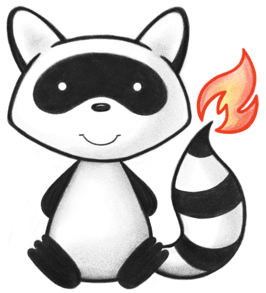
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A report of inventory or stock items. 052 */ 053@ResourceDef(name="InventoryReport", profile="http://hl7.org/fhir/StructureDefinition/InventoryReport") 054public class InventoryReport extends DomainResource { 055 056 public enum InventoryCountType { 057 /** 058 * The inventory report is a current absolute snapshot, i.e. it represents the quantities at hand. 059 */ 060 SNAPSHOT, 061 /** 062 * The inventory report is about the difference between a previous count and a current count, i.e. it represents the items that have been added/subtracted from inventory. 063 */ 064 DIFFERENCE, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static InventoryCountType fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("snapshot".equals(codeString)) 073 return SNAPSHOT; 074 if ("difference".equals(codeString)) 075 return DIFFERENCE; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown InventoryCountType code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case SNAPSHOT: return "snapshot"; 084 case DIFFERENCE: return "difference"; 085 case NULL: return null; 086 default: return "?"; 087 } 088 } 089 public String getSystem() { 090 switch (this) { 091 case SNAPSHOT: return "http://hl7.org/fhir/inventoryreport-counttype"; 092 case DIFFERENCE: return "http://hl7.org/fhir/inventoryreport-counttype"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getDefinition() { 098 switch (this) { 099 case SNAPSHOT: return "The inventory report is a current absolute snapshot, i.e. it represents the quantities at hand."; 100 case DIFFERENCE: return "The inventory report is about the difference between a previous count and a current count, i.e. it represents the items that have been added/subtracted from inventory."; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDisplay() { 106 switch (this) { 107 case SNAPSHOT: return "Snapshot"; 108 case DIFFERENCE: return "Difference"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 } 114 115 public static class InventoryCountTypeEnumFactory implements EnumFactory<InventoryCountType> { 116 public InventoryCountType fromCode(String codeString) throws IllegalArgumentException { 117 if (codeString == null || "".equals(codeString)) 118 if (codeString == null || "".equals(codeString)) 119 return null; 120 if ("snapshot".equals(codeString)) 121 return InventoryCountType.SNAPSHOT; 122 if ("difference".equals(codeString)) 123 return InventoryCountType.DIFFERENCE; 124 throw new IllegalArgumentException("Unknown InventoryCountType code '"+codeString+"'"); 125 } 126 public Enumeration<InventoryCountType> fromType(PrimitiveType<?> code) throws FHIRException { 127 if (code == null) 128 return null; 129 if (code.isEmpty()) 130 return new Enumeration<InventoryCountType>(this, InventoryCountType.NULL, code); 131 String codeString = ((PrimitiveType) code).asStringValue(); 132 if (codeString == null || "".equals(codeString)) 133 return new Enumeration<InventoryCountType>(this, InventoryCountType.NULL, code); 134 if ("snapshot".equals(codeString)) 135 return new Enumeration<InventoryCountType>(this, InventoryCountType.SNAPSHOT, code); 136 if ("difference".equals(codeString)) 137 return new Enumeration<InventoryCountType>(this, InventoryCountType.DIFFERENCE, code); 138 throw new FHIRException("Unknown InventoryCountType code '"+codeString+"'"); 139 } 140 public String toCode(InventoryCountType code) { 141 if (code == InventoryCountType.NULL) 142 return null; 143 if (code == InventoryCountType.SNAPSHOT) 144 return "snapshot"; 145 if (code == InventoryCountType.DIFFERENCE) 146 return "difference"; 147 return "?"; 148 } 149 public String toSystem(InventoryCountType code) { 150 return code.getSystem(); 151 } 152 } 153 154 public enum InventoryReportStatus { 155 /** 156 * The existence of the report is registered, but it is still without content or only some preliminary content. 157 */ 158 DRAFT, 159 /** 160 * The inventory report has been requested but there is no data available. 161 */ 162 REQUESTED, 163 /** 164 * This report is submitted as current. 165 */ 166 ACTIVE, 167 /** 168 * The report has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it. 169 */ 170 ENTEREDINERROR, 171 /** 172 * added to help the parsers with the generic types 173 */ 174 NULL; 175 public static InventoryReportStatus fromCode(String codeString) throws FHIRException { 176 if (codeString == null || "".equals(codeString)) 177 return null; 178 if ("draft".equals(codeString)) 179 return DRAFT; 180 if ("requested".equals(codeString)) 181 return REQUESTED; 182 if ("active".equals(codeString)) 183 return ACTIVE; 184 if ("entered-in-error".equals(codeString)) 185 return ENTEREDINERROR; 186 if (Configuration.isAcceptInvalidEnums()) 187 return null; 188 else 189 throw new FHIRException("Unknown InventoryReportStatus code '"+codeString+"'"); 190 } 191 public String toCode() { 192 switch (this) { 193 case DRAFT: return "draft"; 194 case REQUESTED: return "requested"; 195 case ACTIVE: return "active"; 196 case ENTEREDINERROR: return "entered-in-error"; 197 case NULL: return null; 198 default: return "?"; 199 } 200 } 201 public String getSystem() { 202 switch (this) { 203 case DRAFT: return "http://hl7.org/fhir/inventoryreport-status"; 204 case REQUESTED: return "http://hl7.org/fhir/inventoryreport-status"; 205 case ACTIVE: return "http://hl7.org/fhir/inventoryreport-status"; 206 case ENTEREDINERROR: return "http://hl7.org/fhir/inventoryreport-status"; 207 case NULL: return null; 208 default: return "?"; 209 } 210 } 211 public String getDefinition() { 212 switch (this) { 213 case DRAFT: return "The existence of the report is registered, but it is still without content or only some preliminary content."; 214 case REQUESTED: return "The inventory report has been requested but there is no data available."; 215 case ACTIVE: return "This report is submitted as current."; 216 case ENTEREDINERROR: return "The report has been withdrawn following a previous final release. This electronic record should never have existed, though it is possible that real-world decisions were based on it."; 217 case NULL: return null; 218 default: return "?"; 219 } 220 } 221 public String getDisplay() { 222 switch (this) { 223 case DRAFT: return "Draft"; 224 case REQUESTED: return "Requested"; 225 case ACTIVE: return "Active"; 226 case ENTEREDINERROR: return "Entered in Error"; 227 case NULL: return null; 228 default: return "?"; 229 } 230 } 231 } 232 233 public static class InventoryReportStatusEnumFactory implements EnumFactory<InventoryReportStatus> { 234 public InventoryReportStatus fromCode(String codeString) throws IllegalArgumentException { 235 if (codeString == null || "".equals(codeString)) 236 if (codeString == null || "".equals(codeString)) 237 return null; 238 if ("draft".equals(codeString)) 239 return InventoryReportStatus.DRAFT; 240 if ("requested".equals(codeString)) 241 return InventoryReportStatus.REQUESTED; 242 if ("active".equals(codeString)) 243 return InventoryReportStatus.ACTIVE; 244 if ("entered-in-error".equals(codeString)) 245 return InventoryReportStatus.ENTEREDINERROR; 246 throw new IllegalArgumentException("Unknown InventoryReportStatus code '"+codeString+"'"); 247 } 248 public Enumeration<InventoryReportStatus> fromType(PrimitiveType<?> code) throws FHIRException { 249 if (code == null) 250 return null; 251 if (code.isEmpty()) 252 return new Enumeration<InventoryReportStatus>(this, InventoryReportStatus.NULL, code); 253 String codeString = ((PrimitiveType) code).asStringValue(); 254 if (codeString == null || "".equals(codeString)) 255 return new Enumeration<InventoryReportStatus>(this, InventoryReportStatus.NULL, code); 256 if ("draft".equals(codeString)) 257 return new Enumeration<InventoryReportStatus>(this, InventoryReportStatus.DRAFT, code); 258 if ("requested".equals(codeString)) 259 return new Enumeration<InventoryReportStatus>(this, InventoryReportStatus.REQUESTED, code); 260 if ("active".equals(codeString)) 261 return new Enumeration<InventoryReportStatus>(this, InventoryReportStatus.ACTIVE, code); 262 if ("entered-in-error".equals(codeString)) 263 return new Enumeration<InventoryReportStatus>(this, InventoryReportStatus.ENTEREDINERROR, code); 264 throw new FHIRException("Unknown InventoryReportStatus code '"+codeString+"'"); 265 } 266 public String toCode(InventoryReportStatus code) { 267 if (code == InventoryReportStatus.NULL) 268 return null; 269 if (code == InventoryReportStatus.DRAFT) 270 return "draft"; 271 if (code == InventoryReportStatus.REQUESTED) 272 return "requested"; 273 if (code == InventoryReportStatus.ACTIVE) 274 return "active"; 275 if (code == InventoryReportStatus.ENTEREDINERROR) 276 return "entered-in-error"; 277 return "?"; 278 } 279 public String toSystem(InventoryReportStatus code) { 280 return code.getSystem(); 281 } 282 } 283 284 @Block() 285 public static class InventoryReportInventoryListingComponent extends BackboneElement implements IBaseBackboneElement { 286 /** 287 * Location of the inventory items. 