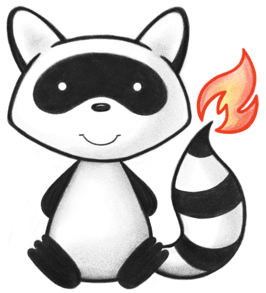
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner. This event can also be used to record waste using a status of not-done and the appropriate statusReason. 052 */ 053@ResourceDef(name="MedicationAdministration", profile="http://hl7.org/fhir/StructureDefinition/MedicationAdministration") 054public class MedicationAdministration extends DomainResource { 055 056 public enum MedicationAdministrationStatusCodes { 057 /** 058 * The administration has started but has not yet completed. 059 */ 060 INPROGRESS, 061 /** 062 * The administration was terminated prior to any impact on the subject (though preparatory actions may have been taken) 063 */ 064 NOTDONE, 065 /** 066 * Actions implied by the administration have been temporarily halted, but are expected to continue later. May also be called 'suspended'. 067 */ 068 ONHOLD, 069 /** 070 * All actions that are implied by the administration have occurred. 071 */ 072 COMPLETED, 073 /** 074 * The administration was entered in error and therefore nullified. 075 */ 076 ENTEREDINERROR, 077 /** 078 * Actions implied by the administration have been permanently halted, before all of them occurred. 079 */ 080 STOPPED, 081 /** 082 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for 'other' - one of the listed statuses is presumed to apply, it's just not known which one. 083 */ 084 UNKNOWN, 085 /** 086 * added to help the parsers with the generic types 087 */ 088 NULL; 089 public static MedicationAdministrationStatusCodes fromCode(String codeString) throws FHIRException { 090 if (codeString == null || "".equals(codeString)) 091 return null; 092 if ("in-progress".equals(codeString)) 093 return INPROGRESS; 094 if ("not-done".equals(codeString)) 095 return NOTDONE; 096 if ("on-hold".equals(codeString)) 097 return ONHOLD; 098 if ("completed".equals(codeString)) 099 return COMPLETED; 100 if ("entered-in-error".equals(codeString)) 101 return ENTEREDINERROR; 102 if ("stopped".equals(codeString)) 103 return STOPPED; 104 if ("unknown".equals(codeString)) 105 return UNKNOWN; 106 if (Configuration.isAcceptInvalidEnums()) 107 return null; 108 else 109 throw new FHIRException("Unknown MedicationAdministrationStatusCodes code '"+codeString+"'"); 110 } 111 public String toCode() { 112 switch (this) { 113 case INPROGRESS: return "in-progress"; 114 case NOTDONE: return "not-done"; 115 case ONHOLD: return "on-hold"; 116 case COMPLETED: return "completed"; 117 case ENTEREDINERROR: return "entered-in-error"; 118 case STOPPED: return "stopped"; 119 case UNKNOWN: return "unknown"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getSystem() { 125 switch (this) { 126 case INPROGRESS: return "http://hl7.org/fhir/CodeSystem/medication-admin-status"; 127 case NOTDONE: return "http://hl7.org/fhir/CodeSystem/medication-admin-status"; 128 case ONHOLD: return "http://hl7.org/fhir/CodeSystem/medication-admin-status"; 129 case COMPLETED: return "http://hl7.org/fhir/CodeSystem/medication-admin-status"; 130 case ENTEREDINERROR: return "http://hl7.org/fhir/CodeSystem/medication-admin-status"; 131 case STOPPED: return "http://hl7.org/fhir/CodeSystem/medication-admin-status"; 132 case UNKNOWN: return "http://hl7.org/fhir/CodeSystem/medication-admin-status"; 133 case NULL: return null; 134 default: return "?"; 135 } 136 } 137 public String getDefinition() { 138 switch (this) { 139 case INPROGRESS: return "The administration has started but has not yet completed."; 140 case NOTDONE: return "The administration was terminated prior to any impact on the subject (though preparatory actions may have been taken)"; 141 case ONHOLD: return "Actions implied by the administration have been temporarily halted, but are expected to continue later. May also be called 'suspended'."; 142 case COMPLETED: return "All actions that are implied by the administration have occurred."; 143 case ENTEREDINERROR: return "The administration was entered in error and therefore nullified."; 144 case STOPPED: return "Actions implied by the administration have been permanently halted, before all of them occurred."; 145 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for 'other' - one of the listed statuses is presumed to apply, it's just not known which one."; 146 case NULL: return null; 147 default: return "?"; 148 } 149 } 150 public String getDisplay() { 151 switch (this) { 152 case INPROGRESS: return "In Progress"; 153 case NOTDONE: return "Not Done"; 154 case ONHOLD: return "On Hold"; 155 case COMPLETED: return "Completed"; 156 case ENTEREDINERROR: return "Entered in Error"; 157 case STOPPED: return "Stopped"; 158 case UNKNOWN: return "Unknown"; 159 case NULL: return null; 160 default: return "?"; 161 } 162 } 163 } 164 165 public static class MedicationAdministrationStatusCodesEnumFactory implements EnumFactory<MedicationAdministrationStatusCodes> { 166 public MedicationAdministrationStatusCodes fromCode(String codeString) throws IllegalArgumentException { 167 if (codeString == null || "".equals(codeString)) 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("in-progress".equals(codeString)) 171 return MedicationAdministrationStatusCodes.INPROGRESS; 172 if ("not-done".equals(codeString)) 173 return MedicationAdministrationStatusCodes.NOTDONE; 174 if ("on-hold".equals(codeString)) 175 return MedicationAdministrationStatusCodes.ONHOLD; 176 if ("completed".equals(codeString)) 177 return MedicationAdministrationStatusCodes.COMPLETED; 178 if ("entered-in-error".equals(codeString)) 179 return MedicationAdministrationStatusCodes.ENTEREDINERROR; 180 if ("stopped".equals(codeString)) 181 return MedicationAdministrationStatusCodes.STOPPED; 182 if ("unknown".equals(codeString)) 183 return MedicationAdministrationStatusCodes.UNKNOWN; 184 throw new IllegalArgumentException("Unknown MedicationAdministrationStatusCodes code '"+codeString+"'"); 185 } 186 public Enumeration<MedicationAdministrationStatusCodes> fromType(PrimitiveType<?> code) throws FHIRException { 187 if (code == null) 188 return null; 189 if (code.isEmpty()) 190 return new Enumeration<MedicationAdministrationStatusCodes>(this, MedicationAdministrationStatusCodes.NULL, code); 191 String codeString = ((PrimitiveType) code).asStringValue(); 192 if (codeString == null || "".equals(codeString)) 193 return new Enumeration<MedicationAdministrationStatusCodes>(this, MedicationAdministrationStatusCodes.NULL, code); 194 if ("in-progress".equals(codeString)) 195 return new Enumeration<MedicationAdministrationStatusCodes>(this, MedicationAdministrationStatusCodes.INPROGRESS, code); 196 if ("not-done".equals(codeString)) 197 return new Enumeration<MedicationAdministrationStatusCodes>(this, MedicationAdministrationStatusCodes.NOTDONE, code); 198 if ("on-hold".equals(codeString)) 199 return new Enumeration<MedicationAdministrationStatusCodes>(this, MedicationAdministrationStatusCodes.ONHOLD, code); 200 if ("completed".equals(codeString)) 201 return new Enumeration<MedicationAdministrationStatusCodes>(this, MedicationAdministrationStatusCodes.COMPLETED, code); 202 if ("entered-in-error".equals(codeString)) 203 return new Enumeration<MedicationAdministrationStatusCodes>(this, MedicationAdministrationStatusCodes.ENTEREDINERROR, code); 204 if ("stopped".equals(codeString)) 205 return new Enumeration<MedicationAdministrationStatusCodes>(this, MedicationAdministrationStatusCodes.STOPPED, code); 206 if ("unknown".equals(codeString)) 207 return new Enumeration<MedicationAdministrationStatusCodes>(this, MedicationAdministrationStatusCodes.UNKNOWN, code); 208 throw new FHIRException("Unknown MedicationAdministrationStatusCodes code '"+codeString+"'"); 209 } 210 public String toCode(MedicationAdministrationStatusCodes code) { 211 if (code == MedicationAdministrationStatusCodes.NULL) 212 return null; 213 if (code == MedicationAdministrationStatusCodes.INPROGRESS) 214 return "in-progress"; 215 if (code == MedicationAdministrationStatusCodes.NOTDONE) 216 return "not-done"; 217 if (code == MedicationAdministrationStatusCodes.ONHOLD) 218 return "on-hold"; 219 if (code == MedicationAdministrationStatusCodes.COMPLETED) 220 return "completed"; 221 if (code == MedicationAdministrationStatusCodes.ENTEREDINERROR) 222 return "entered-in-error"; 223 if (code == MedicationAdministrationStatusCodes.STOPPED) 224 return "stopped"; 225 if (code == MedicationAdministrationStatusCodes.UNKNOWN) 226 return "unknown"; 227 return "?"; 228 } 229 public String toSystem(MedicationAdministrationStatusCodes code) { 230 return code.getSystem(); 231 } 232 } 233 234 @Block() 235 public static class MedicationAdministrationPerformerComponent extends BackboneElement implements IBaseBackboneElement { 236 /** 237 * Distinguishes the type of involvement of the performer in the medication administration. 238 */ 239 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 240 @Description(shortDefinition="Type of performance", formalDefinition="Distinguishes the type of involvement of the performer in the medication administration." ) 241 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/med-admin-perform-function") 242 protected CodeableConcept function; 243 244 /** 245 * Indicates who or what performed the medication administration. 246 */ 247 @Child(name = "actor", type = {CodeableReference.class}, order=2, min=1, max=1, modifier=false, summary=true) 248 @Description(shortDefinition="Who or what performed the medication administration", formalDefinition="Indicates who or what performed the medication administration." ) 249 protected CodeableReference actor; 250 251 private static final long serialVersionUID = -1725418144L; 252 253 /** 254 * Constructor 255 */ 256 public MedicationAdministrationPerformerComponent() { 257 super(); 258 } 259 260 /** 261 * Constructor 262 */ 263 public MedicationAdministrationPerformerComponent(CodeableReference actor) { 264 super(); 265 this.setActor(actor); 266 } 267 268 /** 269 * @return {@link #function} (Distinguishes the type of involvement of the performer in the medication administration.) 270 */ 271 public CodeableConcept getFunction() { 272 if (this.function == null) 273 if (Configuration.errorOnAutoCreate()) 274 throw new Error("Attempt to auto-create MedicationAdministrationPerformerComponent.function"); 275 else if (Configuration.doAutoCreate()) 276 this.function = new CodeableConcept(); // cc 277 return this.function; 278 } 279 280 public boolean hasFunction() { 281 return this.function != null && !this.function.isEmpty(); 282 } 283 284 /** 285 * @param value {@link #function} (Distinguishes the type of involvement of the performer in the medication administration.) 286 */ 287 public MedicationAdministrationPerformerComponent setFunction(CodeableConcept value) { 288 this.function = value; 289 return this; 290 } 291 292 /** 293 * @return {@link #actor} (Indicates who or what performed the medication administration.) 294 */ 295 public CodeableReference getActor() { 296 if (this.actor == null) 297 if (Configuration.errorOnAutoCreate()) 298 throw new Error("Attempt to auto-create MedicationAdministrationPerformerComponent.actor"); 299 else if (Configuration.doAutoCreate()) 300 this.actor = new CodeableReference(); // cc 301 return this.actor; 302 } 303 304 public boolean hasActor() { 305 return this.actor != null && !this.actor.isEmpty(); 306 } 307 308 /** 309 * @param value {@link #actor} (Indicates who or what performed the medication administration.) 310 */ 311 public MedicationAdministrationPerformerComponent setActor(CodeableReference value) { 312 this.actor = value; 313 return this; 314 } 315 316 protected void listChildren(List<Property> children) { 317 super.listChildren(children); 318 children.add(new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the performer in the medication administration.", 0, 1, function)); 319 children.add(new Property("actor", "CodeableReference(Practitioner|PractitionerRole|Patient|RelatedPerson|Device)", "Indicates who or what performed the medication administration.", 0, 1, actor)); 320 } 321 322 @Override 323 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 324 switch (_hash) { 325 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Distinguishes the type of involvement of the performer in the medication administration.", 0, 1, function); 326 case 92645877: /*actor*/ return new Property("actor", "CodeableReference(Practitioner|PractitionerRole|Patient|RelatedPerson|Device)", "Indicates who or what performed the medication administration.", 0, 1, actor); 327 default: return super.getNamedProperty(_hash, _name, _checkValid); 328 } 329 330 } 331 332 @Override 333 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 334 switch (hash) { 335 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 336 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // CodeableReference 337 default: return super.getProperty(hash, name, checkValid); 338 } 339 340 } 341 342 @Override 343 public Base setProperty(int hash, String name, Base value) throws FHIRException { 344 switch (hash) { 345 case 1380938712: // function 346 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 347 return value; 348 case 92645877: // actor 349 this.actor = TypeConvertor.castToCodeableReference(value); // CodeableReference 350 return value; 351 default: return super.setProperty(hash, name, value); 352 } 353 354 } 355 356 @Override 357 public Base setProperty(String name, Base value) throws FHIRException { 358 if (name.equals("function")) { 359 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 360 } else if (name.equals("actor")) { 361 this.actor = TypeConvertor.castToCodeableReference(value); // CodeableReference 362 } else 363 return super.setProperty(name, value); 364 return value; 365 } 366 367 @Override 368 public void removeChild(String name, Base value) throws FHIRException { 369 if (name.equals("function")) { 370 this.function = null; 371 } else if (name.equals("actor")) { 372 this.actor = null; 373 } else 374 super.removeChild(name, value); 375 376 } 377 378 @Override 379 public Base makeProperty(int hash, String name) throws FHIRException { 380 switch (hash) { 381 case 1380938712: return getFunction(); 382 case 92645877: return getActor(); 383 default: return super.makeProperty(hash, name); 384 } 385 386 } 387 388 @Override 389 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 390 switch (hash) { 391 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 392 case 92645877: /*actor*/ return new String[] {"CodeableReference"}; 393 default: return super.getTypesForProperty(hash, name); 394 } 395 396 } 397 398 @Override 399 public Base addChild(String name) throws FHIRException { 400 if (name.equals("function")) { 401 this.function = new CodeableConcept(); 402 return this.function; 403 } 404 else if (name.equals("actor")) { 405 this.actor = new CodeableReference(); 406 return this.actor; 407 } 408 else 409 return super.addChild(name); 410 } 411 412 public MedicationAdministrationPerformerComponent copy() { 413 MedicationAdministrationPerformerComponent dst = new MedicationAdministrationPerformerComponent(); 414 copyValues(dst); 415 return dst; 416 } 417 418 public void copyValues(MedicationAdministrationPerformerComponent dst) { 419 super.copyValues(dst); 420 dst.function = function == null ? null : function.copy(); 421 dst.actor = actor == null ? null : actor.copy(); 422 } 423 424 @Override 425 public boolean equalsDeep(Base other_) { 426 if (!super.equalsDeep(other_)) 427 return false; 428 if (!(other_ instanceof MedicationAdministrationPerformerComponent)) 429 return false; 430 MedicationAdministrationPerformerComponent o = (MedicationAdministrationPerformerComponent) other_; 431 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 432 } 433 434 @Override 435 public boolean equalsShallow(Base other_) { 436 if (!super.equalsShallow(other_)) 437 return false; 438 if (!(other_ instanceof MedicationAdministrationPerformerComponent)) 439 return false; 440 MedicationAdministrationPerformerComponent o = (MedicationAdministrationPerformerComponent) other_; 441 return true; 442 } 443 444 public boolean isEmpty() { 445 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 446 } 447 448 public String fhirType() { 449 return "MedicationAdministration.performer"; 450 451 } 452 453 } 454 455 @Block() 456 public static class MedicationAdministrationDosageComponent extends BackboneElement implements IBaseBackboneElement { 457 /** 458 * Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans. 459 460The dosage instructions should reflect the dosage of the medication that was administered. 461 */ 462 @Child(name = "text", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 463 @Description(shortDefinition="Free text dosage instructions e.g. SIG", formalDefinition="Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans.\r\rThe dosage instructions should reflect the dosage of the medication that was administered." ) 464 protected StringType text; 465 466 /** 467 * A coded specification of the anatomic site where the medication first entered the body. For example, "left arm". 468 */ 469 @Child(name = "site", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 470 @Description(shortDefinition="Body site administered to", formalDefinition="A coded specification of the anatomic site where the medication first entered the body. For example, \"left arm\"." ) 471 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/approach-site-codes") 472 protected CodeableConcept site; 473 474 /** 475 * A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc. 476 */ 477 @Child(name = "route", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 478 @Description(shortDefinition="Path of substance into body", formalDefinition="A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc." ) 479 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/route-codes") 480 protected CodeableConcept route; 481 482 /** 483 * A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV. 484 */ 485 @Child(name = "method", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 486 @Description(shortDefinition="How drug was administered", formalDefinition="A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV." ) 487 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administration-method-codes") 488 protected CodeableConcept method; 489 490 /** 491 * The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection. 