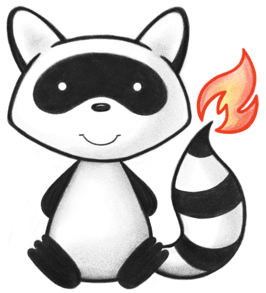
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * A List is a curated collection of resources, for things such as problem lists, allergy lists, facility list, organization list, etc. 052 */ 053@ResourceDef(name="List", profile="http://hl7.org/fhir/StructureDefinition/List") 054public class ListResource extends DomainResource { 055 056 public enum ListStatus { 057 /** 058 * The list is considered to be an active part of the patient's record. 059 */ 060 CURRENT, 061 /** 062 * The list is \"old\" and should no longer be considered accurate or relevant. 063 */ 064 RETIRED, 065 /** 066 * The list was never accurate. It is retained for medico-legal purposes only. 067 */ 068 ENTEREDINERROR, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static ListStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("current".equals(codeString)) 077 return CURRENT; 078 if ("retired".equals(codeString)) 079 return RETIRED; 080 if ("entered-in-error".equals(codeString)) 081 return ENTEREDINERROR; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown ListStatus code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case CURRENT: return "current"; 090 case RETIRED: return "retired"; 091 case ENTEREDINERROR: return "entered-in-error"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case CURRENT: return "http://hl7.org/fhir/list-status"; 099 case RETIRED: return "http://hl7.org/fhir/list-status"; 100 case ENTEREDINERROR: return "http://hl7.org/fhir/list-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case CURRENT: return "The list is considered to be an active part of the patient's record."; 108 case RETIRED: return "The list is \"old\" and should no longer be considered accurate or relevant."; 109 case ENTEREDINERROR: return "The list was never accurate. It is retained for medico-legal purposes only."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case CURRENT: return "Current"; 117 case RETIRED: return "Retired"; 118 case ENTEREDINERROR: return "Entered In Error"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class ListStatusEnumFactory implements EnumFactory<ListStatus> { 126 public ListStatus fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("current".equals(codeString)) 131 return ListStatus.CURRENT; 132 if ("retired".equals(codeString)) 133 return ListStatus.RETIRED; 134 if ("entered-in-error".equals(codeString)) 135 return ListStatus.ENTEREDINERROR; 136 throw new IllegalArgumentException("Unknown ListStatus code '"+codeString+"'"); 137 } 138 public Enumeration<ListStatus> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<ListStatus>(this, ListStatus.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<ListStatus>(this, ListStatus.NULL, code); 146 if ("current".equals(codeString)) 147 return new Enumeration<ListStatus>(this, ListStatus.CURRENT, code); 148 if ("retired".equals(codeString)) 149 return new Enumeration<ListStatus>(this, ListStatus.RETIRED, code); 150 if ("entered-in-error".equals(codeString)) 151 return new Enumeration<ListStatus>(this, ListStatus.ENTEREDINERROR, code); 152 throw new FHIRException("Unknown ListStatus code '"+codeString+"'"); 153 } 154 public String toCode(ListStatus code) { 155 if (code == ListStatus.NULL) 156 return null; 157 if (code == ListStatus.CURRENT) 158 return "current"; 159 if (code == ListStatus.RETIRED) 160 return "retired"; 161 if (code == ListStatus.ENTEREDINERROR) 162 return "entered-in-error"; 163 return "?"; 164 } 165 public String toSystem(ListStatus code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class ListResourceEntryComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * The flag allows the system constructing the list to indicate the role and significance of the item in the list. 174 */ 175 @Child(name = "flag", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 176 @Description(shortDefinition="Status/Workflow information about this item", formalDefinition="The flag allows the system constructing the list to indicate the role and significance of the item in the list." ) 177 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-item-flag") 178 protected CodeableConcept flag; 179 180 /** 181 * True if this item is marked as deleted in the list. 182 */ 183 @Child(name = "deleted", type = {BooleanType.class}, order=2, min=0, max=1, modifier=true, summary=false) 184 @Description(shortDefinition="If this item is actually marked as deleted", formalDefinition="True if this item is marked as deleted in the list." ) 185 protected BooleanType deleted; 186 187 /** 188 * When this item was added to the list. 189 */ 190 @Child(name = "date", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 191 @Description(shortDefinition="When item added to list", formalDefinition="When this item was added to the list." ) 192 protected DateTimeType date; 193 194 /** 195 * A reference to the actual resource from which data was derived. 196 */ 197 @Child(name = "item", type = {Reference.class}, order=4, min=1, max=1, modifier=false, summary=false) 198 @Description(shortDefinition="Actual entry", formalDefinition="A reference to the actual resource from which data was derived." ) 199 protected Reference item; 200 201 private static final long serialVersionUID = -872672029L; 202 203 /** 204 * Constructor 205 */ 206 public ListResourceEntryComponent() { 207 super(); 208 } 209 210 /** 211 * Constructor 212 */ 213 public ListResourceEntryComponent(Reference item) { 214 super(); 215 this.setItem(item); 216 } 217 218 /** 219 * @return {@link #flag} (The flag allows the system constructing the list to indicate the role and significance of the item in the list.) 220 */ 221 public CodeableConcept getFlag() { 222 if (this.flag == null) 223 if (Configuration.errorOnAutoCreate()) 224 throw new Error("Attempt to auto-create ListResourceEntryComponent.flag"); 225 else if (Configuration.doAutoCreate()) 226 this.flag = new CodeableConcept(); // cc 227 return this.flag; 228 } 229 230 public boolean hasFlag() { 231 return this.flag != null && !this.flag.isEmpty(); 232 } 233 234 /** 235 * @param value {@link #flag} (The flag allows the system constructing the list to indicate the role and significance of the item in the list.) 236 */ 237 public ListResourceEntryComponent setFlag(CodeableConcept value) { 238 this.flag = value; 239 return this; 240 } 241 242 /** 243 * @return {@link #deleted} (True if this item is marked as deleted in the list.). This is the underlying object with id, value and extensions. The accessor "getDeleted" gives direct access to the value 244 */ 245 public BooleanType getDeletedElement() { 246 if (this.deleted == null) 247 if (Configuration.errorOnAutoCreate()) 248 throw new Error("Attempt to auto-create ListResourceEntryComponent.deleted"); 249 else if (Configuration.doAutoCreate()) 250 this.deleted = new BooleanType(); // bb 251 return this.deleted; 252 } 253 254 public boolean hasDeletedElement() { 255 return this.deleted != null && !this.deleted.isEmpty(); 256 } 257 258 public boolean hasDeleted() { 259 return this.deleted != null && !this.deleted.isEmpty(); 260 } 261 262 /** 263 * @param value {@link #deleted} (True if this item is marked as deleted in the list.). This is the underlying object with id, value and extensions. The accessor "getDeleted" gives direct access to the value 264 */ 265 public ListResourceEntryComponent setDeletedElement(BooleanType value) { 266 this.deleted = value; 267 return this; 268 } 269 270 /** 271 * @return True if this item is marked as deleted in the list. 272 */ 273 public boolean getDeleted() { 274 return this.deleted == null || this.deleted.isEmpty() ? false : this.deleted.getValue(); 275 } 276 277 /** 278 * @param value True if this item is marked as deleted in the list. 279 */ 280 public ListResourceEntryComponent setDeleted(boolean value) { 281 if (this.deleted == null) 282 this.deleted = new BooleanType(); 283 this.deleted.setValue(value); 284 return this; 285 } 286 287 /** 288 * @return {@link #date} (When this item was added to the list.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 289 */ 290 public DateTimeType getDateElement() { 291 if (this.date == null) 292 if (Configuration.errorOnAutoCreate()) 293 throw new Error("Attempt to auto-create ListResourceEntryComponent.date"); 294 else if (Configuration.doAutoCreate()) 295 this.date = new DateTimeType(); // bb 296 return this.date; 297 } 298 299 public boolean hasDateElement() { 300 return this.date != null && !this.date.isEmpty(); 301 } 302 303 public boolean hasDate() { 304 return this.date != null && !this.date.isEmpty(); 305 } 306 307 /** 308 * @param value {@link #date} (When this item was added to the list.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 309 */ 310 public ListResourceEntryComponent setDateElement(DateTimeType value) { 311 this.date = value; 312 return this; 313 } 314 315 /** 316 * @return When this item was added to the list. 317 */ 318 public Date getDate() { 319 return this.date == null ? null : this.date.getValue(); 320 } 321 322 /** 323 * @param value When this item was added to the list. 324 */ 325 public ListResourceEntryComponent setDate(Date value) { 326 if (value == null) 327 this.date = null; 328 else { 329 if (this.date == null) 330 this.date = new DateTimeType(); 331 this.date.setValue(value); 332 } 333 return this; 334 } 335 336 /** 337 * @return {@link #item} (A reference to the actual resource from which data was derived.) 338 */ 339 public Reference getItem() { 340 if (this.item == null) 341 if (Configuration.errorOnAutoCreate()) 342 throw new Error("Attempt to auto-create ListResourceEntryComponent.item"); 343 else if (Configuration.doAutoCreate()) 344 this.item = new Reference(); // cc 345 return this.item; 346 } 347 348 public boolean hasItem() { 349 return this.item != null && !this.item.isEmpty(); 350 } 351 352 /** 353 * @param value {@link #item} (A reference to the actual resource from which data was derived.) 