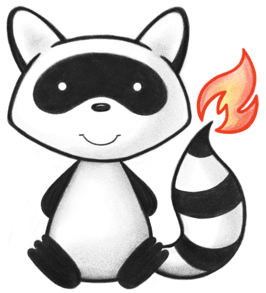
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Information about a medication that is used to support knowledge. 052 */ 053@ResourceDef(name="MedicationKnowledge", profile="http://hl7.org/fhir/StructureDefinition/MedicationKnowledge") 054public class MedicationKnowledge extends DomainResource { 055 056 public enum MedicationKnowledgeStatusCodes { 057 /** 058 * The medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. 059 */ 060 ACTIVE, 061 /** 062 * The medication referred to by this MedicationKnowledge was entered in error within the drug database or inventory system. 063 */ 064 ENTEREDINERROR, 065 /** 066 * The medication referred to by this MedicationKnowledge is not in active use within the drug database or inventory system. 067 */ 068 INACTIVE, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static MedicationKnowledgeStatusCodes fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("entered-in-error".equals(codeString)) 079 return ENTEREDINERROR; 080 if ("inactive".equals(codeString)) 081 return INACTIVE; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown MedicationKnowledgeStatusCodes code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case ACTIVE: return "active"; 090 case ENTEREDINERROR: return "entered-in-error"; 091 case INACTIVE: return "inactive"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case ACTIVE: return "http://hl7.org/fhir/CodeSystem/medicationknowledge-status"; 099 case ENTEREDINERROR: return "http://hl7.org/fhir/CodeSystem/medicationknowledge-status"; 100 case INACTIVE: return "http://hl7.org/fhir/CodeSystem/medicationknowledge-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case ACTIVE: return "The medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system."; 108 case ENTEREDINERROR: return "The medication referred to by this MedicationKnowledge was entered in error within the drug database or inventory system."; 109 case INACTIVE: return "The medication referred to by this MedicationKnowledge is not in active use within the drug database or inventory system."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case ACTIVE: return "Active"; 117 case ENTEREDINERROR: return "Entered in Error"; 118 case INACTIVE: return "Inactive"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class MedicationKnowledgeStatusCodesEnumFactory implements EnumFactory<MedicationKnowledgeStatusCodes> { 126 public MedicationKnowledgeStatusCodes fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("active".equals(codeString)) 131 return MedicationKnowledgeStatusCodes.ACTIVE; 132 if ("entered-in-error".equals(codeString)) 133 return MedicationKnowledgeStatusCodes.ENTEREDINERROR; 134 if ("inactive".equals(codeString)) 135 return MedicationKnowledgeStatusCodes.INACTIVE; 136 throw new IllegalArgumentException("Unknown MedicationKnowledgeStatusCodes code '"+codeString+"'"); 137 } 138 public Enumeration<MedicationKnowledgeStatusCodes> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<MedicationKnowledgeStatusCodes>(this, MedicationKnowledgeStatusCodes.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<MedicationKnowledgeStatusCodes>(this, MedicationKnowledgeStatusCodes.NULL, code); 146 if ("active".equals(codeString)) 147 return new Enumeration<MedicationKnowledgeStatusCodes>(this, MedicationKnowledgeStatusCodes.ACTIVE, code); 148 if ("entered-in-error".equals(codeString)) 149 return new Enumeration<MedicationKnowledgeStatusCodes>(this, MedicationKnowledgeStatusCodes.ENTEREDINERROR, code); 150 if ("inactive".equals(codeString)) 151 return new Enumeration<MedicationKnowledgeStatusCodes>(this, MedicationKnowledgeStatusCodes.INACTIVE, code); 152 throw new FHIRException("Unknown MedicationKnowledgeStatusCodes code '"+codeString+"'"); 153 } 154 public String toCode(MedicationKnowledgeStatusCodes code) { 155 if (code == MedicationKnowledgeStatusCodes.ACTIVE) 156 return "active"; 157 if (code == MedicationKnowledgeStatusCodes.ENTEREDINERROR) 158 return "entered-in-error"; 159 if (code == MedicationKnowledgeStatusCodes.INACTIVE) 160 return "inactive"; 161 return "?"; 162 } 163 public String toSystem(MedicationKnowledgeStatusCodes code) { 164 return code.getSystem(); 165 } 166 } 167 168 @Block() 169 public static class MedicationKnowledgeRelatedMedicationKnowledgeComponent extends BackboneElement implements IBaseBackboneElement { 170 /** 171 * The category of the associated medication knowledge reference. 172 */ 173 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 174 @Description(shortDefinition="Category of medicationKnowledge", formalDefinition="The category of the associated medication knowledge reference." ) 175 protected CodeableConcept type; 176 177 /** 178 * Associated documentation about the associated medication knowledge. 179 */ 180 @Child(name = "reference", type = {MedicationKnowledge.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 181 @Description(shortDefinition="Associated documentation about the associated medication knowledge", formalDefinition="Associated documentation about the associated medication knowledge." ) 182 protected List<Reference> reference; 183 184 private static final long serialVersionUID = 1687147899L; 185 186 /** 187 * Constructor 188 */ 189 public MedicationKnowledgeRelatedMedicationKnowledgeComponent() { 190 super(); 191 } 192 193 /** 194 * Constructor 195 */ 196 public MedicationKnowledgeRelatedMedicationKnowledgeComponent(CodeableConcept type, Reference reference) { 197 super(); 198 this.setType(type); 199 this.addReference(reference); 200 } 201 202 /** 203 * @return {@link #type} (The category of the associated medication knowledge reference.) 204 */ 205 public CodeableConcept getType() { 206 if (this.type == null) 207 if (Configuration.errorOnAutoCreate()) 208 throw new Error("Attempt to auto-create MedicationKnowledgeRelatedMedicationKnowledgeComponent.type"); 209 else if (Configuration.doAutoCreate()) 210 this.type = new CodeableConcept(); // cc 211 return this.type; 212 } 213 214 public boolean hasType() { 215 return this.type != null && !this.type.isEmpty(); 216 } 217 218 /** 219 * @param value {@link #type} (The category of the associated medication knowledge reference.) 220 */ 221 public MedicationKnowledgeRelatedMedicationKnowledgeComponent setType(CodeableConcept value) { 222 this.type = value; 223 return this; 224 } 225 226 /** 227 * @return {@link #reference} (Associated documentation about the associated medication knowledge.) 228 */ 229 public List<Reference> getReference() { 230 if (this.reference == null) 231 this.reference = new ArrayList<Reference>(); 232 return this.reference; 233 } 234 235 /** 236 * @return Returns a reference to <code>this</code> for easy method chaining 237 */ 238 public MedicationKnowledgeRelatedMedicationKnowledgeComponent setReference(List<Reference> theReference) { 239 this.reference = theReference; 240 return this; 241 } 242 243 public boolean hasReference() { 244 if (this.reference == null) 245 return false; 246 for (Reference item : this.reference) 247 if (!item.isEmpty()) 248 return true; 249 return false; 250 } 251 252 public Reference addReference() { //3 253 Reference t = new Reference(); 254 if (this.reference == null) 255 this.reference = new ArrayList<Reference>(); 256 this.reference.add(t); 257 return t; 258 } 259 260 public MedicationKnowledgeRelatedMedicationKnowledgeComponent addReference(Reference t) { //3 261 if (t == null) 262 return this; 263 if (this.reference == null) 264 this.reference = new ArrayList<Reference>(); 265 this.reference.add(t); 266 return this; 267 } 268 269 /** 270 * @return The first repetition of repeating field {@link #reference}, creating it if it does not already exist {3} 271 */ 272 public Reference getReferenceFirstRep() { 273 if (getReference().isEmpty()) { 274 addReference(); 275 } 276 return getReference().get(0); 277 } 278 279 protected void listChildren(List<Property> children) { 280 super.listChildren(children); 281 children.add(new Property("type", "CodeableConcept", "The category of the associated medication knowledge reference.", 0, 1, type)); 282 children.add(new Property("reference", "Reference(MedicationKnowledge)", "Associated documentation about the associated medication knowledge.", 0, java.lang.Integer.MAX_VALUE, reference)); 283 } 284 285 @Override 286 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 287 switch (_hash) { 288 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The category of the associated medication knowledge reference.", 0, 1, type); 289 case -925155509: /*reference*/ return new Property("reference", "Reference(MedicationKnowledge)", "Associated documentation about the associated medication knowledge.", 0, java.lang.Integer.MAX_VALUE, reference); 290 default: return super.getNamedProperty(_hash, _name, _checkValid); 291 } 292 293 } 294 295 @Override 296 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 297 switch (hash) { 298 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 299 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : this.reference.toArray(new Base[this.reference.size()]); // Reference 300 default: return super.getProperty(hash, name, checkValid); 301 } 302 303 } 304 305 @Override 306 public Base setProperty(int hash, String name, Base value) throws FHIRException { 307 switch (hash) { 308 case 3575610: // type 309 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 310 return value; 311 case -925155509: // reference 312 this.getReference().add(TypeConvertor.castToReference(value)); // Reference 313 return value; 314 default: return super.setProperty(hash, name, value); 315 } 316 317 } 318 319 @Override 320 public Base setProperty(String name, Base value) throws FHIRException { 321 if (name.equals("type")) { 322 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 323 } else if (name.equals("reference")) { 324 this.getReference().add(TypeConvertor.castToReference(value)); 325 } else 326 return super.setProperty(name, value); 327 return value; 328 } 329 330 @Override 331 public void removeChild(String name, Base value) throws FHIRException { 332 if (name.equals("type")) { 333 this.type = null; 334 } else if (name.equals("reference")) { 335 this.getReference().remove(value); 336 } else 337 super.removeChild(name, value); 338 339 } 340 341 @Override 342 public Base makeProperty(int hash, String name) throws FHIRException { 343 switch (hash) { 344 case 3575610: return getType(); 345 case -925155509: return addReference(); 346 default: return super.makeProperty(hash, name); 347 } 348 349 } 350 351 @Override 352 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 353 switch (hash) { 354 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 355 case -925155509: /*reference*/ return new String[] {"Reference"}; 356 default: return super.getTypesForProperty(hash, name); 357 } 358 359 } 360 361 @Override 362 public Base addChild(String name) throws FHIRException { 363 if (name.equals("type")) { 364 this.type = new CodeableConcept(); 365 return this.type; 366 } 367 else if (name.equals("reference")) { 368 return addReference(); 369 } 370 else 371 return super.addChild(name); 372 } 373 374 public MedicationKnowledgeRelatedMedicationKnowledgeComponent copy() { 375 MedicationKnowledgeRelatedMedicationKnowledgeComponent dst = new MedicationKnowledgeRelatedMedicationKnowledgeComponent(); 376 copyValues(dst); 377 return dst; 378 } 379 380 public void copyValues(MedicationKnowledgeRelatedMedicationKnowledgeComponent dst) { 381 super.copyValues(dst); 382 dst.type = type == null ? null : type.copy(); 383 if (reference != null) { 384 dst.reference = new ArrayList<Reference>(); 385 for (Reference i : reference) 386 dst.reference.add(i.copy()); 387 }; 388 } 389 390 @Override 391 public boolean equalsDeep(Base other_) { 392 if (!super.equalsDeep(other_)) 393 return false; 394 if (!(other_ instanceof MedicationKnowledgeRelatedMedicationKnowledgeComponent)) 395 return false; 396 MedicationKnowledgeRelatedMedicationKnowledgeComponent o = (MedicationKnowledgeRelatedMedicationKnowledgeComponent) other_; 397 return compareDeep(type, o.type, true) && compareDeep(reference, o.reference, true); 398 } 399 400 @Override 401 public boolean equalsShallow(Base other_) { 402 if (!super.equalsShallow(other_)) 403 return false; 404 if (!(other_ instanceof MedicationKnowledgeRelatedMedicationKnowledgeComponent)) 405 return false; 406 MedicationKnowledgeRelatedMedicationKnowledgeComponent o = (MedicationKnowledgeRelatedMedicationKnowledgeComponent) other_; 407 return true; 408 } 409 410 public boolean isEmpty() { 411 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, reference); 412 } 413 414 public String fhirType() { 415 return "MedicationKnowledge.relatedMedicationKnowledge"; 416 417 } 418 419 } 420 421 @Block() 422 public static class MedicationKnowledgeMonographComponent extends BackboneElement implements IBaseBackboneElement { 423 /** 424 * The category of documentation about the medication. (e.g. professional monograph, patient education monograph). 425 */ 426 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 427 @Description(shortDefinition="The category of medication document", formalDefinition="The category of documentation about the medication. (e.g. professional monograph, patient education monograph)." ) 428 protected CodeableConcept type; 429 430 /** 431 * Associated documentation about the medication. 432 */ 433 @Child(name = "source", type = {DocumentReference.class}, order=2, min=0, max=1, modifier=false, summary=false) 434 @Description(shortDefinition="Associated documentation about the medication", formalDefinition="Associated documentation about the medication." ) 435 protected Reference source; 436 437 private static final long serialVersionUID = -197893751L; 438 439 /** 440 * Constructor 441 */ 442 public MedicationKnowledgeMonographComponent() { 443 super(); 444 } 445 446 /** 447 * @return {@link #type} (The category of documentation about the medication. (e.g. professional monograph, patient education monograph).) 448 */ 449 public CodeableConcept getType() { 450 if (this.type == null) 451 if (Configuration.errorOnAutoCreate()) 452 throw new Error("Attempt to auto-create MedicationKnowledgeMonographComponent.type"); 453 else if (Configuration.doAutoCreate()) 454 this.type = new CodeableConcept(); // cc 455 return this.type; 456 } 457 458 public boolean hasType() { 459 return this.type != null && !this.type.isEmpty(); 460 } 461 462 /** 463 * @param value {@link #type} (The category of documentation about the medication. (e.g. professional monograph, patient education monograph).) 464 */ 465 public MedicationKnowledgeMonographComponent setType(CodeableConcept value) { 466 this.type = value; 467 return this; 468 } 469 470 /** 471 * @return {@link #source} (Associated documentation about the medication.) 472 */ 473 public Reference getSource() { 474 if (this.source == null) 475 if (Configuration.errorOnAutoCreate()) 476 throw new Error("Attempt to auto-create MedicationKnowledgeMonographComponent.source"); 477 else if (Configuration.doAutoCreate()) 478 this.source = new Reference(); // cc 479 return this.source; 480 } 481 482 public boolean hasSource() { 483 return this.source != null && !this.source.isEmpty(); 484 } 485 486 /** 487 * @param value {@link #source} (Associated documentation about the medication.) 488 */ 489 public MedicationKnowledgeMonographComponent setSource(Reference value) { 490 this.source = value; 491 return this; 492 } 493 494 protected void listChildren(List<Property> children) { 495 super.listChildren(children); 496 children.add(new Property("type", "CodeableConcept", "The category of documentation about the medication. (e.g. professional monograph, patient education monograph).", 0, 1, type)); 497 children.add(new Property("source", "Reference(DocumentReference)", "Associated documentation about the medication.", 0, 1, source)); 498 } 499 500 @Override 501 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 502 switch (_hash) { 503 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The category of documentation about the medication. (e.g. professional monograph, patient education monograph).", 0, 1, type); 504 case -896505829: /*source*/ return new Property("source", "Reference(DocumentReference)", "Associated documentation about the medication.", 0, 1, source); 505 default: return super.getNamedProperty(_hash, _name, _checkValid); 506 } 507 508 } 509 510 @Override 511 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 512 switch (hash) { 513 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 514 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // Reference 515 default: return super.getProperty(hash, name, checkValid); 516 } 517 518 } 519 520 @Override 521 public Base setProperty(int hash, String name, Base value) throws FHIRException { 522 switch (hash) { 523 case 3575610: // type 524 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 525 return value; 526 case -896505829: // source 527 this.source = TypeConvertor.castToReference(value); // Reference 528 return value; 529 default: return super.setProperty(hash, name, value); 530 } 531 532 } 533 534 @Override 535 public Base setProperty(String name, Base value) throws FHIRException { 536 if (name.equals("type")) { 537 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 538 } else if (name.equals("source")) { 539 this.source = TypeConvertor.castToReference(value); // Reference 540 } else 541 return super.setProperty(name, value); 542 return value; 543 } 544 545 @Override 546 public void removeChild(String name, Base value) throws FHIRException { 547 if (name.equals("type")) { 548 this.type = null; 549 } else if (name.equals("source")) { 550 this.source = null; 551 } else 552 super.removeChild(name, value); 553 554 } 555 556 @Override 557 public Base makeProperty(int hash, String name) throws FHIRException { 558 switch (hash) { 559 case 3575610: return getType(); 560 case -896505829: return getSource(); 561 default: return super.makeProperty(hash, name); 562 } 563 564 } 565 566 @Override 567 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 568 switch (hash) { 569 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 570 case -896505829: /*source*/ return new String[] {"Reference"}; 571 default: return super.getTypesForProperty(hash, name); 572 } 573 574 } 575 576 @Override 577 public Base addChild(String name) throws FHIRException { 578 if (name.equals("type")) { 579 this.type = new CodeableConcept(); 580 return this.type; 581 } 582 else if (name.equals("source")) { 583 this.source = new Reference(); 584 return this.source; 585 } 586 else 587 return super.addChild(name); 588 } 589 590 public MedicationKnowledgeMonographComponent copy() { 591 MedicationKnowledgeMonographComponent dst = new MedicationKnowledgeMonographComponent(); 592 copyValues(dst); 593 return dst; 594 } 595 596 public void copyValues(MedicationKnowledgeMonographComponent dst) { 597 super.copyValues(dst); 598 dst.type = type == null ? null : type.copy(); 599 dst.source = source == null ? null : source.copy(); 600 } 601 602 @Override 603 public boolean equalsDeep(Base other_) { 604 if (!super.equalsDeep(other_)) 605 return false; 606 if (!(other_ instanceof MedicationKnowledgeMonographComponent)) 607 return false; 608 MedicationKnowledgeMonographComponent o = (MedicationKnowledgeMonographComponent) other_; 609 return compareDeep(type, o.type, true) && compareDeep(source, o.source, true); 610 } 611 612 @Override 613 public boolean equalsShallow(Base other_) { 614 if (!super.equalsShallow(other_)) 615 return false; 616 if (!(other_ instanceof MedicationKnowledgeMonographComponent)) 617 return false; 618 MedicationKnowledgeMonographComponent o = (MedicationKnowledgeMonographComponent) other_; 619 return true; 620 } 621 622 public boolean isEmpty() { 623 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, source); 624 } 625 626 public String fhirType() { 627 return "MedicationKnowledge.monograph"; 628 629 } 630 631 } 632 633 @Block() 634 public static class MedicationKnowledgeCostComponent extends BackboneElement implements IBaseBackboneElement { 635 /** 636 * The date range for which the cost information of the medication is effective. 637 */ 638 @Child(name = "effectiveDate", type = {Period.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 639 @Description(shortDefinition="The date range for which the cost is effective", formalDefinition="The date range for which the cost information of the medication is effective." ) 640 protected List<Period> effectiveDate; 641 642 /** 643 * The category of the cost information. For example, manufacturers' cost, patient cost, claim reimbursement cost, actual acquisition cost. 644 */ 645 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 646 @Description(shortDefinition="The category of the cost information", formalDefinition="The category of the cost information. For example, manufacturers' cost, patient cost, claim reimbursement cost, actual acquisition cost." ) 647 protected CodeableConcept type; 648 649 /** 650 * The source or owner that assigns the price to the medication. 651 */ 652 @Child(name = "source", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 653 @Description(shortDefinition="The source or owner for the price information", formalDefinition="The source or owner that assigns the price to the medication." ) 654 protected StringType source; 655 656 /** 657 * The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication. 658 */ 659 @Child(name = "cost", type = {Money.class, CodeableConcept.class}, order=4, min=1, max=1, modifier=false, summary=false) 660 @Description(shortDefinition="The price or category of the cost of the medication", formalDefinition="The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication." ) 661 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-cost-category") 662 protected DataType cost; 663 664 private static final long serialVersionUID = 747402134L; 665 666 /** 667 * Constructor 668 */ 669 public MedicationKnowledgeCostComponent() { 670 super(); 671 } 672 673 /** 674 * Constructor 675 */ 676 public MedicationKnowledgeCostComponent(CodeableConcept type, DataType cost) { 677 super(); 678 this.setType(type); 679 this.setCost(cost); 680 } 681 682 /** 683 * @return {@link #effectiveDate} (The date range for which the cost information of the medication is effective.) 684 */ 685 public List<Period> getEffectiveDate() { 686 if (this.effectiveDate == null) 687 this.effectiveDate = new ArrayList<Period>(); 688 return this.effectiveDate; 689 } 690 691 /** 692 * @return Returns a reference to <code>this</code> for easy method chaining 693 */ 694 public MedicationKnowledgeCostComponent setEffectiveDate(List<Period> theEffectiveDate) { 695 this.effectiveDate = theEffectiveDate; 696 return this; 697 } 698 699 public boolean hasEffectiveDate() { 700 if (this.effectiveDate == null) 701 return false; 702 for (Period item : this.effectiveDate) 703 if (!item.isEmpty()) 704 return true; 705 return false; 706 } 707 708 public Period addEffectiveDate() { //3 709 Period t = new Period(); 710 if (this.effectiveDate == null) 711 this.effectiveDate = new ArrayList<Period>(); 712 this.effectiveDate.add(t); 713 return t; 714 } 715 716 public MedicationKnowledgeCostComponent addEffectiveDate(Period t) { //3 717 if (t == null) 718 return this; 719 if (this.effectiveDate == null) 720 this.effectiveDate = new ArrayList<Period>(); 721 this.effectiveDate.add(t); 722 return this; 723 } 724 725 /** 726 * @return The first repetition of repeating field {@link #effectiveDate}, creating it if it does not already exist {3} 727 */ 728 public Period getEffectiveDateFirstRep() { 729 if (getEffectiveDate().isEmpty()) { 730 addEffectiveDate(); 731 } 732 return getEffectiveDate().get(0); 733 } 734 735 /** 736 * @return {@link #type} (The category of the cost information. For example, manufacturers' cost, patient cost, claim reimbursement cost, actual acquisition cost.) 737 */ 738 public CodeableConcept getType() { 739 if (this.type == null) 740 if (Configuration.errorOnAutoCreate()) 741 throw new Error("Attempt to auto-create MedicationKnowledgeCostComponent.type"); 742 else if (Configuration.doAutoCreate()) 743 this.type = new CodeableConcept(); // cc 744 return this.type; 745 } 746 747 public boolean hasType() { 748 return this.type != null && !this.type.isEmpty(); 749 } 750 751 /** 752 * @param value {@link #type} (The category of the cost information. For example, manufacturers' cost, patient cost, claim reimbursement cost, actual acquisition cost.) 753 */ 754 public MedicationKnowledgeCostComponent setType(CodeableConcept value) { 755 this.type = value; 756 return this; 757 } 758 759 /** 760 * @return {@link #source} (The source or owner that assigns the price to the medication.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 761 */ 762 public StringType getSourceElement() { 763 if (this.source == null) 764 if (Configuration.errorOnAutoCreate()) 765 throw new Error("Attempt to auto-create MedicationKnowledgeCostComponent.source"); 766 else if (Configuration.doAutoCreate()) 767 this.source = new StringType(); // bb 768 return this.source; 769 } 770 771 public boolean hasSourceElement() { 772 return this.source != null && !this.source.isEmpty(); 773 } 774 775 public boolean hasSource() { 776 return this.source != null && !this.source.isEmpty(); 777 } 778 779 /** 780 * @param value {@link #source} (The source or owner that assigns the price to the medication.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 781 */ 782 public MedicationKnowledgeCostComponent setSourceElement(StringType value) { 783 this.source = value; 784 return this; 785 } 786 787 /** 788 * @return The source or owner that assigns the price to the medication. 789 */ 790 public String getSource() { 791 return this.source == null ? null : this.source.getValue(); 792 } 793 794 /** 795 * @param value The source or owner that assigns the price to the medication. 796 */ 797 public MedicationKnowledgeCostComponent setSource(String value) { 798 if (Utilities.noString(value)) 799 this.source = null; 800 else { 801 if (this.source == null) 802 this.source = new StringType(); 803 this.source.setValue(value); 804 } 805 return this; 806 } 807 808 /** 809 * @return {@link #cost} (The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.) 810 */ 811 public DataType getCost() { 812 return this.cost; 813 } 814 815 /** 816 * @return {@link #cost} (The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.) 817 */ 818 public Money getCostMoney() throws FHIRException { 819 if (this.cost == null) 820 this.cost = new Money(); 821 if (!(this.cost instanceof Money)) 822 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.cost.getClass().getName()+" was encountered"); 823 return (Money) this.cost; 824 } 825 826 public boolean hasCostMoney() { 827 return this != null && this.cost instanceof Money; 828 } 829 830 /** 831 * @return {@link #cost} (The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.) 832 */ 833 public CodeableConcept getCostCodeableConcept() throws FHIRException { 834 if (this.cost == null) 835 this.cost = new CodeableConcept(); 836 if (!(this.cost instanceof CodeableConcept)) 837 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.cost.getClass().getName()+" was encountered"); 838 return (CodeableConcept) this.cost; 839 } 840 841 public boolean hasCostCodeableConcept() { 842 return this != null && this.cost instanceof CodeableConcept; 843 } 844 845 public boolean hasCost() { 846 return this.cost != null && !this.cost.isEmpty(); 847 } 848 849 /** 850 * @param value {@link #cost} (The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.) 851 */ 852 public MedicationKnowledgeCostComponent setCost(DataType value) { 853 if (value != null && !(value instanceof Money || value instanceof CodeableConcept)) 854 throw new FHIRException("Not the right type for MedicationKnowledge.cost.cost[x]: "+value.fhirType()); 855 this.cost = value; 856 return this; 857 } 858 859 protected void listChildren(List<Property> children) { 860 super.listChildren(children); 861 children.add(new Property("effectiveDate", "Period", "The date range for which the cost information of the medication is effective.", 0, java.lang.Integer.MAX_VALUE, effectiveDate)); 862 children.add(new Property("type", "CodeableConcept", "The category of the cost information. For example, manufacturers' cost, patient cost, claim reimbursement cost, actual acquisition cost.", 0, 1, type)); 863 children.add(new Property("source", "string", "The source or owner that assigns the price to the medication.", 0, 1, source)); 864 children.add(new Property("cost[x]", "Money|CodeableConcept", "The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.", 0, 1, cost)); 865 } 866 867 @Override 868 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 869 switch (_hash) { 870 case -930389515: /*effectiveDate*/ return new Property("effectiveDate", "Period", "The date range for which the cost information of the medication is effective.", 0, java.lang.Integer.MAX_VALUE, effectiveDate); 871 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The category of the cost information. For example, manufacturers' cost, patient cost, claim reimbursement cost, actual acquisition cost.", 0, 1, type); 872 case -896505829: /*source*/ return new Property("source", "string", "The source or owner that assigns the price to the medication.", 0, 1, source); 873 case 956138899: /*cost[x]*/ return new Property("cost[x]", "Money|CodeableConcept", "The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.", 0, 1, cost); 874 case 3059661: /*cost*/ return new Property("cost[x]", "Money|CodeableConcept", "The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.", 0, 1, cost); 875 case -286697229: /*costMoney*/ return new Property("cost[x]", "Money", "The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.", 0, 1, cost); 876 case -238369772: /*costCodeableConcept*/ return new Property("cost[x]", "CodeableConcept", "The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.", 0, 1, cost); 877 default: return super.getNamedProperty(_hash, _name, _checkValid); 878 } 879 880 } 881 882 @Override 883 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 884 switch (hash) { 885 case -930389515: /*effectiveDate*/ return this.effectiveDate == null ? new Base[0] : this.effectiveDate.toArray(new Base[this.effectiveDate.size()]); // Period 886 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 887 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // StringType 888 case 3059661: /*cost*/ return this.cost == null ? new Base[0] : new Base[] {this.cost}; // DataType 889 default: return super.getProperty(hash, name, checkValid); 890 } 891 892 } 893 894 @Override 895 public Base setProperty(int hash, String name, Base value) throws FHIRException { 896 switch (hash) { 897 case -930389515: // effectiveDate 898 this.getEffectiveDate().add(TypeConvertor.castToPeriod(value)); // Period 899 return value; 900 case 3575610: // type 901 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 902 return value; 903 case -896505829: // source 904 this.source = TypeConvertor.castToString(value); // StringType 905 return value; 906 case 3059661: // cost 907 this.cost = TypeConvertor.castToType(value); // DataType 908 return value; 909 default: return super.setProperty(hash, name, value); 910 } 911 912 } 913 914 @Override 915 public Base setProperty(String name, Base value) throws FHIRException { 916 if (name.equals("effectiveDate")) { 917 this.getEffectiveDate().add(TypeConvertor.castToPeriod(value)); 918 } else if (name.equals("type")) { 919 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 920 } else if (name.equals("source")) { 921 this.source = TypeConvertor.castToString(value); // StringType 922 } else if (name.equals("cost[x]")) { 923 this.cost = TypeConvertor.castToType(value); // DataType 924 } else 925 return super.setProperty(name, value); 926 return value; 927 } 928 929 @Override 930 public void removeChild(String name, Base value) throws FHIRException { 931 if (name.equals("effectiveDate")) { 932 this.getEffectiveDate().remove(value); 933 } else if (name.equals("type")) { 934 this.type = null; 935 } else if (name.equals("source")) { 936 this.source = null; 937 } else if (name.equals("cost[x]")) { 938 this.cost = null; 939 } else 940 super.removeChild(name, value); 941 942 } 943 944 @Override 945 public Base makeProperty(int hash, String name) throws FHIRException { 946 switch (hash) { 947 case -930389515: return addEffectiveDate(); 948 case 3575610: return getType(); 949 case -896505829: return getSourceElement(); 950 case 956138899: return getCost(); 951 case 3059661: return getCost(); 952 default: return super.makeProperty(hash, name); 953 } 954 955 } 956 957 @Override 958 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 959 switch (hash) { 960 case -930389515: /*effectiveDate*/ return new String[] {"Period"}; 961 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 962 case -896505829: /*source*/ return new String[] {"string"}; 963 case 3059661: /*cost*/ return new String[] {"Money", "CodeableConcept"}; 964 default: return super.getTypesForProperty(hash, name); 965 } 966 967 } 968 969 @Override 970 public Base addChild(String name) throws FHIRException { 971 if (name.equals("effectiveDate")) { 972 return addEffectiveDate(); 973 } 974 else if (name.equals("type")) { 975 this.type = new CodeableConcept(); 976 return this.type; 977 } 978 else if (name.equals("source")) { 979 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.cost.source"); 980 } 981 else if (name.equals("costMoney")) { 982 this.cost = new Money(); 983 return this.cost; 984 } 985 else if (name.equals("costCodeableConcept")) { 986 this.cost = new CodeableConcept(); 987 return this.cost; 988 } 989 else 990 return super.addChild(name); 991 } 992 993 public MedicationKnowledgeCostComponent copy() { 994 MedicationKnowledgeCostComponent dst = new MedicationKnowledgeCostComponent(); 995 copyValues(dst); 996 return dst; 997 } 998 999 public void copyValues(MedicationKnowledgeCostComponent dst) { 1000 super.copyValues(dst); 1001 if (effectiveDate != null) { 1002 dst.effectiveDate = new ArrayList<Period>(); 1003 for (Period i : effectiveDate) 1004 dst.effectiveDate.add(i.copy()); 1005 }; 1006 dst.type = type == null ? null : type.copy(); 1007 dst.source = source == null ? null : source.copy(); 1008 dst.cost = cost == null ? null : cost.copy(); 1009 } 1010 1011 @Override 1012 public boolean equalsDeep(Base other_) { 1013 if (!super.equalsDeep(other_)) 1014 return false; 1015 if (!(other_ instanceof MedicationKnowledgeCostComponent)) 1016 return false; 1017 MedicationKnowledgeCostComponent o = (MedicationKnowledgeCostComponent) other_; 1018 return compareDeep(effectiveDate, o.effectiveDate, true) && compareDeep(type, o.type, true) && compareDeep(source, o.source, true) 1019 && compareDeep(cost, o.cost, true); 1020 } 1021 1022 @Override 1023 public boolean equalsShallow(Base other_) { 1024 if (!super.equalsShallow(other_)) 1025 return false; 1026 if (!(other_ instanceof MedicationKnowledgeCostComponent)) 1027 return false; 1028 MedicationKnowledgeCostComponent o = (MedicationKnowledgeCostComponent) other_; 1029 return compareValues(source, o.source, true); 1030 } 1031 1032 public boolean isEmpty() { 1033 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(effectiveDate, type, source 1034 , cost); 1035 } 1036 1037 public String fhirType() { 1038 return "MedicationKnowledge.cost"; 1039 1040 } 1041 1042 } 1043 1044 @Block() 1045 public static class MedicationKnowledgeMonitoringProgramComponent extends BackboneElement implements IBaseBackboneElement { 1046 /** 1047 * Type of program under which the medication is monitored. 1048 */ 1049 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1050 @Description(shortDefinition="Type of program under which the medication is monitored", formalDefinition="Type of program under which the medication is monitored." ) 1051 protected CodeableConcept type; 1052 1053 /** 1054 * Name of the reviewing program. 1055 */ 1056 @Child(name = "name", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1057 @Description(shortDefinition="Name of the reviewing program", formalDefinition="Name of the reviewing program." ) 1058 protected StringType name; 1059 1060 private static final long serialVersionUID = -280346281L; 1061 1062 /** 1063 * Constructor 1064 */ 1065 public MedicationKnowledgeMonitoringProgramComponent() { 1066 super(); 1067 } 1068 1069 /** 1070 * @return {@link #type} (Type of program under which the medication is monitored.) 1071 */ 1072 public CodeableConcept getType() { 1073 if (this.type == null) 1074 if (Configuration.errorOnAutoCreate()) 1075 throw new Error("Attempt to auto-create MedicationKnowledgeMonitoringProgramComponent.type"); 1076 else if (Configuration.doAutoCreate()) 1077 this.type = new CodeableConcept(); // cc 1078 return this.type; 1079 } 1080 1081 public boolean hasType() { 1082 return this.type != null && !this.type.isEmpty(); 1083 } 1084 1085 /** 1086 * @param value {@link #type} (Type of program under which the medication is monitored.) 1087 */ 1088 public MedicationKnowledgeMonitoringProgramComponent setType(CodeableConcept value) { 1089 this.type = value; 1090 return this; 1091 } 1092 1093 /** 1094 * @return {@link #name} (Name of the reviewing program.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1095 */ 1096 public StringType getNameElement() { 1097 if (this.name == null) 1098 if (Configuration.errorOnAutoCreate()) 1099 throw new Error("Attempt to auto-create MedicationKnowledgeMonitoringProgramComponent.name"); 1100 else if (Configuration.doAutoCreate()) 1101 this.name = new StringType(); // bb 1102 return this.name; 1103 } 1104 1105 public boolean hasNameElement() { 1106 return this.name != null && !this.name.isEmpty(); 1107 } 1108 1109 public boolean hasName() { 1110 return this.name != null && !this.name.isEmpty(); 1111 } 1112 1113 /** 1114 * @param value {@link #name} (Name of the reviewing program.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1115 */ 1116 public MedicationKnowledgeMonitoringProgramComponent setNameElement(StringType value) { 1117 this.name = value; 1118 return this; 1119 } 1120 1121 /** 1122 * @return Name of the reviewing program. 1123 */ 1124 public String getName() { 1125 return this.name == null ? null : this.name.getValue(); 1126 } 1127 1128 /** 1129 * @param value Name of the reviewing program. 