeriesDosesElement(StringType value) { 1171 this.seriesDoses = value; 1172 return this; 1173 } 1174 1175 /** 1176 * @return The recommended number of doses to achieve immunity as intended by the practitioner administering the dose. 1177 */ 1178 public String getSeriesDoses() { 1179 return this.seriesDoses == null ? null : this.seriesDoses.getValue(); 1180 } 1181 1182 /** 1183 * @param value The recommended number of doses to achieve immunity as intended by the practitioner administering the dose. 1184 */ 1185 public ImmunizationProtocolAppliedComponent setSeriesDoses(String value) { 1186 if (Utilities.noString(value)) 1187 this.seriesDoses = null; 1188 else { 1189 if (this.seriesDoses == null) 1190 this.seriesDoses = new StringType(); 1191 this.seriesDoses.setValue(value); 1192 } 1193 return this; 1194 } 1195 1196 protected void listChildren(List<Property> children) { 1197 super.listChildren(children); 1198 children.add(new Property("series", "string", "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1, series)); 1199 children.add(new Property("authority", "Reference(Organization)", "Indicates the authority who published the protocol (e.g. ACIP) that is being followed.", 0, 1, authority)); 1200 children.add(new Property("targetDisease", "CodeableConcept", "The vaccine preventable disease the dose is being administered against.", 0, java.lang.Integer.MAX_VALUE, targetDisease)); 1201 children.add(new Property("doseNumber", "string", "Nominal position in a series as intended by the practitioner administering the dose.", 0, 1, doseNumber)); 1202 children.add(new Property("seriesDoses", "string", "The recommended number of doses to achieve immunity as intended by the practitioner administering the dose.", 0, 1, seriesDoses)); 1203 } 1204 1205 @Override 1206 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1207 switch (_hash) { 1208 case -905838985: /*series*/ return new Property("series", "string", "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1, series); 1209 case 1475610435: /*authority*/ return new Property("authority", "Reference(Organization)", "Indicates the authority who published the protocol (e.g. ACIP) that is being followed.", 0, 1, authority); 1210 case -319593813: /*targetDisease*/ return new Property("targetDisease", "CodeableConcept", "The vaccine preventable disease the dose is being administered against.", 0, java.lang.Integer.MAX_VALUE, targetDisease); 1211 case -887709242: /*doseNumber*/ return new Property("doseNumber", "string", "Nominal position in a series as intended by the practitioner administering the dose.", 0, 1, doseNumber); 1212 case -1936727105: /*seriesDoses*/ return new Property("seriesDoses", "string", "The recommended number of doses to achieve immunity as intended by the practitioner administering the dose.", 0, 1, seriesDoses); 1213 default: return super.getNamedProperty(_hash, _name, _checkValid); 1214 } 1215 1216 } 1217 1218 @Override 1219 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1220 switch (hash) { 1221 case -905838985: /*series*/ return this.series == null ? new Base[0] : new Base[] {this.series}; // StringType 1222 case 1475610435: /*authority*/ return this.authority == null ? new Base[0] : new Base[] {this.authority}; // Reference 1223 case -319593813: /*targetDisease*/ return this.targetDisease == null ? new Base[0] : this.targetDisease.toArray(new Base[this.targetDisease.size()]); // CodeableConcept 1224 case -887709242: /*doseNumber*/ return this.doseNumber == null ? new Base[0] : new Base[] {this.doseNumber}; // StringType 1225 case -1936727105: /*seriesDoses*/ return this.seriesDoses == null ? new Base[0] : new Base[] {this.seriesDoses}; // StringType 1226 default: return super.getProperty(hash, name, checkValid); 1227 } 1228 1229 } 1230 1231 @Override 1232 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1233 switch (hash) { 1234 case -905838985: // series 1235 this.series = TypeConvertor.castToString(value); // StringType 1236 return value; 1237 case 1475610435: // authority 1238 this.authority = TypeConvertor.castToReference(value); // Reference 1239 return value; 1240 case -319593813: // targetDisease 1241 this.getTargetDisease().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1242 return value; 1243 case -887709242: // doseNumber 1244 this.doseNumber = TypeConvertor.castToString(value); // StringType 1245 return value; 1246 case -1936727105: // seriesDoses 1247 this.seriesDoses = TypeConvertor.castToString(value); // StringType 1248 return value; 1249 default: return super.setProperty(hash, name, value); 1250 } 1251 1252 } 1253 1254 @Override 1255 public Base setProperty(String name, Base value) throws FHIRException { 1256 if (name.equals("series")) { 1257 this.series = TypeConvertor.castToString(value); // StringType 1258 } else if (name.equals("authority")) { 1259 this.authority = TypeConvertor.castToReference(value); // Reference 1260 } else if (name.equals("targetDisease")) { 1261 this.getTargetDisease().add(TypeConvertor.castToCodeableConcept(value)); 1262 } else if (name.equals("doseNumber")) { 1263 this.doseNumber = TypeConvertor.castToString(value); // StringType 1264 } else if (name.equals("seriesDoses")) { 1265 this.seriesDoses = TypeConvertor.castToString(value); // StringType 1266 } else 1267 return super.setProperty(name, value); 1268 return value; 1269 } 1270 1271 @Override 1272 public void removeChild(String name, Base value) throws FHIRException { 1273 if (name.equals("series")) { 1274 this.series = null; 1275 } else if (name.equals("authority")) { 1276 this.authority = null; 1277 } else if (name.equals("targetDisease")) { 1278 this.getTargetDisease().remove(value); 1279 } else if (name.equals("doseNumber")) { 1280 this.doseNumber = null; 1281 } else if (name.equals("seriesDoses")) { 1282 this.seriesDoses = null; 1283 } else 1284 super.removeChild(name, value); 1285 1286 } 1287 1288 @Override 1289 public Base makeProperty(int hash, String name) throws FHIRException { 1290 switch (hash) { 1291 case -905838985: return getSeriesElement(); 1292 case 1475610435: return getAuthority(); 1293 case -319593813: return addTargetDisease(); 1294 case -887709242: return getDoseNumberElement(); 1295 case -1936727105: return getSeriesDosesElement(); 1296 default: return super.makeProperty(hash, name); 1297 } 1298 1299 } 1300 1301 @Override 1302 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1303 switch (hash) { 1304 case -905838985: /*series*/ return new String[] {"string"}; 1305 case 1475610435: /*authority*/ return new String[] {"Reference"}; 1306 case -319593813: /*targetDisease*/ return new String[] {"CodeableConcept"}; 1307 case -887709242: /*doseNumber*/ return new String[] {"string"}; 1308 case -1936727105: /*seriesDoses*/ return new String[] {"string"}; 1309 default: return super.getTypesForProperty(hash, name); 1310 } 1311 1312 } 1313 1314 @Override 1315 public Base addChild(String name) throws FHIRException { 1316 if (name.equals("series")) { 1317 throw new FHIRException("Cannot call addChild on a singleton property Immunization.protocolApplied.series"); 1318 } 1319 else if (name.equals("authority")) { 1320 this.authority = new Reference(); 1321 return this.authority; 1322 } 1323 else if (name.equals("targetDisease")) { 1324 return addTargetDisease(); 1325 } 1326 else if (name.equals("doseNumber")) { 1327 throw new FHIRException("Cannot call addChild on a singleton property Immunization.protocolApplied.doseNumber"); 1328 } 1329 else if (name.equals("seriesDoses")) { 1330 throw new FHIRException("Cannot call addChild on a singleton property Immunization.protocolApplied.seriesDoses"); 1331 } 1332 else 1333 return super.addChild(name); 1334 } 1335 1336 public ImmunizationProtocolAppliedComponent copy() { 1337 ImmunizationProtocolAppliedComponent dst = new ImmunizationProtocolAppliedComponent(); 1338 copyValues(dst); 1339 return dst; 1340 } 1341 1342 public void copyValues(ImmunizationProtocolAppliedComponent dst) { 1343 super.copyValues(dst); 1344 dst.series = series == null ? null : series.copy(); 1345 dst.authority = authority == null ? null : authority.copy(); 1346 if (targetDisease != null) { 1347 dst.targetDisease = new ArrayList<CodeableConcept>(); 1348 for (CodeableConcept i : targetDisease) 1349 dst.targetDisease.add(i.copy()); 1350 }; 1351 dst.doseNumber = doseNumber == null ? null : doseNumber.copy(); 1352 dst.seriesDoses = seriesDoses == null ? null : seriesDoses.copy(); 1353 } 1354 1355 @Override 1356 public boolean equalsDeep(Base other_) { 1357 if (!super.equalsDeep(other_)) 1358 return false; 1359 if (!(other_ instanceof ImmunizationProtocolAppliedComponent)) 1360 return false; 1361 ImmunizationProtocolAppliedComponent o = (ImmunizationProtocolAppliedComponent) other_; 1362 return compareDeep(series, o.series, true) && compareDeep(authority, o.authority, true) && compareDeep(targetDisease, o.targetDisease, true) 1363 && compareDeep(doseNumber, o.doseNumber, true) && compareDeep(seriesDoses, o.seriesDoses, true) 1364 ; 1365 } 1366 1367 @Override 1368 public boolean equalsShallow(Base other_) { 1369 if (!super.equalsShallow(other_)) 1370 return false; 1371 if (!(other_ instanceof ImmunizationProtocolAppliedComponent)) 1372 return false; 1373 ImmunizationProtocolAppliedComponent o = (ImmunizationProtocolAppliedComponent) other_; 1374 return compareValues(series, o.series, true) && compareValues(doseNumber, o.doseNumber, true) && compareValues(seriesDoses, o.seriesDoses, true) 1375 ; 1376 } 1377 1378 public boolean isEmpty() { 1379 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(series, authority, targetDisease 1380 , doseNumber, seriesDoses); 1381 } 1382 1383 public String fhirType() { 1384 return "Immunization.protocolApplied"; 1385 1386 } 1387 1388 } 1389 1390 /** 1391 * A unique identifier assigned to this immunization record. 1392 */ 1393 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1394 @Description(shortDefinition="Business identifier", formalDefinition="A unique identifier assigned to this immunization record." ) 1395 protected List<Identifier> identifier; 1396 1397 /** 1398 * A plan, order or recommendation fulfilled in whole or in part by this immunization. 1399 */ 1400 @Child(name = "basedOn", type = {CarePlan.class, MedicationRequest.class, ServiceRequest.class, ImmunizationRecommendation.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1401 @Description(shortDefinition="Authority that the immunization event is based on", formalDefinition="A plan, order or recommendation fulfilled in whole or in part by this immunization." ) 1402 protected List<Reference> basedOn; 1403 1404 /** 1405 * Indicates the current status of the immunization event. 1406 */ 1407 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 1408 @Description(shortDefinition="completed | entered-in-error | not-done", formalDefinition="Indicates the current status of the immunization event." ) 1409 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-status") 1410 protected Enumeration<ImmunizationStatusCodes> status; 1411 1412 /** 1413 * Indicates the reason the immunization event was not performed. 1414 */ 1415 @Child(name = "statusReason", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1416 @Description(shortDefinition="Reason for current status", formalDefinition="Indicates the reason the immunization event was not performed." ) 1417 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-status-reason") 1418 protected CodeableConcept statusReason; 1419 1420 /** 1421 * Vaccine that was administered or was to be administered. 1422 */ 1423 @Child(name = "vaccineCode", type = {CodeableConcept.class}, order=4, min=1, max=1, modifier=false, summary=true) 1424 @Description(shortDefinition="Vaccine administered", formalDefinition="Vaccine that was administered or was to be administered." ) 1425 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vaccine-code") 1426 protected CodeableConcept vaccineCode; 1427 1428 /** 1429 * An indication of which product was administered to the patient. This is typically a more detailed representation of the concept conveyed by the vaccineCode data element. If a Medication resource is referenced, it may be to a stand-alone resource or a contained resource within the Immunization resource. 1430 */ 1431 @Child(name = "administeredProduct", type = {CodeableReference.class}, order=5, min=0, max=1, modifier=false, summary=false) 1432 @Description(shortDefinition="Product that was administered", formalDefinition="An indication of which product was administered to the patient. This is typically a more detailed representation of the concept conveyed by the vaccineCode data element. If a Medication resource is referenced, it may be to a stand-alone resource or a contained resource within the Immunization resource." ) 1433 protected CodeableReference administeredProduct; 1434 1435 /** 1436 * Name of vaccine manufacturer. 1437 */ 1438 @Child(name = "manufacturer", type = {CodeableReference.class}, order=6, min=0, max=1, modifier=false, summary=false) 1439 @Description(shortDefinition="Vaccine manufacturer", formalDefinition="Name of vaccine manufacturer." ) 1440 protected CodeableReference manufacturer; 1441 1442 /** 1443 * Lot number of the vaccine product. 1444 */ 1445 @Child(name = "lotNumber", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 1446 @Description(shortDefinition="Vaccine lot number", formalDefinition="Lot number of the vaccine product." ) 1447 protected StringType lotNumber; 1448 1449 /** 1450 * Date vaccine batch expires. 1451 */ 1452 @Child(name = "expirationDate", type = {DateType.class}, order=8, min=0, max=1, modifier=false, summary=false) 1453 @Description(shortDefinition="Vaccine expiration date", formalDefinition="Date vaccine batch expires." ) 1454 protected DateType expirationDate; 1455 1456 /** 1457 * The patient who either received or did not receive the immunization. 1458 */ 1459 @Child(name = "patient", type = {Patient.class}, order=9, min=1, max=1, modifier=false, summary=true) 1460 @Description(shortDefinition="Who was immunized", formalDefinition="The patient who either received or did not receive the immunization." ) 1461 protected Reference patient; 1462 1463 /** 1464 * The visit or admission or other contact between patient and health care provider the immunization was performed as part of. 1465 */ 1466 @Child(name = "encounter", type = {Encounter.class}, order=10, min=0, max=1, modifier=false, summary=false) 1467 @Description(shortDefinition="Encounter immunization was part of", formalDefinition="The visit or admission or other contact between patient and health care provider the immunization was performed as part of." ) 1468 protected Reference encounter; 1469 1470 /** 1471 * Additional information that is relevant to the immunization (e.g. for a vaccine recipient who is pregnant, the gestational age of the fetus). The reason why a vaccine was given (e.g. occupation, underlying medical condition) should be conveyed in Immunization.reason, not as supporting information. The reason why a vaccine was not given (e.g. contraindication) should be conveyed in Immunization.statusReason, not as supporting information. 1472 */ 1473 @Child(name = "supportingInformation", type = {Reference.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1474 @Description(shortDefinition="Additional information in support of the immunization", formalDefinition="Additional information that is relevant to the immunization (e.g. for a vaccine recipient who is pregnant, the gestational age of the fetus). The reason why a vaccine was given (e.g. occupation, underlying medical condition) should be conveyed in Immunization.reason, not as supporting information. The reason why a vaccine was not given (e.g. contraindication) should be conveyed in Immunization.statusReason, not as supporting information." ) 1475 protected List<Reference> supportingInformation; 1476 1477 /** 1478 * Date vaccine administered or was to be administered. 1479 */ 1480 @Child(name = "occurrence", type = {DateTimeType.class, StringType.class}, order=12, min=1, max=1, modifier=false, summary=true) 1481 @Description(shortDefinition="Vaccine administration date", formalDefinition="Date vaccine administered or was to be administered." ) 1482 protected DataType occurrence; 1483 1484 /** 1485 * Indicates whether the data contained in the resource was captured by the individual/organization which was responsible for the administration of the vaccine rather than as 'secondary reported' data documented by a third party. A value of 'true' means this data originated with the individual/organization which was responsible for the administration of the vaccine. 1486 */ 1487 @Child(name = "primarySource", type = {BooleanType.class}, order=13, min=0, max=1, modifier=false, summary=true) 1488 @Description(shortDefinition="Indicates context the data was captured in", formalDefinition="Indicates whether the data contained in the resource was captured by the individual/organization which was responsible for the administration of the vaccine rather than as 'secondary reported' data documented by a third party. A value of 'true' means this data originated with the individual/organization which was responsible for the administration of the vaccine." ) 1489 protected BooleanType primarySource; 1490 1491 /** 1492 * Typically the source of the data when the report of the immunization event is not based on information from the person who administered the vaccine. 