492 */ 493 @Child(name = "dose", type = {Quantity.class}, order=5, min=0, max=1, modifier=false, summary=false) 494 @Description(shortDefinition="Amount of medication per dose", formalDefinition="The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection." ) 495 protected Quantity dose; 496 497 /** 498 * Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours. 499 */ 500 @Child(name = "rate", type = {Ratio.class, Quantity.class}, order=6, min=0, max=1, modifier=false, summary=false) 501 @Description(shortDefinition="Dose quantity per unit of time", formalDefinition="Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours." ) 502 protected DataType rate; 503 504 private static final long serialVersionUID = -484090956L; 505 506 /** 507 * Constructor 508 */ 509 public MedicationAdministrationDosageComponent() { 510 super(); 511 } 512 513 /** 514 * @return {@link #text} (Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans. 515 516The dosage instructions should reflect the dosage of the medication that was administered.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 517 */ 518 public StringType getTextElement() { 519 if (this.text == null) 520 if (Configuration.errorOnAutoCreate()) 521 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.text"); 522 else if (Configuration.doAutoCreate()) 523 this.text = new StringType(); // bb 524 return this.text; 525 } 526 527 public boolean hasTextElement() { 528 return this.text != null && !this.text.isEmpty(); 529 } 530 531 public boolean hasText() { 532 return this.text != null && !this.text.isEmpty(); 533 } 534 535 /** 536 * @param value {@link #text} (Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans. 537 538The dosage instructions should reflect the dosage of the medication that was administered.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 539 */ 540 public MedicationAdministrationDosageComponent setTextElement(StringType value) { 541 this.text = value; 542 return this; 543 } 544 545 /** 546 * @return Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans. 547 548The dosage instructions should reflect the dosage of the medication that was administered. 549 */ 550 public String getText() { 551 return this.text == null ? null : this.text.getValue(); 552 } 553 554 /** 555 * @param value Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans. 556 557The dosage instructions should reflect the dosage of the medication that was administered. 558 */ 559 public MedicationAdministrationDosageComponent setText(String value) { 560 if (Utilities.noString(value)) 561 this.text = null; 562 else { 563 if (this.text == null) 564 this.text = new StringType(); 565 this.text.setValue(value); 566 } 567 return this; 568 } 569 570 /** 571 * @return {@link #site} (A coded specification of the anatomic site where the medication first entered the body. For example, "left arm".) 572 */ 573 public CodeableConcept getSite() { 574 if (this.site == null) 575 if (Configuration.errorOnAutoCreate()) 576 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.site"); 577 else if (Configuration.doAutoCreate()) 578 this.site = new CodeableConcept(); // cc 579 return this.site; 580 } 581 582 public boolean hasSite() { 583 return this.site != null && !this.site.isEmpty(); 584 } 585 586 /** 587 * @param value {@link #site} (A coded specification of the anatomic site where the medication first entered the body. For example, "left arm".) 588 */ 589 public MedicationAdministrationDosageComponent setSite(CodeableConcept value) { 590 this.site = value; 591 return this; 592 } 593 594 /** 595 * @return {@link #route} (A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.) 596 */ 597 public CodeableConcept getRoute() { 598 if (this.route == null) 599 if (Configuration.errorOnAutoCreate()) 600 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.route"); 601 else if (Configuration.doAutoCreate()) 602 this.route = new CodeableConcept(); // cc 603 return this.route; 604 } 605 606 public boolean hasRoute() { 607 return this.route != null && !this.route.isEmpty(); 608 } 609 610 /** 611 * @param value {@link #route} (A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.) 612 */ 613 public MedicationAdministrationDosageComponent setRoute(CodeableConcept value) { 614 this.route = value; 615 return this; 616 } 617 618 /** 619 * @return {@link #method} (A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.) 620 */ 621 public CodeableConcept getMethod() { 622 if (this.method == null) 623 if (Configuration.errorOnAutoCreate()) 624 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.method"); 625 else if (Configuration.doAutoCreate()) 626 this.method = new CodeableConcept(); // cc 627 return this.method; 628 } 629 630 public boolean hasMethod() { 631 return this.method != null && !this.method.isEmpty(); 632 } 633 634 /** 635 * @param value {@link #method} (A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.) 636 */ 637 public MedicationAdministrationDosageComponent setMethod(CodeableConcept value) { 638 this.method = value; 639 return this; 640 } 641 642 /** 643 * @return {@link #dose} (The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.) 644 */ 645 public Quantity getDose() { 646 if (this.dose == null) 647 if (Configuration.errorOnAutoCreate()) 648 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.dose"); 649 else if (Configuration.doAutoCreate()) 650 this.dose = new Quantity(); // cc 651 return this.dose; 652 } 653 654 public boolean hasDose() { 655 return this.dose != null && !this.dose.isEmpty(); 656 } 657 658 /** 659 * @param value {@link #dose} (The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.) 660 */ 661 public MedicationAdministrationDosageComponent setDose(Quantity value) { 662 this.dose = value; 663 return this; 664 } 665 666 /** 667 * @return {@link #rate} (Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.) 668 */ 669 public DataType getRate() { 670 return this.rate; 671 } 672 673 /** 674 * @return {@link #rate} (Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.) 675 */ 676 public Ratio getRateRatio() throws FHIRException { 677 if (this.rate == null) 678 this.rate = new Ratio(); 679 if (!(this.rate instanceof Ratio)) 680 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.rate.getClass().getName()+" was encountered"); 681 return (Ratio) this.rate; 682 } 683 684 public boolean hasRateRatio() { 685 return this.rate instanceof Ratio; 686 } 687 688 /** 689 * @return {@link #rate} (Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.) 690 */ 691 public Quantity getRateQuantity() throws FHIRException { 692 if (this.rate == null) 693 this.rate = new Quantity(); 694 if (!(this.rate instanceof Quantity)) 695 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.rate.getClass().getName()+" was encountered"); 696 return (Quantity) this.rate; 697 } 698 699 public boolean hasRateQuantity() { 700 return this.rate instanceof Quantity; 701 } 702 703 public boolean hasRate() { 704 return this.rate != null && !this.rate.isEmpty(); 705 } 706 707 /** 708 * @param value {@link #rate} (Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.) 709 */ 710 public MedicationAdministrationDosageComponent setRate(DataType value) { 711 if (value != null && !(value instanceof Ratio || value instanceof Quantity)) 712 throw new FHIRException("Not the right type for MedicationAdministration.dosage.rate[x]: "+value.fhirType()); 713 this.rate = value; 714 return this; 715 } 716 717 protected void listChildren(List<Property> children) { 718 super.listChildren(children); 719 children.add(new Property("text", "string", "Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans.\r\rThe dosage instructions should reflect the dosage of the medication that was administered.", 0, 1, text)); 720 children.add(new Property("site", "CodeableConcept", "A coded specification of the anatomic site where the medication first entered the body. For example, \"left arm\".", 0, 1, site)); 721 children.add(new Property("route", "CodeableConcept", "A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.", 0, 1, route)); 722 children.add(new Property("method", "CodeableConcept", "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.", 0, 1, method)); 723 children.add(new Property("dose", "Quantity", "The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.", 0, 1, dose)); 724 children.add(new Property("rate[x]", "Ratio|Quantity", "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 0, 1, rate)); 725 } 726 727 @Override 728 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 729 switch (_hash) { 730 case 3556653: /*text*/ return new Property("text", "string", "Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans.\r\rThe dosage instructions should reflect the dosage of the medication that was administered.", 0, 1, text); 731 case 3530567: /*site*/ return new Property("site", "CodeableConcept", "A coded specification of the anatomic site where the medication first entered the body. For example, \"left arm\".", 0, 1, site); 732 case 108704329: /*route*/ return new Property("route", "CodeableConcept", "A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.", 0, 1, route); 733 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.", 0, 1, method); 734 case 3089437: /*dose*/ return new Property("dose", "Quantity", "The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.", 0, 1, dose); 735 case 983460768: /*rate[x]*/ return new Property("rate[x]", "Ratio|Quantity", "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 0, 1, rate); 736 case 3493088: /*rate*/ return new Property("rate[x]", "Ratio|Quantity", "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 0, 1, rate); 737 case 204021515: /*rateRatio*/ return new Property("rate[x]", "Ratio", "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 0, 1, rate); 738 case -1085459061: /*rateQuantity*/ return new Property("rate[x]", "Quantity", "Identifies the speed with which the medication was or will be introduced into the patient. Typically, the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time, e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 0, 1, rate); 739 default: return super.getNamedProperty(_hash, _name, _checkValid); 740 } 741 742 } 743 744 @Override 745 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 746 switch (hash) { 747 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 748 case 3530567: /*site*/ return this.site == null ? new Base[0] : new Base[] {this.site}; // CodeableConcept 749 case 108704329: /*route*/ return this.route == null ? new Base[0] : new Base[] {this.route}; // CodeableConcept 750 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 751 case 3089437: /*dose*/ return this.dose == null ? new Base[0] : new Base[] {this.dose}; // Quantity 752 case 3493088: /*rate*/ return this.rate == null ? new Base[0] : new Base[] {this.rate}; // DataType 753 default: return super.getProperty(hash, name, checkValid); 754 } 755 756 } 757 758 @Override 759 public Base setProperty(int hash, String name, Base value) throws FHIRException { 760 switch (hash) { 761 case 3556653: // text 762 this.text = TypeConvertor.castToString(value); // StringType 763 return value; 764 case 3530567: // site 765 this.site = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 766 return value; 767 case 108704329: // route 768 this.route = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 769 return value; 770 case -1077554975: // method 771 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 772 return value; 773 case 3089437: // dose 774 this.dose = TypeConvertor.castToQuantity(value); // Quantity 775 return value; 776 case 3493088: // rate 777 this.rate = TypeConvertor.castToType(value); // DataType 778 return value; 779 default: return super.setProperty(hash, name, value); 780 } 781 782 } 783 784 @Override 785 public Base setProperty(String name, Base value) throws FHIRException { 786 if (name.equals("text")) { 787 this.text = TypeConvertor.castToString(value); // StringType 788 } else if (name.equals("site")) { 789 this.site = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 790 } else if (name.equals("route")) { 791 this.route = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 792 } else if (name.equals("method")) { 793 this.method = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 794 } else if (name.equals("dose")) { 795 this.dose = TypeConvertor.castToQuantity(value); // Quantity 796 } else if (name.equals("rate[x]")) { 797 this.rate = TypeConvertor.castToType(value); // DataType 798 } else 799 return super.setProperty(name, value); 800 return value; 801 } 802 803 @Override 804 public void removeChild(String name, Base value) throws FHIRException { 805 if (name.equals("text")) { 806 this.text = null; 807 } else if (name.equals("site")) { 808 this.site = null; 809 } else if (name.equals("route")) { 810 this.route = null; 811 } else if (name.equals("method")) { 812 this.method = null; 813 } else if (name.equals("dose")) { 814 this.dose = null; 815 } else if (name.equals("rate[x]")) { 816 this.rate = null; 817 } else 818 super.removeChild(name, value); 819 820 } 821 822 @Override 823 public Base makeProperty(int hash, String name) throws FHIRException { 824 switch (hash) { 825 case 3556653: return getTextElement(); 826 case 3530567: return getSite(); 827 case 108704329: return getRoute(); 828 case -1077554975: return getMethod(); 829 case 3089437: return getDose(); 830 case 983460768: return getRate(); 831 case 3493088: return getRate(); 832 default: return super.makeProperty(hash, name); 833 } 834 835 } 836 837 @Override 838 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 839 switch (hash) { 840 case 3556653: /*text*/ return new String[] {"string"}; 841 case 3530567: /*site*/ return new String[] {"CodeableConcept"}; 842 case 108704329: /*route*/ return new String[] {"CodeableConcept"}; 843 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 844 case 3089437: /*dose*/ return new String[] {"Quantity"}; 845 case 3493088: /*rate*/ return new String[] {"Ratio", "Quantity"}; 846 default: return super.getTypesForProperty(hash, name); 847 } 848 849 } 850 851 @Override 852 public Base addChild(String name) throws FHIRException { 853 if (name.equals("text")) { 854 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.dosage.text"); 855 } 856 else if (name.equals("site")) { 857 this.site = new CodeableConcept(); 858 return this.site; 859 } 860 else if (name.equals("route")) { 861 this.route = new CodeableConcept(); 862 return this.route; 863 } 864 else if (name.equals("method")) { 865 this.method = new CodeableConcept(); 866 return this.method; 867 } 868 else if (name.equals("dose")) { 869 this.dose = new Quantity(); 870 return this.dose; 871 } 872 else if (name.equals("rateRatio")) { 873 this.rate = new Ratio(); 874 return this.rate; 875 } 876 else if (name.equals("rateQuantity")) { 877 this.rate = new Quantity(); 878 return this.rate; 879 } 880 else 881 return super.addChild(name); 882 } 883 884 public MedicationAdministrationDosageComponent copy() { 885 MedicationAdministrationDosageComponent dst = new MedicationAdministrationDosageComponent(); 886 copyValues(dst); 887 return dst; 888 } 889 890 public void copyValues(MedicationAdministrationDosageComponent dst) { 891 super.copyValues(dst); 892 dst.text = text == null ? null : text.copy(); 893 dst.site = site == null ? null : site.copy(); 894 dst.route = route == null ? null : route.copy(); 895 dst.method = method == null ? null : method.copy(); 896 dst.dose = dose == null ? null : dose.copy(); 897 dst.rate = rate == null ? null : rate.copy(); 898 } 899 900 @Override 901 public boolean equalsDeep(Base other_) { 902 if (!super.equalsDeep(other_)) 903 return false; 904 if (!(other_ instanceof MedicationAdministrationDosageComponent)) 905 return false; 906 MedicationAdministrationDosageComponent o = (MedicationAdministrationDosageComponent) other_; 907 return compareDeep(text, o.text, true) && compareDeep(site, o.site, true) && compareDeep(route, o.route, true) 908 && compareDeep(method, o.method, true) && compareDeep(dose, o.dose, true) && compareDeep(rate, o.rate, true) 909 ; 910 } 911 912 @Override 913 public boolean equalsShallow(Base other_) { 914 if (!super.equalsShallow(other_)) 915 return false; 916 if (!(other_ instanceof MedicationAdministrationDosageComponent)) 917 return false; 918 MedicationAdministrationDosageComponent o = (MedicationAdministrationDosageComponent) other_; 919 return compareValues(text, o.text, true); 920 } 921 922 public boolean isEmpty() { 923 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(text, site, route, method 924 , dose, rate); 925 } 926 927 public String fhirType() { 928 return "MedicationAdministration.dosage"; 929 930 } 931 932 } 933 934 /** 935 * Identifiers associated with this Medication Administration that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server. 936 */ 937 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 938 @Description(shortDefinition="External identifier", formalDefinition="Identifiers associated with this Medication Administration that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server." ) 939 protected List<Identifier> identifier; 940 941 /** 942 * A plan that is fulfilled in whole or in part by this MedicationAdministration. 943 */ 944 @Child(name = "basedOn", type = {CarePlan.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 945 @Description(shortDefinition="Plan this is fulfilled by this administration", formalDefinition="A plan that is fulfilled in whole or in part by this MedicationAdministration." ) 946 protected List<Reference> basedOn; 947 948 /** 949 * A larger event of which this particular event is a component or step. 950 */ 951 @Child(name = "partOf", type = {MedicationAdministration.class, Procedure.class, MedicationDispense.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 952 @Description(shortDefinition="Part of referenced event", formalDefinition="A larger event of which this particular event is a component or step." ) 953 protected List<Reference> partOf; 954 955 /** 956 * Will generally be set to show that the administration has been completed. For some long running administrations such as infusions, it is possible for an administration to be started but not completed or it may be paused while some other process is under way. 957 */ 958 @Child(name = "status", type = {CodeType.class}, order=3, min=1, max=1, modifier=true, summary=true) 959 @Description(shortDefinition="in-progress | not-done | on-hold | completed | entered-in-error | stopped | unknown", formalDefinition="Will generally be set to show that the administration has been completed. For some long running administrations such as infusions, it is possible for an administration to be started but not completed or it may be paused while some other process is under way." ) 960 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-admin-status") 961 protected Enumeration<MedicationAdministrationStatusCodes> status; 962 963 /** 964 * A code indicating why the administration was not performed. 