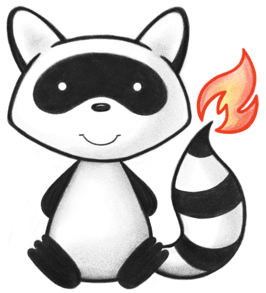
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A report of inventory or stock items. 052 */ 053@ResourceDef(name="InventoryReport", profile="http://hl7.org/fhir/StructureDefinition/InventoryReport") 054public class InventoryReport extends DomainResource { 055 056 public enum InventoryCountType { 057 /** 058 * The inventory report is a current absolute snapshot, i.e. it represents the quantities at hand. 059 */ 060 SNAPSHOT, 061 /** 062 * The inventory report is about the difference between a previous count and a current count, i.e. it represents the items that have been added/subtracted from inventory. 063 */ 064 DIFFERENCE, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static InventoryCountType fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("snapshot".equals(codeString)) 073 return SNAPSHOT; 074 if ("difference".equals(codeString)) 075 return DIFFERENCE; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown InventoryCountType code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case SNAPSHOT: return "snapshot"; 084 case DIFFERENCE: return "difference"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 switch (this) { 091 case SNAPSHOT: return "http://hl7.org/fhir/inventoryreport-counttype"; 092 case DIFFERENCE: return "http://hl7.org/fhir/inventoryreport-counttype"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getDefinition() { 098 switch (this) { 099 case SNAPSHOT: return "The inventory report is a current absolute snapshot, i.e. it represents the quantities at hand."; 100 case DIFFERENCE: return "The inventory report is about the difference between a previous count and a current count, i.e. it represents the items that have been added/subtracted from inventory."; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDisplay() { 106 switch (this) { 107 case SNAPSHOT: return "Snapshot"; 108 case DIFFERENCE: return "Difference"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 } 114 115 public static class InventoryCountTypeEnumFactory implements EnumFactory<InventoryCountType> { 116 public InventoryCountType fromCode(String codeString) throws IllegalArgumentException { 117 if (codeString == null || "".equals(codeString)) 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("snapshot".equals(codeString)) 121 return InventoryCountType.SNAPSHOT; 122 if ("difference".equals(codeString)) 123 return InventoryCountType.DIFFERENCE; 124 throw new IllegalArgumentException("Unknown InventoryCountType code '"+codeString+"'"); 125 } 126 public Enumeration<InventoryCountType> fromType(PrimitiveType<?> code) throws FHIRException { 127 if (code == null) 128 return null; 129 if (code.isEmpty()) 130 return new Enumeration<InventoryCountType>(this, InventoryCountType.NULL, code); 131 String codeString = ((PrimitiveType) code).asStringValue(); 132 if (codeString == null || "".equals(codeString)) 133 return new Enumeration<InventoryCountType>(this, InventoryCountType.NULL, code); 134 if ("snapshot".equals(codeString)) 135 return new Enumeration<InventoryCountType>(this, InventoryCountType.SNAPSHOT, code); 136 if ("difference".equals(codeString)) 137 return new Enumeration<InventoryCountType>(this, InventoryCountType.DIFFERENCE, code); 138 throw new FHIRException("Unknown InventoryCountType code '"+codeString+"'"); 139 } 140 public String toCode(InventoryCountType code) { 141 if (code == InventoryCountType.SNAPSHOT) 142 return "snapshot"; 143 if (code == InventoryCountType.DIFFERENCE) 144 return "difference"; 145 return "?"; 146 } 147 public String toSystem(InventoryCountType code) { 148 return code.getSystem(); 149 } 150 } 151 152 public enum InventoryReportStatus { 153 /** 154 * The existence of the report is registered, but it is still without content or only some preliminary content. 155 */ 156 DRAFT, 157 /** 158 * The inventory report has been requested but there is no data available. 159 */ 160 REQUESTED, 161 /** 162 * This report is submitted as current. 163 */ 164 ACTIVE, 165 /** 166 * The report has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. 167 */ 168 ENTEREDINERROR, 169 /** 170 * added to help the parsers with the generic types 171 */ 172 NULL; 173 public static InventoryReportStatus fromCode(String codeString) throws FHIRException { 174 if (codeString == null || "".equals(codeString)) 175 return null; 176 if ("draft".equals(codeString)) 177 return DRAFT; 178 if ("requested".equals(codeString)) 179 return REQUESTED; 180 if ("active".equals(codeString)) 181 return ACTIVE; 182 if ("entered-in-error".equals(codeString)) 183 return ENTEREDINERROR; 184 if (Configuration.isAcceptInvalidEnums()) 185 return null; 186 else 187 throw new FHIRException("Unknown InventoryReportStatus code '"+codeString+"'"); 188 } 189 public String toCode() { 190 switch (this) { 191 case DRAFT: return "draft"; 192 case REQUESTED: return "requested"; 193 case ACTIVE: return "active"; 194 case ENTEREDINERROR: return "entered-in-error"; 195 case NULL: return null; 196 default: return "?"; 197 } 198 } 199 public String getSystem() { 200 switch (this) { 201 case DRAFT: return "http://hl7.org/fhir/inventoryreport-status"; 202 case REQUESTED: return "http://hl7.org/fhir/inventoryreport-status"; 203 case ACTIVE: return "http://hl7.org/fhir/inventoryreport-status"; 204 case ENTEREDINERROR: return "http://hl7.org/fhir/inventoryreport-status"; 205 case NULL: return null; 206 default: return "?"; 207 } 208 } 209 public String getDefinition() { 210 switch (this) { 211 case DRAFT: return "The existence of the report is registered, but it is still without content or only some preliminary content."; 212 case REQUESTED: return "The inventory report has been requested but there is no data available."; 213 case ACTIVE: return "This report is submitted as current."; 214 case ENTEREDINERROR: return "The report has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it."; 215 case NULL: return null; 216 default: return "?"; 217 } 218 } 219 public String getDisplay() { 220 switch (this) { 221 case DRAFT: return "Draft"; 222 case REQUESTED: return "Requested"; 223 case ACTIVE: return "Active"; 224 case ENTEREDINERROR: return "Entered in Error"; 225 case NULL: return null; 226 default: return "?"; 227 } 228 } 229 } 230 231 public static class InventoryReportStatusEnumFactory implements EnumFactory<InventoryReportStatus> { 232 public InventoryReportStatus fromCode(String codeString) throws IllegalArgumentException { 233 if (codeString == null || "".equals(codeString)) 234 if (codeString == null || "".equals(codeString)) 235 return null; 236 if ("draft".equals(codeString)) 237 return InventoryReportStatus.DRAFT; 238 if ("requested".equals(codeString)) 239 return InventoryReportStatus.REQUESTED; 240 if ("active".equals(codeString)) 241 return InventoryReportStatus.ACTIVE; 242 if ("entered-in-error".equals(codeString)) 243 return InventoryReportStatus.ENTEREDINERROR; 244 throw new IllegalArgumentException("Unknown InventoryReportStatus code '"+codeString+"'"); 245 } 246 public Enumeration<InventoryReportStatus> fromType(PrimitiveType<?> code) throws FHIRException { 247 if (code == null) 248 return null; 249 if (code.isEmpty()) 250 return new Enumeration<InventoryReportStatus>(this, InventoryReportStatus.NULL, code); 251 String codeString = ((PrimitiveType) code).asStringValue(); 252 if (codeString == null || "".equals(codeString)) 253 return new Enumeration<InventoryReportStatus>(this, InventoryReportStatus.NULL, code); 254 if ("draft".equals(codeString)) 255 return new Enumeration<InventoryReportStatus>(this, InventoryReportStatus.DRAFT, code); 256 if ("requested".equals(codeString)) 257 return new Enumeration<InventoryReportStatus>(this, InventoryReportStatus.REQUESTED, code); 258 if ("active".equals(codeString)) 259 return new Enumeration<InventoryReportStatus>(this, InventoryReportStatus.ACTIVE, code); 260 if ("entered-in-error".equals(codeString)) 261 return new Enumeration<InventoryReportStatus>(this, InventoryReportStatus.ENTEREDINERROR, code); 262 throw new FHIRException("Unknown InventoryReportStatus code '"+codeString+"'"); 263 } 264 public String toCode(InventoryReportStatus code) { 265 if (code == InventoryReportStatus.DRAFT) 266 return "draft"; 267 if (code == InventoryReportStatus.REQUESTED) 268 return "requested"; 269 if (code == InventoryReportStatus.ACTIVE) 270 return "active"; 271 if (code == InventoryReportStatus.ENTEREDINERROR) 272 return "entered-in-error"; 273 return "?"; 274 } 275 public String toSystem(InventoryReportStatus code) { 276 return code.getSystem(); 277 } 278 } 279 280 @Block() 281 public static class InventoryReportInventoryListingComponent extends BackboneElement implements IBaseBackboneElement { 282 /** 283 * Location of the inventory items. 