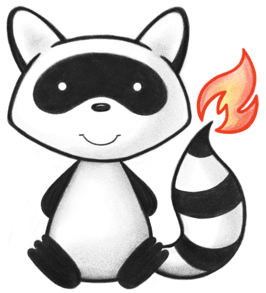
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * An ingredient of a manufactured item or pharmaceutical product. 052 */ 053@ResourceDef(name="Ingredient", profile="http://hl7.org/fhir/StructureDefinition/Ingredient") 054public class Ingredient extends DomainResource { 055 056 public enum IngredientManufacturerRole { 057 /** 058 * 059 */ 060 ALLOWED, 061 /** 062 * 063 */ 064 POSSIBLE, 065 /** 066 * 067 */ 068 ACTUAL, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static IngredientManufacturerRole fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("allowed".equals(codeString)) 077 return ALLOWED; 078 if ("possible".equals(codeString)) 079 return POSSIBLE; 080 if ("actual".equals(codeString)) 081 return ACTUAL; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown IngredientManufacturerRole code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case ALLOWED: return "allowed"; 090 case POSSIBLE: return "possible"; 091 case ACTUAL: return "actual"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case ALLOWED: return "http://hl7.org/fhir/ingredient-manufacturer-role"; 099 case POSSIBLE: return "http://hl7.org/fhir/ingredient-manufacturer-role"; 100 case ACTUAL: return "http://hl7.org/fhir/ingredient-manufacturer-role"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case ALLOWED: return ""; 108 case POSSIBLE: return ""; 109 case ACTUAL: return ""; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case ALLOWED: return "Manufacturer is specifically allowed for this ingredient"; 117 case POSSIBLE: return "Manufacturer is known to make this ingredient in general"; 118 case ACTUAL: return "Manufacturer actually makes this particular ingredient"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class IngredientManufacturerRoleEnumFactory implements EnumFactory<IngredientManufacturerRole> { 126 public IngredientManufacturerRole fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("allowed".equals(codeString)) 131 return IngredientManufacturerRole.ALLOWED; 132 if ("possible".equals(codeString)) 133 return IngredientManufacturerRole.POSSIBLE; 134 if ("actual".equals(codeString)) 135 return IngredientManufacturerRole.ACTUAL; 136 throw new IllegalArgumentException("Unknown IngredientManufacturerRole code '"+codeString+"'"); 137 } 138 public Enumeration<IngredientManufacturerRole> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<IngredientManufacturerRole>(this, IngredientManufacturerRole.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<IngredientManufacturerRole>(this, IngredientManufacturerRole.NULL, code); 146 if ("allowed".equals(codeString)) 147 return new Enumeration<IngredientManufacturerRole>(this, IngredientManufacturerRole.ALLOWED, code); 148 if ("possible".equals(codeString)) 149 return new Enumeration<IngredientManufacturerRole>(this, IngredientManufacturerRole.POSSIBLE, code); 150 if ("actual".equals(codeString)) 151 return new Enumeration<IngredientManufacturerRole>(this, IngredientManufacturerRole.ACTUAL, code); 152 throw new FHIRException("Unknown IngredientManufacturerRole code '"+codeString+"'"); 153 } 154 public String toCode(IngredientManufacturerRole code) { 155 if (code == IngredientManufacturerRole.NULL) 156 return null; 157 if (code == IngredientManufacturerRole.ALLOWED) 158 return "allowed"; 159 if (code == IngredientManufacturerRole.POSSIBLE) 160 return "possible"; 161 if (code == IngredientManufacturerRole.ACTUAL) 162 return "actual"; 163 return "?"; 164 } 165 public String toSystem(IngredientManufacturerRole code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class IngredientManufacturerComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * The way in which this manufacturer is associated with the ingredient. For example whether it is a possible one (others allowed), or an exclusive authorized one for this ingredient. Note that this is not the manufacturing process role. 174 */ 175 @Child(name = "role", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 176 @Description(shortDefinition="allowed | possible | actual", formalDefinition="The way in which this manufacturer is associated with the ingredient. For example whether it is a possible one (others allowed), or an exclusive authorized one for this ingredient. Note that this is not the manufacturing process role." ) 177 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ingredient-manufacturer-role") 178 protected Enumeration<IngredientManufacturerRole> role; 179 180 /** 181 * An organization that manufactures this ingredient. 182 */ 183 @Child(name = "manufacturer", type = {Organization.class}, order=2, min=1, max=1, modifier=false, summary=true) 184 @Description(shortDefinition="An organization that manufactures this ingredient", formalDefinition="An organization that manufactures this ingredient." ) 185 protected Reference manufacturer; 186 187 private static final long serialVersionUID = -1226688097L; 188 189 /** 190 * Constructor 191 */ 192 public IngredientManufacturerComponent() { 193 super(); 194 } 195 196 /** 197 * Constructor 198 */ 199 public IngredientManufacturerComponent(Reference manufacturer) { 200 super(); 201 this.setManufacturer(manufacturer); 202 } 203 204 /** 205 * @return {@link #role} (The way in which this manufacturer is associated with the ingredient. For example whether it is a possible one (others allowed), or an exclusive authorized one for this ingredient. Note that this is not the manufacturing process role.). This is the underlying object with id, value and extensions. The accessor "getRole" gives direct access to the value 206 */ 207 public Enumeration<IngredientManufacturerRole> getRoleElement() { 208 if (this.role == null) 209 if (Configuration.errorOnAutoCreate()) 210 throw new Error("Attempt to auto-create IngredientManufacturerComponent.role"); 211 else if (Configuration.doAutoCreate()) 212 this.role = new Enumeration<IngredientManufacturerRole>(new IngredientManufacturerRoleEnumFactory()); // bb 213 return this.role; 214 } 215 216 public boolean hasRoleElement() { 217 return this.role != null && !this.role.isEmpty(); 218 } 219 220 public boolean hasRole() { 221 return this.role != null && !this.role.isEmpty(); 222 } 223 224 /** 225 * @param value {@link #role} (The way in which this manufacturer is associated with the ingredient. For example whether it is a possible one (others allowed), or an exclusive authorized one for this ingredient. Note that this is not the manufacturing process role.). This is the underlying object with id, value and extensions. The accessor "getRole" gives direct access to the value 226 */ 227 public IngredientManufacturerComponent setRoleElement(Enumeration<IngredientManufacturerRole> value) { 228 this.role = value; 229 return this; 230 } 231 232 /** 233 * @return The way in which this manufacturer is associated with the ingredient. For example whether it is a possible one (others allowed), or an exclusive authorized one for this ingredient. Note that this is not the manufacturing process role. 234 */ 235 public IngredientManufacturerRole getRole() { 236 return this.role == null ? null : this.role.getValue(); 237 } 238 239 /** 240 * @param value The way in which this manufacturer is associated with the ingredient. For example whether it is a possible one (others allowed), or an exclusive authorized one for this ingredient. Note that this is not the manufacturing process role. 241 */ 242 public IngredientManufacturerComponent setRole(IngredientManufacturerRole value) { 243 if (value == null) 244 this.role = null; 245 else { 246 if (this.role == null) 247 this.role = new Enumeration<IngredientManufacturerRole>(new IngredientManufacturerRoleEnumFactory()); 248 this.role.setValue(value); 249 } 250 return this; 251 } 252 253 /** 254 * @return {@link #manufacturer} (An organization that manufactures this ingredient.) 255 */ 256 public Reference getManufacturer() { 257 if (this.manufacturer == null) 258 if (Configuration.errorOnAutoCreate()) 259 throw new Error("Attempt to auto-create IngredientManufacturerComponent.manufacturer"); 260 else if (Configuration.doAutoCreate()) 261 this.manufacturer = new Reference(); // cc 262 return this.manufacturer; 263 } 264 265 public boolean hasManufacturer() { 266 return this.manufacturer != null && !this.manufacturer.isEmpty(); 267 } 268 269 /** 270 * @param value {@link #manufacturer} (An organization that manufactures this ingredient.) 271 */ 272 public IngredientManufacturerComponent setManufacturer(Reference value) { 273 this.manufacturer = value; 274 return this; 275 } 276 277 protected void listChildren(List<Property> children) { 278 super.listChildren(children); 279 children.add(new Property("role", "code", "The way in which this manufacturer is associated with the ingredient. For example whether it is a possible one (others allowed), or an exclusive authorized one for this ingredient. Note that this is not the manufacturing process role.", 0, 1, role)); 280 children.add(new Property("manufacturer", "Reference(Organization)", "An organization that manufactures this ingredient.", 0, 1, manufacturer)); 281 } 282 283 @Override 284 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 285 switch (_hash) { 286 case 3506294: /*role*/ return new Property("role", "code", "The way in which this manufacturer is associated with the ingredient. For example whether it is a possible one (others allowed), or an exclusive authorized one for this ingredient. Note that this is not the manufacturing process role.", 0, 1, role); 287 case -1969347631: /*manufacturer*/ return new Property("manufacturer", "Reference(Organization)", "An organization that manufactures this ingredient.", 0, 1, manufacturer); 288 default: return super.getNamedProperty(_hash, _name, _checkValid); 289 } 290 291 } 292 293 @Override 294 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 295 switch (hash) { 296 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // Enumeration<IngredientManufacturerRole> 297 case -1969347631: /*manufacturer*/ return this.manufacturer == null ? new Base[0] : new Base[] {this.manufacturer}; // Reference 298 default: return super.getProperty(hash, name, checkValid); 299 } 300 301 } 302 303 @Override 304 public Base setProperty(int hash, String name, Base value) throws FHIRException { 305 switch (hash) { 306 case 3506294: // role 307 value = new IngredientManufacturerRoleEnumFactory().fromType(TypeConvertor.castToCode(value)); 308 this.role = (Enumeration) value; // Enumeration<IngredientManufacturerRole> 309 return value; 310 case -1969347631: // manufacturer 311 this.manufacturer = TypeConvertor.castToReference(value); // Reference 312 return value; 313 default: return super.setProperty(hash, name, value); 314 } 315 316 } 317 318 @Override 319 public Base setProperty(String name, Base value) throws FHIRException { 320 if (name.equals("role")) { 321 value = new IngredientManufacturerRoleEnumFactory().fromType(TypeConvertor.castToCode(value)); 322 this.role = (Enumeration) value; // Enumeration<IngredientManufacturerRole> 323 } else if (name.equals("manufacturer")) { 324 this.manufacturer = TypeConvertor.castToReference(value); // Reference 325 } else 326 return super.setProperty(name, value); 327 return value; 328 } 329 330 @Override 331 public void removeChild(String name, Base value) throws FHIRException { 332 if (name.equals("role")) { 333 value = new IngredientManufacturerRoleEnumFactory().fromType(TypeConvertor.castToCode(value)); 334 this.role = (Enumeration) value; // Enumeration<IngredientManufacturerRole> 335 } else if (name.equals("manufacturer")) { 336 this.manufacturer = null; 337 } else 338 super.removeChild(name, value); 339 340 } 341 342 @Override 343 public Base makeProperty(int hash, String name) throws FHIRException { 344 switch (hash) { 345 case 3506294: return getRoleElement(); 346 case -1969347631: return getManufacturer(); 347 default: return super.makeProperty(hash, name); 348 } 349 350 } 351 352 @Override 353 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 354 switch (hash) { 355 case 3506294: /*role*/ return new String[] {"code"}; 356 case -1969347631: /*manufacturer*/ return new String[] {"Reference"}; 357 default: return super.getTypesForProperty(hash, name); 358 } 359 360 } 361 362 @Override 363 public Base addChild(String name) throws FHIRException { 364 if (name.equals("role")) { 365 throw new FHIRException("Cannot call addChild on a singleton property Ingredient.manufacturer.role"); 366 } 367 else if (name.equals("manufacturer")) { 368 this.manufacturer = new Reference(); 369 return this.manufacturer; 370 } 371 else 372 return super.addChild(name); 373 } 374 375 public IngredientManufacturerComponent copy() { 376 IngredientManufacturerComponent dst = new IngredientManufacturerComponent(); 377 copyValues(dst); 378 return dst; 379 } 380 381 public void copyValues(IngredientManufacturerComponent dst) { 382 super.copyValues(dst); 383 dst.role = role == null ? null : role.copy(); 384 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 385 } 386 387 @Override 388 public boolean equalsDeep(Base other_) { 389 if (!super.equalsDeep(other_)) 390 return false; 391 if (!(other_ instanceof IngredientManufacturerComponent)) 392 return false; 393 IngredientManufacturerComponent o = (IngredientManufacturerComponent) other_; 394 return compareDeep(role, o.role, true) && compareDeep(manufacturer, o.manufacturer, true); 395 } 396 397 @Override 398 public boolean equalsShallow(Base other_) { 399 if (!super.equalsShallow(other_)) 400 return false; 401 if (!(other_ instanceof IngredientManufacturerComponent)) 402 return false; 403 IngredientManufacturerComponent o = (IngredientManufacturerComponent) other_; 404 return compareValues(role, o.role, true); 405 } 406 407 public boolean isEmpty() { 408 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, manufacturer); 409 } 410 411 public String fhirType() { 412 return "Ingredient.manufacturer"; 413 414 } 415 416 } 417 418 @Block() 419 public static class IngredientSubstanceComponent extends BackboneElement implements IBaseBackboneElement { 420 /** 421 * A code or full resource that represents the ingredient's substance. 422 */ 423 @Child(name = "code", type = {CodeableReference.class}, order=1, min=1, max=1, modifier=false, summary=true) 424 @Description(shortDefinition="A code or full resource that represents the ingredient substance", formalDefinition="A code or full resource that represents the ingredient's substance." ) 425 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-codes") 426 protected CodeableReference code; 427 428 /** 429 * The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. The allowed repetitions do not represent different strengths, but are different representations - mathematically equivalent - of a single strength. 430 */ 431 @Child(name = "strength", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 432 @Description(shortDefinition="The quantity of substance, per presentation, or per volume or mass, and type of quantity", formalDefinition="The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. The allowed repetitions do not represent different strengths, but are different representations - mathematically equivalent - of a single strength." ) 433 protected List<IngredientSubstanceStrengthComponent> strength; 434 435 private static final long serialVersionUID = 538347209L; 436 437 /** 438 * Constructor 439 */ 440 public IngredientSubstanceComponent() { 441 super(); 442 } 443 444 /** 445 * Constructor 446 */ 447 public IngredientSubstanceComponent(CodeableReference code) { 448 super(); 449 this.setCode(code); 450 } 451 452 /** 453 * @return {@link #code} (A code or full resource that represents the ingredient's substance.) 454 */ 455 public CodeableReference getCode() { 456 if (this.code == null) 457 if (Configuration.errorOnAutoCreate()) 458 throw new Error("Attempt to auto-create IngredientSubstanceComponent.code"); 459 else if (Configuration.doAutoCreate()) 460 this.code = new CodeableReference(); // cc 461 return this.code; 462 } 463 464 public boolean hasCode() { 465 return this.code != null && !this.code.isEmpty(); 466 } 467 468 /** 469 * @param value {@link #code} (A code or full resource that represents the ingredient's substance.) 470 */ 471 public IngredientSubstanceComponent setCode(CodeableReference value) { 472 this.code = value; 473 return this; 474 } 475 476 /** 477 * @return {@link #strength} (The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. The allowed repetitions do not represent different strengths, but are different representations - mathematically equivalent - of a single strength.) 