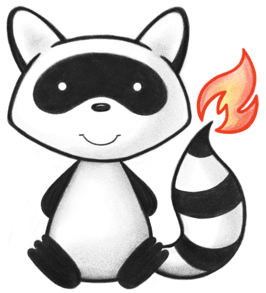
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.utilities.Utilities; 038import org.hl7.fhir.r5.model.Enumerations.*; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.ICompositeType; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.ChildOrder; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.Block; 049 050/** 051 * Information about a medication that is used to support knowledge. 052 */ 053@ResourceDef(name="MedicationKnowledge", profile="http://hl7.org/fhir/StructureDefinition/MedicationKnowledge") 054public class MedicationKnowledge extends DomainResource { 055 056 public enum MedicationKnowledgeStatusCodes { 057 /** 058 * The medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. 059 */ 060 ACTIVE, 061 /** 062 * The medication referred to by this MedicationKnowledge was entered in error within the drug database or inventory system. 063 */ 064 ENTEREDINERROR, 065 /** 066 * The medication referred to by this MedicationKnowledge is not in active use within the drug database or inventory system. 067 */ 068 INACTIVE, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static MedicationKnowledgeStatusCodes fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("entered-in-error".equals(codeString)) 079 return ENTEREDINERROR; 080 if ("inactive".equals(codeString)) 081 return INACTIVE; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown MedicationKnowledgeStatusCodes code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case ACTIVE: return "active"; 090 case ENTEREDINERROR: return "entered-in-error"; 091 case INACTIVE: return "inactive"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case ACTIVE: return "http://hl7.org/fhir/CodeSystem/medicationknowledge-status"; 099 case ENTEREDINERROR: return "http://hl7.org/fhir/CodeSystem/medicationknowledge-status"; 100 case INACTIVE: return "http://hl7.org/fhir/CodeSystem/medicationknowledge-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case ACTIVE: return "The medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system."; 108 case ENTEREDINERROR: return "The medication referred to by this MedicationKnowledge was entered in error within the drug database or inventory system."; 109 case INACTIVE: return "The medication referred to by this MedicationKnowledge is not in active use within the drug database or inventory system."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case ACTIVE: return "Active"; 117 case ENTEREDINERROR: return "Entered in Error"; 118 case INACTIVE: return "Inactive"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class MedicationKnowledgeStatusCodesEnumFactory implements EnumFactory<MedicationKnowledgeStatusCodes> { 126 public MedicationKnowledgeStatusCodes fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("active".equals(codeString)) 131 return MedicationKnowledgeStatusCodes.ACTIVE; 132 if ("entered-in-error".equals(codeString)) 133 return MedicationKnowledgeStatusCodes.ENTEREDINERROR; 134 if ("inactive".equals(codeString)) 135 return MedicationKnowledgeStatusCodes.INACTIVE; 136 throw new IllegalArgumentException("Unknown MedicationKnowledgeStatusCodes code '"+codeString+"'"); 137 } 138 public Enumeration<MedicationKnowledgeStatusCodes> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<MedicationKnowledgeStatusCodes>(this, MedicationKnowledgeStatusCodes.NULL, code); 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return new Enumeration<MedicationKnowledgeStatusCodes>(this, MedicationKnowledgeStatusCodes.NULL, code); 146 if ("active".equals(codeString)) 147 return new Enumeration<MedicationKnowledgeStatusCodes>(this, MedicationKnowledgeStatusCodes.ACTIVE, code); 148 if ("entered-in-error".equals(codeString)) 149 return new Enumeration<MedicationKnowledgeStatusCodes>(this, MedicationKnowledgeStatusCodes.ENTEREDINERROR, code); 150 if ("inactive".equals(codeString)) 151 return new Enumeration<MedicationKnowledgeStatusCodes>(this, MedicationKnowledgeStatusCodes.INACTIVE, code); 152 throw new FHIRException("Unknown MedicationKnowledgeStatusCodes code '"+codeString+"'"); 153 } 154 public String toCode(MedicationKnowledgeStatusCodes code) { 155 if (code == MedicationKnowledgeStatusCodes.NULL) 156 return null; 157 if (code == MedicationKnowledgeStatusCodes.ACTIVE) 158 return "active"; 159 if (code == MedicationKnowledgeStatusCodes.ENTEREDINERROR) 160 return "entered-in-error"; 161 if (code == MedicationKnowledgeStatusCodes.INACTIVE) 162 return "inactive"; 163 return "?"; 164 } 165 public String toSystem(MedicationKnowledgeStatusCodes code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class MedicationKnowledgeRelatedMedicationKnowledgeComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * The category of the associated medication knowledge reference. 174 */ 175 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 176 @Description(shortDefinition="Category of medicationKnowledge", formalDefinition="The category of the associated medication knowledge reference." ) 177 protected CodeableConcept type; 178 179 /** 180 * Associated documentation about the associated medication knowledge. 181 */ 182 @Child(name = "reference", type = {MedicationKnowledge.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 183 @Description(shortDefinition="Associated documentation about the associated medication knowledge", formalDefinition="Associated documentation about the associated medication knowledge." ) 184 protected List<Reference> reference; 185 186 private static final long serialVersionUID = 1687147899L; 187 188 /** 189 * Constructor 190 */ 191 public MedicationKnowledgeRelatedMedicationKnowledgeComponent() { 192 super(); 193 } 194 195 /** 196 * Constructor 197 */ 198 public MedicationKnowledgeRelatedMedicationKnowledgeComponent(CodeableConcept type, Reference reference) { 199 super(); 200 this.setType(type); 201 this.addReference(reference); 202 } 203 204 /** 205 * @return {@link #type} (The category of the associated medication knowledge reference.) 