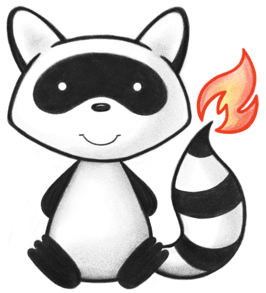
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets. 052 */ 053@ResourceDef(name="Library", profile="http://hl7.org/fhir/StructureDefinition/Library") 054public class Library extends MetadataResource { 055 056 /** 057 * An absolute URI that is used to identify this library when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this library is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the library is stored on different servers. 058 */ 059 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 060 @Description(shortDefinition="Canonical identifier for this library, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this library when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this library is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the library is stored on different servers." ) 061 protected UriType url; 062 063 /** 064 * A formal identifier that is used to identify this library when it is represented in other formats, or referenced in a specification, model, design or an instance. e.g. CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts. 065 */ 066 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 067 @Description(shortDefinition="Additional identifier for the library", formalDefinition="A formal identifier that is used to identify this library when it is represented in other formats, or referenced in a specification, model, design or an instance. e.g. CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts." ) 068 protected List<Identifier> identifier; 069 070 /** 071 * The identifier that is used to identify this version of the library when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the library author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 072 */ 073 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 074 @Description(shortDefinition="Business version of the library", formalDefinition="The identifier that is used to identify this version of the library when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the library author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts." ) 075 protected StringType version; 076 077 /** 078 * Indicates the mechanism used to compare versions to determine which is more current. 079 */ 080 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 081 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 082 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 083 protected DataType versionAlgorithm; 084 085 /** 086 * A natural language name identifying the library. This name should be usable as an identifier for the module by machine processing applications such as code generation. 087 */ 088 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 089 @Description(shortDefinition="Name for this library (computer friendly)", formalDefinition="A natural language name identifying the library. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 090 protected StringType name; 091 092 /** 093 * A short, descriptive, user-friendly title for the library. 094 */ 095 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 096 @Description(shortDefinition="Name for this library (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the library." ) 097 protected StringType title; 098 099 /** 100 * An explanatory or alternate title for the library giving additional information about its content. 101 */ 102 @Child(name = "subtitle", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 103 @Description(shortDefinition="Subordinate title of the library", formalDefinition="An explanatory or alternate title for the library giving additional information about its content." ) 104 protected StringType subtitle; 105 106 /** 107 * The status of this library. Enables tracking the life-cycle of the content. 108 */ 109 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 110 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this library. Enables tracking the life-cycle of the content." ) 111 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 112 protected Enumeration<PublicationStatus> status; 113 114 /** 115 * A Boolean value to indicate that this library is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 116 */ 117 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 118 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this library is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 119 protected BooleanType experimental; 120 121 /** 122 * Identifies the type of library such as a Logic Library, Model Definition, Asset Collection, or Module Definition. 123 */ 124 @Child(name = "type", type = {CodeableConcept.class}, order=9, min=1, max=1, modifier=false, summary=true) 125 @Description(shortDefinition="logic-library | model-definition | asset-collection | module-definition", formalDefinition="Identifies the type of library such as a Logic Library, Model Definition, Asset Collection, or Module Definition." ) 126 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/library-type") 127 protected CodeableConcept type; 128 129 /** 130 * A code or group definition that describes the intended subject of the contents of the library. 131 */ 132 @Child(name = "subject", type = {CodeableConcept.class, Group.class}, order=10, min=0, max=1, modifier=false, summary=false) 133 @Description(shortDefinition="Type of individual the library content is focused on", formalDefinition="A code or group definition that describes the intended subject of the contents of the library." ) 134 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participant-resource-types") 135 protected DataType subject; 136 137 /** 138 * The date (and optionally time) when the library was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the library changes. 139 */ 140 @Child(name = "date", type = {DateTimeType.class}, order=11, min=0, max=1, modifier=false, summary=true) 141 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the library was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the library changes." ) 142 protected DateTimeType date; 143 144 /** 145 * The name of the organization or individual responsible for the release and ongoing maintenance of the library. 146 */ 147 @Child(name = "publisher", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=true) 148 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the library." ) 149 protected StringType publisher; 150 151 /** 152 * Contact details to assist a user in finding and communicating with the publisher. 153 */ 154 @Child(name = "contact", type = {ContactDetail.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 155 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 156 protected List<ContactDetail> contact; 157 158 /** 159 * A free text natural language description of the library from a consumer's perspective. 160 */ 161 @Child(name = "description", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=true) 162 @Description(shortDefinition="Natural language description of the library", formalDefinition="A free text natural language description of the library from a consumer's perspective." ) 163 protected MarkdownType description; 164 165 /** 166 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate library instances. 167 */ 168 @Child(name = "useContext", type = {UsageContext.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 169 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate library instances." ) 170 protected List<UsageContext> useContext; 171 172 /** 173 * A legal or geographic region in which the library is intended to be used. 174 */ 175 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 176 @Description(shortDefinition="Intended jurisdiction for library (if applicable)", formalDefinition="A legal or geographic region in which the library is intended to be used." ) 177 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 178 protected List<CodeableConcept> jurisdiction; 179 180 /** 181 * Explanation of why this library is needed and why it has been designed as it has. 182 */ 183 @Child(name = "purpose", type = {MarkdownType.class}, order=17, min=0, max=1, modifier=false, summary=false) 184 @Description(shortDefinition="Why this library is defined", formalDefinition="Explanation of why this library is needed and why it has been designed as it has." ) 185 protected MarkdownType purpose; 186 187 /** 188 * A detailed description of how the library is used from a clinical perspective. 189 */ 190 @Child(name = "usage", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=false) 191 @Description(shortDefinition="Describes the clinical usage of the library", formalDefinition="A detailed description of how the library is used from a clinical perspective." ) 192 protected MarkdownType usage; 193 194 /** 195 * A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library. 196 */ 197 @Child(name = "copyright", type = {MarkdownType.class}, order=19, min=0, max=1, modifier=false, summary=false) 198 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library." ) 199 protected MarkdownType copyright; 200 201 /** 202 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 203 */ 204 @Child(name = "copyrightLabel", type = {StringType.class}, order=20, min=0, max=1, modifier=false, summary=false) 205 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 206 protected StringType copyrightLabel; 207 208 /** 209 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 210 */ 211 @Child(name = "approvalDate", type = {DateType.class}, order=21, min=0, max=1, modifier=false, summary=false) 212 @Description(shortDefinition="When the library was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 213 protected DateType approvalDate; 214 215 /** 216 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 217 */ 218 @Child(name = "lastReviewDate", type = {DateType.class}, order=22, min=0, max=1, modifier=false, summary=false) 219 @Description(shortDefinition="When the library was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 220 protected DateType lastReviewDate; 221 222 /** 223 * The period during which the library content was or is planned to be in active use. 224 */ 225 @Child(name = "effectivePeriod", type = {Period.class}, order=23, min=0, max=1, modifier=false, summary=true) 226 @Description(shortDefinition="When the library is expected to be used", formalDefinition="The period during which the library content was or is planned to be in active use." ) 227 protected Period effectivePeriod; 228 229 /** 230 * Descriptive topics related to the content of the library. Topics provide a high-level categorization of the library that can be useful for filtering and searching. 231 */ 232 @Child(name = "topic", type = {CodeableConcept.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 233 @Description(shortDefinition="E.g. Education, Treatment, Assessment, etc", formalDefinition="Descriptive topics related to the content of the library. Topics provide a high-level categorization of the library that can be useful for filtering and searching." ) 234 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-topic") 235 protected List<CodeableConcept> topic; 236 237 /** 238 * An individiual or organization primarily involved in the creation and maintenance of the content. 239 */ 240 @Child(name = "author", type = {ContactDetail.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 241 @Description(shortDefinition="Who authored the content", formalDefinition="An individiual or organization primarily involved in the creation and maintenance of the content." ) 242 protected List<ContactDetail> author; 243 244 /** 245 * An individual or organization primarily responsible for internal coherence of the content. 246 */ 247 @Child(name = "editor", type = {ContactDetail.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 248 @Description(shortDefinition="Who edited the content", formalDefinition="An individual or organization primarily responsible for internal coherence of the content." ) 249 protected List<ContactDetail> editor; 250 251 /** 252 * An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content. 253 */ 254 @Child(name = "reviewer", type = {ContactDetail.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 255 @Description(shortDefinition="Who reviewed the content", formalDefinition="An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content." ) 256 protected List<ContactDetail> reviewer; 257 258 /** 259 * An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting. 260 */ 261 @Child(name = "endorser", type = {ContactDetail.class}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 262 @Description(shortDefinition="Who endorsed the content", formalDefinition="An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting." ) 263 protected List<ContactDetail> endorser; 264 265 /** 266 * Related artifacts such as additional documentation, justification, or bibliographic references. 267 */ 268 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 269 @Description(shortDefinition="Additional documentation, citations, etc", formalDefinition="Related artifacts such as additional documentation, justification, or bibliographic references." ) 270 protected List<RelatedArtifact> relatedArtifact; 271 272 /** 273 * The parameter element defines parameters used by the library. 274 */ 275 @Child(name = "parameter", type = {ParameterDefinition.class}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 276 @Description(shortDefinition="Parameters defined by the library", formalDefinition="The parameter element defines parameters used by the library." ) 277 protected List<ParameterDefinition> parameter; 278 279 /** 280 * Describes a set of data that must be provided in order to be able to successfully perform the computations defined by the library. 281 */ 282 @Child(name = "dataRequirement", type = {DataRequirement.class}, order=31, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 283 @Description(shortDefinition="What data is referenced by this library", formalDefinition="Describes a set of data that must be provided in order to be able to successfully perform the computations defined by the library." ) 284 protected List<DataRequirement> dataRequirement; 285 286 /** 287 * The content of the library as an Attachment. The content may be a reference to a url, or may be directly embedded as a base-64 string. Either way, the contentType of the attachment determines how to interpret the content. 288 */ 289 @Child(name = "content", type = {Attachment.class}, order=32, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 290 @Description(shortDefinition="Contents of the library, either embedded or referenced", formalDefinition="The content of the library as an Attachment. The content may be a reference to a url, or may be directly embedded as a base-64 string. Either way, the contentType of the attachment determines how to interpret the content." ) 291 protected List<Attachment> content; 292 293 private static final long serialVersionUID = 1098204872L; 294 295 /** 296 * Constructor 297 */ 298 public Library() { 299 super(); 300 } 301 302 /** 303 * Constructor 304 */ 305 public Library(PublicationStatus status, CodeableConcept type) { 306 super(); 307 this.setStatus(status); 308 this.setType(type); 309 } 310 311 /** 312 * @return {@link #url} (An absolute URI that is used to identify this library when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this library is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the library is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 313 */ 314 public UriType getUrlElement() { 315 if (this.url == null) 316 if (Configuration.errorOnAutoCreate()) 317 throw new Error("Attempt to auto-create Library.url"); 318 else if (Configuration.doAutoCreate()) 319 this.url = new UriType(); // bb 320 return this.url; 321 } 322 323 public boolean hasUrlElement() { 324 return this.url != null && !this.url.isEmpty(); 325 } 326 327 public boolean hasUrl() { 328 return this.url != null && !this.url.isEmpty(); 329 } 330 331 /** 332 * @param value {@link #url} (An absolute URI that is used to identify this library when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this library is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the library is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 333 */ 334 public Library setUrlElement(UriType value) { 335 this.url = value; 336 return this; 337 } 338 339 /** 340 * @return An absolute URI that is used to identify this library when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this library is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the library is stored on different servers. 341 */ 342 public String getUrl() { 343 return this.url == null ? null : this.url.getValue(); 344 } 345 346 /** 347 * @param value An absolute URI that is used to identify this library when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this library is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the library is stored on different servers. 348 */ 349 public Library setUrl(String value) { 350 if (Utilities.noString(value)) 351 this.url = null; 352 else { 353 if (this.url == null) 354 this.url = new UriType(); 355 this.url.setValue(value); 356 } 357 return this; 358 } 359 360 /** 361 * @return {@link #identifier} (A formal identifier that is used to identify this library when it is represented in other formats, or referenced in a specification, model, design or an instance. e.g. CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts.) 362 */ 363 public List<Identifier> getIdentifier() { 364 if (this.identifier == null) 365 this.identifier = new ArrayList<Identifier>(); 366 return this.identifier; 367 } 368 369 /** 370 * @return Returns a reference to <code>this</code> for easy method chaining 371 */ 372 public Library setIdentifier(List<Identifier> theIdentifier) { 373 this.identifier = theIdentifier; 374 return this; 375 } 376 377 public boolean hasIdentifier() { 378 if (this.identifier == null) 379 return false; 380 for (Identifier item : this.identifier) 381 if (!item.isEmpty()) 382 return true; 383 return false; 384 } 385 386 public Identifier addIdentifier() { //3 387 Identifier t = new Identifier(); 388 if (this.identifier == null) 389 this.identifier = new ArrayList<Identifier>(); 390 this.identifier.add(t); 391 return t; 392 } 393 394 public Library addIdentifier(Identifier t) { //3 395 if (t == null) 396 return this; 397 if (this.identifier == null) 398 this.identifier = new ArrayList<Identifier>(); 399 this.identifier.add(t); 400 return this; 401 } 402 403 /** 404 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 405 */ 406 public Identifier getIdentifierFirstRep() { 407 if (getIdentifier().isEmpty()) { 408 addIdentifier(); 409 } 410 return getIdentifier().get(0); 411 } 412 413 /** 414 * @return {@link #version} (The identifier that is used to identify this version of the library when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the library author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 415 */ 416 public StringType getVersionElement() { 417 if (this.version == null) 418 if (Configuration.errorOnAutoCreate()) 419 throw new Error("Attempt to auto-create Library.version"); 420 else if (Configuration.doAutoCreate()) 421 this.version = new StringType(); // bb 422 return this.version; 423 } 424 425 public boolean hasVersionElement() { 426 return this.version != null && !this.version.isEmpty(); 427 } 428 429 public boolean hasVersion() { 430 return this.version != null && !this.version.isEmpty(); 431 } 432 433 /** 434 * @param value {@link #version} (The identifier that is used to identify this version of the library when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the library author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 435 */ 436 public Library setVersionElement(StringType value) { 437 this.version = value; 438 return this; 439 } 440 441 /** 442 * @return The identifier that is used to identify this version of the library when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the library author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 443 */ 444 public String getVersion() { 445 return this.version == null ? null : this.version.getValue(); 446 } 447 448 /** 449 * @param value The identifier that is used to identify this version of the library when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the library author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 450 */ 451 public Library setVersion(String value) { 452 if (Utilities.noString(value)) 453 this.version = null; 454 else { 455 if (this.version == null) 456 this.version = new StringType(); 457 this.version.setValue(value); 458 } 459 return this; 460 } 461 462 /** 463 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 464 */ 465 public DataType getVersionAlgorithm() { 466 return this.versionAlgorithm; 467 } 468 469 /** 470 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 471 */ 472 public StringType getVersionAlgorithmStringType() throws FHIRException { 473 if (this.versionAlgorithm == null) 474 this.versionAlgorithm = new StringType(); 475 if (!(this.versionAlgorithm instanceof StringType)) 476 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 477 return (StringType) this.versionAlgorithm; 478 } 479 480 public boolean hasVersionAlgorithmStringType() { 481 return this != null && this.versionAlgorithm instanceof StringType; 482 } 483 484 /** 485 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 486 */ 487 public Coding getVersionAlgorithmCoding() throws FHIRException { 488 if (this.versionAlgorithm == null) 489 this.versionAlgorithm = new Coding(); 490 if (!(this.versionAlgorithm instanceof Coding)) 491 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 492 return (Coding) this.versionAlgorithm; 493 } 494 495 public boolean hasVersionAlgorithmCoding() { 496 return this != null && this.versionAlgorithm instanceof Coding; 497 } 498 499 public boolean hasVersionAlgorithm() { 500 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 501 } 502 503 /** 504 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 505 */ 506 public Library setVersionAlgorithm(DataType value) { 507 if (value != null && !(value instanceof StringType || value instanceof Coding)) 508 throw new FHIRException("Not the right type for Library.versionAlgorithm[x]: "+value.fhirType()); 509 this.versionAlgorithm = value; 510 return this; 511 } 512 513 /** 514 * @return {@link #name} (A natural language name identifying the library. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 515 */ 516 public StringType getNameElement() { 517 if (this.name == null) 518 if (Configuration.errorOnAutoCreate()) 519 throw new Error("Attempt to auto-create Library.name"); 520 else if (Configuration.doAutoCreate()) 521 this.name = new StringType(); // bb 522 return this.name; 523 } 524 525 public boolean hasNameElement() { 526 return this.name != null && !this.name.isEmpty(); 527 } 528 529 public boolean hasName() { 530 return this.name != null && !this.name.isEmpty(); 531 } 532 533 /** 534 * @param value {@link #name} (A natural language name identifying the library. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 535 */ 536 public Library setNameElement(StringType value) { 537 this.name = value; 538 return this; 539 } 540 541 /** 542 * @return A natural language name identifying the library. This name should be usable as an identifier for the module by machine processing applications such as code generation. 543 */ 544 public String getName() { 545 return this.name == null ? null : this.name.getValue(); 546 } 547 548 /** 549 * @param value A natural language name identifying the library. This name should be usable as an identifier for the module by machine processing applications such as code generation. 550 */ 551 public Library setName(String value) { 552 if (Utilities.noString(value)) 553 this.name = null; 554 else { 555 if (this.name == null) 556 this.name = new StringType(); 557 this.name.setValue(value); 558 } 559 return this; 560 } 561 562 /** 563 * @return {@link #title} (A short, descriptive, user-friendly title for the library.