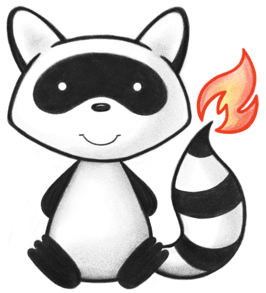
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A set of rules of how a particular interoperability or standards problem is solved - typically through the use of FHIR resources. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts. 052 */ 053@ResourceDef(name="ImplementationGuide", profile="http://hl7.org/fhir/StructureDefinition/ImplementationGuide") 054public class ImplementationGuide extends CanonicalResource { 055 056 public enum GuidePageGeneration { 057 /** 058 * Page is proper xhtml with no templating. Will be brought across unchanged for standard post-processing. 059 */ 060 HTML, 061 /** 062 * Page is markdown with templating. Will use the template to create a file that imports the markdown file prior to post-processing. 063 */ 064 MARKDOWN, 065 /** 066 * Page is xml with templating. Will use the template to create a file that imports the source file and run the nominated XSLT transform (see parameters) if present prior to post-processing. 067 */ 068 XML, 069 /** 070 * Page will be generated by the publication process - no source to bring across. 071 */ 072 GENERATED, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static GuidePageGeneration fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("html".equals(codeString)) 081 return HTML; 082 if ("markdown".equals(codeString)) 083 return MARKDOWN; 084 if ("xml".equals(codeString)) 085 return XML; 086 if ("generated".equals(codeString)) 087 return GENERATED; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown GuidePageGeneration code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case HTML: return "html"; 096 case MARKDOWN: return "markdown"; 097 case XML: return "xml"; 098 case GENERATED: return "generated"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case HTML: return "http://hl7.org/fhir/guide-page-generation"; 106 case MARKDOWN: return "http://hl7.org/fhir/guide-page-generation"; 107 case XML: return "http://hl7.org/fhir/guide-page-generation"; 108 case GENERATED: return "http://hl7.org/fhir/guide-page-generation"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case HTML: return "Page is proper xhtml with no templating. Will be brought across unchanged for standard post-processing."; 116 case MARKDOWN: return "Page is markdown with templating. Will use the template to create a file that imports the markdown file prior to post-processing."; 117 case XML: return "Page is xml with templating. Will use the template to create a file that imports the source file and run the nominated XSLT transform (see parameters) if present prior to post-processing."; 118 case GENERATED: return "Page will be generated by the publication process - no source to bring across."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case HTML: return "HTML"; 126 case MARKDOWN: return "Markdown"; 127 case XML: return "XML"; 128 case GENERATED: return "Generated"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class GuidePageGenerationEnumFactory implements EnumFactory<GuidePageGeneration> { 136 public GuidePageGeneration fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("html".equals(codeString)) 141 return GuidePageGeneration.HTML; 142 if ("markdown".equals(codeString)) 143 return GuidePageGeneration.MARKDOWN; 144 if ("xml".equals(codeString)) 145 return GuidePageGeneration.XML; 146 if ("generated".equals(codeString)) 147 return GuidePageGeneration.GENERATED; 148 throw new IllegalArgumentException("Unknown GuidePageGeneration code '"+codeString+"'"); 149 } 150 public Enumeration<GuidePageGeneration> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<GuidePageGeneration>(this, GuidePageGeneration.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<GuidePageGeneration>(this, GuidePageGeneration.NULL, code); 158 if ("html".equals(codeString)) 159 return new Enumeration<GuidePageGeneration>(this, GuidePageGeneration.HTML, code); 160 if ("markdown".equals(codeString)) 161 return new Enumeration<GuidePageGeneration>(this, GuidePageGeneration.MARKDOWN, code); 162 if ("xml".equals(codeString)) 163 return new Enumeration<GuidePageGeneration>(this, GuidePageGeneration.XML, code); 164 if ("generated".equals(codeString)) 165 return new Enumeration<GuidePageGeneration>(this, GuidePageGeneration.GENERATED, code); 166 throw new FHIRException("Unknown GuidePageGeneration code '"+codeString+"'"); 167 } 168 public String toCode(GuidePageGeneration code) { 169 if (code == GuidePageGeneration.NULL) 170 return null; 171 if (code == GuidePageGeneration.HTML) 172 return "html"; 173 if (code == GuidePageGeneration.MARKDOWN) 174 return "markdown"; 175 if (code == GuidePageGeneration.XML) 176 return "xml"; 177 if (code == GuidePageGeneration.GENERATED) 178 return "generated"; 179 return "?"; 180 } 181 public String toSystem(GuidePageGeneration code) { 182 return code.getSystem(); 183 } 184 } 185 186 public enum SPDXLicense { 187 /** 188 * BSD Zero Clause License 189 */ 190 _0BSD, 191 /** 192 * Attribution Assurance License 193 */ 194 AAL, 195 /** 196 * Abstyles License 197 */ 198 ABSTYLES, 199 /** 200 * AdaCore Doc License 201 */ 202 ADACORE_DOC, 203 /** 204 * Adobe Systems Incorporated Source Code License Agreement 205 */ 206 ADOBE_2006, 207 /** 208 * Adobe Glyph List License 209 */ 210 ADOBE_GLYPH, 211 /** 212 * Amazon Digital Services License 213 */ 214 ADSL, 215 /** 216 * Academic Free License v1.1 217 */ 218 AFL_1_1, 219 /** 220 * Academic Free License v1.2 221 */ 222 AFL_1_2, 223 /** 224 * Academic Free License v2.0 225 */ 226 AFL_2_0, 227 /** 228 * Academic Free License v2.1 229 */ 230 AFL_2_1, 231 /** 232 * Academic Free License v3.0 233 */ 234 AFL_3_0, 235 /** 236 * Afmparse License 237 */ 238 AFMPARSE, 239 /** 240 * Affero General Public License v1.0 241 */ 242 AGPL_1_0, 243 /** 244 * Affero General Public License v1.0 only 245 */ 246 AGPL_1_0_ONLY, 247 /** 248 * Affero General Public License v1.0 or later 249 */ 250 AGPL_1_0_OR_LATER, 251 /** 252 * GNU Affero General Public License v3.0 253 */ 254 AGPL_3_0, 255 /** 256 * GNU Affero General Public License v3.0 only 257 */ 258 AGPL_3_0_ONLY, 259 /** 260 * GNU Affero General Public License v3.0 or later 261 */ 262 AGPL_3_0_OR_LATER, 263 /** 264 * Aladdin Free Public License 265 */ 266 ALADDIN, 267 /** 268 * AMD's plpa_map.c License 269 */ 270 AMDPLPA, 271 /** 272 * Apple MIT License 273 */ 274 AML, 275 /** 276 * Academy of Motion Picture Arts and Sciences BSD 277 */ 278 AMPAS, 279 /** 280 * ANTLR Software Rights Notice 281 */ 282 ANTLR_PD, 283 /** 284 * ANTLR Software Rights Notice with license fallback 285 */ 286 ANTLR_PD_FALLBACK, 287 /** 288 * Apache License 1.0 289 */ 290 APACHE_1_0, 291 /** 292 * Apache License 1.1 293 */ 294 APACHE_1_1, 295 /** 296 * Apache License 2.0 297 */ 298 APACHE_2_0, 299 /** 300 * Adobe Postscript AFM License 301 */ 302 APAFML, 303 /** 304 * Adaptive Public License 1.0 305 */ 306 APL_1_0, 307 /** 308 * App::s2p License 309 */ 310 APP_S2P, 311 /** 312 * Apple Public Source License 1.0 313 */ 314 APSL_1_0, 315 /** 316 * Apple Public Source License 1.1 317 */ 318 APSL_1_1, 319 /** 320 * Apple Public Source License 1.2 321 */ 322 APSL_1_2, 323 /** 324 * Apple Public Source License 2.0 325 */ 326 APSL_2_0, 327 /** 328 * Arphic Public License 329 */ 330 ARPHIC_1999, 331 /** 332 * Artistic License 1.0 333 */ 334 ARTISTIC_1_0, 335 /** 336 * Artistic License 1.0 w/clause 8 337 */ 338 ARTISTIC_1_0_CL8, 339 /** 340 * Artistic License 1.0 (Perl) 341 */ 342 ARTISTIC_1_0_PERL, 343 /** 344 * Artistic License 2.0 345 */ 346 ARTISTIC_2_0, 347 /** 348 * ASWF Digital Assets License version 1.0 349 */ 350 ASWF_DIGITAL_ASSETS_1_0, 351 /** 352 * ASWF Digital Assets License 1.1 353 */ 354 ASWF_DIGITAL_ASSETS_1_1, 355 /** 356 * Baekmuk License 357 */ 358 BAEKMUK, 359 /** 360 * Bahyph License 361 */ 362 BAHYPH, 363 /** 364 * Barr License 365 */ 366 BARR, 367 /** 368 * Beerware License 369 */ 370 BEERWARE, 371 /** 372 * Bitstream Charter Font License 373 */ 374 BITSTREAM_CHARTER, 375 /** 376 * Bitstream Vera Font License 377 */ 378 BITSTREAM_VERA, 379 /** 380 * BitTorrent Open Source License v1.0 381 */ 382 BITTORRENT_1_0, 383 /** 384 * BitTorrent Open Source License v1.1 385 */ 386 BITTORRENT_1_1, 387 /** 388 * SQLite Blessing 389 */ 390 BLESSING, 391 /** 392 * Blue Oak Model License 1.0.0 393 */ 394 BLUEOAK_1_0_0, 395 /** 396 * Boehm-Demers-Weiser GC License 397 */ 398 BOEHM_GC, 399 /** 400 * Borceux license 401 */ 402 BORCEUX, 403 /** 404 * Brian Gladman 3-Clause License 405 */ 406 BRIAN_GLADMAN_3_CLAUSE, 407 /** 408 * BSD 1-Clause License 409 */ 410 BSD_1_CLAUSE, 411 /** 412 * BSD 2-Clause "Simplified" License 413 */ 414 BSD_2_CLAUSE, 415 /** 416 * BSD 2-Clause FreeBSD License 417 */ 418 BSD_2_CLAUSE_FREEBSD, 419 /** 420 * BSD 2-Clause NetBSD License 421 */ 422 BSD_2_CLAUSE_NETBSD, 423 /** 424 * BSD-2-Clause Plus Patent License 425 */ 426 BSD_2_CLAUSE_PATENT, 427 /** 428 * BSD 2-Clause with views sentence 429 */ 430 BSD_2_CLAUSE_VIEWS, 431 /** 432 * BSD 3-Clause "New" or "Revised" License 433 */ 434 BSD_3_CLAUSE, 435 /** 436 * BSD with attribution 437 */ 438 BSD_3_CLAUSE_ATTRIBUTION, 439 /** 440 * BSD 3-Clause Clear License 441 */ 442 BSD_3_CLAUSE_CLEAR, 443 /** 444 * Lawrence Berkeley National Labs BSD variant license 445 */ 446 BSD_3_CLAUSE_LBNL, 447 /** 448 * BSD 3-Clause Modification 449 */ 450 BSD_3_CLAUSE_MODIFICATION, 451 /** 452 * BSD 3-Clause No Military License 453 */ 454 BSD_3_CLAUSE_NO_MILITARY_LICENSE, 455 /** 456 * BSD 3-Clause No Nuclear License 457 */ 458 BSD_3_CLAUSE_NO_NUCLEAR_LICENSE, 459 /** 460 * BSD 3-Clause No Nuclear License 2014 461 */ 462 BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014, 463 /** 464 * BSD 3-Clause No Nuclear Warranty 465 */ 466 BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY, 467 /** 468 * BSD 3-Clause Open MPI variant 469 */ 470 BSD_3_CLAUSE_OPEN_MPI, 471 /** 472 * BSD 4-Clause "Original" or "Old" License 473 */ 474 BSD_4_CLAUSE, 475 /** 476 * BSD 4 Clause Shortened 477 */ 478 BSD_4_CLAUSE_SHORTENED, 479 /** 480 * BSD-4-Clause (University of California-Specific) 481 */ 482 BSD_4_CLAUSE_UC, 483 /** 484 * BSD 4.3 RENO License 485 */ 486 BSD_4_3RENO, 487 /** 488 * BSD 4.3 TAHOE License 489 */ 490 BSD_4_3TAHOE, 491 /** 492 * BSD Advertising Acknowledgement License 493 */ 494 BSD_ADVERTISING_ACKNOWLEDGEMENT, 495 /** 496 * BSD with Attribution and HPND disclaimer 497 */ 498 BSD_ATTRIBUTION_HPND_DISCLAIMER, 499 /** 500 * BSD Protection License 501 */ 502 BSD_PROTECTION, 503 /** 504 * BSD Source Code Attribution 505 */ 506 BSD_SOURCE_CODE, 507 /** 508 * Boost Software License 1.0 509 */ 510 BSL_1_0, 511 /** 512 * Business Source License 1.1 513 */ 514 BUSL_1_1, 515 /** 516 * bzip2 and libbzip2 License v1.0.5 517 */ 518 BZIP2_1_0_5, 519 /** 520 * bzip2 and libbzip2 License v1.0.6 521 */ 522 BZIP2_1_0_6, 523 /** 524 * Computational Use of Data Agreement v1.0 525 */ 526 C_UDA_1_0, 527 /** 528 * Cryptographic Autonomy License 1.0 529 */ 530 CAL_1_0, 531 /** 532 * Cryptographic Autonomy License 1.0 (Combined Work Exception) 533 */ 534 CAL_1_0_COMBINED_WORK_EXCEPTION, 535 /** 536 * Caldera License 537 */ 538 CALDERA, 539 /** 540 * Computer Associates Trusted Open Source License 1.1 541 */ 542 CATOSL_1_1, 543 /** 544 * Creative Commons Attribution 1.0 Generic 545 */ 546 CC_BY_1_0, 547 /** 548 * Creative Commons Attribution 2.0 Generic 549 */ 550 CC_BY_2_0, 551 /** 552 * Creative Commons Attribution 2.5 Generic 553 */ 554 CC_BY_2_5, 555 /** 556 * Creative Commons Attribution 2.5 Australia 557 */ 558 CC_BY_2_5_AU, 559 /** 560 * Creative Commons Attribution 3.0 Unported 561 */ 562 CC_BY_3_0, 563 /** 564 * Creative Commons Attribution 3.0 Austria 565 */ 566 CC_BY_3_0_AT, 567 /** 568 * Creative Commons Attribution 3.0 Germany 569 */ 570 CC_BY_3_0_DE, 571 /** 572 * Creative Commons Attribution 3.0 IGO 573 */ 574 CC_BY_3_0_IGO, 575 /** 576 * Creative Commons Attribution 3.0 Netherlands 577 */ 578 CC_BY_3_0_NL, 579 /** 580 * Creative Commons Attribution 3.0 United States 581 */ 582 CC_BY_3_0_US, 583 /** 584 * Creative Commons Attribution 4.0 International 585 */ 586 CC_BY_4_0, 587 /** 588 * Creative Commons Attribution Non Commercial 1.0 Generic 589 */ 590 CC_BY_NC_1_0, 591 /** 592 * Creative Commons Attribution Non Commercial 2.0 Generic 593 */ 594 CC_BY_NC_2_0, 595 /** 596 * Creative Commons Attribution Non Commercial 2.5 Generic 597 */ 598 CC_BY_NC_2_5, 599 /** 600 * Creative Commons Attribution Non Commercial 3.0 Unported 601 */ 602 CC_BY_NC_3_0, 603 /** 604 * Creative Commons Attribution Non Commercial 3.0 Germany 605 */ 606 CC_BY_NC_3_0_DE, 607 /** 608 * Creative Commons Attribution Non Commercial 4.0 International 609 */ 610 CC_BY_NC_4_0, 611 /** 612 * Creative Commons Attribution Non Commercial No Derivatives 1.0 Generic 613 */ 614 CC_BY_NC_ND_1_0, 615 /** 616 * Creative Commons Attribution Non Commercial No Derivatives 2.0 Generic 617 */ 618 CC_BY_NC_ND_2_0, 619 /** 620 * Creative Commons Attribution Non Commercial No Derivatives 2.5 Generic 621 */ 622 CC_BY_NC_ND_2_5, 623 /** 624 * Creative Commons Attribution Non Commercial No Derivatives 3.0 Unported 625 */ 626 CC_BY_NC_ND_3_0, 627 /** 628 * Creative Commons Attribution Non Commercial No Derivatives 3.0 Germany 629 */ 630 CC_BY_NC_ND_3_0_DE, 631 /** 632 * Creative Commons Attribution Non Commercial No Derivatives 3.0 IGO 633 */ 634 CC_BY_NC_ND_3_0_IGO, 635 /** 636 * Creative Commons Attribution Non Commercial No Derivatives 4.0 International 637 */ 638 CC_BY_NC_ND_4_0, 639 /** 640 * Creative Commons Attribution Non Commercial Share Alike 1.0 Generic 641 */ 642 CC_BY_NC_SA_1_0, 643 /** 644 * Creative Commons Attribution Non Commercial Share Alike 2.0 Generic 645 */ 646 CC_BY_NC_SA_2_0, 647 /** 648 * Creative Commons Attribution Non Commercial Share Alike 2.0 Germany 649 */ 650 CC_BY_NC_SA_2_0_DE, 651 /** 652 * Creative Commons Attribution-NonCommercial-ShareAlike 2.0 France 653 */ 654 CC_BY_NC_SA_2_0_FR, 655 /** 656 * Creative Commons Attribution Non Commercial Share Alike 2.0 England and Wales 657 */ 658 CC_BY_NC_SA_2_0_UK, 659 /** 660 * Creative Commons Attribution Non Commercial Share Alike 2.5 Generic 661 */ 662 CC_BY_NC_SA_2_5, 663 /** 664 * Creative Commons Attribution Non Commercial Share Alike 3.0 Unported 665 */ 666 CC_BY_NC_SA_3_0, 667 /** 668 * Creative Commons Attribution Non Commercial Share Alike 3.0 Germany 669 */ 670 CC_BY_NC_SA_3_0_DE, 671 /** 672 * Creative Commons Attribution Non Commercial Share Alike 3.0 IGO 673 */ 674 CC_BY_NC_SA_3_0_IGO, 675 /** 676 * Creative Commons Attribution Non Commercial Share Alike 4.0 International 677 */ 678 CC_BY_NC_SA_4_0, 679 /** 680 * Creative Commons Attribution No Derivatives 1.0 Generic 681 */ 682 CC_BY_ND_1_0, 683 /** 684 * Creative Commons Attribution No Derivatives 2.0 Generic 685 */ 686 CC_BY_ND_2_0, 687 /** 688 * Creative Commons Attribution No Derivatives 2.5 Generic 689 */ 690 CC_BY_ND_2_5, 691 /** 692 * Creative Commons Attribution No Derivatives 3.0 Unported 693 */ 694 CC_BY_ND_3_0, 695 /** 696 * Creative Commons Attribution No Derivatives 3.0 Germany 697 */ 698 CC_BY_ND_3_0_DE, 699 /** 700 * Creative Commons Attribution No Derivatives 4.0 International 701 */ 702 CC_BY_ND_4_0, 703 /** 704 * Creative Commons Attribution Share Alike 1.0 Generic 705 */ 706 CC_BY_SA_1_0, 707 /** 708 * Creative Commons Attribution Share Alike 2.0 Generic 709 */ 710 CC_BY_SA_2_0, 711 /** 712 * Creative Commons Attribution Share Alike 2.0 England and Wales 713 */ 714 CC_BY_SA_2_0_UK, 715 /** 716 * Creative Commons Attribution Share Alike 2.1 Japan 717 */ 718 CC_BY_SA_2_1_JP, 719 /** 720 * Creative Commons Attribution Share Alike 2.5 Generic 721 */ 722 CC_BY_SA_2_5, 723 /** 724 * Creative Commons Attribution Share Alike 3.0 Unported 725 */ 726 CC_BY_SA_3_0, 727 /** 728 * Creative Commons Attribution Share Alike 3.0 Austria 729 */ 730 CC_BY_SA_3_0_AT, 731 /** 732 * Creative Commons Attribution Share Alike 3.0 Germany 733 */ 734 CC_BY_SA_3_0_DE, 735 /** 736 * Creative Commons Attribution-ShareAlike 3.0 IGO 737 */ 738 CC_BY_SA_3_0_IGO, 739 /** 740 * Creative Commons Attribution Share Alike 4.0 International 741 */ 742 CC_BY_SA_4_0, 743 /** 744 * Creative Commons Public Domain Dedication and Certification 745 */ 746 CC_PDDC, 747 /** 748 * Creative Commons Zero v1.0 Universal 749 */ 750 CC0_1_0, 751 /** 752 * Common Development and Distribution License 1.0 753 */ 754 CDDL_1_0, 755 /** 756 * Common Development and Distribution License 1.1 757 */ 758 CDDL_1_1, 759 /** 760 * Common Documentation License 1.0 761 */ 762 CDL_1_0, 763 /** 764 * Community Data License Agreement Permissive 1.0 765 */ 766 CDLA_PERMISSIVE_1_0, 767 /** 768 * Community Data License Agreement Permissive 2.0 769 */ 770 CDLA_PERMISSIVE_2_0, 771 /** 772 * Community Data License Agreement Sharing 1.0 773 */ 774 CDLA_SHARING_1_0, 775 /** 776 * CeCILL Free Software License Agreement v1.0 777 */ 778 CECILL_1_0, 779 /** 780 * CeCILL Free Software License Agreement v1.1 781 */ 782 CECILL_1_1, 783 /** 784 * CeCILL Free Software License Agreement v2.0 785 */ 786 CECILL_2_0, 787 /** 788 * CeCILL Free Software License Agreement v2.1 789 */ 790 CECILL_2_1, 791 /** 792 * CeCILL-B Free Software License Agreement 793 */ 794 CECILL_B, 795 /** 796 * CeCILL-C Free Software License Agreement 797 */ 798 CECILL_C, 799 /** 800 * CERN Open Hardware Licence v1.1 801 */ 802 CERN_OHL_1_1, 803 /** 804 * CERN Open Hardware Licence v1.2 805 */ 806 CERN_OHL_1_2, 807 /** 808 * CERN Open Hardware Licence Version 2 - Permissive 809 */ 810 CERN_OHL_P_2_0, 811 /** 812 * CERN Open Hardware Licence Version 2 - Strongly Reciprocal 813 */ 814 CERN_OHL_S_2_0, 815 /** 816 * CERN Open Hardware Licence Version 2 - Weakly Reciprocal 817 */ 818 CERN_OHL_W_2_0, 819 /** 820 * CFITSIO License 821 */ 822 CFITSIO, 823 /** 824 * Checkmk License 825 */ 826 CHECKMK, 827 /** 828 * Clarified Artistic License 829 */ 830 CLARTISTIC, 831 /** 832 * Clips License 833 */ 834 CLIPS, 835 /** 836 * CMU Mach License 837 */ 838 CMU_MACH, 839 /** 840 * CNRI Jython License 841 */ 842 CNRI_JYTHON, 843 /** 844 * CNRI Python License 845 */ 846 CNRI_PYTHON, 847 /** 848 * CNRI Python Open Source GPL Compatible License Agreement 849 */ 850 CNRI_PYTHON_GPL_COMPATIBLE, 851 /** 852 * Copyfree Open Innovation License 853 */ 854 COIL_1_0, 855 /** 856 * Community Specification License 1.0 857 */ 858 COMMUNITY_SPEC_1_0, 859 /** 860 * Condor Public License v1.1 861 */ 862 CONDOR_1_1, 863 /** 864 * copyleft-next 0.3.0 865 */ 866 COPYLEFT_NEXT_0_3_0, 867 /** 868 * copyleft-next 0.3.1 869 */ 870 COPYLEFT_NEXT_0_3_1, 871 /** 872 * Cornell Lossless JPEG License 873 */ 874 CORNELL_LOSSLESS_JPEG, 875 /** 876 * Common Public Attribution License 1.0 877 */ 878 CPAL_1_0, 879 /** 880 * Common Public License 1.0 881 */ 882 CPL_1_0, 883 /** 884 * Code Project Open License 1.02 885 */ 886 CPOL_1_02, 887 /** 888 * Crossword License 889 */ 890 CROSSWORD, 891 /** 892 * CrystalStacker License 893 */ 894 CRYSTALSTACKER, 895 /** 896 * CUA Office Public License v1.0 897 */ 898 CUA_OPL_1_0, 899 /** 900 * Cube License 901 */ 902 CUBE, 903 /** 904 * curl License 905 */ 906 CURL, 907 /** 908 * Deutsche Freie Software Lizenz 909 */ 910 D_FSL_1_0, 911 /** 912 * diffmark license 913 */ 914 DIFFMARK, 915 /** 916 * Data licence Germany ? attribution ? version 2.0 917 */ 918 DL_DE_BY_2_0, 919 /** 920 * DOC License 921 */ 922 DOC, 923 /** 924 * Dotseqn License 925 */ 926 DOTSEQN, 927 /** 928 * Detection Rule License 1.0 929 */ 930 DRL_1_0, 931 /** 932 * DSDP License 933 */ 934 DSDP, 935 /** 936 * David M. Gay dtoa License 937 */ 938 DTOA, 939 /** 940 * dvipdfm License 941 */ 942 DVIPDFM, 943 /** 944 * Educational Community License v1.0 945 */ 946 ECL_1_0, 947 /** 948 * Educational Community License v2.0 949 */ 950 ECL_2_0, 951 /** 952 * eCos license version 2.0 953 */ 954 ECOS_2_0, 955 /** 956 * Eiffel Forum License v1.0 957 */ 958 EFL_1_0, 959 /** 960 * Eiffel Forum License v2.0 961 */ 962 EFL_2_0, 963 /** 964 * eGenix.com Public License 1.1.0 965 */ 966 EGENIX, 967 /** 968 * Elastic License 2.0 969 */ 970 ELASTIC_2_0, 971 /** 972 * Entessa Public License v1.0 973 */ 974 ENTESSA, 975 /** 976 * EPICS Open License 977 */ 978 EPICS, 979 /** 980 * Eclipse Public License 1.0 981 */ 982 EPL_1_0, 983 /** 984 * Eclipse Public License 2.0 985 */ 986 EPL_2_0, 987 /** 988 * Erlang Public License v1.1 989 */ 990 ERLPL_1_1, 991 /** 992 * Etalab Open License 2.0 993 */ 994 ETALAB_2_0, 995 /** 996 * EU DataGrid Software License 997 */ 998 EUDATAGRID, 999 /** 1000 * European Union Public License 1.0 1001 */ 1002 EUPL_1_0, 1003 /** 1004 * European Union Public License 1.1 1005 */ 1006 EUPL_1_1, 1007 /** 1008 * European Union Public License 1.2 1009 */ 1010 EUPL_1_2, 1011 /** 1012 * Eurosym License 1013 */ 1014 EUROSYM, 1015 /** 1016 * Fair License 1017 */ 1018 FAIR, 1019 /** 1020 * Fraunhofer FDK AAC Codec Library 1021 */ 1022 FDK_AAC, 1023 /** 1024 * Frameworx Open License 1.0 1025 */ 1026 FRAMEWORX_1_0, 1027 /** 1028 * FreeBSD Documentation License 1029 */ 1030 FREEBSD_DOC, 1031 /** 1032 * FreeImage Public License v1.0 1033 */ 1034 FREEIMAGE, 1035 /** 1036 * FSF All Permissive License 1037 */ 1038 FSFAP, 1039 /** 1040 * FSF Unlimited License 1041 */ 1042 FSFUL, 1043 /** 1044 * FSF Unlimited License (with License Retention) 1045 */ 1046 FSFULLR, 1047 /** 1048 * FSF Unlimited License (With License Retention and Warranty Disclaimer) 1049 */ 1050 FSFULLRWD, 1051 /** 1052 * Freetype Project License 1053 */ 1054 FTL, 1055 /** 1056 * GD License 1057 */ 1058 GD, 1059 /** 1060 * GNU Free Documentation License v1.1 1061 */ 1062 GFDL_1_1, 1063 /** 1064 * GNU Free Documentation License v1.1 only - invariants 1065 */ 1066 GFDL_1_1_INVARIANTS_ONLY, 1067 /** 1068 * GNU Free Documentation License v1.1 or later - invariants 1069 */ 1070 GFDL_1_1_INVARIANTS_OR_LATER, 1071 /** 1072 * GNU Free Documentation License v1.1 only - no invariants 1073 */ 1074 GFDL_1_1_NO_INVARIANTS_ONLY, 1075 /** 1076 * GNU Free Documentation License v1.1 or later - no invariants 1077 */ 1078 GFDL_1_1_NO_INVARIANTS_OR_LATER, 1079 /** 1080 * GNU Free Documentation License v1.1 only 1081 */ 1082 GFDL_1_1_ONLY, 1083 /** 1084 * GNU Free Documentation License v1.1 or later 1085 */ 1086 GFDL_1_1_OR_LATER, 1087 /** 1088 * GNU Free Documentation License v1.2 1089 */ 1090 GFDL_1_2, 1091 /** 1092 * GNU Free Documentation License v1.2 only - invariants 1093 */ 1094 GFDL_1_2_INVARIANTS_ONLY, 1095 /** 1096 * GNU Free Documentation License v1.2 or later - invariants 1097 */ 1098 GFDL_1_2_INVARIANTS_OR_LATER, 1099 /** 1100 * GNU Free Documentation License v1.2 only - no invariants 1101 */ 1102 GFDL_1_2_NO_INVARIANTS_ONLY, 1103 /** 1104 * GNU Free Documentation License v1.2 or later - no invariants 1105 */ 1106 GFDL_1_2_NO_INVARIANTS_OR_LATER, 1107 /** 1108 * GNU Free Documentation License v1.2 only 1109 */ 1110 GFDL_1_2_ONLY, 1111 /** 1112 * GNU Free Documentation License v1.2 or later 1113 */ 1114 GFDL_1_2_OR_LATER, 1115 /** 1116 * GNU Free Documentation License v1.3 1117 */ 1118 GFDL_1_3, 1119 /** 1120 * GNU Free Documentation License v1.3 only - invariants 1121 */ 1122 GFDL_1_3_INVARIANTS_ONLY, 1123 /** 1124 * GNU Free Documentation License v1.3 or later - invariants 1125 */ 1126 GFDL_1_3_INVARIANTS_OR_LATER, 1127 /** 1128 * GNU Free Documentation License v1.3 only - no invariants 1129 */ 1130 GFDL_1_3_NO_INVARIANTS_ONLY, 1131 /** 1132 * GNU Free Documentation License v1.3 or later - no invariants 1133 */ 1134 GFDL_1_3_NO_INVARIANTS_OR_LATER, 1135 /** 1136 * GNU Free Documentation License v1.3 only 1137 */ 1138 GFDL_1_3_ONLY, 1139 /** 1140 * GNU Free Documentation License v1.3 or later 1141 */ 1142 GFDL_1_3_OR_LATER, 1143 /** 1144 * Giftware License 1145 */ 1146 GIFTWARE, 1147 /** 1148 * GL2PS License 1149 */ 1150 GL2PS, 1151 /** 1152 * 3dfx Glide License 1153 */ 1154 GLIDE, 1155 /** 1156 * Glulxe License 1157 */ 1158 GLULXE, 1159 /** 1160 * Good Luck With That Public License 1161 */ 1162 GLWTPL, 1163 /** 1164 * gnuplot License 1165 */ 1166 GNUPLOT, 1167 /** 1168 * GNU General Public License v1.0 only 1169 */ 1170 GPL_1_0, 1171 /** 1172 * GNU General Public License v1.0 or later 1173 */ 1174 GPL_1_0PLUS, 1175 /** 1176 * GNU General Public License v1.0 only 1177 */ 1178 GPL_1_0_ONLY, 1179 /** 1180 * GNU General Public License v1.0 or later 1181 */ 1182 GPL_1_0_OR_LATER, 1183 /** 1184 * GNU General Public License v2.0 only 1185 */ 1186 GPL_2_0, 1187 /** 1188 * GNU General Public License v2.0 or later 1189 */ 1190 GPL_2_0PLUS, 1191 /** 1192 * GNU General Public License v2.0 only 1193 */ 1194 GPL_2_0_ONLY, 1195 /** 1196 * GNU General Public License v2.0 or later 1197 */ 1198 GPL_2_0_OR_LATER, 1199 /** 1200 * GNU General Public License v2.0 w/Autoconf exception 1201 */ 1202 GPL_2_0_WITH_AUTOCONF_EXCEPTION, 1203 /** 1204 * GNU General Public License v2.0 w/Bison exception 1205 */ 1206 GPL_2_0_WITH_BISON_EXCEPTION, 1207 /** 1208 * GNU General Public License v2.0 w/Classpath exception 1209 */ 1210 GPL_2_0_WITH_CLASSPATH_EXCEPTION, 1211 /** 1212 * GNU General Public License v2.0 w/Font exception 1213 */ 1214 GPL_2_0_WITH_FONT_EXCEPTION, 1215 /** 1216 * GNU General Public License v2.0 w/GCC Runtime Library exception 1217 */ 1218 GPL_2_0_WITH_GCC_EXCEPTION, 1219 /** 1220 * GNU General Public License v3.0 only 1221 */ 1222 GPL_3_0, 1223 /** 1224 * GNU General Public License v3.0 or later 1225 */ 1226 GPL_3_0PLUS, 1227 /** 1228 * GNU General Public License v3.0 only 1229 */ 1230 GPL_3_0_ONLY, 1231 /** 1232 * GNU General Public License v3.0 or later 1233 */ 1234 GPL_3_0_OR_LATER, 1235 /** 1236 * GNU General Public License v3.0 w/Autoconf exception 1237 */ 1238 GPL_3_0_WITH_AUTOCONF_EXCEPTION, 1239 /** 1240 * GNU General Public License v3.0 w/GCC Runtime Library exception 1241 */ 1242 GPL_3_0_WITH_GCC_EXCEPTION, 1243 /** 1244 * Graphics Gems License 1245 */ 1246 GRAPHICS_GEMS, 1247 /** 1248 * gSOAP Public License v1.3b 1249 */ 1250 GSOAP_1_3B, 1251 /** 1252 * Haskell Language Report License 1253 */ 1254 HASKELLREPORT, 1255 /** 1256 * Hippocratic License 2.1 1257 */ 1258 HIPPOCRATIC_2_1, 1259 /** 1260 * Hewlett-Packard 1986 License 1261 */ 1262 HP_1986, 1263 /** 1264 * Historical Permission Notice and Disclaimer 1265 */ 1266 HPND, 1267 /** 1268 * HPND with US Government export control warning 1269 */ 1270 HPND_EXPORT_US, 1271 /** 1272 * Historical Permission Notice and Disclaimer - Markus Kuhn variant 1273 */ 1274 HPND_MARKUS_KUHN, 1275 /** 1276 * Historical Permission Notice and Disclaimer - sell variant 1277 */ 1278 HPND_SELL_VARIANT, 1279 /** 1280 * HPND sell variant with MIT disclaimer 1281 */ 1282 HPND_SELL_VARIANT_MIT_DISCLAIMER, 1283 /** 1284 * HTML Tidy License 1285 */ 1286 HTMLTIDY, 1287 /** 1288 * IBM PowerPC Initialization and Boot Software 1289 */ 1290 IBM_PIBS, 1291 /** 1292 * ICU License 1293 */ 1294 ICU, 1295 /** 1296 * IEC Code Components End-user licence agreement 1297 */ 1298 IEC_CODE_COMPONENTS_EULA, 1299 /** 1300 * Independent JPEG Group License 1301 */ 1302 IJG, 1303 /** 1304 * Independent JPEG Group License - short 1305 */ 1306 IJG_SHORT, 1307 /** 1308 * ImageMagick License 1309 */ 1310 IMAGEMAGICK, 1311 /** 1312 * iMatix Standard Function Library Agreement 1313 */ 1314 IMATIX, 1315 /** 1316 * Imlib2 License 1317 */ 1318 IMLIB2, 1319 /** 1320 * Info-ZIP License 1321 */ 1322 INFO_ZIP, 1323 /** 1324 * Inner Net License v2.0 1325 */ 1326 INNER_NET_2_0, 1327 /** 1328 * Intel Open Source License 1329 */ 1330 INTEL, 1331 /** 1332 * Intel ACPI Software License Agreement 1333 */ 1334 INTEL_ACPI, 1335 /** 1336 * Interbase Public License v1.0 1337 */ 1338 INTERBASE_1_0, 1339 /** 1340 * IPA Font License 1341 */ 1342 IPA, 1343 /** 1344 * IBM Public License v1.0 1345 */ 1346 IPL_1_0, 1347 /** 1348 * ISC License 1349 */ 1350 ISC, 1351 /** 1352 * Jam License 1353 */ 1354 JAM, 1355 /** 1356 * JasPer License 1357 */ 1358 JASPER_2_0, 1359 /** 1360 * JPL Image Use Policy 1361 */ 1362 JPL_IMAGE, 1363 /** 1364 * Japan Network Information Center License 1365 */ 1366 JPNIC, 1367 /** 1368 * JSON License 1369 */ 1370 JSON, 1371 /** 1372 * Kazlib License 1373 */ 1374 KAZLIB, 1375 /** 1376 * Knuth CTAN License 1377 */ 1378 KNUTH_CTAN, 1379 /** 1380 * Licence Art Libre 1.2 1381 */ 1382 LAL_1_2, 1383 /** 1384 * Licence Art Libre 1.3 1385 */ 1386 LAL_1_3, 1387 /** 1388 * Latex2e License 1389 */ 1390 LATEX2E, 1391 /** 1392 * Latex2e with translated notice permission 1393 */ 1394 LATEX2E_TRANSLATED_NOTICE, 1395 /** 1396 * Leptonica License 1397 */ 1398 LEPTONICA, 1399 /** 1400 * GNU Library General Public License v2 only 1401 */ 1402 LGPL_2_0, 1403 /** 1404 * GNU Library General Public License v2 or later 1405 */ 1406 LGPL_2_0PLUS, 1407 /** 1408 * GNU Library General Public License v2 only 1409 */ 1410 LGPL_2_0_ONLY, 1411 /** 1412 * GNU Library General Public License v2 or later 1413 */ 1414 LGPL_2_0_OR_LATER, 1415 /** 1416 * GNU Lesser General Public License v2.1 only 1417 */ 1418 LGPL_2_1, 1419 /** 1420 * GNU Lesser General Public License v2.1 or later 1421 */ 1422 LGPL_2_1PLUS, 1423 /** 1424 * GNU Lesser General Public License v2.1 only 1425 */ 1426 LGPL_2_1_ONLY, 1427 /** 1428 * GNU Lesser General Public License v2.1 or later 1429 */ 1430 LGPL_2_1_OR_LATER, 1431 /** 1432 * GNU Lesser General Public License v3.0 only 1433 */ 1434 LGPL_3_0, 1435 /** 1436 * GNU Lesser General Public License v3.0 or later 1437 */ 1438 LGPL_3_0PLUS, 1439 /** 1440 * GNU Lesser General Public License v3.0 only 1441 */ 1442 LGPL_3_0_ONLY, 1443 /** 1444 * GNU Lesser General Public License v3.0 or later 1445 */ 1446 LGPL_3_0_OR_LATER, 1447 /** 1448 * Lesser General Public License For Linguistic Resources 1449 */ 1450 LGPLLR, 1451 /** 1452 * libpng License 1453 */ 1454 LIBPNG, 1455 /** 1456 * PNG Reference Library version 2 1457 */ 1458 LIBPNG_2_0, 1459 /** 1460 * libselinux public domain notice 1461 */ 1462 LIBSELINUX_1_0, 1463 /** 1464 * libtiff License 1465 */ 1466 LIBTIFF, 1467 /** 1468 * libutil David Nugent License 1469 */ 1470 LIBUTIL_DAVID_NUGENT, 1471 /** 1472 * Licence Libre du Québec ? Permissive version 1.1 1473 */ 1474 LILIQ_P_1_1, 1475 /** 1476 * Licence Libre du Québec ? Réciprocité version 1.1 1477 */ 1478 LILIQ_R_1_1, 1479 /** 1480 * Licence Libre du Québec ? Réciprocité forte version 1.1 1481 */ 1482 LILIQ_RPLUS_1_1, 1483 /** 1484 * Linux man-pages - 1 paragraph 1485 */ 1486 LINUX_MAN_PAGES_1_PARA, 1487 /** 1488 * Linux man-pages Copyleft 1489 */ 1490 LINUX_MAN_PAGES_COPYLEFT, 1491 /** 1492 * Linux man-pages Copyleft - 2 paragraphs 1493 */ 1494 LINUX_MAN_PAGES_COPYLEFT_2_PARA, 1495 /** 1496 * Linux man-pages Copyleft Variant 1497 */ 1498 LINUX_MAN_PAGES_COPYLEFT_VAR, 1499 /** 1500 * Linux Kernel Variant of OpenIB.org license 1501 */ 1502 LINUX_OPENIB, 1503 /** 1504 * Common Lisp LOOP License 1505 */ 1506 LOOP, 1507 /** 1508 * Lucent Public License Version 1.0 1509 */ 1510 LPL_1_0, 1511 /** 1512 * Lucent Public License v1.02 1513 */ 1514 LPL_1_02, 1515 /** 1516 * LaTeX Project Public License v1.0 1517 */ 1518 LPPL_1_0, 1519 /** 1520 * LaTeX Project Public License v1.1 1521 */ 1522 LPPL_1_1, 1523 /** 1524 * LaTeX Project Public License v1.2 1525 */ 1526 LPPL_1_2, 1527 /** 1528 * LaTeX Project Public License v1.3a 1529 */ 1530 LPPL_1_3A, 1531 /** 1532 * LaTeX Project Public License v1.3c 1533 */ 1534 LPPL_1_3C, 1535 /** 1536 * LZMA SDK License (versions 9.11 to 9.20) 1537 */ 1538 LZMA_SDK_9_11_TO_9_20, 1539 /** 1540 * LZMA SDK License (versions 9.22 and beyond) 1541 */ 1542 LZMA_SDK_9_22, 1543 /** 1544 * MakeIndex License 1545 */ 1546 MAKEINDEX, 1547 /** 1548 * Martin Birgmeier License 1549 */ 1550 MARTIN_BIRGMEIER, 1551 /** 1552 * metamail License 1553 */ 1554 METAMAIL, 1555 /** 1556 * Minpack License 1557 */ 1558 MINPACK, 1559 /** 1560 * The MirOS Licence 1561 */ 1562 MIROS, 1563 /** 1564 * MIT License 1565 */ 1566 MIT, 1567 /** 1568 * MIT No Attribution 1569 */ 1570 MIT_0, 1571 /** 1572 * Enlightenment License (e16) 1573 */ 1574 MIT_ADVERTISING, 1575 /** 1576 * CMU License 1577 */ 1578 MIT_CMU, 1579 /** 1580 * enna License 1581 */ 1582 MIT_ENNA, 1583 /** 1584 * feh License 1585 */ 1586 MIT_FEH, 1587 /** 1588 * MIT Festival Variant 1589 */ 1590 MIT_FESTIVAL, 1591 /** 1592 * MIT License Modern Variant 1593 */ 1594 MIT_MODERN_VARIANT, 1595 /** 1596 * MIT Open Group variant 1597 */ 1598 MIT_OPEN_GROUP, 1599 /** 1600 * MIT Tom Wu Variant 1601 */ 1602 MIT_WU, 1603 /** 1604 * MIT +no-false-attribs license 1605 */ 1606 MITNFA, 1607 /** 1608 * Motosoto License 1609 */ 1610 MOTOSOTO, 1611 /** 1612 * mpi Permissive License 1613 */ 1614 MPI_PERMISSIVE, 1615 /** 1616 * mpich2 License 1617 */ 1618 MPICH2, 1619 /** 1620 * Mozilla Public License 1.0 1621 */ 1622 MPL_1_0, 1623 /** 1624 * Mozilla Public License 1.1 1625 */ 1626 MPL_1_1, 1627 /** 1628 * Mozilla Public License 2.0 1629 */ 1630 MPL_2_0, 1631 /** 1632 * Mozilla Public License 2.0 (no copyleft exception) 1633 */ 1634 MPL_2_0_NO_COPYLEFT_EXCEPTION, 1635 /** 1636 * mplus Font License 1637 */ 1638 MPLUS, 1639 /** 1640 * Microsoft Limited Public License 1641 */ 1642 MS_LPL, 1643 /** 1644 * Microsoft Public License 1645 */ 1646 MS_PL, 1647 /** 1648 * Microsoft Reciprocal License 1649 */ 1650 MS_RL, 1651 /** 1652 * Matrix Template Library License 1653 */ 1654 MTLL, 1655 /** 1656 * Mulan Permissive Software License, Version 1 1657 */ 1658 MULANPSL_1_0, 1659 /** 1660 * Mulan Permissive Software License, Version 2 1661 */ 1662 MULANPSL_2_0, 1663 /** 1664 * Multics License 1665 */ 1666 MULTICS, 1667 /** 1668 * Mup License 1669 */ 1670 MUP, 1671 /** 1672 * Nara Institute of Science and Technology License (2003) 1673 */ 1674 NAIST_2003, 1675 /** 1676 * NASA Open Source Agreement 1.3 1677 */ 1678 NASA_1_3, 1679 /** 1680 * Naumen Public License 1681 */ 1682 NAUMEN, 1683 /** 1684 * Net Boolean Public License v1 1685 */ 1686 NBPL_1_0, 1687 /** 1688 * Non-Commercial Government Licence 1689 */ 1690 NCGL_UK_2_0, 1691 /** 1692 * University of Illinois/NCSA Open Source License 1693 */ 1694 NCSA, 1695 /** 1696 * Net-SNMP License 1697 */ 1698 NET_SNMP, 1699 /** 1700 * NetCDF license 1701 */ 1702 NETCDF, 1703 /** 1704 * Newsletr License 1705 */ 1706 NEWSLETR, 1707 /** 1708 * Nethack General Public License 1709 */ 1710 NGPL, 1711 /** 1712 * NICTA Public Software License, Version 1.0 1713 */ 1714 NICTA_1_0, 1715 /** 1716 * NIST Public Domain Notice 1717 */ 1718 NIST_PD, 1719 /** 1720 * NIST Public Domain Notice with license fallback 1721 */ 1722 NIST_PD_FALLBACK, 1723 /** 1724 * NIST Software License 1725 */ 1726 NIST_SOFTWARE, 1727 /** 1728 * Norwegian Licence for Open Government Data (NLOD) 1.0 1729 */ 1730 NLOD_1_0, 1731 /** 1732 * Norwegian Licence for Open Government Data (NLOD) 2.0 1733 */ 1734 NLOD_2_0, 1735 /** 1736 * No Limit Public License 1737 */ 1738 NLPL, 1739 /** 1740 * Nokia Open Source License 1741 */ 1742 NOKIA, 1743 /** 1744 * Netizen Open Source License 1745 */ 1746 NOSL, 1747 /** 1748 * Not an open source license. 1749 */ 1750 NOT_OPEN_SOURCE, 1751 /** 1752 * Noweb License 1753 */ 1754 NOWEB, 1755 /** 1756 * Netscape Public License v1.0 1757 */ 1758 NPL_1_0, 1759 /** 1760 * Netscape Public License v1.1 1761 */ 1762 NPL_1_1, 1763 /** 1764 * Non-Profit Open Software License 3.0 1765 */ 1766 NPOSL_3_0, 1767 /** 1768 * NRL License 1769 */ 1770 NRL, 1771 /** 1772 * NTP License 1773 */ 1774 NTP, 1775 /** 1776 * NTP No Attribution 1777 */ 1778 NTP_0, 1779 /** 1780 * Nunit License 1781 */ 1782 NUNIT, 1783 /** 1784 * Open Use of Data Agreement v1.0 1785 */ 1786 O_UDA_1_0, 1787 /** 1788 * Open CASCADE Technology Public License 1789 */ 1790 OCCT_PL, 1791 /** 1792 * OCLC Research Public License 2.0 1793 */ 1794 OCLC_2_0, 1795 /** 1796 * Open Data Commons Open Database License v1.0 1797 */ 1798 ODBL_1_0, 1799 /** 1800 * Open Data Commons Attribution License v1.0 1801 */ 1802 ODC_BY_1_0, 1803 /** 1804 * OFFIS License 1805 */ 1806 OFFIS, 1807 /** 1808 * SIL Open Font License 1.0 1809 */ 1810 OFL_1_0, 1811 /** 1812 * SIL Open Font License 1.0 with no Reserved Font Name 1813 */ 1814 OFL_1_0_NO_RFN, 1815 /** 1816 * SIL Open Font License 1.0 with Reserved Font Name 1817 */ 1818 OFL_1_0_RFN, 1819 /** 1820 * SIL Open Font License 1.1 1821 */ 1822 OFL_1_1, 1823 /** 1824 * SIL Open Font License 1.1 with no Reserved Font Name 1825 */ 1826 OFL_1_1_NO_RFN, 1827 /** 1828 * SIL Open Font License 1.1 with Reserved Font Name 1829 */ 1830 OFL_1_1_RFN, 1831 /** 1832 * OGC Software License, Version 1.0 1833 */ 1834 OGC_1_0, 1835 /** 1836 * Taiwan Open Government Data License, version 1.0 1837 */ 1838 OGDL_TAIWAN_1_0, 1839 /** 1840 * Open Government Licence - Canada 1841 */ 1842 OGL_CANADA_2_0, 1843 /** 1844 * Open Government Licence v1.0 1845 */ 1846 OGL_UK_1_0, 1847 /** 1848 * Open Government Licence v2.0 1849 */ 1850 OGL_UK_2_0, 1851 /** 1852 * Open Government Licence v3.0 1853 */ 1854 OGL_UK_3_0, 1855 /** 1856 * Open Group Test Suite License 1857 */ 1858 OGTSL, 1859 /** 1860 * Open LDAP Public License v1.1 1861 */ 1862 OLDAP_1_1, 1863 /** 1864 * Open LDAP Public License v1.2 1865 */ 1866 OLDAP_1_2, 1867 /** 1868 * Open LDAP Public License v1.3 1869 */ 1870 OLDAP_1_3, 1871 /** 1872 * Open LDAP Public License v1.4 1873 */ 1874 OLDAP_1_4, 1875 /** 1876 * Open LDAP Public License v2.0 (or possibly 2.0A and 2.0B) 1877 */ 1878 OLDAP_2_0, 1879 /** 1880 * Open LDAP Public License v2.0.1 1881 */ 1882 OLDAP_2_0_1, 1883 /** 1884 * Open LDAP Public License v2.1 1885 */ 1886 OLDAP_2_1, 1887 /** 1888 * Open LDAP Public License v2.2 1889 */ 1890 OLDAP_2_2, 1891 /** 1892 * Open LDAP Public License v2.2.1 1893 */ 1894 OLDAP_2_2_1, 1895 /** 1896 * Open LDAP Public License 2.2.2 1897 */ 1898 OLDAP_2_2_2, 1899 /** 1900 * Open LDAP Public License v2.3 1901 */ 1902 OLDAP_2_3, 1903 /** 1904 * Open LDAP Public License v2.4 1905 */ 1906 OLDAP_2_4, 1907 /** 1908 * Open LDAP Public License v2.5 1909 */ 1910 OLDAP_2_5, 1911 /** 1912 * Open LDAP Public License v2.6 1913 */ 1914 OLDAP_2_6, 1915 /** 1916 * Open LDAP Public License v2.7 1917 */ 1918 OLDAP_2_7, 1919 /** 1920 * Open LDAP Public License v2.8 1921 */ 1922 OLDAP_2_8, 1923 /** 1924 * Open Logistics Foundation License Version 1.3 1925 */ 1926 OLFL_1_3, 1927 /** 1928 * Open Market License 1929 */ 1930 OML, 1931 /** 1932 * OpenPBS v2.3 Software License 1933 */ 1934 OPENPBS_2_3, 1935 /** 1936 * OpenSSL License 1937 */ 1938 OPENSSL, 1939 /** 1940 * Open Public License v1.0 1941 */ 1942 OPL_1_0, 1943 /** 1944 * United Kingdom Open Parliament Licence v3.0 1945 */ 1946 OPL_UK_3_0, 1947 /** 1948 * Open Publication License v1.0 1949 */ 1950 OPUBL_1_0, 1951 /** 1952 * OSET Public License version 2.1 1953 */ 1954 OSET_PL_2_1, 1955 /** 1956 * Open Software License 1.0 1957 */ 1958 OSL_1_0, 1959 /** 1960 * Open Software License 1.1 1961 */ 1962 OSL_1_1, 1963 /** 1964 * Open Software License 2.0 1965 */ 1966 OSL_2_0, 1967 /** 1968 * Open Software License 2.1 1969 */ 1970 OSL_2_1, 1971 /** 1972 * Open Software License 3.0 1973 */ 1974 OSL_3_0, 1975 /** 1976 * The Parity Public License 6.0.0 1977 */ 1978 PARITY_6_0_0, 1979 /** 1980 * The Parity Public License 7.0.0 1981 */ 1982 PARITY_7_0_0, 1983 /** 1984 * Open Data Commons Public Domain Dedication & License 1.0 1985 */ 1986 PDDL_1_0, 1987 /** 1988 * PHP License v3.0 1989 */ 1990 PHP_3_0, 1991 /** 1992 * PHP License v3.01 1993 */ 1994 PHP_3_01, 1995 /** 1996 * Plexus Classworlds License 1997 */ 1998 PLEXUS, 1999 /** 2000 * PolyForm Noncommercial License 1.0.0 2001 */ 2002 POLYFORM_NONCOMMERCIAL_1_0_0, 2003 /** 2004 * PolyForm Small Business License 1.0.0 2005 */ 2006 POLYFORM_SMALL_BUSINESS_1_0_0, 2007 /** 2008 * PostgreSQL License 2009 */ 2010 POSTGRESQL, 2011 /** 2012 * Python Software Foundation License 2.0 2013 */ 2014 PSF_2_0, 2015 /** 2016 * psfrag License 2017 */ 2018 PSFRAG, 2019 /** 2020 * psutils License 2021 */ 2022 PSUTILS, 2023 /** 2024 * Python License 2.0 2025 */ 2026 PYTHON_2_0, 2027 /** 2028 * Python License 2.0.1 2029 */ 2030 PYTHON_2_0_1, 2031 /** 2032 * Qhull License 2033 */ 2034 QHULL, 2035 /** 2036 * Q Public License 1.0 2037 */ 2038 QPL_1_0, 2039 /** 2040 * Q Public License 1.0 - INRIA 2004 variant 2041 */ 2042 QPL_1_0_INRIA_2004, 2043 /** 2044 * Rdisc License 2045 */ 2046 RDISC, 2047 /** 2048 * Red Hat eCos Public License v1.1 2049 */ 2050 RHECOS_1_1, 2051 /** 2052 * Reciprocal Public License 1.1 2053 */ 2054 RPL_1_1, 2055 /** 2056 * Reciprocal Public License 1.5 2057 */ 2058 RPL_1_5, 2059 /** 2060 * RealNetworks Public Source License v1.0 2061 */ 2062 RPSL_1_0, 2063 /** 2064 * RSA Message-Digest License 2065 */ 2066 RSA_MD, 2067 /** 2068 * Ricoh Source Code Public License 2069 */ 2070 RSCPL, 2071 /** 2072 * Ruby License 2073 */ 2074 RUBY, 2075 /** 2076 * Sax Public Domain Notice 2077 */ 2078 SAX_PD, 2079 /** 2080 * Saxpath License 2081 */ 2082 SAXPATH, 2083 /** 2084 * SCEA Shared Source License 2085 */ 2086 SCEA, 2087 /** 2088 * Scheme Language Report License 2089 */ 2090 SCHEMEREPORT, 2091 /** 2092 * Sendmail License 2093 */ 2094 SENDMAIL, 2095 /** 2096 * Sendmail License 8.23 2097 */ 2098 SENDMAIL_8_23, 2099 /** 2100 * SGI Free Software License B v1.0 2101 */ 2102 SGI_B_1_0, 2103 /** 2104 * SGI Free Software License B v1.1 2105 */ 2106 SGI_B_1_1, 2107 /** 2108 * SGI Free Software License B v2.0 2109 */ 2110 SGI_B_2_0, 2111 /** 2112 * SGP4 Permission Notice 2113 */ 2114 SGP4, 2115 /** 2116 * Solderpad Hardware License v0.5 2117 */ 2118 SHL_0_5, 2119 /** 2120 * Solderpad Hardware License, Version 0.51 2121 */ 2122 SHL_0_51, 2123 /** 2124 * Simple Public License 2.0 2125 */ 2126 SIMPL_2_0, 2127 /** 2128 * Sun Industry Standards Source License v1.1 2129 */ 2130 SISSL, 2131 /** 2132 * Sun Industry Standards Source License v1.2 2133 */ 2134 SISSL_1_2, 2135 /** 2136 * Sleepycat License 2137 */ 2138 SLEEPYCAT, 2139 /** 2140 * Standard ML of New Jersey License 2141 */ 2142 SMLNJ, 2143 /** 2144 * Secure Messaging Protocol Public License 2145 */ 2146 SMPPL, 2147 /** 2148 * SNIA Public License 1.1 2149 */ 2150 SNIA, 2151 /** 2152 * snprintf License 2153 */ 2154 SNPRINTF, 2155 /** 2156 * Spencer License 86 2157 */ 2158 SPENCER_86, 2159 /** 2160 * Spencer License 94 2161 */ 2162 SPENCER_94, 2163 /** 2164 * Spencer License 99 2165 */ 2166 SPENCER_99, 2167 /** 2168 * Sun Public License v1.0 2169 */ 2170 SPL_1_0, 2171 /** 2172 * SSH OpenSSH license 2173 */ 2174 SSH_OPENSSH, 2175 /** 2176 * SSH short notice 2177 */ 2178 SSH_SHORT, 2179 /** 2180 * Server Side Public License, v 1 2181 */ 2182 SSPL_1_0, 2183 /** 2184 * Standard ML of New Jersey License 2185 */ 2186 STANDARDML_NJ, 2187 /** 2188 * SugarCRM Public License v1.1.3 2189 */ 2190 SUGARCRM_1_1_3, 2191 /** 2192 * SunPro License 2193 */ 2194 SUNPRO, 2195 /** 2196 * Scheme Widget Library (SWL) Software License Agreement 2197 */ 2198 SWL, 2199 /** 2200 * Symlinks License 2201 */ 2202 SYMLINKS, 2203 /** 2204 * TAPR Open Hardware License v1.0 2205 */ 2206 TAPR_OHL_1_0, 2207 /** 2208 * TCL/TK License 2209 */ 2210 TCL, 2211 /** 2212 * TCP Wrappers License 2213 */ 2214 TCP_WRAPPERS, 2215 /** 2216 * TermReadKey License 2217 */ 2218 TERMREADKEY, 2219 /** 2220 * TMate Open Source License 2221 */ 2222 TMATE, 2223 /** 2224 * TORQUE v2.5+ Software License v1.1 2225 */ 2226 TORQUE_1_1, 2227 /** 2228 * Trusster Open Source License 2229 */ 2230 TOSL, 2231 /** 2232 * Time::ParseDate License 2233 */ 2234 TPDL, 2235 /** 2236 * THOR Public License 1.0 2237 */ 2238 TPL_1_0, 2239 /** 2240 * Text-Tabs+Wrap License 2241 */ 2242 TTWL, 2243 /** 2244 * Technische Universitaet Berlin License 1.0 2245 */ 2246 TU_BERLIN_1_0, 2247 /** 2248 * Technische Universitaet Berlin License 2.0 2249 */ 2250 TU_BERLIN_2_0, 2251 /** 2252 * UCAR License 2253 */ 2254 UCAR, 2255 /** 2256 * Upstream Compatibility License v1.0 2257 */ 2258 UCL_1_0, 2259 /** 2260 * Unicode License Agreement - Data Files and Software (2015) 2261 */ 2262 UNICODE_DFS_2015, 2263 /** 2264 * Unicode License Agreement - Data Files and Software (2016) 2265 */ 2266 UNICODE_DFS_2016, 2267 /** 2268 * Unicode Terms of Use 2269 */ 2270 UNICODE_TOU, 2271 /** 2272 * UnixCrypt License 2273 */ 2274 UNIXCRYPT, 2275 /** 2276 * The Unlicense 2277 */ 2278 UNLICENSE, 2279 /** 2280 * Universal Permissive License v1.0 2281 */ 2282 UPL_1_0, 2283 /** 2284 * Vim License 2285 */ 2286 VIM, 2287 /** 2288 * VOSTROM Public License for Open Source 2289 */ 2290 VOSTROM, 2291 /** 2292 * Vovida Software License v1.0 2293 */ 2294 VSL_1_0, 2295 /** 2296 * W3C Software Notice and License (2002-12-31) 2297 */ 2298 W3C, 2299 /** 2300 * W3C Software Notice and License (1998-07-20) 2301 */ 2302 W3C_19980720, 2303 /** 2304 * W3C Software Notice and Document License (2015-05-13) 2305 */ 2306 W3C_20150513, 2307 /** 2308 * w3m License 2309 */ 2310 W3M, 2311 /** 2312 * Sybase Open Watcom Public License 1.0 2313 */ 2314 WATCOM_1_0, 2315 /** 2316 * Widget Workshop License 2317 */ 2318 WIDGET_WORKSHOP, 2319 /** 2320 * Wsuipa License 2321 */ 2322 WSUIPA, 2323 /** 2324 * Do What The F*ck You Want To Public License 2325 */ 2326 WTFPL, 2327 /** 2328 * wxWindows Library License 2329 */ 2330 WXWINDOWS, 2331 /** 2332 * X11 License 2333 */ 2334 X11, 2335 /** 2336 * X11 License Distribution Modification Variant 2337 */ 2338 X11_DISTRIBUTE_MODIFICATIONS_VARIANT, 2339 /** 2340 * Xdebug License v 1.03 2341 */ 2342 XDEBUG_1_03, 2343 /** 2344 * Xerox License 2345 */ 2346 XEROX, 2347 /** 2348 * Xfig License 2349 */ 2350 XFIG, 2351 /** 2352 * XFree86 License 1.1 2353 */ 2354 XFREE86_1_1, 2355 /** 2356 * xinetd License 2357 */ 2358 XINETD, 2359 /** 2360 * xlock License 2361 */ 2362 XLOCK, 2363 /** 2364 * X.Net License 2365 */ 2366 XNET, 2367 /** 2368 * XPP License 2369 */ 2370 XPP, 2371 /** 2372 * XSkat License 2373 */ 2374 XSKAT, 2375 /** 2376 * Yahoo! Public License v1.0 2377 */ 2378 YPL_1_0, 2379 /** 2380 * Yahoo! Public License v1.1 2381 */ 2382 YPL_1_1, 2383 /** 2384 * Zed License 2385 */ 2386 ZED, 2387 /** 2388 * Zend License v2.0 2389 */ 2390 ZEND_2_0, 2391 /** 2392 * Zimbra Public License v1.3 2393 */ 2394 ZIMBRA_1_3, 2395 /** 2396 * Zimbra Public License v1.4 2397 */ 2398 ZIMBRA_1_4, 2399 /** 2400 * zlib License 2401 */ 2402 ZLIB, 2403 /** 2404 * zlib/libpng License with Acknowledgement 2405 */ 2406 ZLIB_ACKNOWLEDGEMENT, 2407 /** 2408 * Zope Public License 1.1 2409 */ 2410 ZPL_1_1, 2411 /** 2412 * Zope Public License 2.0 2413 */ 2414 ZPL_2_0, 2415 /** 2416 * Zope Public License 2.1 2417 */ 2418 ZPL_2_1, 2419 /** 2420 * added to help the parsers 2421 */ 2422 NULL; 2423 public static SPDXLicense fromCode(String codeString) throws FHIRException { 2424 if (codeString == null || "".equals(codeString)) 2425 return null; 2426 if ("0BSD".equals(codeString)) 2427 return _0BSD; 2428 if ("AAL".equals(codeString)) 2429 return AAL; 2430 if ("Abstyles".equals(codeString)) 2431 return ABSTYLES; 2432 if ("AdaCore-doc".equals(codeString)) 2433 return ADACORE_DOC; 2434 if ("Adobe-2006".equals(codeString)) 2435 return ADOBE_2006; 2436 if ("Adobe-Glyph".equals(codeString)) 2437 return ADOBE_GLYPH; 2438 if ("ADSL".equals(codeString)) 2439 return ADSL; 2440 if ("AFL-1.1".equals(codeString)) 2441 return AFL_1_1; 2442 if ("AFL-1.2".equals(codeString)) 2443 return AFL_1_2; 2444 if ("AFL-2.0".equals(codeString)) 2445 return AFL_2_0; 2446 if ("AFL-2.1".equals(codeString)) 2447 return AFL_2_1; 2448 if ("AFL-3.0".equals(codeString)) 2449 return AFL_3_0; 2450 if ("Afmparse".equals(codeString)) 2451 return AFMPARSE; 2452 if ("AGPL-1.0".equals(codeString)) 2453 return AGPL_1_0; 2454 if ("AGPL-1.0-only".equals(codeString)) 2455 return AGPL_1_0_ONLY; 2456 if ("AGPL-1.0-or-later".equals(codeString)) 2457 return AGPL_1_0_OR_LATER; 2458 if ("AGPL-3.0".equals(codeString)) 2459 return AGPL_3_0; 2460 if ("AGPL-3.0-only".equals(codeString)) 2461 return AGPL_3_0_ONLY; 2462 if ("AGPL-3.0-or-later".equals(codeString)) 2463 return AGPL_3_0_OR_LATER; 2464 if ("Aladdin".equals(codeString)) 2465 return ALADDIN; 2466 if ("AMDPLPA".equals(codeString)) 2467 return AMDPLPA; 2468 if ("AML".equals(codeString)) 2469 return AML; 2470 if ("AMPAS".equals(codeString)) 2471 return AMPAS; 2472 if ("ANTLR-PD".equals(codeString)) 2473 return ANTLR_PD; 2474 if ("ANTLR-PD-fallback".equals(codeString)) 2475 return ANTLR_PD_FALLBACK; 2476 if ("Apache-1.0".equals(codeString)) 2477 return APACHE_1_0; 2478 if ("Apache-1.1".equals(codeString)) 2479 return APACHE_1_1; 2480 if ("Apache-2.0".equals(codeString)) 2481 return APACHE_2_0; 2482 if ("APAFML".equals(codeString)) 2483 return APAFML; 2484 if ("APL-1.0".equals(codeString)) 2485 return APL_1_0; 2486 if ("App-s2p".equals(codeString)) 2487 return APP_S2P; 2488 if ("APSL-1.0".equals(codeString)) 2489 return APSL_1_0; 2490 if ("APSL-1.1".equals(codeString)) 2491 return APSL_1_1; 2492 if ("APSL-1.2".equals(codeString)) 2493 return APSL_1_2; 2494 if ("APSL-2.0".equals(codeString)) 2495 return APSL_2_0; 2496 if ("Arphic-1999".equals(codeString)) 2497 return ARPHIC_1999; 2498 if ("Artistic-1.0".equals(codeString)) 2499 return ARTISTIC_1_0; 2500 if ("Artistic-1.0-cl8".equals(codeString)) 2501 return ARTISTIC_1_0_CL8; 2502 if ("Artistic-1.0-Perl".equals(codeString)) 2503 return ARTISTIC_1_0_PERL; 2504 if ("Artistic-2.0".equals(codeString)) 2505 return ARTISTIC_2_0; 2506 if ("ASWF-Digital-Assets-1.0".equals(codeString)) 2507 return ASWF_DIGITAL_ASSETS_1_0; 2508 if ("ASWF-Digital-Assets-1.1".equals(codeString)) 2509 return ASWF_DIGITAL_ASSETS_1_1; 2510 if ("Baekmuk".equals(codeString)) 2511 return BAEKMUK; 2512 if ("Bahyph".equals(codeString)) 2513 return BAHYPH; 2514 if ("Barr".equals(codeString)) 2515 return BARR; 2516 if ("Beerware".equals(codeString)) 2517 return BEERWARE; 2518 if ("Bitstream-Charter".equals(codeString)) 2519 return BITSTREAM_CHARTER; 2520 if ("Bitstream-Vera".equals(codeString)) 2521 return BITSTREAM_VERA; 2522 if ("BitTorrent-1.0".equals(codeString)) 2523 return BITTORRENT_1_0; 2524 if ("BitTorrent-1.1".equals(codeString)) 2525 return BITTORRENT_1_1; 2526 if ("blessing".equals(codeString)) 2527 return BLESSING; 2528 if ("BlueOak-1.0.0".equals(codeString)) 2529 return BLUEOAK_1_0_0; 2530 if ("Boehm-GC".equals(codeString)) 2531 return BOEHM_GC; 2532 if ("Borceux".equals(codeString)) 2533 return BORCEUX; 2534 if ("Brian-Gladman-3-Clause".equals(codeString)) 2535 return BRIAN_GLADMAN_3_CLAUSE; 2536 if ("BSD-1-Clause".equals(codeString)) 2537 return BSD_1_CLAUSE; 2538 if ("BSD-2-Clause".equals(codeString)) 2539 return BSD_2_CLAUSE; 2540 if ("BSD-2-Clause-FreeBSD".equals(codeString)) 2541 return BSD_2_CLAUSE_FREEBSD; 2542 if ("BSD-2-Clause-NetBSD".equals(codeString)) 2543 return BSD_2_CLAUSE_NETBSD; 2544 if ("BSD-2-Clause-Patent".equals(codeString)) 2545 return BSD_2_CLAUSE_PATENT; 2546 if ("BSD-2-Clause-Views".equals(codeString)) 2547 return BSD_2_CLAUSE_VIEWS; 2548 if ("BSD-3-Clause".equals(codeString)) 2549 return BSD_3_CLAUSE; 2550 if ("BSD-3-Clause-Attribution".equals(codeString)) 2551 return BSD_3_CLAUSE_ATTRIBUTION; 2552 if ("BSD-3-Clause-Clear".equals(codeString)) 2553 return BSD_3_CLAUSE_CLEAR; 2554 if ("BSD-3-Clause-LBNL".equals(codeString)) 2555 return BSD_3_CLAUSE_LBNL; 2556 if ("BSD-3-Clause-Modification".equals(codeString)) 2557 return BSD_3_CLAUSE_MODIFICATION; 2558 if ("BSD-3-Clause-No-Military-License".equals(codeString)) 2559 return BSD_3_CLAUSE_NO_MILITARY_LICENSE; 2560 if ("BSD-3-Clause-No-Nuclear-License".equals(codeString)) 2561 return BSD_3_CLAUSE_NO_NUCLEAR_LICENSE; 2562 if ("BSD-3-Clause-No-Nuclear-License-2014".equals(codeString)) 2563 return BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014; 2564 if ("BSD-3-Clause-No-Nuclear-Warranty".equals(codeString)) 2565 return BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY; 2566 if ("BSD-3-Clause-Open-MPI".equals(codeString)) 2567 return BSD_3_CLAUSE_OPEN_MPI; 2568 if ("BSD-4-Clause".equals(codeString)) 2569 return BSD_4_CLAUSE; 2570 if ("BSD-4-Clause-Shortened".equals(codeString)) 2571 return BSD_4_CLAUSE_SHORTENED; 2572 if ("BSD-4-Clause-UC".equals(codeString)) 2573 return BSD_4_CLAUSE_UC; 2574 if ("BSD-4.3RENO".equals(codeString)) 2575 return BSD_4_3RENO; 2576 if ("BSD-4.3TAHOE".equals(codeString)) 2577 return BSD_4_3TAHOE; 2578 if ("BSD-Advertising-Acknowledgement".equals(codeString)) 2579 return BSD_ADVERTISING_ACKNOWLEDGEMENT; 2580 if ("BSD-Attribution-HPND-disclaimer".equals(codeString)) 2581 return BSD_ATTRIBUTION_HPND_DISCLAIMER; 2582 if ("BSD-Protection".equals(codeString)) 2583 return BSD_PROTECTION; 2584 if ("BSD-Source-Code".equals(codeString)) 2585 return BSD_SOURCE_CODE; 2586 if ("BSL-1.0".equals(codeString)) 2587 return BSL_1_0; 2588 if ("BUSL-1.1".equals(codeString)) 2589 return BUSL_1_1; 2590 if ("bzip2-1.0.5".equals(codeString)) 2591 return BZIP2_1_0_5; 2592 if ("bzip2-1.0.6".equals(codeString)) 2593 return BZIP2_1_0_6; 2594 if ("C-UDA-1.0".equals(codeString)) 2595 return C_UDA_1_0; 2596 if ("CAL-1.0".equals(codeString)) 2597 return CAL_1_0; 2598 if ("CAL-1.0-Combined-Work-Exception".equals(codeString)) 2599 return CAL_1_0_COMBINED_WORK_EXCEPTION; 2600 if ("Caldera".equals(codeString)) 2601 return CALDERA; 2602 if ("CATOSL-1.1".equals(codeString)) 2603 return CATOSL_1_1; 2604 if ("CC-BY-1.0".equals(codeString)) 2605 return CC_BY_1_0; 2606 if ("CC-BY-2.0".equals(codeString)) 2607 return CC_BY_2_0; 2608 if ("CC-BY-2.5".equals(codeString)) 2609 return CC_BY_2_5; 2610 if ("CC-BY-2.5-AU".equals(codeString)) 2611 return CC_BY_2_5_AU; 2612 if ("CC-BY-3.0".equals(codeString)) 2613 return CC_BY_3_0; 2614 if ("CC-BY-3.0-AT".equals(codeString)) 2615 return CC_BY_3_0_AT; 2616 if ("CC-BY-3.0-DE".equals(codeString)) 2617 return CC_BY_3_0_DE; 2618 if ("CC-BY-3.0-IGO".equals(codeString)) 2619 return CC_BY_3_0_IGO; 2620 if ("CC-BY-3.0-NL".equals(codeString)) 2621 return CC_BY_3_0_NL; 2622 if ("CC-BY-3.0-US".equals(codeString)) 2623 return CC_BY_3_0_US; 2624 if ("CC-BY-4.0".equals(codeString)) 2625 return CC_BY_4_0; 2626 if ("CC-BY-NC-1.0".equals(codeString)) 2627 return CC_BY_NC_1_0; 2628 if ("CC-BY-NC-2.0".equals(codeString)) 2629 return CC_BY_NC_2_0; 2630 if ("CC-BY-NC-2.5".equals(codeString)) 2631 return CC_BY_NC_2_5; 2632 if ("CC-BY-NC-3.0".equals(codeString)) 2633 return CC_BY_NC_3_0; 2634 if ("CC-BY-NC-3.0-DE".equals(codeString)) 2635 return CC_BY_NC_3_0_DE; 2636 if ("CC-BY-NC-4.0".equals(codeString)) 2637 return CC_BY_NC_4_0; 2638 if ("CC-BY-NC-ND-1.0".equals(codeString)) 2639 return CC_BY_NC_ND_1_0; 2640 if ("CC-BY-NC-ND-2.0".equals(codeString)) 2641 return CC_BY_NC_ND_2_0; 2642 if ("CC-BY-NC-ND-2.5".equals(codeString)) 2643 return CC_BY_NC_ND_2_5; 2644 if ("CC-BY-NC-ND-3.0".equals(codeString)) 2645 return CC_BY_NC_ND_3_0; 2646 if ("CC-BY-NC-ND-3.0-DE".equals(codeString)) 2647 return CC_BY_NC_ND_3_0_DE; 2648 if ("CC-BY-NC-ND-3.0-IGO".equals(codeString)) 2649 return CC_BY_NC_ND_3_0_IGO; 2650 if ("CC-BY-NC-ND-4.0".equals(codeString)) 2651 return CC_BY_NC_ND_4_0; 2652 if ("CC-BY-NC-SA-1.0".equals(codeString)) 2653 return CC_BY_NC_SA_1_0; 2654 if ("CC-BY-NC-SA-2.0".equals(codeString)) 2655 return CC_BY_NC_SA_2_0; 2656 if ("CC-BY-NC-SA-2.0-DE".equals(codeString)) 2657 return CC_BY_NC_SA_2_0_DE; 2658 if ("CC-BY-NC-SA-2.0-FR".equals(codeString)) 2659 return CC_BY_NC_SA_2_0_FR; 2660 if ("CC-BY-NC-SA-2.0-UK".equals(codeString)) 2661 return CC_BY_NC_SA_2_0_UK; 2662 if ("CC-BY-NC-SA-2.5".equals(codeString)) 2663 return CC_BY_NC_SA_2_5; 2664 if ("CC-BY-NC-SA-3.0".equals(codeString)) 2665 return CC_BY_NC_SA_3_0; 2666 if ("CC-BY-NC-SA-3.0-DE".equals(codeString)) 2667 return CC_BY_NC_SA_3_0_DE; 2668 if ("CC-BY-NC-SA-3.0-IGO".equals(codeString)) 2669 return CC_BY_NC_SA_3_0_IGO; 2670 if ("CC-BY-NC-SA-4.0".equals(codeString)) 2671 return CC_BY_NC_SA_4_0; 2672 if ("CC-BY-ND-1.0".equals(codeString)) 2673 return CC_BY_ND_1_0; 2674 if ("CC-BY-ND-2.0".equals(codeString)) 2675 return CC_BY_ND_2_0; 2676 if ("CC-BY-ND-2.5".equals(codeString)) 2677 return CC_BY_ND_2_5; 2678 if ("CC-BY-ND-3.0".equals(codeString)) 2679 return CC_BY_ND_3_0; 2680 if ("CC-BY-ND-3.0-DE".equals(codeString)) 2681 return CC_BY_ND_3_0_DE; 2682 if ("CC-BY-ND-4.0".equals(codeString)) 2683 return CC_BY_ND_4_0; 2684 if ("CC-BY-SA-1.0".equals(codeString)) 2685 return CC_BY_SA_1_0; 2686 if ("CC-BY-SA-2.0".equals(codeString)) 2687 return CC_BY_SA_2_0; 2688 if ("CC-BY-SA-2.0-UK".equals(codeString)) 2689 return CC_BY_SA_2_0_UK; 2690 if ("CC-BY-SA-2.1-JP".equals(codeString)) 2691 return CC_BY_SA_2_1_JP; 2692 if ("CC-BY-SA-2.5".equals(codeString)) 2693 return CC_BY_SA_2_5; 2694 if ("CC-BY-SA-3.0".equals(codeString)) 2695 return CC_BY_SA_3_0; 2696 if ("CC-BY-SA-3.0-AT".equals(codeString)) 2697 return CC_BY_SA_3_0_AT; 2698 if ("CC-BY-SA-3.0-DE".equals(codeString)) 2699 return CC_BY_SA_3_0_DE; 2700 if ("CC-BY-SA-3.0-IGO".equals(codeString)) 2701 return CC_BY_SA_3_0_IGO; 2702 if ("CC-BY-SA-4.0".equals(codeString)) 2703 return CC_BY_SA_4_0; 2704 if ("CC-PDDC".equals(codeString)) 2705 return CC_PDDC; 2706 if ("CC0-1.0".equals(codeString)) 2707 return CC0_1_0; 2708 if ("CDDL-1.0".equals(codeString)) 2709 return CDDL_1_0; 2710 if ("CDDL-1.1".equals(codeString)) 2711 return CDDL_1_1; 2712 if ("CDL-1.0".equals(codeString)) 2713 return CDL_1_0; 2714 if ("CDLA-Permissive-1.0".equals(codeString)) 2715 return CDLA_PERMISSIVE_1_0; 2716 if ("CDLA-Permissive-2.0".equals(codeString)) 2717 return CDLA_PERMISSIVE_2_0; 2718 if ("CDLA-Sharing-1.0".equals(codeString)) 2719 return CDLA_SHARING_1_0; 2720 if ("CECILL-1.0".equals(codeString)) 2721 return CECILL_1_0; 2722 if ("CECILL-1.1".equals(codeString)) 2723 return CECILL_1_1; 2724 if ("CECILL-2.0".equals(codeString)) 2725 return CECILL_2_0; 2726 if ("CECILL-2.1".equals(codeString)) 2727 return CECILL_2_1; 2728 if ("CECILL-B".equals(codeString)) 2729 return CECILL_B; 2730 if ("CECILL-C".equals(codeString)) 2731 return CECILL_C; 2732 if ("CERN-OHL-1.1".equals(codeString)) 2733 return CERN_OHL_1_1; 2734 if ("CERN-OHL-1.2".equals(codeString)) 2735 return CERN_OHL_1_2; 2736 if ("CERN-OHL-P-2.0".equals(codeString)) 2737 return CERN_OHL_P_2_0; 2738 if ("CERN-OHL-S-2.0".equals(codeString)) 2739 return CERN_OHL_S_2_0; 2740 if ("CERN-OHL-W-2.0".equals(codeString)) 2741 return CERN_OHL_W_2_0; 2742 if ("CFITSIO".equals(codeString)) 2743 return CFITSIO; 2744 if ("checkmk".equals(codeString)) 2745 return CHECKMK; 2746 if ("ClArtistic".equals(codeString)) 2747 return CLARTISTIC; 2748 if ("Clips".equals(codeString)) 2749 return CLIPS; 2750 if ("CMU-Mach".equals(codeString)) 2751 return CMU_MACH; 2752 if ("CNRI-Jython".equals(codeString)) 2753 return CNRI_JYTHON; 2754 if ("CNRI-Python".equals(codeString)) 2755 return CNRI_PYTHON; 2756 if ("CNRI-Python-GPL-Compatible".equals(codeString)) 2757 return CNRI_PYTHON_GPL_COMPATIBLE; 2758 if ("COIL-1.0".equals(codeString)) 2759 return COIL_1_0; 2760 if ("Community-Spec-1.0".equals(codeString)) 2761 return COMMUNITY_SPEC_1_0; 2762 if ("Condor-1.1".equals(codeString)) 2763 return CONDOR_1_1; 2764 if ("copyleft-next-0.3.0".equals(codeString)) 2765 return COPYLEFT_NEXT_0_3_0; 2766 if ("copyleft-next-0.3.1".equals(codeString)) 2767 return COPYLEFT_NEXT_0_3_1; 2768 if ("Cornell-Lossless-JPEG".equals(codeString)) 2769 return CORNELL_LOSSLESS_JPEG; 2770 if ("CPAL-1.0".equals(codeString)) 2771 return CPAL_1_0; 2772 if ("CPL-1.0".equals(codeString)) 2773 return CPL_1_0; 2774 if ("CPOL-1.02".equals(codeString)) 2775 return CPOL_1_02; 2776 if ("Crossword".equals(codeString)) 2777 return CROSSWORD; 2778 if ("CrystalStacker".equals(codeString)) 2779 return CRYSTALSTACKER; 2780 if ("CUA-OPL-1.0".equals(codeString)) 2781 return CUA_OPL_1_0; 2782 if ("Cube".equals(codeString)) 2783 return CUBE; 2784 if ("curl".equals(codeString)) 2785 return CURL; 2786 if ("D-FSL-1.0".equals(codeString)) 2787 return D_FSL_1_0; 2788 if ("diffmark".equals(codeString)) 2789 return DIFFMARK; 2790 if ("DL-DE-BY-2.0".equals(codeString)) 2791 return DL_DE_BY_2_0; 2792 if ("DOC".equals(codeString)) 2793 return DOC; 2794 if ("Dotseqn".equals(codeString)) 2795 return DOTSEQN; 2796 if ("DRL-1.0".equals(codeString)) 2797 return DRL_1_0; 2798 if ("DSDP".equals(codeString)) 2799 return DSDP; 2800 if ("dtoa".equals(codeString)) 2801 return DTOA; 2802 if ("dvipdfm".equals(codeString)) 2803 return DVIPDFM; 2804 if ("ECL-1.0".equals(codeString)) 2805 return ECL_1_0; 2806 if ("ECL-2.0".equals(codeString)) 2807 return ECL_2_0; 2808 if ("eCos-2.0".equals(codeString)) 2809 return ECOS_2_0; 2810 if ("EFL-1.0".equals(codeString)) 2811 return EFL_1_0; 2812 if ("EFL-2.0".equals(codeString)) 2813 return EFL_2_0; 2814 if ("eGenix".equals(codeString)) 2815 return EGENIX; 2816 if ("Elastic-2.0".equals(codeString)) 2817 return ELASTIC_2_0; 2818 if ("Entessa".equals(codeString)) 2819 return ENTESSA; 2820 if ("EPICS".equals(codeString)) 2821 return EPICS; 2822 if ("EPL-1.0".equals(codeString)) 2823 return EPL_1_0; 2824 if ("EPL-2.0".equals(codeString)) 2825 return EPL_2_0; 2826 if ("ErlPL-1.1".equals(codeString)) 2827 return ERLPL_1_1; 2828 if ("etalab-2.0".equals(codeString)) 2829 return ETALAB_2_0; 2830 if ("EUDatagrid".equals(codeString)) 2831 return EUDATAGRID; 2832 if ("EUPL-1.0".equals(codeString)) 2833 return EUPL_1_0; 2834 if ("EUPL-1.1".equals(codeString)) 2835 return EUPL_1_1; 2836 if ("EUPL-1.2".equals(codeString)) 2837 return EUPL_1_2; 2838 if ("Eurosym".equals(codeString)) 2839 return EUROSYM; 2840 if ("Fair".equals(codeString)) 2841 return FAIR; 2842 if ("FDK-AAC".equals(codeString)) 2843 return FDK_AAC; 2844 if ("Frameworx-1.0".equals(codeString)) 2845 return FRAMEWORX_1_0; 2846 if ("FreeBSD-DOC".equals(codeString)) 2847 return FREEBSD_DOC; 2848 if ("FreeImage".equals(codeString)) 2849 return FREEIMAGE; 2850 if ("FSFAP".equals(codeString)) 2851 return FSFAP; 2852 if ("FSFUL".equals(codeString)) 2853 return FSFUL; 2854 if ("FSFULLR".equals(codeString)) 2855 return FSFULLR; 2856 if ("FSFULLRWD".equals(codeString)) 2857 return FSFULLRWD; 2858 if ("FTL".equals(codeString)) 2859 return FTL; 2860 if ("GD".equals(codeString)) 2861 return GD; 2862 if ("GFDL-1.1".equals(codeString)) 2863 return GFDL_1_1; 2864 if ("GFDL-1.1-invariants-only".equals(codeString)) 2865 return GFDL_1_1_INVARIANTS_ONLY; 2866 if ("GFDL-1.1-invariants-or-later".equals(codeString)) 2867 return GFDL_1_1_INVARIANTS_OR_LATER; 2868 if ("GFDL-1.1-no-invariants-only".equals(codeString)) 2869 return GFDL_1_1_NO_INVARIANTS_ONLY; 2870 if ("GFDL-1.1-no-invariants-or-later".equals(codeString)) 2871 return GFDL_1_1_NO_INVARIANTS_OR_LATER; 2872 if ("GFDL-1.1-only".equals(codeString)) 2873 return GFDL_1_1_ONLY; 2874 if ("GFDL-1.1-or-later".equals(codeString)) 2875 return GFDL_1_1_OR_LATER; 2876 if ("GFDL-1.2".equals(codeString)) 2877 return GFDL_1_2; 2878 if ("GFDL-1.2-invariants-only".equals(codeString)) 2879 return GFDL_1_2_INVARIANTS_ONLY; 2880 if ("GFDL-1.2-invariants-or-later".equals(codeString)) 2881 return GFDL_1_2_INVARIANTS_OR_LATER; 2882 if ("GFDL-1.2-no-invariants-only".equals(codeString)) 2883 return GFDL_1_2_NO_INVARIANTS_ONLY; 2884 if ("GFDL-1.2-no-invariants-or-later".equals(codeString)) 2885 return GFDL_1_2_NO_INVARIANTS_OR_LATER; 2886 if ("GFDL-1.2-only".equals(codeString)) 2887 return GFDL_1_2_ONLY; 2888 if ("GFDL-1.2-or-later".equals(codeString)) 2889 return GFDL_1_2_OR_LATER; 2890 if ("GFDL-1.3".equals(codeString)) 2891 return GFDL_1_3; 2892 if ("GFDL-1.3-invariants-only".equals(codeString)) 2893 return GFDL_1_3_INVARIANTS_ONLY; 2894 if ("GFDL-1.3-invariants-or-later".equals(codeString)) 2895 return GFDL_1_3_INVARIANTS_OR_LATER; 2896 if ("GFDL-1.3-no-invariants-only".equals(codeString)) 2897 return GFDL_1_3_NO_INVARIANTS_ONLY; 2898 if ("GFDL-1.3-no-invariants-or-later".equals(codeString)) 2899 return GFDL_1_3_NO_INVARIANTS_OR_LATER; 2900 if ("GFDL-1.3-only".equals(codeString)) 2901 return GFDL_1_3_ONLY; 2902 if ("GFDL-1.3-or-later".equals(codeString)) 2903 return GFDL_1_3_OR_LATER; 2904 if ("Giftware".equals(codeString)) 2905 return GIFTWARE; 2906 if ("GL2PS".equals(codeString)) 2907 return GL2PS; 2908 if ("Glide".equals(codeString)) 2909 return GLIDE; 2910 if ("Glulxe".equals(codeString)) 2911 return GLULXE; 2912 if ("GLWTPL".equals(codeString)) 2913 return GLWTPL; 2914 if ("gnuplot".equals(codeString)) 2915 return GNUPLOT; 2916 if ("GPL-1.0".equals(codeString)) 2917 return GPL_1_0; 2918 if ("GPL-1.0+".equals(codeString)) 2919 return GPL_1_0PLUS; 2920 if ("GPL-1.0-only".equals(codeString)) 2921 return GPL_1_0_ONLY; 2922 if ("GPL-1.0-or-later".equals(codeString)) 2923 return GPL_1_0_OR_LATER; 2924 if ("GPL-2.0".equals(codeString)) 2925 return GPL_2_0; 2926 if ("GPL-2.0+".equals(codeString)) 2927 return GPL_2_0PLUS; 2928 if ("GPL-2.0-only".equals(codeString)) 2929 return GPL_2_0_ONLY; 2930 if ("GPL-2.0-or-later".equals(codeString)) 2931 return GPL_2_0_OR_LATER; 2932 if ("GPL-2.0-with-autoconf-exception".equals(codeString)) 2933 return GPL_2_0_WITH_AUTOCONF_EXCEPTION; 2934 if ("GPL-2.0-with-bison-exception".equals(codeString)) 2935 return GPL_2_0_WITH_BISON_EXCEPTION; 2936 if ("GPL-2.0-with-classpath-exception".equals(codeString)) 2937 return GPL_2_0_WITH_CLASSPATH_EXCEPTION; 2938 if ("GPL-2.0-with-font-exception".equals(codeString)) 2939 return GPL_2_0_WITH_FONT_EXCEPTION; 2940 if ("GPL-2.0-with-GCC-exception".equals(codeString)) 2941 return GPL_2_0_WITH_GCC_EXCEPTION; 2942 if ("GPL-3.0".equals(codeString)) 2943 return GPL_3_0; 2944 if ("GPL-3.0+".equals(codeString)) 2945 return GPL_3_0PLUS; 2946 if ("GPL-3.0-only".equals(codeString)) 2947 return GPL_3_0_ONLY; 2948 if ("GPL-3.0-or-later".equals(codeString)) 2949 return GPL_3_0_OR_LATER; 2950 if ("GPL-3.0-with-autoconf-exception".equals(codeString)) 2951 return GPL_3_0_WITH_AUTOCONF_EXCEPTION; 2952 if ("GPL-3.0-with-GCC-exception".equals(codeString)) 2953 return GPL_3_0_WITH_GCC_EXCEPTION; 2954 if ("Graphics-Gems".equals(codeString)) 2955 return GRAPHICS_GEMS; 2956 if ("gSOAP-1.3b".equals(codeString)) 2957 return GSOAP_1_3B; 2958 if ("HaskellReport".equals(codeString)) 2959 return HASKELLREPORT; 2960 if ("Hippocratic-2.1".equals(codeString)) 2961 return HIPPOCRATIC_2_1; 2962 if ("HP-1986".equals(codeString)) 2963 return HP_1986; 2964 if ("HPND".equals(codeString)) 2965 return HPND; 2966 if ("HPND-export-US".equals(codeString)) 2967 return HPND_EXPORT_US; 2968 if ("HPND-Markus-Kuhn".equals(codeString)) 2969 return HPND_MARKUS_KUHN; 2970 if ("HPND-sell-variant".equals(codeString)) 2971 return HPND_SELL_VARIANT; 2972 if ("HPND-sell-variant-MIT-disclaimer".equals(codeString)) 2973 return HPND_SELL_VARIANT_MIT_DISCLAIMER; 2974 if ("HTMLTIDY".equals(codeString)) 2975 return HTMLTIDY; 2976 if ("IBM-pibs".equals(codeString)) 2977 return IBM_PIBS; 2978 if ("ICU".equals(codeString)) 2979 return ICU; 2980 if ("IEC-Code-Components-EULA".equals(codeString)) 2981 return IEC_CODE_COMPONENTS_EULA; 2982 if ("IJG".equals(codeString)) 2983 return IJG; 2984 if ("IJG-short".equals(codeString)) 2985 return IJG_SHORT; 2986 if ("ImageMagick".equals(codeString)) 2987 return IMAGEMAGICK; 2988 if ("iMatix".equals(codeString)) 2989 return IMATIX; 2990 if ("Imlib2".equals(codeString)) 2991 return IMLIB2; 2992 if ("Info-ZIP".equals(codeString)) 2993 return INFO_ZIP; 2994 if ("Inner-Net-2.0".equals(codeString)) 2995 return INNER_NET_2_0; 2996 if ("Intel".equals(codeString)) 2997 return INTEL; 2998 if ("Intel-ACPI".equals(codeString)) 2999 return INTEL_ACPI; 3000 if ("Interbase-1.0".equals(codeString)) 3001 return INTERBASE_1_0; 3002 if ("IPA".equals(codeString)) 3003 return IPA; 3004 if ("IPL-1.0".equals(codeString)) 3005 return IPL_1_0; 3006 if ("ISC".equals(codeString)) 3007 return ISC; 3008 if ("Jam".equals(codeString)) 3009 return JAM; 3010 if ("JasPer-2.0".equals(codeString)) 3011 return JASPER_2_0; 3012 if ("JPL-image".equals(codeString)) 3013 return JPL_IMAGE; 3014 if ("JPNIC".equals(codeString)) 3015 return JPNIC; 3016 if ("JSON".equals(codeString)) 3017 return JSON; 3018 if ("Kazlib".equals(codeString)) 3019 return KAZLIB; 3020 if ("Knuth-CTAN".equals(codeString)) 3021 return KNUTH_CTAN; 3022 if ("LAL-1.2".equals(codeString)) 3023 return LAL_1_2; 3024 if ("LAL-1.3".equals(codeString)) 3025 return LAL_1_3; 3026 if ("Latex2e".equals(codeString)) 3027 return LATEX2E; 3028 if ("Latex2e-translated-notice".equals(codeString)) 3029 return LATEX2E_TRANSLATED_NOTICE; 3030 if ("Leptonica".equals(codeString)) 3031 return LEPTONICA; 3032 if ("LGPL-2.0".equals(codeString)) 3033 return LGPL_2_0; 3034 if ("LGPL-2.0+".equals(codeString)) 3035 return LGPL_2_0PLUS; 3036 if ("LGPL-2.0-only".equals(codeString)) 3037 return LGPL_2_0_ONLY; 3038 if ("LGPL-2.0-or-later".equals(codeString)) 3039 return LGPL_2_0_OR_LATER; 3040 if ("LGPL-2.1".equals(codeString)) 3041 return LGPL_2_1; 3042 if ("LGPL-2.1+".equals(codeString)) 3043 return LGPL_2_1PLUS; 3044 if ("LGPL-2.1-only".equals(codeString)) 3045 return LGPL_2_1_ONLY; 3046 if ("LGPL-2.1-or-later".equals(codeString)) 3047 return LGPL_2_1_OR_LATER; 3048 if ("LGPL-3.0".equals(codeString)) 3049 return LGPL_3_0; 3050 if ("LGPL-3.0+".equals(codeString)) 3051 return LGPL_3_0PLUS; 3052 if ("LGPL-3.0-only".equals(codeString)) 3053 return LGPL_3_0_ONLY; 3054 if ("LGPL-3.0-or-later".equals(codeString)) 3055 return LGPL_3_0_OR_LATER; 3056 if ("LGPLLR".equals(codeString)) 3057 return LGPLLR; 3058 if ("Libpng".equals(codeString)) 3059 return LIBPNG; 3060 if ("libpng-2.0".equals(codeString)) 3061 return LIBPNG_2_0; 3062 if ("libselinux-1.0".equals(codeString)) 3063 return LIBSELINUX_1_0; 3064 if ("libtiff".equals(codeString)) 3065 return LIBTIFF; 3066 if ("libutil-David-Nugent".equals(codeString)) 3067 return LIBUTIL_DAVID_NUGENT; 3068 if ("LiLiQ-P-1.1".equals(codeString)) 3069 return LILIQ_P_1_1; 3070 if ("LiLiQ-R-1.1".equals(codeString)) 3071 return LILIQ_R_1_1; 3072 if ("LiLiQ-Rplus-1.1".equals(codeString)) 3073 return LILIQ_RPLUS_1_1; 3074 if ("Linux-man-pages-1-para".equals(codeString)) 3075 return LINUX_MAN_PAGES_1_PARA; 3076 if ("Linux-man-pages-copyleft".equals(codeString)) 3077 return LINUX_MAN_PAGES_COPYLEFT; 3078 if ("Linux-man-pages-copyleft-2-para".equals(codeString)) 3079 return LINUX_MAN_PAGES_COPYLEFT_2_PARA; 3080 if ("Linux-man-pages-copyleft-var".equals(codeString)) 3081 return LINUX_MAN_PAGES_COPYLEFT_VAR; 3082 if ("Linux-OpenIB".equals(codeString)) 3083 return LINUX_OPENIB; 3084 if ("LOOP".equals(codeString)) 3085 return LOOP; 3086 if ("LPL-1.0".equals(codeString)) 3087 return LPL_1_0; 3088 if ("LPL-1.02".equals(codeString)) 3089 return LPL_1_02; 3090 if ("LPPL-1.0".equals(codeString)) 3091 return LPPL_1_0; 3092 if ("LPPL-1.1".equals(codeString)) 3093 return LPPL_1_1; 3094 if ("LPPL-1.2".equals(codeString)) 3095 return LPPL_1_2; 3096 if ("LPPL-1.3a".equals(codeString)) 3097 return LPPL_1_3A; 3098 if ("LPPL-1.3c".equals(codeString)) 3099 return LPPL_1_3C; 3100 if ("LZMA-SDK-9.11-to-9.20".equals(codeString)) 3101 return LZMA_SDK_9_11_TO_9_20; 3102 if ("LZMA-SDK-9.22".equals(codeString)) 3103 return LZMA_SDK_9_22; 3104 if ("MakeIndex".equals(codeString)) 3105 return MAKEINDEX; 3106 if ("Martin-Birgmeier".equals(codeString)) 3107 return MARTIN_BIRGMEIER; 3108 if ("metamail".equals(codeString)) 3109 return METAMAIL; 3110 if ("Minpack".equals(codeString)) 3111 return MINPACK; 3112 if ("MirOS".equals(codeString)) 3113 return MIROS; 3114 if ("MIT".equals(codeString)) 3115 return MIT; 3116 if ("MIT-0".equals(codeString)) 3117 return MIT_0; 3118 if ("MIT-advertising".equals(codeString)) 3119 return MIT_ADVERTISING; 3120 if ("MIT-CMU".equals(codeString)) 3121 return MIT_CMU; 3122 if ("MIT-enna".equals(codeString)) 3123 return MIT_ENNA; 3124 if ("MIT-feh".equals(codeString)) 3125 return MIT_FEH; 3126 if ("MIT-Festival".equals(codeString)) 3127 return MIT_FESTIVAL; 3128 if ("MIT-Modern-Variant".equals(codeString)) 3129 return MIT_MODERN_VARIANT; 3130 if ("MIT-open-group".equals(codeString)) 3131 return MIT_OPEN_GROUP; 3132 if ("MIT-Wu".equals(codeString)) 3133 return MIT_WU; 3134 if ("MITNFA".equals(codeString)) 3135 return MITNFA; 3136 if ("Motosoto".equals(codeString)) 3137 return MOTOSOTO; 3138 if ("mpi-permissive".equals(codeString)) 3139 return MPI_PERMISSIVE; 3140 if ("mpich2".equals(codeString)) 3141 return MPICH2; 3142 if ("MPL-1.0".equals(codeString)) 3143 return MPL_1_0; 3144 if ("MPL-1.1".equals(codeString)) 3145 return MPL_1_1; 3146 if ("MPL-2.0".equals(codeString)) 3147 return MPL_2_0; 3148 if ("MPL-2.0-no-copyleft-exception".equals(codeString)) 3149 return MPL_2_0_NO_COPYLEFT_EXCEPTION; 3150 if ("mplus".equals(codeString)) 3151 return MPLUS; 3152 if ("MS-LPL".equals(codeString)) 3153 return MS_LPL; 3154 if ("MS-PL".equals(codeString)) 3155 return MS_PL; 3156 if ("MS-RL".equals(codeString)) 3157 return MS_RL; 3158 if ("MTLL".equals(codeString)) 3159 return MTLL; 3160 if ("MulanPSL-1.0".equals(codeString)) 3161 return MULANPSL_1_0; 3162 if ("MulanPSL-2.0".equals(codeString)) 3163 return MULANPSL_2_0; 3164 if ("Multics".equals(codeString)) 3165 return MULTICS; 3166 if ("Mup".equals(codeString)) 3167 return MUP; 3168 if ("NAIST-2003".equals(codeString)) 3169 return NAIST_2003; 3170 if ("NASA-1.3".equals(codeString)) 3171 return NASA_1_3; 3172 if ("Naumen".equals(codeString)) 3173 return NAUMEN; 3174 if ("NBPL-1.0".equals(codeString)) 3175 return NBPL_1_0; 3176 if ("NCGL-UK-2.0".equals(codeString)) 3177 return NCGL_UK_2_0; 3178 if ("NCSA".equals(codeString)) 3179 return NCSA; 3180 if ("Net-SNMP".equals(codeString)) 3181 return NET_SNMP; 3182 if ("NetCDF".equals(codeString)) 3183 return NETCDF; 3184 if ("Newsletr".equals(codeString)) 3185 return NEWSLETR; 3186 if ("NGPL".equals(codeString)) 3187 return NGPL; 3188 if ("NICTA-1.0".equals(codeString)) 3189 return NICTA_1_0; 3190 if ("NIST-PD".equals(codeString)) 3191 return NIST_PD; 3192 if ("NIST-PD-fallback".equals(codeString)) 3193 return NIST_PD_FALLBACK; 3194 if ("NIST-Software".equals(codeString)) 3195 return NIST_SOFTWARE; 3196 if ("NLOD-1.0".equals(codeString)) 3197 return NLOD_1_0; 3198 if ("NLOD-2.0".equals(codeString)) 3199 return NLOD_2_0; 3200 if ("NLPL".equals(codeString)) 3201 return NLPL; 3202 if ("Nokia".equals(codeString)) 3203 return NOKIA; 3204 if ("NOSL".equals(codeString)) 3205 return NOSL; 3206 if ("not-open-source".equals(codeString)) 3207 return NOT_OPEN_SOURCE; 3208 if ("Noweb".equals(codeString)) 3209 return NOWEB; 3210 if ("NPL-1.0".equals(codeString)) 3211 return NPL_1_0; 3212 if ("NPL-1.1".equals(codeString)) 3213 return NPL_1_1; 3214 if ("NPOSL-3.0".equals(codeString)) 3215 return NPOSL_3_0; 3216 if ("NRL".equals(codeString)) 3217 return NRL; 3218 if ("NTP".equals(codeString)) 3219 return NTP; 3220 if ("NTP-0".equals(codeString)) 3221 return NTP_0; 3222 if ("Nunit".equals(codeString)) 3223 return NUNIT; 3224 if ("O-UDA-1.0".equals(codeString)) 3225 return O_UDA_1_0; 3226 if ("OCCT-PL".equals(codeString)) 3227 return OCCT_PL; 3228 if ("OCLC-2.0".equals(codeString)) 3229 return OCLC_2_0; 3230 if ("ODbL-1.0".equals(codeString)) 3231 return ODBL_1_0; 3232 if ("ODC-By-1.0".equals(codeString)) 3233 return ODC_BY_1_0; 3234 if ("OFFIS".equals(codeString)) 3235 return OFFIS; 3236 if ("OFL-1.0".equals(codeString)) 3237 return OFL_1_0; 3238 if ("OFL-1.0-no-RFN".equals(codeString)) 3239 return OFL_1_0_NO_RFN; 3240 if ("OFL-1.0-RFN".equals(codeString)) 3241 return OFL_1_0_RFN; 3242 if ("OFL-1.1".equals(codeString)) 3243 return OFL_1_1; 3244 if ("OFL-1.1-no-RFN".equals(codeString)) 3245 return OFL_1_1_NO_RFN; 3246 if ("OFL-1.1-RFN".equals(codeString)) 3247 return OFL_1_1_RFN; 3248 if ("OGC-1.0".equals(codeString)) 3249 return OGC_1_0; 3250 if ("OGDL-Taiwan-1.0".equals(codeString)) 3251 return OGDL_TAIWAN_1_0; 3252 if ("OGL-Canada-2.0".equals(codeString)) 3253 return OGL_CANADA_2_0; 3254 if ("OGL-UK-1.0".equals(codeString)) 3255 return OGL_UK_1_0; 3256 if ("OGL-UK-2.0".equals(codeString)) 3257 return OGL_UK_2_0; 3258 if ("OGL-UK-3.0".equals(codeString)) 3259 return OGL_UK_3_0; 3260 if ("OGTSL".equals(codeString)) 3261 return OGTSL; 3262 if ("OLDAP-1.1".equals(codeString)) 3263 return OLDAP_1_1; 3264 if ("OLDAP-1.2".equals(codeString)) 3265 return OLDAP_1_2; 3266 if ("OLDAP-1.3".equals(codeString)) 3267 return OLDAP_1_3; 3268 if ("OLDAP-1.4".equals(codeString)) 3269 return OLDAP_1_4; 3270 if ("OLDAP-2.0".equals(codeString)) 3271 return OLDAP_2_0; 3272 if ("OLDAP-2.0.1".equals(codeString)) 3273 return OLDAP_2_0_1; 3274 if ("OLDAP-2.1".equals(codeString)) 3275 return OLDAP_2_1; 3276 if ("OLDAP-2.2".equals(codeString)) 3277 return OLDAP_2_2; 3278 if ("OLDAP-2.2.1".equals(codeString)) 3279 return OLDAP_2_2_1; 3280 if ("OLDAP-2.2.2".equals(codeString)) 3281 return OLDAP_2_2_2; 3282 if ("OLDAP-2.3".equals(codeString)) 3283 return OLDAP_2_3; 3284 if ("OLDAP-2.4".equals(codeString)) 3285 return OLDAP_2_4; 3286 if ("OLDAP-2.5".equals(codeString)) 3287 return OLDAP_2_5; 3288 if ("OLDAP-2.6".equals(codeString)) 3289 return OLDAP_2_6; 3290 if ("OLDAP-2.7".equals(codeString)) 3291 return OLDAP_2_7; 3292 if ("OLDAP-2.8".equals(codeString)) 3293 return OLDAP_2_8; 3294 if ("OLFL-1.3".equals(codeString)) 3295 return OLFL_1_3; 3296 if ("OML".equals(codeString)) 3297 return OML; 3298 if ("OpenPBS-2.3".equals(codeString)) 3299 return OPENPBS_2_3; 3300 if ("OpenSSL".equals(codeString)) 3301 return OPENSSL; 3302 if ("OPL-1.0".equals(codeString)) 3303 return OPL_1_0; 3304 if ("OPL-UK-3.0".equals(codeString)) 3305 return OPL_UK_3_0; 3306 if ("OPUBL-1.0".equals(codeString)) 3307 return OPUBL_1_0; 3308 if ("OSET-PL-2.1".equals(codeString)) 3309 return OSET_PL_2_1; 3310 if ("OSL-1.0".equals(codeString)) 3311 return OSL_1_0; 3312 if ("OSL-1.1".equals(codeString)) 3313 return OSL_1_1; 3314 if ("OSL-2.0".equals(codeString)) 3315 return OSL_2_0; 3316 if ("OSL-2.1".equals(codeString)) 3317 return OSL_2_1; 3318 if ("OSL-3.0".equals(codeString)) 3319 return OSL_3_0; 3320 if ("Parity-6.0.0".equals(codeString)) 3321 return PARITY_6_0_0; 3322 if ("Parity-7.0.0".equals(codeString)) 3323 return PARITY_7_0_0; 3324 if ("PDDL-1.0".equals(codeString)) 3325 return PDDL_1_0; 3326 if ("PHP-3.0".equals(codeString)) 3327 return PHP_3_0; 3328 if ("PHP-3.01".equals(codeString)) 3329 return PHP_3_01; 3330 if ("Plexus".equals(codeString)) 3331 return PLEXUS; 3332 if ("PolyForm-Noncommercial-1.0.0".equals(codeString)) 3333 return POLYFORM_NONCOMMERCIAL_1_0_0; 3334 if ("PolyForm-Small-Business-1.0.0".equals(codeString)) 3335 return POLYFORM_SMALL_BUSINESS_1_0_0; 3336 if ("PostgreSQL".equals(codeString)) 3337 return POSTGRESQL; 3338 if ("PSF-2.0".equals(codeString)) 3339 return PSF_2_0; 3340 if ("psfrag".equals(codeString)) 3341 return PSFRAG; 3342 if ("psutils".equals(codeString)) 3343 return PSUTILS; 3344 if ("Python-2.0".equals(codeString)) 3345 return PYTHON_2_0; 3346 if ("Python-2.0.1".equals(codeString)) 3347 return PYTHON_2_0_1; 3348 if ("Qhull".equals(codeString)) 3349 return QHULL; 3350 if ("QPL-1.0".equals(codeString)) 3351 return QPL_1_0; 3352 if ("QPL-1.0-INRIA-2004".equals(codeString)) 3353 return QPL_1_0_INRIA_2004; 3354 if ("Rdisc".equals(codeString)) 3355 return RDISC; 3356 if ("RHeCos-1.1".equals(codeString)) 3357 return RHECOS_1_1; 3358 if ("RPL-1.1".equals(codeString)) 3359 return RPL_1_1; 3360 if ("RPL-1.5".equals(codeString)) 3361 return RPL_1_5; 3362 if ("RPSL-1.0".equals(codeString)) 3363 return RPSL_1_0; 3364 if ("RSA-MD".equals(codeString)) 3365 return RSA_MD; 3366 if ("RSCPL".equals(codeString)) 3367 return RSCPL; 3368 if ("Ruby".equals(codeString)) 3369 return RUBY; 3370 if ("SAX-PD".equals(codeString)) 3371 return SAX_PD; 3372 if ("Saxpath".equals(codeString)) 3373 return SAXPATH; 3374 if ("SCEA".equals(codeString)) 3375 return SCEA; 3376 if ("SchemeReport".equals(codeString)) 3377 return SCHEMEREPORT; 3378 if ("Sendmail".equals(codeString)) 3379 return SENDMAIL; 3380 if ("Sendmail-8.23".equals(codeString)) 3381 return SENDMAIL_8_23; 3382 if ("SGI-B-1.0".equals(codeString)) 3383 return SGI_B_1_0; 3384 if ("SGI-B-1.1".equals(codeString)) 3385 return SGI_B_1_1; 3386 if ("SGI-B-2.0".equals(codeString)) 3387 return SGI_B_2_0; 3388 if ("SGP4".equals(codeString)) 3389 return SGP4; 3390 if ("SHL-0.5".equals(codeString)) 3391 return SHL_0_5; 3392 if ("SHL-0.51".equals(codeString)) 3393 return SHL_0_51; 3394 if ("SimPL-2.0".equals(codeString)) 3395 return SIMPL_2_0; 3396 if ("SISSL".equals(codeString)) 3397 return SISSL; 3398 if ("SISSL-1.2".equals(codeString)) 3399 return SISSL_1_2; 3400 if ("Sleepycat".equals(codeString)) 3401 return SLEEPYCAT; 3402 if ("SMLNJ".equals(codeString)) 3403 return SMLNJ; 3404 if ("SMPPL".equals(codeString)) 3405 return SMPPL; 3406 if ("SNIA".equals(codeString)) 3407 return SNIA; 3408 if ("snprintf".equals(codeString)) 3409 return SNPRINTF; 3410 if ("Spencer-86".equals(codeString)) 3411 return SPENCER_86; 3412 if ("Spencer-94".equals(codeString)) 3413 return SPENCER_94; 3414 if ("Spencer-99".equals(codeString)) 3415 return SPENCER_99; 3416 if ("SPL-1.0".equals(codeString)) 3417 return SPL_1_0; 3418 if ("SSH-OpenSSH".equals(codeString)) 3419 return SSH_OPENSSH; 3420 if ("SSH-short".equals(codeString)) 3421 return SSH_SHORT; 3422 if ("SSPL-1.0".equals(codeString)) 3423 return SSPL_1_0; 3424 if ("StandardML-NJ".equals(codeString)) 3425 return STANDARDML_NJ; 3426 if ("SugarCRM-1.1.3".equals(codeString)) 3427 return SUGARCRM_1_1_3; 3428 if ("SunPro".equals(codeString)) 3429 return SUNPRO; 3430 if ("SWL".equals(codeString)) 3431 return SWL; 3432 if ("Symlinks".equals(codeString)) 3433 return SYMLINKS; 3434 if ("TAPR-OHL-1.0".equals(codeString)) 3435 return TAPR_OHL_1_0; 3436 if ("TCL".equals(codeString)) 3437 return TCL; 3438 if ("TCP-wrappers".equals(codeString)) 3439 return TCP_WRAPPERS; 3440 if ("TermReadKey".equals(codeString)) 3441 return TERMREADKEY; 3442 if ("TMate".equals(codeString)) 3443 return TMATE; 3444 if ("TORQUE-1.1".equals(codeString)) 3445 return TORQUE_1_1; 3446 if ("TOSL".equals(codeString)) 3447 return TOSL; 3448 if ("TPDL".equals(codeString)) 3449 return TPDL; 3450 if ("TPL-1.0".equals(codeString)) 3451 return TPL_1_0; 3452 if ("TTWL".equals(codeString)) 3453 return TTWL; 3454 if ("TU-Berlin-1.0".equals(codeString)) 3455 return TU_BERLIN_1_0; 3456 if ("TU-Berlin-2.0".equals(codeString)) 3457 return TU_BERLIN_2_0; 3458 if ("UCAR".equals(codeString)) 3459 return UCAR; 3460 if ("UCL-1.0".equals(codeString)) 3461 return UCL_1_0; 3462 if ("Unicode-DFS-2015".equals(codeString)) 3463 return UNICODE_DFS_2015; 3464 if ("Unicode-DFS-2016".equals(codeString)) 3465 return UNICODE_DFS_2016; 3466 if ("Unicode-TOU".equals(codeString)) 3467 return UNICODE_TOU; 3468 if ("UnixCrypt".equals(codeString)) 3469 return UNIXCRYPT; 3470 if ("Unlicense".equals(codeString)) 3471 return UNLICENSE; 3472 if ("UPL-1.0".equals(codeString)) 3473 return UPL_1_0; 3474 if ("Vim".equals(codeString)) 3475 return VIM; 3476 if ("VOSTROM".equals(codeString)) 3477 return VOSTROM; 3478 if ("VSL-1.0".equals(codeString)) 3479 return VSL_1_0; 3480 if ("W3C".equals(codeString)) 3481 return W3C; 3482 if ("W3C-19980720".equals(codeString)) 3483 return W3C_19980720; 3484 if ("W3C-20150513".equals(codeString)) 3485 return W3C_20150513; 3486 if ("w3m".equals(codeString)) 3487 return W3M; 3488 if ("Watcom-1.0".equals(codeString)) 3489 return WATCOM_1_0; 3490 if ("Widget-Workshop".equals(codeString)) 3491 return WIDGET_WORKSHOP; 3492 if ("Wsuipa".equals(codeString)) 3493 return WSUIPA; 3494 if ("WTFPL".equals(codeString)) 3495 return WTFPL; 3496 if ("wxWindows".equals(codeString)) 3497 return WXWINDOWS; 3498 if ("X11".equals(codeString)) 3499 return X11; 3500 if ("X11-distribute-modifications-variant".equals(codeString)) 3501 return X11_DISTRIBUTE_MODIFICATIONS_VARIANT; 3502 if ("Xdebug-1.03".equals(codeString)) 3503 return XDEBUG_1_03; 3504 if ("Xerox".equals(codeString)) 3505 return XEROX; 3506 if ("Xfig".equals(codeString)) 3507 return XFIG; 3508 if ("XFree86-1.1".equals(codeString)) 3509 return XFREE86_1_1; 3510 if ("xinetd".equals(codeString)) 3511 return XINETD; 3512 if ("xlock".equals(codeString)) 3513 return XLOCK; 3514 if ("Xnet".equals(codeString)) 3515 return XNET; 3516 if ("xpp".equals(codeString)) 3517 return XPP; 3518 if ("XSkat".equals(codeString)) 3519 return XSKAT; 3520 if ("YPL-1.0".equals(codeString)) 3521 return YPL_1_0; 3522 if ("YPL-1.1".equals(codeString)) 3523 return YPL_1_1; 3524 if ("Zed".equals(codeString)) 3525 return ZED; 3526 if ("Zend-2.0".equals(codeString)) 3527 return ZEND_2_0; 3528 if ("Zimbra-1.3".equals(codeString)) 3529 return ZIMBRA_1_3; 3530 if ("Zimbra-1.4".equals(codeString)) 3531 return ZIMBRA_1_4; 3532 if ("Zlib".equals(codeString)) 3533 return ZLIB; 3534 if ("zlib-acknowledgement".equals(codeString)) 3535 return ZLIB_ACKNOWLEDGEMENT; 3536 if ("ZPL-1.1".equals(codeString)) 3537 return ZPL_1_1; 3538 if ("ZPL-2.0".equals(codeString)) 3539 return ZPL_2_0; 3540 if ("ZPL-2.1".equals(codeString)) 3541 return ZPL_2_1; 3542 throw new FHIRException("Unknown SPDXLicense code '"+codeString+"'"); 3543 } 3544 public static boolean isValidCode(String codeString) { 3545 if (codeString == null || "".equals(codeString)) 3546 return false; 3547 return Utilities.existsInList(codeString, "0BSD", "AAL", "Abstyles", "AdaCore-doc", "Adobe-2006", "Adobe-Glyph", "ADSL", "AFL-1.1", "AFL-1.2", "AFL-2.0", "AFL-2.1", "AFL-3.0", "Afmparse", "AGPL-1.0", "AGPL-1.0-only", "AGPL-1.0-or-later", "AGPL-3.0", "AGPL-3.0-only", "AGPL-3.0-or-later", "Aladdin", "AMDPLPA", "AML", "AMPAS", "ANTLR-PD", "ANTLR-PD-fallback", "Apache-1.0", "Apache-1.1", "Apache-2.0", "APAFML", "APL-1.0", "App-s2p", "APSL-1.0", "APSL-1.1", "APSL-1.2", "APSL-2.0", "Arphic-1999", "Artistic-1.0", "Artistic-1.0-cl8", "Artistic-1.0-Perl", "Artistic-2.0", "ASWF-Digital-Assets-1.0", "ASWF-Digital-Assets-1.1", "Baekmuk", "Bahyph", "Barr", "Beerware", "Bitstream-Charter", "Bitstream-Vera", "BitTorrent-1.0", "BitTorrent-1.1", "blessing", "BlueOak-1.0.0", "Boehm-GC", "Borceux", "Brian-Gladman-3-Clause", "BSD-1-Clause", "BSD-2-Clause", "BSD-2-Clause-FreeBSD", "BSD-2-Clause-NetBSD", "BSD-2-Clause-Patent", "BSD-2-Clause-Views", "BSD-3-Clause", "BSD-3-Clause-Attribution", "BSD-3-Clause-Clear", "BSD-3-Clause-LBNL", "BSD-3-Clause-Modification", "BSD-3-Clause-No-Military-License", "BSD-3-Clause-No-Nuclear-License", "BSD-3-Clause-No-Nuclear-License-2014", "BSD-3-Clause-No-Nuclear-Warranty", "BSD-3-Clause-Open-MPI", "BSD-4-Clause", "BSD-4-Clause-Shortened", "BSD-4-Clause-UC", "BSD-4.3RENO", "BSD-4.3TAHOE", "BSD-Advertising-Acknowledgement", "BSD-Attribution-HPND-disclaimer", "BSD-Protection", "BSD-Source-Code", "BSL-1.0", "BUSL-1.1", "bzip2-1.0.5", "bzip2-1.0.6", "C-UDA-1.0", "CAL-1.0", "CAL-1.0-Combined-Work-Exception", "Caldera", "CATOSL-1.1", "CC-BY-1.0", "CC-BY-2.0", "CC-BY-2.5", "CC-BY-2.5-AU", "CC-BY-3.0", "CC-BY-3.0-AT", "CC-BY-3.0-DE", "CC-BY-3.0-IGO", "CC-BY-3.0-NL", "CC-BY-3.0-US", "CC-BY-4.0", "CC-BY-NC-1.0", "CC-BY-NC-2.0", "CC-BY-NC-2.5", "CC-BY-NC-3.0", "CC-BY-NC-3.0-DE", "CC-BY-NC-4.0", "CC-BY-NC-ND-1.0", "CC-BY-NC-ND-2.0", "CC-BY-NC-ND-2.5", "CC-BY-NC-ND-3.0", "CC-BY-NC-ND-3.0-DE", "CC-BY-NC-ND-3.0-IGO", "CC-BY-NC-ND-4.0", "CC-BY-NC-SA-1.0", "CC-BY-NC-SA-2.0", "CC-BY-NC-SA-2.0-DE", "CC-BY-NC-SA-2.0-FR", "CC-BY-NC-SA-2.0-UK", "CC-BY-NC-SA-2.5", "CC-BY-NC-SA-3.0", "CC-BY-NC-SA-3.0-DE", "CC-BY-NC-SA-3.0-IGO", "CC-BY-NC-SA-4.0", "CC-BY-ND-1.0", "CC-BY-ND-2.0", "CC-BY-ND-2.5", "CC-BY-ND-3.0", "CC-BY-ND-3.0-DE", "CC-BY-ND-4.0", "CC-BY-SA-1.0", "CC-BY-SA-2.0", "CC-BY-SA-2.0-UK", "CC-BY-SA-2.1-JP", "CC-BY-SA-2.5", "CC-BY-SA-3.0", "CC-BY-SA-3.0-AT", "CC-BY-SA-3.0-DE", "CC-BY-SA-3.0-IGO", "CC-BY-SA-4.0", "CC-PDDC", "CC0-1.0", "CDDL-1.0", "CDDL-1.1", "CDL-1.0", "CDLA-Permissive-1.0", "CDLA-Permissive-2.0", "CDLA-Sharing-1.0", "CECILL-1.0", "CECILL-1.1", "CECILL-2.0", "CECILL-2.1", "CECILL-B", "CECILL-C", "CERN-OHL-1.1", "CERN-OHL-1.2", "CERN-OHL-P-2.0", "CERN-OHL-S-2.0", "CERN-OHL-W-2.0", "CFITSIO", "checkmk", "ClArtistic", "Clips", "CMU-Mach", "CNRI-Jython", "CNRI-Python", "CNRI-Python-GPL-Compatible", "COIL-1.0", "Community-Spec-1.0", "Condor-1.1", "copyleft-next-0.3.0", "copyleft-next-0.3.1", "Cornell-Lossless-JPEG", "CPAL-1.0", "CPL-1.0", "CPOL-1.02", "Crossword", "CrystalStacker", "CUA-OPL-1.0", "Cube", "curl", "D-FSL-1.0", "diffmark", "DL-DE-BY-2.0", "DOC", "Dotseqn", "DRL-1.0", "DSDP", "dtoa", "dvipdfm", "ECL-1.0", "ECL-2.0", "eCos-2.0", "EFL-1.0", "EFL-2.0", "eGenix", "Elastic-2.0", "Entessa", "EPICS", "EPL-1.0", "EPL-2.0", "ErlPL-1.1", "etalab-2.0", "EUDatagrid", "EUPL-1.0", "EUPL-1.1", "EUPL-1.2", "Eurosym", "Fair", "FDK-AAC", "Frameworx-1.0", "FreeBSD-DOC", "FreeImage", "FSFAP", "FSFUL", "FSFULLR", "FSFULLRWD", "FTL", "GD", "GFDL-1.1", "GFDL-1.1-invariants-only", "GFDL-1.1-invariants-or-later", "GFDL-1.1-no-invariants-only", "GFDL-1.1-no-invariants-or-later", "GFDL-1.1-only", "GFDL-1.1-or-later", "GFDL-1.2", "GFDL-1.2-invariants-only", "GFDL-1.2-invariants-or-later", "GFDL-1.2-no-invariants-only", "GFDL-1.2-no-invariants-or-later", "GFDL-1.2-only", "GFDL-1.2-or-later", "GFDL-1.3", "GFDL-1.3-invariants-only", "GFDL-1.3-invariants-or-later", "GFDL-1.3-no-invariants-only", "GFDL-1.3-no-invariants-or-later", "GFDL-1.3-only", "GFDL-1.3-or-later", "Giftware", "GL2PS", "Glide", "Glulxe", "GLWTPL", "gnuplot", "GPL-1.0", "GPL-1.0+", "GPL-1.0-only", "GPL-1.0-or-later", "GPL-2.0", "GPL-2.0+", "GPL-2.0-only", "GPL-2.0-or-later", "GPL-2.0-with-autoconf-exception", "GPL-2.0-with-bison-exception", "GPL-2.0-with-classpath-exception", "GPL-2.0-with-font-exception", "GPL-2.0-with-GCC-exception", "GPL-3.0", "GPL-3.0+", "GPL-3.0-only", "GPL-3.0-or-later", "GPL-3.0-with-autoconf-exception", "GPL-3.0-with-GCC-exception", "Graphics-Gems", "gSOAP-1.3b", "HaskellReport", "Hippocratic-2.1", "HP-1986", "HPND", "HPND-export-US", "HPND-Markus-Kuhn", "HPND-sell-variant", "HPND-sell-variant-MIT-disclaimer", "HTMLTIDY", "IBM-pibs", "ICU", "IEC-Code-Components-EULA", "IJG", "IJG-short", "ImageMagick", "iMatix", "Imlib2", "Info-ZIP", "Inner-Net-2.0", "Intel", "Intel-ACPI", "Interbase-1.0", "IPA", "IPL-1.0", "ISC", "Jam", "JasPer-2.0", "JPL-image", "JPNIC", "JSON", "Kazlib", "Knuth-CTAN", "LAL-1.2", "LAL-1.3", "Latex2e", "Latex2e-translated-notice", "Leptonica", "LGPL-2.0", "LGPL-2.0+", "LGPL-2.0-only", "LGPL-2.0-or-later", "LGPL-2.1", "LGPL-2.1+", "LGPL-2.1-only", "LGPL-2.1-or-later", "LGPL-3.0", "LGPL-3.0+", "LGPL-3.0-only", "LGPL-3.0-or-later", "LGPLLR", "Libpng", "libpng-2.0", "libselinux-1.0", "libtiff", "libutil-David-Nugent", "LiLiQ-P-1.1", "LiLiQ-R-1.1", "LiLiQ-Rplus-1.1", "Linux-man-pages-1-para", "Linux-man-pages-copyleft", "Linux-man-pages-copyleft-2-para", "Linux-man-pages-copyleft-var", "Linux-OpenIB", "LOOP", "LPL-1.0", "LPL-1.02", "LPPL-1.0", "LPPL-1.1", "LPPL-1.2", "LPPL-1.3a", "LPPL-1.3c", "LZMA-SDK-9.11-to-9.20", "LZMA-SDK-9.22", "MakeIndex", "Martin-Birgmeier", "metamail", "Minpack", "MirOS", "MIT", "MIT-0", "MIT-advertising", "MIT-CMU", "MIT-enna", "MIT-feh", "MIT-Festival", "MIT-Modern-Variant", "MIT-open-group", "MIT-Wu", "MITNFA", "Motosoto", "mpi-permissive", "mpich2", "MPL-1.0", "MPL-1.1", "MPL-2.0", "MPL-2.0-no-copyleft-exception", "mplus", "MS-LPL", "MS-PL", "MS-RL", "MTLL", "MulanPSL-1.0", "MulanPSL-2.0", "Multics", "Mup", "NAIST-2003", "NASA-1.3", "Naumen", "NBPL-1.0", "NCGL-UK-2.0", "NCSA", "Net-SNMP", "NetCDF", "Newsletr", "NGPL", "NICTA-1.0", "NIST-PD", "NIST-PD-fallback", "NIST-Software", "NLOD-1.0", "NLOD-2.0", "NLPL", "Nokia", "NOSL", "not-open-source", "Noweb", "NPL-1.0", "NPL-1.1", "NPOSL-3.0", "NRL", "NTP", "NTP-0", "Nunit", "O-UDA-1.0", "OCCT-PL", "OCLC-2.0", "ODbL-1.0", "ODC-By-1.0", "OFFIS", "OFL-1.0", "OFL-1.0-no-RFN", "OFL-1.0-RFN", "OFL-1.1", "OFL-1.1-no-RFN", "OFL-1.1-RFN", "OGC-1.0", "OGDL-Taiwan-1.0", "OGL-Canada-2.0", "OGL-UK-1.0", "OGL-UK-2.0", "OGL-UK-3.0", "OGTSL", "OLDAP-1.1", "OLDAP-1.2", "OLDAP-1.3", "OLDAP-1.4", "OLDAP-2.0", "OLDAP-2.0.1", "OLDAP-2.1", "OLDAP-2.2", "OLDAP-2.2.1", "OLDAP-2.2.2", "OLDAP-2.3", "OLDAP-2.4", "OLDAP-2.5", "OLDAP-2.6", "OLDAP-2.7", "OLDAP-2.8", "OLFL-1.3", "OML", "OpenPBS-2.3", "OpenSSL", "OPL-1.0", "OPL-UK-3.0", "OPUBL-1.0", "OSET-PL-2.1", "OSL-1.0", "OSL-1.1", "OSL-2.0", "OSL-2.1", "OSL-3.0", "Parity-6.0.0", "Parity-7.0.0", "PDDL-1.0", "PHP-3.0", "PHP-3.01", "Plexus", "PolyForm-Noncommercial-1.0.0", "PolyForm-Small-Business-1.0.0", "PostgreSQL", "PSF-2.0", "psfrag", "psutils", "Python-2.0", "Python-2.0.1", "Qhull", "QPL-1.0", "QPL-1.0-INRIA-2004", "Rdisc", "RHeCos-1.1", "RPL-1.1", "RPL-1.5", "RPSL-1.0", "RSA-MD", "RSCPL", "Ruby", "SAX-PD", "Saxpath", "SCEA", "SchemeReport", "Sendmail", "Sendmail-8.23", "SGI-B-1.0", "SGI-B-1.1", "SGI-B-2.0", "SGP4", "SHL-0.5", "SHL-0.51", "SimPL-2.0", "SISSL", "SISSL-1.2", "Sleepycat", "SMLNJ", "SMPPL", "SNIA", "snprintf", "Spencer-86", "Spencer-94", "Spencer-99", "SPL-1.0", "SSH-OpenSSH", "SSH-short", "SSPL-1.0", "StandardML-NJ", "SugarCRM-1.1.3", "SunPro", "SWL", "Symlinks", "TAPR-OHL-1.0", "TCL", "TCP-wrappers", "TermReadKey", "TMate", "TORQUE-1.1", "TOSL", "TPDL", "TPL-1.0", "TTWL", "TU-Berlin-1.0", "TU-Berlin-2.0", "UCAR", "UCL-1.0", "Unicode-DFS-2015", "Unicode-DFS-2016", "Unicode-TOU", "UnixCrypt", "Unlicense", "UPL-1.0", "Vim", "VOSTROM", "VSL-1.0", "W3C", "W3C-19980720", "W3C-20150513", "w3m", "Watcom-1.0", "Widget-Workshop", "Wsuipa", "WTFPL", "wxWindows", "X11", "X11-distribute-modifications-variant", "Xdebug-1.03", "Xerox", "Xfig", "XFree86-1.1", "xinetd", "xlock", "Xnet", "xpp", "XSkat", "YPL-1.0", "YPL-1.1", "Zed", "Zend-2.0", "Zimbra-1.3", "Zimbra-1.4", "Zlib", "zlib-acknowledgement", "ZPL-1.1", "ZPL-2.0", "ZPL-2.1"); 