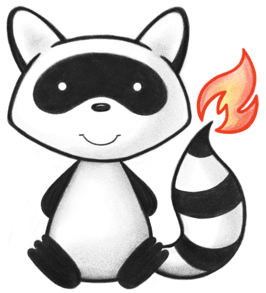
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Details of a Health Insurance product/plan provided by an organization. 052 */ 053@ResourceDef(name="InsurancePlan", profile="http://hl7.org/fhir/StructureDefinition/InsurancePlan") 054public class InsurancePlan extends DomainResource { 055 056 @Block() 057 public static class InsurancePlanCoverageComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * Type of coverage (Medical; Dental; Mental Health; Substance Abuse; Vision; Drug; Short Term; Long Term Care; Hospice; Home Health). 060 */ 061 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="Type of coverage", formalDefinition="Type of coverage (Medical; Dental; Mental Health; Substance Abuse; Vision; Drug; Short Term; Long Term Care; Hospice; Home Health)." ) 063 protected CodeableConcept type; 064 065 /** 066 * Reference to the network that providing the type of coverage. 067 */ 068 @Child(name = "network", type = {Organization.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 069 @Description(shortDefinition="What networks provide coverage", formalDefinition="Reference to the network that providing the type of coverage." ) 070 protected List<Reference> network; 071 072 /** 073 * Specific benefits under this type of coverage. 074 */ 075 @Child(name = "benefit", type = {}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 076 @Description(shortDefinition="List of benefits", formalDefinition="Specific benefits under this type of coverage." ) 077 protected List<CoverageBenefitComponent> benefit; 078 079 private static final long serialVersionUID = 79927205L; 080 081 /** 082 * Constructor 083 */ 084 public InsurancePlanCoverageComponent() { 085 super(); 086 } 087 088 /** 089 * Constructor 090 */ 091 public InsurancePlanCoverageComponent(CodeableConcept type, CoverageBenefitComponent benefit) { 092 super(); 093 this.setType(type); 094 this.addBenefit(benefit); 095 } 096 097 /** 098 * @return {@link #type} (Type of coverage (Medical; Dental; Mental Health; Substance Abuse; Vision; Drug; Short Term; Long Term Care; Hospice; Home Health).) 099 */ 100 public CodeableConcept getType() { 101 if (this.type == null) 102 if (Configuration.errorOnAutoCreate()) 103 throw new Error("Attempt to auto-create InsurancePlanCoverageComponent.type"); 104 else if (Configuration.doAutoCreate()) 105 this.type = new CodeableConcept(); // cc 106 return this.type; 107 } 108 109 public boolean hasType() { 110 return this.type != null && !this.type.isEmpty(); 111 } 112 113 /** 114 * @param value {@link #type} (Type of coverage (Medical; Dental; Mental Health; Substance Abuse; Vision; Drug; Short Term; Long Term Care; Hospice; Home Health).) 115 */ 116 public InsurancePlanCoverageComponent setType(CodeableConcept value) { 117 this.type = value; 118 return this; 119 } 120 121 /** 122 * @return {@link #network} (Reference to the network that providing the type of coverage.) 123 */ 124 public List<Reference> getNetwork() { 125 if (this.network == null) 126 this.network = new ArrayList<Reference>(); 127 return this.network; 128 } 129 130 /** 131 * @return Returns a reference to <code>this</code> for easy method chaining 132 */ 133 public InsurancePlanCoverageComponent setNetwork(List<Reference> theNetwork) { 134 this.network = theNetwork; 135 return this; 136 } 137 138 public boolean hasNetwork() { 139 if (this.network == null) 140 return false; 141 for (Reference item : this.network) 142 if (!item.isEmpty()) 143 return true; 144 return false; 145 } 146 147 public Reference addNetwork() { //3 148 Reference t = new Reference(); 149 if (this.network == null) 150 this.network = new ArrayList<Reference>(); 151 this.network.add(t); 152 return t; 153 } 154 155 public InsurancePlanCoverageComponent addNetwork(Reference t) { //3 156 if (t == null) 157 return this; 158 if (this.network == null) 159 this.network = new ArrayList<Reference>(); 160 this.network.add(t); 161 return this; 162 } 163 164 /** 165 * @return The first repetition of repeating field {@link #network}, creating it if it does not already exist {3} 166 */ 167 public Reference getNetworkFirstRep() { 168 if (getNetwork().isEmpty()) { 169 addNetwork(); 170 } 171 return getNetwork().get(0); 172 } 173 174 /** 175 * @return {@link #benefit} (Specific benefits under this type of coverage.) 176 */ 177 public List<CoverageBenefitComponent> getBenefit() { 178 if (this.benefit == null) 179 this.benefit = new ArrayList<CoverageBenefitComponent>(); 180 return this.benefit; 181 } 182 183 /** 184 * @return Returns a reference to <code>this</code> for easy method chaining 185 */ 186 public InsurancePlanCoverageComponent setBenefit(List<CoverageBenefitComponent> theBenefit) { 187 this.benefit = theBenefit; 188 return this; 189 } 190 191 public boolean hasBenefit() { 192 if (this.benefit == null) 193 return false; 194 for (CoverageBenefitComponent item : this.benefit) 195 if (!item.isEmpty()) 196 return true; 197 return false; 198 } 199 200 public CoverageBenefitComponent addBenefit() { //3 201 CoverageBenefitComponent t = new CoverageBenefitComponent(); 202 if (this.benefit == null) 203 this.benefit = new ArrayList<CoverageBenefitComponent>(); 204 this.benefit.add(t); 205 return t; 206 } 207 208 public InsurancePlanCoverageComponent addBenefit(CoverageBenefitComponent t) { //3 209 if (t == null) 210 return this; 211 if (this.benefit == null) 212 this.benefit = new ArrayList<CoverageBenefitComponent>(); 213 this.benefit.add(t); 214 return this; 215 } 216 217 /** 218 * @return The first repetition of repeating field {@link #benefit}, creating it if it does not already exist {3} 219 */ 220 public CoverageBenefitComponent getBenefitFirstRep() { 221 if (getBenefit().isEmpty()) { 222 addBenefit(); 223 } 224 return getBenefit().get(0); 225 } 226 227 protected void listChildren(List<Property> children) { 228 super.listChildren(children); 229 children.add(new Property("type", "CodeableConcept", "Type of coverage (Medical; Dental; Mental Health; Substance Abuse; Vision; Drug; Short Term; Long Term Care; Hospice; Home Health).", 0, 1, type)); 230 children.add(new Property("network", "Reference(Organization)", "Reference to the network that providing the type of coverage.", 0, java.lang.Integer.MAX_VALUE, network)); 231 children.add(new Property("benefit", "", "Specific benefits under this type of coverage.", 0, java.lang.Integer.MAX_VALUE, benefit)); 232 } 233 234 @Override 235 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 236 switch (_hash) { 237 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of coverage (Medical; Dental; Mental Health; Substance Abuse; Vision; Drug; Short Term; Long Term Care; Hospice; Home Health).", 0, 1, type); 238 case 1843485230: /*network*/ return new Property("network", "Reference(Organization)", "Reference to the network that providing the type of coverage.", 0, java.lang.Integer.MAX_VALUE, network); 239 case -222710633: /*benefit*/ return new Property("benefit", "", "Specific benefits under this type of coverage.", 0, java.lang.Integer.MAX_VALUE, benefit); 240 default: return super.getNamedProperty(_hash, _name, _checkValid); 241 } 242 243 } 244 245 @Override 246 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 247 switch (hash) { 248 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 249 case 1843485230: /*network*/ return this.network == null ? new Base[0] : this.network.toArray(new Base[this.network.size()]); // Reference 250 case -222710633: /*benefit*/ return this.benefit == null ? new Base[0] : this.benefit.toArray(new Base[this.benefit.size()]); // CoverageBenefitComponent 251 default: return super.getProperty(hash, name, checkValid); 252 } 253 254 } 255 256 @Override 257 public Base setProperty(int hash, String name, Base value) throws FHIRException { 258 switch (hash) { 259 case 3575610: // type 260 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 261 return value; 262 case 1843485230: // network 263 this.getNetwork().add(TypeConvertor.castToReference(value)); // Reference 264 return value; 265 case -222710633: // benefit 266 this.getBenefit().add((CoverageBenefitComponent) value); // CoverageBenefitComponent 267 return value; 268 default: return super.setProperty(hash, name, value); 269 } 270 271 } 272 273 @Override 274 public Base setProperty(String name, Base value) throws FHIRException { 275 if (name.equals("type")) { 276 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 277 } else if (name.equals("network")) { 278 this.getNetwork().add(TypeConvertor.castToReference(value)); 279 } else if (name.equals("benefit")) { 280 this.getBenefit().add((CoverageBenefitComponent) value); 281 } else 282 return super.setProperty(name, value); 283 return value; 284 } 285 286 @Override 287 public void removeChild(String name, Base value) throws FHIRException { 288 if (name.equals("type")) { 289 this.type = null; 290 } else if (name.equals("network")) { 291 this.getNetwork().remove(value); 292 } else if (name.equals("benefit")) { 293 this.getBenefit().remove((CoverageBenefitComponent) value); 294 } else 295 super.removeChild(name, value); 296 297 } 298 299 @Override 300 public Base makeProperty(int hash, String name) throws FHIRException { 301 switch (hash) { 302 case 3575610: return getType(); 303 case 1843485230: return addNetwork(); 304 case -222710633: return addBenefit(); 305 default: return super.makeProperty(hash, name); 306 } 307 308 } 309 310 @Override 311 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 312 switch (hash) { 313 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 314 case 1843485230: /*network*/ return new String[] {"Reference"}; 315 case -222710633: /*benefit*/ return new String[] {}; 316 default: return super.getTypesForProperty(hash, name); 317 } 318 319 } 320 321 @Override 322 public Base addChild(String name) throws FHIRException { 323 if (name.equals("type")) { 324 this.type = new CodeableConcept(); 325 return this.type; 326 } 327 else if (name.equals("network")) { 328 return addNetwork(); 329 } 330 else if (name.equals("benefit")) { 331 return addBenefit(); 332 } 333 else 334 return super.addChild(name); 335 } 336 337 public InsurancePlanCoverageComponent copy() { 338 InsurancePlanCoverageComponent dst = new InsurancePlanCoverageComponent(); 339 copyValues(dst); 340 return dst; 341 } 342 343 public void copyValues(InsurancePlanCoverageComponent dst) { 344 super.copyValues(dst); 345 dst.type = type == null ? null : type.copy(); 346 if (network != null) { 347 dst.network = new ArrayList<Reference>(); 348 for (Reference i : network) 349 dst.network.add(i.copy()); 350 }; 351 if (benefit != null) { 352 dst.benefit = new ArrayList<CoverageBenefitComponent>(); 353 for (CoverageBenefitComponent i : benefit) 354 dst.benefit.add(i.copy()); 355 }; 356 } 357 358 @Override 359 public boolean equalsDeep(Base other_) { 360 if (!super.equalsDeep(other_)) 361 return false; 362 if (!(other_ instanceof InsurancePlanCoverageComponent)) 363 return false; 364 InsurancePlanCoverageComponent o = (InsurancePlanCoverageComponent) other_; 365 return compareDeep(type, o.type, true) && compareDeep(network, o.network, true) && compareDeep(benefit, o.benefit, true) 366 ; 367 } 368 369 @Override 370 public boolean equalsShallow(Base other_) { 371 if (!super.equalsShallow(other_)) 372 return false; 373 if (!(other_ instanceof InsurancePlanCoverageComponent)) 374 return false; 375 InsurancePlanCoverageComponent o = (InsurancePlanCoverageComponent) other_; 376 return true; 377 } 378 379 public boolean isEmpty() { 380 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, network, benefit); 381 } 382 383 public String fhirType() { 384 return "InsurancePlan.coverage"; 385 386 } 387 388 } 389 390 @Block() 391 public static class CoverageBenefitComponent extends BackboneElement implements IBaseBackboneElement { 392 /** 393 * Type of benefit (primary care; speciality care; inpatient; outpatient). 394 */ 395 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 396 @Description(shortDefinition="Type of benefit", formalDefinition="Type of benefit (primary care; speciality care; inpatient; outpatient)." ) 397 protected CodeableConcept type; 398 399 /** 400 * The referral requirements to have access/coverage for this benefit. 401 */ 402 @Child(name = "requirement", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 403 @Description(shortDefinition="Referral requirements", formalDefinition="The referral requirements to have access/coverage for this benefit." ) 404 protected StringType requirement; 405 406 /** 407 * The specific limits on the benefit. 408 */ 409 @Child(name = "limit", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 410 @Description(shortDefinition="Benefit limits", formalDefinition="The specific limits on the benefit." ) 411 protected List<CoverageBenefitLimitComponent> limit; 412 413 private static final long serialVersionUID = -113658449L; 414 415 /** 416 * Constructor 417 */ 418 public CoverageBenefitComponent() { 419 super(); 420 } 421 422 /** 423 * Constructor 424 */ 425 public CoverageBenefitComponent(CodeableConcept type) { 426 super(); 427 this.setType(type); 428 } 429 430 /** 431 * @return {@link #type} (Type of benefit (primary care; speciality care; inpatient; outpatient).) 432 */ 433 public CodeableConcept getType() { 434 if (this.type == null) 435 if (Configuration.errorOnAutoCreate()) 436 throw new Error("Attempt to auto-create CoverageBenefitComponent.type"); 437 else if (Configuration.doAutoCreate()) 438 this.type = new CodeableConcept(); // cc 439 return this.type; 440 } 441 442 public boolean hasType() { 443 return this.type != null && !this.type.isEmpty(); 444 } 445 446 /** 447 * @param value {@link #type} (Type of benefit (primary care; speciality care; inpatient; outpatient).) 448 */ 449 public CoverageBenefitComponent setType(CodeableConcept value) { 450 this.type = value; 451 return this; 452 } 453 454 /** 455 * @return {@link #requirement} (The referral requirements to have access/coverage for this benefit.). This is the underlying object with id, value and extensions. The accessor "getRequirement" gives direct access to the value 456 */ 457 public StringType getRequirementElement() { 458 if (this.requirement == null) 459 if (Configuration.errorOnAutoCreate()) 460 throw new Error("Attempt to auto-create CoverageBenefitComponent.requirement"); 461 else if (Configuration.doAutoCreate()) 462 this.requirement = new StringType(); // bb 463 return this.requirement; 464 } 465 466 public boolean hasRequirementElement() { 467 return this.requirement != null && !this.requirement.isEmpty(); 468 } 469 470 public boolean hasRequirement() { 471 return this.requirement != null && !this.requirement.isEmpty(); 472 } 473 474 /** 475 * @param value {@link #requirement} (The referral requirements to have access/coverage for this benefit.). This is the underlying object with id, value and extensions. The accessor "getRequirement" gives direct access to the value 476 */ 477 public CoverageBenefitComponent setRequirementElement(StringType value) { 478 this.requirement = value; 479 return this; 480 } 481 482 /** 483 * @return The referral requirements to have access/coverage for this benefit. 484 */ 485 public String getRequirement() { 486 return this.requirement == null ? null : this.requirement.getValue(); 487 } 488 489 /** 490 * @param value The referral requirements to have access/coverage for this benefit. 491 */ 492 public CoverageBenefitComponent setRequirement(String value) { 493 if (Utilities.noString(value)) 494 this.requirement = null; 495 else { 496 if (this.requirement == null) 497 this.requirement = new StringType(); 498 this.requirement.setValue(value); 499 } 500 return this; 501 } 502 503 /** 504 * @return {@link #limit} (The specific limits on the benefit.) 505 */ 506 public List<CoverageBenefitLimitComponent> getLimit() { 507 if (this.limit == null) 508 this.limit = new ArrayList<CoverageBenefitLimitComponent>(); 509 return this.limit; 510 } 511 512 /** 513 * @return Returns a reference to <code>this</code> for easy method chaining 514 */ 515 public CoverageBenefitComponent setLimit(List<CoverageBenefitLimitComponent> theLimit) { 516 this.limit = theLimit; 517 return this; 518 } 519 520 public boolean hasLimit() { 521 if (this.limit == null) 522 return false; 523 for (CoverageBenefitLimitComponent item : this.limit) 524 if (!item.isEmpty()) 525 return true; 526 return false; 527 } 528 529 public CoverageBenefitLimitComponent addLimit() { //3 530 CoverageBenefitLimitComponent t = new CoverageBenefitLimitComponent(); 531 if (this.limit == null) 532 this.limit = new ArrayList<CoverageBenefitLimitComponent>(); 533 this.limit.add(t); 534 return t; 535 } 536 537 public CoverageBenefitComponent addLimit(CoverageBenefitLimitComponent t) { //3 538 if (t == null) 539 return this; 540 if (this.limit == null) 541 this.limit = new ArrayList<CoverageBenefitLimitComponent>(); 542 this.limit.add(t); 543 return this; 544 } 545 546 /** 547 * @return The first repetition of repeating field {@link #limit}, creating it if it does not already exist {3} 548 */ 549 public CoverageBenefitLimitComponent getLimitFirstRep() { 550 if (getLimit().isEmpty()) { 551 addLimit(); 552 } 553 return getLimit().get(0); 554 } 555 556 protected void listChildren(List<Property> children) { 557 super.listChildren(children); 558 children.add(new Property("type", "CodeableConcept", "Type of benefit (primary care; speciality care; inpatient; outpatient).", 0, 1, type)); 559 children.add(new Property("requirement", "string", "The referral requirements to have access/coverage for this benefit.", 0, 1, requirement)); 560 children.add(new Property("limit", "", "The specific limits on the benefit.", 0, java.lang.Integer.MAX_VALUE, limit)); 561 } 562 563 @Override 564 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 565 switch (_hash) { 566 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of benefit (primary care; speciality care; inpatient; outpatient).", 0, 1, type); 567 case 363387971: /*requirement*/ return new Property("requirement", "string", "The referral requirements to have access/coverage for this benefit.", 0, 1, requirement); 568 case 102976443: /*limit*/ return new Property("limit", "", "The specific limits on the benefit.", 0, java.lang.Integer.MAX_VALUE, limit); 569 default: return super.getNamedProperty(_hash, _name, _checkValid); 570 } 571 572 } 573 574 @Override 575 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 576 switch (hash) { 577 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 578 case 363387971: /*requirement*/ return this.requirement == null ? new Base[0] : new Base[] {this.requirement}; // StringType 579 case 102976443: /*limit*/ return this.limit == null ? new Base[0] : this.limit.toArray(new Base[this.limit.size()]); // CoverageBenefitLimitComponent 580 default: return super.getProperty(hash, name, checkValid); 581 } 582 583 } 584 585 @Override 586 public Base setProperty(int hash, String name, Base value) throws FHIRException { 587 switch (hash) { 588 case 3575610: // type 589 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 590 return value; 