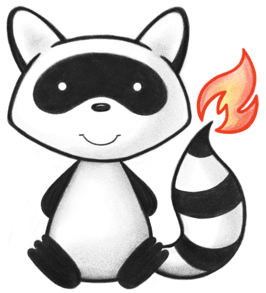
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * functional description of an inventory item used in inventory and supply-related workflows. 052 */ 053@ResourceDef(name="InventoryItem", profile="http://hl7.org/fhir/StructureDefinition/InventoryItem") 054public class InventoryItem extends DomainResource { 055 056 public enum InventoryItemStatusCodes { 057 /** 058 * The item is active and can be referenced. 059 */ 060 ACTIVE, 061 /** 062 * The item is presently inactive - there may be references to it but the item is not expected to be used. 063 */ 064 INACTIVE, 065 /** 066 * The item record was entered in error. 067 */ 068 ENTEREDINERROR, 069 /** 070 * The item status has not been determined. 071 */ 072 UNKNOWN, 073 /** 074 * added to help the parsers with the generic types 075 */ 076 NULL; 077 public static InventoryItemStatusCodes fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("active".equals(codeString)) 081 return ACTIVE; 082 if ("inactive".equals(codeString)) 083 return INACTIVE; 084 if ("entered-in-error".equals(codeString)) 085 return ENTEREDINERROR; 086 if ("unknown".equals(codeString)) 087 return UNKNOWN; 088 if (Configuration.isAcceptInvalidEnums()) 089 return null; 090 else 091 throw new FHIRException("Unknown InventoryItemStatusCodes code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case ACTIVE: return "active"; 096 case INACTIVE: return "inactive"; 097 case ENTEREDINERROR: return "entered-in-error"; 098 case UNKNOWN: return "unknown"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getSystem() { 104 switch (this) { 105 case ACTIVE: return "http://hl7.org/fhir/inventoryitem-status"; 106 case INACTIVE: return "http://hl7.org/fhir/inventoryitem-status"; 107 case ENTEREDINERROR: return "http://hl7.org/fhir/inventoryitem-status"; 108 case UNKNOWN: return "http://hl7.org/fhir/inventoryitem-status"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDefinition() { 114 switch (this) { 115 case ACTIVE: return "The item is active and can be referenced."; 116 case INACTIVE: return "The item is presently inactive - there may be references to it but the item is not expected to be used."; 117 case ENTEREDINERROR: return "The item record was entered in error."; 118 case UNKNOWN: return "The item status has not been determined."; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 public String getDisplay() { 124 switch (this) { 125 case ACTIVE: return "Active"; 126 case INACTIVE: return "Inactive"; 127 case ENTEREDINERROR: return "Entered in Error"; 128 case UNKNOWN: return "Unknown"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 } 134 135 public static class InventoryItemStatusCodesEnumFactory implements EnumFactory<InventoryItemStatusCodes> { 136 public InventoryItemStatusCodes fromCode(String codeString) throws IllegalArgumentException { 137 if (codeString == null || "".equals(codeString)) 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("active".equals(codeString)) 141 return InventoryItemStatusCodes.ACTIVE; 142 if ("inactive".equals(codeString)) 143 return InventoryItemStatusCodes.INACTIVE; 144 if ("entered-in-error".equals(codeString)) 145 return InventoryItemStatusCodes.ENTEREDINERROR; 146 if ("unknown".equals(codeString)) 147 return InventoryItemStatusCodes.UNKNOWN; 148 throw new IllegalArgumentException("Unknown InventoryItemStatusCodes code '"+codeString+"'"); 149 } 150 public Enumeration<InventoryItemStatusCodes> fromType(PrimitiveType<?> code) throws FHIRException { 151 if (code == null) 152 return null; 153 if (code.isEmpty()) 154 return new Enumeration<InventoryItemStatusCodes>(this, InventoryItemStatusCodes.NULL, code); 155 String codeString = ((PrimitiveType) code).asStringValue(); 156 if (codeString == null || "".equals(codeString)) 157 return new Enumeration<InventoryItemStatusCodes>(this, InventoryItemStatusCodes.NULL, code); 158 if ("active".equals(codeString)) 159 return new Enumeration<InventoryItemStatusCodes>(this, InventoryItemStatusCodes.ACTIVE, code); 160 if ("inactive".equals(codeString)) 161 return new Enumeration<InventoryItemStatusCodes>(this, InventoryItemStatusCodes.INACTIVE, code); 162 if ("entered-in-error".equals(codeString)) 163 return new Enumeration<InventoryItemStatusCodes>(this, InventoryItemStatusCodes.ENTEREDINERROR, code); 164 if ("unknown".equals(codeString)) 165 return new Enumeration<InventoryItemStatusCodes>(this, InventoryItemStatusCodes.UNKNOWN, code); 166 throw new FHIRException("Unknown InventoryItemStatusCodes code '"+codeString+"'"); 167 } 168 public String toCode(InventoryItemStatusCodes code) { 169 if (code == InventoryItemStatusCodes.ACTIVE) 170 return "active"; 171 if (code == InventoryItemStatusCodes.INACTIVE) 172 return "inactive"; 173 if (code == InventoryItemStatusCodes.ENTEREDINERROR) 174 return "entered-in-error"; 175 if (code == InventoryItemStatusCodes.UNKNOWN) 176 return "unknown"; 177 return "?"; 178 } 179 public String toSystem(InventoryItemStatusCodes code) { 180 return code.getSystem(); 181 } 182 } 183 184 @Block() 185 public static class InventoryItemNameComponent extends BackboneElement implements IBaseBackboneElement { 186 /** 187 * The type of name e.g. 'brand-name', 'functional-name', 'common-name'. 188 */ 189 @Child(name = "nameType", type = {Coding.class}, order=1, min=1, max=1, modifier=false, summary=true) 190 @Description(shortDefinition="The type of name e.g. 'brand-name', 'functional-name', 'common-name'", formalDefinition="The type of name e.g. 'brand-name', 'functional-name', 'common-name'." ) 191 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/inventoryitem-nametype") 192 protected Coding nameType; 193 194 /** 195 * The language that the item name is expressed in. 196 */ 197 @Child(name = "language", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 198 @Description(shortDefinition="The language used to express the item name", formalDefinition="The language that the item name is expressed in." ) 199 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 200 protected Enumeration<CommonLanguages> language; 201 202 /** 203 * The name or designation that the item is given. 204 */ 205 @Child(name = "name", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=true) 206 @Description(shortDefinition="The name or designation of the item", formalDefinition="The name or designation that the item is given." ) 207 protected StringType name; 208 209 private static final long serialVersionUID = 2074178414L; 210 211 /** 212 * Constructor 213 */ 214 public InventoryItemNameComponent() { 215 super(); 216 } 217 218 /** 219 * Constructor 220 */ 221 public InventoryItemNameComponent(Coding nameType, CommonLanguages language, String name) { 222 super(); 223 this.setNameType(nameType); 224 this.setLanguage(language); 225 this.setName(name); 226 } 227 228 /** 229 * @return {@link #nameType} (The type of name e.g. 'brand-name', 'functional-name', 'common-name'.) 230 */ 231 public Coding getNameType() { 232 if (this.nameType == null) 233 if (Configuration.errorOnAutoCreate()) 234 throw new Error("Attempt to auto-create InventoryItemNameComponent.nameType"); 235 else if (Configuration.doAutoCreate()) 236 this.nameType = new Coding(); // cc 237 return this.nameType; 238 } 239 240 public boolean hasNameType() { 241 return this.nameType != null && !this.nameType.isEmpty(); 242 } 243 244 /** 245 * @param value {@link #nameType} (The type of name e.g. 'brand-name', 'functional-name', 'common-name'.) 246 */ 247 public InventoryItemNameComponent setNameType(Coding value) { 248 this.nameType = value; 249 return this; 250 } 251 252 /** 253 * @return {@link #language} (The language that the item name is expressed in.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 254 */ 255 public Enumeration<CommonLanguages> getLanguageElement() { 256 if (this.language == null) 257 if (Configuration.errorOnAutoCreate()) 258 throw new Error("Attempt to auto-create InventoryItemNameComponent.language"); 259 else if (Configuration.doAutoCreate()) 260 this.language = new Enumeration<CommonLanguages>(new CommonLanguagesEnumFactory()); // bb 261 return this.language; 262 } 263 264 public boolean hasLanguageElement() { 265 return this.language != null && !this.language.isEmpty(); 266 } 267 268 public boolean hasLanguage() { 269 return this.language != null && !this.language.isEmpty(); 270 } 271 272 /** 273 * @param value {@link #language} (The language that the item name is expressed in.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 274 */ 275 public InventoryItemNameComponent setLanguageElement(Enumeration<CommonLanguages> value) { 276 this.language = value; 277 return this; 278 } 279 280 /** 281 * @return The language that the item name is expressed in. 282 */ 283 public CommonLanguages getLanguage() { 284 return this.language == null ? null : this.language.getValue(); 285 } 286 287 /** 288 * @param value The language that the item name is expressed in. 289 */ 290 public InventoryItemNameComponent setLanguage(CommonLanguages value) { 291 if (this.language == null) 292 this.language = new Enumeration<CommonLanguages>(new CommonLanguagesEnumFactory()); 293 this.language.setValue(value); 294 return this; 295 } 296 297 /** 298 * @return {@link #name} (The name or designation that the item is given.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 299 */ 300 public StringType getNameElement() { 301 if (this.name == null) 302 if (Configuration.errorOnAutoCreate()) 303 throw new Error("Attempt to auto-create InventoryItemNameComponent.name"); 304 else if (Configuration.doAutoCreate()) 305 this.name = new StringType(); // bb 306 return this.name; 307 } 308 309 public boolean hasNameElement() { 310 return this.name != null && !this.name.isEmpty(); 311 } 312 313 public boolean hasName() { 314 return this.name != null && !this.name.isEmpty(); 315 } 316 317 /** 318 * @param value {@link #name} (The name or designation that the item is given.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 319 */ 320 public InventoryItemNameComponent setNameElement(StringType value) { 321 this.name = value; 322 return this; 323 } 324 325 /** 326 * @return The name or designation that the item is given. 327 */ 328 public String getName() { 329 return this.name == null ? null : this.name.getValue(); 330 } 331 332 /** 333 * @param value The name or designation that the item is given. 334 */ 335 public InventoryItemNameComponent setName(String value) { 336 if (this.name == null) 337 this.name = new StringType(); 338 this.name.setValue(value); 339 return this; 340 } 341 342 protected void listChildren(List<Property> children) { 343 super.listChildren(children); 344 children.add(new Property("nameType", "Coding", "The type of name e.g. 'brand-name', 'functional-name', 'common-name'.", 0, 1, nameType)); 345 children.add(new Property("language", "code", "The language that the item name is expressed in.", 0, 1, language)); 346 children.add(new Property("name", "string", "The name or designation that the item is given.", 0, 1, name)); 347 } 348 349 @Override 350 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 351 switch (_hash) { 352 case 1840595045: /*nameType*/ return new Property("nameType", "Coding", "The type of name e.g. 'brand-name', 'functional-name', 'common-name'.", 0, 1, nameType); 353 case -1613589672: /*language*/ return new Property("language", "code", "The language that the item name is expressed in.", 0, 1, language); 354 case 3373707: /*name*/ return new Property("name", "string", "The name or designation that the item is given.", 0, 1, name); 355 default: return super.getNamedProperty(_hash, _name, _checkValid); 356 } 357 358 } 359 360 @Override 361 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 362 switch (hash) { 363 case 1840595045: /*nameType*/ return this.nameType == null ? new Base[0] : new Base[] {this.nameType}; // Coding 364 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // Enumeration<CommonLanguages> 365 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 366 default: return super.getProperty(hash, name, checkValid); 367 } 368 369 } 370 371 @Override 372 public Base setProperty(int hash, String name, Base value) throws FHIRException { 373 switch (hash) { 374 case 1840595045: // nameType 375 this.nameType = TypeConvertor.castToCoding(value); // Coding 376 return value; 377 case -1613589672: // language 378 value = new CommonLanguagesEnumFactory().fromType(TypeConvertor.castToCode(value)); 379 this.language = (Enumeration) value; // Enumeration<CommonLanguages> 380 return value; 381 case 3373707: // name 382 this.name = TypeConvertor.castToString(value); // StringType 383 return value; 384 default: return super.setProperty(hash, name, value); 385 } 386 387 } 388 389 @Override 390 public Base setProperty(String name, Base value) throws FHIRException { 391 if (name.equals("nameType")) { 392 this.nameType = TypeConvertor.castToCoding(value); // Coding 393 } else if (name.equals("language")) { 394 value = new CommonLanguagesEnumFactory().fromType(TypeConvertor.castToCode(value)); 395 this.language = (Enumeration) value; // Enumeration<CommonLanguages> 396 } else if (name.equals("name")) { 397 this.name = TypeConvertor.castToString(value); // StringType 398 } else 399 return super.setProperty(name, value); 400 return value; 401 } 402 403 @Override 404 public Base makeProperty(int hash, String name) throws FHIRException { 405 switch (hash) { 406 case 1840595045: return getNameType(); 407 case -1613589672: return getLanguageElement(); 408 case 3373707: return getNameElement(); 409 default: return super.makeProperty(hash, name); 410 } 411 412 } 413 414 @Override 415 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 416 switch (hash) { 417 case 1840595045: /*nameType*/ return new String[] {"Coding"}; 418 case -1613589672: /*language*/ return new String[] {"code"}; 419 case 3373707: /*name*/ return new String[] {"string"}; 420 default: return super.getTypesForProperty(hash, name); 421 } 422 423 } 424 425 @Override 426 public Base addChild(String name) throws FHIRException { 427 if (name.equals("nameType")) { 428 this.nameType = new Coding(); 429 return this.nameType; 430 } 431 else if (name.equals("language")) { 432 throw new FHIRException("Cannot call addChild on a singleton property InventoryItem.name.language"); 433 } 434 else if (name.equals("name")) { 435 throw new FHIRException("Cannot call addChild on a singleton property InventoryItem.name.name"); 436 } 437 else 438 return super.addChild(name); 439 } 440 441 public InventoryItemNameComponent copy() { 442 InventoryItemNameComponent dst = new InventoryItemNameComponent(); 443 copyValues(dst); 444 return dst; 445 } 446 447 public void copyValues(InventoryItemNameComponent dst) { 448 super.copyValues(dst); 449 dst.nameType = nameType == null ? null : nameType.copy(); 450 dst.language = language == null ? null : language.copy(); 451 dst.name = name == null ? null : name.copy(); 452 } 453 454 @Override 455 public boolean equalsDeep(Base other_) { 456 if (!super.equalsDeep(other_)) 457 return false; 458 if (!(other_ instanceof InventoryItemNameComponent)) 459 return false; 460 InventoryItemNameComponent o = (InventoryItemNameComponent) other_; 461 return compareDeep(nameType, o.nameType, true) && compareDeep(language, o.language, true) && compareDeep(name, o.name, true) 462 ; 463 } 464 465 @Override 466 public boolean equalsShallow(Base other_) { 467 if (!super.equalsShallow(other_)) 468 return false; 469 if (!