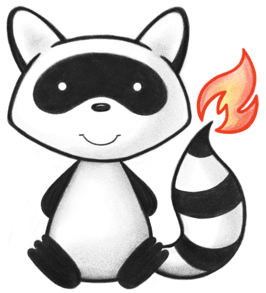
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A patient's point-in-time set of recommendations (i.e. forecasting) according to a published schedule with optional supporting justification. 052 */ 053@ResourceDef(name="ImmunizationRecommendation", profile="http://hl7.org/fhir/StructureDefinition/ImmunizationRecommendation") 054public class ImmunizationRecommendation extends DomainResource { 055 056 @Block() 057 public static class ImmunizationRecommendationRecommendationComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Vaccine(s) or vaccine group that pertain to the recommendation. 060 */ 061 @Child(name = "vaccineCode", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 062 @Description(shortDefinition="Vaccine or vaccine group recommendation applies to", formalDefinition="Vaccine(s) or vaccine group that pertain to the recommendation." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vaccine-code") 064 protected List<CodeableConcept> vaccineCode; 065 066 /** 067 * The targeted disease for the recommendation. 068 */ 069 @Child(name = "targetDisease", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 070 @Description(shortDefinition="Disease to be immunized against", formalDefinition="The targeted disease for the recommendation." ) 071 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-target-disease") 072 protected List<CodeableConcept> targetDisease; 073 074 /** 075 * Vaccine(s) which should not be used to fulfill the recommendation. 076 */ 077 @Child(name = "contraindicatedVaccineCode", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 078 @Description(shortDefinition="Vaccine which is contraindicated to fulfill the recommendation", formalDefinition="Vaccine(s) which should not be used to fulfill the recommendation." ) 079 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vaccine-code") 080 protected List<CodeableConcept> contraindicatedVaccineCode; 081 082 /** 083 * Indicates the patient status with respect to the path to immunity for the target disease. 084 */ 085 @Child(name = "forecastStatus", type = {CodeableConcept.class}, order=4, min=1, max=1, modifier=true, summary=true) 086 @Description(shortDefinition="Vaccine recommendation status", formalDefinition="Indicates the patient status with respect to the path to immunity for the target disease." ) 087 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-recommendation-status") 088 protected CodeableConcept forecastStatus; 089 090 /** 091 * The reason for the assigned forecast status. 092 */ 093 @Child(name = "forecastReason", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 094 @Description(shortDefinition="Vaccine administration status reason", formalDefinition="The reason for the assigned forecast status." ) 095 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-recommendation-reason") 096 protected List<CodeableConcept> forecastReason; 097 098 /** 099 * Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc. 100 */ 101 @Child(name = "dateCriterion", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 102 @Description(shortDefinition="Dates governing proposed immunization", formalDefinition="Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc." ) 103 protected List<ImmunizationRecommendationRecommendationDateCriterionComponent> dateCriterion; 104 105 /** 106 * Contains the description about the protocol under which the vaccine was administered. 107 */ 108 @Child(name = "description", type = {MarkdownType.class}, order=7, min=0, max=1, modifier=false, summary=false) 109 @Description(shortDefinition="Protocol details", formalDefinition="Contains the description about the protocol under which the vaccine was administered." ) 110 protected MarkdownType description; 111 112 /** 113 * One possible path to achieve presumed immunity against a disease - within the context of an authority. 114 */ 115 @Child(name = "series", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=false) 116 @Description(shortDefinition="Name of vaccination series", formalDefinition="One possible path to achieve presumed immunity against a disease - within the context of an authority." ) 117 protected StringType series; 118 119 /** 120 * Nominal position of the recommended dose in a series as determined by the evaluation and forecasting process (e.g. dose 2 is the next recommended dose). 121 */ 122 @Child(name = "doseNumber", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 123 @Description(shortDefinition="Recommended dose number within series", formalDefinition="Nominal position of the recommended dose in a series as determined by the evaluation and forecasting process (e.g. dose 2 is the next recommended dose)." ) 124 protected StringType doseNumber; 125 126 /** 127 * The recommended number of doses to achieve immunity as determined by the evaluation and forecasting process. 128 */ 129 @Child(name = "seriesDoses", type = {StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 130 @Description(shortDefinition="Recommended number of doses for immunity", formalDefinition="The recommended number of doses to achieve immunity as determined by the evaluation and forecasting process." ) 131 protected StringType seriesDoses; 132 133 /** 134 * Immunization event history and/or evaluation that supports the status and recommendation. 135 */ 136 @Child(name = "supportingImmunization", type = {Immunization.class, ImmunizationEvaluation.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 137 @Description(shortDefinition="Past immunizations supporting recommendation", formalDefinition="Immunization event history and/or evaluation that supports the status and recommendation." ) 138 protected List<Reference> supportingImmunization; 139 140 /** 141 * Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information. 142 */ 143 @Child(name = "supportingPatientInformation", type = {Reference.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 144 @Description(shortDefinition="Patient observations supporting recommendation", formalDefinition="Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information." ) 145 protected List<Reference> supportingPatientInformation; 146 147 private static final long serialVersionUID = 1830552442L; 148 149 /** 150 * Constructor 151 */ 152 public ImmunizationRecommendationRecommendationComponent() { 153 super(); 154 } 155 156 /** 157 * Constructor 158 */ 159 public ImmunizationRecommendationRecommendationComponent(CodeableConcept forecastStatus) { 160 super(); 161 this.setForecastStatus(forecastStatus); 162 } 163 164 /** 165 * @return {@link #vaccineCode} (Vaccine(s) or vaccine group that pertain to the recommendation.) 166 */ 167 public List<CodeableConcept> getVaccineCode() { 168 if (this.vaccineCode == null) 169 this.vaccineCode = new ArrayList<CodeableConcept>(); 170 return this.vaccineCode; 171 } 172 173 /** 174 * @return Returns a reference to <code>this</code> for easy method chaining 175 */ 176 public ImmunizationRecommendationRecommendationComponent setVaccineCode(List<CodeableConcept> theVaccineCode) { 177 this.vaccineCode = theVaccineCode; 178 return this; 179 } 180 181 public boolean hasVaccineCode() { 182 if (this.vaccineCode == null) 183 return false; 184 for (CodeableConcept item : this.vaccineCode) 185 if (!item.isEmpty()) 186 return true; 187 return false; 188 } 189 190 public CodeableConcept addVaccineCode() { //3 191 CodeableConcept t = new CodeableConcept(); 192 if (this.vaccineCode == null) 193 this.vaccineCode = new ArrayList<CodeableConcept>(); 194 this.vaccineCode.add(t); 195 return t; 196 } 197 198 public ImmunizationRecommendationRecommendationComponent addVaccineCode(CodeableConcept t) { //3 199 if (t == null) 200 return this; 201 if (this.vaccineCode == null) 202 this.vaccineCode = new ArrayList<CodeableConcept>(); 203 this.vaccineCode.add(t); 204 return this; 205 } 206 207 /** 208 * @return The first repetition of repeating field {@link #vaccineCode}, creating it if it does not already exist {3} 209 */ 210 public CodeableConcept getVaccineCodeFirstRep() { 211 if (getVaccineCode().isEmpty()) { 212 addVaccineCode(); 213 } 214 return getVaccineCode().get(0); 215 } 216 217 /** 218 * @return {@link #targetDisease} (The targeted disease for the recommendation.) 219 */ 220 public List<CodeableConcept> getTargetDisease() { 221 if (this.targetDisease == null) 222 this.targetDisease = new ArrayList<CodeableConcept>(); 223 return this.targetDisease; 224 } 225 226 /** 227 * @return Returns a reference to <code>this</code> for easy method chaining 228 */ 229 public ImmunizationRecommendationRecommendationComponent setTargetDisease(List<CodeableConcept> theTargetDisease) { 230 this.targetDisease = theTargetDisease; 231 return this; 232 } 233 234 public boolean hasTargetDisease() { 235 if (this.targetDisease == null) 236 return false; 237 for (CodeableConcept item : this.targetDisease) 238 if (!item.isEmpty()) 239 return true; 240 return false; 241 } 242 243 public CodeableConcept addTargetDisease() { //3 244 CodeableConcept t = new CodeableConcept(); 245 if (this.targetDisease == null) 246 this.targetDisease = new ArrayList<CodeableConcept>(); 247 this.targetDisease.add(t); 248 return t; 249 } 250 251 public ImmunizationRecommendationRecommendationComponent addTargetDisease(CodeableConcept t) { //3 252 if (t == null) 253 return this; 254 if (this.targetDisease == null) 255 this.targetDisease = new ArrayList<CodeableConcept>(); 256 this.targetDisease.add(t); 257 return this; 258 } 259 260 /** 261 * @return The first repetition of repeating field {@link #targetDisease}, creating it if it does not already exist {3} 262 */ 263 public CodeableConcept getTargetDiseaseFirstRep() { 264 if (getTargetDisease().isEmpty()) { 265 addTargetDisease(); 266 } 267 return getTargetDisease().get(0); 268 } 269 270 /** 271 * @return {@link #contraindicatedVaccineCode} (Vaccine(s) which should not be used to fulfill the recommendation.) 272 */ 273 public List<CodeableConcept> getContraindicatedVaccineCode() { 274 if (this.contraindicatedVaccineCode == null) 275 this.contraindicatedVaccineCode = new ArrayList<CodeableConcept>(); 276 return this.contraindicatedVaccineCode; 277 } 278 279 /** 280 * @return Returns a reference to <code>this</code> for easy method chaining 281 */ 282 public ImmunizationRecommendationRecommendationComponent setContraindicatedVaccineCode(List<CodeableConcept> theContraindicatedVaccineCode) { 283 this.contraindicatedVaccineCode = theContraindicatedVaccineCode; 284 return this; 285 } 286 287 public boolean hasContraindicatedVaccineCode() { 288 if (this.contraindicatedVaccineCode == null) 289 return false; 290 for (CodeableConcept item : this.contraindicatedVaccineCode) 291 if (!item.isEmpty()) 292 return true; 293 return false; 294 } 295 296 public CodeableConcept addContraindicatedVaccineCode() { //3 297 CodeableConcept t = new CodeableConcept(); 298 if (this.contraindicatedVaccineCode == null) 299 this.contraindicatedVaccineCode = new ArrayList<CodeableConcept>(); 300 this.contraindicatedVaccineCode.add(t); 301 return t; 302 } 303 304 public ImmunizationRecommendationRecommendationComponent addContraindicatedVaccineCode(CodeableConcept t) { //3 305 if (t == null) 306 return this; 307 if (this.contraindicatedVaccineCode == null) 308 this.contraindicatedVaccineCode = new ArrayList<CodeableConcept>(); 309 this.contraindicatedVaccineCode.add(t); 310 return this; 311 } 312 313 /** 314 * @return The first repetition of repeating field {@link #contraindicatedVaccineCode}, creating it if it does not already exist {3} 315 */ 316 public CodeableConcept getContraindicatedVaccineCodeFirstRep() { 317 if (getContraindicatedVaccineCode().isEmpty()) { 318 addContraindicatedVaccineCode(); 319 } 320 return getContraindicatedVaccineCode().get(0); 321 } 322 323 /** 324 * @return {@link #forecastStatus} (Indicates the patient status with respect to the path to immunity for the target disease.) 325 */ 326 public CodeableConcept getForecastStatus() { 327 if (this.forecastStatus == null) 328 if (Configuration.errorOnAutoCreate()) 329 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.forecastStatus"); 330 else if (Configuration.doAutoCreate()) 331 this.forecastStatus = new CodeableConcept(); // cc 332 return this.forecastStatus; 333 } 334 335 public boolean hasForecastStatus() { 336 return this.forecastStatus != null && !this.forecastStatus.isEmpty(); 337 } 338 339 /** 340 * @param value {@link #forecastStatus} (Indicates the patient status with respect to the path to immunity for the target disease.) 