1130 */ 1131 public MedicationKnowledgeMonitoringProgramComponent setName(String value) { 1132 if (Utilities.noString(value)) 1133 this.name = null; 1134 else { 1135 if (this.name == null) 1136 this.name = new StringType(); 1137 this.name.setValue(value); 1138 } 1139 return this; 1140 } 1141 1142 protected void listChildren(List<Property> children) { 1143 super.listChildren(children); 1144 children.add(new Property("type", "CodeableConcept", "Type of program under which the medication is monitored.", 0, 1, type)); 1145 children.add(new Property("name", "string", "Name of the reviewing program.", 0, 1, name)); 1146 } 1147 1148 @Override 1149 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1150 switch (_hash) { 1151 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of program under which the medication is monitored.", 0, 1, type); 1152 case 3373707: /*name*/ return new Property("name", "string", "Name of the reviewing program.", 0, 1, name); 1153 default: return super.getNamedProperty(_hash, _name, _checkValid); 1154 } 1155 1156 } 1157 1158 @Override 1159 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1160 switch (hash) { 1161 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1162 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1163 default: return super.getProperty(hash, name, checkValid); 1164 } 1165 1166 } 1167 1168 @Override 1169 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1170 switch (hash) { 1171 case 3575610: // type 1172 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1173 return value; 1174 case 3373707: // name 1175 this.name = TypeConvertor.castToString(value); // StringType 1176 return value; 1177 default: return super.setProperty(hash, name, value); 1178 } 1179 1180 } 1181 1182 @Override 1183 public Base setProperty(String name, Base value) throws FHIRException { 1184 if (name.equals("type")) { 1185 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1186 } else if (name.equals("name")) { 1187 this.name = TypeConvertor.castToString(value); // StringType 1188 } else 1189 return super.setProperty(name, value); 1190 return value; 1191 } 1192 1193 @Override 1194 public void removeChild(String name, Base value) throws FHIRException { 1195 if (name.equals("type")) { 1196 this.type = null; 1197 } else if (name.equals("name")) { 1198 this.name = null; 1199 } else 1200 super.removeChild(name, value); 1201 1202 } 1203 1204 @Override 1205 public Base makeProperty(int hash, String name) throws FHIRException { 1206 switch (hash) { 1207 case 3575610: return getType(); 1208 case 3373707: return getNameElement(); 1209 default: return super.makeProperty(hash, name); 1210 } 1211 1212 } 1213 1214 @Override 1215 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1216 switch (hash) { 1217 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1218 case 3373707: /*name*/ return new String[] {"string"}; 1219 default: return super.getTypesForProperty(hash, name); 1220 } 1221 1222 } 1223 1224 @Override 1225 public Base addChild(String name) throws FHIRException { 1226 if (name.equals("type")) { 1227 this.type = new CodeableConcept(); 1228 return this.type; 1229 } 1230 else if (name.equals("name")) { 1231 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.monitoringProgram.name"); 1232 } 1233 else 1234 return super.addChild(name); 1235 } 1236 1237 public MedicationKnowledgeMonitoringProgramComponent copy() { 1238 MedicationKnowledgeMonitoringProgramComponent dst = new MedicationKnowledgeMonitoringProgramComponent(); 1239 copyValues(dst); 1240 return dst; 1241 } 1242 1243 public void copyValues(MedicationKnowledgeMonitoringProgramComponent dst) { 1244 super.copyValues(dst); 1245 dst.type = type == null ? null : type.copy(); 1246 dst.name = name == null ? null : name.copy(); 1247 } 1248 1249 @Override 1250 public boolean equalsDeep(Base other_) { 1251 if (!super.equalsDeep(other_)) 1252 return false; 1253 if (!(other_ instanceof MedicationKnowledgeMonitoringProgramComponent)) 1254 return false; 1255 MedicationKnowledgeMonitoringProgramComponent o = (MedicationKnowledgeMonitoringProgramComponent) other_; 1256 return compareDeep(type, o.type, true) && compareDeep(name, o.name, true); 1257 } 1258 1259 @Override 1260 public boolean equalsShallow(Base other_) { 1261 if (!super.equalsShallow(other_)) 1262 return false; 1263 if (!(other_ instanceof MedicationKnowledgeMonitoringProgramComponent)) 1264 return false; 1265 MedicationKnowledgeMonitoringProgramComponent o = (MedicationKnowledgeMonitoringProgramComponent) other_; 1266 return compareValues(name, o.name, true); 1267 } 1268 1269 public boolean isEmpty() { 1270 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, name); 1271 } 1272 1273 public String fhirType() { 1274 return "MedicationKnowledge.monitoringProgram"; 1275 1276 } 1277 1278 } 1279 1280 @Block() 1281 public static class MedicationKnowledgeIndicationGuidelineComponent extends BackboneElement implements IBaseBackboneElement { 1282 /** 1283 * Indication or reason for use of the medication that applies to the specific administration guideline. 1284 */ 1285 @Child(name = "indication", type = {CodeableReference.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1286 @Description(shortDefinition="Indication for use that applies to the specific administration guideline", formalDefinition="Indication or reason for use of the medication that applies to the specific administration guideline." ) 1287 protected List<CodeableReference> indication; 1288 1289 /** 1290 * The guidelines for the dosage of the medication for the indication. 1291 */ 1292 @Child(name = "dosingGuideline", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1293 @Description(shortDefinition="Guidelines for dosage of the medication", formalDefinition="The guidelines for the dosage of the medication for the indication." ) 1294 protected List<MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent> dosingGuideline; 1295 1296 private static final long serialVersionUID = 256409722L; 1297 1298 /** 1299 * Constructor 1300 */ 1301 public MedicationKnowledgeIndicationGuidelineComponent() { 1302 super(); 1303 } 1304 1305 /** 1306 * @return {@link #indication} (Indication or reason for use of the medication that applies to the specific administration guideline.) 1307 */ 1308 public List<CodeableReference> getIndication() { 1309 if (this.indication == null) 1310 this.indication = new ArrayList<CodeableReference>(); 1311 return this.indication; 1312 } 1313 1314 /** 1315 * @return Returns a reference to <code>this</code> for easy method chaining 1316 */ 1317 public MedicationKnowledgeIndicationGuidelineComponent setIndication(List<CodeableReference> theIndication) { 1318 this.indication = theIndication; 1319 return this; 1320 } 1321 1322 public boolean hasIndication() { 1323 if (this.indication == null) 1324 return false; 1325 for (CodeableReference item : this.indication) 1326 if (!item.isEmpty()) 1327 return true; 1328 return false; 1329 } 1330 1331 public CodeableReference addIndication() { //3 1332 CodeableReference t = new CodeableReference(); 1333 if (this.indication == null) 1334 this.indication = new ArrayList<CodeableReference>(); 1335 this.indication.add(t); 1336 return t; 1337 } 1338 1339 public MedicationKnowledgeIndicationGuidelineComponent addIndication(CodeableReference t) { //3 1340 if (t == null) 1341 return this; 1342 if (this.indication == null) 1343 this.indication = new ArrayList<CodeableReference>(); 1344 this.indication.add(t); 1345 return this; 1346 } 1347 1348 /** 1349 * @return The first repetition of repeating field {@link #indication}, creating it if it does not already exist {3} 1350 */ 1351 public CodeableReference getIndicationFirstRep() { 1352 if (getIndication().isEmpty()) { 1353 addIndication(); 1354 } 1355 return getIndication().get(0); 1356 } 1357 1358 /** 1359 * @return {@link #dosingGuideline} (The guidelines for the dosage of the medication for the indication.) 1360 */ 1361 public List<MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent> getDosingGuideline() { 1362 if (this.dosingGuideline == null) 1363 this.dosingGuideline = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent>(); 1364 return this.dosingGuideline; 1365 } 1366 1367 /** 1368 * @return Returns a reference to <code>this</code> for easy method chaining 1369 */ 1370 public MedicationKnowledgeIndicationGuidelineComponent setDosingGuideline(List<MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent> theDosingGuideline) { 1371 this.dosingGuideline = theDosingGuideline; 1372 return this; 1373 } 1374 1375 public boolean hasDosingGuideline() { 1376 if (this.dosingGuideline == null) 1377 return false; 1378 for (MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent item : this.dosingGuideline) 1379 if (!item.isEmpty()) 1380 return true; 1381 return false; 1382 } 1383 1384 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent addDosingGuideline() { //3 1385 MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent t = new MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent(); 1386 if (this.dosingGuideline == null) 1387 this.dosingGuideline = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent>(); 1388 this.dosingGuideline.add(t); 1389 return t; 1390 } 1391 1392 public MedicationKnowledgeIndicationGuidelineComponent addDosingGuideline(MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent t) { //3 1393 if (t == null) 1394 return this; 1395 if (this.dosingGuideline == null) 1396 this.dosingGuideline = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent>(); 1397 this.dosingGuideline.add(t); 1398 return this; 1399 } 1400 1401 /** 1402 * @return The first repetition of repeating field {@link #dosingGuideline}, creating it if it does not already exist {3} 1403 */ 1404 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent getDosingGuidelineFirstRep() { 1405 if (getDosingGuideline().isEmpty()) { 1406 addDosingGuideline(); 1407 } 1408 return getDosingGuideline().get(0); 1409 } 1410 1411 protected void listChildren(List<Property> children) { 1412 super.listChildren(children); 1413 children.add(new Property("indication", "CodeableReference(ClinicalUseDefinition)", "Indication or reason for use of the medication that applies to the specific administration guideline.", 0, java.lang.Integer.MAX_VALUE, indication)); 1414 children.add(new Property("dosingGuideline", "", "The guidelines for the dosage of the medication for the indication.", 0, java.lang.Integer.MAX_VALUE, dosingGuideline)); 1415 } 1416 1417 @Override 1418 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1419 switch (_hash) { 1420 case -597168804: /*indication*/ return new Property("indication", "CodeableReference(ClinicalUseDefinition)", "Indication or reason for use of the medication that applies to the specific administration guideline.", 0, java.lang.Integer.MAX_VALUE, indication); 1421 case -1792856970: /*dosingGuideline*/ return new Property("dosingGuideline", "", "The guidelines for the dosage of the medication for the indication.", 0, java.lang.Integer.MAX_VALUE, dosingGuideline); 1422 default: return super.getNamedProperty(_hash, _name, _checkValid); 1423 } 1424 1425 } 1426 1427 @Override 1428 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1429 switch (hash) { 1430 case -597168804: /*indication*/ return this.indication == null ? new Base[0] : this.indication.toArray(new Base[this.indication.size()]); // CodeableReference 1431 case -1792856970: /*dosingGuideline*/ return this.dosingGuideline == null ? new Base[0] : this.dosingGuideline.toArray(new Base[this.dosingGuideline.size()]); // MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent 1432 default: return super.getProperty(hash, name, checkValid); 1433 } 1434 1435 } 1436 1437 @Override 1438 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1439 switch (hash) { 1440 case -597168804: // indication 1441 this.getIndication().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1442 return value; 1443 case -1792856970: // dosingGuideline 1444 this.getDosingGuideline().add((MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent) value); // MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent 1445 return value; 1446 default: return super.setProperty(hash, name, value); 1447 } 1448 1449 } 1450 1451 @Override 1452 public Base setProperty(String name, Base value) throws FHIRException { 1453 if (name.equals("indication")) { 1454 this.getIndication().add(TypeConvertor.castToCodeableReference(value)); 1455 } else if (name.equals("dosingGuideline")) { 1456 this.getDosingGuideline().add((MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent) value); 1457 } else 1458 return super.setProperty(name, value); 1459 return value; 1460 } 1461 1462 @Override 1463 public void removeChild(String name, Base value) throws FHIRException { 1464 if (name.equals("indication")) { 1465 this.getIndication().remove(value); 1466 } else if (name.equals("dosingGuideline")) { 1467 this.getDosingGuideline().remove((MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent) value); 1468 } else 1469 super.removeChild(name, value); 1470 1471 } 1472 1473 @Override 1474 public Base makeProperty(int hash, String name) throws FHIRException { 1475 switch (hash) { 1476 case -597168804: return addIndication(); 1477 case -1792856970: return addDosingGuideline(); 1478 default: return super.makeProperty(hash, name); 1479 } 1480 1481 } 1482 1483 @Override 1484 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1485 switch (hash) { 1486 case -597168804: /*indication*/ return new String[] {"CodeableReference"}; 1487 case -1792856970: /*dosingGuideline*/ return new String[] {}; 1488 default: return super.getTypesForProperty(hash, name); 1489 } 1490 1491 } 1492 1493 @Override 1494 public Base addChild(String name) throws FHIRException { 1495 if (name.equals("indication")) { 1496 return addIndication(); 1497 } 1498 else if (name.equals("dosingGuideline")) { 1499 return addDosingGuideline(); 1500 } 1501 else 1502 return super.addChild(name); 1503 } 1504 1505 public MedicationKnowledgeIndicationGuidelineComponent copy() { 1506 MedicationKnowledgeIndicationGuidelineComponent dst = new MedicationKnowledgeIndicationGuidelineComponent(); 1507 copyValues(dst); 1508 return dst; 1509 } 1510 1511 public void copyValues(MedicationKnowledgeIndicationGuidelineComponent dst) { 1512 super.copyValues(dst); 1513 if (indication != null) { 1514 dst.indication = new ArrayList<CodeableReference>(); 1515 for (CodeableReference i : indication) 1516 dst.indication.add(i.copy()); 1517 }; 1518 if (dosingGuideline != null) { 1519 dst.dosingGuideline = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent>(); 1520 for (MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent i : dosingGuideline) 1521 dst.dosingGuideline.add(i.copy()); 1522 }; 1523 } 1524 1525 @Override 1526 public boolean equalsDeep(Base other_) { 1527 if (!super.equalsDeep(other_)) 1528 return false; 1529 if (!(other_ instanceof MedicationKnowledgeIndicationGuidelineComponent)) 1530 return false; 1531 MedicationKnowledgeIndicationGuidelineComponent o = (MedicationKnowledgeIndicationGuidelineComponent) other_; 1532 return compareDeep(indication, o.indication, true) && compareDeep(dosingGuideline, o.dosingGuideline, true) 1533 ; 1534 } 1535 1536 @Override 1537 public boolean equalsShallow(Base other_) { 1538 if (!super.equalsShallow(other_)) 1539 return false; 1540 if (!(other_ instanceof MedicationKnowledgeIndicationGuidelineComponent)) 1541 return false; 1542 MedicationKnowledgeIndicationGuidelineComponent o = (MedicationKnowledgeIndicationGuidelineComponent) other_; 1543 return true; 1544 } 1545 1546 public boolean isEmpty() { 1547 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(indication, dosingGuideline 1548 ); 1549 } 1550 1551 public String fhirType() { 1552 return "MedicationKnowledge.indicationGuideline"; 1553 1554 } 1555 1556 } 1557 1558 @Block() 1559 public static class MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent extends BackboneElement implements IBaseBackboneElement { 1560 /** 1561 * The overall intention of the treatment, for example, prophylactic, supporative, curative, etc. 1562 */ 1563 @Child(name = "treatmentIntent", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1564 @Description(shortDefinition="Intention of the treatment", formalDefinition="The overall intention of the treatment, for example, prophylactic, supporative, curative, etc." ) 1565 protected CodeableConcept treatmentIntent; 1566 1567 /** 1568 * Dosage for the medication for the specific guidelines. 1569 */ 1570 @Child(name = "dosage", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1571 @Description(shortDefinition="Dosage for the medication for the specific guidelines", formalDefinition="Dosage for the medication for the specific guidelines." ) 1572 protected List<MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent> dosage; 1573 1574 /** 1575 * The type of the treatment that the guideline applies to, for example, long term therapy, first line treatment, etc. 1576 */ 1577 @Child(name = "administrationTreatment", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1578 @Description(shortDefinition="Type of treatment the guideline applies to", formalDefinition="The type of the treatment that the guideline applies to, for example, long term therapy, first line treatment, etc." ) 1579 protected CodeableConcept administrationTreatment; 1580 1581 /** 1582 * Characteristics of the patient that are relevant to the administration guidelines (for example, height, weight, gender, etc.). 1583 */ 1584 @Child(name = "patientCharacteristic", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1585 @Description(shortDefinition="Characteristics of the patient that are relevant to the administration guidelines", formalDefinition="Characteristics of the patient that are relevant to the administration guidelines (for example, height, weight, gender, etc.)." ) 1586 protected List<MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent> patientCharacteristic; 1587 1588 private static final long serialVersionUID = 882198366L; 1589 1590 /** 1591 * Constructor 1592 */ 1593 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent() { 1594 super(); 1595 } 1596 1597 /** 1598 * @return {@link #treatmentIntent} (The overall intention of the treatment, for example, prophylactic, supporative, curative, etc.) 1599 */ 1600 public CodeableConcept getTreatmentIntent() { 1601 if (this.treatmentIntent == null) 1602 if (Configuration.errorOnAutoCreate()) 1603 throw new Error("Attempt to auto-create MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent.treatmentIntent"); 1604 else if (Configuration.doAutoCreate()) 1605 this.treatmentIntent = new CodeableConcept(); // cc 1606 return this.treatmentIntent; 1607 } 1608 1609 public boolean hasTreatmentIntent() { 1610 return this.treatmentIntent != null && !this.treatmentIntent.isEmpty(); 1611 } 1612 1613 /** 1614 * @param value {@link #treatmentIntent} (The overall intention of the treatment, for example, prophylactic, supporative, curative, etc.) 1615 */ 1616 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent setTreatmentIntent(CodeableConcept value) { 1617 this.treatmentIntent = value; 1618 return this; 1619 } 1620 1621 /** 1622 * @return {@link #dosage} (Dosage for the medication for the specific guidelines.) 1623 */ 1624 public List<MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent> getDosage() { 1625 if (this.dosage == null) 1626 this.dosage = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent>(); 1627 return this.dosage; 1628 } 1629 1630 /** 1631 * @return Returns a reference to <code>this</code> for easy method chaining 1632 */ 1633 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent setDosage(List<MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent> theDosage) { 1634 this.dosage = theDosage; 1635 return this; 1636 } 1637 1638 public boolean hasDosage() { 1639 if (this.dosage == null) 1640 return false; 1641 for (MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent item : this.dosage) 1642 if (!item.isEmpty()) 1643 return true; 1644 return false; 1645 } 1646 1647 public MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent addDosage() { //3 1648 MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent t = new MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent(); 1649 if (this.dosage == null) 1650 this.dosage = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent>(); 1651 this.dosage.add(t); 1652 return t; 1653 } 1654 1655 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent addDosage(MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent t) { //3 1656 if (t == null) 1657 return this; 1658 if (this.dosage == null) 1659 this.dosage = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent>(); 1660 this.dosage.add(t); 1661 return this; 1662 } 1663 1664 /** 1665 * @return The first repetition of repeating field {@link #dosage}, creating it if it does not already exist {3} 1666 */ 1667 public MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent getDosageFirstRep() { 1668 if (getDosage().isEmpty()) { 1669 addDosage(); 1670 } 1671 return getDosage().get(0); 1672 } 1673 1674 /** 1675 * @return {@link #administrationTreatment} (The type of the treatment that the guideline applies to, for example, long term therapy, first line treatment, etc.) 1676 */ 1677 public CodeableConcept getAdministrationTreatment() { 1678 if (this.administrationTreatment == null) 1679 if (Configuration.errorOnAutoCreate()) 1680 throw new Error("Attempt to auto-create MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent.administrationTreatment"); 1681 else if (Configuration.doAutoCreate()) 1682 this.administrationTreatment = new CodeableConcept(); // cc 1683 return this.administrationTreatment; 1684 } 1685 1686 public boolean hasAdministrationTreatment() { 1687 return this.administrationTreatment != null && !this.administrationTreatment.isEmpty(); 1688 } 1689 1690 /** 1691 * @param value {@link #administrationTreatment} (The type of the treatment that the guideline applies to, for example, long term therapy, first line treatment, etc.) 1692 */ 1693 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent setAdministrationTreatment(CodeableConcept value) { 1694 this.administrationTreatment = value; 1695 return this; 1696 } 1697 1698 /** 1699 * @return {@link #patientCharacteristic} (Characteristics of the patient that are relevant to the administration guidelines (for example, height, weight, gender, etc.).) 1700 */ 1701 public List<MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent> getPatientCharacteristic() { 1702 if (this.patientCharacteristic == null) 1703 this.patientCharacteristic = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent>(); 1704 return this.patientCharacteristic; 1705 } 1706 1707 /** 1708 * @return Returns a reference to <code>this</code> for easy method chaining 1709 */ 1710 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent setPatientCharacteristic(List<MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent> thePatientCharacteristic) { 1711 this.patientCharacteristic = thePatientCharacteristic; 1712 return this; 1713 } 1714 1715 public boolean hasPatientCharacteristic() { 1716 if (this.patientCharacteristic == null) 1717 return false; 1718 for (MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent item : this.patientCharacteristic) 1719 if (!item.isEmpty()) 1720 return true; 1721 return false; 1722 } 1723 1724 public MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent addPatientCharacteristic() { //3 1725 MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent t = new MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent(); 1726 if (this.patientCharacteristic == null) 1727 this.patientCharacteristic = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent>(); 1728 this.patientCharacteristic.add(t); 1729 return t; 1730 } 1731 1732 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent addPatientCharacteristic(MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent t) { //3 1733 if (t == null) 1734 return this; 1735 if (this.patientCharacteristic == null) 1736 this.patientCharacteristic = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent>(); 1737 this.patientCharacteristic.add(t); 1738 return this; 1739 } 1740 1741 /** 1742 * @return The first repetition of repeating field {@link #patientCharacteristic}, creating it if it does not already exist {3} 1743 */ 1744 public MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent getPatientCharacteristicFirstRep() { 1745 if (getPatientCharacteristic().isEmpty()) { 1746 addPatientCharacteristic(); 1747 } 1748 return getPatientCharacteristic().get(0); 1749 } 1750 1751 protected void listChildren(List<Property> children) { 1752 super.listChildren(children); 1753 children.add(new Property("treatmentIntent", "CodeableConcept", "The overall intention of the treatment, for example, prophylactic, supporative, curative, etc.", 0, 1, treatmentIntent)); 1754 children.add(new Property("dosage", "", "Dosage for the medication for the specific guidelines.", 0, java.lang.Integer.MAX_VALUE, dosage)); 1755 children.add(new Property("administrationTreatment", "CodeableConcept", "The type of the treatment that the guideline applies to, for example, long term therapy, first line treatment, etc.", 0, 1, administrationTreatment)); 1756 children.add(new Property("patientCharacteristic", "", "Characteristics of the patient that are relevant to the administration guidelines (for example, height, weight, gender, etc.).", 0, java.lang.Integer.MAX_VALUE, patientCharacteristic)); 1757 } 1758 1759 @Override 1760 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1761 switch (_hash) { 1762 case 1744920884: /*treatmentIntent*/ return new Property("treatmentIntent", "CodeableConcept", "The overall intention of the treatment, for example, prophylactic, supporative, curative, etc.", 0, 1, treatmentIntent); 1763 case -1326018889: /*dosage*/ return new Property("dosage", "", "Dosage for the medication for the specific guidelines.", 0, java.lang.Integer.MAX_VALUE, dosage); 1764 case 1197121978: /*administrationTreatment*/ return new Property("administrationTreatment", "CodeableConcept", "The type of the treatment that the guideline applies to, for example, long term therapy, first line treatment, etc.", 0, 1, administrationTreatment); 1765 case 1770130432: /*patientCharacteristic*/ return new Property("patientCharacteristic", "", "Characteristics of the patient that are relevant to the administration guidelines (for example, height, weight, gender, etc.).", 0, java.lang.Integer.MAX_VALUE, patientCharacteristic); 1766 default: return super.getNamedProperty(_hash, _name, _checkValid); 1767 } 1768 1769 } 1770 1771 @Override 1772 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1773 switch (hash) { 1774 case 1744920884: /*treatmentIntent*/ return this.treatmentIntent == null ? new Base[0] : new Base[] {this.treatmentIntent}; // CodeableConcept 1775 case -1326018889: /*dosage*/ return this.dosage == null ? new Base[0] : this.dosage.toArray(new Base[this.dosage.size()]); // MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent 1776 case 1197121978: /*administrationTreatment*/ return this.administrationTreatment == null ? new Base[0] : new Base[] {this.administrationTreatment}; // CodeableConcept 1777 case 1770130432: /*patientCharacteristic*/ return this.patientCharacteristic == null ? new Base[0] : this.patientCharacteristic.toArray(new Base[this.patientCharacteristic.size()]); // MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent 1778 default: return super.getProperty(hash, name, checkValid); 1779 } 1780 1781 } 1782 1783 @Override 1784 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1785 switch (hash) { 1786 case 1744920884: // treatmentIntent 1787 this.treatmentIntent = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1788 return value; 1789 case -1326018889: // dosage 1790 this.getDosage().add((MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent) value); // MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent 1791 return value; 1792 case 1197121978: // administrationTreatment 1793 this.administrationTreatment = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1794 return value; 1795 case 1770130432: // patientCharacteristic 1796 this.getPatientCharacteristic().add((MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent) value); // MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent 1797 return value; 1798 default: return super.setProperty(hash, name, value); 1799 } 1800 1801 } 1802 1803 @Override 1804 public Base setProperty(String name, Base value) throws FHIRException { 1805 if (name.equals("treatmentIntent")) { 1806 this.treatmentIntent = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1807 } else if (name.equals("dosage")) { 1808 this.getDosage().add((MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent) value); 1809 } else if (name.equals("administrationTreatment")) { 1810 this.administrationTreatment = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1811 } else if (name.equals("patientCharacteristic")) { 1812 this.getPatientCharacteristic().add((MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent) value); 1813 } else 1814 return super.setProperty(name, value); 1815 return value; 1816 } 1817 1818 @Override 1819 public void removeChild(String name, Base value) throws FHIRException { 1820 if (name.equals("treatmentIntent")) { 1821 this.treatmentIntent = null; 1822 } else if (name.equals("dosage")) { 1823 this.getDosage().remove((MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent) value); 1824 } else if (name.equals("administrationTreatment")) { 1825 this.administrationTreatment = null; 1826 } else if (name.equals("patientCharacteristic")) { 1827 this.getPatientCharacteristic().remove((MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent) value); 1828 } else 1829 super.removeChild(name, value); 1830 1831 } 1832 1833 @Override 1834 public Base makeProperty(int hash, String name) throws FHIRException { 1835 switch (hash) { 1836 case 1744920884: return getTreatmentIntent(); 1837 case -1326018889: return addDosage(); 1838 case 1197121978: return getAdministrationTreatment(); 1839 case 1770130432: return addPatientCharacteristic(); 1840 default: return super.makeProperty(hash, name); 1841 } 1842 1843 } 1844 1845 @Override 1846 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1847 switch (hash) { 1848 case 1744920884: /*treatmentIntent*/ return new String[] {"CodeableConcept"}; 1849 case -1326018889: /*dosage*/ return new String[] {}; 1850 case 1197121978: /*administrationTreatment*/ return new String[] {"CodeableConcept"}; 1851 case 1770130432: /*patientCharacteristic*/ return new String[] {}; 1852 default: return super.getTypesForProperty(hash, name); 1853 } 1854 1855 } 1856 1857 @Override 1858 public Base addChild(String name) throws FHIRException { 1859 if (name.equals("treatmentIntent")) { 1860 this.treatmentIntent = new CodeableConcept(); 1861 return this.treatmentIntent; 1862 } 1863 else if (name.equals("dosage")) { 1864 return addDosage(); 1865 } 1866 else if (name.equals("administrationTreatment")) { 1867 this.administrationTreatment = new CodeableConcept(); 1868 return this.administrationTreatment; 1869 } 1870 else if (name.equals("patientCharacteristic")) { 1871 return addPatientCharacteristic(); 1872 } 1873 else 1874 return super.addChild(name); 1875 } 1876 1877 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent copy() { 1878 MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent dst = new MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent(); 1879 copyValues(dst); 1880 return dst; 1881 } 1882 1883 public void copyValues(MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent dst) { 1884 super.copyValues(dst); 1885 dst.treatmentIntent = treatmentIntent == null ? null : treatmentIntent.copy(); 1886 if (dosage != null) { 1887 dst.dosage = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent>(); 1888 for (MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent i : dosage) 1889 dst.dosage.add(i.copy()); 1890 }; 1891 dst.administrationTreatment = administrationTreatment == null ? null : administrationTreatment.copy(); 1892 if (patientCharacteristic != null) { 1893 dst.patientCharacteristic = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent>(); 1894 for (MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent i : patientCharacteristic) 1895 dst.patientCharacteristic.add(i.copy()); 1896 }; 1897 } 1898 1899 @Override 1900 public boolean equalsDeep(Base other_) { 1901 if (!super.equalsDeep(other_)) 1902 return false; 1903 if (!(other_ instanceof MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent)) 1904 return false; 1905 MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent o = (MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent) other_; 1906 return compareDeep(treatmentIntent, o.treatmentIntent, true) && compareDeep(dosage, o.dosage, true) 1907 && compareDeep(administrationTreatment, o.administrationTreatment, true) && compareDeep(patientCharacteristic, o.patientCharacteristic, true) 1908 ; 1909 } 1910 1911 @Override 1912 public boolean equalsShallow(Base other_) { 1913 if (!super.equalsShallow(other_)) 1914 return false; 1915 if (!(other_ instanceof MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent)) 1916 return false; 1917 MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent o = (MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent) other_; 1918 return true; 1919 } 1920 1921 public boolean isEmpty() { 1922 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(treatmentIntent, dosage, administrationTreatment 1923 , patientCharacteristic); 1924 } 1925 1926 public String fhirType() { 1927 return "MedicationKnowledge.indicationGuideline.dosingGuideline"; 1928 1929 } 1930 1931 } 1932 1933 @Block() 1934 public static class MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent extends BackboneElement implements IBaseBackboneElement { 1935 /** 1936 * The type or category of dosage for a given medication (for example, prophylaxis, maintenance, therapeutic, etc.). 1937 */ 1938 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1939 @Description(shortDefinition="Category of dosage for a medication", formalDefinition="The type or category of dosage for a given medication (for example, prophylaxis, maintenance, therapeutic, etc.)." ) 1940 protected CodeableConcept type; 1941 1942 /** 1943 * Dosage for the medication for the specific guidelines. 1944 */ 1945 @Child(name = "dosage", type = {Dosage.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1946 @Description(shortDefinition="Dosage for the medication for the specific guidelines", formalDefinition="Dosage for the medication for the specific guidelines." ) 1947 protected List<Dosage> dosage; 1948 1949 private static final long serialVersionUID = 1578257961L; 1950 1951 /** 1952 * Constructor 1953 */ 1954 public MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent() { 1955 super(); 1956 } 1957 1958 /** 1959 * Constructor 1960 */ 1961 public MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent(CodeableConcept type, Dosage dosage) { 1962 super(); 1963 this.setType(type); 1964 this.addDosage(dosage); 1965 } 1966 1967 /** 1968 * @return {@link #type} (The type or category of dosage for a given medication (for example, prophylaxis, maintenance, therapeutic, etc.).) 1969 */ 1970 public CodeableConcept getType() { 1971 if (this.type == null) 1972 if (Configuration.errorOnAutoCreate()) 1973 throw new Error("Attempt to auto-create MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent.type"); 1974 else if (Configuration.doAutoCreate()) 1975 this.type = new CodeableConcept(); // cc 1976 return this.type; 1977 } 1978 1979 public boolean hasType() { 1980 return this.type != null && !this.type.isEmpty(); 1981 } 1982 1983 /** 1984 * @param value {@link #type} (The type or category of dosage for a given medication (for example, prophylaxis, maintenance, therapeutic, etc.).) 1985 */ 1986 public MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent setType(CodeableConcept value) { 1987 this.type = value; 1988 return this; 1989 } 1990 1991 /** 1992 * @return {@link #dosage} (Dosage for the medication for the specific guidelines.) 1993 */ 1994 public List<Dosage> getDosage() { 1995 if (this.dosage == null) 1996 this.dosage = new ArrayList<Dosage>(); 1997 return this.dosage; 1998 } 1999 2000 /** 2001 * @return Returns a reference to <code>this</code> for easy method chaining 2002 */ 2003 public MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent setDosage(List<Dosage> theDosage) { 2004 this.dosage = theDosage; 2005 return this; 2006 } 2007 2008 public boolean hasDosage() { 2009 if (this.dosage == null) 2010 return false; 2011 for (Dosage item : this.dosage) 2012 if (!item.isEmpty()) 2013 return true; 2014 return false; 2015 } 2016 2017 public Dosage addDosage() { //3 2018 Dosage t = new Dosage(); 2019 if (this.dosage == null) 2020 this.dosage = new ArrayList<Dosage>(); 2021 this.dosage.add(t); 2022 return t; 2023 } 2024 2025 public MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent addDosage(Dosage t) { //3 2026 if (t == null) 2027 return this; 2028 if (this.dosage == null) 2029 this.dosage = new ArrayList<Dosage>(); 2030 this.dosage.add(t); 2031 return this; 2032 } 2033 2034 /** 2035 * @return The first repetition of repeating field {@link #dosage}, creating it if it does not already exist {3} 2036 */ 2037 public Dosage getDosageFirstRep() { 2038 if (getDosage().isEmpty()) { 2039 addDosage(); 2040 } 2041 return getDosage().get(0); 2042 } 2043 2044 protected void listChildren(List<Property> children) { 2045 super.listChildren(children); 2046 children.add(new Property("type", "CodeableConcept", "The type or category of dosage for a given medication (for example, prophylaxis, maintenance, therapeutic, etc.).", 0, 1, type)); 2047 children.add(new Property("dosage", "Dosage", "Dosage for the medication for the specific guidelines.", 0, java.lang.Integer.MAX_VALUE, dosage)); 2048 } 2049 2050 @Override 2051 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2052 switch (_hash) { 2053 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type or category of dosage for a given medication (for example, prophylaxis, maintenance, therapeutic, etc.).", 0, 1, type); 2054 case -1326018889: /*dosage*/ return new Property("dosage", "Dosage", "Dosage for the medication for the specific guidelines.", 0, java.lang.Integer.MAX_VALUE, dosage); 2055 default: return super.getNamedProperty(_hash, _name, _checkValid); 2056 } 2057 2058 } 2059 2060 @Override 2061 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2062 switch (hash) { 2063 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2064 case -1326018889: /*dosage*/ return this.dosage == null ? new Base[0] : this.dosage.toArray(new Base[this.dosage.size()]); // Dosage 2065 default: return super.getProperty(hash, name, checkValid); 2066 } 2067 2068 } 2069 2070 @Override 2071 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2072 switch (hash) { 2073 case 3575610: // type 2074 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2075 return value; 2076 case -1326018889: // dosage 2077 this.getDosage().add(TypeConvertor.castToDosage(value)); // Dosage 2078 return value; 2079 default: return super.setProperty(hash, name, value); 2080 } 2081 2082 } 2083 2084 @Override 2085 public Base setProperty(String name, Base value) throws FHIRException { 2086 if (name.equals("type")) { 2087 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2088 } else if (name.equals("dosage")) { 2089 this.getDosage().add(TypeConvertor.castToDosage(value)); 2090 } else 2091 return super.setProperty(name, value); 2092 return value; 2093 } 2094 2095 @Override 2096 public void removeChild(String name, Base value) throws FHIRException { 2097 if (name.equals("type")) { 2098 this.type = null; 2099 } else if (name.equals("dosage")) { 2100 this.getDosage().remove(value); 2101 } else 2102 super.removeChild(name, value); 2103 2104 } 2105 2106 @Override 2107 public Base makeProperty(int hash, String name) throws FHIRException { 2108 switch (hash) { 2109 case 3575610: return getType(); 2110 case -1326018889: return addDosage(); 2111 default: return super.makeProperty(hash, name); 2112 } 2113 2114 } 2115 2116 @Override 2117 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2118 switch (hash) { 2119 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2120 case -1326018889: /*dosage*/ return new String[] {"Dosage"}; 2121 default: return super.getTypesForProperty(hash, name); 2122 } 2123 2124 } 2125 2126 @Override 2127 public Base addChild(String name) throws FHIRException { 2128 if (name.equals("type")) { 2129 this.type = new CodeableConcept(); 2130 return this.type; 2131 } 2132 else if (name.equals("dosage")) { 2133 return addDosage(); 2134 } 2135 else 2136 return super.addChild(name); 2137 } 2138 2139 public MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent copy() { 2140 MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent dst = new MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent(); 2141 copyValues(dst); 2142 return dst; 2143 } 2144 2145 public void copyValues(MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent dst) { 2146 super.copyValues(dst); 2147 dst.type = type == null ? null : type.copy(); 2148 if (dosage != null) { 2149 dst.dosage = new ArrayList<Dosage>(); 2150 for (Dosage i : dosage) 2151 dst.dosage.add(i.copy()); 2152 }; 2153 } 2154 2155 @Override 2156 public boolean equalsDeep(Base other_) { 2157 if (!super.equalsDeep(other_)) 2158 return false; 2159 if (!(other_ instanceof MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent)) 2160 return false; 2161 MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent o = (MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent) other_; 2162 return compareDeep(type, o.type, true) && compareDeep(dosage, o.dosage, true); 2163 } 2164 2165 @Override 2166 public boolean equalsShallow(Base other_) { 2167 if (!super.equalsShallow(other_)) 2168 return false; 2169 if (!