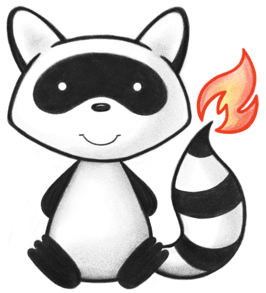
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Identifies two or more records (resource instances) that refer to the same real-world "occurrence". 052 */ 053@ResourceDef(name="Linkage", profile="http://hl7.org/fhir/StructureDefinition/Linkage") 054public class Linkage extends DomainResource { 055 056 public enum LinkageType { 057 /** 058 * The resource represents the \"source of truth\" (from the perspective of this Linkage resource) for the underlying event/condition/etc. 059 */ 060 SOURCE, 061 /** 062 * The resource represents an alternative view of the underlying event/condition/etc. The resource may still be actively maintained, even though it is not considered to be the source of truth. 063 */ 064 ALTERNATE, 065 /** 066 * The resource represents an obsolete record of the underlying event/condition/etc. It is not expected to be actively maintained. 067 */ 068 HISTORICAL, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static LinkageType fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("source".equals(codeString)) 077 return SOURCE; 078 if ("alternate".equals(codeString)) 079 return ALTERNATE; 080 if ("historical".equals(codeString)) 081 return HISTORICAL; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown LinkageType code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case SOURCE: return "source"; 090 case ALTERNATE: return "alternate"; 091 case HISTORICAL: return "historical"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case SOURCE: return "http://hl7.org/fhir/linkage-type"; 099 case ALTERNATE: return "http://hl7.org/fhir/linkage-type"; 100 case HISTORICAL: return "http://hl7.org/fhir/linkage-type"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case SOURCE: return "The resource represents the \"source of truth\" (from the perspective of this Linkage resource) for the underlying event/condition/etc."; 108 case ALTERNATE: return "The resource represents an alternative view of the underlying event/condition/etc. The resource may still be actively maintained, even though it is not considered to be the source of truth."; 109 case HISTORICAL: return "The resource represents an obsolete record of the underlying event/condition/etc. It is not expected to be actively maintained."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case SOURCE: return "Source of Truth"; 117 case ALTERNATE: return "Alternate Record"; 118 case HISTORICAL: return "Historical/Obsolete Record"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class LinkageTypeEnumFactory implements EnumFactory<LinkageType> { 126 public LinkageType fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("source".equals(codeString)) 131 return LinkageType.SOURCE; 132 if ("alternate".equals(codeString)) 133 return LinkageType.ALTERNATE; 134 if ("historical".equals(codeString)) 135 return LinkageType.HISTORICAL; 136 throw new IllegalArgumentException("Unknown LinkageType code '"+codeString+"'"); 137 } 138 public Enumeration<LinkageType> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<LinkageType>(this, LinkageType.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<LinkageType>(this, LinkageType.NULL, code); 146 if ("source".equals(codeString)) 147 return new Enumeration<LinkageType>(this, LinkageType.SOURCE, code); 148 if ("alternate".equals(codeString)) 149 return new Enumeration<LinkageType>(this, LinkageType.ALTERNATE, code); 150 if ("historical".equals(codeString)) 151 return new Enumeration<LinkageType>(this, LinkageType.HISTORICAL, code); 152 throw new FHIRException("Unknown LinkageType code '"+codeString+"'"); 153 } 154 public String toCode(LinkageType code) { 155 if (code == LinkageType.NULL) 156 return null; 157 if (code == LinkageType.SOURCE) 158 return "source"; 159 if (code == LinkageType.ALTERNATE) 160 return "alternate"; 161 if (code == LinkageType.HISTORICAL) 162 return "historical"; 163 return "?"; 164 } 165 public String toSystem(LinkageType code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class LinkageItemComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * Distinguishes which item is "source of truth" (if any) and which items are no longer considered to be current representations. 174 */ 175 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 176 @Description(shortDefinition="source | alternate | historical", formalDefinition="Distinguishes which item is \"source of truth\" (if any) and which items are no longer considered to be current representations." ) 177 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/linkage-type") 178 protected Enumeration<LinkageType> type; 179 180 /** 181 * The resource instance being linked as part of the group. 