591 case 363387971: // requirement 592 this.requirement = TypeConvertor.castToString(value); // StringType 593 return value; 594 case 102976443: // limit 595 this.getLimit().add((CoverageBenefitLimitComponent) value); // CoverageBenefitLimitComponent 596 return value; 597 default: return super.setProperty(hash, name, value); 598 } 599 600 } 601 602 @Override 603 public Base setProperty(String name, Base value) throws FHIRException { 604 if (name.equals("type")) { 605 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 606 } else if (name.equals("requirement")) { 607 this.requirement = TypeConvertor.castToString(value); // StringType 608 } else if (name.equals("limit")) { 609 this.getLimit().add((CoverageBenefitLimitComponent) value); 610 } else 611 return super.setProperty(name, value); 612 return value; 613 } 614 615 @Override 616 public void removeChild(String name, Base value) throws FHIRException { 617 if (name.equals("type")) { 618 this.type = null; 619 } else if (name.equals("requirement")) { 620 this.requirement = null; 621 } else if (name.equals("limit")) { 622 this.getLimit().remove((CoverageBenefitLimitComponent) value); 623 } else 624 super.removeChild(name, value); 625 626 } 627 628 @Override 629 public Base makeProperty(int hash, String name) throws FHIRException { 630 switch (hash) { 631 case 3575610: return getType(); 632 case 363387971: return getRequirementElement(); 633 case 102976443: return addLimit(); 634 default: return super.makeProperty(hash, name); 635 } 636 637 } 638 639 @Override 640 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 641 switch (hash) { 642 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 643 case 363387971: /*requirement*/ return new String[] {"string"}; 644 case 102976443: /*limit*/ return new String[] {}; 645 default: return super.getTypesForProperty(hash, name); 646 } 647 648 } 649 650 @Override 651 public Base addChild(String name) throws FHIRException { 652 if (name.equals("type")) { 653 this.type = new CodeableConcept(); 654 return this.type; 655 } 656 else if (name.equals("requirement")) { 657 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.coverage.benefit.requirement"); 658 } 659 else if (name.equals("limit")) { 660 return addLimit(); 661 } 662 else 663 return super.addChild(name); 664 } 665 666 public CoverageBenefitComponent copy() { 667 CoverageBenefitComponent dst = new CoverageBenefitComponent(); 668 copyValues(dst); 669 return dst; 670 } 671 672 public void copyValues(CoverageBenefitComponent dst) { 673 super.copyValues(dst); 674 dst.type = type == null ? null : type.copy(); 675 dst.requirement = requirement == null ? null : requirement.copy(); 676 if (limit != null) { 677 dst.limit = new ArrayList<CoverageBenefitLimitComponent>(); 678 for (CoverageBenefitLimitComponent i : limit) 679 dst.limit.add(i.copy()); 680 }; 681 } 682 683 @Override 684 public boolean equalsDeep(Base other_) { 685 if (!super.equalsDeep(other_)) 686 return false; 687 if (!(other_ instanceof CoverageBenefitComponent)) 688 return false; 689 CoverageBenefitComponent o = (CoverageBenefitComponent) other_; 690 return compareDeep(type, o.type, true) && compareDeep(requirement, o.requirement, true) && compareDeep(limit, o.limit, true) 691 ; 692 } 693 694 @Override 695 public boolean equalsShallow(Base other_) { 696 if (!super.equalsShallow(other_)) 697 return false; 698 if (!(other_ instanceof CoverageBenefitComponent)) 699 return false; 700 CoverageBenefitComponent o = (CoverageBenefitComponent) other_; 701 return compareValues(requirement, o.requirement, true); 702 } 703 704 public boolean isEmpty() { 705 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, requirement, limit 706 ); 707 } 708 709 public String fhirType() { 710 return "InsurancePlan.coverage.benefit"; 711 712 } 713 714 } 715 716 @Block() 717 public static class CoverageBenefitLimitComponent extends BackboneElement implements IBaseBackboneElement { 718 /** 719 * The maximum amount of a service item a plan will pay for a covered benefit. For examples. wellness visits, or eyeglasses. 720 */ 721 @Child(name = "value", type = {Quantity.class}, order=1, min=0, max=1, modifier=false, summary=false) 722 @Description(shortDefinition="Maximum value allowed", formalDefinition="The maximum amount of a service item a plan will pay for a covered benefit. For examples. wellness visits, or eyeglasses." ) 723 protected Quantity value; 724 725 /** 726 * The specific limit on the benefit. 727 */ 728 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 729 @Description(shortDefinition="Benefit limit details", formalDefinition="The specific limit on the benefit." ) 730 protected CodeableConcept code; 731 732 private static final long serialVersionUID = -304318128L; 733 734 /** 735 * Constructor 736 */ 737 public CoverageBenefitLimitComponent() { 738 super(); 739 } 740 741 /** 742 * @return {@link #value} (The maximum amount of a service item a plan will pay for a covered benefit. For examples. wellness visits, or eyeglasses.) 743 */ 744 public Quantity getValue() { 745 if (this.value == null) 746 if (Configuration.errorOnAutoCreate()) 747 throw new Error("Attempt to auto-create CoverageBenefitLimitComponent.value"); 748 else if (Configuration.doAutoCreate()) 749 this.value = new Quantity(); // cc 750 return this.value; 751 } 752 753 public boolean hasValue() { 754 return this.value != null && !this.value.isEmpty(); 755 } 756 757 /** 758 * @param value {@link #value} (The maximum amount of a service item a plan will pay for a covered benefit. For examples. wellness visits, or eyeglasses.) 759 */ 760 public CoverageBenefitLimitComponent setValue(Quantity value) { 761 this.value = value; 762 return this; 763 } 764 765 /** 766 * @return {@link #code} (The specific limit on the benefit.) 767 */ 768 public CodeableConcept getCode() { 769 if (this.code == null) 770 if (Configuration.errorOnAutoCreate()) 771 throw new Error("Attempt to auto-create CoverageBenefitLimitComponent.code"); 772 else if (Configuration.doAutoCreate()) 773 this.code = new CodeableConcept(); // cc 774 return this.code; 775 } 776 777 public boolean hasCode() { 778 return this.code != null && !this.code.isEmpty(); 779 } 780 781 /** 782 * @param value {@link #code} (The specific limit on the benefit.) 783 */ 784 public CoverageBenefitLimitComponent setCode(CodeableConcept value) { 785 this.code = value; 786 return this; 787 } 788 789 protected void listChildren(List<Property> children) { 790 super.listChildren(children); 791 children.add(new Property("value", "Quantity", "The maximum amount of a service item a plan will pay for a covered benefit. For examples. wellness visits, or eyeglasses.", 0, 1, value)); 792 children.add(new Property("code", "CodeableConcept", "The specific limit on the benefit.", 0, 1, code)); 793 } 794 795 @Override 796 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 797 switch (_hash) { 798 case 111972721: /*value*/ return new Property("value", "Quantity", "The maximum amount of a service item a plan will pay for a covered benefit. For examples. wellness visits, or eyeglasses.", 0, 1, value); 799 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The specific limit on the benefit.", 0, 1, code); 800 default: return super.getNamedProperty(_hash, _name, _checkValid); 801 } 802 803 } 804 805 @Override 806 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 807 switch (hash) { 808 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Quantity 809 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 810 default: return super.getProperty(hash, name, checkValid); 811 } 812 813 } 814 815 @Override 816 public Base setProperty(int hash, String name, Base value) throws FHIRException { 817 switch (hash) { 818 case 111972721: // value 819 this.value = TypeConvertor.castToQuantity(value); // Quantity 820 return value; 821 case 3059181: // code 822 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 823 return value; 824 default: return super.setProperty(hash, name, value); 825 } 826 827 } 828 829 @Override 830 public Base setProperty(String name, Base value) throws FHIRException { 831 if (name.equals("value")) { 832 this.value = TypeConvertor.castToQuantity(value); // Quantity 833 } else if (name.equals("code")) { 834 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 835 } else 836 return super.setProperty(name, value); 837 return value; 838 } 839 840 @Override 841 public void removeChild(String name, Base value) throws FHIRException { 842 if (name.equals("value")) { 843 this.value = null; 844 } else if (name.equals("code")) { 845 this.code = null; 846 } else 847 super.removeChild(name, value); 848 849 } 850 851 @Override 852 public Base makeProperty(int hash, String name) throws FHIRException { 853 switch (hash) { 854 case 111972721: return getValue(); 855 case 3059181: return getCode(); 856 default: return super.makeProperty(hash, name); 857 } 858 859 } 860 861 @Override 862 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 863 switch (hash) { 864 case 111972721: /*value*/ return new String[] {"Quantity"}; 865 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 866 default: return super.getTypesForProperty(hash, name); 867 } 868 869 } 870 871 @Override 872 public Base addChild(String name) throws FHIRException { 873 if (name.equals("value")) { 874 this.value = new Quantity(); 875 return this.value; 876 } 877 else if (name.equals("code")) { 878 this.code = new CodeableConcept(); 879 return this.code; 880 } 881 else 882 return super.addChild(name); 883 } 884 885 public CoverageBenefitLimitComponent copy() { 886 CoverageBenefitLimitComponent dst = new CoverageBenefitLimitComponent(); 887 copyValues(dst); 888 return dst; 889 } 890 891 public void copyValues(CoverageBenefitLimitComponent dst) { 892 super.copyValues(dst); 893 dst.value = value == null ? null : value.copy(); 894 dst.code = code == null ? null : code.copy(); 895 } 896 897 @Override 898 public boolean equalsDeep(Base other_) { 899 if (!super.equalsDeep(other_)) 900 return false; 901 if (!(other_ instanceof CoverageBenefitLimitComponent)) 902 return false; 903 CoverageBenefitLimitComponent o = (CoverageBenefitLimitComponent) other_; 904 return compareDeep(value, o.value, true) && compareDeep(code, o.code, true); 905 } 906 907 @Override 908 public boolean equalsShallow(Base other_) { 909 if (!super.equalsShallow(other_)) 910 return false; 911 if (!(other_ instanceof CoverageBenefitLimitComponent)) 912 return false; 913 CoverageBenefitLimitComponent o = (CoverageBenefitLimitComponent) other_; 914 return true; 915 } 916 917 public boolean isEmpty() { 918 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, code); 919 } 920 921 public String fhirType() { 922 return "InsurancePlan.coverage.benefit.limit"; 923 924 } 925 926 } 927 928 @Block() 929 public static class InsurancePlanPlanComponent extends BackboneElement implements IBaseBackboneElement { 930 /** 931 * Business identifiers assigned to this health insurance plan which remain constant as the resource is updated and propagates from server to server. 932 */ 933 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 934 @Description(shortDefinition="Business Identifier for Product", formalDefinition="Business identifiers assigned to this health insurance plan which remain constant as the resource is updated and propagates from server to server." ) 935 protected List<Identifier> identifier; 936 937 /** 938 * Type of plan. For example, "Platinum" or "High Deductable". 939 */ 940 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 941 @Description(shortDefinition="Type of plan", formalDefinition="Type of plan. For example, \"Platinum\" or \"High Deductable\"." ) 942 protected CodeableConcept type; 943 944 /** 945 * The geographic region in which a health insurance plan's benefits apply. 946 */ 947 @Child(name = "coverageArea", type = {Location.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 948 @Description(shortDefinition="Where product applies", formalDefinition="The geographic region in which a health insurance plan's benefits apply." ) 949 protected List<Reference> coverageArea; 950 951 /** 952 * Reference to the network that providing the type of coverage. 953 */ 954 @Child(name = "network", type = {Organization.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 955 @Description(shortDefinition="What networks provide coverage", formalDefinition="Reference to the network that providing the type of coverage." ) 956 protected List<Reference> network; 957 958 /** 959 * Overall costs associated with the plan. 960 */ 961 @Child(name = "generalCost", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 962 @Description(shortDefinition="Overall costs", formalDefinition="Overall costs associated with the plan." ) 963 protected List<InsurancePlanPlanGeneralCostComponent> generalCost; 964 965 /** 966 * Costs associated with the coverage provided by the product. 967 */ 968 @Child(name = "specificCost", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 969 @Description(shortDefinition="Specific costs", formalDefinition="Costs associated with the coverage provided by the product." ) 970 protected List<InsurancePlanPlanSpecificCostComponent> specificCost; 971 972 private static final long serialVersionUID = -782831938L; 973 974 /** 975 * Constructor 976 */ 977 public InsurancePlanPlanComponent() { 978 super(); 979 } 980 981 /** 982 * @return {@link #identifier} (Business identifiers assigned to this health insurance plan which remain constant as the resource is updated and propagates from server to server.) 983 */ 984 public List<Identifier> getIdentifier() { 985 if (this.identifier == null) 986 this.identifier = new ArrayList<Identifier>(); 987 return this.identifier; 988 } 989 990 /** 991 * @return Returns a reference to <code>this</code> for easy method chaining 992 */ 993 public InsurancePlanPlanComponent setIdentifier(List<Identifier> theIdentifier) { 994 this.identifier = theIdentifier; 995 return this; 996 } 997 998 public boolean hasIdentifier() { 999 if (this.identifier == null) 1000 return false; 1001 for (Identifier item : this.identifier) 1002 if (!item.isEmpty()) 1003 return true; 1004 return false; 1005 } 1006 1007 public Identifier addIdentifier() { //3 1008 Identifier t = new Identifier(); 1009 if (this.identifier == null) 1010 this.identifier = new ArrayList<Identifier>(); 1011 this.identifier.add(t); 1012 return t; 1013 } 1014 1015 public InsurancePlanPlanComponent addIdentifier(Identifier t) { //3 1016 if (t == null) 1017 return this; 1018 if (this.identifier == null) 1019 this.identifier = new ArrayList<Identifier>(); 1020 this.identifier.add(t); 1021 return this; 1022 } 1023 1024 /** 1025 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1026 */ 1027 public Identifier getIdentifierFirstRep() { 1028 if (getIdentifier().isEmpty()) { 1029 addIdentifier(); 1030 } 1031 return getIdentifier().get(0); 1032 } 1033 1034 /** 1035 * @return {@link #type} (Type of plan. For example, "Platinum" or "High Deductable".) 1036 */ 1037 public CodeableConcept getType() { 1038 if (this.type == null) 1039 if (Configuration.errorOnAutoCreate()) 1040 throw new Error("Attempt to auto-create InsurancePlanPlanComponent.type"); 1041 else if (Configuration.doAutoCreate()) 1042 this.type = new CodeableConcept(); // cc 1043 return this.type; 1044 } 1045 1046 public boolean hasType() { 1047 return this.type != null && !this.type.isEmpty(); 1048 } 1049 1050 /** 1051 * @param value {@link #type} (Type of plan. For example, "Platinum" or "High Deductable".) 1052 */ 1053 public InsurancePlanPlanComponent setType(CodeableConcept value) { 1054 this.type = value; 1055 return this; 1056 } 1057 1058 /** 1059 * @return {@link #coverageArea} (The geographic region in which a health insurance plan's benefits apply.) 1060 */ 1061 public List<Reference> getCoverageArea() { 1062 if (this.coverageArea == null) 1063 this.coverageArea = new ArrayList<Reference>(); 1064 return this.coverageArea; 1065 } 1066 1067 /** 1068 * @return Returns a reference to <code>this</code> for easy method chaining 1069 */ 1070 public InsurancePlanPlanComponent setCoverageArea(List<Reference> theCoverageArea) { 1071 this.coverageArea = theCoverageArea; 1072 return this; 1073 } 1074 1075 public boolean hasCoverageArea() { 1076 if (this.coverageArea == null) 1077 return false; 1078 for (Reference item : this.coverageArea) 1079 if (!item.isEmpty()) 1080 return true; 1081 return false; 1082 } 1083 1084 public Reference addCoverageArea() { //3 1085 Reference t = new Reference(); 1086 if (this.coverageArea == null) 1087 this.coverageArea = new ArrayList<Reference>(); 1088 this.coverageArea.add(t); 1089 return t; 1090 } 1091 1092 public InsurancePlanPlanComponent addCoverageArea(Reference t) { //3 1093 if (t == null) 1094 return this; 1095 if (this.coverageArea == null) 1096 this.coverageArea = new ArrayList<Reference>(); 1097 this.coverageArea.add(t); 1098 return this; 1099 } 1100 1101 /** 1102 * @return The first repetition of repeating field {@link #coverageArea}, creating it if it does not already exist {3} 1103 */ 1104 public Reference getCoverageAreaFirstRep() { 1105 if (getCoverageArea().isEmpty()) { 1106 addCoverageArea(); 1107 } 1108 return getCoverageArea().get(0); 1109 } 1110 1111 /** 1112 * @return {@link #network} (Reference to the network that providing the type of coverage.) 1113 */ 1114 public List<Reference> getNetwork() { 1115 if (this.network == null) 1116 this.network = new ArrayList<Reference>(); 1117 return this.network; 1118 } 1119 1120 /** 1121 * @return Returns a reference to <code>this</code> for easy method chaining 1122 */ 1123 public InsurancePlanPlanComponent setNetwork(List<Reference> theNetwork) { 1124 this.network = theNetwork; 1125 return this; 1126 } 1127 1128 public boolean hasNetwork() { 1129 if (this.network == null) 1130 return false; 1131 for (Reference item : this.network) 1132 if (!item.isEmpty()) 1133 return true; 1134 return false; 1135 } 1136 1137 public Reference addNetwork() { //3 1138 Reference t = new Reference(); 1139 if (this.network == null) 1140 this.network = new ArrayList<Reference>(); 1141 this.network.add(t); 1142 return t; 1143 } 1144 1145 public InsurancePlanPlanComponent addNetwork(Reference t) { //3 1146 if (t == null) 1147 return this; 1148 if (this.network == null) 1149 this.network = new ArrayList<Reference>(); 1150 this.network.add(t); 1151 return this; 1152 } 1153 1154 /** 1155 * @return The first repetition of repeating field {@link #network}, creating it if it does not already exist {3} 1156 */ 1157 public Reference getNetworkFirstRep() { 1158 if (getNetwork().isEmpty()) { 1159 addNetwork(); 1160 } 1161 return getNetwork().get(0); 1162 } 1163 1164 /** 1165 * @return {@link #generalCost} (Overall costs associated with the plan.) 1166 */ 1167 public List<InsurancePlanPlanGeneralCostComponent> getGeneralCost() { 1168 if (this.generalCost == null) 1169 this.generalCost = new ArrayList<InsurancePlanPlanGeneralCostComponent>(); 1170 return this.generalCost; 1171 } 1172 1173 /** 1174 * @return Returns a reference to <code>this</code> for easy method chaining 1175 */ 1176 public InsurancePlanPlanComponent setGeneralCost(List<InsurancePlanPlanGeneralCostComponent> theGeneralCost) { 1177 this.generalCost = theGeneralCost; 1178 return this; 1179 } 1180 1181 public boolean hasGeneralCost() { 1182 if (this.generalCost == null) 1183 return false; 1184 for (InsurancePlanPlanGeneralCostComponent item : this.generalCost) 1185 if (!item.isEmpty()) 1186 return true; 1187 return false; 1188 } 1189 1190 public InsurancePlanPlanGeneralCostComponent addGeneralCost() { //3 1191 InsurancePlanPlanGeneralCostComponent t = new InsurancePlanPlanGeneralCostComponent(); 1192 if (this.generalCost == null) 1193 this.generalCost = new ArrayList<InsurancePlanPlanGeneralCostComponent>(); 1194 this.generalCost.add(t); 1195 return t; 1196 } 1197 1198 public InsurancePlanPlanComponent addGeneralCost(InsurancePlanPlanGeneralCostComponent t) { //3 1199 if (t == null) 1200 return this; 1201 if (this.generalCost == null) 1202 this.generalCost = new ArrayList<InsurancePlanPlanGeneralCostComponent>(); 1203 this.generalCost.add(t); 1204 return this; 1205 } 1206 1207 /** 1208 * @return The first repetition of repeating field {@link #generalCost}, creating it if it does not already exist {3} 1209 */ 1210 public InsurancePlanPlanGeneralCostComponent getGeneralCostFirstRep() { 1211 if (getGeneralCost().isEmpty()) { 1212 addGeneralCost(); 1213 } 1214 return getGeneralCost().get(0); 1215 } 1216 1217 /** 1218 * @return {@link #specificCost} (Costs associated with the coverage provided by the product.) 