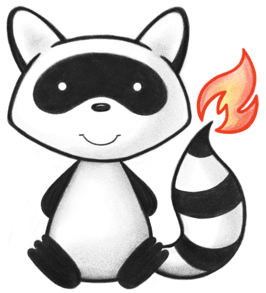
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Identifies two or more records (resource instances) that refer to the same real-world "occurrence". 052 */ 053@ResourceDef(name="Linkage", profile="http://hl7.org/fhir/StructureDefinition/Linkage") 054public class Linkage extends DomainResource { 055 056 public enum LinkageType { 057 /** 058 * The resource represents the \"source of truth\" (from the perspective of this Linkage resource) for the underlying event/condition/etc. 059 */ 060 SOURCE, 061 /** 062 * The resource represents an alternative view of the underlying event/condition/etc. The resource may still be actively maintained, even though it is not considered to be the source of truth. 063 */ 064 ALTERNATE, 065 /** 066 * The resource represents an obsolete record of the underlying event/condition/etc. It is not expected to be actively maintained. 067 */ 068 HISTORICAL, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static LinkageType fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("source".equals(codeString)) 077 return SOURCE; 078 if ("alternate".equals(codeString)) 079 return ALTERNATE; 080 if ("historical".equals(codeString)) 081 return HISTORICAL; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown LinkageType code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case SOURCE: return "source"; 090 case ALTERNATE: return "alternate"; 091 case HISTORICAL: return "historical"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case SOURCE: return "http://hl7.org/fhir/linkage-type"; 099 case ALTERNATE: return "http://hl7.org/fhir/linkage-type"; 100 case HISTORICAL: return "http://hl7.org/fhir/linkage-type"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case SOURCE: return "The resource represents the \"source of truth\" (from the perspective of this Linkage resource) for the underlying event/condition/etc."; 108 case ALTERNATE: return "The resource represents an alternative view of the underlying event/condition/etc. The resource may still be actively maintained, even though it is not considered to be the source of truth."; 109 case HISTORICAL: return "The resource represents an obsolete record of the underlying event/condition/etc. It is not expected to be actively maintained."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case SOURCE: return "Source of Truth"; 117 case ALTERNATE: return "Alternate Record"; 118 case HISTORICAL: return "Historical/Obsolete Record"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class LinkageTypeEnumFactory implements EnumFactory<LinkageType> { 126 public LinkageType fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("source".equals(codeString)) 131 return LinkageType.SOURCE; 132 if ("alternate".equals(codeString)) 133 return LinkageType.ALTERNATE; 134 if ("historical".equals(codeString)) 135 return LinkageType.HISTORICAL; 136 throw new IllegalArgumentException("Unknown LinkageType code '"+codeString+"'"); 137 } 138 public Enumeration<LinkageType> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<LinkageType>(this, LinkageType.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<LinkageType>(this, LinkageType.NULL, code); 146 if ("source".equals(codeString)) 147 return new Enumeration<LinkageType>(this, LinkageType.SOURCE, code); 148 if ("alternate".equals(codeString)) 149 return new Enumeration<LinkageType>(this, LinkageType.ALTERNATE, code); 150 if ("historical".equals(codeString)) 151 return new Enumeration<LinkageType>(this, LinkageType.HISTORICAL, code); 152 throw new FHIRException("Unknown LinkageType code '"+codeString+"'"); 153 } 154 public String toCode(LinkageType code) { 155 if (code == LinkageType.SOURCE) 156 return "source"; 157 if (code == LinkageType.ALTERNATE) 158 return "alternate"; 159 if (code == LinkageType.HISTORICAL) 160 return "historical"; 161 return "?"; 162 } 163 public String toSystem(LinkageType code) { 164 return code.getSystem(); 165 } 166 } 167 168 @Block() 169 public static class LinkageItemComponent extends BackboneElement implements IBaseBackboneElement { 170 /** 171 * Distinguishes which item is "source of truth" (if any) and which items are no longer considered to be current representations. 172 */ 173 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 174 @Description(shortDefinition="source | alternate | historical", formalDefinition="Distinguishes which item is \"source of truth\" (if any) and which items are no longer considered to be current representations." ) 175 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/linkage-type") 176 protected Enumeration<LinkageType> type; 177 178 /** 179 * The resource instance being linked as part of the group. 