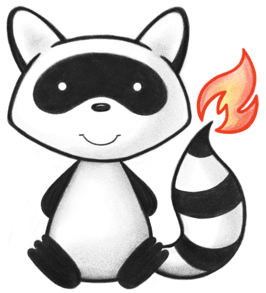
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * functional description of an inventory item used in inventory and supply-related workflows. 052 */ 053@ResourceDef(name="InventoryItem", profile="http://hl7.org/fhir/StructureDefinition/InventoryItem") 054public class InventoryItem extends DomainResource { 055 056 public enum InventoryItemStatusCodes { 057 /** 058 * The item is active and can be referenced. 059 */ 060 ACTIVE, 061 /** 062 * The item is presently inactive - there may be references to it but the item is not expected to be used. 063 */ 064 INACTIVE, 065 /** 066 * The item record was entered in error. 067 */ 068 ENTEREDINERROR, 069 /** 070 * The item status has not been determined. 071 */ 072 UNKNOWN, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static InventoryItemStatusCodes fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("active".equals(codeString)) 081 return ACTIVE; 082 if ("inactive".equals(codeString)) 083 return INACTIVE; 084 if ("entered-in-error".equals(codeString)) 085 return ENTEREDINERROR; 086 if ("unknown".equals(codeString)) 087 return UNKNOWN; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown InventoryItemStatusCodes code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case ACTIVE: return "active"; 096 case INACTIVE: return "inactive"; 097 case ENTEREDINERROR: return "entered-in-error"; 098 case UNKNOWN: return "unknown"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case ACTIVE: return "http://hl7.org/fhir/inventoryitem-status"; 106 case INACTIVE: return "http://hl7.org/fhir/inventoryitem-status"; 107 case ENTEREDINERROR: return "http://hl7.org/fhir/inventoryitem-status"; 108 case UNKNOWN: return "http://hl7.org/fhir/inventoryitem-status"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case ACTIVE: return "The item is active and can be referenced."; 116 case INACTIVE: return "The item is presently inactive - there may be references to it but the item is not expected to be used."; 117 case ENTEREDINERROR: return "The item record was entered in error."; 118 case UNKNOWN: return "The item status has not been determined."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case ACTIVE: return "Active"; 126 case INACTIVE: return "Inactive"; 127 case ENTEREDINERROR: return "Entered in Error"; 128 case UNKNOWN: return "Unknown"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class InventoryItemStatusCodesEnumFactory implements EnumFactory<InventoryItemStatusCodes> { 136 public InventoryItemStatusCodes fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("active".equals(codeString)) 141 return InventoryItemStatusCodes.ACTIVE; 142 if ("inactive".equals(codeString)) 143 return InventoryItemStatusCodes.INACTIVE; 144 if ("entered-in-error".equals(codeString)) 145 return InventoryItemStatusCodes.ENTEREDINERROR; 146 if ("unknown".equals(codeString)) 147 return InventoryItemStatusCodes.UNKNOWN; 148 throw new IllegalArgumentException("Unknown InventoryItemStatusCodes code '"+codeString+"'"); 149 } 150 public Enumeration<InventoryItemStatusCodes> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<InventoryItemStatusCodes>(this, InventoryItemStatusCodes.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<InventoryItemStatusCodes>(this, InventoryItemStatusCodes.NULL, code); 158 if ("active".equals(codeString)) 159 return new Enumeration<InventoryItemStatusCodes>(this, InventoryItemStatusCodes.ACTIVE, code); 160 if ("inactive".equals(codeString)) 161 return new Enumeration<InventoryItemStatusCodes>(this, InventoryItemStatusCodes.INACTIVE, code); 162 if ("entered-in-error".equals(codeString)) 163 return new Enumeration<InventoryItemStatusCodes>(this, InventoryItemStatusCodes.ENTEREDINERROR, code); 164 if ("unknown".equals(codeString)) 165 return new Enumeration<InventoryItemStatusCodes>(this, InventoryItemStatusCodes.UNKNOWN, code); 166 throw new FHIRException("Unknown InventoryItemStatusCodes code '"+codeString+"'"); 167 } 168 public String toCode(InventoryItemStatusCodes code) { 169 if (code == InventoryItemStatusCodes.NULL) 170 return null; 171 if (code == InventoryItemStatusCodes.ACTIVE) 172 return "active"; 173 if (code == InventoryItemStatusCodes.INACTIVE) 174 return "inactive"; 175 if (code == InventoryItemStatusCodes.ENTEREDINERROR) 176 return "entered-in-error"; 177 if (code == InventoryItemStatusCodes.UNKNOWN) 178 return "unknown"; 179 return "?"; 180 } 181 public String toSystem(InventoryItemStatusCodes code) { 182 return code.getSystem(); 183 } 184 } 185 186 @Block() 187 public static class InventoryItemNameComponent extends BackboneElement implements IBaseBackboneElement { 188 /** 189 * The type of name e.g. 'brand-name', 'functional-name', 'common-name'. 190 */ 191 @Child(name = "nameType", type = {Coding.class}, order=1, min=1, max=1, modifier=false, summary=true) 192 @Description(shortDefinition="The type of name e.g. 'brand-name', 'functional-name', 'common-name'", formalDefinition="The type of name e.g. 'brand-name', 'functional-name', 'common-name'." ) 193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/inventoryitem-nametype") 194 protected Coding nameType; 195 196 /** 197 * The language that the item name is expressed in. 198 */ 199 @Child(name = "language", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 200 @Description(shortDefinition="The language used to express the item name", formalDefinition="The language that the item name is expressed in." ) 201 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 202 protected Enumeration<CommonLanguages> language; 203 204 /** 205 * The name or designation that the item is given. 206 */ 207 @Child(name = "name", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=true) 208 @Description(shortDefinition="The name or designation of the item", formalDefinition="The name or designation that the item is given." ) 209 protected StringType name; 210 211 private static final long serialVersionUID = 2074178414L; 212 213 /** 214 * Constructor 215 */ 216 public InventoryItemNameComponent() { 217 super(); 218 } 219 220 /** 221 * Constructor 222 */ 223 public InventoryItemNameComponent(Coding nameType, CommonLanguages language, String name) { 224 super(); 225 this.setNameType(nameType); 226 this.setLanguage(language); 227 this.setName(name); 228 } 229 230 /** 231 * @return {@link #nameType} (The type of name e.g. 'brand-name', 'functional-name', 'common-name'.) 232 */ 233 public Coding getNameType() { 234 if (this.nameType == null) 235 if (Configuration.errorOnAutoCreate()) 236 throw new Error("Attempt to auto-create InventoryItemNameComponent.nameType"); 237 else if (Configuration.doAutoCreate()) 238 this.nameType = new Coding(); // cc 239 return this.nameType; 240 } 241 242 public boolean hasNameType() { 243 return this.nameType != null && !this.nameType.isEmpty(); 244 } 245 246 /** 247 * @param value {@link #nameType} (The type of name e.g. 'brand-name', 'functional-name', 'common-name'.) 248 */ 249 public InventoryItemNameComponent setNameType(Coding value) { 250 this.nameType = value; 251 return this; 252 } 253 254 /** 255 * @return {@link #language} (The language that the item name is expressed in.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 256 */ 257 public Enumeration<CommonLanguages> getLanguageElement() { 258 if (this.language == null) 259 if (Configuration.errorOnAutoCreate()) 260 throw new Error("Attempt to auto-create InventoryItemNameComponent.language"); 261 else if (Configuration.doAutoCreate()) 262 this.language = new Enumeration<CommonLanguages>(new CommonLanguagesEnumFactory()); // bb 263 return this.language; 264 } 265 266 public boolean hasLanguageElement() { 267 return this.language != null && !this.language.isEmpty(); 268 } 269 270 public boolean hasLanguage() { 271 return this.language != null && !this.language.isEmpty(); 272 } 273 274 /** 275 * @param value {@link #language} (The language that the item name is expressed in.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 276 */ 277 public InventoryItemNameComponent setLanguageElement(Enumeration<CommonLanguages> value) { 278 this.language = value; 279 return this; 280 } 281 282 /** 283 * @return The language that the item name is expressed in. 284 */ 285 public CommonLanguages getLanguage() { 286 return this.language == null ? null : this.language.getValue(); 287 } 288 289 /** 290 * @param value The language that the item name is expressed in. 291 */ 292 public InventoryItemNameComponent setLanguage(CommonLanguages value) { 293 if (this.language == null) 294 this.language = new Enumeration<CommonLanguages>(new CommonLanguagesEnumFactory()); 295 this.language.setValue(value); 296 return this; 297 } 298 299 /** 300 * @return {@link #name} (The name or designation that the item is given.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 301 */ 302 public StringType getNameElement() { 303 if (this.name == null) 304 if (Configuration.errorOnAutoCreate()) 305 throw new Error("Attempt to auto-create InventoryItemNameComponent.name"); 306 else if (Configuration.doAutoCreate()) 307 this.name = new StringType(); // bb 308 return this.name; 309 } 310 311 public boolean hasNameElement() { 312 return this.name != null && !this.name.isEmpty(); 313 } 314 315 public boolean hasName() { 316 return this.name != null && !this.name.isEmpty(); 317 } 318 319 /** 320 * @param value {@link #name} (The name or designation that the item is given.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 321 */ 322 public InventoryItemNameComponent setNameElement(StringType value) { 323 this.name = value; 324 return this; 325 } 326 327 /** 328 * @return The name or designation that the item is given. 329 */ 330 public String getName() { 331 return this.name == null ? null : this.name.getValue(); 332 } 333 334 /** 335 * @param value The name or designation that the item is given. 336 */ 337 public InventoryItemNameComponent setName(String value) { 338 if (this.name == null) 339 this.name = new StringType(); 340 this.name.setValue(value); 341 return this; 342 } 343 344 protected void listChildren(List<Property> children) { 345 super.listChildren(children); 346 children.add(new Property("nameType", "Coding", "The type of name e.g. 'brand-name', 'functional-name', 'common-name'.", 0, 1, nameType)); 347 children.add(new Property("language", "code", "The language that the item name is expressed in.", 0, 1, language)); 348 children.add(new Property("name", "string", "The name or designation that the item is given.", 0, 1, name)); 349 } 350 351 @Override 352 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 353 switch (_hash) { 354 case 1840595045: /*nameType*/ return new Property("nameType", "Coding", "The type of name e.g. 'brand-name', 'functional-name', 'common-name'.", 0, 1, nameType); 355 case -1613589672: /*language*/ return new Property("language", "code", "The language that the item name is expressed in.", 0, 1, language); 356 case 3373707: /*name*/ return new Property("name", "string", "The name or designation that the item is given.", 0, 1, name); 357 default: return super.getNamedProperty(_hash, _name, _checkValid); 358 } 359 360 } 361 362 @Override 363 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 364 switch (hash) { 365 case 1840595045: /*nameType*/ return this.nameType == null ? new Base[0] : new Base[] {this.nameType}; // Coding 366 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // Enumeration<CommonLanguages> 367 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 368 default: return super.getProperty(hash, name, checkValid); 369 } 370 371 } 372 373 @Override 374 public Base setProperty(int hash, String name, Base value) throws FHIRException { 375 switch (hash) { 376 case 1840595045: // nameType 377 this.nameType = TypeConvertor.castToCoding(value); // Coding 378 return value; 379 case -1613589672: // language 380 value = new CommonLanguagesEnumFactory().fromType(TypeConvertor.castToCode(value)); 381 this.language = (Enumeration) value; // Enumeration<CommonLanguages> 382 return value; 383 case 3373707: // name 384 this.name = TypeConvertor.castToString(value); // StringType 385 return value; 386 default: return super.setProperty(hash, name, value); 387 } 388 389 } 390 391 @Override 392 public Base setProperty(String name, Base value) throws FHIRException { 393 if (name.equals("nameType")) { 394 this.nameType = TypeConvertor.castToCoding(value); // Coding 395 } else if (name.equals("language")) { 396 value = new CommonLanguagesEnumFactory().fromType(TypeConvertor.castToCode(value)); 397 this.language = (Enumeration) value; // Enumeration<CommonLanguages> 398 } else if (name.equals("name")) { 399 this.name = TypeConvertor.castToString(value); // StringType 400 } else 401 return super.setProperty(name, value); 402 return value; 403 } 404 405 @Override 406 public void removeChild(String name, Base value) throws FHIRException { 407 if (name.equals("nameType")) { 408 this.nameType = null; 409 } else if (name.equals("language")) { 410 value = new CommonLanguagesEnumFactory().fromType(TypeConvertor.castToCode(value)); 411 this.language = (Enumeration) value; // Enumeration<CommonLanguages> 412 } else if (name.equals("name")) { 413 this.name = null; 414 } else 415 super.removeChild(name, value); 416 417 } 418 419 @Override 420 public Base makeProperty(int hash, String name) throws FHIRException { 421 switch (hash) { 422 case 1840595045: return getNameType(); 423 case -1613589672: return getLanguageElement(); 424 case 3373707: return getNameElement(); 425 default: return super.makeProperty(hash, name); 426 } 427 428 } 429 430 @Override 431 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 432 switch (hash) { 433 case 1840595045: /*nameType*/ return new String[] {"Coding"}; 434 case -1613589672: /*language*/ return new String[] {"code"}; 435 case 3373707: /*name*/ return new String[] {"string"}; 436 default: return super.getTypesForProperty(hash, name); 437 } 438 439 } 440 441 @Override 442 public Base addChild(String name) throws FHIRException { 443 if (name.equals("nameType")) { 444 this.nameType = new Coding(); 445 return this.nameType; 446 } 447 else if (name.equals("language")) { 448 throw new FHIRException("Cannot call addChild on a singleton property InventoryItem.name.language"); 449 } 450 else if (name.equals("name")) { 451 throw new FHIRException("Cannot call addChild on a singleton property InventoryItem.name.name"); 452 } 453 else 454 return super.addChild(name); 455 } 456 457 public InventoryItemNameComponent copy() { 458 InventoryItemNameComponent dst = new InventoryItemNameComponent(); 459 copyValues(dst); 460 return dst; 461 } 462 463 public void copyValues(InventoryItemNameComponent dst) { 464 super.copyValues(dst); 465 dst.nameType = nameType == null ? null : nameType.copy(); 466 dst.language = language == null ? null : language.copy(); 467 dst.name = name == null ? null : name.copy(); 468 } 469 470 @Override 471 public boolean equalsDeep(Base other_) { 472 if (!super.equalsDeep(other_)) 473 return false; 474 if (!(other_ instanceof InventoryItemNameComponent)) 475 return false; 476 InventoryItemNameComponent o = (InventoryItemNameComponent) other_; 477 return compareDeep(nameType, o.nameType, true) && compareDeep(language, o.language, true) && compareDeep(name, o.name, true) 478 ; 479 } 480 481 @Override 482 public boolean equalsShallow(Base other_) { 483 if (!super.equalsShallow(other_)) 484 return false; 485 if (!