(other_ instanceof InventoryItemNameComponent)) 470 return false; 471 InventoryItemNameComponent o = (InventoryItemNameComponent) other_; 472 return compareValues(language, o.language, true) && compareValues(name, o.name, true); 473 } 474 475 public boolean isEmpty() { 476 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(nameType, language, name 477 ); 478 } 479 480 public String fhirType() { 481 return "InventoryItem.name"; 482 483 } 484 485 } 486 487 @Block() 488 public static class InventoryItemResponsibleOrganizationComponent extends BackboneElement implements IBaseBackboneElement { 489 /** 490 * The role of the organization e.g. manufacturer, distributor, etc. 491 */ 492 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 493 @Description(shortDefinition="The role of the organization e.g. manufacturer, distributor, or other", formalDefinition="The role of the organization e.g. manufacturer, distributor, etc." ) 494 protected CodeableConcept role; 495 496 /** 497 * An organization that has an association with the item, e.g. manufacturer, distributor, responsible, etc. 498 */ 499 @Child(name = "organization", type = {Organization.class}, order=2, min=1, max=1, modifier=false, summary=false) 500 @Description(shortDefinition="An organization that is associated with the item", formalDefinition="An organization that has an association with the item, e.g. manufacturer, distributor, responsible, etc." ) 501 protected Reference organization; 502 503 private static final long serialVersionUID = -575091539L; 504 505 /** 506 * Constructor 507 */ 508 public InventoryItemResponsibleOrganizationComponent() { 509 super(); 510 } 511 512 /** 513 * Constructor 514 */ 515 public InventoryItemResponsibleOrganizationComponent(CodeableConcept role, Reference organization) { 516 super(); 517 this.setRole(role); 518 this.setOrganization(organization); 519 } 520 521 /** 522 * @return {@link #role} (The role of the organization e.g. manufacturer, distributor, etc.) 523 */ 524 public CodeableConcept getRole() { 525 if (this.role == null) 526 if (Configuration.errorOnAutoCreate()) 527 throw new Error("Attempt to auto-create InventoryItemResponsibleOrganizationComponent.role"); 528 else if (Configuration.doAutoCreate()) 529 this.role = new CodeableConcept(); // cc 530 return this.role; 531 } 532 533 public boolean hasRole() { 534 return this.role != null && !this.role.isEmpty(); 535 } 536 537 /** 538 * @param value {@link #role} (The role of the organization e.g. manufacturer, distributor, etc.) 539 */ 540 public InventoryItemResponsibleOrganizationComponent setRole(CodeableConcept value) { 541 this.role = value; 542 return this; 543 } 544 545 /** 546 * @return {@link #organization} (An organization that has an association with the item, e.g. manufacturer, distributor, responsible, etc.) 547 */ 548 public Reference getOrganization() { 549 if (this.organization == null) 550 if (Configuration.errorOnAutoCreate()) 551 throw new Error("Attempt to auto-create InventoryItemResponsibleOrganizationComponent.organization"); 552 else if (Configuration.doAutoCreate()) 553 this.organization = new Reference(); // cc 554 return this.organization; 555 } 556 557 public boolean hasOrganization() { 558 return this.organization != null && !this.organization.isEmpty(); 559 } 560 561 /** 562 * @param value {@link #organization} (An organization that has an association with the item, e.g. manufacturer, distributor, responsible, etc.) 563 */ 564 public InventoryItemResponsibleOrganizationComponent setOrganization(Reference value) { 565 this.organization = value; 566 return this; 567 } 568 569 protected void listChildren(List<Property> children) { 570 super.listChildren(children); 571 children.add(new Property("role", "CodeableConcept", "The role of the organization e.g. manufacturer, distributor, etc.", 0, 1, role)); 572 children.add(new Property("organization", "Reference(Organization)", "An organization that has an association with the item, e.g. manufacturer, distributor, responsible, etc.", 0, 1, organization)); 573 } 574 575 @Override 576 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 577 switch (_hash) { 578 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The role of the organization e.g. manufacturer, distributor, etc.", 0, 1, role); 579 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "An organization that has an association with the item, e.g. manufacturer, distributor, responsible, etc.", 0, 1, organization); 580 default: return super.getNamedProperty(_hash, _name, _checkValid); 581 } 582 583 } 584 585 @Override 586 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 587 switch (hash) { 588 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 589 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 590 default: return super.getProperty(hash, name, checkValid); 591 } 592 593 } 594 595 @Override 596 public Base setProperty(int hash, String name, Base value) throws FHIRException { 597 switch (hash) { 598 case 3506294: // role 599 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 600 return value; 601 case 1178922291: // organization 602 this.organization = TypeConvertor.castToReference(value); // Reference 603 return value; 604 default: return super.setProperty(hash, name, value); 605 } 606 607 } 608 609 @Override 610 public Base setProperty(String name, Base value) throws FHIRException { 611 if (name.equals("role")) { 612 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 613 } else if (name.equals("organization")) { 614 this.organization = TypeConvertor.castToReference(value); // Reference 615 } else 616 return super.setProperty(name, value); 617 return value; 618 } 619 620 @Override 621 public Base makeProperty(int hash, String name) throws FHIRException { 622 switch (hash) { 623 case 3506294: return getRole(); 624 case 1178922291: return getOrganization(); 625 default: return super.makeProperty(hash, name); 626 } 627 628 } 629 630 @Override 631 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 632 switch (hash) { 633 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 634 case 1178922291: /*organization*/ return new String[] {"Reference"}; 635 default: return super.getTypesForProperty(hash, name); 636 } 637 638 } 639 640 @Override 641 public Base addChild(String name) throws FHIRException { 642 if (name.equals("role")) { 643 this.role = new CodeableConcept(); 644 return this.role; 645 } 646 else if (name.equals("organization")) { 647 this.organization = new Reference(); 648 return this.organization; 649 } 650 else 651 return super.addChild(name); 652 } 653 654 public InventoryItemResponsibleOrganizationComponent copy() { 655 InventoryItemResponsibleOrganizationComponent dst = new InventoryItemResponsibleOrganizationComponent(); 656 copyValues(dst); 657 return dst; 658 } 659 660 public void copyValues(InventoryItemResponsibleOrganizationComponent dst) { 661 super.copyValues(dst); 662 dst.role = role == null ? null : role.copy(); 663 dst.organization = organization == null ? null : organization.copy(); 664 } 665 666 @Override 667 public boolean equalsDeep(Base other_) { 668 if (!super.equalsDeep(other_)) 669 return false; 670 if (!(other_ instanceof InventoryItemResponsibleOrganizationComponent)) 671 return false; 672 InventoryItemResponsibleOrganizationComponent o = (InventoryItemResponsibleOrganizationComponent) other_; 673 return compareDeep(role, o.role, true) && compareDeep(organization, o.organization, true); 674 } 675 676 @Override 677 public boolean equalsShallow(Base other_) { 678 if (!super.equalsShallow(other_)) 679 return false; 680 if (!(other_ instanceof InventoryItemResponsibleOrganizationComponent)) 681 return false; 682 InventoryItemResponsibleOrganizationComponent o = (InventoryItemResponsibleOrganizationComponent) other_; 683 return true; 684 } 685 686 public boolean isEmpty() { 687 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, organization); 688 } 689 690 public String fhirType() { 691 return "InventoryItem.responsibleOrganization"; 692 693 } 694 695 } 696 697 @Block() 698 public static class InventoryItemDescriptionComponent extends BackboneElement implements IBaseBackboneElement { 699 /** 700 * The language for the item description, when an item must be described in different languages and those languages may be authoritative and not translations of a 'main' language. 701 */ 702 @Child(name = "language", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 703 @Description(shortDefinition="The language that is used in the item description", formalDefinition="The language for the item description, when an item must be described in different languages and those languages may be authoritative and not translations of a 'main' language." ) 704 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 705 protected Enumeration<CommonLanguages> language; 706 707 /** 708 * Textual description of the item. 709 */ 710 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 711 @Description(shortDefinition="Textual description of the item", formalDefinition="Textual description of the item." ) 712 protected StringType description; 713 714 private static final long serialVersionUID = -803271414L; 715 716 /** 717 * Constructor 718 */ 719 public InventoryItemDescriptionComponent() { 720 super(); 721 } 722 723 /** 724 * @return {@link #language} (The language for the item description, when an item must be described in different languages and those languages may be authoritative and not translations of a 'main' language.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 725 */ 726 public Enumeration<CommonLanguages> getLanguageElement() { 727 if (this.language == null) 728 if (Configuration.errorOnAutoCreate()) 729 throw new Error("Attempt to auto-create InventoryItemDescriptionComponent.language"); 730 else if (Configuration.doAutoCreate()) 731 this.language = new Enumeration<CommonLanguages>(new CommonLanguagesEnumFactory()); // bb 732 return this.language; 733 } 734 735 public boolean hasLanguageElement() { 736 return this.language != null && !this.language.isEmpty(); 737 } 738 739 public boolean hasLanguage() { 740 return this.language != null && !this.language.isEmpty(); 741 } 742 743 /** 744 * @param value {@link #language} (The language for the item description, when an item must be described in different languages and those languages may be authoritative and not translations of a 'main' language.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 745 */ 746 public InventoryItemDescriptionComponent setLanguageElement(Enumeration<CommonLanguages> value) { 747 this.language = value; 748 return this; 749 } 750 751 /** 752 * @return The language for the item description, when an item must be described in different languages and those languages may be authoritative and not translations of a 'main' language. 753 */ 754 public CommonLanguages getLanguage() { 755 return this.language == null ? null : this.language.getValue(); 756 } 757 758 /** 759 * @param value The language for the item description, when an item must be described in different languages and those languages may be authoritative and not translations of a 'main' language. 760 */ 761 public InventoryItemDescriptionComponent setLanguage(CommonLanguages value) { 762 if (value == null) 763 this.language = null; 764 else { 765 if (this.language == null) 766 this.language = new Enumeration<CommonLanguages>(new CommonLanguagesEnumFactory()); 767 this.language.setValue(value); 768 } 769 return this; 770 } 771 772 /** 773 * @return {@link #description} (Textual description of the item.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 774 */ 775 public StringType getDescriptionElement() { 776 if (this.description == null) 777 if (Configuration.errorOnAutoCreate()) 778 throw new Error("Attempt to auto-create InventoryItemDescriptionComponent.description"); 779 else if (Configuration.doAutoCreate()) 780 this.description = new StringType(); // bb 781 return this.description; 782 } 783 784 public boolean hasDescriptionElement() { 785 return this.description != null && !this.description.isEmpty(); 786 } 787 788 public boolean hasDescription() { 789 return this.description != null && !this.description.isEmpty(); 790 } 791 792 /** 793 * @param value {@link #description} (Textual description of the item.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 794 */ 795 public InventoryItemDescriptionComponent setDescriptionElement(StringType value) { 796 this.description = value; 797 return this; 798 } 799 800 /** 801 * @return Textual description of the item. 802 */ 803 public String getDescription() { 804 return this.description == null ? null : this.description.getValue(); 805 } 806 807 /** 808 * @param value Textual description of the item. 809 */ 810 public InventoryItemDescriptionComponent setDescription(String value) { 811 if (Utilities.noString(value)) 812 this.description = null; 813 else { 814 if (this.description == null) 815 this.description = new StringType(); 816 this.description.setValue(value); 817 } 818 return this; 819 } 820 821 protected void listChildren(List<Property> children) { 822 super.listChildren(children); 823 children.add(new Property("language", "code", "The language for the item description, when an item must be described in different languages and those languages may be authoritative and not translations of a 'main' language.", 0, 1, language)); 824 children.add(new Property("description", "string", "Textual description of the item.", 0, 1, description)); 825 } 826 827 @Override 828 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 829 switch (_hash) { 830 case -1613589672: /*language*/ return new Property("language", "code", "The language for the item description, when an item must be described in different languages and those languages may be authoritative and not translations of a 'main' language.", 0, 1, language); 831 case -1724546052: /*description*/ return new Property("description", "string", "Textual description of the item.", 0, 1, description); 832 default: return super.getNamedProperty(_hash, _name, _checkValid); 833 } 834 835 } 836 837 @Override 838 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 839 switch (hash) { 840 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // Enumeration<CommonLanguages> 841 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 842 default: return super.getProperty(hash, name, checkValid); 843 } 844 845 } 846 847 @Override 848 public Base setProperty(int hash, String name, Base value) throws FHIRException { 849 switch (hash) { 850 case -1613589672: // language 851 value = new CommonLanguagesEnumFactory().fromType(TypeConvertor.castToCode(value)); 852 this.language = (Enumeration) value; // Enumeration<CommonLanguages> 853 return value; 854 case -1724546052: // description 855 this.description = TypeConvertor.castToString(value); // StringType 856 return value; 857 default: return super.setProperty(hash, name, value); 858 } 859 860 } 861 862 @Override 863 public Base setProperty(String name, Base value) throws FHIRException { 864 if (name.equals("language")) { 865 value = new CommonLanguagesEnumFactory().fromType(TypeConvertor.castToCode(value)); 866 this.language = (Enumeration) value; // Enumeration<CommonLanguages> 867 } else if (name.equals("description")) { 868 this.description = TypeConvertor.castToString(value); // StringType 869 } else 870 return super.setProperty(name, value); 871 return value; 872 } 873 874 @Override 875 public Base makeProperty(int hash, String name) throws FHIRException { 876 switch (hash) { 877 case -1613589672: return getLanguageElement(); 878 case -1724546052: return getDescriptionElement(); 879 default: return super.makeProperty(hash, name); 880 } 881 882 } 883 884 @Override 885 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 886 switch (hash) { 887 case -1613589672: /*language*/ return new String[] {"code"}; 888 case -1724546052: /*description*/ return new String[] {"string"}; 889 default: return super.getTypesForProperty(hash, name); 890 } 891 892 } 893 894 @Override 895 public Base addChild(String name) throws FHIRException { 896 if (name.equals("language")) { 897 throw new FHIRException("Cannot call addChild on a singleton property InventoryItem.description.language"); 898 } 899 else if (name.equals("description")) { 900 throw new FHIRException("Cannot call addChild on a singleton property InventoryItem.description.description"); 901 } 902 else 903 return super.addChild(name); 904 } 905 906 public InventoryItemDescriptionComponent copy() { 907 InventoryItemDescriptionComponent dst = new InventoryItemDescriptionComponent(); 908 copyValues(dst); 909 return dst; 910 } 911 912 public void copyValues(InventoryItemDescriptionComponent dst) { 913 super.copyValues(dst); 914 dst.language = language == null ? null : language.copy(); 915 dst.description = description == null ? null : description.copy(); 916 } 917 918 @Override 919 public boolean equalsDeep(Base other_) { 920 if (!super.equalsDeep(other_)) 921 return false; 922 if (!