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 564 */ 565 public StringType getTitleElement() { 566 if (this.title == null) 567 if (Configuration.errorOnAutoCreate()) 568 throw new Error("Attempt to auto-create Library.title"); 569 else if (Configuration.doAutoCreate()) 570 this.title = new StringType(); // bb 571 return this.title; 572 } 573 574 public boolean hasTitleElement() { 575 return this.title != null && !this.title.isEmpty(); 576 } 577 578 public boolean hasTitle() { 579 return this.title != null && !this.title.isEmpty(); 580 } 581 582 /** 583 * @param value {@link #title} (A short, descriptive, user-friendly title for the library.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 584 */ 585 public Library setTitleElement(StringType value) { 586 this.title = value; 587 return this; 588 } 589 590 /** 591 * @return A short, descriptive, user-friendly title for the library. 592 */ 593 public String getTitle() { 594 return this.title == null ? null : this.title.getValue(); 595 } 596 597 /** 598 * @param value A short, descriptive, user-friendly title for the library. 599 */ 600 public Library setTitle(String value) { 601 if (Utilities.noString(value)) 602 this.title = null; 603 else { 604 if (this.title == null) 605 this.title = new StringType(); 606 this.title.setValue(value); 607 } 608 return this; 609 } 610 611 /** 612 * @return {@link #subtitle} (An explanatory or alternate title for the library giving additional information about its content.). This is the underlying object with id, value and extensions. The accessor "getSubtitle" gives direct access to the value 613 */ 614 public StringType getSubtitleElement() { 615 if (this.subtitle == null) 616 if (Configuration.errorOnAutoCreate()) 617 throw new Error("Attempt to auto-create Library.subtitle"); 618 else if (Configuration.doAutoCreate()) 619 this.subtitle = new StringType(); // bb 620 return this.subtitle; 621 } 622 623 public boolean hasSubtitleElement() { 624 return this.subtitle != null && !this.subtitle.isEmpty(); 625 } 626 627 public boolean hasSubtitle() { 628 return this.subtitle != null && !this.subtitle.isEmpty(); 629 } 630 631 /** 632 * @param value {@link #subtitle} (An explanatory or alternate title for the library giving additional information about its content.). This is the underlying object with id, value and extensions. The accessor "getSubtitle" gives direct access to the value 633 */ 634 public Library setSubtitleElement(StringType value) { 635 this.subtitle = value; 636 return this; 637 } 638 639 /** 640 * @return An explanatory or alternate title for the library giving additional information about its content. 641 */ 642 public String getSubtitle() { 643 return this.subtitle == null ? null : this.subtitle.getValue(); 644 } 645 646 /** 647 * @param value An explanatory or alternate title for the library giving additional information about its content. 648 */ 649 public Library setSubtitle(String value) { 650 if (Utilities.noString(value)) 651 this.subtitle = null; 652 else { 653 if (this.subtitle == null) 654 this.subtitle = new StringType(); 655 this.subtitle.setValue(value); 656 } 657 return this; 658 } 659 660 /** 661 * @return {@link #status} (The status of this library. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 662 */ 663 public Enumeration<PublicationStatus> getStatusElement() { 664 if (this.status == null) 665 if (Configuration.errorOnAutoCreate()) 666 throw new Error("Attempt to auto-create Library.status"); 667 else if (Configuration.doAutoCreate()) 668 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 669 return this.status; 670 } 671 672 public boolean hasStatusElement() { 673 return this.status != null && !this.status.isEmpty(); 674 } 675 676 public boolean hasStatus() { 677 return this.status != null && !this.status.isEmpty(); 678 } 679 680 /** 681 * @param value {@link #status} (The status of this library. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 682 */ 683 public Library setStatusElement(Enumeration<PublicationStatus> value) { 684 this.status = value; 685 return this; 686 } 687 688 /** 689 * @return The status of this library. Enables tracking the life-cycle of the content. 690 */ 691 public PublicationStatus getStatus() { 692 return this.status == null ? null : this.status.getValue(); 693 } 694 695 /** 696 * @param value The status of this library. Enables tracking the life-cycle of the content. 697 */ 698 public Library setStatus(PublicationStatus value) { 699 if (this.status == null) 700 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 701 this.status.setValue(value); 702 return this; 703 } 704 705 /** 706 * @return {@link #experimental} (A Boolean value to indicate that this library is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 707 */ 708 public BooleanType getExperimentalElement() { 709 if (this.experimental == null) 710 if (Configuration.errorOnAutoCreate()) 711 throw new Error("Attempt to auto-create Library.experimental"); 712 else if (Configuration.doAutoCreate()) 713 this.experimental = new BooleanType(); // bb 714 return this.experimental; 715 } 716 717 public boolean hasExperimentalElement() { 718 return this.experimental != null && !this.experimental.isEmpty(); 719 } 720 721 public boolean hasExperimental() { 722 return this.experimental != null && !this.experimental.isEmpty(); 723 } 724 725 /** 726 * @param value {@link #experimental} (A Boolean value to indicate that this library is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 727 */ 728 public Library setExperimentalElement(BooleanType value) { 729 this.experimental = value; 730 return this; 731 } 732 733 /** 734 * @return A Boolean value to indicate that this library is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 735 */ 736 public boolean getExperimental() { 737 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 738 } 739 740 /** 741 * @param value A Boolean value to indicate that this library is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 742 */ 743 public Library setExperimental(boolean value) { 744 if (this.experimental == null) 745 this.experimental = new BooleanType(); 746 this.experimental.setValue(value); 747 return this; 748 } 749 750 /** 751 * @return {@link #type} (Identifies the type of library such as a Logic Library, Model Definition, Asset Collection, or Module Definition.) 752 */ 753 public CodeableConcept getType() { 754 if (this.type == null) 755 if (Configuration.errorOnAutoCreate()) 756 throw new Error("Attempt to auto-create Library.type"); 757 else if (Configuration.doAutoCreate()) 758 this.type = new CodeableConcept(); // cc 759 return this.type; 760 } 761 762 public boolean hasType() { 763 return this.type != null && !this.type.isEmpty(); 764 } 765 766 /** 767 * @param value {@link #type} (Identifies the type of library such as a Logic Library, Model Definition, Asset Collection, or Module Definition.) 768 */ 769 public Library setType(CodeableConcept value) { 770 this.type = value; 771 return this; 772 } 773 774 /** 775 * @return {@link #subject} (A code or group definition that describes the intended subject of the contents of the library.) 776 */ 777 public DataType getSubject() { 778 return this.subject; 779 } 780 781 /** 782 * @return {@link #subject} (A code or group definition that describes the intended subject of the contents of the library.) 783 */ 784 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 785 if (this.subject == null) 786 this.subject = new CodeableConcept(); 787 if (!(this.subject instanceof CodeableConcept)) 788 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.subject.getClass().getName()+" was encountered"); 789 return (CodeableConcept) this.subject; 790 } 791 792 public boolean hasSubjectCodeableConcept() { 793 return this != null && this.subject instanceof CodeableConcept; 794 } 795 796 /** 797 * @return {@link #subject} (A code or group definition that describes the intended subject of the contents of the library.) 798 */ 799 public Reference getSubjectReference() throws FHIRException { 800 if (this.subject == null) 801 this.subject = new Reference(); 802 if (!(this.subject instanceof Reference)) 803 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.subject.getClass().getName()+" was encountered"); 804 return (Reference) this.subject; 805 } 806 807 public boolean hasSubjectReference() { 808 return this != null && this.subject instanceof Reference; 809 } 810 811 public boolean hasSubject() { 812 return this.subject != null && !this.subject.isEmpty(); 813 } 814 815 /** 816 * @param value {@link #subject} (A code or group definition that describes the intended subject of the contents of the library.) 817 */ 818 public Library setSubject(DataType value) { 819 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 820 throw new FHIRException("Not the right type for Library.subject[x]: "+value.fhirType()); 821 this.subject = value; 822 return this; 823 } 824 825 /** 826 * @return {@link #date} (The date (and optionally time) when the library was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the library changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 827 */ 828 public DateTimeType getDateElement() { 829 if (this.date == null) 830 if (Configuration.errorOnAutoCreate()) 831 throw new Error("Attempt to auto-create Library.date"); 832 else if (Configuration.doAutoCreate()) 833 this.date = new DateTimeType(); // bb 834 return this.date; 835 } 836 837 public boolean hasDateElement() { 838 return this.date != null && !this.date.isEmpty(); 839 } 840 841 public boolean hasDate() { 842 return this.date != null && !this.date.isEmpty(); 843 } 844 845 /** 846 * @param value {@link #date} (The date (and optionally time) when the library was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the library changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 847 */ 848 public Library setDateElement(DateTimeType value) { 849 this.date = value; 850 return this; 851 } 852 853 /** 854 * @return The date (and optionally time) when the library was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the library changes. 855 */ 856 public Date getDate() { 857 return this.date == null ? null : this.date.getValue(); 858 } 859 860 /** 861 * @param value The date (and optionally time) when the library was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the library changes. 862 */ 863 public Library setDate(Date value) { 864 if (value == null) 865 this.date = null; 866 else { 867 if (this.date == null) 868 this.date = new DateTimeType(); 869 this.date.setValue(value); 870 } 871 return this; 872 } 873 874 /** 875 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the library.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 876 */ 877 public StringType getPublisherElement() { 878 if (this.publisher == null) 879 if (Configuration.errorOnAutoCreate()) 880 throw new Error("Attempt to auto-create Library.publisher"); 881 else if (Configuration.doAutoCreate()) 882 this.publisher = new StringType(); // bb 883 return this.publisher; 884 } 885 886 public boolean hasPublisherElement() { 887 return this.publisher != null && !this.publisher.isEmpty(); 888 } 889 890 public boolean hasPublisher() { 891 return this.publisher != null && !this.publisher.isEmpty(); 892 } 893 894 /** 895 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the library.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 896 */ 897 public Library setPublisherElement(StringType value) { 898 this.publisher = value; 899 return this; 900 } 901 902 /** 903 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the library. 904 */ 905 public String getPublisher() { 906 return this.publisher == null ? null : this.publisher.getValue(); 907 } 908 909 /** 910 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the library. 911 */ 912 public Library setPublisher(String value) { 913 if (Utilities.noString(value)) 914 this.publisher = null; 915 else { 916 if (this.publisher == null) 917 this.publisher = new StringType(); 918 this.publisher.setValue(value); 919 } 920 return this; 921 } 922 923 /** 924 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 925 */ 926 public List<ContactDetail> getContact() { 927 if (this.contact == null) 928 this.contact = new ArrayList<ContactDetail>(); 929 return this.contact; 930 } 931 932 /** 933 * @return Returns a reference to <code>this</code> for easy method chaining 934 */ 935 public Library setContact(List<ContactDetail> theContact) { 936 this.contact = theContact; 937 return this; 938 } 939 940 public boolean hasContact() { 941 if (this.contact == null) 942 return false; 943 for (ContactDetail item : this.contact) 944 if (!item.isEmpty()) 945 return true; 946 return false; 947 } 948 949 public ContactDetail addContact() { //3 950 ContactDetail t = new ContactDetail(); 951 if (this.contact == null) 952 this.contact = new ArrayList<ContactDetail>(); 953 this.contact.add(t); 954 return t; 955 } 956 957 public Library addContact(ContactDetail t) { //3 958 if (t == null) 959 return this; 960 if (this.contact == null) 961 this.contact = new ArrayList<ContactDetail>(); 962 this.contact.add(t); 963 return this; 964 } 965 966 /** 967 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 968 */ 969 public ContactDetail getContactFirstRep() { 970 if (getContact().isEmpty()) { 971 addContact(); 972 } 973 return getContact().get(0); 974 } 975 976 /** 977 * @return {@link #description} (A free text natural language description of the library from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 978 */ 979 public MarkdownType getDescriptionElement() { 980 if (this.description == null) 981 if (Configuration.errorOnAutoCreate()) 982 throw new Error("Attempt to auto-create Library.description"); 983 else if (Configuration.doAutoCreate()) 984 this.description = new MarkdownType(); // bb 985 return this.description; 986 } 987 988 public boolean hasDescriptionElement() { 989 return this.description != null && !this.description.isEmpty(); 990 } 991 992 public boolean hasDescription() { 993 return this.description != null && !this.description.isEmpty(); 994 } 995 996 /** 997 * @param value {@link #description} (A free text natural language description of the library from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 998 */ 999 public Library setDescriptionElement(MarkdownType value) { 1000 this.description = value; 1001 return this; 1002 } 1003 1004 /** 1005 * @return A free text natural language description of the library from a consumer's perspective. 1006 */ 1007 public String getDescription() { 1008 return this.description == null ? null : this.description.getValue(); 1009 } 1010 1011 /** 1012 * @param value A free text natural language description of the library from a consumer's perspective. 1013 */ 1014 public Library setDescription(String value) { 1015 if (Utilities.noString(value)) 1016 this.description = null; 1017 else { 1018 if (this.description == null) 1019 this.description = new MarkdownType(); 1020 this.description.setValue(value); 1021 } 1022 return this; 1023 } 1024 1025 /** 1026 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate library instances.) 1027 */ 1028 public List<UsageContext> getUseContext() { 1029 if (this.useContext == null) 1030 this.useContext = new ArrayList<UsageContext>(); 1031 return this.useContext; 1032 } 1033 1034 /** 1035 * @return Returns a reference to <code>this</code> for easy method chaining 1036 */ 1037 public Library setUseContext(List<UsageContext> theUseContext) { 1038 this.useContext = theUseContext; 1039 return this; 1040 } 1041 1042 public boolean hasUseContext() { 1043 if (this.useContext == null) 1044 return false; 1045 for (UsageContext item : this.useContext) 1046 if (!item.isEmpty()) 1047 return true; 1048 return false; 1049 } 1050 1051 public UsageContext addUseContext() { //3 1052 UsageContext t = new UsageContext(); 1053 if (this.useContext == null) 1054 this.useContext = new ArrayList<UsageContext>(); 1055 this.useContext.add(t); 1056 return t; 1057 } 1058 1059 public Library addUseContext(UsageContext t) { //3 1060 if (t == null) 1061 return this; 1062 if (this.useContext == null) 1063 this.useContext = new ArrayList<UsageContext>(); 1064 this.useContext.add(t); 1065 return this; 1066 } 1067 1068 /** 1069 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 1070 */ 1071 public UsageContext getUseContextFirstRep() { 1072 if (getUseContext().isEmpty()) { 1073 addUseContext(); 1074 } 1075 return getUseContext().get(0); 1076 } 1077 1078 /** 1079 * @return {@link #jurisdiction} (A legal or geographic region in which the library is intended to be used.) 1080 */ 1081 public List<CodeableConcept> getJurisdiction() { 1082 if (this.jurisdiction == null) 1083 this.jurisdiction = new ArrayList<CodeableConcept>(); 1084 return this.jurisdiction; 1085 } 1086 1087 /** 1088 * @return Returns a reference to <code>this</code> for easy method chaining 1089 */ 1090 public Library setJurisdiction(List<CodeableConcept> theJurisdiction) { 1091 this.jurisdiction = theJurisdiction; 1092 return this; 1093 } 1094 1095 public boolean hasJurisdiction() { 1096 if (this.jurisdiction == null) 1097 return false; 1098 for (CodeableConcept item : this.jurisdiction) 1099 if (!item.isEmpty()) 1100 return true; 1101 return false; 1102 } 1103 1104 public CodeableConcept addJurisdiction() { //3 1105 CodeableConcept t = new CodeableConcept(); 1106 if (this.jurisdiction == null) 1107 this.jurisdiction = new ArrayList<CodeableConcept>(); 1108 this.jurisdiction.add(t); 1109 return t; 1110 } 1111 1112 public Library addJurisdiction(CodeableConcept t) { //3 1113 if (t == null) 1114 return this; 1115 if (this.jurisdiction == null) 1116 this.jurisdiction = new ArrayList<CodeableConcept>(); 1117 this.jurisdiction.add(t); 1118 return this; 1119 } 1120 1121 /** 1122 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 1123 */ 1124 public CodeableConcept getJurisdictionFirstRep() { 1125 if (getJurisdiction().isEmpty()) { 1126 addJurisdiction(); 1127 } 1128 return getJurisdiction().get(0); 1129 } 1130 1131 /** 1132 * @return {@link #purpose} (Explanation of why this library is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1133 */ 1134 public MarkdownType getPurposeElement() { 1135 if (this.purpose == null) 1136 if (Configuration.errorOnAutoCreate()) 1137 throw new Error("Attempt to auto-create Library.purpose"); 1138 else if (Configuration.doAutoCreate()) 1139 this.purpose = new MarkdownType(); // bb 1140 return this.purpose; 1141 } 1142 1143 public boolean hasPurposeElement() { 1144 return this.purpose != null && !this.purpose.isEmpty(); 1145 } 1146 1147 public boolean hasPurpose() { 1148 return this.purpose != null && !this.purpose.isEmpty(); 1149 } 1150 1151 /** 1152 * @param value {@link #purpose} (Explanation of why this library is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1153 */ 1154 public Library setPurposeElement(MarkdownType value) { 1155 this.purpose = value; 1156 return this; 1157 } 1158 1159 /** 1160 * @return Explanation of why this library is needed and why it has been designed as it has. 1161 */ 1162 public String getPurpose() { 1163 return this.purpose == null ? null : this.purpose.getValue(); 1164 } 1165 1166 /** 1167 * @param value Explanation of why this library is needed and why it has been designed as it has. 1168 */ 1169 public Library setPurpose(String value) { 1170 if (Utilities.noString(value)) 1171 this.purpose = null; 1172 else { 1173 if (this.purpose == null) 1174 this.purpose = new MarkdownType(); 1175 this.purpose.setValue(value); 1176 } 1177 return this; 1178 } 1179 1180 /** 1181 * @return {@link #usage} (A detailed description of how the library is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 1182 */ 1183 public MarkdownType getUsageElement() { 1184 if (this.usage == null) 1185 if (Configuration.errorOnAutoCreate()) 1186 throw new Error("Attempt to auto-create Library.usage"); 1187 else if (Configuration.doAutoCreate()) 1188 this.usage = new MarkdownType(); // bb 1189 return this.usage; 1190 } 1191 1192 public boolean hasUsageElement() { 1193 return this.usage != null && !this.usage.isEmpty(); 1194 } 1195 1196 public boolean hasUsage() { 1197 return this.usage != null && !this.usage.isEmpty(); 1198 } 1199 1200 /** 1201 * @param value {@link #usage} (A detailed description of how the library is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 1202 */ 1203 public Library setUsageElement(MarkdownType value) { 1204 this.usage = value; 1205 return this; 1206 } 1207 1208 /** 1209 * @return A detailed description of how the library is used from a clinical perspective. 1210 */ 1211 public String getUsage() { 1212 return this.usage == null ? null : this.usage.getValue(); 1213 } 1214 1215 /** 1216 * @param value A detailed description of how the library is used from a clinical perspective. 1217 */ 1218 public Library setUsage(String value) { 1219 if (Utilities.noString(value)) 1220 this.usage = null; 1221 else { 1222 if (this.usage == null) 1223 this.usage = new MarkdownType(); 1224 this.usage.setValue(value); 1225 } 1226 return this; 1227 } 1228 1229 /** 1230 * @return {@link #copyright} (A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1231 */ 1232 public MarkdownType getCopyrightElement() { 1233 if (this.copyright == null) 1234 if (Configuration.errorOnAutoCreate()) 1235 throw new Error("Attempt to auto-create Library.copyright"); 1236 else if (Configuration.doAutoCreate()) 1237 this.copyright = new MarkdownType(); // bb 1238 return this.copyright; 1239 } 1240 1241 public boolean hasCopyrightElement() { 1242 return this.copyright != null && !this.copyright.isEmpty(); 1243 } 1244 1245 public boolean hasCopyright() { 1246 return this.copyright != null && !this.copyright.isEmpty(); 1247 } 1248 1249 /** 1250 * @param value {@link #copyright} (A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1251 */ 1252 public Library setCopyrightElement(MarkdownType value) { 1253 this.copyright = value; 1254 return this; 1255 } 1256 1257 /** 1258 * @return A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library. 1259 */ 1260 public String getCopyright() { 1261 return this.copyright == null ? null : this.copyright.getValue(); 1262 } 1263 1264 /** 1265 * @param value A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library. 