180 */ 181 @Child(name = "resource", type = {Reference.class}, order=2, min=1, max=1, modifier=false, summary=true) 182 @Description(shortDefinition="Resource being linked", formalDefinition="The resource instance being linked as part of the group." ) 183 protected Reference resource; 184 185 private static final long serialVersionUID = 527428511L; 186 187 /** 188 * Constructor 189 */ 190 public LinkageItemComponent() { 191 super(); 192 } 193 194 /** 195 * Constructor 196 */ 197 public LinkageItemComponent(LinkageType type, Reference resource) { 198 super(); 199 this.setType(type); 200 this.setResource(resource); 201 } 202 203 /** 204 * @return {@link #type} (Distinguishes which item is "source of truth" (if any) and which items are no longer considered to be current representations.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 205 */ 206 public Enumeration<LinkageType> getTypeElement() { 207 if (this.type == null) 208 if (Configuration.errorOnAutoCreate()) 209 throw new Error("Attempt to auto-create LinkageItemComponent.type"); 210 else if (Configuration.doAutoCreate()) 211 this.type = new Enumeration<LinkageType>(new LinkageTypeEnumFactory()); // bb 212 return this.type; 213 } 214 215 public boolean hasTypeElement() { 216 return this.type != null && !this.type.isEmpty(); 217 } 218 219 public boolean hasType() { 220 return this.type != null && !this.type.isEmpty(); 221 } 222 223 /** 224 * @param value {@link #type} (Distinguishes which item is "source of truth" (if any) and which items are no longer considered to be current representations.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 225 */ 226 public LinkageItemComponent setTypeElement(Enumeration<LinkageType> value) { 227 this.type = value; 228 return this; 229 } 230 231 /** 232 * @return Distinguishes which item is "source of truth" (if any) and which items are no longer considered to be current representations. 233 */ 234 public LinkageType getType() { 235 return this.type == null ? null : this.type.getValue(); 236 } 237 238 /** 239 * @param value Distinguishes which item is "source of truth" (if any) and which items are no longer considered to be current representations. 240 */ 241 public LinkageItemComponent setType(LinkageType value) { 242 if (this.type == null) 243 this.type = new Enumeration<LinkageType>(new LinkageTypeEnumFactory()); 244 this.type.setValue(value); 245 return this; 246 } 247 248 /** 249 * @return {@link #resource} (The resource instance being linked as part of the group.) 250 */ 251 public Reference getResource() { 252 if (this.resource == null) 253 if (Configuration.errorOnAutoCreate()) 254 throw new Error("Attempt to auto-create LinkageItemComponent.resource"); 255 else if (Configuration.doAutoCreate()) 256 this.resource = new Reference(); // cc 257 return this.resource; 258 } 259 260 public boolean hasResource() { 261 return this.resource != null && !this.resource.isEmpty(); 262 } 263 264 /** 265 * @param value {@link #resource} (The resource instance being linked as part of the group.) 266 */ 267 public LinkageItemComponent setResource(Reference value) { 268 this.resource = value; 269 return this; 270 } 271 272 protected void listChildren(List<Property> children) { 273 super.listChildren(children); 274 children.add(new Property("type", "code", "Distinguishes which item is \"source of truth\" (if any) and which items are no longer considered to be current representations.", 0, 1, type)); 275 children.add(new Property("resource", "Reference(Any)", "The resource instance being linked as part of the group.", 0, 1, resource)); 276 } 277 278 @Override 279 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 280 switch (_hash) { 281 case 3575610: /*type*/ return new Property("type", "code", "Distinguishes which item is \"source of truth\" (if any) and which items are no longer considered to be current representations.", 0, 1, type); 282 case -341064690: /*resource*/ return new Property("resource", "Reference(Any)", "The resource instance being linked as part of the group.", 0, 1, resource); 283 default: return super.getNamedProperty(_hash, _name, _checkValid); 284 } 285 286 } 287 288 @Override 289 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 290 switch (hash) { 291 