(other_ instanceof InventoryItemDescriptionComponent)) 923 return false; 924 InventoryItemDescriptionComponent o = (InventoryItemDescriptionComponent) other_; 925 return compareDeep(language, o.language, true) && compareDeep(description, o.description, true) 926 ; 927 } 928 929 @Override 930 public boolean equalsShallow(Base other_) { 931 if (!super.equalsShallow(other_)) 932 return false; 933 if (!(other_ instanceof InventoryItemDescriptionComponent)) 934 return false; 935 InventoryItemDescriptionComponent o = (InventoryItemDescriptionComponent) other_; 936 return compareValues(language, o.language, true) && compareValues(description, o.description, true) 937 ; 938 } 939 940 public boolean isEmpty() { 941 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, description); 942 } 943 944 public String fhirType() { 945 return "InventoryItem.description"; 946 947 } 948 949 } 950 951 @Block() 952 public static class InventoryItemAssociationComponent extends BackboneElement implements IBaseBackboneElement { 953 /** 954 * This attribute defined the type of association when establishing associations or relations between items, e.g. 'packaged within' or 'used with' or 'to be mixed with. 955 */ 956 @Child(name = "associationType", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 957 @Description(shortDefinition="The type of association between the device and the other item", formalDefinition="This attribute defined the type of association when establishing associations or relations between items, e.g. 'packaged within' or 'used with' or 'to be mixed with." ) 958 protected CodeableConcept associationType; 959 960 /** 961 * The related item or product. 962 */ 963 @Child(name = "relatedItem", type = {InventoryItem.class, Medication.class, MedicationKnowledge.class, Device.class, DeviceDefinition.class, NutritionProduct.class, BiologicallyDerivedProduct.class}, order=2, min=1, max=1, modifier=false, summary=true) 964 @Description(shortDefinition="The related item or product", formalDefinition="The related item or product." ) 965 protected Reference relatedItem; 966 967 /** 968 * The quantity of the related product in this product - Numerator is the quantity of the related product. Denominator is the quantity of the present product. For example a value of 20 means that this product contains 20 units of the related product; a value of 1:20 means the inverse - that the contained product contains 20 units of the present product. 969 */ 970 @Child(name = "quantity", type = {Ratio.class}, order=3, min=1, max=1, modifier=false, summary=true) 971 @Description(shortDefinition="The quantity of the product in this product", formalDefinition="The quantity of the related product in this product - Numerator is the quantity of the related product. Denominator is the quantity of the present product. For example a value of 20 means that this product contains 20 units of the related product; a value of 1:20 means the inverse - that the contained product contains 20 units of the present product." ) 972 protected Ratio quantity; 973 974 private static final long serialVersionUID = -1001386921L; 975 976 /** 977 * Constructor 978 */ 979 public InventoryItemAssociationComponent() { 980 super(); 981 } 982 983 /** 984 * Constructor 985 */ 986 public InventoryItemAssociationComponent(CodeableConcept associationType, Reference relatedItem, Ratio quantity) { 987 super(); 988 this.setAssociationType(associationType); 989 this.setRelatedItem(relatedItem); 990 this.setQuantity(quantity); 991 } 992 993 /** 994 * @return {@link #associationType} (This attribute defined the type of association when establishing associations or relations between items, e.g. 'packaged within' or 'used with' or 'to be mixed with.) 995 */ 996 public CodeableConcept getAssociationType() { 997 if (this.associationType == null) 998 if (Configuration.errorOnAutoCreate()) 999 throw new Error("Attempt to auto-create InventoryItemAssociationComponent.associationType"); 1000 else if (Configuration.doAutoCreate()) 1001 this.associationType = new CodeableConcept(); // cc 1002 return this.associationType; 1003 } 1004 1005 public boolean hasAssociationType() { 1006 return this.associationType != null && !this.associationType.isEmpty(); 1007 } 1008 1009 /** 1010 * @param value {@link #associationType} (This attribute defined the type of association when establishing associations or relations between items, e.g. 'packaged within' or 'used with' or 'to be mixed with.) 1011 */ 1012 public InventoryItemAssociationComponent setAssociationType(CodeableConcept value) { 1013 this.associationType = value; 1014 return this; 1015 } 1016 1017 /** 1018 * @return {@link #relatedItem} (The related item or product.) 1019 */ 1020 public Reference getRelatedItem() { 1021 if (this.relatedItem == null) 1022 if (Configuration.errorOnAutoCreate()) 1023 throw new Error("Attempt to auto-create InventoryItemAssociationComponent.relatedItem"); 1024 else if (Configuration.doAutoCreate()) 1025 this.relatedItem = new Reference(); // cc 1026 return this.relatedItem; 1027 } 1028 1029 public boolean hasRelatedItem() { 1030 return this.relatedItem != null && !this.relatedItem.isEmpty(); 1031 } 1032 1033 /** 1034 * @param value {@link #relatedItem} (The related item or product.) 1035 */ 1036 public InventoryItemAssociationComponent setRelatedItem(Reference value) { 1037 this.relatedItem = value; 1038 return this; 1039 } 1040 1041 /** 1042 * @return {@link #quantity} (The quantity of the related product in this product - Numerator is the quantity of the related product. Denominator is the quantity of the present product. For example a value of 20 means that this product contains 20 units of the related product; a value of 1:20 means the inverse - that the contained product contains 20 units of the present product.) 1043 */ 1044 public Ratio getQuantity() { 1045 if (this.quantity == null) 1046 if (Configuration.errorOnAutoCreate()) 1047 throw new Error("Attempt to auto-create InventoryItemAssociationComponent.quantity"); 1048 else if (Configuration.doAutoCreate()) 1049 this.quantity = new Ratio(); // cc 1050 return this.quantity; 1051 } 1052 1053 public boolean hasQuantity() { 1054 return this.quantity != null && !this.quantity.isEmpty(); 1055 } 1056 1057 /** 1058 * @param value {@link #quantity} (The quantity of the related product in this product - Numerator is the quantity of the related product. Denominator is the quantity of the present product. For example a value of 20 means that this product contains 20 units of the related product; a value of 1:20 means the inverse - that the contained product contains 20 units of the present product.) 1059 */ 1060 public InventoryItemAssociationComponent setQuantity(Ratio value) { 1061 this.quantity = value; 1062 return this; 1063 } 1064 1065 protected void listChildren(List<Property> children) { 1066 super.listChildren(children); 1067 children.add(new Property("associationType", "CodeableConcept", "This attribute defined the type of association when establishing associations or relations between items, e.g. 'packaged within' or 'used with' or 'to be mixed with.", 0, 1, associationType)); 1068 children.add(new Property("relatedItem", "Reference(InventoryItem|Medication|MedicationKnowledge|Device|DeviceDefinition|NutritionProduct|BiologicallyDerivedProduct)", "The related item or product.", 0, 1, relatedItem)); 1069 children.add(new Property("quantity", "Ratio", "The quantity of the related product in this product - Numerator is the quantity of the related product. Denominator is the quantity of the present product. For example a value of 20 means that this product contains 20 units of the related product; a value of 1:20 means the inverse - that the contained product contains 20 units of the present product.", 0, 1, quantity)); 1070 } 1071 1072 @Override 1073 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1074 switch (_hash) { 1075 case 2050799451: /*associationType*/ return new Property("associationType", "CodeableConcept", "This attribute defined the type of association when establishing associations or relations between items, e.g. 'packaged within' or 'used with' or 'to be mixed with.", 0, 1, associationType); 1076 case 1112702430: /*relatedItem*/ return new Property("relatedItem", "Reference(InventoryItem|Medication|MedicationKnowledge|Device|DeviceDefinition|NutritionProduct|BiologicallyDerivedProduct)", "The related item or product.", 0, 1, relatedItem); 1077 case -1285004149: /*quantity*/ return new Property("quantity", "Ratio", "The quantity of the related product in this product - Numerator is the quantity of the related product. Denominator is the quantity of the present product. For example a value of 20 means that this product contains 20 units of the related product; a value of 1:20 means the inverse - that the contained product contains 20 units of the present product.", 0, 1, quantity); 1078 default: return super.getNamedProperty(_hash, _name, _checkValid); 1079 } 1080 1081 } 1082 1083 @Override 1084 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1085 switch (hash) { 1086 case 2050799451: /*associationType*/ return this.associationType == null ? new Base[0] : new Base[] {this.associationType}; // CodeableConcept 1087 case 1112702430: /*relatedItem*/ return this.relatedItem == null ? new Base[0] : new Base[] {this.relatedItem}; // Reference 1088 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Ratio 1089 default: return super.getProperty(hash, name, checkValid); 1090 } 1091 1092 } 1093 1094 @Override 1095 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1096 switch (hash) { 1097 case 2050799451: // associationType 1098 this.associationType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1099 return value; 1100 case 1112702430: // relatedItem 1101 this.relatedItem = TypeConvertor.castToReference(value); // Reference 1102 return value; 1103 case -1285004149: // quantity 1104 this.quantity = TypeConvertor.castToRatio(value); // Ratio 1105 return value; 1106 default: return super.setProperty(hash, name, value); 1107 } 1108 1109 } 1110 1111 @Override 1112 public Base setProperty(String name, Base value) throws FHIRException { 1113 if (name.equals("associationType")) { 1114 this.associationType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1115 } else if (name.equals("relatedItem")) { 1116 this.relatedItem = TypeConvertor.castToReference(value); // Reference 1117 } else if (name.equals("quantity")) { 1118 this.quantity = TypeConvertor.castToRatio(value); // Ratio 1119 } else 1120 return super.setProperty(name, value); 1121 return value; 1122 } 1123 1124 @Override 1125 public Base makeProperty(int hash, String name) throws FHIRException { 1126 switch (hash) { 1127 case 2050799451: return getAssociationType(); 1128 case 1112702430: return getRelatedItem(); 1129 case -1285004149: return getQuantity(); 1130 default: return super.makeProperty(hash, name); 1131 } 1132 1133 } 1134 1135 @Override 1136 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1137 switch (hash) { 1138 case 2050799451: /*associationType*/ return new String[] {"CodeableConcept"}; 1139 case 1112702430: /*relatedItem*/ return new String[] {"Reference"}; 1140 case -1285004149: /*quantity*/ return new String[] {"Ratio"}; 1141 default: return super.getTypesForProperty(hash, name); 1142 } 1143 1144 } 1145 1146 @Override 1147 public Base addChild(String name) throws FHIRException { 1148 if (name.equals("associationType")) { 1149 this.associationType = new CodeableConcept(); 1150 return this.associationType; 1151 } 1152 else if (name.equals("relatedItem")) { 1153 this.relatedItem = new Reference(); 1154 return this.relatedItem; 1155 } 1156 else if (name.equals("quantity")) { 1157 this.quantity = new Ratio(); 1158 return this.quantity; 1159 } 1160 else 1161 return super.addChild(name); 1162 } 1163 1164 public InventoryItemAssociationComponent copy() { 1165 InventoryItemAssociationComponent dst = new InventoryItemAssociationComponent(); 1166 copyValues(dst); 1167 return dst; 1168 } 1169 1170 public void copyValues(InventoryItemAssociationComponent dst) { 1171 super.copyValues(dst); 1172 dst.associationType = associationType == null ? null : associationType.copy(); 1173 dst.relatedItem = relatedItem == null ? null : relatedItem.copy(); 1174 dst.quantity = quantity == null ? null : quantity.copy(); 1175 } 1176 1177 @Override 1178 public boolean equalsDeep(Base other_) { 1179 if (!super.equalsDeep(other_)) 1180 return false; 1181 if (!(other_ instanceof InventoryItemAssociationComponent)) 1182 return false; 1183 InventoryItemAssociationComponent o = (InventoryItemAssociationComponent) other_; 1184 return compareDeep(associationType, o.associationType, true) && compareDeep(relatedItem, o.relatedItem, true) 1185 && compareDeep(quantity, o.quantity, true); 1186 } 1187 1188 @Override 1189 public boolean equalsShallow(Base other_) { 1190 if (!super.equalsShallow(other_)) 1191 return false; 1192 if (!(other_ instanceof InventoryItemAssociationComponent)) 1193 return false; 1194 InventoryItemAssociationComponent o = (InventoryItemAssociationComponent) other_; 1195 return true; 1196 } 1197 1198 public boolean isEmpty() { 1199 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(associationType, relatedItem 1200 , quantity); 1201 } 1202 1203 public String fhirType() { 1204 return "InventoryItem.association"; 1205 1206 } 1207 1208 } 1209 1210 @Block() 1211 public static class InventoryItemCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 1212 /** 1213 * The type of characteristic that is being defined. 1214 */ 1215 @Child(name = "characteristicType", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1216 @Description(shortDefinition="The characteristic that is being defined", formalDefinition="The type of characteristic that is being defined." ) 1217 protected CodeableConcept characteristicType; 1218 1219 /** 1220 * The value of the attribute. 1221 */ 1222 @Child(name = "value", type = {StringType.class, IntegerType.class, DecimalType.class, BooleanType.class, UrlType.class, DateTimeType.class, Quantity.class, Range.class, Ratio.class, Annotation.class, Address.class, Duration.class, CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 1223 @Description(shortDefinition="The value of the attribute", formalDefinition="The value of the attribute." ) 1224 protected DataType value; 1225 1226 private static final long serialVersionUID = -642436065L; 1227 1228 /** 1229 * Constructor 1230 */ 1231 public InventoryItemCharacteristicComponent() { 1232 super(); 1233 } 1234 1235 /** 1236 * Constructor 1237 */ 1238 public InventoryItemCharacteristicComponent(CodeableConcept characteristicType, DataType value) { 1239 super(); 1240 this.setCharacteristicType(characteristicType); 1241 this.setValue(value); 1242 } 1243 1244 /** 1245 * @return {@link #characteristicType} (The type of characteristic that is being defined.) 