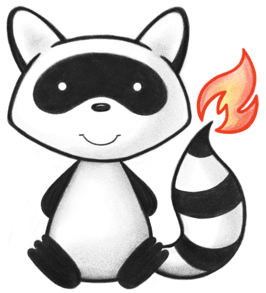
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * This resource is primarily used for the identification and definition of a medication, including ingredients, for the purposes of prescribing, dispensing, and administering a medication as well as for making statements about medication use. 052 */ 053@ResourceDef(name="Medication", profile="http://hl7.org/fhir/StructureDefinition/Medication") 054public class Medication extends DomainResource { 055 056 public enum MedicationStatusCodes { 057 /** 058 * The medication record is current and is appropriate for reference in new instances. 059 */ 060 ACTIVE, 061 /** 062 * The medication record is not current and is not is appropriate for reference in new instances. 063 */ 064 INACTIVE, 065 /** 066 * The medication record was created erroneously and is not appropriated for reference in new instances. 067 */ 068 ENTEREDINERROR, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static MedicationStatusCodes fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("inactive".equals(codeString)) 079 return INACTIVE; 080 if ("entered-in-error".equals(codeString)) 081 return ENTEREDINERROR; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown MedicationStatusCodes code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case ACTIVE: return "active"; 090 case INACTIVE: return "inactive"; 091 case ENTEREDINERROR: return "entered-in-error"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case ACTIVE: return "http://hl7.org/fhir/CodeSystem/medication-status"; 099 case INACTIVE: return "http://hl7.org/fhir/CodeSystem/medication-status"; 100 case ENTEREDINERROR: return "http://hl7.org/fhir/CodeSystem/medication-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case ACTIVE: return "The medication record is current and is appropriate for reference in new instances."; 108 case INACTIVE: return "The medication record is not current and is not is appropriate for reference in new instances."; 109 case ENTEREDINERROR: return "The medication record was created erroneously and is not appropriated for reference in new instances."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case ACTIVE: return "Active"; 117 case INACTIVE: return "Inactive"; 118 case ENTEREDINERROR: return "Entered in Error"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class MedicationStatusCodesEnumFactory implements EnumFactory<MedicationStatusCodes> { 126 public MedicationStatusCodes fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("active".equals(codeString)) 131 return MedicationStatusCodes.ACTIVE; 132 if ("inactive".equals(codeString)) 133 return MedicationStatusCodes.INACTIVE; 134 if ("entered-in-error".equals(codeString)) 135 return MedicationStatusCodes.ENTEREDINERROR; 136 throw new IllegalArgumentException("Unknown MedicationStatusCodes code '"+codeString+"'"); 137 } 138 public Enumeration<MedicationStatusCodes> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<MedicationStatusCodes>(this, MedicationStatusCodes.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<MedicationStatusCodes>(this, MedicationStatusCodes.NULL, code); 146 if ("active".equals(codeString)) 147 return new Enumeration<MedicationStatusCodes>(this, MedicationStatusCodes.ACTIVE, code); 148 if ("inactive".equals(codeString)) 149 return new Enumeration<MedicationStatusCodes>(this, MedicationStatusCodes.INACTIVE, code); 150 if ("entered-in-error".equals(codeString)) 151 return new Enumeration<MedicationStatusCodes>(this, MedicationStatusCodes.ENTEREDINERROR, code); 152 throw new FHIRException("Unknown MedicationStatusCodes code '"+codeString+"'"); 153 } 154 public String toCode(MedicationStatusCodes code) { 155 if (code == MedicationStatusCodes.NULL) 156 return null; 157 if (code == MedicationStatusCodes.ACTIVE) 158 return "active"; 159 if (code == MedicationStatusCodes.INACTIVE) 160 return "inactive"; 161 if (code == MedicationStatusCodes.ENTEREDINERROR) 162 return "entered-in-error"; 163 return "?"; 164 } 165 public String toSystem(MedicationStatusCodes code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class MedicationIngredientComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * The ingredient (substance or medication) that the ingredient.strength relates to. This is represented as a concept from a code system or described in another resource (Substance or Medication). 174 */ 175 @Child(name = "item", type = {CodeableReference.class}, order=1, min=1, max=1, modifier=false, summary=false) 176 @Description(shortDefinition="The ingredient (substance or medication) that the ingredient.strength relates to", formalDefinition="The ingredient (substance or medication) that the ingredient.strength relates to. This is represented as a concept from a code system or described in another resource (Substance or Medication)." ) 177 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 178 protected CodeableReference item; 179 180 /** 181 * Indication of whether this ingredient affects the therapeutic action of the drug. 182 */ 183 @Child(name = "isActive", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 184 @Description(shortDefinition="Active ingredient indicator", formalDefinition="Indication of whether this ingredient affects the therapeutic action of the drug." ) 185 protected BooleanType isActive; 186 187 /** 188 * Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet. 189 */ 190 @Child(name = "strength", type = {Ratio.class, CodeableConcept.class, Quantity.class}, order=3, min=0, max=1, modifier=false, summary=false) 191 @Description(shortDefinition="Quantity of ingredient present", formalDefinition="Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet." ) 192 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-ingredientstrength") 193 protected DataType strength; 194 195 private static final long serialVersionUID = -979760018L; 196 197 /** 198 * Constructor 199 */ 200 public MedicationIngredientComponent() { 201 super(); 202 } 203 204 /** 205 * Constructor 206 */ 207 public MedicationIngredientComponent(CodeableReference item) { 208 super(); 209 this.setItem(item); 210 } 211 212 /** 213 * @return {@link #item} (The ingredient (substance or medication) that the ingredient.strength relates to. This is represented as a concept from a code system or described in another resource (Substance or Medication).) 214 */ 215 public CodeableReference getItem() { 216 if (this.item == null) 217 if (Configuration.errorOnAutoCreate()) 218 throw new Error("Attempt to auto-create MedicationIngredientComponent.item"); 219 else if (Configuration.doAutoCreate()) 220 this.item = new CodeableReference(); // cc 221 return this.item; 222 } 223 224 public boolean hasItem() { 225 return this.item != null && !this.item.isEmpty(); 226 } 227 228 /** 229 * @param value {@link #item} (The ingredient (substance or medication) that the ingredient.strength relates to. This is represented as a concept from a code system or described in another resource (Substance or Medication).) 230 */ 231 public MedicationIngredientComponent setItem(CodeableReference value) { 232 this.item = value; 233 return this; 234 } 235 236 /** 237 * @return {@link #isActive} (Indication of whether this ingredient affects the therapeutic action of the drug.). This is the underlying object with id, value and extensions. The accessor "getIsActive" gives direct access to the value 238 */ 239 public BooleanType getIsActiveElement() { 240 if (this.isActive == null) 241 if (Configuration.errorOnAutoCreate()) 242 throw new Error("Attempt to auto-create MedicationIngredientComponent.isActive"); 243 else if (Configuration.doAutoCreate()) 244 this.isActive = new BooleanType(); // bb 245 return this.isActive; 246 } 247 248 public boolean hasIsActiveElement() { 249 return this.isActive != null && !this.isActive.isEmpty(); 250 } 251 252 public boolean hasIsActive() { 253 return this.isActive != null && !this.isActive.isEmpty(); 254 } 255 256 /** 257 * @param value {@link #isActive} (Indication of whether this ingredient affects the therapeutic action of the drug.). This is the underlying object with id, value and extensions. The accessor "getIsActive" gives direct access to the value 258 */ 259 public MedicationIngredientComponent setIsActiveElement(BooleanType value) { 260 this.isActive = value; 261 return this; 262 } 263 264 /** 265 * @return Indication of whether this ingredient affects the therapeutic action of the drug. 266 */ 267 public boolean getIsActive() { 268 return this.isActive == null || this.isActive.isEmpty() ? false : this.isActive.getValue(); 269 } 270 271 /** 272 * @param value Indication of whether this ingredient affects the therapeutic action of the drug. 273 */ 274 public MedicationIngredientComponent setIsActive(boolean value) { 275 if (this.isActive == null) 276 this.isActive = new BooleanType(); 277 this.isActive.setValue(value); 278 return this; 279 } 280 281 /** 282 * @return {@link #strength} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.) 283 */ 284 public DataType getStrength() { 285 return this.strength; 286 } 287 288 /** 289 * @return {@link #strength} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.) 290 */ 291 public Ratio getStrengthRatio() throws FHIRException { 292 if (this.strength == null) 293 this.strength = new Ratio(); 294 if (!(this.strength instanceof Ratio)) 295 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.strength.getClass().getName()+" was encountered"); 296 return (Ratio) this.strength; 297 } 298 299 public boolean hasStrengthRatio() { 300 return this.strength instanceof Ratio; 301 } 302 303 /** 304 * @return {@link #strength} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.) 