288 */ 289 @Child(name = "location", type = {Location.class}, order=1, min=0, max=1, modifier=false, summary=false) 290 @Description(shortDefinition="Location of the inventory items", formalDefinition="Location of the inventory items." ) 291 protected Reference location; 292 293 /** 294 * The status of the items. 295 */ 296 @Child(name = "itemStatus", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 297 @Description(shortDefinition="The status of the items that are being reported", formalDefinition="The status of the items." ) 298 protected CodeableConcept itemStatus; 299 300 /** 301 * The date and time when the items were counted. 302 */ 303 @Child(name = "countingDateTime", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 304 @Description(shortDefinition="The date and time when the items were counted", formalDefinition="The date and time when the items were counted." ) 305 protected DateTimeType countingDateTime; 306 307 /** 308 * The item or items in this listing. 309 */ 310 @Child(name = "item", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 311 @Description(shortDefinition="The item or items in this listing", formalDefinition="The item or items in this listing." ) 312 protected List<InventoryReportInventoryListingItemComponent> item; 313 314 private static final long serialVersionUID = 1766136476L; 315 316 /** 317 * Constructor 318 */ 319 public InventoryReportInventoryListingComponent() { 320 super(); 321 } 322 323 /** 324 * @return {@link #location} (Location of the inventory items.) 325 */ 326 public Reference getLocation() { 327 if (this.location == null) 328 if (Configuration.errorOnAutoCreate()) 329 throw new Error("Attempt to auto-create InventoryReportInventoryListingComponent.location"); 330 else if (Configuration.doAutoCreate()) 331 this.location = new Reference(); // cc 332 return this.location; 333 } 334 335 public boolean hasLocation() { 336 return this.location != null && !this.location.isEmpty(); 337 } 338 339 /** 340 * @param value {@link #location} (Location of the inventory items.) 341 */ 342 public InventoryReportInventoryListingComponent setLocation(Reference value) { 343 this.location = value; 344 return this; 345 } 346 347 /** 348 * @return {@link #itemStatus} (The status of the items.) 349 */ 350 public CodeableConcept getItemStatus() { 351 if (this.itemStatus == null) 352 if (Configuration.errorOnAutoCreate()) 353 throw new Error("Attempt to auto-create InventoryReportInventoryListingComponent.itemStatus"); 354 else if (Configuration.doAutoCreate()) 355 this.itemStatus = new CodeableConcept(); // cc 356 return this.itemStatus; 357 } 358 359 public boolean hasItemStatus() { 360 return this.itemStatus != null && !this.itemStatus.isEmpty(); 361 } 362 363 /** 364 * @param value {@link #itemStatus} (The status of the items.) 365 */ 366 public InventoryReportInventoryListingComponent setItemStatus(CodeableConcept value) { 367 this.itemStatus = value; 368 return this; 369 } 370 371 /** 372 * @return {@link #countingDateTime} (The date and time when the items were counted.). This is the underlying object with id, value and extensions. The accessor "getCountingDateTime" gives direct access to the value 373 */ 374 public DateTimeType getCountingDateTimeElement() { 375 if (this.countingDateTime == null) 376 if (Configuration.errorOnAutoCreate()) 377 throw new Error("Attempt to auto-create InventoryReportInventoryListingComponent.countingDateTime"); 378 else if (Configuration.doAutoCreate()) 379 this.countingDateTime = new DateTimeType(); // bb 380 return this.countingDateTime; 381 } 382 383 public boolean hasCountingDateTimeElement() { 384 return this.countingDateTime != null && !this.countingDateTime.isEmpty(); 385 } 386 387 public boolean hasCountingDateTime() { 388 return this.countingDateTime != null && !this.countingDateTime.isEmpty(); 389 } 390 391 /** 392 * @param value {@link #countingDateTime} (The date and time when the items were counted.). This is the underlying object with id, value and extensions. The accessor "getCountingDateTime" gives direct access to the value 393 */ 394 public InventoryReportInventoryListingComponent setCountingDateTimeElement(DateTimeType value) { 395 this.countingDateTime = value; 396 return this; 397 } 398 399 /** 400 * @return The date and time when the items were counted. 401 */ 402 public Date getCountingDateTime() { 403 return this.countingDateTime == null ? null : this.countingDateTime.getValue(); 404 } 405 406 /** 407 * @param value The date and time when the items were counted. 408 */ 409 public InventoryReportInventoryListingComponent setCountingDateTime(Date value) { 410 if (value == null) 411 this.countingDateTime = null; 412 else { 413 if (this.countingDateTime == null) 414 this.countingDateTime = new DateTimeType(); 415 this.countingDateTime.setValue(value); 416 } 417 return this; 418 } 419 420 /** 421 * @return {@link #item} (The item or items in this listing.) 422 */ 423 public List<InventoryReportInventoryListingItemComponent> getItem() { 424 if (this.item == null) 425 this.item = new ArrayList<InventoryReportInventoryListingItemComponent>(); 426 return this.item; 427 } 428 429 /** 430 * @return Returns a reference to <code>this</code> for easy method chaining 431 */ 432 public InventoryReportInventoryListingComponent setItem(List<InventoryReportInventoryListingItemComponent> theItem) { 433 this.item = theItem; 434 return this; 435 } 436 437 public boolean hasItem() { 438 if (this.item == null) 439 return false; 440 for (InventoryReportInventoryListingItemComponent item : this.item) 441 if (!item.isEmpty()) 442 return true; 443 return false; 444 } 445 446 public InventoryReportInventoryListingItemComponent addItem() { //3 447 InventoryReportInventoryListingItemComponent t = new InventoryReportInventoryListingItemComponent(); 448 if (this.item == null) 449 this.item = new ArrayList<InventoryReportInventoryListingItemComponent>(); 450 this.item.add(t); 451 return t; 452 } 453 454 public InventoryReportInventoryListingComponent addItem(InventoryReportInventoryListingItemComponent t) { //3 455 if (t == null) 456 return this; 457 if (this.item == null) 458 this.item = new ArrayList<InventoryReportInventoryListingItemComponent>(); 459 this.item.add(t); 460 return this; 461 } 462 463 /** 464 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 465 */ 466 public InventoryReportInventoryListingItemComponent getItemFirstRep() { 467 if (getItem().isEmpty()) { 468 addItem(); 469 } 470 return getItem().get(0); 471 } 472 473 protected void listChildren(List<Property> children) { 474 super.listChildren(children); 475 children.add(new Property("location", "Reference(Location)", "Location of the inventory items.", 0, 1, location)); 476 children.add(new Property("itemStatus", "CodeableConcept", "The status of the items.", 0, 1, itemStatus)); 477 children.add(new Property("countingDateTime", "dateTime", "The date and time when the items were counted.", 0, 1, countingDateTime)); 478 children.add(new Property("item", "", "The item or items in this listing.", 0, java.lang.Integer.MAX_VALUE, item)); 479 } 480 481 @Override 482 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 483 switch (_hash) { 484 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "Location of the inventory items.", 0, 1, location); 485 case 1999789285: /*itemStatus*/ return new Property("itemStatus", "CodeableConcept", "The status of the items.", 0, 1, itemStatus); 486 case -2075203282: /*countingDateTime*/ return new Property("countingDateTime", "dateTime", "The date and time when the items were counted.", 0, 1, countingDateTime); 487 case 3242771: /*item*/ return new Property("item", "", "The item or items in this listing.", 0, java.lang.Integer.MAX_VALUE, item); 488 default: return super.getNamedProperty(_hash, _name, _checkValid); 489 } 490 491 } 492 493 @Override 494 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 495 switch (hash) { 496 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 497 case 1999789285: /*itemStatus*/ return this.itemStatus == null ? new Base[0] : new Base[] {this.itemStatus}; // CodeableConcept 498 case -2075203282: /*countingDateTime*/ return this.countingDateTime == null ? new Base[0] : new Base[] {this.countingDateTime}; // DateTimeType 499 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // InventoryReportInventoryListingItemComponent 500 default: return super.getProperty(hash, name, checkValid); 501 } 502 503 } 504 505 @Override 506 public Base setProperty(int hash, String name, Base value) throws FHIRException { 507 switch (hash) { 508 case 1901043637: // location 509 this.location = TypeConvertor.castToReference(value); // Reference 510 return value; 511 case 1999789285: // itemStatus 512 this.itemStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 513 return value; 514 case -2075203282: // countingDateTime 515 this.countingDateTime = TypeConvertor.castToDateTime(value); // DateTimeType 516 return value; 517 case 3242771: // item 518 this.getItem().add((InventoryReportInventoryListingItemComponent) value); // InventoryReportInventoryListingItemComponent 519 return value; 520 default: return super.setProperty(hash, name, value); 521 } 522 523 } 524 525 @Override 526 public Base setProperty(String name, Base value) throws FHIRException { 527 if (name.equals("location")) { 528 this.location = TypeConvertor.castToReference(value); // Reference 529 } else if (name.equals("itemStatus")) { 530 this.itemStatus = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 531 } else if (name.equals("countingDateTime")) { 532 this.countingDateTime = TypeConvertor.castToDateTime(value); // DateTimeType 533 } else if (name.equals("item")) { 534 this.getItem().add((InventoryReportInventoryListingItemComponent) value); 535 } else 536 return super.setProperty(name, value); 537 return value; 538 } 539 540 @Override 541 public void removeChild(String name, Base value) throws FHIRException { 542 if (name.equals("location")) { 543 this.location = null; 544 } else if (name.equals("itemStatus")) { 545 this.itemStatus = null; 546 } else if (name.equals("countingDateTime")) { 547 this.countingDateTime = null; 548 } else if (name.equals("item")) { 549 this.getItem().remove((InventoryReportInventoryListingItemComponent) value); 550 } else 551 super.removeChild(name, value); 552 553 } 554 555 @Override 556 public Base makeProperty(int hash, String name) throws FHIRException { 557 switch (hash) { 558 case 1901043637: return getLocation(); 559 case 1999789285: return getItemStatus(); 560 case -2075203282: return getCountingDateTimeElement(); 561 case 3242771: return addItem(); 562 default: return super.makeProperty(hash, name); 563 } 564 565 } 566 567 @Override 568 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 569 switch (hash) { 570 case 1901043637: /*location*/ return new String[] {"Reference"}; 571 case 1999789285: /*itemStatus*/ return new String[] {"CodeableConcept"}; 572 case -2075203282: /*countingDateTime*/ return new String[] {"dateTime"}; 573 case 3242771: /*item*/ return new String[] {}; 574 default: return super.getTypesForProperty(hash, name); 575 } 576 577 } 578 579 @Override 580 public Base addChild(String name) throws FHIRException { 581 if (name.equals("location")) { 582 this.location = new Reference(); 583 return this.location; 584 } 585 else if (name.equals("itemStatus")) { 586 this.itemStatus = new CodeableConcept(); 587 return this.itemStatus; 588 } 589 else if (name.equals("countingDateTime")) { 590 throw new FHIRException("Cannot call addChild on a singleton property InventoryReport.inventoryListing.countingDateTime"); 591 } 592 else if (name.equals("item")) { 593 return addItem(); 594 } 595 else 596 return super.addChild(name); 597 } 598 599 public InventoryReportInventoryListingComponent copy() { 600 InventoryReportInventoryListingComponent dst = new InventoryReportInventoryListingComponent(); 601 copyValues(dst); 602 return dst; 603 } 604 605 public void copyValues(InventoryReportInventoryListingComponent dst) { 606 super.copyValues(dst); 607 dst.location = location == null ? null : location.copy(); 608 dst.itemStatus = itemStatus == null ? null : itemStatus.copy(); 609 dst.countingDateTime = countingDateTime == null ? null : countingDateTime.copy(); 610 if (item != null) { 611 dst.item = new ArrayList<InventoryReportInventoryListingItemComponent>(); 612 for (InventoryReportInventoryListingItemComponent i : item) 613 dst.item.add(i.copy()); 614 }; 615 } 616 617 @Override 618 public boolean equalsDeep(Base other_) { 619 if (!super.equalsDeep(other_)) 620 return false; 621 if (!(other_ instanceof InventoryReportInventoryListingComponent)) 622 return false; 623 InventoryReportInventoryListingComponent o = (InventoryReportInventoryListingComponent) other_; 624 return compareDeep(location, o.location, true) && compareDeep(itemStatus, o.itemStatus, true) && compareDeep(countingDateTime, o.countingDateTime, true) 625 && compareDeep(item, o.item, true); 626 } 627 628 @Override 629 public boolean equalsShallow(Base other_) { 630 if (!super.equalsShallow(other_)) 631 return false; 632 if (!(other_ instanceof InventoryReportInventoryListingComponent)) 633 return false; 634 InventoryReportInventoryListingComponent o = (InventoryReportInventoryListingComponent) other_; 635 return compareValues(countingDateTime, o.countingDateTime, true); 636 } 637 638 public boolean isEmpty() { 639 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(location, itemStatus, countingDateTime 640 , item); 641 } 642 643 public String fhirType() { 644 return "InventoryReport.inventoryListing"; 645 646 } 647 648 } 649 650 @Block() 651 public static class InventoryReportInventoryListingItemComponent extends BackboneElement implements IBaseBackboneElement { 652 /** 653 * The inventory category or classification of the items being reported. This is meant not for defining the product, but for inventory categories e.g. 'pending recount' or 'damaged'. 654 */ 655 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 656 @Description(shortDefinition="The inventory category or classification of the items being reported", formalDefinition="The inventory category or classification of the items being reported. This is meant not for defining the product, but for inventory categories e.g. 'pending recount' or 'damaged'." ) 657 protected CodeableConcept category; 658 659 /** 660 * The quantity of the item or items being reported. 661 */ 662 @Child(name = "quantity", type = {Quantity.class}, order=2, min=1, max=1, modifier=false, summary=true) 663 @Description(shortDefinition="The quantity of the item or items being reported", formalDefinition="The quantity of the item or items being reported." ) 664 protected Quantity quantity; 665 666 /** 667 * The code or reference to the item type. 668 */ 669 @Child(name = "item", type = {CodeableReference.class}, order=3, min=1, max=1, modifier=false, summary=true) 670 @Description(shortDefinition="The code or reference to the item type", formalDefinition="The code or reference to the item type." ) 671 protected CodeableReference item; 672 673 private static final long serialVersionUID = 566223460L; 674 675 /** 676 * Constructor 677 */ 678 public InventoryReportInventoryListingItemComponent() { 679 super(); 680 } 681 682 /** 683 * Constructor 684 */ 685 public InventoryReportInventoryListingItemComponent(Quantity quantity, CodeableReference item) { 686 super(); 687 this.setQuantity(quantity); 688 this.setItem(item); 689 } 690 691 /** 692 * @return {@link #category} (The inventory category or classification of the items being reported. This is meant not for defining the product, but for inventory categories e.g. 'pending recount' or 'damaged'.) 693 */ 694 public CodeableConcept getCategory() { 695 if (this.category == null) 696 if (Configuration.errorOnAutoCreate()) 697 throw new Error("Attempt to auto-create InventoryReportInventoryListingItemComponent.category"); 698 else if (Configuration.doAutoCreate()) 699 this.category = new CodeableConcept(); // cc 700 return this.category; 701 } 702 703 public boolean hasCategory() { 704 return this.category != null && !this.category.isEmpty(); 705 } 706 707 /** 708 * @param value {@link #category} (The inventory category or classification of the items being reported. This is meant not for defining the product, but for inventory categories e.g. 'pending recount' or 'damaged'.) 709 */ 710 public InventoryReportInventoryListingItemComponent setCategory(CodeableConcept value) { 711 this.category = value; 712 return this; 713 } 714 715 /** 716 * @return {@link #quantity} (The quantity of the item or items being reported.) 717 */ 718 public Quantity getQuantity() { 719 if (this.quantity == null) 720 if (Configuration.errorOnAutoCreate()) 721 throw new Error("Attempt to auto-create InventoryReportInventoryListingItemComponent.quantity"); 722 else if (Configuration.doAutoCreate()) 723 this.quantity = new Quantity(); // cc 724 return this.quantity; 725 } 726 727 public boolean hasQuantity() { 728 return this.quantity != null && !this.quantity.isEmpty(); 729 } 730 731 /** 732 * @param value {@link #quantity} (The quantity of the item or items being reported.) 