1246 */ 1247 public CodeableConcept getCharacteristicType() { 1248 if (this.characteristicType == null) 1249 if (Configuration.errorOnAutoCreate()) 1250 throw new Error("Attempt to auto-create InventoryItemCharacteristicComponent.characteristicType"); 1251 else if (Configuration.doAutoCreate()) 1252 this.characteristicType = new CodeableConcept(); // cc 1253 return this.characteristicType; 1254 } 1255 1256 public boolean hasCharacteristicType() { 1257 return this.characteristicType != null && !this.characteristicType.isEmpty(); 1258 } 1259 1260 /** 1261 * @param value {@link #characteristicType} (The type of characteristic that is being defined.) 1262 */ 1263 public InventoryItemCharacteristicComponent setCharacteristicType(CodeableConcept value) { 1264 this.characteristicType = value; 1265 return this; 1266 } 1267 1268 /** 1269 * @return {@link #value} (The value of the attribute.) 1270 */ 1271 public DataType getValue() { 1272 return this.value; 1273 } 1274 1275 /** 1276 * @return {@link #value} (The value of the attribute.) 1277 */ 1278 public StringType getValueStringType() throws FHIRException { 1279 if (this.value == null) 1280 this.value = new StringType(); 1281 if (!(this.value instanceof StringType)) 1282 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 1283 return (StringType) this.value; 1284 } 1285 1286 public boolean hasValueStringType() { 1287 return this != null && this.value instanceof StringType; 1288 } 1289 1290 /** 1291 * @return {@link #value} (The value of the attribute.) 1292 */ 1293 public IntegerType getValueIntegerType() throws FHIRException { 1294 if (this.value == null) 1295 this.value = new IntegerType(); 1296 if (!(this.value instanceof IntegerType)) 1297 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.value.getClass().getName()+" was encountered"); 1298 return (IntegerType) this.value; 1299 } 1300 1301 public boolean hasValueIntegerType() { 1302 return this != null && this.value instanceof IntegerType; 1303 } 1304 1305 /** 1306 * @return {@link #value} (The value of the attribute.) 1307 */ 1308 public DecimalType getValueDecimalType() throws FHIRException { 1309 if (this.value == null) 1310 this.value = new DecimalType(); 1311 if (!(this.value instanceof DecimalType)) 1312 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.value.getClass().getName()+" was encountered"); 1313 return (DecimalType) this.value; 1314 } 1315 1316 public boolean hasValueDecimalType() { 1317 return this != null && this.value instanceof DecimalType; 1318 } 1319 1320 /** 1321 * @return {@link #value} (The value of the attribute.) 1322 */ 1323 public BooleanType getValueBooleanType() throws FHIRException { 1324 if (this.value == null) 1325 this.value = new BooleanType(); 1326 if (!(this.value instanceof BooleanType)) 1327 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 1328 return (BooleanType) this.value; 1329 } 1330 1331 public boolean hasValueBooleanType() { 1332 return this != null && this.value instanceof BooleanType; 1333 } 1334 1335 /** 1336 * @return {@link #value} (The value of the attribute.) 1337 */ 1338 public UrlType getValueUrlType() throws FHIRException { 1339 if (this.value == null) 1340 this.value = new UrlType(); 1341 if (!(this.value instanceof UrlType)) 1342 throw new FHIRException("Type mismatch: the type UrlType was expected, but "+this.value.getClass().getName()+" was encountered"); 1343 return (UrlType) this.value; 1344 } 1345 1346 public boolean hasValueUrlType() { 1347 return this != null && this.value instanceof UrlType; 1348 } 1349 1350 /** 1351 * @return {@link #value} (The value of the attribute.) 1352 */ 1353 public DateTimeType getValueDateTimeType() throws FHIRException { 1354 if (this.value == null) 1355 this.value = new DateTimeType(); 1356 if (!(this.value instanceof DateTimeType)) 1357 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.value.getClass().getName()+" was encountered"); 1358 return (DateTimeType) this.value; 1359 } 1360 1361 public boolean hasValueDateTimeType() { 1362 return this != null && this.value instanceof DateTimeType; 1363 } 1364 1365 /** 1366 * @return {@link #value} (The value of the attribute.) 1367 */ 1368 public Quantity getValueQuantity() throws FHIRException { 1369 if (this.value == null) 1370 this.value = new Quantity(); 1371 if (!(this.value instanceof Quantity)) 1372 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1373 return (Quantity) this.value; 1374 } 1375 1376 public boolean hasValueQuantity() { 1377 return this != null && this.value instanceof Quantity; 1378 } 1379 1380 /** 1381 * @return {@link #value} (The value of the attribute.) 1382 */ 1383 public Range getValueRange() throws FHIRException { 1384 if (this.value == null) 1385 this.value = new Range(); 1386 if (!(this.value instanceof Range)) 1387 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 1388 return (Range) this.value; 1389 } 1390 1391 public boolean hasValueRange() { 1392 return this != null && this.value instanceof Range; 1393 } 1394 1395 /** 1396 * @return {@link #value} (The value of the attribute.) 1397 */ 1398 public Ratio getValueRatio() throws FHIRException { 1399 if (this.value == null) 1400 this.value = new Ratio(); 1401 if (!(this.value instanceof Ratio)) 1402 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.value.getClass().getName()+" was encountered"); 1403 return (Ratio) this.value; 1404 } 1405 1406 public boolean hasValueRatio() { 1407 return this != null && this.value instanceof Ratio; 1408 } 1409 1410 /** 1411 * @return {@link #value} (The value of the attribute.) 1412 */ 1413 public Annotation getValueAnnotation() throws FHIRException { 1414 if (this.value == null) 1415 this.value = new Annotation(); 1416 if (!(this.value instanceof Annotation)) 1417 throw new FHIRException("Type mismatch: the type Annotation was expected, but "+this.value.getClass().getName()+" was encountered"); 1418 return (Annotation) this.value; 1419 } 1420 1421 public boolean hasValueAnnotation() { 1422 return this != null && this.value instanceof Annotation; 1423 } 1424 1425 /** 1426 * @return {@link #value} (The value of the attribute.) 1427 */ 1428 public Address getValueAddress() throws FHIRException { 1429 if (this.value == null) 1430 this.value = new Address(); 1431 if (!(this.value instanceof Address)) 1432 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.value.getClass().getName()+" was encountered"); 1433 return (Address) this.value; 1434 } 1435 1436 public boolean hasValueAddress() { 1437 return this != null && this.value instanceof Address; 1438 } 1439 1440 /** 1441 * @return {@link #value} (The value of the attribute.) 1442 */ 1443 public Duration getValueDuration() throws FHIRException { 1444 if (this.value == null) 1445 this.value = new Duration(); 1446 if (!(this.value instanceof Duration)) 1447 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.value.getClass().getName()+" was encountered"); 1448 return (Duration) this.value; 1449 } 1450 1451 public boolean hasValueDuration() { 1452 return this != null && this.value instanceof Duration; 1453 } 1454 1455 /** 1456 * @return {@link #value} (The value of the attribute.) 1457 */ 1458 public CodeableConcept getValueCodeableConcept() throws FHIRException { 1459 if (this.value == null) 1460 this.value = new CodeableConcept(); 1461 if (!(this.value instanceof CodeableConcept)) 1462 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 1463 return (CodeableConcept) this.value; 1464 } 1465 1466 public boolean hasValueCodeableConcept() { 1467 return this != null && this.value instanceof CodeableConcept; 1468 } 1469 1470 public boolean hasValue() { 1471 return this.value != null && !this.value.isEmpty(); 1472 } 1473 1474 /** 1475 * @param value {@link #value} (The value of the attribute.) 1476 */ 1477 public InventoryItemCharacteristicComponent setValue(DataType value) { 1478 if (value != null && !(value instanceof StringType || value instanceof IntegerType || value instanceof DecimalType || value instanceof BooleanType || value instanceof UrlType || value instanceof DateTimeType || value instanceof Quantity || value instanceof Range || value instanceof Ratio || value instanceof Annotation || value instanceof Address || value instanceof Duration || value instanceof CodeableConcept)) 1479 throw new FHIRException("Not the right type for InventoryItem.characteristic.value[x]: "+value.fhirType()); 1480 this.value = value; 1481 return this; 1482 } 1483 1484 protected void listChildren(List<Property> children) { 1485 super.listChildren(children); 1486 children.add(new Property("characteristicType", "CodeableConcept", "The type of characteristic that is being defined.", 0, 1, characteristicType)); 1487 children.add(new Property("value[x]", "string|integer|decimal|boolean|url|dateTime|Quantity|Range|Ratio|Annotation|Address|Duration|CodeableConcept", "The value of the attribute.", 0, 1, value)); 1488 } 1489 1490 @Override 1491 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1492 switch (_hash) { 1493 case 1172127605: /*characteristicType*/ return new Property("characteristicType", "CodeableConcept", "The type of characteristic that is being defined.", 0, 1, characteristicType); 1494 case -1410166417: /*value[x]*/ return new Property("value[x]", "string|integer|decimal|boolean|url|dateTime|Quantity|Range|Ratio|Annotation|Address|Duration|CodeableConcept", "The value of the attribute.", 0, 1, value); 1495 case 111972721: /*value*/ return new Property("value[x]", "string|integer|decimal|boolean|url|dateTime|Quantity|Range|Ratio|Annotation|Address|Duration|CodeableConcept", "The value of the attribute.", 0, 1, value); 1496 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "The value of the attribute.", 0, 1, value); 1497 case -1668204915: /*valueInteger*/ return new Property("value[x]", "integer", "The value of the attribute.", 0, 1, value); 1498 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "decimal", "The value of the attribute.", 0, 1, value); 1499 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value of the attribute.", 0, 1, value); 1500 case -1410172354: /*valueUrl*/ return new Property("value[x]", "url", "The value of the attribute.", 0, 1, value); 1501 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "dateTime", "The value of the attribute.", 0, 1, value); 1502 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The value of the attribute.", 0, 1, value); 1503 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The value of the attribute.", 0, 1, value); 1504 case 2030767386: /*valueRatio*/ return new Property("value[x]", "Ratio", "The value of the attribute.", 0, 1, value); 1505 case -67108992: /*valueAnnotation*/ return new Property("value[x]", "Annotation", "The value of the attribute.", 0, 1, value); 1506 case -478981821: /*valueAddress*/ return new Property("value[x]", "Address", "The value of the attribute.", 0, 1, value); 1507 case 1558135333: /*valueDuration*/ return new Property("value[x]", "Duration", "The value of the attribute.", 0, 1, value); 1508 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The value of the attribute.", 0, 1, value); 1509 default: return super.getNamedProperty(_hash, _name, _checkValid); 1510 } 1511 1512 } 1513 1514 @Override 1515 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1516 switch (hash) { 1517 case 1172127605: /*characteristicType*/ return this.characteristicType == null ? new Base[0] : new Base[] {this.characteristicType}; // CodeableConcept 1518 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 1519 default: return super.getProperty(hash, name, checkValid); 1520 } 1521 1522 } 1523 1524 @Override 1525 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1526 switch (hash) { 1527 case 1172127605: // characteristicType 1528 this.characteristicType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1529 return value; 1530 case 111972721: // value 1531 this.value = TypeConvertor.castToType(value); // DataType 1532 return value; 1533 default: return super.setProperty(hash, name, value); 1534 } 1535 1536 } 1537 1538 @Override 1539 public Base setProperty(String name, Base value) throws FHIRException { 1540 if (name.equals("characteristicType")) { 1541 this.characteristicType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1542 } else if (name.equals("value[x]")) { 1543 this.value = TypeConvertor.castToType(value); // DataType 1544 } else 1545 return super.setProperty(name, value); 1546 return value; 1547 } 1548 1549 @Override 1550 public Base makeProperty(int hash, String name) throws FHIRException { 1551 switch (hash) { 1552 case 1172127605: return getCharacteristicType(); 1553 case -1410166417: return getValue(); 1554 case 111972721: return getValue(); 1555 default: return super.makeProperty(hash, name); 1556 } 1557 1558 } 1559 1560 @Override 1561 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1562 switch (hash) { 1563 case 1172127605: /*characteristicType*/ return new String[] {"CodeableConcept"}; 1564 case 111972721: /*value*/ return new String[] {"string", "integer", "decimal", "boolean", "url", "dateTime", "Quantity", "Range", "Ratio", "Annotation", "Address", "Duration", "CodeableConcept"}; 1565 default: return super.getTypesForProperty(hash, name); 1566 } 1567 1568 } 1569 1570 @Override 1571 public Base addChild(String name) throws FHIRException { 1572 if (name.equals("characteristicType")) { 1573 this.characteristicType = new CodeableConcept(); 1574 return this.characteristicType; 1575 } 1576 else if (name.equals("valueString")) { 1577 this.value = new StringType(); 1578 return this.value; 1579 } 1580 else if (name.equals("valueInteger")) { 1581 this.value = new IntegerType(); 1582 return this.value; 1583 } 1584 else if (name.equals("valueDecimal")) { 1585 this.value = new DecimalType(); 1586 return this.value; 1587 } 1588 else if (name.equals("valueBoolean")) { 1589 this.value = new BooleanType(); 1590 return this.value; 1591 } 1592 else if (name.equals("valueUrl")) { 1593 this.value = new UrlType(); 1594 return this.value; 1595 } 1596 else if (name.equals("valueDateTime")) { 1597 this.value = new DateTimeType(); 1598 return this.value; 1599 } 1600 else if (name.equals("valueQuantity")) { 1601 this.value = new Quantity(); 1602 return this.value; 1603 } 1604 else if (name.equals("valueRange")) { 1605 this.value = new Range(); 1606 return this.value; 1607 } 1608 else if (name.equals("valueRatio")) { 1609 this.value = new Ratio(); 1610 return this.value; 1611 } 1612 else if (name.equals("valueAnnotation")) { 1613 this.value = new Annotation(); 1614 return this.value; 1615 } 1616 else if (name.equals("valueAddress")) { 1617 this.value = new Address(); 1618 return this.value; 1619 } 1620 else if (name.equals("valueDuration")) { 1621 this.value = new Duration(); 1622 return this.value; 1623 } 1624 else if (name.equals("valueCodeableConcept")) { 1625 this.value = new CodeableConcept(); 1626 return this.value; 1627 } 1628 else 1629 return super.addChild(name); 1630 } 1631 1632 public InventoryItemCharacteristicComponent copy() { 1633 InventoryItemCharacteristicComponent dst = new InventoryItemCharacteristicComponent(); 1634 copyValues(dst); 1635 return dst; 1636 } 1637 1638 public void copyValues(InventoryItemCharacteristicComponent dst) { 1639 super.copyValues(dst); 1640 dst.characteristicType = characteristicType == null ? null : characteristicType.copy(); 1641 dst.value = value == null ? null : value.copy(); 1642 } 1643 1644 @Override 1645 public boolean equalsDeep(Base other_) { 1646 if (!super.equalsDeep(other_)) 1647 return false; 1648 if (!