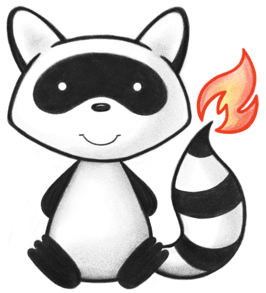
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Invoice containing collected ChargeItems from an Account with calculated individual and total price for Billing purpose. 052 */ 053@ResourceDef(name="Invoice", profile="http://hl7.org/fhir/StructureDefinition/Invoice") 054public class Invoice extends DomainResource { 055 056 public enum InvoiceStatus { 057 /** 058 * the invoice has been prepared but not yet finalized. 059 */ 060 DRAFT, 061 /** 062 * the invoice has been finalized and sent to the recipient. 063 */ 064 ISSUED, 065 /** 066 * the invoice has been balaced / completely paid. 067 */ 068 BALANCED, 069 /** 070 * the invoice was cancelled. 071 */ 072 CANCELLED, 073 /** 074 * the invoice was determined as entered in error before it was issued. 075 */ 076 ENTEREDINERROR, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static InvoiceStatus fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("draft".equals(codeString)) 085 return DRAFT; 086 if ("issued".equals(codeString)) 087 return ISSUED; 088 if ("balanced".equals(codeString)) 089 return BALANCED; 090 if ("cancelled".equals(codeString)) 091 return CANCELLED; 092 if ("entered-in-error".equals(codeString)) 093 return ENTEREDINERROR; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown InvoiceStatus code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case DRAFT: return "draft"; 102 case ISSUED: return "issued"; 103 case BALANCED: return "balanced"; 104 case CANCELLED: return "cancelled"; 105 case ENTEREDINERROR: return "entered-in-error"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case DRAFT: return "http://hl7.org/fhir/invoice-status"; 113 case ISSUED: return "http://hl7.org/fhir/invoice-status"; 114 case BALANCED: return "http://hl7.org/fhir/invoice-status"; 115 case CANCELLED: return "http://hl7.org/fhir/invoice-status"; 116 case ENTEREDINERROR: return "http://hl7.org/fhir/invoice-status"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case DRAFT: return "the invoice has been prepared but not yet finalized."; 124 case ISSUED: return "the invoice has been finalized and sent to the recipient."; 125 case BALANCED: return "the invoice has been balaced / completely paid."; 126 case CANCELLED: return "the invoice was cancelled."; 127 case ENTEREDINERROR: return "the invoice was determined as entered in error before it was issued."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case DRAFT: return "draft"; 135 case ISSUED: return "issued"; 136 case BALANCED: return "balanced"; 137 case CANCELLED: return "cancelled"; 138 case ENTEREDINERROR: return "entered in error"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class InvoiceStatusEnumFactory implements EnumFactory<InvoiceStatus> { 146 public InvoiceStatus fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("draft".equals(codeString)) 151 return InvoiceStatus.DRAFT; 152 if ("issued".equals(codeString)) 153 return InvoiceStatus.ISSUED; 154 if ("balanced".equals(codeString)) 155 return InvoiceStatus.BALANCED; 156 if ("cancelled".equals(codeString)) 157 return InvoiceStatus.CANCELLED; 158 if ("entered-in-error".equals(codeString)) 159 return InvoiceStatus.ENTEREDINERROR; 160 throw new IllegalArgumentException("Unknown InvoiceStatus code '"+codeString+"'"); 161 } 162 public Enumeration<InvoiceStatus> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.NULL, code); 170 if ("draft".equals(codeString)) 171 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.DRAFT, code); 172 if ("issued".equals(codeString)) 173 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.ISSUED, code); 174 if ("balanced".equals(codeString)) 175 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.BALANCED, code); 176 if ("cancelled".equals(codeString)) 177 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.CANCELLED, code); 178 if ("entered-in-error".equals(codeString)) 179 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.ENTEREDINERROR, code); 180 throw new FHIRException("Unknown InvoiceStatus code '"+codeString+"'"); 181 } 182 public String toCode(InvoiceStatus code) { 183 if (code == InvoiceStatus.NULL) 184 return null; 185 if (code == InvoiceStatus.DRAFT) 186 return "draft"; 187 if (code == InvoiceStatus.ISSUED) 188 return "issued"; 189 if (code == InvoiceStatus.BALANCED) 190 return "balanced"; 191 if (code == InvoiceStatus.CANCELLED) 192 return "cancelled"; 193 if (code == InvoiceStatus.ENTEREDINERROR) 194 return "entered-in-error"; 195 return "?"; 196 } 197 public String toSystem(InvoiceStatus code) { 198 return code.getSystem(); 199 } 200 } 201 202 @Block() 203 public static class InvoiceParticipantComponent extends BackboneElement implements IBaseBackboneElement { 204 /** 205 * Describes the type of involvement (e.g. transcriptionist, creator etc.). If the invoice has been created automatically, the Participant may be a billing engine or another kind of device. 206 */ 207 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 208 @Description(shortDefinition="Type of involvement in creation of this Invoice", formalDefinition="Describes the type of involvement (e.g. transcriptionist, creator etc.). If the invoice has been created automatically, the Participant may be a billing engine or another kind of device." ) 209 protected CodeableConcept role; 210 211 /** 212 * The device, practitioner, etc. who performed or participated in the service. 213 */ 214 @Child(name = "actor", type = {Practitioner.class, Organization.class, Patient.class, PractitionerRole.class, Device.class, RelatedPerson.class}, order=2, min=1, max=1, modifier=false, summary=false) 215 @Description(shortDefinition="Individual who was involved", formalDefinition="The device, practitioner, etc. who performed or participated in the service." ) 216 protected Reference actor; 217 218 private static final long serialVersionUID = -1684441509L; 219 220 /** 221 * Constructor 222 */ 223 public InvoiceParticipantComponent() { 224 super(); 225 } 226 227 /** 228 * Constructor 229 */ 230 public InvoiceParticipantComponent(Reference actor) { 231 super(); 232 this.setActor(actor); 233 } 234 235 /** 236 * @return {@link #role} (Describes the type of involvement (e.g. transcriptionist, creator etc.). If the invoice has been created automatically, the Participant may be a billing engine or another kind of device.) 237 */ 238 public CodeableConcept getRole() { 239 if (this.role == null) 240 if (Configuration.errorOnAutoCreate()) 241 throw new Error("Attempt to auto-create InvoiceParticipantComponent.role"); 242 else if (Configuration.doAutoCreate()) 243 this.role = new CodeableConcept(); // cc 244 return this.role; 245 } 246 247 public boolean hasRole() { 248 return this.role != null && !this.role.isEmpty(); 249 } 250 251 /** 252 * @param value {@link #role} (Describes the type of involvement (e.g. transcriptionist, creator etc.). If the invoice has been created automatically, the Participant may be a billing engine or another kind of device.) 253 */ 254 public InvoiceParticipantComponent setRole(CodeableConcept value) { 255 this.role = value; 256 return this; 257 } 258 259 /** 260 * @return {@link #actor} (The device, practitioner, etc. who performed or participated in the service.) 261 */ 262 public Reference getActor() { 263 if (this.actor == null) 264 if (Configuration.errorOnAutoCreate()) 265 throw new Error("Attempt to auto-create InvoiceParticipantComponent.actor"); 266 else if (Configuration.doAutoCreate()) 267 this.actor = new Reference(); // cc 268 return this.actor; 269 } 270 271 public boolean hasActor() { 272 return this.actor != null && !this.actor.isEmpty(); 273 } 274 275 /** 276 * @param value {@link #actor} (The device, practitioner, etc. who performed or participated in the service.) 277 */ 278 public InvoiceParticipantComponent setActor(Reference value) { 279 this.actor = value; 280 return this; 281 } 282 283 protected void listChildren(List<Property> children) { 284 super.listChildren(children); 285 children.add(new Property("role", "CodeableConcept", "Describes the type of involvement (e.g. transcriptionist, creator etc.). If the invoice has been created automatically, the Participant may be a billing engine or another kind of device.", 0, 1, role)); 286 children.add(new Property("actor", "Reference(Practitioner|Organization|Patient|PractitionerRole|Device|RelatedPerson)", "The device, practitioner, etc. who performed or participated in the service.", 0, 1, actor)); 287 } 288 289 @Override 290 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 291 switch (_hash) { 292 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Describes the type of involvement (e.g. transcriptionist, creator etc.). If the invoice has been created automatically, the Participant may be a billing engine or another kind of device.", 0, 1, role); 293 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|Organization|Patient|PractitionerRole|Device|RelatedPerson)", "The device, practitioner, etc. who performed or participated in the service.", 0, 1, actor); 294 default: return super.getNamedProperty(_hash, _name, _checkValid); 295 } 296 297 } 298 299 @Override 300 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 301 switch (hash) { 302 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 303 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 304 default: return super.getProperty(hash, name, checkValid); 305 } 306 307 } 308 309 @Override 310 public Base setProperty(int hash, String name, Base value) throws FHIRException { 311 switch (hash) { 312 case 3506294: // role 313 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 314 return value; 315 case 92645877: // actor 316 this.actor = TypeConvertor.castToReference(value); // Reference 317 return value; 318 default: return super.setProperty(hash, name, value); 319 } 320 321 } 322 323 @Override 324 public Base setProperty(String name, Base value) throws FHIRException { 325 if (name.equals("role")) { 326 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 327 } else if (name.equals("actor")) { 328 this.actor = TypeConvertor.castToReference(value); // Reference 329 } else 330 return super.setProperty(name, value); 331 return value; 332 } 333 334 @Override 335 public void removeChild(String name, Base value) throws FHIRException { 336 if (name.equals("role")) { 337 this.role = null; 338 } else if (name.equals("actor")) { 339 this.actor = null; 340 } else 341 super.removeChild(name, value); 342 343 } 344 345 @Override 346 public Base makeProperty(int hash, String name) throws FHIRException { 347 switch (hash) { 348 case 3506294: return getRole(); 349 case 92645877: return getActor(); 350 default: return super.makeProperty(hash, name); 351 } 352 353 } 354 355 @Override 356 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 357 switch (hash) { 358 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 359 case 92645877: /*actor*/ return new String[] {"Reference"}; 360 default: return super.getTypesForProperty(hash, name); 361 } 362 363 } 364 365 @Override 366 public Base addChild(String name) throws FHIRException { 367 if (name.equals("role")) { 368 this.role = new CodeableConcept(); 369 return this.role; 370 } 371 else if (name.equals("actor")) { 372 this.actor = new Reference(); 373 return this.actor; 374 } 375 else 376 return super.addChild(name); 377 } 378 379 public InvoiceParticipantComponent copy() { 380 InvoiceParticipantComponent dst = new InvoiceParticipantComponent(); 381 copyValues(dst); 382 return dst; 383 } 384 385 public void copyValues(InvoiceParticipantComponent dst) { 386 super.copyValues(dst); 387 dst.role = role == null ? null : role.copy(); 388 dst.actor = actor == null ? null : actor.copy(); 389 } 390 391 @Override 392 public boolean equalsDeep(Base other_) { 393 if (!super.equalsDeep(other_)) 394 return false; 395 if (!(other_ instanceof InvoiceParticipantComponent)) 396 return false; 397 InvoiceParticipantComponent o = (InvoiceParticipantComponent) other_; 398 return compareDeep(role, o.role, true) && compareDeep(actor, o.actor, true); 399 } 400 401 @Override 402 public boolean equalsShallow(Base other_) { 403 if (!super.equalsShallow(other_)) 404 return false; 405 if (!(other_ instanceof InvoiceParticipantComponent)) 406 return false; 407 InvoiceParticipantComponent o = (InvoiceParticipantComponent) other_; 408 return true; 409 } 410 411 public boolean isEmpty() { 412 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, actor); 413 } 414 415 public String fhirType() { 416 return "Invoice.participant"; 417 418 } 419 420 } 421 422 @Block() 423 public static class InvoiceLineItemComponent extends BackboneElement implements IBaseBackboneElement { 424 /** 425 * Sequence in which the items appear on the invoice. 426 */ 427 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 428 @Description(shortDefinition="Sequence number of line item", formalDefinition="Sequence in which the items appear on the invoice." ) 429 protected PositiveIntType sequence; 430 431 /** 432 * Date/time(s) range when this service was delivered or completed. 433 */ 434 @Child(name = "serviced", type = {DateType.class, Period.class}, order=2, min=0, max=1, modifier=false, summary=false) 435 @Description(shortDefinition="Service data or period", formalDefinition="Date/time(s) range when this service was delivered or completed." ) 436 protected DataType serviced; 437 438 /** 439 * The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference. 440 */ 441 @Child(name = "chargeItem", type = {ChargeItem.class, CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=false) 442 @Description(shortDefinition="Reference to ChargeItem containing details of this line item or an inline billing code", formalDefinition="The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference." ) 443 protected DataType chargeItem; 444 445 /** 446 * The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice as to how the prices have been calculated. 447 */ 448 @Child(name = "priceComponent", type = {MonetaryComponent.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 449 @Description(shortDefinition="Components of total line item price", formalDefinition="The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice as to how the prices have been calculated." ) 450 protected List<MonetaryComponent> priceComponent; 451 452 private static final long serialVersionUID = -9393053L; 453 454 /** 455 * Constructor 456 */ 457 public InvoiceLineItemComponent() { 458 super(); 459 } 460 461 /** 462 * Constructor 463 */ 464 public InvoiceLineItemComponent(DataType chargeItem) { 465 super(); 466 this.setChargeItem(chargeItem); 467 } 468 469 /** 470 * @return {@link #sequence} (Sequence in which the items appear on the invoice.