965 */ 966 @Child(name = "statusReason", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 967 @Description(shortDefinition="Reason administration not performed", formalDefinition="A code indicating why the administration was not performed." ) 968 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/reason-medication-not-given-codes") 969 protected List<CodeableConcept> statusReason; 970 971 /** 972 * The type of medication administration (for example, drug classification like ATC, where meds would be administered, legal category of the medication). 973 */ 974 @Child(name = "category", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 975 @Description(shortDefinition="Type of medication administration", formalDefinition="The type of medication administration (for example, drug classification like ATC, where meds would be administered, legal category of the medication)." ) 976 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-admin-location") 977 protected List<CodeableConcept> category; 978 979 /** 980 * Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications. 981 */ 982 @Child(name = "medication", type = {CodeableReference.class}, order=6, min=1, max=1, modifier=false, summary=true) 983 @Description(shortDefinition="What was administered", formalDefinition="Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications." ) 984 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 985 protected CodeableReference medication; 986 987 /** 988 * The person or animal or group receiving the medication. 989 */ 990 @Child(name = "subject", type = {Patient.class, Group.class}, order=7, min=1, max=1, modifier=false, summary=true) 991 @Description(shortDefinition="Who received medication", formalDefinition="The person or animal or group receiving the medication." ) 992 protected Reference subject; 993 994 /** 995 * The visit, admission, or other contact between patient and health care provider during which the medication administration was performed. 996 */ 997 @Child(name = "encounter", type = {Encounter.class}, order=8, min=0, max=1, modifier=false, summary=false) 998 @Description(shortDefinition="Encounter administered as part of", formalDefinition="The visit, admission, or other contact between patient and health care provider during which the medication administration was performed." ) 999 protected Reference encounter; 1000 1001 /** 1002 * Additional information (for example, patient height and weight) that supports the administration of the medication. This attribute can be used to provide documentation of specific characteristics of the patient present at the time of administration. For example, if the dose says "give "x" if the heartrate exceeds "y"", then the heart rate can be included using this attribute. 1003 */ 1004 @Child(name = "supportingInformation", type = {Reference.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1005 @Description(shortDefinition="Additional information to support administration", formalDefinition="Additional information (for example, patient height and weight) that supports the administration of the medication. This attribute can be used to provide documentation of specific characteristics of the patient present at the time of administration. For example, if the dose says \"give \"x\" if the heartrate exceeds \"y\"\", then the heart rate can be included using this attribute." ) 1006 protected List<Reference> supportingInformation; 1007 1008 /** 1009 * A specific date/time or interval of time during which the administration took place (or did not take place). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate. 1010 */ 1011 @Child(name = "occurence", type = {DateTimeType.class, Period.class, Timing.class}, order=10, min=1, max=1, modifier=false, summary=true) 1012 @Description(shortDefinition="Specific date/time or interval of time during which the administration took place (or did not take place)", formalDefinition="A specific date/time or interval of time during which the administration took place (or did not take place). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate." ) 1013 protected DataType occurence; 1014 1015 /** 1016 * The date the occurrence of the MedicationAdministration was first captured in the record - potentially significantly after the occurrence of the event. 1017 */ 1018 @Child(name = "recorded", type = {DateTimeType.class}, order=11, min=0, max=1, modifier=false, summary=true) 1019 @Description(shortDefinition="When the MedicationAdministration was first captured in the subject's record", formalDefinition="The date the occurrence of the MedicationAdministration was first captured in the record - potentially significantly after the occurrence of the event." ) 1020 protected DateTimeType recorded; 1021 1022 /** 1023 * An indication that the full dose was not administered. 1024 */ 1025 @Child(name = "isSubPotent", type = {BooleanType.class}, order=12, min=0, max=1, modifier=false, summary=false) 1026 @Description(shortDefinition="Full dose was not administered", formalDefinition="An indication that the full dose was not administered." ) 1027 protected BooleanType isSubPotent; 1028 1029 /** 1030 * The reason or reasons why the full dose was not administered. 1031 */ 1032 @Child(name = "subPotentReason", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1033 @Description(shortDefinition="Reason full dose was not administered", formalDefinition="The reason or reasons why the full dose was not administered." ) 1034 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administration-subpotent-reason") 1035 protected List<CodeableConcept> subPotentReason; 1036 1037 /** 1038 * The performer of the medication treatment. For devices this is the device that performed the administration of the medication. An IV Pump would be an example of a device that is performing the administration. Both the IV Pump and the practitioner that set the rate or bolus on the pump can be listed as performers. 1039 */ 1040 @Child(name = "performer", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1041 @Description(shortDefinition="Who or what performed the medication administration and what type of performance they did", formalDefinition="The performer of the medication treatment. For devices this is the device that performed the administration of the medication. An IV Pump would be an example of a device that is performing the administration. Both the IV Pump and the practitioner that set the rate or bolus on the pump can be listed as performers." ) 1042 protected List<MedicationAdministrationPerformerComponent> performer; 1043 1044 /** 1045 * A code, Condition or observation that supports why the medication was administered. 1046 */ 1047 @Child(name = "reason", type = {CodeableReference.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1048 @Description(shortDefinition="Concept, condition or observation that supports why the medication was administered", formalDefinition="A code, Condition or observation that supports why the medication was administered." ) 1049 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/reason-medication-given-codes") 1050 protected List<CodeableReference> reason; 1051 1052 /** 1053 * The original request, instruction or authority to perform the administration. 1054 */ 1055 @Child(name = "request", type = {MedicationRequest.class}, order=16, min=0, max=1, modifier=false, summary=false) 1056 @Description(shortDefinition="Request administration performed against", formalDefinition="The original request, instruction or authority to perform the administration." ) 1057 protected Reference request; 1058 1059 /** 1060 * The device that is to be used for the administration of the medication (for example, PCA Pump). 1061 */ 1062 @Child(name = "device", type = {CodeableReference.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1063 @Description(shortDefinition="Device used to administer", formalDefinition="The device that is to be used for the administration of the medication (for example, PCA Pump)." ) 1064 protected List<CodeableReference> device; 1065 1066 /** 1067 * Extra information about the medication administration that is not conveyed by the other attributes. 1068 */ 1069 @Child(name = "note", type = {Annotation.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1070 @Description(shortDefinition="Information about the administration", formalDefinition="Extra information about the medication administration that is not conveyed by the other attributes." ) 1071 protected List<Annotation> note; 1072 1073 /** 1074 * Describes the medication dosage information details e.g. dose, rate, site, route, etc. 1075 */ 1076 @Child(name = "dosage", type = {}, order=19, min=0, max=1, modifier=false, summary=false) 1077 @Description(shortDefinition="Details of how medication was taken", formalDefinition="Describes the medication dosage information details e.g. dose, rate, site, route, etc." ) 1078 protected MedicationAdministrationDosageComponent dosage; 1079 1080 /** 1081 * A summary of the events of interest that have occurred, such as when the administration was verified. 1082 */ 1083 @Child(name = "eventHistory", type = {Provenance.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1084 @Description(shortDefinition="A list of events of interest in the lifecycle", formalDefinition="A summary of the events of interest that have occurred, such as when the administration was verified." ) 1085 protected List<Reference> eventHistory; 1086 1087 private static final long serialVersionUID = 296165909L; 1088 1089 /** 1090 * Constructor 1091 */ 1092 public MedicationAdministration() { 1093 super(); 1094 } 1095 1096 /** 1097 * Constructor 1098 */ 1099 public MedicationAdministration(MedicationAdministrationStatusCodes status, CodeableReference medication, Reference subject, DataType occurence) { 1100 super(); 1101 this.setStatus(status); 1102 this.setMedication(medication); 1103 this.setSubject(subject); 1104 this.setOccurence(occurence); 1105 } 1106 1107 /** 1108 * @return {@link #identifier} (Identifiers associated with this Medication Administration that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.) 1109 */ 1110 public List<Identifier> getIdentifier() { 1111 if (this.identifier == null) 1112 this.identifier = new ArrayList<Identifier>(); 1113 return this.identifier; 1114 } 1115 1116 /** 1117 * @return Returns a reference to <code>this</code> for easy method chaining 1118 */ 1119 public MedicationAdministration setIdentifier(List<Identifier> theIdentifier) { 1120 this.identifier = theIdentifier; 1121 return this; 1122 } 1123 1124 public boolean hasIdentifier() { 1125 if (this.identifier == null) 1126 return false; 1127 for (Identifier item : this.identifier) 1128 if (!item.isEmpty()) 1129 return true; 1130 return false; 1131 } 1132 1133 public Identifier addIdentifier() { //3 1134 Identifier t = new Identifier(); 1135 if (this.identifier == null) 1136 this.identifier = new ArrayList<Identifier>(); 1137 this.identifier.add(t); 1138 return t; 1139 } 1140 1141 public MedicationAdministration addIdentifier(Identifier t) { //3 1142 if (t == null) 1143 return this; 1144 if (this.identifier == null) 1145 this.identifier = new ArrayList<Identifier>(); 1146 this.identifier.add(t); 1147 return this; 1148 } 1149 1150 /** 1151 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1152 */ 1153 public Identifier getIdentifierFirstRep() { 1154 if (getIdentifier().isEmpty()) { 1155 addIdentifier(); 1156 } 1157 return getIdentifier().get(0); 1158 } 1159 1160 /** 1161 * @return {@link #basedOn} (A plan that is fulfilled in whole or in part by this MedicationAdministration.) 1162 */ 1163 public List<Reference> getBasedOn() { 1164 if (this.basedOn == null) 1165 this.basedOn = new ArrayList<Reference>(); 1166 return this.basedOn; 1167 } 1168 1169 /** 1170 * @return Returns a reference to <code>this</code> for easy method chaining 1171 */ 1172 public MedicationAdministration setBasedOn(List<Reference> theBasedOn) { 1173 this.basedOn = theBasedOn; 1174 return this; 1175 } 1176 1177 public boolean hasBasedOn() { 1178 if (this.basedOn == null) 1179 return false; 1180 for (Reference item : this.basedOn) 1181 if (!item.isEmpty()) 1182 return true; 1183 return false; 1184 } 1185 1186 public Reference addBasedOn() { //3 1187 Reference t = new Reference(); 1188 if (this.basedOn == null) 1189 this.basedOn = new ArrayList<Reference>(); 1190 this.basedOn.add(t); 1191 return t; 1192 } 1193 1194 public MedicationAdministration addBasedOn(Reference t) { //3 1195 if (t == null) 1196 return this; 1197 if (this.basedOn == null) 1198 this.basedOn = new ArrayList<Reference>(); 1199 this.basedOn.add(t); 1200 return this; 1201 } 1202 1203 /** 1204 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 1205 */ 1206 public Reference getBasedOnFirstRep() { 1207 if (getBasedOn().isEmpty()) { 1208 addBasedOn(); 1209 } 1210 return getBasedOn().get(0); 1211 } 1212 1213 /** 1214 * @return {@link #partOf} (A larger event of which this particular event is a component or step.) 1215 */ 1216 public List<Reference> getPartOf() { 1217 if (this.partOf == null) 1218 this.partOf = new ArrayList<Reference>(); 1219 return this.partOf; 1220 } 1221 1222 /** 1223 * @return Returns a reference to <code>this</code> for easy method chaining 1224 */ 1225 public MedicationAdministration setPartOf(List<Reference> thePartOf) { 1226 this.partOf = thePartOf; 1227 return this; 1228 } 1229 1230 public boolean hasPartOf() { 1231 if (this.partOf == null) 1232 return false; 1233 for (Reference item : this.partOf) 1234 if (!item.isEmpty()) 1235 return true; 1236 return false; 1237 } 1238 1239 public Reference addPartOf() { //3 1240 Reference t = new Reference(); 1241 if (this.partOf == null) 1242 this.partOf = new ArrayList<Reference>(); 1243 this.partOf.add(t); 1244 return t; 1245 } 1246 1247 public MedicationAdministration addPartOf(Reference t) { //3 1248 if (t == null) 1249 return this; 1250 if (this.partOf == null) 1251 this.partOf = new ArrayList<Reference>(); 1252 this.partOf.add(t); 1253 return this; 1254 } 1255 1256 /** 1257 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 1258 */ 1259 public Reference getPartOfFirstRep() { 1260 if (getPartOf().isEmpty()) { 1261 addPartOf(); 1262 } 1263 return getPartOf().get(0); 1264 } 1265 1266 /** 1267 * @return {@link #status} (Will generally be set to show that the administration has been completed. For some long running administrations such as infusions, it is possible for an administration to be started but not completed or it may be paused while some other process is under way.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1268 */ 1269 public Enumeration<MedicationAdministrationStatusCodes> getStatusElement() { 1270 if (this.status == null) 1271 if (Configuration.errorOnAutoCreate()) 1272 throw new Error("Attempt to auto-create MedicationAdministration.status"); 1273 else if (Configuration.doAutoCreate()) 1274 this.status = new Enumeration<MedicationAdministrationStatusCodes>(new MedicationAdministrationStatusCodesEnumFactory()); // bb 1275 return this.status; 1276 } 1277 1278 public boolean hasStatusElement() { 1279 return this.status != null && !this.status.isEmpty(); 1280 } 1281 1282 public boolean hasStatus() { 1283 return this.status != null && !this.status.isEmpty(); 1284 } 1285 1286 /** 1287 * @param value {@link #status} (Will generally be set to show that the administration has been completed. For some long running administrations such as infusions, it is possible for an administration to be started but not completed or it may be paused while some other process is under way.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1288 */ 1289 public MedicationAdministration setStatusElement(Enumeration<MedicationAdministrationStatusCodes> value) { 1290 this.status = value; 1291 return this; 1292 } 1293 1294 /** 1295 * @return Will generally be set to show that the administration has been completed. For some long running administrations such as infusions, it is possible for an administration to be started but not completed or it may be paused while some other process is under way. 1296 */ 1297 public MedicationAdministrationStatusCodes getStatus() { 1298 return this.status == null ? null : this.status.getValue(); 1299 } 1300 1301 /** 1302 * @param value Will generally be set to show that the administration has been completed. For some long running administrations such as infusions, it is possible for an administration to be started but not completed or it may be paused while some other process is under way. 1303 */ 1304 public MedicationAdministration setStatus(MedicationAdministrationStatusCodes value) { 1305 if (this.status == null) 1306 this.status = new Enumeration<MedicationAdministrationStatusCodes>(new MedicationAdministrationStatusCodesEnumFactory()); 1307 this.status.setValue(value); 1308 return this; 1309 } 1310 1311 /** 1312 * @return {@link #statusReason} (A code indicating why the administration was not performed.) 1313 */ 1314 public List<CodeableConcept> getStatusReason() { 1315 if (this.statusReason == null) 1316 this.statusReason = new ArrayList<CodeableConcept>(); 1317 return this.statusReason; 1318 } 1319 1320 /** 1321 * @return Returns a reference to <code>this</code> for easy method chaining 1322 */ 1323 public MedicationAdministration setStatusReason(List<CodeableConcept> theStatusReason) { 1324 this.statusReason = theStatusReason; 1325 return this; 1326 } 1327 1328 public boolean hasStatusReason() { 1329 if (this.statusReason == null) 1330 return false; 1331 for (CodeableConcept item : this.statusReason) 1332 if (!item.isEmpty()) 1333 return true; 1334 return false; 1335 } 1336 1337 public CodeableConcept addStatusReason() { //3 1338 CodeableConcept t = new CodeableConcept(); 1339 if (this.statusReason == null) 1340 this.statusReason = new ArrayList<CodeableConcept>(); 1341 this.statusReason.add(t); 1342 return t; 1343 } 1344 1345 public MedicationAdministration addStatusReason(CodeableConcept t) { //3 1346 if (t == null) 1347 return this; 1348 if (this.statusReason == null) 1349 this.statusReason = new ArrayList<CodeableConcept>(); 1350 this.statusReason.add(t); 1351 return this; 1352 } 1353 1354 /** 1355 * @return The first repetition of repeating field {@link #statusReason}, creating it if it does not already exist {3} 1356 */ 1357 public CodeableConcept getStatusReasonFirstRep() { 1358 if (getStatusReason().isEmpty()) { 1359 addStatusReason(); 1360 } 1361 return getStatusReason().get(0); 1362 } 1363 1364 /** 1365 * @return {@link #category} (The type of medication administration (for example, drug classification like ATC, where meds would be administered, legal category of the medication).) 