(other_ instanceof MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent)) 2170 return false; 2171 MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent o = (MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent) other_; 2172 return true; 2173 } 2174 2175 public boolean isEmpty() { 2176 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, dosage); 2177 } 2178 2179 public String fhirType() { 2180 return "MedicationKnowledge.indicationGuideline.dosingGuideline.dosage"; 2181 2182 } 2183 2184 } 2185 2186 @Block() 2187 public static class MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 2188 /** 2189 * The categorization of the specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender). 2190 */ 2191 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 2192 @Description(shortDefinition="Categorization of specific characteristic that is relevant to the administration guideline", formalDefinition="The categorization of the specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender)." ) 2193 protected CodeableConcept type; 2194 2195 /** 2196 * The specific characteristic (e.g. height, weight, gender, etc.). 2197 */ 2198 @Child(name = "value", type = {CodeableConcept.class, Quantity.class, Range.class}, order=2, min=0, max=1, modifier=false, summary=false) 2199 @Description(shortDefinition="The specific characteristic", formalDefinition="The specific characteristic (e.g. height, weight, gender, etc.)." ) 2200 protected DataType value; 2201 2202 private static final long serialVersionUID = -1659186716L; 2203 2204 /** 2205 * Constructor 2206 */ 2207 public MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent() { 2208 super(); 2209 } 2210 2211 /** 2212 * Constructor 2213 */ 2214 public MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent(CodeableConcept type) { 2215 super(); 2216 this.setType(type); 2217 } 2218 2219 /** 2220 * @return {@link #type} (The categorization of the specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender).) 2221 */ 2222 public CodeableConcept getType() { 2223 if (this.type == null) 2224 if (Configuration.errorOnAutoCreate()) 2225 throw new Error("Attempt to auto-create MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent.type"); 2226 else if (Configuration.doAutoCreate()) 2227 this.type = new CodeableConcept(); // cc 2228 return this.type; 2229 } 2230 2231 public boolean hasType() { 2232 return this.type != null && !this.type.isEmpty(); 2233 } 2234 2235 /** 2236 * @param value {@link #type} (The categorization of the specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender).) 2237 */ 2238 public MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent setType(CodeableConcept value) { 2239 this.type = value; 2240 return this; 2241 } 2242 2243 /** 2244 * @return {@link #value} (The specific characteristic (e.g. height, weight, gender, etc.).) 2245 */ 2246 public DataType getValue() { 2247 return this.value; 2248 } 2249 2250 /** 2251 * @return {@link #value} (The specific characteristic (e.g. height, weight, gender, etc.).) 2252 */ 2253 public CodeableConcept getValueCodeableConcept() throws FHIRException { 2254 if (this.value == null) 2255 this.value = new CodeableConcept(); 2256 if (!(this.value instanceof CodeableConcept)) 2257 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 2258 return (CodeableConcept) this.value; 2259 } 2260 2261 public boolean hasValueCodeableConcept() { 2262 return this != null && this.value instanceof CodeableConcept; 2263 } 2264 2265 /** 2266 * @return {@link #value} (The specific characteristic (e.g. height, weight, gender, etc.).) 2267 */ 2268 public Quantity getValueQuantity() throws FHIRException { 2269 if (this.value == null) 2270 this.value = new Quantity(); 2271 if (!(this.value instanceof Quantity)) 2272 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 2273 return (Quantity) this.value; 2274 } 2275 2276 public boolean hasValueQuantity() { 2277 return this != null && this.value instanceof Quantity; 2278 } 2279 2280 /** 2281 * @return {@link #value} (The specific characteristic (e.g. height, weight, gender, etc.).) 2282 */ 2283 public Range getValueRange() throws FHIRException { 2284 if (this.value == null) 2285 this.value = new Range(); 2286 if (!(this.value instanceof Range)) 2287 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 2288 return (Range) this.value; 2289 } 2290 2291 public boolean hasValueRange() { 2292 return this != null && this.value instanceof Range; 2293 } 2294 2295 public boolean hasValue() { 2296 return this.value != null && !this.value.isEmpty(); 2297 } 2298 2299 /** 2300 * @param value {@link #value} (The specific characteristic (e.g. height, weight, gender, etc.).) 2301 */ 2302 public MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent setValue(DataType value) { 2303 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity || value instanceof Range)) 2304 throw new FHIRException("Not the right type for MedicationKnowledge.indicationGuideline.dosingGuideline.patientCharacteristic.value[x]: "+value.fhirType()); 2305 this.value = value; 2306 return this; 2307 } 2308 2309 protected void listChildren(List<Property> children) { 2310 super.listChildren(children); 2311 children.add(new Property("type", "CodeableConcept", "The categorization of the specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender).", 0, 1, type)); 2312 children.add(new Property("value[x]", "CodeableConcept|Quantity|Range", "The specific characteristic (e.g. height, weight, gender, etc.).", 0, 1, value)); 2313 } 2314 2315 @Override 2316 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2317 switch (_hash) { 2318 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The categorization of the specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender).", 0, 1, type); 2319 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|Quantity|Range", "The specific characteristic (e.g. height, weight, gender, etc.).", 0, 1, value); 2320 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|Quantity|Range", "The specific characteristic (e.g. height, weight, gender, etc.).", 0, 1, value); 2321 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The specific characteristic (e.g. height, weight, gender, etc.).", 0, 1, value); 2322 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The specific characteristic (e.g. height, weight, gender, etc.).", 0, 1, value); 2323 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The specific characteristic (e.g. height, weight, gender, etc.).", 0, 1, value); 2324 default: return super.getNamedProperty(_hash, _name, _checkValid); 2325 } 2326 2327 } 2328 2329 @Override 2330 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2331 switch (hash) { 2332 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2333 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 2334 default: return super.getProperty(hash, name, checkValid); 2335 } 2336 2337 } 2338 2339 @Override 2340 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2341 switch (hash) { 2342 case 3575610: // type 2343 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2344 return value; 2345 case 111972721: // value 2346 this.value = TypeConvertor.castToType(value); // DataType 2347 return value; 2348 default: return super.setProperty(hash, name, value); 2349 } 2350 2351 } 2352 2353 @Override 2354 public Base setProperty(String name, Base value) throws FHIRException { 2355 if (name.equals("type")) { 2356 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2357 } else if (name.equals("value[x]")) { 2358 this.value = TypeConvertor.castToType(value); // DataType 2359 } else 2360 return super.setProperty(name, value); 2361 return value; 2362 } 2363 2364 @Override 2365 public void removeChild(String name, Base value) throws FHIRException { 2366 if (name.equals("type")) { 2367 this.type = null; 2368 } else if (name.equals("value[x]")) { 2369 this.value = null; 2370 } else 2371 super.removeChild(name, value); 2372 2373 } 2374 2375 @Override 2376 public Base makeProperty(int hash, String name) throws FHIRException { 2377 switch (hash) { 2378 case 3575610: return getType(); 2379 case -1410166417: return getValue(); 2380 case 111972721: return getValue(); 2381 default: return super.makeProperty(hash, name); 2382 } 2383 2384 } 2385 2386 @Override 2387 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2388 switch (hash) { 2389 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2390 case 111972721: /*value*/ return new String[] {"CodeableConcept", "Quantity", "Range"}; 2391 default: return super.getTypesForProperty(hash, name); 2392 } 2393 2394 } 2395 2396 @Override 2397 public Base addChild(String name) throws FHIRException { 2398 if (name.equals("type")) { 2399 this.type = new CodeableConcept(); 2400 return this.type; 2401 } 2402 else if (name.equals("valueCodeableConcept")) { 2403 this.value = new CodeableConcept(); 2404 return this.value; 2405 } 2406 else if (name.equals("valueQuantity")) { 2407 this.value = new Quantity(); 2408 return this.value; 2409 } 2410 else if (name.equals("valueRange")) { 2411 this.value = new Range(); 2412 return this.value; 2413 } 2414 else 2415 return super.addChild(name); 2416 } 2417 2418 public MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent copy() { 2419 MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent dst = new MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent(); 2420 copyValues(dst); 2421 return dst; 2422 } 2423 2424 public void copyValues(MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent dst) { 2425 super.copyValues(dst); 2426 dst.type = type == null ? null : type.copy(); 2427 dst.value = value == null ? null : value.copy(); 2428 } 2429 2430 @Override 2431 public boolean equalsDeep(Base other_) { 2432 if (!super.equalsDeep(other_)) 2433 return false; 2434 if (!(other_ instanceof MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent)) 2435 return false; 2436 MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent o = (MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent) other_; 2437 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 2438 } 2439 2440 @Override 2441 public boolean equalsShallow(Base other_) { 2442 if (!super.equalsShallow(other_)) 2443 return false; 2444 if (!(other_ instanceof MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent)) 2445 return false; 2446 MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent o = (MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent) other_; 2447 return true; 2448 } 2449 2450 public boolean isEmpty() { 2451 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 2452 } 2453 2454 public String fhirType() { 2455 return "MedicationKnowledge.indicationGuideline.dosingGuideline.patientCharacteristic"; 2456 2457 } 2458 2459 } 2460 2461 @Block() 2462 public static class MedicationKnowledgeMedicineClassificationComponent extends BackboneElement implements IBaseBackboneElement { 2463 /** 2464 * The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification). 2465 */ 2466 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 2467 @Description(shortDefinition="The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification)", formalDefinition="The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification)." ) 2468 protected CodeableConcept type; 2469 2470 /** 2471 * Either a textual source of the classification or a reference to an online source. 2472 */ 2473 @Child(name = "source", type = {StringType.class, UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2474 @Description(shortDefinition="The source of the classification", formalDefinition="Either a textual source of the classification or a reference to an online source." ) 2475 protected DataType source; 2476 2477 /** 2478 * Specific category assigned to the medication (e.g. anti-infective, anti-hypertensive, antibiotic, etc.). 2479 */ 2480 @Child(name = "classification", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2481 @Description(shortDefinition="Specific category assigned to the medication", formalDefinition="Specific category assigned to the medication (e.g. anti-infective, anti-hypertensive, antibiotic, etc.)." ) 2482 protected List<CodeableConcept> classification; 2483 2484 private static final long serialVersionUID = 1598220123L; 2485 2486 /** 2487 * Constructor 2488 */ 2489 public MedicationKnowledgeMedicineClassificationComponent() { 2490 super(); 2491 } 2492 2493 /** 2494 * Constructor 2495 */ 2496 public MedicationKnowledgeMedicineClassificationComponent(CodeableConcept type) { 2497 super(); 2498 this.setType(type); 2499 } 2500 2501 /** 2502 * @return {@link #type} (The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification).) 2503 */ 2504 public CodeableConcept getType() { 2505 if (this.type == null) 2506 if (Configuration.errorOnAutoCreate()) 2507 throw new Error("Attempt to auto-create MedicationKnowledgeMedicineClassificationComponent.type"); 2508 else if (Configuration.doAutoCreate()) 2509 this.type = new CodeableConcept(); // cc 2510 return this.type; 2511 } 2512 2513 public boolean hasType() { 2514 return this.type != null && !this.type.isEmpty(); 2515 } 2516 2517 /** 2518 * @param value {@link #type} (The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification).) 2519 */ 2520 public MedicationKnowledgeMedicineClassificationComponent setType(CodeableConcept value) { 2521 this.type = value; 2522 return this; 2523 } 2524 2525 /** 2526 * @return {@link #source} (Either a textual source of the classification or a reference to an online source.) 2527 */ 2528 public DataType getSource() { 2529 return this.source; 2530 } 2531 2532 /** 2533 * @return {@link #source} (Either a textual source of the classification or a reference to an online source.) 2534 */ 2535 public StringType getSourceStringType() throws FHIRException { 2536 if (this.source == null) 2537 this.source = new StringType(); 2538 if (!(this.source instanceof StringType)) 2539 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.source.getClass().getName()+" was encountered"); 2540 return (StringType) this.source; 2541 } 2542 2543 public boolean hasSourceStringType() { 2544 return this != null && this.source instanceof StringType; 2545 } 2546 2547 /** 2548 * @return {@link #source} (Either a textual source of the classification or a reference to an online source.) 2549 */ 2550 public UriType getSourceUriType() throws FHIRException { 2551 if (this.source == null) 2552 this.source = new UriType(); 2553 if (!(this.source instanceof UriType)) 2554 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.source.getClass().getName()+" was encountered"); 2555 return (UriType) this.source; 2556 } 2557 2558 public boolean hasSourceUriType() { 2559 return this != null && this.source instanceof UriType; 2560 } 2561 2562 public boolean hasSource() { 2563 return this.source != null && !this.source.isEmpty(); 2564 } 2565 2566 /** 2567 * @param value {@link #source} (Either a textual source of the classification or a reference to an online source.) 2568 */ 2569 public MedicationKnowledgeMedicineClassificationComponent setSource(DataType value) { 2570 if (value != null && !(value instanceof StringType || value instanceof UriType)) 2571 throw new FHIRException("Not the right type for MedicationKnowledge.medicineClassification.source[x]: "+value.fhirType()); 2572 this.source = value; 2573 return this; 2574 } 2575 2576 /** 2577 * @return {@link #classification} (Specific category assigned to the medication (e.g. anti-infective, anti-hypertensive, antibiotic, etc.).) 2578 */ 2579 public List<CodeableConcept> getClassification() { 2580 if (this.classification == null) 2581 this.classification = new ArrayList<CodeableConcept>(); 2582 return this.classification; 2583 } 2584 2585 /** 2586 * @return Returns a reference to <code>this</code> for easy method chaining 2587 */ 2588 public MedicationKnowledgeMedicineClassificationComponent setClassification(List<CodeableConcept> theClassification) { 2589 this.classification = theClassification; 2590 return this; 2591 } 2592 2593 public boolean hasClassification() { 2594 if (this.classification == null) 2595 return false; 2596 for (CodeableConcept item : this.classification) 2597 if (!item.isEmpty()) 2598 return true; 2599 return false; 2600 } 2601 2602 public CodeableConcept addClassification() { //3 2603 CodeableConcept t = new CodeableConcept(); 2604 if (this.classification == null) 2605 this.classification = new ArrayList<CodeableConcept>(); 2606 this.classification.add(t); 2607 return t; 2608 } 2609 2610 public MedicationKnowledgeMedicineClassificationComponent addClassification(CodeableConcept t) { //3 2611 if (t == null) 2612 return this; 2613 if (this.classification == null) 2614 this.classification = new ArrayList<CodeableConcept>(); 2615 this.classification.add(t); 2616 return this; 2617 } 2618 2619 /** 2620 * @return The first repetition of repeating field {@link #classification}, creating it if it does not already exist {3} 2621 */ 2622 public CodeableConcept getClassificationFirstRep() { 2623 if (getClassification().isEmpty()) { 2624 addClassification(); 2625 } 2626 return getClassification().get(0); 2627 } 2628 2629 protected void listChildren(List<Property> children) { 2630 super.listChildren(children); 2631 children.add(new Property("type", "CodeableConcept", "The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification).", 0, 1, type)); 2632 children.add(new Property("source[x]", "string|uri", "Either a textual source of the classification or a reference to an online source.", 0, 1, source)); 2633 children.add(new Property("classification", "CodeableConcept", "Specific category assigned to the medication (e.g. anti-infective, anti-hypertensive, antibiotic, etc.).", 0, java.lang.Integer.MAX_VALUE, classification)); 2634 } 2635 2636 @Override 2637 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2638 switch (_hash) { 2639 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification).", 0, 1, type); 2640 case -1698413947: /*source[x]*/ return new Property("source[x]", "string|uri", "Either a textual source of the classification or a reference to an online source.", 0, 1, source); 2641 case -896505829: /*source*/ return new Property("source[x]", "string|uri", "Either a textual source of the classification or a reference to an online source.", 0, 1, source); 2642 case 1327821836: /*sourceString*/ return new Property("source[x]", "string", "Either a textual source of the classification or a reference to an online source.", 0, 1, source); 2643 case -1698419887: /*sourceUri*/ return new Property("source[x]", "uri", "Either a textual source of the classification or a reference to an online source.", 0, 1, source); 2644 case 382350310: /*classification*/ return new Property("classification", "CodeableConcept", "Specific category assigned to the medication (e.g. anti-infective, anti-hypertensive, antibiotic, etc.).", 0, java.lang.Integer.MAX_VALUE, classification); 2645 default: return super.getNamedProperty(_hash, _name, _checkValid); 2646 } 2647 2648 } 2649 2650 @Override 2651 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2652 switch (hash) { 2653 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2654 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // DataType 2655 case 382350310: /*classification*/ return this.classification == null ? new Base[0] : this.classification.toArray(new Base[this.classification.size()]); // CodeableConcept 2656 default: return super.getProperty(hash, name, checkValid); 2657 } 2658 2659 } 2660 2661 @Override 2662 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2663 switch (hash) { 2664 case 3575610: // type 2665 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2666 return value; 2667 case -896505829: // source 2668 this.source = TypeConvertor.castToType(value); // DataType 2669 return value; 2670 case 382350310: // classification 2671 this.getClassification().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2672 return value; 2673 default: return super.setProperty(hash, name, value); 2674 } 2675 2676 } 2677 2678 @Override 2679 public Base setProperty(String name, Base value) throws FHIRException { 2680 if (name.equals("type")) { 2681 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2682 } else if (name.equals("source[x]")) { 2683 this.source = TypeConvertor.castToType(value); // DataType 2684 } else if (name.equals("classification")) { 2685 this.getClassification().add(TypeConvertor.castToCodeableConcept(value)); 2686 } else 2687 return super.setProperty(name, value); 2688 return value; 2689 } 2690 2691 @Override 2692 public void removeChild(String name, Base value) throws FHIRException { 2693 if (name.equals("type")) { 2694 this.type = null; 2695 } else if (name.equals("source[x]")) { 2696 this.source = null; 2697 } else if (name.equals("classification")) { 2698 this.getClassification().remove(value); 2699 } else 2700 super.removeChild(name, value); 2701 2702 } 2703 2704 @Override 2705 public Base makeProperty(int hash, String name) throws FHIRException { 2706 switch (hash) { 2707 case 3575610: return getType(); 2708 case -1698413947: return getSource(); 2709 case -896505829: return getSource(); 2710 case 382350310: return addClassification(); 2711 default: return super.makeProperty(hash, name); 2712 } 2713 2714 } 2715 2716 @Override 2717 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2718 switch (hash) { 2719 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2720 case -896505829: /*source*/ return new String[] {"string", "uri"}; 2721 case 382350310: /*classification*/ return new String[] {"CodeableConcept"}; 2722 default: return super.getTypesForProperty(hash, name); 2723 } 2724 2725 } 2726 2727 @Override 2728 public Base addChild(String name) throws FHIRException { 2729 if (name.equals("type")) { 2730 this.type = new CodeableConcept(); 2731 return this.type; 2732 } 2733 else if (name.equals("sourceString")) { 2734 this.source = new StringType(); 2735 return this.source; 2736 } 2737 else if (name.equals("sourceUri")) { 2738 this.source = new UriType(); 2739 return this.source; 2740 } 2741 else if (name.equals("classification")) { 2742 return addClassification(); 2743 } 2744 else 2745 return super.addChild(name); 2746 } 2747 2748 public MedicationKnowledgeMedicineClassificationComponent copy() { 2749 MedicationKnowledgeMedicineClassificationComponent dst = new MedicationKnowledgeMedicineClassificationComponent(); 2750 copyValues(dst); 2751 return dst; 2752 } 2753 2754 public void copyValues(MedicationKnowledgeMedicineClassificationComponent dst) { 2755 super.copyValues(dst); 2756 dst.type = type == null ? null : type.copy(); 2757 dst.source = source == null ? null : source.copy(); 2758 if (classification != null) { 2759 dst.classification = new ArrayList<CodeableConcept>(); 2760 for (CodeableConcept i : classification) 2761 dst.classification.add(i.copy()); 2762 }; 2763 } 2764 2765 @Override 2766 public boolean equalsDeep(Base other_) { 2767 if (!super.equalsDeep(other_)) 2768 return false; 2769 if (!(other_ instanceof MedicationKnowledgeMedicineClassificationComponent)) 2770 return false; 2771 MedicationKnowledgeMedicineClassificationComponent o = (MedicationKnowledgeMedicineClassificationComponent) other_; 2772 return compareDeep(type, o.type, true) && compareDeep(source, o.source, true) && compareDeep(classification, o.classification, true) 2773 ; 2774 } 2775 2776 @Override 2777 public boolean equalsShallow(Base other_) { 2778 if (!super.equalsShallow(other_)) 2779 return false; 2780 if (!(other_ instanceof MedicationKnowledgeMedicineClassificationComponent)) 2781 return false; 2782 MedicationKnowledgeMedicineClassificationComponent o = (MedicationKnowledgeMedicineClassificationComponent) other_; 2783 return true; 2784 } 2785 2786 public boolean isEmpty() { 2787 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, source, classification 2788 ); 2789 } 2790 2791 public String fhirType() { 2792 return "MedicationKnowledge.medicineClassification"; 2793 2794 } 2795 2796 } 2797 2798 @Block() 2799 public static class MedicationKnowledgePackagingComponent extends BackboneElement implements IBaseBackboneElement { 2800 /** 2801 * The cost of the packaged medication. 2802 */ 2803 @Child(name = "cost", type = {MedicationKnowledgeCostComponent.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2804 @Description(shortDefinition="Cost of the packaged medication", formalDefinition="The cost of the packaged medication." ) 2805 protected List<MedicationKnowledgeCostComponent> cost; 2806 2807 /** 2808 * A reference to a PackagedProductDefinition that provides the details of the product that is in the packaging and is being priced. 2809 */ 2810 @Child(name = "packagedProduct", type = {PackagedProductDefinition.class}, order=2, min=0, max=1, modifier=false, summary=false) 2811 @Description(shortDefinition="The packaged medication that is being priced", formalDefinition="A reference to a PackagedProductDefinition that provides the details of the product that is in the packaging and is being priced." ) 2812 protected Reference packagedProduct; 2813 2814 private static final long serialVersionUID = -337249398L; 2815 2816 /** 2817 * Constructor 2818 */ 2819 public MedicationKnowledgePackagingComponent() { 2820 super(); 2821 } 2822 2823 /** 2824 * @return {@link #cost} (The cost of the packaged medication.) 2825 */ 2826 public List<MedicationKnowledgeCostComponent> getCost() { 2827 if (this.cost == null) 2828 this.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 2829 return this.cost; 2830 } 2831 2832 /** 2833 * @return Returns a reference to <code>this</code> for easy method chaining 2834 */ 2835 public MedicationKnowledgePackagingComponent setCost(List<MedicationKnowledgeCostComponent> theCost) { 2836 this.cost = theCost; 2837 return this; 2838 } 2839 2840 public boolean hasCost() { 2841 if (this.cost == null) 2842 return false; 2843 for (MedicationKnowledgeCostComponent item : this.cost) 2844 if (!item.isEmpty()) 2845 return true; 2846 return false; 2847 } 2848 2849 public MedicationKnowledgeCostComponent addCost() { //3 2850 MedicationKnowledgeCostComponent t = new MedicationKnowledgeCostComponent(); 2851 if (this.cost == null) 2852 this.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 2853 this.cost.add(t); 2854 return t; 2855 } 2856 2857 public MedicationKnowledgePackagingComponent addCost(MedicationKnowledgeCostComponent t) { //3 2858 if (t == null) 2859 return this; 2860 if (this.cost == null) 2861 this.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 2862 this.cost.add(t); 2863 return this; 2864 } 2865 2866 /** 2867 * @return The first repetition of repeating field {@link #cost}, creating it if it does not already exist {3} 2868 */ 2869 public MedicationKnowledgeCostComponent getCostFirstRep() { 2870 if (getCost().isEmpty()) { 2871 addCost(); 2872 } 2873 return getCost().get(0); 2874 } 2875 2876 /** 2877 * @return {@link #packagedProduct} (A reference to a PackagedProductDefinition that provides the details of the product that is in the packaging and is being priced.) 2878 */ 2879 public Reference getPackagedProduct() { 2880 if (this.packagedProduct == null) 2881 if (Configuration.errorOnAutoCreate()) 2882 throw new Error("Attempt to auto-create MedicationKnowledgePackagingComponent.packagedProduct"); 2883 else if (Configuration.doAutoCreate()) 2884 this.packagedProduct = new Reference(); // cc 2885 return this.packagedProduct; 2886 } 2887 2888 public boolean hasPackagedProduct() { 2889 return this.packagedProduct != null && !this.packagedProduct.isEmpty(); 2890 } 2891 2892 /** 2893 * @param value {@link #packagedProduct} (A reference to a PackagedProductDefinition that provides the details of the product that is in the packaging and is being priced.) 2894 */ 2895 public MedicationKnowledgePackagingComponent setPackagedProduct(Reference value) { 2896 this.packagedProduct = value; 2897 return this; 2898 } 2899 2900 protected void listChildren(List<Property> children) { 2901 super.listChildren(children); 2902 children.add(new Property("cost", "@MedicationKnowledge.cost", "The cost of the packaged medication.", 0, java.lang.Integer.MAX_VALUE, cost)); 2903 children.add(new Property("packagedProduct", "Reference(PackagedProductDefinition)", "A reference to a PackagedProductDefinition that provides the details of the product that is in the packaging and is being priced.", 0, 1, packagedProduct)); 2904 } 2905 2906 @Override 2907 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2908 switch (_hash) { 2909 case 3059661: /*cost*/ return new Property("cost", "@MedicationKnowledge.cost", "The cost of the packaged medication.", 0, java.lang.Integer.MAX_VALUE, cost); 2910 case 1893956145: /*packagedProduct*/ return new Property("packagedProduct", "Reference(PackagedProductDefinition)", "A reference to a PackagedProductDefinition that provides the details of the product that is in the packaging and is being priced.", 0, 1, packagedProduct); 2911 default: return super.getNamedProperty(_hash, _name, _checkValid); 2912 } 2913 2914 } 2915 2916 @Override 2917 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2918 switch (hash) { 2919 case 3059661: /*cost*/ return this.cost == null ? new Base[0] : this.cost.toArray(new Base[this.cost.size()]); // MedicationKnowledgeCostComponent 2920 case 1893956145: /*packagedProduct*/ return this.packagedProduct == null ? new Base[0] : new Base[] {this.packagedProduct}; // Reference 2921 default: return super.getProperty(hash, name, checkValid); 2922 } 2923 2924 } 2925 2926 @Override 2927 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2928 switch (hash) { 2929 case 3059661: // cost 2930 this.getCost().add((MedicationKnowledgeCostComponent) value); // MedicationKnowledgeCostComponent 2931 return value; 2932 case 1893956145: // packagedProduct 2933 this.packagedProduct = TypeConvertor.castToReference(value); // Reference 2934 return value; 2935 default: return super.setProperty(hash, name, value); 2936 } 2937 2938 } 2939 2940 @Override 2941 public Base setProperty(String name, Base value) throws FHIRException { 2942 if (name.equals("cost")) { 2943 this.getCost().add((MedicationKnowledgeCostComponent) value); 2944 } else if (name.equals("packagedProduct")) { 2945 this.packagedProduct = TypeConvertor.castToReference(value); // Reference 2946 } else 2947 return super.setProperty(name, value); 2948 return value; 2949 } 2950 2951 @Override 2952 public void removeChild(String name, Base value) throws FHIRException { 2953 if (name.equals("cost")) { 2954 this.getCost().remove((MedicationKnowledgeCostComponent) value); 2955 } else if (name.equals("packagedProduct")) { 2956 this.packagedProduct = null; 2957 } else 2958 super.removeChild(name, value); 2959 2960 } 2961 2962 @Override 2963 public Base makeProperty(int hash, String name) throws FHIRException { 2964 switch (hash) { 2965 case 3059661: return addCost(); 2966 case 1893956145: return getPackagedProduct(); 2967 default: return super.makeProperty(hash, name); 2968 } 2969 2970 } 2971 2972 @Override 2973 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2974 switch (hash) { 2975 case 3059661: /*cost*/ return new String[] {"@MedicationKnowledge.cost"}; 2976 case 1893956145: /*packagedProduct*/ return new String[] {"Reference"}; 2977 default: return super.getTypesForProperty(hash, name); 2978 } 2979 2980 } 2981 2982 @Override 2983 public Base addChild(String name) throws FHIRException { 2984 if (name.equals("cost")) { 2985 return addCost(); 2986 } 2987 else if (name.equals("packagedProduct")) { 2988 this.packagedProduct = new Reference(); 2989 return this.packagedProduct; 2990 } 2991 else 2992 return super.addChild(name); 2993 } 2994 2995 public MedicationKnowledgePackagingComponent copy() { 2996 MedicationKnowledgePackagingComponent dst = new MedicationKnowledgePackagingComponent(); 2997 copyValues(dst); 2998 return dst; 2999 } 3000 3001 public void copyValues(MedicationKnowledgePackagingComponent dst) { 3002 super.copyValues(dst); 3003 if (cost != null) { 3004 dst.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 3005 for (MedicationKnowledgeCostComponent i : cost) 3006 dst.cost.add(i.copy()); 3007 }; 3008 dst.packagedProduct = packagedProduct == null ? null : packagedProduct.copy(); 3009 } 3010 3011 @Override 3012 public boolean equalsDeep(Base other_) { 3013 if (!super.equalsDeep(other_)) 3014 return false; 3015 if (!(other_ instanceof MedicationKnowledgePackagingComponent)) 3016 return false; 3017 MedicationKnowledgePackagingComponent o = (MedicationKnowledgePackagingComponent) other_; 3018 return compareDeep(cost, o.cost, true) && compareDeep(packagedProduct, o.packagedProduct, true) 3019 ; 3020 } 3021 3022 @Override 3023 public boolean equalsShallow(Base other_) { 3024 if (!super.equalsShallow(other_)) 3025 return false; 3026 if (!(other_ instanceof MedicationKnowledgePackagingComponent)) 3027 return false; 3028 MedicationKnowledgePackagingComponent o = (MedicationKnowledgePackagingComponent) other_; 3029 return true; 3030 } 3031 3032 public boolean isEmpty() { 3033 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(cost, packagedProduct); 3034 } 3035 3036 public String fhirType() { 3037 return "MedicationKnowledge.packaging"; 3038 3039 } 3040 3041 } 3042 3043 @Block() 3044 public static class MedicationKnowledgeStorageGuidelineComponent extends BackboneElement implements IBaseBackboneElement { 3045 /** 3046 * Reference to additional information about the storage guidelines. 3047 */ 3048 @Child(name = "reference", type = {UriType.class}, order=1, min=0, max=1, modifier=false, summary=false) 3049 @Description(shortDefinition="Reference to additional information", formalDefinition="Reference to additional information about the storage guidelines." ) 3050 protected UriType reference; 3051 3052 /** 3053 * Additional notes about the storage. 3054 */ 3055 @Child(name = "note", type = {Annotation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3056 @Description(shortDefinition="Additional storage notes", formalDefinition="Additional notes about the storage." ) 3057 protected List<Annotation> note; 3058 3059 /** 3060 * Duration that the medication remains stable if the environmentalSetting is respected. 3061 */ 3062 @Child(name = "stabilityDuration", type = {Duration.class}, order=3, min=0, max=1, modifier=false, summary=false) 3063 @Description(shortDefinition="Duration remains stable", formalDefinition="Duration that the medication remains stable if the environmentalSetting is respected." ) 3064 protected Duration stabilityDuration; 3065 3066 /** 3067 * Describes a setting/value on the environment for the adequate storage of the medication and other substances. Environment settings may involve temperature, humidity, or exposure to light. 3068 */ 3069 @Child(name = "environmentalSetting", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3070 @Description(shortDefinition="Setting or value of environment for adequate storage", formalDefinition="Describes a setting/value on the environment for the adequate storage of the medication and other substances. Environment settings may involve temperature, humidity, or exposure to light." ) 3071 protected List<MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent> environmentalSetting; 3072 3073 private static final long serialVersionUID = -304442588L; 3074 3075 /** 3076 * Constructor 3077 */ 3078 public MedicationKnowledgeStorageGuidelineComponent() { 3079 super(); 3080 } 3081 3082 /** 3083 * @return {@link #reference} (Reference to additional information about the storage guidelines.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 3084 */ 3085 public UriType getReferenceElement() { 3086 if (this.reference == null) 3087 if (Configuration.errorOnAutoCreate()) 3088 throw new Error("Attempt to auto-create MedicationKnowledgeStorageGuidelineComponent.reference"); 3089 else if (Configuration.doAutoCreate()) 3090 this.reference = new UriType(); // bb 3091 return this.reference; 3092 } 3093 3094 public boolean hasReferenceElement() { 3095 return this.reference != null && !this.reference.isEmpty(); 3096 } 3097 3098 public boolean hasReference() { 3099 return this.reference != null && !this.reference.isEmpty(); 3100 } 3101 3102 /** 3103 * @param value {@link #reference} (Reference to additional information about the storage guidelines.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 3104 */ 3105 public MedicationKnowledgeStorageGuidelineComponent setReferenceElement(UriType value) { 3106 this.reference = value; 3107 return this; 3108 } 3109 3110 /** 3111 * @return Reference to additional information about the storage guidelines. 3112 */ 3113 public String getReference() { 3114 return this.reference == null ? null : this.reference.getValue(); 3115 } 3116 3117 /** 3118 * @param value Reference to additional information about the storage guidelines. 3119 */ 3120 public MedicationKnowledgeStorageGuidelineComponent setReference(String value) { 3121 if (Utilities.noString(value)) 3122 this.reference = null; 3123 else { 3124 if (this.reference == null) 3125 this.reference = new UriType(); 3126 this.reference.setValue(value); 3127 } 3128 return this; 3129 } 3130 3131 /** 3132 * @return {@link #note} (Additional notes about the storage.) 3133 */ 3134 public List<Annotation> getNote() { 3135 if (this.note == null) 3136 this.note = new ArrayList<Annotation>(); 3137 return this.note; 3138 } 3139 3140 /** 3141 * @return Returns a reference to <code>this</code> for easy method chaining 3142 */ 3143 public MedicationKnowledgeStorageGuidelineComponent setNote(List<Annotation> theNote) { 3144 this.note = theNote; 3145 return this; 3146 } 3147 3148 public boolean hasNote() { 3149 if (this.note == null) 3150 return false; 3151 for (Annotation item : this.note) 3152 if (!item.isEmpty()) 3153 return true; 3154 return false; 3155 } 3156 3157 public Annotation addNote() { //3 3158 Annotation t = new Annotation(); 3159 if (this.note == null) 3160 this.note = new ArrayList<Annotation>(); 3161 this.note.add(t); 3162 return t; 3163 } 3164 3165 public MedicationKnowledgeStorageGuidelineComponent addNote(Annotation t) { //3 3166 if (t == null) 3167 return this; 3168 if (this.note == null) 3169 this.note = new ArrayList<Annotation>(); 3170 this.note.add(t); 3171 return this; 3172 } 3173 3174 /** 3175 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 3176 */ 3177 public Annotation getNoteFirstRep() { 3178 if (getNote().isEmpty()) { 3179 addNote(); 3180 } 3181 return getNote().get(0); 3182 } 3183 3184 /** 3185 * @return {@link #stabilityDuration} (Duration that the medication remains stable if the environmentalSetting is respected.) 3186 */ 3187 public Duration getStabilityDuration() { 3188 if (this.stabilityDuration == null) 3189 if (Configuration.errorOnAutoCreate()) 3190 throw new Error("Attempt to auto-create MedicationKnowledgeStorageGuidelineComponent.stabilityDuration"); 3191 else if (Configuration.doAutoCreate()) 3192 this.stabilityDuration = new Duration(); // cc 3193 return this.stabilityDuration; 3194 } 3195 3196 public boolean hasStabilityDuration() { 3197 return this.stabilityDuration != null && !this.stabilityDuration.isEmpty(); 3198 } 3199 3200 /** 3201 * @param value {@link #stabilityDuration} (Duration that the medication remains stable if the environmentalSetting is respected.) 3202 */ 3203 public MedicationKnowledgeStorageGuidelineComponent setStabilityDuration(Duration value) { 3204 this.stabilityDuration = value; 3205 return this; 3206 } 3207 3208 /** 3209 * @return {@link #environmentalSetting} (Describes a setting/value on the environment for the adequate storage of the medication and other substances. Environment settings may involve temperature, humidity, or exposure to light.) 