(other_ instanceof InventoryItemCharacteristicComponent)) 1649 return false; 1650 InventoryItemCharacteristicComponent o = (InventoryItemCharacteristicComponent) other_; 1651 return compareDeep(characteristicType, o.characteristicType, true) && compareDeep(value, o.value, true) 1652 ; 1653 } 1654 1655 @Override 1656 public boolean equalsShallow(Base other_) { 1657 if (!super.equalsShallow(other_)) 1658 return false; 1659 if (!(other_ instanceof InventoryItemCharacteristicComponent)) 1660 return false; 1661 InventoryItemCharacteristicComponent o = (InventoryItemCharacteristicComponent) other_; 1662 return true; 1663 } 1664 1665 public boolean isEmpty() { 1666 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(characteristicType, value 1667 ); 1668 } 1669 1670 public String fhirType() { 1671 return "InventoryItem.characteristic"; 1672 1673 } 1674 1675 } 1676 1677 @Block() 1678 public static class InventoryItemInstanceComponent extends BackboneElement implements IBaseBackboneElement { 1679 /** 1680 * The identifier for the physical instance, typically a serial number. 1681 */ 1682 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1683 @Description(shortDefinition="The identifier for the physical instance, typically a serial number", formalDefinition="The identifier for the physical instance, typically a serial number." ) 1684 protected List<Identifier> identifier; 1685 1686 /** 1687 * The lot or batch number of the item. 1688 */ 1689 @Child(name = "lotNumber", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1690 @Description(shortDefinition="The lot or batch number of the item", formalDefinition="The lot or batch number of the item." ) 1691 protected StringType lotNumber; 1692 1693 /** 1694 * The expiry date or date and time for the product. 1695 */ 1696 @Child(name = "expiry", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1697 @Description(shortDefinition="The expiry date or date and time for the product", formalDefinition="The expiry date or date and time for the product." ) 1698 protected DateTimeType expiry; 1699 1700 /** 1701 * The subject that the item is associated with. 1702 */ 1703 @Child(name = "subject", type = {Patient.class, Organization.class}, order=4, min=0, max=1, modifier=false, summary=false) 1704 @Description(shortDefinition="The subject that the item is associated with", formalDefinition="The subject that the item is associated with." ) 1705 protected Reference subject; 1706 1707 /** 1708 * The location that the item is associated with. 1709 */ 1710 @Child(name = "location", type = {Location.class}, order=5, min=0, max=1, modifier=false, summary=false) 1711 @Description(shortDefinition="The location that the item is associated with", formalDefinition="The location that the item is associated with." ) 1712 protected Reference location; 1713 1714 private static final long serialVersionUID = 657936980L; 1715 1716 /** 1717 * Constructor 1718 */ 1719 public InventoryItemInstanceComponent() { 1720 super(); 1721 } 1722 1723 /** 1724 * @return {@link #identifier} (The identifier for the physical instance, typically a serial number.) 1725 */ 1726 public List<Identifier> getIdentifier() { 1727 if (this.identifier == null) 1728 this.identifier = new ArrayList<Identifier>(); 1729 return this.identifier; 1730 } 1731 1732 /** 1733 * @return Returns a reference to <code>this</code> for easy method chaining 1734 */ 1735 public InventoryItemInstanceComponent setIdentifier(List<Identifier> theIdentifier) { 1736 this.identifier = theIdentifier; 1737 return this; 1738 } 1739 1740 public boolean hasIdentifier() { 1741 if (this.identifier == null) 1742 return false; 1743 for (Identifier item : this.identifier) 1744 if (!item.isEmpty()) 1745 return true; 1746 return false; 1747 } 1748 1749 public Identifier addIdentifier() { //3 1750 Identifier t = new Identifier(); 1751 if (this.identifier == null) 1752 this.identifier = new ArrayList<Identifier>(); 1753 this.identifier.add(t); 1754 return t; 1755 } 1756 1757 public InventoryItemInstanceComponent addIdentifier(Identifier t) { //3 1758 if (t == null) 1759 return this; 1760 if (this.identifier == null) 1761 this.identifier = new ArrayList<Identifier>(); 1762 this.identifier.add(t); 1763 return this; 1764 } 1765 1766 /** 1767 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1768 */ 1769 public Identifier getIdentifierFirstRep() { 1770 if (getIdentifier().isEmpty()) { 1771 addIdentifier(); 1772 } 1773 return getIdentifier().get(0); 1774 } 1775 1776 /** 1777 * @return {@link #lotNumber} (The lot or batch number of the item.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 1778 */ 1779 public StringType getLotNumberElement() { 1780 if (this.lotNumber == null) 1781 if (Configuration.errorOnAutoCreate()) 1782 throw new Error("Attempt to auto-create InventoryItemInstanceComponent.lotNumber"); 1783 else if (Configuration.doAutoCreate()) 1784 this.lotNumber = new StringType(); // bb 1785 return this.lotNumber; 1786 } 1787 1788 public boolean hasLotNumberElement() { 1789 return this.lotNumber != null && !this.lotNumber.isEmpty(); 1790 } 1791 1792 public boolean hasLotNumber() { 1793 return this.lotNumber != null && !this.lotNumber.isEmpty(); 1794 } 1795 1796 /** 1797 * @param value {@link #lotNumber} (The lot or batch number of the item.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 1798 */ 1799 public InventoryItemInstanceComponent setLotNumberElement(StringType value) { 1800 this.lotNumber = value; 1801 return this; 1802 } 1803 1804 /** 1805 * @return The lot or batch number of the item. 1806 */ 1807 public String getLotNumber() { 1808 return this.lotNumber == null ? null : this.lotNumber.getValue(); 1809 } 1810 1811 /** 1812 * @param value The lot or batch number of the item. 1813 */ 1814 public InventoryItemInstanceComponent setLotNumber(String value) { 1815 if (Utilities.noString(value)) 1816 this.lotNumber = null; 1817 else { 1818 if (this.lotNumber == null) 1819 this.lotNumber = new StringType(); 1820 this.lotNumber.setValue(value); 1821 } 1822 return this; 1823 } 1824 1825 /** 1826 * @return {@link #expiry} (The expiry date or date and time for the product.). This is the underlying object with id, value and extensions. The accessor "getExpiry" gives direct access to the value 1827 */ 1828 public DateTimeType getExpiryElement() { 1829 if (this.expiry == null) 1830 if (Configuration.errorOnAutoCreate()) 1831 throw new Error("Attempt to auto-create InventoryItemInstanceComponent.expiry"); 1832 else if (Configuration.doAutoCreate()) 1833 this.expiry = new DateTimeType(); // bb 1834 return this.expiry; 1835 } 1836 1837 public boolean hasExpiryElement() { 1838 return this.expiry != null && !this.expiry.isEmpty(); 1839 } 1840 1841 public boolean hasExpiry() { 1842 return this.expiry != null && !this.expiry.isEmpty(); 1843 } 1844 1845 /** 1846 * @param value {@link #expiry} (The expiry date or date and time for the product.). This is the underlying object with id, value and extensions. The accessor "getExpiry" gives direct access to the value 1847 */ 1848 public InventoryItemInstanceComponent setExpiryElement(DateTimeType value) { 1849 this.expiry = value; 1850 return this; 1851 } 1852 1853 /** 1854 * @return The expiry date or date and time for the product. 1855 */ 1856 public Date getExpiry() { 1857 return this.expiry == null ? null : this.expiry.getValue(); 1858 } 1859 1860 /** 1861 * @param value The expiry date or date and time for the product. 1862 */ 1863 public InventoryItemInstanceComponent setExpiry(Date value) { 1864 if (value == null) 1865 this.expiry = null; 1866 else { 1867 if (this.expiry == null) 1868 this.expiry = new DateTimeType(); 1869 this.expiry.setValue(value); 1870 } 1871 return this; 1872 } 1873 1874 /** 1875 * @return {@link #subject} (The subject that the item is associated with.) 1876 */ 1877 public Reference getSubject() { 1878 if (this.subject == null) 1879 if (Configuration.errorOnAutoCreate()) 1880 throw new Error("Attempt to auto-create InventoryItemInstanceComponent.subject"); 1881 else if (Configuration.doAutoCreate()) 1882 this.subject = new Reference(); // cc 1883 return this.subject; 1884 } 1885 1886 public boolean hasSubject() { 1887 return this.subject != null && !this.subject.isEmpty(); 1888 } 1889 1890 /** 1891 * @param value {@link #subject} (The subject that the item is associated with.) 1892 */ 1893 public InventoryItemInstanceComponent setSubject(Reference value) { 1894 this.subject = value; 1895 return this; 1896 } 1897 1898 /** 1899 * @return {@link #location} (The location that the item is associated with.) 1900 */ 1901 public Reference getLocation() { 1902 if (this.location == null) 1903 if (Configuration.errorOnAutoCreate()) 1904 throw new Error("Attempt to auto-create InventoryItemInstanceComponent.location"); 1905 else if (Configuration.doAutoCreate()) 1906 this.location = new Reference(); // cc 1907 return this.location; 1908 } 1909 1910 public boolean hasLocation() { 1911 return this.location != null && !this.location.isEmpty(); 1912 } 1913 1914 /** 1915 * @param value {@link #location} (The location that the item is associated with.) 1916 */ 1917 public InventoryItemInstanceComponent setLocation(Reference value) { 1918 this.location = value; 1919 return this; 1920 } 1921 1922 protected void listChildren(List<Property> children) { 1923 super.listChildren(children); 1924 children.add(new Property("identifier", "Identifier", "The identifier for the physical instance, typically a serial number.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1925 children.add(new Property("lotNumber", "string", "The lot or batch number of the item.", 0, 1, lotNumber)); 1926 children.add(new Property("expiry", "dateTime", "The expiry date or date and time for the product.", 0, 1, expiry)); 1927 children.add(new Property("subject", "Reference(Patient|Organization)", "The subject that the item is associated with.", 0, 1, subject)); 1928 children.add(new Property("location", "Reference(Location)", "The location that the item is associated with.", 0, 1, location)); 1929 } 1930 1931 @Override 1932 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1933 switch (_hash) { 1934 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The identifier for the physical instance, typically a serial number.", 0, java.lang.Integer.MAX_VALUE, identifier); 1935 case 462547450: /*lotNumber*/ return new Property("lotNumber", "string", "The lot or batch number of the item.", 0, 1, lotNumber); 1936 case -1289159373: /*expiry*/ return new Property("expiry", "dateTime", "The expiry date or date and time for the product.", 0, 1, expiry); 1937 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Organization)", "The subject that the item is associated with.", 0, 1, subject); 1938 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The location that the item is associated with.", 0, 1, location); 1939 default: return super.getNamedProperty(_hash, _name, _checkValid); 1940 } 1941 1942 } 1943 1944 @Override 1945 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1946 switch (hash) { 1947 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1948 case 462547450: /*lotNumber*/ return this.lotNumber == null ? new Base[0] : new Base[] {this.lotNumber}; // StringType 1949 case -1289159373: /*expiry*/ return this.expiry == null ? new Base[0] : new Base[] {this.expiry}; // DateTimeType 1950 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1951 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 1952 default: return super.getProperty(hash, name, checkValid); 1953 } 1954 1955 } 1956 1957 @Override 1958 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1959 switch (hash) { 1960 case -1618432855: // identifier 1961 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1962 return value; 1963 case 462547450: // lotNumber 1964 this.lotNumber = TypeConvertor.castToString(value); // StringType 1965 return value; 1966 case -1289159373: // expiry 1967 this.expiry = TypeConvertor.castToDateTime(value); // DateTimeType 1968 return value; 1969 case -1867885268: // subject 1970 this.subject = TypeConvertor.castToReference(value); // Reference 1971 return value; 1972 case 1901043637: // location 1973 this.location = TypeConvertor.castToReference(value); // Reference 1974 return value; 1975 default: return super.setProperty(hash, name, value); 1976 } 1977 1978 } 1979 1980 @Override 1981 public Base setProperty(String name, Base value) throws FHIRException { 1982 if (name.equals("identifier")) { 1983 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1984 } else if (name.equals("lotNumber")) { 1985 this.lotNumber = TypeConvertor.castToString(value); // StringType 1986 } else if (name.equals("expiry")) { 1987 this.expiry = TypeConvertor.castToDateTime(value); // DateTimeType 1988 } else if (name.equals("subject")) { 1989 this.subject = TypeConvertor.castToReference(value); // Reference 1990 } else if (name.equals("location")) { 1991 this.location = TypeConvertor.castToReference(value); // Reference 1992 } else 1993 return super.setProperty(name, value); 1994 return value; 1995 } 1996 1997 @Override 1998 public Base makeProperty(int hash, String name) throws FHIRException { 1999 switch (hash) { 2000 case -1618432855: return addIdentifier(); 2001 case 462547450: return getLotNumberElement(); 2002 case -1289159373: return getExpiryElement(); 2003 case -1867885268: return getSubject(); 2004 case 1901043637: return getLocation(); 2005 default: return super.makeProperty(hash, name); 2006 } 2007 2008 } 2009 2010 @Override 2011 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2012 switch (hash) { 2013 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2014 case 462547450: /*lotNumber*/ return new String[] {"string"}; 2015 case -1289159373: /*expiry*/ return new String[] {"dateTime"}; 2016 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2017 case 1901043637: /*location*/ return new String[] {"Reference"}; 2018 default: return super.getTypesForProperty(hash, name); 2019 } 2020 2021 } 2022 2023 @Override 2024 public Base addChild(String name) throws FHIRException { 2025 if (name.equals("identifier")) { 2026 return addIdentifier(); 2027 } 2028 else if (name.equals("lotNumber")) { 2029 throw new FHIRException("Cannot call addChild on a singleton property InventoryItem.instance.lotNumber"); 2030 } 2031 else if (name.equals("expiry")) { 2032 throw new FHIRException("Cannot call addChild on a singleton property InventoryItem.instance.expiry"); 2033 } 2034 else if (name.equals("subject")) { 2035 this.subject = new Reference(); 2036 return this.subject; 2037 } 2038 else if (name.equals("location")) { 2039 this.location = new Reference(); 2040 return this.location; 2041 } 2042 else 2043 return super.addChild(name); 2044 } 2045 2046 public InventoryItemInstanceComponent copy() { 2047 InventoryItemInstanceComponent dst = new InventoryItemInstanceComponent(); 2048 copyValues(dst); 2049 return dst; 2050 } 2051 2052 public void copyValues(InventoryItemInstanceComponent dst) { 2053 super.copyValues(dst); 2054 if (identifier != null) { 2055 dst.identifier = new ArrayList<Identifier>(); 2056 for (Identifier i : identifier) 2057 dst.identifier.add(i.copy()); 2058 }; 2059 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 2060 dst.expiry = expiry == null ? null : expiry.copy(); 2061 dst.subject = subject == null ? null : subject.copy(); 2062 dst.location = location == null ? null : location.copy(); 2063 } 2064 2065 @Override 2066 public boolean equalsDeep(Base other_) { 2067 if (!super.equalsDeep(other_)) 2068 return false; 2069 if (!(other_ instanceof InventoryItemInstanceComponent)) 2070 return false; 2071 InventoryItemInstanceComponent o = (InventoryItemInstanceComponent) other_; 2072 return compareDeep(identifier, o.identifier, true) && compareDeep(lotNumber, o.lotNumber, true) 2073 && compareDeep(expiry, o.expiry, true) && compareDeep(subject, o.subject, true) && compareDeep(location, o.location, true) 2074 ; 2075 } 2076 2077 @Override 2078 public boolean equalsShallow(Base other_) { 2079 if (!super.equalsShallow(other_)) 2080 return false; 2081 if (!