733 */ 734 public InventoryReportInventoryListingItemComponent setQuantity(Quantity value) { 735 this.quantity = value; 736 return this; 737 } 738 739 /** 740 * @return {@link #item} (The code or reference to the item type.) 741 */ 742 public CodeableReference getItem() { 743 if (this.item == null) 744 if (Configuration.errorOnAutoCreate()) 745 throw new Error("Attempt to auto-create InventoryReportInventoryListingItemComponent.item"); 746 else if (Configuration.doAutoCreate()) 747 this.item = new CodeableReference(); // cc 748 return this.item; 749 } 750 751 public boolean hasItem() { 752 return this.item != null && !this.item.isEmpty(); 753 } 754 755 /** 756 * @param value {@link #item} (The code or reference to the item type.) 757 */ 758 public InventoryReportInventoryListingItemComponent setItem(CodeableReference value) { 759 this.item = value; 760 return this; 761 } 762 763 protected void listChildren(List<Property> children) { 764 super.listChildren(children); 765 children.add(new Property("category", "CodeableConcept", "The inventory category or classification of the items being reported. This is meant not for defining the product, but for inventory categories e.g. 'pending recount' or 'damaged'.", 0, 1, category)); 766 children.add(new Property("quantity", "Quantity", "The quantity of the item or items being reported.", 0, 1, quantity)); 767 children.add(new Property("item", "CodeableReference(Medication|Device|Medication|NutritionProduct|InventoryItem|BiologicallyDerivedProduct|InventoryItem)", "The code or reference to the item type.", 0, 1, item)); 768 } 769 770 @Override 771 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 772 switch (_hash) { 773 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The inventory category or classification of the items being reported. This is meant not for defining the product, but for inventory categories e.g. 'pending recount' or 'damaged'.", 0, 1, category); 774 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The quantity of the item or items being reported.", 0, 1, quantity); 775 case 3242771: /*item*/ return new Property("item", "CodeableReference(Medication|Device|Medication|NutritionProduct|InventoryItem|BiologicallyDerivedProduct|InventoryItem)", "The code or reference to the item type.", 0, 1, item); 776 default: return super.getNamedProperty(_hash, _name, _checkValid); 777 } 778 779 } 780 781 @Override 782 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 783 switch (hash) { 784 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 785 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 786 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // CodeableReference 787 default: return super.getProperty(hash, name, checkValid); 788 } 789 790 } 791 792 @Override 793 public Base setProperty(int hash, String name, Base value) throws FHIRException { 794 switch (hash) { 795 case 50511102: // category 796 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 797 return value; 798 case -1285004149: // quantity 799 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 800 return value; 801 case 3242771: // item 802 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 803 return value; 804 default: return super.setProperty(hash, name, value); 805 } 806 807 } 808 809 @Override 810 public Base setProperty(String name, Base value) throws FHIRException { 811 if (name.equals("category")) { 812 this.category = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 813 } else if (name.equals("quantity")) { 814 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 815 } else if (name.equals("item")) { 816 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 817 } else 818 return super.setProperty(name, value); 819 return value; 820 } 821 822 @Override 823 public void removeChild(String name, Base value) throws FHIRException { 824 if (name.equals("category")) { 825 this.category = null; 826 } else if (name.equals("quantity")) { 827 this.quantity = null; 828 } else if (name.equals("item")) { 829 this.item = null; 830 } else 831 super.removeChild(name, value); 832 833 } 834 835 @Override 836 public Base makeProperty(int hash, String name) throws FHIRException { 837 switch (hash) { 838 case 50511102: return getCategory(); 839 case -1285004149: return getQuantity(); 840 case 3242771: return getItem(); 841 default: return super.makeProperty(hash, name); 842 } 843 844 } 845 846 @Override 847 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 848 switch (hash) { 849 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 850 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 851 case 3242771: /*item*/ return new String[] {"CodeableReference"}; 852 default: return super.getTypesForProperty(hash, name); 853 } 854 855 } 856 857 @Override 858 public Base addChild(String name) throws FHIRException { 859 if (name.equals("category")) { 860 this.category = new CodeableConcept(); 861 return this.category; 862 } 863 else if (name.equals("quantity")) { 864 this.quantity = new Quantity(); 865 return this.quantity; 866 } 867 else if (name.equals("item")) { 868 this.item = new CodeableReference(); 869 return this.item; 870 } 871 else 872 return super.addChild(name); 873 } 874 875 public InventoryReportInventoryListingItemComponent copy() { 876 InventoryReportInventoryListingItemComponent dst = new InventoryReportInventoryListingItemComponent(); 877 copyValues(dst); 878 return dst; 879 } 880 881 public void copyValues(InventoryReportInventoryListingItemComponent dst) { 882 super.copyValues(dst); 883 dst.category = category == null ? null : category.copy(); 884 dst.quantity = quantity == null ? null : quantity.copy(); 885 dst.item = item == null ? null : item.copy(); 886 } 887 888 @Override 889 public boolean equalsDeep(Base other_) { 890 if (!super.equalsDeep(other_)) 891 return false; 892 if (!(other_ instanceof InventoryReportInventoryListingItemComponent)) 893 return false; 894 InventoryReportInventoryListingItemComponent o = (InventoryReportInventoryListingItemComponent) other_; 895 return compareDeep(category, o.category, true) && compareDeep(quantity, o.quantity, true) && compareDeep(item, o.item, true) 896 ; 897 } 898 899 @Override 900 public boolean equalsShallow(Base other_) { 901 if (!super.equalsShallow(other_)) 902 return false; 903 if (!(other_ instanceof InventoryReportInventoryListingItemComponent)) 904 return false; 905 InventoryReportInventoryListingItemComponent o = (InventoryReportInventoryListingItemComponent) other_; 906 return true; 907 } 908 909 public boolean isEmpty() { 910 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, quantity, item 911 ); 912 } 913 914 public String fhirType() { 915 return "InventoryReport.inventoryListing.item"; 916 917 } 918 919 } 920 921 /** 922 * Business identifier for the InventoryReport. 923 */ 924 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 925 @Description(shortDefinition="Business identifier for the report", formalDefinition="Business identifier for the InventoryReport." ) 926 protected List<Identifier> identifier; 927 928 /** 929 * The status of the inventory check or notification - whether this is draft (e.g. the report is still pending some updates) or active. 930 */ 931 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 932 @Description(shortDefinition="draft | requested | active | entered-in-error", formalDefinition="The status of the inventory check or notification - whether this is draft (e.g. the report is still pending some updates) or active." ) 933 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/inventoryreport-status") 934 protected Enumeration<InventoryReportStatus> status; 935 936 /** 937 * Whether the report is about the current inventory count (snapshot) or a differential change in inventory (change). 938 */ 939 @Child(name = "countType", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 940 @Description(shortDefinition="snapshot | difference", formalDefinition="Whether the report is about the current inventory count (snapshot) or a differential change in inventory (change)." ) 941 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/inventoryreport-counttype") 942 protected Enumeration<InventoryCountType> countType; 943 944 /** 945 * What type of operation is being performed - addition or subtraction. 946 */ 947 @Child(name = "operationType", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 948 @Description(shortDefinition="addition | subtraction", formalDefinition="What type of operation is being performed - addition or subtraction." ) 949 protected CodeableConcept operationType; 950 951 /** 952 * The reason for this count - regular count, ad-hoc count, new arrivals, etc. 953 */ 954 @Child(name = "operationTypeReason", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 955 @Description(shortDefinition="The reason for this count - regular count, ad-hoc count, new arrivals, etc", formalDefinition="The reason for this count - regular count, ad-hoc count, new arrivals, etc." ) 956 protected CodeableConcept operationTypeReason; 957 958 /** 959 * When the report has been submitted. 