182 */ 183 @Child(name = "resource", type = {Reference.class}, order=2, min=1, max=1, modifier=false, summary=true) 184 @Description(shortDefinition="Resource being linked", formalDefinition="The resource instance being linked as part of the group." ) 185 protected Reference resource; 186 187 private static final long serialVersionUID = 527428511L; 188 189 /** 190 * Constructor 191 */ 192 public LinkageItemComponent() { 193 super(); 194 } 195 196 /** 197 * Constructor 198 */ 199 public LinkageItemComponent(LinkageType type, Reference resource) { 200 super(); 201 this.setType(type); 202 this.setResource(resource); 203 } 204 205 /** 206 * @return {@link #type} (Distinguishes which item is "source of truth" (if any) and which items are no longer considered to be current representations.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 207 */ 208 public Enumeration<LinkageType> getTypeElement() { 209 if (this.type == null) 210 if (Configuration.errorOnAutoCreate()) 211 throw new Error("Attempt to auto-create LinkageItemComponent.type"); 212 else if (Configuration.doAutoCreate()) 213 this.type = new Enumeration<LinkageType>(new LinkageTypeEnumFactory()); // bb 214 return this.type; 215 } 216 217 public boolean hasTypeElement() { 218 return this.type != null && !this.type.isEmpty(); 219 } 220 221 public boolean hasType() { 222 return this.type != null && !this.type.isEmpty(); 223 } 224 225 /** 226 * @param value {@link #type} (Distinguishes which item is "source of truth" (if any) and which items are no longer considered to be current representations.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 227 */ 228 public LinkageItemComponent setTypeElement(Enumeration<LinkageType> value) { 229 this.type = value; 230 return this; 231 } 232 233 /** 234 * @return Distinguishes which item is "source of truth" (if any) and which items are no longer considered to be current representations. 235 */ 236 public LinkageType getType() { 237 return this.type == null ? null : this.type.getValue(); 238 } 239 240 /** 241 * @param value Distinguishes which item is "source of truth" (if any) and which items are no longer considered to be current representations. 242 */ 243 public LinkageItemComponent setType(LinkageType value) { 244 if (this.type == null) 245 this.type = new Enumeration<LinkageType>(new LinkageTypeEnumFactory()); 246 this.type.setValue(value); 247 return this; 248 } 249 250 /** 251 * @return {@link #resource} (The resource instance being linked as part of the group.) 252 */ 253 public Reference getResource() { 254 if (this.resource == null) 255 if (Configuration.errorOnAutoCreate()) 256 throw new Error("Attempt to auto-create LinkageItemComponent.resource"); 257 else if (Configuration.doAutoCreate()) 258 this.resource = new Reference(); // cc 259 return this.resource; 260 } 261 262 public boolean hasResource() { 263 return this.resource != null && !this.resource.isEmpty(); 264 } 265 266 /** 267 * @param value {@link #resource} (The resource instance being linked as part of the group.) 268 */ 269 public LinkageItemComponent setResource(Reference value) { 270 this.resource = value; 271 return this; 272 } 273 274 protected void listChildren(List<Property> children) { 275 super.listChildren(children); 276 children.add(new Property("type", "code", "Distinguishes which item is \"source of truth\" (if any) and which items are no longer considered to be current representations.", 0, 1, type)); 277 children.add(new Property("resource", "Reference(Any)", "The resource instance being linked as part of the group.", 0, 1, resource)); 278 } 279 280 @Override 281 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 282 switch (_hash) { 283 case 3575610: /*type*/ return new Property("type", "code", "Distinguishes which item is \"source of truth\" (if any) and which items are no longer considered to be current representations.", 0, 1, type); 284 case -341064690: /*resource*/ return new Property("resource", "Reference(Any)", "The resource instance being linked as part of the group.", 0, 1, resource); 285 default: return super.getNamedProperty(_hash, _name, _checkValid); 286 } 287 288 } 289 290 @Override 291 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 292 switch (hash) { 293 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<LinkageType> 294 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Reference 295 default: return super.getProperty(hash, name, checkValid); 296 } 297 298 } 299 300 @Override 