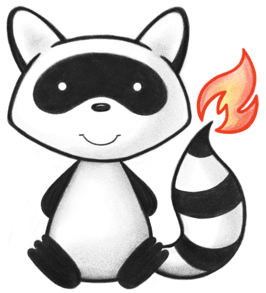
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order. 052 */ 053@ResourceDef(name="MedicationDispense", profile="http://hl7.org/fhir/StructureDefinition/MedicationDispense") 054public class MedicationDispense extends DomainResource { 055 056 public enum MedicationDispenseStatusCodes { 057 /** 058 * The core event has not started yet, but some staging activities have begun (e.g. initial compounding or packaging of medication). Preparation stages may be tracked for billing purposes. 059 */ 060 PREPARATION, 061 /** 062 * The dispensed product is ready for pickup. 063 */ 064 INPROGRESS, 065 /** 066 * The dispensed product was not and will never be picked up by the patient. 067 */ 068 CANCELLED, 069 /** 070 * The dispense process is paused while waiting for an external event to reactivate the dispense. For example, new stock has arrived or the prescriber has called. 071 */ 072 ONHOLD, 073 /** 074 * The dispensed product has been picked up. 075 */ 076 COMPLETED, 077 /** 078 * The dispense was entered in error and therefore nullified. 079 */ 080 ENTEREDINERROR, 081 /** 082 * Actions implied by the dispense have been permanently halted, before all of them occurred. 083 */ 084 STOPPED, 085 /** 086 * The dispense was declined and not performed. 087 */ 088 DECLINED, 089 /** 090 * The authoring system does not know which of the status values applies for this medication dispense. Note: this concept is not to be used for other - one of the listed statuses is presumed to apply, it's just now known which one. 091 */ 092 UNKNOWN, 093 /** 094 * added to help the parsers with the generic types 095 */ 096 NULL; 097 public static MedicationDispenseStatusCodes fromCode(String codeString) throws FHIRException { 098 if (codeString == null || "".equals(codeString)) 099 return null; 100 if ("preparation".equals(codeString)) 101 return PREPARATION; 102 if ("in-progress".equals(codeString)) 103 return INPROGRESS; 104 if ("cancelled".equals(codeString)) 105 return CANCELLED; 106 if ("on-hold".equals(codeString)) 107 return ONHOLD; 108 if ("completed".equals(codeString)) 109 return COMPLETED; 110 if ("entered-in-error".equals(codeString)) 111 return ENTEREDINERROR; 112 if ("stopped".equals(codeString)) 113 return STOPPED; 114 if ("declined".equals(codeString)) 115 return DECLINED; 116 if ("unknown".equals(codeString)) 117 return UNKNOWN; 118 if (Configuration.isAcceptInvalidEnums()) 119 return null; 120 else 121 throw new FHIRException("Unknown MedicationDispenseStatusCodes code '"+codeString+"'"); 122 } 123 public String toCode() { 124 switch (this) { 125 case PREPARATION: return "preparation"; 126 case INPROGRESS: return "in-progress"; 127 case CANCELLED: return "cancelled"; 128 case ONHOLD: return "on-hold"; 129 case COMPLETED: return "completed"; 130 case ENTEREDINERROR: return "entered-in-error"; 131 case STOPPED: return "stopped"; 132 case DECLINED: return "declined"; 133 case UNKNOWN: return "unknown"; 134 case NULL: return null; 135 default: return "?"; 136 } 137 } 138 public String getSystem() { 139 switch (this) { 140 case PREPARATION: return "http://hl7.org/fhir/CodeSystem/medicationdispense-status"; 141 case INPROGRESS: return "http://hl7.org/fhir/CodeSystem/medicationdispense-status"; 142 case CANCELLED: return "http://hl7.org/fhir/CodeSystem/medicationdispense-status"; 143 case ONHOLD: return "http://hl7.org/fhir/CodeSystem/medicationdispense-status"; 144 case COMPLETED: return "http://hl7.org/fhir/CodeSystem/medicationdispense-status"; 145 case ENTEREDINERROR: return "http://hl7.org/fhir/CodeSystem/medicationdispense-status"; 146 case STOPPED: return "http://hl7.org/fhir/CodeSystem/medicationdispense-status"; 147 case DECLINED: return "http://hl7.org/fhir/CodeSystem/medicationdispense-status"; 148 case UNKNOWN: return "http://hl7.org/fhir/CodeSystem/medicationdispense-status"; 149 case NULL: return null; 150 default: return "?"; 151 } 152 } 153 public String getDefinition() { 154 switch (this) { 155 case PREPARATION: return "The core event has not started yet, but some staging activities have begun (e.g. initial compounding or packaging of medication). Preparation stages may be tracked for billing purposes."; 156 case INPROGRESS: return "The dispensed product is ready for pickup."; 157 case CANCELLED: return "The dispensed product was not and will never be picked up by the patient."; 158 case ONHOLD: return "The dispense process is paused while waiting for an external event to reactivate the dispense. For example, new stock has arrived or the prescriber has called."; 159 case COMPLETED: return "The dispensed product has been picked up."; 160 case ENTEREDINERROR: return "The dispense was entered in error and therefore nullified."; 161 case STOPPED: return "Actions implied by the dispense have been permanently halted, before all of them occurred."; 162 case DECLINED: return "The dispense was declined and not performed."; 163 case UNKNOWN: return "The authoring system does not know which of the status values applies for this medication dispense. Note: this concept is not to be used for other - one of the listed statuses is presumed to apply, it's just now known which one."; 164 case NULL: return null; 165 default: return "?"; 166 } 167 } 168 public String getDisplay() { 169 switch (this) { 170 case PREPARATION: return "Preparation"; 171 case INPROGRESS: return "In Progress"; 172 case CANCELLED: return "Cancelled"; 173 case ONHOLD: return "On Hold"; 174 case COMPLETED: return "Completed"; 175 case ENTEREDINERROR: return "Entered in Error"; 176 case STOPPED: return "Stopped"; 177 case DECLINED: return "Declined"; 178 case UNKNOWN: return "Unknown"; 179 case NULL: return null; 180 default: return "?"; 181 } 182 } 183 } 184 185 public static class MedicationDispenseStatusCodesEnumFactory implements EnumFactory<MedicationDispenseStatusCodes> { 186 public MedicationDispenseStatusCodes fromCode(String codeString) throws IllegalArgumentException { 187 if (codeString == null || "".equals(codeString)) 188 if (codeString == null || "".equals(codeString)) 189 return null; 190 if ("preparation".equals(codeString)) 191 return MedicationDispenseStatusCodes.PREPARATION; 192 if ("in-progress".equals(codeString)) 193 return MedicationDispenseStatusCodes.INPROGRESS; 194 if ("cancelled".equals(codeString)) 195 return MedicationDispenseStatusCodes.CANCELLED; 196 if ("on-hold".equals(codeString)) 197 return MedicationDispenseStatusCodes.ONHOLD; 198 if ("completed".equals(codeString)) 199 return MedicationDispenseStatusCodes.COMPLETED; 200 if ("entered-in-error".equals(codeString)) 201 return MedicationDispenseStatusCodes.ENTEREDINERROR; 202 if ("stopped".equals(codeString)) 203 return MedicationDispenseStatusCodes.STOPPED; 204 if ("declined".equals(codeString)) 205 return MedicationDispenseStatusCodes.DECLINED; 206 if ("unknown".equals(codeString)) 207 return MedicationDispenseStatusCodes.UNKNOWN; 208 throw new IllegalArgumentException("Unknown MedicationDispenseStatusCodes code '"+codeString+"'"); 209 } 210 public Enumeration<MedicationDispenseStatusCodes> fromType(PrimitiveType<?> code) throws FHIRException { 211 if (code == null) 212 return null; 213 if (code.isEmpty()) 214 return new Enumeration<MedicationDispenseStatusCodes>(this, MedicationDispenseStatusCodes.NULL, code); 215 String codeString = ((PrimitiveType) code).asStringValue(); 216 if (codeString == null || "".equals(codeString)) 217 return new Enumeration<MedicationDispenseStatusCodes>(this, MedicationDispenseStatusCodes.NULL, code); 218 if ("preparation".equals(codeString)) 219 return new Enumeration<MedicationDispenseStatusCodes>(this, MedicationDispenseStatusCodes.PREPARATION, code); 220 if ("in-progress".equals(codeString)) 221 return new Enumeration<MedicationDispenseStatusCodes>(this, MedicationDispenseStatusCodes.INPROGRESS, code); 222 if ("cancelled".equals(codeString)) 223 return new Enumeration<MedicationDispenseStatusCodes>(this, MedicationDispenseStatusCodes.CANCELLED, code); 224 if ("on-hold".equals(codeString)) 225 return new Enumeration<MedicationDispenseStatusCodes>(this, MedicationDispenseStatusCodes.ONHOLD, code); 226 if ("completed".equals(codeString)) 227 return new Enumeration<MedicationDispenseStatusCodes>(this, MedicationDispenseStatusCodes.COMPLETED, code); 228 if ("entered-in-error".equals(codeString)) 229 return new Enumeration<MedicationDispenseStatusCodes>(this, MedicationDispenseStatusCodes.ENTEREDINERROR, code); 230 if ("stopped".equals(codeString)) 231 return new Enumeration<MedicationDispenseStatusCodes>(this, MedicationDispenseStatusCodes.STOPPED, code); 232 if ("declined".equals(codeString)) 233 return new Enumeration<MedicationDispenseStatusCodes>(this, MedicationDispenseStatusCodes.DECLINED, code); 234 if ("unknown".equals(codeString)) 235 return new Enumeration<MedicationDispenseStatusCodes>(this, MedicationDispenseStatusCodes.UNKNOWN, code); 236 throw new FHIRException("Unknown MedicationDispenseStatusCodes code '"+codeString+"'"); 237 } 238 public String toCode(MedicationDispenseStatusCodes code) { 239 if (code == MedicationDispenseStatusCodes.NULL) 240 return null; 241 if (code == MedicationDispenseStatusCodes.PREPARATION) 242 return "preparation"; 243 if (code == MedicationDispenseStatusCodes.INPROGRESS) 244 return "in-progress"; 245 if (code == MedicationDispenseStatusCodes.CANCELLED) 246 return "cancelled"; 247 if (code == MedicationDispenseStatusCodes.ONHOLD) 248 return "on-hold"; 249 if (code == MedicationDispenseStatusCodes.COMPLETED) 250 return "completed"; 251 if (code == MedicationDispenseStatusCodes.ENTEREDINERROR) 252 return "entered-in-error"; 253 if (code == MedicationDispenseStatusCodes.STOPPED) 254 return "stopped"; 255 if (code == MedicationDispenseStatusCodes.DECLINED) 256 return "declined"; 257 if (code == MedicationDispenseStatusCodes.UNKNOWN) 258 return "unknown"; 259 return "?"; 260 } 261 public String toSystem(MedicationDispenseStatusCodes code) { 262 return code.getSystem(); 263 } 264 } 265 266 @Block() 267 public static class MedicationDispensePerformerComponent extends BackboneElement implements IBaseBackboneElement { 268 /** 269 * Distinguishes the type of performer in the dispense. For example, date enterer, packager, final checker. 270 */ 271 @Child(name = "function", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 272 @Description(shortDefinition="Who performed the dispense and what they did", formalDefinition="Distinguishes the type of performer in the dispense. For example, date enterer, packager, final checker." ) 273 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationdispense-performer-function") 274 protected CodeableConcept function; 275 276 /** 277 * The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication. 278 */ 279 @Child(name = "actor", type = {Practitioner.class, PractitionerRole.class, Organization.class, Patient.class, Device.class, RelatedPerson.class, CareTeam.class}, order=2, min=1, max=1, modifier=false, summary=false) 280 @Description(shortDefinition="Individual who was performing", formalDefinition="The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication." ) 281 protected Reference actor; 282 283 private static final long serialVersionUID = -576943815L; 284 285 /** 286 * Constructor 287 */ 288 public MedicationDispensePerformerComponent() { 289 super(); 290 } 291 292 /** 293 * Constructor 294 */ 295 public MedicationDispensePerformerComponent(Reference actor) { 296 super(); 297 this.setActor(actor); 298 } 299 300 /** 301 * @return {@link #function} (Distinguishes the type of performer in the dispense. For example, date enterer, packager, final checker.) 302 */ 303 public CodeableConcept getFunction() { 304 if (this.function == null) 305 if (Configuration.errorOnAutoCreate()) 306 throw new Error("Attempt to auto-create MedicationDispensePerformerComponent.function"); 307 else if (Configuration.doAutoCreate()) 308 this.function = new CodeableConcept(); // cc 309 return this.function; 310 } 311 312 public boolean hasFunction() { 313 return this.function != null && !this.function.isEmpty(); 314 } 315 316 /** 317 * @param value {@link #function} (Distinguishes the type of performer in the dispense. For example, date enterer, packager, final checker.) 318 */ 319 public MedicationDispensePerformerComponent setFunction(CodeableConcept value) { 320 this.function = value; 321 return this; 322 } 323 324 /** 325 * @return {@link #actor} (The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication.) 326 */ 327 public Reference getActor() { 328 if (this.actor == null) 329 if (Configuration.errorOnAutoCreate()) 330 throw new Error("Attempt to auto-create MedicationDispensePerformerComponent.actor"); 331 else if (Configuration.doAutoCreate()) 332 this.actor = new Reference(); // cc 333 return this.actor; 334 } 335 336 public boolean hasActor() { 337 return this.actor != null && !this.actor.isEmpty(); 338 } 339 340 /** 341 * @param value {@link #actor} (The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication.) 342 */ 343 public MedicationDispensePerformerComponent setActor(Reference value) { 344 this.actor = value; 345 return this; 346 } 347 348 protected void listChildren(List<Property> children) { 349 super.listChildren(children); 350 children.add(new Property("function", "CodeableConcept", "Distinguishes the type of performer in the dispense. For example, date enterer, packager, final checker.", 0, 1, function)); 351 children.add(new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|Patient|Device|RelatedPerson|CareTeam)", "The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication.", 0, 1, actor)); 352 } 353 354 @Override 355 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 356 switch (_hash) { 357 case 1380938712: /*function*/ return new Property("function", "CodeableConcept", "Distinguishes the type of performer in the dispense. For example, date enterer, packager, final checker.", 0, 1, function); 358 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|PractitionerRole|Organization|Patient|Device|RelatedPerson|CareTeam)", "The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication.", 0, 1, actor); 359 default: return super.getNamedProperty(_hash, _name, _checkValid); 360 } 361 362 } 363 364 @Override 365 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 366 switch (hash) { 367 case 1380938712: /*function*/ return this.function == null ? new Base[0] : new Base[] {this.function}; // CodeableConcept 368 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 369 default: return super.getProperty(hash, name, checkValid); 370 } 371 372 } 373 374 @Override 375 public Base setProperty(int hash, String name, Base value) throws FHIRException { 376 switch (hash) { 377 case 1380938712: // function 378 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 379 return value; 380 case 92645877: // actor 381 this.actor = TypeConvertor.castToReference(value); // Reference 382 return value; 383 default: return super.setProperty(hash, name, value); 384 } 385 386 } 387 388 @Override 389 public Base setProperty(String name, Base value) throws FHIRException { 390 if (name.equals("function")) { 391 this.function = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 392 } else if (name.equals("actor")) { 393 this.actor = TypeConvertor.castToReference(value); // Reference 394 } else 395 return super.setProperty(name, value); 396 return value; 397 } 398 399 @Override 400 public void removeChild(String name, Base value) throws FHIRException { 401 if (name.equals("function")) { 402 this.function = null; 403 } else if (name.equals("actor")) { 404 this.actor = null; 405 } else 406 super.removeChild(name, value); 407 408 } 409 410 @Override 411 public Base makeProperty(int hash, String name) throws FHIRException { 412 switch (hash) { 413 case 1380938712: return getFunction(); 414 case 92645877: return getActor(); 415 default: return super.makeProperty(hash, name); 416 } 417 418 } 419 420 @Override 421 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 422 switch (hash) { 423 case 1380938712: /*function*/ return new String[] {"CodeableConcept"}; 424 case 92645877: /*actor*/ return new String[] {"Reference"}; 425 default: return super.getTypesForProperty(hash, name); 426 } 427 428 } 429 430 @Override 431 public Base addChild(String name) throws FHIRException { 432 if (name.equals("function")) { 433 this.function = new CodeableConcept(); 434 return this.function; 435 } 436 else if (name.equals("actor")) { 437 this.actor = new Reference(); 438 return this.actor; 439 } 440 else 441 return super.addChild(name); 442 } 443 444 public MedicationDispensePerformerComponent copy() { 445 MedicationDispensePerformerComponent dst = new MedicationDispensePerformerComponent(); 446 copyValues(dst); 447 return dst; 448 } 449 450 public void copyValues(MedicationDispensePerformerComponent dst) { 451 super.copyValues(dst); 452 dst.function = function == null ? null : function.copy(); 453 dst.actor = actor == null ? null : actor.copy(); 454 } 455 456 @Override 457 public boolean equalsDeep(Base other_) { 458 if (!super.equalsDeep(other_)) 459 return false; 460 if (!(other_ instanceof MedicationDispensePerformerComponent)) 461 return false; 462 MedicationDispensePerformerComponent o = (MedicationDispensePerformerComponent) other_; 463 return compareDeep(function, o.function, true) && compareDeep(actor, o.actor, true); 464 } 465 466 @Override 467 public boolean equalsShallow(Base other_) { 468 if (!super.equalsShallow(other_)) 469 return false; 470 if (!(other_ instanceof MedicationDispensePerformerComponent)) 471 return false; 472 MedicationDispensePerformerComponent o = (MedicationDispensePerformerComponent) other_; 473 return true; 474 } 475 476 public boolean isEmpty() { 477 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(function, actor); 478 } 479 480 public String fhirType() { 481 return "MedicationDispense.performer"; 482 483 } 484 485 } 486 487 @Block() 488 public static class MedicationDispenseSubstitutionComponent extends BackboneElement implements IBaseBackboneElement { 489 /** 490 * True if the dispenser dispensed a different drug or product from what was prescribed. 491 */ 492 @Child(name = "wasSubstituted", type = {BooleanType.class}, order=1, min=1, max=1, modifier=false, summary=false) 493 @Description(shortDefinition="Whether a substitution was or was not performed on the dispense", formalDefinition="True if the dispenser dispensed a different drug or product from what was prescribed." ) 494 protected BooleanType wasSubstituted; 495 496 /** 497 * A code signifying whether a different drug was dispensed from what was prescribed. 498 */ 499 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 500 @Description(shortDefinition="Code signifying whether a different drug was dispensed from what was prescribed", formalDefinition="A code signifying whether a different drug was dispensed from what was prescribed." ) 501 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ActSubstanceAdminSubstitutionCode") 502 protected CodeableConcept type; 503 504 /** 505 * Indicates the reason for the substitution (or lack of substitution) from what was prescribed. 506 */ 507 @Child(name = "reason", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 508 @Description(shortDefinition="Why was substitution made", formalDefinition="Indicates the reason for the substitution (or lack of substitution) from what was prescribed." ) 509 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-SubstanceAdminSubstitutionReason") 510 protected List<CodeableConcept> reason; 511 512 /** 513 * The person or organization that has primary responsibility for the substitution. 514 */ 515 @Child(name = "responsibleParty", type = {Practitioner.class, PractitionerRole.class, Organization.class}, order=4, min=0, max=1, modifier=false, summary=false) 516 @Description(shortDefinition="Who is responsible for the substitution", formalDefinition="The person or organization that has primary responsibility for the substitution." ) 517 protected Reference responsibleParty; 518 519 private static final long serialVersionUID = 431402218L; 520 521 /** 522 * Constructor 523 */ 524 public MedicationDispenseSubstitutionComponent() { 525 super(); 526 } 527 528 /** 529 * Constructor 530 */ 531 public MedicationDispenseSubstitutionComponent(boolean wasSubstituted) { 532 super(); 533 this.setWasSubstituted(wasSubstituted); 534 } 535 536 /** 537 * @return {@link #wasSubstituted} (True if the dispenser dispensed a different drug or product from what was prescribed.). This is the underlying object with id, value and extensions. The accessor "getWasSubstituted" gives direct access to the value 538 */ 539 public BooleanType getWasSubstitutedElement() { 540 if (this.wasSubstituted == null) 541 if (Configuration.errorOnAutoCreate()) 542 throw new Error("Attempt to auto-create MedicationDispenseSubstitutionComponent.wasSubstituted"); 543 else if (Configuration.doAutoCreate()) 544 this.wasSubstituted = new BooleanType(); // bb 545 return this.wasSubstituted; 546 } 547 548 public boolean hasWasSubstitutedElement() { 549 return this.wasSubstituted != null && !this.wasSubstituted.isEmpty(); 550 } 551 552 public boolean hasWasSubstituted() { 553 return this.wasSubstituted != null && !this.wasSubstituted.isEmpty(); 554 } 555 556 /** 557 * @param value {@link #wasSubstituted} (True if the dispenser dispensed a different drug or product from what was prescribed.). This is the underlying object with id, value and extensions. The accessor "getWasSubstituted" gives direct access to the value 558 */ 559 public MedicationDispenseSubstitutionComponent setWasSubstitutedElement(BooleanType value) { 560 this.wasSubstituted = value; 561 return this; 562 } 563 564 /** 565 * @return True if the dispenser dispensed a different drug or product from what was prescribed. 