960 */ 961 @Child(name = "reportedDateTime", type = {DateTimeType.class}, order=5, min=1, max=1, modifier=false, summary=true) 962 @Description(shortDefinition="When the report has been submitted", formalDefinition="When the report has been submitted." ) 963 protected DateTimeType reportedDateTime; 964 965 /** 966 * Who submits the report. 967 */ 968 @Child(name = "reporter", type = {Practitioner.class, Patient.class, RelatedPerson.class, Device.class}, order=6, min=0, max=1, modifier=false, summary=false) 969 @Description(shortDefinition="Who submits the report", formalDefinition="Who submits the report." ) 970 protected Reference reporter; 971 972 /** 973 * The period the report refers to. 974 */ 975 @Child(name = "reportingPeriod", type = {Period.class}, order=7, min=0, max=1, modifier=false, summary=false) 976 @Description(shortDefinition="The period the report refers to", formalDefinition="The period the report refers to." ) 977 protected Period reportingPeriod; 978 979 /** 980 * An inventory listing section (grouped by any of the attributes). 981 */ 982 @Child(name = "inventoryListing", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 983 @Description(shortDefinition="An inventory listing section (grouped by any of the attributes)", formalDefinition="An inventory listing section (grouped by any of the attributes)." ) 984 protected List<InventoryReportInventoryListingComponent> inventoryListing; 985 986 /** 987 * A note associated with the InventoryReport. 988 */ 989 @Child(name = "note", type = {Annotation.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 990 @Description(shortDefinition="A note associated with the InventoryReport", formalDefinition="A note associated with the InventoryReport." ) 991 protected List<Annotation> note; 992 993 private static final long serialVersionUID = 539262671L; 994 995 /** 996 * Constructor 997 */ 998 public InventoryReport() { 999 super(); 1000 } 1001 1002 /** 1003 * Constructor 1004 */ 1005 public InventoryReport(InventoryReportStatus status, InventoryCountType countType, Date reportedDateTime) { 1006 super(); 1007 this.setStatus(status); 1008 this.setCountType(countType); 1009 this.setReportedDateTime(reportedDateTime); 1010 } 1011 1012 /** 1013 * @return {@link #identifier} (Business identifier for the InventoryReport.) 1014 */ 1015 public List<Identifier> getIdentifier() { 1016 if (this.identifier == null) 1017 this.identifier = new ArrayList<Identifier>(); 1018 return this.identifier; 1019 } 1020 1021 /** 1022 * @return Returns a reference to <code>this</code> for easy method chaining 1023 */ 1024 public InventoryReport setIdentifier(List<Identifier> theIdentifier) { 1025 this.identifier = theIdentifier; 1026 return this; 1027 } 1028 1029 public boolean hasIdentifier() { 1030 if (this.identifier == null) 1031 return false; 1032 for (Identifier item : this.identifier) 1033 if (!item.isEmpty()) 1034 return true; 1035 return false; 1036 } 1037 1038 public Identifier addIdentifier() { //3 1039 Identifier t = new Identifier(); 1040 if (this.identifier == null) 1041 this.identifier = new ArrayList<Identifier>(); 1042 this.identifier.add(t); 1043 return t; 1044 } 1045 1046 public InventoryReport addIdentifier(Identifier t) { //3 1047 if (t == null) 1048 return this; 1049 if (this.identifier == null) 1050 this.identifier = new ArrayList<Identifier>(); 1051 this.identifier.add(t); 1052 return this; 1053 } 1054 1055 /** 1056 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1057 */ 1058 public Identifier getIdentifierFirstRep() { 1059 if (getIdentifier().isEmpty()) { 1060 addIdentifier(); 1061 } 1062 return getIdentifier().get(0); 1063 } 1064 1065 /** 1066 * @return {@link #status} (The status of the inventory check or notification - whether this is draft (e.g. the report is still pending some updates) or active.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1067 */ 1068 public Enumeration<InventoryReportStatus> getStatusElement() { 1069 if (this.status == null) 1070 if (Configuration.errorOnAutoCreate()) 1071 throw new Error("Attempt to auto-create InventoryReport.status"); 1072 else if (Configuration.doAutoCreate()) 1073 this.status = new Enumeration<InventoryReportStatus>(new InventoryReportStatusEnumFactory()); // bb 1074 return this.status; 1075 } 1076 1077 public boolean hasStatusElement() { 1078 return this.status != null && !this.status.isEmpty(); 1079 } 1080 1081 public boolean hasStatus() { 1082 return this.status != null && !this.status.isEmpty(); 1083 } 1084 1085 /** 1086 * @param value {@link #status} (The status of the inventory check or notification - whether this is draft (e.g. the report is still pending some updates) or active.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1087 */ 1088 public InventoryReport setStatusElement(Enumeration<InventoryReportStatus> value) { 1089 this.status = value; 1090 return this; 1091 } 1092 1093 /** 1094 * @return The status of the inventory check or notification - whether this is draft (e.g. the report is still pending some updates) or active. 1095 */ 1096 public InventoryReportStatus getStatus() { 1097 return this.status == null ? null : this.status.getValue(); 1098 } 1099 1100 /** 1101 * @param value The status of the inventory check or notification - whether this is draft (e.g. the report is still pending some updates) or active. 1102 */ 1103 public InventoryReport setStatus(InventoryReportStatus value) { 1104 if (this.status == null) 1105 this.status = new Enumeration<InventoryReportStatus>(new InventoryReportStatusEnumFactory()); 1106 this.status.setValue(value); 1107 return this; 1108 } 1109 1110 /** 1111 * @return {@link #countType} (Whether the report is about the current inventory count (snapshot) or a differential change in inventory (change).). This is the underlying object with id, value and extensions. The accessor "getCountType" gives direct access to the value 1112 */ 1113 public Enumeration<InventoryCountType> getCountTypeElement() { 1114 if (this.countType == null) 1115 if (Configuration.errorOnAutoCreate()) 1116 throw new Error("Attempt to auto-create InventoryReport.countType"); 1117 else if (Configuration.doAutoCreate()) 1118 this.countType = new Enumeration<InventoryCountType>(new InventoryCountTypeEnumFactory()); // bb 1119 return this.countType; 1120 } 1121 1122 public boolean hasCountTypeElement() { 1123 return this.countType != null && !this.countType.isEmpty(); 1124 } 1125 1126 public boolean hasCountType() { 1127 return this.countType != null && !this.countType.isEmpty(); 1128 } 1129 1130 /** 1131 * @param value {@link #countType} (Whether the report is about the current inventory count (snapshot) or a differential change in inventory (change).). This is the underlying object with id, value and extensions. The accessor "getCountType" gives direct access to the value 1132 */ 1133 public InventoryReport setCountTypeElement(Enumeration<InventoryCountType> value) { 1134 this.countType = value; 1135 return this; 1136 } 1137 1138 /** 1139 * @return Whether the report is about the current inventory count (snapshot) or a differential change in inventory (change). 1140 */ 1141 public InventoryCountType getCountType() { 1142 return this.countType == null ? null : this.countType.getValue(); 1143 } 1144 1145 /** 1146 * @param value Whether the report is about the current inventory count (snapshot) or a differential change in inventory (change). 1147 */ 1148 public InventoryReport setCountType(InventoryCountType value) { 1149 if (this.countType == null) 1150 this.countType = new Enumeration<InventoryCountType>(new InventoryCountTypeEnumFactory()); 1151 this.countType.setValue(value); 1152 return this; 1153 } 1154 1155 /** 1156 * @return {@link #operationType} (What type of operation is being performed - addition or subtraction.) 1157 */ 1158 public CodeableConcept getOperationType() { 1159 if (this.operationType == null) 1160 if (Configuration.errorOnAutoCreate()) 1161 throw new Error("Attempt to auto-create InventoryReport.operationType"); 1162 else if (Configuration.doAutoCreate()) 1163 this.operationType = new CodeableConcept(); // cc 1164 return this.operationType; 1165 } 1166 1167 public boolean hasOperationType() { 1168 return this.operationType != null && !this.operationType.isEmpty(); 1169 } 1170 1171 /** 1172 * @param value {@link #operationType} (What type of operation is being performed - addition or subtraction.) 