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 471 */ 472 public PositiveIntType getSequenceElement() { 473 if (this.sequence == null) 474 if (Configuration.errorOnAutoCreate()) 475 throw new Error("Attempt to auto-create InvoiceLineItemComponent.sequence"); 476 else if (Configuration.doAutoCreate()) 477 this.sequence = new PositiveIntType(); // bb 478 return this.sequence; 479 } 480 481 public boolean hasSequenceElement() { 482 return this.sequence != null && !this.sequence.isEmpty(); 483 } 484 485 public boolean hasSequence() { 486 return this.sequence != null && !this.sequence.isEmpty(); 487 } 488 489 /** 490 * @param value {@link #sequence} (Sequence in which the items appear on the invoice.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 491 */ 492 public InvoiceLineItemComponent setSequenceElement(PositiveIntType value) { 493 this.sequence = value; 494 return this; 495 } 496 497 /** 498 * @return Sequence in which the items appear on the invoice. 499 */ 500 public int getSequence() { 501 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 502 } 503 504 /** 505 * @param value Sequence in which the items appear on the invoice. 506 */ 507 public InvoiceLineItemComponent setSequence(int value) { 508 if (this.sequence == null) 509 this.sequence = new PositiveIntType(); 510 this.sequence.setValue(value); 511 return this; 512 } 513 514 /** 515 * @return {@link #serviced} (Date/time(s) range when this service was delivered or completed.) 516 */ 517 public DataType getServiced() { 518 return this.serviced; 519 } 520 521 /** 522 * @return {@link #serviced} (Date/time(s) range when this service was delivered or completed.) 523 */ 524 public DateType getServicedDateType() throws FHIRException { 525 if (this.serviced == null) 526 this.serviced = new DateType(); 527 if (!(this.serviced instanceof DateType)) 528 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.serviced.getClass().getName()+" was encountered"); 529 return (DateType) this.serviced; 530 } 531 532 public boolean hasServicedDateType() { 533 return this.serviced instanceof DateType; 534 } 535 536 /** 537 * @return {@link #serviced} (Date/time(s) range when this service was delivered or completed.) 538 */ 539 public Period getServicedPeriod() throws FHIRException { 540 if (this.serviced == null) 541 this.serviced = new Period(); 542 if (!(this.serviced instanceof Period)) 543 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.serviced.getClass().getName()+" was encountered"); 544 return (Period) this.serviced; 545 } 546 547 public boolean hasServicedPeriod() { 548 return this.serviced instanceof Period; 549 } 550 551 public boolean hasServiced() { 552 return this.serviced != null && !this.serviced.isEmpty(); 553 } 554 555 /** 556 * @param value {@link #serviced} (Date/time(s) range when this service was delivered or completed.) 557 */ 558 public InvoiceLineItemComponent setServiced(DataType value) { 559 if (value != null && !(value instanceof DateType || value instanceof Period)) 560 throw new FHIRException("Not the right type for Invoice.lineItem.serviced[x]: "+value.fhirType()); 561 this.serviced = value; 562 return this; 563 } 564 565 /** 566 * @return {@link #chargeItem} (The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.) 567 */ 568 public DataType getChargeItem() { 569 return this.chargeItem; 570 } 571 572 /** 573 * @return {@link #chargeItem} (The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.) 574 */ 575 public Reference getChargeItemReference() throws FHIRException { 576 if (this.chargeItem == null) 577 this.chargeItem = new Reference(); 578 if (!(this.chargeItem instanceof Reference)) 579 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.chargeItem.getClass().getName()+" was encountered"); 580 return (Reference) this.chargeItem; 581 } 582 583 public boolean hasChargeItemReference() { 584 return this.chargeItem instanceof Reference; 585 } 586 587 /** 588 * @return {@link #chargeItem} (The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.) 589 */ 590 public CodeableConcept getChargeItemCodeableConcept() throws FHIRException { 591 if (this.chargeItem == null) 592 this.chargeItem = new CodeableConcept(); 593 if (!(this.chargeItem instanceof CodeableConcept)) 594 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.chargeItem.getClass().getName()+" was encountered"); 595 return (CodeableConcept) this.chargeItem; 596 } 597 598 public boolean hasChargeItemCodeableConcept() { 599 return this.chargeItem instanceof CodeableConcept; 600 } 601 602 public boolean hasChargeItem() { 603 return this.chargeItem != null && !this.chargeItem.isEmpty(); 604 } 605 606 /** 607 * @param value {@link #chargeItem} (The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.) 608 */ 609 public InvoiceLineItemComponent setChargeItem(DataType value) { 610 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 611 throw new FHIRException("Not the right type for Invoice.lineItem.chargeItem[x]: "+value.fhirType()); 612 this.chargeItem = value; 613 return this; 614 } 615 616 /** 617 * @return {@link #priceComponent} (The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice as to how the prices have been calculated.) 618 */ 619 public List<MonetaryComponent> getPriceComponent() { 620 if (this.priceComponent == null) 621 this.priceComponent = new ArrayList<MonetaryComponent>(); 622 return this.priceComponent; 623 } 624 625 /** 626 * @return Returns a reference to <code>this</code> for easy method chaining 627 */ 628 public InvoiceLineItemComponent setPriceComponent(List<MonetaryComponent> thePriceComponent) { 629 this.priceComponent = thePriceComponent; 630 return this; 631 } 632 633 public boolean hasPriceComponent() { 634 if (this.priceComponent == null) 635 return false; 636 for (MonetaryComponent item : this.priceComponent) 637 if (!item.isEmpty()) 638 return true; 639 return false; 640 } 641 642 public MonetaryComponent addPriceComponent() { //3 643 MonetaryComponent t = new MonetaryComponent(); 644 if (this.priceComponent == null) 645 this.priceComponent = new ArrayList<MonetaryComponent>(); 646 this.priceComponent.add(t); 647 return t; 648 } 649 650 public InvoiceLineItemComponent addPriceComponent(MonetaryComponent t) { //3 651 if (t == null) 652 return this; 653 if (this.priceComponent == null) 654 this.priceComponent = new ArrayList<MonetaryComponent>(); 655 this.priceComponent.add(t); 656 return this; 657 } 658 659 /** 660 * @return The first repetition of repeating field {@link #priceComponent}, creating it if it does not already exist {3} 661 */ 662 public MonetaryComponent getPriceComponentFirstRep() { 663 if (getPriceComponent().isEmpty()) { 664 addPriceComponent(); 665 } 666 return getPriceComponent().get(0); 667 } 668 669 protected void listChildren(List<Property> children) { 670 super.listChildren(children); 671 children.add(new Property("sequence", "positiveInt", "Sequence in which the items appear on the invoice.", 0, 1, sequence)); 672 children.add(new Property("serviced[x]", "date|Period", "Date/time(s) range when this service was delivered or completed.", 0, 1, serviced)); 673 children.add(new Property("chargeItem[x]", "Reference(ChargeItem)|CodeableConcept", "The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.", 0, 1, chargeItem)); 674 children.add(new Property("priceComponent", "MonetaryComponent", "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice as to how the prices have been calculated.", 0, java.lang.Integer.MAX_VALUE, priceComponent)); 675 } 676 677 @Override 678 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 679 switch (_hash) { 680 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence in which the items appear on the invoice.", 0, 1, sequence); 681 case -1927922223: /*serviced[x]*/ return new Property("serviced[x]", "date|Period", "Date/time(s) range when this service was delivered or completed.", 0, 1, serviced); 682 case 1379209295: /*serviced*/ return new Property("serviced[x]", "date|Period", "Date/time(s) range when this service was delivered or completed.", 0, 1, serviced); 683 case 363246749: /*servicedDate*/ return new Property("serviced[x]", "date", "Date/time(s) range when this service was delivered or completed.", 0, 1, serviced); 684 case 1534966512: /*servicedPeriod*/ return new Property("serviced[x]", "Period", "Date/time(s) range when this service was delivered or completed.", 0, 1, serviced); 685 case 351104825: /*chargeItem[x]*/ return new Property("chargeItem[x]", "Reference(ChargeItem)|CodeableConcept", "The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.", 0, 1, chargeItem); 686 case 1417779175: /*chargeItem*/ return new Property("chargeItem[x]", "Reference(ChargeItem)|CodeableConcept", "The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.", 0, 1, chargeItem); 687 case 753580836: /*chargeItemReference*/ return new Property("chargeItem[x]", "Reference(ChargeItem)", "The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.", 0, 1, chargeItem); 688 case 1226532026: /*chargeItemCodeableConcept*/ return new Property("chargeItem[x]", "CodeableConcept", "The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.", 0, 1, chargeItem); 689 case 1219095988: /*priceComponent*/ return new Property("priceComponent", "MonetaryComponent", "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice as to how the prices have been calculated.", 0, java.lang.Integer.MAX_VALUE, priceComponent); 690 default: return super.getNamedProperty(_hash, _name, _checkValid); 691 } 692 693 } 694 695 @Override 696 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 697 switch (hash) { 698 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 699 case 1379209295: /*serviced*/ return this.serviced == null ? new Base[0] : new Base[] {this.serviced}; // DataType 700 case 1417779175: /*chargeItem*/ return this.chargeItem == null ? new Base[0] : new Base[] {this.chargeItem}; // DataType 701 case 1219095988: /*priceComponent*/ return this.priceComponent == null ? new Base[0] : this.priceComponent.toArray(new Base[this.priceComponent.size()]); // MonetaryComponent 702 default: return super.getProperty(hash, name, checkValid); 703 } 704 705 } 706 707 @Override 708 public Base setProperty(int hash, String name, Base value) throws FHIRException { 709 switch (hash) { 710 case 1349547969: // sequence 711 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 712 return value; 713 case 1379209295: // serviced 714 this.serviced = TypeConvertor.castToType(value); // DataType 715 return value; 716 case 1417779175: // chargeItem 717 this.chargeItem = TypeConvertor.castToType(value); // DataType 718 return value; 719 case 1219095988: // priceComponent 720 this.getPriceComponent().add(TypeConvertor.castToMonetaryComponent(value)); // MonetaryComponent 721 return value; 722 default: return super.setProperty(hash, name, value); 723 } 724 725 } 726 727 @Override 728 public Base setProperty(String name, Base value) throws FHIRException { 729 if (name.equals("sequence")) { 730 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 731 } else if (name.equals("serviced[x]")) { 732 this.serviced = TypeConvertor.castToType(value); // DataType 733 } else if (name.equals("chargeItem[x]")) { 734 this.chargeItem = TypeConvertor.castToType(value); // DataType 735 } else if (name.equals("priceComponent")) { 736 this.getPriceComponent().add(TypeConvertor.castToMonetaryComponent(value)); 737 } else 738 return super.setProperty(name, value); 739 return value; 740 } 741 742 @Override 743 public void removeChild(String name, Base value) throws FHIRException { 744 if (name.equals("sequence")) { 745 this.sequence = null; 746 } else if (name.equals("serviced[x]")) { 747 this.serviced = null; 748 } else if (name.equals("chargeItem[x]")) { 749 this.chargeItem = null; 750 } else if (name.equals("priceComponent")) { 751 this.getPriceComponent().remove(value); 752 } else 753 super.removeChild(name, value); 754 755 } 756 757 @Override 758 public Base makeProperty(int hash, String name) throws FHIRException { 759 switch (hash) { 760 case 1349547969: return getSequenceElement(); 761 case -1927922223: return getServiced(); 762 case 1379209295: return getServiced(); 763 case 351104825: return getChargeItem(); 764 case 1417779175: return getChargeItem(); 765 case 1219095988: return addPriceComponent(); 766 default: return super.makeProperty(hash, name); 767 } 768 769 } 770 771 @Override 772 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 773 switch (hash) { 774 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 775 case 1379209295: /*serviced*/ return new String[] {"date", "Period"}; 776 case 1417779175: /*chargeItem*/ return new String[] {"Reference", "CodeableConcept"}; 777 case 1219095988: /*priceComponent*/ return new String[] {"MonetaryComponent"}; 778 default: return super.getTypesForProperty(hash, name); 779 } 780 781 } 782 783 @Override 784 public Base addChild(String name) throws FHIRException { 785 if (name.equals("sequence")) { 786 throw new FHIRException("Cannot call addChild on a singleton property Invoice.lineItem.sequence"); 787 } 788 else if (name.equals("servicedDate")) { 789 this.serviced = new DateType(); 790 return this.serviced; 791 } 792 else if (name.equals("servicedPeriod")) { 793 this.serviced = new Period(); 794 return this.serviced; 795 } 796 else if (name.equals("chargeItemReference")) { 797 this.chargeItem = new Reference(); 798 return this.chargeItem; 799 } 800 else if (name.equals("chargeItemCodeableConcept")) { 801 this.chargeItem = new CodeableConcept(); 802 return this.chargeItem; 803 } 804 else if (name.equals("priceComponent")) { 805 return addPriceComponent(); 806 } 807 else 808 return super.addChild(name); 809 } 810 811 public InvoiceLineItemComponent copy() { 812 InvoiceLineItemComponent dst = new InvoiceLineItemComponent(); 813 copyValues(dst); 814 return dst; 815 } 816 817 public void copyValues(InvoiceLineItemComponent dst) { 818 super.copyValues(dst); 819 dst.sequence = sequence == null ? null : sequence.copy(); 820 dst.serviced = serviced == null ? null : serviced.copy(); 821 dst.chargeItem = chargeItem == null ? null : chargeItem.copy(); 822 if (priceComponent != null) { 823 dst.priceComponent = new ArrayList<MonetaryComponent>(); 824 for (MonetaryComponent i : priceComponent) 825 dst.priceComponent.add(i.copy()); 826 }; 827 } 828 829 @Override 830 public boolean equalsDeep(Base other_) { 831 if (!super.equalsDeep(other_)) 832 return false; 833 if (!(other_ instanceof InvoiceLineItemComponent)) 834 return false; 835 InvoiceLineItemComponent o = (InvoiceLineItemComponent) other_; 836 return compareDeep(sequence, o.sequence, true) && compareDeep(serviced, o.serviced, true) && compareDeep(chargeItem, o.chargeItem, true) 837 && compareDeep(priceComponent, o.priceComponent, true); 838 } 839 840 @Override 841 public boolean equalsShallow(Base other_) { 842 if (!super.equalsShallow(other_)) 843 return false; 844 if (!