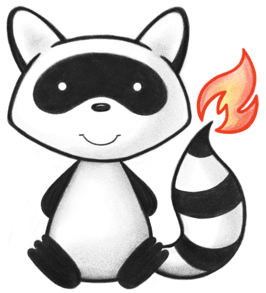
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The Measure resource provides the definition of a quality measure. 052 */ 053@ResourceDef(name="Measure", profile="http://hl7.org/fhir/StructureDefinition/Measure") 054public class Measure extends MetadataResource { 055 056 @Block() 057 public static class MeasureTermComponent extends BackboneElement implements IBaseBackboneElement { 058 /** 059 * A codeable representation of the defined term. 060 */ 061 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="What term?", formalDefinition="A codeable representation of the defined term." ) 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-definition-example") 064 protected CodeableConcept code; 065 066 /** 067 * Provides a definition for the term as used within the measure. 068 */ 069 @Child(name = "definition", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 070 @Description(shortDefinition="Meaning of the term", formalDefinition="Provides a definition for the term as used within the measure." ) 071 protected MarkdownType definition; 072 073 private static final long serialVersionUID = -25931622L; 074 075 /** 076 * Constructor 077 */ 078 public MeasureTermComponent() { 079 super(); 080 } 081 082 /** 083 * @return {@link #code} (A codeable representation of the defined term.) 084 */ 085 public CodeableConcept getCode() { 086 if (this.code == null) 087 if (Configuration.errorOnAutoCreate()) 088 throw new Error("Attempt to auto-create MeasureTermComponent.code"); 089 else if (Configuration.doAutoCreate()) 090 this.code = new CodeableConcept(); // cc 091 return this.code; 092 } 093 094 public boolean hasCode() { 095 return this.code != null && !this.code.isEmpty(); 096 } 097 098 /** 099 * @param value {@link #code} (A codeable representation of the defined term.) 100 */ 101 public MeasureTermComponent setCode(CodeableConcept value) { 102 this.code = value; 103 return this; 104 } 105 106 /** 107 * @return {@link #definition} (Provides a definition for the term as used within the measure.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 108 */ 109 public MarkdownType getDefinitionElement() { 110 if (this.definition == null) 111 if (Configuration.errorOnAutoCreate()) 112 throw new Error("Attempt to auto-create MeasureTermComponent.definition"); 113 else if (Configuration.doAutoCreate()) 114 this.definition = new MarkdownType(); // bb 115 return this.definition; 116 } 117 118 public boolean hasDefinitionElement() { 119 return this.definition != null && !this.definition.isEmpty(); 120 } 121 122 public boolean hasDefinition() { 123 return this.definition != null && !this.definition.isEmpty(); 124 } 125 126 /** 127 * @param value {@link #definition} (Provides a definition for the term as used within the measure.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 128 */ 129 public MeasureTermComponent setDefinitionElement(MarkdownType value) { 130 this.definition = value; 131 return this; 132 } 133 134 /** 135 * @return Provides a definition for the term as used within the measure. 136 */ 137 public String getDefinition() { 138 return this.definition == null ? null : this.definition.getValue(); 139 } 140 141 /** 142 * @param value Provides a definition for the term as used within the measure. 143 */ 144 public MeasureTermComponent setDefinition(String value) { 145 if (Utilities.noString(value)) 146 this.definition = null; 147 else { 148 if (this.definition == null) 149 this.definition = new MarkdownType(); 150 this.definition.setValue(value); 151 } 152 return this; 153 } 154 155 protected void listChildren(List<Property> children) { 156 super.listChildren(children); 157 children.add(new Property("code", "CodeableConcept", "A codeable representation of the defined term.", 0, 1, code)); 158 children.add(new Property("definition", "markdown", "Provides a definition for the term as used within the measure.", 0, 1, definition)); 159 } 160 161 @Override 162 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 163 switch (_hash) { 164 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A codeable representation of the defined term.", 0, 1, code); 165 case -1014418093: /*definition*/ return new Property("definition", "markdown", "Provides a definition for the term as used within the measure.", 0, 1, definition); 166 default: return super.getNamedProperty(_hash, _name, _checkValid); 167 } 168 169 } 170 171 @Override 172 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 173 switch (hash) { 174 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 175 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // MarkdownType 176 default: return super.getProperty(hash, name, checkValid); 177 } 178 179 } 180 181 @Override 182 public Base setProperty(int hash, String name, Base value) throws FHIRException { 183 switch (hash) { 184 case 3059181: // code 185 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 186 return value; 187 case -1014418093: // definition 188 this.definition = TypeConvertor.castToMarkdown(value); // MarkdownType 189 return value; 190 default: return super.setProperty(hash, name, value); 191 } 192 193 } 194 195 @Override 196 public Base setProperty(String name, Base value) throws FHIRException { 197 if (name.equals("code")) { 198 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 199 } else if (name.equals("definition")) { 200 this.definition = TypeConvertor.castToMarkdown(value); // MarkdownType 201 } else 202 return super.setProperty(name, value); 203 return value; 204 } 205 206 @Override 207 public void removeChild(String name, Base value) throws FHIRException { 208 if (name.equals("code")) { 209 this.code = null; 210 } else if (name.equals("definition")) { 211 this.definition = null; 212 } else 213 super.removeChild(name, value); 214 215 } 216 217 @Override 218 public Base makeProperty(int hash, String name) throws FHIRException { 219 switch (hash) { 220 case 3059181: return getCode(); 221 case -1014418093: return getDefinitionElement(); 222 default: return super.makeProperty(hash, name); 223 } 224 225 } 226 227 @Override 228 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 229 switch (hash) { 230 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 231 case -1014418093: /*definition*/ return new String[] {"markdown"}; 232 default: return super.getTypesForProperty(hash, name); 233 } 234 235 } 236 237 @Override 238 public Base addChild(String name) throws FHIRException { 239 if (name.equals("code")) { 240 this.code = new CodeableConcept(); 241 return this.code; 242 } 243 else if (name.equals("definition")) { 244 throw new FHIRException("Cannot call addChild on a singleton property Measure.term.definition"); 245 } 246 else 247 return super.addChild(name); 248 } 249 250 public MeasureTermComponent copy() { 251 MeasureTermComponent dst = new MeasureTermComponent(); 252 copyValues(dst); 253 return dst; 254 } 255 256 public void copyValues(MeasureTermComponent dst) { 257 super.copyValues(dst); 258 dst.code = code == null ? null : code.copy(); 259 dst.definition = definition == null ? null : definition.copy(); 260 } 261 262 @Override 263 public boolean equalsDeep(Base other_) { 264 if (!super.equalsDeep(other_)) 265 return false; 266 if (!(other_ instanceof MeasureTermComponent)) 267 return false; 268 MeasureTermComponent o = (MeasureTermComponent) other_; 269 return compareDeep(code, o.code, true) && compareDeep(definition, o.definition, true); 270 } 271 272 @Override 273 public boolean equalsShallow(Base other_) { 274 if (!super.equalsShallow(other_)) 275 return false; 276 if (!(other_ instanceof MeasureTermComponent)) 277 return false; 278 MeasureTermComponent o = (MeasureTermComponent) other_; 279 return compareValues(definition, o.definition, true); 280 } 281 282 public boolean isEmpty() { 283 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, definition); 284 } 285 286 public String fhirType() { 287 return "Measure.term"; 288 289 } 290 291 } 292 293 @Block() 294 public static class MeasureGroupComponent extends BackboneElement implements IBaseBackboneElement { 295 /** 296 * An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource. 297 */ 298 @Child(name = "linkId", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 299 @Description(shortDefinition="Unique id for group in measure", formalDefinition="An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource." ) 300 protected StringType linkId; 301 302 /** 303 * Indicates a meaning for the group. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing groups to be correlated across measures. 304 */ 305 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 306 @Description(shortDefinition="Meaning of the group", formalDefinition="Indicates a meaning for the group. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing groups to be correlated across measures." ) 307 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-group-example") 308 protected CodeableConcept code; 309 310 /** 311 * The human readable description of this population group. 312 */ 313 @Child(name = "description", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 314 @Description(shortDefinition="Summary description", formalDefinition="The human readable description of this population group." ) 315 protected MarkdownType description; 316 317 /** 318 * Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization. 319 */ 320 @Child(name = "type", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 321 @Description(shortDefinition="process | outcome | structure | patient-reported-outcome | composite", formalDefinition="Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization." ) 322 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-type") 323 protected List<CodeableConcept> type; 324 325 /** 326 * The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything. 327 */ 328 @Child(name = "subject", type = {CodeableConcept.class, Group.class}, order=5, min=0, max=1, modifier=false, summary=false) 329 @Description(shortDefinition="E.g. Patient, Practitioner, RelatedPerson, Organization, Location, Device", formalDefinition="The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything." ) 330 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participant-resource-types") 331 protected DataType subject; 332 333 /** 334 * The population basis specifies the type of elements in the population. For a subject-based measure, this is boolean (because the subject and the population basis are the same, and the population criteria define yes/no values for each individual in the population). For measures that have a population basis that is different than the subject, this element specifies the type of the population basis. For example, an encounter-based measure has a subject of Patient and a population basis of Encounter, and the population criteria all return lists of Encounters. 335 */ 336 @Child(name = "basis", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 337 @Description(shortDefinition="Population basis", formalDefinition="The population basis specifies the type of elements in the population. For a subject-based measure, this is boolean (because the subject and the population basis are the same, and the population criteria define yes/no values for each individual in the population). For measures that have a population basis that is different than the subject, this element specifies the type of the population basis. For example, an encounter-based measure has a subject of Patient and a population basis of Encounter, and the population criteria all return lists of Encounters." ) 338 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fhir-types") 339 protected Enumeration<FHIRTypes> basis; 340 341 /** 342 * Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented. 343 */ 344 @Child(name = "scoring", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 345 @Description(shortDefinition="proportion | ratio | continuous-variable | cohort", formalDefinition="Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented." ) 346 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/measure-scoring") 347 protected CodeableConcept scoring; 348 349 /** 350 * Defines the expected units of measure for the measure score. This element SHOULD be specified as a UCUM unit. 351 */ 352 @Child(name = "scoringUnit", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=true) 353 @Description(shortDefinition="What units?", formalDefinition="Defines the expected units of measure for the measure score. This element SHOULD be specified as a UCUM unit." ) 354 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-scoring-unit") 355 protected CodeableConcept scoringUnit; 356 357 /** 358 * Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result. 359 */ 360 @Child(name = "rateAggregation", type = {MarkdownType.class}, order=9, min=0, max=1, modifier=false, summary=true) 361 @Description(shortDefinition="How is rate aggregation performed for this measure", formalDefinition="Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result." ) 362 protected MarkdownType rateAggregation; 363 364 /** 365 * Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is within a range). 366 */ 367 @Child(name = "improvementNotation", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=true) 368 @Description(shortDefinition="increase | decrease", formalDefinition="Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is within a range)." ) 369 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-improvement-notation") 370 protected CodeableConcept improvementNotation; 371 372 /** 373 * A reference to a Library resource containing the formal logic used by the measure group. 374 */ 375 @Child(name = "library", type = {CanonicalType.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 376 @Description(shortDefinition="Logic used by the measure group", formalDefinition="A reference to a Library resource containing the formal logic used by the measure group." ) 377 protected List<CanonicalType> library; 378 379 /** 380 * A population criteria for the measure. 381 */ 382 @Child(name = "population", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 383 @Description(shortDefinition="Population criteria", formalDefinition="A population criteria for the measure." ) 384 protected List<MeasureGroupPopulationComponent> population; 385 386 /** 387 * The stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library or a valid FHIR Resource Path. 388 */ 389 @Child(name = "stratifier", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 390 @Description(shortDefinition="Stratifier criteria for the measure", formalDefinition="The stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library or a valid FHIR Resource Path." ) 391 protected List<MeasureGroupStratifierComponent> stratifier; 392 393 private static final long serialVersionUID = 1459308752L; 394 395 /** 396 * Constructor 397 */ 398 public MeasureGroupComponent() { 399 super(); 400 } 401 402 /** 403 * @return {@link #linkId} (An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 404 */ 405 public StringType getLinkIdElement() { 406 if (this.linkId == null) 407 if (Configuration.errorOnAutoCreate()) 408 throw new Error("Attempt to auto-create MeasureGroupComponent.linkId"); 409 else if (Configuration.doAutoCreate()) 410 this.linkId = new StringType(); // bb 411 return this.linkId; 412 } 413 414 public boolean hasLinkIdElement() { 415 return this.linkId != null && !this.linkId.isEmpty(); 416 } 417 418 public boolean hasLinkId() { 419 return this.linkId != null && !this.linkId.isEmpty(); 420 } 421 422 /** 423 * @param value {@link #linkId} (An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 424 */ 425 public MeasureGroupComponent setLinkIdElement(StringType value) { 426 this.linkId = value; 427 return this; 428 } 429 430 /** 431 * @return An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource. 432 */ 433 public String getLinkId() { 434 return this.linkId == null ? null : this.linkId.getValue(); 435 } 436 437 /** 438 * @param value An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource. 439 */ 440 public MeasureGroupComponent setLinkId(String value) { 441 if (Utilities.noString(value)) 442 this.linkId = null; 443 else { 444 if (this.linkId == null) 445 this.linkId = new StringType(); 446 this.linkId.setValue(value); 447 } 448 return this; 449 } 450 451 /** 452 * @return {@link #code} (Indicates a meaning for the group. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing groups to be correlated across measures.) 453 */ 454 public CodeableConcept getCode() { 455 if (this.code == null) 456 if (Configuration.errorOnAutoCreate()) 457 throw new Error("Attempt to auto-create MeasureGroupComponent.code"); 458 else if (Configuration.doAutoCreate()) 459 this.code = new CodeableConcept(); // cc 460 return this.code; 461 } 462 463 public boolean hasCode() { 464 return this.code != null && !this.code.isEmpty(); 465 } 466 467 /** 468 * @param value {@link #code} (Indicates a meaning for the group. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing groups to be correlated across measures.) 469 */ 470 public MeasureGroupComponent setCode(CodeableConcept value) { 471 this.code = value; 472 return this; 473 } 474 475 /** 476 * @return {@link #description} (The human readable description of this population group.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 477 */ 478 public MarkdownType getDescriptionElement() { 479 if (this.description == null) 480 if (Configuration.errorOnAutoCreate()) 481 throw new Error("Attempt to auto-create MeasureGroupComponent.description"); 482 else if (Configuration.doAutoCreate()) 483 this.description = new MarkdownType(); // bb 484 return this.description; 485 } 486 487 public boolean hasDescriptionElement() { 488 return this.description != null && !this.description.isEmpty(); 489 } 490 491 public boolean hasDescription() { 492 return this.description != null && !this.description.isEmpty(); 493 } 494 495 /** 496 * @param value {@link #description} (The human readable description of this population group.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 497 */ 498 public MeasureGroupComponent setDescriptionElement(MarkdownType value) { 499 this.description = value; 500 return this; 501 } 502 503 /** 504 * @return The human readable description of this population group. 505 */ 506 public String getDescription() { 507 return this.description == null ? null : this.description.getValue(); 508 } 509 510 /** 511 * @param value The human readable description of this population group. 512 */ 513 public MeasureGroupComponent setDescription(String value) { 514 if (Utilities.noString(value)) 515 this.description = null; 516 else { 517 if (this.description == null) 518 this.description = new MarkdownType(); 519 this.description.setValue(value); 520 } 521 return this; 522 } 523 524 /** 525 * @return {@link #type} (Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization.) 526 */ 527 public List<CodeableConcept> getType() { 528 if (this.type == null) 529 this.type = new ArrayList<CodeableConcept>(); 530 return this.type; 531 } 532 533 /** 534 * @return Returns a reference to <code>this</code> for easy method chaining 535 */ 536 public MeasureGroupComponent setType(List<CodeableConcept> theType) { 537 this.type = theType; 538 return this; 539 } 540 541 public boolean hasType() { 542 if (this.type == null) 543 return false; 544 for (CodeableConcept item : this.type) 545 if (!item.isEmpty()) 546 return true; 547 return false; 548 } 549 550 public CodeableConcept addType() { //3 551 CodeableConcept t = new CodeableConcept(); 552 if (this.type == null) 553 this.type = new ArrayList<CodeableConcept>(); 554 this.type.add(t); 555 return t; 556 } 557 558 public MeasureGroupComponent addType(CodeableConcept t) { //3 559 if (t == null) 560 return this; 561 if (this.type == null) 562 this.type = new ArrayList<CodeableConcept>(); 563 this.type.add(t); 564 return this; 565 } 566 567 /** 568 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 569 */ 570 public CodeableConcept getTypeFirstRep() { 571 if (getType().isEmpty()) { 572 addType(); 573 } 574 return getType().get(0); 575 } 576 577 /** 578 * @return {@link #subject} (The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.) 579 */ 580 public DataType getSubject() { 581 return this.subject; 582 } 583 584 /** 585 * @return {@link #subject} (The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.) 586 */ 587 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 588 if (this.subject == null) 589 this.subject = new CodeableConcept(); 590 if (!(this.subject instanceof CodeableConcept)) 591 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.subject.getClass().getName()+" was encountered"); 592 return (CodeableConcept) this.subject; 593 } 594 595 public boolean hasSubjectCodeableConcept() { 596 return this.subject instanceof CodeableConcept; 597 } 598 599 /** 600 * @return {@link #subject} (The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.) 601 */ 602 public Reference getSubjectReference() throws FHIRException { 603 if (this.subject == null) 604 this.subject = new Reference(); 605 if (!(this.subject instanceof Reference)) 606 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.subject.getClass().getName()+" was encountered"); 607 return (Reference) this.subject; 608 } 609 610 public boolean hasSubjectReference() { 611 return this.subject instanceof Reference; 612 } 613 614 public boolean hasSubject() { 615 return this.subject != null && !this.subject.isEmpty(); 616 } 617 618 /** 619 * @param value {@link #subject} (The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.) 620 */ 621 public MeasureGroupComponent setSubject(DataType value) { 622 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 623 throw new FHIRException("Not the right type for Measure.group.subject[x]: "+value.fhirType()); 624 this.subject = value; 625 return this; 626 } 627 628 /** 629 * @return {@link #basis} (The population basis specifies the type of elements in the population. For a subject-based measure, this is boolean (because the subject and the population basis are the same, and the population criteria define yes/no values for each individual in the population). For measures that have a population basis that is different than the subject, this element specifies the type of the population basis. For example, an encounter-based measure has a subject of Patient and a population basis of Encounter, and the population criteria all return lists of Encounters.). This is the underlying object with id, value and extensions. The accessor "getBasis" gives direct access to the value 630 */ 631 public Enumeration<FHIRTypes> getBasisElement() { 632 if (this.basis == null) 633 if (Configuration.errorOnAutoCreate()) 634 throw new Error("Attempt to auto-create MeasureGroupComponent.basis"); 635 else if (Configuration.doAutoCreate()) 636 this.basis = new Enumeration<FHIRTypes>(new FHIRTypesEnumFactory()); // bb 637 return this.basis; 638 } 639 640 public boolean hasBasisElement() { 641 return this.basis != null && !this.basis.isEmpty(); 642 } 643 644 public boolean hasBasis() { 645 return this.basis != null && !this.basis.isEmpty(); 646 } 647 648 /** 649 * @param value {@link #basis} (The population basis specifies the type of elements in the population. For a subject-based measure, this is boolean (because the subject and the population basis are the same, and the population criteria define yes/no values for each individual in the population). For measures that have a population basis that is different than the subject, this element specifies the type of the population basis. For example, an encounter-based measure has a subject of Patient and a population basis of Encounter, and the population criteria all return lists of Encounters.). This is the underlying object with id, value and extensions. The accessor "getBasis" gives direct access to the value 650 */ 651 public MeasureGroupComponent setBasisElement(Enumeration<FHIRTypes> value) { 652 this.basis = value; 653 return this; 654 } 655 656 /** 657 * @return The population basis specifies the type of elements in the population. For a subject-based measure, this is boolean (because the subject and the population basis are the same, and the population criteria define yes/no values for each individual in the population). For measures that have a population basis that is different than the subject, this element specifies the type of the population basis. For example, an encounter-based measure has a subject of Patient and a population basis of Encounter, and the population criteria all return lists of Encounters. 658 */ 659 public FHIRTypes getBasis() { 660 return this.basis == null ? null : this.basis.getValue(); 661 } 662 663 /** 664 * @param value The population basis specifies the type of elements in the population. For a subject-based measure, this is boolean (because the subject and the population basis are the same, and the population criteria define yes/no values for each individual in the population). For measures that have a population basis that is different than the subject, this element specifies the type of the population basis. For example, an encounter-based measure has a subject of Patient and a population basis of Encounter, and the population criteria all return lists of Encounters. 665 */ 666 public MeasureGroupComponent setBasis(FHIRTypes value) { 667 if (value == null) 668 this.basis = null; 669 else { 670 if (this.basis == null) 671 this.basis = new Enumeration<FHIRTypes>(new FHIRTypesEnumFactory()); 672 this.basis.setValue(value); 673 } 674 return this; 675 } 676 677 /** 678 * @return {@link #scoring} (Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented.) 679 */ 680 public CodeableConcept getScoring() { 681 if (this.scoring == null) 682 if (Configuration.errorOnAutoCreate()) 683 throw new Error("Attempt to auto-create MeasureGroupComponent.scoring"); 684 else if (Configuration.doAutoCreate()) 685 this.scoring = new CodeableConcept(); // cc 686 return this.scoring; 687 } 688 689 public boolean hasScoring() { 690 return this.scoring != null && !this.scoring.isEmpty(); 691 } 692 693 /** 694 * @param value {@link #scoring} (Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented.) 695 */ 696 public MeasureGroupComponent setScoring(CodeableConcept value) { 697 this.scoring = value; 698 return this; 699 } 700 701 /** 702 * @return {@link #scoringUnit} (Defines the expected units of measure for the measure score. This element SHOULD be specified as a UCUM unit.) 703 */ 704 public CodeableConcept getScoringUnit() { 705 if (this.scoringUnit == null) 706 if (Configuration.errorOnAutoCreate()) 707 throw new Error("Attempt to auto-create MeasureGroupComponent.scoringUnit"); 708 else if (Configuration.doAutoCreate()) 709 this.scoringUnit = new CodeableConcept(); // cc 710 return this.scoringUnit; 711 } 712 713 public boolean hasScoringUnit() { 714 return this.scoringUnit != null && !this.scoringUnit.isEmpty(); 715 } 716 717 /** 718 * @param value {@link #scoringUnit} (Defines the expected units of measure for the measure score. This element SHOULD be specified as a UCUM unit.) 719 */ 720 public MeasureGroupComponent setScoringUnit(CodeableConcept value) { 721 this.scoringUnit = value; 722 return this; 723 } 724 725 /** 726 * @return {@link #rateAggregation} (Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result.). This is the underlying object with id, value and extensions. The accessor "getRateAggregation" gives direct access to the value 727 */ 728 public MarkdownType getRateAggregationElement() { 729 if (this.rateAggregation == null) 730 if (Configuration.errorOnAutoCreate()) 731 throw new Error("Attempt to auto-create MeasureGroupComponent.rateAggregation"); 732 else if (Configuration.doAutoCreate()) 733 this.rateAggregation = new MarkdownType(); // bb 734 return this.rateAggregation; 735 } 736 737 public boolean hasRateAggregationElement() { 738 return this.rateAggregation != null && !this.rateAggregation.isEmpty(); 739 } 740 741 public boolean hasRateAggregation() { 742 return this.rateAggregation != null && !this.rateAggregation.isEmpty(); 743 } 744 745 /** 746 * @param value {@link #rateAggregation} (Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result.). This is the underlying object with id, value and extensions. The accessor "getRateAggregation" gives direct access to the value 747 */ 748 public MeasureGroupComponent setRateAggregationElement(MarkdownType value) { 749 this.rateAggregation = value; 750 return this; 751 } 752 753 /** 754 * @return Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result. 755 */ 756 public String getRateAggregation() { 757 return this.rateAggregation == null ? null : this.rateAggregation.getValue(); 758 } 759 760 /** 761 * @param value Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result. 762 */ 763 public MeasureGroupComponent setRateAggregation(String value) { 764 if (Utilities.noString(value)) 765 this.rateAggregation = null; 766 else { 767 if (this.rateAggregation == null) 768 this.rateAggregation = new MarkdownType(); 769 this.rateAggregation.setValue(value); 770 } 771 return this; 772 } 773 774 /** 775 * @return {@link #improvementNotation} (Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is within a range).) 776 */ 777 public CodeableConcept getImprovementNotation() { 778 if (this.improvementNotation == null) 779 if (Configuration.errorOnAutoCreate()) 780 throw new Error("Attempt to auto-create MeasureGroupComponent.improvementNotation"); 781 else if (Configuration.doAutoCreate()) 782 this.improvementNotation = new CodeableConcept(); // cc 783 return this.improvementNotation; 784 } 785 786 public boolean hasImprovementNotation() { 787 return this.improvementNotation != null && !this.improvementNotation.isEmpty(); 788 } 789 790 /** 791 * @param value {@link #improvementNotation} (Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is within a range).) 792 */ 793 public MeasureGroupComponent setImprovementNotation(CodeableConcept value) { 794 this.improvementNotation = value; 795 return this; 796 } 797 798 /** 799 * @return {@link #library} (A reference to a Library resource containing the formal logic used by the measure group.) 800 */ 801 public List<CanonicalType> getLibrary() { 802 if (this.library == null) 803 this.library = new ArrayList<CanonicalType>(); 804 return this.library; 805 } 806 807 /** 808 * @return Returns a reference to <code>this</code> for easy method chaining 809 */ 810 public MeasureGroupComponent setLibrary(List<CanonicalType> theLibrary) { 811 this.library = theLibrary; 812 return this; 813 } 814 815 public boolean hasLibrary() { 816 if (this.library == null) 817 return false; 818 for (CanonicalType item : this.library) 819 if (!item.isEmpty()) 820 return true; 821 return false; 822 } 823 824 /** 825 * @return {@link #library} (A reference to a Library resource containing the formal logic used by the measure group.) 826 */ 827 public CanonicalType addLibraryElement() {//2 828 CanonicalType t = new CanonicalType(); 829 if (this.library == null) 830 this.library = new ArrayList<CanonicalType>(); 831 this.library.add(t); 832 return t; 833 } 834 835 /** 836 * @param value {@link #library} (A reference to a Library resource containing the formal logic used by the measure group.) 837 */ 838 public MeasureGroupComponent addLibrary(String value) { //1 839 CanonicalType t = new CanonicalType(); 840 t.setValue(value); 841 if (this.library == null) 842 this.library = new ArrayList<CanonicalType>(); 843 this.library.add(t); 844 return this; 845 } 846 847 /** 848 * @param value {@link #library} (A reference to a Library resource containing the formal logic used by the measure group.) 849 */ 850 public boolean hasLibrary(String value) { 851 if (this.library == null) 852 return false; 853 for (CanonicalType v : this.library) 854 if (v.getValue().equals(value)) // canonical 855 return true; 856 return false; 857 } 858 859 /** 860 * @return {@link #population} (A population criteria for the measure.) 861 */ 862 public List<MeasureGroupPopulationComponent> getPopulation() { 863 if (this.population == null) 864 this.population = new ArrayList<MeasureGroupPopulationComponent>(); 865 return this.population; 866 } 867 868 /** 869 * @return Returns a reference to <code>this</code> for easy method chaining 870 */ 871 public MeasureGroupComponent setPopulation(List<MeasureGroupPopulationComponent> thePopulation) { 872 this.population = thePopulation; 873 return this; 874 } 875 876 public boolean hasPopulation() { 877 if (this.population == null) 878 return false; 879 for (MeasureGroupPopulationComponent item : this.population) 880 if (!item.isEmpty()) 881 return true; 882 return false; 883 } 884 885 public MeasureGroupPopulationComponent addPopulation() { //3 886 MeasureGroupPopulationComponent t = new MeasureGroupPopulationComponent(); 887 if (this.population == null) 888 this.population = new ArrayList<MeasureGroupPopulationComponent>(); 889 this.population.add(t); 890 return t; 891 } 892 893 public MeasureGroupComponent addPopulation(MeasureGroupPopulationComponent t) { //3 894 if (t == null) 895 return this; 896 if (this.population == null) 897 this.population = new ArrayList<MeasureGroupPopulationComponent>(); 898 this.population.add(t); 899 return this; 900 } 901 902 /** 903 * @return The first repetition of repeating field {@link #population}, creating it if it does not already exist {3} 904 */ 905 public MeasureGroupPopulationComponent getPopulationFirstRep() { 906 if (getPopulation().isEmpty()) { 907 addPopulation(); 908 } 909 return getPopulation().get(0); 910 } 911 912 /** 913 * @return {@link #stratifier} (The stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library or a valid FHIR Resource Path.) 914 */ 915 public List<MeasureGroupStratifierComponent> getStratifier() { 916 if (this.stratifier == null) 917 this.stratifier = new ArrayList<MeasureGroupStratifierComponent>(); 918 return this.stratifier; 919 } 920 921 /** 922 * @return Returns a reference to <code>this</code> for easy method chaining 923 */ 924 public MeasureGroupComponent setStratifier(List<MeasureGroupStratifierComponent> theStratifier) { 925 this.stratifier = theStratifier; 926 return this; 927 } 928 929 public boolean hasStratifier() { 930 if (this.stratifier == null) 931 return false; 932 for (MeasureGroupStratifierComponent item : this.stratifier) 933 if (!item.isEmpty()) 934 return true; 935 return false; 936 } 937 938 public MeasureGroupStratifierComponent addStratifier() { //3 939 MeasureGroupStratifierComponent t = new MeasureGroupStratifierComponent(); 940 if (this.stratifier == null) 941 this.stratifier = new ArrayList<MeasureGroupStratifierComponent>(); 942 this.stratifier.add(t); 943 return t; 944 } 945 946 public MeasureGroupComponent addStratifier(MeasureGroupStratifierComponent t) { //3 947 if (t == null) 948 return this; 949 if (this.stratifier == null) 950 this.stratifier = new ArrayList<MeasureGroupStratifierComponent>(); 951 this.stratifier.add(t); 952 return this; 953 } 954 955 /** 956 * @return The first repetition of repeating field {@link #stratifier}, creating it if it does not already exist {3} 957 */ 958 public MeasureGroupStratifierComponent getStratifierFirstRep() { 959 if (getStratifier().isEmpty()) { 960 addStratifier(); 961 } 962 return getStratifier().get(0); 963 } 964 965 protected void listChildren(List<Property> children) { 966 super.listChildren(children); 967 children.add(new Property("linkId", "string", "An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource.", 0, 1, linkId)); 968 children.add(new Property("code", "CodeableConcept", "Indicates a meaning for the group. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing groups to be correlated across measures.", 0, 1, code)); 969 children.add(new Property("description", "markdown", "The human readable description of this population group.", 0, 1, description)); 970 children.add(new Property("type", "CodeableConcept", "Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization.", 0, java.lang.Integer.MAX_VALUE, type)); 971 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", "The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.", 0, 1, subject)); 972 children.add(new Property("basis", "code", "The population basis specifies the type of elements in the population. For a subject-based measure, this is boolean (because the subject and the population basis are the same, and the population criteria define yes/no values for each individual in the population). For measures that have a population basis that is different than the subject, this element specifies the type of the population basis. For example, an encounter-based measure has a subject of Patient and a population basis of Encounter, and the population criteria all return lists of Encounters.", 0, 1, basis)); 973 children.add(new Property("scoring", "CodeableConcept", "Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented.", 0, 1, scoring)); 974 children.add(new Property("scoringUnit", "CodeableConcept", "Defines the expected units of measure for the measure score. This element SHOULD be specified as a UCUM unit.", 0, 1, scoringUnit)); 975 children.add(new Property("rateAggregation", "markdown", "Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result.", 0, 1, rateAggregation)); 976 children.add(new Property("improvementNotation", "CodeableConcept", "Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is within a range).", 0, 1, improvementNotation)); 977 children.add(new Property("library", "canonical(Library)", "A reference to a Library resource containing the formal logic used by the measure group.", 0, java.lang.Integer.MAX_VALUE, library)); 978 children.add(new Property("population", "", "A population criteria for the measure.", 0, java.lang.Integer.MAX_VALUE, population)); 979 children.add(new Property("stratifier", "", "The stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library or a valid FHIR Resource Path.", 0, java.lang.Integer.MAX_VALUE, stratifier)); 980 } 981 982 @Override 983 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 984 switch (_hash) { 985 case -1102667083: /*linkId*/ return new Property("linkId", "string", "An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource.", 0, 1, linkId); 986 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Indicates a meaning for the group. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing groups to be correlated across measures.", 0, 1, code); 987 case -1724546052: /*description*/ return new Property("description", "markdown", "The human readable description of this population group.", 0, 1, description); 988 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization.", 0, java.lang.Integer.MAX_VALUE, type); 989 case -573640748: /*subject[x]*/ return new Property("subject[x]", "CodeableConcept|Reference(Group)", "The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.", 0, 1, subject); 990 case -1867885268: /*subject*/ return new Property("subject[x]", "CodeableConcept|Reference(Group)", "The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.", 0, 1, subject); 991 case -1257122603: /*subjectCodeableConcept*/ return new Property("subject[x]", "CodeableConcept", "The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.", 0, 1, subject); 992 case 772938623: /*subjectReference*/ return new Property("subject[x]", "Reference(Group)", "The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.", 0, 1, subject); 993 case 93508670: /*basis*/ return new Property("basis", "code", "The population basis specifies the type of elements in the population. For a subject-based measure, this is boolean (because the subject and the population basis are the same, and the population criteria define yes/no values for each individual in the population). For measures that have a population basis that is different than the subject, this element specifies the type of the population basis. For example, an encounter-based measure has a subject of Patient and a population basis of Encounter, and the population criteria all return lists of Encounters.", 0, 1, basis); 994 case 1924005583: /*scoring*/ return new Property("scoring", "CodeableConcept", "Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented.", 0, 1, scoring); 995 case 1527532787: /*scoringUnit*/ return new Property("scoringUnit", "CodeableConcept", "Defines the expected units of measure for the measure score. This element SHOULD be specified as a UCUM unit.", 0, 1, scoringUnit); 996 case 1254503906: /*rateAggregation*/ return new Property("rateAggregation", "markdown", "Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result.", 0, 1, rateAggregation); 997 case -2085456136: /*improvementNotation*/ return new Property("improvementNotation", "CodeableConcept", "Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is within a range).", 0, 1, improvementNotation); 998 case 166208699: /*library*/ return new Property("library", "canonical(Library)", "A reference to a Library resource containing the formal logic used by the measure group.", 0, java.lang.Integer.MAX_VALUE, library); 999 case -2023558323: /*population*/ return new Property("population", "", "A population criteria for the measure.", 0, java.lang.Integer.MAX_VALUE, population); 1000 case 90983669: /*stratifier*/ return new Property("stratifier", "", "The stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library or a valid FHIR Resource Path.", 0, java.lang.Integer.MAX_VALUE, stratifier); 1001 default: return super.getNamedProperty(_hash, _name, _checkValid); 1002 } 1003 1004 } 1005 1006 @Override 1007 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1008 switch (hash) { 1009 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 1010 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1011 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1012 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1013 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // DataType 1014 case 93508670: /*basis*/ return this.basis == null ? new Base[0] : new Base[] {this.basis}; // Enumeration<FHIRTypes> 1015 case 1924005583: /*scoring*/ return this.scoring == null ? new Base[0] : new Base[] {this.scoring}; // CodeableConcept 1016 case 1527532787: /*scoringUnit*/ return this.scoringUnit == null ? new Base[0] : new Base[] {this.scoringUnit}; // CodeableConcept 1017 case 1254503906: /*rateAggregation*/ return this.rateAggregation == null ? new Base[0] : new Base[] {this.rateAggregation}; // MarkdownType 1018 case -2085456136: /*improvementNotation*/ return this.improvementNotation == null ? new Base[0] : new Base[] {this.improvementNotation}; // CodeableConcept 1019 case 166208699: /*library*/ return this.library == null ? new Base[0] : this.library.toArray(new Base[this.library.size()]); // CanonicalType 1020 case -2023558323: /*population*/ return this.population == null ? new Base[0] : this.population.toArray(new Base[this.population.size()]); // MeasureGroupPopulationComponent 1021 case 90983669: /*stratifier*/ return this.stratifier == null ? new Base[0] : this.stratifier.toArray(new Base[this.stratifier.size()]); // MeasureGroupStratifierComponent 1022 default: return super.getProperty(hash, name, checkValid); 1023 } 1024 1025 } 1026 1027 @Override 1028 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1029 switch (hash) { 1030 case -1102667083: // linkId 1031 this.linkId = TypeConvertor.castToString(value); // StringType 1032 return value; 1033 case 3059181: // code 1034 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1035 return value; 1036 case -1724546052: // description 1037 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1038 return value; 1039 case 3575610: // type 1040 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 1041 return value; 1042 case -1867885268: // subject 1043 this.subject = TypeConvertor.castToType(value); // DataType 1044 return value; 1045 case 93508670: // basis 1046 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1047 this.basis = (Enumeration) value; // Enumeration<FHIRTypes> 1048 return value; 1049 case 1924005583: // scoring 1050 this.scoring = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1051 return value; 1052 case 1527532787: // scoringUnit 1053 this.scoringUnit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1054 return value; 1055 case 1254503906: // rateAggregation 1056 this.rateAggregation = TypeConvertor.castToMarkdown(value); // MarkdownType 1057 return value; 1058 case -2085456136: // improvementNotation 1059 this.improvementNotation = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1060 return value; 1061 case 166208699: // library 1062 this.getLibrary().add(TypeConvertor.castToCanonical(value)); // CanonicalType 1063 return value; 1064 case -2023558323: // population 1065 this.getPopulation().add((MeasureGroupPopulationComponent) value); // MeasureGroupPopulationComponent 1066 return value; 1067 case 90983669: // stratifier 1068 this.getStratifier().add((MeasureGroupStratifierComponent) value); // MeasureGroupStratifierComponent 1069 return value; 1070 default: return super.setProperty(hash, name, value); 1071 } 1072 1073 } 1074 1075 @Override 1076 public Base setProperty(String name, Base value) throws FHIRException { 1077 if (name.equals("linkId")) { 1078 this.linkId = TypeConvertor.castToString(value); // StringType 1079 } else if (name.equals("code")) { 1080 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1081 } else if (name.equals("description")) { 1082 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1083 } else if (name.equals("type")) { 1084 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 1085 } else if (name.equals("subject[x]")) { 1086 this.subject = TypeConvertor.castToType(value); // DataType 1087 } else if (name.equals("basis")) { 1088 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1089 this.basis = (Enumeration) value; // Enumeration<FHIRTypes> 1090 } else if (name.equals("scoring")) { 1091 this.scoring = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1092 } else if (name.equals("scoringUnit")) { 1093 this.scoringUnit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1094 } else if (name.equals("rateAggregation")) { 1095 this.rateAggregation = TypeConvertor.castToMarkdown(value); // MarkdownType 1096 } else if (name.equals("improvementNotation")) { 1097 this.improvementNotation = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1098 } else if (name.equals("library")) { 1099 this.getLibrary().add(TypeConvertor.castToCanonical(value)); 1100 } else if (name.equals("population")) { 1101 this.getPopulation().add((MeasureGroupPopulationComponent) value); 1102 } else if (name.equals("stratifier")) { 1103 this.getStratifier().add((MeasureGroupStratifierComponent) value); 1104 } else 1105 return super.setProperty(name, value); 1106 return value; 1107 } 1108 1109 @Override 1110 public void removeChild(String name, Base value) throws FHIRException { 1111 if (name.equals("linkId")) { 1112 this.linkId = null; 1113 } else if (name.equals("code")) { 1114 this.code = null; 1115 } else if (name.equals("description")) { 1116 this.description = null; 1117 } else if (name.equals("type")) { 1118 this.getType().remove(value); 1119 } else if (name.equals("subject[x]")) { 1120 this.subject = null; 1121 } else if (name.equals("basis")) { 1122 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1123 this.basis = (Enumeration) value; // Enumeration<FHIRTypes> 1124 } else if (name.equals("scoring")) { 1125 this.scoring = null; 1126 } else if (name.equals("scoringUnit")) { 1127 this.scoringUnit = null; 1128 } else if (name.equals("rateAggregation")) { 1129 this.rateAggregation = null; 1130 } else if (name.equals("improvementNotation")) { 1131 this.improvementNotation = null; 1132 } else if (name.equals("library")) { 1133 this.getLibrary().remove(value); 1134 } else if (name.equals("population")) { 1135 this.getPopulation().remove((MeasureGroupPopulationComponent) value); 1136 } else if (name.equals("stratifier")) { 1137 this.getStratifier().remove((MeasureGroupStratifierComponent) value); 1138 } else 1139 super.removeChild(name, value); 1140 1141 } 1142 1143 @Override 1144 public Base makeProperty(int hash, String name) throws FHIRException { 1145 switch (hash) { 1146 case -1102667083: return getLinkIdElement(); 1147 case 3059181: return getCode(); 1148 case -1724546052: return getDescriptionElement(); 1149 case 3575610: return addType(); 1150 case -573640748: return getSubject(); 1151 case -1867885268: return getSubject(); 1152 case 93508670: return getBasisElement(); 1153 case 1924005583: return getScoring(); 1154 case 1527532787: return getScoringUnit(); 1155 case 1254503906: return getRateAggregationElement(); 1156 case -2085456136: return getImprovementNotation(); 1157 case 166208699: return addLibraryElement(); 1158 case -2023558323: return addPopulation(); 1159 case 90983669: return addStratifier(); 1160 default: return super.makeProperty(hash, name); 1161 } 1162 1163 } 1164 1165 @Override 1166 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1167 switch (hash) { 1168 case -1102667083: /*linkId*/ return new String[] {"string"}; 1169 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1170 case -1724546052: /*description*/ return new String[] {"markdown"}; 1171 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1172 case -1867885268: /*subject*/ return new String[] {"CodeableConcept", "Reference"}; 1173 case 93508670: /*basis*/ return new String[] {"code"}; 1174 case 1924005583: /*scoring*/ return new String[] {"CodeableConcept"}; 1175 case 1527532787: /*scoringUnit*/ return new String[] {"CodeableConcept"}; 1176 case 1254503906: /*rateAggregation*/ return new String[] {"markdown"}; 1177 case -2085456136: /*improvementNotation*/ return new String[] {"CodeableConcept"}; 1178 case 166208699: /*library*/ return new String[] {"canonical"}; 1179 case -2023558323: /*population*/ return new String[] {}; 1180 case 90983669: /*stratifier*/ return new String[] {}; 1181 default: return super.getTypesForProperty(hash, name); 1182 } 1183 1184 } 1185 1186 @Override 1187 public Base addChild(String name) throws FHIRException { 1188 if (name.equals("linkId")) { 1189 throw new FHIRException("Cannot call addChild on a singleton property Measure.group.linkId"); 1190 } 1191 else if (name.equals("code")) { 1192 this.code = new CodeableConcept(); 1193 return this.code; 1194 } 1195 else if (name.equals("description")) { 1196 throw new FHIRException("Cannot call addChild on a singleton property Measure.group.description"); 1197 } 1198 else if (name.equals("type")) { 1199 return addType(); 1200 } 1201 else if (name.equals("subjectCodeableConcept")) { 1202 this.subject = new CodeableConcept(); 1203 return this.subject; 1204 } 1205 else if (name.equals("subjectReference")) { 1206 this.subject = new Reference(); 1207 return this.subject; 1208 } 1209 else if (name.equals("basis")) { 1210 throw new FHIRException("Cannot call addChild on a singleton property Measure.group.basis"); 1211 } 1212 else if (name.equals("scoring")) { 1213 this.scoring = new CodeableConcept(); 1214 return this.scoring; 1215 } 1216 else if (name.equals("scoringUnit")) { 1217 this.scoringUnit = new CodeableConcept(); 1218 return this.scoringUnit; 1219 } 1220 else if (name.equals("rateAggregation")) { 1221 throw new FHIRException("Cannot call addChild on a singleton property Measure.group.rateAggregation"); 1222 } 1223 else if (name.equals("improvementNotation")) { 1224 this.improvementNotation = new CodeableConcept(); 1225 return this.improvementNotation; 1226 } 1227 else if (name.equals("library")) { 1228 throw new FHIRException("Cannot call addChild on a singleton property Measure.group.library"); 1229 } 1230 else if (name.equals("population")) { 1231 return addPopulation(); 1232 } 1233 else if (name.equals("stratifier")) { 1234 return addStratifier(); 1235 } 1236 else 1237 return super.addChild(name); 1238 } 1239 1240 public MeasureGroupComponent copy() { 1241 MeasureGroupComponent dst = new MeasureGroupComponent(); 1242 copyValues(dst); 1243 return dst; 1244 } 1245 1246 public void copyValues(MeasureGroupComponent dst) { 1247 super.copyValues(dst); 1248 dst.linkId = linkId == null ? null : linkId.copy(); 1249 dst.code = code == null ? null : code.copy(); 1250 dst.description = description == null ? null : description.copy(); 1251 if (type != null) { 1252 dst.type = new ArrayList<CodeableConcept>(); 1253 for (CodeableConcept i : type) 1254 dst.type.add(i.copy()); 1255 }; 1256 dst.subject = subject == null ? null : subject.copy(); 1257 dst.basis = basis == null ? null : basis.copy(); 1258 dst.scoring = scoring == null ? null : scoring.copy(); 1259 dst.scoringUnit = scoringUnit == null ? null : scoringUnit.copy(); 1260 dst.rateAggregation = rateAggregation == null ? null : rateAggregation.copy(); 1261 dst.improvementNotation = improvementNotation == null ? null : improvementNotation.copy(); 1262 if (library != null) { 1263 dst.library = new ArrayList<CanonicalType>(); 1264 for (CanonicalType i : library) 1265 dst.library.add(i.copy()); 1266 }; 1267 if (population != null) { 1268 dst.population = new ArrayList<MeasureGroupPopulationComponent>(); 1269 for (MeasureGroupPopulationComponent i : population) 1270 dst.population.add(i.copy()); 1271 }; 1272 if (stratifier != null) { 1273 dst.stratifier = new ArrayList<MeasureGroupStratifierComponent>(); 1274 for (MeasureGroupStratifierComponent i : stratifier) 1275 dst.stratifier.add(i.copy()); 1276 }; 1277 } 1278 1279 @Override 1280 public boolean equalsDeep(Base other_) { 1281 if (!super.equalsDeep(other_)) 1282 return false; 1283 if (!