1366 */ 1367 public List<CodeableConcept> getCategory() { 1368 if (this.category == null) 1369 this.category = new ArrayList<CodeableConcept>(); 1370 return this.category; 1371 } 1372 1373 /** 1374 * @return Returns a reference to <code>this</code> for easy method chaining 1375 */ 1376 public MedicationAdministration setCategory(List<CodeableConcept> theCategory) { 1377 this.category = theCategory; 1378 return this; 1379 } 1380 1381 public boolean hasCategory() { 1382 if (this.category == null) 1383 return false; 1384 for (CodeableConcept item : this.category) 1385 if (!item.isEmpty()) 1386 return true; 1387 return false; 1388 } 1389 1390 public CodeableConcept addCategory() { //3 1391 CodeableConcept t = new CodeableConcept(); 1392 if (this.category == null) 1393 this.category = new ArrayList<CodeableConcept>(); 1394 this.category.add(t); 1395 return t; 1396 } 1397 1398 public MedicationAdministration addCategory(CodeableConcept t) { //3 1399 if (t == null) 1400 return this; 1401 if (this.category == null) 1402 this.category = new ArrayList<CodeableConcept>(); 1403 this.category.add(t); 1404 return this; 1405 } 1406 1407 /** 1408 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 1409 */ 1410 public CodeableConcept getCategoryFirstRep() { 1411 if (getCategory().isEmpty()) { 1412 addCategory(); 1413 } 1414 return getCategory().get(0); 1415 } 1416 1417 /** 1418 * @return {@link #medication} (Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 1419 */ 1420 public CodeableReference getMedication() { 1421 if (this.medication == null) 1422 if (Configuration.errorOnAutoCreate()) 1423 throw new Error("Attempt to auto-create MedicationAdministration.medication"); 1424 else if (Configuration.doAutoCreate()) 1425 this.medication = new CodeableReference(); // cc 1426 return this.medication; 1427 } 1428 1429 public boolean hasMedication() { 1430 return this.medication != null && !this.medication.isEmpty(); 1431 } 1432 1433 /** 1434 * @param value {@link #medication} (Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 1435 */ 1436 public MedicationAdministration setMedication(CodeableReference value) { 1437 this.medication = value; 1438 return this; 1439 } 1440 1441 /** 1442 * @return {@link #subject} (The person or animal or group receiving the medication.) 1443 */ 1444 public Reference getSubject() { 1445 if (this.subject == null) 1446 if (Configuration.errorOnAutoCreate()) 1447 throw new Error("Attempt to auto-create MedicationAdministration.subject"); 1448 else if (Configuration.doAutoCreate()) 1449 this.subject = new Reference(); // cc 1450 return this.subject; 1451 } 1452 1453 public boolean hasSubject() { 1454 return this.subject != null && !this.subject.isEmpty(); 1455 } 1456 1457 /** 1458 * @param value {@link #subject} (The person or animal or group receiving the medication.) 1459 */ 1460 public MedicationAdministration setSubject(Reference value) { 1461 this.subject = value; 1462 return this; 1463 } 1464 1465 /** 1466 * @return {@link #encounter} (The visit, admission, or other contact between patient and health care provider during which the medication administration was performed.) 1467 */ 1468 public Reference getEncounter() { 1469 if (this.encounter == null) 1470 if (Configuration.errorOnAutoCreate()) 1471 throw new Error("Attempt to auto-create MedicationAdministration.encounter"); 1472 else if (Configuration.doAutoCreate()) 1473 this.encounter = new Reference(); // cc 1474 return this.encounter; 1475 } 1476 1477 public boolean hasEncounter() { 1478 return this.encounter != null && !this.encounter.isEmpty(); 1479 } 1480 1481 /** 1482 * @param value {@link #encounter} (The visit, admission, or other contact between patient and health care provider during which the medication administration was performed.) 1483 */ 1484 public MedicationAdministration setEncounter(Reference value) { 1485 this.encounter = value; 1486 return this; 1487 } 1488 1489 /** 1490 * @return {@link #supportingInformation} (Additional information (for example, patient height and weight) that supports the administration of the medication. This attribute can be used to provide documentation of specific characteristics of the patient present at the time of administration. For example, if the dose says "give "x" if the heartrate exceeds "y"", then the heart rate can be included using this attribute.) 1491 */ 1492 public List<Reference> getSupportingInformation() { 1493 if (this.supportingInformation == null) 1494 this.supportingInformation = new ArrayList<Reference>(); 1495 return this.supportingInformation; 1496 } 1497 1498 /** 1499 * @return Returns a reference to <code>this</code> for easy method chaining 1500 */ 1501 public MedicationAdministration setSupportingInformation(List<Reference> theSupportingInformation) { 1502 this.supportingInformation = theSupportingInformation; 1503 return this; 1504 } 1505 1506 public boolean hasSupportingInformation() { 1507 if (this.supportingInformation == null) 1508 return false; 1509 for (Reference item : this.supportingInformation) 1510 if (!item.isEmpty()) 1511 return true; 1512 return false; 1513 } 1514 1515 public Reference addSupportingInformation() { //3 1516 Reference t = new Reference(); 1517 if (this.supportingInformation == null) 1518 this.supportingInformation = new ArrayList<Reference>(); 1519 this.supportingInformation.add(t); 1520 return t; 1521 } 1522 1523 public MedicationAdministration addSupportingInformation(Reference t) { //3 1524 if (t == null) 1525 return this; 1526 if (this.supportingInformation == null) 1527 this.supportingInformation = new ArrayList<Reference>(); 1528 this.supportingInformation.add(t); 1529 return this; 1530 } 1531 1532 /** 1533 * @return The first repetition of repeating field {@link #supportingInformation}, creating it if it does not already exist {3} 1534 */ 1535 public Reference getSupportingInformationFirstRep() { 1536 if (getSupportingInformation().isEmpty()) { 1537 addSupportingInformation(); 1538 } 1539 return getSupportingInformation().get(0); 1540 } 1541 1542 /** 1543 * @return {@link #occurence} (A specific date/time or interval of time during which the administration took place (or did not take place). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.) 1544 */ 1545 public DataType getOccurence() { 1546 return this.occurence; 1547 } 1548 1549 /** 1550 * @return {@link #occurence} (A specific date/time or interval of time during which the administration took place (or did not take place). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.) 1551 */ 1552 public DateTimeType getOccurenceDateTimeType() throws FHIRException { 1553 if (this.occurence == null) 1554 this.occurence = new DateTimeType(); 1555 if (!(this.occurence instanceof DateTimeType)) 1556 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurence.getClass().getName()+" was encountered"); 1557 return (DateTimeType) this.occurence; 1558 } 1559 1560 public boolean hasOccurenceDateTimeType() { 1561 return this.occurence instanceof DateTimeType; 1562 } 1563 1564 /** 1565 * @return {@link #occurence} (A specific date/time or interval of time during which the administration took place (or did not take place). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.) 1566 */ 1567 public Period getOccurencePeriod() throws FHIRException { 1568 if (this.occurence == null) 1569 this.occurence = new Period(); 1570 if (!(this.occurence instanceof Period)) 1571 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurence.getClass().getName()+" was encountered"); 1572 return (Period) this.occurence; 1573 } 1574 1575 public boolean hasOccurencePeriod() { 1576 return this.occurence instanceof Period; 1577 } 1578 1579 /** 1580 * @return {@link #occurence} (A specific date/time or interval of time during which the administration took place (or did not take place). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.) 1581 */ 1582 public Timing getOccurenceTiming() throws FHIRException { 1583 if (this.occurence == null) 1584 this.occurence = new Timing(); 1585 if (!(this.occurence instanceof Timing)) 1586 throw new FHIRException("Type mismatch: the type Timing was expected, but "+this.occurence.getClass().getName()+" was encountered"); 1587 return (Timing) this.occurence; 1588 } 1589 1590 public boolean hasOccurenceTiming() { 1591 return this.occurence instanceof Timing; 1592 } 1593 1594 public boolean hasOccurence() { 1595 return this.occurence != null && !this.occurence.isEmpty(); 1596 } 1597 1598 /** 1599 * @param value {@link #occurence} (A specific date/time or interval of time during which the administration took place (or did not take place). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.) 1600 */ 1601 public MedicationAdministration setOccurence(DataType value) { 1602 if (value != null && !(value instanceof DateTimeType || value instanceof Period || value instanceof Timing)) 1603 throw new FHIRException("Not the right type for MedicationAdministration.occurence[x]: "+value.fhirType()); 1604 this.occurence = value; 1605 return this; 1606 } 1607 1608 /** 1609 * @return {@link #recorded} (The date the occurrence of the MedicationAdministration was first captured in the record - potentially significantly after the occurrence of the event.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 1610 */ 1611 public DateTimeType getRecordedElement() { 1612 if (this.recorded == null) 1613 if (Configuration.errorOnAutoCreate()) 1614 throw new Error("Attempt to auto-create MedicationAdministration.recorded"); 1615 else if (Configuration.doAutoCreate()) 1616 this.recorded = new DateTimeType(); // bb 1617 return this.recorded; 1618 } 1619 1620 public boolean hasRecordedElement() { 1621 return this.recorded != null && !this.recorded.isEmpty(); 1622 } 1623 1624 public boolean hasRecorded() { 1625 return this.recorded != null && !this.recorded.isEmpty(); 1626 } 1627 1628 /** 1629 * @param value {@link #recorded} (The date the occurrence of the MedicationAdministration was first captured in the record - potentially significantly after the occurrence of the event.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 1630 */ 1631 public MedicationAdministration setRecordedElement(DateTimeType value) { 1632 this.recorded = value; 1633 return this; 1634 } 1635 1636 /** 1637 * @return The date the occurrence of the MedicationAdministration was first captured in the record - potentially significantly after the occurrence of the event. 1638 */ 1639 public Date getRecorded() { 1640 return this.recorded == null ? null : this.recorded.getValue(); 1641 } 1642 1643 /** 1644 * @param value The date the occurrence of the MedicationAdministration was first captured in the record - potentially significantly after the occurrence of the event. 1645 */ 1646 public MedicationAdministration setRecorded(Date value) { 1647 if (value == null) 1648 this.recorded = null; 1649 else { 1650 if (this.recorded == null) 1651 this.recorded = new DateTimeType(); 1652 this.recorded.setValue(value); 1653 } 1654 return this; 1655 } 1656 1657 /** 1658 * @return {@link #isSubPotent} (An indication that the full dose was not administered.). This is the underlying object with id, value and extensions. The accessor "getIsSubPotent" gives direct access to the value 1659 */ 1660 public BooleanType getIsSubPotentElement() { 1661 if (this.isSubPotent == null) 1662 if (Configuration.errorOnAutoCreate()) 1663 throw new Error("Attempt to auto-create MedicationAdministration.isSubPotent"); 1664 else if (Configuration.doAutoCreate()) 1665 this.isSubPotent = new BooleanType(); // bb 1666 return this.isSubPotent; 1667 } 1668 1669 public boolean hasIsSubPotentElement() { 1670 return this.isSubPotent != null && !this.isSubPotent.isEmpty(); 1671 } 1672 1673 public boolean hasIsSubPotent() { 1674 return this.isSubPotent != null && !this.isSubPotent.isEmpty(); 1675 } 1676 1677 /** 1678 * @param value {@link #isSubPotent} (An indication that the full dose was not administered.). This is the underlying object with id, value and extensions. The accessor "getIsSubPotent" gives direct access to the value 1679 */ 1680 public MedicationAdministration setIsSubPotentElement(BooleanType value) { 1681 this.isSubPotent = value; 1682 return this; 1683 } 1684 1685 /** 1686 * @return An indication that the full dose was not administered. 1687 */ 1688 public boolean getIsSubPotent() { 1689 return this.isSubPotent == null || this.isSubPotent.isEmpty() ? false : this.isSubPotent.getValue(); 1690 } 1691 1692 /** 1693 * @param value An indication that the full dose was not administered. 1694 */ 1695 public MedicationAdministration setIsSubPotent(boolean value) { 1696 if (this.isSubPotent == null) 1697 this.isSubPotent = new BooleanType(); 1698 this.isSubPotent.setValue(value); 1699 return this; 1700 } 1701 1702 /** 1703 * @return {@link #subPotentReason} (The reason or reasons why the full dose was not administered.) 1704 */ 1705 public List<CodeableConcept> getSubPotentReason() { 1706 if (this.subPotentReason == null) 1707 this.subPotentReason = new ArrayList<CodeableConcept>(); 1708 return this.subPotentReason; 1709 } 1710 1711 /** 1712 * @return Returns a reference to <code>this</code> for easy method chaining 1713 */ 1714 public MedicationAdministration setSubPotentReason(List<CodeableConcept> theSubPotentReason) { 1715 this.subPotentReason = theSubPotentReason; 1716 return this; 1717 } 1718 1719 public boolean hasSubPotentReason() { 1720 if (this.subPotentReason == null) 1721 return false; 1722 for (CodeableConcept item : this.subPotentReason) 1723 if (!item.isEmpty()) 1724 return true; 1725 return false; 1726 } 1727 1728 public CodeableConcept addSubPotentReason() { //3 1729 CodeableConcept t = new CodeableConcept(); 1730 if (this.subPotentReason == null) 1731 this.subPotentReason = new ArrayList<CodeableConcept>(); 1732 this.subPotentReason.add(t); 1733 return t; 1734 } 1735 1736 public MedicationAdministration addSubPotentReason(CodeableConcept t) { //3 1737 if (t == null) 1738 return this; 1739 if (this.subPotentReason == null) 1740 this.subPotentReason = new ArrayList<CodeableConcept>(); 1741 this.subPotentReason.add(t); 1742 return this; 1743 } 1744 1745 /** 1746 * @return The first repetition of repeating field {@link #subPotentReason}, creating it if it does not already exist {3} 1747 */ 1748 public CodeableConcept getSubPotentReasonFirstRep() { 1749 if (getSubPotentReason().isEmpty()) { 1750 addSubPotentReason(); 1751 } 1752 return getSubPotentReason().get(0); 1753 } 1754 1755 /** 1756 * @return {@link #performer} (The performer of the medication treatment. For devices this is the device that performed the administration of the medication. An IV Pump would be an example of a device that is performing the administration. Both the IV Pump and the practitioner that set the rate or bolus on the pump can be listed as performers.) 1757 */ 1758 public List<MedicationAdministrationPerformerComponent> getPerformer() { 1759 if (this.performer == null) 1760 this.performer = new ArrayList<MedicationAdministrationPerformerComponent>(); 1761 return this.performer; 1762 } 1763 1764 /** 1765 * @return Returns a reference to <code>this</code> for easy method chaining 1766 */ 1767 public MedicationAdministration setPerformer(List<MedicationAdministrationPerformerComponent> thePerformer) { 1768 this.performer = thePerformer; 1769 return this; 1770 } 1771 1772 public boolean hasPerformer() { 1773 if (this.performer == null) 1774 return false; 1775 for (MedicationAdministrationPerformerComponent item : this.performer) 1776 if (!item.isEmpty()) 1777 return true; 1778 return false; 1779 } 1780 1781 public MedicationAdministrationPerformerComponent addPerformer() { //3 1782 MedicationAdministrationPerformerComponent t = new MedicationAdministrationPerformerComponent(); 1783 if (this.performer == null) 1784 this.performer = new ArrayList<MedicationAdministrationPerformerComponent>(); 1785 this.performer.add(t); 1786 return t; 1787 } 1788 1789 public MedicationAdministration addPerformer(MedicationAdministrationPerformerComponent t) { //3 1790 if (t == null) 1791 return this; 1792 if (this.performer == null) 1793 this.performer = new ArrayList<MedicationAdministrationPerformerComponent>(); 1794 this.performer.add(t); 1795 return this; 1796 } 1797 1798 /** 1799 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 1800 */ 1801 public MedicationAdministrationPerformerComponent getPerformerFirstRep() { 1802 if (getPerformer().isEmpty()) { 1803 addPerformer(); 1804 } 1805 return getPerformer().get(0); 1806 } 1807 1808 /** 1809 * @return {@link #reason} (A code, Condition or observation that supports why the medication was administered.) 1810 */ 1811 public List<CodeableReference> getReason() { 1812 if (this.reason == null) 1813 this.reason = new ArrayList<CodeableReference>(); 1814 return this.reason; 1815 } 1816 1817 /** 1818 * @return Returns a reference to <code>this</code> for easy method chaining 1819 */ 1820 public MedicationAdministration setReason(List<CodeableReference> theReason) { 1821 this.reason = theReason; 1822 return this; 1823 } 1824 1825 public boolean hasReason() { 1826 if (this.reason == null) 1827 return false; 1828 for (CodeableReference item : this.reason) 1829 if (!item.isEmpty()) 1830 return true; 1831 return false; 1832 } 1833 1834 public CodeableReference addReason() { //3 1835 CodeableReference t = new CodeableReference(); 1836 if (this.reason == null) 1837 this.reason = new ArrayList<CodeableReference>(); 1838 this.reason.add(t); 1839 return t; 1840 } 1841 1842 public MedicationAdministration addReason(CodeableReference t) { //3 1843 if (t == null) 1844 return this; 1845 if (this.reason == null) 1846 this.reason = new ArrayList<CodeableReference>(); 1847 this.reason.add(t); 1848 return this; 1849 } 1850 1851 /** 1852 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 1853 */ 1854 public CodeableReference getReasonFirstRep() { 1855 if (getReason().isEmpty()) { 1856 addReason(); 1857 } 1858 return getReason().get(0); 1859 } 1860 1861 /** 1862 * @return {@link #request} (The original request, instruction or authority to perform the administration.) 1863 */ 1864 public Reference getRequest() { 1865 if (this.request == null) 1866 if (Configuration.errorOnAutoCreate()) 1867 throw new Error("Attempt to auto-create MedicationAdministration.request"); 1868 else if (Configuration.doAutoCreate()) 1869 this.request = new Reference(); // cc 1870 return this.request; 1871 } 1872 1873 public boolean hasRequest() { 1874 return this.request != null && !this.request.isEmpty(); 1875 } 1876 1877 /** 1878 * @param value {@link #request} (The original request, instruction or authority to perform the administration.) 