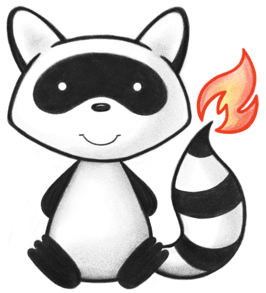
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import java.math.*; 038import org.hl7.fhir.utilities.Utilities; 039import org.hl7.fhir.r5.model.Enumerations.*; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.ICompositeType; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.Block; 050 051/** 052 * Details and position information for a place where services are provided and resources and participants may be stored, found, contained, or accommodated. 053 */ 054@ResourceDef(name="Location", profile="http://hl7.org/fhir/StructureDefinition/Location") 055public class Location extends DomainResource { 056 057 public enum LocationMode { 058 /** 059 * The Location resource represents a specific instance of a location (e.g. Operating Theatre 1A). 060 */ 061 INSTANCE, 062 /** 063 * The Location represents a class of locations (e.g. Any Operating Theatre) although this class of locations could be constrained within a specific boundary (such as organization, or parent location, address etc.). 064 */ 065 KIND, 066 /** 067 * added to help the parsers with the generic types 068 */ 069 NULL; 070 public static LocationMode fromCode(String codeString) throws FHIRException { 071 if (codeString == null || "".equals(codeString)) 072 return null; 073 if ("instance".equals(codeString)) 074 return INSTANCE; 075 if ("kind".equals(codeString)) 076 return KIND; 077 if (Configuration.isAcceptInvalidEnums()) 078 return null; 079 else 080 throw new FHIRException("Unknown LocationMode code '"+codeString+"'"); 081 } 082 public String toCode() { 083 switch (this) { 084 case INSTANCE: return "instance"; 085 case KIND: return "kind"; 086 case NULL: return null; 087 default: return "?"; 088 } 089 } 090 public String getSystem() { 091 switch (this) { 092 case INSTANCE: return "http://hl7.org/fhir/location-mode"; 093 case KIND: return "http://hl7.org/fhir/location-mode"; 094 case NULL: return null; 095 default: return "?"; 096 } 097 } 098 public String getDefinition() { 099 switch (this) { 100 case INSTANCE: return "The Location resource represents a specific instance of a location (e.g. Operating Theatre 1A)."; 101 case KIND: return "The Location represents a class of locations (e.g. Any Operating Theatre) although this class of locations could be constrained within a specific boundary (such as organization, or parent location, address etc.)."; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getDisplay() { 107 switch (this) { 108 case INSTANCE: return "Instance"; 109 case KIND: return "Kind"; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 } 115 116 public static class LocationModeEnumFactory implements EnumFactory<LocationMode> { 117 public LocationMode fromCode(String codeString) throws IllegalArgumentException { 118 if (codeString == null || "".equals(codeString)) 119 if (codeString == null || "".equals(codeString)) 120 return null; 121 if ("instance".equals(codeString)) 122 return LocationMode.INSTANCE; 123 if ("kind".equals(codeString)) 124 return LocationMode.KIND; 125 throw new IllegalArgumentException("Unknown LocationMode code '"+codeString+"'"); 126 } 127 public Enumeration<LocationMode> fromType(PrimitiveType<?> code) throws FHIRException { 128 if (code == null) 129 return null; 130 if (code.isEmpty()) 131 return new Enumeration<LocationMode>(this, LocationMode.NULL, code); 132 String codeString = ((PrimitiveType) code).asStringValue(); 133 if (codeString == null || "".equals(codeString)) 134 return new Enumeration<LocationMode>(this, LocationMode.NULL, code); 135 if ("instance".equals(codeString)) 136 return new Enumeration<LocationMode>(this, LocationMode.INSTANCE, code); 137 if ("kind".equals(codeString)) 138 return new Enumeration<LocationMode>(this, LocationMode.KIND, code); 139 throw new FHIRException("Unknown LocationMode code '"+codeString+"'"); 140 } 141 public String toCode(LocationMode code) { 142 if (code == LocationMode.NULL) 143 return null; 144 if (code == LocationMode.INSTANCE) 145 return "instance"; 146 if (code == LocationMode.KIND) 147 return "kind"; 148 return "?"; 149 } 150 public String toSystem(LocationMode code) { 151 return code.getSystem(); 152 } 153 } 154 155 public enum LocationStatus { 156 /** 157 * The location is operational. 158 */ 159 ACTIVE, 160 /** 161 * The location is temporarily closed. 162 */ 163 SUSPENDED, 164 /** 165 * The location is no longer used. 166 */ 167 INACTIVE, 168 /** 169 * added to help the parsers with the generic types 170 */ 171 NULL; 172 public static LocationStatus fromCode(String codeString) throws FHIRException { 173 if (codeString == null || "".equals(codeString)) 174 return null; 175 if ("active".equals(codeString)) 176 return ACTIVE; 177 if ("suspended".equals(codeString)) 178 return SUSPENDED; 179 if ("inactive".equals(codeString)) 180 return INACTIVE; 181 if (Configuration.isAcceptInvalidEnums()) 182 return null; 183 else 184 throw new FHIRException("Unknown LocationStatus code '"+codeString+"'"); 185 } 186 public String toCode() { 187 switch (this) { 188 case ACTIVE: return "active"; 189 case SUSPENDED: return "suspended"; 190 case INACTIVE: return "inactive"; 191 case NULL: return null; 192 default: return "?"; 193 } 194 } 195 public String getSystem() { 196 switch (this) { 197 case ACTIVE: return "http://hl7.org/fhir/location-status"; 198 case SUSPENDED: return "http://hl7.org/fhir/location-status"; 199 case INACTIVE: return "http://hl7.org/fhir/location-status"; 200 case NULL: return null; 201 default: return "?"; 202 } 203 } 204 public String getDefinition() { 205 switch (this) { 206 case ACTIVE: return "The location is operational."; 207 case SUSPENDED: return "The location is temporarily closed."; 208 case INACTIVE: return "The location is no longer used."; 209 case NULL: return null; 210 default: return "?"; 211 } 212 } 213 public String getDisplay() { 214 switch (this) { 215 case ACTIVE: return "Active"; 216 case SUSPENDED: return "Suspended"; 217 case INACTIVE: return "Inactive"; 218 case NULL: return null; 219 default: return "?"; 220 } 221 } 222 } 223 224 public static class LocationStatusEnumFactory implements EnumFactory<LocationStatus> { 225 public LocationStatus fromCode(String codeString) throws IllegalArgumentException { 226 if (codeString == null || "".equals(codeString)) 227 if (codeString == null || "".equals(codeString)) 228 return null; 229 if ("active".equals(codeString)) 230 return LocationStatus.ACTIVE; 231 if ("suspended".equals(codeString)) 232 return LocationStatus.SUSPENDED; 233 if ("inactive".equals(codeString)) 234 return LocationStatus.INACTIVE; 235 throw new IllegalArgumentException("Unknown LocationStatus code '"+codeString+"'"); 236 } 237 public Enumeration<LocationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 238 if (code == null) 239 return null; 240 if (code.isEmpty()) 241 return new Enumeration<LocationStatus>(this, LocationStatus.NULL, code); 242 String codeString = ((PrimitiveType) code).asStringValue(); 243 if (codeString == null || "".equals(codeString)) 244 return new Enumeration<LocationStatus>(this, LocationStatus.NULL, code); 245 if ("active".equals(codeString)) 246 return new Enumeration<LocationStatus>(this, LocationStatus.ACTIVE, code); 247 if ("suspended".equals(codeString)) 248 return new Enumeration<LocationStatus>(this, LocationStatus.SUSPENDED, code); 249 if ("inactive".equals(codeString)) 250 return new Enumeration<LocationStatus>(this, LocationStatus.INACTIVE, code); 251 throw new FHIRException("Unknown LocationStatus code '"+codeString+"'"); 252 } 253 public String toCode(LocationStatus code) { 254 if (code == LocationStatus.NULL) 255 return null; 256 if (code == LocationStatus.ACTIVE) 257 return "active"; 258 if (code == LocationStatus.SUSPENDED) 259 return "suspended"; 260 if (code == LocationStatus.INACTIVE) 261 return "inactive"; 262 return "?"; 263 } 264 public String toSystem(LocationStatus code) { 265 return code.getSystem(); 266 } 267 } 268 269 @Block() 270 public static class LocationPositionComponent extends BackboneElement implements IBaseBackboneElement { 271 /** 272 * Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes on Location main page). 273 */ 274 @Child(name = "longitude", type = {DecimalType.class}, order=1, min=1, max=1, modifier=false, summary=false) 275 @Description(shortDefinition="Longitude with WGS84 datum", formalDefinition="Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes on Location main page)." ) 276 protected DecimalType longitude; 277 278 /** 279 * Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes on Location main page). 280 */ 281 @Child(name = "latitude", type = {DecimalType.class}, order=2, min=1, max=1, modifier=false, summary=false) 282 @Description(shortDefinition="Latitude with WGS84 datum", formalDefinition="Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes on Location main page)." ) 283 protected DecimalType latitude; 284 285 /** 286 * Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes on Location main page). 287 */ 288 @Child(name = "altitude", type = {DecimalType.class}, order=3, min=0, max=1, modifier=false, summary=false) 289 @Description(shortDefinition="Altitude with WGS84 datum", formalDefinition="Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes on Location main page)." ) 290 protected DecimalType altitude; 291 292 private static final long serialVersionUID = -74276134L; 293 294 /** 295 * Constructor 296 */ 297 public LocationPositionComponent() { 298 super(); 299 } 300 301 /** 302 * Constructor 303 */ 304 public LocationPositionComponent(BigDecimal longitude, BigDecimal latitude) { 305 super(); 306 this.setLongitude(longitude); 307 this.setLatitude(latitude); 308 } 309 310 /** 311 * @return {@link #longitude} (Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes on Location main page).). This is the underlying object with id, value and extensions. The accessor "getLongitude" gives direct access to the value 312 */ 313 public DecimalType getLongitudeElement() { 314 if (this.longitude == null) 315 if (Configuration.errorOnAutoCreate()) 316 throw new Error("Attempt to auto-create LocationPositionComponent.longitude"); 317 else if (Configuration.doAutoCreate()) 318 this.longitude = new DecimalType(); // bb 319 return this.longitude; 320 } 321 322 public boolean hasLongitudeElement() { 323 return this.longitude != null && !this.longitude.isEmpty(); 324 } 325 326 public boolean hasLongitude() { 327 return this.longitude != null && !this.longitude.isEmpty(); 328 } 329 330 /** 331 * @param value {@link #longitude} (Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes on Location main page).). This is the underlying object with id, value and extensions. The accessor "getLongitude" gives direct access to the value 332 */ 333 public LocationPositionComponent setLongitudeElement(DecimalType value) { 334 this.longitude = value; 335 return this; 336 } 337 338 /** 339 * @return Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes on Location main page). 340 */ 341 public BigDecimal getLongitude() { 342 return this.longitude == null ? null : this.longitude.getValue(); 343 } 344 345 /** 346 * @param value Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes on Location main page). 347 */ 348 public LocationPositionComponent setLongitude(BigDecimal value) { 349 if (this.longitude == null) 350 this.longitude = new DecimalType(); 351 this.longitude.setValue(value); 352 return this; 353 } 354 355 /** 356 * @param value Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes on Location main page). 357 */ 358 public LocationPositionComponent setLongitude(long value) { 359 this.longitude = new DecimalType(); 360 this.longitude.setValue(value); 361 return this; 362 } 363 364 /** 365 * @param value Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes on Location main page). 