(other_ instanceof MeasureGroupComponent)) 1284 return false; 1285 MeasureGroupComponent o = (MeasureGroupComponent) other_; 1286 return compareDeep(linkId, o.linkId, true) && compareDeep(code, o.code, true) && compareDeep(description, o.description, true) 1287 && compareDeep(type, o.type, true) && compareDeep(subject, o.subject, true) && compareDeep(basis, o.basis, true) 1288 && compareDeep(scoring, o.scoring, true) && compareDeep(scoringUnit, o.scoringUnit, true) && compareDeep(rateAggregation, o.rateAggregation, true) 1289 && compareDeep(improvementNotation, o.improvementNotation, true) && compareDeep(library, o.library, true) 1290 && compareDeep(population, o.population, true) && compareDeep(stratifier, o.stratifier, true); 1291 } 1292 1293 @Override 1294 public boolean equalsShallow(Base other_) { 1295 if (!super.equalsShallow(other_)) 1296 return false; 1297 if (!(other_ instanceof MeasureGroupComponent)) 1298 return false; 1299 MeasureGroupComponent o = (MeasureGroupComponent) other_; 1300 return compareValues(linkId, o.linkId, true) && compareValues(description, o.description, true) && compareValues(basis, o.basis, true) 1301 && compareValues(rateAggregation, o.rateAggregation, true) && compareValues(library, o.library, true) 1302 ; 1303 } 1304 1305 public boolean isEmpty() { 1306 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, code, description 1307 , type, subject, basis, scoring, scoringUnit, rateAggregation, improvementNotation 1308 , library, population, stratifier); 1309 } 1310 1311 public String fhirType() { 1312 return "Measure.group"; 1313 1314 } 1315 1316 } 1317 1318 @Block() 1319 public static class MeasureGroupPopulationComponent extends BackboneElement implements IBaseBackboneElement { 1320 /** 1321 * An identifier that is unique within the Measure allowing linkage to the equivalent population in a MeasureReport resource. 1322 */ 1323 @Child(name = "linkId", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1324 @Description(shortDefinition="Unique id for population in measure", formalDefinition="An identifier that is unique within the Measure allowing linkage to the equivalent population in a MeasureReport resource." ) 1325 protected StringType linkId; 1326 1327 /** 1328 * The type of population criteria. 1329 */ 1330 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1331 @Description(shortDefinition="initial-population | numerator | numerator-exclusion | denominator | denominator-exclusion | denominator-exception | measure-population | measure-population-exclusion | measure-observation", formalDefinition="The type of population criteria." ) 1332 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-population") 1333 protected CodeableConcept code; 1334 1335 /** 1336 * The human readable description of this population criteria. 1337 */ 1338 @Child(name = "description", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1339 @Description(shortDefinition="The human readable description of this population criteria", formalDefinition="The human readable description of this population criteria." ) 1340 protected MarkdownType description; 1341 1342 /** 1343 * An expression that specifies the criteria for the population, typically the name of an expression in a library. 1344 */ 1345 @Child(name = "criteria", type = {Expression.class}, order=4, min=0, max=1, modifier=false, summary=false) 1346 @Description(shortDefinition="The criteria that defines this population", formalDefinition="An expression that specifies the criteria for the population, typically the name of an expression in a library." ) 1347 protected Expression criteria; 1348 1349 /** 1350 * A Group resource that defines this population as a set of characteristics. 1351 */ 1352 @Child(name = "groupDefinition", type = {Group.class}, order=5, min=0, max=1, modifier=false, summary=false) 1353 @Description(shortDefinition="A group resource that defines this population", formalDefinition="A Group resource that defines this population as a set of characteristics." ) 1354 protected Reference groupDefinition; 1355 1356 /** 1357 * The id of a population element in this measure that provides the input for this population criteria. In most cases, the scoring structure of the measure implies specific relationships (e.g. the Numerator uses the Denominator as the source in a proportion scoring). In some cases, however, multiple possible choices exist and must be resolved explicitly. For example in a ratio measure with multiple initial populations, the denominator must specify which population should be used as the starting point. 1358 */ 1359 @Child(name = "inputPopulationId", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 1360 @Description(shortDefinition="Which population", formalDefinition="The id of a population element in this measure that provides the input for this population criteria. In most cases, the scoring structure of the measure implies specific relationships (e.g. the Numerator uses the Denominator as the source in a proportion scoring). In some cases, however, multiple possible choices exist and must be resolved explicitly. For example in a ratio measure with multiple initial populations, the denominator must specify which population should be used as the starting point." ) 1361 protected StringType inputPopulationId; 1362 1363 /** 1364 * Specifies which method should be used to aggregate measure observation values. For most scoring types, this is implied by scoring (e.g. a proportion measure counts members of the populations). For continuous variables, however, this information must be specified to ensure correct calculation. 1365 */ 1366 @Child(name = "aggregateMethod", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 1367 @Description(shortDefinition="Aggregation method for a measure score (e.g. sum, average, median, minimum, maximum, count)", formalDefinition="Specifies which method should be used to aggregate measure observation values. For most scoring types, this is implied by scoring (e.g. a proportion measure counts members of the populations). For continuous variables, however, this information must be specified to ensure correct calculation." ) 1368 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-aggregate-method") 1369 protected CodeableConcept aggregateMethod; 1370 1371 private static final long serialVersionUID = -1233952485L; 1372 1373 /** 1374 * Constructor 1375 */ 1376 public MeasureGroupPopulationComponent() { 1377 super(); 1378 } 1379 1380 /** 1381 * @return {@link #linkId} (An identifier that is unique within the Measure allowing linkage to the equivalent population in a MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 1382 */ 1383 public StringType getLinkIdElement() { 1384 if (this.linkId == null) 1385 if (Configuration.errorOnAutoCreate()) 1386 throw new Error("Attempt to auto-create MeasureGroupPopulationComponent.linkId"); 1387 else if (Configuration.doAutoCreate()) 1388 this.linkId = new StringType(); // bb 1389 return this.linkId; 1390 } 1391 1392 public boolean hasLinkIdElement() { 1393 return this.linkId != null && !this.linkId.isEmpty(); 1394 } 1395 1396 public boolean hasLinkId() { 1397 return this.linkId != null && !this.linkId.isEmpty(); 1398 } 1399 1400 /** 1401 * @param value {@link #linkId} (An identifier that is unique within the Measure allowing linkage to the equivalent population in a MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 1402 */ 1403 public MeasureGroupPopulationComponent setLinkIdElement(StringType value) { 1404 this.linkId = value; 1405 return this; 1406 } 1407 1408 /** 1409 * @return An identifier that is unique within the Measure allowing linkage to the equivalent population in a MeasureReport resource. 1410 */ 1411 public String getLinkId() { 1412 return this.linkId == null ? null : this.linkId.getValue(); 1413 } 1414 1415 /** 1416 * @param value An identifier that is unique within the Measure allowing linkage to the equivalent population in a MeasureReport resource. 1417 */ 1418 public MeasureGroupPopulationComponent setLinkId(String value) { 1419 if (Utilities.noString(value)) 1420 this.linkId = null; 1421 else { 1422 if (this.linkId == null) 1423 this.linkId = new StringType(); 1424 this.linkId.setValue(value); 1425 } 1426 return this; 1427 } 1428 1429 /** 1430 * @return {@link #code} (The type of population criteria.) 1431 */ 1432 public CodeableConcept getCode() { 1433 if (this.code == null) 1434 if (Configuration.errorOnAutoCreate()) 1435 throw new Error("Attempt to auto-create MeasureGroupPopulationComponent.code"); 1436 else if (Configuration.doAutoCreate()) 1437 this.code = new CodeableConcept(); // cc 1438 return this.code; 1439 } 1440 1441 public boolean hasCode() { 1442 return this.code != null && !this.code.isEmpty(); 1443 } 1444 1445 /** 1446 * @param value {@link #code} (The type of population criteria.) 1447 */ 1448 public MeasureGroupPopulationComponent setCode(CodeableConcept value) { 1449 this.code = value; 1450 return this; 1451 } 1452 1453 /** 1454 * @return {@link #description} (The human readable description of this population criteria.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1455 */ 1456 public MarkdownType getDescriptionElement() { 1457 if (this.description == null) 1458 if (Configuration.errorOnAutoCreate()) 1459 throw new Error("Attempt to auto-create MeasureGroupPopulationComponent.description"); 1460 else if (Configuration.doAutoCreate()) 1461 this.description = new MarkdownType(); // bb 1462 return this.description; 1463 } 1464 1465 public boolean hasDescriptionElement() { 1466 return this.description != null && !this.description.isEmpty(); 1467 } 1468 1469 public boolean hasDescription() { 1470 return this.description != null && !this.description.isEmpty(); 1471 } 1472 1473 /** 1474 * @param value {@link #description} (The human readable description of this population criteria.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1475 */ 1476 public MeasureGroupPopulationComponent setDescriptionElement(MarkdownType value) { 1477 this.description = value; 1478 return this; 1479 } 1480 1481 /** 1482 * @return The human readable description of this population criteria. 1483 */ 1484 public String getDescription() { 1485 return this.description == null ? null : this.description.getValue(); 1486 } 1487 1488 /** 1489 * @param value The human readable description of this population criteria. 1490 */ 1491 public MeasureGroupPopulationComponent setDescription(String value) { 1492 if (Utilities.noString(value)) 1493 this.description = null; 1494 else { 1495 if (this.description == null) 1496 this.description = new MarkdownType(); 1497 this.description.setValue(value); 1498 } 1499 return this; 1500 } 1501 1502 /** 1503 * @return {@link #criteria} (An expression that specifies the criteria for the population, typically the name of an expression in a library.) 1504 */ 1505 public Expression getCriteria() { 1506 if (this.criteria == null) 1507 if (Configuration.errorOnAutoCreate()) 1508 throw new Error("Attempt to auto-create MeasureGroupPopulationComponent.criteria"); 1509 else if (Configuration.doAutoCreate()) 1510 this.criteria = new Expression(); // cc 1511 return this.criteria; 1512 } 1513 1514 public boolean hasCriteria() { 1515 return this.criteria != null && !this.criteria.isEmpty(); 1516 } 1517 1518 /** 1519 * @param value {@link #criteria} (An expression that specifies the criteria for the population, typically the name of an expression in a library.) 1520 */ 1521 public MeasureGroupPopulationComponent setCriteria(Expression value) { 1522 this.criteria = value; 1523 return this; 1524 } 1525 1526 /** 1527 * @return {@link #groupDefinition} (A Group resource that defines this population as a set of characteristics.) 1528 */ 1529 public Reference getGroupDefinition() { 1530 if (this.groupDefinition == null) 1531 if (Configuration.errorOnAutoCreate()) 1532 throw new Error("Attempt to auto-create MeasureGroupPopulationComponent.groupDefinition"); 1533 else if (Configuration.doAutoCreate()) 1534 this.groupDefinition = new Reference(); // cc 1535 return this.groupDefinition; 1536 } 1537 1538 public boolean hasGroupDefinition() { 1539 return this.groupDefinition != null && !this.groupDefinition.isEmpty(); 1540 } 1541 1542 /** 1543 * @param value {@link #groupDefinition} (A Group resource that defines this population as a set of characteristics.) 1544 */ 1545 public MeasureGroupPopulationComponent setGroupDefinition(Reference value) { 1546 this.groupDefinition = value; 1547 return this; 1548 } 1549 1550 /** 1551 * @return {@link #inputPopulationId} (The id of a population element in this measure that provides the input for this population criteria. In most cases, the scoring structure of the measure implies specific relationships (e.g. the Numerator uses the Denominator as the source in a proportion scoring). In some cases, however, multiple possible choices exist and must be resolved explicitly. For example in a ratio measure with multiple initial populations, the denominator must specify which population should be used as the starting point.). This is the underlying object with id, value and extensions. The accessor "getInputPopulationId" gives direct access to the value 1552 */ 1553 public StringType getInputPopulationIdElement() { 1554 if (this.inputPopulationId == null) 1555 if (Configuration.errorOnAutoCreate()) 1556 throw new Error("Attempt to auto-create MeasureGroupPopulationComponent.inputPopulationId"); 1557 else if (Configuration.doAutoCreate()) 1558 this.inputPopulationId = new StringType(); // bb 1559 return this.inputPopulationId; 1560 } 1561 1562 public boolean hasInputPopulationIdElement() { 1563 return this.inputPopulationId != null && !this.inputPopulationId.isEmpty(); 1564 } 1565 1566 public boolean hasInputPopulationId() { 1567 return this.inputPopulationId != null && !this.inputPopulationId.isEmpty(); 1568 } 1569 1570 /** 1571 * @param value {@link #inputPopulationId} (The id of a population element in this measure that provides the input for this population criteria. In most cases, the scoring structure of the measure implies specific relationships (e.g. the Numerator uses the Denominator as the source in a proportion scoring). In some cases, however, multiple possible choices exist and must be resolved explicitly. For example in a ratio measure with multiple initial populations, the denominator must specify which population should be used as the starting point.). This is the underlying object with id, value and extensions. The accessor "getInputPopulationId" gives direct access to the value 1572 */ 1573 public MeasureGroupPopulationComponent setInputPopulationIdElement(StringType value) { 1574 this.inputPopulationId = value; 1575 return this; 1576 } 1577 1578 /** 1579 * @return The id of a population element in this measure that provides the input for this population criteria. In most cases, the scoring structure of the measure implies specific relationships (e.g. the Numerator uses the Denominator as the source in a proportion scoring). In some cases, however, multiple possible choices exist and must be resolved explicitly. For example in a ratio measure with multiple initial populations, the denominator must specify which population should be used as the starting point. 1580 */ 1581 public String getInputPopulationId() { 1582 return this.inputPopulationId == null ? null : this.inputPopulationId.getValue(); 1583 } 1584 1585 /** 1586 * @param value The id of a population element in this measure that provides the input for this population criteria. In most cases, the scoring structure of the measure implies specific relationships (e.g. the Numerator uses the Denominator as the source in a proportion scoring). In some cases, however, multiple possible choices exist and must be resolved explicitly. For example in a ratio measure with multiple initial populations, the denominator must specify which population should be used as the starting point. 1587 */ 1588 public MeasureGroupPopulationComponent setInputPopulationId(String value) { 1589 if (Utilities.noString(value)) 1590 this.inputPopulationId = null; 1591 else { 1592 if (this.inputPopulationId == null) 1593 this.inputPopulationId = new StringType(); 1594 this.inputPopulationId.setValue(value); 1595 } 1596 return this; 1597 } 1598 1599 /** 1600 * @return {@link #aggregateMethod} (Specifies which method should be used to aggregate measure observation values. For most scoring types, this is implied by scoring (e.g. a proportion measure counts members of the populations). For continuous variables, however, this information must be specified to ensure correct calculation.) 1601 */ 1602 public CodeableConcept getAggregateMethod() { 1603 if (this.aggregateMethod == null) 1604 if (Configuration.errorOnAutoCreate()) 1605 throw new Error("Attempt to auto-create MeasureGroupPopulationComponent.aggregateMethod"); 1606 else if (Configuration.doAutoCreate()) 1607 this.aggregateMethod = new CodeableConcept(); // cc 1608 return this.aggregateMethod; 1609 } 1610 1611 public boolean hasAggregateMethod() { 1612 return this.aggregateMethod != null && !this.aggregateMethod.isEmpty(); 1613 } 1614 1615 /** 1616 * @param value {@link #aggregateMethod} (Specifies which method should be used to aggregate measure observation values. For most scoring types, this is implied by scoring (e.g. a proportion measure counts members of the populations). For continuous variables, however, this information must be specified to ensure correct calculation.) 1617 */ 1618 public MeasureGroupPopulationComponent setAggregateMethod(CodeableConcept value) { 1619 this.aggregateMethod = value; 1620 return this; 1621 } 1622 1623 protected void listChildren(List<Property> children) { 1624 super.listChildren(children); 1625 children.add(new Property("linkId", "string", "An identifier that is unique within the Measure allowing linkage to the equivalent population in a MeasureReport resource.", 0, 1, linkId)); 1626 children.add(new Property("code", "CodeableConcept", "The type of population criteria.", 0, 1, code)); 1627 children.add(new Property("description", "markdown", "The human readable description of this population criteria.", 0, 1, description)); 1628 children.add(new Property("criteria", "Expression", "An expression that specifies the criteria for the population, typically the name of an expression in a library.", 0, 1, criteria)); 1629 children.add(new Property("groupDefinition", "Reference(Group)", "A Group resource that defines this population as a set of characteristics.", 0, 1, groupDefinition)); 1630 children.add(new Property("inputPopulationId", "string", "The id of a population element in this measure that provides the input for this population criteria. In most cases, the scoring structure of the measure implies specific relationships (e.g. the Numerator uses the Denominator as the source in a proportion scoring). In some cases, however, multiple possible choices exist and must be resolved explicitly. For example in a ratio measure with multiple initial populations, the denominator must specify which population should be used as the starting point.", 0, 1, inputPopulationId)); 1631 children.add(new Property("aggregateMethod", "CodeableConcept", "Specifies which method should be used to aggregate measure observation values. For most scoring types, this is implied by scoring (e.g. a proportion measure counts members of the populations). For continuous variables, however, this information must be specified to ensure correct calculation.", 0, 1, aggregateMethod)); 1632 } 1633 1634 @Override 1635 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1636 switch (_hash) { 1637 case -1102667083: /*linkId*/ return new Property("linkId", "string", "An identifier that is unique within the Measure allowing linkage to the equivalent population in a MeasureReport resource.", 0, 1, linkId); 1638 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The type of population criteria.", 0, 1, code); 1639 case -1724546052: /*description*/ return new Property("description", "markdown", "The human readable description of this population criteria.", 0, 1, description); 1640 case 1952046943: /*criteria*/ return new Property("criteria", "Expression", "An expression that specifies the criteria for the population, typically the name of an expression in a library.", 0, 1, criteria); 1641 case 158676274: /*groupDefinition*/ return new Property("groupDefinition", "Reference(Group)", "A Group resource that defines this population as a set of characteristics.", 0, 1, groupDefinition); 1642 case -464344526: /*inputPopulationId*/ return new Property("inputPopulationId", "string", "The id of a population element in this measure that provides the input for this population criteria. In most cases, the scoring structure of the measure implies specific relationships (e.g. the Numerator uses the Denominator as the source in a proportion scoring). In some cases, however, multiple possible choices exist and must be resolved explicitly. For example in a ratio measure with multiple initial populations, the denominator must specify which population should be used as the starting point.", 0, 1, inputPopulationId); 1643 case -1036416: /*aggregateMethod*/ return new Property("aggregateMethod", "CodeableConcept", "Specifies which method should be used to aggregate measure observation values. For most scoring types, this is implied by scoring (e.g. a proportion measure counts members of the populations). For continuous variables, however, this information must be specified to ensure correct calculation.", 0, 1, aggregateMethod); 1644 default: return super.getNamedProperty(_hash, _name, _checkValid); 1645 } 1646 1647 } 1648 1649 @Override 1650 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1651 switch (hash) { 1652 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 1653 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1654 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1655 case 1952046943: /*criteria*/ return this.criteria == null ? new Base[0] : new Base[] {this.criteria}; // Expression 1656 case 158676274: /*groupDefinition*/ return this.groupDefinition == null ? new Base[0] : new Base[] {this.groupDefinition}; // Reference 1657 case -464344526: /*inputPopulationId*/ return this.inputPopulationId == null ? new Base[0] : new Base[] {this.inputPopulationId}; // StringType 1658 case -1036416: /*aggregateMethod*/ return this.aggregateMethod == null ? new Base[0] : new Base[] {this.aggregateMethod}; // CodeableConcept 1659 default: return super.getProperty(hash, name, checkValid); 1660 } 1661 1662 } 1663 1664 @Override 1665 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1666 switch (hash) { 1667 case -1102667083: // linkId 1668 this.linkId = TypeConvertor.castToString(value); // StringType 1669 return value; 1670 case 3059181: // code 1671 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1672 return value; 1673 case -1724546052: // description 1674 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1675 return value; 1676 case 1952046943: // criteria 1677 this.criteria = TypeConvertor.castToExpression(value); // Expression 1678 return value; 1679 case 158676274: // groupDefinition 1680 this.groupDefinition = TypeConvertor.castToReference(value); // Reference 1681 return value; 1682 case -464344526: // inputPopulationId 1683 this.inputPopulationId = TypeConvertor.castToString(value); // StringType 1684 return value; 1685 case -1036416: // aggregateMethod 1686 this.aggregateMethod = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1687 return value; 1688 default: return super.setProperty(hash, name, value); 1689 } 1690 1691 } 1692 1693 @Override 1694 public Base setProperty(String name, Base value) throws FHIRException { 1695 if (name.equals("linkId")) { 1696 this.linkId = TypeConvertor.castToString(value); // StringType 1697 } else if (name.equals("code")) { 1698 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1699 } else if (name.equals("description")) { 1700 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 1701 } else if (name.equals("criteria")) { 1702 this.criteria = TypeConvertor.castToExpression(value); // Expression 1703 } else if (name.equals("groupDefinition")) { 1704 this.groupDefinition = TypeConvertor.castToReference(value); // Reference 1705 } else if (name.equals("inputPopulationId")) { 1706 this.inputPopulationId = TypeConvertor.castToString(value); // StringType 1707 } else if (name.equals("aggregateMethod")) { 1708 this.aggregateMethod = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1709 } else 1710 return super.setProperty(name, value); 1711 return value; 1712 } 1713 1714 @Override 1715 public void removeChild(String name, Base value) throws FHIRException { 1716 if (name.equals("linkId")) { 1717 this.linkId = null; 1718 } else if (name.equals("code")) { 1719 this.code = null; 1720 } else if (name.equals("description")) { 1721 this.description = null; 1722 } else if (name.equals("criteria")) { 1723 this.criteria = null; 1724 } else if (name.equals("groupDefinition")) { 1725 this.groupDefinition = null; 1726 } else if (name.equals("inputPopulationId")) { 1727 this.inputPopulationId = null; 1728 } else if (name.equals("aggregateMethod")) { 1729 this.aggregateMethod = null; 1730 } else 1731 super.removeChild(name, value); 1732 1733 } 1734 1735 @Override 1736 public Base makeProperty(int hash, String name) throws FHIRException { 1737 switch (hash) { 1738 case -1102667083: return getLinkIdElement(); 1739 case 3059181: return getCode(); 1740 case -1724546052: return getDescriptionElement(); 1741 case 1952046943: return getCriteria(); 1742 case 158676274: return getGroupDefinition(); 1743 case -464344526: return getInputPopulationIdElement(); 1744 case -1036416: return getAggregateMethod(); 1745 default: return super.makeProperty(hash, name); 1746 } 1747 1748 } 1749 1750 @Override 1751 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1752 switch (hash) { 1753 case -1102667083: /*linkId*/ return new String[] {"string"}; 1754 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1755 case -1724546052: /*description*/ return new String[] {"markdown"}; 1756 case 1952046943: /*criteria*/ return new String[] {"Expression"}; 1757 case 158676274: /*groupDefinition*/ return new String[] {"Reference"}; 1758 case -464344526: /*inputPopulationId*/ return new String[] {"string"}; 1759 case -1036416: /*aggregateMethod*/ return new String[] {"CodeableConcept"}; 1760 default: return super.getTypesForProperty(hash, name); 1761 } 1762 1763 } 1764 1765 @Override 1766 public Base addChild(String name) throws FHIRException { 1767 if (name.equals("linkId")) { 1768 throw new FHIRException("Cannot call addChild on a singleton property Measure.group.population.linkId"); 1769 } 1770 else if (name.equals("code")) { 1771 this.code = new CodeableConcept(); 1772 return this.code; 1773 } 1774 else if (name.equals("description")) { 1775 throw new FHIRException("Cannot call addChild on a singleton property Measure.group.population.description"); 1776 } 1777 else if (name.equals("criteria")) { 1778 this.criteria = new Expression(); 1779 return this.criteria; 1780 } 1781 else if (name.equals("groupDefinition")) { 1782 this.groupDefinition = new Reference(); 1783 return this.groupDefinition; 1784 } 1785 else if (name.equals("inputPopulationId")) { 1786 throw new FHIRException("Cannot call addChild on a singleton property Measure.group.population.inputPopulationId"); 1787 } 1788 else if (name.equals("aggregateMethod")) { 1789 this.aggregateMethod = new CodeableConcept(); 1790 return this.aggregateMethod; 1791 } 1792 else 1793 return super.addChild(name); 1794 } 1795 1796 public MeasureGroupPopulationComponent copy() { 1797 MeasureGroupPopulationComponent dst = new MeasureGroupPopulationComponent(); 1798 copyValues(dst); 1799 return dst; 1800 } 1801 1802 public void copyValues(MeasureGroupPopulationComponent dst) { 1803 super.copyValues(dst); 1804 dst.linkId = linkId == null ? null : linkId.copy(); 1805 dst.code = code == null ? null : code.copy(); 1806 dst.description = description == null ? null : description.copy(); 1807 dst.criteria = criteria == null ? null : criteria.copy(); 1808 dst.groupDefinition = groupDefinition == null ? null : groupDefinition.copy(); 1809 dst.inputPopulationId = inputPopulationId == null ? null : inputPopulationId.copy(); 1810 dst.aggregateMethod = aggregateMethod == null ? null : aggregateMethod.copy(); 1811 } 1812 1813 @Override 1814 public boolean equalsDeep(Base other_) { 1815 if (!super.equalsDeep(other_)) 1816 return false; 1817 if (!(other_ instanceof MeasureGroupPopulationComponent)) 1818 return false; 1819 MeasureGroupPopulationComponent o = (MeasureGroupPopulationComponent) other_; 1820 return compareDeep(linkId, o.linkId, true) && compareDeep(code, o.code, true) && compareDeep(description, o.description, true) 1821 && compareDeep(criteria, o.criteria, true) && compareDeep(groupDefinition, o.groupDefinition, true) 1822 && compareDeep(inputPopulationId, o.inputPopulationId, true) && compareDeep(aggregateMethod, o.aggregateMethod, true) 1823 ; 1824 } 1825 1826 @Override 1827 public boolean equalsShallow(Base other_) { 1828 if (!super.equalsShallow(other_)) 1829 return false; 1830 if (!(other_ instanceof MeasureGroupPopulationComponent)) 1831 return false; 1832 MeasureGroupPopulationComponent o = (MeasureGroupPopulationComponent) other_; 1833 return compareValues(linkId, o.linkId, true) && compareValues(description, o.description, true) && compareValues(inputPopulationId, o.inputPopulationId, true) 1834 ; 1835 } 1836 1837 public boolean isEmpty() { 1838 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, code, description 1839 , criteria, groupDefinition, inputPopulationId, aggregateMethod); 1840 } 1841 1842 public String fhirType() { 1843 return "Measure.group.population"; 1844 1845 } 1846 1847 } 1848 1849 @Block() 1850 public static class MeasureGroupStratifierComponent extends BackboneElement implements IBaseBackboneElement { 1851 /** 1852 * An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource. 1853 */ 1854 @Child(name = "linkId", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1855 @Description(shortDefinition="Unique id for stratifier in measure", formalDefinition="An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource." ) 1856 protected StringType linkId; 1857 1858 /** 1859 * Indicates a meaning for the stratifier. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures. 1860 */ 1861 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1862 @Description(shortDefinition="Meaning of the stratifier", formalDefinition="Indicates a meaning for the stratifier. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures." ) 1863 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-stratifier-example") 1864 protected CodeableConcept code; 1865 1866 /** 1867 * The human readable description of this stratifier criteria. 1868 */ 1869 @Child(name = "description", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1870 @Description(shortDefinition="The human readable description of this stratifier", formalDefinition="The human readable description of this stratifier criteria." ) 1871 protected MarkdownType description; 1872 1873 /** 1874 * An expression that specifies the criteria for the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element. 1875 */ 1876 @Child(name = "criteria", type = {Expression.class}, order=4, min=0, max=1, modifier=false, summary=false) 1877 @Description(shortDefinition="How the measure should be stratified", formalDefinition="An expression that specifies the criteria for the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element." ) 1878 protected Expression criteria; 1879 1880 /** 1881 * A Group resource that defines this population as a set of characteristics. 1882 */ 1883 @Child(name = "groupDefinition", type = {Group.class}, order=5, min=0, max=1, modifier=false, summary=false) 1884 @Description(shortDefinition="A group resource that defines this population", formalDefinition="A Group resource that defines this population as a set of characteristics." ) 1885 protected Reference groupDefinition; 1886 1887 /** 1888 * A component of the stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library or a valid FHIR Resource Path. 1889 */ 1890 @Child(name = "component", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1891 @Description(shortDefinition="Stratifier criteria component for the measure", formalDefinition="A component of the stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library or a valid FHIR Resource Path." ) 1892 protected List<MeasureGroupStratifierComponentComponent> component; 1893 1894 private static final long serialVersionUID = 1169588840L; 1895 1896 /** 1897 * Constructor 1898 */ 1899 public MeasureGroupStratifierComponent() { 1900 super(); 1901 } 1902 1903 /** 1904 * @return {@link #linkId} (An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 1905 */ 1906 public StringType getLinkIdElement() { 1907 if (this.linkId == null) 1908 if (Configuration.errorOnAutoCreate()) 1909 throw new Error("Attempt to auto-create MeasureGroupStratifierComponent.linkId"); 1910 else if (Configuration.doAutoCreate()) 1911 this.linkId = new StringType(); // bb 1912 return this.linkId; 1913 } 1914 1915 public boolean hasLinkIdElement() { 1916 return this.linkId != null && !this.linkId.isEmpty(); 1917 } 1918 1919 public boolean hasLinkId() { 1920 return this.linkId != null && !this.linkId.isEmpty(); 1921 } 1922 1923 /** 1924 * @param value {@link #linkId} (An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 1925 */ 1926 public MeasureGroupStratifierComponent setLinkIdElement(StringType value) { 1927 this.linkId = value; 1928 return this; 1929 } 1930 1931 /** 1932 * @return An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource. 1933 */ 1934 public String getLinkId() { 1935 return this.linkId == null ? null : this.linkId.getValue(); 1936 } 1937 1938 /** 1939 * @param value An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource. 1940 */ 1941 public MeasureGroupStratifierComponent setLinkId(String value) { 1942 if (Utilities.noString(value)) 1943 this.linkId = null; 1944 else { 1945 if (this.linkId == null) 1946 this.linkId = new StringType(); 1947 this.linkId.setValue(value); 1948 } 1949 return this; 1950 } 1951 1952 /** 1953 * @return {@link #code} (Indicates a meaning for the stratifier. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures.) 1954 */ 1955 public CodeableConcept getCode() { 1956 if (this.code == null) 1957 if (Configuration.errorOnAutoCreate()) 1958 throw new Error("Attempt to auto-create MeasureGroupStratifierComponent.code"); 1959 else if (Configuration.doAutoCreate()) 1960 this.code = new CodeableConcept(); // cc 1961 return this.code; 1962 } 1963 1964 public boolean hasCode() { 1965 return this.code != null && !this.code.isEmpty(); 1966 } 1967 1968 /** 1969 * @param value {@link #code} (Indicates a meaning for the stratifier. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures.) 1970 */ 1971 public MeasureGroupStratifierComponent setCode(CodeableConcept value) { 1972 this.code = value; 1973 return this; 1974 } 1975 1976 /** 1977 * @return {@link #description} (The human readable description of this stratifier criteria.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1978 */ 1979 public MarkdownType getDescriptionElement() { 1980 if (this.description == null) 1981 if (Configuration.errorOnAutoCreate()) 1982 throw new Error("Attempt to auto-create MeasureGroupStratifierComponent.description"); 1983 else if (Configuration.doAutoCreate()) 1984 this.description = new MarkdownType(); // bb 1985 return this.description; 1986 } 1987 1988 public boolean hasDescriptionElement() { 1989 return this.description != null && !this.description.isEmpty(); 1990 } 1991 1992 public boolean hasDescription() { 1993 return this.description != null && !this.description.isEmpty(); 1994 } 1995 1996 /** 1997 * @param value {@link #description} (The human readable description of this stratifier criteria.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1998 */ 1999 public MeasureGroupStratifierComponent setDescriptionElement(MarkdownType value) { 2000 this.description = value; 2001 return this; 2002 } 2003 2004 /** 2005 * @return The human readable description of this stratifier criteria. 2006 */ 2007 public String getDescription() { 2008 return this.description == null ? null : this.description.getValue(); 2009 } 2010 2011 /** 2012 * @param value The human readable description of this stratifier criteria. 2013 */ 2014 public MeasureGroupStratifierComponent setDescription(String value) { 2015 if (Utilities.noString(value)) 2016 this.description = null; 2017 else { 2018 if (this.description == null) 2019 this.description = new MarkdownType(); 2020 this.description.setValue(value); 2021 } 2022 return this; 2023 } 2024 2025 /** 2026 * @return {@link #criteria} (An expression that specifies the criteria for the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element.) 2027 */ 2028 public Expression getCriteria() { 2029 if (this.criteria == null) 2030 if (Configuration.errorOnAutoCreate()) 2031 throw new Error("Attempt to auto-create MeasureGroupStratifierComponent.criteria"); 2032 else if (Configuration.doAutoCreate()) 2033 this.criteria = new Expression(); // cc 2034 return this.criteria; 2035 } 2036 2037 public boolean hasCriteria() { 2038 return this.criteria != null && !this.criteria.isEmpty(); 2039 } 2040 2041 /** 2042 * @param value {@link #criteria} (An expression that specifies the criteria for the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element.) 2043 */ 2044 public MeasureGroupStratifierComponent setCriteria(Expression value) { 2045 this.criteria = value; 2046 return this; 2047 } 2048 2049 /** 2050 * @return {@link #groupDefinition} (A Group resource that defines this population as a set of characteristics.) 2051 */ 2052 public Reference getGroupDefinition() { 2053 if (this.groupDefinition == null) 2054 if (Configuration.errorOnAutoCreate()) 2055 throw new Error("Attempt to auto-create MeasureGroupStratifierComponent.groupDefinition"); 2056 else if (Configuration.doAutoCreate()) 2057 this.groupDefinition = new Reference(); // cc 2058 return this.groupDefinition; 2059 } 2060 2061 public boolean hasGroupDefinition() { 2062 return this.groupDefinition != null && !this.groupDefinition.isEmpty(); 2063 } 2064 2065 /** 2066 * @param value {@link #groupDefinition} (A Group resource that defines this population as a set of characteristics.) 2067 */ 2068 public MeasureGroupStratifierComponent setGroupDefinition(Reference value) { 2069 this.groupDefinition = value; 2070 return this; 2071 } 2072 2073 /** 2074 * @return {@link #component} (A component of the stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library or a valid FHIR Resource Path.) 2075 */ 2076 public List<MeasureGroupStratifierComponentComponent> getComponent() { 2077 if (this.component == null) 2078 this.component = new ArrayList<MeasureGroupStratifierComponentComponent>(); 2079 return this.component; 2080 } 2081 2082 /** 2083 * @return Returns a reference to <code>this</code> for easy method chaining 2084 */ 2085 public MeasureGroupStratifierComponent setComponent(List<MeasureGroupStratifierComponentComponent> theComponent) { 2086 this.component = theComponent; 2087 return this; 2088 } 2089 2090 public boolean hasComponent() { 2091 if (this.component == null) 2092 return false; 2093 for (MeasureGroupStratifierComponentComponent item : this.component) 2094 if (!item.isEmpty()) 2095 return true; 2096 return false; 2097 } 2098 2099 public MeasureGroupStratifierComponentComponent addComponent() { //3 2100 MeasureGroupStratifierComponentComponent t = new MeasureGroupStratifierComponentComponent(); 2101 if (this.component == null) 2102 this.component = new ArrayList<MeasureGroupStratifierComponentComponent>(); 2103 this.component.add(t); 2104 return t; 2105 } 2106 2107 public MeasureGroupStratifierComponent addComponent(MeasureGroupStratifierComponentComponent t) { //3 2108 if (t == null) 2109 return this; 2110 if (this.component == null) 2111 this.component = new ArrayList<MeasureGroupStratifierComponentComponent>(); 2112 this.component.add(t); 2113 return this; 2114 } 2115 2116 /** 2117 * @return The first repetition of repeating field {@link #component}, creating it if it does not already exist {3} 2118 */ 2119 public MeasureGroupStratifierComponentComponent getComponentFirstRep() { 2120 if (getComponent().isEmpty()) { 2121 addComponent(); 2122 } 2123 return getComponent().get(0); 2124 } 2125 2126 protected void listChildren(List<Property> children) { 2127 super.listChildren(children); 2128 children.add(new Property("linkId", "string", "An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource.", 0, 1, linkId)); 2129 children.add(new Property("code", "CodeableConcept", "Indicates a meaning for the stratifier. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures.", 0, 1, code)); 2130 children.add(new Property("description", "markdown", "The human readable description of this stratifier criteria.", 0, 1, description)); 2131 children.add(new Property("criteria", "Expression", "An expression that specifies the criteria for the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element.", 0, 1, criteria)); 2132 children.add(new Property("groupDefinition", "Reference(Group)", "A Group resource that defines this population as a set of characteristics.", 0, 1, groupDefinition)); 2133 children.add(new Property("component", "", "A component of the stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library or a valid FHIR Resource Path.", 0, java.lang.Integer.MAX_VALUE, component)); 2134 } 2135 2136 @Override 2137 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2138 switch (_hash) { 2139 case -1102667083: /*linkId*/ return new Property("linkId", "string", "An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource.", 0, 1, linkId); 2140 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Indicates a meaning for the stratifier. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures.", 0, 1, code); 2141 case -1724546052: /*description*/ return new Property("description", "markdown", "The human readable description of this stratifier criteria.", 0, 1, description); 2142 case 1952046943: /*criteria*/ return new Property("criteria", "Expression", "An expression that specifies the criteria for the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element.", 0, 1, criteria); 2143 case 158676274: /*groupDefinition*/ return new Property("groupDefinition", "Reference(Group)", "A Group resource that defines this population as a set of characteristics.", 0, 1, groupDefinition); 2144 case -1399907075: /*component*/ return new Property("component", "", "A component of the stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library or a valid FHIR Resource Path.", 0, java.lang.Integer.MAX_VALUE, component); 2145 default: return super.getNamedProperty(_hash, _name, _checkValid); 2146 } 2147 2148 } 2149 2150 @Override 2151 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2152 switch (hash) { 2153 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 2154 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2155 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2156 case 1952046943: /*criteria*/ return this.criteria == null ? new Base[0] : new Base[] {this.criteria}; // Expression 2157 case 158676274: /*groupDefinition*/ return this.groupDefinition == null ? new Base[0] : new Base[] {this.groupDefinition}; // Reference 2158 case -1399907075: /*component*/ return this.component == null ? new Base[0] : this.component.toArray(new Base[this.component.size()]); // MeasureGroupStratifierComponentComponent 2159 default: return super.getProperty(hash, name, checkValid); 2160 } 2161 2162 } 2163 2164 @Override 2165 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2166 switch (hash) { 2167 case -1102667083: // linkId 2168 this.linkId = TypeConvertor.castToString(value); // StringType 2169 return value; 2170 case 3059181: // code 2171 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2172 return value; 2173 case -1724546052: // description 2174 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2175 return value; 2176 case 1952046943: // criteria 2177 this.criteria = TypeConvertor.castToExpression(value); // Expression 2178 return value; 2179 case 158676274: // groupDefinition 2180 this.groupDefinition = TypeConvertor.castToReference(value); // Reference 2181 return value; 2182 case -1399907075: // component 2183 this.getComponent().add((MeasureGroupStratifierComponentComponent) value); // MeasureGroupStratifierComponentComponent 2184 return value; 2185 default: return super.setProperty(hash, name, value); 2186 } 2187 2188 } 2189 2190 @Override 2191 public Base setProperty(String name, Base value) throws FHIRException { 2192 if (name.equals("linkId")) { 2193 this.linkId = TypeConvertor.castToString(value); // StringType 2194 } else if (name.equals("code")) { 2195 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2196 } else if (name.equals("description")) { 2197 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2198 } else if (name.equals("criteria")) { 2199 this.criteria = TypeConvertor.castToExpression(value); // Expression 2200 } else if (name.equals("groupDefinition")) { 2201 this.groupDefinition = TypeConvertor.castToReference(value); // Reference 2202 } else if (name.equals("component")) { 2203 this.getComponent().add((MeasureGroupStratifierComponentComponent) value); 2204 } else 2205 return super.setProperty(name, value); 2206 return value; 2207 } 2208 2209 @Override 2210 public void removeChild(String name, Base value) throws FHIRException { 2211 if (name.equals("linkId")) { 2212 this.linkId = null; 2213 } else if (name.equals("code")) { 2214 this.code = null; 2215 } else if (name.equals("description")) { 2216 this.description = null; 2217 } else if (name.equals("criteria")) { 2218 this.criteria = null; 2219 } else if (name.equals("groupDefinition")) { 2220 this.groupDefinition = null; 2221 } else if (name.equals("component")) { 2222 this.getComponent().remove((MeasureGroupStratifierComponentComponent) value); 2223 } else 2224 super.removeChild(name, value); 2225 2226 } 2227 2228 @Override 2229 public Base makeProperty(int hash, String name) throws FHIRException { 2230 switch (hash) { 2231 case -1102667083: return getLinkIdElement(); 2232 case 3059181: return getCode(); 2233 case -1724546052: return getDescriptionElement(); 2234 case 1952046943: return getCriteria(); 2235 case 158676274: return getGroupDefinition(); 2236 case -1399907075: return addComponent(); 2237 default: return super.makeProperty(hash, name); 2238 } 2239 2240 } 2241 2242 @Override 2243 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2244 switch (hash) { 2245 case -1102667083: /*linkId*/ return new String[] {"string"}; 2246 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2247 case -1724546052: /*description*/ return new String[] {"markdown"}; 2248 case 1952046943: /*criteria*/ return new String[] {"Expression"}; 2249 case 158676274: /*groupDefinition*/ return new String[] {"Reference"}; 2250 case -1399907075: /*component*/ return new String[] {}; 2251 default: return super.getTypesForProperty(hash, name); 2252 } 2253 2254 } 2255 2256 @Override 2257 public Base addChild(String name) throws FHIRException { 2258 if (name.equals("linkId")) { 2259 throw new FHIRException("Cannot call addChild on a singleton property Measure.group.stratifier.linkId"); 2260 } 2261 else if (name.equals("code")) { 2262 this.code = new CodeableConcept(); 2263 return this.code; 2264 } 2265 else if (name.equals("description")) { 2266 throw new FHIRException("Cannot call addChild on a singleton property Measure.group.stratifier.description"); 2267 } 2268 else if (name.equals("criteria")) { 2269 this.criteria = new Expression(); 2270 return this.criteria; 2271 } 2272 else if (name.equals("groupDefinition")) { 2273 this.groupDefinition = new Reference(); 2274 return this.groupDefinition; 2275 } 2276 else if (name.equals("component")) { 2277 return addComponent(); 2278 } 2279 else 2280 return super.addChild(name); 2281 } 2282 2283 public MeasureGroupStratifierComponent copy() { 2284 MeasureGroupStratifierComponent dst = new MeasureGroupStratifierComponent(); 2285 copyValues(dst); 2286 return dst; 2287 } 2288 2289 public void copyValues(MeasureGroupStratifierComponent dst) { 2290 super.copyValues(dst); 2291 dst.linkId = linkId == null ? null : linkId.copy(); 2292 dst.code = code == null ? null : code.copy(); 2293 dst.description = description == null ? null : description.copy(); 2294 dst.criteria = criteria == null ? null : criteria.copy(); 2295 dst.groupDefinition = groupDefinition == null ? null : groupDefinition.copy(); 2296 if (component != null) { 2297 dst.component = new ArrayList<MeasureGroupStratifierComponentComponent>(); 2298 for (MeasureGroupStratifierComponentComponent i : component) 2299 dst.component.add(i.copy()); 2300 }; 2301 } 2302 2303 @Override 2304 public boolean equalsDeep(Base other_) { 2305 if (!super.equalsDeep(other_)) 2306 return false; 2307 if (!(other_ instanceof MeasureGroupStratifierComponent)) 2308 return false; 2309 MeasureGroupStratifierComponent o = (MeasureGroupStratifierComponent) other_; 2310 return compareDeep(linkId, o.linkId, true) && compareDeep(code, o.code, true) && compareDeep(description, o.description, true) 2311 && compareDeep(criteria, o.criteria, true) && compareDeep(groupDefinition, o.groupDefinition, true) 2312 && compareDeep(component, o.component, true); 2313 } 2314 2315 @Override 2316 public boolean equalsShallow(Base other_) { 2317 if (!super.equalsShallow(other_)) 2318 return false; 2319 if (!(other_ instanceof MeasureGroupStratifierComponent)) 2320 return false; 2321 MeasureGroupStratifierComponent o = (MeasureGroupStratifierComponent) other_; 2322 return compareValues(linkId, o.linkId, true) && compareValues(description, o.description, true); 2323 } 2324 2325 public boolean isEmpty() { 2326 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, code, description 2327 , criteria, groupDefinition, component); 2328 } 2329 2330 public String fhirType() { 2331 return "Measure.group.stratifier"; 2332 2333 } 2334 2335 } 2336 2337 @Block() 2338 public static class MeasureGroupStratifierComponentComponent extends BackboneElement implements IBaseBackboneElement { 2339 /** 2340 * An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource. 2341 */ 2342 @Child(name = "linkId", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2343 @Description(shortDefinition="Unique id for stratifier component in measure", formalDefinition="An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource." ) 2344 protected StringType linkId; 2345 2346 /** 2347 * Indicates a meaning for the stratifier component. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures. 2348 */ 2349 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 2350 @Description(shortDefinition="Meaning of the stratifier component", formalDefinition="Indicates a meaning for the stratifier component. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures." ) 2351 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-stratifier-example") 2352 protected CodeableConcept code; 2353 2354 /** 2355 * The human readable description of this stratifier criteria component. 2356 */ 2357 @Child(name = "description", type = {MarkdownType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2358 @Description(shortDefinition="The human readable description of this stratifier component", formalDefinition="The human readable description of this stratifier criteria component." ) 2359 protected MarkdownType description; 2360 2361 /** 2362 * An expression that specifies the criteria for this component of the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element. 2363 */ 2364 @Child(name = "criteria", type = {Expression.class}, order=4, min=0, max=1, modifier=false, summary=false) 2365 @Description(shortDefinition="Component of how the measure should be stratified", formalDefinition="An expression that specifies the criteria for this component of the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element." ) 2366 protected Expression criteria; 2367 2368 /** 2369 * A Group resource that defines this population as a set of characteristics. 2370 */ 2371 @Child(name = "groupDefinition", type = {Group.class}, order=5, min=0, max=1, modifier=false, summary=false) 2372 @Description(shortDefinition="A group resource that defines this population", formalDefinition="A Group resource that defines this population as a set of characteristics." ) 2373 protected Reference groupDefinition; 2374 2375 private static final long serialVersionUID = 744342583L; 2376 2377 /** 2378 * Constructor 2379 */ 2380 public MeasureGroupStratifierComponentComponent() { 2381 super(); 2382 } 2383 2384 /** 2385 * @return {@link #linkId} (An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 2386 */ 2387 public StringType getLinkIdElement() { 2388 if (this.linkId == null) 2389 if (Configuration.errorOnAutoCreate()) 2390 throw new Error("Attempt to auto-create MeasureGroupStratifierComponentComponent.linkId"); 2391 else if (Configuration.doAutoCreate()) 2392 this.linkId = new StringType(); // bb 2393 return this.linkId; 2394 } 2395 2396 public boolean hasLinkIdElement() { 2397 return this.linkId != null && !this.linkId.isEmpty(); 2398 } 2399 2400 public boolean hasLinkId() { 2401 return this.linkId != null && !this.linkId.isEmpty(); 2402 } 2403 2404 /** 2405 * @param value {@link #linkId} (An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 2406 */ 2407 public MeasureGroupStratifierComponentComponent setLinkIdElement(StringType value) { 2408 this.linkId = value; 2409 return this; 2410 } 2411 2412 /** 2413 * @return An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource. 