(other_ instanceof InvoiceLineItemComponent)) 845 return false; 846 InvoiceLineItemComponent o = (InvoiceLineItemComponent) other_; 847 return compareValues(sequence, o.sequence, true); 848 } 849 850 public boolean isEmpty() { 851 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, serviced, chargeItem 852 , priceComponent); 853 } 854 855 public String fhirType() { 856 return "Invoice.lineItem"; 857 858 } 859 860 } 861 862 /** 863 * Identifier of this Invoice, often used for reference in correspondence about this invoice or for tracking of payments. 864 */ 865 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 866 @Description(shortDefinition="Business Identifier for item", formalDefinition="Identifier of this Invoice, often used for reference in correspondence about this invoice or for tracking of payments." ) 867 protected List<Identifier> identifier; 868 869 /** 870 * The current state of the Invoice. 871 */ 872 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 873 @Description(shortDefinition="draft | issued | balanced | cancelled | entered-in-error", formalDefinition="The current state of the Invoice." ) 874 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/invoice-status") 875 protected Enumeration<InvoiceStatus> status; 876 877 /** 878 * In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.). 879 */ 880 @Child(name = "cancelledReason", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 881 @Description(shortDefinition="Reason for cancellation of this Invoice", formalDefinition="In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.)." ) 882 protected StringType cancelledReason; 883 884 /** 885 * Type of Invoice depending on domain, realm an usage (e.g. internal/external, dental, preliminary). 886 */ 887 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 888 @Description(shortDefinition="Type of Invoice", formalDefinition="Type of Invoice depending on domain, realm an usage (e.g. internal/external, dental, preliminary)." ) 889 protected CodeableConcept type; 890 891 /** 892 * The individual or set of individuals receiving the goods and services billed in this invoice. 893 */ 894 @Child(name = "subject", type = {Patient.class, Group.class}, order=4, min=0, max=1, modifier=false, summary=true) 895 @Description(shortDefinition="Recipient(s) of goods and services", formalDefinition="The individual or set of individuals receiving the goods and services billed in this invoice." ) 896 protected Reference subject; 897 898 /** 899 * The individual or Organization responsible for balancing of this invoice. 900 */ 901 @Child(name = "recipient", type = {Organization.class, Patient.class, RelatedPerson.class}, order=5, min=0, max=1, modifier=false, summary=true) 902 @Description(shortDefinition="Recipient of this invoice", formalDefinition="The individual or Organization responsible for balancing of this invoice." ) 903 protected Reference recipient; 904 905 /** 906 * Depricared by the element below. 907 */ 908 @Child(name = "date", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 909 @Description(shortDefinition="DEPRICATED", formalDefinition="Depricared by the element below." ) 910 protected DateTimeType date; 911 912 /** 913 * Date/time(s) of when this Invoice was posted. 914 */ 915 @Child(name = "creation", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 916 @Description(shortDefinition="When posted", formalDefinition="Date/time(s) of when this Invoice was posted." ) 917 protected DateTimeType creation; 918 919 /** 920 * Date/time(s) range of services included in this invoice. 921 */ 922 @Child(name = "period", type = {DateType.class, Period.class}, order=8, min=0, max=1, modifier=false, summary=true) 923 @Description(shortDefinition="Billing date or period", formalDefinition="Date/time(s) range of services included in this invoice." ) 924 protected DataType period; 925 926 /** 927 * Indicates who or what performed or participated in the charged service. 928 */ 929 @Child(name = "participant", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 930 @Description(shortDefinition="Participant in creation of this Invoice", formalDefinition="Indicates who or what performed or participated in the charged service." ) 931 protected List<InvoiceParticipantComponent> participant; 932 933 /** 934 * The organizationissuing the Invoice. 935 */ 936 @Child(name = "issuer", type = {Organization.class}, order=10, min=0, max=1, modifier=false, summary=false) 937 @Description(shortDefinition="Issuing Organization of Invoice", formalDefinition="The organizationissuing the Invoice." ) 938 protected Reference issuer; 939 940 /** 941 * Account which is supposed to be balanced with this Invoice. 942 */ 943 @Child(name = "account", type = {Account.class}, order=11, min=0, max=1, modifier=false, summary=false) 944 @Description(shortDefinition="Account that is being balanced", formalDefinition="Account which is supposed to be balanced with this Invoice." ) 945 protected Reference account; 946 947 /** 948 * Each line item represents one charge for goods and services rendered. Details such.ofType(date), code and amount are found in the referenced ChargeItem resource. 949 */ 950 @Child(name = "lineItem", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 951 @Description(shortDefinition="Line items of this Invoice", formalDefinition="Each line item represents one charge for goods and services rendered. Details such.ofType(date), code and amount are found in the referenced ChargeItem resource." ) 952 protected List<InvoiceLineItemComponent> lineItem; 953 954 /** 955 * The total amount for the Invoice may be calculated as the sum of the line items with surcharges/deductions that apply in certain conditions. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the total price was calculated. 956 */ 957 @Child(name = "totalPriceComponent", type = {MonetaryComponent.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 958 @Description(shortDefinition="Components of Invoice total", formalDefinition="The total amount for the Invoice may be calculated as the sum of the line items with surcharges/deductions that apply in certain conditions. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the total price was calculated." ) 959 protected List<MonetaryComponent> totalPriceComponent; 960 961 /** 962 * Invoice total , taxes excluded. 963 */ 964 @Child(name = "totalNet", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=true) 965 @Description(shortDefinition="Net total of this Invoice", formalDefinition="Invoice total , taxes excluded." ) 966 protected Money totalNet; 967 968 /** 969 * Invoice total, tax included. 970 */ 971 @Child(name = "totalGross", type = {Money.class}, order=15, min=0, max=1, modifier=false, summary=true) 972 @Description(shortDefinition="Gross total of this Invoice", formalDefinition="Invoice total, tax included." ) 973 protected Money totalGross; 974 975 /** 976 * Payment details such as banking details, period of payment, deductibles, methods of payment. 977 */ 978 @Child(name = "paymentTerms", type = {MarkdownType.class}, order=16, min=0, max=1, modifier=false, summary=false) 979 @Description(shortDefinition="Payment details", formalDefinition="Payment details such as banking details, period of payment, deductibles, methods of payment." ) 980 protected MarkdownType paymentTerms; 981 982 /** 983 * Comments made about the invoice by the issuer, subject, or other participants. 984 */ 985 @Child(name = "note", type = {Annotation.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 986 @Description(shortDefinition="Comments made about the invoice", formalDefinition="Comments made about the invoice by the issuer, subject, or other participants." ) 987 protected List<Annotation> note; 988 989 private static final long serialVersionUID = 6346282L; 990 991 /** 992 * Constructor 993 */ 994 public Invoice() { 995 super(); 996 } 997 998 /** 999 * Constructor 1000 */ 1001 public Invoice(InvoiceStatus status) { 1002 super(); 1003 this.setStatus(status); 1004 } 1005 1006 /** 1007 * @return {@link #identifier} (Identifier of this Invoice, often used for reference in correspondence about this invoice or for tracking of payments.) 1008 */ 1009 public List<Identifier> getIdentifier() { 1010 if (this.identifier == null) 1011 this.identifier = new ArrayList<Identifier>(); 1012 return this.identifier; 1013 } 1014 1015 /** 1016 * @return Returns a reference to <code>this</code> for easy method chaining 1017 */ 1018 public Invoice setIdentifier(List<Identifier> theIdentifier) { 1019 this.identifier = theIdentifier; 1020 return this; 1021 } 1022 1023 public boolean hasIdentifier() { 1024 if (this.identifier == null) 1025 return false; 1026 for (Identifier item : this.identifier) 1027 if (!item.isEmpty()) 1028 return true; 1029 return false; 1030 } 1031 1032 public Identifier addIdentifier() { //3 1033 Identifier t = new Identifier(); 1034 if (this.identifier == null) 1035 this.identifier = new ArrayList<Identifier>(); 1036 this.identifier.add(t); 1037 return t; 1038 } 1039 1040 public Invoice addIdentifier(Identifier t) { //3 1041 if (t == null) 1042 return this; 1043 if (this.identifier == null) 1044 this.identifier = new ArrayList<Identifier>(); 1045 this.identifier.add(t); 1046 return this; 1047 } 1048 1049 /** 1050 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1051 */ 1052 public Identifier getIdentifierFirstRep() { 1053 if (getIdentifier().isEmpty()) { 1054 addIdentifier(); 1055 } 1056 return getIdentifier().get(0); 1057 } 1058 1059 /** 1060 * @return {@link #status} (The current state of the Invoice.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1061 */ 1062 public Enumeration<InvoiceStatus> getStatusElement() { 1063 if (this.status == null) 1064 if (Configuration.errorOnAutoCreate()) 1065 throw new Error("Attempt to auto-create Invoice.status"); 1066 else if (Configuration.doAutoCreate()) 1067 this.status = new Enumeration<InvoiceStatus>(new InvoiceStatusEnumFactory()); // bb 1068 return this.status; 1069 } 1070 1071 public boolean hasStatusElement() { 1072 return this.status != null && !this.status.isEmpty(); 1073 } 1074 1075 public boolean hasStatus() { 1076 return this.status != null && !this.status.isEmpty(); 1077 } 1078 1079 /** 1080 * @param value {@link #status} (The current state of the Invoice.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1081 */ 1082 public Invoice setStatusElement(Enumeration<InvoiceStatus> value) { 1083 this.status = value; 1084 return this; 1085 } 1086 1087 /** 1088 * @return The current state of the Invoice. 1089 */ 1090 public InvoiceStatus getStatus() { 1091 return this.status == null ? null : this.status.getValue(); 1092 } 1093 1094 /** 1095 * @param value The current state of the Invoice. 1096 */ 1097 public Invoice setStatus(InvoiceStatus value) { 1098 if (this.status == null) 1099 this.status = new Enumeration<InvoiceStatus>(new InvoiceStatusEnumFactory()); 1100 this.status.setValue(value); 1101 return this; 1102 } 1103 1104 /** 1105 * @return {@link #cancelledReason} (In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.).). This is the underlying object with id, value and extensions. The accessor "getCancelledReason" gives direct access to the value 1106 */ 1107 public StringType getCancelledReasonElement() { 1108 if (this.cancelledReason == null) 1109 if (Configuration.errorOnAutoCreate()) 1110 throw new Error("Attempt to auto-create Invoice.cancelledReason"); 1111 else if (Configuration.doAutoCreate()) 1112 this.cancelledReason = new StringType(); // bb 1113 return this.cancelledReason; 1114 } 1115 1116 public boolean hasCancelledReasonElement() { 1117 return this.cancelledReason != null && !this.cancelledReason.isEmpty(); 1118 } 1119 1120 public boolean hasCancelledReason() { 1121 return this.cancelledReason != null && !this.cancelledReason.isEmpty(); 1122 } 1123 1124 /** 1125 * @param value {@link #cancelledReason} (In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.).). This is the underlying object with id, value and extensions. The accessor "getCancelledReason" gives direct access to the value 1126 */ 1127 public Invoice setCancelledReasonElement(StringType value) { 1128 this.cancelledReason = value; 1129 return this; 1130 } 1131 1132 /** 1133 * @return In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.). 1134 */ 1135 public String getCancelledReason() { 1136 return this.cancelledReason == null ? null : this.cancelledReason.getValue(); 1137 } 1138 1139 /** 1140 * @param value In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.). 1141 */ 1142 public Invoice setCancelledReason(String value) { 1143 if (Utilities.noString(value)) 1144 this.cancelledReason = null; 1145 else { 1146 if (this.cancelledReason == null) 1147 this.cancelledReason = new StringType(); 1148 this.cancelledReason.setValue(value); 1149 } 1150 return this; 1151 } 1152 1153 /** 1154 * @return {@link #type} (Type of Invoice depending on domain, realm an usage (e.g. internal/external, dental, preliminary).) 1155 */ 1156 public CodeableConcept getType() { 1157 if (this.type == null) 1158 if (Configuration.errorOnAutoCreate()) 1159 throw new Error("Attempt to auto-create Invoice.type"); 1160 else if (Configuration.doAutoCreate()) 1161 this.type = new CodeableConcept(); // cc 1162 return this.type; 1163 } 1164 1165 public boolean hasType() { 1166 return this.type != null && !this.type.isEmpty(); 1167 } 1168 1169 /** 1170 * @param value {@link #type} (Type of Invoice depending on domain, realm an usage (e.g. internal/external, dental, preliminary).) 1171 */ 1172 public Invoice setType(CodeableConcept value) { 1173 this.type = value; 1174 return this; 1175 } 1176 1177 /** 1178 * @return {@link #subject} (The individual or set of individuals receiving the goods and services billed in this invoice.) 1179 */ 1180 public Reference getSubject() { 1181 if (this.subject == null) 1182 if (Configuration.errorOnAutoCreate()) 1183 throw new Error("Attempt to auto-create Invoice.subject"); 1184 else if (Configuration.doAutoCreate()) 1185 this.subject = new Reference(); // cc 1186 return this.subject; 1187 } 1188 1189 public boolean hasSubject() { 1190 return this.subject != null && !this.subject.isEmpty(); 1191 } 1192 1193 /** 1194 * @param value {@link #subject} (The individual or set of individuals receiving the goods and services billed in this invoice.) 1195 */ 1196 public Invoice setSubject(Reference value) { 1197 this.subject = value; 1198 return this; 1199 } 1200 1201 /** 1202 * @return {@link #recipient} (The individual or Organization responsible for balancing of this invoice.) 1203 */ 1204 public Reference getRecipient() { 1205 if (this.recipient == null) 1206 if (Configuration.errorOnAutoCreate()) 1207 throw new Error("Attempt to auto-create Invoice.recipient"); 1208 else if (Configuration.doAutoCreate()) 1209 this.recipient = new Reference(); // cc 1210 return this.recipient; 1211 } 1212 1213 public boolean hasRecipient() { 1214 return this.recipient != null && !this.recipient.isEmpty(); 1215 } 1216 1217 /** 1218 * @param value {@link #recipient} (The individual or Organization responsible for balancing of this invoice.) 