366 */ 367 public LocationPositionComponent setLongitude(double value) { 368 this.longitude = new DecimalType(); 369 this.longitude.setValue(value); 370 return this; 371 } 372 373 /** 374 * @return {@link #latitude} (Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes on Location main page).). This is the underlying object with id, value and extensions. The accessor "getLatitude" gives direct access to the value 375 */ 376 public DecimalType getLatitudeElement() { 377 if (this.latitude == null) 378 if (Configuration.errorOnAutoCreate()) 379 throw new Error("Attempt to auto-create LocationPositionComponent.latitude"); 380 else if (Configuration.doAutoCreate()) 381 this.latitude = new DecimalType(); // bb 382 return this.latitude; 383 } 384 385 public boolean hasLatitudeElement() { 386 return this.latitude != null && !this.latitude.isEmpty(); 387 } 388 389 public boolean hasLatitude() { 390 return this.latitude != null && !this.latitude.isEmpty(); 391 } 392 393 /** 394 * @param value {@link #latitude} (Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes on Location main page).). This is the underlying object with id, value and extensions. The accessor "getLatitude" gives direct access to the value 395 */ 396 public LocationPositionComponent setLatitudeElement(DecimalType value) { 397 this.latitude = value; 398 return this; 399 } 400 401 /** 402 * @return Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes on Location main page). 403 */ 404 public BigDecimal getLatitude() { 405 return this.latitude == null ? null : this.latitude.getValue(); 406 } 407 408 /** 409 * @param value Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes on Location main page). 410 */ 411 public LocationPositionComponent setLatitude(BigDecimal value) { 412 if (this.latitude == null) 413 this.latitude = new DecimalType(); 414 this.latitude.setValue(value); 415 return this; 416 } 417 418 /** 419 * @param value Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes on Location main page). 420 */ 421 public LocationPositionComponent setLatitude(long value) { 422 this.latitude = new DecimalType(); 423 this.latitude.setValue(value); 424 return this; 425 } 426 427 /** 428 * @param value Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes on Location main page). 429 */ 430 public LocationPositionComponent setLatitude(double value) { 431 this.latitude = new DecimalType(); 432 this.latitude.setValue(value); 433 return this; 434 } 435 436 /** 437 * @return {@link #altitude} (Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes on Location main page).). This is the underlying object with id, value and extensions. The accessor "getAltitude" gives direct access to the value 438 */ 439 public DecimalType getAltitudeElement() { 440 if (this.altitude == null) 441 if (Configuration.errorOnAutoCreate()) 442 throw new Error("Attempt to auto-create LocationPositionComponent.altitude"); 443 else if (Configuration.doAutoCreate()) 444 this.altitude = new DecimalType(); // bb 445 return this.altitude; 446 } 447 448 public boolean hasAltitudeElement() { 449 return this.altitude != null && !this.altitude.isEmpty(); 450 } 451 452 public boolean hasAltitude() { 453 return this.altitude != null && !this.altitude.isEmpty(); 454 } 455 456 /** 457 * @param value {@link #altitude} (Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes on Location main page).). This is the underlying object with id, value and extensions. The accessor "getAltitude" gives direct access to the value 458 */ 459 public LocationPositionComponent setAltitudeElement(DecimalType value) { 460 this.altitude = value; 461 return this; 462 } 463 464 /** 465 * @return Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes on Location main page). 466 */ 467 public BigDecimal getAltitude() { 468 return this.altitude == null ? null : this.altitude.getValue(); 469 } 470 471 /** 472 * @param value Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes on Location main page). 473 */ 474 public LocationPositionComponent setAltitude(BigDecimal value) { 475 if (value == null) 476 this.altitude = null; 477 else { 478 if (this.altitude == null) 479 this.altitude = new DecimalType(); 480 this.altitude.setValue(value); 481 } 482 return this; 483 } 484 485 /** 486 * @param value Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes on Location main page). 487 */ 488 public LocationPositionComponent setAltitude(long value) { 489 this.altitude = new DecimalType(); 490 this.altitude.setValue(value); 491 return this; 492 } 493 494 /** 495 * @param value Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes on Location main page). 496 */ 497 public LocationPositionComponent setAltitude(double value) { 498 this.altitude = new DecimalType(); 499 this.altitude.setValue(value); 500 return this; 501 } 502 503 protected void listChildren(List<Property> children) { 504 super.listChildren(children); 505 children.add(new Property("longitude", "decimal", "Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes on Location main page).", 0, 1, longitude)); 506 children.add(new Property("latitude", "decimal", "Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes on Location main page).", 0, 1, latitude)); 507 children.add(new Property("altitude", "decimal", "Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes on Location main page).", 0, 1, altitude)); 508 } 509 510 @Override 511 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 512 switch (_hash) { 513 case 137365935: /*longitude*/ return new Property("longitude", "decimal", "Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes on Location main page).", 0, 1, longitude); 514 case -1439978388: /*latitude*/ return new Property("latitude", "decimal", "Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes on Location main page).", 0, 1, latitude); 515 case 2036550306: /*altitude*/ return new Property("altitude", "decimal", "Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes on Location main page).", 0, 1, altitude); 516 default: return super.getNamedProperty(_hash, _name, _checkValid); 517 } 518 519 } 520 521 @Override 522 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 523 switch (hash) { 524 case 137365935: /*longitude*/ return this.longitude == null ? new Base[0] : new Base[] {this.longitude}; // DecimalType 525 case -1439978388: /*latitude*/ return this.latitude == null ? new Base[0] : new Base[] {this.latitude}; // DecimalType 526 case 2036550306: /*altitude*/ return this.altitude == null ? new Base[0] : new Base[] {this.altitude}; // DecimalType 527 default: return super.getProperty(hash, name, checkValid); 528 } 529 530 } 531 532 @Override 533 public Base setProperty(int hash, String name, Base value) throws FHIRException { 534 switch (hash) { 535 case 137365935: // longitude 536 this.longitude = TypeConvertor.castToDecimal(value); // DecimalType 537 return value; 538 case -1439978388: // latitude 539 this.latitude = TypeConvertor.castToDecimal(value); // DecimalType 540 return value; 541 case 2036550306: // altitude 542 this.altitude = TypeConvertor.castToDecimal(value); // DecimalType 543 return value; 544 default: return super.setProperty(hash, name, value); 545 } 546 547 } 548 549 @Override 550 public Base setProperty(String name, Base value) throws FHIRException { 551 if (name.equals("longitude")) { 552 this.longitude = TypeConvertor.castToDecimal(value); // DecimalType 553 } else if (name.equals("latitude")) { 554 this.latitude = TypeConvertor.castToDecimal(value); // DecimalType 555 } else if (name.equals("altitude")) { 556 this.altitude = TypeConvertor.castToDecimal(value); // DecimalType 557 } else 558 return super.setProperty(name, value); 559 return value; 560 } 561 562 @Override 563 public void removeChild(String name, Base value) throws FHIRException { 564 if (name.equals("longitude")) { 565 this.longitude = null; 566 } else if (name.equals("latitude")) { 567 this.latitude = null; 568 } else if (name.equals("altitude")) { 569 this.altitude = null; 570 } else 571 super.removeChild(name, value); 572 573 } 574 575 @Override 576 public Base makeProperty(int hash, String name) throws FHIRException { 577 switch (hash) { 578 case 137365935: return getLongitudeElement(); 579 case -1439978388: return getLatitudeElement(); 580 case 2036550306: return getAltitudeElement(); 581 default: return super.makeProperty(hash, name); 582 } 583 584 } 585 586 @Override 587 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 588 switch (hash) { 589 case 137365935: /*longitude*/ return new String[] {"decimal"}; 590 case -1439978388: /*latitude*/ return new String[] {"decimal"}; 591 case 2036550306: /*altitude*/ return new String[] {"decimal"}; 592 default: return super.getTypesForProperty(hash, name); 593 } 594 595 } 596 597 @Override 598 public Base addChild(String name) throws FHIRException { 599 if (name.equals("longitude")) { 600 throw new FHIRException("Cannot call addChild on a singleton property Location.position.longitude"); 601 } 602 else if (name.equals("latitude")) { 603 throw new FHIRException("Cannot call addChild on a singleton property Location.position.latitude"); 604 } 605 else if (name.equals("altitude")) { 606 throw new FHIRException("Cannot call addChild on a singleton property Location.position.altitude"); 607 } 608 else 609 return super.addChild(name); 610 } 611 612 public LocationPositionComponent copy() { 613 LocationPositionComponent dst = new LocationPositionComponent(); 614 copyValues(dst); 615 return dst; 616 } 617 618 public void copyValues(LocationPositionComponent dst) { 619 super.copyValues(dst); 620 dst.longitude = longitude == null ? null : longitude.copy(); 621 dst.latitude = latitude == null ? null : latitude.copy(); 622 dst.altitude = altitude == null ? null : altitude.copy(); 623 } 624 625 @Override 626 public boolean equalsDeep(Base other_) { 627 if (!super.equalsDeep(other_)) 628 return false; 629 if (!(other_ instanceof LocationPositionComponent)) 630 return false; 631 LocationPositionComponent o = (LocationPositionComponent) other_; 632 return compareDeep(longitude, o.longitude, true) && compareDeep(latitude, o.latitude, true) && compareDeep(altitude, o.altitude, true) 633 ; 634 } 635 636 @Override 637 public boolean equalsShallow(Base other_) { 638 if (!super.equalsShallow(other_)) 639 return false; 640 if (!(other_ instanceof LocationPositionComponent)) 641 return false; 642 LocationPositionComponent o = (LocationPositionComponent) other_; 643 return compareValues(longitude, o.longitude, true) && compareValues(latitude, o.latitude, true) && compareValues(altitude, o.altitude, true) 644 ; 645 } 646 647 public boolean isEmpty() { 648 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(longitude, latitude, altitude 649 ); 650 } 651 652 public String fhirType() { 653 return "Location.position"; 654 655 } 656 657 } 658 659 /** 660 * Unique code or number identifying the location to its users. 661 */ 662 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 663 @Description(shortDefinition="Unique code or number identifying the location to its users", formalDefinition="Unique code or number identifying the location to its users." ) 664 protected List<Identifier> identifier; 665 666 /** 667 * The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location. 668 */ 669 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 670 @Description(shortDefinition="active | suspended | inactive", formalDefinition="The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location." ) 671 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/location-status") 672 protected Enumeration<LocationStatus> status; 673 674 /** 675 * The operational status covers operation values most relevant to beds (but can also apply to rooms/units/chairs/etc. such as an isolation unit/dialysis chair). This typically covers concepts such as contamination, housekeeping, and other activities like maintenance. 676 */ 677 @Child(name = "operationalStatus", type = {Coding.class}, order=2, min=0, max=1, modifier=false, summary=true) 678 @Description(shortDefinition="The operational status of the location (typically only for a bed/room)", formalDefinition="The operational status covers operation values most relevant to beds (but can also apply to rooms/units/chairs/etc. such as an isolation unit/dialysis chair). This typically covers concepts such as contamination, housekeeping, and other activities like maintenance." ) 679 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v2-0116") 680 protected Coding operationalStatus; 681 682 /** 683 * Name of the location as used by humans. Does not need to be unique. 