2414 */ 2415 public String getLinkId() { 2416 return this.linkId == null ? null : this.linkId.getValue(); 2417 } 2418 2419 /** 2420 * @param value An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource. 2421 */ 2422 public MeasureGroupStratifierComponentComponent setLinkId(String value) { 2423 if (Utilities.noString(value)) 2424 this.linkId = null; 2425 else { 2426 if (this.linkId == null) 2427 this.linkId = new StringType(); 2428 this.linkId.setValue(value); 2429 } 2430 return this; 2431 } 2432 2433 /** 2434 * @return {@link #code} (Indicates a meaning for the stratifier component. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures.) 2435 */ 2436 public CodeableConcept getCode() { 2437 if (this.code == null) 2438 if (Configuration.errorOnAutoCreate()) 2439 throw new Error("Attempt to auto-create MeasureGroupStratifierComponentComponent.code"); 2440 else if (Configuration.doAutoCreate()) 2441 this.code = new CodeableConcept(); // cc 2442 return this.code; 2443 } 2444 2445 public boolean hasCode() { 2446 return this.code != null && !this.code.isEmpty(); 2447 } 2448 2449 /** 2450 * @param value {@link #code} (Indicates a meaning for the stratifier component. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures.) 2451 */ 2452 public MeasureGroupStratifierComponentComponent setCode(CodeableConcept value) { 2453 this.code = value; 2454 return this; 2455 } 2456 2457 /** 2458 * @return {@link #description} (The human readable description of this stratifier criteria component.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2459 */ 2460 public MarkdownType getDescriptionElement() { 2461 if (this.description == null) 2462 if (Configuration.errorOnAutoCreate()) 2463 throw new Error("Attempt to auto-create MeasureGroupStratifierComponentComponent.description"); 2464 else if (Configuration.doAutoCreate()) 2465 this.description = new MarkdownType(); // bb 2466 return this.description; 2467 } 2468 2469 public boolean hasDescriptionElement() { 2470 return this.description != null && !this.description.isEmpty(); 2471 } 2472 2473 public boolean hasDescription() { 2474 return this.description != null && !this.description.isEmpty(); 2475 } 2476 2477 /** 2478 * @param value {@link #description} (The human readable description of this stratifier criteria component.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2479 */ 2480 public MeasureGroupStratifierComponentComponent setDescriptionElement(MarkdownType value) { 2481 this.description = value; 2482 return this; 2483 } 2484 2485 /** 2486 * @return The human readable description of this stratifier criteria component. 2487 */ 2488 public String getDescription() { 2489 return this.description == null ? null : this.description.getValue(); 2490 } 2491 2492 /** 2493 * @param value The human readable description of this stratifier criteria component. 2494 */ 2495 public MeasureGroupStratifierComponentComponent setDescription(String value) { 2496 if (Utilities.noString(value)) 2497 this.description = null; 2498 else { 2499 if (this.description == null) 2500 this.description = new MarkdownType(); 2501 this.description.setValue(value); 2502 } 2503 return this; 2504 } 2505 2506 /** 2507 * @return {@link #criteria} (An expression that specifies the criteria for this component of the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element.) 2508 */ 2509 public Expression getCriteria() { 2510 if (this.criteria == null) 2511 if (Configuration.errorOnAutoCreate()) 2512 throw new Error("Attempt to auto-create MeasureGroupStratifierComponentComponent.criteria"); 2513 else if (Configuration.doAutoCreate()) 2514 this.criteria = new Expression(); // cc 2515 return this.criteria; 2516 } 2517 2518 public boolean hasCriteria() { 2519 return this.criteria != null && !this.criteria.isEmpty(); 2520 } 2521 2522 /** 2523 * @param value {@link #criteria} (An expression that specifies the criteria for this component of the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element.) 2524 */ 2525 public MeasureGroupStratifierComponentComponent setCriteria(Expression value) { 2526 this.criteria = value; 2527 return this; 2528 } 2529 2530 /** 2531 * @return {@link #groupDefinition} (A Group resource that defines this population as a set of characteristics.) 2532 */ 2533 public Reference getGroupDefinition() { 2534 if (this.groupDefinition == null) 2535 if (Configuration.errorOnAutoCreate()) 2536 throw new Error("Attempt to auto-create MeasureGroupStratifierComponentComponent.groupDefinition"); 2537 else if (Configuration.doAutoCreate()) 2538 this.groupDefinition = new Reference(); // cc 2539 return this.groupDefinition; 2540 } 2541 2542 public boolean hasGroupDefinition() { 2543 return this.groupDefinition != null && !this.groupDefinition.isEmpty(); 2544 } 2545 2546 /** 2547 * @param value {@link #groupDefinition} (A Group resource that defines this population as a set of characteristics.) 2548 */ 2549 public MeasureGroupStratifierComponentComponent setGroupDefinition(Reference value) { 2550 this.groupDefinition = value; 2551 return this; 2552 } 2553 2554 protected void listChildren(List<Property> children) { 2555 super.listChildren(children); 2556 children.add(new Property("linkId", "string", "An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource.", 0, 1, linkId)); 2557 children.add(new Property("code", "CodeableConcept", "Indicates a meaning for the stratifier component. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures.", 0, 1, code)); 2558 children.add(new Property("description", "markdown", "The human readable description of this stratifier criteria component.", 0, 1, description)); 2559 children.add(new Property("criteria", "Expression", "An expression that specifies the criteria for this component of the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element.", 0, 1, criteria)); 2560 children.add(new Property("groupDefinition", "Reference(Group)", "A Group resource that defines this population as a set of characteristics.", 0, 1, groupDefinition)); 2561 } 2562 2563 @Override 2564 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2565 switch (_hash) { 2566 case -1102667083: /*linkId*/ return new Property("linkId", "string", "An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource.", 0, 1, linkId); 2567 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Indicates a meaning for the stratifier component. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing stratifiers to be correlated across measures.", 0, 1, code); 2568 case -1724546052: /*description*/ return new Property("description", "markdown", "The human readable description of this stratifier criteria component.", 0, 1, description); 2569 case 1952046943: /*criteria*/ return new Property("criteria", "Expression", "An expression that specifies the criteria for this component of the stratifier. This is typically the name of an expression defined within a referenced library, but it may also be a path to a stratifier element.", 0, 1, criteria); 2570 case 158676274: /*groupDefinition*/ return new Property("groupDefinition", "Reference(Group)", "A Group resource that defines this population as a set of characteristics.", 0, 1, groupDefinition); 2571 default: return super.getNamedProperty(_hash, _name, _checkValid); 2572 } 2573 2574 } 2575 2576 @Override 2577 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2578 switch (hash) { 2579 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 2580 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2581 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2582 case 1952046943: /*criteria*/ return this.criteria == null ? new Base[0] : new Base[] {this.criteria}; // Expression 2583 case 158676274: /*groupDefinition*/ return this.groupDefinition == null ? new Base[0] : new Base[] {this.groupDefinition}; // Reference 2584 default: return super.getProperty(hash, name, checkValid); 2585 } 2586 2587 } 2588 2589 @Override 2590 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2591 switch (hash) { 2592 case -1102667083: // linkId 2593 this.linkId = TypeConvertor.castToString(value); // StringType 2594 return value; 2595 case 3059181: // code 2596 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2597 return value; 2598 case -1724546052: // description 2599 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2600 return value; 2601 case 1952046943: // criteria 2602 this.criteria = TypeConvertor.castToExpression(value); // Expression 2603 return value; 2604 case 158676274: // groupDefinition 2605 this.groupDefinition = TypeConvertor.castToReference(value); // Reference 2606 return value; 2607 default: return super.setProperty(hash, name, value); 2608 } 2609 2610 } 2611 2612 @Override 2613 public Base setProperty(String name, Base value) throws FHIRException { 2614 if (name.equals("linkId")) { 2615 this.linkId = TypeConvertor.castToString(value); // StringType 2616 } else if (name.equals("code")) { 2617 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2618 } else if (name.equals("description")) { 2619 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 2620 } else if (name.equals("criteria")) { 2621 this.criteria = TypeConvertor.castToExpression(value); // Expression 2622 } else if (name.equals("groupDefinition")) { 2623 this.groupDefinition = TypeConvertor.castToReference(value); // Reference 2624 } else 2625 return super.setProperty(name, value); 2626 return value; 2627 } 2628 2629 @Override 2630 public void removeChild(String name, Base value) throws FHIRException { 2631 if (name.equals("linkId")) { 2632 this.linkId = null; 2633 } else if (name.equals("code")) { 2634 this.code = null; 2635 } else if (name.equals("description")) { 2636 this.description = null; 2637 } else if (name.equals("criteria")) { 2638 this.criteria = null; 2639 } else if (name.equals("groupDefinition")) { 2640 this.groupDefinition = null; 2641 } else 2642 super.removeChild(name, value); 2643 2644 } 2645 2646 @Override 2647 public Base makeProperty(int hash, String name) throws FHIRException { 2648 switch (hash) { 2649 case -1102667083: return getLinkIdElement(); 2650 case 3059181: return getCode(); 2651 case -1724546052: return getDescriptionElement(); 2652 case 1952046943: return getCriteria(); 2653 case 158676274: return getGroupDefinition(); 2654 default: return super.makeProperty(hash, name); 2655 } 2656 2657 } 2658 2659 @Override 2660 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2661 switch (hash) { 2662 case -1102667083: /*linkId*/ return new String[] {"string"}; 2663 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2664 case -1724546052: /*description*/ return new String[] {"markdown"}; 2665 case 1952046943: /*criteria*/ return new String[] {"Expression"}; 2666 case 158676274: /*groupDefinition*/ return new String[] {"Reference"}; 2667 default: return super.getTypesForProperty(hash, name); 2668 } 2669 2670 } 2671 2672 @Override 2673 public Base addChild(String name) throws FHIRException { 2674 if (name.equals("linkId")) { 2675 throw new FHIRException("Cannot call addChild on a singleton property Measure.group.stratifier.component.linkId"); 2676 } 2677 else if (name.equals("code")) { 2678 this.code = new CodeableConcept(); 2679 return this.code; 2680 } 2681 else if (name.equals("description")) { 2682 throw new FHIRException("Cannot call addChild on a singleton property Measure.group.stratifier.component.description"); 2683 } 2684 else if (name.equals("criteria")) { 2685 this.criteria = new Expression(); 2686 return this.criteria; 2687 } 2688 else if (name.equals("groupDefinition")) { 2689 this.groupDefinition = new Reference(); 2690 return this.groupDefinition; 2691 } 2692 else 2693 return super.addChild(name); 2694 } 2695 2696 public MeasureGroupStratifierComponentComponent copy() { 2697 MeasureGroupStratifierComponentComponent dst = new MeasureGroupStratifierComponentComponent(); 2698 copyValues(dst); 2699 return dst; 2700 } 2701 2702 public void copyValues(MeasureGroupStratifierComponentComponent dst) { 2703 super.copyValues(dst); 2704 dst.linkId = linkId == null ? null : linkId.copy(); 2705 dst.code = code == null ? null : code.copy(); 2706 dst.description = description == null ? null : description.copy(); 2707 dst.criteria = criteria == null ? null : criteria.copy(); 2708 dst.groupDefinition = groupDefinition == null ? null : groupDefinition.copy(); 2709 } 2710 2711 @Override 2712 public boolean equalsDeep(Base other_) { 2713 if (!super.equalsDeep(other_)) 2714 return false; 2715 if (!(other_ instanceof MeasureGroupStratifierComponentComponent)) 2716 return false; 2717 MeasureGroupStratifierComponentComponent o = (MeasureGroupStratifierComponentComponent) other_; 2718 return compareDeep(linkId, o.linkId, true) && compareDeep(code, o.code, true) && compareDeep(description, o.description, true) 2719 && compareDeep(criteria, o.criteria, true) && compareDeep(groupDefinition, o.groupDefinition, true) 2720 ; 2721 } 2722 2723 @Override 2724 public boolean equalsShallow(Base other_) { 2725 if (!super.equalsShallow(other_)) 2726 return false; 2727 if (!(other_ instanceof MeasureGroupStratifierComponentComponent)) 2728 return false; 2729 MeasureGroupStratifierComponentComponent o = (MeasureGroupStratifierComponentComponent) other_; 2730 return compareValues(linkId, o.linkId, true) && compareValues(description, o.description, true); 2731 } 2732 2733 public boolean isEmpty() { 2734 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, code, description 2735 , criteria, groupDefinition); 2736 } 2737 2738 public String fhirType() { 2739 return "Measure.group.stratifier.component"; 2740 2741 } 2742 2743 } 2744 2745 @Block() 2746 public static class MeasureSupplementalDataComponent extends BackboneElement implements IBaseBackboneElement { 2747 /** 2748 * An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource. 2749 */ 2750 @Child(name = "linkId", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2751 @Description(shortDefinition="Unique id for supplementalData in measure", formalDefinition="An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource." ) 2752 protected StringType linkId; 2753 2754 /** 2755 * Indicates a meaning for the supplemental data. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing supplemental data to be correlated across measures. 2756 */ 2757 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 2758 @Description(shortDefinition="Meaning of the supplemental data", formalDefinition="Indicates a meaning for the supplemental data. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing supplemental data to be correlated across measures." ) 2759 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-supplemental-data-example") 2760 protected CodeableConcept code; 2761 2762 /** 2763 * An indicator of the intended usage for the supplemental data element. Supplemental data indicates the data is additional information requested to augment the measure information. Risk adjustment factor indicates the data is additional information used to calculate risk adjustment factors when applying a risk model to the measure calculation. 2764 */ 2765 @Child(name = "usage", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2766 @Description(shortDefinition="supplemental-data | risk-adjustment-factor", formalDefinition="An indicator of the intended usage for the supplemental data element. Supplemental data indicates the data is additional information requested to augment the measure information. Risk adjustment factor indicates the data is additional information used to calculate risk adjustment factors when applying a risk model to the measure calculation." ) 2767 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-data-usage") 2768 protected List<CodeableConcept> usage; 2769 2770 /** 2771 * The human readable description of this supplemental data. 2772 */ 2773 @Child(name = "description", type = {MarkdownType.class}, order=4, min=0, max=1, modifier=false, summary=false) 2774 @Description(shortDefinition="The human readable description of this supplemental data", formalDefinition="The human readable description of this supplemental data." ) 2775 protected MarkdownType description; 2776 2777 /** 2778 * The criteria for the supplemental data. This is typically the name of a valid expression defined within a referenced library, but it may also be a path to a specific data element. The criteria defines the data to be returned for this element. 2779 */ 2780 @Child(name = "criteria", type = {Expression.class}, order=5, min=1, max=1, modifier=false, summary=false) 2781 @Description(shortDefinition="Expression describing additional data to be reported", formalDefinition="The criteria for the supplemental data. This is typically the name of a valid expression defined within a referenced library, but it may also be a path to a specific data element. The criteria defines the data to be returned for this element." ) 2782 protected Expression criteria; 2783 2784 private static final long serialVersionUID = 745484444L; 2785 2786 /** 2787 * Constructor 2788 */ 2789 public MeasureSupplementalDataComponent() { 2790 super(); 2791 } 2792 2793 /** 2794 * Constructor 2795 */ 2796 public MeasureSupplementalDataComponent(Expression criteria) { 2797 super(); 2798 this.setCriteria(criteria); 2799 } 2800 2801 /** 2802 * @return {@link #linkId} (An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 2803 */ 2804 public StringType getLinkIdElement() { 2805 if (this.linkId == null) 2806 if (Configuration.errorOnAutoCreate()) 2807 throw new Error("Attempt to auto-create MeasureSupplementalDataComponent.linkId"); 2808 else if (Configuration.doAutoCreate()) 2809 this.linkId = new StringType(); // bb 2810 return this.linkId; 2811 } 2812 2813 public boolean hasLinkIdElement() { 2814 return this.linkId != null && !this.linkId.isEmpty(); 2815 } 2816 2817 public boolean hasLinkId() { 2818 return this.linkId != null && !this.linkId.isEmpty(); 2819 } 2820 2821 /** 2822 * @param value {@link #linkId} (An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 2823 */ 2824 public MeasureSupplementalDataComponent setLinkIdElement(StringType value) { 2825 this.linkId = value; 2826 return this; 2827 } 2828 2829 /** 2830 * @return An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource. 2831 */ 2832 public String getLinkId() { 2833 return this.linkId == null ? null : this.linkId.getValue(); 2834 } 2835 2836 /** 2837 * @param value An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource. 2838 */ 2839 public MeasureSupplementalDataComponent setLinkId(String value) { 2840 if (Utilities.noString(value)) 2841 this.linkId = null; 2842 else { 2843 if (this.linkId == null) 2844 this.linkId = new StringType(); 2845 this.linkId.setValue(value); 2846 } 2847 return this; 2848 } 2849 2850 /** 2851 * @return {@link #code} (Indicates a meaning for the supplemental data. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing supplemental data to be correlated across measures.) 2852 */ 2853 public CodeableConcept getCode() { 2854 if (this.code == null) 2855 if (Configuration.errorOnAutoCreate()) 2856 throw new Error("Attempt to auto-create MeasureSupplementalDataComponent.code"); 2857 else if (Configuration.doAutoCreate()) 2858 this.code = new CodeableConcept(); // cc 2859 return this.code; 2860 } 2861 2862 public boolean hasCode() { 2863 return this.code != null && !this.code.isEmpty(); 2864 } 2865 2866 /** 2867 * @param value {@link #code} (Indicates a meaning for the supplemental data. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing supplemental data to be correlated across measures.) 2868 */ 2869 public MeasureSupplementalDataComponent setCode(CodeableConcept value) { 2870 this.code = value; 2871 return this; 2872 } 2873 2874 /** 2875 * @return {@link #usage} (An indicator of the intended usage for the supplemental data element. Supplemental data indicates the data is additional information requested to augment the measure information. Risk adjustment factor indicates the data is additional information used to calculate risk adjustment factors when applying a risk model to the measure calculation.) 2876 */ 2877 public List<CodeableConcept> getUsage() { 2878 if (this.usage == null) 2879 this.usage = new ArrayList<CodeableConcept>(); 2880 return this.usage; 2881 } 2882 2883 /** 2884 * @return Returns a reference to <code>this</code> for easy method chaining 2885 */ 2886 public MeasureSupplementalDataComponent setUsage(List<CodeableConcept> theUsage) { 2887 this.usage = theUsage; 2888 return this; 2889 } 2890 2891 public boolean hasUsage() { 2892 if (this.usage == null) 2893 return false; 2894 for (CodeableConcept item : this.usage) 2895 if (!item.isEmpty()) 2896 return true; 2897 return false; 2898 } 2899 2900 public CodeableConcept addUsage() { //3 2901 CodeableConcept t = new CodeableConcept(); 2902 if (this.usage == null) 2903 this.usage = new ArrayList<CodeableConcept>(); 2904 this.usage.add(t); 2905 return t; 2906 } 2907 2908 public MeasureSupplementalDataComponent addUsage(CodeableConcept t) { //3 2909 if (t == null) 2910 return this; 2911 if (this.usage == null) 2912 this.usage = new ArrayList<CodeableConcept>(); 2913 this.usage.add(t); 2914 return this; 2915 } 2916 2917 /** 2918 * @return The first repetition of repeating field {@link #usage}, creating it if it does not already exist {3} 2919 */ 2920 public CodeableConcept getUsageFirstRep() { 2921 if (getUsage().isEmpty()) { 2922 addUsage(); 2923 } 2924 return getUsage().get(0); 2925 } 2926 2927 /** 2928 * @return {@link #description} (The human readable description of this supplemental data.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2929 */ 2930 public MarkdownType getDescriptionElement() { 2931 if (this.description == null) 2932 if (Configuration.errorOnAutoCreate()) 2933 throw new Error("Attempt to auto-create MeasureSupplementalDataComponent.description"); 2934 else if (Configuration.doAutoCreate()) 2935 this.description = new MarkdownType(); // bb 2936 return this.description; 2937 } 2938 2939 public boolean hasDescriptionElement() { 2940 return this.description != null && !this.description.isEmpty(); 2941 } 2942 2943 public boolean hasDescription() { 2944 return this.description != null && !this.description.isEmpty(); 2945 } 2946 2947 /** 2948 * @param value {@link #description} (The human readable description of this supplemental data.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2949 */ 2950 public MeasureSupplementalDataComponent setDescriptionElement(MarkdownType value) { 2951 this.description = value; 2952 return this; 2953 } 2954 2955 /** 2956 * @return The human readable description of this supplemental data. 2957 */ 2958 public String getDescription() { 2959 return this.description == null ? null : this.description.getValue(); 2960 } 2961 2962 /** 2963 * @param value The human readable description of this supplemental data. 2964 */ 2965 public MeasureSupplementalDataComponent setDescription(String value) { 2966 if (Utilities.noString(value)) 2967 this.description = null; 2968 else { 2969 if (this.description == null) 2970 this.description = new MarkdownType(); 2971 this.description.setValue(value); 2972 } 2973 return this; 2974 } 2975 2976 /** 2977 * @return {@link #criteria} (The criteria for the supplemental data. This is typically the name of a valid expression defined within a referenced library, but it may also be a path to a specific data element. The criteria defines the data to be returned for this element.) 2978 */ 2979 public Expression getCriteria() { 2980 if (this.criteria == null) 2981 if (Configuration.errorOnAutoCreate()) 2982 throw new Error("Attempt to auto-create MeasureSupplementalDataComponent.criteria"); 2983 else if (Configuration.doAutoCreate()) 2984 this.criteria = new Expression(); // cc 2985 return this.criteria; 2986 } 2987 2988 public boolean hasCriteria() { 2989 return this.criteria != null && !this.criteria.isEmpty(); 2990 } 2991 2992 /** 2993 * @param value {@link #criteria} (The criteria for the supplemental data. This is typically the name of a valid expression defined within a referenced library, but it may also be a path to a specific data element. The criteria defines the data to be returned for this element.) 2994 */ 2995 public MeasureSupplementalDataComponent setCriteria(Expression value) { 2996 this.criteria = value; 2997 return this; 2998 } 2999 3000 protected void listChildren(List<Property> children) { 3001 super.listChildren(children); 3002 children.add(new Property("linkId", "string", "An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource.", 0, 1, linkId)); 3003 children.add(new Property("code", "CodeableConcept", "Indicates a meaning for the supplemental data. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing supplemental data to be correlated across measures.", 0, 1, code)); 3004 children.add(new Property("usage", "CodeableConcept", "An indicator of the intended usage for the supplemental data element. Supplemental data indicates the data is additional information requested to augment the measure information. Risk adjustment factor indicates the data is additional information used to calculate risk adjustment factors when applying a risk model to the measure calculation.", 0, java.lang.Integer.MAX_VALUE, usage)); 3005 children.add(new Property("description", "markdown", "The human readable description of this supplemental data.", 0, 1, description)); 3006 children.add(new Property("criteria", "Expression", "The criteria for the supplemental data. This is typically the name of a valid expression defined within a referenced library, but it may also be a path to a specific data element. The criteria defines the data to be returned for this element.", 0, 1, criteria)); 3007 } 3008 3009 @Override 3010 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3011 switch (_hash) { 3012 case -1102667083: /*linkId*/ return new Property("linkId", "string", "An identifier that is unique within the Measure allowing linkage to the equivalent item in a MeasureReport resource.", 0, 1, linkId); 3013 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Indicates a meaning for the supplemental data. This can be as simple as a unique identifier, or it can establish meaning in a broader context by drawing from a terminology, allowing supplemental data to be correlated across measures.", 0, 1, code); 3014 case 111574433: /*usage*/ return new Property("usage", "CodeableConcept", "An indicator of the intended usage for the supplemental data element. Supplemental data indicates the data is additional information requested to augment the measure information. Risk adjustment factor indicates the data is additional information used to calculate risk adjustment factors when applying a risk model to the measure calculation.", 0, java.lang.Integer.MAX_VALUE, usage); 3015 case -1724546052: /*description*/ return new Property("description", "markdown", "The human readable description of this supplemental data.", 0, 1, description); 3016 case 1952046943: /*criteria*/ return new Property("criteria", "Expression", "The criteria for the supplemental data. This is typically the name of a valid expression defined within a referenced library, but it may also be a path to a specific data element. The criteria defines the data to be returned for this element.", 0, 1, criteria); 3017 default: return super.getNamedProperty(_hash, _name, _checkValid); 3018 } 3019 3020 } 3021 3022 @Override 3023 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3024 switch (hash) { 3025 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 3026 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 3027 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : this.usage.toArray(new Base[this.usage.size()]); // CodeableConcept 3028 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3029 case 1952046943: /*criteria*/ return this.criteria == null ? new Base[0] : new Base[] {this.criteria}; // Expression 3030 default: return super.getProperty(hash, name, checkValid); 3031 } 3032 3033 } 3034 3035 @Override 3036 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3037 switch (hash) { 3038 case -1102667083: // linkId 3039 this.linkId = TypeConvertor.castToString(value); // StringType 3040 return value; 3041 case 3059181: // code 3042 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3043 return value; 3044 case 111574433: // usage 3045 this.getUsage().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3046 return value; 3047 case -1724546052: // description 3048 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3049 return value; 3050 case 1952046943: // criteria 3051 this.criteria = TypeConvertor.castToExpression(value); // Expression 3052 return value; 3053 default: return super.setProperty(hash, name, value); 3054 } 3055 3056 } 3057 3058 @Override 3059 public Base setProperty(String name, Base value) throws FHIRException { 3060 if (name.equals("linkId")) { 3061 this.linkId = TypeConvertor.castToString(value); // StringType 3062 } else if (name.equals("code")) { 3063 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3064 } else if (name.equals("usage")) { 3065 this.getUsage().add(TypeConvertor.castToCodeableConcept(value)); 3066 } else if (name.equals("description")) { 3067 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 3068 } else if (name.equals("criteria")) { 3069 this.criteria = TypeConvertor.castToExpression(value); // Expression 3070 } else 3071 return super.setProperty(name, value); 3072 return value; 3073 } 3074 3075 @Override 3076 public void removeChild(String name, Base value) throws FHIRException { 3077 if (name.equals("linkId")) { 3078 this.linkId = null; 3079 } else if (name.equals("code")) { 3080 this.code = null; 3081 } else if (name.equals("usage")) { 3082 this.getUsage().remove(value); 3083 } else if (name.equals("description")) { 3084 this.description = null; 3085 } else if (name.equals("criteria")) { 3086 this.criteria = null; 3087 } else 3088 super.removeChild(name, value); 3089 3090 } 3091 3092 @Override 3093 public Base makeProperty(int hash, String name) throws FHIRException { 3094 switch (hash) { 3095 case -1102667083: return getLinkIdElement(); 3096 case 3059181: return getCode(); 3097 case 111574433: return addUsage(); 3098 case -1724546052: return getDescriptionElement(); 3099 case 1952046943: return getCriteria(); 3100 default: return super.makeProperty(hash, name); 3101 } 3102 3103 } 3104 3105 @Override 3106 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3107 switch (hash) { 3108 case -1102667083: /*linkId*/ return new String[] {"string"}; 3109 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3110 case 111574433: /*usage*/ return new String[] {"CodeableConcept"}; 3111 case -1724546052: /*description*/ return new String[] {"markdown"}; 3112 case 1952046943: /*criteria*/ return new String[] {"Expression"}; 3113 default: return super.getTypesForProperty(hash, name); 3114 } 3115 3116 } 3117 3118 @Override 3119 public Base addChild(String name) throws FHIRException { 3120 if (name.equals("linkId")) { 3121 throw new FHIRException("Cannot call addChild on a singleton property Measure.supplementalData.linkId"); 3122 } 3123 else if (name.equals("code")) { 3124 this.code = new CodeableConcept(); 3125 return this.code; 3126 } 3127 else if (name.equals("usage")) { 3128 return addUsage(); 3129 } 3130 else if (name.equals("description")) { 3131 throw new FHIRException("Cannot call addChild on a singleton property Measure.supplementalData.description"); 3132 } 3133 else if (name.equals("criteria")) { 3134 this.criteria = new Expression(); 3135 return this.criteria; 3136 } 3137 else 3138 return super.addChild(name); 3139 } 3140 3141 public MeasureSupplementalDataComponent copy() { 3142 MeasureSupplementalDataComponent dst = new MeasureSupplementalDataComponent(); 3143 copyValues(dst); 3144 return dst; 3145 } 3146 3147 public void copyValues(MeasureSupplementalDataComponent dst) { 3148 super.copyValues(dst); 3149 dst.linkId = linkId == null ? null : linkId.copy(); 3150 dst.code = code == null ? null : code.copy(); 3151 if (usage != null) { 3152 dst.usage = new ArrayList<CodeableConcept>(); 3153 for (CodeableConcept i : usage) 3154 dst.usage.add(i.copy()); 3155 }; 3156 dst.description = description == null ? null : description.copy(); 3157 dst.criteria = criteria == null ? null : criteria.copy(); 3158 } 3159 3160 @Override 3161 public boolean equalsDeep(Base other_) { 3162 if (!super.equalsDeep(other_)) 3163 return false; 3164 if (!(other_ instanceof MeasureSupplementalDataComponent)) 3165 return false; 3166 MeasureSupplementalDataComponent o = (MeasureSupplementalDataComponent) other_; 3167 return compareDeep(linkId, o.linkId, true) && compareDeep(code, o.code, true) && compareDeep(usage, o.usage, true) 3168 && compareDeep(description, o.description, true) && compareDeep(criteria, o.criteria, true); 3169 } 3170 3171 @Override 3172 public boolean equalsShallow(Base other_) { 3173 if (!super.equalsShallow(other_)) 3174 return false; 3175 if (!(other_ instanceof MeasureSupplementalDataComponent)) 3176 return false; 3177 MeasureSupplementalDataComponent o = (MeasureSupplementalDataComponent) other_; 3178 return compareValues(linkId, o.linkId, true) && compareValues(description, o.description, true); 3179 } 3180 3181 public boolean isEmpty() { 3182 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, code, usage, description 3183 , criteria); 3184 } 3185 3186 public String fhirType() { 3187 return "Measure.supplementalData"; 3188 3189 } 3190 3191 } 3192 3193 /** 3194 * An absolute URI that is used to identify this measure when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this measure is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the measure is stored on different servers. 3195 */ 3196 @Child(name = "url", type = {UriType.class}, order=0, min=0, max=1, modifier=false, summary=true) 3197 @Description(shortDefinition="Canonical identifier for this measure, represented as a URI (globally unique)", formalDefinition="An absolute URI that is used to identify this measure when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this measure is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the measure is stored on different servers." ) 3198 protected UriType url; 3199 3200 /** 3201 * A formal identifier that is used to identify this measure when it is represented in other formats, or referenced in a specification, model, design or an instance. 3202 */ 3203 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3204 @Description(shortDefinition="Additional identifier for the measure", formalDefinition="A formal identifier that is used to identify this measure when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 3205 protected List<Identifier> identifier; 3206 3207 /** 3208 * The identifier that is used to identify this version of the measure when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the measure author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 3209 */ 3210 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 3211 @Description(shortDefinition="Business version of the measure", formalDefinition="The identifier that is used to identify this version of the measure when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the measure author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts." ) 3212 protected StringType version; 3213 3214 /** 3215 * Indicates the mechanism used to compare versions to determine which is more current. 3216 */ 3217 @Child(name = "versionAlgorithm", type = {StringType.class, Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 3218 @Description(shortDefinition="How to compare versions", formalDefinition="Indicates the mechanism used to compare versions to determine which is more current." ) 3219 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/version-algorithm") 3220 protected DataType versionAlgorithm; 3221 3222 /** 3223 * A natural language name identifying the measure. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3224 */ 3225 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 3226 @Description(shortDefinition="Name for this measure (computer friendly)", formalDefinition="A natural language name identifying the measure. This name should be usable as an identifier for the module by machine processing applications such as code generation." ) 3227 protected StringType name; 3228 3229 /** 3230 * A short, descriptive, user-friendly title for the measure. 3231 */ 3232 @Child(name = "title", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 3233 @Description(shortDefinition="Name for this measure (human friendly)", formalDefinition="A short, descriptive, user-friendly title for the measure." ) 3234 protected StringType title; 3235 3236 /** 3237 * An explanatory or alternate title for the measure giving additional information about its content. 3238 */ 3239 @Child(name = "subtitle", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=false) 3240 @Description(shortDefinition="Subordinate title of the measure", formalDefinition="An explanatory or alternate title for the measure giving additional information about its content." ) 3241 protected StringType subtitle; 3242 3243 /** 3244 * The status of this measure. Enables tracking the life-cycle of the content. 3245 */ 3246 @Child(name = "status", type = {CodeType.class}, order=7, min=1, max=1, modifier=true, summary=true) 3247 @Description(shortDefinition="draft | active | retired | unknown", formalDefinition="The status of this measure. Enables tracking the life-cycle of the content." ) 3248 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/publication-status") 3249 protected Enumeration<PublicationStatus> status; 3250 3251 /** 3252 * A Boolean value to indicate that this measure is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 3253 */ 3254 @Child(name = "experimental", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 3255 @Description(shortDefinition="For testing purposes, not real usage", formalDefinition="A Boolean value to indicate that this measure is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage." ) 3256 protected BooleanType experimental; 3257 3258 /** 3259 * The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything. 3260 */ 3261 @Child(name = "subject", type = {CodeableConcept.class, Group.class}, order=9, min=0, max=1, modifier=false, summary=false) 3262 @Description(shortDefinition="E.g. Patient, Practitioner, RelatedPerson, Organization, Location, Device", formalDefinition="The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything." ) 3263 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/participant-resource-types") 3264 protected DataType subject; 3265 3266 /** 3267 * The population basis specifies the type of elements in the population. For a subject-based measure, this is boolean (because the subject and the population basis are the same, and the population criteria define yes/no values for each individual in the population). For measures that have a population basis that is different than the subject, this element specifies the type of the population basis. For example, an encounter-based measure has a subject of Patient and a population basis of Encounter, and the population criteria all return lists of Encounters. 3268 */ 3269 @Child(name = "basis", type = {CodeType.class}, order=10, min=0, max=1, modifier=false, summary=true) 3270 @Description(shortDefinition="Population basis", formalDefinition="The population basis specifies the type of elements in the population. For a subject-based measure, this is boolean (because the subject and the population basis are the same, and the population criteria define yes/no values for each individual in the population). For measures that have a population basis that is different than the subject, this element specifies the type of the population basis. For example, an encounter-based measure has a subject of Patient and a population basis of Encounter, and the population criteria all return lists of Encounters." ) 3271 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fhir-types") 3272 protected Enumeration<FHIRTypes> basis; 3273 3274 /** 3275 * The date (and optionally time) when the measure was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the measure changes. 3276 */ 3277 @Child(name = "date", type = {DateTimeType.class}, order=11, min=0, max=1, modifier=false, summary=true) 3278 @Description(shortDefinition="Date last changed", formalDefinition="The date (and optionally time) when the measure was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the measure changes." ) 3279 protected DateTimeType date; 3280 3281 /** 3282 * The name of the organization or individual responsible for the release and ongoing maintenance of the measure. 3283 */ 3284 @Child(name = "publisher", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=true) 3285 @Description(shortDefinition="Name of the publisher/steward (organization or individual)", formalDefinition="The name of the organization or individual responsible for the release and ongoing maintenance of the measure." ) 3286 protected StringType publisher; 3287 3288 /** 3289 * Contact details to assist a user in finding and communicating with the publisher. 3290 */ 3291 @Child(name = "contact", type = {ContactDetail.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3292 @Description(shortDefinition="Contact details for the publisher", formalDefinition="Contact details to assist a user in finding and communicating with the publisher." ) 3293 protected List<ContactDetail> contact; 3294 3295 /** 3296 * A free text natural language description of the measure from a consumer's perspective. 3297 */ 3298 @Child(name = "description", type = {MarkdownType.class}, order=14, min=0, max=1, modifier=false, summary=true) 3299 @Description(shortDefinition="Natural language description of the measure", formalDefinition="A free text natural language description of the measure from a consumer's perspective." ) 3300 protected MarkdownType description; 3301 3302 /** 3303 * The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate measure instances. 3304 */ 3305 @Child(name = "useContext", type = {UsageContext.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3306 @Description(shortDefinition="The context that the content is intended to support", formalDefinition="The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate measure instances." ) 3307 protected List<UsageContext> useContext; 3308 3309 /** 3310 * A legal or geographic region in which the measure is intended to be used. 3311 */ 3312 @Child(name = "jurisdiction", type = {CodeableConcept.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3313 @Description(shortDefinition="Intended jurisdiction for measure (if applicable)", formalDefinition="A legal or geographic region in which the measure is intended to be used." ) 3314 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/jurisdiction") 3315 protected List<CodeableConcept> jurisdiction; 3316 3317 /** 3318 * Explanation of why this measure is needed and why it has been designed as it has. 3319 */ 3320 @Child(name = "purpose", type = {MarkdownType.class}, order=17, min=0, max=1, modifier=false, summary=false) 3321 @Description(shortDefinition="Why this measure is defined", formalDefinition="Explanation of why this measure is needed and why it has been designed as it has." ) 3322 protected MarkdownType purpose; 3323 3324 /** 3325 * A detailed description, from a clinical perspective, of how the measure is used. 3326 */ 3327 @Child(name = "usage", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=false) 3328 @Description(shortDefinition="Describes the clinical usage of the measure", formalDefinition="A detailed description, from a clinical perspective, of how the measure is used." ) 3329 protected MarkdownType usage; 3330 3331 /** 3332 * A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure. 3333 */ 3334 @Child(name = "copyright", type = {MarkdownType.class}, order=19, min=0, max=1, modifier=false, summary=false) 3335 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure." ) 3336 protected MarkdownType copyright; 3337 3338 /** 3339 * A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 3340 */ 3341 @Child(name = "copyrightLabel", type = {StringType.class}, order=20, min=0, max=1, modifier=false, summary=false) 3342 @Description(shortDefinition="Copyright holder and year(s)", formalDefinition="A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved')." ) 3343 protected StringType copyrightLabel; 3344 3345 /** 3346 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 3347 */ 3348 @Child(name = "approvalDate", type = {DateType.class}, order=21, min=0, max=1, modifier=false, summary=false) 3349 @Description(shortDefinition="When the measure was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 3350 protected DateType approvalDate; 3351 3352 /** 3353 * The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 3354 */ 3355 @Child(name = "lastReviewDate", type = {DateType.class}, order=22, min=0, max=1, modifier=false, summary=false) 3356 @Description(shortDefinition="When the measure was last reviewed by the publisher", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date." ) 3357 protected DateType lastReviewDate; 3358 3359 /** 3360 * The period during which the measure content was or is planned to be in active use. 3361 */ 3362 @Child(name = "effectivePeriod", type = {Period.class}, order=23, min=0, max=1, modifier=false, summary=true) 3363 @Description(shortDefinition="When the measure is expected to be used", formalDefinition="The period during which the measure content was or is planned to be in active use." ) 3364 protected Period effectivePeriod; 3365 3366 /** 3367 * Descriptive topics related to the content of the measure. Topics provide a high-level categorization grouping types of measures that can be useful for filtering and searching. 3368 */ 3369 @Child(name = "topic", type = {CodeableConcept.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3370 @Description(shortDefinition="The category of the measure, such as Education, Treatment, Assessment, etc", formalDefinition="Descriptive topics related to the content of the measure. Topics provide a high-level categorization grouping types of measures that can be useful for filtering and searching." ) 3371 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-topic") 3372 protected List<CodeableConcept> topic; 3373 3374 /** 3375 * An individiual or organization primarily involved in the creation and maintenance of the content. 3376 */ 3377 @Child(name = "author", type = {ContactDetail.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3378 @Description(shortDefinition="Who authored the content", formalDefinition="An individiual or organization primarily involved in the creation and maintenance of the content." ) 3379 protected List<ContactDetail> author; 3380 3381 /** 3382 * An individual or organization primarily responsible for internal coherence of the content. 3383 */ 3384 @Child(name = "editor", type = {ContactDetail.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3385 @Description(shortDefinition="Who edited the content", formalDefinition="An individual or organization primarily responsible for internal coherence of the content." ) 3386 protected List<ContactDetail> editor; 3387 3388 /** 3389 * An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content. 3390 */ 3391 @Child(name = "reviewer", type = {ContactDetail.class}, order=27, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3392 @Description(shortDefinition="Who reviewed the content", formalDefinition="An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content." ) 3393 protected List<ContactDetail> reviewer; 3394 3395 /** 3396 * An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting. 3397 */ 3398 @Child(name = "endorser", type = {ContactDetail.class}, order=28, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3399 @Description(shortDefinition="Who endorsed the content", formalDefinition="An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting." ) 3400 protected List<ContactDetail> endorser; 3401 3402 /** 3403 * Related artifacts such as additional documentation, justification, or bibliographic references. 3404 */ 3405 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=29, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3406 @Description(shortDefinition="Additional documentation, citations, etc", formalDefinition="Related artifacts such as additional documentation, justification, or bibliographic references." ) 3407 protected List<RelatedArtifact> relatedArtifact; 3408 3409 /** 3410 * A reference to a Library resource containing the formal logic used by the measure. 3411 */ 3412 @Child(name = "library", type = {CanonicalType.class}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3413 @Description(shortDefinition="Logic used by the measure", formalDefinition="A reference to a Library resource containing the formal logic used by the measure." ) 3414 protected List<CanonicalType> library; 3415 3416 /** 3417 * Notices and disclaimers regarding the use of the measure or related to intellectual property (such as code systems) referenced by the measure. 3418 */ 3419 @Child(name = "disclaimer", type = {MarkdownType.class}, order=31, min=0, max=1, modifier=false, summary=true) 3420 @Description(shortDefinition="Disclaimer for use of the measure or its referenced content", formalDefinition="Notices and disclaimers regarding the use of the measure or related to intellectual property (such as code systems) referenced by the measure." ) 3421 protected MarkdownType disclaimer; 3422 3423 /** 3424 * Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented. 3425 */ 3426 @Child(name = "scoring", type = {CodeableConcept.class}, order=32, min=0, max=1, modifier=false, summary=true) 3427 @Description(shortDefinition="proportion | ratio | continuous-variable | cohort", formalDefinition="Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented." ) 3428 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/measure-scoring") 3429 protected CodeableConcept scoring; 3430 3431 /** 3432 * Defines the expected units of measure for the measure score. This element SHOULD be specified as a UCUM unit. 3433 */ 3434 @Child(name = "scoringUnit", type = {CodeableConcept.class}, order=33, min=0, max=1, modifier=false, summary=true) 3435 @Description(shortDefinition="What units?", formalDefinition="Defines the expected units of measure for the measure score. This element SHOULD be specified as a UCUM unit." ) 3436 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-scoring-unit") 3437 protected CodeableConcept scoringUnit; 3438 3439 /** 3440 * If this is a composite measure, the scoring method used to combine the component measures to determine the composite score. 3441 */ 3442 @Child(name = "compositeScoring", type = {CodeableConcept.class}, order=34, min=0, max=1, modifier=false, summary=true) 3443 @Description(shortDefinition="opportunity | all-or-nothing | linear | weighted", formalDefinition="If this is a composite measure, the scoring method used to combine the component measures to determine the composite score." ) 3444 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/composite-measure-scoring") 3445 protected CodeableConcept compositeScoring; 3446 3447 /** 3448 * Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization. 3449 */ 3450 @Child(name = "type", type = {CodeableConcept.class}, order=35, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3451 @Description(shortDefinition="process | outcome | structure | patient-reported-outcome | composite", formalDefinition="Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization." ) 3452 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-type") 3453 protected List<CodeableConcept> type; 3454 3455 /** 3456 * A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results. 3457 */ 3458 @Child(name = "riskAdjustment", type = {MarkdownType.class}, order=36, min=0, max=1, modifier=false, summary=true) 3459 @Description(shortDefinition="How risk adjustment is applied for this measure", formalDefinition="A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results." ) 3460 protected MarkdownType riskAdjustment; 3461 3462 /** 3463 * Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result. 3464 */ 3465 @Child(name = "rateAggregation", type = {MarkdownType.class}, order=37, min=0, max=1, modifier=false, summary=true) 3466 @Description(shortDefinition="How is rate aggregation performed for this measure", formalDefinition="Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result." ) 3467 protected MarkdownType rateAggregation; 3468 3469 /** 3470 * Provides a succinct statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence. 3471 */ 3472 @Child(name = "rationale", type = {MarkdownType.class}, order=38, min=0, max=1, modifier=false, summary=true) 3473 @Description(shortDefinition="Detailed description of why the measure exists", formalDefinition="Provides a succinct statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence." ) 3474 protected MarkdownType rationale; 3475 3476 /** 3477 * Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure. 3478 */ 3479 @Child(name = "clinicalRecommendationStatement", type = {MarkdownType.class}, order=39, min=0, max=1, modifier=false, summary=true) 3480 @Description(shortDefinition="Summary of clinical guidelines", formalDefinition="Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure." ) 3481 protected MarkdownType clinicalRecommendationStatement; 3482 3483 /** 3484 * Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is within a range). 