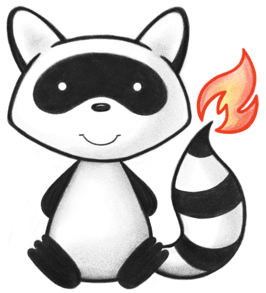
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The definition and characteristics of a medicinal manufactured item, such as a tablet or capsule, as contained in a packaged medicinal product. 052 */ 053@ResourceDef(name="ManufacturedItemDefinition", profile="http://hl7.org/fhir/StructureDefinition/ManufacturedItemDefinition") 054public class ManufacturedItemDefinition extends DomainResource { 055 056 @Block() 057 public static class ManufacturedItemDefinitionPropertyComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * A code expressing the type of characteristic. 060 */ 061 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 062 @Description(shortDefinition="A code expressing the type of characteristic", formalDefinition="A code expressing the type of characteristic." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/product-characteristic-codes") 064 protected CodeableConcept type; 065 066 /** 067 * A value for the characteristic. 068 */ 069 @Child(name = "value", type = {CodeableConcept.class, Quantity.class, DateType.class, BooleanType.class, MarkdownType.class, Attachment.class, Binary.class}, order=2, min=0, max=1, modifier=false, summary=true) 070 @Description(shortDefinition="A value for the characteristic", formalDefinition="A value for the characteristic." ) 071 protected DataType value; 072 073 private static final long serialVersionUID = -1659186716L; 074 075 /** 076 * Constructor 077 */ 078 public ManufacturedItemDefinitionPropertyComponent() { 079 super(); 080 } 081 082 /** 083 * Constructor 084 */ 085 public ManufacturedItemDefinitionPropertyComponent(CodeableConcept type) { 086 super(); 087 this.setType(type); 088 } 089 090 /** 091 * @return {@link #type} (A code expressing the type of characteristic.) 092 */ 093 public CodeableConcept getType() { 094 if (this.type == null) 095 if (Configuration.errorOnAutoCreate()) 096 throw new Error("Attempt to auto-create ManufacturedItemDefinitionPropertyComponent.type"); 097 else if (Configuration.doAutoCreate()) 098 this.type = new CodeableConcept(); // cc 099 return this.type; 100 } 101 102 public boolean hasType() { 103 return this.type != null && !this.type.isEmpty(); 104 } 105 106 /** 107 * @param value {@link #type} (A code expressing the type of characteristic.) 108 */ 109 public ManufacturedItemDefinitionPropertyComponent setType(CodeableConcept value) { 110 this.type = value; 111 return this; 112 } 113 114 /** 115 * @return {@link #value} (A value for the characteristic.) 116 */ 117 public DataType getValue() { 118 return this.value; 119 } 120 121 /** 122 * @return {@link #value} (A value for the characteristic.) 123 */ 124 public CodeableConcept getValueCodeableConcept() throws FHIRException { 125 if (this.value == null) 126 this.value = new CodeableConcept(); 127 if (!(this.value instanceof CodeableConcept)) 128 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 129 return (CodeableConcept) this.value; 130 } 131 132 public boolean hasValueCodeableConcept() { 133 return this.value instanceof CodeableConcept; 134 } 135 136 /** 137 * @return {@link #value} (A value for the characteristic.) 138 */ 139 public Quantity getValueQuantity() throws FHIRException { 140 if (this.value == null) 141 this.value = new Quantity(); 142 if (!(this.value instanceof Quantity)) 143 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 144 return (Quantity) this.value; 145 } 146 147 public boolean hasValueQuantity() { 148 return this.value instanceof Quantity; 149 } 150 151 /** 152 * @return {@link #value} (A value for the characteristic.) 153 */ 154 public DateType getValueDateType() throws FHIRException { 155 if (this.value == null) 156 this.value = new DateType(); 157 if (!(this.value instanceof DateType)) 158 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.value.getClass().getName()+" was encountered"); 159 return (DateType) this.value; 160 } 161 162 public boolean hasValueDateType() { 163 return this.value instanceof DateType; 164 } 165 166 /** 167 * @return {@link #value} (A value for the characteristic.) 168 */ 169 public BooleanType getValueBooleanType() throws FHIRException { 170 if (this.value == null) 171 this.value = new BooleanType(); 172 if (!(this.value instanceof BooleanType)) 173 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 174 return (BooleanType) this.value; 175 } 176 177 public boolean hasValueBooleanType() { 178 return this.value instanceof BooleanType; 179 } 180 181 /** 182 * @return {@link #value} (A value for the characteristic.) 183 */ 184 public MarkdownType getValueMarkdownType() throws FHIRException { 185 if (this.value == null) 186 this.value = new MarkdownType(); 187 if (!(this.value instanceof MarkdownType)) 188 throw new FHIRException("Type mismatch: the type MarkdownType was expected, but "+this.value.getClass().getName()+" was encountered"); 189 return (MarkdownType) this.value; 190 } 191 192 public boolean hasValueMarkdownType() { 193 return this.value instanceof MarkdownType; 194 } 195 196 /** 197 * @return {@link #value} (A value for the characteristic.) 198 */ 199 public Attachment getValueAttachment() throws FHIRException { 200 if (this.value == null) 201 this.value = new Attachment(); 202 if (!(this.value instanceof Attachment)) 203 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 204 return (Attachment) this.value; 205 } 206 207 public boolean hasValueAttachment() { 208 return this.value instanceof Attachment; 209 } 210 211 /** 212 * @return {@link #value} (A value for the characteristic.) 213 */ 214 public Reference getValueReference() throws FHIRException { 215 if (this.value == null) 216 this.value = new Reference(); 217 if (!(this.value instanceof Reference)) 218 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 219 return (Reference) this.value; 220 } 221 222 public boolean hasValueReference() { 223 return this.value instanceof Reference; 224 } 225 226 public boolean hasValue() { 227 return this.value != null && !this.value.isEmpty(); 228 } 229 230 /** 231 * @param value {@link #value} (A value for the characteristic.) 232 */ 233 public ManufacturedItemDefinitionPropertyComponent setValue(DataType value) { 234 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity || value instanceof DateType || value instanceof BooleanType || value instanceof MarkdownType || value instanceof Attachment || value instanceof Reference)) 235 throw new FHIRException("Not the right type for ManufacturedItemDefinition.property.value[x]: "+value.fhirType()); 236 this.value = value; 237 return this; 238 } 239 240 protected void listChildren(List<Property> children) { 241 super.listChildren(children); 242 children.add(new Property("type", "CodeableConcept", "A code expressing the type of characteristic.", 0, 1, type)); 243 children.add(new Property("value[x]", "CodeableConcept|Quantity|date|boolean|markdown|Attachment|Reference(Binary)", "A value for the characteristic.", 0, 1, value)); 244 } 245 246 @Override 247 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 248 switch (_hash) { 249 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A code expressing the type of characteristic.", 0, 1, type); 250 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|Quantity|date|boolean|markdown|Attachment|Reference(Binary)", "A value for the characteristic.", 0, 1, value); 251 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|Quantity|date|boolean|markdown|Attachment|Reference(Binary)", "A value for the characteristic.", 0, 1, value); 252 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "A value for the characteristic.", 0, 1, value); 253 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "A value for the characteristic.", 0, 1, value); 254 case -766192449: /*valueDate*/ return new Property("value[x]", "date", "A value for the characteristic.", 0, 1, value); 255 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "A value for the characteristic.", 0, 1, value); 256 case -497880704: /*valueMarkdown*/ return new Property("value[x]", "markdown", "A value for the characteristic.", 0, 1, value); 257 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "A value for the characteristic.", 0, 1, value); 258 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference(Binary)", "A value for the characteristic.", 0, 1, value); 259 default: return super.getNamedProperty(_hash, _name, _checkValid); 260 } 261 262 } 263 264 @Override 265 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 266 switch (hash) { 267 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 268 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 269 default: return super.getProperty(hash, name, checkValid); 270 } 271 272 } 273 274 @Override 275 public Base setProperty(int hash, String name, Base value) throws FHIRException { 276 switch (hash) { 277 case 3575610: // type 278 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 279 return value; 280 case 111972721: // value 281 this.value = TypeConvertor.castToType(value); // DataType 282 return value; 283 default: return super.setProperty(hash, name, value); 284 } 285 286 } 287 288 @Override 289 public Base setProperty(String name, Base value) throws FHIRException { 290 if (name.equals("type")) { 291 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 292 } else if (name.equals("value[x]")) { 293 this.value = TypeConvertor.castToType(value); // DataType 294 } else 295 return super.setProperty(name, value); 296 return value; 297 } 298 299 @Override 300 public void removeChild(String name, Base value) throws FHIRException { 301 if (name.equals("type")) { 302 this.type = null; 303 } else if (name.equals("value[x]")) { 304 this.value = null; 305 } else 306 super.removeChild(name, value); 307 308 } 309 310 @Override 311 public Base makeProperty(int hash, String name) throws FHIRException { 312 switch (hash) { 313 case 3575610: return getType(); 314 case -1410166417: return getValue(); 315 case 111972721: return getValue(); 316 default: return super.makeProperty(hash, name); 317 } 318 319 } 320 321 @Override 322 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 323 switch (hash) { 324 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 325 case 111972721: /*value*/ return new String[] {"CodeableConcept", "Quantity", "date", "boolean", "markdown", "Attachment", "Reference"}; 326 default: return super.getTypesForProperty(hash, name); 327 } 328 329 } 330 331 @Override 332 public Base addChild(String name) throws FHIRException { 333 if (name.equals("type")) { 334 this.type = new CodeableConcept(); 335 return this.type; 336 } 337 else if (name.equals("valueCodeableConcept")) { 338 this.value = new CodeableConcept(); 339 return this.value; 340 } 341 else if (name.equals("valueQuantity")) { 342 this.value = new Quantity(); 343 return this.value; 344 } 345 else if (name.equals("valueDate")) { 346 this.value = new DateType(); 347 return this.value; 348 } 349 else if (name.equals("valueBoolean")) { 350 this.value = new BooleanType(); 351 return this.value; 352 } 353 else if (name.equals("valueMarkdown")) { 354 this.value = new MarkdownType(); 355 return this.value; 356 } 357 else if (name.equals("valueAttachment")) { 358 this.value = new Attachment(); 359 return this.value; 360 } 361 else if (name.equals("valueReference")) { 362 this.value = new Reference(); 363 return this.value; 364 } 365 else 366 return super.addChild(name); 367 } 368 369 public ManufacturedItemDefinitionPropertyComponent copy() { 370 ManufacturedItemDefinitionPropertyComponent dst = new ManufacturedItemDefinitionPropertyComponent(); 371 copyValues(dst); 372 return dst; 373 } 374 375 public void copyValues(ManufacturedItemDefinitionPropertyComponent dst) { 376 super.copyValues(dst); 377 dst.type = type == null ? null : type.copy(); 378 dst.value = value == null ? null : value.copy(); 379 } 380 381 @Override 382 public boolean equalsDeep(Base other_) { 383 if (!super.equalsDeep(other_)) 384 return false; 385 if (!