3548 } 3549 public String toCode() { 3550 switch (this) { 3551 case _0BSD: return "0BSD"; 3552 case AAL: return "AAL"; 3553 case ABSTYLES: return "Abstyles"; 3554 case ADACORE_DOC: return "AdaCore-doc"; 3555 case ADOBE_2006: return "Adobe-2006"; 3556 case ADOBE_GLYPH: return "Adobe-Glyph"; 3557 case ADSL: return "ADSL"; 3558 case AFL_1_1: return "AFL-1.1"; 3559 case AFL_1_2: return "AFL-1.2"; 3560 case AFL_2_0: return "AFL-2.0"; 3561 case AFL_2_1: return "AFL-2.1"; 3562 case AFL_3_0: return "AFL-3.0"; 3563 case AFMPARSE: return "Afmparse"; 3564 case AGPL_1_0: return "AGPL-1.0"; 3565 case AGPL_1_0_ONLY: return "AGPL-1.0-only"; 3566 case AGPL_1_0_OR_LATER: return "AGPL-1.0-or-later"; 3567 case AGPL_3_0: return "AGPL-3.0"; 3568 case AGPL_3_0_ONLY: return "AGPL-3.0-only"; 3569 case AGPL_3_0_OR_LATER: return "AGPL-3.0-or-later"; 3570 case ALADDIN: return "Aladdin"; 3571 case AMDPLPA: return "AMDPLPA"; 3572 case AML: return "AML"; 3573 case AMPAS: return "AMPAS"; 3574 case ANTLR_PD: return "ANTLR-PD"; 3575 case ANTLR_PD_FALLBACK: return "ANTLR-PD-fallback"; 3576 case APACHE_1_0: return "Apache-1.0"; 3577 case APACHE_1_1: return "Apache-1.1"; 3578 case APACHE_2_0: return "Apache-2.0"; 3579 case APAFML: return "APAFML"; 3580 case APL_1_0: return "APL-1.0"; 3581 case APP_S2P: return "App-s2p"; 3582 case APSL_1_0: return "APSL-1.0"; 3583 case APSL_1_1: return "APSL-1.1"; 3584 case APSL_1_2: return "APSL-1.2"; 3585 case APSL_2_0: return "APSL-2.0"; 3586 case ARPHIC_1999: return "Arphic-1999"; 3587 case ARTISTIC_1_0: return "Artistic-1.0"; 3588 case ARTISTIC_1_0_CL8: return "Artistic-1.0-cl8"; 3589 case ARTISTIC_1_0_PERL: return "Artistic-1.0-Perl"; 3590 case ARTISTIC_2_0: return "Artistic-2.0"; 3591 case ASWF_DIGITAL_ASSETS_1_0: return "ASWF-Digital-Assets-1.0"; 3592 case ASWF_DIGITAL_ASSETS_1_1: return "ASWF-Digital-Assets-1.1"; 3593 case BAEKMUK: return "Baekmuk"; 3594 case BAHYPH: return "Bahyph"; 3595 case BARR: return "Barr"; 3596 case BEERWARE: return "Beerware"; 3597 case BITSTREAM_CHARTER: return "Bitstream-Charter"; 3598 case BITSTREAM_VERA: return "Bitstream-Vera"; 3599 case BITTORRENT_1_0: return "BitTorrent-1.0"; 3600 case BITTORRENT_1_1: return "BitTorrent-1.1"; 3601 case BLESSING: return "blessing"; 3602 case BLUEOAK_1_0_0: return "BlueOak-1.0.0"; 3603 case BOEHM_GC: return "Boehm-GC"; 3604 case BORCEUX: return "Borceux"; 3605 case BRIAN_GLADMAN_3_CLAUSE: return "Brian-Gladman-3-Clause"; 3606 case BSD_1_CLAUSE: return "BSD-1-Clause"; 3607 case BSD_2_CLAUSE: return "BSD-2-Clause"; 3608 case BSD_2_CLAUSE_FREEBSD: return "BSD-2-Clause-FreeBSD"; 3609 case BSD_2_CLAUSE_NETBSD: return "BSD-2-Clause-NetBSD"; 3610 case BSD_2_CLAUSE_PATENT: return "BSD-2-Clause-Patent"; 3611 case BSD_2_CLAUSE_VIEWS: return "BSD-2-Clause-Views"; 3612 case BSD_3_CLAUSE: return "BSD-3-Clause"; 3613 case BSD_3_CLAUSE_ATTRIBUTION: return "BSD-3-Clause-Attribution"; 3614 case BSD_3_CLAUSE_CLEAR: return "BSD-3-Clause-Clear"; 3615 case BSD_3_CLAUSE_LBNL: return "BSD-3-Clause-LBNL"; 3616 case BSD_3_CLAUSE_MODIFICATION: return "BSD-3-Clause-Modification"; 3617 case BSD_3_CLAUSE_NO_MILITARY_LICENSE: return "BSD-3-Clause-No-Military-License"; 3618 case BSD_3_CLAUSE_NO_NUCLEAR_LICENSE: return "BSD-3-Clause-No-Nuclear-License"; 3619 case BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014: return "BSD-3-Clause-No-Nuclear-License-2014"; 3620 case BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY: return "BSD-3-Clause-No-Nuclear-Warranty"; 3621 case BSD_3_CLAUSE_OPEN_MPI: return "BSD-3-Clause-Open-MPI"; 3622 case BSD_4_CLAUSE: return "BSD-4-Clause"; 3623 case BSD_4_CLAUSE_SHORTENED: return "BSD-4-Clause-Shortened"; 3624 case BSD_4_CLAUSE_UC: return "BSD-4-Clause-UC"; 3625 case BSD_4_3RENO: return "BSD-4.3RENO"; 3626 case BSD_4_3TAHOE: return "BSD-4.3TAHOE"; 3627 case BSD_ADVERTISING_ACKNOWLEDGEMENT: return "BSD-Advertising-Acknowledgement"; 3628 case BSD_ATTRIBUTION_HPND_DISCLAIMER: return "BSD-Attribution-HPND-disclaimer"; 3629 case BSD_PROTECTION: return "BSD-Protection"; 3630 case BSD_SOURCE_CODE: return "BSD-Source-Code"; 3631 case BSL_1_0: return "BSL-1.0"; 3632 case BUSL_1_1: return "BUSL-1.1"; 3633 case BZIP2_1_0_5: return "bzip2-1.0.5"; 3634 case BZIP2_1_0_6: return "bzip2-1.0.6"; 3635 case C_UDA_1_0: return "C-UDA-1.0"; 3636 case CAL_1_0: return "CAL-1.0"; 3637 case CAL_1_0_COMBINED_WORK_EXCEPTION: return "CAL-1.0-Combined-Work-Exception"; 3638 case CALDERA: return "Caldera"; 3639 case CATOSL_1_1: return "CATOSL-1.1"; 3640 case CC_BY_1_0: return "CC-BY-1.0"; 3641 case CC_BY_2_0: return "CC-BY-2.0"; 3642 case CC_BY_2_5: return "CC-BY-2.5"; 3643 case CC_BY_2_5_AU: return "CC-BY-2.5-AU"; 3644 case CC_BY_3_0: return "CC-BY-3.0"; 3645 case CC_BY_3_0_AT: return "CC-BY-3.0-AT"; 3646 case CC_BY_3_0_DE: return "CC-BY-3.0-DE"; 3647 case CC_BY_3_0_IGO: return "CC-BY-3.0-IGO"; 3648 case CC_BY_3_0_NL: return "CC-BY-3.0-NL"; 3649 case CC_BY_3_0_US: return "CC-BY-3.0-US"; 3650 case CC_BY_4_0: return "CC-BY-4.0"; 3651 case CC_BY_NC_1_0: return "CC-BY-NC-1.0"; 3652 case CC_BY_NC_2_0: return "CC-BY-NC-2.0"; 3653 case CC_BY_NC_2_5: return "CC-BY-NC-2.5"; 3654 case CC_BY_NC_3_0: return "CC-BY-NC-3.0"; 3655 case CC_BY_NC_3_0_DE: return "CC-BY-NC-3.0-DE"; 3656 case CC_BY_NC_4_0: return "CC-BY-NC-4.0"; 3657 case CC_BY_NC_ND_1_0: return "CC-BY-NC-ND-1.0"; 3658 case CC_BY_NC_ND_2_0: return "CC-BY-NC-ND-2.0"; 3659 case CC_BY_NC_ND_2_5: return "CC-BY-NC-ND-2.5"; 3660 case CC_BY_NC_ND_3_0: return "CC-BY-NC-ND-3.0"; 3661 case CC_BY_NC_ND_3_0_DE: return "CC-BY-NC-ND-3.0-DE"; 3662 case CC_BY_NC_ND_3_0_IGO: return "CC-BY-NC-ND-3.0-IGO"; 3663 case CC_BY_NC_ND_4_0: return "CC-BY-NC-ND-4.0"; 3664 case CC_BY_NC_SA_1_0: return "CC-BY-NC-SA-1.0"; 3665 case CC_BY_NC_SA_2_0: return "CC-BY-NC-SA-2.0"; 3666 case CC_BY_NC_SA_2_0_DE: return "CC-BY-NC-SA-2.0-DE"; 3667 case CC_BY_NC_SA_2_0_FR: return "CC-BY-NC-SA-2.0-FR"; 3668 case CC_BY_NC_SA_2_0_UK: return "CC-BY-NC-SA-2.0-UK"; 3669 case CC_BY_NC_SA_2_5: return "CC-BY-NC-SA-2.5"; 3670 case CC_BY_NC_SA_3_0: return "CC-BY-NC-SA-3.0"; 3671 case CC_BY_NC_SA_3_0_DE: return "CC-BY-NC-SA-3.0-DE"; 3672 case CC_BY_NC_SA_3_0_IGO: return "CC-BY-NC-SA-3.0-IGO"; 3673 case CC_BY_NC_SA_4_0: return "CC-BY-NC-SA-4.0"; 3674 case CC_BY_ND_1_0: return "CC-BY-ND-1.0"; 3675 case CC_BY_ND_2_0: return "CC-BY-ND-2.0"; 3676 case CC_BY_ND_2_5: return "CC-BY-ND-2.5"; 3677 case CC_BY_ND_3_0: return "CC-BY-ND-3.0"; 3678 case CC_BY_ND_3_0_DE: return "CC-BY-ND-3.0-DE"; 3679 case CC_BY_ND_4_0: return "CC-BY-ND-4.0"; 3680 case CC_BY_SA_1_0: return "CC-BY-SA-1.0"; 3681 case CC_BY_SA_2_0: return "CC-BY-SA-2.0"; 3682 case CC_BY_SA_2_0_UK: return "CC-BY-SA-2.0-UK"; 3683 case CC_BY_SA_2_1_JP: return "CC-BY-SA-2.1-JP"; 3684 case CC_BY_SA_2_5: return "CC-BY-SA-2.5"; 3685 case CC_BY_SA_3_0: return "CC-BY-SA-3.0"; 3686 case CC_BY_SA_3_0_AT: return "CC-BY-SA-3.0-AT"; 3687 case CC_BY_SA_3_0_DE: return "CC-BY-SA-3.0-DE"; 3688 case CC_BY_SA_3_0_IGO: return "CC-BY-SA-3.0-IGO"; 3689 case CC_BY_SA_4_0: return "CC-BY-SA-4.0"; 3690 case CC_PDDC: return "CC-PDDC"; 3691 case CC0_1_0: return "CC0-1.0"; 3692 case CDDL_1_0: return "CDDL-1.0"; 3693 case CDDL_1_1: return "CDDL-1.1"; 3694 case CDL_1_0: return "CDL-1.0"; 3695 case CDLA_PERMISSIVE_1_0: return "CDLA-Permissive-1.0"; 3696 case CDLA_PERMISSIVE_2_0: return "CDLA-Permissive-2.0"; 3697 case CDLA_SHARING_1_0: return "CDLA-Sharing-1.0"; 3698 case CECILL_1_0: return "CECILL-1.0"; 3699 case CECILL_1_1: return "CECILL-1.1"; 3700 case CECILL_2_0: return "CECILL-2.0"; 3701 case CECILL_2_1: return "CECILL-2.1"; 3702 case CECILL_B: return "CECILL-B"; 3703 case CECILL_C: return "CECILL-C"; 3704 case CERN_OHL_1_1: return "CERN-OHL-1.1"; 3705 case CERN_OHL_1_2: return "CERN-OHL-1.2"; 3706 case CERN_OHL_P_2_0: return "CERN-OHL-P-2.0"; 3707 case CERN_OHL_S_2_0: return "CERN-OHL-S-2.0"; 3708 case CERN_OHL_W_2_0: return "CERN-OHL-W-2.0"; 3709 case CFITSIO: return "CFITSIO"; 3710 case CHECKMK: return "checkmk"; 3711 case CLARTISTIC: return "ClArtistic"; 3712 case CLIPS: return "Clips"; 3713 case CMU_MACH: return "CMU-Mach"; 3714 case CNRI_JYTHON: return "CNRI-Jython"; 3715 case CNRI_PYTHON: return "CNRI-Python"; 3716 case CNRI_PYTHON_GPL_COMPATIBLE: return "CNRI-Python-GPL-Compatible"; 3717 case COIL_1_0: return "COIL-1.0"; 3718 case COMMUNITY_SPEC_1_0: return "Community-Spec-1.0"; 3719 case CONDOR_1_1: return "Condor-1.1"; 3720 case COPYLEFT_NEXT_0_3_0: return "copyleft-next-0.3.0"; 3721 case COPYLEFT_NEXT_0_3_1: return "copyleft-next-0.3.1"; 3722 case CORNELL_LOSSLESS_JPEG: return "Cornell-Lossless-JPEG"; 3723 case CPAL_1_0: return "CPAL-1.0"; 3724 case CPL_1_0: return "CPL-1.0"; 3725 case CPOL_1_02: return "CPOL-1.02"; 3726 case CROSSWORD: return "Crossword"; 3727 case CRYSTALSTACKER: return "CrystalStacker"; 3728 case CUA_OPL_1_0: return "CUA-OPL-1.0"; 3729 case CUBE: return "Cube"; 3730 case CURL: return "curl"; 3731 case D_FSL_1_0: return "D-FSL-1.0"; 3732 case DIFFMARK: return "diffmark"; 3733 case DL_DE_BY_2_0: return "DL-DE-BY-2.0"; 3734 case DOC: return "DOC"; 3735 case DOTSEQN: return "Dotseqn"; 3736 case DRL_1_0: return "DRL-1.0"; 3737 case DSDP: return "DSDP"; 3738 case DTOA: return "dtoa"; 3739 case DVIPDFM: return "dvipdfm"; 3740 case ECL_1_0: return "ECL-1.0"; 3741 case ECL_2_0: return "ECL-2.0"; 3742 case ECOS_2_0: return "eCos-2.0"; 3743 case EFL_1_0: return "EFL-1.0"; 3744 case EFL_2_0: return "EFL-2.0"; 3745 case EGENIX: return "eGenix"; 3746 case ELASTIC_2_0: return "Elastic-2.0"; 3747 case ENTESSA: return "Entessa"; 3748 case EPICS: return "EPICS"; 3749 case EPL_1_0: return "EPL-1.0"; 3750 case EPL_2_0: return "EPL-2.0"; 3751 case ERLPL_1_1: return "ErlPL-1.1"; 3752 case ETALAB_2_0: return "etalab-2.0"; 3753 case EUDATAGRID: return "EUDatagrid"; 3754 case EUPL_1_0: return "EUPL-1.0"; 3755 case EUPL_1_1: return "EUPL-1.1"; 3756 case EUPL_1_2: return "EUPL-1.2"; 3757 case EUROSYM: return "Eurosym"; 3758 case FAIR: return "Fair"; 3759 case FDK_AAC: return "FDK-AAC"; 3760 case FRAMEWORX_1_0: return "Frameworx-1.0"; 3761 case FREEBSD_DOC: return "FreeBSD-DOC"; 3762 case FREEIMAGE: return "FreeImage"; 3763 case FSFAP: return "FSFAP"; 3764 case FSFUL: return "FSFUL"; 3765 case FSFULLR: return "FSFULLR"; 3766 case FSFULLRWD: return "FSFULLRWD"; 3767 case FTL: return "FTL"; 3768 case GD: return "GD"; 3769 case GFDL_1_1: return "GFDL-1.1"; 3770 case GFDL_1_1_INVARIANTS_ONLY: return "GFDL-1.1-invariants-only"; 3771 case GFDL_1_1_INVARIANTS_OR_LATER: return "GFDL-1.1-invariants-or-later"; 3772 case GFDL_1_1_NO_INVARIANTS_ONLY: return "GFDL-1.1-no-invariants-only"; 3773 case GFDL_1_1_NO_INVARIANTS_OR_LATER: return "GFDL-1.1-no-invariants-or-later"; 3774 case GFDL_1_1_ONLY: return "GFDL-1.1-only"; 3775 case GFDL_1_1_OR_LATER: return "GFDL-1.1-or-later"; 3776 case GFDL_1_2: return "GFDL-1.2"; 3777 case GFDL_1_2_INVARIANTS_ONLY: return "GFDL-1.2-invariants-only"; 3778 case GFDL_1_2_INVARIANTS_OR_LATER: return "GFDL-1.2-invariants-or-later"; 3779 case GFDL_1_2_NO_INVARIANTS_ONLY: return "GFDL-1.2-no-invariants-only"; 3780 case GFDL_1_2_NO_INVARIANTS_OR_LATER: return "GFDL-1.2-no-invariants-or-later"; 3781 case GFDL_1_2_ONLY: return "GFDL-1.2-only"; 3782 case GFDL_1_2_OR_LATER: return "GFDL-1.2-or-later"; 3783 case GFDL_1_3: return "GFDL-1.3"; 3784 case GFDL_1_3_INVARIANTS_ONLY: return "GFDL-1.3-invariants-only"; 3785 case GFDL_1_3_INVARIANTS_OR_LATER: return "GFDL-1.3-invariants-or-later"; 3786 case GFDL_1_3_NO_INVARIANTS_ONLY: return "GFDL-1.3-no-invariants-only"; 3787 case GFDL_1_3_NO_INVARIANTS_OR_LATER: return "GFDL-1.3-no-invariants-or-later"; 3788 case GFDL_1_3_ONLY: return "GFDL-1.3-only"; 3789 case GFDL_1_3_OR_LATER: return "GFDL-1.3-or-later"; 3790 case GIFTWARE: return "Giftware"; 3791 case GL2PS: return "GL2PS"; 3792 case GLIDE: return "Glide"; 3793 case GLULXE: return "Glulxe"; 3794 case GLWTPL: return "GLWTPL"; 3795 case GNUPLOT: return "gnuplot"; 3796 case GPL_1_0: return "GPL-1.0"; 3797 case GPL_1_0PLUS: return "GPL-1.0+"; 3798 case GPL_1_0_ONLY: return "GPL-1.0-only"; 3799 case GPL_1_0_OR_LATER: return "GPL-1.0-or-later"; 3800 case GPL_2_0: return "GPL-2.0"; 3801 case GPL_2_0PLUS: return "GPL-2.0+"; 3802 case GPL_2_0_ONLY: return "GPL-2.0-only"; 3803 case GPL_2_0_OR_LATER: return "GPL-2.0-or-later"; 3804 case GPL_2_0_WITH_AUTOCONF_EXCEPTION: return "GPL-2.0-with-autoconf-exception"; 3805 case GPL_2_0_WITH_BISON_EXCEPTION: return "GPL-2.0-with-bison-exception"; 3806 case GPL_2_0_WITH_CLASSPATH_EXCEPTION: return "GPL-2.0-with-classpath-exception"; 3807 case GPL_2_0_WITH_FONT_EXCEPTION: return "GPL-2.0-with-font-exception"; 3808 case GPL_2_0_WITH_GCC_EXCEPTION: return "GPL-2.0-with-GCC-exception"; 3809 case GPL_3_0: return "GPL-3.0"; 3810 case GPL_3_0PLUS: return "GPL-3.0+"; 3811 case GPL_3_0_ONLY: return "GPL-3.0-only"; 3812 case GPL_3_0_OR_LATER: return "GPL-3.0-or-later"; 3813 case GPL_3_0_WITH_AUTOCONF_EXCEPTION: return "GPL-3.0-with-autoconf-exception"; 3814 case GPL_3_0_WITH_GCC_EXCEPTION: return "GPL-3.0-with-GCC-exception"; 3815 case GRAPHICS_GEMS: return "Graphics-Gems"; 3816 case GSOAP_1_3B: return "gSOAP-1.3b"; 3817 case HASKELLREPORT: return "HaskellReport"; 3818 case HIPPOCRATIC_2_1: return "Hippocratic-2.1"; 3819 case HP_1986: return "HP-1986"; 3820 case HPND: return "HPND"; 3821 case HPND_EXPORT_US: return "HPND-export-US"; 3822 case HPND_MARKUS_KUHN: return "HPND-Markus-Kuhn"; 3823 case HPND_SELL_VARIANT: return "HPND-sell-variant"; 3824 case HPND_SELL_VARIANT_MIT_DISCLAIMER: return "HPND-sell-variant-MIT-disclaimer"; 3825 case HTMLTIDY: return "HTMLTIDY"; 3826 case IBM_PIBS: return "IBM-pibs"; 3827 case ICU: return "ICU"; 3828 case IEC_CODE_COMPONENTS_EULA: return "IEC-Code-Components-EULA"; 3829 case IJG: return "IJG"; 3830 case IJG_SHORT: return "IJG-short"; 3831 case IMAGEMAGICK: return "ImageMagick"; 3832 case IMATIX: return "iMatix"; 3833 case IMLIB2: return "Imlib2"; 3834 case INFO_ZIP: return "Info-ZIP"; 3835 case INNER_NET_2_0: return "Inner-Net-2.0"; 3836 case INTEL: return "Intel"; 3837 case INTEL_ACPI: return "Intel-ACPI"; 3838 case INTERBASE_1_0: return "Interbase-1.0"; 3839 case IPA: return "IPA"; 3840 case IPL_1_0: return "IPL-1.0"; 3841 case ISC: return "ISC"; 3842 case JAM: return "Jam"; 3843 case JASPER_2_0: return "JasPer-2.0"; 3844 case JPL_IMAGE: return "JPL-image"; 3845 case JPNIC: return "JPNIC"; 3846 case JSON: return "JSON"; 3847 case KAZLIB: return "Kazlib"; 3848 case KNUTH_CTAN: return "Knuth-CTAN"; 3849 case LAL_1_2: return "LAL-1.2"; 3850 case LAL_1_3: return "LAL-1.3"; 3851 case LATEX2E: return "Latex2e"; 3852 case LATEX2E_TRANSLATED_NOTICE: return "Latex2e-translated-notice"; 3853 case LEPTONICA: return "Leptonica"; 3854 case LGPL_2_0: return "LGPL-2.0"; 3855 case LGPL_2_0PLUS: return "LGPL-2.0+"; 3856 case LGPL_2_0_ONLY: return "LGPL-2.0-only"; 3857 case LGPL_2_0_OR_LATER: return "LGPL-2.0-or-later"; 3858 case LGPL_2_1: return "LGPL-2.1"; 3859 case LGPL_2_1PLUS: return "LGPL-2.1+"; 3860 case LGPL_2_1_ONLY: return "LGPL-2.1-only"; 3861 case LGPL_2_1_OR_LATER: return "LGPL-2.1-or-later"; 3862 case LGPL_3_0: return "LGPL-3.0"; 3863 case LGPL_3_0PLUS: return "LGPL-3.0+"; 3864 case LGPL_3_0_ONLY: return "LGPL-3.0-only"; 3865 case LGPL_3_0_OR_LATER: return "LGPL-3.0-or-later"; 3866 case LGPLLR: return "LGPLLR"; 3867 case LIBPNG: return "Libpng"; 3868 case LIBPNG_2_0: return "libpng-2.0"; 3869 case LIBSELINUX_1_0: return "libselinux-1.0"; 3870 case LIBTIFF: return "libtiff"; 3871 case LIBUTIL_DAVID_NUGENT: return "libutil-David-Nugent"; 3872 case LILIQ_P_1_1: return "LiLiQ-P-1.1"; 3873 case LILIQ_R_1_1: return "LiLiQ-R-1.1"; 3874 case LILIQ_RPLUS_1_1: return "LiLiQ-Rplus-1.1"; 3875 case LINUX_MAN_PAGES_1_PARA: return "Linux-man-pages-1-para"; 3876 case LINUX_MAN_PAGES_COPYLEFT: return "Linux-man-pages-copyleft"; 3877 case LINUX_MAN_PAGES_COPYLEFT_2_PARA: return "Linux-man-pages-copyleft-2-para"; 3878 case LINUX_MAN_PAGES_COPYLEFT_VAR: return "Linux-man-pages-copyleft-var"; 3879 case LINUX_OPENIB: return "Linux-OpenIB"; 3880 case LOOP: return "LOOP"; 3881 case LPL_1_0: return "LPL-1.0"; 3882 case LPL_1_02: return "LPL-1.02"; 3883 case LPPL_1_0: return "LPPL-1.0"; 3884 case LPPL_1_1: return "LPPL-1.1"; 3885 case LPPL_1_2: return "LPPL-1.2"; 3886 case LPPL_1_3A: return "LPPL-1.3a"; 3887 case LPPL_1_3C: return "LPPL-1.3c"; 3888 case LZMA_SDK_9_11_TO_9_20: return "LZMA-SDK-9.11-to-9.20"; 3889 case LZMA_SDK_9_22: return "LZMA-SDK-9.22"; 3890 case MAKEINDEX: return "MakeIndex"; 3891 case MARTIN_BIRGMEIER: return "Martin-Birgmeier"; 3892 case METAMAIL: return "metamail"; 3893 case MINPACK: return "Minpack"; 3894 case MIROS: return "MirOS"; 3895 case MIT: return "MIT"; 3896 case MIT_0: return "MIT-0"; 3897 case MIT_ADVERTISING: return "MIT-advertising"; 3898 case MIT_CMU: return "MIT-CMU"; 3899 case MIT_ENNA: return "MIT-enna"; 3900 case MIT_FEH: return "MIT-feh"; 3901 case MIT_FESTIVAL: return "MIT-Festival"; 3902 case MIT_MODERN_VARIANT: return "MIT-Modern-Variant"; 3903 case MIT_OPEN_GROUP: return "MIT-open-group"; 3904 case MIT_WU: return "MIT-Wu"; 3905 case MITNFA: return "MITNFA"; 3906 case MOTOSOTO: return "Motosoto"; 3907 case MPI_PERMISSIVE: return "mpi-permissive"; 3908 case MPICH2: return "mpich2"; 3909 case MPL_1_0: return "MPL-1.0"; 3910 case MPL_1_1: return "MPL-1.1"; 3911 case MPL_2_0: return "MPL-2.0"; 3912 case MPL_2_0_NO_COPYLEFT_EXCEPTION: return "MPL-2.0-no-copyleft-exception"; 3913 case MPLUS: return "mplus"; 3914 case MS_LPL: return "MS-LPL"; 3915 case MS_PL: return "MS-PL"; 3916 case MS_RL: return "MS-RL"; 3917 case MTLL: return "MTLL"; 3918 case MULANPSL_1_0: return "MulanPSL-1.0"; 3919 case MULANPSL_2_0: return "MulanPSL-2.0"; 3920 case MULTICS: return "Multics"; 3921 case MUP: return "Mup"; 3922 case NAIST_2003: return "NAIST-2003"; 3923 case NASA_1_3: return "NASA-1.3"; 3924 case NAUMEN: return "Naumen"; 3925 case NBPL_1_0: return "NBPL-1.0"; 3926 case NCGL_UK_2_0: return "NCGL-UK-2.0"; 3927 case NCSA: return "NCSA"; 3928 case NET_SNMP: return "Net-SNMP"; 3929 case NETCDF: return "NetCDF"; 3930 case NEWSLETR: return "Newsletr"; 3931 case NGPL: return "NGPL"; 3932 case NICTA_1_0: return "NICTA-1.0"; 3933 case NIST_PD: return "NIST-PD"; 3934 case NIST_PD_FALLBACK: return "NIST-PD-fallback"; 3935 case NIST_SOFTWARE: return "NIST-Software"; 3936 case NLOD_1_0: return "NLOD-1.0"; 3937 case NLOD_2_0: return "NLOD-2.0"; 3938 case NLPL: return "NLPL"; 3939 case NOKIA: return "Nokia"; 3940 case NOSL: return "NOSL"; 3941 case NOT_OPEN_SOURCE: return "not-open-source"; 3942 case NOWEB: return "Noweb"; 3943 case NPL_1_0: return "NPL-1.0"; 3944 case NPL_1_1: return "NPL-1.1"; 3945 case NPOSL_3_0: return "NPOSL-3.0"; 3946 case NRL: return "NRL"; 3947 case NTP: return "NTP"; 3948 case NTP_0: return "NTP-0"; 3949 case NUNIT: return "Nunit"; 3950 case O_UDA_1_0: return "O-UDA-1.0"; 3951 case OCCT_PL: return "OCCT-PL"; 3952 case OCLC_2_0: return "OCLC-2.0"; 3953 case ODBL_1_0: return "ODbL-1.0"; 3954 case ODC_BY_1_0: return "ODC-By-1.0"; 3955 case OFFIS: return "OFFIS"; 3956 case OFL_1_0: return "OFL-1.0"; 3957 case OFL_1_0_NO_RFN: return "OFL-1.0-no-RFN"; 3958 case OFL_1_0_RFN: return "OFL-1.0-RFN"; 3959 case OFL_1_1: return "OFL-1.1"; 3960 case OFL_1_1_NO_RFN: return "OFL-1.1-no-RFN"; 3961 case OFL_1_1_RFN: return "OFL-1.1-RFN"; 3962 case OGC_1_0: return "OGC-1.0"; 3963 case OGDL_TAIWAN_1_0: return "OGDL-Taiwan-1.0"; 3964 case OGL_CANADA_2_0: return "OGL-Canada-2.0"; 3965 case OGL_UK_1_0: return "OGL-UK-1.0"; 3966 case OGL_UK_2_0: return "OGL-UK-2.0"; 3967 case OGL_UK_3_0: return "OGL-UK-3.0"; 3968 case OGTSL: return "OGTSL"; 3969 case OLDAP_1_1: return "OLDAP-1.1"; 3970 case OLDAP_1_2: return "OLDAP-1.2"; 3971 case OLDAP_1_3: return "OLDAP-1.3"; 3972 case OLDAP_1_4: return "OLDAP-1.4"; 3973 case OLDAP_2_0: return "OLDAP-2.0"; 3974 case OLDAP_2_0_1: return "OLDAP-2.0.1"; 3975 case OLDAP_2_1: return "OLDAP-2.1"; 3976 case OLDAP_2_2: return "OLDAP-2.2"; 3977 case OLDAP_2_2_1: return "OLDAP-2.2.1"; 3978 case OLDAP_2_2_2: return "OLDAP-2.2.2"; 3979 case OLDAP_2_3: return "OLDAP-2.3"; 3980 case OLDAP_2_4: return "OLDAP-2.4"; 3981 case OLDAP_2_5: return "OLDAP-2.5"; 3982 case OLDAP_2_6: return "OLDAP-2.6"; 3983 case OLDAP_2_7: return "OLDAP-2.7"; 3984 case OLDAP_2_8: return "OLDAP-2.8"; 3985 case OLFL_1_3: return "OLFL-1.3"; 3986 case OML: return "OML"; 3987 case OPENPBS_2_3: return "OpenPBS-2.3"; 3988 case OPENSSL: return "OpenSSL"; 3989 case OPL_1_0: return "OPL-1.0"; 3990 case OPL_UK_3_0: return "OPL-UK-3.0"; 3991 case OPUBL_1_0: return "OPUBL-1.0"; 3992 case OSET_PL_2_1: return "OSET-PL-2.1"; 3993 case OSL_1_0: return "OSL-1.0"; 3994 case OSL_1_1: return "OSL-1.1"; 3995 case OSL_2_0: return "OSL-2.0"; 3996 case OSL_2_1: return "OSL-2.1"; 3997 case OSL_3_0: return "OSL-3.0"; 3998 case PARITY_6_0_0: return "Parity-6.0.0"; 3999 case PARITY_7_0_0: return "Parity-7.0.0"; 4000 case PDDL_1_0: return "PDDL-1.0"; 4001 case PHP_3_0: return "PHP-3.0"; 4002 case PHP_3_01: return "PHP-3.01"; 4003 case PLEXUS: return "Plexus"; 4004 case POLYFORM_NONCOMMERCIAL_1_0_0: return "PolyForm-Noncommercial-1.0.0"; 4005 case POLYFORM_SMALL_BUSINESS_1_0_0: return "PolyForm-Small-Business-1.0.0"; 4006 case POSTGRESQL: return "PostgreSQL"; 4007 case PSF_2_0: return "PSF-2.0"; 4008 case PSFRAG: return "psfrag"; 4009 case PSUTILS: return "psutils"; 4010 case PYTHON_2_0: return "Python-2.0"; 4011 case PYTHON_2_0_1: return "Python-2.0.1"; 4012 case QHULL: return "Qhull"; 4013 case QPL_1_0: return "QPL-1.0"; 4014 case QPL_1_0_INRIA_2004: return "QPL-1.0-INRIA-2004"; 4015 case RDISC: return "Rdisc"; 4016 case RHECOS_1_1: return "RHeCos-1.1"; 4017 case RPL_1_1: return "RPL-1.1"; 4018 case RPL_1_5: return "RPL-1.5"; 4019 case RPSL_1_0: return "RPSL-1.0"; 4020 case RSA_MD: return "RSA-MD"; 4021 case RSCPL: return "RSCPL"; 4022 case RUBY: return "Ruby"; 4023 case SAX_PD: return "SAX-PD"; 4024 case SAXPATH: return "Saxpath"; 4025 case SCEA: return "SCEA"; 4026 case SCHEMEREPORT: return "SchemeReport"; 4027 case SENDMAIL: return "Sendmail"; 4028 case SENDMAIL_8_23: return "Sendmail-8.23"; 4029 case SGI_B_1_0: return "SGI-B-1.0"; 4030 case SGI_B_1_1: return "SGI-B-1.1"; 4031 case SGI_B_2_0: return "SGI-B-2.0"; 4032 case SGP4: return "SGP4"; 4033 case SHL_0_5: return "SHL-0.5"; 4034 case SHL_0_51: return "SHL-0.51"; 4035 case SIMPL_2_0: return "SimPL-2.0"; 4036 case SISSL: return "SISSL"; 4037 case SISSL_1_2: return "SISSL-1.2"; 4038 case SLEEPYCAT: return "Sleepycat"; 4039 case SMLNJ: return "SMLNJ"; 4040 case SMPPL: return "SMPPL"; 4041 case SNIA: return "SNIA"; 4042 case SNPRINTF: return "snprintf"; 4043 case SPENCER_86: return "Spencer-86"; 4044 case SPENCER_94: return "Spencer-94"; 4045 case SPENCER_99: return "Spencer-99"; 4046 case SPL_1_0: return "SPL-1.0"; 4047 case SSH_OPENSSH: return "SSH-OpenSSH"; 4048 case SSH_SHORT: return "SSH-short"; 4049 case SSPL_1_0: return "SSPL-1.0"; 4050 case STANDARDML_NJ: return "StandardML-NJ"; 4051 case SUGARCRM_1_1_3: return "SugarCRM-1.1.3"; 4052 case SUNPRO: return "SunPro"; 4053 case SWL: return "SWL"; 4054 case SYMLINKS: return "Symlinks"; 4055 case TAPR_OHL_1_0: return "TAPR-OHL-1.0"; 4056 case TCL: return "TCL"; 4057 case TCP_WRAPPERS: return "TCP-wrappers"; 4058 case TERMREADKEY: return "TermReadKey"; 4059 case TMATE: return "TMate"; 4060 case TORQUE_1_1: return "TORQUE-1.1"; 4061 case TOSL: return "TOSL"; 4062 case TPDL: return "TPDL"; 4063 case TPL_1_0: return "TPL-1.0"; 4064 case TTWL: return "TTWL"; 4065 case TU_BERLIN_1_0: return "TU-Berlin-1.0"; 4066 case TU_BERLIN_2_0: return "TU-Berlin-2.0"; 4067 case UCAR: return "UCAR"; 4068 case UCL_1_0: return "UCL-1.0"; 4069 case UNICODE_DFS_2015: return "Unicode-DFS-2015"; 4070 case UNICODE_DFS_2016: return "Unicode-DFS-2016"; 4071 case UNICODE_TOU: return "Unicode-TOU"; 4072 case UNIXCRYPT: return "UnixCrypt"; 4073 case UNLICENSE: return "Unlicense"; 4074 case UPL_1_0: return "UPL-1.0"; 4075 case VIM: return "Vim"; 4076 case VOSTROM: return "VOSTROM"; 4077 case VSL_1_0: return "VSL-1.0"; 4078 case W3C: return "W3C"; 4079 case W3C_19980720: return "W3C-19980720"; 4080 case W3C_20150513: return "W3C-20150513"; 4081 case W3M: return "w3m"; 4082 case WATCOM_1_0: return "Watcom-1.0"; 4083 case WIDGET_WORKSHOP: return "Widget-Workshop"; 4084 case WSUIPA: return "Wsuipa"; 4085 case WTFPL: return "WTFPL"; 4086 case WXWINDOWS: return "wxWindows"; 4087 case X11: return "X11"; 4088 case X11_DISTRIBUTE_MODIFICATIONS_VARIANT: return "X11-distribute-modifications-variant"; 4089 case XDEBUG_1_03: return "Xdebug-1.03"; 4090 case XEROX: return "Xerox"; 4091 case XFIG: return "Xfig"; 4092 case XFREE86_1_1: return "XFree86-1.1"; 4093 case XINETD: return "xinetd"; 4094 case XLOCK: return "xlock"; 4095 case XNET: return "Xnet"; 4096 case XPP: return "xpp"; 4097 case XSKAT: return "XSkat"; 4098 case YPL_1_0: return "YPL-1.0"; 4099 case YPL_1_1: return "YPL-1.1"; 4100 case ZED: return "Zed"; 4101 case ZEND_2_0: return "Zend-2.0"; 4102 case ZIMBRA_1_3: return "Zimbra-1.3"; 4103 case ZIMBRA_1_4: return "Zimbra-1.4"; 4104 case ZLIB: return "Zlib"; 4105 case ZLIB_ACKNOWLEDGEMENT: return "zlib-acknowledgement"; 4106 case ZPL_1_1: return "ZPL-1.1"; 4107 case ZPL_2_0: return "ZPL-2.0"; 4108 case ZPL_2_1: return "ZPL-2.1"; 4109 case NULL: return null; 4110 default: return "?"; 4111 } 4112 } 4113 public String getSystem() { 4114 switch (this) { 4115 case _0BSD: return "http://hl7.org/fhir/spdx-license"; 4116 case AAL: return "http://hl7.org/fhir/spdx-license"; 4117 case ABSTYLES: return "http://hl7.org/fhir/spdx-license"; 4118 case ADACORE_DOC: return "http://hl7.org/fhir/spdx-license"; 4119 case ADOBE_2006: return "http://hl7.org/fhir/spdx-license"; 4120 case ADOBE_GLYPH: return "http://hl7.org/fhir/spdx-license"; 4121 case ADSL: return "http://hl7.org/fhir/spdx-license"; 4122 case AFL_1_1: return "http://hl7.org/fhir/spdx-license"; 4123 case AFL_1_2: return "http://hl7.org/fhir/spdx-license"; 4124 case AFL_2_0: return "http://hl7.org/fhir/spdx-license"; 4125 case AFL_2_1: return "http://hl7.org/fhir/spdx-license"; 4126 case AFL_3_0: return "http://hl7.org/fhir/spdx-license"; 4127 case AFMPARSE: return "http://hl7.org/fhir/spdx-license"; 4128 case AGPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4129 case AGPL_1_0_ONLY: return "http://hl7.org/fhir/spdx-license"; 4130 case AGPL_1_0_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4131 case AGPL_3_0: return "http://hl7.org/fhir/spdx-license"; 4132 case AGPL_3_0_ONLY: return "http://hl7.org/fhir/spdx-license"; 4133 case AGPL_3_0_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4134 case ALADDIN: return "http://hl7.org/fhir/spdx-license"; 4135 case AMDPLPA: return "http://hl7.org/fhir/spdx-license"; 4136 case AML: return "http://hl7.org/fhir/spdx-license"; 4137 case AMPAS: return "http://hl7.org/fhir/spdx-license"; 4138 case ANTLR_PD: return "http://hl7.org/fhir/spdx-license"; 4139 case ANTLR_PD_FALLBACK: return "http://hl7.org/fhir/spdx-license"; 4140 case APACHE_1_0: return "http://hl7.org/fhir/spdx-license"; 4141 case APACHE_1_1: return "http://hl7.org/fhir/spdx-license"; 4142 case APACHE_2_0: return "http://hl7.org/fhir/spdx-license"; 4143 case APAFML: return "http://hl7.org/fhir/spdx-license"; 4144 case APL_1_0: return "http://hl7.org/fhir/spdx-license"; 4145 case APP_S2P: return "http://hl7.org/fhir/spdx-license"; 4146 case APSL_1_0: return "http://hl7.org/fhir/spdx-license"; 4147 case APSL_1_1: return "http://hl7.org/fhir/spdx-license"; 4148 case APSL_1_2: return "http://hl7.org/fhir/spdx-license"; 4149 case APSL_2_0: return "http://hl7.org/fhir/spdx-license"; 4150 case ARPHIC_1999: return "http://hl7.org/fhir/spdx-license"; 4151 case ARTISTIC_1_0: return "http://hl7.org/fhir/spdx-license"; 4152 case ARTISTIC_1_0_CL8: return "http://hl7.org/fhir/spdx-license"; 4153 case ARTISTIC_1_0_PERL: return "http://hl7.org/fhir/spdx-license"; 4154 case ARTISTIC_2_0: return "http://hl7.org/fhir/spdx-license"; 4155 case ASWF_DIGITAL_ASSETS_1_0: return "http://hl7.org/fhir/spdx-license"; 4156 case ASWF_DIGITAL_ASSETS_1_1: return "http://hl7.org/fhir/spdx-license"; 4157 case BAEKMUK: return "http://hl7.org/fhir/spdx-license"; 4158 case BAHYPH: return "http://hl7.org/fhir/spdx-license"; 4159 case BARR: return "http://hl7.org/fhir/spdx-license"; 4160 case BEERWARE: return "http://hl7.org/fhir/spdx-license"; 4161 case BITSTREAM_CHARTER: return "http://hl7.org/fhir/spdx-license"; 4162 case BITSTREAM_VERA: return "http://hl7.org/fhir/spdx-license"; 4163 case BITTORRENT_1_0: return "http://hl7.org/fhir/spdx-license"; 4164 case BITTORRENT_1_1: return "http://hl7.org/fhir/spdx-license"; 4165 case BLESSING: return "http://hl7.org/fhir/spdx-license"; 4166 case BLUEOAK_1_0_0: return "http://hl7.org/fhir/spdx-license"; 4167 case BOEHM_GC: return "http://hl7.org/fhir/spdx-license"; 4168 case BORCEUX: return "http://hl7.org/fhir/spdx-license"; 4169 case BRIAN_GLADMAN_3_CLAUSE: return "http://hl7.org/fhir/spdx-license"; 4170 case BSD_1_CLAUSE: return "http://hl7.org/fhir/spdx-license"; 4171 case BSD_2_CLAUSE: return "http://hl7.org/fhir/spdx-license"; 4172 case BSD_2_CLAUSE_FREEBSD: return "http://hl7.org/fhir/spdx-license"; 4173 case BSD_2_CLAUSE_NETBSD: return "http://hl7.org/fhir/spdx-license"; 4174 case BSD_2_CLAUSE_PATENT: return "http://hl7.org/fhir/spdx-license"; 4175 case BSD_2_CLAUSE_VIEWS: return "http://hl7.org/fhir/spdx-license"; 4176 case BSD_3_CLAUSE: return "http://hl7.org/fhir/spdx-license"; 4177 case BSD_3_CLAUSE_ATTRIBUTION: return "http://hl7.org/fhir/spdx-license"; 4178 case BSD_3_CLAUSE_CLEAR: return "http://hl7.org/fhir/spdx-license"; 4179 case BSD_3_CLAUSE_LBNL: return "http://hl7.org/fhir/spdx-license"; 4180 case BSD_3_CLAUSE_MODIFICATION: return "http://hl7.org/fhir/spdx-license"; 4181 case BSD_3_CLAUSE_NO_MILITARY_LICENSE: return "http://hl7.org/fhir/spdx-license"; 4182 case BSD_3_CLAUSE_NO_NUCLEAR_LICENSE: return "http://hl7.org/fhir/spdx-license"; 4183 case BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014: return "http://hl7.org/fhir/spdx-license"; 4184 case BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY: return "http://hl7.org/fhir/spdx-license"; 4185 case BSD_3_CLAUSE_OPEN_MPI: return "http://hl7.org/fhir/spdx-license"; 4186 case BSD_4_CLAUSE: return "http://hl7.org/fhir/spdx-license"; 4187 case BSD_4_CLAUSE_SHORTENED: return "http://hl7.org/fhir/spdx-license"; 4188 case BSD_4_CLAUSE_UC: return "http://hl7.org/fhir/spdx-license"; 4189 case BSD_4_3RENO: return "http://hl7.org/fhir/spdx-license"; 4190 case BSD_4_3TAHOE: return "http://hl7.org/fhir/spdx-license"; 4191 case BSD_ADVERTISING_ACKNOWLEDGEMENT: return "http://hl7.org/fhir/spdx-license"; 4192 case BSD_ATTRIBUTION_HPND_DISCLAIMER: return "http://hl7.org/fhir/spdx-license"; 4193 case BSD_PROTECTION: return "http://hl7.org/fhir/spdx-license"; 4194 case BSD_SOURCE_CODE: return "http://hl7.org/fhir/spdx-license"; 4195 case BSL_1_0: return "http://hl7.org/fhir/spdx-license"; 4196 case BUSL_1_1: return "http://hl7.org/fhir/spdx-license"; 4197 case BZIP2_1_0_5: return "http://hl7.org/fhir/spdx-license"; 4198 case BZIP2_1_0_6: return "http://hl7.org/fhir/spdx-license"; 4199 case C_UDA_1_0: return "http://hl7.org/fhir/spdx-license"; 4200 case CAL_1_0: return "http://hl7.org/fhir/spdx-license"; 4201 case CAL_1_0_COMBINED_WORK_EXCEPTION: return "http://hl7.org/fhir/spdx-license"; 4202 case CALDERA: return "http://hl7.org/fhir/spdx-license"; 4203 case CATOSL_1_1: return "http://hl7.org/fhir/spdx-license"; 4204 case CC_BY_1_0: return "http://hl7.org/fhir/spdx-license"; 4205 case CC_BY_2_0: return "http://hl7.org/fhir/spdx-license"; 4206 case CC_BY_2_5: return "http://hl7.org/fhir/spdx-license"; 4207 case CC_BY_2_5_AU: return "http://hl7.org/fhir/spdx-license"; 4208 case CC_BY_3_0: return "http://hl7.org/fhir/spdx-license"; 4209 case CC_BY_3_0_AT: return "http://hl7.org/fhir/spdx-license"; 4210 case CC_BY_3_0_DE: return "http://hl7.org/fhir/spdx-license"; 4211 case CC_BY_3_0_IGO: return "http://hl7.org/fhir/spdx-license"; 4212 case CC_BY_3_0_NL: return "http://hl7.org/fhir/spdx-license"; 4213 case CC_BY_3_0_US: return "http://hl7.org/fhir/spdx-license"; 4214 case CC_BY_4_0: return "http://hl7.org/fhir/spdx-license"; 4215 case CC_BY_NC_1_0: return "http://hl7.org/fhir/spdx-license"; 4216 case CC_BY_NC_2_0: return "http://hl7.org/fhir/spdx-license"; 4217 case CC_BY_NC_2_5: return "http://hl7.org/fhir/spdx-license"; 4218 case CC_BY_NC_3_0: return "http://hl7.org/fhir/spdx-license"; 4219 case CC_BY_NC_3_0_DE: return "http://hl7.org/fhir/spdx-license"; 4220 case CC_BY_NC_4_0: return "http://hl7.org/fhir/spdx-license"; 4221 case CC_BY_NC_ND_1_0: return "http://hl7.org/fhir/spdx-license"; 4222 case CC_BY_NC_ND_2_0: return "http://hl7.org/fhir/spdx-license"; 4223 case CC_BY_NC_ND_2_5: return "http://hl7.org/fhir/spdx-license"; 4224 case CC_BY_NC_ND_3_0: return "http://hl7.org/fhir/spdx-license"; 4225 case CC_BY_NC_ND_3_0_DE: return "http://hl7.org/fhir/spdx-license"; 4226 case CC_BY_NC_ND_3_0_IGO: return "http://hl7.org/fhir/spdx-license"; 4227 case CC_BY_NC_ND_4_0: return "http://hl7.org/fhir/spdx-license"; 4228 case CC_BY_NC_SA_1_0: return "http://hl7.org/fhir/spdx-license"; 4229 case CC_BY_NC_SA_2_0: return "http://hl7.org/fhir/spdx-license"; 4230 case CC_BY_NC_SA_2_0_DE: return "http://hl7.org/fhir/spdx-license"; 4231 case CC_BY_NC_SA_2_0_FR: return "http://hl7.org/fhir/spdx-license"; 4232 case CC_BY_NC_SA_2_0_UK: return "http://hl7.org/fhir/spdx-license"; 4233 case CC_BY_NC_SA_2_5: return "http://hl7.org/fhir/spdx-license"; 4234 case CC_BY_NC_SA_3_0: return "http://hl7.org/fhir/spdx-license"; 4235 case CC_BY_NC_SA_3_0_DE: return "http://hl7.org/fhir/spdx-license"; 4236 case CC_BY_NC_SA_3_0_IGO: return "http://hl7.org/fhir/spdx-license"; 4237 case CC_BY_NC_SA_4_0: return "http://hl7.org/fhir/spdx-license"; 4238 case CC_BY_ND_1_0: return "http://hl7.org/fhir/spdx-license"; 4239 case CC_BY_ND_2_0: return "http://hl7.org/fhir/spdx-license"; 4240 case CC_BY_ND_2_5: return "http://hl7.org/fhir/spdx-license"; 4241 case CC_BY_ND_3_0: return "http://hl7.org/fhir/spdx-license"; 4242 case CC_BY_ND_3_0_DE: return "http://hl7.org/fhir/spdx-license"; 4243 case CC_BY_ND_4_0: return "http://hl7.org/fhir/spdx-license"; 4244 case CC_BY_SA_1_0: return "http://hl7.org/fhir/spdx-license"; 4245 case CC_BY_SA_2_0: return "http://hl7.org/fhir/spdx-license"; 4246 case CC_BY_SA_2_0_UK: return "http://hl7.org/fhir/spdx-license"; 4247 case CC_BY_SA_2_1_JP: return "http://hl7.org/fhir/spdx-license"; 4248 case CC_BY_SA_2_5: return "http://hl7.org/fhir/spdx-license"; 4249 case CC_BY_SA_3_0: return "http://hl7.org/fhir/spdx-license"; 4250 case CC_BY_SA_3_0_AT: return "http://hl7.org/fhir/spdx-license"; 4251 case CC_BY_SA_3_0_DE: return "http://hl7.org/fhir/spdx-license"; 4252 case CC_BY_SA_3_0_IGO: return "http://hl7.org/fhir/spdx-license"; 4253 case CC_BY_SA_4_0: return "http://hl7.org/fhir/spdx-license"; 4254 case CC_PDDC: return "http://hl7.org/fhir/spdx-license"; 4255 case CC0_1_0: return "http://hl7.org/fhir/spdx-license"; 4256 case CDDL_1_0: return "http://hl7.org/fhir/spdx-license"; 4257 case CDDL_1_1: return "http://hl7.org/fhir/spdx-license"; 4258 case CDL_1_0: return "http://hl7.org/fhir/spdx-license"; 4259 case CDLA_PERMISSIVE_1_0: return "http://hl7.org/fhir/spdx-license"; 4260 case CDLA_PERMISSIVE_2_0: return "http://hl7.org/fhir/spdx-license"; 4261 case CDLA_SHARING_1_0: return "http://hl7.org/fhir/spdx-license"; 4262 case CECILL_1_0: return "http://hl7.org/fhir/spdx-license"; 4263 case CECILL_1_1: return "http://hl7.org/fhir/spdx-license"; 4264 case CECILL_2_0: return "http://hl7.org/fhir/spdx-license"; 4265 case CECILL_2_1: return "http://hl7.org/fhir/spdx-license"; 4266 case CECILL_B: return "http://hl7.org/fhir/spdx-license"; 4267 case CECILL_C: return "http://hl7.org/fhir/spdx-license"; 4268 case CERN_OHL_1_1: return "http://hl7.org/fhir/spdx-license"; 4269 case CERN_OHL_1_2: return "http://hl7.org/fhir/spdx-license"; 4270 case CERN_OHL_P_2_0: return "http://hl7.org/fhir/spdx-license"; 4271 case CERN_OHL_S_2_0: return "http://hl7.org/fhir/spdx-license"; 4272 case CERN_OHL_W_2_0: return "http://hl7.org/fhir/spdx-license"; 4273 case CFITSIO: return "http://hl7.org/fhir/spdx-license"; 4274 case CHECKMK: return "http://hl7.org/fhir/spdx-license"; 4275 case CLARTISTIC: return "http://hl7.org/fhir/spdx-license"; 4276 case CLIPS: return "http://hl7.org/fhir/spdx-license"; 4277 case CMU_MACH: return "http://hl7.org/fhir/spdx-license"; 4278 case CNRI_JYTHON: return "http://hl7.org/fhir/spdx-license"; 4279 case CNRI_PYTHON: return "http://hl7.org/fhir/spdx-license"; 4280 case CNRI_PYTHON_GPL_COMPATIBLE: return "http://hl7.org/fhir/spdx-license"; 4281 case COIL_1_0: return "http://hl7.org/fhir/spdx-license"; 4282 case COMMUNITY_SPEC_1_0: return "http://hl7.org/fhir/spdx-license"; 4283 case CONDOR_1_1: return "http://hl7.org/fhir/spdx-license"; 4284 case COPYLEFT_NEXT_0_3_0: return "http://hl7.org/fhir/spdx-license"; 4285 case COPYLEFT_NEXT_0_3_1: return "http://hl7.org/fhir/spdx-license"; 4286 case CORNELL_LOSSLESS_JPEG: return "http://hl7.org/fhir/spdx-license"; 4287 case CPAL_1_0: return "http://hl7.org/fhir/spdx-license"; 4288 case CPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4289 case CPOL_1_02: return "http://hl7.org/fhir/spdx-license"; 4290 case CROSSWORD: return "http://hl7.org/fhir/spdx-license"; 4291 case CRYSTALSTACKER: return "http://hl7.org/fhir/spdx-license"; 4292 case CUA_OPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4293 case CUBE: return "http://hl7.org/fhir/spdx-license"; 4294 case CURL: return "http://hl7.org/fhir/spdx-license"; 4295 case D_FSL_1_0: return "http://hl7.org/fhir/spdx-license"; 4296 case DIFFMARK: return "http://hl7.org/fhir/spdx-license"; 4297 case DL_DE_BY_2_0: return "http://hl7.org/fhir/spdx-license"; 4298 case DOC: return "http://hl7.org/fhir/spdx-license"; 4299 case DOTSEQN: return "http://hl7.org/fhir/spdx-license"; 4300 case DRL_1_0: return "http://hl7.org/fhir/spdx-license"; 4301 case DSDP: return "http://hl7.org/fhir/spdx-license"; 4302 case DTOA: return "http://hl7.org/fhir/spdx-license"; 4303 case DVIPDFM: return "http://hl7.org/fhir/spdx-license"; 4304 case ECL_1_0: return "http://hl7.org/fhir/spdx-license"; 4305 case ECL_2_0: return "http://hl7.org/fhir/spdx-license"; 4306 case ECOS_2_0: return "http://hl7.org/fhir/spdx-license"; 4307 case EFL_1_0: return "http://hl7.org/fhir/spdx-license"; 4308 case EFL_2_0: return "http://hl7.org/fhir/spdx-license"; 4309 case EGENIX: return "http://hl7.org/fhir/spdx-license"; 4310 case ELASTIC_2_0: return "http://hl7.org/fhir/spdx-license"; 4311 case ENTESSA: return "http://hl7.org/fhir/spdx-license"; 4312 case EPICS: return "http://hl7.org/fhir/spdx-license"; 4313 case EPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4314 case EPL_2_0: return "http://hl7.org/fhir/spdx-license"; 4315 case ERLPL_1_1: return "http://hl7.org/fhir/spdx-license"; 4316 case ETALAB_2_0: return "http://hl7.org/fhir/spdx-license"; 4317 case EUDATAGRID: return "http://hl7.org/fhir/spdx-license"; 4318 case EUPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4319 case EUPL_1_1: return "http://hl7.org/fhir/spdx-license"; 4320 case EUPL_1_2: return "http://hl7.org/fhir/spdx-license"; 4321 case EUROSYM: return "http://hl7.org/fhir/spdx-license"; 4322 case FAIR: return "http://hl7.org/fhir/spdx-license"; 4323 case FDK_AAC: return "http://hl7.org/fhir/spdx-license"; 4324 case FRAMEWORX_1_0: return "http://hl7.org/fhir/spdx-license"; 4325 case FREEBSD_DOC: return "http://hl7.org/fhir/spdx-license"; 4326 case FREEIMAGE: return "http://hl7.org/fhir/spdx-license"; 4327 case FSFAP: return "http://hl7.org/fhir/spdx-license"; 4328 case FSFUL: return "http://hl7.org/fhir/spdx-license"; 4329 case FSFULLR: return "http://hl7.org/fhir/spdx-license"; 4330 case FSFULLRWD: return "http://hl7.org/fhir/spdx-license"; 4331 case FTL: return "http://hl7.org/fhir/spdx-license"; 4332 case GD: return "http://hl7.org/fhir/spdx-license"; 4333 case GFDL_1_1: return "http://hl7.org/fhir/spdx-license"; 4334 case GFDL_1_1_INVARIANTS_ONLY: return "http://hl7.org/fhir/spdx-license"; 4335 case GFDL_1_1_INVARIANTS_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4336 case GFDL_1_1_NO_INVARIANTS_ONLY: return "http://hl7.org/fhir/spdx-license"; 4337 case GFDL_1_1_NO_INVARIANTS_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4338 case GFDL_1_1_ONLY: return "http://hl7.org/fhir/spdx-license"; 4339 case GFDL_1_1_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4340 case GFDL_1_2: return "http://hl7.org/fhir/spdx-license"; 4341 case GFDL_1_2_INVARIANTS_ONLY: return "http://hl7.org/fhir/spdx-license"; 4342 case GFDL_1_2_INVARIANTS_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4343 case GFDL_1_2_NO_INVARIANTS_ONLY: return "http://hl7.org/fhir/spdx-license"; 4344 case GFDL_1_2_NO_INVARIANTS_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4345 case GFDL_1_2_ONLY: return "http://hl7.org/fhir/spdx-license"; 4346 case GFDL_1_2_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4347 case GFDL_1_3: return "http://hl7.org/fhir/spdx-license"; 4348 case GFDL_1_3_INVARIANTS_ONLY: return "http://hl7.org/fhir/spdx-license"; 4349 case GFDL_1_3_INVARIANTS_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4350 case GFDL_1_3_NO_INVARIANTS_ONLY: return "http://hl7.org/fhir/spdx-license"; 4351 case GFDL_1_3_NO_INVARIANTS_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4352 case GFDL_1_3_ONLY: return "http://hl7.org/fhir/spdx-license"; 4353 case GFDL_1_3_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4354 case GIFTWARE: return "http://hl7.org/fhir/spdx-license"; 4355 case GL2PS: return "http://hl7.org/fhir/spdx-license"; 4356 case GLIDE: return "http://hl7.org/fhir/spdx-license"; 4357 case GLULXE: return "http://hl7.org/fhir/spdx-license"; 4358 case GLWTPL: return "http://hl7.org/fhir/spdx-license"; 4359 case GNUPLOT: return "http://hl7.org/fhir/spdx-license"; 4360 case GPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4361 case GPL_1_0PLUS: return "http://hl7.org/fhir/spdx-license"; 4362 case GPL_1_0_ONLY: return "http://hl7.org/fhir/spdx-license"; 4363 case GPL_1_0_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4364 case GPL_2_0: return "http://hl7.org/fhir/spdx-license"; 4365 case GPL_2_0PLUS: return "http://hl7.org/fhir/spdx-license"; 4366 case GPL_2_0_ONLY: return "http://hl7.org/fhir/spdx-license"; 4367 case GPL_2_0_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4368 case GPL_2_0_WITH_AUTOCONF_EXCEPTION: return "http://hl7.org/fhir/spdx-license"; 4369 case GPL_2_0_WITH_BISON_EXCEPTION: return "http://hl7.org/fhir/spdx-license"; 4370 case GPL_2_0_WITH_CLASSPATH_EXCEPTION: return "http://hl7.org/fhir/spdx-license"; 4371 case GPL_2_0_WITH_FONT_EXCEPTION: return "http://hl7.org/fhir/spdx-license"; 4372 case GPL_2_0_WITH_GCC_EXCEPTION: return "http://hl7.org/fhir/spdx-license"; 4373 case GPL_3_0: return "http://hl7.org/fhir/spdx-license"; 4374 case GPL_3_0PLUS: return "http://hl7.org/fhir/spdx-license"; 4375 case GPL_3_0_ONLY: return "http://hl7.org/fhir/spdx-license"; 4376 case GPL_3_0_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4377 case GPL_3_0_WITH_AUTOCONF_EXCEPTION: return "http://hl7.org/fhir/spdx-license"; 4378 case GPL_3_0_WITH_GCC_EXCEPTION: return "http://hl7.org/fhir/spdx-license"; 4379 case GRAPHICS_GEMS: return "http://hl7.org/fhir/spdx-license"; 4380 case GSOAP_1_3B: return "http://hl7.org/fhir/spdx-license"; 4381 case HASKELLREPORT: return "http://hl7.org/fhir/spdx-license"; 4382 case HIPPOCRATIC_2_1: return "http://hl7.org/fhir/spdx-license"; 4383 case HP_1986: return "http://hl7.org/fhir/spdx-license"; 4384 case HPND: return "http://hl7.org/fhir/spdx-license"; 4385 case HPND_EXPORT_US: return "http://hl7.org/fhir/spdx-license"; 4386 case HPND_MARKUS_KUHN: return "http://hl7.org/fhir/spdx-license"; 4387 case HPND_SELL_VARIANT: return "http://hl7.org/fhir/spdx-license"; 4388 case HPND_SELL_VARIANT_MIT_DISCLAIMER: return "http://hl7.org/fhir/spdx-license"; 4389 case HTMLTIDY: return "http://hl7.org/fhir/spdx-license"; 4390 case IBM_PIBS: return "http://hl7.org/fhir/spdx-license"; 4391 case ICU: return "http://hl7.org/fhir/spdx-license"; 4392 case IEC_CODE_COMPONENTS_EULA: return "http://hl7.org/fhir/spdx-license"; 4393 case IJG: return "http://hl7.org/fhir/spdx-license"; 4394 case IJG_SHORT: return "http://hl7.org/fhir/spdx-license"; 4395 case IMAGEMAGICK: return "http://hl7.org/fhir/spdx-license"; 4396 case IMATIX: return "http://hl7.org/fhir/spdx-license"; 4397 case IMLIB2: return "http://hl7.org/fhir/spdx-license"; 4398 case INFO_ZIP: return "http://hl7.org/fhir/spdx-license"; 4399 case INNER_NET_2_0: return "http://hl7.org/fhir/spdx-license"; 4400 case INTEL: return "http://hl7.org/fhir/spdx-license"; 4401 case INTEL_ACPI: return "http://hl7.org/fhir/spdx-license"; 4402 case INTERBASE_1_0: return "http://hl7.org/fhir/spdx-license"; 4403 case IPA: return "http://hl7.org/fhir/spdx-license"; 4404 case IPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4405 case ISC: return "http://hl7.org/fhir/spdx-license"; 4406 case JAM: return "http://hl7.org/fhir/spdx-license"; 4407 case JASPER_2_0: return "http://hl7.org/fhir/spdx-license"; 4408 case JPL_IMAGE: return "http://hl7.org/fhir/spdx-license"; 4409 case JPNIC: return "http://hl7.org/fhir/spdx-license"; 4410 case JSON: return "http://hl7.org/fhir/spdx-license"; 4411 case KAZLIB: return "http://hl7.org/fhir/spdx-license"; 4412 case KNUTH_CTAN: return "http://hl7.org/fhir/spdx-license"; 4413 case LAL_1_2: return "http://hl7.org/fhir/spdx-license"; 4414 case LAL_1_3: return "http://hl7.org/fhir/spdx-license"; 4415 case LATEX2E: return "http://hl7.org/fhir/spdx-license"; 4416 case LATEX2E_TRANSLATED_NOTICE: return "http://hl7.org/fhir/spdx-license"; 4417 case LEPTONICA: return "http://hl7.org/fhir/spdx-license"; 4418 case LGPL_2_0: return "http://hl7.org/fhir/spdx-license"; 4419 case LGPL_2_0PLUS: return "http://hl7.org/fhir/spdx-license"; 4420 case LGPL_2_0_ONLY: return "http://hl7.org/fhir/spdx-license"; 4421 case LGPL_2_0_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4422 case LGPL_2_1: return "http://hl7.org/fhir/spdx-license"; 4423 case LGPL_2_1PLUS: return "http://hl7.org/fhir/spdx-license"; 4424 case LGPL_2_1_ONLY: return "http://hl7.org/fhir/spdx-license"; 4425 case LGPL_2_1_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4426 case LGPL_3_0: return "http://hl7.org/fhir/spdx-license"; 4427 case LGPL_3_0PLUS: return "http://hl7.org/fhir/spdx-license"; 4428 case LGPL_3_0_ONLY: return "http://hl7.org/fhir/spdx-license"; 4429 case LGPL_3_0_OR_LATER: return "http://hl7.org/fhir/spdx-license"; 4430 case LGPLLR: return "http://hl7.org/fhir/spdx-license"; 4431 case LIBPNG: return "http://hl7.org/fhir/spdx-license"; 4432 case LIBPNG_2_0: return "http://hl7.org/fhir/spdx-license"; 4433 case LIBSELINUX_1_0: return "http://hl7.org/fhir/spdx-license"; 4434 case LIBTIFF: return "http://hl7.org/fhir/spdx-license"; 4435 case LIBUTIL_DAVID_NUGENT: return "http://hl7.org/fhir/spdx-license"; 4436 case LILIQ_P_1_1: return "http://hl7.org/fhir/spdx-license"; 4437 case LILIQ_R_1_1: return "http://hl7.org/fhir/spdx-license"; 4438 case LILIQ_RPLUS_1_1: return "http://hl7.org/fhir/spdx-license"; 4439 case LINUX_MAN_PAGES_1_PARA: return "http://hl7.org/fhir/spdx-license"; 4440 case LINUX_MAN_PAGES_COPYLEFT: return "http://hl7.org/fhir/spdx-license"; 4441 case LINUX_MAN_PAGES_COPYLEFT_2_PARA: return "http://hl7.org/fhir/spdx-license"; 4442 case LINUX_MAN_PAGES_COPYLEFT_VAR: return "http://hl7.org/fhir/spdx-license"; 4443 case LINUX_OPENIB: return "http://hl7.org/fhir/spdx-license"; 4444 case LOOP: return "http://hl7.org/fhir/spdx-license"; 4445 case LPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4446 case LPL_1_02: return "http://hl7.org/fhir/spdx-license"; 4447 case LPPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4448 case LPPL_1_1: return "http://hl7.org/fhir/spdx-license"; 4449 case LPPL_1_2: return "http://hl7.org/fhir/spdx-license"; 4450 case LPPL_1_3A: return "http://hl7.org/fhir/spdx-license"; 4451 case LPPL_1_3C: return "http://hl7.org/fhir/spdx-license"; 4452 case LZMA_SDK_9_11_TO_9_20: return "http://hl7.org/fhir/spdx-license"; 4453 case LZMA_SDK_9_22: return "http://hl7.org/fhir/spdx-license"; 4454 case MAKEINDEX: return "http://hl7.org/fhir/spdx-license"; 4455 case MARTIN_BIRGMEIER: return "http://hl7.org/fhir/spdx-license"; 4456 case METAMAIL: return "http://hl7.org/fhir/spdx-license"; 4457 case MINPACK: return "http://hl7.org/fhir/spdx-license"; 4458 case MIROS: return "http://hl7.org/fhir/spdx-license"; 4459 case MIT: return "http://hl7.org/fhir/spdx-license"; 4460 case MIT_0: return "http://hl7.org/fhir/spdx-license"; 4461 case MIT_ADVERTISING: return "http://hl7.org/fhir/spdx-license"; 4462 case MIT_CMU: return "http://hl7.org/fhir/spdx-license"; 4463 case MIT_ENNA: return "http://hl7.org/fhir/spdx-license"; 4464 case MIT_FEH: return "http://hl7.org/fhir/spdx-license"; 4465 case MIT_FESTIVAL: return "http://hl7.org/fhir/spdx-license"; 4466 case MIT_MODERN_VARIANT: return "http://hl7.org/fhir/spdx-license"; 4467 case MIT_OPEN_GROUP: return "http://hl7.org/fhir/spdx-license"; 4468 case MIT_WU: return "http://hl7.org/fhir/spdx-license"; 4469 case MITNFA: return "http://hl7.org/fhir/spdx-license"; 4470 case MOTOSOTO: return "http://hl7.org/fhir/spdx-license"; 4471 case MPI_PERMISSIVE: return "http://hl7.org/fhir/spdx-license"; 4472 case MPICH2: return "http://hl7.org/fhir/spdx-license"; 4473 case MPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4474 case MPL_1_1: return "http://hl7.org/fhir/spdx-license"; 4475 case MPL_2_0: return "http://hl7.org/fhir/spdx-license"; 4476 case MPL_2_0_NO_COPYLEFT_EXCEPTION: return "http://hl7.org/fhir/spdx-license"; 4477 case MPLUS: return "http://hl7.org/fhir/spdx-license"; 4478 case MS_LPL: return "http://hl7.org/fhir/spdx-license"; 4479 case MS_PL: return "http://hl7.org/fhir/spdx-license"; 4480 case MS_RL: return "http://hl7.org/fhir/spdx-license"; 4481 case MTLL: return "http://hl7.org/fhir/spdx-license"; 4482 case MULANPSL_1_0: return "http://hl7.org/fhir/spdx-license"; 4483 case MULANPSL_2_0: return "http://hl7.org/fhir/spdx-license"; 4484 case MULTICS: return "http://hl7.org/fhir/spdx-license"; 4485 case MUP: return "http://hl7.org/fhir/spdx-license"; 4486 case NAIST_2003: return "http://hl7.org/fhir/spdx-license"; 4487 case NASA_1_3: return "http://hl7.org/fhir/spdx-license"; 4488 case NAUMEN: return "http://hl7.org/fhir/spdx-license"; 4489 case NBPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4490 case NCGL_UK_2_0: return "http://hl7.org/fhir/spdx-license"; 4491 case NCSA: return "http://hl7.org/fhir/spdx-license"; 4492 case NET_SNMP: return "http://hl7.org/fhir/spdx-license"; 4493 case NETCDF: return "http://hl7.org/fhir/spdx-license"; 4494 case NEWSLETR: return "http://hl7.org/fhir/spdx-license"; 4495 case NGPL: return "http://hl7.org/fhir/spdx-license"; 4496 case NICTA_1_0: return "http://hl7.org/fhir/spdx-license"; 4497 case NIST_PD: return "http://hl7.org/fhir/spdx-license"; 4498 case NIST_PD_FALLBACK: return "http://hl7.org/fhir/spdx-license"; 4499 case NIST_SOFTWARE: return "http://hl7.org/fhir/spdx-license"; 4500 case NLOD_1_0: return "http://hl7.org/fhir/spdx-license"; 4501 case NLOD_2_0: return "http://hl7.org/fhir/spdx-license"; 4502 case NLPL: return "http://hl7.org/fhir/spdx-license"; 4503 case NOKIA: return "http://hl7.org/fhir/spdx-license"; 4504 case NOSL: return "http://hl7.org/fhir/spdx-license"; 4505 case NOT_OPEN_SOURCE: return "http://hl7.org/fhir/spdx-license"; 4506 case NOWEB: return "http://hl7.org/fhir/spdx-license"; 4507 case NPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4508 case NPL_1_1: return "http://hl7.org/fhir/spdx-license"; 4509 case NPOSL_3_0: return "http://hl7.org/fhir/spdx-license"; 4510 case NRL: return "http://hl7.org/fhir/spdx-license"; 4511 case NTP: return "http://hl7.org/fhir/spdx-license"; 4512 case NTP_0: return "http://hl7.org/fhir/spdx-license"; 4513 case NUNIT: return "http://hl7.org/fhir/spdx-license"; 4514 case O_UDA_1_0: return "http://hl7.org/fhir/spdx-license"; 4515 case OCCT_PL: return "http://hl7.org/fhir/spdx-license"; 4516 case OCLC_2_0: return "http://hl7.org/fhir/spdx-license"; 4517 case ODBL_1_0: return "http://hl7.org/fhir/spdx-license"; 4518 case ODC_BY_1_0: return "http://hl7.org/fhir/spdx-license"; 4519 case OFFIS: return "http://hl7.org/fhir/spdx-license"; 4520 case OFL_1_0: return "http://hl7.org/fhir/spdx-license"; 4521 case OFL_1_0_NO_RFN: return "http://hl7.org/fhir/spdx-license"; 4522 case OFL_1_0_RFN: return "http://hl7.org/fhir/spdx-license"; 4523 case OFL_1_1: return "http://hl7.org/fhir/spdx-license"; 4524 case OFL_1_1_NO_RFN: return "http://hl7.org/fhir/spdx-license"; 4525 case OFL_1_1_RFN: return "http://hl7.org/fhir/spdx-license"; 4526 case OGC_1_0: return "http://hl7.org/fhir/spdx-license"; 4527 case OGDL_TAIWAN_1_0: return "http://hl7.org/fhir/spdx-license"; 4528 case OGL_CANADA_2_0: return "http://hl7.org/fhir/spdx-license"; 4529 case OGL_UK_1_0: return "http://hl7.org/fhir/spdx-license"; 4530 case OGL_UK_2_0: return "http://hl7.org/fhir/spdx-license"; 4531 case OGL_UK_3_0: return "http://hl7.org/fhir/spdx-license"; 4532 case OGTSL: return "http://hl7.org/fhir/spdx-license"; 4533 case OLDAP_1_1: return "http://hl7.org/fhir/spdx-license"; 4534 case OLDAP_1_2: return "http://hl7.org/fhir/spdx-license"; 4535 case OLDAP_1_3: return "http://hl7.org/fhir/spdx-license"; 4536 case OLDAP_1_4: return "http://hl7.org/fhir/spdx-license"; 4537 case OLDAP_2_0: return "http://hl7.org/fhir/spdx-license"; 4538 case OLDAP_2_0_1: return "http://hl7.org/fhir/spdx-license"; 4539 case OLDAP_2_1: return "http://hl7.org/fhir/spdx-license"; 4540 case OLDAP_2_2: return "http://hl7.org/fhir/spdx-license"; 4541 case OLDAP_2_2_1: return "http://hl7.org/fhir/spdx-license"; 4542 case OLDAP_2_2_2: return "http://hl7.org/fhir/spdx-license"; 4543 case OLDAP_2_3: return "http://hl7.org/fhir/spdx-license"; 4544 case OLDAP_2_4: return "http://hl7.org/fhir/spdx-license"; 4545 case OLDAP_2_5: return "http://hl7.org/fhir/spdx-license"; 4546 case OLDAP_2_6: return "http://hl7.org/fhir/spdx-license"; 4547 case OLDAP_2_7: return "http://hl7.org/fhir/spdx-license"; 4548 case OLDAP_2_8: return "http://hl7.org/fhir/spdx-license"; 4549 case OLFL_1_3: return "http://hl7.org/fhir/spdx-license"; 4550 case OML: return "http://hl7.org/fhir/spdx-license"; 4551 case OPENPBS_2_3: return "http://hl7.org/fhir/spdx-license"; 4552 case OPENSSL: return "http://hl7.org/fhir/spdx-license"; 4553 case OPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4554 case OPL_UK_3_0: return "http://hl7.org/fhir/spdx-license"; 4555 case OPUBL_1_0: return "http://hl7.org/fhir/spdx-license"; 4556 case OSET_PL_2_1: return "http://hl7.org/fhir/spdx-license"; 4557 case OSL_1_0: return "http://hl7.org/fhir/spdx-license"; 4558 case OSL_1_1: return "http://hl7.org/fhir/spdx-license"; 4559 case OSL_2_0: return "http://hl7.org/fhir/spdx-license"; 4560 case OSL_2_1: return "http://hl7.org/fhir/spdx-license"; 4561 case OSL_3_0: return "http://hl7.org/fhir/spdx-license"; 4562 case PARITY_6_0_0: return "http://hl7.org/fhir/spdx-license"; 4563 case PARITY_7_0_0: return "http://hl7.org/fhir/spdx-license"; 4564 case PDDL_1_0: return "http://hl7.org/fhir/spdx-license"; 4565 case PHP_3_0: return "http://hl7.org/fhir/spdx-license"; 4566 case PHP_3_01: return "http://hl7.org/fhir/spdx-license"; 4567 case PLEXUS: return "http://hl7.org/fhir/spdx-license"; 4568 case POLYFORM_NONCOMMERCIAL_1_0_0: return "http://hl7.org/fhir/spdx-license"; 4569 case POLYFORM_SMALL_BUSINESS_1_0_0: return "http://hl7.org/fhir/spdx-license"; 4570 case POSTGRESQL: return "http://hl7.org/fhir/spdx-license"; 4571 case PSF_2_0: return "http://hl7.org/fhir/spdx-license"; 4572 case PSFRAG: return "http://hl7.org/fhir/spdx-license"; 4573 case PSUTILS: return "http://hl7.org/fhir/spdx-license"; 4574 case PYTHON_2_0: return "http://hl7.org/fhir/spdx-license"; 4575 case PYTHON_2_0_1: return "http://hl7.org/fhir/spdx-license"; 4576 case QHULL: return "http://hl7.org/fhir/spdx-license"; 4577 case QPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4578 case QPL_1_0_INRIA_2004: return "http://hl7.org/fhir/spdx-license"; 4579 case RDISC: return "http://hl7.org/fhir/spdx-license"; 4580 case RHECOS_1_1: return "http://hl7.org/fhir/spdx-license"; 4581 case RPL_1_1: return "http://hl7.org/fhir/spdx-license"; 4582 case RPL_1_5: return "http://hl7.org/fhir/spdx-license"; 4583 case RPSL_1_0: return "http://hl7.org/fhir/spdx-license"; 4584 case RSA_MD: return "http://hl7.org/fhir/spdx-license"; 4585 case RSCPL: return "http://hl7.org/fhir/spdx-license"; 4586 case RUBY: return "http://hl7.org/fhir/spdx-license"; 4587 case SAX_PD: return "http://hl7.org/fhir/spdx-license"; 4588 case SAXPATH: return "http://hl7.org/fhir/spdx-license"; 4589 case SCEA: return "http://hl7.org/fhir/spdx-license"; 4590 case SCHEMEREPORT: return "http://hl7.org/fhir/spdx-license"; 4591 case SENDMAIL: return "http://hl7.org/fhir/spdx-license"; 4592 case SENDMAIL_8_23: return "http://hl7.org/fhir/spdx-license"; 4593 case SGI_B_1_0: return "http://hl7.org/fhir/spdx-license"; 4594 case SGI_B_1_1: return "http://hl7.org/fhir/spdx-license"; 4595 case SGI_B_2_0: return "http://hl7.org/fhir/spdx-license"; 4596 case SGP4: return "http://hl7.org/fhir/spdx-license"; 4597 case SHL_0_5: return "http://hl7.org/fhir/spdx-license"; 4598 case SHL_0_51: return "http://hl7.org/fhir/spdx-license"; 4599 case SIMPL_2_0: return "http://hl7.org/fhir/spdx-license"; 4600 case SISSL: return "http://hl7.org/fhir/spdx-license"; 4601 case SISSL_1_2: return "http://hl7.org/fhir/spdx-license"; 4602 case SLEEPYCAT: return "http://hl7.org/fhir/spdx-license"; 4603 case SMLNJ: return "http://hl7.org/fhir/spdx-license"; 4604 case SMPPL: return "http://hl7.org/fhir/spdx-license"; 4605 case SNIA: return "http://hl7.org/fhir/spdx-license"; 4606 case SNPRINTF: return "http://hl7.org/fhir/spdx-license"; 4607 case SPENCER_86: return "http://hl7.org/fhir/spdx-license"; 4608 case SPENCER_94: return "http://hl7.org/fhir/spdx-license"; 4609 case SPENCER_99: return "http://hl7.org/fhir/spdx-license"; 4610 case SPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4611 case SSH_OPENSSH: return "http://hl7.org/fhir/spdx-license"; 4612 case SSH_SHORT: return "http://hl7.org/fhir/spdx-license"; 4613 case SSPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4614 case STANDARDML_NJ: return "http://hl7.org/fhir/spdx-license"; 4615 case SUGARCRM_1_1_3: return "http://hl7.org/fhir/spdx-license"; 4616 case SUNPRO: return "http://hl7.org/fhir/spdx-license"; 4617 case SWL: return "http://hl7.org/fhir/spdx-license"; 4618 case SYMLINKS: return "http://hl7.org/fhir/spdx-license"; 4619 case TAPR_OHL_1_0: return "http://hl7.org/fhir/spdx-license"; 4620 case TCL: return "http://hl7.org/fhir/spdx-license"; 4621 case TCP_WRAPPERS: return "http://hl7.org/fhir/spdx-license"; 4622 case TERMREADKEY: return "http://hl7.org/fhir/spdx-license"; 4623 case TMATE: return "http://hl7.org/fhir/spdx-license"; 4624 case TORQUE_1_1: return "http://hl7.org/fhir/spdx-license"; 4625 case TOSL: return "http://hl7.org/fhir/spdx-license"; 4626 case TPDL: return "http://hl7.org/fhir/spdx-license"; 4627 case TPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4628 case TTWL: return "http://hl7.org/fhir/spdx-license"; 4629 case TU_BERLIN_1_0: return "http://hl7.org/fhir/spdx-license"; 4630 case TU_BERLIN_2_0: return "http://hl7.org/fhir/spdx-license"; 4631 case UCAR: return "http://hl7.org/fhir/spdx-license"; 4632 case UCL_1_0: return "http://hl7.org/fhir/spdx-license"; 4633 case UNICODE_DFS_2015: return "http://hl7.org/fhir/spdx-license"; 4634 case UNICODE_DFS_2016: return "http://hl7.org/fhir/spdx-license"; 4635 case UNICODE_TOU: return "http://hl7.org/fhir/spdx-license"; 4636 case UNIXCRYPT: return "http://hl7.org/fhir/spdx-license"; 4637 case UNLICENSE: return "http://hl7.org/fhir/spdx-license"; 4638 case UPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4639 case VIM: return "http://hl7.org/fhir/spdx-license"; 4640 case VOSTROM: return "http://hl7.org/fhir/spdx-license"; 4641 case VSL_1_0: return "http://hl7.org/fhir/spdx-license"; 4642 case W3C: return "http://hl7.org/fhir/spdx-license"; 4643 case W3C_19980720: return "http://hl7.org/fhir/spdx-license"; 4644 case W3C_20150513: return "http://hl7.org/fhir/spdx-license"; 4645 case W3M: return "http://hl7.org/fhir/spdx-license"; 4646 case WATCOM_1_0: return "http://hl7.org/fhir/spdx-license"; 4647 case WIDGET_WORKSHOP: return "http://hl7.org/fhir/spdx-license"; 4648 case WSUIPA: return "http://hl7.org/fhir/spdx-license"; 4649 case WTFPL: return "http://hl7.org/fhir/spdx-license"; 4650 case WXWINDOWS: return "http://hl7.org/fhir/spdx-license"; 4651 case X11: return "http://hl7.org/fhir/spdx-license"; 4652 case X11_DISTRIBUTE_MODIFICATIONS_VARIANT: return "http://hl7.org/fhir/spdx-license"; 4653 case XDEBUG_1_03: return "http://hl7.org/fhir/spdx-license"; 4654 case XEROX: return "http://hl7.org/fhir/spdx-license"; 4655 case XFIG: return "http://hl7.org/fhir/spdx-license"; 4656 case XFREE86_1_1: return "http://hl7.org/fhir/spdx-license"; 4657 case XINETD: return "http://hl7.org/fhir/spdx-license"; 4658 case XLOCK: return "http://hl7.org/fhir/spdx-license"; 4659 case XNET: return "http://hl7.org/fhir/spdx-license"; 4660 case XPP: return "http://hl7.org/fhir/spdx-license"; 4661 case XSKAT: return "http://hl7.org/fhir/spdx-license"; 4662 case YPL_1_0: return "http://hl7.org/fhir/spdx-license"; 4663 case YPL_1_1: return "http://hl7.org/fhir/spdx-license"; 4664 case ZED: return "http://hl7.org/fhir/spdx-license"; 4665 case ZEND_2_0: return "http://hl7.org/fhir/spdx-license"; 4666 case ZIMBRA_1_3: return "http://hl7.org/fhir/spdx-license"; 4667 case ZIMBRA_1_4: return "http://hl7.org/fhir/spdx-license"; 4668 case ZLIB: return "http://hl7.org/fhir/spdx-license"; 4669 case ZLIB_ACKNOWLEDGEMENT: return "http://hl7.org/fhir/spdx-license"; 4670 case ZPL_1_1: return "http://hl7.org/fhir/spdx-license"; 4671 case ZPL_2_0: return "http://hl7.org/fhir/spdx-license"; 4672 case ZPL_2_1: return "http://hl7.org/fhir/spdx-license"; 4673 case NULL: return null; 4674 default: return "?"; 4675 } 4676 } 4677 public String getDefinition() { 4678 switch (this) { 4679 case _0BSD: return "BSD Zero Clause License"; 4680 case AAL: return "Attribution Assurance License"; 4681 case ABSTYLES: return "Abstyles License"; 4682 case ADACORE_DOC: return "AdaCore Doc License"; 4683 case ADOBE_2006: return "Adobe Systems Incorporated Source Code License Agreement"; 4684 case ADOBE_GLYPH: return "Adobe Glyph List License"; 4685 case ADSL: return "Amazon Digital Services License"; 4686 case AFL_1_1: return "Academic Free License v1.1"; 4687 case AFL_1_2: return "Academic Free License v1.2"; 4688 case AFL_2_0: return "Academic Free License v2.0"; 4689 case AFL_2_1: return "Academic Free License v2.1"; 4690 case AFL_3_0: return "Academic Free License v3.0"; 4691 case AFMPARSE: return "Afmparse License"; 4692 case AGPL_1_0: return "Affero General Public License v1.0"; 4693 case AGPL_1_0_ONLY: return "Affero General Public License v1.0 only"; 4694 case AGPL_1_0_OR_LATER: return "Affero General Public License v1.0 or later"; 4695 case AGPL_3_0: return "GNU Affero General Public License v3.0"; 4696 case AGPL_3_0_ONLY: return "GNU Affero General Public License v3.0 only"; 4697 case AGPL_3_0_OR_LATER: return "GNU Affero General Public License v3.0 or later"; 4698 case ALADDIN: return "Aladdin Free Public License"; 4699 case AMDPLPA: return "AMD's plpa_map.c License"; 4700 case AML: return "Apple MIT License"; 4701 case AMPAS: return "Academy of Motion Picture Arts and Sciences BSD"; 4702 case ANTLR_PD: return "ANTLR Software Rights Notice"; 4703 case ANTLR_PD_FALLBACK: return "ANTLR Software Rights Notice with license fallback"; 4704 case APACHE_1_0: return "Apache License 1.0"; 4705 case APACHE_1_1: return "Apache License 1.1"; 4706 case APACHE_2_0: return "Apache License 2.0"; 4707 case APAFML: return "Adobe Postscript AFM License"; 4708 case APL_1_0: return "Adaptive Public License 1.0"; 4709 case APP_S2P: return "App::s2p License"; 4710 case APSL_1_0: return "Apple Public Source License 1.0"; 4711 case APSL_1_1: return "Apple Public Source License 1.1"; 4712 case APSL_1_2: return "Apple Public Source License 1.2"; 4713 case APSL_2_0: return "Apple Public Source License 2.0"; 4714 case ARPHIC_1999: return "Arphic Public License"; 4715 case ARTISTIC_1_0: return "Artistic License 1.0"; 4716 case ARTISTIC_1_0_CL8: return "Artistic License 1.0 w/clause 8"; 4717 case ARTISTIC_1_0_PERL: return "Artistic License 1.0 (Perl)"; 4718 case ARTISTIC_2_0: return "Artistic License 2.0"; 4719 case ASWF_DIGITAL_ASSETS_1_0: return "ASWF Digital Assets License version 1.0"; 4720 case ASWF_DIGITAL_ASSETS_1_1: return "ASWF Digital Assets License 1.1"; 4721 case BAEKMUK: return "Baekmuk License"; 4722 case BAHYPH: return "Bahyph License"; 4723 case BARR: return "Barr License"; 4724 case BEERWARE: return "Beerware License"; 4725 case BITSTREAM_CHARTER: return "Bitstream Charter Font License"; 4726 case BITSTREAM_VERA: return "Bitstream Vera Font License"; 4727 case BITTORRENT_1_0: return "BitTorrent Open Source License v1.0"; 4728 case BITTORRENT_1_1: return "BitTorrent Open Source License v1.1"; 4729 case BLESSING: return "SQLite Blessing"; 4730 case BLUEOAK_1_0_0: return "Blue Oak Model License 1.0.0"; 4731 case BOEHM_GC: return "Boehm-Demers-Weiser GC License"; 4732 case BORCEUX: return "Borceux license"; 4733 case BRIAN_GLADMAN_3_CLAUSE: return "Brian Gladman 3-Clause License"; 4734 case BSD_1_CLAUSE: return "BSD 1-Clause License"; 4735 case BSD_2_CLAUSE: return "BSD 2-Clause \"Simplified\" License"; 4736 case BSD_2_CLAUSE_FREEBSD: return "BSD 2-Clause FreeBSD License"; 4737 case BSD_2_CLAUSE_NETBSD: return "BSD 2-Clause NetBSD License"; 4738 case BSD_2_CLAUSE_PATENT: return "BSD-2-Clause Plus Patent License"; 4739 case BSD_2_CLAUSE_VIEWS: return "BSD 2-Clause with views sentence"; 4740 case BSD_3_CLAUSE: return "BSD 3-Clause \"New\" or \"Revised\" License"; 4741 case BSD_3_CLAUSE_ATTRIBUTION: return "BSD with attribution"; 4742 case BSD_3_CLAUSE_CLEAR: return "BSD 3-Clause Clear License"; 4743 case BSD_3_CLAUSE_LBNL: return "Lawrence Berkeley National Labs BSD variant license"; 4744 case BSD_3_CLAUSE_MODIFICATION: return "BSD 3-Clause Modification"; 4745 case BSD_3_CLAUSE_NO_MILITARY_LICENSE: return "BSD 3-Clause No Military License"; 4746 case BSD_3_CLAUSE_NO_NUCLEAR_LICENSE: return "BSD 3-Clause No Nuclear License"; 4747 case BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014: return "BSD 3-Clause No Nuclear License 2014"; 4748 case BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY: return "BSD 3-Clause No Nuclear Warranty"; 4749 case BSD_3_CLAUSE_OPEN_MPI: return "BSD 3-Clause Open MPI variant"; 4750 case BSD_4_CLAUSE: return "BSD 4-Clause \"Original\" or \"Old\" License"; 4751 case BSD_4_CLAUSE_SHORTENED: return "BSD 4 Clause Shortened"; 4752 case BSD_4_CLAUSE_UC: return "BSD-4-Clause (University of California-Specific)"; 4753 case BSD_4_3RENO: return "BSD 4.3 RENO License"; 4754 case BSD_4_3TAHOE: return "BSD 4.3 TAHOE License"; 4755 case BSD_ADVERTISING_ACKNOWLEDGEMENT: return "BSD Advertising Acknowledgement License"; 4756 case BSD_ATTRIBUTION_HPND_DISCLAIMER: return "BSD with Attribution and HPND disclaimer"; 4757 case BSD_PROTECTION: return "BSD Protection License"; 4758 case BSD_SOURCE_CODE: return "BSD Source Code Attribution"; 4759 case BSL_1_0: return "Boost Software License 1.0"; 4760 case BUSL_1_1: return "Business Source License 1.1"; 4761 case BZIP2_1_0_5: return "bzip2 and libbzip2 License v1.0.5"; 4762 case BZIP2_1_0_6: return "bzip2 and libbzip2 License v1.0.6"; 4763 case C_UDA_1_0: return "Computational Use of Data Agreement v1.0"; 4764 case CAL_1_0: return "Cryptographic Autonomy License 1.0"; 4765 case CAL_1_0_COMBINED_WORK_EXCEPTION: return "Cryptographic Autonomy License 1.0 (Combined Work Exception)"; 4766 case CALDERA: return "Caldera License"; 4767 case CATOSL_1_1: return "Computer Associates Trusted Open Source License 1.1"; 4768 case CC_BY_1_0: return "Creative Commons Attribution 1.0 Generic"; 4769 case CC_BY_2_0: return "Creative Commons Attribution 2.0 Generic"; 4770 case CC_BY_2_5: return "Creative Commons Attribution 2.5 Generic"; 4771 case CC_BY_2_5_AU: return "Creative Commons Attribution 2.5 Australia"; 4772 case CC_BY_3_0: return "Creative Commons Attribution 3.0 Unported"; 4773 case CC_BY_3_0_AT: return "Creative Commons Attribution 3.0 Austria"; 4774 case CC_BY_3_0_DE: return "Creative Commons Attribution 3.0 Germany"; 4775 case CC_BY_3_0_IGO: return "Creative Commons Attribution 3.0 IGO"; 4776 case CC_BY_3_0_NL: return "Creative Commons Attribution 3.0 Netherlands"; 4777 case CC_BY_3_0_US: return "Creative Commons Attribution 3.0 United States"; 4778 case CC_BY_4_0: return "Creative Commons Attribution 4.0 International"; 4779 case CC_BY_NC_1_0: return "Creative Commons Attribution Non Commercial 1.0 Generic"; 4780 case CC_BY_NC_2_0: return "Creative Commons Attribution Non Commercial 2.0 Generic"; 4781 case CC_BY_NC_2_5: return "Creative Commons Attribution Non Commercial 2.5 Generic"; 4782 case CC_BY_NC_3_0: return "Creative Commons Attribution Non Commercial 3.0 Unported"; 4783 case CC_BY_NC_3_0_DE: return "Creative Commons Attribution Non Commercial 3.0 Germany"; 4784 case CC_BY_NC_4_0: return "Creative Commons Attribution Non Commercial 4.0 International"; 4785 case CC_BY_NC_ND_1_0: return "Creative Commons Attribution Non Commercial No Derivatives 1.0 Generic"; 4786 case CC_BY_NC_ND_2_0: return "Creative Commons Attribution Non Commercial No Derivatives 2.0 Generic"; 4787 case CC_BY_NC_ND_2_5: return "Creative Commons Attribution Non Commercial No Derivatives 2.5 Generic"; 4788 case CC_BY_NC_ND_3_0: return "Creative Commons Attribution Non Commercial No Derivatives 3.0 Unported"; 4789 case CC_BY_NC_ND_3_0_DE: return "Creative Commons Attribution Non Commercial No Derivatives 3.0 Germany"; 4790 case CC_BY_NC_ND_3_0_IGO: return "Creative Commons Attribution Non Commercial No Derivatives 3.0 IGO"; 4791 case CC_BY_NC_ND_4_0: return "Creative Commons Attribution Non Commercial No Derivatives 4.0 International"; 4792 case CC_BY_NC_SA_1_0: return "Creative Commons Attribution Non Commercial Share Alike 1.0 Generic"; 4793 case CC_BY_NC_SA_2_0: return "Creative Commons Attribution Non Commercial Share Alike 2.0 Generic"; 4794 case CC_BY_NC_SA_2_0_DE: return "Creative Commons Attribution Non Commercial Share Alike 2.0 Germany"; 4795 case CC_BY_NC_SA_2_0_FR: return "Creative Commons Attribution-NonCommercial-ShareAlike 2.0 France"; 4796 case CC_BY_NC_SA_2_0_UK: return "Creative Commons Attribution Non Commercial Share Alike 2.0 England and Wales"; 4797 case CC_BY_NC_SA_2_5: return "Creative Commons Attribution Non Commercial Share Alike 2.5 Generic"; 4798 case CC_BY_NC_SA_3_0: return "Creative Commons Attribution Non Commercial Share Alike 3.0 Unported"; 4799 case CC_BY_NC_SA_3_0_DE: return "Creative Commons Attribution Non Commercial Share Alike 3.0 Germany"; 4800 case CC_BY_NC_SA_3_0_IGO: return "Creative Commons Attribution Non Commercial Share Alike 3.0 IGO"; 4801 case CC_BY_NC_SA_4_0: return "Creative Commons Attribution Non Commercial Share Alike 4.0 International"; 4802 case CC_BY_ND_1_0: return "Creative Commons Attribution No Derivatives 1.0 Generic"; 4803 case CC_BY_ND_2_0: return "Creative Commons Attribution No Derivatives 2.0 Generic"; 4804 case CC_BY_ND_2_5: return "Creative Commons Attribution No Derivatives 2.5 Generic"; 4805 case CC_BY_ND_3_0: return "Creative Commons Attribution No Derivatives 3.0 Unported"; 4806 case CC_BY_ND_3_0_DE: return "Creative Commons Attribution No Derivatives 3.0 Germany"; 4807 case CC_BY_ND_4_0: return "Creative Commons Attribution No Derivatives 4.0 International"; 4808 case CC_BY_SA_1_0: return "Creative Commons Attribution Share Alike 1.0 Generic"; 4809 case CC_BY_SA_2_0: return "Creative Commons Attribution Share Alike 2.0 Generic"; 4810 case CC_BY_SA_2_0_UK: return "Creative Commons Attribution Share Alike 2.0 England and Wales"; 4811 case CC_BY_SA_2_1_JP: return "Creative Commons Attribution Share Alike 2.1 Japan"; 4812 case CC_BY_SA_2_5: return "Creative Commons Attribution Share Alike 2.5 Generic"; 4813 case CC_BY_SA_3_0: return "Creative Commons Attribution Share Alike 3.0 Unported"; 4814 case CC_BY_SA_3_0_AT: return "Creative Commons Attribution Share Alike 3.0 Austria"; 4815 case CC_BY_SA_3_0_DE: return "Creative Commons Attribution Share Alike 3.0 Germany"; 4816 case CC_BY_SA_3_0_IGO: return "Creative Commons Attribution-ShareAlike 3.0 IGO"; 4817 case CC_BY_SA_4_0: return "Creative Commons Attribution Share Alike 4.0 International"; 4818 case CC_PDDC: return "Creative Commons Public Domain Dedication and Certification"; 4819 case CC0_1_0: return "Creative Commons Zero v1.0 Universal"; 4820 case CDDL_1_0: return "Common Development and Distribution License 1.0"; 4821 case CDDL_1_1: return "Common Development and Distribution License 1.1"; 4822 case CDL_1_0: return "Common Documentation License 1.0"; 4823 case CDLA_PERMISSIVE_1_0: return "Community Data License Agreement Permissive 1.0"; 4824 case CDLA_PERMISSIVE_2_0: return "Community Data License Agreement Permissive 2.0"; 4825 case CDLA_SHARING_1_0: return "Community Data License Agreement Sharing 1.0"; 4826 case CECILL_1_0: return "CeCILL Free Software License Agreement v1.0"; 4827 case CECILL_1_1: return "CeCILL Free Software License Agreement v1.1"; 4828 case CECILL_2_0: return "CeCILL Free Software License Agreement v2.0"; 4829 case CECILL_2_1: return "CeCILL Free Software License Agreement v2.1"; 4830 case CECILL_B: return "CeCILL-B Free Software License Agreement"; 4831 case CECILL_C: return "CeCILL-C Free Software License Agreement"; 4832 case CERN_OHL_1_1: return "CERN Open Hardware Licence v1.1"; 4833 case CERN_OHL_1_2: return "CERN Open Hardware Licence v1.2"; 4834 case CERN_OHL_P_2_0: return "CERN Open Hardware Licence Version 2 - Permissive"; 4835 case CERN_OHL_S_2_0: return "CERN Open Hardware Licence Version 2 - Strongly Reciprocal"; 4836 case CERN_OHL_W_2_0: return "CERN Open Hardware Licence Version 2 - Weakly Reciprocal"; 4837 case CFITSIO: return "CFITSIO License"; 4838 case CHECKMK: return "Checkmk License"; 4839 case CLARTISTIC: return "Clarified Artistic License"; 4840 case CLIPS: return "Clips License"; 4841 case CMU_MACH: return "CMU Mach License"; 4842 case CNRI_JYTHON: return "CNRI Jython License"; 4843 case CNRI_PYTHON: return "CNRI Python License"; 4844 case CNRI_PYTHON_GPL_COMPATIBLE: return "CNRI Python Open Source GPL Compatible License Agreement"; 4845 case COIL_1_0: return "Copyfree Open Innovation License"; 4846 case COMMUNITY_SPEC_1_0: return "Community Specification License 1.0"; 4847 case CONDOR_1_1: return "Condor Public License v1.1"; 4848 case COPYLEFT_NEXT_0_3_0: return "copyleft-next 0.3.0"; 4849 case COPYLEFT_NEXT_0_3_1: return "copyleft-next 0.3.1"; 4850 case CORNELL_LOSSLESS_JPEG: return "Cornell Lossless JPEG License"; 4851 case CPAL_1_0: return "Common Public Attribution License 1.0"; 4852 case CPL_1_0: return "Common Public License 1.0"; 4853 case CPOL_1_02: return "Code Project Open License 1.02"; 4854 case CROSSWORD: return "Crossword License"; 4855 case CRYSTALSTACKER: return "CrystalStacker License"; 4856 case CUA_OPL_1_0: return "CUA Office Public License v1.0"; 4857 case CUBE: return "Cube License"; 4858 case CURL: return "curl License"; 4859 case D_FSL_1_0: return "Deutsche Freie Software Lizenz"; 4860 case DIFFMARK: return "diffmark license"; 4861 case DL_DE_BY_2_0: return "Data licence Germany ? attribution ? version 2.0"; 4862 case DOC: return "DOC License"; 4863 case DOTSEQN: return "Dotseqn License"; 4864 case DRL_1_0: return "Detection Rule License 1.0"; 4865 case DSDP: return "DSDP License"; 4866 case DTOA: return "David M. Gay dtoa License"; 4867 case DVIPDFM: return "dvipdfm License"; 4868 case ECL_1_0: return "Educational Community License v1.0"; 4869 case ECL_2_0: return "Educational Community License v2.0"; 4870 case ECOS_2_0: return "eCos license version 2.0"; 4871 case EFL_1_0: return "Eiffel Forum License v1.0"; 4872 case EFL_2_0: return "Eiffel Forum License v2.0"; 4873 case EGENIX: return "eGenix.com Public License 1.1.0"; 4874 case ELASTIC_2_0: return "Elastic License 2.0"; 4875 case ENTESSA: return "Entessa Public License v1.0"; 4876 case EPICS: return "EPICS Open License"; 4877 case EPL_1_0: return "Eclipse Public License 1.0"; 4878 case EPL_2_0: return "Eclipse Public License 2.0"; 4879 case ERLPL_1_1: return "Erlang Public License v1.1"; 4880 case ETALAB_2_0: return "Etalab Open License 2.0"; 4881 case EUDATAGRID: return "EU DataGrid Software License"; 4882 case EUPL_1_0: return "European Union Public License 1.0"; 4883 case EUPL_1_1: return "European Union Public License 1.1"; 4884 case EUPL_1_2: return "European Union Public License 1.2"; 4885 case EUROSYM: return "Eurosym License"; 4886 case FAIR: return "Fair License"; 4887 case FDK_AAC: return "Fraunhofer FDK AAC Codec Library"; 4888 case FRAMEWORX_1_0: return "Frameworx Open License 1.0"; 4889 case FREEBSD_DOC: return "FreeBSD Documentation License"; 4890 case FREEIMAGE: return "FreeImage Public License v1.0"; 4891 case FSFAP: return "FSF All Permissive License"; 4892 case FSFUL: return "FSF Unlimited License"; 4893 case FSFULLR: return "FSF Unlimited License (with License Retention)"; 4894 case FSFULLRWD: return "FSF Unlimited License (With License Retention and Warranty Disclaimer)"; 4895 case FTL: return "Freetype Project License"; 4896 case GD: return "GD License"; 4897 case GFDL_1_1: return "GNU Free Documentation License v1.1"; 4898 case GFDL_1_1_INVARIANTS_ONLY: return "GNU Free Documentation License v1.1 only - invariants"; 4899 case GFDL_1_1_INVARIANTS_OR_LATER: return "GNU Free Documentation License v1.1 or later - invariants"; 4900 case GFDL_1_1_NO_INVARIANTS_ONLY: return "GNU Free Documentation License v1.1 only - no invariants"; 4901 case GFDL_1_1_NO_INVARIANTS_OR_LATER: return "GNU Free Documentation License v1.1 or later - no invariants"; 4902 case GFDL_1_1_ONLY: return "GNU Free Documentation License v1.1 only"; 4903 case GFDL_1_1_OR_LATER: return "GNU Free Documentation License v1.1 or later"; 4904 case GFDL_1_2: return "GNU Free Documentation License v1.2"; 4905 case GFDL_1_2_INVARIANTS_ONLY: return "GNU Free Documentation License v1.2 only - invariants"; 4906 case GFDL_1_2_INVARIANTS_OR_LATER: return "GNU Free Documentation License v1.2 or later - invariants"; 4907 case GFDL_1_2_NO_INVARIANTS_ONLY: return "GNU Free Documentation License v1.2 only - no invariants"; 4908 case GFDL_1_2_NO_INVARIANTS_OR_LATER: return "GNU Free Documentation License v1.2 or later - no invariants"; 4909 case GFDL_1_2_ONLY: return "GNU Free Documentation License v1.2 only"; 4910 case GFDL_1_2_OR_LATER: return "GNU Free Documentation License v1.2 or later"; 4911 case GFDL_1_3: return "GNU Free Documentation License v1.3"; 4912 case GFDL_1_3_INVARIANTS_ONLY: return "GNU Free Documentation License v1.3 only - invariants"; 4913 case GFDL_1_3_INVARIANTS_OR_LATER: return "GNU Free Documentation License v1.3 or later - invariants"; 4914 case GFDL_1_3_NO_INVARIANTS_ONLY: return "GNU Free Documentation License v1.3 only - no invariants"; 4915 case GFDL_1_3_NO_INVARIANTS_OR_LATER: return "GNU Free Documentation License v1.3 or later - no invariants"; 4916 case GFDL_1_3_ONLY: return "GNU Free Documentation License v1.3 only"; 4917 case GFDL_1_3_OR_LATER: return "GNU Free Documentation License v1.3 or later"; 4918 case GIFTWARE: return "Giftware License"; 4919 case GL2PS: return "GL2PS License"; 4920 case GLIDE: return "3dfx Glide License"; 4921 case GLULXE: return "Glulxe License"; 4922 case GLWTPL: return "Good Luck With That Public License"; 4923 case GNUPLOT: return "gnuplot License"; 4924 case GPL_1_0: return "GNU General Public License v1.0 only"; 4925 case GPL_1_0PLUS: return "GNU General Public License v1.0 or later"; 4926 case GPL_1_0_ONLY: return "GNU General Public License v1.0 only"; 4927 case GPL_1_0_OR_LATER: return "GNU General Public License v1.0 or later"; 4928 case GPL_2_0: return "GNU General Public License v2.0 only"; 4929 case GPL_2_0PLUS: return "GNU General Public License v2.0 or later"; 4930 case GPL_2_0_ONLY: return "GNU General Public License v2.0 only"; 4931 case GPL_2_0_OR_LATER: return "GNU General Public License v2.0 or later"; 4932 case GPL_2_0_WITH_AUTOCONF_EXCEPTION: return "GNU General Public License v2.0 w/Autoconf exception"; 4933 case GPL_2_0_WITH_BISON_EXCEPTION: return "GNU General Public License v2.0 w/Bison exception"; 4934 case GPL_2_0_WITH_CLASSPATH_EXCEPTION: return "GNU General Public License v2.0 w/Classpath exception"; 4935 case GPL_2_0_WITH_FONT_EXCEPTION: return "GNU General Public License v2.0 w/Font exception"; 4936 case GPL_2_0_WITH_GCC_EXCEPTION: return "GNU General Public License v2.0 w/GCC Runtime Library exception"; 4937 case GPL_3_0: return "GNU General Public License v3.0 only"; 4938 case GPL_3_0PLUS: return "GNU General Public License v3.0 or later"; 4939 case GPL_3_0_ONLY: return "GNU General Public License v3.0 only"; 4940 case GPL_3_0_OR_LATER: return "GNU General Public License v3.0 or later"; 4941 case GPL_3_0_WITH_AUTOCONF_EXCEPTION: return "GNU General Public License v3.0 w/Autoconf exception"; 4942 case GPL_3_0_WITH_GCC_EXCEPTION: return "GNU General Public License v3.0 w/GCC Runtime Library exception"; 4943 case GRAPHICS_GEMS: return "Graphics Gems License"; 4944 case GSOAP_1_3B: return "gSOAP Public License v1.3b"; 4945 case HASKELLREPORT: return "Haskell Language Report License"; 4946 case HIPPOCRATIC_2_1: return "Hippocratic License 2.1"; 4947 case HP_1986: return "Hewlett-Packard 1986 License"; 4948 case HPND: return "Historical Permission Notice and Disclaimer"; 4949 case HPND_EXPORT_US: return "HPND with US Government export control warning"; 4950 case HPND_MARKUS_KUHN: return "Historical Permission Notice and Disclaimer - Markus Kuhn variant"; 4951 case HPND_SELL_VARIANT: return "Historical Permission Notice and Disclaimer - sell variant"; 4952 case HPND_SELL_VARIANT_MIT_DISCLAIMER: return "HPND sell variant with MIT disclaimer"; 4953 case HTMLTIDY: return "HTML Tidy License"; 4954 case IBM_PIBS: return "IBM PowerPC Initialization and Boot Software"; 4955 case ICU: return "ICU License"; 4956 case IEC_CODE_COMPONENTS_EULA: return "IEC Code Components End-user licence agreement"; 4957 case IJG: return "Independent JPEG Group License"; 4958 case IJG_SHORT: return "Independent JPEG Group License - short"; 4959 case IMAGEMAGICK: return "ImageMagick License"; 4960 case IMATIX: return "iMatix Standard Function Library Agreement"; 4961 case IMLIB2: return "Imlib2 License"; 4962 case INFO_ZIP: return "Info-ZIP License"; 4963 case INNER_NET_2_0: return "Inner Net License v2.0"; 4964 case INTEL: return "Intel Open Source License"; 4965 case INTEL_ACPI: return "Intel ACPI Software License Agreement"; 4966 case INTERBASE_1_0: return "Interbase Public License v1.0"; 4967 case IPA: return "IPA Font License"; 4968 case IPL_1_0: return "IBM Public License v1.0"; 4969 case ISC: return "ISC License"; 4970 case JAM: return "Jam License"; 4971 case JASPER_2_0: return "JasPer License"; 4972 case JPL_IMAGE: return "JPL Image Use Policy"; 4973 case JPNIC: return "Japan Network Information Center License"; 4974 case JSON: return "JSON License"; 4975 case KAZLIB: return "Kazlib License"; 4976 case KNUTH_CTAN: return "Knuth CTAN License"; 4977 case LAL_1_2: return "Licence Art Libre 1.2"; 4978 case LAL_1_3: return "Licence Art Libre 1.3"; 4979 case LATEX2E: return "Latex2e License"; 4980 case LATEX2E_TRANSLATED_NOTICE: return "Latex2e with translated notice permission"; 4981 case LEPTONICA: return "Leptonica License"; 4982 case LGPL_2_0: return "GNU Library General Public License v2 only"; 4983 case LGPL_2_0PLUS: return "GNU Library General Public License v2 or later"; 4984 case LGPL_2_0_ONLY: return "GNU Library General Public License v2 only"; 4985 case LGPL_2_0_OR_LATER: return "GNU Library General Public License v2 or later"; 4986 case LGPL_2_1: return "GNU Lesser General Public License v2.1 only"; 4987 case LGPL_2_1PLUS: return "GNU Lesser General Public License v2.1 or later"; 4988 case LGPL_2_1_ONLY: return "GNU Lesser General Public License v2.1 only"; 4989 case LGPL_2_1_OR_LATER: return "GNU Lesser General Public License v2.1 or later"; 4990 case LGPL_3_0: return "GNU Lesser General Public License v3.0 only"; 4991 case LGPL_3_0PLUS: return "GNU Lesser General Public License v3.0 or later"; 4992 case LGPL_3_0_ONLY: return "GNU Lesser General Public License v3.0 only"; 4993 case LGPL_3_0_OR_LATER: return "GNU Lesser General Public License v3.0 or later"; 4994 case LGPLLR: return "Lesser General Public License For Linguistic Resources"; 4995 case LIBPNG: return "libpng License"; 4996 case LIBPNG_2_0: return "PNG Reference Library version 2"; 4997 case LIBSELINUX_1_0: return "libselinux public domain notice"; 4998 case LIBTIFF: return "libtiff License"; 4999 case LIBUTIL_DAVID_NUGENT: return "libutil David Nugent License"; 5000 case LILIQ_P_1_1: return "Licence Libre du Québec ? Permissive version 1.1"; 5001 case LILIQ_R_1_1: return "Licence Libre du Québec ? Réciprocité version 1.1"; 5002 case LILIQ_RPLUS_1_1: return "Licence Libre du Québec ? Réciprocité forte version 1.1"; 5003 case LINUX_MAN_PAGES_1_PARA: return "Linux man-pages - 1 paragraph"; 5004 case LINUX_MAN_PAGES_COPYLEFT: return "Linux man-pages Copyleft"; 5005 case LINUX_MAN_PAGES_COPYLEFT_2_PARA: return "Linux man-pages Copyleft - 2 paragraphs"; 5006 case LINUX_MAN_PAGES_COPYLEFT_VAR: return "Linux man-pages Copyleft Variant"; 5007 case LINUX_OPENIB: return "Linux Kernel Variant of OpenIB.org license"; 5008 case LOOP: return "Common Lisp LOOP License"; 5009 case LPL_1_0: return "Lucent Public License Version 1.0"; 5010 case LPL_1_02: return "Lucent Public License v1.02"; 5011 case LPPL_1_0: return "LaTeX Project Public License v1.0"; 5012 case LPPL_1_1: return "LaTeX Project Public License v1.1"; 5013 case LPPL_1_2: return "LaTeX Project Public License v1.2"; 5014 case LPPL_1_3A: return "LaTeX Project Public License v1.3a"; 5015 case LPPL_1_3C: return "LaTeX Project Public License v1.3c"; 5016 case LZMA_SDK_9_11_TO_9_20: return "LZMA SDK License (versions 9.11 to 9.20)"; 5017 case LZMA_SDK_9_22: return "LZMA SDK License (versions 9.22 and beyond)"; 5018 case MAKEINDEX: return "MakeIndex License"; 5019 case MARTIN_BIRGMEIER: return "Martin Birgmeier License"; 5020 case METAMAIL: return "metamail License"; 5021 case MINPACK: return "Minpack License"; 5022 case MIROS: return "The MirOS Licence"; 5023 case MIT: return "MIT License"; 5024 case MIT_0: return "MIT No Attribution"; 5025 case MIT_ADVERTISING: return "Enlightenment License (e16)"; 5026 case MIT_CMU: return "CMU License"; 5027 case MIT_ENNA: return "enna License"; 5028 case MIT_FEH: return "feh License"; 5029 case MIT_FESTIVAL: return "MIT Festival Variant"; 5030 case MIT_MODERN_VARIANT: return "MIT License Modern Variant"; 5031 case MIT_OPEN_GROUP: return "MIT Open Group variant"; 5032 case MIT_WU: return "MIT Tom Wu Variant"; 5033 case MITNFA: return "MIT +no-false-attribs license"; 5034 case MOTOSOTO: return "Motosoto License"; 5035 case