305 */ 306 public CodeableConcept getStrengthCodeableConcept() throws FHIRException { 307 if (this.strength == null) 308 this.strength = new CodeableConcept(); 309 if (!(this.strength instanceof CodeableConcept)) 310 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.strength.getClass().getName()+" was encountered"); 311 return (CodeableConcept) this.strength; 312 } 313 314 public boolean hasStrengthCodeableConcept() { 315 return this.strength instanceof CodeableConcept; 316 } 317 318 /** 319 * @return {@link #strength} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.) 320 */ 321 public Quantity getStrengthQuantity() throws FHIRException { 322 if (this.strength == null) 323 this.strength = new Quantity(); 324 if (!(this.strength instanceof Quantity)) 325 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.strength.getClass().getName()+" was encountered"); 326 return (Quantity) this.strength; 327 } 328 329 public boolean hasStrengthQuantity() { 330 return this.strength instanceof Quantity; 331 } 332 333 public boolean hasStrength() { 334 return this.strength != null && !this.strength.isEmpty(); 335 } 336 337 /** 338 * @param value {@link #strength} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.) 339 */ 340 public MedicationIngredientComponent setStrength(DataType value) { 341 if (value != null && !(value instanceof Ratio || value instanceof CodeableConcept || value instanceof Quantity)) 342 throw new FHIRException("Not the right type for Medication.ingredient.strength[x]: "+value.fhirType()); 343 this.strength = value; 344 return this; 345 } 346 347 protected void listChildren(List<Property> children) { 348 super.listChildren(children); 349 children.add(new Property("item", "CodeableReference(Substance|Medication)", "The ingredient (substance or medication) that the ingredient.strength relates to. This is represented as a concept from a code system or described in another resource (Substance or Medication).", 0, 1, item)); 350 children.add(new Property("isActive", "boolean", "Indication of whether this ingredient affects the therapeutic action of the drug.", 0, 1, isActive)); 351 children.add(new Property("strength[x]", "Ratio|CodeableConcept|Quantity", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength)); 352 } 353 354 @Override 355 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 356 switch (_hash) { 357 case 3242771: /*item*/ return new Property("item", "CodeableReference(Substance|Medication)", "The ingredient (substance or medication) that the ingredient.strength relates to. This is represented as a concept from a code system or described in another resource (Substance or Medication).", 0, 1, item); 358 case -748916528: /*isActive*/ return new Property("isActive", "boolean", "Indication of whether this ingredient affects the therapeutic action of the drug.", 0, 1, isActive); 359 case 127377567: /*strength[x]*/ return new Property("strength[x]", "Ratio|CodeableConcept|Quantity", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength); 360 case 1791316033: /*strength*/ return new Property("strength[x]", "Ratio|CodeableConcept|Quantity", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength); 361 case 2141786186: /*strengthRatio*/ return new Property("strength[x]", "Ratio", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength); 362 case -1455903456: /*strengthCodeableConcept*/ return new Property("strength[x]", "CodeableConcept", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength); 363 case -1793570836: /*strengthQuantity*/ return new Property("strength[x]", "Quantity", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength); 364 default: return super.getNamedProperty(_hash, _name, _checkValid); 365 } 366 367 } 368 369 @Override 370 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 371 switch (hash) { 372 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // CodeableReference 373 case -748916528: /*isActive*/ return this.isActive == null ? new Base[0] : new Base[] {this.isActive}; // BooleanType 374 case 1791316033: /*strength*/ return this.strength == null ? new Base[0] : new Base[] {this.strength}; // DataType 375 default: return super.getProperty(hash, name, checkValid); 376 } 377 378 } 379 380 @Override 381 public Base setProperty(int hash, String name, Base value) throws FHIRException { 382 switch (hash) { 383 case 3242771: // item 384 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 385 return value; 386 case -748916528: // isActive 387 this.isActive = TypeConvertor.castToBoolean(value); // BooleanType 388 return value; 389 case 1791316033: // strength 390 this.strength = TypeConvertor.castToType(value); // DataType 391 return value; 392 default: return super.setProperty(hash, name, value); 393 } 394 395 } 396 397 @Override 398 public Base setProperty(String name, Base value) throws FHIRException { 399 if (name.equals("item")) { 400 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 401 } else if (name.equals("isActive")) { 402 this.isActive = TypeConvertor.castToBoolean(value); // BooleanType 403 } else if (name.equals("strength[x]")) { 404 this.strength = TypeConvertor.castToType(value); // DataType 405 } else 406 return super.setProperty(name, value); 407 return value; 408 } 409 410 @Override 411 public void removeChild(String name, Base value) throws FHIRException { 412 if (name.equals("item")) { 413 this.item = null; 414 } else if (name.equals("isActive")) { 415 this.isActive = null; 416 } else if (name.equals("strength[x]")) { 417 this.strength = null; 418 } else 419 super.removeChild(name, value); 420 421 } 422 423 @Override 424 public Base makeProperty(int hash, String name) throws FHIRException { 425 switch (hash) { 426 case 3242771: return getItem(); 427 case -748916528: return getIsActiveElement(); 428 case 127377567: return getStrength(); 429 case 1791316033: return getStrength(); 430 default: return super.makeProperty(hash, name); 431 } 432 433 } 434 435 @Override 436 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 437 switch (hash) { 438 case 3242771: /*item*/ return new String[] {"CodeableReference"}; 439 case -748916528: /*isActive*/ return new String[] {"boolean"}; 440 case 1791316033: /*strength*/ return new String[] {"Ratio", "CodeableConcept", "Quantity"}; 441 default: return super.getTypesForProperty(hash, name); 442 } 443 444 } 445 446 @Override 447 public Base addChild(String name) throws FHIRException { 448 if (name.equals("item")) { 449 this.item = new CodeableReference(); 450 return this.item; 451 } 452 else if (name.equals("isActive")) { 453 throw new FHIRException("Cannot call addChild on a singleton property Medication.ingredient.isActive"); 454 } 455 else if (name.equals("strengthRatio")) { 456 this.strength = new Ratio(); 457 return this.strength; 458 } 459 else if (name.equals("strengthCodeableConcept")) { 460 this.strength = new CodeableConcept(); 461 return this.strength; 462 } 463 else if (name.equals("strengthQuantity")) { 464 this.strength = new Quantity(); 465 return this.strength; 466 } 467 else 468 return super.addChild(name); 469 } 470 471 public MedicationIngredientComponent copy() { 472 MedicationIngredientComponent dst = new MedicationIngredientComponent(); 473 copyValues(dst); 474 return dst; 475 } 476 477 public void copyValues(MedicationIngredientComponent dst) { 478 super.copyValues(dst); 479 dst.item = item == null ? null : item.copy(); 480 dst.isActive = isActive == null ? null : isActive.copy(); 481 dst.strength = strength == null ? null : strength.copy(); 482 } 483 484 @Override 485 public boolean equalsDeep(Base other_) { 486 if (!super.equalsDeep(other_)) 487 return false; 488 if (!(other_ instanceof MedicationIngredientComponent)) 489 return false; 490 MedicationIngredientComponent o = (MedicationIngredientComponent) other_; 491 return compareDeep(item, o.item, true) && compareDeep(isActive, o.isActive, true) && compareDeep(strength, o.strength, true) 492 ; 493 } 494 495 @Override 496 public boolean equalsShallow(Base other_) { 497 if (!super.equalsShallow(other_)) 498 return false; 499 if (!(other_ instanceof MedicationIngredientComponent)) 500 return false; 501 MedicationIngredientComponent o = (MedicationIngredientComponent) other_; 502 return compareValues(isActive, o.isActive, true); 503 } 504 505 public boolean isEmpty() { 506 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item, isActive, strength 507 ); 508 } 509 510 public String fhirType() { 511 return "Medication.ingredient"; 512 513 } 514 515 } 516 517 @Block() 518 public static class MedicationBatchComponent extends BackboneElement implements IBaseBackboneElement { 519 /** 520 * The assigned lot number of a batch of the specified product. 521 */ 522 @Child(name = "lotNumber", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 523 @Description(shortDefinition="Identifier assigned to batch", formalDefinition="The assigned lot number of a batch of the specified product." ) 524 protected StringType lotNumber; 525 526 /** 527 * When this specific batch of product will expire. 528 */ 529 @Child(name = "expirationDate", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 530 @Description(shortDefinition="When batch will expire", formalDefinition="When this specific batch of product will expire." ) 531 protected DateTimeType expirationDate; 532 533 private static final long serialVersionUID = 1982738755L; 534 535 /** 536 * Constructor 537 */ 538 public MedicationBatchComponent() { 539 super(); 540 } 541 542 /** 543 * @return {@link #lotNumber} (The assigned lot number of a batch of the specified product.