354 */ 355 public ListResourceEntryComponent setItem(Reference value) { 356 this.item = value; 357 return this; 358 } 359 360 protected void listChildren(List<Property> children) { 361 super.listChildren(children); 362 children.add(new Property("flag", "CodeableConcept", "The flag allows the system constructing the list to indicate the role and significance of the item in the list.", 0, 1, flag)); 363 children.add(new Property("deleted", "boolean", "True if this item is marked as deleted in the list.", 0, 1, deleted)); 364 children.add(new Property("date", "dateTime", "When this item was added to the list.", 0, 1, date)); 365 children.add(new Property("item", "Reference(Any)", "A reference to the actual resource from which data was derived.", 0, 1, item)); 366 } 367 368 @Override 369 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 370 switch (_hash) { 371 case 3145580: /*flag*/ return new Property("flag", "CodeableConcept", "The flag allows the system constructing the list to indicate the role and significance of the item in the list.", 0, 1, flag); 372 case 1550463001: /*deleted*/ return new Property("deleted", "boolean", "True if this item is marked as deleted in the list.", 0, 1, deleted); 373 case 3076014: /*date*/ return new Property("date", "dateTime", "When this item was added to the list.", 0, 1, date); 374 case 3242771: /*item*/ return new Property("item", "Reference(Any)", "A reference to the actual resource from which data was derived.", 0, 1, item); 375 default: return super.getNamedProperty(_hash, _name, _checkValid); 376 } 377 378 } 379 380 @Override 381 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 382 switch (hash) { 383 case 3145580: /*flag*/ return this.flag == null ? new Base[0] : new Base[] {this.flag}; // CodeableConcept 384 case 1550463001: /*deleted*/ return this.deleted == null ? new Base[0] : new Base[] {this.deleted}; // BooleanType 385 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 386 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // Reference 387 default: return super.getProperty(hash, name, checkValid); 388 } 389 390 } 391 392 @Override 393 public Base setProperty(int hash, String name, Base value) throws FHIRException { 394 switch (hash) { 395 case 3145580: // flag 396 this.flag = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 397 return value; 398 case 1550463001: // deleted 399 this.deleted = TypeConvertor.castToBoolean(value); // BooleanType 400 return value; 401 case 3076014: // date 402 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 403 return value; 404 case 3242771: // item 405 this.item = TypeConvertor.castToReference(value); // Reference 406 return value; 407 default: return super.setProperty(hash, name, value); 408 } 409 410 } 411 412 @Override 413 public Base setProperty(String name, Base value) throws FHIRException { 414 if (name.equals("flag")) { 415 this.flag = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 416 } else if (name.equals("deleted")) { 417 this.deleted = TypeConvertor.castToBoolean(value); // BooleanType 418 } else if (name.equals("date")) { 419 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 420 } else if (name.equals("item")) { 421 this.item = TypeConvertor.castToReference(value); // Reference 422 } else 423 return super.setProperty(name, value); 424 return value; 425 } 426 427 @Override 428 public void removeChild(String name, Base value) throws FHIRException { 429 if (name.equals("flag")) { 430 this.flag = null; 431 } else if (name.equals("deleted")) { 432 this.deleted = null; 433 } else if (name.equals("date")) { 434 this.date = null; 435 } else if (name.equals("item")) { 436 this.item = null; 437 } else 438 super.removeChild(name, value); 439 440 } 441 442 @Override 443 public Base makeProperty(int hash, String name) throws FHIRException { 444 switch (hash) { 445 case 3145580: return getFlag(); 446 case 1550463001: return getDeletedElement(); 447 case 3076014: return getDateElement(); 448 case 3242771: return getItem(); 449 default: return super.makeProperty(hash, name); 450 } 451 452 } 453 454 @Override 455 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 456 switch (hash) { 457 case 3145580: /*flag*/ return new String[] {"CodeableConcept"}; 458 case 1550463001: /*deleted*/ return new String[] {"boolean"}; 459 case 3076014: /*date*/ return new String[] {"dateTime"}; 460 case 3242771: /*item*/ return new String[] {"Reference"}; 461 default: return super.getTypesForProperty(hash, name); 462 } 463 464 } 465 466 @Override 467 public Base addChild(String name) throws FHIRException { 468 if (name.equals("flag")) { 469 this.flag = new CodeableConcept(); 470 return this.flag; 471 } 472 else if (name.equals("deleted")) { 473 throw new FHIRException("Cannot call addChild on a singleton property List.entry.deleted"); 474 } 475 else if (name.equals("date")) { 476 throw new FHIRException("Cannot call addChild on a singleton property List.entry.date"); 477 } 478 else if (name.equals("item")) { 479 this.item = new Reference(); 480 return this.item; 481 } 482 else 483 return super.addChild(name); 484 } 485 486 public ListResourceEntryComponent copy() { 487 ListResourceEntryComponent dst = new ListResourceEntryComponent(); 488 copyValues(dst); 489 return dst; 490 } 491 492 public void copyValues(ListResourceEntryComponent dst) { 493 super.copyValues(dst); 494 dst.flag = flag == null ? null : flag.copy(); 495 dst.deleted = deleted == null ? null : deleted.copy(); 496 dst.date = date == null ? null : date.copy(); 497 dst.item = item == null ? null : item.copy(); 498 } 499 500 @Override 501 public boolean equalsDeep(Base other_) { 502 if (!super.equalsDeep(other_)) 503 return false; 504 if (!(other_ instanceof ListResourceEntryComponent)) 505 return false; 506 ListResourceEntryComponent o = (ListResourceEntryComponent) other_; 507 return compareDeep(flag, o.flag, true) && compareDeep(deleted, o.deleted, true) && compareDeep(date, o.date, true) 508 && compareDeep(item, o.item, true); 509 } 510 511 @Override 512 public boolean equalsShallow(Base other_) { 513 if (!super.equalsShallow(other_)) 514 return false; 515 if (!(other_ instanceof ListResourceEntryComponent)) 516 return false; 517 ListResourceEntryComponent o = (ListResourceEntryComponent) other_; 518 return compareValues(deleted, o.deleted, true) && compareValues(date, o.date, true); 519 } 520 521 public boolean isEmpty() { 522 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(flag, deleted, date, item 523 ); 524 } 525 526 public String fhirType() { 527 return "List.entry"; 528 529 } 530 531 } 532 533 /** 534 * Identifier for the List assigned for business purposes outside the context of FHIR. 535 */ 536 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 537 @Description(shortDefinition="Business identifier", formalDefinition="Identifier for the List assigned for business purposes outside the context of FHIR." ) 538 protected List<Identifier> identifier; 539 540 /** 541 * Indicates the current state of this list. 542 */ 543 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 544 @Description(shortDefinition="current | retired | entered-in-error", formalDefinition="Indicates the current state of this list." ) 545 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-status") 546 protected Enumeration<ListStatus> status; 547 548 /** 549 * How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted. 550 */ 551 @Child(name = "mode", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 552 @Description(shortDefinition="working | snapshot | changes", formalDefinition="How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted." ) 553 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-mode") 554 protected Enumeration<ListMode> mode; 555 556 /** 557 * A label for the list assigned by the author. 558 */ 559 @Child(name = "title", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 560 @Description(shortDefinition="Descriptive name for the list", formalDefinition="A label for the list assigned by the author." ) 561 protected StringType title; 562 563 /** 564 * This code defines the purpose of the list - why it was created. 565 */ 566 @Child(name = "code", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 567 @Description(shortDefinition="What the purpose of this list is", formalDefinition="This code defines the purpose of the list - why it was created." ) 568 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-example-codes") 569 protected CodeableConcept code; 570 571 /** 572 * The common subject(s) (or patient(s)) of the resources that are in the list if there is one (or a set of subjects). 573 */ 574 @Child(name = "subject", type = {Reference.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 575 @Description(shortDefinition="If all resources have the same subject(s)", formalDefinition="The common subject(s) (or patient(s)) of the resources that are in the list if there is one (or a set of subjects)." ) 576 protected List<Reference> subject; 577 578 /** 579 * The encounter that is the context in which this list was created. 580 */ 581 @Child(name = "encounter", type = {Encounter.class}, order=6, min=0, max=1, modifier=false, summary=false) 582 @Description(shortDefinition="Context in which list created", formalDefinition="The encounter that is the context in which this list was created." ) 583 protected Reference encounter; 584 585 /** 586 * Date list was last reviewed/revised and determined to be 'current'. 587 */ 588 @Child(name = "date", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 589 @Description(shortDefinition="When the list was prepared", formalDefinition="Date list was last reviewed/revised and determined to be 'current'." ) 590 protected DateTimeType date; 591 592 /** 593 * The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list. 594 */ 595 @Child(name = "source", type = {Practitioner.class, PractitionerRole.class, Patient.class, Device.class, Organization.class, RelatedPerson.class, CareTeam.class}, order=8, min=0, max=1, modifier=false, summary=true) 596 @Description(shortDefinition="Who and/or what defined the list contents (aka Author)", formalDefinition="The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list." ) 597 protected Reference source; 598 599 /** 600 * What order applies to the items in the list. 601 */ 602 @Child(name = "orderedBy", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=false) 603 @Description(shortDefinition="What order the list has", formalDefinition="What order applies to the items in the list." ) 604 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-order") 605 protected CodeableConcept orderedBy; 606 607 /** 608 * Comments that apply to the overall list. 609 */ 610 @Child(name = "note", type = {Annotation.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 611 @Description(shortDefinition="Comments about the list", formalDefinition="Comments that apply to the overall list." ) 612 protected List<Annotation> note; 613 614 /** 615 * Entries in this list. 616 */ 617 @Child(name = "entry", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 618 @Description(shortDefinition="Entries in the list", formalDefinition="Entries in this list." ) 619 protected List<ListResourceEntryComponent> entry; 620 621 /** 622 * If the list is empty, why the list is empty. 623 */ 624 @Child(name = "emptyReason", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=false) 625 @Description(shortDefinition="Why list is empty", formalDefinition="If the list is empty, why the list is empty." ) 626 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-empty-reason") 627 protected CodeableConcept emptyReason; 628 629 private static final long serialVersionUID = -2099967050L; 630 631 /** 632 * Constructor 633 */ 634 public ListResource() { 635 super(); 636 } 637 638 /** 639 * Constructor 640 */ 641 public ListResource(ListStatus status, ListMode mode) { 642 super(); 643 this.setStatus(status); 644 this.setMode(mode); 645 } 646 647 /** 648 * @return {@link #identifier} (Identifier for the List assigned for business purposes outside the context of FHIR.) 649 */ 650 public List<Identifier> getIdentifier() { 651 if (this.identifier == null) 652 this.identifier = new ArrayList<Identifier>(); 653 return this.identifier; 654 } 655 656 /** 657 * @return Returns a reference to <code>this</code> for easy method chaining 658 */ 659 public ListResource setIdentifier(List<Identifier> theIdentifier) { 660 this.identifier = theIdentifier; 661 return this; 662 } 663 664 public boolean hasIdentifier() { 665 if (this.identifier == null) 666 return false; 667 for (Identifier item : this.identifier) 668 if (!item.isEmpty()) 669 return true; 670 return false; 671 } 672 673 public Identifier addIdentifier() { //3 674 Identifier t = new Identifier(); 675 if (this.identifier == null) 676 this.identifier = new ArrayList<Identifier>(); 677 this.identifier.add(t); 678 return t; 679 } 680 681 public ListResource addIdentifier(Identifier t) { //3 682 if (t == null) 683 return this; 684 if (this.identifier == null) 685 this.identifier = new ArrayList<Identifier>(); 686 this.identifier.add(t); 687 return this; 688 } 689 690 /** 691 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 692 */ 693 public Identifier getIdentifierFirstRep() { 694 if (getIdentifier().isEmpty()) { 695 addIdentifier(); 696 } 697 return getIdentifier().get(0); 698 } 699 700 /** 701 * @return {@link #status} (Indicates the current state of this list.