341 */ 342 public ImmunizationRecommendationRecommendationComponent setForecastStatus(CodeableConcept value) { 343 this.forecastStatus = value; 344 return this; 345 } 346 347 /** 348 * @return {@link #forecastReason} (The reason for the assigned forecast status.) 349 */ 350 public List<CodeableConcept> getForecastReason() { 351 if (this.forecastReason == null) 352 this.forecastReason = new ArrayList<CodeableConcept>(); 353 return this.forecastReason; 354 } 355 356 /** 357 * @return Returns a reference to <code>this</code> for easy method chaining 358 */ 359 public ImmunizationRecommendationRecommendationComponent setForecastReason(List<CodeableConcept> theForecastReason) { 360 this.forecastReason = theForecastReason; 361 return this; 362 } 363 364 public boolean hasForecastReason() { 365 if (this.forecastReason == null) 366 return false; 367 for (CodeableConcept item : this.forecastReason) 368 if (!item.isEmpty()) 369 return true; 370 return false; 371 } 372 373 public CodeableConcept addForecastReason() { //3 374 CodeableConcept t = new CodeableConcept(); 375 if (this.forecastReason == null) 376 this.forecastReason = new ArrayList<CodeableConcept>(); 377 this.forecastReason.add(t); 378 return t; 379 } 380 381 public ImmunizationRecommendationRecommendationComponent addForecastReason(CodeableConcept t) { //3 382 if (t == null) 383 return this; 384 if (this.forecastReason == null) 385 this.forecastReason = new ArrayList<CodeableConcept>(); 386 this.forecastReason.add(t); 387 return this; 388 } 389 390 /** 391 * @return The first repetition of repeating field {@link #forecastReason}, creating it if it does not already exist {3} 392 */ 393 public CodeableConcept getForecastReasonFirstRep() { 394 if (getForecastReason().isEmpty()) { 395 addForecastReason(); 396 } 397 return getForecastReason().get(0); 398 } 399 400 /** 401 * @return {@link #dateCriterion} (Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc.) 402 */ 403 public List<ImmunizationRecommendationRecommendationDateCriterionComponent> getDateCriterion() { 404 if (this.dateCriterion == null) 405 this.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 406 return this.dateCriterion; 407 } 408 409 /** 410 * @return Returns a reference to <code>this</code> for easy method chaining 411 */ 412 public ImmunizationRecommendationRecommendationComponent setDateCriterion(List<ImmunizationRecommendationRecommendationDateCriterionComponent> theDateCriterion) { 413 this.dateCriterion = theDateCriterion; 414 return this; 415 } 416 417 public boolean hasDateCriterion() { 418 if (this.dateCriterion == null) 419 return false; 420 for (ImmunizationRecommendationRecommendationDateCriterionComponent item : this.dateCriterion) 421 if (!item.isEmpty()) 422 return true; 423 return false; 424 } 425 426 public ImmunizationRecommendationRecommendationDateCriterionComponent addDateCriterion() { //3 427 ImmunizationRecommendationRecommendationDateCriterionComponent t = new ImmunizationRecommendationRecommendationDateCriterionComponent(); 428 if (this.dateCriterion == null) 429 this.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 430 this.dateCriterion.add(t); 431 return t; 432 } 433 434 public ImmunizationRecommendationRecommendationComponent addDateCriterion(ImmunizationRecommendationRecommendationDateCriterionComponent t) { //3 435 if (t == null) 436 return this; 437 if (this.dateCriterion == null) 438 this.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 439 this.dateCriterion.add(t); 440 return this; 441 } 442 443 /** 444 * @return The first repetition of repeating field {@link #dateCriterion}, creating it if it does not already exist {3} 445 */ 446 public ImmunizationRecommendationRecommendationDateCriterionComponent getDateCriterionFirstRep() { 447 if (getDateCriterion().isEmpty()) { 448 addDateCriterion(); 449 } 450 return getDateCriterion().get(0); 451 } 452 453 /** 454 * @return {@link #description} (Contains the description about the protocol under which the vaccine was administered.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 455 */ 456 public MarkdownType getDescriptionElement() { 457 if (this.description == null) 458 if (Configuration.errorOnAutoCreate()) 459 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.description"); 460 else if (Configuration.doAutoCreate()) 461 this.description = new MarkdownType(); // bb 462 return this.description; 463 } 464 465 public boolean hasDescriptionElement() { 466 return this.description != null && !this.description.isEmpty(); 467 } 468 469 public boolean hasDescription() { 470 return this.description != null && !this.description.isEmpty(); 471 } 472 473 /** 474 * @param value {@link #description} (Contains the description about the protocol under which the vaccine was administered.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 475 */ 476 public ImmunizationRecommendationRecommendationComponent setDescriptionElement(MarkdownType value) { 477 this.description = value; 478 return this; 479 } 480 481 /** 482 * @return Contains the description about the protocol under which the vaccine was administered. 483 */ 484 public String getDescription() { 485 return this.description == null ? null : this.description.getValue(); 486 } 487 488 /** 489 * @param value Contains the description about the protocol under which the vaccine was administered. 490 */ 491 public ImmunizationRecommendationRecommendationComponent setDescription(String value) { 492 if (Utilities.noString(value)) 493 this.description = null; 494 else { 495 if (this.description == null) 496 this.description = new MarkdownType(); 497 this.description.setValue(value); 498 } 499 return this; 500 } 501 502 /** 503 * @return {@link #series} (One possible path to achieve presumed immunity against a disease - within the context of an authority.). This is the underlying object with id, value and extensions. The accessor "getSeries" gives direct access to the value 504 */ 505 public StringType getSeriesElement() { 506 if (this.series == null) 507 if (Configuration.errorOnAutoCreate()) 508 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.series"); 509 else if (Configuration.doAutoCreate()) 510 this.series = new StringType(); // bb 511 return this.series; 512 } 513 514 public boolean hasSeriesElement() { 515 return this.series != null && !this.series.isEmpty(); 516 } 517 518 public boolean hasSeries() { 519 return this.series != null && !this.series.isEmpty(); 520 } 521 522 /** 523 * @param value {@link #series} (One possible path to achieve presumed immunity against a disease - within the context of an authority.). This is the underlying object with id, value and extensions. The accessor "getSeries" gives direct access to the value 524 */ 525 public ImmunizationRecommendationRecommendationComponent setSeriesElement(StringType value) { 526 this.series = value; 527 return this; 528 } 529 530 /** 531 * @return One possible path to achieve presumed immunity against a disease - within the context of an authority. 532 */ 533 public String getSeries() { 534 return this.series == null ? null : this.series.getValue(); 535 } 536 537 /** 538 * @param value One possible path to achieve presumed immunity against a disease - within the context of an authority. 539 */ 540 public ImmunizationRecommendationRecommendationComponent setSeries(String value) { 541 if (Utilities.noString(value)) 542 this.series = null; 543 else { 544 if (this.series == null) 545 this.series = new StringType(); 546 this.series.setValue(value); 547 } 548 return this; 549 } 550 551 /** 552 * @return {@link #doseNumber} (Nominal position of the recommended dose in a series as determined by the evaluation and forecasting process (e.g. dose 2 is the next recommended dose).). This is the underlying object with id, value and extensions. The accessor "getDoseNumber" gives direct access to the value 553 */ 554 public StringType getDoseNumberElement() { 555 if (this.doseNumber == null) 556 if (Configuration.errorOnAutoCreate()) 557 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.doseNumber"); 558 else if (Configuration.doAutoCreate()) 559 this.doseNumber = new StringType(); // bb 560 return this.doseNumber; 561 } 562 563 public boolean hasDoseNumberElement() { 564 return this.doseNumber != null && !this.doseNumber.isEmpty(); 565 } 566 567 public boolean hasDoseNumber() { 568 return this.doseNumber != null && !this.doseNumber.isEmpty(); 569 } 570 571 /** 572 * @param value {@link #doseNumber} (Nominal position of the recommended dose in a series as determined by the evaluation and forecasting process (e.g. dose 2 is the next recommended dose).). This is the underlying object with id, value and extensions. The accessor "getDoseNumber" gives direct access to the value 573 */ 574 public ImmunizationRecommendationRecommendationComponent setDoseNumberElement(StringType value) { 575 this.doseNumber = value; 576 return this; 577 } 578 579 /** 580 * @return Nominal position of the recommended dose in a series as determined by the evaluation and forecasting process (e.g. dose 2 is the next recommended dose). 581 */ 582 public String getDoseNumber() { 583 return this.doseNumber == null ? null : this.doseNumber.getValue(); 584 } 585 586 /** 587 * @param value Nominal position of the recommended dose in a series as determined by the evaluation and forecasting process (e.g. dose 2 is the next recommended dose). 588 */ 589 public ImmunizationRecommendationRecommendationComponent setDoseNumber(String value) { 590 if (Utilities.noString(value)) 591 this.doseNumber = null; 592 else { 593 if (this.doseNumber == null) 594 this.doseNumber = new StringType(); 595 this.doseNumber.setValue(value); 596 } 597 return this; 598 } 599 600 /** 601 * @return {@link #seriesDoses} (The recommended number of doses to achieve immunity as determined by the evaluation and forecasting process.). This is the underlying object with id, value and extensions. The accessor "getSeriesDoses" gives direct access to the value 602 */ 603 public StringType getSeriesDosesElement() { 604 if (this.seriesDoses == null) 605 if (Configuration.errorOnAutoCreate()) 606 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.seriesDoses"); 607 else if (Configuration.doAutoCreate()) 608 this.seriesDoses = new StringType(); // bb 609 return this.seriesDoses; 610 } 611 612 public boolean hasSeriesDosesElement() { 613 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 614 } 615 616 public boolean hasSeriesDoses() { 617 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 618 } 619 620 /** 621 * @param value {@link #seriesDoses} (The recommended number of doses to achieve immunity as determined by the evaluation and forecasting process.). This is the underlying object with id, value and extensions. The accessor "getSeriesDoses" gives direct access to the value 622 */ 623 public ImmunizationRecommendationRecommendationComponent setSeriesDosesElement(StringType value) { 624 this.seriesDoses = value; 625 return this; 626 } 627 628 /** 629 * @return The recommended number of doses to achieve immunity as determined by the evaluation and forecasting process. 630 */ 631 public String getSeriesDoses() { 632 return this.seriesDoses == null ? null : this.seriesDoses.getValue(); 633 } 634 635 /** 636 * @param value The recommended number of doses to achieve immunity as determined by the evaluation and forecasting process. 637 */ 638 public ImmunizationRecommendationRecommendationComponent setSeriesDoses(String value) { 639 if (Utilities.noString(value)) 640 this.seriesDoses = null; 641 else { 642 if (this.seriesDoses == null) 643 this.seriesDoses = new StringType(); 644 this.seriesDoses.setValue(value); 645 } 646 return this; 647 } 648 649 /** 650 * @return {@link #supportingImmunization} (Immunization event history and/or evaluation that supports the status and recommendation.) 651 */ 652 public List<Reference> getSupportingImmunization() { 653 if (this.supportingImmunization == null) 654 this.supportingImmunization = new ArrayList<Reference>(); 655 return this.supportingImmunization; 656 } 657 658 /** 659 * @return Returns a reference to <code>this</code> for easy method chaining 660 */ 661 public ImmunizationRecommendationRecommendationComponent setSupportingImmunization(List<Reference> theSupportingImmunization) { 662 this.supportingImmunization = theSupportingImmunization; 663 return this; 664 } 665 666 public boolean hasSupportingImmunization() { 667 if (this.supportingImmunization == null) 668 return false; 669 for (Reference item : this.supportingImmunization) 670 if (!item.isEmpty()) 671 return true; 672 return false; 673 } 674 675 public Reference addSupportingImmunization() { //3 676 Reference t = new Reference(); 677 if (this.supportingImmunization == null) 678 this.supportingImmunization = new ArrayList<Reference>(); 679 this.supportingImmunization.add(t); 680 return t; 681 } 682 683 public ImmunizationRecommendationRecommendationComponent addSupportingImmunization(Reference t) { //3 684 if (t == null) 685 return this; 686 if (this.supportingImmunization == null) 687 this.supportingImmunization = new ArrayList<Reference>(); 688 this.supportingImmunization.add(t); 689 return this; 690 } 691 692 /** 693 * @return The first repetition of repeating field {@link #supportingImmunization}, creating it if it does not already exist {3} 694 */ 695 public Reference getSupportingImmunizationFirstRep() { 696 if (getSupportingImmunization().isEmpty()) { 697 addSupportingImmunization(); 698 } 699 return getSupportingImmunization().get(0); 700 } 701 702 /** 703 * @return {@link #supportingPatientInformation} (Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information.) 