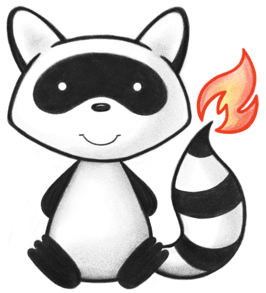
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Describes a comparison of an immunization event against published recommendations to determine if the administration is "valid" in relation to those recommendations. 052 */ 053@ResourceDef(name="ImmunizationEvaluation", profile="http://hl7.org/fhir/StructureDefinition/ImmunizationEvaluation") 054public class ImmunizationEvaluation extends DomainResource { 055 056 public enum ImmunizationEvaluationStatusCodes { 057 /** 058 * All actions that are implied by the administration have occurred. 059 */ 060 COMPLETED, 061 /** 062 * The administration was entered in error and therefore nullified. 063 */ 064 ENTEREDINERROR, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static ImmunizationEvaluationStatusCodes fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("completed".equals(codeString)) 073 return COMPLETED; 074 if ("entered-in-error".equals(codeString)) 075 return ENTEREDINERROR; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown ImmunizationEvaluationStatusCodes code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case COMPLETED: return "completed"; 084 case ENTEREDINERROR: return "entered-in-error"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 switch (this) { 091 case COMPLETED: return "http://hl7.org/fhir/CodeSystem/medication-admin-status"; 092 case ENTEREDINERROR: return "http://hl7.org/fhir/CodeSystem/medication-admin-status"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getDefinition() { 098 switch (this) { 099 case COMPLETED: return "All actions that are implied by the administration have occurred."; 100 case ENTEREDINERROR: return "The administration was entered in error and therefore nullified."; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDisplay() { 106 switch (this) { 107 case COMPLETED: return "Completed"; 108 case ENTEREDINERROR: return "Entered in Error"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 } 114 115 public static class ImmunizationEvaluationStatusCodesEnumFactory implements EnumFactory<ImmunizationEvaluationStatusCodes> { 116 public ImmunizationEvaluationStatusCodes fromCode(String codeString) throws IllegalArgumentException { 117 if (codeString == null || "".equals(codeString)) 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("completed".equals(codeString)) 121 return ImmunizationEvaluationStatusCodes.COMPLETED; 122 if ("entered-in-error".equals(codeString)) 123 return ImmunizationEvaluationStatusCodes.ENTEREDINERROR; 124 throw new IllegalArgumentException("Unknown ImmunizationEvaluationStatusCodes code '"+codeString+"'"); 125 } 126 public Enumeration<ImmunizationEvaluationStatusCodes> fromType(PrimitiveType<?> code) throws FHIRException { 127 if (code == null) 128 return null; 129 if (code.isEmpty()) 130 return new Enumeration<ImmunizationEvaluationStatusCodes>(this, ImmunizationEvaluationStatusCodes.NULL, code); 131 String codeString = ((PrimitiveType) code).asStringValue(); 132 if (codeString == null || "".equals(codeString)) 133 return new Enumeration<ImmunizationEvaluationStatusCodes>(this, ImmunizationEvaluationStatusCodes.NULL, code); 134 if ("completed".equals(codeString)) 135 return new Enumeration<ImmunizationEvaluationStatusCodes>(this, ImmunizationEvaluationStatusCodes.COMPLETED, code); 136 if ("entered-in-error".equals(codeString)) 137 return new Enumeration<ImmunizationEvaluationStatusCodes>(this, ImmunizationEvaluationStatusCodes.ENTEREDINERROR, code); 138 throw new FHIRException("Unknown ImmunizationEvaluationStatusCodes code '"+codeString+"'"); 139 } 140 public String toCode(ImmunizationEvaluationStatusCodes code) { 141 if (code == ImmunizationEvaluationStatusCodes.NULL) 142 return null; 143 if (code == ImmunizationEvaluationStatusCodes.COMPLETED) 144 return "completed"; 145 if (code == ImmunizationEvaluationStatusCodes.ENTEREDINERROR) 146 return "entered-in-error"; 147 return "?"; 148 } 149 public String toSystem(ImmunizationEvaluationStatusCodes code) { 150 return code.getSystem(); 151 } 152 } 153 154 /** 155 * A unique identifier assigned to this immunization evaluation record. 156 */ 157 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 158 @Description(shortDefinition="Business identifier", formalDefinition="A unique identifier assigned to this immunization evaluation record." ) 159 protected List<Identifier> identifier; 160 161 /** 162 * Indicates the current status of the evaluation of the vaccination administration event. 163 */ 164 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 165 @Description(shortDefinition="completed | entered-in-error", formalDefinition="Indicates the current status of the evaluation of the vaccination administration event." ) 166 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-evaluation-status") 167 protected Enumeration<ImmunizationEvaluationStatusCodes> status; 168 169 /** 170 * The individual for whom the evaluation is being done. 171 */ 172 @Child(name = "patient", type = {Patient.class}, order=2, min=1, max=1, modifier=false, summary=true) 173 @Description(shortDefinition="Who this evaluation is for", formalDefinition="The individual for whom the evaluation is being done." ) 174 protected Reference patient; 175 176 /** 177 * The date the evaluation of the vaccine administration event was performed. 178 */ 179 @Child(name = "date", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 180 @Description(shortDefinition="Date evaluation was performed", formalDefinition="The date the evaluation of the vaccine administration event was performed." ) 181 protected DateTimeType date; 182 183 /** 184 * Indicates the authority who published the protocol (e.g. ACIP). 185 */ 186 @Child(name = "authority", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=false) 187 @Description(shortDefinition="Who is responsible for publishing the recommendations", formalDefinition="Indicates the authority who published the protocol (e.g. ACIP)." ) 188 protected Reference authority; 189 190 /** 191 * The vaccine preventable disease the dose is being evaluated against. 192 */ 193 @Child(name = "targetDisease", type = {CodeableConcept.class}, order=5, min=1, max=1, modifier=false, summary=true) 194 @Description(shortDefinition="The vaccine preventable disease schedule being evaluated", formalDefinition="The vaccine preventable disease the dose is being evaluated against." ) 195 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-target-disease") 196 protected CodeableConcept targetDisease; 197 198 /** 199 * The vaccine administration event being evaluated. 200 */ 201 @Child(name = "immunizationEvent", type = {Immunization.class}, order=6, min=1, max=1, modifier=false, summary=true) 202 @Description(shortDefinition="Immunization being evaluated", formalDefinition="The vaccine administration event being evaluated." ) 203 protected Reference immunizationEvent; 204 205 /** 206 * Indicates if the dose is valid or not valid with respect to the published recommendations. 207 */ 208 @Child(name = "doseStatus", type = {CodeableConcept.class}, order=7, min=1, max=1, modifier=false, summary=true) 209 @Description(shortDefinition="Status of the dose relative to published recommendations", formalDefinition="Indicates if the dose is valid or not valid with respect to the published recommendations." ) 210 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-evaluation-dose-status") 211 protected CodeableConcept doseStatus; 212 213 /** 214 * Provides an explanation as to why the vaccine administration event is valid or not relative to the published recommendations. 215 */ 216 @Child(name = "doseStatusReason", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 217 @Description(shortDefinition="Reason why the doese is considered valid, invalid or some other status", formalDefinition="Provides an explanation as to why the vaccine administration event is valid or not relative to the published recommendations." ) 218 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-evaluation-dose-status-reason") 219 protected List<CodeableConcept> doseStatusReason; 220 221 /** 222 * Additional information about the evaluation. 223 */ 224 @Child(name = "description", type = {MarkdownType.class}, order=9, min=0, max=1, modifier=false, summary=false) 225 @Description(shortDefinition="Evaluation notes", formalDefinition="Additional information about the evaluation." ) 226 protected MarkdownType description; 227 228 /** 229 * One possible path to achieve presumed immunity against a disease - within the context of an authority. 230 */ 231 @Child(name = "series", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 232 @Description(shortDefinition="Name of vaccine series", formalDefinition="One possible path to achieve presumed immunity against a disease - within the context of an authority." ) 233 protected StringType series; 234 235 /** 236 * Nominal position in a series as determined by the outcome of the evaluation process. 