684 */ 685 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 686 @Description(shortDefinition="Name of the location as used by humans", formalDefinition="Name of the location as used by humans. Does not need to be unique." ) 687 protected StringType name; 688 689 /** 690 * A list of alternate names that the location is known as, or was known as, in the past. 691 */ 692 @Child(name = "alias", type = {StringType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 693 @Description(shortDefinition="A list of alternate names that the location is known as, or was known as, in the past", formalDefinition="A list of alternate names that the location is known as, or was known as, in the past." ) 694 protected List<StringType> alias; 695 696 /** 697 * Description of the Location, which helps in finding or referencing the place. 698 */ 699 @Child(name = "description", type = {MarkdownType.class}, order=5, min=0, max=1, modifier=false, summary=true) 700 @Description(shortDefinition="Additional details about the location that could be displayed as further information to identify the location beyond its name", formalDefinition="Description of the Location, which helps in finding or referencing the place." ) 701 protected MarkdownType description; 702 703 /** 704 * Indicates whether a resource instance represents a specific location or a class of locations. 705 */ 706 @Child(name = "mode", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 707 @Description(shortDefinition="instance | kind", formalDefinition="Indicates whether a resource instance represents a specific location or a class of locations." ) 708 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/location-mode") 709 protected Enumeration<LocationMode> mode; 710 711 /** 712 * Indicates the type of function performed at the location. 713 */ 714 @Child(name = "type", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 715 @Description(shortDefinition="Type of function performed", formalDefinition="Indicates the type of function performed at the location." ) 716 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ServiceDeliveryLocationRoleType") 717 protected List<CodeableConcept> type; 718 719 /** 720 * The contact details of communication devices available at the location. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites. 721 */ 722 @Child(name = "contact", type = {ExtendedContactDetail.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 723 @Description(shortDefinition="Official contact details for the location", formalDefinition="The contact details of communication devices available at the location. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites." ) 724 protected List<ExtendedContactDetail> contact; 725 726 /** 727 * Physical location. 728 */ 729 @Child(name = "address", type = {Address.class}, order=9, min=0, max=1, modifier=false, summary=false) 730 @Description(shortDefinition="Physical location", formalDefinition="Physical location." ) 731 protected Address address; 732 733 /** 734 * Physical form of the location, e.g. building, room, vehicle, road, virtual. 735 */ 736 @Child(name = "form", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=true) 737 @Description(shortDefinition="Physical form of the location", formalDefinition="Physical form of the location, e.g. building, room, vehicle, road, virtual." ) 738 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/location-form") 739 protected CodeableConcept form; 740 741 /** 742 * The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML). 743 */ 744 @Child(name = "position", type = {}, order=11, min=0, max=1, modifier=false, summary=false) 745 @Description(shortDefinition="The absolute geographic location", formalDefinition="The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML)." ) 746 protected LocationPositionComponent position; 747 748 /** 749 * The organization responsible for the provisioning and upkeep of the location. 750 */ 751 @Child(name = "managingOrganization", type = {Organization.class}, order=12, min=0, max=1, modifier=false, summary=true) 752 @Description(shortDefinition="Organization responsible for provisioning and upkeep", formalDefinition="The organization responsible for the provisioning and upkeep of the location." ) 753 protected Reference managingOrganization; 754 755 /** 756 * Another Location of which this Location is physically a part of. 757 */ 758 @Child(name = "partOf", type = {Location.class}, order=13, min=0, max=1, modifier=false, summary=false) 759 @Description(shortDefinition="Another Location this one is physically a part of", formalDefinition="Another Location of which this Location is physically a part of." ) 760 protected Reference partOf; 761 762 /** 763 * Collection of characteristics (attributes). 764 */ 765 @Child(name = "characteristic", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 766 @Description(shortDefinition="Collection of characteristics (attributes)", formalDefinition="Collection of characteristics (attributes)." ) 767 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/location-characteristic") 768 protected List<CodeableConcept> characteristic; 769 770 /** 771 * What days/times during a week is this location usually open, and any exceptions where the location is not available. 772 */ 773 @Child(name = "hoursOfOperation", type = {Availability.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 774 @Description(shortDefinition="What days/times during a week is this location usually open (including exceptions)", formalDefinition="What days/times during a week is this location usually open, and any exceptions where the location is not available." ) 775 protected List<Availability> hoursOfOperation; 776 777 /** 778 * Connection details of a virtual service (e.g. shared conference call facility with dedicated number/details). 779 */ 780 @Child(name = "virtualService", type = {VirtualServiceDetail.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 781 @Description(shortDefinition="Connection details of a virtual service (e.g. conference call)", formalDefinition="Connection details of a virtual service (e.g. shared conference call facility with dedicated number/details)." ) 782 protected List<VirtualServiceDetail> virtualService; 783 784 /** 785 * Technical endpoints providing access to services operated for the location. 786 */ 787 @Child(name = "endpoint", type = {Endpoint.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 788 @Description(shortDefinition="Technical endpoints providing access to services operated for the location", formalDefinition="Technical endpoints providing access to services operated for the location." ) 789 protected List<Reference> endpoint; 790 791 private static final long serialVersionUID = 1238993068L; 792 793 /** 794 * Constructor 795 */ 796 public Location() { 797 super(); 798 } 799 800 /** 801 * @return {@link #identifier} (Unique code or number identifying the location to its users.) 802 */ 803 public List<Identifier> getIdentifier() { 804 if (this.identifier == null) 805 this.identifier = new ArrayList<Identifier>(); 806 return this.identifier; 807 } 808 809 /** 810 * @return Returns a reference to <code>this</code> for easy method chaining 811 */ 812 public Location setIdentifier(List<Identifier> theIdentifier) { 813 this.identifier = theIdentifier; 814 return this; 815 } 816 817 public boolean hasIdentifier() { 818 if (this.identifier == null) 819 return false; 820 for (Identifier item : this.identifier) 821 if (!item.isEmpty()) 822 return true; 823 return false; 824 } 825 826 public Identifier addIdentifier() { //3 827 Identifier t = new Identifier(); 828 if (this.identifier == null) 829 this.identifier = new ArrayList<Identifier>(); 830 this.identifier.add(t); 831 return t; 832 } 833 834 public Location addIdentifier(Identifier t) { //3 835 if (t == null) 836 return this; 837 if (this.identifier == null) 838 this.identifier = new ArrayList<Identifier>(); 839 this.identifier.add(t); 840 return this; 841 } 842 843 /** 844 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 845 */ 846 public Identifier getIdentifierFirstRep() { 847 if (getIdentifier().isEmpty()) { 848 addIdentifier(); 849 } 850 return getIdentifier().get(0); 851 } 852 853 /** 854 * @return {@link #status} (The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 855 */ 856 public Enumeration<LocationStatus> getStatusElement() { 857 if (this.status == null) 858 if (Configuration.errorOnAutoCreate()) 859 throw new Error("Attempt to auto-create Location.status"); 860 else if (Configuration.doAutoCreate()) 861 this.status = new Enumeration<LocationStatus>(new LocationStatusEnumFactory()); // bb 862 return this.status; 863 } 864 865 public boolean hasStatusElement() { 866 return this.status != null && !this.status.isEmpty(); 867 } 868 869 public boolean hasStatus() { 870 return this.status != null && !this.status.isEmpty(); 871 } 872 873 /** 874 * @param value {@link #status} (The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 875 */ 876 public Location setStatusElement(Enumeration<LocationStatus> value) { 877 this.status = value; 878 return this; 879 } 880 881 /** 882 * @return The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location. 883 */ 884 public LocationStatus getStatus() { 885 return this.status == null ? null : this.status.getValue(); 886 } 887 888 /** 889 * @param value The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location. 890 */ 891 public Location setStatus(LocationStatus value) { 892 if (value == null) 893 this.status = null; 894 else { 895 if (this.status == null) 896 this.status = new Enumeration<LocationStatus>(new LocationStatusEnumFactory()); 897 this.status.setValue(value); 898 } 899 return this; 900 } 901 902 /** 903 * @return {@link #operationalStatus} (The operational status covers operation values most relevant to beds (but can also apply to rooms/units/chairs/etc. such as an isolation unit/dialysis chair). This typically covers concepts such as contamination, housekeeping, and other activities like maintenance.) 904 */ 905 public Coding getOperationalStatus() { 906 if (this.operationalStatus == null) 907 if (Configuration.errorOnAutoCreate()) 908 throw new Error("Attempt to auto-create Location.operationalStatus"); 909 else if (Configuration.doAutoCreate()) 910 this.operationalStatus = new Coding(); // cc 911 return this.operationalStatus; 912 } 913 914 public boolean hasOperationalStatus() { 915 return this.operationalStatus != null && !this.operationalStatus.isEmpty(); 916 } 917 918 /** 919 * @param value {@link #operationalStatus} (The operational status covers operation values most relevant to beds (but can also apply to rooms/units/chairs/etc. such as an isolation unit/dialysis chair). This typically covers concepts such as contamination, housekeeping, and other activities like maintenance.) 920 */ 921 public Location setOperationalStatus(Coding value) { 922 this.operationalStatus = value; 923 return this; 924 } 925 926 /** 927 * @return {@link #name} (Name of the location as used by humans. Does not need to be unique.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 928 */ 929 public StringType getNameElement() { 930 if (this.name == null) 931 if (Configuration.errorOnAutoCreate()) 932 throw new Error("Attempt to auto-create Location.name"); 933 else if (Configuration.doAutoCreate()) 934 this.name = new StringType(); // bb 935 return this.name; 936 } 937 938 public boolean hasNameElement() { 939 return this.name != null && !this.name.isEmpty(); 940 } 941 942 public boolean hasName() { 943 return this.name != null && !this.name.isEmpty(); 944 } 945 946 /** 947 * @param value {@link #name} (Name of the location as used by humans. Does not need to be unique.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 948 */ 949 public Location setNameElement(StringType value) { 950 this.name = value; 951 return this; 952 } 953 954 /** 955 * @return Name of the location as used by humans. Does not need to be unique. 956 */ 957 public String getName() { 958 return this.name == null ? null : this.name.getValue(); 959 } 960 961 /** 962 * @param value Name of the location as used by humans. Does not need to be unique. 