(other_ instanceof InventoryItemNameComponent)) 486 return false; 487 InventoryItemNameComponent o = (InventoryItemNameComponent) other_; 488 return compareValues(language, o.language, true) && compareValues(name, o.name, true); 489 } 490 491 public boolean isEmpty() { 492 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(nameType, language, name 493 ); 494 } 495 496 public String fhirType() { 497 return "InventoryItem.name"; 498 499 } 500 501 } 502 503 @Block() 504 public static class InventoryItemResponsibleOrganizationComponent extends BackboneElement implements IBaseBackboneElement { 505 /** 506 * The role of the organization e.g. manufacturer, distributor, etc. 507 */ 508 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 509 @Description(shortDefinition="The role of the organization e.g. manufacturer, distributor, or other", formalDefinition="The role of the organization e.g. manufacturer, distributor, etc." ) 510 protected CodeableConcept role; 511 512 /** 513 * An organization that has an association with the item, e.g. manufacturer, distributor, responsible, etc. 514 */ 515 @Child(name = "organization", type = {Organization.class}, order=2, min=1, max=1, modifier=false, summary=false) 516 @Description(shortDefinition="An organization that is associated with the item", formalDefinition="An organization that has an association with the item, e.g. manufacturer, distributor, responsible, etc." ) 517 protected Reference organization; 518 519 private static final long serialVersionUID = -575091539L; 520 521 /** 522 * Constructor 523 */ 524 public InventoryItemResponsibleOrganizationComponent() { 525 super(); 526 } 527 528 /** 529 * Constructor 530 */ 531 public InventoryItemResponsibleOrganizationComponent(CodeableConcept role, Reference organization) { 532 super(); 533 this.setRole(role); 534 this.setOrganization(organization); 535 } 536 537 /** 538 * @return {@link #role} (The role of the organization e.g. manufacturer, distributor, etc.) 539 */ 540 public CodeableConcept getRole() { 541 if (this.role == null) 542 if (Configuration.errorOnAutoCreate()) 543 throw new Error("Attempt to auto-create InventoryItemResponsibleOrganizationComponent.role"); 544 else if (Configuration.doAutoCreate()) 545 this.role = new CodeableConcept(); // cc 546 return this.role; 547 } 548 549 public boolean hasRole() { 550 return this.role != null && !this.role.isEmpty(); 551 } 552 553 /** 554 * @param value {@link #role} (The role of the organization e.g. manufacturer, distributor, etc.) 555 */ 556 public InventoryItemResponsibleOrganizationComponent setRole(CodeableConcept value) { 557 this.role = value; 558 return this; 559 } 560 561 /** 562 * @return {@link #organization} (An organization that has an association with the item, e.g. manufacturer, distributor, responsible, etc.) 563 */ 564 public Reference getOrganization() { 565 if (this.organization == null) 566 if (Configuration.errorOnAutoCreate()) 567 throw new Error("Attempt to auto-create InventoryItemResponsibleOrganizationComponent.organization"); 568 else if (Configuration.doAutoCreate()) 569 this.organization = new Reference(); // cc 570 return this.organization; 571 } 572 573 public boolean hasOrganization() { 574 return this.organization != null && !this.organization.isEmpty(); 575 } 576 577 /** 578 * @param value {@link #organization} (An organization that has an association with the item, e.g. manufacturer, distributor, responsible, etc.) 579 */ 580 public InventoryItemResponsibleOrganizationComponent setOrganization(Reference value) { 581 this.organization = value; 582 return this; 583 } 584 585 protected void listChildren(List<Property> children) { 586 super.listChildren(children); 587 children.add(new Property("role", "CodeableConcept", "The role of the organization e.g. manufacturer, distributor, etc.", 0, 1, role)); 588 children.add(new Property("organization", "Reference(Organization)", "An organization that has an association with the item, e.g. manufacturer, distributor, responsible, etc.", 0, 1, organization)); 589 } 590 591 @Override 592 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 593 switch (_hash) { 594 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The role of the organization e.g. manufacturer, distributor, etc.", 0, 1, role); 595 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "An organization that has an association with the item, e.g. manufacturer, distributor, responsible, etc.", 0, 1, organization); 596 default: return super.getNamedProperty(_hash, _name, _checkValid); 597 } 598 599 } 600 601 @Override 602 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 603 switch (hash) { 604 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 605 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 606 default: return super.getProperty(hash, name, checkValid); 607 } 608 609 } 610 611 @Override 612 public Base setProperty(int hash, String name, Base value) throws FHIRException { 613 switch (hash) { 614 case 3506294: // role 615 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 616 return value; 617 case 1178922291: // organization 618 this.organization = TypeConvertor.castToReference(value); // Reference 619 return value; 620 default: return super.setProperty(hash, name, value); 621 } 622 623 } 624 625 @Override 626 public Base setProperty(String name, Base value) throws FHIRException { 627 if (name.equals("role")) { 628 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 629 } else if (name.equals("organization")) { 630 this.organization = TypeConvertor.castToReference(value); // Reference 631 } else 632 return super.setProperty(name, value); 633 return value; 634 } 635 636 @Override 637 public void removeChild(String name, Base value) throws FHIRException { 638 if (name.equals("role")) { 639 this.role = null; 640 } else if (name.equals("organization")) { 641 this.organization = null; 642 } else 643 super.removeChild(name, value); 644 645 } 646 647 @Override 648 public Base makeProperty(int hash, String name) throws FHIRException { 649 switch (hash) { 650 case 3506294: return getRole(); 651 case 1178922291: return getOrganization(); 652 default: return super.makeProperty(hash, name); 653 } 654 655 } 656 657 @Override 658 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 659 switch (hash) { 660 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 661 case 1178922291: /*organization*/ return new String[] {"Reference"}; 662 default: return super.getTypesForProperty(hash, name); 663 } 664 665 } 666 667 @Override 668 public Base addChild(String name) throws FHIRException { 669 if (name.equals("role")) { 670 this.role = new CodeableConcept(); 671 return this.role; 672 } 673 else if (name.equals("organization")) { 674 this.organization = new Reference(); 675 return this.organization; 676 } 677 else 678 return super.addChild(name); 679 } 680 681 public InventoryItemResponsibleOrganizationComponent copy() { 682 InventoryItemResponsibleOrganizationComponent dst = new InventoryItemResponsibleOrganizationComponent(); 683 copyValues(dst); 684 return dst; 685 } 686 687 public void copyValues(InventoryItemResponsibleOrganizationComponent dst) { 688 super.copyValues(dst); 689 dst.role = role == null ? null : role.copy(); 690 dst.organization = organization == null ? null : organization.copy(); 691 } 692 693 @Override 694 public boolean equalsDeep(Base other_) { 695 if (!super.equalsDeep(other_)) 696 return false; 697 if (!(other_ instanceof InventoryItemResponsibleOrganizationComponent)) 698 return false; 699 InventoryItemResponsibleOrganizationComponent o = (InventoryItemResponsibleOrganizationComponent) other_; 700 return compareDeep(role, o.role, true) && compareDeep(organization, o.organization, true); 701 } 702 703 @Override 704 public boolean equalsShallow(Base other_) { 705 if (!super.equalsShallow(other_)) 706 return false; 707 if (!(other_ instanceof InventoryItemResponsibleOrganizationComponent)) 708 return false; 709 InventoryItemResponsibleOrganizationComponent o = (InventoryItemResponsibleOrganizationComponent) other_; 710 return true; 711 } 712 713 public boolean isEmpty() { 714 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, organization); 715 } 716 717 public String fhirType() { 718 return "InventoryItem.responsibleOrganization"; 719 720 } 721 722 } 723 724 @Block() 725 public static class InventoryItemDescriptionComponent extends BackboneElement implements IBaseBackboneElement { 726 /** 727 * The language for the item description, when an item must be described in different languages and those languages may be authoritative and not translations of a 'main' language. 728 */ 729 @Child(name = "language", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 730 @Description(shortDefinition="The language that is used in the item description", formalDefinition="The language for the item description, when an item must be described in different languages and those languages may be authoritative and not translations of a 'main' language." ) 731 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 732 protected Enumeration<CommonLanguages> language; 733 734 /** 735 * Textual description of the item. 736 */ 737 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 738 @Description(shortDefinition="Textual description of the item", formalDefinition="Textual description of the item." ) 739 protected StringType description; 740 741 private static final long serialVersionUID = -803271414L; 742 743 /** 744 * Constructor 745 */ 746 public InventoryItemDescriptionComponent() { 747 super(); 748 } 749 750 /** 751 * @return {@link #language} (The language for the item description, when an item must be described in different languages and those languages may be authoritative and not translations of a 'main' language.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 752 */ 753 public Enumeration<CommonLanguages> getLanguageElement() { 754 if (this.language == null) 755 if (Configuration.errorOnAutoCreate()) 756 throw new Error("Attempt to auto-create InventoryItemDescriptionComponent.language"); 757 else if (Configuration.doAutoCreate()) 758 this.language = new Enumeration<CommonLanguages>(new CommonLanguagesEnumFactory()); // bb 759 return this.language; 760 } 761 762 public boolean hasLanguageElement() { 763 return this.language != null && !this.language.isEmpty(); 764 } 765 766 public boolean hasLanguage() { 767 return this.language != null && !this.language.isEmpty(); 768 } 769 770 /** 771 * @param value {@link #language} (The language for the item description, when an item must be described in different languages and those languages may be authoritative and not translations of a 'main' language.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 772 */ 773 public InventoryItemDescriptionComponent setLanguageElement(Enumeration<CommonLanguages> value) { 774 this.language = value; 775 return this; 776 } 777 778 /** 779 * @return The language for the item description, when an item must be described in different languages and those languages may be authoritative and not translations of a 'main' language. 780 */ 781 public CommonLanguages getLanguage() { 782 return this.language == null ? null : this.language.getValue(); 783 } 784 785 /** 786 * @param value The language for the item description, when an item must be described in different languages and those languages may be authoritative and not translations of a 'main' language. 787 */ 788 public InventoryItemDescriptionComponent setLanguage(CommonLanguages value) { 789 if (value == null) 790 this.language = null; 791 else { 792 if (this.language == null) 793 this.language = new Enumeration<CommonLanguages>(new CommonLanguagesEnumFactory()); 794 this.language.setValue(value); 795 } 796 return this; 797 } 798 799 /** 800 * @return {@link #description} (Textual description of the item.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 801 */ 802 public StringType getDescriptionElement() { 803 if (this.description == null) 804 if (Configuration.errorOnAutoCreate()) 805 throw new Error("Attempt to auto-create InventoryItemDescriptionComponent.description"); 806 else if (Configuration.doAutoCreate()) 807 this.description = new StringType(); // bb 808 return this.description; 809 } 810 811 public boolean hasDescriptionElement() { 812 return this.description != null && !this.description.isEmpty(); 813 } 814 815 public boolean hasDescription() { 816 return this.description != null && !this.description.isEmpty(); 817 } 818 819 /** 820 * @param value {@link #description} (Textual description of the item.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 821 */ 822 public InventoryItemDescriptionComponent setDescriptionElement(StringType value) { 823 this.description = value; 824 return this; 825 } 826 827 /** 828 * @return Textual description of the item. 829 */ 830 public String getDescription() { 831 return this.description == null ? null : this.description.getValue(); 832 } 833 834 /** 835 * @param value Textual description of the item. 836 */ 837 public InventoryItemDescriptionComponent setDescription(String value) { 838 if (Utilities.noString(value)) 839 this.description = null; 840 else { 841 if (this.description == null) 842 this.description = new StringType(); 843 this.description.setValue(value); 844 } 845 return this; 846 } 847 848 protected void listChildren(List<Property> children) { 849 super.listChildren(children); 850 children.add(new Property("language", "code", "The language for the item description, when an item must be described in different languages and those languages may be authoritative and not translations of a 'main' language.", 0, 1, language)); 851 children.add(new Property("description", "string", "Textual description of the item.", 0, 1, description)); 852 } 853 854 @Override 855 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 856 switch (_hash) { 857 case -1613589672: /*language*/ return new Property("language", "code", "The language for the item description, when an item must be described in different languages and those languages may be authoritative and not translations of a 'main' language.", 0, 1, language); 858 case -1724546052: /*description*/ return new Property("description", "string", "Textual description of the item.", 0, 1, description); 859 default: return super.getNamedProperty(_hash, _name, _checkValid); 860 } 861 862 } 863 864 @Override 865 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 866 switch (hash) { 867 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // Enumeration<CommonLanguages> 868 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 869 default: return super.getProperty(hash, name, checkValid); 870 } 871 872 } 873 874 @Override 875 public Base setProperty(int hash, String name, Base value) throws FHIRException { 876 switch (hash) { 877 case -1613589672: // language 878 value = new CommonLanguagesEnumFactory().fromType(TypeConvertor.castToCode(value)); 879 this.language = (Enumeration) value; // Enumeration<CommonLanguages> 880 return value; 881 case -1724546052: // description 882 this.description = TypeConvertor.castToString(value); // StringType 883 return value; 884 default: return super.setProperty(hash, name, value); 885 } 886 887 } 888 889 @Override 890 public Base setProperty(String name, Base value) throws FHIRException { 891 if (name.equals("language")) { 892 value = new CommonLanguagesEnumFactory().fromType(TypeConvertor.castToCode(value)); 893 this.language = (Enumeration) value; // Enumeration<CommonLanguages> 894 } else if (name.equals("description")) { 895 this.description = TypeConvertor.castToString(value); // StringType 896 } else 897 return super.setProperty(name, value); 898 return value; 899 } 900 901 @Override 902 public void removeChild(String name, Base value) throws FHIRException { 903 if (name.equals("language")) { 904 value = new CommonLanguagesEnumFactory().fromType(TypeConvertor.castToCode(value)); 905 this.language = (Enumeration) value; // Enumeration<CommonLanguages> 906 } else if (name.equals("description")) { 907 this.description = null; 908 } else 909 super.removeChild(name, value); 910 911 } 912 913 @Override 914 public Base makeProperty(int hash, String name) throws FHIRException { 915 switch (hash) { 916 case -1613589672: return getLanguageElement(); 917 case -1724546052: return getDescriptionElement(); 918 default: return super.makeProperty(hash, name); 919 } 920 921 } 922 923 @Override 924 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 925 switch (hash) { 926 case -1613589672: /*language*/ return new String[] {"code"}; 927 case -1724546052: /*description*/ return new String[] {"string"}; 928 default: return super.getTypesForProperty(hash, name); 929 } 930 931 } 932 933 @Override 934 public Base addChild(String name) throws FHIRException { 935 if (name.equals("language")) { 936 throw new FHIRException("Cannot call addChild on a singleton property InventoryItem.description.language"); 937 } 938 else if (name.equals("description")) { 939 throw new FHIRException("Cannot call addChild on a singleton property InventoryItem.description.description"); 940 } 941 else 942 return super.addChild(name); 943 } 944 945 public InventoryItemDescriptionComponent copy() { 946 InventoryItemDescriptionComponent dst = new InventoryItemDescriptionComponent(); 947 copyValues(dst); 948 return dst; 949 } 950 951 public void copyValues(InventoryItemDescriptionComponent dst) { 952 super.copyValues(dst); 953 dst.language = language == null ? null : language.copy(); 954 dst.description = description == null ? null : description.copy(); 955 } 956 957 @Override 958 public boolean equalsDeep(Base other_) { 959 if (!super.equalsDeep(other_)) 960 return false; 961 if (!