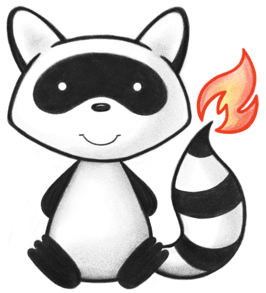
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Invoice containing collected ChargeItems from an Account with calculated individual and total price for Billing purpose. 052 */ 053@ResourceDef(name="Invoice", profile="http://hl7.org/fhir/StructureDefinition/Invoice") 054public class Invoice extends DomainResource { 055 056 public enum InvoiceStatus { 057 /** 058 * the invoice has been prepared but not yet finalized. 059 */ 060 DRAFT, 061 /** 062 * the invoice has been finalized and sent to the recipient. 063 */ 064 ISSUED, 065 /** 066 * the invoice has been balaced / completely paid. 067 */ 068 BALANCED, 069 /** 070 * the invoice was cancelled. 071 */ 072 CANCELLED, 073 /** 074 * the invoice was determined as entered in error before it was issued. 075 */ 076 ENTEREDINERROR, 077 /** 078 * added to help the parsers with the generic types 079 */ 080 NULL; 081 public static InvoiceStatus fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("draft".equals(codeString)) 085 return DRAFT; 086 if ("issued".equals(codeString)) 087 return ISSUED; 088 if ("balanced".equals(codeString)) 089 return BALANCED; 090 if ("cancelled".equals(codeString)) 091 return CANCELLED; 092 if ("entered-in-error".equals(codeString)) 093 return ENTEREDINERROR; 094 if (Configuration.isAcceptInvalidEnums()) 095 return null; 096 else 097 throw new FHIRException("Unknown InvoiceStatus code '"+codeString+"'"); 098 } 099 public String toCode() { 100 switch (this) { 101 case DRAFT: return "draft"; 102 case ISSUED: return "issued"; 103 case BALANCED: return "balanced"; 104 case CANCELLED: return "cancelled"; 105 case ENTEREDINERROR: return "entered-in-error"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getSystem() { 111 switch (this) { 112 case DRAFT: return "http://hl7.org/fhir/invoice-status"; 113 case ISSUED: return "http://hl7.org/fhir/invoice-status"; 114 case BALANCED: return "http://hl7.org/fhir/invoice-status"; 115 case CANCELLED: return "http://hl7.org/fhir/invoice-status"; 116 case ENTEREDINERROR: return "http://hl7.org/fhir/invoice-status"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDefinition() { 122 switch (this) { 123 case DRAFT: return "the invoice has been prepared but not yet finalized."; 124 case ISSUED: return "the invoice has been finalized and sent to the recipient."; 125 case BALANCED: return "the invoice has been balaced / completely paid."; 126 case CANCELLED: return "the invoice was cancelled."; 127 case ENTEREDINERROR: return "the invoice was determined as entered in error before it was issued."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case DRAFT: return "draft"; 135 case ISSUED: return "issued"; 136 case BALANCED: return "balanced"; 137 case CANCELLED: return "cancelled"; 138 case ENTEREDINERROR: return "entered in error"; 139 case NULL: return null; 140 default: return "?"; 141 } 142 } 143 } 144 145 public static class InvoiceStatusEnumFactory implements EnumFactory<InvoiceStatus> { 146 public InvoiceStatus fromCode(String codeString) throws IllegalArgumentException { 147 if (codeString == null || "".equals(codeString)) 148 if (codeString == null || "".equals(codeString)) 149 return null; 150 if ("draft".equals(codeString)) 151 return InvoiceStatus.DRAFT; 152 if ("issued".equals(codeString)) 153 return InvoiceStatus.ISSUED; 154 if ("balanced".equals(codeString)) 155 return InvoiceStatus.BALANCED; 156 if ("cancelled".equals(codeString)) 157 return InvoiceStatus.CANCELLED; 158 if ("entered-in-error".equals(codeString)) 159 return InvoiceStatus.ENTEREDINERROR; 160 throw new IllegalArgumentException("Unknown InvoiceStatus code '"+codeString+"'"); 161 } 162 public Enumeration<InvoiceStatus> fromType(PrimitiveType<?> code) throws FHIRException { 163 if (code == null) 164 return null; 165 if (code.isEmpty()) 166 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.NULL, code); 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.NULL, code); 170 if ("draft".equals(codeString)) 171 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.DRAFT, code); 172 if ("issued".equals(codeString)) 173 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.ISSUED, code); 174 if ("balanced".equals(codeString)) 175 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.BALANCED, code); 176 if ("cancelled".equals(codeString)) 177 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.CANCELLED, code); 178 if ("entered-in-error".equals(codeString)) 179 return new Enumeration<InvoiceStatus>(this, InvoiceStatus.ENTEREDINERROR, code); 180 throw new FHIRException("Unknown InvoiceStatus code '"+codeString+"'"); 181 } 182 public String toCode(InvoiceStatus code) { 183 if (code == InvoiceStatus.DRAFT) 184 return "draft"; 185 if (code == InvoiceStatus.ISSUED) 186 return "issued"; 187 if (code == InvoiceStatus.BALANCED) 188 return "balanced"; 189 if (code == InvoiceStatus.CANCELLED) 190 return "cancelled"; 191 if (code == InvoiceStatus.ENTEREDINERROR) 192 return "entered-in-error"; 193 return "?"; 194 } 195 public String toSystem(InvoiceStatus code) { 196 return code.getSystem(); 197 } 198 } 199 200 @Block() 201 public static class InvoiceParticipantComponent extends BackboneElement implements IBaseBackboneElement { 202 /** 203 * Describes the type of involvement (e.g. transcriptionist, creator etc.). If the invoice has been created automatically, the Participant may be a billing engine or another kind of device. 204 */ 205 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 206 @Description(shortDefinition="Type of involvement in creation of this Invoice", formalDefinition="Describes the type of involvement (e.g. transcriptionist, creator etc.). If the invoice has been created automatically, the Participant may be a billing engine or another kind of device." ) 207 protected CodeableConcept role; 208 209 /** 210 * The device, practitioner, etc. who performed or participated in the service. 211 */ 212 @Child(name = "actor", type = {Practitioner.class, Organization.class, Patient.class, PractitionerRole.class, Device.class, RelatedPerson.class}, order=2, min=1, max=1, modifier=false, summary=false) 213 @Description(shortDefinition="Individual who was involved", formalDefinition="The device, practitioner, etc. who performed or participated in the service." ) 214 protected Reference actor; 215 216 private static final long serialVersionUID = -1684441509L; 217 218 /** 219 * Constructor 220 */ 221 public InvoiceParticipantComponent() { 222 super(); 223 } 224 225 /** 226 * Constructor 227 */ 228 public InvoiceParticipantComponent(Reference actor) { 229 super(); 230 this.setActor(actor); 231 } 232 233 /** 234 * @return {@link #role} (Describes the type of involvement (e.g. transcriptionist, creator etc.). If the invoice has been created automatically, the Participant may be a billing engine or another kind of device.) 235 */ 236 public CodeableConcept getRole() { 237 if (this.role == null) 238 if (Configuration.errorOnAutoCreate()) 239 throw new Error("Attempt to auto-create InvoiceParticipantComponent.role"); 240 else if (Configuration.doAutoCreate()) 241 this.role = new CodeableConcept(); // cc 242 return this.role; 243 } 244 245 public boolean hasRole() { 246 return this.role != null && !this.role.isEmpty(); 247 } 248 249 /** 250 * @param value {@link #role} (Describes the type of involvement (e.g. transcriptionist, creator etc.). If the invoice has been created automatically, the Participant may be a billing engine or another kind of device.) 251 */ 252 public InvoiceParticipantComponent setRole(CodeableConcept value) { 253 this.role = value; 254 return this; 255 } 256 257 /** 258 * @return {@link #actor} (The device, practitioner, etc. who performed or participated in the service.) 259 */ 260 public Reference getActor() { 261 if (this.actor == null) 262 if (Configuration.errorOnAutoCreate()) 263 throw new Error("Attempt to auto-create InvoiceParticipantComponent.actor"); 264 else if (Configuration.doAutoCreate()) 265 this.actor = new Reference(); // cc 266 return this.actor; 267 } 268 269 public boolean hasActor() { 270 return this.actor != null && !this.actor.isEmpty(); 271 } 272 273 /** 274 * @param value {@link #actor} (The device, practitioner, etc. who performed or participated in the service.) 275 */ 276 public InvoiceParticipantComponent setActor(Reference value) { 277 this.actor = value; 278 return this; 279 } 280 281 protected void listChildren(List<Property> children) { 282 super.listChildren(children); 283 children.add(new Property("role", "CodeableConcept", "Describes the type of involvement (e.g. transcriptionist, creator etc.). If the invoice has been created automatically, the Participant may be a billing engine or another kind of device.", 0, 1, role)); 284 children.add(new Property("actor", "Reference(Practitioner|Organization|Patient|PractitionerRole|Device|RelatedPerson)", "The device, practitioner, etc. who performed or participated in the service.", 0, 1, actor)); 285 } 286 287 @Override 288 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 289 switch (_hash) { 290 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Describes the type of involvement (e.g. transcriptionist, creator etc.). If the invoice has been created automatically, the Participant may be a billing engine or another kind of device.", 0, 1, role); 291 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|Organization|Patient|PractitionerRole|Device|RelatedPerson)", "The device, practitioner, etc. who performed or participated in the service.", 0, 1, actor); 292 default: return super.getNamedProperty(_hash, _name, _checkValid); 293 } 294 295 } 296 297 @Override 298 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 299 switch (hash) { 300 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 301 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 302 default: return super.getProperty(hash, name, checkValid); 303 } 304 305 } 306 307 @Override 308 public Base setProperty(int hash, String name, Base value) throws FHIRException { 309 switch (hash) { 310 case 3506294: // role 311 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 312 return value; 313 case 92645877: // actor 314 this.actor = TypeConvertor.castToReference(value); // Reference 315 return value; 316 default: return super.setProperty(hash, name, value); 317 } 318 319 } 320 321 @Override 322 public Base setProperty(String name, Base value) throws FHIRException { 323 if (name.equals("role")) { 324 this.role = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 325 } else if (name.equals("actor")) { 326 this.actor = TypeConvertor.castToReference(value); // Reference 327 } else 328 return super.setProperty(name, value); 329 return value; 330 } 331 332 @Override 333 public void removeChild(String name, Base value) throws FHIRException { 334 if (name.equals("role")) { 335 this.role = null; 336 } else if (name.equals("actor")) { 337 this.actor = null; 338 } else 339 super.removeChild(name, value); 340 341 } 342 343 @Override 344 public Base makeProperty(int hash, String name) throws FHIRException { 345 switch (hash) { 346 case 3506294: return getRole(); 347 case 92645877: return getActor(); 348 default: return super.makeProperty(hash, name); 349 } 350 351 } 352 353 @Override 354 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 355 switch (hash) { 356 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 357 case 92645877: /*actor*/ return new String[] {"Reference"}; 358 default: return super.getTypesForProperty(hash, name); 359 } 360 361 } 362 363 @Override 364 public Base addChild(String name) throws FHIRException { 365 if (name.equals("role")) { 366 this.role = new CodeableConcept(); 367 return this.role; 368 } 369 else if (name.equals("actor")) { 370 this.actor = new Reference(); 371 return this.actor; 372 } 373 else 374 return super.addChild(name); 375 } 376 377 public InvoiceParticipantComponent copy() { 378 InvoiceParticipantComponent dst = new InvoiceParticipantComponent(); 379 copyValues(dst); 380 return dst; 381 } 382 383 public void copyValues(InvoiceParticipantComponent dst) { 384 super.copyValues(dst); 385 dst.role = role == null ? null : role.copy(); 386 dst.actor = actor == null ? null : actor.copy(); 387 } 388 389 @Override 390 public boolean equalsDeep(Base other_) { 391 if (!super.equalsDeep(other_)) 392 return false; 393 if (!(other_ instanceof InvoiceParticipantComponent)) 394 return false; 395 InvoiceParticipantComponent o = (InvoiceParticipantComponent) other_; 396 return compareDeep(role, o.role, true) && compareDeep(actor, o.actor, true); 397 } 398 399 @Override 400 public boolean equalsShallow(Base other_) { 401 if (!super.equalsShallow(other_)) 402 return false; 403 if (!(other_ instanceof InvoiceParticipantComponent)) 404 return false; 405 InvoiceParticipantComponent o = (InvoiceParticipantComponent) other_; 406 return true; 407 } 408 409 public boolean isEmpty() { 410 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, actor); 411 } 412 413 public String fhirType() { 414 return "Invoice.participant"; 415 416 } 417 418 } 419 420 @Block() 421 public static class InvoiceLineItemComponent extends BackboneElement implements IBaseBackboneElement { 422 /** 423 * Sequence in which the items appear on the invoice. 424 */ 425 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 426 @Description(shortDefinition="Sequence number of line item", formalDefinition="Sequence in which the items appear on the invoice." ) 427 protected PositiveIntType sequence; 428 429 /** 430 * Date/time(s) range when this service was delivered or completed. 431 */ 432 @Child(name = "serviced", type = {DateType.class, Period.class}, order=2, min=0, max=1, modifier=false, summary=false) 433 @Description(shortDefinition="Service data or period", formalDefinition="Date/time(s) range when this service was delivered or completed." ) 434 protected DataType serviced; 435 436 /** 437 * The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference. 438 */ 439 @Child(name = "chargeItem", type = {ChargeItem.class, CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=false) 440 @Description(shortDefinition="Reference to ChargeItem containing details of this line item or an inline billing code", formalDefinition="The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference." ) 441 protected DataType chargeItem; 442 443 /** 444 * The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice as to how the prices have been calculated. 445 */ 446 @Child(name = "priceComponent", type = {MonetaryComponent.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 447 @Description(shortDefinition="Components of total line item price", formalDefinition="The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice as to how the prices have been calculated." ) 448 protected List<MonetaryComponent> priceComponent; 449 450 private static final long serialVersionUID = -9393053L; 451 452 /** 453 * Constructor 454 */ 455 public InvoiceLineItemComponent() { 456 super(); 457 } 458 459 /** 460 * Constructor 461 */ 462 public InvoiceLineItemComponent(DataType chargeItem) { 463 super(); 464 this.setChargeItem(chargeItem); 465 } 466 467 /** 468 * @return {@link #sequence} (Sequence in which the items appear on the invoice.