(other_ instanceof InventoryItemInstanceComponent)) 2082 return false; 2083 InventoryItemInstanceComponent o = (InventoryItemInstanceComponent) other_; 2084 return compareValues(lotNumber, o.lotNumber, true) && compareValues(expiry, o.expiry, true); 2085 } 2086 2087 public boolean isEmpty() { 2088 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, lotNumber, expiry 2089 , subject, location); 2090 } 2091 2092 public String fhirType() { 2093 return "InventoryItem.instance"; 2094 2095 } 2096 2097 } 2098 2099 /** 2100 * Business identifier for the inventory item. 2101 */ 2102 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2103 @Description(shortDefinition="Business identifier for the inventory item", formalDefinition="Business identifier for the inventory item." ) 2104 protected List<Identifier> identifier; 2105 2106 /** 2107 * Status of the item entry. 2108 */ 2109 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2110 @Description(shortDefinition="active | inactive | entered-in-error | unknown", formalDefinition="Status of the item entry." ) 2111 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/inventoryitem-status") 2112 protected Enumeration<InventoryItemStatusCodes> status; 2113 2114 /** 2115 * Category or class of the item. 2116 */ 2117 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2118 @Description(shortDefinition="Category or class of the item", formalDefinition="Category or class of the item." ) 2119 protected List<CodeableConcept> category; 2120 2121 /** 2122 * Code designating the specific type of item. 2123 */ 2124 @Child(name = "code", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2125 @Description(shortDefinition="Code designating the specific type of item", formalDefinition="Code designating the specific type of item." ) 2126 protected List<CodeableConcept> code; 2127 2128 /** 2129 * The item name(s) - the brand name, or common name, functional name, generic name. 2130 */ 2131 @Child(name = "name", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2132 @Description(shortDefinition="The item name(s) - the brand name, or common name, functional name, generic name or others", formalDefinition="The item name(s) - the brand name, or common name, functional name, generic name." ) 2133 protected List<InventoryItemNameComponent> name; 2134 2135 /** 2136 * Organization(s) responsible for the product. 2137 */ 2138 @Child(name = "responsibleOrganization", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2139 @Description(shortDefinition="Organization(s) responsible for the product", formalDefinition="Organization(s) responsible for the product." ) 2140 protected List<InventoryItemResponsibleOrganizationComponent> responsibleOrganization; 2141 2142 /** 2143 * The descriptive characteristics of the inventory item. 2144 */ 2145 @Child(name = "description", type = {}, order=6, min=0, max=1, modifier=false, summary=false) 2146 @Description(shortDefinition="Descriptive characteristics of the item", formalDefinition="The descriptive characteristics of the inventory item." ) 2147 protected InventoryItemDescriptionComponent description; 2148 2149 /** 2150 * The usage status e.g. recalled, in use, discarded... This can be used to indicate that the items have been taken out of inventory, or are in use, etc. 2151 */ 2152 @Child(name = "inventoryStatus", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2153 @Description(shortDefinition="The usage status like recalled, in use, discarded", formalDefinition="The usage status e.g. recalled, in use, discarded... This can be used to indicate that the items have been taken out of inventory, or are in use, etc." ) 2154 protected List<CodeableConcept> inventoryStatus; 2155 2156 /** 2157 * The base unit of measure - the unit in which the product is used or counted. 2158 */ 2159 @Child(name = "baseUnit", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=true) 2160 @Description(shortDefinition="The base unit of measure - the unit in which the product is used or counted", formalDefinition="The base unit of measure - the unit in which the product is used or counted." ) 2161 protected CodeableConcept baseUnit; 2162 2163 /** 2164 * Net content or amount present in the item. 2165 */ 2166 @Child(name = "netContent", type = {Quantity.class}, order=9, min=0, max=1, modifier=false, summary=true) 2167 @Description(shortDefinition="Net content or amount present in the item", formalDefinition="Net content or amount present in the item." ) 2168 protected Quantity netContent; 2169 2170 /** 2171 * Association with other items or products. 2172 */ 2173 @Child(name = "association", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2174 @Description(shortDefinition="Association with other items or products", formalDefinition="Association with other items or products." ) 2175 protected List<InventoryItemAssociationComponent> association; 2176 2177 /** 2178 * The descriptive or identifying characteristics of the item. 2179 */ 2180 @Child(name = "characteristic", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2181 @Description(shortDefinition="Characteristic of the item", formalDefinition="The descriptive or identifying characteristics of the item." ) 2182 protected List<InventoryItemCharacteristicComponent> characteristic; 2183 2184 /** 2185 * Instances or occurrences of the product. 2186 */ 2187 @Child(name = "instance", type = {}, order=12, min=0, max=1, modifier=false, summary=false) 2188 @Description(shortDefinition="Instances or occurrences of the product", formalDefinition="Instances or occurrences of the product." ) 2189 protected InventoryItemInstanceComponent instance; 2190 2191 /** 2192 * Link to a product resource used in clinical workflows. 2193 */ 2194 @Child(name = "productReference", type = {Medication.class, Device.class, NutritionProduct.class, BiologicallyDerivedProduct.class}, order=13, min=0, max=1, modifier=false, summary=false) 2195 @Description(shortDefinition="Link to a product resource used in clinical workflows", formalDefinition="Link to a product resource used in clinical workflows." ) 2196 protected Reference productReference; 2197 2198 private static final long serialVersionUID = 2127201564L; 2199 2200 /** 2201 * Constructor 2202 */ 2203 public InventoryItem() { 2204 super(); 2205 } 2206 2207 /** 2208 * Constructor 2209 */ 2210 public InventoryItem(InventoryItemStatusCodes status) { 2211 super(); 2212 this.setStatus(status); 2213 } 2214 2215 /** 2216 * @return {@link #identifier} (Business identifier for the inventory item.) 2217 */ 2218 public List<Identifier> getIdentifier() { 2219 if (this.identifier == null) 2220 this.identifier = new ArrayList<Identifier>(); 2221 return this.identifier; 2222 } 2223 2224 /** 2225 * @return Returns a reference to <code>this</code> for easy method chaining 2226 */ 2227 public InventoryItem setIdentifier(List<Identifier> theIdentifier) { 2228 this.identifier = theIdentifier; 2229 return this; 2230 } 2231 2232 public boolean hasIdentifier() { 2233 if (this.identifier == null) 2234 return false; 2235 for (Identifier item : this.identifier) 2236 if (!item.isEmpty()) 2237 return true; 2238 return false; 2239 } 2240 2241 public Identifier addIdentifier() { //3 2242 Identifier t = new Identifier(); 2243 if (this.identifier == null) 2244 this.identifier = new ArrayList<Identifier>(); 2245 this.identifier.add(t); 2246 return t; 2247 } 2248 2249 public InventoryItem addIdentifier(Identifier t) { //3 2250 if (t == null) 2251 return this; 2252 if (this.identifier == null) 2253 this.identifier = new ArrayList<Identifier>(); 2254 this.identifier.add(t); 2255 return this; 2256 } 2257 2258 /** 2259 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 2260 */ 2261 public Identifier getIdentifierFirstRep() { 2262 if (getIdentifier().isEmpty()) { 2263 addIdentifier(); 2264 } 2265 return getIdentifier().get(0); 2266 } 2267 2268 /** 2269 * @return {@link #status} (Status of the item entry.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2270 */ 2271 public Enumeration<InventoryItemStatusCodes> getStatusElement() { 2272 if (this.status == null) 2273 if (Configuration.errorOnAutoCreate()) 2274 throw new Error("Attempt to auto-create InventoryItem.status"); 2275 else if (Configuration.doAutoCreate()) 2276 this.status = new Enumeration<InventoryItemStatusCodes>(new InventoryItemStatusCodesEnumFactory()); // bb 2277 return this.status; 2278 } 2279 2280 public boolean hasStatusElement() { 2281 return this.status != null && !this.status.isEmpty(); 2282 } 2283 2284 public boolean hasStatus() { 2285 return this.status != null && !this.status.isEmpty(); 2286 } 2287 2288 /** 2289 * @param value {@link #status} (Status of the item entry.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2290 */ 2291 public InventoryItem setStatusElement(Enumeration<InventoryItemStatusCodes> value) { 2292 this.status = value; 2293 return this; 2294 } 2295 2296 /** 2297 * @return Status of the item entry. 2298 */ 2299 public InventoryItemStatusCodes getStatus() { 2300 return this.status == null ? null : this.status.getValue(); 2301 } 2302 2303 /** 2304 * @param value Status of the item entry. 2305 */ 2306 public InventoryItem setStatus(InventoryItemStatusCodes value) { 2307 if (this.status == null) 2308 this.status = new Enumeration<InventoryItemStatusCodes>(new InventoryItemStatusCodesEnumFactory()); 2309 this.status.setValue(value); 2310 return this; 2311 } 2312 2313 /** 2314 * @return {@link #category} (Category or class of the item.) 2315 */ 2316 public List<CodeableConcept> getCategory() { 2317 if (this.category == null) 2318 this.category = new ArrayList<CodeableConcept>(); 2319 return this.category; 2320 } 2321 2322 /** 2323 * @return Returns a reference to <code>this</code> for easy method chaining 2324 */ 2325 public InventoryItem setCategory(List<CodeableConcept> theCategory) { 2326 this.category = theCategory; 2327 return this; 2328 } 2329 2330 public boolean hasCategory() { 2331 if (this.category == null) 2332 return false; 2333 for (CodeableConcept item : this.category) 2334 if (!item.isEmpty()) 2335 return true; 2336 return false; 2337 } 2338 2339 public CodeableConcept addCategory() { //3 2340 CodeableConcept t = new CodeableConcept(); 2341 if (this.category == null) 2342 this.category = new ArrayList<CodeableConcept>(); 2343 this.category.add(t); 2344 return t; 2345 } 2346 2347 public InventoryItem addCategory(CodeableConcept t) { //3 2348 if (t == null) 2349 return this; 2350 if (this.category == null) 2351 this.category = new ArrayList<CodeableConcept>(); 2352 this.category.add(t); 2353 return this; 2354 } 2355 2356 /** 2357 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 2358 */ 2359 public CodeableConcept getCategoryFirstRep() { 2360 if (getCategory().isEmpty()) { 2361 addCategory(); 2362 } 2363 return getCategory().get(0); 2364 } 2365 2366 /** 2367 * @return {@link #code} (Code designating the specific type of item.) 2368 */ 2369 public List<CodeableConcept> getCode() { 2370 if (this.code == null) 2371 this.code = new ArrayList<CodeableConcept>(); 2372 return this.code; 2373 } 2374 2375 /** 2376 * @return Returns a reference to <code>this</code> for easy method chaining 2377 */ 2378 public InventoryItem setCode(List<CodeableConcept> theCode) { 2379 this.code = theCode; 2380 return this; 2381 } 2382 2383 public boolean hasCode() { 2384 if (this.code == null) 2385 return false; 2386 for (CodeableConcept item : this.code) 2387 if (!item.isEmpty()) 2388 return true; 2389 return false; 2390 } 2391 2392 public CodeableConcept addCode() { //3 2393 CodeableConcept t = new CodeableConcept(); 2394 if (this.code == null) 2395 this.code = new ArrayList<CodeableConcept>(); 2396 this.code.add(t); 2397 return t; 2398 } 2399 2400 public InventoryItem addCode(CodeableConcept t) { //3 2401 if (t == null) 2402 return this; 2403 if (this.code == null) 2404 this.code = new ArrayList<CodeableConcept>(); 2405 this.code.add(t); 2406 return this; 2407 } 2408 2409 /** 2410 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist {3} 2411 */ 2412 public CodeableConcept getCodeFirstRep() { 2413 if (getCode().isEmpty()) { 2414 addCode(); 2415 } 2416 return getCode().get(0); 2417 } 2418 2419 /** 2420 * @return {@link #name} (The item name(s) - the brand name, or common name, functional name, generic name.) 2421 */ 2422 public List<InventoryItemNameComponent> getName() { 2423 if (this.name == null) 2424 this.name = new ArrayList<InventoryItemNameComponent>(); 2425 return this.name; 2426 } 2427 2428 /** 2429 * @return Returns a reference to <code>this</code> for easy method chaining 2430 */ 2431 public InventoryItem setName(List<InventoryItemNameComponent> theName) { 2432 this.name = theName; 2433 return this; 2434 } 2435 2436 public boolean hasName() { 2437 if (this.name == null) 2438 return false; 2439 for (InventoryItemNameComponent item : this.name) 2440 if (!item.isEmpty()) 2441 return true; 2442 return false; 2443 } 2444 2445 public InventoryItemNameComponent addName() { //3 2446 InventoryItemNameComponent t = new InventoryItemNameComponent(); 2447 if (this.name == null) 2448 this.name = new ArrayList<InventoryItemNameComponent>(); 2449 this.name.add(t); 2450 return t; 2451 } 2452 2453 public InventoryItem addName(InventoryItemNameComponent t) { //3 2454 if (t == null) 2455 return this; 2456 if (this.name == null) 2457 this.name = new ArrayList<InventoryItemNameComponent>(); 2458 this.name.add(t); 2459 return this; 2460 } 2461 2462 /** 2463 * @return The first repetition of repeating field {@link #name}, creating it if it does not already exist {3} 2464 */ 2465 public InventoryItemNameComponent getNameFirstRep() { 2466 if (getName().isEmpty()) { 2467 addName(); 2468 } 2469 return getName().get(0); 2470 } 2471 2472 /** 2473 * @return {@link #responsibleOrganization} (Organization(s) responsible for the product.) 