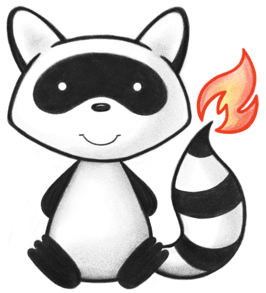
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * The MeasureReport resource contains the results of the calculation of a measure; and optionally a reference to the resources involved in that calculation. 052 */ 053@ResourceDef(name="MeasureReport", profile="http://hl7.org/fhir/StructureDefinition/MeasureReport") 054public class MeasureReport extends DomainResource { 055 056 public enum MeasureReportStatus { 057 /** 058 * The report is complete and ready for use. 059 */ 060 COMPLETE, 061 /** 062 * The report is currently being generated. 063 */ 064 PENDING, 065 /** 066 * An error occurred attempting to generate the report. 067 */ 068 ERROR, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static MeasureReportStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("complete".equals(codeString)) 077 return COMPLETE; 078 if ("pending".equals(codeString)) 079 return PENDING; 080 if ("error".equals(codeString)) 081 return ERROR; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown MeasureReportStatus code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case COMPLETE: return "complete"; 090 case PENDING: return "pending"; 091 case ERROR: return "error"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case COMPLETE: return "http://hl7.org/fhir/measure-report-status"; 099 case PENDING: return "http://hl7.org/fhir/measure-report-status"; 100 case ERROR: return "http://hl7.org/fhir/measure-report-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case COMPLETE: return "The report is complete and ready for use."; 108 case PENDING: return "The report is currently being generated."; 109 case ERROR: return "An error occurred attempting to generate the report."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case COMPLETE: return "Complete"; 117 case PENDING: return "Pending"; 118 case ERROR: return "Error"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class MeasureReportStatusEnumFactory implements EnumFactory<MeasureReportStatus> { 126 public MeasureReportStatus fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("complete".equals(codeString)) 131 return MeasureReportStatus.COMPLETE; 132 if ("pending".equals(codeString)) 133 return MeasureReportStatus.PENDING; 134 if ("error".equals(codeString)) 135 return MeasureReportStatus.ERROR; 136 throw new IllegalArgumentException("Unknown MeasureReportStatus code '"+codeString+"'"); 137 } 138 public Enumeration<MeasureReportStatus> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<MeasureReportStatus>(this, MeasureReportStatus.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<MeasureReportStatus>(this, MeasureReportStatus.NULL, code); 146 if ("complete".equals(codeString)) 147 return new Enumeration<MeasureReportStatus>(this, MeasureReportStatus.COMPLETE, code); 148 if ("pending".equals(codeString)) 149 return new Enumeration<MeasureReportStatus>(this, MeasureReportStatus.PENDING, code); 150 if ("error".equals(codeString)) 151 return new Enumeration<MeasureReportStatus>(this, MeasureReportStatus.ERROR, code); 152 throw new FHIRException("Unknown MeasureReportStatus code '"+codeString+"'"); 153 } 154 public String toCode(MeasureReportStatus code) { 155 if (code == MeasureReportStatus.NULL) 156 return null; 157 if (code == MeasureReportStatus.COMPLETE) 158 return "complete"; 159 if (code == MeasureReportStatus.PENDING) 160 return "pending"; 161 if (code == MeasureReportStatus.ERROR) 162 return "error"; 163 return "?"; 164 } 165 public String toSystem(MeasureReportStatus code) { 166 return code.getSystem(); 167 } 168 } 169 170 public enum MeasureReportType { 171 /** 172 * An individual report that provides information on the performance for a given measure with respect to a single subject. 173 */ 174 INDIVIDUAL, 175 /** 176 * A subject list report that includes a listing of subjects that satisfied each population criteria in the measure. 177 */ 178 SUBJECTLIST, 179 /** 180 * A summary report that returns the number of members in each population criteria for the measure. 181 */ 182 SUMMARY, 183 /** 184 * A data exchange report that contains data-of-interest for the measure (i.e. data that is needed to calculate the measure) 185 */ 186 DATAEXCHANGE, 187 /** 188 * added to help the parsers with the generic types 189 */ 190 NULL; 191 public static MeasureReportType fromCode(String codeString) throws FHIRException { 192 if (codeString == null || "".equals(codeString)) 193 return null; 194 if ("individual".equals(codeString)) 195 return INDIVIDUAL; 196 if ("subject-list".equals(codeString)) 197 return SUBJECTLIST; 198 if ("summary".equals(codeString)) 199 return SUMMARY; 200 if ("data-exchange".equals(codeString)) 201 return DATAEXCHANGE; 202 if (Configuration.isAcceptInvalidEnums()) 203 return null; 204 else 205 throw new FHIRException("Unknown MeasureReportType code '"+codeString+"'"); 206 } 207 public String toCode() { 208 switch (this) { 209 case INDIVIDUAL: return "individual"; 210 case SUBJECTLIST: return "subject-list"; 211 case SUMMARY: return "summary"; 212 case DATAEXCHANGE: return "data-exchange"; 213 case NULL: return null; 214 default: return "?"; 215 } 216 } 217 public String getSystem() { 218 switch (this) { 219 case INDIVIDUAL: return "http://hl7.org/fhir/measure-report-type"; 220 case SUBJECTLIST: return "http://hl7.org/fhir/measure-report-type"; 221 case SUMMARY: return "http://hl7.org/fhir/measure-report-type"; 222 case DATAEXCHANGE: return "http://hl7.org/fhir/measure-report-type"; 223 case NULL: return null; 224 default: return "?"; 225 } 226 } 227 public String getDefinition() { 228 switch (this) { 229 case INDIVIDUAL: return "An individual report that provides information on the performance for a given measure with respect to a single subject."; 230 case SUBJECTLIST: return "A subject list report that includes a listing of subjects that satisfied each population criteria in the measure."; 231 case SUMMARY: return "A summary report that returns the number of members in each population criteria for the measure."; 232 case DATAEXCHANGE: return "A data exchange report that contains data-of-interest for the measure (i.e. data that is needed to calculate the measure)"; 233 case NULL: return null; 234 default: return "?"; 235 } 236 } 237 public String getDisplay() { 238 switch (this) { 239 case INDIVIDUAL: return "Individual"; 240 case SUBJECTLIST: return "Subject List"; 241 case SUMMARY: return "Summary"; 242 case DATAEXCHANGE: return "Data Exchange"; 243 case NULL: return null; 244 default: return "?"; 245 } 246 } 247 } 248 249 public static class MeasureReportTypeEnumFactory implements EnumFactory<MeasureReportType> { 250 public MeasureReportType fromCode(String codeString) throws IllegalArgumentException { 251 if (codeString == null || "".equals(codeString)) 252 if (codeString == null || "".equals(codeString)) 253 return null; 254 if ("individual".equals(codeString)) 255 return MeasureReportType.INDIVIDUAL; 256 if ("subject-list".equals(codeString)) 257 return MeasureReportType.SUBJECTLIST; 258 if ("summary".equals(codeString)) 259 return MeasureReportType.SUMMARY; 260 if ("data-exchange".equals(codeString)) 261 return MeasureReportType.DATAEXCHANGE; 262 throw new IllegalArgumentException("Unknown MeasureReportType code '"+codeString+"'"); 263 } 264 public Enumeration<MeasureReportType> fromType(PrimitiveType<?> code) throws FHIRException { 265 if (code == null) 266 return null; 267 if (code.isEmpty()) 268 return new Enumeration<MeasureReportType>(this, MeasureReportType.NULL, code); 269 String codeString = ((PrimitiveType) code).asStringValue(); 270 if (codeString == null || "".equals(codeString)) 271 return new Enumeration<MeasureReportType>(this, MeasureReportType.NULL, code); 272 if ("individual".equals(codeString)) 273 return new Enumeration<MeasureReportType>(this, MeasureReportType.INDIVIDUAL, code); 274 if ("subject-list".equals(codeString)) 275 return new Enumeration<MeasureReportType>(this, MeasureReportType.SUBJECTLIST, code); 276 if ("summary".equals(codeString)) 277 return new Enumeration<MeasureReportType>(this, MeasureReportType.SUMMARY, code); 278 if ("data-exchange".equals(codeString)) 279 return new Enumeration<MeasureReportType>(this, MeasureReportType.DATAEXCHANGE, code); 280 throw new FHIRException("Unknown MeasureReportType code '"+codeString+"'"); 281 } 282 public String toCode(MeasureReportType code) { 283 if (code == MeasureReportType.NULL) 284 return null; 285 if (code == MeasureReportType.INDIVIDUAL) 286 return "individual"; 287 if (code == MeasureReportType.SUBJECTLIST) 288 return "subject-list"; 289 if (code == MeasureReportType.SUMMARY) 290 return "summary"; 291 if (code == MeasureReportType.DATAEXCHANGE) 292 return "data-exchange"; 293 return "?"; 294 } 295 public String toSystem(MeasureReportType code) { 296 return code.getSystem(); 297 } 298 } 299 300 public enum SubmitDataUpdateType { 301 /** 302 * In contrast to the Snapshot Update, the FHIR Parameters resource used in a Submit Data or the Collect Data scenario contains only the new and updated DEQM and QI Core Profiles since the last transaction. If the Consumer supports incremental updates, the contents of the updated payload updates the previous payload data. 303 */ 304 INCREMENTAL, 305 /** 306 * In contrast to the Incremental Update, the FHIR Parameters resource used in a Submit Data or the Collect Data scenario contains all the DEQM and QI Core Profiles for each transaction. If the Consumer supports snapshot updates, the contents of the updated payload entirely replaces the previous payload 307 */ 308 SNAPSHOT, 309 /** 310 * added to help the parsers with the generic types 311 */ 312 NULL; 313 public static SubmitDataUpdateType fromCode(String codeString) throws FHIRException { 314 if (codeString == null || "".equals(codeString)) 315 return null; 316 if ("incremental".equals(codeString)) 317 return INCREMENTAL; 318 if ("snapshot".equals(codeString)) 319 return SNAPSHOT; 320 if (Configuration.isAcceptInvalidEnums()) 321 return null; 322 else 323 throw new FHIRException("Unknown SubmitDataUpdateType code '"+codeString+"'"); 324 } 325 public String toCode() { 326 switch (this) { 327 case INCREMENTAL: return "incremental"; 328 case SNAPSHOT: return "snapshot"; 329 case NULL: return null; 330 default: return "?"; 331 } 332 } 333 public String getSystem() { 334 switch (this) { 335 case INCREMENTAL: return "http://hl7.org/fhir/CodeSystem/submit-data-update-type"; 336 case SNAPSHOT: return "http://hl7.org/fhir/CodeSystem/submit-data-update-type"; 337 case NULL: return null; 338 default: return "?"; 339 } 340 } 341 public String getDefinition() { 342 switch (this) { 343 case INCREMENTAL: return "In contrast to the Snapshot Update, the FHIR Parameters resource used in a Submit Data or the Collect Data scenario contains only the new and updated DEQM and QI Core Profiles since the last transaction. If the Consumer supports incremental updates, the contents of the updated payload updates the previous payload data."; 344 case SNAPSHOT: return "In contrast to the Incremental Update, the FHIR Parameters resource used in a Submit Data or the Collect Data scenario contains all the DEQM and QI Core Profiles for each transaction. If the Consumer supports snapshot updates, the contents of the updated payload entirely replaces the previous payload"; 345 case NULL: return null; 346 default: return "?"; 347 } 348 } 349 public String getDisplay() { 350 switch (this) { 351 case INCREMENTAL: return "Incremental"; 352 case SNAPSHOT: return "Snapshot"; 353 case NULL: return null; 354 default: return "?"; 355 } 356 } 357 } 358 359 public static class SubmitDataUpdateTypeEnumFactory implements EnumFactory<SubmitDataUpdateType> { 360 public SubmitDataUpdateType fromCode(String codeString) throws IllegalArgumentException { 361 if (codeString == null || "".equals(codeString)) 362 if (codeString == null || "".equals(codeString)) 363 return null; 364 if ("incremental".equals(codeString)) 365 return SubmitDataUpdateType.INCREMENTAL; 366 if ("snapshot".equals(codeString)) 367 return SubmitDataUpdateType.SNAPSHOT; 368 throw new IllegalArgumentException("Unknown SubmitDataUpdateType code '"+codeString+"'"); 369 } 370 public Enumeration<SubmitDataUpdateType> fromType(PrimitiveType<?> code) throws FHIRException { 371 if (code == null) 372 return null; 373 if (code.isEmpty()) 374 return new Enumeration<SubmitDataUpdateType>(this, SubmitDataUpdateType.NULL, code); 375 String codeString = ((PrimitiveType) code).asStringValue(); 376 if (codeString == null || "".equals(codeString)) 377 return new Enumeration<SubmitDataUpdateType>(this, SubmitDataUpdateType.NULL, code); 378 if ("incremental".equals(codeString)) 379 return new Enumeration<SubmitDataUpdateType>(this, SubmitDataUpdateType.INCREMENTAL, code); 380 if ("snapshot".equals(codeString)) 381 return new Enumeration<SubmitDataUpdateType>(this, SubmitDataUpdateType.SNAPSHOT, code); 382 throw new FHIRException("Unknown SubmitDataUpdateType code '"+codeString+"'"); 383 } 384 public String toCode(SubmitDataUpdateType code) { 385 if (code == SubmitDataUpdateType.NULL) 386 return null; 387 if (code == SubmitDataUpdateType.INCREMENTAL) 388 return "incremental"; 389 if (code == SubmitDataUpdateType.SNAPSHOT) 390 return "snapshot"; 391 return "?"; 392 } 393 public String toSystem(SubmitDataUpdateType code) { 394 return code.getSystem(); 395 } 396 } 397 398 @Block() 399 public static class MeasureReportGroupComponent extends BackboneElement implements IBaseBackboneElement { 400 /** 401 * The group from the Measure that corresponds to this group in the MeasureReport resource. 402 */ 403 @Child(name = "linkId", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 404 @Description(shortDefinition="Pointer to specific group from Measure", formalDefinition="The group from the Measure that corresponds to this group in the MeasureReport resource." ) 405 protected StringType linkId; 406 407 /** 408 * The meaning of the population group as defined in the measure definition. 409 */ 410 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 411 @Description(shortDefinition="Meaning of the group", formalDefinition="The meaning of the population group as defined in the measure definition." ) 412 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-group-example") 413 protected CodeableConcept code; 414 415 /** 416 * Optional subject identifying the individual or individuals the report is for. 417 */ 418 @Child(name = "subject", type = {CareTeam.class, Device.class, Group.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=3, min=0, max=1, modifier=false, summary=true) 419 @Description(shortDefinition="What individual(s) the report is for", formalDefinition="Optional subject identifying the individual or individuals the report is for." ) 420 protected Reference subject; 421 422 /** 423 * The populations that make up the population group, one for each type of population appropriate for the measure. 424 */ 425 @Child(name = "population", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 426 @Description(shortDefinition="The populations in the group", formalDefinition="The populations that make up the population group, one for each type of population appropriate for the measure." ) 427 protected List<MeasureReportGroupPopulationComponent> population; 428 429 /** 430 * The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group. 431 */ 432 @Child(name = "measureScore", type = {Quantity.class, DateTimeType.class, CodeableConcept.class, Period.class, Range.class, Duration.class}, order=5, min=0, max=1, modifier=false, summary=true) 433 @Description(shortDefinition="What score this group achieved", formalDefinition="The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group." ) 434 protected DataType measureScore; 435 436 /** 437 * When a measure includes multiple stratifiers, there will be a stratifier group for each stratifier defined by the measure. 438 */ 439 @Child(name = "stratifier", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 440 @Description(shortDefinition="Stratification results", formalDefinition="When a measure includes multiple stratifiers, there will be a stratifier group for each stratifier defined by the measure." ) 441 protected List<MeasureReportGroupStratifierComponent> stratifier; 442 443 private static final long serialVersionUID = 438553747L; 444 445 /** 446 * Constructor 447 */ 448 public MeasureReportGroupComponent() { 449 super(); 450 } 451 452 /** 453 * @return {@link #linkId} (The group from the Measure that corresponds to this group in the MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 454 */ 455 public StringType getLinkIdElement() { 456 if (this.linkId == null) 457 if (Configuration.errorOnAutoCreate()) 458 throw new Error("Attempt to auto-create MeasureReportGroupComponent.linkId"); 459 else if (Configuration.doAutoCreate()) 460 this.linkId = new StringType(); // bb 461 return this.linkId; 462 } 463 464 public boolean hasLinkIdElement() { 465 return this.linkId != null && !this.linkId.isEmpty(); 466 } 467 468 public boolean hasLinkId() { 469 return this.linkId != null && !this.linkId.isEmpty(); 470 } 471 472 /** 473 * @param value {@link #linkId} (The group from the Measure that corresponds to this group in the MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 474 */ 475 public MeasureReportGroupComponent setLinkIdElement(StringType value) { 476 this.linkId = value; 477 return this; 478 } 479 480 /** 481 * @return The group from the Measure that corresponds to this group in the MeasureReport resource. 482 */ 483 public String getLinkId() { 484 return this.linkId == null ? null : this.linkId.getValue(); 485 } 486 487 /** 488 * @param value The group from the Measure that corresponds to this group in the MeasureReport resource. 489 */ 490 public MeasureReportGroupComponent setLinkId(String value) { 491 if (Utilities.noString(value)) 492 this.linkId = null; 493 else { 494 if (this.linkId == null) 495 this.linkId = new StringType(); 496 this.linkId.setValue(value); 497 } 498 return this; 499 } 500 501 /** 502 * @return {@link #code} (The meaning of the population group as defined in the measure definition.) 503 */ 504 public CodeableConcept getCode() { 505 if (this.code == null) 506 if (Configuration.errorOnAutoCreate()) 507 throw new Error("Attempt to auto-create MeasureReportGroupComponent.code"); 508 else if (Configuration.doAutoCreate()) 509 this.code = new CodeableConcept(); // cc 510 return this.code; 511 } 512 513 public boolean hasCode() { 514 return this.code != null && !this.code.isEmpty(); 515 } 516 517 /** 518 * @param value {@link #code} (The meaning of the population group as defined in the measure definition.) 519 */ 520 public MeasureReportGroupComponent setCode(CodeableConcept value) { 521 this.code = value; 522 return this; 523 } 524 525 /** 526 * @return {@link #subject} (Optional subject identifying the individual or individuals the report is for.) 527 */ 528 public Reference getSubject() { 529 if (this.subject == null) 530 if (Configuration.errorOnAutoCreate()) 531 throw new Error("Attempt to auto-create MeasureReportGroupComponent.subject"); 532 else if (Configuration.doAutoCreate()) 533 this.subject = new Reference(); // cc 534 return this.subject; 535 } 536 537 public boolean hasSubject() { 538 return this.subject != null && !this.subject.isEmpty(); 539 } 540 541 /** 542 * @param value {@link #subject} (Optional subject identifying the individual or individuals the report is for.) 543 */ 544 public MeasureReportGroupComponent setSubject(Reference value) { 545 this.subject = value; 546 return this; 547 } 548 549 /** 550 * @return {@link #population} (The populations that make up the population group, one for each type of population appropriate for the measure.) 551 */ 552 public List<MeasureReportGroupPopulationComponent> getPopulation() { 553 if (this.population == null) 554 this.population = new ArrayList<MeasureReportGroupPopulationComponent>(); 555 return this.population; 556 } 557 558 /** 559 * @return Returns a reference to <code>this</code> for easy method chaining 560 */ 561 public MeasureReportGroupComponent setPopulation(List<MeasureReportGroupPopulationComponent> thePopulation) { 562 this.population = thePopulation; 563 return this; 564 } 565 566 public boolean hasPopulation() { 567 if (this.population == null) 568 return false; 569 for (MeasureReportGroupPopulationComponent item : this.population) 570 if (!item.isEmpty()) 571 return true; 572 return false; 573 } 574 575 public MeasureReportGroupPopulationComponent addPopulation() { //3 576 MeasureReportGroupPopulationComponent t = new MeasureReportGroupPopulationComponent(); 577 if (this.population == null) 578 this.population = new ArrayList<MeasureReportGroupPopulationComponent>(); 579 this.population.add(t); 580 return t; 581 } 582 583 public MeasureReportGroupComponent addPopulation(MeasureReportGroupPopulationComponent t) { //3 584 if (t == null) 585 return this; 586 if (this.population == null) 587 this.population = new ArrayList<MeasureReportGroupPopulationComponent>(); 588 this.population.add(t); 589 return this; 590 } 591 592 /** 593 * @return The first repetition of repeating field {@link #population}, creating it if it does not already exist {3} 594 */ 595 public MeasureReportGroupPopulationComponent getPopulationFirstRep() { 596 if (getPopulation().isEmpty()) { 597 addPopulation(); 598 } 599 return getPopulation().get(0); 600 } 601 602 /** 603 * @return {@link #measureScore} (The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.) 604 */ 605 public DataType getMeasureScore() { 606 return this.measureScore; 607 } 608 609 /** 610 * @return {@link #measureScore} (The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.) 611 */ 612 public Quantity getMeasureScoreQuantity() throws FHIRException { 613 if (this.measureScore == null) 614 this.measureScore = new Quantity(); 615 if (!(this.measureScore instanceof Quantity)) 616 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.measureScore.getClass().getName()+" was encountered"); 617 return (Quantity) this.measureScore; 618 } 619 620 public boolean hasMeasureScoreQuantity() { 621 return this != null && this.measureScore instanceof Quantity; 622 } 623 624 /** 625 * @return {@link #measureScore} (The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.) 626 */ 627 public DateTimeType getMeasureScoreDateTimeType() throws FHIRException { 628 if (this.measureScore == null) 629 this.measureScore = new DateTimeType(); 630 if (!(this.measureScore instanceof DateTimeType)) 631 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.measureScore.getClass().getName()+" was encountered"); 632 return (DateTimeType) this.measureScore; 633 } 634 635 public boolean hasMeasureScoreDateTimeType() { 636 return this != null && this.measureScore instanceof DateTimeType; 637 } 638 639 /** 640 * @return {@link #measureScore} (The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.) 641 */ 642 public CodeableConcept getMeasureScoreCodeableConcept() throws FHIRException { 643 if (this.measureScore == null) 644 this.measureScore = new CodeableConcept(); 645 if (!(this.measureScore instanceof CodeableConcept)) 646 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.measureScore.getClass().getName()+" was encountered"); 647 return (CodeableConcept) this.measureScore; 648 } 649 650 public boolean hasMeasureScoreCodeableConcept() { 651 return this != null && this.measureScore instanceof CodeableConcept; 652 } 653 654 /** 655 * @return {@link #measureScore} (The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.) 656 */ 657 public Period getMeasureScorePeriod() throws FHIRException { 658 if (this.measureScore == null) 659 this.measureScore = new Period(); 660 if (!(this.measureScore instanceof Period)) 661 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.measureScore.getClass().getName()+" was encountered"); 662 return (Period) this.measureScore; 663 } 664 665 public boolean hasMeasureScorePeriod() { 666 return this != null && this.measureScore instanceof Period; 667 } 668 669 /** 670 * @return {@link #measureScore} (The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.) 671 */ 672 public Range getMeasureScoreRange() throws FHIRException { 673 if (this.measureScore == null) 674 this.measureScore = new Range(); 675 if (!(this.measureScore instanceof Range)) 676 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.measureScore.getClass().getName()+" was encountered"); 677 return (Range) this.measureScore; 678 } 679 680 public boolean hasMeasureScoreRange() { 681 return this != null && this.measureScore instanceof Range; 682 } 683 684 /** 685 * @return {@link #measureScore} (The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.) 686 */ 687 public Duration getMeasureScoreDuration() throws FHIRException { 688 if (this.measureScore == null) 689 this.measureScore = new Duration(); 690 if (!(this.measureScore instanceof Duration)) 691 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.measureScore.getClass().getName()+" was encountered"); 692 return (Duration) this.measureScore; 693 } 694 695 public boolean hasMeasureScoreDuration() { 696 return this != null && this.measureScore instanceof Duration; 697 } 698 699 public boolean hasMeasureScore() { 700 return this.measureScore != null && !this.measureScore.isEmpty(); 701 } 702 703 /** 704 * @param value {@link #measureScore} (The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.) 705 */ 706 public MeasureReportGroupComponent setMeasureScore(DataType value) { 707 if (value != null && !(value instanceof Quantity || value instanceof DateTimeType || value instanceof CodeableConcept || value instanceof Period || value instanceof Range || value instanceof Duration)) 708 throw new FHIRException("Not the right type for MeasureReport.group.measureScore[x]: "+value.fhirType()); 709 this.measureScore = value; 710 return this; 711 } 712 713 /** 714 * @return {@link #stratifier} (When a measure includes multiple stratifiers, there will be a stratifier group for each stratifier defined by the measure.) 