566 */ 567 public boolean getWasSubstituted() { 568 return this.wasSubstituted == null || this.wasSubstituted.isEmpty() ? false : this.wasSubstituted.getValue(); 569 } 570 571 /** 572 * @param value True if the dispenser dispensed a different drug or product from what was prescribed. 573 */ 574 public MedicationDispenseSubstitutionComponent setWasSubstituted(boolean value) { 575 if (this.wasSubstituted == null) 576 this.wasSubstituted = new BooleanType(); 577 this.wasSubstituted.setValue(value); 578 return this; 579 } 580 581 /** 582 * @return {@link #type} (A code signifying whether a different drug was dispensed from what was prescribed.) 583 */ 584 public CodeableConcept getType() { 585 if (this.type == null) 586 if (Configuration.errorOnAutoCreate()) 587 throw new Error("Attempt to auto-create MedicationDispenseSubstitutionComponent.type"); 588 else if (Configuration.doAutoCreate()) 589 this.type = new CodeableConcept(); // cc 590 return this.type; 591 } 592 593 public boolean hasType() { 594 return this.type != null && !this.type.isEmpty(); 595 } 596 597 /** 598 * @param value {@link #type} (A code signifying whether a different drug was dispensed from what was prescribed.) 599 */ 600 public MedicationDispenseSubstitutionComponent setType(CodeableConcept value) { 601 this.type = value; 602 return this; 603 } 604 605 /** 606 * @return {@link #reason} (Indicates the reason for the substitution (or lack of substitution) from what was prescribed.) 607 */ 608 public List<CodeableConcept> getReason() { 609 if (this.reason == null) 610 this.reason = new ArrayList<CodeableConcept>(); 611 return this.reason; 612 } 613 614 /** 615 * @return Returns a reference to <code>this</code> for easy method chaining 616 */ 617 public MedicationDispenseSubstitutionComponent setReason(List<CodeableConcept> theReason) { 618 this.reason = theReason; 619 return this; 620 } 621 622 public boolean hasReason() { 623 if (this.reason == null) 624 return false; 625 for (CodeableConcept item : this.reason) 626 if (!item.isEmpty()) 627 return true; 628 return false; 629 } 630 631 public CodeableConcept addReason() { //3 632 CodeableConcept t = new CodeableConcept(); 633 if (this.reason == null) 634 this.reason = new ArrayList<CodeableConcept>(); 635 this.reason.add(t); 636 return t; 637 } 638 639 public MedicationDispenseSubstitutionComponent addReason(CodeableConcept t) { //3 640 if (t == null) 641 return this; 642 if (this.reason == null) 643 this.reason = new ArrayList<CodeableConcept>(); 644 this.reason.add(t); 645 return this; 646 } 647 648 /** 649 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist {3} 650 */ 651 public CodeableConcept getReasonFirstRep() { 652 if (getReason().isEmpty()) { 653 addReason(); 654 } 655 return getReason().get(0); 656 } 657 658 /** 659 * @return {@link #responsibleParty} (The person or organization that has primary responsibility for the substitution.) 660 */ 661 public Reference getResponsibleParty() { 662 if (this.responsibleParty == null) 663 if (Configuration.errorOnAutoCreate()) 664 throw new Error("Attempt to auto-create MedicationDispenseSubstitutionComponent.responsibleParty"); 665 else if (Configuration.doAutoCreate()) 666 this.responsibleParty = new Reference(); // cc 667 return this.responsibleParty; 668 } 669 670 public boolean hasResponsibleParty() { 671 return this.responsibleParty != null && !this.responsibleParty.isEmpty(); 672 } 673 674 /** 675 * @param value {@link #responsibleParty} (The person or organization that has primary responsibility for the substitution.) 676 */ 677 public MedicationDispenseSubstitutionComponent setResponsibleParty(Reference value) { 678 this.responsibleParty = value; 679 return this; 680 } 681 682 protected void listChildren(List<Property> children) { 683 super.listChildren(children); 684 children.add(new Property("wasSubstituted", "boolean", "True if the dispenser dispensed a different drug or product from what was prescribed.", 0, 1, wasSubstituted)); 685 children.add(new Property("type", "CodeableConcept", "A code signifying whether a different drug was dispensed from what was prescribed.", 0, 1, type)); 686 children.add(new Property("reason", "CodeableConcept", "Indicates the reason for the substitution (or lack of substitution) from what was prescribed.", 0, java.lang.Integer.MAX_VALUE, reason)); 687 children.add(new Property("responsibleParty", "Reference(Practitioner|PractitionerRole|Organization)", "The person or organization that has primary responsibility for the substitution.", 0, 1, responsibleParty)); 688 } 689 690 @Override 691 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 692 switch (_hash) { 693 case -592113567: /*wasSubstituted*/ return new Property("wasSubstituted", "boolean", "True if the dispenser dispensed a different drug or product from what was prescribed.", 0, 1, wasSubstituted); 694 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A code signifying whether a different drug was dispensed from what was prescribed.", 0, 1, type); 695 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Indicates the reason for the substitution (or lack of substitution) from what was prescribed.", 0, java.lang.Integer.MAX_VALUE, reason); 696 case 1511509392: /*responsibleParty*/ return new Property("responsibleParty", "Reference(Practitioner|PractitionerRole|Organization)", "The person or organization that has primary responsibility for the substitution.", 0, 1, responsibleParty); 697 default: return super.getNamedProperty(_hash, _name, _checkValid); 698 } 699 700 } 701 702 @Override 703 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 704 switch (hash) { 705 case -592113567: /*wasSubstituted*/ return this.wasSubstituted == null ? new Base[0] : new Base[] {this.wasSubstituted}; // BooleanType 706 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 707 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 708 case 1511509392: /*responsibleParty*/ return this.responsibleParty == null ? new Base[0] : new Base[] {this.responsibleParty}; // Reference 709 default: return super.getProperty(hash, name, checkValid); 710 } 711 712 } 713 714 @Override 715 public Base setProperty(int hash, String name, Base value) throws FHIRException { 716 switch (hash) { 717 case -592113567: // wasSubstituted 718 this.wasSubstituted = TypeConvertor.castToBoolean(value); // BooleanType 719 return value; 720 case 3575610: // type 721 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 722 return value; 723 case -934964668: // reason 724 this.getReason().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 725 return value; 726 case 1511509392: // responsibleParty 727 this.responsibleParty = TypeConvertor.castToReference(value); // Reference 728 return value; 729 default: return super.setProperty(hash, name, value); 730 } 731 732 } 733 734 @Override 735 public Base setProperty(String name, Base value) throws FHIRException { 736 if (name.equals("wasSubstituted")) { 737 this.wasSubstituted = TypeConvertor.castToBoolean(value); // BooleanType 738 } else if (name.equals("type")) { 739 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 740 } else if (name.equals("reason")) { 741 this.getReason().add(TypeConvertor.castToCodeableConcept(value)); 742 } else if (name.equals("responsibleParty")) { 743 this.responsibleParty = TypeConvertor.castToReference(value); // Reference 744 } else 745 return super.setProperty(name, value); 746 return value; 747 } 748 749 @Override 750 public void removeChild(String name, Base value) throws FHIRException { 751 if (name.equals("wasSubstituted")) { 752 this.wasSubstituted = null; 753 } else if (name.equals("type")) { 754 this.type = null; 755 } else if (name.equals("reason")) { 756 this.getReason().remove(value); 757 } else if (name.equals("responsibleParty")) { 758 this.responsibleParty = null; 759 } else 760 super.removeChild(name, value); 761 762 } 763 764 @Override 765 public Base makeProperty(int hash, String name) throws FHIRException { 766 switch (hash) { 767 case -592113567: return getWasSubstitutedElement(); 768 case 3575610: return getType(); 769 case -934964668: return addReason(); 770 case 1511509392: return getResponsibleParty(); 771 default: return super.makeProperty(hash, name); 772 } 773 774 } 775 776 @Override 777 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 778 switch (hash) { 779 case -592113567: /*wasSubstituted*/ return new String[] {"boolean"}; 780 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 781 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 782 case 1511509392: /*responsibleParty*/ return new String[] {"Reference"}; 783 default: return super.getTypesForProperty(hash, name); 784 } 785 786 } 787 788 @Override 789 public Base addChild(String name) throws FHIRException { 790 if (name.equals("wasSubstituted")) { 791 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.substitution.wasSubstituted"); 792 } 793 else if (name.equals("type")) { 794 this.type = new CodeableConcept(); 795 return this.type; 796 } 797 else if (name.equals("reason")) { 798 return addReason(); 799 } 800 else if (name.equals("responsibleParty")) { 801 this.responsibleParty = new Reference(); 802 return this.responsibleParty; 803 } 804 else 805 return super.addChild(name); 806 } 807 808 public MedicationDispenseSubstitutionComponent copy() { 809 MedicationDispenseSubstitutionComponent dst = new MedicationDispenseSubstitutionComponent(); 810 copyValues(dst); 811 return dst; 812 } 813 814 public void copyValues(MedicationDispenseSubstitutionComponent dst) { 815 super.copyValues(dst); 816 dst.wasSubstituted = wasSubstituted == null ? null : wasSubstituted.copy(); 817 dst.type = type == null ? null : type.copy(); 818 if (reason != null) { 819 dst.reason = new ArrayList<CodeableConcept>(); 820 for (CodeableConcept i : reason) 821 dst.reason.add(i.copy()); 822 }; 823 dst.responsibleParty = responsibleParty == null ? null : responsibleParty.copy(); 824 } 825 826 @Override 827 public boolean equalsDeep(Base other_) { 828 if (!super.equalsDeep(other_)) 829 return false; 830 if (!(other_ instanceof MedicationDispenseSubstitutionComponent)) 831 return false; 832 MedicationDispenseSubstitutionComponent o = (MedicationDispenseSubstitutionComponent) other_; 833 return compareDeep(wasSubstituted, o.wasSubstituted, true) && compareDeep(type, o.type, true) && compareDeep(reason, o.reason, true) 834 && compareDeep(responsibleParty, o.responsibleParty, true); 835 } 836 837 @Override 838 public boolean equalsShallow(Base other_) { 839 if (!super.equalsShallow(other_)) 840 return false; 841 if (!(other_ instanceof MedicationDispenseSubstitutionComponent)) 842 return false; 843 MedicationDispenseSubstitutionComponent o = (MedicationDispenseSubstitutionComponent) other_; 844 return compareValues(wasSubstituted, o.wasSubstituted, true); 845 } 846 847 public boolean isEmpty() { 848 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(wasSubstituted, type, reason 849 , responsibleParty); 850 } 851 852 public String fhirType() { 853 return "MedicationDispense.substitution"; 854 855 } 856 857 } 858 859 /** 860 * Identifiers associated with this Medication Dispense that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server. 861 */ 862 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 863 @Description(shortDefinition="External identifier", formalDefinition="Identifiers associated with this Medication Dispense that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server." ) 864 protected List<Identifier> identifier; 865 866 /** 867 * A plan that is fulfilled in whole or in part by this MedicationDispense. 868 */ 869 @Child(name = "basedOn", type = {CarePlan.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 870 @Description(shortDefinition="Plan that is fulfilled by this dispense", formalDefinition="A plan that is fulfilled in whole or in part by this MedicationDispense." ) 871 protected List<Reference> basedOn; 872 873 /** 874 * The procedure or medication administration that triggered the dispense. 875 */ 876 @Child(name = "partOf", type = {Procedure.class, MedicationAdministration.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 877 @Description(shortDefinition="Event that dispense is part of", formalDefinition="The procedure or medication administration that triggered the dispense." ) 878 protected List<Reference> partOf; 879 880 /** 881 * A code specifying the state of the set of dispense events. 882 */ 883 @Child(name = "status", type = {CodeType.class}, order=3, min=1, max=1, modifier=true, summary=true) 884 @Description(shortDefinition="preparation | in-progress | cancelled | on-hold | completed | entered-in-error | stopped | declined | unknown", formalDefinition="A code specifying the state of the set of dispense events." ) 885 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationdispense-status") 886 protected Enumeration<MedicationDispenseStatusCodes> status; 887 888 /** 889 * Indicates the reason why a dispense was not performed. 890 */ 891 @Child(name = "notPerformedReason", type = {CodeableReference.class}, order=4, min=0, max=1, modifier=false, summary=false) 892 @Description(shortDefinition="Why a dispense was not performed", formalDefinition="Indicates the reason why a dispense was not performed." ) 893 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationdispense-status-reason") 894 protected CodeableReference notPerformedReason; 895 896 /** 897 * The date (and maybe time) when the status of the dispense record changed. 898 */ 899 @Child(name = "statusChanged", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 900 @Description(shortDefinition="When the status changed", formalDefinition="The date (and maybe time) when the status of the dispense record changed." ) 901 protected DateTimeType statusChanged; 902 903 /** 904 * Indicates the type of medication dispense (for example, drug classification like ATC, where meds would be administered, legal category of the medication.). 905 */ 906 @Child(name = "category", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 907 @Description(shortDefinition="Type of medication dispense", formalDefinition="Indicates the type of medication dispense (for example, drug classification like ATC, where meds would be administered, legal category of the medication.)." ) 908 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationdispense-admin-location") 909 protected List<CodeableConcept> category; 910 911 /** 912 * Identifies the medication supplied. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications. 913 */ 914 @Child(name = "medication", type = {CodeableReference.class}, order=7, min=1, max=1, modifier=false, summary=true) 915 @Description(shortDefinition="What medication was supplied", formalDefinition="Identifies the medication supplied. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications." ) 916 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 917 protected CodeableReference medication; 918 919 /** 920 * A link to a resource representing the person or the group to whom the medication will be given. 921 */ 922 @Child(name = "subject", type = {Patient.class, Group.class}, order=8, min=1, max=1, modifier=false, summary=true) 923 @Description(shortDefinition="Who the dispense is for", formalDefinition="A link to a resource representing the person or the group to whom the medication will be given." ) 924 protected Reference subject; 925 926 /** 927 * The encounter that establishes the context for this event. 928 */ 929 @Child(name = "encounter", type = {Encounter.class}, order=9, min=0, max=1, modifier=false, summary=false) 930 @Description(shortDefinition="Encounter associated with event", formalDefinition="The encounter that establishes the context for this event." ) 931 protected Reference encounter; 932 933 /** 934 * Additional information that supports the medication being dispensed. For example, there may be requirements that a specific lab test has been completed prior to dispensing or the patient's weight at the time of dispensing is documented. 935 */ 936 @Child(name = "supportingInformation", type = {Reference.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 937 @Description(shortDefinition="Information that supports the dispensing of the medication", formalDefinition="Additional information that supports the medication being dispensed. For example, there may be requirements that a specific lab test has been completed prior to dispensing or the patient's weight at the time of dispensing is documented." ) 938 protected List<Reference> supportingInformation; 939 940 /** 941 * Indicates who or what performed the event. 942 */ 943 @Child(name = "performer", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 944 @Description(shortDefinition="Who performed event", formalDefinition="Indicates who or what performed the event." ) 945 protected List<MedicationDispensePerformerComponent> performer; 946 947 /** 948 * The principal physical location where the dispense was performed. 949 */ 950 @Child(name = "location", type = {Location.class}, order=12, min=0, max=1, modifier=false, summary=false) 951 @Description(shortDefinition="Where the dispense occurred", formalDefinition="The principal physical location where the dispense was performed." ) 952 protected Reference location; 953 954 /** 955 * Indicates the medication order that is being dispensed against. 956 */ 957 @Child(name = "authorizingPrescription", type = {MedicationRequest.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 958 @Description(shortDefinition="Medication order that authorizes the dispense", formalDefinition="Indicates the medication order that is being dispensed against." ) 959 protected List<Reference> authorizingPrescription; 960 961 /** 962 * Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc. 963 */ 964 @Child(name = "type", type = {CodeableConcept.class}, order=14, min=0, max=1, modifier=false, summary=false) 965 @Description(shortDefinition="Trial fill, partial fill, emergency fill, etc", formalDefinition="Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc." ) 966 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-ActPharmacySupplyType") 967 protected CodeableConcept type; 968 969 /** 970 * The amount of medication that has been dispensed. Includes unit of measure. 971 */ 972 @Child(name = "quantity", type = {Quantity.class}, order=15, min=0, max=1, modifier=false, summary=false) 973 @Description(shortDefinition="Amount dispensed", formalDefinition="The amount of medication that has been dispensed. Includes unit of measure." ) 974 protected Quantity quantity; 975 976 /** 977 * The amount of medication expressed as a timing amount. 978 */ 979 @Child(name = "daysSupply", type = {Quantity.class}, order=16, min=0, max=1, modifier=false, summary=false) 980 @Description(shortDefinition="Amount of medication expressed as a timing amount", formalDefinition="The amount of medication expressed as a timing amount." ) 981 protected Quantity daysSupply; 982 983 /** 984 * The date (and maybe time) when the dispense activity started if whenPrepared or whenHandedOver is not populated. 985 */ 986 @Child(name = "recorded", type = {DateTimeType.class}, order=17, min=0, max=1, modifier=false, summary=false) 987 @Description(shortDefinition="When the recording of the dispense started", formalDefinition="The date (and maybe time) when the dispense activity started if whenPrepared or whenHandedOver is not populated." ) 988 protected DateTimeType recorded; 989 990 /** 991 * The time when the dispensed product was packaged and reviewed. 992 */ 993 @Child(name = "whenPrepared", type = {DateTimeType.class}, order=18, min=0, max=1, modifier=false, summary=true) 994 @Description(shortDefinition="When product was packaged and reviewed", formalDefinition="The time when the dispensed product was packaged and reviewed." ) 995 protected DateTimeType whenPrepared; 996 997 /** 998 * The time the dispensed product was provided to the patient or their representative. 999 */ 1000 @Child(name = "whenHandedOver", type = {DateTimeType.class}, order=19, min=0, max=1, modifier=false, summary=false) 1001 @Description(shortDefinition="When product was given out", formalDefinition="The time the dispensed product was provided to the patient or their representative." ) 1002 protected DateTimeType whenHandedOver; 1003 1004 /** 1005 * Identification of the facility/location where the medication was/will be shipped to, as part of the dispense event. 