(other_ instanceof InventoryItemDescriptionComponent)) 962 return false; 963 InventoryItemDescriptionComponent o = (InventoryItemDescriptionComponent) other_; 964 return compareDeep(language, o.language, true) && compareDeep(description, o.description, true) 965 ; 966 } 967 968 @Override 969 public boolean equalsShallow(Base other_) { 970 if (!super.equalsShallow(other_)) 971 return false; 972 if (!(other_ instanceof InventoryItemDescriptionComponent)) 973 return false; 974 InventoryItemDescriptionComponent o = (InventoryItemDescriptionComponent) other_; 975 return compareValues(language, o.language, true) && compareValues(description, o.description, true) 976 ; 977 } 978 979 public boolean isEmpty() { 980 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, description); 981 } 982 983 public String fhirType() { 984 return "InventoryItem.description"; 985 986 } 987 988 } 989 990 @Block() 991 public static class InventoryItemAssociationComponent extends BackboneElement implements IBaseBackboneElement { 992 /** 993 * This attribute defined the type of association when establishing associations or relations between items, e.g. 'packaged within' or 'used with' or 'to be mixed with. 994 */ 995 @Child(name = "associationType", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 996 @Description(shortDefinition="The type of association between the device and the other item", formalDefinition="This attribute defined the type of association when establishing associations or relations between items, e.g. 'packaged within' or 'used with' or 'to be mixed with." ) 997 protected CodeableConcept associationType; 998 999 /** 1000 * The related item or product. 1001 */ 1002 @Child(name = "relatedItem", type = {InventoryItem.class, Medication.class, MedicationKnowledge.class, Device.class, DeviceDefinition.class, NutritionProduct.class, BiologicallyDerivedProduct.class}, order=2, min=1, max=1, modifier=false, summary=true) 1003 @Description(shortDefinition="The related item or product", formalDefinition="The related item or product." ) 1004 protected Reference relatedItem; 1005 1006 /** 1007 * The quantity of the related product in this product - Numerator is the quantity of the related product. Denominator is the quantity of the present product. For example a value of 20 means that this product contains 20 units of the related product; a value of 1:20 means the inverse - that the contained product contains 20 units of the present product. 1008 */ 1009 @Child(name = "quantity", type = {Ratio.class}, order=3, min=1, max=1, modifier=false, summary=true) 1010 @Description(shortDefinition="The quantity of the product in this product", formalDefinition="The quantity of the related product in this product - Numerator is the quantity of the related product. Denominator is the quantity of the present product. For example a value of 20 means that this product contains 20 units of the related product; a value of 1:20 means the inverse - that the contained product contains 20 units of the present product." ) 1011 protected Ratio quantity; 1012 1013 private static final long serialVersionUID = -1001386921L; 1014 1015 /** 1016 * Constructor 1017 */ 1018 public InventoryItemAssociationComponent() { 1019 super(); 1020 } 1021 1022 /** 1023 * Constructor 1024 */ 1025 public InventoryItemAssociationComponent(CodeableConcept associationType, Reference relatedItem, Ratio quantity) { 1026 super(); 1027 this.setAssociationType(associationType); 1028 this.setRelatedItem(relatedItem); 1029 this.setQuantity(quantity); 1030 } 1031 1032 /** 1033 * @return {@link #associationType} (This attribute defined the type of association when establishing associations or relations between items, e.g. 'packaged within' or 'used with' or 'to be mixed with.) 1034 */ 1035 public CodeableConcept getAssociationType() { 1036 if (this.associationType == null) 1037 if (Configuration.errorOnAutoCreate()) 1038 throw new Error("Attempt to auto-create InventoryItemAssociationComponent.associationType"); 1039 else if (Configuration.doAutoCreate()) 1040 this.associationType = new CodeableConcept(); // cc 1041 return this.associationType; 1042 } 1043 1044 public boolean hasAssociationType() { 1045 return this.associationType != null && !this.associationType.isEmpty(); 1046 } 1047 1048 /** 1049 * @param value {@link #associationType} (This attribute defined the type of association when establishing associations or relations between items, e.g. 'packaged within' or 'used with' or 'to be mixed with.) 1050 */ 1051 public InventoryItemAssociationComponent setAssociationType(CodeableConcept value) { 1052 this.associationType = value; 1053 return this; 1054 } 1055 1056 /** 1057 * @return {@link #relatedItem} (The related item or product.) 1058 */ 1059 public Reference getRelatedItem() { 1060 if (this.relatedItem == null) 1061 if (Configuration.errorOnAutoCreate()) 1062 throw new Error("Attempt to auto-create InventoryItemAssociationComponent.relatedItem"); 1063 else if (Configuration.doAutoCreate()) 1064 this.relatedItem = new Reference(); // cc 1065 return this.relatedItem; 1066 } 1067 1068 public boolean hasRelatedItem() { 1069 return this.relatedItem != null && !this.relatedItem.isEmpty(); 1070 } 1071 1072 /** 1073 * @param value {@link #relatedItem} (The related item or product.) 1074 */ 1075 public InventoryItemAssociationComponent setRelatedItem(Reference value) { 1076 this.relatedItem = value; 1077 return this; 1078 } 1079 1080 /** 1081 * @return {@link #quantity} (The quantity of the related product in this product - Numerator is the quantity of the related product. Denominator is the quantity of the present product. For example a value of 20 means that this product contains 20 units of the related product; a value of 1:20 means the inverse - that the contained product contains 20 units of the present product.) 1082 */ 1083 public Ratio getQuantity() { 1084 if (this.quantity == null) 1085 if (Configuration.errorOnAutoCreate()) 1086 throw new Error("Attempt to auto-create InventoryItemAssociationComponent.quantity"); 1087 else if (Configuration.doAutoCreate()) 1088 this.quantity = new Ratio(); // cc 1089 return this.quantity; 1090 } 1091 1092 public boolean hasQuantity() { 1093 return this.quantity != null && !this.quantity.isEmpty(); 1094 } 1095 1096 /** 1097 * @param value {@link #quantity} (The quantity of the related product in this product - Numerator is the quantity of the related product. Denominator is the quantity of the present product. For example a value of 20 means that this product contains 20 units of the related product; a value of 1:20 means the inverse - that the contained product contains 20 units of the present product.) 1098 */ 1099 public InventoryItemAssociationComponent setQuantity(Ratio value) { 1100 this.quantity = value; 1101 return this; 1102 } 1103 1104 protected void listChildren(List<Property> children) { 1105 super.listChildren(children); 1106 children.add(new Property("associationType", "CodeableConcept", "This attribute defined the type of association when establishing associations or relations between items, e.g. 'packaged within' or 'used with' or 'to be mixed with.", 0, 1, associationType)); 1107 children.add(new Property("relatedItem", "Reference(InventoryItem|Medication|MedicationKnowledge|Device|DeviceDefinition|NutritionProduct|BiologicallyDerivedProduct)", "The related item or product.", 0, 1, relatedItem)); 1108 children.add(new Property("quantity", "Ratio", "The quantity of the related product in this product - Numerator is the quantity of the related product. Denominator is the quantity of the present product. For example a value of 20 means that this product contains 20 units of the related product; a value of 1:20 means the inverse - that the contained product contains 20 units of the present product.", 0, 1, quantity)); 1109 } 1110 1111 @Override 1112 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1113 switch (_hash) { 1114 case 2050799451: /*associationType*/ return new Property("associationType", "CodeableConcept", "This attribute defined the type of association when establishing associations or relations between items, e.g. 'packaged within' or 'used with' or 'to be mixed with.", 0, 1, associationType); 1115 case 1112702430: /*relatedItem*/ return new Property("relatedItem", "Reference(InventoryItem|Medication|MedicationKnowledge|Device|DeviceDefinition|NutritionProduct|BiologicallyDerivedProduct)", "The related item or product.", 0, 1, relatedItem); 1116 case -1285004149: /*quantity*/ return new Property("quantity", "Ratio", "The quantity of the related product in this product - Numerator is the quantity of the related product. Denominator is the quantity of the present product. For example a value of 20 means that this product contains 20 units of the related product; a value of 1:20 means the inverse - that the contained product contains 20 units of the present product.", 0, 1, quantity); 1117 default: return super.getNamedProperty(_hash, _name, _checkValid); 1118 } 1119 1120 } 1121 1122 @Override 1123 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1124 switch (hash) { 1125 case 2050799451: /*associationType*/ return this.associationType == null ? new Base[0] : new Base[] {this.associationType}; // CodeableConcept 1126 case 1112702430: /*relatedItem*/ return this.relatedItem == null ? new Base[0] : new Base[] {this.relatedItem}; // Reference 1127 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Ratio 1128 default: return super.getProperty(hash, name, checkValid); 1129 } 1130 1131 } 1132 1133 @Override 1134 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1135 switch (hash) { 1136 case 2050799451: // associationType 1137 this.associationType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1138 return value; 1139 case 1112702430: // relatedItem 1140 this.relatedItem = TypeConvertor.castToReference(value); // Reference 1141 return value; 1142 case -1285004149: // quantity 1143 this.quantity = TypeConvertor.castToRatio(value); // Ratio 1144 return value; 1145 default: return super.setProperty(hash, name, value); 1146 } 1147 1148 } 1149 1150 @Override 1151 public Base setProperty(String name, Base value) throws FHIRException { 1152 if (name.equals("associationType")) { 1153 this.associationType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1154 } else if (name.equals("relatedItem")) { 1155 this.relatedItem = TypeConvertor.castToReference(value); // Reference 1156 } else if (name.equals("quantity")) { 1157 this.quantity = TypeConvertor.castToRatio(value); // Ratio 1158 } else 1159 return super.setProperty(name, value); 1160 return value; 1161 } 1162 1163 @Override 1164 public void removeChild(String name, Base value) throws FHIRException { 1165 if (name.equals("associationType")) { 1166 this.associationType = null; 1167 } else if (name.equals("relatedItem")) { 1168 this.relatedItem = null; 1169 } else if (name.equals("quantity")) { 1170 this.quantity = null; 1171 } else 1172 super.removeChild(name, value); 1173 1174 } 1175 1176 @Override 1177 public Base makeProperty(int hash, String name) throws FHIRException { 1178 switch (hash) { 1179 case 2050799451: return getAssociationType(); 1180 case 1112702430: return getRelatedItem(); 1181 case -1285004149: return getQuantity(); 1182 default: return super.makeProperty(hash, name); 1183 } 1184 1185 } 1186 1187 @Override 1188 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1189 switch (hash) { 1190 case 2050799451: /*associationType*/ return new String[] {"CodeableConcept"}; 1191 case 1112702430: /*relatedItem*/ return new String[] {"Reference"}; 1192 case -1285004149: /*quantity*/ return new String[] {"Ratio"}; 1193 default: return super.getTypesForProperty(hash, name); 1194 } 1195 1196 } 1197 1198 @Override 1199 public Base addChild(String name) throws FHIRException { 1200 if (name.equals("associationType")) { 1201 this.associationType = new CodeableConcept(); 1202 return this.associationType; 1203 } 1204 else if (name.equals("relatedItem")) { 1205 this.relatedItem = new Reference(); 1206 return this.relatedItem; 1207 } 1208 else if (name.equals("quantity")) { 1209 this.quantity = new Ratio(); 1210 return this.quantity; 1211 } 1212 else 1213 return super.addChild(name); 1214 } 1215 1216 public InventoryItemAssociationComponent copy() { 1217 InventoryItemAssociationComponent dst = new InventoryItemAssociationComponent(); 1218 copyValues(dst); 1219 return dst; 1220 } 1221 1222 public void copyValues(InventoryItemAssociationComponent dst) { 1223 super.copyValues(dst); 1224 dst.associationType = associationType == null ? null : associationType.copy(); 1225 dst.relatedItem = relatedItem == null ? null : relatedItem.copy(); 1226 dst.quantity = quantity == null ? null : quantity.copy(); 1227 } 1228 1229 @Override 1230 public boolean equalsDeep(Base other_) { 1231 if (!super.equalsDeep(other_)) 1232 return false; 1233 if (!(other_ instanceof InventoryItemAssociationComponent)) 1234 return false; 1235 InventoryItemAssociationComponent o = (InventoryItemAssociationComponent) other_; 1236 return compareDeep(associationType, o.associationType, true) && compareDeep(relatedItem, o.relatedItem, true) 1237 && compareDeep(quantity, o.quantity, true); 1238 } 1239 1240 @Override 1241 public boolean equalsShallow(Base other_) { 1242 if (!super.equalsShallow(other_)) 1243 return false; 1244 if (!(other_ instanceof InventoryItemAssociationComponent)) 1245 return false; 1246 InventoryItemAssociationComponent o = (InventoryItemAssociationComponent) other_; 1247 return true; 1248 } 1249 1250 public boolean isEmpty() { 1251 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(associationType, relatedItem 1252 , quantity); 1253 } 1254 1255 public String fhirType() { 1256 return "InventoryItem.association"; 1257 1258 } 1259 1260 } 1261 1262 @Block() 1263 public static class InventoryItemCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 1264 /** 1265 * The type of characteristic that is being defined. 1266 */ 1267 @Child(name = "characteristicType", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1268 @Description(shortDefinition="The characteristic that is being defined", formalDefinition="The type of characteristic that is being defined." ) 1269 protected CodeableConcept characteristicType; 1270 1271 /** 1272 * The value of the attribute. 1273 */ 1274 @Child(name = "value", type = {StringType.class, IntegerType.class, DecimalType.class, BooleanType.class, UrlType.class, DateTimeType.class, Quantity.class, Range.class, Ratio.class, Annotation.class, Address.class, Duration.class, CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 1275 @Description(shortDefinition="The value of the attribute", formalDefinition="The value of the attribute." ) 1276 protected DataType value; 1277 1278 private static final long serialVersionUID = -642436065L; 1279 1280 /** 1281 * Constructor 1282 */ 1283 public InventoryItemCharacteristicComponent() { 1284 super(); 1285 } 1286 1287 /** 1288 * Constructor 1289 */ 1290 public InventoryItemCharacteristicComponent(CodeableConcept characteristicType, DataType value) { 1291 super(); 1292 this.setCharacteristicType(characteristicType); 1293 this.setValue(value); 1294 } 1295 1296 /** 1297 * @return {@link #characteristicType} (The type of characteristic that is being defined.) 