1219 */ 1220 public List<InsurancePlanPlanSpecificCostComponent> getSpecificCost() { 1221 if (this.specificCost == null) 1222 this.specificCost = new ArrayList<InsurancePlanPlanSpecificCostComponent>(); 1223 return this.specificCost; 1224 } 1225 1226 /** 1227 * @return Returns a reference to <code>this</code> for easy method chaining 1228 */ 1229 public InsurancePlanPlanComponent setSpecificCost(List<InsurancePlanPlanSpecificCostComponent> theSpecificCost) { 1230 this.specificCost = theSpecificCost; 1231 return this; 1232 } 1233 1234 public boolean hasSpecificCost() { 1235 if (this.specificCost == null) 1236 return false; 1237 for (InsurancePlanPlanSpecificCostComponent item : this.specificCost) 1238 if (!item.isEmpty()) 1239 return true; 1240 return false; 1241 } 1242 1243 public InsurancePlanPlanSpecificCostComponent addSpecificCost() { //3 1244 InsurancePlanPlanSpecificCostComponent t = new InsurancePlanPlanSpecificCostComponent(); 1245 if (this.specificCost == null) 1246 this.specificCost = new ArrayList<InsurancePlanPlanSpecificCostComponent>(); 1247 this.specificCost.add(t); 1248 return t; 1249 } 1250 1251 public InsurancePlanPlanComponent addSpecificCost(InsurancePlanPlanSpecificCostComponent t) { //3 1252 if (t == null) 1253 return this; 1254 if (this.specificCost == null) 1255 this.specificCost = new ArrayList<InsurancePlanPlanSpecificCostComponent>(); 1256 this.specificCost.add(t); 1257 return this; 1258 } 1259 1260 /** 1261 * @return The first repetition of repeating field {@link #specificCost}, creating it if it does not already exist {3} 1262 */ 1263 public InsurancePlanPlanSpecificCostComponent getSpecificCostFirstRep() { 1264 if (getSpecificCost().isEmpty()) { 1265 addSpecificCost(); 1266 } 1267 return getSpecificCost().get(0); 1268 } 1269 1270 protected void listChildren(List<Property> children) { 1271 super.listChildren(children); 1272 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this health insurance plan which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1273 children.add(new Property("type", "CodeableConcept", "Type of plan. For example, \"Platinum\" or \"High Deductable\".", 0, 1, type)); 1274 children.add(new Property("coverageArea", "Reference(Location)", "The geographic region in which a health insurance plan's benefits apply.", 0, java.lang.Integer.MAX_VALUE, coverageArea)); 1275 children.add(new Property("network", "Reference(Organization)", "Reference to the network that providing the type of coverage.", 0, java.lang.Integer.MAX_VALUE, network)); 1276 children.add(new Property("generalCost", "", "Overall costs associated with the plan.", 0, java.lang.Integer.MAX_VALUE, generalCost)); 1277 children.add(new Property("specificCost", "", "Costs associated with the coverage provided by the product.", 0, java.lang.Integer.MAX_VALUE, specificCost)); 1278 } 1279 1280 @Override 1281 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1282 switch (_hash) { 1283 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this health insurance plan which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 1284 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of plan. For example, \"Platinum\" or \"High Deductable\".", 0, 1, type); 1285 case -1532328299: /*coverageArea*/ return new Property("coverageArea", "Reference(Location)", "The geographic region in which a health insurance plan's benefits apply.", 0, java.lang.Integer.MAX_VALUE, coverageArea); 1286 case 1843485230: /*network*/ return new Property("network", "Reference(Organization)", "Reference to the network that providing the type of coverage.", 0, java.lang.Integer.MAX_VALUE, network); 1287 case 878344405: /*generalCost*/ return new Property("generalCost", "", "Overall costs associated with the plan.", 0, java.lang.Integer.MAX_VALUE, generalCost); 1288 case -1205656545: /*specificCost*/ return new Property("specificCost", "", "Costs associated with the coverage provided by the product.", 0, java.lang.Integer.MAX_VALUE, specificCost); 1289 default: return super.getNamedProperty(_hash, _name, _checkValid); 1290 } 1291 1292 } 1293 1294 @Override 1295 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1296 switch (hash) { 1297 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1298 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1299 case -1532328299: /*coverageArea*/ return this.coverageArea == null ? new Base[0] : this.coverageArea.toArray(new Base[this.coverageArea.size()]); // Reference 1300 case 1843485230: /*network*/ return this.network == null ? new Base[0] : this.network.toArray(new Base[this.network.size()]); // Reference 1301 case 878344405: /*generalCost*/ return this.generalCost == null ? new Base[0] : this.generalCost.toArray(new Base[this.generalCost.size()]); // InsurancePlanPlanGeneralCostComponent 1302 case -1205656545: /*specificCost*/ return this.specificCost == null ? new Base[0] : this.specificCost.toArray(new Base[this.specificCost.size()]); // InsurancePlanPlanSpecificCostComponent 1303 default: return super.getProperty(hash, name, checkValid); 1304 } 1305 1306 } 1307 1308 @Override 1309 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1310 switch (hash) { 1311 case -1618432855: // identifier 1312 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1313 return value; 1314 case 3575610: // type 1315 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1316 return value; 1317 case -1532328299: // coverageArea 1318 this.getCoverageArea().add(TypeConvertor.castToReference(value)); // Reference 1319 return value; 1320 case 1843485230: // network 1321 this.getNetwork().add(TypeConvertor.castToReference(value)); // Reference 1322 return value; 1323 case 878344405: // generalCost 1324 this.getGeneralCost().add((InsurancePlanPlanGeneralCostComponent) value); // InsurancePlanPlanGeneralCostComponent 1325 return value; 1326 case -1205656545: // specificCost 1327 this.getSpecificCost().add((InsurancePlanPlanSpecificCostComponent) value); // InsurancePlanPlanSpecificCostComponent 1328 return value; 1329 default: return super.setProperty(hash, name, value); 1330 } 1331 1332 } 1333 1334 @Override 1335 public Base setProperty(String name, Base value) throws FHIRException { 1336 if (name.equals("identifier")) { 1337 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1338 } else if (name.equals("type")) { 1339 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1340 } else if (name.equals("coverageArea")) { 1341 this.getCoverageArea().add(TypeConvertor.castToReference(value)); 1342 } else if (name.equals("network")) { 1343 this.getNetwork().add(TypeConvertor.castToReference(value)); 1344 } else if (name.equals("generalCost")) { 1345 this.getGeneralCost().add((InsurancePlanPlanGeneralCostComponent) value); 1346 } else if (name.equals("specificCost")) { 1347 this.getSpecificCost().add((InsurancePlanPlanSpecificCostComponent) value); 1348 } else 1349 return super.setProperty(name, value); 1350 return value; 1351 } 1352 1353 @Override 1354 public void removeChild(String name, Base value) throws FHIRException { 1355 if (name.equals("identifier")) { 1356 this.getIdentifier().remove(value); 1357 } else if (name.equals("type")) { 1358 this.type = null; 1359 } else if (name.equals("coverageArea")) { 1360 this.getCoverageArea().remove(value); 1361 } else if (name.equals("network")) { 1362 this.getNetwork().remove(value); 1363 } else if (name.equals("generalCost")) { 1364 this.getGeneralCost().remove((InsurancePlanPlanGeneralCostComponent) value); 1365 } else if (name.equals("specificCost")) { 1366 this.getSpecificCost().remove((InsurancePlanPlanSpecificCostComponent) value); 1367 } else 1368 super.removeChild(name, value); 1369 1370 } 1371 1372 @Override 1373 public Base makeProperty(int hash, String name) throws FHIRException { 1374 switch (hash) { 1375 case -1618432855: return addIdentifier(); 1376 case 3575610: return getType(); 1377 case -1532328299: return addCoverageArea(); 1378 case 1843485230: return addNetwork(); 1379 case 878344405: return addGeneralCost(); 1380 case -1205656545: return addSpecificCost(); 1381 default: return super.makeProperty(hash, name); 1382 } 1383 1384 } 1385 1386 @Override 1387 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1388 switch (hash) { 1389 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1390 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1391 case -1532328299: /*coverageArea*/ return new String[] {"Reference"}; 1392 case 1843485230: /*network*/ return new String[] {"Reference"}; 1393 case 878344405: /*generalCost*/ return new String[] {}; 1394 case -1205656545: /*specificCost*/ return new String[] {}; 1395 default: return super.getTypesForProperty(hash, name); 1396 } 1397 1398 } 1399 1400 @Override 1401 public Base addChild(String name) throws FHIRException { 1402 if (name.equals("identifier")) { 1403 return addIdentifier(); 1404 } 1405 else if (name.equals("type")) { 1406 this.type = new CodeableConcept(); 1407 return this.type; 1408 } 1409 else if (name.equals("coverageArea")) { 1410 return addCoverageArea(); 1411 } 1412 else if (name.equals("network")) { 1413 return addNetwork(); 1414 } 1415 else if (name.equals("generalCost")) { 1416 return addGeneralCost(); 1417 } 1418 else if (name.equals("specificCost")) { 1419 return addSpecificCost(); 1420 } 1421 else 1422 return super.addChild(name); 1423 } 1424 1425 public InsurancePlanPlanComponent copy() { 1426 InsurancePlanPlanComponent dst = new InsurancePlanPlanComponent(); 1427 copyValues(dst); 1428 return dst; 1429 } 1430 1431 public void copyValues(InsurancePlanPlanComponent dst) { 1432 super.copyValues(dst); 1433 if (identifier != null) { 1434 dst.identifier = new ArrayList<Identifier>(); 1435 for (Identifier i : identifier) 1436 dst.identifier.add(i.copy()); 1437 }; 1438 dst.type = type == null ? null : type.copy(); 1439 if (coverageArea != null) { 1440 dst.coverageArea = new ArrayList<Reference>(); 1441 for (Reference i : coverageArea) 1442 dst.coverageArea.add(i.copy()); 1443 }; 1444 if (network != null) { 1445 dst.network = new ArrayList<Reference>(); 1446 for (Reference i : network) 1447 dst.network.add(i.copy()); 1448 }; 1449 if (generalCost != null) { 1450 dst.generalCost = new ArrayList<InsurancePlanPlanGeneralCostComponent>(); 1451 for (InsurancePlanPlanGeneralCostComponent i : generalCost) 1452 dst.generalCost.add(i.copy()); 1453 }; 1454 if (specificCost != null) { 1455 dst.specificCost = new ArrayList<InsurancePlanPlanSpecificCostComponent>(); 1456 for (InsurancePlanPlanSpecificCostComponent i : specificCost) 1457 dst.specificCost.add(i.copy()); 1458 }; 1459 } 1460 1461 @Override 1462 public boolean equalsDeep(Base other_) { 1463 if (!super.equalsDeep(other_)) 1464 return false; 1465 if (!(other_ instanceof InsurancePlanPlanComponent)) 1466 return false; 1467 InsurancePlanPlanComponent o = (InsurancePlanPlanComponent) other_; 1468 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(coverageArea, o.coverageArea, true) 1469 && compareDeep(network, o.network, true) && compareDeep(generalCost, o.generalCost, true) && compareDeep(specificCost, o.specificCost, true) 1470 ; 1471 } 1472 1473 @Override 1474 public boolean equalsShallow(Base other_) { 1475 if (!super.equalsShallow(other_)) 1476 return false; 1477 if (!(other_ instanceof InsurancePlanPlanComponent)) 1478 return false; 1479 InsurancePlanPlanComponent o = (InsurancePlanPlanComponent) other_; 1480 return true; 1481 } 1482 1483 public boolean isEmpty() { 1484 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, coverageArea 1485 , network, generalCost, specificCost); 1486 } 1487 1488 public String fhirType() { 1489 return "InsurancePlan.plan"; 1490 1491 } 1492 1493 } 1494 1495 @Block() 1496 public static class InsurancePlanPlanGeneralCostComponent extends BackboneElement implements IBaseBackboneElement { 1497 /** 1498 * Type of cost. 1499 */ 1500 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1501 @Description(shortDefinition="Type of cost", formalDefinition="Type of cost." ) 1502 protected CodeableConcept type; 1503 1504 /** 1505 * Number of participants enrolled in the plan. 1506 */ 1507 @Child(name = "groupSize", type = {PositiveIntType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1508 @Description(shortDefinition="Number of enrollees", formalDefinition="Number of participants enrolled in the plan." ) 1509 protected PositiveIntType groupSize; 1510 1511 /** 1512 * Value of the cost. 1513 */ 1514 @Child(name = "cost", type = {Money.class}, order=3, min=0, max=1, modifier=false, summary=false) 1515 @Description(shortDefinition="Cost value", formalDefinition="Value of the cost." ) 1516 protected Money cost; 1517 1518 /** 1519 * Additional information about the general costs associated with this plan. 1520 */ 1521 @Child(name = "comment", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1522 @Description(shortDefinition="Additional cost information", formalDefinition="Additional information about the general costs associated with this plan." ) 1523 protected StringType comment; 1524 1525 private static final long serialVersionUID = 1563949866L; 1526 1527 /** 1528 * Constructor 1529 */ 1530 public InsurancePlanPlanGeneralCostComponent() { 1531 super(); 1532 } 1533 1534 /** 1535 * @return {@link #type} (Type of cost.) 1536 */ 1537 public CodeableConcept getType() { 1538 if (this.type == null) 1539 if (Configuration.errorOnAutoCreate()) 1540 throw new Error("Attempt to auto-create InsurancePlanPlanGeneralCostComponent.type"); 1541 else if (Configuration.doAutoCreate()) 1542 this.type = new CodeableConcept(); // cc 1543 return this.type; 1544 } 1545 1546 public boolean hasType() { 1547 return this.type != null && !this.type.isEmpty(); 1548 } 1549 1550 /** 1551 * @param value {@link #type} (Type of cost.) 1552 */ 1553 public InsurancePlanPlanGeneralCostComponent setType(CodeableConcept value) { 1554 this.type = value; 1555 return this; 1556 } 1557 1558 /** 1559 * @return {@link #groupSize} (Number of participants enrolled in the plan.). This is the underlying object with id, value and extensions. The accessor "getGroupSize" gives direct access to the value 1560 */ 1561 public PositiveIntType getGroupSizeElement() { 1562 if (this.groupSize == null) 1563 if (Configuration.errorOnAutoCreate()) 1564 throw new Error("Attempt to auto-create InsurancePlanPlanGeneralCostComponent.groupSize"); 1565 else if (Configuration.doAutoCreate()) 1566 this.groupSize = new PositiveIntType(); // bb 1567 return this.groupSize; 1568 } 1569 1570 public boolean hasGroupSizeElement() { 1571 return this.groupSize != null && !this.groupSize.isEmpty(); 1572 } 1573 1574 public boolean hasGroupSize() { 1575 return this.groupSize != null && !this.groupSize.isEmpty(); 1576 } 1577 1578 /** 1579 * @param value {@link #groupSize} (Number of participants enrolled in the plan.). This is the underlying object with id, value and extensions. The accessor "getGroupSize" gives direct access to the value 1580 */ 1581 public InsurancePlanPlanGeneralCostComponent setGroupSizeElement(PositiveIntType value) { 1582 this.groupSize = value; 1583 return this; 1584 } 1585 1586 /** 1587 * @return Number of participants enrolled in the plan. 1588 */ 1589 public int getGroupSize() { 1590 return this.groupSize == null || this.groupSize.isEmpty() ? 0 : this.groupSize.getValue(); 1591 } 1592 1593 /** 1594 * @param value Number of participants enrolled in the plan. 1595 */ 1596 public InsurancePlanPlanGeneralCostComponent setGroupSize(int value) { 1597 if (this.groupSize == null) 1598 this.groupSize = new PositiveIntType(); 1599 this.groupSize.setValue(value); 1600 return this; 1601 } 1602 1603 /** 1604 * @return {@link #cost} (Value of the cost.) 1605 */ 1606 public Money getCost() { 1607 if (this.cost == null) 1608 if (Configuration.errorOnAutoCreate()) 1609 throw new Error("Attempt to auto-create InsurancePlanPlanGeneralCostComponent.cost"); 1610 else if (Configuration.doAutoCreate()) 1611 this.cost = new Money(); // cc 1612 return this.cost; 1613 } 1614 1615 public boolean hasCost() { 1616 return this.cost != null && !this.cost.isEmpty(); 1617 } 1618 1619 /** 1620 * @param value {@link #cost} (Value of the cost.) 1621 */ 1622 public InsurancePlanPlanGeneralCostComponent setCost(Money value) { 1623 this.cost = value; 1624 return this; 1625 } 1626 1627 /** 1628 * @return {@link #comment} (Additional information about the general costs associated with this plan.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 1629 */ 1630 public StringType getCommentElement() { 1631 if (this.comment == null) 1632 if (Configuration.errorOnAutoCreate()) 1633 throw new Error("Attempt to auto-create InsurancePlanPlanGeneralCostComponent.comment"); 1634 else if (Configuration.doAutoCreate()) 1635 this.comment = new StringType(); // bb 1636 return this.comment; 1637 } 1638 1639 public boolean hasCommentElement() { 1640 return this.comment != null && !this.comment.isEmpty(); 1641 } 1642 1643 public boolean hasComment() { 1644 return this.comment != null && !this.comment.isEmpty(); 1645 } 1646 1647 /** 1648 * @param value {@link #comment} (Additional information about the general costs associated with this plan.