MPI_PERMISSIVE: return "mpi Permissive License"; 5036 case MPICH2: return "mpich2 License"; 5037 case MPL_1_0: return "Mozilla Public License 1.0"; 5038 case MPL_1_1: return "Mozilla Public License 1.1"; 5039 case MPL_2_0: return "Mozilla Public License 2.0"; 5040 case MPL_2_0_NO_COPYLEFT_EXCEPTION: return "Mozilla Public License 2.0 (no copyleft exception)"; 5041 case MPLUS: return "mplus Font License"; 5042 case MS_LPL: return "Microsoft Limited Public License"; 5043 case MS_PL: return "Microsoft Public License"; 5044 case MS_RL: return "Microsoft Reciprocal License"; 5045 case MTLL: return "Matrix Template Library License"; 5046 case MULANPSL_1_0: return "Mulan Permissive Software License, Version 1"; 5047 case MULANPSL_2_0: return "Mulan Permissive Software License, Version 2"; 5048 case MULTICS: return "Multics License"; 5049 case MUP: return "Mup License"; 5050 case NAIST_2003: return "Nara Institute of Science and Technology License (2003)"; 5051 case NASA_1_3: return "NASA Open Source Agreement 1.3"; 5052 case NAUMEN: return "Naumen Public License"; 5053 case NBPL_1_0: return "Net Boolean Public License v1"; 5054 case NCGL_UK_2_0: return "Non-Commercial Government Licence"; 5055 case NCSA: return "University of Illinois/NCSA Open Source License"; 5056 case NET_SNMP: return "Net-SNMP License"; 5057 case NETCDF: return "NetCDF license"; 5058 case NEWSLETR: return "Newsletr License"; 5059 case NGPL: return "Nethack General Public License"; 5060 case NICTA_1_0: return "NICTA Public Software License, Version 1.0"; 5061 case NIST_PD: return "NIST Public Domain Notice"; 5062 case NIST_PD_FALLBACK: return "NIST Public Domain Notice with license fallback"; 5063 case NIST_SOFTWARE: return "NIST Software License"; 5064 case NLOD_1_0: return "Norwegian Licence for Open Government Data (NLOD) 1.0"; 5065 case NLOD_2_0: return "Norwegian Licence for Open Government Data (NLOD) 2.0"; 5066 case NLPL: return "No Limit Public License"; 5067 case NOKIA: return "Nokia Open Source License"; 5068 case NOSL: return "Netizen Open Source License"; 5069 case NOT_OPEN_SOURCE: return "Not an open source license."; 5070 case NOWEB: return "Noweb License"; 5071 case NPL_1_0: return "Netscape Public License v1.0"; 5072 case NPL_1_1: return "Netscape Public License v1.1"; 5073 case NPOSL_3_0: return "Non-Profit Open Software License 3.0"; 5074 case NRL: return "NRL License"; 5075 case NTP: return "NTP License"; 5076 case NTP_0: return "NTP No Attribution"; 5077 case NUNIT: return "Nunit License"; 5078 case O_UDA_1_0: return "Open Use of Data Agreement v1.0"; 5079 case OCCT_PL: return "Open CASCADE Technology Public License"; 5080 case OCLC_2_0: return "OCLC Research Public License 2.0"; 5081 case ODBL_1_0: return "Open Data Commons Open Database License v1.0"; 5082 case ODC_BY_1_0: return "Open Data Commons Attribution License v1.0"; 5083 case OFFIS: return "OFFIS License"; 5084 case OFL_1_0: return "SIL Open Font License 1.0"; 5085 case OFL_1_0_NO_RFN: return "SIL Open Font License 1.0 with no Reserved Font Name"; 5086 case OFL_1_0_RFN: return "SIL Open Font License 1.0 with Reserved Font Name"; 5087 case OFL_1_1: return "SIL Open Font License 1.1"; 5088 case OFL_1_1_NO_RFN: return "SIL Open Font License 1.1 with no Reserved Font Name"; 5089 case OFL_1_1_RFN: return "SIL Open Font License 1.1 with Reserved Font Name"; 5090 case OGC_1_0: return "OGC Software License, Version 1.0"; 5091 case OGDL_TAIWAN_1_0: return "Taiwan Open Government Data License, version 1.0"; 5092 case OGL_CANADA_2_0: return "Open Government Licence - Canada"; 5093 case OGL_UK_1_0: return "Open Government Licence v1.0"; 5094 case OGL_UK_2_0: return "Open Government Licence v2.0"; 5095 case OGL_UK_3_0: return "Open Government Licence v3.0"; 5096 case OGTSL: return "Open Group Test Suite License"; 5097 case OLDAP_1_1: return "Open LDAP Public License v1.1"; 5098 case OLDAP_1_2: return "Open LDAP Public License v1.2"; 5099 case OLDAP_1_3: return "Open LDAP Public License v1.3"; 5100 case OLDAP_1_4: return "Open LDAP Public License v1.4"; 5101 case OLDAP_2_0: return "Open LDAP Public License v2.0 (or possibly 2.0A and 2.0B)"; 5102 case OLDAP_2_0_1: return "Open LDAP Public License v2.0.1"; 5103 case OLDAP_2_1: return "Open LDAP Public License v2.1"; 5104 case OLDAP_2_2: return "Open LDAP Public License v2.2"; 5105 case OLDAP_2_2_1: return "Open LDAP Public License v2.2.1"; 5106 case OLDAP_2_2_2: return "Open LDAP Public License 2.2.2"; 5107 case OLDAP_2_3: return "Open LDAP Public License v2.3"; 5108 case OLDAP_2_4: return "Open LDAP Public License v2.4"; 5109 case OLDAP_2_5: return "Open LDAP Public License v2.5"; 5110 case OLDAP_2_6: return "Open LDAP Public License v2.6"; 5111 case OLDAP_2_7: return "Open LDAP Public License v2.7"; 5112 case OLDAP_2_8: return "Open LDAP Public License v2.8"; 5113 case OLFL_1_3: return "Open Logistics Foundation License Version 1.3"; 5114 case OML: return "Open Market License"; 5115 case OPENPBS_2_3: return "OpenPBS v2.3 Software License"; 5116 case OPENSSL: return "OpenSSL License"; 5117 case OPL_1_0: return "Open Public License v1.0"; 5118 case OPL_UK_3_0: return "United Kingdom Open Parliament Licence v3.0"; 5119 case OPUBL_1_0: return "Open Publication License v1.0"; 5120 case OSET_PL_2_1: return "OSET Public License version 2.1"; 5121 case OSL_1_0: return "Open Software License 1.0"; 5122 case OSL_1_1: return "Open Software License 1.1"; 5123 case OSL_2_0: return "Open Software License 2.0"; 5124 case OSL_2_1: return "Open Software License 2.1"; 5125 case OSL_3_0: return "Open Software License 3.0"; 5126 case PARITY_6_0_0: return "The Parity Public License 6.0.0"; 5127 case PARITY_7_0_0: return "The Parity Public License 7.0.0"; 5128 case PDDL_1_0: return "Open Data Commons Public Domain Dedication & License 1.0"; 5129 case PHP_3_0: return "PHP License v3.0"; 5130 case PHP_3_01: return "PHP License v3.01"; 5131 case PLEXUS: return "Plexus Classworlds License"; 5132 case POLYFORM_NONCOMMERCIAL_1_0_0: return "PolyForm Noncommercial License 1.0.0"; 5133 case POLYFORM_SMALL_BUSINESS_1_0_0: return "PolyForm Small Business License 1.0.0"; 5134 case POSTGRESQL: return "PostgreSQL License"; 5135 case PSF_2_0: return "Python Software Foundation License 2.0"; 5136 case PSFRAG: return "psfrag License"; 5137 case PSUTILS: return "psutils License"; 5138 case PYTHON_2_0: return "Python License 2.0"; 5139 case PYTHON_2_0_1: return "Python License 2.0.1"; 5140 case QHULL: return "Qhull License"; 5141 case QPL_1_0: return "Q Public License 1.0"; 5142 case QPL_1_0_INRIA_2004: return "Q Public License 1.0 - INRIA 2004 variant"; 5143 case RDISC: return "Rdisc License"; 5144 case RHECOS_1_1: return "Red Hat eCos Public License v1.1"; 5145 case RPL_1_1: return "Reciprocal Public License 1.1"; 5146 case RPL_1_5: return "Reciprocal Public License 1.5"; 5147 case RPSL_1_0: return "RealNetworks Public Source License v1.0"; 5148 case RSA_MD: return "RSA Message-Digest License"; 5149 case RSCPL: return "Ricoh Source Code Public License"; 5150 case RUBY: return "Ruby License"; 5151 case SAX_PD: return "Sax Public Domain Notice"; 5152 case SAXPATH: return "Saxpath License"; 5153 case SCEA: return "SCEA Shared Source License"; 5154 case SCHEMEREPORT: return "Scheme Language Report License"; 5155 case SENDMAIL: return "Sendmail License"; 5156 case SENDMAIL_8_23: return "Sendmail License 8.23"; 5157 case SGI_B_1_0: return "SGI Free Software License B v1.0"; 5158 case SGI_B_1_1: return "SGI Free Software License B v1.1"; 5159 case SGI_B_2_0: return "SGI Free Software License B v2.0"; 5160 case SGP4: return "SGP4 Permission Notice"; 5161 case SHL_0_5: return "Solderpad Hardware License v0.5"; 5162 case SHL_0_51: return "Solderpad Hardware License, Version 0.51"; 5163 case SIMPL_2_0: return "Simple Public License 2.0"; 5164 case SISSL: return "Sun Industry Standards Source License v1.1"; 5165 case SISSL_1_2: return "Sun Industry Standards Source License v1.2"; 5166 case SLEEPYCAT: return "Sleepycat License"; 5167 case SMLNJ: return "Standard ML of New Jersey License"; 5168 case SMPPL: return "Secure Messaging Protocol Public License"; 5169 case SNIA: return "SNIA Public License 1.1"; 5170 case SNPRINTF: return "snprintf License"; 5171 case SPENCER_86: return "Spencer License 86"; 5172 case SPENCER_94: return "Spencer License 94"; 5173 case SPENCER_99: return "Spencer License 99"; 5174 case SPL_1_0: return "Sun Public License v1.0"; 5175 case SSH_OPENSSH: return "SSH OpenSSH license"; 5176 case SSH_SHORT: return "SSH short notice"; 5177 case SSPL_1_0: return "Server Side Public License, v 1"; 5178 case STANDARDML_NJ: return "Standard ML of New Jersey License"; 5179 case SUGARCRM_1_1_3: return "SugarCRM Public License v1.1.3"; 5180 case SUNPRO: return "SunPro License"; 5181 case SWL: return "Scheme Widget Library (SWL) Software License Agreement"; 5182 case SYMLINKS: return "Symlinks License"; 5183 case TAPR_OHL_1_0: return "TAPR Open Hardware License v1.0"; 5184 case TCL: return "TCL/TK License"; 5185 case TCP_WRAPPERS: return "TCP Wrappers License"; 5186 case TERMREADKEY: return "TermReadKey License"; 5187 case TMATE: return "TMate Open Source License"; 5188 case TORQUE_1_1: return "TORQUE v2.5+ Software License v1.1"; 5189 case TOSL: return "Trusster Open Source License"; 5190 case TPDL: return "Time::ParseDate License"; 5191 case TPL_1_0: return "THOR Public License 1.0"; 5192 case TTWL: return "Text-Tabs+Wrap License"; 5193 case TU_BERLIN_1_0: return "Technische Universitaet Berlin License 1.0"; 5194 case TU_BERLIN_2_0: return "Technische Universitaet Berlin License 2.0"; 5195 case UCAR: return "UCAR License"; 5196 case UCL_1_0: return "Upstream Compatibility License v1.0"; 5197 case UNICODE_DFS_2015: return "Unicode License Agreement - Data Files and Software (2015)"; 5198 case UNICODE_DFS_2016: return "Unicode License Agreement - Data Files and Software (2016)"; 5199 case UNICODE_TOU: return "Unicode Terms of Use"; 5200 case UNIXCRYPT: return "UnixCrypt License"; 5201 case UNLICENSE: return "The Unlicense"; 5202 case UPL_1_0: return "Universal Permissive License v1.0"; 5203 case VIM: return "Vim License"; 5204 case VOSTROM: return "VOSTROM Public License for Open Source"; 5205 case VSL_1_0: return "Vovida Software License v1.0"; 5206 case W3C: return "W3C Software Notice and License (2002-12-31)"; 5207 case W3C_19980720: return "W3C Software Notice and License (1998-07-20)"; 5208 case W3C_20150513: return "W3C Software Notice and Document License (2015-05-13)"; 5209 case W3M: return "w3m License"; 5210 case WATCOM_1_0: return "Sybase Open Watcom Public License 1.0"; 5211 case WIDGET_WORKSHOP: return "Widget Workshop License"; 5212 case WSUIPA: return "Wsuipa License"; 5213 case WTFPL: return "Do What The F*ck You Want To Public License"; 5214 case WXWINDOWS: return "wxWindows Library License"; 5215 case X11: return "X11 License"; 5216 case X11_DISTRIBUTE_MODIFICATIONS_VARIANT: return "X11 License Distribution Modification Variant"; 5217 case XDEBUG_1_03: return "Xdebug License v 1.03"; 5218 case XEROX: return "Xerox License"; 5219 case XFIG: return "Xfig License"; 5220 case XFREE86_1_1: return "XFree86 License 1.1"; 5221 case XINETD: return "xinetd License"; 5222 case XLOCK: return "xlock License"; 5223 case XNET: return "X.Net License"; 5224 case XPP: return "XPP License"; 5225 case XSKAT: return "XSkat License"; 5226 case YPL_1_0: return "Yahoo! Public License v1.0"; 5227 case YPL_1_1: return "Yahoo! Public License v1.1"; 5228 case ZED: return "Zed License"; 5229 case ZEND_2_0: return "Zend License v2.0"; 5230 case ZIMBRA_1_3: return "Zimbra Public License v1.3"; 5231 case ZIMBRA_1_4: return "Zimbra Public License v1.4"; 5232 case ZLIB: return "zlib License"; 5233 case ZLIB_ACKNOWLEDGEMENT: return "zlib/libpng License with Acknowledgement"; 5234 case ZPL_1_1: return "Zope Public License 1.1"; 5235 case ZPL_2_0: return "Zope Public License 2.0"; 5236 case ZPL_2_1: return "Zope Public License 2.1"; 5237 case NULL: return null; 5238 default: return "?"; 5239 } 5240 } 5241 public String getDisplay() { 5242 switch (this) { 5243 case _0BSD: return "BSD Zero Clause License"; 5244 case AAL: return "Attribution Assurance License"; 5245 case ABSTYLES: return "Abstyles License"; 5246 case ADACORE_DOC: return "AdaCore Doc License"; 5247 case ADOBE_2006: return "Adobe Systems Incorporated Source Code License Agreement"; 5248 case ADOBE_GLYPH: return "Adobe Glyph List License"; 5249 case ADSL: return "Amazon Digital Services License"; 5250 case AFL_1_1: return "Academic Free License v1.1"; 5251 case AFL_1_2: return "Academic Free License v1.2"; 5252 case AFL_2_0: return "Academic Free License v2.0"; 5253 case AFL_2_1: return "Academic Free License v2.1"; 5254 case AFL_3_0: return "Academic Free License v3.0"; 5255 case AFMPARSE: return "Afmparse License"; 5256 case AGPL_1_0: return "Affero General Public License v1.0"; 5257 case AGPL_1_0_ONLY: return "Affero General Public License v1.0 only"; 5258 case AGPL_1_0_OR_LATER: return "Affero General Public License v1.0 or later"; 5259 case AGPL_3_0: return "GNU Affero General Public License v3.0"; 5260 case AGPL_3_0_ONLY: return "GNU Affero General Public License v3.0 only"; 5261 case AGPL_3_0_OR_LATER: return "GNU Affero General Public License v3.0 or later"; 5262 case ALADDIN: return "Aladdin Free Public License"; 5263 case AMDPLPA: return "AMD's plpa_map.c License"; 5264 case AML: return "Apple MIT License"; 5265 case AMPAS: return "Academy of Motion Picture Arts and Sciences BSD"; 5266 case ANTLR_PD: return "ANTLR Software Rights Notice"; 5267 case ANTLR_PD_FALLBACK: return "ANTLR Software Rights Notice with license fallback"; 5268 case APACHE_1_0: return "Apache License 1.0"; 5269 case APACHE_1_1: return "Apache License 1.1"; 5270 case APACHE_2_0: return "Apache License 2.0"; 5271 case APAFML: return "Adobe Postscript AFM License"; 5272 case APL_1_0: return "Adaptive Public License 1.0"; 5273 case APP_S2P: return "App::s2p License"; 5274 case APSL_1_0: return "Apple Public Source License 1.0"; 5275 case APSL_1_1: return "Apple Public Source License 1.1"; 5276 case APSL_1_2: return "Apple Public Source License 1.2"; 5277 case APSL_2_0: return "Apple Public Source License 2.0"; 5278 case ARPHIC_1999: return "Arphic Public License"; 5279 case ARTISTIC_1_0: return "Artistic License 1.0"; 5280 case ARTISTIC_1_0_CL8: return "Artistic License 1.0 w/clause 8"; 5281 case ARTISTIC_1_0_PERL: return "Artistic License 1.0 (Perl)"; 5282 case ARTISTIC_2_0: return "Artistic License 2.0"; 5283 case ASWF_DIGITAL_ASSETS_1_0: return "ASWF Digital Assets License version 1.0"; 5284 case ASWF_DIGITAL_ASSETS_1_1: return "ASWF Digital Assets License 1.1"; 5285 case BAEKMUK: return "Baekmuk License"; 5286 case BAHYPH: return "Bahyph License"; 5287 case BARR: return "Barr License"; 5288 case BEERWARE: return "Beerware License"; 5289 case BITSTREAM_CHARTER: return "Bitstream Charter Font License"; 5290 case BITSTREAM_VERA: return "Bitstream Vera Font License"; 5291 case BITTORRENT_1_0: return "BitTorrent Open Source License v1.0"; 5292 case BITTORRENT_1_1: return "BitTorrent Open Source License v1.1"; 5293 case BLESSING: return "SQLite Blessing"; 5294 case BLUEOAK_1_0_0: return "Blue Oak Model License 1.0.0"; 5295 case BOEHM_GC: return "Boehm-Demers-Weiser GC License"; 5296 case BORCEUX: return "Borceux license"; 5297 case BRIAN_GLADMAN_3_CLAUSE: return "Brian Gladman 3-Clause License"; 5298 case BSD_1_CLAUSE: return "BSD 1-Clause License"; 5299 case BSD_2_CLAUSE: return "BSD 2-Clause \"Simplified\" License"; 5300 case BSD_2_CLAUSE_FREEBSD: return "BSD 2-Clause FreeBSD License"; 5301 case BSD_2_CLAUSE_NETBSD: return "BSD 2-Clause NetBSD License"; 5302 case BSD_2_CLAUSE_PATENT: return "BSD-2-Clause Plus Patent License"; 5303 case BSD_2_CLAUSE_VIEWS: return "BSD 2-Clause with views sentence"; 5304 case BSD_3_CLAUSE: return "BSD 3-Clause \"New\" or \"Revised\" License"; 5305 case BSD_3_CLAUSE_ATTRIBUTION: return "BSD with attribution"; 5306 case BSD_3_CLAUSE_CLEAR: return "BSD 3-Clause Clear License"; 5307 case BSD_3_CLAUSE_LBNL: return "Lawrence Berkeley National Labs BSD variant license"; 5308 case BSD_3_CLAUSE_MODIFICATION: return "BSD 3-Clause Modification"; 5309 case BSD_3_CLAUSE_NO_MILITARY_LICENSE: return "BSD 3-Clause No Military License"; 5310 case BSD_3_CLAUSE_NO_NUCLEAR_LICENSE: return "BSD 3-Clause No Nuclear License"; 5311 case BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014: return "BSD 3-Clause No Nuclear License 2014"; 5312 case BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY: return "BSD 3-Clause No Nuclear Warranty"; 5313 case BSD_3_CLAUSE_OPEN_MPI: return "BSD 3-Clause Open MPI variant"; 5314 case BSD_4_CLAUSE: return "BSD 4-Clause \"Original\" or \"Old\" License"; 5315 case BSD_4_CLAUSE_SHORTENED: return "BSD 4 Clause Shortened"; 5316 case BSD_4_CLAUSE_UC: return "BSD-4-Clause (University of California-Specific)"; 5317 case BSD_4_3RENO: return "BSD 4.3 RENO License"; 5318 case BSD_4_3TAHOE: return "BSD 4.3 TAHOE License"; 5319 case BSD_ADVERTISING_ACKNOWLEDGEMENT: return "BSD Advertising Acknowledgement License"; 5320 case BSD_ATTRIBUTION_HPND_DISCLAIMER: return "BSD with Attribution and HPND disclaimer"; 5321 case BSD_PROTECTION: return "BSD Protection License"; 5322 case BSD_SOURCE_CODE: return "BSD Source Code Attribution"; 5323 case BSL_1_0: return "Boost Software License 1.0"; 5324 case BUSL_1_1: return "Business Source License 1.1"; 5325 case BZIP2_1_0_5: return "bzip2 and libbzip2 License v1.0.5"; 5326 case BZIP2_1_0_6: return "bzip2 and libbzip2 License v1.0.6"; 5327 case C_UDA_1_0: return "Computational Use of Data Agreement v1.0"; 5328 case CAL_1_0: return "Cryptographic Autonomy License 1.0"; 5329 case CAL_1_0_COMBINED_WORK_EXCEPTION: return "Cryptographic Autonomy License 1.0 (Combined Work Exception)"; 5330 case CALDERA: return "Caldera License"; 5331 case CATOSL_1_1: return "Computer Associates Trusted Open Source License 1.1"; 5332 case CC_BY_1_0: return "Creative Commons Attribution 1.0 Generic"; 5333 case CC_BY_2_0: return "Creative Commons Attribution 2.0 Generic"; 5334 case CC_BY_2_5: return "Creative Commons Attribution 2.5 Generic"; 5335 case CC_BY_2_5_AU: return "Creative Commons Attribution 2.5 Australia"; 5336 case CC_BY_3_0: return "Creative Commons Attribution 3.0 Unported"; 5337 case CC_BY_3_0_AT: return "Creative Commons Attribution 3.0 Austria"; 5338 case CC_BY_3_0_DE: return "Creative Commons Attribution 3.0 Germany"; 5339 case CC_BY_3_0_IGO: return "Creative Commons Attribution 3.0 IGO"; 5340 case CC_BY_3_0_NL: return "Creative Commons Attribution 3.0 Netherlands"; 5341 case CC_BY_3_0_US: return "Creative Commons Attribution 3.0 United States"; 5342 case CC_BY_4_0: return "Creative Commons Attribution 4.0 International"; 5343 case CC_BY_NC_1_0: return "Creative Commons Attribution Non Commercial 1.0 Generic"; 5344 case CC_BY_NC_2_0: return "Creative Commons Attribution Non Commercial 2.0 Generic"; 5345 case CC_BY_NC_2_5: return "Creative Commons Attribution Non Commercial 2.5 Generic"; 5346 case CC_BY_NC_3_0: return "Creative Commons Attribution Non Commercial 3.0 Unported"; 5347 case CC_BY_NC_3_0_DE: return "Creative Commons Attribution Non Commercial 3.0 Germany"; 5348 case CC_BY_NC_4_0: return "Creative Commons Attribution Non Commercial 4.0 International"; 5349 case CC_BY_NC_ND_1_0: return "Creative Commons Attribution Non Commercial No Derivatives 1.0 Generic"; 5350 case CC_BY_NC_ND_2_0: return "Creative Commons Attribution Non Commercial No Derivatives 2.0 Generic"; 5351 case CC_BY_NC_ND_2_5: return "Creative Commons Attribution Non Commercial No Derivatives 2.5 Generic"; 5352 case CC_BY_NC_ND_3_0: return "Creative Commons Attribution Non Commercial No Derivatives 3.0 Unported"; 5353 case CC_BY_NC_ND_3_0_DE: return "Creative Commons Attribution Non Commercial No Derivatives 3.0 Germany"; 5354 case CC_BY_NC_ND_3_0_IGO: return "Creative Commons Attribution Non Commercial No Derivatives 3.0 IGO"; 5355 case CC_BY_NC_ND_4_0: return "Creative Commons Attribution Non Commercial No Derivatives 4.0 International"; 5356 case CC_BY_NC_SA_1_0: return "Creative Commons Attribution Non Commercial Share Alike 1.0 Generic"; 5357 case CC_BY_NC_SA_2_0: return "Creative Commons Attribution Non Commercial Share Alike 2.0 Generic"; 5358 case CC_BY_NC_SA_2_0_DE: return "Creative Commons Attribution Non Commercial Share Alike 2.0 Germany"; 5359 case CC_BY_NC_SA_2_0_FR: return "Creative Commons Attribution-NonCommercial-ShareAlike 2.0 France"; 5360 case CC_BY_NC_SA_2_0_UK: return "Creative Commons Attribution Non Commercial Share Alike 2.0 England and Wales"; 5361 case CC_BY_NC_SA_2_5: return "Creative Commons Attribution Non Commercial Share Alike 2.5 Generic"; 5362 case CC_BY_NC_SA_3_0: return "Creative Commons Attribution Non Commercial Share Alike 3.0 Unported"; 5363 case CC_BY_NC_SA_3_0_DE: return "Creative Commons Attribution Non Commercial Share Alike 3.0 Germany"; 5364 case CC_BY_NC_SA_3_0_IGO: return "Creative Commons Attribution Non Commercial Share Alike 3.0 IGO"; 5365 case CC_BY_NC_SA_4_0: return "Creative Commons Attribution Non Commercial Share Alike 4.0 International"; 5366 case CC_BY_ND_1_0: return "Creative Commons Attribution No Derivatives 1.0 Generic"; 5367 case CC_BY_ND_2_0: return "Creative Commons Attribution No Derivatives 2.0 Generic"; 5368 case CC_BY_ND_2_5: return "Creative Commons Attribution No Derivatives 2.5 Generic"; 5369 case CC_BY_ND_3_0: return "Creative Commons Attribution No Derivatives 3.0 Unported"; 5370 case CC_BY_ND_3_0_DE: return "Creative Commons Attribution No Derivatives 3.0 Germany"; 5371 case CC_BY_ND_4_0: return "Creative Commons Attribution No Derivatives 4.0 International"; 5372 case CC_BY_SA_1_0: return "Creative Commons Attribution Share Alike 1.0 Generic"; 5373 case CC_BY_SA_2_0: return "Creative Commons Attribution Share Alike 2.0 Generic"; 5374 case CC_BY_SA_2_0_UK: return "Creative Commons Attribution Share Alike 2.0 England and Wales"; 5375 case CC_BY_SA_2_1_JP: return "Creative Commons Attribution Share Alike 2.1 Japan"; 5376 case CC_BY_SA_2_5: return "Creative Commons Attribution Share Alike 2.5 Generic"; 5377 case CC_BY_SA_3_0: return "Creative Commons Attribution Share Alike 3.0 Unported"; 5378 case CC_BY_SA_3_0_AT: return "Creative Commons Attribution Share Alike 3.0 Austria"; 5379 case CC_BY_SA_3_0_DE: return "Creative Commons Attribution Share Alike 3.0 Germany"; 5380 case CC_BY_SA_3_0_IGO: return "Creative Commons Attribution-ShareAlike 3.0 IGO"; 5381 case CC_BY_SA_4_0: return "Creative Commons Attribution Share Alike 4.0 International"; 5382 case CC_PDDC: return "Creative Commons Public Domain Dedication and Certification"; 5383 case CC0_1_0: return "Creative Commons Zero v1.0 Universal"; 5384 case CDDL_1_0: return "Common Development and Distribution License 1.0"; 5385 case CDDL_1_1: return "Common Development and Distribution License 1.1"; 5386 case CDL_1_0: return "Common Documentation License 1.0"; 5387 case CDLA_PERMISSIVE_1_0: return "Community Data License Agreement Permissive 1.0"; 5388 case CDLA_PERMISSIVE_2_0: return "Community Data License Agreement Permissive 2.0"; 5389 case CDLA_SHARING_1_0: return "Community Data License Agreement Sharing 1.0"; 5390 case CECILL_1_0: return "CeCILL Free Software License Agreement v1.0"; 5391 case CECILL_1_1: return "CeCILL Free Software License Agreement v1.1"; 5392 case CECILL_2_0: return "CeCILL Free Software License Agreement v2.0"; 5393 case CECILL_2_1: return "CeCILL Free Software License Agreement v2.1"; 5394 case CECILL_B: return "CeCILL-B Free Software License Agreement"; 5395 case CECILL_C: return "CeCILL-C Free Software License Agreement"; 5396 case CERN_OHL_1_1: return "CERN Open Hardware Licence v1.1"; 5397 case CERN_OHL_1_2: return "CERN Open Hardware Licence v1.2"; 5398 case CERN_OHL_P_2_0: return "CERN Open Hardware Licence Version 2 - Permissive"; 5399 case CERN_OHL_S_2_0: return "CERN Open Hardware Licence Version 2 - Strongly Reciprocal"; 5400 case CERN_OHL_W_2_0: return "CERN Open Hardware Licence Version 2 - Weakly Reciprocal"; 5401 case CFITSIO: return "CFITSIO License"; 5402 case CHECKMK: return "Checkmk License"; 5403 case CLARTISTIC: return "Clarified Artistic License"; 5404 case CLIPS: return "Clips License"; 5405 case CMU_MACH: return "CMU Mach License"; 5406 case CNRI_JYTHON: return "CNRI Jython License"; 5407 case CNRI_PYTHON: return "CNRI Python License"; 5408 case CNRI_PYTHON_GPL_COMPATIBLE: return "CNRI Python Open Source GPL Compatible License Agreement"; 5409 case COIL_1_0: return "Copyfree Open Innovation License"; 5410 case COMMUNITY_SPEC_1_0: return "Community Specification License 1.0"; 5411 case CONDOR_1_1: return "Condor Public License v1.1"; 5412 case COPYLEFT_NEXT_0_3_0: return "copyleft-next 0.3.0"; 5413 case COPYLEFT_NEXT_0_3_1: return "copyleft-next 0.3.1"; 5414 case CORNELL_LOSSLESS_JPEG: return "Cornell Lossless JPEG License"; 5415 case CPAL_1_0: return "Common Public Attribution License 1.0"; 5416 case CPL_1_0: return "Common Public License 1.0"; 5417 case CPOL_1_02: return "Code Project Open License 1.02"; 5418 case CROSSWORD: return "Crossword License"; 5419 case CRYSTALSTACKER: return "CrystalStacker License"; 5420 case CUA_OPL_1_0: return "CUA Office Public License v1.0"; 5421 case CUBE: return "Cube License"; 5422 case CURL: return "curl License"; 5423 case D_FSL_1_0: return "Deutsche Freie Software Lizenz"; 5424 case DIFFMARK: return "diffmark license"; 5425 case DL_DE_BY_2_0: return "Data licence Germany ? attribution ? version 2.0"; 5426 case DOC: return "DOC License"; 5427 case DOTSEQN: return "Dotseqn License"; 5428 case DRL_1_0: return "Detection Rule License 1.0"; 5429 case DSDP: return "DSDP License"; 5430 case DTOA: return "David M. Gay dtoa License"; 5431 case DVIPDFM: return "dvipdfm License"; 5432 case ECL_1_0: return "Educational Community License v1.0"; 5433 case ECL_2_0: return "Educational Community License v2.0"; 5434 case ECOS_2_0: return "eCos license version 2.0"; 5435 case EFL_1_0: return "Eiffel Forum License v1.0"; 5436 case EFL_2_0: return "Eiffel Forum License v2.0"; 5437 case EGENIX: return "eGenix.com Public License 1.1.0"; 5438 case ELASTIC_2_0: return "Elastic License 2.0"; 5439 case ENTESSA: return "Entessa Public License v1.0"; 5440 case EPICS: return "EPICS Open License"; 5441 case EPL_1_0: return "Eclipse Public License 1.0"; 5442 case EPL_2_0: return "Eclipse Public License 2.0"; 5443 case ERLPL_1_1: return "Erlang Public License v1.1"; 5444 case ETALAB_2_0: return "Etalab Open License 2.0"; 5445 case EUDATAGRID: return "EU DataGrid Software License"; 5446 case EUPL_1_0: return "European Union Public License 1.0"; 5447 case EUPL_1_1: return "European Union Public License 1.1"; 5448 case EUPL_1_2: return "European Union Public License 1.2"; 5449 case EUROSYM: return "Eurosym License"; 5450 case FAIR: return "Fair License"; 5451 case FDK_AAC: return "Fraunhofer FDK AAC Codec Library"; 5452 case FRAMEWORX_1_0: return "Frameworx Open License 1.0"; 5453 case FREEBSD_DOC: return "FreeBSD Documentation License"; 5454 case FREEIMAGE: return "FreeImage Public License v1.0"; 5455 case FSFAP: return "FSF All Permissive License"; 5456 case FSFUL: return "FSF Unlimited License"; 5457 case FSFULLR: return "FSF Unlimited License (with License Retention)"; 5458 case FSFULLRWD: return "FSF Unlimited License (With License Retention and Warranty Disclaimer)"; 5459 case FTL: return "Freetype Project License"; 5460 case GD: return "GD License"; 5461 case GFDL_1_1: return "GNU Free Documentation License v1.1"; 5462 case GFDL_1_1_INVARIANTS_ONLY: return "GNU Free Documentation License v1.1 only - invariants"; 5463 case GFDL_1_1_INVARIANTS_OR_LATER: return "GNU Free Documentation License v1.1 or later - invariants"; 5464 case GFDL_1_1_NO_INVARIANTS_ONLY: return "GNU Free Documentation License v1.1 only - no invariants"; 5465 case GFDL_1_1_NO_INVARIANTS_OR_LATER: return "GNU Free Documentation License v1.1 or later - no invariants"; 5466 case GFDL_1_1_ONLY: return "GNU Free Documentation License v1.1 only"; 5467 case GFDL_1_1_OR_LATER: return "GNU Free Documentation License v1.1 or later"; 5468 case GFDL_1_2: return "GNU Free Documentation License v1.2"; 5469 case GFDL_1_2_INVARIANTS_ONLY: return "GNU Free Documentation License v1.2 only - invariants"; 5470 case GFDL_1_2_INVARIANTS_OR_LATER: return "GNU Free Documentation License v1.2 or later - invariants"; 5471 case GFDL_1_2_NO_INVARIANTS_ONLY: return "GNU Free Documentation License v1.2 only - no invariants"; 5472 case GFDL_1_2_NO_INVARIANTS_OR_LATER: return "GNU Free Documentation License v1.2 or later - no invariants"; 5473 case GFDL_1_2_ONLY: return "GNU Free Documentation License v1.2 only"; 5474 case GFDL_1_2_OR_LATER: return "GNU Free Documentation License v1.2 or later"; 5475 case GFDL_1_3: return "GNU Free Documentation License v1.3"; 5476 case GFDL_1_3_INVARIANTS_ONLY: return "GNU Free Documentation License v1.3 only - invariants"; 5477 case GFDL_1_3_INVARIANTS_OR_LATER: return "GNU Free Documentation License v1.3 or later - invariants"; 5478 case GFDL_1_3_NO_INVARIANTS_ONLY: return "GNU Free Documentation License v1.3 only - no invariants"; 5479 case GFDL_1_3_NO_INVARIANTS_OR_LATER: return "GNU Free Documentation License v1.3 or later - no invariants"; 5480 case GFDL_1_3_ONLY: return "GNU Free Documentation License v1.3 only"; 5481 case GFDL_1_3_OR_LATER: return "GNU Free Documentation License v1.3 or later"; 5482 case GIFTWARE: return "Giftware License"; 5483 case GL2PS: return "GL2PS License"; 5484 case GLIDE: return "3dfx Glide License"; 5485 case GLULXE: return "Glulxe License"; 5486 case GLWTPL: return "Good Luck With That Public License"; 5487 case GNUPLOT: return "gnuplot License"; 5488 case GPL_1_0: return "GNU General Public License v1.0 only"; 5489 case GPL_1_0PLUS: return "GNU General Public License v1.0 or later"; 5490 case GPL_1_0_ONLY: return "GNU General Public License v1.0 only"; 5491 case GPL_1_0_OR_LATER: return "GNU General Public License v1.0 or later"; 5492 case GPL_2_0: return "GNU General Public License v2.0 only"; 5493 case GPL_2_0PLUS: return "GNU General Public License v2.0 or later"; 5494 case GPL_2_0_ONLY: return "GNU General Public License v2.0 only"; 5495 case GPL_2_0_OR_LATER: return "GNU General Public License v2.0 or later"; 5496 case GPL_2_0_WITH_AUTOCONF_EXCEPTION: return "GNU General Public License v2.0 w/Autoconf exception"; 5497 case GPL_2_0_WITH_BISON_EXCEPTION: return "GNU General Public License v2.0 w/Bison exception"; 5498 case GPL_2_0_WITH_CLASSPATH_EXCEPTION: return "GNU General Public License v2.0 w/Classpath exception"; 5499 case GPL_2_0_WITH_FONT_EXCEPTION: return "GNU General Public License v2.0 w/Font exception"; 5500 case GPL_2_0_WITH_GCC_EXCEPTION: return "GNU General Public License v2.0 w/GCC Runtime Library exception"; 5501 case GPL_3_0: return "GNU General Public License v3.0 only"; 5502 case GPL_3_0PLUS: return "GNU General Public License v3.0 or later"; 5503 case GPL_3_0_ONLY: return "GNU General Public License v3.0 only"; 5504 case GPL_3_0_OR_LATER: return "GNU General Public License v3.0 or later"; 5505 case GPL_3_0_WITH_AUTOCONF_EXCEPTION: return "GNU General Public License v3.0 w/Autoconf exception"; 5506 case GPL_3_0_WITH_GCC_EXCEPTION: return "GNU General Public License v3.0 w/GCC Runtime Library exception"; 5507 case GRAPHICS_GEMS: return "Graphics Gems License"; 5508 case GSOAP_1_3B: return "gSOAP Public License v1.3b"; 5509 case HASKELLREPORT: return "Haskell Language Report License"; 5510 case HIPPOCRATIC_2_1: return "Hippocratic License 2.1"; 5511 case HP_1986: return "Hewlett-Packard 1986 License"; 5512 case HPND: return "Historical Permission Notice and Disclaimer"; 5513 case HPND_EXPORT_US: return "HPND with US Government export control warning"; 5514 case HPND_MARKUS_KUHN: return "Historical Permission Notice and Disclaimer - Markus Kuhn variant"; 5515 case HPND_SELL_VARIANT: return "Historical Permission Notice and Disclaimer - sell variant"; 5516 case HPND_SELL_VARIANT_MIT_DISCLAIMER: return "HPND sell variant with MIT disclaimer"; 5517 case HTMLTIDY: return "HTML Tidy License"; 5518 case IBM_PIBS: return "IBM PowerPC Initialization and Boot Software"; 5519 case ICU: return "ICU License"; 5520 case IEC_CODE_COMPONENTS_EULA: return "IEC Code Components End-user licence agreement"; 5521 case IJG: return "Independent JPEG Group License"; 5522 case IJG_SHORT: return "Independent JPEG Group License - short"; 5523 case IMAGEMAGICK: return "ImageMagick License"; 5524 case IMATIX: return "iMatix Standard Function Library Agreement"; 5525 case IMLIB2: return "Imlib2 License"; 5526 case INFO_ZIP: return "Info-ZIP License"; 5527 case INNER_NET_2_0: return "Inner Net License v2.0"; 5528 case INTEL: return "Intel Open Source License"; 5529 case INTEL_ACPI: return "Intel ACPI Software License Agreement"; 5530 case INTERBASE_1_0: return "Interbase Public License v1.0"; 5531 case IPA: return "IPA Font License"; 5532 case IPL_1_0: return "IBM Public License v1.0"; 5533 case ISC: return "ISC License"; 5534 case JAM: return "Jam License"; 5535 case JASPER_2_0: return "JasPer License"; 5536 case JPL_IMAGE: return "JPL Image Use Policy"; 5537 case JPNIC: return "Japan Network Information Center License"; 5538 case JSON: return "JSON License"; 5539 case KAZLIB: return "Kazlib License"; 5540 case KNUTH_CTAN: return "Knuth CTAN License"; 5541 case LAL_1_2: return "Licence Art Libre 1.2"; 5542 case LAL_1_3: return "Licence Art Libre 1.3"; 5543 case LATEX2E: return "Latex2e License"; 5544 case LATEX2E_TRANSLATED_NOTICE: return "Latex2e with translated notice permission"; 5545 case LEPTONICA: return "Leptonica License"; 5546 case LGPL_2_0: return "GNU Library General Public License v2 only"; 5547 case LGPL_2_0PLUS: return "GNU Library General Public License v2 or later"; 5548 case LGPL_2_0_ONLY: return "GNU Library General Public License v2 only"; 5549 case LGPL_2_0_OR_LATER: return "GNU Library General Public License v2 or later"; 5550 case LGPL_2_1: return "GNU Lesser General Public License v2.1 only"; 5551 case LGPL_2_1PLUS: return "GNU Lesser General Public License v2.1 or later"; 5552 case LGPL_2_1_ONLY: return "GNU Lesser General Public License v2.1 only"; 5553 case LGPL_2_1_OR_LATER: return "GNU Lesser General Public License v2.1 or later"; 5554 case LGPL_3_0: return "GNU Lesser General Public License v3.0 only"; 5555 case LGPL_3_0PLUS: return "GNU Lesser General Public License v3.0 or later"; 5556 case LGPL_3_0_ONLY: return "GNU Lesser General Public License v3.0 only"; 5557 case LGPL_3_0_OR_LATER: return "GNU Lesser General Public License v3.0 or later"; 5558 case LGPLLR: return "Lesser General Public License For Linguistic Resources"; 5559 case LIBPNG: return "libpng License"; 5560 case LIBPNG_2_0: return "PNG Reference Library version 2"; 5561 case LIBSELINUX_1_0: return "libselinux public domain notice"; 5562 case LIBTIFF: return "libtiff License"; 5563 case LIBUTIL_DAVID_NUGENT: return "libutil David Nugent License"; 5564 case LILIQ_P_1_1: return "Licence Libre du Québec ? Permissive version 1.1"; 5565 case LILIQ_R_1_1: return "Licence Libre du Québec ? Réciprocité version 1.1"; 5566 case LILIQ_RPLUS_1_1: return "Licence Libre du Québec ? Réciprocité forte version 1.1"; 5567 case LINUX_MAN_PAGES_1_PARA: return "Linux man-pages - 1 paragraph"; 5568 case LINUX_MAN_PAGES_COPYLEFT: return "Linux man-pages Copyleft"; 5569 case LINUX_MAN_PAGES_COPYLEFT_2_PARA: return "Linux man-pages Copyleft - 2 paragraphs"; 5570 case LINUX_MAN_PAGES_COPYLEFT_VAR: return "Linux man-pages Copyleft Variant"; 5571 case LINUX_OPENIB: return "Linux Kernel Variant of OpenIB.org license"; 5572 case LOOP: return "Common Lisp LOOP License"; 5573 case LPL_1_0: return "Lucent Public License Version 1.0"; 5574 case LPL_1_02: return "Lucent Public License v1.02"; 5575 case LPPL_1_0: return "LaTeX Project Public License v1.0"; 5576 case LPPL_1_1: return "LaTeX Project Public License v1.1"; 5577 case LPPL_1_2: return "LaTeX Project Public License v1.2"; 5578 case LPPL_1_3A: return "LaTeX Project Public License v1.3a"; 5579 case LPPL_1_3C: return "LaTeX Project Public License v1.3c"; 5580 case LZMA_SDK_9_11_TO_9_20: return "LZMA SDK License (versions 9.11 to 9.20)"; 5581 case LZMA_SDK_9_22: return "LZMA SDK License (versions 9.22 and beyond)"; 5582 case MAKEINDEX: return "MakeIndex License"; 5583 case MARTIN_BIRGMEIER: return "Martin Birgmeier License"; 5584 case METAMAIL: return "metamail License"; 5585 case MINPACK: return "Minpack License"; 5586 case MIROS: return "The MirOS Licence"; 5587 case MIT: return "MIT License"; 5588 case MIT_0: return "MIT No Attribution"; 5589 case MIT_ADVERTISING: return "Enlightenment License (e16)"; 5590 case MIT_CMU: return "CMU License"; 5591 case MIT_ENNA: return "enna License"; 5592 case MIT_FEH: return "feh License"; 5593 case MIT_FESTIVAL: return "MIT Festival Variant"; 5594 case MIT_MODERN_VARIANT: return "MIT License Modern Variant"; 5595 case MIT_OPEN_GROUP: return "MIT Open Group variant"; 5596 case MIT_WU: return "MIT Tom Wu Variant"; 5597 case MITNFA: return "MIT +no-false-attribs license"; 5598 case MOTOSOTO: return "Motosoto License"; 5599 case MPI_PERMISSIVE: return "mpi Permissive License"; 5600 case MPICH2: return "mpich2 License"; 5601 case MPL_1_0: return "Mozilla Public License 1.0"; 5602 case MPL_1_1: return "Mozilla Public License 1.1"; 5603 case MPL_2_0: return "Mozilla Public License 2.0"; 5604 case MPL_2_0_NO_COPYLEFT_EXCEPTION: return "Mozilla Public License 2.0 (no copyleft exception)"; 5605 case MPLUS: return "mplus Font License"; 5606 case MS_LPL: return "Microsoft Limited Public License"; 5607 case MS_PL: return "Microsoft Public License"; 5608 case MS_RL: return "Microsoft Reciprocal License"; 5609 case MTLL: return "Matrix Template Library License"; 5610 case MULANPSL_1_0: return "Mulan Permissive Software License, Version 1"; 5611 case MULANPSL_2_0: return "Mulan Permissive Software License, Version 2"; 5612 case MULTICS: return "Multics License"; 5613 case MUP: return "Mup License"; 5614 case NAIST_2003: return "Nara Institute of Science and Technology License (2003)"; 5615 case NASA_1_3: return "NASA Open Source Agreement 1.3"; 5616 case NAUMEN: return "Naumen Public License"; 5617 case NBPL_1_0: return "Net Boolean Public License v1"; 5618 case NCGL_UK_2_0: return "Non-Commercial Government Licence"; 5619 case NCSA: return "University of Illinois/NCSA Open Source License"; 5620 case NET_SNMP: return "Net-SNMP License"; 5621 case NETCDF: return "NetCDF license"; 5622 case NEWSLETR: return "Newsletr License"; 5623 case NGPL: return "Nethack General Public License"; 5624 case NICTA_1_0: return "NICTA Public Software License, Version 1.0"; 5625 case NIST_PD: return "NIST Public Domain Notice"; 5626 case NIST_PD_FALLBACK: return "NIST Public Domain Notice with license fallback"; 5627 case NIST_SOFTWARE: return "NIST Software License"; 5628 case NLOD_1_0: return "Norwegian Licence for Open Government Data (NLOD) 1.0"; 5629 case NLOD_2_0: return "Norwegian Licence for Open Government Data (NLOD) 2.0"; 5630 case NLPL: return "No Limit Public License"; 5631 case NOKIA: return "Nokia Open Source License"; 5632 case NOSL: return "Netizen Open Source License"; 5633 case NOT_OPEN_SOURCE: return "Not open source"; 5634 case NOWEB: return "Noweb License"; 5635 case NPL_1_0: return "Netscape Public License v1.0"; 5636 case NPL_1_1: return "Netscape Public License v1.1"; 5637 case NPOSL_3_0: return "Non-Profit Open Software License 3.0"; 5638 case NRL: return "NRL License"; 5639 case NTP: return "NTP License"; 5640 case NTP_0: return "NTP No Attribution"; 5641 case NUNIT: return "Nunit License"; 5642 case O_UDA_1_0: return "Open Use of Data Agreement v1.0"; 5643 case OCCT_PL: return "Open CASCADE Technology Public License"; 5644 case OCLC_2_0: return "OCLC Research Public License 2.0"; 5645 case ODBL_1_0: return "Open Data Commons Open Database License v1.0"; 5646 case ODC_BY_1_0: return "Open Data Commons Attribution License v1.0"; 5647 case OFFIS: return "OFFIS License"; 5648 case OFL_1_0: return "SIL Open Font License 1.0"; 5649 case OFL_1_0_NO_RFN: return "SIL Open Font License 1.0 with no Reserved Font Name"; 5650 case OFL_1_0_RFN: return "SIL Open Font License 1.0 with Reserved Font Name"; 5651 case OFL_1_1: return "SIL Open Font License 1.1"; 5652 case OFL_1_1_NO_RFN: return "SIL Open Font License 1.1 with no Reserved Font Name"; 5653 case OFL_1_1_RFN: return "SIL Open Font License 1.1 with Reserved Font Name"; 5654 case OGC_1_0: return "OGC Software License, Version 1.0"; 5655 case OGDL_TAIWAN_1_0: return "Taiwan Open Government Data License, version 1.0"; 5656 case OGL_CANADA_2_0: return "Open Government Licence - Canada"; 5657 case OGL_UK_1_0: return "Open Government Licence v1.0"; 5658 case OGL_UK_2_0: return "Open Government Licence v2.0"; 5659 case OGL_UK_3_0: return "Open Government Licence v3.0"; 5660 case OGTSL: return "Open Group Test Suite License"; 5661 case OLDAP_1_1: return "Open LDAP Public License v1.1"; 5662 case OLDAP_1_2: return "Open LDAP Public License v1.2"; 5663 case OLDAP_1_3: return "Open LDAP Public License v1.3"; 5664 case OLDAP_1_4: return "Open LDAP Public License v1.4"; 5665 case OLDAP_2_0: return "Open LDAP Public License v2.0 (or possibly 2.0A and 2.0B)"; 5666 case OLDAP_2_0_1: return "Open LDAP Public License v2.0.1"; 5667 case OLDAP_2_1: return "Open LDAP Public License v2.1"; 5668 case OLDAP_2_2: return "Open LDAP Public License v2.2"; 5669 case OLDAP_2_2_1: return "Open LDAP Public License v2.2.1"; 5670 case OLDAP_2_2_2: return "Open LDAP Public License 2.2.2"; 5671 case OLDAP_2_3: return "Open LDAP Public License v2.3"; 5672 case OLDAP_2_4: return "Open LDAP Public License v2.4"; 5673 case OLDAP_2_5: return "Open LDAP Public License v2.5"; 5674 case OLDAP_2_6: return "Open LDAP Public License v2.6"; 5675 case OLDAP_2_7: return "Open LDAP Public License v2.7"; 5676 case OLDAP_2_8: return "Open LDAP Public License v2.8"; 5677 case OLFL_1_3: return "Open Logistics Foundation License Version 1.3"; 5678 case OML: return "Open Market License"; 5679 case OPENPBS_2_3: return "OpenPBS v2.3 Software License"; 5680 case OPENSSL: return "OpenSSL License"; 5681 case OPL_1_0: return "Open Public License v1.0"; 5682 case OPL_UK_3_0: return "United Kingdom Open Parliament Licence v3.0"; 5683 case OPUBL_1_0: return "Open Publication License v1.0"; 5684 case OSET_PL_2_1: return "OSET Public License version 2.1"; 5685 case OSL_1_0: return "Open Software License 1.0"; 5686 case OSL_1_1: return "Open Software License 1.1"; 5687 case OSL_2_0: return "Open Software License 2.0"; 5688 case OSL_2_1: return "Open Software License 2.1"; 5689 case OSL_3_0: return "Open Software License 3.0"; 5690 case PARITY_6_0_0: return "The Parity Public License 6.0.0"; 5691 case PARITY_7_0_0: return "The Parity Public License 7.0.0"; 5692 case PDDL_1_0: return "Open Data Commons Public Domain Dedication & License 1.0"; 5693 case PHP_3_0: return "PHP License v3.0"; 5694 case PHP_3_01: return "PHP License v3.01"; 5695 case PLEXUS: return "Plexus Classworlds License"; 5696 case POLYFORM_NONCOMMERCIAL_1_0_0: return "PolyForm Noncommercial License 1.0.0"; 5697 case POLYFORM_SMALL_BUSINESS_1_0_0: return "PolyForm Small Business License 1.0.0"; 5698 case POSTGRESQL: return "PostgreSQL License"; 5699 case PSF_2_0: return "Python Software Foundation License 2.0"; 5700 case PSFRAG: return "psfrag License"; 5701 case PSUTILS: return "psutils License"; 5702 case PYTHON_2_0: return "Python License 2.0"; 5703 case PYTHON_2_0_1: return "Python License 2.0.1"; 5704 case QHULL: return "Qhull License"; 5705 case QPL_1_0: return "Q Public License 1.0"; 5706 case QPL_1_0_INRIA_2004: return "Q Public License 1.0 - INRIA 2004 variant"; 5707 case RDISC: return "Rdisc License"; 5708 case RHECOS_1_1: return "Red Hat eCos Public License v1.1"; 5709 case RPL_1_1: return "Reciprocal Public License 1.1"; 5710 case RPL_1_5: return "Reciprocal Public License 1.5"; 5711 case RPSL_1_0: return "RealNetworks Public Source License v1.0"; 5712 case RSA_MD: return "RSA Message-Digest License"; 5713 case RSCPL: return "Ricoh Source Code Public License"; 5714 case RUBY: return "Ruby License"; 5715 case SAX_PD: return "Sax Public Domain Notice"; 5716 case SAXPATH: return "Saxpath License"; 5717 case SCEA: return "SCEA Shared Source License"; 5718 case SCHEMEREPORT: return "Scheme Language Report License"; 5719 case SENDMAIL: return "Sendmail License"; 5720 case SENDMAIL_8_23: return "Sendmail License 8.23"; 5721 case SGI_B_1_0: return "SGI Free Software License B v1.0"; 5722 case SGI_B_1_1: return "SGI Free Software License B v1.1"; 5723 case SGI_B_2_0: return "SGI Free Software License B v2.0"; 5724 case SGP4: return "SGP4 Permission Notice"; 5725 case SHL_0_5: return "Solderpad Hardware License v0.5"; 5726 case SHL_0_51: return "Solderpad Hardware License, Version 0.51"; 5727 case SIMPL_2_0: return "Simple Public License 2.0"; 5728 case SISSL: return "Sun Industry Standards Source License v1.1"; 5729 case SISSL_1_2: return "Sun Industry Standards Source License v1.2"; 5730 case SLEEPYCAT: return "Sleepycat License"; 5731 case SMLNJ: return "Standard ML of New Jersey License"; 5732 case SMPPL: return "Secure Messaging Protocol Public License"; 5733 case SNIA: return "SNIA Public License 1.1"; 5734 case SNPRINTF: return "snprintf License"; 5735 case SPENCER_86: return "Spencer License 86"; 5736 case SPENCER_94: return "Spencer License 94"; 5737 case SPENCER_99: return "Spencer License 99"; 5738 case SPL_1_0: return "Sun Public License v1.0"; 5739 case SSH_OPENSSH: return "SSH OpenSSH license"; 5740 case SSH_SHORT: return "SSH short notice"; 5741 case SSPL_1_0: return "Server Side Public License, v 1"; 5742 case STANDARDML_NJ: return "Standard ML of New Jersey License"; 5743 case SUGARCRM_1_1_3: return "SugarCRM Public License v1.1.3"; 5744 case SUNPRO: return "SunPro License"; 5745 case SWL: return "Scheme Widget Library (SWL) Software License Agreement"; 5746 case SYMLINKS: return "Symlinks License"; 5747 case TAPR_OHL_1_0: return "TAPR Open Hardware License v1.0"; 5748 case TCL: return "TCL/TK License"; 5749 case TCP_WRAPPERS: return "TCP Wrappers License"; 5750 case TERMREADKEY: return "TermReadKey License"; 5751 case TMATE: return "TMate Open Source License"; 5752 case TORQUE_1_1: return "TORQUE v2.5+ Software License v1.1"; 5753 case TOSL: return "Trusster Open Source License"; 5754 case TPDL: return "Time::ParseDate License"; 5755 case TPL_1_0: return "THOR Public License 1.0"; 5756 case TTWL: return "Text-Tabs+Wrap License"; 5757 case TU_BERLIN_1_0: return "Technische Universitaet Berlin License 1.0"; 5758 case TU_BERLIN_2_0: return "Technische Universitaet Berlin License 2.0"; 5759 case UCAR: return "UCAR License"; 5760 case UCL_1_0: return "Upstream Compatibility License v1.0"; 5761 case UNICODE_DFS_2015: return "Unicode License Agreement - Data Files and Software (2015)"; 5762 case UNICODE_DFS_2016: return "Unicode License Agreement - Data Files and Software (2016)"; 5763 case UNICODE_TOU: return "Unicode Terms of Use"; 5764 case UNIXCRYPT: return "UnixCrypt License"; 5765 case UNLICENSE: return "The Unlicense"; 5766 case UPL_1_0: return "Universal Permissive License v1.0"; 5767 case VIM: return "Vim License"; 5768 case VOSTROM: return "VOSTROM Public License for Open Source"; 5769 case VSL_1_0: return "Vovida Software License v1.0"; 5770 case W3C: return "W3C Software Notice and License (2002-12-31)"; 5771 case W3C_19980720: return "W3C Software Notice and License (1998-07-20)"; 5772 case W3C_20150513: return "W3C Software Notice and Document License (2015-05-13)"; 5773 case W3M: return "w3m License"; 5774 case WATCOM_1_0: return "Sybase Open Watcom Public License 1.0"; 5775 case WIDGET_WORKSHOP: return "Widget Workshop License"; 5776 case WSUIPA: return "Wsuipa License"; 5777 case WTFPL: return "Do What The F*ck You Want To Public License"; 5778 case WXWINDOWS: return "wxWindows Library License"; 5779 case X11: return "X11 License"; 5780 case X11_DISTRIBUTE_MODIFICATIONS_VARIANT: return "X11 License Distribution Modification Variant"; 5781 case XDEBUG_1_03: return "Xdebug License v 1.03"; 5782 case XEROX: return "Xerox License"; 5783 case XFIG: return "Xfig License"; 5784 case XFREE86_1_1: return "XFree86 License 1.1"; 5785 case XINETD: return "xinetd License"; 5786 case XLOCK: return "xlock License"; 5787 case XNET: return "X.Net License"; 5788 case XPP: return "XPP License"; 5789 case XSKAT: return "XSkat License"; 5790 case YPL_1_0: return "Yahoo! Public License v1.0"; 5791 case YPL_1_1: return "Yahoo! Public License v1.1"; 5792 case ZED: return "Zed License"; 5793 case ZEND_2_0: return "Zend License v2.0"; 5794 case ZIMBRA_1_3: return "Zimbra Public License v1.3"; 5795 case ZIMBRA_1_4: return "Zimbra Public License v1.4"; 5796 case ZLIB: return "zlib License"; 5797 case ZLIB_ACKNOWLEDGEMENT: return "zlib/libpng License with Acknowledgement"; 5798 case ZPL_1_1: return "Zope Public License 1.1"; 5799 case ZPL_2_0: return "Zope Public License 2.0"; 5800 case ZPL_2_1: return "Zope Public License 2.1"; 5801 case NULL: return null; 5802 default: return "?"; 5803 } 5804 } 5805 } 5806 5807 public static class SPDXLicenseEnumFactory implements EnumFactory<SPDXLicense> { 5808 public SPDXLicense fromCode(String codeString) throws IllegalArgumentException { 5809 if (codeString == null || "".equals(codeString)) 5810 if (codeString == null || "".equals(codeString)) 5811 return null; 5812 if ("0BSD".equals(codeString)) 5813 return SPDXLicense._0BSD; 5814 if ("AAL".equals(codeString)) 5815 return SPDXLicense.AAL; 5816 if ("Abstyles".equals(codeString)) 5817 return SPDXLicense.ABSTYLES; 5818 if ("AdaCore-doc".equals(codeString)) 5819 return SPDXLicense.ADACORE_DOC; 5820 if ("Adobe-2006".equals(codeString)) 5821 return SPDXLicense.ADOBE_2006; 5822 if ("Adobe-Glyph".equals(codeString)) 5823 return SPDXLicense.ADOBE_GLYPH; 5824 if ("ADSL".equals(codeString)) 5825 return SPDXLicense.ADSL; 5826 if ("AFL-1.1".equals(codeString)) 5827 return SPDXLicense.AFL_1_1; 5828 if ("AFL-1.2".equals(codeString)) 5829 return SPDXLicense.AFL_1_2; 5830 if ("AFL-2.0".equals(codeString)) 5831 return SPDXLicense.AFL_2_0; 5832 if ("AFL-2.1".equals(codeString)) 5833 return SPDXLicense.AFL_2_1; 5834 if ("AFL-3.0".equals(codeString)) 5835 return SPDXLicense.AFL_3_0; 5836 if ("Afmparse".equals(codeString)) 5837 return SPDXLicense.AFMPARSE; 5838 if ("AGPL-1.0".equals(codeString)) 5839 return SPDXLicense.AGPL_1_0; 5840 if ("AGPL-1.0-only".equals(codeString)) 5841 return SPDXLicense.AGPL_1_0_ONLY; 5842 if ("AGPL-1.0-or-later".equals(codeString)) 5843 return SPDXLicense.AGPL_1_0_OR_LATER; 5844 if ("AGPL-3.0".equals(codeString)) 5845 return SPDXLicense.AGPL_3_0; 5846 if ("AGPL-3.0-only".equals(codeString)) 5847 return SPDXLicense.AGPL_3_0_ONLY; 5848 if ("AGPL-3.0-or-later".equals(codeString)) 5849 return SPDXLicense.AGPL_3_0_OR_LATER; 5850 if ("Aladdin".equals(codeString)) 5851 return SPDXLicense.ALADDIN; 5852 if ("AMDPLPA".equals(codeString)) 5853 return SPDXLicense.AMDPLPA; 5854 if ("AML".equals(codeString)) 5855 return SPDXLicense.AML; 5856 if ("AMPAS".equals(codeString)) 5857 return SPDXLicense.AMPAS; 5858 if ("ANTLR-PD".equals(codeString)) 5859 return SPDXLicense.ANTLR_PD; 5860 if ("ANTLR-PD-fallback".equals(codeString)) 5861 return SPDXLicense.ANTLR_PD_FALLBACK; 5862 if ("Apache-1.0".equals(codeString)) 5863 return SPDXLicense.APACHE_1_0; 5864 if ("Apache-1.1".equals(codeString)) 5865 return SPDXLicense.APACHE_1_1; 5866 if ("Apache-2.0".equals(codeString)) 5867 return SPDXLicense.APACHE_2_0; 5868 if ("APAFML".equals(codeString)) 5869 return SPDXLicense.APAFML; 5870 if ("APL-1.0".equals(codeString)) 5871 return SPDXLicense.APL_1_0; 5872 if ("App-s2p".equals(codeString)) 5873 return SPDXLicense.APP_S2P; 5874 if ("APSL-1.0".equals(codeString)) 5875 return SPDXLicense.APSL_1_0; 5876 if ("APSL-1.1".equals(codeString)) 5877 return SPDXLicense.APSL_1_1; 5878 if ("APSL-1.2".equals(codeString)) 5879 return SPDXLicense.APSL_1_2; 5880 if ("APSL-2.0".equals(codeString)) 5881 return SPDXLicense.APSL_2_0; 5882 if ("Arphic-1999".equals(codeString)) 5883 return SPDXLicense.ARPHIC_1999; 5884 if ("Artistic-1.0".equals(codeString)) 5885 return SPDXLicense.ARTISTIC_1_0; 5886 if ("Artistic-1.0-cl8".equals(codeString)) 5887 return SPDXLicense.ARTISTIC_1_0_CL8; 5888 if ("Artistic-1.0-Perl".equals(codeString)) 5889 return SPDXLicense.ARTISTIC_1_0_PERL; 5890 if ("Artistic-2.0".equals(codeString)) 5891 return SPDXLicense.ARTISTIC_2_0; 5892 if ("ASWF-Digital-Assets-1.0".equals(codeString)) 5893 return SPDXLicense.ASWF_DIGITAL_ASSETS_1_0; 5894 if ("ASWF-Digital-Assets-1.1".equals(codeString)) 5895 return SPDXLicense.ASWF_DIGITAL_ASSETS_1_1; 5896 if ("Baekmuk".equals(codeString)) 5897 return SPDXLicense.BAEKMUK; 5898 if ("Bahyph".equals(codeString)) 5899 return SPDXLicense.BAHYPH; 5900 if ("Barr".equals(codeString)) 5901 return SPDXLicense.BARR; 5902 if ("Beerware".equals(codeString)) 5903 return SPDXLicense.BEERWARE; 5904 if ("Bitstream-Charter".equals(codeString)) 5905 return SPDXLicense.BITSTREAM_CHARTER; 5906 if ("Bitstream-Vera".equals(codeString)) 5907 return SPDXLicense.BITSTREAM_VERA; 5908 if ("BitTorrent-1.0".equals(codeString)) 5909 return SPDXLicense.BITTORRENT_1_0; 5910 if ("BitTorrent-1.1".equals(codeString)) 5911 return SPDXLicense.BITTORRENT_1_1; 5912 if ("blessing".equals(codeString)) 5913 return SPDXLicense.BLESSING; 5914 if ("BlueOak-1.0.0".equals(codeString)) 5915 return SPDXLicense.BLUEOAK_1_0_0; 5916 if ("Boehm-GC".equals(codeString)) 5917 return SPDXLicense.BOEHM_GC; 5918 if ("Borceux".equals(codeString)) 5919 return SPDXLicense.BORCEUX; 5920 if ("Brian-Gladman-3-Clause".equals(codeString)) 5921 return SPDXLicense.BRIAN_GLADMAN_3_CLAUSE; 5922 if ("BSD-1-Clause".equals(codeString)) 5923 return SPDXLicense.BSD_1_CLAUSE; 5924 if ("BSD-2-Clause".equals(codeString)) 5925 return SPDXLicense.BSD_2_CLAUSE; 5926 if ("BSD-2-Clause-FreeBSD".equals(codeString)) 5927 return SPDXLicense.BSD_2_CLAUSE_FREEBSD; 5928 if ("BSD-2-Clause-NetBSD".equals(codeString)) 5929 return SPDXLicense.BSD_2_CLAUSE_NETBSD; 5930 if ("BSD-2-Clause-Patent".equals(codeString)) 5931 return SPDXLicense.BSD_2_CLAUSE_PATENT; 5932 if ("BSD-2-Clause-Views".equals(codeString)) 5933 return SPDXLicense.BSD_2_CLAUSE_VIEWS; 5934 if ("BSD-3-Clause".equals(codeString)) 5935 return SPDXLicense.BSD_3_CLAUSE; 5936 if ("BSD-3-Clause-Attribution".equals(codeString)) 5937 return SPDXLicense.BSD_3_CLAUSE_ATTRIBUTION; 5938 if ("BSD-3-Clause-Clear".equals(codeString)) 5939 return SPDXLicense.BSD_3_CLAUSE_CLEAR; 5940 if ("BSD-3-Clause-LBNL".equals(codeString)) 5941 return SPDXLicense.BSD_3_CLAUSE_LBNL; 5942 if ("BSD-3-Clause-Modification".equals(codeString)) 5943 return SPDXLicense.BSD_3_CLAUSE_MODIFICATION; 5944 if ("BSD-3-Clause-No-Military-License".equals(codeString)) 5945 return SPDXLicense.BSD_3_CLAUSE_NO_MILITARY_LICENSE; 5946 if ("BSD-3-Clause-No-Nuclear-License".equals(codeString)) 5947 return SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_LICENSE; 5948 if ("BSD-3-Clause-No-Nuclear-License-2014".equals(codeString)) 5949 return SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014; 5950 if ("BSD-3-Clause-No-Nuclear-Warranty".equals(codeString)) 5951 return SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY; 5952 if ("BSD-3-Clause-Open-MPI".equals(codeString)) 5953 return SPDXLicense.BSD_3_CLAUSE_OPEN_MPI; 5954 if ("BSD-4-Clause".equals(codeString)) 5955 return SPDXLicense.BSD_4_CLAUSE; 5956 if ("BSD-4-Clause-Shortened".equals(codeString)) 5957 return SPDXLicense.BSD_4_CLAUSE_SHORTENED; 5958 if ("BSD-4-Clause-UC".equals(codeString)) 5959 return SPDXLicense.BSD_4_CLAUSE_UC; 5960 if ("BSD-4.3RENO".equals(codeString)) 5961 return SPDXLicense.BSD_4_3RENO; 5962 if ("BSD-4.3TAHOE".equals(codeString)) 5963 return SPDXLicense.BSD_4_3TAHOE; 5964 if ("BSD-Advertising-Acknowledgement".equals(codeString)) 5965 return SPDXLicense.BSD_ADVERTISING_ACKNOWLEDGEMENT; 5966 if ("BSD-Attribution-HPND-disclaimer".equals(codeString)) 5967 return SPDXLicense.BSD_ATTRIBUTION_HPND_DISCLAIMER; 5968 if ("BSD-Protection".equals(codeString)) 5969 return SPDXLicense.BSD_PROTECTION; 5970 if ("BSD-Source-Code".equals(codeString)) 5971 return SPDXLicense.BSD_SOURCE_CODE; 5972 if ("BSL-1.0".equals(codeString)) 5973 return SPDXLicense.BSL_1_0; 5974 if ("BUSL-1.1".equals(codeString)) 5975 return SPDXLicense.BUSL_1_1; 5976 if ("bzip2-1.0.5".equals(codeString)) 5977 return SPDXLicense.BZIP2_1_0_5; 5978 if ("bzip2-1.0.6".equals(codeString)) 5979 return SPDXLicense.BZIP2_1_0_6; 5980 if ("C-UDA-1.0".equals(codeString)) 5981 return SPDXLicense.C_UDA_1_0; 5982 if ("CAL-1.0".equals(codeString)) 5983 return SPDXLicense.CAL_1_0; 5984 if ("CAL-1.0-Combined-Work-Exception".equals(codeString)) 5985 return SPDXLicense.CAL_1_0_COMBINED_WORK_EXCEPTION; 5986 if ("Caldera".equals(codeString)) 5987 return SPDXLicense.CALDERA; 5988 if ("CATOSL-1.1".equals(codeString)) 5989 return SPDXLicense.CATOSL_1_1; 5990 if ("CC-BY-1.0".equals(codeString)) 5991 return SPDXLicense.CC_BY_1_0; 5992 if ("CC-BY-2.0".equals(codeString)) 5993 return SPDXLicense.CC_BY_2_0; 5994 if ("CC-BY-2.5".equals(codeString)) 5995 return SPDXLicense.CC_BY_2_5; 5996 if ("CC-BY-2.5-AU".equals(codeString)) 5997 return SPDXLicense.CC_BY_2_5_AU; 5998 if ("CC-BY-3.0".equals(codeString)) 5999 return SPDXLicense.CC_BY_3_0; 6000 if ("CC-BY-3.0-AT".equals(codeString)) 6001 return SPDXLicense.CC_BY_3_0_AT; 6002 if ("CC-BY-3.0-DE".equals(codeString)) 6003 return SPDXLicense.CC_BY_3_0_DE; 6004 if ("CC-BY-3.0-IGO".equals(codeString)) 6005 return SPDXLicense.CC_BY_3_0_IGO; 6006 if ("CC-BY-3.0-NL".equals(codeString)) 6007 return SPDXLicense.CC_BY_3_0_NL; 6008 if ("CC-BY-3.0-US".equals(codeString)) 6009 return SPDXLicense.CC_BY_3_0_US; 6010 if ("CC-BY-4.0".equals(codeString)) 6011 return SPDXLicense.CC_BY_4_0; 6012 if ("CC-BY-NC-1.0".equals(codeString)) 6013 return SPDXLicense.CC_BY_NC_1_0; 6014 if ("CC-BY-NC-2.0".equals(codeString)) 6015 return SPDXLicense.CC_BY_NC_2_0; 6016 if ("CC-BY-NC-2.5".equals(codeString)) 6017 return SPDXLicense.CC_BY_NC_2_5; 6018 if ("CC-BY-NC-3.0".equals(codeString)) 6019 return SPDXLicense.CC_BY_NC_3_0; 6020 if ("CC-BY-NC-3.0-DE".equals(codeString)) 6021 return SPDXLicense.CC_BY_NC_3_0_DE; 6022 if ("CC-BY-NC-4.0".equals(codeString)) 6023 return SPDXLicense.CC_BY_NC_4_0; 6024 if ("CC-BY-NC-ND-1.0".equals(codeString)) 6025 return SPDXLicense.CC_BY_NC_ND_1_0; 6026 if ("CC-BY-NC-ND-2.0".equals(codeString)) 6027 return SPDXLicense.CC_BY_NC_ND_2_0; 6028 if ("CC-BY-NC-ND-2.5".equals(codeString)) 6029 return SPDXLicense.CC_BY_NC_ND_2_5; 6030 if ("CC-BY-NC-ND-3.0".equals(codeString)) 6031 return SPDXLicense.CC_BY_NC_ND_3_0; 6032 if ("CC-BY-NC-ND-3.0-DE".equals(codeString)) 6033 return SPDXLicense.CC_BY_NC_ND_3_0_DE; 6034 if ("CC-BY-NC-ND-3.0-IGO".equals(codeString)) 6035 return SPDXLicense.CC_BY_NC_ND_3_0_IGO; 6036 if ("CC-BY-NC-ND-4.0".equals(codeString)) 6037 return SPDXLicense.CC_BY_NC_ND_4_0; 6038 if ("CC-BY-NC-SA-1.0".equals(codeString)) 6039 return SPDXLicense.CC_BY_NC_SA_1_0; 6040 if ("CC-BY-NC-SA-2.0".equals(codeString)) 6041 return SPDXLicense.CC_BY_NC_SA_2_0; 6042 if ("CC-BY-NC-SA-2.0-DE".equals(codeString)) 6043 return SPDXLicense.CC_BY_NC_SA_2_0_DE; 6044 if ("CC-BY-NC-SA-2.0-FR".equals(codeString)) 6045 return SPDXLicense.CC_BY_NC_SA_2_0_FR; 6046 if ("CC-BY-NC-SA-2.0-UK".equals(codeString)) 6047 return SPDXLicense.CC_BY_NC_SA_2_0_UK; 6048 if ("CC-BY-NC-SA-2.5".equals(codeString)) 6049 return SPDXLicense.CC_BY_NC_SA_2_5; 6050 if ("CC-BY-NC-SA-3.0".equals(codeString)) 6051 return SPDXLicense.CC_BY_NC_SA_3_0; 6052 if ("CC-BY-NC-SA-3.0-DE".equals(codeString)) 6053 return SPDXLicense.CC_BY_NC_SA_3_0_DE; 6054 if ("CC-BY-NC-SA-3.0-IGO".equals(codeString)) 6055 return SPDXLicense.CC_BY_NC_SA_3_0_IGO; 6056 if ("CC-BY-NC-SA-4.0".equals(codeString)) 6057 return SPDXLicense.CC_BY_NC_SA_4_0; 6058 if ("CC-BY-ND-1.0".equals(codeString)) 6059 return SPDXLicense.CC_BY_ND_1_0; 6060 if ("CC-BY-ND-2.0".equals(codeString)) 6061 return SPDXLicense.CC_BY_ND_2_0; 6062 if ("CC-BY-ND-2.5".equals(codeString)) 6063 return SPDXLicense.CC_BY_ND_2_5; 6064 if ("CC-BY-ND-3.0".equals(codeString)) 6065 return SPDXLicense.CC_BY_ND_3_0; 6066 if ("CC-BY-ND-3.0-DE".equals(codeString)) 6067 return SPDXLicense.CC_BY_ND_3_0_DE; 6068 if ("CC-BY-ND-4.0".equals(codeString)) 6069 return SPDXLicense.CC_BY_ND_4_0; 6070 if ("CC-BY-SA-1.0".equals(codeString)) 6071 return SPDXLicense.CC_BY_SA_1_0; 6072 if ("CC-BY-SA-2.0".equals(codeString)) 6073 return SPDXLicense.CC_BY_SA_2_0; 6074 if ("CC-BY-SA-2.0-UK".equals(codeString)) 6075 return SPDXLicense.CC_BY_SA_2_0_UK; 6076 if ("CC-BY-SA-2.1-JP".equals(codeString)) 6077 return SPDXLicense.CC_BY_SA_2_1_JP; 6078 if ("CC-BY-SA-2.5".equals(codeString)) 6079 return SPDXLicense.CC_BY_SA_2_5; 6080 if ("CC-BY-SA-3.0".equals(codeString)) 6081 return SPDXLicense.CC_BY_SA_3_0; 6082 if ("CC-BY-SA-3.0-AT".equals(codeString)) 6083 return SPDXLicense.CC_BY_SA_3_0_AT; 6084 if ("CC-BY-SA-3.0-DE".equals(codeString)) 6085 return SPDXLicense.CC_BY_SA_3_0_DE; 6086 if ("CC-BY-SA-3.0-IGO".equals(codeString)) 6087 return SPDXLicense.CC_BY_SA_3_0_IGO; 6088 if ("CC-BY-SA-4.0".equals(codeString)) 6089 return SPDXLicense.CC_BY_SA_4_0; 6090 if ("CC-PDDC".equals(codeString)) 6091 return SPDXLicense.CC_PDDC; 6092 if ("CC0-1.0".equals(codeString)) 6093 return SPDXLicense.CC0_1_0; 6094 if ("CDDL-1.0".equals(codeString)) 6095 return SPDXLicense.CDDL_1_0; 6096 if ("CDDL-1.1".equals(codeString)) 6097 return SPDXLicense.CDDL_1_1; 6098 if ("CDL-1.0".equals(codeString)) 6099 return SPDXLicense.CDL_1_0; 6100 if ("CDLA-Permissive-1.0".equals(codeString)) 6101 return SPDXLicense.CDLA_PERMISSIVE_1_0; 6102 if ("CDLA-Permissive-2.0".equals(codeString)) 6103 return SPDXLicense.CDLA_PERMISSIVE_2_0; 6104 if ("CDLA-Sharing-1.0".equals(codeString)) 6105 return SPDXLicense.CDLA_SHARING_1_0; 6106 if ("CECILL-1.0".equals(codeString)) 6107 return SPDXLicense.CECILL_1_0; 6108 if ("CECILL-1.1".equals(codeString)) 6109 return SPDXLicense.CECILL_1_1; 6110 if ("CECILL-2.0".equals(codeString)) 6111 return SPDXLicense.CECILL_2_0; 6112 if ("CECILL-2.1".equals(codeString)) 6113 return SPDXLicense.CECILL_2_1; 6114 if ("CECILL-B".equals(codeString)) 6115 return SPDXLicense.CECILL_B; 6116 if ("CECILL-C".equals(codeString)) 6117 return SPDXLicense.CECILL_C; 6118 if ("CERN-OHL-1.1".equals(codeString)) 6119 return SPDXLicense.CERN_OHL_1_1; 6120 if ("CERN-OHL-1.2".equals(codeString)) 6121 return SPDXLicense.CERN_OHL_1_2; 6122 if ("CERN-OHL-P-2.0".equals(codeString)) 6123 return SPDXLicense.CERN_OHL_P_2_0; 6124 if ("CERN-OHL-S-2.0".equals(codeString)) 6125 return SPDXLicense.CERN_OHL_S_2_0; 6126 if ("CERN-OHL-W-2.0".equals(codeString)) 6127 return SPDXLicense.CERN_OHL_W_2_0; 6128 if ("CFITSIO".equals(codeString)) 6129 return SPDXLicense.CFITSIO; 6130 if ("checkmk".equals(codeString)) 6131 return SPDXLicense.CHECKMK; 6132 if ("ClArtistic".equals(codeString)) 6133 return SPDXLicense.CLARTISTIC; 6134 if ("Clips".equals(codeString)) 6135 return SPDXLicense.CLIPS; 6136 if ("CMU-Mach".equals(codeString)) 6137 return SPDXLicense.CMU_MACH; 6138 if ("CNRI-Jython".equals(codeString)) 6139 return SPDXLicense.CNRI_JYTHON; 6140 if ("CNRI-Python".equals(codeString)) 6141 return SPDXLicense.CNRI_PYTHON; 6142 if ("CNRI-Python-GPL-Compatible".equals(codeString)) 6143 return SPDXLicense.CNRI_PYTHON_GPL_COMPATIBLE; 6144 if ("COIL-1.0".equals(codeString)) 6145 return SPDXLicense.COIL_1_0; 6146 if ("Community-Spec-1.0".equals(codeString)) 6147 return SPDXLicense.COMMUNITY_SPEC_1_0; 6148 if ("Condor-1.1".equals(codeString)) 6149 return SPDXLicense.CONDOR_1_1; 6150 if ("copyleft-next-0.3.0".equals(codeString)) 6151 return SPDXLicense.COPYLEFT_NEXT_0_3_0; 6152 if ("copyleft-next-0.3.1".equals(codeString)) 6153 return SPDXLicense.COPYLEFT_NEXT_0_3_1; 6154 if ("Cornell-Lossless-JPEG".equals(codeString)) 6155 return SPDXLicense.CORNELL_LOSSLESS_JPEG; 6156 if ("CPAL-1.0".equals(codeString)) 6157 return SPDXLicense.CPAL_1_0; 6158 if ("CPL-1.0".equals(codeString)) 6159 return SPDXLicense.CPL_1_0; 6160 if ("CPOL-1.02".equals(codeString)) 6161 return SPDXLicense.CPOL_1_02; 6162 if ("Crossword".equals(codeString)) 6163 return SPDXLicense.CROSSWORD; 6164 if ("CrystalStacker".equals(codeString)) 6165 return SPDXLicense.CRYSTALSTACKER; 6166 if ("CUA-OPL-1.0".equals(codeString)) 6167 return SPDXLicense.CUA_OPL_1_0; 6168 if ("Cube".equals(codeString)) 6169 return SPDXLicense.CUBE; 6170 if ("curl".equals(codeString)) 6171 return SPDXLicense.CURL; 6172 if ("D-FSL-1.0".equals(codeString)) 6173 return SPDXLicense.D_FSL_1_0; 6174 if ("diffmark".equals(codeString)) 6175 return SPDXLicense.DIFFMARK; 6176 if ("DL-DE-BY-2.0".equals(codeString)) 6177 return SPDXLicense.DL_DE_BY_2_0; 6178 if ("DOC".equals(codeString)) 6179 return SPDXLicense.DOC; 6180 if ("Dotseqn".equals(codeString)) 6181 return SPDXLicense.DOTSEQN; 6182 if ("DRL-1.0".equals(codeString)) 6183 return SPDXLicense.DRL_1_0; 6184 if ("DSDP".equals(codeString)) 6185 return SPDXLicense.DSDP; 6186 if ("dtoa".equals(codeString)) 6187 return SPDXLicense.DTOA; 6188 if ("dvipdfm".equals(codeString)) 6189 return SPDXLicense.DVIPDFM; 6190 if ("ECL-1.0".equals(codeString)) 6191 return SPDXLicense.ECL_1_0; 6192 if ("ECL-2.0".equals(codeString)) 6193 return SPDXLicense.ECL_2_0; 6194 if ("eCos-2.0".equals(codeString)) 6195 return SPDXLicense.ECOS_2_0; 6196 if ("EFL-1.0".equals(codeString)) 6197 return SPDXLicense.EFL_1_0; 6198 if ("EFL-2.0".equals(codeString)) 6199 return SPDXLicense.EFL_2_0; 6200 if ("eGenix".equals(codeString)) 6201 return SPDXLicense.EGENIX; 6202 if ("Elastic-2.0".equals(codeString)) 6203 return SPDXLicense.ELASTIC_2_0; 6204 if ("Entessa".equals(codeString)) 6205 return SPDXLicense.ENTESSA; 6206 if ("EPICS".equals(codeString)) 6207 return SPDXLicense.EPICS; 6208 if ("EPL-1.0".equals(codeString)) 6209 return SPDXLicense.EPL_1_0; 6210 if ("EPL-2.0".equals(codeString)) 6211 return SPDXLicense.EPL_2_0; 6212 if ("ErlPL-1.1".equals(codeString)) 6213 return SPDXLicense.ERLPL_1_1; 6214 if ("etalab-2.0".equals(codeString)) 6215 return SPDXLicense.ETALAB_2_0; 6216 if ("EUDatagrid".equals(codeString)) 6217 return SPDXLicense.EUDATAGRID; 6218 if ("EUPL-1.0".equals(codeString)) 6219 return SPDXLicense.EUPL_1_0; 6220 if ("EUPL-1.1".equals(codeString)) 6221 return SPDXLicense.EUPL_1_1; 6222 if ("EUPL-1.2".equals(codeString)) 6223 return SPDXLicense.EUPL_1_2; 6224 if ("Eurosym".equals(codeString)) 6225 return SPDXLicense.EUROSYM; 6226 if ("Fair".equals(codeString)) 6227 return SPDXLicense.FAIR; 6228 if ("FDK-AAC".equals(codeString)) 6229 return SPDXLicense.FDK_AAC; 6230 if ("Frameworx-1.0".equals(codeString)) 6231 return SPDXLicense.FRAMEWORX_1_0; 6232 if ("FreeBSD-DOC".equals(codeString)) 6233 return SPDXLicense.FREEBSD_DOC; 6234 if ("FreeImage".equals(codeString)) 6235 return SPDXLicense.FREEIMAGE; 6236 if ("FSFAP".equals(codeString)) 6237 return SPDXLicense.FSFAP; 6238 if ("FSFUL".equals(codeString)) 6239 return SPDXLicense.FSFUL; 6240 if ("FSFULLR".equals(codeString)) 6241 return SPDXLicense.FSFULLR; 6242 if ("FSFULLRWD".equals(codeString)) 6243 return SPDXLicense.FSFULLRWD; 6244 if ("FTL".equals(codeString)) 6245 return SPDXLicense.FTL; 6246 if ("GD".equals(codeString)) 6247 return SPDXLicense.GD; 6248 if ("GFDL-1.1".equals(codeString)) 6249 return SPDXLicense.GFDL_1_1; 6250 if ("GFDL-1.1-invariants-only".equals(codeString)) 6251 return SPDXLicense.GFDL_1_1_INVARIANTS_ONLY; 6252 if ("GFDL-1.1-invariants-or-later".equals(codeString)) 6253 return SPDXLicense.GFDL_1_1_INVARIANTS_OR_LATER; 6254 if ("GFDL-1.1-no-invariants-only".equals(codeString)) 6255 return SPDXLicense.GFDL_1_1_NO_INVARIANTS_ONLY; 6256 if ("GFDL-1.1-no-invariants-or-later".equals(codeString)) 6257 return SPDXLicense.GFDL_1_1_NO_INVARIANTS_OR_LATER; 6258 if ("GFDL-1.1-only".equals(codeString)) 6259 return SPDXLicense.GFDL_1_1_ONLY; 6260 if ("GFDL-1.1-or-later".equals(codeString)) 6261 return SPDXLicense.GFDL_1_1_OR_LATER; 6262 if ("GFDL-1.2".equals(codeString)) 6263 return SPDXLicense.GFDL_1_2; 6264 if ("GFDL-1.2-invariants-only".equals(codeString)) 6265 return SPDXLicense.GFDL_1_2_INVARIANTS_ONLY; 6266 if ("GFDL-1.2-invariants-or-later".equals(codeString)) 6267 return SPDXLicense.GFDL_1_2_INVARIANTS_OR_LATER; 6268 if ("GFDL-1.2-no-invariants-only".equals(codeString)) 6269 return SPDXLicense.GFDL_1_2_NO_INVARIANTS_ONLY; 6270 if ("GFDL-1.2-no-invariants-or-later".equals(codeString)) 6271 return SPDXLicense.GFDL_1_2_NO_INVARIANTS_OR_LATER; 6272 if ("GFDL-1.2-only".equals(codeString)) 6273 return SPDXLicense.GFDL_1_2_ONLY; 6274 if ("GFDL-1.2-or-later".equals(codeString)) 6275 return SPDXLicense.GFDL_1_2_OR_LATER; 6276 if ("GFDL-1.3".equals(codeString)) 6277 return SPDXLicense.GFDL_1_3; 6278 if ("GFDL-1.3-invariants-only".equals(codeString)) 6279 return SPDXLicense.GFDL_1_3_INVARIANTS_ONLY; 6280 if ("GFDL-1.3-invariants-or-later".equals(codeString)) 6281 return SPDXLicense.GFDL_1_3_INVARIANTS_OR_LATER; 6282 if ("GFDL-1.3-no-invariants-only".equals(codeString)) 6283 return SPDXLicense.GFDL_1_3_NO_INVARIANTS_ONLY; 6284 if ("GFDL-1.3-no-invariants-or-later".equals(codeString)) 6285 return SPDXLicense.GFDL_1_3_NO_INVARIANTS_OR_LATER; 6286 if ("GFDL-1.3-only".equals(codeString)) 6287 return SPDXLicense.GFDL_1_3_ONLY; 6288 if ("GFDL-1.3-or-later".equals(codeString)) 6289 return SPDXLicense.GFDL_1_3_OR_LATER; 6290 if ("Giftware".equals(codeString)) 6291 return SPDXLicense.GIFTWARE; 6292 if ("GL2PS".equals(codeString)) 6293 return SPDXLicense.GL2PS; 6294 if ("Glide".equals(codeString)) 6295 return SPDXLicense.GLIDE; 6296 if ("Glulxe".equals(codeString)) 6297 return SPDXLicense.GLULXE; 6298 if ("GLWTPL".equals(codeString)) 6299 return SPDXLicense.GLWTPL; 6300 if ("gnuplot".equals(codeString)) 6301 return SPDXLicense.GNUPLOT; 6302 if ("GPL-1.0".equals(codeString)) 6303 return SPDXLicense.GPL_1_0; 6304 if ("GPL-1.0+".equals(codeString)) 6305 return SPDXLicense.GPL_1_0PLUS; 6306 if ("GPL-1.0-only".equals(codeString)) 6307 return SPDXLicense.GPL_1_0_ONLY; 6308 if ("GPL-1.0-or-later".equals(codeString)) 6309 return SPDXLicense.GPL_1_0_OR_LATER; 6310 if ("GPL-2.0".equals(codeString)) 6311 return SPDXLicense.GPL_2_0; 6312 if ("GPL-2.0+".equals(codeString)) 6313 return SPDXLicense.GPL_2_0PLUS; 6314 if ("GPL-2.0-only".equals(codeString)) 6315 return SPDXLicense.GPL_2_0_ONLY; 6316 if ("GPL-2.0-or-later".equals(codeString)) 6317 return SPDXLicense.GPL_2_0_OR_LATER; 6318 if ("GPL-2.0-with-autoconf-exception".equals(codeString)) 6319 return SPDXLicense.GPL_2_0_WITH_AUTOCONF_EXCEPTION; 6320 if ("GPL-2.0-with-bison-exception".equals(codeString)) 6321 return SPDXLicense.GPL_2_0_WITH_BISON_EXCEPTION; 6322 if ("GPL-2.0-with-classpath-exception".equals(codeString)) 6323 return SPDXLicense.GPL_2_0_WITH_CLASSPATH_EXCEPTION; 6324 if ("GPL-2.0-with-font-exception".equals(codeString)) 6325 return SPDXLicense.GPL_2_0_WITH_FONT_EXCEPTION; 6326 if ("GPL-2.0-with-GCC-exception".equals(codeString)) 6327 return SPDXLicense.GPL_2_0_WITH_GCC_EXCEPTION; 6328 if ("GPL-3.0".equals(codeString)) 6329 return SPDXLicense.GPL_3_0; 6330 if ("GPL-3.0+".equals(codeString)) 6331 return SPDXLicense.GPL_3_0PLUS; 6332 if ("GPL-3.0-only".equals(codeString)) 6333 return SPDXLicense.GPL_3_0_ONLY; 6334 if ("GPL-3.0-or-later".equals(codeString)) 6335 return SPDXLicense.GPL_3_0_OR_LATER; 6336 if ("GPL-3.0-with-autoconf-exception".equals(codeString)) 6337 return SPDXLicense.GPL_3_0_WITH_AUTOCONF_EXCEPTION; 6338 if ("GPL-3.0-with-GCC-exception".equals(codeString)) 6339 return SPDXLicense.GPL_3_0_WITH_GCC_EXCEPTION; 6340 if ("Graphics-Gems".equals(codeString)) 6341 return SPDXLicense.GRAPHICS_GEMS; 6342 if ("gSOAP-1.3b".equals(codeString)) 6343 return SPDXLicense.GSOAP_1_3B; 6344 if ("HaskellReport".equals(codeString)) 6345 return SPDXLicense.HASKELLREPORT; 6346 if ("Hippocratic-2.1".equals(codeString)) 6347 return SPDXLicense.HIPPOCRATIC_2_1; 6348 if ("HP-1986".equals(codeString)) 6349 return SPDXLicense.HP_1986; 6350 if ("HPND".equals(codeString)) 6351 return SPDXLicense.HPND; 6352 if ("HPND-export-US".equals(codeString)) 6353 return SPDXLicense.HPND_EXPORT_US; 6354 if ("HPND-Markus-Kuhn".equals(codeString)) 6355 return SPDXLicense.HPND_MARKUS_KUHN; 6356 if ("HPND-sell-variant".equals(codeString)) 6357 return SPDXLicense.HPND_SELL_VARIANT; 6358 if ("HPND-sell-variant-MIT-disclaimer".equals(codeString)) 6359 return SPDXLicense.HPND_SELL_VARIANT_MIT_DISCLAIMER; 6360 if ("HTMLTIDY".equals(codeString)) 6361 return SPDXLicense.HTMLTIDY; 6362 if ("IBM-pibs".equals(codeString)) 6363 return SPDXLicense.IBM_PIBS; 6364 if ("ICU".equals(codeString)) 6365 return SPDXLicense.ICU; 6366 if ("IEC-Code-Components-EULA".equals(codeString)) 6367 return SPDXLicense.IEC_CODE_COMPONENTS_EULA; 6368 if ("IJG".equals(codeString)) 6369 return SPDXLicense.IJG; 6370 if ("IJG-short".equals(codeString)) 6371 return SPDXLicense.IJG_SHORT; 6372 if ("ImageMagick".equals(codeString)) 6373 return SPDXLicense.IMAGEMAGICK; 6374 if ("iMatix".equals(codeString)) 6375 return SPDXLicense.IMATIX; 6376 if ("Imlib2".equals(codeString)) 6377 return SPDXLicense.IMLIB2; 6378 if ("Info-ZIP".equals(codeString)) 6379 return SPDXLicense.INFO_ZIP; 6380 if ("Inner-Net-2.0".equals(codeString)) 6381 return SPDXLicense.INNER_NET_2_0; 6382 if ("Intel".equals(codeString)) 6383 return SPDXLicense.INTEL; 6384 if ("Intel-ACPI".equals(codeString)) 6385 return SPDXLicense.INTEL_ACPI; 6386 if ("Interbase-1.0".equals(codeString)) 6387 return SPDXLicense.INTERBASE_1_0; 6388 if ("IPA".equals(codeString)) 6389 return SPDXLicense.IPA; 6390 if ("IPL-1.0".equals(codeString)) 6391 return SPDXLicense.IPL_1_0; 6392 if ("ISC".equals(codeString)) 6393 return SPDXLicense.ISC; 6394 if ("Jam".equals(codeString)) 6395 return SPDXLicense.JAM; 6396 if ("JasPer-2.0".equals(codeString)) 6397 return SPDXLicense.JASPER_2_0; 6398 if ("JPL-image".equals(codeString)) 6399 return SPDXLicense.JPL_IMAGE; 6400 if ("JPNIC".equals(codeString)) 6401 return SPDXLicense.JPNIC; 6402 if ("JSON".equals(codeString)) 6403 return SPDXLicense.JSON; 6404 if ("Kazlib".equals(codeString)) 6405 return SPDXLicense.KAZLIB; 6406 if ("Knuth-CTAN".equals(codeString)) 6407 return SPDXLicense.KNUTH_CTAN; 6408 if ("LAL-1.2".equals(codeString)) 6409 return SPDXLicense.LAL_1_2; 6410 if ("LAL-1.3".equals(codeString)) 6411 return SPDXLicense.LAL_1_3; 6412 if ("Latex2e".equals(codeString)) 6413 return SPDXLicense.LATEX2E; 6414 if ("Latex2e-translated-notice".equals(codeString)) 6415 return SPDXLicense.LATEX2E_TRANSLATED_NOTICE; 6416 if ("Leptonica".equals(codeString)) 6417 return SPDXLicense.LEPTONICA; 6418 if ("LGPL-2.0".equals(codeString)) 6419 return SPDXLicense.LGPL_2_0; 6420 if ("LGPL-2.0+".equals(codeString)) 6421 return SPDXLicense.LGPL_2_0PLUS; 6422 if ("LGPL-2.0-only".equals(codeString)) 6423 return SPDXLicense.LGPL_2_0_ONLY; 6424 if ("LGPL-2.0-or-later".equals(codeString)) 6425 return SPDXLicense.LGPL_2_0_OR_LATER; 6426 if ("LGPL-2.1".equals(codeString)) 6427 return SPDXLicense.LGPL_2_1; 6428 if ("LGPL-2.1+".equals(codeString)) 6429 return SPDXLicense.LGPL_2_1PLUS; 6430 if ("LGPL-2.1-only".equals(codeString)) 6431 return SPDXLicense.LGPL_2_1_ONLY; 6432 if ("LGPL-2.1-or-later".equals(codeString)) 6433 return SPDXLicense.LGPL_2_1_OR_LATER; 6434 if ("LGPL-3.0".equals(codeString)) 6435 return SPDXLicense.LGPL_3_0; 6436 if ("LGPL-3.0+".equals(codeString)) 6437 return SPDXLicense.LGPL_3_0PLUS; 6438 if ("LGPL-3.0-only".equals(codeString)) 6439 return SPDXLicense.LGPL_3_0_ONLY; 6440 if ("LGPL-3.0-or-later".equals(codeString)) 6441 return SPDXLicense.LGPL_3_0_OR_LATER; 6442 if ("LGPLLR".equals(codeString)) 6443 return SPDXLicense.LGPLLR; 6444 if ("Libpng".equals(codeString)) 6445 return SPDXLicense.LIBPNG; 6446 if ("libpng-2.0".equals(codeString)) 6447 return SPDXLicense.LIBPNG_2_0; 6448 if ("libselinux-1.0".equals(codeString)) 6449 return SPDXLicense.LIBSELINUX_1_0; 6450 if ("libtiff".equals(codeString)) 6451 return SPDXLicense.LIBTIFF; 6452 if ("libutil-David-Nugent".equals(codeString)) 6453 return SPDXLicense.LIBUTIL_DAVID_NUGENT; 6454 if ("LiLiQ-P-1.1".equals(codeString)) 6455 return SPDXLicense.LILIQ_P_1_1; 6456 if ("LiLiQ-R-1.1".equals(codeString)) 6457 return SPDXLicense.LILIQ_R_1_1; 6458 if ("LiLiQ-Rplus-1.1".equals(codeString)) 6459 return SPDXLicense.LILIQ_RPLUS_1_1; 6460 if ("Linux-man-pages-1-para".equals(codeString)) 6461 return SPDXLicense.LINUX_MAN_PAGES_1_PARA; 6462 if ("Linux-man-pages-copyleft".equals(codeString)) 6463 return SPDXLicense.LINUX_MAN_PAGES_COPYLEFT; 6464 if ("Linux-man-pages-copyleft-2-para".equals(codeString)) 6465 return SPDXLicense.LINUX_MAN_PAGES_COPYLEFT_2_PARA; 6466 if ("Linux-man-pages-copyleft-var".equals(codeString)) 6467 return SPDXLicense.LINUX_MAN_PAGES_COPYLEFT_VAR; 6468 if ("Linux-OpenIB".equals(codeString)) 6469 return SPDXLicense.LINUX_OPENIB; 6470 if ("LOOP".equals(codeString)) 6471 return SPDXLicense.LOOP; 6472 if ("LPL-1.0".equals(codeString)) 6473 return SPDXLicense.LPL_1_0; 6474 if ("LPL-1.02".equals(codeString)) 6475 return SPDXLicense.LPL_1_02; 6476 if ("LPPL-1.0".equals(codeString)) 6477 return SPDXLicense.LPPL_1_0; 6478 if ("LPPL-1.1".equals(codeString)) 6479 return SPDXLicense.LPPL_1_1; 6480 if ("LPPL-1.2".equals(codeString)) 6481 return SPDXLicense.LPPL_1_2; 6482 if ("LPPL-1.3a".equals(codeString)) 6483 return SPDXLicense.LPPL_1_3A; 6484 if ("LPPL-1.3c".equals(codeString)) 6485 return SPDXLicense.LPPL_1_3C; 6486 if ("LZMA-SDK-9.11-to-9.20".equals(codeString)) 6487 return SPDXLicense.LZMA_SDK_9_11_TO_9_20; 6488 if ("LZMA-SDK-9.22".equals(codeString)) 6489 return SPDXLicense.LZMA_SDK_9_22; 6490 if ("MakeIndex".equals(codeString)) 6491 return SPDXLicense.MAKEINDEX; 6492 if ("Martin-Birgmeier".equals(codeString)) 6493 return SPDXLicense.MARTIN_BIRGMEIER; 6494 if ("metamail".equals(codeString)) 6495 return SPDXLicense.METAMAIL; 6496 if ("Minpack".equals(codeString)) 6497 return SPDXLicense.MINPACK; 6498 if ("MirOS".equals(codeString)) 6499 return SPDXLicense.MIROS; 6500 if ("MIT".equals(codeString)) 6501 return SPDXLicense.MIT; 6502 if ("MIT-0".equals(codeString)) 6503 return SPDXLicense.MIT_0; 6504 if ("MIT-advertising".equals(codeString)) 6505 return SPDXLicense.MIT_ADVERTISING; 6506 if ("MIT-CMU".equals(codeString)) 6507 return SPDXLicense.MIT_CMU; 6508 if ("MIT-enna".equals(codeString)) 6509 return SPDXLicense.MIT_ENNA; 6510 if ("MIT-feh".equals(codeString)) 6511 return SPDXLicense.MIT_FEH; 6512 if ("MIT-Festival".equals(codeString)) 6513 return SPDXLicense.MIT_FESTIVAL; 6514 if ("MIT-Modern-Variant".equals(codeString)) 6515 return SPDXLicense.MIT_MODERN_VARIANT; 6516 if ("MIT-open-group".equals(codeString)) 6517 return SPDXLicense.MIT_OPEN_GROUP; 6518 if ("MIT-Wu".equals(codeString)) 6519 return SPDXLicense.MIT_WU; 6520 if ("MITNFA".equals(codeString)) 6521 return SPDXLicense.MITNFA; 6522 if ("Motosoto".equals(codeString)) 6523 return SPDXLicense.MOTOSOTO; 6524 if ("mpi-permissive".equals(codeString)) 6525 return SPDXLicense.MPI_PERMISSIVE; 6526 if ("mpich2".equals(codeString)) 6527 return SPDXLicense.MPICH2; 6528 if ("MPL-1.0".equals(codeString)) 6529 return SPDXLicense.MPL_1_0; 6530 if ("MPL-1.1".equals(codeString)) 6531 return SPDXLicense.MPL_1_1; 6532 if ("MPL-2.0".equals(codeString)) 6533 return SPDXLicense.MPL_2_0; 6534 if ("MPL-2.0-no-copyleft-exception".equals(codeString)) 6535 return SPDXLicense.MPL_2_0_NO_COPYLEFT_EXCEPTION; 6536 if ("mplus".equals(codeString)) 6537 return SPDXLicense.MPLUS; 6538 if ("MS-LPL".equals(codeString)) 6539 return SPDXLicense.MS_LPL; 6540 if ("MS-PL".equals(codeString)) 6541 return SPDXLicense.MS_PL; 6542 if ("MS-RL".equals(codeString)) 6543 return SPDXLicense.MS_RL; 6544 if ("MTLL".equals(codeString)) 6545 return SPDXLicense.MTLL; 6546 if ("MulanPSL-1.0".equals(codeString)) 6547 return SPDXLicense.MULANPSL_1_0; 6548 if ("MulanPSL-2.0".equals(codeString)) 6549 return SPDXLicense.MULANPSL_2_0; 6550 if ("Multics".equals(codeString)) 6551 return SPDXLicense.MULTICS; 6552 if ("Mup".equals(codeString)) 6553 return SPDXLicense.MUP; 6554 if ("NAIST-2003".equals(codeString)) 6555 return SPDXLicense.NAIST_2003; 6556 if ("NASA-1.3".equals(codeString)) 6557 return SPDXLicense.NASA_1_3; 6558 if ("Naumen".equals(codeString)) 6559 return SPDXLicense.NAUMEN; 6560 if ("NBPL-1.0".equals(codeString)) 6561 return SPDXLicense.NBPL_1_0; 6562 if ("NCGL-UK-2.0".equals(codeString)) 6563 return SPDXLicense.NCGL_UK_2_0; 6564 if ("NCSA".equals(codeString)) 6565 return SPDXLicense.NCSA; 6566 if ("Net-SNMP".equals(codeString)) 6567 return SPDXLicense.NET_SNMP; 6568 if ("NetCDF".equals(codeString)) 6569 return SPDXLicense.NETCDF; 6570 if ("Newsletr".equals(codeString)) 6571 return SPDXLicense.NEWSLETR; 6572 if ("NGPL".equals(codeString)) 6573 return SPDXLicense.NGPL; 6574 if ("NICTA-1.0".equals(codeString)) 6575 return SPDXLicense.NICTA_1_0; 6576 if ("NIST-PD".equals(codeString)) 6577 return SPDXLicense.NIST_PD; 6578 if ("NIST-PD-fallback".equals(codeString)) 6579 return SPDXLicense.NIST_PD_FALLBACK; 6580 if ("NIST-Software".equals(codeString)) 6581 return SPDXLicense.NIST_SOFTWARE; 6582 if ("NLOD-1.0".equals(codeString)) 6583 return SPDXLicense.NLOD_1_0; 6584 if ("NLOD-2.0".equals(codeString)) 6585 return SPDXLicense.NLOD_2_0; 6586 if ("NLPL".equals(codeString)) 6587 return SPDXLicense.NLPL; 6588 if ("Nokia".equals(codeString)) 6589 return SPDXLicense.NOKIA; 6590 if ("NOSL".equals(codeString)) 6591 return SPDXLicense.NOSL; 6592 if ("not-open-source".equals(codeString)) 6593 return SPDXLicense.NOT_OPEN_SOURCE; 6594 if ("Noweb".equals(codeString)) 6595 return SPDXLicense.NOWEB; 6596 if ("NPL-1.0".equals(codeString)) 6597 return SPDXLicense.NPL_1_0; 6598 if ("NPL-1.1".equals(codeString)) 6599 return SPDXLicense.NPL_1_1; 6600 if ("NPOSL-3.0".equals(codeString)) 6601 return SPDXLicense.NPOSL_3_0; 6602 if ("NRL".equals(codeString)) 6603 return SPDXLicense.NRL; 6604 if ("NTP".equals(codeString)) 6605 return SPDXLicense.NTP; 6606 if ("NTP-0".equals(codeString)) 6607 return SPDXLicense.NTP_0; 6608 if ("Nunit".equals(codeString)) 6609 return SPDXLicense.NUNIT; 6610 if ("O-UDA-1.0".equals(codeString)) 6611 return SPDXLicense.O_UDA_1_0; 6612 if ("OCCT-PL".equals(codeString)) 6613 return SPDXLicense.OCCT_PL; 6614 if ("OCLC-2.0".equals(codeString)) 6615 return SPDXLicense.OCLC_2_0; 6616 if ("ODbL-1.0".equals(codeString)) 6617 return SPDXLicense.ODBL_1_0; 6618 if ("ODC-By-1.0".equals(codeString)) 6619 return SPDXLicense.ODC_BY_1_0; 6620 if ("OFFIS".equals(codeString)) 6621 return SPDXLicense.OFFIS; 6622 if ("OFL-1.0".equals(codeString)) 6623 return SPDXLicense.OFL_1_0; 6624 if ("OFL-1.0-no-RFN".equals(codeString)) 6625 return SPDXLicense.OFL_1_0_NO_RFN; 6626 if ("OFL-1.0-RFN".equals(codeString)) 6627 return SPDXLicense.OFL_1_0_RFN; 6628 if ("OFL-1.1".equals(codeString)) 6629 return SPDXLicense.OFL_1_1; 6630 if ("OFL-1.1-no-RFN".equals(codeString)) 6631 return SPDXLicense.OFL_1_1_NO_RFN; 6632 if ("OFL-1.1-RFN".equals(codeString)) 6633 return SPDXLicense.OFL_1_1_RFN; 6634 if ("OGC-1.0".equals(codeString)) 6635 return SPDXLicense.OGC_1_0; 6636 if ("OGDL-Taiwan-1.0".equals(codeString)) 6637 return SPDXLicense.OGDL_TAIWAN_1_0; 6638 if ("OGL-Canada-2.0".equals(codeString)) 6639 return SPDXLicense.OGL_CANADA_2_0; 6640 if ("OGL-UK-1.0".equals(codeString)) 6641 return SPDXLicense.OGL_UK_1_0; 6642 if ("OGL-UK-2.0".equals(codeString)) 6643 return SPDXLicense.OGL_UK_2_0; 6644 if ("OGL-UK-3.0".equals(codeString)) 6645 return SPDXLicense.OGL_UK_3_0; 6646 if ("OGTSL".equals(codeString)) 6647 return SPDXLicense.OGTSL; 6648 if ("OLDAP-1.1".equals(codeString)) 6649 return SPDXLicense.OLDAP_1_1; 6650 if ("OLDAP-1.2".equals(codeString)) 6651 return SPDXLicense.OLDAP_1_2; 6652 if ("OLDAP-1.3".equals(codeString)) 6653 return SPDXLicense.OLDAP_1_3; 6654 if ("OLDAP-1.4".equals(codeString)) 6655 return SPDXLicense.OLDAP_1_4; 6656 if ("OLDAP-2.0".equals(codeString)) 6657 return SPDXLicense.OLDAP_2_0; 6658 if ("OLDAP-2.0.1".equals(codeString)) 6659 return SPDXLicense.OLDAP_2_0_1; 6660 if ("OLDAP-2.1".equals(codeString)) 6661 return SPDXLicense.OLDAP_2_1; 6662 if ("OLDAP-2.2".equals(codeString)) 6663 return SPDXLicense.OLDAP_2_2; 6664 if ("OLDAP-2.2.1".equals(codeString)) 6665 return SPDXLicense.OLDAP_2_2_1; 6666 if ("OLDAP-2.2.2".equals(codeString)) 6667 return SPDXLicense.OLDAP_2_2_2; 6668 if ("OLDAP-2.3".equals(codeString)) 6669 return SPDXLicense.OLDAP_2_3; 6670 if ("OLDAP-2.4".equals(codeString)) 6671 return SPDXLicense.OLDAP_2_4; 6672 if ("OLDAP-2.5".equals(codeString)) 6673 return SPDXLicense.OLDAP_2_5; 6674 if ("OLDAP-2.6".equals(codeString)) 6675 return SPDXLicense.OLDAP_2_6; 6676 if ("OLDAP-2.7".equals(codeString)) 6677 return SPDXLicense.OLDAP_2_7; 6678 if ("OLDAP-2.8".equals(codeString)) 6679 return SPDXLicense.OLDAP_2_8; 6680 if ("OLFL-1.3".equals(codeString)) 6681 return SPDXLicense.OLFL_1_3; 6682 if ("OML".equals(codeString)) 6683 return SPDXLicense.OML; 6684 if ("OpenPBS-2.3".equals(codeString)) 6685 return SPDXLicense.OPENPBS_2_3; 6686 if ("OpenSSL".equals(codeString)) 6687 return SPDXLicense.OPENSSL; 6688 if ("OPL-1.0".equals(codeString)) 6689 return SPDXLicense.OPL_1_0; 6690 if ("OPL-UK-3.0".equals(codeString)) 6691 return SPDXLicense.OPL_UK_3_0; 6692 if ("OPUBL-1.0".equals(codeString)) 6693 return SPDXLicense.OPUBL_1_0; 6694 if ("OSET-PL-2.1".equals(codeString)) 6695 return SPDXLicense.OSET_PL_2_1; 6696 if ("OSL-1.0".equals(codeString)) 6697 return SPDXLicense.OSL_1_0; 6698 if ("OSL-1.1".equals(codeString)) 6699 return SPDXLicense.OSL_1_1; 6700 if ("OSL-2.0".equals(codeString)) 6701 return SPDXLicense.OSL_2_0; 6702 if ("OSL-2.1".equals(codeString)) 6703 return SPDXLicense.OSL_2_1; 6704 if ("OSL-3.0".equals(codeString)) 6705 return SPDXLicense.OSL_3_0; 6706 if ("Parity-6.0.0".equals(codeString)) 6707 return SPDXLicense.PARITY_6_0_0; 6708 if ("Parity-7.0.0".equals(codeString)) 6709 return SPDXLicense.PARITY_7_0_0; 6710 if ("PDDL-1.0".equals(codeString)) 6711 return SPDXLicense.PDDL_1_0; 6712 if ("PHP-3.0".equals(codeString)) 6713 return SPDXLicense.PHP_3_0; 6714 if ("PHP-3.01".equals(codeString)) 6715 return SPDXLicense.PHP_3_01; 6716 if ("Plexus".equals(codeString)) 6717 return SPDXLicense.PLEXUS; 6718 if ("PolyForm-Noncommercial-1.0.0".equals(codeString)) 6719 return SPDXLicense.POLYFORM_NONCOMMERCIAL_1_0_0; 6720 if ("PolyForm-Small-Business-1.0.0".equals(codeString)) 6721 return SPDXLicense.POLYFORM_SMALL_BUSINESS_1_0_0; 6722 if ("PostgreSQL".equals(codeString)) 6723 return SPDXLicense.POSTGRESQL; 6724 if ("PSF-2.0".equals(codeString)) 6725 return SPDXLicense.PSF_2_0; 6726 if ("psfrag".equals(codeString)) 6727 return SPDXLicense.PSFRAG; 6728 if ("psutils".equals(codeString)) 6729 return SPDXLicense.PSUTILS; 6730 if ("Python-2.0".equals(codeString)) 6731 return SPDXLicense.PYTHON_2_0; 6732 if ("Python-2.0.1".equals(codeString)) 6733 return SPDXLicense.PYTHON_2_0_1; 6734 if ("Qhull".equals(codeString)) 6735 return SPDXLicense.QHULL; 6736 if ("QPL-1.0".equals(codeString)) 6737 return SPDXLicense.QPL_1_0; 6738 if ("QPL-1.0-INRIA-2004".equals(codeString)) 6739 return SPDXLicense.QPL_1_0_INRIA_2004; 6740 if ("Rdisc".equals(codeString)) 6741 return SPDXLicense.RDISC; 6742 if ("RHeCos-1.1".equals(codeString)) 6743 return SPDXLicense.RHECOS_1_1; 6744 if ("RPL-1.1".equals(codeString)) 6745 return SPDXLicense.RPL_1_1; 6746 if ("RPL-1.5".equals(codeString)) 6747 return SPDXLicense.RPL_1_5; 6748 if ("RPSL-1.0".equals(codeString)) 6749 return SPDXLicense.RPSL_1_0; 6750 if ("RSA-MD".equals(codeString)) 6751 return SPDXLicense.RSA_MD; 6752 if ("RSCPL".equals(codeString)) 6753 return SPDXLicense.RSCPL; 6754 if ("Ruby".equals(codeString)) 6755 return SPDXLicense.RUBY; 6756 if ("SAX-PD".equals(codeString)) 6757 return SPDXLicense.SAX_PD; 6758 if ("Saxpath".equals(codeString)) 6759 return SPDXLicense.SAXPATH; 6760 if ("SCEA".equals(codeString)) 6761 return SPDXLicense.SCEA; 6762 if ("SchemeReport".equals(codeString)) 6763 return SPDXLicense.SCHEMEREPORT; 6764 if ("Sendmail".equals(codeString)) 6765 return SPDXLicense.SENDMAIL; 6766 if ("Sendmail-8.23".equals(codeString)) 6767 return SPDXLicense.SENDMAIL_8_23; 6768 if ("SGI-B-1.0".equals(codeString)) 6769 return SPDXLicense.SGI_B_1_0; 6770 if ("SGI-B-1.1".equals(codeString)) 6771 return SPDXLicense.SGI_B_1_1; 6772 if ("SGI-B-2.0".equals(codeString)) 6773 return SPDXLicense.SGI_B_2_0; 6774 if ("SGP4".equals(codeString)) 6775 return SPDXLicense.SGP4; 6776 if ("SHL-0.5".equals(codeString)) 6777 return SPDXLicense.SHL_0_5; 6778 if ("SHL-0.51".equals(codeString)) 6779 return SPDXLicense.SHL_0_51; 6780 if ("SimPL-2.0".equals(codeString)) 6781 return SPDXLicense.SIMPL_2_0; 6782 if ("SISSL".equals(codeString)) 6783 return SPDXLicense.SISSL; 6784 if ("SISSL-1.2".equals(codeString)) 6785 return SPDXLicense.SISSL_1_2; 6786 if ("Sleepycat".equals(codeString)) 6787 return SPDXLicense.SLEEPYCAT; 6788 if ("SMLNJ".equals(codeString)) 6789 return SPDXLicense.SMLNJ; 6790 if ("SMPPL".equals(codeString)) 6791 return SPDXLicense.SMPPL; 6792 if ("SNIA".equals(codeString)) 6793 return SPDXLicense.SNIA; 6794 if ("snprintf".equals(codeString)) 6795 return SPDXLicense.SNPRINTF; 6796 if ("Spencer-86".equals(codeString)) 6797 return SPDXLicense.SPENCER_86; 6798 if ("Spencer-94".equals(codeString)) 6799 return SPDXLicense.SPENCER_94; 6800 if ("Spencer-99".equals(codeString)) 6801 return SPDXLicense.SPENCER_99; 6802 if ("SPL-1.0".equals(codeString)) 6803 return SPDXLicense.SPL_1_0; 6804 if ("SSH-OpenSSH".equals(codeString)) 6805 return SPDXLicense.SSH_OPENSSH; 6806 if ("SSH-short".equals(codeString)) 6807 return SPDXLicense.SSH_SHORT; 6808 if ("SSPL-1.0".equals(codeString)) 6809 return SPDXLicense.SSPL_1_0; 6810 if ("StandardML-NJ".equals(codeString)) 6811 return SPDXLicense.STANDARDML_NJ; 6812 if ("SugarCRM-1.1.3".equals(codeString)) 6813 return SPDXLicense.SUGARCRM_1_1_3; 6814 if ("SunPro".equals(codeString)) 6815 return SPDXLicense.SUNPRO; 6816 if ("SWL".equals(codeString)) 6817 return SPDXLicense.SWL; 6818 if ("Symlinks".equals(codeString)) 6819 return SPDXLicense.SYMLINKS; 6820 if ("TAPR-OHL-1.0".equals(codeString)) 6821 return SPDXLicense.TAPR_OHL_1_0; 6822 if ("TCL".equals(codeString)) 6823 return SPDXLicense.TCL; 6824 if ("TCP-wrappers".equals(codeString)) 6825 return SPDXLicense.TCP_WRAPPERS; 6826 if ("TermReadKey".equals(codeString)) 6827 return SPDXLicense.TERMREADKEY; 6828 if ("TMate".equals(codeString)) 6829 return SPDXLicense.TMATE; 6830 if ("TORQUE-1.1".equals(codeString)) 6831 return SPDXLicense.TORQUE_1_1; 6832 if ("TOSL".equals(codeString)) 6833 return SPDXLicense.TOSL; 6834 if ("TPDL".equals(codeString)) 6835 return SPDXLicense.TPDL; 6836 if ("TPL-1.0".equals(codeString)) 6837 return SPDXLicense.TPL_1_0; 6838 if ("TTWL".equals(codeString)) 6839 return SPDXLicense.TTWL; 6840 if ("TU-Berlin-1.0".equals(codeString)) 6841 return SPDXLicense.TU_BERLIN_1_0; 6842 if ("TU-Berlin-2.0".equals(codeString)) 6843 return SPDXLicense.TU_BERLIN_2_0; 6844 if ("UCAR".equals(codeString)) 6845 return SPDXLicense.UCAR; 6846 if ("UCL-1.0".equals(codeString)) 6847 return SPDXLicense.UCL_1_0; 6848 if ("Unicode-DFS-2015".equals(codeString)) 6849 return SPDXLicense.UNICODE_DFS_2015; 6850 if ("Unicode-DFS-2016".equals(codeString)) 6851 return SPDXLicense.UNICODE_DFS_2016; 6852 if ("Unicode-TOU".equals(codeString)) 6853 return SPDXLicense.UNICODE_TOU; 6854 if ("UnixCrypt".equals(codeString)) 6855 return SPDXLicense.UNIXCRYPT; 6856 if ("Unlicense".equals(codeString)) 6857 return SPDXLicense.UNLICENSE; 6858 if ("UPL-1.0".equals(codeString)) 6859 return SPDXLicense.UPL_1_0; 6860 if ("Vim".equals(codeString)) 6861 return SPDXLicense.VIM; 6862 if ("VOSTROM".equals(codeString)) 6863 return SPDXLicense.VOSTROM; 6864 if ("VSL-1.0".equals(codeString)) 6865 return SPDXLicense.VSL_1_0; 6866 if ("W3C".equals(codeString)) 6867 return SPDXLicense.W3C; 6868 if ("W3C-19980720".equals(codeString)) 6869 return SPDXLicense.W3C_19980720; 6870 if ("W3C-20150513".equals(codeString)) 6871 return SPDXLicense.W3C_20150513; 6872 if ("w3m".equals(codeString)) 6873 return SPDXLicense.W3M; 6874 if ("Watcom-1.0".equals(codeString)) 6875 return SPDXLicense.WATCOM_1_0; 6876 if ("Widget-Workshop".equals(codeString)) 6877 return SPDXLicense.WIDGET_WORKSHOP; 6878 if ("Wsuipa".equals(codeString)) 6879 return SPDXLicense.WSUIPA; 6880 if ("WTFPL".equals(codeString)) 6881 return SPDXLicense.WTFPL; 6882 if ("wxWindows".equals(codeString)) 6883 return SPDXLicense.WXWINDOWS; 6884 if ("X11".equals(codeString)) 6885 return SPDXLicense.X11; 6886 if ("X11-distribute-modifications-variant".equals(codeString)) 6887 return SPDXLicense.X11_DISTRIBUTE_MODIFICATIONS_VARIANT; 6888 if ("Xdebug-1.03".equals(codeString)) 6889 return SPDXLicense.XDEBUG_1_03; 6890 if ("Xerox".equals(codeString)) 6891 return SPDXLicense.XEROX; 6892 if ("Xfig".equals(codeString)) 6893 return SPDXLicense.XFIG; 6894 if ("XFree86-1.1".equals(codeString)) 6895 return SPDXLicense.XFREE86_1_1; 6896 if ("xinetd".equals(codeString)) 6897 return SPDXLicense.XINETD; 6898 if ("xlock".equals(codeString)) 6899 return SPDXLicense.XLOCK; 6900 if ("Xnet".equals(codeString)) 6901 return SPDXLicense.XNET; 6902 if ("xpp".equals(codeString)) 6903 return SPDXLicense.XPP; 6904 if ("XSkat".equals(codeString)) 6905 return SPDXLicense.XSKAT; 6906 if ("YPL-1.0".equals(codeString)) 6907 return SPDXLicense.YPL_1_0; 6908 if ("YPL-1.1".equals(codeString)) 6909 return SPDXLicense.YPL_1_1; 6910 if ("Zed".equals(codeString)) 6911 return SPDXLicense.ZED; 6912 if ("Zend-2.0".equals(codeString)) 6913 return SPDXLicense.ZEND_2_0; 6914 if ("Zimbra-1.3".equals(codeString)) 6915 return SPDXLicense.ZIMBRA_1_3; 6916 if ("Zimbra-1.4".equals(codeString)) 6917 return SPDXLicense.ZIMBRA_1_4; 6918 if ("Zlib".equals(codeString)) 6919 return SPDXLicense.ZLIB; 6920 if ("zlib-acknowledgement".equals(codeString)) 6921 return SPDXLicense.ZLIB_ACKNOWLEDGEMENT; 6922 if ("ZPL-1.1".equals(codeString)) 6923 return SPDXLicense.ZPL_1_1; 6924 if ("ZPL-2.0".equals(codeString)) 6925 return SPDXLicense.ZPL_2_0; 6926 if ("ZPL-2.1".equals(codeString)) 6927 return SPDXLicense.ZPL_2_1; 6928 throw new IllegalArgumentException("Unknown SPDXLicense code '"+codeString+"'"); 6929 } 6930 6931 public Enumeration<SPDXLicense> fromType(PrimitiveType<?