1298 */ 1299 public CodeableConcept getCharacteristicType() { 1300 if (this.characteristicType == null) 1301 if (Configuration.errorOnAutoCreate()) 1302 throw new Error("Attempt to auto-create InventoryItemCharacteristicComponent.characteristicType"); 1303 else if (Configuration.doAutoCreate()) 1304 this.characteristicType = new CodeableConcept(); // cc 1305 return this.characteristicType; 1306 } 1307 1308 public boolean hasCharacteristicType() { 1309 return this.characteristicType != null && !this.characteristicType.isEmpty(); 1310 } 1311 1312 /** 1313 * @param value {@link #characteristicType} (The type of characteristic that is being defined.) 1314 */ 1315 public InventoryItemCharacteristicComponent setCharacteristicType(CodeableConcept value) { 1316 this.characteristicType = value; 1317 return this; 1318 } 1319 1320 /** 1321 * @return {@link #value} (The value of the attribute.) 1322 */ 1323 public DataType getValue() { 1324 return this.value; 1325 } 1326 1327 /** 1328 * @return {@link #value} (The value of the attribute.) 1329 */ 1330 public StringType getValueStringType() throws FHIRException { 1331 if (this.value == null) 1332 this.value = new StringType(); 1333 if (!(this.value instanceof StringType)) 1334 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 1335 return (StringType) this.value; 1336 } 1337 1338 public boolean hasValueStringType() { 1339 return this.value instanceof StringType; 1340 } 1341 1342 /** 1343 * @return {@link #value} (The value of the attribute.) 1344 */ 1345 public IntegerType getValueIntegerType() throws FHIRException { 1346 if (this.value == null) 1347 this.value = new IntegerType(); 1348 if (!(this.value instanceof IntegerType)) 1349 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 1350 return (IntegerType) this.value; 1351 } 1352 1353 public boolean hasValueIntegerType() { 1354 return this.value instanceof IntegerType; 1355 } 1356 1357 /** 1358 * @return {@link #value} (The value of the attribute.) 1359 */ 1360 public DecimalType getValueDecimalType() throws FHIRException { 1361 if (this.value == null) 1362 this.value = new DecimalType(); 1363 if (!(this.value instanceof DecimalType)) 1364 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 1365 return (DecimalType) this.value; 1366 } 1367 1368 public boolean hasValueDecimalType() { 1369 return this.value instanceof DecimalType; 1370 } 1371 1372 /** 1373 * @return {@link #value} (The value of the attribute.) 1374 */ 1375 public BooleanType getValueBooleanType() throws FHIRException { 1376 if (this.value == null) 1377 this.value = new BooleanType(); 1378 if (!(this.value instanceof BooleanType)) 1379 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 1380 return (BooleanType) this.value; 1381 } 1382 1383 public boolean hasValueBooleanType() { 1384 return this.value instanceof BooleanType; 1385 } 1386 1387 /** 1388 * @return {@link #value} (The value of the attribute.) 1389 */ 1390 public UrlType getValueUrlType() throws FHIRException { 1391 if (this.value == null) 1392 this.value = new UrlType(); 1393 if (!(this.value instanceof UrlType)) 1394 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.value.getClass().getName()+" was encountered"); 1395 return (UrlType) this.value; 1396 } 1397 1398 public boolean hasValueUrlType() { 1399 return this.value instanceof UrlType; 1400 } 1401 1402 /** 1403 * @return {@link #value} (The value of the attribute.) 1404 */ 1405 public DateTimeType getValueDateTimeType() throws FHIRException { 1406 if (this.value == null) 1407 this.value = new DateTimeType(); 1408 if (!(this.value instanceof DateTimeType)) 1409 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 1410 return (DateTimeType) this.value; 1411 } 1412 1413 public boolean hasValueDateTimeType() { 1414 return this.value instanceof DateTimeType; 1415 } 1416 1417 /** 1418 * @return {@link #value} (The value of the attribute.) 1419 */ 1420 public Quantity getValueQuantity() throws FHIRException { 1421 if (this.value == null) 1422 this.value = new Quantity(); 1423 if (!(this.value instanceof Quantity)) 1424 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1425 return (Quantity) this.value; 1426 } 1427 1428 public boolean hasValueQuantity() { 1429 return this.value instanceof Quantity; 1430 } 1431 1432 /** 1433 * @return {@link #value} (The value of the attribute.) 1434 */ 1435 public Range getValueRange() throws FHIRException { 1436 if (this.value == null) 1437 this.value = new Range(); 1438 if (!(this.value instanceof Range)) 1439 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 1440 return (Range) this.value; 1441 } 1442 1443 public boolean hasValueRange() { 1444 return this.value instanceof Range; 1445 } 1446 1447 /** 1448 * @return {@link #value} (The value of the attribute.) 1449 */ 1450 public Ratio getValueRatio() throws FHIRException { 1451 if (this.value == null) 1452 this.value = new Ratio(); 1453 if (!(this.value instanceof Ratio)) 1454 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 1455 return (Ratio) this.value; 1456 } 1457 1458 public boolean hasValueRatio() { 1459 return this.value instanceof Ratio; 1460 } 1461 1462 /** 1463 * @return {@link #value} (The value of the attribute.) 1464 */ 1465 public Annotation getValueAnnotation() throws FHIRException { 1466 if (this.value == null) 1467 this.value = new Annotation(); 1468 if (!(this.value instanceof Annotation)) 1469 throw new FHIRException("Type mismatch: the type Annotation was expected, but "+this.value.getClass().getName()+" was encountered"); 1470 return (Annotation) this.value; 1471 } 1472 1473 public boolean hasValueAnnotation() { 1474 return this.value instanceof Annotation; 1475 } 1476 1477 /** 1478 * @return {@link #value} (The value of the attribute.) 1479 */ 1480 public Address getValueAddress() throws FHIRException { 1481 if (this.value == null) 1482 this.value = new Address(); 1483 if (!(this.value instanceof Address)) 1484 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.value.getClass().getName()+" was encountered"); 1485 return (Address) this.value; 1486 } 1487 1488 public boolean hasValueAddress() { 1489 return this.value instanceof Address; 1490 } 1491 1492 /** 1493 * @return {@link #value} (The value of the attribute.) 1494 */ 1495 public Duration getValueDuration() throws FHIRException { 1496 if (this.value == null) 1497 this.value = new Duration(); 1498 if (!(this.value instanceof Duration)) 1499 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.value.getClass().getName()+" was encountered"); 1500 return (Duration) this.value; 1501 } 1502 1503 public boolean hasValueDuration() { 1504 return this.value instanceof Duration; 1505 } 1506 1507 /** 1508 * @return {@link #value} (The value of the attribute.) 1509 */ 1510 public CodeableConcept getValueCodeableConcept() throws FHIRException { 1511 if (this.value == null) 1512 this.value = new CodeableConcept(); 1513 if (!(this.value instanceof CodeableConcept)) 1514 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 1515 return (CodeableConcept) this.value; 1516 } 1517 1518 public boolean hasValueCodeableConcept() { 1519 return this.value instanceof CodeableConcept; 1520 } 1521 1522 public boolean hasValue() { 1523 return this.value != null && !this.value.isEmpty(); 1524 } 1525 1526 /** 1527 * @param value {@link #value} (The value of the attribute.) 1528 */ 1529 public InventoryItemCharacteristicComponent setValue(DataType value) { 1530 if (value != null && !(value instanceof StringType || value instanceof IntegerType || value instanceof DecimalType || value instanceof BooleanType || value instanceof UrlType || value instanceof DateTimeType || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof Annotation || value instanceof Address || value instanceof Duration || value instanceof CodeableConcept)) 1531 throw new FHIRException("Not the right type for InventoryItem.characteristic.value[x]: "+value.fhirType()); 1532 this.value = value; 1533 return this; 1534 } 1535 1536 protected void listChildren(List<Property> children) { 1537 super.listChildren(children); 1538 children.add(new Property("characteristicType", "CodeableConcept", "The type of characteristic that is being defined.", 0, 1, characteristicType)); 1539 children.add(new Property("value[x]", "string|integer|decimal|boolean|url|dateTime|Quantity|Range|Ratio|Annotation|Address|Duration|CodeableConcept", "The value of the attribute.", 0, 1, value)); 1540 } 1541 1542 @Override 1543 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1544 switch (_hash) { 1545 case 1172127605: /*characteristicType*/ return new Property("characteristicType", "CodeableConcept", "The type of characteristic that is being defined.", 0, 1, characteristicType); 1546 case -1410166417: /*value[x]*/ return new Property("value[x]", "string|integer|decimal|boolean|url|dateTime|Quantity|Range|Ratio|Annotation|Address|Duration|CodeableConcept", "The value of the attribute.", 0, 1, value); 1547 case 111972721: /*value*/ return new Property("value[x]", "string|integer|decimal|boolean|url|dateTime|Quantity|Range|Ratio|Annotation|Address|Duration|CodeableConcept", "The value of the attribute.", 0, 1, value); 1548 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The value of the attribute.", 0, 1, value); 1549 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The value of the attribute.", 0, 1, value); 1550 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The value of the attribute.", 0, 1, value); 1551 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of the attribute.", 0, 1, value); 1552 case -1410172354: /*valueUrl*/ return new Property("value[x]", "url", "The value of the attribute.", 0, 1, value); 1553 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The value of the attribute.", 0, 1, value); 1554 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The value of the attribute.", 0, 1, value); 1555 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The value of the attribute.", 0, 1, value); 1556 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "The value of the attribute.", 0, 1, value); 1557 case -67108992: /*valueAnnotation*/ return new Property("value[x]", "Annotation", "The value of the attribute.", 0, 1, value); 1558 case -478981821: /*valueAddress*/ return new Property("value[x]", "Address", "The value of the attribute.", 0, 1, value); 1559 case 1558135333: /*valueDuration*/ return new Property("value[x]", "Duration", "The value of the attribute.", 0, 1, value); 1560 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The value of the attribute.", 0, 1, value); 1561 default: return super.getNamedProperty(_hash, _name, _checkValid); 1562 } 1563 1564 } 1565 1566 @Override 1567 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1568 switch (hash) { 1569 case 1172127605: /*characteristicType*/ return this.characteristicType == null ? new Base[0] : new Base[] {this.characteristicType}; // CodeableConcept 1570 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1571 default: return super.getProperty(hash, name, checkValid); 1572 } 1573 1574 } 1575 1576 @Override 1577 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1578 switch (hash) { 1579 case 1172127605: // characteristicType 1580 this.characteristicType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1581 return value; 1582 case 111972721: // value 1583 this.value = TypeConvertor.castToType(value); // DataType 1584 return value; 1585 default: return super.setProperty(hash, name, value); 1586 } 1587 1588 } 1589 1590 @Override 1591 public Base setProperty(String name, Base value) throws FHIRException { 1592 if (name.equals("characteristicType")) { 1593 this.characteristicType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1594 } else if (name.equals("value[x]")) { 1595 this.value = TypeConvertor.castToType(value); // DataType 1596 } else 1597 return super.setProperty(name, value); 1598 return value; 1599 } 1600 1601 @Override 1602 public void removeChild(String name, Base value) throws FHIRException { 1603 if (name.equals("characteristicType")) { 1604 this.characteristicType = null; 1605 } else if (name.equals("value[x]")) { 1606 this.value = null; 1607 } else 1608 super.removeChild(name, value); 1609 1610 } 1611 1612 @Override 1613 public Base makeProperty(int hash, String name) throws FHIRException { 1614 switch (hash) { 1615 case 1172127605: return getCharacteristicType(); 1616 case -1410166417: return getValue(); 1617 case 111972721: return getValue(); 1618 default: return super.makeProperty(hash, name); 1619 } 1620 1621 } 1622 1623 @Override 1624 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1625 switch (hash) { 1626 case 1172127605: /*characteristicType*/ return new String[] {"CodeableConcept"}; 1627 case 111972721: /*value*/ return new String[] {"string", "integer", "decimal", "boolean", "url", "dateTime", "Quantity", "Range", "Ratio", "Annotation", "Address", "Duration", "CodeableConcept"}; 1628 default: return super.getTypesForProperty(hash, name); 1629 } 1630 1631 } 1632 1633 @Override 1634 public Base addChild(String name) throws FHIRException { 1635 if (name.equals("characteristicType")) { 1636 this.characteristicType = new CodeableConcept(); 1637 return this.characteristicType; 1638 } 1639 else if (name.equals("valueString")) { 1640 this.value = new StringType(); 1641 return this.value; 1642 } 1643 else if (name.equals("valueInteger")) { 1644 this.value = new IntegerType(); 1645 return this.value; 1646 } 1647 else if (name.equals("valueDecimal")) { 1648 this.value = new DecimalType(); 1649 return this.value; 1650 } 1651 else if (name.equals("valueBoolean")) { 1652 this.value = new BooleanType(); 1653 return this.value; 1654 } 1655 else if (name.equals("valueUrl")) { 1656 this.value = new UrlType(); 1657 return this.value; 1658 } 1659 else if (name.equals("valueDateTime")) { 1660 this.value = new DateTimeType(); 1661 return this.value; 1662 } 1663 else if (name.equals("valueQuantity")) { 1664 this.value = new Quantity(); 1665 return this.value; 1666 } 1667 else if (name.equals("valueRange")) { 1668 this.value = new Range(); 1669 return this.value; 1670 } 1671 else if (name.equals("valueRatio")) { 1672 this.value = new Ratio(); 1673 return this.value; 1674 } 1675 else if (name.equals("valueAnnotation")) { 1676 this.value = new Annotation(); 1677 return this.value; 1678 } 1679 else if (name.equals("valueAddress")) { 1680 this.value = new Address(); 1681 return this.value; 1682 } 1683 else if (name.equals("valueDuration")) { 1684 this.value = new Duration(); 1685 return this.value; 1686 } 1687 else if (name.equals("valueCodeableConcept")) { 1688 this.value = new CodeableConcept(); 1689 return this.value; 1690 } 1691 else 1692 return super.addChild(name); 1693 } 1694 1695 public InventoryItemCharacteristicComponent copy() { 1696 InventoryItemCharacteristicComponent dst = new InventoryItemCharacteristicComponent(); 1697 copyValues(dst); 1698 return dst; 1699 } 1700 1701 public void copyValues(InventoryItemCharacteristicComponent dst) { 1702 super.copyValues(dst); 1703 dst.characteristicType = characteristicType == null ? null : characteristicType.copy(); 1704 dst.value = value == null ? null : value.copy(); 1705 } 1706 1707 @Override 1708 public boolean equalsDeep(Base other_) { 1709 if (!super.equalsDeep(other_)) 1710 return false; 1711 if (!(other_ instanceof InventoryItemCharacteristicComponent)) 1712 return false; 1713 InventoryItemCharacteristicComponent o = (InventoryItemCharacteristicComponent) other_; 1714 return compareDeep(characteristicType, o.characteristicType, true) && compareDeep(value, o.value, true) 1715 ; 1716 } 1717 1718 @Override 1719 public boolean equalsShallow(Base other_) { 1720 if (!super.equalsShallow(other_)) 1721 return false; 1722 if (!(other_ instanceof InventoryItemCharacteristicComponent)) 1723 return false; 1724 InventoryItemCharacteristicComponent o = (InventoryItemCharacteristicComponent) other_; 1725 return true; 1726 } 1727 1728 public boolean isEmpty() { 1729 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(characteristicType, value 1730 ); 1731 } 1732 1733 public String fhirType() { 1734 return "InventoryItem.characteristic"; 1735 1736 } 1737 1738 } 1739 1740 @Block() 1741 public static class InventoryItemInstanceComponent extends BackboneElement implements IBaseBackboneElement { 1742 /** 1743 * The identifier for the physical instance, typically a serial number. 