284 */ 285 @Child(name = "location", type = {Location.class}, order=1, min=0, max=1, modifier=false, summary=false) 286 @Description(shortDefinition="Location of the inventory items", formalDefinition="Location of the inventory items." ) 287 protected Reference location; 288 289 /** 290 * The status of the items. 291 */ 292 @Child(name = "itemStatus", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 293 @Description(shortDefinition="The status of the items that are being reported", formalDefinition="The status of the items." ) 294 protected CodeableConcept itemStatus; 295 296 /** 297 * The date and time when the items were counted. 298 */ 299 @Child(name = "countingDateTime", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 300 @Description(shortDefinition="The date and time when the items were counted", formalDefinition="The date and time when the items were counted." ) 301 protected DateTimeType countingDateTime; 302 303 /** 304 * The item or items in this listing. 305 */ 306 @Child(name = "item", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 307 @Description(shortDefinition="The item or items in this listing", formalDefinition="The item or items in this listing." ) 308 protected List<InventoryReportInventoryListingItemComponent> item; 309 310 private static final long serialVersionUID = 1766136476L; 311 312 /** 313 * Constructor 314 */ 315 public InventoryReportInventoryListingComponent() { 316 super(); 317 } 318 319 /** 320 * @return {@link #location} (Location of the inventory items.) 321 */ 322 public Reference getLocation() { 323 if (this.location == null) 324 if (Configuration.errorOnAutoCreate()) 325 throw new Error("Attempt to auto-create InventoryReportInventoryListingComponent.location"); 326 else if (Configuration.doAutoCreate()) 327 this.location = new Reference(); // cc 328 return this.location; 329 } 330 331 public boolean hasLocation() { 332 return this.location != null && !this.location.isEmpty(); 333 } 334 335 /** 336 * @param value {@link #location} (Location of the inventory items.) 337 */ 338 public InventoryReportInventoryListingComponent setLocation(Reference value) { 339 this.location = value; 340 return this; 341 } 342 343 /** 344 * @return {@link #itemStatus} (The status of the items.) 345 */ 346 public CodeableConcept getItemStatus() { 347 if (this.itemStatus == null) 348 if (Configuration.errorOnAutoCreate()) 349 throw new Error("Attempt to auto-create InventoryReportInventoryListingComponent.itemStatus"); 350 else if (Configuration.doAutoCreate()) 351 this.itemStatus = new CodeableConcept(); // cc 352 return this.itemStatus; 353 } 354 355 public boolean hasItemStatus() { 356 return this.itemStatus != null && !this.itemStatus.isEmpty(); 357 } 358 359 /** 360 * @param value {@link #itemStatus} (The status of the items.) 361 */ 362 public InventoryReportInventoryListingComponent setItemStatus(CodeableConcept value) { 363 this.itemStatus = value; 364 return this; 365 } 366 367 /** 368 * @return {@link #countingDateTime} (The date and time when the items were counted.). This is the underlying object with id, value and extensions. The accessor "getCountingDateTime" gives direct access to the value 369 */ 370 public DateTimeType getCountingDateTimeElement() { 371 if (this.countingDateTime == null) 372 if (Configuration.errorOnAutoCreate()) 373 throw new Error("Attempt to auto-create InventoryReportInventoryListingComponent.countingDateTime"); 374 else if (Configuration.doAutoCreate()) 375 this.countingDateTime = new DateTimeType(); // bb 376 return this.countingDateTime; 377 } 378 379 public boolean hasCountingDateTimeElement() { 380 return this.countingDateTime != null && !this.countingDateTime.isEmpty(); 381 } 382 383 public boolean hasCountingDateTime() { 384 return this.countingDateTime != null && !this.countingDateTime.isEmpty(); 385 } 386 387 /** 388 * @param value {@link #countingDateTime} (The date and time when the items were counted.). This is the underlying object with id, value and extensions. The accessor "getCountingDateTime" gives direct access to the value 389 */ 390 public InventoryReportInventoryListingComponent setCountingDateTimeElement(DateTimeType value) { 391 this.countingDateTime = value; 392 return this; 393 } 394 395 /** 396 * @return The date and time when the items were counted. 397 */ 398 public Date getCountingDateTime() { 399 return this.countingDateTime == null ? null : this.countingDateTime.getValue(); 400 } 401 402 /** 403 * @param value The date and time when the items were counted. 404 */ 405 public InventoryReportInventoryListingComponent setCountingDateTime(Date value) { 406 if (value == null) 407 this.countingDateTime = null; 408 else { 409 if (this.countingDateTime == null) 410 this.countingDateTime = new DateTimeType(); 411 this.countingDateTime.setValue(value); 412 } 413 return this; 414 } 415 416 /** 417 * @return {@link #item} (The item or items in this listing.) 418 */ 419 public List<InventoryReportInventoryListingItemComponent> getItem() { 420 if (this.item == null) 421 this.item = new ArrayList<InventoryReportInventoryListingItemComponent>(); 422 return this.item; 423 } 424 425 /** 426 * @return Returns a reference to <code>this</code> for easy method chaining 427 */ 428 public InventoryReportInventoryListingComponent setItem(List<InventoryReportInventoryListingItemComponent> theItem) { 429 this.item = theItem; 430 return this; 431 } 432 433 public boolean hasItem() { 434 if (this.item == null) 435 return false; 436 for (InventoryReportInventoryListingItemComponent item : this.item) 437 if (!item.isEmpty()) 438 return true; 439 return false; 440 } 441 442 public InventoryReportInventoryListingItemComponent addItem() { //3 443 InventoryReportInventoryListingItemComponent t = new InventoryReportInventoryListingItemComponent(); 444 if (this.item == null) 445 this.item = new ArrayList<InventoryReportInventoryListingItemComponent>(); 446 this.item.add(t); 447 return t; 448 } 449 450 public InventoryReportInventoryListingComponent addItem(InventoryReportInventoryListingItemComponent t) { //3 451 if (t == null) 452 return this; 453 if (this.item == null) 454 this.item = new ArrayList<InventoryReportInventoryListingItemComponent>(); 455 this.item.add(t); 456 return this; 457 } 458 459 /** 460 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 461 */ 462 public InventoryReportInventoryListingItemComponent getItemFirstRep() { 463 if (getItem().isEmpty()) { 464 addItem(); 465 } 466 return getItem().get(0); 467 } 468 469 protected void listChildren(List<Property> children) { 470 super.listChildren(children); 471 children.add(new Property("location", "Reference(Location)", "Location of the inventory items.", 0, 1, location)); 472 children.add(new Property("itemStatus", "CodeableConcept", "The status of the items.", 0, 1, itemStatus)); 473 children.add(new Property("countingDateTime", "dateTime", "The date and time when the items were counted.", 0, 1, countingDateTime)); 474 children.add(new Property("item", "", "The item or items in this listing.", 0, java.lang.Integer.MAX_VALUE, item)); 475 } 476 477 @Override 478 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 479 switch (_hash) { 480 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "Location of the inventory items.", 0, 1, location); 481 case 1999789285: /*itemStatus*/ return new Property("itemStatus", "CodeableConcept", "The status of the items.", 0, 1, itemStatus); 482 case -2075203282: /*countingDateTime*/ return new Property("countingDateTime", "dateTime", "The date and time when the items were counted.", 0, 1, countingDateTime); 483 case 3242771: /*item*/ return new Property("item", "", "The item or items in this listing.", 0, java.lang.Integer.MAX_VALUE, item); 484 default: return super.getNamedProperty(_hash, _name, _checkValid); 485 } 486 487 } 488 489 @Override 490 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 491 switch (hash) { 492 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 493 case 1999789285: /*itemStatus*/ return this.itemStatus == null ? new Base[0] : new Base[] {this.itemStatus}; // CodeableConcept 494 case -2075203282: /*countingDateTime*/ return this.countingDateTime == null ? new Base[0] : new Base[] {this.countingDateTime}; // DateTimeType 495 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // InventoryReportInventoryListingItemComponent 496 default: return super.getProperty(hash, name, checkValid); 497 } 498 499 } 500 501 @Override 502 public Base setProperty(int hash, String name, Base value) throws FHIRException { 503 switch (hash) { 504 case 1901043637: // location 505 this.location = TypeConvertor.castToReference(value); // Reference 506 return value; 507 case 1999789285: // itemStatus 508 this.itemStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 