2474 */ 2475 public List<InventoryItemResponsibleOrganizationComponent> getResponsibleOrganization() { 2476 if (this.responsibleOrganization == null) 2477 this.responsibleOrganization = new ArrayList<InventoryItemResponsibleOrganizationComponent>(); 2478 return this.responsibleOrganization; 2479 } 2480 2481 /** 2482 * @return Returns a reference to <code>this</code> for easy method chaining 2483 */ 2484 public InventoryItem setResponsibleOrganization(List<InventoryItemResponsibleOrganizationComponent> theResponsibleOrganization) { 2485 this.responsibleOrganization = theResponsibleOrganization; 2486 return this; 2487 } 2488 2489 public boolean hasResponsibleOrganization() { 2490 if (this.responsibleOrganization == null) 2491 return false; 2492 for (InventoryItemResponsibleOrganizationComponent item : this.responsibleOrganization) 2493 if (!item.isEmpty()) 2494 return true; 2495 return false; 2496 } 2497 2498 public InventoryItemResponsibleOrganizationComponent addResponsibleOrganization() { //3 2499 InventoryItemResponsibleOrganizationComponent t = new InventoryItemResponsibleOrganizationComponent(); 2500 if (this.responsibleOrganization == null) 2501 this.responsibleOrganization = new ArrayList<InventoryItemResponsibleOrganizationComponent>(); 2502 this.responsibleOrganization.add(t); 2503 return t; 2504 } 2505 2506 public InventoryItem addResponsibleOrganization(InventoryItemResponsibleOrganizationComponent t) { //3 2507 if (t == null) 2508 return this; 2509 if (this.responsibleOrganization == null) 2510 this.responsibleOrganization = new ArrayList<InventoryItemResponsibleOrganizationComponent>(); 2511 this.responsibleOrganization.add(t); 2512 return this; 2513 } 2514 2515 /** 2516 * @return The first repetition of repeating field {@link #responsibleOrganization}, creating it if it does not already exist {3} 2517 */ 2518 public InventoryItemResponsibleOrganizationComponent getResponsibleOrganizationFirstRep() { 2519 if (getResponsibleOrganization().isEmpty()) { 2520 addResponsibleOrganization(); 2521 } 2522 return getResponsibleOrganization().get(0); 2523 } 2524 2525 /** 2526 * @return {@link #description} (The descriptive characteristics of the inventory item.) 2527 */ 2528 public InventoryItemDescriptionComponent getDescription() { 2529 if (this.description == null) 2530 if (Configuration.errorOnAutoCreate()) 2531 throw new Error("Attempt to auto-create InventoryItem.description"); 2532 else if (Configuration.doAutoCreate()) 2533 this.description = new InventoryItemDescriptionComponent(); // cc 2534 return this.description; 2535 } 2536 2537 public boolean hasDescription() { 2538 return this.description != null && !this.description.isEmpty(); 2539 } 2540 2541 /** 2542 * @param value {@link #description} (The descriptive characteristics of the inventory item.) 2543 */ 2544 public InventoryItem setDescription(InventoryItemDescriptionComponent value) { 2545 this.description = value; 2546 return this; 2547 } 2548 2549 /** 2550 * @return {@link #inventoryStatus} (The usage status e.g. recalled, in use, discarded... This can be used to indicate that the items have been taken out of inventory, or are in use, etc.) 2551 */ 2552 public List<CodeableConcept> getInventoryStatus() { 2553 if (this.inventoryStatus == null) 2554 this.inventoryStatus = new ArrayList<CodeableConcept>(); 2555 return this.inventoryStatus; 2556 } 2557 2558 /** 2559 * @return Returns a reference to <code>this</code> for easy method chaining 2560 */ 2561 public InventoryItem setInventoryStatus(List<CodeableConcept> theInventoryStatus) { 2562 this.inventoryStatus = theInventoryStatus; 2563 return this; 2564 } 2565 2566 public boolean hasInventoryStatus() { 2567 if (this.inventoryStatus == null) 2568 return false; 2569 for (CodeableConcept item : this.inventoryStatus) 2570 if (!item.isEmpty()) 2571 return true; 2572 return false; 2573 } 2574 2575 public CodeableConcept addInventoryStatus() { //3 2576 CodeableConcept t = new CodeableConcept(); 2577 if (this.inventoryStatus == null) 2578 this.inventoryStatus = new ArrayList<CodeableConcept>(); 2579 this.inventoryStatus.add(t); 2580 return t; 2581 } 2582 2583 public InventoryItem addInventoryStatus(CodeableConcept t) { //3 2584 if (t == null) 2585 return this; 2586 if (this.inventoryStatus == null) 2587 this.inventoryStatus = new ArrayList<CodeableConcept>(); 2588 this.inventoryStatus.add(t); 2589 return this; 2590 } 2591 2592 /** 2593 * @return The first repetition of repeating field {@link #inventoryStatus}, creating it if it does not already exist {3} 2594 */ 2595 public CodeableConcept getInventoryStatusFirstRep() { 2596 if (getInventoryStatus().isEmpty()) { 2597 addInventoryStatus(); 2598 } 2599 return getInventoryStatus().get(0); 2600 } 2601 2602 /** 2603 * @return {@link #baseUnit} (The base unit of measure - the unit in which the product is used or counted.) 2604 */ 2605 public CodeableConcept getBaseUnit() { 2606 if (this.baseUnit == null) 2607 if (Configuration.errorOnAutoCreate()) 2608 throw new Error("Attempt to auto-create InventoryItem.baseUnit"); 2609 else if (Configuration.doAutoCreate()) 2610 this.baseUnit = new CodeableConcept(); // cc 2611 return this.baseUnit; 2612 } 2613 2614 public boolean hasBaseUnit() { 2615 return this.baseUnit != null && !this.baseUnit.isEmpty(); 2616 } 2617 2618 /** 2619 * @param value {@link #baseUnit} (The base unit of measure - the unit in which the product is used or counted.) 2620 */ 2621 public InventoryItem setBaseUnit(CodeableConcept value) { 2622 this.baseUnit = value; 2623 return this; 2624 } 2625 2626 /** 2627 * @return {@link #netContent} (Net content or amount present in the item.) 2628 */ 2629 public Quantity getNetContent() { 2630 if (this.netContent == null) 2631 if (Configuration.errorOnAutoCreate()) 2632 throw new Error("Attempt to auto-create InventoryItem.netContent"); 2633 else if (Configuration.doAutoCreate()) 2634 this.netContent = new Quantity(); // cc 2635 return this.netContent; 2636 } 2637 2638 public boolean hasNetContent() { 2639 return this.netContent != null && !this.netContent.isEmpty(); 2640 } 2641 2642 /** 2643 * @param value {@link #netContent} (Net content or amount present in the item.) 2644 */ 2645 public InventoryItem setNetContent(Quantity value) { 2646 this.netContent = value; 2647 return this; 2648 } 2649 2650 /** 2651 * @return {@link #association} (Association with other items or products.) 2652 */ 2653 public List<InventoryItemAssociationComponent> getAssociation() { 2654 if (this.association == null) 2655 this.association = new ArrayList<InventoryItemAssociationComponent>(); 2656 return this.association; 2657 } 2658 2659 /** 2660 * @return Returns a reference to <code>this</code> for easy method chaining 2661 */ 2662 public InventoryItem setAssociation(List<InventoryItemAssociationComponent> theAssociation) { 2663 this.association = theAssociation; 2664 return this; 2665 } 2666 2667 public boolean hasAssociation() { 2668 if (this.association == null) 2669 return false; 2670 for (InventoryItemAssociationComponent item : this.association) 2671 if (!item.isEmpty()) 2672 return true; 2673 return false; 2674 } 2675 2676 public InventoryItemAssociationComponent addAssociation() { //3 2677 InventoryItemAssociationComponent t = new InventoryItemAssociationComponent(); 2678 if (this.association == null) 2679 this.association = new ArrayList<InventoryItemAssociationComponent>(); 2680 this.association.add(t); 2681 return t; 2682 } 2683 2684 public InventoryItem addAssociation(InventoryItemAssociationComponent t) { //3 2685 if (t == null) 2686 return this; 2687 if (this.association == null) 2688 this.association = new ArrayList<InventoryItemAssociationComponent>(); 2689 this.association.add(t); 2690 return this; 2691 } 2692 2693 /** 2694 * @return The first repetition of repeating field {@link #association}, creating it if it does not already exist {3} 2695 */ 2696 public InventoryItemAssociationComponent getAssociationFirstRep() { 2697 if (getAssociation().isEmpty()) { 2698 addAssociation(); 2699 } 2700 return getAssociation().get(0); 2701 } 2702 2703 /** 2704 * @return {@link #characteristic} (The descriptive or identifying characteristics of the item.) 2705 */ 2706 public List<InventoryItemCharacteristicComponent> getCharacteristic() { 2707 if (this.characteristic == null) 2708 this.characteristic = new ArrayList<InventoryItemCharacteristicComponent>(); 2709 return this.characteristic; 2710 } 2711 2712 /** 2713 * @return Returns a reference to <code>this</code> for easy method chaining 2714 */ 2715 public InventoryItem setCharacteristic(List<InventoryItemCharacteristicComponent> theCharacteristic) { 2716 this.characteristic = theCharacteristic; 2717 return this; 2718 } 2719 2720 public boolean hasCharacteristic() { 2721 if (this.characteristic == null) 2722 return false; 2723 for (InventoryItemCharacteristicComponent item : this.characteristic) 2724 if (!item.isEmpty()) 2725 return true; 2726 return false; 2727 } 2728 2729 public InventoryItemCharacteristicComponent addCharacteristic() { //3 2730 InventoryItemCharacteristicComponent t = new InventoryItemCharacteristicComponent(); 2731 if (this.characteristic == null) 2732 this.characteristic = new ArrayList<InventoryItemCharacteristicComponent>(); 2733 this.characteristic.add(t); 2734 return t; 2735 } 2736 2737 public InventoryItem addCharacteristic(InventoryItemCharacteristicComponent t) { //3 2738 if (t == null) 2739 return this; 2740 if (this.characteristic == null) 2741 this.characteristic = new ArrayList<InventoryItemCharacteristicComponent>(); 2742 this.characteristic.add(t); 2743 return this; 2744 } 2745 2746 /** 2747 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist {3} 2748 */ 2749 public InventoryItemCharacteristicComponent getCharacteristicFirstRep() { 2750 if (getCharacteristic().isEmpty()) { 2751 addCharacteristic(); 2752 } 2753 return getCharacteristic().get(0); 2754 } 2755 2756 /** 2757 * @return {@link #instance} (Instances or occurrences of the product.) 2758 */ 2759 public InventoryItemInstanceComponent getInstance() { 2760 if (this.instance == null) 2761 if (Configuration.errorOnAutoCreate()) 2762 throw new Error("Attempt to auto-create InventoryItem.instance"); 2763 else if (Configuration.doAutoCreate()) 2764 this.instance = new InventoryItemInstanceComponent(); // cc 2765 return this.instance; 2766 } 2767 2768 public boolean hasInstance() { 2769 return this.instance != null && !this.instance.isEmpty(); 2770 } 2771 2772 /** 2773 * @param value {@link #instance} (Instances or occurrences of the product.) 2774 */ 2775 public InventoryItem setInstance(InventoryItemInstanceComponent value) { 2776 this.instance = value; 2777 return this; 2778 } 2779 2780 /** 2781 * @return {@link #productReference} (Link to a product resource used in clinical workflows.) 2782 */ 2783 public Reference getProductReference() { 2784 if (this.productReference == null) 2785 if (Configuration.errorOnAutoCreate()) 2786 throw new Error("Attempt to auto-create InventoryItem.productReference"); 2787 else if (Configuration.doAutoCreate()) 2788 this.productReference = new Reference(); // cc 2789 return this.productReference; 2790 } 2791 2792 public boolean hasProductReference() { 2793 return this.productReference != null && !this.productReference.isEmpty(); 2794 } 2795 2796 /** 2797 * @param value {@link #productReference} (Link to a product resource used in clinical workflows.) 2798 */ 2799 public InventoryItem setProductReference(Reference value) { 2800 this.productReference = value; 2801 return this; 2802 } 2803 2804 protected void listChildren(List<Property> children) { 2805 super.listChildren(children); 2806 children.add(new Property("identifier", "Identifier", "Business identifier for the inventory item.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2807 children.add(new Property("status", "code", "Status of the item entry.", 0, 1, status)); 2808 children.add(new Property("category", "CodeableConcept", "Category or class of the item.", 0, java.lang.Integer.MAX_VALUE, category)); 2809 children.add(new Property("code", "CodeableConcept", "Code designating the specific type of item.", 0, java.lang.Integer.MAX_VALUE, code)); 2810 children.add(new Property("name", "", "The item name(s) - the brand name, or common name, functional name, generic name.", 0, java.lang.Integer.MAX_VALUE, name)); 2811 children.add(new Property("responsibleOrganization", "", "Organization(s) responsible for the product.", 0, java.lang.Integer.MAX_VALUE, responsibleOrganization)); 2812 children.add(new Property("description", "", "The descriptive characteristics of the inventory item.", 0, 1, description)); 2813 children.add(new Property("inventoryStatus", "CodeableConcept", "The usage status e.g. recalled, in use, discarded... This can be used to indicate that the items have been taken out of inventory, or are in use, etc.", 0, java.lang.Integer.MAX_VALUE, inventoryStatus)); 2814 children.add(new Property("baseUnit", "CodeableConcept", "The base unit of measure - the unit in which the product is used or counted.", 0, 1, baseUnit)); 2815 children.add(new Property("netContent", "Quantity", "Net content or amount present in the item.", 0, 1, netContent)); 2816 children.add(new Property("association", "", "Association with other items or products.", 0, java.lang.Integer.MAX_VALUE, association)); 2817 children.add(new Property("characteristic", "", "The descriptive or identifying characteristics of the item.", 0, java.lang.Integer.MAX_VALUE, characteristic)); 2818 children.add(new Property("instance", "", "Instances or occurrences of the product.", 0, 1, instance)); 2819 children.add(new Property("productReference", "Reference(Medication|Device|NutritionProduct|BiologicallyDerivedProduct)", "Link to a product resource used in clinical workflows.", 0, 1, productReference)); 2820 } 2821 2822 @Override 2823 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2824 switch (_hash) { 2825 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier for the inventory item.", 0, java.lang.Integer.MAX_VALUE, identifier); 2826 case -892481550: /*status*/ return new Property("status", "code", "Status of the item entry.", 0, 1, status); 2827 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Category or class of the item.", 0, java.lang.Integer.MAX_VALUE, category); 2828 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Code designating the specific type of item.", 0, java.lang.Integer.MAX_VALUE, code); 2829 case 3373707: /*name*/ return new Property("name", "", "The item name(s) - the brand name, or common name, functional name, generic name.", 0, java.lang.Integer.MAX_VALUE, name); 2830 case -1704933559: /*responsibleOrganization*/ return new Property("responsibleOrganization", "", "Organization(s) responsible for the product.", 0, java.lang.Integer.MAX_VALUE, responsibleOrganization); 2831 case -1724546052: /*description*/ return new Property("description", "", "The descriptive characteristics of the inventory item.", 0, 1, description); 2832 case -1370922898: /*inventoryStatus*/ return new Property("inventoryStatus", "CodeableConcept", "The usage status e.g. recalled, in use, discarded... This can be used to indicate that the items have been taken out of inventory, or are in use, etc.", 0, java.lang.Integer.MAX_VALUE, inventoryStatus); 2833 case -1721465867: /*baseUnit*/ return new Property("baseUnit", "CodeableConcept", "The base unit of measure - the unit in which the product is used or counted.", 0, 1, baseUnit); 2834 case 612796444: /*netContent*/ return new Property("netContent", "Quantity", "Net content or amount present in the item.", 0, 1, netContent); 2835 case -87499647: /*association*/ return new Property("association", "", "Association with other items or products.", 0, java.lang.Integer.MAX_VALUE, association); 2836 case 366313883: /*characteristic*/ return new Property("characteristic", "", "The descriptive or identifying characteristics of the item.", 0, java.lang.Integer.MAX_VALUE, characteristic); 2837 case 555127957: /*instance*/ return new Property("instance", "", "Instances or occurrences of the product.", 0, 1, instance); 2838 case -669667556: /*productReference*/ return new Property("productReference", "Reference(Medication|Device|NutritionProduct|BiologicallyDerivedProduct)", "Link to a product resource used in clinical workflows.", 0, 1, productReference); 2839 default: return super.getNamedProperty(_hash, _name, _checkValid); 2840 } 2841 2842 } 2843 2844 @Override 2845 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2846 switch (hash) { 2847 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2848 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<InventoryItemStatusCodes> 2849 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2850 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // CodeableConcept 2851 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // InventoryItemNameComponent 2852 case -1704933559: /*responsibleOrganization*/ return this.responsibleOrganization == null ? new Base[0] : this.responsibleOrganization.toArray(new Base[this.responsibleOrganization.size()]); // InventoryItemResponsibleOrganizationComponent 2853 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // InventoryItemDescriptionComponent 2854 case -1370922898: /*inventoryStatus*/ return this.inventoryStatus == null ? new Base[0] : this.inventoryStatus.toArray(new Base[this.inventoryStatus.size()]); // CodeableConcept 2855 case -1721465867: /*baseUnit*/ return this.baseUnit == null ? new Base[0] : new Base[] {this.baseUnit}; // CodeableConcept 2856 case 612796444: /*netContent*/ return this.netContent == null ? new Base[0] : new Base[] {this.netContent}; // Quantity 2857 case -87499647: /*association*/ return this.association == null ? new Base[0] : this.association.toArray(new Base[this.association.size()]); // InventoryItemAssociationComponent 2858 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // InventoryItemCharacteristicComponent 2859 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : new Base[] {this.instance}; // InventoryItemInstanceComponent 2860 case -669667556: /*productReference*/ return this.productReference == null ? new Base[0] : new Base[] {this.productReference}; // Reference 2861 default: return super.getProperty(hash, name, checkValid); 2862 } 2863 2864 } 2865 2866 @Override 2867 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2868 switch (hash) { 2869 case -1618432855: // identifier 2870 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2871 return value; 2872 case -892481550: // status 2873 value = new InventoryItemStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2874 this.status = (Enumeration) value; // Enumeration<InventoryItemStatusCodes> 2875 return value; 2876 case 50511102: // category 2877 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2878 return value; 2879 case 3059181: // code 2880 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2881 return value; 2882 case 3373707: // name 2883 this.getName().add((InventoryItemNameComponent) value); // InventoryItemNameComponent 2884 return value; 2885 case -1704933559: // responsibleOrganization 2886 this.getResponsibleOrganization().add((InventoryItemResponsibleOrganizationComponent) value); // InventoryItemResponsibleOrganizationComponent 2887 return value; 2888 case -1724546052: // description 2889 this.description = (InventoryItemDescriptionComponent) value; // InventoryItemDescriptionComponent 2890 return value; 2891 case -1370922898: // inventoryStatus 2892 this.getInventoryStatus().