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<LinkageType> 292 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Reference 293 default: return super.getProperty(hash, name, checkValid); 294 } 295 296 } 297 298 @Override 299 public Base setProperty(int hash, String name, Base value) throws FHIRException { 300 switch (hash) { 301 case 3575610: // type 302 value = new LinkageTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 303 this.type = (Enumeration) value; // Enumeration<LinkageType> 304 return value; 305 case -341064690: // resource 306 this.resource = TypeConvertor.castToReference(value); // Reference 307 return value; 308 default: return super.setProperty(hash, name, value); 309 } 310 311 } 312 313 @Override 314 public Base setProperty(String name, Base value) throws FHIRException { 315 if (name.equals("type")) { 316 value = new LinkageTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 317 this.type = (Enumeration) value; // Enumeration<LinkageType> 318 } else if (name.equals("resource")) { 319 this.resource = TypeConvertor.castToReference(value); // Reference 320 } else 321 return super.setProperty(name, value); 322 return value; 323 } 324 325 @Override 326 public Base makeProperty(int hash, String name) throws FHIRException { 327 switch (hash) { 328 case 3575610: return getTypeElement(); 329 case -341064690: return getResource(); 330 default: return super.makeProperty(hash, name); 331 } 332 333 } 334 335 @Override 336 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 337 switch (hash) { 338 case 3575610: /*type*/ return new String[] {"code"}; 339 case -341064690: /*resource*/ return new String[] {"Reference"}; 340 default: return super.getTypesForProperty(hash, name); 341 } 342 343 } 344 345 @Override 346 public Base addChild(String name) throws FHIRException { 347 if (name.equals("type")) { 348 throw new FHIRException("Cannot call addChild on a singleton property Linkage.item.type"); 349 } 350 else if (name.equals("resource")) { 351 this.resource = new Reference(); 352 return this.resource; 353 } 354 else 355 return super.addChild(name); 356 } 357 358 public LinkageItemComponent copy() { 359 LinkageItemComponent dst = new LinkageItemComponent(); 360 copyValues(dst); 361 return dst; 362 } 363 364 public void copyValues(LinkageItemComponent dst) { 365 super.copyValues(dst); 366 dst.type = type == null ? null : type.copy(); 367 dst.resource = resource == null ? null : resource.copy(); 368 } 369 370 @Override 371 public boolean equalsDeep(Base other_) { 372 if (!super.equalsDeep(other_)) 373 return false; 374 if (!(other_ instanceof LinkageItemComponent)) 375 return false; 376 LinkageItemComponent o = (LinkageItemComponent) other_; 377 return compareDeep(type, o.type, true) && compareDeep(resource, o.resource, true); 378 } 379 380 @Override 381 public boolean equalsShallow(Base other_) { 382 if (!super.equalsShallow(other_)) 383 return false; 384 if (!(other_ instanceof LinkageItemComponent)) 385 return false; 386 LinkageItemComponent o = (LinkageItemComponent) other_; 387 return compareValues(type, o.type, true); 388 } 389 390 public boolean isEmpty() { 391 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, resource); 392 } 393 394 public String fhirType() { 395 return "Linkage.item"; 396 397 } 398 399 } 400 401 /** 402 * Indicates whether the asserted set of linkages are considered to be "in effect". 403 */ 404 @Child(name = "active", type = {BooleanType.class}, order=0, min=0, max=1, modifier=false, summary=true) 405 @Description(shortDefinition="Whether this linkage assertion is active or not", formalDefinition="Indicates whether the asserted set of linkages are considered to be \"in effect\"." ) 406 protected BooleanType active; 407 408 /** 409 * Identifies the user or organization responsible for asserting the linkages as well as the user or organization who establishes the context in which the nature of each linkage is evaluated. 410 */ 411 @Child(name = "author", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=1, min=0, max=1, modifier=false, summary=true) 412 @Description(shortDefinition="Who is responsible for linkages", formalDefinition="Identifies the user or organization responsible for asserting the linkages as well as the user or organization who establishes the context in which the nature of each linkage is evaluated." ) 413 protected Reference author; 414 415 /** 416 * Identifies which record considered as the reference to the same real-world occurrence as well as how the items should be evaluated within the collection of linked items. 