704 */ 705 public List<Reference> getSupportingPatientInformation() { 706 if (this.supportingPatientInformation == null) 707 this.supportingPatientInformation = new ArrayList<Reference>(); 708 return this.supportingPatientInformation; 709 } 710 711 /** 712 * @return Returns a reference to <code>this</code> for easy method chaining 713 */ 714 public ImmunizationRecommendationRecommendationComponent setSupportingPatientInformation(List<Reference> theSupportingPatientInformation) { 715 this.supportingPatientInformation = theSupportingPatientInformation; 716 return this; 717 } 718 719 public boolean hasSupportingPatientInformation() { 720 if (this.supportingPatientInformation == null) 721 return false; 722 for (Reference item : this.supportingPatientInformation) 723 if (!item.isEmpty()) 724 return true; 725 return false; 726 } 727 728 public Reference addSupportingPatientInformation() { //3 729 Reference t = new Reference(); 730 if (this.supportingPatientInformation == null) 731 this.supportingPatientInformation = new ArrayList<Reference>(); 732 this.supportingPatientInformation.add(t); 733 return t; 734 } 735 736 public ImmunizationRecommendationRecommendationComponent addSupportingPatientInformation(Reference t) { //3 737 if (t == null) 738 return this; 739 if (this.supportingPatientInformation == null) 740 this.supportingPatientInformation = new ArrayList<Reference>(); 741 this.supportingPatientInformation.add(t); 742 return this; 743 } 744 745 /** 746 * @return The first repetition of repeating field {@link #supportingPatientInformation}, creating it if it does not already exist {3} 747 */ 748 public Reference getSupportingPatientInformationFirstRep() { 749 if (getSupportingPatientInformation().isEmpty()) { 750 addSupportingPatientInformation(); 751 } 752 return getSupportingPatientInformation().get(0); 753 } 754 755 protected void listChildren(List<Property> children) { 756 super.listChildren(children); 757 children.add(new Property("vaccineCode", "CodeableConcept", "Vaccine(s) or vaccine group that pertain to the recommendation.", 0, java.lang.Integer.MAX_VALUE, vaccineCode)); 758 children.add(new Property("targetDisease", "CodeableConcept", "The targeted disease for the recommendation.", 0, java.lang.Integer.MAX_VALUE, targetDisease)); 759 children.add(new Property("contraindicatedVaccineCode", "CodeableConcept", "Vaccine(s) which should not be used to fulfill the recommendation.", 0, java.lang.Integer.MAX_VALUE, contraindicatedVaccineCode)); 760 children.add(new Property("forecastStatus", "CodeableConcept", "Indicates the patient status with respect to the path to immunity for the target disease.", 0, 1, forecastStatus)); 761 children.add(new Property("forecastReason", "CodeableConcept", "The reason for the assigned forecast status.", 0, java.lang.Integer.MAX_VALUE, forecastReason)); 762 children.add(new Property("dateCriterion", "", "Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc.", 0, java.lang.Integer.MAX_VALUE, dateCriterion)); 763 children.add(new Property("description", "markdown", "Contains the description about the protocol under which the vaccine was administered.", 0, 1, description)); 764 children.add(new Property("series", "string", "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1, series)); 765 children.add(new Property("doseNumber", "string", "Nominal position of the recommended dose in a series as determined by the evaluation and forecasting process (e.g. dose 2 is the next recommended dose).", 0, 1, doseNumber)); 766 children.add(new Property("seriesDoses", "string", "The recommended number of doses to achieve immunity as determined by the evaluation and forecasting process.", 0, 1, seriesDoses)); 767 children.add(new Property("supportingImmunization", "Reference(Immunization|ImmunizationEvaluation)", "Immunization event history and/or evaluation that supports the status and recommendation.", 0, java.lang.Integer.MAX_VALUE, supportingImmunization)); 768 children.add(new Property("supportingPatientInformation", "Reference(Any)", "Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information.", 0, java.lang.Integer.MAX_VALUE, supportingPatientInformation)); 769 } 770 771 @Override 772 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 773 switch (_hash) { 774 case 664556354: /*vaccineCode*/ return new Property("vaccineCode", "CodeableConcept", "Vaccine(s) or vaccine group that pertain to the recommendation.", 0, java.lang.Integer.MAX_VALUE, vaccineCode); 775 case -319593813: /*targetDisease*/ return new Property("targetDisease", "CodeableConcept", "The targeted disease for the recommendation.", 0, java.lang.Integer.MAX_VALUE, targetDisease); 776 case 571105240: /*contraindicatedVaccineCode*/ return new Property("contraindicatedVaccineCode", "CodeableConcept", "Vaccine(s) which should not be used to fulfill the recommendation.", 0, java.lang.Integer.MAX_VALUE, contraindicatedVaccineCode); 777 case 1904598477: /*forecastStatus*/ return new Property("forecastStatus", "CodeableConcept", "Indicates the patient status with respect to the path to immunity for the target disease.", 0, 1, forecastStatus); 778 case 1862115359: /*forecastReason*/ return new Property("forecastReason", "CodeableConcept", "The reason for the assigned forecast status.", 0, java.lang.Integer.MAX_VALUE, forecastReason); 779 case 2087518867: /*dateCriterion*/ return new Property("dateCriterion", "", "Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc.", 0, java.lang.Integer.MAX_VALUE, dateCriterion); 780 case -1724546052: /*description*/ return new Property("description", "markdown", "Contains the description about the protocol under which the vaccine was administered.", 0, 1, description); 781 case -905838985: /*series*/ return new Property("series", "string", "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1, series); 782 case -887709242: /*doseNumber*/ return new Property("doseNumber", "string", "Nominal position of the recommended dose in a series as determined by the evaluation and forecasting process (e.g. dose 2 is the next recommended dose).", 0, 1, doseNumber); 783 case -1936727105: /*seriesDoses*/ return new Property("seriesDoses", "string", "The recommended number of doses to achieve immunity as determined by the evaluation and forecasting process.", 0, 1, seriesDoses); 784 case 1171592021: /*supportingImmunization*/ return new Property("supportingImmunization", "Reference(Immunization|ImmunizationEvaluation)", "Immunization event history and/or evaluation that supports the status and recommendation.", 0, java.lang.Integer.MAX_VALUE, supportingImmunization); 785 case -1234160646: /*supportingPatientInformation*/ return new Property("supportingPatientInformation", "Reference(Any)", "Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information.", 0, java.lang.Integer.MAX_VALUE, supportingPatientInformation); 786 default: return super.getNamedProperty(_hash, _name, _checkValid); 787 } 788 789 } 790 791 @Override 792 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 793 switch (hash) { 794 case 664556354: /*vaccineCode*/ return this.vaccineCode == null ? new Base[0] : this.vaccineCode.toArray(new Base[this.vaccineCode.size()]); // CodeableConcept 795 case -319593813: /*targetDisease*/ return this.targetDisease == null ? new Base[0] : this.targetDisease.toArray(new Base[this.targetDisease.size()]); // CodeableConcept 796 case 571105240: /*contraindicatedVaccineCode*/ return this.contraindicatedVaccineCode == null ? new Base[0] : this.contraindicatedVaccineCode.toArray(new Base[this.contraindicatedVaccineCode.size()]); // CodeableConcept 797 case 1904598477: /*forecastStatus*/ return this.forecastStatus == null ? new Base[0] : new Base[] {this.forecastStatus}; // CodeableConcept 798 case 1862115359: /*forecastReason*/ return this.forecastReason == null ? new Base[0] : this.forecastReason.toArray(new Base[this.forecastReason.size()]); // CodeableConcept 799 case 2087518867: /*dateCriterion*/ return this.dateCriterion == null ? new Base[0] : this.dateCriterion.toArray(new Base[this.dateCriterion.size()]); // ImmunizationRecommendationRecommendationDateCriterionComponent 800 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 801 case -905838985: /*series*/ return this.series == null ? new Base[0] : new Base[] {this.series}; // StringType 802 case -887709242: /*doseNumber*/ return this.doseNumber == null ? new Base[0] : new Base[] {this.doseNumber}; // StringType 803 case -1936727105: /*seriesDoses*/ return this.seriesDoses == null ? new Base[0] : new Base[] {this.seriesDoses}; // StringType 804 case 1171592021: /*supportingImmunization*/ return this.supportingImmunization == null ? new Base[0] : this.supportingImmunization.toArray(new Base[this.supportingImmunization.size()]); // Reference 805 case -1234160646: /*supportingPatientInformation*/ return this.supportingPatientInformation == null ? new Base[0] : this.supportingPatientInformation.toArray(new Base[this.supportingPatientInformation.size()]); // Reference 806 default: return super.getProperty(hash, name, checkValid); 807 } 808 809 } 810 811 @Override 812 public Base setProperty(int hash, String name, Base value) throws FHIRException { 813 switch (hash) { 814 case 664556354: // vaccineCode 815 this.getVaccineCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 816 return value; 817 case -319593813: // targetDisease 818 this.getTargetDisease().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 819 return value; 820 case 571105240: // contraindicatedVaccineCode 821 this.getContraindicatedVaccineCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 822 return value; 823 case 1904598477: // forecastStatus 824 this.forecastStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 825 return value; 826 case 1862115359: // forecastReason 827 this.getForecastReason().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 828 return value; 829 case 2087518867: // dateCriterion 830 this.getDateCriterion().add((ImmunizationRecommendationRecommendationDateCriterionComponent) value); // ImmunizationRecommendationRecommendationDateCriterionComponent 831 return value; 832 case -1724546052: // description 833 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 834 return value; 835 case -905838985: // series 836 this.series = TypeConvertor.castToString(value); // StringType 837 return value; 838 case -887709242: // doseNumber 839 this.doseNumber = TypeConvertor.castToString(value); // StringType 840 return value; 841 case -1936727105: // seriesDoses 842 this.seriesDoses = TypeConvertor.castToString(value); // StringType 843 return value; 844 case 1171592021: // supportingImmunization 845 this.getSupportingImmunization().add(TypeConvertor.castToReference(value)); // Reference 846 return value; 847 case -1234160646: // supportingPatientInformation 848 this.getSupportingPatientInformation().add(TypeConvertor.castToReference(value)); // Reference 849 return value; 850 default: return super.setProperty(hash, name, value); 851 } 852 853 } 854 855 @Override 856 public Base setProperty(String name, Base value) throws FHIRException { 857 if (name.equals("vaccineCode")) { 858 this.getVaccineCode().add(TypeConvertor.castToCodeableConcept(value)); 859 } else if (name.equals("targetDisease")) { 860 this.getTargetDisease().add(TypeConvertor.castToCodeableConcept(value)); 861 } else if (name.equals("contraindicatedVaccineCode")) { 862 this.getContraindicatedVaccineCode().add(TypeConvertor.castToCodeableConcept(value)); 863 } else if (name.equals("forecastStatus")) { 864 this.forecastStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 865 } else if (name.equals("forecastReason")) { 866 this.getForecastReason().add(TypeConvertor.castToCodeableConcept(value)); 867 } else if (name.equals("dateCriterion")) { 868 