> code) throws FHIRException { 6932 if (code == null) 6933 return null; 6934 if (code.isEmpty()) 6935 return new Enumeration<SPDXLicense>(this, SPDXLicense.NULL, code); 6936 String codeString = ((PrimitiveType) code).asStringValue(); 6937 if (codeString == null || "".equals(codeString)) 6938 return new Enumeration<SPDXLicense>(this, SPDXLicense.NULL, code); 6939 if ("0BSD".equals(codeString)) 6940 return new Enumeration<SPDXLicense>(this, SPDXLicense._0BSD, code); 6941 if ("AAL".equals(codeString)) 6942 return new Enumeration<SPDXLicense>(this, SPDXLicense.AAL, code); 6943 if ("Abstyles".equals(codeString)) 6944 return new Enumeration<SPDXLicense>(this, SPDXLicense.ABSTYLES, code); 6945 if ("AdaCore-doc".equals(codeString)) 6946 return new Enumeration<SPDXLicense>(this, SPDXLicense.ADACORE_DOC, code); 6947 if ("Adobe-2006".equals(codeString)) 6948 return new Enumeration<SPDXLicense>(this, SPDXLicense.ADOBE_2006, code); 6949 if ("Adobe-Glyph".equals(codeString)) 6950 return new Enumeration<SPDXLicense>(this, SPDXLicense.ADOBE_GLYPH, code); 6951 if ("ADSL".equals(codeString)) 6952 return new Enumeration<SPDXLicense>(this, SPDXLicense.ADSL, code); 6953 if ("AFL-1.1".equals(codeString)) 6954 return new Enumeration<SPDXLicense>(this, SPDXLicense.AFL_1_1, code); 6955 if ("AFL-1.2".equals(codeString)) 6956 return new Enumeration<SPDXLicense>(this, SPDXLicense.AFL_1_2, code); 6957 if ("AFL-2.0".equals(codeString)) 6958 return new Enumeration<SPDXLicense>(this, SPDXLicense.AFL_2_0, code); 6959 if ("AFL-2.1".equals(codeString)) 6960 return new Enumeration<SPDXLicense>(this, SPDXLicense.AFL_2_1, code); 6961 if ("AFL-3.0".equals(codeString)) 6962 return new Enumeration<SPDXLicense>(this, SPDXLicense.AFL_3_0, code); 6963 if ("Afmparse".equals(codeString)) 6964 return new Enumeration<SPDXLicense>(this, SPDXLicense.AFMPARSE, code); 6965 if ("AGPL-1.0".equals(codeString)) 6966 return new Enumeration<SPDXLicense>(this, SPDXLicense.AGPL_1_0, code); 6967 if ("AGPL-1.0-only".equals(codeString)) 6968 return new Enumeration<SPDXLicense>(this, SPDXLicense.AGPL_1_0_ONLY, code); 6969 if ("AGPL-1.0-or-later".equals(codeString)) 6970 return new Enumeration<SPDXLicense>(this, SPDXLicense.AGPL_1_0_OR_LATER, code); 6971 if ("AGPL-3.0".equals(codeString)) 6972 return new Enumeration<SPDXLicense>(this, SPDXLicense.AGPL_3_0, code); 6973 if ("AGPL-3.0-only".equals(codeString)) 6974 return new Enumeration<SPDXLicense>(this, SPDXLicense.AGPL_3_0_ONLY, code); 6975 if ("AGPL-3.0-or-later".equals(codeString)) 6976 return new Enumeration<SPDXLicense>(this, SPDXLicense.AGPL_3_0_OR_LATER, code); 6977 if ("Aladdin".equals(codeString)) 6978 return new Enumeration<SPDXLicense>(this, SPDXLicense.ALADDIN, code); 6979 if ("AMDPLPA".equals(codeString)) 6980 return new Enumeration<SPDXLicense>(this, SPDXLicense.AMDPLPA, code); 6981 if ("AML".equals(codeString)) 6982 return new Enumeration<SPDXLicense>(this, SPDXLicense.AML, code); 6983 if ("AMPAS".equals(codeString)) 6984 return new Enumeration<SPDXLicense>(this, SPDXLicense.AMPAS, code); 6985 if ("ANTLR-PD".equals(codeString)) 6986 return new Enumeration<SPDXLicense>(this, SPDXLicense.ANTLR_PD, code); 6987 if ("ANTLR-PD-fallback".equals(codeString)) 6988 return new Enumeration<SPDXLicense>(this, SPDXLicense.ANTLR_PD_FALLBACK, code); 6989 if ("Apache-1.0".equals(codeString)) 6990 return new Enumeration<SPDXLicense>(this, SPDXLicense.APACHE_1_0, code); 6991 if ("Apache-1.1".equals(codeString)) 6992 return new Enumeration<SPDXLicense>(this, SPDXLicense.APACHE_1_1, code); 6993 if ("Apache-2.0".equals(codeString)) 6994 return new Enumeration<SPDXLicense>(this, SPDXLicense.APACHE_2_0, code); 6995 if ("APAFML".equals(codeString)) 6996 return new Enumeration<SPDXLicense>(this, SPDXLicense.APAFML, code); 6997 if ("APL-1.0".equals(codeString)) 6998 return new Enumeration<SPDXLicense>(this, SPDXLicense.APL_1_0, code); 6999 if ("App-s2p".equals(codeString)) 7000 return new Enumeration<SPDXLicense>(this, SPDXLicense.APP_S2P, code); 7001 if ("APSL-1.0".equals(codeString)) 7002 return new Enumeration<SPDXLicense>(this, SPDXLicense.APSL_1_0, code); 7003 if ("APSL-1.1".equals(codeString)) 7004 return new Enumeration<SPDXLicense>(this, SPDXLicense.APSL_1_1, code); 7005 if ("APSL-1.2".equals(codeString)) 7006 return new Enumeration<SPDXLicense>(this, SPDXLicense.APSL_1_2, code); 7007 if ("APSL-2.0".equals(codeString)) 7008 return new Enumeration<SPDXLicense>(this, SPDXLicense.APSL_2_0, code); 7009 if ("Arphic-1999".equals(codeString)) 7010 return new Enumeration<SPDXLicense>(this, SPDXLicense.ARPHIC_1999, code); 7011 if ("Artistic-1.0".equals(codeString)) 7012 return new Enumeration<SPDXLicense>(this, SPDXLicense.ARTISTIC_1_0, code); 7013 if ("Artistic-1.0-cl8".equals(codeString)) 7014 return new Enumeration<SPDXLicense>(this, SPDXLicense.ARTISTIC_1_0_CL8, code); 7015 if ("Artistic-1.0-Perl".equals(codeString)) 7016 return new Enumeration<SPDXLicense>(this, SPDXLicense.ARTISTIC_1_0_PERL, code); 7017 if ("Artistic-2.0".equals(codeString)) 7018 return new Enumeration<SPDXLicense>(this, SPDXLicense.ARTISTIC_2_0, code); 7019 if ("ASWF-Digital-Assets-1.0".equals(codeString)) 7020 return new Enumeration<SPDXLicense>(this, SPDXLicense.ASWF_DIGITAL_ASSETS_1_0, code); 7021 if ("ASWF-Digital-Assets-1.1".equals(codeString)) 7022 return new Enumeration<SPDXLicense>(this, SPDXLicense.ASWF_DIGITAL_ASSETS_1_1, code); 7023 if ("Baekmuk".equals(codeString)) 7024 return new Enumeration<SPDXLicense>(this, SPDXLicense.BAEKMUK, code); 7025 if ("Bahyph".equals(codeString)) 7026 return new Enumeration<SPDXLicense>(this, SPDXLicense.BAHYPH, code); 7027 if ("Barr".equals(codeString)) 7028 return new Enumeration<SPDXLicense>(this, SPDXLicense.BARR, code); 7029 if ("Beerware".equals(codeString)) 7030 return new Enumeration<SPDXLicense>(this, SPDXLicense.BEERWARE, code); 7031 if ("Bitstream-Charter".equals(codeString)) 7032 return new Enumeration<SPDXLicense>(this, SPDXLicense.BITSTREAM_CHARTER, code); 7033 if ("Bitstream-Vera".equals(codeString)) 7034 return new Enumeration<SPDXLicense>(this, SPDXLicense.BITSTREAM_VERA, code); 7035 if ("BitTorrent-1.0".equals(codeString)) 7036 return new Enumeration<SPDXLicense>(this, SPDXLicense.BITTORRENT_1_0, code); 7037 if ("BitTorrent-1.1".equals(codeString)) 7038 return new Enumeration<SPDXLicense>(this, SPDXLicense.BITTORRENT_1_1, code); 7039 if ("blessing".equals(codeString)) 7040 return new Enumeration<SPDXLicense>(this, SPDXLicense.BLESSING, code); 7041 if ("BlueOak-1.0.0".equals(codeString)) 7042 return new Enumeration<SPDXLicense>(this, SPDXLicense.BLUEOAK_1_0_0, code); 7043 if ("Boehm-GC".equals(codeString)) 7044 return new Enumeration<SPDXLicense>(this, SPDXLicense.BOEHM_GC, code); 7045 if ("Borceux".equals(codeString)) 7046 return new Enumeration<SPDXLicense>(this, SPDXLicense.BORCEUX, code); 7047 if ("Brian-Gladman-3-Clause".equals(codeString)) 7048 return new Enumeration<SPDXLicense>(this, SPDXLicense.BRIAN_GLADMAN_3_CLAUSE, code); 7049 if ("BSD-1-Clause".equals(codeString)) 7050 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_1_CLAUSE, code); 7051 if ("BSD-2-Clause".equals(codeString)) 7052 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_2_CLAUSE, code); 7053 if ("BSD-2-Clause-FreeBSD".equals(codeString)) 7054 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_2_CLAUSE_FREEBSD, code); 7055 if ("BSD-2-Clause-NetBSD".equals(codeString)) 7056 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_2_CLAUSE_NETBSD, code); 7057 if ("BSD-2-Clause-Patent".equals(codeString)) 7058 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_2_CLAUSE_PATENT, code); 7059 if ("BSD-2-Clause-Views".equals(codeString)) 7060 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_2_CLAUSE_VIEWS, code); 7061 if ("BSD-3-Clause".equals(codeString)) 7062 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE, code); 7063 if ("BSD-3-Clause-Attribution".equals(codeString)) 7064 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_ATTRIBUTION, code); 7065 if ("BSD-3-Clause-Clear".equals(codeString)) 7066 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_CLEAR, code); 7067 if ("BSD-3-Clause-LBNL".equals(codeString)) 7068 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_LBNL, code); 7069 if ("BSD-3-Clause-Modification".equals(codeString)) 7070 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_MODIFICATION, code); 7071 if ("BSD-3-Clause-No-Military-License".equals(codeString)) 7072 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_NO_MILITARY_LICENSE, code); 7073 if ("BSD-3-Clause-No-Nuclear-License".equals(codeString)) 7074 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_LICENSE, code); 7075 if ("BSD-3-Clause-No-Nuclear-License-2014".equals(codeString)) 7076 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014, code); 7077 if ("BSD-3-Clause-No-Nuclear-Warranty".equals(codeString)) 7078 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY, code); 7079 if ("BSD-3-Clause-Open-MPI".equals(codeString)) 7080 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_3_CLAUSE_OPEN_MPI, code); 7081 if ("BSD-4-Clause".equals(codeString)) 7082 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_4_CLAUSE, code); 7083 if ("BSD-4-Clause-Shortened".equals(codeString)) 7084 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_4_CLAUSE_SHORTENED, code); 7085 if ("BSD-4-Clause-UC".equals(codeString)) 7086 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_4_CLAUSE_UC, code); 7087 if ("BSD-4.3RENO".equals(codeString)) 7088 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_4_3RENO, code); 7089 if ("BSD-4.3TAHOE".equals(codeString)) 7090 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_4_3TAHOE, code); 7091 if ("BSD-Advertising-Acknowledgement".equals(codeString)) 7092 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_ADVERTISING_ACKNOWLEDGEMENT, code); 7093 if ("BSD-Attribution-HPND-disclaimer".equals(codeString)) 7094 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_ATTRIBUTION_HPND_DISCLAIMER, code); 7095 if ("BSD-Protection".equals(codeString)) 7096 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_PROTECTION, code); 7097 if ("BSD-Source-Code".equals(codeString)) 7098 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSD_SOURCE_CODE, code); 7099 if ("BSL-1.0".equals(codeString)) 7100 return new Enumeration<SPDXLicense>(this, SPDXLicense.BSL_1_0, code); 7101 if ("BUSL-1.1".equals(codeString)) 7102 return new Enumeration<SPDXLicense>(this, SPDXLicense.BUSL_1_1, code); 7103 if ("bzip2-1.0.5".equals(codeString)) 7104 return new Enumeration<SPDXLicense>(this, SPDXLicense.BZIP2_1_0_5, code); 7105 if ("bzip2-1.0.6".equals(codeString)) 7106 return new Enumeration<SPDXLicense>(this, SPDXLicense.BZIP2_1_0_6, code); 7107 if ("C-UDA-1.0".equals(codeString)) 7108 return new Enumeration<SPDXLicense>(this, SPDXLicense.C_UDA_1_0, code); 7109 if ("CAL-1.0".equals(codeString)) 7110 return new Enumeration<SPDXLicense>(this, SPDXLicense.CAL_1_0, code); 7111 if ("CAL-1.0-Combined-Work-Exception".equals(codeString)) 7112 return new Enumeration<SPDXLicense>(this, SPDXLicense.CAL_1_0_COMBINED_WORK_EXCEPTION, code); 7113 if ("Caldera".equals(codeString)) 7114 return new Enumeration<SPDXLicense>(this, SPDXLicense.CALDERA, code); 7115 if ("CATOSL-1.1".equals(codeString)) 7116 return new Enumeration<SPDXLicense>(this, SPDXLicense.CATOSL_1_1, code); 7117 if ("CC-BY-1.0".equals(codeString)) 7118 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_1_0, code); 7119 if ("CC-BY-2.0".equals(codeString)) 7120 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_2_0, code); 7121 if ("CC-BY-2.5".equals(codeString)) 7122 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_2_5, code); 7123 if ("CC-BY-2.5-AU".equals(codeString)) 7124 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_2_5_AU, code); 7125 if ("CC-BY-3.0".equals(codeString)) 7126 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_3_0, code); 7127 if ("CC-BY-3.0-AT".equals(codeString)) 7128 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_3_0_AT, code); 7129 if ("CC-BY-3.0-DE".equals(codeString)) 7130 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_3_0_DE, code); 7131 if ("CC-BY-3.0-IGO".equals(codeString)) 7132 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_3_0_IGO, code); 7133 if ("CC-BY-3.0-NL".equals(codeString)) 7134 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_3_0_NL, code); 7135 if ("CC-BY-3.0-US".equals(codeString)) 7136 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_3_0_US, code); 7137 if ("CC-BY-4.0".equals(codeString)) 7138 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_4_0, code); 7139 if ("CC-BY-NC-1.0".equals(codeString)) 7140 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_1_0, code); 7141 if ("CC-BY-NC-2.0".equals(codeString)) 7142 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_2_0, code); 7143 if ("CC-BY-NC-2.5".equals(codeString)) 7144 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_2_5, code); 7145 if ("CC-BY-NC-3.0".equals(codeString)) 7146 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_3_0, code); 7147 if ("CC-BY-NC-3.0-DE".equals(codeString)) 7148 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_3_0_DE, code); 7149 if ("CC-BY-NC-4.0".equals(codeString)) 7150 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_4_0, code); 7151 if ("CC-BY-NC-ND-1.0".equals(codeString)) 7152 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_ND_1_0, code); 7153 if ("CC-BY-NC-ND-2.0".equals(codeString)) 7154 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_ND_2_0, code); 7155 if ("CC-BY-NC-ND-2.5".equals(codeString)) 7156 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_ND_2_5, code); 7157 if ("CC-BY-NC-ND-3.0".equals(codeString)) 7158 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_ND_3_0, code); 7159 if ("CC-BY-NC-ND-3.0-DE".equals(codeString)) 7160 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_ND_3_0_DE, code); 7161 if ("CC-BY-NC-ND-3.0-IGO".equals(codeString)) 7162 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_ND_3_0_IGO, code); 7163 if ("CC-BY-NC-ND-4.0".equals(codeString)) 7164 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_ND_4_0, code); 7165 if ("CC-BY-NC-SA-1.0".equals(codeString)) 7166 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_1_0, code); 7167 if ("CC-BY-NC-SA-2.0".equals(codeString)) 7168 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_2_0, code); 7169 if ("CC-BY-NC-SA-2.0-DE".equals(codeString)) 7170 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_2_0_DE, code); 7171 if ("CC-BY-NC-SA-2.0-FR".equals(codeString)) 7172 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_2_0_FR, code); 7173 if ("CC-BY-NC-SA-2.0-UK".equals(codeString)) 7174 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_2_0_UK, code); 7175 if ("CC-BY-NC-SA-2.5".equals(codeString)) 7176 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_2_5, code); 7177 if ("CC-BY-NC-SA-3.0".equals(codeString)) 7178 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_3_0, code); 7179 if ("CC-BY-NC-SA-3.0-DE".equals(codeString)) 7180 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_3_0_DE, code); 7181 if ("CC-BY-NC-SA-3.0-IGO".equals(codeString)) 7182 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_3_0_IGO, code); 7183 if ("CC-BY-NC-SA-4.0".equals(codeString)) 7184 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_NC_SA_4_0, code); 7185 if ("CC-BY-ND-1.0".equals(codeString)) 7186 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_ND_1_0, code); 7187 if ("CC-BY-ND-2.0".equals(codeString)) 7188 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_ND_2_0, code); 7189 if ("CC-BY-ND-2.5".equals(codeString)) 7190 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_ND_2_5, code); 7191 if ("CC-BY-ND-3.0".equals(codeString)) 7192 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_ND_3_0, code); 7193 if ("CC-BY-ND-3.0-DE".equals(codeString)) 7194 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_ND_3_0_DE, code); 7195 if ("CC-BY-ND-4.0".equals(codeString)) 7196 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_ND_4_0, code); 7197 if ("CC-BY-SA-1.0".equals(codeString)) 7198 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_1_0, code); 7199 if ("CC-BY-SA-2.0".equals(codeString)) 7200 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_2_0, code); 7201 if ("CC-BY-SA-2.0-UK".equals(codeString)) 7202 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_2_0_UK, code); 7203 if ("CC-BY-SA-2.1-JP".equals(codeString)) 7204 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_2_1_JP, code); 7205 if ("CC-BY-SA-2.5".equals(codeString)) 7206 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_2_5, code); 7207 if ("CC-BY-SA-3.0".equals(codeString)) 7208 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_3_0, code); 7209 if ("CC-BY-SA-3.0-AT".equals(codeString)) 7210 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_3_0_AT, code); 7211 if ("CC-BY-SA-3.0-DE".equals(codeString)) 7212 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_3_0_DE, code); 7213 if ("CC-BY-SA-3.0-IGO".equals(codeString)) 7214 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_3_0_IGO, code); 7215 if ("CC-BY-SA-4.0".equals(codeString)) 7216 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_BY_SA_4_0, code); 7217 if ("CC-PDDC".equals(codeString)) 7218 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC_PDDC, code); 7219 if ("CC0-1.0".equals(codeString)) 7220 return new Enumeration<SPDXLicense>(this, SPDXLicense.CC0_1_0, code); 7221 if ("CDDL-1.0".equals(codeString)) 7222 return new Enumeration<SPDXLicense>(this, SPDXLicense.CDDL_1_0, code); 7223 if ("CDDL-1.1".equals(codeString)) 7224 return new Enumeration<SPDXLicense>(this, SPDXLicense.CDDL_1_1, code); 7225 if ("CDL-1.0".equals(codeString)) 7226 return new Enumeration<SPDXLicense>(this, SPDXLicense.CDL_1_0, code); 7227 if ("CDLA-Permissive-1.0".equals(codeString)) 7228 return new Enumeration<SPDXLicense>(this, SPDXLicense.CDLA_PERMISSIVE_1_0, code); 7229 if ("CDLA-Permissive-2.0".equals(codeString)) 7230 return new Enumeration<SPDXLicense>(this, SPDXLicense.CDLA_PERMISSIVE_2_0, code); 7231 if ("CDLA-Sharing-1.0".equals(codeString)) 7232 return new Enumeration<SPDXLicense>(this, SPDXLicense.CDLA_SHARING_1_0, code); 7233 if ("CECILL-1.0".equals(codeString)) 7234 return new Enumeration<SPDXLicense>(this, SPDXLicense.CECILL_1_0, code); 7235 if ("CECILL-1.1".equals(codeString)) 7236 return new Enumeration<SPDXLicense>(this, SPDXLicense.CECILL_1_1, code); 7237 if ("CECILL-2.0".equals(codeString)) 7238 return new Enumeration<SPDXLicense>(this, SPDXLicense.CECILL_2_0, code); 7239 if ("CECILL-2.1".equals(codeString)) 7240 return new Enumeration<SPDXLicense>(this, SPDXLicense.CECILL_2_1, code); 7241 if ("CECILL-B".equals(codeString)) 7242 return new Enumeration<SPDXLicense>(this, SPDXLicense.CECILL_B, code); 7243 if ("CECILL-C".equals(codeString)) 7244 return new Enumeration<SPDXLicense>(this, SPDXLicense.CECILL_C, code); 7245 if ("CERN-OHL-1.1".equals(codeString)) 7246 return new Enumeration<SPDXLicense>(this, SPDXLicense.CERN_OHL_1_1, code); 7247 if ("CERN-OHL-1.2".equals(codeString)) 7248 return new Enumeration<SPDXLicense>(this, SPDXLicense.CERN_OHL_1_2, code); 7249 if ("CERN-OHL-P-2.0".equals(codeString)) 7250 return new Enumeration<SPDXLicense>(this, SPDXLicense.CERN_OHL_P_2_0, code); 7251 if ("CERN-OHL-S-2.0".equals(codeString)) 7252 return new Enumeration<SPDXLicense>(this, SPDXLicense.CERN_OHL_S_2_0, code); 7253 if ("CERN-OHL-W-2.0".equals(codeString)) 7254 return new Enumeration<SPDXLicense>(this, SPDXLicense.CERN_OHL_W_2_0, code); 7255 if ("CFITSIO".equals(codeString)) 7256 return new Enumeration<SPDXLicense>(this, SPDXLicense.CFITSIO, code); 7257 if ("checkmk".equals(codeString)) 7258 return new Enumeration<SPDXLicense>(this, SPDXLicense.CHECKMK, code); 7259 if ("ClArtistic".equals(codeString)) 7260 return new Enumeration<SPDXLicense>(this, SPDXLicense.CLARTISTIC, code); 7261 if ("Clips".equals(codeString)) 7262 return new Enumeration<SPDXLicense>(this, SPDXLicense.CLIPS, code); 7263 if ("CMU-Mach".equals(codeString)) 7264 return new Enumeration<SPDXLicense>(this, SPDXLicense.CMU_MACH, code); 7265 if ("CNRI-Jython".equals(codeString)) 7266 return new Enumeration<SPDXLicense>(this, SPDXLicense.CNRI_JYTHON, code); 7267 if ("CNRI-Python".equals(codeString)) 7268 return new Enumeration<SPDXLicense>(this, SPDXLicense.CNRI_PYTHON, code); 7269 if ("CNRI-Python-GPL-Compatible".equals(codeString)) 7270 return new Enumeration<SPDXLicense>(this, SPDXLicense.CNRI_PYTHON_GPL_COMPATIBLE, code); 7271 if ("COIL-1.0".equals(codeString)) 7272 return new Enumeration<SPDXLicense>(this, SPDXLicense.COIL_1_0, code); 7273 if ("Community-Spec-1.0".equals(codeString)) 7274 return new Enumeration<SPDXLicense>(this, SPDXLicense.COMMUNITY_SPEC_1_0, code); 7275 if ("Condor-1.1".equals(codeString)) 7276 return new Enumeration<SPDXLicense>(this, SPDXLicense.CONDOR_1_1, code); 7277 if ("copyleft-next-0.3.0".equals(codeString)) 7278 return new Enumeration<SPDXLicense>(this, SPDXLicense.COPYLEFT_NEXT_0_3_0, code); 7279 if ("copyleft-next-0.3.1".equals(codeString)) 7280 return new Enumeration<SPDXLicense>(this, SPDXLicense.COPYLEFT_NEXT_0_3_1, code); 7281 if ("Cornell-Lossless-JPEG".equals(codeString)) 7282 return new Enumeration<SPDXLicense>(this, SPDXLicense.CORNELL_LOSSLESS_JPEG, code); 7283 if ("CPAL-1.0".equals(codeString)) 7284 return new Enumeration<SPDXLicense>(this, SPDXLicense.CPAL_1_0, code); 7285 if ("CPL-1.0".equals(codeString)) 7286 return new Enumeration<SPDXLicense>(this, SPDXLicense.CPL_1_0, code); 7287 if ("CPOL-1.02".equals(codeString)) 7288 return new Enumeration<SPDXLicense>(this, SPDXLicense.CPOL_1_02, code); 7289 if ("Crossword".equals(codeString)) 7290 return new Enumeration<SPDXLicense>(this, SPDXLicense.CROSSWORD, code); 7291 if ("CrystalStacker".equals(codeString)) 7292 return new Enumeration<SPDXLicense>(this, SPDXLicense.CRYSTALSTACKER, code); 7293 if ("CUA-OPL-1.0".equals(codeString)) 7294 return new Enumeration<SPDXLicense>(this, SPDXLicense.CUA_OPL_1_0, code); 7295 if ("Cube".equals(codeString)) 7296 return new Enumeration<SPDXLicense>(this, SPDXLicense.CUBE, code); 7297 if ("curl".equals(codeString)) 7298 return new Enumeration<SPDXLicense>(this, SPDXLicense.CURL, code); 7299 if ("D-FSL-1.0".equals(codeString)) 7300 return new Enumeration<SPDXLicense>(this, SPDXLicense.D_FSL_1_0, code); 7301 if ("diffmark".equals(codeString)) 7302 return new Enumeration<SPDXLicense>(this, SPDXLicense.DIFFMARK, code); 7303 if ("DL-DE-BY-2.0".equals(codeString)) 7304 return new Enumeration<SPDXLicense>(this, SPDXLicense.DL_DE_BY_2_0, code); 7305 if ("DOC".equals(codeString)) 7306 return new Enumeration<SPDXLicense>(this, SPDXLicense.DOC, code); 7307 if ("Dotseqn".equals(codeString)) 7308 return new Enumeration<SPDXLicense>(this, SPDXLicense.DOTSEQN, code); 7309 if ("DRL-1.0".equals(codeString)) 7310 return new Enumeration<SPDXLicense>(this, SPDXLicense.DRL_1_0, code); 7311 if ("DSDP".equals(codeString)) 7312 return new Enumeration<SPDXLicense>(this, SPDXLicense.DSDP, code); 7313 if ("dtoa".equals(codeString)) 7314 return new Enumeration<SPDXLicense>(this, SPDXLicense.DTOA, code); 7315 if ("dvipdfm".equals(codeString)) 7316 return new Enumeration<SPDXLicense>(this, SPDXLicense.DVIPDFM, code); 7317 if ("ECL-1.0".equals(codeString)) 7318 return new Enumeration<SPDXLicense>(this, SPDXLicense.ECL_1_0, code); 7319 if ("ECL-2.0".equals(codeString)) 7320 return new Enumeration<SPDXLicense>(this, SPDXLicense.ECL_2_0, code); 7321 if ("eCos-2.0".equals(codeString)) 7322 return new Enumeration<SPDXLicense>(this, SPDXLicense.ECOS_2_0, code); 7323 if ("EFL-1.0".equals(codeString)) 7324 return new Enumeration<SPDXLicense>(this, SPDXLicense.EFL_1_0, code); 7325 if ("EFL-2.0".equals(codeString)) 7326 return new Enumeration<SPDXLicense>(this, SPDXLicense.EFL_2_0, code); 7327 if ("eGenix".equals(codeString)) 7328 return new Enumeration<SPDXLicense>(this, SPDXLicense.EGENIX, code); 7329 if ("Elastic-2.0".equals(codeString)) 7330 return new Enumeration<SPDXLicense>(this, SPDXLicense.ELASTIC_2_0, code); 7331 if ("Entessa".equals(codeString)) 7332 return new Enumeration<SPDXLicense>(this, SPDXLicense.ENTESSA, code); 7333 if ("EPICS".equals(codeString)) 7334 return new Enumeration<SPDXLicense>(this, SPDXLicense.EPICS, code); 7335 if ("EPL-1.0".equals(codeString)) 7336 return new Enumeration<SPDXLicense>(this, SPDXLicense.EPL_1_0, code); 7337 if ("EPL-2.0".equals(codeString)) 7338 return new Enumeration<SPDXLicense>(this, SPDXLicense.EPL_2_0, code); 7339 if ("ErlPL-1.1".equals(codeString)) 7340 return new Enumeration<SPDXLicense>(this, SPDXLicense.ERLPL_1_1, code); 7341 if ("etalab-2.0".equals(codeString)) 7342 return new Enumeration<SPDXLicense>(this, SPDXLicense.ETALAB_2_0, code); 7343 if ("EUDatagrid".equals(codeString)) 7344 return new Enumeration<SPDXLicense>(this, SPDXLicense.EUDATAGRID, code); 7345 if ("EUPL-1.0".equals(codeString)) 7346 return new Enumeration<SPDXLicense>(this, SPDXLicense.EUPL_1_0, code); 7347 if ("EUPL-1.1".equals(codeString)) 7348 return new Enumeration<SPDXLicense>(this, SPDXLicense.EUPL_1_1, code); 7349 if ("EUPL-1.2".equals(codeString)) 7350 return new Enumeration<SPDXLicense>(this, SPDXLicense.EUPL_1_2, code); 7351 if ("Eurosym".equals(codeString)) 7352 return new Enumeration<SPDXLicense>(this, SPDXLicense.EUROSYM, code); 7353 if ("Fair".equals(codeString)) 7354 return new Enumeration<SPDXLicense>(this, SPDXLicense.FAIR, code); 7355 if ("FDK-AAC".equals(codeString)) 7356 return new Enumeration<SPDXLicense>(this, SPDXLicense.FDK_AAC, code); 7357 if ("Frameworx-1.0".equals(codeString)) 7358 return new Enumeration<SPDXLicense>(this, SPDXLicense.FRAMEWORX_1_0, code); 7359 if ("FreeBSD-DOC".equals(codeString)) 7360 return new Enumeration<SPDXLicense>(this, SPDXLicense.FREEBSD_DOC, code); 7361 if ("FreeImage".equals(codeString)) 7362 return new Enumeration<SPDXLicense>(this, SPDXLicense.FREEIMAGE, code); 7363 if ("FSFAP".equals(codeString)) 7364 return new Enumeration<SPDXLicense>(this, SPDXLicense.FSFAP, code); 7365 if ("FSFUL".equals(codeString)) 7366 return new Enumeration<SPDXLicense>(this, SPDXLicense.FSFUL, code); 7367 if ("FSFULLR".equals(codeString)) 7368 return new Enumeration<SPDXLicense>(this, SPDXLicense.FSFULLR, code); 7369 if ("FSFULLRWD".equals(codeString)) 7370 return new Enumeration<SPDXLicense>(this, SPDXLicense.FSFULLRWD, code); 7371 if ("FTL".equals(codeString)) 7372 return new Enumeration<SPDXLicense>(this, SPDXLicense.FTL, code); 7373 if ("GD".equals(codeString)) 7374 return new Enumeration<SPDXLicense>(this, SPDXLicense.GD, code); 7375 if ("GFDL-1.1".equals(codeString)) 7376 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_1, code); 7377 if ("GFDL-1.1-invariants-only".equals(codeString)) 7378 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_1_INVARIANTS_ONLY, code); 7379 if ("GFDL-1.1-invariants-or-later".equals(codeString)) 7380 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_1_INVARIANTS_OR_LATER, code); 7381 if ("GFDL-1.1-no-invariants-only".equals(codeString)) 7382 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_1_NO_INVARIANTS_ONLY, code); 7383 if ("GFDL-1.1-no-invariants-or-later".equals(codeString)) 7384 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_1_NO_INVARIANTS_OR_LATER, code); 7385 if ("GFDL-1.1-only".equals(codeString)) 7386 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_1_ONLY, code); 7387 if ("GFDL-1.1-or-later".equals(codeString)) 7388 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_1_OR_LATER, code); 7389 if ("GFDL-1.2".equals(codeString)) 7390 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_2, code); 7391 if ("GFDL-1.2-invariants-only".equals(codeString)) 7392 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_2_INVARIANTS_ONLY, code); 7393 if ("GFDL-1.2-invariants-or-later".equals(codeString)) 7394 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_2_INVARIANTS_OR_LATER, code); 7395 if ("GFDL-1.2-no-invariants-only".equals(codeString)) 7396 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_2_NO_INVARIANTS_ONLY, code); 7397 if ("GFDL-1.2-no-invariants-or-later".equals(codeString)) 7398 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_2_NO_INVARIANTS_OR_LATER, code); 7399 if ("GFDL-1.2-only".equals(codeString)) 7400 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_2_ONLY, code); 7401 if ("GFDL-1.2-or-later".equals(codeString)) 7402 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_2_OR_LATER, code); 7403 if ("GFDL-1.3".equals(codeString)) 7404 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_3, code); 7405 if ("GFDL-1.3-invariants-only".equals(codeString)) 7406 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_3_INVARIANTS_ONLY, code); 7407 if ("GFDL-1.3-invariants-or-later".equals(codeString)) 7408 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_3_INVARIANTS_OR_LATER, code); 7409 if ("GFDL-1.3-no-invariants-only".equals(codeString)) 7410 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_3_NO_INVARIANTS_ONLY, code); 7411 if ("GFDL-1.3-no-invariants-or-later".equals(codeString)) 7412 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_3_NO_INVARIANTS_OR_LATER, code); 7413 if ("GFDL-1.3-only".equals(codeString)) 7414 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_3_ONLY, code); 7415 if ("GFDL-1.3-or-later".equals(codeString)) 7416 return new Enumeration<SPDXLicense>(this, SPDXLicense.GFDL_1_3_OR_LATER, code); 7417 if ("Giftware".equals(codeString)) 7418 return new Enumeration<SPDXLicense>(this, SPDXLicense.GIFTWARE, code); 7419 if ("GL2PS".equals(codeString)) 7420 return new Enumeration<SPDXLicense>(this, SPDXLicense.GL2PS, code); 7421 if ("Glide".equals(codeString)) 7422 return new Enumeration<SPDXLicense>(this, SPDXLicense.GLIDE, code); 7423 if ("Glulxe".equals(codeString)) 7424 return new Enumeration<SPDXLicense>(this, SPDXLicense.GLULXE, code); 7425 if ("GLWTPL".equals(codeString)) 7426 return new Enumeration<SPDXLicense>(this, SPDXLicense.GLWTPL, code); 7427 if ("gnuplot".equals(codeString)) 7428 return new Enumeration<SPDXLicense>(this, SPDXLicense.GNUPLOT, code); 7429 if ("GPL-1.0".equals(codeString)) 7430 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_1_0, code); 7431 if ("GPL-1.0+".equals(codeString)) 7432 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_1_0PLUS, code); 7433 if ("GPL-1.0-only".equals(codeString)) 7434 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_1_0_ONLY, code); 7435 if ("GPL-1.0-or-later".equals(codeString)) 7436 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_1_0_OR_LATER, code); 7437 if ("GPL-2.0".equals(codeString)) 7438 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0, code); 7439 if ("GPL-2.0+".equals(codeString)) 7440 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0PLUS, code); 7441 if ("GPL-2.0-only".equals(codeString)) 7442 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0_ONLY, code); 7443 if ("GPL-2.0-or-later".equals(codeString)) 7444 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0_OR_LATER, code); 7445 if ("GPL-2.0-with-autoconf-exception".equals(codeString)) 7446 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0_WITH_AUTOCONF_EXCEPTION, code); 7447 if ("GPL-2.0-with-bison-exception".equals(codeString)) 7448 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0_WITH_BISON_EXCEPTION, code); 7449 if ("GPL-2.0-with-classpath-exception".equals(codeString)) 7450 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0_WITH_CLASSPATH_EXCEPTION, code); 7451 if ("GPL-2.0-with-font-exception".equals(codeString)) 7452 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0_WITH_FONT_EXCEPTION, code); 7453 if ("GPL-2.0-with-GCC-exception".equals(codeString)) 7454 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_2_0_WITH_GCC_EXCEPTION, code); 7455 if ("GPL-3.0".equals(codeString)) 7456 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_3_0, code); 7457 if ("GPL-3.0+".equals(codeString)) 7458 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_3_0PLUS, code); 7459 if ("GPL-3.0-only".equals(codeString)) 7460 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_3_0_ONLY, code); 7461 if ("GPL-3.0-or-later".equals(codeString)) 7462 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_3_0_OR_LATER, code); 7463 if ("GPL-3.0-with-autoconf-exception".equals(codeString)) 7464 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_3_0_WITH_AUTOCONF_EXCEPTION, code); 7465 if ("GPL-3.0-with-GCC-exception".equals(codeString)) 7466 return new Enumeration<SPDXLicense>(this, SPDXLicense.GPL_3_0_WITH_GCC_EXCEPTION, code); 7467 if ("Graphics-Gems".equals(codeString)) 7468 return new Enumeration<SPDXLicense>(this, SPDXLicense.GRAPHICS_GEMS, code); 7469 if ("gSOAP-1.3b".equals(codeString)) 7470 return new Enumeration<SPDXLicense>(this, SPDXLicense.GSOAP_1_3B, code); 7471 if ("HaskellReport".equals(codeString)) 7472 return new Enumeration<SPDXLicense>(this, SPDXLicense.HASKELLREPORT, code); 7473 if ("Hippocratic-2.1".equals(codeString)) 7474 return new Enumeration<SPDXLicense>(this, SPDXLicense.HIPPOCRATIC_2_1, code); 7475 if ("HP-1986".equals(codeString)) 7476 return new Enumeration<SPDXLicense>(this, SPDXLicense.HP_1986, code); 7477 if ("HPND".equals(codeString)) 7478 return new Enumeration<SPDXLicense>(this, SPDXLicense.HPND, code); 7479 if ("HPND-export-US".equals(codeString)) 7480 return new Enumeration<SPDXLicense>(this, SPDXLicense.HPND_EXPORT_US, code); 7481 if ("HPND-Markus-Kuhn".equals(codeString)) 7482 return new Enumeration<SPDXLicense>(this, SPDXLicense.HPND_MARKUS_KUHN, code); 7483 if ("HPND-sell-variant".equals(codeString)) 7484 return new Enumeration<SPDXLicense>(this, SPDXLicense.HPND_SELL_VARIANT, code); 7485 if ("HPND-sell-variant-MIT-disclaimer".equals(codeString)) 7486 return new Enumeration<SPDXLicense>(this, SPDXLicense.HPND_SELL_VARIANT_MIT_DISCLAIMER, code); 7487 if ("HTMLTIDY".equals(codeString)) 7488 return new Enumeration<SPDXLicense>(this, SPDXLicense.HTMLTIDY, code); 7489 if ("IBM-pibs".equals(codeString)) 7490 return new Enumeration<SPDXLicense>(this, SPDXLicense.IBM_PIBS, code); 7491 if ("ICU".equals(codeString)) 7492 return new Enumeration<SPDXLicense>(this, SPDXLicense.ICU, code); 7493 if ("IEC-Code-Components-EULA".equals(codeString)) 7494 return new Enumeration<SPDXLicense>(this, SPDXLicense.IEC_CODE_COMPONENTS_EULA, code); 7495 if ("IJG".equals(codeString)) 7496 return new Enumeration<SPDXLicense>(this, SPDXLicense.IJG, code); 7497 if ("IJG-short".equals(codeString)) 7498 return new Enumeration<SPDXLicense>(this, SPDXLicense.IJG_SHORT, code); 7499 if ("ImageMagick".equals(codeString)) 7500 return new Enumeration<SPDXLicense>(this, SPDXLicense.IMAGEMAGICK, code); 7501 if ("iMatix".equals(codeString)) 7502 return new Enumeration<SPDXLicense>(this, SPDXLicense.IMATIX, code); 7503 if ("Imlib2".equals(codeString)) 7504 return new Enumeration<SPDXLicense>(this, SPDXLicense.IMLIB2, code); 7505 if ("Info-ZIP".equals(codeString)) 7506 return new Enumeration<SPDXLicense>(this, SPDXLicense.INFO_ZIP, code); 7507 if ("Inner-Net-2.0".equals(codeString)) 7508 return new Enumeration<SPDXLicense>(this, SPDXLicense.INNER_NET_2_0, code); 7509 if ("Intel".equals(codeString)) 7510 return new Enumeration<SPDXLicense>(this, SPDXLicense.INTEL, code); 7511 if ("Intel-ACPI".equals(codeString)) 7512 return new Enumeration<SPDXLicense>(this, SPDXLicense.INTEL_ACPI, code); 7513 if ("Interbase-1.0".equals(codeString)) 7514 return new Enumeration<SPDXLicense>(this, SPDXLicense.INTERBASE_1_0, code); 7515 if ("IPA".equals(codeString)) 7516 return new Enumeration<SPDXLicense>(this, SPDXLicense.IPA, code); 7517 if ("IPL-1.0".equals(codeString)) 7518 return new Enumeration<SPDXLicense>(this, SPDXLicense.IPL_1_0, code); 7519 if ("ISC".equals(codeString)) 7520 return new Enumeration<SPDXLicense>(this, SPDXLicense.ISC, code); 7521 if ("Jam".equals(codeString)) 7522 return new Enumeration<SPDXLicense>(this, SPDXLicense.JAM, code); 7523 if ("JasPer-2.0".equals(codeString)) 7524 return new Enumeration<SPDXLicense>(this, SPDXLicense.JASPER_2_0, code); 7525 if ("JPL-image".equals(codeString)) 7526 return new Enumeration<SPDXLicense>(this, SPDXLicense.JPL_IMAGE, code); 7527 if ("JPNIC".equals(codeString)) 7528 return new Enumeration<SPDXLicense>(this, SPDXLicense.JPNIC, code); 7529 if ("JSON".equals(codeString)) 7530 return new Enumeration<SPDXLicense>(this, SPDXLicense.JSON, code); 7531 if ("Kazlib".equals(codeString)) 7532 return new Enumeration<SPDXLicense>(this, SPDXLicense.KAZLIB, code); 7533 if ("Knuth-CTAN".equals(codeString)) 7534 return new Enumeration<SPDXLicense>(this, SPDXLicense.KNUTH_CTAN, code); 7535 if ("LAL-1.2".equals(codeString)) 7536 return new Enumeration<SPDXLicense>(this, SPDXLicense.LAL_1_2, code); 7537 if ("LAL-1.3".equals(codeString)) 7538 return new Enumeration<SPDXLicense>(this, SPDXLicense.LAL_1_3, code); 7539 if ("Latex2e".equals(codeString)) 7540 return new Enumeration<SPDXLicense>(this, SPDXLicense.LATEX2E, code); 7541 if ("Latex2e-translated-notice".equals(codeString)) 7542 return new Enumeration<SPDXLicense>(this, SPDXLicense.LATEX2E_TRANSLATED_NOTICE, code); 7543 if ("Leptonica".equals(codeString)) 7544 return new Enumeration<SPDXLicense>(this, SPDXLicense.LEPTONICA, code); 7545 if ("LGPL-2.0".equals(codeString)) 7546 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_2_0, code); 7547 if ("LGPL-2.0+".equals(codeString)) 7548 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_2_0PLUS, code); 7549 if ("LGPL-2.0-only".equals(codeString)) 7550 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_2_0_ONLY, code); 7551 if ("LGPL-2.0-or-later".equals(codeString)) 7552 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_2_0_OR_LATER, code); 7553 if ("LGPL-2.1".equals(codeString)) 7554 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_2_1, code); 7555 if ("LGPL-2.1+".equals(codeString)) 7556 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_2_1PLUS, code); 7557 if ("LGPL-2.1-only".equals(codeString)) 7558 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_2_1_ONLY, code); 7559 if ("LGPL-2.1-or-later".equals(codeString)) 7560 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_2_1_OR_LATER, code); 7561 if ("LGPL-3.0".equals(codeString)) 7562 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_3_0, code); 7563 if ("LGPL-3.0+".equals(codeString)) 7564 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_3_0PLUS, code); 7565 if ("LGPL-3.0-only".equals(codeString)) 7566 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_3_0_ONLY, code); 7567 if ("LGPL-3.0-or-later".equals(codeString)) 7568 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPL_3_0_OR_LATER, code); 7569 if ("LGPLLR".equals(codeString)) 7570 return new Enumeration<SPDXLicense>(this, SPDXLicense.LGPLLR, code); 7571 if ("Libpng".equals(codeString)) 7572 return new Enumeration<SPDXLicense>(this, SPDXLicense.LIBPNG, code); 7573 if ("libpng-2.0".equals(codeString)) 7574 return new Enumeration<SPDXLicense>(this, SPDXLicense.LIBPNG_2_0, code); 7575 if ("libselinux-1.0".equals(codeString)) 7576 return new Enumeration<SPDXLicense>(this, SPDXLicense.LIBSELINUX_1_0, code); 7577 if ("libtiff".equals(codeString)) 7578 return new Enumeration<SPDXLicense>(this, SPDXLicense.LIBTIFF, code); 7579 if ("libutil-David-Nugent".equals(codeString)) 7580 return new Enumeration<SPDXLicense>(this, SPDXLicense.LIBUTIL_DAVID_NUGENT, code); 7581 if ("LiLiQ-P-1.1".equals(codeString)) 7582 return new Enumeration<SPDXLicense>(this, SPDXLicense.LILIQ_P_1_1, code); 7583 if ("LiLiQ-R-1.1".equals(codeString)) 7584 return new Enumeration<SPDXLicense>(this, SPDXLicense.LILIQ_R_1_1, code); 7585 if ("LiLiQ-Rplus-1.1".equals(codeString)) 7586 return new Enumeration<SPDXLicense>(this, SPDXLicense.LILIQ_RPLUS_1_1, code); 7587 if ("Linux-man-pages-1-para".equals(codeString)) 7588 return new Enumeration<SPDXLicense>(this, SPDXLicense.LINUX_MAN_PAGES_1_PARA, code); 7589 if ("Linux-man-pages-copyleft".equals(codeString)) 7590 return new Enumeration<SPDXLicense>(this, SPDXLicense.LINUX_MAN_PAGES_COPYLEFT, code); 7591 if ("Linux-man-pages-copyleft-2-para".equals(codeString)) 7592 return new Enumeration<SPDXLicense>(this, SPDXLicense.LINUX_MAN_PAGES_COPYLEFT_2_PARA, code); 7593 if ("Linux-man-pages-copyleft-var".equals(codeString)) 7594 return new Enumeration<SPDXLicense>(this, SPDXLicense.LINUX_MAN_PAGES_COPYLEFT_VAR, code); 7595 if ("Linux-OpenIB".equals(codeString)) 7596 return new Enumeration<SPDXLicense>(this, SPDXLicense.LINUX_OPENIB, code); 7597 if ("LOOP".equals(codeString)) 7598 return new Enumeration<SPDXLicense>(this, SPDXLicense.LOOP, code); 7599 if ("LPL-1.0".equals(codeString)) 7600 return new Enumeration<SPDXLicense>(this, SPDXLicense.LPL_1_0, code); 7601 if ("LPL-1.02".equals(codeString)) 7602 return new Enumeration<SPDXLicense>(this, SPDXLicense.LPL_1_02, code); 7603 if ("LPPL-1.0".equals(codeString)) 7604 return new Enumeration<SPDXLicense>(this, SPDXLicense.LPPL_1_0, code); 7605 if ("LPPL-1.1".equals(codeString)) 7606 return new Enumeration<SPDXLicense>(this, SPDXLicense.LPPL_1_1, code); 7607 if ("LPPL-1.2".equals(codeString)) 7608 return new Enumeration<SPDXLicense>(this, SPDXLicense.LPPL_1_2, code); 7609 if ("LPPL-1.3a".equals(codeString)) 7610 return new Enumeration<SPDXLicense>(this, SPDXLicense.LPPL_1_3A, code); 7611 if ("LPPL-1.3c".equals(codeString)) 7612 return new Enumeration<SPDXLicense>(this, SPDXLicense.LPPL_1_3C, code); 7613 if ("LZMA-SDK-9.11-to-9.20".equals(codeString)) 7614 return new Enumeration<SPDXLicense>(this, SPDXLicense.LZMA_SDK_9_11_TO_9_20, code); 7615 if ("LZMA-SDK-9.22".equals(codeString)) 7616 return new Enumeration<SPDXLicense>(this, SPDXLicense.LZMA_SDK_9_22, code); 7617 if ("MakeIndex".equals(codeString)) 7618 return new Enumeration<SPDXLicense>(this, SPDXLicense.MAKEINDEX, code); 7619 if ("Martin-Birgmeier".equals(codeString)) 7620 return new Enumeration<SPDXLicense>(this, SPDXLicense.MARTIN_BIRGMEIER, code); 7621 if ("metamail".equals(codeString)) 7622 return new Enumeration<SPDXLicense>(this, SPDXLicense.METAMAIL, code); 7623 if ("Minpack".equals(codeString)) 7624 return new Enumeration<SPDXLicense>(this, SPDXLicense.MINPACK, code); 7625 if ("MirOS".equals(codeString)) 7626 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIROS, code); 7627 if ("MIT".equals(codeString)) 7628 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT, code); 7629 if ("MIT-0".equals(codeString)) 7630 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_0, code); 7631 if ("MIT-advertising".equals(codeString)) 7632 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_ADVERTISING, code); 7633 if ("MIT-CMU".equals(codeString)) 7634 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_CMU, code); 7635 if ("MIT-enna".equals(codeString)) 7636 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_ENNA, code); 7637 if ("MIT-feh".equals(codeString)) 7638 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_FEH, code); 7639 if ("MIT-Festival".equals(codeString)) 7640 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_FESTIVAL, code); 7641 if ("MIT-Modern-Variant".equals(codeString)) 7642 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_MODERN_VARIANT, code); 7643 if ("MIT-open-group".equals(codeString)) 7644 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_OPEN_GROUP, code); 7645 if ("MIT-Wu".equals(codeString)) 7646 return new Enumeration<SPDXLicense>(this, SPDXLicense.MIT_WU, code); 7647 if ("MITNFA".equals(codeString)) 7648 return new Enumeration<SPDXLicense>(this, SPDXLicense.MITNFA, code); 7649 if ("Motosoto".equals(codeString)) 7650 return new Enumeration<SPDXLicense>(this, SPDXLicense.MOTOSOTO, code); 7651 if ("mpi-permissive".equals(codeString)) 7652 return new Enumeration<SPDXLicense>(this, SPDXLicense.MPI_PERMISSIVE, code); 7653 if ("mpich2".equals(codeString)) 7654 return new Enumeration<SPDXLicense>(this, SPDXLicense.MPICH2, code); 7655 if ("MPL-1.0".equals(codeString)) 7656 return new Enumeration<SPDXLicense>(this, SPDXLicense.MPL_1_0, code); 7657 if ("MPL-1.1".equals(codeString)) 7658 return new Enumeration<SPDXLicense>(this, SPDXLicense.MPL_1_1, code); 7659 if ("MPL-2.0".equals(codeString)) 7660 return new Enumeration<SPDXLicense>(this, SPDXLicense.MPL_2_0, code); 7661 if ("MPL-2.0-no-copyleft-exception".equals(codeString)) 7662 return new Enumeration<SPDXLicense>(this, SPDXLicense.MPL_2_0_NO_COPYLEFT_EXCEPTION, code); 7663 if ("mplus".equals(codeString)) 7664 return new Enumeration<SPDXLicense>(this, SPDXLicense.MPLUS, code); 7665 if ("MS-LPL".equals(codeString)) 7666 return new Enumeration<SPDXLicense>(this, SPDXLicense.MS_LPL, code); 7667 if ("MS-PL".equals(codeString)) 7668 return new Enumeration<SPDXLicense>(this, SPDXLicense.MS_PL, code); 7669 if ("MS-RL".equals(codeString)) 7670 return new Enumeration<SPDXLicense>(this, SPDXLicense.MS_RL, code); 7671 if ("MTLL".equals(codeString)) 7672 return new Enumeration<SPDXLicense>(this, SPDXLicense.MTLL, code); 7673 if ("MulanPSL-1.0".equals(codeString)) 7674 return new Enumeration<SPDXLicense>(this, SPDXLicense.MULANPSL_1_0, code); 7675 if ("MulanPSL-2.0".equals(codeString)) 7676 return new Enumeration<SPDXLicense>(this, SPDXLicense.MULANPSL_2_0, code); 7677 if ("Multics".equals(codeString)) 7678 return new Enumeration<SPDXLicense>(this, SPDXLicense.MULTICS, code); 7679 if ("Mup".equals(codeString)) 7680 return new Enumeration<SPDXLicense>(this, SPDXLicense.MUP, code); 7681 if ("NAIST-2003".equals(codeString)) 7682 return new Enumeration<SPDXLicense>(this, SPDXLicense.NAIST_2003, code); 7683 if ("NASA-1.3".equals(codeString)) 7684 return new Enumeration<SPDXLicense>(this, SPDXLicense.NASA_1_3, code); 7685 if ("Naumen".equals(codeString)) 7686 return new Enumeration<SPDXLicense>(this, SPDXLicense.NAUMEN, code); 7687 if ("NBPL-1.0".equals(codeString)) 7688 return new Enumeration<SPDXLicense>(this, SPDXLicense.NBPL_1_0, code); 7689 if ("NCGL-UK-2.0".equals(codeString)) 7690 return new Enumeration<SPDXLicense>(this, SPDXLicense.NCGL_UK_2_0, code); 7691 if ("NCSA".equals(codeString)) 7692 return new Enumeration<SPDXLicense>(this, SPDXLicense.NCSA, code); 7693 if ("Net-SNMP".equals(codeString)) 7694 return new Enumeration<SPDXLicense>(this, SPDXLicense.NET_SNMP, code); 7695 if ("NetCDF".equals(codeString)) 7696 return new Enumeration<SPDXLicense>(this, SPDXLicense.NETCDF, code); 7697 if ("Newsletr".equals(codeString)) 7698 return new Enumeration<SPDXLicense>(this, SPDXLicense.NEWSLETR, code); 7699 if ("NGPL".equals(codeString)) 7700 return new Enumeration<SPDXLicense>(this, SPDXLicense.NGPL, code); 7701 if ("NICTA-1.0".equals(codeString)) 7702 return new Enumeration<SPDXLicense>(this, SPDXLicense.NICTA_1_0, code); 7703 if ("NIST-PD".equals(codeString)) 7704 return new Enumeration<SPDXLicense>(this, SPDXLicense.NIST_PD, code); 7705 if ("NIST-PD-fallback".equals(codeString)) 7706 return new Enumeration<SPDXLicense>(this, SPDXLicense.NIST_PD_FALLBACK, code); 7707 if ("NIST-Software".equals(codeString)) 7708 return new Enumeration<SPDXLicense>(this, SPDXLicense.NIST_SOFTWARE, code); 7709 if ("NLOD-1.0".equals(codeString)) 7710 return new Enumeration<SPDXLicense>(this, SPDXLicense.NLOD_1_0, code); 7711 if ("NLOD-2.0".equals(codeString)) 7712 return new Enumeration<SPDXLicense>(this, SPDXLicense.NLOD_2_0, code); 7713 if ("NLPL".equals(codeString)) 7714 return new Enumeration<SPDXLicense>(this, SPDXLicense.NLPL, code); 7715 if ("Nokia".equals(codeString)) 7716 return new Enumeration<SPDXLicense>(this, SPDXLicense.NOKIA, code); 7717 if ("NOSL".equals(codeString)) 7718 return new Enumeration<SPDXLicense>(this, SPDXLicense.NOSL, code); 7719 if ("not-open-source".equals(codeString)) 7720 return new Enumeration<SPDXLicense>(this, SPDXLicense.NOT_OPEN_SOURCE, code); 7721 if ("Noweb".equals(codeString)) 7722 return new Enumeration<SPDXLicense>(this, SPDXLicense.NOWEB, code); 7723 if ("NPL-1.0".equals(codeString)) 7724 return new Enumeration<SPDXLicense>(this, SPDXLicense.NPL_1_0, code); 7725 if ("NPL-1.1".equals(codeString)) 7726 return new Enumeration<SPDXLicense>(this, SPDXLicense.NPL_1_1, code); 7727 if ("NPOSL-3.0".equals(codeString)) 7728 return new Enumeration<SPDXLicense>(this, SPDXLicense.NPOSL_3_0, code); 7729 if ("NRL".equals(codeString)) 7730 return new Enumeration<SPDXLicense>(this, SPDXLicense.NRL, code); 7731 if ("NTP".equals(codeString)) 7732 return new Enumeration<SPDXLicense>(this, SPDXLicense.NTP, code); 7733 if ("NTP-0".equals(codeString)) 7734 return new Enumeration<SPDXLicense>(this, SPDXLicense.NTP_0, code); 7735 if ("Nunit".equals(codeString)) 7736 return new Enumeration<SPDXLicense>(this, SPDXLicense.NUNIT, code); 7737 if ("O-UDA-1.0".equals(codeString)) 7738 return new Enumeration<SPDXLicense>(this, SPDXLicense.O_UDA_1_0, code); 7739 if ("OCCT-PL".equals(codeString)) 7740 return new Enumeration<SPDXLicense>(this, SPDXLicense.OCCT_PL, code); 7741 if ("OCLC-2.0".equals(codeString)) 7742 return new Enumeration<SPDXLicense>(this, SPDXLicense.OCLC_2_0, code); 7743 if ("ODbL-1.0".equals(codeString)) 7744 return new Enumeration<SPDXLicense>(this, SPDXLicense.ODBL_1_0, code); 7745 if ("ODC-By-1.0".equals(codeString)) 7746 return new Enumeration<SPDXLicense>(this, SPDXLicense.ODC_BY_1_0, code); 7747 if ("OFFIS".equals(codeString)) 7748 return new Enumeration<SPDXLicense>(this, SPDXLicense.OFFIS, code); 7749 if ("OFL-1.0".equals(codeString)) 7750 return new Enumeration<SPDXLicense>(this, SPDXLicense.OFL_1_0, code); 7751 if ("OFL-1.0-no-RFN".equals(codeString)) 7752 return new Enumeration<SPDXLicense>(this, SPDXLicense.OFL_1_0_NO_RFN, code); 7753 if ("OFL-1.0-RFN".equals(codeString)) 7754 return new Enumeration<SPDXLicense>(this, SPDXLicense.OFL_1_0_RFN, code); 7755 if ("OFL-1.1".equals(codeString)) 7756 return new Enumeration<SPDXLicense>(this, SPDXLicense.OFL_1_1, code); 7757 if ("OFL-1.1-no-RFN".equals(codeString)) 7758 return new Enumeration<SPDXLicense>(this, SPDXLicense.OFL_1_1_NO_RFN, code); 7759 if ("OFL-1.1-RFN".equals(codeString)) 7760 return new Enumeration<SPDXLicense>(this, SPDXLicense.OFL_1_1_RFN, code); 7761 if ("OGC-1.0".equals(codeString)) 7762 return new Enumeration<SPDXLicense>(this, SPDXLicense.OGC_1_0, code); 7763 if ("OGDL-Taiwan-1.0".equals(codeString)) 7764 return new Enumeration<SPDXLicense>(this, SPDXLicense.OGDL_TAIWAN_1_0, code); 7765 if ("OGL-Canada-2.0".equals(codeString)) 7766 return new Enumeration<SPDXLicense>(this, SPDXLicense.OGL_CANADA_2_0, code); 7767 if ("OGL-UK-1.0".equals(codeString)) 7768 return new Enumeration<SPDXLicense>(this, SPDXLicense.OGL_UK_1_0, code); 7769 if ("OGL-UK-2.0".equals(codeString)) 7770 return new Enumeration<SPDXLicense>(this, SPDXLicense.OGL_UK_2_0, code); 7771 if ("OGL-UK-3.0".equals(codeString)) 7772 return new Enumeration<SPDXLicense>(this, SPDXLicense.OGL_UK_3_0, code); 7773 if ("OGTSL".equals(codeString)) 7774 return new Enumeration<SPDXLicense>(this, SPDXLicense.OGTSL, code); 7775 if ("OLDAP-1.1".equals(codeString)) 7776 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_1_1, code); 7777 if ("OLDAP-1.2".equals(codeString)) 7778 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_1_2, code); 7779 if ("OLDAP-1.3".equals(codeString)) 7780 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_1_3, code); 7781 if ("OLDAP-1.4".equals(codeString)) 7782 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_1_4, code); 7783 if ("OLDAP-2.0".equals(codeString)) 7784 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_0, code); 7785 if ("OLDAP-2.0.1".equals(codeString)) 7786 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_0_1, code); 7787 if ("OLDAP-2.1".equals(codeString)) 7788 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_1, code); 7789 if ("OLDAP-2.2".equals(codeString)) 7790 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_2, code); 7791 if ("OLDAP-2.2.1".equals(codeString)) 7792 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_2_1, code); 7793 if ("OLDAP-2.2.2".equals(codeString)) 7794 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_2_2, code); 7795 if ("OLDAP-2.3".equals(codeString)) 7796 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_3, code); 7797 if ("OLDAP-2.4".equals(codeString)) 7798 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_4, code); 7799 if ("OLDAP-2.5".equals(codeString)) 7800 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_5, code); 7801 if ("OLDAP-2.6".equals(codeString)) 7802 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_6, code); 7803 if ("OLDAP-2.7".equals(codeString)) 7804 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_7, code); 7805 if ("OLDAP-2.8".equals(codeString)) 7806 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLDAP_2_8, code); 7807 if ("OLFL-1.3".equals(codeString)) 7808 return new Enumeration<SPDXLicense>(this, SPDXLicense.OLFL_1_3, code); 7809 if ("OML".equals(codeString)) 7810 return new Enumeration<SPDXLicense>(this, SPDXLicense.OML, code); 7811 if ("OpenPBS-2.3".equals(codeString)) 7812 return new Enumeration<SPDXLicense>(this, SPDXLicense.OPENPBS_2_3, code); 7813 if ("OpenSSL".equals(codeString)) 7814 return new Enumeration<SPDXLicense>(this, SPDXLicense.OPENSSL, code); 7815 if ("OPL-1.0".equals(codeString)) 7816 return new Enumeration<SPDXLicense>(this, SPDXLicense.OPL_1_0, code); 7817 if ("OPL-UK-3.0".equals(codeString)) 7818 return new Enumeration<SPDXLicense>(this, SPDXLicense.OPL_UK_3_0, code); 7819 if ("OPUBL-1.0".equals(codeString)) 7820 return new Enumeration<SPDXLicense>(this, SPDXLicense.OPUBL_1_0, code); 7821 if ("OSET-PL-2.1".equals(codeString)) 7822 return new Enumeration<SPDXLicense>(this, SPDXLicense.OSET_PL_2_1, code); 7823 if ("OSL-1.0".equals(codeString)) 7824 return new Enumeration<SPDXLicense>(this, SPDXLicense.OSL_1_0, code); 7825 if ("OSL-1.1".equals(codeString)) 7826 return new Enumeration<SPDXLicense>(this, SPDXLicense.OSL_1_1, code); 7827 if ("OSL-2.0".equals(codeString)) 7828 return new Enumeration<SPDXLicense>(this, SPDXLicense.OSL_2_0, code); 7829 if ("OSL-2.1".equals(codeString)) 7830 return new Enumeration<SPDXLicense>(this, SPDXLicense.OSL_2_1, code); 7831 if ("OSL-3.0".equals(codeString)) 7832 return new Enumeration<SPDXLicense>(this, SPDXLicense.OSL_3_0, code); 7833 if ("Parity-6.0.0".equals(codeString)) 7834 return new Enumeration<SPDXLicense>(this, SPDXLicense.PARITY_6_0_0, code); 7835 if ("Parity-7.0.0".equals(codeString)) 7836 return new Enumeration<SPDXLicense>(this, SPDXLicense.PARITY_7_0_0, code); 7837 if ("PDDL-1.0".equals(codeString)) 7838 return new Enumeration<SPDXLicense>(this, SPDXLicense.PDDL_1_0, code); 7839 if ("PHP-3.0".equals(codeString)) 7840 return new Enumeration<SPDXLicense>(this, SPDXLicense.PHP_3_0, code); 7841 if ("PHP-3.01".equals(codeString)) 7842 return new Enumeration<SPDXLicense>(this, SPDXLicense.PHP_3_01, code); 7843 if ("Plexus".equals(codeString)) 7844 return new Enumeration<SPDXLicense>(this, SPDXLicense.PLEXUS, code); 7845 if ("PolyForm-Noncommercial-1.0.0".equals(codeString)) 7846 return new Enumeration<SPDXLicense>(this, SPDXLicense.POLYFORM_NONCOMMERCIAL_1_0_0, code); 7847 if ("PolyForm-Small-Business-1.0.0".equals(codeString)) 7848 return new Enumeration<SPDXLicense>(this, SPDXLicense.POLYFORM_SMALL_BUSINESS_1_0_0, code); 7849 if ("PostgreSQL".equals(codeString)) 7850 return new Enumeration<SPDXLicense>(this, SPDXLicense.POSTGRESQL, code); 7851 if ("PSF-2.0".equals(codeString)) 7852 return new Enumeration<SPDXLicense>(this, SPDXLicense.PSF_2_0, code); 7853 if ("psfrag".equals(codeString)) 7854 return new Enumeration<SPDXLicense>(this, SPDXLicense.PSFRAG, code); 7855 if ("psutils".equals(codeString)) 7856 return new Enumeration<SPDXLicense>(this, SPDXLicense.PSUTILS, code); 7857 if ("Python-2.0".equals(codeString)) 7858 return new Enumeration<SPDXLicense>(this, SPDXLicense.PYTHON_2_0, code); 7859 if ("Python-2.0.1".equals(codeString)) 7860 return new Enumeration<SPDXLicense>(this, SPDXLicense.PYTHON_2_0_1, code); 7861 if ("Qhull".equals(codeString)) 7862 return new Enumeration<SPDXLicense>(this, SPDXLicense.QHULL, code); 7863 if ("QPL-1.0".equals(codeString)) 7864 return new Enumeration<SPDXLicense>(this, SPDXLicense.QPL_1_0, code); 7865 if ("QPL-1.0-INRIA-2004".equals(codeString)) 7866 return new Enumeration<SPDXLicense>(this, SPDXLicense.QPL_1_0_INRIA_2004, code); 7867 if ("Rdisc".equals(codeString)) 7868 return new Enumeration<SPDXLicense>(this, SPDXLicense.RDISC, code); 7869 if ("RHeCos-1.1".equals(codeString)) 7870 return new Enumeration<SPDXLicense>(this, SPDXLicense.RHECOS_1_1, code); 7871 if ("RPL-1.1".equals(codeString)) 7872 return new Enumeration<SPDXLicense>(this, SPDXLicense.RPL_1_1, code); 7873 if ("RPL-1.5".equals(codeString)) 7874 return new Enumeration<SPDXLicense>(this, SPDXLicense.RPL_1_5, code); 7875 if ("RPSL-1.0".equals(codeString)) 7876 return new Enumeration<SPDXLicense>(this, SPDXLicense.RPSL_1_0, code); 7877 if ("RSA-MD".equals(codeString)) 7878 return new Enumeration<SPDXLicense>(this, SPDXLicense.RSA_MD, code); 7879 if ("RSCPL".equals(codeString)) 7880 return new Enumeration<SPDXLicense>(this, SPDXLicense.RSCPL, code); 7881 if ("Ruby".equals(codeString)) 7882 return new Enumeration<SPDXLicense>(this, SPDXLicense.RUBY, code); 7883 if ("SAX-PD".equals(codeString)) 7884 return new Enumeration<SPDXLicense>(this, SPDXLicense.SAX_PD, code); 7885 if ("Saxpath".equals(codeString)) 7886 return new Enumeration<SPDXLicense>(this, SPDXLicense.SAXPATH, code); 7887 if ("SCEA".equals(codeString)) 7888 return new Enumeration<SPDXLicense>(this, SPDXLicense.SCEA, code); 7889 if ("SchemeReport".equals(codeString)) 7890 return new Enumeration<SPDXLicense>(this, SPDXLicense.SCHEMEREPORT, code); 7891 if ("Sendmail".equals(codeString)) 7892 return new Enumeration<SPDXLicense>(this, SPDXLicense.SENDMAIL, code); 7893 if ("Sendmail-8.23".equals(codeString)) 7894 return new Enumeration<SPDXLicense>(this, SPDXLicense.SENDMAIL_8_23, code); 7895 if ("SGI-B-1.0".equals(codeString)) 7896 return new Enumeration<SPDXLicense>(this, SPDXLicense.SGI_B_1_0, code); 7897 if ("SGI-B-1.1".equals(codeString)) 7898 return new Enumeration<SPDXLicense>(this, SPDXLicense.SGI_B_1_1, code); 7899 if ("SGI-B-2.0".equals(codeString)) 7900 return new Enumeration<SPDXLicense>(this, SPDXLicense.SGI_B_2_0, code); 7901 if ("SGP4".equals(codeString)) 7902 return new Enumeration<SPDXLicense>(this, SPDXLicense.SGP4, code); 7903 if ("SHL-0.5".equals(codeString)) 7904 return new Enumeration<SPDXLicense>(this, SPDXLicense.SHL_0_5, code); 7905 if ("SHL-0.51".equals(codeString)) 7906 return new Enumeration<SPDXLicense>(this, SPDXLicense.SHL_0_51, code); 7907 if ("SimPL-2.0".equals(codeString)) 7908 return new Enumeration<SPDXLicense>(this, SPDXLicense.SIMPL_2_0, code); 7909 if ("SISSL".equals(codeString)) 7910 return new Enumeration<SPDXLicense>(this, SPDXLicense.SISSL, code); 7911 if ("SISSL-1.2".equals(codeString)) 7912 return new Enumeration<SPDXLicense>(this, SPDXLicense.SISSL_1_2, code); 7913 if ("Sleepycat".equals(codeString)) 7914 return new Enumeration<SPDXLicense>(this, SPDXLicense.SLEEPYCAT, code); 7915 if ("SMLNJ".equals(codeString)) 7916 return new Enumeration<SPDXLicense>(this, SPDXLicense.SMLNJ, code); 7917 if ("SMPPL".equals(codeString)) 7918 return new Enumeration<SPDXLicense>(this, SPDXLicense.SMPPL, code); 7919 if ("SNIA".equals(codeString)) 7920 return new Enumeration<SPDXLicense>(this, SPDXLicense.SNIA, code); 7921 if ("snprintf".equals(codeString)) 7922 return new Enumeration<SPDXLicense>(this, SPDXLicense.SNPRINTF, code); 7923 if ("Spencer-86".equals(codeString)) 7924 return new Enumeration<SPDXLicense>(this, SPDXLicense.SPENCER_86, code); 7925 if ("Spencer-94".equals(codeString)) 7926 return new Enumeration<SPDXLicense>(this, SPDXLicense.SPENCER_94, code); 7927 if ("Spencer-99".equals(codeString)) 7928 return new Enumeration<SPDXLicense>(this, SPDXLicense.SPENCER_99, code); 7929 if ("SPL-1.0".equals(codeString)) 7930 return new Enumeration<SPDXLicense>(this, SPDXLicense.SPL_1_0, code); 7931 if ("SSH-OpenSSH".equals(codeString)) 7932 return new Enumeration<SPDXLicense>(this, SPDXLicense.SSH_OPENSSH, code); 7933 if ("SSH-short".equals(codeString)) 7934 return new Enumeration<SPDXLicense>(this, SPDXLicense.SSH_SHORT, code); 7935 if ("SSPL-1.0".equals(codeString)) 7936 return new Enumeration<SPDXLicense>(this, SPDXLicense.SSPL_1_0, code); 7937 if ("StandardML-NJ".equals(codeString)) 7938 return new Enumeration<SPDXLicense>(this, SPDXLicense.STANDARDML_NJ, code); 7939 if ("SugarCRM-1.1.3".equals(codeString)) 7940 return new Enumeration<SPDXLicense>(this, SPDXLicense.SUGARCRM_1_1_3, code); 7941 if ("SunPro".equals(codeString)) 7942 return new Enumeration<SPDXLicense>(this, SPDXLicense.SUNPRO, code); 7943 if ("SWL".equals(codeString)) 7944 return new Enumeration<SPDXLicense>(this, SPDXLicense.SWL, code); 7945 if ("Symlinks".equals(codeString)) 7946 return new Enumeration<SPDXLicense>(this, SPDXLicense.SYMLINKS, code); 7947 if ("TAPR-OHL-1.0".equals(codeString)) 7948 return new Enumeration<SPDXLicense>(this, SPDXLicense.TAPR_OHL_1_0, code); 7949 if ("TCL".equals(codeString)) 7950 return new Enumeration<SPDXLicense>(this, SPDXLicense.TCL, code); 7951 if ("TCP-wrappers".equals(codeString)) 7952 return new Enumeration<SPDXLicense>(this, SPDXLicense.TCP_WRAPPERS, code); 7953 if ("TermReadKey".equals(codeString)) 7954 return new Enumeration<SPDXLicense>(this, SPDXLicense.TERMREADKEY, code); 7955 if ("TMate".equals(codeString)) 7956 return new Enumeration<SPDXLicense>(this, SPDXLicense.TMATE, code); 7957 if ("TORQUE-1.1".equals(codeString)) 7958 return new Enumeration<SPDXLicense>(this, SPDXLicense.TORQUE_1_1, code); 7959 if ("TOSL".equals(codeString)) 7960 return new Enumeration<SPDXLicense>(this, SPDXLicense.TOSL, code); 7961 if ("TPDL".equals(codeString)) 7962 return new Enumeration<SPDXLicense>(this, SPDXLicense.TPDL, code); 7963 if ("TPL-1.0".equals(codeString)) 7964 return new Enumeration<SPDXLicense>(this, SPDXLicense.TPL_1_0, code); 7965 if ("TTWL".equals(codeString)) 7966 return new Enumeration<SPDXLicense>(this, SPDXLicense.TTWL, code); 7967 if ("TU-Berlin-1.0".equals(codeString)) 7968 return new Enumeration<SPDXLicense>(this, SPDXLicense.TU_BERLIN_1_0, code); 7969 if ("TU-Berlin-2.0".equals(codeString)) 7970 return new Enumeration<SPDXLicense>(this, SPDXLicense.TU_BERLIN_2_0, code); 7971 if ("UCAR".equals(codeString)) 7972 return new Enumeration<SPDXLicense>(this, SPDXLicense.UCAR, code); 7973 if ("UCL-1.0".equals(codeString)) 7974 return new Enumeration<SPDXLicense>(this, SPDXLicense.UCL_1_0, code); 7975 if ("Unicode-DFS-2015".equals(codeString)) 7976 return new Enumeration<SPDXLicense>(this, SPDXLicense.UNICODE_DFS_2015, code); 7977 if ("Unicode-DFS-2016".equals(codeString)) 7978 return new Enumeration<SPDXLicense>(this, SPDXLicense.UNICODE_DFS_2016, code); 7979 if ("Unicode-TOU".equals(codeString)) 7980 return new Enumeration<SPDXLicense>(this, SPDXLicense.UNICODE_TOU, code); 7981 if ("UnixCrypt".equals(codeString)) 7982 return new Enumeration<SPDXLicense>(this, SPDXLicense.UNIXCRYPT, code); 7983 if ("Unlicense".equals(codeString)) 7984 return new Enumeration<SPDXLicense>(this, SPDXLicense.UNLICENSE, code); 7985 if ("UPL-1.0".equals(codeString)) 7986 return new Enumeration<SPDXLicense>(this, SPDXLicense.UPL_1_0, code); 7987 if ("Vim".equals(codeString)) 7988 return new Enumeration<SPDXLicense>(this, SPDXLicense.VIM, code); 7989 if ("VOSTROM".equals(codeString)) 7990 return new Enumeration<SPDXLicense>(this, SPDXLicense.VOSTROM, code); 7991 if ("VSL-1.0".equals(codeString)) 7992 return new Enumeration<SPDXLicense>(this, SPDXLicense.VSL_1_0, code); 7993 if ("W3C".equals(codeString)) 7994 return new Enumeration<SPDXLicense>(this, SPDXLicense.W3C, code); 7995 if ("W3C-19980720".equals(codeString)) 7996 return new Enumeration<SPDXLicense>(this, SPDXLicense.W3C_19980720, code); 7997 if ("W3C-20150513".equals(codeString)) 7998 return new Enumeration<SPDXLicense>(this, SPDXLicense.W3C_20150513, code); 7999 if ("w3m".equals(codeString)) 8000 return new Enumeration<SPDXLicense>(this, SPDXLicense.W3M, code); 8001 if ("Watcom-1.0".equals(codeString)) 8002 return new Enumeration<SPDXLicense>(this, SPDXLicense.WATCOM_1_0, code); 8003 if ("Widget-Workshop".equals(codeString)) 8004 return new Enumeration<SPDXLicense>(this, SPDXLicense.WIDGET_WORKSHOP, code); 8005 if ("Wsuipa".equals(codeString)) 8006 return new Enumeration<SPDXLicense>(this, SPDXLicense.WSUIPA, code); 8007 if ("WTFPL".equals(codeString)) 8008 return new Enumeration<SPDXLicense>(this, SPDXLicense.WTFPL, code); 8009 if ("wxWindows".equals(codeString)) 8010 return new Enumeration<SPDXLicense>(this, SPDXLicense.WXWINDOWS, code); 8011 if ("X11".equals(codeString)) 8012 return new Enumeration<SPDXLicense>(this, SPDXLicense.X11, code); 8013 if ("X11-distribute-modifications-variant".equals(codeString)) 8014 return new Enumeration<SPDXLicense>(this, SPDXLicense.X11_DISTRIBUTE_MODIFICATIONS_VARIANT, code); 8015 if ("Xdebug-1.03".equals(codeString)) 8016 return new Enumeration<SPDXLicense>(this, SPDXLicense.XDEBUG_1_03, code); 8017 if ("Xerox".equals(codeString)) 8018 return new Enumeration<SPDXLicense>(this, SPDXLicense.XEROX, code); 8019 if ("Xfig".equals(codeString)) 8020 return new Enumeration<SPDXLicense>(this, SPDXLicense.XFIG, code); 8021 if ("XFree86-1.1".equals(codeString)) 8022 return new Enumeration<SPDXLicense>(this, SPDXLicense.XFREE86_1_1, code); 8023 if ("xinetd".equals(codeString)) 8024 return new Enumeration<SPDXLicense>(this, SPDXLicense.XINETD, code); 8025 if ("xlock".equals(codeString)) 8026 return new Enumeration<SPDXLicense>(this, SPDXLicense.XLOCK, code); 8027 if ("Xnet".equals(codeString)) 8028 return new Enumeration<SPDXLicense>(this, SPDXLicense.XNET, code); 8029 if ("xpp".equals(codeString)) 8030 return new Enumeration<SPDXLicense>(this, SPDXLicense.XPP, code); 8031 if ("XSkat".equals(codeString)) 8032 return new Enumeration<SPDXLicense>(this, SPDXLicense.XSKAT, code); 8033 if ("YPL-1.0".equals(codeString)) 8034 return new Enumeration<SPDXLicense>(this, SPDXLicense.YPL_1_0, code); 8035 if ("YPL-1.1".equals(codeString)) 8036 return new Enumeration<SPDXLicense>(this, SPDXLicense.YPL_1_1, code); 8037 if ("Zed".equals(codeString)) 8038 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZED, code); 8039 if ("Zend-2.0".equals(codeString)) 8040 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZEND_2_0, code); 8041 if ("Zimbra-1.3".equals(codeString)) 8042 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZIMBRA_1_3, code); 8043 if ("Zimbra-1.4".equals(codeString)) 8044 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZIMBRA_1_4, code); 8045 if ("Zlib".equals(codeString)) 8046 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZLIB, code); 8047 if ("zlib-acknowledgement".equals(codeString)) 8048 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZLIB_ACKNOWLEDGEMENT, code); 8049 if ("ZPL-1.1".equals(codeString)) 8050 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZPL_1_1, code); 8051 if ("ZPL-2.0".equals(codeString)) 8052 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZPL_2_0, code); 8053 if ("ZPL-2.1".equals(codeString)) 8054 return new Enumeration<SPDXLicense>(this, SPDXLicense.ZPL_2_1, code); 8055 throw new FHIRException("Unknown SPDXLicense code '"+codeString+"'"); 8056 } 8057 public String toCode(SPDXLicense code) { 8058 if (code == SPDXLicense.NULL) 8059 return null; 8060 if (code == SPDXLicense._0BSD) 8061 return "0BSD"; 8062 if (code == SPDXLicense.AAL) 8063 return "AAL"; 8064 if (code == SPDXLicense.ABSTYLES) 8065 return "Abstyles"; 8066 if (code == SPDXLicense.ADACORE_DOC) 8067 return "AdaCore-doc"; 8068 if (code == SPDXLicense.ADOBE_2006) 8069 return "Adobe-2006"; 8070 if (code == SPDXLicense.ADOBE_GLYPH) 8071 return "Adobe-Glyph"; 8072 if (code == SPDXLicense.ADSL) 8073 return "ADSL"; 8074 if (code == SPDXLicense.AFL_1_1) 8075 return "AFL-1.1"; 8076 if (code == SPDXLicense.AFL_1_2) 8077 return "AFL-1.2"; 8078 if (code == SPDXLicense.AFL_2_0) 8079 return "AFL-2.0"; 8080 if (code == SPDXLicense.AFL_2_1) 8081 return "AFL-2.1"; 8082 if (code == SPDXLicense.AFL_3_0) 8083 return "AFL-3.0"; 8084 if (code == SPDXLicense.AFMPARSE) 8085 return "Afmparse"; 8086 if (code == SPDXLicense.AGPL_1_0) 8087 return "AGPL-1.0"; 8088 if (code == SPDXLicense.AGPL_1_0_ONLY) 8089 return "AGPL-1.0-only"; 8090 if (code == SPDXLicense.AGPL_1_0_OR_LATER) 8091 return "AGPL-1.0-or-later"; 8092 if (code == SPDXLicense.AGPL_3_0) 8093 return "AGPL-3.0"; 8094 if (code == SPDXLicense.AGPL_3_0_ONLY) 8095 return "AGPL-3.0-only"; 8096 if (code == SPDXLicense.AGPL_3_0_OR_LATER) 8097 return "AGPL-3.0-or-later"; 8098 if (code == SPDXLicense.ALADDIN) 8099 return "Aladdin"; 8100 if (code == SPDXLicense.AMDPLPA) 8101 return "AMDPLPA"; 8102 if (code == SPDXLicense.AML) 8103 return "AML"; 8104 if (code == SPDXLicense.AMPAS) 8105 return "AMPAS"; 8106 if (code == SPDXLicense.ANTLR_PD) 8107 return "ANTLR-PD"; 8108 if (code == SPDXLicense.ANTLR_PD_FALLBACK) 8109 return "ANTLR-PD-fallback"; 8110 if (code == SPDXLicense.APACHE_1_0) 8111 return "Apache-1.0"; 8112 if (code == SPDXLicense.APACHE_1_1) 8113 return "Apache-1.1"; 8114 if (code == SPDXLicense.APACHE_2_0) 8115 return "Apache-2.0"; 8116 if (code == SPDXLicense.APAFML) 8117 return "APAFML"; 8118 if (code == SPDXLicense.APL_1_0) 8119 return "APL-1.0"; 8120 if (code == SPDXLicense.APP_S2P) 8121 return "App-s2p"; 8122 if (code == SPDXLicense.APSL_1_0) 8123 return "APSL-1.0"; 8124 if (code == SPDXLicense.APSL_1_1) 8125 return "APSL-1.1"; 8126 if (code == SPDXLicense.APSL_1_2) 8127 return "APSL-1.2"; 8128 if (code == SPDXLicense.APSL_2_0) 8129 return "APSL-2.0"; 8130 if (code == SPDXLicense.ARPHIC_1999) 8131 return "Arphic-1999"; 8132 if (code == SPDXLicense.ARTISTIC_1_0) 8133 return "Artistic-1.0"; 8134 if (code == SPDXLicense.ARTISTIC_1_0_CL8) 8135 return "Artistic-1.0-cl8"; 8136 if (code == SPDXLicense.ARTISTIC_1_0_PERL) 8137 return "Artistic-1.0-Perl"; 8138 if (code == SPDXLicense.ARTISTIC_2_0) 8139 return "Artistic-2.0"; 8140 if (code == SPDXLicense.ASWF_DIGITAL_ASSETS_1_0) 8141 return "ASWF-Digital-Assets-1.0"; 8142 if (code == SPDXLicense.ASWF_DIGITAL_ASSETS_1_1) 8143 return "ASWF-Digital-Assets-1.1"; 8144 if (code == SPDXLicense.BAEKMUK) 8145 return "Baekmuk"; 8146 if (code == SPDXLicense.BAHYPH) 8147 return "Bahyph"; 8148 if (code == SPDXLicense.BARR) 8149 return "Barr"; 8150 if (code == SPDXLicense.BEERWARE) 8151 return "Beerware"; 8152 if (code == SPDXLicense.BITSTREAM_CHARTER) 8153 return "Bitstream-Charter"; 8154 if (code == SPDXLicense.BITSTREAM_VERA) 8155 return "Bitstream-Vera"; 8156 if (code == SPDXLicense.BITTORRENT_1_0) 8157 return "BitTorrent-1.0"; 8158 if (code == SPDXLicense.BITTORRENT_1_1) 8159 return "BitTorrent-1.1"; 8160 if (code == SPDXLicense.BLESSING) 8161 return "blessing"; 8162 if (code == SPDXLicense.BLUEOAK_1_0_0) 8163 return "BlueOak-1.0.0"; 8164 if (code == SPDXLicense.BOEHM_GC) 8165 return "Boehm-GC"; 8166 if (code == SPDXLicense.BORCEUX) 8167 return "Borceux"; 8168 if (code == SPDXLicense.BRIAN_GLADMAN_3_CLAUSE) 8169 return "Brian-Gladman-3-Clause"; 8170 if (code == SPDXLicense.BSD_1_CLAUSE) 8171 return "BSD-1-Clause"; 8172 if (code == SPDXLicense.BSD_2_CLAUSE) 8173 return "BSD-2-Clause"; 8174 if (code == SPDXLicense.BSD_2_CLAUSE_FREEBSD) 8175 return "BSD-2-Clause-FreeBSD"; 8176 if (code == SPDXLicense.BSD_2_CLAUSE_NETBSD) 8177 return "BSD-2-Clause-NetBSD"; 8178 if (code == SPDXLicense.BSD_2_CLAUSE_PATENT) 8179 return "BSD-2-Clause-Patent"; 8180 if (code == SPDXLicense.BSD_2_CLAUSE_VIEWS) 8181 return "BSD-2-Clause-Views"; 8182 if (code == SPDXLicense.BSD_3_CLAUSE) 8183 return "BSD-3-Clause"; 8184 if (code == SPDXLicense.BSD_3_CLAUSE_ATTRIBUTION) 8185 return "BSD-3-Clause-Attribution"; 8186 if (code == SPDXLicense.BSD_3_CLAUSE_CLEAR) 8187 return "BSD-3-Clause-Clear"; 8188 if (code == SPDXLicense.BSD_3_CLAUSE_LBNL) 8189 return "BSD-3-Clause-LBNL"; 8190 if (code == SPDXLicense.BSD_3_CLAUSE_MODIFICATION) 8191 return "BSD-3-Clause-Modification"; 8192 if (code == SPDXLicense.BSD_3_CLAUSE_NO_MILITARY_LICENSE) 8193 return "BSD-3-Clause-No-Military-License"; 8194 if (code == SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_LICENSE) 8195 return "BSD-3-Clause-No-Nuclear-License"; 8196 if (code == SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_LICENSE_2014) 8197 return "BSD-3-Clause-No-Nuclear-License-2014"; 8198 if (code == SPDXLicense.BSD_3_CLAUSE_NO_NUCLEAR_WARRANTY) 8199 return "BSD-3-Clause-No-Nuclear-Warranty"; 8200 if (code == SPDXLicense.BSD_3_CLAUSE_OPEN_MPI) 8201 return "BSD-3-Clause-Open-MPI"; 8202 if (code == SPDXLicense.BSD_4_CLAUSE) 8203 return "BSD-4-Clause"; 8204 if (code == SPDXLicense.BSD_4_CLAUSE_SHORTENED) 8205 return "BSD-4-Clause-Shortened"; 8206 if (code == SPDXLicense.BSD_4_CLAUSE_UC) 8207 return "BSD-4-Clause-UC"; 8208 if (code == SPDXLicense.BSD_4_3RENO) 8209 return "BSD-4.3RENO"; 8210 if (code == SPDXLicense.BSD_4_3TAHOE) 8211 return "BSD-4.3TAHOE"; 8212 if (code == SPDXLicense.BSD_ADVERTISING_ACKNOWLEDGEMENT) 8213 return "BSD-Advertising-Acknowledgement"; 8214 if (code == SPDXLicense.BSD_ATTRIBUTION_HPND_DISCLAIMER) 8215 return "BSD-Attribution-HPND-disclaimer"; 8216 if (code == SPDXLicense.BSD_PROTECTION) 8217 return "BSD-Protection"; 8218 if (code == SPDXLicense.BSD_SOURCE_CODE) 8219 return "BSD-Source-Code"; 8220 if (code == SPDXLicense.BSL_1_0) 8221 return "BSL-1.0"; 8222 if (code == SPDXLicense.BUSL_1_1) 8223 return "BUSL-1.1"; 8224 if (code == SPDXLicense.BZIP2_1_0_5) 8225 return "bzip2-1.0.5"; 8226 if (code == SPDXLicense.BZIP2_1_0_6) 8227 return "bzip2-1.0.6"; 8228 if (code == SPDXLicense.C_UDA_1_0) 8229 return "C-UDA-1.0"; 8230 if (code == SPDXLicense.CAL_1_0) 8231 return "CAL-1.0"; 8232 if (code == SPDXLicense.CAL_1_0_COMBINED_WORK_EXCEPTION) 8233 return "CAL-1.0-Combined-Work-Exception"; 8234 if (code == SPDXLicense.CALDERA) 8235 return "Caldera"; 8236 if (code == SPDXLicense.CATOSL_1_1) 8237 return "CATOSL-1.1"; 8238 if (code == SPDXLicense.CC_BY_1_0) 8239 return "CC-BY-1.0"; 8240 if (code == SPDXLicense.CC_BY_2_0) 8241 return "CC-BY-2.0"; 8242 if (code == SPDXLicense.CC_BY_2_5) 8243 return "CC-BY-2.5"; 8244 if (code == SPDXLicense.CC_BY_2_5_AU) 8245 return "CC-BY-2.5-AU"; 8246 if (code == SPDXLicense.CC_BY_3_0) 8247 return "CC-BY-3.0"; 8248 if (code == SPDXLicense.CC_BY_3_0_AT) 8249 return "CC-BY-3.0-AT"; 8250 if (code == SPDXLicense.CC_BY_3_0_DE) 8251 return "CC-BY-3.0-DE"; 8252 if (code == SPDXLicense.CC_BY_3_0_IGO) 8253 return "CC-BY-3.0-IGO"; 8254 if (code == SPDXLicense.CC_BY_3_0_NL) 8255 return "CC-BY-3.0-NL"; 8256 if (code == SPDXLicense.CC_BY_3_0_US) 8257 return "CC-BY-3.0-US"; 8258 if (code == SPDXLicense.CC_BY_4_0) 8259 return "CC-BY-4.0"; 8260 if (code == SPDXLicense.CC_BY_NC_1_0) 8261 return "CC-BY-NC-1.0"; 8262 if (code == SPDXLicense.CC_BY_NC_2_0) 8263 return "CC-BY-NC-2.0"; 8264 if (code == SPDXLicense.CC_BY_NC_2_5) 8265 return "CC-BY-NC-2.5"; 8266 if (code == SPDXLicense.CC_BY_NC_3_0) 8267 return "CC-BY-NC-3.0"; 8268 if (code == SPDXLicense.CC_BY_NC_3_0_DE) 8269 return "CC-BY-NC-3.0-DE"; 8270 if (code == SPDXLicense.CC_BY_NC_4_0) 8271 return "CC-BY-NC-4.0"; 8272 if (code == SPDXLicense.CC_BY_NC_ND_1_0) 8273 return "CC-BY-NC-ND-1.0"; 8274 if (code == SPDXLicense.CC_BY_NC_ND_2_0) 8275 return "CC-BY-NC-ND-2.0"; 8276 if (code == SPDXLicense.CC_BY_NC_ND_2_5) 8277 return "CC-BY-NC-ND-2.5"; 8278 if (code == SPDXLicense.CC_BY_NC_ND_3_0) 8279 return "CC-BY-NC-ND-3.0"; 8280 if (code == SPDXLicense.CC_BY_NC_ND_3_0_DE) 8281 return "CC-BY-NC-ND-3.0-DE"; 8282 if (code == SPDXLicense.CC_BY_NC_ND_3_0_IGO) 8283 return "CC-BY-NC-ND-3.0-IGO"; 8284 if (code == SPDXLicense.CC_BY_NC_ND_4_0) 8285 return "CC-BY-NC-ND-4.0"; 8286 if (code == SPDXLicense.CC_BY_NC_SA_1_0) 8287 return "CC-BY-NC-SA-1.0"; 8288 if (code == SPDXLicense.CC_BY_NC_SA_2_0) 8289 return "CC-BY-NC-SA-2.0"; 8290 if (code == SPDXLicense.CC_BY_NC_SA_2_0_DE) 8291 return "CC-BY-NC-SA-2.0-DE"; 8292 if (code == SPDXLicense.CC_BY_NC_SA_2_0_FR) 8293 return "CC-BY-NC-SA-2.0-FR"; 8294 if (code == SPDXLicense.CC_BY_NC_SA_2_0_UK) 8295 return "CC-BY-NC-SA-2.0-UK"; 8296 if (code == SPDXLicense.CC_BY_NC_SA_2_5) 8297 return "CC-BY-NC-SA-2.5"; 8298 if (code == SPDXLicense.CC_BY_NC_SA_3_0) 8299 return "CC-BY-NC-SA-3.0"; 8300 if (code == SPDXLicense.CC_BY_NC_SA_3_0_DE) 8301 return "CC-BY-NC-SA-3.0-DE"; 8302 if (code == SPDXLicense.CC_BY_NC_SA_3_0_IGO) 8303 return "CC-BY-NC-SA-3.0-IGO"; 8304 if (code == SPDXLicense.CC_BY_NC_SA_4_0) 8305 return "CC-BY-NC-SA-4.0"; 8306 if (code == SPDXLicense.CC_BY_ND_1_0) 8307 return "CC-BY-ND-1.0"; 8308 if (code == SPDXLicense.CC_BY_ND_2_0) 8309 return "CC-BY-ND-2.0"; 8310 if (code == SPDXLicense.CC_BY_ND_2_5) 8311 return "CC-BY-ND-2.5"; 8312 if (code == SPDXLicense.CC_BY_ND_3_0) 8313 return "CC-BY-ND-3.0"; 8314 if (code == SPDXLicense.CC_BY_ND_3_0_DE) 8315 return "CC-BY-ND-3.0-DE"; 8316 if (code == SPDXLicense.CC_BY_ND_4_0) 8317 return "CC-BY-ND-4.0"; 8318 if (code == SPDXLicense.CC_BY_SA_1_0) 8319 return "CC-BY-SA-1.0"; 8320 if (code == SPDXLicense.CC_BY_SA_2_0) 8321 return "CC-BY-SA-2.0"; 8322 if (code == SPDXLicense.CC_BY_SA_2_0_UK) 8323 return "CC-BY-SA-2.0-UK"; 8324 if (code == SPDXLicense.CC_BY_SA_2_1_JP) 8325 return "CC-BY-SA-2.1-JP"; 8326 if (code == SPDXLicense.CC_BY_SA_2_5) 8327 return "CC-BY-SA-2.5"; 8328 if (code == SPDXLicense.CC_BY_SA_3_0) 8329 return "CC-BY-SA-3.0"; 8330 if (code == SPDXLicense.CC_BY_SA_3_0_AT) 8331 return "CC-BY-SA-3.0-AT"; 8332 if (code == SPDXLicense.CC_BY_SA_3_0_DE) 8333 return "CC-BY-SA-3.0-DE"; 8334 if (code == SPDXLicense.CC_BY_SA_3_0_IGO) 8335 return "CC-BY-SA-3.0-IGO"; 8336 if (code == SPDXLicense.CC_BY_SA_4_0) 8337 return "CC-BY-SA-4.0"; 8338 if (code == SPDXLicense.CC_PDDC) 8339 return "CC-PDDC"; 8340 if (code == SPDXLicense.CC0_1_0) 8341 return "CC0-1.0"; 8342 if (code == SPDXLicense.CDDL_1_0) 8343 return "CDDL-1.0"; 8344 if (code == SPDXLicense.CDDL_1_1) 8345 return "CDDL-1.1"; 8346 if (code == SPDXLicense.CDL_1_0) 8347 return "CDL-1.0"; 8348 if (code == SPDXLicense.CDLA_PERMISSIVE_1_0) 8349 return "CDLA-Permissive-1.0"; 8350 if (code == SPDXLicense.CDLA_PERMISSIVE_2_0) 8351 return "CDLA-Permissive-2.0"; 8352 if (code == SPDXLicense.CDLA_SHARING_1_0) 8353 return "CDLA-Sharing-1.0"; 8354 if (code == SPDXLicense.CECILL_1_0) 8355 return "CECILL-1.0"; 8356 if (code == SPDXLicense.CECILL_1_1) 8357 return "CECILL-1.1"; 8358 if (code == SPDXLicense.CECILL_2_0) 8359 return "CECILL-2.0"; 8360 if (code == SPDXLicense.CECILL_2_1) 8361 return "CECILL-2.1"; 8362 if (code == SPDXLicense.CECILL_B) 8363 return "CECILL-B"; 8364 if (code == SPDXLicense.CECILL_C) 8365 return "CECILL-C"; 8366 if (code == SPDXLicense.CERN_OHL_1_1) 8367 return "CERN-OHL-1.1"; 8368 if (code == SPDXLicense.CERN_OHL_1_2) 8369 return "CERN-OHL-1.2"; 8370 if (code == SPDXLicense.CERN_OHL_P_2_0) 8371 return "CERN-OHL-P-2.0"; 8372 if (code == SPDXLicense.CERN_OHL_S_2_0) 8373 return "CERN-OHL-S-2.0"; 8374 if (code == SPDXLicense.CERN_OHL_W_2_0) 8375 return "CERN-OHL-W-2.0"; 8376 if (code == SPDXLicense.CFITSIO) 8377 return "CFITSIO"; 8378 if (code == SPDXLicense.CHECKMK) 8379 return "checkmk"; 8380 if (code == SPDXLicense.CLARTISTIC) 8381 return "ClArtistic"; 8382 if (code == SPDXLicense.CLIPS) 8383 return "Clips"; 8384 if (code == SPDXLicense.CMU_MACH) 8385 return "CMU-Mach"; 8386 if (code == SPDXLicense.CNRI_JYTHON) 8387 return "CNRI-Jython"; 8388 if (code == SPDXLicense.CNRI_PYTHON) 8389 return "CNRI-Python"; 8390 if (code == SPDXLicense.CNRI_PYTHON_GPL_COMPATIBLE) 8391 return "CNRI-Python-GPL-Compatible"; 8392 if (code == SPDXLicense.COIL_1_0) 8393 return "COIL-1.0"; 8394 if (code == SPDXLicense.COMMUNITY_SPEC_1_0) 8395 return "Community-Spec-1.0"; 8396 if (code == SPDXLicense.CONDOR_1_1) 8397 return "Condor-1.1"; 8398 if (code == SPDXLicense.COPYLEFT_NEXT_0_3_0) 8399 return "copyleft-next-0.3.0"; 8400 if (code == SPDXLicense.COPYLEFT_NEXT_0_3_1) 8401 return "copyleft-next-0.3.1"; 8402 if (code == SPDXLicense.CORNELL_LOSSLESS_JPEG) 8403 return "Cornell-Lossless-JPEG"; 8404 if (code == SPDXLicense.CPAL_1_0) 8405 return "CPAL-1.0"; 8406 if (code == SPDXLicense.CPL_1_0) 8407 return "CPL-1.0"; 8408 if (code == SPDXLicense.CPOL_1_02) 8409 return "CPOL-1.02"; 8410 if (code == SPDXLicense.CROSSWORD) 8411 return "Crossword"; 8412 if (code == SPDXLicense.CRYSTALSTACKER) 8413 return "CrystalStacker"; 8414 if (code == SPDXLicense.CUA_OPL_1_0) 8415 return "CUA-OPL-1.0"; 8416 if (code == SPDXLicense.CUBE) 8417 return "Cube"; 8418 if (code == SPDXLicense.CURL) 8419 return "curl"; 8420 if (code == SPDXLicense.D_FSL_1_0) 8421 return "D-FSL-1.0"; 8422 if (code == SPDXLicense.DIFFMARK) 8423 return "diffmark"; 8424 if (code == SPDXLicense.DL_DE_BY_2_0) 8425 return "DL-DE-BY-2.0"; 8426 if (code == SPDXLicense.DOC) 8427 return "DOC"; 8428 if (code == SPDXLicense.DOTSEQN) 8429 return "Dotseqn"; 8430 if (code == SPDXLicense.DRL_1_0) 8431 return "DRL-1.0"; 8432 if (code == SPDXLicense.DSDP) 8433 return "DSDP"; 8434 if (code == SPDXLicense.DTOA) 8435 return "dtoa"; 8436 if (code == SPDXLicense.DVIPDFM) 8437 return "dvipdfm"; 8438 if (code == SPDXLicense.ECL_1_0) 8439 return "ECL-1.0"; 8440 if (code == SPDXLicense.ECL_2_0) 8441 return "ECL-2.0"; 8442 if (code == SPDXLicense.ECOS_2_0) 8443 return "eCos-2.0"; 8444 if (code == SPDXLicense.EFL_1_0) 8445 return "EFL-1.0"; 8446 if (code == SPDXLicense.EFL_2_0) 8447 return "EFL-2.0"; 8448 if (code == SPDXLicense.EGENIX) 8449 return "eGenix"; 8450 if (code == SPDXLicense.ELASTIC_2_0) 8451 return "Elastic-2.0"; 8452 if (code == SPDXLicense.ENTESSA) 8453 return "Entessa"; 8454 if (code == SPDXLicense.EPICS) 8455 return "EPICS"; 8456 if (code == SPDXLicense.EPL_1_0) 8457 return "EPL-1.0"; 8458 if (code == SPDXLicense.EPL_2_0) 8459 return "EPL-2.0"; 8460 if (code == SPDXLicense.ERLPL_1_1) 8461 return "ErlPL-1.1"; 8462 if (code == SPDXLicense.ETALAB_2_0) 8463 return "etalab-2.0"; 8464 if (code == SPDXLicense.EUDATAGRID) 8465 return "EUDatagrid"; 8466 if (code == SPDXLicense.EUPL_1_0) 8467 return "EUPL-1.0"; 8468 if (code == SPDXLicense.EUPL_1_1) 8469 return "EUPL-1.1"; 8470 if (code == SPDXLicense.EUPL_1_2) 8471 return "EUPL-1.2"; 8472 if (code == SPDXLicense.EUROSYM) 8473 return "Eurosym"; 8474 if (code == SPDXLicense.FAIR) 8475 return "Fair"; 8476 if (code == SPDXLicense.FDK_AAC) 8477 return "FDK-AAC"; 8478 if (code == SPDXLicense.FRAMEWORX_1_0) 8479 return "Frameworx-1.0"; 8480 if (code == SPDXLicense.FREEBSD_DOC) 8481 return "FreeBSD-DOC"; 8482 if (code == SPDXLicense.FREEIMAGE) 8483 return "FreeImage"; 8484 if (code == SPDXLicense.FSFAP) 8485 return "FSFAP"; 8486 if (code == SPDXLicense.FSFUL) 8487 return "FSFUL"; 8488 if (code == SPDXLicense.FSFULLR) 8489 return "FSFULLR"; 8490 if (code == SPDXLicense.FSFULLRWD) 8491 return "FSFULLRWD"; 8492 if (code == SPDXLicense.FTL) 8493 return "FTL"; 8494 if (code == SPDXLicense.GD) 8495 return "GD"; 8496 if (code == SPDXLicense.GFDL_1_1) 8497 return "GFDL-1.1"; 8498 if (code == SPDXLicense.GFDL_1_1_INVARIANTS_ONLY) 8499 return "GFDL-1.1-invariants-only"; 8500 if (code == SPDXLicense.GFDL_1_1_INVARIANTS_OR_LATER) 8501 return "GFDL-1.1-invariants-or-later"; 8502 if (code == SPDXLicense.GFDL_1_1_NO_INVARIANTS_ONLY) 8503 return "GFDL-1.1-no-invariants-only"; 8504 if (code == SPDXLicense.GFDL_1_1_NO_INVARIANTS_OR_LATER) 8505 return "GFDL-1.1-no-invariants-or-later"; 8506 if (code == SPDXLicense.GFDL_1_1_ONLY) 8507 return "GFDL-1.1-only"; 8508 if (code == SPDXLicense.GFDL_1_1_OR_LATER) 8509 return "GFDL-1.1-or-later"; 8510 if (code == SPDXLicense.GFDL_1_2) 8511 return "GFDL-1.2"; 8512 if (code == SPDXLicense.GFDL_1_2_INVARIANTS_ONLY) 8513 return "GFDL-1.2-invariants-only"; 8514 if (code == SPDXLicense.GFDL_1_2_INVARIANTS_OR_LATER) 8515 return "GFDL-1.2-invariants-or-later"; 8516 if (code == SPDXLicense.GFDL_1_2_NO_INVARIANTS_ONLY) 8517 return "GFDL-1.2-no-invariants-only"; 8518 if (code == SPDXLicense.GFDL_1_2_NO_INVARIANTS_OR_LATER) 8519 return "GFDL-1.2-no-invariants-or-later"; 8520 if (code == SPDXLicense.GFDL_1_2_ONLY) 8521 return "GFDL-1.2-only"; 8522 if (code == SPDXLicense.GFDL_1_2_OR_LATER) 8523 return "GFDL-1.2-or-later"; 8524 if (code == SPDXLicense.GFDL_1_3) 8525 return "GFDL-1.3"; 8526 if (code == SPDXLicense.GFDL_1_3_INVARIANTS_ONLY) 8527 return "GFDL-1.3-invariants-only"; 8528 if (code == SPDXLicense.GFDL_1_3_INVARIANTS_OR_LATER) 8529 return "GFDL-1.3-invariants-or-later"; 8530 if (code == SPDXLicense.GFDL_1_3_NO_INVARIANTS_ONLY) 8531 return "GFDL-1.3-no-invariants-only"; 8532 if (code == SPDXLicense.GFDL_1_3_NO_INVARIANTS_OR_LATER) 8533 return "GFDL-1.3-no-invariants-or-later"; 8534 if (code == SPDXLicense.GFDL_1_3_ONLY) 8535 return "GFDL-1.3-only"; 8536 if (code == SPDXLicense.GFDL_1_3_OR_LATER) 8537 return "GFDL-1.3-or-later"; 8538 if (code == SPDXLicense.GIFTWARE) 8539 return "Giftware"; 8540 if (code == SPDXLicense.GL2PS) 8541 return "GL2PS"; 8542 if (code == SPDXLicense.GLIDE) 8543 return "Glide"; 8544 if (code == SPDXLicense.GLULXE) 8545 return "Glulxe"; 8546 if (code == SPDXLicense.GLWTPL) 8547 return "GLWTPL"; 8548 if (code == SPDXLicense.GNUPLOT) 8549 return "gnuplot"; 8550 if (code == SPDXLicense.GPL_1_0) 8551 return "GPL-1.0"; 8552 if (code == SPDXLicense.GPL_1_0PLUS) 8553 return "GPL-1.0+"; 8554 if (code == SPDXLicense.GPL_1_0_ONLY) 8555 return "GPL-1.0-only"; 8556 if (code == SPDXLicense.GPL_1_0_OR_LATER) 8557 return "GPL-1.0-or-later"; 8558 if (code == SPDXLicense.GPL_2_0) 8559 return "GPL-2.0"; 8560 if (code == SPDXLicense.GPL_2_0PLUS) 8561 return "GPL-2.0+"; 8562 if (code == SPDXLicense.GPL_2_0_ONLY) 8563 return "GPL-2.0-only"; 8564 if (code == SPDXLicense.GPL_2_0_OR_LATER) 8565 return "GPL-2.0-or-later"; 8566 if (code == SPDXLicense.GPL_2_0_WITH_AUTOCONF_EXCEPTION) 8567 return "GPL-2.0-with-autoconf-exception"; 8568 if (code == SPDXLicense.GPL_2_0_WITH_BISON_EXCEPTION) 8569 return "GPL-2.0-with-bison-exception"; 8570 if (code == SPDXLicense.GPL_2_0_WITH_CLASSPATH_EXCEPTION) 8571 return "GPL-2.0-with-classpath-exception"; 8572 if (code == SPDXLicense.GPL_2_0_WITH_FONT_EXCEPTION) 8573 return "GPL-2.0-with-font-exception"; 8574 if (code == SPDXLicense.GPL_2_0_WITH_GCC_EXCEPTION) 8575 return "GPL-2.0-with-GCC-exception"; 8576 if (code == SPDXLicense.GPL_3_0) 8577 return "GPL-3.0"; 8578 if (code == SPDXLicense.GPL_3_0PLUS) 8579 return "GPL-3.0+"; 8580 if (code == SPDXLicense.GPL_3_0_ONLY) 8581 return "GPL-3.0-only"; 8582 if (code == SPDXLicense.GPL_3_0_OR_LATER) 8583 return "GPL-3.0-or-later"; 8584 if (code == SPDXLicense.GPL_3_0_WITH_AUTOCONF_EXCEPTION) 8585 return "GPL-3.0-with-autoconf-exception"; 8586 if (code == SPDXLicense.GPL_3_0_WITH_GCC_EXCEPTION) 8587 return "GPL-3.0-with-GCC-exception"; 8588 if (code == SPDXLicense.GRAPHICS_GEMS) 8589 return "Graphics-Gems"; 8590 if (code == SPDXLicense.GSOAP_1_3B) 8591 return "gSOAP-1.3b"; 8592 if (code == SPDXLicense.HASKELLREPORT) 8593 return "HaskellReport"; 8594 if (code == SPDXLicense.HIPPOCRATIC_2_1) 8595 return "Hippocratic-2.1"; 8596 if (code == SPDXLicense.HP_1986) 8597 return "HP-1986"; 8598 if (code == SPDXLicense.HPND) 8599 return "HPND"; 8600 if (code == SPDXLicense.HPND_EXPORT_US) 8601 return "HPND-export-US"; 8602 if (code == SPDXLicense.HPND_MARKUS_KUHN) 8603 return "HPND-Markus-Kuhn"; 8604 if (code == SPDXLicense.HPND_SELL_VARIANT) 8605 return "HPND-sell-variant"; 8606 if (code == SPDXLicense.HPND_SELL_VARIANT_MIT_DISCLAIMER) 8607 return "HPND-sell-variant-MIT-disclaimer"; 8608 if (code == SPDXLicense.HTMLTIDY) 8609 return "HTMLTIDY"; 8610 if (code == SPDXLicense.IBM_PIBS) 8611 return "IBM-pibs"; 8612 if (code == SPDXLicense.ICU) 8613 return "ICU"; 8614 if (code == SPDXLicense.IEC_CODE_COMPONENTS_EULA) 8615 return "IEC-Code-Components-EULA"; 8616 if (code == SPDXLicense.IJG) 8617 return "IJG"; 8618 if (code == SPDXLicense.IJG_SHORT) 8619 return "IJG-short"; 8620 if (code == SPDXLicense.IMAGEMAGICK) 8621 return "ImageMagick"; 8622 if (code == SPDXLicense.IMATIX) 8623 return "iMatix"; 8624 if (code == SPDXLicense.IMLIB2) 8625 return "Imlib2"; 8626 if (code == SPDXLicense.INFO_ZIP) 8627 return "Info-ZIP"; 8628 if (code == SPDXLicense.INNER_NET_2_0) 8629 return "Inner-Net-2.0"; 8630 if (code == SPDXLicense.INTEL) 8631 return "Intel"; 8632 if (code == SPDXLicense.INTEL_ACPI) 8633 return "Intel-ACPI"; 8634 if (code == SPDXLicense.INTERBASE_1_0) 8635 return "Interbase-1.0"; 8636 if (code == SPDXLicense.IPA) 8637 return "IPA"; 8638 if (code == SPDXLicense.IPL_1_0) 8639 return "IPL-1.0"; 8640 if (code == SPDXLicense.ISC) 8641 return "ISC"; 8642 if (code == SPDXLicense.JAM) 8643 return "Jam"; 8644 if (code == SPDXLicense.JASPER_2_0) 8645 return "JasPer-2.0"; 8646 if (code == SPDXLicense.JPL_IMAGE) 8647 return "JPL-image"; 8648 if (code == SPDXLicense.JPNIC) 8649 return "JPNIC"; 8650 if (code == SPDXLicense.JSON) 8651 return "JSON"; 8652 if (code == SPDXLicense.KAZLIB) 8653 return "Kazlib"; 8654 if (code == SPDXLicense.KNUTH_CTAN) 8655 return "Knuth-CTAN"; 8656 if (code == SPDXLicense.LAL_1_2) 8657 return "LAL-1.2"; 8658 if (code == SPDXLicense.LAL_1_3) 8659 return "LAL-1.3"; 8660 if (code == SPDXLicense.LATEX2E) 8661 return "Latex2e"; 8662 if (code == SPDXLicense.LATEX2E_TRANSLATED_NOTICE) 8663 return "Latex2e-translated-notice"; 8664 if (code == SPDXLicense.LEPTONICA) 8665 return "Leptonica"; 8666 if (code == SPDXLicense.LGPL_2_0) 8667 return "LGPL-2.0"; 8668 if (code == SPDXLicense.LGPL_2_0PLUS) 8669 return "LGPL-2.0+"; 8670 if (code == SPDXLicense.LGPL_2_0_ONLY) 8671 return "LGPL-2.0-only"; 8672 if (code == SPDXLicense.LGPL_2_0_OR_LATER) 8673 return "LGPL-2.0-or-later"; 8674 if (code == SPDXLicense.LGPL_2_1) 8675 return "LGPL-2.1"; 8676 if (code == SPDXLicense.LGPL_2_1PLUS) 8677 return "LGPL-2.1+"; 8678 if (code == SPDXLicense.LGPL_2_1_ONLY) 8679 return "LGPL-2.1-only"; 8680 if (code == SPDXLicense.LGPL_2_1_OR_LATER) 8681 return "LGPL-2.1-or-later"; 8682 if (code == SPDXLicense.LGPL_3_0) 8683 return "LGPL-3.0"; 8684 if (code == SPDXLicense.LGPL_3_0PLUS) 8685 return "LGPL-3.0+"; 8686 if (code == SPDXLicense.LGPL_3_0_ONLY) 8687 return "LGPL-3.0-only"; 8688 if (code == SPDXLicense.LGPL_3_0_OR_LATER) 8689 return "LGPL-3.0-or-later"; 8690 if (code == SPDXLicense.LGPLLR) 8691 return "LGPLLR"; 8692 if (code == SPDXLicense.LIBPNG) 8693 return "Libpng"; 8694 if (code == SPDXLicense.LIBPNG_2_0) 8695 return "libpng-2.0"; 8696 if (code == SPDXLicense.LIBSELINUX_1_0) 8697 return "libselinux-1.0"; 8698 if (code == SPDXLicense.LIBTIFF) 8699 return "libtiff"; 8700 if (code == SPDXLicense.LIBUTIL_DAVID_NUGENT) 8701 return "libutil-David-Nugent"; 8702 if (code == SPDXLicense.LILIQ_P_1_1) 8703 return "LiLiQ-P-1.1"; 8704 if (code == SPDXLicense.LILIQ_R_1_1) 8705 return "LiLiQ-R-1.1"; 8706 if (code == SPDXLicense.LILIQ_RPLUS_1_1) 8707 return "LiLiQ-Rplus-1.1"; 8708 if (code == SPDXLicense.LINUX_MAN_PAGES_1_PARA) 8709 return "Linux-man-pages-1-para"; 8710 if (code == SPDXLicense.LINUX_MAN_PAGES_COPYLEFT) 8711 return "Linux-man-pages-copyleft"; 8712 if (code == SPDXLicense.LINUX_MAN_PAGES_COPYLEFT_2_PARA) 8713 return "Linux-man-pages-copyleft-2-para"; 8714 if (code == SPDXLicense.LINUX_MAN_PAGES_COPYLEFT_VAR) 8715 return "Linux-man-pages-copyleft-var"; 8716 if (code == SPDXLicense.LINUX_OPENIB) 8717 return "Linux-OpenIB"; 8718 if (code == SPDXLicense.LOOP) 8719 return "LOOP"; 8720 if (code == SPDXLicense.LPL_1_0) 8721 return "LPL-1.0"; 8722 if (code == SPDXLicense.LPL_1_02) 8723 return "LPL-1.02"; 8724 if (code == SPDXLicense.LPPL_1_0) 8725 return "LPPL-1.0"; 8726 if (code == SPDXLicense.LPPL_1_1) 8727 return "LPPL-1.1"; 8728 if (code == SPDXLicense.LPPL_1_2) 8729 return "LPPL-1.2"; 8730 if (code == SPDXLicense.LPPL_1_3A) 8731 return "LPPL-1.3a"; 8732 if (code == SPDXLicense.LPPL_1_3C) 8733 return "LPPL-1.3c"; 8734 if (code == SPDXLicense.LZMA_SDK_9_11_TO_9_20) 8735 return "LZMA-SDK-9.11-to-9.20"; 8736 if (code == SPDXLicense.LZMA_SDK_9_22) 8737 return "LZMA-SDK-9.22"; 8738 if (code == SPDXLicense.MAKEINDEX) 8739 return "MakeIndex"; 8740 if (code == SPDXLicense.MARTIN_BIRGMEIER) 8741 return "Martin-Birgmeier"; 8742 if (code == SPDXLicense.METAMAIL) 8743 return "metamail"; 8744 if (code == SPDXLicense.MINPACK) 8745 return "Minpack"; 8746 if (code == SPDXLicense.MIROS) 8747 return "MirOS"; 8748 if (code == SPDXLicense.MIT) 8749 return "MIT"; 8750 if (code == SPDXLicense.MIT_0) 8751 return "MIT-0"; 8752 if (code == SPDXLicense.MIT_ADVERTISING) 8753 return "MIT-advertising"; 8754 if (code == SPDXLicense.MIT_CMU) 8755 return "MIT-CMU"; 8756 if (code == SPDXLicense.MIT_ENNA) 8757 return "MIT-enna"; 8758 if (code == SPDXLicense.MIT_FEH) 8759 return "MIT-feh"; 8760 if (code == SPDXLicense.MIT_FESTIVAL) 8761 return "MIT-Festival"; 8762 if (code == SPDXLicense.MIT_MODERN_VARIANT) 8763 return "MIT-Modern-Variant"; 8764 if (code == SPDXLicense.MIT_OPEN_GROUP) 8765 return "MIT-open-group"; 8766 if (code == SPDXLicense.MIT_WU) 8767 return "MIT-Wu"; 8768 if (code == SPDXLicense.MITNFA) 8769 return "MITNFA"; 8770 if (code == SPDXLicense.MOTOSOTO) 8771 return "Motosoto"; 8772 if (code == SPDXLicense.MPI_PERMISSIVE) 8773 return "mpi-permissive"; 8774 if (code == SPDXLicense.MPICH2) 8775 return "mpich2"; 8776 if (code == SPDXLicense.MPL_1_0) 8777 return "MPL-1.0"; 8778 if (code == SPDXLicense.MPL_1_1) 8779 return "MPL-1.1"; 8780 if (code == SPDXLicense.MPL_2_0) 8781 return "MPL-2.0"; 8782 if (code == SPDXLicense.MPL_2_0_NO_COPYLEFT_EXCEPTION) 8783 return "MPL-2.0-no-copyleft-exception"; 8784 if (code == SPDXLicense.MPLUS) 8785 return "mplus"; 8786 if (code == SPDXLicense.MS_LPL) 8787 return "MS-LPL"; 8788 if (code == SPDXLicense.MS_PL) 8789 return "MS-PL"; 8790 if (code == SPDXLicense.MS_RL) 8791 return "MS-RL"; 8792 if (code == SPDXLicense.MTLL) 8793 return "MTLL"; 8794 if (code == SPDXLicense.MULANPSL_1_0) 8795 return "MulanPSL-1.0"; 8796 if (code == SPDXLicense.MULANPSL_2_0) 8797 return "MulanPSL-2.0"; 8798 if (code == SPDXLicense.MULTICS) 8799 return "Multics"; 8800 if (code == SPDXLicense.MUP) 8801 return "Mup"; 8802 if (code == SPDXLicense.NAIST_2003) 8803 return "NAIST-2003"; 8804 if (code == SPDXLicense.NASA_1_3) 8805 return "NASA-1.3"; 8806 if (code == SPDXLicense.NAUMEN) 8807 return "Naumen"; 8808 if (code == SPDXLicense.NBPL_1_0) 8809 return "NBPL-1.0"; 8810 if (code == SPDXLicense.NCGL_UK_2_0) 8811 return "NCGL-UK-2.0"; 8812 if (code == SPDXLicense.NCSA) 8813 return "NCSA"; 8814 if (code == SPDXLicense.NET_SNMP) 8815 return "Net-SNMP"; 8816 if (code == SPDXLicense.NETCDF) 8817 return "NetCDF"; 8818 if (code == SPDXLicense.NEWSLETR) 8819 return "Newsletr"; 8820 if (code == SPDXLicense.NGPL) 8821 return "NGPL"; 8822 if (code == SPDXLicense.NICTA_1_0) 8823 return "NICTA-1.0"; 8824 if (code == SPDXLicense.NIST_PD) 8825 return "NIST-PD"; 8826 if (code == SPDXLicense.NIST_PD_FALLBACK) 8827 return "NIST-PD-fallback"; 8828 if (code == SPDXLicense.NIST_SOFTWARE) 8829 return "NIST-Software"; 8830 if (code == SPDXLicense.NLOD_1_0) 8831 return "NLOD-1.0"; 8832 if (code == SPDXLicense.NLOD_2_0) 8833 return "NLOD-2.0"; 8834 if (code == SPDXLicense.NLPL) 8835 return "NLPL"; 8836 if (code == SPDXLicense.NOKIA) 8837 return "Nokia"; 8838 if (code == SPDXLicense.NOSL) 8839 return "NOSL"; 8840 if (code == SPDXLicense.NOT_OPEN_SOURCE) 8841 return "not-open-source"; 8842 if (code == SPDXLicense.NOWEB) 8843 return "Noweb"; 8844 if (code == SPDXLicense.NPL_1_0) 8845 return "NPL-1.0"; 8846 if (code == SPDXLicense.NPL_1_1) 8847 return "NPL-1.1"; 8848 if (code == SPDXLicense.NPOSL_3_0) 8849 return "NPOSL-3.0"; 8850 if (code == SPDXLicense.NRL) 8851 return "NRL"; 8852 if (code == SPDXLicense.NTP) 8853 return "NTP"; 8854 if (code == SPDXLicense.NTP_0) 8855 return "NTP-0"; 8856 if (code == SPDXLicense.NUNIT) 8857 return "Nunit"; 8858 if (code == SPDXLicense.O_UDA_1_0) 8859 return "O-UDA-1.0"; 8860 if (code == SPDXLicense.OCCT_PL) 8861 return "OCCT-PL"; 8862 if (code == SPDXLicense.OCLC_2_0) 8863 return "OCLC-2.0"; 8864 if (code == SPDXLicense.ODBL_1_0) 8865 return "ODbL-1.0"; 8866 if (code == SPDXLicense.ODC_BY_1_0) 8867 return "ODC-By-1.0"; 8868 if (code == SPDXLicense.OFFIS) 8869 return "OFFIS"; 8870 if (code == SPDXLicense.OFL_1_0) 8871 return "OFL-1.0"; 8872 if (code == SPDXLicense.OFL_1_0_NO_RFN) 8873 return "OFL-1.0-no-RFN"; 8874 if (code == SPDXLicense.OFL_1_0_RFN) 8875 return "OFL-1.0-RFN"; 8876 if (code == SPDXLicense.OFL_1_1) 8877 return "OFL-1.1"; 8878 if (code == SPDXLicense.OFL_1_1_NO_RFN) 8879 return "OFL-1.1-no-RFN"; 8880 if (code == SPDXLicense.OFL_1_1_RFN) 8881 return "OFL-1.1-RFN"; 8882 if (code == SPDXLicense.OGC_1_0) 8883 return "OGC-1.0"; 8884 if (code == SPDXLicense.OGDL_TAIWAN_1_0) 8885 return "OGDL-Taiwan-1.0"; 8886 if (code == SPDXLicense.OGL_CANADA_2_0) 8887 return "OGL-Canada-2.0"; 8888 if (code == SPDXLicense.OGL_UK_1_0) 8889 return "OGL-UK-1.0"; 8890 if (code == SPDXLicense.OGL_UK_2_0) 8891 return "OGL-UK-2.0"; 8892 if (code == SPDXLicense.OGL_UK_3_0) 8893 return "OGL-UK-3.0"; 8894 if (code == SPDXLicense.OGTSL) 8895 return "OGTSL"; 8896 if (code == SPDXLicense.OLDAP_1_1) 8897 return "OLDAP-1.1"; 8898 if (code == SPDXLicense.OLDAP_1_2) 8899 return "OLDAP-1.2"; 8900 if (code == SPDXLicense.OLDAP_1_3) 8901 return "OLDAP-1.3"; 8902 if (code == SPDXLicense.OLDAP_1_4) 8903 return "OLDAP-1.4"; 8904 if (code == SPDXLicense.OLDAP_2_0) 8905 return "OLDAP-2.0"; 8906 if (code == SPDXLicense.OLDAP_2_0_1) 8907 return "OLDAP-2.0.1"; 8908 if (code == SPDXLicense.OLDAP_2_1) 8909 return "OLDAP-2.1"; 8910 if (code == SPDXLicense.OLDAP_2_2) 8911 return "OLDAP-2.2"; 8912 if (code == SPDXLicense.OLDAP_2_2_1) 8913 return "OLDAP-2.2.1"; 8914 if (code == SPDXLicense.OLDAP_2_2_2) 8915 return "OLDAP-2.2.2"; 8916 if (code == SPDXLicense.OLDAP_2_3) 8917 return "OLDAP-2.3"; 8918 if (code == SPDXLicense.OLDAP_2_4) 8919 return "OLDAP-2.4"; 8920 if (code == SPDXLicense.OLDAP_2_5) 8921 return "OLDAP-2.5"; 8922 if (code == SPDXLicense.OLDAP_2_6) 8923 return "OLDAP-2.6"; 8924 if (code == SPDXLicense.OLDAP_2_7) 8925 return "OLDAP-2.7"; 8926 if (code == SPDXLicense.OLDAP_2_8) 8927 return "OLDAP-2.8"; 8928 if (code == SPDXLicense.OLFL_1_3) 8929 return "OLFL-1.3"; 8930 if (code == SPDXLicense.OML) 8931 return "OML"; 8932 if (code == SPDXLicense.OPENPBS_2_3) 8933 return "OpenPBS-2.3"; 8934 if (code == SPDXLicense.OPENSSL) 8935 return "OpenSSL"; 8936 if (code == SPDXLicense.OPL_1_0) 8937 return "OPL-1.0"; 8938 if (code == SPDXLicense.OPL_UK_3_0) 8939 return "OPL-UK-3.0"; 8940 if (code == SPDXLicense.OPUBL_1_0) 8941 return "OPUBL-1.0"; 8942 if (code == SPDXLicense.OSET_PL_2_1) 8943 return "OSET-PL-2.1"; 8944 if (code == SPDXLicense.OSL_1_0) 8945 return "OSL-1.0"; 8946 if (code == SPDXLicense.OSL_1_1) 8947 return "OSL-1.1"; 8948 if (code == SPDXLicense.OSL_2_0) 8949 return "OSL-2.0"; 8950 if (code == SPDXLicense.OSL_2_1) 8951 return "OSL-2.1"; 8952 if (code == SPDXLicense.OSL_3_0) 8953 return "OSL-3.0"; 8954 if (code == SPDXLicense.PARITY_6_0_0) 8955 return "Parity-6.0.0"; 8956 if (code == SPDXLicense.PARITY_7_0_0) 8957 return "Parity-7.0.0"; 8958 if (code == SPDXLicense.PDDL_1_0) 8959 return "PDDL-1.0"; 8960 if (code == SPDXLicense.PHP_3_0) 8961 return "PHP-3.0"; 8962 if (code == SPDXLicense.PHP_3_01) 8963 return "PHP-3.01"; 8964 if (code == SPDXLicense.PLEXUS) 8965 return "Plexus"; 8966 if (code == SPDXLicense.POLYFORM_NONCOMMERCIAL_1_0_0) 8967 return "PolyForm-Noncommercial-1.0.0"; 8968 if (code == SPDXLicense.POLYFORM_SMALL_BUSINESS_1_0_0) 8969 return "PolyForm-Small-Business-1.0.0"; 8970 if (code == SPDXLicense.POSTGRESQL) 8971 return "PostgreSQL"; 8972 if (code == SPDXLicense.PSF_2_0) 8973 return "PSF-2.0"; 8974 if (code == SPDXLicense.PSFRAG) 8975 return "psfrag"; 8976 if (code == SPDXLicense.PSUTILS) 8977 return "psutils"; 8978 if (code == SPDXLicense.PYTHON_2_0) 8979 return "Python-2.0"; 8980 if (code == SPDXLicense.PYTHON_2_0_1) 8981 return "Python-2.0.1"; 8982 if (code == SPDXLicense.QHULL) 8983 return "Qhull"; 8984 if (code == SPDXLicense.QPL_1_0) 8985 return "QPL-1.0"; 8986 if (code == SPDXLicense.QPL_1_0_INRIA_2004) 8987 return "QPL-1.0-INRIA-2004"; 8988 if (code == SPDXLicense.RDISC) 8989 return "Rdisc"; 8990 if (code == SPDXLicense.RHECOS_1_1) 8991 return "RHeCos-1.1"; 8992 if (code == SPDXLicense.RPL_1_1) 8993 return "RPL-1.1"; 8994 if (code == SPDXLicense.RPL_1_5) 8995 return "RPL-1.5"; 8996 if (code == SPDXLicense.RPSL_1_0) 8997 return "RPSL-1.0"; 8998 if (code == SPDXLicense.RSA_MD) 8999 return "RSA-MD"; 9000 if (code == SPDXLicense.RSCPL) 9001 return "RSCPL"; 9002 if (code == SPDXLicense.RUBY) 9003 return "Ruby"; 9004 if (code == SPDXLicense.SAX_PD) 9005 return "SAX-PD"; 9006 if (code == SPDXLicense.SAXPATH) 9007 return "Saxpath"; 9008 if (code == SPDXLicense.SCEA) 9009 return "SCEA"; 9010 if (code == SPDXLicense.SCHEMEREPORT) 9011 return "SchemeReport"; 9012 if (code == SPDXLicense.SENDMAIL) 9013 return "Sendmail"; 9014 if (code == SPDXLicense.SENDMAIL_8_23) 9015 return "Sendmail-8.23"; 9016 if (code == SPDXLicense.SGI_B_1_0) 9017 return "SGI-B-1.0"; 9018 if (code == SPDXLicense.SGI_B_1_1) 9019 return "SGI-B-1.1"; 9020 if (code == SPDXLicense.SGI_B_2_0) 9021 return "SGI-B-2.0"; 9022 if (code == SPDXLicense.SGP4) 9023 return "SGP4"; 9024 if (code == SPDXLicense.SHL_0_5) 9025 return "SHL-0.5"; 9026 if (code == SPDXLicense.SHL_0_51) 9027 return "SHL-0.51"; 9028 if (code == SPDXLicense.SIMPL_2_0) 9029 return "SimPL-2.0"; 9030 if (code == SPDXLicense.SISSL) 9031 return "SISSL"; 9032 if (code == SPDXLicense.SISSL_1_2) 9033 return "SISSL-1.2"; 9034 if (code == SPDXLicense.SLEEPYCAT) 9035 return "Sleepycat"; 9036 if (code == SPDXLicense.SMLNJ) 9037 return "SMLNJ"; 9038 if (code == SPDXLicense.SMPPL) 9039 return "SMPPL"; 9040 if (code == SPDXLicense.SNIA) 9041 return "SNIA"; 9042 if (code == SPDXLicense.SNPRINTF) 9043 return "snprintf"; 9044 if (code == SPDXLicense.SPENCER_86) 9045 return "Spencer-86"; 9046 if (code == SPDXLicense.SPENCER_94) 9047 return "Spencer-94"; 9048 if (code == SPDXLicense.SPENCER_99) 9049 return "Spencer-99"; 9050 if (code == SPDXLicense.SPL_1_0) 9051 return "SPL-1.0"; 9052 if (code == SPDXLicense.SSH_OPENSSH) 9053 return "SSH-OpenSSH"; 9054 if (code == SPDXLicense.SSH_SHORT) 9055 return "SSH-short"; 9056 if (code == SPDXLicense.SSPL_1_0) 9057 return "SSPL-1.0"; 9058 if (code == SPDXLicense.STANDARDML_NJ) 9059 return "StandardML-NJ"; 9060 if (code == SPDXLicense.SUGARCRM_1_1_3) 9061 return "SugarCRM-1.1.3"; 9062 if (code == SPDXLicense.SUNPRO) 9063 return "SunPro"; 9064 if (code == SPDXLicense.SWL) 9065 return "SWL"; 9066 if (code == SPDXLicense.SYMLINKS) 9067 return "Symlinks"; 9068 if (code == SPDXLicense.TAPR_OHL_1_0) 9069 return "TAPR-OHL-1.0"; 9070 if (code == SPDXLicense.TCL) 9071 return "TCL"; 9072 if (code == SPDXLicense.TCP_WRAPPERS) 9073 return "TCP-wrappers"; 9074 if (code == SPDXLicense.TERMREADKEY) 9075 return "TermReadKey"; 9076 if (code == SPDXLicense.TMATE) 9077 return "TMate"; 9078 if (code == SPDXLicense.TORQUE_1_1) 9079 return "TORQUE-1.1"; 9080 if (code == SPDXLicense.TOSL) 9081 return "TOSL"; 9082 if (code == SPDXLicense.TPDL) 9083 return "TPDL"; 9084 if (code == SPDXLicense.TPL_1_0) 9085 return "TPL-1.0"; 9086 if (code == SPDXLicense.TTWL) 9087 return "TTWL"; 9088 if (code == SPDXLicense.TU_BERLIN_1_0) 9089 return "TU-Berlin-1.0"; 9090 if (code == SPDXLicense.TU_BERLIN_2_0) 9091 return "TU-Berlin-2.0"; 9092 if (code == SPDXLicense.UCAR) 9093 return "UCAR"; 9094 if (code == SPDXLicense.UCL_1_0) 9095 return "UCL-1.0"; 9096 if (code == SPDXLicense.UNICODE_DFS_2015) 9097 return "Unicode-DFS-2015"; 9098 if (code == SPDXLicense.UNICODE_DFS_2016) 9099 return "Unicode-DFS-2016"; 9100 if (code == SPDXLicense.UNICODE_TOU) 9101 return "Unicode-TOU"; 9102 if (code == SPDXLicense.UNIXCRYPT) 9103 return "UnixCrypt"; 9104 if (code == SPDXLicense.UNLICENSE) 9105 return "Unlicense"; 9106 if (code == SPDXLicense.UPL_1_0) 9107 return "UPL-1.0"; 9108 if (code == SPDXLicense.VIM) 9109 return "Vim"; 9110 if (code == SPDXLicense.VOSTROM) 9111 return "VOSTROM"; 9112 if (code == SPDXLicense.VSL_1_0) 9113 return "VSL-1.0"; 9114 if (code == SPDXLicense.W3C) 9115 return "W3C"; 9116 if (code == SPDXLicense.W3C_19980720) 9117 return "W3C-19980720"; 9118 if (code == SPDXLicense.W3C_20150513) 9119 return "W3C-20150513"; 9120 if (code == SPDXLicense.W3M) 9121 return "w3m"; 9122 if (code == SPDXLicense.WATCOM_1_0) 9123 return "Watcom-1.0"; 9124 if (code == SPDXLicense.WIDGET_WORKSHOP) 9125 return "Widget-Workshop"; 9126 if (code == SPDXLicense.WSUIPA) 9127 return "Wsuipa"; 9128 if (code == SPDXLicense.WTFPL) 9129 return "WTFPL"; 9130 if (code == SPDXLicense.WXWINDOWS) 9131 return "wxWindows"; 9132 if (code == SPDXLicense.X11) 9133 return "X11"; 9134 if (code == SPDXLicense.X11_DISTRIBUTE_MODIFICATIONS_VARIANT) 9135 return "X11-distribute-modifications-variant"; 9136 if (code == SPDXLicense.XDEBUG_1_03) 9137 return "Xdebug-1.03"; 9138 if (code == SPDXLicense.XEROX) 9139 return "Xerox"; 9140 if (code == SPDXLicense.XFIG) 9141 return "Xfig"; 9142 if (code == SPDXLicense.XFREE86_1_1) 9143 return "XFree86-1.1"; 9144 if (code == SPDXLicense.XINETD) 9145 return "xinetd"; 9146 if (code == SPDXLicense.XLOCK) 9147 return "xlock"; 9148 if (code == SPDXLicense.XNET) 9149 return "Xnet"; 9150 if (code == SPDXLicense.XPP) 9151 return "xpp"; 9152 if (code == SPDXLicense.XSKAT) 9153 return "XSkat"; 9154 if (code == SPDXLicense.YPL_1_0) 9155 return "YPL-1.0"; 9156 if (code == SPDXLicense.YPL_1_1) 9157 return "YPL-1.1"; 9158 if (code == SPDXLicense.ZED) 9159 return "Zed"; 9160 if (code == SPDXLicense.ZEND_2_0) 9161 return "Zend-2.0"; 9162 if (code == SPDXLicense.ZIMBRA_1_3) 9163 return "Zimbra-1.3"; 9164 if (code == SPDXLicense.ZIMBRA_1_4) 9165 return "Zimbra-1.4"; 9166 if (code == SPDXLicense.ZLIB) 9167 return "Zlib"; 9168 if (code == SPDXLicense.ZLIB_ACKNOWLEDGEMENT) 9169 return "zlib-acknowledgement"; 9170 if (code == SPDXLicense.ZPL_1_1) 9171 return "ZPL-1.1"; 9172 if (code == SPDXLicense.ZPL_2_0) 9173 return "ZPL-2.0"; 9174 if (code == SPDXLicense.ZPL_2_1) 9175 return "ZPL-2.1"; 9176 return "?"; 9177 } 9178 public String toSystem(SPDXLicense code) { 9179 return code.getSystem(); 9180 } 9181 } 9182 9183 9184 9185 @Block() 9186 public static class ImplementationGuideDependsOnComponent extends BackboneElement implements IBaseBackboneElement { 9187 /** 9188 * A canonical reference to the Implementation guide for the dependency. 9189 */ 9190 @Child(name = "uri", type = {CanonicalType.class}, order=1, min=1, max=1, modifier=false, summary=true) 9191 @Description(shortDefinition="Identity of the IG that this depends on", formalDefinition="A canonical reference to the Implementation guide for the dependency." ) 9192 protected CanonicalType uri; 9193 9194 /** 9195 * The NPM package name for the Implementation Guide that this IG depends on. 9196 */ 9197 @Child(name = "packageId", type = {IdType.class}, order=2, min=0, max=1, modifier=false, summary=true) 9198 @Description(shortDefinition="NPM Package name for IG this depends on", formalDefinition="The NPM package name for the Implementation Guide that this IG depends on." ) 9199 protected IdType packageId; 9200 9201 /** 9202 * The version of the IG that is depended on, when the correct version is required to understand the IG correctly. 9203 */ 9204 @Child(name = "version", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 9205 @Description(shortDefinition="Version of the IG", formalDefinition="The version of the IG that is depended on, when the correct version is required to understand the IG correctly." ) 9206 protected StringType version; 9207 9208 /** 9209 * A description explaining the nature of the dependency on the listed IG. 9210 */ 9211 @Child(name = "reason", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=false) 9212 @Description(shortDefinition="Why dependency exists", formalDefinition="A description explaining the nature of the dependency on the listed IG." ) 9213 protected MarkdownType reason; 9214 9215 private static final long serialVersionUID = 487374450L; 9216 9217 /** 9218 * Constructor 9219 */ 9220 public ImplementationGuideDependsOnComponent() { 9221 super(); 9222 } 9223 9224 /** 9225 * Constructor 9226 */ 9227 public ImplementationGuideDependsOnComponent(String uri) { 9228 super(); 9229 this.setUri(uri); 9230 } 9231 9232 /** 9233 * @return {@link #uri} (A canonical reference to the Implementation guide for the dependency.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 9234 */ 9235 public CanonicalType getUriElement() { 9236 if (this.uri == null) 9237 if (Configuration.errorOnAutoCreate()) 9238 throw new Error("Attempt to auto-create ImplementationGuideDependsOnComponent.uri"); 9239 else if (Configuration.doAutoCreate()) 9240 this.uri = new CanonicalType(); // bb 9241 return this.uri; 9242 } 9243 9244 public boolean hasUriElement() { 9245 return this.uri != null && !this.uri.isEmpty(); 9246 } 9247 9248 public boolean hasUri() { 9249 return this.uri != null && !this.uri.isEmpty(); 9250 } 9251 9252 /** 9253 * @param value {@link #uri} (A canonical reference to the Implementation guide for the dependency.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 9254 */ 9255 public ImplementationGuideDependsOnComponent setUriElement(CanonicalType value) { 9256 this.uri = value; 9257 return this; 9258 } 9259 9260 /** 9261 * @return A canonical reference to the Implementation guide for the dependency. 9262 */ 9263 public String getUri() { 9264 return this.uri == null ? null : this.uri.getValue(); 9265 } 9266 9267 /** 9268 * @param value A canonical reference to the Implementation guide for the dependency. 9269 */ 9270 public ImplementationGuideDependsOnComponent setUri(String value) { 9271 if (this.uri == null) 9272 this.uri = new CanonicalType(); 9273 this.uri.setValue(value); 9274 return this; 9275 } 9276 9277 /** 9278 * @return {@link #packageId} (The NPM package name for the Implementation Guide that this IG depends on.). This is the underlying object with id, value and extensions. The accessor "getPackageId" gives direct access to the value 9279 */ 9280 public IdType getPackageIdElement() { 9281 if (this.packageId == null) 9282 if (Configuration.errorOnAutoCreate()) 9283 throw new Error("Attempt to auto-create ImplementationGuideDependsOnComponent.packageId"); 9284 else if (Configuration.doAutoCreate()) 9285 this.packageId = new IdType(); // bb 9286 return this.packageId; 9287 } 9288 9289 public boolean hasPackageIdElement() { 9290 return this.packageId != null && !this.packageId.isEmpty(); 9291 } 9292 9293 public boolean hasPackageId() { 9294 return this.packageId != null && !this.packageId.isEmpty(); 9295 } 9296 9297 /** 9298 * @param value {@link #packageId} (The NPM package name for the Implementation Guide that this IG depends on.). This is the underlying object with id, value and extensions. The accessor "getPackageId" gives direct access to the value 9299 */ 9300 public ImplementationGuideDependsOnComponent setPackageIdElement(IdType value) { 9301 this.packageId = value; 9302 return this; 9303 } 9304 9305 /** 9306 * @return The NPM package name for the Implementation Guide that this IG depends on. 9307 */ 9308 public String getPackageId() { 9309 return this.packageId == null ? null : this.packageId.getValue(); 9310 } 9311 9312 /** 9313 * @param value The NPM package name for the Implementation Guide that this IG depends on. 9314 */ 9315 public ImplementationGuideDependsOnComponent setPackageId(String value) { 9316 if (Utilities.noString(value)) 9317 this.packageId = null; 9318 else { 9319 if (this.packageId == null) 9320 this.packageId = new IdType(); 9321 this.packageId.setValue(value); 9322 } 9323 return this; 9324 } 9325 9326 /** 9327 * @return {@link #version} (The version of the IG that is depended on, when the correct version is required to understand the IG correctly.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 9328 */ 9329 public StringType getVersionElement() { 9330 if (this.version == null) 9331 if (Configuration.errorOnAutoCreate()) 9332 throw new Error("Attempt to auto-create ImplementationGuideDependsOnComponent.version"); 9333 else if (Configuration.doAutoCreate()) 9334 this.version = new StringType(); // bb 9335 return this.version; 9336 } 9337 9338 public boolean hasVersionElement() { 9339 return this.version != null && !this.version.isEmpty(); 9340 } 9341 9342 public boolean hasVersion() { 9343 return this.version != null && !this.version.isEmpty(); 9344 } 9345 9346 /** 9347 * @param value {@link #version} (The version of the IG that is depended on, when the correct version is required to understand the IG correctly.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 9348 */ 9349 public ImplementationGuideDependsOnComponent setVersionElement(StringType value) { 9350 this.version = value; 9351 return this; 9352 } 9353 9354 /** 9355 * @return The version of the IG that is depended on, when the correct version is required to understand the IG correctly. 9356 */ 9357 public String getVersion() { 9358 return this.version == null ? null : this.version.getValue(); 9359 } 9360 9361 /** 9362 * @param value The version of the IG that is depended on, when the correct version is required to understand the IG correctly. 9363 */ 9364 public ImplementationGuideDependsOnComponent setVersion(String value) { 9365 if (Utilities.noString(value)) 9366 this.version = null; 9367 else { 9368 if (this.version == null) 9369 this.version = new StringType(); 9370 this.version.setValue(value); 9371 } 9372 return this; 9373 } 9374 9375 /** 9376 * @return {@link #reason} (A description explaining the nature of the dependency on the listed IG.). This is the underlying object with id, value and extensions. The accessor "getReason" gives direct access to the value 9377 */ 9378 public MarkdownType getReasonElement() { 9379 if (this.reason == null) 9380 if (Configuration.errorOnAutoCreate()) 9381 throw new Error("Attempt to auto-create ImplementationGuideDependsOnComponent.reason"); 9382 else if (Configuration.doAutoCreate()) 9383 this.reason = new MarkdownType(); // bb 9384 return this.reason; 9385 } 9386 9387 public boolean hasReasonElement() { 9388 return this.reason != null && !this.reason.isEmpty(); 9389 } 9390 9391 public boolean hasReason() { 9392 return this.reason != null && !this.reason.isEmpty(); 9393 } 9394 9395 /** 9396 * @param value {@link #reason} (A description explaining the nature of the dependency on the listed IG.). This is the underlying object with id, value and extensions. The accessor "getReason" gives direct access to the value 9397 */ 9398 public ImplementationGuideDependsOnComponent setReasonElement(MarkdownType value) { 9399 this.reason = value; 9400 return this; 9401 } 9402 9403 /** 9404 * @return A description explaining the nature of the dependency on the listed IG. 9405 */ 9406 public String getReason() { 9407 return this.reason == null ? null : this.reason.getValue(); 9408 } 9409 9410 /** 9411 * @param value A description explaining the nature of the dependency on the listed IG. 9412 */ 9413 public ImplementationGuideDependsOnComponent setReason(String value) { 9414 if (Utilities.noString(value)) 9415 this.reason = null; 9416 else { 9417 if (this.reason == null) 9418 this.reason = new MarkdownType(); 9419 this.reason.setValue(value); 9420 } 9421 return this; 9422 } 9423 9424 protected void listChildren(List<Property> children) { 9425 super.listChildren(children); 9426 children.add(new Property("uri", "canonical(ImplementationGuide)", "A canonical reference to the Implementation guide for the dependency.", 0, 1, uri)); 9427 children.add(new Property("packageId", "id", "The NPM package name for the Implementation Guide that this IG depends on.", 0, 1, packageId)); 9428 children.add(new Property("version", "string", "The version of the IG that is depended on, when the correct version is required to understand the IG correctly.", 0, 1, version)); 9429 children.add(new Property("reason", "markdown", "A description explaining the nature of the dependency on the listed IG.", 0, 1, reason)); 9430 } 9431 9432 @Override 9433 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9434 switch (_hash) { 9435 case 116076: /*uri*/ return new Property("uri", "canonical(ImplementationGuide)", "A canonical reference to the Implementation guide for the dependency.", 0, 1, uri); 9436 case 1802060801: /*packageId*/ return new Property("packageId", "id", "The NPM package name for the Implementation Guide that this IG depends on.", 0, 1, packageId); 9437 case 351608024: /*version*/ return new Property("version", "string", "The version of the IG that is depended on, when the correct version is required to understand the IG correctly.", 0, 1, version); 9438 case -934964668: /*reason*/ return new Property("reason", "markdown", "A description explaining the nature of the dependency on the listed IG.", 0, 1, reason); 9439 default: return super.getNamedProperty(_hash, _name, _checkValid); 9440 } 9441 9442 } 9443 9444 @Override 9445 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9446 switch (hash) { 9447 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // CanonicalType 9448 case 1802060801: /*packageId*/ return this.packageId == null ? new Base[0] : new Base[] {this.packageId}; // IdType 9449 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 9450 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // MarkdownType 9451 default: return super.getProperty(hash, name, checkValid); 9452 } 9453 9454 } 9455 9456 @Override 9457 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9458 switch (hash) { 9459 case 116076: // uri 9460 this.uri = TypeConvertor.castToCanonical(value); // CanonicalType 9461 return value; 9462 case 1802060801: // packageId 9463 this.packageId = TypeConvertor.castToId(value); // IdType 9464 return value; 9465 case 351608024: // version 9466 this.version = TypeConvertor.castToString(value); // StringType 9467 return value; 9468 case -934964668: // reason 9469 this.reason = TypeConvertor.castToMarkdown(value); // MarkdownType 9470 return value; 9471 default: return super.setProperty(hash, name, value); 9472 } 9473 9474 } 9475 9476 @Override 9477 public Base setProperty(String name, Base value) throws FHIRException { 9478 if (name.equals("uri")) { 9479 this.uri = TypeConvertor.castToCanonical(value); // CanonicalType 9480 } else if (name.equals("packageId")) { 9481 this.packageId = TypeConvertor.castToId(value); // IdType 9482 } else if (name.equals("version")) { 9483 this.version = TypeConvertor.castToString(value); // StringType 9484 } else if (name.equals("reason")) { 9485 this.reason = TypeConvertor.castToMarkdown(value); // MarkdownType 9486 } else 9487 return super.setProperty(name, value); 9488 return value; 9489 } 9490 9491 @Override 9492 public void removeChild(String name, Base value) throws FHIRException { 9493 if (name.equals("uri")) { 9494 this.uri = null; 9495 } else if (name.equals("packageId")) { 9496 this.packageId = null; 9497 } else if (name.equals("version")) { 9498 this.version = null; 9499 } else if (name.equals("reason")) { 9500 this.reason = null; 9501 } else 9502 super.removeChild(name, value); 9503 9504 } 9505 9506 @Override 9507 public Base makeProperty(int hash, String name) throws FHIRException { 9508 switch (hash) { 9509 case 116076: return getUriElement(); 9510 case 1802060801: return getPackageIdElement(); 9511 case 351608024: return getVersionElement(); 9512 case -934964668: return getReasonElement(); 9513 default: return super.makeProperty(hash, name); 9514 } 9515 9516 } 9517 9518 @Override 9519 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9520 switch (hash) { 9521 case 116076: /*uri*/ return new String[] {"canonical"}; 9522 case 1802060801: /*packageId*/ return new String[] {"id"}; 9523 case 351608024: /*version*/ return new String[] {"string"}; 9524 case -934964668: /*reason*/ return new String[] {"markdown"}; 9525 default: return super.getTypesForProperty(hash, name); 9526 } 9527 9528 } 9529 9530 @Override 9531 public Base addChild(String name) throws FHIRException { 9532 if (name.equals("uri")) { 9533 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.dependsOn.uri"); 9534 } 9535 else if (name.equals("packageId")) { 9536 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.dependsOn.packageId"); 9537 } 9538 else if (name.equals("version")) { 9539 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.dependsOn.version"); 9540 } 9541 else if (name.equals("reason")) { 9542 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.dependsOn.reason"); 9543 } 9544 else 9545 return super.addChild(name); 9546 } 9547 9548 public ImplementationGuideDependsOnComponent copy() { 9549 ImplementationGuideDependsOnComponent dst = new ImplementationGuideDependsOnComponent(); 9550 copyValues(dst); 9551 return dst; 9552 } 9553 9554 public void copyValues(ImplementationGuideDependsOnComponent dst) { 9555 super.copyValues(dst); 9556 dst.uri = uri == null ? null : uri.copy(); 9557 dst.packageId = packageId == null ? null : packageId.copy(); 9558 dst.version = version == null ? null : version.copy(); 9559 dst.reason = reason == null ? null : reason.copy(); 9560 } 9561 9562 @Override 9563 public boolean equalsDeep(Base other_) { 9564 if (!super.equalsDeep(other_)) 9565 return false; 9566 if (!(other_ instanceof ImplementationGuideDependsOnComponent)) 9567 return false; 9568 ImplementationGuideDependsOnComponent o = (ImplementationGuideDependsOnComponent) other_; 9569 return compareDeep(uri, o.uri, true) && compareDeep(packageId, o.packageId, true) && compareDeep(version, o.version, true) 9570 && compareDeep(reason, o.reason, true); 9571 } 9572 9573 @Override 9574 public boolean equalsShallow(Base other_) { 9575 if (!super.equalsShallow(other_)) 9576 return false; 9577 if (!(other_ instanceof ImplementationGuideDependsOnComponent)) 9578 return false; 9579 ImplementationGuideDependsOnComponent o = (ImplementationGuideDependsOnComponent) other_; 9580 return compareValues(uri, o.uri, true) && compareValues(packageId, o.packageId, true) && compareValues(version, o.version, true) 9581 && compareValues(reason, o.reason, true); 9582 } 9583 9584 public boolean isEmpty() { 9585 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(uri, packageId, version 9586 , reason); 9587 } 9588 9589 public String fhirType() { 9590 return "ImplementationGuide.dependsOn"; 9591 9592 } 9593 9594 } 9595 9596 @Block() 9597 public static class ImplementationGuideGlobalComponent extends BackboneElement implements IBaseBackboneElement { 9598 /** 9599 * The type of resource that all instances must conform to. 9600 */ 9601 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 9602 @Description(shortDefinition="Type this profile applies to", formalDefinition="The type of resource that all instances must conform to." ) 9603 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 9604 protected CodeType type; 9605 9606 /** 9607 * A reference to the profile that all instances must conform to. 9608 */ 9609 @Child(name = "profile", type = {CanonicalType.class}, order=2, min=1, max=1, modifier=false, summary=true) 9610 @Description(shortDefinition="Profile that all resources must conform to", formalDefinition="A reference to the profile that all instances must conform to." ) 9611 protected CanonicalType profile; 9612 9613 private static final long serialVersionUID = 33894666L; 9614 9615 /** 9616 * Constructor 9617 */ 9618 public ImplementationGuideGlobalComponent() { 9619 super(); 9620 } 9621 9622 /** 9623 * Constructor 9624 */ 9625 public ImplementationGuideGlobalComponent(String type, String profile) { 9626 super(); 9627 this.setType(type); 9628 this.setProfile(profile); 9629 } 9630 9631 /** 9632 * @return {@link #type} (The type of resource that all instances must conform to.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 9633 */ 9634 public CodeType getTypeElement() { 9635 if (this.type == null) 9636 if (Configuration.errorOnAutoCreate()) 9637 throw new Error("Attempt to auto-create ImplementationGuideGlobalComponent.type"); 9638 else if (Configuration.doAutoCreate()) 9639 this.type = new CodeType(); // bb 9640 return this.type; 9641 } 9642 9643 public boolean hasTypeElement() { 9644 return this.type != null && !this.type.isEmpty(); 9645 } 9646 9647 public boolean hasType() { 9648 return this.type != null && !this.type.isEmpty(); 9649 } 9650 9651 /** 9652 * @param value {@link #type} (The type of resource that all instances must conform to.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 9653 */ 9654 public ImplementationGuideGlobalComponent setTypeElement(CodeType value) { 9655 this.type = value; 9656 return this; 9657 } 9658 9659 /** 9660 * @return The type of resource that all instances must conform to. 9661 */ 9662 public String getType() { 9663 return this.type == null ? null : this.type.getValue(); 9664 } 9665 9666 /** 9667 * @param value The type of resource that all instances must conform to. 9668 */ 9669 public ImplementationGuideGlobalComponent setType(String value) { 9670 if (this.type == null) 9671 this.type = new CodeType(); 9672 this.type.setValue(value); 9673 return this; 9674 } 9675 9676 /** 9677 * @return {@link #profile} (A reference to the profile that all instances must conform to.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 9678 */ 9679 public CanonicalType getProfileElement() { 9680 if (this.profile == null) 9681 if (Configuration.errorOnAutoCreate()) 9682 throw new Error("Attempt to auto-create ImplementationGuideGlobalComponent.profile"); 9683 else if (Configuration.doAutoCreate()) 9684 this.profile = new CanonicalType(); // bb 9685 return this.profile; 9686 } 9687 9688 public boolean hasProfileElement() { 9689 return this.profile != null && !this.profile.isEmpty(); 9690 } 9691 9692 public boolean hasProfile() { 9693 return this.profile != null && !this.profile.isEmpty(); 9694 } 9695 9696 /** 9697 * @param value {@link #profile} (A reference to the profile that all instances must conform to.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 9698 */ 9699 public ImplementationGuideGlobalComponent setProfileElement(CanonicalType value) { 9700 this.profile = value; 9701 return this; 9702 } 9703 9704 /** 9705 * @return A reference to the profile that all instances must conform to. 9706 */ 9707 public String getProfile() { 9708 return this.profile == null ? null : this.profile.getValue(); 9709 } 9710 9711 /** 9712 * @param value A reference to the profile that all instances must conform to. 9713 */ 9714 public ImplementationGuideGlobalComponent setProfile(String value) { 9715 if (this.profile == null) 9716 this.profile = new CanonicalType(); 9717 this.profile.setValue(value); 9718 return this; 9719 } 9720 9721 protected void listChildren(List<Property> children) { 9722 super.listChildren(children); 9723 children.add(new Property("type", "code", "The type of resource that all instances must conform to.", 0, 1, type)); 9724 children.add(new Property("profile", "canonical(StructureDefinition)", "A reference to the profile that all instances must conform to.", 0, 1, profile)); 9725 } 9726 9727 @Override 9728 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9729 switch (_hash) { 9730 case 3575610: /*type*/ return new Property("type", "code", "The type of resource that all instances must conform to.", 0, 1, type); 9731 case -309425751: /*profile*/ return new Property("profile", "canonical(StructureDefinition)", "A reference to the profile that all instances must conform to.", 0, 1, profile); 9732 default: return super.getNamedProperty(_hash, _name, _checkValid); 9733 } 9734 9735 } 9736 9737 @Override 9738 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9739 switch (hash) { 9740 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeType 9741 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // CanonicalType 9742 default: return super.getProperty(hash, name, checkValid); 9743 } 9744 9745 } 9746 9747 @Override 9748 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9749 switch (hash) { 9750 case 3575610: // type 9751 this.type = TypeConvertor.castToCode(value); // CodeType 9752 return value; 9753 case -309425751: // profile 9754 this.profile = TypeConvertor.castToCanonical(value); // CanonicalType 9755 return value; 9756 default: return super.setProperty(hash, name, value); 9757 } 9758 9759 } 9760 9761 @Override 9762 public Base setProperty(String name, Base value) throws FHIRException { 9763 if (name.equals("type")) { 9764 this.type = TypeConvertor.castToCode(value); // CodeType 9765 } else if (name.equals("profile")) { 9766 this.profile = TypeConvertor.castToCanonical(value); // CanonicalType 9767 } else 9768 return super.setProperty(name, value); 9769 return value; 9770 } 9771 9772 @Override 9773 public void removeChild(String name, Base value) throws FHIRException { 9774 if (name.equals("type")) { 9775 this.type = null; 9776 } else if (name.equals("profile")) { 9777 this.profile = null; 9778 } else 9779 super.removeChild(name, value); 9780 9781 } 9782 9783 @Override 9784 public Base makeProperty(int hash, String name) throws FHIRException { 9785 switch (hash) { 9786 case 3575610: return getTypeElement(); 9787 case -309425751: return getProfileElement(); 9788 default: return super.makeProperty(hash, name); 9789 } 9790 9791 } 9792 9793 @Override 9794 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9795 switch (hash) { 9796 case 3575610: /*type*/ return new String[] {"code"}; 9797 case -309425751: /*profile*/ return new String[] {"canonical"}; 9798 default: return super.getTypesForProperty(hash, name); 9799 } 9800 9801 } 9802 9803 @Override 9804 public Base addChild(String name) throws FHIRException { 9805 if (name.equals("type")) { 9806 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.global.type"); 9807 } 9808 else if (name.equals("profile")) { 9809 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.global.profile"); 9810 } 9811 else 9812 return super.addChild(name); 9813 } 9814 9815 public ImplementationGuideGlobalComponent copy() { 9816 ImplementationGuideGlobalComponent dst = new ImplementationGuideGlobalComponent(); 9817 copyValues(dst); 9818 return dst; 9819 } 9820 9821 public void copyValues(ImplementationGuideGlobalComponent dst) { 9822 super.copyValues(dst); 9823 dst.type = type == null ? null : type.copy(); 9824 dst.profile = profile == null ? null : profile.copy(); 9825 } 9826 9827 @Override 9828 public boolean equalsDeep(Base other_) { 9829 if (!super.equalsDeep(other_)) 9830 return false; 9831 if (!(other_ instanceof ImplementationGuideGlobalComponent)) 9832 return false; 9833 ImplementationGuideGlobalComponent o = (ImplementationGuideGlobalComponent) other_; 9834 return compareDeep(type, o.type, true) && compareDeep(profile, o.profile, true); 9835 } 9836 9837 @Override 9838 public boolean equalsShallow(Base other_) { 9839 if (!super.equalsShallow(other_)) 9840 return false; 9841 if (!(other_ instanceof ImplementationGuideGlobalComponent)) 9842 return false; 9843 ImplementationGuideGlobalComponent o = (ImplementationGuideGlobalComponent) other_; 9844 return compareValues(type, o.type, true) && compareValues(profile, o.profile, true); 9845 } 9846 9847 public boolean isEmpty() { 9848 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, profile); 9849 } 9850 9851 public String fhirType() { 9852 return "ImplementationGuide.global"; 9853 9854 } 9855 9856 } 9857 9858 @Block() 9859 public static class ImplementationGuideDefinitionComponent extends BackboneElement implements IBaseBackboneElement { 9860 /** 9861 * A logical group of resources. Logical groups can be used when building pages. 9862 */ 9863 @Child(name = "grouping", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9864 @Description(shortDefinition="Grouping used to present related resources in the IG", formalDefinition="A logical group of resources. Logical groups can be used when building pages." ) 9865 protected List<ImplementationGuideDefinitionGroupingComponent> grouping; 9866 9867 /** 9868 * A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource. 9869 */ 9870 @Child(name = "resource", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9871 @Description(shortDefinition="Resource in the implementation guide", formalDefinition="A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource." ) 9872 protected List<ImplementationGuideDefinitionResourceComponent> resource; 9873 9874 /** 9875 * A page / section in the implementation guide. The root page is the implementation guide home page. 9876 */ 9877 @Child(name = "page", type = {}, order=3, min=0, max=1, modifier=false, summary=false) 9878 @Description(shortDefinition="Page/Section in the Guide", formalDefinition="A page / section in the implementation guide. The root page is the implementation guide home page." ) 9879 protected ImplementationGuideDefinitionPageComponent page; 9880 9881 /** 9882 * A set of parameters that defines how the implementation guide is built. The parameters are defined by the relevant tools that build the implementation guides. 9883 */ 9884 @Child(name = "parameter", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9885 @Description(shortDefinition="Defines how IG is built by tools", formalDefinition="A set of parameters that defines how the implementation guide is built. The parameters are defined by the relevant tools that build the implementation guides." ) 9886 protected List<ImplementationGuideDefinitionParameterComponent> parameter; 9887 9888 /** 9889 * A template for building resources. 9890 */ 9891 @Child(name = "template", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9892 @Description(shortDefinition="A template for building resources", formalDefinition="A template for building resources." ) 9893 protected List<ImplementationGuideDefinitionTemplateComponent> template; 9894 9895 private static final long serialVersionUID = 179051968L; 9896 9897 /** 9898 * Constructor 9899 */ 9900 public ImplementationGuideDefinitionComponent() { 9901 super(); 9902 } 9903 9904 /** 9905 * @return {@link #grouping} (A logical group of resources. Logical groups can be used when building pages.) 9906 */ 9907 public List<ImplementationGuideDefinitionGroupingComponent> getGrouping() { 9908 if (this.grouping == null) 9909 this.grouping = new ArrayList<ImplementationGuideDefinitionGroupingComponent>(); 9910 return this.grouping; 9911 } 9912 9913 /** 9914 * @return Returns a reference to <code>this</code> for easy method chaining 9915 */ 9916 public ImplementationGuideDefinitionComponent setGrouping(List<ImplementationGuideDefinitionGroupingComponent> theGrouping) { 9917 this.grouping = theGrouping; 9918 return this; 9919 } 9920 9921 public boolean hasGrouping() { 9922 if (this.grouping == null) 9923 return false; 9924 for (ImplementationGuideDefinitionGroupingComponent item : this.grouping) 9925 if (!item.isEmpty()) 9926 return true; 9927 return false; 9928 } 9929 9930 public ImplementationGuideDefinitionGroupingComponent addGrouping() { //3 9931 ImplementationGuideDefinitionGroupingComponent t = new ImplementationGuideDefinitionGroupingComponent(); 9932 if (this.grouping == null) 9933 this.grouping = new ArrayList<ImplementationGuideDefinitionGroupingComponent>(); 9934 this.grouping.add(t); 9935 return t; 9936 } 9937 9938 public ImplementationGuideDefinitionComponent addGrouping(ImplementationGuideDefinitionGroupingComponent t) { //3 9939 if (t == null) 9940 return this; 9941 if (this.grouping == null) 9942 this.grouping = new ArrayList<ImplementationGuideDefinitionGroupingComponent>(); 9943 this.grouping.add(t); 9944 return this; 9945 } 9946 9947 /** 9948 * @return The first repetition of repeating field {@link #grouping}, creating it if it does not already exist {3} 9949 */ 9950 public ImplementationGuideDefinitionGroupingComponent getGroupingFirstRep() { 9951 if (getGrouping().isEmpty()) { 9952 addGrouping(); 9953 } 9954 return getGrouping().get(0); 9955 } 9956 9957 /** 9958 * @return {@link #resource} (A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.) 9959 */ 9960 public List<ImplementationGuideDefinitionResourceComponent> getResource() { 9961 if (this.resource == null) 9962 this.resource = new ArrayList<ImplementationGuideDefinitionResourceComponent>(); 9963 return this.resource; 9964 } 9965 9966 /** 9967 * @return Returns a reference to <code>this</code> for easy method chaining 9968 */ 9969 public ImplementationGuideDefinitionComponent setResource(List<ImplementationGuideDefinitionResourceComponent> theResource) { 9970 this.resource = theResource; 9971 return this; 9972 } 9973 9974 public boolean hasResource() { 9975 if (this.resource == null) 9976 return false; 9977 for (ImplementationGuideDefinitionResourceComponent item : this.resource) 9978 if (!item.isEmpty()) 9979 return true; 9980 return false; 9981 } 9982 9983 public ImplementationGuideDefinitionResourceComponent addResource() { //3 9984 ImplementationGuideDefinitionResourceComponent t = new ImplementationGuideDefinitionResourceComponent(); 9985 if (this.resource == null) 9986 this.resource = new ArrayList<ImplementationGuideDefinitionResourceComponent>(); 9987 this.resource.add(t); 9988 return t; 9989 } 9990 9991 public ImplementationGuideDefinitionComponent addResource(ImplementationGuideDefinitionResourceComponent t) { //3 9992 if (t == null) 9993 return this; 9994 if (this.resource == null) 9995 this.resource = new ArrayList<ImplementationGuideDefinitionResourceComponent>(); 9996 this.resource.add(t); 9997 return this; 9998 } 9999 10000 /** 10001 * @return The first repetition of repeating field {@link #resource}, creating it if it does not already exist {3} 10002 */ 10003 public ImplementationGuideDefinitionResourceComponent getResourceFirstRep() { 10004 if (getResource().isEmpty()) { 10005 addResource(); 10006 } 10007 return getResource().get(0); 10008 } 10009 10010 /** 10011 * @return {@link #page} (A page / section in the implementation guide. The root page is the implementation guide home page.) 10012 */ 10013 public ImplementationGuideDefinitionPageComponent getPage() { 10014 if (this.page == null) 10015 if (Configuration.errorOnAutoCreate()) 10016 throw new Error("Attempt to auto-create ImplementationGuideDefinitionComponent.page"); 10017 else if (Configuration.doAutoCreate()) 10018 this.page = new ImplementationGuideDefinitionPageComponent(); // cc 10019 return this.page; 10020 } 10021 10022 public boolean hasPage() { 10023 return this.page != null && !this.page.isEmpty(); 10024 } 10025 10026 /** 10027 * @param value {@link #page} (A page / section in the implementation guide. The root page is the implementation guide home page.) 10028 */ 10029 public ImplementationGuideDefinitionComponent setPage(ImplementationGuideDefinitionPageComponent value) { 10030 this.page = value; 10031 return this; 10032 } 10033 10034 /** 10035 * @return {@link #parameter} (A set of parameters that defines how the implementation guide is built. The parameters are defined by the relevant tools that build the implementation guides.) 10036 */ 10037 public List<ImplementationGuideDefinitionParameterComponent> getParameter() { 10038 if (this.parameter == null) 10039 this.parameter = new ArrayList<ImplementationGuideDefinitionParameterComponent>(); 10040 return this.parameter; 10041 } 10042 10043 /** 10044 * @return Returns a reference to <code>this</code> for easy method chaining 10045 */ 10046 public ImplementationGuideDefinitionComponent setParameter(List<ImplementationGuideDefinitionParameterComponent> theParameter) { 10047 this.parameter = theParameter; 10048 return this; 10049 } 10050 10051 public boolean hasParameter() { 10052 if (this.parameter == null) 10053 return false; 10054 for (ImplementationGuideDefinitionParameterComponent item : this.parameter) 10055 if (!item.isEmpty()) 10056 return true; 10057 return false; 10058 } 10059 10060 public ImplementationGuideDefinitionParameterComponent addParameter() { //3 10061 ImplementationGuideDefinitionParameterComponent t = new ImplementationGuideDefinitionParameterComponent(); 10062 if (this.parameter == null) 10063 this.parameter = new ArrayList<ImplementationGuideDefinitionParameterComponent>(); 10064 this.parameter.add(t); 10065 return t; 10066 } 10067 10068 public ImplementationGuideDefinitionComponent addParameter(ImplementationGuideDefinitionParameterComponent t) { //3 10069 if (t == null) 10070 return this; 10071 if (this.parameter == null) 10072 this.parameter = new ArrayList<ImplementationGuideDefinitionParameterComponent>(); 10073 this.parameter.add(t); 10074 return this; 10075 } 10076 10077 /** 10078 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist {3} 10079 */ 10080 public ImplementationGuideDefinitionParameterComponent getParameterFirstRep() { 10081 if (getParameter().isEmpty()) { 10082 addParameter(); 10083 } 10084 return getParameter().get(0); 10085 } 10086 10087 /** 10088 * @return {@link #template} (A template for building resources.) 10089 */ 10090 public List<ImplementationGuideDefinitionTemplateComponent> getTemplate() { 10091 if (this.template == null) 10092 this.template = new ArrayList<ImplementationGuideDefinitionTemplateComponent>(); 10093 return this.template; 10094 } 10095 10096 /** 10097 * @return Returns a reference to <code>this</code> for easy method chaining 10098 */ 10099 public ImplementationGuideDefinitionComponent setTemplate(List<ImplementationGuideDefinitionTemplateComponent> theTemplate) { 10100 this.template = theTemplate; 10101 return this; 10102 } 10103 10104 public boolean hasTemplate() { 10105 if (this.template == null) 10106 return false; 10107 for (ImplementationGuideDefinitionTemplateComponent item : this.template) 10108 if (!item.isEmpty()) 10109 return true; 10110 return false; 10111 } 10112 10113 public ImplementationGuideDefinitionTemplateComponent addTemplate() { //3 10114 ImplementationGuideDefinitionTemplateComponent t = new ImplementationGuideDefinitionTemplateComponent(); 10115 if (this.template == null) 10116 this.template = new ArrayList<ImplementationGuideDefinitionTemplateComponent>(); 10117 this.template.add(t); 10118 return t; 10119 } 10120 10121 public ImplementationGuideDefinitionComponent addTemplate(ImplementationGuideDefinitionTemplateComponent t) { //3 10122 if (t == null) 10123 return this; 10124 if (this.template == null) 10125 this.template = new ArrayList<ImplementationGuideDefinitionTemplateComponent>(); 10126 this.template.add(t); 10127 return this; 10128 } 10129 10130 /** 10131 * @return The first repetition of repeating field {@link #template}, creating it if it does not already exist {3} 10132 */ 10133 public ImplementationGuideDefinitionTemplateComponent getTemplateFirstRep() { 10134 if (getTemplate().isEmpty()) { 10135 addTemplate(); 10136 } 10137 return getTemplate().get(0); 10138 } 10139 10140 protected void listChildren(List<Property> children) { 10141 super.listChildren(children); 10142 children.add(new Property("grouping", "", "A logical group of resources. Logical groups can be used when building pages.", 0, java.lang.Integer.MAX_VALUE, grouping)); 10143 children.add(new Property("resource", "", "A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.", 0, java.lang.Integer.MAX_VALUE, resource)); 10144 children.add(new Property("page", "", "A page / section in the implementation guide. The root page is the implementation guide home page.", 0, 1, page)); 10145 children.add(new Property("parameter", "", "A set of parameters that defines how the implementation guide is built. The parameters are defined by the relevant tools that build the implementation guides.", 0, java.lang.Integer.MAX_VALUE, parameter)); 10146 children.add(new Property("template", "", "A template for building resources.", 0, java.lang.Integer.MAX_VALUE, template)); 10147 } 10148 10149 @Override 10150 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10151 switch (_hash) { 10152 case 506371331: /*grouping*/ return new Property("grouping", "", "A logical group of resources. Logical groups can be used when building pages.", 0, java.lang.Integer.MAX_VALUE, grouping); 10153 case -341064690: /*resource*/ return new Property("resource", "", "A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.", 0, java.lang.Integer.MAX_VALUE, resource); 10154 case 3433103: /*page*/ return new Property("page", "", "A page / section in the implementation guide. The root page is the implementation guide home page.", 0, 1, page); 10155 case 1954460585: /*parameter*/ return new Property("parameter", "", "A set of parameters that defines how the implementation guide is built. The parameters are defined by the relevant tools that build the implementation guides.", 0, java.lang.Integer.MAX_VALUE, parameter); 10156 case -1321546630: /*template*/ return new Property("template", "", "A template for building resources.", 0, java.lang.Integer.MAX_VALUE, template); 10157 default: return super.getNamedProperty(_hash, _name, _checkValid); 10158 } 10159 10160 } 10161 10162 @Override 10163 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10164 switch (hash) { 10165 case 506371331: /*grouping*/ return this.grouping == null ? new Base[0] : this.grouping.toArray(new Base[this.grouping.size()]); // ImplementationGuideDefinitionGroupingComponent 10166 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : this.resource.toArray(new Base[this.resource.size()]); // ImplementationGuideDefinitionResourceComponent 10167 case 3433103: /*page*/ return this.page == null ? new Base[0] : new Base[] {this.page}; // ImplementationGuideDefinitionPageComponent 10168 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // ImplementationGuideDefinitionParameterComponent 10169 case -1321546630: /*template*/ return this.template == null ? new Base[0] : this.template.toArray(new Base[this.template.size()]); // ImplementationGuideDefinitionTemplateComponent 10170 default: return super.getProperty(hash, name, checkValid); 10171 } 10172 10173 } 10174 10175 @Override 10176 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10177 switch (hash) { 10178 case 506371331: // grouping 10179 this.getGrouping().add((ImplementationGuideDefinitionGroupingComponent) value); // ImplementationGuideDefinitionGroupingComponent 10180 return value; 10181 case -341064690: // resource 10182 this.getResource().add((ImplementationGuideDefinitionResourceComponent) value); // ImplementationGuideDefinitionResourceComponent 10183 return value; 10184 case 3433103: // page 10185 this.page = (ImplementationGuideDefinitionPageComponent) value; // ImplementationGuideDefinitionPageComponent 10186 return value; 10187 case 1954460585: // parameter 10188 this.getParameter().add((ImplementationGuideDefinitionParameterComponent) value); // ImplementationGuideDefinitionParameterComponent 10189 return value; 10190 case -1321546630: // template 10191 this.getTemplate().add((ImplementationGuideDefinitionTemplateComponent) value); // ImplementationGuideDefinitionTemplateComponent 10192 return value; 10193 default: return super.setProperty(hash, name, value); 10194 } 10195 10196 } 10197 10198 @Override 10199 public Base setProperty(String name, Base value) throws FHIRException { 10200 if (name.equals("grouping")) { 10201 this.getGrouping().add((ImplementationGuideDefinitionGroupingComponent) value); 10202 } else if (name.equals("resource")) { 10203 this.getResource().add((ImplementationGuideDefinitionResourceComponent) value); 10204 } else if (name.equals("page")) { 10205 this.page = (ImplementationGuideDefinitionPageComponent) value; // ImplementationGuideDefinitionPageComponent 10206 } else if (name.equals("parameter")) { 10207 this.getParameter().add((ImplementationGuideDefinitionParameterComponent) value); 10208 } else if (name.equals("template")) { 10209 this.getTemplate().add((ImplementationGuideDefinitionTemplateComponent) value); 10210 } else 10211 return super.setProperty(name, value); 10212 return value; 10213 } 10214 10215 @Override 10216 public void removeChild(String name, Base value) throws FHIRException { 10217 if (name.equals("grouping")) { 10218 this.getGrouping().remove((ImplementationGuideDefinitionGroupingComponent) value); 10219 } else if (name.equals("resource")) { 10220 this.getResource().remove((ImplementationGuideDefinitionResourceComponent) value); 10221 } else if (name.equals("page")) { 10222 this.page = (ImplementationGuideDefinitionPageComponent) value; // ImplementationGuideDefinitionPageComponent 10223 } else if (name.equals("parameter")) { 10224 this.getParameter().remove((ImplementationGuideDefinitionParameterComponent) value); 10225 } else if (name.equals("template")) { 10226 this.getTemplate().remove((ImplementationGuideDefinitionTemplateComponent) value); 10227 } else 10228 super.removeChild(name, value); 10229 10230 } 10231 10232 @Override 10233 public Base makeProperty(int hash, String name) throws FHIRException { 10234 switch (hash) { 10235 case 506371331: return addGrouping(); 10236 case -341064690: return addResource(); 10237 case 3433103: return getPage(); 10238 case 1954460585: return addParameter(); 10239 case -1321546630: return addTemplate(); 10240 default: return super.makeProperty(hash, name); 10241 } 10242 10243 } 10244 10245 @Override 10246 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10247 switch (hash) { 10248 case 506371331: /*grouping*/ return new String[] {}; 10249 case -341064690: /*resource*/ return new String[] {}; 10250 case 3433103: /*page*/ return new String[] {}; 10251 case 1954460585: /*parameter*/ return new String[] {}; 10252 case -1321546630: /*template*/ return new String[] {}; 10253 default: return super.getTypesForProperty(hash, name); 10254 } 10255 10256 } 10257 10258 @Override 10259 public Base addChild(String name) throws FHIRException { 10260 if (name.equals("grouping")) { 10261 return addGrouping(); 10262 } 10263 else if (name.equals("resource")) { 10264 return addResource(); 10265 } 10266 else if (name.equals("page")) { 10267 this.page = new ImplementationGuideDefinitionPageComponent(); 10268 return this.page; 10269 } 10270 else if (name.equals("parameter")) { 10271 return addParameter(); 10272 } 10273 else if (name.equals("template")) { 10274 return addTemplate(); 10275 } 10276 else 10277 return super.addChild(name); 10278 } 10279 10280 public ImplementationGuideDefinitionComponent copy() { 10281 ImplementationGuideDefinitionComponent dst = new ImplementationGuideDefinitionComponent(); 10282 copyValues(dst); 10283 return dst; 10284 } 10285 10286 public void copyValues(ImplementationGuideDefinitionComponent dst) { 10287 super.copyValues(dst); 10288 if (grouping != null) { 10289 dst.grouping = new ArrayList<ImplementationGuideDefinitionGroupingComponent>(); 10290 for (ImplementationGuideDefinitionGroupingComponent i : grouping) 10291 dst.grouping.add(i.copy()); 10292 }; 10293 if (resource != null) { 10294 dst.resource = new ArrayList<ImplementationGuideDefinitionResourceComponent>(); 10295 for (ImplementationGuideDefinitionResourceComponent i : resource) 10296 dst.resource.add(i.copy()); 10297 }; 10298 dst.page = page == null ? null : page.copy(); 10299 if (parameter != null) { 10300 dst.parameter = new ArrayList<ImplementationGuideDefinitionParameterComponent>(); 10301 for (ImplementationGuideDefinitionParameterComponent i : parameter) 10302 dst.parameter.add(i.copy()); 10303 }; 10304 if (template != null) { 10305 dst.template = new ArrayList<ImplementationGuideDefinitionTemplateComponent>(); 10306 for (ImplementationGuideDefinitionTemplateComponent i : template) 10307 dst.template.add(i.copy()); 10308 }; 10309 } 10310 10311 @Override 10312 public boolean equalsDeep(Base other_) { 10313 if (!super.equalsDeep(other_)) 10314 return false; 10315 if (!(other_ instanceof ImplementationGuideDefinitionComponent)) 10316 return false; 10317 ImplementationGuideDefinitionComponent o = (ImplementationGuideDefinitionComponent) other_; 10318 return compareDeep(grouping, o.grouping, true) && compareDeep(resource, o.resource, true) && compareDeep(page, o.page, true) 10319 && compareDeep(parameter, o.parameter, true) && compareDeep(template, o.template, true); 10320 } 10321 10322 @Override 10323 public boolean equalsShallow(Base other_) { 10324 if (!super.equalsShallow(other_)) 10325 return false; 10326 if (!(other_ instanceof ImplementationGuideDefinitionComponent)) 10327 return false; 10328 ImplementationGuideDefinitionComponent o = (ImplementationGuideDefinitionComponent) other_; 10329 return true; 10330 } 10331 10332 public boolean isEmpty() { 10333 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(grouping, resource, page 10334 , parameter, template); 10335 } 10336 10337 public String fhirType() { 10338 return "ImplementationGuide.definition"; 10339 10340 } 10341 10342 } 10343 10344 @Block() 10345 public static class ImplementationGuideDefinitionGroupingComponent extends BackboneElement implements IBaseBackboneElement { 10346 /** 10347 * The human-readable title to display for the package of resources when rendering the implementation guide. 10348 */ 10349 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 10350 @Description(shortDefinition="Descriptive name for the package", formalDefinition="The human-readable title to display for the package of resources when rendering the implementation guide." ) 10351 protected StringType name; 10352 10353 /** 10354 * Human readable text describing the package. 10355 */ 10356 @Child(name = "description", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 10357 @Description(shortDefinition="Human readable text describing the package", formalDefinition="Human readable text describing the package." ) 10358 protected MarkdownType description; 10359 10360 private static final long serialVersionUID = 2116554295L; 10361 10362 /** 10363 * Constructor 10364 */ 10365 public ImplementationGuideDefinitionGroupingComponent() { 10366 super(); 10367 } 10368 10369 /** 10370 * Constructor 10371 */ 10372 public ImplementationGuideDefinitionGroupingComponent(String name) { 10373 super(); 10374 this.setName(name); 10375 } 10376 10377 /** 10378 * @return {@link #name} (The human-readable title to display for the package of resources when rendering the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 10379 */ 10380 public StringType getNameElement() { 10381 if (this.name == null) 10382 if (Configuration.errorOnAutoCreate()) 10383 throw new Error("Attempt to auto-create ImplementationGuideDefinitionGroupingComponent.name"); 10384 else if (Configuration.doAutoCreate()) 10385 this.name = new StringType(); // bb 10386 return this.name; 10387 } 10388 10389 public boolean hasNameElement() { 10390 return this.name != null && !this.name.isEmpty(); 10391 } 10392 10393 public boolean hasName() { 10394 return this.name != null && !this.name.isEmpty(); 10395 } 10396 10397 /** 10398 * @param value {@link #name} (The human-readable title to display for the package of resources when rendering the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 10399 */ 10400 public ImplementationGuideDefinitionGroupingComponent setNameElement(StringType value) { 10401 this.name = value; 10402 return this; 10403 } 10404 10405 /** 10406 * @return The human-readable title to display for the package of resources when rendering the implementation guide. 10407 */ 10408 public String getName() { 10409 return this.name == null ? null : this.name.getValue(); 10410 } 10411 10412 /** 10413 * @param value The human-readable title to display for the package of resources when rendering the implementation guide. 10414 */ 10415 public ImplementationGuideDefinitionGroupingComponent setName(String value) { 10416 if (this.name == null) 10417 this.name = new StringType(); 10418 this.name.setValue(value); 10419 return this; 10420 } 10421 10422 /** 10423 * @return {@link #description} (Human readable text describing the package.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 10424 */ 10425 public MarkdownType getDescriptionElement() { 10426 if (this.description == null) 10427 if (Configuration.errorOnAutoCreate()) 10428 throw new Error("Attempt to auto-create ImplementationGuideDefinitionGroupingComponent.description"); 10429 else if (Configuration.doAutoCreate()) 10430 this.description = new MarkdownType(); // bb 10431 return this.description; 10432 } 10433 10434 public boolean hasDescriptionElement() { 10435 return this.description != null && !this.description.isEmpty(); 10436 } 10437 10438 public boolean hasDescription() { 10439 return this.description != null && !this.description.isEmpty(); 10440 } 10441 10442 /** 10443 * @param value {@link #description} (Human readable text describing the package.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 10444 */ 10445 public ImplementationGuideDefinitionGroupingComponent setDescriptionElement(MarkdownType value) { 10446 this.description = value; 10447 return this; 10448 } 10449 10450 /** 10451 * @return Human readable text describing the package. 10452 */ 10453 public String getDescription() { 10454 return this.description == null ? null : this.description.getValue(); 10455 } 10456 10457 /** 10458 * @param value Human readable text describing the package. 10459 */ 10460 public ImplementationGuideDefinitionGroupingComponent setDescription(String value) { 10461 if (Utilities.noString(value)) 10462 this.description = null; 10463 else { 10464 if (this.description == null) 10465 this.description = new MarkdownType(); 10466 this.description.setValue(value); 10467 } 10468 return this; 10469 } 10470 10471 protected void listChildren(List<Property> children) { 10472 super.listChildren(children); 10473 children.add(new Property("name", "string", "The human-readable title to display for the package of resources when rendering the implementation guide.", 0, 1, name)); 10474 children.add(new Property("description", "markdown", "Human readable text describing the package.", 0, 1, description)); 10475 } 10476 10477 @Override 10478 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10479 switch (_hash) { 10480 case 3373707: /*name*/ return new Property("name", "string", "The human-readable title to display for the package of resources when rendering the implementation guide.", 0, 1, name); 10481 case -1724546052: /*description*/ return new Property("description", "markdown", "Human readable text describing the package.", 0, 1, description); 10482 default: return super.getNamedProperty(_hash, _name, _checkValid); 10483 } 10484 10485 } 10486 10487 @Override 10488 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10489 switch (hash) { 10490 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 10491 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 10492 default: return super.getProperty(hash, name, checkValid); 10493 } 10494 10495 } 10496 10497 @Override 10498 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10499 switch (hash) { 10500 case 3373707: // name 10501 this.name = TypeConvertor.castToString(value); // StringType 10502 return value; 10503 case -1724546052: // description 10504 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 10505 return value; 10506 default: return super.setProperty(hash, name, value); 10507 } 10508 10509 } 10510 10511 @Override 10512 public Base setProperty(String name, Base value) throws FHIRException { 10513 if (name.equals("name")) { 10514 this.name = TypeConvertor.castToString(value); // StringType 10515 } else if (name.equals("description")) { 10516 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 10517 } else 10518 return super.setProperty(name, value); 10519 return value; 10520 } 10521 10522 @Override 10523 public void removeChild(String name, Base value) throws FHIRException { 10524 if (name.equals("name")) { 10525 this.name = null; 10526 } else if (name.equals("description")) { 10527 this.description = null; 10528 } else 10529 super.removeChild(name, value); 10530 10531 } 10532 10533 @Override 10534 public Base makeProperty(int hash, String name) throws FHIRException { 10535 switch (hash) { 10536 case 3373707: return getNameElement(); 10537 case -1724546052: return getDescriptionElement(); 10538 default: return super.makeProperty(hash, name); 10539 } 10540 10541 } 10542 10543 @Override 10544 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10545 switch (hash) { 10546 case 3373707: /*name*/ return new String[] {"string"}; 10547 case -1724546052: /*description*/ return new String[] {"markdown"}; 10548 default: return super.getTypesForProperty(hash, name); 10549 } 10550 10551 } 10552 10553 @Override 10554 public Base addChild(String name) throws FHIRException { 10555 if (name.equals("name")) { 10556 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.definition.grouping.name"); 10557 } 10558 else if (name.equals("description")) { 10559 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.definition.grouping.description"); 10560 } 10561 else 10562 return super.addChild(name); 10563 } 10564 10565 public ImplementationGuideDefinitionGroupingComponent copy() { 10566 ImplementationGuideDefinitionGroupingComponent dst = new ImplementationGuideDefinitionGroupingComponent(); 10567 copyValues(dst); 10568 return dst; 10569 } 10570 10571 public void copyValues(ImplementationGuideDefinitionGroupingComponent dst) { 10572 super.copyValues(dst); 10573 dst.name = name == null ? null : name.copy(); 10574 dst.description = description == null ? null : description.copy(); 10575 } 10576 10577 @Override 10578 public boolean equalsDeep(Base other_) { 10579 if (!super.equalsDeep(other_)) 10580 return false; 10581 if (!(other_ instanceof ImplementationGuideDefinitionGroupingComponent)) 10582 return false; 10583 ImplementationGuideDefinitionGroupingComponent o = (ImplementationGuideDefinitionGroupingComponent) other_; 10584 return compareDeep(name, o.name, true) && compareDeep(description, o.description, true); 10585 } 10586 10587 @Override 10588 public boolean equalsShallow(Base other_) { 10589 if (!super.equalsShallow(other_)) 10590 return false; 10591 if (!(other_ instanceof ImplementationGuideDefinitionGroupingComponent)) 10592 return false; 10593 ImplementationGuideDefinitionGroupingComponent o = (ImplementationGuideDefinitionGroupingComponent) other_; 10594 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 10595 } 10596 10597 public boolean isEmpty() { 10598 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, description); 10599 } 10600 10601 public String fhirType() { 10602 return "ImplementationGuide.definition.grouping"; 10603 10604 } 10605 10606 } 10607 10608 @Block() 10609 public static class ImplementationGuideDefinitionResourceComponent extends BackboneElement implements IBaseBackboneElement { 10610 /** 10611 * Where this resource is found. 10612 */ 10613 @Child(name = "reference", type = {Reference.class}, order=1, min=1, max=1, modifier=false, summary=false) 10614 @Description(shortDefinition="Location of the resource", formalDefinition="Where this resource is found." ) 10615 protected Reference reference; 10616 10617 @Override 10618 public String toString() { 10619 return "ImplementationGuideDefinitionResourceComponent [name=" + name + ", reference=" + reference 10620 + ", profile=" + profile + "]"; 10621 } 10622 10623 /** 10624 * Indicates the FHIR Version(s) this artifact is intended to apply to. If no versions are specified, the resource is assumed to apply to all the versions stated in ImplementationGuide.fhirVersion. 10625 */ 10626 @Child(name = "fhirVersion", type = {CodeType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10627 @Description(shortDefinition="Versions this applies to (if different to IG)", formalDefinition="Indicates the FHIR Version(s) this artifact is intended to apply to. If no versions are specified, the resource is assumed to apply to all the versions stated in ImplementationGuide.fhirVersion." ) 10628 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/FHIR-version") 10629 protected List<Enumeration<FHIRVersion>> fhirVersion; 10630 10631 /** 10632 * A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name). 10633 */ 10634 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 10635 @Description(shortDefinition="Human readable name for the resource", formalDefinition="A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name)." ) 10636 protected StringType name; 10637 10638 /** 10639 * A description of the reason that a resource has been included in the implementation guide. 10640 */ 10641 @Child(name = "description", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=false) 10642 @Description(shortDefinition="Reason why included in guide", formalDefinition="A description of the reason that a resource has been included in the implementation guide." ) 10643 protected MarkdownType description; 10644 10645 /** 10646 * If true, indicates the resource is an example instance. 10647 */ 10648 @Child(name = "isExample", type = {BooleanType.class}, order=5, min=0, max=1, modifier=false, summary=false) 10649 @Description(shortDefinition="Is this an example", formalDefinition="If true, indicates the resource is an example instance." ) 10650 protected BooleanType isExample; 10651 10652 /** 10653 * If present, indicates profile(s) the instance is valid against. 10654 */ 10655 @Child(name = "profile", type = {CanonicalType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10656 @Description(shortDefinition="Profile(s) this is an example of", formalDefinition="If present, indicates profile(s) the instance is valid against." ) 10657 protected List<CanonicalType> profile; 10658 10659 /** 10660 * Reference to the id of the grouping this resource appears in. 10661 */ 10662 @Child(name = "groupingId", type = {IdType.class}, order=7, min=0, max=1, modifier=false, summary=false) 10663 @Description(shortDefinition="Grouping this is part of", formalDefinition="Reference to the id of the grouping this resource appears in." ) 10664 protected IdType groupingId; 10665 10666 private static final long serialVersionUID = 804050536L; 10667 10668 /** 10669 * Constructor 10670 */ 10671 public ImplementationGuideDefinitionResourceComponent() { 10672 super(); 10673 } 10674 10675 /** 10676 * Constructor 10677 */ 10678 public ImplementationGuideDefinitionResourceComponent(Reference reference) { 10679 super(); 10680 this.setReference(reference); 10681 } 10682 10683 /** 10684 * @return {@link #reference} (Where this resource is found.) 10685 */ 10686 public Reference getReference() { 10687 if (this.reference == null) 10688 if (Configuration.errorOnAutoCreate()) 10689 throw new Error("Attempt to auto-create ImplementationGuideDefinitionResourceComponent.reference"); 10690 else if (Configuration.doAutoCreate()) 10691 this.reference = new Reference(); // cc 10692 return this.reference; 10693 } 10694 10695 public boolean hasReference() { 10696 return this.reference != null && !this.reference.isEmpty(); 10697 } 10698 10699 /** 10700 * @param value {@link #reference} (Where this resource is found.) 10701 */ 10702 public ImplementationGuideDefinitionResourceComponent setReference(Reference value) { 10703 this.reference = value; 10704 return this; 10705 } 10706 10707 /** 10708 * @return {@link #fhirVersion} (Indicates the FHIR Version(s) this artifact is intended to apply to. If no versions are specified, the resource is assumed to apply to all the versions stated in ImplementationGuide.fhirVersion.) 10709 */ 10710 public List<Enumeration<FHIRVersion>> getFhirVersion() { 10711 if (this.fhirVersion == null) 10712 this.fhirVersion = new ArrayList<Enumeration<FHIRVersion>>(); 10713 return this.fhirVersion; 10714 } 10715 10716 /** 10717 * @return Returns a reference to <code>this</code> for easy method chaining 10718 */ 10719 public ImplementationGuideDefinitionResourceComponent setFhirVersion(List<Enumeration<FHIRVersion>> theFhirVersion) { 10720 this.fhirVersion = theFhirVersion; 10721 return this; 10722 } 10723 10724 public boolean hasFhirVersion() { 10725 if (this.fhirVersion == null) 10726 return false; 10727 for (Enumeration<FHIRVersion> item : this.fhirVersion) 10728 if (!item.isEmpty()) 10729 return true; 10730 return false; 10731 } 10732 10733 /** 10734 * @return {@link #fhirVersion} (Indicates the FHIR Version(s) this artifact is intended to apply to. If no versions are specified, the resource is assumed to apply to all the versions stated in ImplementationGuide.fhirVersion.) 10735 */ 10736 public Enumeration<FHIRVersion> addFhirVersionElement() {//2 10737 Enumeration<FHIRVersion> t = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); 10738 if (this.fhirVersion == null) 10739 this.fhirVersion = new ArrayList<Enumeration<FHIRVersion>>(); 10740 this.fhirVersion.add(t); 10741 return t; 10742 } 10743 10744 /** 10745 * @param value {@link #fhirVersion} (Indicates the FHIR Version(s) this artifact is intended to apply to. If no versions are specified, the resource is assumed to apply to all the versions stated in ImplementationGuide.fhirVersion.) 10746 */ 10747 public ImplementationGuideDefinitionResourceComponent addFhirVersion(FHIRVersion value) { //1 10748 Enumeration<FHIRVersion> t = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); 10749 t.setValue(value); 10750 if (this.fhirVersion == null) 10751 this.fhirVersion = new ArrayList<Enumeration<FHIRVersion>>(); 10752 this.fhirVersion.add(t); 10753 return this; 10754 } 10755 10756 /** 10757 * @param value {@link #fhirVersion} (Indicates the FHIR Version(s) this artifact is intended to apply to. If no versions are specified, the resource is assumed to apply to all the versions stated in ImplementationGuide.fhirVersion.) 10758 */ 10759 public boolean hasFhirVersion(FHIRVersion value) { 10760 if (this.fhirVersion == null) 10761 return false; 10762 for (Enumeration<FHIRVersion> v : this.fhirVersion) 10763 if (v.getValue().equals(value)) // code 10764 return true; 10765 return false; 10766 } 10767 10768 /** 10769 * @return {@link #name} (A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name).). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 10770 */ 10771 public StringType getNameElement() { 10772 if (this.name == null) 10773 if (Configuration.errorOnAutoCreate()) 10774 throw new Error("Attempt to auto-create ImplementationGuideDefinitionResourceComponent.name"); 10775 else if (Configuration.doAutoCreate()) 10776 this.name = new StringType(); // bb 10777 return this.name; 10778 } 10779 10780 public boolean hasNameElement() { 10781 return this.name != null && !this.name.isEmpty(); 10782 } 10783 10784 public boolean hasName() { 10785 return this.name != null && !this.name.isEmpty(); 10786 } 10787 10788 /** 10789 * @param value {@link #name} (A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name).). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 10790 */ 10791 public ImplementationGuideDefinitionResourceComponent setNameElement(StringType value) { 10792 this.name = value; 10793 return this; 10794 } 10795 10796 /** 10797 * @return A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name). 10798 */ 10799 public String getName() { 10800 return this.name == null ? null : this.name.getValue(); 10801 } 10802 10803 /** 10804 * @param value A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name). 10805 */ 10806 public ImplementationGuideDefinitionResourceComponent setName(String value) { 10807 if (Utilities.noString(value)) 10808 this.name = null; 10809 else { 10810 if (this.name == null) 10811 this.name = new StringType(); 10812 this.name.setValue(value); 10813 } 10814 return this; 10815 } 10816 10817 /** 10818 * @return {@link #description} (A description of the reason that a resource has been included in the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 10819 */ 10820 public MarkdownType getDescriptionElement() { 10821 if (this.description == null) 10822 if (Configuration.errorOnAutoCreate()) 10823 throw new Error("Attempt to auto-create ImplementationGuideDefinitionResourceComponent.description"); 10824 else if (Configuration.doAutoCreate()) 10825 this.description = new MarkdownType(); // bb 10826 return this.description; 10827 } 10828 10829 public boolean hasDescriptionElement() { 10830 return this.description != null && !this.description.isEmpty(); 10831 } 10832 10833 public boolean hasDescription() { 10834 return this.description != null && !this.description.isEmpty(); 10835 } 10836 10837 /** 10838 * @param value {@link #description} (A description of the reason that a resource has been included in the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 10839 */ 10840 public ImplementationGuideDefinitionResourceComponent setDescriptionElement(MarkdownType value) { 10841 this.description = value; 10842 return this; 10843 } 10844 10845 /** 10846 * @return A description of the reason that a resource has been included in the implementation guide. 10847 */ 10848 public String getDescription() { 10849 return this.description == null ? null : this.description.getValue(); 10850 } 10851 10852 /** 10853 * @param value A description of the reason that a resource has been included in the implementation guide. 10854 */ 10855 public ImplementationGuideDefinitionResourceComponent setDescription(String value) { 10856 if (Utilities.noString(value)) 10857 this.description = null; 10858 else { 10859 if (this.description == null) 10860 this.description = new MarkdownType(); 10861 this.description.setValue(value); 10862 } 10863 return this; 10864 } 10865 10866 /** 10867 * @return {@link #isExample} (If true, indicates the resource is an example instance.). This is the underlying object with id, value and extensions. The accessor "getIsExample" gives direct access to the value 10868 */ 10869 public BooleanType getIsExampleElement() { 10870 if (this.isExample == null) 10871 if (Configuration.errorOnAutoCreate()) 10872 throw new Error("Attempt to auto-create ImplementationGuideDefinitionResourceComponent.isExample"); 10873 else if (Configuration.doAutoCreate()) 10874 this.isExample = new BooleanType(); // bb 10875 return this.isExample; 10876 } 10877 10878 public boolean hasIsExampleElement() { 10879 return this.isExample != null && !this.isExample.isEmpty(); 10880 } 10881 10882 public boolean hasIsExample() { 10883 return this.isExample != null && !this.isExample.isEmpty(); 10884 } 10885 10886 /** 10887 * @param value {@link #isExample} (If true, indicates the resource is an example instance.). This is the underlying object with id, value and extensions. The accessor "getIsExample" gives direct access to the value 10888 */ 10889 public ImplementationGuideDefinitionResourceComponent setIsExampleElement(BooleanType value) { 10890 this.isExample = value; 10891 return this; 10892 } 10893 10894 /** 10895 * @return If true, indicates the resource is an example instance. 10896 */ 10897 public boolean getIsExample() { 10898 return this.isExample == null || this.isExample.isEmpty() ? false : this.isExample.getValue(); 10899 } 10900 10901 /** 10902 * @param value If true, indicates the resource is an example instance. 10903 */ 10904 public ImplementationGuideDefinitionResourceComponent setIsExample(boolean value) { 10905 if (this.isExample == null) 10906 this.isExample = new BooleanType(); 10907 this.isExample.setValue(value); 10908 return this; 10909 } 10910 10911 /** 10912 * @return {@link #profile} (If present, indicates profile(s) the instance is valid against.) 10913 */ 10914 public List<CanonicalType> getProfile() { 10915 if (this.profile == null) 10916 this.profile = new ArrayList<CanonicalType>(); 10917 return this.profile; 10918 } 10919 10920 /** 10921 * @return Returns a reference to <code>this</code> for easy method chaining 10922 */ 10923 public ImplementationGuideDefinitionResourceComponent setProfile(List<CanonicalType> theProfile) { 10924 this.profile = theProfile; 10925 return this; 10926 } 10927 10928 public boolean hasProfile() { 10929 if (this.profile == null) 10930 return false; 10931 for (CanonicalType item : this.profile) 10932 if (!item.isEmpty()) 10933 return true; 10934 return false; 10935 } 10936 10937 /** 10938 * @return {@link #profile} (If present, indicates profile(s) the instance is valid against.) 10939 */ 10940 public CanonicalType addProfileElement() {//2 10941 CanonicalType t = new CanonicalType(); 10942 if (this.profile == null) 10943 this.profile = new ArrayList<CanonicalType>(); 10944 this.profile.add(t); 10945 return t; 10946 } 10947 10948 /** 10949 * @param value {@link #profile} (If present, indicates profile(s) the instance is valid against.) 