715 */ 716 public List<MeasureReportGroupStratifierComponent> getStratifier() { 717 if (this.stratifier == null) 718 this.stratifier = new ArrayList<MeasureReportGroupStratifierComponent>(); 719 return this.stratifier; 720 } 721 722 /** 723 * @return Returns a reference to <code>this</code> for easy method chaining 724 */ 725 public MeasureReportGroupComponent setStratifier(List<MeasureReportGroupStratifierComponent> theStratifier) { 726 this.stratifier = theStratifier; 727 return this; 728 } 729 730 public boolean hasStratifier() { 731 if (this.stratifier == null) 732 return false; 733 for (MeasureReportGroupStratifierComponent item : this.stratifier) 734 if (!item.isEmpty()) 735 return true; 736 return false; 737 } 738 739 public MeasureReportGroupStratifierComponent addStratifier() { //3 740 MeasureReportGroupStratifierComponent t = new MeasureReportGroupStratifierComponent(); 741 if (this.stratifier == null) 742 this.stratifier = new ArrayList<MeasureReportGroupStratifierComponent>(); 743 this.stratifier.add(t); 744 return t; 745 } 746 747 public MeasureReportGroupComponent addStratifier(MeasureReportGroupStratifierComponent t) { //3 748 if (t == null) 749 return this; 750 if (this.stratifier == null) 751 this.stratifier = new ArrayList<MeasureReportGroupStratifierComponent>(); 752 this.stratifier.add(t); 753 return this; 754 } 755 756 /** 757 * @return The first repetition of repeating field {@link #stratifier}, creating it if it does not already exist {3} 758 */ 759 public MeasureReportGroupStratifierComponent getStratifierFirstRep() { 760 if (getStratifier().isEmpty()) { 761 addStratifier(); 762 } 763 return getStratifier().get(0); 764 } 765 766 protected void listChildren(List<Property> children) { 767 super.listChildren(children); 768 children.add(new Property("linkId", "string", "The group from the Measure that corresponds to this group in the MeasureReport resource.", 0, 1, linkId)); 769 children.add(new Property("code", "CodeableConcept", "The meaning of the population group as defined in the measure definition.", 0, 1, code)); 770 children.add(new Property("subject", "Reference(CareTeam|Device|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Optional subject identifying the individual or individuals the report is for.", 0, 1, subject)); 771 children.add(new Property("population", "", "The populations that make up the population group, one for each type of population appropriate for the measure.", 0, java.lang.Integer.MAX_VALUE, population)); 772 children.add(new Property("measureScore[x]", "Quantity|dateTime|CodeableConcept|Period|Range|Duration", "The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.", 0, 1, measureScore)); 773 children.add(new Property("stratifier", "", "When a measure includes multiple stratifiers, there will be a stratifier group for each stratifier defined by the measure.", 0, java.lang.Integer.MAX_VALUE, stratifier)); 774 } 775 776 @Override 777 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 778 switch (_hash) { 779 case -1102667083: /*linkId*/ return new Property("linkId", "string", "The group from the Measure that corresponds to this group in the MeasureReport resource.", 0, 1, linkId); 780 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The meaning of the population group as defined in the measure definition.", 0, 1, code); 781 case -1867885268: /*subject*/ return new Property("subject", "Reference(CareTeam|Device|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Optional subject identifying the individual or individuals the report is for.", 0, 1, subject); 782 case -2023558323: /*population*/ return new Property("population", "", "The populations that make up the population group, one for each type of population appropriate for the measure.", 0, java.lang.Integer.MAX_VALUE, population); 783 case 1854115884: /*measureScore[x]*/ return new Property("measureScore[x]", "Quantity|dateTime|CodeableConcept|Period|Range|Duration", "The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.", 0, 1, measureScore); 784 case -386313260: /*measureScore*/ return new Property("measureScore[x]", "Quantity|dateTime|CodeableConcept|Period|Range|Duration", "The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.", 0, 1, measureScore); 785 case -1880815489: /*measureScoreQuantity*/ return new Property("measureScore[x]", "Quantity", "The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.", 0, 1, measureScore); 786 case 1196938127: /*measureScoreDateTime*/ return new Property("measureScore[x]", "dateTime", "The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.", 0, 1, measureScore); 787 case -1193234131: /*measureScoreCodeableConcept*/ return new Property("measureScore[x]", "CodeableConcept", "The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.", 0, 1, measureScore); 788 case -1939831115: /*measureScorePeriod*/ return new Property("measureScore[x]", "Period", "The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.", 0, 1, measureScore); 789 case -615040567: /*measureScoreRange*/ return new Property("measureScore[x]", "Range", "The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.", 0, 1, measureScore); 790 case 1707143560: /*measureScoreDuration*/ return new Property("measureScore[x]", "Duration", "The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.", 0, 1, measureScore); 791 case 90983669: /*stratifier*/ return new Property("stratifier", "", "When a measure includes multiple stratifiers, there will be a stratifier group for each stratifier defined by the measure.", 0, java.lang.Integer.MAX_VALUE, stratifier); 792 default: return super.getNamedProperty(_hash, _name, _checkValid); 793 } 794 795 } 796 797 @Override 798 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 799 switch (hash) { 800 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 801 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 802 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 803 case -2023558323: /*population*/ return this.population == null ? new Base[0] : this.population.toArray(new Base[this.population.size()]); // MeasureReportGroupPopulationComponent 804 case -386313260: /*measureScore*/ return this.measureScore == null ? new Base[0] : new Base[] {this.measureScore}; // DataType 805 case 90983669: /*stratifier*/ return this.stratifier == null ? new Base[0] : this.stratifier.toArray(new Base[this.stratifier.size()]); // MeasureReportGroupStratifierComponent 806 default: return super.getProperty(hash, name, checkValid); 807 } 808 809 } 810 811 @Override 812 public Base setProperty(int hash, String name, Base value) throws FHIRException { 813 switch (hash) { 814 case -1102667083: // linkId 815 this.linkId = TypeConvertor.castToString(value); // StringType 816 return value; 817 case 3059181: // code 818 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 819 return value; 820 case -1867885268: // subject 821 this.subject = TypeConvertor.castToReference(value); // Reference 822 return value; 823 case -2023558323: // population 824 this.getPopulation().add((MeasureReportGroupPopulationComponent) value); // MeasureReportGroupPopulationComponent 825 return value; 826 case -386313260: // measureScore 827 this.measureScore = TypeConvertor.castToType(value); // DataType 828 return value; 829 case 90983669: // stratifier 830 this.getStratifier().add((MeasureReportGroupStratifierComponent) value); // MeasureReportGroupStratifierComponent 831 return value; 832 default: return super.setProperty(hash, name, value); 833 } 834 835 } 836 837 @Override 838 public Base setProperty(String name, Base value) throws FHIRException { 839 if (name.equals("linkId")) { 840 this.linkId = TypeConvertor.castToString(value); // StringType 841 } else if (name.equals("code")) { 842 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 843 } else if (name.equals("subject")) { 844 this.subject = TypeConvertor.castToReference(value); // Reference 845 } else if (name.equals("population")) { 846 this.getPopulation().add((MeasureReportGroupPopulationComponent) value); 847 } else if (name.equals("measureScore[x]")) { 848 this.measureScore = TypeConvertor.castToType(value); // DataType 849 } else if (name.equals("stratifier")) { 850 this.getStratifier().add((MeasureReportGroupStratifierComponent) value); 851 } else 852 return super.setProperty(name, value); 853 return value; 854 } 855 856 @Override 857 public void removeChild(String name, Base value) throws FHIRException { 858 if (name.equals("linkId")) { 859 this.linkId = null; 860 } else if (name.equals("code")) { 861 this.code = null; 862 } else if (name.equals("subject")) { 863 this.subject = null; 864 } else if (name.equals("population")) { 865 this.getPopulation().remove((MeasureReportGroupPopulationComponent) value); 866 } else if (name.equals("measureScore[x]")) { 867 this.measureScore = null; 868 } else if (name.equals("stratifier")) { 869 this.getStratifier().remove((MeasureReportGroupStratifierComponent) value); 870 } else 871 super.removeChild(name, value); 872 873 } 874 875 @Override 876 public Base makeProperty(int hash, String name) throws FHIRException { 877 switch (hash) { 878 case -1102667083: return getLinkIdElement(); 879 case 3059181: return getCode(); 880 case -1867885268: return getSubject(); 881 case -2023558323: return addPopulation(); 882 case 1854115884: return getMeasureScore(); 883 case -386313260: return getMeasureScore(); 884 case 90983669: return addStratifier(); 885 default: return super.makeProperty(hash, name); 886 } 887 888 } 889 890 @Override 891 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 892 switch (hash) { 893 case -1102667083: /*linkId*/ return new String[] {"string"}; 894 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 895 case -1867885268: /*subject*/ return new String[] {"Reference"}; 896 case -2023558323: /*population*/ return new String[] {}; 897 case -386313260: /*measureScore*/ return new String[] {"Quantity", "dateTime", "CodeableConcept", "Period", "Range", "Duration"}; 898 case 90983669: /*stratifier*/ return new String[] {}; 899 default: return super.getTypesForProperty(hash, name); 900 } 901 902 } 903 904 @Override 905 public Base addChild(String name) throws FHIRException { 906 if (name.equals("linkId")) { 907 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.group.linkId"); 908 } 909 else if (name.equals("code")) { 910 this.code = new CodeableConcept(); 911 return this.code; 912 } 913 else if (name.equals("subject")) { 914 this.subject = new Reference(); 915 return this.subject; 916 } 917 else if (name.equals("population")) { 918 return addPopulation(); 919 } 920 else if (name.equals("measureScoreQuantity")) { 921 this.measureScore = new Quantity(); 922 return this.measureScore; 923 } 924 else if (name.equals("measureScoreDateTime")) { 925 this.measureScore = new DateTimeType(); 926 return this.measureScore; 927 } 928 else if (name.equals("measureScoreCodeableConcept")) { 929 this.measureScore = new CodeableConcept(); 930 return this.measureScore; 931 } 932 else if (name.equals("measureScorePeriod")) { 933 this.measureScore = new Period(); 934 return this.measureScore; 935 } 936 else if (name.equals("measureScoreRange")) { 937 this.measureScore = new Range(); 938 return this.measureScore; 939 } 940 else if (name.equals("measureScoreDuration")) { 941 this.measureScore = new Duration(); 942 return this.measureScore; 943 } 944 else if (name.equals("stratifier")) { 945 return addStratifier(); 946 } 947 else 948 return super.addChild(name); 949 } 950 951 public MeasureReportGroupComponent copy() { 952 MeasureReportGroupComponent dst = new MeasureReportGroupComponent(); 953 copyValues(dst); 954 return dst; 955 } 956 957 public void copyValues(MeasureReportGroupComponent dst) { 958 super.copyValues(dst); 959 dst.linkId = linkId == null ? null : linkId.copy(); 960 dst.code = code == null ? null : code.copy(); 961 dst.subject = subject == null ? null : subject.copy(); 962 if (population != null) { 963 dst.population = new ArrayList<MeasureReportGroupPopulationComponent>(); 964 for (MeasureReportGroupPopulationComponent i : population) 965 dst.population.add(i.copy()); 966 }; 967 dst.measureScore = measureScore == null ? null : measureScore.copy(); 968 if (stratifier != null) { 969 dst.stratifier = new ArrayList<MeasureReportGroupStratifierComponent>(); 970 for (MeasureReportGroupStratifierComponent i : stratifier) 971 dst.stratifier.add(i.copy()); 972 }; 973 } 974 975 @Override 976 public boolean equalsDeep(Base other_) { 977 if (!super.equalsDeep(other_)) 978 return false; 979 if (!(other_ instanceof MeasureReportGroupComponent)) 980 return false; 981 MeasureReportGroupComponent o = (MeasureReportGroupComponent) other_; 982 return compareDeep(linkId, o.linkId, true) && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 983 && compareDeep(population, o.population, true) && compareDeep(measureScore, o.measureScore, true) 984 && compareDeep(stratifier, o.stratifier, true); 985 } 986 987 @Override 988 public boolean equalsShallow(Base other_) { 989 if (!super.equalsShallow(other_)) 990 return false; 991 if (!(other_ instanceof MeasureReportGroupComponent)) 992 return false; 993 MeasureReportGroupComponent o = (MeasureReportGroupComponent) other_; 994 return compareValues(linkId, o.linkId, true); 995 } 996 997 public boolean isEmpty() { 998 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, code, subject, population 999 , measureScore, stratifier); 1000 } 1001 1002 public String fhirType() { 1003 return "MeasureReport.group"; 1004 1005 } 1006 1007 } 1008 1009 @Block() 1010 public static class MeasureReportGroupPopulationComponent extends BackboneElement implements IBaseBackboneElement { 1011 /** 1012 * The population from the Measure that corresponds to this population in the MeasureReport resource. 1013 */ 1014 @Child(name = "linkId", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1015 @Description(shortDefinition="Pointer to specific population from Measure", formalDefinition="The population from the Measure that corresponds to this population in the MeasureReport resource." ) 1016 protected StringType linkId; 1017 1018 /** 1019 * The type of the population. 1020 */ 1021 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1022 @Description(shortDefinition="initial-population | numerator | numerator-exclusion | denominator | denominator-exclusion | denominator-exception | measure-population | measure-population-exclusion | measure-observation", formalDefinition="The type of the population." ) 1023 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-population") 1024 protected CodeableConcept code; 1025 1026 /** 1027 * The number of members of the population. 1028 */ 1029 @Child(name = "count", type = {IntegerType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1030 @Description(shortDefinition="Size of the population", formalDefinition="The number of members of the population." ) 1031 protected IntegerType count; 1032 1033 /** 1034 * This element refers to a List of individual level MeasureReport resources, one for each subject in this population. 1035 */ 1036 @Child(name = "subjectResults", type = {ListResource.class}, order=4, min=0, max=1, modifier=false, summary=false) 1037 @Description(shortDefinition="For subject-list reports, the subject results in this population", formalDefinition="This element refers to a List of individual level MeasureReport resources, one for each subject in this population." ) 1038 protected Reference subjectResults; 1039 1040 /** 1041 * A reference to an individual level MeasureReport resource for a member of the population. 1042 */ 1043 @Child(name = "subjectReport", type = {MeasureReport.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1044 @Description(shortDefinition="For subject-list reports, a subject result in this population", formalDefinition="A reference to an individual level MeasureReport resource for a member of the population." ) 1045 protected List<Reference> subjectReport; 1046 1047 /** 1048 * Optional Group identifying the individuals that make up the population. 1049 */ 1050 @Child(name = "subjects", type = {Group.class}, order=6, min=0, max=1, modifier=false, summary=false) 1051 @Description(shortDefinition="What individual(s) in the population", formalDefinition="Optional Group identifying the individuals that make up the population." ) 1052 protected Reference subjects; 1053 1054 private static final long serialVersionUID = -203678073L; 1055 1056 /** 1057 * Constructor 1058 */ 1059 public MeasureReportGroupPopulationComponent() { 1060 super(); 1061 } 1062 1063 /** 1064 * @return {@link #linkId} (The population from the Measure that corresponds to this population in the MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 1065 */ 1066 public StringType getLinkIdElement() { 1067 if (this.linkId == null) 1068 if (Configuration.errorOnAutoCreate()) 1069 throw new Error("Attempt to auto-create MeasureReportGroupPopulationComponent.linkId"); 1070 else if (Configuration.doAutoCreate()) 1071 this.linkId = new StringType(); // bb 1072 return this.linkId; 1073 } 1074 1075 public boolean hasLinkIdElement() { 1076 return this.linkId != null && !this.linkId.isEmpty(); 1077 } 1078 1079 public boolean hasLinkId() { 1080 return this.linkId != null && !this.linkId.isEmpty(); 1081 } 1082 1083 /** 1084 * @param value {@link #linkId} (The population from the Measure that corresponds to this population in the MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 1085 */ 1086 public MeasureReportGroupPopulationComponent setLinkIdElement(StringType value) { 1087 this.linkId = value; 1088 return this; 1089 } 1090 1091 /** 1092 * @return The population from the Measure that corresponds to this population in the MeasureReport resource. 1093 */ 1094 public String getLinkId() { 1095 return this.linkId == null ? null : this.linkId.getValue(); 1096 } 1097 1098 /** 1099 * @param value The population from the Measure that corresponds to this population in the MeasureReport resource. 1100 */ 1101 public MeasureReportGroupPopulationComponent setLinkId(String value) { 1102 if (Utilities.noString(value)) 1103 this.linkId = null; 1104 else { 1105 if (this.linkId == null) 1106 this.linkId = new StringType(); 1107 this.linkId.setValue(value); 1108 } 1109 return this; 1110 } 1111 1112 /** 1113 * @return {@link #code} (The type of the population.) 1114 */ 1115 public CodeableConcept getCode() { 1116 if (this.code == null) 1117 if (Configuration.errorOnAutoCreate()) 1118 throw new Error("Attempt to auto-create MeasureReportGroupPopulationComponent.code"); 1119 else if (Configuration.doAutoCreate()) 1120 this.code = new CodeableConcept(); // cc 1121 return this.code; 1122 } 1123 1124 public boolean hasCode() { 1125 return this.code != null && !this.code.isEmpty(); 1126 } 1127 1128 /** 1129 * @param value {@link #code} (The type of the population.) 1130 */ 1131 public MeasureReportGroupPopulationComponent setCode(CodeableConcept value) { 1132 this.code = value; 1133 return this; 1134 } 1135 1136 /** 1137 * @return {@link #count} (The number of members of the population.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 1138 */ 1139 public IntegerType getCountElement() { 1140 if (this.count == null) 1141 if (Configuration.errorOnAutoCreate()) 1142 throw new Error("Attempt to auto-create MeasureReportGroupPopulationComponent.count"); 1143 else if (Configuration.doAutoCreate()) 1144 this.count = new IntegerType(); // bb 1145 return this.count; 1146 } 1147 1148 public boolean hasCountElement() { 1149 return this.count != null && !this.count.isEmpty(); 1150 } 1151 1152 public boolean hasCount() { 1153 return this.count != null && !this.count.isEmpty(); 1154 } 1155 1156 /** 1157 * @param value {@link #count} (The number of members of the population.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 1158 */ 1159 public MeasureReportGroupPopulationComponent setCountElement(IntegerType value) { 1160 this.count = value; 1161 return this; 1162 } 1163 1164 /** 1165 * @return The number of members of the population. 1166 */ 1167 public int getCount() { 1168 return this.count == null || this.count.isEmpty() ? 0 : this.count.getValue(); 1169 } 1170 1171 /** 1172 * @param value The number of members of the population. 1173 */ 1174 public MeasureReportGroupPopulationComponent setCount(int value) { 1175 if (this.count == null) 1176 this.count = new IntegerType(); 1177 this.count.setValue(value); 1178 return this; 1179 } 1180 1181 /** 1182 * @return {@link #subjectResults} (This element refers to a List of individual level MeasureReport resources, one for each subject in this population.) 1183 */ 1184 public Reference getSubjectResults() { 1185 if (this.subjectResults == null) 1186 if (Configuration.errorOnAutoCreate()) 1187 throw new Error("Attempt to auto-create MeasureReportGroupPopulationComponent.subjectResults"); 1188 else if (Configuration.doAutoCreate()) 1189 this.subjectResults = new Reference(); // cc 1190 return this.subjectResults; 1191 } 1192 1193 public boolean hasSubjectResults() { 1194 return this.subjectResults != null && !this.subjectResults.isEmpty(); 1195 } 1196 1197 /** 1198 * @param value {@link #subjectResults} (This element refers to a List of individual level MeasureReport resources, one for each subject in this population.) 1199 */ 1200 public MeasureReportGroupPopulationComponent setSubjectResults(Reference value) { 1201 this.subjectResults = value; 1202 return this; 1203 } 1204 1205 /** 1206 * @return {@link #subjectReport} (A reference to an individual level MeasureReport resource for a member of the population.) 1207 */ 1208 public List<Reference> getSubjectReport() { 1209 if (this.subjectReport == null) 1210 this.subjectReport = new ArrayList<Reference>(); 1211 return this.subjectReport; 1212 } 1213 1214 /** 1215 * @return Returns a reference to <code>this</code> for easy method chaining 1216 */ 1217 public MeasureReportGroupPopulationComponent setSubjectReport(List<Reference> theSubjectReport) { 1218 this.subjectReport = theSubjectReport; 1219 return this; 1220 } 1221 1222 public boolean hasSubjectReport() { 1223 if (this.subjectReport == null) 1224 return false; 1225 for (Reference item : this.subjectReport) 1226 if (!item.isEmpty()) 1227 return true; 1228 return false; 1229 } 1230 1231 public Reference addSubjectReport() { //3 1232 Reference t = new Reference(); 1233 if (this.subjectReport == null) 1234 this.subjectReport = new ArrayList<Reference>(); 1235 this.subjectReport.add(t); 1236 return t; 1237 } 1238 1239 public MeasureReportGroupPopulationComponent addSubjectReport(Reference t) { //3 1240 if (t == null) 1241 return this; 1242 if (this.subjectReport == null) 1243 this.subjectReport = new ArrayList<Reference>(); 1244 this.subjectReport.add(t); 1245 return this; 1246 } 1247 1248 /** 1249 * @return The first repetition of repeating field {@link #subjectReport}, creating it if it does not already exist {3} 1250 */ 1251 public Reference getSubjectReportFirstRep() { 1252 if (getSubjectReport().isEmpty()) { 1253 addSubjectReport(); 1254 } 1255 return getSubjectReport().get(0); 1256 } 1257 1258 /** 1259 * @return {@link #subjects} (Optional Group identifying the individuals that make up the population.) 1260 */ 1261 public Reference getSubjects() { 1262 if (this.subjects == null) 1263 if (Configuration.errorOnAutoCreate()) 1264 throw new Error("Attempt to auto-create MeasureReportGroupPopulationComponent.subjects"); 1265 else if (Configuration.doAutoCreate()) 1266 this.subjects = new Reference(); // cc 1267 return this.subjects; 1268 } 1269 1270 public boolean hasSubjects() { 1271 return this.subjects != null && !this.subjects.isEmpty(); 1272 } 1273 1274 /** 1275 * @param value {@link #subjects} (Optional Group identifying the individuals that make up the population.) 1276 */ 1277 public MeasureReportGroupPopulationComponent setSubjects(Reference value) { 1278 this.subjects = value; 1279 return this; 1280 } 1281 1282 protected void listChildren(List<Property> children) { 1283 super.listChildren(children); 1284 children.add(new Property("linkId", "string", "The population from the Measure that corresponds to this population in the MeasureReport resource.", 0, 1, linkId)); 1285 children.add(new Property("code", "CodeableConcept", "The type of the population.", 0, 1, code)); 1286 children.add(new Property("count", "integer", "The number of members of the population.", 0, 1, count)); 1287 children.add(new Property("subjectResults", "Reference(List)", "This element refers to a List of individual level MeasureReport resources, one for each subject in this population.", 0, 1, subjectResults)); 1288 children.add(new Property("subjectReport", "Reference(MeasureReport)", "A reference to an individual level MeasureReport resource for a member of the population.", 0, java.lang.Integer.MAX_VALUE, subjectReport)); 1289 children.add(new Property("subjects", "Reference(Group)", "Optional Group identifying the individuals that make up the population.", 0, 1, subjects)); 1290 } 1291 1292 @Override 1293 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1294 switch (_hash) { 1295 case -1102667083: /*linkId*/ return new Property("linkId", "string", "The population from the Measure that corresponds to this population in the MeasureReport resource.", 0, 1, linkId); 1296 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The type of the population.", 0, 1, code); 1297 case 94851343: /*count*/ return new Property("count", "integer", "The number of members of the population.", 0, 1, count); 1298 case 2136184106: /*subjectResults*/ return new Property("subjectResults", "Reference(List)", "This element refers to a List of individual level MeasureReport resources, one for each subject in this population.", 0, 1, subjectResults); 1299 case 68814208: /*subjectReport*/ return new Property("subjectReport", "Reference(MeasureReport)", "A reference to an individual level MeasureReport resource for a member of the population.", 0, java.lang.Integer.MAX_VALUE, subjectReport); 1300 case -2069868345: /*subjects*/ return new Property("subjects", "Reference(Group)", "Optional Group identifying the individuals that make up the population.", 0, 1, subjects); 1301 default: return super.getNamedProperty(_hash, _name, _checkValid); 1302 } 1303 1304 } 1305 1306 @Override 1307 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1308 switch (hash) { 1309 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 1310 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1311 case 94851343: /*count*/ return this.count == null ? new Base[0] : new Base[] {this.count}; // IntegerType 1312 case 2136184106: /*subjectResults*/ return this.subjectResults == null ? new Base[0] : new Base[] {this.subjectResults}; // Reference 1313 case 68814208: /*subjectReport*/ return this.subjectReport == null ? new Base[0] : this.subjectReport.toArray(new Base[this.subjectReport.size()]); // Reference 1314 case -2069868345: /*subjects*/ return this.subjects == null ? new Base[0] : new Base[] {this.subjects}; // Reference 1315 default: return super.getProperty(hash, name, checkValid); 1316 } 1317 1318 } 1319 1320 @Override 1321 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1322 switch (hash) { 1323 case -1102667083: // linkId 1324 this.linkId = TypeConvertor.castToString(value); // StringType 1325 return value; 1326 case 3059181: // code 1327 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1328 return value; 1329 case 94851343: // count 1330 this.count = TypeConvertor.castToInteger(value); // IntegerType 1331 return value; 1332 case 2136184106: // subjectResults 1333 this.subjectResults = TypeConvertor.castToReference(value); // Reference 1334 return value; 1335 case 68814208: // subjectReport 1336 this.getSubjectReport().add(TypeConvertor.castToReference(value)); // Reference 1337 return value; 1338 case -2069868345: // subjects 1339 this.subjects = TypeConvertor.castToReference(value); // Reference 1340 return value; 1341 default: return super.setProperty(hash, name, value); 1342 } 1343 1344 } 1345 1346 @Override 1347 public Base setProperty(String name, Base value) throws FHIRException { 1348 if (name.equals("linkId")) { 1349 this.linkId = TypeConvertor.castToString(value); // StringType 1350 } else if (name.equals("code")) { 1351 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1352 } else if (name.equals("count")) { 1353 this.count = TypeConvertor.castToInteger(value); // IntegerType 1354 } else if (name.equals("subjectResults")) { 1355 this.subjectResults = TypeConvertor.castToReference(value); // Reference 1356 } else if (name.equals("subjectReport")) { 1357 this.getSubjectReport().add(TypeConvertor.castToReference(value)); 1358 } else if (name.equals("subjects")) { 1359 this.subjects = TypeConvertor.castToReference(value); // Reference 1360 } else 1361 return super.setProperty(name, value); 1362 return value; 1363 } 1364 1365 @Override 1366 public void removeChild(String name, Base value) throws FHIRException { 1367 if (name.equals("linkId")) { 1368 this.linkId = null; 1369 } else if (name.equals("code")) { 1370 this.code = null; 1371 } else if (name.equals("count")) { 1372 this.count = null; 1373 } else if (name.equals("subjectResults")) { 1374 this.subjectResults = null; 1375 } else if (name.equals("subjectReport")) { 1376 this.getSubjectReport().remove(value); 1377 } else if (name.equals("subjects")) { 1378 this.subjects = null; 1379 } else 1380 super.removeChild(name, value); 1381 1382 } 1383 1384 @Override 1385 public Base makeProperty(int hash, String name) throws FHIRException { 1386 switch (hash) { 1387 case -1102667083: return getLinkIdElement(); 1388 case 3059181: return getCode(); 1389 case 94851343: return getCountElement(); 1390 case 2136184106: return getSubjectResults(); 1391 case 68814208: return addSubjectReport(); 1392 case -2069868345: return getSubjects(); 1393 default: return super.makeProperty(hash, name); 1394 } 1395 1396 } 1397 1398 @Override 1399 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1400 switch (hash) { 1401 case -1102667083: /*linkId*/ return new String[] {"string"}; 1402 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1403 case 94851343: /*count*/ return new String[] {"integer"}; 1404 case 2136184106: /*subjectResults*/ return new String[] {"Reference"}; 1405 case 68814208: /*subjectReport*/ return new String[] {"Reference"}; 1406 case -2069868345: /*subjects*/ return new String[] {"Reference"}; 1407 default: return super.getTypesForProperty(hash, name); 1408 } 1409 1410 } 1411 1412 @Override 1413 public Base addChild(String name) throws FHIRException { 1414 if (name.equals("linkId")) { 1415 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.group.population.linkId"); 1416 } 1417 else if (name.equals("code")) { 1418 this.code = new CodeableConcept(); 1419 return this.code; 1420 } 1421 else if (name.equals("count")) { 1422 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.group.population.count"); 1423 } 1424 else if (name.equals("subjectResults")) { 1425 this.subjectResults = new Reference(); 1426 return this.subjectResults; 1427 } 1428 else if (name.equals("subjectReport")) { 1429 return addSubjectReport(); 1430 } 1431 else if (name.equals("subjects")) { 1432 this.subjects = new Reference(); 1433 return this.subjects; 1434 } 1435 else 1436 return super.addChild(name); 1437 } 1438 1439 public MeasureReportGroupPopulationComponent copy() { 1440 MeasureReportGroupPopulationComponent dst = new MeasureReportGroupPopulationComponent(); 1441 copyValues(dst); 1442 return dst; 1443 } 1444 1445 public void copyValues(MeasureReportGroupPopulationComponent dst) { 1446 super.copyValues(dst); 1447 dst.linkId = linkId == null ? null : linkId.copy(); 1448 dst.code = code == null ? null : code.copy(); 1449 dst.count = count == null ? null : count.copy(); 1450 dst.subjectResults = subjectResults == null ? null : subjectResults.copy(); 1451 if (subjectReport != null) { 1452 dst.subjectReport = new ArrayList<Reference>(); 1453 for (Reference i : subjectReport) 1454 dst.subjectReport.add(i.copy()); 1455 }; 1456 dst.subjects = subjects == null ? null : subjects.copy(); 1457 } 1458 1459 @Override 1460 public boolean equalsDeep(Base other_) { 1461 if (!super.equalsDeep(other_)) 1462 return false; 1463 if (!