3210 */ 3211 public List<MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent> getEnvironmentalSetting() { 3212 if (this.environmentalSetting == null) 3213 this.environmentalSetting = new ArrayList<MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent>(); 3214 return this.environmentalSetting; 3215 } 3216 3217 /** 3218 * @return Returns a reference to <code>this</code> for easy method chaining 3219 */ 3220 public MedicationKnowledgeStorageGuidelineComponent setEnvironmentalSetting(List<MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent> theEnvironmentalSetting) { 3221 this.environmentalSetting = theEnvironmentalSetting; 3222 return this; 3223 } 3224 3225 public boolean hasEnvironmentalSetting() { 3226 if (this.environmentalSetting == null) 3227 return false; 3228 for (MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent item : this.environmentalSetting) 3229 if (!item.isEmpty()) 3230 return true; 3231 return false; 3232 } 3233 3234 public MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent addEnvironmentalSetting() { //3 3235 MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent t = new MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent(); 3236 if (this.environmentalSetting == null) 3237 this.environmentalSetting = new ArrayList<MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent>(); 3238 this.environmentalSetting.add(t); 3239 return t; 3240 } 3241 3242 public MedicationKnowledgeStorageGuidelineComponent addEnvironmentalSetting(MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent t) { //3 3243 if (t == null) 3244 return this; 3245 if (this.environmentalSetting == null) 3246 this.environmentalSetting = new ArrayList<MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent>(); 3247 this.environmentalSetting.add(t); 3248 return this; 3249 } 3250 3251 /** 3252 * @return The first repetition of repeating field {@link #environmentalSetting}, creating it if it does not already exist {3} 3253 */ 3254 public MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent getEnvironmentalSettingFirstRep() { 3255 if (getEnvironmentalSetting().isEmpty()) { 3256 addEnvironmentalSetting(); 3257 } 3258 return getEnvironmentalSetting().get(0); 3259 } 3260 3261 protected void listChildren(List<Property> children) { 3262 super.listChildren(children); 3263 children.add(new Property("reference", "uri", "Reference to additional information about the storage guidelines.", 0, 1, reference)); 3264 children.add(new Property("note", "Annotation", "Additional notes about the storage.", 0, java.lang.Integer.MAX_VALUE, note)); 3265 children.add(new Property("stabilityDuration", "Duration", "Duration that the medication remains stable if the environmentalSetting is respected.", 0, 1, stabilityDuration)); 3266 children.add(new Property("environmentalSetting", "", "Describes a setting/value on the environment for the adequate storage of the medication and other substances. Environment settings may involve temperature, humidity, or exposure to light.", 0, java.lang.Integer.MAX_VALUE, environmentalSetting)); 3267 } 3268 3269 @Override 3270 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3271 switch (_hash) { 3272 case -925155509: /*reference*/ return new Property("reference", "uri", "Reference to additional information about the storage guidelines.", 0, 1, reference); 3273 case 3387378: /*note*/ return new Property("note", "Annotation", "Additional notes about the storage.", 0, java.lang.Integer.MAX_VALUE, note); 3274 case 1823268957: /*stabilityDuration*/ return new Property("stabilityDuration", "Duration", "Duration that the medication remains stable if the environmentalSetting is respected.", 0, 1, stabilityDuration); 3275 case 105846514: /*environmentalSetting*/ return new Property("environmentalSetting", "", "Describes a setting/value on the environment for the adequate storage of the medication and other substances. Environment settings may involve temperature, humidity, or exposure to light.", 0, java.lang.Integer.MAX_VALUE, environmentalSetting); 3276 default: return super.getNamedProperty(_hash, _name, _checkValid); 3277 } 3278 3279 } 3280 3281 @Override 3282 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3283 switch (hash) { 3284 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // UriType 3285 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3286 case 1823268957: /*stabilityDuration*/ return this.stabilityDuration == null ? new Base[0] : new Base[] {this.stabilityDuration}; // Duration 3287 case 105846514: /*environmentalSetting*/ return this.environmentalSetting == null ? new Base[0] : this.environmentalSetting.toArray(new Base[this.environmentalSetting.size()]); // MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent 3288 default: return super.getProperty(hash, name, checkValid); 3289 } 3290 3291 } 3292 3293 @Override 3294 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3295 switch (hash) { 3296 case -925155509: // reference 3297 this.reference = TypeConvertor.castToUri(value); // UriType 3298 return value; 3299 case 3387378: // note 3300 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 3301 return value; 3302 case 1823268957: // stabilityDuration 3303 this.stabilityDuration = TypeConvertor.castToDuration(value); // Duration 3304 return value; 3305 case 105846514: // environmentalSetting 3306 this.getEnvironmentalSetting().add((MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent) value); // MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent 3307 return value; 3308 default: return super.setProperty(hash, name, value); 3309 } 3310 3311 } 3312 3313 @Override 3314 public Base setProperty(String name, Base value) throws FHIRException { 3315 if (name.equals("reference")) { 3316 this.reference = TypeConvertor.castToUri(value); // UriType 3317 } else if (name.equals("note")) { 3318 this.getNote().add(TypeConvertor.castToAnnotation(value)); 3319 } else if (name.equals("stabilityDuration")) { 3320 this.stabilityDuration = TypeConvertor.castToDuration(value); // Duration 3321 } else if (name.equals("environmentalSetting")) { 3322 this.getEnvironmentalSetting().add((MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent) value); 3323 } else 3324 return super.setProperty(name, value); 3325 return value; 3326 } 3327 3328 @Override 3329 public void removeChild(String name, Base value) throws FHIRException { 3330 if (name.equals("reference")) { 3331 this.reference = null; 3332 } else if (name.equals("note")) { 3333 this.getNote().remove(value); 3334 } else if (name.equals("stabilityDuration")) { 3335 this.stabilityDuration = null; 3336 } else if (name.equals("environmentalSetting")) { 3337 this.getEnvironmentalSetting().remove((MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent) value); 3338 } else 3339 super.removeChild(name, value); 3340 3341 } 3342 3343 @Override 3344 public Base makeProperty(int hash, String name) throws FHIRException { 3345 switch (hash) { 3346 case -925155509: return getReferenceElement(); 3347 case 3387378: return addNote(); 3348 case 1823268957: return getStabilityDuration(); 3349 case 105846514: return addEnvironmentalSetting(); 3350 default: return super.makeProperty(hash, name); 3351 } 3352 3353 } 3354 3355 @Override 3356 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3357 switch (hash) { 3358 case -925155509: /*reference*/ return new String[] {"uri"}; 3359 case 3387378: /*note*/ return new String[] {"Annotation"}; 3360 case 1823268957: /*stabilityDuration*/ return new String[] {"Duration"}; 3361 case 105846514: /*environmentalSetting*/ return new String[] {}; 3362 default: return super.getTypesForProperty(hash, name); 3363 } 3364 3365 } 3366 3367 @Override 3368 public Base addChild(String name) throws FHIRException { 3369 if (name.equals("reference")) { 3370 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.storageGuideline.reference"); 3371 } 3372 else if (name.equals("note")) { 3373 return addNote(); 3374 } 3375 else if (name.equals("stabilityDuration")) { 3376 this.stabilityDuration = new Duration(); 3377 return this.stabilityDuration; 3378 } 3379 else if (name.equals("environmentalSetting")) { 3380 return addEnvironmentalSetting(); 3381 } 3382 else 3383 return super.addChild(name); 3384 } 3385 3386 public MedicationKnowledgeStorageGuidelineComponent copy() { 3387 MedicationKnowledgeStorageGuidelineComponent dst = new MedicationKnowledgeStorageGuidelineComponent(); 3388 copyValues(dst); 3389 return dst; 3390 } 3391 3392 public void copyValues(MedicationKnowledgeStorageGuidelineComponent dst) { 3393 super.copyValues(dst); 3394 dst.reference = reference == null ? null : reference.copy(); 3395 if (note != null) { 3396 dst.note = new ArrayList<Annotation>(); 3397 for (Annotation i : note) 3398 dst.note.add(i.copy()); 3399 }; 3400 dst.stabilityDuration = stabilityDuration == null ? null : stabilityDuration.copy(); 3401 if (environmentalSetting != null) { 3402 dst.environmentalSetting = new ArrayList<MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent>(); 3403 for (MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent i : environmentalSetting) 3404 dst.environmentalSetting.add(i.copy()); 3405 }; 3406 } 3407 3408 @Override 3409 public boolean equalsDeep(Base other_) { 3410 if (!super.equalsDeep(other_)) 3411 return false; 3412 if (!(other_ instanceof MedicationKnowledgeStorageGuidelineComponent)) 3413 return false; 3414 MedicationKnowledgeStorageGuidelineComponent o = (MedicationKnowledgeStorageGuidelineComponent) other_; 3415 return compareDeep(reference, o.reference, true) && compareDeep(note, o.note, true) && compareDeep(stabilityDuration, o.stabilityDuration, true) 3416 && compareDeep(environmentalSetting, o.environmentalSetting, true); 3417 } 3418 3419 @Override 3420 public boolean equalsShallow(Base other_) { 3421 if (!super.equalsShallow(other_)) 3422 return false; 3423 if (!(other_ instanceof MedicationKnowledgeStorageGuidelineComponent)) 3424 return false; 3425 MedicationKnowledgeStorageGuidelineComponent o = (MedicationKnowledgeStorageGuidelineComponent) other_; 3426 return compareValues(reference, o.reference, true); 3427 } 3428 3429 public boolean isEmpty() { 3430 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, note, stabilityDuration 3431 , environmentalSetting); 3432 } 3433 3434 public String fhirType() { 3435 return "MedicationKnowledge.storageGuideline"; 3436 3437 } 3438 3439 } 3440 3441 @Block() 3442 public static class MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent extends BackboneElement implements IBaseBackboneElement { 3443 /** 3444 * Identifies the category or type of setting (e.g., type of location, temperature, humidity). 3445 */ 3446 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 3447 @Description(shortDefinition="Categorization of the setting", formalDefinition="Identifies the category or type of setting (e.g., type of location, temperature, humidity)." ) 3448 protected CodeableConcept type; 3449 3450 /** 3451 * Value associated to the setting. E.g., 40° ? 50°F for temperature. 3452 */ 3453 @Child(name = "value", type = {Quantity.class, Range.class, CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 3454 @Description(shortDefinition="Value of the setting", formalDefinition="Value associated to the setting. E.g., 40° ? 50°F for temperature." ) 3455 protected DataType value; 3456 3457 private static final long serialVersionUID = -1659186716L; 3458 3459 /** 3460 * Constructor 3461 */ 3462 public MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent() { 3463 super(); 3464 } 3465 3466 /** 3467 * Constructor 3468 */ 3469 public MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent(CodeableConcept type, DataType value) { 3470 super(); 3471 this.setType(type); 3472 this.setValue(value); 3473 } 3474 3475 /** 3476 * @return {@link #type} (Identifies the category or type of setting (e.g., type of location, temperature, humidity).) 3477 */ 3478 public CodeableConcept getType() { 3479 if (this.type == null) 3480 if (Configuration.errorOnAutoCreate()) 3481 throw new Error("Attempt to auto-create MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent.type"); 3482 else if (Configuration.doAutoCreate()) 3483 this.type = new CodeableConcept(); // cc 3484 return this.type; 3485 } 3486 3487 public boolean hasType() { 3488 return this.type != null && !this.type.isEmpty(); 3489 } 3490 3491 /** 3492 * @param value {@link #type} (Identifies the category or type of setting (e.g., type of location, temperature, humidity).) 3493 */ 3494 public MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent setType(CodeableConcept value) { 3495 this.type = value; 3496 return this; 3497 } 3498 3499 /** 3500 * @return {@link #value} (Value associated to the setting. E.g., 40° ? 50°F for temperature.) 3501 */ 3502 public DataType getValue() { 3503 return this.value; 3504 } 3505 3506 /** 3507 * @return {@link #value} (Value associated to the setting. E.g., 40° ? 50°F for temperature.) 3508 */ 3509 public Quantity getValueQuantity() throws FHIRException { 3510 if (this.value == null) 3511 this.value = new Quantity(); 3512 if (!(this.value instanceof Quantity)) 3513 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 3514 return (Quantity) this.value; 3515 } 3516 3517 public boolean hasValueQuantity() { 3518 return this != null && this.value instanceof Quantity; 3519 } 3520 3521 /** 3522 * @return {@link #value} (Value associated to the setting. E.g., 40° ? 50°F for temperature.) 3523 */ 3524 public Range getValueRange() throws FHIRException { 3525 if (this.value == null) 3526 this.value = new Range(); 3527 if (!(this.value instanceof Range)) 3528 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 3529 return (Range) this.value; 3530 } 3531 3532 public boolean hasValueRange() { 3533 return this != null && this.value instanceof Range; 3534 } 3535 3536 /** 3537 * @return {@link #value} (Value associated to the setting. E.g., 40° ? 50°F for temperature.) 3538 */ 3539 public CodeableConcept getValueCodeableConcept() throws FHIRException { 3540 if (this.value == null) 3541 this.value = new CodeableConcept(); 3542 if (!(this.value instanceof CodeableConcept)) 3543 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 3544 return (CodeableConcept) this.value; 3545 } 3546 3547 public boolean hasValueCodeableConcept() { 3548 return this != null && this.value instanceof CodeableConcept; 3549 } 3550 3551 public boolean hasValue() { 3552 return this.value != null && !this.value.isEmpty(); 3553 } 3554 3555 /** 3556 * @param value {@link #value} (Value associated to the setting. E.g., 40° ? 50°F for temperature.) 3557 */ 3558 public MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent setValue(DataType value) { 3559 if (value != null && !(value instanceof Quantity || value instanceof Range || value instanceof CodeableConcept)) 3560 throw new FHIRException("Not the right type for MedicationKnowledge.storageGuideline.environmentalSetting.value[x]: "+value.fhirType()); 3561 this.value = value; 3562 return this; 3563 } 3564 3565 protected void listChildren(List<Property> children) { 3566 super.listChildren(children); 3567 children.add(new Property("type", "CodeableConcept", "Identifies the category or type of setting (e.g., type of location, temperature, humidity).", 0, 1, type)); 3568 children.add(new Property("value[x]", "Quantity|Range|CodeableConcept", "Value associated to the setting. E.g., 40° ? 50°F for temperature.", 0, 1, value)); 3569 } 3570 3571 @Override 3572 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3573 switch (_hash) { 3574 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Identifies the category or type of setting (e.g., type of location, temperature, humidity).", 0, 1, type); 3575 case -1410166417: /*value[x]*/ return new Property("value[x]", "Quantity|Range|CodeableConcept", "Value associated to the setting. E.g., 40° ? 50°F for temperature.", 0, 1, value); 3576 case 111972721: /*value*/ return new Property("value[x]", "Quantity|Range|CodeableConcept", "Value associated to the setting. E.g., 40° ? 50°F for temperature.", 0, 1, value); 3577 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Value associated to the setting. E.g., 40° ? 50°F for temperature.", 0, 1, value); 3578 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "Value associated to the setting. E.g., 40° ? 50°F for temperature.", 0, 1, value); 3579 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "Value associated to the setting. E.g., 40° ? 50°F for temperature.", 0, 1, value); 3580 default: return super.getNamedProperty(_hash, _name, _checkValid); 3581 } 3582 3583 } 3584 3585 @Override 3586 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3587 switch (hash) { 3588 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3589 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 3590 default: return super.getProperty(hash, name, checkValid); 3591 } 3592 3593 } 3594 3595 @Override 3596 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3597 switch (hash) { 3598 case 3575610: // type 3599 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3600 return value; 3601 case 111972721: // value 3602 this.value = TypeConvertor.castToType(value); // DataType 3603 return value; 3604 default: return super.setProperty(hash, name, value); 3605 } 3606 3607 } 3608 3609 @Override 3610 public Base setProperty(String name, Base value) throws FHIRException { 3611 if (name.equals("type")) { 3612 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3613 } else if (name.equals("value[x]")) { 3614 this.value = TypeConvertor.castToType(value); // DataType 3615 } else 3616 return super.setProperty(name, value); 3617 return value; 3618 } 3619 3620 @Override 3621 public void removeChild(String name, Base value) throws FHIRException { 3622 if (name.equals("type")) { 3623 this.type = null; 3624 } else if (name.equals("value[x]")) { 3625 this.value = null; 3626 } else 3627 super.removeChild(name, value); 3628 3629 } 3630 3631 @Override 3632 public Base makeProperty(int hash, String name) throws FHIRException { 3633 switch (hash) { 3634 case 3575610: return getType(); 3635 case -1410166417: return getValue(); 3636 case 111972721: return getValue(); 3637 default: return super.makeProperty(hash, name); 3638 } 3639 3640 } 3641 3642 @Override 3643 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3644 switch (hash) { 3645 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3646 case 111972721: /*value*/ return new String[] {"Quantity", "Range", "CodeableConcept"}; 3647 default: return super.getTypesForProperty(hash, name); 3648 } 3649 3650 } 3651 3652 @Override 3653 public Base addChild(String name) throws FHIRException { 3654 if (name.equals("type")) { 3655 this.type = new CodeableConcept(); 3656 return this.type; 3657 } 3658 else if (name.equals("valueQuantity")) { 3659 this.value = new Quantity(); 3660 return this.value; 3661 } 3662 else if (name.equals("valueRange")) { 3663 this.value = new Range(); 3664 return this.value; 3665 } 3666 else if (name.equals("valueCodeableConcept")) { 3667 this.value = new CodeableConcept(); 3668 return this.value; 3669 } 3670 else 3671 return super.addChild(name); 3672 } 3673 3674 public MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent copy() { 3675 MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent dst = new MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent(); 3676 copyValues(dst); 3677 return dst; 3678 } 3679 3680 public void copyValues(MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent dst) { 3681 super.copyValues(dst); 3682 dst.type = type == null ? null : type.copy(); 3683 dst.value = value == null ? null : value.copy(); 3684 } 3685 3686 @Override 3687 public boolean equalsDeep(Base other_) { 3688 if (!super.equalsDeep(other_)) 3689 return false; 3690 if (!(other_ instanceof MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent)) 3691 return false; 3692 MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent o = (MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent) other_; 3693 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 3694 } 3695 3696 @Override 3697 public boolean equalsShallow(Base other_) { 3698 if (!super.equalsShallow(other_)) 3699 return false; 3700 if (!(other_ instanceof MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent)) 3701 return false; 3702 MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent o = (MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent) other_; 3703 return true; 3704 } 3705 3706 public boolean isEmpty() { 3707 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 3708 } 3709 3710 public String fhirType() { 3711 return "MedicationKnowledge.storageGuideline.environmentalSetting"; 3712 3713 } 3714 3715 } 3716 3717 @Block() 3718 public static class MedicationKnowledgeRegulatoryComponent extends BackboneElement implements IBaseBackboneElement { 3719 /** 3720 * The authority that is specifying the regulations. 3721 */ 3722 @Child(name = "regulatoryAuthority", type = {Organization.class}, order=1, min=1, max=1, modifier=false, summary=false) 3723 @Description(shortDefinition="Specifies the authority of the regulation", formalDefinition="The authority that is specifying the regulations." ) 3724 protected Reference regulatoryAuthority; 3725 3726 /** 3727 * Specifies if changes are allowed when dispensing a medication from a regulatory perspective. 3728 */ 3729 @Child(name = "substitution", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3730 @Description(shortDefinition="Specifies if changes are allowed when dispensing a medication from a regulatory perspective", formalDefinition="Specifies if changes are allowed when dispensing a medication from a regulatory perspective." ) 3731 protected List<MedicationKnowledgeRegulatorySubstitutionComponent> substitution; 3732 3733 /** 3734 * Specifies the schedule of a medication in jurisdiction. 3735 */ 3736 @Child(name = "schedule", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3737 @Description(shortDefinition="Specifies the schedule of a medication in jurisdiction", formalDefinition="Specifies the schedule of a medication in jurisdiction." ) 3738 protected List<CodeableConcept> schedule; 3739 3740 /** 3741 * The maximum number of units of the medication that can be dispensed in a period. 3742 */ 3743 @Child(name = "maxDispense", type = {}, order=4, min=0, max=1, modifier=false, summary=false) 3744 @Description(shortDefinition="The maximum number of units of the medication that can be dispensed in a period", formalDefinition="The maximum number of units of the medication that can be dispensed in a period." ) 3745 protected MedicationKnowledgeRegulatoryMaxDispenseComponent maxDispense; 3746 3747 private static final long serialVersionUID = -2005823416L; 3748 3749 /** 3750 * Constructor 3751 */ 3752 public MedicationKnowledgeRegulatoryComponent() { 3753 super(); 3754 } 3755 3756 /** 3757 * Constructor 3758 */ 3759 public MedicationKnowledgeRegulatoryComponent(Reference regulatoryAuthority) { 3760 super(); 3761 this.setRegulatoryAuthority(regulatoryAuthority); 3762 } 3763 3764 /** 3765 * @return {@link #regulatoryAuthority} (The authority that is specifying the regulations.) 3766 */ 3767 public Reference getRegulatoryAuthority() { 3768 if (this.regulatoryAuthority == null) 3769 if (Configuration.errorOnAutoCreate()) 3770 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatoryComponent.regulatoryAuthority"); 3771 else if (Configuration.doAutoCreate()) 3772 this.regulatoryAuthority = new Reference(); // cc 3773 return this.regulatoryAuthority; 3774 } 3775 3776 public boolean hasRegulatoryAuthority() { 3777 return this.regulatoryAuthority != null && !this.regulatoryAuthority.isEmpty(); 3778 } 3779 3780 /** 3781 * @param value {@link #regulatoryAuthority} (The authority that is specifying the regulations.) 3782 */ 3783 public MedicationKnowledgeRegulatoryComponent setRegulatoryAuthority(Reference value) { 3784 this.regulatoryAuthority = value; 3785 return this; 3786 } 3787 3788 /** 3789 * @return {@link #substitution} (Specifies if changes are allowed when dispensing a medication from a regulatory perspective.) 3790 */ 3791 public List<MedicationKnowledgeRegulatorySubstitutionComponent> getSubstitution() { 3792 if (this.substitution == null) 3793 this.substitution = new ArrayList<MedicationKnowledgeRegulatorySubstitutionComponent>(); 3794 return this.substitution; 3795 } 3796 3797 /** 3798 * @return Returns a reference to <code>this</code> for easy method chaining 3799 */ 3800 public MedicationKnowledgeRegulatoryComponent setSubstitution(List<MedicationKnowledgeRegulatorySubstitutionComponent> theSubstitution) { 3801 this.substitution = theSubstitution; 3802 return this; 3803 } 3804 3805 public boolean hasSubstitution() { 3806 if (this.substitution == null) 3807 return false; 3808 for (MedicationKnowledgeRegulatorySubstitutionComponent item : this.substitution) 3809 if (!item.isEmpty()) 3810 return true; 3811 return false; 3812 } 3813 3814 public MedicationKnowledgeRegulatorySubstitutionComponent addSubstitution() { //3 3815 MedicationKnowledgeRegulatorySubstitutionComponent t = new MedicationKnowledgeRegulatorySubstitutionComponent(); 3816 if (this.substitution == null) 3817 this.substitution = new ArrayList<MedicationKnowledgeRegulatorySubstitutionComponent>(); 3818 this.substitution.add(t); 3819 return t; 3820 } 3821 3822 public MedicationKnowledgeRegulatoryComponent addSubstitution(MedicationKnowledgeRegulatorySubstitutionComponent t) { //3 3823 if (t == null) 3824 return this; 3825 if (this.substitution == null) 3826 this.substitution = new ArrayList<MedicationKnowledgeRegulatorySubstitutionComponent>(); 3827 this.substitution.add(t); 3828 return this; 3829 } 3830 3831 /** 3832 * @return The first repetition of repeating field {@link #substitution}, creating it if it does not already exist {3} 3833 */ 3834 public MedicationKnowledgeRegulatorySubstitutionComponent getSubstitutionFirstRep() { 3835 if (getSubstitution().isEmpty()) { 3836 addSubstitution(); 3837 } 3838 return getSubstitution().get(0); 3839 } 3840 3841 /** 3842 * @return {@link #schedule} (Specifies the schedule of a medication in jurisdiction.) 3843 */ 3844 public List<CodeableConcept> getSchedule() { 3845 if (this.schedule == null) 3846 this.schedule = new ArrayList<CodeableConcept>(); 3847 return this.schedule; 3848 } 3849 3850 /** 3851 * @return Returns a reference to <code>this</code> for easy method chaining 3852 */ 3853 public MedicationKnowledgeRegulatoryComponent setSchedule(List<CodeableConcept> theSchedule) { 3854 this.schedule = theSchedule; 3855 return this; 3856 } 3857 3858 public boolean hasSchedule() { 3859 if (this.schedule == null) 3860 return false; 3861 for (CodeableConcept item : this.schedule) 3862 if (!item.isEmpty()) 3863 return true; 3864 return false; 3865 } 3866 3867 public CodeableConcept addSchedule() { //3 3868 CodeableConcept t = new CodeableConcept(); 3869 if (this.schedule == null) 3870 this.schedule = new ArrayList<CodeableConcept>(); 3871 this.schedule.add(t); 3872 return t; 3873 } 3874 3875 public MedicationKnowledgeRegulatoryComponent addSchedule(CodeableConcept t) { //3 3876 if (t == null) 3877 return this; 3878 if (this.schedule == null) 3879 this.schedule = new ArrayList<CodeableConcept>(); 3880 this.schedule.add(t); 3881 return this; 3882 } 3883 3884 /** 3885 * @return The first repetition of repeating field {@link #schedule}, creating it if it does not already exist {3} 3886 */ 3887 public CodeableConcept getScheduleFirstRep() { 3888 if (getSchedule().isEmpty()) { 3889 addSchedule(); 3890 } 3891 return getSchedule().get(0); 3892 } 3893 3894 /** 3895 * @return {@link #maxDispense} (The maximum number of units of the medication that can be dispensed in a period.) 3896 */ 3897 public MedicationKnowledgeRegulatoryMaxDispenseComponent getMaxDispense() { 3898 if (this.maxDispense == null) 3899 if (Configuration.errorOnAutoCreate()) 3900 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatoryComponent.maxDispense"); 3901 else if (Configuration.doAutoCreate()) 3902 this.maxDispense = new MedicationKnowledgeRegulatoryMaxDispenseComponent(); // cc 3903 return this.maxDispense; 3904 } 3905 3906 public boolean hasMaxDispense() { 3907 return this.maxDispense != null && !this.maxDispense.isEmpty(); 3908 } 3909 3910 /** 3911 * @param value {@link #maxDispense} (The maximum number of units of the medication that can be dispensed in a period.) 3912 */ 3913 public MedicationKnowledgeRegulatoryComponent setMaxDispense(MedicationKnowledgeRegulatoryMaxDispenseComponent value) { 3914 this.maxDispense = value; 3915 return this; 3916 } 3917 3918 protected void listChildren(List<Property> children) { 3919 super.listChildren(children); 3920 children.add(new Property("regulatoryAuthority", "Reference(Organization)", "The authority that is specifying the regulations.", 0, 1, regulatoryAuthority)); 3921 children.add(new Property("substitution", "", "Specifies if changes are allowed when dispensing a medication from a regulatory perspective.", 0, java.lang.Integer.MAX_VALUE, substitution)); 3922 children.add(new Property("schedule", "CodeableConcept", "Specifies the schedule of a medication in jurisdiction.", 0, java.lang.Integer.MAX_VALUE, schedule)); 3923 children.add(new Property("maxDispense", "", "The maximum number of units of the medication that can be dispensed in a period.", 0, 1, maxDispense)); 3924 } 3925 3926 @Override 3927 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3928 switch (_hash) { 3929 case 711233419: /*regulatoryAuthority*/ return new Property("regulatoryAuthority", "Reference(Organization)", "The authority that is specifying the regulations.", 0, 1, regulatoryAuthority); 3930 case 826147581: /*substitution*/ return new Property("substitution", "", "Specifies if changes are allowed when dispensing a medication from a regulatory perspective.", 0, java.lang.Integer.MAX_VALUE, substitution); 3931 case -697920873: /*schedule*/ return new Property("schedule", "CodeableConcept", "Specifies the schedule of a medication in jurisdiction.", 0, java.lang.Integer.MAX_VALUE, schedule); 3932 case -1977784607: /*maxDispense*/ return new Property("maxDispense", "", "The maximum number of units of the medication that can be dispensed in a period.", 0, 1, maxDispense); 3933 default: return super.getNamedProperty(_hash, _name, _checkValid); 3934 } 3935 3936 } 3937 3938 @Override 3939 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3940 switch (hash) { 3941 case 711233419: /*regulatoryAuthority*/ return this.regulatoryAuthority == null ? new Base[0] : new Base[] {this.regulatoryAuthority}; // Reference 3942 case 826147581: /*substitution*/ return this.substitution == null ? new Base[0] : this.substitution.toArray(new Base[this.substitution.size()]); // MedicationKnowledgeRegulatorySubstitutionComponent 3943 case -697920873: /*schedule*/ return this.schedule == null ? new Base[0] : this.schedule.toArray(new Base[this.schedule.size()]); // CodeableConcept 3944 case -1977784607: /*maxDispense*/ return this.maxDispense == null ? new Base[0] : new Base[] {this.maxDispense}; // MedicationKnowledgeRegulatoryMaxDispenseComponent 3945 default: return super.getProperty(hash, name, checkValid); 3946 } 3947 3948 } 3949 3950 @Override 3951 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3952 switch (hash) { 3953 case 711233419: // regulatoryAuthority 3954 this.regulatoryAuthority = TypeConvertor.castToReference(value); // Reference 3955 return value; 3956 case 826147581: // substitution 3957 this.getSubstitution().add((MedicationKnowledgeRegulatorySubstitutionComponent) value); // MedicationKnowledgeRegulatorySubstitutionComponent 3958 return value; 3959 case -697920873: // schedule 3960 this.getSchedule().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3961 return value; 3962 case -1977784607: // maxDispense 3963 this.maxDispense = (MedicationKnowledgeRegulatoryMaxDispenseComponent) value; // MedicationKnowledgeRegulatoryMaxDispenseComponent 3964 return value; 3965 default: return super.setProperty(hash, name, value); 3966 } 3967 3968 } 3969 3970 @Override 3971 public Base setProperty(String name, Base value) throws FHIRException { 3972 if (name.equals("regulatoryAuthority")) { 3973 this.regulatoryAuthority = TypeConvertor.castToReference(value); // Reference 3974 } else if (name.equals("substitution")) { 3975 this.getSubstitution().add((MedicationKnowledgeRegulatorySubstitutionComponent) value); 3976 } else if (name.equals("schedule")) { 3977 this.getSchedule().add(TypeConvertor.castToCodeableConcept(value)); 3978 } else if (name.equals("maxDispense")) { 3979 this.maxDispense = (MedicationKnowledgeRegulatoryMaxDispenseComponent) value; // MedicationKnowledgeRegulatoryMaxDispenseComponent 3980 } else 3981 return super.setProperty(name, value); 3982 return value; 3983 } 3984 3985 @Override 3986 public void removeChild(String name, Base value) throws FHIRException { 3987 if (name.equals("regulatoryAuthority")) { 3988 this.regulatoryAuthority = null; 3989 } else if (name.equals("substitution")) { 3990 this.getSubstitution().remove((MedicationKnowledgeRegulatorySubstitutionComponent) value); 3991 } else if (name.equals("schedule")) { 3992 this.getSchedule().remove(value); 3993 } else if (name.equals("maxDispense")) { 3994 this.maxDispense = (MedicationKnowledgeRegulatoryMaxDispenseComponent) value; // MedicationKnowledgeRegulatoryMaxDispenseComponent 3995 } else 3996 super.removeChild(name, value); 3997 3998 } 3999 4000 @Override 4001 public Base makeProperty(int hash, String name) throws FHIRException { 4002 switch (hash) { 4003 case 711233419: return getRegulatoryAuthority(); 4004 case 826147581: return addSubstitution(); 4005 case -697920873: return addSchedule(); 4006 case -1977784607: return getMaxDispense(); 4007 default: return super.makeProperty(hash, name); 4008 } 4009 4010 } 4011 4012 @Override 4013 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4014 switch (hash) { 4015 case 711233419: /*regulatoryAuthority*/ return new String[] {"Reference"}; 4016 case 826147581: /*substitution*/ return new String[] {}; 4017 case -697920873: /*schedule*/ return new String[] {"CodeableConcept"}; 4018 case -1977784607: /*maxDispense*/ return new String[] {}; 4019 default: return super.getTypesForProperty(hash, name); 4020 } 4021 4022 } 4023 4024 @Override 4025 public Base addChild(String name) throws FHIRException { 4026 if (name.equals("regulatoryAuthority")) { 4027 this.regulatoryAuthority = new Reference(); 4028 return this.regulatoryAuthority; 4029 } 4030 else if (name.equals("substitution")) { 4031 return addSubstitution(); 4032 } 4033 else if (name.equals("schedule")) { 4034 return addSchedule(); 4035 } 4036 else if (name.equals("maxDispense")) { 4037 this.maxDispense = new MedicationKnowledgeRegulatoryMaxDispenseComponent(); 4038 return this.maxDispense; 4039 } 4040 else 4041 return super.addChild(name); 4042 } 4043 4044 public MedicationKnowledgeRegulatoryComponent copy() { 4045 MedicationKnowledgeRegulatoryComponent dst = new MedicationKnowledgeRegulatoryComponent(); 4046 copyValues(dst); 4047 return dst; 4048 } 4049 4050 public void copyValues(MedicationKnowledgeRegulatoryComponent dst) { 4051 super.copyValues(dst); 4052 dst.regulatoryAuthority = regulatoryAuthority == null ? null : regulatoryAuthority.copy(); 4053 if (substitution != null) { 4054 dst.substitution = new ArrayList<MedicationKnowledgeRegulatorySubstitutionComponent>(); 4055 for (MedicationKnowledgeRegulatorySubstitutionComponent i : substitution) 4056 dst.substitution.add(i.copy()); 4057 }; 4058 if (schedule != null) { 4059 dst.schedule = new ArrayList<CodeableConcept>(); 4060 for (CodeableConcept i : schedule) 4061 dst.schedule.add(i.copy()); 4062 }; 4063 dst.maxDispense = maxDispense == null ? null : maxDispense.copy(); 4064 } 4065 4066 @Override 4067 public boolean equalsDeep(Base other_) { 4068 if (!super.equalsDeep(other_)) 4069 return false; 4070 if (!(other_ instanceof MedicationKnowledgeRegulatoryComponent)) 4071 return false; 4072 MedicationKnowledgeRegulatoryComponent o = (MedicationKnowledgeRegulatoryComponent) other_; 4073 return compareDeep(regulatoryAuthority, o.regulatoryAuthority, true) && compareDeep(substitution, o.substitution, true) 4074 && compareDeep(schedule, o.schedule, true) && compareDeep(maxDispense, o.maxDispense, true); 4075 } 4076 4077 @Override 4078 public boolean equalsShallow(Base other_) { 4079 if (!super.equalsShallow(other_)) 4080 return false; 4081 if (!(other_ instanceof MedicationKnowledgeRegulatoryComponent)) 4082 return false; 4083 MedicationKnowledgeRegulatoryComponent o = (MedicationKnowledgeRegulatoryComponent) other_; 4084 return true; 4085 } 4086 4087 public boolean isEmpty() { 4088 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(regulatoryAuthority, substitution 4089 , schedule, maxDispense); 4090 } 4091 4092 public String fhirType() { 4093 return "MedicationKnowledge.regulatory"; 4094 4095 } 4096 4097 } 4098 4099 @Block() 4100 public static class MedicationKnowledgeRegulatorySubstitutionComponent extends BackboneElement implements IBaseBackboneElement { 4101 /** 4102 * Specifies the type of substitution allowed. 4103 */ 4104 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 4105 @Description(shortDefinition="Specifies the type of substitution allowed", formalDefinition="Specifies the type of substitution allowed." ) 4106 protected CodeableConcept type; 4107 4108 /** 4109 * Specifies if regulation allows for changes in the medication when dispensing. 4110 */ 4111 @Child(name = "allowed", type = {BooleanType.class}, order=2, min=1, max=1, modifier=false, summary=false) 4112 @Description(shortDefinition="Specifies if regulation allows for changes in the medication when dispensing", formalDefinition="Specifies if regulation allows for changes in the medication when dispensing." ) 4113 protected BooleanType allowed; 4114 4115 private static final long serialVersionUID = 396354861L; 4116 4117 /** 4118 * Constructor 4119 */ 4120 public MedicationKnowledgeRegulatorySubstitutionComponent() { 4121 super(); 4122 } 4123 4124 /** 4125 * Constructor 4126 */ 4127 public MedicationKnowledgeRegulatorySubstitutionComponent(CodeableConcept type, boolean allowed) { 4128 super(); 4129 this.setType(type); 4130 this.setAllowed(allowed); 4131 } 4132 4133 /** 4134 * @return {@link #type} (Specifies the type of substitution allowed.) 4135 */ 4136 public CodeableConcept getType() { 4137 if (this.type == null) 4138 if (Configuration.errorOnAutoCreate()) 4139 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatorySubstitutionComponent.type"); 4140 else if (Configuration.doAutoCreate()) 4141 this.type = new CodeableConcept(); // cc 4142 return this.type; 4143 } 4144 4145 public boolean hasType() { 4146 return this.type != null && !this.type.isEmpty(); 4147 } 4148 4149 /** 4150 * @param value {@link #type} (Specifies the type of substitution allowed.) 4151 */ 4152 public MedicationKnowledgeRegulatorySubstitutionComponent setType(CodeableConcept value) { 4153 this.type = value; 4154 return this; 4155 } 4156 4157 /** 4158 * @return {@link #allowed} (Specifies if regulation allows for changes in the medication when dispensing.). This is the underlying object with id, value and extensions. The accessor "getAllowed" gives direct access to the value 4159 */ 4160 public BooleanType getAllowedElement() { 4161 if (this.allowed == null) 4162 if (Configuration.errorOnAutoCreate()) 4163 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatorySubstitutionComponent.allowed"); 4164 else if (Configuration.doAutoCreate()) 4165 this.allowed = new BooleanType(); // bb 4166 return this.allowed; 4167 } 4168 4169 public boolean hasAllowedElement() { 4170 return this.allowed != null && !this.allowed.isEmpty(); 4171 } 4172 4173 public boolean hasAllowed() { 4174 return this.allowed != null && !this.allowed.isEmpty(); 4175 } 4176 4177 /** 4178 * @param value {@link #allowed} (Specifies if regulation allows for changes in the medication when dispensing.). This is the underlying object with id, value and extensions. The accessor "getAllowed" gives direct access to the value 4179 */ 4180 public MedicationKnowledgeRegulatorySubstitutionComponent setAllowedElement(BooleanType value) { 4181 this.allowed = value; 4182 return this; 4183 } 4184 4185 /** 4186 * @return Specifies if regulation allows for changes in the medication when dispensing. 4187 */ 4188 public boolean getAllowed() { 4189 return this.allowed == null || this.allowed.isEmpty() ? false : this.allowed.getValue(); 4190 } 4191 4192 /** 4193 * @param value Specifies if regulation allows for changes in the medication when dispensing. 