1173 */ 1174 public InventoryReport setOperationType(CodeableConcept value) { 1175 this.operationType = value; 1176 return this; 1177 } 1178 1179 /** 1180 * @return {@link #operationTypeReason} (The reason for this count - regular count, ad-hoc count, new arrivals, etc.) 1181 */ 1182 public CodeableConcept getOperationTypeReason() { 1183 if (this.operationTypeReason == null) 1184 if (Configuration.errorOnAutoCreate()) 1185 throw new Error("Attempt to auto-create InventoryReport.operationTypeReason"); 1186 else if (Configuration.doAutoCreate()) 1187 this.operationTypeReason = new CodeableConcept(); // cc 1188 return this.operationTypeReason; 1189 } 1190 1191 public boolean hasOperationTypeReason() { 1192 return this.operationTypeReason != null && !this.operationTypeReason.isEmpty(); 1193 } 1194 1195 /** 1196 * @param value {@link #operationTypeReason} (The reason for this count - regular count, ad-hoc count, new arrivals, etc.) 1197 */ 1198 public InventoryReport setOperationTypeReason(CodeableConcept value) { 1199 this.operationTypeReason = value; 1200 return this; 1201 } 1202 1203 /** 1204 * @return {@link #reportedDateTime} (When the report has been submitted.). This is the underlying object with id, value and extensions. The accessor "getReportedDateTime" gives direct access to the value 1205 */ 1206 public DateTimeType getReportedDateTimeElement() { 1207 if (this.reportedDateTime == null) 1208 if (Configuration.errorOnAutoCreate()) 1209 throw new Error("Attempt to auto-create InventoryReport.reportedDateTime"); 1210 else if (Configuration.doAutoCreate()) 1211 this.reportedDateTime = new DateTimeType(); // bb 1212 return this.reportedDateTime; 1213 } 1214 1215 public boolean hasReportedDateTimeElement() { 1216 return this.reportedDateTime != null && !this.reportedDateTime.isEmpty(); 1217 } 1218 1219 public boolean hasReportedDateTime() { 1220 return this.reportedDateTime != null && !this.reportedDateTime.isEmpty(); 1221 } 1222 1223 /** 1224 * @param value {@link #reportedDateTime} (When the report has been submitted.). This is the underlying object with id, value and extensions. The accessor "getReportedDateTime" gives direct access to the value 1225 */ 1226 public InventoryReport setReportedDateTimeElement(DateTimeType value) { 1227 this.reportedDateTime = value; 1228 return this; 1229 } 1230 1231 /** 1232 * @return When the report has been submitted. 1233 */ 1234 public Date getReportedDateTime() { 1235 return this.reportedDateTime == null ? null : this.reportedDateTime.getValue(); 1236 } 1237 1238 /** 1239 * @param value When the report has been submitted. 1240 */ 1241 public InventoryReport setReportedDateTime(Date value) { 1242 if (this.reportedDateTime == null) 1243 this.reportedDateTime = new DateTimeType(); 1244 this.reportedDateTime.setValue(value); 1245 return this; 1246 } 1247 1248 /** 1249 * @return {@link #reporter} (Who submits the report.) 1250 */ 1251 public Reference getReporter() { 1252 if (this.reporter == null) 1253 if (Configuration.errorOnAutoCreate()) 1254 throw new Error("Attempt to auto-create InventoryReport.reporter"); 1255 else if (Configuration.doAutoCreate()) 1256 this.reporter = new Reference(); // cc 1257 return this.reporter; 1258 } 1259 1260 public boolean hasReporter() { 1261 return this.reporter != null && !this.reporter.isEmpty(); 1262 } 1263 1264 /** 1265 * @param value {@link #reporter} (Who submits the report.) 1266 */ 1267 public InventoryReport setReporter(Reference value) { 1268 this.reporter = value; 1269 return this; 1270 } 1271 1272 /** 1273 * @return {@link #reportingPeriod} (The period the report refers to.) 1274 */ 1275 public Period getReportingPeriod() { 1276 if (this.reportingPeriod == null) 1277 if (Configuration.errorOnAutoCreate()) 1278 throw new Error("Attempt to auto-create InventoryReport.reportingPeriod"); 1279 else if (Configuration.doAutoCreate()) 1280 this.reportingPeriod = new Period(); // cc 1281 return this.reportingPeriod; 1282 } 1283 1284 public boolean hasReportingPeriod() { 1285 return this.reportingPeriod != null && !this.reportingPeriod.isEmpty(); 1286 } 1287 1288 /** 1289 * @param value {@link #reportingPeriod} (The period the report refers to.) 1290 */ 1291 public InventoryReport setReportingPeriod(Period value) { 1292 this.reportingPeriod = value; 1293 return this; 1294 } 1295 1296 /** 1297 * @return {@link #inventoryListing} (An inventory listing section (grouped by any of the attributes).) 1298 */ 1299 public List<InventoryReportInventoryListingComponent> getInventoryListing() { 1300 if (this.inventoryListing == null) 1301 this.inventoryListing = new ArrayList<InventoryReportInventoryListingComponent>(); 1302 return this.inventoryListing; 1303 } 1304 1305 /** 1306 * @return Returns a reference to <code>this</code> for easy method chaining 1307 */ 1308 public InventoryReport setInventoryListing(List<InventoryReportInventoryListingComponent> theInventoryListing) { 1309 this.inventoryListing = theInventoryListing; 1310 return this; 1311 } 1312 1313 public boolean hasInventoryListing() { 1314 if (this.inventoryListing == null) 1315 return false; 1316 for (InventoryReportInventoryListingComponent item : this.inventoryListing) 1317 if (!item.isEmpty()) 1318 return true; 1319 return false; 1320 } 1321 1322 public InventoryReportInventoryListingComponent addInventoryListing() { //3 1323 InventoryReportInventoryListingComponent t = new InventoryReportInventoryListingComponent(); 1324 if (this.inventoryListing == null) 1325 this.inventoryListing = new ArrayList<InventoryReportInventoryListingComponent>(); 1326 this.inventoryListing.add(t); 1327 return t; 1328 } 1329 1330 public InventoryReport addInventoryListing(InventoryReportInventoryListingComponent t) { //3 1331 if (t == null) 1332 return this; 1333 if (this.inventoryListing == null) 1334 this.inventoryListing = new ArrayList<InventoryReportInventoryListingComponent>(); 1335 this.inventoryListing.add(t); 1336 return this; 1337 } 1338 1339 /** 1340 * @return The first repetition of repeating field {@link #inventoryListing}, creating it if it does not already exist {3} 1341 */ 1342 public InventoryReportInventoryListingComponent getInventoryListingFirstRep() { 1343 if (getInventoryListing().isEmpty()) { 1344 addInventoryListing(); 1345 } 1346 return getInventoryListing().get(0); 1347 } 1348 1349 /** 1350 * @return {@link #note} (A note associated with the InventoryReport.) 1351 */ 1352 public List<Annotation> getNote() { 1353 if (this.note == null) 1354 this.note = new ArrayList<Annotation>(); 1355 return this.note; 1356 } 1357 1358 /** 1359 * @return Returns a reference to <code>this</code> for easy method chaining 1360 */ 1361 public InventoryReport setNote(List<Annotation> theNote) { 1362 this.note = theNote; 1363 return this; 1364 } 1365 1366 public boolean hasNote() { 1367 if (this.note == null) 1368 return false; 1369 for (Annotation item : this.note) 1370 if (!item.isEmpty()) 1371 return true; 1372 return false; 1373 } 1374 1375 public Annotation addNote() { //3 1376 Annotation t = new Annotation(); 1377 if (this.note == null) 1378 this.note = new ArrayList<Annotation>(); 1379 this.note.add(t); 1380 return t; 1381 } 1382 1383 public InventoryReport addNote(Annotation t) { //3 1384 if (t == null) 1385 return this; 1386 if (this.note == null) 1387 this.note = new ArrayList<Annotation>(); 1388 this.note.add(t); 1389 return this; 1390 } 1391 1392 /** 1393 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1394 */ 1395 public Annotation getNoteFirstRep() { 1396 if (getNote().isEmpty()) { 1397 addNote(); 1398 } 1399 return getNote().get(0); 1400 } 1401 1402 protected void listChildren(List<Property> children) { 1403 super.listChildren(children); 1404 children.add(new Property("identifier", "Identifier", "Business identifier for the InventoryReport.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1405 children.add(new Property("status", "code", "The status of the inventory check or notification - whether this is draft (e.g. the report is still pending some updates) or active.", 0, 1, status)); 1406 children.add(new Property("countType", "code", "Whether the report is about the current inventory count (snapshot) or a differential change in inventory (change).", 0, 1, countType)); 1407 children.add(new Property("operationType", "CodeableConcept", "What type of operation is being performed - addition or subtraction.", 0, 1, operationType)); 1408 children.add(new Property("operationTypeReason", "CodeableConcept", "The reason for this count - regular count, ad-hoc count, new arrivals, etc.", 0, 1, operationTypeReason)); 1409 children.add(new Property("reportedDateTime", "dateTime", "When the report has been submitted.", 0, 1, reportedDateTime)); 1410 children.add(new Property("reporter", "Reference(Practitioner|Patient|RelatedPerson|Device)", "Who submits the report.", 0, 1, reporter)); 1411 children.add(new Property("reportingPeriod", "Period", "The period the report refers to.", 0, 1, reportingPeriod)); 1412 children.add(new Property("inventoryListing", "", "An inventory listing section (grouped by any of the attributes).", 0, java.lang.Integer.MAX_VALUE, inventoryListing)); 