1006 */ 1007 @Child(name = "destination", type = {Location.class}, order=20, min=0, max=1, modifier=false, summary=false) 1008 @Description(shortDefinition="Where the medication was/will be sent", formalDefinition="Identification of the facility/location where the medication was/will be shipped to, as part of the dispense event." ) 1009 protected Reference destination; 1010 1011 /** 1012 * Identifies the person who picked up the medication or the location of where the medication was delivered. This will usually be a patient or their caregiver, but some cases exist where it can be a healthcare professional or a location. 1013 */ 1014 @Child(name = "receiver", type = {Patient.class, Practitioner.class, RelatedPerson.class, Location.class, PractitionerRole.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1015 @Description(shortDefinition="Who collected the medication or where the medication was delivered", formalDefinition="Identifies the person who picked up the medication or the location of where the medication was delivered. This will usually be a patient or their caregiver, but some cases exist where it can be a healthcare professional or a location." ) 1016 protected List<Reference> receiver; 1017 1018 /** 1019 * Extra information about the dispense that could not be conveyed in the other attributes. 1020 */ 1021 @Child(name = "note", type = {Annotation.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1022 @Description(shortDefinition="Information about the dispense", formalDefinition="Extra information about the dispense that could not be conveyed in the other attributes." ) 1023 protected List<Annotation> note; 1024 1025 /** 1026 * The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses. 1027 */ 1028 @Child(name = "renderedDosageInstruction", type = {MarkdownType.class}, order=23, min=0, max=1, modifier=false, summary=false) 1029 @Description(shortDefinition="Full representation of the dosage instructions", formalDefinition="The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses." ) 1030 protected MarkdownType renderedDosageInstruction; 1031 1032 /** 1033 * Indicates how the medication is to be used by the patient. 1034 */ 1035 @Child(name = "dosageInstruction", type = {Dosage.class}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1036 @Description(shortDefinition="How the medication is to be used by the patient or administered by the caregiver", formalDefinition="Indicates how the medication is to be used by the patient." ) 1037 protected List<Dosage> dosageInstruction; 1038 1039 /** 1040 * Indicates whether or not substitution was made as part of the dispense. In some cases, substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why. If nothing is specified, substitution was not done. 1041 */ 1042 @Child(name = "substitution", type = {}, order=25, min=0, max=1, modifier=false, summary=false) 1043 @Description(shortDefinition="Whether a substitution was performed on the dispense", formalDefinition="Indicates whether or not substitution was made as part of the dispense. In some cases, substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why. If nothing is specified, substitution was not done." ) 1044 protected MedicationDispenseSubstitutionComponent substitution; 1045 1046 /** 1047 * A summary of the events of interest that have occurred, such as when the dispense was verified. 1048 */ 1049 @Child(name = "eventHistory", type = {Provenance.class}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1050 @Description(shortDefinition="A list of relevant lifecycle events", formalDefinition="A summary of the events of interest that have occurred, such as when the dispense was verified." ) 1051 protected List<Reference> eventHistory; 1052 1053 private static final long serialVersionUID = 88138059L; 1054 1055 /** 1056 * Constructor 1057 */ 1058 public MedicationDispense() { 1059 super(); 1060 } 1061 1062 /** 1063 * Constructor 1064 */ 1065 public MedicationDispense(MedicationDispenseStatusCodes status, CodeableReference medication, Reference subject) { 1066 super(); 1067 this.setStatus(status); 1068 this.setMedication(medication); 1069 this.setSubject(subject); 1070 } 1071 1072 /** 1073 * @return {@link #identifier} (Identifiers associated with this Medication Dispense that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.) 1074 */ 1075 public List<Identifier> getIdentifier() { 1076 if (this.identifier == null) 1077 this.identifier = new ArrayList<Identifier>(); 1078 return this.identifier; 1079 } 1080 1081 /** 1082 * @return Returns a reference to <code>this</code> for easy method chaining 1083 */ 1084 public MedicationDispense setIdentifier(List<Identifier> theIdentifier) { 1085 this.identifier = theIdentifier; 1086 return this; 1087 } 1088 1089 public boolean hasIdentifier() { 1090 if (this.identifier == null) 1091 return false; 1092 for (Identifier item : this.identifier) 1093 if (!item.isEmpty()) 1094 return true; 1095 return false; 1096 } 1097 1098 public Identifier addIdentifier() { //3 1099 Identifier t = new Identifier(); 1100 if (this.identifier == null) 1101 this.identifier = new ArrayList<Identifier>(); 1102 this.identifier.add(t); 1103 return t; 1104 } 1105 1106 public MedicationDispense addIdentifier(Identifier t) { //3 1107 if (t == null) 1108 return this; 1109 if (this.identifier == null) 1110 this.identifier = new ArrayList<Identifier>(); 1111 this.identifier.add(t); 1112 return this; 1113 } 1114 1115 /** 1116 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 1117 */ 1118 public Identifier getIdentifierFirstRep() { 1119 if (getIdentifier().isEmpty()) { 1120 addIdentifier(); 1121 } 1122 return getIdentifier().get(0); 1123 } 1124 1125 /** 1126 * @return {@link #basedOn} (A plan that is fulfilled in whole or in part by this MedicationDispense.) 1127 */ 1128 public List<Reference> getBasedOn() { 1129 if (this.basedOn == null) 1130 this.basedOn = new ArrayList<Reference>(); 1131 return this.basedOn; 1132 } 1133 1134 /** 1135 * @return Returns a reference to <code>this</code> for easy method chaining 1136 */ 1137 public MedicationDispense setBasedOn(List<Reference> theBasedOn) { 1138 this.basedOn = theBasedOn; 1139 return this; 1140 } 1141 1142 public boolean hasBasedOn() { 1143 if (this.basedOn == null) 1144 return false; 1145 for (Reference item : this.basedOn) 1146 if (!item.isEmpty()) 1147 return true; 1148 return false; 1149 } 1150 1151 public Reference addBasedOn() { //3 1152 Reference t = new Reference(); 1153 if (this.basedOn == null) 1154 this.basedOn = new ArrayList<Reference>(); 1155 this.basedOn.add(t); 1156 return t; 1157 } 1158 1159 public MedicationDispense addBasedOn(Reference t) { //3 1160 if (t == null) 1161 return this; 1162 if (this.basedOn == null) 1163 this.basedOn = new ArrayList<Reference>(); 1164 this.basedOn.add(t); 1165 return this; 1166 } 1167 1168 /** 1169 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist {3} 1170 */ 1171 public Reference getBasedOnFirstRep() { 1172 if (getBasedOn().isEmpty()) { 1173 addBasedOn(); 1174 } 1175 return getBasedOn().get(0); 1176 } 1177 1178 /** 1179 * @return {@link #partOf} (The procedure or medication administration that triggered the dispense.) 1180 */ 1181 public List<Reference> getPartOf() { 1182 if (this.partOf == null) 1183 this.partOf = new ArrayList<Reference>(); 1184 return this.partOf; 1185 } 1186 1187 /** 1188 * @return Returns a reference to <code>this</code> for easy method chaining 1189 */ 1190 public MedicationDispense setPartOf(List<Reference> thePartOf) { 1191 this.partOf = thePartOf; 1192 return this; 1193 } 1194 1195 public boolean hasPartOf() { 1196 if (this.partOf == null) 1197 return false; 1198 for (Reference item : this.partOf) 1199 if (!item.isEmpty()) 1200 return true; 1201 return false; 1202 } 1203 1204 public Reference addPartOf() { //3 1205 Reference t = new Reference(); 1206 if (this.partOf == null) 1207 this.partOf = new ArrayList<Reference>(); 1208 this.partOf.add(t); 1209 return t; 1210 } 1211 1212 public MedicationDispense addPartOf(Reference t) { //3 1213 if (t == null) 1214 return this; 1215 if (this.partOf == null) 1216 this.partOf = new ArrayList<Reference>(); 1217 this.partOf.add(t); 1218 return this; 1219 } 1220 1221 /** 1222 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist {3} 1223 */ 1224 public Reference getPartOfFirstRep() { 1225 if (getPartOf().isEmpty()) { 1226 addPartOf(); 1227 } 1228 return getPartOf().get(0); 1229 } 1230 1231 /** 1232 * @return {@link #status} (A code specifying the state of the set of dispense events.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1233 */ 1234 public Enumeration<MedicationDispenseStatusCodes> getStatusElement() { 1235 if (this.status == null) 1236 if (Configuration.errorOnAutoCreate()) 1237 throw new Error("Attempt to auto-create MedicationDispense.status"); 1238 else if (Configuration.doAutoCreate()) 1239 this.status = new Enumeration<MedicationDispenseStatusCodes>(new MedicationDispenseStatusCodesEnumFactory()); // bb 1240 return this.status; 1241 } 1242 1243 public boolean hasStatusElement() { 1244 return this.status != null && !this.status.isEmpty(); 1245 } 1246 1247 public boolean hasStatus() { 1248 return this.status != null && !this.status.isEmpty(); 1249 } 1250 1251 /** 1252 * @param value {@link #status} (A code specifying the state of the set of dispense events.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1253 */ 1254 public MedicationDispense setStatusElement(Enumeration<MedicationDispenseStatusCodes> value) { 1255 this.status = value; 1256 return this; 1257 } 1258 1259 /** 1260 * @return A code specifying the state of the set of dispense events. 1261 */ 1262 public MedicationDispenseStatusCodes getStatus() { 1263 return this.status == null ? null : this.status.getValue(); 1264 } 1265 1266 /** 1267 * @param value A code specifying the state of the set of dispense events. 1268 */ 1269 public MedicationDispense setStatus(MedicationDispenseStatusCodes value) { 1270 if (this.status == null) 1271 this.status = new Enumeration<MedicationDispenseStatusCodes>(new MedicationDispenseStatusCodesEnumFactory()); 1272 this.status.setValue(value); 1273 return this; 1274 } 1275 1276 /** 1277 * @return {@link #notPerformedReason} (Indicates the reason why a dispense was not performed.) 1278 */ 1279 public CodeableReference getNotPerformedReason() { 1280 if (this.notPerformedReason == null) 1281 if (Configuration.errorOnAutoCreate()) 1282 throw new Error("Attempt to auto-create MedicationDispense.notPerformedReason"); 1283 else if (Configuration.doAutoCreate()) 1284 this.notPerformedReason = new CodeableReference(); // cc 1285 return this.notPerformedReason; 1286 } 1287 1288 public boolean hasNotPerformedReason() { 1289 return this.notPerformedReason != null && !this.notPerformedReason.isEmpty(); 1290 } 1291 1292 /** 1293 * @param value {@link #notPerformedReason} (Indicates the reason why a dispense was not performed.) 1294 */ 1295 public MedicationDispense setNotPerformedReason(CodeableReference value) { 1296 this.notPerformedReason = value; 1297 return this; 1298 } 1299 1300 /** 1301 * @return {@link #statusChanged} (The date (and maybe time) when the status of the dispense record changed.). This is the underlying object with id, value and extensions. The accessor "getStatusChanged" gives direct access to the value 1302 */ 1303 public DateTimeType getStatusChangedElement() { 1304 if (this.statusChanged == null) 1305 if (Configuration.errorOnAutoCreate()) 1306 throw new Error("Attempt to auto-create MedicationDispense.statusChanged"); 1307 else if (Configuration.doAutoCreate()) 1308 this.statusChanged = new DateTimeType(); // bb 1309 return this.statusChanged; 1310 } 1311 1312 public boolean hasStatusChangedElement() { 1313 return this.statusChanged != null && !this.statusChanged.isEmpty(); 1314 } 1315 1316 public boolean hasStatusChanged() { 1317 return this.statusChanged != null && !this.statusChanged.isEmpty(); 1318 } 1319 1320 /** 1321 * @param value {@link #statusChanged} (The date (and maybe time) when the status of the dispense record changed.). This is the underlying object with id, value and extensions. The accessor "getStatusChanged" gives direct access to the value 1322 */ 1323 public MedicationDispense setStatusChangedElement(DateTimeType value) { 1324 this.statusChanged = value; 1325 return this; 1326 } 1327 1328 /** 1329 * @return The date (and maybe time) when the status of the dispense record changed. 1330 */ 1331 public Date getStatusChanged() { 1332 return this.statusChanged == null ? null : this.statusChanged.getValue(); 1333 } 1334 1335 /** 1336 * @param value The date (and maybe time) when the status of the dispense record changed. 1337 */ 1338 public MedicationDispense setStatusChanged(Date value) { 1339 if (value == null) 1340 this.statusChanged = null; 1341 else { 1342 if (this.statusChanged == null) 1343 this.statusChanged = new DateTimeType(); 1344 this.statusChanged.setValue(value); 1345 } 1346 return this; 1347 } 1348 1349 /** 1350 * @return {@link #category} (Indicates the type of medication dispense (for example, drug classification like ATC, where meds would be administered, legal category of the medication.).) 1351 */ 1352 public List<CodeableConcept> getCategory() { 1353 if (this.category == null) 1354 this.category = new ArrayList<CodeableConcept>(); 1355 return this.category; 1356 } 1357 1358 /** 1359 * @return Returns a reference to <code>this</code> for easy method chaining 1360 */ 1361 public MedicationDispense setCategory(List<CodeableConcept> theCategory) { 1362 this.category = theCategory; 1363 return this; 1364 } 1365 1366 public boolean hasCategory() { 1367 if (this.category == null) 1368 return false; 1369 for (CodeableConcept item : this.category) 1370 if (!item.isEmpty()) 1371 return true; 1372 return false; 1373 } 1374 1375 public CodeableConcept addCategory() { //3 1376 CodeableConcept t = new CodeableConcept(); 1377 if (this.category == null) 1378 this.category = new ArrayList<CodeableConcept>(); 1379 this.category.add(t); 1380 return t; 1381 } 1382 1383 public MedicationDispense addCategory(CodeableConcept t) { //3 1384 if (t == null) 1385 return this; 1386 if (this.category == null) 1387 this.category = new ArrayList<CodeableConcept>(); 1388 this.category.add(t); 1389 return this; 1390 } 1391 1392 /** 1393 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist {3} 1394 */ 1395 public CodeableConcept getCategoryFirstRep() { 1396 if (getCategory().isEmpty()) { 1397 addCategory(); 1398 } 1399 return getCategory().get(0); 1400 } 1401 1402 /** 1403 * @return {@link #medication} (Identifies the medication supplied. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 1404 */ 1405 public CodeableReference getMedication() { 1406 if (this.medication == null) 1407 if (Configuration.errorOnAutoCreate()) 1408 throw new Error("Attempt to auto-create MedicationDispense.medication"); 1409 else if (Configuration.doAutoCreate()) 1410 this.medication = new CodeableReference(); // cc 1411 return this.medication; 1412 } 1413 1414 public boolean hasMedication() { 1415 return this.medication != null && !this.medication.isEmpty(); 1416 } 1417 1418 /** 1419 * @param value {@link #medication} (Identifies the medication supplied. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 1420 */ 1421 public MedicationDispense setMedication(CodeableReference value) { 1422 this.medication = value; 1423 return this; 1424 } 1425 1426 /** 1427 * @return {@link #subject} (A link to a resource representing the person or the group to whom the medication will be given.) 1428 */ 1429 public Reference getSubject() { 1430 if (this.subject == null) 1431 if (Configuration.errorOnAutoCreate()) 1432 throw new Error("Attempt to auto-create MedicationDispense.subject"); 1433 else if (Configuration.doAutoCreate()) 1434 this.subject = new Reference(); // cc 1435 return this.subject; 1436 } 1437 1438 public boolean hasSubject() { 1439 return this.subject != null && !this.subject.isEmpty(); 1440 } 1441 1442 /** 1443 * @param value {@link #subject} (A link to a resource representing the person or the group to whom the medication will be given.) 1444 */ 1445 public MedicationDispense setSubject(Reference value) { 1446 this.subject = value; 1447 return this; 1448 } 1449 1450 /** 1451 * @return {@link #encounter} (The encounter that establishes the context for this event.) 1452 */ 1453 public Reference getEncounter() { 1454 if (this.encounter == null) 1455 if (Configuration.errorOnAutoCreate()) 1456 throw new Error("Attempt to auto-create MedicationDispense.encounter"); 1457 else if (Configuration.doAutoCreate()) 1458 this.encounter = new Reference(); // cc 1459 return this.encounter; 1460 } 1461 1462 public boolean hasEncounter() { 1463 return this.encounter != null && !this.encounter.isEmpty(); 1464 } 1465 1466 /** 1467 * @param value {@link #encounter} (The encounter that establishes the context for this event.) 1468 */ 1469 public MedicationDispense setEncounter(Reference value) { 1470 this.encounter = value; 1471 return this; 1472 } 1473 1474 /** 1475 * @return {@link #supportingInformation} (Additional information that supports the medication being dispensed. For example, there may be requirements that a specific lab test has been completed prior to dispensing or the patient's weight at the time of dispensing is documented.) 1476 */ 1477 public List<Reference> getSupportingInformation() { 1478 if (this.supportingInformation == null) 1479 this.supportingInformation = new ArrayList<Reference>(); 1480 return this.supportingInformation; 1481 } 1482 1483 /** 1484 * @return Returns a reference to <code>this</code> for easy method chaining 1485 */ 1486 public MedicationDispense setSupportingInformation(List<Reference> theSupportingInformation) { 1487 this.supportingInformation = theSupportingInformation; 1488 return this; 1489 } 1490 1491 public boolean hasSupportingInformation() { 1492 if (this.supportingInformation == null) 1493 return false; 1494 for (Reference item : this.supportingInformation) 1495 if (!item.isEmpty()) 1496 return true; 1497 return false; 1498 } 1499 1500 public Reference addSupportingInformation() { //3 1501 Reference t = new Reference(); 1502 if (this.supportingInformation == null) 1503 this.supportingInformation = new ArrayList<Reference>(); 1504 this.supportingInformation.add(t); 1505 return t; 1506 } 1507 1508 public MedicationDispense addSupportingInformation(Reference t) { //3 1509 if (t == null) 1510 return this; 1511 if (this.supportingInformation == null) 1512 this.supportingInformation = new ArrayList<Reference>(); 1513 this.supportingInformation.add(t); 1514 return this; 1515 } 1516 1517 /** 1518 * @return The first repetition of repeating field {@link #supportingInformation}, creating it if it does not already exist {3} 1519 */ 1520 public Reference getSupportingInformationFirstRep() { 1521 if (getSupportingInformation().isEmpty()) { 1522 addSupportingInformation(); 1523 } 1524 return getSupportingInformation().get(0); 1525 } 1526 1527 /** 1528 * @return {@link #performer} (Indicates who or what performed the event.) 1529 */ 1530 public List<MedicationDispensePerformerComponent> getPerformer() { 1531 if (this.performer == null) 1532 this.performer = new ArrayList<MedicationDispensePerformerComponent>(); 1533 return this.performer; 1534 } 1535 1536 /** 1537 * @return Returns a reference to <code>this</code> for easy method chaining 1538 */ 1539 public MedicationDispense setPerformer(List<MedicationDispensePerformerComponent> thePerformer) { 1540 this.performer = thePerformer; 1541 return this; 1542 } 1543 1544 public boolean hasPerformer() { 1545 if (this.performer == null) 1546 return false; 1547 for (MedicationDispensePerformerComponent item : this.performer) 1548 if (!item.isEmpty()) 1549 return true; 1550 return false; 1551 } 1552 1553 public MedicationDispensePerformerComponent addPerformer() { //3 1554 MedicationDispensePerformerComponent t = new MedicationDispensePerformerComponent(); 1555 if (this.performer == null) 1556 this.performer = new ArrayList<MedicationDispensePerformerComponent>(); 1557 this.performer.add(t); 1558 return t; 1559 } 1560 1561 public MedicationDispense addPerformer(MedicationDispensePerformerComponent t) { //3 1562 if (t == null) 1563 return this; 1564 if (this.performer == null) 1565 this.performer = new ArrayList<MedicationDispensePerformerComponent>(); 1566 this.performer.add(t); 1567 return this; 1568 } 1569 1570 /** 1571 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist {3} 1572 */ 1573 public MedicationDispensePerformerComponent getPerformerFirstRep() { 1574 if (getPerformer().isEmpty()) { 1575 addPerformer(); 1576 } 1577 return getPerformer().get(0); 1578 } 1579 1580 /** 1581 * @return {@link #location} (The principal physical location where the dispense was performed.) 1582 */ 1583 public Reference getLocation() { 1584 if (this.location == null) 1585 if (Configuration.errorOnAutoCreate()) 1586 throw new Error("Attempt to auto-create MedicationDispense.location"); 1587 else if (Configuration.doAutoCreate()) 1588 this.location = new Reference(); // cc 1589 return this.location; 1590 } 1591 1592 public boolean hasLocation() { 1593 return this.location != null && !this.location.isEmpty(); 1594 } 1595 1596 /** 1597 * @param value {@link #location} (The principal physical location where the dispense was performed.) 1598 */ 1599 public MedicationDispense setLocation(Reference value) { 1600 this.location = value; 1601 return this; 1602 } 1603 1604 /** 1605 * @return {@link #authorizingPrescription} (Indicates the medication order that is being dispensed against.) 