4194 */ 4195 public MedicationKnowledgeRegulatorySubstitutionComponent setAllowed(boolean value) { 4196 if (this.allowed == null) 4197 this.allowed = new BooleanType(); 4198 this.allowed.setValue(value); 4199 return this; 4200 } 4201 4202 protected void listChildren(List<Property> children) { 4203 super.listChildren(children); 4204 children.add(new Property("type", "CodeableConcept", "Specifies the type of substitution allowed.", 0, 1, type)); 4205 children.add(new Property("allowed", "boolean", "Specifies if regulation allows for changes in the medication when dispensing.", 0, 1, allowed)); 4206 } 4207 4208 @Override 4209 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4210 switch (_hash) { 4211 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Specifies the type of substitution allowed.", 0, 1, type); 4212 case -911343192: /*allowed*/ return new Property("allowed", "boolean", "Specifies if regulation allows for changes in the medication when dispensing.", 0, 1, allowed); 4213 default: return super.getNamedProperty(_hash, _name, _checkValid); 4214 } 4215 4216 } 4217 4218 @Override 4219 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4220 switch (hash) { 4221 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 4222 case -911343192: /*allowed*/ return this.allowed == null ? new Base[0] : new Base[] {this.allowed}; // BooleanType 4223 default: return super.getProperty(hash, name, checkValid); 4224 } 4225 4226 } 4227 4228 @Override 4229 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4230 switch (hash) { 4231 case 3575610: // type 4232 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4233 return value; 4234 case -911343192: // allowed 4235 this.allowed = TypeConvertor.castToBoolean(value); // BooleanType 4236 return value; 4237 default: return super.setProperty(hash, name, value); 4238 } 4239 4240 } 4241 4242 @Override 4243 public Base setProperty(String name, Base value) throws FHIRException { 4244 if (name.equals("type")) { 4245 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4246 } else if (name.equals("allowed")) { 4247 this.allowed = TypeConvertor.castToBoolean(value); // BooleanType 4248 } else 4249 return super.setProperty(name, value); 4250 return value; 4251 } 4252 4253 @Override 4254 public void removeChild(String name, Base value) throws FHIRException { 4255 if (name.equals("type")) { 4256 this.type = null; 4257 } else if (name.equals("allowed")) { 4258 this.allowed = null; 4259 } else 4260 super.removeChild(name, value); 4261 4262 } 4263 4264 @Override 4265 public Base makeProperty(int hash, String name) throws FHIRException { 4266 switch (hash) { 4267 case 3575610: return getType(); 4268 case -911343192: return getAllowedElement(); 4269 default: return super.makeProperty(hash, name); 4270 } 4271 4272 } 4273 4274 @Override 4275 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4276 switch (hash) { 4277 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 4278 case -911343192: /*allowed*/ return new String[] {"boolean"}; 4279 default: return super.getTypesForProperty(hash, name); 4280 } 4281 4282 } 4283 4284 @Override 4285 public Base addChild(String name) throws FHIRException { 4286 if (name.equals("type")) { 4287 this.type = new CodeableConcept(); 4288 return this.type; 4289 } 4290 else if (name.equals("allowed")) { 4291 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.regulatory.substitution.allowed"); 4292 } 4293 else 4294 return super.addChild(name); 4295 } 4296 4297 public MedicationKnowledgeRegulatorySubstitutionComponent copy() { 4298 MedicationKnowledgeRegulatorySubstitutionComponent dst = new MedicationKnowledgeRegulatorySubstitutionComponent(); 4299 copyValues(dst); 4300 return dst; 4301 } 4302 4303 public void copyValues(MedicationKnowledgeRegulatorySubstitutionComponent dst) { 4304 super.copyValues(dst); 4305 dst.type = type == null ? null : type.copy(); 4306 dst.allowed = allowed == null ? null : allowed.copy(); 4307 } 4308 4309 @Override 4310 public boolean equalsDeep(Base other_) { 4311 if (!super.equalsDeep(other_)) 4312 return false; 4313 if (!(other_ instanceof MedicationKnowledgeRegulatorySubstitutionComponent)) 4314 return false; 4315 MedicationKnowledgeRegulatorySubstitutionComponent o = (MedicationKnowledgeRegulatorySubstitutionComponent) other_; 4316 return compareDeep(type, o.type, true) && compareDeep(allowed, o.allowed, true); 4317 } 4318 4319 @Override 4320 public boolean equalsShallow(Base other_) { 4321 if (!super.equalsShallow(other_)) 4322 return false; 4323 if (!(other_ instanceof MedicationKnowledgeRegulatorySubstitutionComponent)) 4324 return false; 4325 MedicationKnowledgeRegulatorySubstitutionComponent o = (MedicationKnowledgeRegulatorySubstitutionComponent) other_; 4326 return compareValues(allowed, o.allowed, true); 4327 } 4328 4329 public boolean isEmpty() { 4330 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, allowed); 4331 } 4332 4333 public String fhirType() { 4334 return "MedicationKnowledge.regulatory.substitution"; 4335 4336 } 4337 4338 } 4339 4340 @Block() 4341 public static class MedicationKnowledgeRegulatoryMaxDispenseComponent extends BackboneElement implements IBaseBackboneElement { 4342 /** 4343 * The maximum number of units of the medication that can be dispensed. 4344 */ 4345 @Child(name = "quantity", type = {Quantity.class}, order=1, min=1, max=1, modifier=false, summary=false) 4346 @Description(shortDefinition="The maximum number of units of the medication that can be dispensed", formalDefinition="The maximum number of units of the medication that can be dispensed." ) 4347 protected Quantity quantity; 4348 4349 /** 4350 * The period that applies to the maximum number of units. 4351 */ 4352 @Child(name = "period", type = {Duration.class}, order=2, min=0, max=1, modifier=false, summary=false) 4353 @Description(shortDefinition="The period that applies to the maximum number of units", formalDefinition="The period that applies to the maximum number of units." ) 4354 protected Duration period; 4355 4356 private static final long serialVersionUID = -441724185L; 4357 4358 /** 4359 * Constructor 4360 */ 4361 public MedicationKnowledgeRegulatoryMaxDispenseComponent() { 4362 super(); 4363 } 4364 4365 /** 4366 * Constructor 4367 */ 4368 public MedicationKnowledgeRegulatoryMaxDispenseComponent(Quantity quantity) { 4369 super(); 4370 this.setQuantity(quantity); 4371 } 4372 4373 /** 4374 * @return {@link #quantity} (The maximum number of units of the medication that can be dispensed.) 4375 */ 4376 public Quantity getQuantity() { 4377 if (this.quantity == null) 4378 if (Configuration.errorOnAutoCreate()) 4379 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatoryMaxDispenseComponent.quantity"); 4380 else if (Configuration.doAutoCreate()) 4381 this.quantity = new Quantity(); // cc 4382 return this.quantity; 4383 } 4384 4385 public boolean hasQuantity() { 4386 return this.quantity != null && !this.quantity.isEmpty(); 4387 } 4388 4389 /** 4390 * @param value {@link #quantity} (The maximum number of units of the medication that can be dispensed.) 4391 */ 4392 public MedicationKnowledgeRegulatoryMaxDispenseComponent setQuantity(Quantity value) { 4393 this.quantity = value; 4394 return this; 4395 } 4396 4397 /** 4398 * @return {@link #period} (The period that applies to the maximum number of units.) 4399 */ 4400 public Duration getPeriod() { 4401 if (this.period == null) 4402 if (Configuration.errorOnAutoCreate()) 4403 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatoryMaxDispenseComponent.period"); 4404 else if (Configuration.doAutoCreate()) 4405 this.period = new Duration(); // cc 4406 return this.period; 4407 } 4408 4409 public boolean hasPeriod() { 4410 return this.period != null && !this.period.isEmpty(); 4411 } 4412 4413 /** 4414 * @param value {@link #period} (The period that applies to the maximum number of units.) 4415 */ 4416 public MedicationKnowledgeRegulatoryMaxDispenseComponent setPeriod(Duration value) { 4417 this.period = value; 4418 return this; 4419 } 4420 4421 protected void listChildren(List<Property> children) { 4422 super.listChildren(children); 4423 children.add(new Property("quantity", "Quantity", "The maximum number of units of the medication that can be dispensed.", 0, 1, quantity)); 4424 children.add(new Property("period", "Duration", "The period that applies to the maximum number of units.", 0, 1, period)); 4425 } 4426 4427 @Override 4428 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4429 switch (_hash) { 4430 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The maximum number of units of the medication that can be dispensed.", 0, 1, quantity); 4431 case -991726143: /*period*/ return new Property("period", "Duration", "The period that applies to the maximum number of units.", 0, 1, period); 4432 default: return super.getNamedProperty(_hash, _name, _checkValid); 4433 } 4434 4435 } 4436 4437 @Override 4438 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4439 switch (hash) { 4440 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 4441 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Duration 4442 default: return super.getProperty(hash, name, checkValid); 4443 } 4444 4445 } 4446 4447 @Override 4448 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4449 switch (hash) { 4450 case -1285004149: // quantity 4451 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 4452 return value; 4453 case -991726143: // period 4454 this.period = TypeConvertor.castToDuration(value); // Duration 4455 return value; 4456 default: return super.setProperty(hash, name, value); 4457 } 4458 4459 } 4460 4461 @Override 4462 public Base setProperty(String name, Base value) throws FHIRException { 4463 if (name.equals("quantity")) { 4464 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 4465 } else if (name.equals("period")) { 4466 this.period = TypeConvertor.castToDuration(value); // Duration 4467 } else 4468 return super.setProperty(name, value); 4469 return value; 4470 } 4471 4472 @Override 4473 public void removeChild(String name, Base value) throws FHIRException { 4474 if (name.equals("quantity")) { 4475 this.quantity = null; 4476 } else if (name.equals("period")) { 4477 this.period = null; 4478 } else 4479 super.removeChild(name, value); 4480 4481 } 4482 4483 @Override 4484 public Base makeProperty(int hash, String name) throws FHIRException { 4485 switch (hash) { 4486 case -1285004149: return getQuantity(); 4487 case -991726143: return getPeriod(); 4488 default: return super.makeProperty(hash, name); 4489 } 4490 4491 } 4492 4493 @Override 4494 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4495 switch (hash) { 4496 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 4497 case -991726143: /*period*/ return new String[] {"Duration"}; 4498 default: return super.getTypesForProperty(hash, name); 4499 } 4500 4501 } 4502 4503 @Override 4504 public Base addChild(String name) throws FHIRException { 4505 if (name.equals("quantity")) { 4506 this.quantity = new Quantity(); 4507 return this.quantity; 4508 } 4509 else if (name.equals("period")) { 4510 this.period = new Duration(); 4511 return this.period; 4512 } 4513 else 4514 return super.addChild(name); 4515 } 4516 4517 public MedicationKnowledgeRegulatoryMaxDispenseComponent copy() { 4518 MedicationKnowledgeRegulatoryMaxDispenseComponent dst = new MedicationKnowledgeRegulatoryMaxDispenseComponent(); 4519 copyValues(dst); 4520 return dst; 4521 } 4522 4523 public void copyValues(MedicationKnowledgeRegulatoryMaxDispenseComponent dst) { 4524 super.copyValues(dst); 4525 dst.quantity = quantity == null ? null : quantity.copy(); 4526 dst.period = period == null ? null : period.copy(); 4527 } 4528 4529 @Override 4530 public boolean equalsDeep(Base other_) { 4531 if (!super.equalsDeep(other_)) 4532 return false; 4533 if (!(other_ instanceof MedicationKnowledgeRegulatoryMaxDispenseComponent)) 4534 return false; 4535 MedicationKnowledgeRegulatoryMaxDispenseComponent o = (MedicationKnowledgeRegulatoryMaxDispenseComponent) other_; 4536 return compareDeep(quantity, o.quantity, true) && compareDeep(period, o.period, true); 4537 } 4538 4539 @Override 4540 public boolean equalsShallow(Base other_) { 4541 if (!super.equalsShallow(other_)) 4542 return false; 4543 if (!(other_ instanceof MedicationKnowledgeRegulatoryMaxDispenseComponent)) 4544 return false; 4545 MedicationKnowledgeRegulatoryMaxDispenseComponent o = (MedicationKnowledgeRegulatoryMaxDispenseComponent) other_; 4546 return true; 4547 } 4548 4549 public boolean isEmpty() { 4550 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, period); 4551 } 4552 4553 public String fhirType() { 4554 return "MedicationKnowledge.regulatory.maxDispense"; 4555 4556 } 4557 4558 } 4559 4560 @Block() 4561 public static class MedicationKnowledgeDefinitionalComponent extends BackboneElement implements IBaseBackboneElement { 4562 /** 4563 * Associated definitions for this medication. 4564 */ 4565 @Child(name = "definition", type = {MedicinalProductDefinition.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4566 @Description(shortDefinition="Definitional resources that provide more information about this medication", formalDefinition="Associated definitions for this medication." ) 4567 protected List<Reference> definition; 4568 4569 /** 4570 * Describes the form of the item. Powder; tablets; capsule. 4571 */ 4572 @Child(name = "doseForm", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 4573 @Description(shortDefinition="powder | tablets | capsule +", formalDefinition="Describes the form of the item. Powder; tablets; capsule." ) 4574 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-form-codes") 4575 protected CodeableConcept doseForm; 4576 4577 /** 4578 * The intended or approved route of administration. 4579 */ 4580 @Child(name = "intendedRoute", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4581 @Description(shortDefinition="The intended or approved route of administration", formalDefinition="The intended or approved route of administration." ) 4582 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/route-codes") 4583 protected List<CodeableConcept> intendedRoute; 4584 4585 /** 4586 * Identifies a particular constituent of interest in the product. 4587 */ 4588 @Child(name = "ingredient", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4589 @Description(shortDefinition="Active or inactive ingredient", formalDefinition="Identifies a particular constituent of interest in the product." ) 4590 protected List<MedicationKnowledgeDefinitionalIngredientComponent> ingredient; 4591 4592 /** 4593 * Specifies descriptive properties of the medicine, such as color, shape, imprints, etc. 4594 */ 4595 @Child(name = "drugCharacteristic", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4596 @Description(shortDefinition="Specifies descriptive properties of the medicine", formalDefinition="Specifies descriptive properties of the medicine, such as color, shape, imprints, etc." ) 4597 protected List<MedicationKnowledgeDefinitionalDrugCharacteristicComponent> drugCharacteristic; 4598 4599 private static final long serialVersionUID = 2050532775L; 4600 4601 /** 4602 * Constructor 4603 */ 4604 public MedicationKnowledgeDefinitionalComponent() { 4605 super(); 4606 } 4607 4608 /** 4609 * @return {@link #definition} (Associated definitions for this medication.) 4610 */ 4611 public List<Reference> getDefinition() { 4612 if (this.definition == null) 4613 this.definition = new ArrayList<Reference>(); 4614 return this.definition; 4615 } 4616 4617 /** 4618 * @return Returns a reference to <code>this</code> for easy method chaining 4619 */ 4620 public MedicationKnowledgeDefinitionalComponent setDefinition(List<Reference> theDefinition) { 4621 this.definition = theDefinition; 4622 return this; 4623 } 4624 4625 public boolean hasDefinition() { 4626 if (this.definition == null) 4627 return false; 4628 for (Reference item : this.definition) 4629 if (!item.isEmpty()) 4630 return true; 4631 return false; 4632 } 4633 4634 public Reference addDefinition() { //3 4635 Reference t = new Reference(); 4636 if (this.definition == null) 4637 this.definition = new ArrayList<Reference>(); 4638 this.definition.add(t); 4639 return t; 4640 } 4641 4642 public MedicationKnowledgeDefinitionalComponent addDefinition(Reference t) { //3 4643 if (t == null) 4644 return this; 4645 if (this.definition == null) 4646 this.definition = new ArrayList<Reference>(); 4647 this.definition.add(t); 4648 return this; 4649 } 4650 4651 /** 4652 * @return The first repetition of repeating field {@link #definition}, creating it if it does not already exist {3} 4653 */ 4654 public Reference getDefinitionFirstRep() { 4655 if (getDefinition().isEmpty()) { 4656 addDefinition(); 4657 } 4658 return getDefinition().get(0); 4659 } 4660 4661 /** 4662 * @return {@link #doseForm} (Describes the form of the item. Powder; tablets; capsule.) 4663 */ 4664 public CodeableConcept getDoseForm() { 4665 if (this.doseForm == null) 4666 if (Configuration.errorOnAutoCreate()) 4667 throw new Error("Attempt to auto-create MedicationKnowledgeDefinitionalComponent.doseForm"); 4668 else if (Configuration.doAutoCreate()) 4669 this.doseForm = new CodeableConcept(); // cc 4670 return this.doseForm; 4671 } 4672 4673 public boolean hasDoseForm() { 4674 return this.doseForm != null && !this.doseForm.isEmpty(); 4675 } 4676 4677 /** 4678 * @param value {@link #doseForm} (Describes the form of the item. Powder; tablets; capsule.) 4679 */ 4680 public MedicationKnowledgeDefinitionalComponent setDoseForm(CodeableConcept value) { 4681 this.doseForm = value; 4682 return this; 4683 } 4684 4685 /** 4686 * @return {@link #intendedRoute} (The intended or approved route of administration.) 4687 */ 4688 public List<CodeableConcept> getIntendedRoute() { 4689 if (this.intendedRoute == null) 4690 this.intendedRoute = new ArrayList<CodeableConcept>(); 4691 return this.intendedRoute; 4692 } 4693 4694 /** 4695 * @return Returns a reference to <code>this</code> for easy method chaining 4696 */ 4697 public MedicationKnowledgeDefinitionalComponent setIntendedRoute(List<CodeableConcept> theIntendedRoute) { 4698 this.intendedRoute = theIntendedRoute; 4699 return this; 4700 } 4701 4702 public boolean hasIntendedRoute() { 4703 if (this.intendedRoute == null) 4704 return false; 4705 for (CodeableConcept item : this.intendedRoute) 4706 if (!item.isEmpty()) 4707 return true; 4708 return false; 4709 } 4710 4711 public CodeableConcept addIntendedRoute() { //3 4712 CodeableConcept t = new CodeableConcept(); 4713 if (this.intendedRoute == null) 4714 this.intendedRoute = new ArrayList<CodeableConcept>(); 4715 this.intendedRoute.add(t); 4716 return t; 4717 } 4718 4719 public MedicationKnowledgeDefinitionalComponent addIntendedRoute(CodeableConcept t) { //3 4720 if (t == null) 4721 return this; 4722 if (this.intendedRoute == null) 4723 this.intendedRoute = new ArrayList<CodeableConcept>(); 4724 this.intendedRoute.add(t); 4725 return this; 4726 } 4727 4728 /** 4729 * @return The first repetition of repeating field {@link #intendedRoute}, creating it if it does not already exist {3} 4730 */ 4731 public CodeableConcept getIntendedRouteFirstRep() { 4732 if (getIntendedRoute().isEmpty()) { 4733 addIntendedRoute(); 4734 } 4735 return getIntendedRoute().get(0); 4736 } 4737 4738 /** 4739 * @return {@link #ingredient} (Identifies a particular constituent of interest in the product.) 4740 */ 4741 public List<MedicationKnowledgeDefinitionalIngredientComponent> getIngredient() { 4742 if (this.ingredient == null) 4743 this.ingredient = new ArrayList<MedicationKnowledgeDefinitionalIngredientComponent>(); 4744 return this.ingredient; 4745 } 4746 4747 /** 4748 * @return Returns a reference to <code>this</code> for easy method chaining 4749 */ 4750 public MedicationKnowledgeDefinitionalComponent setIngredient(List<MedicationKnowledgeDefinitionalIngredientComponent> theIngredient) { 4751 this.ingredient = theIngredient; 4752 return this; 4753 } 4754 4755 public boolean hasIngredient() { 4756 if (this.ingredient == null) 4757 return false; 4758 for (MedicationKnowledgeDefinitionalIngredientComponent item : this.ingredient) 4759 if (!item.isEmpty()) 4760 return true; 4761 return false; 4762 } 4763 4764 public MedicationKnowledgeDefinitionalIngredientComponent addIngredient() { //3 4765 MedicationKnowledgeDefinitionalIngredientComponent t = new MedicationKnowledgeDefinitionalIngredientComponent(); 4766 if (this.ingredient == null) 4767 this.ingredient = new ArrayList<MedicationKnowledgeDefinitionalIngredientComponent>(); 4768 this.ingredient.add(t); 4769 return t; 4770 } 4771 4772 public MedicationKnowledgeDefinitionalComponent addIngredient(MedicationKnowledgeDefinitionalIngredientComponent t) { //3 4773 if (t == null) 4774 return this; 4775 if (this.ingredient == null) 4776 this.ingredient = new ArrayList<MedicationKnowledgeDefinitionalIngredientComponent>(); 4777 this.ingredient.add(t); 4778 return this; 4779 } 4780 4781 /** 4782 * @return The first repetition of repeating field {@link #ingredient}, creating it if it does not already exist {3} 4783 */ 4784 public MedicationKnowledgeDefinitionalIngredientComponent getIngredientFirstRep() { 4785 if (getIngredient().isEmpty()) { 4786 addIngredient(); 4787 } 4788 return getIngredient().get(0); 4789 } 4790 4791 /** 4792 * @return {@link #drugCharacteristic} (Specifies descriptive properties of the medicine, such as color, shape, imprints, etc.) 4793 */ 4794 public List<MedicationKnowledgeDefinitionalDrugCharacteristicComponent> getDrugCharacteristic() { 4795 if (this.drugCharacteristic == null) 4796 this.drugCharacteristic = new ArrayList<MedicationKnowledgeDefinitionalDrugCharacteristicComponent>(); 4797 return this.drugCharacteristic; 4798 } 4799 4800 /** 4801 * @return Returns a reference to <code>this</code> for easy method chaining 4802 */ 4803 public MedicationKnowledgeDefinitionalComponent setDrugCharacteristic(List<MedicationKnowledgeDefinitionalDrugCharacteristicComponent> theDrugCharacteristic) { 4804 this.drugCharacteristic = theDrugCharacteristic; 4805 return this; 4806 } 4807 4808 public boolean hasDrugCharacteristic() { 4809 if (this.drugCharacteristic == null) 4810 return false; 4811 for (MedicationKnowledgeDefinitionalDrugCharacteristicComponent item : this.drugCharacteristic) 4812 if (!item.isEmpty()) 4813 return true; 4814 return false; 4815 } 4816 4817 public MedicationKnowledgeDefinitionalDrugCharacteristicComponent addDrugCharacteristic() { //3 4818 MedicationKnowledgeDefinitionalDrugCharacteristicComponent t = new MedicationKnowledgeDefinitionalDrugCharacteristicComponent(); 4819 if (this.drugCharacteristic == null) 4820 this.drugCharacteristic = new ArrayList<MedicationKnowledgeDefinitionalDrugCharacteristicComponent>(); 4821 this.drugCharacteristic.add(t); 4822 return t; 4823 } 4824 4825 public MedicationKnowledgeDefinitionalComponent addDrugCharacteristic(MedicationKnowledgeDefinitionalDrugCharacteristicComponent t) { //3 4826 if (t == null) 4827 return this; 4828 if (this.drugCharacteristic == null) 4829 this.drugCharacteristic = new ArrayList<MedicationKnowledgeDefinitionalDrugCharacteristicComponent>(); 4830 this.drugCharacteristic.add(t); 4831 return this; 4832 } 4833 4834 /** 4835 * @return The first repetition of repeating field {@link #drugCharacteristic}, creating it if it does not already exist {3} 4836 */ 4837 public MedicationKnowledgeDefinitionalDrugCharacteristicComponent getDrugCharacteristicFirstRep() { 4838 if (getDrugCharacteristic().isEmpty()) { 4839 addDrugCharacteristic(); 4840 } 4841 return getDrugCharacteristic().get(0); 4842 } 4843 4844 protected void listChildren(List<Property> children) { 4845 super.listChildren(children); 4846 children.add(new Property("definition", "Reference(MedicinalProductDefinition)", "Associated definitions for this medication.", 0, java.lang.Integer.MAX_VALUE, definition)); 4847 children.add(new Property("doseForm", "CodeableConcept", "Describes the form of the item. Powder; tablets; capsule.", 0, 1, doseForm)); 4848 children.add(new Property("intendedRoute", "CodeableConcept", "The intended or approved route of administration.", 0, java.lang.Integer.MAX_VALUE, intendedRoute)); 4849 children.add(new Property("ingredient", "", "Identifies a particular constituent of interest in the product.", 0, java.lang.Integer.MAX_VALUE, ingredient)); 4850 children.add(new Property("drugCharacteristic", "", "Specifies descriptive properties of the medicine, such as color, shape, imprints, etc.", 0, java.lang.Integer.MAX_VALUE, drugCharacteristic)); 4851 } 4852 4853 @Override 4854 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4855 switch (_hash) { 4856 case -1014418093: /*definition*/ return new Property("definition", "Reference(MedicinalProductDefinition)", "Associated definitions for this medication.", 0, java.lang.Integer.MAX_VALUE, definition); 4857 case 1303858817: /*doseForm*/ return new Property("doseForm", "CodeableConcept", "Describes the form of the item. Powder; tablets; capsule.", 0, 1, doseForm); 4858 case -767798050: /*intendedRoute*/ return new Property("intendedRoute", "CodeableConcept", "The intended or approved route of administration.", 0, java.lang.Integer.MAX_VALUE, intendedRoute); 4859 case -206409263: /*ingredient*/ return new Property("ingredient", "", "Identifies a particular constituent of interest in the product.", 0, java.lang.Integer.MAX_VALUE, ingredient); 4860 case -844126885: /*drugCharacteristic*/ return new Property("drugCharacteristic", "", "Specifies descriptive properties of the medicine, such as color, shape, imprints, etc.", 0, java.lang.Integer.MAX_VALUE, drugCharacteristic); 4861 default: return super.getNamedProperty(_hash, _name, _checkValid); 4862 } 4863 4864 } 4865 4866 @Override 4867 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4868 switch (hash) { 4869 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // Reference 4870 case 1303858817: /*doseForm*/ return this.doseForm == null ? new Base[0] : new Base[] {this.doseForm}; // CodeableConcept 4871 case -767798050: /*intendedRoute*/ return this.intendedRoute == null ? new Base[0] : this.intendedRoute.toArray(new Base[this.intendedRoute.size()]); // CodeableConcept 4872 case -206409263: /*ingredient*/ return this.ingredient == null ? new Base[0] : this.ingredient.toArray(new Base[this.ingredient.size()]); // MedicationKnowledgeDefinitionalIngredientComponent 4873 case -844126885: /*drugCharacteristic*/ return this.drugCharacteristic == null ? new Base[0] : this.drugCharacteristic.toArray(new Base[this.drugCharacteristic.size()]); // MedicationKnowledgeDefinitionalDrugCharacteristicComponent 4874 default: return super.getProperty(hash, name, checkValid); 4875 } 4876 4877 } 4878 4879 @Override 4880 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4881 switch (hash) { 4882 case -1014418093: // definition 4883 this.getDefinition().add(TypeConvertor.castToReference(value)); // Reference 4884 return value; 4885 case 1303858817: // doseForm 4886 this.doseForm = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4887 return value; 4888 case -767798050: // intendedRoute 4889 this.getIntendedRoute().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4890 return value; 4891 case -206409263: // ingredient 4892 this.getIngredient().add((MedicationKnowledgeDefinitionalIngredientComponent) value); // MedicationKnowledgeDefinitionalIngredientComponent 4893 return value; 4894 case -844126885: // drugCharacteristic 4895 this.getDrugCharacteristic().add((MedicationKnowledgeDefinitionalDrugCharacteristicComponent) value); // MedicationKnowledgeDefinitionalDrugCharacteristicComponent 4896 return value; 4897 default: return super.setProperty(hash, name, value); 4898 } 4899 4900 } 4901 4902 @Override 4903 public Base setProperty(String name, Base value) throws FHIRException { 4904 if (name.equals("definition")) { 4905 this.getDefinition().add(TypeConvertor.castToReference(value)); 4906 } else if (name.equals("doseForm")) { 4907 this.doseForm = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4908 } else if (name.equals("intendedRoute")) { 4909 this.getIntendedRoute().add(TypeConvertor.castToCodeableConcept(value)); 4910 } else if (name.equals("ingredient")) { 4911 this.getIngredient().add((MedicationKnowledgeDefinitionalIngredientComponent) value); 4912 } else if (name.equals("drugCharacteristic")) { 4913 this.getDrugCharacteristic().add((MedicationKnowledgeDefinitionalDrugCharacteristicComponent) value); 4914 } else 4915 return super.setProperty(name, value); 4916 return value; 4917 } 4918 4919 @Override 4920 public void removeChild(String name, Base value) throws FHIRException { 4921 if (name.equals("definition")) { 4922 this.getDefinition().remove(value); 4923 } else if (name.equals("doseForm")) { 4924 this.doseForm = null; 4925 } else if (name.equals("intendedRoute")) { 4926 this.getIntendedRoute().remove(value); 4927 } else if (name.equals("ingredient")) { 4928 this.getIngredient().remove((MedicationKnowledgeDefinitionalIngredientComponent) value); 4929 } else if (name.equals("drugCharacteristic")) { 4930 this.getDrugCharacteristic().remove((MedicationKnowledgeDefinitionalDrugCharacteristicComponent) value); 4931 } else 4932 super.removeChild(name, value); 4933 4934 } 4935 4936 @Override 4937 public Base makeProperty(int hash, String name) throws FHIRException { 4938 switch (hash) { 4939 case -1014418093: return addDefinition(); 4940 case 1303858817: return getDoseForm(); 4941 case -767798050: return addIntendedRoute(); 4942 case -206409263: return addIngredient(); 4943 case -844126885: return addDrugCharacteristic(); 4944 default: return super.makeProperty(hash, name); 4945 } 4946 4947 } 4948 4949 @Override 4950 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4951 switch (hash) { 4952 case -1014418093: /*definition*/ return new String[] {"Reference"}; 4953 case 1303858817: /*doseForm*/ return new String[] {"CodeableConcept"}; 4954 case -767798050: /*intendedRoute*/ return new String[] {"CodeableConcept"}; 4955 case -206409263: /*ingredient*/ return new String[] {}; 4956 case -844126885: /*drugCharacteristic*/ return new String[] {}; 4957 default: return super.getTypesForProperty(hash, name); 4958 } 4959 4960 } 4961 4962 @Override 4963 public Base addChild(String name) throws FHIRException { 4964 if (name.equals("definition")) { 4965 return addDefinition(); 4966 } 4967 else if (name.equals("doseForm")) { 4968 this.doseForm = new CodeableConcept(); 4969 return this.doseForm; 4970 } 4971 else if (name.equals("intendedRoute")) { 4972 return addIntendedRoute(); 4973 } 4974 else if (name.equals("ingredient")) { 4975 return addIngredient(); 4976 } 4977 else if (name.equals("drugCharacteristic")) { 4978 return addDrugCharacteristic(); 4979 } 4980 else 4981 return super.addChild(name); 4982 } 4983 4984 public MedicationKnowledgeDefinitionalComponent copy() { 4985 MedicationKnowledgeDefinitionalComponent dst = new MedicationKnowledgeDefinitionalComponent(); 4986 copyValues(dst); 4987 return dst; 4988 } 4989 4990 public void copyValues(MedicationKnowledgeDefinitionalComponent dst) { 4991 super.copyValues(dst); 4992 if (definition != null) { 4993 dst.definition = new ArrayList<Reference>(); 4994 for (Reference i : definition) 4995 dst.definition.add(i.copy()); 4996 }; 4997 dst.doseForm = doseForm == null ? null : doseForm.copy(); 4998 if (intendedRoute != null) { 4999 dst.intendedRoute = new ArrayList<CodeableConcept>(); 5000 for (CodeableConcept i : intendedRoute) 5001 dst.intendedRoute.add(i.copy()); 5002 }; 5003 if (ingredient != null) { 5004 dst.ingredient = new ArrayList<MedicationKnowledgeDefinitionalIngredientComponent>(); 5005 for (MedicationKnowledgeDefinitionalIngredientComponent i : ingredient) 5006 dst.ingredient.add(i.copy()); 5007 }; 5008 if (drugCharacteristic != null) { 5009 dst.drugCharacteristic = new ArrayList<MedicationKnowledgeDefinitionalDrugCharacteristicComponent>(); 5010 for (MedicationKnowledgeDefinitionalDrugCharacteristicComponent i : drugCharacteristic) 5011 dst.drugCharacteristic.add(i.copy()); 5012 }; 5013 } 5014 5015 @Override 5016 public boolean equalsDeep(Base other_) { 5017 if (!super.equalsDeep(other_)) 5018 return false; 5019 if (!(other_ instanceof MedicationKnowledgeDefinitionalComponent)) 5020 return false; 5021 MedicationKnowledgeDefinitionalComponent o = (MedicationKnowledgeDefinitionalComponent) other_; 5022 return compareDeep(definition, o.definition, true) && compareDeep(doseForm, o.doseForm, true) && compareDeep(intendedRoute, o.intendedRoute, true) 5023 && compareDeep(ingredient, o.ingredient, true) && compareDeep(drugCharacteristic, o.drugCharacteristic, true) 5024 ; 5025 } 5026 5027 @Override 5028 public boolean equalsShallow(Base other_) { 5029 if (!super.equalsShallow(other_)) 5030 return false; 5031 if (!(other_ instanceof MedicationKnowledgeDefinitionalComponent)) 5032 return false; 5033 MedicationKnowledgeDefinitionalComponent o = (MedicationKnowledgeDefinitionalComponent) other_; 5034 return true; 5035 } 5036 5037 public boolean isEmpty() { 5038 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(definition, doseForm, intendedRoute 5039 , ingredient, drugCharacteristic); 5040 } 5041 5042 public String fhirType() { 5043 return "MedicationKnowledge.definitional"; 5044 5045 } 5046 5047 } 5048 5049 @Block() 5050 public static class MedicationKnowledgeDefinitionalIngredientComponent extends BackboneElement implements IBaseBackboneElement { 5051 /** 5052 * A reference to the resource that provides information about the ingredient. 5053 */ 5054 @Child(name = "item", type = {CodeableReference.class}, order=1, min=1, max=1, modifier=false, summary=true) 5055 @Description(shortDefinition="Substances contained in the medication", formalDefinition="A reference to the resource that provides information about the ingredient." ) 5056 protected CodeableReference item; 5057 5058 /** 5059 * Indication of whether this ingredient affects the therapeutic action of the drug. 5060 */ 5061 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 5062 @Description(shortDefinition="A code that defines the type of ingredient, active, base, etc", formalDefinition="Indication of whether this ingredient affects the therapeutic action of the drug." ) 5063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-RoleClassIngredientEntity") 5064 protected CodeableConcept type; 5065 5066 /** 5067 * Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet. 5068 */ 5069 @Child(name = "strength", type = {Ratio.class, CodeableConcept.class, Quantity.class}, order=3, min=0, max=1, modifier=false, summary=false) 5070 @Description(shortDefinition="Quantity of ingredient present", formalDefinition="Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet." ) 5071 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-ingredientstrength") 5072 protected DataType strength; 5073 5074 private static final long serialVersionUID = 1772676131L; 5075 5076 /** 5077 * Constructor 5078 */ 5079 public MedicationKnowledgeDefinitionalIngredientComponent() { 5080 super(); 5081 } 5082 5083 /** 5084 * Constructor 5085 */ 5086 public MedicationKnowledgeDefinitionalIngredientComponent(CodeableReference item) { 5087 super(); 5088 this.setItem(item); 5089 } 5090 5091 /** 5092 * @return {@link #item} (A reference to the resource that provides information about the ingredient.) 5093 */ 5094 public CodeableReference getItem() { 5095 if (this.item == null) 5096 if (Configuration.errorOnAutoCreate()) 5097 throw new Error("Attempt to auto-create MedicationKnowledgeDefinitionalIngredientComponent.item"); 5098 else if (Configuration.doAutoCreate()) 5099 this.item = new CodeableReference(); // cc 5100 return this.item; 5101 } 5102 5103 public boolean hasItem() { 5104 return this.item != null && !this.item.isEmpty(); 5105 } 5106 5107 /** 5108 * @param value {@link #item} (A reference to the resource that provides information about the ingredient.) 5109 */ 5110 public MedicationKnowledgeDefinitionalIngredientComponent setItem(CodeableReference value) { 5111 this.item = value; 5112 return this; 5113 } 5114 5115 /** 5116 * @return {@link #type} (Indication of whether this ingredient affects the therapeutic action of the drug.) 5117 */ 5118 public CodeableConcept getType() { 5119 if (this.type == null) 5120 if (Configuration.errorOnAutoCreate()) 5121 throw new Error("Attempt to auto-create MedicationKnowledgeDefinitionalIngredientComponent.type"); 5122 else if (Configuration.doAutoCreate()) 5123 this.type = new CodeableConcept(); // cc 5124 return this.type; 5125 } 5126 5127 public boolean hasType() { 5128 return this.type != null && !this.type.isEmpty(); 5129 } 5130 5131 /** 5132 * @param value {@link #type} (Indication of whether this ingredient affects the therapeutic action of the drug.) 5133 */ 5134 public MedicationKnowledgeDefinitionalIngredientComponent setType(CodeableConcept value) { 5135 this.type = value; 5136 return this; 5137 } 5138 5139 /** 5140 * @return {@link #strength} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.) 5141 */ 5142 public DataType getStrength() { 5143 return this.strength; 5144 } 5145 5146 /** 5147 * @return {@link #strength} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.) 5148 */ 5149 public Ratio getStrengthRatio() throws FHIRException { 5150 if (this.strength == null) 5151 this.strength = new Ratio(); 5152 if (!(this.strength instanceof Ratio)) 5153 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.strength.getClass().getName()+" was encountered"); 5154 return (Ratio) this.strength; 5155 } 5156 5157 public boolean hasStrengthRatio() { 5158 return this != null && this.strength instanceof Ratio; 5159 } 5160 5161 /** 5162 * @return {@link #strength} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.) 5163 */ 5164 public CodeableConcept getStrengthCodeableConcept() throws FHIRException { 5165 if (this.strength == null) 5166 this.strength = new CodeableConcept(); 5167 if (!(this.strength instanceof CodeableConcept)) 5168 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.strength.getClass().getName()+" was encountered"); 5169 return (CodeableConcept) this.strength; 5170 } 5171 5172 public boolean hasStrengthCodeableConcept() { 5173 return this != null && this.strength instanceof CodeableConcept; 5174 } 5175 5176 /** 5177 * @return {@link #strength} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.) 5178 */ 5179 public Quantity getStrengthQuantity() throws FHIRException { 5180 if (this.strength == null) 5181 this.strength = new Quantity(); 5182 if (!(this.strength instanceof Quantity)) 5183 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.strength.getClass().getName()+" was encountered"); 5184 return (Quantity) this.strength; 5185 } 5186 5187 public boolean hasStrengthQuantity() { 5188 return this != null && this.strength instanceof Quantity; 5189 } 5190 5191 public boolean hasStrength() { 5192 return this.strength != null && !this.strength.isEmpty(); 5193 } 5194 5195 /** 5196 * @param value {@link #strength} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.) 5197 */ 5198 public MedicationKnowledgeDefinitionalIngredientComponent setStrength(DataType value) { 5199 if (value != null && !