237 */ 238 @Child(name = "doseNumber", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=false) 239 @Description(shortDefinition="Dose number within series", formalDefinition="Nominal position in a series as determined by the outcome of the evaluation process." ) 240 protected StringType doseNumber; 241 242 /** 243 * The recommended number of doses to achieve immunity as determined by the outcome of the evaluation process. 244 */ 245 @Child(name = "seriesDoses", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=false) 246 @Description(shortDefinition="Recommended number of doses for immunity", formalDefinition="The recommended number of doses to achieve immunity as determined by the outcome of the evaluation process." ) 247 protected StringType seriesDoses; 248 249 private static final long serialVersionUID = 1336970813L; 250 251 /** 252 * Constructor 253 */ 254 public ImmunizationEvaluation() { 255 super(); 256 } 257 258 /** 259 * Constructor 260 */ 261 public ImmunizationEvaluation(ImmunizationEvaluationStatusCodes status, Reference patient, CodeableConcept targetDisease, Reference immunizationEvent, CodeableConcept doseStatus) { 262 super(); 263 this.setStatus(status); 264 this.setPatient(patient); 265 this.setTargetDisease(targetDisease); 266 this.setImmunizationEvent(immunizationEvent); 267 this.setDoseStatus(doseStatus); 268 } 269 270 /** 271 * @return {@link #identifier} (A unique identifier assigned to this immunization evaluation record.) 272 */ 273 public List<Identifier> getIdentifier() { 274 if (this.identifier == null) 275 this.identifier = new ArrayList<Identifier>(); 276 return this.identifier; 277 } 278 279 /** 280 * @return Returns a reference to <code>this</code> for easy method chaining 281 */ 282 public ImmunizationEvaluation setIdentifier(List<Identifier> theIdentifier) { 283 this.identifier = theIdentifier; 284 return this; 285 } 286 287 public boolean hasIdentifier() { 288 if (this.identifier == null) 289 return false; 290 for (Identifier item : this.identifier) 291 if (!item.isEmpty()) 292 return true; 293 return false; 294 } 295 296 public Identifier addIdentifier() { //3 297 Identifier t = new Identifier(); 298 if (this.identifier == null) 299 this.identifier = new ArrayList<Identifier>(); 300 this.identifier.add(t); 301 return t; 302 } 303 304 public ImmunizationEvaluation addIdentifier(Identifier t) { //3 305 if (t == null) 306 return this; 307 if (this.identifier == null) 308 this.identifier = new ArrayList<Identifier>(); 309 this.identifier.add(t); 310 return this; 311 } 312 313 /** 314 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 315 */ 316 public Identifier getIdentifierFirstRep() { 317 if (getIdentifier().isEmpty()) { 318 addIdentifier(); 319 } 320 return getIdentifier().get(0); 321 } 322 323 /** 324 * @return {@link #status} (Indicates the current status of the evaluation of the vaccination administration event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 325 */ 326 public Enumeration<ImmunizationEvaluationStatusCodes> getStatusElement() { 327 if (this.status == null) 328 if (Configuration.errorOnAutoCreate()) 329 throw new Error("Attempt to auto-create ImmunizationEvaluation.status"); 330 else if (Configuration.doAutoCreate()) 331 this.status = new Enumeration<ImmunizationEvaluationStatusCodes>(new ImmunizationEvaluationStatusCodesEnumFactory()); // bb 332 return this.status; 333 } 334 335 public boolean hasStatusElement() { 336 return this.status != null && !this.status.isEmpty(); 337 } 338 339 public boolean hasStatus() { 340 return this.status != null && !this.status.isEmpty(); 341 } 342 343 /** 344 * @param value {@link #status} (Indicates the current status of the evaluation of the vaccination administration event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 345 */ 346 public ImmunizationEvaluation setStatusElement(Enumeration<ImmunizationEvaluationStatusCodes> value) { 347 this.status = value; 348 return this; 349 } 350 351 /** 352 * @return Indicates the current status of the evaluation of the vaccination administration event. 353 */ 354 public ImmunizationEvaluationStatusCodes getStatus() { 355 return this.status == null ? null : this.status.getValue(); 356 } 357 358 /** 359 * @param value Indicates the current status of the evaluation of the vaccination administration event. 360 */ 361 public ImmunizationEvaluation setStatus(ImmunizationEvaluationStatusCodes value) { 362 if (this.status == null) 363 this.status = new Enumeration<ImmunizationEvaluationStatusCodes>(new ImmunizationEvaluationStatusCodesEnumFactory()); 364 this.status.setValue(value); 365 return this; 366 } 367 368 /** 369 * @return {@link #patient} (The individual for whom the evaluation is being done.) 370 */ 371 public Reference getPatient() { 372 if (this.patient == null) 373 if (Configuration.errorOnAutoCreate()) 374 throw new Error("Attempt to auto-create ImmunizationEvaluation.patient"); 375 else if (Configuration.doAutoCreate()) 376 this.patient = new Reference(); // cc 377 return this.patient; 378 } 379 380 public boolean hasPatient() { 381 return this.patient != null && !this.patient.isEmpty(); 382 } 383 384 /** 385 * @param value {@link #patient} (The individual for whom the evaluation is being done.) 386 */ 387 public ImmunizationEvaluation setPatient(Reference value) { 388 this.patient = value; 389 return this; 390 } 391 392 /** 393 * @return {@link #date} (The date the evaluation of the vaccine administration event was performed.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 394 */ 395 public DateTimeType getDateElement() { 396 if (this.date == null) 397 if (Configuration.errorOnAutoCreate()) 398 throw new Error("Attempt to auto-create ImmunizationEvaluation.date"); 399 else if (Configuration.doAutoCreate()) 400 this.date = new DateTimeType(); // bb 401 return this.date; 402 } 403 404 public boolean hasDateElement() { 405 return this.date != null && !this.date.isEmpty(); 406 } 407 408 public boolean hasDate() { 409 return this.date != null && !this.date.isEmpty(); 410 } 411 412 /** 413 * @param value {@link #date} (The date the evaluation of the vaccine administration event was performed.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 414 */ 415 public ImmunizationEvaluation setDateElement(DateTimeType value) { 416 this.date = value; 417 return this; 418 } 419 420 /** 421 * @return The date the evaluation of the vaccine administration event was performed. 422 */ 423 public Date getDate() { 424 return this.date == null ? null : this.date.getValue(); 425 } 426 427 /** 428 * @param value The date the evaluation of the vaccine administration event was performed. 429 */ 430 public ImmunizationEvaluation setDate(Date value) { 431 if (value == null) 432 this.date = null; 433 else { 434 if (this.date == null) 435 this.date = new DateTimeType(); 436 this.date.setValue(value); 437 } 438 return this; 439 } 440 441 /** 442 * @return {@link #authority} (Indicates the authority who published the protocol (e.g. ACIP).) 443 */ 444 public Reference getAuthority() { 445 if (this.authority == null) 446 if (Configuration.errorOnAutoCreate()) 447 throw new Error("Attempt to auto-create ImmunizationEvaluation.authority"); 448 else if (Configuration.doAutoCreate()) 449 this.authority = new Reference(); // cc 450 return this.authority; 451 } 452 453 public boolean hasAuthority() { 454 return this.authority != null && !this.authority.isEmpty(); 455 } 456 457 /** 458 * @param value {@link #authority} (Indicates the authority who published the protocol (e.g. ACIP).) 459 */ 460 public ImmunizationEvaluation setAuthority(Reference value) { 461 this.authority = value; 462 return this; 463 } 464 465 /** 466 * @return {@link #targetDisease} (The vaccine preventable disease the dose is being evaluated against.) 467 */ 468 public CodeableConcept getTargetDisease() { 469 if (this.targetDisease == null) 470 if (Configuration.errorOnAutoCreate()) 471 throw new Error("Attempt to auto-create ImmunizationEvaluation.targetDisease"); 472 else if (Configuration.doAutoCreate()) 473 this.targetDisease = new CodeableConcept(); // cc 474 return this.targetDisease; 475 } 476 477 public boolean hasTargetDisease() { 478 return this.targetDisease != null && !this.targetDisease.isEmpty(); 479 } 480 481 /** 482 * @param value {@link #targetDisease} (The vaccine preventable disease the dose is being evaluated against.) 483 */ 484 public ImmunizationEvaluation setTargetDisease(CodeableConcept value) { 485 this.targetDisease = value; 486 return this; 487 } 488 489 /** 490 * @return {@link #immunizationEvent} (The vaccine administration event being evaluated.) 491 */ 492 public Reference getImmunizationEvent() { 493 if (this.immunizationEvent == null) 494 if (Configuration.errorOnAutoCreate()) 495 throw new Error("Attempt to auto-create ImmunizationEvaluation.immunizationEvent"); 496 else if (Configuration.doAutoCreate()) 497 this.immunizationEvent = new Reference(); // cc 498 return this.immunizationEvent; 499 } 500 501 public boolean hasImmunizationEvent() { 502 return this.immunizationEvent != null && !this.immunizationEvent.isEmpty(); 503 } 504 505 /** 506 * @param value {@link #immunizationEvent} (The vaccine administration event being evaluated.) 