1493 */ 1494 @Child(name = "informationSource", type = {CodeableReference.class}, order=14, min=0, max=1, modifier=false, summary=false) 1495 @Description(shortDefinition="Indicates the source of a reported record", formalDefinition="Typically the source of the data when the report of the immunization event is not based on information from the person who administered the vaccine." ) 1496 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-origin") 1497 protected CodeableReference informationSource; 1498 1499 /** 1500 * The service delivery location where the vaccine administration occurred. 1501 */ 1502 @Child(name = "location", type = {Location.class}, order=15, min=0, max=1, modifier=false, summary=false) 1503 @Description(shortDefinition="Where immunization occurred", formalDefinition="The service delivery location where the vaccine administration occurred." ) 1504 protected Reference location; 1505 1506 /** 1507 * Body site where vaccine was administered. 1508 */ 1509 @Child(name = "site", type = {CodeableConcept.class}, order=16, min=0, max=1, modifier=false, summary=false) 1510 @Description(shortDefinition="Body site vaccine was administered", formalDefinition="Body site where vaccine was administered." ) 1511 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-site") 1512 protected CodeableConcept site; 1513 1514 /** 1515 * The path by which the vaccine product is taken into the body. 1516 */ 1517 @Child(name = "route", type = {CodeableConcept.class}, order=17, min=0, max=1, modifier=false, summary=false) 1518 @Description(shortDefinition="How vaccine entered body", formalDefinition="The path by which the vaccine product is taken into the body." ) 1519 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-route") 1520 protected CodeableConcept route; 1521 1522 /** 1523 * The quantity of vaccine product that was administered. 1524 */ 1525 @Child(name = "doseQuantity", type = {Quantity.class}, order=18, min=0, max=1, modifier=false, summary=false) 1526 @Description(shortDefinition="Amount of vaccine administered", formalDefinition="The quantity of vaccine product that was administered." ) 1527 protected Quantity doseQuantity; 1528 1529 /** 1530 * Indicates who performed the immunization event. 1531 */ 1532 @Child(name = "performer", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1533 @Description(shortDefinition="Who performed event", formalDefinition="Indicates who performed the immunization event." ) 1534 protected List<ImmunizationPerformerComponent> performer; 1535 1536 /** 1537 * Extra information about the immunization that is not conveyed by the other attributes. 1538 */ 1539 @Child(name = "note", type = {Annotation.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1540 @Description(shortDefinition="Additional immunization notes", formalDefinition="Extra information about the immunization that is not conveyed by the other attributes." ) 1541 protected List<Annotation> note; 1542 1543 /** 1544 * Describes why the immunization occurred in coded or textual form, or Indicates another resource (Condition, Observation or DiagnosticReport) whose existence justifies this immunization. 1545 */ 1546 @Child(name = "reason", type = {CodeableReference.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1547 @Description(shortDefinition="Why immunization occurred", formalDefinition="Describes why the immunization occurred in coded or textual form, or Indicates another resource (Condition, Observation or DiagnosticReport) whose existence justifies this immunization." ) 1548 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-reason") 1549 protected List<CodeableReference> reason; 1550 1551 /** 1552 * Indication if a dose is considered to be subpotent. By default, a dose should be considered to be potent. 1553 */ 1554 @Child(name = "isSubpotent", type = {BooleanType.class}, order=22, min=0, max=1, modifier=true, summary=true) 1555 @Description(shortDefinition="Dose potency", formalDefinition="Indication if a dose is considered to be subpotent. By default, a dose should be considered to be potent." ) 1556 protected BooleanType isSubpotent; 1557 1558 /** 1559 * Reason why a dose is considered to be subpotent. 1560 */ 1561 @Child(name = "subpotentReason", type = {CodeableConcept.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1562 @Description(shortDefinition="Reason for being subpotent", formalDefinition="Reason why a dose is considered to be subpotent." ) 1563 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-subpotent-reason") 1564 protected List<CodeableConcept> subpotentReason; 1565 1566 /** 1567 * Indicates a patient's eligibility for a funding program. 1568 */ 1569 @Child(name = "programEligibility", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1570 @Description(shortDefinition="Patient eligibility for a specific vaccination program", formalDefinition="Indicates a patient's eligibility for a funding program." ) 1571 protected List<ImmunizationProgramEligibilityComponent> programEligibility; 1572 1573 /** 1574 * Indicates the source of the vaccine actually administered. This may be different than the patient eligibility (e.g. the patient may be eligible for a publically purchased vaccine but due to inventory issues, vaccine purchased with private funds was actually administered). 1575 */ 1576 @Child(name = "fundingSource", type = {CodeableConcept.class}, order=25, min=0, max=1, modifier=false, summary=false) 1577 @Description(shortDefinition="Funding source for the vaccine", formalDefinition="Indicates the source of the vaccine actually administered. This may be different than the patient eligibility (e.g. the patient may be eligible for a publically purchased vaccine but due to inventory issues, vaccine purchased with private funds was actually administered)." ) 1578 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-funding-source") 1579 protected CodeableConcept fundingSource; 1580 1581 /** 1582 * Categorical data indicating that an adverse event is associated in time to an immunization. 1583 */ 1584 @Child(name = "reaction", type = {}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1585 @Description(shortDefinition="Details of a reaction that follows immunization", formalDefinition="Categorical data indicating that an adverse event is associated in time to an immunization." ) 1586 protected List<ImmunizationReactionComponent> reaction; 1587 1588 /** 1589 * The protocol (set of recommendations) being followed by the provider who administered the dose. 1590 */ 1591 @Child(name = "protocolApplied", type = {}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1592 @Description(shortDefinition="Protocol followed by the provider", formalDefinition="The protocol (set of recommendations) being followed by the provider who administered the dose." ) 1593 protected List<ImmunizationProtocolAppliedComponent> protocolApplied; 1594 1595 private static final long serialVersionUID = 425193818L; 1596 1597 /** 1598 * Constructor 1599 */ 1600 public Immunization() { 1601 super(); 1602 } 1603 1604 /** 1605 * Constructor 1606 */ 1607 public Immunization(ImmunizationStatusCodes status, CodeableConcept vaccineCode, Reference patient, DataType occurrence) { 1608 super(); 1609 this.setStatus(status); 1610 this.setVaccineCode(vaccineCode); 1611 this.setPatient(patient); 1612 this.setOccurrence(occurrence); 1613 } 1614 1615 /** 1616 * @return {@link #identifier} (A unique identifier assigned to this immunization record.) 1617 */ 1618 public List<Identifier> getIdentifier() { 1619 if (this.identifier == null) 1620 this.identifier = new ArrayList<Identifier>(); 1621 return this.identifier; 1622 } 1623 1624 /** 1625 * @return Returns a reference to <code>this</code> for easy method chaining 1626 */ 1627 public Immunization setIdentifier(List<Identifier> theIdentifier) { 1628 this.identifier = theIdentifier; 1629 return this; 1630 } 1631 1632 public boolean hasIdentifier() { 1633 if (this.identifier == null) 1634 return false; 1635 for (Identifier item : this.identifier) 1636 if (!item.isEmpty()) 1637 return true; 1638 return false; 1639 } 1640 1641 public Identifier addIdentifier() { //3 1642 Identifier t = new Identifier(); 1643 if (this.identifier == null) 1644 this.identifier = new ArrayList<Identifier>(); 1645 this.identifier.add(t); 1646 return t; 1647 } 1648 1649 public Immunization addIdentifier(Identifier t) { //3 1650 if (t == null) 1651 return this; 1652 if (this.identifier == null) 1653 this.identifier = new ArrayList<Identifier>(); 1654 this.identifier.add(t); 1655 return this; 1656 } 1657 1658 /** 1659 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1660 */ 1661 public Identifier getIdentifierFirstRep() { 1662 if (getIdentifier().isEmpty()) { 1663 addIdentifier(); 1664 } 1665 return getIdentifier().get(0); 1666 } 1667 1668 /** 1669 * @return {@link #basedOn} (A plan, order or recommendation fulfilled in whole or in part by this immunization.) 1670 */ 1671 public List<Reference> getBasedOn() { 1672 if (this.basedOn == null) 1673 this.basedOn = new ArrayList<Reference>(); 1674 return this.basedOn; 1675 } 1676 1677 /** 1678 * @return Returns a reference to <code>this</code> for easy method chaining 1679 */ 1680 public Immunization setBasedOn(List<Reference> theBasedOn) { 1681 this.basedOn = theBasedOn; 1682 return this; 1683 } 1684 1685 public boolean hasBasedOn() { 1686 if (this.basedOn == null) 1687 return false; 1688 for (Reference item : this.basedOn) 1689 if (!item.isEmpty()) 1690 return true; 1691 return false; 1692 } 1693 1694 public Reference addBasedOn() { //3 1695 Reference t = new Reference(); 1696 if (this.basedOn == null) 1697 this.basedOn = new ArrayList<Reference>(); 1698 this.basedOn.add(t); 1699 return t; 1700 } 1701 1702 public Immunization addBasedOn(Reference t) { //3 1703 if (t == null) 1704 return this; 1705 if (this.basedOn == null) 1706 this.basedOn = new ArrayList<Reference>(); 1707 this.basedOn.add(t); 1708 return this; 1709 } 1710 1711 /** 1712 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 1713 */ 1714 public Reference getBasedOnFirstRep() { 1715 if (getBasedOn().isEmpty()) { 1716 addBasedOn(); 1717 } 1718 return getBasedOn().get(0); 1719 } 1720 1721 /** 1722 * @return {@link #status} (Indicates the current status of the immunization event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1723 */ 1724 public Enumeration<ImmunizationStatusCodes> getStatusElement() { 1725 if (this.status == null) 1726 if (Configuration.errorOnAutoCreate()) 1727 throw new Error("Attempt to auto-create Immunization.status"); 1728 else if (Configuration.doAutoCreate()) 1729 this.status = new Enumeration<ImmunizationStatusCodes>(new ImmunizationStatusCodesEnumFactory()); // bb 1730 return this.status; 1731 } 1732 1733 public boolean hasStatusElement() { 1734 return this.status != null && !this.status.isEmpty(); 1735 } 1736 1737 public boolean hasStatus() { 1738 return this.status != null && !this.status.isEmpty(); 1739 } 1740 1741 /** 1742 * @param value {@link #status} (Indicates the current status of the immunization event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1743 */ 1744 public Immunization setStatusElement(Enumeration<ImmunizationStatusCodes> value) { 1745 this.status = value; 1746 return this; 1747 } 1748 1749 /** 1750 * @return Indicates the current status of the immunization event. 1751 */ 1752 public ImmunizationStatusCodes getStatus() { 1753 return this.status == null ? null : this.status.getValue(); 1754 } 1755 1756 /** 1757 * @param value Indicates the current status of the immunization event. 1758 */ 1759 public Immunization setStatus(ImmunizationStatusCodes value) { 1760 if (this.status == null) 1761 this.status = new Enumeration<ImmunizationStatusCodes>(new ImmunizationStatusCodesEnumFactory()); 1762 this.status.setValue(value); 1763 return this; 1764 } 1765 1766 /** 1767 * @return {@link #statusReason} (Indicates the reason the immunization event was not performed.) 1768 */ 1769 public CodeableConcept getStatusReason() { 1770 if (this.statusReason == null) 1771 if (Configuration.errorOnAutoCreate()) 1772 throw new Error("Attempt to auto-create Immunization.statusReason"); 1773 else if (Configuration.doAutoCreate()) 1774 this.statusReason = new CodeableConcept(); // cc 1775 return this.statusReason; 1776 } 1777 1778 public boolean hasStatusReason() { 1779 return this.statusReason != null && !this.statusReason.isEmpty(); 1780 } 1781 1782 /** 1783 * @param value {@link #statusReason} (Indicates the reason the immunization event was not performed.) 1784 */ 1785 public Immunization setStatusReason(CodeableConcept value) { 1786 this.statusReason = value; 1787 return this; 1788 } 1789 1790 /** 1791 * @return {@link #vaccineCode} (Vaccine that was administered or was to be administered.) 1792 */ 1793 public CodeableConcept getVaccineCode() { 1794 if (this.vaccineCode == null) 1795 if (Configuration.errorOnAutoCreate()) 1796 throw new Error("Attempt to auto-create Immunization.vaccineCode"); 1797 else if (Configuration.doAutoCreate()) 1798 this.vaccineCode = new CodeableConcept(); // cc 1799 return this.vaccineCode; 1800 } 1801 1802 public boolean hasVaccineCode() { 1803 return this.vaccineCode != null && !this.vaccineCode.isEmpty(); 1804 } 1805 1806 /** 1807 * @param value {@link #vaccineCode} (Vaccine that was administered or was to be administered.) 1808 */ 1809 public Immunization setVaccineCode(CodeableConcept value) { 1810 this.vaccineCode = value; 1811 return this; 1812 } 1813 1814 /** 1815 * @return {@link #administeredProduct} (An indication of which product was administered to the patient. This is typically a more detailed representation of the concept conveyed by the vaccineCode data element. If a Medication resource is referenced, it may be to a stand-alone resource or a contained resource within the Immunization resource.) 1816 */ 1817 public CodeableReference getAdministeredProduct() { 1818 if (this.administeredProduct == null) 1819 if (Configuration.errorOnAutoCreate()) 1820 throw new Error("Attempt to auto-create Immunization.administeredProduct"); 1821 else if (Configuration.doAutoCreate()) 1822 this.administeredProduct = new CodeableReference(); // cc 1823 return this.administeredProduct; 1824 } 1825 1826 public boolean hasAdministeredProduct() { 1827 return this.administeredProduct != null && !this.administeredProduct.isEmpty(); 1828 } 1829 1830 /** 1831 * @param value {@link #administeredProduct} (An indication of which product was administered to the patient. This is typically a more detailed representation of the concept conveyed by the vaccineCode data element. If a Medication resource is referenced, it may be to a stand-alone resource or a contained resource within the Immunization resource.) 1832 */ 1833 public Immunization setAdministeredProduct(CodeableReference value) { 1834 this.administeredProduct = value; 1835 return this; 1836 } 1837 1838 /** 1839 * @return {@link #manufacturer} (Name of vaccine manufacturer.) 1840 */ 1841 public CodeableReference getManufacturer() { 1842 if (this.manufacturer == null) 1843 if (Configuration.errorOnAutoCreate()) 1844 throw new Error("Attempt to auto-create Immunization.manufacturer"); 1845 else if (Configuration.doAutoCreate()) 1846 this.manufacturer = new CodeableReference(); // cc 1847 return this.manufacturer; 1848 } 1849 1850 public boolean hasManufacturer() { 1851 return this.manufacturer != null && !this.manufacturer.isEmpty(); 1852 } 1853 1854 /** 1855 * @param value {@link #manufacturer} (Name of vaccine manufacturer.) 1856 */ 1857 public Immunization setManufacturer(CodeableReference value) { 1858 this.manufacturer = value; 1859 return this; 1860 } 1861 1862 /** 1863 * @return {@link #lotNumber} (Lot number of the vaccine product.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 1864 */ 1865 public StringType getLotNumberElement() { 1866 if (this.lotNumber == null) 1867 if (Configuration.errorOnAutoCreate()) 1868 throw new Error("Attempt to auto-create Immunization.lotNumber"); 1869 else if (Configuration.doAutoCreate()) 1870 this.lotNumber = new StringType(); // bb 1871 return this.lotNumber; 1872 } 1873 1874 public boolean hasLotNumberElement() { 1875 return this.lotNumber != null && !this.lotNumber.isEmpty(); 1876 } 1877 1878 public boolean hasLotNumber() { 1879 return this.lotNumber != null && !this.lotNumber.isEmpty(); 1880 } 1881 1882 /** 1883 * @param value {@link #lotNumber} (Lot number of the vaccine product.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 1884 */ 1885 public Immunization setLotNumberElement(StringType value) { 1886 this.lotNumber = value; 1887 return this; 1888 } 1889 1890 /** 1891 * @return Lot number of the vaccine product. 1892 */ 1893 public String getLotNumber() { 1894 return this.lotNumber == null ? null : this.lotNumber.getValue(); 1895 } 1896 1897 /** 1898 * @param value Lot number of the vaccine product. 1899 */ 1900 public Immunization setLotNumber(String value) { 1901 if (Utilities.noString(value)) 1902 this.lotNumber = null; 1903 else { 1904 if (this.lotNumber == null) 1905 this.lotNumber = new StringType(); 1906 this.lotNumber.setValue(value); 1907 } 1908 return this; 1909 } 1910 1911 /** 1912 * @return {@link #expirationDate} (Date vaccine batch expires.). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 1913 */ 1914 public DateType getExpirationDateElement() { 1915 if (this.expirationDate == null) 1916 if (Configuration.errorOnAutoCreate()) 1917 throw new Error("Attempt to auto-create Immunization.expirationDate"); 1918 else if (Configuration.doAutoCreate()) 1919 this.expirationDate = new DateType(); // bb 1920 return this.expirationDate; 1921 } 1922 1923 public boolean hasExpirationDateElement() { 1924 return this.expirationDate != null && !this.expirationDate.isEmpty(); 1925 } 1926 1927 public boolean hasExpirationDate() { 1928 return this.expirationDate != null && !this.expirationDate.isEmpty(); 1929 } 1930 1931 /** 1932 * @param value {@link #expirationDate} (Date vaccine batch expires.). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 1933 */ 1934 public Immunization setExpirationDateElement(DateType value) { 1935 this.expirationDate = value; 1936 return this; 1937 } 1938 1939 /** 1940 * @return Date vaccine batch expires. 1941 */ 1942 public Date getExpirationDate() { 1943 return this.expirationDate == null ? null : this.expirationDate.getValue(); 1944 } 1945 1946 /** 1947 * @param value Date vaccine batch expires. 1948 */ 1949 public Immunization setExpirationDate(Date value) { 1950 if (value == null) 1951 this.expirationDate = null; 1952 else { 1953 if (this.expirationDate == null) 1954 this.expirationDate = new DateType(); 1955 this.expirationDate.setValue(value); 1956 } 1957 return this; 1958 } 1959 1960 /** 1961 * @return {@link #patient} (The patient who either received or did not receive the immunization.) 1962 */ 1963 public Reference getPatient() { 1964 if (this.patient == null) 1965 if (Configuration.errorOnAutoCreate()) 1966 throw new Error("Attempt to auto-create Immunization.patient"); 1967 else if (Configuration.doAutoCreate()) 1968 this.patient = new Reference(); // cc 1969 return this.patient; 1970 } 1971 1972 public boolean hasPatient() { 1973 return this.patient != null && !this.patient.isEmpty(); 1974 } 1975 1976 /** 1977 * @param value {@link #patient} (The patient who either received or did not receive the immunization.) 1978 */ 1979 public Immunization setPatient(Reference value) { 1980 this.patient = value; 1981 return this; 1982 } 1983 1984 /** 1985 * @return {@link #encounter} (The visit or admission or other contact between patient and health care provider the immunization was performed as part of.) 1986 */ 1987 public Reference getEncounter() { 1988 if (this.encounter == null) 1989 if (Configuration.errorOnAutoCreate()) 1990 throw new Error("Attempt to auto-create Immunization.encounter"); 1991 else if (Configuration.doAutoCreate()) 1992 this.encounter = new Reference(); // cc 1993 return this.encounter; 1994 } 1995 1996 public boolean hasEncounter() { 1997 return this.encounter != null && !this.encounter.isEmpty(); 1998 } 1999 2000 /** 2001 * @param value {@link #encounter} (The visit or admission or other contact between patient and health care provider the immunization was performed as part of.) 2002 */ 2003 public Immunization setEncounter(Reference value) { 2004 this.encounter = value; 2005 return this; 2006 } 2007 2008 /** 2009 * @return {@link #supportingInformation} (Additional information that is relevant to the immunization (e.g. for a vaccine recipient who is pregnant, the gestational age of the fetus). The reason why a vaccine was given (e.g. occupation, underlying medical condition) should be conveyed in Immunization.reason, not as supporting information. The reason why a vaccine was not given (e.g. contraindication) should be conveyed in Immunization.statusReason, not as supporting information.) 2010 */ 2011 public List<Reference> getSupportingInformation() { 2012 if (this.supportingInformation == null) 2013 this.supportingInformation = new ArrayList<Reference>(); 2014 return this.supportingInformation; 2015 } 2016 2017 /** 2018 * @return Returns a reference to <code>this</code> for easy method chaining 2019 */ 2020 public Immunization setSupportingInformation(List<Reference> theSupportingInformation) { 2021 this.supportingInformation = theSupportingInformation; 2022 return this; 2023 } 2024 2025 public boolean hasSupportingInformation() { 2026 if (this.supportingInformation == null) 2027 return false; 2028 for (Reference item : this.supportingInformation) 2029 if (!item.isEmpty()) 2030 return true; 2031 return false; 2032 } 2033 2034 public Reference addSupportingInformation() { //3 2035 Reference t = new Reference(); 2036 if (this.supportingInformation == null) 2037 this.supportingInformation = new ArrayList<Reference>(); 2038 this.supportingInformation.add(t); 2039 return t; 2040 } 2041 2042 public Immunization addSupportingInformation(Reference t) { //3 2043 if (t == null) 2044 return this; 2045 if (this.supportingInformation == null) 2046 this.supportingInformation = new ArrayList<Reference>(); 2047 this.supportingInformation.add(t); 2048 return this; 2049 } 2050 2051 /** 2052 * @return The first repetition of repeating field {@link #supportingInformation}, creating it if it does not already exist {3} 2053 */ 2054 public Reference getSupportingInformationFirstRep() { 2055 if (getSupportingInformation().isEmpty()) { 2056 addSupportingInformation(); 2057 } 2058 return getSupportingInformation().get(0); 2059 } 2060 2061 /** 2062 * @return {@link #occurrence} (Date vaccine administered or was to be administered.) 2063 */ 2064 public DataType getOccurrence() { 2065 return this.occurrence; 2066 } 2067 2068 /** 2069 * @return {@link #occurrence} (Date vaccine administered or was to be administered.) 2070 */ 2071 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 2072 if (this.occurrence == null) 2073 this.occurrence = new DateTimeType(); 2074 if (!(this.occurrence instanceof DateTimeType)) 2075 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 2076 return (DateTimeType) this.occurrence; 2077 } 2078 2079 public boolean hasOccurrenceDateTimeType() { 2080 return this != null && this.occurrence instanceof DateTimeType; 2081 } 2082 2083 /** 2084 * @return {@link #occurrence} (Date vaccine administered or was to be administered.) 2085 */ 2086 public StringType getOccurrenceStringType() throws FHIRException { 2087 if (this.occurrence == null) 2088 this.occurrence = new StringType(); 2089 if (!(this.occurrence instanceof StringType)) 2090 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 2091 return (StringType) this.occurrence; 2092 } 2093 2094 public boolean hasOccurrenceStringType() { 2095 return this != null && this.occurrence instanceof StringType; 2096 } 2097 2098 public boolean hasOccurrence() { 2099 return this.occurrence != null && !this.occurrence.isEmpty(); 2100 } 2101 2102 /** 2103 * @param value {@link #occurrence} (Date vaccine administered or was to be administered.) 2104 */ 2105 public Immunization setOccurrence(DataType value) { 2106 if (value != null && !(value instanceof DateTimeType || value instanceof StringType)) 2107 throw new FHIRException("Not the right type for Immunization.occurrence[x]: "+value.fhirType()); 2108 this.occurrence = value; 2109 return this; 2110 } 2111 2112 /** 2113 * @return {@link #primarySource} (Indicates whether the data contained in the resource was captured by the individual/organization which was responsible for the administration of the vaccine rather than as 'secondary reported' data documented by a third party. A value of 'true' means this data originated with the individual/organization which was responsible for the administration of the vaccine.). This is the underlying object with id, value and extensions. The accessor "getPrimarySource" gives direct access to the value 2114 */ 2115 public BooleanType getPrimarySourceElement() { 2116 if (this.primarySource == null) 2117 if (Configuration.errorOnAutoCreate()) 2118 throw new Error("Attempt to auto-create Immunization.primarySource"); 2119 else if (Configuration.doAutoCreate()) 2120 this.primarySource = new BooleanType(); // bb 2121 return this.primarySource; 2122 } 2123 2124 public boolean hasPrimarySourceElement() { 2125 return this.primarySource != null && !this.primarySource.isEmpty(); 2126 } 2127 2128 public boolean hasPrimarySource() { 2129 return this.primarySource != null && !this.primarySource.isEmpty(); 2130 } 2131 2132 /** 2133 * @param value {@link #primarySource} (Indicates whether the data contained in the resource was captured by the individual/organization which was responsible for the administration of the vaccine rather than as 'secondary reported' data documented by a third party. A value of 'true' means this data originated with the individual/organization which was responsible for the administration of the vaccine.). This is the underlying object with id, value and extensions. The accessor "getPrimarySource" gives direct access to the value 2134 */ 2135 public Immunization setPrimarySourceElement(BooleanType value) { 2136 this.primarySource = value; 2137 return this; 2138 } 2139 2140 /** 2141 * @return Indicates whether the data contained in the resource was captured by the individual/organization which was responsible for the administration of the vaccine rather than as 'secondary reported' data documented by a third party. A value of 'true' means this data originated with the individual/organization which was responsible for the administration of the vaccine. 2142 */ 2143 public boolean getPrimarySource() { 2144 return this.primarySource == null || this.primarySource.isEmpty() ? false : this.primarySource.getValue(); 2145 } 2146 2147 /** 2148 * @param value Indicates whether the data contained in the resource was captured by the individual/organization which was responsible for the administration of the vaccine rather than as 'secondary reported' data documented by a third party. A value of 'true' means this data originated with the individual/organization which was responsible for the administration of the vaccine. 2149 */ 2150 public Immunization setPrimarySource(boolean value) { 2151 if (this.primarySource == null) 2152 this.primarySource = new BooleanType(); 2153 this.primarySource.setValue(value); 2154 return this; 2155 } 2156 2157 /** 2158 * @return {@link #informationSource} (Typically the source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.) 2159 */ 2160 public CodeableReference getInformationSource() { 2161 if (this.informationSource == null) 2162 if (Configuration.errorOnAutoCreate()) 2163 throw new Error("Attempt to auto-create Immunization.informationSource"); 2164 else if (Configuration.doAutoCreate()) 2165 this.informationSource = new CodeableReference(); // cc 2166 return this.informationSource; 2167 } 2168 2169 public boolean hasInformationSource() { 2170 return this.informationSource != null && !this.informationSource.isEmpty(); 2171 } 2172 2173 /** 2174 * @param value {@link #informationSource} (Typically the source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.) 2175 */ 2176 public Immunization setInformationSource(CodeableReference value) { 2177 this.informationSource = value; 2178 return this; 2179 } 2180 2181 /** 2182 * @return {@link #location} (The service delivery location where the vaccine administration occurred.) 2183 */ 2184 public Reference getLocation() { 2185 if (this.location == null) 2186 if (Configuration.errorOnAutoCreate()) 2187 throw new Error("Attempt to auto-create Immunization.location"); 2188 else if (Configuration.doAutoCreate()) 2189 this.location = new Reference(); // cc 2190 return this.location; 2191 } 2192 2193 public boolean hasLocation() { 2194 return this.location != null && !this.location.isEmpty(); 2195 } 2196 2197 /** 2198 * @param value {@link #location} (The service delivery location where the vaccine administration occurred.) 2199 */ 2200 public Immunization setLocation(Reference value) { 2201 this.location = value; 2202 return this; 2203 } 2204 2205 /** 2206 * @return {@link #site} (Body site where vaccine was administered.) 2207 */ 2208 public CodeableConcept getSite() { 2209 if (this.site == null) 2210 if (Configuration.errorOnAutoCreate()) 2211 throw new Error("Attempt to auto-create Immunization.site"); 2212 else if (Configuration.doAutoCreate()) 2213 this.site = new CodeableConcept(); // cc 2214 return this.site; 2215 } 2216 2217 public boolean hasSite() { 2218 return this.site != null && !this.site.isEmpty(); 2219 } 2220 2221 /** 2222 * @param value {@link #site} (Body site where vaccine was administered.) 2223 */ 2224 public Immunization setSite(CodeableConcept value) { 2225 this.site = value; 2226 return this; 2227 } 2228 2229 /** 2230 * @return {@link #route} (The path by which the vaccine product is taken into the body.) 2231 */ 2232 public CodeableConcept getRoute() { 2233 if (this.route == null) 2234 if (Configuration.errorOnAutoCreate()) 2235 throw new Error("Attempt to auto-create Immunization.route"); 2236 else if (Configuration.doAutoCreate()) 2237 this.route = new CodeableConcept(); // cc 2238 return this.route; 2239 } 2240 2241 public boolean hasRoute() { 2242 return this.route != null && !this.route.isEmpty(); 2243 } 2244 2245 /** 2246 * @param value {@link #route} (The path by which the vaccine product is taken into the body.) 2247 */ 2248 public Immunization setRoute(CodeableConcept value) { 2249 this.route = value; 2250 return this; 2251 } 2252 2253 /** 2254 * @return {@link #doseQuantity} (The quantity of vaccine product that was administered.) 2255 */ 2256 public Quantity getDoseQuantity() { 2257 if (this.doseQuantity == null) 2258 if (Configuration.errorOnAutoCreate()) 2259 throw new Error("Attempt to auto-create Immunization.doseQuantity"); 2260 else if (Configuration.doAutoCreate()) 2261 this.doseQuantity = new Quantity(); // cc 2262 return this.doseQuantity; 2263 } 2264 2265 public boolean hasDoseQuantity() { 2266 return this.doseQuantity != null && !this.doseQuantity.isEmpty(); 2267 } 2268 2269 /** 2270 * @param value {@link #doseQuantity} (The quantity of vaccine product that was administered.) 2271 */ 2272 public Immunization setDoseQuantity(Quantity value) { 2273 this.doseQuantity = value; 2274 return this; 2275 } 2276 2277 /** 2278 * @return {@link #performer} (Indicates who performed the immunization event.) 2279 */ 2280 public List<ImmunizationPerformerComponent> getPerformer() { 2281 if (this.performer == null) 2282 this.performer = new ArrayList<ImmunizationPerformerComponent>(); 2283 return this.performer; 2284 } 2285 2286 /** 2287 * @return Returns a reference to <code>this</code> for easy method chaining 2288 */ 2289 public Immunization setPerformer(List<ImmunizationPerformerComponent> thePerformer) { 2290 this.performer = thePerformer; 2291 return this; 2292 } 2293 2294 public boolean hasPerformer() { 2295 if (this.performer == null) 2296 return false; 2297 for (ImmunizationPerformerComponent item : this.performer) 2298 if (!item.isEmpty()) 2299 return true; 2300 return false; 2301 } 2302 2303 public ImmunizationPerformerComponent addPerformer() { //3 2304 ImmunizationPerformerComponent t = new ImmunizationPerformerComponent(); 2305 if (this.performer == null) 2306 this.performer = new ArrayList<ImmunizationPerformerComponent>(); 2307 this.performer.add(t); 2308 return t; 2309 } 2310 2311 public Immunization addPerformer(ImmunizationPerformerComponent t) { //3 2312 if (t == null) 2313 return this; 2314 if (this.performer == null) 2315 this.performer = new ArrayList<ImmunizationPerformerComponent>(); 2316 this.performer.add(t); 2317 return this; 2318 } 2319 2320 /** 2321 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 2322 */ 2323 public ImmunizationPerformerComponent getPerformerFirstRep() { 2324 if (getPerformer().isEmpty()) { 2325 addPerformer(); 2326 } 2327 return getPerformer().get(0); 2328 } 2329 2330 /** 2331 * @return {@link #note} (Extra information about the immunization that is not conveyed by the other attributes.) 2332 */ 2333 public List<Annotation> getNote() { 2334 if (this.note == null) 2335 this.note = new ArrayList<Annotation>(); 2336 return this.note; 2337 } 2338 2339 /** 2340 * @return Returns a reference to <code>this</code> for easy method chaining 2341 */ 2342 public Immunization setNote(List<Annotation> theNote) { 2343 this.note = theNote; 2344 return this; 2345 } 2346 2347 public boolean hasNote() { 2348 if (this.note == null) 2349 return false; 2350 for (Annotation item : this.note) 2351 if (!item.isEmpty()) 2352 return true; 2353 return false; 2354 } 2355 2356 public Annotation addNote() { //3 2357 Annotation t = new Annotation(); 2358 if (this.note == null) 2359 this.note = new ArrayList<Annotation>(); 2360 this.note.add(t); 2361 return t; 2362 } 2363 2364 public Immunization addNote(Annotation t) { //3 2365 if (t == null) 2366 return this; 2367 if (this.note == null) 2368 this.note = new ArrayList<Annotation>(); 2369 this.note.add(t); 2370 return this; 2371 } 2372 2373 /** 2374 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 2375 */ 2376 public Annotation getNoteFirstRep() { 2377 if (getNote().isEmpty()) { 2378 addNote(); 2379 } 2380 return getNote().get(0); 2381 } 2382 2383 /** 2384 * @return {@link #reason} (Describes why the immunization occurred in coded or textual form, or Indicates another resource (Condition, Observation or DiagnosticReport) whose existence justifies this immunization.) 