(other_ instanceof MeasureReportGroupPopulationComponent)) 1464 return false; 1465 MeasureReportGroupPopulationComponent o = (MeasureReportGroupPopulationComponent) other_; 1466 return compareDeep(linkId, o.linkId, true) && compareDeep(code, o.code, true) && compareDeep(count, o.count, true) 1467 && compareDeep(subjectResults, o.subjectResults, true) && compareDeep(subjectReport, o.subjectReport, true) 1468 && compareDeep(subjects, o.subjects, true); 1469 } 1470 1471 @Override 1472 public boolean equalsShallow(Base other_) { 1473 if (!super.equalsShallow(other_)) 1474 return false; 1475 if (!(other_ instanceof MeasureReportGroupPopulationComponent)) 1476 return false; 1477 MeasureReportGroupPopulationComponent o = (MeasureReportGroupPopulationComponent) other_; 1478 return compareValues(linkId, o.linkId, true) && compareValues(count, o.count, true); 1479 } 1480 1481 public boolean isEmpty() { 1482 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, code, count, subjectResults 1483 , subjectReport, subjects); 1484 } 1485 1486 public String fhirType() { 1487 return "MeasureReport.group.population"; 1488 1489 } 1490 1491 } 1492 1493 @Block() 1494 public static class MeasureReportGroupStratifierComponent extends BackboneElement implements IBaseBackboneElement { 1495 /** 1496 * The stratifier from the Measure that corresponds to this stratifier in the MeasureReport resource. 1497 */ 1498 @Child(name = "linkId", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1499 @Description(shortDefinition="Pointer to specific stratifier from Measure", formalDefinition="The stratifier from the Measure that corresponds to this stratifier in the MeasureReport resource." ) 1500 protected StringType linkId; 1501 1502 /** 1503 * The meaning of this stratifier, as defined in the measure definition. 1504 */ 1505 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1506 @Description(shortDefinition="What stratifier of the group", formalDefinition="The meaning of this stratifier, as defined in the measure definition." ) 1507 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-stratifier-example") 1508 protected CodeableConcept code; 1509 1510 /** 1511 * This element contains the results for a single stratum within the stratifier. For example, when stratifying on administrative gender, there will be four strata, one for each possible gender value. 1512 */ 1513 @Child(name = "stratum", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1514 @Description(shortDefinition="Stratum results, one for each unique value, or set of values, in the stratifier, or stratifier components", formalDefinition="This element contains the results for a single stratum within the stratifier. For example, when stratifying on administrative gender, there will be four strata, one for each possible gender value." ) 1515 protected List<StratifierGroupComponent> stratum; 1516 1517 private static final long serialVersionUID = 1021076195L; 1518 1519 /** 1520 * Constructor 1521 */ 1522 public MeasureReportGroupStratifierComponent() { 1523 super(); 1524 } 1525 1526 /** 1527 * @return {@link #linkId} (The stratifier from the Measure that corresponds to this stratifier in the MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 1528 */ 1529 public StringType getLinkIdElement() { 1530 if (this.linkId == null) 1531 if (Configuration.errorOnAutoCreate()) 1532 throw new Error("Attempt to auto-create MeasureReportGroupStratifierComponent.linkId"); 1533 else if (Configuration.doAutoCreate()) 1534 this.linkId = new StringType(); // bb 1535 return this.linkId; 1536 } 1537 1538 public boolean hasLinkIdElement() { 1539 return this.linkId != null && !this.linkId.isEmpty(); 1540 } 1541 1542 public boolean hasLinkId() { 1543 return this.linkId != null && !this.linkId.isEmpty(); 1544 } 1545 1546 /** 1547 * @param value {@link #linkId} (The stratifier from the Measure that corresponds to this stratifier in the MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 1548 */ 1549 public MeasureReportGroupStratifierComponent setLinkIdElement(StringType value) { 1550 this.linkId = value; 1551 return this; 1552 } 1553 1554 /** 1555 * @return The stratifier from the Measure that corresponds to this stratifier in the MeasureReport resource. 1556 */ 1557 public String getLinkId() { 1558 return this.linkId == null ? null : this.linkId.getValue(); 1559 } 1560 1561 /** 1562 * @param value The stratifier from the Measure that corresponds to this stratifier in the MeasureReport resource. 1563 */ 1564 public MeasureReportGroupStratifierComponent setLinkId(String value) { 1565 if (Utilities.noString(value)) 1566 this.linkId = null; 1567 else { 1568 if (this.linkId == null) 1569 this.linkId = new StringType(); 1570 this.linkId.setValue(value); 1571 } 1572 return this; 1573 } 1574 1575 /** 1576 * @return {@link #code} (The meaning of this stratifier, as defined in the measure definition.) 1577 */ 1578 public CodeableConcept getCode() { 1579 if (this.code == null) 1580 if (Configuration.errorOnAutoCreate()) 1581 throw new Error("Attempt to auto-create MeasureReportGroupStratifierComponent.code"); 1582 else if (Configuration.doAutoCreate()) 1583 this.code = new CodeableConcept(); // cc 1584 return this.code; 1585 } 1586 1587 public boolean hasCode() { 1588 return this.code != null && !this.code.isEmpty(); 1589 } 1590 1591 /** 1592 * @param value {@link #code} (The meaning of this stratifier, as defined in the measure definition.) 1593 */ 1594 public MeasureReportGroupStratifierComponent setCode(CodeableConcept value) { 1595 this.code = value; 1596 return this; 1597 } 1598 1599 /** 1600 * @return {@link #stratum} (This element contains the results for a single stratum within the stratifier. For example, when stratifying on administrative gender, there will be four strata, one for each possible gender value.) 1601 */ 1602 public List<StratifierGroupComponent> getStratum() { 1603 if (this.stratum == null) 1604 this.stratum = new ArrayList<StratifierGroupComponent>(); 1605 return this.stratum; 1606 } 1607 1608 /** 1609 * @return Returns a reference to <code>this</code> for easy method chaining 1610 */ 1611 public MeasureReportGroupStratifierComponent setStratum(List<StratifierGroupComponent> theStratum) { 1612 this.stratum = theStratum; 1613 return this; 1614 } 1615 1616 public boolean hasStratum() { 1617 if (this.stratum == null) 1618 return false; 1619 for (StratifierGroupComponent item : this.stratum) 1620 if (!item.isEmpty()) 1621 return true; 1622 return false; 1623 } 1624 1625 public StratifierGroupComponent addStratum() { //3 1626 StratifierGroupComponent t = new StratifierGroupComponent(); 1627 if (this.stratum == null) 1628 this.stratum = new ArrayList<StratifierGroupComponent>(); 1629 this.stratum.add(t); 1630 return t; 1631 } 1632 1633 public MeasureReportGroupStratifierComponent addStratum(StratifierGroupComponent t) { //3 1634 if (t == null) 1635 return this; 1636 if (this.stratum == null) 1637 this.stratum = new ArrayList<StratifierGroupComponent>(); 1638 this.stratum.add(t); 1639 return this; 1640 } 1641 1642 /** 1643 * @return The first repetition of repeating field {@link #stratum}, creating it if it does not already exist {3} 1644 */ 1645 public StratifierGroupComponent getStratumFirstRep() { 1646 if (getStratum().isEmpty()) { 1647 addStratum(); 1648 } 1649 return getStratum().get(0); 1650 } 1651 1652 protected void listChildren(List<Property> children) { 1653 super.listChildren(children); 1654 children.add(new Property("linkId", "string", "The stratifier from the Measure that corresponds to this stratifier in the MeasureReport resource.", 0, 1, linkId)); 1655 children.add(new Property("code", "CodeableConcept", "The meaning of this stratifier, as defined in the measure definition.", 0, 1, code)); 1656 children.add(new Property("stratum", "", "This element contains the results for a single stratum within the stratifier. For example, when stratifying on administrative gender, there will be four strata, one for each possible gender value.", 0, java.lang.Integer.MAX_VALUE, stratum)); 1657 } 1658 1659 @Override 1660 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1661 switch (_hash) { 1662 case -1102667083: /*linkId*/ return new Property("linkId", "string", "The stratifier from the Measure that corresponds to this stratifier in the MeasureReport resource.", 0, 1, linkId); 1663 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The meaning of this stratifier, as defined in the measure definition.", 0, 1, code); 1664 case -1881991236: /*stratum*/ return new Property("stratum", "", "This element contains the results for a single stratum within the stratifier. For example, when stratifying on administrative gender, there will be four strata, one for each possible gender value.", 0, java.lang.Integer.MAX_VALUE, stratum); 1665 default: return super.getNamedProperty(_hash, _name, _checkValid); 1666 } 1667 1668 } 1669 1670 @Override 1671 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1672 switch (hash) { 1673 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 1674 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1675 case -1881991236: /*stratum*/ return this.stratum == null ? new Base[0] : this.stratum.toArray(new Base[this.stratum.size()]); // StratifierGroupComponent 1676 default: return super.getProperty(hash, name, checkValid); 1677 } 1678 1679 } 1680 1681 @Override 1682 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1683 switch (hash) { 1684 case -1102667083: // linkId 1685 this.linkId = TypeConvertor.castToString(value); // StringType 1686 return value; 1687 case 3059181: // code 1688 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1689 return value; 1690 case -1881991236: // stratum 1691 this.getStratum().add((StratifierGroupComponent) value); // StratifierGroupComponent 1692 return value; 1693 default: return super.setProperty(hash, name, value); 1694 } 1695 1696 } 1697 1698 @Override 1699 public Base setProperty(String name, Base value) throws FHIRException { 1700 if (name.equals("linkId")) { 1701 this.linkId = TypeConvertor.castToString(value); // StringType 1702 } else if (name.equals("code")) { 1703 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1704 } else if (name.equals("stratum")) { 1705 this.getStratum().add((StratifierGroupComponent) value); 1706 } else 1707 return super.setProperty(name, value); 1708 return value; 1709 } 1710 1711 @Override 1712 public void removeChild(String name, Base value) throws FHIRException { 1713 if (name.equals("linkId")) { 1714 this.linkId = null; 1715 } else if (name.equals("code")) { 1716 this.code = null; 1717 } else if (name.equals("stratum")) { 1718 this.getStratum().remove((StratifierGroupComponent) value); 1719 } else 1720 super.removeChild(name, value); 1721 1722 } 1723 1724 @Override 1725 public Base makeProperty(int hash, String name) throws FHIRException { 1726 switch (hash) { 1727 case -1102667083: return getLinkIdElement(); 1728 case 3059181: return getCode(); 1729 case -1881991236: return addStratum(); 1730 default: return super.makeProperty(hash, name); 1731 } 1732 1733 } 1734 1735 @Override 1736 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1737 switch (hash) { 1738 case -1102667083: /*linkId*/ return new String[] {"string"}; 1739 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1740 case -1881991236: /*stratum*/ return new String[] {}; 1741 default: return super.getTypesForProperty(hash, name); 1742 } 1743 1744 } 1745 1746 @Override 1747 public Base addChild(String name) throws FHIRException { 1748 if (name.equals("linkId")) { 1749 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.group.stratifier.linkId"); 1750 } 1751 else if (name.equals("code")) { 1752 this.code = new CodeableConcept(); 1753 return this.code; 1754 } 1755 else if (name.equals("stratum")) { 1756 return addStratum(); 1757 } 1758 else 1759 return super.addChild(name); 1760 } 1761 1762 public MeasureReportGroupStratifierComponent copy() { 1763 MeasureReportGroupStratifierComponent dst = new MeasureReportGroupStratifierComponent(); 1764 copyValues(dst); 1765 return dst; 1766 } 1767 1768 public void copyValues(MeasureReportGroupStratifierComponent dst) { 1769 super.copyValues(dst); 1770 dst.linkId = linkId == null ? null : linkId.copy(); 1771 dst.code = code == null ? null : code.copy(); 1772 if (stratum != null) { 1773 dst.stratum = new ArrayList<StratifierGroupComponent>(); 1774 for (StratifierGroupComponent i : stratum) 1775 dst.stratum.add(i.copy()); 1776 }; 1777 } 1778 1779 @Override 1780 public boolean equalsDeep(Base other_) { 1781 if (!super.equalsDeep(other_)) 1782 return false; 1783 if (!(other_ instanceof MeasureReportGroupStratifierComponent)) 1784 return false; 1785 MeasureReportGroupStratifierComponent o = (MeasureReportGroupStratifierComponent) other_; 1786 return compareDeep(linkId, o.linkId, true) && compareDeep(code, o.code, true) && compareDeep(stratum, o.stratum, true) 1787 ; 1788 } 1789 1790 @Override 1791 public boolean equalsShallow(Base other_) { 1792 if (!super.equalsShallow(other_)) 1793 return false; 1794 if (!(other_ instanceof MeasureReportGroupStratifierComponent)) 1795 return false; 1796 MeasureReportGroupStratifierComponent o = (MeasureReportGroupStratifierComponent) other_; 1797 return compareValues(linkId, o.linkId, true); 1798 } 1799 1800 public boolean isEmpty() { 1801 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, code, stratum); 1802 } 1803 1804 public String fhirType() { 1805 return "MeasureReport.group.stratifier"; 1806 1807 } 1808 1809 } 1810 1811 @Block() 1812 public static class StratifierGroupComponent extends BackboneElement implements IBaseBackboneElement { 1813 /** 1814 * The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique. 1815 */ 1816 @Child(name = "value", type = {CodeableConcept.class, BooleanType.class, Quantity.class, Range.class, Reference.class}, order=1, min=0, max=1, modifier=false, summary=false) 1817 @Description(shortDefinition="The stratum value, e.g. male", formalDefinition="The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique." ) 1818 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measurereport-stratifier-value-example") 1819 protected DataType value; 1820 1821 /** 1822 * A stratifier component value. 1823 */ 1824 @Child(name = "component", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1825 @Description(shortDefinition="Stratifier component values", formalDefinition="A stratifier component value." ) 1826 protected List<StratifierGroupComponentComponent> component; 1827 1828 /** 1829 * The populations that make up the stratum, one for each type of population appropriate to the measure. 1830 */ 1831 @Child(name = "population", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1832 @Description(shortDefinition="Population results in this stratum", formalDefinition="The populations that make up the stratum, one for each type of population appropriate to the measure." ) 1833 protected List<StratifierGroupPopulationComponent> population; 1834 1835 /** 1836 * The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum. 1837 */ 1838 @Child(name = "measureScore", type = {Quantity.class, DateTimeType.class, CodeableConcept.class, Period.class, Range.class, Duration.class}, order=4, min=0, max=1, modifier=false, summary=false) 1839 @Description(shortDefinition="What score this stratum achieved", formalDefinition="The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum." ) 1840 protected DataType measureScore; 1841 1842 private static final long serialVersionUID = -1713783491L; 1843 1844 /** 1845 * Constructor 1846 */ 1847 public StratifierGroupComponent() { 1848 super(); 1849 } 1850 1851 /** 1852 * @return {@link #value} (The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.) 1853 */ 1854 public DataType getValue() { 1855 return this.value; 1856 } 1857 1858 /** 1859 * @return {@link #value} (The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.) 1860 */ 1861 public CodeableConcept getValueCodeableConcept() throws FHIRException { 1862 if (this.value == null) 1863 this.value = new CodeableConcept(); 1864 if (!(this.value instanceof CodeableConcept)) 1865 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 1866 return (CodeableConcept) this.value; 1867 } 1868 1869 public boolean hasValueCodeableConcept() { 1870 return this != null && this.value instanceof CodeableConcept; 1871 } 1872 1873 /** 1874 * @return {@link #value} (The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.) 1875 */ 1876 public BooleanType getValueBooleanType() throws FHIRException { 1877 if (this.value == null) 1878 this.value = new BooleanType(); 1879 if (!(this.value instanceof BooleanType)) 1880 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 1881 return (BooleanType) this.value; 1882 } 1883 1884 public boolean hasValueBooleanType() { 1885 return this != null && this.value instanceof BooleanType; 1886 } 1887 1888 /** 1889 * @return {@link #value} (The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.) 1890 */ 1891 public Quantity getValueQuantity() throws FHIRException { 1892 if (this.value == null) 1893 this.value = new Quantity(); 1894 if (!(this.value instanceof Quantity)) 1895 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 1896 return (Quantity) this.value; 1897 } 1898 1899 public boolean hasValueQuantity() { 1900 return this != null && this.value instanceof Quantity; 1901 } 1902 1903 /** 1904 * @return {@link #value} (The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.) 1905 */ 1906 public Range getValueRange() throws FHIRException { 1907 if (this.value == null) 1908 this.value = new Range(); 1909 if (!(this.value instanceof Range)) 1910 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 1911 return (Range) this.value; 1912 } 1913 1914 public boolean hasValueRange() { 1915 return this != null && this.value instanceof Range; 1916 } 1917 1918 /** 1919 * @return {@link #value} (The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.) 1920 */ 1921 public Reference getValueReference() throws FHIRException { 1922 if (this.value == null) 1923 this.value = new Reference(); 1924 if (!(this.value instanceof Reference)) 1925 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 1926 return (Reference) this.value; 1927 } 1928 1929 public boolean hasValueReference() { 1930 return this != null && this.value instanceof Reference; 1931 } 1932 1933 public boolean hasValue() { 1934 return this.value != null && !this.value.isEmpty(); 1935 } 1936 1937 /** 1938 * @param value {@link #value} (The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.) 1939 */ 1940 public StratifierGroupComponent setValue(DataType value) { 1941 if (value != null && !(value instanceof CodeableConcept || value instanceof BooleanType || value instanceof Quantity || value instanceof Range || value instanceof Reference)) 1942 throw new FHIRException("Not the right type for MeasureReport.group.stratifier.stratum.value[x]: "+value.fhirType()); 1943 this.value = value; 1944 return this; 1945 } 1946 1947 /** 1948 * @return {@link #component} (A stratifier component value.) 1949 */ 1950 public List<StratifierGroupComponentComponent> getComponent() { 1951 if (this.component == null) 1952 this.component = new ArrayList<StratifierGroupComponentComponent>(); 1953 return this.component; 1954 } 1955 1956 /** 1957 * @return Returns a reference to <code>this</code> for easy method chaining 1958 */ 1959 public StratifierGroupComponent setComponent(List<StratifierGroupComponentComponent> theComponent) { 1960 this.component = theComponent; 1961 return this; 1962 } 1963 1964 public boolean hasComponent() { 1965 if (this.component == null) 1966 return false; 1967 for (StratifierGroupComponentComponent item : this.component) 1968 if (!item.isEmpty()) 1969 return true; 1970 return false; 1971 } 1972 1973 public StratifierGroupComponentComponent addComponent() { //3 1974 StratifierGroupComponentComponent t = new StratifierGroupComponentComponent(); 1975 if (this.component == null) 1976 this.component = new ArrayList<StratifierGroupComponentComponent>(); 1977 this.component.add(t); 1978 return t; 1979 } 1980 1981 public StratifierGroupComponent addComponent(StratifierGroupComponentComponent t) { //3 1982 if (t == null) 1983 return this; 1984 if (this.component == null) 1985 this.component = new ArrayList<StratifierGroupComponentComponent>(); 1986 this.component.add(t); 1987 return this; 1988 } 1989 1990 /** 1991 * @return The first repetition of repeating field {@link #component}, creating it if it does not already exist {3} 1992 */ 1993 public StratifierGroupComponentComponent getComponentFirstRep() { 1994 if (getComponent().isEmpty()) { 1995 addComponent(); 1996 } 1997 return getComponent().get(0); 1998 } 1999 2000 /** 2001 * @return {@link #population} (The populations that make up the stratum, one for each type of population appropriate to the measure.) 2002 */ 2003 public List<StratifierGroupPopulationComponent> getPopulation() { 2004 if (this.population == null) 2005 this.population = new ArrayList<StratifierGroupPopulationComponent>(); 2006 return this.population; 2007 } 2008 2009 /** 2010 * @return Returns a reference to <code>this</code> for easy method chaining 2011 */ 2012 public StratifierGroupComponent setPopulation(List<StratifierGroupPopulationComponent> thePopulation) { 2013 this.population = thePopulation; 2014 return this; 2015 } 2016 2017 public boolean hasPopulation() { 2018 if (this.population == null) 2019 return false; 2020 for (StratifierGroupPopulationComponent item : this.population) 2021 if (!item.isEmpty()) 2022 return true; 2023 return false; 2024 } 2025 2026 public StratifierGroupPopulationComponent addPopulation() { //3 2027 StratifierGroupPopulationComponent t = new StratifierGroupPopulationComponent(); 2028 if (this.population == null) 2029 this.population = new ArrayList<StratifierGroupPopulationComponent>(); 2030 this.population.add(t); 2031 return t; 2032 } 2033 2034 public StratifierGroupComponent addPopulation(StratifierGroupPopulationComponent t) { //3 2035 if (t == null) 2036 return this; 2037 if (this.population == null) 2038 this.population = new ArrayList<StratifierGroupPopulationComponent>(); 2039 this.population.add(t); 2040 return this; 2041 } 2042 2043 /** 2044 * @return The first repetition of repeating field {@link #population}, creating it if it does not already exist {3} 2045 */ 2046 public StratifierGroupPopulationComponent getPopulationFirstRep() { 2047 if (getPopulation().isEmpty()) { 2048 addPopulation(); 2049 } 2050 return getPopulation().get(0); 2051 } 2052 2053 /** 2054 * @return {@link #measureScore} (The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.) 2055 */ 2056 public DataType getMeasureScore() { 2057 return this.measureScore; 2058 } 2059 2060 /** 2061 * @return {@link #measureScore} (The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.) 2062 */ 2063 public Quantity getMeasureScoreQuantity() throws FHIRException { 2064 if (this.measureScore == null) 2065 this.measureScore = new Quantity(); 2066 if (!(this.measureScore instanceof Quantity)) 2067 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.measureScore.getClass().getName()+" was encountered"); 2068 return (Quantity) this.measureScore; 2069 } 2070 2071 public boolean hasMeasureScoreQuantity() { 2072 return this != null && this.measureScore instanceof Quantity; 2073 } 2074 2075 /** 2076 * @return {@link #measureScore} (The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.) 2077 */ 2078 public DateTimeType getMeasureScoreDateTimeType() throws FHIRException { 2079 if (this.measureScore == null) 2080 this.measureScore = new DateTimeType(); 2081 if (!(this.measureScore instanceof DateTimeType)) 2082 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.measureScore.getClass().getName()+" was encountered"); 2083 return (DateTimeType) this.measureScore; 2084 } 2085 2086 public boolean hasMeasureScoreDateTimeType() { 2087 return this != null && this.measureScore instanceof DateTimeType; 2088 } 2089 2090 /** 2091 * @return {@link #measureScore} (The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.) 2092 */ 2093 public CodeableConcept getMeasureScoreCodeableConcept() throws FHIRException { 2094 if (this.measureScore == null) 2095 this.measureScore = new CodeableConcept(); 2096 if (!(this.measureScore instanceof CodeableConcept)) 2097 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.measureScore.getClass().getName()+" was encountered"); 2098 return (CodeableConcept) this.measureScore; 2099 } 2100 2101 public boolean hasMeasureScoreCodeableConcept() { 2102 return this != null && this.measureScore instanceof CodeableConcept; 2103 } 2104 2105 /** 2106 * @return {@link #measureScore} (The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.) 2107 */ 2108 public Period getMeasureScorePeriod() throws FHIRException { 2109 if (this.measureScore == null) 2110 this.measureScore = new Period(); 2111 if (!(this.measureScore instanceof Period)) 2112 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.measureScore.getClass().getName()+" was encountered"); 2113 return (Period) this.measureScore; 2114 } 2115 2116 public boolean hasMeasureScorePeriod() { 2117 return this != null && this.measureScore instanceof Period; 2118 } 2119 2120 /** 2121 * @return {@link #measureScore} (The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.) 2122 */ 2123 public Range getMeasureScoreRange() throws FHIRException { 2124 if (this.measureScore == null) 2125 this.measureScore = new Range(); 2126 if (!(this.measureScore instanceof Range)) 2127 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.measureScore.getClass().getName()+" was encountered"); 2128 return (Range) this.measureScore; 2129 } 2130 2131 public boolean hasMeasureScoreRange() { 2132 return this != null && this.measureScore instanceof Range; 2133 } 2134 2135 /** 2136 * @return {@link #measureScore} (The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.) 2137 */ 2138 public Duration getMeasureScoreDuration() throws FHIRException { 2139 if (this.measureScore == null) 2140 this.measureScore = new Duration(); 2141 if (!(this.measureScore instanceof Duration)) 2142 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.measureScore.getClass().getName()+" was encountered"); 2143 return (Duration) this.measureScore; 2144 } 2145 2146 public boolean hasMeasureScoreDuration() { 2147 return this != null && this.measureScore instanceof Duration; 2148 } 2149 2150 public boolean hasMeasureScore() { 2151 return this.measureScore != null && !this.measureScore.isEmpty(); 2152 } 2153 2154 /** 2155 * @param value {@link #measureScore} (The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.) 2156 */ 2157 public StratifierGroupComponent setMeasureScore(DataType value) { 2158 if (value != null && !