507 */ 508 public ImmunizationEvaluation setImmunizationEvent(Reference value) { 509 this.immunizationEvent = value; 510 return this; 511 } 512 513 /** 514 * @return {@link #doseStatus} (Indicates if the dose is valid or not valid with respect to the published recommendations.) 515 */ 516 public CodeableConcept getDoseStatus() { 517 if (this.doseStatus == null) 518 if (Configuration.errorOnAutoCreate()) 519 throw new Error("Attempt to auto-create ImmunizationEvaluation.doseStatus"); 520 else if (Configuration.doAutoCreate()) 521 this.doseStatus = new CodeableConcept(); // cc 522 return this.doseStatus; 523 } 524 525 public boolean hasDoseStatus() { 526 return this.doseStatus != null && !this.doseStatus.isEmpty(); 527 } 528 529 /** 530 * @param value {@link #doseStatus} (Indicates if the dose is valid or not valid with respect to the published recommendations.) 531 */ 532 public ImmunizationEvaluation setDoseStatus(CodeableConcept value) { 533 this.doseStatus = value; 534 return this; 535 } 536 537 /** 538 * @return {@link #doseStatusReason} (Provides an explanation as to why the vaccine administration event is valid or not relative to the published recommendations.) 539 */ 540 public List<CodeableConcept> getDoseStatusReason() { 541 if (this.doseStatusReason == null) 542 this.doseStatusReason = new ArrayList<CodeableConcept>(); 543 return this.doseStatusReason; 544 } 545 546 /** 547 * @return Returns a reference to <code>this</code> for easy method chaining 548 */ 549 public ImmunizationEvaluation setDoseStatusReason(List<CodeableConcept> theDoseStatusReason) { 550 this.doseStatusReason = theDoseStatusReason; 551 return this; 552 } 553 554 public boolean hasDoseStatusReason() { 555 if (this.doseStatusReason == null) 556 return false; 557 for (CodeableConcept item : this.doseStatusReason) 558 if (!item.isEmpty()) 559 return true; 560 return false; 561 } 562 563 public CodeableConcept addDoseStatusReason() { //3 564 CodeableConcept t = new CodeableConcept(); 565 if (this.doseStatusReason == null) 566 this.doseStatusReason = new ArrayList<CodeableConcept>(); 567 this.doseStatusReason.add(t); 568 return t; 569 } 570 571 public ImmunizationEvaluation addDoseStatusReason(CodeableConcept t) { //3 572 if (t == null) 573 return this; 574 if (this.doseStatusReason == null) 575 this.doseStatusReason = new ArrayList<CodeableConcept>(); 576 this.doseStatusReason.add(t); 577 return this; 578 } 579 580 /** 581 * @return The first repetition of repeating field {@link #doseStatusReason}, creating it if it does not already exist {3} 582 */ 583 public CodeableConcept getDoseStatusReasonFirstRep() { 584 if (getDoseStatusReason().isEmpty()) { 585 addDoseStatusReason(); 586 } 587 return getDoseStatusReason().get(0); 588 } 589 590 /** 591 * @return {@link #description} (Additional information about the evaluation.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 592 */ 593 public MarkdownType getDescriptionElement() { 594 if (this.description == null) 595 if (Configuration.errorOnAutoCreate()) 596 throw new Error("Attempt to auto-create ImmunizationEvaluation.description"); 597 else if (Configuration.doAutoCreate()) 598 this.description = new MarkdownType(); // bb 599 return this.description; 600 } 601 602 public boolean hasDescriptionElement() { 603 return this.description != null && !this.description.isEmpty(); 604 } 605 606 public boolean hasDescription() { 607 return this.description != null && !this.description.isEmpty(); 608 } 609 610 /** 611 * @param value {@link #description} (Additional information about the evaluation.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 612 */ 613 public ImmunizationEvaluation setDescriptionElement(MarkdownType value) { 614 this.description = value; 615 return this; 616 } 617 618 /** 619 * @return Additional information about the evaluation. 620 */ 621 public String getDescription() { 622 return this.description == null ? null : this.description.getValue(); 623 } 624 625 /** 626 * @param value Additional information about the evaluation. 627 */ 628 public ImmunizationEvaluation setDescription(String value) { 629 if (Utilities.noString(value)) 630 this.description = null; 631 else { 632 if (this.description == null) 633 this.description = new MarkdownType(); 634 this.description.setValue(value); 635 } 636 return this; 637 } 638 639 /** 640 * @return {@link #series} (One possible path to achieve presumed immunity against a disease - within the context of an authority.). This is the underlying object with id, value and extensions. The accessor "getSeries" gives direct access to the value 641 */ 642 public StringType getSeriesElement() { 643 if (this.series == null) 644 if (Configuration.errorOnAutoCreate()) 645 throw new Error("Attempt to auto-create ImmunizationEvaluation.series"); 646 else if (Configuration.doAutoCreate()) 647 this.series = new StringType(); // bb 648 return this.series; 649 } 650 651 public boolean hasSeriesElement() { 652 return this.series != null && !this.series.isEmpty(); 653 } 654 655 public boolean hasSeries() { 656 return this.series != null && !this.series.isEmpty(); 657 } 658 659 /** 660 * @param value {@link #series} (One possible path to achieve presumed immunity against a disease - within the context of an authority.). This is the underlying object with id, value and extensions. The accessor "getSeries" gives direct access to the value 661 */ 662 public ImmunizationEvaluation setSeriesElement(StringType value) { 663 this.series = value; 664 return this; 665 } 666 667 /** 668 * @return One possible path to achieve presumed immunity against a disease - within the context of an authority. 669 */ 670 public String getSeries() { 671 return this.series == null ? null : this.series.getValue(); 672 } 673 674 /** 675 * @param value One possible path to achieve presumed immunity against a disease - within the context of an authority. 676 */ 677 public ImmunizationEvaluation setSeries(String value) { 678 if (Utilities.noString(value)) 679 this.series = null; 680 else { 681 if (this.series == null) 682 this.series = new StringType(); 683 this.series.setValue(value); 684 } 685 return this; 686 } 687 688 /** 689 * @return {@link #doseNumber} (Nominal position in a series as determined by the outcome of the evaluation process.). This is the underlying object with id, value and extensions. The accessor "getDoseNumber" gives direct access to the value 690 */ 691 public StringType getDoseNumberElement() { 692 if (this.doseNumber == null) 693 if (Configuration.errorOnAutoCreate()) 694 throw new Error("Attempt to auto-create ImmunizationEvaluation.doseNumber"); 695 else if (Configuration.doAutoCreate()) 696 this.doseNumber = new StringType(); // bb 697 return this.doseNumber; 698 } 699 700 public boolean hasDoseNumberElement() { 701 return this.doseNumber != null && !this.doseNumber.isEmpty(); 702 } 703 704 public boolean hasDoseNumber() { 705 return this.doseNumber != null && !this.doseNumber.isEmpty(); 706 } 707 708 /** 709 * @param value {@link #doseNumber} (Nominal position in a series as determined by the outcome of the evaluation process.). This is the underlying object with id, value and extensions. The accessor "getDoseNumber" gives direct access to the value 710 */ 711 public ImmunizationEvaluation setDoseNumberElement(StringType value) { 712 this.doseNumber = value; 713 return this; 714 } 715 716 /** 717 * @return Nominal position in a series as determined by the outcome of the evaluation process. 718 */ 719 public String getDoseNumber() { 720 return this.doseNumber == null ? null : this.doseNumber.getValue(); 721 } 722 723 /** 724 * @param value Nominal position in a series as determined by the outcome of the evaluation process. 725 */ 726 public ImmunizationEvaluation setDoseNumber(String value) { 727 if (Utilities.noString(value)) 728 this.doseNumber = null; 729 else { 730 if (this.doseNumber == null) 731 this.doseNumber = new StringType(); 732 this.doseNumber.setValue(value); 733 } 734 return this; 735 } 736 737 /** 738 * @return {@link #seriesDoses} (The recommended number of doses to achieve immunity as determined by the outcome of the evaluation process.). This is the underlying object with id, value and extensions. The accessor "getSeriesDoses" gives direct access to the value 739 */ 740 public StringType getSeriesDosesElement() { 741 if (this.seriesDoses == null) 742 if (Configuration.errorOnAutoCreate()) 743 throw new Error("Attempt to auto-create ImmunizationEvaluation.seriesDoses"); 744 else if (Configuration.doAutoCreate()) 745 this.seriesDoses = new StringType(); // bb 746 return this.seriesDoses; 747 } 748 749 public boolean hasSeriesDosesElement() { 750 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 751 } 752 753 public boolean hasSeriesDoses() { 754 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 755 } 756 757 /** 758 * @param value {@link #seriesDoses} (The recommended number of doses to achieve immunity as determined by the outcome of the evaluation process.). This is the underlying object with id, value and extensions. The accessor "getSeriesDoses" gives direct access to the value 759 */ 760 public ImmunizationEvaluation setSeriesDosesElement(StringType value) { 761 this.seriesDoses = value; 762 return this; 763 } 764 765 /** 766 * @return The recommended number of doses to achieve immunity as determined by the outcome of the evaluation process. 