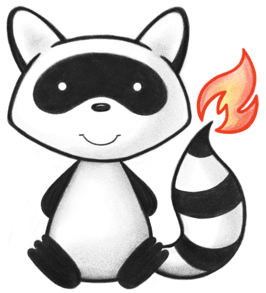
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.ChildOrder; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.Block; 046 047/** 048 * MarketingStatus Type: The marketing status describes the date when a medicinal product is actually put on the market or the date as of which it is no longer available. 049 */ 050@DatatypeDef(name="MarketingStatus") 051public class MarketingStatus extends BackboneType implements ICompositeType { 052 053 /** 054 * The country in which the marketing authorization has been granted shall be specified It should be specified using the ISO 3166 ? 1 alpha-2 code elements. 055 */ 056 @Child(name = "country", type = {CodeableConcept.class}, order=0, min=0, max=1, modifier=false, summary=true) 057 @Description(shortDefinition="The country in which the marketing authorization has been granted shall be specified It should be specified using the ISO 3166 ? 1 alpha-2 code elements", formalDefinition="The country in which the marketing authorization has been granted shall be specified It should be specified using the ISO 3166 ? 1 alpha-2 code elements." ) 058 protected CodeableConcept country; 059 060 /** 061 * Where a Medicines Regulatory Agency has granted a marketing authorization for which specific provisions within a jurisdiction apply, the jurisdiction can be specified using an appropriate controlled terminology The controlled term and the controlled term identifier shall be specified. 062 */ 063 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 064 @Description(shortDefinition="Where a Medicines Regulatory Agency has granted a marketing authorization for which specific provisions within a jurisdiction apply, the jurisdiction can be specified using an appropriate controlled terminology The controlled term and the controlled term identifier shall be specified", formalDefinition="Where a Medicines Regulatory Agency has granted a marketing authorization for which specific provisions within a jurisdiction apply, the jurisdiction can be specified using an appropriate controlled terminology The controlled term and the controlled term identifier shall be specified." ) 065 protected CodeableConcept jurisdiction; 066 067 /** 068 * This attribute provides information on the status of the marketing of the medicinal product See ISO/TS 20443 for more information and examples. 069 */ 070 @Child(name = "status", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=true) 071 @Description(shortDefinition="This attribute provides information on the status of the marketing of the medicinal product See ISO/TS 20443 for more information and examples", formalDefinition="This attribute provides information on the status of the marketing of the medicinal product See ISO/TS 20443 for more information and examples." ) 072 protected CodeableConcept status; 073 074 /** 075 * The date when the Medicinal Product is placed on the market by the Marketing Authorization Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain. 076 */ 077 @Child(name = "dateRange", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=true) 078 @Description(shortDefinition="The date when the Medicinal Product is placed on the market by the Marketing Authorization Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain", formalDefinition="The date when the Medicinal Product is placed on the market by the Marketing Authorization Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain." ) 079 protected Period dateRange; 080 081 /** 082 * The date when the Medicinal Product is placed on the market by the Marketing Authorization Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain. 083 */ 084 @Child(name = "restoreDate", type = {DateTimeType.class}, order=4, min=0, max=1, modifier=false, summary=true) 085 @Description(shortDefinition="The date when the Medicinal Product is placed on the market by the Marketing Authorization Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain", formalDefinition="The date when the Medicinal Product is placed on the market by the Marketing Authorization Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain." ) 086 protected DateTimeType restoreDate; 087 088 private static final long serialVersionUID = -1445736863L; 089 090 /** 091 * Constructor 092 */ 093 public MarketingStatus() { 094 super(); 095 } 096 097 /** 098 * Constructor 099 */ 100 public MarketingStatus(CodeableConcept status) { 101 super(); 102 this.setStatus(status); 103 } 104 105 /** 106 * @return {@link #country} (The country in which the marketing authorization has been granted shall be specified It should be specified using the ISO 3166 ? 1 alpha-2 code elements.) 107 */ 108 public CodeableConcept getCountry() { 109 if (this.country == null) 110 if (Configuration.errorOnAutoCreate()) 111 throw new Error("Attempt to auto-create MarketingStatus.country"); 112 else if (Configuration.doAutoCreate()) 113 this.country = new CodeableConcept(); // cc 114 return this.country; 115 } 116 117 public boolean hasCountry() { 118 return this.country != null && !this.country.isEmpty(); 119 } 120 121 /** 122 * @param value {@link #country} (The country in which the marketing authorization has been granted shall be specified It should be specified using the ISO 3166 ? 