509 return value; 510 case -2075203282: // countingDateTime 511 this.countingDateTime = TypeConvertor.castToDateTime(value); // DateTimeType 512 return value; 513 case 3242771: // item 514 this.getItem().add((InventoryReportInventoryListingItemComponent) value); // InventoryReportInventoryListingItemComponent 515 return value; 516 default: return super.setProperty(hash, name, value); 517 } 518 519 } 520 521 @Override 522 public Base setProperty(String name, Base value) throws FHIRException { 523 if (name.equals("location")) { 524 this.location = TypeConvertor.castToReference(value); // Reference 525 } else if (name.equals("itemStatus")) { 526 this.itemStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 527 } else if (name.equals("countingDateTime")) { 528 this.countingDateTime = TypeConvertor.castToDateTime(value); // DateTimeType 529 } else if (name.equals("item")) { 530 this.getItem().add((InventoryReportInventoryListingItemComponent) value); 531 } else 532 return super.setProperty(name, value); 533 return value; 534 } 535 536 @Override 537 public void removeChild(String name, Base value) throws FHIRException { 538 if (name.equals("location")) { 539 this.location = null; 540 } else if (name.equals("itemStatus")) { 541 this.itemStatus = null; 542 } else if (name.equals("countingDateTime")) { 543 this.countingDateTime = null; 544 } else if (name.equals("item")) { 545 this.getItem().remove((InventoryReportInventoryListingItemComponent) value); 546 } else 547 super.removeChild(name, value); 548 549 } 550 551 @Override 552 public Base makeProperty(int hash, String name) throws FHIRException { 553 switch (hash) { 554 case 1901043637: return getLocation(); 555 case 1999789285: return getItemStatus(); 556 case -2075203282: return getCountingDateTimeElement(); 557 case 3242771: return addItem(); 558 default: return super.makeProperty(hash, name); 559 } 560 561 } 562 563 @Override 564 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 565 switch (hash) { 566 case 1901043637: /*location*/ return new String[] {"Reference"}; 567 case 1999789285: /*itemStatus*/ return new String[] {"CodeableConcept"}; 568 case -2075203282: /*countingDateTime*/ return new String[] {"dateTime"}; 569 case 3242771: /*item*/ return new String[] {}; 570 default: return super.getTypesForProperty(hash, name); 571 } 572 573 } 574 575 @Override 576 public Base addChild(String name) throws FHIRException { 577 if (name.equals("location")) { 578 this.location = new Reference(); 579 return this.location; 580 } 581 else if (name.equals("itemStatus")) { 582 this.itemStatus = new CodeableConcept(); 583 return this.itemStatus; 584 } 585 else if (name.equals("countingDateTime")) { 586 throw new FHIRException("Cannot call addChild on a singleton property InventoryReport.inventoryListing.countingDateTime"); 587 } 588 else if (name.equals("item")) { 589 return addItem(); 590 } 591 else 592 return super.addChild(name); 593 } 594 595 public InventoryReportInventoryListingComponent copy() { 596 InventoryReportInventoryListingComponent dst = new InventoryReportInventoryListingComponent(); 597 copyValues(dst); 598 return dst; 599 } 600 601 public void copyValues(InventoryReportInventoryListingComponent dst) { 602 super.copyValues(dst); 603 dst.location = location == null ? null : location.copy(); 604 dst.itemStatus = itemStatus == null ? null : itemStatus.copy(); 605 dst.countingDateTime = countingDateTime == null ? null : countingDateTime.copy(); 606 if (item != null) { 607 dst.item = new ArrayList<InventoryReportInventoryListingItemComponent>(); 608 for (InventoryReportInventoryListingItemComponent i : item) 609 dst.item.add(i.copy()); 610 }; 611 } 612 613 @Override 614 public boolean equalsDeep(Base other_) { 615 if (!super.equalsDeep(other_)) 616 return false; 617 if (!(other_ instanceof InventoryReportInventoryListingComponent)) 618 return false; 619 InventoryReportInventoryListingComponent o = (InventoryReportInventoryListingComponent) other_; 620 return compareDeep(location, o.location, true) && compareDeep(itemStatus, o.itemStatus, true) && compareDeep(countingDateTime, o.countingDateTime, true) 621 && compareDeep(item, o.item, true); 622 } 623 624 @Override 625 public boolean equalsShallow(Base other_) { 626 if (!super.equalsShallow(other_)) 627 return false; 628 if (!(other_ instanceof InventoryReportInventoryListingComponent)) 629 return false; 630 InventoryReportInventoryListingComponent o = (InventoryReportInventoryListingComponent) other_; 631 return compareValues(countingDateTime, o.countingDateTime, true); 632 } 633 634 public boolean isEmpty() { 635 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(location, itemStatus, countingDateTime 636 , item); 637 } 638 639 public String fhirType() { 640 return "InventoryReport.inventoryListing"; 641 642 } 643 644 } 645 646 @Block() 647 public static class InventoryReportInventoryListingItemComponent extends BackboneElement implements IBaseBackboneElement { 648 /** 649 * The inventory category or classification of the items being reported. This is meant not for defining the product, but for inventory categories e.g. 'pending recount' or 'damaged'. 650 */ 651 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 652 @Description(shortDefinition="The inventory category or classification of the items being reported", formalDefinition="The inventory category or classification of the items being reported. This is meant not for defining the product, but for inventory categories e.g. 'pending recount' or 'damaged'." ) 653 protected CodeableConcept category; 654 655 /** 656 * The quantity of the item or items being reported. 657 */ 658 @Child(name = "quantity", type = {Quantity.class}, order=2, min=1, max=1, modifier=false, summary=true) 659 @Description(shortDefinition="The quantity of the item or items being reported", formalDefinition="The quantity of the item or items being reported." ) 660 protected Quantity quantity; 661 662 /** 663 * The code or reference to the item type. 664 */ 665 @Child(name = "item", type = {CodeableReference.class}, order=3, min=1, max=1, modifier=false, summary=true) 666 @Description(shortDefinition="The code or reference to the item type", formalDefinition="The code or reference to the item type." ) 667 protected CodeableReference item; 668 669 private static final long serialVersionUID = 566223460L; 670 671 /** 672 * Constructor 673 */ 674 public InventoryReportInventoryListingItemComponent() { 675 super(); 676 } 677 678 /** 679 * Constructor 680 */ 681 public InventoryReportInventoryListingItemComponent(Quantity quantity, CodeableReference item) { 682 super(); 683 this.setQuantity(quantity); 684 this.setItem(item); 685 } 686 687 /** 688 * @return {@link #category} (The inventory category or classification of the items being reported. This is meant not for defining the product, but for inventory categories e.g. 'pending recount' or 'damaged'.) 689 */ 690 public CodeableConcept getCategory() { 691 if (this.category == null) 692 if (Configuration.errorOnAutoCreate()) 693 throw new Error("Attempt to auto-create InventoryReportInventoryListingItemComponent.category"); 694 else if (Configuration.doAutoCreate()) 695 this.category = new CodeableConcept(); // cc 696 return this.category; 697 } 698 699 public boolean hasCategory() { 700 return this.category != null && !this.category.isEmpty(); 701 } 702 703 /** 704 * @param value {@link #category} (The inventory category or classification of the items being reported. This is meant not for defining the product, but for inventory categories e.g. 'pending recount' or 'damaged'.) 705 */ 706 public InventoryReportInventoryListingItemComponent setCategory(CodeableConcept value) { 707 this.category = value; 708 return this; 709 } 710 711 /** 712 * @return {@link #quantity} (The quantity of the item or items being reported.) 713 */ 714 public Quantity getQuantity() { 715 if (this.quantity == null) 716 if (Configuration.errorOnAutoCreate()) 717 throw new Error("Attempt to auto-create InventoryReportInventoryListingItemComponent.quantity"); 718 else if (Configuration.doAutoCreate()) 719 this.quantity = new Quantity(); // cc 720 return this.quantity; 721 } 722 723 public boolean hasQuantity() { 724 return this.quantity != null && !this.quantity.isEmpty(); 725 } 726 727 /** 728 * @param value {@link #quantity} (The quantity of the item or items being reported.) 