1606 */ 1607 public List<Reference> getAuthorizingPrescription() { 1608 if (this.authorizingPrescription == null) 1609 this.authorizingPrescription = new ArrayList<Reference>(); 1610 return this.authorizingPrescription; 1611 } 1612 1613 /** 1614 * @return Returns a reference to <code>this</code> for easy method chaining 1615 */ 1616 public MedicationDispense setAuthorizingPrescription(List<Reference> theAuthorizingPrescription) { 1617 this.authorizingPrescription = theAuthorizingPrescription; 1618 return this; 1619 } 1620 1621 public boolean hasAuthorizingPrescription() { 1622 if (this.authorizingPrescription == null) 1623 return false; 1624 for (Reference item : this.authorizingPrescription) 1625 if (!item.isEmpty()) 1626 return true; 1627 return false; 1628 } 1629 1630 public Reference addAuthorizingPrescription() { //3 1631 Reference t = new Reference(); 1632 if (this.authorizingPrescription == null) 1633 this.authorizingPrescription = new ArrayList<Reference>(); 1634 this.authorizingPrescription.add(t); 1635 return t; 1636 } 1637 1638 public MedicationDispense addAuthorizingPrescription(Reference t) { //3 1639 if (t == null) 1640 return this; 1641 if (this.authorizingPrescription == null) 1642 this.authorizingPrescription = new ArrayList<Reference>(); 1643 this.authorizingPrescription.add(t); 1644 return this; 1645 } 1646 1647 /** 1648 * @return The first repetition of repeating field {@link #authorizingPrescription}, creating it if it does not already exist {3} 1649 */ 1650 public Reference getAuthorizingPrescriptionFirstRep() { 1651 if (getAuthorizingPrescription().isEmpty()) { 1652 addAuthorizingPrescription(); 1653 } 1654 return getAuthorizingPrescription().get(0); 1655 } 1656 1657 /** 1658 * @return {@link #type} (Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.) 1659 */ 1660 public CodeableConcept getType() { 1661 if (this.type == null) 1662 if (Configuration.errorOnAutoCreate()) 1663 throw new Error("Attempt to auto-create MedicationDispense.type"); 1664 else if (Configuration.doAutoCreate()) 1665 this.type = new CodeableConcept(); // cc 1666 return this.type; 1667 } 1668 1669 public boolean hasType() { 1670 return this.type != null && !this.type.isEmpty(); 1671 } 1672 1673 /** 1674 * @param value {@link #type} (Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.) 1675 */ 1676 public MedicationDispense setType(CodeableConcept value) { 1677 this.type = value; 1678 return this; 1679 } 1680 1681 /** 1682 * @return {@link #quantity} (The amount of medication that has been dispensed. Includes unit of measure.) 1683 */ 1684 public Quantity getQuantity() { 1685 if (this.quantity == null) 1686 if (Configuration.errorOnAutoCreate()) 1687 throw new Error("Attempt to auto-create MedicationDispense.quantity"); 1688 else if (Configuration.doAutoCreate()) 1689 this.quantity = new Quantity(); // cc 1690 return this.quantity; 1691 } 1692 1693 public boolean hasQuantity() { 1694 return this.quantity != null && !this.quantity.isEmpty(); 1695 } 1696 1697 /** 1698 * @param value {@link #quantity} (The amount of medication that has been dispensed. Includes unit of measure.) 1699 */ 1700 public MedicationDispense setQuantity(Quantity value) { 1701 this.quantity = value; 1702 return this; 1703 } 1704 1705 /** 1706 * @return {@link #daysSupply} (The amount of medication expressed as a timing amount.) 1707 */ 1708 public Quantity getDaysSupply() { 1709 if (this.daysSupply == null) 1710 if (Configuration.errorOnAutoCreate()) 1711 throw new Error("Attempt to auto-create MedicationDispense.daysSupply"); 1712 else if (Configuration.doAutoCreate()) 1713 this.daysSupply = new Quantity(); // cc 1714 return this.daysSupply; 1715 } 1716 1717 public boolean hasDaysSupply() { 1718 return this.daysSupply != null && !this.daysSupply.isEmpty(); 1719 } 1720 1721 /** 1722 * @param value {@link #daysSupply} (The amount of medication expressed as a timing amount.) 1723 */ 1724 public MedicationDispense setDaysSupply(Quantity value) { 1725 this.daysSupply = value; 1726 return this; 1727 } 1728 1729 /** 1730 * @return {@link #recorded} (The date (and maybe time) when the dispense activity started if whenPrepared or whenHandedOver is not populated.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 1731 */ 1732 public DateTimeType getRecordedElement() { 1733 if (this.recorded == null) 1734 if (Configuration.errorOnAutoCreate()) 1735 throw new Error("Attempt to auto-create MedicationDispense.recorded"); 1736 else if (Configuration.doAutoCreate()) 1737 this.recorded = new DateTimeType(); // bb 1738 return this.recorded; 1739 } 1740 1741 public boolean hasRecordedElement() { 1742 return this.recorded != null && !this.recorded.isEmpty(); 1743 } 1744 1745 public boolean hasRecorded() { 1746 return this.recorded != null && !this.recorded.isEmpty(); 1747 } 1748 1749 /** 1750 * @param value {@link #recorded} (The date (and maybe time) when the dispense activity started if whenPrepared or whenHandedOver is not populated.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 1751 */ 1752 public MedicationDispense setRecordedElement(DateTimeType value) { 1753 this.recorded = value; 1754 return this; 1755 } 1756 1757 /** 1758 * @return The date (and maybe time) when the dispense activity started if whenPrepared or whenHandedOver is not populated. 1759 */ 1760 public Date getRecorded() { 1761 return this.recorded == null ? null : this.recorded.getValue(); 1762 } 1763 1764 /** 1765 * @param value The date (and maybe time) when the dispense activity started if whenPrepared or whenHandedOver is not populated. 1766 */ 1767 public MedicationDispense setRecorded(Date value) { 1768 if (value == null) 1769 this.recorded = null; 1770 else { 1771 if (this.recorded == null) 1772 this.recorded = new DateTimeType(); 1773 this.recorded.setValue(value); 1774 } 1775 return this; 1776 } 1777 1778 /** 1779 * @return {@link #whenPrepared} (The time when the dispensed product was packaged and reviewed.). This is the underlying object with id, value and extensions. The accessor "getWhenPrepared" gives direct access to the value 1780 */ 1781 public DateTimeType getWhenPreparedElement() { 1782 if (this.whenPrepared == null) 1783 if (Configuration.errorOnAutoCreate()) 1784 throw new Error("Attempt to auto-create MedicationDispense.whenPrepared"); 1785 else if (Configuration.doAutoCreate()) 1786 this.whenPrepared = new DateTimeType(); // bb 1787 return this.whenPrepared; 1788 } 1789 1790 public boolean hasWhenPreparedElement() { 1791 return this.whenPrepared != null && !this.whenPrepared.isEmpty(); 1792 } 1793 1794 public boolean hasWhenPrepared() { 1795 return this.whenPrepared != null && !this.whenPrepared.isEmpty(); 1796 } 1797 1798 /** 1799 * @param value {@link #whenPrepared} (The time when the dispensed product was packaged and reviewed.). This is the underlying object with id, value and extensions. The accessor "getWhenPrepared" gives direct access to the value 1800 */ 1801 public MedicationDispense setWhenPreparedElement(DateTimeType value) { 1802 this.whenPrepared = value; 1803 return this; 1804 } 1805 1806 /** 1807 * @return The time when the dispensed product was packaged and reviewed. 1808 */ 1809 public Date getWhenPrepared() { 1810 return this.whenPrepared == null ? null : this.whenPrepared.getValue(); 1811 } 1812 1813 /** 1814 * @param value The time when the dispensed product was packaged and reviewed. 1815 */ 1816 public MedicationDispense setWhenPrepared(Date value) { 1817 if (value == null) 1818 this.whenPrepared = null; 1819 else { 1820 if (this.whenPrepared == null) 1821 this.whenPrepared = new DateTimeType(); 1822 this.whenPrepared.setValue(value); 1823 } 1824 return this; 1825 } 1826 1827 /** 1828 * @return {@link #whenHandedOver} (The time the dispensed product was provided to the patient or their representative.). This is the underlying object with id, value and extensions. The accessor "getWhenHandedOver" gives direct access to the value 1829 */ 1830 public DateTimeType getWhenHandedOverElement() { 1831 if (this.whenHandedOver == null) 1832 if (Configuration.errorOnAutoCreate()) 1833 throw new Error("Attempt to auto-create MedicationDispense.whenHandedOver"); 1834 else if (Configuration.doAutoCreate()) 1835 this.whenHandedOver = new DateTimeType(); // bb 1836 return this.whenHandedOver; 1837 } 1838 1839 public boolean hasWhenHandedOverElement() { 1840 return this.whenHandedOver != null && !this.whenHandedOver.isEmpty(); 1841 } 1842 1843 public boolean hasWhenHandedOver() { 1844 return this.whenHandedOver != null && !this.whenHandedOver.isEmpty(); 1845 } 1846 1847 /** 1848 * @param value {@link #whenHandedOver} (The time the dispensed product was provided to the patient or their representative.). This is the underlying object with id, value and extensions. The accessor "getWhenHandedOver" gives direct access to the value 1849 */ 1850 public MedicationDispense setWhenHandedOverElement(DateTimeType value) { 1851 this.whenHandedOver = value; 1852 return this; 1853 } 1854 1855 /** 1856 * @return The time the dispensed product was provided to the patient or their representative. 1857 */ 1858 public Date getWhenHandedOver() { 1859 return this.whenHandedOver == null ? null : this.whenHandedOver.getValue(); 1860 } 1861 1862 /** 1863 * @param value The time the dispensed product was provided to the patient or their representative. 1864 */ 1865 public MedicationDispense setWhenHandedOver(Date value) { 1866 if (value == null) 1867 this.whenHandedOver = null; 1868 else { 1869 if (this.whenHandedOver == null) 1870 this.whenHandedOver = new DateTimeType(); 1871 this.whenHandedOver.setValue(value); 1872 } 1873 return this; 1874 } 1875 1876 /** 1877 * @return {@link #destination} (Identification of the facility/location where the medication was/will be shipped to, as part of the dispense event.) 1878 */ 1879 public Reference getDestination() { 1880 if (this.destination == null) 1881 if (Configuration.errorOnAutoCreate()) 1882 throw new Error("Attempt to auto-create MedicationDispense.destination"); 1883 else if (Configuration.doAutoCreate()) 1884 this.destination = new Reference(); // cc 1885 return this.destination; 1886 } 1887 1888 public boolean hasDestination() { 1889 return this.destination != null && !this.destination.isEmpty(); 1890 } 1891 1892 /** 1893 * @param value {@link #destination} (Identification of the facility/location where the medication was/will be shipped to, as part of the dispense event.) 1894 */ 1895 public MedicationDispense setDestination(Reference value) { 1896 this.destination = value; 1897 return this; 1898 } 1899 1900 /** 1901 * @return {@link #receiver} (Identifies the person who picked up the medication or the location of where the medication was delivered. This will usually be a patient or their caregiver, but some cases exist where it can be a healthcare professional or a location.) 1902 */ 1903 public List<Reference> getReceiver() { 1904 if (this.receiver == null) 1905 this.receiver = new ArrayList<Reference>(); 1906 return this.receiver; 1907 } 1908 1909 /** 1910 * @return Returns a reference to <code>this</code> for easy method chaining 1911 */ 1912 public MedicationDispense setReceiver(List<Reference> theReceiver) { 1913 this.receiver = theReceiver; 1914 return this; 1915 } 1916 1917 public boolean hasReceiver() { 1918 if (this.receiver == null) 1919 return false; 1920 for (Reference item : this.receiver) 1921 if (!item.isEmpty()) 1922 return true; 1923 return false; 1924 } 1925 1926 public Reference addReceiver() { //3 1927 Reference t = new Reference(); 1928 if (this.receiver == null) 1929 this.receiver = new ArrayList<Reference>(); 1930 this.receiver.add(t); 1931 return t; 1932 } 1933 1934 public MedicationDispense addReceiver(Reference t) { //3 1935 if (t == null) 1936 return this; 1937 if (this.receiver == null) 1938 this.receiver = new ArrayList<Reference>(); 1939 this.receiver.add(t); 1940 return this; 1941 } 1942 1943 /** 1944 * @return The first repetition of repeating field {@link #receiver}, creating it if it does not already exist {3} 1945 */ 1946 public Reference getReceiverFirstRep() { 1947 if (getReceiver().isEmpty()) { 1948 addReceiver(); 1949 } 1950 return getReceiver().get(0); 1951 } 1952 1953 /** 1954 * @return {@link #note} (Extra information about the dispense that could not be conveyed in the other attributes.) 1955 */ 1956 public List<Annotation> getNote() { 1957 if (this.note == null) 1958 this.note = new ArrayList<Annotation>(); 1959 return this.note; 1960 } 1961 1962 /** 1963 * @return Returns a reference to <code>this</code> for easy method chaining 1964 */ 1965 public MedicationDispense setNote(List<Annotation> theNote) { 1966 this.note = theNote; 1967 return this; 1968 } 1969 1970 public boolean hasNote() { 1971 if (this.note == null) 1972 return false; 1973 for (Annotation item : this.note) 1974 if (!item.isEmpty()) 1975 return true; 1976 return false; 1977 } 1978 1979 public Annotation addNote() { //3 1980 Annotation t = new Annotation(); 1981 if (this.note == null) 1982 this.note = new ArrayList<Annotation>(); 1983 this.note.add(t); 1984 return t; 1985 } 1986 1987 public MedicationDispense addNote(Annotation t) { //3 1988 if (t == null) 1989 return this; 1990 if (this.note == null) 1991 this.note = new ArrayList<Annotation>(); 1992 this.note.add(t); 1993 return this; 1994 } 1995 1996 /** 1997 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 1998 */ 1999 public Annotation getNoteFirstRep() { 2000 if (getNote().isEmpty()) { 2001 addNote(); 2002 } 2003 return getNote().get(0); 2004 } 2005 2006 /** 2007 * @return {@link #renderedDosageInstruction} (The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.). This is the underlying object with id, value and extensions. The accessor "getRenderedDosageInstruction" gives direct access to the value 2008 */ 2009 public MarkdownType getRenderedDosageInstructionElement() { 2010 if (this.renderedDosageInstruction == null) 2011 if (Configuration.errorOnAutoCreate()) 2012 throw new Error("Attempt to auto-create MedicationDispense.renderedDosageInstruction"); 2013 else if (Configuration.doAutoCreate()) 2014 this.renderedDosageInstruction = new MarkdownType(); // bb 2015 return this.renderedDosageInstruction; 2016 } 2017 2018 public boolean hasRenderedDosageInstructionElement() { 2019 return this.renderedDosageInstruction != null && !this.renderedDosageInstruction.isEmpty(); 2020 } 2021 2022 public boolean hasRenderedDosageInstruction() { 2023 return this.renderedDosageInstruction != null && !this.renderedDosageInstruction.isEmpty(); 2024 } 2025 2026 /** 2027 * @param value {@link #renderedDosageInstruction} (The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.). This is the underlying object with id, value and extensions. The accessor "getRenderedDosageInstruction" gives direct access to the value 2028 */ 2029 public MedicationDispense setRenderedDosageInstructionElement(MarkdownType value) { 2030 this.renderedDosageInstruction = value; 2031 return this; 2032 } 2033 2034 /** 2035 * @return The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses. 2036 */ 2037 public String getRenderedDosageInstruction() { 2038 return this.renderedDosageInstruction == null ? null : this.renderedDosageInstruction.getValue(); 2039 } 2040 2041 /** 2042 * @param value The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses. 2043 */ 2044 public MedicationDispense setRenderedDosageInstruction(String value) { 2045 if (Utilities.noString(value)) 2046 this.renderedDosageInstruction = null; 2047 else { 2048 if (this.renderedDosageInstruction == null) 2049 this.renderedDosageInstruction = new MarkdownType(); 2050 this.renderedDosageInstruction.setValue(value); 2051 } 2052 return this; 2053 } 2054 2055 /** 2056 * @return {@link #dosageInstruction} (Indicates how the medication is to be used by the patient.) 2057 */ 2058 public List<Dosage> getDosageInstruction() { 2059 if (this.dosageInstruction == null) 2060 this.dosageInstruction = new ArrayList<Dosage>(); 2061 return this.dosageInstruction; 2062 } 2063 2064 /** 2065 * @return Returns a reference to <code>this</code> for easy method chaining 2066 */ 2067 public MedicationDispense setDosageInstruction(List<Dosage> theDosageInstruction) { 2068 this.dosageInstruction = theDosageInstruction; 2069 return this; 2070 } 2071 2072 public boolean hasDosageInstruction() { 2073 if (this.dosageInstruction == null) 2074 return false; 2075 for (Dosage item : this.dosageInstruction) 2076 if (!item.isEmpty()) 2077 return true; 2078 return false; 2079 } 2080 2081 public Dosage addDosageInstruction() { //3 2082 Dosage t = new Dosage(); 2083 if (this.dosageInstruction == null) 2084 this.dosageInstruction = new ArrayList<Dosage>(); 2085 this.dosageInstruction.add(t); 2086 return t; 2087 } 2088 2089 public MedicationDispense addDosageInstruction(Dosage t) { //3 2090 if (t == null) 2091 return this; 2092 if (this.dosageInstruction == null) 2093 this.dosageInstruction = new ArrayList<Dosage>(); 2094 this.dosageInstruction.add(t); 2095 return this; 2096 } 2097 2098 /** 2099 * @return The first repetition of repeating field {@link #dosageInstruction}, creating it if it does not already exist {3} 2100 */ 2101 public Dosage getDosageInstructionFirstRep() { 2102 if (getDosageInstruction().isEmpty()) { 2103 addDosageInstruction(); 2104 } 2105 return getDosageInstruction().get(0); 2106 } 2107 2108 /** 2109 * @return {@link #substitution} (Indicates whether or not substitution was made as part of the dispense. In some cases, substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why. If nothing is specified, substitution was not done.) 2110 */ 2111 public MedicationDispenseSubstitutionComponent getSubstitution() { 2112 if (this.substitution == null) 2113 if (Configuration.errorOnAutoCreate()) 2114 throw new Error("Attempt to auto-create MedicationDispense.substitution"); 2115 else if (Configuration.doAutoCreate()) 2116 this.substitution = new MedicationDispenseSubstitutionComponent(); // cc 2117 return this.substitution; 2118 } 2119 2120 public boolean hasSubstitution() { 2121 return this.substitution != null && !this.substitution.isEmpty(); 2122 } 2123 2124 /** 2125 * @param value {@link #substitution} (Indicates whether or not substitution was made as part of the dispense. In some cases, substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why. If nothing is specified, substitution was not done.) 2126 */ 2127 public MedicationDispense setSubstitution(MedicationDispenseSubstitutionComponent value) { 2128 this.substitution = value; 2129 return this; 2130 } 2131 2132 /** 2133 * @return {@link #eventHistory} (A summary of the events of interest that have occurred, such as when the dispense was verified.) 2134 */ 2135 public List<Reference> getEventHistory() { 2136 if (this.eventHistory == null) 2137 this.eventHistory = new ArrayList<Reference>(); 2138 return this.eventHistory; 2139 } 2140 2141 /** 2142 * @return Returns a reference to <code>this</code> for easy method chaining 2143 */ 2144 public MedicationDispense setEventHistory(List<Reference> theEventHistory) { 2145 this.eventHistory = theEventHistory; 2146 return this; 2147 } 2148 2149 public boolean hasEventHistory() { 2150 if (this.eventHistory == null) 2151 return false; 2152 for (Reference item : this.eventHistory) 2153 if (!item.isEmpty()) 2154 return true; 2155 return false; 2156 } 2157 2158 public Reference addEventHistory() { //3 2159 Reference t = new Reference(); 2160 if (this.eventHistory == null) 2161 this.eventHistory = new ArrayList<Reference>(); 2162 this.eventHistory.add(t); 2163 return t; 2164 } 2165 2166 public MedicationDispense addEventHistory(Reference t) { //3 2167 if (t == null) 2168 return this; 2169 if (this.eventHistory == null) 2170 this.eventHistory = new ArrayList<Reference>(); 2171 this.eventHistory.add(t); 2172 return this; 2173 } 2174 2175 /** 2176 * @return The first repetition of repeating field {@link #eventHistory}, creating it if it does not already exist {3} 2177 */ 2178 public Reference getEventHistoryFirstRep() { 2179 if (getEventHistory().isEmpty()) { 2180 addEventHistory(); 2181 } 2182 return getEventHistory().get(0); 2183 } 2184 2185 protected void listChildren(List<Property> children) { 2186 super.listChildren(children); 2187 children.add(new Property("identifier", "Identifier", "Identifiers associated with this Medication Dispense that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2188 children.add(new Property("basedOn", "Reference(CarePlan)", "A plan that is fulfilled in whole or in part by this MedicationDispense.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2189 children.add(new Property("partOf", "Reference(Procedure|MedicationAdministration)", "The procedure or medication administration that triggered the dispense.", 0, java.lang.Integer.MAX_VALUE, partOf)); 2190 children.add(new Property("status", "code", "A code specifying the state of the set of dispense events.", 0, 1, status)); 2191 children.add(new Property("notPerformedReason", "CodeableReference(DetectedIssue)", "Indicates the reason why a dispense was not performed.", 0, 1, notPerformedReason)); 2192 children.add(new Property("statusChanged", "dateTime", "The date (and maybe time) when the status of the dispense record changed.", 0, 1, statusChanged)); 2193 children.add(new Property("category", "CodeableConcept", "Indicates the type of medication dispense (for example, drug classification like ATC, where meds would be administered, legal category of the medication.).", 0, java.lang.Integer.MAX_VALUE, category)); 2194 children.add(new Property("medication", "CodeableReference(Medication)", "Identifies the medication supplied. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication)); 2195 children.add(new Property("subject", "Reference(Patient|Group)", "A link to a resource representing the person or the group to whom the medication will be given.", 0, 1, subject)); 2196 children.add(new Property("encounter", "Reference(Encounter)", "The encounter that establishes the context for this event.", 0, 1, encounter)); 2197 children.add(new Property("supportingInformation", "Reference(Any)", "Additional information that supports the medication being dispensed. For example, there may be requirements that a specific lab test has been completed prior to dispensing or the patient's weight at the time of dispensing is documented.", 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 2198 children.add(new Property("performer", "", "Indicates who or what performed the event.", 0, java.lang.Integer.MAX_VALUE, performer)); 2199 children.add(new Property("location", "Reference(Location)", "The principal physical location where the dispense was performed.", 0, 1, location)); 2200 children.add(new Property("authorizingPrescription", "Reference(MedicationRequest)", "Indicates the medication order that is being dispensed against.", 0, java.lang.Integer.MAX_VALUE, authorizingPrescription)); 2201 children.add(new Property("type", "CodeableConcept", "Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.", 0, 1, type)); 2202 children.add(new Property("quantity", "Quantity", "The amount of medication that has been dispensed. Includes unit of measure.", 0, 1, quantity)); 2203 children.add(new Property("daysSupply", "Quantity", "The amount of medication expressed as a timing amount.", 0, 1, daysSupply)); 2204 children.add(new Property("recorded", "dateTime", "The date (and maybe time) when the dispense activity started if whenPrepared or whenHandedOver is not populated.", 0, 1, recorded)); 2205 children.add(new Property("whenPrepared", "dateTime", "The time when the dispensed product was packaged and reviewed.", 0, 1, whenPrepared)); 2206 children.add(new Property("whenHandedOver", "dateTime", "The time the dispensed product was provided to the patient or their representative.", 0, 1, whenHandedOver)); 2207 children.add(new Property("destination", "Reference(Location)", "Identification of the facility/location where the medication was/will be shipped to, as part of the dispense event.", 0, 1, destination)); 2208 children.add(new Property("receiver", "Reference(Patient|Practitioner|RelatedPerson|Location|PractitionerRole)", "Identifies the person who picked up the medication or the location of where the medication was delivered. This will usually be a patient or their caregiver, but some cases exist where it can be a healthcare professional or a location.", 0, java.lang.Integer.MAX_VALUE, receiver)); 2209 children.add(new Property("note", "Annotation", "Extra information about the dispense that could not be conveyed in the other attributes.", 0, java.lang.Integer.MAX_VALUE, note)); 2210 children.add(new Property("renderedDosageInstruction", "markdown", "The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.", 0, 1, renderedDosageInstruction)); 2211 children.add(new Property("dosageInstruction", "Dosage", "Indicates how the medication is to be used by the patient.", 0, java.lang.Integer.MAX_VALUE, dosageInstruction)); 2212 children.add(new Property("substitution", "", "Indicates whether or not substitution was made as part of the dispense. In some cases, substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why. If nothing is specified, substitution was not done.", 0, 1, substitution)); 2213 children.add(new Property("eventHistory", "Reference(Provenance)", "A summary of the events of interest that have occurred, such as when the dispense was verified.", 0, java.lang.Integer.MAX_VALUE, eventHistory)); 2214 } 2215 2216 @Override 2217 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2218 switch (_hash) { 2219 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers associated with this Medication Dispense that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. They are business identifiers assigned to this resource by the performer or other systems and remain constant as the resource is updated and propagates from server to server.", 0, java.lang.Integer.MAX_VALUE, identifier); 2220 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan)", "A plan that is fulfilled in whole or in part by this MedicationDispense.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2221 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Procedure|MedicationAdministration)", "The procedure or medication administration that triggered the dispense.", 0, java.lang.Integer.MAX_VALUE, partOf); 2222 case -892481550: /*status*/ return new Property("status", "code", "A code specifying the state of the set of dispense events.", 0, 1, status); 2223 case -820839727: /*notPerformedReason*/ return new Property("notPerformedReason", "CodeableReference(DetectedIssue)", "Indicates the reason why a dispense was not performed.", 0, 1, notPerformedReason); 2224 case -1174686110: /*statusChanged*/ return new Property("statusChanged", "dateTime", "The date (and maybe time) when the status of the dispense record changed.", 0, 1, statusChanged); 2225 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Indicates the type of medication dispense (for example, drug classification like ATC, where meds would be administered, legal category of the medication.).", 0, java.lang.Integer.MAX_VALUE, category); 2226 case 1998965455: /*medication*/ return new Property("medication", "CodeableReference(Medication)", "Identifies the medication supplied. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 2227 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "A link to a resource representing the person or the group to whom the medication will be given.", 0, 1, subject); 2228 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The encounter that establishes the context for this event.", 0, 1, encounter); 2229 case -1248768647: /*supportingInformation*/ return new Property("supportingInformation", "Reference(Any)", "Additional information that supports the medication being dispensed. For example, there may be requirements that a specific lab test has been completed prior to dispensing or the patient's weight at the time of dispensing is documented.", 0, java.lang.Integer.MAX_VALUE, supportingInformation); 2230 case 481140686: /*performer*/ return new Property("performer", "", "Indicates who or what performed the event.", 0, java.lang.Integer.MAX_VALUE, performer); 2231 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The principal physical location where the dispense was performed.", 0, 1, location); 2232 case -1237557856: /*authorizingPrescription*/ return new Property("authorizingPrescription", "Reference(MedicationRequest)", "Indicates the medication order that is being dispensed against.", 0, java.lang.Integer.MAX_VALUE, authorizingPrescription); 2233 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.", 0, 1, type); 2234 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The amount of medication that has been dispensed. Includes unit of measure.", 0, 1, quantity); 2235 case 197175334: /*daysSupply*/ return new Property("daysSupply", "Quantity", "The amount of medication expressed as a timing amount.", 0, 1, daysSupply); 2236 case -799233872: /*recorded*/ return new Property("recorded", "dateTime", "The date (and maybe time) when the dispense activity started if whenPrepared or whenHandedOver is not populated.", 0, 1, recorded); 2237 case -562837097: /*whenPrepared*/ return new Property("whenPrepared", "dateTime", "The time when the dispensed product was packaged and reviewed.", 0, 1, whenPrepared); 2238 case -940241380: /*whenHandedOver*/ return new Property("whenHandedOver", "dateTime", "The time the dispensed product was provided to the patient or their representative.", 0, 1, whenHandedOver); 2239 case -1429847026: /*destination*/ return new Property("destination", "Reference(Location)", "Identification of the facility/location where the medication was/will be shipped to, as part of the dispense event.", 0, 1, destination); 2240 case -808719889: /*receiver*/ return new Property("receiver", "Reference(Patient|Practitioner|RelatedPerson|Location|PractitionerRole)", "Identifies the person who picked up the medication or the location of where the medication was delivered. This will usually be a patient or their caregiver, but some cases exist where it can be a healthcare professional or a location.", 0, java.lang.Integer.MAX_VALUE, receiver); 2241 case 3387378: /*note*/ return new Property("note", "Annotation", "Extra information about the dispense that could not be conveyed in the other attributes.", 0, java.lang.Integer.MAX_VALUE, note); 2242 case 1718902050: /*renderedDosageInstruction*/ return new Property("renderedDosageInstruction", "markdown", "The full representation of the dose of the medication included in all dosage instructions. To be used when multiple dosage instructions are included to represent complex dosing such as increasing or tapering doses.", 0, 1, renderedDosageInstruction); 2243 case -1201373865: /*dosageInstruction*/ return new Property("dosageInstruction", "Dosage", "Indicates how the medication is to be used by the patient.", 0, java.lang.Integer.MAX_VALUE, dosageInstruction); 2244 case 826147581: /*substitution*/ return new Property("substitution", "", "Indicates whether or not substitution was made as part of the dispense. In some cases, substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why. If nothing is specified, substitution was not done.", 0, 1, substitution); 2245 case 1835190426: /*eventHistory*/ return new Property("eventHistory", "Reference(Provenance)", "A summary of the events of interest that have occurred, such as when the dispense was verified.", 0, java.lang.Integer.MAX_VALUE, eventHistory); 2246 default: return super.getNamedProperty(_hash, _name, _checkValid); 2247 } 2248 2249 } 2250 2251 @Override 2252 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2253 switch (hash) { 2254 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2255 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2256 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2257 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MedicationDispenseStatusCodes> 2258 case -820839727: /*notPerformedReason*/ return this.notPerformedReason == null ? new Base[0] : new Base[] {this.notPerformedReason}; // CodeableReference 2259 case -1174686110: /*statusChanged*/ return this.statusChanged == null ? new Base[0] : new Base[] {this.statusChanged}; // DateTimeType 2260 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 2261 case 1998965455: /*medication*/ return this.medication == null ? new Base[0] : new Base[] {this.medication}; // CodeableReference 2262 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2263 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 2264 case -1248768647: /*supportingInformation*/ return this.supportingInformation == null ? new Base[0] : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 2265 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // MedicationDispensePerformerComponent 2266 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 2267 case -1237557856: /*authorizingPrescription*/ return this.authorizingPrescription == null ? new Base[0] : this.authorizingPrescription.toArray(new Base[this.authorizingPrescription.size()]); // Reference 2268 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2269 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 2270 case 197175334: /*daysSupply*/ return this.daysSupply == null ? new Base[0] : new Base[] {this.daysSupply}; // Quantity 2271 case -799233872: /*recorded*/ return this.recorded == null ? new Base[0] : new Base[] {this.recorded}; // DateTimeType 2272 case -562837097: /*whenPrepared*/ return this.whenPrepared == null ? new Base[0] : new Base[] {this.whenPrepared}; // DateTimeType 2273 case -940241380: /*whenHandedOver*/ return this.whenHandedOver == null ? new Base[0] : new Base[] {this.whenHandedOver}; // DateTimeType 2274 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : new Base[] {this.destination}; // Reference 2275 case -808719889: /*receiver*/ return this.receiver == null ? new Base[0] : this.receiver.toArray(new Base[this.receiver.size()]); // Reference 2276 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2277 case 1718902050: /*renderedDosageInstruction*/ return this.renderedDosageInstruction == null ? new Base[0] : new Base[] {this.renderedDosageInstruction}; // MarkdownType 2278 case -1201373865: /*dosageInstruction*/ return this.dosageInstruction == null ? new Base[0] : this.dosageInstruction.toArray(new Base[this.dosageInstruction.size()]); // Dosage 2279 case 826147581: /*substitution*/ return this.substitution == null ? new Base[0] : new Base[] {this.substitution}; // MedicationDispenseSubstitutionComponent 2280 case 1835190426: /*eventHistory*/ return this.eventHistory == null ? new Base[0] : this.eventHistory.toArray(new Base[this.eventHistory.size()]); // Reference 2281 default: return super.getProperty(hash, name, checkValid); 2282 } 2283 2284 } 2285 2286 @Override 2287 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2288 switch (hash) { 2289 case -1618432855: // identifier 2290 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 2291 return value; 2292 case -332612366: // basedOn 2293 this.getBasedOn().add(TypeConvertor.castToReference(value)); // Reference 2294 return value; 2295 case -995410646: // partOf 2296 this.getPartOf().add(TypeConvertor.castToReference(value)); // Reference 2297 return value; 2298 case -892481550: // status 2299 value = new MedicationDispenseStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2300 this.status = (Enumeration) value; // Enumeration<MedicationDispenseStatusCodes> 2301 return value; 2302 case -820839727: // notPerformedReason 2303 this.notPerformedReason = TypeConvertor.castToCodeableReference(value); // CodeableReference 2304 return value; 2305 case -1174686110: // statusChanged 2306 this.statusChanged = TypeConvertor.castToDateTime(value); // DateTimeType 2307 return value; 2308 case 50511102: // category 2309 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2310 return value; 2311 case 1998965455: // medication 2312 this.medication = TypeConvertor.castToCodeableReference(value); // CodeableReference 2313 return value; 2314 case -1867885268: // subject 2315 this.subject = TypeConvertor.castToReference(value); // Reference 2316 return value; 2317 case 1524132147: // encounter 2318 this.encounter = TypeConvertor.castToReference(value); // Reference 2319 return value; 2320 case -1248768647: // supportingInformation 2321 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); // Reference 2322 return value; 2323 case 481140686: // performer 2324 this.getPerformer().add((MedicationDispensePerformerComponent) value); // MedicationDispensePerformerComponent 2325 return value; 2326 case 1901043637: // location 2327 this.location = TypeConvertor.castToReference(value); // Reference 2328 return value; 2329 case -1237557856: // authorizingPrescription 2330 this.getAuthorizingPrescription().add(TypeConvertor.castToReference(value)); // Reference 2331 return value; 2332 case 3575610: // type 2333 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2334 return value; 2335 case -1285004149: // quantity 2336 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 2337 return value; 2338 case 197175334: // daysSupply 2339 this.daysSupply = TypeConvertor.castToQuantity(value); // Quantity 2340 return value; 2341 case -799233872: // recorded 2342 this.recorded = TypeConvertor.castToDateTime(value); // DateTimeType 2343 return value; 2344 case -562837097: // whenPrepared 2345 this.whenPrepared = TypeConvertor.castToDateTime(value); // DateTimeType 2346 return value; 2347 case -940241380: // whenHandedOver 2348 this.whenHandedOver = TypeConvertor.castToDateTime(value); // DateTimeType 2349 return value; 2350 case -1429847026: // destination 2351 this.destination = TypeConvertor.castToReference(value); // Reference 2352 return value; 2353 case -808719889: // receiver 2354 this.getReceiver().add(TypeConvertor.castToReference(value)); // Reference 2355 return value; 2356 case 3387378: // note 2357 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 2358 return value; 2359 case 1718902050: // renderedDosageInstruction 2360 this.renderedDosageInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 2361 return value; 2362 case -1201373865: // dosageInstruction 2363 this.getDosageInstruction().add(TypeConvertor.castToDosage(value)); // Dosage 2364 return value; 2365 case 826147581: // substitution 2366 this.substitution = (MedicationDispenseSubstitutionComponent) value; // MedicationDispenseSubstitutionComponent 2367 return value; 2368 case 1835190426: // eventHistory 2369 this.getEventHistory().add(TypeConvertor.castToReference(value)); // Reference 2370 return value; 2371 default: return super.setProperty(hash, name, value); 2372 } 2373 2374 } 2375 2376 @Override 2377 public Base setProperty(String name, Base value) throws FHIRException { 2378 if (name.equals("identifier")) { 2379 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 2380 } else if (name.equals("basedOn")) { 2381 this.getBasedOn().add(TypeConvertor.castToReference(value)); 2382 } else if (name.equals("partOf")) { 2383 this.getPartOf().add(TypeConvertor.castToReference(value)); 2384 } else if (name.equals("status")) { 2385 value = new MedicationDispenseStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2386 this.status = (Enumeration) value; // Enumeration<MedicationDispenseStatusCodes> 2387 } else if (name.equals("notPerformedReason")) { 2388 this.notPerformedReason = TypeConvertor.castToCodeableReference(value); // CodeableReference 2389 } else if (name.equals("statusChanged")) { 2390 this.statusChanged = TypeConvertor.castToDateTime(value); // DateTimeType 2391 } else if (name.equals("category")) { 2392 this.getCategory().add(TypeConvertor.castToCodeableConcept(value)); 2393 } else if (name.equals("medication")) { 2394 this.medication = TypeConvertor.castToCodeableReference(value); // CodeableReference 2395 } else if (name.equals("subject")) { 2396 this.subject = TypeConvertor.castToReference(value); // Reference 2397 } else if (name.equals("encounter")) { 2398 this.encounter = TypeConvertor.castToReference(value); // Reference 2399 } else if (name.equals("supportingInformation")) { 2400 this.getSupportingInformation().add(TypeConvertor.castToReference(value)); 2401 } else if (name.equals("performer")) { 2402 this.getPerformer().add((MedicationDispensePerformerComponent) value); 2403 } else if (name.equals("location")) { 2404 this.location = TypeConvertor.castToReference(value); // Reference 2405 } else if (name.equals("authorizingPrescription")) { 2406 this.getAuthorizingPrescription().add(TypeConvertor.castToReference(value)); 2407 } else if (name.equals("type")) { 2408 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2409 } else if (name.equals("quantity")) { 2410 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 2411 } else if (name.equals("daysSupply")) { 2412 this.daysSupply = TypeConvertor.castToQuantity(value); // Quantity 2413 } else if (name.equals("recorded")) { 2414 this.recorded = TypeConvertor.castToDateTime(value); // DateTimeType 2415 } else if (name.equals("whenPrepared")) { 2416 this.whenPrepared = TypeConvertor.castToDateTime(value); // DateTimeType 2417 } else if (name.equals("whenHandedOver")) { 2418 this.whenHandedOver = TypeConvertor.castToDateTime(value); // DateTimeType 2419 } else if (name.equals("destination")) { 2420 this.destination = TypeConvertor.castToReference(value); // Reference 2421 } else if (name.equals("receiver")) { 2422 this.getReceiver().add(TypeConvertor.castToReference(value)); 2423 } else if (name.equals("note")) { 2424 this.getNote().add(TypeConvertor.castToAnnotation(value)); 2425 } else if (name.equals("renderedDosageInstruction")) { 2426 this.renderedDosageInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 2427 } else if (name.equals("dosageInstruction")) { 2428 this.getDosageInstruction().add(TypeConvertor.castToDosage(value)); 2429 } else if (name.equals("substitution")) { 2430 this.substitution = (MedicationDispenseSubstitutionComponent) value; // MedicationDispenseSubstitutionComponent 2431 } else if (name.equals("eventHistory")) { 2432 this.getEventHistory().add(TypeConvertor.castToReference(value)); 2433 } else 2434 return super.setProperty(name, value); 2435 return value; 2436 } 2437 2438 @Override 2439 public void removeChild(String name, Base value) throws FHIRException { 2440 if (name.equals("identifier")) { 2441 this.getIdentifier().remove(value); 2442 } else if (name.equals("basedOn")) { 2443 