10950 */ 10951 public ImplementationGuideDefinitionResourceComponent addProfile(String value) { //1 10952 CanonicalType t = new CanonicalType(); 10953 t.setValue(value); 10954 if (this.profile == null) 10955 this.profile = new ArrayList<CanonicalType>(); 10956 this.profile.add(t); 10957 return this; 10958 } 10959 10960 /** 10961 * @param value {@link #profile} (If present, indicates profile(s) the instance is valid against.) 10962 */ 10963 public boolean hasProfile(String value) { 10964 if (this.profile == null) 10965 return false; 10966 for (CanonicalType v : this.profile) 10967 if (v.getValue().equals(value)) // canonical 10968 return true; 10969 return false; 10970 } 10971 10972 /** 10973 * @return {@link #groupingId} (Reference to the id of the grouping this resource appears in.). This is the underlying object with id, value and extensions. The accessor "getGroupingId" gives direct access to the value 10974 */ 10975 public IdType getGroupingIdElement() { 10976 if (this.groupingId == null) 10977 if (Configuration.errorOnAutoCreate()) 10978 throw new Error("Attempt to auto-create ImplementationGuideDefinitionResourceComponent.groupingId"); 10979 else if (Configuration.doAutoCreate()) 10980 this.groupingId = new IdType(); // bb 10981 return this.groupingId; 10982 } 10983 10984 public boolean hasGroupingIdElement() { 10985 return this.groupingId != null && !this.groupingId.isEmpty(); 10986 } 10987 10988 public boolean hasGroupingId() { 10989 return this.groupingId != null && !this.groupingId.isEmpty(); 10990 } 10991 10992 /** 10993 * @param value {@link #groupingId} (Reference to the id of the grouping this resource appears in.). This is the underlying object with id, value and extensions. The accessor "getGroupingId" gives direct access to the value 10994 */ 10995 public ImplementationGuideDefinitionResourceComponent setGroupingIdElement(IdType value) { 10996 this.groupingId = value; 10997 return this; 10998 } 10999 11000 /** 11001 * @return Reference to the id of the grouping this resource appears in. 11002 */ 11003 public String getGroupingId() { 11004 return this.groupingId == null ? null : this.groupingId.getValue(); 11005 } 11006 11007 /** 11008 * @param value Reference to the id of the grouping this resource appears in. 11009 */ 11010 public ImplementationGuideDefinitionResourceComponent setGroupingId(String value) { 11011 if (Utilities.noString(value)) 11012 this.groupingId = null; 11013 else { 11014 if (this.groupingId == null) 11015 this.groupingId = new IdType(); 11016 this.groupingId.setValue(value); 11017 } 11018 return this; 11019 } 11020 11021 protected void listChildren(List<Property> children) { 11022 super.listChildren(children); 11023 children.add(new Property("reference", "Reference(Any)", "Where this resource is found.", 0, 1, reference)); 11024 children.add(new Property("fhirVersion", "code", "Indicates the FHIR Version(s) this artifact is intended to apply to. If no versions are specified, the resource is assumed to apply to all the versions stated in ImplementationGuide.fhirVersion.", 0, java.lang.Integer.MAX_VALUE, fhirVersion)); 11025 children.add(new Property("name", "string", "A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name).", 0, 1, name)); 11026 children.add(new Property("description", "markdown", "A description of the reason that a resource has been included in the implementation guide.", 0, 1, description)); 11027 children.add(new Property("isExample", "boolean", "If true, indicates the resource is an example instance.", 0, 1, isExample)); 11028 children.add(new Property("profile", "canonical(StructureDefinition)", "If present, indicates profile(s) the instance is valid against.", 0, java.lang.Integer.MAX_VALUE, profile)); 11029 children.add(new Property("groupingId", "id", "Reference to the id of the grouping this resource appears in.", 0, 1, groupingId)); 11030 } 11031 11032 @Override 11033 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 11034 switch (_hash) { 11035 case -925155509: /*reference*/ return new Property("reference", "Reference(Any)", "Where this resource is found.", 0, 1, reference); 11036 case 461006061: /*fhirVersion*/ return new Property("fhirVersion", "code", "Indicates the FHIR Version(s) this artifact is intended to apply to. If no versions are specified, the resource is assumed to apply to all the versions stated in ImplementationGuide.fhirVersion.", 0, java.lang.Integer.MAX_VALUE, fhirVersion); 11037 case 3373707: /*name*/ return new Property("name", "string", "A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name).", 0, 1, name); 11038 case -1724546052: /*description*/ return new Property("description", "markdown", "A description of the reason that a resource has been included in the implementation guide.", 0, 1, description); 11039 case -1902749472: /*isExample*/ return new Property("isExample", "boolean", "If true, indicates the resource is an example instance.", 0, 1, isExample); 11040 case -309425751: /*profile*/ return new Property("profile", "canonical(StructureDefinition)", "If present, indicates profile(s) the instance is valid against.", 0, java.lang.Integer.MAX_VALUE, profile); 11041 case 1291547006: /*groupingId*/ return new Property("groupingId", "id", "Reference to the id of the grouping this resource appears in.", 0, 1, groupingId); 11042 default: return super.getNamedProperty(_hash, _name, _checkValid); 11043 } 11044 11045 } 11046 11047 @Override 11048 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 11049 switch (hash) { 11050 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 11051 case 461006061: /*fhirVersion*/ return this.fhirVersion == null ? new Base[0] : this.fhirVersion.toArray(new Base[this.fhirVersion.size()]); // Enumeration<FHIRVersion> 11052 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 11053 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 11054 case -1902749472: /*isExample*/ return this.isExample == null ? new Base[0] : new Base[] {this.isExample}; // BooleanType 11055 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // CanonicalType 11056 case 1291547006: /*groupingId*/ return this.groupingId == null ? new Base[0] : new Base[] {this.groupingId}; // IdType 11057 default: return super.getProperty(hash, name, checkValid); 11058 } 11059 11060 } 11061 11062 @Override 11063 public Base setProperty(int hash, String name, Base value) throws FHIRException { 11064 switch (hash) { 11065 case -925155509: // reference 11066 this.reference = TypeConvertor.castToReference(value); // Reference 11067 return value; 11068 case 461006061: // fhirVersion 11069 value = new FHIRVersionEnumFactory().fromType(TypeConvertor.castToCode(value)); 11070 this.getFhirVersion().add((Enumeration) value); // Enumeration<FHIRVersion> 11071 return value; 11072 case 3373707: // name 11073 this.name = TypeConvertor.castToString(value); // StringType 11074 return value; 11075 case -1724546052: // description 11076 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 11077 return value; 11078 case -1902749472: // isExample 11079 this.isExample = TypeConvertor.castToBoolean(value); // BooleanType 11080 return value; 11081 case -309425751: // profile 11082 this.getProfile().add(TypeConvertor.castToCanonical(value)); // CanonicalType 11083 return value; 11084 case 1291547006: // groupingId 11085 this.groupingId = TypeConvertor.castToId(value); // IdType 11086 return value; 11087 default: return super.setProperty(hash, name, value); 11088 } 11089 11090 } 11091 11092 @Override 11093 public Base setProperty(String name, Base value) throws FHIRException { 11094 if (name.equals("reference")) { 11095 this.reference = TypeConvertor.castToReference(value); // Reference 11096 } else if (name.equals("fhirVersion")) { 11097 value = new FHIRVersionEnumFactory().fromType(TypeConvertor.castToCode(value)); 11098 this.getFhirVersion().add((Enumeration) value); 11099 } else if (name.equals("name")) { 11100 this.name = TypeConvertor.castToString(value); // StringType 11101 } else if (name.equals("description")) { 11102 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 11103 } else if (name.equals("isExample")) { 11104 this.isExample = TypeConvertor.castToBoolean(value); // BooleanType 11105 } else if (name.equals("profile")) { 11106 this.getProfile().add(TypeConvertor.castToCanonical(value)); 11107 } else if (name.equals("groupingId")) { 11108 this.groupingId = TypeConvertor.castToId(value); // IdType 11109 } else 11110 return super.setProperty(name, value); 11111 return value; 11112 } 11113 11114 @Override 11115 public void removeChild(String name, Base value) throws FHIRException { 11116 if (name.equals("reference")) { 11117 this.reference = null; 11118 } else if (name.equals("fhirVersion")) { 11119 value = new FHIRVersionEnumFactory().fromType(TypeConvertor.castToCode(value)); 11120 this.getFhirVersion().remove((Enumeration) value); 11121 } else if (name.equals("name")) { 11122 this.name = null; 11123 } else if (name.equals("description")) { 11124 this.description = null; 11125 } else if (name.equals("isExample")) { 11126 this.isExample = null; 11127 } else if (name.equals("profile")) { 11128 this.getProfile().remove(value); 11129 } else if (name.equals("groupingId")) { 11130 this.groupingId = null; 11131 } else 11132 super.removeChild(name, value); 11133 11134 } 11135 11136 @Override 11137 public Base makeProperty(int hash, String name) throws FHIRException { 11138 switch (hash) { 11139 case -925155509: return getReference(); 11140 case 461006061: return addFhirVersionElement(); 11141 case 3373707: return getNameElement(); 11142 case -1724546052: return getDescriptionElement(); 11143 case -1902749472: return getIsExampleElement(); 11144 case -309425751: return addProfileElement(); 11145 case 1291547006: return getGroupingIdElement(); 11146 default: return super.makeProperty(hash, name); 11147 } 11148 11149 } 11150 11151 @Override 11152 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 11153 switch (hash) { 11154 case -925155509: /*reference*/ return new String[] {"Reference"}; 11155 case 461006061: /*fhirVersion*/ return new String[] {"code"}; 11156 case 3373707: /*name*/ return new String[] {"string"}; 11157 case -1724546052: /*description*/ return new String[] {"markdown"}; 11158 case -1902749472: /*isExample*/ return new String[] {"boolean"}; 11159 case -309425751: /*profile*/ return new String[] {"canonical"}; 11160 case 1291547006: /*groupingId*/ return new String[] {"id"}; 11161 default: return super.getTypesForProperty(hash, name); 11162 } 11163 11164 } 11165 11166 @Override 11167 public Base addChild(String name) throws FHIRException { 11168 if (name.equals("reference")) { 11169 this.reference = new Reference(); 11170 return this.reference; 11171 } 11172 else if (name.equals("fhirVersion")) { 11173 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.definition.resource.fhirVersion"); 11174 } 11175 else if (name.equals("name")) { 11176 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.definition.resource.name"); 11177 } 11178 else if (name.equals("description")) { 11179 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.definition.resource.description"); 11180 } 11181 else if (name.equals("isExample")) { 11182 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.definition.resource.isExample"); 11183 } 11184 else if (name.equals("profile")) { 11185 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.definition.resource.profile"); 11186 } 11187 else if (name.equals("groupingId")) { 11188 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.definition.resource.groupingId"); 11189 } 11190 else 11191 return super.addChild(name); 11192 } 11193 11194 public ImplementationGuideDefinitionResourceComponent copy() { 11195 ImplementationGuideDefinitionResourceComponent dst = new ImplementationGuideDefinitionResourceComponent(); 11196 copyValues(dst); 11197 return dst; 11198 } 11199 11200 public void copyValues(ImplementationGuideDefinitionResourceComponent dst) { 11201 super.copyValues(dst); 11202 dst.reference = reference == null ? null : reference.copy(); 11203 if (fhirVersion != null) { 11204 dst.fhirVersion = new ArrayList<Enumeration<FHIRVersion>>(); 11205 for (Enumeration<FHIRVersion> i : fhirVersion) 11206 dst.fhirVersion.add(i.copy()); 11207 }; 11208 dst.name = name == null ? null : name.copy(); 11209 dst.description = description == null ? null : description.copy(); 11210 dst.isExample = isExample == null ? null : isExample.copy(); 11211 if (profile != null) { 11212 dst.profile = new ArrayList<CanonicalType>(); 11213 for (CanonicalType i : profile) 11214 dst.profile.add(i.copy()); 11215 }; 11216 dst.groupingId = groupingId == null ? null : groupingId.copy(); 11217 } 11218 11219 @Override 11220 public boolean equalsDeep(Base other_) { 11221 if (!super.equalsDeep(other_)) 11222 return false; 11223 if (!(other_ instanceof ImplementationGuideDefinitionResourceComponent)) 11224 return false; 11225 ImplementationGuideDefinitionResourceComponent o = (ImplementationGuideDefinitionResourceComponent) other_; 11226 return compareDeep(reference, o.reference, true) && compareDeep(fhirVersion, o.fhirVersion, true) 11227 && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) && compareDeep(isExample, o.isExample, true) 11228 && compareDeep(profile, o.profile, true) && compareDeep(groupingId, o.groupingId, true); 11229 } 11230 11231 @Override 11232 public boolean equalsShallow(Base other_) { 11233 if (!super.equalsShallow(other_)) 11234 return false; 11235 if (!(other_ instanceof ImplementationGuideDefinitionResourceComponent)) 11236 return false; 11237 ImplementationGuideDefinitionResourceComponent o = (ImplementationGuideDefinitionResourceComponent) other_; 11238 return compareValues(fhirVersion, o.fhirVersion, true) && compareValues(name, o.name, true) && compareValues(description, o.description, true) 11239 && compareValues(isExample, o.isExample, true) && compareValues(profile, o.profile, true) && compareValues(groupingId, o.groupingId, true) 11240 ; 11241 } 11242 11243 public boolean isEmpty() { 11244 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, fhirVersion, name 11245 , description, isExample, profile, groupingId); 11246 } 11247 11248 public String fhirType() { 11249 return "ImplementationGuide.definition.resource"; 11250 11251 } 11252 11253 // added from java-adornments.txt: 11254 11255 // end addition 11256 } 11257 11258 @Block() 11259 public static class ImplementationGuideDefinitionPageComponent extends BackboneElement implements IBaseBackboneElement { 11260 /** 11261 * Indicates the URL or the actual content to provide for the page. 11262 */ 11263 @Child(name = "source", type = {UrlType.class, StringType.class, MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 11264 @Description(shortDefinition="Source for page", formalDefinition="Indicates the URL or the actual content to provide for the page." ) 11265 protected DataType source; 11266 11267 /** 11268 * The url by which the page should be known when published. 11269 */ 11270 @Child(name = "name", type = {UrlType.class}, order=2, min=1, max=1, modifier=false, summary=false) 11271 @Description(shortDefinition="Name of the page when published", formalDefinition="The url by which the page should be known when published." ) 11272 protected UrlType name; 11273 11274 /** 11275 * A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc. 11276 */ 11277 @Child(name = "title", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=false) 11278 @Description(shortDefinition="Short title shown for navigational assistance", formalDefinition="A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc." ) 11279 protected StringType title; 11280 11281 /** 11282 * A code that indicates how the page is generated. 11283 */ 11284 @Child(name = "generation", type = {CodeType.class}, order=4, min=1, max=1, modifier=false, summary=false) 11285 @Description(shortDefinition="html | markdown | xml | generated", formalDefinition="A code that indicates how the page is generated." ) 11286 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/guide-page-generation") 11287 protected Enumeration<GuidePageGeneration> generation; 11288 11289 /** 11290 * Nested Pages/Sections under this page. 11291 */ 11292 @Child(name = "page", type = {ImplementationGuideDefinitionPageComponent.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 11293 @Description(shortDefinition="Nested Pages / Sections", formalDefinition="Nested Pages/Sections under this page." ) 11294 protected List<ImplementationGuideDefinitionPageComponent> page; 11295 11296 private static final long serialVersionUID = 57473246L; 11297 11298 /** 11299 * Constructor 11300 */ 11301 public ImplementationGuideDefinitionPageComponent() { 11302 super(); 11303 } 11304 11305 /** 11306 * Constructor 11307 */ 11308 public ImplementationGuideDefinitionPageComponent(String name, String title, GuidePageGeneration generation) { 11309 super(); 11310 this.setName(name); 11311 this.setTitle(title); 11312 this.setGeneration(generation); 11313 } 11314 11315 /** 11316 * @return {@link #source} (Indicates the URL or the actual content to provide for the page.) 11317 */ 11318 public DataType getSource() { 11319 return this.source; 11320 } 11321 11322 /** 11323 * @return {@link #source} (Indicates the URL or the actual content to provide for the page.) 11324 */ 11325 public UrlType getSourceUrlType() throws FHIRException { 11326 if (this.source == null) 11327 this.source = new UrlType(); 11328 if (!(this.source instanceof UrlType)) 11329 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.source.getClass().getName()+" was encountered"); 11330 return (UrlType) this.source; 11331 } 11332 11333 public boolean hasSourceUrlType() { 11334 return this != null && this.source instanceof UrlType; 11335 } 11336 11337 /** 11338 * @return {@link #source} (Indicates the URL or the actual content to provide for the page.) 11339 */ 11340 public StringType getSourceStringType() throws FHIRException { 11341 if (this.source == null) 11342 this.source = new StringType(); 11343 if (!(this.source instanceof StringType)) 11344 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.source.getClass().getName()+" was encountered"); 11345 return (StringType) this.source; 11346 } 11347 11348 public boolean hasSourceStringType() { 11349 return this != null && this.source instanceof StringType; 11350 } 11351 11352 /** 11353 * @return {@link #source} (Indicates the URL or the actual content to provide for the page.) 11354 */ 11355 public MarkdownType getSourceMarkdownType() throws FHIRException { 11356 if (this.source == null) 11357 this.source = new MarkdownType(); 11358 if (!(this.source instanceof MarkdownType)) 11359 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.source.getClass().getName()+" was encountered"); 11360 return (MarkdownType) this.source; 11361 } 11362 11363 public boolean hasSourceMarkdownType() { 11364 return this != null && this.source instanceof MarkdownType; 11365 } 11366 11367 public boolean hasSource() { 11368 return this.source != null && !this.source.isEmpty(); 11369 } 11370 11371 /** 11372 * @param value {@link #source} (Indicates the URL or the actual content to provide for the page.) 11373 */ 11374 public ImplementationGuideDefinitionPageComponent setSource(DataType value) { 11375 if (value != null && !(value instanceof UrlType || value instanceof StringType || value instanceof MarkdownType)) 11376 throw new FHIRException("Not the right type for ImplementationGuide.definition.page.source[x]: "+value.fhirType()); 11377 this.source = value; 11378 return this; 11379 } 11380 11381 /** 11382 * @return {@link #name} (The url by which the page should be known when published.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 11383 */ 11384 public UrlType getNameElement() { 11385 if (this.name == null) 11386 if (Configuration.errorOnAutoCreate()) 11387 throw new Error("Attempt to auto-create ImplementationGuideDefinitionPageComponent.name"); 11388 else if (Configuration.doAutoCreate()) 11389 this.name = new UrlType(); // bb 11390 return this.name; 11391 } 11392 11393 public boolean hasNameElement() { 11394 return this.name != null && !this.name.isEmpty(); 11395 } 11396 11397 public boolean hasName() { 11398 return this.name != null && !this.name.isEmpty(); 11399 } 11400 11401 /** 11402 * @param value {@link #name} (The url by which the page should be known when published.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 11403 */ 11404 public ImplementationGuideDefinitionPageComponent setNameElement(UrlType value) { 11405 this.name = value; 11406 return this; 11407 } 11408 11409 /** 11410 * @return The url by which the page should be known when published. 11411 */ 11412 public String getName() { 11413 return this.name == null ? null : this.name.getValue(); 11414 } 11415 11416 /** 11417 * @param value The url by which the page should be known when published. 11418 */ 11419 public ImplementationGuideDefinitionPageComponent setName(String value) { 11420 if (this.name == null) 11421 this.name = new UrlType(); 11422 this.name.setValue(value); 11423 return this; 11424 } 11425 11426 /** 11427 * @return {@link #title} (A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 11428 */ 11429 public StringType getTitleElement() { 11430 if (this.title == null) 11431 if (Configuration.errorOnAutoCreate()) 11432 throw new Error("Attempt to auto-create ImplementationGuideDefinitionPageComponent.title"); 11433 else if (Configuration.doAutoCreate()) 11434 this.title = new StringType(); // bb 11435 return this.title; 11436 } 11437 11438 public boolean hasTitleElement() { 11439 return this.title != null && !this.title.isEmpty(); 11440 } 11441 11442 public boolean hasTitle() { 11443 return this.title != null && !this.title.isEmpty(); 11444 } 11445 11446 /** 11447 * @param value {@link #title} (A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 11448 */ 11449 public ImplementationGuideDefinitionPageComponent setTitleElement(StringType value) { 11450 this.title = value; 11451 return this; 11452 } 11453 11454 /** 11455 * @return A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc. 11456 */ 11457 public String getTitle() { 11458 return this.title == null ? null : this.title.getValue(); 11459 } 11460 11461 /** 11462 * @param value A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc. 11463 */ 11464 public ImplementationGuideDefinitionPageComponent setTitle(String value) { 11465 if (this.title == null) 11466 this.title = new StringType(); 11467 this.title.setValue(value); 11468 return this; 11469 } 11470 11471 /** 11472 * @return {@link #generation} (A code that indicates how the page is generated.). This is the underlying object with id, value and extensions. The accessor "getGeneration" gives direct access to the value 11473 */ 11474 public Enumeration<GuidePageGeneration> getGenerationElement() { 11475 if (this.generation == null) 11476 if (Configuration.errorOnAutoCreate()) 11477 throw new Error("Attempt to auto-create ImplementationGuideDefinitionPageComponent.generation"); 11478 else if (Configuration.doAutoCreate()) 11479 this.generation = new Enumeration<GuidePageGeneration>(new GuidePageGenerationEnumFactory()); // bb 11480 return this.generation; 11481 } 11482 11483 public boolean hasGenerationElement() { 11484 return this.generation != null && !this.generation.isEmpty(); 11485 } 11486 11487 public boolean hasGeneration() { 11488 return this.generation != null && !this.generation.isEmpty(); 11489 } 11490 11491 /** 11492 * @param value {@link #generation} (A code that indicates how the page is generated.). This is the underlying object with id, value and extensions. The accessor "getGeneration" gives direct access to the value 11493 */ 11494 public ImplementationGuideDefinitionPageComponent setGenerationElement(Enumeration<GuidePageGeneration> value) { 11495 this.generation = value; 11496 return this; 11497 } 11498 11499 /** 11500 * @return A code that indicates how the page is generated. 11501 */ 11502 public GuidePageGeneration getGeneration() { 11503 return this.generation == null ? null : this.generation.getValue(); 11504 } 11505 11506 /** 11507 * @param value A code that indicates how the page is generated. 11508 */ 11509 public ImplementationGuideDefinitionPageComponent setGeneration(GuidePageGeneration value) { 11510 if (this.generation == null) 11511 this.generation = new Enumeration<GuidePageGeneration>(new GuidePageGenerationEnumFactory()); 11512 this.generation.setValue(value); 11513 return this; 11514 } 11515 11516 /** 11517 * @return {@link #page} (Nested Pages/Sections under this page.) 11518 */ 11519 public List<ImplementationGuideDefinitionPageComponent> getPage() { 11520 if (this.page == null) 11521 this.page = new ArrayList<ImplementationGuideDefinitionPageComponent>(); 11522 return this.page; 11523 } 11524 11525 /** 11526 * @return Returns a reference to <code>this</code> for easy method chaining 11527 */ 11528 public ImplementationGuideDefinitionPageComponent setPage(List<ImplementationGuideDefinitionPageComponent> thePage) { 11529 this.page = thePage; 11530 return this; 11531 } 11532 11533 public boolean hasPage() { 11534 if (this.page == null) 11535 return false; 11536 for (ImplementationGuideDefinitionPageComponent item : this.page) 11537 if (!item.isEmpty()) 11538 return true; 11539 return false; 11540 } 11541 11542 public ImplementationGuideDefinitionPageComponent addPage() { //3 11543 ImplementationGuideDefinitionPageComponent t = new ImplementationGuideDefinitionPageComponent(); 11544 if (this.page == null) 11545 this.page = new ArrayList<ImplementationGuideDefinitionPageComponent>(); 11546 this.page.add(t); 11547 return t; 11548 } 11549 11550 public ImplementationGuideDefinitionPageComponent addPage(ImplementationGuideDefinitionPageComponent t) { //3 11551 if (t == null) 11552 return this; 11553 if (this.page == null) 11554 this.page = new ArrayList<ImplementationGuideDefinitionPageComponent>(); 11555 this.page.add(t); 11556 return this; 11557 } 11558 11559 /** 11560 * @return The first repetition of repeating field {@link #page}, creating it if it does not already exist {3} 11561 */ 11562 public ImplementationGuideDefinitionPageComponent getPageFirstRep() { 11563 if (getPage().isEmpty()) { 11564 addPage(); 11565 } 11566 return getPage().get(0); 11567 } 11568 11569 protected void listChildren(List<Property> children) { 11570 super.listChildren(children); 11571 children.add(new Property("source[x]", "url|string|markdown", "Indicates the URL or the actual content to provide for the page.", 0, 1, source)); 11572 children.add(new Property("name", "url", "The url by which the page should be known when published.", 0, 1, name)); 11573 children.add(new Property("title", "string", "A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc.", 0, 1, title)); 11574 children.add(new Property("generation", "code", "A code that indicates how the page is generated.", 0, 1, generation)); 11575 children.add(new Property("page", "@ImplementationGuide.definition.page", "Nested Pages/Sections under this page.", 0, java.lang.Integer.MAX_VALUE, page)); 11576 } 11577 11578 @Override 11579 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 11580 switch (_hash) { 11581 case -1698413947: /*source[x]*/ return new Property("source[x]", "url|string|markdown", "Indicates the URL or the actual content to provide for the page.", 0, 1, source); 11582 case -896505829: /*source*/ return new Property("source[x]", "url|string|markdown", "Indicates the URL or the actual content to provide for the page.", 0, 1, source); 11583 case -1698419884: /*sourceUrl*/ return new Property("source[x]", "url", "Indicates the URL or the actual content to provide for the page.", 0, 1, source); 11584 case 1327821836: /*sourceString*/ return new Property("source[x]", "string", "Indicates the URL or the actual content to provide for the page.", 0, 1, source); 11585 case -1116570070: /*sourceMarkdown*/ return new Property("source[x]", "markdown", "Indicates the URL or the actual content to provide for the page.", 0, 1, source); 11586 case 3373707: /*name*/ return new Property("name", "url", "The url by which the page should be known when published.", 0, 1, name); 11587 case 110371416: /*title*/ return new Property("title", "string", "A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc.", 0, 1, title); 11588 case 305703192: /*generation*/ return new Property("generation", "code", "A code that indicates how the page is generated.", 0, 1, generation); 11589 case 3433103: /*page*/ return new Property("page", "@ImplementationGuide.definition.page", "Nested Pages/Sections under this page.", 0, java.lang.Integer.MAX_VALUE, page); 11590 default: return super.getNamedProperty(_hash, _name, _checkValid); 11591 } 11592 11593 } 11594 11595 @Override 11596 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 11597 switch (hash) { 11598 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // DataType 11599 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // UrlType 11600 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 11601 case 305703192: /*generation*/ return this.generation == null ? new Base[0] : new Base[] {this.generation}; // Enumeration<GuidePageGeneration> 11602 case 3433103: /*page*/ return this.page == null ? new Base[0] : this.page.toArray(new Base[this.page.size()]); // ImplementationGuideDefinitionPageComponent 11603 default: return super.getProperty(hash, name, checkValid); 11604 } 11605 11606 } 11607 11608 @Override 11609 public Base setProperty(int hash, String name, Base value) throws FHIRException { 11610 switch (hash) { 11611 case -896505829: // source 11612 this.source = TypeConvertor.castToType(value); // DataType 11613 return value; 11614 case 3373707: // name 11615 this.name = TypeConvertor.castToUrl(value); // UrlType 11616 return value; 11617 case 110371416: // title 11618 this.title = TypeConvertor.castToString(value); // StringType 11619 return value; 11620 case 305703192: // generation 11621 value = new GuidePageGenerationEnumFactory().fromType(TypeConvertor.castToCode(value)); 11622 this.generation = (Enumeration) value; // Enumeration<GuidePageGeneration> 11623 return value; 11624 case 3433103: // page 11625 this.getPage().add((ImplementationGuideDefinitionPageComponent) value); // ImplementationGuideDefinitionPageComponent 11626 return value; 11627 default: return super.setProperty(hash, name, value); 11628 } 11629 11630 } 11631 11632 @Override 11633 public Base setProperty(String name, Base value) throws FHIRException { 11634 if (name.equals("source[x]")) { 11635 this.source = TypeConvertor.castToType(value); // DataType 11636 } else if (name.equals("name")) { 11637 this.name = TypeConvertor.castToUrl(value); // UrlType 11638 } else if (name.equals("title")) { 11639 this.title = TypeConvertor.castToString(value); // StringType 11640 } else if (name.equals("generation")) { 11641 value = new GuidePageGenerationEnumFactory().fromType(TypeConvertor.castToCode(value)); 11642 this.generation = (Enumeration) value; // Enumeration<GuidePageGeneration> 11643 } else if (name.equals("page")) { 11644 this.getPage().add((ImplementationGuideDefinitionPageComponent) value); 11645 } else 11646 return super.setProperty(name, value); 11647 return value; 11648 } 11649 11650 @Override 11651 public void removeChild(String name, Base value) throws FHIRException { 11652 if (name.equals("source[x]")) { 11653 this.source = null; 11654 } else if (name.equals("name")) { 11655 this.name = null; 11656 } else if (name.equals("title")) { 11657 this.title = null; 11658 } else if (name.equals("generation")) { 11659 value = new GuidePageGenerationEnumFactory().fromType(TypeConvertor.castToCode(value)); 11660 this.generation = (Enumeration) value; // Enumeration<GuidePageGeneration> 11661 } else if (name.equals("page")) { 11662 this.getPage().remove((ImplementationGuideDefinitionPageComponent) value); 11663 } else 11664 super.removeChild(name, value); 11665 11666 } 11667 11668 @Override 11669 public Base makeProperty(int hash, String name) throws FHIRException { 11670 switch (hash) { 11671 case -1698413947: return getSource(); 11672 case -896505829: return getSource(); 11673 case 3373707: return getNameElement(); 11674 case 110371416: return getTitleElement(); 11675 case 305703192: return getGenerationElement(); 11676 case 3433103: return addPage(); 11677 default: return super.makeProperty(hash, name); 11678 } 11679 11680 } 11681 11682 @Override 11683 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 11684 switch (hash) { 11685 case -896505829: /*source*/ return new String[] {"url", "string", "markdown"}; 11686 case 3373707: /*name*/ return new String[] {"url"}; 11687 case 110371416: /*title*/ return new String[] {"string"}; 11688 case 305703192: /*generation*/ return new String[] {"code"}; 11689 case 3433103: /*page*/ return new String[] {"@ImplementationGuide.definition.page"}; 11690 default: return super.getTypesForProperty(hash, name); 11691 } 11692 11693 } 11694 11695 @Override 11696 public Base addChild(String name) throws FHIRException { 11697 if (name.equals("sourceUrl")) { 11698 this.source = new UrlType(); 11699 return this.source; 11700 } 11701 else if (name.equals("sourceString")) { 11702 this.source = new StringType(); 11703 return this.source; 11704 } 11705 else if (name.equals("sourceMarkdown")) { 11706 this.source = new MarkdownType(); 11707 return this.source; 11708 } 11709 else if (name.equals("name")) { 11710 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.definition.page.name"); 11711 } 11712 else if (name.equals("title")) { 11713 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.definition.page.title"); 11714 } 11715 else if (name.equals("generation")) { 11716 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.definition.page.generation"); 11717 } 11718 else if (name.equals("page")) { 11719 return addPage(); 11720 } 11721 else 11722 return super.addChild(name); 11723 } 11724 11725 public ImplementationGuideDefinitionPageComponent copy() { 11726 ImplementationGuideDefinitionPageComponent dst = new ImplementationGuideDefinitionPageComponent(); 11727 copyValues(dst); 11728 return dst; 11729 } 11730 11731 public void copyValues(ImplementationGuideDefinitionPageComponent dst) { 11732 super.copyValues(dst); 11733 dst.source = source == null ? null : source.copy(); 11734 dst.name = name == null ? null : name.copy(); 11735 dst.title = title == null ? null : title.copy(); 11736 dst.generation = generation == null ? null : generation.copy(); 11737 if (page != null) { 11738 dst.page = new ArrayList<ImplementationGuideDefinitionPageComponent>(); 11739 for (ImplementationGuideDefinitionPageComponent i : page) 11740 dst.page.add(i.copy()); 11741 }; 11742 } 11743 11744 @Override 11745 public boolean equalsDeep(Base other_) { 11746 if (!super.equalsDeep(other_)) 11747 return false; 11748 if (!(other_ instanceof ImplementationGuideDefinitionPageComponent)) 11749 return false; 11750 ImplementationGuideDefinitionPageComponent o = (ImplementationGuideDefinitionPageComponent) other_; 11751 return compareDeep(source, o.source, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 11752 && compareDeep(generation, o.generation, true) && compareDeep(page, o.page, true); 11753 } 11754 11755 @Override 11756 public boolean equalsShallow(Base other_) { 11757 if (!super.equalsShallow(other_)) 11758 return false; 11759 if (!(other_ instanceof ImplementationGuideDefinitionPageComponent)) 11760 return false; 11761 ImplementationGuideDefinitionPageComponent o = (ImplementationGuideDefinitionPageComponent) other_; 11762 return compareValues(name, o.name, true) && compareValues(title, o.title, true) && compareValues(generation, o.generation, true) 11763 ; 11764 } 11765 11766 public boolean isEmpty() { 11767 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(source, name, title, generation 11768 , page); 11769 } 11770 11771 public String fhirType() { 11772 return "ImplementationGuide.definition.page"; 11773 11774 } 11775 11776 } 11777 11778 @Block() 11779 public static class ImplementationGuideDefinitionParameterComponent extends BackboneElement implements IBaseBackboneElement { 11780 /** 11781 * A tool-specific code that defines the parameter. 11782 */ 11783 @Child(name = "code", type = {Coding.class}, order=1, min=1, max=1, modifier=false, summary=false) 11784 @Description(shortDefinition="Code that identifies parameter", formalDefinition="A tool-specific code that defines the parameter." ) 11785 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/guide-parameter-code") 11786 protected Coding code; 11787 11788 /** 11789 * Value for named type. 11790 */ 11791 @Child(name = "value", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 11792 @Description(shortDefinition="Value for named type", formalDefinition="Value for named type." ) 11793 protected StringType value; 11794 11795 private static final long serialVersionUID = -1728909245L; 11796 11797 /** 11798 * Constructor 11799 */ 11800 public ImplementationGuideDefinitionParameterComponent() { 11801 super(); 11802 } 11803 11804 /** 11805 * Constructor 11806 */ 11807 public ImplementationGuideDefinitionParameterComponent(Coding code, String value) { 11808 super(); 11809 this.setCode(code); 11810 this.setValue(value); 11811 } 11812 11813 /** 11814 * @return {@link #code} (A tool-specific code that defines the parameter.) 11815 */ 11816 public Coding getCode() { 11817 if (this.code == null) 11818 if (Configuration.errorOnAutoCreate()) 11819 throw new Error("Attempt to auto-create ImplementationGuideDefinitionParameterComponent.code"); 11820 else if (Configuration.doAutoCreate()) 11821 this.code = new Coding(); // cc 11822 return this.code; 11823 } 11824 11825 public boolean hasCode() { 11826 return this.code != null && !this.code.isEmpty(); 11827 } 11828 11829 /** 11830 * @param value {@link #code} (A tool-specific code that defines the parameter.) 11831 */ 11832 public ImplementationGuideDefinitionParameterComponent setCode(Coding value) { 11833 this.code = value; 11834 return this; 11835 } 11836 11837 /** 11838 * @return {@link #value} (Value for named type.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 11839 */ 11840 public StringType getValueElement() { 11841 if (this.value == null) 11842 if (Configuration.errorOnAutoCreate()) 11843 throw new Error("Attempt to auto-create ImplementationGuideDefinitionParameterComponent.value"); 11844 else if (Configuration.doAutoCreate()) 11845 this.value = new StringType(); // bb 11846 return this.value; 11847 } 11848 11849 public boolean hasValueElement() { 11850 return this.value != null && !this.value.isEmpty(); 11851 } 11852 11853 public boolean hasValue() { 11854 return this.value != null && !this.value.isEmpty(); 11855 } 11856 11857 /** 11858 * @param value {@link #value} (Value for named type.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 11859 */ 11860 public ImplementationGuideDefinitionParameterComponent setValueElement(StringType value) { 11861 this.value = value; 11862 return this; 11863 } 11864 11865 /** 11866 * @return Value for named type. 11867 */ 11868 public String getValue() { 11869 return this.value == null ? null : this.value.getValue(); 11870 } 11871 11872 /** 11873 * @param value Value for named type. 11874 */ 11875 public ImplementationGuideDefinitionParameterComponent setValue(String value) { 11876 if (this.value == null) 11877 this.value = new StringType(); 11878 this.value.setValue(value); 11879 return this; 11880 } 11881 11882 protected void listChildren(List<Property> children) { 11883 super.listChildren(children); 11884 children.add(new Property("code", "Coding", "A tool-specific code that defines the parameter.", 0, 1, code)); 11885 children.add(new Property("value", "string", "Value for named type.", 0, 1, value)); 11886 } 11887 11888 @Override 11889 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 11890 switch (_hash) { 11891 case 3059181: /*code*/ return new Property("code", "Coding", "A tool-specific code that defines the parameter.", 0, 1, code); 11892 case 111972721: /*value*/ return new Property("value", "string", "Value for named type.", 0, 1, value); 11893 default: return super.getNamedProperty(_hash, _name, _checkValid); 11894 } 11895 11896 } 11897 11898 @Override 11899 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 11900 switch (hash) { 11901 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Coding 11902 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 11903 default: return super.getProperty(hash, name, checkValid); 11904 } 11905 11906 } 11907 11908 @Override 11909 public Base setProperty(int hash, String name, Base value) throws FHIRException { 11910 switch (hash) { 11911 case 3059181: // code 11912 this.code = TypeConvertor.castToCoding(value); // Coding 11913 return value; 11914 case 111972721: // value 11915 this.value = TypeConvertor.castToString(value); // StringType 11916 return value; 11917 default: return super.setProperty(hash, name, value); 11918 } 11919 11920 } 11921 11922 @Override 11923 public Base setProperty(String name, Base value) throws FHIRException { 11924 if (name.equals("code")) { 11925 this.code = TypeConvertor.castToCoding(value); // Coding 11926 } else if (name.equals("value")) { 11927 this.value = TypeConvertor.castToString(value); // StringType 11928 } else 11929 return super.setProperty(name, value); 11930 return value; 11931 } 11932 11933 @Override 11934 public void removeChild(String name, Base value) throws FHIRException { 11935 if (name.equals("code")) { 11936 this.code = null; 11937 } else if (name.equals("value")) { 11938 this.value = null; 11939 } else 11940 super.removeChild(name, value); 11941 11942 } 11943 11944 @Override 11945 public Base makeProperty(int hash, String name) throws FHIRException { 11946 switch (hash) { 11947 case 3059181: return getCode(); 11948 case 111972721: return getValueElement(); 11949 default: return super.makeProperty(hash, name); 11950 } 11951 11952 } 11953 11954 @Override 11955 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 11956 switch (hash) { 11957 case 3059181: /*code*/ return new String[] {"Coding"}; 11958 case 111972721: /*value*/ return new String[] {"string"}; 11959 default: return super.getTypesForProperty(hash, name); 11960 } 11961 11962 } 11963 11964 @Override 11965 public Base addChild(String name) throws FHIRException { 11966 if (name.equals("code")) { 11967 this.code = new Coding(); 11968 return this.code; 11969 } 11970 else if (name.equals("value")) { 11971 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.definition.parameter.value"); 11972 } 11973 else 11974 return super.addChild(name); 11975 } 11976 11977 public ImplementationGuideDefinitionParameterComponent copy() { 11978 ImplementationGuideDefinitionParameterComponent dst = new ImplementationGuideDefinitionParameterComponent(); 11979 copyValues(dst); 11980 return dst; 11981 } 11982 11983 public void copyValues(ImplementationGuideDefinitionParameterComponent dst) { 11984 super.copyValues(dst); 11985 dst.code = code == null ? null : code.copy(); 11986 dst.value = value == null ? null : value.copy(); 11987 } 11988 11989 @Override 11990 public boolean equalsDeep(Base other_) { 11991 if (!super.equalsDeep(other_)) 11992 return false; 11993 if (!(other_ instanceof ImplementationGuideDefinitionParameterComponent)) 11994 return false; 11995 ImplementationGuideDefinitionParameterComponent o = (ImplementationGuideDefinitionParameterComponent) other_; 11996 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 11997 } 11998 11999 @Override 12000 public boolean equalsShallow(Base other_) { 12001 if (!super.equalsShallow(other_)) 12002 return false; 12003 if (!(other_ instanceof ImplementationGuideDefinitionParameterComponent)) 12004 return false; 12005 ImplementationGuideDefinitionParameterComponent o = (ImplementationGuideDefinitionParameterComponent) other_; 12006 return compareValues(value, o.value, true); 12007 } 12008 12009 public boolean isEmpty() { 12010 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 12011 } 12012 12013 public String fhirType() { 12014 return "ImplementationGuide.definition.parameter"; 12015 12016 } 12017 12018 } 12019 12020 @Block() 12021 public static class ImplementationGuideDefinitionTemplateComponent extends BackboneElement implements IBaseBackboneElement { 12022 /** 12023 * Type of template specified. 12024 */ 12025 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 12026 @Description(shortDefinition="Type of template specified", formalDefinition="Type of template specified." ) 12027 protected CodeType code; 12028 12029 /** 12030 * The source location for the template. 12031 */ 12032 @Child(name = "source", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=false) 12033 @Description(shortDefinition="The source location for the template", formalDefinition="The source location for the template." ) 12034 protected StringType source; 12035 12036 /** 12037 * The scope in which the template applies. 12038 */ 12039 @Child(name = "scope", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 12040 @Description(shortDefinition="The scope in which the template applies", formalDefinition="The scope in which the template applies." ) 12041 protected StringType scope; 12042 12043 private static final long serialVersionUID = 923832457L; 12044 12045 /** 12046 * Constructor 12047 */ 12048 public ImplementationGuideDefinitionTemplateComponent() { 12049 super(); 12050 } 12051 12052 /** 12053 * Constructor 12054 */ 12055 public ImplementationGuideDefinitionTemplateComponent(String code, String source) { 12056 super(); 12057 this.setCode(code); 12058 this.setSource(source); 12059 } 12060 12061 /** 12062 * @return {@link #code} (Type of template specified.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 12063 */ 12064 public CodeType getCodeElement() { 12065 if (this.code == null) 12066 if (Configuration.errorOnAutoCreate()) 12067 throw new Error("Attempt to auto-create ImplementationGuideDefinitionTemplateComponent.code"); 12068 else if (Configuration.doAutoCreate()) 12069 this.code = new CodeType(); // bb 12070 return this.code; 12071 } 12072 12073 public boolean hasCodeElement() { 12074 return this.code != null && !this.code.isEmpty(); 12075 } 12076 12077 public boolean hasCode() { 12078 return this.code != null && !this.code.isEmpty(); 12079 } 12080 12081 /** 12082 * @param value {@link #code} (Type of template specified.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 12083 */ 12084 public ImplementationGuideDefinitionTemplateComponent setCodeElement(CodeType value) { 12085 this.code = value; 12086 return this; 12087 } 12088 12089 /** 12090 * @return Type of template specified. 12091 */ 12092 public String getCode() { 12093 return this.code == null ? null : this.code.getValue(); 12094 } 12095 12096 /** 12097 * @param value Type of template specified. 12098 */ 12099 public ImplementationGuideDefinitionTemplateComponent setCode(String value) { 12100 if (this.code == null) 12101 this.code = new CodeType(); 12102 this.code.setValue(value); 12103 return this; 12104 } 12105 12106 /** 12107 * @return {@link #source} (The source location for the template.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 12108 */ 12109 public StringType getSourceElement() { 12110 if (this.source == null) 12111 if (Configuration.errorOnAutoCreate()) 12112 throw new Error("Attempt to auto-create ImplementationGuideDefinitionTemplateComponent.source"); 12113 else if (Configuration.doAutoCreate()) 12114 this.source = new StringType(); // bb 12115 return this.source; 12116 } 12117 12118 public boolean hasSourceElement() { 12119 return this.source != null && !this.source.isEmpty(); 12120 } 12121 12122 public boolean hasSource() { 12123 return this.source != null && !this.source.isEmpty(); 12124 } 12125 12126 /** 12127 * @param value {@link #source} (The source location for the template.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 12128 */ 12129 public ImplementationGuideDefinitionTemplateComponent setSourceElement(StringType value) { 12130 this.source = value; 12131 return this; 12132 } 12133 12134 /** 12135 * @return The source location for the template. 12136 */ 12137 public String getSource() { 12138 return this.source == null ? null : this.source.getValue(); 12139 } 12140 12141 /** 12142 * @param value The source location for the template. 12143 */ 12144 public ImplementationGuideDefinitionTemplateComponent setSource(String value) { 12145 if (this.source == null) 12146 this.source = new StringType(); 12147 this.source.setValue(value); 12148 return this; 12149 } 12150 12151 /** 12152 * @return {@link #scope} (The scope in which the template applies.). This is the underlying object with id, value and extensions. The accessor "getScope" gives direct access to the value 12153 */ 12154 public StringType getScopeElement() { 12155 if (this.scope == null) 12156 if (Configuration.errorOnAutoCreate()) 12157 throw new Error("Attempt to auto-create ImplementationGuideDefinitionTemplateComponent.scope"); 12158 else if (Configuration.doAutoCreate()) 12159 this.scope = new StringType(); // bb 12160 return this.scope; 12161 } 12162 12163 public boolean hasScopeElement() { 12164 return this.scope != null && !this.scope.isEmpty(); 12165 } 12166 12167 public boolean hasScope() { 12168 return this.scope != null && !this.scope.isEmpty(); 12169 } 12170 12171 /** 12172 * @param value {@link #scope} (The scope in which the template applies.). This is the underlying object with id, value and extensions. The accessor "getScope" gives direct access to the value 12173 */ 12174 public ImplementationGuideDefinitionTemplateComponent setScopeElement(StringType value) { 12175 this.scope = value; 12176 return this; 12177 } 12178 12179 /** 12180 * @return The scope in which the template applies. 12181 */ 12182 public String getScope() { 12183 return this.scope == null ? null : this.scope.getValue(); 12184 } 12185 12186 /** 12187 * @param value The scope in which the template applies. 12188 */ 12189 public ImplementationGuideDefinitionTemplateComponent setScope(String value) { 12190 if (Utilities.noString(value)) 12191 this.scope = null; 12192 else { 12193 if (this.scope == null) 12194 this.scope = new StringType(); 12195 this.scope.setValue(value); 12196 } 12197 return this; 12198 } 12199 12200 protected void listChildren(List<Property> children) { 12201 super.listChildren(children); 12202 children.add(new Property("code", "code", "Type of template specified.", 0, 1, code)); 12203 children.add(new Property("source", "string", "The source location for the template.", 0, 1, source)); 12204 children.add(new Property("scope", "string", "The scope in which the template applies.", 0, 1, scope)); 12205 } 12206 12207 @Override 12208 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 12209 switch (_hash) { 12210 case 3059181: /*code*/ return new Property("code", "code", "Type of template specified.", 0, 1, code); 12211 case -896505829: /*source*/ return new Property("source", "string", "The source location for the template.", 0, 1, source); 12212 case 109264468: /*scope*/ return new Property("scope", "string", "The scope in which the template applies.", 0, 1, scope); 12213 default: return super.getNamedProperty(_hash, _name, _checkValid); 12214 } 12215 12216 } 12217 12218 @Override 12219 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 12220 switch (hash) { 12221 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 12222 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // StringType 12223 case 109264468: /*scope*/ return this.scope == null ? new Base[0] : new Base[] {this.scope}; // StringType 12224 default: return super.getProperty(hash, name, checkValid); 12225 } 12226 12227 } 12228 12229 @Override 12230 public Base setProperty(int hash, String name, Base value) throws FHIRException { 12231 switch (hash) { 12232 case 3059181: // code 12233 this.code = TypeConvertor.castToCode(value); // CodeType 12234 return value; 12235 case -896505829: // source 12236 this.source = TypeConvertor.castToString(value); // StringType 12237 return value; 12238 case 109264468: // scope 12239 this.scope = TypeConvertor.castToString(value); // StringType 12240 return value; 12241 default: return super.setProperty(hash, name, value); 12242 } 12243 12244 } 12245 12246 @Override 12247 public Base setProperty(String name, Base value) throws FHIRException { 12248 if (name.equals("code")) { 12249 this.code = TypeConvertor.castToCode(value); // CodeType 12250 } else if (name.equals("source")) { 12251 this.source = TypeConvertor.castToString(value); // StringType 12252 } else if (name.equals("scope")) { 12253 this.scope = TypeConvertor.castToString(value); // StringType 12254 } else 12255 return super.setProperty(name, value); 12256 return value; 12257 } 12258 12259 @Override 12260 public void removeChild(String name, Base value) throws FHIRException { 12261 if (name.equals("code")) { 12262 this.code = null; 12263 } else if (name.equals("source")) { 12264 this.source = null; 12265 } else if (name.equals("scope")) { 12266 this.scope = null; 12267 } else 12268 super.removeChild(name, value); 12269 12270 } 12271 12272 @Override 12273 public Base makeProperty(int hash, String name) throws FHIRException { 12274 switch (hash) { 12275 case 3059181: return getCodeElement(); 12276 case -896505829: return getSourceElement(); 12277 case 109264468: return getScopeElement(); 12278 default: return super.makeProperty(hash, name); 12279 } 12280 12281 } 12282 12283 @Override 12284 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 12285 switch (hash) { 12286 case 3059181: /*code*/ return new String[] {"code"}; 12287 case -896505829: /*source*/ return new String[] {"string"}; 12288 case 109264468: /*scope*/ return new String[] {"string"}; 12289 default: return super.getTypesForProperty(hash, name); 12290 } 12291 12292 } 12293 12294 @Override 12295 public Base addChild(String name) throws FHIRException { 12296 if (name.equals("code")) { 12297 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.definition.template.code"); 12298 } 12299 else if (name.equals("source")) { 12300 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.definition.template.source"); 12301 } 12302 else if (name.equals("scope")) { 12303 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.definition.template.scope"); 12304 } 12305 else 12306 return super.addChild(name); 12307 } 12308 12309 public ImplementationGuideDefinitionTemplateComponent copy() { 12310 ImplementationGuideDefinitionTemplateComponent dst = new ImplementationGuideDefinitionTemplateComponent(); 12311 copyValues(dst); 12312 return dst; 12313 } 12314 12315 public void copyValues(ImplementationGuideDefinitionTemplateComponent dst) { 12316 super.copyValues(dst); 12317 dst.code = code == null ? null : code.copy(); 12318 dst.source = source == null ? null : source.copy(); 12319 dst.scope = scope == null ? null : scope.copy(); 12320 } 12321 12322 @Override 12323 public boolean equalsDeep(Base other_) { 12324 if (!super.equalsDeep(other_)) 12325 return false; 12326 if (!(other_ instanceof ImplementationGuideDefinitionTemplateComponent)) 12327 return false; 12328 ImplementationGuideDefinitionTemplateComponent o = (ImplementationGuideDefinitionTemplateComponent) other_; 12329 return compareDeep(code, o.code, true) && compareDeep(source, o.source, true) && compareDeep(scope, o.scope, true) 12330 ; 12331 } 12332 12333 @Override 12334 public boolean equalsShallow(Base other_) { 12335 if (!super.equalsShallow(other_)) 12336 return false; 12337 if (!(other_ instanceof ImplementationGuideDefinitionTemplateComponent)) 12338 return false; 12339 ImplementationGuideDefinitionTemplateComponent o = (ImplementationGuideDefinitionTemplateComponent) other_; 12340 return compareValues(code, o.code, true) && compareValues(source, o.source, true) && compareValues(scope, o.scope, true) 12341 ; 12342 } 12343 12344 public boolean isEmpty() { 12345 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, source, scope); 12346 } 12347 12348 public String fhirType() { 12349 return "ImplementationGuide.definition.template"; 12350 12351 } 12352 12353 } 12354 12355 @Block() 12356 public static class ImplementationGuideManifestComponent extends BackboneElement implements IBaseBackboneElement { 12357 /** 12358 * A pointer to official web page, PDF or other rendering of the implementation guide. 12359 */ 12360 @Child(name = "rendering", type = {UrlType.class}, order=1, min=0, max=1, modifier=false, summary=true) 12361 @Description(shortDefinition="Location of rendered implementation guide", formalDefinition="A pointer to official web page, PDF or other rendering of the implementation guide." ) 12362 protected UrlType rendering; 12363 12364 /** 12365 * A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource. 12366 */ 12367 @Child(name = "resource", type = {}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 12368 @Description(shortDefinition="Resource in the implementation guide", formalDefinition="A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource." ) 12369 protected List<ManifestResourceComponent> resource; 12370 12371 /** 12372 * Information about a page within the IG. 12373 */ 12374 @Child(name = "page", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 12375 @Description(shortDefinition="HTML page within the parent IG", formalDefinition="Information about a page within the IG." ) 12376 protected List<ManifestPageComponent> page; 12377 12378 /** 12379 * Indicates a relative path to an image that exists within the IG. 12380 */ 12381 @Child(name = "image", type = {StringType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 12382 @Description(shortDefinition="Image within the IG", formalDefinition="Indicates a relative path to an image that exists within the IG." ) 12383 protected List<StringType> image; 12384 12385 /** 12386 * Indicates the relative path of an additional non-page, non-image file that is part of the IG - e.g. zip, jar and similar files that could be the target of a hyperlink in a derived IG. 12387 */ 12388 @Child(name = "other", type = {StringType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 12389 @Description(shortDefinition="Additional linkable file in IG", formalDefinition="Indicates the relative path of an additional non-page, non-image file that is part of the IG - e.g. zip, jar and similar files that could be the target of a hyperlink in a derived IG." ) 12390 protected List<StringType> other; 12391 12392 private static final long serialVersionUID = 1881327712L; 12393 12394 /** 12395 * Constructor 12396 */ 12397 public ImplementationGuideManifestComponent() { 12398 super(); 12399 } 12400 12401 /** 12402 * Constructor 12403 */ 12404 public ImplementationGuideManifestComponent(ManifestResourceComponent resource) { 12405 super(); 12406 this.addResource(resource); 12407 } 12408 12409 /** 12410 * @return {@link #rendering} (A pointer to official web page, PDF or other rendering of the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getRendering" gives direct access to the value 12411 */ 12412 public UrlType getRenderingElement() { 12413 if (this.rendering == null) 12414 if (Configuration.errorOnAutoCreate()) 12415 throw new Error("Attempt to auto-create ImplementationGuideManifestComponent.rendering"); 12416 else if (Configuration.doAutoCreate()) 12417 this.rendering = new UrlType(); // bb 12418 return this.rendering; 12419 } 12420 12421 public boolean hasRenderingElement() { 12422 return this.rendering != null && !this.rendering.isEmpty(); 12423 } 12424 12425 public boolean hasRendering() { 12426 return this.rendering != null && !this.rendering.isEmpty(); 12427 } 12428 12429 /** 12430 * @param value {@link #rendering} (A pointer to official web page, PDF or other rendering of the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getRendering" gives direct access to the value 12431 */ 12432 public ImplementationGuideManifestComponent setRenderingElement(UrlType value) { 12433 this.rendering = value; 12434 return this; 12435 } 12436 12437 /** 12438 * @return A pointer to official web page, PDF or other rendering of the implementation guide. 12439 */ 12440 public String getRendering() { 12441 return this.rendering == null ? null : this.rendering.getValue(); 12442 } 12443 12444 /** 12445 * @param value A pointer to official web page, PDF or other rendering of the implementation guide. 12446 */ 12447 public ImplementationGuideManifestComponent setRendering(String value) { 12448 if (Utilities.noString(value)) 12449 this.rendering = null; 12450 else { 12451 if (this.rendering == null) 12452 this.rendering = new UrlType(); 12453 this.rendering.setValue(value); 12454 } 12455 return this; 12456 } 12457 12458 /** 12459 * @return {@link #resource} (A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.) 12460 */ 12461 public List<ManifestResourceComponent> getResource() { 12462 if (this.resource == null) 12463 this.resource = new ArrayList<ManifestResourceComponent>(); 12464 return this.resource; 12465 } 12466 12467 /** 12468 * @return Returns a reference to <code>this</code> for easy method chaining 12469 */ 12470 public ImplementationGuideManifestComponent setResource(List<ManifestResourceComponent> theResource) { 12471 this.resource = theResource; 12472 return this; 12473 } 12474 12475 public boolean hasResource() { 12476 if (this.resource == null) 12477 return false; 12478 for (ManifestResourceComponent item : this.resource) 12479 if (!item.isEmpty()) 12480 return true; 12481 return false; 12482 } 12483 12484 public ManifestResourceComponent addResource() { //3 12485 ManifestResourceComponent t = new ManifestResourceComponent(); 12486 if (this.resource == null) 12487 this.resource = new ArrayList<ManifestResourceComponent>(); 12488 this.resource.add(t); 12489 return t; 12490 } 12491 12492 public ImplementationGuideManifestComponent addResource(ManifestResourceComponent t) { //3 12493 if (t == null) 12494 return this; 12495 if (this.resource == null) 12496 this.resource = new ArrayList<ManifestResourceComponent>(); 12497 this.resource.add(t); 12498 return this; 12499 } 12500 12501 /** 12502 * @return The first repetition of repeating field {@link #resource}, creating it if it does not already exist {3} 12503 */ 12504 public ManifestResourceComponent getResourceFirstRep() { 12505 if (getResource().isEmpty()) { 12506 addResource(); 12507 } 12508 return getResource().get(0); 12509 } 12510 12511 /** 12512 * @return {@link #page} (Information about a page within the IG.) 12513 */ 12514 public List<ManifestPageComponent> getPage() { 12515 if (this.page == null) 12516 this.page = new ArrayList<ManifestPageComponent>(); 12517 return this.page; 12518 } 12519 12520 /** 12521 * @return Returns a reference to <code>this</code> for easy method chaining 12522 */ 12523 public ImplementationGuideManifestComponent setPage(List<ManifestPageComponent> thePage) { 12524 this.page = thePage; 12525 return this; 12526 } 12527 12528 public boolean hasPage() { 12529 if (this.page == null) 12530 return false; 12531 for (ManifestPageComponent item : this.page) 12532 if (!item.isEmpty()) 12533 return true; 12534 return false; 12535 } 12536 12537 public ManifestPageComponent addPage() { //3 12538 ManifestPageComponent t = new ManifestPageComponent(); 12539 if (this.page == null) 12540 this.page = new ArrayList<ManifestPageComponent>(); 12541 this.page.add(t); 12542 return t; 12543 } 12544 12545 public ImplementationGuideManifestComponent addPage(ManifestPageComponent t) { //3 12546 if (t == null) 12547 return this; 12548 if (this.page == null) 12549 this.page = new ArrayList<ManifestPageComponent>(); 12550 this.page.add(t); 12551 return this; 12552 } 12553 12554 /** 12555 * @return The first repetition of repeating field {@link #page}, creating it if it does not already exist {3} 12556 */ 12557 public ManifestPageComponent getPageFirstRep() { 12558 if (getPage().isEmpty()) { 12559 addPage(); 12560 } 12561 return getPage().get(0); 12562 } 12563 12564 /** 12565 * @return {@link #image} (Indicates a relative path to an image that exists within the IG.) 12566 */ 12567 public List<StringType> getImage() { 12568 if (this.image == null) 12569 this.image = new ArrayList<StringType>(); 12570 return this.image; 12571 } 12572 12573 /** 12574 * @return Returns a reference to <code>this</code> for easy method chaining 12575 */ 12576 public ImplementationGuideManifestComponent setImage(List<StringType> theImage) { 12577 this.image = theImage; 12578 return this; 12579 } 12580 12581 public boolean hasImage() { 12582 if (this.image == null) 12583 return false; 12584 for (StringType item : this.image) 12585 if (!item.isEmpty()) 12586 return true; 12587 return false; 12588 } 12589 12590 /** 12591 * @return {@link #image} (Indicates a relative path to an image that exists within the IG.) 12592 */ 12593 public StringType addImageElement() {//2 12594 StringType t = new StringType(); 12595 if (this.image == null) 12596 this.image = new ArrayList<StringType>(); 12597 this.image.add(t); 12598 return t; 12599 } 12600 12601 /** 12602 * @param value {@link #image} (Indicates a relative path to an image that exists within the IG.) 12603 */ 12604 public ImplementationGuideManifestComponent addImage(String value) { //1 12605 StringType t = new StringType(); 12606 t.setValue(value); 12607 if (this.image == null) 12608 this.image = new ArrayList<StringType>(); 12609 this.image.add(t); 12610 return this; 12611 } 12612 12613 /** 12614 * @param value {@link #image} (Indicates a relative path to an image that exists within the IG.) 12615 */ 12616 public boolean hasImage(String value) { 12617 if (this.image == null) 12618 return false; 12619 for (StringType v : this.image) 12620 if (v.getValue().equals(value)) // string 12621 return true; 12622 return false; 12623 } 12624 12625 /** 12626 * @return {@link #other} (Indicates the relative path of an additional non-page, non-image file that is part of the IG - e.g. zip, jar and similar files that could be the target of a hyperlink in a derived IG.) 12627 */ 12628 public List<StringType> getOther() { 12629 if (this.other == null) 12630 this.other = new ArrayList<StringType>(); 12631 return this.other; 12632 } 12633 12634 /** 12635 * @return Returns a reference to <code>this</code> for easy method chaining 12636 */ 12637 public ImplementationGuideManifestComponent setOther(List<StringType> theOther) { 12638 this.other = theOther; 12639 return this; 12640 } 12641 12642 public boolean hasOther() { 12643 if (this.other == null) 12644 return false; 12645 for (StringType item : this.other) 12646 if (!item.isEmpty()) 12647 return true; 12648 return false; 12649 } 12650 12651 /** 12652 * @return {@link #other} (Indicates the relative path of an additional non-page, non-image file that is part of the IG - e.g. zip, jar and similar files that could be the target of a hyperlink in a derived IG.) 12653 */ 12654 public StringType addOtherElement() {//2 12655 StringType t = new StringType(); 12656 if (this.other == null) 12657 this.other = new ArrayList<StringType>(); 12658 this.other.add(t); 12659 return t; 12660 } 12661 12662 /** 12663 * @param value {@link #other} (Indicates the relative path of an additional non-page, non-image file that is part of the IG - e.g. zip, jar and similar files that could be the target of a hyperlink in a derived IG.) 12664 */ 12665 public ImplementationGuideManifestComponent addOther(String value) { //1 12666 StringType t = new StringType(); 12667 t.setValue(value); 12668 if (this.other == null) 12669 this.other = new ArrayList<StringType>(); 12670 this.other.add(t); 12671 return this; 12672 } 12673 12674 /** 12675 * @param value {@link #other} (Indicates the relative path of an additional non-page, non-image file that is part of the IG - e.g. zip, jar and similar files that could be the target of a hyperlink in a derived IG.) 12676 */ 12677 public boolean hasOther(String value) { 12678 if (this.other == null) 12679 return false; 12680 for (StringType v : this.other) 12681 if (v.getValue().equals(value)) // string 12682 return true; 12683 return false; 12684 } 12685 12686 protected void listChildren(List<Property> children) { 12687 super.listChildren(children); 12688 children.add(new Property("rendering", "url", "A pointer to official web page, PDF or other rendering of the implementation guide.", 0, 1, rendering)); 12689 children.add(new Property("resource", "", "A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.", 0, java.lang.Integer.MAX_VALUE, resource)); 12690 children.add(new Property("page", "", "Information about a page within the IG.", 0, java.lang.Integer.MAX_VALUE, page)); 12691 children.add(new Property("image", "string", "Indicates a relative path to an image that exists within the IG.", 0, java.lang.Integer.MAX_VALUE, image)); 12692 children.add(new Property("other", "string", "Indicates the relative path of an additional non-page, non-image file that is part of the IG - e.g. zip, jar and similar files that could be the target of a hyperlink in a derived IG.", 0, java.lang.Integer.MAX_VALUE, other)); 12693 } 12694 12695 @Override 12696 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 12697 switch (_hash) { 12698 case 1839654540: /*rendering*/ return new Property("rendering", "url", "A pointer to official web page, PDF or other rendering of the implementation guide.", 0, 1, rendering); 12699 case -341064690: /*resource*/ return new Property("resource", "", "A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.", 0, java.lang.Integer.MAX_VALUE, resource); 12700 case 3433103: /*page*/ return new Property("page", "", "Information about a page within the IG.", 0, java.lang.Integer.MAX_VALUE, page); 12701 case 100313435: /*image*/ return new Property("image", "string", "Indicates a relative path to an image that exists within the IG.", 0, java.lang.Integer.MAX_VALUE, image); 12702 case 106069776: /*other*/ return new Property("other", "string", "Indicates the relative path of an additional non-page, non-image file that is part of the IG - e.g. zip, jar and similar files that could be the target of a hyperlink in a derived IG.", 0, java.lang.Integer.MAX_VALUE, other); 12703 default: return super.getNamedProperty(_hash, _name, _checkValid); 12704 } 12705 12706 } 12707 12708 @Override 12709 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 12710 switch (hash) { 12711 case 1839654540: /*rendering*/ return this.rendering == null ? new Base[0] : new Base[] {this.rendering}; // UrlType 12712 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : this.resource.toArray(new Base[this.resource.size()]); // ManifestResourceComponent 12713 case 3433103: /*page*/ return this.page == null ? new Base[0] : this.page.toArray(new Base[this.page.size()]); // ManifestPageComponent 12714 case 100313435: /*image*/ return this.image == null ? new Base[0] : this.image.toArray(new Base[this.image.size()]); // StringType 12715 case 106069776: /*other*/ return this.other == null ? new Base[0] : this.other.toArray(new Base[this.other.size()]); // StringType 12716 default: return super.getProperty(hash, name, checkValid); 12717 } 12718 12719 } 12720 12721 @Override 12722 public Base setProperty(int hash, String name, Base value) throws FHIRException { 12723 switch (hash) { 12724 case 1839654540: // rendering 12725 this.rendering = TypeConvertor.castToUrl(value); // UrlType 12726 return value; 12727 case -341064690: // resource 12728 this.getResource().add((ManifestResourceComponent) value); // ManifestResourceComponent 12729 return value; 12730 case 3433103: // page 12731 this.getPage().add((ManifestPageComponent) value); // ManifestPageComponent 12732 return value; 12733 case 100313435: // image 12734 this.getImage().add(TypeConvertor.castToString(value)); // StringType 12735 return value; 12736 case 106069776: // other 12737 this.getOther().add(TypeConvertor.castToString(value)); // StringType 12738 return value; 12739 default: return super.setProperty(hash, name, value); 12740 } 12741 12742 } 12743 12744 @Override 12745 public Base setProperty(String name, Base value) throws FHIRException { 12746 if (name.equals("rendering")) { 12747 this.rendering = TypeConvertor.castToUrl(value); // UrlType 12748 } else if (name.equals("resource")) { 12749 this.getResource().add((ManifestResourceComponent) value); 12750 } else if (name.equals("page")) { 12751 this.getPage().add((ManifestPageComponent) value); 12752 } else if (name.equals("image")) { 12753 this.getImage().add(TypeConvertor.castToString(value)); 12754 } else if (name.equals("other")) { 12755 this.getOther().add(TypeConvertor.castToString(value)); 12756 } else 12757 return super.setProperty(name, value); 12758 return value; 12759 } 12760 12761 @Override 12762 public void removeChild(String name, Base value) throws FHIRException { 12763 if (name.equals("rendering")) { 12764 this.rendering = null; 12765 } else if (name.equals("resource")) { 12766 this.getResource().remove((ManifestResourceComponent) value); 12767 } else if (name.equals("page")) { 12768 this.getPage().remove((ManifestPageComponent) value); 12769 } else if (name.equals("image")) { 12770 this.getImage().remove(value); 12771 } else if (name.equals("other")) { 12772 this.getOther().remove(value); 12773 } else 12774 super.removeChild(name, value); 12775 12776 } 12777 12778 @Override 12779 public Base makeProperty(int hash, String name) throws FHIRException { 12780 switch (hash) { 12781 case 1839654540: return getRenderingElement(); 12782 case -341064690: return addResource(); 12783 case 3433103: return addPage(); 12784 case 100313435: return addImageElement(); 12785 case 106069776: return addOtherElement(); 12786 default: return super.makeProperty(hash, name); 12787 } 12788 12789 } 12790 12791 @Override 12792 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 12793 switch (hash) { 12794 case 1839654540: /*rendering*/ return new String[] {"url"}; 12795 case -341064690: /*resource*/ return new String[] {}; 12796 case 3433103: /*page*/ return new String[] {}; 12797 case 100313435: /*image*/ return new String[] {"string"}; 12798 case 106069776: /*other*/ return new String[] {"string"}; 12799 default: return super.getTypesForProperty(hash, name); 12800 } 12801 12802 } 12803 12804 @Override 12805 public Base addChild(String name) throws FHIRException { 12806 if (name.equals("rendering")) { 12807 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.manifest.rendering"); 12808 } 12809 else if (name.equals("resource")) { 12810 return addResource(); 12811 } 12812 else if (name.equals("page")) { 12813 return addPage(); 12814 } 12815 else if (name.equals("image")) { 12816 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.manifest.image"); 12817 } 12818 else if (name.equals("other")) { 12819 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.manifest.other"); 12820 } 12821 else 12822 return super.addChild(name); 12823 } 12824 12825 public ImplementationGuideManifestComponent copy() { 12826 ImplementationGuideManifestComponent dst = new ImplementationGuideManifestComponent(); 12827 copyValues(dst); 12828 return dst; 12829 } 12830 12831 public void copyValues(ImplementationGuideManifestComponent dst) { 12832 super.copyValues(dst); 12833 dst.rendering = rendering == null ? null : rendering.copy(); 12834 if (resource != null) { 12835 dst.resource = new ArrayList<ManifestResourceComponent>(); 12836 for (ManifestResourceComponent i : resource) 12837 dst.resource.add(i.copy()); 12838 }; 12839 if (page != null) { 12840 dst.page = new ArrayList<ManifestPageComponent>(); 12841 for (ManifestPageComponent i : page) 12842 dst.page.add(i.copy()); 12843 }; 12844 if (image != null) { 12845 dst.image = new ArrayList<StringType>(); 12846 for (StringType i : image) 12847 dst.image.add(i.copy()); 12848 }; 12849 if (other != null) { 12850 dst.other = new ArrayList<StringType>(); 12851 for (StringType i : other) 12852 dst.other.add(i.copy()); 12853 }; 12854 } 12855 12856 @Override 12857 public boolean equalsDeep(Base other_) { 12858 if (!super.equalsDeep(other_)) 12859 return false; 12860 if (!(other_ instanceof ImplementationGuideManifestComponent)) 12861 return false; 12862 ImplementationGuideManifestComponent o = (ImplementationGuideManifestComponent) other_; 12863 return compareDeep(rendering, o.rendering, true) && compareDeep(resource, o.resource, true) && compareDeep(page, o.page, true) 12864 && compareDeep(image, o.image, true) && compareDeep(other, o.other, true); 12865 } 12866 12867 @Override 12868 public boolean equalsShallow(Base other_) { 12869 if (!super.equalsShallow(other_)) 12870 return false; 12871 if (!(other_ instanceof ImplementationGuideManifestComponent)) 12872 return false; 12873 ImplementationGuideManifestComponent o = (ImplementationGuideManifestComponent) other_; 12874 return compareValues(rendering, o.rendering, true) && compareValues(image, o.image, true) && compareValues(other, o.other, true) 12875 ; 12876 } 12877 12878 public boolean isEmpty() { 12879 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(rendering, resource, page 12880 , image, other); 12881 } 12882 12883 public String fhirType() { 12884 return "ImplementationGuide.manifest"; 12885 12886 } 12887 12888 } 12889 12890 @Block() 12891 public static class ManifestResourceComponent extends BackboneElement implements IBaseBackboneElement { 12892 /** 12893 * Where this resource is found. 12894 */ 12895 @Child(name = "reference", type = {Reference.class}, order=1, min=1, max=1, modifier=false, summary=true) 12896 @Description(shortDefinition="Location of the resource", formalDefinition="Where this resource is found." ) 12897 protected Reference reference; 12898 12899 /** 12900 * If true, indicates the resource is an example instance. 12901 */ 12902 @Child(name = "isExample", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 12903 @Description(shortDefinition="Is this an example", formalDefinition="If true, indicates the resource is an example instance." ) 12904 protected BooleanType isExample; 12905 12906 /** 12907 * If present, indicates profile(s) the instance is valid against. 12908 */ 12909 @Child(name = "profile", type = {CanonicalType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 12910 @Description(shortDefinition="Profile(s) this is an example of", formalDefinition="If present, indicates profile(s) the instance is valid against." ) 12911 protected List<CanonicalType> profile; 12912 12913 /** 12914 * The relative path for primary page for this resource within the IG. 12915 */ 12916 @Child(name = "relativePath", type = {UrlType.class}, order=4, min=0, max=1, modifier=false, summary=false) 12917 @Description(shortDefinition="Relative path for page in IG", formalDefinition="The relative path for primary page for this resource within the IG." ) 12918 protected UrlType relativePath; 12919 12920 private static final long serialVersionUID = 66726063L; 12921 12922 /** 12923 * Constructor 12924 */ 12925 public ManifestResourceComponent() { 12926 super(); 12927 } 12928 12929 /** 12930 * Constructor 12931 */ 12932 public ManifestResourceComponent(Reference reference) { 12933 super(); 12934 this.setReference(reference); 12935 } 12936 12937 /** 12938 * @return {@link #reference} (Where this resource is found.) 12939 */ 12940 public Reference getReference() { 12941 if (this.reference == null) 12942 if (Configuration.errorOnAutoCreate()) 12943 throw new Error("Attempt to auto-create ManifestResourceComponent.reference"); 12944 else if (Configuration.doAutoCreate()) 12945 this.reference = new Reference(); // cc 12946 return this.reference; 12947 } 12948 12949 public boolean hasReference() { 12950 return this.reference != null && !this.reference.isEmpty(); 12951 } 12952 12953 /** 12954 * @param value {@link #reference} (Where this resource is found.) 12955 */ 12956 public ManifestResourceComponent setReference(Reference value) { 12957 this.reference = value; 12958 return this; 12959 } 12960 12961 /** 12962 * @return {@link #isExample} (If true, indicates the resource is an example instance.). This is the underlying object with id, value and extensions. The accessor "getIsExample" gives direct access to the value 12963 */ 12964 public BooleanType getIsExampleElement() { 12965 if (this.isExample == null) 12966 if (Configuration.errorOnAutoCreate()) 12967 throw new Error("Attempt to auto-create ManifestResourceComponent.isExample"); 12968 else if (Configuration.doAutoCreate()) 12969 this.isExample = new BooleanType(); // bb 12970 return this.isExample; 12971 } 12972 12973 public boolean hasIsExampleElement() { 12974 return this.isExample != null && !this.isExample.isEmpty(); 12975 } 12976 12977 public boolean hasIsExample() { 12978 return this.isExample != null && !this.isExample.isEmpty(); 12979 } 12980 12981 /** 12982 * @param value {@link #isExample} (If true, indicates the resource is an example instance.). This is the underlying object with id, value and extensions. The accessor "getIsExample" gives direct access to the value 12983 */ 12984 public ManifestResourceComponent setIsExampleElement(BooleanType value) { 12985 this.isExample = value; 12986 return this; 12987 } 12988 12989 /** 12990 * @return If true, indicates the resource is an example instance. 12991 */ 12992 public boolean getIsExample() { 12993 return this.isExample == null || this.isExample.isEmpty() ? false : this.isExample.getValue(); 12994 } 12995 12996 /** 12997 * @param value If true, indicates the resource is an example instance. 12998 */ 12999 public ManifestResourceComponent setIsExample(boolean value) { 13000 if (this.isExample == null) 13001 this.isExample = new BooleanType(); 13002 this.isExample.setValue(value); 13003 return this; 13004 } 13005 13006 /** 13007 * @return {@link #profile} (If present, indicates profile(s) the instance is valid against.) 13008 */ 13009 public List<CanonicalType> getProfile() { 13010 if (this.profile == null) 13011 this.profile = new ArrayList<CanonicalType>(); 13012 return this.profile; 13013 } 13014 13015 /** 13016 * @return Returns a reference to <code>this</code> for easy method chaining 13017 */ 13018 public ManifestResourceComponent setProfile(List<CanonicalType> theProfile) { 13019 this.profile = theProfile; 13020 return this; 13021 } 13022 13023 public boolean hasProfile() { 13024 if (this.profile == null) 13025 return false; 13026 for (CanonicalType item : this.profile) 13027 if (!item.isEmpty()) 13028 return true; 13029 return false; 13030 } 13031 13032 /** 13033 * @return {@link #profile} (If present, indicates profile(s) the instance is valid against.) 13034 */ 13035 public CanonicalType addProfileElement() {//2 13036 CanonicalType t = new CanonicalType(); 13037 if (this.profile == null) 13038 this.profile = new ArrayList<CanonicalType>(); 13039 this.profile.add(t); 13040 return t; 13041 } 13042 13043 /** 13044 * @param value {@link #profile} (If present, indicates profile(s) the instance is valid against.) 13045 */ 13046 public ManifestResourceComponent addProfile(String value) { //1 13047 CanonicalType t = new CanonicalType(); 13048 t.setValue(value); 13049 if (this.profile == null) 13050 this.profile = new ArrayList<CanonicalType>(); 13051 this.profile.add(t); 13052 return this; 13053 } 13054 13055 /** 13056 * @param value {@link #profile} (If present, indicates profile(s) the instance is valid against.) 13057 */ 13058 public boolean hasProfile(String value) { 13059 if (this.profile == null) 13060 return false; 13061 for (CanonicalType v : this.profile) 13062 if (v.getValue().equals(value)) // canonical 13063 return true; 13064 return false; 13065 } 13066 13067 /** 13068 * @return {@link #relativePath} (The relative path for primary page for this resource within the IG.). This is the underlying object with id, value and extensions. The accessor "getRelativePath" gives direct access to the value 13069 */ 13070 public UrlType getRelativePathElement() { 13071 if (this.relativePath == null) 13072 if (Configuration.errorOnAutoCreate()) 13073 throw new Error("Attempt to auto-create ManifestResourceComponent.relativePath"); 13074 else if (Configuration.doAutoCreate()) 13075 this.relativePath = new UrlType(); // bb 13076 return this.relativePath; 13077 } 13078 13079 public boolean hasRelativePathElement() { 13080 return this.relativePath != null && !this.relativePath.isEmpty(); 13081 } 13082 13083 public boolean hasRelativePath() { 13084 return this.relativePath != null && !this.relativePath.isEmpty(); 13085 } 13086 13087 /** 13088 * @param value {@link #relativePath} (The relative path for primary page for this resource within the IG.). This is the underlying object with id, value and extensions. The accessor "getRelativePath" gives direct access to the value 13089 */ 13090 public ManifestResourceComponent setRelativePathElement(UrlType value) { 13091 this.relativePath = value; 13092 return this; 13093 } 13094 13095 /** 13096 * @return The relative path for primary page for this resource within the IG. 13097 */ 13098 public String getRelativePath() { 13099 return this.relativePath == null ? null : this.relativePath.getValue(); 13100 } 13101 13102 /** 13103 * @param value The relative path for primary page for this resource within the IG. 13104 */ 13105 public ManifestResourceComponent setRelativePath(String value) { 13106 if (Utilities.noString(value)) 13107 this.relativePath = null; 13108 else { 13109 if (this.relativePath == null) 13110 this.relativePath = new UrlType(); 13111 this.relativePath.setValue(value); 13112 } 13113 return this; 13114 } 13115 13116 protected void listChildren(List<Property> children) { 13117 super.listChildren(children); 13118 children.add(new Property("reference", "Reference(Any)", "Where this resource is found.", 0, 1, reference)); 13119 children.add(new Property("isExample", "boolean", "If true, indicates the resource is an example instance.", 0, 1, isExample)); 13120 children.add(new Property("profile", "canonical(StructureDefinition)", "If present, indicates profile(s) the instance is valid against.", 0, java.lang.Integer.MAX_VALUE, profile)); 13121 children.add(new Property("relativePath", "url", "The relative path for primary page for this resource within the IG.", 0, 1, relativePath)); 13122 } 13123 13124 @Override 13125 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 13126 switch (_hash) { 13127 case -925155509: /*reference*/ return new Property("reference", "Reference(Any)", "Where this resource is found.", 0, 1, reference); 13128 case -1902749472: /*isExample*/ return new Property("isExample", "boolean", "If true, indicates the resource is an example instance.", 0, 1, isExample); 13129 case -309425751: /*profile*/ return new Property("profile", "canonical(StructureDefinition)", "If present, indicates profile(s) the instance is valid against.", 0, java.lang.Integer.MAX_VALUE, profile); 13130 case -70808303: /*relativePath*/ return new Property("relativePath", "url", "The relative path for primary page for this resource within the IG.", 0, 1, relativePath); 13131 default: return super.getNamedProperty(_hash, _name, _checkValid); 13132 } 13133 13134 } 13135 13136 @Override 13137 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 13138 switch (hash) { 13139 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Reference 13140 case -1902749472: /*isExample*/ return this.isExample == null ? new Base[0] : new Base[] {this.isExample}; // BooleanType 13141 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : this.profile.toArray(new Base[this.profile.size()]); // CanonicalType 13142 case -70808303: /*relativePath*/ return this.relativePath == null ? new Base[0] : new Base[] {this.relativePath}; // UrlType 13143 default: return super.getProperty(hash, name, checkValid); 13144 } 13145 13146 } 13147 13148 @Override 13149 public Base setProperty(int hash, String name, Base value) throws FHIRException { 13150 switch (hash) { 13151 case -925155509: // reference 13152 this.reference = TypeConvertor.castToReference(value); // Reference 13153 return value; 13154 case -1902749472: // isExample 13155 this.isExample = TypeConvertor.castToBoolean(value); // BooleanType 13156 return value; 13157 case -309425751: // profile 13158 this.getProfile().add(TypeConvertor.castToCanonical(value)); // CanonicalType 13159 return value; 13160 case -70808303: // relativePath 13161 this.relativePath = TypeConvertor.castToUrl(value); // UrlType 13162 return value; 13163 default: return super.setProperty(hash, name, value); 13164 } 13165 13166 } 13167 13168 @Override 13169 public Base setProperty(String name, Base value) throws FHIRException { 13170 if (name.equals("reference")) { 13171 this.reference = TypeConvertor.castToReference(value); // Reference 13172 } else if (name.equals("isExample")) { 13173 this.isExample = TypeConvertor.castToBoolean(value); // BooleanType 13174 } else if (name.equals("profile")) { 13175 this.getProfile().add(TypeConvertor.castToCanonical(value)); 13176 } else if (name.equals("relativePath")) { 13177 this.relativePath = TypeConvertor.castToUrl(value); // UrlType 13178 } else 13179 return super.setProperty(name, value); 13180 return value; 13181 } 13182 13183 @Override 13184 public void removeChild(String name, Base value) throws FHIRException { 13185 if (name.equals("reference")) { 13186 this.reference = null; 13187 } else if (name.equals("isExample")) { 13188 this.isExample = null; 13189 } else if (name.equals("profile")) { 13190 this.getProfile().remove(value); 13191 } else if (name.equals("relativePath")) { 13192 this.relativePath = null; 13193 } else 13194 super.removeChild(name, value); 13195 13196 } 13197 13198 @Override 13199 public Base makeProperty(int hash, String name) throws FHIRException { 13200 switch (hash) { 13201 case -925155509: return getReference(); 13202 case -1902749472: return getIsExampleElement(); 13203 case -309425751: return addProfileElement(); 13204 case -70808303: return getRelativePathElement(); 13205 default: return super.makeProperty(hash, name); 13206 } 13207 13208 } 13209 13210 @Override 13211 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 13212 switch (hash) { 13213 case -925155509: /*reference*/ return new String[] {"Reference"}; 13214 case -1902749472: /*isExample*/ return new String[] {"boolean"}; 13215 case -309425751: /*profile*/ return new String[] {"canonical"}; 13216 case -70808303: /*relativePath*/ return new String[] {"url"}; 13217 default: return super.getTypesForProperty(hash, name); 13218 } 13219 13220 } 13221 13222 @Override 13223 public Base addChild(String name) throws FHIRException { 13224 if (name.equals("reference")) { 13225 this.reference = new Reference(); 13226 return this.reference; 13227 } 13228 else if (name.equals("isExample")) { 13229 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.manifest.resource.isExample"); 13230 } 13231 else if (name.equals("profile")) { 13232 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.manifest.resource.profile"); 13233 } 13234 else if (name.equals("relativePath")) { 13235 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.manifest.resource.relativePath"); 13236 } 13237 else 13238 return super.addChild(name); 13239 } 13240 13241 public ManifestResourceComponent copy() { 13242 ManifestResourceComponent dst = new ManifestResourceComponent(); 13243 copyValues(dst); 13244 return dst; 13245 } 13246 13247 public void copyValues(ManifestResourceComponent dst) { 13248 super.copyValues(dst); 13249 dst.reference = reference == null ? null : reference.copy(); 13250 dst.isExample = isExample == null ? null : isExample.copy(); 13251 if (profile != null) { 13252 dst.profile = new ArrayList<CanonicalType>(); 13253 for (CanonicalType i : profile) 13254 dst.profile.add(i.copy()); 13255 }; 13256 dst.relativePath = relativePath == null ? null : relativePath.copy(); 13257 } 13258 13259 @Override 13260 public boolean equalsDeep(Base other_) { 13261 if (!super.equalsDeep(other_)) 13262 return false; 13263 if (!