1266 */ 1267 public Library setCopyright(String value) { 1268 if (Utilities.noString(value)) 1269 this.copyright = null; 1270 else { 1271 if (this.copyright == null) 1272 this.copyright = new MarkdownType(); 1273 this.copyright.setValue(value); 1274 } 1275 return this; 1276 } 1277 1278 /** 1279 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 1280 */ 1281 public StringType getCopyrightLabelElement() { 1282 if (this.copyrightLabel == null) 1283 if (Configuration.errorOnAutoCreate()) 1284 throw new Error("Attempt to auto-create Library.copyrightLabel"); 1285 else if (Configuration.doAutoCreate()) 1286 this.copyrightLabel = new StringType(); // bb 1287 return this.copyrightLabel; 1288 } 1289 1290 public boolean hasCopyrightLabelElement() { 1291 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 1292 } 1293 1294 public boolean hasCopyrightLabel() { 1295 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 1296 } 1297 1298 /** 1299 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 1300 */ 1301 public Library setCopyrightLabelElement(StringType value) { 1302 this.copyrightLabel = value; 1303 return this; 1304 } 1305 1306 /** 1307 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1308 */ 1309 public String getCopyrightLabel() { 1310 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 1311 } 1312 1313 /** 1314 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 1315 */ 1316 public Library setCopyrightLabel(String value) { 1317 if (Utilities.noString(value)) 1318 this.copyrightLabel = null; 1319 else { 1320 if (this.copyrightLabel == null) 1321 this.copyrightLabel = new StringType(); 1322 this.copyrightLabel.setValue(value); 1323 } 1324 return this; 1325 } 1326 1327 /** 1328 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 1329 */ 1330 public DateType getApprovalDateElement() { 1331 if (this.approvalDate == null) 1332 if (Configuration.errorOnAutoCreate()) 1333 throw new Error("Attempt to auto-create Library.approvalDate"); 1334 else if (Configuration.doAutoCreate()) 1335 this.approvalDate = new DateType(); // bb 1336 return this.approvalDate; 1337 } 1338 1339 public boolean hasApprovalDateElement() { 1340 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1341 } 1342 1343 public boolean hasApprovalDate() { 1344 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1345 } 1346 1347 /** 1348 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 1349 */ 1350 public Library setApprovalDateElement(DateType value) { 1351 this.approvalDate = value; 1352 return this; 1353 } 1354 1355 /** 1356 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1357 */ 1358 public Date getApprovalDate() { 1359 return this.approvalDate == null ? null : this.approvalDate.getValue(); 1360 } 1361 1362 /** 1363 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1364 */ 1365 public Library setApprovalDate(Date value) { 1366 if (value == null) 1367 this.approvalDate = null; 1368 else { 1369 if (this.approvalDate == null) 1370 this.approvalDate = new DateType(); 1371 this.approvalDate.setValue(value); 1372 } 1373 return this; 1374 } 1375 1376 /** 1377 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 1378 */ 1379 public DateType getLastReviewDateElement() { 1380 if (this.lastReviewDate == null) 1381 if (Configuration.errorOnAutoCreate()) 1382 throw new Error("Attempt to auto-create Library.lastReviewDate"); 1383 else if (Configuration.doAutoCreate()) 1384 this.lastReviewDate = new DateType(); // bb 1385 return this.lastReviewDate; 1386 } 1387 1388 public boolean hasLastReviewDateElement() { 1389 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1390 } 1391 1392 public boolean hasLastReviewDate() { 1393 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1394 } 1395 1396 /** 1397 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 1398 */ 1399 public Library setLastReviewDateElement(DateType value) { 1400 this.lastReviewDate = value; 1401 return this; 1402 } 1403 1404 /** 1405 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 1406 */ 1407 public Date getLastReviewDate() { 1408 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 1409 } 1410 1411 /** 1412 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 1413 */ 1414 public Library setLastReviewDate(Date value) { 1415 if (value == null) 1416 this.lastReviewDate = null; 1417 else { 1418 if (this.lastReviewDate == null) 1419 this.lastReviewDate = new DateType(); 1420 this.lastReviewDate.setValue(value); 1421 } 1422 return this; 1423 } 1424 1425 /** 1426 * @return {@link #effectivePeriod} (The period during which the library content was or is planned to be in active use.) 1427 */ 1428 public Period getEffectivePeriod() { 1429 if (this.effectivePeriod == null) 1430 if (Configuration.errorOnAutoCreate()) 1431 throw new Error("Attempt to auto-create Library.effectivePeriod"); 1432 else if (Configuration.doAutoCreate()) 1433 this.effectivePeriod = new Period(); // cc 1434 return this.effectivePeriod; 1435 } 1436 1437 public boolean hasEffectivePeriod() { 1438 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 1439 } 1440 1441 /** 1442 * @param value {@link #effectivePeriod} (The period during which the library content was or is planned to be in active use.) 1443 */ 1444 public Library setEffectivePeriod(Period value) { 1445 this.effectivePeriod = value; 1446 return this; 1447 } 1448 1449 /** 1450 * @return {@link #topic} (Descriptive topics related to the content of the library. Topics provide a high-level categorization of the library that can be useful for filtering and searching.) 1451 */ 1452 public List<CodeableConcept> getTopic() { 1453 if (this.topic == null) 1454 this.topic = new ArrayList<CodeableConcept>(); 1455 return this.topic; 1456 } 1457 1458 /** 1459 * @return Returns a reference to <code>this</code> for easy method chaining 1460 */ 1461 public Library setTopic(List<CodeableConcept> theTopic) { 1462 this.topic = theTopic; 1463 return this; 1464 } 1465 1466 public boolean hasTopic() { 1467 if (this.topic == null) 1468 return false; 1469 for (CodeableConcept item : this.topic) 1470 if (!item.isEmpty()) 1471 return true; 1472 return false; 1473 } 1474 1475 public CodeableConcept addTopic() { //3 1476 CodeableConcept t = new CodeableConcept(); 1477 if (this.topic == null) 1478 this.topic = new ArrayList<CodeableConcept>(); 1479 this.topic.add(t); 1480 return t; 1481 } 1482 1483 public Library addTopic(CodeableConcept t) { //3 1484 if (t == null) 1485 return this; 1486 if (this.topic == null) 1487 this.topic = new ArrayList<CodeableConcept>(); 1488 this.topic.add(t); 1489 return this; 1490 } 1491 1492 /** 1493 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {3} 1494 */ 1495 public CodeableConcept getTopicFirstRep() { 1496 if (getTopic().isEmpty()) { 1497 addTopic(); 1498 } 1499 return getTopic().get(0); 1500 } 1501 1502 /** 1503 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the content.) 1504 */ 1505 public List<ContactDetail> getAuthor() { 1506 if (this.author == null) 1507 this.author = new ArrayList<ContactDetail>(); 1508 return this.author; 1509 } 1510 1511 /** 1512 * @return Returns a reference to <code>this</code> for easy method chaining 1513 */ 1514 public Library setAuthor(List<ContactDetail> theAuthor) { 1515 this.author = theAuthor; 1516 return this; 1517 } 1518 1519 public boolean hasAuthor() { 1520 if (this.author == null) 1521 return false; 1522 for (ContactDetail item : this.author) 1523 if (!item.isEmpty()) 1524 return true; 1525 return false; 1526 } 1527 1528 public ContactDetail addAuthor() { //3 1529 ContactDetail t = new ContactDetail(); 1530 if (this.author == null) 1531 this.author = new ArrayList<ContactDetail>(); 1532 this.author.add(t); 1533 return t; 1534 } 1535 1536 public Library addAuthor(ContactDetail t) { //3 1537 if (t == null) 1538 return this; 1539 if (this.author == null) 1540 this.author = new ArrayList<ContactDetail>(); 1541 this.author.add(t); 1542 return this; 1543 } 1544 1545 /** 1546 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 1547 */ 1548 public ContactDetail getAuthorFirstRep() { 1549 if (getAuthor().isEmpty()) { 1550 addAuthor(); 1551 } 1552 return getAuthor().get(0); 1553 } 1554 1555 /** 1556 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the content.) 1557 */ 1558 public List<ContactDetail> getEditor() { 1559 if (this.editor == null) 1560 this.editor = new ArrayList<ContactDetail>(); 1561 return this.editor; 1562 } 1563 1564 /** 1565 * @return Returns a reference to <code>this</code> for easy method chaining 1566 */ 1567 public Library setEditor(List<ContactDetail> theEditor) { 1568 this.editor = theEditor; 1569 return this; 1570 } 1571 1572 public boolean hasEditor() { 1573 if (this.editor == null) 1574 return false; 1575 for (ContactDetail item : this.editor) 1576 if (!item.isEmpty()) 1577 return true; 1578 return false; 1579 } 1580 1581 public ContactDetail addEditor() { //3 1582 ContactDetail t = new ContactDetail(); 1583 if (this.editor == null) 1584 this.editor = new ArrayList<ContactDetail>(); 1585 this.editor.add(t); 1586 return t; 1587 } 1588 1589 public Library addEditor(ContactDetail t) { //3 1590 if (t == null) 1591 return this; 1592 if (this.editor == null) 1593 this.editor = new ArrayList<ContactDetail>(); 1594 this.editor.add(t); 1595 return this; 1596 } 1597 1598 /** 1599 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {3} 1600 */ 1601 public ContactDetail getEditorFirstRep() { 1602 if (getEditor().isEmpty()) { 1603 addEditor(); 1604 } 1605 return getEditor().get(0); 1606 } 1607 1608 /** 1609 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.) 1610 */ 1611 public List<ContactDetail> getReviewer() { 1612 if (this.reviewer == null) 1613 this.reviewer = new ArrayList<ContactDetail>(); 1614 return this.reviewer; 1615 } 1616 1617 /** 1618 * @return Returns a reference to <code>this</code> for easy method chaining 1619 */ 1620 public Library setReviewer(List<ContactDetail> theReviewer) { 1621 this.reviewer = theReviewer; 1622 return this; 1623 } 1624 1625 public boolean hasReviewer() { 1626 if (this.reviewer == null) 1627 return false; 1628 for (ContactDetail item : this.reviewer) 1629 if (!item.isEmpty()) 1630 return true; 1631 return false; 1632 } 1633 1634 public ContactDetail addReviewer() { //3 1635 ContactDetail t = new ContactDetail(); 1636 if (this.reviewer == null) 1637 this.reviewer = new ArrayList<ContactDetail>(); 1638 this.reviewer.add(t); 1639 return t; 1640 } 1641 1642 public Library addReviewer(ContactDetail t) { //3 1643 if (t == null) 1644 return this; 1645 if (this.reviewer == null) 1646 this.reviewer = new ArrayList<ContactDetail>(); 1647 this.reviewer.add(t); 1648 return this; 1649 } 1650 1651 /** 1652 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {3} 1653 */ 1654 public ContactDetail getReviewerFirstRep() { 1655 if (getReviewer().isEmpty()) { 1656 addReviewer(); 1657 } 1658 return getReviewer().get(0); 1659 } 1660 1661 /** 1662 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.) 1663 */ 1664 public List<ContactDetail> getEndorser() { 1665 if (this.endorser == null) 1666 this.endorser = new ArrayList<ContactDetail>(); 1667 return this.endorser; 1668 } 1669 1670 /** 1671 * @return Returns a reference to <code>this</code> for easy method chaining 1672 */ 1673 public Library setEndorser(List<ContactDetail> theEndorser) { 1674 this.endorser = theEndorser; 1675 return this; 1676 } 1677 1678 public boolean hasEndorser() { 1679 if (this.endorser == null) 1680 return false; 1681 for (ContactDetail item : this.endorser) 1682 if (!item.isEmpty()) 1683 return true; 1684 return false; 1685 } 1686 1687 public ContactDetail addEndorser() { //3 1688 ContactDetail t = new ContactDetail(); 1689 if (this.endorser == null) 1690 this.endorser = new ArrayList<ContactDetail>(); 1691 this.endorser.add(t); 1692 return t; 1693 } 1694 1695 public Library addEndorser(ContactDetail t) { //3 1696 if (t == null) 1697 return this; 1698 if (this.endorser == null) 1699 this.endorser = new ArrayList<ContactDetail>(); 1700 this.endorser.add(t); 1701 return this; 1702 } 1703 1704 /** 1705 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {3} 1706 */ 1707 public ContactDetail getEndorserFirstRep() { 1708 if (getEndorser().isEmpty()) { 1709 addEndorser(); 1710 } 1711 return getEndorser().get(0); 1712 } 1713 1714 /** 1715 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, or bibliographic references.) 1716 */ 1717 public List<RelatedArtifact> getRelatedArtifact() { 1718 if (this.relatedArtifact == null) 1719 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1720 return this.relatedArtifact; 1721 } 1722 1723 /** 1724 * @return Returns a reference to <code>this</code> for easy method chaining 1725 */ 1726 public Library setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 1727 this.relatedArtifact = theRelatedArtifact; 1728 return this; 1729 } 1730 1731 public boolean hasRelatedArtifact() { 1732 if (this.relatedArtifact == null) 1733 return false; 1734 for (RelatedArtifact item : this.relatedArtifact) 1735 if (!item.isEmpty()) 1736 return true; 1737 return false; 1738 } 1739 1740 public RelatedArtifact addRelatedArtifact() { //3 1741 RelatedArtifact t = new RelatedArtifact(); 1742 if (this.relatedArtifact == null) 1743 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1744 this.relatedArtifact.add(t); 1745 return t; 1746 } 1747 1748 public Library addRelatedArtifact(RelatedArtifact t) { //3 1749 if (t == null) 1750 return this; 1751 if (this.relatedArtifact == null) 1752 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1753 this.relatedArtifact.add(t); 1754 return this; 1755 } 1756 1757 /** 1758 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 1759 */ 1760 public RelatedArtifact getRelatedArtifactFirstRep() { 1761 if (getRelatedArtifact().isEmpty()) { 1762 addRelatedArtifact(); 1763 } 1764 return getRelatedArtifact().get(0); 1765 } 1766 1767 /** 1768 * @return {@link #parameter} (The parameter element defines parameters used by the library.) 1769 */ 1770 public List<ParameterDefinition> getParameter() { 1771 if (this.parameter == null) 1772 this.parameter = new ArrayList<ParameterDefinition>(); 1773 return this.parameter; 1774 } 1775 1776 /** 1777 * @return Returns a reference to <code>this</code> for easy method chaining 1778 */ 1779 public Library setParameter(List<ParameterDefinition> theParameter) { 1780 this.parameter = theParameter; 1781 return this; 1782 } 1783 1784 public boolean hasParameter() { 1785 if (this.parameter == null) 1786 return false; 1787 for (ParameterDefinition item : this.parameter) 1788 if (!item.isEmpty()) 1789 return true; 1790 return false; 1791 } 1792 1793 public ParameterDefinition addParameter() { //3 1794 ParameterDefinition t = new ParameterDefinition(); 1795 if (this.parameter == null) 1796 this.parameter = new ArrayList<ParameterDefinition>(); 1797 this.parameter.add(t); 1798 return t; 1799 } 1800 1801 public Library addParameter(ParameterDefinition t) { //3 1802 if (t == null) 1803 return this; 1804 if (this.parameter == null) 1805 this.parameter = new ArrayList<ParameterDefinition>(); 1806 this.parameter.add(t); 1807 return this; 1808 } 1809 1810 /** 1811 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist {3} 1812 */ 1813 public ParameterDefinition getParameterFirstRep() { 1814 if (getParameter().isEmpty()) { 1815 addParameter(); 1816 } 1817 return getParameter().get(0); 1818 } 1819 1820 /** 1821 * @return {@link #dataRequirement} (Describes a set of data that must be provided in order to be able to successfully perform the computations defined by the library.) 1822 */ 1823 public List<DataRequirement> getDataRequirement() { 1824 if (this.dataRequirement == null) 1825 this.dataRequirement = new ArrayList<DataRequirement>(); 1826 return this.dataRequirement; 1827 } 1828 1829 /** 1830 * @return Returns a reference to <code>this</code> for easy method chaining 1831 */ 1832 public Library setDataRequirement(List<DataRequirement> theDataRequirement) { 1833 this.dataRequirement = theDataRequirement; 1834 return this; 1835 } 1836 1837 public boolean hasDataRequirement() { 1838 if (this.dataRequirement == null) 1839 return false; 1840 for (DataRequirement item : this.dataRequirement) 1841 if (!item.isEmpty()) 1842 return true; 1843 return false; 1844 } 1845 1846 public DataRequirement addDataRequirement() { //3 1847 DataRequirement t = new DataRequirement(); 1848 if (this.dataRequirement == null) 1849 this.dataRequirement = new ArrayList<DataRequirement>(); 1850 this.dataRequirement.add(t); 1851 return t; 1852 } 1853 1854 public Library addDataRequirement(DataRequirement t) { //3 1855 if (t == null) 1856 return this; 1857 if (this.dataRequirement == null) 1858 this.dataRequirement = new ArrayList<DataRequirement>(); 1859 this.dataRequirement.add(t); 1860 return this; 1861 } 1862 1863 /** 1864 * @return The first repetition of repeating field {@link #dataRequirement}, creating it if it does not already exist {3} 1865 */ 1866 public DataRequirement getDataRequirementFirstRep() { 1867 if (getDataRequirement().isEmpty()) { 1868 addDataRequirement(); 1869 } 1870 return getDataRequirement().get(0); 1871 } 1872 1873 /** 1874 * @return {@link #content} (The content of the library as an Attachment. The content may be a reference to a url, or may be directly embedded as a base-64 string. Either way, the contentType of the attachment determines how to interpret the content.) 1875 */ 1876 public List<Attachment> getContent() { 1877 if (this.content == null) 1878 this.content = new ArrayList<Attachment>(); 1879 return this.content; 1880 } 1881 1882 /** 1883 * @return Returns a reference to <code>this</code> for easy method chaining 1884 */ 1885 public Library setContent(List<Attachment> theContent) { 1886 this.content = theContent; 1887 return this; 1888 } 1889 1890 public boolean hasContent() { 1891 if (this.content == null) 1892 return false; 1893 for (Attachment item : this.content) 1894 if (!item.isEmpty()) 1895 return true; 1896 return false; 1897 } 1898 1899 public Attachment addContent() { //3 1900 Attachment t = new Attachment(); 1901 if (this.content == null) 1902 this.content = new ArrayList<Attachment>(); 1903 this.content.add(t); 1904 return t; 1905 } 1906 1907 public Library addContent(Attachment t) { //3 1908 if (t == null) 1909 return this; 1910 if (this.content == null) 1911 this.content = new ArrayList<Attachment>(); 1912 this.content.add(t); 1913 return this; 1914 } 1915 1916 /** 1917 * @return The first repetition of repeating field {@link #content}, creating it if it does not already exist {3} 1918 */ 1919 public Attachment getContentFirstRep() { 1920 if (getContent().isEmpty()) { 1921 addContent(); 1922 } 1923 return getContent().get(0); 1924 } 1925 1926 protected void listChildren(List<Property> children) { 1927 super.listChildren(children); 1928 children.add(new Property("url", "uri", "An absolute URI that is used to identify this library when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this library is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the library is stored on different servers.", 0, 1, url)); 1929 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this library when it is represented in other formats, or referenced in a specification, model, design or an instance. e.g. CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1930 children.add(new Property("version", "string", "The identifier that is used to identify this version of the library when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the library author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 0, 1, version)); 1931 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 1932 children.add(new Property("name", "string", "A natural language name identifying the library. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 1933 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the library.", 0, 1, title)); 1934 children.add(new Property("subtitle", "string", "An explanatory or alternate title for the library giving additional information about its content.", 0, 1, subtitle)); 1935 children.add(new Property("status", "code", "The status of this library. Enables tracking the life-cycle of the content.", 0, 1, status)); 1936 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this library is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 1937 children.add(new Property("type", "CodeableConcept", "Identifies the type of library such as a Logic Library, Model Definition, Asset Collection, or Module Definition.", 0, 1, type)); 1938 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", "A code or group definition that describes the intended subject of the contents of the library.", 0, 1, subject)); 1939 children.add(new Property("date", "dateTime", "The date (and optionally time) when the library was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the library changes.", 0, 1, date)); 1940 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the library.", 0, 1, publisher)); 1941 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 1942 children.add(new Property("description", "markdown", "A free text natural language description of the library from a consumer's perspective.", 0, 1, description)); 1943 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate library instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 1944 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the library is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 1945 children.add(new Property("purpose", "markdown", "Explanation of why this library is needed and why it has been designed as it has.", 0, 1, purpose)); 1946 children.add(new Property("usage", "markdown", "A detailed description of how the library is used from a clinical perspective.", 0, 1, usage)); 1947 children.add(new Property("copyright", "markdown", "A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library.", 0, 1, copyright)); 1948 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 1949 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 1950 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 1951 children.add(new Property("effectivePeriod", "Period", "The period during which the library content was or is planned to be in active use.", 0, 1, effectivePeriod)); 1952 children.add(new Property("topic", "CodeableConcept", "Descriptive topics related to the content of the library. Topics provide a high-level categorization of the library that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic)); 1953 children.add(new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author)); 1954 children.add(new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor)); 1955 children.add(new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer)); 1956 children.add(new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser)); 1957 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 1958 children.add(new Property("parameter", "ParameterDefinition", "The parameter element defines parameters used by the library.", 0, java.lang.Integer.MAX_VALUE, parameter)); 1959 children.add(new Property("dataRequirement", "DataRequirement", "Describes a set of data that must be provided in order to be able to successfully perform the computations defined by the library.", 0, java.lang.Integer.MAX_VALUE, dataRequirement)); 1960 children.add(new Property("content", "Attachment", "The content of the library as an Attachment. The content may be a reference to a url, or may be directly embedded as a base-64 string. Either way, the contentType of the attachment determines how to interpret the content.", 0, java.lang.Integer.MAX_VALUE, content)); 1961 } 1962 1963 @Override 1964 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1965 switch (_hash) { 1966 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this library when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this library is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the library is stored on different servers.", 0, 1, url); 1967 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this library when it is represented in other formats, or referenced in a specification, model, design or an instance. e.g. CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts.", 0, java.lang.Integer.MAX_VALUE, identifier); 1968 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the library when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the library author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 0, 1, version); 1969 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 1970 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 1971 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 1972 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 1973 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the library. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 1974 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the library.", 0, 1, title); 1975 case -2060497896: /*subtitle*/ return new Property("subtitle", "string", "An explanatory or alternate title for the library giving additional information about its content.", 0, 1, subtitle); 1976 case -892481550: /*status*/ return new Property("status", "code", "The status of this library. Enables tracking the life-cycle of the content.", 0, 1, status); 1977 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this library is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 1978 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Identifies the type of library such as a Logic Library, Model Definition, Asset Collection, or Module Definition.", 0, 1, type); 1979 case -573640748: /*subject[x]*/ return new Property("subject[x]", "CodeableConcept|Reference(Group)", "A code or group definition that describes the intended subject of the contents of the library.", 0, 1, subject); 1980 case -1867885268: /*subject*/ return new Property("subject[x]", "CodeableConcept|Reference(Group)", "A code or group definition that describes the intended subject of the contents of the library.", 0, 1, subject); 1981 case -1257122603: /*subjectCodeableConcept*/ return new Property("subject[x]", "CodeableConcept", "A code or group definition that describes the intended subject of the contents of the library.", 0, 1, subject); 1982 case 772938623: /*subjectReference*/ return new Property("subject[x]", "Reference(Group)", "A code or group definition that describes the intended subject of the contents of the library.", 0, 1, subject); 1983 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the library was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the library changes.", 0, 1, date); 1984 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the library.", 0, 1, publisher); 1985 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 1986 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the library from a consumer's perspective.", 0, 1, description); 1987 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate library instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 1988 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the library is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 1989 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this library is needed and why it has been designed as it has.", 0, 1, purpose); 1990 case 111574433: /*usage*/ return new Property("usage", "markdown", "A detailed description of how the library is used from a clinical perspective.", 0, 1, usage); 1991 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library.", 0, 1, copyright); 1992 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 1993 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 1994 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 1995 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the library content was or is planned to be in active use.", 0, 1, effectivePeriod); 1996 case 110546223: /*topic*/ return new Property("topic", "CodeableConcept", "Descriptive topics related to the content of the library. Topics provide a high-level categorization of the library that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic); 1997 case -1406328437: /*author*/ return new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author); 1998 case -1307827859: /*editor*/ return new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor); 1999 case -261190139: /*reviewer*/ return new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer); 2000 case 1740277666: /*endorser*/ return new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser); 2001 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 2002 case 1954460585: /*parameter*/ return new Property("parameter", "ParameterDefinition", "The parameter element defines parameters used by the library.", 0, java.lang.Integer.MAX_VALUE, parameter); 2003 case 629147193: /*dataRequirement*/ return new Property("dataRequirement", "DataRequirement", "Describes a set of data that must be provided in order to be able to successfully perform the computations defined by the library.", 0, java.lang.Integer.MAX_VALUE, dataRequirement); 2004 case 951530617: /*content*/ return new Property("content", "Attachment", "The content of the library as an Attachment. The content may be a reference to a url, or may be directly embedded as a base-64 string. Either way, the contentType of the attachment determines how to interpret the content.", 0, java.lang.Integer.MAX_VALUE, content); 2005 default: return super.getNamedProperty(_hash, _name, _checkValid); 2006 } 2007 2008 } 2009 2010 @Override 2011 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2012 switch (hash) { 2013 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2014 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2015 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2016 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 2017 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2018 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2019 case -2060497896: /*subtitle*/ return this.subtitle == null ? new Base[0] : new Base[] {this.subtitle}; // StringType 2020 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2021 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2022 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2023 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // DataType 2024 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2025 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2026 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2027 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2028 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2029 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2030 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2031 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : new Base[] {this.usage}; // MarkdownType 2032 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 2033 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 2034 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 2035 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 2036 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 2037 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 2038 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 2039 case -1307827859: /*editor*/ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 2040 case -261190139: /*reviewer*/ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 2041 case 1740277666: /*endorser*/ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 2042 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 2043 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // ParameterDefinition 2044 case 629147193: /*dataRequirement*/ return this.dataRequirement == null ? new Base[0] : this.dataRequirement.toArray(new Base[this.dataRequirement.size()]); // DataRequirement 2045 case 951530617: /*content*/ return this.content == null ? new Base[0] : this.content.toArray(new Base[this.content.size()]); // Attachment 2046 default: return super.getProperty(hash, name, checkValid); 2047 } 2048 2049 } 2050 2051 @Override 2052 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2053 switch (hash) { 2054 case 116079: // url 2055 this.url = TypeConvertor.castToUri(value); // UriType 2056 return value; 2057 case -1618432855: // identifier 2058 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2059 return value; 2060 case 351608024: // version 2061 this.version = TypeConvertor.castToString(value); // StringType 2062 return value; 2063 case 1508158071: // versionAlgorithm 2064 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2065 return value; 2066 case 3373707: // name 2067 this.name = TypeConvertor.castToString(value); // StringType 2068 return value; 2069 case 110371416: // title 2070 this.title = TypeConvertor.castToString(value); // StringType 2071 return value; 2072 case -2060497896: // subtitle 2073 this.subtitle = TypeConvertor.castToString(value); // StringType 2074 return value; 2075 case -892481550: // status 2076 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2077 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2078 return value; 2079 case -404562712: // experimental 2080 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2081 return value; 2082 case 3575610: // type 2083 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2084 return value; 2085 case -1867885268: // subject 2086 this.subject = TypeConvertor.castToType(value); // DataType 2087 return value; 2088 case 3076014: // date 2089 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2090 return value; 2091 case 1447404028: // publisher 2092 this.publisher = TypeConvertor.castToString(value); // StringType 2093 return value; 2094 case 951526432: // contact 2095 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2096 return value; 2097 case -1724546052: // description 2098 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2099 return value; 2100 case -669707736: // useContext 2101 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 2102 return value; 2103 case -507075711: // jurisdiction 2104 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2105 return value; 2106 case -220463842: // purpose 2107 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2108 return value; 2109 case 111574433: // usage 2110 this.usage = TypeConvertor.castToMarkdown(value); // MarkdownType 2111 return value; 2112 case 1522889671: // copyright 2113 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2114 return value; 2115 case 765157229: // copyrightLabel 2116 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2117 return value; 2118 case 223539345: // approvalDate 2119 this.approvalDate = TypeConvertor.castToDate(value); // DateType 2120 return value; 2121 case -1687512484: // lastReviewDate 2122 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 2123 return value; 2124 case -403934648: // effectivePeriod 2125 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 2126 return value; 2127 case 110546223: // topic 2128 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2129 return value; 2130 case -1406328437: // author 2131 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2132 return value; 2133 case -1307827859: // editor 2134 this.getEditor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2135 return value; 2136 case -261190139: // reviewer 2137 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2138 return value; 2139 case 1740277666: // endorser 2140 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 2141 return value; 2142 case 666807069: // relatedArtifact 2143 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 2144 return value; 2145 case 1954460585: // parameter 2146 this.getParameter().add(TypeConvertor.castToParameterDefinition(value)); // ParameterDefinition 2147 return value; 2148 case 629147193: // dataRequirement 2149 this.getDataRequirement().add(TypeConvertor.castToDataRequirement(value)); // DataRequirement 2150 return value; 2151 case 951530617: // content 2152 this.getContent().add(TypeConvertor.castToAttachment(value)); // Attachment 2153 return value; 2154 default: return super.setProperty(hash, name, value); 2155 } 2156 2157 } 2158 2159 @Override 2160 public Base setProperty(String name, Base value) throws FHIRException { 2161 if (name.equals("url")) { 2162 this.url = TypeConvertor.castToUri(value); // UriType 2163 } else if (name.equals("identifier")) { 2164 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2165 } else if (name.equals("version")) { 2166 this.version = TypeConvertor.castToString(value); // StringType 2167 } else if (name.equals("versionAlgorithm[x]")) { 2168 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 2169 } else if (name.equals("name")) { 2170 this.name = TypeConvertor.castToString(value); // StringType 2171 } else if (name.equals("title")) { 2172 this.title = TypeConvertor.castToString(value); // StringType 2173 } else if (name.equals("subtitle")) { 2174 this.subtitle = TypeConvertor.castToString(value); // StringType 2175 } else if (name.equals("status")) { 2176 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2177 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2178 } else if (name.equals("experimental")) { 2179 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 2180 } else if (name.equals("type")) { 2181 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2182 } else if (name.equals("subject[x]")) { 2183 this.subject = TypeConvertor.castToType(value); // DataType 2184 } else if (name.equals("date")) { 2185 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 2186 } else if (name.equals("publisher")) { 2187 this.publisher = TypeConvertor.castToString(value); // StringType 2188 } else if (name.equals("contact")) { 2189 this.getContact().add(TypeConvertor.castToContactDetail(value)); 2190 } else if (name.equals("description")) { 2191 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2192 } else if (name.equals("useContext")) { 2193 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 2194 } else if (name.equals("jurisdiction")) { 2195 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 2196 } else if (name.equals("purpose")) { 2197 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 2198 } else if (name.equals("usage")) { 2199 this.usage = TypeConvertor.castToMarkdown(value); // MarkdownType 2200 } else if (name.equals("copyright")) { 2201 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 2202 } else if (name.equals("copyrightLabel")) { 2203 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 2204 } else if (name.equals("approvalDate")) { 2205 this.approvalDate = TypeConvertor.castToDate(value); // DateType 2206 } else if (name.equals("lastReviewDate")) { 2207 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 2208 } else if (name.equals("effectivePeriod")) { 2209 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 2210 } else if (name.equals("topic")) { 2211 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); 2212 } else if (name.equals("author")) { 2213 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); 2214 } else if (name.equals("editor")) { 2215 this.getEditor().add(TypeConvertor.castToContactDetail(value)); 2216 } else if (name.equals("reviewer")) { 2217 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); 2218 } else if (name.equals("endorser")) { 2219 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); 2220 } else if (name.equals("relatedArtifact")) { 2221 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 2222 } else if (name.equals("parameter")) { 2223 this.getParameter().add(TypeConvertor.castToParameterDefinition(value)); 2224 } else if (name.equals("dataRequirement")) { 2225 this.getDataRequirement().add(TypeConvertor.castToDataRequirement(value)); 2226 } else if (name.equals("content")) { 2227 this.getContent().add(TypeConvertor.castToAttachment(value)); 2228 } else 2229 return super.setProperty(name, value); 2230 return value; 2231 } 2232 2233 @Override 2234 public void removeChild(String name, Base value) throws FHIRException { 2235 if (name.equals("url")) { 2236 this.url = null; 2237 } else if (name.equals("identifier")) { 2238 this.getIdentifier().remove(value); 2239 } else if (name.equals("version")) { 2240 this.version = null; 2241 } else if (name.equals("versionAlgorithm[x]")) { 2242 this.versionAlgorithm = null; 2243 } else if (name.equals("name")) { 2244 this.name = null; 2245 } else if (name.equals("title")) { 2246 this.title = null; 2247 } else if (name.equals("subtitle")) { 2248 this.subtitle = null; 2249 } else if (name.equals("status")) { 2250 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2251 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2252 } else if (name.equals("experimental")) { 2253 this.experimental = null; 2254 } else if (name.equals("type")) { 2255 this.type = null; 2256 } else if (name.equals("subject[x]")) { 2257 this.subject = null; 2258 } else if (name.equals("date")) { 2259 this.date = null; 2260 } else if (name.equals("publisher")) { 2261 this.publisher = null; 2262 } else if (name.equals("contact")) { 2263 this.getContact().remove(value); 2264 } else if (name.equals("description")) { 2265 this.description = null; 2266 } else if (name.equals("useContext")) { 2267 this.getUseContext().remove(value); 2268 } else if (name.equals("jurisdiction")) { 2269 this.getJurisdiction().remove(value); 2270 } else if (name.equals("purpose")) { 2271 this.purpose = null; 2272 } else if (name.equals("usage")) { 2273 this.usage = null; 2274 } else if (name.equals("copyright")) { 2275 this.copyright = null; 2276 } else if (name.equals("copyrightLabel")) { 2277 this.copyrightLabel = null; 2278 } else if (name.equals("approvalDate")) { 2279 this.approvalDate = null; 2280 } else if (name.equals("lastReviewDate")) { 2281 this.lastReviewDate = null; 2282 } else if (name.equals("effectivePeriod")) { 2283 this.effectivePeriod = null; 2284 } else if (name.equals("topic")) { 2285 this.getTopic().remove(value); 2286 } else if (name.equals("author")) { 2287 this.getAuthor().remove(value); 2288 } else if (name.equals("editor")) { 2289 this.getEditor().remove(value); 2290 } else if (name.equals("reviewer")) { 2291 this.getReviewer().remove(value); 2292 } else if (name.equals("endorser")) { 2293 this.getEndorser().remove(value); 2294 } else if (name.equals("relatedArtifact")) { 2295 this.getRelatedArtifact().remove(value); 2296 } else if (name.equals("parameter")) { 2297 this.getParameter().remove(value); 2298 } else if (name.equals("dataRequirement")) { 2299 this.getDataRequirement().remove(value); 2300 } else if (name.equals("content")) { 2301 this.getContent().remove(value); 2302 } else 2303 super.removeChild(name, value); 2304 2305 } 2306 2307 @Override 2308 public Base makeProperty(int hash, String name) throws FHIRException { 2309 switch (hash) { 2310 case 116079: return getUrlElement(); 2311 case -1618432855: return addIdentifier(); 2312 case 351608024: return getVersionElement(); 2313 case -115699031: return getVersionAlgorithm(); 2314 case 1508158071: return getVersionAlgorithm(); 2315 case 3373707: return getNameElement(); 2316 case 110371416: return getTitleElement(); 2317 case -2060497896: return getSubtitleElement(); 2318 case -892481550: return getStatusElement(); 2319 case -404562712: return getExperimentalElement(); 2320 case 3575610: return getType(); 2321 case -573640748: return getSubject(); 2322 case -1867885268: return getSubject(); 2323 case 3076014: return getDateElement(); 2324 case 1447404028: return getPublisherElement(); 2325 case 951526432: return addContact(); 2326 case -1724546052: return getDescriptionElement(); 2327 case -669707736: return addUseContext(); 2328 case -507075711: return addJurisdiction(); 2329 case -220463842: return getPurposeElement(); 2330 case 111574433: return getUsageElement(); 2331 case 1522889671: return getCopyrightElement(); 2332 case 765157229: return getCopyrightLabelElement(); 2333 case 223539345: return getApprovalDateElement(); 2334 case -1687512484: return getLastReviewDateElement(); 2335 case -403934648: return getEffectivePeriod(); 2336 case 110546223: return addTopic(); 2337 case -1406328437: return addAuthor(); 2338 case -1307827859: return addEditor(); 2339 case -261190139: return addReviewer(); 2340 case 1740277666: return addEndorser(); 2341 case 666807069: return addRelatedArtifact(); 2342 case 1954460585: return addParameter(); 2343 case 629147193: return addDataRequirement(); 2344 case 951530617: return addContent(); 2345 default: return super.makeProperty(hash, name); 2346 } 2347 2348 } 2349 2350 @Override 2351 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2352 switch (hash) { 2353 case 116079: /*url*/ return new String[] {"uri"}; 2354 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2355 case 351608024: /*version*/ return new String[] {"string"}; 2356 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 2357 case 3373707: /*name*/ return new String[] {"string"}; 2358 case 110371416: /*title*/ return new String[] {"string"}; 2359 case -2060497896: /*subtitle*/ return new String[] {"string"}; 2360 case -892481550: /*status*/ return new String[] {"code"}; 2361 case -404562712: /*experimental*/ return new String[] {"boolean"}; 2362 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2363 case -1867885268: /*subject*/ return new String[] {"CodeableConcept", "Reference"}; 2364 case 3076014: /*date*/ return new String[] {"dateTime"}; 2365 case 1447404028: /*publisher*/ return new String[] {"string"}; 2366 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 2367 case -1724546052: /*description*/ return new String[] {"markdown"}; 2368 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 2369 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 2370 case -220463842: /*purpose*/ return new String[] {"markdown"}; 2371 case 111574433: /*usage*/ return new String[] {"markdown"}; 2372 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 2373 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 2374 case 223539345: /*approvalDate*/ return new String[] {"date"}; 2375 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 2376 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 2377 case 110546223: /*topic*/ return new String[] {"CodeableConcept"}; 2378 case -1406328437: /*author*/ return new String[] {"ContactDetail"}; 2379 case -1307827859: /*editor*/ return new String[] {"ContactDetail"}; 2380 case -261190139: /*reviewer*/ return new String[] {"ContactDetail"}; 2381 case 1740277666: /*endorser*/ return new String[] {"ContactDetail"}; 2382 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 2383 case 1954460585: /*parameter*/ return new String[] {"ParameterDefinition"}; 2384 case 629147193: /*dataRequirement*/ return new String[] {"DataRequirement"}; 2385 case 951530617: /*content*/ return new String[] {"Attachment"}; 2386 default: return super.getTypesForProperty(hash, name); 2387 } 2388 2389 } 2390 2391 @Override 2392 public Base addChild(String name) throws FHIRException { 2393 if (name.equals("url")) { 2394 throw new FHIRException("Cannot call addChild on a singleton property Library.url"); 2395 } 2396 else if (name.equals("identifier")) { 2397 return addIdentifier(); 2398 } 2399 else if (name.equals("version")) { 2400 throw new FHIRException("Cannot call addChild on a singleton property Library.version"); 2401 } 2402 else if (name.equals("versionAlgorithmString")) { 2403 this.versionAlgorithm = new StringType(); 2404 return this.versionAlgorithm; 2405 } 2406 else if (name.equals("versionAlgorithmCoding")) { 2407 this.versionAlgorithm = new Coding(); 2408 return this.versionAlgorithm; 2409 } 2410 else if (name.equals("name")) { 2411 throw new FHIRException("Cannot call addChild on a singleton property Library.name"); 2412 } 2413 else if (name.equals("title")) { 2414 throw new FHIRException("Cannot call addChild on a singleton property Library.title"); 2415 } 2416 else if (name.equals("subtitle")) { 2417 throw new FHIRException("Cannot call addChild on a singleton property Library.subtitle"); 2418 } 2419 else if (name.equals("status")) { 2420 throw new FHIRException("Cannot call addChild on a singleton property Library.status"); 2421 } 2422 else if (name.equals("experimental")) { 2423 throw new FHIRException("Cannot call addChild on a singleton property Library.experimental"); 2424 } 2425 else if (name.equals("type")) { 2426 this.type = new CodeableConcept(); 2427 return this.type; 2428 } 2429 else if (name.equals("subjectCodeableConcept")) { 2430 this.subject = new CodeableConcept(); 2431 return this.subject; 2432 } 2433 else if (name.equals("subjectReference")) { 2434 this.subject = new Reference(); 2435 return this.subject; 2436 } 2437 else if (name.equals("date")) { 2438 throw new FHIRException("Cannot call addChild on a singleton property Library.date"); 2439 } 2440 else if (name.equals("publisher")) { 2441 throw new FHIRException("Cannot call addChild on a singleton property Library.publisher"); 2442 } 2443 else if (name.equals("contact")) { 2444 return addContact(); 2445 } 2446 else if (name.equals("description")) { 2447 throw new FHIRException("Cannot call addChild on a singleton property Library.description"); 2448 } 2449 else if (name.equals("useContext")) { 2450 return addUseContext(); 2451 } 2452 else if (name.equals("jurisdiction")) { 2453 return addJurisdiction(); 2454 } 2455 else if (name.equals("purpose")) { 2456 throw new FHIRException("Cannot call addChild on a singleton property Library.purpose"); 2457 } 2458 else if (name.equals("usage")) { 2459 throw new FHIRException("Cannot call addChild on a singleton property Library.usage"); 2460 } 2461 else if (name.equals("copyright")) { 2462 throw new