301 public Base setProperty(int hash, String name, Base value) throws FHIRException { 302 switch (hash) { 303 case 3575610: // type 304 value = new LinkageTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 305 this.type = (Enumeration) value; // Enumeration<LinkageType> 306 return value; 307 case -341064690: // resource 308 this.resource = TypeConvertor.castToReference(value); // Reference 309 return value; 310 default: return super.setProperty(hash, name, value); 311 } 312 313 } 314 315 @Override 316 public Base setProperty(String name, Base value) throws FHIRException { 317 if (name.equals("type")) { 318 value = new LinkageTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 319 this.type = (Enumeration) value; // Enumeration<LinkageType> 320 } else if (name.equals("resource")) { 321 this.resource = TypeConvertor.castToReference(value); // Reference 322 } else 323 return super.setProperty(name, value); 324 return value; 325 } 326 327 @Override 328 public void removeChild(String name, Base value) throws FHIRException { 329 if (name.equals("type")) { 330 value = new LinkageTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 331 this.type = (Enumeration) value; // Enumeration<LinkageType> 332 } else if (name.equals("resource")) { 333 this.resource = null; 334 } else 335 super.removeChild(name, value); 336 337 } 338 339 @Override 340 public Base makeProperty(int hash, String name) throws FHIRException { 341 switch (hash) { 342 case 3575610: return getTypeElement(); 343 case -341064690: return getResource(); 344 default: return super.makeProperty(hash, name); 345 } 346 347 } 348 349 @Override 350 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 351 switch (hash) { 352 case 3575610: /*type*/ return new String[] {"code"}; 353 case -341064690: /*resource*/ return new String[] {"Reference"}; 354 default: return super.getTypesForProperty(hash, name); 355 } 356 357 } 358 359 @Override 360 public Base addChild(String name) throws FHIRException { 361 if (name.equals("type")) { 362 throw new FHIRException("Cannot call addChild on a singleton property Linkage.item.type"); 363 } 364 else if (name.equals("resource")) { 365 this.resource = new Reference(); 366 return this.resource; 367 } 368 else 369 return super.addChild(name); 370 } 371 372 public LinkageItemComponent copy() { 373 LinkageItemComponent dst = new LinkageItemComponent(); 374 copyValues(dst); 375 return dst; 376 } 377 378 public void copyValues(LinkageItemComponent dst) { 379 super.copyValues(dst); 380 dst.type = type == null ? null : type.copy(); 381 dst.resource = resource == null ? null : resource.copy(); 382 } 383 384 @Override 385 public boolean equalsDeep(Base other_) { 386 if (!super.equalsDeep(other_)) 387 return false; 388 if (!(other_ instanceof LinkageItemComponent)) 389 return false; 390 LinkageItemComponent o = (LinkageItemComponent) other_; 391 return compareDeep(type, o.type, true) && compareDeep(resource, o.resource, true); 392 } 393 394 @Override 395 public boolean equalsShallow(Base other_) { 396 if (!super.equalsShallow(other_)) 397 return false; 398 if (!(other_ instanceof LinkageItemComponent)) 399 return false; 400 LinkageItemComponent o = (LinkageItemComponent) other_; 401 return compareValues(type, o.type, true); 402 } 403 404 public boolean isEmpty() { 405 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, resource); 406 } 407 408 public String fhirType() { 409 return "Linkage.item"; 410 411 } 412 413 } 414 415 /** 416 * Indicates whether the asserted set of linkages are considered to be "in effect". 417 */ 418 @Child(name = "active", type = {BooleanType.class}, order=0, min=0, max=1, modifier=false, summary=true) 419 @Description(shortDefinition="Whether this linkage assertion is active or not", formalDefinition="Indicates whether the asserted set of linkages are considered to be \"in effect\"." ) 420 protected BooleanType active; 421 422 /** 423 * Identifies the user or organization responsible for asserting the linkages as well as the user or organization who establishes the context in which the nature of each linkage is evaluated. 424 */ 425 @Child(name = "author", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=1, min=0, max=1, modifier=false, summary=true) 426 @Description(shortDefinition="Who is responsible for linkages", formalDefinition="Identifies the user or organization responsible for asserting the linkages as well as the user or organization who establishes the context in which the nature of each linkage is evaluated." ) 427 protected Reference author; 428 429 /** 430 * Identifies which record considered as the reference to the same real-world occurrence as well as how the items should be evaluated within the collection of linked items. 