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 544 */ 545 public StringType getLotNumberElement() { 546 if (this.lotNumber == null) 547 if (Configuration.errorOnAutoCreate()) 548 throw new Error("Attempt to auto-create MedicationBatchComponent.lotNumber"); 549 else if (Configuration.doAutoCreate()) 550 this.lotNumber = new StringType(); // bb 551 return this.lotNumber; 552 } 553 554 public boolean hasLotNumberElement() { 555 return this.lotNumber != null && !this.lotNumber.isEmpty(); 556 } 557 558 public boolean hasLotNumber() { 559 return this.lotNumber != null && !this.lotNumber.isEmpty(); 560 } 561 562 /** 563 * @param value {@link #lotNumber} (The assigned lot number of a batch of the specified product.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 564 */ 565 public MedicationBatchComponent setLotNumberElement(StringType value) { 566 this.lotNumber = value; 567 return this; 568 } 569 570 /** 571 * @return The assigned lot number of a batch of the specified product. 572 */ 573 public String getLotNumber() { 574 return this.lotNumber == null ? null : this.lotNumber.getValue(); 575 } 576 577 /** 578 * @param value The assigned lot number of a batch of the specified product. 579 */ 580 public MedicationBatchComponent setLotNumber(String value) { 581 if (Utilities.noString(value)) 582 this.lotNumber = null; 583 else { 584 if (this.lotNumber == null) 585 this.lotNumber = new StringType(); 586 this.lotNumber.setValue(value); 587 } 588 return this; 589 } 590 591 /** 592 * @return {@link #expirationDate} (When this specific batch of product will expire.). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 593 */ 594 public DateTimeType getExpirationDateElement() { 595 if (this.expirationDate == null) 596 if (Configuration.errorOnAutoCreate()) 597 throw new Error("Attempt to auto-create MedicationBatchComponent.expirationDate"); 598 else if (Configuration.doAutoCreate()) 599 this.expirationDate = new DateTimeType(); // bb 600 return this.expirationDate; 601 } 602 603 public boolean hasExpirationDateElement() { 604 return this.expirationDate != null && !this.expirationDate.isEmpty(); 605 } 606 607 public boolean hasExpirationDate() { 608 return this.expirationDate != null && !this.expirationDate.isEmpty(); 609 } 610 611 /** 612 * @param value {@link #expirationDate} (When this specific batch of product will expire.). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 613 */ 614 public MedicationBatchComponent setExpirationDateElement(DateTimeType value) { 615 this.expirationDate = value; 616 return this; 617 } 618 619 /** 620 * @return When this specific batch of product will expire. 621 */ 622 public Date getExpirationDate() { 623 return this.expirationDate == null ? null : this.expirationDate.getValue(); 624 } 625 626 /** 627 * @param value When this specific batch of product will expire. 628 */ 629 public MedicationBatchComponent setExpirationDate(Date value) { 630 if (value == null) 631 this.expirationDate = null; 632 else { 633 if (this.expirationDate == null) 634 this.expirationDate = new DateTimeType(); 635 this.expirationDate.setValue(value); 636 } 637 return this; 638 } 639 640 protected void listChildren(List<Property> children) { 641 super.listChildren(children); 642 children.add(new Property("lotNumber", "string", "The assigned lot number of a batch of the specified product.", 0, 1, lotNumber)); 643 children.add(new Property("expirationDate", "dateTime", "When this specific batch of product will expire.", 0, 1, expirationDate)); 644 } 645 646 @Override 647 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 648 switch (_hash) { 649 case 462547450: /*lotNumber*/ return new Property("lotNumber", "string", "The assigned lot number of a batch of the specified product.", 0, 1, lotNumber); 650 case -668811523: /*expirationDate*/ return new Property("expirationDate", "dateTime", "When this specific batch of product will expire.", 0, 1, expirationDate); 651 default: return super.getNamedProperty(_hash, _name, _checkValid); 652 } 653 654 } 655 656 @Override 657 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 658 switch (hash) { 659 case 462547450: /*lotNumber*/ return this.lotNumber == null ? new Base[0] : new Base[] {this.lotNumber}; // StringType 660 case -668811523: /*expirationDate*/ return this.expirationDate == null ? new Base[0] : new Base[] {this.expirationDate}; // DateTimeType 661 default: return super.getProperty(hash, name, checkValid); 662 } 663 664 } 665 666 @Override 667 public Base setProperty(int hash, String name, Base value) throws FHIRException { 668 switch (hash) { 669 case 462547450: // lotNumber 670 this.lotNumber = TypeConvertor.castToString(value); // StringType 671 return value; 672 case -668811523: // expirationDate 673 this.expirationDate = TypeConvertor.castToDateTime(value); // DateTimeType 674 return value; 675 default: return super.setProperty(hash, name, value); 676 } 677 678 } 679 680 @Override 681 public Base setProperty(String name, Base value) throws FHIRException { 682 if (name.equals("lotNumber")) { 683 this.lotNumber = TypeConvertor.castToString(value); // StringType 684 } else if (name.equals("expirationDate")) { 685 this.expirationDate = TypeConvertor.castToDateTime(value); // DateTimeType 686 } else 687 return super.setProperty(name, value); 688 return value; 689 } 690 691 @Override 692 public void removeChild(String name, Base value) throws FHIRException { 693 if (name.equals("lotNumber")) { 694 this.lotNumber = null; 695 } else if (name.equals("expirationDate")) { 696 this.expirationDate = null; 697 } else 698 super.removeChild(name, value); 699 700 } 701 702 @Override 703 public Base makeProperty(int hash, String name) throws FHIRException { 704 switch (hash) { 705 case 462547450: return getLotNumberElement(); 706 case -668811523: return getExpirationDateElement(); 707 default: return super.makeProperty(hash, name); 708 } 709 710 } 711 712 @Override 713 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 714 switch (hash) { 715 case 462547450: /*lotNumber*/ return new String[] {"string"}; 716 case -668811523: /*expirationDate*/ return new String[] {"dateTime"}; 717 default: return super.getTypesForProperty(hash, name); 718 } 719 720 } 721 722 @Override 723 public Base addChild(String name) throws FHIRException { 724 if (name.equals("lotNumber")) { 725 throw new FHIRException("Cannot call addChild on a singleton property Medication.batch.lotNumber"); 726 } 727 else if (name.equals("expirationDate")) { 728 throw new FHIRException("Cannot call addChild on a singleton property Medication.batch.expirationDate"); 729 } 730 else 731 return super.addChild(name); 732 } 733 734 public MedicationBatchComponent copy() { 735 MedicationBatchComponent dst = new MedicationBatchComponent(); 736 copyValues(dst); 737 return dst; 738 } 739 740 public void copyValues(MedicationBatchComponent dst) { 741 super.copyValues(dst); 742 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 743 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 744 } 745 746 @Override 747 public boolean equalsDeep(Base other_) { 748 if (!super.equalsDeep(other_)) 749 return false; 750 if (!(other_ instanceof MedicationBatchComponent)) 751 return false; 752 MedicationBatchComponent o = (MedicationBatchComponent) other_; 753 return compareDeep(lotNumber, o.lotNumber, true) && compareDeep(expirationDate, o.expirationDate, true) 754 ; 755 } 756 757 @Override 758 public boolean equalsShallow(Base other_) { 759 if (!super.equalsShallow(other_)) 760 return false; 761 if (!(other_ instanceof MedicationBatchComponent)) 762 return false; 763 MedicationBatchComponent o = (MedicationBatchComponent) other_; 764 return compareValues(lotNumber, o.lotNumber, true) && compareValues(expirationDate, o.expirationDate, true) 765 ; 766 } 767 768 public boolean isEmpty() { 769 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(lotNumber, expirationDate 770 ); 771 } 772 773 public String fhirType() { 774 return "Medication.batch"; 775 776 } 777 778 } 779 780 /** 781 * Business identifier for this medication. 782 */ 783 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 784 @Description(shortDefinition="Business identifier for this medication", formalDefinition="Business identifier for this medication." ) 785 protected List<Identifier> identifier; 786 787 /** 788 * A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems. 789 */ 790 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 791 @Description(shortDefinition="Codes that identify this medication", formalDefinition="A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems." ) 792 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 793 protected CodeableConcept code; 794 795 /** 796 * A code to indicate if the medication is in active use. 797 */ 798 @Child(name = "status", type = {CodeType.class}, order=2, min=0, max=1, modifier=true, summary=true) 799 @Description(shortDefinition="active | inactive | entered-in-error", formalDefinition="A code to indicate if the medication is in active use." ) 800 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-status") 801 protected Enumeration<MedicationStatusCodes> status; 802 803 /** 804 * The company or other legal entity that has authorization, from the appropriate drug regulatory authority, to market a medicine in one or more jurisdictions. Typically abbreviated MAH.Note: The MAH may manufacture the product and may also contract the manufacturing of the product to one or more companies (organizations). 