1219 */ 1220 public Invoice setRecipient(Reference value) { 1221 this.recipient = value; 1222 return this; 1223 } 1224 1225 /** 1226 * @return {@link #date} (Depricared by the element below.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1227 */ 1228 public DateTimeType getDateElement() { 1229 if (this.date == null) 1230 if (Configuration.errorOnAutoCreate()) 1231 throw new Error("Attempt to auto-create Invoice.date"); 1232 else if (Configuration.doAutoCreate()) 1233 this.date = new DateTimeType(); // bb 1234 return this.date; 1235 } 1236 1237 public boolean hasDateElement() { 1238 return this.date != null && !this.date.isEmpty(); 1239 } 1240 1241 public boolean hasDate() { 1242 return this.date != null && !this.date.isEmpty(); 1243 } 1244 1245 /** 1246 * @param value {@link #date} (Depricared by the element below.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1247 */ 1248 public Invoice setDateElement(DateTimeType value) { 1249 this.date = value; 1250 return this; 1251 } 1252 1253 /** 1254 * @return Depricared by the element below. 1255 */ 1256 public Date getDate() { 1257 return this.date == null ? null : this.date.getValue(); 1258 } 1259 1260 /** 1261 * @param value Depricared by the element below. 1262 */ 1263 public Invoice setDate(Date value) { 1264 if (value == null) 1265 this.date = null; 1266 else { 1267 if (this.date == null) 1268 this.date = new DateTimeType(); 1269 this.date.setValue(value); 1270 } 1271 return this; 1272 } 1273 1274 /** 1275 * @return {@link #creation} (Date/time(s) of when this Invoice was posted.). This is the underlying object with id, value and extensions. The accessor "getCreation" gives direct access to the value 1276 */ 1277 public DateTimeType getCreationElement() { 1278 if (this.creation == null) 1279 if (Configuration.errorOnAutoCreate()) 1280 throw new Error("Attempt to auto-create Invoice.creation"); 1281 else if (Configuration.doAutoCreate()) 1282 this.creation = new DateTimeType(); // bb 1283 return this.creation; 1284 } 1285 1286 public boolean hasCreationElement() { 1287 return this.creation != null && !this.creation.isEmpty(); 1288 } 1289 1290 public boolean hasCreation() { 1291 return this.creation != null && !this.creation.isEmpty(); 1292 } 1293 1294 /** 1295 * @param value {@link #creation} (Date/time(s) of when this Invoice was posted.). This is the underlying object with id, value and extensions. The accessor "getCreation" gives direct access to the value 1296 */ 1297 public Invoice setCreationElement(DateTimeType value) { 1298 this.creation = value; 1299 return this; 1300 } 1301 1302 /** 1303 * @return Date/time(s) of when this Invoice was posted. 1304 */ 1305 public Date getCreation() { 1306 return this.creation == null ? null : this.creation.getValue(); 1307 } 1308 1309 /** 1310 * @param value Date/time(s) of when this Invoice was posted. 1311 */ 1312 public Invoice setCreation(Date value) { 1313 if (value == null) 1314 this.creation = null; 1315 else { 1316 if (this.creation == null) 1317 this.creation = new DateTimeType(); 1318 this.creation.setValue(value); 1319 } 1320 return this; 1321 } 1322 1323 /** 1324 * @return {@link #period} (Date/time(s) range of services included in this invoice.) 1325 */ 1326 public DataType getPeriod() { 1327 return this.period; 1328 } 1329 1330 /** 1331 * @return {@link #period} (Date/time(s) range of services included in this invoice.) 1332 */ 1333 public DateType getPeriodDateType() throws FHIRException { 1334 if (this.period == null) 1335 this.period = new DateType(); 1336 if (!(this.period instanceof DateType)) 1337 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.period.getClass().getName()+" was encountered"); 1338 return (DateType) this.period; 1339 } 1340 1341 public boolean hasPeriodDateType() { 1342 return this.period instanceof DateType; 1343 } 1344 1345 /** 1346 * @return {@link #period} (Date/time(s) range of services included in this invoice.) 1347 */ 1348 public Period getPeriodPeriod() throws FHIRException { 1349 if (this.period == null) 1350 this.period = new Period(); 1351 if (!(this.period instanceof Period)) 1352 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.period.getClass().getName()+" was encountered"); 1353 return (Period) this.period; 1354 } 1355 1356 public boolean hasPeriodPeriod() { 1357 return this.period instanceof Period; 1358 } 1359 1360 public boolean hasPeriod() { 1361 return this.period != null && !this.period.isEmpty(); 1362 } 1363 1364 /** 1365 * @param value {@link #period} (Date/time(s) range of services included in this invoice.) 1366 */ 1367 public Invoice setPeriod(DataType value) { 1368 if (value != null && !(value instanceof DateType || value instanceof Period)) 1369 throw new FHIRException("Not the right type for Invoice.period[x]: "+value.fhirType()); 1370 this.period = value; 1371 return this; 1372 } 1373 1374 /** 1375 * @return {@link #participant} (Indicates who or what performed or participated in the charged service.) 1376 */ 1377 public List<InvoiceParticipantComponent> getParticipant() { 1378 if (this.participant == null) 1379 this.participant = new ArrayList<InvoiceParticipantComponent>(); 1380 return this.participant; 1381 } 1382 1383 /** 1384 * @return Returns a reference to <code>this</code> for easy method chaining 1385 */ 1386 public Invoice setParticipant(List<InvoiceParticipantComponent> theParticipant) { 1387 this.participant = theParticipant; 1388 return this; 1389 } 1390 1391 public boolean hasParticipant() { 1392 if (this.participant == null) 1393 return false; 1394 for (InvoiceParticipantComponent item : this.participant) 1395 if (!item.isEmpty()) 1396 return true; 1397 return false; 1398 } 1399 1400 public InvoiceParticipantComponent addParticipant() { //3 1401 InvoiceParticipantComponent t = new InvoiceParticipantComponent(); 1402 if (this.participant == null) 1403 this.participant = new ArrayList<InvoiceParticipantComponent>(); 1404 this.participant.add(t); 1405 return t; 1406 } 1407 1408 public Invoice addParticipant(InvoiceParticipantComponent t) { //3 1409 if (t == null) 1410 return this; 1411 if (this.participant == null) 1412 this.participant = new ArrayList<InvoiceParticipantComponent>(); 1413 this.participant.add(t); 1414 return this; 1415 } 1416 1417 /** 1418 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist {3} 1419 */ 1420 public InvoiceParticipantComponent getParticipantFirstRep() { 1421 if (getParticipant().isEmpty()) { 1422 addParticipant(); 1423 } 1424 return getParticipant().get(0); 1425 } 1426 1427 /** 1428 * @return {@link #issuer} (The organizationissuing the Invoice.) 1429 */ 1430 public Reference getIssuer() { 1431 if (this.issuer == null) 1432 if (Configuration.errorOnAutoCreate()) 1433 throw new Error("Attempt to auto-create Invoice.issuer"); 1434 else if (Configuration.doAutoCreate()) 1435 this.issuer = new Reference(); // cc 1436 return this.issuer; 1437 } 1438 1439 public boolean hasIssuer() { 1440 return this.issuer != null && !this.issuer.isEmpty(); 1441 } 1442 1443 /** 1444 * @param value {@link #issuer} (The organizationissuing the Invoice.) 1445 */ 1446 public Invoice setIssuer(Reference value) { 1447 this.issuer = value; 1448 return this; 1449 } 1450 1451 /** 1452 * @return {@link #account} (Account which is supposed to be balanced with this Invoice.) 1453 */ 1454 public Reference getAccount() { 1455 if (this.account == null) 1456 if (Configuration.errorOnAutoCreate()) 1457 throw new Error("Attempt to auto-create Invoice.account"); 1458 else if (Configuration.doAutoCreate()) 1459 this.account = new Reference(); // cc 1460 return this.account; 1461 } 1462 1463 public boolean hasAccount() { 1464 return this.account != null && !this.account.isEmpty(); 1465 } 1466 1467 /** 1468 * @param value {@link #account} (Account which is supposed to be balanced with this Invoice.) 1469 */ 1470 public Invoice setAccount(Reference value) { 1471 this.account = value; 1472 return this; 1473 } 1474 1475 /** 1476 * @return {@link #lineItem} (Each line item represents one charge for goods and services rendered. Details such.ofType(date), code and amount are found in the referenced ChargeItem resource.) 1477 */ 1478 public List<InvoiceLineItemComponent> getLineItem() { 1479 if (this.lineItem == null) 1480 this.lineItem = new ArrayList<InvoiceLineItemComponent>(); 1481 return this.lineItem; 1482 } 1483 1484 /** 1485 * @return Returns a reference to <code>this</code> for easy method chaining 1486 */ 1487 public Invoice setLineItem(List<InvoiceLineItemComponent> theLineItem) { 1488 this.lineItem = theLineItem; 1489 return this; 1490 } 1491 1492 public boolean hasLineItem() { 1493 if (this.lineItem == null) 1494 return false; 1495 for (InvoiceLineItemComponent item : this.lineItem) 1496 if (!item.isEmpty()) 1497 return true; 1498 return false; 1499 } 1500 1501 public InvoiceLineItemComponent addLineItem() { //3 1502 InvoiceLineItemComponent t = new InvoiceLineItemComponent(); 1503 if (this.lineItem == null) 1504 this.lineItem = new ArrayList<InvoiceLineItemComponent>(); 1505 this.lineItem.add(t); 1506 return t; 1507 } 1508 1509 public Invoice addLineItem(InvoiceLineItemComponent t) { //3 1510 if (t == null) 1511 return this; 1512 if (this.lineItem == null) 1513 this.lineItem = new ArrayList<InvoiceLineItemComponent>(); 1514 this.lineItem.add(t); 1515 return this; 1516 } 1517 1518 /** 1519 * @return The first repetition of repeating field {@link #lineItem}, creating it if it does not already exist {3} 1520 */ 1521 public InvoiceLineItemComponent getLineItemFirstRep() { 1522 if (getLineItem().isEmpty()) { 1523 addLineItem(); 1524 } 1525 return getLineItem().get(0); 1526 } 1527 1528 /** 1529 * @return {@link #totalPriceComponent} (The total amount for the Invoice may be calculated as the sum of the line items with surcharges/deductions that apply in certain conditions. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the total price was calculated.) 1530 */ 1531 public List<MonetaryComponent> getTotalPriceComponent() { 1532 if (this.totalPriceComponent == null) 1533 this.totalPriceComponent = new ArrayList<MonetaryComponent>(); 1534 return this.totalPriceComponent; 1535 } 1536 1537 /** 1538 * @return Returns a reference to <code>this</code> for easy method chaining 1539 */ 1540 public Invoice setTotalPriceComponent(List<MonetaryComponent> theTotalPriceComponent) { 1541 this.totalPriceComponent = theTotalPriceComponent; 1542 return this; 1543 } 1544 1545 public boolean hasTotalPriceComponent() { 1546 if (this.totalPriceComponent == null) 1547 return false; 1548 for (MonetaryComponent item : this.totalPriceComponent) 1549 if (!item.isEmpty()) 1550 return true; 1551 return false; 1552 } 1553 1554 public MonetaryComponent addTotalPriceComponent() { //3 1555 MonetaryComponent t = new MonetaryComponent(); 1556 if (this.totalPriceComponent == null) 1557 this.totalPriceComponent = new ArrayList<MonetaryComponent>(); 1558 this.totalPriceComponent.add(t); 1559 return t; 1560 } 1561 1562 public Invoice addTotalPriceComponent(MonetaryComponent t) { //3 1563 if (t == null) 1564 return this; 1565 if (this.totalPriceComponent == null) 1566 this.totalPriceComponent = new ArrayList<MonetaryComponent>(); 1567 this.totalPriceComponent.add(t); 1568 return this; 1569 } 1570 1571 /** 1572 * @return The first repetition of repeating field {@link #totalPriceComponent}, creating it if it does not already exist {3} 1573 */ 1574 public MonetaryComponent getTotalPriceComponentFirstRep() { 1575 if (getTotalPriceComponent().isEmpty()) { 1576 addTotalPriceComponent(); 1577 } 1578 return getTotalPriceComponent().get(0); 1579 } 1580 1581 /** 1582 * @return {@link #totalNet} (Invoice total , taxes excluded.) 1583 */ 1584 public Money getTotalNet() { 1585 if (this.totalNet == null) 1586 if (Configuration.errorOnAutoCreate()) 1587 throw new Error("Attempt to auto-create Invoice.totalNet"); 1588 else if (Configuration.doAutoCreate()) 1589 this.totalNet = new Money(); // cc 1590 return this.totalNet; 1591 } 1592 1593 public boolean hasTotalNet() { 1594 return this.totalNet != null && !this.totalNet.isEmpty(); 1595 } 1596 1597 /** 1598 * @param value {@link #totalNet} (Invoice total , taxes excluded.) 1599 */ 1600 public Invoice setTotalNet(Money value) { 1601 this.totalNet = value; 1602 return this; 1603 } 1604 1605 /** 1606 * @return {@link #totalGross} (Invoice total, tax included.) 1607 */ 1608 public Money getTotalGross() { 1609 if (this.totalGross == null) 1610 if (Configuration.errorOnAutoCreate()) 1611 throw new Error("Attempt to auto-create Invoice.totalGross"); 1612 else if (Configuration.doAutoCreate()) 1613 this.totalGross = new Money(); // cc 1614 return this.totalGross; 1615 } 1616 1617 public boolean hasTotalGross() { 1618 return this.totalGross != null && !this.totalGross.isEmpty(); 1619 } 1620 1621 /** 1622 * @param value {@link #totalGross} (Invoice total, tax included.) 1623 */ 1624 public Invoice setTotalGross(Money value) { 1625 this.totalGross = value; 1626 return this; 1627 } 1628 1629 /** 1630 * @return {@link #paymentTerms} (Payment details such as banking details, period of payment, deductibles, methods of payment.). This is the underlying object with id, value and extensions. The accessor "getPaymentTerms" gives direct access to the value 1631 */ 1632 public MarkdownType getPaymentTermsElement() { 1633 if (this.paymentTerms == null) 1634 if (Configuration.errorOnAutoCreate()) 1635 throw new Error("Attempt to auto-create Invoice.paymentTerms"); 1636 else if (Configuration.doAutoCreate()) 1637 this.paymentTerms = new MarkdownType(); // bb 1638 return this.paymentTerms; 1639 } 1640 1641 public boolean hasPaymentTermsElement() { 1642 return this.paymentTerms != null && !this.paymentTerms.isEmpty(); 1643 } 1644 1645 public boolean hasPaymentTerms() { 1646 return this.paymentTerms != null && !this.paymentTerms.isEmpty(); 1647 } 1648 1649 /** 1650 * @param value {@link #paymentTerms} (Payment details such as banking details, period of payment, deductibles, methods of payment.). This is the underlying object with id, value and extensions. The accessor "getPaymentTerms" gives direct access to the value 1651 */ 1652 public Invoice setPaymentTermsElement(MarkdownType value) { 1653 this.paymentTerms = value; 1654 return this; 1655 } 1656 1657 /** 1658 * @return Payment details such as banking details, period of payment, deductibles, methods of payment. 1659 */ 1660 public String getPaymentTerms() { 1661 return this.paymentTerms == null ? null : this.paymentTerms.getValue(); 1662 } 1663 1664 /** 1665 * @param value Payment details such as banking details, period of payment, deductibles, methods of payment. 