729 */ 730 public InventoryReportInventoryListingItemComponent setQuantity(Quantity value) { 731 this.quantity = value; 732 return this; 733 } 734 735 /** 736 * @return {@link #item} (The code or reference to the item type.) 737 */ 738 public CodeableReference getItem() { 739 if (this.item == null) 740 if (Configuration.errorOnAutoCreate()) 741 throw new Error("Attempt to auto-create InventoryReportInventoryListingItemComponent.item"); 742 else if (Configuration.doAutoCreate()) 743 this.item = new CodeableReference(); // cc 744 return this.item; 745 } 746 747 public boolean hasItem() { 748 return this.item != null && !this.item.isEmpty(); 749 } 750 751 /** 752 * @param value {@link #item} (The code or reference to the item type.) 753 */ 754 public InventoryReportInventoryListingItemComponent setItem(CodeableReference value) { 755 this.item = value; 756 return this; 757 } 758 759 protected void listChildren(List<Property> children) { 760 super.listChildren(children); 761 children.add(new Property("category", "CodeableConcept", "The inventory category or classification of the items being reported. This is meant not for defining the product, but for inventory categories e.g. 'pending recount' or 'damaged'.", 0, 1, category)); 762 children.add(new Property("quantity", "Quantity", "The quantity of the item or items being reported.", 0, 1, quantity)); 763 children.add(new Property("item", "CodeableReference(Medication|Device|Medication|NutritionProduct|InventoryItem|BiologicallyDerivedProduct|InventoryItem)", "The code or reference to the item type.", 0, 1, item)); 764 } 765 766 @Override 767 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 768 switch (_hash) { 769 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The inventory category or classification of the items being reported. This is meant not for defining the product, but for inventory categories e.g. 'pending recount' or 'damaged'.", 0, 1, category); 770 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The quantity of the item or items being reported.", 0, 1, quantity); 771 case 3242771: /*item*/ return new Property("item", "CodeableReference(Medication|Device|Medication|NutritionProduct|InventoryItem|BiologicallyDerivedProduct|InventoryItem)", "The code or reference to the item type.", 0, 1, item); 772 default: return super.getNamedProperty(_hash, _name, _checkValid); 773 } 774 775 } 776 777 @Override 778 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 779 switch (hash) { 780 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 781 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 782 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // CodeableReference 783 default: return super.getProperty(hash, name, checkValid); 784 } 785 786 } 787 788 @Override 789 public Base setProperty(int hash, String name, Base value) throws FHIRException { 790 switch (hash) { 791 case 50511102: // category 792 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 793 return value; 794 case -1285004149: // quantity 795 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 796 return value; 797 case 3242771: // item 798 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 799 return value; 800 default: return super.setProperty(hash, name, value); 801 } 802 803 } 804 805 @Override 806 public Base setProperty(String name, Base value) throws FHIRException { 807 if (name.equals("category")) { 808 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 809 } else if (name.equals("quantity")) { 810 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 811 } else if (name.equals("item")) { 812 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 813 } else 814 return super.setProperty(name, value); 815 return value; 816 } 817 818 @Override 819 public void removeChild(String name, Base value) throws FHIRException { 820 if (name.equals("category")) { 821 this.category = null; 822 } else if (name.equals("quantity")) { 823 this.quantity = null; 824 } else if (name.equals("item")) { 825 this.item = null; 826 } else 827 super.removeChild(name, value); 828 829 } 830 831 @Override 832 public Base makeProperty(int hash, String name) throws FHIRException { 833 switch (hash) { 834 case 50511102: return getCategory(); 835 case -1285004149: return getQuantity(); 836 case 3242771: return getItem(); 837 default: return super.makeProperty(hash, name); 838 } 839 840 } 841 842 @Override 843 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 844 switch (hash) { 845 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 846 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 847 case 3242771: /*item*/ return new String[] {"CodeableReference"}; 848 default: return super.getTypesForProperty(hash, name); 849 } 850 851 } 852 853 @Override 854 public Base addChild(String name) throws FHIRException { 855 if (name.equals("category")) { 856 this.category = new CodeableConcept(); 857 return this.category; 858 } 859 else if (name.equals("quantity")) { 860 this.quantity = new Quantity(); 861 return this.quantity; 862 } 863 else if (name.equals("item")) { 864 this.item = new CodeableReference(); 865 return this.item; 866 } 867 else 868 return super.addChild(name); 869 } 870 871 public InventoryReportInventoryListingItemComponent copy() { 872 InventoryReportInventoryListingItemComponent dst = new InventoryReportInventoryListingItemComponent(); 873 copyValues(dst); 874 return dst; 875 } 876 877 public void copyValues(InventoryReportInventoryListingItemComponent dst) { 878 super.copyValues(dst); 879 dst.category = category == null ? null : category.copy(); 880 dst.quantity = quantity == null ? null : quantity.copy(); 881 dst.item = item == null ? null : item.copy(); 882 } 883 884 @Override 885 public boolean equalsDeep(Base other_) { 886 if (!super.equalsDeep(other_)) 887 return false; 888 if (!(other_ instanceof InventoryReportInventoryListingItemComponent)) 889 return false; 890 InventoryReportInventoryListingItemComponent o = (InventoryReportInventoryListingItemComponent) other_; 891 return compareDeep(category, o.category, true) && compareDeep(quantity, o.quantity, true) && compareDeep(item, o.item, true) 892 ; 893 } 894 895 @Override 896 public boolean equalsShallow(Base other_) { 897 if (!super.equalsShallow(other_)) 898 return false; 899 if (!(other_ instanceof InventoryReportInventoryListingItemComponent)) 900 return false; 901 InventoryReportInventoryListingItemComponent o = (InventoryReportInventoryListingItemComponent) other_; 902 return true; 903 } 904 905 public boolean isEmpty() { 906 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, quantity, item 907 ); 908 } 909 910 public String fhirType() { 911 return "InventoryReport.inventoryListing.item"; 912 913 } 914 915 } 916 917 /** 918 * Business identifier for the InventoryReport. 919 */ 920 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 921 @Description(shortDefinition="Business identifier for the report", formalDefinition="Business identifier for the InventoryReport." ) 922 protected List<Identifier> identifier; 923 924 /** 925 * The status of the inventory check or notification - whether this is draft (e.g. the report is still pending some updates) or active. 926 */ 927 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 928 @Description(shortDefinition="draft | requested | active | entered-in-error", formalDefinition="The status of the inventory check or notification - whether this is draft (e.g. the report is still pending some updates) or active." ) 929 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/inventoryreport-status") 930 protected Enumeration<InventoryReportStatus> status; 931 932 /** 933 * Whether the report is about the current inventory count (snapshot) or a differential change in inventory (change). 934 */ 935 @Child(name = "countType", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 936 @Description(shortDefinition="snapshot | difference", formalDefinition="Whether the report is about the current inventory count (snapshot) or a differential change in inventory (change)." ) 937 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/inventoryreport-counttype") 938 protected Enumeration<InventoryCountType> countType; 939 940 /** 941 * What type of operation is being performed - addition or subtraction. 942 */ 943 @Child(name = "operationType", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 944 @Description(shortDefinition="addition | subtraction", formalDefinition="What type of operation is being performed - addition or subtraction." ) 945 protected CodeableConcept operationType; 946 947 /** 948 * The reason for this count - regular count, ad-hoc count, new arrivals, etc. 949 */ 950 @Child(name = "operationTypeReason", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 951 @Description(shortDefinition="The reason for this count - regular count, ad-hoc count, new arrivals, etc", formalDefinition="The reason for this count - regular count, ad-hoc count, new arrivals, etc." ) 952 protected CodeableConcept operationTypeReason; 953 954 /** 955 * When the report has been submitted. 