(other_ instanceof ManifestResourceComponent)) 13264 return false; 13265 ManifestResourceComponent o = (ManifestResourceComponent) other_; 13266 return compareDeep(reference, o.reference, true) && compareDeep(isExample, o.isExample, true) && compareDeep(profile, o.profile, true) 13267 && compareDeep(relativePath, o.relativePath, true); 13268 } 13269 13270 @Override 13271 public boolean equalsShallow(Base other_) { 13272 if (!super.equalsShallow(other_)) 13273 return false; 13274 if (!(other_ instanceof ManifestResourceComponent)) 13275 return false; 13276 ManifestResourceComponent o = (ManifestResourceComponent) other_; 13277 return compareValues(isExample, o.isExample, true) && compareValues(profile, o.profile, true) && compareValues(relativePath, o.relativePath, true) 13278 ; 13279 } 13280 13281 public boolean isEmpty() { 13282 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, isExample, profile 13283 , relativePath); 13284 } 13285 13286 public String fhirType() { 13287 return "ImplementationGuide.manifest.resource"; 13288 13289 } 13290 13291 // added from java-adornments.txt: 13292 13293 // end addition 13294 } 13295 13296 @Block() 13297 public static class ManifestPageComponent extends BackboneElement implements IBaseBackboneElement { 13298 /** 13299 * Relative path to the page. 13300 */ 13301 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 13302 @Description(shortDefinition="HTML page name", formalDefinition="Relative path to the page." ) 13303 protected StringType name; 13304 13305 /** 13306 * Label for the page intended for human display. 13307 */ 13308 @Child(name = "title", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 13309 @Description(shortDefinition="Title of the page, for references", formalDefinition="Label for the page intended for human display." ) 13310 protected StringType title; 13311 13312 /** 13313 * The name of an anchor available on the page. 13314 */ 13315 @Child(name = "anchor", type = {StringType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 13316 @Description(shortDefinition="Anchor available on the page", formalDefinition="The name of an anchor available on the page." ) 13317 protected List<StringType> anchor; 13318 13319 private static final long serialVersionUID = 1920576611L; 13320 13321 /** 13322 * Constructor 13323 */ 13324 public ManifestPageComponent() { 13325 super(); 13326 } 13327 13328 /** 13329 * Constructor 13330 */ 13331 public ManifestPageComponent(String name) { 13332 super(); 13333 this.setName(name); 13334 } 13335 13336 /** 13337 * @return {@link #name} (Relative path to the page.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 13338 */ 13339 public StringType getNameElement() { 13340 if (this.name == null) 13341 if (Configuration.errorOnAutoCreate()) 13342 throw new Error("Attempt to auto-create ManifestPageComponent.name"); 13343 else if (Configuration.doAutoCreate()) 13344 this.name = new StringType(); // bb 13345 return this.name; 13346 } 13347 13348 public boolean hasNameElement() { 13349 return this.name != null && !this.name.isEmpty(); 13350 } 13351 13352 public boolean hasName() { 13353 return this.name != null && !this.name.isEmpty(); 13354 } 13355 13356 /** 13357 * @param value {@link #name} (Relative path to the page.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 13358 */ 13359 public ManifestPageComponent setNameElement(StringType value) { 13360 this.name = value; 13361 return this; 13362 } 13363 13364 /** 13365 * @return Relative path to the page. 13366 */ 13367 public String getName() { 13368 return this.name == null ? null : this.name.getValue(); 13369 } 13370 13371 /** 13372 * @param value Relative path to the page. 13373 */ 13374 public ManifestPageComponent setName(String value) { 13375 if (this.name == null) 13376 this.name = new StringType(); 13377 this.name.setValue(value); 13378 return this; 13379 } 13380 13381 /** 13382 * @return {@link #title} (Label for the page intended for human display.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 13383 */ 13384 public StringType getTitleElement() { 13385 if (this.title == null) 13386 if (Configuration.errorOnAutoCreate()) 13387 throw new Error("Attempt to auto-create ManifestPageComponent.title"); 13388 else if (Configuration.doAutoCreate()) 13389 this.title = new StringType(); // bb 13390 return this.title; 13391 } 13392 13393 public boolean hasTitleElement() { 13394 return this.title != null && !this.title.isEmpty(); 13395 } 13396 13397 public boolean hasTitle() { 13398 return this.title != null && !this.title.isEmpty(); 13399 } 13400 13401 /** 13402 * @param value {@link #title} (Label for the page intended for human display.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 13403 */ 13404 public ManifestPageComponent setTitleElement(StringType value) { 13405 this.title = value; 13406 return this; 13407 } 13408 13409 /** 13410 * @return Label for the page intended for human display. 13411 */ 13412 public String getTitle() { 13413 return this.title == null ? null : this.title.getValue(); 13414 } 13415 13416 /** 13417 * @param value Label for the page intended for human display. 13418 */ 13419 public ManifestPageComponent setTitle(String value) { 13420 if (Utilities.noString(value)) 13421 this.title = null; 13422 else { 13423 if (this.title == null) 13424 this.title = new StringType(); 13425 this.title.setValue(value); 13426 } 13427 return this; 13428 } 13429 13430 /** 13431 * @return {@link #anchor} (The name of an anchor available on the page.) 13432 */ 13433 public List<StringType> getAnchor() { 13434 if (this.anchor == null) 13435 this.anchor = new ArrayList<StringType>(); 13436 return this.anchor; 13437 } 13438 13439 /** 13440 * @return Returns a reference to <code>this</code> for easy method chaining 13441 */ 13442 public ManifestPageComponent setAnchor(List<StringType> theAnchor) { 13443 this.anchor = theAnchor; 13444 return this; 13445 } 13446 13447 public boolean hasAnchor() { 13448 if (this.anchor == null) 13449 return false; 13450 for (StringType item : this.anchor) 13451 if (!item.isEmpty()) 13452 return true; 13453 return false; 13454 } 13455 13456 /** 13457 * @return {@link #anchor} (The name of an anchor available on the page.) 13458 */ 13459 public StringType addAnchorElement() {//2 13460 StringType t = new StringType(); 13461 if (this.anchor == null) 13462 this.anchor = new ArrayList<StringType>(); 13463 this.anchor.add(t); 13464 return t; 13465 } 13466 13467 /** 13468 * @param value {@link #anchor} (The name of an anchor available on the page.) 13469 */ 13470 public ManifestPageComponent addAnchor(String value) { //1 13471 StringType t = new StringType(); 13472 t.setValue(value); 13473 if (this.anchor == null) 13474 this.anchor = new ArrayList<StringType>(); 13475 this.anchor.add(t); 13476 return this; 13477 } 13478 13479 /** 13480 * @param value {@link #anchor} (The name of an anchor available on the page.) 13481 */ 13482 public boolean hasAnchor(String value) { 13483 if (this.anchor == null) 13484 return false; 13485 for (StringType v : this.anchor) 13486 if (v.getValue().equals(value)) // string 13487 return true; 13488 return false; 13489 } 13490 13491 protected void listChildren(List<Property> children) { 13492 super.listChildren(children); 13493 children.add(new Property("name", "string", "Relative path to the page.", 0, 1, name)); 13494 children.add(new Property("title", "string", "Label for the page intended for human display.", 0, 1, title)); 13495 children.add(new Property("anchor", "string", "The name of an anchor available on the page.", 0, java.lang.Integer.MAX_VALUE, anchor)); 13496 } 13497 13498 @Override 13499 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 13500 switch (_hash) { 13501 case 3373707: /*name*/ return new Property("name", "string", "Relative path to the page.", 0, 1, name); 13502 case 110371416: /*title*/ return new Property("title", "string", "Label for the page intended for human display.", 0, 1, title); 13503 case -1413299531: /*anchor*/ return new Property("anchor", "string", "The name of an anchor available on the page.", 0, java.lang.Integer.MAX_VALUE, anchor); 13504 default: return super.getNamedProperty(_hash, _name, _checkValid); 13505 } 13506 13507 } 13508 13509 @Override 13510 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 13511 switch (hash) { 13512 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 13513 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 13514 case -1413299531: /*anchor*/ return this.anchor == null ? new Base[0] : this.anchor.toArray(new Base[this.anchor.size()]); // StringType 13515 default: return super.getProperty(hash, name, checkValid); 13516 } 13517 13518 } 13519 13520 @Override 13521 public Base setProperty(int hash, String name, Base value) throws FHIRException { 13522 switch (hash) { 13523 case 3373707: // name 13524 this.name = TypeConvertor.castToString(value); // StringType 13525 return value; 13526 case 110371416: // title 13527 this.title = TypeConvertor.castToString(value); // StringType 13528 return value; 13529 case -1413299531: // anchor 13530 this.getAnchor().add(TypeConvertor.castToString(value)); // StringType 13531 return value; 13532 default: return super.setProperty(hash, name, value); 13533 } 13534 13535 } 13536 13537 @Override 13538 public Base setProperty(String name, Base value) throws FHIRException { 13539 if (name.equals("name")) { 13540 this.name = TypeConvertor.castToString(value); // StringType 13541 } else if (name.equals("title")) { 13542 this.title = TypeConvertor.castToString(value); // StringType 13543 } else if (name.equals("anchor")) { 13544 this.getAnchor().add(TypeConvertor.castToString(value)); 13545 } else 13546 return super.setProperty(name, value); 13547 return value; 13548 } 13549 13550 @Override 13551 public void removeChild(String name, Base value) throws FHIRException { 13552 if (name.equals("name")) { 13553 this.name = null; 13554 } else if (name.equals("title")) { 13555 this.title = null; 13556 } else if (name.equals("anchor")) { 13557 this.getAnchor().remove(value); 13558 } else 13559 super.removeChild(name, value); 13560 13561 } 13562 13563 @Override 13564 public Base makeProperty(int hash, String name) throws FHIRException { 13565 switch (hash) { 13566 case 3373707: return getNameElement(); 13567 case 110371416: return getTitleElement(); 13568 case -1413299531: return addAnchorElement(); 13569 default: return super.makeProperty(hash, name); 13570 } 13571 13572 } 13573 13574 @Override 13575 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 13576 switch (hash) { 13577 case 3373707: /*name*/ return new String[] {"string"}; 13578 case 110371416: /*title*/ return new String[] {"string"}; 13579 case -1413299531: /*anchor*/ return new String[] {"string"}; 13580 default: return super.getTypesForProperty(hash, name); 13581 } 13582 13583 } 13584 13585 @Override 13586 public Base addChild(String name) throws FHIRException { 13587 if (name.equals("name")) { 13588 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.manifest.page.name"); 13589 } 13590 else if (name.equals("title")) { 13591 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.manifest.page.title"); 13592 } 13593 else if (name.equals("anchor")) { 13594 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.manifest.page.anchor"); 13595 } 13596 else 13597 return super.addChild(name); 13598 } 13599 13600 public ManifestPageComponent copy() { 13601 ManifestPageComponent dst = new ManifestPageComponent(); 13602 copyValues(dst); 13603 return dst; 13604 } 13605 13606 public void copyValues(ManifestPageComponent dst) { 13607 super.copyValues(dst); 13608 dst.name = name == null ? null : name.copy(); 13609 dst.title = title == null ? null : title.copy(); 13610 if (anchor != null) { 13611 dst.anchor = new ArrayList<StringType>(); 13612 for (StringType i : anchor) 13613 dst.anchor.add(i.copy()); 13614 }; 13615 } 13616 13617 @Override 13618 public boolean equalsDeep(Base other_) { 13619 if (!super.equalsDeep(other_)) 13620 return false; 13621 if (!(other_ instanceof ManifestPageComponent)) 13622 return false; 13623 ManifestPageComponent o = (ManifestPageComponent) other_; 13624 return compareDeep(name, o.name, true) && compareDeep(title, o.title, true) && compareDeep(anchor, o.anchor, true) 13625 ; 13626 } 13627 13628 @Override 13629 public boolean equalsShallow(Base other_) { 13630 if (!super.equalsShallow(other_)) 13631 return false; 13632 if (!(other_ instanceof ManifestPageComponent)) 13633 return false; 13634 ManifestPageComponent o = (ManifestPageComponent) other_; 13635 return compareValues(name, o.name, true) && compareValues(title, o.title, true) && compareValues(anchor, o.anchor, true) 13636 ; 13637 } 13638 13639 public boolean isEmpty() { 13640 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, title, anchor); 13641 } 13642 13643 public String fhirType() { 13644 return "ImplementationGuide.manifest.page"; 13645 13646 } 13647 13648 } 13649 13650 /** 13651 * An absolute URI that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this implementation guide is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the implementation guide is stored on different servers. 13652 */ 13653 @Child(name = "url", type = {UriType.class}, order=0, min=1, max=1, modifier=false, summary=true) 13654 @Description(shortDefinition="Canonical identifier for this implementation guide, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this implementation guide is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the implementation guide is stored on different servers." ) 13655 protected UriType url; 13656 13657 /** 13658 * A formal identifier that is used to identify this implementation guide when it is represented in other formats, or referenced in a specification, model, design or an instance. 13659 */ 13660 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 13661 @Description(shortDefinition="Additional identifier for the implementation guide (business identifier)", formalDefinition="A formal identifier that is used to identify this implementation guide when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 13662 protected List<Identifier> identifier; 13663 13664 /** 13665 * The identifier that is used to identify this version of the implementation guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the implementation guide author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 13666 */ 13667 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 13668 @Description(shortDefinition="Business version of the implementation guide", formalDefinition="The identifier that is used to identify this version of the implementation guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the implementation guide author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence." ) 13669 protected StringType version; 13670 13671 /** 13672 * Indicates the mechanism used to compare versions to determine which is more current. 13673 */ 13674 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 13675 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 13676 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 13677 protected DataType versionAlgorithm; 13678 13679 /** 13680 * A natural language name identifying the implementation guide. This name should be usable as an identifier for the module by machine processing applications such as code generation. 13681 */ 13682 @Child(name = "name", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=true) 13683 @Description(shortDefinition="Name for this implementation guide (computer friendly)", formalDefinition="A natural language name identifying the implementation guide. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 13684 protected StringType name; 13685 13686 /** 13687 * A short, descriptive, user-friendly title for the implementation guide. 13688 */ 13689 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 13690 @Description(shortDefinition="Name for this implementation guide (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the implementation guide." ) 13691 protected StringType title; 13692 13693 /** 13694 * The status of this implementation guide. Enables tracking the life-cycle of the content. 13695 */ 13696 @Child(name = "status", type = {CodeType.class}, order=6, min=1, max=1, modifier=true, summary=true) 13697 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this implementation guide. Enables tracking the life-cycle of the content." ) 13698 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 13699 protected Enumeration<PublicationStatus> status; 13700 13701 /** 13702 * A Boolean value to indicate that this implementation guide is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 13703 */ 13704 @Child(name = "experimental", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 13705 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this implementation guide is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 13706 protected BooleanType experimental; 13707 13708 /** 13709 * The date (and optionally time) when the implementation guide was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes. 13710 */ 13711 @Child(name = "date", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 13712 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the implementation guide was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes." ) 13713 protected DateTimeType date; 13714 13715 /** 13716 * The name of the organization or individual responsible for the release and ongoing maintenance of the implementation guide. 13717 */ 13718 @Child(name = "publisher", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=true) 13719 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the implementation guide." ) 13720 protected StringType publisher; 13721 13722 /** 13723 * Contact details to assist a user in finding and communicating with the publisher. 13724 */ 13725 @Child(name = "contact", type = {ContactDetail.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 13726 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 13727 protected List<ContactDetail> contact; 13728 13729 /** 13730 * A free text natural language description of the implementation guide from a consumer's perspective. 13731 */ 13732 @Child(name = "description", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=false) 13733 @Description(shortDefinition="Natural language description of the implementation guide", formalDefinition="A free text natural language description of the implementation guide from a consumer's perspective." ) 13734 protected MarkdownType description; 13735 13736 /** 13737 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate implementation guide instances. 13738 */ 13739 @Child(name = "useContext", type = {UsageContext.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 13740 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate implementation guide instances." ) 13741 protected List<UsageContext> useContext; 13742 13743 /** 13744 * A legal or geographic region in which the implementation guide is intended to be used. 13745 */ 13746 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 13747 @Description(shortDefinition="Intended jurisdiction for implementation guide (if applicable)", formalDefinition="A legal or geographic region in which the implementation guide is intended to be used." ) 13748 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 13749 protected List<CodeableConcept> jurisdiction; 13750 13751 /** 13752 * Explanation of why this implementation guide is needed and why it has been designed as it has. 13753 */ 13754 @Child(name = "purpose", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=false) 13755 @Description(shortDefinition="Why this implementation guide is defined", formalDefinition="Explanation of why this implementation guide is needed and why it has been designed as it has." ) 13756 protected MarkdownType purpose; 13757 13758 /** 13759 * A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide. 13760 */ 13761 @Child(name = "copyright", type = {MarkdownType.class}, order=15, min=0, max=1, modifier=false, summary=false) 13762 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide." ) 13763 protected MarkdownType copyright; 13764 13765 /** 13766 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 13767 */ 13768 @Child(name = "copyrightLabel", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 13769 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 13770 protected StringType copyrightLabel; 13771 13772 /** 13773 * The NPM package name for this Implementation Guide, used in the NPM package distribution, which is the primary mechanism by which FHIR based tooling manages IG dependencies. This value must be globally unique, and should be assigned with care. 13774 */ 13775 @Child(name = "packageId", type = {IdType.class}, order=17, min=1, max=1, modifier=false, summary=true) 13776 @Description(shortDefinition="NPM Package name for IG", formalDefinition="The NPM package name for this Implementation Guide, used in the NPM package distribution, which is the primary mechanism by which FHIR based tooling manages IG dependencies. This value must be globally unique, and should be assigned with care." ) 13777 protected IdType packageId; 13778 13779 /** 13780 * The license that applies to this Implementation Guide, using an SPDX license code, or 'not-open-source'. 13781 */ 13782 @Child(name = "license", type = {CodeType.class}, order=18, min=0, max=1, modifier=false, summary=true) 13783 @Description(shortDefinition="SPDX license code for this IG (or not-open-source)", formalDefinition="The license that applies to this Implementation Guide, using an SPDX license code, or 'not-open-source'." ) 13784 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/spdx-license") 13785 protected Enumeration<SPDXLicense> license; 13786 13787 /** 13788 * The version(s) of the FHIR specification that this ImplementationGuide targets - e.g. describes how to use. The value of this element is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version. 13789 */ 13790 @Child(name = "fhirVersion", type = {CodeType.class}, order=19, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 13791 @Description(shortDefinition="FHIR Version(s) this Implementation Guide targets", formalDefinition="The version(s) of the FHIR specification that this ImplementationGuide targets - e.g. describes how to use. The value of this element is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version." ) 13792 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/FHIR-version") 13793 protected List<Enumeration<FHIRVersion>> fhirVersion; 13794 13795 /** 13796 * Another implementation guide that this implementation depends on. Typically, an implementation guide uses value sets, profiles etc.defined in other implementation guides. 13797 */ 13798 @Child(name = "dependsOn", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 13799 @Description(shortDefinition="Another Implementation guide this depends on", formalDefinition="Another implementation guide that this implementation depends on. Typically, an implementation guide uses value sets, profiles etc.defined in other implementation guides." ) 13800 protected List<ImplementationGuideDependsOnComponent> dependsOn; 13801 13802 /** 13803 * A set of profiles that all resources covered by this implementation guide must conform to. 13804 */ 13805 @Child(name = "global", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 13806 @Description(shortDefinition="Profiles that apply globally", formalDefinition="A set of profiles that all resources covered by this implementation guide must conform to." ) 13807 protected List<ImplementationGuideGlobalComponent> global; 13808 13809 /** 13810 * The information needed by an IG publisher tool to publish the whole implementation guide. 13811 */ 13812 @Child(name = "definition", type = {}, order=22, min=0, max=1, modifier=false, summary=false) 13813 @Description(shortDefinition="Information needed to build the IG", formalDefinition="The information needed by an IG publisher tool to publish the whole implementation guide." ) 13814 protected ImplementationGuideDefinitionComponent definition; 13815 13816 /** 13817 * Information about an assembled implementation guide, created by the publication tooling. 13818 */ 13819 @Child(name = "manifest", type = {}, order=23, min=0, max=1, modifier=false, summary=false) 13820 @Description(shortDefinition="Information about an assembled IG", formalDefinition="Information about an assembled implementation guide, created by the publication tooling." ) 13821 protected ImplementationGuideManifestComponent manifest; 13822 13823 private static final long serialVersionUID = 183592979L; 13824 13825 /** 13826 * Constructor 13827 */ 13828 public ImplementationGuide() { 13829 super(); 13830 } 13831 13832 /** 13833 * Constructor 13834 */ 13835 public ImplementationGuide(String url, String name, PublicationStatus status, String packageId, FHIRVersion fhirVersion) { 13836 super(); 13837 this.setUrl(url); 13838 this.setName(name); 13839 this.setStatus(status); 13840 this.setPackageId(packageId); 13841 this.addFhirVersion(fhirVersion); 13842 } 13843 13844 /** 13845 * @return {@link #url} (An absolute URI that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this implementation guide is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the implementation guide is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 13846 */ 13847 public UriType getUrlElement() { 13848 if (this.url == null) 13849 if (Configuration.errorOnAutoCreate()) 13850 throw new Error("Attempt to auto-create ImplementationGuide.url"); 13851 else if (Configuration.doAutoCreate()) 13852 this.url = new UriType(); // bb 13853 return this.url; 13854 } 13855 13856 public boolean hasUrlElement() { 13857 return this.url != null && !this.url.isEmpty(); 13858 } 13859 13860 public boolean hasUrl() { 13861 return this.url != null && !this.url.isEmpty(); 13862 } 13863 13864 /** 13865 * @param value {@link #url} (An absolute URI that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this implementation guide is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the implementation guide is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 13866 */ 13867 public ImplementationGuide setUrlElement(UriType value) { 13868 this.url = value; 13869 return this; 13870 } 13871 13872 /** 13873 * @return An absolute URI that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this implementation guide is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the implementation guide is stored on different servers. 13874 */ 13875 public String getUrl() { 13876 return this.url == null ? null : this.url.getValue(); 13877 } 13878 13879 /** 13880 * @param value An absolute URI that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this implementation guide is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the implementation guide is stored on different servers. 13881 */ 13882 public ImplementationGuide setUrl(String value) { 13883 if (this.url == null) 13884 this.url = new UriType(); 13885 this.url.setValue(value); 13886 return this; 13887 } 13888 13889 /** 13890 * @return {@link #identifier} (A formal identifier that is used to identify this implementation guide when it is represented in other formats, or referenced in a specification, model, design or an instance.) 13891 */ 13892 public List<Identifier> getIdentifier() { 13893 if (this.identifier == null) 13894 this.identifier = new ArrayList<Identifier>(); 13895 return this.identifier; 13896 } 13897 13898 /** 13899 * @return Returns a reference to <code>this</code> for easy method chaining 13900 */ 13901 public ImplementationGuide setIdentifier(List<Identifier> theIdentifier) { 13902 this.identifier = theIdentifier; 13903 return this; 13904 } 13905 13906 public boolean hasIdentifier() { 13907 if (this.identifier == null) 13908 return false; 13909 for (Identifier item : this.identifier) 13910 if (!item.isEmpty()) 13911 return true; 13912 return false; 13913 } 13914 13915 public Identifier addIdentifier() { //3 13916 Identifier t = new Identifier(); 13917 if (this.identifier == null) 13918 this.identifier = new ArrayList<Identifier>(); 13919 this.identifier.add(t); 13920 return t; 13921 } 13922 13923 public ImplementationGuide addIdentifier(Identifier t) { //3 13924 if (t == null) 13925 return this; 13926 if (this.identifier == null) 13927 this.identifier = new ArrayList<Identifier>(); 13928 this.identifier.add(t); 13929 return this; 13930 } 13931 13932 /** 13933 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 13934 */ 13935 public Identifier getIdentifierFirstRep() { 13936 if (getIdentifier().isEmpty()) { 13937 addIdentifier(); 13938 } 13939 return getIdentifier().get(0); 13940 } 13941 13942 /** 13943 * @return {@link #version} (The identifier that is used to identify this version of the implementation guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the implementation guide author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 13944 */ 13945 public StringType getVersionElement() { 13946 if (this.version == null) 13947 if (Configuration.errorOnAutoCreate()) 13948 throw new Error("Attempt to auto-create ImplementationGuide.version"); 13949 else if (Configuration.doAutoCreate()) 13950 this.version = new StringType(); // bb 13951 return this.version; 13952 } 13953 13954 public boolean hasVersionElement() { 13955 return this.version != null && !this.version.isEmpty(); 13956 } 13957 13958 public boolean hasVersion() { 13959 return this.version != null && !this.version.isEmpty(); 13960 } 13961 13962 /** 13963 * @param value {@link #version} (The identifier that is used to identify this version of the implementation guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the implementation guide author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 13964 */ 13965 public ImplementationGuide setVersionElement(StringType value) { 13966 this.version = value; 13967 return this; 13968 } 13969 13970 /** 13971 * @return The identifier that is used to identify this version of the implementation guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the implementation guide author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 13972 */ 13973 public String getVersion() { 13974 return this.version == null ? null : this.version.getValue(); 13975 } 13976 13977 /** 13978 * @param value The identifier that is used to identify this version of the implementation guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the implementation guide author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 13979 */ 13980 public ImplementationGuide setVersion(String value) { 13981 if (Utilities.noString(value)) 13982 this.version = null; 13983 else { 13984 if (this.version == null) 13985 this.version = new StringType(); 13986 this.version.setValue(value); 13987 } 13988 return this; 13989 } 13990 13991 /** 13992 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 13993 */ 13994 public DataType getVersionAlgorithm() { 13995 return this.versionAlgorithm; 13996 } 13997 13998 /** 13999 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 14000 */ 14001 public StringType getVersionAlgorithmStringType() throws FHIRException { 14002 if (this.versionAlgorithm == null) 14003 this.versionAlgorithm = new StringType(); 14004 if (!(this.versionAlgorithm instanceof StringType)) 14005 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 14006 return (StringType) this.versionAlgorithm; 14007 } 14008 14009 public boolean hasVersionAlgorithmStringType() { 14010 return this != null && this.versionAlgorithm instanceof StringType; 14011 } 14012 14013 /** 14014 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 14015 */ 14016 public Coding getVersionAlgorithmCoding() throws FHIRException { 14017 if (this.versionAlgorithm == null) 14018 this.versionAlgorithm = new Coding(); 14019 if (!(this.versionAlgorithm instanceof Coding)) 14020 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 14021 return (Coding) this.versionAlgorithm; 14022 } 14023 14024 public boolean hasVersionAlgorithmCoding() { 14025 return this != null && this.versionAlgorithm instanceof Coding; 14026 } 14027 14028 public boolean hasVersionAlgorithm() { 14029 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 14030 } 14031 14032 /** 14033 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 14034 */ 14035 public ImplementationGuide setVersionAlgorithm(DataType value) { 14036 if (value != null && !(value instanceof StringType || value instanceof Coding)) 14037 throw new FHIRException("Not the right type for ImplementationGuide.versionAlgorithm[x]: "+value.fhirType()); 14038 this.versionAlgorithm = value; 14039 return this; 14040 } 14041 14042 /** 14043 * @return {@link #name} (A natural language name identifying the implementation guide. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 14044 */ 14045 public StringType getNameElement() { 14046 if (this.name == null) 14047 if (Configuration.errorOnAutoCreate()) 14048 throw new Error("Attempt to auto-create ImplementationGuide.name"); 14049 else if (Configuration.doAutoCreate()) 14050 this.name = new StringType(); // bb 14051 return this.name; 14052 } 14053 14054 public boolean hasNameElement() { 14055 return this.name != null && !this.name.isEmpty(); 14056 } 14057 14058 public boolean hasName() { 14059 return this.name != null && !this.name.isEmpty(); 14060 } 14061 14062 /** 14063 * @param value {@link #name} (A natural language name identifying the implementation guide. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 14064 */ 14065 public ImplementationGuide setNameElement(StringType value) { 14066 this.name = value; 14067 return this; 14068 } 14069 14070 /** 14071 * @return A natural language name identifying the implementation guide. This name should be usable as an identifier for the module by machine processing applications such as code generation. 14072 */ 14073 public String getName() { 14074 return this.name == null ? null : this.name.getValue(); 14075 } 14076 14077 /** 14078 * @param value A natural language name identifying the implementation guide. This name should be usable as an identifier for the module by machine processing applications such as code generation. 14079 */ 14080 public ImplementationGuide setName(String value) { 14081 if (this.name == null) 14082 this.name = new StringType(); 14083 this.name.setValue(value); 14084 return this; 14085 } 14086 14087 /** 14088 * @return {@link #title} (A short, descriptive, user-friendly title for the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 14089 */ 14090 public StringType getTitleElement() { 14091 if (this.title == null) 14092 if (Configuration.errorOnAutoCreate()) 14093 throw new Error("Attempt to auto-create ImplementationGuide.title"); 14094 else if (Configuration.doAutoCreate()) 14095 this.title = new StringType(); // bb 14096 return this.title; 14097 } 14098 14099 public boolean hasTitleElement() { 14100 return this.title != null && !this.title.isEmpty(); 14101 } 14102 14103 public boolean hasTitle() { 14104 return this.title != null && !this.title.isEmpty(); 14105 } 14106 14107 /** 14108 * @param value {@link #title} (A short, descriptive, user-friendly title for the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 14109 */ 14110 public ImplementationGuide setTitleElement(StringType value) { 14111 this.title = value; 14112 return this; 14113 } 14114 14115 /** 14116 * @return A short, descriptive, user-friendly title for the implementation guide. 14117 */ 14118 public String getTitle() { 14119 return this.title == null ? null : this.title.getValue(); 14120 } 14121 14122 /** 14123 * @param value A short, descriptive, user-friendly title for the implementation guide. 14124 */ 14125 public ImplementationGuide setTitle(String value) { 14126 if (Utilities.noString(value)) 14127 this.title = null; 14128 else { 14129 if (this.title == null) 14130 this.title = new StringType(); 14131 this.title.setValue(value); 14132 } 14133 return this; 14134 } 14135 14136 /** 14137 * @return {@link #status} (The status of this implementation guide. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 14138 */ 14139 public Enumeration<PublicationStatus> getStatusElement() { 14140 if (this.status == null) 14141 if (Configuration.errorOnAutoCreate()) 14142 throw new Error("Attempt to auto-create ImplementationGuide.status"); 14143 else if (Configuration.doAutoCreate()) 14144 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 14145 return this.status; 14146 } 14147 14148 public boolean hasStatusElement() { 14149 return this.status != null && !this.status.isEmpty(); 14150 } 14151 14152 public boolean hasStatus() { 14153 return this.status != null && !this.status.isEmpty(); 14154 } 14155 14156 /** 14157 * @param value {@link #status} (The status of this implementation guide. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 14158 */ 14159 public ImplementationGuide setStatusElement(Enumeration<PublicationStatus> value) { 14160 this.status = value; 14161 return this; 14162 } 14163 14164 /** 14165 * @return The status of this implementation guide. Enables tracking the life-cycle of the content. 14166 */ 14167 public PublicationStatus getStatus() { 14168 return this.status == null ? null : this.status.getValue(); 14169 } 14170 14171 /** 14172 * @param value The status of this implementation guide. Enables tracking the life-cycle of the content. 14173 */ 14174 public ImplementationGuide setStatus(PublicationStatus value) { 14175 if (this.status == null) 14176 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 14177 this.status.setValue(value); 14178 return this; 14179 } 14180 14181 /** 14182 * @return {@link #experimental} (A Boolean value to indicate that this implementation guide is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 14183 */ 14184 public BooleanType getExperimentalElement() { 14185 if (this.experimental == null) 14186 if (Configuration.errorOnAutoCreate()) 14187 throw new Error("Attempt to auto-create ImplementationGuide.experimental"); 14188 else if (Configuration.doAutoCreate()) 14189 this.experimental = new BooleanType(); // bb 14190 return this.experimental; 14191 } 14192 14193 public boolean hasExperimentalElement() { 14194 return this.experimental != null && !this.experimental.isEmpty(); 14195 } 14196 14197 public boolean hasExperimental() { 14198 return this.experimental != null && !this.experimental.isEmpty(); 14199 } 14200 14201 /** 14202 * @param value {@link #experimental} (A Boolean value to indicate that this implementation guide is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 14203 */ 14204 public ImplementationGuide setExperimentalElement(BooleanType value) { 14205 this.experimental = value; 14206 return this; 14207 } 14208 14209 /** 14210 * @return A Boolean value to indicate that this implementation guide is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 14211 */ 14212 public boolean getExperimental() { 14213 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 14214 } 14215 14216 /** 14217 * @param value A Boolean value to indicate that this implementation guide is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 14218 */ 14219 public ImplementationGuide setExperimental(boolean value) { 14220 if (this.experimental == null) 14221 this.experimental = new BooleanType(); 14222 this.experimental.setValue(value); 14223 return this; 14224 } 14225 14226 /** 14227 * @return {@link #date} (The date (and optionally time) when the implementation guide was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 14228 */ 14229 public DateTimeType getDateElement() { 14230 if (this.date == null) 14231 if (Configuration.errorOnAutoCreate()) 14232 throw new Error("Attempt to auto-create ImplementationGuide.date"); 14233 else if (Configuration.doAutoCreate()) 14234 this.date = new DateTimeType(); // bb 14235 return this.date; 14236 } 14237 14238 public boolean hasDateElement() { 14239 return this.date != null && !this.date.isEmpty(); 14240 } 14241 14242 public boolean hasDate() { 14243 return this.date != null && !this.date.isEmpty(); 14244 } 14245 14246 /** 14247 * @param value {@link #date} (The date (and optionally time) when the implementation guide was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 14248 */ 14249 public ImplementationGuide setDateElement(DateTimeType value) { 14250 this.date = value; 14251 return this; 14252 } 14253 14254 /** 14255 * @return The date (and optionally time) when the implementation guide was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes. 14256 */ 14257 public Date getDate() { 14258 return this.date == null ? null : this.date.getValue(); 14259 } 14260 14261 /** 14262 * @param value The date (and optionally time) when the implementation guide was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes. 14263 */ 14264 public ImplementationGuide setDate(Date value) { 14265 if (value == null) 14266 this.date = null; 14267 else { 14268 if (this.date == null) 14269 this.date = new DateTimeType(); 14270 this.date.setValue(value); 14271 } 14272 return this; 14273 } 14274 14275 /** 14276 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 14277 */ 14278 public StringType getPublisherElement() { 14279 if (this.publisher == null) 14280 if (Configuration.errorOnAutoCreate()) 14281 throw new Error("Attempt to auto-create ImplementationGuide.publisher"); 14282 else if (Configuration.doAutoCreate()) 14283 this.publisher = new StringType(); // bb 14284 return this.publisher; 14285 } 14286 14287 public boolean hasPublisherElement() { 14288 return this.publisher != null && !this.publisher.isEmpty(); 14289 } 14290 14291 public boolean hasPublisher() { 14292 return this.publisher != null && !this.publisher.isEmpty(); 14293 } 14294 14295 /** 14296 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 14297 */ 14298 public ImplementationGuide setPublisherElement(StringType value) { 14299 this.publisher = value; 14300 return this; 14301 } 14302 14303 /** 14304 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the implementation guide. 14305 */ 14306 public String getPublisher() { 14307 return this.publisher == null ? null : this.publisher.getValue(); 14308 } 14309 14310 /** 14311 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the implementation guide. 14312 */ 14313 public ImplementationGuide setPublisher(String value) { 14314 if (Utilities.noString(value)) 14315 this.publisher = null; 14316 else { 14317 if (this.publisher == null) 14318 this.publisher = new StringType(); 14319 this.publisher.setValue(value); 14320 } 14321 return this; 14322 } 14323 14324 /** 14325 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 14326 */ 14327 public List<ContactDetail> getContact() { 14328 if (this.contact == null) 14329 this.contact = new ArrayList<ContactDetail>(); 14330 return this.contact; 14331 } 14332 14333 /** 14334 * @return Returns a reference to <code>this</code> for easy method chaining 14335 */ 14336 public ImplementationGuide setContact(List<ContactDetail> theContact) { 14337 this.contact = theContact; 14338 return this; 14339 } 14340 14341 public boolean hasContact() { 14342 if (this.contact == null) 14343 return false; 14344 for (ContactDetail item : this.contact) 14345 if (!item.isEmpty()) 14346 return true; 14347 return false; 14348 } 14349 14350 public ContactDetail addContact() { //3 14351 ContactDetail t = new ContactDetail(); 14352 if (this.contact == null) 14353 this.contact = new ArrayList<ContactDetail>(); 14354 this.contact.add(t); 14355 return t; 14356 } 14357 14358 public ImplementationGuide addContact(ContactDetail t) { //3 14359 if (t == null) 14360 return this; 14361 if (this.contact == null) 14362 this.contact = new ArrayList<ContactDetail>(); 14363 this.contact.add(t); 14364 return this; 14365 } 14366 14367 /** 14368 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 14369 */ 14370 public ContactDetail getContactFirstRep() { 14371 if (getContact().isEmpty()) { 14372 addContact(); 14373 } 14374 return getContact().get(0); 14375 } 14376 14377 /** 14378 * @return {@link #description} (A free text natural language description of the implementation guide from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 14379 */ 14380 public MarkdownType getDescriptionElement() { 14381 if (this.description == null) 14382 if (Configuration.errorOnAutoCreate()) 14383 throw new Error("Attempt to auto-create ImplementationGuide.description"); 14384 else if (Configuration.doAutoCreate()) 14385 this.description = new MarkdownType(); // bb 14386 return this.description; 14387 } 14388 14389 public boolean hasDescriptionElement() { 14390 return this.description != null && !this.description.isEmpty(); 14391 } 14392 14393 public boolean hasDescription() { 14394 return this.description != null && !this.description.isEmpty(); 14395 } 14396 14397 /** 14398 * @param value {@link #description} (A free text natural language description of the implementation guide from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 14399 */ 14400 public ImplementationGuide setDescriptionElement(MarkdownType value) { 14401 this.description = value; 14402 return this; 14403 } 14404 14405 /** 14406 * @return A free text natural language description of the implementation guide from a consumer's perspective. 14407 */ 14408 public String getDescription() { 14409 return this.description == null ? null : this.description.getValue(); 14410 } 14411 14412 /** 14413 * @param value A free text natural language description of the implementation guide from a consumer's perspective. 14414 */ 14415 public ImplementationGuide setDescription(String value) { 14416 if (Utilities.noString(value)) 14417 this.description = null; 14418 else { 14419 if (this.description == null) 14420 this.description = new MarkdownType(); 14421 this.description.setValue(value); 14422 } 14423 return this; 14424 } 14425 14426 /** 14427 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate implementation guide instances.) 14428 */ 14429 public List<UsageContext> getUseContext() { 14430 if (this.useContext == null) 14431 this.useContext = new ArrayList<UsageContext>(); 14432 return this.useContext; 14433 } 14434 14435 /** 14436 * @return Returns a reference to <code>this</code> for easy method chaining 14437 */ 14438 public ImplementationGuide setUseContext(List<UsageContext> theUseContext) { 14439 this.useContext = theUseContext; 14440 return this; 14441 } 14442 14443 public boolean hasUseContext() { 14444 if (this.useContext == null) 14445 return false; 14446 for (UsageContext item : this.useContext) 14447 if (!item.isEmpty()) 14448 return true; 14449 return false; 14450 } 14451 14452 public UsageContext addUseContext() { //3 14453 UsageContext t = new UsageContext(); 14454 if (this.useContext == null) 14455 this.useContext = new ArrayList<UsageContext>(); 14456 this.useContext.add(t); 14457 return t; 14458 } 14459 14460 public ImplementationGuide addUseContext(UsageContext t) { //3 14461 if (t == null) 14462 return this; 14463 if (this.useContext == null) 14464 this.useContext = new ArrayList<UsageContext>(); 14465 this.useContext.add(t); 14466 return this; 14467 } 14468 14469 /** 14470 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 14471 */ 14472 public UsageContext getUseContextFirstRep() { 14473 if (getUseContext().isEmpty()) { 14474 addUseContext(); 14475 } 14476 return getUseContext().get(0); 14477 } 14478 14479 /** 14480 * @return {@link #jurisdiction} (A legal or geographic region in which the implementation guide is intended to be used.) 14481 */ 14482 public List<CodeableConcept> getJurisdiction() { 14483 if (this.jurisdiction == null) 14484 this.jurisdiction = new ArrayList<CodeableConcept>(); 14485 return this.jurisdiction; 14486 } 14487 14488 /** 14489 * @return Returns a reference to <code>this</code> for easy method chaining 14490 */ 14491 public ImplementationGuide setJurisdiction(List<CodeableConcept> theJurisdiction) { 14492 this.jurisdiction = theJurisdiction; 14493 return this; 14494 } 14495 14496 public boolean hasJurisdiction() { 14497 if (this.jurisdiction == null) 14498 return false; 14499 for (CodeableConcept item : this.jurisdiction) 14500 if (!item.isEmpty()) 14501 return true; 14502 return false; 14503 } 14504 14505 public CodeableConcept addJurisdiction() { //3 14506 CodeableConcept t = new CodeableConcept(); 14507 if (this.jurisdiction == null) 14508 this.jurisdiction = new ArrayList<CodeableConcept>(); 14509 this.jurisdiction.add(t); 14510 return t; 14511 } 14512 14513 public ImplementationGuide addJurisdiction(CodeableConcept t) { //3 14514 if (t == null) 14515 return this; 14516 if (this.jurisdiction == null) 14517 this.jurisdiction = new ArrayList<CodeableConcept>(); 14518 this.jurisdiction.add(t); 14519 return this; 14520 } 14521 14522 /** 14523 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 14524 */ 14525 public CodeableConcept getJurisdictionFirstRep() { 14526 if (getJurisdiction().isEmpty()) { 14527 addJurisdiction(); 14528 } 14529 return getJurisdiction().get(0); 14530 } 14531 14532 /** 14533 * @return {@link #purpose} (Explanation of why this implementation guide is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 14534 */ 14535 public MarkdownType getPurposeElement() { 14536 if (this.purpose == null) 14537 if (Configuration.errorOnAutoCreate()) 14538 throw new Error("Attempt to auto-create ImplementationGuide.purpose"); 14539 else if (Configuration.doAutoCreate()) 14540 this.purpose = new MarkdownType(); // bb 14541 return this.purpose; 14542 } 14543 14544 public boolean hasPurposeElement() { 14545 return this.purpose != null && !this.purpose.isEmpty(); 14546 } 14547 14548 public boolean hasPurpose() { 14549 return this.purpose != null && !this.purpose.isEmpty(); 14550 } 14551 14552 /** 14553 * @param value {@link #purpose} (Explanation of why this implementation guide is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 14554 */ 14555 public ImplementationGuide setPurposeElement(MarkdownType value) { 14556 this.purpose = value; 14557 return this; 14558 } 14559 14560 /** 14561 * @return Explanation of why this implementation guide is needed and why it has been designed as it has. 14562 */ 14563 public String getPurpose() { 14564 return this.purpose == null ? null : this.purpose.getValue(); 14565 } 14566 14567 /** 14568 * @param value Explanation of why this implementation guide is needed and why it has been designed as it has. 14569 */ 14570 public ImplementationGuide setPurpose(String value) { 14571 if (Utilities.noString(value)) 14572 this.purpose = null; 14573 else { 14574 if (this.purpose == null) 14575 this.purpose = new MarkdownType(); 14576 this.purpose.setValue(value); 14577 } 14578 return this; 14579 } 14580 14581 /** 14582 * @return {@link #copyright} (A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 14583 */ 14584 public MarkdownType getCopyrightElement() { 14585 if (this.copyright == null) 14586 if (Configuration.errorOnAutoCreate()) 14587 throw new Error("Attempt to auto-create ImplementationGuide.copyright"); 14588 else if (Configuration.doAutoCreate()) 14589 this.copyright = new MarkdownType(); // bb 14590 return this.copyright; 14591 } 14592 14593 public boolean hasCopyrightElement() { 14594 return this.copyright != null && !this.copyright.isEmpty(); 14595 } 14596 14597 public boolean hasCopyright() { 14598 return this.copyright != null && !this.copyright.isEmpty(); 14599 } 14600 14601 /** 14602 * @param value {@link #copyright} (A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 14603 */ 14604 public ImplementationGuide setCopyrightElement(MarkdownType value) { 14605 this.copyright = value; 14606 return this; 14607 } 14608 14609 /** 14610 * @return A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide. 14611 */ 14612 public String getCopyright() { 14613 return this.copyright == null ? null : this.copyright.getValue(); 14614 } 14615 14616 /** 14617 * @param value A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide. 14618 */ 14619 public ImplementationGuide setCopyright(String value) { 14620 if (Utilities.noString(value)) 14621 this.copyright = null; 14622 else { 14623 if (this.copyright == null) 14624 this.copyright = new MarkdownType(); 14625 this.copyright.setValue(value); 14626 } 14627 return this; 14628 } 14629 14630 /** 14631 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 14632 */ 14633 public StringType getCopyrightLabelElement() { 14634 if (this.copyrightLabel == null) 14635 if (Configuration.errorOnAutoCreate()) 14636 throw new Error("Attempt to auto-create ImplementationGuide.copyrightLabel"); 14637 else if (Configuration.doAutoCreate()) 14638 this.copyrightLabel = new StringType(); // bb 14639 return this.copyrightLabel; 14640 } 14641 14642 public boolean hasCopyrightLabelElement() { 14643 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 14644 } 14645 14646 public boolean hasCopyrightLabel() { 14647 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 14648 } 14649 14650 /** 14651 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 14652 */ 14653 public ImplementationGuide setCopyrightLabelElement(StringType value) { 14654 this.copyrightLabel = value; 14655 return this; 14656 } 14657 14658 /** 14659 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 14660 */ 14661 public String getCopyrightLabel() { 14662 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 14663 } 14664 14665 /** 14666 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 14667 */ 14668 public ImplementationGuide setCopyrightLabel(String value) { 14669 if (Utilities.noString(value)) 14670 this.copyrightLabel = null; 14671 else { 14672 if (this.copyrightLabel == null) 14673 this.copyrightLabel = new StringType(); 14674 this.copyrightLabel.setValue(value); 14675 } 14676 return this; 14677 } 14678 14679 /** 14680 * @return {@link #packageId} (The NPM package name for this Implementation Guide, used in the NPM package distribution, which is the primary mechanism by which FHIR based tooling manages IG dependencies. This value must be globally unique, and should be assigned with care.). This is the underlying object with id, value and extensions. The accessor "getPackageId" gives direct access to the value 14681 */ 14682 public IdType getPackageIdElement() { 14683 if (this.packageId == null) 14684 if (Configuration.errorOnAutoCreate()) 14685 throw new Error("Attempt to auto-create ImplementationGuide.packageId"); 14686 else if (Configuration.doAutoCreate()) 14687 this.packageId = new IdType(); // bb 14688 return this.packageId; 14689 } 14690 14691 public boolean hasPackageIdElement() { 14692 return this.packageId != null && !this.packageId.isEmpty(); 14693 } 14694 14695 public boolean hasPackageId() { 14696 return this.packageId != null && !this.packageId.isEmpty(); 14697 } 14698 14699 /** 14700 * @param value {@link #packageId} (The NPM package name for this Implementation Guide, used in the NPM package distribution, which is the primary mechanism by which FHIR based tooling manages IG dependencies. This value must be globally unique, and should be assigned with care.). This is the underlying object with id, value and extensions. The accessor "getPackageId" gives direct access to the value 14701 */ 14702 public ImplementationGuide setPackageIdElement(IdType value) { 14703 this.packageId = value; 14704 return this; 14705 } 14706 14707 /** 14708 * @return The NPM package name for this Implementation Guide, used in the NPM package distribution, which is the primary mechanism by which FHIR based tooling manages IG dependencies. This value must be globally unique, and should be assigned with care. 14709 */ 14710 public String getPackageId() { 14711 return this.packageId == null ? null : this.packageId.getValue(); 14712 } 14713 14714 /** 14715 * @param value The NPM package name for this Implementation Guide, used in the NPM package distribution, which is the primary mechanism by which FHIR based tooling manages IG dependencies. This value must be globally unique, and should be assigned with care. 14716 */ 14717 public ImplementationGuide setPackageId(String value) { 14718 if (this.packageId == null) 14719 this.packageId = new IdType(); 14720 this.packageId.setValue(value); 14721 return this; 14722 } 14723 14724 /** 14725 * @return {@link #license} (The license that applies to this Implementation Guide, using an SPDX license code, or 'not-open-source'.). This is the underlying object with id, value and extensions. The accessor "getLicense" gives direct access to the value 14726 */ 14727 public Enumeration<SPDXLicense> getLicenseElement() { 14728 if (this.license == null) 14729 if (Configuration.errorOnAutoCreate()) 14730 throw new Error("Attempt to auto-create ImplementationGuide.license"); 14731 else if (Configuration.doAutoCreate()) 14732 this.license = new Enumeration<SPDXLicense>(new SPDXLicenseEnumFactory()); // bb 14733 return this.license; 14734 } 14735 14736 public boolean hasLicenseElement() { 14737 return this.license != null && !this.license.isEmpty(); 14738 } 14739 14740 public boolean hasLicense() { 14741 return this.license != null && !this.license.isEmpty(); 14742 } 14743 14744 /** 14745 * @param value {@link #license} (The license that applies to this Implementation Guide, using an SPDX license code, or 'not-open-source'.). This is the underlying object with id, value and extensions. The accessor "getLicense" gives direct access to the value 14746 */ 14747 public ImplementationGuide setLicenseElement(Enumeration<SPDXLicense> value) { 14748 this.license = value; 14749 return this; 14750 } 14751 14752 /** 14753 * @return The license that applies to this Implementation Guide, using an SPDX license code, or 'not-open-source'. 14754 */ 14755 public SPDXLicense getLicense() { 14756 return this.license == null ? null : this.license.getValue(); 14757 } 14758 14759 /** 14760 * @param value The license that applies to this Implementation Guide, using an SPDX license code, or 'not-open-source'. 14761 */ 14762 public ImplementationGuide setLicense(SPDXLicense value) { 14763 if (value == null) 14764 this.license = null; 14765 else { 14766 if (this.license == null) 14767 this.license = new Enumeration<SPDXLicense>(new SPDXLicenseEnumFactory()); 14768 this.license.setValue(value); 14769 } 14770 return this; 14771 } 14772 14773 /** 14774 * @return {@link #fhirVersion} (The version(s) of the FHIR specification that this ImplementationGuide targets - e.g. describes how to use. The value of this element is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version.) 14775 */ 14776 public List<Enumeration<FHIRVersion>> getFhirVersion() { 14777 if (this.fhirVersion == null) 14778 this.fhirVersion = new ArrayList<Enumeration<FHIRVersion>>(); 14779 return this.fhirVersion; 14780 } 14781 14782 /** 14783 * @return Returns a reference to <code>this</code> for easy method chaining 14784 */ 14785 public ImplementationGuide setFhirVersion(List<Enumeration<FHIRVersion>> theFhirVersion) { 14786 this.fhirVersion = theFhirVersion; 14787 return this; 14788 } 14789 14790 public boolean hasFhirVersion() { 14791 if (this.fhirVersion == null) 14792 return false; 14793 for (Enumeration<FHIRVersion> item : this.fhirVersion) 14794 if (!item.isEmpty()) 14795 return true; 14796 return false; 14797 } 14798 14799 /** 14800 * @return {@link #fhirVersion} (The version(s) of the FHIR specification that this ImplementationGuide targets - e.g. describes how to use. The value of this element is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version.) 14801 */ 14802 public Enumeration<FHIRVersion> addFhirVersionElement() {//2 14803 Enumeration<FHIRVersion> t = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); 14804 if (this.fhirVersion == null) 14805 this.fhirVersion = new ArrayList<Enumeration<FHIRVersion>>(); 14806 this.fhirVersion.add(t); 14807 return t; 14808 } 14809 14810 /** 14811 * @param value {@link #fhirVersion} (The version(s) of the FHIR specification that this ImplementationGuide targets - e.g. describes how to use. The value of this element is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version.) 14812 */ 14813 public ImplementationGuide addFhirVersion(FHIRVersion value) { //1 14814 Enumeration<FHIRVersion> t = new Enumeration<FHIRVersion>(new FHIRVersionEnumFactory()); 14815 t.setValue(value); 14816 if (this.fhirVersion == null) 14817 this.fhirVersion = new ArrayList<Enumeration<FHIRVersion>>(); 14818 this.fhirVersion.add(t); 14819 return this; 14820 } 14821 14822 /** 14823 * @param value {@link #fhirVersion} (The version(s) of the FHIR specification that this ImplementationGuide targets - e.g. describes how to use. The value of this element is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version.) 14824 */ 14825 public boolean hasFhirVersion(FHIRVersion value) { 14826 if (this.fhirVersion == null) 14827 return false; 14828 for (Enumeration<FHIRVersion> v : this.fhirVersion) 14829 if (v.getValue().equals(value)) // code 14830 return true; 14831 return false; 14832 } 14833 14834 /** 14835 * @return {@link #dependsOn} (Another implementation guide that this implementation depends on. Typically, an implementation guide uses value sets, profiles etc.defined in other implementation guides.) 14836 */ 14837 public List<ImplementationGuideDependsOnComponent> getDependsOn() { 14838 if (this.dependsOn == null) 14839 this.dependsOn = new ArrayList<ImplementationGuideDependsOnComponent>(); 14840 return this.dependsOn; 14841 } 14842 14843 /** 14844 * @return Returns a reference to <code>this</code> for easy method chaining 14845 */ 14846 public ImplementationGuide setDependsOn(List<ImplementationGuideDependsOnComponent> theDependsOn) { 14847 this.dependsOn = theDependsOn; 14848 return this; 14849 } 14850 14851 public boolean hasDependsOn() { 14852 if (this.dependsOn == null) 14853 return false; 14854 for (ImplementationGuideDependsOnComponent item : this.dependsOn) 14855 if (!item.isEmpty()) 14856 return true; 14857 return false; 14858 } 14859 14860 public ImplementationGuideDependsOnComponent addDependsOn() { //3 14861 ImplementationGuideDependsOnComponent t = new ImplementationGuideDependsOnComponent(); 14862 if (this.dependsOn == null) 14863 this.dependsOn = new ArrayList<ImplementationGuideDependsOnComponent>(); 14864 this.dependsOn.add(t); 14865 return t; 14866 } 14867 14868 public ImplementationGuide addDependsOn(ImplementationGuideDependsOnComponent t) { //3 14869 if (t == null) 14870 return this; 14871 if (this.dependsOn == null) 14872 this.dependsOn = new ArrayList<ImplementationGuideDependsOnComponent>(); 14873 this.dependsOn.add(t); 14874 return this; 14875 } 14876 14877 /** 14878 * @return The first repetition of repeating field {@link #dependsOn}, creating it if it does not already exist {3} 14879 */ 14880 public ImplementationGuideDependsOnComponent getDependsOnFirstRep() { 14881 if (getDependsOn().isEmpty()) { 14882 addDependsOn(); 14883 } 14884 return getDependsOn().get(0); 14885 } 14886 14887 /** 14888 * @return {@link #global} (A set of profiles that all resources covered by this implementation guide must conform to.) 14889 */ 14890 public List<ImplementationGuideGlobalComponent> getGlobal() { 14891 if (this.global == null) 14892 this.global = new ArrayList<ImplementationGuideGlobalComponent>(); 14893 return this.global; 14894 } 14895 14896 /** 14897 * @return Returns a reference to <code>this</code> for easy method chaining 14898 */ 14899 public ImplementationGuide setGlobal(List<ImplementationGuideGlobalComponent> theGlobal) { 14900 this.global = theGlobal; 14901 return this; 14902 } 14903 14904 public boolean hasGlobal() { 14905 if (this.global == null) 14906 return false; 14907 for (ImplementationGuideGlobalComponent item : this.global) 14908 if (!item.isEmpty()) 14909 return true; 14910 return false; 14911 } 14912 14913 public ImplementationGuideGlobalComponent addGlobal() { //3 14914 ImplementationGuideGlobalComponent t = new ImplementationGuideGlobalComponent(); 14915 if (this.global == null) 14916 this.global = new ArrayList<ImplementationGuideGlobalComponent>(); 14917 this.global.add(t); 14918 return t; 14919 } 14920 14921 public ImplementationGuide addGlobal(ImplementationGuideGlobalComponent t) { //3 14922 if (t == null) 14923 return this; 14924 if (this.global == null) 14925 this.global = new ArrayList<ImplementationGuideGlobalComponent>(); 14926 this.global.add(t); 14927 return this; 14928 } 14929 14930 /** 14931 * @return The first repetition of repeating field {@link #global}, creating it if it does not already exist {3} 14932 */ 14933 public ImplementationGuideGlobalComponent getGlobalFirstRep() { 14934 if (getGlobal().isEmpty()) { 14935 addGlobal(); 14936 } 14937 return getGlobal().get(0); 14938 } 14939 14940 /** 14941 * @return {@link #definition} (The information needed by an IG publisher tool to publish the whole implementation guide.) 14942 */ 14943 public ImplementationGuideDefinitionComponent getDefinition() { 14944 if (this.definition == null) 14945 if (Configuration.errorOnAutoCreate()) 14946 throw new Error("Attempt to auto-create ImplementationGuide.definition"); 14947 else if (Configuration.doAutoCreate()) 14948 this.definition = new ImplementationGuideDefinitionComponent(); // cc 14949 return this.definition; 14950 } 14951 14952 public boolean hasDefinition() { 14953 return this.definition != null && !this.definition.isEmpty(); 14954 } 14955 14956 /** 14957 * @param value {@link #definition} (The information needed by an IG publisher tool to publish the whole implementation guide.) 14958 */ 14959 public ImplementationGuide setDefinition(ImplementationGuideDefinitionComponent value) { 14960 this.definition = value; 14961 return this; 14962 } 14963 14964 /** 14965 * @return {@link #manifest} (Information about an assembled implementation guide, created by the publication tooling.) 14966 */ 14967 public ImplementationGuideManifestComponent getManifest() { 14968 if (this.manifest == null) 14969 if (Configuration.errorOnAutoCreate()) 14970 throw new Error("Attempt to auto-create ImplementationGuide.manifest"); 14971 else if (Configuration.doAutoCreate()) 14972 this.manifest = new ImplementationGuideManifestComponent(); // cc 14973 return this.manifest; 14974 } 14975 14976 public boolean hasManifest() { 14977 return this.manifest != null && !this.manifest.isEmpty(); 14978 } 14979 14980 /** 14981 * @param value {@link #manifest} (Information about an assembled implementation guide, created by the publication tooling.) 14982 */ 14983 public ImplementationGuide setManifest(ImplementationGuideManifestComponent value) { 14984 this.manifest = value; 14985 return this; 14986 } 14987 14988 protected void listChildren(List<Property> children) { 14989 super.listChildren(children); 14990 children.add(new Property("url", "uri", "An absolute URI that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this implementation guide is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the implementation guide is stored on different servers.", 0, 1, url)); 14991 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this implementation guide when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 14992 children.add(new Property("version", "string", "The identifier that is used to identify this version of the implementation guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the implementation guide author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 14993 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 14994 children.add(new Property("name", "string", "A natural language name identifying the implementation guide. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 14995 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the implementation guide.", 0, 1, title)); 14996 children.add(new Property("status", "code", "The status of this implementation guide. Enables tracking the life-cycle of the content.", 0, 1, status)); 14997 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this implementation guide is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 14998 children.add(new Property("date", "dateTime", "The date (and optionally time) when the implementation guide was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes.", 0, 1, date)); 14999 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the implementation guide.", 0, 1, publisher)); 15000 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 15001 children.add(new Property("description", "markdown", "A free text natural language description of the implementation guide from a consumer's perspective.", 0, 1, description)); 15002 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate implementation guide instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 15003 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the implementation guide is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 15004 children.add(new Property("purpose", "markdown", "Explanation of why this implementation guide is needed and why it has been designed as it has.", 0, 1, purpose)); 15005 children.add(new Property("copyright", "markdown", "A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide.", 0, 1, copyright)); 15006 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 15007 children.add(new Property("packageId", "id", "The NPM package name for this Implementation Guide, used in the NPM package distribution, which is the primary mechanism by which FHIR based tooling manages IG dependencies. This value must be globally unique, and should be assigned with care.", 0, 1, packageId)); 15008 children.add(new Property("license", "code", "The license that applies to this Implementation Guide, using an SPDX license code, or 'not-open-source'.", 0, 1, license)); 15009 children.add(new Property("fhirVersion", "code", "The version(s) of the FHIR specification that this ImplementationGuide targets - e.g. describes how to use. The value of this element is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version.", 0, java.lang.Integer.MAX_VALUE, fhirVersion)); 15010 children.add(new Property("dependsOn", "", "Another implementation guide that this implementation depends on. Typically, an implementation guide uses value sets, profiles etc.defined in other implementation guides.", 0, java.lang.Integer.MAX_VALUE, dependsOn)); 15011 children.add(new Property("global", "", "A set of profiles that all resources covered by this implementation guide must conform to.", 0, java.lang.Integer.MAX_VALUE, global)); 15012 children.add(new Property("definition", "", "The information needed by an IG publisher tool to publish the whole implementation guide.", 0, 1, definition)); 15013 children.add(new Property("manifest", "", "Information about an assembled implementation guide, created by the publication tooling.", 0, 1, manifest)); 15014 } 15015 15016 @Override 15017 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 15018 switch (_hash) { 15019 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this implementation guide is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the implementation guide is stored on different servers.", 0, 1, url); 15020 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this implementation guide when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 15021 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the implementation guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the implementation guide author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 15022 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 15023 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 15024 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 15025 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 15026 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the implementation guide. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 15027 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the implementation guide.", 0, 1, title); 15028 case -892481550: /*status*/ return new Property("status", "code", "The status of this implementation guide. Enables tracking the life-cycle of the content.", 0, 1, status); 15029 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this implementation guide is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 15030 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the implementation guide was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes.", 0, 1, date); 15031 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the implementation guide.", 0, 1, publisher); 15032 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 15033 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the implementation guide from a consumer's perspective.", 0, 1, description); 15034 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate implementation guide instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 15035 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the implementation guide is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 15036 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this implementation guide is needed and why it has been designed as it has.", 0, 1, purpose); 15037 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide.", 0, 1, copyright); 15038 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 15039 case 1802060801: /*packageId*/ return new Property("packageId", "id", "The NPM package name for this Implementation Guide, used in the NPM package distribution, which is the primary mechanism by which FHIR based tooling manages IG dependencies. This value must be globally unique, and should be assigned with care.", 0, 1, packageId); 15040 case 166757441: /*license*/ return new Property("license", "code", "The license that applies to this Implementation Guide, using an SPDX license code, or 'not-open-source'.", 0, 1, license); 15041 case 461006061: /*fhirVersion*/ return new Property("fhirVersion", "code", "The version(s) of the FHIR specification that this ImplementationGuide targets - e.g. describes how to use. The value of this element is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 4.6.0. for this version.", 0, java.lang.Integer.MAX_VALUE, fhirVersion); 15042 case -1109214266: /*dependsOn*/ return new Property("dependsOn", "", "Another implementation guide that this implementation depends on. Typically, an implementation guide uses value sets, profiles etc.defined in other implementation guides.", 0, java.lang.Integer.MAX_VALUE, dependsOn); 15043 case -1243020381: /*global*/ return new Property("global", "", "A set of profiles that all resources covered by this implementation guide must conform to.", 0, java.lang.Integer.MAX_VALUE, global); 15044 case -1014418093: /*definition*/ return new Property("definition", "", "The information needed by an IG publisher tool to publish the whole implementation guide.", 0, 1, definition); 15045 case 130625071: /*manifest*/ return new Property("manifest", "", "Information about an assembled implementation guide, created by the publication tooling.", 0, 1, manifest); 15046 default: return super.getNamedProperty(_hash, _name, _checkValid); 15047 } 15048 15049 } 15050 15051 @Override 15052 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 15053 switch (hash) { 15054 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 15055 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 15056 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 15057 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 15058 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 15059 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 15060 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 15061 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 15062 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 15063 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 15064 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 15065 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 15066 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 15067 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 15068 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 15069 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 15070 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 15071 case 1802060801: /*packageId*/ return this.packageId == null ? new Base[0] : new Base[] {this.packageId}; // IdType 15072 case 166757441: /*license*/ return this.license == null ? new Base[0] : new Base[] {this.license}; // Enumeration<SPDXLicense> 15073 case 461006061: /*fhirVersion*/ return this.fhirVersion == null ? new Base[0] : this.fhirVersion.toArray(new Base[this.fhirVersion.size()]); // Enumeration<FHIRVersion> 15074 case -1109214266: /*dependsOn*/ return this.dependsOn == null ? new Base[0] : this.dependsOn.toArray(new Base[this.dependsOn.size()]); // ImplementationGuideDependsOnComponent 15075 case -1243020381: /*global*/ return this.global == null ? new Base[0] : this.global.toArray(new Base[this.global.size()]); // ImplementationGuideGlobalComponent 15076 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // ImplementationGuideDefinitionComponent 15077 case 130625071: /*manifest*/ return this.manifest == null ? new Base[0] : new Base[] {this.manifest}; // ImplementationGuideManifestComponent 15078 default: return super.getProperty(hash, name, checkValid); 15079 } 15080 15081 } 15082 15083 @Override 15084 public Base setProperty(int hash, String name, Base value) throws FHIRException { 15085 switch (hash) { 15086 case 116079: // url 15087 this.url = TypeConvertor.castToUri(value); // UriType 15088 return value; 15089 case -1618432855: // identifier 15090 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 15091 return value; 15092 case 351608024: // version 15093 this.version = TypeConvertor.castToString(value); // StringType 15094 return value; 15095 case 1508158071: // versionAlgorithm 15096 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 15097 return value; 15098 case 3373707: // name 15099 this.name = TypeConvertor.castToString(value); // StringType 15100 return value; 15101 case 110371416: // title 15102 this.title = TypeConvertor.castToString(value); // StringType 15103 return value; 15104 case -892481550: // status 15105 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 15106 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 15107 return value; 15108 case -404562712: // experimental 15109 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 15110 return value; 15111 case 3076014: // date 15112 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 15113 return value; 15114 case 1447404028: // publisher 15115 this.publisher = TypeConvertor.castToString(value); // StringType 15116 return value; 15117 case 951526432: // contact 15118 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 15119 return value; 15120 case -1724546052: // description 15121 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 15122 return value; 15123 case -669707736: // useContext 15124 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 15125 return value; 15126 case -507075711: // jurisdiction 15127 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 15128 return value; 15129 case -220463842: // purpose 15130 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 15131 return value; 15132 case 1522889671: // copyright 15133 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 15134 return value; 15135 case 765157229: // copyrightLabel 15136 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 15137 return value; 15138 case 1802060801: // packageId 15139 this.packageId = TypeConvertor.castToId(value); // IdType 15140 return value; 15141 case 166757441: // license 15142 value = new SPDXLicenseEnumFactory().fromType(TypeConvertor.castToCode(value)); 15143 this.license = (Enumeration) value; // Enumeration<SPDXLicense> 15144 return value; 15145 case 461006061: // fhirVersion 15146 value = new FHIRVersionEnumFactory().fromType(TypeConvertor.castToCode(value)); 15147 this.getFhirVersion().add((Enumeration) value); // Enumeration<FHIRVersion> 15148 return value; 15149 case -1109214266: // dependsOn 15150 this.getDependsOn().add((ImplementationGuideDependsOnComponent) value); // ImplementationGuideDependsOnComponent 15151 return value; 15152 case -1243020381: // global 15153 this.getGlobal().add((ImplementationGuideGlobalComponent) value); // ImplementationGuideGlobalComponent 15154 return value; 15155 case -1014418093: // definition 15156 this.definition = (ImplementationGuideDefinitionComponent) value; // ImplementationGuideDefinitionComponent 15157 return value; 15158 case 130625071: // manifest 15159 this.manifest = (ImplementationGuideManifestComponent) value; // ImplementationGuideManifestComponent 15160 return value; 15161 default: return super.setProperty(hash, name, value); 15162 } 15163 15164 } 15165 15166 @Override 15167 public Base setProperty(String name, Base value) throws FHIRException { 15168 if (name.equals("url")) { 15169 this.url = TypeConvertor.castToUri(value); // UriType 15170 } else if (name.equals("identifier")) { 15171 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 15172 } else if (name.equals("version")) { 15173 this.version = TypeConvertor.castToString(value); // StringType 15174 } else if (name.equals("versionAlgorithm[x]")) { 15175 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 15176 } else if (name.equals("name")) { 15177 this.name = TypeConvertor.castToString(value); // StringType 15178 } else if (name.equals("title")) { 15179 this.title = TypeConvertor.castToString(value); // StringType 15180 } else if (name.equals("status")) { 15181 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 15182 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 15183 } else if (name.equals("experimental")) { 15184 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 15185 } else if (name.equals("date")) { 15186 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 15187 } else if (name.equals("publisher")) { 15188 this.publisher = TypeConvertor.castToString(value); // StringType 15189 } else if (name.equals("contact")) { 15190 this.getContact().add(TypeConvertor.castToContactDetail(value)); 15191 } else if (name.equals("description")) { 15192 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 15193 } else if (name.equals("useContext")) { 15194 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 15195 } else if (name.equals("jurisdiction")) { 15196 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 15197 } else if (name.equals("purpose")) { 15198 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 15199 } else if (name.equals("copyright")) { 15200 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 15201 } else if (name.equals("copyrightLabel")) { 15202 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 15203 } else if (name.equals("packageId")) { 15204 this.packageId = TypeConvertor.castToId(value); // IdType 15205 } else if (name.equals("license")) { 15206 value = new SPDXLicenseEnumFactory().fromType(TypeConvertor.castToCode(value)); 15207 this.license = (Enumeration) value; // Enumeration<SPDXLicense> 15208 } else if (name.equals("fhirVersion")) { 15209 value = new FHIRVersionEnumFactory().fromType(TypeConvertor.castToCode(value)); 15210 this.getFhirVersion().add((Enumeration) value); 15211 } else if (name.equals("dependsOn")) { 15212 this.getDependsOn().add((ImplementationGuideDependsOnComponent) value); 15213 } else if (name.equals("global")) { 15214 this.getGlobal().add((ImplementationGuideGlobalComponent) value); 15215 } else if (name.equals("definition")) { 15216 this.definition = (ImplementationGuideDefinitionComponent) value; // ImplementationGuideDefinitionComponent 15217 } else if (name.equals("manifest")) { 15218 this.manifest = (ImplementationGuideManifestComponent) value; // ImplementationGuideManifestComponent 15219 } else 15220 return super.setProperty(name, value); 15221 return value; 15222 } 15223 15224 @Override 15225 public void removeChild(String name, Base value) throws FHIRException { 15226 if (name.equals("url")) { 15227 this.url = null; 15228 } else if (name.equals("identifier")) { 15229 this.getIdentifier().remove(value); 15230 } else if (name.equals("version")) { 15231 this.version = null; 15232 } else if (name.equals("versionAlgorithm[x]")) { 15233 this.versionAlgorithm = null; 15234 } else if (name.equals("name")) { 15235 this.name = null; 15236 } else if (name.equals("title")) { 15237 this.title = null; 15238 } else if (name.equals("status")) { 15239 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 15240 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 15241 } else if (name.equals("experimental")) { 15242 this.experimental = null; 15243 } else if (name.equals("date")) { 15244 this.date = null; 15245 } else if (name.equals("publisher")) { 15246 this.publisher = null; 15247 } else if (name.equals("contact")) { 15248 this.getContact().remove(value); 15249 } else if (name.equals("description")) { 15250 this.description = null; 15251 } else if (name.equals("useContext")) { 15252 this.getUseContext().remove(value); 15253 } else if (name.equals("jurisdiction")) { 15254 this.getJurisdiction().remove(value); 15255 } else if (name.equals("purpose")) { 15256 this.purpose = null; 15257 } else if (name.equals("copyright")) { 15258 this.copyright = null; 15259 } else if (name.equals("copyrightLabel")) { 15260 this.copyrightLabel = null; 15261 } else if (name.equals("packageId")) { 15262 this.packageId = null; 15263 } else if (name.equals("license")) { 15264 value = new SPDXLicenseEnumFactory().fromType(TypeConvertor.castToCode(value)); 15265 this.license = (Enumeration) value; // Enumeration<SPDXLicense> 15266 } else if (name.equals("fhirVersion")) { 15267 value = new FHIRVersionEnumFactory().fromType(TypeConvertor.castToCode(value)); 15268 this.getFhirVersion().remove((Enumeration) value); 15269 } else if (name.equals("dependsOn")) { 15270 this.getDependsOn().remove((ImplementationGuideDependsOnComponent) value); 15271 } else if (name.equals("global")) { 15272 this.getGlobal().remove((ImplementationGuideGlobalComponent) value); 15273 } else if (name.equals("definition")) { 15274 this.definition = (ImplementationGuideDefinitionComponent) value; // ImplementationGuideDefinitionComponent 15275 } else if (name.equals("manifest")) { 15276 this.manifest = (ImplementationGuideManifestComponent) value; // ImplementationGuideManifestComponent 15277 } else 15278 super.removeChild(name, value); 15279 15280 } 15281 15282 @Override 15283 public Base makeProperty(int hash, String name) throws FHIRException { 15284 switch (hash) { 15285 case 116079: return getUrlElement(); 15286 case -1618432855: return addIdentifier(); 15287 case 351608024: return getVersionElement(); 15288 case -115699031: return getVersionAlgorithm(); 15289 case 1508158071: return getVersionAlgorithm(); 15290 case 3373707: return getNameElement(); 15291 case 110371416: return getTitleElement(); 15292 case -892481550: return getStatusElement(); 15293 case -404562712: return getExperimentalElement(); 15294 case 3076014: return getDateElement(); 15295 case 1447404028: return getPublisherElement(); 15296 case 951526432: return addContact(); 15297 case -1724546052: return getDescriptionElement(); 15298 case -669707736: return addUseContext(); 15299 case -507075711: return addJurisdiction(); 15300 case -220463842: return getPurposeElement(); 15301 case 1522889671: return getCopyrightElement(); 15302 case 765157229: return getCopyrightLabelElement(); 15303 case 1802060801: return getPackageIdElement(); 15304 case 166757441: return getLicenseElement(); 15305 case 461006061: return addFhirVersionElement(); 15306 case -1109214266: return addDependsOn(); 15307 case -1243020381: return addGlobal(); 15308 case -1014418093: return getDefinition(); 15309 case 130625071: return getManifest(); 15310 default: return super.makeProperty(hash, name); 15311 } 15312 15313 } 15314 15315 @Override 15316 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 15317 switch (hash) { 15318 case 116079: /*url*/ return new String[] {"uri"}; 15319 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 15320 case 351608024: /*version*/ return new String[] {"string"}; 15321 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 15322 case 3373707: /*name*/ return new String[] {"string"}; 15323 case 110371416: /*title*/ return new String[] {"string"}; 15324 case -892481550: /*status*/ return new String[] {"code"}; 15325 case -404562712: /*experimental*/ return new String[] {"boolean"}; 15326 case 3076014: /*date*/ return new String[] {"dateTime"}; 15327 case 1447404028: /*publisher*/ return new String[] {"string"}; 15328 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 15329 case -1724546052: /*description*/ return new String[] {"markdown"}; 15330 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 15331 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 15332 case -220463842: /*purpose*/ return new String[] {"markdown"}; 15333 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 15334 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 15335 case 1802060801: /*packageId*/ return new String[] {"id"}; 15336 case 166757441: /*license*/ return new String[] {"code"}; 15337 case 461006061: /*fhirVersion*/ return new String[] {"code"}; 15338 case -1109214266: /*dependsOn*/ return new String[] {}; 15339 case -1243020381: /*global*/ return new String[] {}; 15340 case -1014418093: /*definition*/ return new String[] {}; 15341 case 130625071: /*manifest*/ return new String[] {}; 15342 default: return super.getTypesForProperty(hash, name); 15343 } 15344 15345 } 15346 15347 @Override 15348 public Base addChild(String name) throws FHIRException { 15349 if (name.equals("url")) { 15350 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.url"); 15351 } 15352 else if (name.equals("identifier")) { 15353 return addIdentifier(); 15354 } 15355 else if (name.equals("version")) { 15356 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.version"); 15357 } 15358 else if (name.equals("versionAlgorithmString")) { 15359 this.versionAlgorithm = new StringType(); 15360 return this.versionAlgorithm; 15361 } 15362 else if (name.equals("versionAlgorithmCoding")) { 15363 this.versionAlgorithm = new Coding(); 15364 return this.versionAlgorithm; 15365 } 15366 else if (name.equals("name")) { 15367 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.name"); 15368 } 15369 else if (name.equals("title")) { 15370 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.title"); 15371 } 15372 else if (name.equals("status")) { 15373 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.status"); 15374 } 15375 else if (name.equals("experimental")) { 15376 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.experimental"); 15377 } 15378 else if (name.equals("date")) { 15379 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.date"); 15380 } 15381 else if (name.equals("publisher")) { 15382 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.publisher"); 15383 } 15384 else if (name.equals("contact")) { 15385 return addContact(); 15386 } 15387 else if (name.equals("description")) { 15388 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.description"); 15389 } 15390 else if (name.equals("useContext")) { 15391 return addUseContext(); 15392 } 15393 else if (name.equals("jurisdiction")) { 15394 return addJurisdiction(); 15395 } 15396 else if (name.equals("purpose")) { 15397 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.purpose"); 15398 } 15399 else if (name.equals("copyright")) { 15400 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.copyright"); 15401 } 15402 else if (name.equals("copyrightLabel")) { 15403 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.copyrightLabel"); 15404 } 15405 else if (name.equals("packageId")) { 15406 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.packageId"); 15407 } 15408 else if (name.equals("license")) { 15409 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.license"); 15410 } 15411 else if (name.equals("fhirVersion")) { 15412 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.fhirVersion"); 15413 } 15414 else if (name.equals("dependsOn")) { 15415 return addDependsOn(); 15416 } 15417 else if (name.equals("global")) { 15418 return addGlobal(); 15419 } 15420 else if (name.equals("definition")) { 15421 this.definition = new ImplementationGuideDefinitionComponent(); 15422 return this.definition; 15423 } 15424 else if (name.equals("manifest")) { 15425 this.manifest = new ImplementationGuideManifestComponent(); 15426 return this.manifest; 15427 } 15428 else 15429 return super.addChild(name); 15430 } 15431 15432 public String fhirType() { 15433 return "ImplementationGuide"; 15434 15435 } 15436 15437 public ImplementationGuide copy() { 15438 ImplementationGuide dst = new ImplementationGuide(); 15439 copyValues(dst); 15440 return dst; 15441 } 15442 15443 public void copyValues(ImplementationGuide dst) { 15444 super.copyValues(dst); 15445 dst.url = url == null ? null : url.copy(); 15446 if (identifier != null) { 15447 dst.identifier = new ArrayList<Identifier>(); 15448 for (Identifier i : identifier) 15449 dst.identifier.add(i.copy()); 15450 }; 15451 dst.version = version == null ? null : version.copy(); 15452 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 15453 dst.name = name == null ? null : name.copy(); 15454 dst.title = title == null ? null : title.copy(); 15455 dst.status = status == null ? null : status.copy(); 15456 dst.experimental = experimental == null ? null : experimental.copy(); 15457 dst.date = date == null ? null : date.copy(); 15458 dst.publisher = publisher == null ? null : publisher.copy(); 15459 if (contact != null) { 15460 dst.contact = new ArrayList<ContactDetail>(); 15461 for (ContactDetail i : contact) 15462 dst.contact.add(i.copy()); 15463 }; 15464 dst.description = description == null ? null : description.copy(); 15465 if (useContext != null) { 15466 dst.useContext = new ArrayList<UsageContext>(); 15467 for (UsageContext i : useContext) 15468 dst.useContext.add(i.copy()); 15469 }; 15470 if (jurisdiction != null) { 15471 dst.jurisdiction = new ArrayList<CodeableConcept>(); 15472 for (CodeableConcept i : jurisdiction) 15473 dst.jurisdiction.add(i.copy()); 15474 }; 15475 dst.purpose = purpose == null ? null : purpose.copy(); 15476 dst.copyright = copyright == null ? null : copyright.copy(); 15477 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 15478 dst.packageId = packageId == null ? null : packageId.copy(); 15479 dst.license = license == null ? null : license.copy(); 15480 if (fhirVersion != null) { 15481 dst.fhirVersion = new ArrayList<Enumeration<FHIRVersion>>(); 15482 for (Enumeration<FHIRVersion> i : fhirVersion) 15483 dst.fhirVersion.add(i.copy()); 15484 }; 15485 if (dependsOn != null) { 15486 dst.dependsOn = new ArrayList<ImplementationGuideDependsOnComponent>(); 15487 for (ImplementationGuideDependsOnComponent i : dependsOn) 15488 dst.dependsOn.add(i.copy()); 15489 }; 15490 if (global != null) { 15491 dst.global = new ArrayList<ImplementationGuideGlobalComponent>(); 15492 for (ImplementationGuideGlobalComponent i : global) 15493 dst.global.add(i.copy()); 15494 }; 15495 dst.definition = definition == null ? null : definition.copy(); 15496 dst.manifest = manifest == null ? null : manifest.copy(); 15497 } 15498 15499 protected ImplementationGuide typedCopy() { 15500 return copy(); 15501 } 15502 15503 @Override 15504 public boolean equalsDeep(Base other_) { 15505 if (!super.equalsDeep(other_)) 15506 return false; 15507 if (!