805 */ 806 @Child(name = "marketingAuthorizationHolder", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=true) 807 @Description(shortDefinition="Organization that has authorization to market medication", formalDefinition="The company or other legal entity that has authorization, from the appropriate drug regulatory authority, to market a medicine in one or more jurisdictions. Typically abbreviated MAH.Note: The MAH may manufacture the product and may also contract the manufacturing of the product to one or more companies (organizations)." ) 808 protected Reference marketingAuthorizationHolder; 809 810 /** 811 * Describes the form of the item. Powder; tablets; capsule. 812 */ 813 @Child(name = "doseForm", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 814 @Description(shortDefinition="powder | tablets | capsule +", formalDefinition="Describes the form of the item. Powder; tablets; capsule." ) 815 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-form-codes") 816 protected CodeableConcept doseForm; 817 818 /** 819 * When the specified product code does not infer a package size, this is the specific amount of drug in the product. For example, when specifying a product that has the same strength (For example, Insulin glargine 100 unit per mL solution for injection), this attribute provides additional clarification of the package amount (For example, 3 mL, 10mL, etc.). 820 */ 821 @Child(name = "totalVolume", type = {Quantity.class}, order=5, min=0, max=1, modifier=false, summary=true) 822 @Description(shortDefinition="When the specified product code does not infer a package size, this is the specific amount of drug in the product", formalDefinition="When the specified product code does not infer a package size, this is the specific amount of drug in the product. For example, when specifying a product that has the same strength (For example, Insulin glargine 100 unit per mL solution for injection), this attribute provides additional clarification of the package amount (For example, 3 mL, 10mL, etc.)." ) 823 protected Quantity totalVolume; 824 825 /** 826 * Identifies a particular constituent of interest in the product. 827 */ 828 @Child(name = "ingredient", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 829 @Description(shortDefinition="Active or inactive ingredient", formalDefinition="Identifies a particular constituent of interest in the product." ) 830 protected List<MedicationIngredientComponent> ingredient; 831 832 /** 833 * Information that only applies to packages (not products). 834 */ 835 @Child(name = "batch", type = {}, order=7, min=0, max=1, modifier=false, summary=false) 836 @Description(shortDefinition="Details about packaged medications", formalDefinition="Information that only applies to packages (not products)." ) 837 protected MedicationBatchComponent batch; 838 839 /** 840 * A reference to a knowledge resource that provides more information about this medication. 841 */ 842 @Child(name = "definition", type = {MedicationKnowledge.class}, order=8, min=0, max=1, modifier=false, summary=false) 843 @Description(shortDefinition="Knowledge about this medication", formalDefinition="A reference to a knowledge resource that provides more information about this medication." ) 844 protected Reference definition; 845 846 private static final long serialVersionUID = 603813239L; 847 848 /** 849 * Constructor 850 */ 851 public Medication() { 852 super(); 853 } 854 855 /** 856 * @return {@link #identifier} (Business identifier for this medication.) 857 */ 858 public List<Identifier> getIdentifier() { 859 if (this.identifier == null) 860 this.identifier = new ArrayList<Identifier>(); 861 return this.identifier; 862 } 863 864 /** 865 * @return Returns a reference to <code>this</code> for easy method chaining 866 */ 867 public Medication setIdentifier(List<Identifier> theIdentifier) { 868 this.identifier = theIdentifier; 869 return this; 870 } 871 872 public boolean hasIdentifier() { 873 if (this.identifier == null) 874 return false; 875 for (Identifier item : this.identifier) 876 if (!item.isEmpty()) 877 return true; 878 return false; 879 } 880 881 public Identifier addIdentifier() { //3 882 Identifier t = new Identifier(); 883 if (this.identifier == null) 884 this.identifier = new ArrayList<Identifier>(); 885 this.identifier.add(t); 886 return t; 887 } 888 889 public Medication addIdentifier(Identifier t) { //3 890 if (t == null) 891 return this; 892 if (this.identifier == null) 893 this.identifier = new ArrayList<Identifier>(); 894 this.identifier.add(t); 895 return this; 896 } 897 898 /** 899 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 900 */ 901 public Identifier getIdentifierFirstRep() { 902 if (getIdentifier().isEmpty()) { 903 addIdentifier(); 904 } 905 return getIdentifier().get(0); 906 } 907 908 /** 909 * @return {@link #code} (A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.) 910 */ 911 public CodeableConcept getCode() { 912 if (this.code == null) 913 if (Configuration.errorOnAutoCreate()) 914 throw new Error("Attempt to auto-create Medication.code"); 915 else if (Configuration.doAutoCreate()) 916 this.code = new CodeableConcept(); // cc 917 return this.code; 918 } 919 920 public boolean hasCode() { 921 return this.code != null && !this.code.isEmpty(); 922 } 923 924 /** 925 * @param value {@link #code} (A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.) 926 */ 927 public Medication setCode(CodeableConcept value) { 928 this.code = value; 929 return this; 930 } 931 932 /** 933 * @return {@link #status} (A code to indicate if the medication is in active use.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 934 */ 935 public Enumeration<MedicationStatusCodes> getStatusElement() { 936 if (this.status == null) 937 if (Configuration.errorOnAutoCreate()) 938 throw new Error("Attempt to auto-create Medication.status"); 939 else if (Configuration.doAutoCreate()) 940 this.status = new Enumeration<MedicationStatusCodes>(new MedicationStatusCodesEnumFactory()); // bb 941 return this.status; 942 } 943 944 public boolean hasStatusElement() { 945 return this.status != null && !this.status.isEmpty(); 946 } 947 948 public boolean hasStatus() { 949 return this.status != null && !this.status.isEmpty(); 950 } 951 952 /** 953 * @param value {@link #status} (A code to indicate if the medication is in active use.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 954 */ 955 public Medication setStatusElement(Enumeration<MedicationStatusCodes> value) { 956 this.status = value; 957 return this; 958 } 959 960 /** 961 * @return A code to indicate if the medication is in active use. 962 */ 963 public MedicationStatusCodes getStatus() { 964 return this.status == null ? null : this.status.getValue(); 965 } 966 967 /** 968 * @param value A code to indicate if the medication is in active use. 969 */ 970 public Medication setStatus(MedicationStatusCodes value) { 971 if (value == null) 972 this.status = null; 973 else { 974 if (this.status == null) 975 this.status = new Enumeration<MedicationStatusCodes>(new MedicationStatusCodesEnumFactory()); 976 this.status.setValue(value); 977 } 978 return this; 979 } 980 981 /** 982 * @return {@link #marketingAuthorizationHolder} (The company or other legal entity that has authorization, from the appropriate drug regulatory authority, to market a medicine in one or more jurisdictions. Typically abbreviated MAH.Note: The MAH may manufacture the product and may also contract the manufacturing of the product to one or more companies (organizations).) 983 */ 984 public Reference getMarketingAuthorizationHolder() { 985 if (this.marketingAuthorizationHolder == null) 986 if (Configuration.errorOnAutoCreate()) 987 throw new Error("Attempt to auto-create Medication.marketingAuthorizationHolder"); 988 else if (Configuration.doAutoCreate()) 989 this.marketingAuthorizationHolder = new Reference(); // cc 990 return this.marketingAuthorizationHolder; 991 } 992 993 public boolean hasMarketingAuthorizationHolder() { 994 return this.marketingAuthorizationHolder != null && !this.marketingAuthorizationHolder.isEmpty(); 995 } 996 997 /** 998 * @param value {@link #marketingAuthorizationHolder} (The company or other legal entity that has authorization, from the appropriate drug regulatory authority, to market a medicine in one or more jurisdictions. Typically abbreviated MAH.Note: The MAH may manufacture the product and may also contract the manufacturing of the product to one or more companies (organizations).) 999 */ 1000 public Medication setMarketingAuthorizationHolder(Reference value) { 1001 this.marketingAuthorizationHolder = value; 1002 return this; 1003 } 1004 1005 /** 1006 * @return {@link #doseForm} (Describes the form of the item. Powder; tablets; capsule.) 1007 */ 1008 public CodeableConcept getDoseForm() { 1009 if (this.doseForm == null) 1010 if (Configuration.errorOnAutoCreate()) 1011 throw new Error("Attempt to auto-create Medication.doseForm"); 1012 else if (Configuration.doAutoCreate()) 1013 this.doseForm = new CodeableConcept(); // cc 1014 return this.doseForm; 1015 } 1016 1017 public boolean hasDoseForm() { 1018 return this.doseForm != null && !this.doseForm.isEmpty(); 1019 } 1020 1021 /** 1022 * @param value {@link #doseForm} (Describes the form of the item. Powder; tablets; capsule.) 1023 */ 1024 public Medication setDoseForm(CodeableConcept value) { 1025 this.doseForm = value; 1026 return this; 1027 } 1028 1029 /** 1030 * @return {@link #totalVolume} (When the specified product code does not infer a package size, this is the specific amount of drug in the product. For example, when specifying a product that has the same strength (For example, Insulin glargine 100 unit per mL solution for injection), this attribute provides additional clarification of the package amount (For example, 3 mL, 10mL, etc.).) 