1666 */ 1667 public Invoice setPaymentTerms(String value) { 1668 if (Utilities.noString(value)) 1669 this.paymentTerms = null; 1670 else { 1671 if (this.paymentTerms == null) 1672 this.paymentTerms = new MarkdownType(); 1673 this.paymentTerms.setValue(value); 1674 } 1675 return this; 1676 } 1677 1678 /** 1679 * @return {@link #note} (Comments made about the invoice by the issuer, subject, or other participants.) 1680 */ 1681 public List<Annotation> getNote() { 1682 if (this.note == null) 1683 this.note = new ArrayList<Annotation>(); 1684 return this.note; 1685 } 1686 1687 /** 1688 * @return Returns a reference to <code>this</code> for easy method chaining 1689 */ 1690 public Invoice setNote(List<Annotation> theNote) { 1691 this.note = theNote; 1692 return this; 1693 } 1694 1695 public boolean hasNote() { 1696 if (this.note == null) 1697 return false; 1698 for (Annotation item : this.note) 1699 if (!item.isEmpty()) 1700 return true; 1701 return false; 1702 } 1703 1704 public Annotation addNote() { //3 1705 Annotation t = new Annotation(); 1706 if (this.note == null) 1707 this.note = new ArrayList<Annotation>(); 1708 this.note.add(t); 1709 return t; 1710 } 1711 1712 public Invoice addNote(Annotation t) { //3 1713 if (t == null) 1714 return this; 1715 if (this.note == null) 1716 this.note = new ArrayList<Annotation>(); 1717 this.note.add(t); 1718 return this; 1719 } 1720 1721 /** 1722 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1723 */ 1724 public Annotation getNoteFirstRep() { 1725 if (getNote().isEmpty()) { 1726 addNote(); 1727 } 1728 return getNote().get(0); 1729 } 1730 1731 protected void listChildren(List<Property> children) { 1732 super.listChildren(children); 1733 children.add(new Property("identifier", "Identifier", "Identifier of this Invoice, often used for reference in correspondence about this invoice or for tracking of payments.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1734 children.add(new Property("status", "code", "The current state of the Invoice.", 0, 1, status)); 1735 children.add(new Property("cancelledReason", "string", "In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.).", 0, 1, cancelledReason)); 1736 children.add(new Property("type", "CodeableConcept", "Type of Invoice depending on domain, realm an usage (e.g. internal/external, dental, preliminary).", 0, 1, type)); 1737 children.add(new Property("subject", "Reference(Patient|Group)", "The individual or set of individuals receiving the goods and services billed in this invoice.", 0, 1, subject)); 1738 children.add(new Property("recipient", "Reference(Organization|Patient|RelatedPerson)", "The individual or Organization responsible for balancing of this invoice.", 0, 1, recipient)); 1739 children.add(new Property("date", "dateTime", "Depricared by the element below.", 0, 1, date)); 1740 children.add(new Property("creation", "dateTime", "Date/time(s) of when this Invoice was posted.", 0, 1, creation)); 1741 children.add(new Property("period[x]", "date|Period", "Date/time(s) range of services included in this invoice.", 0, 1, period)); 1742 children.add(new Property("participant", "", "Indicates who or what performed or participated in the charged service.", 0, java.lang.Integer.MAX_VALUE, participant)); 1743 children.add(new Property("issuer", "Reference(Organization)", "The organizationissuing the Invoice.", 0, 1, issuer)); 1744 children.add(new Property("account", "Reference(Account)", "Account which is supposed to be balanced with this Invoice.", 0, 1, account)); 1745 children.add(new Property("lineItem", "", "Each line item represents one charge for goods and services rendered. Details such.ofType(date), code and amount are found in the referenced ChargeItem resource.", 0, java.lang.Integer.MAX_VALUE, lineItem)); 1746 children.add(new Property("totalPriceComponent", "MonetaryComponent", "The total amount for the Invoice may be calculated as the sum of the line items with surcharges/deductions that apply in certain conditions. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the total price was calculated.", 0, java.lang.Integer.MAX_VALUE, totalPriceComponent)); 1747 children.add(new Property("totalNet", "Money", "Invoice total , taxes excluded.", 0, 1, totalNet)); 1748 children.add(new Property("totalGross", "Money", "Invoice total, tax included.", 0, 1, totalGross)); 1749 children.add(new Property("paymentTerms", "markdown", "Payment details such as banking details, period of payment, deductibles, methods of payment.", 0, 1, paymentTerms)); 1750 children.add(new Property("note", "Annotation", "Comments made about the invoice by the issuer, subject, or other participants.", 0, java.lang.Integer.MAX_VALUE, note)); 1751 } 1752 1753 @Override 1754 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1755 switch (_hash) { 1756 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier of this Invoice, often used for reference in correspondence about this invoice or for tracking of payments.", 0, java.lang.Integer.MAX_VALUE, identifier); 1757 case -892481550: /*status*/ return new Property("status", "code", "The current state of the Invoice.", 0, 1, status); 1758 case 1550362357: /*cancelledReason*/ return new Property("cancelledReason", "string", "In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.).", 0, 1, cancelledReason); 1759 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of Invoice depending on domain, realm an usage (e.g. internal/external, dental, preliminary).", 0, 1, type); 1760 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The individual or set of individuals receiving the goods and services billed in this invoice.", 0, 1, subject); 1761 case 820081177: /*recipient*/ return new Property("recipient", "Reference(Organization|Patient|RelatedPerson)", "The individual or Organization responsible for balancing of this invoice.", 0, 1, recipient); 1762 case 3076014: /*date*/ return new Property("date", "dateTime", "Depricared by the element below.", 0, 1, date); 1763 case 1820421855: /*creation*/ return new Property("creation", "dateTime", "Date/time(s) of when this Invoice was posted.", 0, 1, creation); 1764 case 566594335: /*period[x]*/ return new Property("period[x]", "date|Period", "Date/time(s) range of services included in this invoice.", 0, 1, period); 1765 case -991726143: /*period*/ return new Property("period[x]", "date|Period", "Date/time(s) range of services included in this invoice.", 0, 1, period); 1766 case 383848719: /*periodDate*/ return new Property("period[x]", "date", "Date/time(s) range of services included in this invoice.", 0, 1, period); 1767 case -141376798: /*periodPeriod*/ return new Property("period[x]", "Period", "Date/time(s) range of services included in this invoice.", 0, 1, period); 1768 case 767422259: /*participant*/ return new Property("participant", "", "Indicates who or what performed or participated in the charged service.", 0, java.lang.Integer.MAX_VALUE, participant); 1769 case -1179159879: /*issuer*/ return new Property("issuer", "Reference(Organization)", "The organizationissuing the Invoice.", 0, 1, issuer); 1770 case -1177318867: /*account*/ return new Property("account", "Reference(Account)", "Account which is supposed to be balanced with this Invoice.", 0, 1, account); 1771 case 1188332839: /*lineItem*/ return new Property("lineItem", "", "Each line item represents one charge for goods and services rendered. Details such.ofType(date), code and amount are found in the referenced ChargeItem resource.", 0, java.lang.Integer.MAX_VALUE, lineItem); 1772 case 1731497496: /*totalPriceComponent*/ return new Property("totalPriceComponent", "MonetaryComponent", "The total amount for the Invoice may be calculated as the sum of the line items with surcharges/deductions that apply in certain conditions. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the total price was calculated.", 0, java.lang.Integer.MAX_VALUE, totalPriceComponent); 1773 case -849911879: /*totalNet*/ return new Property("totalNet", "Money", "Invoice total , taxes excluded.", 0, 1, totalNet); 1774 case -727607968: /*totalGross*/ return new Property("totalGross", "Money", "Invoice total, tax included.", 0, 1, totalGross); 1775 case -507544799: /*paymentTerms*/ return new Property("paymentTerms", "markdown", "Payment details such as banking details, period of payment, deductibles, methods of payment.", 0, 1, paymentTerms); 1776 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the invoice by the issuer, subject, or other participants.", 0, java.lang.Integer.MAX_VALUE, note); 1777 default: return super.getNamedProperty(_hash, _name, _checkValid); 1778 } 1779 1780 } 1781 1782 @Override 1783 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1784 switch (hash) { 1785 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1786 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<InvoiceStatus> 1787 case 1550362357: /*cancelledReason*/ return this.cancelledReason == null ? new Base[0] : new Base[] {this.cancelledReason}; // StringType 1788 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1789 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1790 case 820081177: /*recipient*/ return this.recipient == null ? new Base[0] : new Base[] {this.recipient}; // Reference 1791 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1792 case 1820421855: /*creation*/ return this.creation == null ? new Base[0] : new Base[] {this.creation}; // DateTimeType 1793 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // DataType 1794 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // InvoiceParticipantComponent 1795 case -1179159879: /*issuer*/ return this.issuer == null ? new Base[0] : new Base[] {this.issuer}; // Reference 1796 case -1177318867: /*account*/ return this.account == null ? new Base[0] : new Base[] {this.account}; // Reference 1797 case 1188332839: /*lineItem*/ return this.lineItem == null ? new Base[0] : this.lineItem.toArray(new Base[this.lineItem.size()]); // InvoiceLineItemComponent 1798 case 1731497496: /*totalPriceComponent*/ return this.totalPriceComponent == null ? new Base[0] : this.totalPriceComponent.toArray(new Base[this.totalPriceComponent.size()]); // MonetaryComponent 1799 case -849911879: /*totalNet*/ return this.totalNet == null ? new Base[0] : new Base[] {this.totalNet}; // Money 1800 case -727607968: /*totalGross*/ return this.totalGross == null ? new Base[0] : new Base[] {this.totalGross}; // Money 1801 case -507544799: /*paymentTerms*/ return this.paymentTerms == null ? new Base[0] : new Base[] {this.paymentTerms}; // MarkdownType 1802 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1803 default: return super.getProperty(hash, name, checkValid); 1804 } 1805 1806 } 1807 1808 @Override 1809 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1810 switch (hash) { 1811 case -1618432855: // identifier 1812 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1813 return value; 1814 case -892481550: // status 1815 value = new InvoiceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1816 this.status = (Enumeration) value; // Enumeration<InvoiceStatus> 1817 return value; 1818 case 1550362357: // cancelledReason 1819 this.cancelledReason = TypeConvertor.castToString(value); // StringType 1820 return value; 1821 case 3575610: // type 1822 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1823 return value; 1824 case -1867885268: // subject 1825 this.subject = TypeConvertor.castToReference(value); // Reference 1826 return value; 1827 case 820081177: // recipient 1828 this.recipient = TypeConvertor.castToReference(value); // Reference 1829 return value; 1830 case 3076014: // date 1831 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1832 return value; 1833 case 1820421855: // creation 1834 this.creation = TypeConvertor.castToDateTime(value); // DateTimeType 1835 return value; 1836 case -991726143: // period 1837 this.period = TypeConvertor.castToType(value); // DataType 1838 return value; 1839 case 767422259: // participant 1840 this.getParticipant().add((InvoiceParticipantComponent) value); // InvoiceParticipantComponent 1841 return value; 1842 case -1179159879: // issuer 1843 this.issuer = TypeConvertor.castToReference(value); // Reference 1844 return value; 1845 case -1177318867: // account 1846 this.account = TypeConvertor.castToReference(value); // Reference 1847 return value; 1848 case 1188332839: // lineItem 1849 this.getLineItem().add((InvoiceLineItemComponent) value); // InvoiceLineItemComponent 1850 return value; 1851 case 1731497496: // totalPriceComponent 1852 this.getTotalPriceComponent().add(TypeConvertor.castToMonetaryComponent(value)); // MonetaryComponent 1853 return value; 1854 case -849911879: // totalNet 1855 this.totalNet = TypeConvertor.castToMoney(value); // Money 1856 return value; 1857 case -727607968: // totalGross 1858 this.totalGross = TypeConvertor.castToMoney(value); // Money 1859 return value; 1860 case -507544799: // paymentTerms 1861 this.paymentTerms = TypeConvertor.castToMarkdown(value); // MarkdownType 1862 return value; 1863 case 3387378: // note 1864 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1865 return value; 1866 default: return super.setProperty(hash, name, value); 1867 } 1868 1869 } 1870 1871 @Override 1872 public Base setProperty(String name, Base value) throws FHIRException { 1873 if (name.equals("identifier")) { 1874 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1875 } else if (name.equals("status")) { 1876 value = new InvoiceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1877 this.status = (Enumeration) value; // Enumeration<InvoiceStatus> 1878 } else if (name.equals("cancelledReason")) { 1879 this.cancelledReason = TypeConvertor.castToString(value); // StringType 1880 } else if (name.equals("type")) { 1881 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1882 } else if (name.equals("subject")) { 1883 this.subject = TypeConvertor.castToReference(value); // Reference 1884 } else if (name.equals("recipient")) { 1885 this.recipient = TypeConvertor.castToReference(value); // Reference 1886 } else if (name.equals("date")) { 1887 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1888 } else if (name.equals("creation")) { 1889 this.creation = TypeConvertor.castToDateTime(value); // DateTimeType 1890 } else if (name.equals("period[x]")) { 1891 this.period = TypeConvertor.castToType(value); // DataType 1892 } else if (name.equals("participant")) { 1893 this.getParticipant().add((InvoiceParticipantComponent) value); 1894 } else if (name.equals("issuer")) { 1895 this.issuer = TypeConvertor.castToReference(value); // Reference 1896 } else if (name.equals("account")) { 1897 this.account = TypeConvertor.castToReference(value); // Reference 1898 } else if (name.equals("lineItem")) { 1899 this.getLineItem().add((InvoiceLineItemComponent) value); 1900 } else if (name.equals("totalPriceComponent")) { 1901 this.getTotalPriceComponent().add(TypeConvertor.castToMonetaryComponent(value)); 