3485 */ 3486 @Child(name = "improvementNotation", type = {CodeableConcept.class}, order=40, min=0, max=1, modifier=false, summary=true) 3487 @Description(shortDefinition="increase | decrease", formalDefinition="Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is within a range)." ) 3488 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-improvement-notation") 3489 protected CodeableConcept improvementNotation; 3490 3491 /** 3492 * Provides a description of an individual term used within the measure. 3493 */ 3494 @Child(name = "term", type = {}, order=41, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3495 @Description(shortDefinition="Defined terms used in the measure documentation", formalDefinition="Provides a description of an individual term used within the measure." ) 3496 protected List<MeasureTermComponent> term; 3497 3498 /** 3499 * Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure. 3500 */ 3501 @Child(name = "guidance", type = {MarkdownType.class}, order=42, min=0, max=1, modifier=false, summary=true) 3502 @Description(shortDefinition="Additional guidance for implementers (deprecated)", formalDefinition="Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure." ) 3503 protected MarkdownType guidance; 3504 3505 /** 3506 * A group of population criteria for the measure. 3507 */ 3508 @Child(name = "group", type = {}, order=43, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3509 @Description(shortDefinition="Population criteria group", formalDefinition="A group of population criteria for the measure." ) 3510 protected List<MeasureGroupComponent> group; 3511 3512 /** 3513 * The supplemental data criteria for the measure report, specified as either the name of a valid CQL expression within a referenced library, or a valid FHIR Resource Path. 3514 */ 3515 @Child(name = "supplementalData", type = {}, order=44, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3516 @Description(shortDefinition="What other data should be reported with the measure", formalDefinition="The supplemental data criteria for the measure report, specified as either the name of a valid CQL expression within a referenced library, or a valid FHIR Resource Path." ) 3517 protected List<MeasureSupplementalDataComponent> supplementalData; 3518 3519 private static final long serialVersionUID = -1907068641L; 3520 3521 /** 3522 * Constructor 3523 */ 3524 public Measure() { 3525 super(); 3526 } 3527 3528 /** 3529 * Constructor 3530 */ 3531 public Measure(PublicationStatus status) { 3532 super(); 3533 this.setStatus(status); 3534 } 3535 3536 /** 3537 * @return {@link #url} (An absolute URI that is used to identify this measure when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this measure is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the measure is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3538 */ 3539 public UriType getUrlElement() { 3540 if (this.url == null) 3541 if (Configuration.errorOnAutoCreate()) 3542 throw new Error("Attempt to auto-create Measure.url"); 3543 else if (Configuration.doAutoCreate()) 3544 this.url = new UriType(); // bb 3545 return this.url; 3546 } 3547 3548 public boolean hasUrlElement() { 3549 return this.url != null && !this.url.isEmpty(); 3550 } 3551 3552 public boolean hasUrl() { 3553 return this.url != null && !this.url.isEmpty(); 3554 } 3555 3556 /** 3557 * @param value {@link #url} (An absolute URI that is used to identify this measure when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this measure is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the measure is stored on different servers.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 3558 */ 3559 public Measure setUrlElement(UriType value) { 3560 this.url = value; 3561 return this; 3562 } 3563 3564 /** 3565 * @return An absolute URI that is used to identify this measure when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this measure is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the measure is stored on different servers. 3566 */ 3567 public String getUrl() { 3568 return this.url == null ? null : this.url.getValue(); 3569 } 3570 3571 /** 3572 * @param value An absolute URI that is used to identify this measure when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this measure is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the measure is stored on different servers. 3573 */ 3574 public Measure setUrl(String value) { 3575 if (Utilities.noString(value)) 3576 this.url = null; 3577 else { 3578 if (this.url == null) 3579 this.url = new UriType(); 3580 this.url.setValue(value); 3581 } 3582 return this; 3583 } 3584 3585 /** 3586 * @return {@link #identifier} (A formal identifier that is used to identify this measure when it is represented in other formats, or referenced in a specification, model, design or an instance.) 3587 */ 3588 public List<Identifier> getIdentifier() { 3589 if (this.identifier == null) 3590 this.identifier = new ArrayList<Identifier>(); 3591 return this.identifier; 3592 } 3593 3594 /** 3595 * @return Returns a reference to <code>this</code> for easy method chaining 3596 */ 3597 public Measure setIdentifier(List<Identifier> theIdentifier) { 3598 this.identifier = theIdentifier; 3599 return this; 3600 } 3601 3602 public boolean hasIdentifier() { 3603 if (this.identifier == null) 3604 return false; 3605 for (Identifier item : this.identifier) 3606 if (!item.isEmpty()) 3607 return true; 3608 return false; 3609 } 3610 3611 public Identifier addIdentifier() { //3 3612 Identifier t = new Identifier(); 3613 if (this.identifier == null) 3614 this.identifier = new ArrayList<Identifier>(); 3615 this.identifier.add(t); 3616 return t; 3617 } 3618 3619 public Measure addIdentifier(Identifier t) { //3 3620 if (t == null) 3621 return this; 3622 if (this.identifier == null) 3623 this.identifier = new ArrayList<Identifier>(); 3624 this.identifier.add(t); 3625 return this; 3626 } 3627 3628 /** 3629 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 3630 */ 3631 public Identifier getIdentifierFirstRep() { 3632 if (getIdentifier().isEmpty()) { 3633 addIdentifier(); 3634 } 3635 return getIdentifier().get(0); 3636 } 3637 3638 /** 3639 * @return {@link #version} (The identifier that is used to identify this version of the measure when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the measure author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3640 */ 3641 public StringType getVersionElement() { 3642 if (this.version == null) 3643 if (Configuration.errorOnAutoCreate()) 3644 throw new Error("Attempt to auto-create Measure.version"); 3645 else if (Configuration.doAutoCreate()) 3646 this.version = new StringType(); // bb 3647 return this.version; 3648 } 3649 3650 public boolean hasVersionElement() { 3651 return this.version != null && !this.version.isEmpty(); 3652 } 3653 3654 public boolean hasVersion() { 3655 return this.version != null && !this.version.isEmpty(); 3656 } 3657 3658 /** 3659 * @param value {@link #version} (The identifier that is used to identify this version of the measure when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the measure author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 3660 */ 3661 public Measure setVersionElement(StringType value) { 3662 this.version = value; 3663 return this; 3664 } 3665 3666 /** 3667 * @return The identifier that is used to identify this version of the measure when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the measure author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 3668 */ 3669 public String getVersion() { 3670 return this.version == null ? null : this.version.getValue(); 3671 } 3672 3673 /** 3674 * @param value The identifier that is used to identify this version of the measure when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the measure author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 3675 */ 3676 public Measure setVersion(String value) { 3677 if (Utilities.noString(value)) 3678 this.version = null; 3679 else { 3680 if (this.version == null) 3681 this.version = new StringType(); 3682 this.version.setValue(value); 3683 } 3684 return this; 3685 } 3686 3687 /** 3688 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3689 */ 3690 public DataType getVersionAlgorithm() { 3691 return this.versionAlgorithm; 3692 } 3693 3694 /** 3695 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3696 */ 3697 public StringType getVersionAlgorithmStringType() throws FHIRException { 3698 if (this.versionAlgorithm == null) 3699 this.versionAlgorithm = new StringType(); 3700 if (!(this.versionAlgorithm instanceof StringType)) 3701 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 3702 return (StringType) this.versionAlgorithm; 3703 } 3704 3705 public boolean hasVersionAlgorithmStringType() { 3706 return this.versionAlgorithm instanceof StringType; 3707 } 3708 3709 /** 3710 * @return {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3711 */ 3712 public Coding getVersionAlgorithmCoding() throws FHIRException { 3713 if (this.versionAlgorithm == null) 3714 this.versionAlgorithm = new Coding(); 3715 if (!(this.versionAlgorithm instanceof Coding)) 3716 throw new FHIRException("Type mismatch: the type Coding was expected, but "+this.versionAlgorithm.getClass().getName()+" was encountered"); 3717 return (Coding) this.versionAlgorithm; 3718 } 3719 3720 public boolean hasVersionAlgorithmCoding() { 3721 return this.versionAlgorithm instanceof Coding; 3722 } 3723 3724 public boolean hasVersionAlgorithm() { 3725 return this.versionAlgorithm != null && !this.versionAlgorithm.isEmpty(); 3726 } 3727 3728 /** 3729 * @param value {@link #versionAlgorithm} (Indicates the mechanism used to compare versions to determine which is more current.) 3730 */ 3731 public Measure setVersionAlgorithm(DataType value) { 3732 if (value != null && !(value instanceof StringType || value instanceof Coding)) 3733 throw new FHIRException("Not the right type for Measure.versionAlgorithm[x]: "+value.fhirType()); 3734 this.versionAlgorithm = value; 3735 return this; 3736 } 3737 3738 /** 3739 * @return {@link #name} (A natural language name identifying the measure. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3740 */ 3741 public StringType getNameElement() { 3742 if (this.name == null) 3743 if (Configuration.errorOnAutoCreate()) 3744 throw new Error("Attempt to auto-create Measure.name"); 3745 else if (Configuration.doAutoCreate()) 3746 this.name = new StringType(); // bb 3747 return this.name; 3748 } 3749 3750 public boolean hasNameElement() { 3751 return this.name != null && !this.name.isEmpty(); 3752 } 3753 3754 public boolean hasName() { 3755 return this.name != null && !this.name.isEmpty(); 3756 } 3757 3758 /** 3759 * @param value {@link #name} (A natural language name identifying the measure. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 3760 */ 3761 public Measure setNameElement(StringType value) { 3762 this.name = value; 3763 return this; 3764 } 3765 3766 /** 3767 * @return A natural language name identifying the measure. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3768 */ 3769 public String getName() { 3770 return this.name == null ? null : this.name.getValue(); 3771 } 3772 3773 /** 3774 * @param value A natural language name identifying the measure. This name should be usable as an identifier for the module by machine processing applications such as code generation. 3775 */ 3776 public Measure setName(String value) { 3777 if (Utilities.noString(value)) 3778 this.name = null; 3779 else { 3780 if (this.name == null) 3781 this.name = new StringType(); 3782 this.name.setValue(value); 3783 } 3784 return this; 3785 } 3786 3787 /** 3788 * @return {@link #title} (A short, descriptive, user-friendly title for the measure.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3789 */ 3790 public StringType getTitleElement() { 3791 if (this.title == null) 3792 if (Configuration.errorOnAutoCreate()) 3793 throw new Error("Attempt to auto-create Measure.title"); 3794 else if (Configuration.doAutoCreate()) 3795 this.title = new StringType(); // bb 3796 return this.title; 3797 } 3798 3799 public boolean hasTitleElement() { 3800 return this.title != null && !this.title.isEmpty(); 3801 } 3802 3803 public boolean hasTitle() { 3804 return this.title != null && !this.title.isEmpty(); 3805 } 3806 3807 /** 3808 * @param value {@link #title} (A short, descriptive, user-friendly title for the measure.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 3809 */ 3810 public Measure setTitleElement(StringType value) { 3811 this.title = value; 3812 return this; 3813 } 3814 3815 /** 3816 * @return A short, descriptive, user-friendly title for the measure. 3817 */ 3818 public String getTitle() { 3819 return this.title == null ? null : this.title.getValue(); 3820 } 3821 3822 /** 3823 * @param value A short, descriptive, user-friendly title for the measure. 3824 */ 3825 public Measure setTitle(String value) { 3826 if (Utilities.noString(value)) 3827 this.title = null; 3828 else { 3829 if (this.title == null) 3830 this.title = new StringType(); 3831 this.title.setValue(value); 3832 } 3833 return this; 3834 } 3835 3836 /** 3837 * @return {@link #subtitle} (An explanatory or alternate title for the measure giving additional information about its content.). This is the underlying object with id, value and extensions. The accessor "getSubtitle" gives direct access to the value 3838 */ 3839 public StringType getSubtitleElement() { 3840 if (this.subtitle == null) 3841 if (Configuration.errorOnAutoCreate()) 3842 throw new Error("Attempt to auto-create Measure.subtitle"); 3843 else if (Configuration.doAutoCreate()) 3844 this.subtitle = new StringType(); // bb 3845 return this.subtitle; 3846 } 3847 3848 public boolean hasSubtitleElement() { 3849 return this.subtitle != null && !this.subtitle.isEmpty(); 3850 } 3851 3852 public boolean hasSubtitle() { 3853 return this.subtitle != null && !this.subtitle.isEmpty(); 3854 } 3855 3856 /** 3857 * @param value {@link #subtitle} (An explanatory or alternate title for the measure giving additional information about its content.). This is the underlying object with id, value and extensions. The accessor "getSubtitle" gives direct access to the value 3858 */ 3859 public Measure setSubtitleElement(StringType value) { 3860 this.subtitle = value; 3861 return this; 3862 } 3863 3864 /** 3865 * @return An explanatory or alternate title for the measure giving additional information about its content. 3866 */ 3867 public String getSubtitle() { 3868 return this.subtitle == null ? null : this.subtitle.getValue(); 3869 } 3870 3871 /** 3872 * @param value An explanatory or alternate title for the measure giving additional information about its content. 3873 */ 3874 public Measure setSubtitle(String value) { 3875 if (Utilities.noString(value)) 3876 this.subtitle = null; 3877 else { 3878 if (this.subtitle == null) 3879 this.subtitle = new StringType(); 3880 this.subtitle.setValue(value); 3881 } 3882 return this; 3883 } 3884 3885 /** 3886 * @return {@link #status} (The status of this measure. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3887 */ 3888 public Enumeration<PublicationStatus> getStatusElement() { 3889 if (this.status == null) 3890 if (Configuration.errorOnAutoCreate()) 3891 throw new Error("Attempt to auto-create Measure.status"); 3892 else if (Configuration.doAutoCreate()) 3893 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 3894 return this.status; 3895 } 3896 3897 public boolean hasStatusElement() { 3898 return this.status != null && !this.status.isEmpty(); 3899 } 3900 3901 public boolean hasStatus() { 3902 return this.status != null && !this.status.isEmpty(); 3903 } 3904 3905 /** 3906 * @param value {@link #status} (The status of this measure. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3907 */ 3908 public Measure setStatusElement(Enumeration<PublicationStatus> value) { 3909 this.status = value; 3910 return this; 3911 } 3912 3913 /** 3914 * @return The status of this measure. Enables tracking the life-cycle of the content. 3915 */ 3916 public PublicationStatus getStatus() { 3917 return this.status == null ? null : this.status.getValue(); 3918 } 3919 3920 /** 3921 * @param value The status of this measure. Enables tracking the life-cycle of the content. 3922 */ 3923 public Measure setStatus(PublicationStatus value) { 3924 if (this.status == null) 3925 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 3926 this.status.setValue(value); 3927 return this; 3928 } 3929 3930 /** 3931 * @return {@link #experimental} (A Boolean value to indicate that this measure is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3932 */ 3933 public BooleanType getExperimentalElement() { 3934 if (this.experimental == null) 3935 if (Configuration.errorOnAutoCreate()) 3936 throw new Error("Attempt to auto-create Measure.experimental"); 3937 else if (Configuration.doAutoCreate()) 3938 this.experimental = new BooleanType(); // bb 3939 return this.experimental; 3940 } 3941 3942 public boolean hasExperimentalElement() { 3943 return this.experimental != null && !this.experimental.isEmpty(); 3944 } 3945 3946 public boolean hasExperimental() { 3947 return this.experimental != null && !this.experimental.isEmpty(); 3948 } 3949 3950 /** 3951 * @param value {@link #experimental} (A Boolean value to indicate that this measure is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 3952 */ 3953 public Measure setExperimentalElement(BooleanType value) { 3954 this.experimental = value; 3955 return this; 3956 } 3957 3958 /** 3959 * @return A Boolean value to indicate that this measure is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 3960 */ 3961 public boolean getExperimental() { 3962 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 3963 } 3964 3965 /** 3966 * @param value A Boolean value to indicate that this measure is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage. 3967 */ 3968 public Measure setExperimental(boolean value) { 3969 if (this.experimental == null) 3970 this.experimental = new BooleanType(); 3971 this.experimental.setValue(value); 3972 return this; 3973 } 3974 3975 /** 3976 * @return {@link #subject} (The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.) 3977 */ 3978 public DataType getSubject() { 3979 return this.subject; 3980 } 3981 3982 /** 3983 * @return {@link #subject} (The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.) 3984 */ 3985 public CodeableConcept getSubjectCodeableConcept() throws FHIRException { 3986 if (this.subject == null) 3987 this.subject = new CodeableConcept(); 3988 if (!(this.subject instanceof CodeableConcept)) 3989 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.subject.getClass().getName()+" was encountered"); 3990 return (CodeableConcept) this.subject; 3991 } 3992 3993 public boolean hasSubjectCodeableConcept() { 3994 return this.subject instanceof CodeableConcept; 3995 } 3996 3997 /** 3998 * @return {@link #subject} (The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.) 3999 */ 4000 public Reference getSubjectReference() throws FHIRException { 4001 if (this.subject == null) 4002 this.subject = new Reference(); 4003 if (!(this.subject instanceof Reference)) 4004 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.subject.getClass().getName()+" was encountered"); 4005 return (Reference) this.subject; 4006 } 4007 4008 public boolean hasSubjectReference() { 4009 return this.subject instanceof Reference; 4010 } 4011 4012 public boolean hasSubject() { 4013 return this.subject != null && !this.subject.isEmpty(); 4014 } 4015 4016 /** 4017 * @param value {@link #subject} (The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.) 4018 */ 4019 public Measure setSubject(DataType value) { 4020 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 4021 throw new FHIRException("Not the right type for Measure.subject[x]: "+value.fhirType()); 4022 this.subject = value; 4023 return this; 4024 } 4025 4026 /** 4027 * @return {@link #basis} (The population basis specifies the type of elements in the population. For a subject-based measure, this is boolean (because the subject and the population basis are the same, and the population criteria define yes/no values for each individual in the population). For measures that have a population basis that is different than the subject, this element specifies the type of the population basis. For example, an encounter-based measure has a subject of Patient and a population basis of Encounter, and the population criteria all return lists of Encounters.). This is the underlying object with id, value and extensions. The accessor "getBasis" gives direct access to the value 4028 */ 4029 public Enumeration<FHIRTypes> getBasisElement() { 4030 if (this.basis == null) 4031 if (Configuration.errorOnAutoCreate()) 4032 throw new Error("Attempt to auto-create Measure.basis"); 4033 else if (Configuration.doAutoCreate()) 4034 this.basis = new Enumeration<FHIRTypes>(new FHIRTypesEnumFactory()); // bb 4035 return this.basis; 4036 } 4037 4038 public boolean hasBasisElement() { 4039 return this.basis != null && !this.basis.isEmpty(); 4040 } 4041 4042 public boolean hasBasis() { 4043 return this.basis != null && !this.basis.isEmpty(); 4044 } 4045 4046 /** 4047 * @param value {@link #basis} (The population basis specifies the type of elements in the population. For a subject-based measure, this is boolean (because the subject and the population basis are the same, and the population criteria define yes/no values for each individual in the population). For measures that have a population basis that is different than the subject, this element specifies the type of the population basis. For example, an encounter-based measure has a subject of Patient and a population basis of Encounter, and the population criteria all return lists of Encounters.). This is the underlying object with id, value and extensions. The accessor "getBasis" gives direct access to the value 4048 */ 4049 public Measure setBasisElement(Enumeration<FHIRTypes> value) { 4050 this.basis = value; 4051 return this; 4052 } 4053 4054 /** 4055 * @return The population basis specifies the type of elements in the population. For a subject-based measure, this is boolean (because the subject and the population basis are the same, and the population criteria define yes/no values for each individual in the population). For measures that have a population basis that is different than the subject, this element specifies the type of the population basis. For example, an encounter-based measure has a subject of Patient and a population basis of Encounter, and the population criteria all return lists of Encounters. 4056 */ 4057 public FHIRTypes getBasis() { 4058 return this.basis == null ? null : this.basis.getValue(); 4059 } 4060 4061 /** 4062 * @param value The population basis specifies the type of elements in the population. For a subject-based measure, this is boolean (because the subject and the population basis are the same, and the population criteria define yes/no values for each individual in the population). For measures that have a population basis that is different than the subject, this element specifies the type of the population basis. For example, an encounter-based measure has a subject of Patient and a population basis of Encounter, and the population criteria all return lists of Encounters. 4063 */ 4064 public Measure setBasis(FHIRTypes value) { 4065 if (value == null) 4066 this.basis = null; 4067 else { 4068 if (this.basis == null) 4069 this.basis = new Enumeration<FHIRTypes>(new FHIRTypesEnumFactory()); 4070 this.basis.setValue(value); 4071 } 4072 return this; 4073 } 4074 4075 /** 4076 * @return {@link #date} (The date (and optionally time) when the measure was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the measure changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 4077 */ 4078 public DateTimeType getDateElement() { 4079 if (this.date == null) 4080 if (Configuration.errorOnAutoCreate()) 4081 throw new Error("Attempt to auto-create Measure.date"); 4082 else if (Configuration.doAutoCreate()) 4083 this.date = new DateTimeType(); // bb 4084 return this.date; 4085 } 4086 4087 public boolean hasDateElement() { 4088 return this.date != null && !this.date.isEmpty(); 4089 } 4090 4091 public boolean hasDate() { 4092 return this.date != null && !this.date.isEmpty(); 4093 } 4094 4095 /** 4096 * @param value {@link #date} (The date (and optionally time) when the measure was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the measure changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 4097 */ 4098 public Measure setDateElement(DateTimeType value) { 4099 this.date = value; 4100 return this; 4101 } 4102 4103 /** 4104 * @return The date (and optionally time) when the measure was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the measure changes. 4105 */ 4106 public Date getDate() { 4107 return this.date == null ? null : this.date.getValue(); 4108 } 4109 4110 /** 4111 * @param value The date (and optionally time) when the measure was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the measure changes. 4112 */ 4113 public Measure setDate(Date value) { 4114 if (value == null) 4115 this.date = null; 4116 else { 4117 if (this.date == null) 4118 this.date = new DateTimeType(); 4119 this.date.setValue(value); 4120 } 4121 return this; 4122 } 4123 4124 /** 4125 * @return {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the measure.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 4126 */ 4127 public StringType getPublisherElement() { 4128 if (this.publisher == null) 4129 if (Configuration.errorOnAutoCreate()) 4130 throw new Error("Attempt to auto-create Measure.publisher"); 4131 else if (Configuration.doAutoCreate()) 4132 this.publisher = new StringType(); // bb 4133 return this.publisher; 4134 } 4135 4136 public boolean hasPublisherElement() { 4137 return this.publisher != null && !this.publisher.isEmpty(); 4138 } 4139 4140 public boolean hasPublisher() { 4141 return this.publisher != null && !this.publisher.isEmpty(); 4142 } 4143 4144 /** 4145 * @param value {@link #publisher} (The name of the organization or individual responsible for the release and ongoing maintenance of the measure.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 4146 */ 4147 public Measure setPublisherElement(StringType value) { 4148 this.publisher = value; 4149 return this; 4150 } 4151 4152 /** 4153 * @return The name of the organization or individual responsible for the release and ongoing maintenance of the measure. 4154 */ 4155 public String getPublisher() { 4156 return this.publisher == null ? null : this.publisher.getValue(); 4157 } 4158 4159 /** 4160 * @param value The name of the organization or individual responsible for the release and ongoing maintenance of the measure. 4161 */ 4162 public Measure setPublisher(String value) { 4163 if (Utilities.noString(value)) 4164 this.publisher = null; 4165 else { 4166 if (this.publisher == null) 4167 this.publisher = new StringType(); 4168 this.publisher.setValue(value); 4169 } 4170 return this; 4171 } 4172 4173 /** 4174 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 4175 */ 4176 public List<ContactDetail> getContact() { 4177 if (this.contact == null) 4178 this.contact = new ArrayList<ContactDetail>(); 4179 return this.contact; 4180 } 4181 4182 /** 4183 * @return Returns a reference to <code>this</code> for easy method chaining 4184 */ 4185 public Measure setContact(List<ContactDetail> theContact) { 4186 this.contact = theContact; 4187 return this; 4188 } 4189 4190 public boolean hasContact() { 4191 if (this.contact == null) 4192 return false; 4193 for (ContactDetail item : this.contact) 4194 if (!item.isEmpty()) 4195 return true; 4196 return false; 4197 } 4198 4199 public ContactDetail addContact() { //3 4200 ContactDetail t = new ContactDetail(); 4201 if (this.contact == null) 4202 this.contact = new ArrayList<ContactDetail>(); 4203 this.contact.add(t); 4204 return t; 4205 } 4206 4207 public Measure addContact(ContactDetail t) { //3 4208 if (t == null) 4209 return this; 4210 if (this.contact == null) 4211 this.contact = new ArrayList<ContactDetail>(); 4212 this.contact.add(t); 4213 return this; 4214 } 4215 4216 /** 4217 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist {3} 4218 */ 4219 public ContactDetail getContactFirstRep() { 4220 if (getContact().isEmpty()) { 4221 addContact(); 4222 } 4223 return getContact().get(0); 4224 } 4225 4226 /** 4227 * @return {@link #description} (A free text natural language description of the measure from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4228 */ 4229 public MarkdownType getDescriptionElement() { 4230 if (this.description == null) 4231 if (Configuration.errorOnAutoCreate()) 4232 throw new Error("Attempt to auto-create Measure.description"); 4233 else if (Configuration.doAutoCreate()) 4234 this.description = new MarkdownType(); // bb 4235 return this.description; 4236 } 4237 4238 public boolean hasDescriptionElement() { 4239 return this.description != null && !this.description.isEmpty(); 4240 } 4241 4242 public boolean hasDescription() { 4243 return this.description != null && !this.description.isEmpty(); 4244 } 4245 4246 /** 4247 * @param value {@link #description} (A free text natural language description of the measure from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 4248 */ 4249 public Measure setDescriptionElement(MarkdownType value) { 4250 this.description = value; 4251 return this; 4252 } 4253 4254 /** 4255 * @return A free text natural language description of the measure from a consumer's perspective. 4256 */ 4257 public String getDescription() { 4258 return this.description == null ? null : this.description.getValue(); 4259 } 4260 4261 /** 4262 * @param value A free text natural language description of the measure from a consumer's perspective. 4263 */ 4264 public Measure setDescription(String value) { 4265 if (Utilities.noString(value)) 4266 this.description = null; 4267 else { 4268 if (this.description == null) 4269 this.description = new MarkdownType(); 4270 this.description.setValue(value); 4271 } 4272 return this; 4273 } 4274 4275 /** 4276 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate measure instances.) 4277 */ 4278 public List<UsageContext> getUseContext() { 4279 if (this.useContext == null) 4280 this.useContext = new ArrayList<UsageContext>(); 4281 return this.useContext; 4282 } 4283 4284 /** 4285 * @return Returns a reference to <code>this</code> for easy method chaining 4286 */ 4287 public Measure setUseContext(List<UsageContext> theUseContext) { 4288 this.useContext = theUseContext; 4289 return this; 4290 } 4291 4292 public boolean hasUseContext() { 4293 if (this.useContext == null) 4294 return false; 4295 for (UsageContext item : this.useContext) 4296 if (!item.isEmpty()) 4297 return true; 4298 return false; 4299 } 4300 4301 public UsageContext addUseContext() { //3 4302 UsageContext t = new UsageContext(); 4303 if (this.useContext == null) 4304 this.useContext = new ArrayList<UsageContext>(); 4305 this.useContext.add(t); 4306 return t; 4307 } 4308 4309 public Measure addUseContext(UsageContext t) { //3 4310 if (t == null) 4311 return this; 4312 if (this.useContext == null) 4313 this.useContext = new ArrayList<UsageContext>(); 4314 this.useContext.add(t); 4315 return this; 4316 } 4317 4318 /** 4319 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist {3} 4320 */ 4321 public UsageContext getUseContextFirstRep() { 4322 if (getUseContext().isEmpty()) { 4323 addUseContext(); 4324 } 4325 return getUseContext().get(0); 4326 } 4327 4328 /** 4329 * @return {@link #jurisdiction} (A legal or geographic region in which the measure is intended to be used.) 4330 */ 4331 public List<CodeableConcept> getJurisdiction() { 4332 if (this.jurisdiction == null) 4333 this.jurisdiction = new ArrayList<CodeableConcept>(); 4334 return this.jurisdiction; 4335 } 4336 4337 /** 4338 * @return Returns a reference to <code>this</code> for easy method chaining 4339 */ 4340 public Measure setJurisdiction(List<CodeableConcept> theJurisdiction) { 4341 this.jurisdiction = theJurisdiction; 4342 return this; 4343 } 4344 4345 public boolean hasJurisdiction() { 4346 if (this.jurisdiction == null) 4347 return false; 4348 for (CodeableConcept item : this.jurisdiction) 4349 if (!item.isEmpty()) 4350 return true; 4351 return false; 4352 } 4353 4354 public CodeableConcept addJurisdiction() { //3 4355 CodeableConcept t = new CodeableConcept(); 4356 if (this.jurisdiction == null) 4357 this.jurisdiction = new ArrayList<CodeableConcept>(); 4358 this.jurisdiction.add(t); 4359 return t; 4360 } 4361 4362 public Measure addJurisdiction(CodeableConcept t) { //3 4363 if (t == null) 4364 return this; 4365 if (this.jurisdiction == null) 4366 this.jurisdiction = new ArrayList<CodeableConcept>(); 4367 this.jurisdiction.add(t); 4368 return this; 4369 } 4370 4371 /** 4372 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist {3} 4373 */ 4374 public CodeableConcept getJurisdictionFirstRep() { 4375 if (getJurisdiction().isEmpty()) { 4376 addJurisdiction(); 4377 } 4378 return getJurisdiction().get(0); 4379 } 4380 4381 /** 4382 * @return {@link #purpose} (Explanation of why this measure is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 4383 */ 4384 public MarkdownType getPurposeElement() { 4385 if (this.purpose == null) 4386 if (Configuration.errorOnAutoCreate()) 4387 throw new Error("Attempt to auto-create Measure.purpose"); 4388 else if (Configuration.doAutoCreate()) 4389 this.purpose = new MarkdownType(); // bb 4390 return this.purpose; 4391 } 4392 4393 public boolean hasPurposeElement() { 4394 return this.purpose != null && !this.purpose.isEmpty(); 4395 } 4396 4397 public boolean hasPurpose() { 4398 return this.purpose != null && !this.purpose.isEmpty(); 4399 } 4400 4401 /** 4402 * @param value {@link #purpose} (Explanation of why this measure is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 4403 */ 4404 public Measure setPurposeElement(MarkdownType value) { 4405 this.purpose = value; 4406 return this; 4407 } 4408 4409 /** 4410 * @return Explanation of why this measure is needed and why it has been designed as it has. 4411 */ 4412 public String getPurpose() { 4413 return this.purpose == null ? null : this.purpose.getValue(); 4414 } 4415 4416 /** 4417 * @param value Explanation of why this measure is needed and why it has been designed as it has. 4418 */ 4419 public Measure setPurpose(String value) { 4420 if (Utilities.noString(value)) 4421 this.purpose = null; 4422 else { 4423 if (this.purpose == null) 4424 this.purpose = new MarkdownType(); 4425 this.purpose.setValue(value); 4426 } 4427 return this; 4428 } 4429 4430 /** 4431 * @return {@link #usage} (A detailed description, from a clinical perspective, of how the measure is used.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 4432 */ 4433 public MarkdownType getUsageElement() { 4434 if (this.usage == null) 4435 if (Configuration.errorOnAutoCreate()) 4436 throw new Error("Attempt to auto-create Measure.usage"); 4437 else if (Configuration.doAutoCreate()) 4438 this.usage = new MarkdownType(); // bb 4439 return this.usage; 4440 } 4441 4442 public boolean hasUsageElement() { 4443 return this.usage != null && !this.usage.isEmpty(); 4444 } 4445 4446 public boolean hasUsage() { 4447 return this.usage != null && !this.usage.isEmpty(); 4448 } 4449 4450 /** 4451 * @param value {@link #usage} (A detailed description, from a clinical perspective, of how the measure is used.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 4452 */ 4453 public Measure setUsageElement(MarkdownType value) { 4454 this.usage = value; 4455 return this; 4456 } 4457 4458 /** 4459 * @return A detailed description, from a clinical perspective, of how the measure is used. 4460 */ 4461 public String getUsage() { 4462 return this.usage == null ? null : this.usage.getValue(); 4463 } 4464 4465 /** 4466 * @param value A detailed description, from a clinical perspective, of how the measure is used. 4467 */ 4468 public Measure setUsage(String value) { 4469 if (Utilities.noString(value)) 4470 this.usage = null; 4471 else { 4472 if (this.usage == null) 4473 this.usage = new MarkdownType(); 4474 this.usage.setValue(value); 4475 } 4476 return this; 4477 } 4478 4479 /** 4480 * @return {@link #copyright} (A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 4481 */ 4482 public MarkdownType getCopyrightElement() { 4483 if (this.copyright == null) 4484 if (Configuration.errorOnAutoCreate()) 4485 throw new Error("Attempt to auto-create Measure.copyright"); 4486 else if (Configuration.doAutoCreate()) 4487 this.copyright = new MarkdownType(); // bb 4488 return this.copyright; 4489 } 4490 4491 public boolean hasCopyrightElement() { 4492 return this.copyright != null && !this.copyright.isEmpty(); 4493 } 4494 4495 public boolean hasCopyright() { 4496 return this.copyright != null && !this.copyright.isEmpty(); 4497 } 4498 4499 /** 4500 * @param value {@link #copyright} (A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 4501 */ 4502 public Measure setCopyrightElement(MarkdownType value) { 4503 this.copyright = value; 4504 return this; 4505 } 4506 4507 /** 4508 * @return A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure. 4509 */ 4510 public String getCopyright() { 4511 return this.copyright == null ? null : this.copyright.getValue(); 4512 } 4513 4514 /** 4515 * @param value A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure. 4516 */ 4517 public Measure setCopyright(String value) { 4518 if (Utilities.noString(value)) 4519 this.copyright = null; 4520 else { 4521 if (this.copyright == null) 4522 this.copyright = new MarkdownType(); 4523 this.copyright.setValue(value); 4524 } 4525 return this; 4526 } 4527 4528 /** 4529 * @return {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 4530 */ 4531 public StringType getCopyrightLabelElement() { 4532 if (this.copyrightLabel == null) 4533 if (Configuration.errorOnAutoCreate()) 4534 throw new Error("Attempt to auto-create Measure.copyrightLabel"); 4535 else if (Configuration.doAutoCreate()) 4536 this.copyrightLabel = new StringType(); // bb 4537 return this.copyrightLabel; 4538 } 4539 4540 public boolean hasCopyrightLabelElement() { 4541 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 4542 } 4543 4544 public boolean hasCopyrightLabel() { 4545 return this.copyrightLabel != null && !this.copyrightLabel.isEmpty(); 4546 } 4547 4548 /** 4549 * @param value {@link #copyrightLabel} (A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').). This is the underlying object with id, value and extensions. The accessor "getCopyrightLabel" gives direct access to the value 4550 */ 4551 public Measure setCopyrightLabelElement(StringType value) { 4552 this.copyrightLabel = value; 4553 return this; 4554 } 4555 4556 /** 4557 * @return A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 4558 */ 4559 public String getCopyrightLabel() { 4560 return this.copyrightLabel == null ? null : this.copyrightLabel.getValue(); 4561 } 4562 4563 /** 4564 * @param value A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved'). 4565 */ 4566 public Measure setCopyrightLabel(String value) { 4567 if (Utilities.noString(value)) 4568 this.copyrightLabel = null; 4569 else { 4570 if (this.copyrightLabel == null) 4571 this.copyrightLabel = new StringType(); 4572 this.copyrightLabel.setValue(value); 4573 } 4574 return this; 4575 } 4576 4577 /** 4578 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 4579 */ 4580 public DateType getApprovalDateElement() { 4581 if (this.approvalDate == null) 4582 if (Configuration.errorOnAutoCreate()) 4583 throw new Error("Attempt to auto-create Measure.approvalDate"); 4584 else if (Configuration.doAutoCreate()) 4585 this.approvalDate = new DateType(); // bb 4586 return this.approvalDate; 4587 } 4588 4589 public boolean hasApprovalDateElement() { 4590 return this.approvalDate != null && !this.approvalDate.isEmpty(); 4591 } 4592 4593 public boolean hasApprovalDate() { 4594 return this.approvalDate != null && !this.approvalDate.isEmpty(); 4595 } 4596 4597 /** 4598 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 4599 */ 4600 public Measure setApprovalDateElement(DateType value) { 4601 this.approvalDate = value; 4602 return this; 4603 } 4604 4605 /** 4606 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 4607 */ 4608 public Date getApprovalDate() { 4609 return this.approvalDate == null ? null : this.approvalDate.getValue(); 4610 } 4611 4612 /** 4613 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 4614 */ 4615 public Measure setApprovalDate(Date value) { 4616 if (value == null) 4617 this.approvalDate = null; 4618 else { 4619 if (this.approvalDate == null) 4620 this.approvalDate = new DateType(); 4621 this.approvalDate.setValue(value); 4622 } 4623 return this; 4624 } 4625 4626 /** 4627 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 4628 */ 4629 public DateType getLastReviewDateElement() { 4630 if (this.lastReviewDate == null) 4631 if (Configuration.errorOnAutoCreate()) 4632 throw new Error("Attempt to auto-create Measure.lastReviewDate"); 4633 else if (Configuration.doAutoCreate()) 4634 this.lastReviewDate = new DateType(); // bb 4635 return this.lastReviewDate; 4636 } 4637 4638 public boolean hasLastReviewDateElement() { 4639 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 4640 } 4641 4642 public boolean hasLastReviewDate() { 4643 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 4644 } 4645 4646 /** 4647 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 4648 */ 4649 public Measure setLastReviewDateElement(DateType value) { 4650 this.lastReviewDate = value; 4651 return this; 4652 } 4653 4654 /** 4655 * @return The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 4656 */ 4657 public Date getLastReviewDate() { 4658 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 4659 } 4660 4661 /** 4662 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date. 4663 */ 4664 public Measure setLastReviewDate(Date value) { 4665 if (value == null) 4666 this.lastReviewDate = null; 4667 else { 4668 if (this.lastReviewDate == null) 4669 this.lastReviewDate = new DateType(); 4670 this.lastReviewDate.setValue(value); 4671 } 4672 return this; 4673 } 4674 4675 /** 4676 * @return {@link #effectivePeriod} (The period during which the measure content was or is planned to be in active use.) 4677 */ 4678 public Period getEffectivePeriod() { 4679 if (this.effectivePeriod == null) 4680 if (Configuration.errorOnAutoCreate()) 4681 throw new Error("Attempt to auto-create Measure.effectivePeriod"); 4682 else if (Configuration.doAutoCreate()) 4683 this.effectivePeriod = new Period(); // cc 4684 return this.effectivePeriod; 4685 } 4686 4687 public boolean hasEffectivePeriod() { 4688 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 4689 } 4690 4691 /** 4692 * @param value {@link #effectivePeriod} (The period during which the measure content was or is planned to be in active use.) 4693 */ 4694 public Measure setEffectivePeriod(Period value) { 4695 this.effectivePeriod = value; 4696 return this; 4697 } 4698 4699 /** 4700 * @return {@link #topic} (Descriptive topics related to the content of the measure. Topics provide a high-level categorization grouping types of measures that can be useful for filtering and searching.) 4701 */ 4702 public List<CodeableConcept> getTopic() { 4703 if (this.topic == null) 4704 this.topic = new ArrayList<CodeableConcept>(); 4705 return this.topic; 4706 } 4707 4708 /** 4709 * @return Returns a reference to <code>this</code> for easy method chaining 4710 */ 4711 public Measure setTopic(List<CodeableConcept> theTopic) { 4712 this.topic = theTopic; 4713 return this; 4714 } 4715 4716 public boolean hasTopic() { 4717 if (this.topic == null) 4718 return false; 4719 for (CodeableConcept item : this.topic) 4720 if (!item.isEmpty()) 4721 return true; 4722 return false; 4723 } 4724 4725 public CodeableConcept addTopic() { //3 4726 CodeableConcept t = new CodeableConcept(); 4727 if (this.topic == null) 4728 this.topic = new ArrayList<CodeableConcept>(); 4729 this.topic.add(t); 4730 return t; 4731 } 4732 4733 public Measure addTopic(CodeableConcept t) { //3 4734 if (t == null) 4735 return this; 4736 if (this.topic == null) 4737 this.topic = new ArrayList<CodeableConcept>(); 4738 this.topic.add(t); 4739 return this; 4740 } 4741 4742 /** 4743 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist {3} 4744 */ 4745 public CodeableConcept getTopicFirstRep() { 4746 if (getTopic().isEmpty()) { 4747 addTopic(); 4748 } 4749 return getTopic().get(0); 4750 } 4751 4752 /** 4753 * @return {@link #author} (An individiual or organization primarily involved in the creation and maintenance of the content.) 4754 */ 4755 public List<ContactDetail> getAuthor() { 4756 if (this.author == null) 4757 this.author = new ArrayList<ContactDetail>(); 4758 return this.author; 4759 } 4760 4761 /** 4762 * @return Returns a reference to <code>this</code> for easy method chaining 4763 */ 4764 public Measure setAuthor(List<ContactDetail> theAuthor) { 4765 this.author = theAuthor; 4766 return this; 4767 } 4768 4769 public boolean hasAuthor() { 4770 if (this.author == null) 4771 return false; 4772 for (ContactDetail item : this.author) 4773 if (!item.isEmpty()) 4774 return true; 4775 return false; 4776 } 4777 4778 public ContactDetail addAuthor() { //3 4779 ContactDetail t = new ContactDetail(); 4780 if (this.author == null) 4781 this.author = new ArrayList<ContactDetail>(); 4782 this.author.add(t); 4783 return t; 4784 } 4785 4786 public Measure addAuthor(ContactDetail t) { //3 4787 if (t == null) 4788 return this; 4789 if (this.author == null) 4790 this.author = new ArrayList<ContactDetail>(); 4791 this.author.add(t); 4792 return this; 4793 } 4794 4795 /** 4796 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist {3} 4797 */ 4798 public ContactDetail getAuthorFirstRep() { 4799 if (getAuthor().isEmpty()) { 4800 addAuthor(); 4801 } 4802 return getAuthor().get(0); 4803 } 4804 4805 /** 4806 * @return {@link #editor} (An individual or organization primarily responsible for internal coherence of the content.) 4807 */ 4808 public List<ContactDetail> getEditor() { 4809 if (this.editor == null) 4810 this.editor = new ArrayList<ContactDetail>(); 4811 return this.editor; 4812 } 4813 4814 /** 4815 * @return Returns a reference to <code>this</code> for easy method chaining 4816 */ 4817 public Measure setEditor(List<ContactDetail> theEditor) { 4818 this.editor = theEditor; 4819 return this; 4820 } 4821 4822 public boolean hasEditor() { 4823 if (this.editor == null) 4824 return false; 4825 for (ContactDetail item : this.editor) 4826 if (!item.isEmpty()) 4827 return true; 4828 return false; 4829 } 4830 4831 public ContactDetail addEditor() { //3 4832 ContactDetail t = new ContactDetail(); 4833 if (this.editor == null) 4834 this.editor = new ArrayList<ContactDetail>(); 4835 this.editor.add(t); 4836 return t; 4837 } 4838 4839 public Measure addEditor(ContactDetail t) { //3 4840 if (t == null) 4841 return this; 4842 if (this.editor == null) 4843 this.editor = new ArrayList<ContactDetail>(); 4844 this.editor.add(t); 4845 return this; 4846 } 4847 4848 /** 4849 * @return The first repetition of repeating field {@link #editor}, creating it if it does not already exist {3} 4850 */ 4851 public ContactDetail getEditorFirstRep() { 4852 if (getEditor().isEmpty()) { 4853 addEditor(); 4854 } 4855 return getEditor().get(0); 4856 } 4857 4858 /** 4859 * @return {@link #reviewer} (An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.) 4860 */ 4861 public List<ContactDetail> getReviewer() { 4862 if (this.reviewer == null) 4863 this.reviewer = new ArrayList<ContactDetail>(); 4864 return this.reviewer; 4865 } 4866 4867 /** 4868 * @return Returns a reference to <code>this</code> for easy method chaining 4869 */ 4870 public Measure setReviewer(List<ContactDetail> theReviewer) { 4871 this.reviewer = theReviewer; 4872 return this; 4873 } 4874 4875 public boolean hasReviewer() { 4876 if (this.reviewer == null) 4877 return false; 4878 for (ContactDetail item : this.reviewer) 4879 if (!item.isEmpty()) 4880 return true; 4881 return false; 4882 } 4883 4884 public ContactDetail addReviewer() { //3 4885 ContactDetail t = new ContactDetail(); 4886 if (this.reviewer == null) 4887 this.reviewer = new ArrayList<ContactDetail>(); 4888 this.reviewer.add(t); 4889 return t; 4890 } 4891 4892 public Measure addReviewer(ContactDetail t) { //3 4893 if (t == null) 4894 return this; 4895 if (this.reviewer == null) 4896 this.reviewer = new ArrayList<ContactDetail>(); 4897 this.reviewer.add(t); 4898 return this; 4899 } 4900 4901 /** 4902 * @return The first repetition of repeating field {@link #reviewer}, creating it if it does not already exist {3} 4903 */ 4904 public ContactDetail getReviewerFirstRep() { 4905 if (getReviewer().isEmpty()) { 4906 addReviewer(); 4907 } 4908 return getReviewer().get(0); 4909 } 4910 4911 /** 4912 * @return {@link #endorser} (An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.) 4913 */ 4914 public List<ContactDetail> getEndorser() { 4915 if (this.endorser == null) 4916 this.endorser = new ArrayList<ContactDetail>(); 4917 return this.endorser; 4918 } 4919 4920 /** 4921 * @return Returns a reference to <code>this</code> for easy method chaining 4922 */ 4923 public Measure setEndorser(List<ContactDetail> theEndorser) { 4924 this.endorser = theEndorser; 4925 return this; 4926 } 4927 4928 public boolean hasEndorser() { 4929 if (this.endorser == null) 4930 return false; 4931 for (ContactDetail item : this.endorser) 4932 if (!item.isEmpty()) 4933 return true; 4934 return false; 4935 } 4936 4937 public ContactDetail addEndorser() { //3 4938 ContactDetail t = new ContactDetail(); 4939 if (this.endorser == null) 4940 this.endorser = new ArrayList<ContactDetail>(); 4941 this.endorser.add(t); 4942 return t; 4943 } 4944 4945 public Measure addEndorser(ContactDetail t) { //3 4946 if (t == null) 4947 return this; 4948 if (this.endorser == null) 4949 this.endorser = new ArrayList<ContactDetail>(); 4950 this.endorser.add(t); 4951 return this; 4952 } 4953 4954 /** 4955 * @return The first repetition of repeating field {@link #endorser}, creating it if it does not already exist {3} 4956 */ 4957 public ContactDetail getEndorserFirstRep() { 4958 if (getEndorser().isEmpty()) { 4959 addEndorser(); 4960 } 4961 return getEndorser().get(0); 4962 } 4963 4964 /** 4965 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, or bibliographic references.) 4966 */ 4967 public List<RelatedArtifact> getRelatedArtifact() { 4968 if (this.relatedArtifact == null) 4969 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4970 return this.relatedArtifact; 4971 } 4972 4973 /** 4974 * @return Returns a reference to <code>this</code> for easy method chaining 4975 */ 4976 public Measure setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 4977 this.relatedArtifact = theRelatedArtifact; 4978 return this; 4979 } 4980 4981 public boolean hasRelatedArtifact() { 4982 if (this.relatedArtifact == null) 4983 return false; 4984 for (RelatedArtifact item : this.relatedArtifact) 4985 if (!item.isEmpty()) 4986 return true; 4987 return false; 4988 } 4989 4990 public RelatedArtifact addRelatedArtifact() { //3 4991 RelatedArtifact t = new RelatedArtifact(); 4992 if (this.relatedArtifact == null) 4993 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 4994 this.relatedArtifact.add(t); 4995 return t; 4996 } 4997 4998 public Measure addRelatedArtifact(RelatedArtifact t) { //3 4999 if (t == null) 5000 return this; 5001 if (this.relatedArtifact == null) 5002 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 5003 this.relatedArtifact.add(t); 5004 return this; 5005 } 5006 5007 /** 5008 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist {3} 5009 */ 5010 public RelatedArtifact getRelatedArtifactFirstRep() { 5011 if (getRelatedArtifact().isEmpty()) { 5012 addRelatedArtifact(); 5013 } 5014 return getRelatedArtifact().get(0); 5015 } 5016 5017 /** 5018 * @return {@link #library} (A reference to a Library resource containing the formal logic used by the measure.) 5019 */ 5020 public List<CanonicalType> getLibrary() { 5021 if (this.library == null) 5022 this.library = new ArrayList<CanonicalType>(); 5023 return this.library; 5024 } 5025 5026 /** 5027 * @return Returns a reference to <code>this</code> for easy method chaining 5028 */ 5029 public Measure setLibrary(List<CanonicalType> theLibrary) { 5030 this.library = theLibrary; 5031 return this; 5032 } 5033 5034 public boolean hasLibrary() { 5035 if (this.library == null) 5036 return false; 5037 for (CanonicalType item : this.library) 5038 if (!item.isEmpty()) 5039 return true; 5040 return false; 5041 } 5042 5043 /** 5044 * @return {@link #library} (A reference to a Library resource containing the formal logic used by the measure.) 5045 */ 5046 public CanonicalType addLibraryElement() {//2 5047 CanonicalType t = new CanonicalType(); 5048 if (this.library == null) 5049 this.library = new ArrayList<CanonicalType>(); 5050 this.library.add(t); 5051 return t; 5052 } 5053 5054 /** 5055 * @param value {@link #library} (A reference to a Library resource containing the formal logic used by the measure.) 