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 469 */ 470 public PositiveIntType getSequenceElement() { 471 if (this.sequence == null) 472 if (Configuration.errorOnAutoCreate()) 473 throw new Error("Attempt to auto-create InvoiceLineItemComponent.sequence"); 474 else if (Configuration.doAutoCreate()) 475 this.sequence = new PositiveIntType(); // bb 476 return this.sequence; 477 } 478 479 public boolean hasSequenceElement() { 480 return this.sequence != null && !this.sequence.isEmpty(); 481 } 482 483 public boolean hasSequence() { 484 return this.sequence != null && !this.sequence.isEmpty(); 485 } 486 487 /** 488 * @param value {@link #sequence} (Sequence in which the items appear on the invoice.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 489 */ 490 public InvoiceLineItemComponent setSequenceElement(PositiveIntType value) { 491 this.sequence = value; 492 return this; 493 } 494 495 /** 496 * @return Sequence in which the items appear on the invoice. 497 */ 498 public int getSequence() { 499 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 500 } 501 502 /** 503 * @param value Sequence in which the items appear on the invoice. 504 */ 505 public InvoiceLineItemComponent setSequence(int value) { 506 if (this.sequence == null) 507 this.sequence = new PositiveIntType(); 508 this.sequence.setValue(value); 509 return this; 510 } 511 512 /** 513 * @return {@link #serviced} (Date/time(s) range when this service was delivered or completed.) 514 */ 515 public DataType getServiced() { 516 return this.serviced; 517 } 518 519 /** 520 * @return {@link #serviced} (Date/time(s) range when this service was delivered or completed.) 521 */ 522 public DateType getServicedDateType() throws FHIRException { 523 if (this.serviced == null) 524 this.serviced = new DateType(); 525 if (!(this.serviced instanceof DateType)) 526 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.serviced.getClass().getName()+" was encountered"); 527 return (DateType) this.serviced; 528 } 529 530 public boolean hasServicedDateType() { 531 return this != null && this.serviced instanceof DateType; 532 } 533 534 /** 535 * @return {@link #serviced} (Date/time(s) range when this service was delivered or completed.) 536 */ 537 public Period getServicedPeriod() throws FHIRException { 538 if (this.serviced == null) 539 this.serviced = new Period(); 540 if (!(this.serviced instanceof Period)) 541 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.serviced.getClass().getName()+" was encountered"); 542 return (Period) this.serviced; 543 } 544 545 public boolean hasServicedPeriod() { 546 return this != null && this.serviced instanceof Period; 547 } 548 549 public boolean hasServiced() { 550 return this.serviced != null && !this.serviced.isEmpty(); 551 } 552 553 /** 554 * @param value {@link #serviced} (Date/time(s) range when this service was delivered or completed.) 555 */ 556 public InvoiceLineItemComponent setServiced(DataType value) { 557 if (value != null && !(value instanceof DateType || value instanceof Period)) 558 throw new FHIRException("Not the right type for Invoice.lineItem.serviced[x]: "+value.fhirType()); 559 this.serviced = value; 560 return this; 561 } 562 563 /** 564 * @return {@link #chargeItem} (The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.) 565 */ 566 public DataType getChargeItem() { 567 return this.chargeItem; 568 } 569 570 /** 571 * @return {@link #chargeItem} (The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.) 572 */ 573 public Reference getChargeItemReference() throws FHIRException { 574 if (this.chargeItem == null) 575 this.chargeItem = new Reference(); 576 if (!(this.chargeItem instanceof Reference)) 577 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.chargeItem.getClass().getName()+" was encountered"); 578 return (Reference) this.chargeItem; 579 } 580 581 public boolean hasChargeItemReference() { 582 return this != null && this.chargeItem instanceof Reference; 583 } 584 585 /** 586 * @return {@link #chargeItem} (The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.) 587 */ 588 public CodeableConcept getChargeItemCodeableConcept() throws FHIRException { 589 if (this.chargeItem == null) 590 this.chargeItem = new CodeableConcept(); 591 if (!(this.chargeItem instanceof CodeableConcept)) 592 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.chargeItem.getClass().getName()+" was encountered"); 593 return (CodeableConcept) this.chargeItem; 594 } 595 596 public boolean hasChargeItemCodeableConcept() { 597 return this != null && this.chargeItem instanceof CodeableConcept; 598 } 599 600 public boolean hasChargeItem() { 601 return this.chargeItem != null && !this.chargeItem.isEmpty(); 602 } 603 604 /** 605 * @param value {@link #chargeItem} (The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.) 606 */ 607 public InvoiceLineItemComponent setChargeItem(DataType value) { 608 if (value != null && !(value instanceof Reference || value instanceof CodeableConcept)) 609 throw new FHIRException("Not the right type for Invoice.lineItem.chargeItem[x]: "+value.fhirType()); 610 this.chargeItem = value; 611 return this; 612 } 613 614 /** 615 * @return {@link #priceComponent} (The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice as to how the prices have been calculated.) 616 */ 617 public List<MonetaryComponent> getPriceComponent() { 618 if (this.priceComponent == null) 619 this.priceComponent = new ArrayList<MonetaryComponent>(); 620 return this.priceComponent; 621 } 622 623 /** 624 * @return Returns a reference to <code>this</code> for easy method chaining 625 */ 626 public InvoiceLineItemComponent setPriceComponent(List<MonetaryComponent> thePriceComponent) { 627 this.priceComponent = thePriceComponent; 628 return this; 629 } 630 631 public boolean hasPriceComponent() { 632 if (this.priceComponent == null) 633 return false; 634 for (MonetaryComponent item : this.priceComponent) 635 if (!item.isEmpty()) 636 return true; 637 return false; 638 } 639 640 public MonetaryComponent addPriceComponent() { //3 641 MonetaryComponent t = new MonetaryComponent(); 642 if (this.priceComponent == null) 643 this.priceComponent = new ArrayList<MonetaryComponent>(); 644 this.priceComponent.add(t); 645 return t; 646 } 647 648 public InvoiceLineItemComponent addPriceComponent(MonetaryComponent t) { //3 649 if (t == null) 650 return this; 651 if (this.priceComponent == null) 652 this.priceComponent = new ArrayList<MonetaryComponent>(); 653 this.priceComponent.add(t); 654 return this; 655 } 656 657 /** 658 * @return The first repetition of repeating field {@link #priceComponent}, creating it if it does not already exist {3} 659 */ 660 public MonetaryComponent getPriceComponentFirstRep() { 661 if (getPriceComponent().isEmpty()) { 662 addPriceComponent(); 663 } 664 return getPriceComponent().get(0); 665 } 666 667 protected void listChildren(List<Property> children) { 668 super.listChildren(children); 669 children.add(new Property("sequence", "positiveInt", "Sequence in which the items appear on the invoice.", 0, 1, sequence)); 670 children.add(new Property("serviced[x]", "date|Period", "Date/time(s) range when this service was delivered or completed.", 0, 1, serviced)); 671 children.add(new Property("chargeItem[x]", "Reference(ChargeItem)|CodeableConcept", "The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.", 0, 1, chargeItem)); 672 children.add(new Property("priceComponent", "MonetaryComponent", "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice as to how the prices have been calculated.", 0, java.lang.Integer.MAX_VALUE, priceComponent)); 673 } 674 675 @Override 676 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 677 switch (_hash) { 678 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence in which the items appear on the invoice.", 0, 1, sequence); 679 case -1927922223: /*serviced[x]*/ return new Property("serviced[x]", "date|Period", "Date/time(s) range when this service was delivered or completed.", 0, 1, serviced); 680 case 1379209295: /*serviced*/ return new Property("serviced[x]", "date|Period", "Date/time(s) range when this service was delivered or completed.", 0, 1, serviced); 681 case 363246749: /*servicedDate*/ return new Property("serviced[x]", "date", "Date/time(s) range when this service was delivered or completed.", 0, 1, serviced); 682 case 1534966512: /*servicedPeriod*/ return new Property("serviced[x]", "Period", "Date/time(s) range when this service was delivered or completed.", 0, 1, serviced); 683 case 351104825: /*chargeItem[x]*/ return new Property("chargeItem[x]", "Reference(ChargeItem)|CodeableConcept", "The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.", 0, 1, chargeItem); 684 case 1417779175: /*chargeItem*/ return new Property("chargeItem[x]", "Reference(ChargeItem)|CodeableConcept", "The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.", 0, 1, chargeItem); 685 case 753580836: /*chargeItemReference*/ return new Property("chargeItem[x]", "Reference(ChargeItem)", "The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.", 0, 1, chargeItem); 686 case 1226532026: /*chargeItemCodeableConcept*/ return new Property("chargeItem[x]", "CodeableConcept", "The ChargeItem contains information such as the billing code, date, amount etc. If no further details are required for the lineItem, inline billing codes can be added using the CodeableConcept data type instead of the Reference.", 0, 1, chargeItem); 687 case 1219095988: /*priceComponent*/ return new Property("priceComponent", "MonetaryComponent", "The price for a ChargeItem may be calculated as a base price with surcharges/deductions that apply in certain conditions. A ChargeItemDefinition resource that defines the prices, factors and conditions that apply to a billing code is currently under development. The priceComponent element can be used to offer transparency to the recipient of the Invoice as to how the prices have been calculated.", 0, java.lang.Integer.MAX_VALUE, priceComponent); 688 default: return super.getNamedProperty(_hash, _name, _checkValid); 689 } 690 691 } 692 693 @Override 694 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 695 switch (hash) { 696 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 697 case 1379209295: /*serviced*/ return this.serviced == null ? new Base[0] : new Base[] {this.serviced}; // DataType 698 case 1417779175: /*chargeItem*/ return this.chargeItem == null ? new Base[0] : new Base[] {this.chargeItem}; // DataType 699 case 1219095988: /*priceComponent*/ return this.priceComponent == null ? new Base[0] : this.priceComponent.toArray(new Base[this.priceComponent.size()]); // MonetaryComponent 700 default: return super.getProperty(hash, name, checkValid); 701 } 702 703 } 704 705 @Override 706 public Base setProperty(int hash, String name, Base value) throws FHIRException { 707 switch (hash) { 708 case 1349547969: // sequence 709 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 710 return value; 711 case 1379209295: // serviced 712 this.serviced = TypeConvertor.castToType(value); // DataType 713 return value; 714 case 1417779175: // chargeItem 715 this.chargeItem = TypeConvertor.castToType(value); // DataType 716 return value; 717 case 1219095988: // priceComponent 718 this.getPriceComponent().add(TypeConvertor.castToMonetaryComponent(value)); // MonetaryComponent 719 return value; 720 default: return super.setProperty(hash, name, value); 721 } 722 723 } 724 725 @Override 726 public Base setProperty(String name, Base value) throws FHIRException { 727 if (name.equals("sequence")) { 728 this.sequence = TypeConvertor.castToPositiveInt(value); // PositiveIntType 729 } else if (name.equals("serviced[x]")) { 730 this.serviced = TypeConvertor.castToType(value); // DataType 731 } else if (name.equals("chargeItem[x]")) { 732 this.chargeItem = TypeConvertor.castToType(value); // DataType 733 } else if (name.equals("priceComponent")) { 734 this.getPriceComponent().add(TypeConvertor.castToMonetaryComponent(value)); 735 } else 736 return super.setProperty(name, value); 737 return value; 738 } 739 740 @Override 741 public void removeChild(String name, Base value) throws FHIRException { 742 if (name.equals("sequence")) { 743 this.sequence = null; 744 } else if (name.equals("serviced[x]")) { 745 this.serviced = null; 746 } else if (name.equals("chargeItem[x]")) { 747 this.chargeItem = null; 748 } else if (name.equals("priceComponent")) { 749 this.getPriceComponent().remove(value); 750 } else 751 super.removeChild(name, value); 752 753 } 754 755 @Override 756 public Base makeProperty(int hash, String name) throws FHIRException { 757 switch (hash) { 758 case 1349547969: return getSequenceElement(); 759 case -1927922223: return getServiced(); 760 case 1379209295: return getServiced(); 761 case 351104825: return getChargeItem(); 762 case 1417779175: return getChargeItem(); 763 case 1219095988: return addPriceComponent(); 764 default: return super.makeProperty(hash, name); 765 } 766 767 } 768 769 @Override 770 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 771 switch (hash) { 772 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 773 case 1379209295: /*serviced*/ return new String[] {"date", "Period"}; 774 case 1417779175: /*chargeItem*/ return new String[] {"Reference", "CodeableConcept"}; 775 case 1219095988: /*priceComponent*/ return new String[] {"MonetaryComponent"}; 776 default: return super.getTypesForProperty(hash, name); 777 } 778 779 } 780 781 @Override 782 public Base addChild(String name) throws FHIRException { 783 if (name.equals("sequence")) { 784 throw new FHIRException("Cannot call addChild on a singleton property Invoice.lineItem.sequence"); 785 } 786 else if (name.equals("servicedDate")) { 787 this.serviced = new DateType(); 788 return this.serviced; 789 } 790 else if (name.equals("servicedPeriod")) { 791 this.serviced = new Period(); 792 return this.serviced; 793 } 794 else if (name.equals("chargeItemReference")) { 795 this.chargeItem = new Reference(); 796 return this.chargeItem; 797 } 798 else if (name.equals("chargeItemCodeableConcept")) { 799 this.chargeItem = new CodeableConcept(); 800 return this.chargeItem; 801 } 802 else if (name.equals("priceComponent")) { 803 return addPriceComponent(); 804 } 805 else 806 return super.addChild(name); 807 } 808 809 public InvoiceLineItemComponent copy() { 810 InvoiceLineItemComponent dst = new InvoiceLineItemComponent(); 811 copyValues(dst); 812 return dst; 813 } 814 815 public void copyValues(InvoiceLineItemComponent dst) { 816 super.copyValues(dst); 817 dst.sequence = sequence == null ? null : sequence.copy(); 818 dst.serviced = serviced == null ? null : serviced.copy(); 819 dst.chargeItem = chargeItem == null ? null : chargeItem.copy(); 820 if (priceComponent != null) { 821 dst.priceComponent = new ArrayList<MonetaryComponent>(); 822 for (MonetaryComponent i : priceComponent) 823 dst.priceComponent.add(i.copy()); 824 }; 825 } 826 827 @Override 828 public boolean equalsDeep(Base other_) { 829 if (!super.equalsDeep(other_)) 830 return false; 831 if (!