1902 } else if (name.equals("totalNet")) { 1903 this.totalNet = TypeConvertor.castToMoney(value); // Money 1904 } else if (name.equals("totalGross")) { 1905 this.totalGross = TypeConvertor.castToMoney(value); // Money 1906 } else if (name.equals("paymentTerms")) { 1907 this.paymentTerms = TypeConvertor.castToMarkdown(value); // MarkdownType 1908 } else if (name.equals("note")) { 1909 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1910 } else 1911 return super.setProperty(name, value); 1912 return value; 1913 } 1914 1915 @Override 1916 public void removeChild(String name, Base value) throws FHIRException { 1917 if (name.equals("identifier")) { 1918 this.getIdentifier().remove(value); 1919 } else if (name.equals("status")) { 1920 value = new InvoiceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1921 this.status = (Enumeration) value; // Enumeration<InvoiceStatus> 1922 } else if (name.equals("cancelledReason")) { 1923 this.cancelledReason = null; 1924 } else if (name.equals("type")) { 1925 this.type = null; 1926 } else if (name.equals("subject")) { 1927 this.subject = null; 1928 } else if (name.equals("recipient")) { 1929 this.recipient = null; 1930 } else if (name.equals("date")) { 1931 this.date = null; 1932 } else if (name.equals("creation")) { 1933 this.creation = null; 1934 } else if (name.equals("period[x]")) { 1935 this.period = null; 1936 } else if (name.equals("participant")) { 1937 this.getParticipant().remove((InvoiceParticipantComponent) value); 1938 } else if (name.equals("issuer")) { 1939 this.issuer = null; 1940 } else if (name.equals("account")) { 1941 this.account = null; 1942 } else if (name.equals("lineItem")) { 1943 this.getLineItem().remove((InvoiceLineItemComponent) value); 1944 } else if (name.equals("totalPriceComponent")) { 1945 this.getTotalPriceComponent().remove(value); 1946 } else if (name.equals("totalNet")) { 1947 this.totalNet = null; 1948 } else if (name.equals("totalGross")) { 1949 this.totalGross = null; 1950 } else if (name.equals("paymentTerms")) { 1951 this.paymentTerms = null; 1952 } else if (name.equals("note")) { 1953 this.getNote().remove(value); 1954 } else 1955 super.removeChild(name, value); 1956 1957 } 1958 1959 @Override 1960 public Base makeProperty(int hash, String name) throws FHIRException { 1961 switch (hash) { 1962 case -1618432855: return addIdentifier(); 1963 case -892481550: return getStatusElement(); 1964 case 1550362357: return getCancelledReasonElement(); 1965 case 3575610: return getType(); 1966 case -1867885268: return getSubject(); 1967 case 820081177: return getRecipient(); 1968 case 3076014: return getDateElement(); 1969 case 1820421855: return getCreationElement(); 1970 case 566594335: return getPeriod(); 1971 case -991726143: return getPeriod(); 1972 case 767422259: return addParticipant(); 1973 case -1179159879: return getIssuer(); 1974 case -1177318867: return getAccount(); 1975 case 1188332839: return addLineItem(); 1976 case 1731497496: return addTotalPriceComponent(); 1977 case -849911879: return getTotalNet(); 1978 case -727607968: return getTotalGross(); 1979 case -507544799: return getPaymentTermsElement(); 1980 case 3387378: return addNote(); 1981 default: return super.makeProperty(hash, name); 1982 } 1983 1984 } 1985 1986 @Override 1987 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1988 switch (hash) { 1989 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1990 case -892481550: /*status*/ return new String[] {"code"}; 1991 case 1550362357: /*cancelledReason*/ return new String[] {"string"}; 1992 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1993 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1994 case 820081177: /*recipient*/ return new String[] {"Reference"}; 1995 case 3076014: /*date*/ return new String[] {"dateTime"}; 1996 case 1820421855: /*creation*/ return new String[] {"dateTime"}; 1997 case -991726143: /*period*/ return new String[] {"date", "Period"}; 1998 case 767422259: /*participant*/ return new String[] {}; 1999 case -1179159879: /*issuer*/ return new String[] {"Reference"}; 2000 case -1177318867: /*account*/ return new String[] {"Reference"}; 2001 case 1188332839: /*lineItem*/ return new String[] {}; 2002 case 1731497496: /*totalPriceComponent*/ return new String[] {"MonetaryComponent"}; 2003 case -849911879: /*totalNet*/ return new String[] {"Money"}; 2004 case -727607968: /*totalGross*/ return new String[] {"Money"}; 2005 case -507544799: /*paymentTerms*/ return new String[] {"markdown"}; 2006 case 3387378: /*note*/ return new String[] {"Annotation"}; 2007 default: return super.getTypesForProperty(hash, name); 2008 } 2009 2010 } 2011 2012 @Override 2013 public Base addChild(String name) throws FHIRException { 2014 if (name.equals("identifier")) { 2015 return addIdentifier(); 2016 } 2017 else if (name.equals("status")) { 2018 throw new FHIRException("Cannot call addChild on a singleton property Invoice.status"); 2019 } 2020 else if (name.equals("cancelledReason")) { 2021 throw new FHIRException("Cannot call addChild on a singleton property Invoice.cancelledReason"); 2022 } 2023 else if (name.equals("type")) { 2024 this.type = new CodeableConcept(); 2025 return this.type; 2026 } 2027 else if (name.equals("subject")) { 2028 this.subject = new Reference(); 2029 return this.subject; 2030 } 2031 else if (name.equals("recipient")) { 2032 this.recipient = new Reference(); 2033 return this.recipient; 2034 } 2035 else if (name.equals("date")) { 2036 throw new FHIRException("Cannot call addChild on a singleton property Invoice.date"); 2037 } 2038 else if (name.equals("creation")) { 2039 throw new FHIRException("Cannot call addChild on a singleton property Invoice.creation"); 2040 } 2041 else if (name.equals("periodDate")) { 2042 this.period = new DateType(); 2043 return this.period; 2044 } 2045 else if (name.equals("periodPeriod")) { 2046 this.period = new Period(); 2047 return this.period; 2048 } 2049 else if (name.equals("participant")) { 2050 return addParticipant(); 2051 } 2052 else if (name.equals("issuer")) { 2053 this.issuer = new Reference(); 2054 return this.issuer; 2055 } 2056 else if (name.equals("account")) { 2057 this.account = new Reference(); 2058 return this.account; 2059 } 2060 else if (name.equals("lineItem")) { 2061 return addLineItem(); 2062 } 2063 else if (name.equals("totalPriceComponent")) { 2064 return addTotalPriceComponent(); 2065 } 2066 else if (name.equals("totalNet")) { 2067 this.totalNet = new Money(); 2068 return this.totalNet; 2069 } 2070 else if (name.equals("totalGross")) { 2071 this.totalGross = new Money(); 2072 return this.totalGross; 2073 } 2074 else if (name.equals("paymentTerms")) { 2075 throw new FHIRException("Cannot call addChild on a singleton property Invoice.paymentTerms"); 2076 } 2077 else if (name.equals("note")) { 2078 return addNote(); 2079 } 2080 else 2081 return super.addChild(name); 2082 } 2083 2084 public String fhirType() { 2085 return "Invoice"; 2086 2087 } 2088 2089 public Invoice copy() { 2090 Invoice dst = new Invoice(); 2091 copyValues(dst); 2092 return dst; 2093 } 2094 2095 public void copyValues(Invoice dst) { 2096 super.copyValues(dst); 2097 if (identifier != null) { 2098 dst.identifier = new ArrayList<Identifier>(); 2099 for (Identifier i : identifier) 2100 dst.identifier.add(i.copy()); 2101 }; 2102 dst.status = status == null ? null : status.copy(); 2103 dst.cancelledReason = cancelledReason == null ? null : cancelledReason.copy(); 2104 dst.type = type == null ? null : type.copy(); 2105 dst.subject = subject == null ? null : subject.copy(); 2106 dst.recipient = recipient == null ? null : recipient.copy(); 2107 dst.date = date == null ? null : date.copy(); 2108 dst.creation = creation == null ? null : creation.copy(); 2109 dst.period = period == null ? null : period.copy(); 2110 if (participant != null) { 2111 dst.participant = new ArrayList<InvoiceParticipantComponent>(); 2112 for (InvoiceParticipantComponent i : participant) 2113 dst.participant.add(i.copy()); 2114 }; 2115 dst.issuer = issuer == null ? null : issuer.copy(); 2116 dst.account = account == null ? null : account.copy(); 2117 if (lineItem != null) { 2118 dst.lineItem = new ArrayList<InvoiceLineItemComponent>(); 2119 for (InvoiceLineItemComponent i : lineItem) 2120 dst.lineItem.add(i.copy()); 2121 }; 2122 if (totalPriceComponent != null) { 2123 dst.totalPriceComponent = new ArrayList<MonetaryComponent>(); 2124 for (MonetaryComponent i : totalPriceComponent) 2125 dst.totalPriceComponent.add(i.copy()); 2126 }; 2127 dst.totalNet = totalNet == null ? null : totalNet.copy(); 2128 dst.totalGross = totalGross == null ? null : totalGross.copy(); 2129 dst.paymentTerms = paymentTerms == null ? null : paymentTerms.copy(); 2130 if (note != null) { 2131 dst.note = new ArrayList<Annotation>(); 2132 for (Annotation i : note) 2133 dst.note.add(i.copy()); 2134 }; 2135 } 2136 2137 protected Invoice typedCopy() { 2138 return copy(); 2139 } 2140 2141 @Override 2142 public boolean equalsDeep(Base other_) { 2143 if (!super.equalsDeep(other_)) 2144 return false; 2145 if (!(other_ instanceof Invoice)) 2146 return false; 2147 Invoice o = (Invoice) other_; 2148 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(cancelledReason, o.cancelledReason, true) 2149 && compareDeep(type, o.type, true) && compareDeep(subject, o.subject, true) && compareDeep(recipient, o.recipient, true) 2150 && compareDeep(date, o.date, true) && compareDeep(creation, o.creation, true) && compareDeep(period, o.period, true) 2151 && compareDeep(participant, o.participant, true) && compareDeep(issuer, o.issuer, true) && compareDeep(account, o.account, true) 2152 && compareDeep(lineItem, o.lineItem, true) && compareDeep(totalPriceComponent, o.totalPriceComponent, true) 2153 && compareDeep(totalNet, o.totalNet, true) && compareDeep(totalGross, o.totalGross, true) && compareDeep(paymentTerms, o.paymentTerms, true) 2154 && compareDeep(note, o.note, true); 2155 } 2156 2157 @Override 2158 public boolean equalsShallow(Base other_) { 2159 if (!super.equalsShallow(other_)) 2160 return false; 2161 if (!(other_ instanceof Invoice)) 2162 return false; 2163 Invoice o = (Invoice) other_; 2164 return compareValues(status, o.status, true) && compareValues(cancelledReason, o.cancelledReason, true) 2165 && compareValues(date, o.date, true) && compareValues(creation, o.creation, true) && compareValues(paymentTerms, o.paymentTerms, true) 2166 ; 2167 } 2168 2169 public boolean isEmpty() { 2170 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, cancelledReason 2171 , type, subject, recipient, date, creation, period, participant, issuer, account 2172 , lineItem, totalPriceComponent, totalNet, totalGross, paymentTerms, note); 2173 } 2174 2175 @Override 2176 public ResourceType getResourceType() { 2177 return ResourceType.Invoice; 2178 } 2179 2180 /** 2181 * Search parameter: <b>account</b> 2182 * <p> 2183 * Description: <b>Account that is being balanced</b><br> 2184 * Type: <b>reference</b><br> 2185 * Path: <b>Invoice.account</b><br> 2186 * </p> 2187 */ 2188 @SearchParamDefinition(name="account", path="Invoice.account", description="Account that is being balanced", type="reference", target={Account.class } ) 2189 public static final String SP_ACCOUNT = "account"; 2190 /** 2191 * <b>Fluent Client</b> search parameter constant for <b>account</b> 2192 * <p> 2193 * Description: <b>Account that is being balanced</b><br> 2194 * Type: <b>reference</b><br> 2195 * Path: <b>Invoice.account</b><br> 2196 * </p> 2197 */ 2198 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACCOUNT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACCOUNT); 2199 2200/** 2201 * Constant for fluent queries to be used to add include statements. Specifies 2202 * the path value of "<b>Invoice:account</b>". 2203 */ 2204 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACCOUNT = new ca.uhn.fhir.model.api.Include("Invoice:account").toLocked(); 2205 2206 /** 2207 * Search parameter: <b>issuer</b> 2208 * <p> 2209 * Description: <b>Issuing Organization of Invoice</b><br> 2210 * Type: <b>reference</b><br> 2211 * Path: <b>Invoice.issuer</b><br> 2212 * </p> 2213 */ 2214 @SearchParamDefinition(name="issuer", path="Invoice.issuer", description="Issuing Organization of Invoice", type="reference", target={Organization.class } ) 2215 public static final String SP_ISSUER = "issuer"; 2216 /** 2217 * <b>Fluent Client</b> search parameter constant for <b>issuer</b> 2218 * <p> 2219 * Description: <b>Issuing Organization of Invoice</b><br> 2220 * Type: <b>reference</b><br> 2221 * Path: <b>Invoice.issuer</b><br> 2222 * </p> 2223 */ 2224 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ISSUER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ISSUER); 2225 2226/** 2227 * Constant for fluent queries to be used to add include statements. Specifies 2228 * the path value of "<b>Invoice:issuer</b>". 2229 */ 2230 public static final ca.uhn.fhir.model.api.Include INCLUDE_ISSUER = new ca.uhn.fhir.model.api.Include("Invoice:issuer").toLocked(); 2231 2232 /** 2233 * Search parameter: <b>participant-role</b> 2234 * <p> 2235 * Description: <b>Type of involvement in creation of this Invoice</b><br> 2236 * Type: <b>token</b><br> 2237 * Path: <b>Invoice.participant.role</b><br> 2238 * </p> 2239 */ 2240 @SearchParamDefinition(name="participant-role", path="Invoice.participant.role", description="Type of involvement in creation of this Invoice", type="token" ) 2241 public static final String SP_PARTICIPANT_ROLE = "participant-role"; 2242 /** 2243 * <b>Fluent Client</b> search parameter constant for <b>participant-role</b> 2244 * <p> 2245 * Description: <b>Type of involvement in creation of this Invoice</b><br> 2246 * Type: <b>token</b><br> 2247 * Path: <b>Invoice.participant.role</b><br> 2248 * </p> 2249 */ 2250 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PARTICIPANT_ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PARTICIPANT_ROLE); 2251 2252 /** 2253 * Search parameter: <b>participant</b> 2254 * <p> 2255 * Description: <b>Individual who was involved</b><br> 2256 * Type: <b>reference</b><br> 2257 * Path: <b>Invoice.participant.actor</b><br> 2258 * </p> 2259 */ 2260 @SearchParamDefinition(name="participant", path="Invoice.participant.actor", description="Individual who was involved", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2261 public static final String SP_PARTICIPANT = "participant"; 2262 /** 2263 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 2264 * <p> 2265 * Description: <b>Individual who was involved</b><br> 2266 * Type: <b>reference</b><br> 2267 * Path: <b>Invoice.participant.actor</b><br> 2268 * </p> 2269 */ 2270 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTICIPANT); 2271 2272/** 2273 * Constant for fluent queries to be used to add include statements. Specifies 2274 * the path value of "<b>Invoice:participant</b>". 