963 */ 964 public Location setName(String value) { 965 if (Utilities.noString(value)) 966 this.name = null; 967 else { 968 if (this.name == null) 969 this.name = new StringType(); 970 this.name.setValue(value); 971 } 972 return this; 973 } 974 975 /** 976 * @return {@link #alias} (A list of alternate names that the location is known as, or was known as, in the past.) 977 */ 978 public List<StringType> getAlias() { 979 if (this.alias == null) 980 this.alias = new ArrayList<StringType>(); 981 return this.alias; 982 } 983 984 /** 985 * @return Returns a reference to <code>this</code> for easy method chaining 986 */ 987 public Location setAlias(List<StringType> theAlias) { 988 this.alias = theAlias; 989 return this; 990 } 991 992 public boolean hasAlias() { 993 if (this.alias == null) 994 return false; 995 for (StringType item : this.alias) 996 if (!item.isEmpty()) 997 return true; 998 return false; 999 } 1000 1001 /** 1002 * @return {@link #alias} (A list of alternate names that the location is known as, or was known as, in the past.) 1003 */ 1004 public StringType addAliasElement() {//2 1005 StringType t = new StringType(); 1006 if (this.alias == null) 1007 this.alias = new ArrayList<StringType>(); 1008 this.alias.add(t); 1009 return t; 1010 } 1011 1012 /** 1013 * @param value {@link #alias} (A list of alternate names that the location is known as, or was known as, in the past.) 1014 */ 1015 public Location addAlias(String value) { //1 1016 StringType t = new StringType(); 1017 t.setValue(value); 1018 if (this.alias == null) 1019 this.alias = new ArrayList<StringType>(); 1020 this.alias.add(t); 1021 return this; 1022 } 1023 1024 /** 1025 * @param value {@link #alias} (A list of alternate names that the location is known as, or was known as, in the past.) 1026 */ 1027 public boolean hasAlias(String value) { 1028 if (this.alias == null) 1029 return false; 1030 for (StringType v : this.alias) 1031 if (v.getValue().equals(value)) // string 1032 return true; 1033 return false; 1034 } 1035 1036 /** 1037 * @return {@link #description} (Description of the Location, which helps in finding or referencing the place.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1038 */ 1039 public MarkdownType getDescriptionElement() { 1040 if (this.description == null) 1041 if (Configuration.errorOnAutoCreate()) 1042 throw new Error("Attempt to auto-create Location.description"); 1043 else if (Configuration.doAutoCreate()) 1044 this.description = new MarkdownType(); // bb 1045 return this.description; 1046 } 1047 1048 public boolean hasDescriptionElement() { 1049 return this.description != null && !this.description.isEmpty(); 1050 } 1051 1052 public boolean hasDescription() { 1053 return this.description != null && !this.description.isEmpty(); 1054 } 1055 1056 /** 1057 * @param value {@link #description} (Description of the Location, which helps in finding or referencing the place.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1058 */ 1059 public Location setDescriptionElement(MarkdownType value) { 1060 this.description = value; 1061 return this; 1062 } 1063 1064 /** 1065 * @return Description of the Location, which helps in finding or referencing the place. 1066 */ 1067 public String getDescription() { 1068 return this.description == null ? null : this.description.getValue(); 1069 } 1070 1071 /** 1072 * @param value Description of the Location, which helps in finding or referencing the place. 1073 */ 1074 public Location setDescription(String value) { 1075 if (Utilities.noString(value)) 1076 this.description = null; 1077 else { 1078 if (this.description == null) 1079 this.description = new MarkdownType(); 1080 this.description.setValue(value); 1081 } 1082 return this; 1083 } 1084 1085 /** 1086 * @return {@link #mode} (Indicates whether a resource instance represents a specific location or a class of locations.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1087 */ 1088 public Enumeration<LocationMode> getModeElement() { 1089 if (this.mode == null) 1090 if (Configuration.errorOnAutoCreate()) 1091 throw new Error("Attempt to auto-create Location.mode"); 1092 else if (Configuration.doAutoCreate()) 1093 this.mode = new Enumeration<LocationMode>(new LocationModeEnumFactory()); // bb 1094 return this.mode; 1095 } 1096 1097 public boolean hasModeElement() { 1098 return this.mode != null && !this.mode.isEmpty(); 1099 } 1100 1101 public boolean hasMode() { 1102 return this.mode != null && !this.mode.isEmpty(); 1103 } 1104 1105 /** 1106 * @param value {@link #mode} (Indicates whether a resource instance represents a specific location or a class of locations.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1107 */ 1108 public Location setModeElement(Enumeration<LocationMode> value) { 1109 this.mode = value; 1110 return this; 1111 } 1112 1113 /** 1114 * @return Indicates whether a resource instance represents a specific location or a class of locations. 1115 */ 1116 public LocationMode getMode() { 1117 return this.mode == null ? null : this.mode.getValue(); 1118 } 1119 1120 /** 1121 * @param value Indicates whether a resource instance represents a specific location or a class of locations. 1122 */ 1123 public Location setMode(LocationMode value) { 1124 if (value == null) 1125 this.mode = null; 1126 else { 1127 if (this.mode == null) 1128 this.mode = new Enumeration<LocationMode>(new LocationModeEnumFactory()); 1129 this.mode.setValue(value); 1130 } 1131 return this; 1132 } 1133 1134 /** 1135 * @return {@link #type} (Indicates the type of function performed at the location.) 1136 */ 1137 public List<CodeableConcept> getType() { 1138 if (this.type == null) 1139 this.type = new ArrayList<CodeableConcept>(); 1140 return this.type; 1141 } 1142 1143 /** 1144 * @return Returns a reference to <code>this</code> for easy method chaining 1145 */ 1146 public Location setType(List<CodeableConcept> theType) { 1147 this.type = theType; 1148 return this; 1149 } 1150 1151 public boolean hasType() { 1152 if (this.type == null) 1153 return false; 1154 for (CodeableConcept item : this.type) 1155 if (!item.isEmpty()) 1156 return true; 1157 return false; 1158 } 1159 1160 public CodeableConcept addType() { //3 1161 CodeableConcept t = new CodeableConcept(); 1162 if (this.type == null) 1163 this.type = new ArrayList<CodeableConcept>(); 1164 this.type.add(t); 1165 return t; 1166 } 1167 1168 public Location addType(CodeableConcept t) { //3 1169 if (t == null) 1170 return this; 1171 if (this.type == null) 1172 this.type = new ArrayList<CodeableConcept>(); 1173 this.type.add(t); 1174 return this; 1175 } 1176 1177 /** 1178 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 1179 */ 1180 public CodeableConcept getTypeFirstRep() { 1181 if (getType().isEmpty()) { 1182 addType(); 1183 } 1184 return getType().get(0); 1185 } 1186 1187 /** 1188 * @return {@link #contact} (The contact details of communication devices available at the location. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites.) 1189 */ 1190 public List<ExtendedContactDetail> getContact() { 1191 if (this.contact == null) 1192 this.contact = new ArrayList<ExtendedContactDetail>(); 1193 return this.contact; 1194 } 1195 1196 /** 1197 * @return Returns a reference to <code>this</code> for easy method chaining 1198 */ 1199 public Location setContact(List<ExtendedContactDetail> theContact) { 1200 this.contact = theContact; 1201 return this; 1202 } 1203 1204 public boolean hasContact() { 1205 if (this.contact == null) 1206 return false; 1207 for (ExtendedContactDetail item : this.contact) 1208 if (!item.isEmpty()) 1209 return true; 1210 return false; 1211 } 1212 1213 public ExtendedContactDetail addContact() { //3 1214 ExtendedContactDetail t = new ExtendedContactDetail(); 1215 if (this.contact == null) 1216 this.contact = new ArrayList<ExtendedContactDetail>(); 1217 this.contact.add(t); 1218 return t; 1219 } 1220 1221 public Location addContact(ExtendedContactDetail t) { //3 1222 if (t == null) 1223 return this; 1224 if (this.contact == null) 1225 this.contact = new ArrayList<ExtendedContactDetail>(); 1226 this.contact.add(t); 1227 return this; 1228 } 1229 1230 /** 1231 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 1232 */ 1233 public ExtendedContactDetail getContactFirstRep() { 1234 if (getContact().isEmpty()) { 1235 addContact(); 1236 } 1237 return getContact().get(0); 1238 } 1239 1240 /** 1241 * @return {@link #address} (Physical location.) 1242 */ 1243 public Address getAddress() { 1244 if (this.address == null) 1245 if (Configuration.errorOnAutoCreate()) 1246 throw new Error("Attempt to auto-create Location.address"); 1247 else if (Configuration.doAutoCreate()) 1248 this.address = new Address(); // cc 1249 return this.address; 1250 } 1251 1252 public boolean hasAddress() { 1253 return this.address != null && !this.address.isEmpty(); 1254 } 1255 1256 /** 1257 * @param value {@link #address} (Physical location.) 1258 */ 1259 public Location setAddress(Address value) { 1260 this.address = value; 1261 return this; 1262 } 1263 1264 /** 1265 * @return {@link #form} (Physical form of the location, e.g. building, room, vehicle, road, virtual.) 1266 */ 1267 public CodeableConcept getForm() { 1268 if (this.form == null) 1269 if (Configuration.errorOnAutoCreate()) 1270 throw new Error("Attempt to auto-create Location.form"); 1271 else if (Configuration.doAutoCreate()) 1272 this.form = new CodeableConcept(); // cc 1273 return this.form; 1274 } 1275 1276 public boolean hasForm() { 1277 return this.form != null && !this.form.isEmpty(); 1278 } 1279 1280 /** 1281 * @param value {@link #form} (Physical form of the location, e.g. building, room, vehicle, road, virtual.) 1282 */ 1283 public Location setForm(CodeableConcept value) { 1284 this.form = value; 1285 return this; 1286 } 1287 1288 /** 1289 * @return {@link #position} (The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML).) 1290 */ 1291 public LocationPositionComponent getPosition() { 1292 if (this.position == null) 1293 if (Configuration.errorOnAutoCreate()) 1294 throw new Error("Attempt to auto-create Location.position"); 1295 else if (Configuration.doAutoCreate()) 1296 this.position = new LocationPositionComponent(); // cc 1297 return this.position; 1298 } 1299 1300 public boolean hasPosition() { 1301 return this.position != null && !this.position.isEmpty(); 1302 } 1303 1304 /** 1305 * @param value {@link #position} (The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML).) 1306 */ 1307 public Location setPosition(LocationPositionComponent value) { 1308 this.position = value; 1309 return this; 1310 } 1311 1312 /** 1313 * @return {@link #managingOrganization} (The organization responsible for the provisioning and upkeep of the location.) 1314 */ 1315 public Reference getManagingOrganization() { 1316 if (this.managingOrganization == null) 1317 if (Configuration.errorOnAutoCreate()) 1318 throw new Error("Attempt to auto-create Location.managingOrganization"); 1319 else if (Configuration.doAutoCreate()) 1320 this.managingOrganization = new Reference(); // cc 1321 return this.managingOrganization; 1322 } 1323 1324 public boolean hasManagingOrganization() { 1325 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 1326 } 1327 1328 /** 1329 * @param value {@link #managingOrganization} (The organization responsible for the provisioning and upkeep of the location.) 1330 */ 1331 public Location setManagingOrganization(Reference value) { 1332 this.managingOrganization = value; 1333 return this; 1334 } 1335 1336 /** 1337 * @return {@link #partOf} (Another Location of which this Location is physically a part of.) 1338 */ 1339 public Reference getPartOf() { 1340 if (this.partOf == null) 1341 if (Configuration.errorOnAutoCreate()) 1342 throw new Error("Attempt to auto-create Location.partOf"); 1343 else if (Configuration.doAutoCreate()) 1344 this.partOf = new Reference(); // cc 1345 return this.partOf; 1346 } 1347 1348 public boolean hasPartOf() { 1349 return this.partOf != null && !this.partOf.isEmpty(); 1350 } 1351 1352 /** 1353 * @param value {@link #partOf} (Another Location of which this Location is physically a part of.) 1354 */ 1355 public Location setPartOf(Reference value) { 1356 this.partOf = value; 1357 return this; 1358 } 1359 1360 /** 1361 * @return {@link #characteristic} (Collection of characteristics (attributes).) 