1879 */ 1880 public MedicationAdministration setRequest(Reference value) { 1881 this.request = value; 1882 return this; 1883 } 1884 1885 /** 1886 * @return {@link #device} (The device that is to be used for the administration of the medication (for example, PCA Pump).) 1887 */ 1888 public List<CodeableReference> getDevice() { 1889 if (this.device == null) 1890 this.device = new ArrayList<CodeableReference>(); 1891 return this.device; 1892 } 1893 1894 /** 1895 * @return Returns a reference to <code>this</code> for easy method chaining 1896 */ 1897 public MedicationAdministration setDevice(List<CodeableReference> theDevice) { 1898 this.device = theDevice; 1899 return this; 1900 } 1901 1902 public boolean hasDevice() { 1903 if (this.device == null) 1904 return false; 1905 for (CodeableReference item : this.device) 1906 if (!item.isEmpty()) 1907 return true; 1908 return false; 1909 } 1910 1911 public CodeableReference addDevice() { //3 1912 CodeableReference t = new CodeableReference(); 1913 if (this.device == null) 1914 this.device = new ArrayList<CodeableReference>(); 1915 this.device.add(t); 1916 return t; 1917 } 1918 1919 public MedicationAdministration addDevice(CodeableReference t) { //3 1920 if (t == null) 1921 return this; 1922 if (this.device == null) 1923 this.device = new ArrayList<CodeableReference>(); 1924 this.device.add(t); 1925 return this; 1926 } 1927 1928 /** 1929 * @return The first repetition of repeating field {@link #device}, creating it if it does not already exist {3} 1930 */ 1931 public CodeableReference getDeviceFirstRep() { 1932 if (getDevice().isEmpty()) { 1933 addDevice(); 1934 } 1935 return getDevice().get(0); 1936 } 1937 1938 /** 1939 * @return {@link #note} (Extra information about the medication administration that is not conveyed by the other attributes.) 1940 */ 1941 public List<Annotation> getNote() { 1942 if (this.note == null) 1943 this.note = new ArrayList<Annotation>(); 1944 return this.note; 1945 } 1946 1947 /** 1948 * @return Returns a reference to <code>this</code> for easy method chaining 1949 */ 1950 public MedicationAdministration setNote(List<Annotation> theNote) { 1951 this.note = theNote; 1952 return this; 1953 } 1954 1955 public boolean hasNote() { 1956 if (this.note == null) 1957 return false; 1958 for (Annotation item : this.note) 1959 if (!item.isEmpty()) 1960 return true; 1961 return false; 1962 } 1963 1964 public Annotation addNote() { //3 1965 Annotation t = new Annotation(); 1966 if (this.note == null) 1967 this.note = new ArrayList<Annotation>(); 1968 this.note.add(t); 1969 return t; 1970 } 1971 1972 public MedicationAdministration addNote(Annotation t) { //3 1973 if (t == null) 1974 return this; 1975 if (this.note == null) 1976 this.note = new ArrayList<Annotation>(); 1977 this.note.add(t); 1978 return this; 1979 } 1980 1981 /** 1982 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1983 */ 1984 public Annotation getNoteFirstRep() { 1985 if (getNote().isEmpty()) { 1986 addNote(); 1987 } 1988 return getNote().get(0); 1989 } 1990 1991 /** 1992 * @return {@link #dosage} (Describes the medication dosage information details e.g. dose, rate, site, route, etc.) 1993 */ 1994 public MedicationAdministrationDosageComponent getDosage() { 1995 if (this.dosage == null) 1996 if (Configuration.errorOnAutoCreate()) 1997 throw new Error("Attempt to auto-create MedicationAdministration.dosage"); 1998 else if (Configuration.doAutoCreate()) 1999 this.dosage = new MedicationAdministrationDosageComponent(); // cc 2000 return this.dosage; 2001 } 2002 2003 public boolean hasDosage() { 2004 return this.dosage != null && !this.dosage.isEmpty(); 2005 } 2006 2007 /** 2008 * @param value {@link #dosage} (Describes the medication dosage information details e.g. dose, rate, site, route, etc.) 2009 */ 2010 public MedicationAdministration setDosage(MedicationAdministrationDosageComponent value) { 2011 this.dosage = value; 2012 return this; 2013 } 2014 2015 /** 2016 * @return {@link #eventHistory} (A summary of the events of interest that have occurred, such as when the administration was verified.) 2017 */ 2018 public List<Reference> getEventHistory() { 2019 if (this.eventHistory == null) 2020 this.eventHistory = new ArrayList<Reference>(); 2021 return this.eventHistory; 2022 } 2023 2024 /** 2025 * @return Returns a reference to <code>this</code> for easy method chaining 2026 */ 2027 public MedicationAdministration setEventHistory(List<Reference> theEventHistory) { 2028 this.eventHistory = theEventHistory; 2029 return this; 2030 } 2031 2032 public boolean hasEventHistory() { 2033 if (this.eventHistory == null) 2034 return false; 2035 for (Reference item : this.eventHistory) 2036 if (!item.isEmpty()) 2037 return true; 2038 return false; 2039 } 2040 2041 public Reference addEventHistory() { //3 2042 Reference t = new Reference(); 2043 if (this.eventHistory == null) 2044 this.eventHistory = new ArrayList<Reference>(); 2045 this.eventHistory.add(t); 2046 return t; 2047 } 2048 2049 public MedicationAdministration addEventHistory(Reference t) { //3 2050 if (t == null) 2051 return this; 2052 if (this.eventHistory == null) 2053 this.eventHistory = new ArrayList<Reference>(); 2054 this.eventHistory.add(t); 2055 return this; 2056 } 2057 2058 /** 2059 * @return The first repetition of repeating field {@link #eventHistory}, creating it if it does not already exist {3} 2060 */ 2061 public Reference getEventHistoryFirstRep() { 2062 if (getEventHistory().isEmpty()) { 2063 addEventHistory(); 2064 } 2065 return getEventHistory().get(0); 2066 } 2067 2068 protected void listChildren(List<Property> children) { 2069 super.listChildren(children); 2070 children.add(new Property("identifier", "Identifier", "Identifiers associated with this Medication Administration that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2071 children.add(new Property("basedOn", "Reference(CarePlan)", "A plan that is fulfilled in whole or in part by this MedicationAdministration.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2072 children.add(new Property("partOf", "Reference(MedicationAdministration|Procedure|MedicationDispense)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 2073 children.add(new Property("status", "code", "Will generally be set to show that the administration has been completed. For some long running administrations such as infusions, it is possible for an administration to be started but not completed or it may be paused while some other process is under way.", 0, 1, status)); 2074 children.add(new Property("statusReason", "CodeableConcept", "A code indicating why the administration was not performed.", 0, java.lang.Integer.MAX_VALUE, statusReason)); 2075 children.add(new Property("category", "CodeableConcept", "The type of medication administration (for example, drug classification like ATC, where meds would be administered, legal category of the medication).", 0, java.lang.Integer.MAX_VALUE, category)); 2076 children.add(new Property("medication", "CodeableReference(Medication)", "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication)); 2077 children.add(new Property("subject", "Reference(Patient|Group)", "The person or animal or group receiving the medication.", 0, 1, subject)); 2078 children.add(new Property("encounter", "Reference(Encounter)", "The visit, admission, or other contact between patient and health care provider during which the medication administration was performed.", 0, 1, encounter)); 2079 children.add(new Property("supportingInformation", "Reference(Any)", "Additional information (for example, patient height and weight) that supports the administration of the medication. This attribute can be used to provide documentation of specific characteristics of the patient present at the time of administration. For example, if the dose says \"give \"x\" if the heartrate exceeds \"y\"\", then the heart rate can be included using this attribute.", 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 2080 children.add(new Property("occurence[x]", "dateTime|Period|Timing", "A specific date/time or interval of time during which the administration took place (or did not take place). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 0, 1, occurence)); 2081 children.add(new Property("recorded", "dateTime", "The date the occurrence of the MedicationAdministration was first captured in the record - potentially significantly after the occurrence of the event.", 0, 1, recorded)); 2082 children.add(new Property("isSubPotent", "boolean", "An indication that the full dose was not administered.", 0, 1, isSubPotent)); 2083 children.add(new Property("subPotentReason", "CodeableConcept", "The reason or reasons why the full dose was not administered.", 0, java.lang.Integer.MAX_VALUE, subPotentReason)); 2084 children.add(new Property("performer", "", "The performer of the medication treatment. For devices this is the device that performed the administration of the medication. An IV Pump would be an example of a device that is performing the administration. Both the IV Pump and the practitioner that set the rate or bolus on the pump can be listed as performers.", 0, java.lang.Integer.MAX_VALUE, performer)); 2085 children.add(new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport)", "A code, Condition or observation that supports why the medication was administered.", 0, java.lang.Integer.MAX_VALUE, reason)); 2086 children.add(new Property("request", "Reference(MedicationRequest)", "The original request, instruction or authority to perform the administration.", 0, 1, request)); 2087 children.add(new Property("device", "CodeableReference(Device)", "The device that is to be used for the administration of the medication (for example, PCA Pump).", 0, java.lang.Integer.MAX_VALUE, device)); 2088 children.add(new Property("note", "Annotation", "Extra information about the medication administration that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note)); 2089 children.add(new Property("dosage", "", "Describes the medication dosage information details e.g. dose, rate, site, route, etc.", 0, 1, dosage)); 2090 children.add(new Property("eventHistory", "Reference(Provenance)", "A summary of the events of interest that have occurred, such as when the administration was verified.", 0, java.lang.Integer.MAX_VALUE, eventHistory)); 2091 } 2092 2093 @Override 2094 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2095 switch (_hash) { 2096 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers associated with this Medication Administration that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 2097 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan)", "A plan that is fulfilled in whole or in part by this MedicationAdministration.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2098 case -995410646: /*partOf*/ return new Property("partOf", "Reference(MedicationAdministration|Procedure|MedicationDispense)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 2099 case -892481550: /*status*/ return new Property("status", "code", "Will generally be set to show that the administration has been completed. For some long running administrations such as infusions, it is possible for an administration to be started but not completed or it may be paused while some other process is under way.", 0, 1, status); 2100 case 2051346646: /*statusReason*/ return new Property("statusReason", "CodeableConcept", "A code indicating why the administration was not performed.", 0, java.lang.Integer.MAX_VALUE, statusReason); 2101 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The type of medication administration (for example, drug classification like ATC, where meds would be administered, legal category of the medication).", 0, java.lang.Integer.MAX_VALUE, category); 2102 case 1998965455: /*medication*/ return new Property("medication", "CodeableReference(Medication)", "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 2103 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The person or animal or group receiving the medication.", 0, 1, subject); 2104 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The visit, admission, or other contact between patient and health care provider during which the medication administration was performed.", 0, 1, encounter); 2105 case -1248768647: /*supportingInformation*/ return new Property("supportingInformation", "Reference(Any)", "Additional information (for example, patient height and weight) that supports the administration of the medication. This attribute can be used to provide documentation of specific characteristics of the patient present at the time of administration. For example, if the dose says \"give \"x\" if the heartrate exceeds \"y\"\", then the heart rate can be included using this attribute.", 0, java.lang.Integer.MAX_VALUE, supportingInformation); 2106 case 144188521: /*occurence[x]*/ return new Property("occurence[x]", "dateTime|Period|Timing", "A specific date/time or interval of time during which the administration took place (or did not take place). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 0, 1, occurence); 2107 case -1192857417: /*occurence*/ return new Property("occurence[x]", "dateTime|Period|Timing", "A specific date/time or interval of time during which the administration took place (or did not take place). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 0, 1, occurence); 2108 case -820552334: /*occurenceDateTime*/ return new Property("occurence[x]", "dateTime", "A specific date/time or interval of time during which the administration took place (or did not take place). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 0, 1, occurence); 2109 case 221195608: /*occurencePeriod*/ return new Property("occurence[x]", "Period", "A specific date/time or interval of time during which the administration took place (or did not take place). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 0, 1, occurence); 2110 case 339257313: /*occurenceTiming*/ return new Property("occurence[x]", "Timing", "A specific date/time or interval of time during which the administration took place (or did not take place). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 0, 1, occurence); 2111 case -799233872: /*recorded*/ return new Property("recorded", "dateTime", "The date the occurrence of the MedicationAdministration was first captured in the record - potentially significantly after the occurrence of the event.", 0, 1, recorded); 2112 case 702379724: /*isSubPotent*/ return new Property("isSubPotent", "boolean", "An indication that the full dose was not administered.", 0, 1, isSubPotent); 2113 case 969489082: /*subPotentReason*/ return new Property("subPotentReason", "CodeableConcept", "The reason or reasons why the full dose was not administered.", 0, java.lang.Integer.MAX_VALUE, subPotentReason); 2114 case 481140686: /*performer*/ return new Property("performer", "", "The performer of the medication treatment. For devices this is the device that performed the administration of the medication. An IV Pump would be an example of a device that is performing the administration. Both the IV Pump and the practitioner that set the rate or bolus on the pump can be listed as performers.", 0, java.lang.Integer.MAX_VALUE, performer); 2115 case -934964668: /*reason*/ return new Property("reason", "CodeableReference(Condition|Observation|DiagnosticReport)", "A code, Condition or observation that supports why the medication was administered.", 0, java.lang.Integer.MAX_VALUE, reason); 2116 case 1095692943: /*request*/ return new Property("request", "Reference(MedicationRequest)", "The original request, instruction or authority to perform the administration.", 0, 1, request); 2117 case -1335157162: /*device*/ return new Property("device", "CodeableReference(Device)", "The device that is to be used for the administration of the medication (for example, PCA Pump).", 0, java.lang.Integer.MAX_VALUE, device); 2118 case 3387378: /*note*/ return new Property("note", "Annotation", "Extra information about the medication administration that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note); 2119 case -1326018889: /*dosage*/ return new Property("dosage", "", "Describes the medication dosage information details e.g. dose, rate, site, route, etc.", 0, 1, dosage); 2120 case 1835190426: /*eventHistory*/ return new Property("eventHistory", "Reference(Provenance)", "A summary of the events of interest that have occurred, such as when the administration was verified.", 0, java.lang.Integer.MAX_VALUE, eventHistory); 2121 default: return super.getNamedProperty(_hash, _name, _checkValid); 2122 } 2123 2124 } 2125 2126 @Override 2127 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2128 switch (hash) { 2129 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2130 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2131 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2132 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MedicationAdministrationStatusCodes> 2133 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : this.statusReason.toArray(new Base[this.statusReason.size()]); // CodeableConcept 2134 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2135 case 1998965455: /*medication*/ return this.medication == null ? new Base[0] : new Base[] {this.medication}; // CodeableReference 2136 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2137 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 2138 case -1248768647: /*supportingInformation*/ return this.supportingInformation == null ? new Base[0] : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 2139 case -1192857417: /*occurence*/ return this.occurence == null ? new Base[0] : new Base[] {this.occurence}; // DataType 2140 case -799233872: /*recorded*/ return this.recorded == null ? new Base[0] : new Base[] {this.recorded}; // DateTimeType 2141 case 702379724: /*isSubPotent*/ return this.isSubPotent == null ? new Base[0] : new Base[] {this.isSubPotent}; // BooleanType 2142 case 969489082: /*subPotentReason*/ return this.subPotentReason == null ? new Base[0] : this.subPotentReason.toArray(new Base[this.subPotentReason.size()]); // CodeableConcept 2143 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // MedicationAdministrationPerformerComponent 2144 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableReference 2145 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // Reference 2146 case -1335157162: /*device*/ return this.device == null ? new Base[0] : this.device.toArray(new Base[this.device.size()]); // CodeableReference 2147 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2148 case -1326018889: /*dosage*/ return this.dosage == null ? new Base[0] : new Base[] {this.dosage}; // MedicationAdministrationDosageComponent 2149 case 1835190426: /*eventHistory*/ return this.eventHistory == null ? new Base[0] : this.eventHistory.toArray(new Base[this.eventHistory.size()]); // Reference 2150 default: return super.getProperty(hash, name, checkValid); 2151 } 2152 2153 } 2154 2155 @Override 2156 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2157 switch (hash) { 2158 case -1618432855: // identifier 2159 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2160 return value; 2161 case -332612366: // basedOn 2162 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 2163 return value; 2164 case -995410646: // partOf 2165 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 2166 return value; 2167 case -892481550: // status 2168 value = new MedicationAdministrationStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2169 this.status = (Enumeration) value; // Enumeration<MedicationAdministrationStatusCodes> 2170 return value; 2171 case 2051346646: // statusReason 2172 this.getStatusReason().