2275 */ 2276 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include("Invoice:participant").toLocked(); 2277 2278 /** 2279 * Search parameter: <b>recipient</b> 2280 * <p> 2281 * Description: <b>Recipient of this invoice</b><br> 2282 * Type: <b>reference</b><br> 2283 * Path: <b>Invoice.recipient</b><br> 2284 * </p> 2285 */ 2286 @SearchParamDefinition(name="recipient", path="Invoice.recipient", description="Recipient of this invoice", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Organization.class, Patient.class, RelatedPerson.class } ) 2287 public static final String SP_RECIPIENT = "recipient"; 2288 /** 2289 * <b>Fluent Client</b> search parameter constant for <b>recipient</b> 2290 * <p> 2291 * Description: <b>Recipient of this invoice</b><br> 2292 * Type: <b>reference</b><br> 2293 * Path: <b>Invoice.recipient</b><br> 2294 * </p> 2295 */ 2296 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECIPIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECIPIENT); 2297 2298/** 2299 * Constant for fluent queries to be used to add include statements. Specifies 2300 * the path value of "<b>Invoice:recipient</b>". 2301 */ 2302 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECIPIENT = new ca.uhn.fhir.model.api.Include("Invoice:recipient").toLocked(); 2303 2304 /** 2305 * Search parameter: <b>status</b> 2306 * <p> 2307 * Description: <b>draft | issued | balanced | cancelled | entered-in-error</b><br> 2308 * Type: <b>token</b><br> 2309 * Path: <b>Invoice.status</b><br> 2310 * </p> 2311 */ 2312 @SearchParamDefinition(name="status", path="Invoice.status", description="draft | issued | balanced | cancelled | entered-in-error", type="token" ) 2313 public static final String SP_STATUS = "status"; 2314 /** 2315 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2316 * <p> 2317 * Description: <b>draft | issued | balanced | cancelled | entered-in-error</b><br> 2318 * Type: <b>token</b><br> 2319 * Path: <b>Invoice.status</b><br> 2320 * </p> 2321 */ 2322 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2323 2324 /** 2325 * Search parameter: <b>subject</b> 2326 * <p> 2327 * Description: <b>Recipient(s) of goods and services</b><br> 2328 * Type: <b>reference</b><br> 2329 * Path: <b>Invoice.subject</b><br> 2330 * </p> 2331 */ 2332 @SearchParamDefinition(name="subject", path="Invoice.subject", description="Recipient(s) of goods and services", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class } ) 2333 public static final String SP_SUBJECT = "subject"; 2334 /** 2335 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2336 * <p> 2337 * Description: <b>Recipient(s) of goods and services</b><br> 2338 * Type: <b>reference</b><br> 2339 * Path: <b>Invoice.subject</b><br> 2340 * </p> 2341 */ 2342 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2343 2344/** 2345 * Constant for fluent queries to be used to add include statements. Specifies 2346 * the path value of "<b>Invoice:subject</b>". 2347 */ 2348 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Invoice:subject").toLocked(); 2349 2350 /** 2351 * Search parameter: <b>totalgross</b> 2352 * <p> 2353 * Description: <b>Gross total of this Invoice</b><br> 2354 * Type: <b>quantity</b><br> 2355 * Path: <b>Invoice.totalGross</b><br> 2356 * </p> 2357 */ 2358 @SearchParamDefinition(name="totalgross", path="Invoice.totalGross", description="Gross total of this Invoice", type="quantity" ) 2359 public static final String SP_TOTALGROSS = "totalgross"; 2360 /** 2361 * <b>Fluent Client</b> search parameter constant for <b>totalgross</b> 2362 * <p> 2363 * Description: <b>Gross total of this Invoice</b><br> 2364 * Type: <b>quantity</b><br> 2365 * Path: <b>Invoice.totalGross</b><br> 2366 * </p> 2367 */ 2368 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam TOTALGROSS = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_TOTALGROSS); 2369 2370 /** 2371 * Search parameter: <b>totalnet</b> 2372 * <p> 2373 * Description: <b>Net total of this Invoice</b><br> 2374 * Type: <b>quantity</b><br> 2375 * Path: <b>Invoice.totalNet</b><br> 2376 * </p> 2377 */ 2378 @SearchParamDefinition(name="totalnet", path="Invoice.totalNet", description="Net total of this Invoice", type="quantity" ) 2379 public static final String SP_TOTALNET = "totalnet"; 2380 /** 2381 * <b>Fluent Client</b> search parameter constant for <b>totalnet</b> 2382 * <p> 2383 * Description: <b>Net total of this Invoice</b><br> 2384 * Type: <b>quantity</b><br> 2385 * Path: <b>Invoice.totalNet</b><br> 2386 * </p> 2387 */ 2388 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam TOTALNET = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_TOTALNET); 2389 2390 /** 2391 * Search parameter: <b>date</b> 2392 * <p> 2393 * Description: <b>Multiple Resources: 2394 2395* [AdverseEvent](adverseevent.html): When the event occurred 2396* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 2397* [Appointment](appointment.html): Appointment date/time. 2398* [AuditEvent](auditevent.html): Time when the event was recorded 2399* [CarePlan](careplan.html): Time period plan covers 2400* [CareTeam](careteam.html): A date within the coverage time period. 2401* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 2402* [Composition](composition.html): Composition editing time 2403* [Consent](consent.html): When consent was agreed to 2404* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 2405* [DocumentReference](documentreference.html): When this document reference was created 2406* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 2407* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 2408* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 2409* [Flag](flag.html): Time period when flag is active 2410* [Immunization](immunization.html): Vaccination (non)-Administration Date 2411* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 2412* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 2413* [Invoice](invoice.html): Invoice date / posting date 2414* [List](list.html): When the list was prepared 2415* [MeasureReport](measurereport.html): The date of the measure report 2416* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 2417* [Observation](observation.html): Clinically relevant time/time-period for observation 2418* [Procedure](procedure.html): When the procedure occurred or is occurring 2419* [ResearchSubject](researchsubject.html): Start and end of participation 2420* [RiskAssessment](riskassessment.html): When was assessment made? 2421* [SupplyRequest](supplyrequest.html): When the request was made 2422</b><br> 2423 * Type: <b>date</b><br> 2424 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 2425 * </p> 2426 */ 2427 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 2428 public static final String SP_DATE = "date"; 2429 /** 2430 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2431 * <p> 2432 * Description: <b>Multiple Resources: 2433 2434* [AdverseEvent](adverseevent.html): When the event occurred 2435* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 2436* [Appointment](appointment.html): Appointment date/time. 2437* [AuditEvent](auditevent.html): Time when the event was recorded 2438* [CarePlan](careplan.html): Time period plan covers 2439* [CareTeam](careteam.html): A date within the coverage time period. 2440* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 2441* [Composition](composition.html): Composition editing time 2442* [Consent](consent.html): When consent was agreed to 2443* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 2444* [DocumentReference](documentreference.html): When this document reference was created 2445* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 2446* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 2447* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 2448* [Flag](flag.html): Time period when flag is active 2449* [Immunization](immunization.html): Vaccination (non)-Administration Date 2450* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 2451* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 2452* [Invoice](invoice.html): Invoice date / posting date 2453* [List](list.html): When the list was prepared 2454* [MeasureReport](measurereport.html): The date of the measure report 2455* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 2456* [Observation](observation.html): Clinically relevant time/time-period for observation 2457* [Procedure](procedure.html): When the procedure occurred or is occurring 2458* [ResearchSubject](researchsubject.html): Start and end of participation 2459* [RiskAssessment](riskassessment.html): When was assessment made? 2460* [SupplyRequest](supplyrequest.html): When the request was made 2461</b><br> 2462 * Type: <b>date</b><br> 2463 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 2464 * </p> 2465 */ 2466 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2467 2468 /** 2469 * Search parameter: <b>identifier</b> 2470 * <p> 2471 * Description: <b>Multiple Resources: 2472 2473* [Account](account.html): Account number 2474* [AdverseEvent](adverseevent.html): Business identifier for the event 2475* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2476* [Appointment](appointment.html): An Identifier of the Appointment 2477* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2478* [Basic](basic.html): Business identifier 2479* [BodyStructure](bodystructure.html): Bodystructure identifier 2480* [CarePlan](careplan.html): External Ids for this plan 2481* [CareTeam](careteam.html): External Ids for this team 2482* [ChargeItem](chargeitem.html): Business Identifier for item 2483* [Claim](claim.html): The primary identifier of the financial resource 2484* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2485* [ClinicalImpression](clinicalimpression.html): Business identifier 2486* [Communication](communication.html): Unique identifier 2487* [CommunicationRequest](communicationrequest.html): Unique identifier 2488* [Composition](composition.html): Version-independent identifier for the Composition 2489* [Condition](condition.html): A unique identifier of the condition record 2490* [Consent](consent.html): Identifier for this record (external references) 2491* [Contract](contract.html): The identity of the contract 2492* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2493* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2494* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2495* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2496* [DeviceRequest](devicerequest.html): Business identifier for request/order 2497* [DeviceUsage](deviceusage.html): Search by identifier 2498* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2499* [DocumentReference](documentreference.html): Identifier of the attachment binary 2500* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2501* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2502* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2503* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2504* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2505* [Flag](flag.html): Business identifier 2506* [Goal](goal.html): External Ids for this goal 2507* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2508* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2509* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2510* [Immunization](immunization.html): Business identifier 2511* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2512* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2513* [Invoice](invoice.html): Business Identifier for item 2514* [List](list.html): Business identifier 2515* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2516* [Medication](medication.html): Returns medications with this external identifier 2517* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2518* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2519* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2520* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2521* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2522* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2523* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2524* [Observation](observation.html): The unique id for a particular observation 2525* [Person](person.html): A person Identifier 2526* [Procedure](procedure.html): A unique identifier for a procedure 2527* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2528* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2529* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2530* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2531* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2532* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2533* [Specimen](specimen.html): The unique identifier associated with the specimen 2534* [SupplyDelivery](supplydelivery.html): External identifier 2535* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2536* [Task](task.html): Search for a task instance by its business identifier 2537* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2538</b><br> 2539 * Type: <b>token</b><br> 2540 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2541 * </p> 2542 */ 2543 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2544 public static final String SP_IDENTIFIER = "identifier"; 2545 /** 2546 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2547 * <p> 2548 * Description: <b>Multiple Resources: 2549 2550* [Account](account.html): Account number 2551* [AdverseEvent](adverseevent.html): Business identifier for the event 2552* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2553* [Appointment](appointment.html): An Identifier of the Appointment 2554* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2555* [Basic](basic.html): Business identifier 2556* [BodyStructure](bodystructure.html): Bodystructure identifier 2557* [CarePlan](careplan.html): External Ids for this plan 2558* [CareTeam](careteam.html): External Ids for this team 2559* [ChargeItem](chargeitem.html): Business Identifier for item 2560* [Claim](claim.html): The primary identifier of the financial resource 2561* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2562* [ClinicalImpression](clinicalimpression.html): Business identifier 2563* [Communication](communication.html): Unique identifier 2564* [CommunicationRequest](communicationrequest.html): Unique identifier 2565* [Composition](composition.html): Version-independent identifier for the Composition 2566* [Condition](condition.html): A unique identifier of the condition record 2567* [Consent](consent.html): Identifier for this record (external references) 2568* [Contract](contract.html): The identity of the contract 2569* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2570* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2571* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2572* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2573* [DeviceRequest](devicerequest.html): Business