(other_ instanceof ManufacturedItemDefinitionPropertyComponent)) 386 return false; 387 ManufacturedItemDefinitionPropertyComponent o = (ManufacturedItemDefinitionPropertyComponent) other_; 388 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 389 } 390 391 @Override 392 public boolean equalsShallow(Base other_) { 393 if (!super.equalsShallow(other_)) 394 return false; 395 if (!(other_ instanceof ManufacturedItemDefinitionPropertyComponent)) 396 return false; 397 ManufacturedItemDefinitionPropertyComponent o = (ManufacturedItemDefinitionPropertyComponent) other_; 398 return true; 399 } 400 401 public boolean isEmpty() { 402 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 403 } 404 405 public String fhirType() { 406 return "ManufacturedItemDefinition.property"; 407 408 } 409 410 } 411 412 @Block() 413 public static class ManufacturedItemDefinitionComponentComponent extends BackboneElement implements IBaseBackboneElement { 414 /** 415 * Defining type of the component e.g. shell, layer, ink. 416 */ 417 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 418 @Description(shortDefinition="Defining type of the component e.g. shell, layer, ink", formalDefinition="Defining type of the component e.g. shell, layer, ink." ) 419 protected CodeableConcept type; 420 421 /** 422 * The function of this component within the item e.g. delivers active ingredient, masks taste. 423 */ 424 @Child(name = "function", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 425 @Description(shortDefinition="The function of this component within the item e.g. delivers active ingredient, masks taste", formalDefinition="The function of this component within the item e.g. delivers active ingredient, masks taste." ) 426 protected List<CodeableConcept> function; 427 428 /** 429 * The measurable amount of total quantity of all substances in the component, expressable in different ways (e.g. by mass or volume). 430 */ 431 @Child(name = "amount", type = {Quantity.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 432 @Description(shortDefinition="The measurable amount of total quantity of all substances in the component, expressable in different ways (e.g. by mass or volume)", formalDefinition="The measurable amount of total quantity of all substances in the component, expressable in different ways (e.g. by mass or volume)." ) 433 protected List<Quantity> amount; 434 435 /** 436 * A reference to a constituent of the manufactured item as a whole, linked here so that its component location within the item can be indicated. This not where the item's ingredient are primarily stated (for which see Ingredient.for or ManufacturedItemDefinition.ingredient). 437 */ 438 @Child(name = "constituent", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 439 @Description(shortDefinition="A reference to a constituent of the manufactured item as a whole, linked here so that its component location within the item can be indicated. This not where the item's ingredient are primarily stated (for which see Ingredient.for or ManufacturedItemDefinition.ingredient)", formalDefinition="A reference to a constituent of the manufactured item as a whole, linked here so that its component location within the item can be indicated. This not where the item's ingredient are primarily stated (for which see Ingredient.for or ManufacturedItemDefinition.ingredient)." ) 440 protected List<ManufacturedItemDefinitionComponentConstituentComponent> constituent; 441 442 /** 443 * General characteristics of this component. 444 */ 445 @Child(name = "property", type = {ManufacturedItemDefinitionPropertyComponent.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 446 @Description(shortDefinition="General characteristics of this component", formalDefinition="General characteristics of this component." ) 447 protected List<ManufacturedItemDefinitionPropertyComponent> property; 448 449 /** 450 * A component that this component contains or is made from. 451 */ 452 @Child(name = "component", type = {ManufacturedItemDefinitionComponentComponent.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 453 @Description(shortDefinition="A component that this component contains or is made from", formalDefinition="A component that this component contains or is made from." ) 454 protected List<ManufacturedItemDefinitionComponentComponent> component; 455 456 private static final long serialVersionUID = 537950590L; 457 458 /** 459 * Constructor 460 */ 461 public ManufacturedItemDefinitionComponentComponent() { 462 super(); 463 } 464 465 /** 466 * Constructor 467 */ 468 public ManufacturedItemDefinitionComponentComponent(CodeableConcept type) { 469 super(); 470 this.setType(type); 471 } 472 473 /** 474 * @return {@link #type} (Defining type of the component e.g. shell, layer, ink.) 475 */ 476 public CodeableConcept getType() { 477 if (this.type == null) 478 if (Configuration.errorOnAutoCreate()) 479 throw new Error("Attempt to auto-create ManufacturedItemDefinitionComponentComponent.type"); 480 else if (Configuration.doAutoCreate()) 481 this.type = new CodeableConcept(); // cc 482 return this.type; 483 } 484 485 public boolean hasType() { 486 return this.type != null && !this.type.isEmpty(); 487 } 488 489 /** 490 * @param value {@link #type} (Defining type of the component e.g. shell, layer, ink.) 491 */ 492 public ManufacturedItemDefinitionComponentComponent setType(CodeableConcept value) { 493 this.type = value; 494 return this; 495 } 496 497 /** 498 * @return {@link #function} (The function of this component within the item e.g. delivers active ingredient, masks taste.) 499 */ 500 public List<CodeableConcept> getFunction() { 501 if (this.function == null) 502 this.function = new ArrayList<CodeableConcept>(); 503 return this.function; 504 } 505 506 /** 507 * @return Returns a reference to <code>this</code> for easy method chaining 508 */ 509 public ManufacturedItemDefinitionComponentComponent setFunction(List<CodeableConcept> theFunction) { 510 this.function = theFunction; 511 return this; 512 } 513 514 public boolean hasFunction() { 515 if (this.function == null) 516 return false; 517 for (CodeableConcept item : this.function) 518 if (!item.isEmpty()) 519 return true; 520 return false; 521 } 522 523 public CodeableConcept addFunction() { //3 524 CodeableConcept t = new CodeableConcept(); 525 if (this.function == null) 526 this.function = new ArrayList<CodeableConcept>(); 527 this.function.add(t); 528 return t; 529 } 530 531 public ManufacturedItemDefinitionComponentComponent addFunction(CodeableConcept t) { //3 532 if (t == null) 533 return this; 534 if (this.function == null) 535 this.function = new ArrayList<CodeableConcept>(); 536 this.function.add(t); 537 return this; 538 } 539 540 /** 541 * @return The first repetition of repeating field {@link #function}, creating it if it does not already exist {3} 542 */ 543 public CodeableConcept getFunctionFirstRep() { 544 if (getFunction().isEmpty()) { 545 addFunction(); 546 } 547 return getFunction().get(0); 548 } 549 550 /** 551 * @return {@link #amount} (The measurable amount of total quantity of all substances in the component, expressable in different ways (e.g. by mass or volume).) 552 */ 553 public List<Quantity> getAmount() { 554 if (this.amount == null) 555 this.amount = new ArrayList<Quantity>(); 556 return this.amount; 557 } 558 559 /** 560 * @return Returns a reference to <code>this</code> for easy method chaining 561 */ 562 public ManufacturedItemDefinitionComponentComponent setAmount(List<Quantity> theAmount) { 563 this.amount = theAmount; 564 return this; 565 } 566 567 public boolean hasAmount() { 568 if (this.amount == null) 569 return false; 570 for (Quantity item : this.amount) 571 if (!item.isEmpty()) 572 return true; 573 return false; 574 } 575 576 public Quantity addAmount() { //3 577 Quantity t = new Quantity(); 578 if (this.amount == null) 579 this.amount = new ArrayList<Quantity>(); 580 this.amount.add(t); 581 return t; 582 } 583 584 public ManufacturedItemDefinitionComponentComponent addAmount(Quantity t) { //3 585 if (t == null) 586 return this; 587 if (this.amount == null) 588 this.amount = new ArrayList<Quantity>(); 589 this.amount.add(t); 590 return this; 591 } 592 593 /** 594 * @return The first repetition of repeating field {@link #amount}, creating it if it does not already exist {3} 595 */ 596 public Quantity getAmountFirstRep() { 597 if (getAmount().isEmpty()) { 598 addAmount(); 599 } 600 return getAmount().get(0); 601 } 602 603 /** 604 * @return {@link #constituent} (A reference to a constituent of the manufactured item as a whole, linked here so that its component location within the item can be indicated. This not where the item's ingredient are primarily stated (for which see Ingredient.for or ManufacturedItemDefinition.ingredient).) 605 */ 606 public List<ManufacturedItemDefinitionComponentConstituentComponent> getConstituent() { 607 if (this.constituent == null) 608 this.constituent = new ArrayList<ManufacturedItemDefinitionComponentConstituentComponent>(); 609 return this.constituent; 610 } 611 612 /** 613 * @return Returns a reference to <code>this</code> for easy method chaining 614 */ 615 public ManufacturedItemDefinitionComponentComponent setConstituent(List<ManufacturedItemDefinitionComponentConstituentComponent> theConstituent) { 616 this.constituent = theConstituent; 617 return this; 618 } 619 620 public boolean hasConstituent() { 621 if (this.constituent == null) 622 return false; 623 for (ManufacturedItemDefinitionComponentConstituentComponent item : this.constituent) 624 if (!item.isEmpty()) 625 return true; 626 return false; 627 } 628 629 public ManufacturedItemDefinitionComponentConstituentComponent addConstituent() { //3 630 ManufacturedItemDefinitionComponentConstituentComponent t = new ManufacturedItemDefinitionComponentConstituentComponent(); 631 if (this.constituent == null) 632 this.constituent = new ArrayList<ManufacturedItemDefinitionComponentConstituentComponent>(); 633 this.constituent.add(t); 634 return t; 635 } 636 637 public ManufacturedItemDefinitionComponentComponent addConstituent(ManufacturedItemDefinitionComponentConstituentComponent t) { //3 638 if (t == null) 639 return this; 640 if (this.constituent == null) 641 this.constituent = new ArrayList<ManufacturedItemDefinitionComponentConstituentComponent>(); 642 this.constituent.add(t); 643 return this; 644 } 645 646 /** 647 * @return The first repetition of repeating field {@link #constituent}, creating it if it does not already exist {3} 648 */ 649 public ManufacturedItemDefinitionComponentConstituentComponent getConstituentFirstRep() { 650 if (getConstituent().isEmpty()) { 651 addConstituent(); 652 } 653 return getConstituent().get(0); 654 } 655 656 /** 657 * @return {@link #property} (General characteristics of this component.) 