(other_ instanceof ImplementationGuide)) 15508 return false; 15509 ImplementationGuide o = (ImplementationGuide) other_; 15510 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 15511 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 15512 && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) && compareDeep(date, o.date, true) 15513 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 15514 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 15515 && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) && compareDeep(copyrightLabel, o.copyrightLabel, true) 15516 && compareDeep(packageId, o.packageId, true) && compareDeep(license, o.license, true) && compareDeep(fhirVersion, o.fhirVersion, true) 15517 && compareDeep(dependsOn, o.dependsOn, true) && compareDeep(global, o.global, true) && compareDeep(definition, o.definition, true) 15518 && compareDeep(manifest, o.manifest, true); 15519 } 15520 15521 @Override 15522 public boolean equalsShallow(Base other_) { 15523 if (!super.equalsShallow(other_)) 15524 return false; 15525 if (!(other_ instanceof ImplementationGuide)) 15526 return false; 15527 ImplementationGuide o = (ImplementationGuide) other_; 15528 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 15529 && compareValues(title, o.title, true) && compareValues(status, o.status, true) && compareValues(experimental, o.experimental, true) 15530 && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) 15531 && compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 15532 && compareValues(packageId, o.packageId, true) && compareValues(license, o.license, true) && compareValues(fhirVersion, o.fhirVersion, true) 15533 ; 15534 } 15535 15536 public boolean isEmpty() { 15537 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 15538 , versionAlgorithm, name, title, status, experimental, date, publisher, contact 15539 , description, useContext, jurisdiction, purpose, copyright, copyrightLabel, packageId 15540 , license, fhirVersion, dependsOn, global, definition, manifest); 15541 } 15542 15543 @Override 15544 public ResourceType getResourceType() { 15545 return ResourceType.ImplementationGuide; 15546 } 15547 15548 /** 15549 * Search parameter: <b>context-quantity</b> 15550 * <p> 15551 * Description: <b>Multiple Resources: 15552 15553 * [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 15554 * [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 15555 * [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 15556 * [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 15557 * [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 15558 * [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 15559 * [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 15560 * [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 15561 * [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 15562 * [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 15563 * [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 15564 * [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 15565 * [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 15566 * [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 15567 * [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 15568 * [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 15569 * [Library](library.html): A quantity- or range-valued use context assigned to the library 15570 * [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 15571 * [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 15572 * [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 15573 * [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 15574 * [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 15575 * [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 15576 * [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 15577 * [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 15578 * [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 15579 * [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 15580 * [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 15581 * [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 15582 * [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 15583</b><br> 15584 * Type: <b>quantity</b><br> 15585 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 15586 * </p> 15587 */ 15588 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 15589 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 15590 /** 15591 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 15592 * <p> 15593 * Description: <b>Multiple Resources: 15594 15595 * [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 15596 * [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 15597 * [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 15598 * [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 15599 * [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 15600 * [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 15601 * [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 15602 * [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 15603 * [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 15604 * [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 15605 * [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 15606 * [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 15607 * [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 15608 * [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 15609 * [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 15610 * [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 15611 * [Library](library.html): A quantity- or range-valued use context assigned to the library 15612 * [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 15613 * [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 15614 * [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 15615 * [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 15616 * [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 15617 * [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 15618 * [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 15619 * [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 15620 * [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 15621 * [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 15622 * [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 15623 * [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 15624 * [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 15625</b><br> 15626 * Type: <b>quantity</b><br> 15627 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 15628 * </p> 15629 */ 15630 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 15631 15632 /** 15633 * Search parameter: <b>context-type-quantity</b> 15634 * <p> 15635 * Description: <b>Multiple Resources: 15636 15637 * [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 15638 * [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 15639 * [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 15640 * [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 15641 * [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 15642 * [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 15643 * [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 15644 * [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 15645 * [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 15646 * [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 15647 * [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 15648 * [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 15649 * [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 15650 * [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 15651 * [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 15652 * [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 15653 * [Library](library.html): A use context type and quantity- or range-based value assigned to the library 15654 * [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 15655 * [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 15656 * [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 15657 * [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 15658 * [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 15659 * [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 15660 * [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 15661 * [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 15662 * [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 15663 * [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 15664 * [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 15665 * [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 15666 * [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 15667</b><br> 15668 * Type: <b>composite</b><br> 15669 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 15670 * </p> 15671 */ 15672 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 15673 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 15674 /** 15675 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 15676 * <p> 15677 * Description: <b>Multiple Resources: 15678 15679 * [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 15680 * [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 15681 * [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 15682 * [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 15683 * [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 15684 * [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 15685 * [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 15686 * [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 15687 * [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 15688 * [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 15689 * [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 15690 * [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 15691 * [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 15692 * [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 15693 * [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 15694 * [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 15695 * [Library](library.html): A use context type and quantity- or range-based value assigned to the library 15696 * [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 15697 * [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 15698 * [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 15699 * [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 15700 * [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 15701 * [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 15702 * [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 15703 * [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 15704 * [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 15705 * [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 15706 * [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 15707 * [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 15708 * [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 15709</b><br> 15710 * Type: <b>composite</b><br> 15711 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 15712 * </p> 15713 */ 15714 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 15715 15716 /** 15717 * Search parameter: <b>context-type-value</b> 15718 * <p> 15719 * Description: <b>Multiple Resources: 15720 15721 * [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 15722 * [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 15723 * [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 15724 * [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 15725 * [Citation](citation.html): A use context type and value assigned to the citation 15726 * [CodeSystem](codesystem.html): A use context type and value assigned to the code system 15727 * [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 15728 * [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 15729 * [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 15730 * [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 15731 * [Evidence](evidence.html): A use context type and value assigned to the evidence 15732 * [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 15733 * [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 15734 * [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 15735 * [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 15736 * [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 15737 * [Library](library.html): A use context type and value assigned to the library 15738 * [Measure](measure.html): A use context type and value assigned to the measure 15739 * [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 15740 * [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 15741 * [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 15742 * [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 15743 * [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 15744 * [Requirements](requirements.html): A use context type and value assigned to the requirements 15745 * [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 15746 * [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 15747 * [StructureMap](structuremap.html): A use context type and value assigned to the structure map 15748 * [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 15749 * [TestScript](testscript.html): A use context type and value assigned to the test script 15750 * [ValueSet](valueset.html): A use context type and value assigned to the value set 15751</b><br> 15752 * Type: <b>composite</b><br> 15753 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 15754 * </p> 15755 */ 15756 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 15757 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 15758 /** 15759 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 15760 * <p> 15761 * Description: <b>Multiple Resources: 15762 15763 * [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 15764 * [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 15765 * [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 15766 * [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 15767 * [Citation](citation.html): A use context type and value assigned to the citation 15768 * [CodeSystem](codesystem.html): A use context type and value assigned to the code system 15769 * [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 15770 * [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 15771 * [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 15772 * [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 15773 * [Evidence](evidence.html): A use context type and value assigned to the evidence 15774 * [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 15775 * [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 15776 * [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 15777 * [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 15778 * [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 15779 * [Library](library.html): A use context type and value assigned to the library 15780 * [Measure](measure.html): A use context type and value assigned to the measure 15781 * [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 15782 * [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 15783 * [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 15784 * [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 15785 * [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 15786 * [Requirements](requirements.html): A use context type and value assigned to the requirements 15787 * [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 15788 * [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 15789 * [StructureMap](structuremap.html): A use context type and value assigned to the structure map 15790 * [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 15791 * [TestScript](testscript.html): A use context type and value assigned to the test script 15792 * [ValueSet](valueset.html): A use context type and value assigned to the value set 15793</b><br> 15794 * Type: <b>composite</b><br> 15795 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 15796 * </p> 15797 */ 15798 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 15799 15800 /** 15801 * Search parameter: <b>context-type</b> 15802 * <p> 15803 * Description: <b>Multiple Resources: 15804 15805 * [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 15806 * [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 15807 * [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 15808 * [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 15809 * [Citation](citation.html): A type of use context assigned to the citation 15810 * [CodeSystem](codesystem.html): A type of use context assigned to the code system 15811 * [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 15812 * [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 15813 * [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 15814 * [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 15815 * [Evidence](evidence.html): A type of use context assigned to the evidence 15816 * [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 15817 * [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 15818 * [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 15819 * [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 15820 * [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 15821 * [Library](library.html): A type of use context assigned to the library 15822 * [Measure](measure.html): A type of use context assigned to the measure 15823 * [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 15824 * [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 15825 * [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 15826 * [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 15827 * [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 15828 * [Requirements](requirements.html): A type of use context assigned to the requirements 15829 * [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 15830 * [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 15831 * [StructureMap](structuremap.html): A type of use context assigned to the structure map 15832 * [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 15833 * [TestScript](testscript.html): A type of use context assigned to the test script 15834 * [ValueSet](valueset.html): A type of use context assigned to the value set 15835</b><br> 15836 * Type: <b>token</b><br> 15837 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 15838 * </p> 15839 */ 15840 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 15841 public static final String SP_CONTEXT_TYPE = "context-type"; 15842 /** 15843 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 15844 * <p> 15845 * Description: <b>Multiple Resources: 15846 15847 * [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 15848 * [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 15849 * [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 15850 * [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 15851 * [Citation](citation.html): A type of use context assigned to the citation 15852 * [CodeSystem](codesystem.html): A type of use context assigned to the code system 15853 * [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 15854 * [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 15855 * [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 15856 * [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 15857 * [Evidence](evidence.html): A type of use context assigned to the evidence 15858 * [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 15859 * [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 15860 * [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 15861 * [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 15862 * [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 15863 * [Library](library.html): A type of use context assigned to the library 15864 * [Measure](measure.html): A type of use context assigned to the measure 15865 * [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 15866 * [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 15867 * [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 15868 * [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 15869 * [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 15870 * [Requirements](requirements.html): A type of use context assigned to the requirements 15871 * [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 15872 * [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 15873 * [StructureMap](structuremap.html): A type of use context assigned to the structure map 15874 * [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 15875 * [TestScript](testscript.html): A type of use context assigned to the test script 15876 * [ValueSet](valueset.html): A type of use context assigned to the value set 15877</b><br> 15878 * Type: <b>token</b><br> 15879 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 15880 * </p> 15881 */ 15882 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 15883 15884 /** 15885 * Search parameter: <b>context</b> 15886 * <p> 15887 * Description: <b>Multiple Resources: 15888 15889 * [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 15890 * [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 15891 * [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 15892 * [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 15893 * [Citation](citation.html): A use context assigned to the citation 15894 * [CodeSystem](codesystem.html): A use context assigned to the code system 15895 * [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 15896 * [ConceptMap](conceptmap.html): A use context assigned to the concept map 15897 * [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 15898 * [EventDefinition](eventdefinition.html): A use context assigned to the event definition 15899 * [Evidence](evidence.html): A use context assigned to the evidence 15900 * [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 15901 * [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 15902 * [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 15903 * [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 15904 * [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 15905 * [Library](library.html): A use context assigned to the library 15906 * [Measure](measure.html): A use context assigned to the measure 15907 * [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 15908 * [NamingSystem](namingsystem.html): A use context assigned to the naming system 15909 * [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 15910 * [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 15911 * [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 15912 * [Requirements](requirements.html): A use context assigned to the requirements 15913 * [SearchParameter](searchparameter.html): A use context assigned to the search parameter 15914 * [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 15915 * [StructureMap](structuremap.html): A use context assigned to the structure map 15916 * [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 15917 * [TestScript](testscript.html): A use context assigned to the test script 15918 * [ValueSet](valueset.html): A use context assigned to the value set 15919</b><br> 15920 * Type: <b>token</b><br> 15921 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 15922 * </p> 15923 */ 15924 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 15925 public static final String SP_CONTEXT = "context"; 15926 /** 15927 * <b>Fluent Client</b> search parameter constant for <b>context</b> 15928 * <p> 15929 * Description: <b>Multiple Resources: 15930 15931 * [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 15932 * [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 15933 * [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 15934 * [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 15935 * [Citation](citation.html): A use context assigned to the citation 15936 * [CodeSystem](codesystem.html): A use context assigned to the code system 15937 * [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 15938 * [ConceptMap](conceptmap.html): A use context assigned to the concept map 15939 * [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 15940 * [EventDefinition](eventdefinition.html): A use context assigned to the event definition 15941 * [Evidence](evidence.html): A use context assigned to the evidence 15942 * [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 15943 * [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 15944 * [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 15945 * [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 15946 * [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 15947 * [Library](library.html): A use context assigned to the library 15948 * [Measure](measure.html): A use context assigned to the measure 15949 * [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 15950 * [NamingSystem](namingsystem.html): A use context assigned to the naming system 15951 * [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 15952 * [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 15953 * [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 15954 * [Requirements](requirements.html): A use context assigned to the requirements 15955 * [SearchParameter](searchparameter.html): A use context assigned to the search parameter 15956 * [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 15957 * [StructureMap](structuremap.html): A use context assigned to the structure map 15958 * [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 15959 * [TestScript](testscript.html): A use context assigned to the test script 15960 * [ValueSet](valueset.html): A use context assigned to the value set 15961</b><br> 15962 * Type: <b>token</b><br> 15963 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 15964 * </p> 15965 */ 15966 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 15967 15968 /** 15969 * Search parameter: <b>date</b> 15970 * <p> 15971 * Description: <b>Multiple Resources: 15972 15973 * [ActivityDefinition](activitydefinition.html): The activity definition publication date 15974 * [ActorDefinition](actordefinition.html): The Actor Definition publication date 15975 * [CapabilityStatement](capabilitystatement.html): The capability statement publication date 15976 * [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 15977 * [Citation](citation.html): The citation publication date 15978 * [CodeSystem](codesystem.html): The code system publication date 15979 * [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 15980 * [ConceptMap](conceptmap.html): The concept map publication date 15981 * [ConditionDefinition](conditiondefinition.html): The condition definition publication date 15982 * [EventDefinition](eventdefinition.html): The event definition publication date 15983 * [Evidence](evidence.html): The evidence publication date 15984 * [EvidenceVariable](evidencevariable.html): The evidence variable publication date 15985 * [ExampleScenario](examplescenario.html): The example scenario publication date 15986 * [GraphDefinition](graphdefinition.html): The graph definition publication date 15987 * [ImplementationGuide](implementationguide.html): The implementation guide publication date 15988 * [Library](library.html): The library publication date 15989 * [Measure](measure.html): The measure publication date 15990 * [MessageDefinition](messagedefinition.html): The message definition publication date 15991 * [NamingSystem](namingsystem.html): The naming system publication date 15992 * [OperationDefinition](operationdefinition.html): The operation definition publication date 15993 * [PlanDefinition](plandefinition.html): The plan definition publication date 15994 * [Questionnaire](questionnaire.html): The questionnaire publication date 15995 * [Requirements](requirements.html): The requirements publication date 15996 * [SearchParameter](searchparameter.html): The search parameter publication date 15997 * [StructureDefinition](structuredefinition.html): The structure definition publication date 15998 * [StructureMap](structuremap.html): The structure map publication date 15999 * [SubscriptionTopic](subscriptiontopic.html): Date status first applied 16000 * [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 16001 * [TestScript](testscript.html): The test script publication date 16002 * [ValueSet](valueset.html): The value set publication date 16003</b><br> 16004 * Type: <b>date</b><br> 16005 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 16006 * </p> 16007 */ 16008 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 16009 public static final String SP_DATE = "date"; 16010 /** 16011 * <b>Fluent Client</b> search parameter constant for <b>date</b> 16012 * <p> 16013 * Description: <b>Multiple Resources: 16014 16015 * [ActivityDefinition](activitydefinition.html): The activity definition publication date 16016 * [ActorDefinition](actordefinition.html): The Actor Definition publication date 16017 * [CapabilityStatement](capabilitystatement.html): The capability statement publication date 16018 * [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 16019 * [Citation](citation.html): The citation publication date 16020 * [CodeSystem](codesystem.html): The code system publication date 16021 * [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 16022 * [ConceptMap](conceptmap.html): The concept map publication date 16023 * [ConditionDefinition](conditiondefinition.html): The condition definition publication date 16024 * [EventDefinition](eventdefinition.html): The event definition publication date 16025 * [Evidence](evidence.html): The evidence publication date 16026 * [EvidenceVariable](evidencevariable.html): The evidence variable publication date 16027 * [ExampleScenario](examplescenario.html): The example scenario publication date 16028 * [GraphDefinition](graphdefinition.html): The graph definition publication date 16029 * [ImplementationGuide](implementationguide.html): The implementation guide publication date 16030 * [Library](library.html): The library publication date 16031 * [Measure](measure.html): The measure publication date 16032 * [MessageDefinition](messagedefinition.html): The message definition publication date 16033 * [NamingSystem](namingsystem.html): The naming system publication date 16034 * [OperationDefinition](operationdefinition.html): The operation definition publication date 16035 * [PlanDefinition](plandefinition.html): The plan definition publication date 16036 * [Questionnaire](questionnaire.html): The questionnaire publication date 16037 * [Requirements](requirements.html): The requirements publication date 16038 * [SearchParameter](searchparameter.html): The search parameter publication date 16039 * [StructureDefinition](structuredefinition.html): The structure definition publication date 16040 * [StructureMap](structuremap.html): The structure map publication date 16041 * [SubscriptionTopic](subscriptiontopic.html): Date status first applied 16042 * [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 16043 * [TestScript](testscript.html): The test script publication date 16044 * [ValueSet](valueset.html): The value set publication date 16045</b><br> 16046 * Type: <b>date</b><br> 16047 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 16048 * </p> 16049 */ 16050 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 16051 16052 /** 16053 * Search parameter: <b>description</b> 16054 * <p> 16055 * Description: <b>Multiple Resources: 16056 16057 * [ActivityDefinition](activitydefinition.html): The description of the activity definition 16058 * [ActorDefinition](actordefinition.html): The description of the Actor Definition 16059 * [CapabilityStatement](capabilitystatement.html): The description of the capability statement 16060 * [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 16061 * [Citation](citation.html): The description of the citation 16062 * [CodeSystem](codesystem.html): The description of the code system 16063 * [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 16064 * [ConceptMap](conceptmap.html): The description of the concept map 16065 * [ConditionDefinition](conditiondefinition.html): The description of the condition definition 16066 * [EventDefinition](eventdefinition.html): The description of the event definition 16067 * [Evidence](evidence.html): The description of the evidence 16068 * [EvidenceVariable](evidencevariable.html): The description of the evidence variable 16069 * [GraphDefinition](graphdefinition.html): The description of the graph definition 16070 * [ImplementationGuide](implementationguide.html): The description of the implementation guide 16071 * [Library](library.html): The description of the library 16072 * [Measure](measure.html): The description of the measure 16073 * [MessageDefinition](messagedefinition.html): The description of the message definition 16074 * [NamingSystem](namingsystem.html): The description of the naming system 16075 * [OperationDefinition](operationdefinition.html): The description of the operation definition 16076 * [PlanDefinition](plandefinition.html): The description of the plan definition 16077 * [Questionnaire](questionnaire.html): The description of the questionnaire 16078 * [Requirements](requirements.html): The description of the requirements 16079 * [SearchParameter](searchparameter.html): The description of the search parameter 16080 * [StructureDefinition](structuredefinition.html): The description of the structure definition 16081 * [StructureMap](structuremap.html): The description of the structure map 16082 * [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 16083 * [TestScript](testscript.html): The description of the test script 16084 * [ValueSet](valueset.html): The description of the value set 16085</b><br> 16086 * Type: <b>string</b><br> 16087 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 16088 * </p> 16089 */ 16090 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 16091 public static final String SP_DESCRIPTION = "description"; 16092 /** 16093 * <b>Fluent Client</b> search parameter constant for <b>description</b> 16094 * <p> 16095 * Description: <b>Multiple Resources: 16096 16097 * [ActivityDefinition](activitydefinition.html): The description of the activity definition 16098 * [ActorDefinition](actordefinition.html): The description of the Actor Definition 16099 * [CapabilityStatement](capabilitystatement.html): The description of the capability statement 16100 * [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 16101 * [Citation](citation.html): The description of the citation 16102 * [CodeSystem](codesystem.html): The description of the code system 16103 * [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 16104 * [ConceptMap](conceptmap.html): The description of the concept map 16105 * [ConditionDefinition](conditiondefinition.html): The description of the condition definition 16106 * [EventDefinition](eventdefinition.html): The description of the event definition 16107 * [Evidence](evidence.html): The description of the evidence 16108 * [EvidenceVariable](evidencevariable.html): The description of the evidence variable 16109 * [GraphDefinition](graphdefinition.html): The description of the graph definition 16110 * [ImplementationGuide](implementationguide.html): The description of the implementation guide 16111 * [Library](library.html): The description of the library 16112 * [Measure](measure.html): The description of the measure 16113 * [MessageDefinition](messagedefinition.html): The description of the message definition 16114 * [NamingSystem](namingsystem.html): The description of the naming system 16115 * [OperationDefinition](operationdefinition.html): The description of the operation definition 16116 * [PlanDefinition](plandefinition.html): The description of the plan definition 16117 * [Questionnaire](questionnaire.html): The description of the questionnaire 16118 * [Requirements](requirements.html): The description of the requirements 16119 * [SearchParameter](searchparameter.html): The description of the search parameter 16120 * [StructureDefinition](structuredefinition.html): The description of the structure definition 16121 * [StructureMap](structuremap.html): The description of the structure map 16122 * [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 16123 * [TestScript](testscript.html): The description of the test script 16124 * [ValueSet](valueset.html): The description of the value set 16125</b><br> 16126 * Type: <b>string</b><br> 16127 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 16128 * </p> 16129 */ 16130 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 16131 16132 /** 16133 * Search parameter: <b>identifier</b> 16134 * <p> 16135 * Description: <b>Multiple Resources: 16136 16137 * [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 16138 * [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 16139 * [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 16140 * [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 16141 * [Citation](citation.html): External identifier for the citation 16142 * [CodeSystem](codesystem.html): External identifier for the code system 16143 * [ConceptMap](conceptmap.html): External identifier for the concept map 16144 * [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 16145 * [EventDefinition](eventdefinition.html): External identifier for the event definition 16146 * [Evidence](evidence.html): External identifier for the evidence 16147 * [EvidenceReport](evidencereport.html): External identifier for the evidence report 16148 * [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 16149 * [ExampleScenario](examplescenario.html): External identifier for the example scenario 16150 * [GraphDefinition](graphdefinition.html): External identifier for the graph definition 16151 * [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 16152 * [Library](library.html): External identifier for the library 16153 * [Measure](measure.html): External identifier for the measure 16154 * [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 16155 * [MessageDefinition](messagedefinition.html): External identifier for the message definition 16156 * [NamingSystem](namingsystem.html): External identifier for the naming system 16157 * [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 16158 * [OperationDefinition](operationdefinition.html): External identifier for the search parameter 16159 * [PlanDefinition](plandefinition.html): External identifier for the plan definition 16160 * [Questionnaire](questionnaire.html): External identifier for the questionnaire 16161 * [Requirements](requirements.html): External identifier for the requirements 16162 * [SearchParameter](searchparameter.html): External identifier for the search parameter 16163 * [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 16164 * [StructureDefinition](structuredefinition.html): External identifier for the structure definition 16165 * [StructureMap](structuremap.html): External identifier for the structure map 16166 * [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 16167 * [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 16168 * [TestPlan](testplan.html): An identifier for the test plan 16169 * [TestScript](testscript.html): External identifier for the test script 16170 * [ValueSet](valueset.html): External identifier for the value set 16171</b><br> 16172 * Type: <b>token</b><br> 16173 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 16174 * </p> 16175 */ 16176 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 16177 public static final String SP_IDENTIFIER = "identifier"; 16178 /** 16179 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 16180 * <p> 16181 * Description: <b>Multiple Resources: 16182 16183 * [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 16184 * [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 16185 * [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 16186 * [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 16187 * [Citation](citation.html): External identifier for the citation 16188 * [CodeSystem](codesystem.html): External identifier for the code system 16189 * [ConceptMap](conceptmap.html): External identifier for the concept map 16190 * [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 16191 * [EventDefinition](eventdefinition.html): External identifier for the event definition 16192 * [Evidence](evidence.html): External identifier for the evidence 16193 * [EvidenceReport](evidencereport.html): External identifier for the evidence report 16194 * [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 16195 * [ExampleScenario](examplescenario.html): External identifier for the example scenario 16196 * [GraphDefinition](graphdefinition.html): External identifier for the graph definition 16197 * [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 16198 * [Library](library.html): External identifier for the library 16199 * [Measure](measure.html): External identifier for the measure 16200 * [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 16201 * [MessageDefinition](messagedefinition.html): External identifier for the message definition 16202 * [NamingSystem](namingsystem.html): External identifier for the naming system 16203 * [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 16204 * [OperationDefinition](operationdefinition.html): External identifier for the search parameter 16205 * [PlanDefinition](plandefinition.html): External identifier for the plan definition 16206 * [Questionnaire](questionnaire.html): External identifier for the questionnaire 16207 * [Requirements](requirements.html): External identifier for the requirements 16208 * [SearchParameter](searchparameter.html): External identifier for the search parameter 16209 * [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 16210 * [StructureDefinition](structuredefinition.html): External identifier for the structure definition 16211 * [StructureMap](structuremap.html): External identifier for the structure map 16212 * [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 16213 * [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 16214 * [TestPlan](testplan.html): An identifier for the test plan 16215 * [TestScript](testscript.html): External identifier for the test script 16216 * [ValueSet](valueset.html): External identifier for the value set 16217</b><br> 16218 * Type: <b>token</b><br> 16219 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 16220 * </p> 16221 */ 16222 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 16223 16224 /** 16225 * Search parameter: <b>jurisdiction</b> 16226 * <p> 16227 * Description: <b>Multiple Resources: 16228 16229 * [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 16230 * [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 16231 * [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 16232 * [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 16233 * [Citation](citation.html): Intended jurisdiction for the citation 16234 * [CodeSystem](codesystem.html): Intended jurisdiction for the code system 16235 * [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 16236 * [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 16237 * [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 16238 * [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 16239 * [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 16240 * [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 16241 * [Library](library.html): Intended jurisdiction for the library 16242 * [Measure](measure.html): Intended jurisdiction for the measure 16243 * [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 16244 * [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 16245 * [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 16246 * [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 16247 * [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 16248 * [Requirements](requirements.html): Intended jurisdiction for the requirements 16249 * [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 16250 * [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 16251 * [StructureMap](structuremap.html): Intended jurisdiction for the structure map 16252 * [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 16253 * [TestScript](testscript.html): Intended jurisdiction for the test script 16254 * [ValueSet](valueset.html): Intended jurisdiction for the value set 16255</b><br> 16256 * Type: <b>token</b><br> 16257 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 16258 * </p> 16259 */ 16260 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 16261 public static final String SP_JURISDICTION = "jurisdiction"; 16262 /** 16263 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 16264 * <p> 16265 * Description: <b>Multiple Resources: 16266 16267 * [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 16268 * [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 16269 * [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 16270 * [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 16271 * [Citation](citation.html): Intended jurisdiction for the citation 16272 * [CodeSystem](codesystem.html): Intended jurisdiction for the code system 16273 * [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 16274 * [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 16275 * [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 16276 * [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 16277 * [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 16278 * [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 16279 * [Library](library.html): Intended jurisdiction for the library 16280 * [Measure](measure.html): Intended jurisdiction for the measure 16281 * [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 16282 * [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 16283 * [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 16284 * [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 16285 * [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 16286 * [Requirements](requirements.html): Intended jurisdiction for the requirements 16287 * [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 16288 * [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 16289 * [StructureMap](structuremap.html): Intended jurisdiction for the structure map 16290 * [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 16291 * [TestScript](testscript.html): Intended jurisdiction for the test script 16292 * [ValueSet](valueset.html): Intended jurisdiction for the value set 16293</b><br> 16294 * Type: <b>token</b><br> 16295 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 16296 * </p> 16297 */ 16298 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 16299 16300 /** 16301 * Search parameter: <b>name</b> 16302 * <p> 16303 * Description: <b>Multiple Resources: 16304 16305 * [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 16306 * [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 16307 * [Citation](citation.html): Computationally friendly name of the citation 16308 * [CodeSystem](codesystem.html): Computationally friendly name of the code system 16309 * [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 16310 * [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 16311 * [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 16312 * [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 16313 * [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 16314 * [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 16315 * [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 16316 * [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 16317 * [Library](library.html): Computationally friendly name of the library 16318 * [Measure](measure.html): Computationally friendly name of the measure 16319 * [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 16320 * [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 16321 * [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 16322 * [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 16323 * [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 16324 * [Requirements](requirements.html): Computationally friendly name of the requirements 16325 * [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 16326 * [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 16327 * [StructureMap](structuremap.html): Computationally friendly name of the structure map 16328 * [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 16329 * [TestScript](testscript.html): Computationally friendly name of the test script 16330 * [ValueSet](valueset.html): Computationally friendly name of the value set 16331</b><br> 16332 * Type: <b>string</b><br> 16333 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 16334 * </p> 16335 */ 16336 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 16337 public static final String SP_NAME = "name"; 16338 /** 16339 * <b>Fluent Client</b> search parameter constant for <b>name</b> 16340 * <p> 16341 * Description: <b>Multiple Resources: 16342 16343 * [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 16344 * [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 16345 * [Citation](citation.html): Computationally friendly name of the citation 16346 * [CodeSystem](codesystem.html): Computationally friendly name of the code system 16347 * [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 16348 * [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 16349 * [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 16350 * [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 16351 * [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 16352 * [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 16353 * [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 16354 * [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 16355 * [Library](library.html): Computationally friendly name of the library 16356 * [Measure](measure.html): Computationally friendly name of the measure 16357 * [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 16358 * [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 16359 * [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 16360 * [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 16361 * [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 16362 * [Requirements](requirements.html): Computationally friendly name of the requirements 16363 * [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 16364 * [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 16365 * [StructureMap](structuremap.html): Computationally friendly name of the structure map 16366 * [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 16367 * [TestScript](testscript.html): Computationally friendly name of the test script 16368 * [ValueSet](valueset.html): Computationally friendly name of the value set 16369</b><br> 16370 * Type: <b>string</b><br> 16371 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 16372 * </p> 16373 */ 16374 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 16375 16376 /** 16377 * Search parameter: <b>publisher</b> 16378 * <p> 16379 * Description: <b>Multiple Resources: 16380 16381 * [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 16382 * [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 16383 * [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 16384 * [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 16385 * [Citation](citation.html): Name of the publisher of the citation 16386 * [CodeSystem](codesystem.html): Name of the publisher of the code system 16387 * [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 16388 * [ConceptMap](conceptmap.html): Name of the publisher of the concept map 16389 * [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 16390 * [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 16391 * [Evidence](evidence.html): Name of the publisher of the evidence 16392 * [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 16393 * [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 16394 * [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 16395 * [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 16396 * [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 16397 * [Library](library.html): Name of the publisher of the library 16398 * [Measure](measure.html): Name of the publisher of the measure 16399 * [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 16400 * [NamingSystem](namingsystem.html): Name of the publisher of the naming system 16401 * [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 16402 * [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 16403 * [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 16404 * [Requirements](requirements.html): Name of the publisher of the requirements 16405 * [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 16406 * [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 16407 * [StructureMap](structuremap.html): Name of the publisher of the structure map 16408 * [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 16409 * [TestScript](testscript.html): Name of the publisher of the test script 16410 * [ValueSet](valueset.html): Name of the publisher of the value set 16411</b><br> 16412 * Type: <b>string</b><br> 16413 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 16414 * </p> 16415 */ 16416 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 16417 public static final String SP_PUBLISHER = "publisher"; 16418 /** 16419 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 16420 * <p> 16421 * Description: <b>Multiple Resources: 16422 16423 * [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 16424 * [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 16425 * [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 16426 * [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 16427 * [Citation](citation.html): Name of the publisher of the citation 16428 * [CodeSystem](codesystem.html): Name of the publisher of the code system 16429 * [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 16430 * [ConceptMap](conceptmap.html): Name of the publisher of the concept map 16431 * [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 16432 * [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 16433 * [Evidence](evidence.html): Name of the publisher of the evidence 16434 * [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 16435 * [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 16436 * [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 16437 * [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 16438 * [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 16439 * [Library](library.html): Name of the publisher of the library 16440 * [Measure](measure.html): Name of the publisher of the measure 16441 * [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 16442 * [NamingSystem](namingsystem.html): Name of the publisher of the naming system 16443 * [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 16444 * [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 16445 * [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 16446 * [Requirements](requirements.html): Name of the publisher of the requirements 16447 * [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 16448 * [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 16449 * [StructureMap](structuremap.html): Name of the publisher of the structure map 16450 * [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 16451 * [TestScript](testscript.html): Name of the publisher of the test script 16452 * [ValueSet](valueset.html): Name of the publisher of the value set 16453</b><br> 16454 * Type: <b>string</b><br> 16455 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 16456 * </p> 16457 */ 16458 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 16459 16460 /** 16461 * Search parameter: <b>status</b> 16462 * <p> 16463 * Description: <b>Multiple Resources: 16464 16465 * [ActivityDefinition](activitydefinition.html): The current status of the activity definition 16466 * [ActorDefinition](actordefinition.html): The current status of the Actor Definition 16467 * [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 16468 * [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 16469 * [Citation](citation.html): The current status of the citation 16470 * [CodeSystem](codesystem.html): The current status of the code system 16471 * [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 16472 * [ConceptMap](conceptmap.html): The current status of the concept map 16473 * [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 16474 * [EventDefinition](eventdefinition.html): The current status of the event definition 16475 * [Evidence](evidence.html): The current status of the evidence 16476 * [EvidenceReport](evidencereport.html): The current status of the evidence report 16477 * [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 16478 * [ExampleScenario](examplescenario.html): The current status of the example scenario 16479 * [GraphDefinition](graphdefinition.html): The current status of the graph definition 16480 * [ImplementationGuide](implementationguide.html): The current status of the implementation guide 16481 * [Library](library.html): The current status of the library 16482 * [Measure](measure.html): The current status of the measure 16483 * [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 16484 * [MessageDefinition](messagedefinition.html): The current status of the message definition 16485 * [NamingSystem](namingsystem.html): The current status of the naming system 16486 * [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 16487 * [OperationDefinition](operationdefinition.html): The current status of the operation definition 16488 * [PlanDefinition](plandefinition.html): The current status of the plan definition 16489 * [Questionnaire](questionnaire.html): The current status of the questionnaire 16490 * [Requirements](requirements.html): The current status of the requirements 16491 * [SearchParameter](searchparameter.html): The current status of the search parameter 16492 * [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 16493 * [StructureDefinition](structuredefinition.html): The current status of the structure definition 16494 * [StructureMap](structuremap.html): The current status of the structure map 16495 * [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 16496 * [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 16497 * [TestPlan](testplan.html): The current status of the test plan 16498 * [TestScript](testscript.html): The current status of the test script 16499 * [ValueSet](valueset.html): The current status of the value set 16500</b><br> 16501 * Type: <b>token</b><br> 16502 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 16503 * </p> 16504 */ 16505 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 16506 public static final String SP_STATUS = "status"; 16507 /** 16508 * <b>Fluent Client</b> search parameter constant for <b>status</b> 16509 * <p> 16510 * Description: <b>Multiple Resources: 16511 16512 * [ActivityDefinition](activitydefinition.html): The current status of the activity definition 16513 * [ActorDefinition](actordefinition.html): The current status of the Actor Definition 16514 * [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 16515 * [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 16516 * [Citation](citation.html): The current status of the citation 16517 * [CodeSystem](codesystem.html): The current status of the code system 16518 * [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 16519 * [ConceptMap](conceptmap.html): The current status of the concept map 16520 * [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 16521 * [EventDefinition](eventdefinition.html): The current status of the event definition 16522 * [Evidence](evidence.html): The current status of the evidence 16523 * [EvidenceReport](evidencereport.html): The current status of the evidence report 16524 * [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 16525 * [ExampleScenario](examplescenario.html): The current status of the example scenario 16526 * [GraphDefinition](graphdefinition.html): The current status of the graph definition 16527 * [ImplementationGuide](implementationguide.html): The current status of the implementation guide 16528 * [Library](library.html): The current status of the library 16529 * [Measure](measure.html): The current status of the measure 16530 * [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 16531 * [MessageDefinition](messagedefinition.html): The current status of the message definition 16532 * [NamingSystem](namingsystem.html): The current status of the naming system 16533 * [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 16534 * [OperationDefinition](operationdefinition.html): The current status of the operation definition 16535 * [PlanDefinition](plandefinition.html): The current status of the plan definition 16536 * [Questionnaire](questionnaire.html): The current status of the questionnaire 16537 * [Requirements](requirements.html): The current status of the requirements 16538 * [SearchParameter](searchparameter.html): The current status of the search parameter 16539 * [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 16540 * [StructureDefinition](structuredefinition.html): The current status of the structure definition 16541 * [StructureMap](structuremap.html): The current status of the structure map 16542 * [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 16543 * [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 16544 * [TestPlan](testplan.html): The current status of the test plan 16545 * [TestScript](testscript.html): The current status of the test script 16546 * [ValueSet](valueset.html): The current status of the value set 16547</b><br> 16548 * Type: <b>token</b><br> 16549 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 16550 * </p> 16551 */ 16552 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 16553 16554 /** 16555 * Search parameter: <b>title</b> 16556 * <p> 16557 * Description: <b>Multiple Resources: 16558 16559 * [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 16560 * [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 16561 * [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 16562 * [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 16563 * [Citation](citation.html): The human-friendly name of the citation 16564 * [CodeSystem](codesystem.html): The human-friendly name of the code system 16565 * [ConceptMap](conceptmap.html): The human-friendly name of the concept map 16566 * [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 16567 * [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 16568 * [Evidence](evidence.html): The human-friendly name of the evidence 16569 * [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 16570 * [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 16571 * [Library](library.html): The human-friendly name of the library 16572 * [Measure](measure.html): The human-friendly name of the measure 16573 * [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 16574 * [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 16575 * [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 16576 * [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 16577 * [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 16578 * [Requirements](requirements.html): The human-friendly name of the requirements 16579 * [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 16580 * [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 16581 * [StructureMap](structuremap.html): The human-friendly name of the structure map 16582 * [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 16583 * [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 16584 * [TestScript](testscript.html): The human-friendly name of the test script 16585 * [ValueSet](valueset.html): The human-friendly name of the value set 16586</b><br> 16587 * Type: <b>string</b><br> 16588 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 16589 * </p> 16590 */ 16591 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 16592 public static final String SP_TITLE = "title"; 16593 /** 16594 * <b>Fluent Client</b> search parameter constant for <b>title</b> 16595 * <p> 16596 * Description: <b>Multiple Resources: 16597 16598 * [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 16599 * [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 16600 * [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 16601 * [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 16602 * [Citation](citation.html): The human-friendly name of the citation 16603 * [CodeSystem](codesystem.html): The human-friendly name of the code system 16604 * [ConceptMap](conceptmap.html): The human-friendly name of the concept map 16605 * [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 16606 * [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 16607 * [Evidence](evidence.html): The human-friendly name of the evidence 16608 * [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 16609 * [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 16610 * [Library](library.html): The human-friendly name of the library 16611 * [Measure](measure.html): The human-friendly name of the measure 16612 * [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 16613 * [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 16614 * [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 16615 * [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 16616 * [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 16617 * [Requirements](requirements.html): The human-friendly name of the requirements 16618 * [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 16619 * [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 16620 * [StructureMap](structuremap.html): The human-friendly name of the structure map 16621 * [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 16622 * [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 16623 * [TestScript](testscript.html): The human-friendly name of the test script 16624 * [ValueSet](valueset.html): The human-friendly name of the value set 16625</b><br> 16626 * Type: <b>string</b><br> 16627 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 16628 * </p> 16629 */ 16630 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 16631 16632 /** 16633 * Search parameter: <b>url</b> 16634 * <p> 16635 * Description: <b>Multiple Resources: 16636 16637 * [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 16638 * [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 16639 * [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 16640 * [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 16641 * [Citation](citation.html): The uri that identifies the citation 16642 * [CodeSystem](codesystem.html): The uri that identifies the code system 16643 * [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 16644 * [ConceptMap](conceptmap.html): The URI that identifies the concept map 16645 * [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 16646 * [EventDefinition](eventdefinition.html): The uri that identifies the event definition 16647 * [Evidence](evidence.html): The uri that identifies the evidence 16648 * [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 16649 * [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 16650 * [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 16651 * [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 16652 * [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 16653 * [Library](library.html): The uri that identifies the library 16654 * [Measure](measure.html): The uri that identifies the measure 16655 * [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 16656 * [NamingSystem](namingsystem.html): The uri that identifies the naming system 16657 * [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 16658 * [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 16659 * [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 16660 * [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 16661 * [Requirements](requirements.html): The uri that identifies the requirements 16662 * [SearchParameter](searchparameter.html): The uri that identifies the search parameter 16663 * [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 16664 * [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 16665 * [StructureMap](structuremap.html): The uri that identifies the structure map 16666 * [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 16667 * [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 16668 * [TestPlan](testplan.html): The uri that identifies the test plan 16669 * [TestScript](testscript.html): The uri that identifies the test script 16670 * [ValueSet](valueset.html): The uri that identifies the value set 16671</b><br> 16672 * Type: <b>uri</b><br> 16673 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 16674 * </p> 16675 */ 16676 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 16677 public static final String SP_URL = "url"; 16678 /** 16679 * <b>Fluent Client</b> search parameter constant for <b>url</b> 16680 * <p> 16681 * Description: <b>Multiple Resources: 16682 16683 * [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 16684 * [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 16685 * [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 16686 * [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 16687 * [Citation](citation.html): The uri that identifies the citation 16688 * [CodeSystem](codesystem.html): The uri that identifies the code system 16689 * [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 16690 * [ConceptMap](conceptmap.html): The URI that identifies the concept map 16691 * [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 16692 * [EventDefinition](eventdefinition.html): The uri that identifies the event definition 16693 * [Evidence](evidence.html): The uri that identifies the evidence 16694 * [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 16695 * [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 16696 * [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 16697 * [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 16698 * [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 16699 * [Library](library.html): The uri that identifies the library 16700 * [Measure](measure.html): The uri that identifies the measure 16701 * [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 16702 * [NamingSystem](namingsystem.html): The uri that identifies the naming system 16703 * [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 16704 * [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 16705 * [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 16706 * [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 16707 * [Requirements](requirements.html): The uri that identifies the requirements 16708 * [SearchParameter](searchparameter.html): The uri that identifies the search parameter 16709 * [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 16710 * [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 16711 * [StructureMap](structuremap.html): The uri that identifies the structure map 16712 * [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 16713 * [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 16714 * [TestPlan](testplan.html): The uri that identifies the test plan 16715 * [TestScript](testscript.html): The uri that identifies the test script 16716 * [ValueSet](valueset.html): The uri that identifies the value set 16717</b><br> 16718 * Type: <b>uri</b><br> 16719 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 16720 * </p> 16721 */ 16722 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 16723 16724 /** 16725 * Search parameter: <b>version</b> 16726 * <p> 16727 * Description: <b>Multiple Resources: 16728 16729 * [ActivityDefinition](activitydefinition.html): The business version of the activity definition 16730 * [ActorDefinition](actordefinition.html): The business version of the Actor Definition 16731 * [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 16732 * [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 16733 * [Citation](citation.html): The business version of the citation 16734 * [CodeSystem](codesystem.html): The business version of the code system 16735 * [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 16736 * [ConceptMap](conceptmap.html): The business version of the concept map 16737 * [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 16738 * [EventDefinition](eventdefinition.html): The business version of the event definition 16739 * [Evidence](evidence.html): The business version of the evidence 16740 * [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 16741 * [ExampleScenario](examplescenario.html): The business version of the example scenario 16742 * [GraphDefinition](graphdefinition.html): The business version of the graph definition 16743 * [ImplementationGuide](implementationguide.html): The business version of the implementation guide 16744 * [Library](library.html): The business version of the library 16745 * [Measure](measure.html): The business version of the measure 16746 * [MessageDefinition](messagedefinition.html): The business version of the message definition 16747 * [NamingSystem](namingsystem.html): The business version of the naming system 16748 * [OperationDefinition](operationdefinition.html): The business version of the operation definition 16749 * [PlanDefinition](plandefinition.html): The business version of the plan definition 16750 * [Questionnaire](questionnaire.html): The business version of the questionnaire 16751 * [Requirements](requirements.html): The business version of the requirements 16752 * [SearchParameter](searchparameter.html): The business version of the search parameter 16753 * [StructureDefinition](structuredefinition.html): The business version of the structure definition 16754 * [StructureMap](structuremap.html): The business version of the structure map 16755 * [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 16756 * [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 16757 * [TestScript](testscript.html): The business version of the test script 16758 * [ValueSet](valueset.html): The business version of the value set 16759</b><br> 16760 * Type: <b>token</b><br> 16761 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 16762 * </p> 16763 */ 16764 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 16765 public static final String SP_VERSION = "version"; 16766 /** 16767 * <b>Fluent Client</b> search parameter constant for <b>version</b> 16768 * <p> 16769 * Description: <b>Multiple Resources: 16770 16771 * [ActivityDefinition](activitydefinition.html): The business version of the activity definition 16772 * [ActorDefinition](actordefinition.html): The business version of the Actor Definition 16773 * [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 16774 * [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 16775 * [Citation](citation.html): The business version of the citation 16776 * [CodeSystem](codesystem.html): The business version of the code system 16777 * [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 16778 * [ConceptMap](conceptmap.html): The business version of the concept map 16779 * [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 16780 * [EventDefinition](eventdefinition.html): The business version of the event definition 16781 * [Evidence](evidence.html): The business version of the evidence 16782 * [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 16783 * [ExampleScenario](examplescenario.html): The business version of the example scenario 16784 * [GraphDefinition](graphdefinition.html): The business version of the graph definition 16785 * [ImplementationGuide](implementationguide.html): The business version of the implementation guide 16786 * [Library](library.html): The business version of the library 16787 * [Measure](measure.html): The business version of the measure 16788 * [MessageDefinition](messagedefinition.html): The business version of the message definition 16789 * [NamingSystem](namingsystem.html): The business version of the naming system 16790 * [OperationDefinition](operationdefinition.html): The business version of the operation definition 16791 * [PlanDefinition](plandefinition.html): The business version of the plan definition 16792 * [Questionnaire](questionnaire.html): The business version of the questionnaire 16793 * [Requirements](requirements.html): The business version of the requirements 16794 * [SearchParameter](searchparameter.html): The business version of the search parameter 16795 * [StructureDefinition](structuredefinition.html): The business version of the structure definition 16796 * [StructureMap](structuremap.html): The business version of the structure map 16797 * [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 16798 * [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 16799 * [TestScript](testscript.html): The business version of the test script 16800 * [ValueSet](valueset.html): The business version of the value set 16801</b><br> 16802 * Type: <b>token</b><br> 16803 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 16804 * </p> 16805 */ 16806 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 16807 16808 /** 16809 * Search parameter: <b>depends-on</b> 16810 * <p> 16811 * Description: <b>Identity of the IG that this depends on</b><br> 16812 * Type: <b>reference</b><br> 16813 * Path: <b>ImplementationGuide.dependsOn.uri</b><br> 16814 * </p> 16815 */ 16816 @SearchParamDefinition(name="depends-on", path="ImplementationGuide.dependsOn.uri", description="Identity of the IG that this depends on", type="reference", target={ImplementationGuide.class } ) 16817 public static final String SP_DEPENDS_ON = "depends-on"; 16818 /** 16819 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 16820 * <p> 16821 * Description: <b>Identity of the IG that this depends on</b><br> 16822 * Type: <b>reference</b><br> 16823 * Path: <b>ImplementationGuide.dependsOn.uri</b><br> 16824 * </p> 16825 */ 16826 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEPENDS_ON); 16827 16828 /** 16829 * Constant for fluent queries to be used to add include statements. Specifies 16830 * the path value of "<b>ImplementationGuide:depends-on</b>". 16831 */ 16832 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include("ImplementationGuide:depends-on").toLocked(); 16833 16834 /** 16835 * Search parameter: <b>experimental</b> 16836 * <p> 16837 * Description: <b>For testing purposes, not real usage</b><br> 16838 * Type: <b>token</b><br> 16839 * Path: <b>ImplementationGuide.experimental</b><br> 16840 * </p> 16841 */ 16842 @SearchParamDefinition(name="experimental", path="ImplementationGuide.experimental", description="For testing purposes, not real usage", type="token" ) 16843 public static final String SP_EXPERIMENTAL = "experimental"; 16844 /** 16845 * <b>Fluent Client</b> search parameter constant for <b>experimental</b> 16846 * <p> 16847 * Description: <b>For testing purposes, not real usage</b><br> 16848 * Type: <b>token</b><br> 16849 * Path: <b>ImplementationGuide.experimental</b><br> 16850 * </p> 16851 */ 16852 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXPERIMENTAL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EXPERIMENTAL); 16853 16854 /** 16855 * Search parameter: <b>global</b> 16856 * <p> 16857 * Description: <b>Profile that all resources must conform to</b><br> 16858 * Type: <b>reference</b><br> 16859 * Path: <b>ImplementationGuide.global.profile</b><br> 16860 * </p> 16861 */ 16862 @SearchParamDefinition(name="global", path="ImplementationGuide.global.profile", description="Profile that all resources must conform to", type="reference", target={StructureDefinition.class } ) 16863 public static final String SP_GLOBAL = "global"; 16864 /** 16865 * <b>Fluent Client</b> search parameter constant for <b>global</b> 16866 * <p> 16867 * Description: <b>Profile that all resources must conform to</b><br> 16868 * Type: <b>reference</b><br> 16869 * Path: <b>ImplementationGuide.global.profile</b><br> 16870 * </p> 16871 */ 16872 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam GLOBAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_GLOBAL); 16873 16874 /** 16875 * Constant for fluent queries to be used to add include statements. Specifies 16876 * the path value of "<b>ImplementationGuide:global</b>". 16877 */ 16878 public static final ca.uhn.fhir.model.api.Include INCLUDE_GLOBAL = new ca.uhn.fhir.model.api.Include("ImplementationGuide:global").toLocked(); 16879 16880 /** 16881 * Search parameter: <b>resource</b> 16882 * <p> 16883 * Description: <b>Location of the resource</b><br> 16884 * Type: <b>reference</b><br> 16885 * Path: <b>ImplementationGuide.definition.resource.reference</b><br> 16886 * </p> 16887 */ 16888 @SearchParamDefinition(name="resource", path="ImplementationGuide.definition.resource.reference", description="Location of the resource", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 16889 public static final String SP_RESOURCE = "resource"; 16890 /** 16891 * <b>Fluent Client</b> search parameter constant for <b>resource</b> 16892 * <p> 16893 * Description: <b>Location of the resource</b><br> 16894 * Type: <b>reference</b><br> 16895 * Path: <b>ImplementationGuide.definition.resource.reference</b><br> 16896 * </p> 16897 */ 16898 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RESOURCE); 16899 16900 /** 16901 * Constant for fluent queries to be used to add include statements. Specifies 16902 * the path value of "<b>ImplementationGuide:resource</b>". 16903 */ 16904 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESOURCE = new ca.uhn.fhir.model.api.Include("ImplementationGuide:resource").toLocked(); 16905 16906 public ImplementationGuideDefinitionPageComponent getPageByName(String name) { 16907 if (!hasDefinition() || !getDefinition().hasPage()) { 16908 return null; 16909 } 16910 return getPageByName(getDefinition().getPage(), name); 16911 } 16912 16913 private ImplementationGuideDefinitionPageComponent getPageByName(ImplementationGuideDefinitionPageComponent page, String name) { 16914 if (name.equals(page.getName())) { 16915 return page; 16916 } 16917 for (ImplementationGuideDefinitionPageComponent t : page.getPage()) { 16918 ImplementationGuideDefinitionPageComponent r = getPageByName(t, name); 16919 if (r != null) { 16920 return r; 16921 } 16922 } 16923 return null; 16924 } 16925 16926 16927} 16928