(value instanceof Quantity || value instanceof DateTimeType || value instanceof CodeableConcept || value instanceof Period || value instanceof Range || value instanceof Duration)) 2159 throw new FHIRException("Not the right type for MeasureReport.group.stratifier.stratum.measureScore[x]: "+value.fhirType()); 2160 this.measureScore = value; 2161 return this; 2162 } 2163 2164 protected void listChildren(List<Property> children) { 2165 super.listChildren(children); 2166 children.add(new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", "The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.", 0, 1, value)); 2167 children.add(new Property("component", "", "A stratifier component value.", 0, java.lang.Integer.MAX_VALUE, component)); 2168 children.add(new Property("population", "", "The populations that make up the stratum, one for each type of population appropriate to the measure.", 0, java.lang.Integer.MAX_VALUE, population)); 2169 children.add(new Property("measureScore[x]", "Quantity|dateTime|CodeableConcept|Period|Range|Duration", "The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.", 0, 1, measureScore)); 2170 } 2171 2172 @Override 2173 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2174 switch (_hash) { 2175 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", "The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.", 0, 1, value); 2176 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", "The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.", 0, 1, value); 2177 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.", 0, 1, value); 2178 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.", 0, 1, value); 2179 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.", 0, 1, value); 2180 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.", 0, 1, value); 2181 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference", "The value for this stratum, expressed as a CodeableConcept. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.", 0, 1, value); 2182 case -1399907075: /*component*/ return new Property("component", "", "A stratifier component value.", 0, java.lang.Integer.MAX_VALUE, component); 2183 case -2023558323: /*population*/ return new Property("population", "", "The populations that make up the stratum, one for each type of population appropriate to the measure.", 0, java.lang.Integer.MAX_VALUE, population); 2184 case 1854115884: /*measureScore[x]*/ return new Property("measureScore[x]", "Quantity|dateTime|CodeableConcept|Period|Range|Duration", "The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.", 0, 1, measureScore); 2185 case -386313260: /*measureScore*/ return new Property("measureScore[x]", "Quantity|dateTime|CodeableConcept|Period|Range|Duration", "The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.", 0, 1, measureScore); 2186 case -1880815489: /*measureScoreQuantity*/ return new Property("measureScore[x]", "Quantity", "The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.", 0, 1, measureScore); 2187 case 1196938127: /*measureScoreDateTime*/ return new Property("measureScore[x]", "dateTime", "The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.", 0, 1, measureScore); 2188 case -1193234131: /*measureScoreCodeableConcept*/ return new Property("measureScore[x]", "CodeableConcept", "The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.", 0, 1, measureScore); 2189 case -1939831115: /*measureScorePeriod*/ return new Property("measureScore[x]", "Period", "The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.", 0, 1, measureScore); 2190 case -615040567: /*measureScoreRange*/ return new Property("measureScore[x]", "Range", "The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.", 0, 1, measureScore); 2191 case 1707143560: /*measureScoreDuration*/ return new Property("measureScore[x]", "Duration", "The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.", 0, 1, measureScore); 2192 default: return super.getNamedProperty(_hash, _name, _checkValid); 2193 } 2194 2195 } 2196 2197 @Override 2198 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2199 switch (hash) { 2200 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 2201 case -1399907075: /*component*/ return this.component == null ? new Base[0] : this.component.toArray(new Base[this.component.size()]); // StratifierGroupComponentComponent 2202 case -2023558323: /*population*/ return this.population == null ? new Base[0] : this.population.toArray(new Base[this.population.size()]); // StratifierGroupPopulationComponent 2203 case -386313260: /*measureScore*/ return this.measureScore == null ? new Base[0] : new Base[] {this.measureScore}; // DataType 2204 default: return super.getProperty(hash, name, checkValid); 2205 } 2206 2207 } 2208 2209 @Override 2210 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2211 switch (hash) { 2212 case 111972721: // value 2213 this.value = TypeConvertor.castToType(value); // DataType 2214 return value; 2215 case -1399907075: // component 2216 this.getComponent().add((StratifierGroupComponentComponent) value); // StratifierGroupComponentComponent 2217 return value; 2218 case -2023558323: // population 2219 this.getPopulation().add((StratifierGroupPopulationComponent) value); // StratifierGroupPopulationComponent 2220 return value; 2221 case -386313260: // measureScore 2222 this.measureScore = TypeConvertor.castToType(value); // DataType 2223 return value; 2224 default: return super.setProperty(hash, name, value); 2225 } 2226 2227 } 2228 2229 @Override 2230 public Base setProperty(String name, Base value) throws FHIRException { 2231 if (name.equals("value[x]")) { 2232 this.value = TypeConvertor.castToType(value); // DataType 2233 } else if (name.equals("component")) { 2234 this.getComponent().add((StratifierGroupComponentComponent) value); 2235 } else if (name.equals("population")) { 2236 this.getPopulation().add((StratifierGroupPopulationComponent) value); 2237 } else if (name.equals("measureScore[x]")) { 2238 this.measureScore = TypeConvertor.castToType(value); // DataType 2239 } else 2240 return super.setProperty(name, value); 2241 return value; 2242 } 2243 2244 @Override 2245 public void removeChild(String name, Base value) throws FHIRException { 2246 if (name.equals("value[x]")) { 2247 this.value = null; 2248 } else if (name.equals("component")) { 2249 this.getComponent().remove((StratifierGroupComponentComponent) value); 2250 } else if (name.equals("population")) { 2251 this.getPopulation().remove((StratifierGroupPopulationComponent) value); 2252 } else if (name.equals("measureScore[x]")) { 2253 this.measureScore = null; 2254 } else 2255 super.removeChild(name, value); 2256 2257 } 2258 2259 @Override 2260 public Base makeProperty(int hash, String name) throws FHIRException { 2261 switch (hash) { 2262 case -1410166417: return getValue(); 2263 case 111972721: return getValue(); 2264 case -1399907075: return addComponent(); 2265 case -2023558323: return addPopulation(); 2266 case 1854115884: return getMeasureScore(); 2267 case -386313260: return getMeasureScore(); 2268 default: return super.makeProperty(hash, name); 2269 } 2270 2271 } 2272 2273 @Override 2274 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2275 switch (hash) { 2276 case 111972721: /*value*/ return new String[] {"CodeableConcept", "boolean", "Quantity", "Range", "Reference"}; 2277 case -1399907075: /*component*/ return new String[] {}; 2278 case -2023558323: /*population*/ return new String[] {}; 2279 case -386313260: /*measureScore*/ return new String[] {"Quantity", "dateTime", "CodeableConcept", "Period", "Range", "Duration"}; 2280 default: return super.getTypesForProperty(hash, name); 2281 } 2282 2283 } 2284 2285 @Override 2286 public Base addChild(String name) throws FHIRException { 2287 if (name.equals("valueCodeableConcept")) { 2288 this.value = new CodeableConcept(); 2289 return this.value; 2290 } 2291 else if (name.equals("valueBoolean")) { 2292 this.value = new BooleanType(); 2293 return this.value; 2294 } 2295 else if (name.equals("valueQuantity")) { 2296 this.value = new Quantity(); 2297 return this.value; 2298 } 2299 else if (name.equals("valueRange")) { 2300 this.value = new Range(); 2301 return this.value; 2302 } 2303 else if (name.equals("valueReference")) { 2304 this.value = new Reference(); 2305 return this.value; 2306 } 2307 else if (name.equals("component")) { 2308 return addComponent(); 2309 } 2310 else if (name.equals("population")) { 2311 return addPopulation(); 2312 } 2313 else if (name.equals("measureScoreQuantity")) { 2314 this.measureScore = new Quantity(); 2315 return this.measureScore; 2316 } 2317 else if (name.equals("measureScoreDateTime")) { 2318 this.measureScore = new DateTimeType(); 2319 return this.measureScore; 2320 } 2321 else if (name.equals("measureScoreCodeableConcept")) { 2322 this.measureScore = new CodeableConcept(); 2323 return this.measureScore; 2324 } 2325 else if (name.equals("measureScorePeriod")) { 2326 this.measureScore = new Period(); 2327 return this.measureScore; 2328 } 2329 else if (name.equals("measureScoreRange")) { 2330 this.measureScore = new Range(); 2331 return this.measureScore; 2332 } 2333 else if (name.equals("measureScoreDuration")) { 2334 this.measureScore = new Duration(); 2335 return this.measureScore; 2336 } 2337 else 2338 return super.addChild(name); 2339 } 2340 2341 public StratifierGroupComponent copy() { 2342 StratifierGroupComponent dst = new StratifierGroupComponent(); 2343 copyValues(dst); 2344 return dst; 2345 } 2346 2347 public void copyValues(StratifierGroupComponent dst) { 2348 super.copyValues(dst); 2349 dst.value = value == null ? null : value.copy(); 2350 if (component != null) { 2351 dst.component = new ArrayList<StratifierGroupComponentComponent>(); 2352 for (StratifierGroupComponentComponent i : component) 2353 dst.component.add(i.copy()); 2354 }; 2355 if (population != null) { 2356 dst.population = new ArrayList<StratifierGroupPopulationComponent>(); 2357 for (StratifierGroupPopulationComponent i : population) 2358 dst.population.add(i.copy()); 2359 }; 2360 dst.measureScore = measureScore == null ? null : measureScore.copy(); 2361 } 2362 2363 @Override 2364 public boolean equalsDeep(Base other_) { 2365 if (!super.equalsDeep(other_)) 2366 return false; 2367 if (!(other_ instanceof StratifierGroupComponent)) 2368 return false; 2369 StratifierGroupComponent o = (StratifierGroupComponent) other_; 2370 return compareDeep(value, o.value, true) && compareDeep(component, o.component, true) && compareDeep(population, o.population, true) 2371 && compareDeep(measureScore, o.measureScore, true); 2372 } 2373 2374 @Override 2375 public boolean equalsShallow(Base other_) { 2376 if (!super.equalsShallow(other_)) 2377 return false; 2378 if (!(other_ instanceof StratifierGroupComponent)) 2379 return false; 2380 StratifierGroupComponent o = (StratifierGroupComponent) other_; 2381 return true; 2382 } 2383 2384 public boolean isEmpty() { 2385 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, component, population 2386 , measureScore); 2387 } 2388 2389 public String fhirType() { 2390 return "MeasureReport.group.stratifier.stratum"; 2391 2392 } 2393 2394 } 2395 2396 @Block() 2397 public static class StratifierGroupComponentComponent extends BackboneElement implements IBaseBackboneElement { 2398 /** 2399 * The stratifier component from the Measure that corresponds to this stratifier component in the MeasureReport resource. 2400 */ 2401 @Child(name = "linkId", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2402 @Description(shortDefinition="Pointer to specific stratifier component from Measure", formalDefinition="The stratifier component from the Measure that corresponds to this stratifier component in the MeasureReport resource." ) 2403 protected StringType linkId; 2404 2405 /** 2406 * The code for the stratum component value. 2407 */ 2408 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 2409 @Description(shortDefinition="What stratifier component of the group", formalDefinition="The code for the stratum component value." ) 2410 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-stratifier-example") 2411 protected CodeableConcept code; 2412 2413 /** 2414 * The stratum component value. 2415 */ 2416 @Child(name = "value", type = {CodeableConcept.class, BooleanType.class, Quantity.class, Range.class, Reference.class}, order=3, min=1, max=1, modifier=false, summary=false) 2417 @Description(shortDefinition="The stratum component value, e.g. male", formalDefinition="The stratum component value." ) 2418 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measurereport-stratifier-value-example") 2419 protected DataType value; 2420 2421 private static final long serialVersionUID = 368088311L; 2422 2423 /** 2424 * Constructor 2425 */ 2426 public StratifierGroupComponentComponent() { 2427 super(); 2428 } 2429 2430 /** 2431 * Constructor 2432 */ 2433 public StratifierGroupComponentComponent(CodeableConcept code, DataType value) { 2434 super(); 2435 this.setCode(code); 2436 this.setValue(value); 2437 } 2438 2439 /** 2440 * @return {@link #linkId} (The stratifier component from the Measure that corresponds to this stratifier component in the MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 2441 */ 2442 public StringType getLinkIdElement() { 2443 if (this.linkId == null) 2444 if (Configuration.errorOnAutoCreate()) 2445 throw new Error("Attempt to auto-create StratifierGroupComponentComponent.linkId"); 2446 else if (Configuration.doAutoCreate()) 2447 this.linkId = new StringType(); // bb 2448 return this.linkId; 2449 } 2450 2451 public boolean hasLinkIdElement() { 2452 return this.linkId != null && !this.linkId.isEmpty(); 2453 } 2454 2455 public boolean hasLinkId() { 2456 return this.linkId != null && !this.linkId.isEmpty(); 2457 } 2458 2459 /** 2460 * @param value {@link #linkId} (The stratifier component from the Measure that corresponds to this stratifier component in the MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 2461 */ 2462 public StratifierGroupComponentComponent setLinkIdElement(StringType value) { 2463 this.linkId = value; 2464 return this; 2465 } 2466 2467 /** 2468 * @return The stratifier component from the Measure that corresponds to this stratifier component in the MeasureReport resource. 2469 */ 2470 public String getLinkId() { 2471 return this.linkId == null ? null : this.linkId.getValue(); 2472 } 2473 2474 /** 2475 * @param value The stratifier component from the Measure that corresponds to this stratifier component in the MeasureReport resource. 2476 */ 2477 public StratifierGroupComponentComponent setLinkId(String value) { 2478 if (Utilities.noString(value)) 2479 this.linkId = null; 2480 else { 2481 if (this.linkId == null) 2482 this.linkId = new StringType(); 2483 this.linkId.setValue(value); 2484 } 2485 return this; 2486 } 2487 2488 /** 2489 * @return {@link #code} (The code for the stratum component value.) 2490 */ 2491 public CodeableConcept getCode() { 2492 if (this.code == null) 2493 if (Configuration.errorOnAutoCreate()) 2494 throw new Error("Attempt to auto-create StratifierGroupComponentComponent.code"); 2495 else if (Configuration.doAutoCreate()) 2496 this.code = new CodeableConcept(); // cc 2497 return this.code; 2498 } 2499 2500 public boolean hasCode() { 2501 return this.code != null && !this.code.isEmpty(); 2502 } 2503 2504 /** 2505 * @param value {@link #code} (The code for the stratum component value.) 2506 */ 2507 public StratifierGroupComponentComponent setCode(CodeableConcept value) { 2508 this.code = value; 2509 return this; 2510 } 2511 2512 /** 2513 * @return {@link #value} (The stratum component value.) 2514 */ 2515 public DataType getValue() { 2516 return this.value; 2517 } 2518 2519 /** 2520 * @return {@link #value} (The stratum component value.) 2521 */ 2522 public CodeableConcept getValueCodeableConcept() throws FHIRException { 2523 if (this.value == null) 2524 this.value = new CodeableConcept(); 2525 if (!(this.value instanceof CodeableConcept)) 2526 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 2527 return (CodeableConcept) this.value; 2528 } 2529 2530 public boolean hasValueCodeableConcept() { 2531 return this != null && this.value instanceof CodeableConcept; 2532 } 2533 2534 /** 2535 * @return {@link #value} (The stratum component value.) 2536 */ 2537 public BooleanType getValueBooleanType() throws FHIRException { 2538 if (this.value == null) 2539 this.value = new BooleanType(); 2540 if (!(this.value instanceof BooleanType)) 2541 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 2542 return (BooleanType) this.value; 2543 } 2544 2545 public boolean hasValueBooleanType() { 2546 return this != null && this.value instanceof BooleanType; 2547 } 2548 2549 /** 2550 * @return {@link #value} (The stratum component value.) 2551 */ 2552 public Quantity getValueQuantity() throws FHIRException { 2553 if (this.value == null) 2554 this.value = new Quantity(); 2555 if (!(this.value instanceof Quantity)) 2556 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 2557 return (Quantity) this.value; 2558 } 2559 2560 public boolean hasValueQuantity() { 2561 return this != null && this.value instanceof Quantity; 2562 } 2563 2564 /** 2565 * @return {@link #value} (The stratum component value.) 2566 */ 2567 public Range getValueRange() throws FHIRException { 2568 if (this.value == null) 2569 this.value = new Range(); 2570 if (!(this.value instanceof Range)) 2571 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 2572 return (Range) this.value; 2573 } 2574 2575 public boolean hasValueRange() { 2576 return this != null && this.value instanceof Range; 2577 } 2578 2579 /** 2580 * @return {@link #value} (The stratum component value.) 2581 */ 2582 public Reference getValueReference() throws FHIRException { 2583 if (this.value == null) 2584 this.value = new Reference(); 2585 if (!(this.value instanceof Reference)) 2586 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 2587 return (Reference) this.value; 2588 } 2589 2590 public boolean hasValueReference() { 2591 return this != null && this.value instanceof Reference; 2592 } 2593 2594 public boolean hasValue() { 2595 return this.value != null && !this.value.isEmpty(); 2596 } 2597 2598 /** 2599 * @param value {@link #value} (The stratum component value.) 2600 */ 2601 public StratifierGroupComponentComponent setValue(DataType value) { 2602 if (value != null && !(value instanceof CodeableConcept || value instanceof BooleanType || value instanceof Quantity || value instanceof Range || value instanceof Reference)) 2603 throw new FHIRException("Not the right type for MeasureReport.group.stratifier.stratum.component.value[x]: "+value.fhirType()); 2604 this.value = value; 2605 return this; 2606 } 2607 2608 protected void listChildren(List<Property> children) { 2609 super.listChildren(children); 2610 children.add(new Property("linkId", "string", "The stratifier component from the Measure that corresponds to this stratifier component in the MeasureReport resource.", 0, 1, linkId)); 2611 children.add(new Property("code", "CodeableConcept", "The code for the stratum component value.", 0, 1, code)); 2612 children.add(new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", "The stratum component value.", 0, 1, value)); 2613 } 2614 2615 @Override 2616 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2617 switch (_hash) { 2618 case -1102667083: /*linkId*/ return new Property("linkId", "string", "The stratifier component from the Measure that corresponds to this stratifier component in the MeasureReport resource.", 0, 1, linkId); 2619 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The code for the stratum component value.", 0, 1, code); 2620 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", "The stratum component value.", 0, 1, value); 2621 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range|Reference", "The stratum component value.", 0, 1, value); 2622 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The stratum component value.", 0, 1, value); 2623 case 733421943: /*valueBoolean*/ return new Property("value[x]", "boolean", "The stratum component value.", 0, 1, value); 2624 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The stratum component value.", 0, 1, value); 2625 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The stratum component value.", 0, 1, value); 2626 case 1755241690: /*valueReference*/ return new Property("value[x]", "Reference", "The stratum component value.", 0, 1, value); 2627 default: return super.getNamedProperty(_hash, _name, _checkValid); 2628 } 2629 2630 } 2631 2632 @Override 2633 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2634 switch (hash) { 2635 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 2636 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 2637 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 2638 default: return super.getProperty(hash, name, checkValid); 2639 } 2640 2641 } 2642 2643 @Override 2644 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2645 switch (hash) { 2646 case -1102667083: // linkId 2647 this.linkId = TypeConvertor.castToString(value); // StringType 2648 return value; 2649 case 3059181: // code 2650 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2651 return value; 2652 case 111972721: // value 2653 this.value = TypeConvertor.castToType(value); // DataType 2654 return value; 2655 default: return super.setProperty(hash, name, value); 2656 } 2657 2658 } 2659 2660 @Override 2661 public Base setProperty(String name, Base value) throws FHIRException { 2662 if (name.equals("linkId")) { 2663 this.linkId = TypeConvertor.castToString(value); // StringType 2664 } else if (name.equals("code")) { 2665 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2666 } else if (name.equals("value[x]")) { 2667 this.value = TypeConvertor.castToType(value); // DataType 2668 } else 2669 return super.setProperty(name, value); 2670 return value; 2671 } 2672 2673 @Override 2674 public void removeChild(String name, Base value) throws FHIRException { 2675 if (name.equals("linkId")) { 2676 this.linkId = null; 2677 } else if (name.equals("code")) { 2678 this.code = null; 2679 } else if (name.equals("value[x]")) { 2680 this.value = null; 2681 } else 2682 super.removeChild(name, value); 2683 2684 } 2685 2686 @Override 2687 public Base makeProperty(int hash, String name) throws FHIRException { 2688 switch (hash) { 2689 case -1102667083: return getLinkIdElement(); 2690 case 3059181: return getCode(); 2691 case -1410166417: return getValue(); 2692 case 111972721: return getValue(); 2693 default: return super.makeProperty(hash, name); 2694 } 2695 2696 } 2697 2698 @Override 2699 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2700 switch (hash) { 2701 case -1102667083: /*linkId*/ return new String[] {"string"}; 2702 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 2703 case 111972721: /*value*/ return new String[] {"CodeableConcept", "boolean", "Quantity", "Range", "Reference"}; 2704 default: return super.getTypesForProperty(hash, name); 2705 } 2706 2707 } 2708 2709 @Override 2710 public Base addChild(String name) throws FHIRException { 2711 if (name.equals("linkId")) { 2712 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.group.stratifier.stratum.component.linkId"); 2713 } 2714 else if (name.equals("code")) { 2715 this.code = new CodeableConcept(); 2716 return this.code; 2717 } 2718 else if (name.equals("valueCodeableConcept")) { 2719 this.value = new CodeableConcept(); 2720 return this.value; 2721 } 2722 else if (name.equals("valueBoolean")) { 2723 this.value = new BooleanType(); 2724 return this.value; 2725 } 2726 else if (name.equals("valueQuantity")) { 2727 this.value = new Quantity(); 2728 return this.value; 2729 } 2730 else if (name.equals("valueRange")) { 2731 this.value = new Range(); 2732 return this.value; 2733 } 2734 else if (name.equals("valueReference")) { 2735 this.value = new Reference(); 2736 return this.value; 2737 } 2738 else 2739 return super.addChild(name); 2740 } 2741 2742 public StratifierGroupComponentComponent copy() { 2743 StratifierGroupComponentComponent dst = new StratifierGroupComponentComponent(); 2744 copyValues(dst); 2745 return dst; 2746 } 2747 2748 public void copyValues(StratifierGroupComponentComponent dst) { 2749 super.copyValues(dst); 2750 dst.linkId = linkId == null ? null : linkId.copy(); 2751 dst.code = code == null ? null : code.copy(); 2752 dst.value = value == null ? null : value.copy(); 2753 } 2754 2755 @Override 2756 public boolean equalsDeep(Base other_) { 2757 if (!super.equalsDeep(other_)) 2758 return false; 2759 if (!(other_ instanceof StratifierGroupComponentComponent)) 2760 return false; 2761 StratifierGroupComponentComponent o = (StratifierGroupComponentComponent) other_; 2762 return compareDeep(linkId, o.linkId, true) && compareDeep(code, o.code, true) && compareDeep(value, o.value, true) 2763 ; 2764 } 2765 2766 @Override 2767 public boolean equalsShallow(Base other_) { 2768 if (!super.equalsShallow(other_)) 2769 return false; 2770 if (!(other_ instanceof StratifierGroupComponentComponent)) 2771 return false; 2772 StratifierGroupComponentComponent o = (StratifierGroupComponentComponent) other_; 2773 return compareValues(linkId, o.linkId, true); 2774 } 2775 2776 public boolean isEmpty() { 2777 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, code, value); 2778 } 2779 2780 public String fhirType() { 2781 return "MeasureReport.group.stratifier.stratum.component"; 2782 2783 } 2784 2785 } 2786 2787 @Block() 2788 public static class StratifierGroupPopulationComponent extends BackboneElement implements IBaseBackboneElement { 2789 /** 2790 * The population from the Measure that corresponds to this population in the MeasureReport resource. 2791 */ 2792 @Child(name = "linkId", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2793 @Description(shortDefinition="Pointer to specific population from Measure", formalDefinition="The population from the Measure that corresponds to this population in the MeasureReport resource." ) 2794 protected StringType linkId; 2795 2796 /** 2797 * The type of the population. 2798 */ 2799 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 2800 @Description(shortDefinition="initial-population | numerator | numerator-exclusion | denominator | denominator-exclusion | denominator-exception | measure-population | measure-population-exclusion | measure-observation", formalDefinition="The type of the population." ) 2801 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-population") 2802 protected CodeableConcept code; 2803 2804 /** 2805 * The number of members of the population in this stratum. 2806 */ 2807 @Child(name = "count", type = {IntegerType.class}, order=3, min=0, max=1, modifier=false, summary=false) 2808 @Description(shortDefinition="Size of the population", formalDefinition="The number of members of the population in this stratum." ) 2809 protected IntegerType count; 2810 2811 /** 2812 * This element refers to a List of individual level MeasureReport resources, one for each subject in this population in this stratum. 2813 */ 2814 @Child(name = "subjectResults", type = {ListResource.class}, order=4, min=0, max=1, modifier=false, summary=false) 2815 @Description(shortDefinition="For subject-list reports, the subject results in this population", formalDefinition="This element refers to a List of individual level MeasureReport resources, one for each subject in this population in this stratum." ) 2816 protected Reference subjectResults; 2817 2818 /** 2819 * A reference to an individual level MeasureReport resource for a member of the population. 2820 */ 2821 @Child(name = "subjectReport", type = {MeasureReport.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2822 @Description(shortDefinition="For subject-list reports, a subject result in this population", formalDefinition="A reference to an individual level MeasureReport resource for a member of the population." ) 2823 protected List<Reference> subjectReport; 2824 2825 /** 2826 * Optional Group identifying the individuals that make up the population. 