this.getDateCriterion().add((ImmunizationRecommendationRecommendationDateCriterionComponent) value); 869 } else if (name.equals("description")) { 870 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 871 } else if (name.equals("series")) { 872 this.series = TypeConvertor.castToString(value); // StringType 873 } else if (name.equals("doseNumber")) { 874 this.doseNumber = TypeConvertor.castToString(value); // StringType 875 } else if (name.equals("seriesDoses")) { 876 this.seriesDoses = TypeConvertor.castToString(value); // StringType 877 } else if (name.equals("supportingImmunization")) { 878 this.getSupportingImmunization().add(TypeConvertor.castToReference(value)); 879 } else if (name.equals("supportingPatientInformation")) { 880 this.getSupportingPatientInformation().add(TypeConvertor.castToReference(value)); 881 } else 882 return super.setProperty(name, value); 883 return value; 884 } 885 886 @Override 887 public void removeChild(String name, Base value) throws FHIRException { 888 if (name.equals("vaccineCode")) { 889 this.getVaccineCode().remove(value); 890 } else if (name.equals("targetDisease")) { 891 this.getTargetDisease().remove(value); 892 } else if (name.equals("contraindicatedVaccineCode")) { 893 this.getContraindicatedVaccineCode().remove(value); 894 } else if (name.equals("forecastStatus")) { 895 this.forecastStatus = null; 896 } else if (name.equals("forecastReason")) { 897 this.getForecastReason().remove(value); 898 } else if (name.equals("dateCriterion")) { 899 this.getDateCriterion().remove((ImmunizationRecommendationRecommendationDateCriterionComponent) value); 900 } else if (name.equals("description")) { 901 this.description = null; 902 } else if (name.equals("series")) { 903 this.series = null; 904 } else if (name.equals("doseNumber")) { 905 this.doseNumber = null; 906 } else if (name.equals("seriesDoses")) { 907 this.seriesDoses = null; 908 } else if (name.equals("supportingImmunization")) { 909 this.getSupportingImmunization().remove(value); 910 } else if (name.equals("supportingPatientInformation")) { 911 this.getSupportingPatientInformation().remove(value); 912 } else 913 super.removeChild(name, value); 914 915 } 916 917 @Override 918 public Base makeProperty(int hash, String name) throws FHIRException { 919 switch (hash) { 920 case 664556354: return addVaccineCode(); 921 case -319593813: return addTargetDisease(); 922 case 571105240: return addContraindicatedVaccineCode(); 923 case 1904598477: return getForecastStatus(); 924 case 1862115359: return addForecastReason(); 925 case 2087518867: return addDateCriterion(); 926 case -1724546052: return getDescriptionElement(); 927 case -905838985: return getSeriesElement(); 928 case -887709242: return getDoseNumberElement(); 929 case -1936727105: return getSeriesDosesElement(); 930 case 1171592021: return addSupportingImmunization(); 931 case -1234160646: return addSupportingPatientInformation(); 932 default: return super.makeProperty(hash, name); 933 } 934 935 } 936 937 @Override 938 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 939 switch (hash) { 940 case 664556354: /*vaccineCode*/ return new String[] {"CodeableConcept"}; 941 case -319593813: /*targetDisease*/ return new String[] {"CodeableConcept"}; 942 case 571105240: /*contraindicatedVaccineCode*/ return new String[] {"CodeableConcept"}; 943 case 1904598477: /*forecastStatus*/ return new String[] {"CodeableConcept"}; 944 case 1862115359: /*forecastReason*/ return new String[] {"CodeableConcept"}; 945 case 2087518867: /*dateCriterion*/ return new String[] {}; 946 case -1724546052: /*description*/ return new String[] {"markdown"}; 947 case -905838985: /*series*/ return new String[] {"string"}; 948 case -887709242: /*doseNumber*/ return new String[] {"string"}; 949 case -1936727105: /*seriesDoses*/ return new String[] {"string"}; 950 case 1171592021: /*supportingImmunization*/ return new String[] {"Reference"}; 951 case -1234160646: /*supportingPatientInformation*/ return new String[] {"Reference"}; 952 default: return super.getTypesForProperty(hash, name); 953 } 954 955 } 956 957 @Override 958 public Base addChild(String name) throws FHIRException { 959 if (name.equals("vaccineCode")) { 960 return addVaccineCode(); 961 } 962 else if (name.equals("targetDisease")) { 963 return addTargetDisease(); 964 } 965 else if (name.equals("contraindicatedVaccineCode")) { 966 return addContraindicatedVaccineCode(); 967 } 968 else if (name.equals("forecastStatus")) { 969 this.forecastStatus = new CodeableConcept(); 970 return this.forecastStatus; 971 } 972 else if (name.equals("forecastReason")) { 973 return addForecastReason(); 974 } 975 else if (name.equals("dateCriterion")) { 976 return addDateCriterion(); 977 } 978 else if (name.equals("description")) { 979 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.recommendation.description"); 980 } 981 else if (name.equals("series")) { 982 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.recommendation.series"); 983 } 984 else if (name.equals("doseNumber")) { 985 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.recommendation.doseNumber"); 986 } 987 else if (name.equals("seriesDoses")) { 988 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.recommendation.seriesDoses"); 989 } 990 else if (name.equals("supportingImmunization")) { 991 return addSupportingImmunization(); 992 } 993 else if (name.equals("supportingPatientInformation")) { 994 return addSupportingPatientInformation(); 995 } 996 else 997 return super.addChild(name); 998 } 999 1000 public ImmunizationRecommendationRecommendationComponent copy() { 1001 ImmunizationRecommendationRecommendationComponent dst = new ImmunizationRecommendationRecommendationComponent(); 1002 copyValues(dst); 1003 return dst; 1004 } 1005 1006 public void copyValues(ImmunizationRecommendationRecommendationComponent dst) { 1007 super.copyValues(dst); 1008 if (vaccineCode != null) { 1009 dst.vaccineCode = new ArrayList<CodeableConcept>(); 1010 for (CodeableConcept i : vaccineCode) 1011 dst.vaccineCode.add(i.copy()); 1012 }; 1013 if (targetDisease != null) { 1014 dst.targetDisease = new ArrayList<CodeableConcept>(); 1015 for (CodeableConcept i : targetDisease) 1016 dst.targetDisease.add(i.copy()); 1017 }; 1018 if (contraindicatedVaccineCode != null) { 1019 dst.contraindicatedVaccineCode = new ArrayList<CodeableConcept>(); 1020 for (CodeableConcept i : contraindicatedVaccineCode) 1021 dst.contraindicatedVaccineCode.add(i.copy()); 1022 }; 1023 dst.forecastStatus = forecastStatus == null ? null : forecastStatus.copy(); 1024 if (forecastReason != null) { 1025 dst.forecastReason = new ArrayList<CodeableConcept>(); 1026 for (CodeableConcept i : forecastReason) 1027 dst.forecastReason.add(i.copy()); 1028 }; 1029 if (dateCriterion != null) { 1030 dst.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 1031 for (ImmunizationRecommendationRecommendationDateCriterionComponent i : dateCriterion) 1032 dst.dateCriterion.add(i.copy()); 1033 }; 1034 dst.description = description == null ? null : description.copy(); 1035 dst.series = series == null ? null : series.copy(); 1036 dst.doseNumber = doseNumber == null ? null : doseNumber.copy(); 1037 dst.seriesDoses = seriesDoses == null ? null : seriesDoses.copy(); 1038 if (supportingImmunization != null) { 1039 dst.supportingImmunization = new ArrayList<Reference>(); 1040 for (Reference i : supportingImmunization) 1041 dst.supportingImmunization.add(i.copy()); 1042 }; 1043 if (supportingPatientInformation != null) { 1044 dst.supportingPatientInformation = new ArrayList<Reference>(); 1045 for (Reference i : supportingPatientInformation) 1046 dst.supportingPatientInformation.add(i.copy()); 1047 }; 1048 } 1049 1050 @Override 1051 public boolean equalsDeep(Base other_) { 1052 if (!super.equalsDeep(other_)) 1053 return false; 1054 if (!(other_ instanceof ImmunizationRecommendationRecommendationComponent)) 1055 return false; 1056 ImmunizationRecommendationRecommendationComponent o = (ImmunizationRecommendationRecommendationComponent) other_; 1057 return compareDeep(vaccineCode, o.vaccineCode, true) && compareDeep(targetDisease, o.targetDisease, true) 1058 && compareDeep(contraindicatedVaccineCode, o.contraindicatedVaccineCode, true) && compareDeep(forecastStatus, o.forecastStatus, true) 1059 && compareDeep(forecastReason, o.forecastReason, true) && compareDeep(dateCriterion, o.dateCriterion, true) 1060 && compareDeep(description, o.description, true) && compareDeep(series, o.series, true) && compareDeep(doseNumber, o.doseNumber, true) 1061 && compareDeep(seriesDoses, o.seriesDoses, true) && compareDeep(supportingImmunization, o.supportingImmunization, true) 1062 && compareDeep(supportingPatientInformation, o.supportingPatientInformation, true); 1063 } 1064 1065 @Override 1066 public boolean equalsShallow(Base other_) { 1067 if (!super.equalsShallow(other_)) 1068 return false; 1069 if (!(other_ instanceof ImmunizationRecommendationRecommendationComponent)) 1070 return false; 1071 ImmunizationRecommendationRecommendationComponent o = (ImmunizationRecommendationRecommendationComponent) other_; 1072 return compareValues(description, o.description, true) && compareValues(series, o.series, true) && compareValues(doseNumber, o.doseNumber, true) 1073 && compareValues(seriesDoses, o.seriesDoses, true); 1074 } 1075 1076 public boolean isEmpty() { 1077 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(vaccineCode, targetDisease 1078 , contraindicatedVaccineCode, forecastStatus, forecastReason, dateCriterion, description 1079 , series, doseNumber, seriesDoses, supportingImmunization, supportingPatientInformation 1080 ); 1081 } 1082 1083 public String fhirType() { 1084 return "ImmunizationRecommendation.recommendation"; 1085 1086 } 1087 1088 } 1089 1090 @Block() 1091 public static class ImmunizationRecommendationRecommendationDateCriterionComponent extends BackboneElement implements IBaseBackboneElement { 1092 /** 1093 * Date classification of recommendation. For example, earliest date to give, latest date to give, etc. 1094 */ 1095 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1096 @Description(shortDefinition="Type of date", formalDefinition="Date classification of recommendation. For example, earliest date to give, latest date to give, etc." ) 1097 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-recommendation-date-criterion") 1098 protected CodeableConcept code; 1099 1100 /** 1101 * The date whose meaning is specified by dateCriterion.code. 1102 */ 1103 @Child(name = "value", type = {DateTimeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 1104 @Description(shortDefinition="Recommended date", formalDefinition="The date whose meaning is specified by dateCriterion.code." ) 1105 protected DateTimeType value; 1106 1107 private static final long serialVersionUID = 1036994566L; 1108 1109 /** 1110 * Constructor 1111 */ 1112 public ImmunizationRecommendationRecommendationDateCriterionComponent() { 1113 super(); 1114 } 1115 1116 /** 1117 * Constructor 1118 */ 1119 public ImmunizationRecommendationRecommendationDateCriterionComponent(CodeableConcept code, Date value) { 1120 super(); 1121 this.setCode(code); 1122 this.setValue(value); 1123 } 1124 1125 /** 1126 * @return {@link #code} (Date classification of recommendation. For example, earliest date to give, latest date to give, etc.) 1127 */ 1128 public CodeableConcept getCode() { 1129 if (this.code == null) 1130 if (Configuration.errorOnAutoCreate()) 1131 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationDateCriterionComponent.code"); 1132 else if (Configuration.doAutoCreate()) 1133 this.code = new CodeableConcept(); // cc 1134 return this.code; 1135 } 1136 1137 public boolean hasCode() { 1138 return this.code != null && !this.code.isEmpty(); 1139 } 1140 1141 /** 1142 * @param value {@link #code} (Date classification of recommendation. For example, earliest date to give, latest date to give, etc.) 1143 */ 1144 public ImmunizationRecommendationRecommendationDateCriterionComponent setCode(CodeableConcept value) { 1145 this.code = value; 1146 return this; 1147 } 1148 1149 /** 1150 * @return {@link #value} (The date whose meaning is specified by dateCriterion.code.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1151 */ 1152 public DateTimeType getValueElement() { 1153 if (this.value == null) 1154 if (Configuration.errorOnAutoCreate()) 1155 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationDateCriterionComponent.value"); 1156 else if (Configuration.doAutoCreate()) 1157 this.value = new DateTimeType(); // bb 1158 return this.value; 1159 } 1160 1161 public boolean hasValueElement() { 1162 return this.value != null && !this.value.isEmpty(); 1163 } 1164 1165 public boolean hasValue() { 1166 return this.value != null && !this.value.isEmpty(); 1167 } 1168 1169 /** 1170 * @param value {@link #value} (The date whose meaning is specified by dateCriterion.code.