identifier for request/order 2574* [DeviceUsage](deviceusage.html): Search by identifier 2575* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2576* [DocumentReference](documentreference.html): Identifier of the attachment binary 2577* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2578* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2579* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2580* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2581* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2582* [Flag](flag.html): Business identifier 2583* [Goal](goal.html): External Ids for this goal 2584* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2585* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2586* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2587* [Immunization](immunization.html): Business identifier 2588* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2589* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2590* [Invoice](invoice.html): Business Identifier for item 2591* [List](list.html): Business identifier 2592* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2593* [Medication](medication.html): Returns medications with this external identifier 2594* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2595* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2596* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2597* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2598* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2599* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2600* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2601* [Observation](observation.html): The unique id for a particular observation 2602* [Person](person.html): A person Identifier 2603* [Procedure](procedure.html): A unique identifier for a procedure 2604* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2605* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2606* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2607* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2608* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2609* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2610* [Specimen](specimen.html): The unique identifier associated with the specimen 2611* [SupplyDelivery](supplydelivery.html): External identifier 2612* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2613* [Task](task.html): Search for a task instance by its business identifier 2614* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2615</b><br> 2616 * Type: <b>token</b><br> 2617 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2618 * </p> 2619 */ 2620 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2621 2622 /** 2623 * Search parameter: <b>patient</b> 2624 * <p> 2625 * Description: <b>Multiple Resources: 2626 2627* [Account](account.html): The entity that caused the expenses 2628* [AdverseEvent](adverseevent.html): Subject impacted by event 2629* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2630* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2631* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2632* [AuditEvent](auditevent.html): Where the activity involved patient data 2633* [Basic](basic.html): Identifies the focus of this resource 2634* [BodyStructure](bodystructure.html): Who this is about 2635* [CarePlan](careplan.html): Who the care plan is for 2636* [CareTeam](careteam.html): Who care team is for 2637* [ChargeItem](chargeitem.html): Individual service was done for/to 2638* [Claim](claim.html): Patient receiving the products or services 2639* [ClaimResponse](claimresponse.html): The subject of care 2640* [ClinicalImpression](clinicalimpression.html): Patient assessed 2641* [Communication](communication.html): Focus of message 2642* [CommunicationRequest](communicationrequest.html): Focus of message 2643* [Composition](composition.html): Who and/or what the composition is about 2644* [Condition](condition.html): Who has the condition? 2645* [Consent](consent.html): Who the consent applies to 2646* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2647* [Coverage](coverage.html): Retrieve coverages for a patient 2648* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2649* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2650* [DetectedIssue](detectedissue.html): Associated patient 2651* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2652* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2653* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2654* [DocumentReference](documentreference.html): Who/what is the subject of the document 2655* [Encounter](encounter.html): The patient present at the encounter 2656* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2657* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2658* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2659* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2660* [Flag](flag.html): The identity of a subject to list flags for 2661* [Goal](goal.html): Who this goal is intended for 2662* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2663* [ImagingSelection](imagingselection.html): Who the study is about 2664* [ImagingStudy](imagingstudy.html): Who the study is about 2665* [Immunization](immunization.html): The patient for the vaccination record 2666* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2667* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2668* [Invoice](invoice.html): Recipient(s) of goods and services 2669* [List](list.html): If all resources have the same subject 2670* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2671* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2672* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2673* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2674* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2675* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2676* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2677* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2678* [Observation](observation.html): The subject that the observation is about (if patient) 2679* [Person](person.html): The Person links to this Patient 2680* [Procedure](procedure.html): Search by subject - a patient 2681* [Provenance](provenance.html): Where the activity involved patient data 2682* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2683* [RelatedPerson](relatedperson.html): The patient this related person is related to 2684* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2685* [ResearchSubject](researchsubject.html): Who or what is part of study 2686* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2687* [ServiceRequest](servicerequest.html): Search by subject - a patient 2688* [Specimen](specimen.html): The patient the specimen comes from 2689* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2690* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2691* [Task](task.html): Search by patient 2692* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2693</b><br> 2694 * Type: <b>reference</b><br> 2695 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2696 * </p> 2697 */ 2698 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 2699 public static final String SP_PATIENT = "patient"; 2700 /** 2701 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2702 * <p> 2703 * Description: <b>Multiple Resources: 2704 2705* [Account](account.html): The entity that caused the expenses 2706* [AdverseEvent](adverseevent.html): Subject impacted by event 2707* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2708* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2709* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2710* [AuditEvent](auditevent.html): Where the activity involved patient data 2711* [Basic](basic.html): Identifies the focus of this resource 2712* [BodyStructure](bodystructure.html): Who this is about 2713* [CarePlan](careplan.html): Who the care plan is for 2714* [CareTeam](careteam.html): Who care team is for 2715* [ChargeItem](chargeitem.html): Individual service was done for/to 2716* [Claim](claim.html): Patient receiving the products or services 2717* [ClaimResponse](claimresponse.html): The subject of care 2718* [ClinicalImpression](clinicalimpression.html): Patient assessed 2719* [Communication](communication.html): Focus of message 2720* [CommunicationRequest](communicationrequest.html): Focus of message 2721* [Composition](composition.html): Who and/or what the composition is about 2722* [Condition](condition.html): Who has the condition? 2723* [Consent](consent.html): Who the consent applies to 2724* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2725* [Coverage](coverage.html): Retrieve coverages for a patient 2726* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2727* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2728* [DetectedIssue](detectedissue.html): Associated patient 2729* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2730* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2731* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2732* [DocumentReference](documentreference.html): Who/what is the subject of the document 2733* [Encounter](encounter.html): The patient present at the encounter 2734* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2735* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2736* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2737* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2738* [Flag](flag.html): The identity of a subject to list flags for 2739* [Goal](goal.html): Who this goal is intended for 2740* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2741* [ImagingSelection](imagingselection.html): Who the study is about 2742* [ImagingStudy](imagingstudy.html): Who the study is about 2743* [Immunization](immunization.html): The patient for the vaccination record 2744* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2745* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2746* [Invoice](invoice.html): Recipient(s) of goods and services 2747* [List](list.html): If all resources have the same subject 2748* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2749* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2750* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2751* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2752* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2753* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2754* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2755* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2756* [Observation](observation.html): The subject that the observation is about (if patient) 2757* [Person](person.html): The Person links to this Patient 2758* [Procedure](procedure.html): Search by subject - a patient 2759* [Provenance](provenance.html): Where the activity involved patient data 2760* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2761* [RelatedPerson](relatedperson.html): The patient this related person is related to 2762* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2763* [ResearchSubject](researchsubject.html): Who or what is part of study 2764* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2765* [ServiceRequest](servicerequest.html): Search by subject - a patient 2766* [Specimen](specimen.html): The patient the specimen comes from 2767* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2768* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2769* [Task](task.html): Search by patient 2770* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2771</b><br> 2772 * Type: <b>reference</b><br> 2773 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2774 * </p> 2775 */ 2776 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2777 2778/** 2779 * Constant for fluent queries to be used to add include statements. Specifies 2780 * the path value of "<b>Invoice:patient</b>". 2781 */ 2782 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Invoice:patient").toLocked(); 2783 2784 /** 2785 * Search parameter: <b>type</b> 2786 * <p> 2787 * Description: <b>Multiple Resources: 2788 2789* [Account](account.html): E.g. patient, expense, depreciation 2790* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 2791* [Composition](composition.html): Kind of composition (LOINC if possible) 2792* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 2793* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 2794* [Encounter](encounter.html): Specific type of encounter 2795* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 2796* [Invoice](invoice.html): Type of Invoice 2797* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 2798* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 2799* [Specimen](specimen.html): The specimen type 2800</b><br> 2801 * Type: <b>token</b><br> 2802 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 2803 * </p> 2804 */ 2805 @SearchParamDefinition(name="type", path="Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type", description="Multiple Resources: \r\n\r\n* [Account](account.html): E.g. patient, expense, depreciation\r\n* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known)\r\n* [Composition](composition.html): Kind of composition (LOINC if possible)\r\n* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation)\r\n* [DocumentReference](documentreference.html): Kind of document (LOINC if possible)\r\n* [Encounter](encounter.html): Specific type of encounter\r\n* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management\r\n* [Invoice](invoice.html): Type of Invoice\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type\r\n* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence\r\n* [Specimen](specimen.html): The specimen type\r\n", type="token" ) 2806 public static final String SP_TYPE = "type"; 2807 /** 2808 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2809 * <p> 2810 * Description: <b>Multiple Resources: 2811 2812* [Account](account.html): E.g. patient, expense, depreciation 2813* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 2814* [Composition](composition.html): Kind of composition (LOINC if possible) 2815* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 2816* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 2817* [Encounter](encounter.html): Specific type of encounter 2818* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 2819* [Invoice](invoice.html): Type of Invoice 2820* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 2821* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 2822* [Specimen](specimen.html): The specimen type 2823</b><br> 2824 * Type: <b>token</b><br> 2825 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 2826 * </p> 2827 */ 2828 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2829 2830 2831} 2832