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 1649 */ 1650 public InsurancePlanPlanGeneralCostComponent setCommentElement(StringType value) { 1651 this.comment = value; 1652 return this; 1653 } 1654 1655 /** 1656 * @return Additional information about the general costs associated with this plan. 1657 */ 1658 public String getComment() { 1659 return this.comment == null ? null : this.comment.getValue(); 1660 } 1661 1662 /** 1663 * @param value Additional information about the general costs associated with this plan. 1664 */ 1665 public InsurancePlanPlanGeneralCostComponent setComment(String value) { 1666 if (Utilities.noString(value)) 1667 this.comment = null; 1668 else { 1669 if (this.comment == null) 1670 this.comment = new StringType(); 1671 this.comment.setValue(value); 1672 } 1673 return this; 1674 } 1675 1676 protected void listChildren(List<Property> children) { 1677 super.listChildren(children); 1678 children.add(new Property("type", "CodeableConcept", "Type of cost.", 0, 1, type)); 1679 children.add(new Property("groupSize", "positiveInt", "Number of participants enrolled in the plan.", 0, 1, groupSize)); 1680 children.add(new Property("cost", "Money", "Value of the cost.", 0, 1, cost)); 1681 children.add(new Property("comment", "string", "Additional information about the general costs associated with this plan.", 0, 1, comment)); 1682 } 1683 1684 @Override 1685 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1686 switch (_hash) { 1687 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of cost.", 0, 1, type); 1688 case -1483017440: /*groupSize*/ return new Property("groupSize", "positiveInt", "Number of participants enrolled in the plan.", 0, 1, groupSize); 1689 case 3059661: /*cost*/ return new Property("cost", "Money", "Value of the cost.", 0, 1, cost); 1690 case 950398559: /*comment*/ return new Property("comment", "string", "Additional information about the general costs associated with this plan.", 0, 1, comment); 1691 default: return super.getNamedProperty(_hash, _name, _checkValid); 1692 } 1693 1694 } 1695 1696 @Override 1697 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1698 switch (hash) { 1699 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1700 case -1483017440: /*groupSize*/ return this.groupSize == null ? new Base[0] : new Base[] {this.groupSize}; // PositiveIntType 1701 case 3059661: /*cost*/ return this.cost == null ? new Base[0] : new Base[] {this.cost}; // Money 1702 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 1703 default: return super.getProperty(hash, name, checkValid); 1704 } 1705 1706 } 1707 1708 @Override 1709 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1710 switch (hash) { 1711 case 3575610: // type 1712 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1713 return value; 1714 case -1483017440: // groupSize 1715 this.groupSize = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1716 return value; 1717 case 3059661: // cost 1718 this.cost = TypeConvertor.castToMoney(value); // Money 1719 return value; 1720 case 950398559: // comment 1721 this.comment = TypeConvertor.castToString(value); // StringType 1722 return value; 1723 default: return super.setProperty(hash, name, value); 1724 } 1725 1726 } 1727 1728 @Override 1729 public Base setProperty(String name, Base value) throws FHIRException { 1730 if (name.equals("type")) { 1731 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1732 } else if (name.equals("groupSize")) { 1733 this.groupSize = TypeConvertor.castToPositiveInt(value); // PositiveIntType 1734 } else if (name.equals("cost")) { 1735 this.cost = TypeConvertor.castToMoney(value); // Money 1736 } else if (name.equals("comment")) { 1737 this.comment = TypeConvertor.castToString(value); // StringType 1738 } else 1739 return super.setProperty(name, value); 1740 return value; 1741 } 1742 1743 @Override 1744 public void removeChild(String name, Base value) throws FHIRException { 1745 if (name.equals("type")) { 1746 this.type = null; 1747 } else if (name.equals("groupSize")) { 1748 this.groupSize = null; 1749 } else if (name.equals("cost")) { 1750 this.cost = null; 1751 } else if (name.equals("comment")) { 1752 this.comment = null; 1753 } else 1754 super.removeChild(name, value); 1755 1756 } 1757 1758 @Override 1759 public Base makeProperty(int hash, String name) throws FHIRException { 1760 switch (hash) { 1761 case 3575610: return getType(); 1762 case -1483017440: return getGroupSizeElement(); 1763 case 3059661: return getCost(); 1764 case 950398559: return getCommentElement(); 1765 default: return super.makeProperty(hash, name); 1766 } 1767 1768 } 1769 1770 @Override 1771 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1772 switch (hash) { 1773 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1774 case -1483017440: /*groupSize*/ return new String[] {"positiveInt"}; 1775 case 3059661: /*cost*/ return new String[] {"Money"}; 1776 case 950398559: /*comment*/ return new String[] {"string"}; 1777 default: return super.getTypesForProperty(hash, name); 1778 } 1779 1780 } 1781 1782 @Override 1783 public Base addChild(String name) throws FHIRException { 1784 if (name.equals("type")) { 1785 this.type = new CodeableConcept(); 1786 return this.type; 1787 } 1788 else if (name.equals("groupSize")) { 1789 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.plan.generalCost.groupSize"); 1790 } 1791 else if (name.equals("cost")) { 1792 this.cost = new Money(); 1793 return this.cost; 1794 } 1795 else if (name.equals("comment")) { 1796 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.plan.generalCost.comment"); 1797 } 1798 else 1799 return super.addChild(name); 1800 } 1801 1802 public InsurancePlanPlanGeneralCostComponent copy() { 1803 InsurancePlanPlanGeneralCostComponent dst = new InsurancePlanPlanGeneralCostComponent(); 1804 copyValues(dst); 1805 return dst; 1806 } 1807 1808 public void copyValues(InsurancePlanPlanGeneralCostComponent dst) { 1809 super.copyValues(dst); 1810 dst.type = type == null ? null : type.copy(); 1811 dst.groupSize = groupSize == null ? null : groupSize.copy(); 1812 dst.cost = cost == null ? null : cost.copy(); 1813 dst.comment = comment == null ? null : comment.copy(); 1814 } 1815 1816 @Override 1817 public boolean equalsDeep(Base other_) { 1818 if (!super.equalsDeep(other_)) 1819 return false; 1820 if (!(other_ instanceof InsurancePlanPlanGeneralCostComponent)) 1821 return false; 1822 InsurancePlanPlanGeneralCostComponent o = (InsurancePlanPlanGeneralCostComponent) other_; 1823 return compareDeep(type, o.type, true) && compareDeep(groupSize, o.groupSize, true) && compareDeep(cost, o.cost, true) 1824 && compareDeep(comment, o.comment, true); 1825 } 1826 1827 @Override 1828 public boolean equalsShallow(Base other_) { 1829 if (!super.equalsShallow(other_)) 1830 return false; 1831 if (!(other_ instanceof InsurancePlanPlanGeneralCostComponent)) 1832 return false; 1833 InsurancePlanPlanGeneralCostComponent o = (InsurancePlanPlanGeneralCostComponent) other_; 1834 return compareValues(groupSize, o.groupSize, true) && compareValues(comment, o.comment, true); 1835 } 1836 1837 public boolean isEmpty() { 1838 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, groupSize, cost, comment 1839 ); 1840 } 1841 1842 public String fhirType() { 1843 return "InsurancePlan.plan.generalCost"; 1844 1845 } 1846 1847 } 1848 1849 @Block() 1850 public static class InsurancePlanPlanSpecificCostComponent extends BackboneElement implements IBaseBackboneElement { 1851 /** 1852 * General category of benefit (Medical; Dental; Vision; Drug; Mental Health; Substance Abuse; Hospice, Home Health). 1853 */ 1854 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1855 @Description(shortDefinition="General category of benefit", formalDefinition="General category of benefit (Medical; Dental; Vision; Drug; Mental Health; Substance Abuse; Hospice, Home Health)." ) 1856 protected CodeableConcept category; 1857 1858 /** 1859 * List of the specific benefits under this category of benefit. 1860 */ 1861 @Child(name = "benefit", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1862 @Description(shortDefinition="Benefits list", formalDefinition="List of the specific benefits under this category of benefit." ) 1863 protected List<PlanBenefitComponent> benefit; 1864 1865 private static final long serialVersionUID = 922585525L; 1866 1867 /** 1868 * Constructor 1869 */ 1870 public InsurancePlanPlanSpecificCostComponent() { 1871 super(); 1872 } 1873 1874 /** 1875 * Constructor 1876 */ 1877 public InsurancePlanPlanSpecificCostComponent(CodeableConcept category) { 1878 super(); 1879 this.setCategory(category); 1880 } 1881 1882 /** 1883 * @return {@link #category} (General category of benefit (Medical; Dental; Vision; Drug; Mental Health; Substance Abuse; Hospice, Home Health).) 1884 */ 1885 public CodeableConcept getCategory() { 1886 if (this.category == null) 1887 if (Configuration.errorOnAutoCreate()) 1888 throw new Error("Attempt to auto-create InsurancePlanPlanSpecificCostComponent.category"); 1889 else if (Configuration.doAutoCreate()) 1890 this.category = new CodeableConcept(); // cc 1891 return this.category; 1892 } 1893 1894 public boolean hasCategory() { 1895 return this.category != null && !this.category.isEmpty(); 1896 } 1897 1898 /** 1899 * @param value {@link #category} (General category of benefit (Medical; Dental; Vision; Drug; Mental Health; Substance Abuse; Hospice, Home Health).) 1900 */ 1901 public InsurancePlanPlanSpecificCostComponent setCategory(CodeableConcept value) { 1902 this.category = value; 1903 return this; 1904 } 1905 1906 /** 1907 * @return {@link #benefit} (List of the specific benefits under this category of benefit.) 1908 */ 1909 public List<PlanBenefitComponent> getBenefit() { 1910 if (this.benefit == null) 1911 this.benefit = new ArrayList<PlanBenefitComponent>(); 1912 return this.benefit; 1913 } 1914 1915 /** 1916 * @return Returns a reference to <code>this</code> for easy method chaining 1917 */ 1918 public InsurancePlanPlanSpecificCostComponent setBenefit(List<PlanBenefitComponent> theBenefit) { 1919 this.benefit = theBenefit; 1920 return this; 1921 } 1922 1923 public boolean hasBenefit() { 1924 if (this.benefit == null) 1925 return false; 1926 for (PlanBenefitComponent item : this.benefit) 1927 if (!item.isEmpty()) 1928 return true; 1929 return false; 1930 } 1931 1932 public PlanBenefitComponent addBenefit() { //3 1933 PlanBenefitComponent t = new PlanBenefitComponent(); 1934 if (this.benefit == null) 1935 this.benefit = new ArrayList<PlanBenefitComponent>(); 1936 this.benefit.add(t); 1937 return t; 1938 } 1939 1940 public InsurancePlanPlanSpecificCostComponent addBenefit(PlanBenefitComponent t) { //3 1941 if (t == null) 1942 return this; 1943 if (this.benefit == null) 1944 this.benefit = new ArrayList<PlanBenefitComponent>(); 1945 this.benefit.add(t); 1946 return this; 1947 } 1948 1949 /** 1950 * @return The first repetition of repeating field {@link #benefit}, creating it if it does not already exist {3} 1951 */ 1952 public PlanBenefitComponent getBenefitFirstRep() { 1953 if (getBenefit().isEmpty()) { 1954 addBenefit(); 1955 } 1956 return getBenefit().get(0); 1957 } 1958 1959 protected void listChildren(List<Property> children) { 1960 super.listChildren(children); 1961 children.add(new Property("category", "CodeableConcept", "General category of benefit (Medical; Dental; Vision; Drug; Mental Health; Substance Abuse; Hospice, Home Health).", 0, 1, category)); 1962 children.add(new Property("benefit", "", "List of the specific benefits under this category of benefit.", 0, java.lang.Integer.MAX_VALUE, benefit)); 1963 } 1964 1965 @Override 1966 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1967 switch (_hash) { 1968 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "General category of benefit (Medical; Dental; Vision; Drug; Mental Health; Substance Abuse; Hospice, Home Health).", 0, 1, category); 1969 case -222710633: /*benefit*/ return new Property("benefit", "", "List of the specific benefits under this category of benefit.", 0, java.lang.Integer.MAX_VALUE, benefit); 1970 default: return super.getNamedProperty(_hash, _name, _checkValid); 1971 } 1972 1973 } 1974 1975 @Override 1976 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1977 switch (hash) { 1978 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1979 case -222710633: /*benefit*/ return this.benefit == null ? new Base[0] : this.benefit.toArray(new Base[this.benefit.size()]); // PlanBenefitComponent 1980 default: return super.getProperty(hash, name, checkValid); 1981 } 1982 1983 } 1984 1985 @Override 1986 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1987 switch (hash) { 1988 case 50511102: // category 1989 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1990 return value; 1991 case -222710633: // benefit 1992 this.getBenefit().add((PlanBenefitComponent) value); // PlanBenefitComponent 1993 return value; 1994 default: return super.setProperty(hash, name, value); 1995 } 1996 1997 } 1998 1999 @Override 2000 public Base setProperty(String name, Base value) throws FHIRException { 2001 if (name.equals("category")) { 2002 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2003 } else if (name.equals("benefit")) { 2004 this.getBenefit().add((PlanBenefitComponent) value); 2005 } else 2006 return super.setProperty(name, value); 2007 return value; 2008 } 2009 2010 @Override 2011 public void removeChild(String name, Base value) throws FHIRException { 2012 if (name.equals("category")) { 2013 this.category = null; 2014 } else if (name.equals("benefit")) { 2015 this.getBenefit().remove((PlanBenefitComponent) value); 2016 } else 2017 super.removeChild(name, value); 2018 2019 } 2020 2021 @Override 2022 public Base makeProperty(int hash, String name) throws FHIRException { 2023 switch (hash) { 2024 case 50511102: return getCategory(); 2025 case -222710633: return addBenefit(); 2026 default: return super.makeProperty(hash, name); 2027 } 2028 2029 } 2030 2031 @Override 2032 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2033 switch (hash) { 2034 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2035 case -222710633: /*benefit*/ return new String[] {}; 2036 default: return super.getTypesForProperty(hash, name); 2037 } 2038 2039 } 2040 2041 @Override 2042 public Base addChild(String name) throws FHIRException { 2043 if (name.equals("category")) { 2044 this.category = new CodeableConcept(); 2045 return this.category; 2046 } 2047 else if (name.equals("benefit")) { 2048 return addBenefit(); 2049 } 2050 else 2051 return super.addChild(name); 2052 } 2053 2054 public InsurancePlanPlanSpecificCostComponent copy() { 2055 InsurancePlanPlanSpecificCostComponent dst = new InsurancePlanPlanSpecificCostComponent(); 2056 copyValues(dst); 2057 return dst; 2058 } 2059 2060 public void copyValues(InsurancePlanPlanSpecificCostComponent dst) { 2061 super.copyValues(dst); 2062 dst.category = category == null ? null : category.copy(); 2063 if (benefit != null) { 2064 dst.benefit = new ArrayList<PlanBenefitComponent>(); 2065 for (PlanBenefitComponent i : benefit) 2066 dst.benefit.add(i.copy()); 2067 }; 2068 } 2069 2070 @Override 2071 public boolean equalsDeep(Base other_) { 2072 if (!super.equalsDeep(other_)) 2073 return false; 2074 if (!(other_ instanceof InsurancePlanPlanSpecificCostComponent)) 2075 return false; 2076 InsurancePlanPlanSpecificCostComponent o = (InsurancePlanPlanSpecificCostComponent) other_; 2077 return compareDeep(category, o.category, true) && compareDeep(benefit, o.benefit, true); 2078 } 2079 2080 @Override 2081 public boolean equalsShallow(Base other_) { 2082 if (!super.equalsShallow(other_)) 2083 return false; 2084 if (!(other_ instanceof InsurancePlanPlanSpecificCostComponent)) 2085 return false; 2086 InsurancePlanPlanSpecificCostComponent o = (InsurancePlanPlanSpecificCostComponent) other_; 2087 return true; 2088 } 2089 2090 public boolean isEmpty() { 2091 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, benefit); 2092 } 2093 2094 public String fhirType() { 2095 return "InsurancePlan.plan.specificCost"; 2096 2097 } 2098 2099 } 2100 2101 @Block() 2102 public static class PlanBenefitComponent extends BackboneElement implements IBaseBackboneElement { 2103 /** 2104 * Type of specific benefit (preventative; primary care office visit; speciality office visit; hospitalization; emergency room; urgent care). 2105 */ 2106 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 2107 @Description(shortDefinition="Type of specific benefit", formalDefinition="Type of specific benefit (preventative; primary care office visit; speciality office visit; hospitalization; emergency room; urgent care)." ) 2108 protected CodeableConcept type; 2109 2110 /** 2111 * List of the costs associated with a specific benefit. 2112 */ 2113 @Child(name = "cost", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2114 @Description(shortDefinition="List of the costs", formalDefinition="List of the costs associated with a specific benefit." ) 2115 protected List<PlanBenefitCostComponent> cost; 2116 2117 private static final long serialVersionUID = 792296200L; 2118 2119 /** 2120 * Constructor 2121 */ 2122 public PlanBenefitComponent() { 2123 super(); 2124 } 2125 2126 /** 2127 * Constructor 2128 */ 2129 public PlanBenefitComponent(CodeableConcept type) { 2130 super(); 2131 this.setType(type); 2132 } 2133 2134 /** 2135 * @return {@link #type} (Type of specific benefit (preventative; primary care office visit; speciality office visit; hospitalization; emergency room; urgent care).) 2136 */ 2137 public CodeableConcept getType() { 2138 if (this.type == null) 2139 if (Configuration.errorOnAutoCreate()) 2140 throw new Error("Attempt to auto-create PlanBenefitComponent.type"); 2141 else if (Configuration.doAutoCreate()) 2142 this.type = new CodeableConcept(); // cc 2143 return this.type; 2144 } 2145 2146 public boolean hasType() { 2147 return this.type != null && !this.type.isEmpty(); 2148 } 2149 2150 /** 2151 * @param value {@link #type} (Type of specific benefit (preventative; primary care office visit; speciality office visit; hospitalization; emergency room; urgent care).) 2152 */ 2153 public PlanBenefitComponent setType(CodeableConcept value) { 2154 this.type = value; 2155 return this; 2156 } 2157 2158 /** 2159 * @return {@link #cost} (List of the costs associated with a specific benefit.) 