1031 */ 1032 public Quantity getTotalVolume() { 1033 if (this.totalVolume == null) 1034 if (Configuration.errorOnAutoCreate()) 1035 throw new Error("Attempt to auto-create Medication.totalVolume"); 1036 else if (Configuration.doAutoCreate()) 1037 this.totalVolume = new Quantity(); // cc 1038 return this.totalVolume; 1039 } 1040 1041 public boolean hasTotalVolume() { 1042 return this.totalVolume != null && !this.totalVolume.isEmpty(); 1043 } 1044 1045 /** 1046 * @param value {@link #totalVolume} (When the specified product code does not infer a package size, this is the specific amount of drug in the product. For example, when specifying a product that has the same strength (For example, Insulin glargine 100 unit per mL solution for injection), this attribute provides additional clarification of the package amount (For example, 3 mL, 10mL, etc.).) 1047 */ 1048 public Medication setTotalVolume(Quantity value) { 1049 this.totalVolume = value; 1050 return this; 1051 } 1052 1053 /** 1054 * @return {@link #ingredient} (Identifies a particular constituent of interest in the product.) 1055 */ 1056 public List<MedicationIngredientComponent> getIngredient() { 1057 if (this.ingredient == null) 1058 this.ingredient = new ArrayList<MedicationIngredientComponent>(); 1059 return this.ingredient; 1060 } 1061 1062 /** 1063 * @return Returns a reference to <code>this</code> for easy method chaining 1064 */ 1065 public Medication setIngredient(List<MedicationIngredientComponent> theIngredient) { 1066 this.ingredient = theIngredient; 1067 return this; 1068 } 1069 1070 public boolean hasIngredient() { 1071 if (this.ingredient == null) 1072 return false; 1073 for (MedicationIngredientComponent item : this.ingredient) 1074 if (!item.isEmpty()) 1075 return true; 1076 return false; 1077 } 1078 1079 public MedicationIngredientComponent addIngredient() { //3 1080 MedicationIngredientComponent t = new MedicationIngredientComponent(); 1081 if (this.ingredient == null) 1082 this.ingredient = new ArrayList<MedicationIngredientComponent>(); 1083 this.ingredient.add(t); 1084 return t; 1085 } 1086 1087 public Medication addIngredient(MedicationIngredientComponent t) { //3 1088 if (t == null) 1089 return this; 1090 if (this.ingredient == null) 1091 this.ingredient = new ArrayList<MedicationIngredientComponent>(); 1092 this.ingredient.add(t); 1093 return this; 1094 } 1095 1096 /** 1097 * @return The first repetition of repeating field {@link #ingredient}, creating it if it does not already exist {3} 1098 */ 1099 public MedicationIngredientComponent getIngredientFirstRep() { 1100 if (getIngredient().isEmpty()) { 1101 addIngredient(); 1102 } 1103 return getIngredient().get(0); 1104 } 1105 1106 /** 1107 * @return {@link #batch} (Information that only applies to packages (not products).) 1108 */ 1109 public MedicationBatchComponent getBatch() { 1110 if (this.batch == null) 1111 if (Configuration.errorOnAutoCreate()) 1112 throw new Error("Attempt to auto-create Medication.batch"); 1113 else if (Configuration.doAutoCreate()) 1114 this.batch = new MedicationBatchComponent(); // cc 1115 return this.batch; 1116 } 1117 1118 public boolean hasBatch() { 1119 return this.batch != null && !this.batch.isEmpty(); 1120 } 1121 1122 /** 1123 * @param value {@link #batch} (Information that only applies to packages (not products).) 1124 */ 1125 public Medication setBatch(MedicationBatchComponent value) { 1126 this.batch = value; 1127 return this; 1128 } 1129 1130 /** 1131 * @return {@link #definition} (A reference to a knowledge resource that provides more information about this medication.) 1132 */ 1133 public Reference getDefinition() { 1134 if (this.definition == null) 1135 if (Configuration.errorOnAutoCreate()) 1136 throw new Error("Attempt to auto-create Medication.definition"); 1137 else if (Configuration.doAutoCreate()) 1138 this.definition = new Reference(); // cc 1139 return this.definition; 1140 } 1141 1142 public boolean hasDefinition() { 1143 return this.definition != null && !this.definition.isEmpty(); 1144 } 1145 1146 /** 1147 * @param value {@link #definition} (A reference to a knowledge resource that provides more information about this medication.) 1148 */ 1149 public Medication setDefinition(Reference value) { 1150 this.definition = value; 1151 return this; 1152 } 1153 1154 protected void listChildren(List<Property> children) { 1155 super.listChildren(children); 1156 children.add(new Property("identifier", "Identifier", "Business identifier for this medication.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1157 children.add(new Property("code", "CodeableConcept", "A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.", 0, 1, code)); 1158 children.add(new Property("status", "code", "A code to indicate if the medication is in active use.", 0, 1, status)); 1159 children.add(new Property("marketingAuthorizationHolder", "Reference(Organization)", "The company or other legal entity that has authorization, from the appropriate drug regulatory authority, to market a medicine in one or more jurisdictions. Typically abbreviated MAH.Note: The MAH may manufacture the product and may also contract the manufacturing of the product to one or more companies (organizations).", 0, 1, marketingAuthorizationHolder)); 1160 children.add(new Property("doseForm", "CodeableConcept", "Describes the form of the item. Powder; tablets; capsule.", 0, 1, doseForm)); 1161 children.add(new Property("totalVolume", "Quantity", "When the specified product code does not infer a package size, this is the specific amount of drug in the product. For example, when specifying a product that has the same strength (For example, Insulin glargine 100 unit per mL solution for injection), this attribute provides additional clarification of the package amount (For example, 3 mL, 10mL, etc.).", 0, 1, totalVolume)); 1162 children.add(new Property("ingredient", "", "Identifies a particular constituent of interest in the product.", 0, java.lang.Integer.MAX_VALUE, ingredient)); 1163 children.add(new Property("batch", "", "Information that only applies to packages (not products).", 0, 1, batch)); 1164 children.add(new Property("definition", "Reference(MedicationKnowledge)", "A reference to a knowledge resource that provides more information about this medication.", 0, 1, definition)); 1165 } 1166 1167 @Override 1168 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1169 switch (_hash) { 1170 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier for this medication.", 0, java.lang.Integer.MAX_VALUE, identifier); 1171 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.", 0, 1, code); 1172 case -892481550: /*status*/ return new Property("status", "code", "A code to indicate if the medication is in active use.", 0, 1, status); 1173 case -1565971585: /*marketingAuthorizationHolder*/ return new Property("marketingAuthorizationHolder", "Reference(Organization)", "The company or other legal entity that has authorization, from the appropriate drug regulatory authority, to market a medicine in one or more jurisdictions. Typically abbreviated MAH.Note: The MAH may manufacture the product and may also contract the manufacturing of the product to one or more companies (organizations).", 0, 1, marketingAuthorizationHolder); 1174 case 1303858817: /*doseForm*/ return new Property("doseForm", "CodeableConcept", "Describes the form of the item. Powder; tablets; capsule.", 0, 1, doseForm); 1175 case -654431362: /*totalVolume*/ return new Property("totalVolume", "Quantity", "When the specified product code does not infer a package size, this is the specific amount of drug in the product. For example, when specifying a product that has the same strength (For example, Insulin glargine 100 unit per mL solution for injection), this attribute provides additional clarification of the package amount (For example, 3 mL, 10mL, etc.).", 0, 1, totalVolume); 1176 case -206409263: /*ingredient*/ return new Property("ingredient", "", "Identifies a particular constituent of interest in the product.", 0, java.lang.Integer.MAX_VALUE, ingredient); 1177 case 93509434: /*batch*/ return new Property("batch", "", "Information that only applies to packages (not products).", 0, 1, batch); 1178 case -1014418093: /*definition*/ return new Property("definition", "Reference(MedicationKnowledge)", "A reference to a knowledge resource that provides more information about this medication.", 0, 1, definition); 1179 default: return super.getNamedProperty(_hash, _name, _checkValid); 1180 } 1181 1182 } 1183 1184 @Override 1185 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1186 switch (hash) { 1187 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1188 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1189 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MedicationStatusCodes> 1190 case -1565971585: /*marketingAuthorizationHolder*/ return this.marketingAuthorizationHolder == null ? new Base[0] : new Base[] {this.marketingAuthorizationHolder}; // Reference 1191 case 1303858817: /*doseForm*/ return this.doseForm == null ? new Base[0] : new Base[] {this.doseForm}; // CodeableConcept 1192 case -654431362: /*totalVolume*/ return this.totalVolume == null ? new Base[0] : new Base[] {this.totalVolume}; // Quantity 1193 case -206409263: /*ingredient*/ return this.ingredient == null ? new Base[0] : this.ingredient.toArray(new Base[this.ingredient.size()]); // MedicationIngredientComponent 1194 case 93509434: /*batch*/ return this.batch == null ? new Base[0] : new Base[] {this.batch}; // MedicationBatchComponent 1195 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // Reference 1196 default: return super.getProperty(hash, name, checkValid); 1197 } 1198 1199 } 1200 1201 @Override 1202 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1203 switch (hash) { 1204 