767 */ 768 public String getSeriesDoses() { 769 return this.seriesDoses == null ? null : this.seriesDoses.getValue(); 770 } 771 772 /** 773 * @param value The recommended number of doses to achieve immunity as determined by the outcome of the evaluation process. 774 */ 775 public ImmunizationEvaluation setSeriesDoses(String value) { 776 if (Utilities.noString(value)) 777 this.seriesDoses = null; 778 else { 779 if (this.seriesDoses == null) 780 this.seriesDoses = new StringType(); 781 this.seriesDoses.setValue(value); 782 } 783 return this; 784 } 785 786 protected void listChildren(List<Property> children) { 787 super.listChildren(children); 788 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this immunization evaluation record.", 0, java.lang.Integer.MAX_VALUE, identifier)); 789 children.add(new Property("status", "code", "Indicates the current status of the evaluation of the vaccination administration event.", 0, 1, status)); 790 children.add(new Property("patient", "Reference(Patient)", "The individual for whom the evaluation is being done.", 0, 1, patient)); 791 children.add(new Property("date", "dateTime", "The date the evaluation of the vaccine administration event was performed.", 0, 1, date)); 792 children.add(new Property("authority", "Reference(Organization)", "Indicates the authority who published the protocol (e.g. ACIP).", 0, 1, authority)); 793 children.add(new Property("targetDisease", "CodeableConcept", "The vaccine preventable disease the dose is being evaluated against.", 0, 1, targetDisease)); 794 children.add(new Property("immunizationEvent", "Reference(Immunization)", "The vaccine administration event being evaluated.", 0, 1, immunizationEvent)); 795 children.add(new Property("doseStatus", "CodeableConcept", "Indicates if the dose is valid or not valid with respect to the published recommendations.", 0, 1, doseStatus)); 796 children.add(new Property("doseStatusReason", "CodeableConcept", "Provides an explanation as to why the vaccine administration event is valid or not relative to the published recommendations.", 0, java.lang.Integer.MAX_VALUE, doseStatusReason)); 797 children.add(new Property("description", "markdown", "Additional information about the evaluation.", 0, 1, description)); 798 children.add(new Property("series", "string", "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1, series)); 799 children.add(new Property("doseNumber", "string", "Nominal position in a series as determined by the outcome of the evaluation process.", 0, 1, doseNumber)); 800 children.add(new Property("seriesDoses", "string", "The recommended number of doses to achieve immunity as determined by the outcome of the evaluation process.", 0, 1, seriesDoses)); 801 } 802 803 @Override 804 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 805 switch (_hash) { 806 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this immunization evaluation record.", 0, java.lang.Integer.MAX_VALUE, identifier); 807 case -892481550: /*status*/ return new Property("status", "code", "Indicates the current status of the evaluation of the vaccination administration event.", 0, 1, status); 808 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The individual for whom the evaluation is being done.", 0, 1, patient); 809 case 3076014: /*date*/ return new Property("date", "dateTime", "The date the evaluation of the vaccine administration event was performed.", 0, 1, date); 810 case 1475610435: /*authority*/ return new Property("authority", "Reference(Organization)", "Indicates the authority who published the protocol (e.g. ACIP).", 0, 1, authority); 811 case -319593813: /*targetDisease*/ return new Property("targetDisease", "CodeableConcept", "The vaccine preventable disease the dose is being evaluated against.", 0, 1, targetDisease); 812 case 1081446840: /*immunizationEvent*/ return new Property("immunizationEvent", "Reference(Immunization)", "The vaccine administration event being evaluated.", 0, 1, immunizationEvent); 813 case -745826705: /*doseStatus*/ return new Property("doseStatus", "CodeableConcept", "Indicates if the dose is valid or not valid with respect to the published recommendations.", 0, 1, doseStatus); 814 case 662783379: /*doseStatusReason*/ return new Property("doseStatusReason", "CodeableConcept", "Provides an explanation as to why the vaccine administration event is valid or not relative to the published recommendations.", 0, java.lang.Integer.MAX_VALUE, doseStatusReason); 815 case -1724546052: /*description*/ return new Property("description", "markdown", "Additional information about the evaluation.", 0, 1, description); 816 case -905838985: /*series*/ return new Property("series", "string", "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1, series); 817 case -887709242: /*doseNumber*/ return new Property("doseNumber", "string", "Nominal position in a series as determined by the outcome of the evaluation process.", 0, 1, doseNumber); 818 case -1936727105: /*seriesDoses*/ return new Property("seriesDoses", "string", "The recommended number of doses to achieve immunity as determined by the outcome of the evaluation process.", 0, 1, seriesDoses); 819 default: return super.getNamedProperty(_hash, _name, _checkValid); 820 } 821 822 } 823 824 @Override 825 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 826 switch (hash) { 827 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 828 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ImmunizationEvaluationStatusCodes> 829 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 830 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 831 case 1475610435: /*authority*/ return this.authority == null ? new Base[0] : new Base[] {this.authority}; // Reference 832 case -319593813: /*targetDisease*/ return this.targetDisease == null ? new Base[0] : new Base[] {this.targetDisease}; // CodeableConcept 833 case 1081446840: /*immunizationEvent*/ return this.immunizationEvent == null ? new Base[0] : new Base[] {this.immunizationEvent}; // Reference 834 case -745826705: /*doseStatus*/ return this.doseStatus == null ? new Base[0] : new Base[] {this.doseStatus}; // CodeableConcept 835 case 662783379: /*doseStatusReason*/ return this.doseStatusReason == null ? new Base[0] : this.doseStatusReason.toArray(new Base[this.doseStatusReason.size()]); // CodeableConcept 836 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 837 case -905838985: /*series*/ return this.series == null ? new Base[0] : new Base[] {this.series}; // StringType 838 case -887709242: /*doseNumber*/ return this.doseNumber == null ? new Base[0] : new Base[] {this.doseNumber}; // StringType 839 case -1936727105: /*seriesDoses*/ return this.seriesDoses == null ? new Base[0] : new Base[] {this.seriesDoses}; // StringType 840 default: return super.getProperty(hash, name, checkValid); 841 } 842 843 } 844 845 @Override 846 public Base setProperty(int hash, String name, Base value) throws FHIRException { 847 switch (hash) { 848 case -1618432855: // identifier 849 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 850 return value; 851 case -892481550: // status 852 value = new ImmunizationEvaluationStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 853 this.status = (Enumeration) value; // Enumeration<ImmunizationEvaluationStatusCodes> 854 return value; 855 case -791418107: // patient 856 this.patient = TypeConvertor.castToReference(value); // Reference 857 return value; 858 case 3076014: // date 859 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 860 return value; 861 case 1475610435: // authority 862 this.authority = TypeConvertor.castToReference(value); // Reference 863 return value; 864 case -319593813: // targetDisease 865 this.targetDisease = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 866 return value; 867 case 1081446840: // immunizationEvent 868 this.immunizationEvent = TypeConvertor.castToReference(value); // Reference 869 return value; 870 case -745826705: // doseStatus 871 this.doseStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 872 return value; 873 case 662783379: // doseStatusReason 874 this.getDoseStatusReason().