1362 */ 1363 public List<CodeableConcept> getCharacteristic() { 1364 if (this.characteristic == null) 1365 this.characteristic = new ArrayList<CodeableConcept>(); 1366 return this.characteristic; 1367 } 1368 1369 /** 1370 * @return Returns a reference to <code>this</code> for easy method chaining 1371 */ 1372 public Location setCharacteristic(List<CodeableConcept> theCharacteristic) { 1373 this.characteristic = theCharacteristic; 1374 return this; 1375 } 1376 1377 public boolean hasCharacteristic() { 1378 if (this.characteristic == null) 1379 return false; 1380 for (CodeableConcept item : this.characteristic) 1381 if (!item.isEmpty()) 1382 return true; 1383 return false; 1384 } 1385 1386 public CodeableConcept addCharacteristic() { //3 1387 CodeableConcept t = new CodeableConcept(); 1388 if (this.characteristic == null) 1389 this.characteristic = new ArrayList<CodeableConcept>(); 1390 this.characteristic.add(t); 1391 return t; 1392 } 1393 1394 public Location addCharacteristic(CodeableConcept t) { //3 1395 if (t == null) 1396 return this; 1397 if (this.characteristic == null) 1398 this.characteristic = new ArrayList<CodeableConcept>(); 1399 this.characteristic.add(t); 1400 return this; 1401 } 1402 1403 /** 1404 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist {3} 1405 */ 1406 public CodeableConcept getCharacteristicFirstRep() { 1407 if (getCharacteristic().isEmpty()) { 1408 addCharacteristic(); 1409 } 1410 return getCharacteristic().get(0); 1411 } 1412 1413 /** 1414 * @return {@link #hoursOfOperation} (What days/times during a week is this location usually open, and any exceptions where the location is not available.) 1415 */ 1416 public List<Availability> getHoursOfOperation() { 1417 if (this.hoursOfOperation == null) 1418 this.hoursOfOperation = new ArrayList<Availability>(); 1419 return this.hoursOfOperation; 1420 } 1421 1422 /** 1423 * @return Returns a reference to <code>this</code> for easy method chaining 1424 */ 1425 public Location setHoursOfOperation(List<Availability> theHoursOfOperation) { 1426 this.hoursOfOperation = theHoursOfOperation; 1427 return this; 1428 } 1429 1430 public boolean hasHoursOfOperation() { 1431 if (this.hoursOfOperation == null) 1432 return false; 1433 for (Availability item : this.hoursOfOperation) 1434 if (!item.isEmpty()) 1435 return true; 1436 return false; 1437 } 1438 1439 public Availability addHoursOfOperation() { //3 1440 Availability t = new Availability(); 1441 if (this.hoursOfOperation == null) 1442 this.hoursOfOperation = new ArrayList<Availability>(); 1443 this.hoursOfOperation.add(t); 1444 return t; 1445 } 1446 1447 public Location addHoursOfOperation(Availability t) { //3 1448 if (t == null) 1449 return this; 1450 if (this.hoursOfOperation == null) 1451 this.hoursOfOperation = new ArrayList<Availability>(); 1452 this.hoursOfOperation.add(t); 1453 return this; 1454 } 1455 1456 /** 1457 * @return The first repetition of repeating field {@link #hoursOfOperation}, creating it if it does not already exist {3} 1458 */ 1459 public Availability getHoursOfOperationFirstRep() { 1460 if (getHoursOfOperation().isEmpty()) { 1461 addHoursOfOperation(); 1462 } 1463 return getHoursOfOperation().get(0); 1464 } 1465 1466 /** 1467 * @return {@link #virtualService} (Connection details of a virtual service (e.g. shared conference call facility with dedicated number/details).) 1468 */ 1469 public List<VirtualServiceDetail> getVirtualService() { 1470 if (this.virtualService == null) 1471 this.virtualService = new ArrayList<VirtualServiceDetail>(); 1472 return this.virtualService; 1473 } 1474 1475 /** 1476 * @return Returns a reference to <code>this</code> for easy method chaining 1477 */ 1478 public Location setVirtualService(List<VirtualServiceDetail> theVirtualService) { 1479 this.virtualService = theVirtualService; 1480 return this; 1481 } 1482 1483 public boolean hasVirtualService() { 1484 if (this.virtualService == null) 1485 return false; 1486 for (VirtualServiceDetail item : this.virtualService) 1487 if (!item.isEmpty()) 1488 return true; 1489 return false; 1490 } 1491 1492 public VirtualServiceDetail addVirtualService() { //3 1493 VirtualServiceDetail t = new VirtualServiceDetail(); 1494 if (this.virtualService == null) 1495 this.virtualService = new ArrayList<VirtualServiceDetail>(); 1496 this.virtualService.add(t); 1497 return t; 1498 } 1499 1500 public Location addVirtualService(VirtualServiceDetail t) { //3 1501 if (t == null) 1502 return this; 1503 if (this.virtualService == null) 1504 this.virtualService = new ArrayList<VirtualServiceDetail>(); 1505 this.virtualService.add(t); 1506 return this; 1507 } 1508 1509 /** 1510 * @return The first repetition of repeating field {@link #virtualService}, creating it if it does not already exist {3} 1511 */ 1512 public VirtualServiceDetail getVirtualServiceFirstRep() { 1513 if (getVirtualService().isEmpty()) { 1514 addVirtualService(); 1515 } 1516 return getVirtualService().get(0); 1517 } 1518 1519 /** 1520 * @return {@link #endpoint} (Technical endpoints providing access to services operated for the location.) 1521 */ 1522 public List<Reference> getEndpoint() { 1523 if (this.endpoint == null) 1524 this.endpoint = new ArrayList<Reference>(); 1525 return this.endpoint; 1526 } 1527 1528 /** 1529 * @return Returns a reference to <code>this</code> for easy method chaining 1530 */ 1531 public Location setEndpoint(List<Reference> theEndpoint) { 1532 this.endpoint = theEndpoint; 1533 return this; 1534 } 1535 1536 public boolean hasEndpoint() { 1537 if (this.endpoint == null) 1538 return false; 1539 for (Reference item : this.endpoint) 1540 if (!item.isEmpty()) 1541 return true; 1542 return false; 1543 } 1544 1545 public Reference addEndpoint() { //3 1546 Reference t = new Reference(); 1547 if (this.endpoint == null) 1548 this.endpoint = new ArrayList<Reference>(); 1549 this.endpoint.add(t); 1550 return t; 1551 } 1552 1553 public Location addEndpoint(Reference t) { //3 1554 if (t == null) 1555 return this; 1556 if (this.endpoint == null) 1557 this.endpoint = new ArrayList<Reference>(); 1558 this.endpoint.add(t); 1559 return this; 1560 } 1561 1562 /** 1563 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist {3} 1564 */ 1565 public Reference getEndpointFirstRep() { 1566 if (getEndpoint().isEmpty()) { 1567 addEndpoint(); 1568 } 1569 return getEndpoint().get(0); 1570 } 1571 1572 protected void listChildren(List<Property> children) { 1573 super.listChildren(children); 1574 children.add(new Property("identifier", "Identifier", "Unique code or number identifying the location to its users.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1575 children.add(new Property("status", "code", "The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location.", 0, 1, status)); 1576 children.add(new Property("operationalStatus", "Coding", "The operational status covers operation values most relevant to beds (but can also apply to rooms/units/chairs/etc. such as an isolation unit/dialysis chair). This typically covers concepts such as contamination, housekeeping, and other activities like maintenance.", 0, 1, operationalStatus)); 1577 children.add(new Property("name", "string", "Name of the location as used by humans. Does not need to be unique.", 0, 1, name)); 1578 children.add(new Property("alias", "string", "A list of alternate names that the location is known as, or was known as, in the past.", 0, java.lang.Integer.MAX_VALUE, alias)); 1579 children.add(new Property("description", "markdown", "Description of the Location, which helps in finding or referencing the place.", 0, 1, description)); 1580 children.add(new Property("mode", "code", "Indicates whether a resource instance represents a specific location or a class of locations.", 0, 1, mode)); 1581 children.add(new Property("type", "CodeableConcept", "Indicates the type of function performed at the location.", 0, java.lang.Integer.MAX_VALUE, type)); 1582 children.add(new Property("contact", "ExtendedContactDetail", "The contact details of communication devices available at the location. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 0, java.lang.Integer.MAX_VALUE, contact)); 1583 children.add(new Property("address", "Address", "Physical location.", 0, 1, address)); 1584 children.add(new Property("form", "CodeableConcept", "Physical form of the location, e.g. building, room, vehicle, road, virtual.", 0, 1, form)); 1585 children.add(new Property("position", "", "The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML).", 0, 1, position)); 1586 children.add(new Property("managingOrganization", "Reference(Organization)", "The organization responsible for the provisioning and upkeep of the location.", 0, 1, managingOrganization)); 1587 children.add(new Property("partOf", "Reference(Location)", "Another Location of which this Location is physically a part of.", 0, 1, partOf)); 1588 children.add(new Property("characteristic", "CodeableConcept", "Collection of characteristics (attributes).", 0, java.lang.Integer.MAX_VALUE, characteristic)); 1589 children.add(new Property("hoursOfOperation", "Availability", "What days/times during a week is this location usually open, and any exceptions where the location is not available.", 0, java.lang.Integer.MAX_VALUE, hoursOfOperation)); 1590 children.add(new Property("virtualService", "VirtualServiceDetail", "Connection details of a virtual service (e.g. shared conference call facility with dedicated number/details).", 0, java.lang.Integer.MAX_VALUE, virtualService)); 1591 children.add(new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for the location.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 1592 } 1593 1594 @Override 1595 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1596 switch (_hash) { 1597 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique code or number identifying the location to its users.", 0, java.lang.Integer.MAX_VALUE, identifier); 1598 case -892481550: /*status*/ return new Property("status", "code", "The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location.", 0, 1, status); 1599 case -2103166364: /*operationalStatus*/ return new Property("operationalStatus", "Coding", "The operational status covers operation values most relevant to beds (but can also apply to rooms/units/chairs/etc. such as an isolation unit/dialysis chair). This typically covers concepts such as contamination, housekeeping, and other activities like maintenance.", 0, 1, operationalStatus); 1600 case 3373707: /*name*/ return new Property("name", "string", "Name of the location as used by humans. Does not need to be unique.", 0, 1, name); 1601 case 92902992: /*alias*/ return new Property("alias", "string", "A list of alternate names that the location is known as, or was known as, in the past.", 0, java.lang.Integer.MAX_VALUE, alias); 1602 case -1724546052: /*description*/ return new Property("description", "markdown", "Description of the Location, which helps in finding or referencing the place.", 0, 1, description); 1603 case 3357091: /*mode*/ return new Property("mode", "code", "Indicates whether a resource instance represents a specific location or a class of locations.", 0, 1, mode); 1604 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Indicates the type of function performed at the location.", 0, java.lang.Integer.MAX_VALUE, type); 1605 case 951526432: /*contact*/ return new Property("contact", "ExtendedContactDetail", "The contact details of communication devices available at the location. This can include addresses, phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 0, java.lang.Integer.MAX_VALUE, contact); 1606 case -1147692044: /*address*/ return new Property("address", "Address", "Physical location.", 0, 1, address); 1607 case 3148996: /*form*/ return new Property("form", "CodeableConcept", "Physical form of the location, e.g. building, room, vehicle, road, virtual.", 0, 1, form); 1608 case 747804969: /*position*/ return new Property("position", "", "The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML).", 0, 1, position); 1609 case -2058947787: /*managingOrganization*/ return new Property("managingOrganization", "Reference(Organization)", "The organization responsible for the provisioning and upkeep of the location.", 0, 1, managingOrganization); 1610 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Location)", "Another Location of which this Location is physically a part of.", 0, 1, partOf); 1611 case 366313883: /*characteristic*/ return new Property("characteristic", "CodeableConcept", "Collection of characteristics (attributes).", 0, java.lang.Integer.MAX_VALUE, characteristic); 1612 case -1588872511: /*hoursOfOperation*/ return new Property("hoursOfOperation", "Availability", "What days/times during a week is this location usually open, and any exceptions where the location is not available.", 0, java.lang.Integer.MAX_VALUE, hoursOfOperation); 1613 case 1420774698: /*virtualService*/ return new Property("virtualService", "VirtualServiceDetail", "Connection details of a virtual service (e.g. shared conference call facility with dedicated number/details).", 0, java.lang.Integer.MAX_VALUE, virtualService); 1614 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for the location.", 0, java.lang.Integer.MAX_VALUE, endpoint); 1615 default: return super.getNamedProperty(_hash, _name, _checkValid); 1616 } 1617 1618 } 1619 1620 @Override 1621 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1622 switch (hash) { 1623 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1624 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<LocationStatus> 1625 case -2103166364: /*operationalStatus*/ return this.operationalStatus == null ? new Base[0] : new Base[] {this.operationalStatus}; // Coding 1626 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1627 case 92902992: /*alias*/ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 1628 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1629 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<LocationMode> 1630 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1631 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ExtendedContactDetail 1632 case -1147692044: /*address*/ return this.address == null ? new Base[0] : new Base[] {this.address}; // Address 1633 case 3148996: /*form*/ return this.form == null ? new Base[0] : new Base[] {this.form}; // CodeableConcept 1634 case 747804969: /*position*/ return this.position == null ? new Base[0] : new Base[] {this.position}; // LocationPositionComponent 1635 case -2058947787: /*managingOrganization*/ return this.managingOrganization == null ? new Base[0] : new Base[] {this.managingOrganization}; // Reference 1636 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : new Base[] {this.partOf}; // Reference 1637 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // CodeableConcept 1638 case -1588872511: /*hoursOfOperation*/ return this.hoursOfOperation == null ? new Base[0] : this.hoursOfOperation.toArray(new Base[this.hoursOfOperation.size()]); // Availability 1639 case 1420774698: /*virtualService*/ return this.virtualService == null ? new Base[0] : this.virtualService.toArray(new Base[this.virtualService.size()]); // VirtualServiceDetail 1640 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 1641 default: return super.getProperty(hash, name, checkValid); 1642 } 1643 1644 } 1645 1646 @Override 1647 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1648 switch (hash) { 1649 case -1618432855: // identifier 1650 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1651 return value; 1652 case -892481550: // status 1653 value = new LocationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1654 this.status = (Enumeration) value; // Enumeration<LocationStatus> 1655 return value; 1656 case -2103166364: // operationalStatus 1657 this.operationalStatus = TypeConvertor.castToCoding(value); // Coding 1658 return value; 1659 case 3373707: // name 1660 this.name = TypeConvertor.castToString(value); // StringType 1661 return value; 1662 case 92902992: // alias 1663 this.getAlias().add(TypeConvertor.castToString(value)); // StringType 1664 return value; 1665 case -1724546052: // description 1666 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1667 return value; 1668 case 3357091: // mode 1669 value = new LocationModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1670 this.mode = (Enumeration) value; // Enumeration<LocationMode> 1671 return value; 1672 case 3575610: // type 1673 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1674 return value; 1675 case 951526432: // contact 1676 this.getContact().add(TypeConvertor.castToExtendedContactDetail(value)); // ExtendedContactDetail 1677 return value; 1678 case -1147692044: // address 1679 this.address = TypeConvertor.castToAddress(value); // Address 1680 return value; 1681 case 3148996: // form 1682 this.form = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1683 return value; 1684 case 747804969: // position 1685 this.position = (LocationPositionComponent) value; // LocationPositionComponent 1686 return value; 1687 case -2058947787: // managingOrganization 1688 this.managingOrganization = TypeConvertor.castToReference(value); // Reference 1689 return value; 1690 case -995410646: // partOf 1691 this.partOf = TypeConvertor.castToReference(value); // Reference 1692 return value; 1693 case 366313883: // characteristic 1694 this.getCharacteristic().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1695 return value; 1696 case -1588872511: // hoursOfOperation 1697 this.getHoursOfOperation().add(TypeConvertor.castToAvailability(value)); // Availability 1698 return value; 1699 case 1420774698: // virtualService 1700 this.getVirtualService().add(TypeConvertor.castToVirtualServiceDetail(value)); // VirtualServiceDetail 1701 return value; 1702 case 1741102485: // endpoint 1703 this.getEndpoint().add(TypeConvertor.castToReference(value)); // Reference 1704 return value; 1705 default: return super.setProperty(hash, name, value); 1706 } 1707 1708 } 1709 1710 @Override 1711 public Base setProperty(String name, Base value) throws FHIRException { 1712 if (name.equals("identifier")) { 1713 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1714 } else if (name.equals("status")) { 1715 value = new LocationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1716 this.status = (Enumeration) value; // Enumeration<LocationStatus> 1717 } else if (name.equals("operationalStatus")) { 1718 this.operationalStatus = TypeConvertor.castToCoding(value); // Coding 1719 } else if (name.equals("name")) { 1720 this.name = TypeConvertor.castToString(value); // StringType 1721 } else if (name.equals("alias")) { 1722 this.getAlias().add(TypeConvertor.castToString(value)); 1723 } else if (name.equals("description")) { 1724 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1725 } else if (name.equals("mode")) { 1726 value = new LocationModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1727 this.mode = (Enumeration) value; // Enumeration<LocationMode> 1728 } else if (name.equals("type")) { 1729 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 1730 } else if (name.equals("contact")) { 1731 this.getContact().add(TypeConvertor.castToExtendedContactDetail(value)); 1732 } else if (name.equals("address")) { 1733 this.address = TypeConvertor.castToAddress(value); // Address 1734 } else if (name.equals("form")) { 1735 this.form = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1736 } else if (name.equals("position")) { 1737 this.position = (LocationPositionComponent) value; // LocationPositionComponent 1738 } else if (name.equals("managingOrganization")) { 1739 this.managingOrganization = TypeConvertor.castToReference(value); // Reference 1740 } else if (name.equals("partOf")) { 1741 this.partOf = TypeConvertor.castToReference(value); // Reference 1742 } else if (name.equals("characteristic")) { 1743 this.getCharacteristic().add(TypeConvertor.castToCodeableConcept(value)); 1744 } else if (name.equals("hoursOfOperation")) { 1745 this.getHoursOfOperation().add(TypeConvertor.castToAvailability(value)); 1746 } else if (name.equals("virtualService")) { 1747 this.getVirtualService().add(TypeConvertor.castToVirtualServiceDetail(value)); 1748 } else if (name.equals("endpoint")) { 1749 this.getEndpoint().add(TypeConvertor.castToReference(value)); 1750 } else 1751 return super.setProperty(name, value); 1752 return value; 1753 } 1754 1755 @Override 1756 public void removeChild(String name, Base value) throws FHIRException { 1757 if (name.equals("identifier")) { 1758 this.getIdentifier().remove(value); 1759 } else if (name.equals("status")) { 1760 value = new LocationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 1761 this.status = (Enumeration) value; // Enumeration<LocationStatus> 1762 } else if (name.equals("operationalStatus")) { 1763 this.operationalStatus = null; 1764 } else if (name.equals("name")) { 1765 this.name = null; 1766 } else if (name.equals("alias")) { 1767 this.getAlias().remove(value); 1768 } else if (name.equals("description")) { 1769 this.description = null; 1770 } else if (name.equals("mode")) { 1771 value = new LocationModeEnumFactory().fromType(TypeConvertor.castToCode(value)); 1772 this.mode = (Enumeration) value; // Enumeration<LocationMode> 1773 } else if (name.equals("type")) { 1774 this.getType().remove(value); 1775 } else if (name.equals("contact")) { 1776 this.getContact().remove(value); 1777 } else if (name.equals("address")) { 1778 this.address = null; 1779 } else if (name.equals("form")) { 1780 this.form = null; 1781 } else if (name.equals("position")) { 1782 this.position = (LocationPositionComponent) value; // LocationPositionComponent 1783 } else if (name.equals("managingOrganization")) { 1784 this.managingOrganization = null; 1785 } else if (name.equals("partOf")) { 1786 this.partOf = null; 1787 } else if (name.equals("characteristic")) { 1788 this.getCharacteristic().remove(value); 1789 } else if (name.equals("hoursOfOperation")) { 1790 this.getHoursOfOperation().remove(value); 1791 } else if (name.equals("virtualService")) { 1792 this.getVirtualService().remove(value); 1793 } else if (name.equals("endpoint")) { 1794 this.getEndpoint().remove(value); 1795 } else 1796 super.removeChild(name, value); 1797 1798 } 1799 1800 @Override 1801 public Base makeProperty(int hash, String name) throws FHIRException { 1802 switch (hash) { 1803 case -1618432855: return addIdentifier(); 1804 case -892481550: return getStatusElement(); 1805 case -2103166364: return getOperationalStatus(); 1806 case 3373707: return getNameElement(); 1807 case 92902992: return addAliasElement(); 1808 case -1724546052: return getDescriptionElement(); 1809 case 3357091: return getModeElement(); 1810 case 3575610: return addType(); 1811 case 951526432: return addContact(); 1812 case -1147692044: return getAddress(); 1813 case 3148996: return getForm(); 1814 case 747804969: return getPosition(); 1815 case -2058947787: return getManagingOrganization(); 1816 case -995410646: return getPartOf(); 1817 case 366313883: return addCharacteristic(); 1818 case -1588872511: return addHoursOfOperation(); 1819 case 1420774698: return addVirtualService(); 1820 case 1741102485: return addEndpoint(); 1821 default: return super.makeProperty(hash, name); 1822 } 1823 1824 } 1825 1826 @Override 1827 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1828 switch (hash) { 1829 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1830 case -892481550: /*status*/ return new String[] {"code"}; 1831 case -2103166364: /*operationalStatus*/ return new String[] {"Coding"}; 1832 case 3373707: /*name*/ return new String[] {"string"}; 1833 case 92902992: /*alias*/ return new String[] {"string"}; 1834 case -1724546052: /*description*/ return new String[] {"markdown"}; 1835 case 3357091: /*mode*/ return new String[] {"code"}; 1836 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1837 case 951526432: /*contact*/ return new String[] {"ExtendedContactDetail"}; 1838 case -1147692044: /*address*/ return new String[] {"Address"}; 1839 case 3148996: /*form*/ return new String[] {"CodeableConcept"}; 1840 case 747804969: /*position*/ return new String[] {}; 1841 case -2058947787: /*managingOrganization*/ return new String[] {"Reference"}; 1842 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1843 case 366313883: /*characteristic*/ return new String[] {"CodeableConcept"}; 1844 case -1588872511: /*hoursOfOperation*/ return new String[] {"Availability"}; 1845 case 1420774698: /*virtualService*/ return new String[] {"VirtualServiceDetail"}; 1846 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 1847 default: return super.getTypesForProperty(hash, name); 1848 } 1849 1850 } 1851 1852 @Override 1853 public Base addChild(String name) throws FHIRException { 1854 if (name.equals("identifier")) { 1855 return addIdentifier(); 1856 } 1857 else if (name.equals("status")) { 1858 throw new FHIRException("Cannot call addChild on a