2827 */ 2828 @Child(name = "subjects", type = {Group.class}, order=6, min=0, max=1, modifier=false, summary=false) 2829 @Description(shortDefinition="What individual(s) in the population", formalDefinition="Optional Group identifying the individuals that make up the population." ) 2830 protected Reference subjects; 2831 2832 private static final long serialVersionUID = -203678073L; 2833 2834 /** 2835 * Constructor 2836 */ 2837 public StratifierGroupPopulationComponent() { 2838 super(); 2839 } 2840 2841 /** 2842 * @return {@link #linkId} (The population from the Measure that corresponds to this population in the MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 2843 */ 2844 public StringType getLinkIdElement() { 2845 if (this.linkId == null) 2846 if (Configuration.errorOnAutoCreate()) 2847 throw new Error("Attempt to auto-create StratifierGroupPopulationComponent.linkId"); 2848 else if (Configuration.doAutoCreate()) 2849 this.linkId = new StringType(); // bb 2850 return this.linkId; 2851 } 2852 2853 public boolean hasLinkIdElement() { 2854 return this.linkId != null && !this.linkId.isEmpty(); 2855 } 2856 2857 public boolean hasLinkId() { 2858 return this.linkId != null && !this.linkId.isEmpty(); 2859 } 2860 2861 /** 2862 * @param value {@link #linkId} (The population from the Measure that corresponds to this population in the MeasureReport resource.). This is the underlying object with id, value and extensions. The accessor "getLinkId" gives direct access to the value 2863 */ 2864 public StratifierGroupPopulationComponent setLinkIdElement(StringType value) { 2865 this.linkId = value; 2866 return this; 2867 } 2868 2869 /** 2870 * @return The population from the Measure that corresponds to this population in the MeasureReport resource. 2871 */ 2872 public String getLinkId() { 2873 return this.linkId == null ? null : this.linkId.getValue(); 2874 } 2875 2876 /** 2877 * @param value The population from the Measure that corresponds to this population in the MeasureReport resource. 2878 */ 2879 public StratifierGroupPopulationComponent setLinkId(String value) { 2880 if (Utilities.noString(value)) 2881 this.linkId = null; 2882 else { 2883 if (this.linkId == null) 2884 this.linkId = new StringType(); 2885 this.linkId.setValue(value); 2886 } 2887 return this; 2888 } 2889 2890 /** 2891 * @return {@link #code} (The type of the population.) 2892 */ 2893 public CodeableConcept getCode() { 2894 if (this.code == null) 2895 if (Configuration.errorOnAutoCreate()) 2896 throw new Error("Attempt to auto-create StratifierGroupPopulationComponent.code"); 2897 else if (Configuration.doAutoCreate()) 2898 this.code = new CodeableConcept(); // cc 2899 return this.code; 2900 } 2901 2902 public boolean hasCode() { 2903 return this.code != null && !this.code.isEmpty(); 2904 } 2905 2906 /** 2907 * @param value {@link #code} (The type of the population.) 2908 */ 2909 public StratifierGroupPopulationComponent setCode(CodeableConcept value) { 2910 this.code = value; 2911 return this; 2912 } 2913 2914 /** 2915 * @return {@link #count} (The number of members of the population in this stratum.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 2916 */ 2917 public IntegerType getCountElement() { 2918 if (this.count == null) 2919 if (Configuration.errorOnAutoCreate()) 2920 throw new Error("Attempt to auto-create StratifierGroupPopulationComponent.count"); 2921 else if (Configuration.doAutoCreate()) 2922 this.count = new IntegerType(); // bb 2923 return this.count; 2924 } 2925 2926 public boolean hasCountElement() { 2927 return this.count != null && !this.count.isEmpty(); 2928 } 2929 2930 public boolean hasCount() { 2931 return this.count != null && !this.count.isEmpty(); 2932 } 2933 2934 /** 2935 * @param value {@link #count} (The number of members of the population in this stratum.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 2936 */ 2937 public StratifierGroupPopulationComponent setCountElement(IntegerType value) { 2938 this.count = value; 2939 return this; 2940 } 2941 2942 /** 2943 * @return The number of members of the population in this stratum. 2944 */ 2945 public int getCount() { 2946 return this.count == null || this.count.isEmpty() ? 0 : this.count.getValue(); 2947 } 2948 2949 /** 2950 * @param value The number of members of the population in this stratum. 2951 */ 2952 public StratifierGroupPopulationComponent setCount(int value) { 2953 if (this.count == null) 2954 this.count = new IntegerType(); 2955 this.count.setValue(value); 2956 return this; 2957 } 2958 2959 /** 2960 * @return {@link #subjectResults} (This element refers to a List of individual level MeasureReport resources, one for each subject in this population in this stratum.) 2961 */ 2962 public Reference getSubjectResults() { 2963 if (this.subjectResults == null) 2964 if (Configuration.errorOnAutoCreate()) 2965 throw new Error("Attempt to auto-create StratifierGroupPopulationComponent.subjectResults"); 2966 else if (Configuration.doAutoCreate()) 2967 this.subjectResults = new Reference(); // cc 2968 return this.subjectResults; 2969 } 2970 2971 public boolean hasSubjectResults() { 2972 return this.subjectResults != null && !this.subjectResults.isEmpty(); 2973 } 2974 2975 /** 2976 * @param value {@link #subjectResults} (This element refers to a List of individual level MeasureReport resources, one for each subject in this population in this stratum.) 2977 */ 2978 public StratifierGroupPopulationComponent setSubjectResults(Reference value) { 2979 this.subjectResults = value; 2980 return this; 2981 } 2982 2983 /** 2984 * @return {@link #subjectReport} (A reference to an individual level MeasureReport resource for a member of the population.) 2985 */ 2986 public List<Reference> getSubjectReport() { 2987 if (this.subjectReport == null) 2988 this.subjectReport = new ArrayList<Reference>(); 2989 return this.subjectReport; 2990 } 2991 2992 /** 2993 * @return Returns a reference to <code>this</code> for easy method chaining 2994 */ 2995 public StratifierGroupPopulationComponent setSubjectReport(List<Reference> theSubjectReport) { 2996 this.subjectReport = theSubjectReport; 2997 return this; 2998 } 2999 3000 public boolean hasSubjectReport() { 3001 if (this.subjectReport == null) 3002 return false; 3003 for (Reference item : this.subjectReport) 3004 if (!item.isEmpty()) 3005 return true; 3006 return false; 3007 } 3008 3009 public Reference addSubjectReport() { //3 3010 Reference t = new Reference(); 3011 if (this.subjectReport == null) 3012 this.subjectReport = new ArrayList<Reference>(); 3013 this.subjectReport.add(t); 3014 return t; 3015 } 3016 3017 public StratifierGroupPopulationComponent addSubjectReport(Reference t) { //3 3018 if (t == null) 3019 return this; 3020 if (this.subjectReport == null) 3021 this.subjectReport = new ArrayList<Reference>(); 3022 this.subjectReport.add(t); 3023 return this; 3024 } 3025 3026 /** 3027 * @return The first repetition of repeating field {@link #subjectReport}, creating it if it does not already exist {3} 3028 */ 3029 public Reference getSubjectReportFirstRep() { 3030 if (getSubjectReport().isEmpty()) { 3031 addSubjectReport(); 3032 } 3033 return getSubjectReport().get(0); 3034 } 3035 3036 /** 3037 * @return {@link #subjects} (Optional Group identifying the individuals that make up the population.) 3038 */ 3039 public Reference getSubjects() { 3040 if (this.subjects == null) 3041 if (Configuration.errorOnAutoCreate()) 3042 throw new Error("Attempt to auto-create StratifierGroupPopulationComponent.subjects"); 3043 else if (Configuration.doAutoCreate()) 3044 this.subjects = new Reference(); // cc 3045 return this.subjects; 3046 } 3047 3048 public boolean hasSubjects() { 3049 return this.subjects != null && !this.subjects.isEmpty(); 3050 } 3051 3052 /** 3053 * @param value {@link #subjects} (Optional Group identifying the individuals that make up the population.) 3054 */ 3055 public StratifierGroupPopulationComponent setSubjects(Reference value) { 3056 this.subjects = value; 3057 return this; 3058 } 3059 3060 protected void listChildren(List<Property> children) { 3061 super.listChildren(children); 3062 children.add(new Property("linkId", "string", "The population from the Measure that corresponds to this population in the MeasureReport resource.", 0, 1, linkId)); 3063 children.add(new Property("code", "CodeableConcept", "The type of the population.", 0, 1, code)); 3064 children.add(new Property("count", "integer", "The number of members of the population in this stratum.", 0, 1, count)); 3065 children.add(new Property("subjectResults", "Reference(List)", "This element refers to a List of individual level MeasureReport resources, one for each subject in this population in this stratum.", 0, 1, subjectResults)); 3066 children.add(new Property("subjectReport", "Reference(MeasureReport)", "A reference to an individual level MeasureReport resource for a member of the population.", 0, java.lang.Integer.MAX_VALUE, subjectReport)); 3067 children.add(new Property("subjects", "Reference(Group)", "Optional Group identifying the individuals that make up the population.", 0, 1, subjects)); 3068 } 3069 3070 @Override 3071 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3072 switch (_hash) { 3073 case -1102667083: /*linkId*/ return new Property("linkId", "string", "The population from the Measure that corresponds to this population in the MeasureReport resource.", 0, 1, linkId); 3074 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The type of the population.", 0, 1, code); 3075 case 94851343: /*count*/ return new Property("count", "integer", "The number of members of the population in this stratum.", 0, 1, count); 3076 case 2136184106: /*subjectResults*/ return new Property("subjectResults", "Reference(List)", "This element refers to a List of individual level MeasureReport resources, one for each subject in this population in this stratum.", 0, 1, subjectResults); 3077 case 68814208: /*subjectReport*/ return new Property("subjectReport", "Reference(MeasureReport)", "A reference to an individual level MeasureReport resource for a member of the population.", 0, java.lang.Integer.MAX_VALUE, subjectReport); 3078 case -2069868345: /*subjects*/ return new Property("subjects", "Reference(Group)", "Optional Group identifying the individuals that make up the population.", 0, 1, subjects); 3079 default: return super.getNamedProperty(_hash, _name, _checkValid); 3080 } 3081 3082 } 3083 3084 @Override 3085 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3086 switch (hash) { 3087 case -1102667083: /*linkId*/ return this.linkId == null ? new Base[0] : new Base[] {this.linkId}; // StringType 3088 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 3089 case 94851343: /*count*/ return this.count == null ? new Base[0] : new Base[] {this.count}; // IntegerType 3090 case 2136184106: /*subjectResults*/ return this.subjectResults == null ? new Base[0] : new Base[] {this.subjectResults}; // Reference 3091 case 68814208: /*subjectReport*/ return this.subjectReport == null ? new Base[0] : this.subjectReport.toArray(new Base[this.subjectReport.size()]); // Reference 3092 case -2069868345: /*subjects*/ return this.subjects == null ? new Base[0] : new Base[] {this.subjects}; // Reference 3093 default: return super.getProperty(hash, name, checkValid); 3094 } 3095 3096 } 3097 3098 @Override 3099 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3100 switch (hash) { 3101 case -1102667083: // linkId 3102 this.linkId = TypeConvertor.castToString(value); // StringType 3103 return value; 3104 case 3059181: // code 3105 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3106 return value; 3107 case 94851343: // count 3108 this.count = TypeConvertor.castToInteger(value); // IntegerType 3109 return value; 3110 case 2136184106: // subjectResults 3111 this.subjectResults = TypeConvertor.castToReference(value); // Reference 3112 return value; 3113 case 68814208: // subjectReport 3114 this.getSubjectReport().add(TypeConvertor.castToReference(value)); // Reference 3115 return value; 3116 case -2069868345: // subjects 3117 this.subjects = TypeConvertor.castToReference(value); // Reference 3118 return value; 3119 default: return super.setProperty(hash, name, value); 3120 } 3121 3122 } 3123 3124 @Override 3125 public Base setProperty(String name, Base value) throws FHIRException { 3126 if (name.equals("linkId")) { 3127 this.linkId = TypeConvertor.castToString(value); // StringType 3128 } else if (name.equals("code")) { 3129 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3130 } else if (name.equals("count")) { 3131 this.count = TypeConvertor.castToInteger(value); // IntegerType 3132 } else if (name.equals("subjectResults")) { 3133 this.subjectResults = TypeConvertor.castToReference(value); // Reference 3134 } else if (name.equals("subjectReport")) { 3135 this.getSubjectReport().add(TypeConvertor.castToReference(value)); 3136 } else if (name.equals("subjects")) { 3137 this.subjects = TypeConvertor.castToReference(value); // Reference 3138 } else 3139 return super.setProperty(name, value); 3140 return value; 3141 } 3142 3143 @Override 3144 public void removeChild(String name, Base value) throws FHIRException { 3145 if (name.equals("linkId")) { 3146 this.linkId = null; 3147 } else if (name.equals("code")) { 3148 this.code = null; 3149 } else if (name.equals("count")) { 3150 this.count = null; 3151 } else if (name.equals("subjectResults")) { 3152 this.subjectResults = null; 3153 } else if (name.equals("subjectReport")) { 3154 this.getSubjectReport().remove(value); 3155 } else if (name.equals("subjects")) { 3156 this.subjects = null; 3157 } else 3158 super.removeChild(name, value); 3159 3160 } 3161 3162 @Override 3163 public Base makeProperty(int hash, String name) throws FHIRException { 3164 switch (hash) { 3165 case -1102667083: return getLinkIdElement(); 3166 case 3059181: return getCode(); 3167 case 94851343: return getCountElement(); 3168 case 2136184106: return getSubjectResults(); 3169 case 68814208: return addSubjectReport(); 3170 case -2069868345: return getSubjects(); 3171 default: return super.makeProperty(hash, name); 3172 } 3173 3174 } 3175 3176 @Override 3177 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3178 switch (hash) { 3179 case -1102667083: /*linkId*/ return new String[] {"string"}; 3180 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 3181 case 94851343: /*count*/ return new String[] {"integer"}; 3182 case 2136184106: /*subjectResults*/ return new String[] {"Reference"}; 3183 case 68814208: /*subjectReport*/ return new String[] {"Reference"}; 3184 case -2069868345: /*subjects*/ return new String[] {"Reference"}; 3185 default: return super.getTypesForProperty(hash, name); 3186 } 3187 3188 } 3189 3190 @Override 3191 public Base addChild(String name) throws FHIRException { 3192 if (name.equals("linkId")) { 3193 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.group.stratifier.stratum.population.linkId"); 3194 } 3195 else if (name.equals("code")) { 3196 this.code = new CodeableConcept(); 3197 return this.code; 3198 } 3199 else if (name.equals("count")) { 3200 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.group.stratifier.stratum.population.count"); 3201 } 3202 else if (name.equals("subjectResults")) { 3203 this.subjectResults = new Reference(); 3204 return this.subjectResults; 3205 } 3206 else if (name.equals("subjectReport")) { 3207 return addSubjectReport(); 3208 } 3209 else if (name.equals("subjects")) { 3210 this.subjects = new Reference(); 3211 return this.subjects; 3212 } 3213 else 3214 return super.addChild(name); 3215 } 3216 3217 public StratifierGroupPopulationComponent copy() { 3218 StratifierGroupPopulationComponent dst = new StratifierGroupPopulationComponent(); 3219 copyValues(dst); 3220 return dst; 3221 } 3222 3223 public void copyValues(StratifierGroupPopulationComponent dst) { 3224 super.copyValues(dst); 3225 dst.linkId = linkId == null ? null : linkId.copy(); 3226 dst.code = code == null ? null : code.copy(); 3227 dst.count = count == null ? null : count.copy(); 3228 dst.subjectResults = subjectResults == null ? null : subjectResults.copy(); 3229 if (subjectReport != null) { 3230 dst.subjectReport = new ArrayList<Reference>(); 3231 for (Reference i : subjectReport) 3232 dst.subjectReport.add(i.copy()); 3233 }; 3234 dst.subjects = subjects == null ? null : subjects.copy(); 3235 } 3236 3237 @Override 3238 public boolean equalsDeep(Base other_) { 3239 if (!super.equalsDeep(other_)) 3240 return false; 3241 if (!(other_ instanceof StratifierGroupPopulationComponent)) 3242 return false; 3243 StratifierGroupPopulationComponent o = (StratifierGroupPopulationComponent) other_; 3244 return compareDeep(linkId, o.linkId, true) && compareDeep(code, o.code, true) && compareDeep(count, o.count, true) 3245 && compareDeep(subjectResults, o.subjectResults, true) && compareDeep(subjectReport, o.subjectReport, true) 3246 && compareDeep(subjects, o.subjects, true); 3247 } 3248 3249 @Override 3250 public boolean equalsShallow(Base other_) { 3251 if (!super.equalsShallow(other_)) 3252 return false; 3253 if (!(other_ instanceof StratifierGroupPopulationComponent)) 3254 return false; 3255 StratifierGroupPopulationComponent o = (StratifierGroupPopulationComponent) other_; 3256 return compareValues(linkId, o.linkId, true) && compareValues(count, o.count, true); 3257 } 3258 3259 public boolean isEmpty() { 3260 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(linkId, code, count, subjectResults 3261 , subjectReport, subjects); 3262 } 3263 3264 public String fhirType() { 3265 return "MeasureReport.group.stratifier.stratum.population"; 3266 3267 } 3268 3269 } 3270 3271 /** 3272 * A formal identifier that is used to identify this MeasureReport when it is represented in other formats or referenced in a specification, model, design or an instance. 3273 */ 3274 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3275 @Description(shortDefinition="Additional identifier for the MeasureReport", formalDefinition="A formal identifier that is used to identify this MeasureReport when it is represented in other formats or referenced in a specification, model, design or an instance." ) 3276 protected List<Identifier> identifier; 3277 3278 /** 3279 * The MeasureReport status. No data will be available until the MeasureReport status is complete. 3280 */ 3281 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 3282 @Description(shortDefinition="complete | pending | error", formalDefinition="The MeasureReport status. No data will be available until the MeasureReport status is complete." ) 3283 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-report-status") 3284 protected Enumeration<MeasureReportStatus> status; 3285 3286 /** 3287 * The type of measure report. This may be an individual report, which provides the score for the measure for an individual member of the population; a subject-listing, which returns the list of members that meet the various criteria in the measure; a summary report, which returns a population count for each of the criteria in the measure; or a data-collection, which enables the MeasureReport to be used to exchange the data-of-interest for a quality measure. 3288 */ 3289 @Child(name = "type", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 3290 @Description(shortDefinition="individual | subject-list | summary | data-exchange", formalDefinition="The type of measure report. This may be an individual report, which provides the score for the measure for an individual member of the population; a subject-listing, which returns the list of members that meet the various criteria in the measure; a summary report, which returns a population count for each of the criteria in the measure; or a data-collection, which enables the MeasureReport to be used to exchange the data-of-interest for a quality measure." ) 3291 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-report-type") 3292 protected Enumeration<MeasureReportType> type; 3293 3294 /** 3295 * Indicates whether the data submitted in a data-exchange report represents a snapshot or incremental update. A snapshot update replaces all previously submitted data for the receiver, whereas an incremental update represents only updated and/or changed data and should be applied as a differential update to the existing submitted data for the receiver. 3296 */ 3297 @Child(name = "dataUpdateType", type = {CodeType.class}, order=3, min=0, max=1, modifier=true, summary=true) 3298 @Description(shortDefinition="incremental | snapshot", formalDefinition="Indicates whether the data submitted in a data-exchange report represents a snapshot or incremental update. A snapshot update replaces all previously submitted data for the receiver, whereas an incremental update represents only updated and/or changed data and should be applied as a differential update to the existing submitted data for the receiver." ) 3299 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/submit-data-update-type") 3300 protected Enumeration<SubmitDataUpdateType> dataUpdateType; 3301 3302 /** 3303 * A reference to the Measure that was calculated to produce this report. 3304 */ 3305 @Child(name = "measure", type = {CanonicalType.class}, order=4, min=0, max=1, modifier=false, summary=true) 3306 @Description(shortDefinition="What measure was calculated", formalDefinition="A reference to the Measure that was calculated to produce this report." ) 3307 protected CanonicalType measure; 3308 3309 /** 3310 * Optional subject identifying the individual or individuals the report is for. 3311 */ 3312 @Child(name = "subject", type = {CareTeam.class, Device.class, Group.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class}, order=5, min=0, max=1, modifier=false, summary=true) 3313 @Description(shortDefinition="What individual(s) the report is for", formalDefinition="Optional subject identifying the individual or individuals the report is for." ) 3314 protected Reference subject; 3315 3316 /** 3317 * The date this measure was calculated. 3318 */ 3319 @Child(name = "date", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 3320 @Description(shortDefinition="When the measure was calculated", formalDefinition="The date this measure was calculated." ) 3321 protected DateTimeType date; 3322 3323 /** 3324 * The individual or organization that is reporting the data. 3325 */ 3326 @Child(name = "reporter", type = {Practitioner.class, PractitionerRole.class, Organization.class, Group.class}, order=7, min=0, max=1, modifier=false, summary=true) 3327 @Description(shortDefinition="Who is reporting the data", formalDefinition="The individual or organization that is reporting the data." ) 3328 protected Reference reporter; 3329 3330 /** 3331 * A reference to the vendor who queried the data, calculated results and/or generated the report. The ?reporting vendor? is intended to represent the submitting entity when it is not the same as the reporting entity. This extension is used when the Receiver is interested in getting vendor information in the report. 3332 */ 3333 @Child(name = "reportingVendor", type = {Organization.class}, order=8, min=0, max=1, modifier=false, summary=false) 3334 @Description(shortDefinition="What vendor prepared the data", formalDefinition="A reference to the vendor who queried the data, calculated results and/or generated the report. The ?reporting vendor? is intended to represent the submitting entity when it is not the same as the reporting entity. This extension is used when the Receiver is interested in getting vendor information in the report." ) 3335 protected Reference reportingVendor; 3336 3337 /** 3338 * A reference to the location for which the data is being reported. 3339 */ 3340 @Child(name = "location", type = {Location.class}, order=9, min=0, max=1, modifier=false, summary=false) 3341 @Description(shortDefinition="Where the reported data is from", formalDefinition="A reference to the location for which the data is being reported." ) 3342 protected Reference location; 3343 3344 /** 3345 * The reporting period for which the report was calculated. 3346 */ 3347 @Child(name = "period", type = {Period.class}, order=10, min=1, max=1, modifier=false, summary=true) 3348 @Description(shortDefinition="What period the report covers", formalDefinition="The reporting period for which the report was calculated." ) 3349 protected Period period; 3350 3351 /** 3352 * A reference to a Parameters resource (typically represented using a contained resource) that represents any input parameters that were provided to the operation that generated the report. 3353 */ 3354 @Child(name = "inputParameters", type = {Parameters.class}, order=11, min=0, max=1, modifier=false, summary=false) 3355 @Description(shortDefinition="What parameters were provided to the report", formalDefinition="A reference to a Parameters resource (typically represented using a contained resource) that represents any input parameters that were provided to the operation that generated the report." ) 3356 protected Reference inputParameters; 3357 3358 /** 3359 * Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented. It is expected to be the same as the scoring element on the referenced Measure. 3360 */ 3361 @Child(name = "scoring", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=true, summary=true) 3362 @Description(shortDefinition="What scoring method (e.g. proportion, ratio, continuous-variable)", formalDefinition="Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented. It is expected to be the same as the scoring element on the referenced Measure." ) 3363 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/measure-scoring") 3364 protected CodeableConcept scoring; 3365 3366 /** 3367 * Whether improvement in the measure is noted by an increase or decrease in the measure score. 3368 */ 3369 @Child(name = "improvementNotation", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=true, summary=true) 3370 @Description(shortDefinition="increase | decrease", formalDefinition="Whether improvement in the measure is noted by an increase or decrease in the measure score." ) 3371 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-improvement-notation") 3372 protected CodeableConcept improvementNotation; 3373 3374 /** 3375 * The results of the calculation, one for each population group in the measure. 3376 */ 3377 @Child(name = "group", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3378 @Description(shortDefinition="Measure results for each group", formalDefinition="The results of the calculation, one for each population group in the measure." ) 3379 protected List<MeasureReportGroupComponent> group; 3380 3381 /** 3382 * A reference to a Resource that represents additional information collected for the report. If the value of the supplemental data is not a Resource (i.e. evaluating the supplementalData expression for this case in the measure results in a value that is not a FHIR Resource), it is reported as a reference to a contained Observation resource. 3383 */ 3384 @Child(name = "supplementalData", type = {Reference.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3385 @Description(shortDefinition="Additional information collected for the report", formalDefinition="A reference to a Resource that represents additional information collected for the report. If the value of the supplemental data is not a Resource (i.e. evaluating the supplementalData expression for this case in the measure results in a value that is not a FHIR Resource), it is reported as a reference to a contained Observation resource." ) 3386 protected List<Reference> supplementalData; 3387 3388 /** 3389 * Evaluated resources are used to capture what data was involved in the calculation of a measure. This usage is only allowed for individual reports to ensure that the size of the MeasureReport resource is bounded. 3390 */ 3391 @Child(name = "evaluatedResource", type = {Reference.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3392 @Description(shortDefinition="What data was used to calculate the measure score", formalDefinition="Evaluated resources are used to capture what data was involved in the calculation of a measure. This usage is only allowed for individual reports to ensure that the size of the MeasureReport resource is bounded." ) 3393 protected List<Reference> evaluatedResource; 3394 3395 private static final long serialVersionUID = 962133374L; 3396 3397 /** 3398 * Constructor 3399 */ 3400 public MeasureReport() { 3401 super(); 3402 } 3403 3404 /** 3405 * Constructor 3406 */ 3407 public MeasureReport(MeasureReportStatus status, MeasureReportType type, Period period) { 3408 super(); 3409 this.setStatus(status); 3410 this.setType(type); 3411 this.setPeriod(period); 3412 } 3413 3414 /** 3415 * @return {@link #identifier} (A formal identifier that is used to identify this MeasureReport when it is represented in other formats or referenced in a specification, model, design or an instance.) 