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 702 */ 703 public Enumeration<ListStatus> getStatusElement() { 704 if (this.status == null) 705 if (Configuration.errorOnAutoCreate()) 706 throw new Error("Attempt to auto-create ListResource.status"); 707 else if (Configuration.doAutoCreate()) 708 this.status = new Enumeration<ListStatus>(new ListStatusEnumFactory()); // bb 709 return this.status; 710 } 711 712 public boolean hasStatusElement() { 713 return this.status != null && !this.status.isEmpty(); 714 } 715 716 public boolean hasStatus() { 717 return this.status != null && !this.status.isEmpty(); 718 } 719 720 /** 721 * @param value {@link #status} (Indicates the current state of this list.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 722 */ 723 public ListResource setStatusElement(Enumeration<ListStatus> value) { 724 this.status = value; 725 return this; 726 } 727 728 /** 729 * @return Indicates the current state of this list. 730 */ 731 public ListStatus getStatus() { 732 return this.status == null ? null : this.status.getValue(); 733 } 734 735 /** 736 * @param value Indicates the current state of this list. 737 */ 738 public ListResource setStatus(ListStatus value) { 739 if (this.status == null) 740 this.status = new Enumeration<ListStatus>(new ListStatusEnumFactory()); 741 this.status.setValue(value); 742 return this; 743 } 744 745 /** 746 * @return {@link #mode} (How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 747 */ 748 public Enumeration<ListMode> getModeElement() { 749 if (this.mode == null) 750 if (Configuration.errorOnAutoCreate()) 751 throw new Error("Attempt to auto-create ListResource.mode"); 752 else if (Configuration.doAutoCreate()) 753 this.mode = new Enumeration<ListMode>(new ListModeEnumFactory()); // bb 754 return this.mode; 755 } 756 757 public boolean hasModeElement() { 758 return this.mode != null && !this.mode.isEmpty(); 759 } 760 761 public boolean hasMode() { 762 return this.mode != null && !this.mode.isEmpty(); 763 } 764 765 /** 766 * @param value {@link #mode} (How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 767 */ 768 public ListResource setModeElement(Enumeration<ListMode> value) { 769 this.mode = value; 770 return this; 771 } 772 773 /** 774 * @return How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted. 775 */ 776 public ListMode getMode() { 777 return this.mode == null ? null : this.mode.getValue(); 778 } 779 780 /** 781 * @param value How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted. 782 */ 783 public ListResource setMode(ListMode value) { 784 if (this.mode == null) 785 this.mode = new Enumeration<ListMode>(new ListModeEnumFactory()); 786 this.mode.setValue(value); 787 return this; 788 } 789 790 /** 791 * @return {@link #title} (A label for the list assigned by the author.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 792 */ 793 public StringType getTitleElement() { 794 if (this.title == null) 795 if (Configuration.errorOnAutoCreate()) 796 throw new Error("Attempt to auto-create ListResource.title"); 797 else if (Configuration.doAutoCreate()) 798 this.title = new StringType(); // bb 799 return this.title; 800 } 801 802 public boolean hasTitleElement() { 803 return this.title != null && !this.title.isEmpty(); 804 } 805 806 public boolean hasTitle() { 807 return this.title != null && !this.title.isEmpty(); 808 } 809 810 /** 811 * @param value {@link #title} (A label for the list assigned by the author.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 812 */ 813 public ListResource setTitleElement(StringType value) { 814 this.title = value; 815 return this; 816 } 817 818 /** 819 * @return A label for the list assigned by the author. 820 */ 821 public String getTitle() { 822 return this.title == null ? null : this.title.getValue(); 823 } 824 825 /** 826 * @param value A label for the list assigned by the author. 827 */ 828 public ListResource setTitle(String value) { 829 if (Utilities.noString(value)) 830 this.title = null; 831 else { 832 if (this.title == null) 833 this.title = new StringType(); 834 this.title.setValue(value); 835 } 836 return this; 837 } 838 839 /** 840 * @return {@link #code} (This code defines the purpose of the list - why it was created.) 841 */ 842 public CodeableConcept getCode() { 843 if (this.code == null) 844 if (Configuration.errorOnAutoCreate()) 845 throw new Error("Attempt to auto-create ListResource.code"); 846 else if (Configuration.doAutoCreate()) 847 this.code = new CodeableConcept(); // cc 848 return this.code; 849 } 850 851 public boolean hasCode() { 852 return this.code != null && !this.code.isEmpty(); 853 } 854 855 /** 856 * @param value {@link #code} (This code defines the purpose of the list - why it was created.) 857 */ 858 public ListResource setCode(CodeableConcept value) { 859 this.code = value; 860 return this; 861 } 862 863 /** 864 * @return {@link #subject} (The common subject(s) (or patient(s)) of the resources that are in the list if there is one (or a set of subjects).) 865 */ 866 public List<Reference> getSubject() { 867 if (this.subject == null) 868 this.subject = new ArrayList<Reference>(); 869 return this.subject; 870 } 871 872 /** 873 * @return Returns a reference to <code>this</code> for easy method chaining 874 */ 875 public ListResource setSubject(List<Reference> theSubject) { 876 this.subject = theSubject; 877 return this; 878 } 879 880 public boolean hasSubject() { 881 if (this.subject == null) 882 return false; 883 for (Reference item : this.subject) 884 if (!item.isEmpty()) 885 return true; 886 return false; 887 } 888 889 public Reference addSubject() { //3 890 Reference t = new Reference(); 891 if (this.subject == null) 892 this.subject = new ArrayList<Reference>(); 893 this.subject.add(t); 894 return t; 895 } 896 897 public ListResource addSubject(Reference t) { //3 898 if (t == null) 899 return this; 900 if (this.subject == null) 901 this.subject = new ArrayList<Reference>(); 902 this.subject.add(t); 903 return this; 904 } 905 906 /** 907 * @return The first repetition of repeating field {@link #subject}, creating it if it does not already exist {3} 908 */ 909 public Reference getSubjectFirstRep() { 910 if (getSubject().isEmpty()) { 911 addSubject(); 912 } 913 return getSubject().get(0); 914 } 915 916 /** 917 * @return {@link #encounter} (The encounter that is the context in which this list was created.) 918 */ 919 public Reference getEncounter() { 920 if (this.encounter == null) 921 if (Configuration.errorOnAutoCreate()) 922 throw new Error("Attempt to auto-create ListResource.encounter"); 923 else if (Configuration.doAutoCreate()) 924 this.encounter = new Reference(); // cc 925 return this.encounter; 926 } 927 928 public boolean hasEncounter() { 929 return this.encounter != null && !this.encounter.isEmpty(); 930 } 931 932 /** 933 * @param value {@link #encounter} (The encounter that is the context in which this list was created.) 934 */ 935 public ListResource setEncounter(Reference value) { 936 this.encounter = value; 937 return this; 938 } 939 940 /** 941 * @return {@link #date} (Date list was last reviewed/revised and determined to be 'current'.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 942 */ 943 public DateTimeType getDateElement() { 944 if (this.date == null) 945 if (Configuration.errorOnAutoCreate()) 946 throw new Error("Attempt to auto-create ListResource.date"); 947 else if (Configuration.doAutoCreate()) 948 this.date = new DateTimeType(); // bb 949 return this.date; 950 } 951 952 public boolean hasDateElement() { 953 return this.date != null && !this.date.isEmpty(); 954 } 955 956 public boolean hasDate() { 957 return this.date != null && !this.date.isEmpty(); 958 } 959 960 /** 961 * @param value {@link #date} (Date list was last reviewed/revised and determined to be 'current'.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 962 */ 963 public ListResource setDateElement(DateTimeType value) { 964 this.date = value; 965 return this; 966 } 967 968 /** 969 * @return Date list was last reviewed/revised and determined to be 'current'. 970 */ 971 public Date getDate() { 972 return this.date == null ? null : this.date.getValue(); 973 } 974 975 /** 976 * @param value Date list was last reviewed/revised and determined to be 'current'. 977 */ 978 public ListResource setDate(Date value) { 979 if (value == null) 980 this.date = null; 981 else { 982 if (this.date == null) 983 this.date = new DateTimeType(); 984 this.date.setValue(value); 985 } 986 return this; 987 } 988 989 /** 990 * @return {@link #source} (The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.) 991 */ 992 public Reference getSource() { 993 if (this.source == null) 994 if (Configuration.errorOnAutoCreate()) 995 throw new Error("Attempt to auto-create ListResource.source"); 996 else if (Configuration.doAutoCreate()) 997 this.source = new Reference(); // cc 998 return this.source; 999 } 1000 1001 public boolean hasSource() { 1002 return this.source != null && !this.source.isEmpty(); 1003 } 1004 1005 /** 1006 * @param value {@link #source} (The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.) 