case -1618432855: // identifier 1205 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 1206 return value; 1207 case 3059181: // code 1208 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1209 return value; 1210 case -892481550: // status 1211 value = new MedicationStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1212 this.status = (Enumeration) value; // Enumeration<MedicationStatusCodes> 1213 return value; 1214 case -1565971585: // marketingAuthorizationHolder 1215 this.marketingAuthorizationHolder = TypeConvertor.castToReference(value); // Reference 1216 return value; 1217 case 1303858817: // doseForm 1218 this.doseForm = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1219 return value; 1220 case -654431362: // totalVolume 1221 this.totalVolume = TypeConvertor.castToQuantity(value); // Quantity 1222 return value; 1223 case -206409263: // ingredient 1224 this.getIngredient().add((MedicationIngredientComponent) value); // MedicationIngredientComponent 1225 return value; 1226 case 93509434: // batch 1227 this.batch = (MedicationBatchComponent) value; // MedicationBatchComponent 1228 return value; 1229 case -1014418093: // definition 1230 this.definition = TypeConvertor.castToReference(value); // Reference 1231 return value; 1232 default: return super.setProperty(hash, name, value); 1233 } 1234 1235 } 1236 1237 @Override 1238 public Base setProperty(String name, Base value) throws FHIRException { 1239 if (name.equals("identifier")) { 1240 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 1241 } else if (name.equals("code")) { 1242 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1243 } else if (name.equals("status")) { 1244 value = new MedicationStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1245 this.status = (Enumeration) value; // Enumeration<MedicationStatusCodes> 1246 } else if (name.equals("marketingAuthorizationHolder")) { 1247 this.marketingAuthorizationHolder = TypeConvertor.castToReference(value); // Reference 1248 } else if (name.equals("doseForm")) { 1249 this.doseForm = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1250 } else if (name.equals("totalVolume")) { 1251 this.totalVolume = TypeConvertor.castToQuantity(value); // Quantity 1252 } else if (name.equals("ingredient")) { 1253 this.getIngredient().add((MedicationIngredientComponent) value); 1254 } else if (name.equals("batch")) { 1255 this.batch = (MedicationBatchComponent) value; // MedicationBatchComponent 1256 } else if (name.equals("definition")) { 1257 this.definition = TypeConvertor.castToReference(value); // Reference 1258 } else 1259 return super.setProperty(name, value); 1260 return value; 1261 } 1262 1263 @Override 1264 public void removeChild(String name, Base value) throws FHIRException { 1265 if (name.equals("identifier")) { 1266 this.getIdentifier().remove(value); 1267 } else if (name.equals("code")) { 1268 this.code = null; 1269 } else if (name.equals("status")) { 1270 value = new MedicationStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 1271 this.status = (Enumeration) value; // Enumeration<MedicationStatusCodes> 1272 } else if (name.equals("marketingAuthorizationHolder")) { 1273 this.marketingAuthorizationHolder = null; 1274 } else if (name.equals("doseForm")) { 1275 this.doseForm = null; 1276 } else if (name.equals("totalVolume")) { 1277 this.totalVolume = null; 1278 } else if (name.equals("ingredient")) { 1279 this.getIngredient().remove((MedicationIngredientComponent) value); 1280 } else if (name.equals("batch")) { 1281 this.batch = (MedicationBatchComponent) value; // MedicationBatchComponent 1282 } else if (name.equals("definition")) { 1283 this.definition = null; 1284 } else 1285 super.removeChild(name, value); 1286 1287 } 1288 1289 @Override 1290 public Base makeProperty(int hash, String name) throws FHIRException { 1291 switch (hash) { 1292 case -1618432855: return addIdentifier(); 1293 case 3059181: return getCode(); 1294 case -892481550: return getStatusElement(); 1295 case -1565971585: return getMarketingAuthorizationHolder(); 1296 case 1303858817: return getDoseForm(); 1297 case -654431362: return getTotalVolume(); 1298 case -206409263: return addIngredient(); 1299 case 93509434: return getBatch(); 1300 case -1014418093: return getDefinition(); 1301 default: return super.makeProperty(hash, name); 1302 } 1303 1304 } 1305 1306 @Override 1307 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1308 switch (hash) { 1309 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1310 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1311 case -892481550: /*status*/ return new String[] {"code"}; 1312 case -1565971585: /*marketingAuthorizationHolder*/ return new String[] {"Reference"}; 1313 case 1303858817: /*doseForm*/ return new String[] {"CodeableConcept"}; 1314 case -654431362: /*totalVolume*/ return new String[] {"Quantity"}; 1315 case -206409263: /*ingredient*/ return new String[] {}; 1316 case 93509434: /*batch*/ return new String[] {}; 1317 case -1014418093: /*definition*/ return new String[] {"Reference"}; 1318 default: return super.getTypesForProperty(hash, name); 1319 } 1320 1321 } 1322 1323 @Override 1324 public Base addChild(String name) throws FHIRException { 1325 if (name.equals("identifier")) { 1326 return addIdentifier(); 1327 } 1328 else if (name.equals("code")) { 1329 this.code = new CodeableConcept(); 1330 return this.code; 1331 } 1332 else if (name.equals("status")) { 1333 throw new FHIRException("Cannot call addChild on a singleton property Medication.status"); 1334 } 1335 else if (name.equals("marketingAuthorizationHolder")) { 1336 this.marketingAuthorizationHolder = new Reference(); 1337 return this.marketingAuthorizationHolder; 1338 } 1339 else if (name.equals("doseForm")) { 1340 this.doseForm = new CodeableConcept(); 1341 return this.doseForm; 1342 } 1343 else if (name.equals("totalVolume")) { 1344 this.totalVolume = new Quantity(); 1345 return this.totalVolume; 1346 } 1347 else if (name.equals("ingredient")) { 1348 return addIngredient(); 1349 } 1350 else if (name.equals("batch")) { 1351 this.batch = new MedicationBatchComponent(); 1352 return this.batch; 1353 } 1354 else if (name.equals("definition")) { 1355 this.definition = new Reference(); 1356 return this.definition; 1357 } 1358 else 1359 return super.addChild(name); 1360 } 1361 1362 public String fhirType() { 1363 return "Medication"; 1364 1365 } 1366 1367 public Medication copy() { 1368 Medication dst = new Medication(); 1369 copyValues(dst); 1370 return dst; 1371 } 1372 1373 public void copyValues(Medication dst) { 1374 super.copyValues(dst); 1375 if (identifier != null) { 1376 dst.identifier = new ArrayList<Identifier>(); 1377 for (Identifier i : identifier) 1378 dst.identifier.add(i.copy()); 1379 }; 1380 dst.code = code == null ? null : code.copy(); 1381 dst.status = status == null ? null : status.copy(); 1382 dst.marketingAuthorizationHolder = marketingAuthorizationHolder == null ? null : marketingAuthorizationHolder.copy(); 1383 dst.doseForm = doseForm == null ? null : doseForm.copy(); 1384 dst.totalVolume = totalVolume == null ? null : totalVolume.copy(); 1385 if (ingredient != null) { 1386 dst.ingredient = new ArrayList<MedicationIngredientComponent>(); 1387 for (MedicationIngredientComponent i : ingredient) 1388 dst.ingredient.add(i.copy()); 1389 }; 1390 dst.batch = batch == null ? null : batch.copy(); 1391 dst.definition = definition == null ? null : definition.copy(); 1392 } 1393 1394 protected Medication typedCopy() { 1395 return copy(); 1396 } 1397 1398 @Override 1399 public boolean equalsDeep(Base other_) { 1400 if (!super.equalsDeep(other_)) 1401 return false; 1402 if (!(other_ instanceof Medication)) 1403 return false; 1404 Medication o = (Medication) other_; 1405 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) && compareDeep(status, o.status, true) 1406 && compareDeep(marketingAuthorizationHolder, o.marketingAuthorizationHolder, true) && compareDeep(doseForm, o.doseForm, true) 1407 && compareDeep(totalVolume, o.totalVolume, true) && compareDeep(ingredient, o.ingredient, true) 1408 && compareDeep(batch, o.batch, true) && compareDeep(definition, o.definition, true); 1409 } 1410 1411 @Override 1412 public boolean equalsShallow(Base other_) { 1413 if (!super.equalsShallow(other_)) 1414 return false; 1415 if (!