431 */ 432 @Child(name = "item", type = {}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 433 @Description(shortDefinition="Item to be linked", formalDefinition="Identifies which record considered as the reference to the same real-world occurrence as well as how the items should be evaluated within the collection of linked items." ) 434 protected List<LinkageItemComponent> item; 435 436 private static final long serialVersionUID = 810520886L; 437 438 /** 439 * Constructor 440 */ 441 public Linkage() { 442 super(); 443 } 444 445 /** 446 * Constructor 447 */ 448 public Linkage(LinkageItemComponent item) { 449 super(); 450 this.addItem(item); 451 } 452 453 /** 454 * @return {@link #active} (Indicates whether the asserted set of linkages are considered to be "in effect".). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 455 */ 456 public BooleanType getActiveElement() { 457 if (this.active == null) 458 if (Configuration.errorOnAutoCreate()) 459 throw new Error("Attempt to auto-create Linkage.active"); 460 else if (Configuration.doAutoCreate()) 461 this.active = new BooleanType(); // bb 462 return this.active; 463 } 464 465 public boolean hasActiveElement() { 466 return this.active != null && !this.active.isEmpty(); 467 } 468 469 public boolean hasActive() { 470 return this.active != null && !this.active.isEmpty(); 471 } 472 473 /** 474 * @param value {@link #active} (Indicates whether the asserted set of linkages are considered to be "in effect".). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 475 */ 476 public Linkage setActiveElement(BooleanType value) { 477 this.active = value; 478 return this; 479 } 480 481 /** 482 * @return Indicates whether the asserted set of linkages are considered to be "in effect". 483 */ 484 public boolean getActive() { 485 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 486 } 487 488 /** 489 * @param value Indicates whether the asserted set of linkages are considered to be "in effect". 490 */ 491 public Linkage setActive(boolean value) { 492 if (this.active == null) 493 this.active = new BooleanType(); 494 this.active.setValue(value); 495 return this; 496 } 497 498 /** 499 * @return {@link #author} (Identifies the user or organization responsible for asserting the linkages as well as the user or organization who establishes the context in which the nature of each linkage is evaluated.) 500 */ 501 public Reference getAuthor() { 502 if (this.author == null) 503 if (Configuration.errorOnAutoCreate()) 504 throw new Error("Attempt to auto-create Linkage.author"); 505 else if (Configuration.doAutoCreate()) 506 this.author = new Reference(); // cc 507 return this.author; 508 } 509 510 public boolean hasAuthor() { 511 return this.author != null && !this.author.isEmpty(); 512 } 513 514 /** 515 * @param value {@link #author} (Identifies the user or organization responsible for asserting the linkages as well as the user or organization who establishes the context in which the nature of each linkage is evaluated.) 516 */ 517 public Linkage setAuthor(Reference value) { 518 this.author = value; 519 return this; 520 } 521 522 /** 523 * @return {@link #item} (Identifies which record considered as the reference to the same real-world occurrence as well as how the items should be evaluated within the collection of linked items.) 524 */ 525 public List<LinkageItemComponent> getItem() { 526 if (this.item == null) 527 this.item = new ArrayList<LinkageItemComponent>(); 528 return this.item; 529 } 530 531 /** 532 * @return Returns a reference to <code>this</code> for easy method chaining 533 */ 534 public Linkage setItem(List<LinkageItemComponent> theItem) { 535 this.item = theItem; 536 return this; 537 } 538 539 public boolean hasItem() { 540 if (this.item == null) 541 return false; 542 for (LinkageItemComponent item : this.item) 543 if (!item.isEmpty()) 544 return true; 545 return false; 546 } 547 548 public LinkageItemComponent addItem() { //3 549 LinkageItemComponent t = new LinkageItemComponent(); 550 if (this.item == null) 551 this.item = new ArrayList<LinkageItemComponent>(); 552 this.item.add(t); 553 return t; 554 } 555 556 public Linkage addItem(LinkageItemComponent t) { //3 557 if (t == null) 558 return this; 559 if (this.item == null) 560 this.item = new ArrayList<LinkageItemComponent>(); 561 this.item.add(t); 562 return this; 563 } 564 565 /** 566 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 567 */ 568 public