1007 */ 1008 public ListResource setSource(Reference value) { 1009 this.source = value; 1010 return this; 1011 } 1012 1013 /** 1014 * @return {@link #orderedBy} (What order applies to the items in the list.) 1015 */ 1016 public CodeableConcept getOrderedBy() { 1017 if (this.orderedBy == null) 1018 if (Configuration.errorOnAutoCreate()) 1019 throw new Error("Attempt to auto-create ListResource.orderedBy"); 1020 else if (Configuration.doAutoCreate()) 1021 this.orderedBy = new CodeableConcept(); // cc 1022 return this.orderedBy; 1023 } 1024 1025 public boolean hasOrderedBy() { 1026 return this.orderedBy != null && !this.orderedBy.isEmpty(); 1027 } 1028 1029 /** 1030 * @param value {@link #orderedBy} (What order applies to the items in the list.) 1031 */ 1032 public ListResource setOrderedBy(CodeableConcept value) { 1033 this.orderedBy = value; 1034 return this; 1035 } 1036 1037 /** 1038 * @return {@link #note} (Comments that apply to the overall list.) 1039 */ 1040 public List<Annotation> getNote() { 1041 if (this.note == null) 1042 this.note = new ArrayList<Annotation>(); 1043 return this.note; 1044 } 1045 1046 /** 1047 * @return Returns a reference to <code>this</code> for easy method chaining 1048 */ 1049 public ListResource setNote(List<Annotation> theNote) { 1050 this.note = theNote; 1051 return this; 1052 } 1053 1054 public boolean hasNote() { 1055 if (this.note == null) 1056 return false; 1057 for (Annotation item : this.note) 1058 if (!item.isEmpty()) 1059 return true; 1060 return false; 1061 } 1062 1063 public Annotation addNote() { //3 1064 Annotation t = new Annotation(); 1065 if (this.note == null) 1066 this.note = new ArrayList<Annotation>(); 1067 this.note.add(t); 1068 return t; 1069 } 1070 1071 public ListResource addNote(Annotation t) { //3 1072 if (t == null) 1073 return this; 1074 if (this.note == null) 1075 this.note = new ArrayList<Annotation>(); 1076 this.note.add(t); 1077 return this; 1078 } 1079 1080 /** 1081 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1082 */ 1083 public Annotation getNoteFirstRep() { 1084 if (getNote().isEmpty()) { 1085 addNote(); 1086 } 1087 return getNote().get(0); 1088 } 1089 1090 /** 1091 * @return {@link #entry} (Entries in this list.) 1092 */ 1093 public List<ListResourceEntryComponent> getEntry() { 1094 if (this.entry == null) 1095 this.entry = new ArrayList<ListResourceEntryComponent>(); 1096 return this.entry; 1097 } 1098 1099 /** 1100 * @return Returns a reference to <code>this</code> for easy method chaining 1101 */ 1102 public ListResource setEntry(List<ListResourceEntryComponent> theEntry) { 1103 this.entry = theEntry; 1104 return this; 1105 } 1106 1107 public boolean hasEntry() { 1108 if (this.entry == null) 1109 return false; 1110 for (ListResourceEntryComponent item : this.entry) 1111 if (!item.isEmpty()) 1112 return true; 1113 return false; 1114 } 1115 1116 public ListResourceEntryComponent addEntry() { //3 1117 ListResourceEntryComponent t = new ListResourceEntryComponent(); 1118 if (this.entry == null) 1119 this.entry = new ArrayList<ListResourceEntryComponent>(); 1120 this.entry.add(t); 1121 return t; 1122 } 1123 1124 public ListResource addEntry(ListResourceEntryComponent t) { //3 1125 if (t == null) 1126 return this; 1127 if (this.entry == null) 1128 this.entry = new ArrayList<ListResourceEntryComponent>(); 1129 this.entry.add(t); 1130 return this; 1131 } 1132 1133 /** 1134 * @return The first repetition of repeating field {@link #entry}, creating it if it does not already exist {3} 1135 */ 1136 public ListResourceEntryComponent getEntryFirstRep() { 1137 if (getEntry().isEmpty()) { 1138 addEntry(); 1139 } 1140 return getEntry().get(0); 1141 } 1142 1143 /** 1144 * @return {@link #emptyReason} (If the list is empty, why the list is empty.) 1145 */ 1146 public CodeableConcept getEmptyReason() { 1147 if (this.emptyReason == null) 1148 if (Configuration.errorOnAutoCreate()) 1149 throw new Error("Attempt to auto-create ListResource.emptyReason"); 1150 else if (Configuration.doAutoCreate()) 1151 this.emptyReason = new CodeableConcept(); // cc 1152 return this.emptyReason; 1153 } 1154 1155 public boolean hasEmptyReason() { 1156 return this.emptyReason != null && !this.emptyReason.isEmpty(); 1157 } 1158 1159 /** 1160 * @param value {@link #emptyReason} (If the list is empty, why the list is empty.) 1161 */ 1162 public ListResource setEmptyReason(CodeableConcept value) { 1163 this.emptyReason = value; 1164 return this; 1165 } 1166 1167 protected void listChildren(List<Property> children) { 1168 super.listChildren(children); 1169 children.add(new Property("identifier", "Identifier", "Identifier for the List assigned for business purposes outside the context of FHIR.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1170 children.add(new Property("status", "code", "Indicates the current state of this list.", 0, 1, status)); 1171 children.add(new Property("mode", "code", "How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 0, 1, mode)); 1172 children.add(new Property("title", "string", "A label for the list assigned by the author.", 0, 1, title)); 1173 children.add(new Property("code", "CodeableConcept", "This code defines the purpose of the list - why it was created.", 0, 1, code)); 1174 children.add(new Property("subject", "Reference(Any)", "The common subject(s) (or patient(s)) of the resources that are in the list if there is one (or a set of subjects).", 0, java.lang.Integer.MAX_VALUE, subject)); 1175 children.add(new Property("encounter", "Reference(Encounter)", "The encounter that is the context in which this list was created.", 0, 1, encounter)); 1176 children.add(new Property("date", "dateTime", "Date list was last reviewed/revised and determined to be 'current'.", 0, 1, date)); 1177 children.add(new Property("source", "Reference(Practitioner|PractitionerRole|Patient|Device|Organization|RelatedPerson|CareTeam)", "The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.", 0, 1, source)); 1178 children.add(new Property("orderedBy", "CodeableConcept", "What order applies to the items in the list.", 0, 1, orderedBy)); 1179 children.add(new Property("note", "Annotation", "Comments that apply to the overall list.", 0, java.lang.Integer.MAX_VALUE, note)); 1180 children.add(new Property("entry", "", "Entries in this list.", 0, java.lang.Integer.MAX_VALUE, entry)); 1181 children.add(new Property("emptyReason", "CodeableConcept", "If the list is empty, why the list is empty.", 0, 1, emptyReason)); 1182 } 1183 1184 @Override 1185 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1186 switch (_hash) { 1187 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for the List assigned for business purposes outside the context of FHIR.", 0, java.lang.Integer.MAX_VALUE, identifier); 1188 case -892481550: /*status*/ return new Property("status", "code", "Indicates the current state of this list.", 0, 1, status); 1189 case 3357091: /*mode*/ return new Property("mode", "code", "How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 0, 1, mode); 1190 case 110371416: /*title*/ return new Property("title", "string", "A label for the list assigned by the author.", 0, 1, title); 1191 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "This code defines the purpose of the list - why it was created.", 0, 1, code); 1192 case -1867885268: /*subject*/ return new Property("subject", "Reference(Any)", "The common subject(s) (or patient(s)) of the resources that are in the list if there is one (or a set of subjects).", 0, java.lang.Integer.MAX_VALUE, subject); 1193 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The encounter that is the context in which this list was created.", 0, 1, encounter); 1194 case 3076014: /*date*/ return new Property("date", "dateTime", "Date list was last reviewed/revised and determined to be 'current'.", 0, 1, date); 1195 case -896505829: /*source*/ return new Property("source", "Reference(Practitioner|PractitionerRole|Patient|Device|Organization|RelatedPerson|CareTeam)", "The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.", 0, 1, source); 1196 case -391079516: /*orderedBy*/ return new Property("orderedBy", "CodeableConcept", "What order applies to the items in the list.", 0, 1, orderedBy); 1197 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments that apply to the overall list.", 0, java.lang.Integer.MAX_VALUE, note); 1198 case 96667762: /*entry*/ return new Property("entry", "", "Entries in this list.", 0, java.lang.Integer.MAX_VALUE, entry); 1199 case 1140135409: /*emptyReason*/ return new Property("emptyReason", "CodeableConcept", "If the list is empty, why the list is empty.", 0, 1, emptyReason); 1200 default: return super.getNamedProperty(_hash, _name, _checkValid); 1201 } 1202 1203 } 1204 1205 @Override 1206 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1207 switch (hash) { 1208 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1209 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ListStatus> 1210 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<ListMode> 1211 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1212 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1213 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 1214 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1215 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1216 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // Reference 1217 case -391079516: /*orderedBy*/ return this.orderedBy == null ? new Base[0] : new Base[] {this.orderedBy}; // CodeableConcept 1218 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1219 case 96667762: /*entry*/ return this.entry == null ? new Base[0] : this.entry.toArray(new Base[this.entry.size()]); // ListResourceEntryComponent 1220 case 1140135409: /*emptyReason*/ return this.emptyReason == null ? new Base[0] : new Base[] {this.emptyReason}; // CodeableConcept 1221 default: return super.getProperty(hash, name, checkValid); 1222 } 1223 1224 } 1225 1226 @Override 1227 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1228 switch (hash) { 1229 case -1618432855: // identifier 1230 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1231 return value; 1232 case -892481550: // status 1233 value = new ListStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1234 this.status = (Enumeration) value; // Enumeration<ListStatus> 1235 return value; 1236 case 3357091: // mode 1237 value = new ListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1238 this.mode = (Enumeration) value; // Enumeration<ListMode> 1239 return value; 1240 case 110371416: // title 1241 this.title = TypeConvertor.castToString(value); // StringType 1242 return value; 1243 case 3059181: // code 1244 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1245 return value; 1246 case -1867885268: // subject 1247 this.getSubject().add(TypeConvertor.castToReference(value)); // Reference 1248 return value; 1249 case 1524132147: // encounter 1250 this.encounter = TypeConvertor.castToReference(value); // Reference 1251 return value; 1252 case 3076014: // date 1253 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1254 return value; 1255 case -896505829: // source 1256 this.source = TypeConvertor.castToReference(value); // Reference 1257 return value; 1258 case -391079516: // orderedBy 1259 this.orderedBy = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1260 return value; 1261 case 3387378: // note 1262 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 1263 return value; 1264 case 96667762: // entry 1265 this.getEntry().add((ListResourceEntryComponent) value); // ListResourceEntryComponent 1266 return value; 1267 case 1140135409: // emptyReason 1268 this.emptyReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1269 return value; 1270 default: return super.setProperty(hash, name, value); 1271 } 1272 1273 } 1274 1275 @Override 1276 public Base setProperty(String name, Base value) throws FHIRException { 1277 if (name.equals("identifier")) { 1278 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1279 } else if (name.equals("status")) { 1280 value = new ListStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1281 this.status = (Enumeration) value; // Enumeration<ListStatus> 1282 } else if (name.equals("mode")) { 1283 value = new ListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1284 this.mode = (Enumeration) value; // Enumeration<ListMode> 1285 } else if (name.equals("title")) { 1286 this.title = TypeConvertor.castToString(value); // StringType 1287 } else if (name.equals("code")) { 1288 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1289 } else if (name.equals("subject")) { 1290 