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2173 return value; 2174 case 50511102: // category 2175 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2176 return value; 2177 case 1998965455: // medication 2178 this.medication = TypeConvertor.castToCodeableReference(value); // CodeableReference 2179 return value; 2180 case -1867885268: // subject 2181 this.subject = TypeConvertor.castToReference(value); // Reference 2182 return value; 2183 case 1524132147: // encounter 2184 this.encounter = TypeConvertor.castToReference(value); // Reference 2185 return value; 2186 case -1248768647: // supportingInformation 2187 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); // Reference 2188 return value; 2189 case -1192857417: // occurence 2190 this.occurence = TypeConvertor.castToType(value); // DataType 2191 return value; 2192 case -799233872: // recorded 2193 this.recorded = TypeConvertor.castToDateTime(value); // DateTimeType 2194 return value; 2195 case 702379724: // isSubPotent 2196 this.isSubPotent = TypeConvertor.castToBoolean(value); // BooleanType 2197 return value; 2198 case 969489082: // subPotentReason 2199 this.getSubPotentReason().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2200 return value; 2201 case 481140686: // performer 2202 this.getPerformer().add((MedicationAdministrationPerformerComponent) value); // MedicationAdministrationPerformerComponent 2203 return value; 2204 case -934964668: // reason 2205 this.getReason().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2206 return value; 2207 case 1095692943: // request 2208 this.request = TypeConvertor.castToReference(value); // Reference 2209 return value; 2210 case -1335157162: // device 2211 this.getDevice().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 2212 return value; 2213 case 3387378: // note 2214 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2215 return value; 2216 case -1326018889: // dosage 2217 this.dosage = (MedicationAdministrationDosageComponent) value; // MedicationAdministrationDosageComponent 2218 return value; 2219 case 1835190426: // eventHistory 2220 this.getEventHistory().add(TypeConvertor.castToReference(value)); // Reference 2221 return value; 2222 default: return super.setProperty(hash, name, value); 2223 } 2224 2225 } 2226 2227 @Override 2228 public Base setProperty(String name, Base value) throws FHIRException { 2229 if (name.equals("identifier")) { 2230 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2231 } else if (name.equals("basedOn")) { 2232 this.getBasedOn().add(TypeConvertor.castToReference(value)); 2233 } else if (name.equals("partOf")) { 2234 this.getPartOf().add(TypeConvertor.castToReference(value)); 2235 } else if (name.equals("status")) { 2236 value = new MedicationAdministrationStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2237 this.status = (Enumeration) value; // Enumeration<MedicationAdministrationStatusCodes> 2238 } else if (name.equals("statusReason")) { 2239 this.getStatusReason().add(TypeConvertor.castToCodeableConcept(value)); 2240 } else if (name.equals("category")) { 2241 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 2242 } else if (name.equals("medication")) { 2243 this.medication = TypeConvertor.castToCodeableReference(value); // CodeableReference 2244 } else if (name.equals("subject")) { 2245 this.subject = TypeConvertor.castToReference(value); // Reference 2246 } else if (name.equals("encounter")) { 2247 this.encounter = TypeConvertor.castToReference(value); // Reference 2248 } else if (name.equals("supportingInformation")) { 2249 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); 2250 } else if (name.equals("occurence[x]")) { 2251 this.occurence = TypeConvertor.castToType(value); // DataType 2252 } else if (name.equals("recorded")) { 2253 this.recorded = TypeConvertor.castToDateTime(value); // DateTimeType 2254 } else if (name.equals("isSubPotent")) { 2255 this.isSubPotent = TypeConvertor.castToBoolean(value); // BooleanType 2256 } else if (name.equals("subPotentReason")) { 2257 this.getSubPotentReason().add(TypeConvertor.castToCodeableConcept(value)); 2258 } else if (name.equals("performer")) { 2259 this.getPerformer().add((MedicationAdministrationPerformerComponent) value); 2260 } else if (name.equals("reason")) { 2261 this.getReason().add(TypeConvertor.castToCodeableReference(value)); 2262 } else if (name.equals("request")) { 2263 this.request = TypeConvertor.castToReference(value); // Reference 2264 } else if (name.equals("device")) { 2265 this.getDevice().add(TypeConvertor.castToCodeableReference(value)); 2266 } else if (name.equals("note")) { 2267 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2268 } else if (name.equals("dosage")) { 2269 this.dosage = (MedicationAdministrationDosageComponent) value; // MedicationAdministrationDosageComponent 2270 } else if (name.equals("eventHistory")) { 2271 this.getEventHistory().add(TypeConvertor.castToReference(value)); 2272 } else 2273 return super.setProperty(name, value); 2274 return value; 2275 } 2276 2277 @Override 2278 public void removeChild(String name, Base value) throws FHIRException { 2279 if (name.equals("identifier")) { 2280 this.getIdentifier().remove(value); 2281 } else if (name.equals("basedOn")) { 2282 this.getBasedOn().remove(value); 2283 } else if (name.equals("partOf")) { 2284 this.getPartOf().remove(value); 2285 } else if (name.equals("status")) { 2286 value = new MedicationAdministrationStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2287 this.status = (Enumeration) value; // Enumeration<MedicationAdministrationStatusCodes> 2288 } else if (name.equals("statusReason")) { 2289 this.getStatusReason().remove(value); 2290 } else if (name.equals("category")) { 2291 this.getCategory().remove(value); 2292 } else if (name.equals("medication")) { 2293 this.medication = null; 2294 } else if (name.equals("subject")) { 2295 this.subject = null; 2296 } else if (name.equals("encounter")) { 2297 this.encounter = null; 2298 } else if (name.equals("supportingInformation")) { 2299 this.getSupportingInformation().remove(value); 2300 } else if (name.equals("occurence[x]")) { 2301 this.occurence = null; 2302 } else if (name.equals("recorded")) { 2303 this.recorded = null; 2304 } else if (name.equals("isSubPotent")) { 2305 this.isSubPotent = null; 2306 } else if (name.equals("subPotentReason")) { 2307 this.getSubPotentReason().remove(value); 2308 } else if (name.equals("performer")) { 2309 this.getPerformer().remove((MedicationAdministrationPerformerComponent) value); 2310 } else if (name.equals("reason")) { 2311 this.getReason().remove(value); 2312 } else if (name.equals("request")) { 2313 this.request = null; 2314 } else if (name.equals("device")) { 2315 this.getDevice().remove(value); 2316 } else if (name.equals("note")) { 2317 this.getNote().remove(value); 2318 } else if (name.equals("dosage")) { 2319 this.dosage = (MedicationAdministrationDosageComponent) value; // MedicationAdministrationDosageComponent 2320 } else if (name.equals("eventHistory")) { 2321 this.getEventHistory().remove(value); 2322 } else 2323 super.removeChild(name, value); 2324 2325 } 2326 2327 @Override 2328 public Base makeProperty(int hash, String name) throws FHIRException { 2329 switch (hash) { 2330 case -1618432855: return addIdentifier(); 2331 case -332612366: return addBasedOn(); 2332 case -995410646: return addPartOf(); 2333 case -892481550: return getStatusElement(); 2334 case 2051346646: return addStatusReason(); 2335 case 50511102: return addCategory(); 2336 case 1998965455: return getMedication(); 2337 case -1867885268: return getSubject(); 2338 case 1524132147: return getEncounter(); 2339 case -1248768647: return addSupportingInformation(); 2340 case 144188521: return getOccurence(); 2341 case -1192857417: return getOccurence(); 2342 case -799233872: return getRecordedElement(); 2343 case 702379724: return getIsSubPotentElement(); 2344 case 969489082: return addSubPotentReason(); 2345 case 481140686: return addPerformer(); 2346 case -934964668: return addReason(); 2347 case 1095692943: return getRequest(); 2348 case -1335157162: return addDevice(); 2349 case 3387378: return addNote(); 2350 case -1326018889: return getDosage(); 2351 case 1835190426: return addEventHistory(); 2352 default: return super.makeProperty(hash, name); 2353 } 2354 2355 } 2356 2357 @Override 2358 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2359 switch (hash) { 2360 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2361 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2362 case -995410646: /*partOf*/ return new String[] {"Reference"}; 2363 case -892481550: /*status*/ return new String[] {"code"}; 2364 case 2051346646: /*statusReason*/ return new String[] {"CodeableConcept"}; 2365 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2366 case 1998965455: /*medication*/ return new String[] {"CodeableReference"}; 2367 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2368 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 2369 case -1248768647: /*supportingInformation*/ return new String[] {"Reference"}; 2370 case -1192857417: /*occurence*/ return new String[] {"dateTime", "Period", "Timing"}; 2371 case -799233872: /*recorded*/ return new String[] {"dateTime"}; 2372 case 702379724: /*isSubPotent*/ return new String[] {"boolean"}; 2373 case 969489082: /*subPotentReason*/ return new String[] {"CodeableConcept"}; 2374 case 481140686: /*performer*/ return new String[] {}; 2375 case -934964668: /*reason*/ return new String[] {"CodeableReference"}; 2376 case 1095692943: /*request*/ return new String[] {"Reference"}; 2377 case -1335157162: /*device*/ return new String[] {"CodeableReference"}; 2378 case 3387378: /*note*/ return new String[] {"Annotation"}; 2379 case -1326018889: /*dosage*/ return new String[] {}; 2380 case 1835190426: /*eventHistory*/ return new String[] {"Reference"}; 2381 default: return super.getTypesForProperty(hash, name); 2382 } 2383 2384 } 2385 2386 @Override 2387 public Base addChild(String name) throws FHIRException { 2388 if (name.equals("identifier")) { 2389 return addIdentifier(); 2390 } 2391 else if (name.equals("basedOn")) { 2392 return addBasedOn(); 2393 } 2394 else if (name.equals("partOf")) { 2395 return addPartOf(); 2396 } 2397 else if (name.equals("status")) { 2398 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.status"); 2399 } 2400 else if (name.equals("statusReason")) { 2401 return addStatusReason(); 2402 } 2403 else if (name.equals("category")) { 2404 return addCategory(); 2405 } 2406 else if (name.equals("medication")) { 2407 this.medication = new CodeableReference(); 2408 return this.medication; 2409 } 2410 else if (name.equals("subject")) { 2411 this.subject = new Reference(); 2412 return this.subject; 2413 } 2414 else if (name.equals("encounter")) { 2415 this.encounter = new Reference(); 2416 return this.encounter; 2417 } 2418 else if (name.equals("supportingInformation")) { 2419 return addSupportingInformation(); 2420 } 2421 else if (name.equals("occurenceDateTime")) { 2422 this.occurence = new DateTimeType(); 2423 return this.occurence; 2424 } 2425 else if (name.equals("occurencePeriod")) { 2426 this.occurence = new Period(); 2427 return this.occurence; 2428 } 2429 else if (name.equals("occurenceTiming")) { 2430 this.occurence = new Timing(); 2431 return this.occurence; 2432 } 2433 else if (name.equals("recorded")) { 2434 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.recorded"); 2435 } 2436 else if (name.equals("isSubPotent")) { 2437 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.isSubPotent"); 2438 } 2439 else if (name.equals("subPotentReason")) { 2440 return addSubPotentReason(); 2441 } 2442 else if (name.equals("performer")) { 2443 return addPerformer(); 2444 } 2445 else if (name.equals("reason")) { 2446 return addReason(); 2447 } 2448 else if (name.equals("request")) { 2449 this.request = new Reference(); 2450 return this.request; 2451 } 2452 else if (name.equals("device")) { 2453 return addDevice(); 2454 } 2455 else if (name.equals("note")) { 2456 return addNote(); 2457 } 2458 else if (name.equals("dosage")) { 2459 this.dosage = new MedicationAdministrationDosageComponent(); 2460 return this.dosage; 2461 } 2462 else if (name.equals("eventHistory")) { 2463 return addEventHistory(); 2464 } 2465 else 2466 return super.addChild(name); 2467 } 2468 2469 public String fhirType() { 2470 return "MedicationAdministration"; 2471 2472 } 2473 2474 public MedicationAdministration copy() { 2475 MedicationAdministration dst = new MedicationAdministration(); 2476 copyValues(dst); 2477 return dst; 2478 } 2479 2480 public void copyValues(MedicationAdministration dst) { 2481 super.copyValues(dst); 2482 if (identifier != null) { 2483 dst.identifier = new ArrayList<Identifier>(); 2484 for (Identifier i : identifier) 2485 dst.identifier.add(i.copy()); 2486 }; 2487 if (basedOn != null) { 2488 dst.basedOn = new ArrayList<Reference>(); 2489 for (Reference i : basedOn) 2490 dst.basedOn.add(i.copy()); 2491 }; 2492 if (partOf != null) { 2493 dst.partOf = new ArrayList<Reference>(); 2494 for (Reference i : partOf) 2495 dst.partOf.add(i.copy()); 2496 }; 2497 dst.status = status == null ? null : status.copy(); 2498 if (statusReason != null) { 2499 dst.statusReason = new ArrayList<CodeableConcept>(); 2500 for (CodeableConcept i : statusReason) 2501 dst.statusReason.add(i.copy()); 2502 }; 2503 if (category != null) { 2504 dst.category = new ArrayList<CodeableConcept>(); 2505 for (CodeableConcept i : category) 2506 dst.category.add(i.copy()); 2507 }; 2508 dst.medication = medication == null ? null : medication.copy(); 2509 dst.subject = subject == null ? null : subject.copy(); 2510 dst.encounter = encounter == null ? null : encounter.copy(); 2511 if (supportingInformation != null) { 2512 dst.supportingInformation = new ArrayList<Reference>(); 2513 for (Reference i : supportingInformation) 2514 dst.supportingInformation.add(i.copy()); 2515 }; 2516 dst.occurence = occurence == null ? null : occurence.copy(); 2517 dst.recorded = recorded == null ? null : recorded.copy(); 2518 dst.isSubPotent = isSubPotent == null ? null : isSubPotent.copy(); 2519 if (subPotentReason != null) { 2520 dst.subPotentReason = new ArrayList<CodeableConcept>(); 2521 for (CodeableConcept i : subPotentReason) 2522 dst.subPotentReason.add(i.copy()); 2523 }; 2524 if (performer != null) { 2525 dst.performer = new ArrayList<MedicationAdministrationPerformerComponent>(); 2526 for (MedicationAdministrationPerformerComponent i : performer) 2527 dst.performer.add(i.copy()); 2528 }; 2529 if (reason != null) { 2530 dst.reason = new ArrayList<CodeableReference>(); 2531 for (CodeableReference i : reason) 2532 dst.reason.add(i.copy()); 2533 }; 2534 dst.request = request == null ? null : request.copy(); 2535 if (device != null) { 2536 dst.device = new ArrayList<CodeableReference>(); 2537 for (CodeableReference i : device) 2538 dst.device.add(i.copy()); 2539 }; 2540 if (note != null) { 2541 dst.note = new ArrayList<Annotation>(); 2542 for (Annotation i : note) 2543 dst.note.add(i.copy()); 2544 }; 2545 dst.dosage = dosage == null ? null : dosage.copy(); 2546 if (eventHistory != null) { 2547 dst.eventHistory = new ArrayList<Reference>(); 2548 for (Reference i : eventHistory) 2549 dst.eventHistory.add(i.copy()); 2550 }; 2551 } 2552 2553 protected MedicationAdministration typedCopy() { 2554 return copy(); 2555 } 2556 2557 @Override 2558 public boolean equalsDeep(Base other_) { 2559 if (!super.equalsDeep(other_)) 2560 return false; 2561 if (!(other_ instanceof MedicationAdministration)) 2562 return false; 2563 MedicationAdministration o = (MedicationAdministration) other_; 2564 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(partOf, o.partOf, true) 2565 && compareDeep(status, o.status, true) && compareDeep(statusReason, o.statusReason, true) && compareDeep(category, o.category, true) 2566 && compareDeep(medication, o.medication, true) && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 2567 && compareDeep(supportingInformation, o.supportingInformation, true) && compareDeep(occurence, o.occurence, true) 2568 && compareDeep(recorded, o.recorded, true) && compareDeep(isSubPotent, o.isSubPotent, true) && compareDeep(subPotentReason, o.subPotentReason, true) 2569 && compareDeep(performer, o.performer, true) && compareDeep(reason, o.reason, true) && compareDeep(request, o.request, true) 2570 && compareDeep(device, o.device, true) && compareDeep(note, o.note, true) && compareDeep(dosage, o.dosage, true) 2571 && compareDeep(eventHistory, o.eventHistory, true); 2572 } 2573 2574 @Override 2575 public boolean equalsShallow(Base other_) { 2576 if (!super.equalsShallow(other_)) 2577 return false; 2578 if (!