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1171 */ 1172 public ImmunizationRecommendationRecommendationDateCriterionComponent setValueElement(DateTimeType value) { 1173 this.value = value; 1174 return this; 1175 } 1176 1177 /** 1178 * @return The date whose meaning is specified by dateCriterion.code. 1179 */ 1180 public Date getValue() { 1181 return this.value == null ? null : this.value.getValue(); 1182 } 1183 1184 /** 1185 * @param value The date whose meaning is specified by dateCriterion.code. 1186 */ 1187 public ImmunizationRecommendationRecommendationDateCriterionComponent setValue(Date value) { 1188 if (this.value == null) 1189 this.value = new DateTimeType(); 1190 this.value.setValue(value); 1191 return this; 1192 } 1193 1194 protected void listChildren(List<Property> children) { 1195 super.listChildren(children); 1196 children.add(new Property("code", "CodeableConcept", "Date classification of recommendation. For example, earliest date to give, latest date to give, etc.", 0, 1, code)); 1197 children.add(new Property("value", "dateTime", "The date whose meaning is specified by dateCriterion.code.", 0, 1, value)); 1198 } 1199 1200 @Override 1201 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1202 switch (_hash) { 1203 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Date classification of recommendation. For example, earliest date to give, latest date to give, etc.", 0, 1, code); 1204 case 111972721: /*value*/ return new Property("value", "dateTime", "The date whose meaning is specified by dateCriterion.code.", 0, 1, value); 1205 default: return super.getNamedProperty(_hash, _name, _checkValid); 1206 } 1207 1208 } 1209 1210 @Override 1211 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1212 switch (hash) { 1213 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1214 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DateTimeType 1215 default: return super.getProperty(hash, name, checkValid); 1216 } 1217 1218 } 1219 1220 @Override 1221 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1222 switch (hash) { 1223 case 3059181: // code 1224 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1225 return value; 1226 case 111972721: // value 1227 this.value = TypeConvertor.castToDateTime(value); // DateTimeType 1228 return value; 1229 default: return super.setProperty(hash, name, value); 1230 } 1231 1232 } 1233 1234 @Override 1235 public Base setProperty(String name, Base value) throws FHIRException { 1236 if (name.equals("code")) { 1237 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1238 } else if (name.equals("value")) { 1239 this.value = TypeConvertor.castToDateTime(value); // DateTimeType 1240 } else 1241 return super.setProperty(name, value); 1242 return value; 1243 } 1244 1245 @Override 1246 public void removeChild(String name, Base value) throws FHIRException { 1247 if (name.equals("code")) { 1248 this.code = null; 1249 } else if (name.equals("value")) { 1250 this.value = null; 1251 } else 1252 super.removeChild(name, value); 1253 1254 } 1255 1256 @Override 1257 public Base makeProperty(int hash, String name) throws FHIRException { 1258 switch (hash) { 1259 case 3059181: return getCode(); 1260 case 111972721: return getValueElement(); 1261 default: return super.makeProperty(hash, name); 1262 } 1263 1264 } 1265 1266 @Override 1267 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1268 switch (hash) { 1269 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1270 case 111972721: /*value*/ return new String[] {"dateTime"}; 1271 default: return super.getTypesForProperty(hash, name); 1272 } 1273 1274 } 1275 1276 @Override 1277 public Base addChild(String name) throws FHIRException { 1278 if (name.equals("code")) { 1279 this.code = new CodeableConcept(); 1280 return this.code; 1281 } 1282 else if (name.equals("value")) { 1283 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.recommendation.dateCriterion.value"); 1284 } 1285 else 1286 return super.addChild(name); 1287 } 1288 1289 public ImmunizationRecommendationRecommendationDateCriterionComponent copy() { 1290 ImmunizationRecommendationRecommendationDateCriterionComponent dst = new ImmunizationRecommendationRecommendationDateCriterionComponent(); 1291 copyValues(dst); 1292 return dst; 1293 } 1294 1295 public void copyValues(ImmunizationRecommendationRecommendationDateCriterionComponent dst) { 1296 super.copyValues(dst); 1297 dst.code = code == null ? null : code.copy(); 1298 dst.value = value == null ? null : value.copy(); 1299 } 1300 1301 @Override 1302 public boolean equalsDeep(Base other_) { 1303 if (!super.equalsDeep(other_)) 1304 return false; 1305 if (!(other_ instanceof ImmunizationRecommendationRecommendationDateCriterionComponent)) 1306 return false; 1307 ImmunizationRecommendationRecommendationDateCriterionComponent o = (ImmunizationRecommendationRecommendationDateCriterionComponent) other_; 1308 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 1309 } 1310 1311 @Override 1312 public boolean equalsShallow(Base other_) { 1313 if (!super.equalsShallow(other_)) 1314 return false; 1315 if (!(other_ instanceof ImmunizationRecommendationRecommendationDateCriterionComponent)) 1316 return false; 1317 ImmunizationRecommendationRecommendationDateCriterionComponent o = (ImmunizationRecommendationRecommendationDateCriterionComponent) other_; 1318 return compareValues(value, o.value, true); 1319 } 1320 1321 public boolean isEmpty() { 1322 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 1323 } 1324 1325 public String fhirType() { 1326 return "ImmunizationRecommendation.recommendation.dateCriterion"; 1327 1328 } 1329 1330 } 1331 1332 /** 1333 * A unique identifier assigned to this particular recommendation record. 1334 */ 1335 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1336 @Description(shortDefinition="Business identifier", formalDefinition="A unique identifier assigned to this particular recommendation record." ) 1337 protected List<Identifier> identifier; 1338 1339 /** 1340 * The patient the recommendation(s) are for. 1341 */ 1342 @Child(name = "patient", type = {Patient.class}, order=1, min=1, max=1, modifier=false, summary=true) 1343 @Description(shortDefinition="Who this profile is for", formalDefinition="The patient the recommendation(s) are for." ) 1344 protected Reference patient; 1345 1346 /** 1347 * The date the immunization recommendation(s) were created. 1348 */ 1349 @Child(name = "date", type = {DateTimeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1350 @Description(shortDefinition="Date recommendation(s) created", formalDefinition="The date the immunization recommendation(s) were created." ) 1351 protected DateTimeType date; 1352 1353 /** 1354 * Indicates the authority who published the protocol (e.g. ACIP). 1355 */ 1356 @Child(name = "authority", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=false) 1357 @Description(shortDefinition="Who is responsible for protocol", formalDefinition="Indicates the authority who published the protocol (e.g. ACIP)." ) 1358 protected Reference authority; 1359 1360 /** 1361 * Vaccine administration recommendations. 1362 */ 1363 @Child(name = "recommendation", type = {}, order=4, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1364 @Description(shortDefinition="Vaccine administration recommendations", formalDefinition="Vaccine administration recommendations." ) 1365 protected List<ImmunizationRecommendationRecommendationComponent> recommendation; 1366 1367 private static final long serialVersionUID = 534427937L; 1368 1369 /** 1370 * Constructor 1371 */ 1372 public ImmunizationRecommendation() { 1373 super(); 1374 } 1375 1376 /** 1377 * Constructor 1378 */ 1379 public ImmunizationRecommendation(Reference patient, Date date, ImmunizationRecommendationRecommendationComponent recommendation) { 1380 super(); 1381 this.setPatient(patient); 1382 this.setDate(date); 1383 this.addRecommendation(recommendation); 1384 } 1385 1386 /** 1387 * @return {@link #identifier} (A unique identifier assigned to this particular recommendation record.) 1388 */ 1389 public List<Identifier> getIdentifier() { 1390 if (this.identifier == null) 1391 this.identifier = new ArrayList<Identifier>(); 1392 return this.identifier; 1393 } 1394 1395 /** 1396 * @return Returns a reference to <code>this</code> for easy method chaining 1397 */ 1398 public ImmunizationRecommendation setIdentifier(List<Identifier> theIdentifier) { 1399 this.identifier = theIdentifier; 1400 return this; 1401 } 1402 1403 public boolean hasIdentifier() { 1404 if (this.identifier == null) 1405 return false; 1406 for (Identifier item : this.identifier) 1407 if (!item.isEmpty()) 1408 return true; 1409 return false; 1410 } 1411 1412 public Identifier addIdentifier() { //3 1413 Identifier t = new Identifier(); 1414 if (this.identifier == null) 1415 this.identifier = new ArrayList<Identifier>(); 1416 this.identifier.add(t); 1417 return t; 1418 } 1419 1420 public ImmunizationRecommendation addIdentifier(Identifier t) { //3 1421 if (t == null) 1422 return this; 1423 if (this.identifier == null) 1424 this.identifier = new ArrayList<Identifier>(); 1425 this.identifier.add(t); 1426 return this; 1427 } 1428 1429 /** 1430 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1431 */ 1432 public Identifier getIdentifierFirstRep() { 1433 if (getIdentifier().isEmpty()) { 1434 addIdentifier(); 1435 } 1436 return getIdentifier().get(0); 1437 } 1438 1439 /** 1440 * @return {@link #patient} (The patient the recommendation(s) are for.) 1441 */ 1442 public Reference getPatient() { 1443 if (this.patient == null) 1444 if (Configuration.errorOnAutoCreate()) 1445 throw new Error("Attempt to auto-create ImmunizationRecommendation.patient"); 1446 else if (Configuration.doAutoCreate()) 1447 this.patient = new Reference(); // cc 1448 return this.patient; 1449 } 1450 1451 public boolean hasPatient() { 1452 return this.patient != null && !this.patient.isEmpty(); 1453 } 1454 1455 /** 1456 * @param value {@link #patient} (The patient the recommendation(s) are for.) 1457 */ 1458 public ImmunizationRecommendation setPatient(Reference value) { 1459 this.patient = value; 1460 return this; 1461 } 1462 1463 /** 1464 * @return {@link #date} (The date the immunization recommendation(s) were created.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1465 */ 1466 public DateTimeType getDateElement() { 1467 if (this.date == null) 1468 if (Configuration.errorOnAutoCreate()) 1469 throw new Error("Attempt to auto-create ImmunizationRecommendation.date"); 1470 else if (Configuration.doAutoCreate()) 1471 this.date = new DateTimeType(); // bb 1472 return this.date; 1473 } 1474 1475 public boolean hasDateElement() { 1476 return this.date != null && !this.date.isEmpty(); 1477 } 1478 1479 public boolean hasDate() { 1480 return this.date != null && !this.date.isEmpty(); 1481 } 1482 1483 /** 1484 * @param value {@link #date} (The date the immunization recommendation(s) were created.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1485 */ 1486 public ImmunizationRecommendation setDateElement(DateTimeType value) { 1487 this.date = value; 1488 return this; 1489 } 1490 1491 /** 1492 * @return The date the immunization recommendation(s) were created. 1493 */ 1494 public Date getDate() { 1495 return this.date == null ? null : this.date.getValue(); 1496 } 1497 1498 /** 1499 * @param value The date the immunization recommendation(s) were created. 1500 */ 1501 public ImmunizationRecommendation setDate(Date value) { 1502 if (this.date == null) 1503 this.date = new DateTimeType(); 1504 this.date.setValue(value); 1505 return this; 1506 } 1507 1508 /** 1509 * @return {@link #authority} (Indicates the authority who published the protocol (e.g. ACIP).) 1510 */ 1511 public Reference getAuthority() { 1512 if (this.authority == null) 1513 if (Configuration.errorOnAutoCreate()) 1514 throw new Error("Attempt to auto-create ImmunizationRecommendation.authority"); 1515 else if (Configuration.doAutoCreate()) 1516 this.authority = new Reference(); // cc 1517 return this.authority; 1518 } 1519 1520 public boolean hasAuthority() { 1521 return this.authority != null && !this.authority.isEmpty(); 1522 } 1523 1524 /** 1525 * @param value {@link #authority} (Indicates the authority who published the protocol (e.g. ACIP).) 1526 */ 1527 public ImmunizationRecommendation setAuthority(Reference value) { 1528 this.authority = value; 1529 return this; 1530 } 1531 1532 /** 1533 * @return {@link #recommendation} (Vaccine administration recommendations.) 