658 */ 659 public List<ManufacturedItemDefinitionPropertyComponent> getProperty() { 660 if (this.property == null) 661 this.property = new ArrayList<ManufacturedItemDefinitionPropertyComponent>(); 662 return this.property; 663 } 664 665 /** 666 * @return Returns a reference to <code>this</code> for easy method chaining 667 */ 668 public ManufacturedItemDefinitionComponentComponent setProperty(List<ManufacturedItemDefinitionPropertyComponent> theProperty) { 669 this.property = theProperty; 670 return this; 671 } 672 673 public boolean hasProperty() { 674 if (this.property == null) 675 return false; 676 for (ManufacturedItemDefinitionPropertyComponent item : this.property) 677 if (!item.isEmpty()) 678 return true; 679 return false; 680 } 681 682 public ManufacturedItemDefinitionPropertyComponent addProperty() { //3 683 ManufacturedItemDefinitionPropertyComponent t = new ManufacturedItemDefinitionPropertyComponent(); 684 if (this.property == null) 685 this.property = new ArrayList<ManufacturedItemDefinitionPropertyComponent>(); 686 this.property.add(t); 687 return t; 688 } 689 690 public ManufacturedItemDefinitionComponentComponent addProperty(ManufacturedItemDefinitionPropertyComponent t) { //3 691 if (t == null) 692 return this; 693 if (this.property == null) 694 this.property = new ArrayList<ManufacturedItemDefinitionPropertyComponent>(); 695 this.property.add(t); 696 return this; 697 } 698 699 /** 700 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist {3} 701 */ 702 public ManufacturedItemDefinitionPropertyComponent getPropertyFirstRep() { 703 if (getProperty().isEmpty()) { 704 addProperty(); 705 } 706 return getProperty().get(0); 707 } 708 709 /** 710 * @return {@link #component} (A component that this component contains or is made from.) 711 */ 712 public List<ManufacturedItemDefinitionComponentComponent> getComponent() { 713 if (this.component == null) 714 this.component = new ArrayList<ManufacturedItemDefinitionComponentComponent>(); 715 return this.component; 716 } 717 718 /** 719 * @return Returns a reference to <code>this</code> for easy method chaining 720 */ 721 public ManufacturedItemDefinitionComponentComponent setComponent(List<ManufacturedItemDefinitionComponentComponent> theComponent) { 722 this.component = theComponent; 723 return this; 724 } 725 726 public boolean hasComponent() { 727 if (this.component == null) 728 return false; 729 for (ManufacturedItemDefinitionComponentComponent item : this.component) 730 if (!item.isEmpty()) 731 return true; 732 return false; 733 } 734 735 public ManufacturedItemDefinitionComponentComponent addComponent() { //3 736 ManufacturedItemDefinitionComponentComponent t = new ManufacturedItemDefinitionComponentComponent(); 737 if (this.component == null) 738 this.component = new ArrayList<ManufacturedItemDefinitionComponentComponent>(); 739 this.component.add(t); 740 return t; 741 } 742 743 public ManufacturedItemDefinitionComponentComponent addComponent(ManufacturedItemDefinitionComponentComponent t) { //3 744 if (t == null) 745 return this; 746 if (this.component == null) 747 this.component = new ArrayList<ManufacturedItemDefinitionComponentComponent>(); 748 this.component.add(t); 749 return this; 750 } 751 752 /** 753 * @return The first repetition of repeating field {@link #component}, creating it if it does not already exist {3} 754 */ 755 public ManufacturedItemDefinitionComponentComponent getComponentFirstRep() { 756 if (getComponent().isEmpty()) { 757 addComponent(); 758 } 759 return getComponent().get(0); 760 } 761 762 protected void listChildren(List<Property> children) { 763 super.listChildren(children); 764 children.add(new Property("type", "CodeableConcept", "Defining type of the component e.g. shell, layer, ink.", 0, 1, type)); 765 children.add(new Property("function", "CodeableConcept", "The function of this component within the item e.g. delivers active ingredient, masks taste.", 0, java.lang.Integer.MAX_VALUE, function)); 766 children.add(new Property("amount", "Quantity", "The measurable amount of total quantity of all substances in the component, expressable in different ways (e.g. by mass or volume).", 0, java.lang.Integer.MAX_VALUE, amount)); 767 children.add(new Property("constituent", "", "A reference to a constituent of the manufactured item as a whole, linked here so that its component location within the item can be indicated. This not where the item's ingredient are primarily stated (for which see Ingredient.for or ManufacturedItemDefinition.ingredient).", 0, java.lang.Integer.MAX_VALUE, constituent)); 768 children.add(new Property("property", "@ManufacturedItemDefinition.property", "General characteristics of this component.", 0, java.lang.Integer.MAX_VALUE, property)); 769 children.add(new Property("component", "@ManufacturedItemDefinition.component", "A component that this component contains or is made from.", 0, java.lang.Integer.MAX_VALUE, component)); 770 } 771 772 @Override 773 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 774 switch (_hash) { 775 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Defining type of the component e.g. shell, layer, ink.", 0, 1, type); 776 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "The function of this component within the item e.g. delivers active ingredient, masks taste.", 0, java.lang.Integer.MAX_VALUE, function); 777 case -1413853096: /*amount*/ return new Property("amount", "Quantity", "The measurable amount of total quantity of all substances in the component, expressable in different ways (e.g. by mass or volume).", 0, java.lang.Integer.MAX_VALUE, amount); 778 case -1846470364: /*constituent*/ return new Property("constituent", "", "A reference to a constituent of the manufactured item as a whole, linked here so that its component location within the item can be indicated. This not where the item's ingredient are primarily stated (for which see Ingredient.for or ManufacturedItemDefinition.ingredient).", 0, java.lang.Integer.MAX_VALUE, constituent); 779 case -993141291: /*property*/ return new Property("property", "@ManufacturedItemDefinition.property", "General characteristics of this component.", 0, java.lang.Integer.MAX_VALUE, property); 780 case -1399907075: /*component*/ return new Property("component", "@ManufacturedItemDefinition.component", "A component that this component contains or is made from.", 0, java.lang.Integer.MAX_VALUE, component); 781 default: return super.getNamedProperty(_hash, _name, _checkValid); 782 } 783 784 } 785 786 @Override 787 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 788 switch (hash) { 789 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 790 case 1380938712: /*function*/ return this.function == null ? new Base[0] : this.function.toArray(new Base[this.function.size()]); // CodeableConcept 791 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : this.amount.toArray(new Base[this.amount.size()]); // Quantity 792 case -1846470364: /*constituent*/ return this.constituent == null ? new Base[0] : this.constituent.toArray(new Base[this.constituent.size()]); // ManufacturedItemDefinitionComponentConstituentComponent 793 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // ManufacturedItemDefinitionPropertyComponent 794 case -1399907075: /*component*/ return this.component == null ? new Base[0] : this.component.toArray(new Base[this.component.size()]); // ManufacturedItemDefinitionComponentComponent 795 default: return super.getProperty(hash, name, checkValid); 796 } 797 798 } 799 800 @Override 801 public Base setProperty(int hash, String name, Base value) throws FHIRException { 802 switch (hash) { 803 case 3575610: // type 804 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 805 return value; 806 case 1380938712: // function 807 this.getFunction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 808 return value; 809 case -1413853096: // amount 810 this.getAmount().add(TypeConvertor.castToQuantity(value)); // Quantity 811 return value; 812 case -1846470364: // constituent 813 this.getConstituent().add((ManufacturedItemDefinitionComponentConstituentComponent) value); // ManufacturedItemDefinitionComponentConstituentComponent 814 return value; 815 case -993141291: // property 816 this.getProperty().add((ManufacturedItemDefinitionPropertyComponent) value); // ManufacturedItemDefinitionPropertyComponent 817 return value; 818 case -1399907075: // component 819 this.getComponent().add((ManufacturedItemDefinitionComponentComponent) value); // ManufacturedItemDefinitionComponentComponent 820 return value; 821 default: return super.setProperty(hash, name, value); 822 } 823 824 } 825 826 @Override 827 public Base setProperty(String name, Base value) throws FHIRException { 828 if (name.equals("type")) { 829 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 830 } else if (name.equals("function")) { 831 this.getFunction().add(TypeConvertor.castToCodeableConcept(value)); 832 } else if (name.equals("amount")) { 833 this.getAmount().add(TypeConvertor.castToQuantity(value)); 834 } else if (name.equals("constituent")) { 835 this.getConstituent().add((ManufacturedItemDefinitionComponentConstituentComponent) value); 836 } else if (name.equals("property")) { 837 this.getProperty().add((ManufacturedItemDefinitionPropertyComponent) value); 838 } else if (name.equals("component")) { 839 this.getComponent().add((ManufacturedItemDefinitionComponentComponent) value); 840 } else 841 return super.setProperty(name, value); 842 return value; 843 } 844 845 @Override 846 public void removeChild(String name, Base value) throws FHIRException { 847 if (name.equals("type")) { 848 this.type = null; 849 } else if (name.equals("function")) { 850 this.getFunction().remove(value); 851 } else if (name.equals("amount")) { 852 this.getAmount().remove(value); 853 } else if (name.equals("constituent")) { 854 this.getConstituent().remove((ManufacturedItemDefinitionComponentConstituentComponent) value); 855 } else if (name.equals("property")) { 856 this.getProperty().remove((ManufacturedItemDefinitionPropertyComponent) value); 857 } else if (name.equals("component")) { 858 this.getComponent().remove((ManufacturedItemDefinitionComponentComponent) value); 859 } else 860 super.removeChild(name, value); 861 862 } 863 864 @Override 865 public Base makeProperty(int hash, String name) throws FHIRException { 866 switch (hash) { 867 case 3575610: return getType(); 868 case 1380938712: return addFunction(); 869 case -1413853096: return addAmount(); 870 case -1846470364: return addConstituent(); 871 case -993141291: return addProperty(); 872 case -1399907075: return addComponent(); 873 default: return super.makeProperty(hash, name); 874 } 875 876 } 877 878 @Override 879 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 880 switch (hash) { 881 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 882 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 883 case -1413853096: /*amount*/ return new String[] {"Quantity"}; 884 case -1846470364: /*constituent*/ return new String[] {}; 885 case -993141291: /*property*/ return new String[] {"@ManufacturedItemDefinition.property"}; 886 case -1399907075: /*component*/ return new String[] {"@ManufacturedItemDefinition.component"}; 887 default: return super.getTypesForProperty(hash, name); 888 } 889 890 } 891 892 @Override 893 public Base addChild(String name) throws FHIRException { 894 if (name.equals("type")) { 895 this.type = new CodeableConcept(); 896 return this.type; 897 } 898 else if (name.equals("function")) { 899 return addFunction(); 900 } 901 else if (name.equals("amount")) { 902 return addAmount(); 903 } 904 else if (name.equals("constituent")) { 905 return addConstituent(); 906 } 907 else if (name.equals("property")) { 908 return addProperty(); 909 } 910 else if (name.equals("component")) { 911 return addComponent(); 912 } 913 else 914 return super.addChild(name); 915 } 916 917 public ManufacturedItemDefinitionComponentComponent copy() { 918 ManufacturedItemDefinitionComponentComponent dst = new ManufacturedItemDefinitionComponentComponent(); 919 copyValues(dst); 920 return dst; 921 } 922 923 public void copyValues(ManufacturedItemDefinitionComponentComponent dst) { 924 super.copyValues(dst); 925 dst.type = type == null ? null : type.copy(); 926 if (function != null) { 927 dst.function = new ArrayList<CodeableConcept>(); 928 for (CodeableConcept i : function) 929 dst.function.add(i.copy()); 930 }; 931 if (amount != null) { 932 dst.amount = new ArrayList<Quantity>(); 933 for (Quantity i : amount) 934 dst.amount.add(i.copy()); 935 }; 936 if (constituent != null) { 937 dst.constituent = new ArrayList<ManufacturedItemDefinitionComponentConstituentComponent>(); 938 for (ManufacturedItemDefinitionComponentConstituentComponent i : constituent) 939 dst.constituent.add(i.copy()); 940 }; 941 if (property != null) { 942 dst.property = new ArrayList<ManufacturedItemDefinitionPropertyComponent>(); 943 for (ManufacturedItemDefinitionPropertyComponent i : property) 944 dst.property.add(i.copy()); 945 }; 946 if (component != null) { 947 dst.component = new ArrayList<ManufacturedItemDefinitionComponentComponent>(); 948 for (ManufacturedItemDefinitionComponentComponent i : component) 949 dst.component.add(i.copy()); 950 }; 951 } 952 953 @Override 954 public boolean equalsDeep(Base other_) { 955 if (!super.equalsDeep(other_)) 956 return false; 957 if (!