478 */ 479 public List<IngredientSubstanceStrengthComponent> getStrength() { 480 if (this.strength == null) 481 this.strength = new ArrayList<IngredientSubstanceStrengthComponent>(); 482 return this.strength; 483 } 484 485 /** 486 * @return Returns a reference to <code>this</code> for easy method chaining 487 */ 488 public IngredientSubstanceComponent setStrength(List<IngredientSubstanceStrengthComponent> theStrength) { 489 this.strength = theStrength; 490 return this; 491 } 492 493 public boolean hasStrength() { 494 if (this.strength == null) 495 return false; 496 for (IngredientSubstanceStrengthComponent item : this.strength) 497 if (!item.isEmpty()) 498 return true; 499 return false; 500 } 501 502 public IngredientSubstanceStrengthComponent addStrength() { //3 503 IngredientSubstanceStrengthComponent t = new IngredientSubstanceStrengthComponent(); 504 if (this.strength == null) 505 this.strength = new ArrayList<IngredientSubstanceStrengthComponent>(); 506 this.strength.add(t); 507 return t; 508 } 509 510 public IngredientSubstanceComponent addStrength(IngredientSubstanceStrengthComponent t) { //3 511 if (t == null) 512 return this; 513 if (this.strength == null) 514 this.strength = new ArrayList<IngredientSubstanceStrengthComponent>(); 515 this.strength.add(t); 516 return this; 517 } 518 519 /** 520 * @return The first repetition of repeating field {@link #strength}, creating it if it does not already exist {3} 521 */ 522 public IngredientSubstanceStrengthComponent getStrengthFirstRep() { 523 if (getStrength().isEmpty()) { 524 addStrength(); 525 } 526 return getStrength().get(0); 527 } 528 529 protected void listChildren(List<Property> children) { 530 super.listChildren(children); 531 children.add(new Property("code", "CodeableReference(SubstanceDefinition)", "A code or full resource that represents the ingredient's substance.", 0, 1, code)); 532 children.add(new Property("strength", "", "The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. The allowed repetitions do not represent different strengths, but are different representations - mathematically equivalent - of a single strength.", 0, java.lang.Integer.MAX_VALUE, strength)); 533 } 534 535 @Override 536 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 537 switch (_hash) { 538 case 3059181: /*code*/ return new Property("code", "CodeableReference(SubstanceDefinition)", "A code or full resource that represents the ingredient's substance.", 0, 1, code); 539 case 1791316033: /*strength*/ return new Property("strength", "", "The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. The allowed repetitions do not represent different strengths, but are different representations - mathematically equivalent - of a single strength.", 0, java.lang.Integer.MAX_VALUE, strength); 540 default: return super.getNamedProperty(_hash, _name, _checkValid); 541 } 542 543 } 544 545 @Override 546 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 547 switch (hash) { 548 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableReference 549 case 1791316033: /*strength*/ return this.strength == null ? new Base[0] : this.strength.toArray(new Base[this.strength.size()]); // IngredientSubstanceStrengthComponent 550 default: return super.getProperty(hash, name, checkValid); 551 } 552 553 } 554 555 @Override 556 public Base setProperty(int hash, String name, Base value) throws FHIRException { 557 switch (hash) { 558 case 3059181: // code 559 this.code = TypeConvertor.castToCodeableReference(value); // CodeableReference 560 return value; 561 case 1791316033: // strength 562 this.getStrength().add((IngredientSubstanceStrengthComponent) value); // IngredientSubstanceStrengthComponent 563 return value; 564 default: return super.setProperty(hash, name, value); 565 } 566 567 } 568 569 @Override 570 public Base setProperty(String name, Base value) throws FHIRException { 571 if (name.equals("code")) { 572 this.code = TypeConvertor.castToCodeableReference(value); // CodeableReference 573 } else if (name.equals("strength")) { 574 this.getStrength().add((IngredientSubstanceStrengthComponent) value); 575 } else 576 return super.setProperty(name, value); 577 return value; 578 } 579 580 @Override 581 public void removeChild(String name, Base value) throws FHIRException { 582 if (name.equals("code")) { 583 this.code = null; 584 } else if (name.equals("strength")) { 585 this.getStrength().remove((IngredientSubstanceStrengthComponent) value); 586 } else 587 super.removeChild(name, value); 588 589 } 590 591 @Override 592 public Base makeProperty(int hash, String name) throws FHIRException { 593 switch (hash) { 594 case 3059181: return getCode(); 595 case 1791316033: return addStrength(); 596 default: return super.makeProperty(hash, name); 597 } 598 599 } 600 601 @Override 602 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 603 switch (hash) { 604 case 3059181: /*code*/ return new String[] {"CodeableReference"}; 605 case 1791316033: /*strength*/ return new String[] {}; 606 default: return super.getTypesForProperty(hash, name); 607 } 608 609 } 610 611 @Override 612 public Base addChild(String name) throws FHIRException { 613 if (name.equals("code")) { 614 this.code = new CodeableReference(); 615 return this.code; 616 } 617 else if (name.equals("strength")) { 618 return addStrength(); 619 } 620 else 621 return super.addChild(name); 622 } 623 624 public IngredientSubstanceComponent copy() { 625 IngredientSubstanceComponent dst = new IngredientSubstanceComponent(); 626 copyValues(dst); 627 return dst; 628 } 629 630 public void copyValues(IngredientSubstanceComponent dst) { 631 super.copyValues(dst); 632 dst.code = code == null ? null : code.copy(); 633 if (strength != null) { 634 dst.strength = new ArrayList<IngredientSubstanceStrengthComponent>(); 635 for (IngredientSubstanceStrengthComponent i : strength) 636 dst.strength.add(i.copy()); 637 }; 638 } 639 640 @Override 641 public boolean equalsDeep(Base other_) { 642 if (!super.equalsDeep(other_)) 643 return false; 644 if (!(other_ instanceof IngredientSubstanceComponent)) 645 return false; 646 IngredientSubstanceComponent o = (IngredientSubstanceComponent) other_; 647 return compareDeep(code, o.code, true) && compareDeep(strength, o.strength, true); 648 } 649 650 @Override 651 public boolean equalsShallow(Base other_) { 652 if (!super.equalsShallow(other_)) 653 return false; 654 if (!(other_ instanceof IngredientSubstanceComponent)) 655 return false; 656 IngredientSubstanceComponent o = (IngredientSubstanceComponent) other_; 657 return true; 658 } 659 660 public boolean isEmpty() { 661 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, strength); 662 } 663 664 public String fhirType() { 665 return "Ingredient.substance"; 666 667 } 668 669 } 670 671 @Block() 672 public static class IngredientSubstanceStrengthComponent extends BackboneElement implements IBaseBackboneElement { 673 /** 674 * The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. Unit of presentation refers to the quantity that the item occurs in e.g. a strength per tablet size, perhaps 'per 20mg' (the size of the tablet). It is not generally normalized as a unitary unit, which would be 'per mg'). 675 */ 676 @Child(name = "presentation", type = {Ratio.class, RatioRange.class, CodeableConcept.class, Quantity.class}, order=1, min=0, max=1, modifier=false, summary=true) 677 @Description(shortDefinition="The quantity of substance in the unit of presentation", formalDefinition="The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. Unit of presentation refers to the quantity that the item occurs in e.g. a strength per tablet size, perhaps 'per 20mg' (the size of the tablet). It is not generally normalized as a unitary unit, which would be 'per mg')." ) 678 protected DataType presentation; 679 680 /** 681 * A textual represention of either the whole of the presentation strength or a part of it - with the rest being in Strength.presentation as a ratio. 682 */ 683 @Child(name = "textPresentation", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 684 @Description(shortDefinition="Text of either the whole presentation strength or a part of it (rest being in Strength.presentation as a ratio)", formalDefinition="A textual represention of either the whole of the presentation strength or a part of it - with the rest being in Strength.presentation as a ratio." ) 685 protected StringType textPresentation; 686 687 /** 688 * The strength per unitary volume (or mass). 689 */ 690 @Child(name = "concentration", type = {Ratio.class, RatioRange.class, CodeableConcept.class, Quantity.class}, order=3, min=0, max=1, modifier=false, summary=true) 691 @Description(shortDefinition="The strength per unitary volume (or mass)", formalDefinition="The strength per unitary volume (or mass)." ) 692 protected DataType concentration; 693 694 /** 695 * A textual represention of either the whole of the concentration strength or a part of it - with the rest being in Strength.concentration as a ratio. 696 */ 697 @Child(name = "textConcentration", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 698 @Description(shortDefinition="Text of either the whole concentration strength or a part of it (rest being in Strength.concentration as a ratio)", formalDefinition="A textual represention of either the whole of the concentration strength or a part of it - with the rest being in Strength.concentration as a ratio." ) 699 protected StringType textConcentration; 700 701 /** 702 * A code that indicates if the strength is, for example, based on the ingredient substance as stated or on the substance base (when the ingredient is a salt). 703 */ 704 @Child(name = "basis", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 705 @Description(shortDefinition="A code that indicates if the strength is, for example, based on the ingredient substance as stated or on the substance base (when the ingredient is a salt)", formalDefinition="A code that indicates if the strength is, for example, based on the ingredient substance as stated or on the substance base (when the ingredient is a salt)." ) 706 protected CodeableConcept basis; 707 708 /** 709 * For when strength is measured at a particular point or distance. There are products where strength is measured at a particular point. For example, the strength of the ingredient in some inhalers is measured at a particular position relative to the point of aerosolization. 710 */ 711 @Child(name = "measurementPoint", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 712 @Description(shortDefinition="When strength is measured at a particular point or distance", formalDefinition="For when strength is measured at a particular point or distance. There are products where strength is measured at a particular point. For example, the strength of the ingredient in some inhalers is measured at a particular position relative to the point of aerosolization." ) 713 protected StringType measurementPoint; 714 715 /** 716 * The country or countries for which the strength range applies. 717 */ 718 @Child(name = "country", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 719 @Description(shortDefinition="Where the strength range applies", formalDefinition="The country or countries for which the strength range applies." ) 720 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/country") 721 protected List<CodeableConcept> country; 722 723 /** 724 * Strength expressed in terms of a reference substance. For when the ingredient strength is additionally expressed as equivalent to the strength of some other closely related substance (e.g. salt vs. base). Reference strength represents the strength (quantitative composition) of the active moiety of the active substance. There are situations when the active substance and active moiety are different, therefore both a strength and a reference strength are needed. 725 */ 726 @Child(name = "referenceStrength", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 727 @Description(shortDefinition="Strength expressed in terms of a reference substance", formalDefinition="Strength expressed in terms of a reference substance. For when the ingredient strength is additionally expressed as equivalent to the strength of some other closely related substance (e.g. salt vs. base). Reference strength represents the strength (quantitative composition) of the active moiety of the active substance. There are situations when the active substance and active moiety are different, therefore both a strength and a reference strength are needed." ) 728 protected List<IngredientSubstanceStrengthReferenceStrengthComponent> referenceStrength; 729 730 private static final long serialVersionUID = 1409093088L; 731 732 /** 733 * Constructor 734 */ 735 public IngredientSubstanceStrengthComponent() { 736 super(); 737 } 738 739 /** 740 * @return {@link #presentation} (The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. Unit of presentation refers to the quantity that the item occurs in e.g. a strength per tablet size, perhaps 'per 20mg' (the size of the tablet). It is not generally normalized as a unitary unit, which would be 'per mg').) 741 */ 742 public DataType getPresentation() { 743 return this.presentation; 744 } 745 746 /** 747 * @return {@link #presentation} (The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. Unit of presentation refers to the quantity that the item occurs in e.g. a strength per tablet size, perhaps 'per 20mg' (the size of the tablet). It is not generally normalized as a unitary unit, which would be 'per mg').) 748 */ 749 public Ratio getPresentationRatio() throws FHIRException { 750 if (this.presentation == null) 751 this.presentation = new Ratio(); 752 if (!(this.presentation instanceof Ratio)) 753 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.presentation.getClass().getName()+" was encountered"); 754 return (Ratio) this.presentation; 755 } 756 757 public boolean hasPresentationRatio() { 758 return this.presentation instanceof Ratio; 759 } 760 761 /** 762 * @return {@link #presentation} (The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. Unit of presentation refers to the quantity that the item occurs in e.g. a strength per tablet size, perhaps 'per 20mg' (the size of the tablet). It is not generally normalized as a unitary unit, which would be 'per mg').) 763 */ 764 public RatioRange getPresentationRatioRange() throws FHIRException { 765 if (this.presentation == null) 766 this.presentation = new RatioRange(); 767 if (!(this.presentation instanceof RatioRange)) 768 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.presentation.getClass().getName()+" was encountered"); 769 return (RatioRange) this.presentation; 770 } 771 772 public boolean hasPresentationRatioRange() { 773 return this.presentation instanceof RatioRange; 774 } 775 776 /** 777 * @return {@link #presentation} (The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. Unit of presentation refers to the quantity that the item occurs in e.g. a strength per tablet size, perhaps 'per 20mg' (the size of the tablet). It is not generally normalized as a unitary unit, which would be 'per mg').) 778 */ 779 public CodeableConcept getPresentationCodeableConcept() throws FHIRException { 780 if (this.presentation == null) 781 this.presentation = new CodeableConcept(); 782 if (!(this.presentation instanceof CodeableConcept)) 783 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.presentation.getClass().getName()+" was encountered"); 784 return (CodeableConcept) this.presentation; 785 } 786 787 public boolean hasPresentationCodeableConcept() { 788 return this.presentation instanceof CodeableConcept; 789 } 790 791 /** 792 * @return {@link #presentation} (The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. Unit of presentation refers to the quantity that the item occurs in e.g. a strength per tablet size, perhaps 'per 20mg' (the size of the tablet). It is not generally normalized as a unitary unit, which would be 'per mg').) 793 */ 794 public Quantity getPresentationQuantity() throws FHIRException { 795 if (this.presentation == null) 796 this.presentation = new Quantity(); 797 if (!(this.presentation instanceof Quantity)) 798 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.presentation.getClass().getName()+" was encountered"); 799 return (Quantity) this.presentation; 800 } 801 802 public boolean hasPresentationQuantity() { 803 return this.presentation instanceof Quantity; 804 } 805 806 public boolean hasPresentation() { 807 return this.presentation != null && !this.presentation.isEmpty(); 808 } 809 810 /** 811 * @param value {@link #presentation} (The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. Unit of presentation refers to the quantity that the item occurs in e.g. a strength per tablet size, perhaps 'per 20mg' (the size of the tablet). It is not generally normalized as a unitary unit, which would be 'per mg').) 812 */ 813 public IngredientSubstanceStrengthComponent setPresentation(DataType value) { 814 if (value != null && !