1534 */ 1535 public List<ImmunizationRecommendationRecommendationComponent> getRecommendation() { 1536 if (this.recommendation == null) 1537 this.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1538 return this.recommendation; 1539 } 1540 1541 /** 1542 * @return Returns a reference to <code>this</code> for easy method chaining 1543 */ 1544 public ImmunizationRecommendation setRecommendation(List<ImmunizationRecommendationRecommendationComponent> theRecommendation) { 1545 this.recommendation = theRecommendation; 1546 return this; 1547 } 1548 1549 public boolean hasRecommendation() { 1550 if (this.recommendation == null) 1551 return false; 1552 for (ImmunizationRecommendationRecommendationComponent item : this.recommendation) 1553 if (!item.isEmpty()) 1554 return true; 1555 return false; 1556 } 1557 1558 public ImmunizationRecommendationRecommendationComponent addRecommendation() { //3 1559 ImmunizationRecommendationRecommendationComponent t = new ImmunizationRecommendationRecommendationComponent(); 1560 if (this.recommendation == null) 1561 this.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1562 this.recommendation.add(t); 1563 return t; 1564 } 1565 1566 public ImmunizationRecommendation addRecommendation(ImmunizationRecommendationRecommendationComponent t) { //3 1567 if (t == null) 1568 return this; 1569 if (this.recommendation == null) 1570 this.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1571 this.recommendation.add(t); 1572 return this; 1573 } 1574 1575 /** 1576 * @return The first repetition of repeating field {@link #recommendation}, creating it if it does not already exist {3} 1577 */ 1578 public ImmunizationRecommendationRecommendationComponent getRecommendationFirstRep() { 1579 if (getRecommendation().isEmpty()) { 1580 addRecommendation(); 1581 } 1582 return getRecommendation().get(0); 1583 } 1584 1585 protected void listChildren(List<Property> children) { 1586 super.listChildren(children); 1587 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this particular recommendation record.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1588 children.add(new Property("patient", "Reference(Patient)", "The patient the recommendation(s) are for.", 0, 1, patient)); 1589 children.add(new Property("date", "dateTime", "The date the immunization recommendation(s) were created.", 0, 1, date)); 1590 children.add(new Property("authority", "Reference(Organization)", "Indicates the authority who published the protocol (e.g. ACIP).", 0, 1, authority)); 1591 children.add(new Property("recommendation", "", "Vaccine administration recommendations.", 0, java.lang.Integer.MAX_VALUE, recommendation)); 1592 } 1593 1594 @Override 1595 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1596 switch (_hash) { 1597 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this particular recommendation record.", 0, java.lang.Integer.MAX_VALUE, identifier); 1598 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient the recommendation(s) are for.", 0, 1, patient); 1599 case 3076014: /*date*/ return new Property("date", "dateTime", "The date the immunization recommendation(s) were created.", 0, 1, date); 1600 case 1475610435: /*authority*/ return new Property("authority", "Reference(Organization)", "Indicates the authority who published the protocol (e.g. ACIP).", 0, 1, authority); 1601 case -1028636743: /*recommendation*/ return new Property("recommendation", "", "Vaccine administration recommendations.", 0, java.lang.Integer.MAX_VALUE, recommendation); 1602 default: return super.getNamedProperty(_hash, _name, _checkValid); 1603 } 1604 1605 } 1606 1607 @Override 1608 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1609 switch (hash) { 1610 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1611 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1612 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1613 case 1475610435: /*authority*/ return this.authority == null ? new Base[0] : new Base[] {this.authority}; // Reference 1614 case -1028636743: /*recommendation*/ return this.recommendation == null ? new Base[0] : this.recommendation.toArray(new Base[this.recommendation.size()]); // ImmunizationRecommendationRecommendationComponent 1615 default: return super.getProperty(hash, name, checkValid); 1616 } 1617 1618 } 1619 1620 @Override 1621 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1622 switch (hash) { 1623 case -1618432855: // identifier 1624 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1625 return value; 1626 case -791418107: // patient 1627 this.patient = TypeConvertor.castToReference(value); // Reference 1628 return value; 1629 case 3076014: // date 1630 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1631 return value; 1632 case 1475610435: // authority 1633 this.authority = TypeConvertor.castToReference(value); // Reference 1634 return value; 1635 case -1028636743: // recommendation 1636 this.getRecommendation().add((ImmunizationRecommendationRecommendationComponent) value); // ImmunizationRecommendationRecommendationComponent 1637 return value; 1638 default: return super.setProperty(hash, name, value); 1639 } 1640 1641 } 1642 1643 @Override 1644 public Base setProperty(String name, Base value) throws FHIRException { 1645 if (name.equals("identifier")) { 1646 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1647 } else if (name.equals("patient")) { 1648 this.patient = TypeConvertor.castToReference(value); // Reference 1649 } else if (name.equals("date")) { 1650 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1651 } else if (name.equals("authority")) { 1652 this.authority = TypeConvertor.castToReference(value); // Reference 1653 } else if (name.equals("recommendation")) { 1654 this.getRecommendation().add((ImmunizationRecommendationRecommendationComponent) value); 1655 } else 1656 return super.setProperty(name, value); 1657 return value; 1658 } 1659 1660 @Override 1661 public void removeChild(String name, Base value) throws FHIRException { 1662 if (name.equals("identifier")) { 1663 this.getIdentifier().remove(value); 1664 } else if (name.equals("patient")) { 1665 this.patient = null; 1666 } else if (name.equals("date")) { 1667 this.date = null; 1668 } else if (name.equals("authority")) { 1669 this.authority = null; 1670 } else if (name.equals("recommendation")) { 1671 this.getRecommendation().remove((ImmunizationRecommendationRecommendationComponent) value); 1672 } else 1673 super.removeChild(name, value); 1674 1675 } 1676 1677 @Override 1678 public Base makeProperty(int hash, String name) throws FHIRException { 1679 switch (hash) { 1680 case -1618432855: return addIdentifier(); 1681 case -791418107: return getPatient(); 1682 case 3076014: return getDateElement(); 1683 case 1475610435: return getAuthority(); 1684 case -1028636743: return addRecommendation(); 1685 default: return super.makeProperty(hash, name); 1686 } 1687 1688 } 1689 1690 @Override 1691 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1692 switch (hash) { 1693 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1694 case -791418107: /*patient*/ return new String[] {"Reference"}; 1695 case 3076014: /*date*/ return new String[] {"dateTime"}; 1696 case 1475610435: /*authority*/ return new String[] {"Reference"}; 1697 case -1028636743: /*recommendation*/ return new String[] {}; 1698 default: return super.getTypesForProperty(hash, name); 1699 } 1700 1701 } 1702 1703 @Override 1704 public Base addChild(String name) throws FHIRException { 1705 if (name.equals("identifier")) { 1706 return addIdentifier(); 1707 } 1708 else if (name.equals("patient")) { 1709 this.patient = new Reference(); 1710 return this.patient; 1711 } 1712 else if (name.equals("date")) { 1713 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.date"); 1714 } 1715 else if (name.equals("authority")) { 1716 this.authority = new Reference(); 1717 return this.authority; 1718 } 1719 else if (name.equals("recommendation")) { 1720 return addRecommendation(); 1721 } 1722 else 1723 return super.addChild(name); 1724 } 1725 1726 public String fhirType() { 1727 return "ImmunizationRecommendation"; 1728 1729 } 1730 1731 public ImmunizationRecommendation copy() { 1732 ImmunizationRecommendation dst = new ImmunizationRecommendation(); 1733 copyValues(dst); 1734 return dst; 1735 } 1736 1737 public void copyValues(ImmunizationRecommendation dst) { 1738 super.copyValues(dst); 1739 if (identifier != null) { 1740 dst.identifier = new ArrayList<Identifier>(); 1741 for (Identifier i : identifier) 1742 dst.identifier.add(i.copy()); 1743 }; 1744 dst.patient = patient == null ? null : patient.copy(); 1745 dst.date = date == null ? null : date.copy(); 1746 dst.authority = authority == null ? null : authority.copy(); 1747 if (recommendation != null) { 1748 dst.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1749 for (ImmunizationRecommendationRecommendationComponent i : recommendation) 1750 dst.recommendation.add(i.copy()); 1751 }; 1752 } 1753 1754 protected ImmunizationRecommendation typedCopy() { 1755 return copy(); 1756 } 1757 1758 @Override 1759 public boolean equalsDeep(Base other_) { 1760 if (!super.equalsDeep(other_)) 1761 return false; 1762 if (!(other_ instanceof ImmunizationRecommendation)) 1763 return false; 1764 ImmunizationRecommendation o = (ImmunizationRecommendation) other_; 1765 return compareDeep(identifier, o.identifier, true) && compareDeep(patient, o.patient, true) && compareDeep(date, o.date, true) 1766 && compareDeep(authority, o.authority, true) && compareDeep(recommendation, o.recommendation, true) 1767 ; 1768 } 1769 1770 @Override 1771 public boolean equalsShallow(Base other_) { 1772 if (!super.equalsShallow(other_)) 1773 return false; 1774 if (!(other_ instanceof ImmunizationRecommendation)) 1775 return false; 1776 ImmunizationRecommendation o = (ImmunizationRecommendation) other_; 1777 return compareValues(date, o.date, true); 1778 } 1779 1780 public boolean isEmpty() { 1781 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, patient, date 1782 , authority, recommendation); 1783 } 1784 1785 @Override 1786 public ResourceType getResourceType() { 1787 return ResourceType.ImmunizationRecommendation; 1788 } 1789 1790 /** 1791 * Search parameter: <b>information</b> 1792 * <p> 1793 * Description: <b>Patient observations supporting recommendation</b><br> 1794 * Type: <b>reference</b><br> 1795 * Path: <b>ImmunizationRecommendation.recommendation.supportingPatientInformation</b><br> 1796 * </p> 1797 */ 1798 @SearchParamDefinition(name="information", path="ImmunizationRecommendation.recommendation.supportingPatientInformation", description="Patient observations supporting recommendation", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 1799 public static final String SP_INFORMATION = "information"; 1800 /** 1801 * <b>Fluent Client</b> search parameter constant for <b>information</b> 1802 * <p> 1803 * Description: <b>Patient observations supporting recommendation</b><br> 1804 * Type: <b>reference</b><br> 1805 * Path: <b>ImmunizationRecommendation.recommendation.supportingPatientInformation</b><br> 1806 * </p> 1807 */ 1808 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INFORMATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INFORMATION); 1809 1810/** 1811 * Constant for fluent queries to be used to add include statements. Specifies 1812 * the path value of "<b>ImmunizationRecommendation:information</b>". 1813 */ 1814 public static final ca.uhn.fhir.model.api.Include INCLUDE_INFORMATION = new ca.uhn.fhir.model.api.Include("ImmunizationRecommendation:information").toLocked(); 1815 1816 /** 1817 * Search parameter: <b>status</b> 1818 * <p> 1819 * Description: <b>Vaccine recommendation status</b><br> 1820 * Type: <b>token</b><br> 1821 * Path: <b>ImmunizationRecommendation.recommendation.forecastStatus</b><br> 1822 * </p> 1823 */ 1824 @SearchParamDefinition(name="status", path="ImmunizationRecommendation.recommendation.forecastStatus", description="Vaccine recommendation status", type="token" ) 1825 public static final String SP_STATUS = "status"; 1826 /** 1827 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1828 * <p> 1829 * Description: <b>Vaccine recommendation status</b><br> 1830 * Type: <b>token</b><br> 1831 * Path: <b>ImmunizationRecommendation.recommendation.forecastStatus</b><br> 1832 * </p> 1833 */ 1834 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1835 1836 /** 1837 * Search parameter: <b>support</b> 1838 * <p> 1839 * Description: <b>Past immunizations supporting recommendation</b><br> 1840 * Type: <b>reference</b><br> 1841 * Path: <b>ImmunizationRecommendation.recommendation.supportingImmunization</b><br> 1842 * </p> 1843 */ 1844 @SearchParamDefinition(name="support", path="ImmunizationRecommendation.recommendation.supportingImmunization", description="Past immunizations supporting recommendation", type="reference", target={Immunization.class, ImmunizationEvaluation.class } ) 1845 public static final String SP_SUPPORT = "support"; 1846 /** 1847 * <b>Fluent Client</b> search parameter constant for <b>support</b> 1848 * <p> 1849 * Description: <b>Past immunizations supporting recommendation</b><br> 1850 * Type: <b>reference</b><br> 1851 * Path: <b>ImmunizationRecommendation.recommendation.supportingImmunization</b><br> 1852 * </p> 1853 */ 1854 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPORT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUPPORT); 1855 1856/** 1857 * Constant for fluent queries to be used to add include statements. Specifies 1858 * the path value of "<b>ImmunizationRecommendation:support</b>". 