(other_ instanceof ManufacturedItemDefinitionComponentComponent)) 958 return false; 959 ManufacturedItemDefinitionComponentComponent o = (ManufacturedItemDefinitionComponentComponent) other_; 960 return compareDeep(type, o.type, true) && compareDeep(function, o.function, true) && compareDeep(amount, o.amount, true) 961 && compareDeep(constituent, o.constituent, true) && compareDeep(property, o.property, true) && compareDeep(component, o.component, true) 962 ; 963 } 964 965 @Override 966 public boolean equalsShallow(Base other_) { 967 if (!super.equalsShallow(other_)) 968 return false; 969 if (!(other_ instanceof ManufacturedItemDefinitionComponentComponent)) 970 return false; 971 ManufacturedItemDefinitionComponentComponent o = (ManufacturedItemDefinitionComponentComponent) other_; 972 return true; 973 } 974 975 public boolean isEmpty() { 976 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, function, amount, constituent 977 , property, component); 978 } 979 980 public String fhirType() { 981 return "ManufacturedItemDefinition.component"; 982 983 } 984 985 } 986 987 @Block() 988 public static class ManufacturedItemDefinitionComponentConstituentComponent extends BackboneElement implements IBaseBackboneElement { 989 /** 990 * The measurable amount of the substance, expressable in different ways (e.g. by mass or volume). 991 */ 992 @Child(name = "amount", type = {Quantity.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 993 @Description(shortDefinition="The measurable amount of the substance, expressable in different ways (e.g. by mass or volume)", formalDefinition="The measurable amount of the substance, expressable in different ways (e.g. by mass or volume)." ) 994 protected List<Quantity> amount; 995 996 /** 997 * The physical location of the constituent/ingredient within the component. Example ? if the component is the bead in the capsule, then the location would be where the ingredient resides within the product part ? intragranular, extra-granular, etc. 998 */ 999 @Child(name = "location", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1000 @Description(shortDefinition="The physical location of the constituent/ingredient within the component", formalDefinition="The physical location of the constituent/ingredient within the component. Example ? if the component is the bead in the capsule, then the location would be where the ingredient resides within the product part ? intragranular, extra-granular, etc." ) 1001 protected List<CodeableConcept> location; 1002 1003 /** 1004 * The function of this constituent within the component e.g. binder. 1005 */ 1006 @Child(name = "function", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1007 @Description(shortDefinition="The function of this constituent within the component e.g. binder", formalDefinition="The function of this constituent within the component e.g. binder." ) 1008 protected List<CodeableConcept> function; 1009 1010 /** 1011 * The ingredient that is the constituent of the given component. 1012 */ 1013 @Child(name = "hasIngredient", type = {CodeableReference.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1014 @Description(shortDefinition="The ingredient that is the constituent of the given component", formalDefinition="The ingredient that is the constituent of the given component." ) 1015 protected List<CodeableReference> hasIngredient; 1016 1017 private static final long serialVersionUID = -708786069L; 1018 1019 /** 1020 * Constructor 1021 */ 1022 public ManufacturedItemDefinitionComponentConstituentComponent() { 1023 super(); 1024 } 1025 1026 /** 1027 * @return {@link #amount} (The measurable amount of the substance, expressable in different ways (e.g. by mass or volume).) 1028 */ 1029 public List<Quantity> getAmount() { 1030 if (this.amount == null) 1031 this.amount = new ArrayList<Quantity>(); 1032 return this.amount; 1033 } 1034 1035 /** 1036 * @return Returns a reference to <code>this</code> for easy method chaining 1037 */ 1038 public ManufacturedItemDefinitionComponentConstituentComponent setAmount(List<Quantity> theAmount) { 1039 this.amount = theAmount; 1040 return this; 1041 } 1042 1043 public boolean hasAmount() { 1044 if (this.amount == null) 1045 return false; 1046 for (Quantity item : this.amount) 1047 if (!item.isEmpty()) 1048 return true; 1049 return false; 1050 } 1051 1052 public Quantity addAmount() { //3 1053 Quantity t = new Quantity(); 1054 if (this.amount == null) 1055 this.amount = new ArrayList<Quantity>(); 1056 this.amount.add(t); 1057 return t; 1058 } 1059 1060 public ManufacturedItemDefinitionComponentConstituentComponent addAmount(Quantity t) { //3 1061 if (t == null) 1062 return this; 1063 if (this.amount == null) 1064 this.amount = new ArrayList<Quantity>(); 1065 this.amount.add(t); 1066 return this; 1067 } 1068 1069 /** 1070 * @return The first repetition of repeating field {@link #amount}, creating it if it does not already exist {3} 1071 */ 1072 public Quantity getAmountFirstRep() { 1073 if (getAmount().isEmpty()) { 1074 addAmount(); 1075 } 1076 return getAmount().get(0); 1077 } 1078 1079 /** 1080 * @return {@link #location} (The physical location of the constituent/ingredient within the component. Example ? if the component is the bead in the capsule, then the location would be where the ingredient resides within the product part ? intragranular, extra-granular, etc.) 1081 */ 1082 public List<CodeableConcept> getLocation() { 1083 if (this.location == null) 1084 this.location = new ArrayList<CodeableConcept>(); 1085 return this.location; 1086 } 1087 1088 /** 1089 * @return Returns a reference to <code>this</code> for easy method chaining 1090 */ 1091 public ManufacturedItemDefinitionComponentConstituentComponent setLocation(List<CodeableConcept> theLocation) { 1092 this.location = theLocation; 1093 return this; 1094 } 1095 1096 public boolean hasLocation() { 1097 if (this.location == null) 1098 return false; 1099 for (CodeableConcept item : this.location) 1100 if (!item.isEmpty()) 1101 return true; 1102 return false; 1103 } 1104 1105 public CodeableConcept addLocation() { //3 1106 CodeableConcept t = new CodeableConcept(); 1107 if (this.location == null) 1108 this.location = new ArrayList<CodeableConcept>(); 1109 this.location.add(t); 1110 return t; 1111 } 1112 1113 public ManufacturedItemDefinitionComponentConstituentComponent addLocation(CodeableConcept t) { //3 1114 if (t == null) 1115 return this; 1116 if (this.location == null) 1117 this.location = new ArrayList<CodeableConcept>(); 1118 this.location.add(t); 1119 return this; 1120 } 1121 1122 /** 1123 * @return The first repetition of repeating field {@link #location}, creating it if it does not already exist {3} 1124 */ 1125 public CodeableConcept getLocationFirstRep() { 1126 if (getLocation().isEmpty()) { 1127 addLocation(); 1128 } 1129 return getLocation().get(0); 1130 } 1131 1132 /** 1133 * @return {@link #function} (The function of this constituent within the component e.g. binder.) 1134 */ 1135 public List<CodeableConcept> getFunction() { 1136 if (this.function == null) 1137 this.function = new ArrayList<CodeableConcept>(); 1138 return this.function; 1139 } 1140 1141 /** 1142 * @return Returns a reference to <code>this</code> for easy method chaining 1143 */ 1144 public ManufacturedItemDefinitionComponentConstituentComponent setFunction(List<CodeableConcept> theFunction) { 1145 this.function = theFunction; 1146 return this; 1147 } 1148 1149 public boolean hasFunction() { 1150 if (this.function == null) 1151 return false; 1152 for (CodeableConcept item : this.function) 1153 if (!item.isEmpty()) 1154 return true; 1155 return false; 1156 } 1157 1158 public CodeableConcept addFunction() { //3 1159 CodeableConcept t = new CodeableConcept(); 1160 if (this.function == null) 1161 this.function = new ArrayList<CodeableConcept>(); 1162 this.function.add(t); 1163 return t; 1164 } 1165 1166 public ManufacturedItemDefinitionComponentConstituentComponent addFunction(CodeableConcept t) { //3 1167 if (t == null) 1168 return this; 1169 if (this.function == null) 1170 this.function = new ArrayList<CodeableConcept>(); 1171 this.function.add(t); 1172 return this; 1173 } 1174 1175 /** 1176 * @return The first repetition of repeating field {@link #function}, creating it if it does not already exist {3} 1177 */ 1178 public CodeableConcept getFunctionFirstRep() { 1179 if (getFunction().isEmpty()) { 1180 addFunction(); 1181 } 1182 return getFunction().get(0); 1183 } 1184 1185 /** 1186 * @return {@link #hasIngredient} (The ingredient that is the constituent of the given component.) 1187 */ 1188 public List<CodeableReference> getHasIngredient() { 1189 if (this.hasIngredient == null) 1190 this.hasIngredient = new ArrayList<CodeableReference>(); 1191 return this.hasIngredient; 1192 } 1193 1194 /** 1195 * @return Returns a reference to <code>this</code> for easy method chaining 1196 */ 1197 public ManufacturedItemDefinitionComponentConstituentComponent setHasIngredient(List<CodeableReference> theHasIngredient) { 1198 this.hasIngredient = theHasIngredient; 1199 return this; 1200 } 1201 1202 public boolean hasHasIngredient() { 1203 if (this.hasIngredient == null) 1204 return false; 1205 for (CodeableReference item : this.hasIngredient) 1206 if (!item.isEmpty()) 1207 return true; 1208 return false; 1209 } 1210 1211 public CodeableReference addHasIngredient() { //3 1212 CodeableReference t = new CodeableReference(); 1213 if (this.hasIngredient == null) 1214 this.hasIngredient = new ArrayList<CodeableReference>(); 1215 this.hasIngredient.add(t); 1216 return t; 1217 } 1218 1219 public ManufacturedItemDefinitionComponentConstituentComponent addHasIngredient(CodeableReference t) { //3 1220 if (t == null) 1221 return this; 1222 if (this.hasIngredient == null) 1223 this.hasIngredient = new ArrayList<CodeableReference>(); 1224 this.hasIngredient.add(t); 1225 return this; 1226 } 1227 1228 /** 1229 * @return The first repetition of repeating field {@link #hasIngredient}, creating it if it does not already exist {3} 1230 */ 1231 public CodeableReference getHasIngredientFirstRep() { 1232 if (getHasIngredient().isEmpty()) { 1233 addHasIngredient(); 1234 } 1235 return getHasIngredient().get(0); 1236 } 1237 1238 protected void listChildren(List<Property> children) { 1239 super.listChildren(children); 1240 children.add(new Property("amount", "Quantity", "The measurable amount of the substance, expressable in different ways (e.g. by mass or volume).", 0, java.lang.Integer.MAX_VALUE, amount)); 1241 children.add(new Property("location", "CodeableConcept", "The physical location of the constituent/ingredient within the component. Example ? if the component is the bead in the capsule, then the location would be where the ingredient resides within the product part ? intragranular, extra-granular, etc.", 0, java.lang.Integer.MAX_VALUE, location)); 1242 children.add(new Property("function", "CodeableConcept", "The function of this constituent within the component e.g. binder.", 0, java.lang.Integer.MAX_VALUE, function)); 1243 children.add(new Property("hasIngredient", "CodeableReference(Ingredient)", "The ingredient that is the constituent of the given component.", 0, java.lang.Integer.MAX_VALUE, hasIngredient)); 1244 } 1245 1246 @Override 1247 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1248 switch (_hash) { 1249 case -1413853096: /*amount*/ return new Property("amount", "Quantity", "The measurable amount of the substance, expressable in different ways (e.g. by mass or volume).", 0, java.lang.Integer.MAX_VALUE, amount); 1250 case 1901043637: /*location*/ return new Property("location", "CodeableConcept", "The physical location of the constituent/ingredient within the component. Example ? if the component is the bead in the capsule, then the location would be where the ingredient resides within the product part ? intragranular, extra-granular, etc.", 0, java.lang.Integer.MAX_VALUE, location); 1251 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "The function of this constituent within the component e.g. binder.", 0, java.lang.Integer.MAX_VALUE, function); 1252 case 483059723: /*hasIngredient*/ return new Property("hasIngredient", "CodeableReference(Ingredient)", "The ingredient that is the constituent of the given component.", 0, java.lang.Integer.MAX_VALUE, hasIngredient); 1253 default: return super.getNamedProperty(_hash, _name, _checkValid); 1254 } 1255 1256 } 1257 1258 @Override 1259 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1260 switch (hash) { 1261 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : this.amount.toArray(new Base[this.amount.size()]); // Quantity 1262 case 1901043637: /*location*/ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // CodeableConcept 1263 case 1380938712: /*function*/ return this.function == null ? new Base[0] : this.function.toArray(new Base[this.function.size()]); // CodeableConcept 1264 case 483059723: /*hasIngredient*/ return this.hasIngredient == null ? new Base[0] : this.hasIngredient.toArray(new Base[this.hasIngredient.size()]); // CodeableReference 1265 default: return super.getProperty(hash, name, checkValid); 1266 } 1267 1268 } 1269 1270 @Override 1271 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1272 switch (hash) { 1273 case -1413853096: // amount 1274 this.getAmount().add(TypeConvertor.castToQuantity(value)); // Quantity 1275 return value; 1276 case 1901043637: // location 1277 this.getLocation().