(value instanceof Ratio || value instanceof RatioRange || value instanceof CodeableConcept || value instanceof Quantity)) 815 throw new FHIRException("Not the right type for Ingredient.substance.strength.presentation[x]: "+value.fhirType()); 816 this.presentation = value; 817 return this; 818 } 819 820 /** 821 * @return {@link #textPresentation} (A textual represention of either the whole of the presentation strength or a part of it - with the rest being in Strength.presentation as a ratio.). This is the underlying object with id, value and extensions. The accessor "getTextPresentation" gives direct access to the value 822 */ 823 public StringType getTextPresentationElement() { 824 if (this.textPresentation == null) 825 if (Configuration.errorOnAutoCreate()) 826 throw new Error("Attempt to auto-create IngredientSubstanceStrengthComponent.textPresentation"); 827 else if (Configuration.doAutoCreate()) 828 this.textPresentation = new StringType(); // bb 829 return this.textPresentation; 830 } 831 832 public boolean hasTextPresentationElement() { 833 return this.textPresentation != null && !this.textPresentation.isEmpty(); 834 } 835 836 public boolean hasTextPresentation() { 837 return this.textPresentation != null && !this.textPresentation.isEmpty(); 838 } 839 840 /** 841 * @param value {@link #textPresentation} (A textual represention of either the whole of the presentation strength or a part of it - with the rest being in Strength.presentation as a ratio.). This is the underlying object with id, value and extensions. The accessor "getTextPresentation" gives direct access to the value 842 */ 843 public IngredientSubstanceStrengthComponent setTextPresentationElement(StringType value) { 844 this.textPresentation = value; 845 return this; 846 } 847 848 /** 849 * @return A textual represention of either the whole of the presentation strength or a part of it - with the rest being in Strength.presentation as a ratio. 850 */ 851 public String getTextPresentation() { 852 return this.textPresentation == null ? null : this.textPresentation.getValue(); 853 } 854 855 /** 856 * @param value A textual represention of either the whole of the presentation strength or a part of it - with the rest being in Strength.presentation as a ratio. 857 */ 858 public IngredientSubstanceStrengthComponent setTextPresentation(String value) { 859 if (Utilities.noString(value)) 860 this.textPresentation = null; 861 else { 862 if (this.textPresentation == null) 863 this.textPresentation = new StringType(); 864 this.textPresentation.setValue(value); 865 } 866 return this; 867 } 868 869 /** 870 * @return {@link #concentration} (The strength per unitary volume (or mass).) 871 */ 872 public DataType getConcentration() { 873 return this.concentration; 874 } 875 876 /** 877 * @return {@link #concentration} (The strength per unitary volume (or mass).) 878 */ 879 public Ratio getConcentrationRatio() throws FHIRException { 880 if (this.concentration == null) 881 this.concentration = new Ratio(); 882 if (!(this.concentration instanceof Ratio)) 883 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.concentration.getClass().getName()+" was encountered"); 884 return (Ratio) this.concentration; 885 } 886 887 public boolean hasConcentrationRatio() { 888 return this.concentration instanceof Ratio; 889 } 890 891 /** 892 * @return {@link #concentration} (The strength per unitary volume (or mass).) 893 */ 894 public RatioRange getConcentrationRatioRange() throws FHIRException { 895 if (this.concentration == null) 896 this.concentration = new RatioRange(); 897 if (!(this.concentration instanceof RatioRange)) 898 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.concentration.getClass().getName()+" was encountered"); 899 return (RatioRange) this.concentration; 900 } 901 902 public boolean hasConcentrationRatioRange() { 903 return this.concentration instanceof RatioRange; 904 } 905 906 /** 907 * @return {@link #concentration} (The strength per unitary volume (or mass).) 908 */ 909 public CodeableConcept getConcentrationCodeableConcept() throws FHIRException { 910 if (this.concentration == null) 911 this.concentration = new CodeableConcept(); 912 if (!(this.concentration instanceof CodeableConcept)) 913 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.concentration.getClass().getName()+" was encountered"); 914 return (CodeableConcept) this.concentration; 915 } 916 917 public boolean hasConcentrationCodeableConcept() { 918 return this.concentration instanceof CodeableConcept; 919 } 920 921 /** 922 * @return {@link #concentration} (The strength per unitary volume (or mass).) 923 */ 924 public Quantity getConcentrationQuantity() throws FHIRException { 925 if (this.concentration == null) 926 this.concentration = new Quantity(); 927 if (!(this.concentration instanceof Quantity)) 928 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.concentration.getClass().getName()+" was encountered"); 929 return (Quantity) this.concentration; 930 } 931 932 public boolean hasConcentrationQuantity() { 933 return this.concentration instanceof Quantity; 934 } 935 936 public boolean hasConcentration() { 937 return this.concentration != null && !this.concentration.isEmpty(); 938 } 939 940 /** 941 * @param value {@link #concentration} (The strength per unitary volume (or mass).) 942 */ 943 public IngredientSubstanceStrengthComponent setConcentration(DataType value) { 944 if (value != null && !(value instanceof Ratio || value instanceof RatioRange || value instanceof CodeableConcept || value instanceof Quantity)) 945 throw new FHIRException("Not the right type for Ingredient.substance.strength.concentration[x]: "+value.fhirType()); 946 this.concentration = value; 947 return this; 948 } 949 950 /** 951 * @return {@link #textConcentration} (A textual represention of either the whole of the concentration strength or a part of it - with the rest being in Strength.concentration as a ratio.). This is the underlying object with id, value and extensions. The accessor "getTextConcentration" gives direct access to the value 952 */ 953 public StringType getTextConcentrationElement() { 954 if (this.textConcentration == null) 955 if (Configuration.errorOnAutoCreate()) 956 throw new Error("Attempt to auto-create IngredientSubstanceStrengthComponent.textConcentration"); 957 else if (Configuration.doAutoCreate()) 958 this.textConcentration = new StringType(); // bb 959 return this.textConcentration; 960 } 961 962 public boolean hasTextConcentrationElement() { 963 return this.textConcentration != null && !this.textConcentration.isEmpty(); 964 } 965 966 public boolean hasTextConcentration() { 967 return this.textConcentration != null && !this.textConcentration.isEmpty(); 968 } 969 970 /** 971 * @param value {@link #textConcentration} (A textual represention of either the whole of the concentration strength or a part of it - with the rest being in Strength.concentration as a ratio.). This is the underlying object with id, value and extensions. The accessor "getTextConcentration" gives direct access to the value 972 */ 973 public IngredientSubstanceStrengthComponent setTextConcentrationElement(StringType value) { 974 this.textConcentration = value; 975 return this; 976 } 977 978 /** 979 * @return A textual represention of either the whole of the concentration strength or a part of it - with the rest being in Strength.concentration as a ratio. 980 */ 981 public String getTextConcentration() { 982 return this.textConcentration == null ? null : this.textConcentration.getValue(); 983 } 984 985 /** 986 * @param value A textual represention of either the whole of the concentration strength or a part of it - with the rest being in Strength.concentration as a ratio. 987 */ 988 public IngredientSubstanceStrengthComponent setTextConcentration(String value) { 989 if (Utilities.noString(value)) 990 this.textConcentration = null; 991 else { 992 if (this.textConcentration == null) 993 this.textConcentration = new StringType(); 994 this.textConcentration.setValue(value); 995 } 996 return this; 997 } 998 999 /** 1000 * @return {@link #basis} (A code that indicates if the strength is, for example, based on the ingredient substance as stated or on the substance base (when the ingredient is a salt).) 1001 */ 1002 public CodeableConcept getBasis() { 1003 if (this.basis == null) 1004 if (Configuration.errorOnAutoCreate()) 1005 throw new Error("Attempt to auto-create IngredientSubstanceStrengthComponent.basis"); 1006 else if (Configuration.doAutoCreate()) 1007 this.basis = new CodeableConcept(); // cc 1008 return this.basis; 1009 } 1010 1011 public boolean hasBasis() { 1012 return this.basis != null && !this.basis.isEmpty(); 1013 } 1014 1015 /** 1016 * @param value {@link #basis} (A code that indicates if the strength is, for example, based on the ingredient substance as stated or on the substance base (when the ingredient is a salt).) 1017 */ 1018 public IngredientSubstanceStrengthComponent setBasis(CodeableConcept value) { 1019 this.basis = value; 1020 return this; 1021 } 1022 1023 /** 1024 * @return {@link #measurementPoint} (For when strength is measured at a particular point or distance. There are products where strength is measured at a particular point. For example, the strength of the ingredient in some inhalers is measured at a particular position relative to the point of aerosolization.). This is the underlying object with id, value and extensions. The accessor "getMeasurementPoint" gives direct access to the value 1025 */ 1026 public StringType getMeasurementPointElement() { 1027 if (this.measurementPoint == null) 1028 if (Configuration.errorOnAutoCreate()) 1029 throw new Error("Attempt to auto-create IngredientSubstanceStrengthComponent.measurementPoint"); 1030 else if (Configuration.doAutoCreate()) 1031 this.measurementPoint = new StringType(); // bb 1032 return this.measurementPoint; 1033 } 1034 1035 public boolean hasMeasurementPointElement() { 1036 return this.measurementPoint != null && !this.measurementPoint.isEmpty(); 1037 } 1038 1039 public boolean hasMeasurementPoint() { 1040 return this.measurementPoint != null && !this.measurementPoint.isEmpty(); 1041 } 1042 1043 /** 1044 * @param value {@link #measurementPoint} (For when strength is measured at a particular point or distance. There are products where strength is measured at a particular point. For example, the strength of the ingredient in some inhalers is measured at a particular position relative to the point of aerosolization.). This is the underlying object with id, value and extensions. The accessor "getMeasurementPoint" gives direct access to the value 1045 */ 1046 public IngredientSubstanceStrengthComponent setMeasurementPointElement(StringType value) { 1047 this.measurementPoint = value; 1048 return this; 1049 } 1050 1051 /** 1052 * @return For when strength is measured at a particular point or distance. There are products where strength is measured at a particular point. For example, the strength of the ingredient in some inhalers is measured at a particular position relative to the point of aerosolization. 1053 */ 1054 public String getMeasurementPoint() { 1055 return this.measurementPoint == null ? null : this.measurementPoint.getValue(); 1056 } 1057 1058 /** 1059 * @param value For when strength is measured at a particular point or distance. There are products where strength is measured at a particular point. For example, the strength of the ingredient in some inhalers is measured at a particular position relative to the point of aerosolization. 1060 */ 1061 public IngredientSubstanceStrengthComponent setMeasurementPoint(String value) { 1062 if (Utilities.noString(value)) 1063 this.measurementPoint = null; 1064 else { 1065 if (this.measurementPoint == null) 1066 this.measurementPoint = new StringType(); 1067 this.measurementPoint.setValue(value); 1068 } 1069 return this; 1070 } 1071 1072 /** 1073 * @return {@link #country} (The country or countries for which the strength range applies.) 1074 */ 1075 public List<CodeableConcept> getCountry() { 1076 if (this.country == null) 1077 this.country = new ArrayList<CodeableConcept>(); 1078 return this.country; 1079 } 1080 1081 /** 1082 * @return Returns a reference to <code>this</code> for easy method chaining 1083 */ 1084 public IngredientSubstanceStrengthComponent setCountry(List<CodeableConcept> theCountry) { 1085 this.country = theCountry; 1086 return this; 1087 } 1088 1089 public boolean hasCountry() { 1090 if (this.country == null) 1091 return false; 1092 for (CodeableConcept item : this.country) 1093 if (!item.isEmpty()) 1094 return true; 1095 return false; 1096 } 1097 1098 public CodeableConcept addCountry() { //3 1099 CodeableConcept t = new CodeableConcept(); 1100 if (this.country == null) 1101 this.country = new ArrayList<CodeableConcept>(); 1102 this.country.add(t); 1103 return t; 1104 } 1105 1106 public IngredientSubstanceStrengthComponent addCountry(CodeableConcept t) { //3 1107 if (t == null) 1108 return this; 1109 if (this.country == null) 1110 this.country = new ArrayList<CodeableConcept>(); 1111 this.country.add(t); 1112 return this; 1113 } 1114 1115 /** 1116 * @return The first repetition of repeating field {@link #country}, creating it if it does not already exist {3} 1117 */ 1118 public CodeableConcept getCountryFirstRep() { 1119 if (getCountry().isEmpty()) { 1120 addCountry(); 1121 } 1122 return getCountry().get(0); 1123 } 1124 1125 /** 1126 * @return {@link #referenceStrength} (Strength expressed in terms of a reference substance. For when the ingredient strength is additionally expressed as equivalent to the strength of some other closely related substance (e.g. salt vs. base). Reference strength represents the strength (quantitative composition) of the active moiety of the active substance. There are situations when the active substance and active moiety are different, therefore both a strength and a reference strength are needed.) 1127 */ 1128 public List<IngredientSubstanceStrengthReferenceStrengthComponent> getReferenceStrength() { 1129 if (this.referenceStrength == null) 1130 this.referenceStrength = new ArrayList<IngredientSubstanceStrengthReferenceStrengthComponent>(); 1131 return this.referenceStrength; 1132 } 1133 1134 /** 1135 * @return Returns a reference to <code>this</code> for easy method chaining 1136 */ 1137 public IngredientSubstanceStrengthComponent setReferenceStrength(List<IngredientSubstanceStrengthReferenceStrengthComponent> theReferenceStrength) { 1138 this.referenceStrength = theReferenceStrength; 1139 return this; 1140 } 1141 1142 public boolean hasReferenceStrength() { 1143 if (this.referenceStrength == null) 1144 return false; 1145 for (IngredientSubstanceStrengthReferenceStrengthComponent item : this.referenceStrength) 1146 if (!item.isEmpty()) 1147 return true; 1148 return false; 1149 } 1150 1151 public IngredientSubstanceStrengthReferenceStrengthComponent addReferenceStrength() { //3 1152 IngredientSubstanceStrengthReferenceStrengthComponent t = new IngredientSubstanceStrengthReferenceStrengthComponent(); 1153 if (this.referenceStrength == null) 1154 this.referenceStrength = new ArrayList<IngredientSubstanceStrengthReferenceStrengthComponent>(); 1155 this.referenceStrength.add(t); 1156 return t; 1157 } 1158 1159 public IngredientSubstanceStrengthComponent addReferenceStrength(IngredientSubstanceStrengthReferenceStrengthComponent t) { //3 1160 if (t == null) 1161 return this; 1162 if (this.referenceStrength == null) 1163 this.referenceStrength = new ArrayList<IngredientSubstanceStrengthReferenceStrengthComponent>(); 1164 this.referenceStrength.add(t); 1165 return this; 1166 } 1167 1168 /** 1169 * @return The first repetition of repeating field {@link #referenceStrength}, creating it if it does not already exist {3} 1170 */ 1171 public IngredientSubstanceStrengthReferenceStrengthComponent getReferenceStrengthFirstRep() { 1172 if (getReferenceStrength().isEmpty()) { 1173 addReferenceStrength(); 1174 } 1175 return getReferenceStrength().get(0); 1176 } 1177 1178 protected void listChildren(List<Property> children) { 1179 super.listChildren(children); 1180 children.add(new Property("presentation[x]", "Ratio|RatioRange|CodeableConcept|Quantity", "The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. Unit of presentation refers to the quantity that the item occurs in e.g. a strength per tablet size, perhaps 'per 20mg' (the size of the tablet). It is not generally normalized as a unitary unit, which would be 'per mg').", 0, 1, presentation)); 1181 children.add(new Property("textPresentation", "string", "A textual represention of either the whole of the presentation strength or a part of it - with the rest being in Strength.presentation as a ratio.", 0, 1, textPresentation)); 1182 children.add(new Property("concentration[x]", "Ratio|RatioRange|CodeableConcept|Quantity", "The strength per unitary volume (or mass).", 0, 1, concentration)); 1183 children.add(new Property("textConcentration", "string", "A textual represention of either the whole of the concentration strength or a part of it - with the rest being in Strength.concentration as a ratio.", 0, 1, textConcentration)); 1184 children.add(new Property("basis", "CodeableConcept", "A code that indicates if the strength is, for example, based on