this.getBasedOn().remove(value); 2444 } else if (name.equals("partOf")) { 2445 this.getPartOf().remove(value); 2446 } else if (name.equals("status")) { 2447 value = new MedicationDispenseStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 2448 this.status = (Enumeration) value; // Enumeration<MedicationDispenseStatusCodes> 2449 } else if (name.equals("notPerformedReason")) { 2450 this.notPerformedReason = null; 2451 } else if (name.equals("statusChanged")) { 2452 this.statusChanged = null; 2453 } else if (name.equals("category")) { 2454 this.getCategory().remove(value); 2455 } else if (name.equals("medication")) { 2456 this.medication = null; 2457 } else if (name.equals("subject")) { 2458 this.subject = null; 2459 } else if (name.equals("encounter")) { 2460 this.encounter = null; 2461 } else if (name.equals("supportingInformation")) { 2462 this.getSupportingInformation().remove(value); 2463 } else if (name.equals("performer")) { 2464 this.getPerformer().remove((MedicationDispensePerformerComponent) value); 2465 } else if (name.equals("location")) { 2466 this.location = null; 2467 } else if (name.equals("authorizingPrescription")) { 2468 this.getAuthorizingPrescription().remove(value); 2469 } else if (name.equals("type")) { 2470 this.type = null; 2471 } else if (name.equals("quantity")) { 2472 this.quantity = null; 2473 } else if (name.equals("daysSupply")) { 2474 this.daysSupply = null; 2475 } else if (name.equals("recorded")) { 2476 this.recorded = null; 2477 } else if (name.equals("whenPrepared")) { 2478 this.whenPrepared = null; 2479 } else if (name.equals("whenHandedOver")) { 2480 this.whenHandedOver = null; 2481 } else if (name.equals("destination")) { 2482 this.destination = null; 2483 } else if (name.equals("receiver")) { 2484 this.getReceiver().remove(value); 2485 } else if (name.equals("note")) { 2486 this.getNote().remove(value); 2487 } else if (name.equals("renderedDosageInstruction")) { 2488 this.renderedDosageInstruction = null; 2489 } else if (name.equals("dosageInstruction")) { 2490 this.getDosageInstruction().remove(value); 2491 } else if (name.equals("substitution")) { 2492 this.substitution = (MedicationDispenseSubstitutionComponent) value; // MedicationDispenseSubstitutionComponent 2493 } else if (name.equals("eventHistory")) { 2494 this.getEventHistory().remove(value); 2495 } else 2496 super.removeChild(name, value); 2497 2498 } 2499 2500 @Override 2501 public Base makeProperty(int hash, String name) throws FHIRException { 2502 switch (hash) { 2503 case -1618432855: return addIdentifier(); 2504 case -332612366: return addBasedOn(); 2505 case -995410646: return addPartOf(); 2506 case -892481550: return getStatusElement(); 2507 case -820839727: return getNotPerformedReason(); 2508 case -1174686110: return getStatusChangedElement(); 2509 case 50511102: return addCategory(); 2510 case 1998965455: return getMedication(); 2511 case -1867885268: return getSubject(); 2512 case 1524132147: return getEncounter(); 2513 case -1248768647: return addSupportingInformation(); 2514 case 481140686: return addPerformer(); 2515 case 1901043637: return getLocation(); 2516 case -1237557856: return addAuthorizingPrescription(); 2517 case 3575610: return getType(); 2518 case -1285004149: return getQuantity(); 2519 case 197175334: return getDaysSupply(); 2520 case -799233872: return getRecordedElement(); 2521 case -562837097: return getWhenPreparedElement(); 2522 case -940241380: return getWhenHandedOverElement(); 2523 case -1429847026: return getDestination(); 2524 case -808719889: return addReceiver(); 2525 case 3387378: return addNote(); 2526 case 1718902050: return getRenderedDosageInstructionElement(); 2527 case -1201373865: return addDosageInstruction(); 2528 case 826147581: return getSubstitution(); 2529 case 1835190426: return addEventHistory(); 2530 default: return super.makeProperty(hash, name); 2531 } 2532 2533 } 2534 2535 @Override 2536 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2537 switch (hash) { 2538 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2539 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2540 case -995410646: /*partOf*/ return new String[] {"Reference"}; 2541 case -892481550: /*status*/ return new String[] {"code"}; 2542 case -820839727: /*notPerformedReason*/ return new String[] {"CodeableReference"}; 2543 case -1174686110: /*statusChanged*/ return new String[] {"dateTime"}; 2544 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2545 case 1998965455: /*medication*/ return new String[] {"CodeableReference"}; 2546 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2547 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 2548 case -1248768647: /*supportingInformation*/ return new String[] {"Reference"}; 2549 case 481140686: /*performer*/ return new String[] {}; 2550 case 1901043637: /*location*/ return new String[] {"Reference"}; 2551 case -1237557856: /*authorizingPrescription*/ return new String[] {"Reference"}; 2552 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2553 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 2554 case 197175334: /*daysSupply*/ return new String[] {"Quantity"}; 2555 case -799233872: /*recorded*/ return new String[] {"dateTime"}; 2556 case -562837097: /*whenPrepared*/ return new String[] {"dateTime"}; 2557 case -940241380: /*whenHandedOver*/ return new String[] {"dateTime"}; 2558 case -1429847026: /*destination*/ return new String[] {"Reference"}; 2559 case -808719889: /*receiver*/ return new String[] {"Reference"}; 2560 case 3387378: /*note*/ return new String[] {"Annotation"}; 2561 case 1718902050: /*renderedDosageInstruction*/ return new String[] {"markdown"}; 2562 case -1201373865: /*dosageInstruction*/ return new String[] {"Dosage"}; 2563 case 826147581: /*substitution*/ return new String[] {}; 2564 case 1835190426: /*eventHistory*/ return new String[] {"Reference"}; 2565 default: return super.getTypesForProperty(hash, name); 2566 } 2567 2568 } 2569 2570 @Override 2571 public Base addChild(String name) throws FHIRException { 2572 if (name.equals("identifier")) { 2573 return addIdentifier(); 2574 } 2575 else if (name.equals("basedOn")) { 2576 return addBasedOn(); 2577 } 2578 else if (name.equals("partOf")) { 2579 return addPartOf(); 2580 } 2581 else if (name.equals("status")) { 2582 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.status"); 2583 } 2584 else if (name.equals("notPerformedReason")) { 2585 this.notPerformedReason = new CodeableReference(); 2586 return this.notPerformedReason; 2587 } 2588 else if (name.equals("statusChanged")) { 2589 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.statusChanged"); 2590 } 2591 else if (name.equals("category")) { 2592 return addCategory(); 2593 } 2594 else if (name.equals("medication")) { 2595 this.medication = new CodeableReference(); 2596 return this.medication; 2597 } 2598 else if (name.equals("subject")) { 2599 this.subject = new Reference(); 2600 return this.subject; 2601 } 2602 else if (name.equals("encounter")) { 2603 this.encounter = new Reference(); 2604 return this.encounter; 2605 } 2606 else if (name.equals("supportingInformation")) { 2607 return addSupportingInformation(); 2608 } 2609 else if (name.equals("performer")) { 2610 return addPerformer(); 2611 } 2612 else if (name.equals("location")) { 2613 this.location = new Reference(); 2614 return this.location; 2615 } 2616 else if (name.equals("authorizingPrescription")) { 2617 return addAuthorizingPrescription(); 2618 } 2619 else if (name.equals("type")) { 2620 this.type = new CodeableConcept(); 2621 return this.type; 2622 } 2623 else if (name.equals("quantity")) { 2624 this.quantity = new Quantity(); 2625 return this.quantity; 2626 } 2627 else if (name.equals("daysSupply")) { 2628 this.daysSupply = new Quantity(); 2629 return this.daysSupply; 2630 } 2631 else if (name.equals("recorded")) { 2632 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.recorded"); 2633 } 2634 else if (name.equals("whenPrepared")) { 2635 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.whenPrepared"); 2636 } 2637 else if (name.equals("whenHandedOver")) { 2638 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.whenHandedOver"); 2639 } 2640 else if (name.equals("destination")) { 2641 this.destination = new Reference(); 2642 return this.destination; 2643 } 2644 else if (name.equals("receiver")) { 2645 return addReceiver(); 2646 } 2647 else if (name.equals("note")) { 2648 return addNote(); 2649 } 2650 else if (name.equals("renderedDosageInstruction")) { 2651 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.renderedDosageInstruction"); 2652 } 2653 else if (name.equals("dosageInstruction")) { 2654 return addDosageInstruction(); 2655 } 2656 else if (name.equals("substitution")) { 2657 this.substitution = new MedicationDispenseSubstitutionComponent(); 2658 return this.substitution; 2659 } 2660 else if (name.equals("eventHistory")) { 2661 return addEventHistory(); 2662 } 2663 else 2664 return super.addChild(name); 2665 } 2666 2667 public String fhirType() { 2668 return "MedicationDispense"; 2669 2670 } 2671 2672 public MedicationDispense copy() { 2673 MedicationDispense dst = new MedicationDispense(); 2674 copyValues(dst); 2675 return dst; 2676 } 2677 2678 public void copyValues(MedicationDispense dst) { 2679 super.copyValues(dst); 2680 if (identifier != null) { 2681 dst.identifier = new ArrayList<Identifier>(); 2682 for (Identifier i : identifier) 2683 dst.identifier.add(i.copy()); 2684 }; 2685 if (basedOn != null) { 2686 dst.basedOn = new ArrayList<Reference>(); 2687 for (Reference i : basedOn) 2688 dst.basedOn.add(i.copy()); 2689 }; 2690 if (partOf != null) { 2691 dst.partOf = new ArrayList<Reference>(); 2692 for (Reference i : partOf) 2693 dst.partOf.add(i.copy()); 2694 }; 2695 dst.status = status == null ? null : status.copy(); 2696 dst.notPerformedReason = notPerformedReason == null ? null : notPerformedReason.copy(); 2697 dst.statusChanged = statusChanged == null ? null : statusChanged.copy(); 2698 if (category != null) { 2699 dst.category = new ArrayList<CodeableConcept>(); 2700 for (CodeableConcept i : category) 2701 dst.category.add(i.copy()); 2702 }; 2703 dst.medication = medication == null ? null : medication.copy(); 2704 dst.subject = subject == null ? null : subject.copy(); 2705 dst.encounter = encounter == null ? null : encounter.copy(); 2706 if (supportingInformation != null) { 2707 dst.supportingInformation = new ArrayList<Reference>(); 2708 for (Reference i : supportingInformation) 2709 dst.supportingInformation.add(i.copy()); 2710 }; 2711 if (performer != null) { 2712 dst.performer = new ArrayList<MedicationDispensePerformerComponent>(); 2713 for (MedicationDispensePerformerComponent i : performer) 2714 dst.performer.add(i.copy()); 2715 }; 2716 dst.location = location == null ? null : location.copy(); 2717 if (authorizingPrescription != null) { 2718 dst.authorizingPrescription = new ArrayList<Reference>(); 2719 for (Reference i : authorizingPrescription) 2720 dst.authorizingPrescription.add(i.copy()); 2721 }; 2722 dst.type = type == null ? null : type.copy(); 2723 dst.quantity = quantity == null ? null : quantity.copy(); 2724 dst.daysSupply = daysSupply == null ? null : daysSupply.copy(); 2725 dst.recorded = recorded == null ? null : recorded.copy(); 2726 dst.whenPrepared = whenPrepared == null ? null : whenPrepared.copy(); 2727 dst.whenHandedOver = whenHandedOver == null ? null : whenHandedOver.copy(); 2728 dst.destination = destination == null ? null : destination.copy(); 2729 if (receiver != null) { 2730 dst.receiver = new ArrayList<Reference>(); 2731 for (Reference i : receiver) 2732 dst.receiver.add(i.copy()); 2733 }; 2734 if (note != null) { 2735 dst.note = new ArrayList<Annotation>(); 2736 for (Annotation i : note) 2737 dst.note.add(i.copy()); 2738 }; 2739 dst.renderedDosageInstruction = renderedDosageInstruction == null ? null : renderedDosageInstruction.copy(); 2740 if (dosageInstruction != null) { 2741 dst.dosageInstruction = new ArrayList<Dosage>(); 2742 for (Dosage i : dosageInstruction) 2743 dst.dosageInstruction.add(i.copy()); 2744 }; 2745 dst.substitution = substitution == null ? null : substitution.copy(); 2746 if (eventHistory != null) { 2747 dst.eventHistory = new ArrayList<Reference>(); 2748 for (Reference i : eventHistory) 2749 dst.eventHistory.add(i.copy()); 2750 }; 2751 } 2752 2753 protected MedicationDispense typedCopy() { 2754 return copy(); 2755 } 2756 2757 @Override 2758 public boolean equalsDeep(Base other_) { 2759 if (!super.equalsDeep(other_)) 2760 return false; 2761 if (!(other_ instanceof MedicationDispense)) 2762 return false; 2763 MedicationDispense o = (MedicationDispense) other_; 2764 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(partOf, o.partOf, true) 2765 && compareDeep(status, o.status, true) && compareDeep(notPerformedReason, o.notPerformedReason, true) 2766 && compareDeep(statusChanged, o.statusChanged, true) && compareDeep(category, o.category, true) 2767 && compareDeep(medication, o.medication, true) && compareDeep(subject, o.subject, true) && compareDeep(encounter, o.encounter, true) 2768 && compareDeep(supportingInformation, o.supportingInformation, true) && compareDeep(performer, o.performer, true) 2769 && compareDeep(location, o.location, true) && compareDeep(authorizingPrescription, o.authorizingPrescription, true) 2770 && compareDeep(type, o.type, true) && compareDeep(quantity, o.quantity, true) && compareDeep(daysSupply, o.daysSupply, true) 2771 && compareDeep(recorded, o.recorded, true) && compareDeep(whenPrepared, o.whenPrepared, true) && compareDeep(whenHandedOver, o.whenHandedOver, true) 2772 && compareDeep(destination, o.destination, true) && compareDeep(receiver, o.receiver, true) && compareDeep(note, o.note, true) 2773 && compareDeep(renderedDosageInstruction, o.renderedDosageInstruction, true) && compareDeep(dosageInstruction, o.dosageInstruction, true) 2774 && compareDeep(substitution, o.substitution, true) && compareDeep(eventHistory, o.eventHistory, true) 2775 ; 2776 } 2777 2778 @Override 2779 public boolean equalsShallow(Base other_) { 2780 if (!super.equalsShallow(other_)) 2781 return false; 2782 if (!(other_ instanceof MedicationDispense)) 2783 return false; 2784 MedicationDispense o = (MedicationDispense) other_; 2785 return compareValues(status, o.status, true) && compareValues(statusChanged, o.statusChanged, true) 2786 && compareValues(recorded, o.recorded, true) && compareValues(whenPrepared, o.whenPrepared, true) && compareValues(whenHandedOver, o.whenHandedOver, true) 2787 && compareValues(renderedDosageInstruction, o.renderedDosageInstruction, true); 2788 } 2789 2790 public boolean isEmpty() { 2791 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf 2792 , status, notPerformedReason, statusChanged, category, medication, subject, encounter 2793 , supportingInformation, performer, location, authorizingPrescription, type, quantity 2794 , daysSupply, recorded, whenPrepared, whenHandedOver, destination, receiver, note 2795 , renderedDosageInstruction, dosageInstruction, substitution, eventHistory); 2796 } 2797 2798 @Override 2799 public ResourceType getResourceType() { 2800 return ResourceType.MedicationDispense; 2801 } 2802 2803 /** 2804 * Search parameter: <b>destination</b> 2805 * <p> 2806 * Description: <b>Returns dispenses that should be sent to a specific destination</b><br> 2807 * Type: <b>reference</b><br> 2808 * Path: <b>MedicationDispense.destination</b><br> 2809 * </p> 2810 */ 2811 @SearchParamDefinition(name="destination", path="MedicationDispense.destination", description="Returns dispenses that should be sent to a specific destination", type="reference", target={Location.class } ) 2812 public static final String SP_DESTINATION = "destination"; 2813 /** 2814 * <b>Fluent Client</b> search parameter constant for <b>destination</b> 2815 * <p> 2816 * Description: <b>Returns dispenses that should be sent to a specific destination</b><br> 2817 * Type: <b>reference</b><br> 2818 * Path: <b>MedicationDispense.destination</b><br> 2819 * </p> 2820 */ 2821 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DESTINATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DESTINATION); 2822 2823/** 2824 * Constant for fluent queries to be used to add include statements. Specifies 2825 * the path value of "<b>MedicationDispense:destination</b>". 2826 */ 2827 public static final ca.uhn.fhir.model.api.Include INCLUDE_DESTINATION = new ca.uhn.fhir.model.api.Include("MedicationDispense:destination").toLocked(); 2828 2829 /** 2830 * Search parameter: <b>location</b> 2831 * <p> 2832 * Description: <b>Returns dispense for a given location</b><br> 2833 * Type: <b>reference</b><br> 2834 * Path: <b>MedicationDispense.location</b><br> 2835 * </p> 2836 */ 2837 @SearchParamDefinition(name="location", path="MedicationDispense.location", description="Returns dispense for a given location", type="reference", target={Location.class } ) 2838 public static final String SP_LOCATION = "location"; 2839 /** 2840 * <b>Fluent Client</b> search parameter constant for <b>location</b> 2841 * <p> 2842 * Description: <b>Returns dispense for a given location</b><br> 2843 * Type: <b>reference</b><br> 2844 * Path: <b>MedicationDispense.location</b><br> 2845 * </p> 2846 */ 2847 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 2848 2849/** 2850 * Constant for fluent queries to be used to add include statements. Specifies 2851 * the path value of "<b>MedicationDispense:location</b>". 2852 */ 2853 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("MedicationDispense:location").toLocked(); 2854 2855 /** 2856 * Search parameter: <b>performer</b> 2857 * <p> 2858 * Description: <b>Returns dispenses performed by a specific individual</b><br> 2859 * Type: <b>reference</b><br> 2860 * Path: <b>MedicationDispense.performer.actor</b><br> 2861 * </p> 2862 */ 2863 @SearchParamDefinition(name="performer", path="MedicationDispense.performer.actor", description="Returns dispenses performed by a specific individual", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={CareTeam.class, Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2864 public static final String SP_PERFORMER = "performer"; 2865 /** 2866 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2867 * <p> 2868 * Description: <b>Returns dispenses performed by a specific individual</b><br> 2869 * Type: <b>reference</b><br> 2870 * Path: <b>MedicationDispense.performer.actor</b><br> 2871 * </p> 2872 */ 2873 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 2874 2875/** 2876 * Constant for fluent queries to be used to add include statements. Specifies 2877 * the path value of "<b>MedicationDispense:performer</b>". 2878 */ 2879 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("MedicationDispense:performer").toLocked(); 2880 2881 /** 2882 * Search parameter: <b>receiver</b> 2883 * <p> 2884 * Description: <b>The identity of a receiver to list dispenses for</b><br> 2885 * Type: <b>reference</b><br> 2886 * Path: <b>MedicationDispense.receiver</b><br> 2887 * </p> 2888 */ 2889 @SearchParamDefinition(name="receiver", path="MedicationDispense.receiver", description="The identity of a receiver to list dispenses for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Location.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 2890 public static final String SP_RECEIVER = "receiver"; 2891 /** 2892 * <b>Fluent Client</b> search parameter constant for <b>receiver</b> 2893 * <p> 2894 * Description: <b>The identity of a receiver to list dispenses for</b><br> 2895 * Type: <b>reference</b><br> 2896 * Path: <b>MedicationDispense.receiver</b><br> 2897 * </p> 2898 */ 2899 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECEIVER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECEIVER); 2900 2901/** 2902 * Constant for fluent queries to be used to add include statements. Specifies 2903 * the path value of "<b>MedicationDispense:receiver</b>". 2904 */ 2905 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECEIVER = new ca.uhn.fhir.model.api.Include("MedicationDispense:receiver").toLocked(); 2906 2907 /** 2908 * Search parameter: <b>recorded</b> 2909 * <p> 2910 * Description: <b>Returns dispenses where dispensing activity began on this date</b><br> 2911 * Type: <b>date</b><br> 2912 * Path: <b>MedicationDispense.recorded</b><br> 2913 * </p> 2914 */ 2915 @SearchParamDefinition(name="recorded", path="MedicationDispense.recorded", description="Returns dispenses where dispensing activity began on this date", type="date" ) 2916 public static final String SP_RECORDED = "recorded"; 2917 /** 2918 * <b>Fluent Client</b> search parameter constant for <b>recorded</b> 2919 * <p> 2920 * Description: <b>Returns dispenses where dispensing activity began on this date</b><br> 2921 * Type: <b>date</b><br> 2922 * Path: <b>MedicationDispense.recorded</b><br> 2923 * </p> 2924 */ 2925 public static final ca.uhn.fhir.rest.gclient.DateClientParam RECORDED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_RECORDED); 2926 2927 /** 2928 * Search parameter: <b>responsibleparty</b> 2929 * <p> 2930 * Description: <b>Returns dispenses with the specified responsible party</b><br> 2931 * Type: <b>reference</b><br> 2932 * Path: <b>MedicationDispense.substitution.responsibleParty</b><br> 2933 * </p> 2934 */ 2935 @SearchParamDefinition(name="responsibleparty", path="MedicationDispense.substitution.responsibleParty", description="Returns dispenses with the specified responsible party", type="reference", target={Organization.class, Practitioner.class, PractitionerRole.class } ) 2936 public static final String SP_RESPONSIBLEPARTY = "responsibleparty"; 2937 /** 2938 * <b>Fluent Client</b> search parameter constant for <b>responsibleparty</b> 2939 * <p> 2940 * Description: <b>Returns dispenses with the specified responsible party</b><br> 2941 * Type: <b>reference</b><br> 2942 * Path: <b>MedicationDispense.substitution.responsibleParty</b><br> 2943 * </p> 2944 */ 2945 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESPONSIBLEPARTY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RESPONSIBLEPARTY); 2946 2947/** 2948 * Constant for fluent queries to be used to add include statements. Specifies 2949 * the path value of "<b>MedicationDispense:responsibleparty</b>". 