1744 */ 1745 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1746 @Description(shortDefinition="The identifier for the physical instance, typically a serial number", formalDefinition="The identifier for the physical instance, typically a serial number." ) 1747 protected List<Identifier> identifier; 1748 1749 /** 1750 * The lot or batch number of the item. 1751 */ 1752 @Child(name = "lotNumber", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1753 @Description(shortDefinition="The lot or batch number of the item", formalDefinition="The lot or batch number of the item." ) 1754 protected StringType lotNumber; 1755 1756 /** 1757 * The expiry date or date and time for the product. 1758 */ 1759 @Child(name = "expiry", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1760 @Description(shortDefinition="The expiry date or date and time for the product", formalDefinition="The expiry date or date and time for the product." ) 1761 protected DateTimeType expiry; 1762 1763 /** 1764 * The subject that the item is associated with. 1765 */ 1766 @Child(name = "subject", type = {Patient.class, Organization.class}, order=4, min=0, max=1, modifier=false, summary=false) 1767 @Description(shortDefinition="The subject that the item is associated with", formalDefinition="The subject that the item is associated with." ) 1768 protected Reference subject; 1769 1770 /** 1771 * The location that the item is associated with. 1772 */ 1773 @Child(name = "location", type = {Location.class}, order=5, min=0, max=1, modifier=false, summary=false) 1774 @Description(shortDefinition="The location that the item is associated with", formalDefinition="The location that the item is associated with." ) 1775 protected Reference location; 1776 1777 private static final long serialVersionUID = 657936980L; 1778 1779 /** 1780 * Constructor 1781 */ 1782 public InventoryItemInstanceComponent() { 1783 super(); 1784 } 1785 1786 /** 1787 * @return {@link #identifier} (The identifier for the physical instance, typically a serial number.) 1788 */ 1789 public List<Identifier> getIdentifier() { 1790 if (this.identifier == null) 1791 this.identifier = new ArrayList<Identifier>(); 1792 return this.identifier; 1793 } 1794 1795 /** 1796 * @return Returns a reference to <code>this</code> for easy method chaining 1797 */ 1798 public InventoryItemInstanceComponent setIdentifier(List<Identifier> theIdentifier) { 1799 this.identifier = theIdentifier; 1800 return this; 1801 } 1802 1803 public boolean hasIdentifier() { 1804 if (this.identifier == null) 1805 return false; 1806 for (Identifier item : this.identifier) 1807 if (!item.isEmpty()) 1808 return true; 1809 return false; 1810 } 1811 1812 public Identifier addIdentifier() { //3 1813 Identifier t = new Identifier(); 1814 if (this.identifier == null) 1815 this.identifier = new ArrayList<Identifier>(); 1816 this.identifier.add(t); 1817 return t; 1818 } 1819 1820 public InventoryItemInstanceComponent addIdentifier(Identifier t) { //3 1821 if (t == null) 1822 return this; 1823 if (this.identifier == null) 1824 this.identifier = new ArrayList<Identifier>(); 1825 this.identifier.add(t); 1826 return this; 1827 } 1828 1829 /** 1830 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1831 */ 1832 public Identifier getIdentifierFirstRep() { 1833 if (getIdentifier().isEmpty()) { 1834 addIdentifier(); 1835 } 1836 return getIdentifier().get(0); 1837 } 1838 1839 /** 1840 * @return {@link #lotNumber} (The lot or batch number of the item.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 1841 */ 1842 public StringType getLotNumberElement() { 1843 if (this.lotNumber == null) 1844 if (Configuration.errorOnAutoCreate()) 1845 throw new Error("Attempt to auto-create InventoryItemInstanceComponent.lotNumber"); 1846 else if (Configuration.doAutoCreate()) 1847 this.lotNumber = new StringType(); // bb 1848 return this.lotNumber; 1849 } 1850 1851 public boolean hasLotNumberElement() { 1852 return this.lotNumber != null && !this.lotNumber.isEmpty(); 1853 } 1854 1855 public boolean hasLotNumber() { 1856 return this.lotNumber != null && !this.lotNumber.isEmpty(); 1857 } 1858 1859 /** 1860 * @param value {@link #lotNumber} (The lot or batch number of the item.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 1861 */ 1862 public InventoryItemInstanceComponent setLotNumberElement(StringType value) { 1863 this.lotNumber = value; 1864 return this; 1865 } 1866 1867 /** 1868 * @return The lot or batch number of the item. 1869 */ 1870 public String getLotNumber() { 1871 return this.lotNumber == null ? null : this.lotNumber.getValue(); 1872 } 1873 1874 /** 1875 * @param value The lot or batch number of the item. 1876 */ 1877 public InventoryItemInstanceComponent setLotNumber(String value) { 1878 if (Utilities.noString(value)) 1879 this.lotNumber = null; 1880 else { 1881 if (this.lotNumber == null) 1882 this.lotNumber = new StringType(); 1883 this.lotNumber.setValue(value); 1884 } 1885 return this; 1886 } 1887 1888 /** 1889 * @return {@link #expiry} (The expiry date or date and time for the product.). This is the underlying object with id, value and extensions. The accessor "getExpiry" gives direct access to the value 1890 */ 1891 public DateTimeType getExpiryElement() { 1892 if (this.expiry == null) 1893 if (Configuration.errorOnAutoCreate()) 1894 throw new Error("Attempt to auto-create InventoryItemInstanceComponent.expiry"); 1895 else if (Configuration.doAutoCreate()) 1896 this.expiry = new DateTimeType(); // bb 1897 return this.expiry; 1898 } 1899 1900 public boolean hasExpiryElement() { 1901 return this.expiry != null && !this.expiry.isEmpty(); 1902 } 1903 1904 public boolean hasExpiry() { 1905 return this.expiry != null && !this.expiry.isEmpty(); 1906 } 1907 1908 /** 1909 * @param value {@link #expiry} (The expiry date or date and time for the product.). This is the underlying object with id, value and extensions. The accessor "getExpiry" gives direct access to the value 1910 */ 1911 public InventoryItemInstanceComponent setExpiryElement(DateTimeType value) { 1912 this.expiry = value; 1913 return this; 1914 } 1915 1916 /** 1917 * @return The expiry date or date and time for the product. 1918 */ 1919 public Date getExpiry() { 1920 return this.expiry == null ? null : this.expiry.getValue(); 1921 } 1922 1923 /** 1924 * @param value The expiry date or date and time for the product. 1925 */ 1926 public InventoryItemInstanceComponent setExpiry(Date value) { 1927 if (value == null) 1928 this.expiry = null; 1929 else { 1930 if (this.expiry == null) 1931 this.expiry = new DateTimeType(); 1932 this.expiry.setValue(value); 1933 } 1934 return this; 1935 } 1936 1937 /** 1938 * @return {@link #subject} (The subject that the item is associated with.) 1939 */ 1940 public Reference getSubject() { 1941 if (this.subject == null) 1942 if (Configuration.errorOnAutoCreate()) 1943 throw new Error("Attempt to auto-create InventoryItemInstanceComponent.subject"); 1944 else if (Configuration.doAutoCreate()) 1945 this.subject = new Reference(); // cc 1946 return this.subject; 1947 } 1948 1949 public boolean hasSubject() { 1950 return this.subject != null && !this.subject.isEmpty(); 1951 } 1952 1953 /** 1954 * @param value {@link #subject} (The subject that the item is associated with.) 1955 */ 1956 public InventoryItemInstanceComponent setSubject(Reference value) { 1957 this.subject = value; 1958 return this; 1959 } 1960 1961 /** 1962 * @return {@link #location} (The location that the item is associated with.) 1963 */ 1964 public Reference getLocation() { 1965 if (this.location == null) 1966 if (Configuration.errorOnAutoCreate()) 1967 throw new Error("Attempt to auto-create InventoryItemInstanceComponent.location"); 1968 else if (Configuration.doAutoCreate()) 1969 this.location = new Reference(); // cc 1970 return this.location; 1971 } 1972 1973 public boolean hasLocation() { 1974 return this.location != null && !this.location.isEmpty(); 1975 } 1976 1977 /** 1978 * @param value {@link #location} (The location that the item is associated with.) 1979 */ 1980 public InventoryItemInstanceComponent setLocation(Reference value) { 1981 this.location = value; 1982 return this; 1983 } 1984 1985 protected void listChildren(List<Property> children) { 1986 super.listChildren(children); 1987 children.add(new Property("identifier", "Identifier", "The identifier for the physical instance, typically a serial number.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1988 children.add(new Property("lotNumber", "string", "The lot or batch number of the item.", 0, 1, lotNumber)); 1989 children.add(new Property("expiry", "dateTime", "The expiry date or date and time for the product.", 0, 1, expiry)); 1990 children.add(new Property("subject", "Reference(Patient|Organization)", "The subject that the item is associated with.", 0, 1, subject)); 1991 children.add(new Property("location", "Reference(Location)", "The location that the item is associated with.", 0, 1, location)); 1992 } 1993 1994 @Override 1995 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1996 switch (_hash) { 1997 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The identifier for the physical instance, typically a serial number.", 0, java.lang.Integer.MAX_VALUE, identifier); 1998 case 462547450: /*lotNumber*/ return new Property("lotNumber", "string", "The lot or batch number of the item.", 0, 1, lotNumber); 1999 case -1289159373: /*expiry*/ return new Property("expiry", "dateTime", "The expiry date or date and time for the product.", 0, 1, expiry); 2000 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Organization)", "The subject that the item is associated with.", 0, 1, subject); 2001 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The location that the item is associated with.", 0, 1, location); 2002 default: return super.getNamedProperty(_hash, _name, _checkValid); 2003 } 2004 2005 } 2006 2007 @Override 2008 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2009 switch (hash) { 2010 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2011 case 462547450: /*lotNumber*/ return this.lotNumber == null ? new Base[0] : new Base[] {this.lotNumber}; // StringType 2012 case -1289159373: /*expiry*/ return this.expiry == null ? new Base[0] : new Base[] {this.expiry}; // DateTimeType 2013 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2014 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 2015 default: return super.getProperty(hash, name, checkValid); 2016 } 2017 2018 } 2019 2020 @Override 2021 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2022 switch (hash) { 2023 case -1618432855: // identifier 2024 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2025 return value; 2026 case 462547450: // lotNumber 2027 this.lotNumber = TypeConvertor.castToString(value); // StringType 2028 return value; 2029 case -1289159373: // expiry 2030 this.expiry = TypeConvertor.castToDateTime(value); // DateTimeType 2031 return value; 2032 case -1867885268: // subject 2033 this.subject = TypeConvertor.castToReference(value); // Reference 2034 return value; 2035 case 1901043637: // location 2036 this.location = TypeConvertor.castToReference(value); // Reference 2037 return value; 2038 default: return super.setProperty(hash, name, value); 2039 } 2040 2041 } 2042 2043 @Override 2044 public Base setProperty(String name, Base value) throws FHIRException { 2045 if (name.equals("identifier")) { 2046 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2047 } else if (name.equals("lotNumber")) { 2048 this.lotNumber = TypeConvertor.castToString(value); // StringType 2049 } else if (name.equals("expiry")) { 2050 this.expiry = TypeConvertor.castToDateTime(value); // DateTimeType 2051 } else if (name.equals("subject")) { 2052 this.subject = TypeConvertor.castToReference(value); // Reference 2053 } else if (name.equals("location")) { 2054 this.location = TypeConvertor.castToReference(value); // Reference 2055 } else 2056 return super.setProperty(name, value); 2057 return value; 2058 } 2059 2060 @Override 2061 public void removeChild(String name, Base value) throws FHIRException { 2062 if (name.equals("identifier")) { 2063 this.getIdentifier().remove(value); 2064 } else if (name.equals("lotNumber")) { 2065 this.lotNumber = null; 2066 } else if (name.equals("expiry")) { 2067 this.expiry = null; 2068 } else if (name.equals("subject")) { 2069 this.subject = null; 2070 } else if (name.equals("location")) { 2071 this.location = null; 2072 } else 2073 super.removeChild(name, value); 2074 2075 } 2076 2077 @Override 2078 public Base makeProperty(int hash, String name) throws FHIRException { 2079 switch (hash) { 2080 case -1618432855: return addIdentifier(); 2081 case 462547450: return getLotNumberElement(); 2082 case -1289159373: return getExpiryElement(); 2083 case -1867885268: return getSubject(); 2084 case 1901043637: return getLocation(); 2085 default: return super.makeProperty(hash, name); 2086 } 2087 2088 } 2089 2090 @Override 2091 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2092 switch (hash) { 2093 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2094 case 462547450: /*lotNumber*/ return new String[] {"string"}; 2095 case -1289159373: /*expiry*/ return new String[] {"dateTime"}; 2096 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2097 case 1901043637: /*location*/ return new String[] {"Reference"}; 2098 default: return super.getTypesForProperty(hash, name); 2099 } 2100 2101 } 2102 2103 @Override 2104 public Base addChild(String name) throws FHIRException { 2105 if (name.equals("identifier")) { 2106 return addIdentifier(); 2107 } 2108 else if (name.equals("lotNumber")) { 2109 throw new FHIRException("Cannot call addChild on a singleton property InventoryItem.instance.lotNumber"); 2110 } 2111 else if (name.equals("expiry")) { 2112 throw new FHIRException("Cannot call addChild on a singleton property InventoryItem.instance.expiry"); 2113 } 2114 else if (name.equals("subject")) { 2115 this.subject = new Reference(); 2116 return this.subject; 2117 } 2118 else if (name.equals("location")) { 2119 this.location = new Reference(); 2120 return this.location; 2121 } 2122 else 2123 return super.addChild(name); 2124 } 2125 2126 public InventoryItemInstanceComponent copy() { 2127 InventoryItemInstanceComponent dst = new InventoryItemInstanceComponent(); 2128 copyValues(dst); 2129 return dst; 2130 } 2131 2132 public void copyValues(InventoryItemInstanceComponent dst) { 2133 super.copyValues(dst); 2134 if (identifier != null) { 2135 dst.identifier = new ArrayList<Identifier>(); 2136 for (Identifier i : identifier) 2137 dst.identifier.add(i.copy()); 2138 }; 2139 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 2140 dst.expiry = expiry == null ? null : expiry.copy(); 2141 dst.subject = subject == null ? null : subject.copy(); 2142 dst.location = location == null ? null : location.copy(); 2143 } 2144 2145 @Override 2146 public boolean equalsDeep(Base other_) { 2147 if (!super.equalsDeep(other_)) 2148 return false; 2149 if (!(other_ instanceof InventoryItemInstanceComponent)) 2150 return false; 2151 InventoryItemInstanceComponent o = (InventoryItemInstanceComponent) other_; 2152 return compareDeep(identifier, o.identifier, true) && compareDeep(lotNumber, o.lotNumber, true) 2153 && compareDeep(expiry, o.expiry, true) && compareDeep(subject, o.subject, true) && compareDeep(location, o.location, true) 2154 ; 2155 } 2156 2157 @Override 2158 public boolean equalsShallow(Base other_) { 2159 if (!super.equalsShallow(other_)) 2160 return false; 2161 if (!