the ingredient substance as stated or on the substance base (when the ingredient is a salt).", 0, 1, basis)); 1185 children.add(new Property("measurementPoint", "string", "For when strength is measured at a particular point or distance. There are products where strength is measured at a particular point. For example, the strength of the ingredient in some inhalers is measured at a particular position relative to the point of aerosolization.", 0, 1, measurementPoint)); 1186 children.add(new Property("country", "CodeableConcept", "The country or countries for which the strength range applies.", 0, java.lang.Integer.MAX_VALUE, country)); 1187 children.add(new Property("referenceStrength", "", "Strength expressed in terms of a reference substance. For when the ingredient strength is additionally expressed as equivalent to the strength of some other closely related substance (e.g. salt vs. base). Reference strength represents the strength (quantitative composition) of the active moiety of the active substance. There are situations when the active substance and active moiety are different, therefore both a strength and a reference strength are needed.", 0, java.lang.Integer.MAX_VALUE, referenceStrength)); 1188 } 1189 1190 @Override 1191 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1192 switch (_hash) { 1193 case 1714280230: /*presentation[x]*/ return new Property("presentation[x]", "Ratio|RatioRange|CodeableConcept|Quantity", "The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. Unit of presentation refers to the quantity that the item occurs in e.g. a strength per tablet size, perhaps 'per 20mg' (the size of the tablet). It is not generally normalized as a unitary unit, which would be 'per mg').", 0, 1, presentation); 1194 case 696975130: /*presentation*/ return new Property("presentation[x]", "Ratio|RatioRange|CodeableConcept|Quantity", "The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. Unit of presentation refers to the quantity that the item occurs in e.g. a strength per tablet size, perhaps 'per 20mg' (the size of the tablet). It is not generally normalized as a unitary unit, which would be 'per mg').", 0, 1, presentation); 1195 case -1853112047: /*presentationRatio*/ return new Property("presentation[x]", "Ratio", "The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. Unit of presentation refers to the quantity that the item occurs in e.g. a strength per tablet size, perhaps 'per 20mg' (the size of the tablet). It is not generally normalized as a unitary unit, which would be 'per mg').", 0, 1, presentation); 1196 case 643336876: /*presentationRatioRange*/ return new Property("presentation[x]", "RatioRange", "The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. Unit of presentation refers to the quantity that the item occurs in e.g. a strength per tablet size, perhaps 'per 20mg' (the size of the tablet). It is not generally normalized as a unitary unit, which would be 'per mg').", 0, 1, presentation); 1197 case 1095127335: /*presentationCodeableConcept*/ return new Property("presentation[x]", "CodeableConcept", "The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. Unit of presentation refers to the quantity that the item occurs in e.g. a strength per tablet size, perhaps 'per 20mg' (the size of the tablet). It is not generally normalized as a unitary unit, which would be 'per mg').", 0, 1, presentation); 1198 case -263057979: /*presentationQuantity*/ return new Property("presentation[x]", "Quantity", "The quantity of substance in the unit of presentation, or in the volume (or mass) of the single pharmaceutical product or manufactured item. Unit of presentation refers to the quantity that the item occurs in e.g. a strength per tablet size, perhaps 'per 20mg' (the size of the tablet). It is not generally normalized as a unitary unit, which would be 'per mg').", 0, 1, presentation); 1199 case -799720217: /*textPresentation*/ return new Property("textPresentation", "string", "A textual represention of either the whole of the presentation strength or a part of it - with the rest being in Strength.presentation as a ratio.", 0, 1, textPresentation); 1200 case 1153502451: /*concentration[x]*/ return new Property("concentration[x]", "Ratio|RatioRange|CodeableConcept|Quantity", "The strength per unitary volume (or mass).", 0, 1, concentration); 1201 case -410557331: /*concentration*/ return new Property("concentration[x]", "Ratio|RatioRange|CodeableConcept|Quantity", "The strength per unitary volume (or mass).", 0, 1, concentration); 1202 case 405321630: /*concentrationRatio*/ return new Property("concentration[x]", "Ratio", "The strength per unitary volume (or mass).", 0, 1, concentration); 1203 case 436249663: /*concentrationRatioRange*/ return new Property("concentration[x]", "RatioRange", "The strength per unitary volume (or mass).", 0, 1, concentration); 1204 case -90293388: /*concentrationCodeableConcept*/ return new Property("concentration[x]", "CodeableConcept", "The strength per unitary volume (or mass).", 0, 1, concentration); 1205 case 71921688: /*concentrationQuantity*/ return new Property("concentration[x]", "Quantity", "The strength per unitary volume (or mass).", 0, 1, concentration); 1206 case 436527168: /*textConcentration*/ return new Property("textConcentration", "string", "A textual represention of either the whole of the concentration strength or a part of it - with the rest being in Strength.concentration as a ratio.", 0, 1, textConcentration); 1207 case 93508670: /*basis*/ return new Property("basis", "CodeableConcept", "A code that indicates if the strength is, for example, based on the ingredient substance as stated or on the substance base (when the ingredient is a salt).", 0, 1, basis); 1208 case 235437876: /*measurementPoint*/ return new Property("measurementPoint", "string", "For when strength is measured at a particular point or distance. There are products where strength is measured at a particular point. For example, the strength of the ingredient in some inhalers is measured at a particular position relative to the point of aerosolization.", 0, 1, measurementPoint); 1209 case 957831062: /*country*/ return new Property("country", "CodeableConcept", "The country or countries for which the strength range applies.", 0, java.lang.Integer.MAX_VALUE, country); 1210 case 1943566508: /*referenceStrength*/ return new Property("referenceStrength", "", "Strength expressed in terms of a reference substance. For when the ingredient strength is additionally expressed as equivalent to the strength of some other closely related substance (e.g. salt vs. base). Reference strength represents the strength (quantitative composition) of the active moiety of the active substance. There are situations when the active substance and active moiety are different, therefore both a strength and a reference strength are needed.", 0, java.lang.Integer.MAX_VALUE, referenceStrength); 1211 default: return super.getNamedProperty(_hash, _name, _checkValid); 1212 } 1213 1214 } 1215 1216 @Override 1217 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1218 switch (hash) { 1219 case 696975130: /*presentation*/ return this.presentation == null ? new Base[0] : new Base[] {this.presentation}; // DataType 1220 case -799720217: /*textPresentation*/ return this.textPresentation == null ? new Base[0] : new Base[] {this.textPresentation}; // StringType 1221 case -410557331: /*concentration*/ return this.concentration == null ? new Base[0] : new Base[] {this.concentration}; // DataType 1222 case 436527168: /*textConcentration*/ return this.textConcentration == null ? new Base[0] : new Base[] {this.textConcentration}; // StringType 1223 case 93508670: /*basis*/ return this.basis == null ? new Base[0] : new Base[] {this.basis}; // CodeableConcept 1224 case 235437876: /*measurementPoint*/ return this.measurementPoint == null ? new Base[0] : new Base[] {this.measurementPoint}; // StringType 1225 case 957831062: /*country*/ return this.country == null ? new Base[0] : this.country.toArray(new Base[this.country.size()]); // CodeableConcept 1226 case 1943566508: /*referenceStrength*/ return this.referenceStrength == null ? new Base[0] : this.referenceStrength.toArray(new Base[this.referenceStrength.size()]); // IngredientSubstanceStrengthReferenceStrengthComponent 1227 default: return super.getProperty(hash, name, checkValid); 1228 } 1229 1230 } 1231 1232 @Override 1233 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1234 switch (hash) { 1235 case 696975130: // presentation 1236 this.presentation = TypeConvertor.castToType(value); // DataType 1237 return value; 1238 case -799720217: // textPresentation 1239 this.textPresentation = TypeConvertor.castToString(value); // StringType 1240 return value; 1241 case -410557331: // concentration 1242 this.concentration = TypeConvertor.castToType(value); // DataType 1243 return value; 1244 case 436527168: // textConcentration 1245 this.textConcentration = TypeConvertor.castToString(value); // StringType 1246 return value; 1247 case 93508670: // basis 1248 this.basis = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1249 return value; 1250 case 235437876: // measurementPoint 1251 this.measurementPoint = TypeConvertor.castToString(value); // StringType 1252 return value; 1253 case 957831062: // country 1254 this.getCountry().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1255 return value; 1256 case 1943566508: // referenceStrength 1257 this.getReferenceStrength().add((IngredientSubstanceStrengthReferenceStrengthComponent) value); // IngredientSubstanceStrengthReferenceStrengthComponent 1258 return value; 1259 default: return super.setProperty(hash, name, value); 1260 } 1261 1262 } 1263 1264 @Override 1265 public Base setProperty(String name, Base value) throws FHIRException { 1266 if (name.equals("presentation[x]")) { 1267 this.presentation = TypeConvertor.castToType(value); // DataType 1268 } else if (name.equals("textPresentation")) { 1269 this.textPresentation = TypeConvertor.castToString(value); // StringType 1270 } else if (name.equals("concentration[x]")) { 1271 this.concentration = TypeConvertor.castToType(value); // DataType 1272 } else if (name.equals("textConcentration")) { 1273 this.textConcentration = TypeConvertor.castToString(value); // StringType 1274 } else if (name.equals("basis")) { 1275 this.basis = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1276 } else if (name.equals("measurementPoint")) { 1277 this.measurementPoint = TypeConvertor.castToString(value); // StringType 1278 } else if (name.equals("country")) { 1279 this.getCountry().add(TypeConvertor.castToCodeableConcept(value)); 1280 } else if (name.equals("referenceStrength")) { 1281 this.getReferenceStrength().add((IngredientSubstanceStrengthReferenceStrengthComponent) value); 1282 } else 1283 return super.setProperty(name, value); 1284 return value; 1285 } 1286 1287 @Override 1288 public void removeChild(String name, Base value) throws FHIRException { 1289 if (name.equals("presentation[x]")) { 1290 this.presentation = null; 1291 } else if (name.equals("textPresentation")) { 1292 this.textPresentation = null; 1293 } else if (name.equals("concentration[x]")) { 1294 this.concentration = null; 1295 } else if (name.equals("textConcentration")) { 1296 this.textConcentration = null; 1297 } else if (name.equals("basis")) { 1298 this.basis = null; 1299 } else if (name.equals("measurementPoint")) { 1300 this.measurementPoint = null; 1301 } else if (name.equals("country")) { 1302 this.getCountry().remove(value); 1303 } else if (name.equals("referenceStrength")) { 1304 this.getReferenceStrength().remove((IngredientSubstanceStrengthReferenceStrengthComponent) value); 1305 } else 1306 super.removeChild(name, value); 1307 1308 } 1309 1310 @Override 1311 public Base makeProperty(int hash, String name) throws FHIRException { 1312 switch (hash) { 1313 case 1714280230: return getPresentation(); 1314 case 696975130: return getPresentation(); 1315 case -799720217: return getTextPresentationElement(); 1316 case 1153502451: return getConcentration(); 1317 case -410557331: return getConcentration(); 1318 case 436527168: return getTextConcentrationElement(); 1319 case 93508670: return getBasis(); 1320 case 235437876: return getMeasurementPointElement(); 1321 case 957831062: return addCountry(); 1322 case 1943566508: return addReferenceStrength(); 1323 default: return super.makeProperty(hash, name); 1324 } 1325 1326 } 1327 1328 @Override 1329 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1330 switch (hash) { 1331 case 696975130: /*presentation*/ return new String[] {"Ratio", "RatioRange", "CodeableConcept", "Quantity"}; 1332 case -799720217: /*textPresentation*/ return new String[] {"string"}; 1333 case -410557331: /*concentration*/ return new String[] {"Ratio", "RatioRange", "CodeableConcept", "Quantity"}; 1334 case 436527168: /*textConcentration*/ return new String[] {"string"}; 1335 case 93508670: /*basis*/ return new String[] {"CodeableConcept"}; 1336 case 235437876: /*measurementPoint*/ return new String[] {"string"}; 1337 case 957831062: /*country*/ return new String[] {"CodeableConcept"}; 1338 case 1943566508: /*referenceStrength*/ return new String[] {}; 1339 default: return super.getTypesForProperty(hash, name); 1340 } 1341 1342 } 1343 1344 @Override 1345 public Base addChild(String name) throws FHIRException { 1346 if (name.equals("presentationRatio")) { 1347 this.presentation = new Ratio(); 1348 return this.presentation; 1349 } 1350 else if (name.equals("presentationRatioRange")) { 1351 this.presentation = new RatioRange(); 1352 return this.presentation; 1353 } 1354 else if (name.equals("presentationCodeableConcept")) { 1355 this.presentation = new CodeableConcept(); 1356 return this.presentation; 1357 } 1358 else if (name.equals("presentationQuantity")) { 1359 this.presentation = new Quantity(); 1360 return this.presentation; 1361 } 1362 else if (name.equals("textPresentation")) { 1363 throw new FHIRException("Cannot call addChild on a singleton property Ingredient.substance.strength.textPresentation"); 1364 } 1365 else if (name.equals("concentrationRatio")) { 1366 this.concentration = new Ratio(); 1367 return this.concentration; 1368 } 1369 else if (name.equals("concentrationRatioRange")) { 1370 this.concentration = new RatioRange(); 1371 return this.concentration; 1372 } 1373 else if (name.equals("concentrationCodeableConcept")) { 1374 this.concentration = new CodeableConcept(); 1375 return this.concentration; 1376 } 1377 else if (name.equals("concentrationQuantity")) { 1378 this.concentration = new Quantity(); 1379 return this.concentration; 1380 } 1381 else if (name.equals("textConcentration")) { 1382 throw new FHIRException("Cannot call addChild on a singleton property Ingredient.substance.strength.textConcentration"); 1383 } 1384 else if (name.equals("basis")) { 1385 this.basis = new CodeableConcept(); 1386 return this.basis; 1387 } 1388 else if (name.equals("measurementPoint")) { 1389 throw new FHIRException("Cannot call addChild on a singleton property Ingredient.substance.strength.measurementPoint"); 1390 } 1391 else if (name.equals("country")) { 1392 return addCountry(); 1393 } 1394 else if (name.equals("referenceStrength")) { 1395 return addReferenceStrength(); 1396 } 1397 else 1398 return super.addChild(name); 1399 } 1400 1401 public IngredientSubstanceStrengthComponent copy() { 1402 IngredientSubstanceStrengthComponent dst = new IngredientSubstanceStrengthComponent(); 1403 copyValues(dst); 1404 return dst; 1405 } 1406 1407 public void copyValues(IngredientSubstanceStrengthComponent dst) { 1408 super.copyValues(dst); 1409 dst.presentation = presentation == null ? null : presentation.copy(); 1410 dst.textPresentation = textPresentation == null ? null : textPresentation.copy(); 1411 dst.concentration = concentration == null ? null : concentration.copy(); 1412 dst.textConcentration = textConcentration == null ? null : textConcentration.copy(); 1413 dst.basis = basis == null ? null : basis.copy(); 1414 dst.measurementPoint = measurementPoint == null ? null : measurementPoint.copy(); 1415 if (country != null) { 1416 dst.country = new ArrayList<CodeableConcept>(); 1417 for (CodeableConcept i : country) 1418 dst.country.add(i.copy()); 1419 }; 1420 if (referenceStrength != null) { 1421 dst.referenceStrength = new ArrayList<IngredientSubstanceStrengthReferenceStrengthComponent>(); 1422 for (IngredientSubstanceStrengthReferenceStrengthComponent i : referenceStrength) 1423 dst.referenceStrength.add(i.copy()); 1424 }; 1425 } 1426 1427 @Override 1428 public boolean equalsDeep(Base other_) { 1429 if (!super.equalsDeep(other_)) 1430 return false; 1431 if (!