(other_ instanceof InvoiceLineItemComponent)) 832 return false; 833 InvoiceLineItemComponent o = (InvoiceLineItemComponent) other_; 834 return compareDeep(sequence, o.sequence, true) && compareDeep(serviced, o.serviced, true) && compareDeep(chargeItem, o.chargeItem, true) 835 && compareDeep(priceComponent, o.priceComponent, true); 836 } 837 838 @Override 839 public boolean equalsShallow(Base other_) { 840 if (!super.equalsShallow(other_)) 841 return false; 842 if (!(other_ instanceof InvoiceLineItemComponent)) 843 return false; 844 InvoiceLineItemComponent o = (InvoiceLineItemComponent) other_; 845 return compareValues(sequence, o.sequence, true); 846 } 847 848 public boolean isEmpty() { 849 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, serviced, chargeItem 850 , priceComponent); 851 } 852 853 public String fhirType() { 854 return "Invoice.lineItem"; 855 856 } 857 858 } 859 860 /** 861 * Identifier of this Invoice, often used for reference in correspondence about this invoice or for tracking of payments. 862 */ 863 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 864 @Description(shortDefinition="Business Identifier for item", formalDefinition="Identifier of this Invoice, often used for reference in correspondence about this invoice or for tracking of payments." ) 865 protected List<Identifier> identifier; 866 867 /** 868 * The current state of the Invoice. 869 */ 870 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 871 @Description(shortDefinition="draft | issued | balanced | cancelled | entered-in-error", formalDefinition="The current state of the Invoice." ) 872 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/invoice-status") 873 protected Enumeration<InvoiceStatus> status; 874 875 /** 876 * In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.). 877 */ 878 @Child(name = "cancelledReason", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 879 @Description(shortDefinition="Reason for cancellation of this Invoice", formalDefinition="In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.)." ) 880 protected StringType cancelledReason; 881 882 /** 883 * Type of Invoice depending on domain, realm an usage (e.g. internal/external, dental, preliminary). 884 */ 885 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 886 @Description(shortDefinition="Type of Invoice", formalDefinition="Type of Invoice depending on domain, realm an usage (e.g. internal/external, dental, preliminary)." ) 887 protected CodeableConcept type; 888 889 /** 890 * The individual or set of individuals receiving the goods and services billed in this invoice. 891 */ 892 @Child(name = "subject", type = {Patient.class, Group.class}, order=4, min=0, max=1, modifier=false, summary=true) 893 @Description(shortDefinition="Recipient(s) of goods and services", formalDefinition="The individual or set of individuals receiving the goods and services billed in this invoice." ) 894 protected Reference subject; 895 896 /** 897 * The individual or Organization responsible for balancing of this invoice. 898 */ 899 @Child(name = "recipient", type = {Organization.class, Patient.class, RelatedPerson.class}, order=5, min=0, max=1, modifier=false, summary=true) 900 @Description(shortDefinition="Recipient of this invoice", formalDefinition="The individual or Organization responsible for balancing of this invoice." ) 901 protected Reference recipient; 902 903 /** 904 * Depricared by the element below. 905 */ 906 @Child(name = "date", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 907 @Description(shortDefinition="DEPRICATED", formalDefinition="Depricared by the element below." ) 908 protected DateTimeType date; 909 910 /** 911 * Date/time(s) of when this Invoice was posted. 912 */ 913 @Child(name = "creation", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 914 @Description(shortDefinition="When posted", formalDefinition="Date/time(s) of when this Invoice was posted." ) 915 protected DateTimeType creation; 916 917 /** 918 * Date/time(s) range of services included in this invoice. 919 */ 920 @Child(name = "period", type = {DateType.class, Period.class}, order=8, min=0, max=1, modifier=false, summary=true) 921 @Description(shortDefinition="Billing date or period", formalDefinition="Date/time(s) range of services included in this invoice." ) 922 protected DataType period; 923 924 /** 925 * Indicates who or what performed or participated in the charged service. 926 */ 927 @Child(name = "participant", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 928 @Description(shortDefinition="Participant in creation of this Invoice", formalDefinition="Indicates who or what performed or participated in the charged service." ) 929 protected List<InvoiceParticipantComponent> participant; 930 931 /** 932 * The organizationissuing the Invoice. 933 */ 934 @Child(name = "issuer", type = {Organization.class}, order=10, min=0, max=1, modifier=false, summary=false) 935 @Description(shortDefinition="Issuing Organization of Invoice", formalDefinition="The organizationissuing the Invoice." ) 936 protected Reference issuer; 937 938 /** 939 * Account which is supposed to be balanced with this Invoice. 940 */ 941 @Child(name = "account", type = {Account.class}, order=11, min=0, max=1, modifier=false, summary=false) 942 @Description(shortDefinition="Account that is being balanced", formalDefinition="Account which is supposed to be balanced with this Invoice." ) 943 protected Reference account; 944 945 /** 946 * Each line item represents one charge for goods and services rendered. Details such.ofType(date), code and amount are found in the referenced ChargeItem resource. 947 */ 948 @Child(name = "lineItem", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 949 @Description(shortDefinition="Line items of this Invoice", formalDefinition="Each line item represents one charge for goods and services rendered. Details such.ofType(date), code and amount are found in the referenced ChargeItem resource." ) 950 protected List<InvoiceLineItemComponent> lineItem; 951 952 /** 953 * The total amount for the Invoice may be calculated as the sum of the line items with surcharges/deductions that apply in certain conditions. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the total price was calculated. 954 */ 955 @Child(name = "totalPriceComponent", type = {MonetaryComponent.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 956 @Description(shortDefinition="Components of Invoice total", formalDefinition="The total amount for the Invoice may be calculated as the sum of the line items with surcharges/deductions that apply in certain conditions. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the total price was calculated." ) 957 protected List<MonetaryComponent> totalPriceComponent; 958 959 /** 960 * Invoice total , taxes excluded. 961 */ 962 @Child(name = "totalNet", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=true) 963 @Description(shortDefinition="Net total of this Invoice", formalDefinition="Invoice total , taxes excluded." ) 964 protected Money totalNet; 965 966 /** 967 * Invoice total, tax included. 968 */ 969 @Child(name = "totalGross", type = {Money.class}, order=15, min=0, max=1, modifier=false, summary=true) 970 @Description(shortDefinition="Gross total of this Invoice", formalDefinition="Invoice total, tax included." ) 971 protected Money totalGross; 972 973 /** 974 * Payment details such as banking details, period of payment, deductibles, methods of payment. 975 */ 976 @Child(name = "paymentTerms", type = {MarkdownType.class}, order=16, min=0, max=1, modifier=false, summary=false) 977 @Description(shortDefinition="Payment details", formalDefinition="Payment details such as banking details, period of payment, deductibles, methods of payment." ) 978 protected MarkdownType paymentTerms; 979 980 /** 981 * Comments made about the invoice by the issuer, subject, or other participants. 982 */ 983 @Child(name = "note", type = {Annotation.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 984 @Description(shortDefinition="Comments made about the invoice", formalDefinition="Comments made about the invoice by the issuer, subject, or other participants." ) 985 protected List<Annotation> note; 986 987 private static final long serialVersionUID = 6346282L; 988 989 /** 990 * Constructor 991 */ 992 public Invoice() { 993 super(); 994 } 995 996 /** 997 * Constructor 998 */ 999 public Invoice(InvoiceStatus status) { 1000 super(); 1001 this.setStatus(status); 1002 } 1003 1004 /** 1005 * @return {@link #identifier} (Identifier of this Invoice, often used for reference in correspondence about this invoice or for tracking of payments.) 1006 */ 1007 public List<Identifier> getIdentifier() { 1008 if (this.identifier == null) 1009 this.identifier = new ArrayList<Identifier>(); 1010 return this.identifier; 1011 } 1012 1013 /** 1014 * @return Returns a reference to <code>this</code> for easy method chaining 1015 */ 1016 public Invoice setIdentifier(List<Identifier> theIdentifier) { 1017 this.identifier = theIdentifier; 1018 return this; 1019 } 1020 1021 public boolean hasIdentifier() { 1022 if (this.identifier == null) 1023 return false; 1024 for (Identifier item : this.identifier) 1025 if (!item.isEmpty()) 1026 return true; 1027 return false; 1028 } 1029 1030 public Identifier addIdentifier() { //3 1031 Identifier t = new Identifier(); 1032 if (this.identifier == null) 1033 this.identifier = new ArrayList<Identifier>(); 1034 this.identifier.add(t); 1035 return t; 1036 } 1037 1038 public Invoice addIdentifier(Identifier t) { //3 1039 if (t == null) 1040 return this; 1041 if (this.identifier == null) 1042 this.identifier = new ArrayList<Identifier>(); 1043 this.identifier.add(t); 1044 return this; 1045 } 1046 1047 /** 1048 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1049 */ 1050 public Identifier getIdentifierFirstRep() { 1051 if (getIdentifier().isEmpty()) { 1052 addIdentifier(); 1053 } 1054 return getIdentifier().get(0); 1055 } 1056 1057 /** 1058 * @return {@link #status} (The current state of the Invoice.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1059 */ 1060 public Enumeration<InvoiceStatus> getStatusElement() { 1061 if (this.status == null) 1062 if (Configuration.errorOnAutoCreate()) 1063 throw new Error("Attempt to auto-create Invoice.status"); 1064 else if (Configuration.doAutoCreate()) 1065 this.status = new Enumeration<InvoiceStatus>(new InvoiceStatusEnumFactory()); // bb 1066 return this.status; 1067 } 1068 1069 public boolean hasStatusElement() { 1070 return this.status != null && !this.status.isEmpty(); 1071 } 1072 1073 public boolean hasStatus() { 1074 return this.status != null && !this.status.isEmpty(); 1075 } 1076 1077 /** 1078 * @param value {@link #status} (The current state of the Invoice.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1079 */ 1080 public Invoice setStatusElement(Enumeration<InvoiceStatus> value) { 1081 this.status = value; 1082 return this; 1083 } 1084 1085 /** 1086 * @return The current state of the Invoice. 1087 */ 1088 public InvoiceStatus getStatus() { 1089 return this.status == null ? null : this.status.getValue(); 1090 } 1091 1092 /** 1093 * @param value The current state of the Invoice. 1094 */ 1095 public Invoice setStatus(InvoiceStatus value) { 1096 if (this.status == null) 1097 this.status = new Enumeration<InvoiceStatus>(new InvoiceStatusEnumFactory()); 1098 this.status.setValue(value); 1099 return this; 1100 } 1101 1102 /** 1103 * @return {@link #cancelledReason} (In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.).). This is the underlying object with id, value and extensions. The accessor "getCancelledReason" gives direct access to the value 1104 */ 1105 public StringType getCancelledReasonElement() { 1106 if (this.cancelledReason == null) 1107 if (Configuration.errorOnAutoCreate()) 1108 throw new Error("Attempt to auto-create Invoice.cancelledReason"); 1109 else if (Configuration.doAutoCreate()) 1110 this.cancelledReason = new StringType(); // bb 1111 return this.cancelledReason; 1112 } 1113 1114 public boolean hasCancelledReasonElement() { 1115 return this.cancelledReason != null && !this.cancelledReason.isEmpty(); 1116 } 1117 1118 public boolean hasCancelledReason() { 1119 return this.cancelledReason != null && !this.cancelledReason.isEmpty(); 1120 } 1121 1122 /** 1123 * @param value {@link #cancelledReason} (In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.).). This is the underlying object with id, value and extensions. The accessor "getCancelledReason" gives direct access to the value 1124 */ 1125 public Invoice setCancelledReasonElement(StringType value) { 1126 this.cancelledReason = value; 1127 return this; 1128 } 1129 1130 /** 1131 * @return In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.). 1132 */ 1133 public String getCancelledReason() { 1134 return this.cancelledReason == null ? null : this.cancelledReason.getValue(); 1135 } 1136 1137 /** 1138 * @param value In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.). 1139 */ 1140 public Invoice setCancelledReason(String value) { 1141 if (Utilities.noString(value)) 1142 this.cancelledReason = null; 1143 else { 1144 if (this.cancelledReason == null) 1145 this.cancelledReason = new StringType(); 1146 this.cancelledReason.setValue(value); 1147 } 1148 return this; 1149 } 1150 1151 /** 1152 * @return {@link #type} (Type of Invoice depending on domain, realm an usage (e.g. internal/external, dental, preliminary).) 1153 */ 1154 public CodeableConcept getType() { 1155 if (this.type == null) 1156 if (Configuration.errorOnAutoCreate()) 1157 throw new Error("Attempt to auto-create Invoice.type"); 1158 else if (Configuration.doAutoCreate()) 1159 this.type = new CodeableConcept(); // cc 1160 return this.type; 1161 } 1162 1163 public boolean hasType() { 1164 return this.type != null && !this.type.isEmpty(); 1165 } 1166 1167 /** 1168 * @param value {@link #type} (Type of Invoice depending on domain, realm an usage (e.g. internal/external, dental, preliminary).) 1169 */ 1170 public Invoice setType(CodeableConcept value) { 1171 this.type = value; 1172 return this; 1173 } 1174 1175 /** 1176 * @return {@link #subject} (The individual or set of individuals receiving the goods and services billed in this invoice.) 1177 */ 1178 public Reference getSubject() { 1179 if (this.subject == null) 1180 if (Configuration.errorOnAutoCreate()) 1181 throw new Error("Attempt to auto-create Invoice.subject"); 1182 else if (Configuration.doAutoCreate()) 1183 this.subject = new Reference(); // cc 1184 return this.subject; 1185 } 1186 1187 public boolean hasSubject() { 1188 return this.subject != null && !this.subject.isEmpty(); 1189 } 1190 1191 /** 1192 * @param value {@link #subject} (The individual or set of individuals receiving the goods and services billed in this invoice.) 