(other_ instanceof InventoryItemInstanceComponent)) 2162 return false; 2163 InventoryItemInstanceComponent o = (InventoryItemInstanceComponent) other_; 2164 return compareValues(lotNumber, o.lotNumber, true) && compareValues(expiry, o.expiry, true); 2165 } 2166 2167 public boolean isEmpty() { 2168 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, lotNumber, expiry 2169 , subject, location); 2170 } 2171 2172 public String fhirType() { 2173 return "InventoryItem.instance"; 2174 2175 } 2176 2177 } 2178 2179 /** 2180 * Business identifier for the inventory item. 2181 */ 2182 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2183 @Description(shortDefinition="Business identifier for the inventory item", formalDefinition="Business identifier for the inventory item." ) 2184 protected List<Identifier> identifier; 2185 2186 /** 2187 * Status of the item entry. 2188 */ 2189 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2190 @Description(shortDefinition="active | inactive | entered-in-error | unknown", formalDefinition="Status of the item entry." ) 2191 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/inventoryitem-status") 2192 protected Enumeration<InventoryItemStatusCodes> status; 2193 2194 /** 2195 * Category or class of the item. 2196 */ 2197 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2198 @Description(shortDefinition="Category or class of the item", formalDefinition="Category or class of the item." ) 2199 protected List<CodeableConcept> category; 2200 2201 /** 2202 * Code designating the specific type of item. 2203 */ 2204 @Child(name = "code", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2205 @Description(shortDefinition="Code designating the specific type of item", formalDefinition="Code designating the specific type of item." ) 2206 protected List<CodeableConcept> code; 2207 2208 /** 2209 * The item name(s) - the brand name, or common name, functional name, generic name. 2210 */ 2211 @Child(name = "name", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2212 @Description(shortDefinition="The item name(s) - the brand name, or common name, functional name, generic name or others", formalDefinition="The item name(s) - the brand name, or common name, functional name, generic name." ) 2213 protected List<InventoryItemNameComponent> name; 2214 2215 /** 2216 * Organization(s) responsible for the product. 2217 */ 2218 @Child(name = "responsibleOrganization", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2219 @Description(shortDefinition="Organization(s) responsible for the product", formalDefinition="Organization(s) responsible for the product." ) 2220 protected List<InventoryItemResponsibleOrganizationComponent> responsibleOrganization; 2221 2222 /** 2223 * The descriptive characteristics of the inventory item. 2224 */ 2225 @Child(name = "description", type = {}, order=6, min=0, max=1, modifier=false, summary=false) 2226 @Description(shortDefinition="Descriptive characteristics of the item", formalDefinition="The descriptive characteristics of the inventory item." ) 2227 protected InventoryItemDescriptionComponent description; 2228 2229 /** 2230 * The usage status e.g. recalled, in use, discarded... This can be used to indicate that the items have been taken out of inventory, or are in use, etc. 2231 */ 2232 @Child(name = "inventoryStatus", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2233 @Description(shortDefinition="The usage status like recalled, in use, discarded", formalDefinition="The usage status e.g. recalled, in use, discarded... This can be used to indicate that the items have been taken out of inventory, or are in use, etc." ) 2234 protected List<CodeableConcept> inventoryStatus; 2235 2236 /** 2237 * The base unit of measure - the unit in which the product is used or counted. 2238 */ 2239 @Child(name = "baseUnit", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=true) 2240 @Description(shortDefinition="The base unit of measure - the unit in which the product is used or counted", formalDefinition="The base unit of measure - the unit in which the product is used or counted." ) 2241 protected CodeableConcept baseUnit; 2242 2243 /** 2244 * Net content or amount present in the item. 2245 */ 2246 @Child(name = "netContent", type = {Quantity.class}, order=9, min=0, max=1, modifier=false, summary=true) 2247 @Description(shortDefinition="Net content or amount present in the item", formalDefinition="Net content or amount present in the item." ) 2248 protected Quantity netContent; 2249 2250 /** 2251 * Association with other items or products. 2252 */ 2253 @Child(name = "association", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2254 @Description(shortDefinition="Association with other items or products", formalDefinition="Association with other items or products." ) 2255 protected List<InventoryItemAssociationComponent> association; 2256 2257 /** 2258 * The descriptive or identifying characteristics of the item. 2259 */ 2260 @Child(name = "characteristic", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2261 @Description(shortDefinition="Characteristic of the item", formalDefinition="The descriptive or identifying characteristics of the item." ) 2262 protected List<InventoryItemCharacteristicComponent> characteristic; 2263 2264 /** 2265 * Instances or occurrences of the product. 2266 */ 2267 @Child(name = "instance", type = {}, order=12, min=0, max=1, modifier=false, summary=false) 2268 @Description(shortDefinition="Instances or occurrences of the product", formalDefinition="Instances or occurrences of the product." ) 2269 protected InventoryItemInstanceComponent instance; 2270 2271 /** 2272 * Link to a product resource used in clinical workflows. 2273 */ 2274 @Child(name = "productReference", type = {Medication.class, Device.class, NutritionProduct.class, BiologicallyDerivedProduct.class}, order=13, min=0, max=1, modifier=false, summary=false) 2275 @Description(shortDefinition="Link to a product resource used in clinical workflows", formalDefinition="Link to a product resource used in clinical workflows." ) 2276 protected Reference productReference; 2277 2278 private static final long serialVersionUID = 2127201564L; 2279 2280 /** 2281 * Constructor 2282 */ 2283 public InventoryItem() { 2284 super(); 2285 } 2286 2287 /** 2288 * Constructor 2289 */ 2290 public InventoryItem(InventoryItemStatusCodes status) { 2291 super(); 2292 this.setStatus(status); 2293 } 2294 2295 /** 2296 * @return {@link #identifier} (Business identifier for the inventory item.) 2297 */ 2298 public List<Identifier> getIdentifier() { 2299 if (this.identifier == null) 2300 this.identifier = new ArrayList<Identifier>(); 2301 return this.identifier; 2302 } 2303 2304 /** 2305 * @return Returns a reference to <code>this</code> for easy method chaining 2306 */ 2307 public InventoryItem setIdentifier(List<Identifier> theIdentifier) { 2308 this.identifier = theIdentifier; 2309 return this; 2310 } 2311 2312 public boolean hasIdentifier() { 2313 if (this.identifier == null) 2314 return false; 2315 for (Identifier item : this.identifier) 2316 if (!item.isEmpty()) 2317 return true; 2318 return false; 2319 } 2320 2321 public Identifier addIdentifier() { //3 2322 Identifier t = new Identifier(); 2323 if (this.identifier == null) 2324 this.identifier = new ArrayList<Identifier>(); 2325 this.identifier.add(t); 2326 return t; 2327 } 2328 2329 public InventoryItem addIdentifier(Identifier t) { //3 2330 if (t == null) 2331 return this; 2332 if (this.identifier == null) 2333 this.identifier = new ArrayList<Identifier>(); 2334 this.identifier.add(t); 2335 return this; 2336 } 2337 2338 /** 2339 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2340 */ 2341 public Identifier getIdentifierFirstRep() { 2342 if (getIdentifier().isEmpty()) { 2343 addIdentifier(); 2344 } 2345 return getIdentifier().get(0); 2346 } 2347 2348 /** 2349 * @return {@link #status} (Status of the item entry.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2350 */ 2351 public Enumeration<InventoryItemStatusCodes> getStatusElement() { 2352 if (this.status == null) 2353 if (Configuration.errorOnAutoCreate()) 2354 throw new Error("Attempt to auto-create InventoryItem.status"); 2355 else if (Configuration.doAutoCreate()) 2356 this.status = new Enumeration<InventoryItemStatusCodes>(new InventoryItemStatusCodesEnumFactory()); // bb 2357 return this.status; 2358 } 2359 2360 public boolean hasStatusElement() { 2361 return this.status != null && !this.status.isEmpty(); 2362 } 2363 2364 public boolean hasStatus() { 2365 return this.status != null && !this.status.isEmpty(); 2366 } 2367 2368 /** 2369 * @param value {@link #status} (Status of the item entry.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2370 */ 2371 public InventoryItem setStatusElement(Enumeration<InventoryItemStatusCodes> value) { 2372 this.status = value; 2373 return this; 2374 } 2375 2376 /** 2377 * @return Status of the item entry. 2378 */ 2379 public InventoryItemStatusCodes getStatus() { 2380 return this.status == null ? null : this.status.getValue(); 2381 } 2382 2383 /** 2384 * @param value Status of the item entry. 2385 */ 2386 public InventoryItem setStatus(InventoryItemStatusCodes value) { 2387 if (this.status == null) 2388 this.status = new Enumeration<InventoryItemStatusCodes>(new InventoryItemStatusCodesEnumFactory()); 2389 this.status.setValue(value); 2390 return this; 2391 } 2392 2393 /** 2394 * @return {@link #category} (Category or class of the item.) 2395 */ 2396 public List<CodeableConcept> getCategory() { 2397 if (this.category == null) 2398 this.category = new ArrayList<CodeableConcept>(); 2399 return this.category; 2400 } 2401 2402 /** 2403 * @return Returns a reference to <code>this</code> for easy method chaining 2404 */ 2405 public InventoryItem setCategory(List<CodeableConcept> theCategory) { 2406 this.category = theCategory; 2407 return this; 2408 } 2409 2410 public boolean hasCategory() { 2411 if (this.category == null) 2412 return false; 2413 for (CodeableConcept item : this.category) 2414 if (!item.isEmpty()) 2415 return true; 2416 return false; 2417 } 2418 2419 public CodeableConcept addCategory() { //3 2420 CodeableConcept t = new CodeableConcept(); 2421 if (this.category == null) 2422 this.category = new ArrayList<CodeableConcept>(); 2423 this.category.add(t); 2424 return t; 2425 } 2426 2427 public InventoryItem addCategory(CodeableConcept t) { //3 2428 if (t == null) 2429 return this; 2430 if (this.category == null) 2431 this.category = new ArrayList<CodeableConcept>(); 2432 this.category.add(t); 2433 return this; 2434 } 2435 2436 /** 2437 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 2438 */ 2439 public CodeableConcept getCategoryFirstRep() { 2440 if (getCategory().isEmpty()) { 2441 addCategory(); 2442 } 2443 return getCategory().get(0); 2444 } 2445 2446 /** 2447 * @return {@link #code} (Code designating the specific type of item.) 2448 */ 2449 public List<CodeableConcept> getCode() { 2450 if (this.code == null) 2451 this.code = new ArrayList<CodeableConcept>(); 2452 return this.code; 2453 } 2454 2455 /** 2456 * @return Returns a reference to <code>this</code> for easy method chaining 2457 */ 2458 public InventoryItem setCode(List<CodeableConcept> theCode) { 2459 this.code = theCode; 2460 return this; 2461 } 2462 2463 public boolean hasCode() { 2464 if (this.code == null) 2465 return false; 2466 for (CodeableConcept item : this.code) 2467 if (!item.isEmpty()) 2468 return true; 2469 return false; 2470 } 2471 2472 public CodeableConcept addCode() { //3 2473 CodeableConcept t = new CodeableConcept(); 2474 if (this.code == null) 2475 this.code = new ArrayList<CodeableConcept>(); 2476 this.code.add(t); 2477 return t; 2478 } 2479 2480 public InventoryItem addCode(CodeableConcept t) { //3 2481 if (t == null) 2482 return this; 2483 if (this.code == null) 2484 this.code = new ArrayList<CodeableConcept>(); 2485 this.code.add(t); 2486 return this; 2487 } 2488 2489 /** 2490 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist {3} 2491 */ 2492 public CodeableConcept getCodeFirstRep() { 2493 if (getCode().isEmpty()) { 2494 addCode(); 2495 } 2496 return getCode().get(0); 2497 } 2498 2499 /** 2500 * @return {@link #name} (The item name(s) - the brand name, or common name, functional name, generic name.) 2501 */ 2502 public List<InventoryItemNameComponent> getName() { 2503 if (this.name == null) 2504 this.name = new ArrayList<InventoryItemNameComponent>(); 2505 return this.name; 2506 } 2507 2508 /** 2509 * @return Returns a reference to <code>this</code> for easy method chaining 2510 */ 2511 public InventoryItem setName(List<InventoryItemNameComponent> theName) { 2512 this.name = theName; 2513 return this; 2514 } 2515 2516 public boolean hasName() { 2517 if (this.name == null) 2518 return false; 2519 for (InventoryItemNameComponent item : this.name) 2520 if (!item.isEmpty()) 2521 return true; 2522 return false; 2523 } 2524 2525 public InventoryItemNameComponent addName() { //3 2526 InventoryItemNameComponent t = new InventoryItemNameComponent(); 2527 if (this.name == null) 2528 this.name = new ArrayList<InventoryItemNameComponent>(); 2529 this.name.add(t); 2530 return t; 2531 } 2532 2533 public InventoryItem addName(InventoryItemNameComponent t) { //3 2534 if (t == null) 2535 return this; 2536 if (this.name == null) 2537 this.name = new ArrayList<InventoryItemNameComponent>(); 2538 this.name.add(t); 2539 return this; 2540 } 2541 2542 /** 2543 * @return The first repetition of repeating field {@link #name}, creating it if it does not already exist {3} 2544 */ 2545 public InventoryItemNameComponent getNameFirstRep() { 2546 if (getName().isEmpty()) { 2547 addName(); 2548 } 2549 return getName().get(0); 2550 } 2551 2552 /** 2553 * @return {@link #responsibleOrganization} (Organization(s) responsible for the product.) 2554 */ 2555 public List<InventoryItemResponsibleOrganizationComponent> getResponsibleOrganization() { 2556 if (this.responsibleOrganization == null) 2557 this.responsibleOrganization = new ArrayList<InventoryItemResponsibleOrganizationComponent>(); 2558 return this.responsibleOrganization; 2559 } 2560 2561 /** 2562 * @return Returns a reference to <code>this</code> for easy method chaining 2563 */ 2564 public InventoryItem setResponsibleOrganization(List<InventoryItemResponsibleOrganizationComponent> theResponsibleOrganization) { 2565 this.responsibleOrganization = theResponsibleOrganization; 2566 return this; 2567 } 2568 2569 public boolean hasResponsibleOrganization() { 2570 if (this.responsibleOrganization == null) 2571 return false; 2572 for (InventoryItemResponsibleOrganizationComponent item : this.responsibleOrganization) 2573 if (!item.isEmpty()) 2574 return true; 2575 return false; 2576 } 2577 2578 public InventoryItemResponsibleOrganizationComponent addResponsibleOrganization() { //3 2579 InventoryItemResponsibleOrganizationComponent t = new InventoryItemResponsibleOrganizationComponent(); 2580 if (this.responsibleOrganization == null) 2581 this.responsibleOrganization = new ArrayList<InventoryItemResponsibleOrganizationComponent>(); 2582 this.responsibleOrganization.add(t); 2583 return t; 2584 } 2585 2586 public InventoryItem addResponsibleOrganization(InventoryItemResponsibleOrganizationComponent t) { //3 2587 if (t == null) 2588 return this; 2589 if (this.responsibleOrganization == null) 2590 this.responsibleOrganization = new ArrayList<InventoryItemResponsibleOrganizationComponent>(); 2591 this.responsibleOrganization.add(t); 2592 return this; 2593 } 2594 2595 /** 2596 * @return The first repetition of repeating field {@link #responsibleOrganization}, creating it if it does not already exist {3} 2597 */ 2598 public InventoryItemResponsibleOrganizationComponent getResponsibleOrganizationFirstRep() { 2599 if (getResponsibleOrganization().isEmpty()) { 2600 addResponsibleOrganization(); 2601 } 2602 return getResponsibleOrganization().get(0); 2603 } 2604 2605 /** 2606 * @return {@link #description} (The descriptive characteristics of the inventory item.) 2607 */ 2608 public InventoryItemDescriptionComponent getDescription() { 2609 if (this.description == null) 2610 if (Configuration.errorOnAutoCreate()) 2611 throw new Error("Attempt to auto-create InventoryItem.description"); 2612 else if (Configuration.doAutoCreate()) 2613 this.description = new InventoryItemDescriptionComponent(); // cc 2614 return this.description; 2615 } 2616 2617 public boolean hasDescription() { 2618 return this.description != null && !this.description.isEmpty(); 2619 } 2620 2621 /** 2622 * @param value {@link #description} (The descriptive characteristics of the inventory item.) 2623 */ 2624 public InventoryItem setDescription(InventoryItemDescriptionComponent value) { 2625 this.description = value; 2626 return this; 2627 } 2628 2629 /** 2630 * @return {@link #inventoryStatus} (The usage status e.g. recalled, in use, discarded... This can be used to indicate that the items have been taken out of inventory, or are in use, etc.) 2631 */ 2632 public List<CodeableConcept> getInventoryStatus() { 2633 if (this.inventoryStatus == null) 2634 this.inventoryStatus = new ArrayList<CodeableConcept>(); 2635 return this.inventoryStatus; 2636 } 2637 2638 /** 2639 * @return Returns a reference to <code>this</code> for easy method chaining 2640 */ 2641 public InventoryItem setInventoryStatus(List<CodeableConcept> theInventoryStatus) { 2642 this.inventoryStatus = theInventoryStatus; 2643 return this; 2644 } 2645 2646 public boolean hasInventoryStatus() { 2647 if (this.inventoryStatus == null) 2648 return false; 2649 for (CodeableConcept item : this.inventoryStatus) 2650 if (!item.isEmpty()) 2651 return true; 2652 return false; 2653 } 2654 2655 public CodeableConcept addInventoryStatus() { //3 2656 CodeableConcept t = new CodeableConcept(); 2657 if (this.inventoryStatus == null) 2658 this.inventoryStatus = new ArrayList<CodeableConcept>(); 2659 this.inventoryStatus.add(t); 2660 return t; 2661 } 2662 2663 public InventoryItem addInventoryStatus(CodeableConcept t) { //3 2664 if (t == null) 2665 return this; 2666 if (this.inventoryStatus == null) 2667 this.inventoryStatus = new ArrayList<CodeableConcept>(); 2668 this.inventoryStatus.add(t); 2669 return this; 2670 } 2671 2672 /** 2673 * @return The first repetition of repeating field {@link #inventoryStatus}, creating it if it does not already exist {3} 2674 */ 2675 public CodeableConcept getInventoryStatusFirstRep() { 2676 if (getInventoryStatus().isEmpty()) { 2677 addInventoryStatus(); 2678 } 2679 return getInventoryStatus().get(0); 2680 } 2681 2682 /** 2683 * @return {@link #baseUnit} (The base unit of measure - the unit in which the product is used or counted.) 