5056 */ 5057 public Measure addLibrary(String value) { //1 5058 CanonicalType t = new CanonicalType(); 5059 t.setValue(value); 5060 if (this.library == null) 5061 this.library = new ArrayList<CanonicalType>(); 5062 this.library.add(t); 5063 return this; 5064 } 5065 5066 /** 5067 * @param value {@link #library} (A reference to a Library resource containing the formal logic used by the measure.) 5068 */ 5069 public boolean hasLibrary(String value) { 5070 if (this.library == null) 5071 return false; 5072 for (CanonicalType v : this.library) 5073 if (v.getValue().equals(value)) // canonical 5074 return true; 5075 return false; 5076 } 5077 5078 /** 5079 * @return {@link #disclaimer} (Notices and disclaimers regarding the use of the measure or related to intellectual property (such as code systems) referenced by the measure.). This is the underlying object with id, value and extensions. The accessor "getDisclaimer" gives direct access to the value 5080 */ 5081 public MarkdownType getDisclaimerElement() { 5082 if (this.disclaimer == null) 5083 if (Configuration.errorOnAutoCreate()) 5084 throw new Error("Attempt to auto-create Measure.disclaimer"); 5085 else if (Configuration.doAutoCreate()) 5086 this.disclaimer = new MarkdownType(); // bb 5087 return this.disclaimer; 5088 } 5089 5090 public boolean hasDisclaimerElement() { 5091 return this.disclaimer != null && !this.disclaimer.isEmpty(); 5092 } 5093 5094 public boolean hasDisclaimer() { 5095 return this.disclaimer != null && !this.disclaimer.isEmpty(); 5096 } 5097 5098 /** 5099 * @param value {@link #disclaimer} (Notices and disclaimers regarding the use of the measure or related to intellectual property (such as code systems) referenced by the measure.). This is the underlying object with id, value and extensions. The accessor "getDisclaimer" gives direct access to the value 5100 */ 5101 public Measure setDisclaimerElement(MarkdownType value) { 5102 this.disclaimer = value; 5103 return this; 5104 } 5105 5106 /** 5107 * @return Notices and disclaimers regarding the use of the measure or related to intellectual property (such as code systems) referenced by the measure. 5108 */ 5109 public String getDisclaimer() { 5110 return this.disclaimer == null ? null : this.disclaimer.getValue(); 5111 } 5112 5113 /** 5114 * @param value Notices and disclaimers regarding the use of the measure or related to intellectual property (such as code systems) referenced by the measure. 5115 */ 5116 public Measure setDisclaimer(String value) { 5117 if (Utilities.noString(value)) 5118 this.disclaimer = null; 5119 else { 5120 if (this.disclaimer == null) 5121 this.disclaimer = new MarkdownType(); 5122 this.disclaimer.setValue(value); 5123 } 5124 return this; 5125 } 5126 5127 /** 5128 * @return {@link #scoring} (Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented.) 5129 */ 5130 public CodeableConcept getScoring() { 5131 if (this.scoring == null) 5132 if (Configuration.errorOnAutoCreate()) 5133 throw new Error("Attempt to auto-create Measure.scoring"); 5134 else if (Configuration.doAutoCreate()) 5135 this.scoring = new CodeableConcept(); // cc 5136 return this.scoring; 5137 } 5138 5139 public boolean hasScoring() { 5140 return this.scoring != null && !this.scoring.isEmpty(); 5141 } 5142 5143 /** 5144 * @param value {@link #scoring} (Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented.) 5145 */ 5146 public Measure setScoring(CodeableConcept value) { 5147 this.scoring = value; 5148 return this; 5149 } 5150 5151 /** 5152 * @return {@link #scoringUnit} (Defines the expected units of measure for the measure score. This element SHOULD be specified as a UCUM unit.) 5153 */ 5154 public CodeableConcept getScoringUnit() { 5155 if (this.scoringUnit == null) 5156 if (Configuration.errorOnAutoCreate()) 5157 throw new Error("Attempt to auto-create Measure.scoringUnit"); 5158 else if (Configuration.doAutoCreate()) 5159 this.scoringUnit = new CodeableConcept(); // cc 5160 return this.scoringUnit; 5161 } 5162 5163 public boolean hasScoringUnit() { 5164 return this.scoringUnit != null && !this.scoringUnit.isEmpty(); 5165 } 5166 5167 /** 5168 * @param value {@link #scoringUnit} (Defines the expected units of measure for the measure score. This element SHOULD be specified as a UCUM unit.) 5169 */ 5170 public Measure setScoringUnit(CodeableConcept value) { 5171 this.scoringUnit = value; 5172 return this; 5173 } 5174 5175 /** 5176 * @return {@link #compositeScoring} (If this is a composite measure, the scoring method used to combine the component measures to determine the composite score.) 5177 */ 5178 public CodeableConcept getCompositeScoring() { 5179 if (this.compositeScoring == null) 5180 if (Configuration.errorOnAutoCreate()) 5181 throw new Error("Attempt to auto-create Measure.compositeScoring"); 5182 else if (Configuration.doAutoCreate()) 5183 this.compositeScoring = new CodeableConcept(); // cc 5184 return this.compositeScoring; 5185 } 5186 5187 public boolean hasCompositeScoring() { 5188 return this.compositeScoring != null && !this.compositeScoring.isEmpty(); 5189 } 5190 5191 /** 5192 * @param value {@link #compositeScoring} (If this is a composite measure, the scoring method used to combine the component measures to determine the composite score.) 5193 */ 5194 public Measure setCompositeScoring(CodeableConcept value) { 5195 this.compositeScoring = value; 5196 return this; 5197 } 5198 5199 /** 5200 * @return {@link #type} (Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization.) 5201 */ 5202 public List<CodeableConcept> getType() { 5203 if (this.type == null) 5204 this.type = new ArrayList<CodeableConcept>(); 5205 return this.type; 5206 } 5207 5208 /** 5209 * @return Returns a reference to <code>this</code> for easy method chaining 5210 */ 5211 public Measure setType(List<CodeableConcept> theType) { 5212 this.type = theType; 5213 return this; 5214 } 5215 5216 public boolean hasType() { 5217 if (this.type == null) 5218 return false; 5219 for (CodeableConcept item : this.type) 5220 if (!item.isEmpty()) 5221 return true; 5222 return false; 5223 } 5224 5225 public CodeableConcept addType() { //3 5226 CodeableConcept t = new CodeableConcept(); 5227 if (this.type == null) 5228 this.type = new ArrayList<CodeableConcept>(); 5229 this.type.add(t); 5230 return t; 5231 } 5232 5233 public Measure addType(CodeableConcept t) { //3 5234 if (t == null) 5235 return this; 5236 if (this.type == null) 5237 this.type = new ArrayList<CodeableConcept>(); 5238 this.type.add(t); 5239 return this; 5240 } 5241 5242 /** 5243 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist {3} 5244 */ 5245 public CodeableConcept getTypeFirstRep() { 5246 if (getType().isEmpty()) { 5247 addType(); 5248 } 5249 return getType().get(0); 5250 } 5251 5252 /** 5253 * @return {@link #riskAdjustment} (A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results.). This is the underlying object with id, value and extensions. The accessor "getRiskAdjustment" gives direct access to the value 5254 */ 5255 public MarkdownType getRiskAdjustmentElement() { 5256 if (this.riskAdjustment == null) 5257 if (Configuration.errorOnAutoCreate()) 5258 throw new Error("Attempt to auto-create Measure.riskAdjustment"); 5259 else if (Configuration.doAutoCreate()) 5260 this.riskAdjustment = new MarkdownType(); // bb 5261 return this.riskAdjustment; 5262 } 5263 5264 public boolean hasRiskAdjustmentElement() { 5265 return this.riskAdjustment != null && !this.riskAdjustment.isEmpty(); 5266 } 5267 5268 public boolean hasRiskAdjustment() { 5269 return this.riskAdjustment != null && !this.riskAdjustment.isEmpty(); 5270 } 5271 5272 /** 5273 * @param value {@link #riskAdjustment} (A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results.). This is the underlying object with id, value and extensions. The accessor "getRiskAdjustment" gives direct access to the value 5274 */ 5275 public Measure setRiskAdjustmentElement(MarkdownType value) { 5276 this.riskAdjustment = value; 5277 return this; 5278 } 5279 5280 /** 5281 * @return A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results. 5282 */ 5283 public String getRiskAdjustment() { 5284 return this.riskAdjustment == null ? null : this.riskAdjustment.getValue(); 5285 } 5286 5287 /** 5288 * @param value A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results. 5289 */ 5290 public Measure setRiskAdjustment(String value) { 5291 if (Utilities.noString(value)) 5292 this.riskAdjustment = null; 5293 else { 5294 if (this.riskAdjustment == null) 5295 this.riskAdjustment = new MarkdownType(); 5296 this.riskAdjustment.setValue(value); 5297 } 5298 return this; 5299 } 5300 5301 /** 5302 * @return {@link #rateAggregation} (Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result.). This is the underlying object with id, value and extensions. The accessor "getRateAggregation" gives direct access to the value 5303 */ 5304 public MarkdownType getRateAggregationElement() { 5305 if (this.rateAggregation == null) 5306 if (Configuration.errorOnAutoCreate()) 5307 throw new Error("Attempt to auto-create Measure.rateAggregation"); 5308 else if (Configuration.doAutoCreate()) 5309 this.rateAggregation = new MarkdownType(); // bb 5310 return this.rateAggregation; 5311 } 5312 5313 public boolean hasRateAggregationElement() { 5314 return this.rateAggregation != null && !this.rateAggregation.isEmpty(); 5315 } 5316 5317 public boolean hasRateAggregation() { 5318 return this.rateAggregation != null && !this.rateAggregation.isEmpty(); 5319 } 5320 5321 /** 5322 * @param value {@link #rateAggregation} (Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result.). This is the underlying object with id, value and extensions. The accessor "getRateAggregation" gives direct access to the value 5323 */ 5324 public Measure setRateAggregationElement(MarkdownType value) { 5325 this.rateAggregation = value; 5326 return this; 5327 } 5328 5329 /** 5330 * @return Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result. 5331 */ 5332 public String getRateAggregation() { 5333 return this.rateAggregation == null ? null : this.rateAggregation.getValue(); 5334 } 5335 5336 /** 5337 * @param value Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result. 5338 */ 5339 public Measure setRateAggregation(String value) { 5340 if (Utilities.noString(value)) 5341 this.rateAggregation = null; 5342 else { 5343 if (this.rateAggregation == null) 5344 this.rateAggregation = new MarkdownType(); 5345 this.rateAggregation.setValue(value); 5346 } 5347 return this; 5348 } 5349 5350 /** 5351 * @return {@link #rationale} (Provides a succinct statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence.). This is the underlying object with id, value and extensions. The accessor "getRationale" gives direct access to the value 5352 */ 5353 public MarkdownType getRationaleElement() { 5354 if (this.rationale == null) 5355 if (Configuration.errorOnAutoCreate()) 5356 throw new Error("Attempt to auto-create Measure.rationale"); 5357 else if (Configuration.doAutoCreate()) 5358 this.rationale = new MarkdownType(); // bb 5359 return this.rationale; 5360 } 5361 5362 public boolean hasRationaleElement() { 5363 return this.rationale != null && !this.rationale.isEmpty(); 5364 } 5365 5366 public boolean hasRationale() { 5367 return this.rationale != null && !this.rationale.isEmpty(); 5368 } 5369 5370 /** 5371 * @param value {@link #rationale} (Provides a succinct statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence.). This is the underlying object with id, value and extensions. The accessor "getRationale" gives direct access to the value 5372 */ 5373 public Measure setRationaleElement(MarkdownType value) { 5374 this.rationale = value; 5375 return this; 5376 } 5377 5378 /** 5379 * @return Provides a succinct statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence. 5380 */ 5381 public String getRationale() { 5382 return this.rationale == null ? null : this.rationale.getValue(); 5383 } 5384 5385 /** 5386 * @param value Provides a succinct statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence. 5387 */ 5388 public Measure setRationale(String value) { 5389 if (Utilities.noString(value)) 5390 this.rationale = null; 5391 else { 5392 if (this.rationale == null) 5393 this.rationale = new MarkdownType(); 5394 this.rationale.setValue(value); 5395 } 5396 return this; 5397 } 5398 5399 /** 5400 * @return {@link #clinicalRecommendationStatement} (Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure.). This is the underlying object with id, value and extensions. The accessor "getClinicalRecommendationStatement" gives direct access to the value 5401 */ 5402 public MarkdownType getClinicalRecommendationStatementElement() { 5403 if (this.clinicalRecommendationStatement == null) 5404 if (Configuration.errorOnAutoCreate()) 5405 throw new Error("Attempt to auto-create Measure.clinicalRecommendationStatement"); 5406 else if (Configuration.doAutoCreate()) 5407 this.clinicalRecommendationStatement = new MarkdownType(); // bb 5408 return this.clinicalRecommendationStatement; 5409 } 5410 5411 public boolean hasClinicalRecommendationStatementElement() { 5412 return this.clinicalRecommendationStatement != null && !this.clinicalRecommendationStatement.isEmpty(); 5413 } 5414 5415 public boolean hasClinicalRecommendationStatement() { 5416 return this.clinicalRecommendationStatement != null && !this.clinicalRecommendationStatement.isEmpty(); 5417 } 5418 5419 /** 5420 * @param value {@link #clinicalRecommendationStatement} (Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure.). This is the underlying object with id, value and extensions. The accessor "getClinicalRecommendationStatement" gives direct access to the value 5421 */ 5422 public Measure setClinicalRecommendationStatementElement(MarkdownType value) { 5423 this.clinicalRecommendationStatement = value; 5424 return this; 5425 } 5426 5427 /** 5428 * @return Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure. 5429 */ 5430 public String getClinicalRecommendationStatement() { 5431 return this.clinicalRecommendationStatement == null ? null : this.clinicalRecommendationStatement.getValue(); 5432 } 5433 5434 /** 5435 * @param value Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure. 5436 */ 5437 public Measure setClinicalRecommendationStatement(String value) { 5438 if (Utilities.noString(value)) 5439 this.clinicalRecommendationStatement = null; 5440 else { 5441 if (this.clinicalRecommendationStatement == null) 5442 this.clinicalRecommendationStatement = new MarkdownType(); 5443 this.clinicalRecommendationStatement.setValue(value); 5444 } 5445 return this; 5446 } 5447 5448 /** 5449 * @return {@link #improvementNotation} (Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is within a range).) 5450 */ 5451 public CodeableConcept getImprovementNotation() { 5452 if (this.improvementNotation == null) 5453 if (Configuration.errorOnAutoCreate()) 5454 throw new Error("Attempt to auto-create Measure.improvementNotation"); 5455 else if (Configuration.doAutoCreate()) 5456 this.improvementNotation = new CodeableConcept(); // cc 5457 return this.improvementNotation; 5458 } 5459 5460 public boolean hasImprovementNotation() { 5461 return this.improvementNotation != null && !this.improvementNotation.isEmpty(); 5462 } 5463 5464 /** 5465 * @param value {@link #improvementNotation} (Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is within a range).) 5466 */ 5467 public Measure setImprovementNotation(CodeableConcept value) { 5468 this.improvementNotation = value; 5469 return this; 5470 } 5471 5472 /** 5473 * @return {@link #term} (Provides a description of an individual term used within the measure.) 5474 */ 5475 public List<MeasureTermComponent> getTerm() { 5476 if (this.term == null) 5477 this.term = new ArrayList<MeasureTermComponent>(); 5478 return this.term; 5479 } 5480 5481 /** 5482 * @return Returns a reference to <code>this</code> for easy method chaining 5483 */ 5484 public Measure setTerm(List<MeasureTermComponent> theTerm) { 5485 this.term = theTerm; 5486 return this; 5487 } 5488 5489 public boolean hasTerm() { 5490 if (this.term == null) 5491 return false; 5492 for (MeasureTermComponent item : this.term) 5493 if (!item.isEmpty()) 5494 return true; 5495 return false; 5496 } 5497 5498 public MeasureTermComponent addTerm() { //3 5499 MeasureTermComponent t = new MeasureTermComponent(); 5500 if (this.term == null) 5501 this.term = new ArrayList<MeasureTermComponent>(); 5502 this.term.add(t); 5503 return t; 5504 } 5505 5506 public Measure addTerm(MeasureTermComponent t) { //3 5507 if (t == null) 5508 return this; 5509 if (this.term == null) 5510 this.term = new ArrayList<MeasureTermComponent>(); 5511 this.term.add(t); 5512 return this; 5513 } 5514 5515 /** 5516 * @return The first repetition of repeating field {@link #term}, creating it if it does not already exist {3} 5517 */ 5518 public MeasureTermComponent getTermFirstRep() { 5519 if (getTerm().isEmpty()) { 5520 addTerm(); 5521 } 5522 return getTerm().get(0); 5523 } 5524 5525 /** 5526 * @return {@link #guidance} (Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure.). This is the underlying object with id, value and extensions. The accessor "getGuidance" gives direct access to the value 5527 */ 5528 public MarkdownType getGuidanceElement() { 5529 if (this.guidance == null) 5530 if (Configuration.errorOnAutoCreate()) 5531 throw new Error("Attempt to auto-create Measure.guidance"); 5532 else if (Configuration.doAutoCreate()) 5533 this.guidance = new MarkdownType(); // bb 5534 return this.guidance; 5535 } 5536 5537 public boolean hasGuidanceElement() { 5538 return this.guidance != null && !this.guidance.isEmpty(); 5539 } 5540 5541 public boolean hasGuidance() { 5542 return this.guidance != null && !this.guidance.isEmpty(); 5543 } 5544 5545 /** 5546 * @param value {@link #guidance} (Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure.). This is the underlying object with id, value and extensions. The accessor "getGuidance" gives direct access to the value 5547 */ 5548 public Measure setGuidanceElement(MarkdownType value) { 5549 this.guidance = value; 5550 return this; 5551 } 5552 5553 /** 5554 * @return Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure. 5555 */ 5556 public String getGuidance() { 5557 return this.guidance == null ? null : this.guidance.getValue(); 5558 } 5559 5560 /** 5561 * @param value Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure. 5562 */ 5563 public Measure setGuidance(String value) { 5564 if (Utilities.noString(value)) 5565 this.guidance = null; 5566 else { 5567 if (this.guidance == null) 5568 this.guidance = new MarkdownType(); 5569 this.guidance.setValue(value); 5570 } 5571 return this; 5572 } 5573 5574 /** 5575 * @return {@link #group} (A group of population criteria for the measure.) 5576 */ 5577 public List<MeasureGroupComponent> getGroup() { 5578 if (this.group == null) 5579 this.group = new ArrayList<MeasureGroupComponent>(); 5580 return this.group; 5581 } 5582 5583 /** 5584 * @return Returns a reference to <code>this</code> for easy method chaining 5585 */ 5586 public Measure setGroup(List<MeasureGroupComponent> theGroup) { 5587 this.group = theGroup; 5588 return this; 5589 } 5590 5591 public boolean hasGroup() { 5592 if (this.group == null) 5593 return false; 5594 for (MeasureGroupComponent item : this.group) 5595 if (!item.isEmpty()) 5596 return true; 5597 return false; 5598 } 5599 5600 public MeasureGroupComponent addGroup() { //3 5601 MeasureGroupComponent t = new MeasureGroupComponent(); 5602 if (this.group == null) 5603 this.group = new ArrayList<MeasureGroupComponent>(); 5604 this.group.add(t); 5605 return t; 5606 } 5607 5608 public Measure addGroup(MeasureGroupComponent t) { //3 5609 if (t == null) 5610 return this; 5611 if (this.group == null) 5612 this.group = new ArrayList<MeasureGroupComponent>(); 5613 this.group.add(t); 5614 return this; 5615 } 5616 5617 /** 5618 * @return The first repetition of repeating field {@link #group}, creating it if it does not already exist {3} 5619 */ 5620 public MeasureGroupComponent getGroupFirstRep() { 5621 if (getGroup().isEmpty()) { 5622 addGroup(); 5623 } 5624 return getGroup().get(0); 5625 } 5626 5627 /** 5628 * @return {@link #supplementalData} (The supplemental data criteria for the measure report, specified as either the name of a valid CQL expression within a referenced library, or a valid FHIR Resource Path.) 5629 */ 5630 public List<MeasureSupplementalDataComponent> getSupplementalData() { 5631 if (this.supplementalData == null) 5632 this.supplementalData = new ArrayList<MeasureSupplementalDataComponent>(); 5633 return this.supplementalData; 5634 } 5635 5636 /** 5637 * @return Returns a reference to <code>this</code> for easy method chaining 5638 */ 5639 public Measure setSupplementalData(List<MeasureSupplementalDataComponent> theSupplementalData) { 5640 this.supplementalData = theSupplementalData; 5641 return this; 5642 } 5643 5644 public boolean hasSupplementalData() { 5645 if (this.supplementalData == null) 5646 return false; 5647 for (MeasureSupplementalDataComponent item : this.supplementalData) 5648 if (!item.isEmpty()) 5649 return true; 5650 return false; 5651 } 5652 5653 public MeasureSupplementalDataComponent addSupplementalData() { //3 5654 MeasureSupplementalDataComponent t = new MeasureSupplementalDataComponent(); 5655 if (this.supplementalData == null) 5656 this.supplementalData = new ArrayList<MeasureSupplementalDataComponent>(); 5657 this.supplementalData.add(t); 5658 return t; 5659 } 5660 5661 public Measure addSupplementalData(MeasureSupplementalDataComponent t) { //3 5662 if (t == null) 5663 return this; 5664 if (this.supplementalData == null) 5665 this.supplementalData = new ArrayList<MeasureSupplementalDataComponent>(); 5666 this.supplementalData.add(t); 5667 return this; 5668 } 5669 5670 /** 5671 * @return The first repetition of repeating field {@link #supplementalData}, creating it if it does not already exist {3} 5672 */ 5673 public MeasureSupplementalDataComponent getSupplementalDataFirstRep() { 5674 if (getSupplementalData().isEmpty()) { 5675 addSupplementalData(); 5676 } 5677 return getSupplementalData().get(0); 5678 } 5679 5680 protected void listChildren(List<Property> children) { 5681 super.listChildren(children); 5682 children.add(new Property("url", "uri", "An absolute URI that is used to identify this measure when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this measure is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the measure is stored on different servers.", 0, 1, url)); 5683 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this measure when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 5684 children.add(new Property("version", "string", "The identifier that is used to identify this version of the measure when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the measure author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 0, 1, version)); 5685 children.add(new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm)); 5686 children.add(new Property("name", "string", "A natural language name identifying the measure. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 5687 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the measure.", 0, 1, title)); 5688 children.add(new Property("subtitle", "string", "An explanatory or alternate title for the measure giving additional information about its content.", 0, 1, subtitle)); 5689 children.add(new Property("status", "code", "The status of this measure. Enables tracking the life-cycle of the content.", 0, 1, status)); 5690 children.add(new Property("experimental", "boolean", "A Boolean value to indicate that this measure is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental)); 5691 children.add(new Property("subject[x]", "CodeableConcept|Reference(Group)", "The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.", 0, 1, subject)); 5692 children.add(new Property("basis", "code", "The population basis specifies the type of elements in the population. For a subject-based measure, this is boolean (because the subject and the population basis are the same, and the population criteria define yes/no values for each individual in the population). For measures that have a population basis that is different than the subject, this element specifies the type of the population basis. For example, an encounter-based measure has a subject of Patient and a population basis of Encounter, and the population criteria all return lists of Encounters.", 0, 1, basis)); 5693 children.add(new Property("date", "dateTime", "The date (and optionally time) when the measure was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the measure changes.", 0, 1, date)); 5694 children.add(new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the measure.", 0, 1, publisher)); 5695 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 5696 children.add(new Property("description", "markdown", "A free text natural language description of the measure from a consumer's perspective.", 0, 1, description)); 5697 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate measure instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 5698 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the measure is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 5699 children.add(new Property("purpose", "markdown", "Explanation of why this measure is needed and why it has been designed as it has.", 0, 1, purpose)); 5700 children.add(new Property("usage", "markdown", "A detailed description, from a clinical perspective, of how the measure is used.", 0, 1, usage)); 5701 children.add(new Property("copyright", "markdown", "A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure.", 0, 1, copyright)); 5702 children.add(new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel)); 5703 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 5704 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate)); 5705 children.add(new Property("effectivePeriod", "Period", "The period during which the measure content was or is planned to be in active use.", 0, 1, effectivePeriod)); 5706 children.add(new Property("topic", "CodeableConcept", "Descriptive topics related to the content of the measure. Topics provide a high-level categorization grouping types of measures that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic)); 5707 children.add(new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author)); 5708 children.add(new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor)); 5709 children.add(new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer)); 5710 children.add(new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser)); 5711 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 5712 children.add(new Property("library", "canonical(Library)", "A reference to a Library resource containing the formal logic used by the measure.", 0, java.lang.Integer.MAX_VALUE, library)); 5713 children.add(new Property("disclaimer", "markdown", "Notices and disclaimers regarding the use of the measure or related to intellectual property (such as code systems) referenced by the measure.", 0, 1, disclaimer)); 5714 children.add(new Property("scoring", "CodeableConcept", "Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented.", 0, 1, scoring)); 5715 children.add(new Property("scoringUnit", "CodeableConcept", "Defines the expected units of measure for the measure score. This element SHOULD be specified as a UCUM unit.", 0, 1, scoringUnit)); 5716 children.add(new Property("compositeScoring", "CodeableConcept", "If this is a composite measure, the scoring method used to combine the component measures to determine the composite score.", 0, 1, compositeScoring)); 5717 children.add(new Property("type", "CodeableConcept", "Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization.", 0, java.lang.Integer.MAX_VALUE, type)); 5718 children.add(new Property("riskAdjustment", "markdown", "A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results.", 0, 1, riskAdjustment)); 5719 children.add(new Property("rateAggregation", "markdown", "Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result.", 0, 1, rateAggregation)); 5720 children.add(new Property("rationale", "markdown", "Provides a succinct statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence.", 0, 1, rationale)); 5721 children.add(new Property("clinicalRecommendationStatement", "markdown", "Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure.", 0, 1, clinicalRecommendationStatement)); 5722 children.add(new Property("improvementNotation", "CodeableConcept", "Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is within a range).", 0, 1, improvementNotation)); 5723 children.add(new Property("term", "", "Provides a description of an individual term used within the measure.", 0, java.lang.Integer.MAX_VALUE, term)); 5724 children.add(new Property("guidance", "markdown", "Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure.", 0, 1, guidance)); 5725 children.add(new Property("group", "", "A group of population criteria for the measure.", 0, java.lang.Integer.MAX_VALUE, group)); 5726 children.add(new Property("supplementalData", "", "The supplemental data criteria for the measure report, specified as either the name of a valid CQL expression within a referenced library, or a valid FHIR Resource Path.", 0, java.lang.Integer.MAX_VALUE, supplementalData)); 5727 } 5728 5729 @Override 5730 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5731 switch (_hash) { 5732 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this measure when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which an authoritative instance of this measure is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the measure is stored on different servers.", 0, 1, url); 5733 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this measure when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 5734 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the measure when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the measure author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 0, 1, version); 5735 case -115699031: /*versionAlgorithm[x]*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 5736 case 1508158071: /*versionAlgorithm*/ return new Property("versionAlgorithm[x]", "string|Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 5737 case 1836908904: /*versionAlgorithmString*/ return new Property("versionAlgorithm[x]", "string", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 5738 case 1373807809: /*versionAlgorithmCoding*/ return new Property("versionAlgorithm[x]", "Coding", "Indicates the mechanism used to compare versions to determine which is more current.", 0, 1, versionAlgorithm); 5739 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the measure. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 5740 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the measure.", 0, 1, title); 5741 case -2060497896: /*subtitle*/ return new Property("subtitle", "string", "An explanatory or alternate title for the measure giving additional information about its content.", 0, 1, subtitle); 5742 case -892481550: /*status*/ return new Property("status", "code", "The status of this measure. Enables tracking the life-cycle of the content.", 0, 1, status); 5743 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A Boolean value to indicate that this measure is authored for testing purposes (or education/evaluation/marketing) and is not intended to be used for genuine usage.", 0, 1, experimental); 5744 case -573640748: /*subject[x]*/ return new Property("subject[x]", "CodeableConcept|Reference(Group)", "The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.", 0, 1, subject); 5745 case -1867885268: /*subject*/ return new Property("subject[x]", "CodeableConcept|Reference(Group)", "The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.", 0, 1, subject); 5746 case -1257122603: /*subjectCodeableConcept*/ return new Property("subject[x]", "CodeableConcept", "The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.", 0, 1, subject); 5747 case 772938623: /*subjectReference*/ return new Property("subject[x]", "Reference(Group)", "The intended subjects for the measure. If this element is not provided, a Patient subject is assumed, but the subject of the measure can be anything.", 0, 1, subject); 5748 case 93508670: /*basis*/ return new Property("basis", "code", "The population basis specifies the type of elements in the population. For a subject-based measure, this is boolean (because the subject and the population basis are the same, and the population criteria define yes/no values for each individual in the population). For measures that have a population basis that is different than the subject, this element specifies the type of the population basis. For example, an encounter-based measure has a subject of Patient and a population basis of Encounter, and the population criteria all return lists of Encounters.", 0, 1, basis); 5749 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the measure was last significantly changed. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the measure changes.", 0, 1, date); 5750 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the organization or individual responsible for the release and ongoing maintenance of the measure.", 0, 1, publisher); 5751 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 5752 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the measure from a consumer's perspective.", 0, 1, description); 5753 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate measure instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 5754 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the measure is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 5755 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explanation of why this measure is needed and why it has been designed as it has.", 0, 1, purpose); 5756 case 111574433: /*usage*/ return new Property("usage", "markdown", "A detailed description, from a clinical perspective, of how the measure is used.", 0, 1, usage); 5757 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure.", 0, 1, copyright); 5758 case 765157229: /*copyrightLabel*/ return new Property("copyrightLabel", "string", "A short string (<50 characters), suitable for inclusion in a page footer that identifies the copyright holder, effective period, and optionally whether rights are resctricted. (e.g. 'All rights reserved', 'Some rights reserved').", 0, 1, copyrightLabel); 5759 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 5760 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 0, 1, lastReviewDate); 5761 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the measure content was or is planned to be in active use.", 0, 1, effectivePeriod); 5762 case 110546223: /*topic*/ return new Property("topic", "CodeableConcept", "Descriptive topics related to the content of the measure. Topics provide a high-level categorization grouping types of measures that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic); 5763 case -1406328437: /*author*/ return new Property("author", "ContactDetail", "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, java.lang.Integer.MAX_VALUE, author); 5764 case -1307827859: /*editor*/ return new Property("editor", "ContactDetail", "An individual or organization primarily responsible for internal coherence of the content.", 0, java.lang.Integer.MAX_VALUE, editor); 5765 case -261190139: /*reviewer*/ return new Property("reviewer", "ContactDetail", "An individual or organization asserted by the publisher to be primarily responsible for review of some aspect of the content.", 0, java.lang.Integer.MAX_VALUE, reviewer); 5766 case 1740277666: /*endorser*/ return new Property("endorser", "ContactDetail", "An individual or organization asserted by the publisher to be responsible for officially endorsing the content for use in some setting.", 0, java.lang.Integer.MAX_VALUE, endorser); 5767 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 5768 case 166208699: /*library*/ return new Property("library", "canonical(Library)", "A reference to a Library resource containing the formal logic used by the measure.", 0, java.lang.Integer.MAX_VALUE, library); 5769 case 432371099: /*disclaimer*/ return new Property("disclaimer", "markdown", "Notices and disclaimers regarding the use of the measure or related to intellectual property (such as code systems) referenced by the measure.", 0, 1, disclaimer); 5770 case 1924005583: /*scoring*/ return new Property("scoring", "CodeableConcept", "Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented.", 0, 1, scoring); 5771 case 1527532787: /*scoringUnit*/ return new Property("scoringUnit", "CodeableConcept", "Defines the expected units of measure for the measure score. This element SHOULD be specified as a UCUM unit.", 0, 1, scoringUnit); 5772 case 569347656: /*compositeScoring*/ return new Property("compositeScoring", "CodeableConcept", "If this is a composite measure, the scoring method used to combine the component measures to determine the composite score.", 0, 1, compositeScoring); 5773 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization.", 0, java.lang.Integer.MAX_VALUE, type); 5774 case 93273500: /*riskAdjustment*/ return new Property("riskAdjustment", "markdown", "A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results.", 0, 1, riskAdjustment); 5775 case 1254503906: /*rateAggregation*/ return new Property("rateAggregation", "markdown", "Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result.", 0, 1, rateAggregation); 5776 case 345689335: /*rationale*/ return new Property("rationale", "markdown", "Provides a succinct statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence.", 0, 1, rationale); 5777 case -18631389: /*clinicalRecommendationStatement*/ return new Property("clinicalRecommendationStatement", "markdown", "Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure.", 0, 1, clinicalRecommendationStatement); 5778 case -2085456136: /*improvementNotation*/ return new Property("improvementNotation", "CodeableConcept", "Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is within a range).", 0, 1, improvementNotation); 5779 case 3556460: /*term*/ return new Property("term", "", "Provides a description of an individual term used within the measure.", 0, java.lang.Integer.MAX_VALUE, term); 5780 case -1314002088: /*guidance*/ return new Property("guidance", "markdown", "Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure.", 0, 1, guidance); 5781 case 98629247: /*group*/ return new Property("group", "", "A group of population criteria for the measure.", 0, java.lang.Integer.MAX_VALUE, group); 5782 case 1447496814: /*supplementalData*/ return new Property("supplementalData", "", "The supplemental data criteria for the measure report, specified as either the name of a valid CQL expression within a referenced library, or a valid FHIR Resource Path.", 0, java.lang.Integer.MAX_VALUE, supplementalData); 5783 default: return super.getNamedProperty(_hash, _name, _checkValid); 5784 } 5785 5786 } 5787 5788 @Override 5789 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5790 switch (hash) { 5791 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 5792 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 5793 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 5794 case 1508158071: /*versionAlgorithm*/ return this.versionAlgorithm == null ? new Base[0] : new Base[] {this.versionAlgorithm}; // DataType 5795 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 5796 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 5797 case -2060497896: /*subtitle*/ return this.subtitle == null ? new Base[0] : new Base[] {this.subtitle}; // StringType 5798 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 5799 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 5800 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // DataType 5801 case 93508670: /*basis*/ return this.basis == null ? new Base[0] : new Base[] {this.basis}; // Enumeration<FHIRTypes> 5802 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 5803 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 5804 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 5805 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 5806 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 5807 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 5808 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 5809 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : new Base[] {this.usage}; // MarkdownType 5810 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 5811 case 765157229: /*copyrightLabel*/ return this.copyrightLabel == null ? new Base[0] : new Base[] {this.copyrightLabel}; // StringType 5812 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 5813 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 5814 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 5815 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 5816 case -1406328437: /*author*/ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 5817 case -1307827859: /*editor*/ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 5818 case -261190139: /*reviewer*/ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 5819 case 1740277666: /*endorser*/ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 5820 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 5821 case 166208699: /*library*/ return this.library == null ? new Base[0] : this.library.toArray(new Base[this.library.size()]); // CanonicalType 5822 case 432371099: /*disclaimer*/ return this.disclaimer == null ? new Base[0] : new Base[] {this.disclaimer}; // MarkdownType 5823 case 1924005583: /*scoring*/ return this.scoring == null ? new Base[0] : new Base[] {this.scoring}; // CodeableConcept 5824 case 1527532787: /*scoringUnit*/ return this.scoringUnit == null ? new Base[0] : new Base[] {this.scoringUnit}; // CodeableConcept 5825 case 569347656: /*compositeScoring*/ return this.compositeScoring == null ? new Base[0] : new Base[] {this.compositeScoring}; // CodeableConcept 5826 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 5827 case 93273500: /*riskAdjustment*/ return this.riskAdjustment == null ? new Base[0] : new Base[] {this.riskAdjustment}; // MarkdownType 5828 case 1254503906: /*rateAggregation*/ return this.rateAggregation == null ? new Base[0] : new Base[] {this.rateAggregation}; // MarkdownType 5829 case 345689335: /*rationale*/ return this.rationale == null ? new Base[0] : new Base[] {this.rationale}; // MarkdownType 5830 case -18631389: /*clinicalRecommendationStatement*/ return this.clinicalRecommendationStatement == null ? new Base[0] : new Base[] {this.clinicalRecommendationStatement}; // MarkdownType 5831 case -2085456136: /*improvementNotation*/ return this.improvementNotation == null ? new Base[0] : new Base[] {this.improvementNotation}; // CodeableConcept 5832 case 3556460: /*term*/ return this.term == null ? new Base[0] : this.term.toArray(new Base[this.term.size()]); // MeasureTermComponent 5833 case -1314002088: /*guidance*/ return this.guidance == null ? new Base[0] : new Base[] {this.guidance}; // MarkdownType 5834 case 98629247: /*group*/ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // MeasureGroupComponent 5835 case 1447496814: /*supplementalData*/ return this.supplementalData == null ? new Base[0] : this.supplementalData.toArray(new Base[this.supplementalData.size()]); // MeasureSupplementalDataComponent 5836 default: return super.getProperty(hash, name, checkValid); 5837 } 5838 5839 } 5840 5841 @Override 5842 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5843 switch (hash) { 5844 case 116079: // url 5845 this.url = TypeConvertor.castToUri(value); // UriType 5846 return value; 5847 case -1618432855: // identifier 5848 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 5849 return value; 5850 case 351608024: // version 5851 this.version = TypeConvertor.castToString(value); // StringType 5852 return value; 5853 case 1508158071: // versionAlgorithm 5854 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 5855 return value; 5856 case 3373707: // name 5857 this.name = TypeConvertor.castToString(value); // StringType 5858 return value; 5859 case 110371416: // title 5860 this.title = TypeConvertor.castToString(value); // StringType 5861 return value; 5862 case -2060497896: // subtitle 5863 this.subtitle = TypeConvertor.castToString(value); // StringType 5864 return value; 5865 case -892481550: // status 5866 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 5867 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 5868 return value; 5869 case -404562712: // experimental 5870 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 5871 return value; 5872 case -1867885268: // subject 5873 this.subject = TypeConvertor.castToType(value); // DataType 5874 return value; 5875 case 93508670: // basis 5876 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 5877 this.basis = (Enumeration) value; // Enumeration<FHIRTypes> 5878 return value; 5879 case 3076014: // date 5880 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 5881 return value; 5882 case 1447404028: // publisher 5883 this.publisher = TypeConvertor.castToString(value); // StringType 5884 return value; 5885 case 951526432: // contact 5886 this.getContact().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 5887 return value; 5888 case -1724546052: // description 5889 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 5890 return value; 5891 case -669707736: // useContext 5892 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); // UsageContext 5893 return value; 5894 case -507075711: // jurisdiction 5895 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5896 return value; 5897 case -220463842: // purpose 5898 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 5899 return value; 5900 case 111574433: // usage 5901 this.usage = TypeConvertor.castToMarkdown(value); // MarkdownType 5902 return value; 5903 case 1522889671: // copyright 5904 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 5905 return value; 5906 case 765157229: // copyrightLabel 5907 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 5908 return value; 5909 case 223539345: // approvalDate 5910 this.approvalDate = TypeConvertor.castToDate(value); // DateType 5911 return value; 5912 case -1687512484: // lastReviewDate 5913 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 5914 return value; 5915 case -403934648: // effectivePeriod 5916 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 5917 return value; 5918 case 110546223: // topic 5919 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5920 return value; 5921 case -1406328437: // author 5922 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 5923 return value; 5924 case -1307827859: // editor 5925 this.getEditor().