1413 children.add(new Property("note", "Annotation", "A note associated with the InventoryReport.", 0, java.lang.Integer.MAX_VALUE, note)); 1414 } 1415 1416 @Override 1417 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1418 switch (_hash) { 1419 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier for the InventoryReport.", 0, java.lang.Integer.MAX_VALUE, identifier); 1420 case -892481550: /*status*/ return new Property("status", "code", "The status of the inventory check or notification - whether this is draft (e.g. the report is still pending some updates) or active.", 0, 1, status); 1421 case 1351759081: /*countType*/ return new Property("countType", "code", "Whether the report is about the current inventory count (snapshot) or a differential change in inventory (change).", 0, 1, countType); 1422 case 91999553: /*operationType*/ return new Property("operationType", "CodeableConcept", "What type of operation is being performed - addition or subtraction.", 0, 1, operationType); 1423 case 449681125: /*operationTypeReason*/ return new Property("operationTypeReason", "CodeableConcept", "The reason for this count - regular count, ad-hoc count, new arrivals, etc.", 0, 1, operationTypeReason); 1424 case -1048250994: /*reportedDateTime*/ return new Property("reportedDateTime", "dateTime", "When the report has been submitted.", 0, 1, reportedDateTime); 1425 case -427039519: /*reporter*/ return new Property("reporter", "Reference(Practitioner|Patient|RelatedPerson|Device)", "Who submits the report.", 0, 1, reporter); 1426 case 409685391: /*reportingPeriod*/ return new Property("reportingPeriod", "Period", "The period the report refers to.", 0, 1, reportingPeriod); 1427 case -1764804216: /*inventoryListing*/ return new Property("inventoryListing", "", "An inventory listing section (grouped by any of the attributes).", 0, java.lang.Integer.MAX_VALUE, inventoryListing); 1428 case 3387378: /*note*/ return new Property("note", "Annotation", "A note associated with the InventoryReport.", 0, java.lang.Integer.MAX_VALUE, note); 1429 default: return super.getNamedProperty(_hash, _name, _checkValid); 1430 } 1431 1432 } 1433 1434 @Override 1435 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1436 switch (hash) { 1437 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1438 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<InventoryReportStatus> 1439 case 1351759081: /*countType*/ return this.countType == null ? new Base[0] : new Base[] {this.countType}; // Enumeration<InventoryCountType> 1440 case 91999553: /*operationType*/ return this.operationType == null ? new Base[0] : new Base[] {this.operationType}; // CodeableConcept 1441 case 449681125: /*operationTypeReason*/ return this.operationTypeReason == null ? new Base[0] : new Base[] {this.operationTypeReason}; // CodeableConcept 1442 case -1048250994: /*reportedDateTime*/ return this.reportedDateTime == null ? new Base[0] : new Base[] {this.reportedDateTime}; // DateTimeType 1443 case -427039519: /*reporter*/ return this.reporter == null ? new Base[0] : new Base[] {this.reporter}; // Reference 1444 case 409685391: /*reportingPeriod*/ return this.reportingPeriod == null ? new Base[0] : new Base[] {this.reportingPeriod}; // Period 1445 case -1764804216: /*inventoryListing*/ return this.inventoryListing == null ? new Base[0] : this.inventoryListing.toArray(new Base[this.inventoryListing.size()]); // InventoryReportInventoryListingComponent 1446 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1447 default: return super.getProperty(hash, name, checkValid); 1448 } 1449 1450 } 1451 1452 @Override 1453 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1454 switch (hash) { 1455 case -1618432855: // identifier 1456 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1457 return value; 1458 case -892481550: // status 1459 value = new InventoryReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1460 this.status = (Enumeration) value; // Enumeration<InventoryReportStatus> 1461 return value; 1462 case 1351759081: // countType 1463 value = new InventoryCountTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1464 this.countType = (Enumeration) value; // Enumeration<InventoryCountType> 1465 return value; 1466 case 91999553: // operationType 1467 this.operationType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1468 return value; 1469 case 449681125: // operationTypeReason 1470 this.operationTypeReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1471 return value; 1472 case -1048250994: // reportedDateTime 1473 this.reportedDateTime = TypeConvertor.castToDateTime(value); // DateTimeType 1474 return value; 1475 case -427039519: // reporter 1476 this.reporter = TypeConvertor.castToReference(value); // Reference 1477 return value; 1478 case 409685391: // reportingPeriod 1479 this.reportingPeriod = TypeConvertor.castToPeriod(value); // Period 1480 return value; 1481 case -1764804216: // inventoryListing 1482 this.getInventoryListing().add((InventoryReportInventoryListingComponent) value); // InventoryReportInventoryListingComponent 1483 return value; 1484 case 3387378: // note 1485 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1486 return value; 1487 default: return super.setProperty(hash, name, value); 1488 } 1489 1490 } 1491 1492 @Override 1493 public Base setProperty(String name, Base value) throws FHIRException { 1494 if (name.equals("identifier")) { 1495 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1496 } else if (name.equals("status")) { 1497 value = new InventoryReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1498 this.status = (Enumeration) value; // Enumeration<InventoryReportStatus> 1499 } else if (name.equals("countType")) { 1500 value = new InventoryCountTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1501 this.countType = (Enumeration) value; // Enumeration<InventoryCountType> 1502 } else if (name.equals("operationType")) { 1503 this.operationType = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1504 } else if (name.equals("operationTypeReason")) { 1505 this.operationTypeReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1506 } else if (name.equals("reportedDateTime")) { 1507 this.reportedDateTime = TypeConvertor.castToDateTime(value); // DateTimeType 1508 } else if (name.equals("reporter")) { 1509 this.reporter = TypeConvertor.castToReference(value); // Reference 1510 } else if (name.equals("reportingPeriod")) { 1511 this.reportingPeriod = TypeConvertor.castToPeriod(value); // Period 1512 } else if (name.equals("inventoryListing")) { 1513 this.getInventoryListing().add((InventoryReportInventoryListingComponent) value); 1514 } else if (name.equals("note")) { 1515 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1516 } else 1517 return super.setProperty(name, value); 1518 return value; 1519 } 1520 1521 @Override 1522 public void removeChild(String name, Base value) throws FHIRException { 1523 if (name.equals("identifier")) { 1524 this.getIdentifier().remove(value); 1525 } else if (name.equals("status")) { 1526 value = new InventoryReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1527 this.status = (Enumeration) value; // Enumeration<InventoryReportStatus> 1528 } else if (name.equals("countType")) { 1529 value = new InventoryCountTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1530 this.countType = (Enumeration) value; // Enumeration<InventoryCountType> 1531 } else if (name.equals("operationType")) { 1532 this.operationType = null; 1533 } else if (name.equals("operationTypeReason")) { 1534 this.operationTypeReason = null; 1535 } else if (name.equals("reportedDateTime")) { 1536 this.reportedDateTime = null; 1537 } else if (name.equals("reporter")) { 1538 this.reporter = null; 1539 } else if (name.equals("reportingPeriod")) { 1540 this.reportingPeriod = null; 1541 } else if (name.equals("inventoryListing")) { 1542 this.getInventoryListing().remove((InventoryReportInventoryListingComponent) value); 1543 } else if (name.equals("note")) { 1544 this.getNote().remove(value); 1545 } else 1546 super.removeChild(name, value); 1547 1548 } 1549 1550 @Override 1551 public Base makeProperty(int hash, String name) throws FHIRException { 1552 switch (hash) { 1553 case -1618432855: return addIdentifier(); 1554 case -892481550: return getStatusElement(); 1555 case 1351759081: return getCountTypeElement(); 1556 case 91999553: return getOperationType(); 1557 case 449681125: return getOperationTypeReason(); 1558 case -1048250994: return getReportedDateTimeElement(); 1559 case -427039519: return getReporter(); 1560 case 409685391: return getReportingPeriod(); 1561 case -1764804216: return addInventoryListing(); 1562 case 3387378: return addNote(); 1563 default: return super.makeProperty(hash, name); 1564 } 1565 1566 } 1567 1568 @Override 1569 