2684 */ 2685 public CodeableConcept getBaseUnit() { 2686 if (this.baseUnit == null) 2687 if (Configuration.errorOnAutoCreate()) 2688 throw new Error("Attempt to auto-create InventoryItem.baseUnit"); 2689 else if (Configuration.doAutoCreate()) 2690 this.baseUnit = new CodeableConcept(); // cc 2691 return this.baseUnit; 2692 } 2693 2694 public boolean hasBaseUnit() { 2695 return this.baseUnit != null && !this.baseUnit.isEmpty(); 2696 } 2697 2698 /** 2699 * @param value {@link #baseUnit} (The base unit of measure - the unit in which the product is used or counted.) 2700 */ 2701 public InventoryItem setBaseUnit(CodeableConcept value) { 2702 this.baseUnit = value; 2703 return this; 2704 } 2705 2706 /** 2707 * @return {@link #netContent} (Net content or amount present in the item.) 2708 */ 2709 public Quantity getNetContent() { 2710 if (this.netContent == null) 2711 if (Configuration.errorOnAutoCreate()) 2712 throw new Error("Attempt to auto-create InventoryItem.netContent"); 2713 else if (Configuration.doAutoCreate()) 2714 this.netContent = new Quantity(); // cc 2715 return this.netContent; 2716 } 2717 2718 public boolean hasNetContent() { 2719 return this.netContent != null && !this.netContent.isEmpty(); 2720 } 2721 2722 /** 2723 * @param value {@link #netContent} (Net content or amount present in the item.) 2724 */ 2725 public InventoryItem setNetContent(Quantity value) { 2726 this.netContent = value; 2727 return this; 2728 } 2729 2730 /** 2731 * @return {@link #association} (Association with other items or products.) 2732 */ 2733 public List<InventoryItemAssociationComponent> getAssociation() { 2734 if (this.association == null) 2735 this.association = new ArrayList<InventoryItemAssociationComponent>(); 2736 return this.association; 2737 } 2738 2739 /** 2740 * @return Returns a reference to <code>this</code> for easy method chaining 2741 */ 2742 public InventoryItem setAssociation(List<InventoryItemAssociationComponent> theAssociation) { 2743 this.association = theAssociation; 2744 return this; 2745 } 2746 2747 public boolean hasAssociation() { 2748 if (this.association == null) 2749 return false; 2750 for (InventoryItemAssociationComponent item : this.association) 2751 if (!item.isEmpty()) 2752 return true; 2753 return false; 2754 } 2755 2756 public InventoryItemAssociationComponent addAssociation() { //3 2757 InventoryItemAssociationComponent t = new InventoryItemAssociationComponent(); 2758 if (this.association == null) 2759 this.association = new ArrayList<InventoryItemAssociationComponent>(); 2760 this.association.add(t); 2761 return t; 2762 } 2763 2764 public InventoryItem addAssociation(InventoryItemAssociationComponent t) { //3 2765 if (t == null) 2766 return this; 2767 if (this.association == null) 2768 this.association = new ArrayList<InventoryItemAssociationComponent>(); 2769 this.association.add(t); 2770 return this; 2771 } 2772 2773 /** 2774 * @return The first repetition of repeating field {@link #association}, creating it if it does not already exist {3} 2775 */ 2776 public InventoryItemAssociationComponent getAssociationFirstRep() { 2777 if (getAssociation().isEmpty()) { 2778 addAssociation(); 2779 } 2780 return getAssociation().get(0); 2781 } 2782 2783 /** 2784 * @return {@link #characteristic} (The descriptive or identifying characteristics of the item.) 2785 */ 2786 public List<InventoryItemCharacteristicComponent> getCharacteristic() { 2787 if (this.characteristic == null) 2788 this.characteristic = new ArrayList<InventoryItemCharacteristicComponent>(); 2789 return this.characteristic; 2790 } 2791 2792 /** 2793 * @return Returns a reference to <code>this</code> for easy method chaining 2794 */ 2795 public InventoryItem setCharacteristic(List<InventoryItemCharacteristicComponent> theCharacteristic) { 2796 this.characteristic = theCharacteristic; 2797 return this; 2798 } 2799 2800 public boolean hasCharacteristic() { 2801 if (this.characteristic == null) 2802 return false; 2803 for (InventoryItemCharacteristicComponent item : this.characteristic) 2804 if (!item.isEmpty()) 2805 return true; 2806 return false; 2807 } 2808 2809 public InventoryItemCharacteristicComponent addCharacteristic() { //3 2810 InventoryItemCharacteristicComponent t = new InventoryItemCharacteristicComponent(); 2811 if (this.characteristic == null) 2812 this.characteristic = new ArrayList<InventoryItemCharacteristicComponent>(); 2813 this.characteristic.add(t); 2814 return t; 2815 } 2816 2817 public InventoryItem addCharacteristic(InventoryItemCharacteristicComponent t) { //3 2818 if (t == null) 2819 return this; 2820 if (this.characteristic == null) 2821 this.characteristic = new ArrayList<InventoryItemCharacteristicComponent>(); 2822 this.characteristic.add(t); 2823 return this; 2824 } 2825 2826 /** 2827 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist {3} 2828 */ 2829 public InventoryItemCharacteristicComponent getCharacteristicFirstRep() { 2830 if (getCharacteristic().isEmpty()) { 2831 addCharacteristic(); 2832 } 2833 return getCharacteristic().get(0); 2834 } 2835 2836 /** 2837 * @return {@link #instance} (Instances or occurrences of the product.) 2838 */ 2839 public InventoryItemInstanceComponent getInstance() { 2840 if (this.instance == null) 2841 if (Configuration.errorOnAutoCreate()) 2842 throw new Error("Attempt to auto-create InventoryItem.instance"); 2843 else if (Configuration.doAutoCreate()) 2844 this.instance = new InventoryItemInstanceComponent(); // cc 2845 return this.instance; 2846 } 2847 2848 public boolean hasInstance() { 2849 return this.instance != null && !this.instance.isEmpty(); 2850 } 2851 2852 /** 2853 * @param value {@link #instance} (Instances or occurrences of the product.) 2854 */ 2855 public InventoryItem setInstance(InventoryItemInstanceComponent value) { 2856 this.instance = value; 2857 return this; 2858 } 2859 2860 /** 2861 * @return {@link #productReference} (Link to a product resource used in clinical workflows.) 2862 */ 2863 public Reference getProductReference() { 2864 if (this.productReference == null) 2865 if (Configuration.errorOnAutoCreate()) 2866 throw new Error("Attempt to auto-create InventoryItem.productReference"); 2867 else if (Configuration.doAutoCreate()) 2868 this.productReference = new Reference(); // cc 2869 return this.productReference; 2870 } 2871 2872 public boolean hasProductReference() { 2873 return this.productReference != null && !this.productReference.isEmpty(); 2874 } 2875 2876 /** 2877 * @param value {@link #productReference} (Link to a product resource used in clinical workflows.) 2878 */ 2879 public InventoryItem setProductReference(Reference value) { 2880 this.productReference = value; 2881 return this; 2882 } 2883 2884 protected void listChildren(List<Property> children) { 2885 super.listChildren(children); 2886 children.add(new Property("identifier", "Identifier", "Business identifier for the inventory item.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2887 children.add(new Property("status", "code", "Status of the item entry.", 0, 1, status)); 2888 children.add(new Property("category", "CodeableConcept", "Category or class of the item.", 0, java.lang.Integer.MAX_VALUE, category)); 2889 children.add(new Property("code", "CodeableConcept", "Code designating the specific type of item.", 0, java.lang.Integer.MAX_VALUE, code)); 2890 children.add(new Property("name", "", "The item name(s) - the brand name, or common name, functional name, generic name.", 0, java.lang.Integer.MAX_VALUE, name)); 2891 children.add(new Property("responsibleOrganization", "", "Organization(s) responsible for the product.", 0, java.lang.Integer.MAX_VALUE, responsibleOrganization)); 2892 children.add(new Property("description", "", "The descriptive characteristics of the inventory item.", 0, 1, description)); 2893 children.add(new Property("inventoryStatus", "CodeableConcept", "The usage status e.g. recalled, in use, discarded... This can be used to indicate that the items have been taken out of inventory, or are in use, etc.", 0, java.lang.Integer.MAX_VALUE, inventoryStatus)); 2894 children.add(new Property("baseUnit", "CodeableConcept", "The base unit of measure - the unit in which the product is used or counted.", 0, 1, baseUnit)); 2895 children.add(new Property("netContent", "Quantity", "Net content or amount present in the item.", 0, 1, netContent)); 2896 children.add(new Property("association", "", "Association with other items or products.", 0, java.lang.Integer.MAX_VALUE, association)); 2897 children.add(new Property("characteristic", "", "The descriptive or identifying characteristics of the item.", 0, java.lang.Integer.MAX_VALUE, characteristic)); 2898 children.add(new Property("instance", "", "Instances or occurrences of the product.", 0, 1, instance)); 2899 children.add(new Property("productReference", "Reference(Medication|Device|NutritionProduct|BiologicallyDerivedProduct)", "Link to a product resource used in clinical workflows.", 0, 1, productReference)); 2900 } 2901 2902 @Override 2903 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2904 switch (_hash) { 2905 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier for the inventory item.", 0, java.lang.Integer.MAX_VALUE, identifier); 2906 case -892481550: /*status*/ return new Property("status", "code", "Status of the item entry.", 0, 1, status); 2907 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Category or class of the item.", 0, java.lang.Integer.MAX_VALUE, category); 2908 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Code designating the specific type of item.", 0, java.lang.Integer.MAX_VALUE, code); 2909 case 3373707: /*name*/ return new Property("name", "", "The item name(s) - the brand name, or common name, functional name, generic name.", 0, java.lang.Integer.MAX_VALUE, name); 2910 case -1704933559: /*responsibleOrganization*/ return new Property("responsibleOrganization", "", "Organization(s) responsible for the product.", 0, java.lang.Integer.MAX_VALUE, responsibleOrganization); 2911 case -1724546052: /*description*/ return new Property("description", "", "The descriptive characteristics of the inventory item.", 0, 1, description); 2912 case -1370922898: /*inventoryStatus*/ return new Property("inventoryStatus", "CodeableConcept", "The usage status e.g. recalled, in use, discarded... This can be used to indicate that the items have been taken out of inventory, or are in use, etc.", 0, java.lang.Integer.MAX_VALUE, inventoryStatus); 2913 case -1721465867: /*baseUnit*/ return new Property("baseUnit", "CodeableConcept", "The base unit of measure - the unit in which the product is used or counted.", 0, 1, baseUnit); 2914 case 612796444: /*netContent*/ return new Property("netContent", "Quantity", "Net content or amount present in the item.", 0, 1, netContent); 2915 case -87499647: /*association*/ return new Property("association", "", "Association with other items or products.", 0, java.lang.Integer.MAX_VALUE, association); 2916 case 366313883: /*characteristic*/ return new Property("characteristic", "", "The descriptive or identifying characteristics of the item.", 0, java.lang.Integer.MAX_VALUE, characteristic); 2917 case 555127957: /*instance*/ return new Property("instance", "", "Instances or occurrences of the product.", 0, 1, instance); 2918 case -669667556: /*productReference*/ return new Property("productReference", "Reference(Medication|Device|NutritionProduct|BiologicallyDerivedProduct)", "Link to a product resource used in clinical workflows.", 0, 1, productReference); 2919 default: return super.getNamedProperty(_hash, _name, _checkValid); 2920 } 2921 2922 } 2923 2924 @Override 2925 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2926 switch (hash) { 2927 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2928 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<InventoryItemStatusCodes> 2929 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2930 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 2931 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // InventoryItemNameComponent 2932 case -1704933559: /*responsibleOrganization*/ return this.responsibleOrganization == null ? new Base[0] : this.responsibleOrganization.toArray(new Base[this.responsibleOrganization.size()]); // InventoryItemResponsibleOrganizationComponent 2933 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // InventoryItemDescriptionComponent 2934 case -1370922898: /*inventoryStatus*/ return this.inventoryStatus == null ? new Base[0] : this.inventoryStatus.toArray(new Base[this.inventoryStatus.size()]); // CodeableConcept 2935 case -1721465867: /*baseUnit*/ return this.baseUnit == null ? new Base[0] : new Base[] {this.baseUnit}; // CodeableConcept 2936 case 612796444: /*netContent*/ return this.netContent == null ? new Base[0] : new Base[] {this.netContent}; // Quantity 2937 case -87499647: /*association*/ return this.association == null ? new Base[0] : this.association.toArray(new Base[this.association.size()]); // InventoryItemAssociationComponent 2938 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // InventoryItemCharacteristicComponent 2939 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : new Base[] {this.instance}; // InventoryItemInstanceComponent 2940 case -669667556: /*productReference*/ return this.productReference == null ? new Base[0] : new Base[] {this.productReference}; // Reference 2941 default: return super.getProperty(hash, name, checkValid); 2942 } 2943 2944 } 2945 2946 @Override 2947 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2948 switch (hash) { 2949 case -1618432855: // identifier 2950 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2951 return value; 2952 case -892481550: // status 2953 value = new InventoryItemStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2954 this.status = (Enumeration) value; // Enumeration<InventoryItemStatusCodes> 2955 return value; 2956 case 50511102: // category 2957 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2958 return value; 2959 case 3059181: // code 2960 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2961 return value; 2962 case 3373707: // name 2963 this.getName().add((InventoryItemNameComponent) value); // InventoryItemNameComponent 2964 return value; 2965 case -1704933559: // responsibleOrganization 2966 this.getResponsibleOrganization().add((InventoryItemResponsibleOrganizationComponent) value); // InventoryItemResponsibleOrganizationComponent 2967 return value; 2968 case -1724546052: // description 2969 this.description = (InventoryItemDescriptionComponent) value; // InventoryItemDescriptionComponent 2970 return value; 2971 case -1370922898: // inventoryStatus 2972 this.getInventoryStatus().