1 alpha-2 code elements.) 123 */ 124 public MarketingStatus setCountry(CodeableConcept value) { 125 this.country = value; 126 return this; 127 } 128 129 /** 130 * @return {@link #jurisdiction} (Where a Medicines Regulatory Agency has granted a marketing authorization for which specific provisions within a jurisdiction apply, the jurisdiction can be specified using an appropriate controlled terminology The controlled term and the controlled term identifier shall be specified.) 131 */ 132 public CodeableConcept getJurisdiction() { 133 if (this.jurisdiction == null) 134 if (Configuration.errorOnAutoCreate()) 135 throw new Error("Attempt to auto-create MarketingStatus.jurisdiction"); 136 else if (Configuration.doAutoCreate()) 137 this.jurisdiction = new CodeableConcept(); // cc 138 return this.jurisdiction; 139 } 140 141 public boolean hasJurisdiction() { 142 return this.jurisdiction != null && !this.jurisdiction.isEmpty(); 143 } 144 145 /** 146 * @param value {@link #jurisdiction} (Where a Medicines Regulatory Agency has granted a marketing authorization for which specific provisions within a jurisdiction apply, the jurisdiction can be specified using an appropriate controlled terminology The controlled term and the controlled term identifier shall be specified.) 147 */ 148 public MarketingStatus setJurisdiction(CodeableConcept value) { 149 this.jurisdiction = value; 150 return this; 151 } 152 153 /** 154 * @return {@link #status} (This attribute provides information on the status of the marketing of the medicinal product See ISO/TS 20443 for more information and examples.) 155 */ 156 public CodeableConcept getStatus() { 157 if (this.status == null) 158 if (Configuration.errorOnAutoCreate()) 159 throw new Error("Attempt to auto-create MarketingStatus.status"); 160 else if (Configuration.doAutoCreate()) 161 this.status = new CodeableConcept(); // cc 162 return this.status; 163 } 164 165 public boolean hasStatus() { 166 return this.status != null && !this.status.isEmpty(); 167 } 168 169 /** 170 * @param value {@link #status} (This attribute provides information on the status of the marketing of the medicinal product See ISO/TS 20443 for more information and examples.) 171 */ 172 public MarketingStatus setStatus(CodeableConcept value) { 173 this.status = value; 174 return this; 175 } 176 177 /** 178 * @return {@link #dateRange} (The date when the Medicinal Product is placed on the market by the Marketing Authorization Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain.) 179 */ 180 public Period getDateRange() { 181 if (this.dateRange == null) 182 if (Configuration.errorOnAutoCreate()) 183 throw new Error("Attempt to auto-create MarketingStatus.dateRange"); 184 else if (Configuration.doAutoCreate()) 185 this.dateRange = new Period(); // cc 186 return this.dateRange; 187 } 188 189 public boolean hasDateRange() { 190 return this.dateRange != null && !this.dateRange.isEmpty(); 191 } 192 193 /** 194 * @param value {@link #dateRange} (The date when the Medicinal Product is placed on the market by the Marketing Authorization Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain.) 195 */ 196 public MarketingStatus setDateRange(Period value) { 197 this.dateRange = value; 198 return this; 199 } 200 201 /** 202 * @return {@link #restoreDate} (The date when the Medicinal Product is placed on the market by the Marketing Authorization Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain.). This is the underlying object with id, value and extensions. The accessor "getRestoreDate" gives direct access to the value 203 */ 204 public DateTimeType getRestoreDateElement() { 205 if (this.restoreDate == null) 206 if (Configuration.errorOnAutoCreate()) 207 throw new Error("Attempt to auto-create MarketingStatus.restoreDate"); 208 else if (Configuration.doAutoCreate()) 209 this.restoreDate = new DateTimeType(); // bb 210 return this.restoreDate; 211 } 212 213 public boolean hasRestoreDateElement() { 214 return this.restoreDate != null && !this.restoreDate.isEmpty(); 215 } 216 217 public boolean hasRestoreDate() { 218 return this.restoreDate != null && !this.restoreDate.isEmpty(); 219 } 220 221 /** 222 * @param value {@link #restoreDate} (The date when the Medicinal Product is placed on the market by the Marketing Authorization Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain.). This is the underlying object with id, value and extensions. The accessor "getRestoreDate" gives direct access to the value 223 */ 224 public MarketingStatus setRestoreDateElement(DateTimeType value) { 225 this.restoreDate = value; 226 return this; 227 } 228 229 /** 230 * @return The date when the Medicinal Product is placed