(other_ instanceof IngredientSubstanceStrengthComponent)) 1432 return false; 1433 IngredientSubstanceStrengthComponent o = (IngredientSubstanceStrengthComponent) other_; 1434 return compareDeep(presentation, o.presentation, true) && compareDeep(textPresentation, o.textPresentation, true) 1435 && compareDeep(concentration, o.concentration, true) && compareDeep(textConcentration, o.textConcentration, true) 1436 && compareDeep(basis, o.basis, true) && compareDeep(measurementPoint, o.measurementPoint, true) 1437 && compareDeep(country, o.country, true) && compareDeep(referenceStrength, o.referenceStrength, true) 1438 ; 1439 } 1440 1441 @Override 1442 public boolean equalsShallow(Base other_) { 1443 if (!super.equalsShallow(other_)) 1444 return false; 1445 if (!(other_ instanceof IngredientSubstanceStrengthComponent)) 1446 return false; 1447 IngredientSubstanceStrengthComponent o = (IngredientSubstanceStrengthComponent) other_; 1448 return compareValues(textPresentation, o.textPresentation, true) && compareValues(textConcentration, o.textConcentration, true) 1449 && compareValues(measurementPoint, o.measurementPoint, true); 1450 } 1451 1452 public boolean isEmpty() { 1453 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(presentation, textPresentation 1454 , concentration, textConcentration, basis, measurementPoint, country, referenceStrength 1455 ); 1456 } 1457 1458 public String fhirType() { 1459 return "Ingredient.substance.strength"; 1460 1461 } 1462 1463 } 1464 1465 @Block() 1466 public static class IngredientSubstanceStrengthReferenceStrengthComponent extends BackboneElement implements IBaseBackboneElement { 1467 /** 1468 * Relevant reference substance. 1469 */ 1470 @Child(name = "substance", type = {CodeableReference.class}, order=1, min=1, max=1, modifier=false, summary=true) 1471 @Description(shortDefinition="Relevant reference substance", formalDefinition="Relevant reference substance." ) 1472 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-codes") 1473 protected CodeableReference substance; 1474 1475 /** 1476 * Strength expressed in terms of a reference substance. 1477 */ 1478 @Child(name = "strength", type = {Ratio.class, RatioRange.class, Quantity.class}, order=2, min=1, max=1, modifier=false, summary=true) 1479 @Description(shortDefinition="Strength expressed in terms of a reference substance", formalDefinition="Strength expressed in terms of a reference substance." ) 1480 protected DataType strength; 1481 1482 /** 1483 * For when strength is measured at a particular point or distance. 1484 */ 1485 @Child(name = "measurementPoint", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1486 @Description(shortDefinition="When strength is measured at a particular point or distance", formalDefinition="For when strength is measured at a particular point or distance." ) 1487 protected StringType measurementPoint; 1488 1489 /** 1490 * The country or countries for which the strength range applies. 1491 */ 1492 @Child(name = "country", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1493 @Description(shortDefinition="Where the strength range applies", formalDefinition="The country or countries for which the strength range applies." ) 1494 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/country") 1495 protected List<CodeableConcept> country; 1496 1497 private static final long serialVersionUID = 1700529245L; 1498 1499 /** 1500 * Constructor 1501 */ 1502 public IngredientSubstanceStrengthReferenceStrengthComponent() { 1503 super(); 1504 } 1505 1506 /** 1507 * Constructor 1508 */ 1509 public IngredientSubstanceStrengthReferenceStrengthComponent(CodeableReference substance, DataType strength) { 1510 super(); 1511 this.setSubstance(substance); 1512 this.setStrength(strength); 1513 } 1514 1515 /** 1516 * @return {@link #substance} (Relevant reference substance.) 1517 */ 1518 public CodeableReference getSubstance() { 1519 if (this.substance == null) 1520 if (Configuration.errorOnAutoCreate()) 1521 throw new Error("Attempt to auto-create IngredientSubstanceStrengthReferenceStrengthComponent.substance"); 1522 else if (Configuration.doAutoCreate()) 1523 this.substance = new CodeableReference(); // cc 1524 return this.substance; 1525 } 1526 1527 public boolean hasSubstance() { 1528 return this.substance != null && !this.substance.isEmpty(); 1529 } 1530 1531 /** 1532 * @param value {@link #substance} (Relevant reference substance.) 1533 */ 1534 public IngredientSubstanceStrengthReferenceStrengthComponent setSubstance(CodeableReference value) { 1535 this.substance = value; 1536 return this; 1537 } 1538 1539 /** 1540 * @return {@link #strength} (Strength expressed in terms of a reference substance.) 1541 */ 1542 public DataType getStrength() { 1543 return this.strength; 1544 } 1545 1546 /** 1547 * @return {@link #strength} (Strength expressed in terms of a reference substance.) 1548 */ 1549 public Ratio getStrengthRatio() throws FHIRException { 1550 if (this.strength == null) 1551 this.strength = new Ratio(); 1552 if (!(this.strength instanceof Ratio)) 1553 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.strength.getClass().getName()+" was encountered"); 1554 return (Ratio) this.strength; 1555 } 1556 1557 public boolean hasStrengthRatio() { 1558 return this.strength instanceof Ratio; 1559 } 1560 1561 /** 1562 * @return {@link #strength} (Strength expressed in terms of a reference substance.) 1563 */ 1564 public RatioRange getStrengthRatioRange() throws FHIRException { 1565 if (this.strength == null) 1566 this.strength = new RatioRange(); 1567 if (!(this.strength instanceof RatioRange)) 1568 throw new FHIRException("Type mismatch: the type RatioRange was expected, but "+this.strength.getClass().getName()+" was encountered"); 1569 return (RatioRange) this.strength; 1570 } 1571 1572 public boolean hasStrengthRatioRange() { 1573 return this.strength instanceof RatioRange; 1574 } 1575 1576 /** 1577 * @return {@link #strength} (Strength expressed in terms of a reference substance.) 1578 */ 1579 public Quantity getStrengthQuantity() throws FHIRException { 1580 if (this.strength == null) 1581 this.strength = new Quantity(); 1582 if (!(this.strength instanceof Quantity)) 1583 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.strength.getClass().getName()+" was encountered"); 1584 return (Quantity) this.strength; 1585 } 1586 1587 public boolean hasStrengthQuantity() { 1588 return this.strength instanceof Quantity; 1589 } 1590 1591 public boolean hasStrength() { 1592 return this.strength != null && !this.strength.isEmpty(); 1593 } 1594 1595 /** 1596 * @param value {@link #strength} (Strength expressed in terms of a reference substance.) 1597 */ 1598 public IngredientSubstanceStrengthReferenceStrengthComponent setStrength(DataType value) { 1599 if (value != null && !(value instanceof Ratio || value instanceof RatioRange || value instanceof Quantity)) 1600 throw new FHIRException("Not the right type for Ingredient.substance.strength.referenceStrength.strength[x]: "+value.fhirType()); 1601 this.strength = value; 1602 return this; 1603 } 1604 1605 /** 1606 * @return {@link #measurementPoint} (For when strength is measured at a particular point or distance.). This is the underlying object with id, value and extensions. The accessor "getMeasurementPoint" gives direct access to the value 1607 */ 1608 public StringType getMeasurementPointElement() { 1609 if (this.measurementPoint == null) 1610 if (Configuration.errorOnAutoCreate()) 1611 throw new Error("Attempt to auto-create IngredientSubstanceStrengthReferenceStrengthComponent.measurementPoint"); 1612 else if (Configuration.doAutoCreate()) 1613 this.measurementPoint = new StringType(); // bb 1614 return this.measurementPoint; 1615 } 1616 1617 public boolean hasMeasurementPointElement() { 1618 return this.measurementPoint != null && !this.measurementPoint.isEmpty(); 1619 } 1620 1621 public boolean hasMeasurementPoint() { 1622 return this.measurementPoint != null && !this.measurementPoint.isEmpty(); 1623 } 1624 1625 /** 1626 * @param value {@link #measurementPoint} (For when strength is measured at a particular point or distance.). This is the underlying object with id, value and extensions. The accessor "getMeasurementPoint" gives direct access to the value 1627 */ 1628 public IngredientSubstanceStrengthReferenceStrengthComponent setMeasurementPointElement(StringType value) { 1629 this.measurementPoint = value; 1630 return this; 1631 } 1632 1633 /** 1634 * @return For when strength is measured at a particular point or distance. 1635 */ 1636 public String getMeasurementPoint() { 1637 return this.measurementPoint == null ? null : this.measurementPoint.getValue(); 1638 } 1639 1640 /** 1641 * @param value For when strength is measured at a particular point or distance. 1642 */ 1643 public IngredientSubstanceStrengthReferenceStrengthComponent setMeasurementPoint(String value) { 1644 if (Utilities.noString(value)) 1645 this.measurementPoint = null; 1646 else { 1647 if (this.measurementPoint == null) 1648 this.measurementPoint = new StringType(); 1649 this.measurementPoint.setValue(value); 1650 } 1651 return this; 1652 } 1653 1654 /** 1655 * @return {@link #country} (The country or countries for which the strength range applies.) 1656 */ 1657 public List<CodeableConcept> getCountry() { 1658 if (this.country == null) 1659 this.country = new ArrayList<CodeableConcept>(); 1660 return this.country; 1661 } 1662 1663 /** 1664 * @return Returns a reference to <code>this</code> for easy method chaining 1665 */ 1666 public IngredientSubstanceStrengthReferenceStrengthComponent setCountry(List<CodeableConcept> theCountry) { 1667 this.country = theCountry; 1668 return this; 1669 } 1670 1671 public boolean hasCountry() { 1672 if (this.country == null) 1673 return false; 1674 for (CodeableConcept item : this.country) 1675 if (!item.isEmpty()) 1676 return true; 1677 return false; 1678 } 1679 1680 public CodeableConcept addCountry() { //3 1681 CodeableConcept t = new CodeableConcept(); 1682 if (this.country == null) 1683 this.country = new ArrayList<CodeableConcept>(); 1684 this.country.add(t); 1685 return t; 1686 } 1687 1688 public IngredientSubstanceStrengthReferenceStrengthComponent addCountry(CodeableConcept t) { //3 1689 if (t == null) 1690 return this; 1691 if (this.country == null) 1692 this.country = new ArrayList<CodeableConcept>(); 1693 this.country.add(t); 1694 return this; 1695 } 1696 1697 /** 1698 * @return The first repetition of repeating field {@link #country}, creating it if it does not already exist {3} 1699 */ 1700 public CodeableConcept getCountryFirstRep() { 1701 if (getCountry().isEmpty()) { 1702 addCountry(); 1703 } 1704 return getCountry().get(0); 1705 } 1706 1707 protected void listChildren(List<Property> children) { 1708 super.listChildren(children); 1709 children.add(new Property("substance", "CodeableReference(SubstanceDefinition)", "Relevant reference substance.", 0, 1, substance)); 1710 children.add(new Property("strength[x]", "Ratio|RatioRange|Quantity", "Strength expressed in terms of a reference substance.", 0, 1, strength)); 1711 children.add(new Property("measurementPoint", "string", "For when strength is measured at a particular point or distance.", 0, 1, measurementPoint)); 1712 children.add(new Property("country", "CodeableConcept", "The country or countries for which the strength range applies.", 0, java.lang.Integer.MAX_VALUE, country)); 1713 } 1714 1715 @Override 1716 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1717 switch (_hash) { 1718 case 530040176: /*substance*/ return new Property("substance", "CodeableReference(SubstanceDefinition)", "Relevant reference substance.", 0, 1, substance); 1719 case 127377567: /*strength[x]*/ return new Property("strength[x]", "Ratio|RatioRange|Quantity", "Strength expressed in terms of a reference substance.", 0, 1, strength); 1720 case 1791316033: /*strength*/ return new Property("strength[x]", "Ratio|RatioRange|Quantity", "Strength expressed in terms of a reference substance.", 0, 1, strength); 1721 case 2141786186: /*strengthRatio*/ return new Property("strength[x]", "Ratio", "Strength expressed in terms of a reference substance.", 0, 1, strength); 1722 case -1300703469: /*strengthRatioRange*/ return new Property("strength[x]", "RatioRange", "Strength expressed in terms of a reference substance.", 0, 1, strength); 1723 case -1793570836: /*strengthQuantity*/ return new Property("strength[x]", "Quantity", "Strength expressed in terms of a reference substance.", 0, 1, strength); 1724 case 235437876: /*measurementPoint*/ return new Property("measurementPoint", "string", "For when strength is measured at a particular point or distance.", 0, 1, measurementPoint); 1725 case 957831062: /*country*/ return new Property("country", "CodeableConcept", "The country or countries for which the strength range applies.", 0, java.lang.Integer.MAX_VALUE, country); 1726 default: return super.getNamedProperty(_hash, _name, _checkValid); 1727 } 1728 1729 } 1730 1731 @Override 1732 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1733 switch (hash) { 1734 case 530040176: /*substance*/ return this.substance == null ? new Base[0] : new Base[] {this.substance}; // CodeableReference 1735 case 1791316033: /*strength*/ return this.strength == null ? new Base[0] : new Base[] {this.strength}; // DataType 1736 case 235437876: /*measurementPoint*/ return this.measurementPoint == null ? new Base[0] : new Base[] {this.measurementPoint}; // StringType 1737 case 957831062: /*country*/ return this.country == null ? new Base[0] : this.country.toArray(new Base[this.country.size()]); // CodeableConcept 1738 default: return super.getProperty(hash, name, checkValid); 1739 } 1740 1741 } 1742 1743 @Override 1744 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1745 switch (hash) { 1746 case 530040176: // substance 1747 this.substance = TypeConvertor.castToCodeableReference(value); // CodeableReference 1748 return value; 1749 case 1791316033: // strength 1750 this.strength = TypeConvertor.castToType(value); // DataType 1751 return value; 1752 case 235437876: // measurementPoint 1753 this.measurementPoint = TypeConvertor.castToString(value); // StringType 1754 return value; 1755 case 957831062: // country 1756 this.getCountry().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1757 return value; 1758 default: return super.setProperty(hash, name, value); 1759 } 1760 1761 } 1762 1763 @Override 1764 public Base setProperty(String name, Base value) throws FHIRException { 1765 if (name.equals("substance")) { 1766 this.substance = TypeConvertor.castToCodeableReference(value); // CodeableReference 1767 } else if (name.equals("strength[x]")) { 1768 this.strength = TypeConvertor.castToType(value); // DataType 1769 } else if (name.equals("measurementPoint")) { 1770 this.measurementPoint = TypeConvertor.castToString(value); // StringType 1771 } else if (name.equals("country")) { 1772 this.getCountry().add(TypeConvertor.castToCodeableConcept(value)); 1773 } else 1774 return super.setProperty(name, value); 1775 return value; 1776 } 1777 1778 @Override 1779 public void removeChild(String name, Base value) throws FHIRException { 1780 if (name.equals("substance")) { 1781 this.substance = null; 1782 } else if (name.equals("strength[x]")) { 1783 this.strength = null; 1784 } else if (name.equals("measurementPoint")) { 1785 this.measurementPoint = null; 1786 } else if (name.equals("country")) { 1787 this.getCountry().remove(value); 1788 } else 1789 super.removeChild(name, value); 1790 1791 } 1792 1793 @Override 1794 public Base makeProperty(int hash, String name) throws FHIRException { 1795 switch (hash) { 1796 case 530040176: return getSubstance(); 1797 case 127377567: return getStrength(); 1798 case 1791316033: return getStrength(); 1799 case 235437876: return getMeasurementPointElement(); 1800 case 957831062: return addCountry(); 1801 default: return super.makeProperty(hash, name); 1802 } 1803 1804 } 1805 1806 @Override 1807 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1808 switch (hash) { 1809 case 530040176: /*substance*/ return new String[] {"CodeableReference"}; 1810 case 1791316033: /*strength*/ return new String[] {"Ratio", "RatioRange", "Quantity"}; 1811 case 235437876: /*measurementPoint*/ return new String[] {"string"}; 1812 case 957831062: /*country*/ return new String[] {"CodeableConcept"}; 1813 default: return super.getTypesForProperty(hash, name); 1814 } 1815 1816 } 1817 1818 @Override 1819 public Base addChild(String name) throws FHIRException { 1820 if (name.equals("substance")) { 1821 this.substance = new CodeableReference(); 1822 return this.substance; 1823 } 1824 else if (name.equals("strengthRatio")) { 1825 this.strength = new Ratio(); 1826 return this.strength; 1827 } 1828 else if (name.equals("strengthRatioRange")) { 1829 this.strength = new RatioRange(); 1830 return this.strength; 1831 } 1832 else if (name.equals("strengthQuantity")) { 1833 this.strength = new Quantity(); 1834 return this.strength; 1835 } 1836 else if (name.equals("measurementPoint")) { 1837 throw new FHIRException("Cannot call addChild on a singleton property Ingredient.substance.strength.referenceStrength.measurementPoint"); 1838 } 1839 else if (name.equals("country")) { 1840 return addCountry(); 1841 } 1842 else 1843 return super.addChild(name); 1844 } 1845 1846 public IngredientSubstanceStrengthReferenceStrengthComponent copy() { 1847 IngredientSubstanceStrengthReferenceStrengthComponent dst = new IngredientSubstanceStrengthReferenceStrengthComponent(); 1848 copyValues(dst); 1849 return dst; 1850 } 1851 1852 public void copyValues(IngredientSubstanceStrengthReferenceStrengthComponent dst) { 1853 super.copyValues(dst); 1854 dst.substance = substance == null ? null : substance.copy(); 1855 dst.strength = strength == null ? null : strength.copy(); 1856 dst.measurementPoint = measurementPoint == null ? null : measurementPoint.copy(); 1857 if (country != null) { 1858 dst.country = new ArrayList<CodeableConcept>(); 1859 for (CodeableConcept i : country) 1860 dst.country.add(i.copy()); 1861 }; 1862 } 1863 1864 @Override 1865 public boolean equalsDeep(Base other_) { 1866 if (!super.equalsDeep(other_)) 1867 return false; 1868 if (!