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2973 return value; 2974 case -1721465867: // baseUnit 2975 this.baseUnit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2976 return value; 2977 case 612796444: // netContent 2978 this.netContent = TypeConvertor.castToQuantity(value); // Quantity 2979 return value; 2980 case -87499647: // association 2981 this.getAssociation().add((InventoryItemAssociationComponent) value); // InventoryItemAssociationComponent 2982 return value; 2983 case 366313883: // characteristic 2984 this.getCharacteristic().add((InventoryItemCharacteristicComponent) value); // InventoryItemCharacteristicComponent 2985 return value; 2986 case 555127957: // instance 2987 this.instance = (InventoryItemInstanceComponent) value; // InventoryItemInstanceComponent 2988 return value; 2989 case -669667556: // productReference 2990 this.productReference = TypeConvertor.castToReference(value); // Reference 2991 return value; 2992 default: return super.setProperty(hash, name, value); 2993 } 2994 2995 } 2996 2997 @Override 2998 public Base setProperty(String name, Base value) throws FHIRException { 2999 if (name.equals("identifier")) { 3000 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 3001 } else if (name.equals("status")) { 3002 value = new InventoryItemStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 3003 this.status = (Enumeration) value; // Enumeration<InventoryItemStatusCodes> 3004 } else if (name.equals("category")) { 3005 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 3006 } else if (name.equals("code")) { 3007 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); 3008 } else if (name.equals("name")) { 3009 this.getName().add((InventoryItemNameComponent) value); 3010 } else if (name.equals("responsibleOrganization")) { 3011 this.getResponsibleOrganization().add((InventoryItemResponsibleOrganizationComponent) value); 3012 } else if (name.equals("description")) { 3013 this.description = (InventoryItemDescriptionComponent) value; // InventoryItemDescriptionComponent 3014 } else if (name.equals("inventoryStatus")) { 3015 this.getInventoryStatus().add(TypeConvertor.castToCodeableConcept(value)); 3016 } else if (name.equals("baseUnit")) { 3017 this.baseUnit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3018 } else if (name.equals("netContent")) { 3019 this.netContent = TypeConvertor.castToQuantity(value); // Quantity 3020 } else if (name.equals("association")) { 3021 this.getAssociation().add((InventoryItemAssociationComponent) value); 3022 } else if (name.equals("characteristic")) { 3023 this.getCharacteristic().add((InventoryItemCharacteristicComponent) value); 3024 } else if (name.equals("instance")) { 3025 this.instance = (InventoryItemInstanceComponent) value; // InventoryItemInstanceComponent 3026 } else if (name.equals("productReference")) { 3027 this.productReference = TypeConvertor.castToReference(value); // Reference 3028 } else 3029 return super.setProperty(name, value); 3030 return value; 3031 } 3032 3033 @Override 3034 public void removeChild(String name, Base value) throws FHIRException { 3035 if (name.equals("identifier")) { 3036 this.getIdentifier().remove(value); 3037 } else if (name.equals("status")) { 3038 value = new InventoryItemStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 3039 this.status = (Enumeration) value; // Enumeration<InventoryItemStatusCodes> 3040 } else if (name.equals("category")) { 3041 this.getCategory().remove(value); 3042 } else if (name.equals("code")) { 3043 this.getCode().remove(value); 3044 } else if (name.equals("name")) { 3045 this.getName().remove((InventoryItemNameComponent) value); 3046 } else if (name.equals("responsibleOrganization")) { 3047 this.getResponsibleOrganization().remove((InventoryItemResponsibleOrganizationComponent) value); 3048 } else if (name.equals("description")) { 3049 this.description = (InventoryItemDescriptionComponent) value; // InventoryItemDescriptionComponent 3050 } else if (name.equals("inventoryStatus")) { 3051 this.getInventoryStatus().remove(value); 3052 } else if (name.equals("baseUnit")) { 3053 this.baseUnit = null; 3054 } else if (name.equals("netContent")) { 3055 this.netContent = null; 3056 } else if (name.equals("association")) { 3057 this.getAssociation().remove((InventoryItemAssociationComponent) value); 3058 } else if (name.equals("characteristic")) { 3059 this.getCharacteristic().remove((InventoryItemCharacteristicComponent) value); 3060 } else if (name.equals("instance")) { 3061 this.instance = (InventoryItemInstanceComponent) value; // InventoryItemInstanceComponent 3062 } else if (name.equals("productReference")) { 3063 this.productReference = null; 3064 } else 3065 super.removeChild(name, value); 3066 3067 } 3068 3069 @Override 3070 public Base makeProperty(int hash, String name) throws FHIRException { 3071 switch (hash) { 3072 case -1618432855: return addIdentifier(); 3073 case -892481550: return getStatusElement(); 3074 case 50511102: return addCategory(); 3075 case 3059181: return addCode(); 3076 case 3373707: return addName(); 3077 case -1704933559: return addResponsibleOrganization(); 3078 case -1724546052: return getDescription(); 3079 case -1370922898: return addInventoryStatus(); 3080 case -1721465867: return getBaseUnit(); 3081 case 612796444: return getNetContent(); 3082 case -87499647: return addAssociation(); 3083 case 366313883: return addCharacteristic(); 3084 case 555127957: return getInstance(); 3085 case -669667556: return getProductReference(); 3086 default: return super.makeProperty(hash, name); 3087 } 3088 3089 } 3090 3091 @Override 3092 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3093 switch (hash) { 3094 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3095 case -892481550: /*status*/ return new String[] {"code"}; 3096 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3097 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3098 case 3373707: /*name*/ return new String[] {}; 3099 case -1704933559: /*responsibleOrganization*/ return new String[] {}; 3100 case -1724546052: /*description*/ return new String[] {}; 3101 case -1370922898: /*inventoryStatus*/ return new String[] {"CodeableConcept"}; 3102 case -1721465867: /*baseUnit*/ return new String[] {"CodeableConcept"}; 3103 case 612796444: /*netContent*/ return new String[] {"Quantity"}; 3104 case -87499647: /*association*/ return new String[] {}; 3105 case 366313883: /*characteristic*/ return new String[] {}; 3106 case 555127957: /*instance*/ return new String[] {}; 3107 case -669667556: /*productReference*/ return new String[] {"Reference"}; 3108 default: return super.getTypesForProperty(hash, name); 3109 } 3110 3111 } 3112 3113 @Override 3114 public Base addChild(String name) throws FHIRException { 3115 if (name.equals("identifier")) { 3116 return addIdentifier(); 3117 } 3118 else if (name.equals("status")) { 3119 throw new FHIRException("Cannot call addChild on a singleton property InventoryItem.status"); 3120 } 3121 else if (name.equals("category")) { 3122 return addCategory(); 3123 } 3124 else if (name.equals("code")) { 3125 return addCode(); 3126 } 3127 else if (name.equals("name")) { 3128 return addName(); 3129 } 3130 else if (name.equals("responsibleOrganization")) { 3131 return addResponsibleOrganization(); 3132 } 3133 else if (name.equals("description")) { 3134 this.description = new InventoryItemDescriptionComponent(); 3135 return this.description; 3136 } 3137 else if (name.equals("inventoryStatus")) { 3138 return addInventoryStatus(); 3139 } 3140 else if (name.equals("baseUnit")) { 3141 this.baseUnit = new CodeableConcept(); 3142 return this.baseUnit; 3143 } 3144 else if (name.equals("netContent")) { 3145 this.netContent = new Quantity(); 3146 return this.netContent; 3147 } 3148 else if (name.equals("association")) { 3149 return addAssociation(); 3150 } 3151 else if (name.equals("characteristic")) { 3152 return addCharacteristic(); 3153 } 3154 else if (name.equals("instance")) { 3155 this.instance = new InventoryItemInstanceComponent(); 3156 return this.instance; 3157 } 3158 else if (name.equals("productReference")) { 3159 this.productReference = new Reference(); 3160 return this.productReference; 3161 } 3162 else 3163 return super.addChild(name); 3164 } 3165 3166 public String fhirType() { 3167 return "InventoryItem"; 3168 3169 } 3170 3171 public InventoryItem copy() { 3172 InventoryItem dst = new InventoryItem(); 3173 copyValues(dst); 3174 return dst; 3175 } 3176 3177 public void copyValues(InventoryItem dst) { 3178 super.copyValues(dst); 3179 if (identifier != null) { 3180 dst.identifier = new ArrayList<Identifier>(); 3181 for (Identifier i : identifier) 3182 dst.identifier.add(i.copy()); 3183 }; 3184 dst.status = status == null ? null : status.copy(); 3185 if (category != null) { 3186 dst.category = new ArrayList<CodeableConcept>(); 3187 for (CodeableConcept i : category) 3188 dst.category.add(i.copy()); 3189 }; 3190 if (code != null) { 3191 dst.code = new ArrayList<CodeableConcept>(); 3192 for (CodeableConcept i : code) 3193 dst.code.add(i.copy()); 3194 }; 3195 if (name != null) { 3196 dst.name = new ArrayList<InventoryItemNameComponent>(); 3197 for (InventoryItemNameComponent i : name) 3198 dst.name.add(i.copy()); 3199 }; 3200 if (responsibleOrganization != null) { 3201 dst.responsibleOrganization = new ArrayList<InventoryItemResponsibleOrganizationComponent>(); 3202 for (InventoryItemResponsibleOrganizationComponent i : responsibleOrganization) 3203 dst.responsibleOrganization.add(i.copy()); 3204 }; 3205 dst.description = description == null ? null : description.copy(); 3206 if (inventoryStatus != null) { 3207 dst.inventoryStatus = new ArrayList<CodeableConcept>(); 3208 for (CodeableConcept i : inventoryStatus) 3209 dst.inventoryStatus.add(i.copy()); 3210 }; 3211 dst.baseUnit = baseUnit == null ? null : baseUnit.copy(); 3212 dst.netContent = netContent == null ? null : netContent.copy(); 3213 if (association != null) { 3214 dst.association = new ArrayList<InventoryItemAssociationComponent>(); 3215 for (InventoryItemAssociationComponent i : association) 3216 dst.association.add(i.copy()); 3217 }; 3218 if (characteristic != null) { 3219 dst.characteristic = new ArrayList<InventoryItemCharacteristicComponent>(); 3220 for (InventoryItemCharacteristicComponent i : characteristic) 3221 dst.characteristic.add(i.copy()); 3222 }; 3223 dst.instance = instance == null ? null : instance.copy(); 3224 dst.productReference = productReference == null ? null : productReference.copy(); 3225 } 3226 3227 protected InventoryItem typedCopy() { 3228 return copy(); 3229 } 3230 3231 @Override 3232 public boolean equalsDeep(Base other_) { 3233 if (!super.equalsDeep(other_)) 3234 return false; 3235 if (!(other_ instanceof InventoryItem)) 3236 return false; 3237 InventoryItem o = (InventoryItem) other_; 3238 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 3239 && compareDeep(code, o.code, true) && compareDeep(name, o.name, true) && compareDeep(responsibleOrganization, o.responsibleOrganization, true) 3240 && compareDeep(description, o.description, true) && compareDeep(inventoryStatus, o.inventoryStatus, true) 3241 && compareDeep(baseUnit, o.baseUnit, true) && compareDeep(netContent, o.netContent, true) && compareDeep(association, o.association, true) 3242 && compareDeep(characteristic, o.characteristic, true) && compareDeep(instance, o.instance, true) 3243 && compareDeep(productReference, o.productReference, true); 3244 } 3245 3246 @Override 3247 public boolean equalsShallow(Base other_) { 3248 if (!super.equalsShallow(other_)) 3249 return false; 3250 if (!(other_ instanceof InventoryItem)) 3251 return false; 3252 InventoryItem o = (InventoryItem) other_; 3253 return compareValues(status, o.status, true); 3254 } 3255 3256 public boolean isEmpty() { 3257 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 3258 , code, name, responsibleOrganization, description, inventoryStatus, baseUnit, netContent 3259 , association, characteristic, instance, productReference); 3260 } 3261 3262 @Override 3263 public ResourceType getResourceType() { 3264 return ResourceType.InventoryItem; 3265 } 3266 3267 /** 3268 * Search parameter: <b>code</b> 3269 * <p> 3270 * Description: <b>Search for products that match this code</b><br> 3271 * Type: <b>token</b><br> 3272 * Path: <b>InventoryItem.code</b><br> 3273 * </p> 3274 */ 3275 @SearchParamDefinition(name="code", path="InventoryItem.code", description="Search for products that match this code", type="token" ) 3276 public static final String SP_CODE = "code"; 3277 /** 3278 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3279 * <p> 3280 * Description: <b>Search for products that match this code</b><br> 3281 * Type: <b>token</b><br> 3282 * Path: <b>InventoryItem.code</b><br> 3283 * </p> 3284 */ 3285 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 3286 3287 /** 3288 * Search parameter: <b>identifier</b> 3289 * <p> 3290 * Description: <b>The identifier of the item</b><br> 3291 * Type: <b>token</b><br> 3292 * Path: <b>InventoryItem.identifier</b><br> 3293 * </p> 3294 */ 3295 @SearchParamDefinition(name="identifier", path="InventoryItem.identifier", description="The identifier of the item", type="token" ) 3296 public static final String SP_IDENTIFIER = "identifier"; 3297 /** 3298 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3299 * <p> 3300 * Description: <b>The identifier of the item</b><br> 3301 * Type: <b>token</b><br> 3302 * Path: <b>InventoryItem.identifier</b><br> 3303 * </p> 3304 */ 3305 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3306 3307 /** 3308 * Search parameter: <b>status</b> 3309 * <p> 3310 * Description: <b>The status of the item</b><br> 3311 * Type: <b>token</b><br> 3312 * Path: <b>InventoryItem.status</b><br> 3313 * </p> 3314 */ 3315 @SearchParamDefinition(name="status", path="InventoryItem.status", description="The status of the item", type="token" ) 3316 public static final String SP_STATUS = "status"; 3317 /** 3318 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3319 * <p> 3320 * Description: <b>The status of the item</b><br> 3321 * Type: <b>token</b><br> 3322 * Path: <b>InventoryItem.status</b><br> 3323 * </p> 3324 */ 3325 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3326 3327 /** 3328 * Search parameter: <b>subject</b> 3329 * <p> 3330 * Description: <b>The identity of a patient for whom to list associations</b><br> 3331 * Type: <b>reference</b><br> 3332 * Path: <b>InventoryItem.instance.subject</b><br> 3333 * </p> 3334 */ 3335 @SearchParamDefinition(name="subject", path="InventoryItem.instance.subject", description="The identity of a patient for whom to list associations", type="reference", target={Organization.class, Patient.class } ) 3336 public static final String SP_SUBJECT = "subject"; 3337 /** 3338 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3339 * <p> 3340 * Description: <b>The identity of a patient for whom to list associations</b><br> 3341 * Type: <b>reference</b><br> 3342 * Path: <b>InventoryItem.instance.subject</b><br> 3343 * </p> 3344 */ 3345 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3346 3347/** 3348 * Constant for fluent queries to be used to add include statements. Specifies 3349 * the path value of "<b>InventoryItem:subject</b>". 3350 */ 3351 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("InventoryItem:subject").toLocked(); 3352 3353 3354} 3355