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1278 return value; 1279 case 1380938712: // function 1280 this.getFunction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1281 return value; 1282 case 483059723: // hasIngredient 1283 this.getHasIngredient().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1284 return value; 1285 default: return super.setProperty(hash, name, value); 1286 } 1287 1288 } 1289 1290 @Override 1291 public Base setProperty(String name, Base value) throws FHIRException { 1292 if (name.equals("amount")) { 1293 this.getAmount().add(TypeConvertor.castToQuantity(value)); 1294 } else if (name.equals("location")) { 1295 this.getLocation().add(TypeConvertor.castToCodeableConcept(value)); 1296 } else if (name.equals("function")) { 1297 this.getFunction().add(TypeConvertor.castToCodeableConcept(value)); 1298 } else if (name.equals("hasIngredient")) { 1299 this.getHasIngredient().add(TypeConvertor.castToCodeableReference(value)); 1300 } else 1301 return super.setProperty(name, value); 1302 return value; 1303 } 1304 1305 @Override 1306 public void removeChild(String name, Base value) throws FHIRException { 1307 if (name.equals("amount")) { 1308 this.getAmount().remove(value); 1309 } else if (name.equals("location")) { 1310 this.getLocation().remove(value); 1311 } else if (name.equals("function")) { 1312 this.getFunction().remove(value); 1313 } else if (name.equals("hasIngredient")) { 1314 this.getHasIngredient().remove(value); 1315 } else 1316 super.removeChild(name, value); 1317 1318 } 1319 1320 @Override 1321 public Base makeProperty(int hash, String name) throws FHIRException { 1322 switch (hash) { 1323 case -1413853096: return addAmount(); 1324 case 1901043637: return addLocation(); 1325 case 1380938712: return addFunction(); 1326 case 483059723: return addHasIngredient(); 1327 default: return super.makeProperty(hash, name); 1328 } 1329 1330 } 1331 1332 @Override 1333 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1334 switch (hash) { 1335 case -1413853096: /*amount*/ return new String[] {"Quantity"}; 1336 case 1901043637: /*location*/ return new String[] {"CodeableConcept"}; 1337 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 1338 case 483059723: /*hasIngredient*/ return new String[] {"CodeableReference"}; 1339 default: return super.getTypesForProperty(hash, name); 1340 } 1341 1342 } 1343 1344 @Override 1345 public Base addChild(String name) throws FHIRException { 1346 if (name.equals("amount")) { 1347 return addAmount(); 1348 } 1349 else if (name.equals("location")) { 1350 return addLocation(); 1351 } 1352 else if (name.equals("function")) { 1353 return addFunction(); 1354 } 1355 else if (name.equals("hasIngredient")) { 1356 return addHasIngredient(); 1357 } 1358 else 1359 return super.addChild(name); 1360 } 1361 1362 public ManufacturedItemDefinitionComponentConstituentComponent copy() { 1363 ManufacturedItemDefinitionComponentConstituentComponent dst = new ManufacturedItemDefinitionComponentConstituentComponent(); 1364 copyValues(dst); 1365 return dst; 1366 } 1367 1368 public void copyValues(ManufacturedItemDefinitionComponentConstituentComponent dst) { 1369 super.copyValues(dst); 1370 if (amount != null) { 1371 dst.amount = new ArrayList<Quantity>(); 1372 for (Quantity i : amount) 1373 dst.amount.add(i.copy()); 1374 }; 1375 if (location != null) { 1376 dst.location = new ArrayList<CodeableConcept>(); 1377 for (CodeableConcept i : location) 1378 dst.location.add(i.copy()); 1379 }; 1380 if (function != null) { 1381 dst.function = new ArrayList<CodeableConcept>(); 1382 for (CodeableConcept i : function) 1383 dst.function.add(i.copy()); 1384 }; 1385 if (hasIngredient != null) { 1386 dst.hasIngredient = new ArrayList<CodeableReference>(); 1387 for (CodeableReference i : hasIngredient) 1388 dst.hasIngredient.add(i.copy()); 1389 }; 1390 } 1391 1392 @Override 1393 public boolean equalsDeep(Base other_) { 1394 if (!super.equalsDeep(other_)) 1395 return false; 1396 if (!(other_ instanceof ManufacturedItemDefinitionComponentConstituentComponent)) 1397 return false; 1398 ManufacturedItemDefinitionComponentConstituentComponent o = (ManufacturedItemDefinitionComponentConstituentComponent) other_; 1399 return compareDeep(amount, o.amount, true) && compareDeep(location, o.location, true) && compareDeep(function, o.function, true) 1400 && compareDeep(hasIngredient, o.hasIngredient, true); 1401 } 1402 1403 @Override 1404 public boolean equalsShallow(Base other_) { 1405 if (!super.equalsShallow(other_)) 1406 return false; 1407 if (!(other_ instanceof ManufacturedItemDefinitionComponentConstituentComponent)) 1408 return false; 1409 ManufacturedItemDefinitionComponentConstituentComponent o = (ManufacturedItemDefinitionComponentConstituentComponent) other_; 1410 return true; 1411 } 1412 1413 public boolean isEmpty() { 1414 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(amount, location, function 1415 , hasIngredient); 1416 } 1417 1418 public String fhirType() { 1419 return "ManufacturedItemDefinition.component.constituent"; 1420 1421 } 1422 1423 } 1424 1425 /** 1426 * Unique identifier. 1427 */ 1428 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1429 @Description(shortDefinition="Unique identifier", formalDefinition="Unique identifier." ) 1430 protected List<Identifier> identifier; 1431 1432 /** 1433 * The status of this item. Enables tracking the life-cycle of the content. 1434 */ 1435 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 1436 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this item. Enables tracking the life-cycle of the content." ) 1437 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 1438 protected Enumeration<PublicationStatus> status; 1439 1440 /** 1441 * A descriptive name applied to this item. 1442 */ 1443 @Child(name = "name", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1444 @Description(shortDefinition="A descriptive name applied to this item", formalDefinition="A descriptive name applied to this item." ) 1445 protected StringType name; 1446 1447 /** 1448 * Dose form as manufactured and before any transformation into the pharmaceutical product. 1449 */ 1450 @Child(name = "manufacturedDoseForm", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 1451 @Description(shortDefinition="Dose form as manufactured (before any necessary transformation)", formalDefinition="Dose form as manufactured and before any transformation into the pharmaceutical product." ) 1452 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/manufactured-dose-form") 1453 protected CodeableConcept manufacturedDoseForm; 1454 1455 /** 1456 * The ?real-world? units in which the quantity of the manufactured item is described. 1457 */ 1458 @Child(name = "unitOfPresentation", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 1459 @Description(shortDefinition="The ?real-world? units in which the quantity of the item is described", formalDefinition="The ?real-world? units in which the quantity of the manufactured item is described." ) 1460 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/unit-of-presentation") 1461 protected CodeableConcept unitOfPresentation; 1462 1463 /** 1464 * Manufacturer of the item, one of several possible. 1465 */ 1466 @Child(name = "manufacturer", type = {Organization.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1467 @Description(shortDefinition="Manufacturer of the item, one of several possible", formalDefinition="Manufacturer of the item, one of several possible." ) 1468 protected List<Reference> manufacturer; 1469 1470 /** 1471 * Allows specifying that an item is on the market for sale, or that it is not available, and the dates and locations associated. 1472 */ 1473 @Child(name = "marketingStatus", type = {MarketingStatus.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1474 @Description(shortDefinition="Allows specifying that an item is on the market for sale, or that it is not available, and the dates and locations associated", formalDefinition="Allows specifying that an item is on the market for sale, or that it is not available, and the dates and locations associated." ) 1475 protected List<MarketingStatus> marketingStatus; 1476 1477 /** 1478 * The ingredients of this manufactured item. This is only needed if the ingredients are not specified by incoming references from the Ingredient resource. 1479 */ 1480 @Child(name = "ingredient", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1481 @Description(shortDefinition="The ingredients of this manufactured item. Only needed if these are not specified by incoming references from the Ingredient resource", formalDefinition="The ingredients of this manufactured item. This is only needed if the ingredients are not specified by incoming references from the Ingredient resource." ) 1482 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/substance-codes") 1483 protected List<CodeableConcept> ingredient; 1484 1485 /** 1486 * General characteristics of this item. 1487 */ 1488 @Child(name = "property", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1489 @Description(shortDefinition="General characteristics of this item", formalDefinition="General characteristics of this item." ) 1490 protected List<ManufacturedItemDefinitionPropertyComponent> property; 1491 1492 /** 1493 * Physical parts of the manufactured item, that it is intrisically made from. This is distinct from the ingredients that are part of its chemical makeup. 1494 */ 1495 @Child(name = "component", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1496 @Description(shortDefinition="Physical parts of the manufactured item, that it is intrisically made from. This is distinct from the ingredients that are part of its chemical makeup", formalDefinition="Physical parts of the manufactured item, that it is intrisically made from. This is distinct from the ingredients that are part of its chemical makeup." ) 1497 protected List<ManufacturedItemDefinitionComponentComponent> component; 1498 1499 private static final long serialVersionUID = 516510494L; 1500 1501 /** 1502 * Constructor 1503 */ 1504 public ManufacturedItemDefinition() { 1505 super(); 1506 } 1507 1508 /** 1509 * Constructor 1510 */ 1511 public ManufacturedItemDefinition(PublicationStatus status, CodeableConcept manufacturedDoseForm) { 1512 super(); 1513 this.setStatus(status); 1514 this.setManufacturedDoseForm(manufacturedDoseForm); 1515 } 1516 1517 /** 1518 * @return {@link #identifier} (Unique identifier.) 