(other_ instanceof MedicationAdministration)) 2579 return false; 2580 MedicationAdministration o = (MedicationAdministration) other_; 2581 return compareValues(status, o.status, true) && compareValues(recorded, o.recorded, true) && compareValues(isSubPotent, o.isSubPotent, true) 2582 ; 2583 } 2584 2585 public boolean isEmpty() { 2586 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf 2587 , status, statusReason, category, medication, subject, encounter, supportingInformation 2588 , occurence, recorded, isSubPotent, subPotentReason, performer, reason, request 2589 , device, note, dosage, eventHistory); 2590 } 2591 2592 @Override 2593 public ResourceType getResourceType() { 2594 return ResourceType.MedicationAdministration; 2595 } 2596 2597 /** 2598 * Search parameter: <b>device</b> 2599 * <p> 2600 * Description: <b>Return administrations with this administration device identity</b><br> 2601 * Type: <b>reference</b><br> 2602 * Path: <b>MedicationAdministration.device.reference</b><br> 2603 * </p> 2604 */ 2605 @SearchParamDefinition(name="device", path="MedicationAdministration.device.reference", description="Return administrations with this administration device identity", type="reference", target={Device.class } ) 2606 public static final String SP_DEVICE = "device"; 2607 /** 2608 * <b>Fluent Client</b> search parameter constant for <b>device</b> 2609 * <p> 2610 * Description: <b>Return administrations with this administration device identity</b><br> 2611 * Type: <b>reference</b><br> 2612 * Path: <b>MedicationAdministration.device.reference</b><br> 2613 * </p> 2614 */ 2615 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEVICE); 2616 2617/** 2618 * Constant for fluent queries to be used to add include statements. Specifies 2619 * the path value of "<b>MedicationAdministration:device</b>". 2620 */ 2621 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("MedicationAdministration:device").toLocked(); 2622 2623 /** 2624 * Search parameter: <b>performer-device-code</b> 2625 * <p> 2626 * Description: <b>The identity of the individual who administered the medication</b><br> 2627 * Type: <b>token</b><br> 2628 * Path: <b>MedicationAdministration.performer.actor.concept</b><br> 2629 * </p> 2630 */ 2631 @SearchParamDefinition(name="performer-device-code", path="MedicationAdministration.performer.actor.concept", description="The identity of the individual who administered the medication", type="token" ) 2632 public static final String SP_PERFORMER_DEVICE_CODE = "performer-device-code"; 2633 /** 2634 * <b>Fluent Client</b> search parameter constant for <b>performer-device-code</b> 2635 * <p> 2636 * Description: <b>The identity of the individual who administered the medication</b><br> 2637 * Type: <b>token</b><br> 2638 * Path: <b>MedicationAdministration.performer.actor.concept</b><br> 2639 * </p> 2640 */ 2641 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PERFORMER_DEVICE_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PERFORMER_DEVICE_CODE); 2642 2643 /** 2644 * Search parameter: <b>performer</b> 2645 * <p> 2646 * Description: <b>The identity of the individual who administered the medication</b><br> 2647 * Type: <b>reference</b><br> 2648 * Path: <b>MedicationAdministration.performer.actor.reference</b><br> 2649 * </p> 2650 */ 2651 @SearchParamDefinition(name="performer", path="MedicationAdministration.performer.actor.reference", description="The identity of the individual who administered the medication", type="reference", target={Device.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2652 public static final String SP_PERFORMER = "performer"; 2653 /** 2654 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2655 * <p> 2656 * Description: <b>The identity of the individual who administered the medication</b><br> 2657 * Type: <b>reference</b><br> 2658 * Path: <b>MedicationAdministration.performer.actor.reference</b><br> 2659 * </p> 2660 */ 2661 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 2662 2663/** 2664 * Constant for fluent queries to be used to add include statements. Specifies 2665 * the path value of "<b>MedicationAdministration:performer</b>". 2666 */ 2667 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("MedicationAdministration:performer").toLocked(); 2668 2669 /** 2670 * Search parameter: <b>reason-given-code</b> 2671 * <p> 2672 * Description: <b>Reasons for administering the medication</b><br> 2673 * Type: <b>token</b><br> 2674 * Path: <b>MedicationAdministration.reason.concept</b><br> 2675 * </p> 2676 */ 2677 @SearchParamDefinition(name="reason-given-code", path="MedicationAdministration.reason.concept", description="Reasons for administering the medication", type="token" ) 2678 public static final String SP_REASON_GIVEN_CODE = "reason-given-code"; 2679 /** 2680 * <b>Fluent Client</b> search parameter constant for <b>reason-given-code</b> 2681 * <p> 2682 * Description: <b>Reasons for administering the medication</b><br> 2683 * Type: <b>token</b><br> 2684 * Path: <b>MedicationAdministration.reason.concept</b><br> 2685 * </p> 2686 */ 2687 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_GIVEN_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REASON_GIVEN_CODE); 2688 2689 /** 2690 * Search parameter: <b>reason-given</b> 2691 * <p> 2692 * Description: <b>Reference to a resource (by instance)</b><br> 2693 * Type: <b>reference</b><br> 2694 * Path: <b>MedicationAdministration.reason.reference</b><br> 2695 * </p> 2696 */ 2697 @SearchParamDefinition(name="reason-given", path="MedicationAdministration.reason.reference", description="Reference to a resource (by instance)", type="reference", target={Condition.class, DiagnosticReport.class, Observation.class } ) 2698 public static final String SP_REASON_GIVEN = "reason-given"; 2699 /** 2700 * <b>Fluent Client</b> search parameter constant for <b>reason-given</b> 2701 * <p> 2702 * Description: <b>Reference to a resource (by instance)</b><br> 2703 * Type: <b>reference</b><br> 2704 * Path: <b>MedicationAdministration.reason.reference</b><br> 2705 * </p> 2706 */ 2707 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REASON_GIVEN = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REASON_GIVEN); 2708 2709/** 2710 * Constant for fluent queries to be used to add include statements. Specifies 2711 * the path value of "<b>MedicationAdministration:reason-given</b>". 2712 */ 2713 public static final ca.uhn.fhir.model.api.Include INCLUDE_REASON_GIVEN = new ca.uhn.fhir.model.api.Include("MedicationAdministration:reason-given").toLocked(); 2714 2715 /** 2716 * Search parameter: <b>reason-not-given</b> 2717 * <p> 2718 * Description: <b>Reasons for not administering the medication</b><br> 2719 * Type: <b>token</b><br> 2720 * Path: <b>MedicationAdministration.statusReason</b><br> 2721 * </p> 2722 */ 2723 @SearchParamDefinition(name="reason-not-given", path="MedicationAdministration.statusReason", description="Reasons for not administering the medication", type="token" ) 2724 public static final String SP_REASON_NOT_GIVEN = "reason-not-given"; 2725 /** 2726 * <b>Fluent Client</b> search parameter constant for <b>reason-not-given</b> 2727 * <p> 2728 * Description: <b>Reasons for not administering the medication</b><br> 2729 * Type: <b>token</b><br> 2730 * Path: <b>MedicationAdministration.statusReason</b><br> 2731 * </p> 2732 */ 2733 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_NOT_GIVEN = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REASON_NOT_GIVEN); 2734 2735 /** 2736 * Search parameter: <b>request</b> 2737 * <p> 2738 * Description: <b>The identity of a request to list administrations from</b><br> 2739 * Type: <b>reference</b><br> 2740 * Path: <b>MedicationAdministration.request</b><br> 2741 * </p> 2742 */ 2743 @SearchParamDefinition(name="request", path="MedicationAdministration.request", description="The identity of a request to list administrations from", type="reference", target={MedicationRequest.class } ) 2744 public static final String SP_REQUEST = "request"; 2745 /** 2746 * <b>Fluent Client</b> search parameter constant for <b>request</b> 2747 * <p> 2748 * Description: <b>The identity of a request to list administrations from</b><br> 2749 * Type: <b>reference</b><br> 2750 * Path: <b>MedicationAdministration.request</b><br> 2751 * </p> 2752 */ 2753 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST); 2754 2755/** 2756 * Constant for fluent queries to be used to add include statements. Specifies 2757 * the path value of "<b>MedicationAdministration:request</b>". 2758 */ 2759 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include("MedicationAdministration:request").toLocked(); 2760 2761 /** 2762 * Search parameter: <b>subject</b> 2763 * <p> 2764 * Description: <b>The identity of the individual or group to list administrations for</b><br> 2765 * Type: <b>reference</b><br> 2766 * Path: <b>MedicationAdministration.subject</b><br> 2767 * </p> 2768 */ 2769 @SearchParamDefinition(name="subject", path="MedicationAdministration.subject", description="The identity of the individual or group to list administrations for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class } ) 2770 public static final String SP_SUBJECT = "subject"; 2771 /** 2772 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2773 * <p> 2774 * Description: <b>The identity of the individual or group to list administrations for</b><br> 2775 * Type: <b>reference</b><br> 2776 * Path: <b>MedicationAdministration.subject</b><br> 2777 * </p> 2778 */ 2779 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2780 2781/** 2782 * Constant for fluent queries to be used to add include statements. Specifies 2783 * the path value of "<b>MedicationAdministration:subject</b>". 2784 */ 2785 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("MedicationAdministration:subject").toLocked(); 2786 2787 /** 2788 * Search parameter: <b>code</b> 2789 * <p> 2790 * Description: <b>Multiple Resources: 2791 2792* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 2793* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 2794* [AuditEvent](auditevent.html): More specific code for the event 2795* [Basic](basic.html): Kind of Resource 2796* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 2797* [Condition](condition.html): Code for the condition 2798* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 2799* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 2800* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 2801* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 2802* [ImagingSelection](imagingselection.html): The imaging selection status 2803* [List](list.html): What the purpose of this list is 2804* [Medication](medication.html): Returns medications for a specific code 2805* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 2806* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 2807* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 2808* [MedicationStatement](medicationstatement.html): Return statements of this medication code 2809* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 2810* [Observation](observation.html): The code of the observation type 2811* [Procedure](procedure.html): A code to identify a procedure 2812* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 2813* [Task](task.html): Search by task code 2814</b><br> 2815 * Type: <b>token</b><br> 2816 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 2817 * </p> 2818 */ 2819 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 2820 public static final String SP_CODE = "code"; 2821 /** 2822 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2823 * <p> 2824 * Description: <b>Multiple Resources: 2825 2826* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 2827* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 2828* [AuditEvent](auditevent.html): More specific code for the event 2829* [Basic](basic.html): Kind of Resource 2830* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 2831* [Condition](condition.html): Code for the condition 2832* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 2833* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 2834* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 2835* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 2836* [ImagingSelection](imagingselection.html): The imaging selection status 2837* [List](list.html): What the purpose of this list is 2838* [Medication](medication.html): Returns medications for a specific code 2839* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 2840* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 2841* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 2842* [MedicationStatement](medicationstatement.html): Return statements of this medication code 2843* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 2844* [Observation](observation.html): The code of the observation type 2845* [Procedure](procedure.html): A code to identify a procedure 2846* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 2847* [Task](task.html): Search by task code 2848</b><br> 2849 * Type: <b>token</b><br> 2850 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 2851 * </p> 2852 */ 2853 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2854 2855 /** 2856 * Search parameter: <b>identifier</b> 2857 * <p> 2858 * Description: <b>Multiple Resources: 2859 2860* [Account](account.html): Account number 2861* [AdverseEvent](adverseevent.html): Business identifier for the event 2862* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2863* [Appointment](appointment.html): An Identifier of the Appointment 2864* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2865* [Basic](basic.html): Business identifier 2866* [BodyStructure](bodystructure.html): Bodystructure identifier 2867* [CarePlan](careplan.html): External Ids for this plan 2868* [CareTeam](careteam.html): External Ids for this team 2869* [ChargeItem](chargeitem.html): Business Identifier for item 2870* [Claim](claim.html): The primary identifier of the financial resource 2871* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2872* [ClinicalImpression](clinicalimpression.html): Business identifier 2873* [Communication](communication.html): Unique identifier 2874* [CommunicationRequest](communicationrequest.html): Unique identifier 2875* [Composition](composition.html): Version-independent identifier for the Composition 2876* [Condition](condition.html): A unique identifier of the condition record 2877* [Consent](consent.html): Identifier for this record (external references) 2878* [Contract](contract.html): The identity of the contract 2879* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2880* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2881* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2882* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2883* [DeviceRequest](devicerequest.html): Business identifier for request/order 2884* [DeviceUsage](deviceusage.html): Search by identifier 2885* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2886* [DocumentReference](documentreference.html): Identifier of the attachment binary 2887* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2888* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2889* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2890* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2891* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2892* [Flag](flag.html): Business identifier 2893* [Goal](goal.html): External Ids for this goal 2894* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2895* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2896* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2897* [Immunization](immunization.html): Business identifier 2898* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2899* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2900* [Invoice](invoice.html): Business Identifier for item 2901* [List](list.html): Business identifier 2902* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2903* [Medication](medication.html): Returns medications with this external identifier 2904* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2905* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2906* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2907* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2908* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2909* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2910* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2911* [Observation](observation.html): The unique id for a particular observation 2912* [Person](person.html): A person Identifier 2913* [Procedure](procedure.html): A unique identifier for a procedure 2914* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2915* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2916* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2917* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2918* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2919* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2920* [Specimen](specimen.html): The unique identifier associated with the specimen 2921* [SupplyDelivery](supplydelivery.html): External identifier 2922* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 2923* [Task](task.html): Search for a task instance by its business identifier 2924* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 2925</b><br> 2926 * Type: <b>token</b><br> 2927 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 2928 * </p> 2929 */ 2930 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 2931 public static final String SP_IDENTIFIER = "identifier"; 2932 /** 2933 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2934 * <p> 2935 * Description: <b>Multiple Resources: 2936 2937* [Account](account.html): Account number 2938* [AdverseEvent](adverseevent.html): Business identifier for the event 2939* [AllergyIntolerance](allergyintolerance.html): External ids for this item 2940* [Appointment](appointment.html): An Identifier of the Appointment 2941* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 2942* [Basic](basic.html): Business identifier 2943* [BodyStructure](bodystructure.html): Bodystructure identifier 2944* [CarePlan](careplan.html): External Ids for this plan 2945* [CareTeam](careteam.html): External Ids for this team 2946* [ChargeItem](chargeitem.html): Business Identifier for item 2947* [Claim](claim.html): The primary identifier of the financial resource 2948* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 2949* [ClinicalImpression](clinicalimpression.html): Business identifier 2950* [Communication](communication.html): Unique identifier 2951* [CommunicationRequest](communicationrequest.html): Unique identifier 2952* [Composition](composition.html): Version-independent identifier for the Composition 2953* [Condition](condition.html): A unique identifier of the condition record 2954* [Consent](consent.html): Identifier for this record (external references) 2955* [Contract](contract.html): The identity of the contract 2956* [Coverage](coverage.html): The primary identifier of the insured and the coverage 2957* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 2958* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 2959* [DetectedIssue](detectedissue.html): Unique id for the detected issue 2960* [DeviceRequest](devicerequest.html): Business identifier for request/order 