1859 */ 1860 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPORT = new ca.uhn.fhir.model.api.Include("ImmunizationRecommendation:support").toLocked(); 1861 1862 /** 1863 * Search parameter: <b>target-disease</b> 1864 * <p> 1865 * Description: <b>Disease to be immunized against</b><br> 1866 * Type: <b>token</b><br> 1867 * Path: <b>ImmunizationRecommendation.recommendation.targetDisease</b><br> 1868 * </p> 1869 */ 1870 @SearchParamDefinition(name="target-disease", path="ImmunizationRecommendation.recommendation.targetDisease", description="Disease to be immunized against", type="token" ) 1871 public static final String SP_TARGET_DISEASE = "target-disease"; 1872 /** 1873 * <b>Fluent Client</b> search parameter constant for <b>target-disease</b> 1874 * <p> 1875 * Description: <b>Disease to be immunized against</b><br> 1876 * Type: <b>token</b><br> 1877 * Path: <b>ImmunizationRecommendation.recommendation.targetDisease</b><br> 1878 * </p> 1879 */ 1880 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_DISEASE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TARGET_DISEASE); 1881 1882 /** 1883 * Search parameter: <b>vaccine-type</b> 1884 * <p> 1885 * Description: <b>Vaccine or vaccine group recommendation applies to</b><br> 1886 * Type: <b>token</b><br> 1887 * Path: <b>ImmunizationRecommendation.recommendation.vaccineCode</b><br> 1888 * </p> 1889 */ 1890 @SearchParamDefinition(name="vaccine-type", path="ImmunizationRecommendation.recommendation.vaccineCode", description="Vaccine or vaccine group recommendation applies to", type="token" ) 1891 public static final String SP_VACCINE_TYPE = "vaccine-type"; 1892 /** 1893 * <b>Fluent Client</b> search parameter constant for <b>vaccine-type</b> 1894 * <p> 1895 * Description: <b>Vaccine or vaccine group recommendation applies to</b><br> 1896 * Type: <b>token</b><br> 1897 * Path: <b>ImmunizationRecommendation.recommendation.vaccineCode</b><br> 1898 * </p> 1899 */ 1900 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VACCINE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VACCINE_TYPE); 1901 1902 /** 1903 * Search parameter: <b>date</b> 1904 * <p> 1905 * Description: <b>Multiple Resources: 1906 1907* [AdverseEvent](adverseevent.html): When the event occurred 1908* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 1909* [Appointment](appointment.html): Appointment date/time. 1910* [AuditEvent](auditevent.html): Time when the event was recorded 1911* [CarePlan](careplan.html): Time period plan covers 1912* [CareTeam](careteam.html): A date within the coverage time period. 1913* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 1914* [Composition](composition.html): Composition editing time 1915* [Consent](consent.html): When consent was agreed to 1916* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 1917* [DocumentReference](documentreference.html): When this document reference was created 1918* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 1919* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 1920* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 1921* [Flag](flag.html): Time period when flag is active 1922* [Immunization](immunization.html): Vaccination (non)-Administration Date 1923* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 1924* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 1925* [Invoice](invoice.html): Invoice date / posting date 1926* [List](list.html): When the list was prepared 1927* [MeasureReport](measurereport.html): The date of the measure report 1928* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 1929* [Observation](observation.html): Clinically relevant time/time-period for observation 1930* [Procedure](procedure.html): When the procedure occurred or is occurring 1931* [ResearchSubject](researchsubject.html): Start and end of participation 1932* [RiskAssessment](riskassessment.html): When was assessment made? 1933* [SupplyRequest](supplyrequest.html): When the request was made 1934</b><br> 1935 * Type: <b>date</b><br> 1936 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 1937 * </p> 1938 */ 1939 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 1940 public static final String SP_DATE = "date"; 1941 /** 1942 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1943 * <p> 1944 * Description: <b>Multiple Resources: 1945 1946* [AdverseEvent](adverseevent.html): When the event occurred 1947* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 1948* [Appointment](appointment.html): Appointment date/time. 1949* [AuditEvent](auditevent.html): Time when the event was recorded 1950* [CarePlan](careplan.html): Time period plan covers 1951* [CareTeam](careteam.html): A date within the coverage time period. 1952* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 1953* [Composition](composition.html): Composition editing time 1954* [Consent](consent.html): When consent was agreed to 1955* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 1956* [DocumentReference](documentreference.html): When this document reference was created 1957* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 1958* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 1959* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 1960* [Flag](flag.html): Time period when flag is active 1961* [Immunization](immunization.html): Vaccination (non)-Administration Date 1962* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 1963* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 1964* [Invoice](invoice.html): Invoice date / posting date 1965* [List](list.html): When the list was prepared 1966* [MeasureReport](measurereport.html): The date of the measure report 1967* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 1968* [Observation](observation.html): Clinically relevant time/time-period for observation 1969* [Procedure](procedure.html): When the procedure occurred or is occurring 1970* [ResearchSubject](researchsubject.html): Start and end of participation 1971* [RiskAssessment](riskassessment.html): When was assessment made? 1972* [SupplyRequest](supplyrequest.html): When the request was made 1973</b><br> 1974 * Type: <b>date</b><br> 1975 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 1976 * </p> 1977 */ 1978 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1979 1980 /** 1981 * Search parameter: <b>identifier</b> 1982 * <p> 1983 * Description: <b>Multiple Resources: 1984 1985* [Account](account.html): Account number 1986* [AdverseEvent](adverseevent.html): Business identifier for the event 1987* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1988* [Appointment](appointment.html): An Identifier of the Appointment 1989* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1990* [Basic](basic.html): Business identifier 1991* [BodyStructure](bodystructure.html): Bodystructure identifier 1992* [CarePlan](careplan.html): External Ids for this plan 1993* [CareTeam](careteam.html): External Ids for this team 1994* [ChargeItem](chargeitem.html): Business Identifier for item 1995* [Claim](claim.html): The primary identifier of the financial resource 1996* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1997* [ClinicalImpression](clinicalimpression.html): Business identifier 1998* [Communication](communication.html): Unique identifier 1999* [CommunicationRequest](communicationrequest.html): Unique identifier 2000* [Composition](composition.html): Version-independent identifier for the Composition 2001* [Condition](condition.html): A unique identifier of the condition record 2002* [Consent](consent.html): Identifier for this record (external references) 2003* [Contract](contract.html): The identity of the contract 2004* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2005* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2006* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2007* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2008* [DeviceRequest](devicerequest.html): Business identifier for request/order 2009* [DeviceUsage](deviceusage.html): Search by identifier 2010* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2011* [DocumentReference](documentreference.html): Identifier of the attachment binary 2012* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2013* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2014* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2015* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2016* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2017* [Flag](flag.html): Business identifier 2018* [Goal](goal.html): External Ids for this goal 2019* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2020* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2021* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2022* [Immunization](immunization.html): Business identifier 2023* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2024* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2025* [Invoice](invoice.html): Business Identifier for item 2026* [List](list.html): Business identifier 2027* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2028* [Medication](medication.html): Returns medications with this external identifier 2029* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2030* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2031* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2032* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2033* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2034* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2035* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2036* [Observation](observation.html): The unique id for a particular observation 2037* [Person](person.html): A person Identifier 2038* [Procedure](procedure.html): A unique identifier for a procedure 2039* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2040* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2041* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2042* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2043* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2044* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2045* [Specimen](specimen.html): The unique identifier associated with the specimen 2046* [SupplyDelivery](supplydelivery.html): External identifier 2047* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2048* [Task](task.html): Search for a task instance by its business identifier 2049* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2050</b><br> 2051 * Type: <b>token</b><br> 2052 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2053 * </p> 2054 */ 2055 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2056 public static final String SP_IDENTIFIER = "identifier"; 2057 /** 2058 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2059 * <p> 2060 * Description: <b>Multiple Resources: 2061 2062* [Account](account.html): Account number 2063* [AdverseEvent](adverseevent.html): Business identifier for the event 2064* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2065* [Appointment](appointment.html): An Identifier of the Appointment 2066* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2067* [Basic](basic.html): Business identifier 2068* [BodyStructure](bodystructure.html): Bodystructure identifier 2069* [CarePlan](careplan.html): External Ids for this plan 2070* [CareTeam](careteam.html): External Ids for this team 2071* [ChargeItem](chargeitem.html): Business Identifier for item 2072* [Claim](claim.html): The primary identifier of the financial resource 2073* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2074* [ClinicalImpression](clinicalimpression.html): Business identifier 2075* [Communication](communication.html): Unique identifier 2076* [CommunicationRequest](communicationrequest.html): Unique