this.getSubject().add(TypeConvertor.castToReference(value)); 1291 } else if (name.equals("encounter")) { 1292 this.encounter = TypeConvertor.castToReference(value); // Reference 1293 } else if (name.equals("date")) { 1294 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 1295 } else if (name.equals("source")) { 1296 this.source = TypeConvertor.castToReference(value); // Reference 1297 } else if (name.equals("orderedBy")) { 1298 this.orderedBy = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1299 } else if (name.equals("note")) { 1300 this.getNote().add(TypeConvertor.castToAnnotation(value)); 1301 } else if (name.equals("entry")) { 1302 this.getEntry().add((ListResourceEntryComponent) value); 1303 } else if (name.equals("emptyReason")) { 1304 this.emptyReason = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1305 } else 1306 return super.setProperty(name, value); 1307 return value; 1308 } 1309 1310 @Override 1311 public void removeChild(String name, Base value) throws FHIRException { 1312 if (name.equals("identifier")) { 1313 this.getIdentifier().remove(value); 1314 } else if (name.equals("status")) { 1315 value = new ListStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1316 this.status = (Enumeration) value; // Enumeration<ListStatus> 1317 } else if (name.equals("mode")) { 1318 value = new ListModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1319 this.mode = (Enumeration) value; // Enumeration<ListMode> 1320 } else if (name.equals("title")) { 1321 this.title = null; 1322 } else if (name.equals("code")) { 1323 this.code = null; 1324 } else if (name.equals("subject")) { 1325 this.getSubject().remove(value); 1326 } else if (name.equals("encounter")) { 1327 this.encounter = null; 1328 } else if (name.equals("date")) { 1329 this.date = null; 1330 } else if (name.equals("source")) { 1331 this.source = null; 1332 } else if (name.equals("orderedBy")) { 1333 this.orderedBy = null; 1334 } else if (name.equals("note")) { 1335 this.getNote().remove(value); 1336 } else if (name.equals("entry")) { 1337 this.getEntry().remove((ListResourceEntryComponent) value); 1338 } else if (name.equals("emptyReason")) { 1339 this.emptyReason = null; 1340 } else 1341 super.removeChild(name, value); 1342 1343 } 1344 1345 @Override 1346 public Base makeProperty(int hash, String name) throws FHIRException { 1347 switch (hash) { 1348 case -1618432855: return addIdentifier(); 1349 case -892481550: return getStatusElement(); 1350 case 3357091: return getModeElement(); 1351 case 110371416: return getTitleElement(); 1352 case 3059181: return getCode(); 1353 case -1867885268: return addSubject(); 1354 case 1524132147: return getEncounter(); 1355 case 3076014: return getDateElement(); 1356 case -896505829: return getSource(); 1357 case -391079516: return getOrderedBy(); 1358 case 3387378: return addNote(); 1359 case 96667762: return addEntry(); 1360 case 1140135409: return getEmptyReason(); 1361 default: return super.makeProperty(hash, name); 1362 } 1363 1364 } 1365 1366 @Override 1367 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1368 switch (hash) { 1369 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1370 case -892481550: /*status*/ return new String[] {"code"}; 1371 case 3357091: /*mode*/ return new String[] {"code"}; 1372 case 110371416: /*title*/ return new String[] {"string"}; 1373 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1374 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1375 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1376 case 3076014: /*date*/ return new String[] {"dateTime"}; 1377 case -896505829: /*source*/ return new String[] {"Reference"}; 1378 case -391079516: /*orderedBy*/ return new String[] {"CodeableConcept"}; 1379 case 3387378: /*note*/ return new String[] {"Annotation"}; 1380 case 96667762: /*entry*/ return new String[] {}; 1381 case 1140135409: /*emptyReason*/ return new String[] {"CodeableConcept"}; 1382 default: return super.getTypesForProperty(hash, name); 1383 } 1384 1385 } 1386 1387 @Override 1388 public Base addChild(String name) throws FHIRException { 1389 if (name.equals("identifier")) { 1390 return addIdentifier(); 1391 } 1392 else if (name.equals("status")) { 1393 throw new FHIRException("Cannot call addChild on a singleton property List.status"); 1394 } 1395 else if (name.equals("mode")) { 1396 throw new FHIRException("Cannot call addChild on a singleton property List.mode"); 1397 } 1398 else if (name.equals("title")) { 1399 throw new FHIRException("Cannot call addChild on a singleton property List.title"); 1400 } 1401 else if (name.equals("code")) { 1402 this.code = new CodeableConcept(); 1403 return this.code; 1404 } 1405 else if (name.equals("subject")) { 1406 return addSubject(); 1407 } 1408 else if (name.equals("encounter")) { 1409 this.encounter = new Reference(); 1410 return this.encounter; 1411 } 1412 else if (name.equals("date")) { 1413 throw new FHIRException("Cannot call addChild on a singleton property List.date"); 1414 } 1415 else if (name.equals("source")) { 1416 this.source = new Reference(); 1417 return this.source; 1418 } 1419 else if (name.equals("orderedBy")) { 1420 this.orderedBy = new CodeableConcept(); 1421 return this.orderedBy; 1422 } 1423 else if (name.equals("note")) { 1424 return addNote(); 1425 } 1426 else if (name.equals("entry")) { 1427 return addEntry(); 1428 } 1429 else if (name.equals("emptyReason")) { 1430 this.emptyReason = new CodeableConcept(); 1431 return this.emptyReason; 1432 } 1433 else 1434 return super.addChild(name); 1435 } 1436 1437 public String fhirType() { 1438 return "List"; 1439 1440 } 1441 1442 public ListResource copy() { 1443 ListResource dst = new ListResource(); 1444 copyValues(dst); 1445 return dst; 1446 } 1447 1448 public void copyValues(ListResource dst) { 1449 super.copyValues(dst); 1450 if (identifier != null) { 1451 dst.identifier = new ArrayList<Identifier>(); 1452 for (Identifier i : identifier) 1453 dst.identifier.add(i.copy()); 1454 }; 1455 dst.status = status == null ? null : status.copy(); 1456 dst.mode = mode == null ? null : mode.copy(); 1457 dst.title = title == null ? null : title.copy(); 1458 dst.code = code == null ? null : code.copy(); 1459 if (subject != null) { 1460 dst.subject = new ArrayList<Reference>(); 1461 for (Reference i : subject) 1462 dst.subject.add(i.copy()); 1463 }; 1464 dst.encounter = encounter == null ? null : encounter.copy(); 1465 dst.date = date == null ? null : date.copy(); 1466 dst.source = source == null ? null : source.copy(); 1467 dst.orderedBy = orderedBy == null ? null : orderedBy.copy(); 1468 if (note != null) { 1469 dst.note = new ArrayList<Annotation>(); 1470 for (Annotation i : note) 1471 dst.note.add(i.copy()); 1472 }; 1473 if (entry != null) { 1474 dst.entry = new ArrayList<ListResourceEntryComponent>(); 1475 for (ListResourceEntryComponent i : entry) 1476 dst.entry.add(i.copy()); 1477 }; 1478 dst.emptyReason = emptyReason == null ? null : emptyReason.copy(); 1479 } 1480 1481 protected ListResource typedCopy() { 1482 return copy(); 1483 } 1484 1485 @Override 1486 public boolean equalsDeep(Base other_) { 1487 if (!super.equalsDeep(other_)) 1488 return false; 1489 if (!(other_ instanceof ListResource)) 1490 return false; 1491 ListResource o = (ListResource) other_; 1492 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(mode, o.mode, true) 1493 && compareDeep(title, o.title, true) && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 1494 && compareDeep(encounter, o.encounter, true) && compareDeep(date, o.date, true) && compareDeep(source, o.source, true) 1495 && compareDeep(orderedBy, o.orderedBy, true) && compareDeep(note, o.note, true) && compareDeep(entry, o.entry, true) 1496 && compareDeep(emptyReason, o.emptyReason, true); 1497 } 1498 1499 @Override 1500 public boolean equalsShallow(Base other_) { 1501 if (!super.equalsShallow(other_)) 1502 return false; 1503 if (!(other_ instanceof ListResource)) 1504 return false; 1505 ListResource o = (ListResource) other_; 1506 return compareValues(status, o.status, true) && compareValues(mode, o.mode, true) && compareValues(title, o.title, true) 1507 && compareValues(date, o.date, true); 1508 } 1509 1510 public boolean isEmpty() { 1511 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, mode 1512 , title, code, subject, encounter, date, source, orderedBy, note, entry, emptyReason 1513 ); 1514 } 1515 1516 @Override 1517 public ResourceType getResourceType() { 1518 return ResourceType.List; 1519 } 1520 1521 /** 1522 * Search parameter: <b>empty-reason</b> 1523 * <p> 1524 * Description: <b>Why list is empty</b><br> 1525 * Type: <b>token</b><br> 1526 * Path: <b>List.emptyReason</b><br> 1527 * </p> 1528 */ 1529 @SearchParamDefinition(name="empty-reason", path="List.emptyReason", description="Why list is empty", type="token" ) 1530 public static final String SP_EMPTY_REASON = "empty-reason"; 1531 /** 1532 * <b>Fluent Client</b> search parameter constant for <b>empty-reason</b> 1533 * <p> 1534 * Description: <b>Why list is empty</b><br> 1535 * Type: <b>token</b><br> 1536 * Path: <b>List.emptyReason</b><br> 1537 * </p> 1538 */ 1539 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMPTY_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EMPTY_REASON); 1540 1541 /** 1542 * Search parameter: <b>item</b> 1543 * <p> 1544 * Description: <b>Actual entry</b><br> 1545 * Type: <b>reference</b><br> 1546 * Path: <b>List.entry.item</b><br> 1547 * </p> 1548 */ 1549 @SearchParamDefinition(name="item", path="List.entry.item", description="Actual entry", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 1550 public static final String SP_ITEM = "item"; 1551 /** 1552 * <b>Fluent Client</b> search parameter constant for <b>item</b> 1553 * <p> 1554 * Description: <b>Actual entry</b><br> 1555 * Type: <b>reference</b><br> 1556 * Path: <b>List.entry.item</b><br> 1557 * </p> 1558 */ 1559 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ITEM); 1560 1561/** 1562 * Constant for fluent queries to be used to add include statements. Specifies 1563 * the path value of "<b>List:item</b>". 1564 */ 1565 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM = new ca.uhn.fhir.model.api.Include("List:item").toLocked(); 1566 1567 /** 1568 * Search parameter: <b>notes</b> 1569 * <p> 1570 * Description: <b>The annotation - text content (as markdown)</b><br> 1571 * Type: <b>string</b><br> 1572 * Path: <b>List.note.text</b><br> 1573 * </p> 1574 */ 1575 @SearchParamDefinition(name="notes", path="List.note.text", description="The annotation - text content (as markdown)", type="string" ) 1576 public static final String SP_NOTES = "notes"; 1577 /** 1578 * <b>Fluent Client</b> search parameter constant for <b>notes</b> 1579 * <p> 1580 * Description: <b>The annotation - text content (as markdown)</b><br> 1581 * Type: <b>string</b><br> 1582 * Path: <b>List.note.text</b><br> 1583 * </p> 1584 */ 1585 public static final ca.uhn.fhir.rest.gclient.StringClientParam NOTES = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NOTES); 1586 1587 /** 1588 * Search parameter: <b>source</b> 1589 * <p> 1590 * Description: <b>Who and/or what defined the list contents (aka Author)</b><br> 1591 * Type: <b>reference</b><br> 1592 * Path: <b>List.source</b><br> 1593 * </p> 1594 */ 1595 @SearchParamDefinition(name="source", path="List.source", description="Who and/or what defined the list contents (aka Author)", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={CareTeam.class, Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 1596 public static final String SP_SOURCE = "source"; 1597 /** 1598 * <b>Fluent Client</b> search parameter constant for <b>source</b> 1599 * <p> 1600 * Description: <b>Who and/or what defined the list contents (aka Author)</b><br> 1601 * Type: <b>reference</b><br> 1602 * Path: <b>List.source</b><br> 1603 * </p> 1604 */ 1605 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 1606 1607/** 1608 * Constant for fluent queries to be used to add include statements. Specifies 1609 * the path value of "<b>List:source</b>". 