956 */ 957 @Child(name = "reportedDateTime", type = {DateTimeType.class}, order=5, min=1, max=1, modifier=false, summary=true) 958 @Description(shortDefinition="When the report has been submitted", formalDefinition="When the report has been submitted." ) 959 protected DateTimeType reportedDateTime; 960 961 /** 962 * Who submits the report. 963 */ 964 @Child(name = "reporter", type = {Practitioner.class, Patient.class, RelatedPerson.class, Device.class}, order=6, min=0, max=1, modifier=false, summary=false) 965 @Description(shortDefinition="Who submits the report", formalDefinition="Who submits the report." ) 966 protected Reference reporter; 967 968 /** 969 * The period the report refers to. 970 */ 971 @Child(name = "reportingPeriod", type = {Period.class}, order=7, min=0, max=1, modifier=false, summary=false) 972 @Description(shortDefinition="The period the report refers to", formalDefinition="The period the report refers to." ) 973 protected Period reportingPeriod; 974 975 /** 976 * An inventory listing section (grouped by any of the attributes). 977 */ 978 @Child(name = "inventoryListing", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 979 @Description(shortDefinition="An inventory listing section (grouped by any of the attributes)", formalDefinition="An inventory listing section (grouped by any of the attributes)." ) 980 protected List<InventoryReportInventoryListingComponent> inventoryListing; 981 982 /** 983 * A note associated with the InventoryReport. 984 */ 985 @Child(name = "note", type = {Annotation.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 986 @Description(shortDefinition="A note associated with the InventoryReport", formalDefinition="A note associated with the InventoryReport." ) 987 protected List<Annotation> note; 988 989 private static final long serialVersionUID = 539262671L; 990 991 /** 992 * Constructor 993 */ 994 public InventoryReport() { 995 super(); 996 } 997 998 /** 999 * Constructor 1000 */ 1001 public InventoryReport(InventoryReportStatus status, InventoryCountType countType, Date reportedDateTime) { 1002 super(); 1003 this.setStatus(status); 1004 this.setCountType(countType); 1005 this.setReportedDateTime(reportedDateTime); 1006 } 1007 1008 /** 1009 * @return {@link #identifier} (Business identifier for the InventoryReport.) 1010 */ 1011 public List<Identifier> getIdentifier() { 1012 if (this.identifier == null) 1013 this.identifier = new ArrayList<Identifier>(); 1014 return this.identifier; 1015 } 1016 1017 /** 1018 * @return Returns a reference to <code>this</code> for easy method chaining 1019 */ 1020 public InventoryReport setIdentifier(List<Identifier> theIdentifier) { 1021 this.identifier = theIdentifier; 1022 return this; 1023 } 1024 1025 public boolean hasIdentifier() { 1026 if (this.identifier == null) 1027 return false; 1028 for (Identifier item : this.identifier) 1029 if (!item.isEmpty()) 1030 return true; 1031 return false; 1032 } 1033 1034 public Identifier addIdentifier() { //3 1035 Identifier t = new Identifier(); 1036 if (this.identifier == null) 1037 this.identifier = new ArrayList<Identifier>(); 1038 this.identifier.add(t); 1039 return t; 1040 } 1041 1042 public InventoryReport addIdentifier(Identifier t) { //3 1043 if (t == null) 1044 return this; 1045 if (this.identifier == null) 1046 this.identifier = new ArrayList<Identifier>(); 1047 this.identifier.add(t); 1048 return this; 1049 } 1050 1051 /** 1052 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1053 */ 1054 public Identifier getIdentifierFirstRep() { 1055 if (getIdentifier().isEmpty()) { 1056 addIdentifier(); 1057 } 1058 return getIdentifier().get(0); 1059 } 1060 1061 /** 1062 * @return {@link #status} (The status of the inventory check or notification - whether this is draft (e.g. the report is still pending some updates) or active.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1063 */ 1064 public Enumeration<InventoryReportStatus> getStatusElement() { 1065 if (this.status == null) 1066 if (Configuration.errorOnAutoCreate()) 1067 throw new Error("Attempt to auto-create InventoryReport.status"); 1068 else if (Configuration.doAutoCreate()) 1069 this.status = new Enumeration<InventoryReportStatus>(new InventoryReportStatusEnumFactory()); // bb 1070 return this.status; 1071 } 1072 1073 public boolean hasStatusElement() { 1074 return this.status != null && !this.status.isEmpty(); 1075 } 1076 1077 public boolean hasStatus() { 1078 return this.status != null && !this.status.isEmpty(); 1079 } 1080 1081 /** 1082 * @param value {@link #status} (The status of the inventory check or notification - whether this is draft (e.g. the report is still pending some updates) or active.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1083 */ 1084 public InventoryReport setStatusElement(Enumeration<InventoryReportStatus> value) { 1085 this.status = value; 1086 return this; 1087 } 1088 1089 /** 1090 * @return The status of the inventory check or notification - whether this is draft (e.g. the report is still pending some updates) or active. 1091 */ 1092 public InventoryReportStatus getStatus() { 1093 return this.status == null ? null : this.status.getValue(); 1094 } 1095 1096 /** 1097 * @param value The status of the inventory check or notification - whether this is draft (e.g. the report is still pending some updates) or active. 1098 */ 1099 public InventoryReport setStatus(InventoryReportStatus value) { 1100 if (this.status == null) 1101 this.status = new Enumeration<InventoryReportStatus>(new InventoryReportStatusEnumFactory()); 1102 this.status.setValue(value); 1103 return this; 1104 } 1105 1106 /** 1107 * @return {@link #countType} (Whether the report is about the current inventory count (snapshot) or a differential change in inventory (change).). This is the underlying object with id, value and extensions. The accessor "getCountType" gives direct access to the value 1108 */ 1109 public Enumeration<InventoryCountType> getCountTypeElement() { 1110 if (this.countType == null) 1111 if (Configuration.errorOnAutoCreate()) 1112 throw new Error("Attempt to auto-create InventoryReport.countType"); 1113 else if (Configuration.doAutoCreate()) 1114 this.countType = new Enumeration<InventoryCountType>(new InventoryCountTypeEnumFactory()); // bb 1115 return this.countType; 1116 } 1117 1118 public boolean hasCountTypeElement() { 1119 return this.countType != null && !this.countType.isEmpty(); 1120 } 1121 1122 public boolean hasCountType() { 1123 return this.countType != null && !this.countType.isEmpty(); 1124 } 1125 1126 /** 1127 * @param value {@link #countType} (Whether the report is about the current inventory count (snapshot) or a differential change in inventory (change).). This is the underlying object with id, value and extensions. The accessor "getCountType" gives direct access to the value 1128 */ 1129 public InventoryReport setCountTypeElement(Enumeration<InventoryCountType> value) { 1130 this.countType = value; 1131 return this; 1132 } 1133 1134 /** 1135 * @return Whether the report is about the current inventory count (snapshot) or a differential change in inventory (change). 1136 */ 1137 public InventoryCountType getCountType() { 1138 return this.countType == null ? null : this.countType.getValue(); 1139 } 1140 1141 /** 1142 * @param value Whether the report is about the current inventory count (snapshot) or a differential change in inventory (change). 1143 */ 1144 public InventoryReport setCountType(InventoryCountType value) { 1145 if (this.countType == null) 1146 this.countType = new Enumeration<InventoryCountType>(new InventoryCountTypeEnumFactory()); 1147 this.countType.setValue(value); 1148 return this; 1149 } 1150 1151 /** 1152 * @return {@link #operationType} (What type of operation is being performed - addition or subtraction.) 1153 */ 1154 public CodeableConcept getOperationType() { 1155 if (this.operationType == null) 1156 if (Configuration.errorOnAutoCreate()) 1157 throw new Error("Attempt to auto-create InventoryReport.operationType"); 1158 else if (Configuration.doAutoCreate()) 1159 this.operationType = new CodeableConcept(); // cc 1160 return this.operationType; 1161 } 1162 1163 public boolean hasOperationType() { 1164 return this.operationType != null && !this.operationType.isEmpty(); 1165 } 1166 1167 /** 1168 * @param value {@link #operationType} (What type of operation is being performed - addition or subtraction.) 