2385 */ 2386 public List<CodeableReference> getReason() { 2387 if (this.reason == null) 2388 this.reason = new ArrayList<CodeableReference>(); 2389 return this.reason; 2390 } 2391 2392 /** 2393 * @return Returns a reference to <code>this</code> for easy method chaining 2394 */ 2395 public Immunization setReason(List<CodeableReference> theReason) { 2396 this.reason = theReason; 2397 return this; 2398 } 2399 2400 public boolean hasReason() { 2401 if (this.reason == null) 2402 return false; 2403 for (CodeableReference item : this.reason) 2404 if (!item.isEmpty()) 2405 return true; 2406 return false; 2407 } 2408 2409 public CodeableReference addReason() { //3 2410 CodeableReference t = new CodeableReference(); 2411 if (this.reason == null) 2412 this.reason = new ArrayList<CodeableReference>(); 2413 this.reason.add(t); 2414 return t; 2415 } 2416 2417 public Immunization addReason(CodeableReference t) { //3 2418 if (t == null) 2419 return this; 2420 if (this.reason == null) 2421 this.reason = new ArrayList<CodeableReference>(); 2422 this.reason.add(t); 2423 return this; 2424 } 2425 2426 /** 2427 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 2428 */ 2429 public CodeableReference getReasonFirstRep() { 2430 if (getReason().isEmpty()) { 2431 addReason(); 2432 } 2433 return getReason().get(0); 2434 } 2435 2436 /** 2437 * @return {@link #isSubpotent} (Indication if a dose is considered to be subpotent. By default, a dose should be considered to be potent.). This is the underlying object with id, value and extensions. The accessor "getIsSubpotent" gives direct access to the value 2438 */ 2439 public BooleanType getIsSubpotentElement() { 2440 if (this.isSubpotent == null) 2441 if (Configuration.errorOnAutoCreate()) 2442 throw new Error("Attempt to auto-create Immunization.isSubpotent"); 2443 else if (Configuration.doAutoCreate()) 2444 this.isSubpotent = new BooleanType(); // bb 2445 return this.isSubpotent; 2446 } 2447 2448 public boolean hasIsSubpotentElement() { 2449 return this.isSubpotent != null && !this.isSubpotent.isEmpty(); 2450 } 2451 2452 public boolean hasIsSubpotent() { 2453 return this.isSubpotent != null && !this.isSubpotent.isEmpty(); 2454 } 2455 2456 /** 2457 * @param value {@link #isSubpotent} (Indication if a dose is considered to be subpotent. By default, a dose should be considered to be potent.). This is the underlying object with id, value and extensions. The accessor "getIsSubpotent" gives direct access to the value 2458 */ 2459 public Immunization setIsSubpotentElement(BooleanType value) { 2460 this.isSubpotent = value; 2461 return this; 2462 } 2463 2464 /** 2465 * @return Indication if a dose is considered to be subpotent. By default, a dose should be considered to be potent. 2466 */ 2467 public boolean getIsSubpotent() { 2468 return this.isSubpotent == null || this.isSubpotent.isEmpty() ? false : this.isSubpotent.getValue(); 2469 } 2470 2471 /** 2472 * @param value Indication if a dose is considered to be subpotent. By default, a dose should be considered to be potent. 2473 */ 2474 public Immunization setIsSubpotent(boolean value) { 2475 if (this.isSubpotent == null) 2476 this.isSubpotent = new BooleanType(); 2477 this.isSubpotent.setValue(value); 2478 return this; 2479 } 2480 2481 /** 2482 * @return {@link #subpotentReason} (Reason why a dose is considered to be subpotent.) 2483 */ 2484 public List<CodeableConcept> getSubpotentReason() { 2485 if (this.subpotentReason == null) 2486 this.subpotentReason = new ArrayList<CodeableConcept>(); 2487 return this.subpotentReason; 2488 } 2489 2490 /** 2491 * @return Returns a reference to <code>this</code> for easy method chaining 2492 */ 2493 public Immunization setSubpotentReason(List<CodeableConcept> theSubpotentReason) { 2494 this.subpotentReason = theSubpotentReason; 2495 return this; 2496 } 2497 2498 public boolean hasSubpotentReason() { 2499 if (this.subpotentReason == null) 2500 return false; 2501 for (CodeableConcept item : this.subpotentReason) 2502 if (!item.isEmpty()) 2503 return true; 2504 return false; 2505 } 2506 2507 public CodeableConcept addSubpotentReason() { //3 2508 CodeableConcept t = new CodeableConcept(); 2509 if (this.subpotentReason == null) 2510 this.subpotentReason = new ArrayList<CodeableConcept>(); 2511 this.subpotentReason.add(t); 2512 return t; 2513 } 2514 2515 public Immunization addSubpotentReason(CodeableConcept t) { //3 2516 if (t == null) 2517 return this; 2518 if (this.subpotentReason == null) 2519 this.subpotentReason = new ArrayList<CodeableConcept>(); 2520 this.subpotentReason.add(t); 2521 return this; 2522 } 2523 2524 /** 2525 * @return The first repetition of repeating field {@link #subpotentReason}, creating it if it does not already exist {3} 2526 */ 2527 public CodeableConcept getSubpotentReasonFirstRep() { 2528 if (getSubpotentReason().isEmpty()) { 2529 addSubpotentReason(); 2530 } 2531 return getSubpotentReason().get(0); 2532 } 2533 2534 /** 2535 * @return {@link #programEligibility} (Indicates a patient's eligibility for a funding program.) 2536 */ 2537 public List<ImmunizationProgramEligibilityComponent> getProgramEligibility() { 2538 if (this.programEligibility == null) 2539 this.programEligibility = new ArrayList<ImmunizationProgramEligibilityComponent>(); 2540 return this.programEligibility; 2541 } 2542 2543 /** 2544 * @return Returns a reference to <code>this</code> for easy method chaining 2545 */ 2546 public Immunization setProgramEligibility(List<ImmunizationProgramEligibilityComponent> theProgramEligibility) { 2547 this.programEligibility = theProgramEligibility; 2548 return this; 2549 } 2550 2551 public boolean hasProgramEligibility() { 2552 if (this.programEligibility == null) 2553 return false; 2554 for (ImmunizationProgramEligibilityComponent item : this.programEligibility) 2555 if (!item.isEmpty()) 2556 return true; 2557 return false; 2558 } 2559 2560 public ImmunizationProgramEligibilityComponent addProgramEligibility() { //3 2561 ImmunizationProgramEligibilityComponent t = new ImmunizationProgramEligibilityComponent(); 2562 if (this.programEligibility == null) 2563 this.programEligibility = new ArrayList<ImmunizationProgramEligibilityComponent>(); 2564 this.programEligibility.add(t); 2565 return t; 2566 } 2567 2568 public Immunization addProgramEligibility(ImmunizationProgramEligibilityComponent t) { //3 2569 if (t == null) 2570 return this; 2571 if (this.programEligibility == null) 2572 this.programEligibility = new ArrayList<ImmunizationProgramEligibilityComponent>(); 2573 this.programEligibility.add(t); 2574 return this; 2575 } 2576 2577 /** 2578 * @return The first repetition of repeating field {@link #programEligibility}, creating it if it does not already exist {3} 2579 */ 2580 public ImmunizationProgramEligibilityComponent getProgramEligibilityFirstRep() { 2581 if (getProgramEligibility().isEmpty()) { 2582 addProgramEligibility(); 2583 } 2584 return getProgramEligibility().get(0); 2585 } 2586 2587 /** 2588 * @return {@link #fundingSource} (Indicates the source of the vaccine actually administered. This may be different than the patient eligibility (e.g. the patient may be eligible for a publically purchased vaccine but due to inventory issues, vaccine purchased with private funds was actually administered).) 2589 */ 2590 public CodeableConcept getFundingSource() { 2591 if (this.fundingSource == null) 2592 if (Configuration.errorOnAutoCreate()) 2593 throw new Error("Attempt to auto-create Immunization.fundingSource"); 2594 else if (Configuration.doAutoCreate()) 2595 this.fundingSource = new CodeableConcept(); // cc 2596 return this.fundingSource; 2597 } 2598 2599 public boolean hasFundingSource() { 2600 return this.fundingSource != null && !this.fundingSource.isEmpty(); 2601 } 2602 2603 /** 2604 * @param value {@link #fundingSource} (Indicates the source of the vaccine actually administered. This may be different than the patient eligibility (e.g. the patient may be eligible for a publically purchased vaccine but due to inventory issues, vaccine purchased with private funds was actually administered).) 2605 */ 2606 public Immunization setFundingSource(CodeableConcept value) { 2607 this.fundingSource = value; 2608 return this; 2609 } 2610 2611 /** 2612 * @return {@link #reaction} (Categorical data indicating that an adverse event is associated in time to an immunization.) 2613 */ 2614 public List<ImmunizationReactionComponent> getReaction() { 2615 if (this.reaction == null) 2616 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 2617 return this.reaction; 2618 } 2619 2620 /** 2621 * @return Returns a reference to <code>this</code> for easy method chaining 2622 */ 2623 public Immunization setReaction(List<ImmunizationReactionComponent> theReaction) { 2624 this.reaction = theReaction; 2625 return this; 2626 } 2627 2628 public boolean hasReaction() { 2629 if (this.reaction == null) 2630 return false; 2631 for (ImmunizationReactionComponent item : this.reaction) 2632 if (!item.isEmpty()) 2633 return true; 2634 return false; 2635 } 2636 2637 public ImmunizationReactionComponent addReaction() { //3 2638 ImmunizationReactionComponent t = new ImmunizationReactionComponent(); 2639 if (this.reaction == null) 2640 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 2641 this.reaction.add(t); 2642 return t; 2643 } 2644 2645 public Immunization addReaction(ImmunizationReactionComponent t) { //3 2646 if (t == null) 2647 return this; 2648 if (this.reaction == null) 2649 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 2650 this.reaction.add(t); 2651 return this; 2652 } 2653 2654 /** 2655 * @return The first repetition of repeating field {@link #reaction}, creating it if it does not already exist {3} 2656 */ 2657 public ImmunizationReactionComponent getReactionFirstRep() { 2658 if (getReaction().isEmpty()) { 2659 addReaction(); 2660 } 2661 return getReaction().get(0); 2662 } 2663 2664 /** 2665 * @return {@link #protocolApplied} (The protocol (set of recommendations) being followed by the provider who administered the dose.) 2666 */ 2667 public List<ImmunizationProtocolAppliedComponent> getProtocolApplied() { 2668 if (this.protocolApplied == null) 2669 this.protocolApplied = new ArrayList<ImmunizationProtocolAppliedComponent>(); 2670 return this.protocolApplied; 2671 } 2672 2673 /** 2674 * @return Returns a reference to <code>this</code> for easy method chaining 2675 */ 2676 public Immunization setProtocolApplied(List<ImmunizationProtocolAppliedComponent> theProtocolApplied) { 2677 this.protocolApplied = theProtocolApplied; 2678 return this; 2679 } 2680 2681 public boolean hasProtocolApplied() { 2682 if (this.protocolApplied == null) 2683 return false; 2684 for (ImmunizationProtocolAppliedComponent item : this.protocolApplied) 2685 if (!item.isEmpty()) 2686 return true; 2687 return false; 2688 } 2689 2690 public ImmunizationProtocolAppliedComponent addProtocolApplied() { //3 2691 ImmunizationProtocolAppliedComponent t = new ImmunizationProtocolAppliedComponent(); 2692 if (this.protocolApplied == null) 2693 this.protocolApplied = new ArrayList<ImmunizationProtocolAppliedComponent>(); 2694 this.protocolApplied.add(t); 2695 return t; 2696 } 2697 2698 public Immunization addProtocolApplied(ImmunizationProtocolAppliedComponent t) { //3 2699 if (t == null) 2700 return this; 2701 if (this.protocolApplied == null) 2702 this.protocolApplied = new ArrayList<ImmunizationProtocolAppliedComponent>(); 2703 this.protocolApplied.add(t); 2704 return this; 2705 } 2706 2707 /** 2708 * @return The first repetition of repeating field {@link #protocolApplied}, creating it if it does not already exist {3} 2709 */ 2710 public ImmunizationProtocolAppliedComponent getProtocolAppliedFirstRep() { 2711 if (getProtocolApplied().isEmpty()) { 2712 addProtocolApplied(); 2713 } 2714 return getProtocolApplied().get(0); 2715 } 2716 2717 protected void listChildren(List<Property> children) { 2718 super.listChildren(children); 2719 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this immunization record.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2720 children.add(new Property("basedOn", "Reference(CarePlan|MedicationRequest|ServiceRequest|ImmunizationRecommendation)", "A plan, order or recommendation fulfilled in whole or in part by this immunization.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2721 children.add(new Property("status", "code", "Indicates the current status of the immunization event.", 0, 1, status)); 2722 children.add(new Property("statusReason", "CodeableConcept", "Indicates the reason the immunization event was not performed.", 0, 1, statusReason)); 2723 children.add(new Property("vaccineCode", "CodeableConcept", "Vaccine that was administered or was to be administered.", 0, 1, vaccineCode)); 2724 children.add(new Property("administeredProduct", "CodeableReference(Medication)", "An indication of which product was administered to the patient. This is typically a more detailed representation of the concept conveyed by the vaccineCode data element. If a Medication resource is referenced, it may be to a stand-alone resource or a contained resource within the Immunization resource.", 0, 1, administeredProduct)); 2725 children.add(new Property("manufacturer", "CodeableReference(Organization)", "Name of vaccine manufacturer.", 0, 1, manufacturer)); 2726 children.add(new Property("lotNumber", "string", "Lot number of the vaccine product.", 0, 1, lotNumber)); 2727 children.add(new Property("expirationDate", "date", "Date vaccine batch expires.", 0, 1, expirationDate)); 2728 children.add(new Property("patient", "Reference(Patient)", "The patient who either received or did not receive the immunization.", 0, 1, patient)); 2729 children.add(new Property("encounter", "Reference(Encounter)", "The visit or admission or other contact between patient and health care provider the immunization was performed as part of.", 0, 1, encounter)); 2730 children.add(new Property("supportingInformation", "Reference(Any)", "Additional information that is relevant to the immunization (e.g. for a vaccine recipient who is pregnant, the gestational age of the fetus). The reason why a vaccine was given (e.g. occupation, underlying medical condition) should be conveyed in Immunization.reason, not as supporting information. The reason why a vaccine was not given (e.g. contraindication) should be conveyed in Immunization.statusReason, not as supporting information.", 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 2731 children.add(new Property("occurrence[x]", "dateTime|string", "Date vaccine administered or was to be administered.", 0, 1, occurrence)); 2732 children.add(new Property("primarySource", "boolean", "Indicates whether the data contained in the resource was captured by the individual/organization which was responsible for the administration of the vaccine rather than as 'secondary reported' data documented by a third party. A value of 'true' means this data originated with the individual/organization which was responsible for the administration of the vaccine.", 0, 1, primarySource)); 2733 children.add(new Property("informationSource", "CodeableReference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", "Typically the source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.", 0, 1, informationSource)); 2734 children.add(new Property("location", "Reference(Location)", "The service delivery location where the vaccine administration occurred.", 0, 1, location)); 2735 children.add(new Property("site", "CodeableConcept", "Body site where vaccine was administered.", 0, 1, site)); 2736 children.add(new Property("route", "CodeableConcept", "The path by which the vaccine product is taken into the body.", 0, 1, route)); 2737 children.add(new Property("doseQuantity", "Quantity", "The quantity of vaccine product that was administered.", 0, 1, doseQuantity)); 2738 children.add(new Property("performer", "", "Indicates who performed the immunization event.", 0, java.lang.Integer.MAX_VALUE, performer)); 2739 children.add(new Property("note", "Annotation", "Extra information about the immunization that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note)); 2740 children.add(new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport)", "Describes why the immunization occurred in coded or textual form, or Indicates another resource (Condition, Observation or DiagnosticReport) whose existence justifies this immunization.", 0, java.lang.Integer.MAX_VALUE, reason)); 2741 children.add(new Property("isSubpotent", "boolean", "Indication if a dose is considered to be subpotent. By default, a dose should be considered to be potent.", 0, 1, isSubpotent)); 2742 children.add(new Property("subpotentReason", "CodeableConcept", "Reason why a dose is considered to be subpotent.", 0, java.lang.Integer.MAX_VALUE, subpotentReason)); 2743 children.add(new Property("programEligibility", "", "Indicates a patient's eligibility for a funding program.", 0, java.lang.Integer.MAX_VALUE, programEligibility)); 2744 children.add(new Property("fundingSource", "CodeableConcept", "Indicates the source of the vaccine actually administered. This may be different than the patient eligibility (e.g. the patient may be eligible for a publically purchased vaccine but due to inventory issues, vaccine purchased with private funds was actually administered).", 0, 1, fundingSource)); 2745 children.add(new Property("reaction", "", "Categorical data indicating that an adverse event is associated in time to an immunization.", 0, java.lang.Integer.MAX_VALUE, reaction)); 2746 children.add(new Property("protocolApplied", "", "The protocol (set of recommendations) being followed by the provider who administered the dose.", 0, java.lang.Integer.MAX_VALUE, protocolApplied)); 2747 } 2748 2749 @Override 2750 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2751 switch (_hash) { 2752 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this immunization record.", 0, java.lang.Integer.MAX_VALUE, identifier); 2753 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|MedicationRequest|ServiceRequest|ImmunizationRecommendation)", "A plan, order or recommendation fulfilled in whole or in part by this immunization.