1519 */ 1520 public List<Identifier> getIdentifier() { 1521 if (this.identifier == null) 1522 this.identifier = new ArrayList<Identifier>(); 1523 return this.identifier; 1524 } 1525 1526 /** 1527 * @return Returns a reference to <code>this</code> for easy method chaining 1528 */ 1529 public ManufacturedItemDefinition setIdentifier(List<Identifier> theIdentifier) { 1530 this.identifier = theIdentifier; 1531 return this; 1532 } 1533 1534 public boolean hasIdentifier() { 1535 if (this.identifier == null) 1536 return false; 1537 for (Identifier item : this.identifier) 1538 if (!item.isEmpty()) 1539 return true; 1540 return false; 1541 } 1542 1543 public Identifier addIdentifier() { //3 1544 Identifier t = new Identifier(); 1545 if (this.identifier == null) 1546 this.identifier = new ArrayList<Identifier>(); 1547 this.identifier.add(t); 1548 return t; 1549 } 1550 1551 public ManufacturedItemDefinition addIdentifier(Identifier t) { //3 1552 if (t == null) 1553 return this; 1554 if (this.identifier == null) 1555 this.identifier = new ArrayList<Identifier>(); 1556 this.identifier.add(t); 1557 return this; 1558 } 1559 1560 /** 1561 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1562 */ 1563 public Identifier getIdentifierFirstRep() { 1564 if (getIdentifier().isEmpty()) { 1565 addIdentifier(); 1566 } 1567 return getIdentifier().get(0); 1568 } 1569 1570 /** 1571 * @return {@link #status} (The status of this item. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1572 */ 1573 public Enumeration<PublicationStatus> getStatusElement() { 1574 if (this.status == null) 1575 if (Configuration.errorOnAutoCreate()) 1576 throw new Error("Attempt to auto-create ManufacturedItemDefinition.status"); 1577 else if (Configuration.doAutoCreate()) 1578 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1579 return this.status; 1580 } 1581 1582 public boolean hasStatusElement() { 1583 return this.status != null && !this.status.isEmpty(); 1584 } 1585 1586 public boolean hasStatus() { 1587 return this.status != null && !this.status.isEmpty(); 1588 } 1589 1590 /** 1591 * @param value {@link #status} (The status of this item. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1592 */ 1593 public ManufacturedItemDefinition setStatusElement(Enumeration<PublicationStatus> value) { 1594 this.status = value; 1595 return this; 1596 } 1597 1598 /** 1599 * @return The status of this item. Enables tracking the life-cycle of the content. 1600 */ 1601 public PublicationStatus getStatus() { 1602 return this.status == null ? null : this.status.getValue(); 1603 } 1604 1605 /** 1606 * @param value The status of this item. Enables tracking the life-cycle of the content. 1607 */ 1608 public ManufacturedItemDefinition setStatus(PublicationStatus value) { 1609 if (this.status == null) 1610 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1611 this.status.setValue(value); 1612 return this; 1613 } 1614 1615 /** 1616 * @return {@link #name} (A descriptive name applied to this item.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1617 */ 1618 public StringType getNameElement() { 1619 if (this.name == null) 1620 if (Configuration.errorOnAutoCreate()) 1621 throw new Error("Attempt to auto-create ManufacturedItemDefinition.name"); 1622 else if (Configuration.doAutoCreate()) 1623 this.name = new StringType(); // bb 1624 return this.name; 1625 } 1626 1627 public boolean hasNameElement() { 1628 return this.name != null && !this.name.isEmpty(); 1629 } 1630 1631 public boolean hasName() { 1632 return this.name != null && !this.name.isEmpty(); 1633 } 1634 1635 /** 1636 * @param value {@link #name} (A descriptive name applied to this item.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1637 */ 1638 public ManufacturedItemDefinition setNameElement(StringType value) { 1639 this.name = value; 1640 return this; 1641 } 1642 1643 /** 1644 * @return A descriptive name applied to this item. 1645 */ 1646 public String getName() { 1647 return this.name == null ? null : this.name.getValue(); 1648 } 1649 1650 /** 1651 * @param value A descriptive name applied to this item. 1652 */ 1653 public ManufacturedItemDefinition setName(String value) { 1654 if (Utilities.noString(value)) 1655 this.name = null; 1656 else { 1657 if (this.name == null) 1658 this.name = new StringType(); 1659 this.name.setValue(value); 1660 } 1661 return this; 1662 } 1663 1664 /** 1665 * @return {@link #manufacturedDoseForm} (Dose form as manufactured and before any transformation into the pharmaceutical product.) 1666 */ 1667 public CodeableConcept getManufacturedDoseForm() { 1668 if (this.manufacturedDoseForm == null) 1669 if (Configuration.errorOnAutoCreate()) 1670 throw new Error("Attempt to auto-create ManufacturedItemDefinition.manufacturedDoseForm"); 1671 else if (Configuration.doAutoCreate()) 1672 this.manufacturedDoseForm = new CodeableConcept(); // cc 1673 return this.manufacturedDoseForm; 1674 } 1675 1676 public boolean hasManufacturedDoseForm() { 1677 return this.manufacturedDoseForm != null && !this.manufacturedDoseForm.isEmpty(); 1678 } 1679 1680 /** 1681 * @param value {@link #manufacturedDoseForm} (Dose form as manufactured and before any transformation into the pharmaceutical product.) 1682 */ 1683 public ManufacturedItemDefinition setManufacturedDoseForm(CodeableConcept value) { 1684 this.manufacturedDoseForm = value; 1685 return this; 1686 } 1687 1688 /** 1689 * @return {@link #unitOfPresentation} (The ?real-world? units in which the quantity of the manufactured item is described.) 1690 */ 1691 public CodeableConcept getUnitOfPresentation() { 1692 if (this.unitOfPresentation == null) 1693 if (Configuration.errorOnAutoCreate()) 1694 throw new Error("Attempt to auto-create ManufacturedItemDefinition.unitOfPresentation"); 1695 else if (Configuration.doAutoCreate()) 1696 this.unitOfPresentation = new CodeableConcept(); // cc 1697 return this.unitOfPresentation; 1698 } 1699 1700 public boolean hasUnitOfPresentation() { 1701 return this.unitOfPresentation != null && !this.unitOfPresentation.isEmpty(); 1702 } 1703 1704 /** 1705 * @param value {@link #unitOfPresentation} (The ?real-world? units in which the quantity of the manufactured item is described.) 1706 */ 1707 public ManufacturedItemDefinition setUnitOfPresentation(CodeableConcept value) { 1708 this.unitOfPresentation = value; 1709 return this; 1710 } 1711 1712 /** 1713 * @return {@link #manufacturer} (Manufacturer of the item, one of several possible.) 1714 */ 1715 public List<Reference> getManufacturer() { 1716 if (this.manufacturer == null) 1717 this.manufacturer = new ArrayList<Reference>(); 1718 return this.manufacturer; 1719 } 1720 1721 /** 1722 * @return Returns a reference to <code>this</code> for easy method chaining 1723 */ 1724 public ManufacturedItemDefinition setManufacturer(List<Reference> theManufacturer) { 1725 this.manufacturer = theManufacturer; 1726 return this; 1727 } 1728 1729 public boolean hasManufacturer() { 1730 if (this.manufacturer == null) 1731 return false; 1732 for (Reference item : this.manufacturer) 1733 if (!item.isEmpty()) 1734 return true; 1735 return false; 1736 } 1737 1738 public Reference addManufacturer() { //3 1739 Reference t = new Reference(); 1740 if (this.manufacturer == null) 1741 this.manufacturer = new ArrayList<Reference>(); 1742 this.manufacturer.add(t); 1743 return t; 1744 } 1745 1746 public ManufacturedItemDefinition addManufacturer(Reference t) { //3 1747 if (t == null) 1748 return this; 1749 if (this.manufacturer == null) 1750 this.manufacturer = new ArrayList<Reference>(); 1751 this.manufacturer.add(t); 1752 return this; 1753 } 1754 1755 /** 1756 * @return The first repetition of repeating field {@link #manufacturer}, creating it if it does not already exist {3} 1757 */ 1758 public Reference getManufacturerFirstRep() { 1759 if (getManufacturer().isEmpty()) { 1760 addManufacturer(); 1761 } 1762 return getManufacturer().get(0); 1763 } 1764 1765 /** 1766 * @return {@link #marketingStatus} (Allows specifying that an item is on the market for sale, or that it is not available, and the dates and locations associated.) 1767 */ 1768 public List<MarketingStatus> getMarketingStatus() { 1769 if (this.marketingStatus == null) 1770 this.marketingStatus = new ArrayList<MarketingStatus>(); 1771 return this.marketingStatus; 1772 } 1773 1774 /** 1775 * @return Returns a reference to <code>this</code> for easy method chaining 1776 */ 1777 public ManufacturedItemDefinition setMarketingStatus(List<MarketingStatus> theMarketingStatus) { 1778 this.marketingStatus = theMarketingStatus; 1779 return this; 1780 } 1781 1782 public boolean hasMarketingStatus() { 1783 if (this.marketingStatus == null) 1784 return false; 1785 for (MarketingStatus item : this.marketingStatus) 1786 if (!item.isEmpty()) 1787 return true; 1788 return false; 1789 } 1790 1791 public MarketingStatus addMarketingStatus() { //3 1792 MarketingStatus t = new MarketingStatus(); 1793 if (this.marketingStatus == null) 1794 this.marketingStatus = new ArrayList<MarketingStatus>(); 1795 this.marketingStatus.add(t); 1796 return t; 1797 } 1798 1799 public ManufacturedItemDefinition addMarketingStatus(MarketingStatus t) { //3 1800 if (t == null) 1801 return this; 1802 if (this.marketingStatus == null) 1803 this.marketingStatus = new ArrayList<MarketingStatus>(); 1804 this.marketingStatus.add(t); 1805 return this; 1806 } 1807 1808 /** 1809 * @return The first repetition of repeating field {@link #marketingStatus}, creating it if it does not already exist {3} 1810 */ 1811 public MarketingStatus getMarketingStatusFirstRep() { 1812 if (getMarketingStatus().isEmpty()) { 1813 addMarketingStatus(); 1814 } 1815 return getMarketingStatus().get(0); 1816 } 1817 1818 /** 1819 * @return {@link #ingredient} (The ingredients of this manufactured item. This is only needed if the ingredients are not specified by incoming references from the Ingredient resource.) 1820 */ 1821 public List<CodeableConcept> getIngredient() { 1822 if (this.ingredient == null) 1823 this.ingredient = new ArrayList<CodeableConcept>(); 1824 return this.ingredient; 1825 } 1826 1827 /** 1828 * @return Returns a reference to <code>this</code> for easy method chaining 1829 */ 1830 public ManufacturedItemDefinition setIngredient(List<CodeableConcept> theIngredient) { 1831 this.ingredient = theIngredient; 1832 return this; 1833 } 1834 1835 public boolean hasIngredient() { 1836 if (this.ingredient == null) 1837 return false; 1838 for (CodeableConcept item : this.ingredient) 1839 if (!item.isEmpty()) 1840 return true; 1841 return false; 1842 } 1843 1844 public CodeableConcept addIngredient() { //3 1845 CodeableConcept t = new CodeableConcept(); 1846 if (this.ingredient == null) 1847 this.ingredient = new ArrayList<CodeableConcept>(); 1848 this.ingredient.add(t); 1849 return t; 1850 } 1851 1852 public ManufacturedItemDefinition addIngredient(CodeableConcept t) { //3 1853 if (t == null) 1854 return this; 1855 if (this.ingredient == null) 1856 this.ingredient = new ArrayList<CodeableConcept>(); 1857 this.ingredient.add(t); 1858 return this; 1859 } 1860 1861 /** 1862 * @return The first repetition of repeating field {@link #ingredient}, creating it if it does not already exist {3} 1863 */ 1864 public CodeableConcept getIngredientFirstRep() { 1865 if (getIngredient().isEmpty()) { 1866 addIngredient(); 1867 } 1868 return getIngredient().get(0); 1869 } 1870 1871 /** 1872 * @return {@link #property} (General characteristics of this item.) 1873 */ 1874 public List<ManufacturedItemDefinitionPropertyComponent> getProperty() { 1875 if (this.property == null) 1876 this.property = new ArrayList<ManufacturedItemDefinitionPropertyComponent>(); 1877 return this.property; 1878 } 1879 1880 /** 1881 * @return Returns a reference to <code>this</code> for easy method chaining 1882 */ 1883 public ManufacturedItemDefinition setProperty(List<ManufacturedItemDefinitionPropertyComponent> theProperty) { 1884 this.property = theProperty; 1885 return this; 1886 } 1887 1888 public boolean hasProperty() { 1889 if (this.property == null) 1890 return false; 1891 for (ManufacturedItemDefinitionPropertyComponent item : this.property) 1892 if (!item.isEmpty()) 1893 return true; 1894 return false; 1895 } 1896 1897 public ManufacturedItemDefinitionPropertyComponent addProperty() { //3 1898 ManufacturedItemDefinitionPropertyComponent t = new ManufacturedItemDefinitionPropertyComponent(); 1899 if (this.property == null) 1900 this.property = new ArrayList<ManufacturedItemDefinitionPropertyComponent>(); 1901 this.property.add(t); 1902 return t; 1903 } 1904 1905 public ManufacturedItemDefinition addProperty(ManufacturedItemDefinitionPropertyComponent t) { //3 1906 if (t == null) 1907 return this; 1908 if (this.property == null) 1909 this.property = new ArrayList<ManufacturedItemDefinitionPropertyComponent>(); 1910 this.property.add(t); 1911 return this; 1912 } 1913 1914 /** 1915 * @return The first repetition of repeating field {@link #property}, creating it if it does not already exist {3} 1916 */ 1917 public ManufacturedItemDefinitionPropertyComponent getPropertyFirstRep() { 1918 if (getProperty().isEmpty()) { 1919 addProperty(); 1920 } 1921 return getProperty().get(0); 1922 } 1923 1924 /** 1925 * @return {@link #component} (Physical parts of the manufactured item, that it is intrisically made from. This is distinct from the ingredients that are part of its chemical makeup.) 