1193 */ 1194 public Invoice setSubject(Reference value) { 1195 this.subject = value; 1196 return this; 1197 } 1198 1199 /** 1200 * @return {@link #recipient} (The individual or Organization responsible for balancing of this invoice.) 1201 */ 1202 public Reference getRecipient() { 1203 if (this.recipient == null) 1204 if (Configuration.errorOnAutoCreate()) 1205 throw new Error("Attempt to auto-create Invoice.recipient"); 1206 else if (Configuration.doAutoCreate()) 1207 this.recipient = new Reference(); // cc 1208 return this.recipient; 1209 } 1210 1211 public boolean hasRecipient() { 1212 return this.recipient != null && !this.recipient.isEmpty(); 1213 } 1214 1215 /** 1216 * @param value {@link #recipient} (The individual or Organization responsible for balancing of this invoice.) 1217 */ 1218 public Invoice setRecipient(Reference value) { 1219 this.recipient = value; 1220 return this; 1221 } 1222 1223 /** 1224 * @return {@link #date} (Depricared by the element below.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1225 */ 1226 public DateTimeType getDateElement() { 1227 if (this.date == null) 1228 if (Configuration.errorOnAutoCreate()) 1229 throw new Error("Attempt to auto-create Invoice.date"); 1230 else if (Configuration.doAutoCreate()) 1231 this.date = new DateTimeType(); // bb 1232 return this.date; 1233 } 1234 1235 public boolean hasDateElement() { 1236 return this.date != null && !this.date.isEmpty(); 1237 } 1238 1239 public boolean hasDate() { 1240 return this.date != null && !this.date.isEmpty(); 1241 } 1242 1243 /** 1244 * @param value {@link #date} (Depricared by the element below.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1245 */ 1246 public Invoice setDateElement(DateTimeType value) { 1247 this.date = value; 1248 return this; 1249 } 1250 1251 /** 1252 * @return Depricared by the element below. 1253 */ 1254 public Date getDate() { 1255 return this.date == null ? null : this.date.getValue(); 1256 } 1257 1258 /** 1259 * @param value Depricared by the element below. 1260 */ 1261 public Invoice setDate(Date value) { 1262 if (value == null) 1263 this.date = null; 1264 else { 1265 if (this.date == null) 1266 this.date = new DateTimeType(); 1267 this.date.setValue(value); 1268 } 1269 return this; 1270 } 1271 1272 /** 1273 * @return {@link #creation} (Date/time(s) of when this Invoice was posted.). This is the underlying object with id, value and extensions. The accessor "getCreation" gives direct access to the value 1274 */ 1275 public DateTimeType getCreationElement() { 1276 if (this.creation == null) 1277 if (Configuration.errorOnAutoCreate()) 1278 throw new Error("Attempt to auto-create Invoice.creation"); 1279 else if (Configuration.doAutoCreate()) 1280 this.creation = new DateTimeType(); // bb 1281 return this.creation; 1282 } 1283 1284 public boolean hasCreationElement() { 1285 return this.creation != null && !this.creation.isEmpty(); 1286 } 1287 1288 public boolean hasCreation() { 1289 return this.creation != null && !this.creation.isEmpty(); 1290 } 1291 1292 /** 1293 * @param value {@link #creation} (Date/time(s) of when this Invoice was posted.). This is the underlying object with id, value and extensions. The accessor "getCreation" gives direct access to the value 1294 */ 1295 public Invoice setCreationElement(DateTimeType value) { 1296 this.creation = value; 1297 return this; 1298 } 1299 1300 /** 1301 * @return Date/time(s) of when this Invoice was posted. 1302 */ 1303 public Date getCreation() { 1304 return this.creation == null ? null : this.creation.getValue(); 1305 } 1306 1307 /** 1308 * @param value Date/time(s) of when this Invoice was posted. 1309 */ 1310 public Invoice setCreation(Date value) { 1311 if (value == null) 1312 this.creation = null; 1313 else { 1314 if (this.creation == null) 1315 this.creation = new DateTimeType(); 1316 this.creation.setValue(value); 1317 } 1318 return this; 1319 } 1320 1321 /** 1322 * @return {@link #period} (Date/time(s) range of services included in this invoice.) 1323 */ 1324 public DataType getPeriod() { 1325 return this.period; 1326 } 1327 1328 /** 1329 * @return {@link #period} (Date/time(s) range of services included in this invoice.) 1330 */ 1331 public DateType getPeriodDateType() throws FHIRException { 1332 if (this.period == null) 1333 this.period = new DateType(); 1334 if (!(this.period instanceof DateType)) 1335 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.period.getClass().getName()+" was encountered"); 1336 return (DateType) this.period; 1337 } 1338 1339 public boolean hasPeriodDateType() { 1340 return this != null && this.period instanceof DateType; 1341 } 1342 1343 /** 1344 * @return {@link #period} (Date/time(s) range of services included in this invoice.) 1345 */ 1346 public Period getPeriodPeriod() throws FHIRException { 1347 if (this.period == null) 1348 this.period = new Period(); 1349 if (!(this.period instanceof Period)) 1350 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.period.getClass().getName()+" was encountered"); 1351 return (Period) this.period; 1352 } 1353 1354 public boolean hasPeriodPeriod() { 1355 return this != null && this.period instanceof Period; 1356 } 1357 1358 public boolean hasPeriod() { 1359 return this.period != null && !this.period.isEmpty(); 1360 } 1361 1362 /** 1363 * @param value {@link #period} (Date/time(s) range of services included in this invoice.) 1364 */ 1365 public Invoice setPeriod(DataType value) { 1366 if (value != null && !(value instanceof DateType || value instanceof Period)) 1367 throw new FHIRException("Not the right type for Invoice.period[x]: "+value.fhirType()); 1368 this.period = value; 1369 return this; 1370 } 1371 1372 /** 1373 * @return {@link #participant} (Indicates who or what performed or participated in the charged service.) 1374 */ 1375 public List<InvoiceParticipantComponent> getParticipant() { 1376 if (this.participant == null) 1377 this.participant = new ArrayList<InvoiceParticipantComponent>(); 1378 return this.participant; 1379 } 1380 1381 /** 1382 * @return Returns a reference to <code>this</code> for easy method chaining 1383 */ 1384 public Invoice setParticipant(List<InvoiceParticipantComponent> theParticipant) { 1385 this.participant = theParticipant; 1386 return this; 1387 } 1388 1389 public boolean hasParticipant() { 1390 if (this.participant == null) 1391 return false; 1392 for (InvoiceParticipantComponent item : this.participant) 1393 if (!item.isEmpty()) 1394 return true; 1395 return false; 1396 } 1397 1398 public InvoiceParticipantComponent addParticipant() { //3 1399 InvoiceParticipantComponent t = new InvoiceParticipantComponent(); 1400 if (this.participant == null) 1401 this.participant = new ArrayList<InvoiceParticipantComponent>(); 1402 this.participant.add(t); 1403 return t; 1404 } 1405 1406 public Invoice addParticipant(InvoiceParticipantComponent t) { //3 1407 if (t == null) 1408 return this; 1409 if (this.participant == null) 1410 this.participant = new ArrayList<InvoiceParticipantComponent>(); 1411 this.participant.add(t); 1412 return this; 1413 } 1414 1415 /** 1416 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist {3} 1417 */ 1418 public InvoiceParticipantComponent getParticipantFirstRep() { 1419 if (getParticipant().isEmpty()) { 1420 addParticipant(); 1421 } 1422 return getParticipant().get(0); 1423 } 1424 1425 /** 1426 * @return {@link #issuer} (The organizationissuing the Invoice.) 1427 */ 1428 public Reference getIssuer() { 1429 if (this.issuer == null) 1430 if (Configuration.errorOnAutoCreate()) 1431 throw new Error("Attempt to auto-create Invoice.issuer"); 1432 else if (Configuration.doAutoCreate()) 1433 this.issuer = new Reference(); // cc 1434 return this.issuer; 1435 } 1436 1437 public boolean hasIssuer() { 1438 return this.issuer != null && !this.issuer.isEmpty(); 1439 } 1440 1441 /** 1442 * @param value {@link #issuer} (The organizationissuing the Invoice.) 1443 */ 1444 public Invoice setIssuer(Reference value) { 1445 this.issuer = value; 1446 return this; 1447 } 1448 1449 /** 1450 * @return {@link #account} (Account which is supposed to be balanced with this Invoice.) 1451 */ 1452 public Reference getAccount() { 1453 if (this.account == null) 1454 if (Configuration.errorOnAutoCreate()) 1455 throw new Error("Attempt to auto-create Invoice.account"); 1456 else if (Configuration.doAutoCreate()) 1457 this.account = new Reference(); // cc 1458 return this.account; 1459 } 1460 1461 public boolean hasAccount() { 1462 return this.account != null && !this.account.isEmpty(); 1463 } 1464 1465 /** 1466 * @param value {@link #account} (Account which is supposed to be balanced with this Invoice.) 1467 */ 1468 public Invoice setAccount(Reference value) { 1469 this.account = value; 1470 return this; 1471 } 1472 1473 /** 1474 * @return {@link #lineItem} (Each line item represents one charge for goods and services rendered. Details such.ofType(date), code and amount are found in the referenced ChargeItem resource.) 1475 */ 1476 public List<InvoiceLineItemComponent> getLineItem() { 1477 if (this.lineItem == null) 1478 this.lineItem = new ArrayList<InvoiceLineItemComponent>(); 1479 return this.lineItem; 1480 } 1481 1482 /** 1483 * @return Returns a reference to <code>this</code> for easy method chaining 1484 */ 1485 public Invoice setLineItem(List<InvoiceLineItemComponent> theLineItem) { 1486 this.lineItem = theLineItem; 1487 return this; 1488 } 1489 1490 public boolean hasLineItem() { 1491 if (this.lineItem == null) 1492 return false; 1493 for (InvoiceLineItemComponent item : this.lineItem) 1494 if (!item.isEmpty()) 1495 return true; 1496 return false; 1497 } 1498 1499 public InvoiceLineItemComponent addLineItem() { //3 1500 InvoiceLineItemComponent t = new InvoiceLineItemComponent(); 1501 if (this.lineItem == null) 1502 this.lineItem = new ArrayList<InvoiceLineItemComponent>(); 1503 this.lineItem.add(t); 1504 return t; 1505 } 1506 1507 public Invoice addLineItem(InvoiceLineItemComponent t) { //3 1508 if (t == null) 1509 return this; 1510 if (this.lineItem == null) 1511 this.lineItem = new ArrayList<InvoiceLineItemComponent>(); 1512 this.lineItem.add(t); 1513 return this; 1514 } 1515 1516 /** 1517 * @return The first repetition of repeating field {@link #lineItem}, creating it if it does not already exist {3} 1518 */ 1519 public InvoiceLineItemComponent getLineItemFirstRep() { 1520 if (getLineItem().isEmpty()) { 1521 addLineItem(); 1522 } 1523 return getLineItem().get(0); 1524 } 1525 1526 /** 1527 * @return {@link #totalPriceComponent} (The total amount for the Invoice may be calculated as the sum of the line items with surcharges/deductions that apply in certain conditions. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the total price was calculated.) 1528 */ 1529 public List<MonetaryComponent> getTotalPriceComponent() { 1530 if (this.totalPriceComponent == null) 1531 this.totalPriceComponent = new ArrayList<MonetaryComponent>(); 1532 return this.totalPriceComponent; 1533 } 1534 1535 /** 1536 * @return Returns a reference to <code>this</code> for easy method chaining 1537 */ 1538 public Invoice setTotalPriceComponent(List<MonetaryComponent> theTotalPriceComponent) { 1539 this.totalPriceComponent = theTotalPriceComponent; 1540 return this; 1541 } 1542 1543 public boolean hasTotalPriceComponent() { 1544 if (this.totalPriceComponent == null) 1545 return false; 1546 for (MonetaryComponent item : this.totalPriceComponent) 1547 if (!item.isEmpty()) 1548 return true; 1549 return false; 1550 } 1551 1552 public MonetaryComponent addTotalPriceComponent() { //3 1553 MonetaryComponent t = new MonetaryComponent(); 1554 if (this.totalPriceComponent == null) 1555 this.totalPriceComponent = new ArrayList<MonetaryComponent>(); 1556 this.totalPriceComponent.add(t); 1557 return t; 1558 } 1559 1560 public Invoice addTotalPriceComponent(MonetaryComponent t) { //3 1561 if (t == null) 1562 return this; 1563 if (this.totalPriceComponent == null) 1564 this.totalPriceComponent = new ArrayList<MonetaryComponent>(); 1565 this.totalPriceComponent.add(t); 1566 return this; 1567 } 1568 1569 /** 1570 * @return The first repetition of repeating field {@link #totalPriceComponent}, creating it if it does not already exist {3} 1571 */ 1572 public MonetaryComponent getTotalPriceComponentFirstRep() { 1573 if (getTotalPriceComponent().isEmpty()) { 1574 addTotalPriceComponent(); 1575 } 1576 return getTotalPriceComponent().get(0); 1577 } 1578 1579 /** 1580 * @return {@link #totalNet} (Invoice total , taxes excluded.) 1581 */ 1582 public Money getTotalNet() { 1583 if (this.totalNet == null) 1584 if (Configuration.errorOnAutoCreate()) 1585 throw new Error("Attempt to auto-create Invoice.totalNet"); 1586 else if (Configuration.doAutoCreate()) 1587 this.totalNet = new Money(); // cc 1588 return this.totalNet; 1589 } 1590 1591 public boolean hasTotalNet() { 1592 return this.totalNet != null && !this.totalNet.isEmpty(); 1593 } 1594 1595 /** 1596 * @param value {@link #totalNet} (Invoice total , taxes excluded.) 1597 */ 1598 public Invoice setTotalNet(Money value) { 1599 this.totalNet = value; 1600 return this; 1601 } 1602 1603 /** 1604 * @return {@link #totalGross} (Invoice total, tax included.) 1605 */ 1606 public Money getTotalGross() { 1607 if (this.totalGross == null) 1608 if (Configuration.errorOnAutoCreate()) 1609 throw new Error("Attempt to auto-create Invoice.totalGross"); 1610 else if (Configuration.doAutoCreate()) 1611 this.totalGross = new Money(); // cc 1612 return this.totalGross; 1613 } 1614 1615 public boolean hasTotalGross() { 1616 return this.totalGross != null && !this.totalGross.isEmpty(); 1617 } 1618 1619 /** 1620 * @param value {@link #totalGross} (Invoice total, tax included.) 1621 */ 1622 public Invoice setTotalGross(Money value) { 1623 this.totalGross = value; 1624 return this; 1625 } 1626 1627 /** 1628 * @return {@link #paymentTerms} (Payment details such as banking details, period of payment, deductibles, methods of payment.). This is the underlying object with id, value and extensions. The accessor "getPaymentTerms" gives direct access to the value 1629 */ 1630 public MarkdownType getPaymentTermsElement() { 1631 if (this.paymentTerms == null) 1632 if (Configuration.errorOnAutoCreate()) 1633 throw new Error("Attempt to auto-create Invoice.paymentTerms"); 1634 else if (Configuration.doAutoCreate()) 1635 this.paymentTerms = new MarkdownType(); // bb 1636 return this.paymentTerms; 1637 } 1638 1639 public boolean hasPaymentTermsElement() { 1640 return this.paymentTerms != null && !this.paymentTerms.isEmpty(); 1641 } 1642 1643 public boolean hasPaymentTerms() { 1644 return this.paymentTerms != null && !this.paymentTerms.isEmpty(); 1645 } 1646 1647 /** 1648 * @param value {@link #paymentTerms} (Payment details such as banking details, period of payment, deductibles, methods of payment.). This is the underlying object with id, value and extensions. The accessor "getPaymentTerms" gives direct access to the value 1649 */ 1650 public Invoice setPaymentTermsElement(MarkdownType value) { 1651 this.paymentTerms = value; 1652 return this; 1653 } 1654 1655 /** 1656 * @return Payment details such as banking details, period of payment, deductibles, methods of payment. 