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2754 case -892481550: /*status*/ return new Property("status", "code", "Indicates the current status of the immunization event.", 0, 1, status); 2755 case 2051346646: /*statusReason*/ return new Property("statusReason", "CodeableConcept", "Indicates the reason the immunization event was not performed.", 0, 1, statusReason); 2756 case 664556354: /*vaccineCode*/ return new Property("vaccineCode", "CodeableConcept", "Vaccine that was administered or was to be administered.", 0, 1, vaccineCode); 2757 case 1610069896: /*administeredProduct*/ return new Property("administeredProduct", "CodeableReference(Medication)", "An indication of which product was administered to the patient. This is typically a more detailed representation of the concept conveyed by the vaccineCode data element. If a Medication resource is referenced, it may be to a stand-alone resource or a contained resource within the Immunization resource.", 0, 1, administeredProduct); 2758 case -1969347631: /*manufacturer*/ return new Property("manufacturer", "CodeableReference(Organization)", "Name of vaccine manufacturer.", 0, 1, manufacturer); 2759 case 462547450: /*lotNumber*/ return new Property("lotNumber", "string", "Lot number of the vaccine product.", 0, 1, lotNumber); 2760 case -668811523: /*expirationDate*/ return new Property("expirationDate", "date", "Date vaccine batch expires.", 0, 1, expirationDate); 2761 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient who either received or did not receive the immunization.", 0, 1, patient); 2762 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The visit or admission or other contact between patient and health care provider the immunization was performed as part of.", 0, 1, encounter); 2763 case -1248768647: /*supportingInformation*/ return new Property("supportingInformation", "Reference(Any)", "Additional information that is relevant to the immunization (e.g. for a vaccine recipient who is pregnant, the gestational age of the fetus). The reason why a vaccine was given (e.g. occupation, underlying medical condition) should be conveyed in Immunization.reason, not as supporting information. The reason why a vaccine was not given (e.g. contraindication) should be conveyed in Immunization.statusReason, not as supporting information.", 0, java.lang.Integer.MAX_VALUE, supportingInformation); 2764 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|string", "Date vaccine administered or was to be administered.", 0, 1, occurrence); 2765 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|string", "Date vaccine administered or was to be administered.", 0, 1, occurrence); 2766 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime", "Date vaccine administered or was to be administered.", 0, 1, occurrence); 2767 case 1496896834: /*occurrenceString*/ return new Property("occurrence[x]", "string", "Date vaccine administered or was to be administered.", 0, 1, occurrence); 2768 case -528721731: /*primarySource*/ return new Property("primarySource", "boolean", "Indicates whether the data contained in the resource was captured by the individual/organization which was responsible for the administration of the vaccine rather than as 'secondary reported' data documented by a third party. A value of 'true' means this data originated with the individual/organization which was responsible for the administration of the vaccine.", 0, 1, primarySource); 2769 case -2123220889: /*informationSource*/ return new Property("informationSource", "CodeableReference(Patient|Practitioner|PractitionerRole|RelatedPerson|Organization)", "Typically the source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.", 0, 1, informationSource); 2770 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The service delivery location where the vaccine administration occurred.", 0, 1, location); 2771 case 3530567: /*site*/ return new Property("site", "CodeableConcept", "Body site where vaccine was administered.", 0, 1, site); 2772 case 108704329: /*route*/ return new Property("route", "CodeableConcept", "The path by which the vaccine product is taken into the body.", 0, 1, route); 2773 case -2083618872: /*doseQuantity*/ return new Property("doseQuantity", "Quantity", "The quantity of vaccine product that was administered.", 0, 1, doseQuantity); 2774 case 481140686: /*performer*/ return new Property("performer", "", "Indicates who performed the immunization event.", 0, java.lang.Integer.MAX_VALUE, performer); 2775 case 3387378: /*note*/ return new Property("note", "Annotation", "Extra information about the immunization that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note); 2776 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport)", "Describes why the immunization occurred in coded or textual form, or Indicates another resource (Condition, Observation or DiagnosticReport) whose existence justifies this immunization.", 0, java.lang.Integer.MAX_VALUE, reason); 2777 case 1618512556: /*isSubpotent*/ return new Property("isSubpotent", "boolean", "Indication if a dose is considered to be subpotent. By default, a dose should be considered to be potent.", 0, 1, isSubpotent); 2778 case 805168794: /*subpotentReason*/ return new Property("subpotentReason", "CodeableConcept", "Reason why a dose is considered to be subpotent.", 0, java.lang.Integer.MAX_VALUE, subpotentReason); 2779 case 1207530089: /*programEligibility*/ return new Property("programEligibility", "", "Indicates a patient's eligibility for a funding program.", 0, java.lang.Integer.MAX_VALUE, programEligibility); 2780 case 1120150904: /*fundingSource*/ return new Property("fundingSource", "CodeableConcept", "Indicates the source of the vaccine actually administered. This may be different than the patient eligibility (e.g. the patient may be eligible for a publically purchased vaccine but due to inventory issues, vaccine purchased with private funds was actually administered).", 0, 1, fundingSource); 2781 case -867509719: /*reaction*/ return new Property("reaction", "", "Categorical data indicating that an adverse event is associated in time to an immunization.", 0, java.lang.Integer.MAX_VALUE, reaction); 2782 case 607985349: /*protocolApplied*/ return new Property("protocolApplied", "", "The protocol (set of recommendations) being followed by the provider who administered the dose.", 0, java.lang.Integer.MAX_VALUE, protocolApplied); 2783 default: return super.getNamedProperty(_hash, _name, _checkValid); 2784 } 2785 2786 } 2787 2788 @Override 2789 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2790 switch (hash) { 2791 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2792 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2793 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ImmunizationStatusCodes> 2794 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : new Base[] {this.statusReason}; // CodeableConcept 2795 case 664556354: /*vaccineCode*/ return this.vaccineCode == null ? new Base[0] : new Base[] {this.vaccineCode}; // CodeableConcept 2796 case 1610069896: /*administeredProduct*/ return this.administeredProduct == null ? new Base[0] : new Base[] {this.administeredProduct}; // CodeableReference 2797 case -1969347631: /*manufacturer*/ return this.manufacturer == null ? new Base[0] : new Base[] {this.manufacturer}; // CodeableReference 2798 case 462547450: /*lotNumber*/ return this.lotNumber == null ? new Base[0] : new Base[] {this.lotNumber}; // StringType 2799 case -668811523: /*expirationDate*/ return this.expirationDate == null ? new Base[0] : new Base[] {this.expirationDate}; // DateType 2800 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 2801 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 2802 case -1248768647: /*supportingInformation*/ return this.supportingInformation == null ? new Base[0] : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 2803 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // DataType 2804 case -528721731: /*primarySource*/ return this.primarySource == null ? new Base[0] : new Base[] {this.primarySource}; // BooleanType 2805 case -2123220889: /*informationSource*/ return this.informationSource == null ? new Base[0] : new Base[] {this.informationSource}; // CodeableReference 2806 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 2807 case 3530567: /*site*/ return this.site == null ? new Base[0] : new Base[] {this.site}; // CodeableConcept 2808 case 108704329: /*route*/ return this.route == null ? new Base[0] : new Base[] {this.route}; // CodeableConcept 2809 case -2083618872: /*doseQuantity*/ return this.doseQuantity == null ? new Base[0] : new Base[] {this.doseQuantity}; // Quantity 2810 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // ImmunizationPerformerComponent 2811 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2812 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 2813 case 1618512556: /*isSubpotent*/ return this.isSubpotent == null ? new Base[0] : new Base[] {this.isSubpotent}; // BooleanType 2814 case 805168794: /*subpotentReason*/ return this.subpotentReason == null ? new Base[0] : this.subpotentReason.toArray(new Base[this.subpotentReason.size()]); // CodeableConcept 2815 case 1207530089: /*programEligibility*/ return this.programEligibility == null ? new Base[0] : this.programEligibility.toArray(new Base[this.programEligibility.size()]); // ImmunizationProgramEligibilityComponent 2816 case 1120150904: /*fundingSource*/ return this.fundingSource == null ? new Base[0] : new Base[] {this.fundingSource}; // CodeableConcept 2817 case -867509719: /*reaction*/ return this.reaction == null ? new Base[0] : this.reaction.toArray(new Base[this.reaction.size()]); // ImmunizationReactionComponent 2818 case 607985349: /*protocolApplied*/ return this.protocolApplied == null ? new Base[0] : this.protocolApplied.toArray(new Base[this.protocolApplied.size()]); // ImmunizationProtocolAppliedComponent 2819 default: return super.getProperty(hash, name, checkValid); 2820 } 2821 2822 } 2823 2824 @Override 2825 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2826 switch (hash) { 2827 case -1618432855: // identifier 2828 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2829 return value; 2830 case -332612366: // basedOn 2831 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 2832 return value; 2833 case -892481550: // status 2834 value = new ImmunizationStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2835 this.status = (Enumeration) value; // Enumeration<ImmunizationStatusCodes> 2836 return value; 2837 case 2051346646: // statusReason 2838 this.statusReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2839 return value; 2840 case 664556354: // vaccineCode 2841 this.vaccineCode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2842 return value; 2843 case 1610069896: // administeredProduct 2844 this.administeredProduct = TypeConvertor.castToCodeableReference(value); // CodeableReference 2845 return value; 2846 case -1969347631: // manufacturer 2847 this.manufacturer = TypeConvertor.castToCodeableReference(value); // CodeableReference 2848 return value; 2849 case 462547450: // lotNumber 2850 this.lotNumber = TypeConvertor.castToString(value); // StringType 2851 return value; 2852 case -668811523: // expirationDate 2853 this.expirationDate = TypeConvertor.castToDate(value); // DateType 2854 return value; 2855 case -791418107: // patient 2856 this.patient = TypeConvertor.castToReference(value); // Reference 2857 return value; 2858 case 1524132147: // encounter 2859 this.encounter = TypeConvertor.castToReference(value); // Reference 2860 return value; 2861 case -1248768647: // supportingInformation 2862 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); // Reference 2863 return value; 2864 case 1687874001: // occurrence 2865 this.occurrence = TypeConvertor.castToType(value); // DataType 2866 return value; 2867 case -528721731: // primarySource 2868 this.primarySource = TypeConvertor.castToBoolean(value); // BooleanType 2869 return value; 2870 case -2123220889: // informationSource 2871 this.informationSource = TypeConvertor.castToCodeableReference(value); // CodeableReference 2872 return value; 2873 case 1901043637: // location 2874 this.location = TypeConvertor.castToReference(value); // Reference 2875 return value; 2876 case 3530567: // site 2877 this.site = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2878 return value; 2879 case 108704329: // route 2880 this.route = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2881 return value; 2882 case -2083618872: // doseQuantity 2883 this.doseQuantity = TypeConvertor.castToQuantity(value); // Quantity 2884 return value; 2885 case 481140686: // performer 2886 this.getPerformer().add((ImmunizationPerformerComponent) value); // ImmunizationPerformerComponent 2887 return value; 2888 case 3387378: // note 2889 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2890 return value; 2891 case -934964668: // reason 2892 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2893 return value; 2894 case 1618512556: // isSubpotent 2895 this.isSubpotent = TypeConvertor.castToBoolean(value); // BooleanType 2896 return value; 2897 case 805168794: // subpotentReason 2898 this.getSubpotentReason().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2899 return value; 2900 case 1207530089: // programEligibility 2901 this.getProgramEligibility().add((ImmunizationProgramEligibilityComponent) value); // ImmunizationProgramEligibilityComponent 2902 return value; 2903 case 1120150904: // fundingSource 2904 this.fundingSource = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2905 return value; 2906 case -867509719: // reaction 2907 this.getReaction().add((ImmunizationReactionComponent) value); // ImmunizationReactionComponent 2908 return value; 2909 case 607985349: // protocolApplied 2910 this.getProtocolApplied().add((ImmunizationProtocolAppliedComponent) value); // ImmunizationProtocolAppliedComponent 2911 return value; 2912 default: return super.setProperty(hash, name, value); 2913 } 2914 2915 } 2916 2917 @Override 2918 public Base setProperty(String name, Base value) throws FHIRException { 2919 if (name.equals("identifier")) { 2920 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2921 } else if (name.equals("basedOn")) { 2922 this.getBasedOn().add(TypeConvertor.castToReference(value)); 2923 } else if (name.equals("status")) { 2924 value = new ImmunizationStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2925 this.status = (Enumeration) value; // Enumeration<ImmunizationStatusCodes> 2926 } else if (name.equals("statusReason")) { 2927 this.statusReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2928 } else if (name.equals("vaccineCode")) { 2929 this.vaccineCode = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2930 } else if (name.equals("administeredProduct")) { 2931 this.administeredProduct = TypeConvertor.castToCodeableReference(value); // CodeableReference 2932 } else if (name.equals("manufacturer")) { 2933 this.manufacturer = TypeConvertor.castToCodeableReference(value); // CodeableReference 2934 } else if (name.equals("lotNumber")) { 2935 this.lotNumber = TypeConvertor.castToString(value); // StringType 2936 } else if (name.equals("expirationDate")) { 2937 this.expirationDate = TypeConvertor.castToDate(value); // DateType 2938 } else if (name.equals("patient")) { 2939 this.patient = TypeConvertor.castToReference(value); // Reference 2940 } else if (name.equals("encounter")) { 2941 this.encounter = TypeConvertor.castToReference(value); // Reference 2942 } else if (name.equals("supportingInformation")) { 2943 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); 2944 } else if (name.equals("occurrence[x]")) { 2945 this.occurrence = TypeConvertor.castToType(value); // DataType 2946 } else if (name.equals("primarySource")) { 2947 this.primarySource = TypeConvertor.castToBoolean(value); // BooleanType 2948 } else if (name.equals("informationSource")) { 2949 this.informationSource = TypeConvertor.castToCodeableReference(value); // CodeableReference 2950 } else if (name.equals("location")) { 2951 this.location = TypeConvertor.castToReference(value); // Reference 2952 } else if (name.equals("site")) { 2953 this.site = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2954 } else if (name.equals("route")) { 2955 this.route = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2956 } else if (name.equals("doseQuantity")) { 2957 this.doseQuantity = TypeConvertor.castToQuantity(value); // Quantity 2958 } else if (name.equals("performer")) { 2959 this.getPerformer().add((ImmunizationPerformerComponent) value); 2960 } else if (name.equals("note")) { 2961 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2962 } else if (name.equals("reason")) { 2963 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 2964 } else if (name.equals("isSubpotent")) { 2965 this.isSubpotent = TypeConvertor.castToBoolean(value); // BooleanType 2966 } else if (name.equals("subpotentReason")) { 2967 this.getSubpotentReason().add(TypeConvertor.castToCodeableConcept(value)); 2968 } else if (name.equals("programEligibility")) { 2969 this.getProgramEligibility().add((ImmunizationProgramEligibilityComponent) value); 2970 } else if (name.equals("fundingSource")) { 2971 this.fundingSource = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2972 } else if (name.equals("reaction")) { 2973 this.getReaction().add((ImmunizationReactionComponent) value); 2974 } else if (name.equals("protocolApplied")) { 2975 this.getProtocolApplied().add((ImmunizationProtocolAppliedComponent) value); 2976 } else 2977 return super.setProperty(name, value); 2978 return value; 2979 } 2980 2981 @Override 2982 public void removeChild(String name, Base value) throws FHIRException { 2983 if (name.equals("identifier")) { 2984 this.getIdentifier().remove(value); 2985 } else if (name.equals("basedOn")) { 2986 this.getBasedOn().remove(value); 2987 } else if (name.equals("status")) { 2988 value = new ImmunizationStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2989 this.status = (Enumeration) value; // Enumeration<ImmunizationStatusCodes> 2990 } else if (name.equals("statusReason")) { 2991 this.statusReason = null; 2992 } else if (name.equals("vaccineCode")) { 2993 this.vaccineCode = null; 2994 } else if (name.equals("administeredProduct")) { 2995 this.administeredProduct = null; 2996 } else if (name.equals("manufacturer")) { 2997 this.manufacturer = null; 2998 } else if (name.equals("lotNumber")) { 2999 this.lotNumber = null; 3000 } else if (name.equals("expirationDate")) { 3001 this.expirationDate = null; 3002 } else if (name.equals("patient")) { 3003 this.patient = null; 3004 } else if (name.equals("encounter")) { 3005 this.encounter = null; 3006 } else if (name.equals("supportingInformation")) { 3007 this.getSupportingInformation().remove(value); 3008 } else if (name.equals("occurrence[x]")) { 3009 this.occurrence = null; 3010 } else if (name.equals("primarySource")) { 3011 this.primarySource = null; 3012 } else if (name.equals("informationSource")) { 3013 this.informationSource = null; 3014 } else if (name.equals("location")) { 3015 this.location = null; 3016 } else if (name.equals("site")) { 3017 this.site = null; 3018 } else if (name.equals("route")) { 3019 this.route = null; 3020 } else if (name.equals("doseQuantity")) { 3021 this.doseQuantity = null; 3022 } else if (name.equals("performer")) { 3023 this.getPerformer().remove((ImmunizationPerformerComponent) value); 3024 } else if (name.equals("note")) { 3025 this.getNote().remove(value); 3026 } else if (name.equals("reason")) { 3027 this.getReason().remove(value); 3028 } else if (name.equals("isSubpotent")) { 3029 this.isSubpotent = null; 3030 } else if (name.equals("subpotentReason")) { 3031 this.getSubpotentReason().remove(value); 3032 } else if (name.equals("programEligibility")) { 3033 this.getProgramEligibility().remove((ImmunizationProgramEligibilityComponent) value); 3034 } else if (name.equals("fundingSource")) { 3035 this.fundingSource = null; 3036 } else if (name.equals("reaction")) { 3037 this.getReaction().remove((ImmunizationReactionComponent) value); 3038 } else if (name.equals("protocolApplied")) { 3039 this.getProtocolApplied().remove((ImmunizationProtocolAppliedComponent) value); 3040 } else 3041 super.removeChild(name, value); 3042 3043 } 3044 3045 @Override 3046 public Base makeProperty(int hash, String name) throws FHIRException { 3047 switch (hash) { 3048 case -1618432855: return addIdentifier(); 3049 case -332612366: return addBasedOn(); 3050 case -892481550: return getStatusElement(); 3051 case 2051346646: return getStatusReason(); 3052 case 664556354: return getVaccineCode(); 3053 case 1610069896: return getAdministeredProduct(); 3054 case -1969347631: return getManufacturer(); 3055 case 462547450: return getLotNumberElement(); 3056 case -668811523: return getExpirationDateElement(); 3057 case -791418107: return getPatient(); 3058 case 1524132147: return getEncounter(); 3059 case -1248768647: return addSupportingInformation(); 3060 case -2022646513: return getOccurrence(); 3061 case 1687874001: return getOccurrence(); 3062 case -528721731: return getPrimarySourceElement(); 3063 case -2123220889: return getInformationSource(); 3064 case 1901043637: return getLocation(); 3065 case 3530567: return getSite(); 3066 case 108704329: return getRoute(); 3067 case -2083618872: return getDoseQuantity(); 3068 case 481140686: return addPerformer(); 3069 case 3387378: return addNote(); 3070 case -934964668: return addReason(); 3071 case 1618512556: return getIsSubpotentElement(); 3072 case 805168794: return addSubpotentReason(); 3073 case 1207530089: return addProgramEligibility(); 3074 case 1120150904: return getFundingSource(); 3075 case -867509719: return addReaction(); 3076 case 607985349: return addProtocolApplied(); 3077 default: return super.makeProperty(hash, name); 3078 } 3079 3080 } 3081 3082 @Override 3083 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3084 switch (hash) { 3085 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3086 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 3087 case -892481550: /*status*/ return new String[] {"code"}; 3088 case 2051346646: /*statusReason*/ return new String[] {"CodeableConcept"}; 3089 case 664556354: /*vaccineCode*/ return new String[] {"CodeableConcept"}; 3090 case 1610069896: /*administeredProduct*/ return new String[] {"CodeableReference"}; 3091 case -1969347631: /*manufacturer*/ return new String[] {"CodeableReference"}; 3092 case 462547450: /*lotNumber*/ return new String[] {"string"}; 3093 case -668811523: /*expirationDate*/ return new String[] {"date"}; 3094 case -791418107: /*patient*/ return new String[] {"Reference"}; 3095 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 3096 case -1248768647: /*supportingInformation*/ return new String[] {"Reference"}; 3097 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "string"}; 3098 case -528721731: /*primarySource*/ return new String[] {"boolean"}; 3099 case -2123220889: /*informationSource*/ return new String[] {"CodeableReference"}; 3100 case 1901043637: /*location*/ return new String[] {"Reference"}; 3101 case 3530567: /*site*/ return new String[] {"CodeableConcept"}; 3102 case 108704329: /*route*/ return new String[] {"CodeableConcept"}; 3103 case -2083618872: /*doseQuantity*/ return new String[] {"Quantity"}; 3104 case 481140686: /*performer*/ return new String[] {}; 3105 case 3387378: /*note*/ return new String[] {"Annotation"}; 3106 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 3107 case 1618512556: /*isSubpotent*/ return new String[] {"boolean"}; 3108 case 805168794: /*subpotentReason*/ return new String[] {"CodeableConcept"}; 3109 case 1207530089: /*programEligibility*/ return new String[] {}; 3110 case 1120150904: /*fundingSource*/ return new String[] {"CodeableConcept"}; 3111 case -867509719: /*reaction*/ return new String[] {}; 3112 case 607985349: /*protocolApplied*/ return new String[] {}; 3113 default: return super.getTypesForProperty(hash, name); 3114 } 3115 3116 } 3117 3118 @Override 3119 public Base addChild(String name) throws FHIRException { 3120 if (name.equals("identifier")) { 3121 return addIdentifier(); 3122 } 3123 else if (name.equals("basedOn")) { 3124 return addBasedOn(); 3125 } 3126 else if (name.equals("status")) { 3127 throw new FHIRException("Cannot call addChild on a singleton property Immunization.status"); 3128 } 3129 else if (name.equals("statusReason")) { 3130 this.statusReason = new CodeableConcept(); 3131 return this.statusReason; 3132 } 3133 else if (name.equals("vaccineCode")) { 3134 this.vaccineCode = new CodeableConcept(); 3135 return this.vaccineCode; 3136 } 3137 else if (name.equals("administeredProduct")) { 3138 this.administeredProduct = new CodeableReference(); 3139 return this.administeredProduct; 3140 } 3141 else if (name.equals("manufacturer")) { 3142 this.manufacturer = new CodeableReference(); 3143 return this.manufacturer; 3144 } 3145 else if (name.equals("lotNumber")) { 3146 throw new FHIRException("Cannot call addChild on a singleton property Immunization.lotNumber"); 3147 } 3148 else if (name.equals("expirationDate")) { 3149 throw new FHIRException("Cannot call addChild on a singleton property Immunization.expirationDate"); 3150 } 3151 else if (name.equals("patient")) { 3152 this.patient = new Reference(); 3153 return this.patient; 3154 } 3155 else if (name.equals("encounter")) { 3156 this.encounter = new Reference(); 3157 return this.encounter; 3158 } 3159 else if (name.equals("supportingInformation")) { 3160 return addSupportingInformation(); 3161 } 3162 else if (name.equals("occurrenceDateTime")) { 3163 this.occurrence = new DateTimeType(); 3164 return this.occurrence; 3165 } 3166 else if (name.equals("occurrenceString")) { 3167 this.occurrence = new StringType(); 3168 return this.occurrence; 3169 } 3170 else if (name.equals("primarySource")) { 3171 throw new FHIRException("Cannot call addChild on a singleton property Immunization.primarySource"); 3172 } 3173 else if (name.equals("informationSource")) { 3174 this.informationSource = new CodeableReference(); 3175 return this.informationSource; 3176 } 3177 else if (name.equals("location")) { 3178 this.location = new Reference(); 3179 return this.location; 3180 } 3181 else if (name.equals("site")) { 3182 this.site = new CodeableConcept(); 3183 return this.site; 3184 } 3185 else if (name.equals("route")) { 3186 this.route = new CodeableConcept(); 3187 return this.route; 3188 } 3189 else if (name.equals("doseQuantity")) { 3190 this.doseQuantity = new Quantity(); 3191 return this.doseQuantity; 3192 } 3193 else if (name.equals("performer")) { 3194 return addPerformer(); 3195 } 3196 else if (name.equals("note")) { 3197 return addNote(); 3198 } 3199 else if (name.equals("reason")) { 3200 return addReason(); 3201 } 3202 else if (name.equals("isSubpotent")) { 3203 throw new FHIRException("Cannot call addChild on a singleton property Immunization.isSubpotent"); 3204 } 3205 else if (name.equals("subpotentReason")) { 3206 return addSubpotentReason(); 3207 } 3208 else if (name.equals("programEligibility")) { 3209 return addProgramEligibility(); 3210 } 3211 else if (name.equals("fundingSource")) { 3212 this.fundingSource = new CodeableConcept(); 3213 return this.fundingSource; 3214 } 3215 else if (name.equals("reaction")) { 3216 return addReaction(); 3217 } 3218 else if (name.equals("protocolApplied")) { 3219 return addProtocolApplied(); 3220 } 3221 else 3222 return super.addChild(name); 3223 } 3224 3225 public String fhirType() { 3226 return "Immunization"; 3227 3228 } 3229 3230 public Immunization copy() { 3231 Immunization dst = new Immunization(); 3232 copyValues(dst); 3233 return dst; 3234 } 3235 3236 public void copyValues(Immunization dst) { 3237 super.copyValues(dst); 3238 if (identifier != null) { 3239 dst.identifier = new ArrayList<Identifier>(); 3240 for (Identifier i : identifier) 3241 dst.identifier.add(i.copy()); 3242 }; 3243 if (basedOn != null) { 3244 dst.basedOn = new ArrayList<Reference>(); 3245 for (Reference i : basedOn) 3246 dst.basedOn.add(i.copy()); 3247 }; 3248 dst.status = status == null ? null : status.copy(); 3249 dst.statusReason = statusReason == null ? null : statusReason.copy(); 3250 dst.vaccineCode = vaccineCode == null ? null : vaccineCode.copy(); 3251 dst.administeredProduct = administeredProduct == null ? null : administeredProduct.copy(); 3252 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 3253 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 3254 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 3255 dst.patient = patient == null ? null : patient.copy(); 3256 dst.encounter = encounter == null ? null : encounter.copy(); 3257 if (supportingInformation != null) { 3258 dst.supportingInformation = new ArrayList<Reference>(); 3259 for (Reference i : supportingInformation) 3260 dst.supportingInformation.add(i.copy()); 3261 }; 3262 dst.occurrence = occurrence == null ? null : occurrence.copy(); 3263 dst.primarySource = primarySource == null ? null : primarySource.copy(); 3264 dst.informationSource = informationSource == null ? null : informationSource.copy(); 3265 dst.location = location == null ? null : location.copy(); 3266 dst.site = site == null ? null : site.copy(); 3267 dst.route = route == null ? null : route.copy(); 3268 dst.doseQuantity = doseQuantity == null ? null : doseQuantity.copy(); 3269 if (performer != null) { 3270 dst.performer = new ArrayList<ImmunizationPerformerComponent>(); 3271 for (ImmunizationPerformerComponent i : performer) 3272 dst.performer.add(i.copy()); 3273 }; 3274 if (note != null) { 3275 dst.note = new ArrayList<Annotation>(); 3276 for (Annotation i : note) 3277 dst.note.add(i.copy()); 3278 }; 3279 if (reason != null) { 3280 dst.reason = new ArrayList<CodeableReference>(); 3281 for (CodeableReference i : reason) 3282 dst.reason.add(i.copy()); 3283 }; 3284 dst.isSubpotent = isSubpotent == null ? null : isSubpotent.copy(); 3285 if (subpotentReason != null) { 3286 dst.subpotentReason = new ArrayList<CodeableConcept>(); 3287 for (CodeableConcept i : subpotentReason) 3288 dst.subpotentReason.add(i.copy()); 3289 }; 3290 if (programEligibility != null) { 3291 dst.programEligibility = new ArrayList<ImmunizationProgramEligibilityComponent>(); 3292 for (ImmunizationProgramEligibilityComponent i : programEligibility) 3293 dst.programEligibility.add(i.copy()); 3294 }; 3295 dst.fundingSource = fundingSource == null ? null : fundingSource.copy(); 3296 if (reaction != null) { 3297 dst.reaction = new ArrayList<ImmunizationReactionComponent>(); 3298 for (ImmunizationReactionComponent i : reaction) 3299 dst.reaction.add(i.copy()); 3300 }; 3301 if (protocolApplied != null) { 3302 dst.protocolApplied = new ArrayList<ImmunizationProtocolAppliedComponent>(); 3303 for (ImmunizationProtocolAppliedComponent i : protocolApplied) 3304 dst.protocolApplied.add(i.copy()); 3305 }; 3306 } 3307 3308 protected Immunization typedCopy() { 3309 return copy(); 3310 } 3311 3312 @Override 3313 public boolean equalsDeep(Base other_) { 3314 if (!super.equalsDeep(other_)) 3315 return false; 3316 if (!(other_ instanceof Immunization)) 3317 return false; 3318 Immunization o = (Immunization) other_; 3319 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(status, o.status, true) 3320 && compareDeep(statusReason, o.statusReason, true) && compareDeep(vaccineCode, o.vaccineCode, true) 3321 && compareDeep(administeredProduct, o.administeredProduct, true) && compareDeep(manufacturer, o.manufacturer, true) 3322 && compareDeep(lotNumber, o.lotNumber, true) && compareDeep(expirationDate, o.expirationDate, true) 3323 && compareDeep(patient, o.patient, true) && compareDeep(encounter, o.encounter, true) && compareDeep(supportingInformation, o.supportingInformation, true) 3324 && compareDeep(occurrence, o.occurrence, true) && compareDeep(primarySource, o.primarySource, true) 3325 && compareDeep(informationSource, o.informationSource, true) && compareDeep(location, o.location, true) 3326 && compareDeep(site, o.site, true) && compareDeep(route, o.route, true) && compareDeep(doseQuantity, o.doseQuantity, true) 3327 && compareDeep(performer, o.performer, true) && compareDeep(note, o.note, true) && compareDeep(reason, o.reason, true) 3328 && compareDeep(isSubpotent, o.isSubpotent, true) && compareDeep(subpotentReason, o.subpotentReason, true) 3329 && compareDeep(programEligibility, o.programEligibility, true) && compareDeep(fundingSource, o.fundingSource, true) 3330 && compareDeep(reaction, o.reaction, true) && compareDeep(protocolApplied, o.protocolApplied, true) 3331 ; 3332 } 3333 3334 @Override 3335 public boolean equalsShallow(Base other_) { 3336 if (!super.equalsShallow(other_)) 3337 return false; 3338 if (!(other_ instanceof Immunization)) 3339 return false; 3340 Immunization o = (Immunization) other_; 3341 return compareValues(status, o.status, true) && compareValues(lotNumber, o.lotNumber, true) && compareValues(expirationDate, o.expirationDate, true) 3342 && compareValues(primarySource, o.primarySource, true) && compareValues(isSubpotent, o.isSubpotent, true) 3343 ; 3344 } 3345 3346 public boolean isEmpty() { 3347 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, status 3348 , statusReason, vaccineCode, administeredProduct, manufacturer, lotNumber, expirationDate 3349 , patient, encounter, supportingInformation, occurrence, primarySource, informationSource 3350 , location, site, route, doseQuantity, performer, note, reason, isSubpotent 3351 , subpotentReason, programEligibility, fundingSource, reaction, protocolApplied); 3352 } 3353 3354 @Override 3355 public ResourceType getResourceType() { 3356 return ResourceType.Immunization; 3357 } 3358 3359 /** 3360 * Search parameter: <b>location</b> 3361 * <p> 3362 * Description: <b>The service delivery location or facility in which the vaccine was / was to be administered</b><br> 3363 * Type: <b>reference</b><br> 3364 * Path: <b>Immunization.location</b><br> 3365 * </p> 3366 */ 3367 @SearchParamDefinition(name="location", path="Immunization.location", description="The service delivery location or facility in which the vaccine was / was to be administered", type="reference", target={Location.class } ) 3368 public static final String SP_LOCATION = "location"; 3369 /** 3370 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3371 * <p> 3372 * Description: <b>The service delivery location or facility in which the vaccine was / was to be administered</b><br> 3373 * Type: <b>reference</b><br> 3374 * Path: <b>Immunization.location</b><br> 3375 * </p> 3376 */ 3377 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 3378 3379/** 3380 * Constant for fluent queries to be used to add include statements. Specifies 3381 * the path value of "<b>Immunization:location</b>". 