1610 */ 1611 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("List:source").toLocked(); 1612 1613 /** 1614 * Search parameter: <b>status</b> 1615 * <p> 1616 * Description: <b>current | retired | entered-in-error</b><br> 1617 * Type: <b>token</b><br> 1618 * Path: <b>List.status</b><br> 1619 * </p> 1620 */ 1621 @SearchParamDefinition(name="status", path="List.status", description="current | retired | entered-in-error", type="token" ) 1622 public static final String SP_STATUS = "status"; 1623 /** 1624 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1625 * <p> 1626 * Description: <b>current | retired | entered-in-error</b><br> 1627 * Type: <b>token</b><br> 1628 * Path: <b>List.status</b><br> 1629 * </p> 1630 */ 1631 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1632 1633 /** 1634 * Search parameter: <b>subject</b> 1635 * <p> 1636 * Description: <b>If all resources have the same subject</b><br> 1637 * Type: <b>reference</b><br> 1638 * Path: <b>List.subject</b><br> 1639 * </p> 1640 */ 1641 @SearchParamDefinition(name="subject", path="List.subject", description="If all resources have the same subject", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 1642 public static final String SP_SUBJECT = "subject"; 1643 /** 1644 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1645 * <p> 1646 * Description: <b>If all resources have the same subject</b><br> 1647 * Type: <b>reference</b><br> 1648 * Path: <b>List.subject</b><br> 1649 * </p> 1650 */ 1651 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1652 1653/** 1654 * Constant for fluent queries to be used to add include statements. Specifies 1655 * the path value of "<b>List:subject</b>". 1656 */ 1657 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("List:subject").toLocked(); 1658 1659 /** 1660 * Search parameter: <b>title</b> 1661 * <p> 1662 * Description: <b>Descriptive name for the list</b><br> 1663 * Type: <b>string</b><br> 1664 * Path: <b>List.title</b><br> 1665 * </p> 1666 */ 1667 @SearchParamDefinition(name="title", path="List.title", description="Descriptive name for the list", type="string" ) 1668 public static final String SP_TITLE = "title"; 1669 /** 1670 * <b>Fluent Client</b> search parameter constant for <b>title</b> 1671 * <p> 1672 * Description: <b>Descriptive name for the list</b><br> 1673 * Type: <b>string</b><br> 1674 * Path: <b>List.title</b><br> 1675 * </p> 1676 */ 1677 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 1678 1679 /** 1680 * Search parameter: <b>code</b> 1681 * <p> 1682 * Description: <b>Multiple Resources: 1683 1684* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 1685* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 1686* [AuditEvent](auditevent.html): More specific code for the event 1687* [Basic](basic.html): Kind of Resource 1688* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 1689* [Condition](condition.html): Code for the condition 1690* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 1691* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 1692* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 1693* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 1694* [ImagingSelection](imagingselection.html): The imaging selection status 1695* [List](list.html): What the purpose of this list is 1696* [Medication](medication.html): Returns medications for a specific code 1697* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 1698* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 1699* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 1700* [MedicationStatement](medicationstatement.html): Return statements of this medication code 1701* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 1702* [Observation](observation.html): The code of the observation type 1703* [Procedure](procedure.html): A code to identify a procedure 1704* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 1705* [Task](task.html): Search by task code 1706</b><br> 1707 * Type: <b>token</b><br> 1708 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 1709 * </p> 1710 */ 1711 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 1712 public static final String SP_CODE = "code"; 1713 /** 1714 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1715 * <p> 1716 * Description: <b>Multiple Resources: 1717 1718* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 1719* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 1720* [AuditEvent](auditevent.html): More specific code for the event 1721* [Basic](basic.html): Kind of Resource 1722* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 1723* [Condition](condition.html): Code for the condition 1724* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 1725* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 1726* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 1727* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 1728* [ImagingSelection](imagingselection.html): The imaging selection status 1729* [List](list.html): What the purpose of this list is 1730* [Medication](medication.html): Returns medications for a specific code 1731* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 1732* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 1733* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 1734* [MedicationStatement](medicationstatement.html): Return statements of this medication code 1735* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 1736* [Observation](observation.html): The code of the observation type 1737* [Procedure](procedure.html): A code to identify a procedure 1738* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 1739* [Task](task.html): Search by task code 1740</b><br> 1741 * Type: <b>token</b><br> 1742 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 1743 * </p> 1744 */ 1745 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 1746 1747 /** 1748 * Search parameter: <b>date</b> 1749 * <p> 1750 * Description: <b>Multiple Resources: 1751 1752* [AdverseEvent](adverseevent.html): When the event occurred 1753* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 1754* [Appointment](appointment.html): Appointment date/time. 1755* [AuditEvent](auditevent.html): Time when the event was recorded 1756* [CarePlan](careplan.html): Time period plan covers 1757* [CareTeam](careteam.html): A date within the coverage time period. 1758* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 1759* [Composition](composition.html): Composition editing time 1760* [Consent](consent.html): When consent was agreed to 1761* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 1762* [DocumentReference](documentreference.html): When this document reference was created 1763* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 1764* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 1765* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 1766* [Flag](flag.html): Time period when flag is active 1767* [Immunization](immunization.html): Vaccination (non)-Administration Date 1768* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 1769* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 1770* [Invoice](invoice.html): Invoice date / posting date 1771* [List](list.html): When the list was prepared 1772* [MeasureReport](measurereport.html): The date of the measure report 1773* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 1774* [Observation](observation.html): Clinically relevant time/time-period for observation 1775* [Procedure](procedure.html): When the procedure occurred or is occurring 1776* [ResearchSubject](researchsubject.html): Start and end of participation 1777* [RiskAssessment](riskassessment.html): When was assessment made? 1778* [SupplyRequest](supplyrequest.html): When the request was made 1779</b><br> 1780 * Type: <b>date</b><br> 1781 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 1782 * </p> 1783 */ 1784 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 1785 public static final String SP_DATE = "date"; 1786 /** 1787 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1788 * <p> 1789 * Description: <b>Multiple Resources: 1790 1791* [AdverseEvent](adverseevent.html): When the event occurred 1792* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 1793* [Appointment](appointment.html): Appointment date/time. 1794* [AuditEvent](auditevent.html): Time when the event was recorded 1795* [CarePlan](careplan.html): Time period plan covers 1796* [CareTeam](careteam.html): A date within the coverage time period. 1797* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 1798* [Composition](composition.html): Composition editing time 1799* [Consent](consent.html): When consent was agreed to 1800* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 1801* [DocumentReference](documentreference.html): When this document reference was created 1802* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 1803* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 1804* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 1805* [Flag](flag.html): Time period when flag is active 1806* [Immunization](immunization.html): Vaccination (non)-Administration Date 1807* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 1808* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 1809* [Invoice](invoice.html): Invoice date / posting date 1810* [List](list.html): When the list was prepared 1811* [MeasureReport](measurereport.html): The date of the measure report 1812* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 1813* [Observation](observation.html): Clinically relevant time/time-period for observation 1814* [Procedure](procedure.html): When the procedure occurred or is occurring 1815* [ResearchSubject](researchsubject.html): Start and end of participation 1816* [RiskAssessment](riskassessment.html): When was assessment made? 1817* [SupplyRequest](supplyrequest.html): When the request was made 1818</b><br> 1819 * Type: <b>date</b><br> 1820 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 1821 * </p> 1822 */ 1823 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1824 1825 /** 1826 * Search parameter: <b>encounter</b> 1827 * <p> 1828 * Description: <b>Multiple Resources: 1829 1830* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 1831* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 1832* [ChargeItem](chargeitem.html): Encounter associated with event 1833* [Claim](claim.html): Encounters associated with a billed line item 1834* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 1835* [Communication](communication.html): The Encounter during which this Communication was created 1836* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 1837* [Composition](composition.html): Context of the Composition 1838* [Condition](condition.html): The Encounter during which this Condition was created 1839* [DeviceRequest](devicerequest.html): Encounter during which request was created 1840* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 1841* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 1842* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 1843* [Flag](flag.html): Alert relevant during encounter 1844* [ImagingStudy](imagingstudy.html): The context of the study 1845* [List](list.html): Context in which list created 1846* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 1847* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 1848* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 1849* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 1850* [Observation](observation.html): Encounter related to the observation 1851* [Procedure](procedure.html): The Encounter during which this Procedure was created 1852* [Provenance](provenance.html): Encounter related to the Provenance 1853* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 1854* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 1855* [RiskAssessment](riskassessment.html): Where was assessment performed? 