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 875 return value; 876 case -1724546052: // description 877 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 878 return value; 879 case -905838985: // series 880 this.series = TypeConvertor.castToString(value); // StringType 881 return value; 882 case -887709242: // doseNumber 883 this.doseNumber = TypeConvertor.castToString(value); // StringType 884 return value; 885 case -1936727105: // seriesDoses 886 this.seriesDoses = TypeConvertor.castToString(value); // StringType 887 return value; 888 default: return super.setProperty(hash, name, value); 889 } 890 891 } 892 893 @Override 894 public Base setProperty(String name, Base value) throws FHIRException { 895 if (name.equals("identifier")) { 896 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 897 } else if (name.equals("status")) { 898 value = new ImmunizationEvaluationStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 899 this.status = (Enumeration) value; // Enumeration<ImmunizationEvaluationStatusCodes> 900 } else if (name.equals("patient")) { 901 this.patient = TypeConvertor.castToReference(value); // Reference 902 } else if (name.equals("date")) { 903 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 904 } else if (name.equals("authority")) { 905 this.authority = TypeConvertor.castToReference(value); // Reference 906 } else if (name.equals("targetDisease")) { 907 this.targetDisease = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 908 } else if (name.equals("immunizationEvent")) { 909 this.immunizationEvent = TypeConvertor.castToReference(value); // Reference 910 } else if (name.equals("doseStatus")) { 911 this.doseStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 912 } else if (name.equals("doseStatusReason")) { 913 this.getDoseStatusReason().add(TypeConvertor.castToCodeableConcept(value)); 914 } else if (name.equals("description")) { 915 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 916 } else if (name.equals("series")) { 917 this.series = TypeConvertor.castToString(value); // StringType 918 } else if (name.equals("doseNumber")) { 919 this.doseNumber = TypeConvertor.castToString(value); // StringType 920 } else if (name.equals("seriesDoses")) { 921 this.seriesDoses = TypeConvertor.castToString(value); // StringType 922 } else 923 return super.setProperty(name, value); 924 return value; 925 } 926 927 @Override 928 public void removeChild(String name, Base value) throws FHIRException { 929 if (name.equals("identifier")) { 930 this.getIdentifier().remove(value); 931 } else if (name.equals("status")) { 932 value = new ImmunizationEvaluationStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 933 this.status = (Enumeration) value; // Enumeration<ImmunizationEvaluationStatusCodes> 934 } else if (name.equals("patient")) { 935 this.patient = null; 936 } else if (name.equals("date")) { 937 this.date = null; 938 } else if (name.equals("authority")) { 939 this.authority = null; 940 } else if (name.equals("targetDisease")) { 941 this.targetDisease = null; 942 } else if (name.equals("immunizationEvent")) { 943 this.immunizationEvent = null; 944 } else if (name.equals("doseStatus")) { 945 this.doseStatus = null; 946 } else if (name.equals("doseStatusReason")) { 947 this.getDoseStatusReason().remove(value); 948 } else if (name.equals("description")) { 949 this.description = null; 950 } else if (name.equals("series")) { 951 this.series = null; 952 } else if (name.equals("doseNumber")) { 953 this.doseNumber = null; 954 } else if (name.equals("seriesDoses")) { 955 this.seriesDoses = null; 956 } else 957 super.removeChild(name, value); 958 959 } 960 961 @Override 962 public Base makeProperty(int hash, String name) throws FHIRException { 963 switch (hash) { 964 case -1618432855: return addIdentifier(); 965 case -892481550: return getStatusElement(); 966 case -791418107: return getPatient(); 967 case 3076014: return getDateElement(); 968 case 1475610435: return getAuthority(); 969 case -319593813: return getTargetDisease(); 970 case 1081446840: return getImmunizationEvent(); 971 case -745826705: return getDoseStatus(); 972 case 662783379: return addDoseStatusReason(); 973 case -1724546052: return getDescriptionElement(); 974 case -905838985: return getSeriesElement(); 975 case -887709242: return getDoseNumberElement(); 976 case -1936727105: return getSeriesDosesElement(); 977 default: return super.makeProperty(hash, name); 978 } 979 980 } 981 982 @Override 983 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 984 switch (hash) { 985 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 986 case -892481550: /*status*/ return new String[] {"code"}; 987 case -791418107: /*patient*/ return new String[] {"Reference"}; 988 case 3076014: /*date*/ return new String[] {"dateTime"}; 989 case 1475610435: /*authority*/ return new String[] {"Reference"}; 990 case -319593813: /*targetDisease*/ return new String[] {"CodeableConcept"}; 991 case 1081446840: /*immunizationEvent*/ return new String[] {"Reference"}; 992 case -745826705: /*doseStatus*/ return new String[] {"CodeableConcept"}; 993 case 662783379: /*doseStatusReason*/ return new String[] {"CodeableConcept"}; 994 case -1724546052: /*description*/ return new String[] {"markdown"}; 995 case -905838985: /*series*/ return new String[] {"string"}; 996 case -887709242: /*doseNumber*/ return new String[] {"string"}; 997 case -1936727105: /*seriesDoses*/ return new String[] {"string"}; 998 default: return super.getTypesForProperty(hash, name); 999 } 1000 1001 } 1002 1003 @Override 1004 public Base addChild(String name) throws FHIRException { 1005 if (name.equals("identifier")) { 1006 return addIdentifier(); 1007 } 1008 else if (name.equals("status")) { 1009 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationEvaluation.status"); 1010 } 1011 else if (name.equals("patient")) { 1012 this.patient = new Reference(); 1013 return this.patient; 1014 } 1015 else if (name.equals("date")) { 1016 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationEvaluation.date"); 1017 } 1018 else if (name.equals("authority")) { 1019 this.authority = new Reference(); 1020 return this.authority; 1021 } 1022 else if (name.equals("targetDisease")) { 1023 this.targetDisease = new CodeableConcept(); 1024 return this.targetDisease; 1025 } 1026 else if (name.equals("immunizationEvent")) { 1027 this.immunizationEvent = new Reference(); 1028 return this.immunizationEvent; 1029 } 1030 else if (name.equals("doseStatus")) { 1031 this.doseStatus = new CodeableConcept(); 1032 return this.doseStatus; 1033 } 1034 else if (name.equals("doseStatusReason")) { 1035 return addDoseStatusReason(); 1036 } 1037 else if (name.equals("description")) { 1038 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationEvaluation.description"); 1039 } 1040 else if (name.equals("series")) { 1041 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationEvaluation.series"); 1042 } 1043 else if (name.equals("doseNumber")) { 1044 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationEvaluation.doseNumber"); 1045 } 1046 else if (name.equals("seriesDoses")) { 1047 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationEvaluation.seriesDoses"); 1048 } 1049 else 1050 return super.addChild(name); 1051 } 1052 1053 public String fhirType() { 1054 return "ImmunizationEvaluation"; 1055 1056 } 1057 1058 public ImmunizationEvaluation copy() { 1059 ImmunizationEvaluation dst = new ImmunizationEvaluation(); 1060 copyValues(dst); 1061 return dst; 1062 } 1063 1064 public void copyValues(ImmunizationEvaluation dst) { 1065 super.copyValues(dst); 1066 if (identifier != null) { 1067 dst.identifier = new ArrayList<Identifier>(); 1068 for (Identifier i : identifier) 1069 dst.identifier.add(i.copy()); 1070 }; 1071 dst.status = status == null ? null : status.copy(); 1072 dst.patient = patient == null ? null : patient.copy(); 1073 dst.date = date == null ? null : date.copy(); 1074 dst.authority = authority == null ? null : authority.copy(); 1075 dst.targetDisease = targetDisease == null ? null : targetDisease.copy(); 1076 dst.immunizationEvent = immunizationEvent == null ? null : immunizationEvent.copy(); 1077 dst.doseStatus = doseStatus == null ? null : doseStatus.copy(); 1078 if (doseStatusReason != null) { 1079 dst.doseStatusReason = new ArrayList<CodeableConcept>(); 1080 for (CodeableConcept i : doseStatusReason) 1081 dst.doseStatusReason.add(i.copy()); 1082 }; 1083 dst.description = description == null ? null : description.copy(); 1084 dst.series = series == null ? null : series.copy(); 1085 dst.doseNumber = doseNumber == null ? null : doseNumber.copy(); 1086 dst.seriesDoses = seriesDoses == null ? null : seriesDoses.copy(); 1087 } 1088 1089 protected ImmunizationEvaluation typedCopy() { 1090 return copy(); 1091 } 1092 1093 @Override 1094 public boolean equalsDeep(Base other_) { 1095 if (!super.equalsDeep(other_)) 1096 return false; 1097 if (!(other_ instanceof ImmunizationEvaluation)) 1098 return false; 1099 ImmunizationEvaluation o = (ImmunizationEvaluation) other_; 1100 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(patient, o.patient, true) 1101 && compareDeep(date, o.date, true) && compareDeep(authority, o.authority, true) && compareDeep(targetDisease, o.targetDisease, true) 1102 && compareDeep(immunizationEvent, o.immunizationEvent, true) && compareDeep(doseStatus, o.doseStatus, true) 1103 && compareDeep(doseStatusReason, o.doseStatusReason, true) && compareDeep(description, o.description, true) 1104 && compareDeep(series, o.series, true) && compareDeep(doseNumber, o.doseNumber, true) && compareDeep(seriesDoses, o.seriesDoses, true) 1105 ; 1106 } 1107 1108 @Override 1109 public boolean equalsShallow(Base other_) { 1110 if (!super.equalsShallow(other_)) 1111 return false; 1112 if (!