3382 */ 3383 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("Immunization:location").toLocked(); 3384 3385 /** 3386 * Search parameter: <b>lot-number</b> 3387 * <p> 3388 * Description: <b>Vaccine Lot Number</b><br> 3389 * Type: <b>string</b><br> 3390 * Path: <b>Immunization.lotNumber</b><br> 3391 * </p> 3392 */ 3393 @SearchParamDefinition(name="lot-number", path="Immunization.lotNumber", description="Vaccine Lot Number", type="string" ) 3394 public static final String SP_LOT_NUMBER = "lot-number"; 3395 /** 3396 * <b>Fluent Client</b> search parameter constant for <b>lot-number</b> 3397 * <p> 3398 * Description: <b>Vaccine Lot Number</b><br> 3399 * Type: <b>string</b><br> 3400 * Path: <b>Immunization.lotNumber</b><br> 3401 * </p> 3402 */ 3403 public static final ca.uhn.fhir.rest.gclient.StringClientParam LOT_NUMBER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_LOT_NUMBER); 3404 3405 /** 3406 * Search parameter: <b>manufacturer</b> 3407 * <p> 3408 * Description: <b>Vaccine Manufacturer</b><br> 3409 * Type: <b>reference</b><br> 3410 * Path: <b>Immunization.manufacturer.reference</b><br> 3411 * </p> 3412 */ 3413 @SearchParamDefinition(name="manufacturer", path="Immunization.manufacturer.reference", description="Vaccine Manufacturer", type="reference", target={Organization.class } ) 3414 public static final String SP_MANUFACTURER = "manufacturer"; 3415 /** 3416 * <b>Fluent Client</b> search parameter constant for <b>manufacturer</b> 3417 * <p> 3418 * Description: <b>Vaccine Manufacturer</b><br> 3419 * Type: <b>reference</b><br> 3420 * Path: <b>Immunization.manufacturer.reference</b><br> 3421 * </p> 3422 */ 3423 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANUFACTURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MANUFACTURER); 3424 3425/** 3426 * Constant for fluent queries to be used to add include statements. Specifies 3427 * the path value of "<b>Immunization:manufacturer</b>". 3428 */ 3429 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANUFACTURER = new ca.uhn.fhir.model.api.Include("Immunization:manufacturer").toLocked(); 3430 3431 /** 3432 * Search parameter: <b>performer</b> 3433 * <p> 3434 * Description: <b>The practitioner, individual or organization who played a role in the vaccination</b><br> 3435 * Type: <b>reference</b><br> 3436 * Path: <b>Immunization.performer.actor</b><br> 3437 * </p> 3438 */ 3439 @SearchParamDefinition(name="performer", path="Immunization.performer.actor", description="The practitioner, individual or organization who played a role in the vaccination", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 3440 public static final String SP_PERFORMER = "performer"; 3441 /** 3442 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 3443 * <p> 3444 * Description: <b>The practitioner, individual or organization who played a role in the vaccination</b><br> 3445 * Type: <b>reference</b><br> 3446 * Path: <b>Immunization.performer.actor</b><br> 3447 * </p> 3448 */ 3449 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 3450 3451/** 3452 * Constant for fluent queries to be used to add include statements. Specifies 3453 * the path value of "<b>Immunization:performer</b>". 3454 */ 3455 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("Immunization:performer").toLocked(); 3456 3457 /** 3458 * Search parameter: <b>reaction-date</b> 3459 * <p> 3460 * Description: <b>When reaction started</b><br> 3461 * Type: <b>date</b><br> 3462 * Path: <b>Immunization.reaction.date</b><br> 3463 * </p> 3464 */ 3465 @SearchParamDefinition(name="reaction-date", path="Immunization.reaction.date", description="When reaction started", type="date" ) 3466 public static final String SP_REACTION_DATE = "reaction-date"; 3467 /** 3468 * <b>Fluent Client</b> search parameter constant for <b>reaction-date</b> 3469 * <p> 3470 * Description: <b>When reaction started</b><br> 3471 * Type: <b>date</b><br> 3472 * Path: <b>Immunization.reaction.date</b><br> 3473 * </p> 3474 */ 3475 public static final ca.uhn.fhir.rest.gclient.DateClientParam REACTION_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_REACTION_DATE); 3476 3477 /** 3478 * Search parameter: <b>reaction</b> 3479 * <p> 3480 * Description: <b>Additional information on reaction</b><br> 3481 * Type: <b>reference</b><br> 3482 * Path: <b>Immunization.reaction.manifestation.reference</b><br> 3483 * </p> 3484 */ 3485 @SearchParamDefinition(name="reaction", path="Immunization.reaction.manifestation.reference", description="Additional information on reaction", type="reference", target={Observation.class } ) 3486 public static final String SP_REACTION = "reaction"; 3487 /** 3488 * <b>Fluent Client</b> search parameter constant for <b>reaction</b> 3489 * <p> 3490 * Description: <b>Additional information on reaction</b><br> 3491 * Type: <b>reference</b><br> 3492 * Path: <b>Immunization.reaction.manifestation.reference</b><br> 3493 * </p> 3494 */ 3495 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REACTION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REACTION); 3496 3497/** 3498 * Constant for fluent queries to be used to add include statements. Specifies 3499 * the path value of "<b>Immunization:reaction</b>". 3500 */ 3501 public static final ca.uhn.fhir.model.api.Include INCLUDE_REACTION = new ca.uhn.fhir.model.api.Include("Immunization:reaction").toLocked(); 3502 3503 /** 3504 * Search parameter: <b>reason-code</b> 3505 * <p> 3506 * Description: <b>Reason why the vaccine was administered</b><br> 3507 * Type: <b>token</b><br> 3508 * Path: <b>Immunization.reason.concept</b><br> 3509 * </p> 3510 */ 3511 @SearchParamDefinition(name="reason-code", path="Immunization.reason.concept", description="Reason why the vaccine was administered", type="token" ) 3512 public static final String SP_REASON_CODE = "reason-code"; 3513 /** 3514 * <b>Fluent Client</b> search parameter constant for <b>reason-code</b> 3515 * <p> 3516 * Description: <b>Reason why the vaccine was administered</b><br> 3517 * Type: <b>token</b><br> 3518 * Path: <b>Immunization.reason.concept</b><br> 3519 * </p> 3520 */ 3521 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REASON_CODE); 3522 3523 /** 3524 * Search parameter: <b>reason-reference</b> 3525 * <p> 3526 * Description: <b>Reference to a resource (by instance)</b><br> 3527 * Type: <b>reference</b><br> 3528 * Path: <b>Immunization.reason.reference</b><br> 3529 * </p> 3530 */ 3531 @SearchParamDefinition(name="reason-reference", path="Immunization.reason.reference", description="Reference to a resource (by instance)", type="reference", target={Condition.class, DiagnosticReport.class, Observation.class } ) 3532 public static final String SP_REASON_REFERENCE = "reason-reference"; 3533 /** 3534 * <b>Fluent Client</b> search parameter constant for <b>reason-reference</b> 3535 * <p> 3536 * Description: <b>Reference to a resource (by instance)</b><br> 3537 * Type: <b>reference</b><br> 3538 * Path: <b>Immunization.reason.reference</b><br> 3539 * </p> 3540 */ 3541 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REASON_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REASON_REFERENCE); 3542 3543/** 3544 * Constant for fluent queries to be used to add include statements. Specifies 3545 * the path value of "<b>Immunization:reason-reference</b>". 3546 */ 3547 public static final ca.uhn.fhir.model.api.Include INCLUDE_REASON_REFERENCE = new ca.uhn.fhir.model.api.Include("Immunization:reason-reference").toLocked(); 3548 3549 /** 3550 * Search parameter: <b>series</b> 3551 * <p> 3552 * Description: <b>The series being followed by the provider</b><br> 3553 * Type: <b>string</b><br> 3554 * Path: <b>Immunization.protocolApplied.series</b><br> 3555 * </p> 3556 */ 3557 @SearchParamDefinition(name="series", path="Immunization.protocolApplied.series", description="The series being followed by the provider", type="string" ) 3558 public static final String SP_SERIES = "series"; 3559 /** 3560 * <b>Fluent Client</b> search parameter constant for <b>series</b> 3561 * <p> 3562 * Description: <b>The series being followed by the provider</b><br> 3563 * Type: <b>string</b><br> 3564 * Path: <b>Immunization.protocolApplied.series</b><br> 3565 * </p> 3566 */ 3567 public static final ca.uhn.fhir.rest.gclient.StringClientParam SERIES = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_SERIES); 3568 3569 /** 3570 * Search parameter: <b>status-reason</b> 3571 * <p> 3572 * Description: <b>Reason why the vaccine was not administered</b><br> 3573 * Type: <b>token</b><br> 3574 * Path: <b>Immunization.statusReason</b><br> 3575 * </p> 3576 */ 3577 @SearchParamDefinition(name="status-reason", path="Immunization.statusReason", description="Reason why the vaccine was not administered", type="token" ) 3578 public static final String SP_STATUS_REASON = "status-reason"; 3579 /** 3580 * <b>Fluent Client</b> search parameter constant for <b>status-reason</b> 3581 * <p> 3582 * Description: <b>Reason why the vaccine was not administered</b><br> 3583 * Type: <b>token</b><br> 3584 * Path: <b>Immunization.statusReason</b><br> 3585 * </p> 3586 */ 3587 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS_REASON); 3588 3589 /** 3590 * Search parameter: <b>status</b> 3591 * <p> 3592 * Description: <b>Immunization event status</b><br> 3593 * Type: <b>token</b><br> 3594 * Path: <b>Immunization.status</b><br> 3595 * </p> 3596 */ 3597 @SearchParamDefinition(name="status", path="Immunization.status", description="Immunization event status", type="token" ) 3598 public static final String SP_STATUS = "status"; 3599 /** 3600 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3601 * <p> 3602 * Description: <b>Immunization event status</b><br> 3603 * Type: <b>token</b><br> 3604 * Path: <b>Immunization.status</b><br> 3605 * </p> 3606 */ 3607 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3608 3609 /** 3610 * Search parameter: <b>target-disease</b> 3611 * <p> 3612 * Description: <b>The target disease the dose is being administered against</b><br> 3613 * Type: <b>token</b><br> 3614 * Path: <b>Immunization.protocolApplied.targetDisease</b><br> 3615 * </p> 3616 */ 3617 @SearchParamDefinition(name="target-disease", path="Immunization.protocolApplied.targetDisease", description="The target disease the dose is being administered against", type="token" ) 3618 public static final String SP_TARGET_DISEASE = "target-disease"; 3619 /** 3620 * <b>Fluent Client</b> search parameter constant for <b>target-disease</b> 3621 * <p> 3622 * Description: <b>The target disease the dose is being administered against</b><br> 3623 * Type: <b>token</b><br> 3624 * Path: <b>Immunization.protocolApplied.targetDisease</b><br> 3625 * </p> 3626 */ 3627 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_DISEASE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TARGET_DISEASE); 3628 3629 /** 3630 * Search parameter: <b>vaccine-code</b> 3631 * <p> 3632 * Description: <b>Vaccine Product Administered</b><br> 3633 * Type: <b>token</b><br> 3634 * Path: <b>Immunization.vaccineCode</b><br> 3635 * </p> 3636 */ 3637 @SearchParamDefinition(name="vaccine-code", path="Immunization.vaccineCode", description="Vaccine Product Administered", type="token" ) 3638 public static final String SP_VACCINE_CODE = "vaccine-code"; 3639 /** 3640 * <b>Fluent Client</b> search parameter constant for <b>vaccine-code</b> 3641 * <p> 3642 * Description: <b>Vaccine Product Administered</b><br> 3643 * Type: <b>token</b><br> 3644 * Path: <b>Immunization.vaccineCode</b><br> 3645 * </p> 3646 */ 3647 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VACCINE_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VACCINE_CODE); 3648 3649 /** 3650 * Search parameter: <b>date</b> 3651 * <p> 3652 * Description: <b>Multiple Resources: 3653 3654* [AdverseEvent](adverseevent.html): When the event occurred 3655* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 3656* [Appointment](appointment.html): Appointment date/time. 3657* [AuditEvent](auditevent.html): Time when the event was recorded 3658* [CarePlan](careplan.html): Time period plan covers 3659* [CareTeam](careteam.html): A date within the coverage time period. 3660* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 3661* [Composition](composition.html): Composition editing time 3662* [Consent](consent.html): When consent was agreed to 3663* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 3664* [DocumentReference](documentreference.html): When this document reference was created 3665* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 3666* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 3667* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 3668* [Flag](flag.html): Time period when flag is active 3669* [Immunization](immunization.html): Vaccination (non)-Administration Date 3670* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 3671* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 3672* [Invoice](invoice.html): Invoice date / posting date 3673* [List](list.html): When the list was prepared 3674* [MeasureReport](measurereport.html): The date of the measure report 3675* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 3676* [Observation](observation.html): Clinically relevant time/time-period for observation 3677* [Procedure](procedure.html): When the procedure occurred or is occurring 3678* [ResearchSubject](researchsubject.html): Start and end of participation 3679* [RiskAssessment](riskassessment.html): When was assessment made? 3680* [SupplyRequest](supplyrequest.html): When the request was made 3681</b><br> 3682 * Type: <b>date</b><br> 3683 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 3684 * </p> 3685 */ 3686 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 3687 public static final String SP_DATE = "date"; 3688 /** 3689 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3690 * <p> 3691 * Description: <b>Multiple Resources: 3692 3693* [AdverseEvent](adverseevent.html): When the event occurred 3694* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 3695* [Appointment](appointment.html): Appointment date/time. 3696* [AuditEvent](auditevent.html): Time when the event was recorded 3697* [CarePlan](careplan.html): Time period plan covers 3698* [CareTeam](careteam.html): A date within the coverage time period. 