2160 */ 2161 public List<PlanBenefitCostComponent> getCost() { 2162 if (this.cost == null) 2163 this.cost = new ArrayList<PlanBenefitCostComponent>(); 2164 return this.cost; 2165 } 2166 2167 /** 2168 * @return Returns a reference to <code>this</code> for easy method chaining 2169 */ 2170 public PlanBenefitComponent setCost(List<PlanBenefitCostComponent> theCost) { 2171 this.cost = theCost; 2172 return this; 2173 } 2174 2175 public boolean hasCost() { 2176 if (this.cost == null) 2177 return false; 2178 for (PlanBenefitCostComponent item : this.cost) 2179 if (!item.isEmpty()) 2180 return true; 2181 return false; 2182 } 2183 2184 public PlanBenefitCostComponent addCost() { //3 2185 PlanBenefitCostComponent t = new PlanBenefitCostComponent(); 2186 if (this.cost == null) 2187 this.cost = new ArrayList<PlanBenefitCostComponent>(); 2188 this.cost.add(t); 2189 return t; 2190 } 2191 2192 public PlanBenefitComponent addCost(PlanBenefitCostComponent t) { //3 2193 if (t == null) 2194 return this; 2195 if (this.cost == null) 2196 this.cost = new ArrayList<PlanBenefitCostComponent>(); 2197 this.cost.add(t); 2198 return this; 2199 } 2200 2201 /** 2202 * @return The first repetition of repeating field {@link #cost}, creating it if it does not already exist {3} 2203 */ 2204 public PlanBenefitCostComponent getCostFirstRep() { 2205 if (getCost().isEmpty()) { 2206 addCost(); 2207 } 2208 return getCost().get(0); 2209 } 2210 2211 protected void listChildren(List<Property> children) { 2212 super.listChildren(children); 2213 children.add(new Property("type", "CodeableConcept", "Type of specific benefit (preventative; primary care office visit; speciality office visit; hospitalization; emergency room; urgent care).", 0, 1, type)); 2214 children.add(new Property("cost", "", "List of the costs associated with a specific benefit.", 0, java.lang.Integer.MAX_VALUE, cost)); 2215 } 2216 2217 @Override 2218 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2219 switch (_hash) { 2220 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of specific benefit (preventative; primary care office visit; speciality office visit; hospitalization; emergency room; urgent care).", 0, 1, type); 2221 case 3059661: /*cost*/ return new Property("cost", "", "List of the costs associated with a specific benefit.", 0, java.lang.Integer.MAX_VALUE, cost); 2222 default: return super.getNamedProperty(_hash, _name, _checkValid); 2223 } 2224 2225 } 2226 2227 @Override 2228 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2229 switch (hash) { 2230 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2231 case 3059661: /*cost*/ return this.cost == null ? new Base[0] : this.cost.toArray(new Base[this.cost.size()]); // PlanBenefitCostComponent 2232 default: return super.getProperty(hash, name, checkValid); 2233 } 2234 2235 } 2236 2237 @Override 2238 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2239 switch (hash) { 2240 case 3575610: // type 2241 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2242 return value; 2243 case 3059661: // cost 2244 this.getCost().add((PlanBenefitCostComponent) value); // PlanBenefitCostComponent 2245 return value; 2246 default: return super.setProperty(hash, name, value); 2247 } 2248 2249 } 2250 2251 @Override 2252 public Base setProperty(String name, Base value) throws FHIRException { 2253 if (name.equals("type")) { 2254 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2255 } else if (name.equals("cost")) { 2256 this.getCost().add((PlanBenefitCostComponent) value); 2257 } else 2258 return super.setProperty(name, value); 2259 return value; 2260 } 2261 2262 @Override 2263 public void removeChild(String name, Base value) throws FHIRException { 2264 if (name.equals("type")) { 2265 this.type = null; 2266 } else if (name.equals("cost")) { 2267 this.getCost().remove((PlanBenefitCostComponent) value); 2268 } else 2269 super.removeChild(name, value); 2270 2271 } 2272 2273 @Override 2274 public Base makeProperty(int hash, String name) throws FHIRException { 2275 switch (hash) { 2276 case 3575610: return getType(); 2277 case 3059661: return addCost(); 2278 default: return super.makeProperty(hash, name); 2279 } 2280 2281 } 2282 2283 @Override 2284 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2285 switch (hash) { 2286 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2287 case 3059661: /*cost*/ return new String[] {}; 2288 default: return super.getTypesForProperty(hash, name); 2289 } 2290 2291 } 2292 2293 @Override 2294 public Base addChild(String name) throws FHIRException { 2295 if (name.equals("type")) { 2296 this.type = new CodeableConcept(); 2297 return this.type; 2298 } 2299 else if (name.equals("cost")) { 2300 return addCost(); 2301 } 2302 else 2303 return super.addChild(name); 2304 } 2305 2306 public PlanBenefitComponent copy() { 2307 PlanBenefitComponent dst = new PlanBenefitComponent(); 2308 copyValues(dst); 2309 return dst; 2310 } 2311 2312 public void copyValues(PlanBenefitComponent dst) { 2313 super.copyValues(dst); 2314 dst.type = type == null ? null : type.copy(); 2315 if (cost != null) { 2316 dst.cost = new ArrayList<PlanBenefitCostComponent>(); 2317 for (PlanBenefitCostComponent i : cost) 2318 dst.cost.add(i.copy()); 2319 }; 2320 } 2321 2322 @Override 2323 public boolean equalsDeep(Base other_) { 2324 if (!super.equalsDeep(other_)) 2325 return false; 2326 if (!(other_ instanceof PlanBenefitComponent)) 2327 return false; 2328 PlanBenefitComponent o = (PlanBenefitComponent) other_; 2329 return compareDeep(type, o.type, true) && compareDeep(cost, o.cost, true); 2330 } 2331 2332 @Override 2333 public boolean equalsShallow(Base other_) { 2334 if (!super.equalsShallow(other_)) 2335 return false; 2336 if (!(other_ instanceof PlanBenefitComponent)) 2337 return false; 2338 PlanBenefitComponent o = (PlanBenefitComponent) other_; 2339 return true; 2340 } 2341 2342 public boolean isEmpty() { 2343 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, cost); 2344 } 2345 2346 public String fhirType() { 2347 return "InsurancePlan.plan.specificCost.benefit"; 2348 2349 } 2350 2351 } 2352 2353 @Block() 2354 public static class PlanBenefitCostComponent extends BackboneElement implements IBaseBackboneElement { 2355 /** 2356 * Type of cost (copay; individual cap; family cap; coinsurance; deductible). 2357 */ 2358 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 2359 @Description(shortDefinition="Type of cost", formalDefinition="Type of cost (copay; individual cap; family cap; coinsurance; deductible)." ) 2360 protected CodeableConcept type; 2361 2362 /** 2363 * Whether the cost applies to in-network or out-of-network providers (in-network; out-of-network; other). 2364 */ 2365 @Child(name = "applicability", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 2366 @Description(shortDefinition="in-network | out-of-network | other", formalDefinition="Whether the cost applies to in-network or out-of-network providers (in-network; out-of-network; other)." ) 2367 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/insuranceplan-applicability") 2368 protected CodeableConcept applicability; 2369 2370 /** 2371 * Additional information about the cost, such as information about funding sources (e.g. HSA, HRA, FSA, RRA). 2372 */ 2373 @Child(name = "qualifiers", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2374 @Description(shortDefinition="Additional information about the cost", formalDefinition="Additional information about the cost, such as information about funding sources (e.g. HSA, HRA, FSA, RRA)." ) 2375 protected List<CodeableConcept> qualifiers; 2376 2377 /** 2378 * The actual cost value. (some of the costs may be represented as percentages rather than currency, e.g. 10% coinsurance). 2379 */ 2380 @Child(name = "value", type = {Quantity.class}, order=4, min=0, max=1, modifier=false, summary=false) 2381 @Description(shortDefinition="The actual cost value", formalDefinition="The actual cost value. (some of the costs may be represented as percentages rather than currency, e.g. 10% coinsurance)." ) 2382 protected Quantity value; 2383 2384 private static final long serialVersionUID = -340688733L; 2385 2386 /** 2387 * Constructor 2388 */ 2389 public PlanBenefitCostComponent() { 2390 super(); 2391 } 2392 2393 /** 2394 * Constructor 2395 */ 2396 public PlanBenefitCostComponent(CodeableConcept type) { 2397 super(); 2398 this.setType(type); 2399 } 2400 2401 /** 2402 * @return {@link #type} (Type of cost (copay; individual cap; family cap; coinsurance; deductible).) 2403 */ 2404 public CodeableConcept getType() { 2405 if (this.type == null) 2406 if (Configuration.errorOnAutoCreate()) 2407 throw new Error("Attempt to auto-create PlanBenefitCostComponent.type"); 2408 else if (Configuration.doAutoCreate()) 2409 this.type = new CodeableConcept(); // cc 2410 return this.type; 2411 } 2412 2413 public boolean hasType() { 2414 return this.type != null && !this.type.isEmpty(); 2415 } 2416 2417 /** 2418 * @param value {@link #type} (Type of cost (copay; individual cap; family cap; coinsurance; deductible).) 2419 */ 2420 public PlanBenefitCostComponent setType(CodeableConcept value) { 2421 this.type = value; 2422 return this; 2423 } 2424 2425 /** 2426 * @return {@link #applicability} (Whether the cost applies to in-network or out-of-network providers (in-network; out-of-network; other).) 2427 */ 2428 public CodeableConcept getApplicability() { 2429 if (this.applicability == null) 2430 if (Configuration.errorOnAutoCreate()) 2431 throw new Error("Attempt to auto-create PlanBenefitCostComponent.applicability"); 2432 else if (Configuration.doAutoCreate()) 2433 this.applicability = new CodeableConcept(); // cc 2434 return this.applicability; 2435 } 2436 2437 public boolean hasApplicability() { 2438 return this.applicability != null && !this.applicability.isEmpty(); 2439 } 2440 2441 /** 2442 * @param value {@link #applicability} (Whether the cost applies to in-network or out-of-network providers (in-network; out-of-network; other).) 2443 */ 2444 public PlanBenefitCostComponent setApplicability(CodeableConcept value) { 2445 this.applicability = value; 2446 return this; 2447 } 2448 2449 /** 2450 * @return {@link #qualifiers} (Additional information about the cost, such as information about funding sources (e.g. HSA, HRA, FSA, RRA).) 2451 */ 2452 public List<CodeableConcept> getQualifiers() { 2453 if (this.qualifiers == null) 2454 this.qualifiers = new ArrayList<CodeableConcept>(); 2455 return this.qualifiers; 2456 } 2457 2458 /** 2459 * @return Returns a reference to <code>this</code> for easy method chaining 2460 */ 2461 public PlanBenefitCostComponent setQualifiers(List<CodeableConcept> theQualifiers) { 2462 this.qualifiers = theQualifiers; 2463 return this; 2464 } 2465 2466 public boolean hasQualifiers() { 2467 if (this.qualifiers == null) 2468 return false; 2469 for (CodeableConcept item : this.qualifiers) 2470 if (!item.isEmpty()) 2471 return true; 2472 return false; 2473 } 2474 2475 public CodeableConcept addQualifiers() { //3 2476 CodeableConcept t = new CodeableConcept(); 2477 if (this.qualifiers == null) 2478 this.qualifiers = new ArrayList<CodeableConcept>(); 2479 this.qualifiers.add(t); 2480 return t; 2481 } 2482 2483 public PlanBenefitCostComponent addQualifiers(CodeableConcept t) { //3 2484 if (t == null) 2485 return this; 2486 if (this.qualifiers == null) 2487 this.qualifiers = new ArrayList<CodeableConcept>(); 2488 this.qualifiers.add(t); 2489 return this; 2490 } 2491 2492 /** 2493 * @return The first repetition of repeating field {@link #qualifiers}, creating it if it does not already exist {3} 2494 */ 2495 public CodeableConcept getQualifiersFirstRep() { 2496 if (getQualifiers().isEmpty()) { 2497 addQualifiers(); 2498 } 2499 return getQualifiers().get(0); 2500 } 2501 2502 /** 2503 * @return {@link #value} (The actual cost value. (some of the costs may be represented as percentages rather than currency, e.g. 10% coinsurance).) 2504 */ 2505 public Quantity getValue() { 2506 if (this.value == null) 2507 if (Configuration.errorOnAutoCreate()) 2508 throw new Error("Attempt to auto-create PlanBenefitCostComponent.value"); 2509 else if (Configuration.doAutoCreate()) 2510 this.value = new Quantity(); // cc 2511 return this.value; 2512 } 2513 2514 public boolean hasValue() { 2515 return this.value != null && !this.value.isEmpty(); 2516 } 2517 2518 /** 2519 * @param value {@link #value} (The actual cost value. (some of the costs may be represented as percentages rather than currency, e.g. 10% coinsurance).) 2520 */ 2521 public PlanBenefitCostComponent setValue(Quantity value) { 2522 this.value = value; 2523 return this; 2524 } 2525 2526 protected void listChildren(List<Property> children) { 2527 super.listChildren(children); 2528 children.add(new Property("type", "CodeableConcept", "Type of cost (copay; individual cap; family cap; coinsurance; deductible).", 0, 1, type)); 2529 children.add(new Property("applicability", "CodeableConcept", "Whether the cost applies to in-network or out-of-network providers (in-network; out-of-network; other).", 0, 1, applicability)); 2530 children.add(new Property("qualifiers", "CodeableConcept", "Additional information about the cost, such as information about funding sources (e.g. HSA, HRA, FSA, RRA).", 0, java.lang.Integer.MAX_VALUE, qualifiers)); 2531 children.add(new Property("value", "Quantity", "The actual cost value. (some of the costs may be represented as percentages rather than currency, e.g. 10% coinsurance).", 0, 1, value)); 2532 } 2533 2534 @Override 2535 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2536 switch (_hash) { 2537 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of cost (copay; individual cap; family cap; coinsurance; deductible).", 0, 1, type); 2538 case -1526770491: /*applicability*/ return new Property("applicability", "CodeableConcept", "Whether the cost applies to in-network or out-of-network providers (in-network; out-of-network; other).", 0, 1, applicability); 2539 case -31447799: /*qualifiers*/ return new Property("qualifiers", "CodeableConcept", "Additional information about the cost, such as information about funding sources (e.g. HSA, HRA, FSA, RRA).", 0, java.lang.Integer.MAX_VALUE, qualifiers); 2540 case 111972721: /*value*/ return new Property("value", "Quantity", "The actual cost value. (some of the costs may be represented as percentages rather than currency, e.g. 10% coinsurance).", 0, 1, value); 2541 default: return super.getNamedProperty(_hash, _name, _checkValid); 2542 } 2543 2544 } 2545 2546 @Override 2547 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2548 switch (hash) { 2549 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2550 case -1526770491: /*applicability*/ return this.applicability == null ? new Base[0] : new Base[] {this.applicability}; // CodeableConcept 2551 case -31447799: /*qualifiers*/ return this.qualifiers == null ? new Base[0] : this.qualifiers.toArray(new Base[this.qualifiers.size()]); // CodeableConcept 2552 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Quantity 2553 default: return super.getProperty(hash, name, checkValid); 2554 } 2555 2556 } 2557 2558 @Override 2559 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2560 switch (hash) { 2561 case 3575610: // type 2562 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2563 return value; 2564 case -1526770491: // applicability 2565 this.applicability = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2566 return value; 2567 case -31447799: // qualifiers 2568 this.getQualifiers().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2569 return value; 2570 case 111972721: // value 2571 this.value = TypeConvertor.castToQuantity(value); // Quantity 2572 return value; 2573 default: return super.setProperty(hash, name, value); 2574 } 2575 2576 } 2577 2578 @Override 2579 public Base setProperty(String name, Base value) throws FHIRException { 2580 if (name.equals("type")) { 2581 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2582 } else if (name.equals("applicability")) { 2583 this.applicability = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2584 } else if (name.equals("qualifiers")) { 2585 this.getQualifiers().add(TypeConvertor.castToCodeableConcept(value)); 2586 } else if (name.equals("value")) { 2587 this.value = TypeConvertor.castToQuantity(value); // Quantity 2588 } else 2589 return super.setProperty(name, value); 2590 return value; 2591 } 2592 2593 @Override 2594 public void removeChild(String name, Base value) throws FHIRException { 2595 if (name.equals("type")) { 2596 this.type = null; 2597 } else if (name.equals("applicability")) { 2598 this.applicability = null; 2599 } else if (name.equals("qualifiers")) { 2600 this.getQualifiers().remove(value); 2601 } else if (name.equals("value")) { 2602 this.value = null; 2603 } else 2604 super.removeChild(name, value); 2605 2606 } 2607 2608 @Override 2609 public Base makeProperty(int hash, String name) throws FHIRException { 2610 switch (hash) { 2611 case 3575610: return getType(); 2612 case -1526770491: return getApplicability(); 2613 case -31447799: return addQualifiers(); 2614 case 111972721: return getValue(); 2615 default: return super.makeProperty(hash, name); 2616 } 2617 2618 } 2619 2620 @Override 2621 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2622 switch (hash) { 2623 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2624 case -1526770491: /*applicability*/ return new String[] {"CodeableConcept"}; 2625 case -31447799: /*qualifiers*/ return new String[] {"CodeableConcept"}; 2626 case 111972721: /*value*/ return new String[] {"Quantity"}; 2627 default: return super.getTypesForProperty(hash, name); 2628 } 2629 2630 } 2631 2632 @Override 2633 public Base addChild(String name) throws FHIRException { 2634 if (name.equals("type")) { 2635 this.type = new CodeableConcept(); 2636 return this.type; 2637 } 2638 else if (name.equals("applicability")) { 2639 this.applicability = new CodeableConcept(); 2640 return this.applicability; 2641 } 2642 else if (name.equals("qualifiers")) { 2643 return addQualifiers(); 2644 } 2645 else if (name.equals("value")) { 2646 this.value = new Quantity(); 2647 return this.value; 2648 } 2649 else 2650 return super.addChild(name); 2651 } 2652 2653 public PlanBenefitCostComponent copy() { 2654 PlanBenefitCostComponent dst = new PlanBenefitCostComponent(); 2655 copyValues(dst); 2656 return dst; 2657 } 2658 2659 public void copyValues(PlanBenefitCostComponent dst) { 2660 super.copyValues(dst); 2661 dst.type = type == null ? null : type.copy(); 2662 dst.applicability = applicability == null ? null : applicability.copy(); 2663 if (qualifiers != null) { 2664 dst.qualifiers = new ArrayList<CodeableConcept>(); 2665 for (CodeableConcept i : qualifiers) 2666 dst.qualifiers.add(i.copy()); 2667 }; 2668 dst.value = value == null ? null : value.copy(); 2669 } 2670 2671 @Override 2672 public boolean equalsDeep(Base other_) { 2673 if (!super.equalsDeep(other_)) 2674 return false; 2675 if (!(other_ instanceof PlanBenefitCostComponent)) 2676 return false; 2677 PlanBenefitCostComponent o = (PlanBenefitCostComponent) other_; 2678 return compareDeep(type, o.type, true) && compareDeep(applicability, o.applicability, true) && compareDeep(qualifiers, o.qualifiers, true) 2679 && compareDeep(value, o.value, true); 2680 } 2681 2682 @Override 2683 public boolean equalsShallow(Base other_) { 2684 if (!super.equalsShallow(other_)) 2685 return false; 2686 if (!(other_ instanceof PlanBenefitCostComponent)) 2687 return false; 2688 PlanBenefitCostComponent o = (PlanBenefitCostComponent) other_; 2689 return true; 2690 } 2691 2692 public boolean isEmpty() { 2693 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, applicability, qualifiers 2694 , value); 2695 } 2696 2697 public String fhirType() { 2698 return "InsurancePlan.plan.specificCost.benefit.cost"; 2699 2700 } 2701 2702 } 2703 2704 /** 2705 * Business identifiers assigned to this health insurance product which remain constant as the resource is updated and propagates from server to server. 2706 */ 2707 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2708 @Description(shortDefinition="Business Identifier for Product", formalDefinition="Business identifiers assigned to this health insurance product which remain constant as the resource is updated and propagates from server to server." ) 2709 protected List<Identifier> identifier; 2710 2711 /** 2712 * The current state of the health insurance product. 