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 5926 return value; 5927 case -261190139: // reviewer 5928 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 5929 return value; 5930 case 1740277666: // endorser 5931 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); // ContactDetail 5932 return value; 5933 case 666807069: // relatedArtifact 5934 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); // RelatedArtifact 5935 return value; 5936 case 166208699: // library 5937 this.getLibrary().add(TypeConvertor.castToCanonical(value)); // CanonicalType 5938 return value; 5939 case 432371099: // disclaimer 5940 this.disclaimer = TypeConvertor.castToMarkdown(value); // MarkdownType 5941 return value; 5942 case 1924005583: // scoring 5943 this.scoring = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5944 return value; 5945 case 1527532787: // scoringUnit 5946 this.scoringUnit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5947 return value; 5948 case 569347656: // compositeScoring 5949 this.compositeScoring = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5950 return value; 5951 case 3575610: // type 5952 this.getType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 5953 return value; 5954 case 93273500: // riskAdjustment 5955 this.riskAdjustment = TypeConvertor.castToMarkdown(value); // MarkdownType 5956 return value; 5957 case 1254503906: // rateAggregation 5958 this.rateAggregation = TypeConvertor.castToMarkdown(value); // MarkdownType 5959 return value; 5960 case 345689335: // rationale 5961 this.rationale = TypeConvertor.castToMarkdown(value); // MarkdownType 5962 return value; 5963 case -18631389: // clinicalRecommendationStatement 5964 this.clinicalRecommendationStatement = TypeConvertor.castToMarkdown(value); // MarkdownType 5965 return value; 5966 case -2085456136: // improvementNotation 5967 this.improvementNotation = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5968 return value; 5969 case 3556460: // term 5970 this.getTerm().add((MeasureTermComponent) value); // MeasureTermComponent 5971 return value; 5972 case -1314002088: // guidance 5973 this.guidance = TypeConvertor.castToMarkdown(value); // MarkdownType 5974 return value; 5975 case 98629247: // group 5976 this.getGroup().add((MeasureGroupComponent) value); // MeasureGroupComponent 5977 return value; 5978 case 1447496814: // supplementalData 5979 this.getSupplementalData().add((MeasureSupplementalDataComponent) value); // MeasureSupplementalDataComponent 5980 return value; 5981 default: return super.setProperty(hash, name, value); 5982 } 5983 5984 } 5985 5986 @Override 5987 public Base setProperty(String name, Base value) throws FHIRException { 5988 if (name.equals("url")) { 5989 this.url = TypeConvertor.castToUri(value); // UriType 5990 } else if (name.equals("identifier")) { 5991 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 5992 } else if (name.equals("version")) { 5993 this.version = TypeConvertor.castToString(value); // StringType 5994 } else if (name.equals("versionAlgorithm[x]")) { 5995 this.versionAlgorithm = TypeConvertor.castToType(value); // DataType 5996 } else if (name.equals("name")) { 5997 this.name = TypeConvertor.castToString(value); // StringType 5998 } else if (name.equals("title")) { 5999 this.title = TypeConvertor.castToString(value); // StringType 6000 } else if (name.equals("subtitle")) { 6001 this.subtitle = TypeConvertor.castToString(value); // StringType 6002 } else if (name.equals("status")) { 6003 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 6004 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 6005 } else if (name.equals("experimental")) { 6006 this.experimental = TypeConvertor.castToBoolean(value); // BooleanType 6007 } else if (name.equals("subject[x]")) { 6008 this.subject = TypeConvertor.castToType(value); // DataType 6009 } else if (name.equals("basis")) { 6010 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 6011 this.basis = (Enumeration) value; // Enumeration<FHIRTypes> 6012 } else if (name.equals("date")) { 6013 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 6014 } else if (name.equals("publisher")) { 6015 this.publisher = TypeConvertor.castToString(value); // StringType 6016 } else if (name.equals("contact")) { 6017 this.getContact().add(TypeConvertor.castToContactDetail(value)); 6018 } else if (name.equals("description")) { 6019 this.description = TypeConvertor.castToMarkdown(value); // MarkdownType 6020 } else if (name.equals("useContext")) { 6021 this.getUseContext().add(TypeConvertor.castToUsageContext(value)); 6022 } else if (name.equals("jurisdiction")) { 6023 this.getJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 6024 } else if (name.equals("purpose")) { 6025 this.purpose = TypeConvertor.castToMarkdown(value); // MarkdownType 6026 } else if (name.equals("usage")) { 6027 this.usage = TypeConvertor.castToMarkdown(value); // MarkdownType 6028 } else if (name.equals("copyright")) { 6029 this.copyright = TypeConvertor.castToMarkdown(value); // MarkdownType 6030 } else if (name.equals("copyrightLabel")) { 6031 this.copyrightLabel = TypeConvertor.castToString(value); // StringType 6032 } else if (name.equals("approvalDate")) { 6033 this.approvalDate = TypeConvertor.castToDate(value); // DateType 6034 } else if (name.equals("lastReviewDate")) { 6035 this.lastReviewDate = TypeConvertor.castToDate(value); // DateType 6036 } else if (name.equals("effectivePeriod")) { 6037 this.effectivePeriod = TypeConvertor.castToPeriod(value); // Period 6038 } else if (name.equals("topic")) { 6039 this.getTopic().add(TypeConvertor.castToCodeableConcept(value)); 6040 } else if (name.equals("author")) { 6041 this.getAuthor().add(TypeConvertor.castToContactDetail(value)); 6042 } else if (name.equals("editor")) { 6043 this.getEditor().add(TypeConvertor.castToContactDetail(value)); 6044 } else if (name.equals("reviewer")) { 6045 this.getReviewer().add(TypeConvertor.castToContactDetail(value)); 6046 } else if (name.equals("endorser")) { 6047 this.getEndorser().add(TypeConvertor.castToContactDetail(value)); 6048 } else if (name.equals("relatedArtifact")) { 6049 this.getRelatedArtifact().add(TypeConvertor.castToRelatedArtifact(value)); 6050 } else if (name.equals("library")) { 6051 this.getLibrary().add(TypeConvertor.castToCanonical(value)); 6052 } else if (name.equals("disclaimer")) { 6053 this.disclaimer = TypeConvertor.castToMarkdown(value); // MarkdownType 6054 } else if (name.equals("scoring")) { 6055 this.scoring = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6056 } else if (name.equals("scoringUnit")) { 6057 this.scoringUnit = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6058 } else if (name.equals("compositeScoring")) { 6059 this.compositeScoring = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6060 } else if (name.equals("type")) { 6061 this.getType().add(TypeConvertor.castToCodeableConcept(value)); 6062 } else if (name.equals("riskAdjustment")) { 6063 this.riskAdjustment = TypeConvertor.castToMarkdown(value); // MarkdownType 6064 } else if (name.equals("rateAggregation")) { 6065 this.rateAggregation = TypeConvertor.castToMarkdown(value); // MarkdownType 6066 } else if (name.equals("rationale")) { 6067 this.rationale = TypeConvertor.castToMarkdown(value); // MarkdownType 6068 } else if (name.equals("clinicalRecommendationStatement")) { 6069 this.clinicalRecommendationStatement = TypeConvertor.castToMarkdown(value); // MarkdownType 6070 } else if (name.equals("improvementNotation")) { 6071 this.improvementNotation = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6072 } else if (name.equals("term")) { 6073 this.getTerm().add((MeasureTermComponent) value); 6074 } else if (name.equals("guidance")) { 6075 this.guidance = TypeConvertor.castToMarkdown(value); // MarkdownType 6076 } else if (name.equals("group")) { 6077 this.getGroup().add((MeasureGroupComponent) value); 6078 } else if (name.equals("supplementalData")) { 6079 this.getSupplementalData().add((MeasureSupplementalDataComponent) value); 6080 } else 6081 return super.setProperty(name, value); 6082 return value; 6083 } 6084 6085 @Override 6086 public void removeChild(String name, Base value) throws FHIRException { 6087 if (name.equals("url")) { 6088 this.url = null; 6089 } else if (name.equals("identifier")) { 6090 this.getIdentifier().remove(value); 6091 } else if (name.equals("version")) { 6092 this.version = null; 6093 } else if (name.equals("versionAlgorithm[x]")) { 6094 this.versionAlgorithm = null; 6095 } else if (name.equals("name")) { 6096 this.name = null; 6097 } else if (name.equals("title")) { 6098 this.title = null; 6099 } else if (name.equals("subtitle")) { 6100 this.subtitle = null; 6101 } else if (name.equals("status")) { 6102 value = new PublicationStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 6103 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 6104 } else if (name.equals("experimental")) { 6105 this.experimental = null; 6106 } else if (name.equals("subject[x]")) { 6107 this.subject = null; 6108 } else if (name.equals("basis")) { 6109 value = new FHIRTypesEnumFactory().fromType(TypeConvertor.castToCode(value)); 6110 this.basis = (Enumeration) value; // Enumeration<FHIRTypes> 6111 } else if (name.equals("date")) { 6112 this.date = null; 6113 } else if (name.equals("publisher")) { 6114 this.publisher = null; 6115 } else if (name.equals("contact")) { 6116 this.getContact().remove(value); 6117 } else if (name.equals("description")) { 6118 this.description = null; 6119 } else if (name.equals("useContext")) { 6120 this.getUseContext().remove(value); 6121 } else if (name.equals("jurisdiction")) { 6122 this.getJurisdiction().remove(value); 6123 } else if (name.equals("purpose")) { 6124 this.purpose = null; 6125 } else if (name.equals("usage")) { 6126 this.usage = null; 6127 } else if (name.equals("copyright")) { 6128 this.copyright = null; 6129 } else if (name.equals("copyrightLabel")) { 6130 this.copyrightLabel = null; 6131 } else if (name.equals("approvalDate")) { 6132 this.approvalDate = null; 6133 } else if (name.equals("lastReviewDate")) { 6134 this.lastReviewDate = null; 6135 } else if (name.equals("effectivePeriod")) { 6136 this.effectivePeriod = null; 6137 } else if (name.equals("topic")) { 6138 this.getTopic().remove(value); 6139 } else if (name.equals("author")) { 6140 this.getAuthor().remove(value); 6141 } else if (name.equals("editor")) { 6142 this.getEditor().remove(value); 6143 } else if (name.equals("reviewer")) { 6144 this.getReviewer().remove(value); 6145 } else if (name.equals("endorser")) { 6146 this.getEndorser().remove(value); 6147 } else if (name.equals("relatedArtifact")) { 6148 this.getRelatedArtifact().remove(value); 6149 } else if (name.equals("library")) { 6150 this.getLibrary().remove(value); 6151 } else if (name.equals("disclaimer")) { 6152 this.disclaimer = null; 6153 } else if (name.equals("scoring")) { 6154 this.scoring = null; 6155 } else if (name.equals("scoringUnit")) { 6156 this.scoringUnit = null; 6157 } else if (name.equals("compositeScoring")) { 6158 this.compositeScoring = null; 6159 } else if (name.equals("type")) { 6160 this.getType().remove(value); 6161 } else if (name.equals("riskAdjustment")) { 6162 this.riskAdjustment = null; 6163 } else if (name.equals("rateAggregation")) { 6164 this.rateAggregation = null; 6165 } else if (name.equals("rationale")) { 6166 this.rationale = null; 6167 } else if (name.equals("clinicalRecommendationStatement")) { 6168 this.clinicalRecommendationStatement = null; 6169 } else if (name.equals("improvementNotation")) { 6170 this.improvementNotation = null; 6171 } else if (name.equals("term")) { 6172 this.getTerm().remove((MeasureTermComponent) value); 6173 } else if (name.equals("guidance")) { 6174 this.guidance = null; 6175 } else if (name.equals("group")) { 6176 this.getGroup().remove((MeasureGroupComponent) value); 6177 } else if (name.equals("supplementalData")) { 6178 this.getSupplementalData().remove((MeasureSupplementalDataComponent) value); 6179 } else 6180 super.removeChild(name, value); 6181 6182 } 6183 6184 @Override 6185 public Base makeProperty(int hash, String name) throws FHIRException { 6186 switch (hash) { 6187 case 116079: return getUrlElement(); 6188 case -1618432855: return addIdentifier(); 6189 case 351608024: return getVersionElement(); 6190 case -115699031: return getVersionAlgorithm(); 6191 case 1508158071: return getVersionAlgorithm(); 6192 case 3373707: return getNameElement(); 6193 case 110371416: return getTitleElement(); 6194 case -2060497896: return getSubtitleElement(); 6195 case -892481550: return getStatusElement(); 6196 case -404562712: return getExperimentalElement(); 6197 case -573640748: return getSubject(); 6198 case -1867885268: return getSubject(); 6199 case 93508670: return getBasisElement(); 6200 case 3076014: return getDateElement(); 6201 case 1447404028: return getPublisherElement(); 6202 case 951526432: return addContact(); 6203 case -1724546052: return getDescriptionElement(); 6204 case -669707736: return addUseContext(); 6205 case -507075711: return addJurisdiction(); 6206 case -220463842: return getPurposeElement(); 6207 case 111574433: return getUsageElement(); 6208 case 1522889671: return getCopyrightElement(); 6209 case 765157229: return getCopyrightLabelElement(); 6210 case 223539345: return getApprovalDateElement(); 6211 case -1687512484: return getLastReviewDateElement(); 6212 case -403934648: return getEffectivePeriod(); 6213 case 110546223: return addTopic(); 6214 case -1406328437: return addAuthor(); 6215 case -1307827859: return addEditor(); 6216 case -261190139: return addReviewer(); 6217 case 1740277666: return addEndorser(); 6218 case 666807069: return addRelatedArtifact(); 6219 case 166208699: return addLibraryElement(); 6220 case 432371099: return getDisclaimerElement(); 6221 case 1924005583: return getScoring(); 6222 case 1527532787: return getScoringUnit(); 6223 case 569347656: return getCompositeScoring(); 6224 case 3575610: return addType(); 6225 case 93273500: return getRiskAdjustmentElement(); 6226 case 1254503906: return getRateAggregationElement(); 6227 case 345689335: return getRationaleElement(); 6228 case -18631389: return getClinicalRecommendationStatementElement(); 6229 case -2085456136: return getImprovementNotation(); 6230 case 3556460: return addTerm(); 6231 case -1314002088: return getGuidanceElement(); 6232 case 98629247: return addGroup(); 6233 case 1447496814: return addSupplementalData(); 6234 default: return super.makeProperty(hash, name); 6235 } 6236 6237 } 6238 6239 @Override 6240 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6241 switch (hash) { 6242 case 116079: /*url*/ return new String[] {"uri"}; 6243 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 6244 case 351608024: /*version*/ return new String[] {"string"}; 6245 case 1508158071: /*versionAlgorithm*/ return new String[] {"string", "Coding"}; 6246 case 3373707: /*name*/ return new String[] {"string"}; 6247 case 110371416: /*title*/ return new String[] {"string"}; 6248 case -2060497896: /*subtitle*/ return new String[] {"string"}; 6249 case -892481550: /*status*/ return new String[] {"code"}; 6250 case -404562712: /*experimental*/ return new String[] {"boolean"}; 6251 case -1867885268: /*subject*/ return new String[] {"CodeableConcept", "Reference"}; 6252 case 93508670: /*basis*/ return new String[] {"code"}; 6253 case 3076014: /*date*/ return new String[] {"dateTime"}; 6254 case 1447404028: /*publisher*/ return new String[] {"string"}; 6255 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 6256 case -1724546052: /*description*/ return new String[] {"markdown"}; 6257 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 6258 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 6259 case -220463842: /*purpose*/ return new String[] {"markdown"}; 6260 case 111574433: /*usage*/ return new String[] {"markdown"}; 6261 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 6262 case 765157229: /*copyrightLabel*/ return new String[] {"string"}; 6263 case 223539345: /*approvalDate*/ return new String[] {"date"}; 6264 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 6265 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 6266 case 110546223: /*topic*/ return new String[] {"CodeableConcept"}; 6267 case -1406328437: /*author*/ return new String[] {"ContactDetail"}; 6268 case -1307827859: /*editor*/ return new String[] {"ContactDetail"}; 6269 case -261190139: /*reviewer*/ return new String[] {"ContactDetail"}; 6270 case 1740277666: /*endorser*/ return new String[] {"ContactDetail"}; 6271 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 6272 case 166208699: /*library*/ return new String[] {"canonical"}; 6273 case 432371099: /*disclaimer*/ return new String[] {"markdown"}; 6274 case 1924005583: /*scoring*/ return new String[] {"CodeableConcept"}; 6275 case 1527532787: /*scoringUnit*/ return new String[] {"CodeableConcept"}; 6276 case 569347656: /*compositeScoring*/ return new String[] {"CodeableConcept"}; 6277 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 6278 case 93273500: /*riskAdjustment*/ return new String[] {"markdown"}; 6279 case 1254503906: /*rateAggregation*/ return new String[] {"markdown"}; 6280 case 345689335: /*rationale*/ return new String[] {"markdown"}; 6281 case -18631389: /*clinicalRecommendationStatement*/ return new String[] {"markdown"}; 6282 case -2085456136: /*improvementNotation*/ return new String[] {"CodeableConcept"}; 6283 case 3556460: /*term*/ return new String[] {}; 6284 case -1314002088: /*guidance*/ return new String[] {"markdown"}; 6285 case 98629247: /*group*/ return new String[] {}; 6286 case 1447496814: /*supplementalData*/ return new String[] {}; 6287 default: return super.getTypesForProperty(hash, name); 6288 } 6289 6290 } 6291 6292 @Override 6293 public Base addChild(String name) throws FHIRException { 6294 if (name.equals("url")) { 6295 throw new FHIRException("Cannot call addChild on a singleton property Measure.url"); 6296 } 6297 else if (name.equals("identifier")) { 6298 return addIdentifier(); 6299 } 6300 else if (name.equals("version")) { 6301 throw new FHIRException("Cannot call addChild on a singleton property Measure.version"); 6302 } 6303 else if (name.equals("versionAlgorithmString")) { 6304 this.versionAlgorithm = new StringType(); 6305 return this.versionAlgorithm; 6306 } 6307 else if (name.equals("versionAlgorithmCoding")) { 6308 this.versionAlgorithm = new Coding(); 6309 return this.versionAlgorithm; 6310 } 6311 else if (name.equals("name")) { 6312 throw new FHIRException("Cannot call addChild on a singleton property Measure.name"); 6313 } 6314 else if (name.equals("title")) { 6315 throw new FHIRException("Cannot call addChild on a singleton property Measure.title"); 6316 } 6317 else if (name.equals("subtitle")) { 6318 throw new FHIRException("Cannot call addChild on a singleton property Measure.subtitle"); 6319 } 6320 else if (name.equals("status")) { 6321 throw new FHIRException("Cannot call addChild on a singleton property Measure.status"); 6322 } 6323 else if (name.equals("experimental")) { 6324 throw new FHIRException("Cannot call addChild on a singleton property Measure.experimental"); 6325 } 6326 else if (name.equals("subjectCodeableConcept")) { 6327 this.subject = new CodeableConcept(); 6328 return this.subject; 6329 } 6330 else if (name.equals("subjectReference")) { 6331 this.subject = new Reference(); 6332 return this.subject; 6333 } 6334 else if (name.equals("basis")) { 6335 throw new FHIRException("Cannot call addChild on a singleton property Measure.basis"); 6336 } 6337 else if (name.equals("date")) { 6338 throw new FHIRException("Cannot call addChild on a singleton property Measure.date"); 6339 } 6340 else if (name.equals("publisher")) { 6341 throw new FHIRException("Cannot call addChild on a singleton property Measure.publisher"); 6342 } 6343 else if (name.equals("contact")) { 6344 return addContact(); 6345 } 6346 else if (name.equals("description")) { 6347 throw new FHIRException("Cannot call addChild on a singleton property Measure.description"); 6348 } 6349 else if (name.equals("useContext")) { 6350 return addUseContext(); 6351 } 6352 else if (name.equals("jurisdiction")) { 6353 return addJurisdiction(); 6354 } 6355 else if (name.equals("purpose")) { 6356 throw new FHIRException("Cannot call addChild on a singleton property Measure.purpose"); 6357 } 6358 else if (name.equals("usage")) { 6359 throw new FHIRException("Cannot call addChild on a singleton property Measure.usage"); 6360 } 6361 else if (name.equals("copyright")) { 6362 throw new FHIRException("Cannot call addChild on a singleton property Measure.copyright"); 6363 } 6364 else if (name.equals("copyrightLabel")) { 6365 throw new FHIRException("Cannot call addChild on a singleton property Measure.copyrightLabel"); 6366 } 6367 else if (name.equals("approvalDate")) { 6368 throw new FHIRException("Cannot call addChild on a singleton property Measure.approvalDate"); 6369 } 6370 else if (name.equals("lastReviewDate")) { 6371 throw new FHIRException("Cannot call addChild on a singleton property Measure.lastReviewDate"); 6372 } 6373 else if (name.equals("effectivePeriod")) { 6374 this.effectivePeriod = new Period(); 6375 return this.effectivePeriod; 6376 } 6377 else if (name.equals("topic")) { 6378 return addTopic(); 6379 } 6380 else if (name.equals("author")) { 6381 return addAuthor(); 6382 } 6383 else if (name.equals("editor")) { 6384 return addEditor(); 6385 } 6386 else if (name.equals("reviewer")) { 6387 return addReviewer(); 6388 } 6389 else if (name.equals("endorser")) { 6390 return addEndorser(); 6391 } 6392 else if (name.equals("relatedArtifact")) { 6393 return addRelatedArtifact(); 6394 } 6395 else if (name.equals("library")) { 6396 throw new FHIRException("Cannot call addChild on a singleton property Measure.library"); 6397 } 6398 else if (name.equals("disclaimer")) { 6399 throw new FHIRException("Cannot call addChild on a singleton property Measure.disclaimer"); 6400 } 6401 else if (name.equals("scoring")) { 6402 this.scoring = new CodeableConcept(); 6403 return this.scoring; 6404 } 6405 else if (name.equals("scoringUnit")) { 6406 this.scoringUnit = new CodeableConcept(); 6407 return this.scoringUnit; 6408 } 6409 else if (name.equals("compositeScoring")) { 6410 this.compositeScoring = new CodeableConcept(); 6411 return this.compositeScoring; 6412 } 6413 else if (name.equals("type")) { 6414 return addType(); 6415 } 6416 else if (name.equals("riskAdjustment")) { 6417 throw new FHIRException("Cannot call addChild on a singleton property Measure.riskAdjustment"); 6418 } 6419 else if (name.equals("rateAggregation")) { 6420 throw new FHIRException("Cannot call addChild on a singleton property Measure.rateAggregation"); 6421 } 6422 else if (name.equals("rationale")) { 6423 throw new FHIRException("Cannot call addChild on a singleton property Measure.rationale"); 6424 } 6425 else if (name.equals("clinicalRecommendationStatement")) { 6426 throw new FHIRException("Cannot call addChild on a singleton property Measure.clinicalRecommendationStatement"); 6427 } 6428 else if (name.equals("improvementNotation")) { 6429 this.improvementNotation = new CodeableConcept(); 6430 return this.improvementNotation; 6431 } 6432 else if (name.equals("term")) { 6433 return addTerm(); 6434 } 6435 else if (name.equals("guidance")) { 6436 throw new FHIRException("Cannot call addChild on a singleton property Measure.guidance"); 6437 } 6438 else if (name.equals("group")) { 6439 return addGroup(); 6440 } 6441 else if (name.equals("supplementalData")) { 6442 return addSupplementalData(); 6443 } 6444 else 6445 return super.addChild(name); 6446 } 6447 6448 public String fhirType() { 6449 return "Measure"; 6450 6451 } 6452 6453 public Measure copy() { 6454 Measure dst = new Measure(); 6455 copyValues(dst); 6456 return dst; 6457 } 6458 6459 public void copyValues(Measure dst) { 6460 super.copyValues(dst); 6461 dst.url = url == null ? null : url.copy(); 6462 if (identifier != null) { 6463 dst.identifier = new ArrayList<Identifier>(); 6464 for (Identifier i : identifier) 6465 dst.identifier.add(i.copy()); 6466 }; 6467 dst.version = version == null ? null : version.copy(); 6468 dst.versionAlgorithm = versionAlgorithm == null ? null : versionAlgorithm.copy(); 6469 dst.name = name == null ? null : name.copy(); 6470 dst.title = title == null ? null : title.copy(); 6471 dst.subtitle = subtitle == null ? null : subtitle.copy(); 6472 dst.status = status == null ? null : status.copy(); 6473 dst.experimental = experimental == null ? null : experimental.copy(); 6474 dst.subject = subject == null ? null : subject.copy(); 6475 dst.basis = basis == null ? null : basis.copy(); 6476 dst.date = date == null ? null : date.copy(); 6477 dst.publisher = publisher == null ? null : publisher.copy(); 6478 if (contact != null) { 6479 dst.contact = new ArrayList<ContactDetail>(); 6480 for (ContactDetail i : contact) 6481 dst.contact.add(i.copy()); 6482 }; 6483 dst.description = description == null ? null : description.copy(); 6484 if (useContext != null) { 6485 dst.useContext = new ArrayList<UsageContext>(); 6486 for (UsageContext i : useContext) 6487 dst.useContext.add(i.copy()); 6488 }; 6489 if (jurisdiction != null) { 6490 dst.jurisdiction = new ArrayList<CodeableConcept>(); 6491 for (CodeableConcept i : jurisdiction) 6492 dst.jurisdiction.add(i.copy()); 6493 }; 6494 dst.purpose = purpose == null ? null : purpose.copy(); 6495 dst.usage = usage == null ? null : usage.copy(); 6496 dst.copyright = copyright == null ? null : copyright.copy(); 6497 dst.copyrightLabel = copyrightLabel == null ? null : copyrightLabel.copy(); 6498 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 6499 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 6500 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 6501 if (topic != null) { 6502 dst.topic = new ArrayList<CodeableConcept>(); 6503 for (CodeableConcept i : topic) 6504 dst.topic.add(i.copy()); 6505 }; 6506 if (author != null) { 6507 dst.author = new ArrayList<ContactDetail>(); 6508 for (ContactDetail i : author) 6509 dst.author.add(i.copy()); 6510 }; 6511 if (editor != null) { 6512 dst.editor = new ArrayList<ContactDetail>(); 6513 for (ContactDetail i : editor) 6514 dst.editor.add(i.copy()); 6515 }; 6516 if (reviewer != null) { 6517 dst.reviewer = new ArrayList<ContactDetail>(); 6518 for (ContactDetail i : reviewer) 6519 dst.reviewer.add(i.copy()); 6520 }; 6521 if (endorser != null) { 6522 dst.endorser = new ArrayList<ContactDetail>(); 6523 for (ContactDetail i : endorser) 6524 dst.endorser.add(i.copy()); 6525 }; 6526 if (relatedArtifact != null) { 6527 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 6528 for (RelatedArtifact i : relatedArtifact) 6529 dst.relatedArtifact.add(i.copy()); 6530 }; 6531 if (library != null) { 6532 dst.library = new ArrayList<CanonicalType>(); 6533 for (CanonicalType i : library) 6534 dst.library.add(i.copy()); 6535 }; 6536 dst.disclaimer = disclaimer == null ? null : disclaimer.copy(); 6537 dst.scoring = scoring == null ? null : scoring.copy(); 6538 dst.scoringUnit = scoringUnit == null ? null : scoringUnit.copy(); 6539 dst.compositeScoring = compositeScoring == null ? null : compositeScoring.copy(); 6540 if (type != null) { 6541 dst.type = new ArrayList<CodeableConcept>(); 6542 for (CodeableConcept i : type) 6543 dst.type.add(i.copy()); 6544 }; 6545 dst.riskAdjustment = riskAdjustment == null ? null : riskAdjustment.copy(); 6546 dst.rateAggregation = rateAggregation == null ? null : rateAggregation.copy(); 6547 dst.rationale = rationale == null ? null : rationale.copy(); 6548 dst.clinicalRecommendationStatement = clinicalRecommendationStatement == null ? null : clinicalRecommendationStatement.copy(); 6549 dst.improvementNotation = improvementNotation == null ? null : improvementNotation.copy(); 6550 if (term != null) { 6551 dst.term = new ArrayList<MeasureTermComponent>(); 6552 for (MeasureTermComponent i : term) 6553 dst.term.add(i.copy()); 6554 }; 6555 dst.guidance = guidance == null ? null : guidance.copy(); 6556 if (group != null) { 6557 dst.group = new ArrayList<MeasureGroupComponent>(); 6558 for (MeasureGroupComponent i : group) 6559 dst.group.add(i.copy()); 6560 }; 6561 if (supplementalData != null) { 6562 dst.supplementalData = new ArrayList<MeasureSupplementalDataComponent>(); 6563 for (MeasureSupplementalDataComponent i : supplementalData) 6564 dst.supplementalData.add(i.copy()); 6565 }; 6566 } 6567 6568 protected Measure typedCopy() { 6569 return copy(); 6570 } 6571 6572 @Override 6573 public boolean equalsDeep(Base other_) { 6574 if (!super.equalsDeep(other_)) 6575 return false; 6576 if (!(other_ instanceof Measure)) 6577 return false; 6578 Measure o = (Measure) other_; 6579 return compareDeep(url, o.url, true) && compareDeep(identifier, o.identifier, true) && compareDeep(version, o.version, true) 6580 && compareDeep(versionAlgorithm, o.versionAlgorithm, true) && compareDeep(name, o.name, true) && compareDeep(title, o.title, true) 6581 && compareDeep(subtitle, o.subtitle, true) && compareDeep(status, o.status, true) && compareDeep(experimental, o.experimental, true) 6582 && compareDeep(subject, o.subject, true) && compareDeep(basis, o.basis, true) && compareDeep(date, o.date, true) 6583 && compareDeep(publisher, o.publisher, true) && compareDeep(contact, o.contact, true) && compareDeep(description, o.description, true) 6584 && compareDeep(useContext, o.useContext, true) && compareDeep(jurisdiction, o.jurisdiction, true) 6585 && compareDeep(purpose, o.purpose, true) && compareDeep(usage, o.usage, true) && compareDeep(copyright, o.copyright, true) 6586 && compareDeep(copyrightLabel, o.copyrightLabel, true) && compareDeep(approvalDate, o.approvalDate, true) 6587 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 6588 && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) && compareDeep(editor, o.editor, true) 6589 && compareDeep(reviewer, o.reviewer, true) && compareDeep(endorser, o.endorser, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 6590 && compareDeep(library, o.library, true) && compareDeep(disclaimer, o.disclaimer, true) && compareDeep(scoring, o.scoring, true) 6591 && compareDeep(scoringUnit, o.scoringUnit, true) && compareDeep(compositeScoring, o.compositeScoring, true) 6592 && compareDeep(type, o.type, true) && compareDeep(riskAdjustment, o.riskAdjustment, true) && compareDeep(rateAggregation, o.rateAggregation, true) 6593 && compareDeep(rationale, o.rationale, true) && compareDeep(clinicalRecommendationStatement, o.clinicalRecommendationStatement, true) 6594 && compareDeep(improvementNotation, o.improvementNotation, true) && compareDeep(term, o.term, true) 6595 && compareDeep(guidance, o.guidance, true) && compareDeep(group, o.group, true) && compareDeep(supplementalData, o.supplementalData, true) 6596 ; 6597 } 6598 6599 @Override 6600 public boolean equalsShallow(Base other_) { 6601 if (!super.equalsShallow(other_)) 6602 return false; 6603 if (!(other_ instanceof Measure)) 6604 return false; 6605 Measure o = (Measure) other_; 6606 return compareValues(url, o.url, true) && compareValues(version, o.version, true) && compareValues(name, o.name, true) 6607 && compareValues(title, o.title, true) && compareValues(subtitle, o.subtitle, true) && compareValues(status, o.status, true) 6608 && compareValues(experimental, o.experimental, true) && compareValues(basis, o.basis, true) && compareValues(date, o.date, true) 6609 && compareValues(publisher, o.publisher, true) && compareValues(description, o.description, true) && compareValues(purpose, o.purpose, true) 6610 && compareValues(usage, o.usage, true) && compareValues(copyright, o.copyright, true) && compareValues(copyrightLabel, o.copyrightLabel, true) 6611 && compareValues(approvalDate, o.approvalDate, true) && compareValues(lastReviewDate, o.lastReviewDate, true) 6612 && compareValues(library, o.library, true) && compareValues(disclaimer, o.disclaimer, true) && compareValues(riskAdjustment, o.riskAdjustment, true) 6613 && compareValues(rateAggregation, o.rateAggregation, true) && compareValues(rationale, o.rationale, true) 6614 && compareValues(clinicalRecommendationStatement, o.clinicalRecommendationStatement, true) && compareValues(guidance, o.guidance, true) 6615 ; 6616 } 6617 6618 public boolean isEmpty() { 6619 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, identifier, version 6620 , versionAlgorithm, name, title, subtitle, status, experimental, subject, basis 6621 , date, publisher, contact, description, useContext, jurisdiction, purpose, usage 6622 , copyright, copyrightLabel, approvalDate, lastReviewDate, effectivePeriod, topic 6623 , author, editor, reviewer, endorser, relatedArtifact, library, disclaimer, scoring 6624 , scoringUnit, compositeScoring, type, riskAdjustment, rateAggregation, rationale 6625 , clinicalRecommendationStatement, improvementNotation, term, guidance, group, supplementalData 6626 ); 6627 } 6628 6629 @Override 6630 public ResourceType getResourceType() { 6631 return ResourceType.Measure; 6632 } 6633 6634 /** 6635 * Search parameter: <b>context-quantity</b> 6636 * <p> 6637 * Description: <b>Multiple Resources: 6638 6639* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 6640* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 6641* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 6642* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 6643* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 6644* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 6645* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 6646* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 6647* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 6648* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 6649* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 6650* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 6651* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 6652* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 6653* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 6654* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 6655* [Library](library.html): A quantity- or range-valued use context assigned to the library 6656* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 6657* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 6658* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 6659* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 6660* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 6661* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 6662* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 6663* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 6664* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 6665* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 6666* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 6667* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 6668* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 6669</b><br> 6670 * Type: <b>quantity</b><br> 6671 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 6672 * </p> 6673 */ 6674 @SearchParamDefinition(name="context-quantity", path="(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition\r\n* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition\r\n* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide\r\n* [Library](library.html): A quantity- or range-valued use context assigned to the library\r\n* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script\r\n* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set\r\n", type="quantity" ) 6675 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 6676 /** 6677 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 6678 * <p> 6679 * Description: <b>Multiple Resources: 6680 6681* [ActivityDefinition](activitydefinition.html): A quantity- or range-valued use context assigned to the activity definition 6682* [ActorDefinition](actordefinition.html): A quantity- or range-valued use context assigned to the Actor Definition 6683* [CapabilityStatement](capabilitystatement.html): A quantity- or range-valued use context assigned to the capability statement 6684* [ChargeItemDefinition](chargeitemdefinition.html): A quantity- or range-valued use context assigned to the charge item definition 6685* [Citation](citation.html): A quantity- or range-valued use context assigned to the citation 6686* [CodeSystem](codesystem.html): A quantity- or range-valued use context assigned to the code system 6687* [CompartmentDefinition](compartmentdefinition.html): A quantity- or range-valued use context assigned to the compartment definition 6688* [ConceptMap](conceptmap.html): A quantity- or range-valued use context assigned to the concept map 6689* [ConditionDefinition](conditiondefinition.html): A quantity- or range-valued use context assigned to the condition definition 6690* [EventDefinition](eventdefinition.html): A quantity- or range-valued use context assigned to the event definition 6691* [Evidence](evidence.html): A quantity- or range-valued use context assigned to the evidence 6692* [EvidenceReport](evidencereport.html): A quantity- or range-valued use context assigned to the evidence report 6693* [EvidenceVariable](evidencevariable.html): A quantity- or range-valued use context assigned to the evidence variable 6694* [ExampleScenario](examplescenario.html): A quantity- or range-valued use context assigned to the example scenario 6695* [GraphDefinition](graphdefinition.html): A quantity- or range-valued use context assigned to the graph definition 6696* [ImplementationGuide](implementationguide.html): A quantity- or range-valued use context assigned to the implementation guide 6697* [Library](library.html): A quantity- or range-valued use context assigned to the library 6698* [Measure](measure.html): A quantity- or range-valued use context assigned to the measure 6699* [MessageDefinition](messagedefinition.html): A quantity- or range-valued use context assigned to the message definition 6700* [NamingSystem](namingsystem.html): A quantity- or range-valued use context assigned to the naming system 6701* [OperationDefinition](operationdefinition.html): A quantity- or range-valued use context assigned to the operation definition 6702* [PlanDefinition](plandefinition.html): A quantity- or range-valued use context assigned to the plan definition 6703* [Questionnaire](questionnaire.html): A quantity- or range-valued use context assigned to the questionnaire 6704* [Requirements](requirements.html): A quantity- or range-valued use context assigned to the requirements 6705* [SearchParameter](searchparameter.html): A quantity- or range-valued use context assigned to the search parameter 6706* [StructureDefinition](structuredefinition.html): A quantity- or range-valued use context assigned to the structure definition 6707* [StructureMap](structuremap.html): A quantity- or range-valued use context assigned to the structure map 6708* [TerminologyCapabilities](terminologycapabilities.html): A quantity- or range-valued use context assigned to the terminology capabilities 6709* [TestScript](testscript.html): A quantity- or range-valued use context assigned to the test script 6710* [ValueSet](valueset.html): A quantity- or range-valued use context assigned to the value set 6711</b><br> 6712 * Type: <b>quantity</b><br> 6713 * Path: <b>(ActivityDefinition.useContext.value.ofType(Quantity)) | (ActivityDefinition.useContext.value.ofType(Range)) | (ActorDefinition.useContext.value.ofType(Quantity)) | (ActorDefinition.useContext.value.ofType(Range)) | (CapabilityStatement.useContext.value.ofType(Quantity)) | (CapabilityStatement.useContext.value.ofType(Range)) | (ChargeItemDefinition.useContext.value.ofType(Quantity)) | (ChargeItemDefinition.useContext.value.ofType(Range)) | (Citation.useContext.value.ofType(Quantity)) | (Citation.useContext.value.ofType(Range)) | (CodeSystem.useContext.value.ofType(Quantity)) | (CodeSystem.useContext.value.ofType(Range)) | (CompartmentDefinition.useContext.value.ofType(Quantity)) | (CompartmentDefinition.useContext.value.ofType(Range)) | (ConceptMap.useContext.value.ofType(Quantity)) | (ConceptMap.useContext.value.ofType(Range)) | (ConditionDefinition.useContext.value.ofType(Quantity)) | (ConditionDefinition.useContext.value.ofType(Range)) | (EventDefinition.useContext.value.ofType(Quantity)) | (EventDefinition.useContext.value.ofType(Range)) | (Evidence.useContext.value.ofType(Quantity)) | (Evidence.useContext.value.ofType(Range)) | (EvidenceReport.useContext.value.ofType(Quantity)) | (EvidenceReport.useContext.value.ofType(Range)) | (EvidenceVariable.useContext.value.ofType(Quantity)) | (EvidenceVariable.useContext.value.ofType(Range)) | (ExampleScenario.useContext.value.ofType(Quantity)) | (ExampleScenario.useContext.value.ofType(Range)) | (GraphDefinition.useContext.value.ofType(Quantity)) | (GraphDefinition.useContext.value.ofType(Range)) | (ImplementationGuide.useContext.value.ofType(Quantity)) | (ImplementationGuide.useContext.value.ofType(Range)) | (Library.useContext.value.ofType(Quantity)) | (Library.useContext.value.ofType(Range)) | (Measure.useContext.value.ofType(Quantity)) | (Measure.useContext.value.ofType(Range)) | (MessageDefinition.useContext.value.ofType(Quantity)) | (MessageDefinition.useContext.value.ofType(Range)) | (NamingSystem.useContext.value.ofType(Quantity)) | (NamingSystem.useContext.value.ofType(Range)) | (OperationDefinition.useContext.value.ofType(Quantity)) | (OperationDefinition.useContext.value.ofType(Range)) | (PlanDefinition.useContext.value.ofType(Quantity)) | (PlanDefinition.useContext.value.ofType(Range)) | (Questionnaire.useContext.value.ofType(Quantity)) | (Questionnaire.useContext.value.ofType(Range)) | (Requirements.useContext.value.ofType(Quantity)) | (Requirements.useContext.value.ofType(Range)) | (SearchParameter.useContext.value.ofType(Quantity)) | (SearchParameter.useContext.value.ofType(Range)) | (StructureDefinition.useContext.value.ofType(Quantity)) | (StructureDefinition.useContext.value.ofType(Range)) | (StructureMap.useContext.value.ofType(Quantity)) | (StructureMap.useContext.value.ofType(Range)) | (TerminologyCapabilities.useContext.value.ofType(Quantity)) | (TerminologyCapabilities.useContext.value.ofType(Range)) | (TestScript.useContext.value.ofType(Quantity)) | (TestScript.useContext.value.ofType(Range)) | (ValueSet.useContext.value.ofType(Quantity)) | (ValueSet.useContext.value.ofType(Range))</b><br> 6714 * </p> 6715 */ 6716 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_CONTEXT_QUANTITY); 6717 6718 /** 6719 * Search parameter: <b>context-type-quantity</b> 6720 * <p> 6721 * Description: <b>Multiple Resources: 6722 6723* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 6724* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 6725* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 6726* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 6727* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 6728* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 6729* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 6730* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 6731* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 6732* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 6733* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 6734* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 6735* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 6736* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 6737* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 6738* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 6739* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 6740* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 6741* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 6742* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 6743* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 6744* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 6745* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 6746* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 6747* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 6748* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 6749* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 6750* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 6751* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 6752* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 6753</b><br> 6754 * Type: <b>composite</b><br> 6755 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 6756 * </p> 6757 */ 6758 @SearchParamDefinition(name="context-type-quantity", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide\r\n* [Library](library.html): A use context type and quantity- or range-based value assigned to the library\r\n* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context-quantity"} ) 6759 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 6760 /** 6761 * <b>Fluent Client</b> search parameter constant for <b>context-type-quantity</b> 6762 * <p> 6763 * Description: <b>Multiple Resources: 6764 6765* [ActivityDefinition](activitydefinition.html): A use context type and quantity- or range-based value assigned to the activity definition 6766* [ActorDefinition](actordefinition.html): A use context type and quantity- or range-based value assigned to the Actor Definition 6767* [CapabilityStatement](capabilitystatement.html): A use context type and quantity- or range-based value assigned to the capability statement 6768* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and quantity- or range-based value assigned to the charge item definition 6769* [Citation](citation.html): A use context type and quantity- or range-based value assigned to the citation 6770* [CodeSystem](codesystem.html): A use context type and quantity- or range-based value assigned to the code system 6771* [CompartmentDefinition](compartmentdefinition.html): A use context type and quantity- or range-based value assigned to the compartment definition 6772* [ConceptMap](conceptmap.html): A use context type and quantity- or range-based value assigned to the concept map 6773* [ConditionDefinition](conditiondefinition.html): A use context type and quantity- or range-based value assigned to the condition definition 6774* [EventDefinition](eventdefinition.html): A use context type and quantity- or range-based value assigned to the event definition 6775* [Evidence](evidence.html): A use context type and quantity- or range-based value assigned to the evidence 6776* [EvidenceReport](evidencereport.html): A use context type and quantity- or range-based value assigned to the evidence report 6777* [EvidenceVariable](evidencevariable.html): A use context type and quantity- or range-based value assigned to the evidence variable 6778* [ExampleScenario](examplescenario.html): A use context type and quantity- or range-based value assigned to the example scenario 6779* [GraphDefinition](graphdefinition.html): A use context type and quantity- or range-based value assigned to the graph definition 6780* [ImplementationGuide](implementationguide.html): A use context type and quantity- or range-based value assigned to the implementation guide 6781* [Library](library.html): A use context type and quantity- or range-based value assigned to the library 6782* [Measure](measure.html): A use context type and quantity- or range-based value assigned to the measure 6783* [MessageDefinition](messagedefinition.html): A use context type and quantity- or range-based value assigned to the message definition 6784* [NamingSystem](namingsystem.html): A use context type and quantity- or range-based value assigned to the naming system 6785* [OperationDefinition](operationdefinition.html): A use context type and quantity- or range-based value assigned to the operation definition 6786* [PlanDefinition](plandefinition.html): A use context type and quantity- or range-based value assigned to the plan definition 6787* [Questionnaire](questionnaire.html): A use context type and quantity- or range-based value assigned to the questionnaire 6788* [Requirements](requirements.html): A use context type and quantity- or range-based value assigned to the requirements 6789* [SearchParameter](searchparameter.html): A use context type and quantity- or range-based value assigned to the search parameter 6790* [StructureDefinition](structuredefinition.html): A use context type and quantity- or range-based value assigned to the structure definition 6791* [StructureMap](structuremap.html): A use context type and quantity- or range-based value assigned to the structure map 6792* [TerminologyCapabilities](terminologycapabilities.html): A use context type and quantity- or range-based value assigned to the terminology capabilities 6793* [TestScript](testscript.html): A use context type and quantity- or range-based value assigned to the test script 6794* [ValueSet](valueset.html): A use context type and quantity- or range-based value assigned to the value set 6795</b><br> 6796 * Type: <b>composite</b><br> 6797 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 6798 * </p> 6799 */ 6800 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>(SP_CONTEXT_TYPE_QUANTITY); 6801 6802 /** 6803 * Search parameter: <b>context-type-value</b> 6804 * <p> 6805 * Description: <b>Multiple Resources: 6806 6807* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 6808* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 6809* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 6810* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 6811* [Citation](citation.html): A use context type and value assigned to the citation 6812* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 6813* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 6814* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 6815* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 6816* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 6817* [Evidence](evidence.html): A use context type and value assigned to the evidence 6818* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 6819* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 6820* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 6821* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 6822* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 6823* [Library](library.html): A use context type and value assigned to the library 6824* [Measure](measure.html): A use context type and value assigned to the measure 6825* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 6826* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 6827* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 6828* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 6829* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 6830* [Requirements](requirements.html): A use context type and value assigned to the requirements 6831* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 6832* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 6833* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 6834* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 6835* [TestScript](testscript.html): A use context type and value assigned to the test script 6836* [ValueSet](valueset.html): A use context type and value assigned to the value set 6837</b><br> 6838 * Type: <b>composite</b><br> 6839 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 6840 * </p> 6841 */ 6842 @SearchParamDefinition(name="context-type-value", path="ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition\r\n* [Citation](citation.html): A use context type and value assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context type and value assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition\r\n* [Evidence](evidence.html): A use context type and value assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide\r\n* [Library](library.html): A use context type and value assigned to the library\r\n* [Measure](measure.html): A use context type and value assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context type and value assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context type and value assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context type and value assigned to the test script\r\n* [ValueSet](valueset.html): A use context type and value assigned to the value set\r\n", type="composite", compositeOf={"context-type", "context"} ) 6843 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 6844 /** 6845 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 6846 * <p> 6847 * Description: <b>Multiple Resources: 6848 6849* [ActivityDefinition](activitydefinition.html): A use context type and value assigned to the activity definition 6850* [ActorDefinition](actordefinition.html): A use context type and value assigned to the Actor Definition 6851* [CapabilityStatement](capabilitystatement.html): A use context type and value assigned to the capability statement 6852* [ChargeItemDefinition](chargeitemdefinition.html): A use context type and value assigned to the charge item definition 6853* [Citation](citation.html): A use context type and value assigned to the citation 6854* [CodeSystem](codesystem.html): A use context type and value assigned to the code system 6855* [CompartmentDefinition](compartmentdefinition.html): A use context type and value assigned to the compartment definition 6856* [ConceptMap](conceptmap.html): A use context type and value assigned to the concept map 6857* [ConditionDefinition](conditiondefinition.html): A use context type and value assigned to the condition definition 6858* [EventDefinition](eventdefinition.html): A use context type and value assigned to the event definition 6859* [Evidence](evidence.html): A use context type and value assigned to the evidence 6860* [EvidenceReport](evidencereport.html): A use context type and value assigned to the evidence report 6861* [EvidenceVariable](evidencevariable.html): A use context type and value assigned to the evidence variable 6862* [ExampleScenario](examplescenario.html): A use context type and value assigned to the example scenario 6863* [GraphDefinition](graphdefinition.html): A use context type and value assigned to the graph definition 6864* [ImplementationGuide](implementationguide.html): A use context type and value assigned to the implementation guide 6865* [Library](library.html): A use context type and value assigned to the library 6866* [Measure](measure.html): A use context type and value assigned to the measure 6867* [MessageDefinition](messagedefinition.html): A use context type and value assigned to the message definition 6868* [NamingSystem](namingsystem.html): A use context type and value assigned to the naming system 6869* [OperationDefinition](operationdefinition.html): A use context type and value assigned to the operation definition 6870* [PlanDefinition](plandefinition.html): A use context type and value assigned to the plan definition 6871* [Questionnaire](questionnaire.html): A use context type and value assigned to the questionnaire 6872* [Requirements](requirements.html): A use context type and value assigned to the requirements 6873* [SearchParameter](searchparameter.html): A use context type and value assigned to the search parameter 6874* [StructureDefinition](structuredefinition.html): A use context type and value assigned to the structure definition 6875* [StructureMap](structuremap.html): A use context type and value assigned to the structure map 6876* [TerminologyCapabilities](terminologycapabilities.html): A use context type and value assigned to the terminology capabilities 6877* [TestScript](testscript.html): A use context type and value assigned to the test script 6878* [ValueSet](valueset.html): A use context type and value assigned to the value set 6879</b><br> 6880 * Type: <b>composite</b><br> 6881 * Path: <b>ActivityDefinition.useContext | ActorDefinition.useContext | CapabilityStatement.useContext | ChargeItemDefinition.useContext | Citation.useContext | CodeSystem.useContext | CompartmentDefinition.useContext | ConceptMap.useContext | ConditionDefinition.useContext | EventDefinition.useContext | Evidence.useContext | EvidenceReport.useContext | EvidenceVariable.useContext | ExampleScenario.useContext | GraphDefinition.useContext | ImplementationGuide.useContext | Library.useContext | Measure.useContext | MessageDefinition.useContext | NamingSystem.useContext | OperationDefinition.useContext | PlanDefinition.useContext | Questionnaire.useContext | Requirements.useContext | SearchParameter.useContext | StructureDefinition.useContext | StructureMap.useContext | TerminologyCapabilities.useContext | TestScript.useContext | ValueSet.useContext</b><br> 6882 * </p> 6883 */ 6884 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CONTEXT_TYPE_VALUE); 6885 6886 /** 6887 * Search parameter: <b>context-type</b> 6888 * <p> 6889 * Description: <b>Multiple Resources: 6890 6891* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 6892* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 6893* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 6894* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 6895* [Citation](citation.html): A type of use context assigned to the citation 6896* [CodeSystem](codesystem.html): A type of use context assigned to the code system 6897* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 6898* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 6899* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 6900* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 6901* [Evidence](evidence.html): A type of use context assigned to the evidence 6902* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 6903* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 6904* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 6905* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 6906* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 6907* [Library](library.html): A type of use context assigned to the library 6908* [Measure](measure.html): A type of use context assigned to the measure 6909* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 6910* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 6911* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 6912* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 6913* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 6914* [Requirements](requirements.html): A type of use context assigned to the requirements 6915* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 6916* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 6917* [StructureMap](structuremap.html): A type of use context assigned to the structure map 6918* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 6919* [TestScript](testscript.html): A type of use context assigned to the test script 6920* [ValueSet](valueset.html): A type of use context assigned to the value set 6921</b><br> 6922 * Type: <b>token</b><br> 6923 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 6924 * </p> 6925 */ 6926 @SearchParamDefinition(name="context-type", path="ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition\r\n* [Citation](citation.html): A type of use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A type of use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition\r\n* [Evidence](evidence.html): A type of use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide\r\n* [Library](library.html): A type of use context assigned to the library\r\n* [Measure](measure.html): A type of use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A type of use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A type of use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A type of use context assigned to the test script\r\n* [ValueSet](valueset.html): A type of use context assigned to the value set\r\n", type="token" ) 6927 public static final String SP_CONTEXT_TYPE = "context-type"; 6928 /** 6929 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 6930 * <p> 6931 * Description: <b>Multiple Resources: 6932 