3699* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 3700* [Composition](composition.html): Composition editing time 3701* [Consent](consent.html): When consent was agreed to 3702* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 3703* [DocumentReference](documentreference.html): When this document reference was created 3704* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 3705* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 3706* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 3707* [Flag](flag.html): Time period when flag is active 3708* [Immunization](immunization.html): Vaccination (non)-Administration Date 3709* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 3710* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 3711* [Invoice](invoice.html): Invoice date / posting date 3712* [List](list.html): When the list was prepared 3713* [MeasureReport](measurereport.html): The date of the measure report 3714* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 3715* [Observation](observation.html): Clinically relevant time/time-period for observation 3716* [Procedure](procedure.html): When the procedure occurred or is occurring 3717* [ResearchSubject](researchsubject.html): Start and end of participation 3718* [RiskAssessment](riskassessment.html): When was assessment made? 3719* [SupplyRequest](supplyrequest.html): When the request was made 3720</b><br> 3721 * Type: <b>date</b><br> 3722 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 3723 * </p> 3724 */ 3725 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3726 3727 /** 3728 * Search parameter: <b>identifier</b> 3729 * <p> 3730 * Description: <b>Multiple Resources: 3731 3732* [Account](account.html): Account number 3733* [AdverseEvent](adverseevent.html): Business identifier for the event 3734* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3735* [Appointment](appointment.html): An Identifier of the Appointment 3736* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3737* [Basic](basic.html): Business identifier 3738* [BodyStructure](bodystructure.html): Bodystructure identifier 3739* [CarePlan](careplan.html): External Ids for this plan 3740* [CareTeam](careteam.html): External Ids for this team 3741* [ChargeItem](chargeitem.html): Business Identifier for item 3742* [Claim](claim.html): The primary identifier of the financial resource 3743* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3744* [ClinicalImpression](clinicalimpression.html): Business identifier 3745* [Communication](communication.html): Unique identifier 3746* [CommunicationRequest](communicationrequest.html): Unique identifier 3747* [Composition](composition.html): Version-independent identifier for the Composition 3748* [Condition](condition.html): A unique identifier of the condition record 3749* [Consent](consent.html): Identifier for this record (external references) 3750* [Contract](contract.html): The identity of the contract 3751* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3752* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3753* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3754* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3755* [DeviceRequest](devicerequest.html): Business identifier for request/order 3756* [DeviceUsage](deviceusage.html): Search by identifier 3757* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3758* [DocumentReference](documentreference.html): Identifier of the attachment binary 3759* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3760* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3761* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3762* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3763* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3764* [Flag](flag.html): Business identifier 3765* [Goal](goal.html): External Ids for this goal 3766* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3767* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3768* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3769* [Immunization](immunization.html): Business identifier 3770* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3771* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3772* [Invoice](invoice.html): Business Identifier for item 3773* [List](list.html): Business identifier 3774* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3775* [Medication](medication.html): Returns medications with this external identifier 3776* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3777* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3778* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3779* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3780* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3781* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3782* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3783* [Observation](observation.html): The unique id for a particular observation 3784* [Person](person.html): A person Identifier 3785* [Procedure](procedure.html): A unique identifier for a procedure 3786* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3787* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3788* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3789* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3790* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3791* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3792* [Specimen](specimen.html): The unique identifier associated with the specimen 3793* [SupplyDelivery](supplydelivery.html): External identifier 3794* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3795* [Task](task.html): Search for a task instance by its business identifier 3796* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3797</b><br> 3798 * Type: <b>token</b><br> 3799 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3800 * </p> 3801 */ 3802 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 3803 public static final String SP_IDENTIFIER = "identifier"; 3804 /** 3805 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3806 * <p> 3807 * Description: <b>Multiple Resources: 3808 3809* [Account](account.html): Account number 3810* [AdverseEvent](adverseevent.html): Business identifier for the event 3811* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3812* [Appointment](appointment.html): An Identifier of the Appointment 3813* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3814* [Basic](basic.html): Business identifier 3815* [BodyStructure](bodystructure.html): Bodystructure identifier 3816* [CarePlan](careplan.html): External Ids for this plan 3817* [CareTeam](careteam.html): External Ids for this team 3818* [ChargeItem](chargeitem.html): Business Identifier for item 3819* [Claim](claim.html): The primary identifier of the financial resource 3820* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3821* [ClinicalImpression](clinicalimpression.html): Business identifier 3822* [Communication](communication.html): Unique identifier 3823* [CommunicationRequest](communicationrequest.html): Unique identifier 3824* [Composition](composition.html): Version-independent identifier for the Composition 3825* [Condition](condition.html): A unique identifier of the condition record 3826* [Consent](consent.html): Identifier for this record (external references) 3827* [Contract](contract.html): The identity of the contract 3828* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3829* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3830* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3831* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3832* [DeviceRequest](devicerequest.html): Business identifier for request/order 3833* [DeviceUsage](deviceusage.html): Search by identifier 3834* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3835* [DocumentReference](documentreference.html): Identifier of the attachment binary 3836* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3837* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3838* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3839* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3840* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3841* [Flag](flag.html): Business identifier 3842* [Goal](goal.html): External Ids for this goal 3843* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3844* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3845* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3846* [Immunization](immunization.html): Business identifier 3847* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3848* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3849* [Invoice](invoice.html): Business Identifier for item 3850* [List](list.html): Business identifier 3851* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3852* [Medication](medication.html): Returns medications with this external identifier 3853* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3854* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3855* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3856* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3857* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3858* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3859* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3860* [Observation](observation.html): The unique id for a particular observation 3861* [Person](person.html): A person Identifier 3862* [Procedure](procedure.html): A unique identifier for a procedure 3863* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3864* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3865* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3866* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3867* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3868* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3869* [Specimen](specimen.html): The unique identifier associated with the specimen 3870* [SupplyDelivery](supplydelivery.html): External identifier 3871* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3872* [Task](task.html): Search for a task instance by its business identifier 3873* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3874</b><br> 3875 * Type: <b>token</b><br> 3876 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3877 * </p> 3878 */ 3879 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3880 3881 /** 3882 * Search parameter: <b>patient</b> 3883 * <p> 3884 * Description: <b>Multiple Resources: 3885 3886* [Account](account.html): The entity that caused the expenses 3887* [AdverseEvent](adverseevent.html): Subject impacted by event 3888* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3889* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3890* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3891* [AuditEvent](auditevent.html): Where the activity involved patient data 3892* [Basic](basic.html): Identifies the focus of this resource 3893* [BodyStructure](bodystructure.html): Who this is about 3894* [CarePlan](careplan.html): Who the care plan is for 3895* [CareTeam](careteam.html): Who care team is for 3896* [ChargeItem](chargeitem.html): Individual service was done for/to 3897* [Claim](claim.html): Patient receiving the products or services 3898* [ClaimResponse](claimresponse.html): The subject of care 3899* [ClinicalImpression](clinicalimpression.html): Patient assessed 3900* [Communication](communication.html): Focus of message 3901* [CommunicationRequest](communicationrequest.html): Focus of message 3902* [Composition](composition.html): Who and/or what the composition is about 3903* [Condition](condition.html): Who has the condition? 3904* [Consent](consent.html): Who the consent applies to 3905* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3906* [Coverage](coverage.html): Retrieve coverages for a patient 3907* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3908* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3909* [DetectedIssue](detectedissue.html): Associated patient 3910* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3911* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3912* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3913* [DocumentReference](documentreference.html): Who/what is the subject of the document 3914* [Encounter](encounter.html): The patient present at the encounter 3915* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3916* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3917* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3918* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3919* [Flag](flag.html): The identity of a subject to list flags for 3920* [Goal](goal.html): Who this goal is intended for 3921* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3922* [ImagingSelection](imagingselection.html): Who the study is about 3923* [ImagingStudy](imagingstudy.html): Who the study is about 3924* [Immunization](immunization.html): The patient for the vaccination record 3925* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3926* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3927* [Invoice](invoice.html): Recipient(s) of goods and services 3928* [List](list.html): If all resources have the same subject 3929* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3930* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3931* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3932* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3933* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3934* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3935* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3936* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3937* [Observation](observation.html): The subject that the observation is about (if patient) 3938* [Person](person.html): The Person links to this Patient 3939* [Procedure](procedure.html): Search by subject - a patient 3940* [Provenance](provenance.html): Where the activity involved patient data 3941* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3942* [RelatedPerson](relatedperson.html): The patient this related person is related to 3943* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3944* [ResearchSubject](researchsubject.html): Who or what is part of study 3945* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3946* [ServiceRequest](servicerequest.html): Search by subject - a patient 3947* [Specimen](specimen.html): The patient the specimen comes from 3948* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3949* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3950* [Task](task.html): Search by patient 3951* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3952</b><br> 3953 * Type: <b>reference</b><br> 3954 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3955 * </p> 3956 */ 3957 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 3958 public static final String SP_PATIENT = "patient"; 3959 /** 3960 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3961 * <p> 3962 * Description: <b>Multiple Resources: 3963 3964* [Account](account.html): The entity that caused the expenses 3965* [AdverseEvent](adverseevent.html): Subject impacted by event 3966* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3967* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3968* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3969* [AuditEvent](auditevent.html): Where the activity involved patient data 3970* [Basic](basic.html): Identifies the focus of this resource 3971* [BodyStructure](bodystructure.html): Who this is about 3972* [CarePlan](careplan.html): Who the care plan is for 3973* [CareTeam](careteam.html): Who care team is for 3974* [ChargeItem](chargeitem.html): Individual service was done for/to 3975* [Claim](claim.html): Patient receiving the products or services 3976* [ClaimResponse](claimresponse.html): The subject of care 3977* [ClinicalImpression](clinicalimpression.html): Patient assessed 3978* [Communication](communication.html): Focus of message 3979* [CommunicationRequest](communicationrequest.html): Focus of message 3980* [Composition](composition.html): Who and/or what the composition is about 3981* [Condition](condition.html): Who has the condition? 3982* [Consent](consent.html): Who the consent applies to 3983* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3984* [Coverage](coverage.html): Retrieve coverages for a patient 3985* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3986* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3987* [DetectedIssue](detectedissue.html): Associated patient 3988* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3989* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3990* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3991* [DocumentReference](documentreference.html): Who/what is the subject of the document 3992* [Encounter](encounter.html): The patient present at the encounter 3993* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3994* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3995* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3996* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3997* [Flag](flag.html): The identity of a subject to list flags for 3998* [Goal](goal.html): Who this goal is intended for 3999* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4000* [ImagingSelection](imagingselection.html): Who the study is about 4001* [ImagingStudy](imagingstudy.html): Who the study is about 4002* [Immunization](immunization.html): The patient for the vaccination record 4003* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4004* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4005* [Invoice](invoice.html): Recipient(s) of goods and services 4006* [List](list.html): If all resources have the same subject 4007* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4008* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4009* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4010* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4011* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4012* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4013* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4014* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4015* [Observation](observation.html): The subject that the observation is about (if patient) 4016* [Person](person.html): The Person links to this Patient 4017* [Procedure](procedure.html): Search by subject - a patient 4018* [Provenance](provenance.html): Where the activity involved patient data 4019* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4020* [RelatedPerson](relatedperson.html): The patient this related person is related to 4021* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4022* [ResearchSubject](researchsubject.html): Who or what is part of study 4023* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4024* [ServiceRequest](servicerequest.html): Search by subject - a patient 4025* [Specimen](specimen.html): The patient the specimen comes from 4026* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4027* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4028* [Task](task.html): Search by patient 4029* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4030</b><br> 4031 * Type: <b>reference</b><br> 4032 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4033 * </p> 4034 */ 4035 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4036 4037/** 4038 * Constant for fluent queries to be used to add include statements. Specifies 4039 * the path value of "<b>Immunization:patient</b>". 4040 */ 4041 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Immunization:patient").toLocked(); 4042 4043 4044} 4045