1169 */ 1170 public InventoryReport setOperationType(CodeableConcept value) { 1171 this.operationType = value; 1172 return this; 1173 } 1174 1175 /** 1176 * @return {@link #operationTypeReason} (The reason for this count - regular count, ad-hoc count, new arrivals, etc.) 1177 */ 1178 public CodeableConcept getOperationTypeReason() { 1179 if (this.operationTypeReason == null) 1180 if (Configuration.errorOnAutoCreate()) 1181 throw new Error("Attempt to auto-create InventoryReport.operationTypeReason"); 1182 else if (Configuration.doAutoCreate()) 1183 this.operationTypeReason = new CodeableConcept(); // cc 1184 return this.operationTypeReason; 1185 } 1186 1187 public boolean hasOperationTypeReason() { 1188 return this.operationTypeReason != null && !this.operationTypeReason.isEmpty(); 1189 } 1190 1191 /** 1192 * @param value {@link #operationTypeReason} (The reason for this count - regular count, ad-hoc count, new arrivals, etc.) 1193 */ 1194 public InventoryReport setOperationTypeReason(CodeableConcept value) { 1195 this.operationTypeReason = value; 1196 return this; 1197 } 1198 1199 /** 1200 * @return {@link #reportedDateTime} (When the report has been submitted.). This is the underlying object with id, value and extensions. The accessor "getReportedDateTime" gives direct access to the value 1201 */ 1202 public DateTimeType getReportedDateTimeElement() { 1203 if (this.reportedDateTime == null) 1204 if (Configuration.errorOnAutoCreate()) 1205 throw new Error("Attempt to auto-create InventoryReport.reportedDateTime"); 1206 else if (Configuration.doAutoCreate()) 1207 this.reportedDateTime = new DateTimeType(); // bb 1208 return this.reportedDateTime; 1209 } 1210 1211 public boolean hasReportedDateTimeElement() { 1212 return this.reportedDateTime != null && !this.reportedDateTime.isEmpty(); 1213 } 1214 1215 public boolean hasReportedDateTime() { 1216 return this.reportedDateTime != null && !this.reportedDateTime.isEmpty(); 1217 } 1218 1219 /** 1220 * @param value {@link #reportedDateTime} (When the report has been submitted.). This is the underlying object with id, value and extensions. The accessor "getReportedDateTime" gives direct access to the value 1221 */ 1222 public InventoryReport setReportedDateTimeElement(DateTimeType value) { 1223 this.reportedDateTime = value; 1224 return this; 1225 } 1226 1227 /** 1228 * @return When the report has been submitted. 1229 */ 1230 public Date getReportedDateTime() { 1231 return this.reportedDateTime == null ? null : this.reportedDateTime.getValue(); 1232 } 1233 1234 /** 1235 * @param value When the report has been submitted. 1236 */ 1237 public InventoryReport setReportedDateTime(Date value) { 1238 if (this.reportedDateTime == null) 1239 this.reportedDateTime = new DateTimeType(); 1240 this.reportedDateTime.setValue(value); 1241 return this; 1242 } 1243 1244 /** 1245 * @return {@link #reporter} (Who submits the report.) 1246 */ 1247 public Reference getReporter() { 1248 if (this.reporter == null) 1249 if (Configuration.errorOnAutoCreate()) 1250 throw new Error("Attempt to auto-create InventoryReport.reporter"); 1251 else if (Configuration.doAutoCreate()) 1252 this.reporter = new Reference(); // cc 1253 return this.reporter; 1254 } 1255 1256 public boolean hasReporter() { 1257 return this.reporter != null && !this.reporter.isEmpty(); 1258 } 1259 1260 /** 1261 * @param value {@link #reporter} (Who submits the report.) 1262 */ 1263 public InventoryReport setReporter(Reference value) { 1264 this.reporter = value; 1265 return this; 1266 } 1267 1268 /** 1269 * @return {@link #reportingPeriod} (The period the report refers to.) 1270 */ 1271 public Period getReportingPeriod() { 1272 if (this.reportingPeriod == null) 1273 if (Configuration.errorOnAutoCreate()) 1274 throw new Error("Attempt to auto-create InventoryReport.reportingPeriod"); 1275 else if (Configuration.doAutoCreate()) 1276 this.reportingPeriod = new Period(); // cc 1277 return this.reportingPeriod; 1278 } 1279 1280 public boolean hasReportingPeriod() { 1281 return this.reportingPeriod != null && !this.reportingPeriod.isEmpty(); 1282 } 1283 1284 /** 1285 * @param value {@link #reportingPeriod} (The period the report refers to.) 1286 */ 1287 public InventoryReport setReportingPeriod(Period value) { 1288 this.reportingPeriod = value; 1289 return this; 1290 } 1291 1292 /** 1293 * @return {@link #inventoryListing} (An inventory listing section (grouped by any of the attributes).) 1294 */ 1295 public List<InventoryReportInventoryListingComponent> getInventoryListing() { 1296 if (this.inventoryListing == null) 1297 this.inventoryListing = new ArrayList<InventoryReportInventoryListingComponent>(); 1298 return this.inventoryListing; 1299 } 1300 1301 /** 1302 * @return Returns a reference to <code>this</code> for easy method chaining 1303 */ 1304 public InventoryReport setInventoryListing(List<InventoryReportInventoryListingComponent> theInventoryListing) { 1305 this.inventoryListing = theInventoryListing; 1306 return this; 1307 } 1308 1309 public boolean hasInventoryListing() { 1310 if (this.inventoryListing == null) 1311 return false; 1312 for (InventoryReportInventoryListingComponent item : this.inventoryListing) 1313 if (!item.isEmpty()) 1314 return true; 1315 return false; 1316 } 1317 1318 public InventoryReportInventoryListingComponent addInventoryListing() { //3 1319 InventoryReportInventoryListingComponent t = new InventoryReportInventoryListingComponent(); 1320 if (this.inventoryListing == null) 1321 this.inventoryListing = new ArrayList<InventoryReportInventoryListingComponent>(); 1322 this.inventoryListing.add(t); 1323 return t; 1324 } 1325 1326 public InventoryReport addInventoryListing(InventoryReportInventoryListingComponent t) { //3 1327 if (t == null) 1328 return this; 1329 if (this.inventoryListing == null) 1330 this.inventoryListing = new ArrayList<InventoryReportInventoryListingComponent>(); 1331 this.inventoryListing.add(t); 1332 return this; 1333 } 1334 1335 /** 1336 * @return The first repetition of repeating field {@link #inventoryListing}, creating it if it does not already exist {3} 1337 */ 1338 public InventoryReportInventoryListingComponent getInventoryListingFirstRep() { 1339 if (getInventoryListing().isEmpty()) { 1340 addInventoryListing(); 1341 } 1342 return getInventoryListing().get(0); 1343 } 1344 1345 /** 1346 * @return {@link #note} (A note associated with the InventoryReport.) 1347 */ 1348 public List<Annotation> getNote() { 1349 if (this.note == null) 1350 this.note = new ArrayList<Annotation>(); 1351 return this.note; 1352 } 1353 1354 /** 1355 * @return Returns a reference to <code>this</code> for easy method chaining 1356 */ 1357 public InventoryReport setNote(List<Annotation> theNote) { 1358 this.note = theNote; 1359 return this; 1360 } 1361 1362 public boolean hasNote() { 1363 if (this.note == null) 1364 return false; 1365 for (Annotation item : this.note) 1366 if (!item.isEmpty()) 1367 return true; 1368 return false; 1369 } 1370 1371 public Annotation addNote() { //3 1372 Annotation t = new Annotation(); 1373 if (this.note == null) 1374 this.note = new ArrayList<Annotation>(); 1375 this.note.add(t); 1376 return t; 1377 } 1378 1379 public InventoryReport addNote(Annotation t) { //3 1380 if (t == null) 1381 return this; 1382 if (this.note == null) 1383 this.note = new ArrayList<Annotation>(); 1384 this.note.add(t); 1385 return this; 1386 } 1387 1388 /** 1389 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1390 */ 1391 public Annotation getNoteFirstRep() { 1392 if (getNote().isEmpty()) { 1393 addNote(); 1394 } 1395 return getNote().get(0); 1396 } 1397 1398 protected void listChildren(List<Property> children) { 1399 super.listChildren(children); 1400 children.add(new Property("identifier", "Identifier", "Business identifier for the InventoryReport.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1401 children.add(new Property("status", "code", "The status of the inventory check or notification - whether this is draft (e.g. the report is still pending some updates) or active.", 0, 1, status)); 1402 children.add(new Property("countType", "code", "Whether the report is about the current inventory count (snapshot) or a differential change in inventory (change).", 0, 1, countType)); 1403 children.add(new Property("operationType", "CodeableConcept", "What type of operation is being performed - addition or subtraction.", 0, 1, operationType)); 1404 children.add(new Property("operationTypeReason", "CodeableConcept", "The reason for this count - regular count, ad-hoc count, new arrivals, etc.", 0, 1, operationTypeReason)); 1405 children.add(new Property("reportedDateTime", "dateTime", "When the report has been submitted.", 0, 1, reportedDateTime)); 1406 children.add(new Property("reporter", "Reference(Practitioner|Patient|RelatedPerson|Device)", "Who submits the report.", 0, 1, reporter)); 1407 children.add(new Property("reportingPeriod", "Period", "The period the report refers to.", 0, 1, reportingPeriod)); 1408 children.add(new Property("inventoryListing", "", "An inventory listing section (grouped by any of the attributes).", 0, java.lang.Integer.MAX_VALUE, inventoryListing)); 1409 children.add(new