1856* [ServiceRequest](servicerequest.html): An encounter in which this request is made 1857* [Task](task.html): Search by encounter 1858* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 1859</b><br> 1860 * Type: <b>reference</b><br> 1861 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 1862 * </p> 1863 */ 1864 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", target={Encounter.class } ) 1865 public static final String SP_ENCOUNTER = "encounter"; 1866 /** 1867 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 1868 * <p> 1869 * Description: <b>Multiple Resources: 1870 1871* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 1872* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 1873* [ChargeItem](chargeitem.html): Encounter associated with event 1874* [Claim](claim.html): Encounters associated with a billed line item 1875* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 1876* [Communication](communication.html): The Encounter during which this Communication was created 1877* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 1878* [Composition](composition.html): Context of the Composition 1879* [Condition](condition.html): The Encounter during which this Condition was created 1880* [DeviceRequest](devicerequest.html): Encounter during which request was created 1881* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 1882* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 1883* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 1884* [Flag](flag.html): Alert relevant during encounter 1885* [ImagingStudy](imagingstudy.html): The context of the study 1886* [List](list.html): Context in which list created 1887* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 1888* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 1889* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 1890* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 1891* [Observation](observation.html): Encounter related to the observation 1892* [Procedure](procedure.html): The Encounter during which this Procedure was created 1893* [Provenance](provenance.html): Encounter related to the Provenance 1894* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 1895* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 1896* [RiskAssessment](riskassessment.html): Where was assessment performed? 1897* [ServiceRequest](servicerequest.html): An encounter in which this request is made 1898* [Task](task.html): Search by encounter 1899* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 1900</b><br> 1901 * Type: <b>reference</b><br> 1902 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 1903 * </p> 1904 */ 1905 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 1906 1907/** 1908 * Constant for fluent queries to be used to add include statements. Specifies 1909 * the path value of "<b>List:encounter</b>". 1910 */ 1911 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("List:encounter").toLocked(); 1912 1913 /** 1914 * Search parameter: <b>identifier</b> 1915 * <p> 1916 * Description: <b>Multiple Resources: 1917 1918* [Account](account.html): Account number 1919* [AdverseEvent](adverseevent.html): Business identifier for the event 1920* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1921* [Appointment](appointment.html): An Identifier of the Appointment 1922* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1923* [Basic](basic.html): Business identifier 1924* [BodyStructure](bodystructure.html): Bodystructure identifier 1925* [CarePlan](careplan.html): External Ids for this plan 1926* [CareTeam](careteam.html): External Ids for this team 1927* [ChargeItem](chargeitem.html): Business Identifier for item 1928* [Claim](claim.html): The primary identifier of the financial resource 1929* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1930* [ClinicalImpression](clinicalimpression.html): Business identifier 1931* [Communication](communication.html): Unique identifier 1932* [CommunicationRequest](communicationrequest.html): Unique identifier 1933* [Composition](composition.html): Version-independent identifier for the Composition 1934* [Condition](condition.html): A unique identifier of the condition record 1935* [Consent](consent.html): Identifier for this record (external references) 1936* [Contract](contract.html): The identity of the contract 1937* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1938* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1939* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1940* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1941* [DeviceRequest](devicerequest.html): Business identifier for request/order 1942* [DeviceUsage](deviceusage.html): Search by identifier 1943* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1944* [DocumentReference](documentreference.html): Identifier of the attachment binary 1945* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1946* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1947* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1948* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1949* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1950* [Flag](flag.html): Business identifier 1951* [Goal](goal.html): External Ids for this goal 1952* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1953* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1954* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1955* [Immunization](immunization.html): Business identifier 1956* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1957* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1958* [Invoice](invoice.html): Business Identifier for item 1959* [List](list.html): Business identifier 1960* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1961* [Medication](medication.html): Returns medications with this external identifier 1962* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1963* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1964* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1965* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1966* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1967* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1968* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1969* [Observation](observation.html): The unique id for a particular observation 1970* [Person](person.html): A person Identifier 1971* [Procedure](procedure.html): A unique identifier for a procedure 1972* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1973* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1974* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1975* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1976* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1977* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1978* [Specimen](specimen.html): The unique identifier associated with the specimen 1979* [SupplyDelivery](supplydelivery.html): External identifier 1980* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1981* [Task](task.html): Search for a task instance by its business identifier 1982* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1983</b><br> 1984 * Type: <b>token</b><br> 1985 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1986 * </p> 1987 */ 1988 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 1989 public static final String SP_IDENTIFIER = "identifier"; 1990 /** 1991 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1992 * <p> 1993 * Description: <b>Multiple Resources: 1994 1995* [Account](account.html): Account number 1996* [AdverseEvent](adverseevent.html): Business identifier for the event 1997* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1998* [Appointment](appointment.html): An Identifier of the Appointment 1999* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2000* [Basic](basic.html): Business identifier 2001* [BodyStructure](bodystructure.html): Bodystructure identifier 2002* [CarePlan](careplan.html): External Ids for this plan 2003* [CareTeam](careteam.html): External Ids for this team 2004* [ChargeItem](chargeitem.html): Business Identifier for item 2005* [Claim](claim.html): The primary identifier of the financial resource 2006* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2007* [ClinicalImpression](clinicalimpression.html): Business identifier 2008* [Communication](communication.html): Unique identifier 2009* [CommunicationRequest](communicationrequest.html): Unique identifier 2010* [Composition](composition.html): Version-independent identifier for the Composition 2011* [Condition](condition.html): A unique identifier of the condition record 2012* [Consent](consent.html): Identifier for this record (external references) 2013* [Contract](contract.html): The identity of the contract 2014* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2015* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2016* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2017* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2018* [DeviceRequest](devicerequest.html): Business identifier for request/order 2019* [DeviceUsage](deviceusage.html): Search by identifier 2020* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2021* [DocumentReference](documentreference.html): Identifier of the attachment binary 2022* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2023* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2024* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2025* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2026* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2027* [Flag](flag.html): Business identifier 2028* [Goal](goal.html): External Ids for this goal 2029* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2030* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2031* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2032* [Immunization](immunization.html): Business identifier 