1657 */ 1658 public String getPaymentTerms() { 1659 return this.paymentTerms == null ? null : this.paymentTerms.getValue(); 1660 } 1661 1662 /** 1663 * @param value Payment details such as banking details, period of payment, deductibles, methods of payment. 1664 */ 1665 public Invoice setPaymentTerms(String value) { 1666 if (Utilities.noString(value)) 1667 this.paymentTerms = null; 1668 else { 1669 if (this.paymentTerms == null) 1670 this.paymentTerms = new MarkdownType(); 1671 this.paymentTerms.setValue(value); 1672 } 1673 return this; 1674 } 1675 1676 /** 1677 * @return {@link #note} (Comments made about the invoice by the issuer, subject, or other participants.) 1678 */ 1679 public List<Annotation> getNote() { 1680 if (this.note == null) 1681 this.note = new ArrayList<Annotation>(); 1682 return this.note; 1683 } 1684 1685 /** 1686 * @return Returns a reference to <code>this</code> for easy method chaining 1687 */ 1688 public Invoice setNote(List<Annotation> theNote) { 1689 this.note = theNote; 1690 return this; 1691 } 1692 1693 public boolean hasNote() { 1694 if (this.note == null) 1695 return false; 1696 for (Annotation item : this.note) 1697 if (!item.isEmpty()) 1698 return true; 1699 return false; 1700 } 1701 1702 public Annotation addNote() { //3 1703 Annotation t = new Annotation(); 1704 if (this.note == null) 1705 this.note = new ArrayList<Annotation>(); 1706 this.note.add(t); 1707 return t; 1708 } 1709 1710 public Invoice addNote(Annotation t) { //3 1711 if (t == null) 1712 return this; 1713 if (this.note == null) 1714 this.note = new ArrayList<Annotation>(); 1715 this.note.add(t); 1716 return this; 1717 } 1718 1719 /** 1720 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1721 */ 1722 public Annotation getNoteFirstRep() { 1723 if (getNote().isEmpty()) { 1724 addNote(); 1725 } 1726 return getNote().get(0); 1727 } 1728 1729 protected void listChildren(List<Property> children) { 1730 super.listChildren(children); 1731 children.add(new Property("identifier", "Identifier", "Identifier of this Invoice, often used for reference in correspondence about this invoice or for tracking of payments.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1732 children.add(new Property("status", "code", "The current state of the Invoice.", 0, 1, status)); 1733 children.add(new Property("cancelledReason", "string", "In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.).", 0, 1, cancelledReason)); 1734 children.add(new Property("type", "CodeableConcept", "Type of Invoice depending on domain, realm an usage (e.g. internal/external, dental, preliminary).", 0, 1, type)); 1735 children.add(new Property("subject", "Reference(Patient|Group)", "The individual or set of individuals receiving the goods and services billed in this invoice.", 0, 1, subject)); 1736 children.add(new Property("recipient", "Reference(Organization|Patient|RelatedPerson)", "The individual or Organization responsible for balancing of this invoice.", 0, 1, recipient)); 1737 children.add(new Property("date", "dateTime", "Depricared by the element below.", 0, 1, date)); 1738 children.add(new Property("creation", "dateTime", "Date/time(s) of when this Invoice was posted.", 0, 1, creation)); 1739 children.add(new Property("period[x]", "date|Period", "Date/time(s) range of services included in this invoice.", 0, 1, period)); 1740 children.add(new Property("participant", "", "Indicates who or what performed or participated in the charged service.", 0, java.lang.Integer.MAX_VALUE, participant)); 1741 children.add(new Property("issuer", "Reference(Organization)", "The organizationissuing the Invoice.", 0, 1, issuer)); 1742 children.add(new Property("account", "Reference(Account)", "Account which is supposed to be balanced with this Invoice.", 0, 1, account)); 1743 children.add(new Property("lineItem", "", "Each line item represents one charge for goods and services rendered. Details such.ofType(date), code and amount are found in the referenced ChargeItem resource.", 0, java.lang.Integer.MAX_VALUE, lineItem)); 1744 children.add(new Property("totalPriceComponent", "MonetaryComponent", "The total amount for the Invoice may be calculated as the sum of the line items with surcharges/deductions that apply in certain conditions. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the total price was calculated.", 0, java.lang.Integer.MAX_VALUE, totalPriceComponent)); 1745 children.add(new Property("totalNet", "Money", "Invoice total , taxes excluded.", 0, 1, totalNet)); 1746 children.add(new Property("totalGross", "Money", "Invoice total, tax included.", 0, 1, totalGross)); 1747 children.add(new Property("paymentTerms", "markdown", "Payment details such as banking details, period of payment, deductibles, methods of payment.", 0, 1, paymentTerms)); 1748 children.add(new Property("note", "Annotation", "Comments made about the invoice by the issuer, subject, or other participants.", 0, java.lang.Integer.MAX_VALUE, note)); 1749 } 1750 1751 @Override 1752 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1753 switch (_hash) { 1754 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier of this Invoice, often used for reference in correspondence about this invoice or for tracking of payments.", 0, java.lang.Integer.MAX_VALUE, identifier); 1755 case -892481550: /*status*/ return new Property("status", "code", "The current state of the Invoice.", 0, 1, status); 1756 case 1550362357: /*cancelledReason*/ return new Property("cancelledReason", "string", "In case of Invoice cancellation a reason must be given (entered in error, superseded by corrected invoice etc.).", 0, 1, cancelledReason); 1757 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of Invoice depending on domain, realm an usage (e.g. internal/external, dental, preliminary).", 0, 1, type); 1758 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The individual or set of individuals receiving the goods and services billed in this invoice.", 0, 1, subject); 1759 case 820081177: /*recipient*/ return new Property("recipient", "Reference(Organization|Patient|RelatedPerson)", "The individual or Organization responsible for balancing of this invoice.", 0, 1, recipient); 1760 case 3076014: /*date*/ return new Property("date", "dateTime", "Depricared by the element below.", 0, 1, date); 1761 case 1820421855: /*creation*/ return new Property("creation", "dateTime", "Date/time(s) of when this Invoice was posted.", 0, 1, creation); 1762 case 566594335: /*period[x]*/ return new Property("period[x]", "date|Period", "Date/time(s) range of services included in this invoice.", 0, 1, period); 1763 case -991726143: /*period*/ return new Property("period[x]", "date|Period", "Date/time(s) range of services included in this invoice.", 0, 1, period); 1764 case 383848719: /*periodDate*/ return new Property("period[x]", "date", "Date/time(s) range of services included in this invoice.", 0, 1, period); 1765 case -141376798: /*periodPeriod*/ return new Property("period[x]", "Period", "Date/time(s) range of services included in this invoice.", 0, 1, period); 1766 case 767422259: /*participant*/ return new Property("participant", "", "Indicates who or what performed or participated in the charged service.", 0, java.lang.Integer.MAX_VALUE, participant); 1767 case -1179159879: /*issuer*/ return new Property("issuer", "Reference(Organization)", "The organizationissuing the Invoice.", 0, 1, issuer); 1768 case -1177318867: /*account*/ return new Property("account", "Reference(Account)", "Account which is supposed to be balanced with this Invoice.", 0, 1, account); 1769 case 1188332839: /*lineItem*/ return new Property("lineItem", "", "Each line item represents one charge for goods and services rendered. Details such.ofType(date), code and amount are found in the referenced ChargeItem resource.", 0, java.lang.Integer.MAX_VALUE, lineItem); 1770 case 1731497496: /*totalPriceComponent*/ return new Property("totalPriceComponent", "MonetaryComponent", "The total amount for the Invoice may be calculated as the sum of the line items with surcharges/deductions that apply in certain conditions. The priceComponent element can be used to offer transparency to the recipient of the Invoice of how the total price was calculated.", 0, java.lang.Integer.MAX_VALUE, totalPriceComponent); 1771 case -849911879: /*totalNet*/ return new Property("totalNet", "Money", "Invoice total , taxes excluded.", 0, 1, totalNet); 1772 case -727607968: /*totalGross*/ return new Property("totalGross", "Money", "Invoice total, tax included.", 0, 1, totalGross); 1773 case -507544799: /*paymentTerms*/ return new Property("paymentTerms", "markdown", "Payment details such as banking details, period of payment, deductibles, methods of payment.", 0, 1, paymentTerms); 1774 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the invoice by the issuer, subject, or other participants.", 0, java.lang.Integer.MAX_VALUE, note); 1775 default: return super.getNamedProperty(_hash, _name, _checkValid); 1776 } 1777 1778 } 1779 1780 @Override 1781 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1782 switch (hash) { 1783 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1784 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<InvoiceStatus> 1785 case 1550362357: /*cancelledReason*/ return this.cancelledReason == null ? new Base[0] : new Base[] {this.cancelledReason}; // StringType 1786 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1787 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1788 case 820081177: /*recipient*/ return this.recipient == null ? new Base[0] : new Base[] {this.recipient}; // Reference 1789 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1790 case 1820421855: /*creation*/ return this.creation == null ? new Base[0] : new Base[] {this.creation}; // DateTimeType 1791 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // DataType 1792 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // InvoiceParticipantComponent 1793 case -1179159879: /*issuer*/ return this.issuer == null ? new Base[0] : new Base[] {this.issuer}; // Reference 1794 case -1177318867: /*account*/ return this.account == null ? new Base[0] : new Base[] {this.account}; // Reference 1795 case 1188332839: /*lineItem*/ return this.lineItem == null ? new Base[0] : this.lineItem.toArray(new Base[this.lineItem.size()]); // InvoiceLineItemComponent 1796 case 1731497496: /*totalPriceComponent*/ return this.totalPriceComponent == null ? new Base[0] : this.totalPriceComponent.toArray(new Base[this.totalPriceComponent.size()]); // MonetaryComponent 1797 case -849911879: /*totalNet*/ return this.totalNet == null ? new Base[0] : new Base[] {this.totalNet}; // Money 1798 case -727607968: /*totalGross*/ return this.totalGross == null ? new Base[0] : new Base[] {this.totalGross}; // Money 1799 case -507544799: /*paymentTerms*/ return this.paymentTerms == null ? new Base[0] : new Base[] {this.paymentTerms}; // MarkdownType 1800 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1801 default: return super.getProperty(hash, name, checkValid); 1802 } 1803 1804 } 1805 1806 @Override 1807 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1808 switch (hash) { 1809 case -1618432855: // identifier 1810 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1811 return value; 1812 case -892481550: // status 1813 value = new InvoiceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1814 this.status = (Enumeration) value; // Enumeration<InvoiceStatus> 1815 return value; 1816 case 1550362357: // cancelledReason 1817 this.cancelledReason = TypeConvertor.castToString(value); // StringType 1818 return value; 1819 case 3575610: // type 1820 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1821 return value; 1822 case -1867885268: // subject 1823 this.subject = TypeConvertor.castToReference(value); // Reference 1824 return value; 1825 case 820081177: // recipient 1826 this.recipient = TypeConvertor.castToReference(value); // Reference 1827 return value; 1828 case 3076014: // date 1829 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1830 return value; 1831 case 1820421855: // creation 1832 this.creation = TypeConvertor.castToDateTime(value); // DateTimeType 1833 return value; 1834 case -991726143: // period 1835 this.period = TypeConvertor.castToType(value); // DataType 1836 return value; 1837 case 767422259: // participant 1838 this.getParticipant().add((InvoiceParticipantComponent) value); // InvoiceParticipantComponent 1839 return value; 1840 case -1179159879: // issuer 1841 this.issuer = TypeConvertor.castToReference(value); // Reference 1842 return value; 1843 case -1177318867: // account 1844 this.account = TypeConvertor.castToReference(value); // Reference 1845 return value; 1846 case 1188332839: // lineItem 1847 this.getLineItem().add((InvoiceLineItemComponent) value); // InvoiceLineItemComponent 1848 return value; 1849 case 1731497496: // totalPriceComponent 1850 this.getTotalPriceComponent().add(TypeConvertor.castToMonetaryComponent(value)); // MonetaryComponent 1851 return value; 1852 case -849911879: // totalNet 1853 this.totalNet = TypeConvertor.castToMoney(value); // Money 1854 return value; 1855 case -727607968: // totalGross 1856 this.totalGross = TypeConvertor.castToMoney(value); // Money 1857 return value; 1858 case -507544799: // paymentTerms 1859 this.paymentTerms = TypeConvertor.castToMarkdown(value); // MarkdownType 1860 return value; 1861 case 3387378: // note 1862 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1863 return value; 1864 default: return super.setProperty(hash, name, value); 1865 } 1866 1867 } 1868 1869 @Override 1870 public Base setProperty(String name, Base value) throws FHIRException { 1871 if (name.equals("identifier")) { 1872 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1873 } else if (name.equals("status")) { 1874 value = new InvoiceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1875 this.status = (Enumeration) value; // Enumeration<InvoiceStatus> 1876 } else if (name.equals("cancelledReason")) { 1877 this.cancelledReason = TypeConvertor.castToString(value); // StringType 1878 } else if (name.equals("type")) { 1879 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1880 } else if (name.equals("subject")) { 1881 this.subject = TypeConvertor.castToReference(value); // Reference 1882 } else if (name.equals("recipient")) { 1883 this.recipient = TypeConvertor.castToReference(value); // Reference 1884 } else if (name.equals("date")) { 1885 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1886 } else if (name.equals("creation")) { 1887 this.creation = TypeConvertor.castToDateTime(value); // DateTimeType 1888 } else if (name.equals("period[x]")) { 1889 this.period = TypeConvertor.castToType(value); // DataType 1890 } else if (name.equals("participant")) { 1891 this.getParticipant().add((InvoiceParticipantComponent) value); 