417 */ 418 @Child(name = "item", type = {}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 419 @Description(shortDefinition="Item to be linked", formalDefinition="Identifies which record considered as the reference to the same real-world occurrence as well as how the items should be evaluated within the collection of linked items." ) 420 protected List<LinkageItemComponent> item; 421 422 private static final long serialVersionUID = 810520886L; 423 424 /** 425 * Constructor 426 */ 427 public Linkage() { 428 super(); 429 } 430 431 /** 432 * Constructor 433 */ 434 public Linkage(LinkageItemComponent item) { 435 super(); 436 this.addItem(item); 437 } 438 439 /** 440 * @return {@link #active} (Indicates whether the asserted set of linkages are considered to be "in effect".). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 441 */ 442 public BooleanType getActiveElement() { 443 if (this.active == null) 444 if (Configuration.errorOnAutoCreate()) 445 throw new Error("Attempt to auto-create Linkage.active"); 446 else if (Configuration.doAutoCreate()) 447 this.active = new BooleanType(); // bb 448 return this.active; 449 } 450 451 public boolean hasActiveElement() { 452 return this.active != null && !this.active.isEmpty(); 453 } 454 455 public boolean hasActive() { 456 return this.active != null && !this.active.isEmpty(); 457 } 458 459 /** 460 * @param value {@link #active} (Indicates whether the asserted set of linkages are considered to be "in effect".). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 461 */ 462 public Linkage setActiveElement(BooleanType value) { 463 this.active = value; 464 return this; 465 } 466 467 /** 468 * @return Indicates whether the asserted set of linkages are considered to be "in effect". 469 */ 470 public boolean getActive() { 471 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 472 } 473 474 /** 475 * @param value Indicates whether the asserted set of linkages are considered to be "in effect". 476 */ 477 public Linkage setActive(boolean value) { 478 if (this.active == null) 479 this.active = new BooleanType(); 480 this.active.setValue(value); 481 return this; 482 } 483 484 /** 485 * @return {@link #author} (Identifies the user or organization responsible for asserting the linkages as well as the user or organization who establishes the context in which the nature of each linkage is evaluated.) 486 */ 487 public Reference getAuthor() { 488 if (this.author == null) 489 if (Configuration.errorOnAutoCreate()) 490 throw new Error("Attempt to auto-create Linkage.author"); 491 else if (Configuration.doAutoCreate()) 492 this.author = new Reference(); // cc 493 return this.author; 494 } 495 496 public boolean hasAuthor() { 497 return this.author != null && !this.author.isEmpty(); 498 } 499 500 /** 501 * @param value {@link #author} (Identifies the user or organization responsible for asserting the linkages as well as the user or organization who establishes the context in which the nature of each linkage is evaluated.) 502 */ 503 public Linkage setAuthor(Reference value) { 504 this.author = value; 505 return this; 506 } 507 508 /** 509 * @return {@link #item} (Identifies which record considered as the reference to the same real-world occurrence as well as how the items should be evaluated within the collection of linked items.) 510 */ 511 public List<LinkageItemComponent> getItem() { 512 if (this.item == null) 513 this.item = new ArrayList<LinkageItemComponent>(); 514 return this.item; 515 } 516 517 /** 518 * @return Returns a reference to <code>this</code> for easy method chaining 519 */ 520 public Linkage setItem(List<LinkageItemComponent> theItem) { 521 this.item = theItem; 522 return this; 523 } 524 525 public boolean hasItem() { 526 if (this.item == null) 527 return false; 528 for (LinkageItemComponent item : this.item) 529 if (!item.isEmpty()) 530 return true; 531 return false; 532 } 533 534 public LinkageItemComponent addItem() { //3 535 LinkageItemComponent t = new LinkageItemComponent(); 536 if (this.item == null) 537 this.item = new ArrayList<LinkageItemComponent>(); 538 this.item.add(t); 539 return t; 540 } 541 542 public Linkage addItem(LinkageItemComponent t) { //3 543 if (t == null) 544 return this; 545 if (this.item == null) 546 this.item = new ArrayList<LinkageItemComponent>(); 547 this.item.add(t); 548 return this; 549 } 550 551 /** 552 