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1570 switch (hash) { 1571 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1572 case -892481550: /*status*/ return new String[] {"code"}; 1573 case 1351759081: /*countType*/ return new String[] {"code"}; 1574 case 91999553: /*operationType*/ return new String[] {"CodeableConcept"}; 1575 case 449681125: /*operationTypeReason*/ return new String[] {"CodeableConcept"}; 1576 case -1048250994: /*reportedDateTime*/ return new String[] {"dateTime"}; 1577 case -427039519: /*reporter*/ return new String[] {"Reference"}; 1578 case 409685391: /*reportingPeriod*/ return new String[] {"Period"}; 1579 case -1764804216: /*inventoryListing*/ return new String[] {}; 1580 case 3387378: /*note*/ return new String[] {"Annotation"}; 1581 default: return super.getTypesForProperty(hash, name); 1582 } 1583 1584 } 1585 1586 @Override 1587 public Base addChild(String name) throws FHIRException { 1588 if (name.equals("identifier")) { 1589 return addIdentifier(); 1590 } 1591 else if (name.equals("status")) { 1592 throw new FHIRException("Cannot call addChild on a singleton property InventoryReport.status"); 1593 } 1594 else if (name.equals("countType")) { 1595 throw new FHIRException("Cannot call addChild on a singleton property InventoryReport.countType"); 1596 } 1597 else if (name.equals("operationType")) { 1598 this.operationType = new CodeableConcept(); 1599 return this.operationType; 1600 } 1601 else if (name.equals("operationTypeReason")) { 1602 this.operationTypeReason = new CodeableConcept(); 1603 return this.operationTypeReason; 1604 } 1605 else if (name.equals("reportedDateTime")) { 1606 throw new FHIRException("Cannot call addChild on a singleton property InventoryReport.reportedDateTime"); 1607 } 1608 else if (name.equals("reporter")) { 1609 this.reporter = new Reference(); 1610 return this.reporter; 1611 } 1612 else if (name.equals("reportingPeriod")) { 1613 this.reportingPeriod = new Period(); 1614 return this.reportingPeriod; 1615 } 1616 else if (name.equals("inventoryListing")) { 1617 return addInventoryListing(); 1618 } 1619 else if (name.equals("note")) { 1620 return addNote(); 1621 } 1622 else 1623 return super.addChild(name); 1624 } 1625 1626 public String fhirType() { 1627 return "InventoryReport"; 1628 1629 } 1630 1631 public InventoryReport copy() { 1632 InventoryReport dst = new InventoryReport(); 1633 copyValues(dst); 1634 return dst; 1635 } 1636 1637 public void copyValues(InventoryReport dst) { 1638 super.copyValues(dst); 1639 if (identifier != null) { 1640 dst.identifier = new ArrayList<Identifier>(); 1641 for (Identifier i : identifier) 1642 dst.identifier.add(i.copy()); 1643 }; 1644 dst.status = status == null ? null : status.copy(); 1645 dst.countType = countType == null ? null : countType.copy(); 1646 dst.operationType = operationType == null ? null : operationType.copy(); 1647 dst.operationTypeReason = operationTypeReason == null ? null : operationTypeReason.copy(); 1648 dst.reportedDateTime = reportedDateTime == null ? null : reportedDateTime.copy(); 1649 dst.reporter = reporter == null ? null : reporter.copy(); 1650 dst.reportingPeriod = reportingPeriod == null ? null : reportingPeriod.copy(); 1651 if (inventoryListing != null) { 1652 dst.inventoryListing = new ArrayList<InventoryReportInventoryListingComponent>(); 1653 for (InventoryReportInventoryListingComponent i : inventoryListing) 1654 dst.inventoryListing.add(i.copy()); 1655 }; 1656 if (note != null) { 1657 dst.note = new ArrayList<Annotation>(); 1658 for (Annotation i : note) 1659 dst.note.add(i.copy()); 1660 }; 1661 } 1662 1663 protected InventoryReport typedCopy() { 1664 return copy(); 1665 } 1666 1667 @Override 1668 public boolean equalsDeep(Base other_) { 1669 if (!super.equalsDeep(other_)) 1670 return false; 1671 if (!(other_ instanceof InventoryReport)) 1672 return false; 1673 InventoryReport o = (InventoryReport) other_; 1674 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(countType, o.countType, true) 1675 && compareDeep(operationType, o.operationType, true) && compareDeep(operationTypeReason, o.operationTypeReason, true) 1676 && compareDeep(reportedDateTime, o.reportedDateTime, true) && compareDeep(reporter, o.reporter, true) 1677 && compareDeep(reportingPeriod, o.reportingPeriod, true) && compareDeep(inventoryListing, o.inventoryListing, true) 1678 && compareDeep(note, o.note, true); 1679 } 1680 1681 @Override 1682 public boolean equalsShallow(Base other_) { 1683 if (!super.equalsShallow(other_)) 1684 return false; 1685 if (!(other_ instanceof InventoryReport)) 1686 return false; 1687 InventoryReport o = (InventoryReport) other_; 1688 return compareValues(status, o.status, true) && compareValues(countType, o.countType, true) && compareValues(reportedDateTime, o.reportedDateTime, true) 1689 ; 1690 } 1691 1692 public boolean isEmpty() { 1693 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, countType 1694 , operationType, operationTypeReason, reportedDateTime, reporter, reportingPeriod 1695 , inventoryListing, note); 1696 } 1697 1698 @Override 1699 public ResourceType getResourceType() { 1700 return ResourceType.InventoryReport; 1701 } 1702 1703 /** 1704 * Search parameter: <b>identifier</b> 1705 * <p> 1706 * Description: <b>Search by identifier</b><br> 1707 * Type: <b>token</b><br> 1708 * Path: <b>InventoryReport.identifier</b><br> 1709 * </p> 1710 */ 1711 @SearchParamDefinition(name="identifier", path="InventoryReport.identifier", description="Search by identifier", type="token" ) 1712 public static final String SP_IDENTIFIER = "identifier"; 1713 /** 1714 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1715 * <p> 1716 * Description: <b>Search by identifier</b><br> 1717 * Type: <b>token</b><br> 1718 * Path: <b>InventoryReport.identifier</b><br> 1719 * </p> 1720 */ 1721 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1722 1723 /** 1724 * Search parameter: <b>item-reference</b> 1725 * <p> 1726 * Description: <b>Search by items in inventory report</b><br> 1727 * Type: <b>reference</b><br> 1728 * Path: <b>InventoryReport.inventoryListing.item.item.reference</b><br> 1729 * </p> 1730 */ 1731 @SearchParamDefinition(name="item-reference", path="InventoryReport.inventoryListing.item.item.reference", description="Search by items in inventory report", type="reference", target={BiologicallyDerivedProduct.class, Device.class, InventoryItem.class, Medication.class, NutritionProduct.class } ) 1732 public static final String SP_ITEM_REFERENCE = "item-reference"; 1733 /** 1734 * <b>Fluent Client</b> search parameter constant for <b>item-reference</b> 1735 * <p> 1736 * Description: <b>Search by items in inventory report</b><br> 1737 * Type: <b>reference</b><br> 1738 * Path: <b>InventoryReport.inventoryListing.item.item.reference</b><br> 1739 * </p> 1740 */ 1741 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM_REFERENCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ITEM_REFERENCE); 1742 1743/** 1744 * Constant for fluent queries to be used to add include statements. Specifies 1745 * the path value of "<b>InventoryReport:item-reference</b>". 1746 */ 1747 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM_REFERENCE = new ca.uhn.fhir.model.api.Include("InventoryReport:item-reference").toLocked(); 1748 1749 /** 1750 * Search parameter: <b>item</b> 1751 * <p> 1752 * Description: <b>Search by items in inventory report</b><br> 1753 * Type: <b>token</b><br> 1754 * Path: <b>InventoryReport.inventoryListing.item.item.concept</b><br> 1755 * </p> 1756 */ 1757 @SearchParamDefinition(name="item", path="InventoryReport.inventoryListing.item.item.concept", description="Search by items in inventory report", type="token" ) 1758 public static final String SP_ITEM = "item"; 1759 /** 1760 * <b>Fluent Client</b> search parameter constant for <b>item</b> 1761 * <p> 1762 * Description: <b>Search by items in inventory report</b><br> 1763 * Type: <b>token</b><br> 1764 * Path: <b>InventoryReport.inventoryListing.item.item.concept</b><br> 1765 * </p> 1766 */ 1767 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ITEM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ITEM); 1768 1769 /** 1770 * Search parameter: <b>status</b> 1771 * <p> 1772 * Description: <b>Search by status</b><br> 1773 * Type: <b>token</b><br> 1774 * Path: <b>InventoryReport.status</b><br> 1775 * </p> 1776 */ 1777 @SearchParamDefinition(name="status", path="InventoryReport.status", description="Search by status", type="token" ) 1778 public static final String SP_STATUS = "status"; 1779 /** 1780 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1781 * <p> 1782 * Description: <b>Search by status</b><br> 1783 * Type: <b>token</b><br> 1784 * Path: <b>InventoryReport.status</b><br> 1785 * </p> 1786 */ 1787 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1788 1789 1790} 1791