LinkageItemComponent getItemFirstRep() { 569 if (getItem().isEmpty()) { 570 addItem(); 571 } 572 return getItem().get(0); 573 } 574 575 protected void listChildren(List<Property> children) { 576 super.listChildren(children); 577 children.add(new Property("active", "boolean", "Indicates whether the asserted set of linkages are considered to be \"in effect\".", 0, 1, active)); 578 children.add(new Property("author", "Reference(Practitioner|PractitionerRole|Organization)", "Identifies the user or organization responsible for asserting the linkages as well as the user or organization who establishes the context in which the nature of each linkage is evaluated.", 0, 1, author)); 579 children.add(new Property("item", "", "Identifies which record considered as the reference to the same real-world occurrence as well as how the items should be evaluated within the collection of linked items.", 0, java.lang.Integer.MAX_VALUE, item)); 580 } 581 582 @Override 583 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 584 switch (_hash) { 585 case -1422950650: /*active*/ return new Property("active", "boolean", "Indicates whether the asserted set of linkages are considered to be \"in effect\".", 0, 1, active); 586 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|PractitionerRole|Organization)", "Identifies the user or organization responsible for asserting the linkages as well as the user or organization who establishes the context in which the nature of each linkage is evaluated.", 0, 1, author); 587 case 3242771: /*item*/ return new Property("item", "", "Identifies which record considered as the reference to the same real-world occurrence as well as how the items should be evaluated within the collection of linked items.", 0, java.lang.Integer.MAX_VALUE, item); 588 default: return super.getNamedProperty(_hash, _name, _checkValid); 589 } 590 591 } 592 593 @Override 594 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 595 switch (hash) { 596 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 597 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 598 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // LinkageItemComponent 599 default: return super.getProperty(hash, name, checkValid); 600 } 601 602 } 603 604 @Override 605 public Base setProperty(int hash, String name, Base value) throws FHIRException { 606 switch (hash) { 607 case -1422950650: // active 608 this.active = TypeConvertor.castToBoolean(value); // BooleanType 609 return value; 610 case -1406328437: // author 611 this.author = TypeConvertor.castToReference(value); // Reference 612 return value; 613 case 3242771: // item 614 this.getItem().add((LinkageItemComponent) value); // LinkageItemComponent 615 return value; 616 default: return super.setProperty(hash, name, value); 617 } 618 619 } 620 621 @Override 622 public Base setProperty(String name, Base value) throws FHIRException { 623 if (name.equals("active")) { 624 this.active = TypeConvertor.castToBoolean(value); // BooleanType 625 } else if (name.equals("author")) { 626 this.author = TypeConvertor.castToReference(value); // Reference 627 } else if (name.equals("item")) { 628 this.getItem().add((LinkageItemComponent) value); 629 } else 630 return super.setProperty(name, value); 631 return value; 632 } 633 634 @Override 635 public void removeChild(String name, Base value) throws FHIRException { 636 if (name.equals("active")) { 637 this.active = null; 638 } else if (name.equals("author")) { 639 this.author = null; 640 } else if (name.equals("item")) { 641 this.getItem().remove((LinkageItemComponent) value); 642 } else 643 super.removeChild(name, value); 644 645 } 646 647 @Override 648 public Base makeProperty(int hash, String name) throws FHIRException { 649 switch (hash) { 650 case -1422950650: return getActiveElement(); 651 case -1406328437: return getAuthor(); 652 case 3242771: return addItem(); 653 default: return super.makeProperty(hash, name); 654 } 655 656 } 657 658 @Override 659 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 660 switch (hash) { 661 case -1422950650: /*active*/ return new String[] {"boolean"}; 662 case -1406328437: /*author*/ return new String[] {"Reference"}; 663 case 3242771: /*item*/ return new String[] {}; 664 default: return super.getTypesForProperty(hash, name); 665 } 666 667 } 668 669 @Override 670 public Base addChild(String name) throws FHIRException { 671 if (name.equals("active")) { 672 throw new FHIRException("Cannot call addChild on a singleton property Linkage.active"); 673 } 674 else if (name.equals("author")) { 675 this.author = new Reference(); 676 