(other_ instanceof ImmunizationEvaluation)) 1113 return false; 1114 ImmunizationEvaluation o = (ImmunizationEvaluation) other_; 1115 return compareValues(status, o.status, true) && compareValues(date, o.date, true) && compareValues(description, o.description, true) 1116 && compareValues(series, o.series, true) && compareValues(doseNumber, o.doseNumber, true) && compareValues(seriesDoses, o.seriesDoses, true) 1117 ; 1118 } 1119 1120 public boolean isEmpty() { 1121 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, patient 1122 , date, authority, targetDisease, immunizationEvent, doseStatus, doseStatusReason 1123 , description, series, doseNumber, seriesDoses); 1124 } 1125 1126 @Override 1127 public ResourceType getResourceType() { 1128 return ResourceType.ImmunizationEvaluation; 1129 } 1130 1131 /** 1132 * Search parameter: <b>dose-status</b> 1133 * <p> 1134 * Description: <b>The status of the dose relative to published recommendations</b><br> 1135 * Type: <b>token</b><br> 1136 * Path: <b>ImmunizationEvaluation.doseStatus</b><br> 1137 * </p> 1138 */ 1139 @SearchParamDefinition(name="dose-status", path="ImmunizationEvaluation.doseStatus", description="The status of the dose relative to published recommendations", type="token" ) 1140 public static final String SP_DOSE_STATUS = "dose-status"; 1141 /** 1142 * <b>Fluent Client</b> search parameter constant for <b>dose-status</b> 1143 * <p> 1144 * Description: <b>The status of the dose relative to published recommendations</b><br> 1145 * Type: <b>token</b><br> 1146 * Path: <b>ImmunizationEvaluation.doseStatus</b><br> 1147 * </p> 1148 */ 1149 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DOSE_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DOSE_STATUS); 1150 1151 /** 1152 * Search parameter: <b>immunization-event</b> 1153 * <p> 1154 * Description: <b>The vaccine administration event being evaluated</b><br> 1155 * Type: <b>reference</b><br> 1156 * Path: <b>ImmunizationEvaluation.immunizationEvent</b><br> 1157 * </p> 1158 */ 1159 @SearchParamDefinition(name="immunization-event", path="ImmunizationEvaluation.immunizationEvent", description="The vaccine administration event being evaluated", type="reference", target={Immunization.class } ) 1160 public static final String SP_IMMUNIZATION_EVENT = "immunization-event"; 1161 /** 1162 * <b>Fluent Client</b> search parameter constant for <b>immunization-event</b> 1163 * <p> 1164 * Description: <b>The vaccine administration event being evaluated</b><br> 1165 * Type: <b>reference</b><br> 1166 * Path: <b>ImmunizationEvaluation.immunizationEvent</b><br> 1167 * </p> 1168 */ 1169 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam IMMUNIZATION_EVENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_IMMUNIZATION_EVENT); 1170 1171/** 1172 * Constant for fluent queries to be used to add include statements. Specifies 1173 * the path value of "<b>ImmunizationEvaluation:immunization-event</b>". 1174 */ 1175 public static final ca.uhn.fhir.model.api.Include INCLUDE_IMMUNIZATION_EVENT = new ca.uhn.fhir.model.api.Include("ImmunizationEvaluation:immunization-event").toLocked(); 1176 1177 /** 1178 * Search parameter: <b>status</b> 1179 * <p> 1180 * Description: <b>Immunization evaluation status</b><br> 1181 * Type: <b>token</b><br> 1182 * Path: <b>ImmunizationEvaluation.status</b><br> 1183 * </p> 1184 */ 1185 @SearchParamDefinition(name="status", path="ImmunizationEvaluation.status", description="Immunization evaluation status", type="token" ) 1186 public static final String SP_STATUS = "status"; 1187 /** 1188 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1189 * <p> 1190 * Description: <b>Immunization evaluation status</b><br> 1191 * Type: <b>token</b><br> 1192 * Path: <b>ImmunizationEvaluation.status</b><br> 1193 * </p> 1194 */ 1195 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1196 1197 /** 1198 * Search parameter: <b>target-disease</b> 1199 * <p> 1200 * Description: <b>The vaccine preventable disease being evaluated against</b><br> 1201 * Type: <b>token</b><br> 1202 * Path: <b>ImmunizationEvaluation.targetDisease</b><br> 1203 * </p> 1204 */ 1205 @SearchParamDefinition(name="target-disease", path="ImmunizationEvaluation.targetDisease", description="The vaccine preventable disease being evaluated against", type="token" ) 1206 public static final String SP_TARGET_DISEASE = "target-disease"; 1207 /** 1208 * <b>Fluent Client</b> search parameter constant for <b>target-disease</b> 1209 * <p> 1210 * Description: <b>The vaccine preventable disease being evaluated against</b><br> 1211 * Type: <b>token</b><br> 1212 * Path: <b>ImmunizationEvaluation.targetDisease</b><br> 1213 * </p> 1214 */ 1215 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_DISEASE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TARGET_DISEASE); 1216 1217 /** 1218 * Search parameter: <b>date</b> 1219 * <p> 1220 * Description: <b>Multiple Resources: 1221 1222* [AdverseEvent](adverseevent.html): When the event occurred 1223* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 1224* [Appointment](appointment.html): Appointment date/time. 1225* [AuditEvent](auditevent.html): Time when the event was recorded 1226* [CarePlan](careplan.html): Time period plan covers 1227* [CareTeam](careteam.html): A date within the coverage time period. 1228* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 1229* [Composition](composition.html): Composition editing time 1230* [Consent](consent.html): When consent was agreed to 1231* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 1232* [DocumentReference](documentreference.html): When this document reference was created 1233* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 1234* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 1235* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 1236* [Flag](flag.html): Time period when flag is active 1237* [Immunization](immunization.html): Vaccination (non)-Administration Date 1238* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 1239* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 1240* [Invoice](invoice.html): Invoice date / posting date 1241* [List](list.html): When the list was prepared 1242* [MeasureReport](measurereport.html): The date of the measure report 1243* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 1244* [Observation](observation.html): Clinically relevant time/time-period for observation 1245* [Procedure](procedure.html): When the procedure occurred or is occurring 1246* [ResearchSubject](researchsubject.html): Start and end of participation 1247* [RiskAssessment](riskassessment.html): When was assessment made? 1248* [SupplyRequest](supplyrequest.html): When the request was made 1249</b><br> 1250 * Type: <b>date</b><br> 1251 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 1252 * </p> 1253 */ 1254 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 1255 public static final String SP_DATE = "date"; 1256 /** 1257 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1258 * <p> 1259 * Description: <b>Multiple Resources: 1260 1261* [AdverseEvent](adverseevent.html): When the event occurred 1262* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 1263* [Appointment](appointment.html): Appointment date/time. 1264* [AuditEvent](auditevent.html): Time when the event was recorded 1265* [CarePlan](careplan.html): Time period plan covers 1266* [CareTeam](careteam.html): A date within the coverage time period. 1267* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 1268* [Composition](composition.html): Composition editing time 1269* [Consent](consent.html): When consent was agreed to 1270* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 1271* [DocumentReference](documentreference.html): When this document reference was created 1272* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 1273* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 1274* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 1275* [Flag](flag.html): Time period when flag is active 1276* [Immunization](immunization.html): Vaccination (non)-Administration Date 1277* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 1278* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 1279* [Invoice](invoice.html): Invoice date / posting date 1280* [List](list.html): When the list was prepared 1281* [MeasureReport](measurereport.html): The date of the measure report 1282* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 1283* [Observation](observation.html): Clinically relevant time/time-period for observation 1284* [Procedure](procedure.html): When the procedure occurred or is occurring 1285* [ResearchSubject](researchsubject.html): Start and end of participation 1286* [RiskAssessment](riskassessment.html): When was assessment made? 1287* [SupplyRequest](supplyrequest.html): When the request was made 1288</b><br> 1289 * Type: <b>date</b><br> 1290 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 1291 * </p> 1292 */ 1293 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1294 1295 /** 1296 * Search parameter: <b>identifier</b> 1297 * <p> 1298 * Description: <b>Multiple Resources: 1299 1300* [Account](account.html): Account number 1301* [AdverseEvent](adverseevent.html): Business identifier for the event 1302* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1303* [Appointment](appointment.html): An Identifier of the Appointment 1304* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1305* [Basic](basic.html): Business identifier 1306* [BodyStructure](bodystructure.html): Bodystructure identifier 1307* [CarePlan](careplan.html): External Ids for this plan 1308* [CareTeam](careteam.html): External Ids for this team 1309* [ChargeItem](chargeitem.html): Business Identifier for item 1310* [Claim](claim.html): The primary identifier of the financial resource 1311* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1312* [ClinicalImpression](clinicalimpression.html): Business identifier 1313* [Communication](communication.html): Unique identifier 1314* [CommunicationRequest](communicationrequest.html): Unique identifier 1315* [Composition](composition.html): Version-independent identifier for the Composition 1316* [Condition](condition.html): A unique identifier of the condition record 1317* [Consent](consent.html): Identifier for this record (external references) 1318* [Contract](contract.html): The identity of the contract 1319* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1320* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1321* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1322* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1323* [DeviceRequest](devicerequest.html): Business identifier for request/order 1324* [DeviceUsage](deviceusage.html): Search by identifier 1325* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1326* [DocumentReference](documentreference.html): Identifier of the attachment binary 1327* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1328* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1329* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1330* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1331* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1332* [Flag](flag.html): Business identifier 1333* [Goal](goal.html): External Ids for this goal 1334* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1335* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1336* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1337* [Immunization](immunization.html): Business identifier 1338* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1339* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1340* [Invoice](invoice.html): Business Identifier for item 1341* [List](list.html): Business identifier 1342* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1343* [Medication](medication.html): Returns medications with this external identifier 1344* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1345* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1346* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1347* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1348* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1349* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1350* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1351* [Observation](observation.html): The unique id for a particular observation 1352* [Person](person.html): A person Identifier 1353* [Procedure](procedure.html): A unique identifier for a procedure 1354* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1355* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1356* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1357* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1358* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1359* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1360* [Specimen](specimen.html): The unique identifier associated with the specimen 1361* [SupplyDelivery](supplydelivery.html): External identifier 1362* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1363* [Task](task.html): Search for a task instance by its business identifier 1364* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1365</b><br> 1366 * Type: <b>token</b><br> 1367 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1368 * </p> 1369 */ 1370 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 1371 public static final String SP_IDENTIFIER = "identifier"; 1372 /** 1373 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1374 * <p> 1375 * Description: <b>Multiple Resources: 1376 1377* [Account](account.html): Account number 1378* [AdverseEvent](adverseevent.html): Business identifier for the event 1379* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1380* [Appointment](appointment.html): An Identifier of the Appointment 1381* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1382* [Basic](basic.html): Business identifier 1383* [BodyStructure](bodystructure.html): Bodystructure identifier 1384* [CarePlan](careplan.html): External Ids for this plan 1385* [CareTeam](careteam.html): External Ids for this team 1386* [ChargeItem](chargeitem.html): Business Identifier for item 1387* [Claim](claim.html): The primary identifier of the financial resource 1388* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1389* [ClinicalImpression](clinicalimpression.html): Business identifier 1390* [Communication](communication.html): Unique identifier 1391* [CommunicationRequest](communicationrequest.html): Unique identifier 1392* [Composition](composition.html): Version-independent identifier for the Composition 1393* [Condition](condition.html): A unique identifier of the condition record 1394* [Consent](consent.html): Identifier for this record (external references) 1395* [Contract](contract.html): The identity of the contract 1396* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1397* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1398* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1399* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1400* [DeviceRequest](devicerequest.html): Business identifier for request/order 1401* [DeviceUsage](deviceusage.html): Search by identifier 1402* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1403* [DocumentReference](documentreference.html): Identifier of the attachment binary 1404* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1405* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1406* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1407* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1408* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1409* [Flag](flag.html): Business identifier 1410* [Goal](goal.html): External Ids for this goal 1411* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1412* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1413* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1414* [Immunization](immunization.html): Business identifier 1415* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1416* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1417* [Invoice](invoice.html): Business Identifier for item 1418* [List](list.html): Business identifier 1419* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1420* [Medication](medication.html): Returns medications with this external identifier 1421* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1422* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1423* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1424* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1425* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1426* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1427* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1428* [Observation](observation.html): The unique id for a particular observation 1429* [Person](person.html): A person Identifier 1430* [Procedure](procedure.html): A unique identifier for a procedure 1431* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1432* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1433* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1434* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1435* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1436* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1437* [Specimen](specimen.html): The unique identifier associated with the specimen 1438* [SupplyDelivery](supplydelivery.html): External identifier 1439* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1440* [Task](task.html): Search for a task instance by its business identifier 1441* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1442</b><br> 1443 * Type: <b>token</b><br> 1444 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1445 * </p> 1446 */ 1447 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1448 1449 /** 1450 * Search parameter: <b>patient</b> 1451 * <p> 1452 * Description: <b>Multiple Resources: 1453 1454* [Account](account.html): The entity that caused the expenses 1455* [AdverseEvent](adverseevent.html): Subject impacted by event 1456* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1457* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1458* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1459* [AuditEvent](auditevent.html): Where the activity involved patient data 1460* [Basic](basic.html): Identifies the focus of this resource 1461* [BodyStructure](bodystructure.html): Who this is about 1462* [CarePlan](careplan.html): Who the care plan is for 1463* [CareTeam](careteam.html): Who care team is for 1464* [ChargeItem](chargeitem.html): Individual service was done for/to 1465* [Claim](claim.html): Patient receiving the products or services 1466* [ClaimResponse](claimresponse.html): The subject of care 1467* [ClinicalImpression](clinicalimpression.html): Patient assessed 1468* [Communication](communication.html): Focus of message 1469* [CommunicationRequest](communicationrequest.html): Focus of message 1470* [Composition](composition.html): Who and/or what the composition is about 1471* [Condition](condition.html): Who has the condition? 