6933* [ActivityDefinition](activitydefinition.html): A type of use context assigned to the activity definition 6934* [ActorDefinition](actordefinition.html): A type of use context assigned to the Actor Definition 6935* [CapabilityStatement](capabilitystatement.html): A type of use context assigned to the capability statement 6936* [ChargeItemDefinition](chargeitemdefinition.html): A type of use context assigned to the charge item definition 6937* [Citation](citation.html): A type of use context assigned to the citation 6938* [CodeSystem](codesystem.html): A type of use context assigned to the code system 6939* [CompartmentDefinition](compartmentdefinition.html): A type of use context assigned to the compartment definition 6940* [ConceptMap](conceptmap.html): A type of use context assigned to the concept map 6941* [ConditionDefinition](conditiondefinition.html): A type of use context assigned to the condition definition 6942* [EventDefinition](eventdefinition.html): A type of use context assigned to the event definition 6943* [Evidence](evidence.html): A type of use context assigned to the evidence 6944* [EvidenceReport](evidencereport.html): A type of use context assigned to the evidence report 6945* [EvidenceVariable](evidencevariable.html): A type of use context assigned to the evidence variable 6946* [ExampleScenario](examplescenario.html): A type of use context assigned to the example scenario 6947* [GraphDefinition](graphdefinition.html): A type of use context assigned to the graph definition 6948* [ImplementationGuide](implementationguide.html): A type of use context assigned to the implementation guide 6949* [Library](library.html): A type of use context assigned to the library 6950* [Measure](measure.html): A type of use context assigned to the measure 6951* [MessageDefinition](messagedefinition.html): A type of use context assigned to the message definition 6952* [NamingSystem](namingsystem.html): A type of use context assigned to the naming system 6953* [OperationDefinition](operationdefinition.html): A type of use context assigned to the operation definition 6954* [PlanDefinition](plandefinition.html): A type of use context assigned to the plan definition 6955* [Questionnaire](questionnaire.html): A type of use context assigned to the questionnaire 6956* [Requirements](requirements.html): A type of use context assigned to the requirements 6957* [SearchParameter](searchparameter.html): A type of use context assigned to the search parameter 6958* [StructureDefinition](structuredefinition.html): A type of use context assigned to the structure definition 6959* [StructureMap](structuremap.html): A type of use context assigned to the structure map 6960* [TerminologyCapabilities](terminologycapabilities.html): A type of use context assigned to the terminology capabilities 6961* [TestScript](testscript.html): A type of use context assigned to the test script 6962* [ValueSet](valueset.html): A type of use context assigned to the value set 6963</b><br> 6964 * Type: <b>token</b><br> 6965 * Path: <b>ActivityDefinition.useContext.code | ActorDefinition.useContext.code | CapabilityStatement.useContext.code | ChargeItemDefinition.useContext.code | Citation.useContext.code | CodeSystem.useContext.code | CompartmentDefinition.useContext.code | ConceptMap.useContext.code | ConditionDefinition.useContext.code | EventDefinition.useContext.code | Evidence.useContext.code | EvidenceReport.useContext.code | EvidenceVariable.useContext.code | ExampleScenario.useContext.code | GraphDefinition.useContext.code | ImplementationGuide.useContext.code | Library.useContext.code | Measure.useContext.code | MessageDefinition.useContext.code | NamingSystem.useContext.code | OperationDefinition.useContext.code | PlanDefinition.useContext.code | Questionnaire.useContext.code | Requirements.useContext.code | SearchParameter.useContext.code | StructureDefinition.useContext.code | StructureMap.useContext.code | TerminologyCapabilities.useContext.code | TestScript.useContext.code | ValueSet.useContext.code</b><br> 6966 * </p> 6967 */ 6968 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT_TYPE); 6969 6970 /** 6971 * Search parameter: <b>context</b> 6972 * <p> 6973 * Description: <b>Multiple Resources: 6974 6975* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 6976* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 6977* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 6978* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 6979* [Citation](citation.html): A use context assigned to the citation 6980* [CodeSystem](codesystem.html): A use context assigned to the code system 6981* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 6982* [ConceptMap](conceptmap.html): A use context assigned to the concept map 6983* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 6984* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 6985* [Evidence](evidence.html): A use context assigned to the evidence 6986* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 6987* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 6988* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 6989* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 6990* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 6991* [Library](library.html): A use context assigned to the library 6992* [Measure](measure.html): A use context assigned to the measure 6993* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 6994* [NamingSystem](namingsystem.html): A use context assigned to the naming system 6995* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 6996* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 6997* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 6998* [Requirements](requirements.html): A use context assigned to the requirements 6999* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 7000* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 7001* [StructureMap](structuremap.html): A use context assigned to the structure map 7002* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 7003* [TestScript](testscript.html): A use context assigned to the test script 7004* [ValueSet](valueset.html): A use context assigned to the value set 7005</b><br> 7006 * Type: <b>token</b><br> 7007 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 7008 * </p> 7009 */ 7010 @SearchParamDefinition(name="context", path="(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition\r\n* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition\r\n* [Citation](citation.html): A use context assigned to the citation\r\n* [CodeSystem](codesystem.html): A use context assigned to the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition\r\n* [ConceptMap](conceptmap.html): A use context assigned to the concept map\r\n* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition\r\n* [EventDefinition](eventdefinition.html): A use context assigned to the event definition\r\n* [Evidence](evidence.html): A use context assigned to the evidence\r\n* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report\r\n* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable\r\n* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario\r\n* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition\r\n* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide\r\n* [Library](library.html): A use context assigned to the library\r\n* [Measure](measure.html): A use context assigned to the measure\r\n* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition\r\n* [NamingSystem](namingsystem.html): A use context assigned to the naming system\r\n* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition\r\n* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition\r\n* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire\r\n* [Requirements](requirements.html): A use context assigned to the requirements\r\n* [SearchParameter](searchparameter.html): A use context assigned to the search parameter\r\n* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition\r\n* [StructureMap](structuremap.html): A use context assigned to the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities\r\n* [TestScript](testscript.html): A use context assigned to the test script\r\n* [ValueSet](valueset.html): A use context assigned to the value set\r\n", type="token" ) 7011 public static final String SP_CONTEXT = "context"; 7012 /** 7013 * <b>Fluent Client</b> search parameter constant for <b>context</b> 7014 * <p> 7015 * Description: <b>Multiple Resources: 7016 7017* [ActivityDefinition](activitydefinition.html): A use context assigned to the activity definition 7018* [ActorDefinition](actordefinition.html): A use context assigned to the Actor Definition 7019* [CapabilityStatement](capabilitystatement.html): A use context assigned to the capability statement 7020* [ChargeItemDefinition](chargeitemdefinition.html): A use context assigned to the charge item definition 7021* [Citation](citation.html): A use context assigned to the citation 7022* [CodeSystem](codesystem.html): A use context assigned to the code system 7023* [CompartmentDefinition](compartmentdefinition.html): A use context assigned to the compartment definition 7024* [ConceptMap](conceptmap.html): A use context assigned to the concept map 7025* [ConditionDefinition](conditiondefinition.html): A use context assigned to the condition definition 7026* [EventDefinition](eventdefinition.html): A use context assigned to the event definition 7027* [Evidence](evidence.html): A use context assigned to the evidence 7028* [EvidenceReport](evidencereport.html): A use context assigned to the evidence report 7029* [EvidenceVariable](evidencevariable.html): A use context assigned to the evidence variable 7030* [ExampleScenario](examplescenario.html): A use context assigned to the example scenario 7031* [GraphDefinition](graphdefinition.html): A use context assigned to the graph definition 7032* [ImplementationGuide](implementationguide.html): A use context assigned to the implementation guide 7033* [Library](library.html): A use context assigned to the library 7034* [Measure](measure.html): A use context assigned to the measure 7035* [MessageDefinition](messagedefinition.html): A use context assigned to the message definition 7036* [NamingSystem](namingsystem.html): A use context assigned to the naming system 7037* [OperationDefinition](operationdefinition.html): A use context assigned to the operation definition 7038* [PlanDefinition](plandefinition.html): A use context assigned to the plan definition 7039* [Questionnaire](questionnaire.html): A use context assigned to the questionnaire 7040* [Requirements](requirements.html): A use context assigned to the requirements 7041* [SearchParameter](searchparameter.html): A use context assigned to the search parameter 7042* [StructureDefinition](structuredefinition.html): A use context assigned to the structure definition 7043* [StructureMap](structuremap.html): A use context assigned to the structure map 7044* [TerminologyCapabilities](terminologycapabilities.html): A use context assigned to the terminology capabilities 7045* [TestScript](testscript.html): A use context assigned to the test script 7046* [ValueSet](valueset.html): A use context assigned to the value set 7047</b><br> 7048 * Type: <b>token</b><br> 7049 * Path: <b>(ActivityDefinition.useContext.value.ofType(CodeableConcept)) | (ActorDefinition.useContext.value.ofType(CodeableConcept)) | (CapabilityStatement.useContext.value.ofType(CodeableConcept)) | (ChargeItemDefinition.useContext.value.ofType(CodeableConcept)) | (Citation.useContext.value.ofType(CodeableConcept)) | (CodeSystem.useContext.value.ofType(CodeableConcept)) | (CompartmentDefinition.useContext.value.ofType(CodeableConcept)) | (ConceptMap.useContext.value.ofType(CodeableConcept)) | (ConditionDefinition.useContext.value.ofType(CodeableConcept)) | (EventDefinition.useContext.value.ofType(CodeableConcept)) | (Evidence.useContext.value.ofType(CodeableConcept)) | (EvidenceReport.useContext.value.ofType(CodeableConcept)) | (EvidenceVariable.useContext.value.ofType(CodeableConcept)) | (ExampleScenario.useContext.value.ofType(CodeableConcept)) | (GraphDefinition.useContext.value.ofType(CodeableConcept)) | (ImplementationGuide.useContext.value.ofType(CodeableConcept)) | (Library.useContext.value.ofType(CodeableConcept)) | (Measure.useContext.value.ofType(CodeableConcept)) | (MessageDefinition.useContext.value.ofType(CodeableConcept)) | (NamingSystem.useContext.value.ofType(CodeableConcept)) | (OperationDefinition.useContext.value.ofType(CodeableConcept)) | (PlanDefinition.useContext.value.ofType(CodeableConcept)) | (Questionnaire.useContext.value.ofType(CodeableConcept)) | (Requirements.useContext.value.ofType(CodeableConcept)) | (SearchParameter.useContext.value.ofType(CodeableConcept)) | (StructureDefinition.useContext.value.ofType(CodeableConcept)) | (StructureMap.useContext.value.ofType(CodeableConcept)) | (TerminologyCapabilities.useContext.value.ofType(CodeableConcept)) | (TestScript.useContext.value.ofType(CodeableConcept)) | (ValueSet.useContext.value.ofType(CodeableConcept))</b><br> 7050 * </p> 7051 */ 7052 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTEXT); 7053 7054 /** 7055 * Search parameter: <b>date</b> 7056 * <p> 7057 * Description: <b>Multiple Resources: 7058 7059* [ActivityDefinition](activitydefinition.html): The activity definition publication date 7060* [ActorDefinition](actordefinition.html): The Actor Definition publication date 7061* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 7062* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 7063* [Citation](citation.html): The citation publication date 7064* [CodeSystem](codesystem.html): The code system publication date 7065* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 7066* [ConceptMap](conceptmap.html): The concept map publication date 7067* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 7068* [EventDefinition](eventdefinition.html): The event definition publication date 7069* [Evidence](evidence.html): The evidence publication date 7070* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 7071* [ExampleScenario](examplescenario.html): The example scenario publication date 7072* [GraphDefinition](graphdefinition.html): The graph definition publication date 7073* [ImplementationGuide](implementationguide.html): The implementation guide publication date 7074* [Library](library.html): The library publication date 7075* [Measure](measure.html): The measure publication date 7076* [MessageDefinition](messagedefinition.html): The message definition publication date 7077* [NamingSystem](namingsystem.html): The naming system publication date 7078* [OperationDefinition](operationdefinition.html): The operation definition publication date 7079* [PlanDefinition](plandefinition.html): The plan definition publication date 7080* [Questionnaire](questionnaire.html): The questionnaire publication date 7081* [Requirements](requirements.html): The requirements publication date 7082* [SearchParameter](searchparameter.html): The search parameter publication date 7083* [StructureDefinition](structuredefinition.html): The structure definition publication date 7084* [StructureMap](structuremap.html): The structure map publication date 7085* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 7086* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 7087* [TestScript](testscript.html): The test script publication date 7088* [ValueSet](valueset.html): The value set publication date 7089</b><br> 7090 * Type: <b>date</b><br> 7091 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 7092 * </p> 7093 */ 7094 @SearchParamDefinition(name="date", path="ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The activity definition publication date\r\n* [ActorDefinition](actordefinition.html): The Actor Definition publication date\r\n* [CapabilityStatement](capabilitystatement.html): The capability statement publication date\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date\r\n* [Citation](citation.html): The citation publication date\r\n* [CodeSystem](codesystem.html): The code system publication date\r\n* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date\r\n* [ConceptMap](conceptmap.html): The concept map publication date\r\n* [ConditionDefinition](conditiondefinition.html): The condition definition publication date\r\n* [EventDefinition](eventdefinition.html): The event definition publication date\r\n* [Evidence](evidence.html): The evidence publication date\r\n* [EvidenceVariable](evidencevariable.html): The evidence variable publication date\r\n* [ExampleScenario](examplescenario.html): The example scenario publication date\r\n* [GraphDefinition](graphdefinition.html): The graph definition publication date\r\n* [ImplementationGuide](implementationguide.html): The implementation guide publication date\r\n* [Library](library.html): The library publication date\r\n* [Measure](measure.html): The measure publication date\r\n* [MessageDefinition](messagedefinition.html): The message definition publication date\r\n* [NamingSystem](namingsystem.html): The naming system publication date\r\n* [OperationDefinition](operationdefinition.html): The operation definition publication date\r\n* [PlanDefinition](plandefinition.html): The plan definition publication date\r\n* [Questionnaire](questionnaire.html): The questionnaire publication date\r\n* [Requirements](requirements.html): The requirements publication date\r\n* [SearchParameter](searchparameter.html): The search parameter publication date\r\n* [StructureDefinition](structuredefinition.html): The structure definition publication date\r\n* [StructureMap](structuremap.html): The structure map publication date\r\n* [SubscriptionTopic](subscriptiontopic.html): Date status first applied\r\n* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date\r\n* [TestScript](testscript.html): The test script publication date\r\n* [ValueSet](valueset.html): The value set publication date\r\n", type="date" ) 7095 public static final String SP_DATE = "date"; 7096 /** 7097 * <b>Fluent Client</b> search parameter constant for <b>date</b> 7098 * <p> 7099 * Description: <b>Multiple Resources: 7100 7101* [ActivityDefinition](activitydefinition.html): The activity definition publication date 7102* [ActorDefinition](actordefinition.html): The Actor Definition publication date 7103* [CapabilityStatement](capabilitystatement.html): The capability statement publication date 7104* [ChargeItemDefinition](chargeitemdefinition.html): The charge item definition publication date 7105* [Citation](citation.html): The citation publication date 7106* [CodeSystem](codesystem.html): The code system publication date 7107* [CompartmentDefinition](compartmentdefinition.html): The compartment definition publication date 7108* [ConceptMap](conceptmap.html): The concept map publication date 7109* [ConditionDefinition](conditiondefinition.html): The condition definition publication date 7110* [EventDefinition](eventdefinition.html): The event definition publication date 7111* [Evidence](evidence.html): The evidence publication date 7112* [EvidenceVariable](evidencevariable.html): The evidence variable publication date 7113* [ExampleScenario](examplescenario.html): The example scenario publication date 7114* [GraphDefinition](graphdefinition.html): The graph definition publication date 7115* [ImplementationGuide](implementationguide.html): The implementation guide publication date 7116* [Library](library.html): The library publication date 7117* [Measure](measure.html): The measure publication date 7118* [MessageDefinition](messagedefinition.html): The message definition publication date 7119* [NamingSystem](namingsystem.html): The naming system publication date 7120* [OperationDefinition](operationdefinition.html): The operation definition publication date 7121* [PlanDefinition](plandefinition.html): The plan definition publication date 7122* [Questionnaire](questionnaire.html): The questionnaire publication date 7123* [Requirements](requirements.html): The requirements publication date 7124* [SearchParameter](searchparameter.html): The search parameter publication date 7125* [StructureDefinition](structuredefinition.html): The structure definition publication date 7126* [StructureMap](structuremap.html): The structure map publication date 7127* [SubscriptionTopic](subscriptiontopic.html): Date status first applied 7128* [TerminologyCapabilities](terminologycapabilities.html): The terminology capabilities publication date 7129* [TestScript](testscript.html): The test script publication date 7130* [ValueSet](valueset.html): The value set publication date 7131</b><br> 7132 * Type: <b>date</b><br> 7133 * Path: <b>ActivityDefinition.date | ActorDefinition.date | CapabilityStatement.date | ChargeItemDefinition.date | Citation.date | CodeSystem.date | CompartmentDefinition.date | ConceptMap.date | ConditionDefinition.date | EventDefinition.date | Evidence.date | EvidenceVariable.date | ExampleScenario.date | GraphDefinition.date | ImplementationGuide.date | Library.date | Measure.date | MessageDefinition.date | NamingSystem.date | OperationDefinition.date | PlanDefinition.date | Questionnaire.date | Requirements.date | SearchParameter.date | StructureDefinition.date | StructureMap.date | SubscriptionTopic.date | TerminologyCapabilities.date | TestScript.date | ValueSet.date</b><br> 7134 * </p> 7135 */ 7136 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 7137 7138 /** 7139 * Search parameter: <b>description</b> 7140 * <p> 7141 * Description: <b>Multiple Resources: 7142 7143* [ActivityDefinition](activitydefinition.html): The description of the activity definition 7144* [ActorDefinition](actordefinition.html): The description of the Actor Definition 7145* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 7146* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 7147* [Citation](citation.html): The description of the citation 7148* [CodeSystem](codesystem.html): The description of the code system 7149* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 7150* [ConceptMap](conceptmap.html): The description of the concept map 7151* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 7152* [EventDefinition](eventdefinition.html): The description of the event definition 7153* [Evidence](evidence.html): The description of the evidence 7154* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 7155* [GraphDefinition](graphdefinition.html): The description of the graph definition 7156* [ImplementationGuide](implementationguide.html): The description of the implementation guide 7157* [Library](library.html): The description of the library 7158* [Measure](measure.html): The description of the measure 7159* [MessageDefinition](messagedefinition.html): The description of the message definition 7160* [NamingSystem](namingsystem.html): The description of the naming system 7161* [OperationDefinition](operationdefinition.html): The description of the operation definition 7162* [PlanDefinition](plandefinition.html): The description of the plan definition 7163* [Questionnaire](questionnaire.html): The description of the questionnaire 7164* [Requirements](requirements.html): The description of the requirements 7165* [SearchParameter](searchparameter.html): The description of the search parameter 7166* [StructureDefinition](structuredefinition.html): The description of the structure definition 7167* [StructureMap](structuremap.html): The description of the structure map 7168* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 7169* [TestScript](testscript.html): The description of the test script 7170* [ValueSet](valueset.html): The description of the value set 7171</b><br> 7172 * Type: <b>string</b><br> 7173 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 7174 * </p> 7175 */ 7176 @SearchParamDefinition(name="description", path="ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The description of the activity definition\r\n* [ActorDefinition](actordefinition.html): The description of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The description of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition\r\n* [Citation](citation.html): The description of the citation\r\n* [CodeSystem](codesystem.html): The description of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition\r\n* [ConceptMap](conceptmap.html): The description of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The description of the condition definition\r\n* [EventDefinition](eventdefinition.html): The description of the event definition\r\n* [Evidence](evidence.html): The description of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The description of the evidence variable\r\n* [GraphDefinition](graphdefinition.html): The description of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The description of the implementation guide\r\n* [Library](library.html): The description of the library\r\n* [Measure](measure.html): The description of the measure\r\n* [MessageDefinition](messagedefinition.html): The description of the message definition\r\n* [NamingSystem](namingsystem.html): The description of the naming system\r\n* [OperationDefinition](operationdefinition.html): The description of the operation definition\r\n* [PlanDefinition](plandefinition.html): The description of the plan definition\r\n* [Questionnaire](questionnaire.html): The description of the questionnaire\r\n* [Requirements](requirements.html): The description of the requirements\r\n* [SearchParameter](searchparameter.html): The description of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The description of the structure definition\r\n* [StructureMap](structuremap.html): The description of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities\r\n* [TestScript](testscript.html): The description of the test script\r\n* [ValueSet](valueset.html): The description of the value set\r\n", type="string" ) 7177 public static final String SP_DESCRIPTION = "description"; 7178 /** 7179 * <b>Fluent Client</b> search parameter constant for <b>description</b> 7180 * <p> 7181 * Description: <b>Multiple Resources: 7182 7183* [ActivityDefinition](activitydefinition.html): The description of the activity definition 7184* [ActorDefinition](actordefinition.html): The description of the Actor Definition 7185* [CapabilityStatement](capabilitystatement.html): The description of the capability statement 7186* [ChargeItemDefinition](chargeitemdefinition.html): The description of the charge item definition 7187* [Citation](citation.html): The description of the citation 7188* [CodeSystem](codesystem.html): The description of the code system 7189* [CompartmentDefinition](compartmentdefinition.html): The description of the compartment definition 7190* [ConceptMap](conceptmap.html): The description of the concept map 7191* [ConditionDefinition](conditiondefinition.html): The description of the condition definition 7192* [EventDefinition](eventdefinition.html): The description of the event definition 7193* [Evidence](evidence.html): The description of the evidence 7194* [EvidenceVariable](evidencevariable.html): The description of the evidence variable 7195* [GraphDefinition](graphdefinition.html): The description of the graph definition 7196* [ImplementationGuide](implementationguide.html): The description of the implementation guide 7197* [Library](library.html): The description of the library 7198* [Measure](measure.html): The description of the measure 7199* [MessageDefinition](messagedefinition.html): The description of the message definition 7200* [NamingSystem](namingsystem.html): The description of the naming system 7201* [OperationDefinition](operationdefinition.html): The description of the operation definition 7202* [PlanDefinition](plandefinition.html): The description of the plan definition 7203* [Questionnaire](questionnaire.html): The description of the questionnaire 7204* [Requirements](requirements.html): The description of the requirements 7205* [SearchParameter](searchparameter.html): The description of the search parameter 7206* [StructureDefinition](structuredefinition.html): The description of the structure definition 7207* [StructureMap](structuremap.html): The description of the structure map 7208* [TerminologyCapabilities](terminologycapabilities.html): The description of the terminology capabilities 7209* [TestScript](testscript.html): The description of the test script 7210* [ValueSet](valueset.html): The description of the value set 7211</b><br> 7212 * Type: <b>string</b><br> 7213 * Path: <b>ActivityDefinition.description | ActorDefinition.description | CapabilityStatement.description | ChargeItemDefinition.description | Citation.description | CodeSystem.description | CompartmentDefinition.description | ConceptMap.description | ConditionDefinition.description | EventDefinition.description | Evidence.description | EvidenceVariable.description | GraphDefinition.description | ImplementationGuide.description | Library.description | Measure.description | MessageDefinition.description | NamingSystem.description | OperationDefinition.description | PlanDefinition.description | Questionnaire.description | Requirements.description | SearchParameter.description | StructureDefinition.description | StructureMap.description | TerminologyCapabilities.description | TestScript.description | ValueSet.description</b><br> 7214 * </p> 7215 */ 7216 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 7217 7218 /** 7219 * Search parameter: <b>identifier</b> 7220 * <p> 7221 * Description: <b>Multiple Resources: 7222 7223* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 7224* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 7225* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 7226* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 7227* [Citation](citation.html): External identifier for the citation 7228* [CodeSystem](codesystem.html): External identifier for the code system 7229* [ConceptMap](conceptmap.html): External identifier for the concept map 7230* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 7231* [EventDefinition](eventdefinition.html): External identifier for the event definition 7232* [Evidence](evidence.html): External identifier for the evidence 7233* [EvidenceReport](evidencereport.html): External identifier for the evidence report 7234* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 7235* [ExampleScenario](examplescenario.html): External identifier for the example scenario 7236* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 7237* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 7238* [Library](library.html): External identifier for the library 7239* [Measure](measure.html): External identifier for the measure 7240* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 7241* [MessageDefinition](messagedefinition.html): External identifier for the message definition 7242* [NamingSystem](namingsystem.html): External identifier for the naming system 7243* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 7244* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 7245* [PlanDefinition](plandefinition.html): External identifier for the plan definition 7246* [Questionnaire](questionnaire.html): External identifier for the questionnaire 7247* [Requirements](requirements.html): External identifier for the requirements 7248* [SearchParameter](searchparameter.html): External identifier for the search parameter 7249* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 7250* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 7251* [StructureMap](structuremap.html): External identifier for the structure map 7252* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 7253* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 7254* [TestPlan](testplan.html): An identifier for the test plan 7255* [TestScript](testscript.html): External identifier for the test script 7256* [ValueSet](valueset.html): External identifier for the value set 7257</b><br> 7258 * Type: <b>token</b><br> 7259 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 7260 * </p> 7261 */ 7262 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 7263 public static final String SP_IDENTIFIER = "identifier"; 7264 /** 7265 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 7266 * <p> 7267 * Description: <b>Multiple Resources: 7268 7269* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 7270* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 7271* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 7272* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 7273* [Citation](citation.html): External identifier for the citation 7274* [CodeSystem](codesystem.html): External identifier for the code system 7275* [ConceptMap](conceptmap.html): External identifier for the concept map 7276* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 7277* [EventDefinition](eventdefinition.html): External identifier for the event definition 7278* [Evidence](evidence.html): External identifier for the evidence 7279* [EvidenceReport](evidencereport.html): External identifier for the evidence report 7280* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 7281* [ExampleScenario](examplescenario.html): External identifier for the example scenario 7282* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 7283* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 7284* [Library](library.html): External identifier for the library 7285* [Measure](measure.html): External identifier for the measure 7286* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 7287* [MessageDefinition](messagedefinition.html): External identifier for the message definition 7288* [NamingSystem](namingsystem.html): External identifier for the naming system 7289* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 7290* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 7291* [PlanDefinition](plandefinition.html): External identifier for the plan definition 7292* [Questionnaire](questionnaire.html): External identifier for the questionnaire 7293* [Requirements](requirements.html): External identifier for the requirements 7294* [SearchParameter](searchparameter.html): External identifier for the search parameter 7295* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 7296* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 7297* [StructureMap](structuremap.html): External identifier for the structure map 7298* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 7299* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 7300* [TestPlan](testplan.html): An identifier for the test plan 7301* [TestScript](testscript.html): External identifier for the test script 7302* [ValueSet](valueset.html): External identifier for the value set 7303</b><br> 7304 * Type: <b>token</b><br> 7305 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 7306 * </p> 7307 */ 7308 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 7309 7310 /** 7311 * Search parameter: <b>jurisdiction</b> 7312 * <p> 7313 * Description: <b>Multiple Resources: 7314 7315* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 7316* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 7317* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 7318* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 7319* [Citation](citation.html): Intended jurisdiction for the citation 7320* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 7321* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 7322* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 7323* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 7324* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 7325* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 7326* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 7327* [Library](library.html): Intended jurisdiction for the library 7328* [Measure](measure.html): Intended jurisdiction for the measure 7329* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 7330* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 7331* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 7332* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 7333* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 7334* [Requirements](requirements.html): Intended jurisdiction for the requirements 7335* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 7336* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 7337* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 7338* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 7339* [TestScript](testscript.html): Intended jurisdiction for the test script 7340* [ValueSet](valueset.html): Intended jurisdiction for the value set 7341</b><br> 7342 * Type: <b>token</b><br> 7343 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 7344 * </p> 7345 */ 7346 @SearchParamDefinition(name="jurisdiction", path="ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition\r\n* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition\r\n* [Citation](citation.html): Intended jurisdiction for the citation\r\n* [CodeSystem](codesystem.html): Intended jurisdiction for the code system\r\n* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition\r\n* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition\r\n* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario\r\n* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition\r\n* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide\r\n* [Library](library.html): Intended jurisdiction for the library\r\n* [Measure](measure.html): Intended jurisdiction for the measure\r\n* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition\r\n* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system\r\n* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition\r\n* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition\r\n* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire\r\n* [Requirements](requirements.html): Intended jurisdiction for the requirements\r\n* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter\r\n* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition\r\n* [StructureMap](structuremap.html): Intended jurisdiction for the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities\r\n* [TestScript](testscript.html): Intended jurisdiction for the test script\r\n* [ValueSet](valueset.html): Intended jurisdiction for the value set\r\n", type="token" ) 7347 public static final String SP_JURISDICTION = "jurisdiction"; 7348 /** 7349 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 7350 * <p> 7351 * Description: <b>Multiple Resources: 7352 7353* [ActivityDefinition](activitydefinition.html): Intended jurisdiction for the activity definition 7354* [ActorDefinition](actordefinition.html): Intended jurisdiction for the Actor Definition 7355* [CapabilityStatement](capabilitystatement.html): Intended jurisdiction for the capability statement 7356* [ChargeItemDefinition](chargeitemdefinition.html): Intended jurisdiction for the charge item definition 7357* [Citation](citation.html): Intended jurisdiction for the citation 7358* [CodeSystem](codesystem.html): Intended jurisdiction for the code system 7359* [ConceptMap](conceptmap.html): Intended jurisdiction for the concept map 7360* [ConditionDefinition](conditiondefinition.html): Intended jurisdiction for the condition definition 7361* [EventDefinition](eventdefinition.html): Intended jurisdiction for the event definition 7362* [ExampleScenario](examplescenario.html): Intended jurisdiction for the example scenario 7363* [GraphDefinition](graphdefinition.html): Intended jurisdiction for the graph definition 7364* [ImplementationGuide](implementationguide.html): Intended jurisdiction for the implementation guide 7365* [Library](library.html): Intended jurisdiction for the library 7366* [Measure](measure.html): Intended jurisdiction for the measure 7367* [MessageDefinition](messagedefinition.html): Intended jurisdiction for the message definition 7368* [NamingSystem](namingsystem.html): Intended jurisdiction for the naming system 7369* [OperationDefinition](operationdefinition.html): Intended jurisdiction for the operation definition 7370* [PlanDefinition](plandefinition.html): Intended jurisdiction for the plan definition 7371* [Questionnaire](questionnaire.html): Intended jurisdiction for the questionnaire 7372* [Requirements](requirements.html): Intended jurisdiction for the requirements 7373* [SearchParameter](searchparameter.html): Intended jurisdiction for the search parameter 7374* [StructureDefinition](structuredefinition.html): Intended jurisdiction for the structure definition 7375* [StructureMap](structuremap.html): Intended jurisdiction for the structure map 7376* [TerminologyCapabilities](terminologycapabilities.html): Intended jurisdiction for the terminology capabilities 7377* [TestScript](testscript.html): Intended jurisdiction for the test script 7378* [ValueSet](valueset.html): Intended jurisdiction for the value set 7379</b><br> 7380 * Type: <b>token</b><br> 7381 * Path: <b>ActivityDefinition.jurisdiction | ActorDefinition.jurisdiction | CapabilityStatement.jurisdiction | ChargeItemDefinition.jurisdiction | Citation.jurisdiction | CodeSystem.jurisdiction | ConceptMap.jurisdiction | ConditionDefinition.jurisdiction | EventDefinition.jurisdiction | ExampleScenario.jurisdiction | GraphDefinition.jurisdiction | ImplementationGuide.jurisdiction | Library.jurisdiction | Measure.jurisdiction | MessageDefinition.jurisdiction | NamingSystem.jurisdiction | OperationDefinition.jurisdiction | PlanDefinition.jurisdiction | Questionnaire.jurisdiction | Requirements.jurisdiction | SearchParameter.jurisdiction | StructureDefinition.jurisdiction | StructureMap.jurisdiction | TerminologyCapabilities.jurisdiction | TestScript.jurisdiction | ValueSet.jurisdiction</b><br> 7382 * </p> 7383 */ 7384 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 7385 7386 /** 7387 * Search parameter: <b>name</b> 7388 * <p> 7389 * Description: <b>Multiple Resources: 7390 7391* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 7392* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 7393* [Citation](citation.html): Computationally friendly name of the citation 7394* [CodeSystem](codesystem.html): Computationally friendly name of the code system 7395* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 7396* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 7397* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 7398* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 7399* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 7400* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 7401* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 7402* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 7403* [Library](library.html): Computationally friendly name of the library 7404* [Measure](measure.html): Computationally friendly name of the measure 7405* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 7406* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 7407* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 7408* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 7409* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 7410* [Requirements](requirements.html): Computationally friendly name of the requirements 7411* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 7412* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 7413* [StructureMap](structuremap.html): Computationally friendly name of the structure map 7414* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 7415* [TestScript](testscript.html): Computationally friendly name of the test script 7416* [ValueSet](valueset.html): Computationally friendly name of the value set 7417</b><br> 7418 * Type: <b>string</b><br> 7419 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 7420 * </p> 7421 */ 7422 @SearchParamDefinition(name="name", path="ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition\r\n* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement\r\n* [Citation](citation.html): Computationally friendly name of the citation\r\n* [CodeSystem](codesystem.html): Computationally friendly name of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition\r\n* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition\r\n* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide\r\n* [Library](library.html): Computationally friendly name of the library\r\n* [Measure](measure.html): Computationally friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition\r\n* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system\r\n* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire\r\n* [Requirements](requirements.html): Computationally friendly name of the requirements\r\n* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition\r\n* [StructureMap](structuremap.html): Computationally friendly name of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): Computationally friendly name of the test script\r\n* [ValueSet](valueset.html): Computationally friendly name of the value set\r\n", type="string" ) 7423 public static final String SP_NAME = "name"; 7424 /** 7425 * <b>Fluent Client</b> search parameter constant for <b>name</b> 7426 * <p> 7427 * Description: <b>Multiple Resources: 7428 7429* [ActivityDefinition](activitydefinition.html): Computationally friendly name of the activity definition 7430* [CapabilityStatement](capabilitystatement.html): Computationally friendly name of the capability statement 7431* [Citation](citation.html): Computationally friendly name of the citation 7432* [CodeSystem](codesystem.html): Computationally friendly name of the code system 7433* [CompartmentDefinition](compartmentdefinition.html): Computationally friendly name of the compartment definition 7434* [ConceptMap](conceptmap.html): Computationally friendly name of the concept map 7435* [ConditionDefinition](conditiondefinition.html): Computationally friendly name of the condition definition 7436* [EventDefinition](eventdefinition.html): Computationally friendly name of the event definition 7437* [EvidenceVariable](evidencevariable.html): Computationally friendly name of the evidence variable 7438* [ExampleScenario](examplescenario.html): Computationally friendly name of the example scenario 7439* [GraphDefinition](graphdefinition.html): Computationally friendly name of the graph definition 7440* [ImplementationGuide](implementationguide.html): Computationally friendly name of the implementation guide 7441* [Library](library.html): Computationally friendly name of the library 7442* [Measure](measure.html): Computationally friendly name of the measure 7443* [MessageDefinition](messagedefinition.html): Computationally friendly name of the message definition 7444* [NamingSystem](namingsystem.html): Computationally friendly name of the naming system 7445* [OperationDefinition](operationdefinition.html): Computationally friendly name of the operation definition 7446* [PlanDefinition](plandefinition.html): Computationally friendly name of the plan definition 7447* [Questionnaire](questionnaire.html): Computationally friendly name of the questionnaire 7448* [Requirements](requirements.html): Computationally friendly name of the requirements 7449* [SearchParameter](searchparameter.html): Computationally friendly name of the search parameter 7450* [StructureDefinition](structuredefinition.html): Computationally friendly name of the structure definition 7451* [StructureMap](structuremap.html): Computationally friendly name of the structure map 7452* [TerminologyCapabilities](terminologycapabilities.html): Computationally friendly name of the terminology capabilities 7453* [TestScript](testscript.html): Computationally friendly name of the test script 7454* [ValueSet](valueset.html): Computationally friendly name of the value set 7455</b><br> 7456 * Type: <b>string</b><br> 7457 * Path: <b>ActivityDefinition.name | CapabilityStatement.name | Citation.name | CodeSystem.name | CompartmentDefinition.name | ConceptMap.name | ConditionDefinition.name | EventDefinition.name | EvidenceVariable.name | ExampleScenario.name | GraphDefinition.name | ImplementationGuide.name | Library.name | Measure.name | MessageDefinition.name | NamingSystem.name | OperationDefinition.name | PlanDefinition.name | Questionnaire.name | Requirements.name | SearchParameter.name | StructureDefinition.name | StructureMap.name | TerminologyCapabilities.name | TestScript.name | ValueSet.name</b><br> 7458 * </p> 7459 */ 7460 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 7461 7462 /** 7463 * Search parameter: <b>publisher</b> 7464 * <p> 7465 * Description: <b>Multiple Resources: 7466 7467* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 7468* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 7469* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 7470* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 7471* [Citation](citation.html): Name of the publisher of the citation 7472* [CodeSystem](codesystem.html): Name of the publisher of the code system 7473* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 7474* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 7475* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 7476* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 7477* [Evidence](evidence.html): Name of the publisher of the evidence 7478* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 7479* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 7480* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 7481* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 7482* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 7483* [Library](library.html): Name of the publisher of the library 7484* [Measure](measure.html): Name of the publisher of the measure 7485* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 7486* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 7487* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 7488* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 7489* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 7490* [Requirements](requirements.html): Name of the publisher of the requirements 7491* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 7492* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 7493* [StructureMap](structuremap.html): Name of the publisher of the structure map 7494* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 7495* [TestScript](testscript.html): Name of the publisher of the test script 7496* [ValueSet](valueset.html): Name of the publisher of the value set 7497</b><br> 7498 * Type: <b>string</b><br> 7499 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 7500 * </p> 7501 */ 7502 @SearchParamDefinition(name="publisher", path="ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition\r\n* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition\r\n* [Citation](citation.html): Name of the publisher of the citation\r\n* [CodeSystem](codesystem.html): Name of the publisher of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition\r\n* [ConceptMap](conceptmap.html): Name of the publisher of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition\r\n* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition\r\n* [Evidence](evidence.html): Name of the publisher of the evidence\r\n* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable\r\n* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario\r\n* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition\r\n* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide\r\n* [Library](library.html): Name of the publisher of the library\r\n* [Measure](measure.html): Name of the publisher of the measure\r\n* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition\r\n* [NamingSystem](namingsystem.html): Name of the publisher of the naming system\r\n* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition\r\n* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition\r\n* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire\r\n* [Requirements](requirements.html): Name of the publisher of the requirements\r\n* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter\r\n* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition\r\n* [StructureMap](structuremap.html): Name of the publisher of the structure map\r\n* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities\r\n* [TestScript](testscript.html): Name of the publisher of the test script\r\n* [ValueSet](valueset.html): Name of the publisher of the value set\r\n", type="string" ) 7503 public static final String SP_PUBLISHER = "publisher"; 7504 /** 7505 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 7506 * <p> 7507 * Description: <b>Multiple Resources: 7508 7509* [ActivityDefinition](activitydefinition.html): Name of the publisher of the activity definition 7510* [ActorDefinition](actordefinition.html): Name of the publisher of the Actor Definition 7511* [CapabilityStatement](capabilitystatement.html): Name of the publisher of the capability statement 7512* [ChargeItemDefinition](chargeitemdefinition.html): Name of the publisher of the charge item definition 7513* [Citation](citation.html): Name of the publisher of the citation 7514* [CodeSystem](codesystem.html): Name of the publisher of the code system 7515* [CompartmentDefinition](compartmentdefinition.html): Name of the publisher of the compartment definition 7516* [ConceptMap](conceptmap.html): Name of the publisher of the concept map 7517* [ConditionDefinition](conditiondefinition.html): Name