singleton property Location.status"); 1859 } 1860 else if (name.equals("operationalStatus")) { 1861 this.operationalStatus = new Coding(); 1862 return this.operationalStatus; 1863 } 1864 else if (name.equals("name")) { 1865 throw new FHIRException("Cannot call addChild on a singleton property Location.name"); 1866 } 1867 else if (name.equals("alias")) { 1868 throw new FHIRException("Cannot call addChild on a singleton property Location.alias"); 1869 } 1870 else if (name.equals("description")) { 1871 throw new FHIRException("Cannot call addChild on a singleton property Location.description"); 1872 } 1873 else if (name.equals("mode")) { 1874 throw new FHIRException("Cannot call addChild on a singleton property Location.mode"); 1875 } 1876 else if (name.equals("type")) { 1877 return addType(); 1878 } 1879 else if (name.equals("contact")) { 1880 return addContact(); 1881 } 1882 else if (name.equals("address")) { 1883 this.address = new Address(); 1884 return this.address; 1885 } 1886 else if (name.equals("form")) { 1887 this.form = new CodeableConcept(); 1888 return this.form; 1889 } 1890 else if (name.equals("position")) { 1891 this.position = new LocationPositionComponent(); 1892 return this.position; 1893 } 1894 else if (name.equals("managingOrganization")) { 1895 this.managingOrganization = new Reference(); 1896 return this.managingOrganization; 1897 } 1898 else if (name.equals("partOf")) { 1899 this.partOf = new Reference(); 1900 return this.partOf; 1901 } 1902 else if (name.equals("characteristic")) { 1903 return addCharacteristic(); 1904 } 1905 else if (name.equals("hoursOfOperation")) { 1906 return addHoursOfOperation(); 1907 } 1908 else if (name.equals("virtualService")) { 1909 return addVirtualService(); 1910 } 1911 else if (name.equals("endpoint")) { 1912 return addEndpoint(); 1913 } 1914 else 1915 return super.addChild(name); 1916 } 1917 1918 public String fhirType() { 1919 return "Location"; 1920 1921 } 1922 1923 public Location copy() { 1924 Location dst = new Location(); 1925 copyValues(dst); 1926 return dst; 1927 } 1928 1929 public void copyValues(Location dst) { 1930 super.copyValues(dst); 1931 if (identifier != null) { 1932 dst.identifier = new ArrayList<Identifier>(); 1933 for (Identifier i : identifier) 1934 dst.identifier.add(i.copy()); 1935 }; 1936 dst.status = status == null ? null : status.copy(); 1937 dst.operationalStatus = operationalStatus == null ? null : operationalStatus.copy(); 1938 dst.name = name == null ? null : name.copy(); 1939 if (alias != null) { 1940 dst.alias = new ArrayList<StringType>(); 1941 for (StringType i : alias) 1942 dst.alias.add(i.copy()); 1943 }; 1944 dst.description = description == null ? null : description.copy(); 1945 dst.mode = mode == null ? null : mode.copy(); 1946 if (type != null) { 1947 dst.type = new ArrayList<CodeableConcept>(); 1948 for (CodeableConcept i : type) 1949 dst.type.add(i.copy()); 1950 }; 1951 if (contact != null) { 1952 dst.contact = new ArrayList<ExtendedContactDetail>(); 1953 for (ExtendedContactDetail i : contact) 1954 dst.contact.add(i.copy()); 1955 }; 1956 dst.address = address == null ? null : address.copy(); 1957 dst.form = form == null ? null : form.copy(); 1958 dst.position = position == null ? null : position.copy(); 1959 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 1960 dst.partOf = partOf == null ? null : partOf.copy(); 1961 if (characteristic != null) { 1962 dst.characteristic = new ArrayList<CodeableConcept>(); 1963 for (CodeableConcept i : characteristic) 1964 dst.characteristic.add(i.copy()); 1965 }; 1966 if (hoursOfOperation != null) { 1967 dst.hoursOfOperation = new ArrayList<Availability>(); 1968 for (Availability i : hoursOfOperation) 1969 dst.hoursOfOperation.add(i.copy()); 1970 }; 1971 if (virtualService != null) { 1972 dst.virtualService = new ArrayList<VirtualServiceDetail>(); 1973 for (VirtualServiceDetail i : virtualService) 1974 dst.virtualService.add(i.copy()); 1975 }; 1976 if (endpoint != null) { 1977 dst.endpoint = new ArrayList<Reference>(); 1978 for (Reference i : endpoint) 1979 dst.endpoint.add(i.copy()); 1980 }; 1981 } 1982 1983 protected Location typedCopy() { 1984 return copy(); 1985 } 1986 1987 @Override 1988 public boolean equalsDeep(Base other_) { 1989 if (!super.equalsDeep(other_)) 1990 return false; 1991 if (!(other_ instanceof Location)) 1992 return false; 1993 Location o = (Location) other_; 1994 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(operationalStatus, o.operationalStatus, true) 1995 && compareDeep(name, o.name, true) && compareDeep(alias, o.alias, true) && compareDeep(description, o.description, true) 1996 && compareDeep(mode, o.mode, true) && compareDeep(type, o.type, true) && compareDeep(contact, o.contact, true) 1997 && compareDeep(address, o.address, true) && compareDeep(form, o.form, true) && compareDeep(position, o.position, true) 1998 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(partOf, o.partOf, true) 1999 && compareDeep(characteristic, o.characteristic, true) && compareDeep(hoursOfOperation, o.hoursOfOperation, true) 2000 && compareDeep(virtualService, o.virtualService, true) && compareDeep(endpoint, o.endpoint, true) 2001 ; 2002 } 2003 2004 @Override 2005 public boolean equalsShallow(Base other_) { 2006 if (!super.equalsShallow(other_)) 2007 return false; 2008 if (!(other_ instanceof Location)) 2009 return false; 2010 Location o = (Location) other_; 2011 return compareValues(status, o.status, true) && compareValues(name, o.name, true) && compareValues(alias, o.alias, true) 2012 && compareValues(description, o.description, true) && compareValues(mode, o.mode, true); 2013 } 2014 2015 public boolean isEmpty() { 2016 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, operationalStatus 2017 , name, alias, description, mode, type, contact, address, form, position, managingOrganization 2018 , partOf, characteristic, hoursOfOperation, virtualService, endpoint); 2019 } 2020 2021 @Override 2022 public ResourceType getResourceType() { 2023 return ResourceType.Location; 2024 } 2025 2026 /** 2027 * Search parameter: <b>address-city</b> 2028 * <p> 2029 * Description: <b>A city specified in an address</b><br> 2030 * Type: <b>string</b><br> 2031 * Path: <b>Location.address.city</b><br> 2032 * </p> 2033 */ 2034 @SearchParamDefinition(name="address-city", path="Location.address.city", description="A city specified in an address", type="string" ) 2035 public static final String SP_ADDRESS_CITY = "address-city"; 2036 /** 2037 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 2038 * <p> 2039 * Description: <b>A city specified in an address</b><br> 2040 * Type: <b>string</b><br> 2041 * Path: <b>Location.address.city</b><br> 2042 * </p> 2043 */ 2044 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_CITY); 2045 2046 /** 2047 * Search parameter: <b>address-country</b> 2048 * <p> 2049 * Description: <b>A country specified in an address</b><br> 2050 * Type: <b>string</b><br> 2051 * Path: <b>Location.address.country</b><br> 2052 * </p> 2053 */ 2054 @SearchParamDefinition(name="address-country", path="Location.address.country", description="A country specified in an address", type="string" ) 2055 public static final String SP_ADDRESS_COUNTRY = "address-country"; 2056 /** 2057 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 2058 * <p> 2059 * Description: <b>A country specified in an address</b><br> 2060 * Type: <b>string</b><br> 2061 * Path: <b>Location.address.country</b><br> 2062 * </p> 2063 */ 2064 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_COUNTRY); 2065 2066 /** 2067 * Search parameter: <b>address-postalcode</b> 2068 * <p> 2069 * Description: <b>A postal code specified in an address</b><br> 2070 * Type: <b>string</b><br> 2071 * Path: <b>Location.address.postalCode</b><br> 2072 * </p> 2073 */ 2074 @SearchParamDefinition(name="address-postalcode", path="Location.address.postalCode", description="A postal code specified in an address", type="string" ) 2075 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 2076 /** 2077 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 2078 * <p> 2079 * Description: <b>A postal code specified in an address</b><br> 2080 * Type: <b>string</b><br> 2081 * Path: <b>Location.address.postalCode</b><br> 2082 * </p> 2083 */ 2084 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_POSTALCODE); 2085 2086 /** 2087 * Search parameter: <b>address-state</b> 2088 * <p> 2089 * Description: <b>A state specified in an address</b><br> 2090 * Type: <b>string</b><br> 2091 * Path: <b>Location.address.state</b><br> 2092 * </p> 2093 */ 2094 @SearchParamDefinition(name="address-state", path="Location.address.state", description="A state specified in an address", type="string" ) 2095 public static final String SP_ADDRESS_STATE = "address-state"; 2096 /** 2097 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 2098 * <p> 2099 * Description: <b>A state specified in an address</b><br> 2100 * Type: <b>string</b><br> 2101 * Path: <b>Location.address.state</b><br> 2102 * </p> 2103 */ 2104 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_STATE); 2105 2106 /** 2107 * Search parameter: <b>address-use</b> 2108 * <p> 2109 * Description: <b>A use code specified in an address</b><br> 2110 * Type: <b>token</b><br> 2111 * Path: <b>Location.address.use</b><br> 2112 * </p> 2113 */ 2114 @SearchParamDefinition(name="address-use", path="Location.address.use", description="A use code specified in an address", type="token" ) 2115 public static final String SP_ADDRESS_USE = "address-use"; 2116 /** 2117 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 2118 * <p> 2119 * Description: <b>A use code specified in an address</b><br> 2120 * Type: <b>token</b><br> 2121 * Path: <b>Location.address.use</b><br> 2122 * </p> 2123 */ 2124 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADDRESS_USE); 2125 2126 /** 2127 * Search parameter: <b>address</b> 2128 * <p> 2129 * Description: <b>A (part of the) address of the location</b><br> 2130 * Type: <b>string</b><br> 2131 * Path: <b>Location.address</b><br> 2132 * </p> 2133 */ 2134 @SearchParamDefinition(name="address", path="Location.address", description="A (part of the) address of the location", type="string" ) 2135 public static final String SP_ADDRESS = "address"; 2136 /** 2137 * <b>Fluent Client</b> search parameter constant for <b>address</b> 2138 * <p> 2139 * Description: <b>A (part of the) address of the location</b><br> 2140 * Type: <b>string</b><br> 2141 * Path: <b>Location.address</b><br> 2142 * </p> 2143 */ 2144 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS); 2145 2146 /** 2147 * Search parameter: <b>characteristic</b> 2148 * <p> 2149 * Description: <b>One of the Location's characteristics</b><br> 2150 * Type: <b>token</b><br> 2151 * Path: <b>Location.characteristic</b><br> 2152 * </p> 2153 */ 2154 @SearchParamDefinition(name="characteristic", path="Location.characteristic", description="One of the Location's characteristics", type="token" ) 2155 public static final String SP_CHARACTERISTIC = "characteristic"; 2156 /** 2157 * <b>Fluent Client</b> search parameter constant for <b>characteristic</b> 2158 * <p> 2159 * Description: <b>One of the Location's characteristics</b><br> 2160 * Type: <b>token</b><br> 2161 * Path: <b>Location.characteristic</b><br> 2162 * </p> 2163 */ 2164 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHARACTERISTIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CHARACTERISTIC); 2165 2166 /** 2167 * Search parameter: <b>contains</b> 2168 * <p> 2169 * Description: <b>Select locations that contain the specified co-ordinates</b><br> 2170 * Type: <b>special</b><br> 2171 * Path: <b>Location.extension('http://hl7.org/fhir/StructureDefinition/location-boundary-geojson').value</b><br> 2172 * </p> 2173 */ 2174 @SearchParamDefinition(name="contains", path="Location.extension('http://hl7.org/fhir/StructureDefinition/location-boundary-geojson').value", description="Select locations that contain the specified co-ordinates", type="special" ) 2175 public static final String SP_CONTAINS = "contains"; 2176 /** 2177 * <b>Fluent Client</b> search parameter constant for <b>contains</b> 2178 * <p> 2179 * Description: <b>Select locations that contain the specified co-ordinates</b><br> 2180 * Type: <b>special</b><br> 2181 * Path: <b>Location.extension('http://hl7.org/fhir/StructureDefinition/location-boundary-geojson').value</b><br> 2182 * </p> 2183 */ 2184 public static final ca.uhn.fhir.rest.gclient.SpecialClientParam CONTAINS = new ca.uhn.fhir.rest.gclient.SpecialClientParam(SP_CONTAINS); 2185 2186 /** 2187 * Search parameter: <b>endpoint</b> 2188 * <p> 2189 * Description: <b>Technical endpoints providing access to services operated for the location</b><br> 2190 * Type: <b>reference</b><br> 2191 * Path: <b>Location.endpoint</b><br> 2192 * </p> 2193 */ 2194 @SearchParamDefinition(name="endpoint", path="Location.endpoint", description="Technical endpoints providing access to services operated for the location", type="reference", target={Endpoint.class } ) 2195 public static final String SP_ENDPOINT = "endpoint"; 2196 /** 2197 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 2198 * <p> 2199 * Description: <b>Technical endpoints providing access to services operated for the location</b><br> 2200 * Type: <b>reference</b><br> 2201 * Path: <b>Location.endpoint</b><br> 2202 * </p> 2203 */ 2204 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENDPOINT); 2205 2206/** 2207 * Constant for fluent queries to be used to add include statements. Specifies 2208 * the path value of "<b>Location:endpoint</b>". 