(value instanceof Ratio || value instanceof CodeableConcept || value instanceof Quantity)) 5200 throw new FHIRException("Not the right type for MedicationKnowledge.definitional.ingredient.strength[x]: "+value.fhirType()); 5201 this.strength = value; 5202 return this; 5203 } 5204 5205 protected void listChildren(List<Property> children) { 5206 super.listChildren(children); 5207 children.add(new Property("item", "CodeableReference(Substance)", "A reference to the resource that provides information about the ingredient.", 0, 1, item)); 5208 children.add(new Property("type", "CodeableConcept", "Indication of whether this ingredient affects the therapeutic action of the drug.", 0, 1, type)); 5209 children.add(new Property("strength[x]", "Ratio|CodeableConcept|Quantity", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength)); 5210 } 5211 5212 @Override 5213 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5214 switch (_hash) { 5215 case 3242771: /*item*/ return new Property("item", "CodeableReference(Substance)", "A reference to the resource that provides information about the ingredient.", 0, 1, item); 5216 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Indication of whether this ingredient affects the therapeutic action of the drug.", 0, 1, type); 5217 case 127377567: /*strength[x]*/ return new Property("strength[x]", "Ratio|CodeableConcept|Quantity", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength); 5218 case 1791316033: /*strength*/ return new Property("strength[x]", "Ratio|CodeableConcept|Quantity", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength); 5219 case 2141786186: /*strengthRatio*/ return new Property("strength[x]", "Ratio", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength); 5220 case -1455903456: /*strengthCodeableConcept*/ return new Property("strength[x]", "CodeableConcept", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength); 5221 case -1793570836: /*strengthQuantity*/ return new Property("strength[x]", "Quantity", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength); 5222 default: return super.getNamedProperty(_hash, _name, _checkValid); 5223 } 5224 5225 } 5226 5227 @Override 5228 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5229 switch (hash) { 5230 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // CodeableReference 5231 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 5232 case 1791316033: /*strength*/ return this.strength == null ? new Base[0] : new Base[] {this.strength}; // DataType 5233 default: return super.getProperty(hash, name, checkValid); 5234 } 5235 5236 } 5237 5238 @Override 5239 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5240 switch (hash) { 5241 case 3242771: // item 5242 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 5243 return value; 5244 case 3575610: // type 5245 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5246 return value; 5247 case 1791316033: // strength 5248 this.strength = TypeConvertor.castToType(value); // DataType 5249 return value; 5250 default: return super.setProperty(hash, name, value); 5251 } 5252 5253 } 5254 5255 @Override 5256 public Base setProperty(String name, Base value) throws FHIRException { 5257 if (name.equals("item")) { 5258 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 5259 } else if (name.equals("type")) { 5260 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5261 } else if (name.equals("strength[x]")) { 5262 this.strength = TypeConvertor.castToType(value); // DataType 5263 } else 5264 return super.setProperty(name, value); 5265 return value; 5266 } 5267 5268 @Override 5269 public void removeChild(String name, Base value) throws FHIRException { 5270 if (name.equals("item")) { 5271 this.item = null; 5272 } else if (name.equals("type")) { 5273 this.type = null; 5274 } else if (name.equals("strength[x]")) { 5275 this.strength = null; 5276 } else 5277 super.removeChild(name, value); 5278 5279 } 5280 5281 @Override 5282 public Base makeProperty(int hash, String name) throws FHIRException { 5283 switch (hash) { 5284 case 3242771: return getItem(); 5285 case 3575610: return getType(); 5286 case 127377567: return getStrength(); 5287 case 1791316033: return getStrength(); 5288 default: return super.makeProperty(hash, name); 5289 } 5290 5291 } 5292 5293 @Override 5294 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5295 switch (hash) { 5296 case 3242771: /*item*/ return new String[] {"CodeableReference"}; 5297 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 5298 case 1791316033: /*strength*/ return new String[] {"Ratio", "CodeableConcept", "Quantity"}; 5299 default: return super.getTypesForProperty(hash, name); 5300 } 5301 5302 } 5303 5304 @Override 5305 public Base addChild(String name) throws FHIRException { 5306 if (name.equals("item")) { 5307 this.item = new CodeableReference(); 5308 return this.item; 5309 } 5310 else if (name.equals("type")) { 5311 this.type = new CodeableConcept(); 5312 return this.type; 5313 } 5314 else if (name.equals("strengthRatio")) { 5315 this.strength = new Ratio(); 5316 return this.strength; 5317 } 5318 else if (name.equals("strengthCodeableConcept")) { 5319 this.strength = new CodeableConcept(); 5320 return this.strength; 5321 } 5322 else if (name.equals("strengthQuantity")) { 5323 this.strength = new Quantity(); 5324 return this.strength; 5325 } 5326 else 5327 return super.addChild(name); 5328 } 5329 5330 public MedicationKnowledgeDefinitionalIngredientComponent copy() { 5331 MedicationKnowledgeDefinitionalIngredientComponent dst = new MedicationKnowledgeDefinitionalIngredientComponent(); 5332 copyValues(dst); 5333 return dst; 5334 } 5335 5336 public void copyValues(MedicationKnowledgeDefinitionalIngredientComponent dst) { 5337 super.copyValues(dst); 5338 dst.item = item == null ? null : item.copy(); 5339 dst.type = type == null ? null : type.copy(); 5340 dst.strength = strength == null ? null : strength.copy(); 5341 } 5342 5343 @Override 5344 public boolean equalsDeep(Base other_) { 5345 if (!super.equalsDeep(other_)) 5346 return false; 5347 if (!(other_ instanceof MedicationKnowledgeDefinitionalIngredientComponent)) 5348 return false; 5349 MedicationKnowledgeDefinitionalIngredientComponent o = (MedicationKnowledgeDefinitionalIngredientComponent) other_; 5350 return compareDeep(item, o.item, true) && compareDeep(type, o.type, true) && compareDeep(strength, o.strength, true) 5351 ; 5352 } 5353 5354 @Override 5355 public boolean equalsShallow(Base other_) { 5356 if (!super.equalsShallow(other_)) 5357 return false; 5358 if (!(other_ instanceof MedicationKnowledgeDefinitionalIngredientComponent)) 5359 return false; 5360 MedicationKnowledgeDefinitionalIngredientComponent o = (MedicationKnowledgeDefinitionalIngredientComponent) other_; 5361 return true; 5362 } 5363 5364 public boolean isEmpty() { 5365 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item, type, strength); 5366 } 5367 5368 public String fhirType() { 5369 return "MedicationKnowledge.definitional.ingredient"; 5370 5371 } 5372 5373 } 5374 5375 @Block() 5376 public static class MedicationKnowledgeDefinitionalDrugCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 5377 /** 5378 * A code specifying which characteristic of the medicine is being described (for example, colour, shape, imprint). 5379 */ 5380 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 5381 @Description(shortDefinition="Code specifying the type of characteristic of medication", formalDefinition="A code specifying which characteristic of the medicine is being described (for example, colour, shape, imprint)." ) 5382 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationknowledge-characteristic") 5383 protected CodeableConcept type; 5384 5385 /** 5386 * Description of the characteristic. 5387 */ 5388 @Child(name = "value", type = {CodeableConcept.class, StringType.class, Quantity.class, Base64BinaryType.class, Attachment.class}, order=2, min=0, max=1, modifier=false, summary=false) 5389 @Description(shortDefinition="Description of the characteristic", formalDefinition="Description of the characteristic." ) 5390 protected DataType value; 5391 5392 private static final long serialVersionUID = -1659186716L; 5393 5394 /** 5395 * Constructor 5396 */ 5397 public MedicationKnowledgeDefinitionalDrugCharacteristicComponent() { 5398 super(); 5399 } 5400 5401 /** 5402 * @return {@link #type} (A code specifying which characteristic of the medicine is being described (for example, colour, shape, imprint).) 5403 */ 5404 public CodeableConcept getType() { 5405 if (this.type == null) 5406 if (Configuration.errorOnAutoCreate()) 5407 throw new Error("Attempt to auto-create MedicationKnowledgeDefinitionalDrugCharacteristicComponent.type"); 5408 else if (Configuration.doAutoCreate()) 5409 this.type = new CodeableConcept(); // cc 5410 return this.type; 5411 } 5412 5413 public boolean hasType() { 5414 return this.type != null && !this.type.isEmpty(); 5415 } 5416 5417 /** 5418 * @param value {@link #type} (A code specifying which characteristic of the medicine is being described (for example, colour, shape, imprint).) 5419 */ 5420 public MedicationKnowledgeDefinitionalDrugCharacteristicComponent setType(CodeableConcept value) { 5421 this.type = value; 5422 return this; 5423 } 5424 5425 /** 5426 * @return {@link #value} (Description of the characteristic.) 5427 */ 5428 public DataType getValue() { 5429 return this.value; 5430 } 5431 5432 /** 5433 * @return {@link #value} (Description of the characteristic.) 5434 */ 5435 public CodeableConcept getValueCodeableConcept() throws FHIRException { 5436 if (this.value == null) 5437 this.value = new CodeableConcept(); 5438 if (!(this.value instanceof CodeableConcept)) 5439 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 5440 return (CodeableConcept) this.value; 5441 } 5442 5443 public boolean hasValueCodeableConcept() { 5444 return this != null && this.value instanceof CodeableConcept; 5445 } 5446 5447 /** 5448 * @return {@link #value} (Description of the characteristic.) 5449 */ 5450 public StringType getValueStringType() throws FHIRException { 5451 if (this.value == null) 5452 this.value = new StringType(); 5453 if (!(this.value instanceof StringType)) 5454 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 5455 return (StringType) this.value; 5456 } 5457 5458 public boolean hasValueStringType() { 5459 return this != null && this.value instanceof StringType; 5460 } 5461 5462 /** 5463 * @return {@link #value} (Description of the characteristic.) 5464 */ 5465 public Quantity getValueQuantity() throws FHIRException { 5466 if (this.value == null) 5467 this.value = new Quantity(); 5468 if (!(this.value instanceof Quantity)) 5469 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 5470 return (Quantity) this.value; 5471 } 5472 5473 public boolean hasValueQuantity() { 5474 return this != null && this.value instanceof Quantity; 5475 } 5476 5477 /** 5478 * @return {@link #value} (Description of the characteristic.) 5479 */ 5480 public Base64BinaryType getValueBase64BinaryType() throws FHIRException { 5481 if (this.value == null) 5482 this.value = new Base64BinaryType(); 5483 if (!(this.value instanceof Base64BinaryType)) 5484 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.value.getClass().getName()+" was encountered"); 5485 return (Base64BinaryType) this.value; 5486 } 5487 5488 public boolean hasValueBase64BinaryType() { 5489 return this != null && this.value instanceof Base64BinaryType; 5490 } 5491 5492 /** 5493 * @return {@link #value} (Description of the characteristic.) 5494 */ 5495 public Attachment getValueAttachment() throws FHIRException { 5496 if (this.value == null) 5497 this.value = new Attachment(); 5498 if (!(this.value instanceof Attachment)) 5499 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 5500 return (Attachment) this.value; 5501 } 5502 5503 public boolean hasValueAttachment() { 5504 return this != null && this.value instanceof Attachment; 5505 } 5506 5507 public boolean hasValue() { 5508 return this.value != null && !this.value.isEmpty(); 5509 } 5510 5511 /** 5512 * @param value {@link #value} (Description of the characteristic.) 5513 */ 5514 public MedicationKnowledgeDefinitionalDrugCharacteristicComponent setValue(DataType value) { 5515 if (value != null && !(value instanceof CodeableConcept || value instanceof StringType || value instanceof Quantity || value instanceof Base64BinaryType || value instanceof Attachment)) 5516 throw new FHIRException("Not the right type for MedicationKnowledge.definitional.drugCharacteristic.value[x]: "+value.fhirType()); 5517 this.value = value; 5518 return this; 5519 } 5520 5521 protected void listChildren(List<Property> children) { 5522 super.listChildren(children); 5523 children.add(new Property("type", "CodeableConcept", "A code specifying which characteristic of the medicine is being described (for example, colour, shape, imprint).", 0, 1, type)); 5524 children.add(new Property("value[x]", "CodeableConcept|string|Quantity|base64Binary|Attachment", "Description of the characteristic.", 0, 1, value)); 5525 } 5526 5527 @Override 5528 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5529 switch (_hash) { 5530 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A code specifying which characteristic of the medicine is being described (for example, colour, shape, imprint).", 0, 1, type); 5531 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|string|Quantity|base64Binary|Attachment", "Description of the characteristic.", 0, 1, value); 5532 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|string|Quantity|base64Binary|Attachment", "Description of the characteristic.", 0, 1, value); 5533 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "Description of the characteristic.", 0, 1, value); 5534 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "Description of the characteristic.", 0, 1, value); 5535 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Description of the characteristic.", 0, 1, value); 5536 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "base64Binary", "Description of the characteristic.", 0, 1, value); 5537 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "Description of the characteristic.", 0, 1, value); 5538 default: return super.getNamedProperty(_hash, _name, _checkValid); 5539 } 5540 5541 } 5542 5543 @Override 5544 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5545 switch (hash) { 5546 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 5547 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 5548 default: return super.getProperty(hash, name, checkValid); 5549 } 5550 5551 } 5552 5553 @Override 5554 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5555 switch (hash) { 5556 case 3575610: // type 5557 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5558 return value; 5559 case 111972721: // value 5560 this.value = TypeConvertor.castToType(value); // DataType 5561 return value; 5562 default: return super.setProperty(hash, name, value); 5563 } 5564 5565 } 5566 5567 @Override 5568 public Base setProperty(String name, Base value) throws FHIRException { 5569 if (name.equals("type")) { 5570 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5571 } else if (name.equals("value[x]")) { 5572 this.value = TypeConvertor.castToType(value); // DataType 5573 } else 5574 return super.setProperty(name, value); 5575 return value; 5576 } 5577 5578 @Override 5579 public void removeChild(String name, Base value) throws FHIRException { 5580 if (name.equals("type")) { 5581 this.type = null; 5582 } else if (name.equals("value[x]")) { 5583 this.value = null; 5584 } else 5585 super.removeChild(name, value); 5586 5587 } 5588 5589 @Override 5590 public Base makeProperty(int hash, String name) throws FHIRException { 5591 switch (hash) { 5592 case 3575610: return getType(); 5593 case -1410166417: return getValue(); 5594 case 111972721: return getValue(); 5595 default: return super.makeProperty(hash, name); 5596 } 5597 5598 } 5599 5600 @Override 5601 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5602 switch (hash) { 5603 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 5604 case 111972721: /*value*/ return new String[] {"CodeableConcept", "string", "Quantity", "base64Binary", "Attachment"}; 5605 default: return super.getTypesForProperty(hash, name); 5606 } 5607 5608 } 5609 5610 @Override 5611 public Base addChild(String name) throws FHIRException { 5612 if (name.equals("type")) { 5613 this.type = new CodeableConcept(); 5614 return this.type; 5615 } 5616 else if (name.equals("valueCodeableConcept")) { 5617 this.value = new CodeableConcept(); 5618 return this.value; 5619 } 5620 else if (name.equals("valueString")) { 5621 this.value = new StringType(); 5622 return this.value; 5623 } 5624 else if (name.equals("valueQuantity")) { 5625 this.value = new Quantity(); 5626 return this.value; 5627 } 5628 else if (name.equals("valueBase64Binary")) { 5629 this.value = new Base64BinaryType(); 5630 return this.value; 5631 } 5632 else if (name.equals("valueAttachment")) { 5633 this.value = new Attachment(); 5634 return this.value; 5635 } 5636 else 5637 return super.addChild(name); 5638 } 5639 5640 public MedicationKnowledgeDefinitionalDrugCharacteristicComponent copy() { 5641 MedicationKnowledgeDefinitionalDrugCharacteristicComponent dst = new MedicationKnowledgeDefinitionalDrugCharacteristicComponent(); 5642 copyValues(dst); 5643 return dst; 5644 } 5645 5646 public void copyValues(MedicationKnowledgeDefinitionalDrugCharacteristicComponent dst) { 5647 super.copyValues(dst); 5648 dst.type = type == null ? null : type.copy(); 5649 dst.value = value == null ? null : value.copy(); 5650 } 5651 5652 @Override 5653 public boolean equalsDeep(Base other_) { 5654 if (!super.equalsDeep(other_)) 5655 return false; 5656 if (!(other_ instanceof MedicationKnowledgeDefinitionalDrugCharacteristicComponent)) 5657 return false; 5658 MedicationKnowledgeDefinitionalDrugCharacteristicComponent o = (MedicationKnowledgeDefinitionalDrugCharacteristicComponent) other_; 5659 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 5660 } 5661 5662 @Override 5663 public boolean equalsShallow(Base other_) { 5664 if (!super.equalsShallow(other_)) 5665 return false; 5666 if (!(other_ instanceof MedicationKnowledgeDefinitionalDrugCharacteristicComponent)) 5667 return false; 5668 MedicationKnowledgeDefinitionalDrugCharacteristicComponent o = (MedicationKnowledgeDefinitionalDrugCharacteristicComponent) other_; 5669 return true; 5670 } 5671 5672 public boolean isEmpty() { 5673 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 5674 } 5675 5676 public String fhirType() { 5677 return "MedicationKnowledge.definitional.drugCharacteristic"; 5678 5679 } 5680 5681 } 5682 5683 /** 5684 * Business identifier for this medication. 5685 */ 5686 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5687 @Description(shortDefinition="Business identifier for this medication", formalDefinition="Business identifier for this medication." ) 5688 protected List<Identifier> identifier; 5689 5690 /** 5691 * A code that specifies this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems. 5692 */ 5693 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 5694 @Description(shortDefinition="Code that identifies this medication", formalDefinition="A code that specifies this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems." ) 5695 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 5696 protected CodeableConcept code; 5697 5698 /** 5699 * A code to indicate if the medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. The status refers to the validity about the information of the medication and not to its medicinal properties. 5700 */ 5701 @Child(name = "status", type = {CodeType.class}, order=2, min=0, max=1, modifier=true, summary=true) 5702 @Description(shortDefinition="active | entered-in-error | inactive", formalDefinition="A code to indicate if the medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. The status refers to the validity about the information of the medication and not to its medicinal properties." ) 5703 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationknowledge-status") 5704 protected Enumeration<MedicationKnowledgeStatusCodes> status; 5705 5706 /** 5707 * The creator or owner of the knowledge or information about the medication. 5708 */ 5709 @Child(name = "author", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=false) 5710 @Description(shortDefinition="Creator or owner of the knowledge or information about the medication", formalDefinition="The creator or owner of the knowledge or information about the medication." ) 5711 protected Reference author; 5712 5713 /** 5714 * Lists the jurisdictions that this medication knowledge was written for. 5715 */ 5716 @Child(name = "intendedJurisdiction", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5717 @Description(shortDefinition="Codes that identify the different jurisdictions for which the information of this resource was created", formalDefinition="Lists the jurisdictions that this medication knowledge was written for." ) 5718 protected List<CodeableConcept> intendedJurisdiction; 5719 5720 /** 5721 * All of the names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol. 5722 */ 5723 @Child(name = "name", type = {StringType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5724 @Description(shortDefinition="A name associated with the medication being described", formalDefinition="All of the names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol." ) 5725 protected List<StringType> name; 5726 5727 /** 5728 * Associated or related medications. For example, if the medication is a branded product (e.g. Crestor), this is the Therapeutic Moeity (e.g. Rosuvastatin) or if this is a generic medication (e.g. Rosuvastatin), this would link to a branded product (e.g. Crestor. 5729 */ 5730 @Child(name = "relatedMedicationKnowledge", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5731 @Description(shortDefinition="Associated or related medication information", formalDefinition="Associated or related medications. For example, if the medication is a branded product (e.g. Crestor), this is the Therapeutic Moeity (e.g. Rosuvastatin) or if this is a generic medication (e.g. Rosuvastatin), this would link to a branded product (e.g. Crestor." ) 5732 protected List<MedicationKnowledgeRelatedMedicationKnowledgeComponent> relatedMedicationKnowledge; 5733 5734 /** 5735 * Links to associated medications that could be prescribed, dispensed or administered. 5736 */ 5737 @Child(name = "associatedMedication", type = {Medication.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5738 @Description(shortDefinition="The set of medication resources that are associated with this medication", formalDefinition="Links to associated medications that could be prescribed, dispensed or administered." ) 5739 protected List<Reference> associatedMedication; 5740 5741 /** 5742 * Category of the medication or product (e.g. branded product, therapeutic moeity, generic product, innovator product, etc.). 5743 */ 5744 @Child(name = "productType", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5745 @Description(shortDefinition="Category of the medication or product", formalDefinition="Category of the medication or product (e.g. branded product, therapeutic moeity, generic product, innovator product, etc.)." ) 5746 protected List<CodeableConcept> productType; 5747 5748 /** 5749 * Associated documentation about the medication. 5750 */ 5751 @Child(name = "monograph", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5752 @Description(shortDefinition="Associated documentation about the medication", formalDefinition="Associated documentation about the medication." ) 5753 protected List<MedicationKnowledgeMonographComponent> monograph; 5754 5755 /** 5756 * The instructions for preparing the medication. 5757 */ 5758 @Child(name = "preparationInstruction", type = {MarkdownType.class}, order=10, min=0, max=1, modifier=false, summary=false) 5759 @Description(shortDefinition="The instructions for preparing the medication", formalDefinition="The instructions for preparing the medication." ) 5760 protected MarkdownType preparationInstruction; 5761 5762 /** 5763 * The price of the medication. 5764 */ 5765 @Child(name = "cost", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5766 @Description(shortDefinition="The pricing of the medication", formalDefinition="The price of the medication." ) 5767 protected List<MedicationKnowledgeCostComponent> cost; 5768 5769 /** 5770 * The program under which the medication is reviewed. 5771 */ 5772 @Child(name = "monitoringProgram", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5773 @Description(shortDefinition="Program under which a medication is reviewed", formalDefinition="The program under which the medication is reviewed." ) 5774 protected List<MedicationKnowledgeMonitoringProgramComponent> monitoringProgram; 5775 5776 /** 5777 * Guidelines or protocols that are applicable for the administration of the medication based on indication. 5778 */ 5779 @Child(name = "indicationGuideline", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5780 @Description(shortDefinition="Guidelines or protocols for administration of the medication for an indication", formalDefinition="Guidelines or protocols that are applicable for the administration of the medication based on indication." ) 5781 protected List<MedicationKnowledgeIndicationGuidelineComponent> indicationGuideline; 5782 5783 /** 5784 * Categorization of the medication within a formulary or classification system. 5785 */ 5786 @Child(name = "medicineClassification", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5787 @Description(shortDefinition="Categorization of the medication within a formulary or classification system", formalDefinition="Categorization of the medication within a formulary or classification system." ) 5788 protected List<MedicationKnowledgeMedicineClassificationComponent> medicineClassification; 5789 5790 /** 5791 * Information that only applies to packages (not products). 5792 */ 5793 @Child(name = "packaging", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5794 @Description(shortDefinition="Details about packaged medications", formalDefinition="Information that only applies to packages (not products)." ) 5795 protected List<MedicationKnowledgePackagingComponent> packaging; 5796 5797 /** 5798 * Potential clinical issue with or between medication(s) (for example, drug-drug interaction, drug-disease contraindication, drug-allergy interaction, etc.). 5799 */ 5800 @Child(name = "clinicalUseIssue", type = {ClinicalUseDefinition.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5801 @Description(shortDefinition="Potential clinical issue with or between medication(s)", formalDefinition="Potential clinical issue with or between medication(s) (for example, drug-drug interaction, drug-disease contraindication, drug-allergy interaction, etc.)." ) 5802 protected List<Reference> clinicalUseIssue; 5803 5804 /** 5805 * Information on how the medication should be stored, for example, refrigeration temperatures and length of stability at a given temperature. 5806 */ 5807 @Child(name = "storageGuideline", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5808 @Description(shortDefinition="How the medication should be stored", formalDefinition="Information on how the medication should be stored, for example, refrigeration temperatures and length of stability at a given temperature." ) 5809 protected List<MedicationKnowledgeStorageGuidelineComponent> storageGuideline; 5810 5811 /** 5812 * Regulatory information about a medication. 5813 */ 5814 @Child(name = "regulatory", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5815 @Description(shortDefinition="Regulatory information about a medication", formalDefinition="Regulatory information about a medication." ) 5816 protected List<MedicationKnowledgeRegulatoryComponent> regulatory; 5817 5818 /** 5819 * Along with the link to a Medicinal Product Definition resource, this information provides common definitional elements that are needed to understand the specific medication that is being described. 5820 */ 5821 @Child(name = "definitional", type = {}, order=19, min=0, max=1, modifier=false, summary=false) 5822 @Description(shortDefinition="Minimal definition information about the medication", formalDefinition="Along with the link to a Medicinal Product Definition resource, this information provides common definitional elements that are needed to understand the specific medication that is being described." ) 5823 protected MedicationKnowledgeDefinitionalComponent definitional; 5824 5825 private static final long serialVersionUID = -814493741L; 5826 5827 /** 5828 * Constructor 5829 */ 5830 public MedicationKnowledge() { 5831 super(); 5832 } 5833 5834 /** 5835 * @return {@link #identifier} (Business identifier for this medication.) 5836 */ 5837 public List<Identifier> getIdentifier() { 5838 if (this.identifier == null) 5839 this.identifier = new ArrayList<Identifier>(); 5840 return this.identifier; 5841 } 5842 5843 /** 5844 * @return Returns a reference to <code>this</code> for easy method chaining 5845 */ 5846 public MedicationKnowledge setIdentifier(List<Identifier> theIdentifier) { 5847 this.identifier = theIdentifier; 5848 return this; 5849 } 5850 5851 public boolean hasIdentifier() { 5852 if (this.identifier == null) 5853 return false; 5854 for (Identifier item : this.identifier) 5855 if (!item.isEmpty()) 5856 return true; 5857 return false; 5858 } 5859 5860 public Identifier addIdentifier() { //3 5861 Identifier t = new Identifier(); 5862 if (this.identifier == null) 5863 this.identifier = new ArrayList<Identifier>(); 5864 this.identifier.add(t); 5865 return t; 5866 } 5867 5868 public MedicationKnowledge addIdentifier(Identifier t) { //3 5869 if (t == null) 5870 return this; 5871 if (this.identifier == null) 5872 this.identifier = new ArrayList<Identifier>(); 5873 this.identifier.add(t); 5874 return this; 5875 } 5876 5877 /** 5878 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 5879 */ 5880 public Identifier getIdentifierFirstRep() { 5881 if (getIdentifier().isEmpty()) { 5882 addIdentifier(); 5883 } 5884 return getIdentifier().get(0); 5885 } 5886 5887 /** 5888 * @return {@link #code} (A code that specifies this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.) 5889 */ 5890 public CodeableConcept getCode() { 5891 if (this.code == null) 5892 if (Configuration.errorOnAutoCreate()) 5893 throw new Error("Attempt to auto-create MedicationKnowledge.code"); 5894 else if (Configuration.doAutoCreate()) 5895 this.code = new CodeableConcept(); // cc 5896 return this.code; 5897 } 5898 5899 public boolean hasCode() { 5900 return this.code != null && !this.code.isEmpty(); 5901 } 5902 5903 /** 5904 * @param value {@link #code} (A code that specifies this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.) 5905 */ 5906 public MedicationKnowledge setCode(CodeableConcept value) { 5907 this.code = value; 5908 return this; 5909 } 5910 5911 /** 5912 * @return {@link #status} (A code to indicate if the medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. The status refers to the validity about the information of the medication and not to its medicinal properties.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 5913 */ 5914 public Enumeration<MedicationKnowledgeStatusCodes> getStatusElement() { 5915 if (this.status == null) 5916 if (Configuration.errorOnAutoCreate()) 5917 throw new Error("Attempt to auto-create MedicationKnowledge.status"); 5918 else if (Configuration.doAutoCreate()) 5919 this.status = new Enumeration<MedicationKnowledgeStatusCodes>(new MedicationKnowledgeStatusCodesEnumFactory()); // bb 5920 return this.status; 5921 } 5922 5923 public boolean hasStatusElement() { 5924 return this.status != null && !this.status.isEmpty(); 5925 } 5926 5927 public boolean hasStatus() { 5928 return this.status != null && !this.status.isEmpty(); 5929 } 5930 5931 /** 5932 * @param value {@link #status} (A code to indicate if the medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. The status refers to the validity about the information of the medication and not to its medicinal properties.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 5933 */ 5934 public MedicationKnowledge setStatusElement(Enumeration<MedicationKnowledgeStatusCodes> value) { 5935 this.status = value; 5936 return this; 5937 } 5938 5939 /** 5940 * @return A code to indicate if the medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. The status refers to the validity about the information of the medication and not to its medicinal properties. 5941 */ 5942 public MedicationKnowledgeStatusCodes getStatus() { 5943 return this.status == null ? null : this.status.getValue(); 5944 } 5945 5946 /** 5947 * @param value A code to indicate if the medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. The status refers to the validity about the information of the medication and not to its medicinal properties. 5948 */ 5949 public MedicationKnowledge setStatus(MedicationKnowledgeStatusCodes value) { 5950 if (value == null) 5951 this.status = null; 5952 else { 5953 if (this.status == null) 5954 this.status = new Enumeration<MedicationKnowledgeStatusCodes>(new MedicationKnowledgeStatusCodesEnumFactory()); 5955 this.status.setValue(value); 5956 } 5957 return this; 5958 } 5959 5960 /** 5961 * @return {@link #author} (The creator or owner of the knowledge or information about the medication.) 5962 */ 5963 public Reference getAuthor() { 5964 if (this.author == null) 5965 if (Configuration.errorOnAutoCreate()) 5966 throw new Error("Attempt to auto-create MedicationKnowledge.author"); 5967 else if (Configuration.doAutoCreate()) 5968 this.author = new Reference(); // cc 5969 return this.author; 5970 } 5971 5972 public boolean hasAuthor() { 5973 return this.author != null && !this.author.isEmpty(); 5974 } 5975 5976 /** 5977 * @param value {@link #author} (The creator or owner of the knowledge or information about the medication.) 5978 */ 5979 public MedicationKnowledge setAuthor(Reference value) { 5980 this.author = value; 5981 return this; 5982 } 5983 5984 /** 5985 * @return {@link #intendedJurisdiction} (Lists the jurisdictions that this medication knowledge was written for.) 5986 */ 5987 public List<CodeableConcept> getIntendedJurisdiction() { 5988 if (this.intendedJurisdiction == null) 5989 this.intendedJurisdiction = new ArrayList<CodeableConcept>(); 5990 return this.intendedJurisdiction; 5991 } 5992 5993 /** 5994 * @return Returns a reference to <code>this</code> for easy method chaining 5995 */ 5996 public MedicationKnowledge setIntendedJurisdiction(List<CodeableConcept> theIntendedJurisdiction) { 5997 this.intendedJurisdiction = theIntendedJurisdiction; 5998 return this; 5999 } 6000 6001 public boolean hasIntendedJurisdiction() { 6002 if (this.intendedJurisdiction == null) 6003 return false; 6004 for (CodeableConcept item : this.intendedJurisdiction) 6005 if (!item.isEmpty()) 6006 return true; 6007 return false; 6008 } 6009 6010 public CodeableConcept addIntendedJurisdiction() { //3 6011 CodeableConcept t = new CodeableConcept(); 6012 if (this.intendedJurisdiction == null) 6013 this.intendedJurisdiction = new ArrayList<CodeableConcept>(); 6014 this.intendedJurisdiction.add(t); 6015 return t; 6016 } 6017 6018 public MedicationKnowledge addIntendedJurisdiction(CodeableConcept t) { //3 6019 if (t == null) 6020 return this; 6021 if (this.intendedJurisdiction == null) 6022 this.intendedJurisdiction = new ArrayList<CodeableConcept>(); 6023 this.intendedJurisdiction.add(t); 6024 return this; 6025 } 6026 6027 /** 6028 * @return The first repetition of repeating field {@link #intendedJurisdiction}, creating it if it does not already exist {3} 6029 */ 6030 public CodeableConcept getIntendedJurisdictionFirstRep() { 6031 if (getIntendedJurisdiction().isEmpty()) { 6032 addIntendedJurisdiction(); 6033 } 6034 return getIntendedJurisdiction().get(0); 6035 } 6036 6037 /** 6038 * @return {@link #name} (All of the names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol.) 6039 */ 6040 public List<StringType> getName() { 6041 if (this.name == null) 6042 this.name = new ArrayList<StringType>(); 6043 return this.name; 6044 } 6045 6046 /** 6047 * @return Returns a reference to <code>this</code> for easy method chaining 6048 */ 6049 public MedicationKnowledge setName(List<StringType> theName) { 6050 this.name = theName; 6051 return this; 6052 } 6053 6054 public boolean hasName() { 6055 if (this.name == null) 6056 return false; 6057 for (StringType item : this.name) 6058 if (!item.isEmpty()) 6059 return true; 6060 return false; 6061 } 6062 6063 /** 6064 * @return {@link #name} (All of the names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol.) 6065 */ 6066 public StringType addNameElement() {//2 6067 StringType t = new StringType(); 6068 if (this.name == null) 6069 this.name = new ArrayList<StringType>(); 6070 this.name.add(t); 6071 return t; 6072 } 6073 6074 /** 6075 * @param value {@link #name} (All of the names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol.) 6076 */ 6077 public MedicationKnowledge addName(String value) { //1 6078 StringType t = new StringType(); 6079 t.setValue(value); 6080 if (this.name == null) 6081 this.name = new ArrayList<StringType>(); 6082 this.name.add(t); 6083 return this; 6084 } 6085 6086 /** 6087 * @param value {@link #name} (All of the names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol.) 6088 */ 6089 public boolean hasName(String value) { 6090 if (this.name == null) 6091 return false; 6092 for (StringType v : this.name) 6093 if (v.getValue().equals(value)) // string 6094 return true; 6095 return false; 6096 } 6097 6098 /** 6099 * @return {@link #relatedMedicationKnowledge} (Associated or related medications. For example, if the medication is a branded product (e.g. Crestor), this is the Therapeutic Moeity (e.g. Rosuvastatin) or if this is a generic medication (e.g. Rosuvastatin), this would link to a branded product (e.g. Crestor.) 6100 */ 6101 public List<MedicationKnowledgeRelatedMedicationKnowledgeComponent> getRelatedMedicationKnowledge() { 6102 if (this.relatedMedicationKnowledge == null) 6103 this.relatedMedicationKnowledge = new ArrayList<MedicationKnowledgeRelatedMedicationKnowledgeComponent>(); 6104 return this.relatedMedicationKnowledge; 6105 } 6106 6107 /** 6108 * @return Returns a reference to <code>this</code> for easy method chaining 6109 */ 6110 public MedicationKnowledge setRelatedMedicationKnowledge(List<MedicationKnowledgeRelatedMedicationKnowledgeComponent> theRelatedMedicationKnowledge) { 6111 this.relatedMedicationKnowledge = theRelatedMedicationKnowledge; 6112 return this; 6113 } 6114 6115 public boolean hasRelatedMedicationKnowledge() { 6116 if (this.relatedMedicationKnowledge == null) 6117 return false; 6118 for (MedicationKnowledgeRelatedMedicationKnowledgeComponent item : this.relatedMedicationKnowledge) 6119 if (!item.isEmpty()) 6120 return true; 6121 return false; 6122 } 6123 6124 public MedicationKnowledgeRelatedMedicationKnowledgeComponent addRelatedMedicationKnowledge() { //3 6125 MedicationKnowledgeRelatedMedicationKnowledgeComponent t = new MedicationKnowledgeRelatedMedicationKnowledgeComponent(); 6126 if (this.relatedMedicationKnowledge == null) 6127 this.relatedMedicationKnowledge = new ArrayList<MedicationKnowledgeRelatedMedicationKnowledgeComponent>(); 6128 this.relatedMedicationKnowledge.add(t); 6129 return t; 6130 } 6131 6132 public MedicationKnowledge addRelatedMedicationKnowledge(MedicationKnowledgeRelatedMedicationKnowledgeComponent t) { //3 6133 if (t == null) 6134 return this; 6135 if (this.relatedMedicationKnowledge == null) 6136 this.relatedMedicationKnowledge = new ArrayList<MedicationKnowledgeRelatedMedicationKnowledgeComponent>(); 6137 this.relatedMedicationKnowledge.add(t); 6138 return this; 6139 } 6140 6141 /** 6142 * @return The first repetition of repeating field {@link #relatedMedicationKnowledge}, creating it if it does not already exist {3} 6143 */ 6144 public MedicationKnowledgeRelatedMedicationKnowledgeComponent getRelatedMedicationKnowledgeFirstRep() { 6145 if (getRelatedMedicationKnowledge().isEmpty()) { 6146 addRelatedMedicationKnowledge(); 6147 } 6148 return getRelatedMedicationKnowledge().get(0); 6149 } 6150 6151 /** 6152 * @return {@link #associatedMedication} (Links to associated medications that could be prescribed, dispensed or administered.) 