(other_ instanceof IngredientSubstanceStrengthReferenceStrengthComponent)) 1869 return false; 1870 IngredientSubstanceStrengthReferenceStrengthComponent o = (IngredientSubstanceStrengthReferenceStrengthComponent) other_; 1871 return compareDeep(substance, o.substance, true) && compareDeep(strength, o.strength, true) && compareDeep(measurementPoint, o.measurementPoint, true) 1872 && compareDeep(country, o.country, true); 1873 } 1874 1875 @Override 1876 public boolean equalsShallow(Base other_) { 1877 if (!super.equalsShallow(other_)) 1878 return false; 1879 if (!(other_ instanceof IngredientSubstanceStrengthReferenceStrengthComponent)) 1880 return false; 1881 IngredientSubstanceStrengthReferenceStrengthComponent o = (IngredientSubstanceStrengthReferenceStrengthComponent) other_; 1882 return compareValues(measurementPoint, o.measurementPoint, true); 1883 } 1884 1885 public boolean isEmpty() { 1886 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(substance, strength, measurementPoint 1887 , country); 1888 } 1889 1890 public String fhirType() { 1891 return "Ingredient.substance.strength.referenceStrength"; 1892 1893 } 1894 1895 } 1896 1897 /** 1898 * The identifier(s) of this Ingredient that are assigned by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. 1899 */ 1900 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 1901 @Description(shortDefinition="An identifier or code by which the ingredient can be referenced", formalDefinition="The identifier(s) of this Ingredient that are assigned by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate." ) 1902 protected Identifier identifier; 1903 1904 /** 1905 * The status of this ingredient. Enables tracking the life-cycle of the content. 1906 */ 1907 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 1908 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this ingredient. Enables tracking the life-cycle of the content." ) 1909 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 1910 protected Enumeration<PublicationStatus> status; 1911 1912 /** 1913 * The product which this ingredient is a constituent part of. 1914 */ 1915 @Child(name = "for", type = {MedicinalProductDefinition.class, AdministrableProductDefinition.class, ManufacturedItemDefinition.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1916 @Description(shortDefinition="The product which this ingredient is a constituent part of", formalDefinition="The product which this ingredient is a constituent part of." ) 1917 protected List<Reference> for_; 1918 1919 /** 1920 * A classification of the ingredient identifying its purpose within the product, e.g. active, inactive. 1921 */ 1922 @Child(name = "role", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 1923 @Description(shortDefinition="Purpose of the ingredient within the product, e.g. active, inactive", formalDefinition="A classification of the ingredient identifying its purpose within the product, e.g. active, inactive." ) 1924 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ingredient-role") 1925 protected CodeableConcept role; 1926 1927 /** 1928 * A classification of the ingredient identifying its precise purpose(s) in the drug product. This extends the Ingredient.role to add more detail. Example: antioxidant, alkalizing agent. 1929 */ 1930 @Child(name = "function", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1931 @Description(shortDefinition="Precise action within the drug product, e.g. antioxidant, alkalizing agent", formalDefinition="A classification of the ingredient identifying its precise purpose(s) in the drug product. This extends the Ingredient.role to add more detail. Example: antioxidant, alkalizing agent." ) 1932 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ingredient-function") 1933 protected List<CodeableConcept> function; 1934 1935 /** 1936 * A classification of the ingredient according to where in the physical item it tends to be used, such the outer shell of a tablet, inner body or ink. 1937 */ 1938 @Child(name = "group", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 1939 @Description(shortDefinition="A classification of the ingredient according to where in the physical item it tends to be used, such the outer shell of a tablet, inner body or ink", formalDefinition="A classification of the ingredient according to where in the physical item it tends to be used, such the outer shell of a tablet, inner body or ink." ) 1940 protected CodeableConcept group; 1941 1942 /** 1943 * If the ingredient is a known or suspected allergen. Note that this is a property of the substance, so if a reference to a SubstanceDefinition is used to decribe that (rather than just a code), the allergen information should go there, not here. 1944 */ 1945 @Child(name = "allergenicIndicator", type = {BooleanType.class}, order=6, min=0, max=1, modifier=false, summary=true) 1946 @Description(shortDefinition="If the ingredient is a known or suspected allergen", formalDefinition="If the ingredient is a known or suspected allergen. Note that this is a property of the substance, so if a reference to a SubstanceDefinition is used to decribe that (rather than just a code), the allergen information should go there, not here." ) 1947 protected BooleanType allergenicIndicator; 1948 1949 /** 1950 * A place for providing any notes that are relevant to the component, e.g. removed during process, adjusted for loss on drying. 1951 */ 1952 @Child(name = "comment", type = {MarkdownType.class}, order=7, min=0, max=1, modifier=false, summary=false) 1953 @Description(shortDefinition="A place for providing any notes that are relevant to the component, e.g. removed during process, adjusted for loss on drying", formalDefinition="A place for providing any notes that are relevant to the component, e.g. removed during process, adjusted for loss on drying." ) 1954 protected MarkdownType comment; 1955 1956 /** 1957 * The organization(s) that manufacture this ingredient. Can be used to indicate: 1) Organizations we are aware of that manufacture this ingredient 2) Specific Manufacturer(s) currently being used 3) Set of organisations allowed to manufacture this ingredient for this product Users must be clear on the application of context relevant to their use case. 1958 */ 1959 @Child(name = "manufacturer", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1960 @Description(shortDefinition="An organization that manufactures this ingredient", formalDefinition="The organization(s) that manufacture this ingredient. Can be used to indicate: 1) Organizations we are aware of that manufacture this ingredient 2) Specific Manufacturer(s) currently being used 3) Set of organisations allowed to manufacture this ingredient for this product Users must be clear on the application of context relevant to their use case." ) 1961 protected List<IngredientManufacturerComponent> manufacturer; 1962 1963 /** 1964 * The substance that comprises this ingredient. 1965 */ 1966 @Child(name = "substance", type = {}, order=9, min=1, max=1, modifier=false, summary=true) 1967 @Description(shortDefinition="The substance that comprises this ingredient", formalDefinition="The substance that comprises this ingredient." ) 1968 protected IngredientSubstanceComponent substance; 1969 1970 private static final long serialVersionUID = 701648703L; 1971 1972 /** 1973 * Constructor 1974 */ 1975 public Ingredient() { 1976 super(); 1977 } 1978 1979 /** 1980 * Constructor 1981 */ 1982 public Ingredient(PublicationStatus status, CodeableConcept role, IngredientSubstanceComponent substance) { 1983 super(); 1984 this.setStatus(status); 1985 this.setRole(role); 1986 this.setSubstance(substance); 1987 } 1988 1989 /** 1990 * @return {@link #identifier} (The identifier(s) of this Ingredient that are assigned by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate.) 1991 */ 1992 public Identifier getIdentifier() { 1993 if (this.identifier == null) 1994 if (Configuration.errorOnAutoCreate()) 1995 throw new Error("Attempt to auto-create Ingredient.identifier"); 1996 else if (Configuration.doAutoCreate()) 1997 this.identifier = new Identifier(); // cc 1998 return this.identifier; 1999 } 2000 2001 public boolean hasIdentifier() { 2002 return this.identifier != null && !this.identifier.isEmpty(); 2003 } 2004 2005 /** 2006 * @param value {@link #identifier} (The identifier(s) of this Ingredient that are assigned by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate.) 2007 */ 2008 public Ingredient setIdentifier(Identifier value) { 2009 this.identifier = value; 2010 return this; 2011 } 2012 2013 /** 2014 * @return {@link #status} (The status of this ingredient. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2015 */ 2016 public Enumeration<PublicationStatus> getStatusElement() { 2017 if (this.status == null) 2018 if (Configuration.errorOnAutoCreate()) 2019 throw new Error("Attempt to auto-create Ingredient.status"); 2020 else if (Configuration.doAutoCreate()) 2021 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2022 return this.status; 2023 } 2024 2025 public boolean hasStatusElement() { 2026 return this.status != null && !this.status.isEmpty(); 2027 } 2028 2029 public boolean hasStatus() { 2030 return this.status != null && !this.status.isEmpty(); 2031 } 2032 2033 /** 2034 * @param value {@link #status} (The status of this ingredient. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2035 */ 2036 public Ingredient setStatusElement(Enumeration<PublicationStatus> value) { 2037 this.status = value; 2038 return this; 2039 } 2040 2041 /** 2042 * @return The status of this ingredient. Enables tracking the life-cycle of the content. 2043 */ 2044 public PublicationStatus getStatus() { 2045 return this.status == null ? null : this.status.getValue(); 2046 } 2047 2048 /** 2049 * @param value The status of this ingredient. Enables tracking the life-cycle of the content. 2050 */ 2051 public Ingredient setStatus(PublicationStatus value) { 2052 if (this.status == null) 2053 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2054 this.status.setValue(value); 2055 return this; 2056 } 2057 2058 /** 2059 * @return {@link #for_} (The product which this ingredient is a constituent part of.) 2060 */ 2061 public List<Reference> getFor() { 2062 if (this.for_ == null) 2063 this.for_ = new ArrayList<Reference>(); 2064 return this.for_; 2065 } 2066 2067 /** 2068 * @return Returns a reference to <code>this</code> for easy method chaining 2069 */ 2070 public Ingredient setFor(List<Reference> theFor) { 2071 this.for_ = theFor; 2072 return this; 2073 } 2074 2075 public boolean hasFor() { 2076 if (this.for_ == null) 2077 return false; 2078 for (Reference item : this.for_) 2079 if (!item.isEmpty()) 2080 return true; 2081 return false; 2082 } 2083 2084 public Reference addFor() { //3 2085 Reference t = new Reference(); 2086 if (this.for_ == null) 2087 this.for_ = new ArrayList<Reference>(); 2088 this.for_.add(t); 2089 return t; 2090 } 2091 2092 public Ingredient addFor(Reference t) { //3 2093 if (t == null) 2094 return this; 2095 if (this.for_ == null) 2096 this.for_ = new ArrayList<Reference>(); 2097 this.for_.add(t); 2098 return this; 2099 } 2100 2101 /** 2102 * @return The first repetition of repeating field {@link #for_}, creating it if it does not already exist {3} 2103 */ 2104 public Reference getForFirstRep() { 2105 if (getFor().isEmpty()) { 2106 addFor(); 2107 } 2108 return getFor().get(0); 2109 } 2110 2111 /** 2112 * @return {@link #role} (A classification of the ingredient identifying its purpose within the product, e.g. active, inactive.) 2113 */ 2114 public CodeableConcept getRole() { 2115 if (this.role == null) 2116 if (Configuration.errorOnAutoCreate()) 2117 throw new Error("Attempt to auto-create Ingredient.role"); 2118 else if (Configuration.doAutoCreate()) 2119 this.role = new CodeableConcept(); // cc 2120 return this.role; 2121 } 2122 2123 public boolean hasRole() { 2124 return this.role != null && !this.role.isEmpty(); 2125 } 2126 2127 /** 2128 * @param value {@link #role} (A classification of the ingredient identifying its purpose within the product, e.g. active, inactive.) 2129 */ 2130 public Ingredient setRole(CodeableConcept value) { 2131 this.role = value; 2132 return this; 2133 } 2134 2135 /** 2136 * @return {@link #function} (A classification of the ingredient identifying its precise purpose(s) in the drug product. This extends the Ingredient.role to add more detail. Example: antioxidant, alkalizing agent.) 2137 */ 2138 public List<CodeableConcept> getFunction() { 2139 if (this.function == null) 2140 this.function = new ArrayList<CodeableConcept>(); 2141 return this.function; 2142 } 2143 2144 /** 2145 * @return Returns a reference to <code>this</code> for easy method chaining 2146 */ 2147 public Ingredient setFunction(List<CodeableConcept> theFunction) { 2148 this.function = theFunction; 2149 return this; 2150 } 2151 2152 public boolean hasFunction() { 2153 if (this.function == null) 2154 return false; 2155 for (CodeableConcept item : this.function) 2156 if (!item.isEmpty()) 2157 return true; 2158 return false; 2159 } 2160 2161 public CodeableConcept addFunction() { //3 2162 CodeableConcept t = new CodeableConcept(); 2163 if (this.function == null) 2164 this.function = new ArrayList<CodeableConcept>(); 2165 this.function.add(t); 2166 return t; 2167 } 2168 2169 public Ingredient addFunction(CodeableConcept t) { //3 2170 if (t == null) 2171 return this; 2172 if (this.function == null) 2173 this.function = new ArrayList<CodeableConcept>(); 2174 this.function.add(t); 2175 return this; 2176 } 2177 2178 /** 2179 * @return The first repetition of repeating field {@link #function}, creating it if it does not already exist {3} 2180 */ 2181 public CodeableConcept getFunctionFirstRep() { 2182 if (getFunction().isEmpty()) { 2183 addFunction(); 2184 } 2185 return getFunction().get(0); 2186 } 2187 2188 /** 2189 * @return {@link #group} (A classification of the ingredient according to where in the physical item it tends to be used, such the outer shell of a tablet, inner body or ink.) 2190 */ 2191 public CodeableConcept getGroup() { 2192 if (this.group == null) 2193 if (Configuration.errorOnAutoCreate()) 2194 throw new Error("Attempt to auto-create Ingredient.group"); 2195 else if (Configuration.doAutoCreate()) 2196 this.group = new CodeableConcept(); // cc 2197 return this.group; 2198 } 2199 2200 public boolean hasGroup() { 2201 return this.group != null && !this.group.isEmpty(); 2202 } 2203 2204 /** 2205 * @param value {@link #group} (A classification of the ingredient according to where in the physical item it tends to be used, such the outer shell of a tablet, inner body or ink.) 2206 */ 2207 public Ingredient setGroup(CodeableConcept value) { 2208 this.group = value; 2209 return this; 2210 } 2211 2212 /** 2213 * @return {@link #allergenicIndicator} (If the ingredient is a known or suspected allergen. Note that this is a property of the substance, so if a reference to a SubstanceDefinition is used to decribe that (rather than just a code), the allergen information should go there, not here.). This is the underlying object with id, value and extensions. The accessor "getAllergenicIndicator" gives direct access to the value 2214 */ 2215 public BooleanType getAllergenicIndicatorElement() { 2216 if (this.allergenicIndicator == null) 2217 if (Configuration.errorOnAutoCreate()) 2218 throw new Error("Attempt to auto-create Ingredient.allergenicIndicator"); 2219 else if (Configuration.doAutoCreate()) 2220 this.allergenicIndicator = new BooleanType(); // bb 2221 return this.allergenicIndicator; 2222 } 2223 2224 public boolean hasAllergenicIndicatorElement() { 2225 return this.allergenicIndicator != null && !this.allergenicIndicator.isEmpty(); 2226 } 2227 2228 public boolean hasAllergenicIndicator() { 2229 return this.allergenicIndicator != null && !this.allergenicIndicator.isEmpty(); 2230 } 2231 2232 /** 2233 * @param value {@link #allergenicIndicator} (If the ingredient is a known or suspected allergen. Note that this is a property of the substance, so if a reference to a SubstanceDefinition is used to decribe that (rather than just a code), the allergen information should go there, not here.). This is the underlying object with id, value and extensions. The accessor "getAllergenicIndicator" gives direct access to the value 2234 */ 2235 public Ingredient setAllergenicIndicatorElement(BooleanType value) { 2236 this.allergenicIndicator = value; 2237 return this; 2238 } 2239 2240 /** 2241 * @return If the ingredient is a known or suspected allergen. Note that this is a property of the substance, so if a reference to a SubstanceDefinition is used to decribe that (rather than just a code), the allergen information should go there, not here. 2242 */ 2243 public boolean getAllergenicIndicator() { 2244 return this.allergenicIndicator == null || this.allergenicIndicator.isEmpty() ? false : this.allergenicIndicator.getValue(); 2245 } 2246 2247 /** 2248 * @param value If the ingredient is a known or suspected allergen. Note that this is a property of the substance, so if a reference to a SubstanceDefinition is used to decribe that (rather than just a code), the allergen information should go there, not here. 2249 */ 2250 public Ingredient setAllergenicIndicator(boolean value) { 2251 if (this.allergenicIndicator == null) 2252 this.allergenicIndicator = new BooleanType(); 2253 this.allergenicIndicator.setValue(value); 2254 return this; 2255 } 2256 2257 /** 2258 * @return {@link #comment} (A place for providing any notes that are relevant to the component, e.g. removed during process, adjusted for loss on drying.