2033* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2034* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2035* [Invoice](invoice.html): Business Identifier for item 2036* [List](list.html): Business identifier 2037* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2038* [Medication](medication.html): Returns medications with this external identifier 2039* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2040* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2041* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2042* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2043* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2044* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2045* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2046* [Observation](observation.html): The unique id for a particular observation 2047* [Person](person.html): A person Identifier 2048* [Procedure](procedure.html): A unique identifier for a procedure 2049* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2050* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2051* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2052* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2053* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2054* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2055* [Specimen](specimen.html): The unique identifier associated with the specimen 2056* [SupplyDelivery](supplydelivery.html): External identifier 2057* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2058* [Task](task.html): Search for a task instance by its business identifier 2059* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2060</b><br> 2061 * Type: <b>token</b><br> 2062 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2063 * </p> 2064 */ 2065 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2066 2067 /** 2068 * Search parameter: <b>patient</b> 2069 * <p> 2070 * Description: <b>Multiple Resources: 2071 2072* [Account](account.html): The entity that caused the expenses 2073* [AdverseEvent](adverseevent.html): Subject impacted by event 2074* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2075* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2076* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2077* [AuditEvent](auditevent.html): Where the activity involved patient data 2078* [Basic](basic.html): Identifies the focus of this resource 2079* [BodyStructure](bodystructure.html): Who this is about 2080* [CarePlan](careplan.html): Who the care plan is for 2081* [CareTeam](careteam.html): Who care team is for 2082* [ChargeItem](chargeitem.html): Individual service was done for/to 2083* [Claim](claim.html): Patient receiving the products or services 2084* [ClaimResponse](claimresponse.html): The subject of care 2085* [ClinicalImpression](clinicalimpression.html): Patient assessed 2086* [Communication](communication.html): Focus of message 2087* [CommunicationRequest](communicationrequest.html): Focus of message 2088* [Composition](composition.html): Who and/or what the composition is about 2089* [Condition](condition.html): Who has the condition? 2090* [Consent](consent.html): Who the consent applies to 2091* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2092* [Coverage](coverage.html): Retrieve coverages for a patient 2093* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2094* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2095* [DetectedIssue](detectedissue.html): Associated patient 2096* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2097* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2098* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2099* [DocumentReference](documentreference.html): Who/what is the subject of the document 2100* [Encounter](encounter.html): The patient present at the encounter 2101* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2102* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2103* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2104* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2105* [Flag](flag.html): The identity of a subject to list flags for 2106* [Goal](goal.html): Who this goal is intended for 2107* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2108* [ImagingSelection](imagingselection.html): Who the study is about 2109* [ImagingStudy](imagingstudy.html): Who the study is about 2110* [Immunization](immunization.html): The patient for the vaccination record 2111* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2112* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2113* [Invoice](invoice.html): Recipient(s) of goods and services 2114* [List](list.html): If all resources have the same subject 2115* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2116* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2117* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2118* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2119* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2120* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2121* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2122* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2123* [Observation](observation.html): The subject that the observation is about (if patient) 2124* [Person](person.html): The Person links to this Patient 2125* [Procedure](procedure.html): Search by subject - a patient 2126* [Provenance](provenance.html): Where the activity involved patient data 2127* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2128* [RelatedPerson](relatedperson.html): The patient this related person is related to 2129* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2130* [ResearchSubject](researchsubject.html): Who or what is part of study 2131* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2132* [ServiceRequest](servicerequest.html): Search by subject - a patient 2133* [Specimen](specimen.html): The patient the specimen comes from 2134* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2135* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2136* [Task](task.html): Search by patient 2137* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2138</b><br> 2139 * Type: <b>reference</b><br> 2140 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2141 * </p> 2142 */ 2143 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", target={Patient.class } ) 2144 public static final String SP_PATIENT = "patient"; 2145 /** 2146 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2147 * <p> 2148 * Description: <b>Multiple Resources: 2149 2150* [Account](account.html): The entity that caused the expenses 2151* [AdverseEvent](adverseevent.html): Subject impacted by event 2152* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 2153* [Appointment](appointment.html): One of the individuals of the appointment is this patient 2154* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 2155* [AuditEvent](auditevent.html): Where the activity involved patient data 2156* [Basic](basic.html): Identifies the focus of this resource 2157* [BodyStructure](bodystructure.html): Who this is about 2158* [CarePlan](careplan.html): Who the care plan is for 2159* [CareTeam](careteam.html): Who care team is for 2160* [ChargeItem](chargeitem.html): Individual service was done for/to 2161* [Claim](claim.html): Patient receiving the products or services 2162* [ClaimResponse](claimresponse.html): The subject of care 2163* [ClinicalImpression](clinicalimpression.html): Patient assessed 2164* [Communication](communication.html): Focus of message 2165* [CommunicationRequest](communicationrequest.html): Focus of message 2166* [Composition](composition.html): Who and/or what the composition is about 2167* [Condition](condition.html): Who has the condition? 2168* [Consent](consent.html): Who the consent applies to 2169* [Contract](contract.html): The identity of the subject of the contract (if a patient) 2170* [Coverage](coverage.html): Retrieve coverages for a patient 2171* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 2172* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 2173* [DetectedIssue](detectedissue.html): Associated patient 2174* [DeviceRequest](devicerequest.html): Individual the service is ordered for 2175* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 2176* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 2177* [DocumentReference](documentreference.html): Who/what is the subject of the document 2178* [Encounter](encounter.html): The patient present at the encounter 2179* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 2180* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 2181* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 2182* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 2183* [Flag](flag.html): The identity of a subject to list flags for 2184* [Goal](goal.html): Who this goal is intended for 2185* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 2186* [ImagingSelection](imagingselection.html): Who the study is about 2187* [ImagingStudy](imagingstudy.html): Who the study is about 2188* [Immunization](immunization.html): The patient for the vaccination record 2189* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 2190* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 2191* [Invoice](invoice.html): Recipient(s) of goods and services 2192* [List](list.html): If all resources have the same subject 2193* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 2194* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 2195* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 2196* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 2197* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 2198* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 2199* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 2200* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 2201* [Observation](observation.html): The subject that the observation is about (if patient) 2202* [Person](person.html): The Person links to this Patient 2203* [Procedure](procedure.html): Search by subject - a patient 2204* [Provenance](provenance.html): Where the activity involved patient data 2205* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 2206* [RelatedPerson](relatedperson.html): The patient this related person is related to 2207* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 2208* [ResearchSubject](researchsubject.html): Who or what is part of study 2209* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 2210* [ServiceRequest](servicerequest.html): Search by subject - a patient 2211* [Specimen](specimen.html): The patient the specimen comes from 2212* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 2213* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 2214* [Task](task.html): Search by patient 2215* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 2216</b><br> 2217 * Type: <b>reference</b><br> 2218 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 2219 * </p> 2220 */ 2221 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2222 2223/** 2224 * Constant for fluent queries to be used to add include statements. Specifies 2225 * the path value of "<b>List:patient</b>". 2226 */ 2227 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("List:patient").toLocked(); 2228 2229 2230} 2231