on the market by the Marketing Authorization Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain. 231 */ 232 public Date getRestoreDate() { 233 return this.restoreDate == null ? null : this.restoreDate.getValue(); 234 } 235 236 /** 237 * @param value The date when the Medicinal Product is placed on the market by the Marketing Authorization Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain. 238 */ 239 public MarketingStatus setRestoreDate(Date value) { 240 if (value == null) 241 this.restoreDate = null; 242 else { 243 if (this.restoreDate == null) 244 this.restoreDate = new DateTimeType(); 245 this.restoreDate.setValue(value); 246 } 247 return this; 248 } 249 250 protected void listChildren(List<Property> children) { 251 super.listChildren(children); 252 children.add(new Property("country", "CodeableConcept", "The country in which the marketing authorization has been granted shall be specified It should be specified using the ISO 3166 ? 1 alpha-2 code elements.", 0, 1, country)); 253 children.add(new Property("jurisdiction", "CodeableConcept", "Where a Medicines Regulatory Agency has granted a marketing authorization for which specific provisions within a jurisdiction apply, the jurisdiction can be specified using an appropriate controlled terminology The controlled term and the controlled term identifier shall be specified.", 0, 1, jurisdiction)); 254 children.add(new Property("status", "CodeableConcept", "This attribute provides information on the status of the marketing of the medicinal product See ISO/TS 20443 for more information and examples.", 0, 1, status)); 255 children.add(new Property("dateRange", "Period", "The date when the Medicinal Product is placed on the market by the Marketing Authorization Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain.", 0, 1, dateRange)); 256 children.add(new Property("restoreDate", "dateTime", "The date when the Medicinal Product is placed on the market by the Marketing Authorization Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain.", 0, 1, restoreDate)); 257 } 258 259 @Override 260 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 261 switch (_hash) { 262 case 957831062: /*country*/ return new Property("country", "CodeableConcept", "The country in which the marketing authorization has been granted shall be specified It should be specified using the ISO 3166 ? 1 alpha-2 code elements.", 0, 1, country); 263 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "Where a Medicines Regulatory Agency has granted a marketing authorization for which specific provisions within a jurisdiction apply, the jurisdiction can be specified using an appropriate controlled terminology The controlled term and the controlled term identifier shall be specified.", 0, 1, jurisdiction); 264 case -892481550: /*status*/ return new Property("status", "CodeableConcept", "This attribute provides information on the status of the marketing of the medicinal product See ISO/TS 20443 for more information and examples.", 0, 1, status); 265 case -261425617: /*dateRange*/ return new Property("dateRange", "Period", "The date when the Medicinal Product is placed on the market by the Marketing Authorization Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain.", 0, 1, dateRange); 266 case 329465692: /*restoreDate*/ return new Property("restoreDate", "dateTime", "The date when the Medicinal Product is placed on the market by the Marketing Authorization Holder (or where applicable, the manufacturer/distributor) in a country and/or jurisdiction shall be provided A complete date consisting of day, month and year shall be specified using the ISO 8601 date format NOTE ?Placed on the market? refers to the release of the Medicinal Product into the distribution chain.", 0, 1, restoreDate); 267 default: return super.getNamedProperty(_hash, _name, _checkValid); 268 } 269 270 } 271 272 @Override 273 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 274 switch (hash) { 275 case 957831062: /*country*/ return this.country == null ? new Base[0] : new Base[] {this.country}; // CodeableConcept 276 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : new Base[] {this.jurisdiction}; // CodeableConcept 277 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // CodeableConcept 278 case -261425617: /*dateRange*/ return this.dateRange == null ? new Base[0] : new Base[] {this.dateRange}; // Period 279 case 329465692: /*restoreDate*/ return this.restoreDate == null ? new Base[0] : new Base[] {this.restoreDate}; // DateTimeType 280 default: return super.getProperty(hash, name, checkValid); 281 } 282 283 } 284 285 @Override 286 public Base setProperty(int hash, String name, Base value) throws FHIRException { 287 switch (hash) { 288 case 957831062: // country 289 this.country = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 290 return value; 291 case -507075711: // jurisdiction 292 this.jurisdiction = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 293 return