6153 */ 6154 public List<Reference> getAssociatedMedication() { 6155 if (this.associatedMedication == null) 6156 this.associatedMedication = new ArrayList<Reference>(); 6157 return this.associatedMedication; 6158 } 6159 6160 /** 6161 * @return Returns a reference to <code>this</code> for easy method chaining 6162 */ 6163 public MedicationKnowledge setAssociatedMedication(List<Reference> theAssociatedMedication) { 6164 this.associatedMedication = theAssociatedMedication; 6165 return this; 6166 } 6167 6168 public boolean hasAssociatedMedication() { 6169 if (this.associatedMedication == null) 6170 return false; 6171 for (Reference item : this.associatedMedication) 6172 if (!item.isEmpty()) 6173 return true; 6174 return false; 6175 } 6176 6177 public Reference addAssociatedMedication() { //3 6178 Reference t = new Reference(); 6179 if (this.associatedMedication == null) 6180 this.associatedMedication = new ArrayList<Reference>(); 6181 this.associatedMedication.add(t); 6182 return t; 6183 } 6184 6185 public MedicationKnowledge addAssociatedMedication(Reference t) { //3 6186 if (t == null) 6187 return this; 6188 if (this.associatedMedication == null) 6189 this.associatedMedication = new ArrayList<Reference>(); 6190 this.associatedMedication.add(t); 6191 return this; 6192 } 6193 6194 /** 6195 * @return The first repetition of repeating field {@link #associatedMedication}, creating it if it does not already exist {3} 6196 */ 6197 public Reference getAssociatedMedicationFirstRep() { 6198 if (getAssociatedMedication().isEmpty()) { 6199 addAssociatedMedication(); 6200 } 6201 return getAssociatedMedication().get(0); 6202 } 6203 6204 /** 6205 * @return {@link #productType} (Category of the medication or product (e.g. branded product, therapeutic moeity, generic product, innovator product, etc.).) 6206 */ 6207 public List<CodeableConcept> getProductType() { 6208 if (this.productType == null) 6209 this.productType = new ArrayList<CodeableConcept>(); 6210 return this.productType; 6211 } 6212 6213 /** 6214 * @return Returns a reference to <code>this</code> for easy method chaining 6215 */ 6216 public MedicationKnowledge setProductType(List<CodeableConcept> theProductType) { 6217 this.productType = theProductType; 6218 return this; 6219 } 6220 6221 public boolean hasProductType() { 6222 if (this.productType == null) 6223 return false; 6224 for (CodeableConcept item : this.productType) 6225 if (!item.isEmpty()) 6226 return true; 6227 return false; 6228 } 6229 6230 public CodeableConcept addProductType() { //3 6231 CodeableConcept t = new CodeableConcept(); 6232 if (this.productType == null) 6233 this.productType = new ArrayList<CodeableConcept>(); 6234 this.productType.add(t); 6235 return t; 6236 } 6237 6238 public MedicationKnowledge addProductType(CodeableConcept t) { //3 6239 if (t == null) 6240 return this; 6241 if (this.productType == null) 6242 this.productType = new ArrayList<CodeableConcept>(); 6243 this.productType.add(t); 6244 return this; 6245 } 6246 6247 /** 6248 * @return The first repetition of repeating field {@link #productType}, creating it if it does not already exist {3} 6249 */ 6250 public CodeableConcept getProductTypeFirstRep() { 6251 if (getProductType().isEmpty()) { 6252 addProductType(); 6253 } 6254 return getProductType().get(0); 6255 } 6256 6257 /** 6258 * @return {@link #monograph} (Associated documentation about the medication.) 6259 */ 6260 public List<MedicationKnowledgeMonographComponent> getMonograph() { 6261 if (this.monograph == null) 6262 this.monograph = new ArrayList<MedicationKnowledgeMonographComponent>(); 6263 return this.monograph; 6264 } 6265 6266 /** 6267 * @return Returns a reference to <code>this</code> for easy method chaining 6268 */ 6269 public MedicationKnowledge setMonograph(List<MedicationKnowledgeMonographComponent> theMonograph) { 6270 this.monograph = theMonograph; 6271 return this; 6272 } 6273 6274 public boolean hasMonograph() { 6275 if (this.monograph == null) 6276 return false; 6277 for (MedicationKnowledgeMonographComponent item : this.monograph) 6278 if (!item.isEmpty()) 6279 return true; 6280 return false; 6281 } 6282 6283 public MedicationKnowledgeMonographComponent addMonograph() { //3 6284 MedicationKnowledgeMonographComponent t = new MedicationKnowledgeMonographComponent(); 6285 if (this.monograph == null) 6286 this.monograph = new ArrayList<MedicationKnowledgeMonographComponent>(); 6287 this.monograph.add(t); 6288 return t; 6289 } 6290 6291 public MedicationKnowledge addMonograph(MedicationKnowledgeMonographComponent t) { //3 6292 if (t == null) 6293 return this; 6294 if (this.monograph == null) 6295 this.monograph = new ArrayList<MedicationKnowledgeMonographComponent>(); 6296 this.monograph.add(t); 6297 return this; 6298 } 6299 6300 /** 6301 * @return The first repetition of repeating field {@link #monograph}, creating it if it does not already exist {3} 6302 */ 6303 public MedicationKnowledgeMonographComponent getMonographFirstRep() { 6304 if (getMonograph().isEmpty()) { 6305 addMonograph(); 6306 } 6307 return getMonograph().get(0); 6308 } 6309 6310 /** 6311 * @return {@link #preparationInstruction} (The instructions for preparing the medication.). This is the underlying object with id, value and extensions. The accessor "getPreparationInstruction" gives direct access to the value 6312 */ 6313 public MarkdownType getPreparationInstructionElement() { 6314 if (this.preparationInstruction == null) 6315 if (Configuration.errorOnAutoCreate()) 6316 throw new Error("Attempt to auto-create MedicationKnowledge.preparationInstruction"); 6317 else if (Configuration.doAutoCreate()) 6318 this.preparationInstruction = new MarkdownType(); // bb 6319 return this.preparationInstruction; 6320 } 6321 6322 public boolean hasPreparationInstructionElement() { 6323 return this.preparationInstruction != null && !this.preparationInstruction.isEmpty(); 6324 } 6325 6326 public boolean hasPreparationInstruction() { 6327 return this.preparationInstruction != null && !this.preparationInstruction.isEmpty(); 6328 } 6329 6330 /** 6331 * @param value {@link #preparationInstruction} (The instructions for preparing the medication.). This is the underlying object with id, value and extensions. The accessor "getPreparationInstruction" gives direct access to the value 6332 */ 6333 public MedicationKnowledge setPreparationInstructionElement(MarkdownType value) { 6334 this.preparationInstruction = value; 6335 return this; 6336 } 6337 6338 /** 6339 * @return The instructions for preparing the medication. 6340 */ 6341 public String getPreparationInstruction() { 6342 return this.preparationInstruction == null ? null : this.preparationInstruction.getValue(); 6343 } 6344 6345 /** 6346 * @param value The instructions for preparing the medication. 6347 */ 6348 public MedicationKnowledge setPreparationInstruction(String value) { 6349 if (Utilities.noString(value)) 6350 this.preparationInstruction = null; 6351 else { 6352 if (this.preparationInstruction == null) 6353 this.preparationInstruction = new MarkdownType(); 6354 this.preparationInstruction.setValue(value); 6355 } 6356 return this; 6357 } 6358 6359 /** 6360 * @return {@link #cost} (The price of the medication.) 6361 */ 6362 public List<MedicationKnowledgeCostComponent> getCost() { 6363 if (this.cost == null) 6364 this.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 6365 return this.cost; 6366 } 6367 6368 /** 6369 * @return Returns a reference to <code>this</code> for easy method chaining 6370 */ 6371 public MedicationKnowledge setCost(List<MedicationKnowledgeCostComponent> theCost) { 6372 this.cost = theCost; 6373 return this; 6374 } 6375 6376 public boolean hasCost() { 6377 if (this.cost == null) 6378 return false; 6379 for (MedicationKnowledgeCostComponent item : this.cost) 6380 if (!item.isEmpty()) 6381 return true; 6382 return false; 6383 } 6384 6385 public MedicationKnowledgeCostComponent addCost() { //3 6386 MedicationKnowledgeCostComponent t = new MedicationKnowledgeCostComponent(); 6387 if (this.cost == null) 6388 this.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 6389 this.cost.add(t); 6390 return t; 6391 } 6392 6393 public MedicationKnowledge addCost(MedicationKnowledgeCostComponent t) { //3 6394 if (t == null) 6395 return this; 6396 if (this.cost == null) 6397 this.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 6398 this.cost.add(t); 6399 return this; 6400 } 6401 6402 /** 6403 * @return The first repetition of repeating field {@link #cost}, creating it if it does not already exist {3} 6404 */ 6405 public MedicationKnowledgeCostComponent getCostFirstRep() { 6406 if (getCost().isEmpty()) { 6407 addCost(); 6408 } 6409 return getCost().get(0); 6410 } 6411 6412 /** 6413 * @return {@link #monitoringProgram} (The program under which the medication is reviewed.) 6414 */ 6415 public List<MedicationKnowledgeMonitoringProgramComponent> getMonitoringProgram() { 6416 if (this.monitoringProgram == null) 6417 this.monitoringProgram = new ArrayList<MedicationKnowledgeMonitoringProgramComponent>(); 6418 return this.monitoringProgram; 6419 } 6420 6421 /** 6422 * @return Returns a reference to <code>this</code> for easy method chaining 6423 */ 6424 public MedicationKnowledge setMonitoringProgram(List<MedicationKnowledgeMonitoringProgramComponent> theMonitoringProgram) { 6425 this.monitoringProgram = theMonitoringProgram; 6426 return this; 6427 } 6428 6429 public boolean hasMonitoringProgram() { 6430 if (this.monitoringProgram == null) 6431 return false; 6432 for (MedicationKnowledgeMonitoringProgramComponent item : this.monitoringProgram) 6433 if (!item.isEmpty()) 6434 return true; 6435 return false; 6436 } 6437 6438 public MedicationKnowledgeMonitoringProgramComponent addMonitoringProgram() { //3 6439 MedicationKnowledgeMonitoringProgramComponent t = new MedicationKnowledgeMonitoringProgramComponent(); 6440 if (this.monitoringProgram == null) 6441 this.monitoringProgram = new ArrayList<MedicationKnowledgeMonitoringProgramComponent>(); 6442 this.monitoringProgram.add(t); 6443 return t; 6444 } 6445 6446 public MedicationKnowledge addMonitoringProgram(MedicationKnowledgeMonitoringProgramComponent t) { //3 6447 if (t == null) 6448 return this; 6449 if (this.monitoringProgram == null) 6450 this.monitoringProgram = new ArrayList<MedicationKnowledgeMonitoringProgramComponent>(); 6451 this.monitoringProgram.add(t); 6452 return this; 6453 } 6454 6455 /** 6456 * @return The first repetition of repeating field {@link #monitoringProgram}, creating it if it does not already exist {3} 6457 */ 6458 public MedicationKnowledgeMonitoringProgramComponent getMonitoringProgramFirstRep() { 6459 if (getMonitoringProgram().isEmpty()) { 6460 addMonitoringProgram(); 6461 } 6462 return getMonitoringProgram().get(0); 6463 } 6464 6465 /** 6466 * @return {@link #indicationGuideline} (Guidelines or protocols that are applicable for the administration of the medication based on indication.) 6467 */ 6468 public List<MedicationKnowledgeIndicationGuidelineComponent> getIndicationGuideline() { 6469 if (this.indicationGuideline == null) 6470 this.indicationGuideline = new ArrayList<MedicationKnowledgeIndicationGuidelineComponent>(); 6471 return this.indicationGuideline; 6472 } 6473 6474 /** 6475 * @return Returns a reference to <code>this</code> for easy method chaining 6476 */ 6477 public MedicationKnowledge setIndicationGuideline(List<MedicationKnowledgeIndicationGuidelineComponent> theIndicationGuideline) { 6478 this.indicationGuideline = theIndicationGuideline; 6479 return this; 6480 } 6481 6482 public boolean hasIndicationGuideline() { 6483 if (this.indicationGuideline == null) 6484 return false; 6485 for (MedicationKnowledgeIndicationGuidelineComponent item : this.indicationGuideline) 6486 if (!item.isEmpty()) 6487 return true; 6488 return false; 6489 } 6490 6491 public MedicationKnowledgeIndicationGuidelineComponent addIndicationGuideline() { //3 6492 MedicationKnowledgeIndicationGuidelineComponent t = new MedicationKnowledgeIndicationGuidelineComponent(); 6493 if (this.indicationGuideline == null) 6494 this.indicationGuideline = new ArrayList<MedicationKnowledgeIndicationGuidelineComponent>(); 6495 this.indicationGuideline.add(t); 6496 return t; 6497 } 6498 6499 public MedicationKnowledge addIndicationGuideline(MedicationKnowledgeIndicationGuidelineComponent t) { //3 6500 if (t == null) 6501 return this; 6502 if (this.indicationGuideline == null) 6503 this.indicationGuideline = new ArrayList<MedicationKnowledgeIndicationGuidelineComponent>(); 6504 this.indicationGuideline.add(t); 6505 return this; 6506 } 6507 6508 /** 6509 * @return The first repetition of repeating field {@link #indicationGuideline}, creating it if it does not already exist {3} 6510 */ 6511 public MedicationKnowledgeIndicationGuidelineComponent getIndicationGuidelineFirstRep() { 6512 if (getIndicationGuideline().isEmpty()) { 6513 addIndicationGuideline(); 6514 } 6515 return getIndicationGuideline().get(0); 6516 } 6517 6518 /** 6519 * @return {@link #medicineClassification} (Categorization of the medication within a formulary or classification system.) 6520 */ 6521 public List<MedicationKnowledgeMedicineClassificationComponent> getMedicineClassification() { 6522 if (this.medicineClassification == null) 6523 this.medicineClassification = new ArrayList<MedicationKnowledgeMedicineClassificationComponent>(); 6524 return this.medicineClassification; 6525 } 6526 6527 /** 6528 * @return Returns a reference to <code>this</code> for easy method chaining 6529 */ 6530 public MedicationKnowledge setMedicineClassification(List<MedicationKnowledgeMedicineClassificationComponent> theMedicineClassification) { 6531 this.medicineClassification = theMedicineClassification; 6532 return this; 6533 } 6534 6535 public boolean hasMedicineClassification() { 6536 if (this.medicineClassification == null) 6537 return false; 6538 for (MedicationKnowledgeMedicineClassificationComponent item : this.medicineClassification) 6539 if (!item.isEmpty()) 6540 return true; 6541 return false; 6542 } 6543 6544 public MedicationKnowledgeMedicineClassificationComponent addMedicineClassification() { //3 6545 MedicationKnowledgeMedicineClassificationComponent t = new MedicationKnowledgeMedicineClassificationComponent(); 6546 if (this.medicineClassification == null) 6547 this.medicineClassification = new ArrayList<MedicationKnowledgeMedicineClassificationComponent>(); 6548 this.medicineClassification.add(t); 6549 return t; 6550 } 6551 6552 public MedicationKnowledge addMedicineClassification(MedicationKnowledgeMedicineClassificationComponent t) { //3 6553 if (t == null) 6554 return this; 6555 if (this.medicineClassification == null) 6556 this.medicineClassification = new ArrayList<MedicationKnowledgeMedicineClassificationComponent>(); 6557 this.medicineClassification.add(t); 6558 return this; 6559 } 6560 6561 /** 6562 * @return The first repetition of repeating field {@link #medicineClassification}, creating it if it does not already exist {3} 6563 */ 6564 public MedicationKnowledgeMedicineClassificationComponent getMedicineClassificationFirstRep() { 6565 if (getMedicineClassification().isEmpty()) { 6566 addMedicineClassification(); 6567 } 6568 return getMedicineClassification().get(0); 6569 } 6570 6571 /** 6572 * @return {@link #packaging} (Information that only applies to packages (not products).) 6573 */ 6574 public List<MedicationKnowledgePackagingComponent> getPackaging() { 6575 if (this.packaging == null) 6576 this.packaging = new ArrayList<MedicationKnowledgePackagingComponent>(); 6577 return this.packaging; 6578 } 6579 6580 /** 6581 * @return Returns a reference to <code>this</code> for easy method chaining 6582 */ 6583 public MedicationKnowledge setPackaging(List<MedicationKnowledgePackagingComponent> thePackaging) { 6584 this.packaging = thePackaging; 6585 return this; 6586 } 6587 6588 public boolean hasPackaging() { 6589 if (this.packaging == null) 6590 return false; 6591 for (MedicationKnowledgePackagingComponent item : this.packaging) 6592 if (!item.isEmpty()) 6593 return true; 6594 return false; 6595 } 6596 6597 public MedicationKnowledgePackagingComponent addPackaging() { //3 6598 MedicationKnowledgePackagingComponent t = new MedicationKnowledgePackagingComponent(); 6599 if (this.packaging == null) 6600 this.packaging = new ArrayList<MedicationKnowledgePackagingComponent>(); 6601 this.packaging.add(t); 6602 return t; 6603 } 6604 6605 public MedicationKnowledge addPackaging(MedicationKnowledgePackagingComponent t) { //3 6606 if (t == null) 6607 return this; 6608 if (this.packaging == null) 6609 this.packaging = new ArrayList<MedicationKnowledgePackagingComponent>(); 6610 this.packaging.add(t); 6611 return this; 6612 } 6613 6614 /** 6615 * @return The first repetition of repeating field {@link #packaging}, creating it if it does not already exist {3} 6616 */ 6617 public MedicationKnowledgePackagingComponent getPackagingFirstRep() { 6618 if (getPackaging().isEmpty()) { 6619 addPackaging(); 6620 } 6621 return getPackaging().get(0); 6622 } 6623 6624 /** 6625 * @return {@link #clinicalUseIssue} (Potential clinical issue with or between medication(s) (for example, drug-drug interaction, drug-disease contraindication, drug-allergy interaction, etc.).) 6626 */ 6627 public List<Reference> getClinicalUseIssue() { 6628 if (this.clinicalUseIssue == null) 6629 this.clinicalUseIssue = new ArrayList<Reference>(); 6630 return this.clinicalUseIssue; 6631 } 6632 6633 /** 6634 * @return Returns a reference to <code>this</code> for easy method chaining 6635 */ 6636 public MedicationKnowledge setClinicalUseIssue(List<Reference> theClinicalUseIssue) { 6637 this.clinicalUseIssue = theClinicalUseIssue; 6638 return this; 6639 } 6640 6641 public boolean hasClinicalUseIssue() { 6642 if (this.clinicalUseIssue == null) 6643 return false; 6644 for (Reference item : this.clinicalUseIssue) 6645 if (!item.isEmpty()) 6646 return true; 6647 return false; 6648 } 6649 6650 public Reference addClinicalUseIssue() { //3 6651 Reference t = new Reference(); 6652 if (this.clinicalUseIssue == null) 6653 this.clinicalUseIssue = new ArrayList<Reference>(); 6654 this.clinicalUseIssue.add(t); 6655 return t; 6656 } 6657 6658 public MedicationKnowledge addClinicalUseIssue(Reference t) { //3 6659 if (t == null) 6660 return this; 6661 if (this.clinicalUseIssue == null) 6662 this.clinicalUseIssue = new ArrayList<Reference>(); 6663 this.clinicalUseIssue.add(t); 6664 return this; 6665 } 6666 6667 /** 6668 * @return The first repetition of repeating field {@link #clinicalUseIssue}, creating it if it does not already exist {3} 6669 */ 6670 public Reference getClinicalUseIssueFirstRep() { 6671 if (getClinicalUseIssue().isEmpty()) { 6672 addClinicalUseIssue(); 6673 } 6674 return getClinicalUseIssue().get(0); 6675 } 6676 6677 /** 6678 * @return {@link #storageGuideline} (Information on how the medication should be stored, for example, refrigeration temperatures and length of stability at a given temperature.) 6679 */ 6680 public List<MedicationKnowledgeStorageGuidelineComponent> getStorageGuideline() { 6681 if (this.storageGuideline == null) 6682 this.storageGuideline = new ArrayList<MedicationKnowledgeStorageGuidelineComponent>(); 6683 return this.storageGuideline; 6684 } 6685 6686 /** 6687 * @return Returns a reference to <code>this</code> for easy method chaining 6688 */ 6689 public MedicationKnowledge setStorageGuideline(List<MedicationKnowledgeStorageGuidelineComponent> theStorageGuideline) { 6690 this.storageGuideline = theStorageGuideline; 6691 return this; 6692 } 6693 6694 public boolean hasStorageGuideline() { 6695 if (this.storageGuideline == null) 6696 return false; 6697 for (MedicationKnowledgeStorageGuidelineComponent item : this.storageGuideline) 6698 if (!item.isEmpty()) 6699 return true; 6700 return false; 6701 } 6702 6703 public MedicationKnowledgeStorageGuidelineComponent addStorageGuideline() { //3 6704 MedicationKnowledgeStorageGuidelineComponent t = new MedicationKnowledgeStorageGuidelineComponent(); 6705 if (this.storageGuideline == null) 6706 this.storageGuideline = new ArrayList<MedicationKnowledgeStorageGuidelineComponent>(); 6707 this.storageGuideline.add(t); 6708 return t; 6709 } 6710 6711 public MedicationKnowledge addStorageGuideline(MedicationKnowledgeStorageGuidelineComponent t) { //3 6712 if (t == null) 6713 return this; 6714 if (this.storageGuideline == null) 6715 this.storageGuideline = new ArrayList<MedicationKnowledgeStorageGuidelineComponent>(); 6716 this.storageGuideline.add(t); 6717 return this; 6718 } 6719 6720 /** 6721 * @return The first repetition of repeating field {@link #storageGuideline}, creating it if it does not already exist {3} 6722 */ 6723 public MedicationKnowledgeStorageGuidelineComponent getStorageGuidelineFirstRep() { 6724 if (getStorageGuideline().isEmpty()) { 6725 addStorageGuideline(); 6726 } 6727 return getStorageGuideline().get(0); 6728 } 6729 6730 /** 6731 * @return {@link #regulatory} (Regulatory information about a medication.) 6732 */ 6733 public List<MedicationKnowledgeRegulatoryComponent> getRegulatory() { 6734 if (this.regulatory == null) 6735 this.regulatory = new ArrayList<MedicationKnowledgeRegulatoryComponent>(); 6736 return this.regulatory; 6737 } 6738 6739 /** 6740 * @return Returns a reference to <code>this</code> for easy method chaining 6741 */ 6742 public MedicationKnowledge setRegulatory(List<MedicationKnowledgeRegulatoryComponent> theRegulatory) { 6743 this.regulatory = theRegulatory; 6744 return this; 6745 } 6746 6747 public boolean hasRegulatory() { 6748 if (this.regulatory == null) 6749 return false; 6750 for (MedicationKnowledgeRegulatoryComponent item : this.regulatory) 6751 if (!item.isEmpty()) 6752 return true; 6753 return false; 6754 } 6755 6756 public MedicationKnowledgeRegulatoryComponent addRegulatory() { //3 6757 MedicationKnowledgeRegulatoryComponent t = new MedicationKnowledgeRegulatoryComponent(); 6758 if (this.regulatory == null) 6759 this.regulatory = new ArrayList<MedicationKnowledgeRegulatoryComponent>(); 6760 this.regulatory.add(t); 6761 return t; 6762 } 6763 6764 public MedicationKnowledge addRegulatory(MedicationKnowledgeRegulatoryComponent t) { //3 6765 if (t == null) 6766 return this; 6767 if (this.regulatory == null) 6768 this.regulatory = new ArrayList<MedicationKnowledgeRegulatoryComponent>(); 6769 this.regulatory.add(t); 6770 return this; 6771 } 6772 6773 /** 6774 * @return The first repetition of repeating field {@link #regulatory}, creating it if it does not already exist {3} 6775 */ 6776 public MedicationKnowledgeRegulatoryComponent getRegulatoryFirstRep() { 6777 if (getRegulatory().isEmpty()) { 6778 addRegulatory(); 6779 } 6780 return getRegulatory().get(0); 6781 } 6782 6783 /** 6784 * @return {@link #definitional} (Along with the link to a Medicinal Product Definition resource, this information provides common definitional elements that are needed to understand the specific medication that is being described.) 6785 */ 6786 public MedicationKnowledgeDefinitionalComponent getDefinitional() { 6787 if (this.definitional == null) 6788 if (Configuration.errorOnAutoCreate()) 6789 throw new Error("Attempt to auto-create MedicationKnowledge.definitional"); 6790 else if (Configuration.doAutoCreate()) 6791 this.definitional = new MedicationKnowledgeDefinitionalComponent(); // cc 6792 return this.definitional; 6793 } 6794 6795 public boolean hasDefinitional() { 6796 return this.definitional != null && !this.definitional.isEmpty(); 6797 } 6798 6799 /** 6800 * @param value {@link #definitional} (Along with the link to a Medicinal Product Definition resource, this information provides common definitional elements that are needed to understand the specific medication that is being described.) 6801 */ 6802 public MedicationKnowledge setDefinitional(MedicationKnowledgeDefinitionalComponent value) { 6803 this.definitional = value; 6804 return this; 6805 } 6806 6807 protected void listChildren(List<Property> children) { 6808 super.listChildren(children); 6809 children.add(new Property("identifier", "Identifier", "Business identifier for this medication.", 0, java.lang.Integer.MAX_VALUE, identifier)); 6810 children.add(new Property("code", "CodeableConcept", "A code that specifies this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.", 0, 1, code)); 6811 children.add(new Property("status", "code", "A code to indicate if the medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. The status refers to the validity about the information of the medication and not to its medicinal properties.", 0, 1, status)); 6812 children.add(new Property("author", "Reference(Organization)", "The creator or owner of the knowledge or information about the medication.", 0, 1, author)); 6813 children.add(new Property("intendedJurisdiction", "CodeableConcept", "Lists the jurisdictions that this medication knowledge was written for.", 0, java.lang.Integer.MAX_VALUE, intendedJurisdiction)); 6814 children.add(new Property("name", "string", "All of the names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol.", 0, java.lang.Integer.MAX_VALUE, name)); 6815 children.add(new Property("relatedMedicationKnowledge", "", "Associated or related medications. For example, if the medication is a branded product (e.g. Crestor), this is the Therapeutic Moeity (e.g. Rosuvastatin) or if this is a generic medication (e.g. Rosuvastatin), this would link to a branded product (e.g. Crestor.", 0, java.lang.Integer.MAX_VALUE, relatedMedicationKnowledge)); 6816 children.add(new Property("associatedMedication", "Reference(Medication)", "Links to associated medications that could be prescribed, dispensed or administered.", 0, java.lang.Integer.MAX_VALUE, associatedMedication)); 6817 children.add(new Property("productType", "CodeableConcept", "Category of the medication or product (e.g. branded product, therapeutic moeity, generic product, innovator product, etc.).", 0, java.lang.Integer.MAX_VALUE, productType)); 6818 children.add(new Property("monograph", "", "Associated documentation about the medication.", 0, java.lang.Integer.MAX_VALUE, monograph)); 6819 children.add(new Property("preparationInstruction", "markdown", "The instructions for preparing the medication.", 0, 1, preparationInstruction)); 6820 children.add(new Property("cost", "", "The price of the medication.", 0, java.lang.Integer.MAX_VALUE, cost)); 6821 children.add(new Property("monitoringProgram", "", "The program under which the medication is reviewed.", 0, java.lang.Integer.MAX_VALUE, monitoringProgram)); 6822 children.add(new Property("indicationGuideline", "", "Guidelines or protocols that are applicable for the administration of the medication based on indication.", 0, java.lang.Integer.MAX_VALUE, indicationGuideline)); 6823 children.add(new Property("medicineClassification", "", "Categorization of the medication within a formulary or classification system.", 0, java.lang.Integer.MAX_VALUE, medicineClassification)); 6824 children.add(new Property("packaging", "", "Information that only applies to packages (not products).", 0, java.lang.Integer.MAX_VALUE, packaging)); 6825 children.add(new Property("clinicalUseIssue", "Reference(ClinicalUseDefinition)", "Potential clinical issue with or between medication(s) (for example, drug-drug interaction, drug-disease contraindication, drug-allergy interaction, etc.).", 0, java.lang.Integer.MAX_VALUE, clinicalUseIssue)); 6826 children.add(new Property("storageGuideline", "", "Information on how the medication should be stored, for example, refrigeration temperatures and length of stability at a given temperature.", 0, java.lang.Integer.MAX_VALUE, storageGuideline)); 6827 children.add(new Property("regulatory", "", "Regulatory information about a medication.", 0, java.lang.Integer.MAX_VALUE, regulatory)); 6828 children.add(new Property("definitional", "", "Along with the link to a Medicinal Product Definition resource, this information provides common definitional elements that are needed to understand the specific medication that is being described.", 0, 1, definitional)); 6829 } 6830 6831 @Override 6832 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6833 switch (_hash) { 6834 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier for this medication.", 0, java.lang.Integer.MAX_VALUE, identifier); 6835 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code that specifies this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.", 0, 1, code); 6836 case -892481550: /*status*/ return new Property("status", "code", "A code to indicate if the medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. The status refers to the validity about the information of the medication and not to its medicinal properties.", 0, 1, status); 6837 case -1406328437: /*author*/ return new Property("author", "Reference(Organization)", "The creator or owner of the knowledge or information about the medication.", 0, 1, author); 6838 case 2136596300: /*intendedJurisdiction*/ return new Property("intendedJurisdiction", "CodeableConcept", "Lists the jurisdictions that this medication knowledge was written for.", 0, java.lang.Integer.MAX_VALUE, intendedJurisdiction); 6839 case 3373707: /*name*/ return new Property("name", "string", "All of the names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol.", 0, java.lang.Integer.MAX_VALUE, name); 6840 case 723067972: /*relatedMedicationKnowledge*/ return new Property("relatedMedicationKnowledge", "", "Associated or related medications. For example, if the medication is a branded product (e.g. Crestor), this is the Therapeutic Moeity (e.g. Rosuvastatin) or if this is a generic medication (e.g. Rosuvastatin), this would link to a branded product (e.g. Crestor.", 0, java.lang.Integer.MAX_VALUE, relatedMedicationKnowledge); 6841 case 1312779381: /*associatedMedication*/ return new Property("associatedMedication", "Reference(Medication)", "Links to associated medications that could be prescribed, dispensed or administered.", 0, java.lang.Integer.MAX_VALUE, associatedMedication); 6842 case -1491615543: /*productType*/ return new Property("productType", "CodeableConcept", "Category of the medication or product (e.g. branded product, therapeutic moeity, generic product, innovator product, etc.).", 0, java.lang.Integer.MAX_VALUE, productType); 6843 case -1442980789: /*monograph*/ return new Property("monograph", "", "Associated documentation about the medication.", 0, java.lang.Integer.MAX_VALUE, monograph); 6844 case 1025456503: /*preparationInstruction*/ return new Property("preparationInstruction", "markdown", "The instructions for preparing the medication.", 0, 1, preparationInstruction); 6845 case 3059661: /*cost*/ return new Property("cost", "", "The price of the medication.", 0, java.lang.Integer.MAX_VALUE, cost); 6846 case 569848092: /*monitoringProgram*/ return new Property("monitoringProgram", "", "The program under which the medication is reviewed.", 0, java.lang.Integer.MAX_VALUE, monitoringProgram); 6847 case -347044108: /*indicationGuideline*/ return new Property("indicationGuideline", "", "Guidelines or protocols that are applicable for the administration of the medication based on indication.", 0, java.lang.Integer.MAX_VALUE, indicationGuideline); 6848 case 1791551680: /*medicineClassification*/ return new Property("medicineClassification", "", "Categorization of the medication within a formulary or classification system.", 0, java.lang.Integer.MAX_VALUE, medicineClassification); 6849 case 1802065795: /*packaging*/ return new Property("packaging", "", "Information that only applies to packages (not products).", 0, java.lang.Integer.MAX_VALUE, packaging); 6850 case 251885509: /*clinicalUseIssue*/ return new Property("clinicalUseIssue", "Reference(ClinicalUseDefinition)", "Potential clinical issue with or between medication(s) (for example, drug-drug interaction, drug-disease contraindication, drug-allergy interaction, etc.).", 0, java.lang.Integer.MAX_VALUE, clinicalUseIssue); 6851 case 1618773173: /*storageGuideline*/ return new Property("storageGuideline", "", "Information on how the medication should be stored, for example, refrigeration temperatures and length of stability at a given temperature.", 0, java.lang.Integer.MAX_VALUE, storageGuideline); 6852 case -27327848: /*regulatory*/ return new Property("regulatory", "", "Regulatory information about a medication.", 0, java.lang.Integer.MAX_VALUE, regulatory); 6853 case 101791934: /*definitional*/ return new Property("definitional", "", "Along with the link to a Medicinal Product Definition resource, this information provides common definitional elements that are needed to understand the specific medication that is being described.", 0, 1, definitional); 6854 default: return super.getNamedProperty(_hash, _name, _checkValid); 6855 } 6856 6857 } 6858 6859 @Override 6860 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6861 switch (hash) { 6862 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 6863 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 6864 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MedicationKnowledgeStatusCodes> 6865 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 6866 case 2136596300: /*intendedJurisdiction*/ return this.intendedJurisdiction == null ? new Base[0] : this.intendedJurisdiction.toArray(new Base[this.intendedJurisdiction.size()]); // CodeableConcept 6867 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // StringType 6868 case 723067972: /*relatedMedicationKnowledge*/ return this.relatedMedicationKnowledge == null ? new Base[0] : this.relatedMedicationKnowledge.toArray(new Base[this.relatedMedicationKnowledge.size()]); // MedicationKnowledgeRelatedMedicationKnowledgeComponent 6869 case 1312779381: /*associatedMedication*/ return this.associatedMedication == null ? new Base[0] : this.associatedMedication.toArray(new Base[this.associatedMedication.size()]); // Reference 6870 case -1491615543: /*productType*/ return this.productType == null ? new Base[0] : this.productType.toArray(new Base[this.productType.size()]); // CodeableConcept 6871 case -1442980789: /*monograph*/ return this.monograph == null ? new Base[0] : this.monograph.toArray(new Base[this.monograph.size()]); // MedicationKnowledgeMonographComponent 6872 case 1025456503: /*preparationInstruction*/ return this.preparationInstruction == null ? new Base[0] : new Base[] {this.preparationInstruction}; // MarkdownType 6873 case 3059661: /*cost*/ return this.cost == null ? new Base[0] : this.cost.toArray(new Base[this.cost.size()]); // MedicationKnowledgeCostComponent 6874 case 569848092: /*monitoringProgram*/ return this.monitoringProgram == null ? new Base[0] : this.monitoringProgram.toArray(new Base[this.monitoringProgram.size()]); // MedicationKnowledgeMonitoringProgramComponent 6875 case -347044108: /*indicationGuideline*/ return this.indicationGuideline == null ? new Base[0] : this.indicationGuideline.toArray(new Base[this.indicationGuideline.size()]); // MedicationKnowledgeIndicationGuidelineComponent 6876 case 1791551680: /*medicineClassification*/ return this.medicineClassification == null ? new Base[0] : this.medicineClassification.toArray(new Base[this.medicineClassification.size()]); // MedicationKnowledgeMedicineClassificationComponent 6877 case 1802065795: /*packaging*/ return this.packaging == null ? new Base[0] : this.packaging.toArray(new Base[this.packaging.size()]); // MedicationKnowledgePackagingComponent 6878 case 251885509: /*clinicalUseIssue*/ return this.clinicalUseIssue == null ? new Base[0] : this.clinicalUseIssue.toArray(new Base[this.clinicalUseIssue.size()]); // Reference 6879 case 1618773173: /*storageGuideline*/ return this.storageGuideline == null ? new Base[0] : this.storageGuideline.toArray(new Base[this.storageGuideline.size()]); // MedicationKnowledgeStorageGuidelineComponent 6880 case -27327848: /*regulatory*/ return this.regulatory == null ? new Base[0] : this.regulatory.toArray(new Base[this.regulatory.size()]); // MedicationKnowledgeRegulatoryComponent 6881 case 101791934: /*definitional*/ return this.definitional == null ? new Base[0] : new Base[] {this.definitional}; // MedicationKnowledgeDefinitionalComponent 6882 default: return super.getProperty(hash, name, checkValid); 6883 } 6884 6885 } 6886 6887 @Override 6888 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6889 switch (hash) { 6890 case -1618432855: // identifier 6891 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 6892 return value; 6893 case 3059181: // code 6894 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6895 return value; 6896 case -892481550: // status 6897 value = new MedicationKnowledgeStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 6898 this.status = (Enumeration) value; // Enumeration<MedicationKnowledgeStatusCodes> 6899 return value; 6900 case -1406328437: // author 6901 this.author = TypeConvertor.castToReference(value); // Reference 6902 return value; 6903 case 2136596300: // intendedJurisdiction 6904 this.getIntendedJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6905 return value; 6906 case 3373707: // name 6907 this.getName().add(TypeConvertor.castToString(value)); // StringType 6908 return value; 6909 case 723067972: // relatedMedicationKnowledge 6910 this.getRelatedMedicationKnowledge().add((MedicationKnowledgeRelatedMedicationKnowledgeComponent) value); // MedicationKnowledgeRelatedMedicationKnowledgeComponent 6911 return value; 6912 case 1312779381: // associatedMedication 6913 this.getAssociatedMedication().add(TypeConvertor.castToReference(value)); // Reference 6914 return value; 6915 case -1491615543: // productType 6916 this.getProductType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6917 return value; 6918 case -1442980789: // monograph 6919 this.getMonograph().add((MedicationKnowledgeMonographComponent) value); // MedicationKnowledgeMonographComponent 6920 return value; 6921 case 1025456503: // preparationInstruction 6922 this.preparationInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 6923 return value; 6924 case 3059661: // cost 6925 this.getCost().add((MedicationKnowledgeCostComponent) value); // MedicationKnowledgeCostComponent 6926 return value; 6927 case 569848092: // monitoringProgram 6928 this.getMonitoringProgram().add((MedicationKnowledgeMonitoringProgramComponent) value); // MedicationKnowledgeMonitoringProgramComponent 6929 return value; 6930 case -347044108: // indicationGuideline 6931 this.getIndicationGuideline().add((MedicationKnowledgeIndicationGuidelineComponent) value); // MedicationKnowledgeIndicationGuidelineComponent 6932 return value; 6933 case 1791551680: // medicineClassification 6934 this.getMedicineClassification().add((MedicationKnowledgeMedicineClassificationComponent) value); // MedicationKnowledgeMedicineClassificationComponent 6935 return value; 6936 case 1802065795: // packaging 6937 this.getPackaging().add((MedicationKnowledgePackagingComponent) value); // MedicationKnowledgePackagingComponent 6938 return value; 6939 case 251885509: // clinicalUseIssue 6940 this.getClinicalUseIssue().add(TypeConvertor.castToReference(value)); // Reference 6941 return value; 6942 case 1618773173: // storageGuideline 6943 this.getStorageGuideline().add((MedicationKnowledgeStorageGuidelineComponent) value); // MedicationKnowledgeStorageGuidelineComponent 6944 return value; 6945 case -27327848: // regulatory 6946 this.getRegulatory().add((MedicationKnowledgeRegulatoryComponent) value); // MedicationKnowledgeRegulatoryComponent 6947 return value; 6948 case 101791934: // definitional 6949 this.definitional = (MedicationKnowledgeDefinitionalComponent) value; // MedicationKnowledgeDefinitionalComponent 6950 return value; 6951 default: return super.setProperty(hash, name, value); 6952 } 6953 6954 } 6955 6956 @Override 6957 public Base setProperty(String name, Base value) throws FHIRException { 6958 if (name.equals("identifier")) { 6959 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 6960 } else if (name.equals("code")) { 6961 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6962 } else if (name.equals("status")) { 6963 value = new MedicationKnowledgeStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 6964 this.status = (Enumeration) value; // Enumeration<MedicationKnowledgeStatusCodes> 6965 } else if (name.equals("author")) { 6966 this.author = TypeConvertor.castToReference(value); // Reference 6967 } else if (name.equals("intendedJurisdiction")) { 6968 this.getIntendedJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 6969 } else if (name.equals("name")) { 6970 this.getName().add(TypeConvertor.castToString(value)); 6971 } else if (name.equals("relatedMedicationKnowledge")) { 6972 this.getRelatedMedicationKnowledge().add((MedicationKnowledgeRelatedMedicationKnowledgeComponent) value); 6973 } else if (name.equals("associatedMedication")) { 6974 this.getAssociatedMedication().add(TypeConvertor.castToReference(value)); 6975 } else if (name.equals("productType")) { 6976 this.getProductType().add(TypeConvertor.castToCodeableConcept(value)); 