identifier 2077* [Composition](composition.html): Version-independent identifier for the Composition 2078* [Condition](condition.html): A unique identifier of the condition record 2079* [Consent](consent.html): Identifier for this record (external references) 2080* [Contract](contract.html): The identity of the contract 2081* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2082* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2083* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2084* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2085* [DeviceRequest](devicerequest.html): Business identifier for request/order 2086* [DeviceUsage](deviceusage.html): Search by identifier 2087* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2088* [DocumentReference](documentreference.html): Identifier of the attachment binary 2089* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2090* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2091* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2092* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2093* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2094* [Flag](flag.html): Business identifier 2095* [Goal](goal.html): External Ids for this goal 2096* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2097* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2098* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2099* [Immunization](immunization.html): Business identifier 2100* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2101* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2102* [Invoice](invoice.html): Business Identifier for item 2103* [List](list.html): Business identifier 2104* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2105* [Medication](medication.html): Returns medications with this external identifier 2106* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2107* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2108* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2109* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2110* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2111* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2112* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2113* [Observation](observation.html): The unique id for a particular observation 2114* [Person](person.html): A person Identifier 2115* [Procedure](procedure.html): A unique identifier for a procedure 2116* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2117* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2118* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2119* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2120* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2121* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2122* [Specimen](specimen.html): The unique identifier associated with the specimen 2123* [SupplyDelivery](supplydelivery.html): External identifier 2124* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2125* [Task](task.html): Search for a task instance by its business identifier 2126* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2127</b><br> 2128 * Type: <b>token</b><br> 2129 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2130 * </p> 2131 */ 2132 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2133 2134 /** 2135 * Search parameter: <b>patient</b> 2136 * <p> 2137 * Description: <b>Multiple Resources: 2138 2139* [Account](account.html): The entity that caused the expenses 2140* [AdverseEvent](adverseevent.html): Subject impacted by event 2141* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2142* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2143* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2144* [AuditEvent](auditevent.html): Where the activity involved patient data 2145* [Basic](basic.html): Identifies the focus of this resource 2146* [BodyStructure](bodystructure.html): Who this is about 2147* [CarePlan](careplan.html): Who the care plan is for 2148* [CareTeam](careteam.html): Who care team is for 2149* [ChargeItem](chargeitem.html): Individual service was done for/to 2150* [Claim](claim.html): Patient receiving the products or services 2151* [ClaimResponse](claimresponse.html): The subject of care 2152* [ClinicalImpression](clinicalimpression.html): Patient assessed 2153* [Communication](communication.html): Focus of message 2154* [CommunicationRequest](communicationrequest.html): Focus of message 2155* [Composition](composition.html): Who and/or what the composition is about 2156* [Condition](condition.html): Who has the condition? 2157* [Consent](consent.html): Who the consent applies to 2158* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2159* [Coverage](coverage.html): Retrieve coverages for a patient 2160* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2161* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2162* [DetectedIssue](detectedissue.html): Associated patient 2163* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2164* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2165* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2166* [DocumentReference](documentreference.html): Who/what is the subject of the document 2167* [Encounter](encounter.html): The patient present at the encounter 2168* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2169* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2170* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2171* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2172* [Flag](flag.html): The identity of a subject to list flags for 2173* [Goal](goal.html): Who this goal is intended for 2174* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2175* [ImagingSelection](imagingselection.html): Who the study is about 2176* [ImagingStudy](imagingstudy.html): Who the study is about 2177* [Immunization](immunization.html): The patient for the vaccination record 2178* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2179* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2180* [Invoice](invoice.html): Recipient(s) of goods and services 2181* [List](list.html): If all resources have the same subject 2182* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2183* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2184* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2185* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2186* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2187* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2188* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2189* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2190* [Observation](observation.html): The subject that the observation is about (if patient) 2191* [Person](person.html): The Person links to this Patient 2192* [Procedure](procedure.html): Search by subject - a patient 2193* [Provenance](provenance.html): Where the activity involved patient data 2194* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2195* [RelatedPerson](relatedperson.html): The patient this related person is related to 2196* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2197* [ResearchSubject](researchsubject.html): Who or what is part of study 2198* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2199* [ServiceRequest](servicerequest.html): Search by subject - a patient 2200* [Specimen](specimen.html): The patient the specimen comes from 2201* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2202* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2203* [Task](task.html): Search by patient 2204* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2205</b><br> 2206 * Type: <b>reference</b><br> 2207 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2208 * </p> 2209 */ 2210 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 2211 public static final String SP_PATIENT = "patient"; 2212 /** 2213 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2214 * <p> 2215 * Description: <b>Multiple Resources: 2216 2217* [Account](account.html): The entity that caused the expenses 2218* [AdverseEvent](adverseevent.html): Subject impacted by event 2219* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2220* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2221* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2222* [AuditEvent](auditevent.html): Where the activity involved patient data 2223* [Basic](basic.html): Identifies the focus of this resource 2224* [BodyStructure](bodystructure.html): Who this is about 2225* [CarePlan](careplan.html): Who the care plan is for 2226* [CareTeam](careteam.html): Who care team is for 2227* [ChargeItem](chargeitem.html): Individual service was done for/to 2228* [Claim](claim.html): Patient receiving the products or services 2229* [ClaimResponse](claimresponse.html): The subject of care 2230* [ClinicalImpression](clinicalimpression.html): Patient assessed 2231* [Communication](communication.html): Focus of message 2232* [CommunicationRequest](communicationrequest.html): Focus of message 2233* [Composition](composition.html): Who and/or what the composition is about 2234* [Condition](condition.html): Who has the condition? 2235* [Consent](consent.html): Who the consent applies to 2236* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2237* [Coverage](coverage.html): Retrieve coverages for a patient 2238* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2239* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2240* [DetectedIssue](detectedissue.html): Associated patient 2241* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2242* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2243* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2244* [DocumentReference](documentreference.html): Who/what is the subject of the document 2245* [Encounter](encounter.html): The patient present at the encounter 2246* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2247* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2248* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2249* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2250* [Flag](flag.html): The identity of a subject to list flags for 2251* [Goal](goal.html): Who this goal is intended for 2252* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2253* [ImagingSelection](imagingselection.html): Who the study is about 2254* [ImagingStudy](imagingstudy.html): Who the study is about 2255* [Immunization](immunization.html): The patient for the vaccination record 2256* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2257* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2258* [Invoice](invoice.html): Recipient(s) of goods and services 2259* [List](list.html): If all resources have the same subject 2260* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2261* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2262* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2263* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2264* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2265* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2266* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2267* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2268* [Observation](observation.html): The subject that the observation is about (if patient) 2269* [Person](person.html): The Person links to this Patient 2270* [Procedure](procedure.html): Search by subject - a patient 2271* [Provenance](provenance.html): Where the activity involved patient data 2272* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2273* [RelatedPerson](relatedperson.html): The patient this related person is related to 2274* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2275* [ResearchSubject](researchsubject.html): Who or what is part of study 2276* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2277* [ServiceRequest](servicerequest.html): Search by subject - a patient 2278* [Specimen](specimen.html): The patient the specimen comes from 2279* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2280* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2281* [Task](task.html): Search by patient 2282* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2283</b><br> 2284 * Type: <b>reference</b><br> 2285 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2286 * </p> 2287 */ 2288 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2289 2290/** 2291 * Constant for fluent queries to be used to add include statements. Specifies 2292 * the path value of "<b>ImmunizationRecommendation:patient</b>". 2293 */ 2294 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ImmunizationRecommendation:patient").toLocked(); 2295 2296 2297} 2298