1892 } else if (name.equals("issuer")) { 1893 this.issuer = TypeConvertor.castToReference(value); // Reference 1894 } else if (name.equals("account")) { 1895 this.account = TypeConvertor.castToReference(value); // Reference 1896 } else if (name.equals("lineItem")) { 1897 this.getLineItem().add((InvoiceLineItemComponent) value); 1898 } else if (name.equals("totalPriceComponent")) { 1899 this.getTotalPriceComponent().add(TypeConvertor.castToMonetaryComponent(value)); 1900 } else if (name.equals("totalNet")) { 1901 this.totalNet = TypeConvertor.castToMoney(value); // Money 1902 } else if (name.equals("totalGross")) { 1903 this.totalGross = TypeConvertor.castToMoney(value); // Money 1904 } else if (name.equals("paymentTerms")) { 1905 this.paymentTerms = TypeConvertor.castToMarkdown(value); // MarkdownType 1906 } else if (name.equals("note")) { 1907 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1908 } else 1909 return super.setProperty(name, value); 1910 return value; 1911 } 1912 1913 @Override 1914 public void removeChild(String name, Base value) throws FHIRException { 1915 if (name.equals("identifier")) { 1916 this.getIdentifier().remove(value); 1917 } else if (name.equals("status")) { 1918 value = new InvoiceStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1919 this.status = (Enumeration) value; // Enumeration<InvoiceStatus> 1920 } else if (name.equals("cancelledReason")) { 1921 this.cancelledReason = null; 1922 } else if (name.equals("type")) { 1923 this.type = null; 1924 } else if (name.equals("subject")) { 1925 this.subject = null; 1926 } else if (name.equals("recipient")) { 1927 this.recipient = null; 1928 } else if (name.equals("date")) { 1929 this.date = null; 1930 } else if (name.equals("creation")) { 1931 this.creation = null; 1932 } else if (name.equals("period[x]")) { 1933 this.period = null; 1934 } else if (name.equals("participant")) { 1935 this.getParticipant().remove((InvoiceParticipantComponent) value); 1936 } else if (name.equals("issuer")) { 1937 this.issuer = null; 1938 } else if (name.equals("account")) { 1939 this.account = null; 1940 } else if (name.equals("lineItem")) { 1941 this.getLineItem().remove((InvoiceLineItemComponent) value); 1942 } else if (name.equals("totalPriceComponent")) { 1943 this.getTotalPriceComponent().remove(value); 1944 } else if (name.equals("totalNet")) { 1945 this.totalNet = null; 1946 } else if (name.equals("totalGross")) { 1947 this.totalGross = null; 1948 } else if (name.equals("paymentTerms")) { 1949 this.paymentTerms = null; 1950 } else if (name.equals("note")) { 1951 this.getNote().remove(value); 1952 } else 1953 super.removeChild(name, value); 1954 1955 } 1956 1957 @Override 1958 public Base makeProperty(int hash, String name) throws FHIRException { 1959 switch (hash) { 1960 case -1618432855: return addIdentifier(); 1961 case -892481550: return getStatusElement(); 1962 case 1550362357: return getCancelledReasonElement(); 1963 case 3575610: return getType(); 1964 case -1867885268: return getSubject(); 1965 case 820081177: return getRecipient(); 1966 case 3076014: return getDateElement(); 1967 case 1820421855: return getCreationElement(); 1968 case 566594335: return getPeriod(); 1969 case -991726143: return getPeriod(); 1970 case 767422259: return addParticipant(); 1971 case -1179159879: return getIssuer(); 1972 case -1177318867: return getAccount(); 1973 case 1188332839: return addLineItem(); 1974 case 1731497496: return addTotalPriceComponent(); 1975 case -849911879: return getTotalNet(); 1976 case -727607968: return getTotalGross(); 1977 case -507544799: return getPaymentTermsElement(); 1978 case 3387378: return addNote(); 1979 default: return super.makeProperty(hash, name); 1980 } 1981 1982 } 1983 1984 @Override 1985 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1986 switch (hash) { 1987 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1988 case -892481550: /*status*/ return new String[] {"code"}; 1989 case 1550362357: /*cancelledReason*/ return new String[] {"string"}; 1990 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1991 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1992 case 820081177: /*recipient*/ return new String[] {"Reference"}; 1993 case 3076014: /*date*/ return new String[] {"dateTime"}; 1994 case 1820421855: /*creation*/ return new String[] {"dateTime"}; 1995 case -991726143: /*period*/ return new String[] {"date", "Period"}; 1996 case 767422259: /*participant*/ return new String[] {}; 1997 case -1179159879: /*issuer*/ return new String[] {"Reference"}; 1998 case -1177318867: /*account*/ return new String[] {"Reference"}; 1999 case 1188332839: /*lineItem*/ return new String[] {}; 2000 case 1731497496: /*totalPriceComponent*/ return new String[] {"MonetaryComponent"}; 2001 case -849911879: /*totalNet*/ return new String[] {"Money"}; 2002 case -727607968: /*totalGross*/ return new String[] {"Money"}; 2003 case -507544799: /*paymentTerms*/ return new String[] {"markdown"}; 2004 case 3387378: /*note*/ return new String[] {"Annotation"}; 2005 default: return super.getTypesForProperty(hash, name); 2006 } 2007 2008 } 2009 2010 @Override 2011 public Base addChild(String name) throws FHIRException { 2012 if (name.equals("identifier")) { 2013 return addIdentifier(); 2014 } 2015 else if (name.equals("status")) { 2016 throw new FHIRException("Cannot call addChild on a singleton property Invoice.status"); 2017 } 2018 else if (name.equals("cancelledReason")) { 2019 throw new FHIRException("Cannot call addChild on a singleton property Invoice.cancelledReason"); 2020 } 2021 else if (name.equals("type")) { 2022 this.type = new CodeableConcept(); 2023 return this.type; 2024 } 2025 else if (name.equals("subject")) { 2026 this.subject = new Reference(); 2027 return this.subject; 2028 } 2029 else if (name.equals("recipient")) { 2030 this.recipient = new Reference(); 2031 return this.recipient; 2032 } 2033 else if (name.equals("date")) { 2034 throw new FHIRException("Cannot call addChild on a singleton property Invoice.date"); 2035 } 2036 else if (name.equals("creation")) { 2037 throw new FHIRException("Cannot call addChild on a singleton property Invoice.creation"); 2038 } 2039 else if (name.equals("periodDate")) { 2040 this.period = new DateType(); 2041 return this.period; 2042 } 2043 else if (name.equals("periodPeriod")) { 2044 this.period = new Period(); 2045 return this.period; 2046 } 2047 else if (name.equals("participant")) { 2048 return addParticipant(); 2049 } 2050 else if (name.equals("issuer")) { 2051 this.issuer = new Reference(); 2052 return this.issuer; 2053 } 2054 else if (name.equals("account")) { 2055 this.account = new Reference(); 2056 return this.account; 2057 } 2058 else if (name.equals("lineItem")) { 2059 return addLineItem(); 2060 } 2061 else if (name.equals("totalPriceComponent")) { 2062 return addTotalPriceComponent(); 2063 } 2064 else if (name.equals("totalNet")) { 2065 this.totalNet = new Money(); 2066 return this.totalNet; 2067 } 2068 else if (name.equals("totalGross")) { 2069 this.totalGross = new Money(); 2070 return this.totalGross; 2071 } 2072 else if (name.equals("paymentTerms")) { 2073 throw new FHIRException("Cannot call addChild on a singleton property Invoice.paymentTerms"); 2074 } 2075 else if (name.equals("note")) { 2076 return addNote(); 2077 } 2078 else 2079 return super.addChild(name); 2080 } 2081 2082 public String fhirType() { 2083 return "Invoice"; 2084 2085 } 2086 2087 public Invoice copy() { 2088 Invoice dst = new Invoice(); 2089 copyValues(dst); 2090 return dst; 2091 } 2092 2093 public void copyValues(Invoice dst) { 2094 super.copyValues(dst); 2095 if (identifier != null) { 2096 dst.identifier = new ArrayList<Identifier>(); 2097 for (Identifier i : identifier) 2098 dst.identifier.add(i.copy()); 2099 }; 2100 dst.status = status == null ? null : status.copy(); 2101 dst.cancelledReason = cancelledReason == null ? null : cancelledReason.copy(); 2102 dst.type = type == null ? null : type.copy(); 2103 dst.subject = subject == null ? null : subject.copy(); 2104 dst.recipient = recipient == null ? null : recipient.copy(); 2105 dst.date = date == null ? null : date.copy(); 2106 dst.creation = creation == null ? null : creation.copy(); 2107 dst.period = period == null ? null : period.copy(); 2108 if (participant != null) { 2109 dst.participant = new ArrayList<InvoiceParticipantComponent>(); 2110 for (InvoiceParticipantComponent i : participant) 2111 dst.participant.add(i.copy()); 2112 }; 2113 dst.issuer = issuer == null ? null : issuer.copy(); 2114 dst.account = account == null ? null : account.copy(); 2115 if (lineItem != null) { 2116 dst.lineItem = new ArrayList<InvoiceLineItemComponent>(); 2117 for (InvoiceLineItemComponent i : lineItem) 2118 dst.lineItem.add(i.copy()); 2119 }; 2120 if (totalPriceComponent != null) { 2121 dst.totalPriceComponent = new ArrayList<MonetaryComponent>(); 2122 for (MonetaryComponent i : totalPriceComponent) 2123 dst.totalPriceComponent.add(i.copy()); 2124 }; 2125 dst.totalNet = totalNet == null ? null : totalNet.copy(); 2126 dst.totalGross = totalGross == null ? null : totalGross.copy(); 2127 dst.paymentTerms = paymentTerms == null ? null : paymentTerms.copy(); 2128 if (note != null) { 2129 dst.note = new ArrayList<Annotation>(); 2130 for (Annotation i : note) 2131 dst.note.add(i.copy()); 2132 }; 2133 } 2134 2135 protected Invoice typedCopy() { 2136 return copy(); 2137 } 2138 2139 @Override 2140 public boolean equalsDeep(Base other_) { 2141 if (!super.equalsDeep(other_)) 2142 return false; 2143 if (!(other_ instanceof Invoice)) 2144 return false; 2145 Invoice o = (Invoice) other_; 2146 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(cancelledReason, o.cancelledReason, true) 2147 && compareDeep(type, o.type, true) && compareDeep(subject, o.subject, true) && compareDeep(recipient, o.recipient, true) 2148 && compareDeep(date, o.date, true) && compareDeep(creation, o.creation, true) && compareDeep(period, o.period, true) 2149 && compareDeep(participant, o.participant, true) && compareDeep(issuer, o.issuer, true) && compareDeep(account, o.account, true) 2150 && compareDeep(lineItem, o.lineItem, true) && compareDeep(totalPriceComponent, o.totalPriceComponent, true) 2151 && compareDeep(totalNet, o.totalNet, true) && compareDeep(totalGross, o.totalGross, true) && compareDeep(paymentTerms, o.paymentTerms, true) 2152 && compareDeep(note, o.note, true); 2153 } 2154 2155 @Override 2156 public boolean equalsShallow(Base other_) { 2157 if (!super.equalsShallow(other_)) 2158 return false; 2159 if (!(other_ instanceof Invoice)) 2160 return false; 2161 Invoice o = (Invoice) other_; 2162 return compareValues(status, o.status, true) && compareValues(cancelledReason, o.cancelledReason, true) 2163 && compareValues(date, o.date, true) && compareValues(creation, o.creation, true) && compareValues(paymentTerms, o.paymentTerms, true) 2164 ; 2165 } 2166 2167 public boolean isEmpty() { 2168 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, cancelledReason 2169 , type, subject, recipient, date, creation, period, participant, issuer, account 2170 , lineItem, totalPriceComponent, totalNet, totalGross, paymentTerms, note); 2171 } 2172 2173 @Override 2174 public ResourceType getResourceType() { 2175 return ResourceType.Invoice; 2176 } 2177 2178 /** 2179 * Search parameter: <b>account</b> 2180 * <p> 2181 * Description: <b>Account that is being balanced</b><br> 2182 * Type: <b>reference</b><br> 2183 * Path: <b>Invoice.account</b><br> 2184 * </p> 2185 */ 2186 @SearchParamDefinition(name="account", path="Invoice.account", description="Account that is being balanced", type="reference", target={Account.class } ) 2187 public static final String SP_ACCOUNT = "account"; 2188 /** 2189 * <b>Fluent Client</b> search parameter constant for <b>account</b> 2190 * <p> 2191 * Description: <b>Account that is being balanced</b><br> 2192 * Type: <b>reference</b><br> 2193 * Path: <b>Invoice.account</b><br> 2194 * </p> 2195 */ 2196 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACCOUNT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ACCOUNT); 2197 2198/** 2199 * Constant for fluent queries to be used to add include statements. Specifies 2200 * the path value of "<b>Invoice:account</b>". 2201 */ 2202 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACCOUNT = new ca.uhn.fhir.model.api.Include("Invoice:account").toLocked(); 2203 2204 /** 2205 * Search parameter: <b>issuer</b> 2206 * <p> 2207 * Description: <b>Issuing Organization of Invoice</b><br> 2208 * Type: <b>reference</b><br> 2209 * Path: <b>Invoice.issuer</b><br> 2210 * </p> 2211 */ 2212 @SearchParamDefinition(name="issuer", path="Invoice.issuer", description="Issuing Organization of Invoice", type="reference", target={Organization.class } ) 2213 public static final String SP_ISSUER = "issuer"; 2214 /** 2215 * <b>Fluent Client</b> search parameter constant for <b>issuer</b> 2216 * <p> 2217 * Description: <b>Issuing Organization of Invoice</b><br> 2218 * Type: <b>reference</b><br> 2219 * Path: <b>Invoice.issuer</b><br> 2220 * </p> 2221 */ 2222 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ISSUER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ISSUER); 2223 2224/** 2225 * Constant for fluent queries to be used to add include statements. Specifies 2226 * the path value of "<b>Invoice:issuer</b>". 2227 */ 2228 public static final ca.uhn.fhir.model.api.Include INCLUDE_ISSUER = new ca.uhn.fhir.model.api.Include("Invoice:issuer").toLocked(); 2229 2230 /** 2231 * Search parameter: <b>participant-role</b> 2232 * <p> 2233 * Description: <b>Type of involvement in creation of this Invoice</b><br> 2234 * Type: <b>token</b><br> 2235 * Path: <b>Invoice.participant.role</b><br> 2236 * </p> 2237 */ 2238 @SearchParamDefinition(name="participant-role", path="Invoice.participant.role", description="Type of involvement in creation of this Invoice", type="token" ) 2239 public static final String SP_PARTICIPANT_ROLE = "participant-role"; 2240 /** 2241 * <b>Fluent Client</b> search parameter constant for <b>participant-role</b> 2242 * <p> 2243 * Description: <b>Type of involvement in creation of this Invoice</b><br> 2244 * Type: <b>token</b><br> 2245 * Path: <b>Invoice.participant.role</b><br> 2246 * </p> 2247 */ 2248 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PARTICIPANT_ROLE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PARTICIPANT_ROLE); 2249 2250 /** 2251 * Search parameter: <b>participant</b> 2252 * <p> 2253 * Description: <b>Individual who was involved</b><br> 2254 * Type: <b>reference</b><br> 2255 * Path: <b>Invoice.participant.actor</b><br> 2256 * </p> 2257 */ 2258 @SearchParamDefinition(name="participant", path="Invoice.participant.actor", description="Individual who was involved", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2259 public static final String SP_PARTICIPANT = "participant"; 2260 /** 2261 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 2262 * <p> 2263 * Description: <b>Individual who was involved</b><br> 2264 * Type: <b>reference</b><br> 2265 * Path: <b>Invoice.participant.actor</b><br> 2266 * </p> 2267 */ 2268 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTICIPANT); 2269 2270/** 2271 * Constant for fluent queries to be used to add include statements. Specifies 2272 * the path value of "<b>Invoice:participant</b>". 