of the publisher of the condition definition 7518* [EventDefinition](eventdefinition.html): Name of the publisher of the event definition 7519* [Evidence](evidence.html): Name of the publisher of the evidence 7520* [EvidenceReport](evidencereport.html): Name of the publisher of the evidence report 7521* [EvidenceVariable](evidencevariable.html): Name of the publisher of the evidence variable 7522* [ExampleScenario](examplescenario.html): Name of the publisher of the example scenario 7523* [GraphDefinition](graphdefinition.html): Name of the publisher of the graph definition 7524* [ImplementationGuide](implementationguide.html): Name of the publisher of the implementation guide 7525* [Library](library.html): Name of the publisher of the library 7526* [Measure](measure.html): Name of the publisher of the measure 7527* [MessageDefinition](messagedefinition.html): Name of the publisher of the message definition 7528* [NamingSystem](namingsystem.html): Name of the publisher of the naming system 7529* [OperationDefinition](operationdefinition.html): Name of the publisher of the operation definition 7530* [PlanDefinition](plandefinition.html): Name of the publisher of the plan definition 7531* [Questionnaire](questionnaire.html): Name of the publisher of the questionnaire 7532* [Requirements](requirements.html): Name of the publisher of the requirements 7533* [SearchParameter](searchparameter.html): Name of the publisher of the search parameter 7534* [StructureDefinition](structuredefinition.html): Name of the publisher of the structure definition 7535* [StructureMap](structuremap.html): Name of the publisher of the structure map 7536* [TerminologyCapabilities](terminologycapabilities.html): Name of the publisher of the terminology capabilities 7537* [TestScript](testscript.html): Name of the publisher of the test script 7538* [ValueSet](valueset.html): Name of the publisher of the value set 7539</b><br> 7540 * Type: <b>string</b><br> 7541 * Path: <b>ActivityDefinition.publisher | ActorDefinition.publisher | CapabilityStatement.publisher | ChargeItemDefinition.publisher | Citation.publisher | CodeSystem.publisher | CompartmentDefinition.publisher | ConceptMap.publisher | ConditionDefinition.publisher | EventDefinition.publisher | Evidence.publisher | EvidenceReport.publisher | EvidenceVariable.publisher | ExampleScenario.publisher | GraphDefinition.publisher | ImplementationGuide.publisher | Library.publisher | Measure.publisher | MessageDefinition.publisher | NamingSystem.publisher | OperationDefinition.publisher | PlanDefinition.publisher | Questionnaire.publisher | Requirements.publisher | SearchParameter.publisher | StructureDefinition.publisher | StructureMap.publisher | TerminologyCapabilities.publisher | TestScript.publisher | ValueSet.publisher</b><br> 7542 * </p> 7543 */ 7544 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 7545 7546 /** 7547 * Search parameter: <b>status</b> 7548 * <p> 7549 * Description: <b>Multiple Resources: 7550 7551* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 7552* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 7553* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 7554* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 7555* [Citation](citation.html): The current status of the citation 7556* [CodeSystem](codesystem.html): The current status of the code system 7557* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 7558* [ConceptMap](conceptmap.html): The current status of the concept map 7559* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 7560* [EventDefinition](eventdefinition.html): The current status of the event definition 7561* [Evidence](evidence.html): The current status of the evidence 7562* [EvidenceReport](evidencereport.html): The current status of the evidence report 7563* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 7564* [ExampleScenario](examplescenario.html): The current status of the example scenario 7565* [GraphDefinition](graphdefinition.html): The current status of the graph definition 7566* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 7567* [Library](library.html): The current status of the library 7568* [Measure](measure.html): The current status of the measure 7569* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 7570* [MessageDefinition](messagedefinition.html): The current status of the message definition 7571* [NamingSystem](namingsystem.html): The current status of the naming system 7572* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 7573* [OperationDefinition](operationdefinition.html): The current status of the operation definition 7574* [PlanDefinition](plandefinition.html): The current status of the plan definition 7575* [Questionnaire](questionnaire.html): The current status of the questionnaire 7576* [Requirements](requirements.html): The current status of the requirements 7577* [SearchParameter](searchparameter.html): The current status of the search parameter 7578* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 7579* [StructureDefinition](structuredefinition.html): The current status of the structure definition 7580* [StructureMap](structuremap.html): The current status of the structure map 7581* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 7582* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 7583* [TestPlan](testplan.html): The current status of the test plan 7584* [TestScript](testscript.html): The current status of the test script 7585* [ValueSet](valueset.html): The current status of the value set 7586</b><br> 7587 * Type: <b>token</b><br> 7588 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 7589 * </p> 7590 */ 7591 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 7592 public static final String SP_STATUS = "status"; 7593 /** 7594 * <b>Fluent Client</b> search parameter constant for <b>status</b> 7595 * <p> 7596 * Description: <b>Multiple Resources: 7597 7598* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 7599* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 7600* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 7601* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 7602* [Citation](citation.html): The current status of the citation 7603* [CodeSystem](codesystem.html): The current status of the code system 7604* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 7605* [ConceptMap](conceptmap.html): The current status of the concept map 7606* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 7607* [EventDefinition](eventdefinition.html): The current status of the event definition 7608* [Evidence](evidence.html): The current status of the evidence 7609* [EvidenceReport](evidencereport.html): The current status of the evidence report 7610* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 7611* [ExampleScenario](examplescenario.html): The current status of the example scenario 7612* [GraphDefinition](graphdefinition.html): The current status of the graph definition 7613* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 7614* [Library](library.html): The current status of the library 7615* [Measure](measure.html): The current status of the measure 7616* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 7617* [MessageDefinition](messagedefinition.html): The current status of the message definition 7618* [NamingSystem](namingsystem.html): The current status of the naming system 7619* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 7620* [OperationDefinition](operationdefinition.html): The current status of the operation definition 7621* [PlanDefinition](plandefinition.html): The current status of the plan definition 7622* [Questionnaire](questionnaire.html): The current status of the questionnaire 7623* [Requirements](requirements.html): The current status of the requirements 7624* [SearchParameter](searchparameter.html): The current status of the search parameter 7625* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 7626* [StructureDefinition](structuredefinition.html): The current status of the structure definition 7627* [StructureMap](structuremap.html): The current status of the structure map 7628* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 7629* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 7630* [TestPlan](testplan.html): The current status of the test plan 7631* [TestScript](testscript.html): The current status of the test script 7632* [ValueSet](valueset.html): The current status of the value set 7633</b><br> 7634 * Type: <b>token</b><br> 7635 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 7636 * </p> 7637 */ 7638 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 7639 7640 /** 7641 * Search parameter: <b>title</b> 7642 * <p> 7643 * Description: <b>Multiple Resources: 7644 7645* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 7646* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 7647* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 7648* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 7649* [Citation](citation.html): The human-friendly name of the citation 7650* [CodeSystem](codesystem.html): The human-friendly name of the code system 7651* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 7652* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 7653* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 7654* [Evidence](evidence.html): The human-friendly name of the evidence 7655* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 7656* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 7657* [Library](library.html): The human-friendly name of the library 7658* [Measure](measure.html): The human-friendly name of the measure 7659* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 7660* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 7661* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 7662* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 7663* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 7664* [Requirements](requirements.html): The human-friendly name of the requirements 7665* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 7666* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 7667* [StructureMap](structuremap.html): The human-friendly name of the structure map 7668* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 7669* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 7670* [TestScript](testscript.html): The human-friendly name of the test script 7671* [ValueSet](valueset.html): The human-friendly name of the value set 7672</b><br> 7673 * Type: <b>string</b><br> 7674 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 7675 * </p> 7676 */ 7677 @SearchParamDefinition(name="title", path="ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition\r\n* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition\r\n* [Citation](citation.html): The human-friendly name of the citation\r\n* [CodeSystem](codesystem.html): The human-friendly name of the code system\r\n* [ConceptMap](conceptmap.html): The human-friendly name of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition\r\n* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition\r\n* [Evidence](evidence.html): The human-friendly name of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable\r\n* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide\r\n* [Library](library.html): The human-friendly name of the library\r\n* [Measure](measure.html): The human-friendly name of the measure\r\n* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition\r\n* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition\r\n* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition\r\n* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition\r\n* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire\r\n* [Requirements](requirements.html): The human-friendly name of the requirements\r\n* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition\r\n* [StructureMap](structuremap.html): The human-friendly name of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities\r\n* [TestScript](testscript.html): The human-friendly name of the test script\r\n* [ValueSet](valueset.html): The human-friendly name of the value set\r\n", type="string" ) 7678 public static final String SP_TITLE = "title"; 7679 /** 7680 * <b>Fluent Client</b> search parameter constant for <b>title</b> 7681 * <p> 7682 * Description: <b>Multiple Resources: 7683 7684* [ActivityDefinition](activitydefinition.html): The human-friendly name of the activity definition 7685* [ActorDefinition](actordefinition.html): The human-friendly name of the Actor Definition 7686* [CapabilityStatement](capabilitystatement.html): The human-friendly name of the capability statement 7687* [ChargeItemDefinition](chargeitemdefinition.html): The human-friendly name of the charge item definition 7688* [Citation](citation.html): The human-friendly name of the citation 7689* [CodeSystem](codesystem.html): The human-friendly name of the code system 7690* [ConceptMap](conceptmap.html): The human-friendly name of the concept map 7691* [ConditionDefinition](conditiondefinition.html): The human-friendly name of the condition definition 7692* [EventDefinition](eventdefinition.html): The human-friendly name of the event definition 7693* [Evidence](evidence.html): The human-friendly name of the evidence 7694* [EvidenceVariable](evidencevariable.html): The human-friendly name of the evidence variable 7695* [ImplementationGuide](implementationguide.html): The human-friendly name of the implementation guide 7696* [Library](library.html): The human-friendly name of the library 7697* [Measure](measure.html): The human-friendly name of the measure 7698* [MessageDefinition](messagedefinition.html): The human-friendly name of the message definition 7699* [ObservationDefinition](observationdefinition.html): Human-friendly name of the ObservationDefinition 7700* [OperationDefinition](operationdefinition.html): The human-friendly name of the operation definition 7701* [PlanDefinition](plandefinition.html): The human-friendly name of the plan definition 7702* [Questionnaire](questionnaire.html): The human-friendly name of the questionnaire 7703* [Requirements](requirements.html): The human-friendly name of the requirements 7704* [SpecimenDefinition](specimendefinition.html): Human-friendly name of the SpecimenDefinition 7705* [StructureDefinition](structuredefinition.html): The human-friendly name of the structure definition 7706* [StructureMap](structuremap.html): The human-friendly name of the structure map 7707* [SubscriptionTopic](subscriptiontopic.html): Name for this SubscriptionTopic (Human friendly) 7708* [TerminologyCapabilities](terminologycapabilities.html): The human-friendly name of the terminology capabilities 7709* [TestScript](testscript.html): The human-friendly name of the test script 7710* [ValueSet](valueset.html): The human-friendly name of the value set 7711</b><br> 7712 * Type: <b>string</b><br> 7713 * Path: <b>ActivityDefinition.title | ActorDefinition.title | CapabilityStatement.title | ChargeItemDefinition.title | Citation.title | CodeSystem.title | ConceptMap.title | ConditionDefinition.title | EventDefinition.title | Evidence.title | EvidenceVariable.title | ImplementationGuide.title | Library.title | Measure.title | MessageDefinition.title | ObservationDefinition.title | OperationDefinition.title | PlanDefinition.title | Questionnaire.title | Requirements.title | SpecimenDefinition.title | StructureDefinition.title | StructureMap.title | SubscriptionTopic.title | TerminologyCapabilities.title | TestScript.title | ValueSet.title</b><br> 7714 * </p> 7715 */ 7716 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 7717 7718 /** 7719 * Search parameter: <b>url</b> 7720 * <p> 7721 * Description: <b>Multiple Resources: 7722 7723* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 7724* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 7725* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 7726* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 7727* [Citation](citation.html): The uri that identifies the citation 7728* [CodeSystem](codesystem.html): The uri that identifies the code system 7729* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 7730* [ConceptMap](conceptmap.html): The URI that identifies the concept map 7731* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 7732* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 7733* [Evidence](evidence.html): The uri that identifies the evidence 7734* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 7735* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 7736* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 7737* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 7738* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 7739* [Library](library.html): The uri that identifies the library 7740* [Measure](measure.html): The uri that identifies the measure 7741* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 7742* [NamingSystem](namingsystem.html): The uri that identifies the naming system 7743* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 7744* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 7745* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 7746* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 7747* [Requirements](requirements.html): The uri that identifies the requirements 7748* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 7749* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 7750* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 7751* [StructureMap](structuremap.html): The uri that identifies the structure map 7752* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 7753* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 7754* [TestPlan](testplan.html): The uri that identifies the test plan 7755* [TestScript](testscript.html): The uri that identifies the test script 7756* [ValueSet](valueset.html): The uri that identifies the value set 7757</b><br> 7758 * Type: <b>uri</b><br> 7759 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 7760 * </p> 7761 */ 7762 @SearchParamDefinition(name="url", path="ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition\r\n* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition\r\n* [Citation](citation.html): The uri that identifies the citation\r\n* [CodeSystem](codesystem.html): The uri that identifies the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition\r\n* [ConceptMap](conceptmap.html): The URI that identifies the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition\r\n* [EventDefinition](eventdefinition.html): The uri that identifies the event definition\r\n* [Evidence](evidence.html): The uri that identifies the evidence\r\n* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable\r\n* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario\r\n* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition\r\n* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide\r\n* [Library](library.html): The uri that identifies the library\r\n* [Measure](measure.html): The uri that identifies the measure\r\n* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition\r\n* [NamingSystem](namingsystem.html): The uri that identifies the naming system\r\n* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition\r\n* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition\r\n* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition\r\n* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire\r\n* [Requirements](requirements.html): The uri that identifies the requirements\r\n* [SearchParameter](searchparameter.html): The uri that identifies the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition\r\n* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition\r\n* [StructureMap](structuremap.html): The uri that identifies the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique)\r\n* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities\r\n* [TestPlan](testplan.html): The uri that identifies the test plan\r\n* [TestScript](testscript.html): The uri that identifies the test script\r\n* [ValueSet](valueset.html): The uri that identifies the value set\r\n", type="uri" ) 7763 public static final String SP_URL = "url"; 7764 /** 7765 * <b>Fluent Client</b> search parameter constant for <b>url</b> 7766 * <p> 7767 * Description: <b>Multiple Resources: 7768 7769* [ActivityDefinition](activitydefinition.html): The uri that identifies the activity definition 7770* [ActorDefinition](actordefinition.html): The uri that identifies the Actor Definition 7771* [CapabilityStatement](capabilitystatement.html): The uri that identifies the capability statement 7772* [ChargeItemDefinition](chargeitemdefinition.html): The uri that identifies the charge item definition 7773* [Citation](citation.html): The uri that identifies the citation 7774* [CodeSystem](codesystem.html): The uri that identifies the code system 7775* [CompartmentDefinition](compartmentdefinition.html): The uri that identifies the compartment definition 7776* [ConceptMap](conceptmap.html): The URI that identifies the concept map 7777* [ConditionDefinition](conditiondefinition.html): The uri that identifies the condition definition 7778* [EventDefinition](eventdefinition.html): The uri that identifies the event definition 7779* [Evidence](evidence.html): The uri that identifies the evidence 7780* [EvidenceReport](evidencereport.html): The uri that identifies the evidence report 7781* [EvidenceVariable](evidencevariable.html): The uri that identifies the evidence variable 7782* [ExampleScenario](examplescenario.html): The uri that identifies the example scenario 7783* [GraphDefinition](graphdefinition.html): The uri that identifies the graph definition 7784* [ImplementationGuide](implementationguide.html): The uri that identifies the implementation guide 7785* [Library](library.html): The uri that identifies the library 7786* [Measure](measure.html): The uri that identifies the measure 7787* [MessageDefinition](messagedefinition.html): The uri that identifies the message definition 7788* [NamingSystem](namingsystem.html): The uri that identifies the naming system 7789* [ObservationDefinition](observationdefinition.html): The uri that identifies the observation definition 7790* [OperationDefinition](operationdefinition.html): The uri that identifies the operation definition 7791* [PlanDefinition](plandefinition.html): The uri that identifies the plan definition 7792* [Questionnaire](questionnaire.html): The uri that identifies the questionnaire 7793* [Requirements](requirements.html): The uri that identifies the requirements 7794* [SearchParameter](searchparameter.html): The uri that identifies the search parameter 7795* [SpecimenDefinition](specimendefinition.html): The uri that identifies the specimen definition 7796* [StructureDefinition](structuredefinition.html): The uri that identifies the structure definition 7797* [StructureMap](structuremap.html): The uri that identifies the structure map 7798* [SubscriptionTopic](subscriptiontopic.html): Logical canonical URL to reference this SubscriptionTopic (globally unique) 7799* [TerminologyCapabilities](terminologycapabilities.html): The uri that identifies the terminology capabilities 7800* [TestPlan](testplan.html): The uri that identifies the test plan 7801* [TestScript](testscript.html): The uri that identifies the test script 7802* [ValueSet](valueset.html): The uri that identifies the value set 7803</b><br> 7804 * Type: <b>uri</b><br> 7805 * Path: <b>ActivityDefinition.url | ActorDefinition.url | CapabilityStatement.url | ChargeItemDefinition.url | Citation.url | CodeSystem.url | CompartmentDefinition.url | ConceptMap.url | ConditionDefinition.url | EventDefinition.url | Evidence.url | EvidenceReport.url | EvidenceVariable.url | ExampleScenario.url | GraphDefinition.url | ImplementationGuide.url | Library.url | Measure.url | MessageDefinition.url | NamingSystem.url | ObservationDefinition.url | OperationDefinition.url | PlanDefinition.url | Questionnaire.url | Requirements.url | SearchParameter.url | SpecimenDefinition.url | StructureDefinition.url | StructureMap.url | SubscriptionTopic.url | TerminologyCapabilities.url | TestPlan.url | TestScript.url | ValueSet.url</b><br> 7806 * </p> 7807 */ 7808 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 7809 7810 /** 7811 * Search parameter: <b>version</b> 7812 * <p> 7813 * Description: <b>Multiple Resources: 7814 7815* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 7816* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 7817* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 7818* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 7819* [Citation](citation.html): The business version of the citation 7820* [CodeSystem](codesystem.html): The business version of the code system 7821* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 7822* [ConceptMap](conceptmap.html): The business version of the concept map 7823* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 7824* [EventDefinition](eventdefinition.html): The business version of the event definition 7825* [Evidence](evidence.html): The business version of the evidence 7826* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 7827* [ExampleScenario](examplescenario.html): The business version of the example scenario 7828* [GraphDefinition](graphdefinition.html): The business version of the graph definition 7829* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 7830* [Library](library.html): The business version of the library 7831* [Measure](measure.html): The business version of the measure 7832* [MessageDefinition](messagedefinition.html): The business version of the message definition 7833* [NamingSystem](namingsystem.html): The business version of the naming system 7834* [OperationDefinition](operationdefinition.html): The business version of the operation definition 7835* [PlanDefinition](plandefinition.html): The business version of the plan definition 7836* [Questionnaire](questionnaire.html): The business version of the questionnaire 7837* [Requirements](requirements.html): The business version of the requirements 7838* [SearchParameter](searchparameter.html): The business version of the search parameter 7839* [StructureDefinition](structuredefinition.html): The business version of the structure definition 7840* [StructureMap](structuremap.html): The business version of the structure map 7841* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 7842* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 7843* [TestScript](testscript.html): The business version of the test script 7844* [ValueSet](valueset.html): The business version of the value set 7845</b><br> 7846 * Type: <b>token</b><br> 7847 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 7848 * </p> 7849 */ 7850 @SearchParamDefinition(name="version", path="ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The business version of the activity definition\r\n* [ActorDefinition](actordefinition.html): The business version of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition\r\n* [Citation](citation.html): The business version of the citation\r\n* [CodeSystem](codesystem.html): The business version of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition\r\n* [ConceptMap](conceptmap.html): The business version of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition\r\n* [EventDefinition](eventdefinition.html): The business version of the event definition\r\n* [Evidence](evidence.html): The business version of the evidence\r\n* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The business version of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The business version of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The business version of the implementation guide\r\n* [Library](library.html): The business version of the library\r\n* [Measure](measure.html): The business version of the measure\r\n* [MessageDefinition](messagedefinition.html): The business version of the message definition\r\n* [NamingSystem](namingsystem.html): The business version of the naming system\r\n* [OperationDefinition](operationdefinition.html): The business version of the operation definition\r\n* [PlanDefinition](plandefinition.html): The business version of the plan definition\r\n* [Questionnaire](questionnaire.html): The business version of the questionnaire\r\n* [Requirements](requirements.html): The business version of the requirements\r\n* [SearchParameter](searchparameter.html): The business version of the search parameter\r\n* [StructureDefinition](structuredefinition.html): The business version of the structure definition\r\n* [StructureMap](structuremap.html): The business version of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities\r\n* [TestScript](testscript.html): The business version of the test script\r\n* [ValueSet](valueset.html): The business version of the value set\r\n", type="token" ) 7851 public static final String SP_VERSION = "version"; 7852 /** 7853 * <b>Fluent Client</b> search parameter constant for <b>version</b> 7854 * <p> 7855 * Description: <b>Multiple Resources: 7856 7857* [ActivityDefinition](activitydefinition.html): The business version of the activity definition 7858* [ActorDefinition](actordefinition.html): The business version of the Actor Definition 7859* [CapabilityStatement](capabilitystatement.html): The business version of the capability statement 7860* [ChargeItemDefinition](chargeitemdefinition.html): The business version of the charge item definition 7861* [Citation](citation.html): The business version of the citation 7862* [CodeSystem](codesystem.html): The business version of the code system 7863* [CompartmentDefinition](compartmentdefinition.html): The business version of the compartment definition 7864* [ConceptMap](conceptmap.html): The business version of the concept map 7865* [ConditionDefinition](conditiondefinition.html): The business version of the condition definition 7866* [EventDefinition](eventdefinition.html): The business version of the event definition 7867* [Evidence](evidence.html): The business version of the evidence 7868* [EvidenceVariable](evidencevariable.html): The business version of the evidence variable 7869* [ExampleScenario](examplescenario.html): The business version of the example scenario 7870* [GraphDefinition](graphdefinition.html): The business version of the graph definition 7871* [ImplementationGuide](implementationguide.html): The business version of the implementation guide 7872* [Library](library.html): The business version of the library 7873* [Measure](measure.html): The business version of the measure 7874* [MessageDefinition](messagedefinition.html): The business version of the message definition 7875* [NamingSystem](namingsystem.html): The business version of the naming system 7876* [OperationDefinition](operationdefinition.html): The business version of the operation definition 7877* [PlanDefinition](plandefinition.html): The business version of the plan definition 7878* [Questionnaire](questionnaire.html): The business version of the questionnaire 7879* [Requirements](requirements.html): The business version of the requirements 7880* [SearchParameter](searchparameter.html): The business version of the search parameter 7881* [StructureDefinition](structuredefinition.html): The business version of the structure definition 7882* [StructureMap](structuremap.html): The business version of the structure map 7883* [SubscriptionTopic](subscriptiontopic.html): Business version of the SubscriptionTopic 7884* [TerminologyCapabilities](terminologycapabilities.html): The business version of the terminology capabilities 7885* [TestScript](testscript.html): The business version of the test script 7886* [ValueSet](valueset.html): The business version of the value set 7887</b><br> 7888 * Type: <b>token</b><br> 7889 * Path: <b>ActivityDefinition.version | ActorDefinition.version | CapabilityStatement.version | ChargeItemDefinition.version | Citation.version | CodeSystem.version | CompartmentDefinition.version | ConceptMap.version | ConditionDefinition.version | EventDefinition.version | Evidence.version | EvidenceVariable.version | ExampleScenario.version | GraphDefinition.version | ImplementationGuide.version | Library.version | Measure.version | MessageDefinition.version | NamingSystem.version | OperationDefinition.version | PlanDefinition.version | Questionnaire.version | Requirements.version | SearchParameter.version | StructureDefinition.version | StructureMap.version | SubscriptionTopic.version | TerminologyCapabilities.version | TestScript.version | ValueSet.version</b><br> 7890 * </p> 7891 */ 7892 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 7893 7894 /** 7895 * Search parameter: <b>composed-of</b> 7896 * <p> 7897 * Description: <b>Multiple Resources: 7898 7899* [ActivityDefinition](activitydefinition.html): What resource is being referenced 7900* [EventDefinition](eventdefinition.html): What resource is being referenced 7901* [EvidenceVariable](evidencevariable.html): What resource is being referenced 7902* [Library](library.html): What resource is being referenced 7903* [Measure](measure.html): What resource is being referenced 7904* [PlanDefinition](plandefinition.html): What resource is being referenced 7905</b><br> 7906 * Type: <b>reference</b><br> 7907 * Path: <b>ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource</b><br> 7908 * </p> 7909 */ 7910 @SearchParamDefinition(name="composed-of", path="ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 7911 public static final String SP_COMPOSED_OF = "composed-of"; 7912 /** 7913 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 7914 * <p> 7915 * Description: <b>Multiple Resources: 7916 7917* [ActivityDefinition](activitydefinition.html): What resource is being referenced 7918* [EventDefinition](eventdefinition.html): What resource is being referenced 7919* [EvidenceVariable](evidencevariable.html): What resource is being referenced 7920* [Library](library.html): What resource is being referenced 7921* [Measure](measure.html): What resource is being referenced 7922* [PlanDefinition](plandefinition.html): What resource is being referenced 7923</b><br> 7924 * Type: <b>reference</b><br> 7925 * Path: <b>ActivityDefinition.relatedArtifact.where(type='composed-of').resource | EventDefinition.relatedArtifact.where(type='composed-of').resource | EvidenceVariable.relatedArtifact.where(type='composed-of').resource | Library.relatedArtifact.where(type='composed-of').resource | Measure.relatedArtifact.where(type='composed-of').resource | PlanDefinition.relatedArtifact.where(type='composed-of').resource</b><br> 7926 * </p> 7927 */ 7928 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPOSED_OF); 7929 7930/** 7931 * Constant for fluent queries to be used to add include statements. Specifies 7932 * the path value of "<b>Measure:composed-of</b>". 7933 */ 7934 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include("Measure:composed-of").toLocked(); 7935 7936 /** 7937 * Search parameter: <b>depends-on</b> 7938 * <p> 7939 * Description: <b>Multiple Resources: 7940 7941* [ActivityDefinition](activitydefinition.html): What resource is being referenced 7942* [EventDefinition](eventdefinition.html): What resource is being referenced 7943* [EvidenceVariable](evidencevariable.html): What resource is being referenced 7944* [Library](library.html): What resource is being referenced 7945* [Measure](measure.html): What resource is being referenced 7946* [PlanDefinition](plandefinition.html): What resource is being referenced 7947</b><br> 7948 * Type: <b>reference</b><br> 7949 * Path: <b>ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library</b><br> 7950 * </p> 7951 */ 7952 @SearchParamDefinition(name="depends-on", path="ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 7953 public static final String SP_DEPENDS_ON = "depends-on"; 7954 /** 7955 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 7956 * <p> 7957 * Description: <b>Multiple Resources: 7958 7959* [ActivityDefinition](activitydefinition.html): What resource is being referenced 7960* [EventDefinition](eventdefinition.html): What resource is being referenced 7961* [EvidenceVariable](evidencevariable.html): What resource is being referenced 7962* [Library](library.html): What resource is being referenced 7963* [Measure](measure.html): What resource is being referenced 7964* [PlanDefinition](plandefinition.html): What resource is being referenced 7965</b><br> 7966 * Type: <b>reference</b><br> 7967 * Path: <b>ActivityDefinition.relatedArtifact.where(type='depends-on').resource | ActivityDefinition.library | EventDefinition.relatedArtifact.where(type='depends-on').resource | EvidenceVariable.relatedArtifact.where(type='depends-on').resource | Library.relatedArtifact.where(type='depends-on').resource | Measure.relatedArtifact.where(type='depends-on').resource | Measure.library | PlanDefinition.relatedArtifact.where(type='depends-on').resource | PlanDefinition.library</b><br> 7968 * </p> 7969 */ 7970 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEPENDS_ON); 7971 7972/** 7973 * Constant for fluent queries to be used to add include statements. Specifies 7974 * the path value of "<b>Measure:depends-on</b>". 7975 */ 7976 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include("Measure:depends-on").toLocked(); 7977 7978 /** 7979 * Search parameter: <b>derived-from</b> 7980 * <p> 7981 * Description: <b>Multiple Resources: 7982 7983* [ActivityDefinition](activitydefinition.html): What resource is being referenced 7984* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 7985* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 7986* [EventDefinition](eventdefinition.html): What resource is being referenced 7987* [EvidenceVariable](evidencevariable.html): What resource is being referenced 7988* [Library](library.html): What resource is being referenced 7989* [Measure](measure.html): What resource is being referenced 7990* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 7991* [PlanDefinition](plandefinition.html): What resource is being referenced 7992* [ValueSet](valueset.html): A resource that the ValueSet is derived from 7993</b><br> 7994 * Type: <b>reference</b><br> 7995 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 7996 * </p> 7997 */ 7998 @SearchParamDefinition(name="derived-from", path="ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from\r\n* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): A resource that the ValueSet is derived from\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 7999 public static final String SP_DERIVED_FROM = "derived-from"; 8000 /** 8001 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 8002 * <p> 8003 * Description: <b>Multiple Resources: 8004 8005* [ActivityDefinition](activitydefinition.html): What resource is being referenced 8006* [CodeSystem](codesystem.html): A resource that the CodeSystem is derived from 8007* [ConceptMap](conceptmap.html): A resource that the ConceptMap is derived from 8008* [EventDefinition](eventdefinition.html): What resource is being referenced 8009* [EvidenceVariable](evidencevariable.html): What resource is being referenced 8010* [Library](library.html): What resource is being referenced 8011* [Measure](measure.html): What resource is being referenced 8012* [NamingSystem](namingsystem.html): A resource that the NamingSystem is derived from 8013* [PlanDefinition](plandefinition.html): What resource is being referenced 8014* [ValueSet](valueset.html): A resource that the ValueSet is derived from 8015</b><br> 8016 * Type: <b>reference</b><br> 8017 * Path: <b>ActivityDefinition.relatedArtifact.where(type='derived-from').resource | CodeSystem.relatedArtifact.where(type='derived-from').resource | ConceptMap.relatedArtifact.where(type='derived-from').resource | EventDefinition.relatedArtifact.where(type='derived-from').resource | EvidenceVariable.relatedArtifact.where(type='derived-from').resource | Library.relatedArtifact.where(type='derived-from').resource | Measure.relatedArtifact.where(type='derived-from').resource | NamingSystem.relatedArtifact.where(type='derived-from').resource | PlanDefinition.relatedArtifact.where(type='derived-from').resource | ValueSet.relatedArtifact.where(type='derived-from').resource</b><br> 8018 * </p> 8019 */ 8020 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 8021 8022/** 8023 * Constant for fluent queries to be used to add include statements. Specifies 8024 * the path value of "<b>Measure:derived-from</b>". 8025 */ 8026 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("Measure:derived-from").toLocked(); 8027 8028 /** 8029 * Search parameter: <b>effective</b> 8030 * <p> 8031 * Description: <b>Multiple Resources: 8032 8033* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 8034* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 8035* [Citation](citation.html): The time during which the citation is intended to be in use 8036* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 8037* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 8038* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 8039* [Library](library.html): The time during which the library is intended to be in use 8040* [Measure](measure.html): The time during which the measure is intended to be in use 8041* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 8042* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 8043* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 8044* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 8045</b><br> 8046 * Type: <b>date</b><br> 8047 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 8048 * </p> 8049 */ 8050 @SearchParamDefinition(name="effective", path="ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use\r\n* [Citation](citation.html): The time during which the citation is intended to be in use\r\n* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use\r\n* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use\r\n* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use\r\n* [Library](library.html): The time during which the library is intended to be in use\r\n* [Measure](measure.html): The time during which the measure is intended to be in use\r\n* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use\r\n* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use\r\n* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use\r\n* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use\r\n", type="date" ) 8051 public static final String SP_EFFECTIVE = "effective"; 8052 /** 8053 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 8054 * <p> 8055 * Description: <b>Multiple Resources: 8056 8057* [ActivityDefinition](activitydefinition.html): The time during which the activity definition is intended to be in use 8058* [ChargeItemDefinition](chargeitemdefinition.html): The time during which the charge item definition is intended to be in use 8059* [Citation](citation.html): The time during which the citation is intended to be in use 8060* [CodeSystem](codesystem.html): The time during which the CodeSystem is intended to be in use 8061* [ConceptMap](conceptmap.html): The time during which the ConceptMap is intended to be in use 8062* [EventDefinition](eventdefinition.html): The time during which the event definition is intended to be in use 8063* [Library](library.html): The time during which the library is intended to be in use 8064* [Measure](measure.html): The time during which the measure is intended to be in use 8065* [NamingSystem](namingsystem.html): The time during which the NamingSystem is intended to be in use 8066* [PlanDefinition](plandefinition.html): The time during which the plan definition is intended to be in use 8067* [Questionnaire](questionnaire.html): The time during which the questionnaire is intended to be in use 8068* [ValueSet](valueset.html): The time during which the ValueSet is intended to be in use 8069</b><br> 8070 * Type: <b>date</b><br> 8071 * Path: <b>ActivityDefinition.effectivePeriod | ChargeItemDefinition.applicability.effectivePeriod | Citation.effectivePeriod | CodeSystem.effectivePeriod | ConceptMap.effectivePeriod | EventDefinition.effectivePeriod | Library.effectivePeriod | Measure.effectivePeriod | NamingSystem.effectivePeriod | PlanDefinition.effectivePeriod | Questionnaire.effectivePeriod | ValueSet.effectivePeriod</b><br> 8072 * </p> 8073 */ 8074 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 8075 8076 /** 8077 * Search parameter: <b>predecessor</b> 8078 * <p> 8079 * Description: <b>Multiple Resources: 8080 8081* [ActivityDefinition](activitydefinition.html): What resource is being referenced 8082* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 8083* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 8084* [EventDefinition](eventdefinition.html): What resource is being referenced 8085* [EvidenceVariable](evidencevariable.html): What resource is being referenced 8086* [Library](library.html): What resource is being referenced 8087* [Measure](measure.html): What resource is being referenced 8088* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 8089* [PlanDefinition](plandefinition.html): What resource is being referenced 8090* [ValueSet](valueset.html): The predecessor of the ValueSet 8091</b><br> 8092 * Type: <b>reference</b><br> 8093 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 8094 * </p> 8095 */ 8096 @SearchParamDefinition(name="predecessor", path="ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [CodeSystem](codesystem.html): The predecessor of the CodeSystem\r\n* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n* [ValueSet](valueset.html): The predecessor of the ValueSet\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 8097 public static final String SP_PREDECESSOR = "predecessor"; 8098 /** 8099 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 8100 * <p> 8101 * Description: <b>Multiple Resources: 8102 8103* [ActivityDefinition](activitydefinition.html): What resource is being referenced 8104* [CodeSystem](codesystem.html): The predecessor of the CodeSystem 8105* [ConceptMap](conceptmap.html): The predecessor of the ConceptMap 8106* [EventDefinition](eventdefinition.html): What resource is being referenced 8107* [EvidenceVariable](evidencevariable.html): What resource is being referenced 8108* [Library](library.html): What resource is being referenced 8109* [Measure](measure.html): What resource is being referenced 8110* [NamingSystem](namingsystem.html): The predecessor of the NamingSystem 8111* [PlanDefinition](plandefinition.html): What resource is being referenced 8112* [ValueSet](valueset.html): The predecessor of the ValueSet 8113</b><br> 8114 * Type: <b>reference</b><br> 8115 * Path: <b>ActivityDefinition.relatedArtifact.where(type='predecessor').resource | CodeSystem.relatedArtifact.where(type='predecessor').resource | ConceptMap.relatedArtifact.where(type='predecessor').resource | EventDefinition.relatedArtifact.where(type='predecessor').resource | EvidenceVariable.relatedArtifact.where(type='predecessor').resource | Library.relatedArtifact.where(type='predecessor').resource | Measure.relatedArtifact.where(type='predecessor').resource | NamingSystem.relatedArtifact.where(type='predecessor').resource | PlanDefinition.relatedArtifact.where(type='predecessor').resource | ValueSet.relatedArtifact.where(type='predecessor').resource</b><br> 8116 * </p> 8117 */ 8118 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 8119 8120/** 8121 * Constant for fluent queries to be used to add include statements. Specifies 8122 * the path value of "<b>Measure:predecessor</b>". 8123 */ 8124 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("Measure:predecessor").toLocked(); 8125 8126 /** 8127 * Search parameter: <b>successor</b> 8128 * <p> 8129 * Description: <b>Multiple Resources: 8130 8131* [ActivityDefinition](activitydefinition.html): What resource is being referenced 8132* [EventDefinition](eventdefinition.html): What resource is being referenced 8133* [EvidenceVariable](evidencevariable.html): What resource is being referenced 8134* [Library](library.html): What resource is being referenced 8135* [Measure](measure.html): What resource is being referenced 8136* [PlanDefinition](plandefinition.html): What resource is being referenced 8137</b><br> 8138 * Type: <b>reference</b><br> 8139 * Path: <b>ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource</b><br> 8140 * </p> 8141 */ 8142 @SearchParamDefinition(name="successor", path="ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): What resource is being referenced\r\n* [EventDefinition](eventdefinition.html): What resource is being referenced\r\n* [EvidenceVariable](evidencevariable.html): What resource is being referenced\r\n* [Library](library.html): What resource is being referenced\r\n* [Measure](measure.html): What resource is being referenced\r\n* [PlanDefinition](plandefinition.html): What resource is being referenced\r\n", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 8143 public static final String SP_SUCCESSOR = "successor"; 8144 /** 8145 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 8146 * <p> 8147 * Description: <b>Multiple Resources: 8148 8149* [ActivityDefinition](activitydefinition.html): What resource is being referenced 8150* [EventDefinition](eventdefinition.html): What resource is being referenced 8151* [EvidenceVariable](evidencevariable.html): What resource is being referenced 8152* [Library](library.html): What resource is being referenced 8153* [Measure](measure.html): What resource is being referenced 8154* [PlanDefinition](plandefinition.html): What resource is being referenced 8155</b><br> 8156 * Type: <b>reference</b><br> 8157 * Path: <b>ActivityDefinition.relatedArtifact.where(type='successor').resource | EventDefinition.relatedArtifact.where(type='successor').resource | EvidenceVariable.relatedArtifact.where(type='successor').resource | Library.relatedArtifact.where(type='successor').resource | Measure.relatedArtifact.where(type='successor').resource | PlanDefinition.relatedArtifact.where(type='successor').resource</b><br> 8158 * </p> 8159 */ 8160 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUCCESSOR); 8161 8162/** 8163 * Constant for fluent queries to be used to add include statements. Specifies 8164 * the path value of "<b>Measure:successor</b>". 8165 */ 8166 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include("Measure:successor").toLocked(); 8167 8168 /** 8169 * Search parameter: <b>topic</b> 8170 * <p> 8171 * Description: <b>Multiple Resources: 8172 8173* [ActivityDefinition](activitydefinition.html): Topics associated with the module 8174* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 8175* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 8176* [EventDefinition](eventdefinition.html): Topics associated with the module 8177* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 8178* [Library](library.html): Topics associated with the module 8179* [Measure](measure.html): Topics associated with the measure 8180* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 8181* [PlanDefinition](plandefinition.html): Topics associated with the module 8182* [ValueSet](valueset.html): Topics associated with the ValueSet 8183</b><br> 8184 * Type: <b>token</b><br> 8185 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 8186 * </p> 8187 */ 8188 @SearchParamDefinition(name="topic", path="ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): Topics associated with the module\r\n* [CodeSystem](codesystem.html): Topics associated with the CodeSystem\r\n* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap\r\n* [EventDefinition](eventdefinition.html): Topics associated with the module\r\n* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable\r\n* [Library](library.html): Topics associated with the module\r\n* [Measure](measure.html): Topics associated with the measure\r\n* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem\r\n* [PlanDefinition](plandefinition.html): Topics associated with the module\r\n* [ValueSet](valueset.html): Topics associated with the ValueSet\r\n", type="token" ) 8189 public static final String SP_TOPIC = "topic"; 8190 /** 8191 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 8192 * <p> 8193 * Description: <b>Multiple Resources: 8194 8195* [ActivityDefinition](activitydefinition.html): Topics associated with the module 8196* [CodeSystem](codesystem.html): Topics associated with the CodeSystem 8197* [ConceptMap](conceptmap.html): Topics associated with the ConceptMap 8198* [EventDefinition](eventdefinition.html): Topics associated with the module 8199* [EvidenceVariable](evidencevariable.html): Topics associated with the EvidenceVariable 8200* [Library](library.html): Topics associated with the module 8201* [Measure](measure.html): Topics associated with the measure 8202* [NamingSystem](namingsystem.html): Topics associated with the NamingSystem 8203* [PlanDefinition](plandefinition.html): Topics associated with the module 8204* [ValueSet](valueset.html): Topics associated with the ValueSet 8205</b><br> 8206 * Type: <b>token</b><br> 8207 * Path: <b>ActivityDefinition.topic | CodeSystem.topic | ConceptMap.topic | EventDefinition.topic | Library.topic | Measure.topic | NamingSystem.topic | PlanDefinition.topic | ValueSet.topic</b><br> 8208 * </p> 8209 */ 8210 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 8211 8212 8213} 8214