FHIRException("Cannot call addChild on a singleton property Library.copyright"); 2463 } 2464 else if (name.equals("copyrightLabel")) { 2465 throw new FHIRException("Cannot call addChild on a singleton property Library.copyrightLabel"); 2466 } 2467 else if (name.equals("approvalDate")) { 2468 throw new FHIRException("Cannot call addChild on a singleton property Library.approvalDate"); 2469 } 2470 else if (name.equals("lastReviewDate")) { 2471 throw new FHIRException("Cannot call addChild on a singleton property Library.lastReviewDate"); 2472 } 2473 else if (name.equals("effectivePeriod")) { 2474 this.effectivePeriod = new Period(); 2475 return this.effectivePeriod; 2476 } 2477 else if (name.equals("topic")) { 2478 return addTopic(); 2479 } 2480 else if (name.equals("author")) { 2481 return addAuthor(); 2482 } 2483 else if (name.equals("editor")) { 2484 return addEditor(); 2485 } 2486 else if (name.equals("reviewer")) { 2487 return addReviewer(); 2488 } 2489 else if (name.equals("endorser")) { 2490 return addEndorser(); 2491 } 2492 else if (name.equals("relatedArtifact")) { 2493 return addRelatedArtifact(); 2494 } 2495 else if (name.equals("parameter")) { 2496 return addParameter(); 2497 } 2498 else if (name.equals("dataRequirement")) { 2499 return addDataRequirement(); 2500 } 2501 else if (name.equals("content")) { 2502 return addContent(); 2503 } 2504 else 2505 return super.addChild(name); 2506 } 2507 2508 public String fhirType() { 2509 return "Library"; 2510 2511 } 2512 2513 public Library copy() { 2514 Library dst = new Library(); 2515 copyValues(dst); 2516 return dst; 2517 } 2518 2519 public void copyValues(Library dst) { 2520 super.copyValues(dst); 2521 dst.url = url == null ? null : url.copy(); 2522 if (identifier != null) { 2523 dst.identifier = new ArrayList<Identifier>(); 2524 for (Identifier i : identifier) 2525 dst.identifier.add(i.copy()); 2526 }; 2527 dst.version = version == null ? null : version.copy(); 2528 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 2529 dst.name = name == null ? null : name.copy(); 2530 dst.title = title == null ? null : title.copy(); 2531 dst.subtitle = subtitle == null ? null : subtitle.copy(); 2532 dst.status = status == null ? null : status.copy(); 2533 dst.experimental = experimental == null ? null : experimental.copy(); 2534 dst.type = type == null ? null : type.copy(); 2535 dst.subject = subject == null ? null : subject.copy(); 2536 dst.date = date == null ? null : date.copy(); 2537 dst.publisher = publisher == null ? null : publisher.copy(); 2538 if (contact != null) { 2539 dst.contact = new ArrayList<ContactDetail>(); 2540 for (ContactDetail i : contact) 2541 dst.contact.add(i.copy()); 2542 }; 2543 dst.description = description == null ? null : description.copy(); 2544 if (useContext != null) { 2545 dst.useContext = new ArrayList<UsageContext>(); 2546 for (UsageContext i : useContext) 2547 dst.useContext.add(i.copy()); 2548 }; 2549 if (jurisdiction != null) { 2550 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2551 for (CodeableConcept i : jurisdiction) 2552 dst.jurisdiction.add(i.copy()); 2553 }; 2554 dst.purpose = purpose == null ? null : purpose.copy(); 2555 dst.usage = usage == null ? null : usage.copy(); 2556 dst.copyright = copyright == null ? null : copyright.copy(); 2557 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 2558 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 2559 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 2560 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 2561 if (topic != null) { 2562 dst.topic = new ArrayList<CodeableConcept>(); 2563 for (CodeableConcept i : topic) 2564 dst.topic.add(i.copy()); 2565 }; 2566 if (author != null) { 2567 dst.author = new ArrayList<ContactDetail>(); 2568 for (ContactDetail i : author) 2569 dst.author.add(i.copy()); 2570 }; 2571 if (editor != null) { 2572 dst.editor = new ArrayList<ContactDetail>(); 2573 for (ContactDetail i : editor) 2574 dst.editor.add(i.copy()); 2575 }; 2576 if (reviewer != null) { 2577 dst.reviewer = new ArrayList<ContactDetail>(); 2578 for (ContactDetail i : reviewer) 2579 dst.reviewer.add(i.copy()); 2580 }; 2581 if (endorser != null) { 2582 dst.endorser = new ArrayList<ContactDetail>(); 2583 for (ContactDetail i : endorser) 2584 dst.endorser.add(i.copy()); 2585 }; 2586 if (relatedArtifact != null) { 2587 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 2588 for (RelatedArtifact i : relatedArtifact) 2589 dst.relatedArtifact.add(i.copy()); 2590 }; 2591 if (parameter != null) { 2592 dst.parameter = new ArrayList<ParameterDefinition>(); 2593 for (ParameterDefinition i : parameter) 2594 dst.parameter.add(i.copy()); 2595 }; 2596 if (dataRequirement != null) { 2597 dst.dataRequirement = new ArrayList<DataRequirement>(); 2598 for (DataRequirement i : dataRequirement) 2599 dst.dataRequirement.add(i.copy()); 2600 }; 2601 if (content != null) { 2602 dst.content = new ArrayList<Attachment>(); 2603 for (Attachment i : content) 2604 dst.content.add(i.copy()); 2605 }; 2606 } 2607 2608 protected Library typedCopy() { 2609 return copy(); 2610 } 2611 2612 @Override 2613 public boolean equalsDeep(Base other_) { 2614 if (!super.equalsDeep(other_)) 2615 return false; 2616 if (!(other_ instanceof Library)) 2617 return false; 2618 Library o = (Library) other_; 2619 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 2620 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 2621 && compareDeep(subtitle, o.subtitle, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 2622 && compareDeep(type, o.type, true) && compareDeep(subject, o.subject, true) && compareDeep(date, o.date, true) 2623 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 2624 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 2625 && compareDeep(purpose, o.purpose, true) && compareDeep(usage, o.usage, true) && compareDeep(copyright, o.copyright, true) 2626 && compareDeep(copyrightLabel, o.copyrightLabel, true) && compareDeep(approvalDate, o.approvalDate, true) 2627 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 2628 && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) && compareDeep(editor, o.editor, true) 2629 && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 2630 && compareDeep(parameter, o.parameter, true) && compareDeep(dataRequirement, o.dataRequirement, true) 2631 && compareDeep(content, o.content, true); 2632 } 2633 2634 @Override 2635 public boolean equalsShallow(Base other_) { 2636 if (!super.equalsShallow(other_)) 2637 return false; 2638 if (!(other_ instanceof Library)) 2639 return false; 2640 Library o = (Library) other_; 2641 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 2642 && compareValues(title, o.title, true) && compareValues(subtitle, o.subtitle, true) && compareValues(status, o.status, true) 2643 && compareValues(experimental, o.experimental, true) && compareValues(date, o.date, true) && compareValues(publisher, o.publisher, true) 2644 && compareValues(description, o.description, true) && compareValues(purpose, o.purpose, true) && compareValues(usage, o.usage, true) 2645 && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 2646 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 2647 ; 2648 } 2649 2650 public boolean isEmpty() { 2651 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 2652 , versionAlgorithm, name, title, subtitle, status, experimental, type, subject 2653 , date, publisher, contact, description, useContext, jurisdiction, purpose, usage 2654 , copyright, copyrightLabel, approvalDate, lastReviewDate, effectivePeriod, topic 2655 , author, editor, reviewer, endorser, relatedArtifact, parameter, dataRequirement 2656 , content); 2657 } 2658 2659 @Override 2660 public ResourceType getResourceType() { 2661 return ResourceType.Library; 2662 } 2663 2664 /** 2665 * Search parameter: <b>context-quantity</b> 2666 * <p> 2667 * Description: <b>Multiple Resources: 2668 2669* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2670* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2671* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2672* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2673* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2674* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2675* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2676* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2677* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2678* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2679* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2680* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2681* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2682* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 2683* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 2684* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 2685* [Library](library.html): A quantity- or range-valued use context assigned to the library 2686* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 2687* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 2688* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 2689* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 2690* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 2691* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 2692* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 2693* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 2694* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 2695* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 2696* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 2697* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 2698* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 2699</b><br> 2700 * Type: <b>quantity</b><br> 2701 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 2702 * </p> 2703 */ 2704 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 2705 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 2706 /** 2707 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 2708 * <p> 2709 * Description: <b>Multiple Resources: 2710 2711* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 2712* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 2713* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 2714* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 2715* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 2716* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 2717* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 2718* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 2719* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 2720* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 2721* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 2722* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 2723* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 2724* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 2725* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 2726* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 2727* [Library](library.html): A quantity- or range-valued use context assigned to the library 2728* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 2729* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 2730* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 2731* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 2732* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 2733* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 2734* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 2735* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 2736* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 2737* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 2738* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 2739* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 2740* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 2741</b><br> 2742 * Type: <b>quantity</b><br> 2743 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 2744 * </p> 2745 */ 2746 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 2747 2748 /** 2749 * Search parameter: <b>context-type-quantity</b> 2750 * <p> 2751 * Description: <b>Multiple Resources: 2752 2753* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 2754* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 2755* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 2756* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 2757* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 2758* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 2759* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 2760* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 2761* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 2762* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 2763* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 2764* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 2765* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 2766* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 2767* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 2768* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 2769* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 2770* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 2771* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 2772* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 2773* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 2774* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 2775* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 2776* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 2777* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 2778* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 2779* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 2780* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 2781* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 2782* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 2783</b><br> 2784 * Type: <b>composite</b><br> 2785 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2786 * </p> 2787 */ 2788 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 2789 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 2790 /** 2791 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 2792 * <p> 2793 * Description: <b>Multiple Resources: 2794 2795* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 2796* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 2797* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 2798* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 2799* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 2800* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 2801* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 2802* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 2803* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 2804* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 2805* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 2806* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 2807* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 2808* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 2809* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 2810* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 2811* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 2812* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 2813* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 2814* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 2815* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 2816* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 2817* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 2818* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 2819* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 2820* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 2821* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 2822* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 2823* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 2824* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 2825</b><br> 2826 * Type: <b>composite</b><br> 2827 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2828 * </p> 2829 */ 2830 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 2831 2832 /** 2833 * Search parameter: <b>context-type-value</b> 2834 * <p> 2835 * Description: <b>Multiple Resources: 2836 2837* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 2838* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 2839* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 2840* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 2841* [Citation](citation.html): A use context type and value assigned to the citation 2842* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 2843* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 2844* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 2845* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 2846* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 2847* [Evidence](evidence.html): A use context type and value assigned to the evidence 2848* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 2849* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 2850* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 2851* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 2852* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 2853* [Library](library.html): A use context type and value assigned to the library 2854* [Measure](measure.html): A use context type and value assigned to the measure 2855* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 2856* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 2857* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 2858* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 2859* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 2860* [Requirements](requirements.html): A use context type and value assigned to the requirements 2861* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 2862* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 2863* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 2864* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 2865* [TestScript](testscript.html): A use context type and value assigned to the test script 2866* [ValueSet](valueset.html): A use context type and value assigned to the value set 2867</b><br> 2868 * Type: <b>composite</b><br> 2869 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2870 * </p> 2871 */ 2872 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 2873 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 2874 /** 2875 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 2876 * <p> 2877 * Description: <b>Multiple Resources: 2878 2879* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 2880* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 2881* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 2882* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 2883* [Citation](citation.html): A use context type and value assigned to the citation 2884* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 2885* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 2886* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 2887* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 2888* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 2889* [Evidence](evidence.html): A use context type and value assigned to the evidence 2890* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 2891* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 2892* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 2893* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 2894* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 2895* [Library](library.html): A use context type and value assigned to the library 2896* [Measure](measure.html): A use context type and value assigned to the measure 2897* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 2898* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 2899* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 2900* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 2901* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 2902* [Requirements](requirements.html): A use context type and value assigned to the requirements 2903* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 2904* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 2905* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 2906* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 2907* [TestScript](testscript.html): A use context type and value assigned to the test script 2908* [ValueSet](valueset.html): A use context type and value assigned to the value set 2909</b><br> 2910 * Type: <b>composite</b><br> 2911 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 2912 * </p> 2913 */ 2914 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 2915 2916 /** 2917 * Search parameter: <b>context-type</b> 2918 * <p> 2919 * Description: <b>Multiple Resources: 2920 2921* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 2922* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 2923* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 2924* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 2925* [Citation](citation.html): A type of use context assigned to the citation 2926* [CodeSystem](codesystem.html): A type of use context assigned to the code system 2927* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 2928* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 2929* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 2930* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 2931* [Evidence](evidence.html): A type of use context assigned to the evidence 2932* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 2933* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 2934* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 2935* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 2936* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 2937* [Library](library.html): A type of use context assigned to the library 2938* [Measure](measure.html): A type of use context assigned to the measure 2939* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 2940* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 2941* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 2942* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 2943* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 2944* [Requirements](requirements.html): A type of use context assigned to the requirements 2945* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 2946* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 2947* [StructureMap](structuremap.html): A type of use context assigned to the structure map 2948* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 2949* [TestScript](testscript.html): A type of use context assigned to the test script 2950* [ValueSet](valueset.html): A type of use context assigned to the value set 2951</b><br> 2952 * Type: <b>token</b><br> 2953 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 2954 * </p> 2955 */ 2956 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 2957 public static final String SP_CONTEXT_TYPE = "context-type"; 2958 /** 2959 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 2960 * <p> 2961 * Description: <b>Multiple Resources: 2962 2963* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 2964* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 2965* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 2966* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 2967* [Citation](citation.html): A type of use context assigned to the citation 2968* [CodeSystem](codesystem.html): A type of use context assigned to the code system 2969* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 2970* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 2971* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 2972* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 2973* [Evidence](evidence.html): A