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2893 return value; 2894 case -1721465867: // baseUnit 2895 this.baseUnit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2896 return value; 2897 case 612796444: // netContent 2898 this.netContent = TypeConvertor.castToQuantity(value); // Quantity 2899 return value; 2900 case -87499647: // association 2901 this.getAssociation().add((InventoryItemAssociationComponent) value); // InventoryItemAssociationComponent 2902 return value; 2903 case 366313883: // characteristic 2904 this.getCharacteristic().add((InventoryItemCharacteristicComponent) value); // InventoryItemCharacteristicComponent 2905 return value; 2906 case 555127957: // instance 2907 this.instance = (InventoryItemInstanceComponent) value; // InventoryItemInstanceComponent 2908 return value; 2909 case -669667556: // productReference 2910 this.productReference = TypeConvertor.castToReference(value); // Reference 2911 return value; 2912 default: return super.setProperty(hash, name, value); 2913 } 2914 2915 } 2916 2917 @Override 2918 public Base setProperty(String name, Base value) throws FHIRException { 2919 if (name.equals("identifier")) { 2920 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2921 } else if (name.equals("status")) { 2922 value = new InventoryItemStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2923 this.status = (Enumeration) value; // Enumeration<InventoryItemStatusCodes> 2924 } else if (name.equals("category")) { 2925 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 2926 } else if (name.equals("code")) { 2927 this.getCode().add(TypeConvertor.castToCodeableConcept(value)); 2928 } else if (name.equals("name")) { 2929 this.getName().add((InventoryItemNameComponent) value); 2930 } else if (name.equals("responsibleOrganization")) { 2931 this.getResponsibleOrganization().add((InventoryItemResponsibleOrganizationComponent) value); 2932 } else if (name.equals("description")) { 2933 this.description = (InventoryItemDescriptionComponent) value; // InventoryItemDescriptionComponent 2934 } else if (name.equals("inventoryStatus")) { 2935 this.getInventoryStatus().add(TypeConvertor.castToCodeableConcept(value)); 2936 } else if (name.equals("baseUnit")) { 2937 this.baseUnit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2938 } else if (name.equals("netContent")) { 2939 this.netContent = TypeConvertor.castToQuantity(value); // Quantity 2940 } else if (name.equals("association")) { 2941 this.getAssociation().add((InventoryItemAssociationComponent) value); 2942 } else if (name.equals("characteristic")) { 2943 this.getCharacteristic().add((InventoryItemCharacteristicComponent) value); 2944 } else if (name.equals("instance")) { 2945 this.instance = (InventoryItemInstanceComponent) value; // InventoryItemInstanceComponent 2946 } else if (name.equals("productReference")) { 2947 this.productReference = TypeConvertor.castToReference(value); // Reference 2948 } else 2949 return super.setProperty(name, value); 2950 return value; 2951 } 2952 2953 @Override 2954 public Base makeProperty(int hash, String name) throws FHIRException { 2955 switch (hash) { 2956 case -1618432855: return addIdentifier(); 2957 case -892481550: return getStatusElement(); 2958 case 50511102: return addCategory(); 2959 case 3059181: return addCode(); 2960 case 3373707: return addName(); 2961 case -1704933559: return addResponsibleOrganization(); 2962 case -1724546052: return getDescription(); 2963 case -1370922898: return addInventoryStatus(); 2964 case -1721465867: return getBaseUnit(); 2965 case 612796444: return getNetContent(); 2966 case -87499647: return addAssociation(); 2967 case 366313883: return addCharacteristic(); 2968 case 555127957: return getInstance(); 2969 case -669667556: return getProductReference(); 2970 default: return super.makeProperty(hash, name); 2971 } 2972 2973 } 2974 2975 @Override 2976 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2977 switch (hash) { 2978 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2979 case -892481550: /*status*/ return new String[] {"code"}; 2980 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2981 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2982 case 3373707: /*name*/ return new String[] {}; 2983 case -1704933559: /*responsibleOrganization*/ return new String[] {}; 2984 case -1724546052: /*description*/ return new String[] {}; 2985 case -1370922898: /*inventoryStatus*/ return new String[] {"CodeableConcept"}; 2986 case -1721465867: /*baseUnit*/ return new String[] {"CodeableConcept"}; 2987 case 612796444: /*netContent*/ return new String[] {"Quantity"}; 2988 case -87499647: /*association*/ return new String[] {}; 2989 case 366313883: /*characteristic*/ return new String[] {}; 2990 case 555127957: /*instance*/ return new String[] {}; 2991 case -669667556: /*productReference*/ return new String[] {"Reference"}; 2992 default: return super.getTypesForProperty(hash, name); 2993 } 2994 2995 } 2996 2997 @Override 2998 public Base addChild(String name) throws FHIRException { 2999 if (name.equals("identifier")) { 3000 return addIdentifier(); 3001 } 3002 else if (name.equals("status")) { 3003 throw new FHIRException("Cannot call addChild on a singleton property InventoryItem.status"); 3004 } 3005 else if (name.equals("category")) { 3006 return addCategory(); 3007 } 3008 else if (name.equals("code")) { 3009 return addCode(); 3010 } 3011 else if (name.equals("name")) { 3012 return addName(); 3013 } 3014 else if (name.equals("responsibleOrganization")) { 3015 return addResponsibleOrganization(); 3016 } 3017 else if (name.equals("description")) { 3018 this.description = new InventoryItemDescriptionComponent(); 3019 return this.description; 3020 } 3021 else if (name.equals("inventoryStatus")) { 3022 return addInventoryStatus(); 3023 } 3024 else if (name.equals("baseUnit")) { 3025 this.baseUnit = new CodeableConcept(); 3026 return this.baseUnit; 3027 } 3028 else if (name.equals("netContent")) { 3029 this.netContent = new Quantity(); 3030 return this.netContent; 3031 } 3032 else if (name.equals("association")) { 3033 return addAssociation(); 3034 } 3035 else if (name.equals("characteristic")) { 3036 return addCharacteristic(); 3037 } 3038 else if (name.equals("instance")) { 3039 this.instance = new InventoryItemInstanceComponent(); 3040 return this.instance; 3041 } 3042 else if (name.equals("productReference")) { 3043 this.productReference = new Reference(); 3044 return this.productReference; 3045 } 3046 else 3047 return super.addChild(name); 3048 } 3049 3050 public String fhirType() { 3051 return "InventoryItem"; 3052 3053 } 3054 3055 public InventoryItem copy() { 3056 InventoryItem dst = new InventoryItem(); 3057 copyValues(dst); 3058 return dst; 3059 } 3060 3061 public void copyValues(InventoryItem dst) { 3062 super.copyValues(dst); 3063 if (identifier != null) { 3064 dst.identifier = new ArrayList<Identifier>(); 3065 for (Identifier i : identifier) 3066 dst.identifier.add(i.copy()); 3067 }; 3068 dst.status = status == null ? null : status.copy(); 3069 if (category != null) { 3070 dst.category = new ArrayList<CodeableConcept>(); 3071 for (CodeableConcept i : category) 3072 dst.category.add(i.copy()); 3073 }; 3074 if (code != null) { 3075 dst.code = new ArrayList<CodeableConcept>(); 3076 for (CodeableConcept i : code) 3077 dst.code.add(i.copy()); 3078 }; 3079 if (name != null) { 3080 dst.name = new ArrayList<InventoryItemNameComponent>(); 3081 for (InventoryItemNameComponent i : name) 3082 dst.name.add(i.copy()); 3083 }; 3084 if (responsibleOrganization != null) { 3085 dst.responsibleOrganization = new ArrayList<InventoryItemResponsibleOrganizationComponent>(); 3086 for (InventoryItemResponsibleOrganizationComponent i : responsibleOrganization) 3087 dst.responsibleOrganization.add(i.copy()); 3088 }; 3089 dst.description = description == null ? null : description.copy(); 3090 if (inventoryStatus != null) { 3091 dst.inventoryStatus = new ArrayList<CodeableConcept>(); 3092 for (CodeableConcept i : inventoryStatus) 3093 dst.inventoryStatus.add(i.copy()); 3094 }; 3095 dst.baseUnit = baseUnit == null ? null : baseUnit.copy(); 3096 dst.netContent = netContent == null ? null : netContent.copy(); 3097 if (association != null) { 3098 dst.association = new ArrayList<InventoryItemAssociationComponent>(); 3099 for (InventoryItemAssociationComponent i : association) 3100 dst.association.add(i.copy()); 3101 }; 3102 if (characteristic != null) { 3103 dst.characteristic = new ArrayList<InventoryItemCharacteristicComponent>(); 3104 for (InventoryItemCharacteristicComponent i : characteristic) 3105 dst.characteristic.add(i.copy()); 3106 }; 3107 dst.instance = instance == null ? null : instance.copy(); 3108 dst.productReference = productReference == null ? null : productReference.copy(); 3109 } 3110 3111 protected InventoryItem typedCopy() { 3112 return copy(); 3113 } 3114 3115 @Override 3116 public boolean equalsDeep(Base other_) { 3117 if (!super.equalsDeep(other_)) 3118 return false; 3119 if (!(other_ instanceof InventoryItem)) 3120 return false; 3121 InventoryItem o = (InventoryItem) other_; 3122 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 3123 && compareDeep(code, o.code, true) && compareDeep(name, o.name, true) && compareDeep(responsibleOrganization, o.responsibleOrganization, true) 3124 && compareDeep(description, o.description, true) && compareDeep(inventoryStatus, o.inventoryStatus, true) 3125 && compareDeep(baseUnit, o.baseUnit, true) && compareDeep(netContent, o.netContent, true) && compareDeep(association, o.association, true) 3126 && compareDeep(characteristic, o.characteristic, true) && compareDeep(instance, o.instance, true) 3127 && compareDeep(productReference, o.productReference, true); 3128 } 3129 3130 @Override 3131 public boolean equalsShallow(Base other_) { 3132 if (!super.equalsShallow(other_)) 3133 return false; 3134 if (!(other_ instanceof InventoryItem)) 3135 return false; 3136 InventoryItem o = (InventoryItem) other_; 3137 return compareValues(status, o.status, true); 3138 } 3139 3140 public boolean isEmpty() { 3141 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 3142 , code, name, responsibleOrganization, description, inventoryStatus, baseUnit, netContent 3143 , association, characteristic, instance, productReference); 3144 } 3145 3146 @Override 3147 public ResourceType getResourceType() { 3148 return ResourceType.InventoryItem; 3149 } 3150 3151 /** 3152 * Search parameter: <b>code</b> 3153 * <p> 3154 * Description: <b>Search for products that match this code</b><br> 3155 * Type: <b>token</b><br> 3156 * Path: <b>InventoryItem.code</b><br> 3157 * </p> 3158 */ 3159 @SearchParamDefinition(name="code", path="InventoryItem.code", description="Search for products that match this code", type="token" ) 3160 public static final String SP_CODE = "code"; 3161 /** 3162 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3163 * <p> 3164 * Description: <b>Search for products that match this code</b><br> 3165 * Type: <b>token</b><br> 3166 * Path: <b>InventoryItem.code</b><br> 3167 * </p> 3168 */ 3169 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 3170 3171 /** 3172 * Search parameter: <b>identifier</b> 3173 * <p> 3174 * Description: <b>The identifier of the item</b><br> 3175 * Type: <b>token</b><br> 3176 * Path: <b>InventoryItem.identifier</b><br> 3177 * </p> 3178 */ 3179 @SearchParamDefinition(name="identifier", path="InventoryItem.identifier", description="The identifier of the item", type="token" ) 3180 public static final String SP_IDENTIFIER = "identifier"; 3181 /** 3182 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3183 * <p> 3184 * Description: <b>The identifier of the item</b><br> 3185 * Type: <b>token</b><br> 3186 * Path: <b>InventoryItem.identifier</b><br> 3187 * </p> 3188 */ 3189 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3190 3191 /** 3192 * Search parameter: <b>status</b> 3193 * <p> 3194 * Description: <b>The status of the item</b><br> 3195 * Type: <b>token</b><br> 3196 * Path: <b>InventoryItem.status</b><br> 3197 * </p> 3198 */ 3199 @SearchParamDefinition(name="status", path="InventoryItem.status", description="The status of the item", type="token" ) 3200 public static final String SP_STATUS = "status"; 3201 /** 3202 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3203 * <p> 3204 * Description: <b>The status of the item</b><br> 3205 * Type: <b>token</b><br> 3206 * Path: <b>InventoryItem.status</b><br> 3207 * </p> 3208 */ 3209 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3210 3211 /** 3212 * Search parameter: <b>subject</b> 3213 * <p> 3214 * Description: <b>The identity of a patient for whom to list associations</b><br> 3215 * Type: <b>reference</b><br> 3216 * Path: <b>InventoryItem.instance.subject</b><br> 3217 * </p> 3218 */ 3219 @SearchParamDefinition(name="subject", path="InventoryItem.instance.subject", description="The identity of a patient for whom to list associations", type="reference", target={Organization.class, Patient.class } ) 3220 public static final String SP_SUBJECT = "subject"; 3221 /** 3222 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3223 * <p> 3224 * Description: <b>The identity of a patient for whom to list associations</b><br> 3225 * Type: <b>reference</b><br> 3226 * Path: <b>InventoryItem.instance.subject</b><br> 3227 * </p> 3228 */ 3229 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3230 3231/** 3232 * Constant for fluent queries to be used to add include statements. Specifies 3233 * the path value of "<b>InventoryItem:subject</b>". 3234 */ 3235 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("InventoryItem:subject").toLocked(); 3236 3237 3238} 3239