1926 */ 1927 public List<ManufacturedItemDefinitionComponentComponent> getComponent() { 1928 if (this.component == null) 1929 this.component = new ArrayList<ManufacturedItemDefinitionComponentComponent>(); 1930 return this.component; 1931 } 1932 1933 /** 1934 * @return Returns a reference to <code>this</code> for easy method chaining 1935 */ 1936 public ManufacturedItemDefinition setComponent(List<ManufacturedItemDefinitionComponentComponent> theComponent) { 1937 this.component = theComponent; 1938 return this; 1939 } 1940 1941 public boolean hasComponent() { 1942 if (this.component == null) 1943 return false; 1944 for (ManufacturedItemDefinitionComponentComponent item : this.component) 1945 if (!item.isEmpty()) 1946 return true; 1947 return false; 1948 } 1949 1950 public ManufacturedItemDefinitionComponentComponent addComponent() { //3 1951 ManufacturedItemDefinitionComponentComponent t = new ManufacturedItemDefinitionComponentComponent(); 1952 if (this.component == null) 1953 this.component = new ArrayList<ManufacturedItemDefinitionComponentComponent>(); 1954 this.component.add(t); 1955 return t; 1956 } 1957 1958 public ManufacturedItemDefinition addComponent(ManufacturedItemDefinitionComponentComponent t) { //3 1959 if (t == null) 1960 return this; 1961 if (this.component == null) 1962 this.component = new ArrayList<ManufacturedItemDefinitionComponentComponent>(); 1963 this.component.add(t); 1964 return this; 1965 } 1966 1967 /** 1968 * @return The first repetition of repeating field {@link #component}, creating it if it does not already exist {3} 1969 */ 1970 public ManufacturedItemDefinitionComponentComponent getComponentFirstRep() { 1971 if (getComponent().isEmpty()) { 1972 addComponent(); 1973 } 1974 return getComponent().get(0); 1975 } 1976 1977 protected void listChildren(List<Property> children) { 1978 super.listChildren(children); 1979 children.add(new Property("identifier", "Identifier", "Unique identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1980 children.add(new Property("status", "code", "The status of this item. Enables tracking the life-cycle of the content.", 0, 1, status)); 1981 children.add(new Property("name", "string", "A descriptive name applied to this item.", 0, 1, name)); 1982 children.add(new Property("manufacturedDoseForm", "CodeableConcept", "Dose form as manufactured and before any transformation into the pharmaceutical product.", 0, 1, manufacturedDoseForm)); 1983 children.add(new Property("unitOfPresentation", "CodeableConcept", "The ?real-world? units in which the quantity of the manufactured item is described.", 0, 1, unitOfPresentation)); 1984 children.add(new Property("manufacturer", "Reference(Organization)", "Manufacturer of the item, one of several possible.", 0, java.lang.Integer.MAX_VALUE, manufacturer)); 1985 children.add(new Property("marketingStatus", "MarketingStatus", "Allows specifying that an item is on the market for sale, or that it is not available, and the dates and locations associated.", 0, java.lang.Integer.MAX_VALUE, marketingStatus)); 1986 children.add(new Property("ingredient", "CodeableConcept", "The ingredients of this manufactured item. This is only needed if the ingredients are not specified by incoming references from the Ingredient resource.", 0, java.lang.Integer.MAX_VALUE, ingredient)); 1987 children.add(new Property("property", "", "General characteristics of this item.", 0, java.lang.Integer.MAX_VALUE, property)); 1988 children.add(new Property("component", "", "Physical parts of the manufactured item, that it is intrisically made from. This is distinct from the ingredients that are part of its chemical makeup.", 0, java.lang.Integer.MAX_VALUE, component)); 1989 } 1990 1991 @Override 1992 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1993 switch (_hash) { 1994 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 1995 case -892481550: /*status*/ return new Property("status", "code", "The status of this item. Enables tracking the life-cycle of the content.", 0, 1, status); 1996 case 3373707: /*name*/ return new Property("name", "string", "A descriptive name applied to this item.", 0, 1, name); 1997 case -1451400348: /*manufacturedDoseForm*/ return new Property("manufacturedDoseForm", "CodeableConcept", "Dose form as manufactured and before any transformation into the pharmaceutical product.", 0, 1, manufacturedDoseForm); 1998 case -1427765963: /*unitOfPresentation*/ return new Property("unitOfPresentation", "CodeableConcept", "The ?real-world? units in which the quantity of the manufactured item is described.", 0, 1, unitOfPresentation); 1999 case -1969347631: /*manufacturer*/ return new Property("manufacturer", "Reference(Organization)", "Manufacturer of the item, one of several possible.", 0, java.lang.Integer.MAX_VALUE, manufacturer); 2000 case 70767032: /*marketingStatus*/ return new Property("marketingStatus", "MarketingStatus", "Allows specifying that an item is on the market for sale, or that it is not available, and the dates and locations associated.", 0, java.lang.Integer.MAX_VALUE, marketingStatus); 2001 case -206409263: /*ingredient*/ return new Property("ingredient", "CodeableConcept", "The ingredients of this manufactured item. This is only needed if the ingredients are not specified by incoming references from the Ingredient resource.", 0, java.lang.Integer.MAX_VALUE, ingredient); 2002 case -993141291: /*property*/ return new Property("property", "", "General characteristics of this item.", 0, java.lang.Integer.MAX_VALUE, property); 2003 case -1399907075: /*component*/ return new Property("component", "", "Physical parts of the manufactured item, that it is intrisically made from. This is distinct from the ingredients that are part of its chemical makeup.", 0, java.lang.Integer.MAX_VALUE, component); 2004 default: return super.getNamedProperty(_hash, _name, _checkValid); 2005 } 2006 2007 } 2008 2009 @Override 2010 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2011 switch (hash) { 2012 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2013 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2014 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2015 case -1451400348: /*manufacturedDoseForm*/ return this.manufacturedDoseForm == null ? new Base[0] : new Base[] {this.manufacturedDoseForm}; // CodeableConcept 2016 case -1427765963: /*unitOfPresentation*/ return this.unitOfPresentation == null ? new Base[0] : new Base[] {this.unitOfPresentation}; // CodeableConcept 2017 case -1969347631: /*manufacturer*/ return this.manufacturer == null ? new Base[0] : this.manufacturer.toArray(new Base[this.manufacturer.size()]); // Reference 2018 case 70767032: /*marketingStatus*/ return this.marketingStatus == null ? new Base[0] : this.marketingStatus.toArray(new Base[this.marketingStatus.size()]); // MarketingStatus 2019 case -206409263: /*ingredient*/ return this.ingredient == null ? new Base[0] : this.ingredient.toArray(new Base[this.ingredient.size()]); // CodeableConcept 2020 case -993141291: /*property*/ return this.property == null ? new Base[0] : this.property.toArray(new Base[this.property.size()]); // ManufacturedItemDefinitionPropertyComponent 2021 case -1399907075: /*component*/ return this.component == null ? new Base[0] : this.component.toArray(new Base[this.component.size()]); // ManufacturedItemDefinitionComponentComponent 2022 default: return super.getProperty(hash, name, checkValid); 2023 } 2024 2025 } 2026 2027 @Override 2028 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2029 switch (hash) { 2030 case -1618432855: // identifier 2031 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2032 return value; 2033 case -892481550: // status 2034 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2035 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2036 return value; 2037 case 3373707: // name 2038 this.name = TypeConvertor.castToString(value); // StringType 2039 return value; 2040 case -1451400348: // manufacturedDoseForm 2041 this.manufacturedDoseForm = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2042 return value; 2043 case -1427765963: // unitOfPresentation 2044 this.unitOfPresentation = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2045 return value; 2046 case -1969347631: // manufacturer 2047 this.getManufacturer().add(TypeConvertor.castToReference(value)); // Reference 2048 return value; 2049 case 70767032: // marketingStatus 2050 this.getMarketingStatus().add(TypeConvertor.castToMarketingStatus(value)); // MarketingStatus 2051 return value; 2052 case -206409263: // ingredient 2053 this.getIngredient().