3416 */ 3417 public List<Identifier> getIdentifier() { 3418 if (this.identifier == null) 3419 this.identifier = new ArrayList<Identifier>(); 3420 return this.identifier; 3421 } 3422 3423 /** 3424 * @return Returns a reference to <code>this</code> for easy method chaining 3425 */ 3426 public MeasureReport setIdentifier(List<Identifier> theIdentifier) { 3427 this.identifier = theIdentifier; 3428 return this; 3429 } 3430 3431 public boolean hasIdentifier() { 3432 if (this.identifier == null) 3433 return false; 3434 for (Identifier item : this.identifier) 3435 if (!item.isEmpty()) 3436 return true; 3437 return false; 3438 } 3439 3440 public Identifier addIdentifier() { //3 3441 Identifier t = new Identifier(); 3442 if (this.identifier == null) 3443 this.identifier = new ArrayList<Identifier>(); 3444 this.identifier.add(t); 3445 return t; 3446 } 3447 3448 public MeasureReport addIdentifier(Identifier t) { //3 3449 if (t == null) 3450 return this; 3451 if (this.identifier == null) 3452 this.identifier = new ArrayList<Identifier>(); 3453 this.identifier.add(t); 3454 return this; 3455 } 3456 3457 /** 3458 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 3459 */ 3460 public Identifier getIdentifierFirstRep() { 3461 if (getIdentifier().isEmpty()) { 3462 addIdentifier(); 3463 } 3464 return getIdentifier().get(0); 3465 } 3466 3467 /** 3468 * @return {@link #status} (The MeasureReport status. No data will be available until the MeasureReport status is complete.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3469 */ 3470 public Enumeration<MeasureReportStatus> getStatusElement() { 3471 if (this.status == null) 3472 if (Configuration.errorOnAutoCreate()) 3473 throw new Error("Attempt to auto-create MeasureReport.status"); 3474 else if (Configuration.doAutoCreate()) 3475 this.status = new Enumeration<MeasureReportStatus>(new MeasureReportStatusEnumFactory()); // bb 3476 return this.status; 3477 } 3478 3479 public boolean hasStatusElement() { 3480 return this.status != null && !this.status.isEmpty(); 3481 } 3482 3483 public boolean hasStatus() { 3484 return this.status != null && !this.status.isEmpty(); 3485 } 3486 3487 /** 3488 * @param value {@link #status} (The MeasureReport status. No data will be available until the MeasureReport status is complete.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 3489 */ 3490 public MeasureReport setStatusElement(Enumeration<MeasureReportStatus> value) { 3491 this.status = value; 3492 return this; 3493 } 3494 3495 /** 3496 * @return The MeasureReport status. No data will be available until the MeasureReport status is complete. 3497 */ 3498 public MeasureReportStatus getStatus() { 3499 return this.status == null ? null : this.status.getValue(); 3500 } 3501 3502 /** 3503 * @param value The MeasureReport status. No data will be available until the MeasureReport status is complete. 3504 */ 3505 public MeasureReport setStatus(MeasureReportStatus value) { 3506 if (this.status == null) 3507 this.status = new Enumeration<MeasureReportStatus>(new MeasureReportStatusEnumFactory()); 3508 this.status.setValue(value); 3509 return this; 3510 } 3511 3512 /** 3513 * @return {@link #type} (The type of measure report. This may be an individual report, which provides the score for the measure for an individual member of the population; a subject-listing, which returns the list of members that meet the various criteria in the measure; a summary report, which returns a population count for each of the criteria in the measure; or a data-collection, which enables the MeasureReport to be used to exchange the data-of-interest for a quality measure.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3514 */ 3515 public Enumeration<MeasureReportType> getTypeElement() { 3516 if (this.type == null) 3517 if (Configuration.errorOnAutoCreate()) 3518 throw new Error("Attempt to auto-create MeasureReport.type"); 3519 else if (Configuration.doAutoCreate()) 3520 this.type = new Enumeration<MeasureReportType>(new MeasureReportTypeEnumFactory()); // bb 3521 return this.type; 3522 } 3523 3524 public boolean hasTypeElement() { 3525 return this.type != null && !this.type.isEmpty(); 3526 } 3527 3528 public boolean hasType() { 3529 return this.type != null && !this.type.isEmpty(); 3530 } 3531 3532 /** 3533 * @param value {@link #type} (The type of measure report. This may be an individual report, which provides the score for the measure for an individual member of the population; a subject-listing, which returns the list of members that meet the various criteria in the measure; a summary report, which returns a population count for each of the criteria in the measure; or a data-collection, which enables the MeasureReport to be used to exchange the data-of-interest for a quality measure.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 3534 */ 3535 public MeasureReport setTypeElement(Enumeration<MeasureReportType> value) { 3536 this.type = value; 3537 return this; 3538 } 3539 3540 /** 3541 * @return The type of measure report. This may be an individual report, which provides the score for the measure for an individual member of the population; a subject-listing, which returns the list of members that meet the various criteria in the measure; a summary report, which returns a population count for each of the criteria in the measure; or a data-collection, which enables the MeasureReport to be used to exchange the data-of-interest for a quality measure. 3542 */ 3543 public MeasureReportType getType() { 3544 return this.type == null ? null : this.type.getValue(); 3545 } 3546 3547 /** 3548 * @param value The type of measure report. This may be an individual report, which provides the score for the measure for an individual member of the population; a subject-listing, which returns the list of members that meet the various criteria in the measure; a summary report, which returns a population count for each of the criteria in the measure; or a data-collection, which enables the MeasureReport to be used to exchange the data-of-interest for a quality measure. 3549 */ 3550 public MeasureReport setType(MeasureReportType value) { 3551 if (this.type == null) 3552 this.type = new Enumeration<MeasureReportType>(new MeasureReportTypeEnumFactory()); 3553 this.type.setValue(value); 3554 return this; 3555 } 3556 3557 /** 3558 * @return {@link #dataUpdateType} (Indicates whether the data submitted in a data-exchange report represents a snapshot or incremental update. A snapshot update replaces all previously submitted data for the receiver, whereas an incremental update represents only updated and/or changed data and should be applied as a differential update to the existing submitted data for the receiver.). This is the underlying object with id, value and extensions. The accessor "getDataUpdateType" gives direct access to the value 3559 */ 3560 public Enumeration<SubmitDataUpdateType> getDataUpdateTypeElement() { 3561 if (this.dataUpdateType == null) 3562 if (Configuration.errorOnAutoCreate()) 3563 throw new Error("Attempt to auto-create MeasureReport.dataUpdateType"); 3564 else if (Configuration.doAutoCreate()) 3565 this.dataUpdateType = new Enumeration<SubmitDataUpdateType>(new SubmitDataUpdateTypeEnumFactory()); // bb 3566 return this.dataUpdateType; 3567 } 3568 3569 public boolean hasDataUpdateTypeElement() { 3570 return this.dataUpdateType != null && !this.dataUpdateType.isEmpty(); 3571 } 3572 3573 public boolean hasDataUpdateType() { 3574 return this.dataUpdateType != null && !this.dataUpdateType.isEmpty(); 3575 } 3576 3577 /** 3578 * @param value {@link #dataUpdateType} (Indicates whether the data submitted in a data-exchange report represents a snapshot or incremental update. A snapshot update replaces all previously submitted data for the receiver, whereas an incremental update represents only updated and/or changed data and should be applied as a differential update to the existing submitted data for the receiver.). This is the underlying object with id, value and extensions. The accessor "getDataUpdateType" gives direct access to the value 3579 */ 3580 public MeasureReport setDataUpdateTypeElement(Enumeration<SubmitDataUpdateType> value) { 3581 this.dataUpdateType = value; 3582 return this; 3583 } 3584 3585 /** 3586 * @return Indicates whether the data submitted in a data-exchange report represents a snapshot or incremental update. A snapshot update replaces all previously submitted data for the receiver, whereas an incremental update represents only updated and/or changed data and should be applied as a differential update to the existing submitted data for the receiver. 3587 */ 3588 public SubmitDataUpdateType getDataUpdateType() { 3589 return this.dataUpdateType == null ? null : this.dataUpdateType.getValue(); 3590 } 3591 3592 /** 3593 * @param value Indicates whether the data submitted in a data-exchange report represents a snapshot or incremental update. A snapshot update replaces all previously submitted data for the receiver, whereas an incremental update represents only updated and/or changed data and should be applied as a differential update to the existing submitted data for the receiver. 3594 */ 3595 public MeasureReport setDataUpdateType(SubmitDataUpdateType value) { 3596 if (value == null) 3597 this.dataUpdateType = null; 3598 else { 3599 if (this.dataUpdateType == null) 3600 this.dataUpdateType = new Enumeration<SubmitDataUpdateType>(new SubmitDataUpdateTypeEnumFactory()); 3601 this.dataUpdateType.setValue(value); 3602 } 3603 return this; 3604 } 3605 3606 /** 3607 * @return {@link #measure} (A reference to the Measure that was calculated to produce this report.). This is the underlying object with id, value and extensions. The accessor "getMeasure" gives direct access to the value 3608 */ 3609 public CanonicalType getMeasureElement() { 3610 if (this.measure == null) 3611 if (Configuration.errorOnAutoCreate()) 3612 throw new Error("Attempt to auto-create MeasureReport.measure"); 3613 else if (Configuration.doAutoCreate()) 3614 this.measure = new CanonicalType(); // bb 3615 return this.measure; 3616 } 3617 3618 public boolean hasMeasureElement() { 3619 return this.measure != null && !this.measure.isEmpty(); 3620 } 3621 3622 public boolean hasMeasure() { 3623 return this.measure != null && !this.measure.isEmpty(); 3624 } 3625 3626 /** 3627 * @param value {@link #measure} (A reference to the Measure that was calculated to produce this report.). This is the underlying object with id, value and extensions. The accessor "getMeasure" gives direct access to the value 3628 */ 3629 public MeasureReport setMeasureElement(CanonicalType value) { 3630 this.measure = value; 3631 return this; 3632 } 3633 3634 /** 3635 * @return A reference to the Measure that was calculated to produce this report. 3636 */ 3637 public String getMeasure() { 3638 return this.measure == null ? null : this.measure.getValue(); 3639 } 3640 3641 /** 3642 * @param value A reference to the Measure that was calculated to produce this report. 3643 */ 3644 public MeasureReport setMeasure(String value) { 3645 if (Utilities.noString(value)) 3646 this.measure = null; 3647 else { 3648 if (this.measure == null) 3649 this.measure = new CanonicalType(); 3650 this.measure.setValue(value); 3651 } 3652 return this; 3653 } 3654 3655 /** 3656 * @return {@link #subject} (Optional subject identifying the individual or individuals the report is for.) 3657 */ 3658 public Reference getSubject() { 3659 if (this.subject == null) 3660 if (Configuration.errorOnAutoCreate()) 3661 throw new Error("Attempt to auto-create MeasureReport.subject"); 3662 else if (Configuration.doAutoCreate()) 3663 this.subject = new Reference(); // cc 3664 return this.subject; 3665 } 3666 3667 public boolean hasSubject() { 3668 return this.subject != null && !this.subject.isEmpty(); 3669 } 3670 3671 /** 3672 * @param value {@link #subject} (Optional subject identifying the individual or individuals the report is for.) 3673 */ 3674 public MeasureReport setSubject(Reference value) { 3675 this.subject = value; 3676 return this; 3677 } 3678 3679 /** 3680 * @return {@link #date} (The date this measure was calculated.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3681 */ 3682 public DateTimeType getDateElement() { 3683 if (this.date == null) 3684 if (Configuration.errorOnAutoCreate()) 3685 throw new Error("Attempt to auto-create MeasureReport.date"); 3686 else if (Configuration.doAutoCreate()) 3687 this.date = new DateTimeType(); // bb 3688 return this.date; 3689 } 3690 3691 public boolean hasDateElement() { 3692 return this.date != null && !this.date.isEmpty(); 3693 } 3694 3695 public boolean hasDate() { 3696 return this.date != null && !this.date.isEmpty(); 3697 } 3698 3699 /** 3700 * @param value {@link #date} (The date this measure was calculated.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 3701 */ 3702 public MeasureReport setDateElement(DateTimeType value) { 3703 this.date = value; 3704 return this; 3705 } 3706 3707 /** 3708 * @return The date this measure was calculated. 3709 */ 3710 public Date getDate() { 3711 return this.date == null ? null : this.date.getValue(); 3712 } 3713 3714 /** 3715 * @param value The date this measure was calculated. 3716 */ 3717 public MeasureReport setDate(Date value) { 3718 if (value == null) 3719 this.date = null; 3720 else { 3721 if (this.date == null) 3722 this.date = new DateTimeType(); 3723 this.date.setValue(value); 3724 } 3725 return this; 3726 } 3727 3728 /** 3729 * @return {@link #reporter} (The individual or organization that is reporting the data.) 3730 */ 3731 public Reference getReporter() { 3732 if (this.reporter == null) 3733 if (Configuration.errorOnAutoCreate()) 3734 throw new Error("Attempt to auto-create MeasureReport.reporter"); 3735 else if (Configuration.doAutoCreate()) 3736 this.reporter = new Reference(); // cc 3737 return this.reporter; 3738 } 3739 3740 public boolean hasReporter() { 3741 return this.reporter != null && !this.reporter.isEmpty(); 3742 } 3743 3744 /** 3745 * @param value {@link #reporter} (The individual or organization that is reporting the data.) 3746 */ 3747 public MeasureReport setReporter(Reference value) { 3748 this.reporter = value; 3749 return this; 3750 } 3751 3752 /** 3753 * @return {@link #reportingVendor} (A reference to the vendor who queried the data, calculated results and/or generated the report. The ?reporting vendor? is intended to represent the submitting entity when it is not the same as the reporting entity. This extension is used when the Receiver is interested in getting vendor information in the report.) 3754 */ 3755 public Reference getReportingVendor() { 3756 if (this.reportingVendor == null) 3757 if (Configuration.errorOnAutoCreate()) 3758 throw new Error("Attempt to auto-create MeasureReport.reportingVendor"); 3759 else if (Configuration.doAutoCreate()) 3760 this.reportingVendor = new Reference(); // cc 3761 return this.reportingVendor; 3762 } 3763 3764 public boolean hasReportingVendor() { 3765 return this.reportingVendor != null && !this.reportingVendor.isEmpty(); 3766 } 3767 3768 /** 3769 * @param value {@link #reportingVendor} (A reference to the vendor who queried the data, calculated results and/or generated the report. The ?reporting vendor? is intended to represent the submitting entity when it is not the same as the reporting entity. This extension is used when the Receiver is interested in getting vendor information in the report.) 3770 */ 3771 public MeasureReport setReportingVendor(Reference value) { 3772 this.reportingVendor = value; 3773 return this; 3774 } 3775 3776 /** 3777 * @return {@link #location} (A reference to the location for which the data is being reported.) 3778 */ 3779 public Reference getLocation() { 3780 if (this.location == null) 3781 if (Configuration.errorOnAutoCreate()) 3782 throw new Error("Attempt to auto-create MeasureReport.location"); 3783 else if (Configuration.doAutoCreate()) 3784 this.location = new Reference(); // cc 3785 return this.location; 3786 } 3787 3788 public boolean hasLocation() { 3789 return this.location != null && !this.location.isEmpty(); 3790 } 3791 3792 /** 3793 * @param value {@link #location} (A reference to the location for which the data is being reported.) 3794 */ 3795 public MeasureReport setLocation(Reference value) { 3796 this.location = value; 3797 return this; 3798 } 3799 3800 /** 3801 * @return {@link #period} (The reporting period for which the report was calculated.) 3802 */ 3803 public Period getPeriod() { 3804 if (this.period == null) 3805 if (Configuration.errorOnAutoCreate()) 3806 throw new Error("Attempt to auto-create MeasureReport.period"); 3807 else if (Configuration.doAutoCreate()) 3808 this.period = new Period(); // cc 3809 return this.period; 3810 } 3811 3812 public boolean hasPeriod() { 3813 return this.period != null && !this.period.isEmpty(); 3814 } 3815 3816 /** 3817 * @param value {@link #period} (The reporting period for which the report was calculated.) 3818 */ 3819 public MeasureReport setPeriod(Period value) { 3820 this.period = value; 3821 return this; 3822 } 3823 3824 /** 3825 * @return {@link #inputParameters} (A reference to a Parameters resource (typically represented using a contained resource) that represents any input parameters that were provided to the operation that generated the report.) 3826 */ 3827 public Reference getInputParameters() { 3828 if (this.inputParameters == null) 3829 if (Configuration.errorOnAutoCreate()) 3830 throw new Error("Attempt to auto-create MeasureReport.inputParameters"); 3831 else if (Configuration.doAutoCreate()) 3832 this.inputParameters = new Reference(); // cc 3833 return this.inputParameters; 3834 } 3835 3836 public boolean hasInputParameters() { 3837 return this.inputParameters != null && !this.inputParameters.isEmpty(); 3838 } 3839 3840 /** 3841 * @param value {@link #inputParameters} (A reference to a Parameters resource (typically represented using a contained resource) that represents any input parameters that were provided to the operation that generated the report.) 3842 */ 3843 public MeasureReport setInputParameters(Reference value) { 3844 this.inputParameters = value; 3845 return this; 3846 } 3847 3848 /** 3849 * @return {@link #scoring} (Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented. It is expected to be the same as the scoring element on the referenced Measure.) 3850 */ 3851 public CodeableConcept getScoring() { 3852 if (this.scoring == null) 3853 if (Configuration.errorOnAutoCreate()) 3854 throw new Error("Attempt to auto-create MeasureReport.scoring"); 3855 else if (Configuration.doAutoCreate()) 3856 this.scoring = new CodeableConcept(); // cc 3857 return this.scoring; 3858 } 3859 3860 public boolean hasScoring() { 3861 return this.scoring != null && !this.scoring.isEmpty(); 3862 } 3863 3864 /** 3865 * @param value {@link #scoring} (Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented. It is expected to be the same as the scoring element on the referenced Measure.) 3866 */ 3867 public MeasureReport setScoring(CodeableConcept value) { 3868 this.scoring = value; 3869 return this; 3870 } 3871 3872 /** 3873 * @return {@link #improvementNotation} (Whether improvement in the measure is noted by an increase or decrease in the measure score.) 3874 */ 3875 public CodeableConcept getImprovementNotation() { 3876 if (this.improvementNotation == null) 3877 if (Configuration.errorOnAutoCreate()) 3878 throw new Error("Attempt to auto-create MeasureReport.improvementNotation"); 3879 else if (Configuration.doAutoCreate()) 3880 this.improvementNotation = new CodeableConcept(); // cc 3881 return this.improvementNotation; 3882 } 3883 3884 public boolean hasImprovementNotation() { 3885 return this.improvementNotation != null && !this.improvementNotation.isEmpty(); 3886 } 3887 3888 /** 3889 * @param value {@link #improvementNotation} (Whether improvement in the measure is noted by an increase or decrease in the measure score.) 3890 */ 3891 public MeasureReport setImprovementNotation(CodeableConcept value) { 3892 this.improvementNotation = value; 3893 return this; 3894 } 3895 3896 /** 3897 * @return {@link #group} (The results of the calculation, one for each population group in the measure.) 3898 */ 3899 public List<MeasureReportGroupComponent> getGroup() { 3900 if (this.group == null) 3901 this.group = new ArrayList<MeasureReportGroupComponent>(); 3902 return this.group; 3903 } 3904 3905 /** 3906 * @return Returns a reference to <code>this</code> for easy method chaining 3907 */ 3908 public MeasureReport setGroup(List<MeasureReportGroupComponent> theGroup) { 3909 this.group = theGroup; 3910 return this; 3911 } 3912 3913 public boolean hasGroup() { 3914 if (this.group == null) 3915 return false; 3916 for (MeasureReportGroupComponent item : this.group) 3917 if (!item.isEmpty()) 3918 return true; 3919 return false; 3920 } 3921 3922 public MeasureReportGroupComponent addGroup() { //3 3923 MeasureReportGroupComponent t = new MeasureReportGroupComponent(); 3924 if (this.group == null) 3925 this.group = new ArrayList<MeasureReportGroupComponent>(); 3926 this.group.add(t); 3927 return t; 3928 } 3929 3930 public MeasureReport addGroup(MeasureReportGroupComponent t) { //3 3931 if (t == null) 3932 return this; 3933 if (this.group == null) 3934 this.group = new ArrayList<MeasureReportGroupComponent>(); 3935 this.group.add(t); 3936 return this; 3937 } 3938 3939 /** 3940 * @return The first repetition of repeating field {@link #group}, creating it if it does not already exist {3} 3941 */ 3942 public MeasureReportGroupComponent getGroupFirstRep() { 3943 if (getGroup().isEmpty()) { 3944 addGroup(); 3945 } 3946 return getGroup().get(0); 3947 } 3948 3949 /** 3950 * @return {@link #supplementalData} (A reference to a Resource that represents additional information collected for the report. If the value of the supplemental data is not a Resource (i.e. evaluating the supplementalData expression for this case in the measure results in a value that is not a FHIR Resource), it is reported as a reference to a contained Observation resource.) 3951 */ 3952 public List<Reference> getSupplementalData() { 3953 if (this.supplementalData == null) 3954 this.supplementalData = new ArrayList<Reference>(); 3955 return this.supplementalData; 3956 } 3957 3958 /** 3959 * @return Returns a reference to <code>this</code> for easy method chaining 3960 */ 3961 public MeasureReport setSupplementalData(List<Reference> theSupplementalData) { 3962 this.supplementalData = theSupplementalData; 3963 return this; 3964 } 3965 3966 public boolean hasSupplementalData() { 3967 if (this.supplementalData == null) 3968 return false; 3969 for (Reference item : this.supplementalData) 3970 if (!item.isEmpty()) 3971 return true; 3972 return false; 3973 } 3974 3975 public Reference addSupplementalData() { //3 3976 Reference t = new Reference(); 3977 if (this.supplementalData == null) 3978 this.supplementalData = new ArrayList<Reference>(); 3979 this.supplementalData.add(t); 3980 return t; 3981 } 3982 3983 public MeasureReport addSupplementalData(Reference t) { //3 3984 if (t == null) 3985 return this; 3986 if (this.supplementalData == null) 3987 this.supplementalData = new ArrayList<Reference>(); 3988 this.supplementalData.add(t); 3989 return this; 3990 } 3991 3992 /** 3993 * @return The first repetition of repeating field {@link #supplementalData}, creating it if it does not already exist {3} 3994 */ 3995 public Reference getSupplementalDataFirstRep() { 3996 if (getSupplementalData().isEmpty()) { 3997 addSupplementalData(); 3998 } 3999 return getSupplementalData().get(0); 4000 } 4001 4002 /** 4003 * @return {@link #evaluatedResource} (Evaluated resources are used to capture what data was involved in the calculation of a measure. This usage is only allowed for individual reports to ensure that the size of the MeasureReport resource is bounded.) 4004 */ 4005 public List<Reference> getEvaluatedResource() { 4006 if (this.evaluatedResource == null) 4007 this.evaluatedResource = new ArrayList<Reference>(); 4008 return this.evaluatedResource; 4009 } 4010 4011 /** 4012 * @return Returns a reference to <code>this</code> for easy method chaining 4013 */ 4014 public MeasureReport setEvaluatedResource(List<Reference> theEvaluatedResource) { 4015 this.evaluatedResource = theEvaluatedResource; 4016 return this; 4017 } 4018 4019 public boolean hasEvaluatedResource() { 4020 if (this.evaluatedResource == null) 4021 return false; 4022 for (Reference item : this.evaluatedResource) 4023 if (!item.isEmpty()) 4024 return true; 4025 return false; 4026 } 4027 4028 public Reference addEvaluatedResource() { //3 4029 Reference t = new Reference(); 4030 if (this.evaluatedResource == null) 4031 this.evaluatedResource = new ArrayList<Reference>(); 4032 this.evaluatedResource.add(t); 4033 return t; 4034 } 4035 4036 public MeasureReport addEvaluatedResource(Reference t) { //3 4037 if (t == null) 4038 return this; 4039 if (this.evaluatedResource == null) 4040 this.evaluatedResource = new ArrayList<Reference>(); 4041 this.evaluatedResource.add(t); 4042 return this; 4043 } 4044 4045 /** 4046 * @return The first repetition of repeating field {@link #evaluatedResource}, creating it if it does not already exist {3} 4047 */ 4048 public Reference getEvaluatedResourceFirstRep() { 4049 if (getEvaluatedResource().isEmpty()) { 4050 addEvaluatedResource(); 4051 } 4052 return getEvaluatedResource().get(0); 4053 } 4054 4055 protected void listChildren(List<Property> children) { 4056 super.listChildren(children); 4057 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this MeasureReport when it is represented in other formats or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 4058 children.add(new Property("status", "code", "The MeasureReport status. No data will be available until the MeasureReport status is complete.", 0, 1, status)); 4059 children.add(new Property("type", "code", "The type of measure report. This may be an individual report, which provides the score for the measure for an individual member of the population; a subject-listing, which returns the list of members that meet the various criteria in the measure; a summary report, which returns a population count for each of the criteria in the measure; or a data-collection, which enables the MeasureReport to be used to exchange the data-of-interest for a quality measure.", 0, 1, type)); 4060 children.add(new Property("dataUpdateType", "code", "Indicates whether the data submitted in a data-exchange report represents a snapshot or incremental update. A snapshot update replaces all previously submitted data for the receiver, whereas an incremental update represents only updated and/or changed data and should be applied as a differential update to the existing submitted data for the receiver.", 0, 1, dataUpdateType)); 4061 children.add(new Property("measure", "canonical(Measure)", "A reference to the Measure that was calculated to produce this report.", 0, 1, measure)); 4062 children.add(new Property("subject", "Reference(CareTeam|Device|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Optional subject identifying the individual or individuals the report is for.", 0, 1, subject)); 4063 children.add(new Property("date", "dateTime", "The date this measure was calculated.", 0, 1, date)); 4064 children.add(new Property("reporter", "Reference(Practitioner|PractitionerRole|Organization|Group)", "The individual or organization that is reporting the data.", 0, 1, reporter)); 4065 children.add(new Property("reportingVendor", "Reference(Organization)", "A reference to the vendor who queried the data, calculated results and/or generated the report. The ?reporting vendor? is intended to represent the submitting entity when it is not the same as the reporting entity. This extension is used when the Receiver is interested in getting vendor information in the report.", 0, 1, reportingVendor)); 4066 children.add(new Property("location", "Reference(Location)", "A reference to the location for which the data is being reported.", 0, 1, location)); 4067 children.add(new Property("period", "Period", "The reporting period for which the report was calculated.", 0, 1, period)); 4068 children.add(new Property("inputParameters", "Reference(Parameters)", "A reference to a Parameters resource (typically represented using a contained resource) that represents any input parameters that were provided to the operation that generated the report.", 0, 1, inputParameters)); 4069 children.add(new Property("scoring", "CodeableConcept", "Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented. It is expected to be the same as the scoring element on the referenced Measure.", 0, 1, scoring)); 4070 