2713 */ 2714 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 2715 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The current state of the health insurance product." ) 2716 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 2717 protected Enumeration<PublicationStatus> status; 2718 2719 /** 2720 * The kind of health insurance product. 2721 */ 2722 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2723 @Description(shortDefinition="Kind of product", formalDefinition="The kind of health insurance product." ) 2724 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/insuranceplan-type") 2725 protected List<CodeableConcept> type; 2726 2727 /** 2728 * Official name of the health insurance product as designated by the owner. 2729 */ 2730 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 2731 @Description(shortDefinition="Official name", formalDefinition="Official name of the health insurance product as designated by the owner." ) 2732 protected StringType name; 2733 2734 /** 2735 * A list of alternate names that the product is known as, or was known as in the past. 2736 */ 2737 @Child(name = "alias", type = {StringType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2738 @Description(shortDefinition="Alternate names", formalDefinition="A list of alternate names that the product is known as, or was known as in the past." ) 2739 protected List<StringType> alias; 2740 2741 /** 2742 * The period of time that the health insurance product is available. 2743 */ 2744 @Child(name = "period", type = {Period.class}, order=5, min=0, max=1, modifier=false, summary=false) 2745 @Description(shortDefinition="When the product is available", formalDefinition="The period of time that the health insurance product is available." ) 2746 protected Period period; 2747 2748 /** 2749 * The entity that is providing the health insurance product and underwriting the risk. This is typically an insurance carriers, other third-party payers, or health plan sponsors comonly referred to as 'payers'. 2750 */ 2751 @Child(name = "ownedBy", type = {Organization.class}, order=6, min=0, max=1, modifier=false, summary=true) 2752 @Description(shortDefinition="Product issuer", formalDefinition="The entity that is providing the health insurance product and underwriting the risk. This is typically an insurance carriers, other third-party payers, or health plan sponsors comonly referred to as 'payers'." ) 2753 protected Reference ownedBy; 2754 2755 /** 2756 * An organization which administer other services such as underwriting, customer service and/or claims processing on behalf of the health insurance product owner. 2757 */ 2758 @Child(name = "administeredBy", type = {Organization.class}, order=7, min=0, max=1, modifier=false, summary=true) 2759 @Description(shortDefinition="Product administrator", formalDefinition="An organization which administer other services such as underwriting, customer service and/or claims processing on behalf of the health insurance product owner." ) 2760 protected Reference administeredBy; 2761 2762 /** 2763 * The geographic region in which a health insurance product's benefits apply. 2764 */ 2765 @Child(name = "coverageArea", type = {Location.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2766 @Description(shortDefinition="Where product applies", formalDefinition="The geographic region in which a health insurance product's benefits apply." ) 2767 protected List<Reference> coverageArea; 2768 2769 /** 2770 * The contact details of communication devices available relevant to the specific Insurance Plan/Product. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites. 2771 */ 2772 @Child(name = "contact", type = {ExtendedContactDetail.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2773 @Description(shortDefinition="Official contact details relevant to the health insurance plan/product", formalDefinition="The contact details of communication devices available relevant to the specific Insurance Plan/Product. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites." ) 2774 protected List<ExtendedContactDetail> contact; 2775 2776 /** 2777 * The technical endpoints providing access to services operated for the health insurance product. 2778 */ 2779 @Child(name = "endpoint", type = {Endpoint.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2780 @Description(shortDefinition="Technical endpoint", formalDefinition="The technical endpoints providing access to services operated for the health insurance product." ) 2781 protected List<Reference> endpoint; 2782 2783 /** 2784 * Reference to the network included in the health insurance product. 2785 */ 2786 @Child(name = "network", type = {Organization.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2787 @Description(shortDefinition="What networks are Included", formalDefinition="Reference to the network included in the health insurance product." ) 2788 protected List<Reference> network; 2789 2790 /** 2791 * Details about the coverage offered by the insurance product. 2792 */ 2793 @Child(name = "coverage", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2794 @Description(shortDefinition="Coverage details", formalDefinition="Details about the coverage offered by the insurance product." ) 2795 protected List<InsurancePlanCoverageComponent> coverage; 2796 2797 /** 2798 * Details about an insurance plan. 2799 */ 2800 @Child(name = "plan", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2801 @Description(shortDefinition="Plan details", formalDefinition="Details about an insurance plan." ) 2802 protected List<InsurancePlanPlanComponent> plan; 2803 2804 private static final long serialVersionUID = -292692522L; 2805 2806 /** 2807 * Constructor 2808 */ 2809 public InsurancePlan() { 2810 super(); 2811 } 2812 2813 /** 2814 * @return {@link #identifier} (Business identifiers assigned to this health insurance product which remain constant as the resource is updated and propagates from server to server.) 2815 */ 2816 public List<Identifier> getIdentifier() { 2817 if (this.identifier == null) 2818 this.identifier = new ArrayList<Identifier>(); 2819 return this.identifier; 2820 } 2821 2822 /** 2823 * @return Returns a reference to <code>this</code> for easy method chaining 2824 */ 2825 public InsurancePlan setIdentifier(List<Identifier> theIdentifier) { 2826 this.identifier = theIdentifier; 2827 return this; 2828 } 2829 2830 public boolean hasIdentifier() { 2831 if (this.identifier == null) 2832 return false; 2833 for (Identifier item : this.identifier) 2834 if (!item.isEmpty()) 2835 return true; 2836 return false; 2837 } 2838 2839 public Identifier addIdentifier() { //3 2840 Identifier t = new Identifier(); 2841 if (this.identifier == null) 2842 this.identifier = new ArrayList<Identifier>(); 2843 this.identifier.add(t); 2844 return t; 2845 } 2846 2847 public InsurancePlan addIdentifier(Identifier t) { //3 2848 if (t == null) 2849 return this; 2850 if (this.identifier == null) 2851 this.identifier = new ArrayList<Identifier>(); 2852 this.identifier.add(t); 2853 return this; 2854 } 2855 2856 /** 2857 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2858 */ 2859 public Identifier getIdentifierFirstRep() { 2860 if (getIdentifier().isEmpty()) { 2861 addIdentifier(); 2862 } 2863 return getIdentifier().get(0); 2864 } 2865 2866 /** 2867 * @return {@link #status} (The current state of the health insurance product.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2868 */ 2869 public Enumeration<PublicationStatus> getStatusElement() { 2870 if (this.status == null) 2871 if (Configuration.errorOnAutoCreate()) 2872 throw new Error("Attempt to auto-create InsurancePlan.status"); 2873 else if (Configuration.doAutoCreate()) 2874 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2875 return this.status; 2876 } 2877 2878 public boolean hasStatusElement() { 2879 return this.status != null && !this.status.isEmpty(); 2880 } 2881 2882 public boolean hasStatus() { 2883 return this.status != null && !this.status.isEmpty(); 2884 } 2885 2886 /** 2887 * @param value {@link #status} (The current state of the health insurance product.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2888 */ 2889 public InsurancePlan setStatusElement(Enumeration<PublicationStatus> value) { 2890 this.status = value; 2891 return this; 2892 } 2893 2894 /** 2895 * @return The current state of the health insurance product. 2896 */ 2897 public PublicationStatus getStatus() { 2898 return this.status == null ? null : this.status.getValue(); 2899 } 2900 2901 /** 2902 * @param value The current state of the health insurance product. 2903 */ 2904 public InsurancePlan setStatus(PublicationStatus value) { 2905 if (value == null) 2906 this.status = null; 2907 else { 2908 if (this.status == null) 2909 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2910 this.status.setValue(value); 2911 } 2912 return this; 2913 } 2914 2915 /** 2916 * @return {@link #type} (The kind of health insurance product.) 2917 */ 2918 public List<CodeableConcept> getType() { 2919 if (this.type == null) 2920 this.type = new ArrayList<CodeableConcept>(); 2921 return this.type; 2922 } 2923 2924 /** 2925 * @return Returns a reference to <code>this</code> for easy method chaining 2926 */ 2927 public InsurancePlan setType(List<CodeableConcept> theType) { 2928 this.type = theType; 2929 return this; 2930 } 2931 2932 public boolean hasType() { 2933 if (this.type == null) 2934 return false; 2935 for (CodeableConcept item : this.type) 2936 if (!item.isEmpty()) 2937 return true; 2938 return false; 2939 } 2940 2941 public CodeableConcept addType() { //3 2942 CodeableConcept t = new CodeableConcept(); 2943 if (this.type == null) 2944 this.type = new ArrayList<CodeableConcept>(); 2945 this.type.add(t); 2946 return t; 2947 } 2948 2949 public InsurancePlan addType(CodeableConcept t) { //3 2950 if (t == null) 2951 return this; 2952 if (this.type == null) 2953 this.type = new ArrayList<CodeableConcept>(); 2954 this.type.add(t); 2955 return this; 2956 } 2957 2958 /** 2959 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 2960 */ 2961 public CodeableConcept getTypeFirstRep() { 2962 if (getType().isEmpty()) { 2963 addType(); 2964 } 2965 return getType().get(0); 2966 } 2967 2968 /** 2969 * @return {@link #name} (Official name of the health insurance product as designated by the owner.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2970 */ 2971 public StringType getNameElement() { 2972 if (this.name == null) 2973 if (Configuration.errorOnAutoCreate()) 2974 throw new Error("Attempt to auto-create InsurancePlan.name"); 2975 else if (Configuration.doAutoCreate()) 2976 this.name = new StringType(); // bb 2977 return this.name; 2978 } 2979 2980 public boolean hasNameElement() { 2981 return this.name != null && !this.name.isEmpty(); 2982 } 2983 2984 public boolean hasName() { 2985 return this.name != null && !this.name.isEmpty(); 2986 } 2987 2988 /** 2989 * @param value {@link #name} (Official name of the health insurance product as designated by the owner.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2990 */ 2991 public InsurancePlan setNameElement(StringType value) { 2992 this.name = value; 2993 return this; 2994 } 2995 2996 /** 2997 * @return Official name of the health insurance product as designated by the owner. 2998 */ 2999 public String getName() { 3000 return this.name == null ? null : this.name.getValue(); 3001 } 3002 3003 /** 3004 * @param value Official name of the health insurance product as designated by the owner. 3005 */ 3006 public InsurancePlan setName(String value) { 3007 if (Utilities.noString(value)) 3008 this.name = null; 3009 else { 3010 if (this.name == null) 3011 this.name = new StringType(); 3012 this.name.setValue(value); 3013 } 3014 return this; 3015 } 3016 3017 /** 3018 * @return {@link #alias} (A list of alternate names that the product is known as, or was known as in the past.) 3019 */ 3020 public List<StringType> getAlias() { 3021 if (this.alias == null) 3022 this.alias = new ArrayList<StringType>(); 3023 return this.alias; 3024 } 3025 3026 /** 3027 * @return Returns a reference to <code>this</code> for easy method chaining 3028 */ 3029 public InsurancePlan setAlias(List<StringType> theAlias) { 3030 this.alias = theAlias; 3031 return this; 3032 } 3033 3034 public boolean hasAlias() { 3035 if (this.alias == null) 3036 return false; 3037 for (StringType item : this.alias) 3038 if (!item.isEmpty()) 3039 return true; 3040 return false; 3041 } 3042 3043 /** 3044 * @return {@link #alias} (A list of alternate names that the product is known as, or was known as in the past.) 3045 */ 3046 public StringType addAliasElement() {//2 3047 StringType t = new StringType(); 3048 if (this.alias == null) 3049 this.alias = new ArrayList<StringType>(); 3050 this.alias.add(t); 3051 return t; 3052 } 3053 3054 /** 3055 * @param value {@link #alias} (A list of alternate names that the product is known as, or was known as in the past.) 3056 */ 3057 public InsurancePlan addAlias(String value) { //1 3058 StringType t = new StringType(); 3059 t.setValue(value); 3060 if (this.alias == null) 3061 this.alias = new ArrayList<StringType>(); 3062 this.alias.add(t); 3063 return this; 3064 } 3065 3066 /** 3067 * @param value {@link #alias} (A list of alternate names that the product is known as, or was known as in the past.) 3068 */ 3069 public boolean hasAlias(String value) { 3070 if (this.alias == null) 3071 return false; 3072 for (StringType v : this.alias) 3073 if (v.getValue().equals(value)) // string 3074 return true; 3075 return false; 3076 } 3077 3078 /** 3079 * @return {@link #period} (The period of time that the health insurance product is available.) 3080 */ 3081 public Period getPeriod() { 3082 if (this.period == null) 3083 if (Configuration.errorOnAutoCreate()) 3084 throw new Error("Attempt to auto-create InsurancePlan.period"); 3085 else if (Configuration.doAutoCreate()) 3086 this.period = new Period(); // cc 3087 return this.period; 3088 } 3089 3090 public boolean hasPeriod() { 3091 return this.period != null && !this.period.isEmpty(); 3092 } 3093 3094 /** 3095 * @param value {@link #period} (The period of time that the health insurance product is available.) 3096 */ 3097 public InsurancePlan setPeriod(Period value) { 3098 this.period = value; 3099 return this; 3100 } 3101 3102 /** 3103 * @return {@link #ownedBy} (The entity that is providing the health insurance product and underwriting the risk. This is typically an insurance carriers, other third-party payers, or health plan sponsors comonly referred to as 'payers'.) 3104 */ 3105 public Reference getOwnedBy() { 3106 if (this.ownedBy == null) 3107 if (Configuration.errorOnAutoCreate()) 3108 throw new Error("Attempt to auto-create InsurancePlan.ownedBy"); 3109 else if (Configuration.doAutoCreate()) 3110 this.ownedBy = new Reference(); // cc 3111 return this.ownedBy; 3112 } 3113 3114 public boolean hasOwnedBy() { 3115 return this.ownedBy != null && !this.ownedBy.isEmpty(); 3116 } 3117 3118 /** 3119 * @param value {@link #ownedBy} (The entity that is providing the health insurance product and underwriting the risk. This is typically an insurance carriers, other third-party payers, or health plan sponsors comonly referred to as 'payers'.) 3120 */ 3121 public InsurancePlan setOwnedBy(Reference value) { 3122 this.ownedBy = value; 3123 return this; 3124 } 3125 3126 /** 3127 * @return {@link #administeredBy} (An organization which administer other services such as underwriting, customer service and/or claims processing on behalf of the health insurance product owner.) 3128 */ 3129 public Reference getAdministeredBy() { 3130 if (this.administeredBy == null) 3131 if (Configuration.errorOnAutoCreate()) 3132 throw new Error("Attempt to auto-create InsurancePlan.administeredBy"); 3133 else if (Configuration.doAutoCreate()) 3134 this.administeredBy = new Reference(); // cc 3135 return this.administeredBy; 3136 } 3137 3138 public boolean hasAdministeredBy() { 3139 return this.administeredBy != null && !this.administeredBy.isEmpty(); 3140 } 3141 3142 /** 3143 * @param value {@link #administeredBy} (An organization which administer other services such as underwriting, customer service and/or claims processing on behalf of the health insurance product owner.) 3144 */ 3145 public InsurancePlan setAdministeredBy(Reference value) { 3146 this.administeredBy = value; 3147 return this; 3148 } 3149 3150 /** 3151 * @return {@link #coverageArea} (The geographic region in which a health insurance product's benefits apply.) 3152 */ 3153 public List<Reference> getCoverageArea() { 3154 if (this.coverageArea == null) 3155 this.coverageArea = new ArrayList<Reference>(); 3156 return this.coverageArea; 3157 } 3158 3159 /** 3160 * @return Returns a reference to <code>this</code> for easy method chaining 3161 */ 3162 public InsurancePlan setCoverageArea(List<Reference> theCoverageArea) { 3163 this.coverageArea = theCoverageArea; 3164 return this; 3165 } 3166 3167 public boolean hasCoverageArea() { 3168 if (this.coverageArea == null) 3169 return false; 3170 for (Reference item : this.coverageArea) 3171 if (!item.isEmpty()) 3172 return true; 3173 return false; 3174 } 3175 3176 public Reference addCoverageArea() { //3 3177 Reference t = new Reference(); 3178 if (this.coverageArea == null) 3179 this.coverageArea = new ArrayList<Reference>(); 3180 this.coverageArea.add(t); 3181 return t; 3182 } 3183 3184 public InsurancePlan addCoverageArea(Reference t) { //3 3185 if (t == null) 3186 return this; 3187 if (this.coverageArea == null) 3188 this.coverageArea = new ArrayList<Reference>(); 3189 this.coverageArea.add(t); 3190 return this; 3191 } 3192 3193 /** 3194 * @return The first repetition of repeating field {@link #coverageArea}, creating it if it does not already exist {3} 3195 */ 3196 public Reference getCoverageAreaFirstRep() { 3197 if (getCoverageArea().isEmpty()) { 3198 addCoverageArea(); 3199 } 3200 return getCoverageArea().get(0); 3201 } 3202 3203 /** 3204 * @return {@link #contact} (The contact details of communication devices available relevant to the specific Insurance Plan/Product. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites.) 3205 */ 3206 public List<ExtendedContactDetail> getContact() { 3207 if (this.contact == null) 3208 this.contact = new ArrayList<ExtendedContactDetail>(); 3209 return this.contact; 3210 } 3211 3212 /** 3213 * @return Returns a reference to <code>this</code> for easy method chaining 3214 */ 3215 public InsurancePlan setContact(List<ExtendedContactDetail> theContact) { 3216 this.contact = theContact; 3217 return this; 3218 } 3219 3220 public boolean hasContact() { 3221 if (this.contact == null) 3222 return false; 3223 for (ExtendedContactDetail item : this.contact) 3224 if (!item.isEmpty()) 3225 return true; 3226 return false; 3227 } 3228 3229 public ExtendedContactDetail addContact() { //3 3230 ExtendedContactDetail t = new ExtendedContactDetail(); 3231 if (this.contact == null) 3232 this.contact = new ArrayList<ExtendedContactDetail>(); 3233 this.contact.add(t); 3234 return t; 3235 } 3236 3237 public InsurancePlan addContact(ExtendedContactDetail t) { //3 3238 if (t == null) 3239 return this; 3240 if (this.contact == null) 3241 this.contact = new ArrayList<ExtendedContactDetail>(); 3242 this.contact.add(t); 3243 return this; 3244 } 3245 3246 /** 3247 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 3248 */ 3249 public ExtendedContactDetail getContactFirstRep() { 3250 if (getContact().isEmpty()) { 3251 addContact(); 3252 } 3253 return getContact().get(0); 3254 } 3255 3256 /** 3257 * @return {@link #endpoint} (The technical endpoints providing access to services operated for the health insurance product.) 