2209 */ 2210 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include("Location:endpoint").toLocked(); 2211 2212 /** 2213 * Search parameter: <b>identifier</b> 2214 * <p> 2215 * Description: <b>An identifier for the location</b><br> 2216 * Type: <b>token</b><br> 2217 * Path: <b>Location.identifier</b><br> 2218 * </p> 2219 */ 2220 @SearchParamDefinition(name="identifier", path="Location.identifier", description="An identifier for the location", type="token" ) 2221 public static final String SP_IDENTIFIER = "identifier"; 2222 /** 2223 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2224 * <p> 2225 * Description: <b>An identifier for the location</b><br> 2226 * Type: <b>token</b><br> 2227 * Path: <b>Location.identifier</b><br> 2228 * </p> 2229 */ 2230 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2231 2232 /** 2233 * Search parameter: <b>name</b> 2234 * <p> 2235 * Description: <b>A portion of the location's name or alias</b><br> 2236 * Type: <b>string</b><br> 2237 * Path: <b>Location.name | Location.alias</b><br> 2238 * </p> 2239 */ 2240 @SearchParamDefinition(name="name", path="Location.name | Location.alias", description="A portion of the location's name or alias", type="string" ) 2241 public static final String SP_NAME = "name"; 2242 /** 2243 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2244 * <p> 2245 * Description: <b>A portion of the location's name or alias</b><br> 2246 * Type: <b>string</b><br> 2247 * Path: <b>Location.name | Location.alias</b><br> 2248 * </p> 2249 */ 2250 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 2251 2252 /** 2253 * Search parameter: <b>near</b> 2254 * <p> 2255 * Description: <b>Search for locations where the location.position is near to, or within a specified distance of, the provided coordinates expressed as [latitude]|[longitude]|[distance]|[units] (using the WGS84 datum, see notes). 2256 2257Servers which support the near parameter SHALL support the unit string 'km' for kilometers and SHOULD support '[mi_us]' for miles, support for other units is optional. If the units are omitted, then kms should be assumed. If the distance is omitted, then the server can use its own discretion as to what distances should be considered near (and units are irrelevant). 2258 2259If the server is unable to understand the units (and does support the near search parameter), it MIGHT return an OperationOutcome and fail the search with a http status 400 BadRequest. If the server does not support the near parameter, the parameter MIGHT report the unused parameter in a bundled OperationOutcome and still perform the search ignoring the near parameter. 2260 2261Note: The algorithm to determine the distance is not defined by the specification, and systems might have different engines that calculate things differently. They could consider geographic point to point, or path via road, or including current traffic conditions, or just simple neighboring postcodes/localities if that's all it had access to.</b><br> 2262 * Type: <b>special</b><br> 2263 * Path: <b>Location.position</b><br> 2264 * </p> 2265 */ 2266 @SearchParamDefinition(name="near", path="Location.position", description="Search for locations where the location.position is near to, or within a specified distance of, the provided coordinates expressed as [latitude]|[longitude]|[distance]|[units] (using the WGS84 datum, see notes).\n\nServers which support the near parameter SHALL support the unit string 'km' for kilometers and SHOULD support '[mi_us]' for miles, support for other units is optional. If the units are omitted, then kms should be assumed. If the distance is omitted, then the server can use its own discretion as to what distances should be considered near (and units are irrelevant).\r\rIf the server is unable to understand the units (and does support the near search parameter), it MIGHT return an OperationOutcome and fail the search with a http status 400 BadRequest. If the server does not support the near parameter, the parameter MIGHT report the unused parameter in a bundled OperationOutcome and still perform the search ignoring the near parameter.\n\nNote: The algorithm to determine the distance is not defined by the specification, and systems might have different engines that calculate things differently. They could consider geographic point to point, or path via road, or including current traffic conditions, or just simple neighboring postcodes/localities if that's all it had access to.", type="special" ) 2267 public static final String SP_NEAR = "near"; 2268 /** 2269 * <b>Fluent Client</b> search parameter constant for <b>near</b> 2270 * <p> 2271 * Description: <b>Search for locations where the location.position is near to, or within a specified distance of, the provided coordinates expressed as [latitude]|[longitude]|[distance]|[units] (using the WGS84 datum, see notes). 2272 2273Servers which support the near parameter SHALL support the unit string 'km' for kilometers and SHOULD support '[mi_us]' for miles, support for other units is optional. If the units are omitted, then kms should be assumed. If the distance is omitted, then the server can use its own discretion as to what distances should be considered near (and units are irrelevant). 2274 2275If the server is unable to understand the units (and does support the near search parameter), it MIGHT return an OperationOutcome and fail the search with a http status 400 BadRequest. If the server does not support the near parameter, the parameter MIGHT report the unused parameter in a bundled OperationOutcome and still perform the search ignoring the near parameter. 2276 2277Note: The algorithm to determine the distance is not defined by the specification, and systems might have different engines that calculate things differently. They could consider geographic point to point, or path via road, or including current traffic conditions, or just simple neighboring postcodes/localities if that's all it had access to.</b><br> 2278 * Type: <b>special</b><br> 2279 * Path: <b>Location.position</b><br> 2280 * </p> 2281 */ 2282 public static final ca.uhn.fhir.rest.gclient.SpecialClientParam NEAR = new ca.uhn.fhir.rest.gclient.SpecialClientParam(SP_NEAR); 2283 2284 /** 2285 * Search parameter: <b>operational-status</b> 2286 * <p> 2287 * Description: <b>Searches for locations (typically bed/room) that have an operational status (e.g. contaminated, housekeeping)</b><br> 2288 * Type: <b>token</b><br> 2289 * Path: <b>Location.operationalStatus</b><br> 2290 * </p> 2291 */ 2292 @SearchParamDefinition(name="operational-status", path="Location.operationalStatus", description="Searches for locations (typically bed/room) that have an operational status (e.g. contaminated, housekeeping)", type="token" ) 2293 public static final String SP_OPERATIONAL_STATUS = "operational-status"; 2294 /** 2295 * <b>Fluent Client</b> search parameter constant for <b>operational-status</b> 2296 * <p> 2297 * Description: <b>Searches for locations (typically bed/room) that have an operational status (e.g. contaminated, housekeeping)</b><br> 2298 * Type: <b>token</b><br> 2299 * Path: <b>Location.operationalStatus</b><br> 2300 * </p> 2301 */ 2302 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OPERATIONAL_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_OPERATIONAL_STATUS); 2303 2304 /** 2305 * Search parameter: <b>organization</b> 2306 * <p> 2307 * Description: <b>Searches for locations that are managed by the provided organization</b><br> 2308 * Type: <b>reference</b><br> 2309 * Path: <b>Location.managingOrganization</b><br> 2310 * </p> 2311 */ 2312 @SearchParamDefinition(name="organization", path="Location.managingOrganization", description="Searches for locations that are managed by the provided organization", type="reference", target={Organization.class } ) 2313 public static final String SP_ORGANIZATION = "organization"; 2314 /** 2315 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 2316 * <p> 2317 * Description: <b>Searches for locations that are managed by the provided organization</b><br> 2318 * Type: <b>reference</b><br> 2319 * Path: <b>Location.managingOrganization</b><br> 2320 * </p> 2321 */ 2322 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 2323 2324/** 2325 * Constant for fluent queries to be used to add include statements. Specifies 2326 * the path value of "<b>Location:organization</b>". 2327 */ 2328 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Location:organization").toLocked(); 2329 2330 /** 2331 * Search parameter: <b>partof</b> 2332 * <p> 2333 * Description: <b>A location of which this location is a part</b><br> 2334 * Type: <b>reference</b><br> 2335 * Path: <b>Location.partOf</b><br> 2336 * </p> 2337 */ 2338 @SearchParamDefinition(name="partof", path="Location.partOf", description="A location of which this location is a part", type="reference", target={Location.class } ) 2339 public static final String SP_PARTOF = "partof"; 2340 /** 2341 * <b>Fluent Client</b> search parameter constant for <b>partof</b> 2342 * <p> 2343 * Description: <b>A location of which this location is a part</b><br> 2344 * Type: <b>reference</b><br> 2345 * Path: <b>Location.partOf</b><br> 2346 * </p> 2347 */ 2348 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTOF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTOF); 2349 2350/** 2351 * Constant for fluent queries to be used to add include statements. Specifies 2352 * the path value of "<b>Location:partof</b>". 2353 */ 2354 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTOF = new ca.uhn.fhir.model.api.Include("Location:partof").toLocked(); 2355 2356 /** 2357 * Search parameter: <b>status</b> 2358 * <p> 2359 * Description: <b>Searches for locations with a specific kind of status</b><br> 2360 * Type: <b>token</b><br> 2361 * Path: <b>Location.status</b><br> 2362 * </p> 2363 */ 2364 @SearchParamDefinition(name="status", path="Location.status", description="Searches for locations with a specific kind of status", type="token" ) 2365 public static final String SP_STATUS = "status"; 2366 /** 2367 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2368 * <p> 2369 * Description: <b>Searches for locations with a specific kind of status</b><br> 2370 * Type: <b>token</b><br> 2371 * Path: <b>Location.status</b><br> 2372 * </p> 2373 */ 2374 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2375 2376 /** 2377 * Search parameter: <b>type</b> 2378 * <p> 2379 * Description: <b>A code for the type of location</b><br> 2380 * Type: <b>token</b><br> 2381 * Path: <b>Location.type</b><br> 2382 * </p> 2383 */ 2384 @SearchParamDefinition(name="type", path="Location.type", description="A code for the type of location", type="token" ) 2385 public static final String SP_TYPE = "type"; 2386 /** 2387 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2388 * <p> 2389 * Description: <b>A code for the type of location</b><br> 2390 * Type: <b>token</b><br> 2391 * Path: <b>Location.type</b><br> 2392 * </p> 2393 */ 2394 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2395 2396 2397} 2398