2961* [DeviceUsage](deviceusage.html): Search by identifier 2962* [DiagnosticReport](diagnosticreport.html): An identifier for the report 2963* [DocumentReference](documentreference.html): Identifier of the attachment binary 2964* [Encounter](encounter.html): Identifier(s) by which this encounter is known 2965* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 2966* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 2967* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 2968* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 2969* [Flag](flag.html): Business identifier 2970* [Goal](goal.html): External Ids for this goal 2971* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 2972* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 2973* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 2974* [Immunization](immunization.html): Business identifier 2975* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 2976* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 2977* [Invoice](invoice.html): Business Identifier for item 2978* [List](list.html): Business identifier 2979* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 2980* [Medication](medication.html): Returns medications with this external identifier 2981* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 2982* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 2983* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 2984* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 2985* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 2986* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 2987* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 2988* [Observation](observation.html): The unique id for a particular observation 2989* [Person](person.html): A person Identifier 2990* [Procedure](procedure.html): A unique identifier for a procedure 2991* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 2992* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 2993* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 2994* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 2995* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 2996* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 2997* [Specimen](specimen.html): The unique identifier associated with the specimen 2998* [SupplyDelivery](supplydelivery.html): External identifier 2999* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3000* [Task](task.html): Search for a task instance by its business identifier 3001* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3002</b><br> 3003 * Type: <b>token</b><br> 3004 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3005 * </p> 3006 */ 3007 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3008 3009 /** 3010 * Search parameter: <b>patient</b> 3011 * <p> 3012 * Description: <b>Multiple Resources: 3013 3014* [Account](account.html): The entity that caused the expenses 3015* [AdverseEvent](adverseevent.html): Subject impacted by event 3016* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3017* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3018* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3019* [AuditEvent](auditevent.html): Where the activity involved patient data 3020* [Basic](basic.html): Identifies the focus of this resource 3021* [BodyStructure](bodystructure.html): Who this is about 3022* [CarePlan](careplan.html): Who the care plan is for 3023* [CareTeam](careteam.html): Who care team is for 3024* [ChargeItem](chargeitem.html): Individual service was done for/to 3025* [Claim](claim.html): Patient receiving the products or services 3026* [ClaimResponse](claimresponse.html): The subject of care 3027* [ClinicalImpression](clinicalimpression.html): Patient assessed 3028* [Communication](communication.html): Focus of message 3029* [CommunicationRequest](communicationrequest.html): Focus of message 3030* [Composition](composition.html): Who and/or what the composition is about 3031* [Condition](condition.html): Who has the condition? 3032* [Consent](consent.html): Who the consent applies to 3033* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3034* [Coverage](coverage.html): Retrieve coverages for a patient 3035* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3036* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3037* [DetectedIssue](detectedissue.html): Associated patient 3038* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3039* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3040* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3041* [DocumentReference](documentreference.html): Who/what is the subject of the document 3042* [Encounter](encounter.html): The patient present at the encounter 3043* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3044* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3045* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3046* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3047* [Flag](flag.html): The identity of a subject to list flags for 3048* [Goal](goal.html): Who this goal is intended for 3049* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3050* [ImagingSelection](imagingselection.html): Who the study is about 3051* [ImagingStudy](imagingstudy.html): Who the study is about 3052* [Immunization](immunization.html): The patient for the vaccination record 3053* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3054* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3055* [Invoice](invoice.html): Recipient(s) of goods and services 3056* [List](list.html): If all resources have the same subject 3057* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3058* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3059* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3060* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3061* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3062* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3063* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3064* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3065* [Observation](observation.html): The subject that the observation is about (if patient) 3066* [Person](person.html): The Person links to this Patient 3067* [Procedure](procedure.html): Search by subject - a patient 3068* [Provenance](provenance.html): Where the activity involved patient data 3069* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3070* [RelatedPerson](relatedperson.html): The patient this related person is related to 3071* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3072* [ResearchSubject](researchsubject.html): Who or what is part of study 3073* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3074* [ServiceRequest](servicerequest.html): Search by subject - a patient 3075* [Specimen](specimen.html): The patient the specimen comes from 3076* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3077* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3078* [Task](task.html): Search by patient 3079* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3080</b><br> 3081 * Type: <b>reference</b><br> 3082 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3083 * </p> 3084 */ 3085 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 3086 public static final String SP_PATIENT = "patient"; 3087 /** 3088 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3089 * <p> 3090 * Description: <b>Multiple Resources: 3091 3092* [Account](account.html): The entity that caused the expenses 3093* [AdverseEvent](adverseevent.html): Subject impacted by event 3094* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3095* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3096* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3097* [AuditEvent](auditevent.html): Where the activity involved patient data 3098* [Basic](basic.html): Identifies the focus of this resource 3099* [BodyStructure](bodystructure.html): Who this is about 3100* [CarePlan](careplan.html): Who the care plan is for 3101* [CareTeam](careteam.html): Who care team is for 3102* [ChargeItem](chargeitem.html): Individual service was done for/to 3103* [Claim](claim.html): Patient receiving the products or services 3104* [ClaimResponse](claimresponse.html): The subject of care 3105* [ClinicalImpression](clinicalimpression.html): Patient assessed 3106* [Communication](communication.html): Focus of message 3107* [CommunicationRequest](communicationrequest.html): Focus of message 3108* [Composition](composition.html): Who and/or what the composition is about 3109* [Condition](condition.html): Who has the condition? 3110* [Consent](consent.html): Who the consent applies to 3111* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3112* [Coverage](coverage.html): Retrieve coverages for a patient 3113* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3114* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3115* [DetectedIssue](detectedissue.html): Associated patient 3116* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3117* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3118* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3119* [DocumentReference](documentreference.html): Who/what is the subject of the document 3120* [Encounter](encounter.html): The patient present at the encounter 3121* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3122* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3123* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3124* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3125* [Flag](flag.html): The identity of a subject to list flags for 3126* [Goal](goal.html): Who this goal is intended for 3127* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3128* [ImagingSelection](imagingselection.html): Who the study is about 3129* [ImagingStudy](imagingstudy.html): Who the study is about 3130* [Immunization](immunization.html): The patient for the vaccination record 3131* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3132* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3133* [Invoice](invoice.html): Recipient(s) of goods and services 3134* [List](list.html): If all resources have the same subject 3135* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3136* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3137* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3138* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3139* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3140* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3141* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3142* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3143* [Observation](observation.html): The subject that the observation is about (if patient) 3144* [Person](person.html): The Person links to this Patient 3145* [Procedure](procedure.html): Search by subject - a patient 3146* [Provenance](provenance.html): Where the activity involved patient data 3147* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3148* [RelatedPerson](relatedperson.html): The patient this related person is related to 3149* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3150* [ResearchSubject](researchsubject.html): Who or what is part of study 3151* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3152* [ServiceRequest](servicerequest.html): Search by subject - a patient 3153* [Specimen](specimen.html): The patient the specimen comes from 3154* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3155* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3156* [Task](task.html): Search by patient 3157* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3158</b><br> 3159 * Type: <b>reference</b><br> 3160 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3161 * </p> 3162 */ 3163 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3164 3165/** 3166 * Constant for fluent queries to be used to add include statements. Specifies 3167 * the path value of "<b>MedicationAdministration:patient</b>". 3168 */ 3169 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("MedicationAdministration:patient").toLocked(); 3170 3171 /** 3172 * Search parameter: <b>date</b> 3173 * <p> 3174 * Description: <b>Multiple Resources: 3175 3176* [MedicationAdministration](medicationadministration.html): Date administration happened (or did not happen) 3177</b><br> 3178 * Type: <b>date</b><br> 3179 * Path: <b>MedicationAdministration.occurence.ofType(dateTime) | MedicationAdministration.occurence.ofType(Period)</b><br> 3180 * </p> 3181 */ 3182 @SearchParamDefinition(name="date", path="MedicationAdministration.occurence.ofType(dateTime) | MedicationAdministration.occurence.ofType(Period)", description="Multiple Resources: \r\n\r\n* [MedicationAdministration](medicationadministration.html): Date administration happened (or did not happen)\r\n", type="date" ) 3183 public static final String SP_DATE = "date"; 3184 /** 3185 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3186 * <p> 3187 * Description: <b>Multiple Resources: 3188 3189* [MedicationAdministration](medicationadministration.html): Date administration happened (or did not happen) 3190</b><br> 3191 * Type: <b>date</b><br> 3192 * Path: <b>MedicationAdministration.occurence.ofType(dateTime) | MedicationAdministration.occurence.ofType(Period)</b><br> 3193 * </p> 3194 */ 3195 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3196 3197 /** 3198 * Search parameter: <b>encounter</b> 3199 * <p> 3200 * Description: <b>Multiple Resources: 3201 3202* [MedicationAdministration](medicationadministration.html): Return administrations that share this encounter 3203* [MedicationRequest](medicationrequest.html): Return prescriptions with this encounter identifier 3204</b><br> 3205 * Type: <b>reference</b><br> 3206 * Path: <b>MedicationAdministration.encounter | MedicationRequest.encounter</b><br> 3207 * </p> 3208 */ 3209 @SearchParamDefinition(name="encounter", path="MedicationAdministration.encounter | MedicationRequest.encounter", description="Multiple Resources: \r\n\r\n* [MedicationAdministration](medicationadministration.html): Return administrations that share this encounter\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 3210 public static final String SP_ENCOUNTER = "encounter"; 3211 /** 3212 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3213 * <p> 3214 * Description: <b>Multiple Resources: 3215 3216* [MedicationAdministration](medicationadministration.html): Return administrations that share this encounter 3217* [MedicationRequest](medicationrequest.html): Return prescriptions with this encounter identifier 3218</b><br> 3219 * Type: <b>reference</b><br> 3220 * Path: <b>MedicationAdministration.encounter | MedicationRequest.encounter</b><br> 3221 * </p> 3222 */ 3223 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 3224 3225/** 3226 * Constant for fluent queries to be used to add include statements. Specifies 3227 * the path value of "<b>MedicationAdministration:encounter</b>". 3228 */ 3229 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("MedicationAdministration:encounter").toLocked(); 3230 3231 /** 3232 * Search parameter: <b>medication</b> 3233 * <p> 3234 * Description: <b>Multiple Resources: 3235 3236* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference 3237* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource 3238* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference 3239* [MedicationStatement](medicationstatement.html): Return statements of this medication reference 3240</b><br> 3241 * Type: <b>reference</b><br> 3242 * Path: <b>MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference</b><br> 3243 * </p> 3244 */ 3245 @SearchParamDefinition(name="medication", path="MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference", description="Multiple Resources: \r\n\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication reference\r\n", type="reference", target={Medication.class } ) 3246 public static final String SP_MEDICATION = "medication"; 3247 /** 3248 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 3249 * <p> 3250 * Description: <b>Multiple Resources: 3251 3252* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference 3253* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource 3254* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference 3255* [MedicationStatement](medicationstatement.html): Return statements of this medication reference 3256</b><br> 3257 * Type: <b>reference</b><br> 3258 * Path: <b>MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference</b><br> 3259 * </p> 3260 */ 3261 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MEDICATION); 3262 3263/** 3264 * Constant for fluent queries to be used to add include statements. Specifies 3265 * the path value of "<b>MedicationAdministration:medication</b>". 3266 */ 3267 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include("MedicationAdministration:medication").toLocked(); 3268 3269 /** 3270 * Search parameter: <b>status</b> 3271 * <p> 3272 * Description: <b>Multiple Resources: 3273 3274* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified) 3275* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status 3276* [MedicationRequest](medicationrequest.html): Status of the prescription 3277* [MedicationStatement](medicationstatement.html): Return statements that match the given status 3278</b><br> 3279 * Type: <b>token</b><br> 3280 * Path: <b>MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status</b><br> 3281 * </p> 3282 */ 3283 @SearchParamDefinition(name="status", path="MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status", description="Multiple Resources: \r\n\r\n* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified)\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status\r\n* [MedicationRequest](medicationrequest.html): Status of the prescription\r\n* [MedicationStatement](medicationstatement.html): Return statements that match the given status\r\n", type="token" ) 3284 public static final String SP_STATUS = "status"; 3285 /** 3286 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3287 * <p> 3288 * Description: <b>Multiple Resources: 3289 3290* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified) 3291* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status 3292* [MedicationRequest](medicationrequest.html): Status of the prescription 3293* [MedicationStatement](medicationstatement.html): Return statements that match the given status 3294</b><br> 3295 * Type: <b>token</b><br> 3296 * Path: <b>MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status</b><br> 3297 * </p> 3298 */ 3299 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3300 3301 3302} 3303