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 2259 */ 2260 public MarkdownType getCommentElement() { 2261 if (this.comment == null) 2262 if (Configuration.errorOnAutoCreate()) 2263 throw new Error("Attempt to auto-create Ingredient.comment"); 2264 else if (Configuration.doAutoCreate()) 2265 this.comment = new MarkdownType(); // bb 2266 return this.comment; 2267 } 2268 2269 public boolean hasCommentElement() { 2270 return this.comment != null && !this.comment.isEmpty(); 2271 } 2272 2273 public boolean hasComment() { 2274 return this.comment != null && !this.comment.isEmpty(); 2275 } 2276 2277 /** 2278 * @param value {@link #comment} (A place for providing any notes that are relevant to the component, e.g. removed during process, adjusted for loss on drying.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 2279 */ 2280 public Ingredient setCommentElement(MarkdownType value) { 2281 this.comment = value; 2282 return this; 2283 } 2284 2285 /** 2286 * @return A place for providing any notes that are relevant to the component, e.g. removed during process, adjusted for loss on drying. 2287 */ 2288 public String getComment() { 2289 return this.comment == null ? null : this.comment.getValue(); 2290 } 2291 2292 /** 2293 * @param value A place for providing any notes that are relevant to the component, e.g. removed during process, adjusted for loss on drying. 2294 */ 2295 public Ingredient setComment(String value) { 2296 if (Utilities.noString(value)) 2297 this.comment = null; 2298 else { 2299 if (this.comment == null) 2300 this.comment = new MarkdownType(); 2301 this.comment.setValue(value); 2302 } 2303 return this; 2304 } 2305 2306 /** 2307 * @return {@link #manufacturer} (The organization(s) that manufacture this ingredient. Can be used to indicate: 1) Organizations we are aware of that manufacture this ingredient 2) Specific Manufacturer(s) currently being used 3) Set of organisations allowed to manufacture this ingredient for this product Users must be clear on the application of context relevant to their use case.) 2308 */ 2309 public List<IngredientManufacturerComponent> getManufacturer() { 2310 if (this.manufacturer == null) 2311 this.manufacturer = new ArrayList<IngredientManufacturerComponent>(); 2312 return this.manufacturer; 2313 } 2314 2315 /** 2316 * @return Returns a reference to <code>this</code> for easy method chaining 2317 */ 2318 public Ingredient setManufacturer(List<IngredientManufacturerComponent> theManufacturer) { 2319 this.manufacturer = theManufacturer; 2320 return this; 2321 } 2322 2323 public boolean hasManufacturer() { 2324 if (this.manufacturer == null) 2325 return false; 2326 for (IngredientManufacturerComponent item : this.manufacturer) 2327 if (!item.isEmpty()) 2328 return true; 2329 return false; 2330 } 2331 2332 public IngredientManufacturerComponent addManufacturer() { //3 2333 IngredientManufacturerComponent t = new IngredientManufacturerComponent(); 2334 if (this.manufacturer == null) 2335 this.manufacturer = new ArrayList<IngredientManufacturerComponent>(); 2336 this.manufacturer.add(t); 2337 return t; 2338 } 2339 2340 public Ingredient addManufacturer(IngredientManufacturerComponent t) { //3 2341 if (t == null) 2342 return this; 2343 if (this.manufacturer == null) 2344 this.manufacturer = new ArrayList<IngredientManufacturerComponent>(); 2345 this.manufacturer.add(t); 2346 return this; 2347 } 2348 2349 /** 2350 * @return The first repetition of repeating field {@link #manufacturer}, creating it if it does not already exist {3} 2351 */ 2352 public IngredientManufacturerComponent getManufacturerFirstRep() { 2353 if (getManufacturer().isEmpty()) { 2354 addManufacturer(); 2355 } 2356 return getManufacturer().get(0); 2357 } 2358 2359 /** 2360 * @return {@link #substance} (The substance that comprises this ingredient.) 2361 */ 2362 public IngredientSubstanceComponent getSubstance() { 2363 if (this.substance == null) 2364 if (Configuration.errorOnAutoCreate()) 2365 throw new Error("Attempt to auto-create Ingredient.substance"); 2366 else if (Configuration.doAutoCreate()) 2367 this.substance = new IngredientSubstanceComponent(); // cc 2368 return this.substance; 2369 } 2370 2371 public boolean hasSubstance() { 2372 return this.substance != null && !this.substance.isEmpty(); 2373 } 2374 2375 /** 2376 * @param value {@link #substance} (The substance that comprises this ingredient.) 2377 */ 2378 public Ingredient setSubstance(IngredientSubstanceComponent value) { 2379 this.substance = value; 2380 return this; 2381 } 2382 2383 protected void listChildren(List<Property> children) { 2384 super.listChildren(children); 2385 children.add(new Property("identifier", "Identifier", "The identifier(s) of this Ingredient that are assigned by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate.", 0, 1, identifier)); 2386 children.add(new Property("status", "code", "The status of this ingredient. Enables tracking the life-cycle of the content.", 0, 1, status)); 2387 children.add(new Property("for", "Reference(MedicinalProductDefinition|AdministrableProductDefinition|ManufacturedItemDefinition)", "The product which this ingredient is a constituent part of.", 0, java.lang.Integer.MAX_VALUE, for_)); 2388 children.add(new Property("role", "CodeableConcept", "A classification of the ingredient identifying its purpose within the product, e.g. active, inactive.", 0, 1, role)); 2389 children.add(new Property("function", "CodeableConcept", "A classification of the ingredient identifying its precise purpose(s) in the drug product. This extends the Ingredient.role to add more detail. Example: antioxidant, alkalizing agent.", 0, java.lang.Integer.MAX_VALUE, function)); 2390 children.add(new Property("group", "CodeableConcept", "A classification of the ingredient according to where in the physical item it tends to be used, such the outer shell of a tablet, inner body or ink.", 0, 1, group)); 2391 children.add(new Property("allergenicIndicator", "boolean", "If the ingredient is a known or suspected allergen. Note that this is a property of the substance, so if a reference to a SubstanceDefinition is used to decribe that (rather than just a code), the allergen information should go there, not here.", 0, 1, allergenicIndicator)); 2392 children.add(new Property("comment", "markdown", "A place for providing any notes that are relevant to the component, e.g. removed during process, adjusted for loss on drying.", 0, 1, comment)); 2393 children.add(new Property("manufacturer", "", "The organization(s) that manufacture this ingredient. Can be used to indicate: 1) Organizations we are aware of that manufacture this ingredient 2) Specific Manufacturer(s) currently being used 3) Set of organisations allowed to manufacture this ingredient for this product Users must be clear on the application of context relevant to their use case.", 0, java.lang.Integer.MAX_VALUE, manufacturer)); 2394 children.add(new Property("substance", "", "The substance that comprises this ingredient.", 0, 1, substance)); 2395 } 2396 2397 @Override 2398 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2399 switch (_hash) { 2400 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The identifier(s) of this Ingredient that are assigned by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate.", 0, 1, identifier); 2401 case -892481550: /*status*/ return new Property("status", "code", "The status of this ingredient. Enables tracking the life-cycle of the content.", 0, 1, status); 2402 case 101577: /*for*/ return new Property("for", "Reference(MedicinalProductDefinition|AdministrableProductDefinition|ManufacturedItemDefinition)", "The product which this ingredient is a constituent part of.", 0, java.lang.Integer.MAX_VALUE, for_); 2403 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "A classification of the ingredient identifying its purpose within the product, e.g. active, inactive.", 0, 1, role); 2404 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "A classification of the ingredient identifying its precise purpose(s) in the drug product. This extends the Ingredient.role to add more detail. Example: antioxidant, alkalizing agent.", 0, java.lang.Integer.MAX_VALUE, function); 2405 case 98629247: /*group*/ return new Property("group", "CodeableConcept", "A classification of the ingredient according to where in the physical item it tends to be used, such the outer shell of a tablet, inner body or ink.", 0, 1, group); 2406 case 75406931: /*allergenicIndicator*/ return new Property("allergenicIndicator", "boolean", "If the ingredient is a known or suspected allergen. Note that this is a property of the substance, so if a reference to a SubstanceDefinition is used to decribe that (rather than just a code), the allergen information should go there, not here.", 0, 1, allergenicIndicator); 2407 case 950398559: /*comment*/ return new Property("comment", "markdown", "A place for providing any notes that are relevant to the component, e.g. removed during process, adjusted for loss on drying.", 0, 1, comment); 2408 case -1969347631: /*manufacturer*/ return new Property("manufacturer", "", "The organization(s) that manufacture this ingredient. Can be used to indicate: 1) Organizations we are aware of that manufacture this ingredient 2) Specific Manufacturer(s) currently being used 3) Set of organisations allowed to manufacture this ingredient for this product Users must be clear on the application of context relevant to their use case.", 0, java.lang.Integer.MAX_VALUE, manufacturer); 2409 case 530040176: /*substance*/ return new Property("substance", "", "The substance that comprises this ingredient.", 0, 1, substance); 2410 default: return super.getNamedProperty(_hash, _name, _checkValid); 2411 } 2412 2413 } 2414 2415 @Override 2416 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2417 switch (hash) { 2418 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 2419 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2420 case 101577: /*for*/ return this.for_ == null ? new Base[0] : this.for_.toArray(new Base[this.for_.size()]); // Reference 2421 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 2422 case 1380938712: /*function*/ return this.function == null ? new Base[0] : this.function.toArray(new Base[this.function.size()]); // CodeableConcept 2423 case 98629247: /*group*/ return this.group == null ? new Base[0] : new Base[] {this.group}; // CodeableConcept 2424 case 75406931: /*allergenicIndicator*/ return this.allergenicIndicator == null ? new Base[0] : new Base[] {this.allergenicIndicator}; // BooleanType 2425 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // MarkdownType 2426 case -1969347631: /*manufacturer*/ return this.manufacturer == null ? new Base[0] : this.manufacturer.toArray(new Base[this.manufacturer.size()]); // IngredientManufacturerComponent 2427 case 530040176: /*substance*/ return this.substance == null ? new Base[0] : new Base[] {this.substance}; // IngredientSubstanceComponent 2428 default: return super.getProperty(hash, name, checkValid); 2429 } 2430 2431 } 2432 2433 @Override 2434 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2435 switch (hash) { 2436 case -1618432855: // identifier 2437 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 2438 return value; 2439 case -892481550: // status 2440 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2441 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2442 return value; 2443 case 101577: // for 2444 this.getFor().add(TypeConvertor.castToReference(value)); // Reference 2445 return value; 2446 case 3506294: // role 2447 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2448 return value; 2449 case 1380938712: // function 2450 this.getFunction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2451 return value; 2452 case 98629247: // group 2453 this.group = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2454 return value; 2455 case 75406931: // allergenicIndicator 2456 this.allergenicIndicator = TypeConvertor.castToBoolean(value); // BooleanType 2457 return value; 2458 case 950398559: // comment 2459 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 2460 return value; 2461 case -1969347631: // manufacturer 2462 this.getManufacturer().add((IngredientManufacturerComponent) value); // IngredientManufacturerComponent 2463 return value; 2464 case 530040176: // substance 2465 this.substance = (IngredientSubstanceComponent) value; // IngredientSubstanceComponent 2466 return value; 2467 default: return super.setProperty(hash, name, value); 2468 } 2469 2470 } 2471 2472 @Override 2473 public Base setProperty(String name, Base value) throws FHIRException { 2474 if (name.equals("identifier")) { 2475 this.identifier = TypeConvertor.castToIdentifier(value); // Identifier 2476 } else if (name.equals("status")) { 2477 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2478 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2479 } else if (name.equals("for")) { 2480 this.getFor().add(TypeConvertor.castToReference(value)); 2481 } else if (name.equals("role")) { 2482 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2483 } else if (name.equals("function")) { 2484 this.getFunction().add(TypeConvertor.castToCodeableConcept(value)); 2485 } else if (name.equals("group")) { 2486 this.group = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2487 } else if (name.equals("allergenicIndicator")) { 2488 this.allergenicIndicator = TypeConvertor.castToBoolean(value); // BooleanType 2489 } else if (name.equals("comment")) { 2490 this.comment = TypeConvertor.castToMarkdown(value); // MarkdownType 2491 } else if (name.equals("manufacturer")) { 2492 this.getManufacturer().add((IngredientManufacturerComponent) value); 2493 } else if (name.equals("substance")) { 2494 this.substance = (IngredientSubstanceComponent) value; // IngredientSubstanceComponent 2495 } else 2496 return super.setProperty(name, value); 2497 return value; 2498 } 2499 2500 @Override 2501 public void removeChild(String name, Base value) throws FHIRException { 2502 if (name.equals("identifier")) { 2503 this.identifier = null; 2504 } else if (name.equals("status")) { 2505 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2506 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2507 } else if (name.equals("for")) { 2508 this.getFor().remove(value); 2509 } else if (name.equals("role")) { 2510 this.role = null; 2511 } else if (name.equals("function")) { 2512 this.getFunction().remove(value); 2513 } else if (name.equals("group")) { 2514 this.group = null; 2515 } else if (name.equals("allergenicIndicator")) { 2516 this.allergenicIndicator = null; 2517 } else if (name.equals("comment")) { 2518 this.comment = null; 2519 } else if (name.equals("manufacturer")) { 2520 this.getManufacturer().remove((IngredientManufacturerComponent) value); 2521 } else if (name.equals("substance")) { 2522 this.substance = (IngredientSubstanceComponent) value; // IngredientSubstanceComponent 2523 } else 2524 super.removeChild(name, value); 2525 2526 } 2527 2528 @Override 2529 public Base makeProperty(int hash, String name) throws FHIRException { 2530 switch (hash) { 2531 case -1618432855: return getIdentifier(); 2532 case -892481550: return getStatusElement(); 2533 case 101577: return addFor(); 2534 case 3506294: return getRole(); 2535 case 1380938712: return addFunction(); 2536 case 98629247: return