1472* [Consent](consent.html): Who the consent applies to 1473* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1474* [Coverage](coverage.html): Retrieve coverages for a patient 1475* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1476* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1477* [DetectedIssue](detectedissue.html): Associated patient 1478* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1479* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1480* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1481* [DocumentReference](documentreference.html): Who/what is the subject of the document 1482* [Encounter](encounter.html): The patient present at the encounter 1483* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1484* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1485* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1486* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1487* [Flag](flag.html): The identity of a subject to list flags for 1488* [Goal](goal.html): Who this goal is intended for 1489* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1490* [ImagingSelection](imagingselection.html): Who the study is about 1491* [ImagingStudy](imagingstudy.html): Who the study is about 1492* [Immunization](immunization.html): The patient for the vaccination record 1493* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1494* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1495* [Invoice](invoice.html): Recipient(s) of goods and services 1496* [List](list.html): If all resources have the same subject 1497* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1498* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1499* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1500* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1501* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1502* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1503* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1504* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1505* [Observation](observation.html): The subject that the observation is about (if patient) 1506* [Person](person.html): The Person links to this Patient 1507* [Procedure](procedure.html): Search by subject - a patient 1508* [Provenance](provenance.html): Where the activity involved patient data 1509* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1510* [RelatedPerson](relatedperson.html): The patient this related person is related to 1511* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1512* [ResearchSubject](researchsubject.html): Who or what is part of study 1513* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1514* [ServiceRequest](servicerequest.html): Search by subject - a patient 1515* [Specimen](specimen.html): The patient the specimen comes from 1516* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1517* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1518* [Task](task.html): Search by patient 1519* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1520</b><br> 1521 * Type: <b>reference</b><br> 1522 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1523 * </p> 1524 */ 1525 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 1526 public static final String SP_PATIENT = "patient"; 1527 /** 1528 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1529 * <p> 1530 * Description: <b>Multiple Resources: 1531 1532* [Account](account.html): The entity that caused the expenses 1533* [AdverseEvent](adverseevent.html): Subject impacted by event 1534* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 1535* [Appointment](appointment.html): One of the individuals of the appointment is this patient 1536* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 1537* [AuditEvent](auditevent.html): Where the activity involved patient data 1538* [Basic](basic.html): Identifies the focus of this resource 1539* [BodyStructure](bodystructure.html): Who this is about 1540* [CarePlan](careplan.html): Who the care plan is for 1541* [CareTeam](careteam.html): Who care team is for 1542* [ChargeItem](chargeitem.html): Individual service was done for/to 1543* [Claim](claim.html): Patient receiving the products or services 1544* [ClaimResponse](claimresponse.html): The subject of care 1545* [ClinicalImpression](clinicalimpression.html): Patient assessed 1546* [Communication](communication.html): Focus of message 1547* [CommunicationRequest](communicationrequest.html): Focus of message 1548* [Composition](composition.html): Who and/or what the composition is about 1549* [Condition](condition.html): Who has the condition? 1550* [Consent](consent.html): Who the consent applies to 1551* [Contract](contract.html): The identity of the subject of the contract (if a patient) 1552* [Coverage](coverage.html): Retrieve coverages for a patient 1553* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 1554* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 1555* [DetectedIssue](detectedissue.html): Associated patient 1556* [DeviceRequest](devicerequest.html): Individual the service is ordered for 1557* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 1558* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 1559* [DocumentReference](documentreference.html): Who/what is the subject of the document 1560* [Encounter](encounter.html): The patient present at the encounter 1561* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 1562* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 1563* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 1564* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 1565* [Flag](flag.html): The identity of a subject to list flags for 1566* [Goal](goal.html): Who this goal is intended for 1567* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 1568* [ImagingSelection](imagingselection.html): Who the study is about 1569* [ImagingStudy](imagingstudy.html): Who the study is about 1570* [Immunization](immunization.html): The patient for the vaccination record 1571* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 1572* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 1573* [Invoice](invoice.html): Recipient(s) of goods and services 1574* [List](list.html): If all resources have the same subject 1575* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 1576* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 1577* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 1578* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 1579* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 1580* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 1581* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 1582* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 1583* [Observation](observation.html): The subject that the observation is about (if patient) 1584* [Person](person.html): The Person links to this Patient 1585* [Procedure](procedure.html): Search by subject - a patient 1586* [Provenance](provenance.html): Where the activity involved patient data 1587* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 1588* [RelatedPerson](relatedperson.html): The patient this related person is related to 1589* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 1590* [ResearchSubject](researchsubject.html): Who or what is part of study 1591* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 1592* [ServiceRequest](servicerequest.html): Search by subject - a patient 1593* [Specimen](specimen.html): The patient the specimen comes from 1594* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 1595* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 1596* [Task](task.html): Search by patient 1597* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 1598</b><br> 1599 * Type: <b>reference</b><br> 1600 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 1601 * </p> 1602 */ 1603 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1604 1605/** 1606 * Constant for fluent queries to be used to add include statements. Specifies 1607 * the path value of "<b>ImmunizationEvaluation:patient</b>". 1608 */ 1609 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ImmunizationEvaluation:patient").toLocked(); 1610 1611 1612} 1613