type of use context assigned to the evidence 2974* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 2975* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 2976* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 2977* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 2978* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 2979* [Library](library.html): A type of use context assigned to the library 2980* [Measure](measure.html): A type of use context assigned to the measure 2981* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 2982* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 2983* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 2984* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 2985* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 2986* [Requirements](requirements.html): A type of use context assigned to the requirements 2987* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 2988* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 2989* [StructureMap](structuremap.html): A type of use context assigned to the structure map 2990* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 2991* [TestScript](testscript.html): A type of use context assigned to the test script 2992* [ValueSet](valueset.html): A type of use context assigned to the value set 2993</b><br> 2994 * Type: <b>token</b><br> 2995 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 2996 * </p> 2997 */ 2998 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 2999 3000 /** 3001 * Search parameter: <b>context</b> 3002 * <p> 3003 * Description: <b>Multiple Resources: 3004 3005* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3006* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3007* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3008* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3009* [Citation](citation.html): A use context assigned to the citation 3010* [CodeSystem](codesystem.html): A use context assigned to the code system 3011* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3012* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3013* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3014* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3015* [Evidence](evidence.html): A use context assigned to the evidence 3016* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3017* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3018* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3019* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3020* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3021* [Library](library.html): A use context assigned to the library 3022* [Measure](measure.html): A use context assigned to the measure 3023* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3024* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3025* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3026* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3027* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3028* [Requirements](requirements.html): A use context assigned to the requirements 3029* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3030* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3031* [StructureMap](structuremap.html): A use context assigned to the structure map 3032* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3033* [TestScript](testscript.html): A use context assigned to the test script 3034* [ValueSet](valueset.html): A use context assigned to the value set 3035</b><br> 3036 * Type: <b>token</b><br> 3037 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3038 * </p> 3039 */ 3040 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 3041 public static final String SP_CONTEXT = "context"; 3042 /** 3043 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3044 * <p> 3045 * Description: <b>Multiple Resources: 3046 3047* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 3048* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 3049* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 3050* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 3051* [Citation](citation.html): A use context assigned to the citation 3052* [CodeSystem](codesystem.html): A use context assigned to the code system 3053* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 3054* [ConceptMap](conceptmap.html): A use context assigned to the concept map 3055* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 3056* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 3057* [Evidence](evidence.html): A use context assigned to the evidence 3058* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 3059* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 3060* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 3061* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 3062* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 3063* [Library](library.html): A use context assigned to the library 3064* [Measure](measure.html): A use context assigned to the measure 3065* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 3066* [NamingSystem](namingsystem.html): A use context assigned to the naming system 3067* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 3068* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 3069* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 3070* [Requirements](requirements.html): A use context assigned to the requirements 3071* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 3072* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 3073* [StructureMap](structuremap.html): A use context assigned to the structure map 3074* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 3075* [TestScript](testscript.html): A use context assigned to the test script 3076* [ValueSet](valueset.html): A use context assigned to the value set 3077</b><br> 3078 * Type: <b>token</b><br> 3079 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 3080 * </p> 3081 */ 3082 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 3083 3084 /** 3085 * Search parameter: <b>date</b> 3086 * <p> 3087 * Description: <b>Multiple Resources: 3088 3089* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3090* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3091* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3092* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3093* [Citation](citation.html): The citation publication date 3094* [CodeSystem](codesystem.html): The code system publication date 3095* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3096* [ConceptMap](conceptmap.html): The concept map publication date 3097* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3098* [EventDefinition](eventdefinition.html): The event definition publication date 3099* [Evidence](evidence.html): The evidence publication date 3100* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3101* [ExampleScenario](examplescenario.html): The example scenario publication date 3102* [GraphDefinition](graphdefinition.html): The graph definition publication date 3103* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3104* [Library](library.html): The library publication date 3105* [Measure](measure.html): The measure publication date 3106* [MessageDefinition](messagedefinition.html): The message definition publication date 3107* [NamingSystem](namingsystem.html): The naming system publication date 3108* [OperationDefinition](operationdefinition.html): The operation definition publication date 3109* [PlanDefinition](plandefinition.html): The plan definition publication date 3110* [Questionnaire](questionnaire.html): The questionnaire publication date 3111* [Requirements](requirements.html): The requirements publication date 3112* [SearchParameter](searchparameter.html): The search parameter publication date 3113* [StructureDefinition](structuredefinition.html): The structure definition publication date 3114* [StructureMap](structuremap.html): The structure map publication date 3115* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3116* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3117* [TestScript](testscript.html): The test script publication date 3118* [ValueSet](valueset.html): The value set publication date 3119</b><br> 3120 * Type: <b>date</b><br> 3121 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3122 * </p> 3123 */ 3124 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 3125 public static final String SP_DATE = "date"; 3126 /** 3127 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3128 * <p> 3129 * Description: <b>Multiple Resources: 3130 3131* [ActivityDefinition](activitydefinition.html): The activity definition publication date 3132* [ActorDefinition](actordefinition.html): The Actor Definition publication date 3133* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 3134* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 3135* [Citation](citation.html): The citation publication date 3136* [CodeSystem](codesystem.html): The code system publication date 3137* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 3138* [ConceptMap](conceptmap.html): The concept map publication date 3139* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 3140* [EventDefinition](eventdefinition.html): The event definition publication date 3141* [Evidence](evidence.html): The evidence publication date 3142* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 3143* [ExampleScenario](examplescenario.html): The example scenario publication date 3144* [GraphDefinition](graphdefinition.html): The graph definition publication date 3145* [ImplementationGuide](implementationguide.html): The implementation guide publication date 3146* [Library](library.html): The library publication date 3147* [Measure](measure.html): The measure publication date 3148* [MessageDefinition](messagedefinition.html): The message definition publication date 3149* [NamingSystem](namingsystem.html): The naming system publication date 3150* [OperationDefinition](operationdefinition.html): The operation definition publication date 3151* [PlanDefinition](plandefinition.html): The plan definition publication date 3152* [Questionnaire](questionnaire.html): The questionnaire publication date 3153* [Requirements](requirements.html): The requirements publication date 3154* [SearchParameter](searchparameter.html): The search parameter publication date 3155* [StructureDefinition](structuredefinition.html): The structure definition publication date 3156* [StructureMap](structuremap.html): The structure map publication date 3157* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 3158* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 3159* [TestScript](testscript.html): The test script publication date 3160* [ValueSet](valueset.html): The value set publication date 3161</b><br> 3162 * Type: <b>date</b><br> 3163 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 3164 * </p> 3165 */ 3166 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3167 3168 /** 3169 * Search parameter: <b>description</b> 3170 * <p> 3171 * Description: <b>Multiple Resources: 3172 3173* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3174* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3175* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3176* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3177* [Citation](citation.html): The description of the citation 3178* [CodeSystem](codesystem.html): The description of the code system 3179* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3180* [ConceptMap](conceptmap.html): The description of the concept map 3181* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3182* [EventDefinition](eventdefinition.html): The description of the event definition 3183* [Evidence](evidence.html): The description of the evidence 3184* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3185* [GraphDefinition](graphdefinition.html): The description of the graph definition 3186* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3187* [Library](library.html): The description of the library 3188* [Measure](measure.html): The description of the measure 3189* [MessageDefinition](messagedefinition.html): The description of the message definition 3190* [NamingSystem](namingsystem.html): The description of the naming system 3191* [OperationDefinition](operationdefinition.html): The description of the operation definition 3192* [PlanDefinition](plandefinition.html): The description of the plan definition 3193* [Questionnaire](questionnaire.html): The description of the questionnaire 3194* [Requirements](requirements.html): The description of the requirements 3195* [SearchParameter](searchparameter.html): The description of the search parameter 3196* [StructureDefinition](structuredefinition.html): The description of the structure definition 3197* [StructureMap](structuremap.html): The description of the structure map 3198* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3199* [TestScript](testscript.html): The description of the test script 3200* [ValueSet](valueset.html): The description of the value set 3201</b><br> 3202 * Type: <b>string</b><br> 3203 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3204 * </p> 3205 */ 3206 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 3207 public static final String SP_DESCRIPTION = "description"; 3208 /** 3209 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3210 * <p> 3211 * Description: <b>Multiple Resources: 3212 3213* [ActivityDefinition](activitydefinition.html): The description of the activity definition 3214* [ActorDefinition](actordefinition.html): The description of the Actor Definition 3215* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 3216* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 3217* [Citation](citation.html): The description of the citation 3218* [CodeSystem](codesystem.html): The description of the code system 3219* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 3220* [ConceptMap](conceptmap.html): The description of the concept map 3221* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 3222* [EventDefinition](eventdefinition.html): The description of the event definition 3223* [Evidence](evidence.html): The description of the evidence 3224* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 3225* [GraphDefinition](graphdefinition.html): The description of the graph definition 3226* [ImplementationGuide](implementationguide.html): The description of the implementation guide 3227* [Library](library.html): The description of the library 3228* [Measure](measure.html): The description of the measure 3229* [MessageDefinition](messagedefinition.html): The description of the message definition 3230* [NamingSystem](namingsystem.html): The description of the naming system 3231* [OperationDefinition](operationdefinition.html): The description of the operation definition 3232* [PlanDefinition](plandefinition.html): The description of the plan definition 3233* [Questionnaire](questionnaire.html): The description of the questionnaire 3234* [Requirements](requirements.html): The description of the requirements 3235* [SearchParameter](searchparameter.html): The description of the search parameter 3236* [StructureDefinition](structuredefinition.html): The description of the structure definition 3237* [StructureMap](structuremap.html): The description of the structure map 3238* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 3239* [TestScript](testscript.html): The description of the test script 3240* [ValueSet](valueset.html): The description of the value set 3241</b><br> 3242 * Type: <b>string</b><br> 3243 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 3244 * </p> 3245 */ 3246 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3247 3248 /** 3249 * Search parameter: <b>identifier</b> 3250 * <p> 3251 * Description: <b>Multiple Resources: 3252 3253* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3254* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3255* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3256* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3257* [Citation](citation.html): External identifier for the citation 3258* [CodeSystem](codesystem.html): External identifier for the code system 3259* [ConceptMap](conceptmap.html): External identifier for the concept map 3260* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3261* [EventDefinition](eventdefinition.html): External identifier for the event definition 3262* [Evidence](evidence.html): External identifier for the evidence 3263* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3264* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3265* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3266* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3267* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3268* [Library](library.html): External identifier for the library 3269* [Measure](measure.html): External identifier for the measure 3270* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3271* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3272* [NamingSystem](namingsystem.html): External identifier for the naming system 3273* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3274* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3275* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3276* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3277* [Requirements](requirements.html): External identifier for the requirements 3278* [SearchParameter](searchparameter.html): External identifier for the search parameter 3279* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3280* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3281* [StructureMap](structuremap.html): External identifier for the structure map 3282* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3283* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3284* [TestPlan](testplan.html): An identifier for the test plan 3285* [TestScript](testscript.html): External identifier for the test script 3286* [ValueSet](valueset.html): External identifier for the value set 3287</b><br> 3288 * Type: <b>token</b><br> 3289 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3290 * </p> 3291 */ 3292 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 3293 public static final String SP_IDENTIFIER = "identifier"; 3294 /** 3295 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3296 * <p> 3297 * Description: <b>Multiple Resources: 3298 3299* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 3300* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 3301* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 3302* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 3303* [Citation](citation.html): External identifier for the citation 3304* [CodeSystem](codesystem.html): External identifier for the code system 3305* [ConceptMap](conceptmap.html): External identifier for the concept map 3306* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 3307* [EventDefinition](eventdefinition.html): External identifier for the event definition 3308* [Evidence](evidence.html): External identifier for the evidence 3309* [EvidenceReport](evidencereport.html): External identifier for the evidence report 3310* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 3311* [ExampleScenario](examplescenario.html): External identifier for the example scenario 3312* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 3313* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 3314* [Library](library.html): External identifier for the library 3315* [Measure](measure.html): External identifier for the measure 3316* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 3317* [MessageDefinition](messagedefinition.html): External identifier for the message definition 3318* [NamingSystem](namingsystem.html): External identifier for the naming system 3319* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 3320* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 3321* [PlanDefinition](plandefinition.html): External identifier for the plan definition 3322* [Questionnaire](questionnaire.html): External identifier for the questionnaire 3323* [Requirements](requirements.html): External identifier for the requirements 3324* [SearchParameter](searchparameter.html): External identifier for the search parameter 3325* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 3326* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 3327* [StructureMap](structuremap.html): External identifier for the structure map 3328* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 3329* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 3330* [TestPlan](testplan.html): An identifier for the test plan 3331* [TestScript](testscript.html): External identifier for the test script 3332* [ValueSet](valueset.html): External identifier for the value set 3333</b><br> 3334 * Type: <b>token</b><br> 3335 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 3336 * </p> 3337 */ 3338 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3339 3340 /** 3341 * Search parameter: <b>jurisdiction</b> 3342 * <p> 3343 * Description: <b>Multiple Resources: 3344 3345* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3346* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3347* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3348* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3349* [Citation](citation.html): Intended jurisdiction for the citation 3350* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3351* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3352* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3353* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3354* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3355* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3356* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3357* [Library](library.html): Intended jurisdiction for the library 3358* [Measure](measure.html): Intended jurisdiction for the measure 3359* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3360* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3361* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3362* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3363* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3364* [Requirements](requirements.html): Intended jurisdiction for the requirements 3365* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3366* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3367* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3368* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3369* [TestScript](testscript.html): Intended jurisdiction for the test script 3370* [ValueSet](valueset.html): Intended jurisdiction for the value set 3371</b><br> 3372 * Type: <b>token</b><br> 3373 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3374 * </p> 3375 */ 3376 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 3377 public static final String SP_JURISDICTION = "jurisdiction"; 3378 /** 3379 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3380 * <p> 3381 * Description: <b>Multiple Resources: 3382 3383* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 3384* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 3385* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 3386* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 3387* [Citation](citation.html): Intended jurisdiction for the citation 3388* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 3389* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 3390* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 3391* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 3392* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 3393* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 3394* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 3395* [Library](library.html): Intended jurisdiction for the library 3396* [Measure](measure.html): Intended jurisdiction for the measure 