Property("note", "Annotation", "A note associated with the InventoryReport.", 0, java.lang.Integer.MAX_VALUE, note)); 1410 } 1411 1412 @Override 1413 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1414 switch (_hash) { 1415 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier for the InventoryReport.", 0, java.lang.Integer.MAX_VALUE, identifier); 1416 case -892481550: /*status*/ return new Property("status", "code", "The status of the inventory check or notification - whether this is draft (e.g. the report is still pending some updates) or active.", 0, 1, status); 1417 case 1351759081: /*countType*/ return new Property("countType", "code", "Whether the report is about the current inventory count (snapshot) or a differential change in inventory (change).", 0, 1, countType); 1418 case 91999553: /*operationType*/ return new Property("operationType", "CodeableConcept", "What type of operation is being performed - addition or subtraction.", 0, 1, operationType); 1419 case 449681125: /*operationTypeReason*/ return new Property("operationTypeReason", "CodeableConcept", "The reason for this count - regular count, ad-hoc count, new arrivals, etc.", 0, 1, operationTypeReason); 1420 case -1048250994: /*reportedDateTime*/ return new Property("reportedDateTime", "dateTime", "When the report has been submitted.", 0, 1, reportedDateTime); 1421 case -427039519: /*reporter*/ return new Property("reporter", "Reference(Practitioner|Patient|RelatedPerson|Device)", "Who submits the report.", 0, 1, reporter); 1422 case 409685391: /*reportingPeriod*/ return new Property("reportingPeriod", "Period", "The period the report refers to.", 0, 1, reportingPeriod); 1423 case -1764804216: /*inventoryListing*/ return new Property("inventoryListing", "", "An inventory listing section (grouped by any of the attributes).", 0, java.lang.Integer.MAX_VALUE, inventoryListing); 1424 case 3387378: /*note*/ return new Property("note", "Annotation", "A note associated with the InventoryReport.", 0, java.lang.Integer.MAX_VALUE, note); 1425 default: return super.getNamedProperty(_hash, _name, _checkValid); 1426 } 1427 1428 } 1429 1430 @Override 1431 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1432 switch (hash) { 1433 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1434 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<InventoryReportStatus> 1435 case 1351759081: /*countType*/ return this.countType == null ? new Base[0] : new Base[] {this.countType}; // Enumeration<InventoryCountType> 1436 case 91999553: /*operationType*/ return this.operationType == null ? new Base[0] : new Base[] {this.operationType}; // CodeableConcept 1437 case 449681125: /*operationTypeReason*/ return this.operationTypeReason == null ? new Base[0] : new Base[] {this.operationTypeReason}; // CodeableConcept 1438 case -1048250994: /*reportedDateTime*/ return this.reportedDateTime == null ? new Base[0] : new Base[] {this.reportedDateTime}; // DateTimeType 1439 case -427039519: /*reporter*/ return this.reporter == null ? new Base[0] : new Base[] {this.reporter}; // Reference 1440 case 409685391: /*reportingPeriod*/ return this.reportingPeriod == null ? new Base[0] : new Base[] {this.reportingPeriod}; // Period 1441 case -1764804216: /*inventoryListing*/ return this.inventoryListing == null ? new Base[0] : this.inventoryListing.toArray(new Base[this.inventoryListing.size()]); // InventoryReportInventoryListingComponent 1442 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1443 default: return super.getProperty(hash, name, checkValid); 1444 } 1445 1446 } 1447 1448 @Override 1449 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1450 switch (hash) { 1451 case -1618432855: // identifier 1452 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1453 return value; 1454 case -892481550: // status 1455 value = new InventoryReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1456 this.status = (Enumeration) value; // Enumeration<InventoryReportStatus> 1457 return value; 1458 case 1351759081: // countType 1459 value = new InventoryCountTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1460 this.countType = (Enumeration) value; // Enumeration<InventoryCountType> 1461 return value; 1462 case 91999553: // operationType 1463 this.operationType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1464 return value; 1465 case 449681125: // operationTypeReason 1466 this.operationTypeReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1467 return value; 1468 case -1048250994: // reportedDateTime 1469 this.reportedDateTime = TypeConvertor.castToDateTime(value); // DateTimeType 1470 return value; 1471 case -427039519: // reporter 1472 this.reporter = TypeConvertor.castToReference(value); // Reference 1473 return value; 1474 case 409685391: // reportingPeriod 1475 this.reportingPeriod = TypeConvertor.castToPeriod(value); // Period 1476 return value; 1477 case -1764804216: // inventoryListing 1478 this.getInventoryListing().add((InventoryReportInventoryListingComponent) value); // InventoryReportInventoryListingComponent 1479 return value; 1480 case 3387378: // note 1481 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1482 return value; 1483 default: return super.setProperty(hash, name, value); 1484 } 1485 1486 } 1487 1488 @Override 1489 public Base setProperty(String name, Base value) throws FHIRException { 1490 if (name.equals("identifier")) { 1491 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1492 } else if (name.equals("status")) { 1493 value = new InventoryReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1494 this.status = (Enumeration) value; // Enumeration<InventoryReportStatus> 1495 } else if (name.equals("countType")) { 1496 value = new InventoryCountTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1497 this.countType = (Enumeration) value; // Enumeration<InventoryCountType> 1498 } else if (name.equals("operationType")) { 1499 this.operationType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1500 } else if (name.equals("operationTypeReason")) { 1501 this.operationTypeReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1502 } else if (name.equals("reportedDateTime")) { 1503 this.reportedDateTime = TypeConvertor.castToDateTime(value); // DateTimeType 1504 } else if (name.equals("reporter")) { 1505 this.reporter = TypeConvertor.castToReference(value); // Reference 1506 } else if (name.equals("reportingPeriod")) { 1507 this.reportingPeriod = TypeConvertor.castToPeriod(value); // Period 1508 } else if (name.equals("inventoryListing")) { 1509 this.getInventoryListing().add((InventoryReportInventoryListingComponent) value); 1510 } else if (name.equals("note")) { 1511 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1512 } else 1513 return super.setProperty(name, value); 1514 return value; 1515 } 1516 1517 @Override 1518 public void removeChild(String name, Base value) throws FHIRException { 1519 if (name.equals("identifier")) { 1520 this.getIdentifier().remove(value); 1521 } else if (name.equals("status")) { 1522 value = new InventoryReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1523 this.status = (Enumeration) value; // Enumeration<InventoryReportStatus> 1524 } else if (name.equals("countType")) { 1525 value = new InventoryCountTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1526 this.countType = (Enumeration) value; // Enumeration<InventoryCountType> 1527 } else if (name.equals("operationType")) { 1528 this.operationType = null; 1529 } else if (name.equals("operationTypeReason")) { 1530 this.operationTypeReason = null; 1531 } else if (name.equals("reportedDateTime")) { 1532 this.reportedDateTime = null; 1533 } else if (name.equals("reporter")) { 1534 this.reporter = null; 1535 } else if (name.equals("reportingPeriod")) { 1536 this.reportingPeriod = null; 1537 } else if (name.equals("inventoryListing")) { 1538 this.getInventoryListing().remove((InventoryReportInventoryListingComponent) value); 1539 } else if (name.equals("note")) { 1540 this.getNote().remove(value); 1541 } else 1542 super.removeChild(name, value); 1543 1544 } 1545 1546 @Override 1547 public Base makeProperty(int hash, String name) throws FHIRException { 1548 switch (hash) { 1549 case -1618432855: return addIdentifier(); 1550 case -892481550: return getStatusElement(); 1551 case 1351759081: return getCountTypeElement(); 1552 case 91999553: return getOperationType(); 1553 case 449681125: return getOperationTypeReason(); 1554 case -1048250994: return getReportedDateTimeElement(); 1555 case -427039519: return getReporter(); 1556 case 409685391: return getReportingPeriod(); 1557 case -1764804216: return addInventoryListing(); 1558 case 3387378: return addNote(); 1559 default: return super.makeProperty(hash, name); 1560 } 1561 1562 } 1563 1564 @Override 1565 