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2054 return value; 2055 case -993141291: // property 2056 this.getProperty().add((ManufacturedItemDefinitionPropertyComponent) value); // ManufacturedItemDefinitionPropertyComponent 2057 return value; 2058 case -1399907075: // component 2059 this.getComponent().add((ManufacturedItemDefinitionComponentComponent) value); // ManufacturedItemDefinitionComponentComponent 2060 return value; 2061 default: return super.setProperty(hash, name, value); 2062 } 2063 2064 } 2065 2066 @Override 2067 public Base setProperty(String name, Base value) throws FHIRException { 2068 if (name.equals("identifier")) { 2069 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2070 } else if (name.equals("status")) { 2071 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2072 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2073 } else if (name.equals("name")) { 2074 this.name = TypeConvertor.castToString(value); // StringType 2075 } else if (name.equals("manufacturedDoseForm")) { 2076 this.manufacturedDoseForm = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2077 } else if (name.equals("unitOfPresentation")) { 2078 this.unitOfPresentation = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2079 } else if (name.equals("manufacturer")) { 2080 this.getManufacturer().add(TypeConvertor.castToReference(value)); 2081 } else if (name.equals("marketingStatus")) { 2082 this.getMarketingStatus().add(TypeConvertor.castToMarketingStatus(value)); 2083 } else if (name.equals("ingredient")) { 2084 this.getIngredient().add(TypeConvertor.castToCodeableConcept(value)); 2085 } else if (name.equals("property")) { 2086 this.getProperty().add((ManufacturedItemDefinitionPropertyComponent) value); 2087 } else if (name.equals("component")) { 2088 this.getComponent().add((ManufacturedItemDefinitionComponentComponent) value); 2089 } else 2090 return super.setProperty(name, value); 2091 return value; 2092 } 2093 2094 @Override 2095 public void removeChild(String name, Base value) throws FHIRException { 2096 if (name.equals("identifier")) { 2097 this.getIdentifier().remove(value); 2098 } else if (name.equals("status")) { 2099 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 2100 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2101 } else if (name.equals("name")) { 2102 this.name = null; 2103 } else if (name.equals("manufacturedDoseForm")) { 2104 this.manufacturedDoseForm = null; 2105 } else if (name.equals("unitOfPresentation")) { 2106 this.unitOfPresentation = null; 2107 } else if (name.equals("manufacturer")) { 2108 this.getManufacturer().remove(value); 2109 } else if (name.equals("marketingStatus")) { 2110 this.getMarketingStatus().remove(value); 2111 } else if (name.equals("ingredient")) { 2112 this.getIngredient().remove(value); 2113 } else if (name.equals("property")) { 2114 this.getProperty().remove((ManufacturedItemDefinitionPropertyComponent) value); 2115 } else if (name.equals("component")) { 2116 this.getComponent().remove((ManufacturedItemDefinitionComponentComponent) value); 2117 } else 2118 super.removeChild(name, value); 2119 2120 } 2121 2122 @Override 2123 public Base makeProperty(int hash, String name) throws FHIRException { 2124 switch (hash) { 2125 case -1618432855: return addIdentifier(); 2126 case -892481550: return getStatusElement(); 2127 case 3373707: return getNameElement(); 2128 case -1451400348: return getManufacturedDoseForm(); 2129 case -1427765963: return getUnitOfPresentation(); 2130 case -1969347631: return addManufacturer(); 2131 case 70767032: return addMarketingStatus(); 2132 case -206409263: return addIngredient(); 2133 case -993141291: return addProperty(); 2134 case -1399907075: return addComponent(); 2135 default: return super.makeProperty(hash, name); 2136 } 2137 2138 } 2139 2140 @Override 2141 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2142 switch (hash) { 2143 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2144 case -892481550: /*status*/ return new String[] {"code"}; 2145 case 3373707: /*name*/ return new String[] {"string"}; 2146 case -1451400348: /*manufacturedDoseForm*/ return new String[] {"CodeableConcept"}; 2147 case -1427765963: /*unitOfPresentation*/ return new String[] {"CodeableConcept"}; 2148 case -1969347631: /*manufacturer*/ return new String[] {"Reference"}; 2149 case 70767032: /*marketingStatus*/ return new String[] {"MarketingStatus"}; 2150 case -206409263: /*ingredient*/ return new String[] {"CodeableConcept"}; 2151 case -993141291: /*property*/ return new String[] {}; 2152 case -1399907075: /*component*/ return new String[] {}; 2153 default: return super.getTypesForProperty(hash, name); 2154 } 2155 2156 } 2157 2158 @Override 2159 public Base addChild(String name) throws FHIRException { 2160 if (name.equals("identifier")) { 2161 return addIdentifier(); 2162 } 2163 else if (name.equals("status")) { 2164 throw new FHIRException("Cannot call addChild on a singleton property ManufacturedItemDefinition.status"); 2165 } 2166 else if (name.equals("name")) { 2167 throw new FHIRException("Cannot call addChild on a singleton property ManufacturedItemDefinition.name"); 2168 } 2169 else if (name.equals("manufacturedDoseForm")) { 2170 this.manufacturedDoseForm = new CodeableConcept(); 2171 return this.manufacturedDoseForm; 2172 } 2173 else if (name.equals("unitOfPresentation")) { 2174 this.unitOfPresentation = new CodeableConcept(); 2175 return this.unitOfPresentation; 2176 } 2177 else if (name.equals("manufacturer")) { 2178 return addManufacturer(); 2179 } 2180 else if (name.equals("marketingStatus")) { 2181 return addMarketingStatus(); 2182 } 2183 else if (name.equals("ingredient")) { 2184 return addIngredient(); 2185 } 2186 else if (name.equals("property")) { 2187 return addProperty(); 2188 } 2189 else if (name.equals("component")) { 2190 return addComponent(); 2191 } 2192 else 2193 return super.addChild(name); 2194 } 2195 2196 public String fhirType() { 2197 return "ManufacturedItemDefinition"; 2198 2199 } 2200 2201 public ManufacturedItemDefinition copy() { 2202 ManufacturedItemDefinition dst = new ManufacturedItemDefinition(); 2203 copyValues(dst); 2204 return dst; 2205 } 2206 2207 public void copyValues(ManufacturedItemDefinition dst) { 2208 super.copyValues(dst); 2209 if (identifier != null) { 2210 dst.identifier = new ArrayList<Identifier>(); 2211 for (Identifier i : identifier) 2212 dst.identifier.add(i.copy()); 2213 }; 2214 dst.status = status == null ? null : status.copy(); 2215 dst.name = name == null ? null : name.copy(); 2216 dst.manufacturedDoseForm = manufacturedDoseForm == null ? null : manufacturedDoseForm.copy(); 2217 dst.unitOfPresentation = unitOfPresentation == null ? null : unitOfPresentation.copy(); 2218 if (manufacturer != null) { 2219 dst.manufacturer = new ArrayList<Reference>(); 2220 for (Reference i : manufacturer) 2221 dst.manufacturer.add(i.copy()); 2222 }; 2223 if (marketingStatus != null) { 2224 dst.marketingStatus = new ArrayList<MarketingStatus>(); 2225 for (MarketingStatus i : marketingStatus) 2226 dst.marketingStatus.add(i.copy()); 2227 }; 2228 if (ingredient != null) { 2229 dst.ingredient = new ArrayList<CodeableConcept>(); 2230 for (CodeableConcept i : ingredient) 2231 dst.ingredient.add(i.copy()); 2232 }; 2233 if (property != null) { 2234 dst.property = new ArrayList<ManufacturedItemDefinitionPropertyComponent>(); 2235 for (ManufacturedItemDefinitionPropertyComponent i : property) 2236 dst.property.add(i.copy()); 2237 }; 2238 if (component != null) { 2239 dst.component = new ArrayList<ManufacturedItemDefinitionComponentComponent>(); 2240 for (ManufacturedItemDefinitionComponentComponent i : component) 2241 dst.component.add(i.copy()); 2242 }; 2243 } 2244 2245 protected ManufacturedItemDefinition typedCopy() { 2246 return copy(); 2247 } 2248 2249 @Override 2250 public boolean equalsDeep(Base other_) { 2251 if (!super.equalsDeep(other_)) 2252 return false; 2253 if (!(other_ instanceof ManufacturedItemDefinition)) 2254 return false; 2255 ManufacturedItemDefinition o = (ManufacturedItemDefinition) other_; 2256 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(name, o.name, true) 2257 && compareDeep(manufacturedDoseForm, o.manufacturedDoseForm, true) && compareDeep(unitOfPresentation, o.unitOfPresentation, true) 2258 && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(marketingStatus, o.marketingStatus, true) 2259 && compareDeep(ingredient, o.ingredient, true) && compareDeep(property, o.property, true) && compareDeep(component, o.component, true) 2260 ; 2261 } 2262 2263 @Override 2264 public boolean equalsShallow(Base other_) { 2265 if (!super.equalsShallow(other_)) 2266 return false; 2267 if (!(other_ instanceof ManufacturedItemDefinition)) 2268 return false; 2269 ManufacturedItemDefinition o = (ManufacturedItemDefinition) other_; 2270 return compareValues(status, o.status, true) && compareValues(name, o.name, true); 2271 } 2272 2273 public boolean isEmpty() { 2274 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, name 2275 , manufacturedDoseForm, unitOfPresentation, manufacturer, marketingStatus, ingredient 2276 , property, component); 2277 } 2278 2279 @Override 2280 public ResourceType getResourceType() { 2281 return ResourceType.ManufacturedItemDefinition; 2282 } 2283 2284 /** 2285 * Search parameter: <b>dose-form</b> 2286 * <p> 2287 * Description: <b>Dose form as manufactured and before any transformation into the pharmaceutical product</b><br> 2288 * Type: <b>token</b><br> 2289 * Path: <b>ManufacturedItemDefinition.manufacturedDoseForm</b><br> 2290 * </p> 2291 */ 2292 @SearchParamDefinition(name="dose-form", path="ManufacturedItemDefinition.manufacturedDoseForm", description="Dose form as manufactured and before any transformation into the pharmaceutical product", type="token" ) 2293 public static final String SP_DOSE_FORM = "dose-form"; 2294 /** 2295 * <b>Fluent Client</b> search parameter constant for <b>dose-form</b> 2296 * <p> 2297 * Description: <b>Dose form as manufactured and before any transformation into the pharmaceutical product</b><br> 2298 * Type: <b>token</b><br> 2299 * Path: <b>ManufacturedItemDefinition.manufacturedDoseForm</b><br> 2300 * </p> 2301 */ 2302 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DOSE_FORM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DOSE_FORM); 2303 2304 /** 2305 * Search parameter: <b>identifier</b> 2306 * <p> 2307 * Description: <b>Unique identifier</b><br> 2308 * Type: <b>token</b><br> 2309 * Path: <b>ManufacturedItemDefinition.identifier</b><br> 2310 * </p> 2311 */ 2312 @SearchParamDefinition(name="identifier", path="ManufacturedItemDefinition.identifier", description="Unique identifier", type="token" ) 2313 public static final String SP_IDENTIFIER = "identifier"; 2314 /** 2315 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2316 * <p> 2317 * Description: <b>Unique identifier</b><br> 2318 * Type: <b>token</b><br> 2319 * Path: <b>ManufacturedItemDefinition.identifier</b><br> 2320 * </p> 2321 */ 2322 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2323 2324 /** 2325 * Search parameter: <b>ingredient</b> 2326 * <p> 2327 * Description: <b>An ingredient of this item</b><br> 2328 * Type: <b>token</b><br> 2329 * Path: <b>ManufacturedItemDefinition.ingredient</b><br> 2330 * </p> 2331 */ 2332 @SearchParamDefinition(name="ingredient", path="ManufacturedItemDefinition.ingredient", description="An ingredient of this item", type="token" ) 2333 public static final String SP_INGREDIENT = "ingredient"; 2334 /** 2335 * <b>Fluent Client</b> search parameter constant for <b>ingredient</b> 2336 * <p> 2337 * Description: <b>An ingredient of this item</b><br> 2338 * Type: <b>token</b><br> 2339 * Path: <b>ManufacturedItemDefinition.ingredient</b><br> 2340 * </p> 2341 */ 2342 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INGREDIENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INGREDIENT); 2343 2344 /** 2345 * Search parameter: <b>name</b> 2346 * <p> 2347 * Description: <b>A descriptive name applied to this item</b><br> 2348 * Type: <b>token</b><br> 2349 * Path: <b>ManufacturedItemDefinition.name</b><br> 2350 * </p> 2351 */ 2352 @SearchParamDefinition(name="name", path="ManufacturedItemDefinition.name", description="A descriptive name applied to this item", type="token" ) 2353 public static final String SP_NAME = "name"; 2354 /** 2355 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2356 * <p> 2357 * Description: <b>A descriptive name applied to this item</b><br> 2358 * Type: <b>token</b><br> 2359 * Path: <b>ManufacturedItemDefinition.name</b><br> 2360 * </p> 2361 */ 2362 public static final ca.uhn.fhir.rest.gclient.TokenClientParam NAME = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_NAME); 2363 2364 /** 2365 * Search parameter: <b>status</b> 2366 * <p> 2367 * Description: <b>The status of this item. Enables tracking the life-cycle of the content.</b><br> 2368 * Type: <b>token</b><br> 2369 * Path: <b>ManufacturedItemDefinition.status</b><br> 2370 * </p> 2371 */ 2372 @SearchParamDefinition(name="status", path="ManufacturedItemDefinition.status", description="The status of this item. Enables tracking the life-cycle of the content.", type="token" ) 2373 public static final String SP_STATUS = "status"; 2374 /** 2375 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2376 * <p> 2377 * Description: <b>The status of this item. Enables tracking the life-cycle of the content.</b><br> 2378 * Type: <b>token</b><br> 2379 * Path: <b>ManufacturedItemDefinition.status</b><br> 2380 * </p> 2381 */ 2382 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2383 2384 2385} 2386