3397* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 3398* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 3399* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 3400* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 3401* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 3402* [Requirements](requirements.html): Intended jurisdiction for the requirements 3403* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 3404* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 3405* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 3406* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 3407* [TestScript](testscript.html): Intended jurisdiction for the test script 3408* [ValueSet](valueset.html): Intended jurisdiction for the value set 3409</b><br> 3410 * Type: <b>token</b><br> 3411 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 3412 * </p> 3413 */ 3414 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 3415 3416 /** 3417 * Search parameter: <b>name</b> 3418 * <p> 3419 * Description: <b>Multiple Resources: 3420 3421* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 3422* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 3423* [Citation](citation.html): Computationally friendly name of the citation 3424* [CodeSystem](codesystem.html): Computationally friendly name of the code system 3425* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 3426* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 3427* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 3428* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 3429* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 3430* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 3431* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 3432* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 3433* [Library](library.html): Computationally friendly name of the library 3434* [Measure](measure.html): Computationally friendly name of the measure 3435* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 3436* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 3437* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 3438* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 3439* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 3440* [Requirements](requirements.html): Computationally friendly name of the requirements 3441* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 3442* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 3443* [StructureMap](structuremap.html): Computationally friendly name of the structure map 3444* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 3445* [TestScript](testscript.html): Computationally friendly name of the test script 3446* [ValueSet](valueset.html): Computationally friendly name of the value set 3447</b><br> 3448 * Type: <b>string</b><br> 3449 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 3450 * </p> 3451 */ 3452 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 3453 public static final String SP_NAME = "name"; 3454 /** 3455 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3456 * <p> 3457 * Description: <b>Multiple Resources: 3458 3459* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 3460* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 3461* [Citation](citation.html): Computationally friendly name of the citation 3462* [CodeSystem](codesystem.html): Computationally friendly name of the code system 3463* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 3464* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 3465* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 3466* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 3467* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 3468* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 3469* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 3470* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 3471* [Library](library.html): Computationally friendly name of the library 3472* [Measure](measure.html): Computationally friendly name of the measure 3473* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 3474* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 3475* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 3476* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 3477* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 3478* [Requirements](requirements.html): Computationally friendly name of the requirements 3479* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 3480* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 3481* [StructureMap](structuremap.html): Computationally friendly name of the structure map 3482* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 3483* [TestScript](testscript.html): Computationally friendly name of the test script 3484* [ValueSet](valueset.html): Computationally friendly name of the value set 3485</b><br> 3486 * Type: <b>string</b><br> 3487 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 3488 * </p> 3489 */ 3490 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 3491 3492 /** 3493 * Search parameter: <b>publisher</b> 3494 * <p> 3495 * Description: <b>Multiple Resources: 3496 3497* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3498* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3499* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3500* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3501* [Citation](citation.html): Name of the publisher of the citation 3502* [CodeSystem](codesystem.html): Name of the publisher of the code system 3503* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3504* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3505* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3506* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3507* [Evidence](evidence.html): Name of the publisher of the evidence 3508* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3509* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3510* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3511* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3512* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3513* [Library](library.html): Name of the publisher of the library 3514* [Measure](measure.html): Name of the publisher of the measure 3515* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3516* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3517* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3518* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3519* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3520* [Requirements](requirements.html): Name of the publisher of the requirements 3521* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3522* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3523* [StructureMap](structuremap.html): Name of the publisher of the structure map 3524* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3525* [TestScript](testscript.html): Name of the publisher of the test script 3526* [ValueSet](valueset.html): Name of the publisher of the value set 3527</b><br> 3528 * Type: <b>string</b><br> 3529 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3530 * </p> 3531 */ 3532 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 3533 public static final String SP_PUBLISHER = "publisher"; 3534 /** 3535 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3536 * <p> 3537 * Description: <b>Multiple Resources: 3538 3539* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 3540* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 3541* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 3542* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 3543* [Citation](citation.html): Name of the publisher of the citation 3544* [CodeSystem](codesystem.html): Name of the publisher of the code system 3545* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 3546* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 3547* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 3548* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 3549* [Evidence](evidence.html): Name of the publisher of the evidence 3550* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 3551* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 3552* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 3553* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 3554* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 3555* [Library](library.html): Name of the publisher of the library 3556* [Measure](measure.html): Name of the publisher of the measure 3557* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 3558* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 3559* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 3560* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 3561* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 3562* [Requirements](requirements.html): Name of the publisher of the requirements 3563* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 3564* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 3565* [StructureMap](structuremap.html): Name of the publisher of the structure map 3566* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 3567* [TestScript](testscript.html): Name of the publisher of the test script 3568* [ValueSet](valueset.html): Name of the publisher of the value set 3569</b><br> 3570 * Type: <b>string</b><br> 3571 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 3572 * </p> 3573 */ 3574 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 3575 3576 /** 3577 * Search parameter: <b>status</b> 3578 * <p> 3579 * Description: <b>Multiple Resources: 3580 3581* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3582* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3583* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3584* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3585* [Citation](citation.html): The current status of the citation 3586* [CodeSystem](codesystem.html): The current status of the code system 3587* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3588* [ConceptMap](conceptmap.html): The current status of the concept map 3589* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3590* [EventDefinition](eventdefinition.html): The current status of the event definition 3591* [Evidence](evidence.html): The current status of the evidence 3592* [EvidenceReport](evidencereport.html): The current status of the evidence report 3593* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3594* [ExampleScenario](examplescenario.html): The current status of the example scenario 3595* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3596* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3597* [Library](library.html): The current status of the library 3598* [Measure](measure.html): The current status of the measure 3599* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3600* [MessageDefinition](messagedefinition.html): The current status of the message definition 3601* [NamingSystem](namingsystem.html): The current status of the naming system 3602* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3603* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3604* [PlanDefinition](plandefinition.html): The current status of the plan definition 3605* [Questionnaire](questionnaire.html): The current status of the questionnaire 3606* [Requirements](requirements.html): The current status of the requirements 3607* [SearchParameter](searchparameter.html): The current status of the search parameter 3608* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3609* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3610* [StructureMap](structuremap.html): The current status of the structure map 3611* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3612* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3613* [TestPlan](testplan.html): The current status of the test plan 3614* [TestScript](testscript.html): The current status of the test script 3615* [ValueSet](valueset.html): The current status of the value set 3616</b><br> 3617 * Type: <b>token</b><br> 3618 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3619 * </p> 3620 */ 3621 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 3622 public static final String SP_STATUS = "status"; 3623 /** 3624 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3625 * <p> 3626 * Description: <b>Multiple Resources: 3627 3628* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 3629* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 3630* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 3631* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 3632* [Citation](citation.html): The current status of the citation 3633* [CodeSystem](codesystem.html): The current status of the code system 3634* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 3635* [ConceptMap](conceptmap.html): The current status of the concept map 3636* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 3637* [EventDefinition](eventdefinition.html): The current status of the event definition 3638* [Evidence](evidence.html): The current status of the evidence 3639* [EvidenceReport](evidencereport.html): The current status of the evidence report 3640* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 3641* [ExampleScenario](examplescenario.html): The current status of the example scenario 3642* [GraphDefinition](graphdefinition.html): The current status of the graph definition 3643* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 3644* [Library](library.html): The current status of the library 3645* [Measure](measure.html): The current status of the measure 3646* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 3647* [MessageDefinition](messagedefinition.html): The current status of the message definition 3648* [NamingSystem](namingsystem.html): The current status of the naming system 3649* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 3650* [OperationDefinition](operationdefinition.html): The current status of the operation definition 3651* [PlanDefinition](plandefinition.html): The current status of the plan definition 3652* [Questionnaire](questionnaire.html): The current status of the questionnaire 3653* [Requirements](requirements.html): The current status of the requirements 3654* [SearchParameter](searchparameter.html): The current status of the search parameter 3655* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 3656* [StructureDefinition](structuredefinition.html): The current status of the structure definition 3657* [StructureMap](structuremap.html): The current status of the structure map 3658* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 3659* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 3660* [TestPlan](testplan.html): The current status of the test plan 3661* [TestScript](testscript.html): The current status of the test script 3662* [ValueSet](valueset.html): The current status of the value set 3663</b><br> 3664 * Type: <b>token</b><br> 3665 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 3666 * </p> 3667 */ 3668 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3669 3670 /** 3671 * Search parameter: <b>title</b> 3672 * <p> 3673 * Description: <b>Multiple Resources: 3674 3675* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 3676* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 3677* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 3678* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 3679* [Citation](citation.html): The human-friendly name of the citation 3680* [CodeSystem](codesystem.html): The human-friendly name of the code system 3681* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 3682* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 3683* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 3684* [Evidence](evidence.html): The human-friendly name of the evidence 3685* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 3686* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 3687* [Library](library.html): The human-friendly name of the library 3688* [Measure](measure.html): The human-friendly name of the measure 3689* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 3690* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 3691* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 3692* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 3693* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 3694* [Requirements](requirements.html): The human-friendly name of the requirements 3695* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 3696* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 3697* [StructureMap](structuremap.html): The human-friendly name of the structure map 3698* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 3699* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 3700* [TestScript](testscript.html): The human-friendly name of the test script 3701* [ValueSet](valueset.html): The human-friendly name of the value set 3702</b><br> 3703 * Type: <b>string</b><br> 3704 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 3705 * </p> 3706 */ 3707 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 3708 public static final String SP_TITLE = "title"; 3709 /** 3710 * <b>Fluent Client</b> search parameter constant for <b>title</b> 3711 * <p> 3712 * Description: <b>Multiple Resources: 3713 3714* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 3715* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 3716* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 3717* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 3718* [Citation](citation.html): The human-friendly name of the citation 3719* [CodeSystem](codesystem.html): The human-friendly name of the code system 3720* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 3721* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 3722* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 3723* [Evidence](evidence.html): The human-friendly name of the evidence 3724* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 3725* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 3726* [Library](library.html): The human-friendly name of the library 3727* [Measure](measure.html): The human-friendly name of the measure 3728* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 3729* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 3730* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 3731* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 3732* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 3733* [Requirements](requirements.html): The human-friendly name of the requirements 3734* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 3735* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 3736* [StructureMap](structuremap.html): The human-friendly name of the structure map 3737* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 3738* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 3739* [TestScript](testscript.html): The human-friendly name of the test script 3740* [ValueSet](valueset.html): The human-friendly name of the value set 3741</b><br> 3742 * Type: <b>string</b><br> 3743 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 3744 * </p> 3745 */ 3746 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 3747 3748 /** 3749 * Search parameter: <b>url</b> 3750 * <p> 3751 * Description: <b>Multiple Resources: 3752 3753* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 3754* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 3755* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 3756* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 3757* [Citation](citation.html): The uri that identifies the citation 3758* [CodeSystem](codesystem.html): The uri that identifies the code system 3759* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 3760* [ConceptMap](conceptmap.html): The URI that identifies the concept map 3761* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 3762* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 3763* [Evidence](evidence.html): The uri that identifies the evidence 3764* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 3765* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 3766* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 3767* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 3768* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 3769* [Library](library.html): The uri that identifies the library 3770* [Measure](measure.html): The uri that identifies the measure 3771* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 3772* [NamingSystem](namingsystem.html): The uri that identifies the naming system 3773* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 3774* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 3775* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 3776* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 3777* [Requirements](requirements.html): The uri that identifies the requirements 3778* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 3779* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 3780* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 3781* [StructureMap](structuremap.html): The uri that identifies the structure map 3782* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 3783* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 3784* [TestPlan](testplan.html): The uri that identifies the test plan 3785* [TestScript](testscript.html): The uri that identifies the test script 3786* [ValueSet](valueset.html): The uri that identifies the value set 3787</b><br> 3788 * Type: <b>uri</b><br> 3789 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 3790 * </p> 3791 */ 3792 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 3793 public static final String SP_URL = "url"; 3794 /** 3795 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3796 * <p> 3797 * Description: <b>Multiple Resources: 3798 3799* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 3800* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 3801* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 3802* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 3803* [Citation](citation.html): The uri that identifies the citation 3804* [CodeSystem](codesystem.html): The uri that identifies the code system 3805* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 3806* [ConceptMap](conceptmap.html): The URI that identifies the concept map 3807* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 3808* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 3809* [Evidence](evidence.html): The uri that identifies the evidence 3810* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 3811* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 3812* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 3813* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 3814* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 3815* [Library](library.html): The uri that identifies the library 3816* [Measure](measure.html): The uri that identifies the measure 3817* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 3818* [NamingSystem](namingsystem.html): The uri that identifies the naming system 3819* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 3820* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 3821* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 3822* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 3823* [Requirements](requirements.html): The uri that identifies the requirements 3824* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 3825* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 3826* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 3827* [StructureMap](structuremap.html): The uri that identifies the structure map 3828* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 3829* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 3830* [TestPlan](testplan.html): The uri that identifies the test plan 3831* [TestScript](testscript.html): The