2950 */ 2951 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESPONSIBLEPARTY = new ca.uhn.fhir.model.api.Include("MedicationDispense:responsibleparty").toLocked(); 2952 2953 /** 2954 * Search parameter: <b>subject</b> 2955 * <p> 2956 * Description: <b>The identity of a patient for whom to list dispenses</b><br> 2957 * Type: <b>reference</b><br> 2958 * Path: <b>MedicationDispense.subject</b><br> 2959 * </p> 2960 */ 2961 @SearchParamDefinition(name="subject", path="MedicationDispense.subject", description="The identity of a patient for whom to list dispenses", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Group.class, Patient.class } ) 2962 public static final String SP_SUBJECT = "subject"; 2963 /** 2964 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2965 * <p> 2966 * Description: <b>The identity of a patient for whom to list dispenses</b><br> 2967 * Type: <b>reference</b><br> 2968 * Path: <b>MedicationDispense.subject</b><br> 2969 * </p> 2970 */ 2971 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2972 2973/** 2974 * Constant for fluent queries to be used to add include statements. Specifies 2975 * the path value of "<b>MedicationDispense:subject</b>". 2976 */ 2977 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("MedicationDispense:subject").toLocked(); 2978 2979 /** 2980 * Search parameter: <b>whenhandedover</b> 2981 * <p> 2982 * Description: <b>Returns dispenses handed over on this date</b><br> 2983 * Type: <b>date</b><br> 2984 * Path: <b>MedicationDispense.whenHandedOver</b><br> 2985 * </p> 2986 */ 2987 @SearchParamDefinition(name="whenhandedover", path="MedicationDispense.whenHandedOver", description="Returns dispenses handed over on this date", type="date" ) 2988 public static final String SP_WHENHANDEDOVER = "whenhandedover"; 2989 /** 2990 * <b>Fluent Client</b> search parameter constant for <b>whenhandedover</b> 2991 * <p> 2992 * Description: <b>Returns dispenses handed over on this date</b><br> 2993 * Type: <b>date</b><br> 2994 * Path: <b>MedicationDispense.whenHandedOver</b><br> 2995 * </p> 2996 */ 2997 public static final ca.uhn.fhir.rest.gclient.DateClientParam WHENHANDEDOVER = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_WHENHANDEDOVER); 2998 2999 /** 3000 * Search parameter: <b>whenprepared</b> 3001 * <p> 3002 * Description: <b>Returns dispenses prepared on this date</b><br> 3003 * Type: <b>date</b><br> 3004 * Path: <b>MedicationDispense.whenPrepared</b><br> 3005 * </p> 3006 */ 3007 @SearchParamDefinition(name="whenprepared", path="MedicationDispense.whenPrepared", description="Returns dispenses prepared on this date", type="date" ) 3008 public static final String SP_WHENPREPARED = "whenprepared"; 3009 /** 3010 * <b>Fluent Client</b> search parameter constant for <b>whenprepared</b> 3011 * <p> 3012 * Description: <b>Returns dispenses prepared on this date</b><br> 3013 * Type: <b>date</b><br> 3014 * Path: <b>MedicationDispense.whenPrepared</b><br> 3015 * </p> 3016 */ 3017 public static final ca.uhn.fhir.rest.gclient.DateClientParam WHENPREPARED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_WHENPREPARED); 3018 3019 /** 3020 * Search parameter: <b>code</b> 3021 * <p> 3022 * Description: <b>Multiple Resources: 3023 3024* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 3025* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 3026* [AuditEvent](auditevent.html): More specific code for the event 3027* [Basic](basic.html): Kind of Resource 3028* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 3029* [Condition](condition.html): Code for the condition 3030* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 3031* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 3032* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 3033* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 3034* [ImagingSelection](imagingselection.html): The imaging selection status 3035* [List](list.html): What the purpose of this list is 3036* [Medication](medication.html): Returns medications for a specific code 3037* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 3038* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 3039* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 3040* [MedicationStatement](medicationstatement.html): Return statements of this medication code 3041* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 3042* [Observation](observation.html): The code of the observation type 3043* [Procedure](procedure.html): A code to identify a procedure 3044* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 3045* [Task](task.html): Search by task code 3046</b><br> 3047 * Type: <b>token</b><br> 3048 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 3049 * </p> 3050 */ 3051 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 3052 public static final String SP_CODE = "code"; 3053 /** 3054 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3055 * <p> 3056 * Description: <b>Multiple Resources: 3057 3058* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 3059* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 3060* [AuditEvent](auditevent.html): More specific code for the event 3061* [Basic](basic.html): Kind of Resource 3062* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 3063* [Condition](condition.html): Code for the condition 3064* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 3065* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 3066* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 3067* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 3068* [ImagingSelection](imagingselection.html): The imaging selection status 3069* [List](list.html): What the purpose of this list is 3070* [Medication](medication.html): Returns medications for a specific code 3071* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 3072* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 3073* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 3074* [MedicationStatement](medicationstatement.html): Return statements of this medication code 3075* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 3076* [Observation](observation.html): The code of the observation type 3077* [Procedure](procedure.html): A code to identify a procedure 3078* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 3079* [Task](task.html): Search by task code 3080</b><br> 3081 * Type: <b>token</b><br> 3082 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 3083 * </p> 3084 */ 3085 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 3086 3087 /** 3088 * Search parameter: <b>encounter</b> 3089 * <p> 3090 * Description: <b>Multiple Resources: 3091 3092* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 3093* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 3094* [ChargeItem](chargeitem.html): Encounter associated with event 3095* [Claim](claim.html): Encounters associated with a billed line item 3096* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 3097* [Communication](communication.html): The Encounter during which this Communication was created 3098* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 3099* [Composition](composition.html): Context of the Composition 3100* [Condition](condition.html): The Encounter during which this Condition was created 3101* [DeviceRequest](devicerequest.html): Encounter during which request was created 3102* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 3103* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 3104* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 3105* [Flag](flag.html): Alert relevant during encounter 3106* [ImagingStudy](imagingstudy.html): The context of the study 3107* [List](list.html): Context in which list created 3108* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 3109* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 3110* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 3111* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 3112* [Observation](observation.html): Encounter related to the observation 3113* [Procedure](procedure.html): The Encounter during which this Procedure was created 3114* [Provenance](provenance.html): Encounter related to the Provenance 3115* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 3116* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 3117* [RiskAssessment](riskassessment.html): Where was assessment performed? 3118* [ServiceRequest](servicerequest.html): An encounter in which this request is made 3119* [Task](task.html): Search by encounter 3120* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 3121</b><br> 3122 * Type: <b>reference</b><br> 3123 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 3124 * </p> 3125 */ 3126 @SearchParamDefinition(name="encounter", path="AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter", description="Multiple Resources: \r\n\r\n* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent\r\n* [CarePlan](careplan.html): The Encounter during which this CarePlan was created\r\n* [ChargeItem](chargeitem.html): Encounter associated with event\r\n* [Claim](claim.html): Encounters associated with a billed line item\r\n* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created\r\n* [Communication](communication.html): The Encounter during which this Communication was created\r\n* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created\r\n* [Composition](composition.html): Context of the Composition\r\n* [Condition](condition.html): The Encounter during which this Condition was created\r\n* [DeviceRequest](devicerequest.html): Encounter during which request was created\r\n* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made\r\n* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values\r\n* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item\r\n* [Flag](flag.html): Alert relevant during encounter\r\n* [ImagingStudy](imagingstudy.html): The context of the study\r\n* [List](list.html): Context in which list created\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier\r\n* [Observation](observation.html): Encounter related to the observation\r\n* [Procedure](procedure.html): The Encounter during which this Procedure was created\r\n* [Provenance](provenance.html): Encounter related to the Provenance\r\n* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response\r\n* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to\r\n* [RiskAssessment](riskassessment.html): Where was assessment performed?\r\n* [ServiceRequest](servicerequest.html): An encounter in which this request is made\r\n* [Task](task.html): Search by encounter\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Encounter") }, target={Encounter.class } ) 3127 public static final String SP_ENCOUNTER = "encounter"; 3128 /** 3129 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 3130 * <p> 3131 * Description: <b>Multiple Resources: 3132 3133* [AuditEvent](auditevent.html): Encounter related to the activity recorded in the AuditEvent 3134* [CarePlan](careplan.html): The Encounter during which this CarePlan was created 3135* [ChargeItem](chargeitem.html): Encounter associated with event 3136* [Claim](claim.html): Encounters associated with a billed line item 3137* [ClinicalImpression](clinicalimpression.html): The Encounter during which this ClinicalImpression was created 3138* [Communication](communication.html): The Encounter during which this Communication was created 3139* [CommunicationRequest](communicationrequest.html): The Encounter during which this CommunicationRequest was created 3140* [Composition](composition.html): Context of the Composition 3141* [Condition](condition.html): The Encounter during which this Condition was created 3142* [DeviceRequest](devicerequest.html): Encounter during which request was created 3143* [DiagnosticReport](diagnosticreport.html): The Encounter when the order was made 3144* [EncounterHistory](encounterhistory.html): The Encounter associated with this set of history values 3145* [ExplanationOfBenefit](explanationofbenefit.html): Encounters associated with a billed line item 3146* [Flag](flag.html): Alert relevant during encounter 3147* [ImagingStudy](imagingstudy.html): The context of the study 3148* [List](list.html): Context in which list created 3149* [MedicationDispense](medicationdispense.html): Returns dispenses with a specific encounter 3150* [MedicationStatement](medicationstatement.html): Returns statements for a specific encounter 3151* [NutritionIntake](nutritionintake.html): Returns statements for a specific encounter 3152* [NutritionOrder](nutritionorder.html): Return nutrition orders with this encounter identifier 3153* [Observation](observation.html): Encounter related to the observation 3154* [Procedure](procedure.html): The Encounter during which this Procedure was created 3155* [Provenance](provenance.html): Encounter related to the Provenance 3156* [QuestionnaireResponse](questionnaireresponse.html): Encounter associated with the questionnaire response 3157* [RequestOrchestration](requestorchestration.html): The encounter the request orchestration applies to 3158* [RiskAssessment](riskassessment.html): Where was assessment performed? 3159* [ServiceRequest](servicerequest.html): An encounter in which this request is made 3160* [Task](task.html): Search by encounter 3161* [VisionPrescription](visionprescription.html): Return prescriptions with this encounter identifier 3162</b><br> 3163 * Type: <b>reference</b><br> 3164 * Path: <b>AuditEvent.encounter | CarePlan.encounter | ChargeItem.encounter | Claim.item.encounter | ClinicalImpression.encounter | Communication.encounter | CommunicationRequest.encounter | Composition.encounter | Condition.encounter | DeviceRequest.encounter | DiagnosticReport.encounter | EncounterHistory.encounter | ExplanationOfBenefit.item.encounter | Flag.encounter | ImagingStudy.encounter | List.encounter | MedicationDispense.encounter | MedicationStatement.encounter | NutritionIntake.encounter | NutritionOrder.encounter | Observation.encounter | Procedure.encounter | Provenance.encounter | QuestionnaireResponse.encounter | RequestOrchestration.encounter | RiskAssessment.encounter | ServiceRequest.encounter | Task.encounter | VisionPrescription.encounter</b><br> 3165 * </p> 3166 */ 3167 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 3168 3169/** 3170 * Constant for fluent queries to be used to add include statements. Specifies 3171 * the path value of "<b>MedicationDispense:encounter</b>". 3172 */ 3173 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("MedicationDispense:encounter").toLocked(); 3174 3175 /** 3176 * Search parameter: <b>identifier</b> 3177 * <p> 3178 * Description: <b>Multiple Resources: 3179 3180* [Account](account.html): Account number 3181* [AdverseEvent](adverseevent.html): Business identifier for the event 3182* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3183* [Appointment](appointment.html): An Identifier of the Appointment 3184* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3185* [Basic](basic.html): Business identifier 3186* [BodyStructure](bodystructure.html): Bodystructure identifier 3187* [CarePlan](careplan.html): External Ids for this plan 3188* [CareTeam](careteam.html): External Ids for this team 3189* [ChargeItem](chargeitem.html): Business Identifier for item 3190* [Claim](claim.html): The primary identifier of the financial resource 3191* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3192* [ClinicalImpression](clinicalimpression.html): Business identifier 3193* [Communication](communication.html): Unique identifier 3194* [CommunicationRequest](communicationrequest.html): Unique identifier 3195* [Composition](composition.html): Version-independent identifier for the Composition 3196* [Condition](condition.html): A unique identifier of the condition record 3197* [Consent](consent.html): Identifier for this record (external references) 3198* [Contract](contract.html): The identity of the contract 3199* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3200* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3201* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3202* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3203* [DeviceRequest](devicerequest.html): Business identifier for request/order 3204* [DeviceUsage](deviceusage.html): Search by identifier 3205* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3206* [DocumentReference](documentreference.html): Identifier of the attachment binary 3207* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3208* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3209* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3210* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3211* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3212* [Flag](flag.html): Business identifier 3213* [Goal](goal.html): External Ids for this goal 3214* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3215* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3216* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3217* [Immunization](immunization.html): Business identifier 3218* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3219* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3220* [Invoice](invoice.html): Business Identifier for item 3221* [List](list.html): Business identifier 3222* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3223* [Medication](medication.html): Returns medications with this external identifier 3224* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3225* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3226* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3227* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3228* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3229* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3230* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3231* [Observation](observation.html): The unique id for a particular observation 3232* [Person](person.html): A person Identifier 3233* [Procedure](procedure.html): A unique identifier for a procedure 3234* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3235* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3236* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3237* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3238* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3239* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3240* [Specimen](specimen.html): The unique identifier associated with the specimen 3241* [SupplyDelivery](supplydelivery.html): External identifier 3242* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3243* [Task](task.html): Search for a task instance by its business identifier 3244* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3245</b><br> 3246 * Type: <b>token</b><br> 3247 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3248 * </p> 3249 */ 3250 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 3251 public static final String SP_IDENTIFIER = "identifier"; 3252 /** 3253 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3254 * <p> 3255 * Description: <b>Multiple Resources: 3256 3257* [Account](account.html): Account number 3258* [AdverseEvent](adverseevent.html): Business identifier for the event 3259* [AllergyIntolerance](allergyintolerance.html): External ids for this item 3260* [Appointment](appointment.html): An Identifier of the Appointment 3261* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 3262* [Basic](basic.html): Business identifier 3263* [BodyStructure](bodystructure.html): Bodystructure identifier 3264* [CarePlan](careplan.html): External Ids for this plan 3265* [CareTeam](careteam.html): External Ids for this team 3266* [ChargeItem](chargeitem.html): Business Identifier for item 3267* [Claim](claim.html): The primary identifier of the financial resource 3268* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 3269* [ClinicalImpression](clinicalimpression.html): Business identifier 3270* [Communication](communication.html): Unique identifier 3271* [CommunicationRequest](communicationrequest.html): Unique identifier 3272* [Composition](composition.html): Version-independent identifier for the Composition 3273* [Condition](condition.html): A unique identifier of the condition record 3274* [Consent](consent.html): Identifier for this record (external references) 3275* [Contract](contract.html): The identity of the