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist {3} 553 */ 554 public LinkageItemComponent getItemFirstRep() { 555 if (getItem().isEmpty()) { 556 addItem(); 557 } 558 return getItem().get(0); 559 } 560 561 protected void listChildren(List<Property> children) { 562 super.listChildren(children); 563 children.add(new Property("active", "boolean", "Indicates whether the asserted set of linkages are considered to be \"in effect\".", 0, 1, active)); 564 children.add(new Property("author", "Reference(Practitioner|PractitionerRole|Organization)", "Identifies the user or organization responsible for asserting the linkages as well as the user or organization who establishes the context in which the nature of each linkage is evaluated.", 0, 1, author)); 565 children.add(new Property("item", "", "Identifies which record considered as the reference to the same real-world occurrence as well as how the items should be evaluated within the collection of linked items.", 0, java.lang.Integer.MAX_VALUE, item)); 566 } 567 568 @Override 569 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 570 switch (_hash) { 571 case -1422950650: /*active*/ return new Property("active", "boolean", "Indicates whether the asserted set of linkages are considered to be \"in effect\".", 0, 1, active); 572 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|PractitionerRole|Organization)", "Identifies the user or organization responsible for asserting the linkages as well as the user or organization who establishes the context in which the nature of each linkage is evaluated.", 0, 1, author); 573 case 3242771: /*item*/ return new Property("item", "", "Identifies which record considered as the reference to the same real-world occurrence as well as how the items should be evaluated within the collection of linked items.", 0, java.lang.Integer.MAX_VALUE, item); 574 default: return super.getNamedProperty(_hash, _name, _checkValid); 575 } 576 577 } 578 579 @Override 580 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 581 switch (hash) { 582 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 583 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 584 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // LinkageItemComponent 585 default: return super.getProperty(hash, name, checkValid); 586 } 587 588 } 589 590 @Override 591 public Base setProperty(int hash, String name, Base value) throws FHIRException { 592 switch (hash) { 593 case -1422950650: // active 594 this.active = TypeConvertor.castToBoolean(value); // BooleanType 595 return value; 596 case -1406328437: // author 597 this.author = TypeConvertor.castToReference(value); // Reference 598 return value; 599 case 3242771: // item 600 this.getItem().add((LinkageItemComponent) value); // LinkageItemComponent 601 return value; 602 default: return super.setProperty(hash, name, value); 603 } 604 605 } 606 607 @Override 608 public Base setProperty(String name, Base value) throws FHIRException { 609 if (name.equals("active")) { 610 this.active = TypeConvertor.castToBoolean(value); // BooleanType 611 } else if (name.equals("author")) { 612 this.author = TypeConvertor.castToReference(value); // Reference 613 } else if (name.equals("item")) { 614 this.getItem().add((LinkageItemComponent) value); 615 } else 616 return super.setProperty(name, value); 617 return value; 618 } 619 620 @Override 621 public Base makeProperty(int hash, String name) throws FHIRException { 622 switch (hash) { 623 case -1422950650: return getActiveElement(); 624 case -1406328437: return getAuthor(); 625 case 3242771: return addItem(); 626 default: return super.makeProperty(hash, name); 627 } 628 629 } 630 631 @Override 632 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 633 switch (hash) { 634 case -1422950650: /*active*/ return new String[] {"boolean"}; 635 case -1406328437: /*author*/ return new String[] {"Reference"}; 636 case 3242771: /*item*/ return new String[] {}; 637 default: return super.getTypesForProperty(hash, name); 638 } 639 640 } 641 642 @Override 643 public Base addChild(String name) throws FHIRException { 644 if (name.equals("active")) { 645 throw new FHIRException("Cannot call addChild on a singleton property Linkage.active"); 646 } 647 else if (name.equals("author")) { 648 this.author = new Reference(); 649 return this.author; 650 } 651 else if (name.equals("item")) { 652 return addItem(); 653 } 654 else 655 return super.addChild(name); 656 } 657 658 public String fhirType() { 659 return "Linkage"; 660 661 } 662 663 public Linkage copy() { 664 Linkage dst = new Linkage(); 665 copyValues(dst); 666 return dst; 667 } 668 669 public void copyValues(Linkage dst) { 670 super.copyValues(dst); 671 dst.active = active == null ? null : active.copy(); 672 dst.author = author == null ? null : author.copy(); 673 if (item != null) { 674 dst.item = new ArrayList<LinkageItemComponent>(); 675 for (LinkageItemComponent i : item) 676 dst.item.add(i.copy()); 677 }; 678 } 679 680 protected Linkage typedCopy() { 681 return copy(); 682 } 683 684 @Override 685 public boolean equalsDeep(Base other_) { 686 if (!super.equalsDeep(other_)) 687 return false; 688 if (!