uri that identifies the test script 3832* [ValueSet](valueset.html): The uri that identifies the value set 3833</b><br> 3834 * Type: <b>uri</b><br> 3835 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 3836 * </p> 3837 */ 3838 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3839 3840 /** 3841 * Search parameter: <b>version</b> 3842 * <p> 3843 * Description: <b>Multiple Resources: 3844 3845* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 3846* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 3847* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 3848* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 3849* [Citation](citation.html): The business version of the citation 3850* [CodeSystem](codesystem.html): The business version of the code system 3851* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 3852* [ConceptMap](conceptmap.html): The business version of the concept map 3853* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 3854* [EventDefinition](eventdefinition.html): The business version of the event definition 3855* [Evidence](evidence.html): The business version of the evidence 3856* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 3857* [ExampleScenario](examplescenario.html): The business version of the example scenario 3858* [GraphDefinition](graphdefinition.html): The business version of the graph definition 3859* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 3860* [Library](library.html): The business version of the library 3861* [Measure](measure.html): The business version of the measure 3862* [MessageDefinition](messagedefinition.html): The business version of the message definition 3863* [NamingSystem](namingsystem.html): The business version of the naming system 3864* [OperationDefinition](operationdefinition.html): The business version of the operation definition 3865* [PlanDefinition](plandefinition.html): The business version of the plan definition 3866* [Questionnaire](questionnaire.html): The business version of the questionnaire 3867* [Requirements](requirements.html): The business version of the requirements 3868* [SearchParameter](searchparameter.html): The business version of the search parameter 3869* [StructureDefinition](structuredefinition.html): The business version of the structure definition 3870* [StructureMap](structuremap.html): The business version of the structure map 3871* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 3872* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 3873* [TestScript](testscript.html): The business version of the test script 3874* [ValueSet](valueset.html): The business version of the value set 3875</b><br> 3876 * Type: <b>token</b><br> 3877 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 3878 * </p> 3879 */ 3880 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 3881 public static final String SP_VERSION = "version"; 3882 /** 3883 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3884 * <p> 3885 * Description: <b>Multiple Resources: 3886 3887* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 3888* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 3889* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 3890* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 3891* [Citation](citation.html): The business version of the citation 3892* [CodeSystem](codesystem.html): The business version of the code system 3893* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 3894* [ConceptMap](conceptmap.html): The business version of the concept map 3895* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 3896* [EventDefinition](eventdefinition.html): The business version of the event definition 3897* [Evidence](evidence.html): The business version of the evidence 3898* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 3899* [ExampleScenario](examplescenario.html): The business version of the example scenario 3900* [GraphDefinition](graphdefinition.html): The business version of the graph definition 3901* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 3902* [Library](library.html): The business version of the library 3903* [Measure](measure.html): The business version of the measure 3904* [MessageDefinition](messagedefinition.html): The business version of the message definition 3905* [NamingSystem](namingsystem.html): The business version of the naming system 3906* [OperationDefinition](operationdefinition.html): The business version of the operation definition 3907* [PlanDefinition](plandefinition.html): The business version of the plan definition 3908* [Questionnaire](questionnaire.html): The business version of the questionnaire 3909* [Requirements](requirements.html): The business version of the requirements 3910* [SearchParameter](searchparameter.html): The business version of the search parameter 3911* [StructureDefinition](structuredefinition.html): The business version of the structure definition 3912* [StructureMap](structuremap.html): The business version of the structure map 3913* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 3914* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 3915* [TestScript](testscript.html): The business version of the test script 3916* [ValueSet](valueset.html): The business version of the value set 3917</b><br> 3918 * Type: <b>token</b><br> 3919 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 3920 * </p> 3921 */ 3922 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 3923 3924 /** 3925 * Search parameter: <b>content-type</b> 3926 * <p> 3927 * Description: <b>The type of content in the library (e.g. text/cql)</b><br> 3928 * Type: <b>token</b><br> 3929 * Path: <b>Library.content.contentType</b><br> 3930 * </p> 3931 */ 3932 @SearchParamDefinition(name="content-type", path="Library.content.contentType", description="The type of content in the library (e.g. text/cql)", type="token" ) 3933 public static final String SP_CONTENT_TYPE = "content-type"; 3934 /** 3935 * <b>Fluent Client</b> search parameter constant for <b>content-type</b> 3936 * <p> 3937 * Description: <b>The type of content in the library (e.g. text/cql)</b><br> 3938 * Type: <b>token</b><br> 3939 * Path: <b>Library.content.contentType</b><br> 3940 * </p> 3941 */ 3942 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTENT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTENT_TYPE); 3943 3944 /** 3945 * Search parameter: <b>type</b> 3946 * <p> 3947 * Description: <b>The type of the library (e.g. logic-library, model-definition, asset-collection, module-definition)</b><br> 3948 * Type: <b>token</b><br> 3949 * Path: <b>Library.type</b><br> 3950 * </p> 3951 */ 3952 @SearchParamDefinition(name="type", path="Library.type", description="The type of the library (e.g. logic-library, model-definition, asset-collection, module-definition)", type="token" ) 3953 public static final String SP_TYPE = "type"; 3954 /** 3955 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3956 * <p> 3957 * Description: <b>The type of the library (e.g. logic-library, model-definition, asset-collection, module-definition)</b><br> 3958 * Type: <b>token</b><br> 3959 * Path: <b>Library.type</b><br> 3960 * </p> 3961 */ 3962 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 3963 3964 /** 3965 * Search parameter: <b>composed-of</b> 3966 * <p> 3967 * Description: <b>Multiple Resources: 3968 3969* [ActivityDefinition](activitydefinition.html): What resource is being referenced 3970* [EventDefinition](eventdefinition.html): What resource is being referenced 3971* [EvidenceVariable](evidencevariable.html): What resource is being referenced 3972* [Library](library.html): What resource is being referenced 3973* [Measure](measure.html): What resource is being referenced 3974* [PlanDefinition](plandefinition.html): What resource is being referenced 3975</b><br> 3976 * Type: <b>reference</b><br> 3977 * Path: <b>ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource</b><br> 3978 * </p> 3979 */ 3980 @SearchParamDefinition(name="composed-of", path="ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 3981 public static final String SP_COMPOSED_OF = "composed-of"; 3982 /** 3983 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 3984 * <p> 3985 * Description: <b>Multiple Resources: 3986 3987* [ActivityDefinition](activitydefinition.html): What resource is being referenced 3988* [EventDefinition](eventdefinition.html): What resource is being referenced 3989* [EvidenceVariable](evidencevariable.html): What resource is being referenced 3990* [Library](library.html): What resource is being referenced 3991* [Measure](measure.html): What resource is being referenced 3992* [PlanDefinition](plandefinition.html): What resource is being referenced 3993</b><br> 3994 * Type: <b>reference</b><br> 3995 * Path: <b>ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource</b><br> 3996 * </p> 3997 */ 3998 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPOSED_OF); 3999 4000/** 4001 * Constant for fluent queries to be used to add include statements. Specifies 4002 * the path value of "<b>Library:composed-of</b>". 4003 */ 4004 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include("Library:composed-of").toLocked(); 4005 4006 /** 4007 * Search parameter: <b>depends-on</b> 4008 * <p> 4009 * Description: <b>Multiple Resources: 4010 4011* [ActivityDefinition](activitydefinition.html): What resource is being referenced 4012* [EventDefinition](eventdefinition.html): What resource is being referenced 4013* [EvidenceVariable](evidencevariable.html): What resource is being referenced 4014* [Library](library.html): What resource is being referenced 4015* [Measure](measure.html): What resource is being referenced 4016* [PlanDefinition](plandefinition.html): What resource is being referenced 4017</b><br> 4018 * Type: <b>reference</b><br> 4019 * Path: <b>ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library</b><br> 4020 * </p> 4021 */ 4022 @SearchParamDefinition(name="depends-on", path="ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 4023 public static final String SP_DEPENDS_ON = "depends-on"; 4024 /** 4025 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 4026 * <p> 4027 * Description: <b>Multiple Resources: 4028 4029* [ActivityDefinition](activitydefinition.html): What resource is being referenced 4030* [EventDefinition](eventdefinition.html): What resource is being referenced 4031* [EvidenceVariable](evidencevariable.html): What resource is being referenced 4032* [Library](library.html): What resource is being referenced 4033* [Measure](measure.html): What resource is being referenced 4034* [PlanDefinition](plandefinition.html): What resource is being referenced 4035</b><br> 4036 * Type: <b>reference</b><br> 4037 * Path: <b>ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library</b><br> 4038 * </p> 4039 */ 4040 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEPENDS_ON); 4041 4042/** 4043 * Constant for fluent queries to be used to add include statements. Specifies 4044 * the path value of "<b>Library:depends-on</b>". 4045 */ 4046 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include("Library:depends-on").toLocked(); 4047 4048 /** 4049 * Search parameter: <b>derived-from</b> 4050 * <p> 4051 * Description: <b>Multiple Resources: 4052 4053* [ActivityDefinition](activitydefinition.html): What resource is being referenced 4054* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 4055* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 4056* [EventDefinition](eventdefinition.html): What resource is being referenced 4057* [EvidenceVariable](evidencevariable.html): What resource is being referenced 4058* [Library](library.html): What resource is being referenced 4059* [Measure](measure.html): What resource is being referenced 4060* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 4061* [PlanDefinition](plandefinition.html): What resource is being referenced 4062* [ValueSet](valueset.html): A resource that the ValueSet is derived from 4063</b><br> 4064 * Type: <b>reference</b><br> 4065 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 4066 * </p> 4067 */ 4068 @SearchParamDefinition(name="derived-from", path="ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from\r\n* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): A resource that the ValueSet is derived from\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 4069 public static final String SP_DERIVED_FROM = "derived-from"; 4070 /** 4071 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 4072 * <p> 4073 * Description: <b>Multiple Resources: 4074 4075* [ActivityDefinition](activitydefinition.html): What resource is being referenced 4076* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 4077* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 4078* [EventDefinition](eventdefinition.html): What resource is being referenced 4079* [EvidenceVariable](evidencevariable.html): What resource is being referenced 4080* [Library](library.html): What resource is being referenced 4081* [Measure](measure.html): What resource is being referenced 4082* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 4083* [PlanDefinition](plandefinition.html): What resource is being referenced 4084* [ValueSet](valueset.html): A resource that the ValueSet is derived from 4085</b><br> 4086 * Type: <b>reference</b><br> 4087 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 4088 * </p> 4089 */ 4090 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 4091 4092/** 4093 * Constant for fluent queries to be used to add include statements. Specifies 4094 * the path value of "<b>Library:derived-from</b>". 4095 */ 4096 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("Library:derived-from").toLocked(); 4097 4098 /** 4099 * Search parameter: <b>effective</b> 4100 * <p> 4101 * Description: <b>Multiple Resources: 4102 4103* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 4104* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 4105* [Citation](citation.html): The time during which the citation is intended to be in use 4106* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 4107* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 4108* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 4109* [Library](library.html): The time during which the library is intended to be in use 4110* [Measure](measure.html): The time during which the measure is intended to be in use 4111* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 4112* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 4113* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 4114* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 4115</b><br> 4116 * Type: <b>date</b><br> 4117 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 4118 * </p> 4119 */ 4120 @SearchParamDefinition(name="effective", path="ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use\r\n* [Citation](citation.html): The time during which the citation is intended to be in use\r\n* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use\r\n* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use\r\n* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use\r\n* [Library](library.html): The time during which the library is intended to be in use\r\n* [Measure](measure.html): The time during which the measure is intended to be in use\r\n* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use\r\n* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use\r\n* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use\r\n* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use\r\n", type="date" ) 4121 public static final String SP_EFFECTIVE = "effective"; 4122 /** 4123 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 4124 * <p> 4125 * Description: <b>Multiple Resources: 4126 4127* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 4128* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 4129* [Citation](citation.html): The time during which the citation is intended to be in use 4130* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 4131* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 4132* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 4133* [Library](library.html): The time during which the library is intended to be in use 4134* [Measure](measure.html): The time during which the measure is intended to be in use 4135* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 4136* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 4137* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 4138* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 4139</b><br> 4140 * Type: <b>date</b><br> 4141 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 4142 * </p> 4143 */ 4144 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 4145 4146 /** 4147 * Search parameter: <b>predecessor</b> 4148 * <p> 4149 * Description: <b>Multiple Resources: 4150 4151* [ActivityDefinition](activitydefinition.html): What resource is being referenced 4152* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 4153* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 4154* [EventDefinition](eventdefinition.html): What resource is being referenced 4155* [EvidenceVariable](evidencevariable.html): What resource is being referenced 4156* [Library](library.html): What resource is being referenced 4157* [Measure](measure.html): What resource is being referenced 4158* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 4159* [PlanDefinition](plandefinition.html): What resource is being referenced 4160* [ValueSet](valueset.html): The predecessor of the ValueSet 4161</b><br> 4162 * Type: <b>reference</b><br> 4163 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 4164 * </p> 4165 */ 4166 @SearchParamDefinition(name="predecessor", path="ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): The predecessor of the CodeSystem\r\n* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): The predecessor of the ValueSet\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 4167 public static final String SP_PREDECESSOR = "predecessor"; 4168 /** 4169 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 4170 * <p> 4171 * Description: <b>Multiple Resources: 4172 4173* [ActivityDefinition](activitydefinition.html): What resource is being referenced 4174* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 4175* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 4176* [EventDefinition](eventdefinition.html): What resource is being referenced 4177* [EvidenceVariable](evidencevariable.html): What resource is being referenced 4178* [Library](library.html): What resource is being referenced 4179* [Measure](measure.html): What resource is being referenced 4180* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 4181* [PlanDefinition](plandefinition.html): What resource is being referenced 4182* [ValueSet](valueset.html): The predecessor of the ValueSet 4183</b><br> 4184 * Type: <b>reference</b><br> 4185 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 4186 * </p> 4187 */ 4188 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 4189 4190/** 4191 * Constant for fluent queries to be used to add include statements. Specifies 4192 * the path value of "<b>Library:predecessor</b>". 4193 */ 4194 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("Library:predecessor").toLocked(); 4195 4196 /** 4197 * Search parameter: <b>successor</b> 4198 * <p> 4199 * Description: <b>Multiple Resources: 4200 4201* [ActivityDefinition](activitydefinition.html): What resource is being referenced 4202* [EventDefinition](eventdefinition.html): What resource is being referenced 4203* [EvidenceVariable](evidencevariable.html): What resource is being referenced 4204* [Library](library.html): What resource is being referenced 4205* [Measure](measure.html): What resource is being referenced 4206* [PlanDefinition](plandefinition.html): What resource is being referenced 4207</b><br> 4208 * Type: <b>reference</b><br> 4209 * Path: <b>ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource</b><br> 4210 * </p> 4211 */ 4212 @SearchParamDefinition(name="successor", path="ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 4213 public static final String SP_SUCCESSOR = "successor"; 4214 /** 4215 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 4216 * <p> 4217 * Description: <b>Multiple Resources: 4218 4219* [ActivityDefinition](activitydefinition.html): What resource is being referenced 4220* [EventDefinition](eventdefinition.html): What resource is being referenced 4221* [EvidenceVariable](evidencevariable.html): What resource is being referenced 4222* [Library](library.html): What resource is being referenced 4223* [Measure](measure.html): What resource is being referenced 4224* [PlanDefinition](plandefinition.html): What resource is being referenced 4225</b><br> 4226 * Type: <b>reference</b><br> 4227 * Path: <b>ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource</b><br> 4228 * </p> 4229 */ 4230 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUCCESSOR); 4231 4232/** 4233 * Constant for fluent queries to be used to add include statements. Specifies 4234 * the path value of "<b>Library:successor</b>". 4235 */ 4236 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include("Library:successor").toLocked(); 4237 4238 /** 4239 * Search parameter: <b>topic</b> 4240 * <p> 4241 * Description: <b>Multiple Resources: 4242 4243* [ActivityDefinition](activitydefinition.html): Topics associated with the module 4244* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 4245* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 4246* [EventDefinition](eventdefinition.html): Topics associated with the module 4247* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 4248* [Library](library.html): Topics associated with the module 4249* [Measure](measure.html): Topics associated with the measure 4250* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 4251* [PlanDefinition](plandefinition.html): Topics associated with the module 4252* [ValueSet](valueset.html): Topics associated with the ValueSet 4253</b><br> 4254 * Type: <b>token</b><br> 4255 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 4256 * </p> 4257 */ 4258 @SearchParamDefinition(name="topic", path="ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Topics associated with the module\r\n* [CodeSystem](codesystem.html): Topics associated with the CodeSystem\r\n* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap\r\n* [EventDefinition](eventdefinition.html): Topics associated with the module\r\n* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable\r\n* [Library](library.html): Topics associated with the module\r\n* [Measure](measure.html): Topics associated with the measure\r\n* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem\r\n* [PlanDefinition](plandefinition.html): Topics associated with the module\r\n* [ValueSet](valueset.html): Topics associated with the ValueSet\r\n", type="token" ) 4259 public static final String SP_TOPIC = "topic"; 4260 /** 4261 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 4262 * <p> 4263 * Description: <b>Multiple Resources: 4264 4265* [ActivityDefinition](activitydefinition.html): Topics associated with the module 4266* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 4267* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 4268* [EventDefinition](eventdefinition.html): Topics associated with the module 4269* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 4270* [Library](library.html): Topics associated with the module 4271* [Measure](measure.html): Topics associated with the measure 4272* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 4273* [PlanDefinition](plandefinition.html): Topics associated with the module 4274* [ValueSet](valueset.html): Topics associated with the ValueSet 4275</b><br> 4276 * Type: <b>token</b><br> 4277 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 4278 * </p> 4279 */ 4280 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 4281 4282 4283} 4284