(other_ instanceof Medication)) 1416 return false; 1417 Medication o = (Medication) other_; 1418 return compareValues(status, o.status, true); 1419 } 1420 1421 public boolean isEmpty() { 1422 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, status 1423 , marketingAuthorizationHolder, doseForm, totalVolume, ingredient, batch, definition 1424 ); 1425 } 1426 1427 @Override 1428 public ResourceType getResourceType() { 1429 return ResourceType.Medication; 1430 } 1431 1432 /** 1433 * Search parameter: <b>expiration-date</b> 1434 * <p> 1435 * Description: <b>Returns medications in a batch with this expiration date</b><br> 1436 * Type: <b>date</b><br> 1437 * Path: <b>Medication.batch.expirationDate</b><br> 1438 * </p> 1439 */ 1440 @SearchParamDefinition(name="expiration-date", path="Medication.batch.expirationDate", description="Returns medications in a batch with this expiration date", type="date" ) 1441 public static final String SP_EXPIRATION_DATE = "expiration-date"; 1442 /** 1443 * <b>Fluent Client</b> search parameter constant for <b>expiration-date</b> 1444 * <p> 1445 * Description: <b>Returns medications in a batch with this expiration date</b><br> 1446 * Type: <b>date</b><br> 1447 * Path: <b>Medication.batch.expirationDate</b><br> 1448 * </p> 1449 */ 1450 public static final ca.uhn.fhir.rest.gclient.DateClientParam EXPIRATION_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EXPIRATION_DATE); 1451 1452 /** 1453 * Search parameter: <b>form</b> 1454 * <p> 1455 * Description: <b>Returns medications for a specific dose form</b><br> 1456 * Type: <b>token</b><br> 1457 * Path: <b>null</b><br> 1458 * </p> 1459 */ 1460 @SearchParamDefinition(name="form", path="", description="Returns medications for a specific dose form", type="token" ) 1461 public static final String SP_FORM = "form"; 1462 /** 1463 * <b>Fluent Client</b> search parameter constant for <b>form</b> 1464 * <p> 1465 * Description: <b>Returns medications for a specific dose form</b><br> 1466 * Type: <b>token</b><br> 1467 * Path: <b>null</b><br> 1468 * </p> 1469 */ 1470 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FORM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FORM); 1471 1472 /** 1473 * Search parameter: <b>ingredient-code</b> 1474 * <p> 1475 * Description: <b>Returns medications for this ingredient code</b><br> 1476 * Type: <b>token</b><br> 1477 * Path: <b>Medication.ingredient.item.concept</b><br> 1478 * </p> 1479 */ 1480 @SearchParamDefinition(name="ingredient-code", path="Medication.ingredient.item.concept", description="Returns medications for this ingredient code", type="token" ) 1481 public static final String SP_INGREDIENT_CODE = "ingredient-code"; 1482 /** 1483 * <b>Fluent Client</b> search parameter constant for <b>ingredient-code</b> 1484 * <p> 1485 * Description: <b>Returns medications for this ingredient code</b><br> 1486 * Type: <b>token</b><br> 1487 * Path: <b>Medication.ingredient.item.concept</b><br> 1488 * </p> 1489 */ 1490 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INGREDIENT_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INGREDIENT_CODE); 1491 1492 /** 1493 * Search parameter: <b>ingredient</b> 1494 * <p> 1495 * Description: <b>Returns medications for this ingredient reference</b><br> 1496 * Type: <b>reference</b><br> 1497 * Path: <b>Medication.ingredient.item.reference</b><br> 1498 * </p> 1499 */ 1500 @SearchParamDefinition(name="ingredient", path="Medication.ingredient.item.reference", description="Returns medications for this ingredient reference", type="reference", target={Medication.class, Substance.class } ) 1501 public static final String SP_INGREDIENT = "ingredient"; 1502 /** 1503 * <b>Fluent Client</b> search parameter constant for <b>ingredient</b> 1504 * <p> 1505 * Description: <b>Returns medications for this ingredient reference</b><br> 1506 * Type: <b>reference</b><br> 1507 * Path: <b>Medication.ingredient.item.reference</b><br> 1508 * </p> 1509 */ 1510 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INGREDIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INGREDIENT); 1511 1512/** 1513 * Constant for fluent queries to be used to add include statements. Specifies 1514 * the path value of "<b>Medication:ingredient</b>". 1515 */ 1516 public static final ca.uhn.fhir.model.api.Include INCLUDE_INGREDIENT = new ca.uhn.fhir.model.api.Include("Medication:ingredient").toLocked(); 1517 1518 /** 1519 * Search parameter: <b>lot-number</b> 1520 * <p> 1521 * Description: <b>Returns medications in a batch with this lot number</b><br> 1522 * Type: <b>token</b><br> 1523 * Path: <b>Medication.batch.lotNumber</b><br> 1524 * </p> 1525 */ 1526 @SearchParamDefinition(name="lot-number", path="Medication.batch.lotNumber", description="Returns medications in a batch with this lot number", type="token" ) 1527 public static final String SP_LOT_NUMBER = "lot-number"; 1528 /** 1529 * <b>Fluent Client</b> search parameter constant for <b>lot-number</b> 1530 * <p> 1531 * Description: <b>Returns medications in a batch with this lot number</b><br> 1532 * Type: <b>token</b><br> 1533 * Path: <b>Medication.batch.lotNumber</b><br> 1534 * </p> 1535 */ 1536 public static final ca.uhn.fhir.rest.gclient.TokenClientParam LOT_NUMBER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_LOT_NUMBER); 1537 1538 /** 1539 * Search parameter: <b>marketingauthorizationholder</b> 1540 * <p> 1541 * Description: <b>Returns medications made or sold for this marketing authorization holder</b><br> 1542 * Type: <b>reference</b><br> 1543 * Path: <b>Medication.marketingAuthorizationHolder</b><br> 1544 * </p> 1545 */ 1546 @SearchParamDefinition(name="marketingauthorizationholder", path="Medication.marketingAuthorizationHolder", description="Returns medications made or sold for this marketing authorization holder", type="reference", target={Organization.class } ) 1547 public static final String SP_MARKETINGAUTHORIZATIONHOLDER = "marketingauthorizationholder"; 1548 /** 1549 * <b>Fluent Client</b> search parameter constant for <b>marketingauthorizationholder</b> 1550 * <p> 1551 * Description: <b>Returns medications made or sold for this marketing authorization holder</b><br> 1552 * Type: <b>reference</b><br> 1553 * Path: <b>Medication.marketingAuthorizationHolder</b><br> 1554 * </p> 1555 */ 1556 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MARKETINGAUTHORIZATIONHOLDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MARKETINGAUTHORIZATIONHOLDER); 1557 1558/** 1559 * Constant for fluent queries to be used to add include statements. Specifies 1560 * the path value of "<b>Medication:marketingauthorizationholder</b>". 1561 */ 1562 public static final ca.uhn.fhir.model.api.Include INCLUDE_MARKETINGAUTHORIZATIONHOLDER = new ca.uhn.fhir.model.api.Include("Medication:marketingauthorizationholder").toLocked(); 1563 1564 /** 1565 * Search parameter: <b>serial-number</b> 1566 * <p> 1567 * Description: <b>Returns medications in a batch with this lot number</b><br> 1568 * Type: <b>token</b><br> 1569 * Path: <b>Medication.identifier</b><br> 1570 * </p> 1571 */ 1572 @SearchParamDefinition(name="serial-number", path="Medication.identifier", description="Returns medications in a batch with this lot number", type="token" ) 1573 public static final String SP_SERIAL_NUMBER = "serial-number"; 1574 /** 1575 * <b>Fluent Client</b> search parameter constant for <b>serial-number</b> 1576 * <p> 1577 * Description: <b>Returns medications in a batch with this lot number</b><br> 1578 * Type: <b>token</b><br> 1579 * Path: <b>Medication.identifier</b><br> 1580 * </p> 1581 */ 1582 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERIAL_NUMBER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SERIAL_NUMBER); 1583 1584 /** 1585 * Search parameter: <b>status</b> 1586 * <p> 1587 * Description: <b>Returns medications for this status</b><br> 1588 * Type: <b>token</b><br> 1589 * Path: <b>Medication.status</b><br> 1590 * </p> 1591 */ 1592 @SearchParamDefinition(name="status", path="Medication.status", description="Returns medications for this status", type="token" ) 1593 public static final String SP_STATUS = "status"; 1594 /** 1595 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1596 * <p> 1597 * Description: <b>Returns medications for this status</b><br> 1598 * Type: <b>token</b><br> 1599 * Path: <b>Medication.status</b><br> 1600 * </p> 1601 */ 1602 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1603 1604 /** 1605 * Search parameter: <b>code</b> 1606 * <p> 1607 * Description: <b>Multiple Resources: 1608 1609* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 1610* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 1611* [AuditEvent](auditevent.html): More specific code for the event 1612* [Basic](basic.html): Kind of Resource 1613* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 1614* [Condition](condition.html): Code for the condition 1615* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 1616* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 1617* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 1618* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 1619* [ImagingSelection](imagingselection.html): The imaging selection status 1620* [List](list.html): What the purpose of this list is 1621* [Medication](medication.html): Returns medications for a specific code 1622* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 1623* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 1624* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 1625* [MedicationStatement](medicationstatement.html): Return statements of this medication code 1626* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 1627* [Observation](observation.html): The code of the observation type 1628* [Procedure](procedure.html): A code to identify a procedure 1629* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 1630* [Task](task.html): Search by task code 1631</b><br> 1632 * Type: <b>token</b><br> 1633 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 1634 * </p> 1635 */ 1636 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 1637 public static final String SP_CODE = "code"; 1638 /** 1639 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1640 * <p> 1641 * Description: <b>Multiple Resources: 1642 1643* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 1644* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 1645* [AuditEvent](auditevent.html): More specific code for the event 1646* [Basic](basic.html): Kind of Resource 1647* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 1648* [Condition](condition.html): Code for the condition 1649* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 1650* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 1651* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 1652* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 1653* [ImagingSelection](imagingselection.html): The imaging selection status 1654* [List](list.html): What the purpose of this list is 1655* [Medication](medication.html): Returns medications for a specific code 