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1566 switch (hash) { 1567 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1568 case -892481550: /*status*/ return new String[] {"code"}; 1569 case 1351759081: /*countType*/ return new String[] {"code"}; 1570 case 91999553: /*operationType*/ return new String[] {"CodeableConcept"}; 1571 case 449681125: /*operationTypeReason*/ return new String[] {"CodeableConcept"}; 1572 case -1048250994: /*reportedDateTime*/ return new String[] {"dateTime"}; 1573 case -427039519: /*reporter*/ return new String[] {"Reference"}; 1574 case 409685391: /*reportingPeriod*/ return new String[] {"Period"}; 1575 case -1764804216: /*inventoryListing*/ return new String[] {}; 1576 case 3387378: /*note*/ return new String[] {"Annotation"}; 1577 default: return super.getTypesForProperty(hash, name); 1578 } 1579 1580 } 1581 1582 @Override 1583 public Base addChild(String name) throws FHIRException { 1584 if (name.equals("identifier")) { 1585 return addIdentifier(); 1586 } 1587 else if (name.equals("status")) { 1588 throw new FHIRException("Cannot call addChild on a singleton property InventoryReport.status"); 1589 } 1590 else if (name.equals("countType")) { 1591 throw new FHIRException("Cannot call addChild on a singleton property InventoryReport.countType"); 1592 } 1593 else if (name.equals("operationType")) { 1594 this.operationType = new CodeableConcept(); 1595 return this.operationType; 1596 } 1597 else if (name.equals("operationTypeReason")) { 1598 this.operationTypeReason = new CodeableConcept(); 1599 return this.operationTypeReason; 1600 } 1601 else if (name.equals("reportedDateTime")) { 1602 throw new FHIRException("Cannot call addChild on a singleton property InventoryReport.reportedDateTime"); 1603 } 1604 else if (name.equals("reporter")) { 1605 this.reporter = new Reference(); 1606 return this.reporter; 1607 } 1608 else if (name.equals("reportingPeriod")) { 1609 this.reportingPeriod = new Period(); 1610 return this.reportingPeriod; 1611 } 1612 else if (name.equals("inventoryListing")) { 1613 return addInventoryListing(); 1614 } 1615 else if (name.equals("note")) { 1616 return addNote(); 1617 } 1618 else 1619 return super.addChild(name); 1620 } 1621 1622 public String fhirType() { 1623 return "InventoryReport"; 1624 1625 } 1626 1627 public InventoryReport copy() { 1628 InventoryReport dst = new InventoryReport(); 1629 copyValues(dst); 1630 return dst; 1631 } 1632 1633 public void copyValues(InventoryReport dst) { 1634 super.copyValues(dst); 1635 if (identifier != null) { 1636 dst.identifier = new ArrayList<Identifier>(); 1637 for (Identifier i : identifier) 1638 dst.identifier.add(i.copy()); 1639 }; 1640 dst.status = status == null ? null : status.copy(); 1641 dst.countType = countType == null ? null : countType.copy(); 1642 dst.operationType = operationType == null ? null : operationType.copy(); 1643 dst.operationTypeReason = operationTypeReason == null ? null : operationTypeReason.copy(); 1644 dst.reportedDateTime = reportedDateTime == null ? null : reportedDateTime.copy(); 1645 dst.reporter = reporter == null ? null : reporter.copy(); 1646 dst.reportingPeriod = reportingPeriod == null ? null : reportingPeriod.copy(); 1647 if (inventoryListing != null) { 1648 dst.inventoryListing = new ArrayList<InventoryReportInventoryListingComponent>(); 1649 for (InventoryReportInventoryListingComponent i : inventoryListing) 1650 dst.inventoryListing.add(i.copy()); 1651 }; 1652 if (note != null) { 1653 dst.note = new ArrayList<Annotation>(); 1654 for (Annotation i : note) 1655 dst.note.add(i.copy()); 1656 }; 1657 } 1658 1659 protected InventoryReport typedCopy() { 1660 return copy(); 1661 } 1662 1663 @Override 1664 public boolean equalsDeep(Base other_) { 1665 if (!super.equalsDeep(other_)) 1666 return false; 1667 if (!(other_ instanceof InventoryReport)) 1668 return false; 1669 InventoryReport o = (InventoryReport) other_; 1670 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(countType, o.countType, true) 1671 && compareDeep(operationType, o.operationType, true) && compareDeep(operationTypeReason, o.operationTypeReason, true) 1672 && compareDeep(reportedDateTime, o.reportedDateTime, true) && compareDeep(reporter, o.reporter, true) 1673 && compareDeep(reportingPeriod, o.reportingPeriod, true) && compareDeep(inventoryListing, o.inventoryListing, true) 1674 && compareDeep(note, o.note, true); 1675 } 1676 1677 @Override 1678 public boolean equalsShallow(Base other_) { 1679 if (!super.equalsShallow(other_)) 1680 return false; 1681 if (!(other_ instanceof InventoryReport)) 1682 return false; 1683 InventoryReport o = (InventoryReport) other_; 1684 return compareValues(status, o.status, true) && compareValues(countType, o.countType, true) && compareValues(reportedDateTime, o.reportedDateTime, true) 1685 ; 1686 } 1687 1688 public boolean isEmpty() { 1689 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, countType 1690 , operationType, operationTypeReason, reportedDateTime, reporter, reportingPeriod 1691 , inventoryListing, note); 1692 } 1693 1694 @Override 1695 public ResourceType getResourceType() { 1696 return ResourceType.InventoryReport; 1697 } 1698 1699 /** 1700 * Search parameter: <b>identifier</b> 1701 * <p> 1702 * Description: <b>Search by identifier</b><br> 1703 * Type: <b>token</b><br> 1704 * Path: <b>InventoryReport.identifier</b><br> 1705 * </p> 1706 */ 1707 @SearchParamDefinition(name="identifier", path="InventoryReport.identifier", description="Search by identifier", type="token" ) 1708 public static final String SP_IDENTIFIER = "identifier"; 1709 /** 1710 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1711 * <p> 1712 * Description: <b>Search by identifier</b><br> 1713 * Type: <b>token</b><br> 1714 * Path: <b>InventoryReport.identifier</b><br> 1715 * </p> 1716 */ 1717 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1718 1719 /** 1720 * Search parameter: <b>item-reference</b> 1721 * <p> 1722 * Description: <b>Search by items in inventory report</b><br> 1723 * Type: <b>reference</b><br> 1724 * Path: <b>InventoryReport.inventoryListing.item.item.reference</b><br> 1725 * </p> 1726 */ 1727 @SearchParamDefinition(name="item-reference", path="InventoryReport.inventoryListing.item.item.reference", description="Search by items in inventory report", type="reference", target={BiologicallyDerivedProduct.class, Device.class, InventoryItem.class, Medication.class, NutritionProduct.class } ) 1728 public static final String SP_ITEM_REFERENCE = "item-reference"; 1729 /** 1730 * <b>Fluent Client</b> search parameter constant for <b>item-reference</b> 1731 * <p> 1732 * Description: <b>Search by items in inventory report</b><br> 1733 * Type: <b>reference</b><br> 1734 * Path: <b>InventoryReport.inventoryListing.item.item.reference</b><br> 1735 * </p> 1736 */ 1737 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ITEM_REFERENCE); 1738 1739/** 1740 * Constant for fluent queries to be used to add include statements. Specifies 1741 * the path value of "<b>InventoryReport:item-reference</b>". 1742 */ 1743 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM_REFERENCE = new ca.uhn.fhir.model.api.Include("InventoryReport:item-reference").toLocked(); 1744 1745 /** 1746 * Search parameter: <b>item</b> 1747 * <p> 1748 * Description: <b>Search by items in inventory report</b><br> 1749 * Type: <b>token</b><br> 1750 * Path: <b>InventoryReport.inventoryListing.item.item.concept</b><br> 1751 * </p> 1752 */ 1753 @SearchParamDefinition(name="item", path="InventoryReport.inventoryListing.item.item.concept", description="Search by items in inventory report", type="token" ) 1754 public static final String SP_ITEM = "item"; 1755 /** 1756 * <b>Fluent Client</b> search parameter constant for <b>item</b> 1757 * <p> 1758 * Description: <b>Search by items in inventory report</b><br> 1759 * Type: <b>token</b><br> 1760 * Path: <b>InventoryReport.inventoryListing.item.item.concept</b><br> 1761 * </p> 1762 */ 1763 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ITEM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ITEM); 1764 1765 /** 1766 * Search parameter: <b>status</b> 1767 * <p> 1768 * Description: <b>Search by status</b><br> 1769 * Type: <b>token</b><br> 1770 * Path: <b>InventoryReport.status</b><br> 1771 * </p> 1772 */ 1773 @SearchParamDefinition(name="status", path="InventoryReport.status", description="Search by status", type="token" ) 1774 public static final String SP_STATUS = "status"; 1775 /** 1776 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1777 * <p> 1778 * Description: <b>Search by status</b><br> 1779 * Type: <b>token</b><br> 1780 * Path: <b>InventoryReport.status</b><br> 1781 * </p> 1782 */ 1783 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1784 1785 1786} 1787