3258 */ 3259 public List<Reference> getEndpoint() { 3260 if (this.endpoint == null) 3261 this.endpoint = new ArrayList<Reference>(); 3262 return this.endpoint; 3263 } 3264 3265 /** 3266 * @return Returns a reference to <code>this</code> for easy method chaining 3267 */ 3268 public InsurancePlan setEndpoint(List<Reference> theEndpoint) { 3269 this.endpoint = theEndpoint; 3270 return this; 3271 } 3272 3273 public boolean hasEndpoint() { 3274 if (this.endpoint == null) 3275 return false; 3276 for (Reference item : this.endpoint) 3277 if (!item.isEmpty()) 3278 return true; 3279 return false; 3280 } 3281 3282 public Reference addEndpoint() { //3 3283 Reference t = new Reference(); 3284 if (this.endpoint == null) 3285 this.endpoint = new ArrayList<Reference>(); 3286 this.endpoint.add(t); 3287 return t; 3288 } 3289 3290 public InsurancePlan addEndpoint(Reference t) { //3 3291 if (t == null) 3292 return this; 3293 if (this.endpoint == null) 3294 this.endpoint = new ArrayList<Reference>(); 3295 this.endpoint.add(t); 3296 return this; 3297 } 3298 3299 /** 3300 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist {3} 3301 */ 3302 public Reference getEndpointFirstRep() { 3303 if (getEndpoint().isEmpty()) { 3304 addEndpoint(); 3305 } 3306 return getEndpoint().get(0); 3307 } 3308 3309 /** 3310 * @return {@link #network} (Reference to the network included in the health insurance product.) 3311 */ 3312 public List<Reference> getNetwork() { 3313 if (this.network == null) 3314 this.network = new ArrayList<Reference>(); 3315 return this.network; 3316 } 3317 3318 /** 3319 * @return Returns a reference to <code>this</code> for easy method chaining 3320 */ 3321 public InsurancePlan setNetwork(List<Reference> theNetwork) { 3322 this.network = theNetwork; 3323 return this; 3324 } 3325 3326 public boolean hasNetwork() { 3327 if (this.network == null) 3328 return false; 3329 for (Reference item : this.network) 3330 if (!item.isEmpty()) 3331 return true; 3332 return false; 3333 } 3334 3335 public Reference addNetwork() { //3 3336 Reference t = new Reference(); 3337 if (this.network == null) 3338 this.network = new ArrayList<Reference>(); 3339 this.network.add(t); 3340 return t; 3341 } 3342 3343 public InsurancePlan addNetwork(Reference t) { //3 3344 if (t == null) 3345 return this; 3346 if (this.network == null) 3347 this.network = new ArrayList<Reference>(); 3348 this.network.add(t); 3349 return this; 3350 } 3351 3352 /** 3353 * @return The first repetition of repeating field {@link #network}, creating it if it does not already exist {3} 3354 */ 3355 public Reference getNetworkFirstRep() { 3356 if (getNetwork().isEmpty()) { 3357 addNetwork(); 3358 } 3359 return getNetwork().get(0); 3360 } 3361 3362 /** 3363 * @return {@link #coverage} (Details about the coverage offered by the insurance product.) 3364 */ 3365 public List<InsurancePlanCoverageComponent> getCoverage() { 3366 if (this.coverage == null) 3367 this.coverage = new ArrayList<InsurancePlanCoverageComponent>(); 3368 return this.coverage; 3369 } 3370 3371 /** 3372 * @return Returns a reference to <code>this</code> for easy method chaining 3373 */ 3374 public InsurancePlan setCoverage(List<InsurancePlanCoverageComponent> theCoverage) { 3375 this.coverage = theCoverage; 3376 return this; 3377 } 3378 3379 public boolean hasCoverage() { 3380 if (this.coverage == null) 3381 return false; 3382 for (InsurancePlanCoverageComponent item : this.coverage) 3383 if (!item.isEmpty()) 3384 return true; 3385 return false; 3386 } 3387 3388 public InsurancePlanCoverageComponent addCoverage() { //3 3389 InsurancePlanCoverageComponent t = new InsurancePlanCoverageComponent(); 3390 if (this.coverage == null) 3391 this.coverage = new ArrayList<InsurancePlanCoverageComponent>(); 3392 this.coverage.add(t); 3393 return t; 3394 } 3395 3396 public InsurancePlan addCoverage(InsurancePlanCoverageComponent t) { //3 3397 if (t == null) 3398 return this; 3399 if (this.coverage == null) 3400 this.coverage = new ArrayList<InsurancePlanCoverageComponent>(); 3401 this.coverage.add(t); 3402 return this; 3403 } 3404 3405 /** 3406 * @return The first repetition of repeating field {@link #coverage}, creating it if it does not already exist {3} 3407 */ 3408 public InsurancePlanCoverageComponent getCoverageFirstRep() { 3409 if (getCoverage().isEmpty()) { 3410 addCoverage(); 3411 } 3412 return getCoverage().get(0); 3413 } 3414 3415 /** 3416 * @return {@link #plan} (Details about an insurance plan.) 3417 */ 3418 public List<InsurancePlanPlanComponent> getPlan() { 3419 if (this.plan == null) 3420 this.plan = new ArrayList<InsurancePlanPlanComponent>(); 3421 return this.plan; 3422 } 3423 3424 /** 3425 * @return Returns a reference to <code>this</code> for easy method chaining 3426 */ 3427 public InsurancePlan setPlan(List<InsurancePlanPlanComponent> thePlan) { 3428 this.plan = thePlan; 3429 return this; 3430 } 3431 3432 public boolean hasPlan() { 3433 if (this.plan == null) 3434 return false; 3435 for (InsurancePlanPlanComponent item : this.plan) 3436 if (!item.isEmpty()) 3437 return true; 3438 return false; 3439 } 3440 3441 public InsurancePlanPlanComponent addPlan() { //3 3442 InsurancePlanPlanComponent t = new InsurancePlanPlanComponent(); 3443 if (this.plan == null) 3444 this.plan = new ArrayList<InsurancePlanPlanComponent>(); 3445 this.plan.add(t); 3446 return t; 3447 } 3448 3449 public InsurancePlan addPlan(InsurancePlanPlanComponent t) { //3 3450 if (t == null) 3451 return this; 3452 if (this.plan == null) 3453 this.plan = new ArrayList<InsurancePlanPlanComponent>(); 3454 this.plan.add(t); 3455 return this; 3456 } 3457 3458 /** 3459 * @return The first repetition of repeating field {@link #plan}, creating it if it does not already exist {3} 3460 */ 3461 public InsurancePlanPlanComponent getPlanFirstRep() { 3462 if (getPlan().isEmpty()) { 3463 addPlan(); 3464 } 3465 return getPlan().get(0); 3466 } 3467 3468 protected void listChildren(List<Property> children) { 3469 super.listChildren(children); 3470 children.add(new Property("identifier", "Identifier", "Business identifiers assigned to this health insurance product which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3471 children.add(new Property("status", "code", "The current state of the health insurance product.", 0, 1, status)); 3472 children.add(new Property("type", "CodeableConcept", "The kind of health insurance product.", 0, java.lang.Integer.MAX_VALUE, type)); 3473 children.add(new Property("name", "string", "Official name of the health insurance product as designated by the owner.", 0, 1, name)); 3474 children.add(new Property("alias", "string", "A list of alternate names that the product is known as, or was known as in the past.", 0, java.lang.Integer.MAX_VALUE, alias)); 3475 children.add(new Property("period", "Period", "The period of time that the health insurance product is available.", 0, 1, period)); 3476 children.add(new Property("ownedBy", "Reference(Organization)", "The entity that is providing the health insurance product and underwriting the risk. This is typically an insurance carriers, other third-party payers, or health plan sponsors comonly referred to as 'payers'.", 0, 1, ownedBy)); 3477 children.add(new Property("administeredBy", "Reference(Organization)", "An organization which administer other services such as underwriting, customer service and/or claims processing on behalf of the health insurance product owner.", 0, 1, administeredBy)); 3478 children.add(new Property("coverageArea", "Reference(Location)", "The geographic region in which a health insurance product's benefits apply.", 0, java.lang.Integer.MAX_VALUE, coverageArea)); 3479 children.add(new Property("contact", "ExtendedContactDetail", "The contact details of communication devices available relevant to the specific Insurance Plan/Product. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 0, java.lang.Integer.MAX_VALUE, contact)); 3480 children.add(new Property("endpoint", "Reference(Endpoint)", "The technical endpoints providing access to services operated for the health insurance product.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 3481 children.add(new Property("network", "Reference(Organization)", "Reference to the network included in the health insurance product.", 0, java.lang.Integer.MAX_VALUE, network)); 3482 children.add(new Property("coverage", "", "Details about the coverage offered by the insurance product.", 0, java.lang.Integer.MAX_VALUE, coverage)); 3483 children.add(new Property("plan", "", "Details about an insurance plan.", 0, java.lang.Integer.MAX_VALUE, plan)); 3484 } 3485 3486 @Override 3487 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3488 switch (_hash) { 3489 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifiers assigned to this health insurance product which remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 3490 case -892481550: /*status*/ return new Property("status", "code", "The current state of the health insurance product.", 0, 1, status); 3491 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The kind of health insurance product.", 0, java.lang.Integer.MAX_VALUE, type); 3492 case 3373707: /*name*/ return new Property("name", "string", "Official name of the health insurance product as designated by the owner.", 0, 1, name); 3493 case 92902992: /*alias*/ return new Property("alias", "string", "A list of alternate names that the product is known as, or was known as in the past.", 0, java.lang.Integer.MAX_VALUE, alias); 3494 case -991726143: /*period*/ return new Property("period", "Period", "The period of time that the health insurance product is available.", 0, 1, period); 3495 case -1054743076: /*ownedBy*/ return new Property("ownedBy", "Reference(Organization)", "The entity that is providing the health insurance product and underwriting the risk. This is typically an insurance carriers, other third-party payers, or health plan sponsors comonly referred to as 'payers'.", 0, 1, ownedBy); 3496 case 898770462: /*administeredBy*/ return new Property("administeredBy", "Reference(Organization)", "An organization which administer other services such as underwriting, customer service and/or claims processing on behalf of the health insurance product owner.", 0, 1, administeredBy); 3497 case -1532328299: /*coverageArea*/ return new Property("coverageArea", "Reference(Location)", "The geographic region in which a health insurance product's benefits apply.", 0, java.lang.Integer.MAX_VALUE, coverageArea); 3498 case 951526432: /*contact*/ return new Property("contact", "ExtendedContactDetail", "The contact details of communication devices available relevant to the specific Insurance Plan/Product. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 0, java.lang.Integer.MAX_VALUE, contact); 3499 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "The technical endpoints providing access to services operated for the health insurance product.", 0, java.lang.Integer.MAX_VALUE, endpoint); 3500 case 1843485230: /*network*/ return new Property("network", "Reference(Organization)", "Reference to the network included in the health insurance product.", 0, java.lang.Integer.MAX_VALUE, network); 3501 case -351767064: /*coverage*/ return new Property("coverage", "", "Details about the coverage offered by the insurance product.", 0, java.lang.Integer.MAX_VALUE, coverage); 3502 case 3443497: /*plan*/ return new Property("plan", "", "Details about an insurance plan.", 0, java.lang.Integer.MAX_VALUE, plan); 3503 default: return super.getNamedProperty(_hash, _name, _checkValid); 3504 } 3505 3506 } 3507 3508 @Override 3509 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3510 switch (hash) { 3511 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3512 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 3513 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 3514 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3515 case 92902992: /*alias*/ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 3516 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 3517 case -1054743076: /*ownedBy*/ return this.ownedBy == null ? new Base[0] : new Base[] {this.ownedBy}; // Reference 3518 case 898770462: /*administeredBy*/ return this.administeredBy == null ? new Base[0] : new Base[] {this.administeredBy}; // Reference 3519 case -1532328299: /*coverageArea*/ return this.coverageArea == null ? new Base[0] : this.coverageArea.toArray(new Base[this.coverageArea.size()]); // Reference 3520 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ExtendedContactDetail 3521 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 3522 case 1843485230: /*network*/ return this.network == null ? new Base[0] : this.network.toArray(new Base[this.network.size()]); // Reference 3523 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : this.coverage.toArray(new Base[this.coverage.size()]); // InsurancePlanCoverageComponent 3524 case 3443497: /*plan*/ return this.plan == null ? new Base[0] : this.plan.toArray(new Base[this.plan.size()]); // InsurancePlanPlanComponent 3525 default: return super.getProperty(hash, name, checkValid); 3526 } 3527 3528 } 3529 3530 @Override 3531 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3532 switch (hash) { 3533 case -1618432855: // identifier 3534 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 3535 return value; 3536 case -892481550: // status 3537 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3538 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3539 return value; 3540 case 3575610: // type 3541 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3542 return value; 3543 case 3373707: // name 3544 this.name = TypeConvertor.castToString(value); // StringType 3545 return value; 3546 case 92902992: // alias 3547 this.getAlias().add(TypeConvertor.castToString(value)); // StringType 3548 return value; 3549 case -991726143: // period 3550 this.period = TypeConvertor.castToPeriod(value); // Period 3551 return value; 3552 case -1054743076: // ownedBy 3553 this.ownedBy = TypeConvertor.castToReference(value); // Reference 3554 return value; 3555 case 898770462: // administeredBy 3556 this.administeredBy = TypeConvertor.castToReference(value); // Reference 3557 return value; 3558 case -1532328299: // coverageArea 3559 this.getCoverageArea().add(TypeConvertor.castToReference(value)); // Reference 3560 return value; 3561 case 951526432: // contact 3562 this.getContact().add(TypeConvertor.castToExtendedContactDetail(value)); // ExtendedContactDetail 3563 return value; 3564 case 1741102485: // endpoint 3565 this.getEndpoint().add(TypeConvertor.castToReference(value)); // Reference 3566 return value; 3567 case 1843485230: // network 3568 this.getNetwork().add(TypeConvertor.castToReference(value)); // Reference 3569 return value; 3570 case -351767064: // coverage 3571 this.getCoverage().add((InsurancePlanCoverageComponent) value); // InsurancePlanCoverageComponent 3572 return value; 3573 case 3443497: // plan 3574 this.getPlan().add((InsurancePlanPlanComponent) value); // InsurancePlanPlanComponent 3575 return value; 3576 default: return super.setProperty(hash, name, value); 3577 } 3578 3579 } 3580 3581 @Override 3582 public Base setProperty(String name, Base value) throws FHIRException { 3583 if (name.equals("identifier")) { 3584 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3585 } else if (name.equals("status")) { 3586 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3587 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3588 } else if (name.equals("type")) { 3589 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 3590 } else if (name.equals("name")) { 3591 this.name = TypeConvertor.castToString(value); // StringType 3592 } else if (name.equals("alias")) { 3593 this.getAlias().add(TypeConvertor.castToString(value)); 3594 } else if (name.equals("period")) { 3595 this.period = TypeConvertor.castToPeriod(value); // Period 3596 } else if (name.equals("ownedBy")) { 3597 this.ownedBy = TypeConvertor.castToReference(value); // Reference 3598 } else if (name.equals("administeredBy")) { 3599 this.administeredBy = TypeConvertor.castToReference(value); // Reference 3600 } else if (name.equals("coverageArea")) { 3601 this.getCoverageArea().add(TypeConvertor.castToReference(value)); 3602 } else if (name.equals("contact")) { 3603 this.getContact().add(TypeConvertor.castToExtendedContactDetail(value)); 3604 } else if (name.equals("endpoint")) { 3605 this.getEndpoint().add(TypeConvertor.castToReference(value)); 3606 } else if (name.equals("network")) { 3607 this.getNetwork().add(TypeConvertor.castToReference(value)); 3608 } else if (name.equals("coverage")) { 3609 this.getCoverage().add((InsurancePlanCoverageComponent) value); 3610 } else if (name.equals("plan")) { 3611 this.getPlan().add((InsurancePlanPlanComponent) value); 3612 } else 3613 return super.setProperty(name, value); 3614 return value; 3615 } 3616 3617 @Override 3618 public void removeChild(String name, Base value) throws FHIRException { 3619 if (name.equals("identifier")) { 3620 this.getIdentifier().remove(value); 3621 } else if (name.equals("status")) { 3622 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 3623 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3624 } else if (name.equals("type")) { 3625 this.getType().remove(value); 3626 } else if (name.equals("name")) { 3627 this.name = null; 3628 } else if (name.equals("alias")) { 3629 this.getAlias().remove(value); 3630 } else if (name.equals("period")) { 3631 this.period = null; 3632 } else if (name.equals("ownedBy")) { 3633 this.ownedBy = null; 3634 } else if (name.equals("administeredBy")) { 3635 this.administeredBy = null; 3636 } else if (name.equals("coverageArea")) { 3637 this.getCoverageArea().remove(value); 3638 } else if (name.equals("contact")) { 3639 this.getContact().remove(value); 3640 } else if (name.equals("endpoint")) { 3641 this.getEndpoint().remove(value); 3642 } else if (name.equals("network")) { 3643 this.getNetwork().remove(value); 3644 } else if (name.equals("coverage")) { 3645 this.getCoverage().remove((InsurancePlanCoverageComponent) value); 3646 } else if (name.equals("plan")) { 3647 this.getPlan().remove((InsurancePlanPlanComponent) value); 3648 } else 3649 super.removeChild(name, value); 3650 3651 } 3652 3653 @Override 3654 public Base makeProperty(int hash, String name) throws FHIRException { 3655 switch (hash) { 3656 case -1618432855: return addIdentifier(); 3657 case -892481550: return getStatusElement(); 3658 case 3575610: return