getGroup(); 2537 case 75406931: return getAllergenicIndicatorElement(); 2538 case 950398559: return getCommentElement(); 2539 case -1969347631: return addManufacturer(); 2540 case 530040176: return getSubstance(); 2541 default: return super.makeProperty(hash, name); 2542 } 2543 2544 } 2545 2546 @Override 2547 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2548 switch (hash) { 2549 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2550 case -892481550: /*status*/ return new String[] {"code"}; 2551 case 101577: /*for*/ return new String[] {"Reference"}; 2552 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 2553 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 2554 case 98629247: /*group*/ return new String[] {"CodeableConcept"}; 2555 case 75406931: /*allergenicIndicator*/ return new String[] {"boolean"}; 2556 case 950398559: /*comment*/ return new String[] {"markdown"}; 2557 case -1969347631: /*manufacturer*/ return new String[] {}; 2558 case 530040176: /*substance*/ return new String[] {}; 2559 default: return super.getTypesForProperty(hash, name); 2560 } 2561 2562 } 2563 2564 @Override 2565 public Base addChild(String name) throws FHIRException { 2566 if (name.equals("identifier")) { 2567 this.identifier = new Identifier(); 2568 return this.identifier; 2569 } 2570 else if (name.equals("status")) { 2571 throw new FHIRException("Cannot call addChild on a singleton property Ingredient.status"); 2572 } 2573 else if (name.equals("for")) { 2574 return addFor(); 2575 } 2576 else if (name.equals("role")) { 2577 this.role = new CodeableConcept(); 2578 return this.role; 2579 } 2580 else if (name.equals("function")) { 2581 return addFunction(); 2582 } 2583 else if (name.equals("group")) { 2584 this.group = new CodeableConcept(); 2585 return this.group; 2586 } 2587 else if (name.equals("allergenicIndicator")) { 2588 throw new FHIRException("Cannot call addChild on a singleton property Ingredient.allergenicIndicator"); 2589 } 2590 else if (name.equals("comment")) { 2591 throw new FHIRException("Cannot call addChild on a singleton property Ingredient.comment"); 2592 } 2593 else if (name.equals("manufacturer")) { 2594 return addManufacturer(); 2595 } 2596 else if (name.equals("substance")) { 2597 this.substance = new IngredientSubstanceComponent(); 2598 return this.substance; 2599 } 2600 else 2601 return super.addChild(name); 2602 } 2603 2604 public String fhirType() { 2605 return "Ingredient"; 2606 2607 } 2608 2609 public Ingredient copy() { 2610 Ingredient dst = new Ingredient(); 2611 copyValues(dst); 2612 return dst; 2613 } 2614 2615 public void copyValues(Ingredient dst) { 2616 super.copyValues(dst); 2617 dst.identifier = identifier == null ? null : identifier.copy(); 2618 dst.status = status == null ? null : status.copy(); 2619 if (for_ != null) { 2620 dst.for_ = new ArrayList<Reference>(); 2621 for (Reference i : for_) 2622 dst.for_.add(i.copy()); 2623 }; 2624 dst.role = role == null ? null : role.copy(); 2625 if (function != null) { 2626 dst.function = new ArrayList<CodeableConcept>(); 2627 for (CodeableConcept i : function) 2628 dst.function.add(i.copy()); 2629 }; 2630 dst.group = group == null ? null : group.copy(); 2631 dst.allergenicIndicator = allergenicIndicator == null ? null : allergenicIndicator.copy(); 2632 dst.comment = comment == null ? null : comment.copy(); 2633 if (manufacturer != null) { 2634 dst.manufacturer = new ArrayList<IngredientManufacturerComponent>(); 2635 for (IngredientManufacturerComponent i : manufacturer) 2636 dst.manufacturer.add(i.copy()); 2637 }; 2638 dst.substance = substance == null ? null : substance.copy(); 2639 } 2640 2641 protected Ingredient typedCopy() { 2642 return copy(); 2643 } 2644 2645 @Override 2646 public boolean equalsDeep(Base other_) { 2647 if (!super.equalsDeep(other_)) 2648 return false; 2649 if (!(other_ instanceof Ingredient)) 2650 return false; 2651 Ingredient o = (Ingredient) other_; 2652 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(for_, o.for_, true) 2653 && compareDeep(role, o.role, true) && compareDeep(function, o.function, true) && compareDeep(group, o.group, true) 2654 && compareDeep(allergenicIndicator, o.allergenicIndicator, true) && compareDeep(comment, o.comment, true) 2655 && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(substance, o.substance, true) 2656 ; 2657 } 2658 2659 @Override 2660 public boolean equalsShallow(Base other_) { 2661 if (!super.equalsShallow(other_)) 2662 return false; 2663 if (!(other_ instanceof Ingredient)) 2664 return false; 2665 Ingredient o = (Ingredient) other_; 2666 return compareValues(status, o.status, true) && compareValues(allergenicIndicator, o.allergenicIndicator, true) 2667 && compareValues(comment, o.comment, true); 2668 } 2669 2670 public boolean isEmpty() { 2671 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, for_ 2672 , role, function, group, allergenicIndicator, comment, manufacturer, substance 2673 ); 2674 } 2675 2676 @Override 2677 public ResourceType getResourceType() { 2678 return ResourceType.Ingredient; 2679 } 2680 2681 /** 2682 * Search parameter: <b>for</b> 2683 * <p> 2684 * Description: <b>The product which this ingredient is a constituent part of</b><br> 2685 * Type: <b>reference</b><br> 2686 * Path: <b>Ingredient.for</b><br> 2687 * </p> 2688 */ 2689 @SearchParamDefinition(name="for", path="Ingredient.for", description="The product which this ingredient is a constituent part of", type="reference", target={AdministrableProductDefinition.class, ManufacturedItemDefinition.class, MedicinalProductDefinition.class } ) 2690 public static final String SP_FOR = "for"; 2691 /** 2692 * <b>Fluent Client</b> search parameter constant for <b>for</b> 2693 * <p> 2694 * Description: <b>The product which this ingredient is a constituent part of</b><br> 2695 * Type: <b>reference</b><br> 2696 * Path: <b>Ingredient.for</b><br> 2697 * </p> 2698 */ 2699 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FOR); 2700 2701/** 2702 * Constant for fluent queries to be used to add include statements. Specifies 2703 * the path value of "<b>Ingredient:for</b>". 2704 */ 2705 public static final ca.uhn.fhir.model.api.Include INCLUDE_FOR = new ca.uhn.fhir.model.api.Include("Ingredient:for").toLocked(); 2706 2707 /** 2708 * Search parameter: <b>function</b> 2709 * <p> 2710 * Description: <b>A classification of the ingredient identifying its precise purpose(s) in the drug product. This extends the Ingredient.role to add more detail. Example: Antioxidant, Alkalizing Agent</b><br> 2711 * Type: <b>token</b><br> 2712 * Path: <b>Ingredient.function</b><br> 2713 * </p> 2714 */ 2715 @SearchParamDefinition(name="function", path="Ingredient.function", description="A classification of the ingredient identifying its precise purpose(s) in the drug product. This extends the Ingredient.role to add more detail. Example: Antioxidant, Alkalizing Agent", type="token" ) 2716 public static final String SP_FUNCTION = "function"; 2717 /** 2718 * <b>Fluent Client</b> search parameter constant for <b>function</b> 2719 * <p> 2720 * Description: <b>A classification of the ingredient identifying its precise purpose(s) in the drug product. This extends the Ingredient.role to add more detail. Example: Antioxidant, Alkalizing Agent</b><br> 2721 * Type: <b>token</b><br> 2722 * Path: <b>Ingredient.function</b><br> 2723 * </p> 2724 */ 2725 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FUNCTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FUNCTION); 2726 2727 /** 2728 * Search parameter: <b>identifier</b> 2729 * <p> 2730 * Description: <b>An identifier or code by which the ingredient can be referenced</b><br> 2731 * Type: <b>token</b><br> 2732 * Path: <b>Ingredient.identifier</b><br> 2733 * </p> 2734 */ 2735 @SearchParamDefinition(name="identifier", path="Ingredient.identifier", description="An identifier or code by which the ingredient can be referenced", type="token" ) 2736 public static final String SP_IDENTIFIER = "identifier"; 2737 /** 2738 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2739 * <p> 2740 * Description: <b>An identifier or code by which the ingredient can be referenced</b><br> 2741 * Type: <b>token</b><br> 2742 * Path: <b>Ingredient.identifier</b><br> 2743 * </p> 2744 */ 2745 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2746 2747 /** 2748 * Search parameter: <b>manufacturer</b> 2749 * <p> 2750 * Description: <b>The organization that manufactures this ingredient</b><br> 2751 * Type: <b>reference</b><br> 2752 * Path: <b>Ingredient.manufacturer.manufacturer</b><br> 2753 * </p> 2754 */ 2755 @SearchParamDefinition(name="manufacturer", path="Ingredient.manufacturer.manufacturer", description="The organization that manufactures this ingredient", type="reference", target={Organization.class } ) 2756 public static final String SP_MANUFACTURER = "manufacturer"; 2757 /** 2758 * <b>Fluent Client</b> search parameter constant for <b>manufacturer</b> 2759 * <p> 2760 * Description: <b>The organization that manufactures this ingredient</b><br> 2761 * Type: <b>reference</b><br> 2762 * Path: <b>Ingredient.manufacturer.manufacturer</b><br> 2763 * </p> 2764 */ 2765 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANUFACTURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MANUFACTURER); 2766 2767/** 2768 * Constant for fluent queries to be used to add include statements. Specifies 2769 * the path value of "<b>Ingredient:manufacturer</b>". 2770 */ 2771 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANUFACTURER = new ca.uhn.fhir.model.api.Include("Ingredient:manufacturer").toLocked(); 2772 2773 /** 2774 * Search parameter: <b>role</b> 2775 * <p> 2776 * Description: <b>A classification of the ingredient identifying its purpose within the product, e.g. active, inactive</b><br> 2777 * Type: <b>token</b><br> 2778 * Path: <b>Ingredient.role</b><br> 2779 * </p> 2780 */ 2781 @SearchParamDefinition(name="role", path="Ingredient.role", description="A classification of the ingredient identifying its purpose within the product, e.g. active, inactive", type="token" ) 2782 public static final String SP_ROLE = "role"; 2783 /** 2784 * <b>Fluent Client</b> search parameter constant for <b>role</b> 2785 * <p> 2786 * Description: <b>A classification of the ingredient identifying its purpose within the product, e.g. active, inactive</b><br> 2787 * Type: <b>token</b><br> 2788 * Path: <b>Ingredient.role</b><br> 2789 * </p> 2790 */ 2791 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ROLE); 2792 2793 /** 2794 * Search parameter: <b>status</b> 2795 * <p> 2796 * Description: <b>The status of this ingredient. Enables tracking the life-cycle of the content</b><br> 2797 * Type: <b>token</b><br> 2798 * Path: <b>Ingredient.status</b><br> 2799 * </p> 2800 */ 2801 @SearchParamDefinition(name="status", path="Ingredient.status", description="The status of this ingredient. Enables tracking the life-cycle of the content", type="token" ) 2802 public static final String SP_STATUS = "status"; 2803 /** 2804 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2805 * <p> 2806 * Description: <b>The status of this ingredient. Enables tracking the life-cycle of the content</b><br> 2807 * Type: <b>token</b><br> 2808 * Path: <b>Ingredient.status</b><br> 2809 * </p> 2810 */ 2811 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2812 2813 /** 2814 * Search parameter: <b>strength-concentration-quantity</b> 2815 * <p> 2816 * Description: <b>Ingredient concentration strength as quantity</b><br> 2817 * Type: <b>quantity</b><br> 2818 * Path: <b>Ingredient.substance.strength.concentration.ofType(Quantity)</b><br> 2819 * </p> 2820 */ 2821 @SearchParamDefinition(name="strength-concentration-quantity", path="Ingredient.substance.strength.concentration.ofType(Quantity)", description="Ingredient concentration strength as quantity", type="quantity" ) 2822 public static final String SP_STRENGTH_CONCENTRATION_QUANTITY = "strength-concentration-quantity"; 2823 /** 2824 * <b>Fluent Client</b> search parameter constant for <b>strength-concentration-quantity</b> 2825 * <p> 2826 * Description: <b>Ingredient concentration strength as quantity</b><br> 2827 * Type: <b>quantity</b><br> 2828 * Path: <b>Ingredient.substance.strength.concentration.ofType(Quantity)</b><br> 2829 * </p> 2830 */ 2831 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam STRENGTH_CONCENTRATION_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_STRENGTH_CONCENTRATION_QUANTITY); 2832 2833 /** 2834 * Search parameter: <b>strength-presentation-quantity</b> 2835 * <p> 2836 * Description: <b>Ingredient presentation strength as quantity</b><br> 2837 * Type: <b>quantity</b><br> 2838 * Path: <b>Ingredient.substance.strength.presentation.ofType(Quantity)</b><br> 2839 * </p> 2840 */ 2841 @SearchParamDefinition(name="strength-presentation-quantity", path="Ingredient.substance.strength.presentation.ofType(Quantity)", description="Ingredient presentation strength as quantity", type="quantity" ) 2842 public static final String SP_STRENGTH_PRESENTATION_QUANTITY = "strength-presentation-quantity"; 2843 /** 2844 * <b>Fluent Client</b> search parameter constant for <b>strength-presentation-quantity</b> 2845 * <p> 2846 * Description: <b>Ingredient presentation strength as quantity</b><br> 2847 * Type: <b>quantity</b><br> 2848 * Path: <b>Ingredient.substance.strength.presentation.ofType(Quantity)</b><br> 2849 * </p> 2850 */ 2851 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam STRENGTH_PRESENTATION_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_STRENGTH_PRESENTATION_QUANTITY); 2852 2853 /** 2854 * Search parameter: <b>substance-code</b> 2855 * <p> 2856 * Description: <b>Reference to a concept (by class)</b><br> 2857 * Type: <b>token</b><br> 2858 * Path: <b>Ingredient.substance.code.concept</b><br> 2859 * </p> 2860 */ 2861 @SearchParamDefinition(name="substance-code", path="Ingredient.substance.code.concept", description="Reference to a concept (by class)", type="token" ) 2862 public static final String SP_SUBSTANCE_CODE = "substance-code"; 2863 /** 2864 * <b>Fluent Client</b> search parameter constant for <b>substance-code</b> 2865 * <p> 2866 * Description: <b>Reference to a concept (by class)</b><br> 2867 * Type: <b>token</b><br> 2868 * Path: <b>Ingredient.substance.code.concept</b><br> 2869 * </p> 2870 */ 2871 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SUBSTANCE_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SUBSTANCE_CODE); 2872 2873 /** 2874 * Search parameter: <b>substance-definition</b> 2875 * <p> 2876 * Description: <b>Reference to a resource (by instance)</b><br> 2877 * Type: <b>reference</b><br> 2878 * Path: <b>Ingredient.substance.code.reference</b><br> 2879 * </p> 2880 */ 2881 @SearchParamDefinition(name="substance-definition", path="Ingredient.substance.code.reference", description="Reference to a resource (by instance)", type="reference", target={SubstanceDefinition.class } ) 2882 public static final String SP_SUBSTANCE_DEFINITION = "substance-definition"; 2883 /** 2884 * <b>Fluent Client</b> search parameter constant for <b>substance-definition</b> 2885 * <p> 2886 * Description: <b>Reference to a resource (by instance)</b><br> 2887 * Type: <b>reference</b><br> 2888 * Path: <b>Ingredient.substance.code.reference</b><br> 2889 * </p> 2890 */ 2891 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBSTANCE_DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBSTANCE_DEFINITION); 2892 2893/** 2894 * Constant for fluent queries to be used to add include statements. Specifies 2895 * the path value of "<b>Ingredient:substance-definition</b>". 2896 */ 2897 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBSTANCE_DEFINITION = new ca.uhn.fhir.model.api.Include("Ingredient:substance-definition").toLocked(); 2898 2899 /** 2900 * Search parameter: <b>substance</b> 2901 * <p> 2902 * Description: <b>Reference to a resource (by instance)</b><br> 2903 * Type: <b>reference</b><br> 2904 * Path: <b>Ingredient.substance.code.reference</b><br> 2905 * </p> 2906 */ 2907 @SearchParamDefinition(name="substance", path="Ingredient.substance.code.reference", description="Reference to a resource (by instance)", type="reference", target={SubstanceDefinition.class } ) 2908 public static final String SP_SUBSTANCE = "substance"; 2909 /** 2910 * <b>Fluent Client</b> search parameter constant for <b>substance</b> 2911 * <p> 2912 * Description: <b>Reference to a resource (by instance)</b><br> 2913 * Type: <b>reference</b><br> 2914 * Path: <b>Ingredient.substance.code.reference</b><br> 2915 * </p> 2916 */ 2917 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBSTANCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBSTANCE); 2918 2919/** 2920 * Constant for fluent queries to be used to add include statements. Specifies 2921 * the path value of "<b>Ingredient:substance</b>". 2922 */ 2923 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBSTANCE = new ca.uhn.fhir.model.api.Include("Ingredient:substance").toLocked(); 2924 2925 2926} 2927