value; 294 case -892481550: // status 295 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 296 return value; 297 case -261425617: // dateRange 298 this.dateRange = TypeConvertor.castToPeriod(value); // Period 299 return value; 300 case 329465692: // restoreDate 301 this.restoreDate = TypeConvertor.castToDateTime(value); // DateTimeType 302 return value; 303 default: return super.setProperty(hash, name, value); 304 } 305 306 } 307 308 @Override 309 public Base setProperty(String name, Base value) throws FHIRException { 310 if (name.equals("country")) { 311 this.country = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 312 } else if (name.equals("jurisdiction")) { 313 this.jurisdiction = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 314 } else if (name.equals("status")) { 315 this.status = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 316 } else if (name.equals("dateRange")) { 317 this.dateRange = TypeConvertor.castToPeriod(value); // Period 318 } else if (name.equals("restoreDate")) { 319 this.restoreDate = TypeConvertor.castToDateTime(value); // DateTimeType 320 } else 321 return super.setProperty(name, value); 322 return value; 323 } 324 325 @Override 326 public void removeChild(String name, Base value) throws FHIRException { 327 if (name.equals("country")) { 328 this.country = null; 329 } else if (name.equals("jurisdiction")) { 330 this.jurisdiction = null; 331 } else if (name.equals("status")) { 332 this.status = null; 333 } else if (name.equals("dateRange")) { 334 this.dateRange = null; 335 } else if (name.equals("restoreDate")) { 336 this.restoreDate = null; 337 } else 338 super.removeChild(name, value); 339 340 } 341 342 @Override 343 public Base makeProperty(int hash, String name) throws FHIRException { 344 switch (hash) { 345 case 957831062: return getCountry(); 346 case -507075711: return getJurisdiction(); 347 case -892481550: return getStatus(); 348 case -261425617: return getDateRange(); 349 case 329465692: return getRestoreDateElement(); 350 default: return super.makeProperty(hash, name); 351 } 352 353 } 354 355 @Override 356 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 357 switch (hash) { 358 case 957831062: /*country*/ return new String[] {"CodeableConcept"}; 359 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 360 case -892481550: /*status*/ return new String[] {"CodeableConcept"}; 361 case -261425617: /*dateRange*/ return new String[] {"Period"}; 362 case 329465692: /*restoreDate*/ return new String[] {"dateTime"}; 363 default: return super.getTypesForProperty(hash, name); 364 } 365 366 } 367 368 @Override 369 public Base addChild(String name) throws FHIRException { 370 if (name.equals("country")) { 371 this.country = new CodeableConcept(); 372 return this.country; 373 } 374 else if (name.equals("jurisdiction")) { 375 this.jurisdiction = new CodeableConcept(); 376 return this.jurisdiction; 377 } 378 else if (name.equals("status")) { 379 this.status = new CodeableConcept(); 380 return this.status; 381 } 382 else if (name.equals("dateRange")) { 383 this.dateRange = new Period(); 384 return this.dateRange; 385 } 386 else if (name.equals("restoreDate")) { 387 throw new FHIRException("Cannot call addChild on a singleton property MarketingStatus.restoreDate"); 388 } 389 else 390 return super.addChild(name); 391 } 392 393 public String fhirType() { 394 return "MarketingStatus"; 395 396 } 397 398 public MarketingStatus copy() { 399 MarketingStatus dst = new MarketingStatus(); 400 copyValues(dst); 401 return dst; 402 } 403 404 public void copyValues(MarketingStatus dst) { 405 super.copyValues(dst); 406 dst.country = country == null ? null : country.copy(); 407 dst.jurisdiction = jurisdiction == null ? null : jurisdiction.copy(); 408 dst.status = status == null ? null : status.copy(); 409 dst.dateRange = dateRange == null ? null : dateRange.copy(); 410 dst.restoreDate = restoreDate == null ? null : restoreDate.copy(); 411 } 412 413 protected MarketingStatus typedCopy() { 414 return copy(); 415 } 416 417 @Override 418 public boolean equalsDeep(Base other_) { 419 if (!super.equalsDeep(other_)) 420 return false; 421 if (!(other_ instanceof MarketingStatus)) 422 return false; 423 MarketingStatus o = (MarketingStatus) other_; 424 return compareDeep(country, o.country, true) && compareDeep(jurisdiction, o.jurisdiction, true) 425 && compareDeep(status, o.status, true) && compareDeep(dateRange, o.dateRange, true) && compareDeep(restoreDate, o.restoreDate, true) 426 ; 427 } 428 429 @Override 430 public boolean equalsShallow(Base other_) { 431 if (!super.equalsShallow(other_)) 432 return false; 433 if (!(other_ instanceof MarketingStatus)) 434 return false; 435 MarketingStatus o = (MarketingStatus) other_; 436 return compareValues(restoreDate, o.restoreDate, true); 437 } 438 439 public boolean isEmpty() { 440 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(country, jurisdiction, status 441 , dateRange, restoreDate); 442 } 443 444 445} 446