2273 */ 2274 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include("Invoice:participant").toLocked(); 2275 2276 /** 2277 * Search parameter: <b>recipient</b> 2278 * <p> 2279 * Description: <b>Recipient of this invoice</b><br> 2280 * Type: <b>reference</b><br> 2281 * Path: <b>Invoice.recipient</b><br> 2282 * </p> 2283 */ 2284 @SearchParamDefinition(name="recipient", path="Invoice.recipient", description="Recipient of this invoice", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={Organization.class, Patient.class, RelatedPerson.class } ) 2285 public static final String SP_RECIPIENT = "recipient"; 2286 /** 2287 * <b>Fluent Client</b> search parameter constant for <b>recipient</b> 2288 * <p> 2289 * Description: <b>Recipient of this invoice</b><br> 2290 * Type: <b>reference</b><br> 2291 * Path: <b>Invoice.recipient</b><br> 2292 * </p> 2293 */ 2294 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECIPIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECIPIENT); 2295 2296/** 2297 * Constant for fluent queries to be used to add include statements. Specifies 2298 * the path value of "<b>Invoice:recipient</b>". 2299 */ 2300 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECIPIENT = new ca.uhn.fhir.model.api.Include("Invoice:recipient").toLocked(); 2301 2302 /** 2303 * Search parameter: <b>status</b> 2304 * <p> 2305 * Description: <b>draft | issued | balanced | cancelled | entered-in-error</b><br> 2306 * Type: <b>token</b><br> 2307 * Path: <b>Invoice.status</b><br> 2308 * </p> 2309 */ 2310 @SearchParamDefinition(name="status", path="Invoice.status", description="draft | issued | balanced | cancelled | entered-in-error", type="token" ) 2311 public static final String SP_STATUS = "status"; 2312 /** 2313 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2314 * <p> 2315 * Description: <b>draft | issued | balanced | cancelled | entered-in-error</b><br> 2316 * Type: <b>token</b><br> 2317 * Path: <b>Invoice.status</b><br> 2318 * </p> 2319 */ 2320 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2321 2322 /** 2323 * Search parameter: <b>subject</b> 2324 * <p> 2325 * Description: <b>Recipient(s) of goods and services</b><br> 2326 * Type: <b>reference</b><br> 2327 * Path: <b>Invoice.subject</b><br> 2328 * </p> 2329 */ 2330 @SearchParamDefinition(name="subject", path="Invoice.subject", description="Recipient(s) of goods and services", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class } ) 2331 public static final String SP_SUBJECT = "subject"; 2332 /** 2333 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2334 * <p> 2335 * Description: <b>Recipient(s) of goods and services</b><br> 2336 * Type: <b>reference</b><br> 2337 * Path: <b>Invoice.subject</b><br> 2338 * </p> 2339 */ 2340 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2341 2342/** 2343 * Constant for fluent queries to be used to add include statements. Specifies 2344 * the path value of "<b>Invoice:subject</b>". 2345 */ 2346 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Invoice:subject").toLocked(); 2347 2348 /** 2349 * Search parameter: <b>totalgross</b> 2350 * <p> 2351 * Description: <b>Gross total of this Invoice</b><br> 2352 * Type: <b>quantity</b><br> 2353 * Path: <b>Invoice.totalGross</b><br> 2354 * </p> 2355 */ 2356 @SearchParamDefinition(name="totalgross", path="Invoice.totalGross", description="Gross total of this Invoice", type="quantity" ) 2357 public static final String SP_TOTALGROSS = "totalgross"; 2358 /** 2359 * <b>Fluent Client</b> search parameter constant for <b>totalgross</b> 2360 * <p> 2361 * Description: <b>Gross total of this Invoice</b><br> 2362 * Type: <b>quantity</b><br> 2363 * Path: <b>Invoice.totalGross</b><br> 2364 * </p> 2365 */ 2366 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam TOTALGROSS = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_TOTALGROSS); 2367 2368 /** 2369 * Search parameter: <b>totalnet</b> 2370 * <p> 2371 * Description: <b>Net total of this Invoice</b><br> 2372 * Type: <b>quantity</b><br> 2373 * Path: <b>Invoice.totalNet</b><br> 2374 * </p> 2375 */ 2376 @SearchParamDefinition(name="totalnet", path="Invoice.totalNet", description="Net total of this Invoice", type="quantity" ) 2377 public static final String SP_TOTALNET = "totalnet"; 2378 /** 2379 * <b>Fluent Client</b> search parameter constant for <b>totalnet</b> 2380 * <p> 2381 * Description: <b>Net total of this Invoice</b><br> 2382 * Type: <b>quantity</b><br> 2383 * Path: <b>Invoice.totalNet</b><br> 2384 * </p> 2385 */ 2386 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam TOTALNET = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_TOTALNET); 2387 2388 /** 2389 * Search parameter: <b>date</b> 2390 * <p> 2391 * Description: <b>Multiple Resources: 2392 2393* [AdverseEvent](adverseevent.html): When the event occurred 2394* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 2395* [Appointment](appointment.html): Appointment date/time. 2396* [AuditEvent](auditevent.html): Time when the event was recorded 2397* [CarePlan](careplan.html): Time period plan covers 2398* [CareTeam](careteam.html): A date within the coverage time period. 2399* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 2400* [Composition](composition.html): Composition editing time 2401* [Consent](consent.html): When consent was agreed to 2402* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 2403* [DocumentReference](documentreference.html): When this document reference was created 2404* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 2405* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 2406* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 2407* [Flag](flag.html): Time period when flag is active 2408* [Immunization](immunization.html): Vaccination (non)-Administration Date 2409* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 2410* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 2411* [Invoice](invoice.html): Invoice date / posting date 2412* [List](list.html): When the list was prepared 2413* [MeasureReport](measurereport.html): The date of the measure report 2414* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 2415* [Observation](observation.html): Clinically relevant time/time-period for observation 2416* [Procedure](procedure.html): When the procedure occurred or is occurring 2417* [ResearchSubject](researchsubject.html): Start and end of participation 2418* [RiskAssessment](riskassessment.html): When was assessment made? 2419* [SupplyRequest](supplyrequest.html): When the request was made 2420</b><br> 2421 * Type: <b>date</b><br> 2422 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 2423 * </p> 2424 */ 2425 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 2426 public static final String SP_DATE = "date"; 2427 /** 2428 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2429 * <p> 2430 * Description: <b>Multiple Resources: 2431 2432* [AdverseEvent](adverseevent.html): When the event occurred 2433* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 2434* [Appointment](appointment.html): Appointment date/time. 2435* [AuditEvent](auditevent.html): Time when the event was recorded 2436* [CarePlan](careplan.html): Time period plan covers 2437* [CareTeam](careteam.html): A date within the coverage time period. 2438* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 2439* [Composition](composition.html): Composition editing time 2440* [Consent](consent.html): When consent was agreed to 2441* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 2442* [DocumentReference](documentreference.html): When this document reference was created 2443* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 2444* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 2445* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 2446* [Flag](flag.html): Time period when flag is active 2447* [Immunization](immunization.html): Vaccination (non)-Administration Date 2448* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 2449* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 2450* [Invoice](invoice.html): Invoice date / posting date 2451* [List](list.html): When the list was prepared 2452* [MeasureReport](measurereport.html): The date of the measure report 2453* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 2454* [Observation](observation.html): Clinically relevant time/time-period for observation 2455* [Procedure](procedure.html): When the procedure occurred or is occurring 2456* [ResearchSubject](researchsubject.html): Start and end of participation 2457* [RiskAssessment](riskassessment.html): When was assessment made? 2458* [SupplyRequest](supplyrequest.html): When the request was made 2459</b><br> 2460 * Type: <b>date</b><br> 2461 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 2462 * </p> 2463 */ 2464 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2465 2466 /** 2467 * Search parameter: <b>identifier</b> 2468 * <p> 2469 * Description: <b>Multiple Resources: 2470 2471* [Account](account.html): Account number 2472* [AdverseEvent](adverseevent.html): Business identifier for the event 2473* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2474* [Appointment](appointment.html): An Identifier of the Appointment 2475* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2476* [Basic](basic.html): Business identifier 2477* [BodyStructure](bodystructure.html): Bodystructure identifier 2478* [CarePlan](careplan.html): External Ids for this plan 2479* [CareTeam](careteam.html): External Ids for this team 2480* [ChargeItem](chargeitem.html): Business Identifier for item 2481* [Claim](claim.html): The primary identifier of the financial resource 2482* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2483* [ClinicalImpression](clinicalimpression.html): Business identifier 2484* [Communication](communication.html): Unique identifier 2485* [CommunicationRequest](communicationrequest.html): Unique identifier 2486* [Composition](composition.html): Version-independent identifier for the Composition 2487* [Condition](condition.html): A unique identifier of the condition record 2488* [Consent](consent.html): Identifier for this record (external references) 2489* [Contract](contract.html): The identity of the contract 2490* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2491* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2492* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2493* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2494* [DeviceRequest](devicerequest.html): Business identifier for request/order 2495* [DeviceUsage](deviceusage.html): Search by identifier 2496* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2497* [DocumentReference](documentreference.html): Identifier of the attachment binary 2498* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2499* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2500* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2501* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2502* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2503* [Flag](flag.html): Business identifier 2504* [Goal](goal.html): External Ids for this goal 2505* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2506* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2507* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2508* [Immunization](immunization.html): Business identifier 2509* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2510* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2511* [Invoice](invoice.html): Business Identifier for item 2512* [List](list.html): Business identifier 2513* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2514* [Medication](medication.html): Returns medications with this external identifier 2515* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2516* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2517* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2518* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2519* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2520* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2521* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2522* [Observation](observation.html): The unique id for a particular observation 2523* [Person](person.html): A person Identifier 2524* [Procedure](procedure.html): A unique identifier for a procedure 2525* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2526* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2527* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2528* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2529* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2530* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2531* [Specimen](specimen.html): The unique identifier associated with the specimen 2532* [SupplyDelivery](supplydelivery.html): External identifier 2533* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2534* [Task](task.html): Search for a task instance by its business identifier 2535* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2536</b><br> 2537 * Type: <b>token</b><br> 2538 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2539 * </p> 2540 */ 2541 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2542 public static final String SP_IDENTIFIER = "identifier"; 2543 /** 2544 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2545 * <p> 2546 * Description: <b>Multiple Resources: 2547 2548* [Account](account.html): Account number 2549* [AdverseEvent](adverseevent.html): Business identifier for the event 2550* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2551* [Appointment](appointment.html): An Identifier of the Appointment 2552* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2553* [Basic](basic.html): Business identifier 2554* [BodyStructure](bodystructure.html): Bodystructure identifier 2555* [CarePlan](careplan.html): External Ids for this plan 2556* [CareTeam](careteam.html): External Ids for this team 2557* [ChargeItem](chargeitem.html): Business Identifier for item 2558* [Claim](claim.html): The primary identifier of the financial resource 2559* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2560* [ClinicalImpression](clinicalimpression.html): Business identifier 2561* [Communication](communication.html): Unique identifier 2562* [CommunicationRequest](communicationrequest.html): Unique identifier 2563* [Composition](composition.html): Version-independent identifier for the Composition 2564* [Condition](condition.html): A unique identifier of the condition record 2565* [Consent](consent.html): Identifier for this record (external references) 2566* [Contract](contract.html): The identity of the contract 