(other_ instanceof Linkage)) 689 return false; 690 Linkage o = (Linkage) other_; 691 return compareDeep(active, o.active, true) && compareDeep(author, o.author, true) && compareDeep(item, o.item, true) 692 ; 693 } 694 695 @Override 696 public boolean equalsShallow(Base other_) { 697 if (!super.equalsShallow(other_)) 698 return false; 699 if (!(other_ instanceof Linkage)) 700 return false; 701 Linkage o = (Linkage) other_; 702 return compareValues(active, o.active, true); 703 } 704 705 public boolean isEmpty() { 706 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(active, author, item); 707 } 708 709 @Override 710 public ResourceType getResourceType() { 711 return ResourceType.Linkage; 712 } 713 714 /** 715 * Search parameter: <b>author</b> 716 * <p> 717 * Description: <b>Author of the Linkage</b><br> 718 * Type: <b>reference</b><br> 719 * Path: <b>Linkage.author</b><br> 720 * </p> 721 */ 722 @SearchParamDefinition(name="author", path="Linkage.author", description="Author of the Linkage", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Organization.class, Practitioner.class, PractitionerRole.class } ) 723 public static final String SP_AUTHOR = "author"; 724 /** 725 * <b>Fluent Client</b> search parameter constant for <b>author</b> 726 * <p> 727 * Description: <b>Author of the Linkage</b><br> 728 * Type: <b>reference</b><br> 729 * Path: <b>Linkage.author</b><br> 730 * </p> 731 */ 732 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 733 734/** 735 * Constant for fluent queries to be used to add include statements. Specifies 736 * the path value of "<b>Linkage:author</b>". 737 */ 738 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("Linkage:author").toLocked(); 739 740 /** 741 * Search parameter: <b>item</b> 742 * <p> 743 * Description: <b>Matches on any item in the Linkage</b><br> 744 * Type: <b>reference</b><br> 745 * Path: <b>Linkage.item.resource</b><br> 746 * </p> 747 */ 748 @SearchParamDefinition(name="item", path="Linkage.item.resource", description="Matches on any item in the Linkage", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 749 public static final String SP_ITEM = "item"; 750 /** 751 * <b>Fluent Client</b> search parameter constant for <b>item</b> 752 * <p> 753 * Description: <b>Matches on any item in the Linkage</b><br> 754 * Type: <b>reference</b><br> 755 * Path: <b>Linkage.item.resource</b><br> 756 * </p> 757 */ 758 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ITEM); 759 760/** 761 * Constant for fluent queries to be used to add include statements. Specifies 762 * the path value of "<b>Linkage:item</b>". 763 */ 764 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM = new ca.uhn.fhir.model.api.Include("Linkage:item").toLocked(); 765 766 /** 767 * Search parameter: <b>source</b> 768 * <p> 769 * Description: <b>Matches on any item in the Linkage with a type of 'source'</b><br> 770 * Type: <b>reference</b><br> 771 * Path: <b>Linkage.item.resource</b><br> 772 * </p> 773 */ 774 @SearchParamDefinition(name="source", path="Linkage.item.resource", description="Matches on any item in the Linkage with a type of 'source'", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 775 public static final String SP_SOURCE = "source"; 776 /** 777 * <b>Fluent Client</b> search parameter constant for <b>source</b> 778 * <p> 779 * Description: <b>Matches on any item in the Linkage with a type of 'source'</b><br> 780 * Type: <b>reference</b><br> 781 * Path: <b>Linkage.item.resource</b><br> 782 * </p> 783 */ 784 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 785 786/** 787 * Constant for fluent queries to be used to add include statements. Specifies 788 * the path value of "<b>Linkage:source</b>". 789 */ 790 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("Linkage:source").toLocked(); 791 792 793} 794