contract 3276* [Coverage](coverage.html): The primary identifier of the insured and the coverage 3277* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 3278* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 3279* [DetectedIssue](detectedissue.html): Unique id for the detected issue 3280* [DeviceRequest](devicerequest.html): Business identifier for request/order 3281* [DeviceUsage](deviceusage.html): Search by identifier 3282* [DiagnosticReport](diagnosticreport.html): An identifier for the report 3283* [DocumentReference](documentreference.html): Identifier of the attachment binary 3284* [Encounter](encounter.html): Identifier(s) by which this encounter is known 3285* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 3286* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 3287* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 3288* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 3289* [Flag](flag.html): Business identifier 3290* [Goal](goal.html): External Ids for this goal 3291* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 3292* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 3293* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 3294* [Immunization](immunization.html): Business identifier 3295* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 3296* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 3297* [Invoice](invoice.html): Business Identifier for item 3298* [List](list.html): Business identifier 3299* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 3300* [Medication](medication.html): Returns medications with this external identifier 3301* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 3302* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 3303* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 3304* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 3305* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 3306* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 3307* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 3308* [Observation](observation.html): The unique id for a particular observation 3309* [Person](person.html): A person Identifier 3310* [Procedure](procedure.html): A unique identifier for a procedure 3311* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 3312* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 3313* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 3314* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 3315* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 3316* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 3317* [Specimen](specimen.html): The unique identifier associated with the specimen 3318* [SupplyDelivery](supplydelivery.html): External identifier 3319* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 3320* [Task](task.html): Search for a task instance by its business identifier 3321* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 3322</b><br> 3323 * Type: <b>token</b><br> 3324 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 3325 * </p> 3326 */ 3327 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3328 3329 /** 3330 * Search parameter: <b>patient</b> 3331 * <p> 3332 * Description: <b>Multiple Resources: 3333 3334* [Account](account.html): The entity that caused the expenses 3335* [AdverseEvent](adverseevent.html): Subject impacted by event 3336* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3337* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3338* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3339* [AuditEvent](auditevent.html): Where the activity involved patient data 3340* [Basic](basic.html): Identifies the focus of this resource 3341* [BodyStructure](bodystructure.html): Who this is about 3342* [CarePlan](careplan.html): Who the care plan is for 3343* [CareTeam](careteam.html): Who care team is for 3344* [ChargeItem](chargeitem.html): Individual service was done for/to 3345* [Claim](claim.html): Patient receiving the products or services 3346* [ClaimResponse](claimresponse.html): The subject of care 3347* [ClinicalImpression](clinicalimpression.html): Patient assessed 3348* [Communication](communication.html): Focus of message 3349* [CommunicationRequest](communicationrequest.html): Focus of message 3350* [Composition](composition.html): Who and/or what the composition is about 3351* [Condition](condition.html): Who has the condition? 3352* [Consent](consent.html): Who the consent applies to 3353* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3354* [Coverage](coverage.html): Retrieve coverages for a patient 3355* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3356* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3357* [DetectedIssue](detectedissue.html): Associated patient 3358* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3359* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3360* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3361* [DocumentReference](documentreference.html): Who/what is the subject of the document 3362* [Encounter](encounter.html): The patient present at the encounter 3363* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3364* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3365* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3366* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3367* [Flag](flag.html): The identity of a subject to list flags for 3368* [Goal](goal.html): Who this goal is intended for 3369* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3370* [ImagingSelection](imagingselection.html): Who the study is about 3371* [ImagingStudy](imagingstudy.html): Who the study is about 3372* [Immunization](immunization.html): The patient for the vaccination record 3373* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3374* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3375* [Invoice](invoice.html): Recipient(s) of goods and services 3376* [List](list.html): If all resources have the same subject 3377* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3378* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3379* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3380* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3381* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3382* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3383* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3384* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3385* [Observation](observation.html): The subject that the observation is about (if patient) 3386* [Person](person.html): The Person links to this Patient 3387* [Procedure](procedure.html): Search by subject - a patient 3388* [Provenance](provenance.html): Where the activity involved patient data 3389* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3390* [RelatedPerson](relatedperson.html): The patient this related person is related to 3391* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3392* [ResearchSubject](researchsubject.html): Who or what is part of study 3393* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3394* [ServiceRequest](servicerequest.html): Search by subject - a patient 3395* [Specimen](specimen.html): The patient the specimen comes from 3396* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3397* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3398* [Task](task.html): Search by patient 3399* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3400</b><br> 3401 * Type: <b>reference</b><br> 3402 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3403 * </p> 3404 */ 3405 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 3406 public static final String SP_PATIENT = "patient"; 3407 /** 3408 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3409 * <p> 3410 * Description: <b>Multiple Resources: 3411 3412* [Account](account.html): The entity that caused the expenses 3413* [AdverseEvent](adverseevent.html): Subject impacted by event 3414* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 3415* [Appointment](appointment.html): One of the individuals of the appointment is this patient 3416* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 3417* [AuditEvent](auditevent.html): Where the activity involved patient data 3418* [Basic](basic.html): Identifies the focus of this resource 3419* [BodyStructure](bodystructure.html): Who this is about 3420* [CarePlan](careplan.html): Who the care plan is for 3421* [CareTeam](careteam.html): Who care team is for 3422* [ChargeItem](chargeitem.html): Individual service was done for/to 3423* [Claim](claim.html): Patient receiving the products or services 3424* [ClaimResponse](claimresponse.html): The subject of care 3425* [ClinicalImpression](clinicalimpression.html): Patient assessed 3426* [Communication](communication.html): Focus of message 3427* [CommunicationRequest](communicationrequest.html): Focus of message 3428* [Composition](composition.html): Who and/or what the composition is about 3429* [Condition](condition.html): Who has the condition? 3430* [Consent](consent.html): Who the consent applies to 3431* [Contract](contract.html): The identity of the subject of the contract (if a patient) 3432* [Coverage](coverage.html): Retrieve coverages for a patient 3433* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 3434* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 3435* [DetectedIssue](detectedissue.html): Associated patient 3436* [DeviceRequest](devicerequest.html): Individual the service is ordered for 3437* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 3438* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 3439* [DocumentReference](documentreference.html): Who/what is the subject of the document 3440* [Encounter](encounter.html): The patient present at the encounter 3441* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 3442* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 3443* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 3444* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 3445* [Flag](flag.html): The identity of a subject to list flags for 3446* [Goal](goal.html): Who this goal is intended for 3447* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 3448* [ImagingSelection](imagingselection.html): Who the study is about 3449* [ImagingStudy](imagingstudy.html): Who the study is about 3450* [Immunization](immunization.html): The patient for the vaccination record 3451* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 3452* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 3453* [Invoice](invoice.html): Recipient(s) of goods and services 3454* [List](list.html): If all resources have the same subject 3455* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 3456* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 3457* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 3458* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 3459* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 3460* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 3461* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 3462* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 3463* [Observation](observation.html): The subject that the observation is about (if patient) 3464* [Person](person.html): The Person links to this Patient 3465* [Procedure](procedure.html): Search by subject - a patient 3466* [Provenance](provenance.html): Where the activity involved patient data 3467* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 3468* [RelatedPerson](relatedperson.html): The patient this related person is related to 3469* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 3470* [ResearchSubject](researchsubject.html): Who or what is part of study 3471* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 3472* [ServiceRequest](servicerequest.html): Search by subject - a patient 3473* [Specimen](specimen.html): The patient the specimen comes from 3474* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 3475* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 3476* [Task](task.html): Search by patient 3477* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 3478</b><br> 3479 * Type: <b>reference</b><br> 3480 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 3481 * </p> 3482 */ 3483 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3484 3485/** 3486 * Constant for fluent queries to be used to add include statements. Specifies 3487 * the path value of "<b>MedicationDispense:patient</b>". 3488 */ 3489 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("MedicationDispense:patient").toLocked(); 3490 3491 /** 3492 * Search parameter: <b>type</b> 3493 * <p> 3494 * Description: <b>Multiple Resources: 3495 3496* [Account](account.html): E.g. patient, expense, depreciation 3497* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 3498* [Composition](composition.html): Kind of composition (LOINC if possible) 3499* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 3500* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 3501* [Encounter](encounter.html): Specific type of encounter 3502* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 3503* [Invoice](invoice.html): Type of Invoice 3504* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 3505* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 3506* [Specimen](specimen.html): The specimen type 3507</b><br> 3508 * Type: <b>token</b><br> 3509 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 3510 * </p> 3511 */ 3512 @SearchParamDefinition(name="type", path="Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type", description="Multiple Resources: \r\n\r\n* [Account](account.html): E.g. patient, expense, depreciation\r\n* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known)\r\n* [Composition](composition.html): Kind of composition (LOINC if possible)\r\n* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation)\r\n* [DocumentReference](documentreference.html): Kind of document (LOINC if possible)\r\n* [Encounter](encounter.html): Specific type of encounter\r\n* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management\r\n* [Invoice](invoice.html): Type of Invoice\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type\r\n* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence\r\n* [Specimen](specimen.html): The specimen type\r\n", type="token" ) 3513 public static final String SP_TYPE = "type"; 3514 /** 3515 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3516 * <p> 3517 * Description: <b>Multiple Resources: 3518 3519* [Account](account.html): E.g. patient, expense, depreciation 3520* [AllergyIntolerance](allergyintolerance.html): allergy | intolerance - Underlying mechanism (if known) 3521* [Composition](composition.html): Kind of composition (LOINC if possible) 3522* [Coverage](coverage.html): The kind of coverage (health plan, auto, Workers Compensation) 3523* [DocumentReference](documentreference.html): Kind of document (LOINC if possible) 3524* [Encounter](encounter.html): Specific type of encounter 3525* [EpisodeOfCare](episodeofcare.html): Type/class - e.g. specialist referral, disease management 3526* [Invoice](invoice.html): Type of Invoice 3527* [MedicationDispense](medicationdispense.html): Returns dispenses of a specific type 3528* [MolecularSequence](molecularsequence.html): Amino Acid Sequence/ DNA Sequence / RNA Sequence 3529* [Specimen](specimen.html): The specimen type 3530</b><br> 3531 * Type: <b>token</b><br> 3532 * Path: <b>Account.type | AllergyIntolerance.type | Composition.type | Coverage.type | DocumentReference.type | Encounter.type | EpisodeOfCare.type | Invoice.type | MedicationDispense.type | MolecularSequence.type | Specimen.type</b><br> 3533 * </p> 3534 */ 3535 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 3536 3537 /** 3538 * Search parameter: <b>medication</b> 3539 * <p> 3540 * Description: <b>Multiple Resources: 3541 3542* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference 3543* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource 3544* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference 3545* [MedicationStatement](medicationstatement.html): Return statements of this medication reference 3546</b><br> 3547 * Type: <b>reference</b><br> 3548 * Path: <b>MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference</b><br> 3549 * </p> 3550 */ 3551 @SearchParamDefinition(name="medication", path="MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference", description="Multiple Resources: \r\n\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication reference\r\n", type="reference", target={Medication.class } ) 3552 public static final String SP_MEDICATION = "medication"; 3553 /** 3554 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 3555 * <p> 3556 * Description: <b>Multiple Resources: 3557 3558* [MedicationAdministration](medicationadministration.html): Return administrations of this medication reference 3559* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine resource 3560* [MedicationRequest](medicationrequest.html): Return prescriptions for this medication reference 3561* [MedicationStatement](medicationstatement.html): Return statements of this medication reference 3562</b><br> 3563 * Type: <b>reference</b><br> 3564 * Path: <b>MedicationAdministration.medication.reference | MedicationDispense.medication.reference | MedicationRequest.medication.reference | MedicationStatement.medication.reference</b><br> 3565 * </p> 3566 */ 3567 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MEDICATION); 3568 3569/** 3570 * Constant for fluent queries to be used to add include statements. Specifies 3571 * the path value of "<b>MedicationDispense:medication</b>". 3572 */ 3573 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include("MedicationDispense:medication").toLocked(); 3574 3575 /** 3576 * Search parameter: <b>prescription</b> 3577 * <p> 3578 * Description: <b>Multiple Resources: 3579 3580* [MedicationDispense](medicationdispense.html): The identity of a prescription to list dispenses from 3581</b><br> 3582 * Type: <b>reference</b><br> 3583 * Path: <b>MedicationDispense.authorizingPrescription</b><br> 3584 * </p> 3585 */ 3586 @SearchParamDefinition(name="prescription", path="MedicationDispense.authorizingPrescription", description="Multiple Resources: \r\n\r\n* [MedicationDispense](medicationdispense.html): The identity of a prescription to list dispenses from\r\n", type="reference", target={MedicationRequest.class } ) 3587 public static final String SP_PRESCRIPTION = "prescription"; 3588 /** 3589 * <b>Fluent Client</b> search parameter constant for <b>prescription</b> 3590 * <p> 3591 * Description: <b>Multiple Resources: 3592 3593* [MedicationDispense](medicationdispense.html): The identity of a prescription to list dispenses from 3594</b><br> 3595 * Type: <b>reference</b><br> 3596 * Path: <b>MedicationDispense.authorizingPrescription</b><br> 3597 * </p> 3598 */ 3599 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRESCRIPTION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRESCRIPTION); 3600 3601/** 3602 * Constant for fluent queries to be used to add include statements. Specifies 3603 * the path value of "<b>MedicationDispense:prescription</b>". 3604 */ 3605 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRESCRIPTION = new ca.uhn.fhir.model.api.Include("MedicationDispense:prescription").toLocked(); 3606 3607 /** 3608 * Search parameter: <b>status</b> 3609 * <p> 3610 * Description: <b>Multiple Resources: 3611 3612* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified) 3613* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status 3614* [MedicationRequest](medicationrequest.html): Status of the prescription 3615* [MedicationStatement](medicationstatement.html): Return statements that match the given status 3616</b><br> 3617 * Type: <b>token</b><br> 3618 * Path: <b>MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status</b><br> 3619 * </p> 3620 */ 3621 @SearchParamDefinition(name="status", path="MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status", description="Multiple Resources: \r\n\r\n* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified)\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status\r\n* [MedicationRequest](medicationrequest.html): Status of the prescription\r\n* [MedicationStatement](medicationstatement.html): Return statements that match the given status\r\n", type="token" ) 3622 public static final String SP_STATUS = "status"; 3623 /** 3624 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3625 * <p> 3626 * Description: <b>Multiple Resources: 3627 3628* [MedicationAdministration](medicationadministration.html): MedicationAdministration event status (for example one of active/paused/completed/nullified) 3629* [MedicationDispense](medicationdispense.html): Returns dispenses with a specified dispense status 3630* [MedicationRequest](medicationrequest.html): Status of the prescription 3631* [MedicationStatement](medicationstatement.html): Return statements that match the given status 3632</b><br> 3633 * Type: <b>token</b><br> 3634 * Path: <b>MedicationAdministration.status | MedicationDispense.status | MedicationRequest.status | MedicationStatement.status</b><br> 3635 * </p> 3636 */ 3637 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3638 3639 3640} 3641