return this.author; 677 } 678 else if (name.equals("item")) { 679 return addItem(); 680 } 681 else 682 return super.addChild(name); 683 } 684 685 public String fhirType() { 686 return "Linkage"; 687 688 } 689 690 public Linkage copy() { 691 Linkage dst = new Linkage(); 692 copyValues(dst); 693 return dst; 694 } 695 696 public void copyValues(Linkage dst) { 697 super.copyValues(dst); 698 dst.active = active == null ? null : active.copy(); 699 dst.author = author == null ? null : author.copy(); 700 if (item != null) { 701 dst.item = new ArrayList<LinkageItemComponent>(); 702 for (LinkageItemComponent i : item) 703 dst.item.add(i.copy()); 704 }; 705 } 706 707 protected Linkage typedCopy() { 708 return copy(); 709 } 710 711 @Override 712 public boolean equalsDeep(Base other_) { 713 if (!super.equalsDeep(other_)) 714 return false; 715 if (!(other_ instanceof Linkage)) 716 return false; 717 Linkage o = (Linkage) other_; 718 return compareDeep(active, o.active, true) && compareDeep(author, o.author, true) && compareDeep(item, o.item, true) 719 ; 720 } 721 722 @Override 723 public boolean equalsShallow(Base other_) { 724 if (!super.equalsShallow(other_)) 725 return false; 726 if (!(other_ instanceof Linkage)) 727 return false; 728 Linkage o = (Linkage) other_; 729 return compareValues(active, o.active, true); 730 } 731 732 public boolean isEmpty() { 733 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(active, author, item); 734 } 735 736 @Override 737 public ResourceType getResourceType() { 738 return ResourceType.Linkage; 739 } 740 741 /** 742 * Search parameter: <b>author</b> 743 * <p> 744 * Description: <b>Author of the Linkage</b><br> 745 * Type: <b>reference</b><br> 746 * Path: <b>Linkage.author</b><br> 747 * </p> 748 */ 749 @SearchParamDefinition(name="author", path="Linkage.author", description="Author of the Linkage", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 750 public static final String SP_AUTHOR = "author"; 751 /** 752 * <b>Fluent Client</b> search parameter constant for <b>author</b> 753 * <p> 754 * Description: <b>Author of the Linkage</b><br> 755 * Type: <b>reference</b><br> 756 * Path: <b>Linkage.author</b><br> 757 * </p> 758 */ 759 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 760 761/** 762 * Constant for fluent queries to be used to add include statements. Specifies 763 * the path value of "<b>Linkage:author</b>". 764 */ 765 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("Linkage:author").toLocked(); 766 767 /** 768 * Search parameter: <b>item</b> 769 * <p> 770 * Description: <b>Matches on any item in the Linkage</b><br> 771 * Type: <b>reference</b><br> 772 * Path: <b>Linkage.item.resource</b><br> 773 * </p> 774 */ 775 @SearchParamDefinition(name="item", path="Linkage.item.resource", description="Matches on any item in the Linkage", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 776 public static final String SP_ITEM = "item"; 777 /** 778 * <b>Fluent Client</b> search parameter constant for <b>item</b> 779 * <p> 780 * Description: <b>Matches on any item in the Linkage</b><br> 781 * Type: <b>reference</b><br> 782 * Path: <b>Linkage.item.resource</b><br> 783 * </p> 784 */ 785 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ITEM); 786 787/** 788 * Constant for fluent queries to be used to add include statements. Specifies 789 * the path value of "<b>Linkage:item</b>". 790 */ 791 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM = new ca.uhn.fhir.model.api.Include("Linkage:item").toLocked(); 792 793 /** 794 * Search parameter: <b>source</b> 795 * <p> 796 * Description: <b>Matches on any item in the Linkage with a type of 'source'</b><br> 797 * Type: <b>reference</b><br> 798 * Path: <b>Linkage.item.resource</b><br> 799 * </p> 800 */ 801 @SearchParamDefinition(name="source", path="Linkage.item.resource", description="Matches on any item in the Linkage with a type of 'source'", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 802 public static final String SP_SOURCE = "source"; 803 /** 804 * <b>Fluent Client</b> search parameter constant for <b>source</b> 805 * <p> 806 * Description: <b>Matches on any item in the Linkage with a type of 'source'</b><br> 807 * Type: <b>reference</b><br> 808 * Path: <b>Linkage.item.resource</b><br> 809 * </p> 810 */ 811 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 812 813/** 814 * Constant for fluent queries to be used to add include statements. Specifies 815 * the path value of "<b>Linkage:source</b>". 816 */ 817 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("Linkage:source").toLocked(); 818 819 820} 821