1656* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 1657* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 1658* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 1659* [MedicationStatement](medicationstatement.html): Return statements of this medication code 1660* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 1661* [Observation](observation.html): The code of the observation type 1662* [Procedure](procedure.html): A code to identify a procedure 1663* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 1664* [Task](task.html): Search by task code 1665</b><br> 1666 * Type: <b>token</b><br> 1667 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 1668 * </p> 1669 */ 1670 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 1671 1672 /** 1673 * Search parameter: <b>identifier</b> 1674 * <p> 1675 * Description: <b>Multiple Resources: 1676 1677* [Account](account.html): Account number 1678* [AdverseEvent](adverseevent.html): Business identifier for the event 1679* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1680* [Appointment](appointment.html): An Identifier of the Appointment 1681* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1682* [Basic](basic.html): Business identifier 1683* [BodyStructure](bodystructure.html): Bodystructure identifier 1684* [CarePlan](careplan.html): External Ids for this plan 1685* [CareTeam](careteam.html): External Ids for this team 1686* [ChargeItem](chargeitem.html): Business Identifier for item 1687* [Claim](claim.html): The primary identifier of the financial resource 1688* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1689* [ClinicalImpression](clinicalimpression.html): Business identifier 1690* [Communication](communication.html): Unique identifier 1691* [CommunicationRequest](communicationrequest.html): Unique identifier 1692* [Composition](composition.html): Version-independent identifier for the Composition 1693* [Condition](condition.html): A unique identifier of the condition record 1694* [Consent](consent.html): Identifier for this record (external references) 1695* [Contract](contract.html): The identity of the contract 1696* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1697* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1698* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1699* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1700* [DeviceRequest](devicerequest.html): Business identifier for request/order 1701* [DeviceUsage](deviceusage.html): Search by identifier 1702* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1703* [DocumentReference](documentreference.html): Identifier of the attachment binary 1704* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1705* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1706* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1707* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1708* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1709* [Flag](flag.html): Business identifier 1710* [Goal](goal.html): External Ids for this goal 1711* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1712* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1713* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1714* [Immunization](immunization.html): Business identifier 1715* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1716* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1717* [Invoice](invoice.html): Business Identifier for item 1718* [List](list.html): Business identifier 1719* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1720* [Medication](medication.html): Returns medications with this external identifier 1721* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1722* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1723* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1724* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1725* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1726* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1727* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1728* [Observation](observation.html): The unique id for a particular observation 1729* [Person](person.html): A person Identifier 1730* [Procedure](procedure.html): A unique identifier for a procedure 1731* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1732* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1733* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1734* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1735* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1736* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1737* [Specimen](specimen.html): The unique identifier associated with the specimen 1738* [SupplyDelivery](supplydelivery.html): External identifier 1739* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1740* [Task](task.html): Search for a task instance by its business identifier 1741* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1742</b><br> 1743 * Type: <b>token</b><br> 1744 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1745 * </p> 1746 */ 1747 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 1748 public static final String SP_IDENTIFIER = "identifier"; 1749 /** 1750 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1751 * <p> 1752 * Description: <b>Multiple Resources: 1753 1754* [Account](account.html): Account number 1755* [AdverseEvent](adverseevent.html): Business identifier for the event 1756* [AllergyIntolerance](allergyintolerance.html): External ids for this item 1757* [Appointment](appointment.html): An Identifier of the Appointment 1758* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 1759* [Basic](basic.html): Business identifier 1760* [BodyStructure](bodystructure.html): Bodystructure identifier 1761* [CarePlan](careplan.html): External Ids for this plan 1762* [CareTeam](careteam.html): External Ids for this team 1763* [ChargeItem](chargeitem.html): Business Identifier for item 1764* [Claim](claim.html): The primary identifier of the financial resource 1765* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 1766* [ClinicalImpression](clinicalimpression.html): Business identifier 1767* [Communication](communication.html): Unique identifier 1768* [CommunicationRequest](communicationrequest.html): Unique identifier 1769* [Composition](composition.html): Version-independent identifier for the Composition 1770* [Condition](condition.html): A unique identifier of the condition record 1771* [Consent](consent.html): Identifier for this record (external references) 1772* [Contract](contract.html): The identity of the contract 1773* [Coverage](coverage.html): The primary identifier of the insured and the coverage 1774* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 1775* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 1776* [DetectedIssue](detectedissue.html): Unique id for the detected issue 1777* [DeviceRequest](devicerequest.html): Business identifier for request/order 1778* [DeviceUsage](deviceusage.html): Search by identifier 1779* [DiagnosticReport](diagnosticreport.html): An identifier for the report 1780* [DocumentReference](documentreference.html): Identifier of the attachment binary 1781* [Encounter](encounter.html): Identifier(s) by which this encounter is known 1782* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 1783* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 1784* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 1785* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 1786* [Flag](flag.html): Business identifier 1787* [Goal](goal.html): External Ids for this goal 1788* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 1789* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 1790* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 1791* [Immunization](immunization.html): Business identifier 1792* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 1793* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 1794* [Invoice](invoice.html): Business Identifier for item 1795* [List](list.html): Business identifier 1796* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 1797* [Medication](medication.html): Returns medications with this external identifier 1798* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 1799* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 1800* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 1801* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 1802* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 1803* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 1804* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 1805* [Observation](observation.html): The unique id for a particular observation 1806* [Person](person.html): A person Identifier 1807* [Procedure](procedure.html): A unique identifier for a procedure 1808* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 1809* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 1810* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 1811* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 1812* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 1813* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 1814* [Specimen](specimen.html): The unique identifier associated with the specimen 1815* [SupplyDelivery](supplydelivery.html): External identifier 1816* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 1817* [Task](task.html): Search for a task instance by its business identifier 1818* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 1819</b><br> 1820 * Type: <b>token</b><br> 1821 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 1822 * </p> 1823 */ 1824 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1825 1826 1827} 1828