children.add(new Property("improvementNotation", "CodeableConcept", "Whether improvement in the measure is noted by an increase or decrease in the measure score.", 0, 1, improvementNotation)); 4071 children.add(new Property("group", "", "The results of the calculation, one for each population group in the measure.", 0, java.lang.Integer.MAX_VALUE, group)); 4072 children.add(new Property("supplementalData", "Reference(Any)", "A reference to a Resource that represents additional information collected for the report. If the value of the supplemental data is not a Resource (i.e. evaluating the supplementalData expression for this case in the measure results in a value that is not a FHIR Resource), it is reported as a reference to a contained Observation resource.", 0, java.lang.Integer.MAX_VALUE, supplementalData)); 4073 children.add(new Property("evaluatedResource", "Reference(Any)", "Evaluated resources are used to capture what data was involved in the calculation of a measure. This usage is only allowed for individual reports to ensure that the size of the MeasureReport resource is bounded.", 0, java.lang.Integer.MAX_VALUE, evaluatedResource)); 4074 } 4075 4076 @Override 4077 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4078 switch (_hash) { 4079 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this MeasureReport when it is represented in other formats or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 4080 case -892481550: /*status*/ return new Property("status", "code", "The MeasureReport status. No data will be available until the MeasureReport status is complete.", 0, 1, status); 4081 case 3575610: /*type*/ return new Property("type", "code", "The type of measure report. This may be an individual report, which provides the score for the measure for an individual member of the population; a subject-listing, which returns the list of members that meet the various criteria in the measure; a summary report, which returns a population count for each of the criteria in the measure; or a data-collection, which enables the MeasureReport to be used to exchange the data-of-interest for a quality measure.", 0, 1, type); 4082 case -425890067: /*dataUpdateType*/ return new Property("dataUpdateType", "code", "Indicates whether the data submitted in a data-exchange report represents a snapshot or incremental update. A snapshot update replaces all previously submitted data for the receiver, whereas an incremental update represents only updated and/or changed data and should be applied as a differential update to the existing submitted data for the receiver.", 0, 1, dataUpdateType); 4083 case 938321246: /*measure*/ return new Property("measure", "canonical(Measure)", "A reference to the Measure that was calculated to produce this report.", 0, 1, measure); 4084 case -1867885268: /*subject*/ return new Property("subject", "Reference(CareTeam|Device|Group|HealthcareService|Location|Organization|Patient|Practitioner|PractitionerRole|RelatedPerson)", "Optional subject identifying the individual or individuals the report is for.", 0, 1, subject); 4085 case 3076014: /*date*/ return new Property("date", "dateTime", "The date this measure was calculated.", 0, 1, date); 4086 case -427039519: /*reporter*/ return new Property("reporter", "Reference(Practitioner|PractitionerRole|Organization|Group)", "The individual or organization that is reporting the data.", 0, 1, reporter); 4087 case 581336342: /*reportingVendor*/ return new Property("reportingVendor", "Reference(Organization)", "A reference to the vendor who queried the data, calculated results and/or generated the report. The ?reporting vendor? is intended to represent the submitting entity when it is not the same as the reporting entity. This extension is used when the Receiver is interested in getting vendor information in the report.", 0, 1, reportingVendor); 4088 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "A reference to the location for which the data is being reported.", 0, 1, location); 4089 case -991726143: /*period*/ return new Property("period", "Period", "The reporting period for which the report was calculated.", 0, 1, period); 4090 case -812039852: /*inputParameters*/ return new Property("inputParameters", "Reference(Parameters)", "A reference to a Parameters resource (typically represented using a contained resource) that represents any input parameters that were provided to the operation that generated the report.", 0, 1, inputParameters); 4091 case 1924005583: /*scoring*/ return new Property("scoring", "CodeableConcept", "Indicates how the calculation is performed for the measure, including proportion, ratio, continuous-variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented. It is expected to be the same as the scoring element on the referenced Measure.", 0, 1, scoring); 4092 case -2085456136: /*improvementNotation*/ return new Property("improvementNotation", "CodeableConcept", "Whether improvement in the measure is noted by an increase or decrease in the measure score.", 0, 1, improvementNotation); 4093 case 98629247: /*group*/ return new Property("group", "", "The results of the calculation, one for each population group in the measure.", 0, java.lang.Integer.MAX_VALUE, group); 4094 case 1447496814: /*supplementalData*/ return new Property("supplementalData", "Reference(Any)", "A reference to a Resource that represents additional information collected for the report. If the value of the supplemental data is not a Resource (i.e. evaluating the supplementalData expression for this case in the measure results in a value that is not a FHIR Resource), it is reported as a reference to a contained Observation resource.", 0, java.lang.Integer.MAX_VALUE, supplementalData); 4095 case -1056771047: /*evaluatedResource*/ return new Property("evaluatedResource", "Reference(Any)", "Evaluated resources are used to capture what data was involved in the calculation of a measure. This usage is only allowed for individual reports to ensure that the size of the MeasureReport resource is bounded.", 0, java.lang.Integer.MAX_VALUE, evaluatedResource); 4096 default: return super.getNamedProperty(_hash, _name, _checkValid); 4097 } 4098 4099 } 4100 4101 @Override 4102 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4103 switch (hash) { 4104 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 4105 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MeasureReportStatus> 4106 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<MeasureReportType> 4107 case -425890067: /*dataUpdateType*/ return this.dataUpdateType == null ? new Base[0] : new Base[] {this.dataUpdateType}; // Enumeration<SubmitDataUpdateType> 4108 case 938321246: /*measure*/ return this.measure == null ? new Base[0] : new Base[] {this.measure}; // CanonicalType 4109 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 4110 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 4111 case -427039519: /*reporter*/ return this.reporter == null ? new Base[0] : new Base[] {this.reporter}; // Reference 4112 case 581336342: /*reportingVendor*/ return this.reportingVendor == null ? new Base[0] : new Base[] {this.reportingVendor}; // Reference 4113 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 4114 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 4115 case -812039852: /*inputParameters*/ return this.inputParameters == null ? new Base[0] : new Base[] {this.inputParameters}; // Reference 4116 case 1924005583: /*scoring*/ return this.scoring == null ? new Base[0] : new Base[] {this.scoring}; // CodeableConcept 4117 case -2085456136: /*improvementNotation*/ return this.improvementNotation == null ? new Base[0] : new Base[] {this.improvementNotation}; // CodeableConcept 4118 case 98629247: /*group*/ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // MeasureReportGroupComponent 4119 case 1447496814: /*supplementalData*/ return this.supplementalData == null ? new Base[0] : this.supplementalData.toArray(new Base[this.supplementalData.size()]); // Reference 4120 case -1056771047: /*evaluatedResource*/ return this.evaluatedResource == null ? new Base[0] : this.evaluatedResource.toArray(new Base[this.evaluatedResource.size()]); // Reference 4121 default: return super.getProperty(hash, name, checkValid); 4122 } 4123 4124 } 4125 4126 @Override 4127 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4128 switch (hash) { 4129 case -1618432855: // identifier 4130 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 4131 return value; 4132 case -892481550: // status 4133 value = new MeasureReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4134 this.status = (Enumeration) value; // Enumeration<MeasureReportStatus> 4135 return value; 4136 case 3575610: // type 4137 value = new MeasureReportTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4138 this.type = (Enumeration) value; // Enumeration<MeasureReportType> 4139 return value; 4140 case -425890067: // dataUpdateType 4141 value = new SubmitDataUpdateTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4142 this.dataUpdateType = (Enumeration) value; // Enumeration<SubmitDataUpdateType> 4143 return value; 4144 case 938321246: // measure 4145 this.measure = TypeConvertor.castToCanonical(value); // CanonicalType 4146 return value; 4147 case -1867885268: // subject 4148 this.subject = TypeConvertor.castToReference(value); // Reference 4149 return value; 4150 case 3076014: // date 4151 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4152 return value; 4153 case -427039519: // reporter 4154 this.reporter = TypeConvertor.castToReference(value); // Reference 4155 return value; 4156 case 581336342: // reportingVendor 4157 this.reportingVendor = TypeConvertor.castToReference(value); // Reference 4158 return value; 4159 case 1901043637: // location 4160 this.location = TypeConvertor.castToReference(value); // Reference 4161 return value; 4162 case -991726143: // period 4163 this.period = TypeConvertor.castToPeriod(value); // Period 4164 return value; 4165 case -812039852: // inputParameters 4166 this.inputParameters = TypeConvertor.castToReference(value); // Reference 4167 return value; 4168 case 1924005583: // scoring 4169 this.scoring = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4170 return value; 4171 case -2085456136: // improvementNotation 4172 this.improvementNotation = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4173 return value; 4174 case 98629247: // group 4175 this.getGroup().add((MeasureReportGroupComponent) value); // MeasureReportGroupComponent 4176 return value; 4177 case 1447496814: // supplementalData 4178 this.getSupplementalData().add(TypeConvertor.castToReference(value)); // Reference 4179 return value; 4180 case -1056771047: // evaluatedResource 4181 this.getEvaluatedResource().add(TypeConvertor.castToReference(value)); // Reference 4182 return value; 4183 default: return super.setProperty(hash, name, value); 4184 } 4185 4186 } 4187 4188 @Override 4189 public Base setProperty(String name, Base value) throws FHIRException { 4190 if (name.equals("identifier")) { 4191 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 4192 } else if (name.equals("status")) { 4193 value = new MeasureReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4194 this.status = (Enumeration) value; // Enumeration<MeasureReportStatus> 4195 } else if (name.equals("type")) { 4196 value = new MeasureReportTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4197 this.type = (Enumeration) value; // Enumeration<MeasureReportType> 4198 } else if (name.equals("dataUpdateType")) { 4199 value = new SubmitDataUpdateTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4200 this.dataUpdateType = (Enumeration) value; // Enumeration<SubmitDataUpdateType> 4201 } else if (name.equals("measure")) { 4202 this.measure = TypeConvertor.castToCanonical(value); // CanonicalType 4203 } else if (name.equals("subject")) { 4204 this.subject = TypeConvertor.castToReference(value); // Reference 4205 } else if (name.equals("date")) { 4206 this.date = TypeConvertor.castToDateTime(value); // DateTimeType 4207 } else if (name.equals("reporter")) { 4208 this.reporter = TypeConvertor.castToReference(value); // Reference 4209 } else if (name.equals("reportingVendor")) { 4210 this.reportingVendor = TypeConvertor.castToReference(value); // Reference 4211 } else if (name.equals("location")) { 4212 this.location = TypeConvertor.castToReference(value); // Reference 4213 } else if (name.equals("period")) { 4214 this.period = TypeConvertor.castToPeriod(value); // Period 4215 } else if (name.equals("inputParameters")) { 4216 this.inputParameters = TypeConvertor.castToReference(value); // Reference 4217 } else if (name.equals("scoring")) { 4218 this.scoring = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4219 } else if (name.equals("improvementNotation")) { 4220 this.improvementNotation = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4221 } else if (name.equals("group")) { 4222 this.getGroup().add((MeasureReportGroupComponent) value); 4223 } else if (name.equals("supplementalData")) { 4224 this.getSupplementalData().add(TypeConvertor.castToReference(value)); 4225 } else if (name.equals("evaluatedResource")) { 4226 this.getEvaluatedResource().add(TypeConvertor.castToReference(value)); 4227 } else 4228 return super.setProperty(name, value); 4229 return value; 4230 } 4231 4232 @Override 4233 public void removeChild(String name, Base value) throws FHIRException { 4234 if (name.equals("identifier")) { 4235 this.getIdentifier().remove(value); 4236 } else if (name.equals("status")) { 4237 value = new MeasureReportStatusEnumFactory().fromType(TypeConvertor.castToCode(value)); 4238 this.status = (Enumeration) value; // Enumeration<MeasureReportStatus> 4239 } else if (name.equals("type")) { 4240 value = new MeasureReportTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4241 this.type = (Enumeration) value; // Enumeration<MeasureReportType> 4242 } else if (name.equals("dataUpdateType")) { 4243 value = new SubmitDataUpdateTypeEnumFactory().fromType(TypeConvertor.castToCode(value)); 4244 this.dataUpdateType = (Enumeration) value; // Enumeration<SubmitDataUpdateType> 4245 } else if (name.equals("measure")) { 4246 this.measure = null; 4247 } else if (name.equals("subject")) { 4248 this.subject = null; 4249 } else if (name.equals("date")) { 4250 this.date = null; 4251 } else if (name.equals("reporter")) { 4252 this.reporter = null; 4253 } else if (name.equals("reportingVendor")) { 4254 this.reportingVendor = null; 4255 } else if (name.equals("location")) { 4256 this.location = null; 4257 } else if (name.equals("period")) { 4258 this.period = null; 4259 } else if (name.equals("inputParameters")) { 4260 this.inputParameters = null; 4261 } else if (name.equals("scoring")) { 4262 this.scoring = null; 4263 } else if (name.equals("improvementNotation")) { 4264 this.improvementNotation = null; 4265 } else if (name.equals("group")) { 4266 this.getGroup().remove((MeasureReportGroupComponent) value); 4267 } else if (name.equals("supplementalData")) { 4268 this.getSupplementalData().remove(value); 4269 } else if (name.equals("evaluatedResource")) { 4270 this.getEvaluatedResource().remove(value); 4271 } else 4272 super.removeChild(name, value); 4273 4274 } 4275 4276 @Override 4277 public Base makeProperty(int hash, String name) throws FHIRException { 4278 switch (hash) { 4279 case -1618432855: return addIdentifier(); 4280 case -892481550: return getStatusElement(); 4281 case 3575610: return getTypeElement(); 4282 case -425890067: return getDataUpdateTypeElement(); 4283 case 938321246: return getMeasureElement(); 4284 case -1867885268: return getSubject(); 4285 case 3076014: return getDateElement(); 4286 case -427039519: return getReporter(); 4287 case 581336342: return getReportingVendor(); 4288 case 1901043637: return getLocation(); 4289 case -991726143: return getPeriod(); 4290 case -812039852: return getInputParameters(); 4291 case 1924005583: return getScoring(); 4292 case -2085456136: return getImprovementNotation(); 4293 case 98629247: return addGroup(); 4294 case 1447496814: return addSupplementalData(); 4295 case -1056771047: return addEvaluatedResource(); 4296 default: return super.makeProperty(hash, name); 4297 } 4298 4299 } 4300 4301 @Override 4302 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4303 switch (hash) { 4304 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 4305 case -892481550: /*status*/ return new String[] {"code"}; 4306 case 3575610: /*type*/ return new String[] {"code"}; 4307 case -425890067: /*dataUpdateType*/ return new String[] {"code"}; 4308 case 938321246: /*measure*/ return new String[] {"canonical"}; 4309 case -1867885268: /*subject*/ return new String[] {"Reference"}; 4310 case 3076014: /*date*/ return new String[] {"dateTime"}; 4311 case -427039519: /*reporter*/ return new String[] {"Reference"}; 4312 case 581336342: /*reportingVendor*/ return new String[] {"Reference"}; 4313 case 1901043637: /*location*/ return new String[] {"Reference"}; 4314 case -991726143: /*period*/ return new String[] {"Period"}; 4315 case -812039852: /*inputParameters*/ return new String[] {"Reference"}; 4316 case 1924005583: /*scoring*/ return new String[] {"CodeableConcept"}; 4317 case -2085456136: /*improvementNotation*/ return new String[] {"CodeableConcept"}; 4318 case 98629247: /*group*/ return new String[] {}; 4319 case 1447496814: /*supplementalData*/ return new String[] {"Reference"}; 4320 case -1056771047: /*evaluatedResource*/ return new String[] {"Reference"}; 4321 default: return super.getTypesForProperty(hash, name); 4322 } 4323 4324 } 4325 4326 @Override 4327 public Base addChild(String name) throws FHIRException { 4328 if (name.equals("identifier")) { 4329 return addIdentifier(); 4330 } 4331 else if (name.equals("status")) { 4332 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.status"); 4333 } 4334 else if (name.equals("type")) { 4335 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.type"); 4336 } 4337 else if (name.equals("dataUpdateType")) { 4338 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.dataUpdateType"); 4339 } 4340 else if (name.equals("measure")) { 4341 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.measure"); 4342 } 4343 else if (name.equals("subject")) { 4344 this.subject = new Reference(); 4345 return this.subject; 4346 } 4347 else if (name.equals("date")) { 4348 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.date"); 4349 } 4350 else if (name.equals("reporter")) { 4351 this.reporter = new Reference(); 4352 return this.reporter; 4353 } 4354 else if (name.equals("reportingVendor")) { 4355 this.reportingVendor = new Reference(); 4356 return this.reportingVendor; 4357 } 4358 else if (name.equals("location")) { 4359 this.location = new Reference(); 4360 return this.location; 4361 } 4362 else if (name.equals("period")) { 4363 this.period = new Period(); 4364 return this.period; 4365 } 4366 else if (name.equals("inputParameters")) { 4367 this.inputParameters = new Reference(); 4368 return this.inputParameters; 4369 } 4370 else if (name.equals("scoring")) { 4371 this.scoring = new CodeableConcept(); 4372 return this.scoring; 4373 } 4374 else if (name.equals("improvementNotation")) { 4375 this.improvementNotation = new CodeableConcept(); 4376 return this.improvementNotation; 4377 } 4378 else if (name.equals("group")) { 4379 return addGroup(); 4380 } 4381 else if (name.equals("supplementalData")) { 4382 return addSupplementalData(); 4383 } 4384 else if (name.equals("evaluatedResource")) { 4385 return addEvaluatedResource(); 4386 } 4387 else 4388 return super.addChild(name); 4389 } 4390 4391 public String fhirType() { 4392 return "MeasureReport"; 4393 4394 } 4395 4396 public MeasureReport copy() { 4397 MeasureReport dst = new MeasureReport(); 4398 copyValues(dst); 4399 return dst; 4400 } 4401 4402 public void copyValues(MeasureReport dst) { 4403 super.copyValues(dst); 4404 if (identifier != null) { 4405 dst.identifier = new ArrayList<Identifier>(); 4406 for (Identifier i : identifier) 4407 dst.identifier.add(i.copy()); 4408 }; 4409 dst.status = status == null ? null : status.copy(); 4410 dst.type = type == null ? null : type.copy(); 4411 dst.dataUpdateType = dataUpdateType == null ? null : dataUpdateType.copy(); 4412 dst.measure = measure == null ? null : measure.copy(); 4413 dst.subject = subject == null ? null : subject.copy(); 4414 dst.date = date == null ? null : date.copy(); 4415 dst.reporter = reporter == null ? null : reporter.copy(); 4416 dst.reportingVendor = reportingVendor == null ? null : reportingVendor.copy(); 4417 dst.location = location == null ? null : location.copy(); 4418 dst.period = period == null ? null : period.copy(); 4419 dst.inputParameters = inputParameters == null ? null : inputParameters.copy(); 4420 dst.scoring = scoring == null ? null : scoring.copy(); 4421 dst.improvementNotation = improvementNotation == null ? null : improvementNotation.copy(); 4422 if (group != null) { 4423 dst.group = new ArrayList<MeasureReportGroupComponent>(); 4424 for (MeasureReportGroupComponent i : group) 4425 dst.group.add(i.copy()); 4426 }; 4427 if (supplementalData != null) { 4428 dst.supplementalData = new ArrayList<Reference>(); 4429 for (Reference i : supplementalData) 4430 dst.supplementalData.add(i.copy()); 4431 }; 4432 if (evaluatedResource != null) { 4433 dst.evaluatedResource = new ArrayList<Reference>(); 4434 for (Reference i : evaluatedResource) 4435 dst.evaluatedResource.add(i.copy()); 4436 }; 4437 } 4438 4439 protected MeasureReport typedCopy() { 4440 return copy(); 4441 } 4442 4443 @Override 4444 public boolean equalsDeep(Base other_) { 4445 if (!super.equalsDeep(other_)) 4446 return false; 4447 if (!(other_ instanceof MeasureReport)) 4448 return false; 4449 MeasureReport o = (MeasureReport) other_; 4450 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 4451 && compareDeep(dataUpdateType, o.dataUpdateType, true) && compareDeep(measure, o.measure, true) 4452 && compareDeep(subject, o.subject, true) && compareDeep(date, o.date, true) && compareDeep(reporter, o.reporter, true) 4453 && compareDeep(reportingVendor, o.reportingVendor, true) && compareDeep(location, o.location, true) 4454 && compareDeep(period, o.period, true) && compareDeep(inputParameters, o.inputParameters, true) 4455 && compareDeep(scoring, o.scoring, true) && compareDeep(improvementNotation, o.improvementNotation, true) 4456 && compareDeep(group, o.group, true) && compareDeep(supplementalData, o.supplementalData, true) 4457 && compareDeep(evaluatedResource, o.evaluatedResource, true); 4458 } 4459 4460 @Override 4461 public boolean equalsShallow(Base other_) { 4462 if (!super.equalsShallow(other_)) 4463 return false; 4464 if (!(other_ instanceof MeasureReport)) 4465 return false; 4466 MeasureReport o = (MeasureReport) other_; 4467 return compareValues(status, o.status, true) && compareValues(type, o.type, true) && compareValues(dataUpdateType, o.dataUpdateType, true) 4468 && compareValues(measure, o.measure, true) && compareValues(date, o.date, true); 4469 } 4470 4471 public boolean isEmpty() { 4472 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type 4473 , dataUpdateType, measure, subject, date, reporter, reportingVendor, location 4474 , period, inputParameters, scoring, improvementNotation, group, supplementalData 4475 , evaluatedResource); 4476 } 4477 4478 @Override 4479 public ResourceType getResourceType() { 4480 return ResourceType.MeasureReport; 4481 } 4482 4483 /** 4484 * Search parameter: <b>evaluated-resource</b> 4485 * <p> 4486 * Description: <b>An evaluated resource referenced by the measure report</b><br> 4487 * Type: <b>reference</b><br> 4488 * Path: <b>MeasureReport.evaluatedResource</b><br> 4489 * </p> 4490 */ 4491 @SearchParamDefinition(name="evaluated-resource", path="MeasureReport.evaluatedResource", description="An evaluated resource referenced by the measure report", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 4492 public static final String SP_EVALUATED_RESOURCE = "evaluated-resource"; 4493 /** 4494 * <b>Fluent Client</b> search parameter constant for <b>evaluated-resource</b> 4495 * <p> 4496 * Description: <b>An evaluated resource referenced by the measure report</b><br> 4497 * Type: <b>reference</b><br> 4498 * Path: <b>MeasureReport.evaluatedResource</b><br> 4499 * </p> 4500 */ 4501 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam EVALUATED_RESOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_EVALUATED_RESOURCE); 4502 4503/** 4504 * Constant for fluent queries to be used to add include statements. Specifies 4505 * the path value of "<b>MeasureReport:evaluated-resource</b>". 4506 */ 4507 public static final ca.uhn.fhir.model.api.Include INCLUDE_EVALUATED_RESOURCE = new ca.uhn.fhir.model.api.Include("MeasureReport:evaluated-resource").toLocked(); 4508 4509 /** 4510 * Search parameter: <b>location</b> 4511 * <p> 4512 * Description: <b>The location to return measure report results for</b><br> 4513 * Type: <b>reference</b><br> 4514 * Path: <b>MeasureReport.location</b><br> 4515 * </p> 4516 */ 4517 @SearchParamDefinition(name="location", path="MeasureReport.location", description="The location to return measure report results for", type="reference", target={Location.class } ) 4518 public static final String SP_LOCATION = "location"; 4519 /** 4520 * <b>Fluent Client</b> search parameter constant for <b>location</b> 4521 * <p> 4522 * Description: <b>The location to return measure report results for</b><br> 4523 * Type: <b>reference</b><br> 4524 * Path: <b>MeasureReport.location</b><br> 4525 * </p> 4526 */ 4527 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 4528 4529/** 4530 * Constant for fluent queries to be used to add include statements. Specifies 4531 * the path value of "<b>MeasureReport:location</b>". 4532 */ 4533 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("MeasureReport:location").toLocked(); 4534 4535 /** 4536 * Search parameter: <b>measure</b> 4537 * <p> 4538 * Description: <b>The measure to return measure report results for</b><br> 4539 * Type: <b>reference</b><br> 4540 * Path: <b>MeasureReport.measure</b><br> 4541 * </p> 4542 */ 4543 @SearchParamDefinition(name="measure", path="MeasureReport.measure", description="The measure to return measure report results for", type="reference", target={Measure.class } ) 4544 public static final String SP_MEASURE = "measure"; 4545 /** 4546 * <b>Fluent Client</b> search parameter constant for <b>measure</b> 4547 * <p> 4548 * Description: <b>The measure to return measure report results for</b><br> 4549 * Type: <b>reference</b><br> 4550 * Path: <b>MeasureReport.measure</b><br> 4551 * </p> 4552 */ 4553 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEASURE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MEASURE); 4554 4555/** 4556 * Constant for fluent queries to be used to add include statements. Specifies 4557 * the path value of "<b>MeasureReport:measure</b>". 4558 */ 4559 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEASURE = new ca.uhn.fhir.model.api.Include("MeasureReport:measure").toLocked(); 4560 4561 /** 4562 * Search parameter: <b>period</b> 4563 * <p> 4564 * Description: <b>The period of the measure report</b><br> 4565 * Type: <b>date</b><br> 4566 * Path: <b>MeasureReport.period</b><br> 4567 * </p> 4568 */ 4569 @SearchParamDefinition(name="period", path="MeasureReport.period", description="The period of the measure report", type="date" ) 4570 public static final String SP_PERIOD = "period"; 4571 /** 4572 * <b>Fluent Client</b> search parameter constant for <b>period</b> 4573 * <p> 4574 * Description: <b>The period of the measure report</b><br> 4575 * Type: <b>date</b><br> 4576 * Path: <b>MeasureReport.period</b><br> 4577 * </p> 4578 */ 4579 public static final ca.uhn.fhir.rest.gclient.DateClientParam PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_PERIOD); 4580 4581 /** 4582 * Search parameter: <b>reporter</b> 4583 * <p> 4584 * Description: <b>The reporter to return measure report results for</b><br> 4585 * Type: <b>reference</b><br> 4586 * Path: <b>MeasureReport.reporter</b><br> 4587 * </p> 4588 */ 4589 @SearchParamDefinition(name="reporter", path="MeasureReport.reporter", description="The reporter to return measure report results for", type="reference", target={Group.class, Organization.class, Practitioner.class, PractitionerRole.class } ) 4590 public static final String SP_REPORTER = "reporter"; 4591 /** 4592 * <b>Fluent Client</b> search parameter constant for <b>reporter</b> 4593 * <p> 4594 * Description: <b>The reporter to return measure report results for</b><br> 4595 * Type: <b>reference</b><br> 4596 * Path: <b>MeasureReport.reporter</b><br> 4597 * </p> 4598 */ 4599 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REPORTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REPORTER); 4600 4601/** 4602 * Constant for fluent queries to be used to add include statements. Specifies 4603 * the path value of "<b>MeasureReport:reporter</b>". 