2567* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2568* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2569* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2570* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2571* [DeviceRequest](devicerequest.html): Business identifier for request/order 2572* [DeviceUsage](deviceusage.html): Search by identifier 2573* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2574* [DocumentReference](documentreference.html): Identifier of the attachment binary 2575* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2576* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2577* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2578* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2579* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2580* [Flag](flag.html): Business identifier 2581* [Goal](goal.html): External Ids for this goal 2582* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2583* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2584* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2585* [Immunization](immunization.html): Business identifier 2586* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2587* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2588* [Invoice](invoice.html): Business Identifier for item 2589* [List](list.html): Business identifier 2590* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2591* [Medication](medication.html): Returns medications with this external identifier 2592* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2593* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2594* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2595* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2596* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2597* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2598* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2599* [Observation](observation.html): The unique id for a particular observation 2600* [Person](person.html): A person Identifier 2601* [Procedure](procedure.html): A unique identifier for a procedure 2602* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2603* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2604* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2605* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2606* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2607* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2608* [Specimen](specimen.html): The unique identifier associated with the specimen 2609* [SupplyDelivery](supplydelivery.html): External identifier 2610* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2611* [Task](task.html): Search for a task instance by its business identifier 2612* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2613</b><br> 2614 * Type: <b>token</b><br> 2615 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2616 * </p> 2617 */ 2618 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2619 2620 /** 2621 * Search parameter: <b>patient</b> 2622 * <p> 2623 * Description: <b>Multiple Resources: 2624 2625* [Account](account.html): The entity that caused the expenses 2626* [AdverseEvent](adverseevent.html): Subject impacted by event 2627* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2628* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2629* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2630* [AuditEvent](auditevent.html): Where the activity involved patient data 2631* [Basic](basic.html): Identifies the focus of this resource 2632* [BodyStructure](bodystructure.html): Who this is about 2633* [CarePlan](careplan.html): Who the care plan is for 2634* [CareTeam](careteam.html): Who care team is for 2635* [ChargeItem](chargeitem.html): Individual service was done for/to 2636* [Claim](claim.html): Patient receiving the products or services 2637* [ClaimResponse](claimresponse.html): The subject of care 2638* [ClinicalImpression](clinicalimpression.html): Patient assessed 2639* [Communication](communication.html): Focus of message 2640* [CommunicationRequest](communicationrequest.html): Focus of message 2641* [Composition](composition.html): Who and/or what the composition is about 2642* [Condition](condition.html): Who has the condition? 2643* [Consent](consent.html): Who the consent applies to 2644* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2645* [Coverage](coverage.html): Retrieve coverages for a patient 2646* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2647* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2648* [DetectedIssue](detectedissue.html): Associated patient 2649* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2650* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2651* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2652* [DocumentReference](documentreference.html): Who/what is the subject of the document 2653* [Encounter](encounter.html): The patient present at the encounter 2654* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2655* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2656* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2657* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2658* [Flag](flag.html): The identity of a subject to list flags for 2659* [Goal](goal.html): Who this goal is intended for 2660* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2661* [ImagingSelection](imagingselection.html): Who the study is about 2662* [ImagingStudy](imagingstudy.html): Who the study is about 2663* [Immunization](immunization.html): The patient for the vaccination record 2664* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2665* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2666* [Invoice](invoice.html): Recipient(s) of goods and services 2667* [List](list.html): If all resources have the same subject 2668* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2669* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2670* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2671* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2672* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2673* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2674* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2675* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2676* [Observation](observation.html): The subject that the observation is about (if patient) 2677* [Person](person.html): The Person links to this Patient 2678* [Procedure](procedure.html): Search by subject - a patient 2679* [Provenance](provenance.html): Where the activity involved patient data 2680* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2681* [RelatedPerson](relatedperson.html): The patient this related person is related to 2682* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2683* [ResearchSubject](researchsubject.html): Who or what is part of study 2684* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2685* [ServiceRequest](servicerequest.html): Search by subject - a patient 2686* [Specimen](specimen.html): The patient the specimen comes from 2687* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2688* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2689* [Task](task.html): Search by patient 2690* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2691</b><br> 2692 * Type: <b>reference</b><br> 2693 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2694 * </p> 2695 */ 2696 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 2697 public static final String SP_PATIENT = "patient"; 2698 /** 2699 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2700 * <p> 2701 * Description: <b>Multiple Resources: 2702 2703* [Account](account.html): The entity that caused the expenses 2704* [AdverseEvent](adverseevent.html): Subject impacted by event 2705* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2706* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2707* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2708* [AuditEvent](auditevent.html): Where the activity involved patient data 2709* [Basic](basic.html): Identifies the focus of this resource 2710* [BodyStructure](bodystructure.html): Who this is about 2711* [CarePlan](careplan.html): Who the care plan is for 2712* [CareTeam](careteam.html): Who care team is for 2713* [ChargeItem](chargeitem.html): Individual service was done for/to 2714* [Claim](claim.html): Patient receiving the products or services 2715* [ClaimResponse](claimresponse.html): The subject of care 2716* [ClinicalImpression](clinicalimpression.html): Patient assessed 2717* [Communication](communication.html): Focus of message 2718* [CommunicationRequest](communicationrequest.html): Focus of message 2719* [Composition](composition.html): Who and/or what the composition is about 2720* [Condition](condition.html): Who has the condition? 2721* [Consent](consent.html): Who the consent applies to 2722* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2723* [Coverage](coverage.html): Retrieve coverages for a patient 2724* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2725* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2726* [DetectedIssue](detectedissue.html): Associated patient 2727* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2728* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2729* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2730* [DocumentReference](documentreference.html): Who/what is the subject of the document 2731* [Encounter](encounter.html): The patient present at the encounter 2732* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2733* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2734* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2735* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2736* [Flag](flag.html): The identity of a subject to list flags for 2737* [Goal](goal.html): Who this goal is intended for 2738* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2739* [ImagingSelection](imagingselection.html): Who the study is about 2740* [ImagingStudy](imagingstudy.html): Who the study is about 2741* [Immunization](immunization.html): The patient for the vaccination record 2742* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2743* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2744* [Invoice](invoice.html): Recipient(s) of goods and services 2745* [List](list.html): If all resources have the same subject 2746* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2747* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2748* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2749* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2750* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2751* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2752* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2753* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2754* [Observation](observation.html): The subject that the observation is about (if patient) 2755* [Person](person.html): The Person links to this Patient 2756* [Procedure](procedure.html): Search by subject - a patient 2757* [Provenance](provenance.html): Where the activity involved patient data 2758* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2759* [RelatedPerson](relatedperson.html): The patient this related person is related to 2760* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2761* [ResearchSubject](researchsubject.html): Who or what is part of study 2762* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2763* [ServiceRequest](servicerequest.html): Search by subject - a patient 2764* [Specimen](specimen.html): The patient the specimen comes from 2765* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2766* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2767* [Task](task.html): Search by patient 2768* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2769</b><br> 2770 * Type: <b>reference</b><br> 2771 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2772 * </p> 2773 */ 2774 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2775 2776/** 2777 * Constant for fluent queries to be used to add include statements. Specifies 2778 * the path value of "<b>Invoice:patient</b>". 2779 */ 2780 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Invoice:patient").toLocked(); 2781 2782 /** 2783 * Search parameter: <b>type</b> 2784 * <p> 2785 * Description: <b>Multiple Resources: 2786 2787* [Account](account.html): E.g. patient, expense, depreciation 2788* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 2789* [Composition](composition.html): Kind of composition (LOINC if possible) 2790* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 2791* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 2792* [Encounter](encounter.html): Specific type of encounter 2793* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 2794* [Invoice](invoice.html): Type of Invoice 2795* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 2796* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 2797* [Specimen](specimen.html): The specimen type 2798</b><br> 2799 * Type: <b>token</b><br> 2800 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 2801 * </p> 2802 */ 2803 @SearchParamDefinition(name="type", path="Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type", description="Multiple Resources: \r\n\r\n* [Account](account.html): E.g. patient, expense, depreciation\r\n* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known)\r\n* [Composition](composition.html): Kind of composition (LOINC if possible)\r\n* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation)\r\n* [DocumentReference](documentreference.html): Kind of document (LOINC if possible)\r\n* [Encounter](encounter.html): Specific type of encounter\r\n* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management\r\n* [Invoice](invoice.html): Type of Invoice\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type\r\n* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence\r\n* [Specimen](specimen.html): The specimen type\r\n", type="token" ) 2804 public static final String SP_TYPE = "type"; 2805 /** 2806 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2807 * <p> 2808 * Description: <b>Multiple Resources: 2809 2810* [Account](account.html): E.g. patient, expense, depreciation 2811* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 2812* [Composition](composition.html): Kind of composition (LOINC if possible) 2813* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 2814* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 2815* [Encounter](encounter.html): Specific type of encounter 2816* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 2817* [Invoice](invoice.html): Type of Invoice 2818* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 2819* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 2820* [Specimen](specimen.html): The specimen type 2821</b><br> 2822 * Type: <b>token</b><br> 2823 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 2824 * </p> 2825 */ 2826 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2827 2828 2829} 2830