6977 } else if (name.equals("monograph")) { 6978 this.getMonograph().add((MedicationKnowledgeMonographComponent) value); 6979 } else if (name.equals("preparationInstruction")) { 6980 this.preparationInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 6981 } else if (name.equals("cost")) { 6982 this.getCost().add((MedicationKnowledgeCostComponent) value); 6983 } else if (name.equals("monitoringProgram")) { 6984 this.getMonitoringProgram().add((MedicationKnowledgeMonitoringProgramComponent) value); 6985 } else if (name.equals("indicationGuideline")) { 6986 this.getIndicationGuideline().add((MedicationKnowledgeIndicationGuidelineComponent) value); 6987 } else if (name.equals("medicineClassification")) { 6988 this.getMedicineClassification().add((MedicationKnowledgeMedicineClassificationComponent) value); 6989 } else if (name.equals("packaging")) { 6990 this.getPackaging().add((MedicationKnowledgePackagingComponent) value); 6991 } else if (name.equals("clinicalUseIssue")) { 6992 this.getClinicalUseIssue().add(TypeConvertor.castToReference(value)); 6993 } else if (name.equals("storageGuideline")) { 6994 this.getStorageGuideline().add((MedicationKnowledgeStorageGuidelineComponent) value); 6995 } else if (name.equals("regulatory")) { 6996 this.getRegulatory().add((MedicationKnowledgeRegulatoryComponent) value); 6997 } else if (name.equals("definitional")) { 6998 this.definitional = (MedicationKnowledgeDefinitionalComponent) value; // MedicationKnowledgeDefinitionalComponent 6999 } else 7000 return super.setProperty(name, value); 7001 return value; 7002 } 7003 7004 @Override 7005 public void removeChild(String name, Base value) throws FHIRException { 7006 if (name.equals("identifier")) { 7007 this.getIdentifier().remove(value); 7008 } else if (name.equals("code")) { 7009 this.code = null; 7010 } else if (name.equals("status")) { 7011 value = new MedicationKnowledgeStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 7012 this.status = (Enumeration) value; // Enumeration<MedicationKnowledgeStatusCodes> 7013 } else if (name.equals("author")) { 7014 this.author = null; 7015 } else if (name.equals("intendedJurisdiction")) { 7016 this.getIntendedJurisdiction().remove(value); 7017 } else if (name.equals("name")) { 7018 this.getName().remove(value); 7019 } else if (name.equals("relatedMedicationKnowledge")) { 7020 this.getRelatedMedicationKnowledge().remove((MedicationKnowledgeRelatedMedicationKnowledgeComponent) value); 7021 } else if (name.equals("associatedMedication")) { 7022 this.getAssociatedMedication().remove(value); 7023 } else if (name.equals("productType")) { 7024 this.getProductType().remove(value); 7025 } else if (name.equals("monograph")) { 7026 this.getMonograph().remove((MedicationKnowledgeMonographComponent) value); 7027 } else if (name.equals("preparationInstruction")) { 7028 this.preparationInstruction = null; 7029 } else if (name.equals("cost")) { 7030 this.getCost().remove((MedicationKnowledgeCostComponent) value); 7031 } else if (name.equals("monitoringProgram")) { 7032 this.getMonitoringProgram().remove((MedicationKnowledgeMonitoringProgramComponent) value); 7033 } else if (name.equals("indicationGuideline")) { 7034 this.getIndicationGuideline().remove((MedicationKnowledgeIndicationGuidelineComponent) value); 7035 } else if (name.equals("medicineClassification")) { 7036 this.getMedicineClassification().remove((MedicationKnowledgeMedicineClassificationComponent) value); 7037 } else if (name.equals("packaging")) { 7038 this.getPackaging().remove((MedicationKnowledgePackagingComponent) value); 7039 } else if (name.equals("clinicalUseIssue")) { 7040 this.getClinicalUseIssue().remove(value); 7041 } else if (name.equals("storageGuideline")) { 7042 this.getStorageGuideline().remove((MedicationKnowledgeStorageGuidelineComponent) value); 7043 } else if (name.equals("regulatory")) { 7044 this.getRegulatory().remove((MedicationKnowledgeRegulatoryComponent) value); 7045 } else if (name.equals("definitional")) { 7046 this.definitional = (MedicationKnowledgeDefinitionalComponent) value; // MedicationKnowledgeDefinitionalComponent 7047 } else 7048 super.removeChild(name, value); 7049 7050 } 7051 7052 @Override 7053 public Base makeProperty(int hash, String name) throws FHIRException { 7054 switch (hash) { 7055 case -1618432855: return addIdentifier(); 7056 case 3059181: return getCode(); 7057 case -892481550: return getStatusElement(); 7058 case -1406328437: return getAuthor(); 7059 case 2136596300: return addIntendedJurisdiction(); 7060 case 3373707: return addNameElement(); 7061 case 723067972: return addRelatedMedicationKnowledge(); 7062 case 1312779381: return addAssociatedMedication(); 7063 case -1491615543: return addProductType(); 7064 case -1442980789: return addMonograph(); 7065 case 1025456503: return getPreparationInstructionElement(); 7066 case 3059661: return addCost(); 7067 case 569848092: return addMonitoringProgram(); 7068 case -347044108: return addIndicationGuideline(); 7069 case 1791551680: return addMedicineClassification(); 7070 case 1802065795: return addPackaging(); 7071 case 251885509: return addClinicalUseIssue(); 7072 case 1618773173: return addStorageGuideline(); 7073 case -27327848: return addRegulatory(); 7074 case 101791934: return getDefinitional(); 7075 default: return super.makeProperty(hash, name); 7076 } 7077 7078 } 7079 7080 @Override 7081 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7082 switch (hash) { 7083 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 7084 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 7085 case -892481550: /*status*/ return new String[] {"code"}; 7086 case -1406328437: /*author*/ return new String[] {"Reference"}; 7087 case 2136596300: /*intendedJurisdiction*/ return new String[] {"CodeableConcept"}; 7088 case 3373707: /*name*/ return new String[] {"string"}; 7089 case 723067972: /*relatedMedicationKnowledge*/ return new String[] {}; 7090 case 1312779381: /*associatedMedication*/ return new String[] {"Reference"}; 7091 case -1491615543: /*productType*/ return new String[] {"CodeableConcept"}; 7092 case -1442980789: /*monograph*/ return new String[] {}; 7093 case 1025456503: /*preparationInstruction*/ return new String[] {"markdown"}; 7094 case 3059661: /*cost*/ return new String[] {}; 7095 case 569848092: /*monitoringProgram*/ return new String[] {}; 7096 case -347044108: /*indicationGuideline*/ return new String[] {}; 7097 case 1791551680: /*medicineClassification*/ return new String[] {}; 7098 case 1802065795: /*packaging*/ return new String[] {}; 7099 case 251885509: /*clinicalUseIssue*/ return new String[] {"Reference"}; 7100 case 1618773173: /*storageGuideline*/ return new String[] {}; 7101 case -27327848: /*regulatory*/ return new String[] {}; 7102 case 101791934: /*definitional*/ return new String[] {}; 7103 default: return super.getTypesForProperty(hash, name); 7104 } 7105 7106 } 7107 7108 @Override 7109 public Base addChild(String name) throws FHIRException { 7110 if (name.equals("identifier")) { 7111 return addIdentifier(); 7112 } 7113 else if (name.equals("code")) { 7114 this.code = new CodeableConcept(); 7115 return this.code; 7116 } 7117 else if (name.equals("status")) { 7118 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.status"); 7119 } 7120 else if (name.equals("author")) { 7121 this.author = new Reference(); 7122 return this.author; 7123 } 7124 else if (name.equals("intendedJurisdiction")) { 7125 return addIntendedJurisdiction(); 7126 } 7127 else if (name.equals("name")) { 7128 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.name"); 7129 } 7130 else if (name.equals("relatedMedicationKnowledge")) { 7131 return addRelatedMedicationKnowledge(); 7132 } 7133 else if (name.equals("associatedMedication")) { 7134 return addAssociatedMedication(); 7135 } 7136 else if (name.equals("productType")) { 7137 return addProductType(); 7138 } 7139 else if (name.equals("monograph")) { 7140 return addMonograph(); 7141 } 7142 else if (name.equals("preparationInstruction")) { 7143 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.preparationInstruction"); 7144 } 7145 else if (name.equals("cost")) { 7146 return addCost(); 7147 } 7148 else if (name.equals("monitoringProgram")) { 7149 return addMonitoringProgram(); 7150 } 7151 else if (name.equals("indicationGuideline")) { 7152 return addIndicationGuideline(); 7153 } 7154 else if (name.equals("medicineClassification")) { 7155 return addMedicineClassification(); 7156 } 7157 else if (name.equals("packaging")) { 7158 return addPackaging(); 7159 } 7160 else if (name.equals("clinicalUseIssue")) { 7161 return addClinicalUseIssue(); 7162 } 7163 else if (name.equals("storageGuideline")) { 7164 return addStorageGuideline(); 7165 } 7166 else if (name.equals("regulatory")) { 7167 return addRegulatory(); 7168 } 7169 else if (name.equals("definitional")) { 7170 this.definitional = new MedicationKnowledgeDefinitionalComponent(); 7171 return this.definitional; 7172 } 7173 else 7174 return super.addChild(name); 7175 } 7176 7177 public String fhirType() { 7178 return "MedicationKnowledge"; 7179 7180 } 7181 7182 public MedicationKnowledge copy() { 7183 MedicationKnowledge dst = new MedicationKnowledge(); 7184 copyValues(dst); 7185 return dst; 7186 } 7187 7188 public void copyValues(MedicationKnowledge dst) { 7189 super.copyValues(dst); 7190 if (identifier != null) { 7191 dst.identifier = new ArrayList<Identifier>(); 7192 for (Identifier i : identifier) 7193 dst.identifier.add(i.copy()); 7194 }; 7195 dst.code = code == null ? null : code.copy(); 7196 dst.status = status == null ? null : status.copy(); 7197 dst.author = author == null ? null : author.copy(); 7198 if (intendedJurisdiction != null) { 7199 dst.intendedJurisdiction = new ArrayList<CodeableConcept>(); 7200 for (CodeableConcept i : intendedJurisdiction) 7201 dst.intendedJurisdiction.add(i.copy()); 7202 }; 7203 if (name != null) { 7204 dst.name = new ArrayList<StringType>(); 7205 for (StringType i : name) 7206 dst.name.add(i.copy()); 7207 }; 7208 if (relatedMedicationKnowledge != null) { 7209 dst.relatedMedicationKnowledge = new ArrayList<MedicationKnowledgeRelatedMedicationKnowledgeComponent>(); 7210 for (MedicationKnowledgeRelatedMedicationKnowledgeComponent i : relatedMedicationKnowledge) 7211 dst.relatedMedicationKnowledge.add(i.copy()); 7212 }; 7213 if (associatedMedication != null) { 7214 dst.associatedMedication = new ArrayList<Reference>(); 7215 for (Reference i : associatedMedication) 7216 dst.associatedMedication.add(i.copy()); 7217 }; 7218 if (productType != null) { 7219 dst.productType = new ArrayList<CodeableConcept>(); 7220 for (CodeableConcept i : productType) 7221 dst.productType.add(i.copy()); 7222 }; 7223 if (monograph != null) { 7224 dst.monograph = new ArrayList<MedicationKnowledgeMonographComponent>(); 7225 for (MedicationKnowledgeMonographComponent i : monograph) 7226 dst.monograph.add(i.copy()); 7227 }; 7228 dst.preparationInstruction = preparationInstruction == null ? null : preparationInstruction.copy(); 7229 if (cost != null) { 7230 dst.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 7231 for (MedicationKnowledgeCostComponent i : cost) 7232 dst.cost.add(i.copy()); 7233 }; 7234 if (monitoringProgram != null) { 7235 dst.monitoringProgram = new ArrayList<MedicationKnowledgeMonitoringProgramComponent>(); 7236 for (MedicationKnowledgeMonitoringProgramComponent i : monitoringProgram) 7237 dst.monitoringProgram.add(i.copy()); 7238 }; 7239 if (indicationGuideline != null) { 7240 dst.indicationGuideline = new ArrayList<MedicationKnowledgeIndicationGuidelineComponent>(); 7241 for (MedicationKnowledgeIndicationGuidelineComponent i : indicationGuideline) 7242 dst.indicationGuideline.add(i.copy()); 7243 }; 7244 if (medicineClassification != null) { 7245 dst.medicineClassification = new ArrayList<MedicationKnowledgeMedicineClassificationComponent>(); 7246 for (MedicationKnowledgeMedicineClassificationComponent i : medicineClassification) 7247 dst.medicineClassification.add(i.copy()); 7248 }; 7249 if (packaging != null) { 7250 dst.packaging = new ArrayList<MedicationKnowledgePackagingComponent>(); 7251 for (MedicationKnowledgePackagingComponent i : packaging) 7252 dst.packaging.add(i.copy()); 7253 }; 7254 if (clinicalUseIssue != null) { 7255 dst.clinicalUseIssue = new ArrayList<Reference>(); 7256 for (Reference i : clinicalUseIssue) 7257 dst.clinicalUseIssue.add(i.copy()); 7258 }; 7259 if (storageGuideline != null) { 7260 dst.storageGuideline = new ArrayList<MedicationKnowledgeStorageGuidelineComponent>(); 7261 for (MedicationKnowledgeStorageGuidelineComponent i : storageGuideline) 7262 dst.storageGuideline.add(i.copy()); 7263 }; 7264 if (regulatory != null) { 7265 dst.regulatory = new ArrayList<MedicationKnowledgeRegulatoryComponent>(); 7266 for (MedicationKnowledgeRegulatoryComponent i : regulatory) 7267 dst.regulatory.add(i.copy()); 7268 }; 7269 dst.definitional = definitional == null ? null : definitional.copy(); 7270 } 7271 7272 protected MedicationKnowledge typedCopy() { 7273 return copy(); 7274 } 7275 7276 @Override 7277 public boolean equalsDeep(Base other_) { 7278 if (!super.equalsDeep(other_)) 7279 return false; 7280 if (!(other_ instanceof MedicationKnowledge)) 7281 return false; 7282 MedicationKnowledge o = (MedicationKnowledge) other_; 7283 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) && compareDeep(status, o.status, true) 7284 && compareDeep(author, o.author, true) && compareDeep(intendedJurisdiction, o.intendedJurisdiction, true) 7285 && compareDeep(name, o.name, true) && compareDeep(relatedMedicationKnowledge, o.relatedMedicationKnowledge, true) 7286 && compareDeep(associatedMedication, o.associatedMedication, true) && compareDeep(productType, o.productType, true) 7287 && compareDeep(monograph, o.monograph, true) && compareDeep(preparationInstruction, o.preparationInstruction, true) 7288 && compareDeep(cost, o.cost, true) && compareDeep(monitoringProgram, o.monitoringProgram, true) 7289 && compareDeep(indicationGuideline, o.indicationGuideline, true) && compareDeep(medicineClassification, o.medicineClassification, true) 7290 && compareDeep(packaging, o.packaging, true) && compareDeep(clinicalUseIssue, o.clinicalUseIssue, true) 7291 && compareDeep(storageGuideline, o.storageGuideline, true) && compareDeep(regulatory, o.regulatory, true) 7292 && compareDeep(definitional, o.definitional, true); 7293 } 7294 7295 @Override 7296 public boolean equalsShallow(Base other_) { 7297 if (!super.equalsShallow(other_)) 7298 return false; 7299 if (!(other_ instanceof MedicationKnowledge)) 7300 return false; 7301 MedicationKnowledge o = (MedicationKnowledge) other_; 7302 return compareValues(status, o.status, true) && compareValues(name, o.name, true) && compareValues(preparationInstruction, o.preparationInstruction, true) 7303 ; 7304 } 7305 7306 public boolean isEmpty() { 7307 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, status 7308 , author, intendedJurisdiction, name, relatedMedicationKnowledge, associatedMedication 7309 , productType, monograph, preparationInstruction, cost, monitoringProgram, indicationGuideline 7310 , medicineClassification, packaging, clinicalUseIssue, storageGuideline, regulatory 7311 , definitional); 7312 } 7313 7314 @Override 7315 public ResourceType getResourceType() { 7316 return ResourceType.MedicationKnowledge; 7317 } 7318 7319 /** 7320 * Search parameter: <b>identifier</b> 7321 * <p> 7322 * Description: <b>Multiple Resources: 7323 7324* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 7325* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 7326* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 7327* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 7328* [Citation](citation.html): External identifier for the citation 7329* [CodeSystem](codesystem.html): External identifier for the code system 7330* [ConceptMap](conceptmap.html): External identifier for the concept map 7331* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 7332* [EventDefinition](eventdefinition.html): External identifier for the event definition 7333* [Evidence](evidence.html): External identifier for the evidence 7334* [EvidenceReport](evidencereport.html): External identifier for the evidence report 7335* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 7336* [ExampleScenario](examplescenario.html): External identifier for the example scenario 7337* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 7338* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 7339* [Library](library.html): External identifier for the library 7340* [Measure](measure.html): External identifier for the measure 7341* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 7342* [MessageDefinition](messagedefinition.html): External identifier for the message definition 7343* [NamingSystem](namingsystem.html): External identifier for the naming system 7344* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 7345* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 7346* [PlanDefinition](plandefinition.html): External identifier for the plan definition 7347* [Questionnaire](questionnaire.html): External identifier for the questionnaire 7348* [Requirements](requirements.html): External identifier for the requirements 7349* [SearchParameter](searchparameter.html): External identifier for the search parameter 7350* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 7351* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 7352* [StructureMap](structuremap.html): External identifier for the structure map 7353* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 7354* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 7355* [TestPlan](testplan.html): An identifier for the test plan 7356* [TestScript](testscript.html): External identifier for the test script 7357* [ValueSet](valueset.html): External identifier for the value set 7358</b><br> 7359 * Type: <b>token</b><br> 7360 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 7361 * </p> 7362 */ 7363 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 7364 public static final String SP_IDENTIFIER = "identifier"; 7365 /** 7366 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 7367 * <p> 7368 * Description: <b>Multiple Resources: 7369 7370* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 7371* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 7372* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 7373* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 7374* [Citation](citation.html): External identifier for the citation 7375* [CodeSystem](codesystem.html): External identifier for the code system 7376* [ConceptMap](conceptmap.html): External identifier for the concept map 7377* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 7378* [EventDefinition](eventdefinition.html): External identifier for the event definition 7379* [Evidence](evidence.html): External identifier for the evidence 7380* [EvidenceReport](evidencereport.html): External identifier for the evidence report 7381* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 7382* [ExampleScenario](examplescenario.html): External identifier for the example scenario 7383* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 7384* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 7385* [Library](library.html): External identifier for the library 7386* [Measure](measure.html): External identifier for the measure 7387* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 7388* [MessageDefinition](messagedefinition.html): External identifier for the message definition 7389* [NamingSystem](namingsystem.html): External identifier for the naming system 7390* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 7391* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 7392* [PlanDefinition](plandefinition.html): External identifier for the plan definition 7393* [Questionnaire](questionnaire.html): External identifier for the questionnaire 7394* [Requirements](requirements.html): External identifier for the requirements 7395* [SearchParameter](searchparameter.html): External identifier for the search parameter 7396* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 7397* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 7398* [StructureMap](structuremap.html): External identifier for the structure map 7399* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 7400* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 7401* [TestPlan](testplan.html): An identifier for the test plan 7402* [TestScript](testscript.html): External identifier for the test script 7403* [ValueSet](valueset.html): External identifier for the value set 7404</b><br> 7405 * Type: <b>token</b><br> 7406 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 7407 * </p> 7408 */ 7409 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 7410 7411 /** 7412 * Search parameter: <b>status</b> 7413 * <p> 7414 * Description: <b>Multiple Resources: 7415 7416* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 7417* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 7418* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 7419* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 7420* [Citation](citation.html): The current status of the citation 7421* [CodeSystem](codesystem.html): The current status of the code system 7422* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 7423* [ConceptMap](conceptmap.html): The current status of the concept map 7424* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 7425* [EventDefinition](eventdefinition.html): The current status of the event definition 7426* [Evidence](evidence.html): The current status of the evidence 7427* [EvidenceReport](evidencereport.html): The current status of the evidence report 7428* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 7429* [ExampleScenario](examplescenario.html): The current status of the example scenario 7430* [GraphDefinition](graphdefinition.html): The current status of the graph definition 7431* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 7432* [Library](library.html): The current status of the library 7433* [Measure](measure.html): The current status of the measure 7434* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 7435* [MessageDefinition](messagedefinition.html): The current status of the message definition 7436* [NamingSystem](namingsystem.html): The current status of the naming system 7437* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 7438* [OperationDefinition](operationdefinition.html): The current status of the operation definition 7439* [PlanDefinition](plandefinition.html): The current status of the plan definition 7440* [Questionnaire](questionnaire.html): The current status of the questionnaire 7441* [Requirements](requirements.html): The current status of the requirements 7442* [SearchParameter](searchparameter.html): The current status of the search parameter 7443* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 7444* [StructureDefinition](structuredefinition.html): The current status of the structure definition 7445* [StructureMap](structuremap.html): The current status of the structure map 7446* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 7447* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 7448* [TestPlan](testplan.html): The current status of the test plan 7449* [TestScript](testscript.html): The current status of the test script 7450* [ValueSet](valueset.html): The current status of the value set 7451</b><br> 7452 * Type: <b>token</b><br> 7453 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 7454 * </p> 7455 */ 7456 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 7457 public static final String SP_STATUS = "status"; 7458 /** 7459 * <b>Fluent Client</b> search parameter constant for <b>status</b> 7460 * <p> 7461 * Description: <b>Multiple Resources: 7462 7463* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 7464* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 7465* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 7466* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 7467* [Citation](citation.html): The current status of the citation 7468* [CodeSystem](codesystem.html): The current status of the code system 7469* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 7470* [ConceptMap](conceptmap.html): The current status of the concept map 7471* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 7472* [EventDefinition](eventdefinition.html): The current status of the event definition 7473* [Evidence](evidence.html): The current status of the evidence 7474* [EvidenceReport](evidencereport.html): The current status of the evidence report 7475* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 7476* [ExampleScenario](examplescenario.html): The current status of the example scenario 7477* [GraphDefinition](graphdefinition.html): The current status of the graph definition 7478* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 7479* [Library](library.html): The current status of the library 7480* [Measure](measure.html): The current status of the measure 7481* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 7482* [MessageDefinition](messagedefinition.html): The current status of the message definition 7483* [NamingSystem](namingsystem.html): The current status of the naming system 7484* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 7485* [OperationDefinition](operationdefinition.html): The current status of the operation definition 7486* [PlanDefinition](plandefinition.html): The current status of the plan definition 7487* [Questionnaire](questionnaire.html): The current status of the questionnaire 7488* [Requirements](requirements.html): The current status of the requirements 7489* [SearchParameter](searchparameter.html): The current status of the search parameter 7490* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 7491* [StructureDefinition](structuredefinition.html): The current status of the structure definition 7492* [StructureMap](structuremap.html): The current status of the structure map 7493* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 7494* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 7495* [TestPlan](testplan.html): The current status of the test plan 7496* [TestScript](testscript.html): The current status of the test script 7497* [ValueSet](valueset.html): The current status of the value set 7498</b><br> 7499 * Type: <b>token</b><br> 7500 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 7501 * </p> 7502 */ 7503 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 7504 7505 /** 7506 * Search parameter: <b>classification-type</b> 7507 * <p> 7508 * Description: <b>The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification)</b><br> 7509 * Type: <b>token</b><br> 7510 * Path: <b>MedicationKnowledge.medicineClassification.type</b><br> 7511 * </p> 7512 */ 7513 @SearchParamDefinition(name="classification-type", path="MedicationKnowledge.medicineClassification.type", description="The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification)", type="token" ) 7514 public static final String SP_CLASSIFICATION_TYPE = "classification-type"; 7515 /** 7516 * <b>Fluent Client</b> search parameter constant for <b>classification-type</b> 7517 * <p> 7518 * Description: <b>The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification)</b><br> 7519 * Type: <b>token</b><br> 7520 * Path: <b>MedicationKnowledge.medicineClassification.type</b><br> 7521 * </p> 7522 */ 7523 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASSIFICATION_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLASSIFICATION_TYPE); 7524 7525 /** 7526 * Search parameter: <b>classification</b> 7527 * <p> 7528 * Description: <b>Specific category assigned to the medication</b><br> 7529 * Type: <b>token</b><br> 7530 * Path: <b>MedicationKnowledge.medicineClassification.classification</b><br> 7531 * </p> 7532 */ 7533 @SearchParamDefinition(name="classification", path="MedicationKnowledge.medicineClassification.classification", description="Specific category assigned to the medication", type="token" ) 7534 public static final String SP_CLASSIFICATION = "classification"; 7535 /** 7536 * <b>Fluent Client</b> search parameter constant for <b>classification</b> 7537 * <p> 7538 * Description: <b>Specific category assigned to the medication</b><br> 7539 * Type: <b>token</b><br> 7540 * Path: <b>MedicationKnowledge.medicineClassification.classification</b><br> 7541 * </p> 7542 */ 7543 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASSIFICATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLASSIFICATION); 7544 7545 /** 7546 * Search parameter: <b>code</b> 7547 * <p> 7548 * Description: <b>Code that identifies this medication</b><br> 7549 * Type: <b>token</b><br> 7550 * Path: <b>MedicationKnowledge.code</b><br> 7551 * </p> 7552 */ 7553 @SearchParamDefinition(name="code", path="MedicationKnowledge.code", description="Code that identifies this medication", type="token" ) 7554 public static final String SP_CODE = "code"; 7555 /** 7556 * <b>Fluent Client</b> search parameter constant for <b>code</b> 7557 * <p> 7558 * Description: <b>Code that identifies this medication</b><br> 7559 * Type: <b>token</b><br> 7560 * Path: <b>MedicationKnowledge.code</b><br> 7561 * </p> 7562 */ 7563 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 7564 7565 /** 7566 * Search parameter: <b>doseform</b> 7567 * <p> 7568 * Description: <b>powder | tablets | capsule +</b><br> 7569 * Type: <b>token</b><br> 7570 * Path: <b>MedicationKnowledge.definitional.doseForm</b><br> 7571 * </p> 7572 */ 7573 @SearchParamDefinition(name="doseform", path="MedicationKnowledge.definitional.doseForm", description="powder | tablets | capsule +", type="token" ) 7574 public static final String SP_DOSEFORM = "doseform"; 7575 /** 7576 * <b>Fluent Client</b> search parameter constant for <b>doseform</b> 7577 * <p> 7578 * Description: <b>powder | tablets | capsule +</b><br> 7579 * Type: <b>token</b><br> 7580 * Path: <b>MedicationKnowledge.definitional.doseForm</b><br> 7581 * </p> 7582 */ 7583 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DOSEFORM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DOSEFORM); 7584 7585 /** 7586 * Search parameter: <b>ingredient-code</b> 7587 * <p> 7588 * Description: <b>Reference to a concept (by class)</b><br> 7589 * Type: <b>token</b><br> 7590 * Path: <b>MedicationKnowledge.definitional.ingredient.item.concept</b><br> 7591 * </p> 7592 */ 7593 @SearchParamDefinition(name="ingredient-code", path="MedicationKnowledge.definitional.ingredient.item.concept", description="Reference to a concept (by class)", type="token" ) 7594 public static final String SP_INGREDIENT_CODE = "ingredient-code"; 7595 /** 7596 * <b>Fluent Client</b> search parameter constant for <b>ingredient-code</b> 7597 * <p> 7598 * Description: <b>Reference to a concept (by class)</b><br> 7599 * Type: <b>token</b><br> 7600 * Path: <b>MedicationKnowledge.definitional.ingredient.item.concept</b><br> 7601 * </p> 7602 */ 7603 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INGREDIENT_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INGREDIENT_CODE); 7604 7605 /** 7606 * Search parameter: <b>ingredient</b> 7607 * <p> 7608 * Description: <b>Reference to a resource (by instance)</b><br> 7609 * Type: <b>reference</b><br> 7610 * Path: <b>MedicationKnowledge.definitional.ingredient.item.reference</b><br> 7611 * </p> 7612 */ 7613 @SearchParamDefinition(name="ingredient", path="MedicationKnowledge.definitional.ingredient.item.reference", description="Reference to a resource (by instance)", type="reference", target={Substance.class } ) 7614 public static final String SP_INGREDIENT = "ingredient"; 7615 /** 7616 * <b>Fluent Client</b> search parameter constant for <b>ingredient</b> 7617 * <p> 7618 * Description: <b>Reference to a resource (by instance)</b><br> 7619 * Type: <b>reference</b><br> 7620 * Path: <b>MedicationKnowledge.definitional.ingredient.item.reference</b><br> 7621 * </p> 7622 */ 7623 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INGREDIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INGREDIENT); 7624 7625/** 7626 * Constant for fluent queries to be used to add include statements. Specifies 7627 * the path value of "<b>MedicationKnowledge:ingredient</b>". 7628 */ 7629 public static final ca.uhn.fhir.model.api.Include INCLUDE_INGREDIENT = new ca.uhn.fhir.model.api.Include("MedicationKnowledge:ingredient").toLocked(); 7630 7631 /** 7632 * Search parameter: <b>monitoring-program-name</b> 7633 * <p> 7634 * Description: <b>Name of the reviewing program</b><br> 7635 * Type: <b>token</b><br> 7636 * Path: <b>MedicationKnowledge.monitoringProgram.name</b><br> 7637 * </p> 7638 */ 7639 @SearchParamDefinition(name="monitoring-program-name", path="MedicationKnowledge.monitoringProgram.name", description="Name of the reviewing program", type="token" ) 7640 public static final String SP_MONITORING_PROGRAM_NAME = "monitoring-program-name"; 7641 /** 7642 * <b>Fluent Client</b> search parameter constant for <b>monitoring-program-name</b> 7643 * <p> 7644 * Description: <b>Name of the reviewing program</b><br> 7645 * Type: <b>token</b><br> 7646 * Path: <b>MedicationKnowledge.monitoringProgram.name</b><br> 7647 * </p> 7648 */ 7649 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MONITORING_PROGRAM_NAME = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MONITORING_PROGRAM_NAME); 7650 7651 /** 7652 * Search parameter: <b>monitoring-program-type</b> 7653 * <p> 7654 * Description: <b>Type of program under which the medication is monitored</b><br> 7655 * Type: <b>token</b><br> 7656 * Path: <b>MedicationKnowledge.monitoringProgram.type</b><br> 7657 * </p> 7658 */ 7659 @SearchParamDefinition(name="monitoring-program-type", path="MedicationKnowledge.monitoringProgram.type", description="Type of program under which the medication is monitored", type="token" ) 7660 public static final String SP_MONITORING_PROGRAM_TYPE = "monitoring-program-type"; 7661 /** 7662 * <b>Fluent Client</b> search parameter constant for <b>monitoring-program-type</b> 7663 * <p> 7664 * Description: <b>Type of program under which the medication is monitored</b><br> 7665 * Type: <b>token</b><br> 7666 * Path: <b>MedicationKnowledge.monitoringProgram.type</b><br> 7667 * </p> 7668 */ 7669 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MONITORING_PROGRAM_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MONITORING_PROGRAM_TYPE); 7670 7671 /** 7672 * Search parameter: <b>monograph-type</b> 7673 * <p> 7674 * Description: <b>The category of medication document</b><br> 7675 * Type: <b>token</b><br> 7676 * Path: <b>MedicationKnowledge.monograph.type</b><br> 7677 * </p> 7678 */ 7679 @SearchParamDefinition(name="monograph-type", path="MedicationKnowledge.monograph.type", description="The category of medication document", type="token" ) 7680 public static final String SP_MONOGRAPH_TYPE = "monograph-type"; 7681 /** 7682 * <b>Fluent Client</b> search parameter constant for <b>monograph-type</b> 7683 * <p> 7684 * Description: <b>The category of medication document</b><br> 7685 * Type: <b>token</b><br> 7686 * Path: <b>MedicationKnowledge.monograph.type</b><br> 7687 * </p> 7688 */ 7689 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MONOGRAPH_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MONOGRAPH_TYPE); 7690 7691 /** 7692 * Search parameter: <b>monograph</b> 7693 * <p> 7694 * Description: <b>Associated documentation about the medication</b><br> 7695 * Type: <b>reference</b><br> 7696 * Path: <b>MedicationKnowledge.monograph.source</b><br> 7697 * </p> 7698 */ 7699 @SearchParamDefinition(name="monograph", path="MedicationKnowledge.monograph.source", description="Associated documentation about the medication", type="reference", target={DocumentReference.class } ) 7700 public static final String SP_MONOGRAPH = "monograph"; 7701 /** 7702 * <b>Fluent Client</b> search parameter constant for <b>monograph</b> 7703 * <p> 7704 * Description: <b>Associated documentation about the medication</b><br> 7705 * Type: <b>reference</b><br> 7706 * Path: <b>MedicationKnowledge.monograph.source</b><br> 7707 * </p> 7708 */ 7709 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MONOGRAPH = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MONOGRAPH); 7710 7711/** 7712 * Constant for fluent queries to be used to add include statements. Specifies 7713 * the path value of "<b>MedicationKnowledge:monograph</b>". 7714 */ 7715 public static final ca.uhn.fhir.model.api.Include INCLUDE_MONOGRAPH = new ca.uhn.fhir.model.api.Include("MedicationKnowledge:monograph").toLocked(); 7716 7717 /** 7718 * Search parameter: <b>packaging-cost-concept</b> 7719 * <p> 7720 * Description: <b>The cost of the packaged medication, if the cost is a CodeableConcept</b><br> 7721 * Type: <b>token</b><br> 7722 * Path: <b>null</b><br> 7723 * </p> 7724 */ 7725 @SearchParamDefinition(name="packaging-cost-concept", path="", description="The cost of the packaged medication, if the cost is a CodeableConcept", type="token" ) 7726 public static final String SP_PACKAGING_COST_CONCEPT = "packaging-cost-concept"; 7727 /** 7728 * <b>Fluent Client</b> search parameter constant for <b>packaging-cost-concept</b> 7729 * <p> 7730 * Description: <b>The cost of the packaged medication, if the cost is a CodeableConcept</b><br> 7731 * Type: <b>token</b><br> 7732 * Path: <b>null</b><br> 7733 * </p> 7734 */ 7735 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PACKAGING_COST_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PACKAGING_COST_CONCEPT); 7736 7737 /** 7738 * Search parameter: <b>packaging-cost</b> 7739 * <p> 7740 * Description: <b>The cost of the packaged medication, if the cost is Money</b><br> 7741 * Type: <b>quantity</b><br> 7742 * Path: <b>null</b><br> 7743 * </p> 7744 */ 7745 @SearchParamDefinition(name="packaging-cost", path="", description="The cost of the packaged medication, if the cost is Money", type="quantity" ) 7746 public static final String SP_PACKAGING_COST = "packaging-cost"; 7747 /** 7748 * <b>Fluent Client</b> search parameter constant for <b>packaging-cost</b> 7749 * <p> 7750 * Description: <b>The cost of the packaged medication, if the cost is Money</b><br> 7751 * Type: <b>quantity</b><br> 7752 * Path: <b>null</b><br> 7753 * </p> 7754 */ 7755 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam PACKAGING_COST = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_PACKAGING_COST); 7756 7757 /** 7758 * Search parameter: <b>product-type</b> 7759 * <p> 7760 * Description: <b>Category of the medication or product</b><br> 7761 * Type: <b>token</b><br> 7762 * Path: <b>MedicationKnowledge.productType</b><br> 7763 * </p> 7764 */ 7765 @SearchParamDefinition(name="product-type", path="MedicationKnowledge.productType", description="Category of the medication or product", type="token" ) 7766 public static final String SP_PRODUCT_TYPE = "product-type"; 7767 /** 7768 * <b>Fluent Client</b> search parameter constant for <b>product-type</b> 7769 * <p> 7770 * Description: <b>Category of the medication or product</b><br> 7771 * Type: <b>token</b><br> 7772 * Path: <b>MedicationKnowledge.productType</b><br> 7773 * </p> 7774 */ 7775 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRODUCT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRODUCT_TYPE); 7776 7777 /** 7778 * Search parameter: <b>source-cost</b> 7779 * <p> 7780 * Description: <b>The source or owner for the price information</b><br> 7781 * Type: <b>token</b><br> 7782 * Path: <b>MedicationKnowledge.cost.source</b><br> 7783 * </p> 7784 */ 7785 @SearchParamDefinition(name="source-cost", path="MedicationKnowledge.cost.source", description="The source or owner for the price information", type="token" ) 7786 public static final String SP_SOURCE_COST = "source-cost"; 7787 /** 7788 * <b>Fluent Client</b> search parameter constant for <b>source-cost</b> 7789 * <p> 7790 * Description: <b>The source or owner for the price information</b><br> 7791 * Type: <b>token</b><br> 7792 * Path: <b>MedicationKnowledge.cost.source</b><br> 7793 * </p> 7794 */ 7795 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SOURCE_COST = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SOURCE_COST); 7796 7797 7798} 7799