4604 */ 4605 public static final ca.uhn.fhir.model.api.Include INCLUDE_REPORTER = new ca.uhn.fhir.model.api.Include("MeasureReport:reporter").toLocked(); 4606 4607 /** 4608 * Search parameter: <b>status</b> 4609 * <p> 4610 * Description: <b>The status of the measure report</b><br> 4611 * Type: <b>token</b><br> 4612 * Path: <b>MeasureReport.status</b><br> 4613 * </p> 4614 */ 4615 @SearchParamDefinition(name="status", path="MeasureReport.status", description="The status of the measure report", type="token" ) 4616 public static final String SP_STATUS = "status"; 4617 /** 4618 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4619 * <p> 4620 * Description: <b>The status of the measure report</b><br> 4621 * Type: <b>token</b><br> 4622 * Path: <b>MeasureReport.status</b><br> 4623 * </p> 4624 */ 4625 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4626 4627 /** 4628 * Search parameter: <b>subject</b> 4629 * <p> 4630 * Description: <b>The identity of a subject to search for individual measure report results for</b><br> 4631 * Type: <b>reference</b><br> 4632 * Path: <b>MeasureReport.subject</b><br> 4633 * </p> 4634 */ 4635 @SearchParamDefinition(name="subject", path="MeasureReport.subject", description="The identity of a subject to search for individual measure report results for", type="reference", target={CareTeam.class, Device.class, Group.class, HealthcareService.class, Location.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 4636 public static final String SP_SUBJECT = "subject"; 4637 /** 4638 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4639 * <p> 4640 * Description: <b>The identity of a subject to search for individual measure report results for</b><br> 4641 * Type: <b>reference</b><br> 4642 * Path: <b>MeasureReport.subject</b><br> 4643 * </p> 4644 */ 4645 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 4646 4647/** 4648 * Constant for fluent queries to be used to add include statements. Specifies 4649 * the path value of "<b>MeasureReport:subject</b>". 4650 */ 4651 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("MeasureReport:subject").toLocked(); 4652 4653 /** 4654 * Search parameter: <b>date</b> 4655 * <p> 4656 * Description: <b>Multiple Resources: 4657 4658* [AdverseEvent](adverseevent.html): When the event occurred 4659* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4660* [Appointment](appointment.html): Appointment date/time. 4661* [AuditEvent](auditevent.html): Time when the event was recorded 4662* [CarePlan](careplan.html): Time period plan covers 4663* [CareTeam](careteam.html): A date within the coverage time period. 4664* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4665* [Composition](composition.html): Composition editing time 4666* [Consent](consent.html): When consent was agreed to 4667* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4668* [DocumentReference](documentreference.html): When this document reference was created 4669* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4670* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4671* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4672* [Flag](flag.html): Time period when flag is active 4673* [Immunization](immunization.html): Vaccination (non)-Administration Date 4674* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4675* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4676* [Invoice](invoice.html): Invoice date / posting date 4677* [List](list.html): When the list was prepared 4678* [MeasureReport](measurereport.html): The date of the measure report 4679* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4680* [Observation](observation.html): Clinically relevant time/time-period for observation 4681* [Procedure](procedure.html): When the procedure occurred or is occurring 4682* [ResearchSubject](researchsubject.html): Start and end of participation 4683* [RiskAssessment](riskassessment.html): When was assessment made? 4684* [SupplyRequest](supplyrequest.html): When the request was made 4685</b><br> 4686 * Type: <b>date</b><br> 4687 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4688 * </p> 4689 */ 4690 @SearchParamDefinition(name="date", path="AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): When the event occurred\r\n* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded\r\n* [Appointment](appointment.html): Appointment date/time.\r\n* [AuditEvent](auditevent.html): Time when the event was recorded\r\n* [CarePlan](careplan.html): Time period plan covers\r\n* [CareTeam](careteam.html): A date within the coverage time period.\r\n* [ClinicalImpression](clinicalimpression.html): When the assessment was documented\r\n* [Composition](composition.html): Composition editing time\r\n* [Consent](consent.html): When consent was agreed to\r\n* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report\r\n* [DocumentReference](documentreference.html): When this document reference was created\r\n* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted\r\n* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period\r\n* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated\r\n* [Flag](flag.html): Time period when flag is active\r\n* [Immunization](immunization.html): Vaccination (non)-Administration Date\r\n* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created\r\n* [Invoice](invoice.html): Invoice date / posting date\r\n* [List](list.html): When the list was prepared\r\n* [MeasureReport](measurereport.html): The date of the measure report\r\n* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication\r\n* [Observation](observation.html): Clinically relevant time/time-period for observation\r\n* [Procedure](procedure.html): When the procedure occurred or is occurring\r\n* [ResearchSubject](researchsubject.html): Start and end of participation\r\n* [RiskAssessment](riskassessment.html): When was assessment made?\r\n* [SupplyRequest](supplyrequest.html): When the request was made\r\n", type="date" ) 4691 public static final String SP_DATE = "date"; 4692 /** 4693 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4694 * <p> 4695 * Description: <b>Multiple Resources: 4696 4697* [AdverseEvent](adverseevent.html): When the event occurred 4698* [AllergyIntolerance](allergyintolerance.html): Date first version of the resource instance was recorded 4699* [Appointment](appointment.html): Appointment date/time. 4700* [AuditEvent](auditevent.html): Time when the event was recorded 4701* [CarePlan](careplan.html): Time period plan covers 4702* [CareTeam](careteam.html): A date within the coverage time period. 4703* [ClinicalImpression](clinicalimpression.html): When the assessment was documented 4704* [Composition](composition.html): Composition editing time 4705* [Consent](consent.html): When consent was agreed to 4706* [DiagnosticReport](diagnosticreport.html): The clinically relevant time of the report 4707* [DocumentReference](documentreference.html): When this document reference was created 4708* [Encounter](encounter.html): A date within the actualPeriod the Encounter lasted 4709* [EpisodeOfCare](episodeofcare.html): The provided date search value falls within the episode of care's period 4710* [FamilyMemberHistory](familymemberhistory.html): When history was recorded or last updated 4711* [Flag](flag.html): Time period when flag is active 4712* [Immunization](immunization.html): Vaccination (non)-Administration Date 4713* [ImmunizationEvaluation](immunizationevaluation.html): Date the evaluation was generated 4714* [ImmunizationRecommendation](immunizationrecommendation.html): Date recommendation(s) created 4715* [Invoice](invoice.html): Invoice date / posting date 4716* [List](list.html): When the list was prepared 4717* [MeasureReport](measurereport.html): The date of the measure report 4718* [NutritionIntake](nutritionintake.html): Date when patient was taking (or not taking) the medication 4719* [Observation](observation.html): Clinically relevant time/time-period for observation 4720* [Procedure](procedure.html): When the procedure occurred or is occurring 4721* [ResearchSubject](researchsubject.html): Start and end of participation 4722* [RiskAssessment](riskassessment.html): When was assessment made? 4723* [SupplyRequest](supplyrequest.html): When the request was made 4724</b><br> 4725 * Type: <b>date</b><br> 4726 * Path: <b>AdverseEvent.occurrence.ofType(dateTime) | AdverseEvent.occurrence.ofType(Period) | AdverseEvent.occurrence.ofType(Timing) | AllergyIntolerance.recordedDate | (start | requestedPeriod.start).first() | AuditEvent.recorded | CarePlan.period | ClinicalImpression.date | Composition.date | Consent.date | DiagnosticReport.effective.ofType(dateTime) | DiagnosticReport.effective.ofType(Period) | DocumentReference.date | Encounter.actualPeriod | EpisodeOfCare.period | FamilyMemberHistory.date | Flag.period | (Immunization.occurrence.ofType(dateTime)) | ImmunizationEvaluation.date | ImmunizationRecommendation.date | Invoice.date | List.date | MeasureReport.date | NutritionIntake.occurrence.ofType(dateTime) | NutritionIntake.occurrence.ofType(Period) | Observation.effective.ofType(dateTime) | Observation.effective.ofType(Period) | Observation.effective.ofType(Timing) | Observation.effective.ofType(instant) | Procedure.occurrence.ofType(dateTime) | Procedure.occurrence.ofType(Period) | Procedure.occurrence.ofType(Timing) | ResearchSubject.period | (RiskAssessment.occurrence.ofType(dateTime)) | SupplyRequest.authoredOn</b><br> 4727 * </p> 4728 */ 4729 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4730 4731 /** 4732 * Search parameter: <b>identifier</b> 4733 * <p> 4734 * Description: <b>Multiple Resources: 4735 4736* [Account](account.html): Account number 4737* [AdverseEvent](adverseevent.html): Business identifier for the event 4738* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4739* [Appointment](appointment.html): An Identifier of the Appointment 4740* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4741* [Basic](basic.html): Business identifier 4742* [BodyStructure](bodystructure.html): Bodystructure identifier 4743* [CarePlan](careplan.html): External Ids for this plan 4744* [CareTeam](careteam.html): External Ids for this team 4745* [ChargeItem](chargeitem.html): Business Identifier for item 4746* [Claim](claim.html): The primary identifier of the financial resource 4747* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4748* [ClinicalImpression](clinicalimpression.html): Business identifier 4749* [Communication](communication.html): Unique identifier 4750* [CommunicationRequest](communicationrequest.html): Unique identifier 4751* [Composition](composition.html): Version-independent identifier for the Composition 4752* [Condition](condition.html): A unique identifier of the condition record 4753* [Consent](consent.html): Identifier for this record (external references) 4754* [Contract](contract.html): The identity of the contract 4755* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4756* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4757* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4758* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4759* [DeviceRequest](devicerequest.html): Business identifier for request/order 4760* [DeviceUsage](deviceusage.html): Search by identifier 4761* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4762* [DocumentReference](documentreference.html): Identifier of the attachment binary 4763* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4764* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4765* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4766* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4767* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4768* [Flag](flag.html): Business identifier 4769* [Goal](goal.html): External Ids for this goal 4770* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4771* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4772* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4773* [Immunization](immunization.html): Business identifier 4774* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4775* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4776* [Invoice](invoice.html): Business Identifier for item 4777* [List](list.html): Business identifier 4778* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4779* [Medication](medication.html): Returns medications with this external identifier 4780* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4781* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4782* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4783* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4784* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4785* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4786* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4787* [Observation](observation.html): The unique id for a particular observation 4788* [Person](person.html): A person Identifier 4789* [Procedure](procedure.html): A unique identifier for a procedure 4790* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4791* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4792* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4793* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4794* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4795* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4796* [Specimen](specimen.html): The unique identifier associated with the specimen 4797* [SupplyDelivery](supplydelivery.html): External identifier 4798* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4799* [Task](task.html): Search for a task instance by its business identifier 4800* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4801</b><br> 4802 * Type: <b>token</b><br> 4803 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4804 * </p> 4805 */ 4806 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 4807 public static final String SP_IDENTIFIER = "identifier"; 4808 /** 4809 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4810 * <p> 4811 * Description: <b>Multiple Resources: 4812 4813* [Account](account.html): Account number 4814* [AdverseEvent](adverseevent.html): Business identifier for the event 4815* [AllergyIntolerance](allergyintolerance.html): External ids for this item 4816* [Appointment](appointment.html): An Identifier of the Appointment 4817* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 4818* [Basic](basic.html): Business identifier 4819* [BodyStructure](bodystructure.html): Bodystructure identifier 4820* [CarePlan](careplan.html): External Ids for this plan 4821* [CareTeam](careteam.html): External Ids for this team 4822* [ChargeItem](chargeitem.html): Business Identifier for item 4823* [Claim](claim.html): The primary identifier of the financial resource 4824* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 4825* [ClinicalImpression](clinicalimpression.html): Business identifier 4826* [Communication](communication.html): Unique identifier 4827* [CommunicationRequest](communicationrequest.html): Unique identifier 4828* [Composition](composition.html): Version-independent identifier for the Composition 4829* [Condition](condition.html): A unique identifier of the condition record 4830* [Consent](consent.html): Identifier for this record (external references) 4831* [Contract](contract.html): The identity of the contract 4832* [Coverage](coverage.html): The primary identifier of the insured and the coverage 4833* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 4834* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 4835* [DetectedIssue](detectedissue.html): Unique id for the detected issue 4836* [DeviceRequest](devicerequest.html): Business identifier for request/order 4837* [DeviceUsage](deviceusage.html): Search by identifier 4838* [DiagnosticReport](diagnosticreport.html): An identifier for the report 4839* [DocumentReference](documentreference.html): Identifier of the attachment binary 4840* [Encounter](encounter.html): Identifier(s) by which this encounter is known 4841* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 4842* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 4843* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 4844* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 4845* [Flag](flag.html): Business identifier 4846* [Goal](goal.html): External Ids for this goal 4847* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 4848* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 4849* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 4850* [Immunization](immunization.html): Business identifier 4851* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 4852* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 4853* [Invoice](invoice.html): Business Identifier for item 4854* [List](list.html): Business identifier 4855* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 4856* [Medication](medication.html): Returns medications with this external identifier 4857* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 4858* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 4859* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 4860* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 4861* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 4862* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 4863* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 4864* [Observation](observation.html): The unique id for a particular observation 4865* [Person](person.html): A person Identifier 4866* [Procedure](procedure.html): A unique identifier for a procedure 4867* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 4868* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 4869* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 4870* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 4871* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 4872* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 4873* [Specimen](specimen.html): The unique identifier associated with the specimen 4874* [SupplyDelivery](supplydelivery.html): External identifier 4875* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 4876* [Task](task.html): Search for a task instance by its business identifier 4877* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 4878</b><br> 4879 * Type: <b>token</b><br> 4880 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 4881 * </p> 4882 */ 4883 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4884 4885 /** 4886 * Search parameter: <b>patient</b> 4887 * <p> 4888 * Description: <b>Multiple Resources: 4889 4890* [Account](account.html): The entity that caused the expenses 4891* [AdverseEvent](adverseevent.html): Subject impacted by event 4892* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4893* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4894* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4895* [AuditEvent](auditevent.html): Where the activity involved patient data 4896* [Basic](basic.html): Identifies the focus of this resource 4897* [BodyStructure](bodystructure.html): Who this is about 4898* [CarePlan](careplan.html): Who the care plan is for 4899* [CareTeam](careteam.html): Who care team is for 4900* [ChargeItem](chargeitem.html): Individual service was done for/to 4901* [Claim](claim.html): Patient receiving the products or services 4902* [ClaimResponse](claimresponse.html): The subject of care 4903* [ClinicalImpression](clinicalimpression.html): Patient assessed 4904* [Communication](communication.html): Focus of message 4905* [CommunicationRequest](communicationrequest.html): Focus of message 4906* [Composition](composition.html): Who and/or what the composition is about 4907* [Condition](condition.html): Who has the condition? 4908* [Consent](consent.html): Who the consent applies to 4909* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4910* [Coverage](coverage.html): Retrieve coverages for a patient 4911* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4912* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4913* [DetectedIssue](detectedissue.html): Associated patient 4914* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4915* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4916* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4917* [DocumentReference](documentreference.html): Who/what is the subject of the document 4918* [Encounter](encounter.html): The patient present at the encounter 4919* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4920* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4921* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 4922* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 4923* [Flag](flag.html): The identity of a subject to list flags for 4924* [Goal](goal.html): Who this goal is intended for 4925* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 4926* [ImagingSelection](imagingselection.html): Who the study is about 4927* [ImagingStudy](imagingstudy.html): Who the study is about 4928* [Immunization](immunization.html): The patient for the vaccination record 4929* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 4930* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 4931* [Invoice](invoice.html): Recipient(s) of goods and services 4932* [List](list.html): If all resources have the same subject 4933* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 4934* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 4935* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 4936* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 4937* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 4938* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 4939* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 4940* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 4941* [Observation](observation.html): The subject that the observation is about (if patient) 4942* [Person](person.html): The Person links to this Patient 4943* [Procedure](procedure.html): Search by subject - a patient 4944* [Provenance](provenance.html): Where the activity involved patient data 4945* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 4946* [RelatedPerson](relatedperson.html): The patient this related person is related to 4947* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 4948* [ResearchSubject](researchsubject.html): Who or what is part of study 4949* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 4950* [ServiceRequest](servicerequest.html): Search by subject - a patient 4951* [Specimen](specimen.html): The patient the specimen comes from 4952* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 4953* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 4954* [Task](task.html): Search by patient 4955* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 4956</b><br> 4957 * Type: <b>reference</b><br> 4958 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 4959 * </p> 4960 */ 4961 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 4962 public static final String SP_PATIENT = "patient"; 4963 /** 4964 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4965 * <p> 4966 * Description: <b>Multiple Resources: 4967 4968* [Account](account.html): The entity that caused the expenses 4969* [AdverseEvent](adverseevent.html): Subject impacted by event 4970* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 4971* [Appointment](appointment.html): One of the individuals of the appointment is this patient 4972* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 4973* [AuditEvent](auditevent.html): Where the activity involved patient data 4974* [Basic](basic.html): Identifies the focus of this resource 4975* [BodyStructure](bodystructure.html): Who this is about 4976* [CarePlan](careplan.html): Who the care plan is for 4977* [CareTeam](careteam.html): Who care team is for 4978* [ChargeItem](chargeitem.html): Individual service was done for/to 4979* [Claim](claim.html): Patient receiving the products or services 4980* [ClaimResponse](claimresponse.html): The subject of care 4981* [ClinicalImpression](clinicalimpression.html): Patient assessed 4982* [Communication](communication.html): Focus of message 4983* [CommunicationRequest](communicationrequest.html): Focus of message 4984* [Composition](composition.html): Who and/or what the composition is about 4985* [Condition](condition.html): Who has the condition? 4986* [Consent](consent.html): Who the consent applies to 4987* [Contract](contract.html): The identity of the subject of the contract (if a patient) 4988* [Coverage](coverage.html): Retrieve coverages for a patient 4989* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 4990* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 4991* [DetectedIssue](detectedissue.html): Associated patient 4992* [DeviceRequest](devicerequest.html): Individual the service is ordered for 4993* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 4994* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 4995* [DocumentReference](documentreference.html): Who/what is the subject of the document 4996* [Encounter](encounter.html): The patient present at the encounter 4997* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 4998* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 4999* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 5000* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 5001* [Flag](flag.html): The identity of a subject to list flags for 5002* [Goal](goal.html): Who this goal is intended for 5003* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 5004* [ImagingSelection](imagingselection.html): Who the study is about 5005* [ImagingStudy](imagingstudy.html): Who the study is about 5006* [Immunization](immunization.html): The patient for the vaccination record 5007* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 5008* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 5009* [Invoice](invoice.html): Recipient(s) of goods and services 5010* [List](list.html): If all resources have the same subject 5011* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 5012* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 5013* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 5014* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 5015* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 5016* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 5017* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 5018* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 5019* [Observation](observation.html): The subject that the observation is about (if patient) 5020* [Person](person.html): The Person links to this Patient 5021* [Procedure](procedure.html): Search by subject - a patient 5022* [Provenance](provenance.html): Where the activity involved patient data 5023* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 5024* [RelatedPerson](relatedperson.html): The patient this related person is related to 5025* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 5026* [ResearchSubject](researchsubject.html): Who or what is part of study 5027* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 5028* [ServiceRequest](servicerequest.html): Search by subject - a patient 5029* [Specimen](specimen.html): The patient the specimen comes from 5030* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 5031* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 5032* [Task](task.html): Search by patient 5033* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 5034</b><br> 5035 * Type: <b>reference</b><br> 5036 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 5037 * </p> 5038 */ 5039 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 5040 5041/** 5042 * Constant for fluent queries to be used to add include statements. Specifies 5043 * the path value of "<b>MeasureReport:patient</b>". 5044 */ 5045 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("MeasureReport:patient").toLocked(); 5046 5047 5048} 5049