addType(); 3659 case 3373707: return getNameElement(); 3660 case 92902992: return addAliasElement(); 3661 case -991726143: return getPeriod(); 3662 case -1054743076: return getOwnedBy(); 3663 case 898770462: return getAdministeredBy(); 3664 case -1532328299: return addCoverageArea(); 3665 case 951526432: return addContact(); 3666 case 1741102485: return addEndpoint(); 3667 case 1843485230: return addNetwork(); 3668 case -351767064: return addCoverage(); 3669 case 3443497: return addPlan(); 3670 default: return super.makeProperty(hash, name); 3671 } 3672 3673 } 3674 3675 @Override 3676 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3677 switch (hash) { 3678 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3679 case -892481550: /*status*/ return new String[] {"code"}; 3680 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3681 case 3373707: /*name*/ return new String[] {"string"}; 3682 case 92902992: /*alias*/ return new String[] {"string"}; 3683 case -991726143: /*period*/ return new String[] {"Period"}; 3684 case -1054743076: /*ownedBy*/ return new String[] {"Reference"}; 3685 case 898770462: /*administeredBy*/ return new String[] {"Reference"}; 3686 case -1532328299: /*coverageArea*/ return new String[] {"Reference"}; 3687 case 951526432: /*contact*/ return new String[] {"ExtendedContactDetail"}; 3688 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 3689 case 1843485230: /*network*/ return new String[] {"Reference"}; 3690 case -351767064: /*coverage*/ return new String[] {}; 3691 case 3443497: /*plan*/ return new String[] {}; 3692 default: return super.getTypesForProperty(hash, name); 3693 } 3694 3695 } 3696 3697 @Override 3698 public Base addChild(String name) throws FHIRException { 3699 if (name.equals("identifier")) { 3700 return addIdentifier(); 3701 } 3702 else if (name.equals("status")) { 3703 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.status"); 3704 } 3705 else if (name.equals("type")) { 3706 return addType(); 3707 } 3708 else if (name.equals("name")) { 3709 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.name"); 3710 } 3711 else if (name.equals("alias")) { 3712 throw new FHIRException("Cannot call addChild on a singleton property InsurancePlan.alias"); 3713 } 3714 else if (name.equals("period")) { 3715 this.period = new Period(); 3716 return this.period; 3717 } 3718 else if (name.equals("ownedBy")) { 3719 this.ownedBy = new Reference(); 3720 return this.ownedBy; 3721 } 3722 else if (name.equals("administeredBy")) { 3723 this.administeredBy = new Reference(); 3724 return this.administeredBy; 3725 } 3726 else if (name.equals("coverageArea")) { 3727 return addCoverageArea(); 3728 } 3729 else if (name.equals("contact")) { 3730 return addContact(); 3731 } 3732 else if (name.equals("endpoint")) { 3733 return addEndpoint(); 3734 } 3735 else if (name.equals("network")) { 3736 return addNetwork(); 3737 } 3738 else if (name.equals("coverage")) { 3739 return addCoverage(); 3740 } 3741 else if (name.equals("plan")) { 3742 return addPlan(); 3743 } 3744 else 3745 return super.addChild(name); 3746 } 3747 3748 public String fhirType() { 3749 return "InsurancePlan"; 3750 3751 } 3752 3753 public InsurancePlan copy() { 3754 InsurancePlan dst = new InsurancePlan(); 3755 copyValues(dst); 3756 return dst; 3757 } 3758 3759 public void copyValues(InsurancePlan dst) { 3760 super.copyValues(dst); 3761 if (identifier != null) { 3762 dst.identifier = new ArrayList<Identifier>(); 3763 for (Identifier i : identifier) 3764 dst.identifier.add(i.copy()); 3765 }; 3766 dst.status = status == null ? null : status.copy(); 3767 if (type != null) { 3768 dst.type = new ArrayList<CodeableConcept>(); 3769 for (CodeableConcept i : type) 3770 dst.type.add(i.copy()); 3771 }; 3772 dst.name = name == null ? null : name.copy(); 3773 if (alias != null) { 3774 dst.alias = new ArrayList<StringType>(); 3775 for (StringType i : alias) 3776 dst.alias.add(i.copy()); 3777 }; 3778 dst.period = period == null ? null : period.copy(); 3779 dst.ownedBy = ownedBy == null ? null : ownedBy.copy(); 3780 dst.administeredBy = administeredBy == null ? null : administeredBy.copy(); 3781 if (coverageArea != null) { 3782 dst.coverageArea = new ArrayList<Reference>(); 3783 for (Reference i : coverageArea) 3784 dst.coverageArea.add(i.copy()); 3785 }; 3786 if (contact != null) { 3787 dst.contact = new ArrayList<ExtendedContactDetail>(); 3788 for (ExtendedContactDetail i : contact) 3789 dst.contact.add(i.copy()); 3790 }; 3791 if (endpoint != null) { 3792 dst.endpoint = new ArrayList<Reference>(); 3793 for (Reference i : endpoint) 3794 dst.endpoint.add(i.copy()); 3795 }; 3796 if (network != null) { 3797 dst.network = new ArrayList<Reference>(); 3798 for (Reference i : network) 3799 dst.network.add(i.copy()); 3800 }; 3801 if (coverage != null) { 3802 dst.coverage = new ArrayList<InsurancePlanCoverageComponent>(); 3803 for (InsurancePlanCoverageComponent i : coverage) 3804 dst.coverage.add(i.copy()); 3805 }; 3806 if (plan != null) { 3807 dst.plan = new ArrayList<InsurancePlanPlanComponent>(); 3808 for (InsurancePlanPlanComponent i : plan) 3809 dst.plan.add(i.copy()); 3810 }; 3811 } 3812 3813 protected InsurancePlan typedCopy() { 3814 return copy(); 3815 } 3816 3817 @Override 3818 public boolean equalsDeep(Base other_) { 3819 if (!super.equalsDeep(other_)) 3820 return false; 3821 if (!(other_ instanceof InsurancePlan)) 3822 return false; 3823 InsurancePlan o = (InsurancePlan) other_; 3824 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 3825 && compareDeep(name, o.name, true) && compareDeep(alias, o.alias, true) && compareDeep(period, o.period, true) 3826 && compareDeep(ownedBy, o.ownedBy, true) && compareDeep(administeredBy, o.administeredBy, true) 3827 && compareDeep(coverageArea, o.coverageArea, true) && compareDeep(contact, o.contact, true) && compareDeep(endpoint, o.endpoint, true) 3828 && compareDeep(network, o.network, true) && compareDeep(coverage, o.coverage, true) && compareDeep(plan, o.plan, true) 3829 ; 3830 } 3831 3832 @Override 3833 public boolean equalsShallow(Base other_) { 3834 if (!super.equalsShallow(other_)) 3835 return false; 3836 if (!(other_ instanceof InsurancePlan)) 3837 return false; 3838 InsurancePlan o = (InsurancePlan) other_; 3839 return compareValues(status, o.status, true) && compareValues(name, o.name, true) && compareValues(alias, o.alias, true) 3840 ; 3841 } 3842 3843 public boolean isEmpty() { 3844 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type 3845 , name, alias, period, ownedBy, administeredBy, coverageArea, contact, endpoint 3846 , network, coverage, plan); 3847 } 3848 3849 @Override 3850 public ResourceType getResourceType() { 3851 return ResourceType.InsurancePlan; 3852 } 3853 3854 /** 3855 * Search parameter: <b>address-city</b> 3856 * <p> 3857 * Description: <b>A city specified in an address</b><br> 3858 * Type: <b>string</b><br> 3859 * Path: <b>InsurancePlan.contact.address.city</b><br> 3860 * </p> 3861 */ 3862 @SearchParamDefinition(name="address-city", path="InsurancePlan.contact.address.city", description="A city specified in an address", type="string" ) 3863 public static final String SP_ADDRESS_CITY = "address-city"; 3864 /** 3865 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 3866 * <p> 3867 * Description: <b>A city specified in an address</b><br> 3868 * Type: <b>string</b><br> 3869 * Path: <b>InsurancePlan.contact.address.city</b><br> 3870 * </p> 3871 */ 3872 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_CITY); 3873 3874 /** 3875 * Search parameter: <b>address-country</b> 3876 * <p> 3877 * Description: <b>A country specified in an address</b><br> 3878 * Type: <b>string</b><br> 3879 * Path: <b>InsurancePlan.contact.address.country</b><br> 3880 * </p> 3881 */ 3882 @SearchParamDefinition(name="address-country", path="InsurancePlan.contact.address.country", description="A country specified in an address", type="string" ) 3883 public static final String SP_ADDRESS_COUNTRY = "address-country"; 3884 /** 3885 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 3886 * <p> 3887 * Description: <b>A country specified in an address</b><br> 3888 * Type: <b>string</b><br> 3889 * Path: <b>InsurancePlan.contact.address.country</b><br> 3890 * </p> 3891 */ 3892 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_COUNTRY); 3893 3894 /** 3895 * Search parameter: <b>address-postalcode</b> 3896 * <p> 3897 * Description: <b>A postal code specified in an address</b><br> 3898 * Type: <b>string</b><br> 3899 * Path: <b>InsurancePlan.contact.address.postalCode</b><br> 3900 * </p> 3901 */ 3902 @SearchParamDefinition(name="address-postalcode", path="InsurancePlan.contact.address.postalCode", description="A postal code specified in an address", type="string" ) 3903 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 3904 /** 3905 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 3906 * <p> 3907 * Description: <b>A postal code specified in an address</b><br> 3908 * Type: <b>string</b><br> 3909 * Path: <b>InsurancePlan.contact.address.postalCode</b><br> 3910 * </p> 3911 */ 3912 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_POSTALCODE); 3913 3914 /** 3915 * Search parameter: <b>address-state</b> 3916 * <p> 3917 * Description: <b>A state specified in an address</b><br> 3918 * Type: <b>string</b><br> 3919 * Path: <b>InsurancePlan.contact.address.state</b><br> 3920 * </p> 3921 */ 3922 @SearchParamDefinition(name="address-state", path="InsurancePlan.contact.address.state", description="A state specified in an address", type="string" ) 3923 public static final String SP_ADDRESS_STATE = "address-state"; 3924 /** 3925 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 3926 * <p> 3927 * Description: <b>A state specified in an address</b><br> 3928 * Type: <b>string</b><br> 3929 * Path: <b>InsurancePlan.contact.address.state</b><br> 3930 * </p> 3931 */ 3932 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_STATE); 3933 3934 /** 3935 * Search parameter: <b>address-use</b> 3936 * <p> 3937 * Description: <b>A use code specified in an address</b><br> 3938 * Type: <b>token</b><br> 3939 * Path: <b>InsurancePlan.contact.address.use</b><br> 3940 * </p> 3941 */ 3942 @SearchParamDefinition(name="address-use", path="InsurancePlan.contact.address.use", description="A use code specified in an address", type="token" ) 3943 public static final String SP_ADDRESS_USE = "address-use"; 3944 /** 3945 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 3946 * <p> 3947 * Description: <b>A use code specified in an address</b><br> 3948 * Type: <b>token</b><br> 3949 * Path: <b>InsurancePlan.contact.address.use</b><br> 3950 * </p> 3951 */ 3952 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADDRESS_USE); 3953 3954 /** 3955 * Search parameter: <b>address</b> 3956 * <p> 3957 * Description: <b>A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text</b><br> 3958 * Type: <b>string</b><br> 3959 * Path: <b>InsurancePlan.contact.address</b><br> 3960 * </p> 3961 */ 3962 @SearchParamDefinition(name="address", path="InsurancePlan.contact.address", description="A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text", type="string" ) 3963 public static final String SP_ADDRESS = "address"; 3964 /** 3965 * <b>Fluent Client</b> search parameter constant for <b>address</b> 3966 * <p> 3967 * Description: <b>A server defined search that may match any of the string fields in the Address, including line, city, district, state, country, postalCode, and/or text</b><br> 3968 * Type: <b>string</b><br> 3969 * Path: <b>InsurancePlan.contact.address</b><br> 3970 * </p> 3971 */ 3972 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS); 3973 3974 /** 3975 * Search parameter: <b>administered-by</b> 3976 * <p> 3977 * Description: <b>Product administrator</b><br> 3978 * Type: <b>reference</b><br> 3979 * Path: <b>InsurancePlan.administeredBy</b><br> 3980 * </p> 3981 */ 3982 @SearchParamDefinition(name="administered-by", path="InsurancePlan.administeredBy", description="Product administrator", type="reference", target={Organization.class } ) 3983 public static final String SP_ADMINISTERED_BY = "administered-by"; 3984 /** 3985 * <b>Fluent Client</b> search parameter constant for <b>administered-by</b> 3986 * <p> 3987 * Description: <b>Product administrator</b><br> 3988 * Type: <b>reference</b><br> 3989 * Path: <b>InsurancePlan.administeredBy</b><br> 3990 * </p> 3991 */ 3992 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ADMINISTERED_BY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ADMINISTERED_BY); 3993 3994/** 3995 * Constant for fluent queries to be used to add include statements. Specifies 3996 * the path value of "<b>InsurancePlan:administered-by</b>". 3997 */ 3998 public static final ca.uhn.fhir.model.api.Include INCLUDE_ADMINISTERED_BY = new ca.uhn.fhir.model.api.Include("InsurancePlan:administered-by").toLocked(); 3999 4000 /** 4001 * Search parameter: <b>endpoint</b> 4002 * <p> 4003 * Description: <b>Technical endpoint</b><br> 4004 * Type: <b>reference</b><br> 4005 * Path: <b>InsurancePlan.endpoint</b><br> 4006 * </p> 4007 */ 4008 @SearchParamDefinition(name="endpoint", path="InsurancePlan.endpoint", description="Technical endpoint", type="reference", target={Endpoint.class } ) 4009 public static final String SP_ENDPOINT = "endpoint"; 4010 /** 4011 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 4012 * <p> 4013 * Description: <b>Technical endpoint</b><br> 4014 * Type: <b>reference</b><br> 4015 * Path: <b>InsurancePlan.endpoint</b><br> 4016 * </p> 4017 */ 4018 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENDPOINT); 4019 4020/** 4021 * Constant for fluent queries to be used to add include statements. Specifies 4022 * the path value of "<b>InsurancePlan:endpoint</b>". 4023 */ 4024 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include("InsurancePlan:endpoint").toLocked(); 4025 4026 /** 4027 * Search parameter: <b>identifier</b> 4028 * <p> 4029 * Description: <b>Any identifier for the organization (not the accreditation issuer's identifier)</b><br> 4030 * Type: <b>token</b><br> 4031 * Path: <b>InsurancePlan.identifier</b><br> 4032 * </p> 4033 */ 4034 @SearchParamDefinition(name="identifier", path="InsurancePlan.identifier", description="Any identifier for the organization (not the accreditation issuer's identifier)", type="token" ) 4035 public static final String SP_IDENTIFIER = "identifier"; 4036 /** 4037 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4038 * <p> 4039 * Description: <b>Any identifier for the organization (not the accreditation issuer's identifier)</b><br> 4040 * Type: <b>token</b><br> 4041 * Path: <b>InsurancePlan.identifier</b><br> 4042 * </p> 4043 */ 4044 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4045 4046 /** 4047 * Search parameter: <b>name</b> 4048 * <p> 4049 * Description: <b>A portion of the organization's name or alias</b><br> 4050 * Type: <b>string</b><br> 4051 * Path: <b>InsurancePlan.name | InsurancePlan.alias</b><br> 4052 * </p> 4053 */ 4054 @SearchParamDefinition(name="name", path="InsurancePlan.name | InsurancePlan.alias", description="A portion of the organization's name or alias", type="string" ) 4055 public static final String SP_NAME = "name"; 4056 /** 4057 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4058 * <p> 4059 * Description: <b>A portion of the organization's name or alias</b><br> 4060 * Type: <b>string</b><br> 4061 * Path: <b>InsurancePlan.name | InsurancePlan.alias</b><br> 4062 * </p> 4063 */ 4064 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 4065 4066 /** 4067 * Search parameter: <b>owned-by</b> 4068 * <p> 4069 * Description: <b>An organization of which this organization forms a part</b><br> 4070 * Type: <b>reference</b><br> 4071 * Path: <b>InsurancePlan.ownedBy</b><br> 4072 * </p> 4073 */ 4074 @SearchParamDefinition(name="owned-by", path="InsurancePlan.ownedBy", description="An organization of which this organization forms a part", type="reference", target={Organization.class } ) 4075 public static final String SP_OWNED_BY = "owned-by"; 4076 /** 4077 * <b>Fluent Client</b> search parameter constant for <b>owned-by</b> 4078 * <p> 4079 * Description: <b>An organization of which this organization forms a part</b><br> 4080 * Type: <b>reference</b><br> 4081 * Path: <b>InsurancePlan.ownedBy</b><br> 4082 * </p> 4083 */ 4084 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OWNED_BY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_OWNED_BY); 4085 4086/** 4087 * Constant for fluent queries to be used to add include statements. Specifies 4088 * the path value of "<b>InsurancePlan:owned-by</b>". 4089 */ 4090 public static final ca.uhn.fhir.model.api.Include INCLUDE_OWNED_BY = new ca.uhn.fhir.model.api.Include("InsurancePlan:owned-by").toLocked(); 4091 4092 /** 4093 * Search parameter: <b>phonetic</b> 4094 * <p> 4095 * Description: <b>A portion of the organization's name using some kind of phonetic matching algorithm</b><br> 4096 * Type: <b>string</b><br> 4097 * Path: <b>InsurancePlan.name</b><br> 4098 * </p> 4099 */ 4100 @SearchParamDefinition(name="phonetic", path="InsurancePlan.name", description="A portion of the organization's name using some kind of phonetic matching algorithm", type="string" ) 4101 public static final String SP_PHONETIC = "phonetic"; 4102 /** 4103 * <b>Fluent Client</b> search parameter constant for <b>phonetic</b> 4104 * <p> 4105 * Description: <b>A portion of the organization's name using some kind of phonetic matching algorithm</b><br> 4106 * Type: <b>string</b><br> 4107 * Path: <b>InsurancePlan.name</b><br> 4108 * </p> 4109 */ 4110 public static final ca.uhn.fhir.rest.gclient.StringClientParam PHONETIC = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PHONETIC); 4111 4112 /** 4113 * Search parameter: <b>status</b> 4114 * <p> 4115 * Description: <b>Is the Organization record active</b><br> 4116 * Type: <b>token</b><br> 4117 * Path: <b>InsurancePlan.status</b><br> 4118 * </p> 4119 */ 4120 @SearchParamDefinition(name="status", path="InsurancePlan.status", description="Is the Organization record active", type="token" ) 4121 public static final String SP_STATUS = "status"; 4122 /** 4123 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4124 * <p> 4125 * Description: <b>Is the Organization record active</b><br> 4126 * Type: <b>token</b><br> 4127 * Path: <b>InsurancePlan.status</b><br> 4128 * </p> 4129 */ 4130 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4131 4132 /** 4133 * Search parameter: <b>type</b> 4134 * <p> 4135 * Description: <b>A code for the type of organization</b><br> 4136 * Type: <b>token</b><br> 4137 * Path: <b>InsurancePlan.type</b><br> 4138 * </p> 4139 */ 4140 @SearchParamDefinition(name="type", path="InsurancePlan.type", description="A code for the type of organization", type="token" ) 4141 public static final String SP_TYPE = "type"; 4142 /** 4143 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4144 * <p> 4145 * Description: <b>A code for the type of organization</b><br> 4146 * Type: <b>token</b><br> 4147 * Path: <b>InsurancePlan.type</b><br> 4148 * </p> 4149 */ 4150 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 4151 4152 4153} 4154