206 */ 207 public CodeableConcept getType() { 208 if (this.type == null) 209 if (Configuration.errorOnAutoCreate()) 210 throw new Error("Attempt to auto-create MedicationKnowledgeRelatedMedicationKnowledgeComponent.type"); 211 else if (Configuration.doAutoCreate()) 212 this.type = new CodeableConcept(); // cc 213 return this.type; 214 } 215 216 public boolean hasType() { 217 return this.type != null && !this.type.isEmpty(); 218 } 219 220 /** 221 * @param value {@link #type} (The category of the associated medication knowledge reference.) 222 */ 223 public MedicationKnowledgeRelatedMedicationKnowledgeComponent setType(CodeableConcept value) { 224 this.type = value; 225 return this; 226 } 227 228 /** 229 * @return {@link #reference} (Associated documentation about the associated medication knowledge.) 230 */ 231 public List<Reference> getReference() { 232 if (this.reference == null) 233 this.reference = new ArrayList<Reference>(); 234 return this.reference; 235 } 236 237 /** 238 * @return Returns a reference to <code>this</code> for easy method chaining 239 */ 240 public MedicationKnowledgeRelatedMedicationKnowledgeComponent setReference(List<Reference> theReference) { 241 this.reference = theReference; 242 return this; 243 } 244 245 public boolean hasReference() { 246 if (this.reference == null) 247 return false; 248 for (Reference item : this.reference) 249 if (!item.isEmpty()) 250 return true; 251 return false; 252 } 253 254 public Reference addReference() { //3 255 Reference t = new Reference(); 256 if (this.reference == null) 257 this.reference = new ArrayList<Reference>(); 258 this.reference.add(t); 259 return t; 260 } 261 262 public MedicationKnowledgeRelatedMedicationKnowledgeComponent addReference(Reference t) { //3 263 if (t == null) 264 return this; 265 if (this.reference == null) 266 this.reference = new ArrayList<Reference>(); 267 this.reference.add(t); 268 return this; 269 } 270 271 /** 272 * @return The first repetition of repeating field {@link #reference}, creating it if it does not already exist {3} 273 */ 274 public Reference getReferenceFirstRep() { 275 if (getReference().isEmpty()) { 276 addReference(); 277 } 278 return getReference().get(0); 279 } 280 281 protected void listChildren(List<Property> children) { 282 super.listChildren(children); 283 children.add(new Property("type", "CodeableConcept", "The category of the associated medication knowledge reference.", 0, 1, type)); 284 children.add(new Property("reference", "Reference(MedicationKnowledge)", "Associated documentation about the associated medication knowledge.", 0, java.lang.Integer.MAX_VALUE, reference)); 285 } 286 287 @Override 288 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 289 switch (_hash) { 290 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The category of the associated medication knowledge reference.", 0, 1, type); 291 case -925155509: /*reference*/ return new Property("reference", "Reference(MedicationKnowledge)", "Associated documentation about the associated medication knowledge.", 0, java.lang.Integer.MAX_VALUE, reference); 292 default: return super.getNamedProperty(_hash, _name, _checkValid); 293 } 294 295 } 296 297 @Override 298 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 299 switch (hash) { 300 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 301 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : this.reference.toArray(new Base[this.reference.size()]); // Reference 302 default: return super.getProperty(hash, name, checkValid); 303 } 304 305 } 306 307 @Override 308 public Base setProperty(int hash, String name, Base value) throws FHIRException { 309 switch (hash) { 310 case 3575610: // type 311 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 312 return value; 313 case -925155509: // reference 314 this.getReference().add(TypeConvertor.castToReference(value)); // Reference 315 return value; 316 default: return super.setProperty(hash, name, value); 317 } 318 319 } 320 321 @Override 322 public Base setProperty(String name, Base value) throws FHIRException { 323 if (name.equals("type")) { 324 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 325 } else if (name.equals("reference")) { 326 this.getReference().add(TypeConvertor.castToReference(value)); 327 } else 328 return super.setProperty(name, value); 329 return value; 330 } 331 332 @Override 333 public void removeChild(String name, Base value) throws FHIRException { 334 if (name.equals("type")) { 335 this.type = null; 336 } else if (name.equals("reference")) { 337 this.getReference().remove(value); 338 } else 339 super.removeChild(name, value); 340 341 } 342 343 @Override 344 public Base makeProperty(int hash, String name) throws FHIRException { 345 switch (hash) { 346 case 3575610: return getType(); 347 case -925155509: return addReference(); 348 default: return super.makeProperty(hash, name); 349 } 350 351 } 352 353 @Override 354 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 355 switch (hash) { 356 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 357 case -925155509: /*reference*/ return new String[] {"Reference"}; 358 default: return super.getTypesForProperty(hash, name); 359 } 360 361 } 362 363 @Override 364 public Base addChild(String name) throws FHIRException { 365 if (name.equals("type")) { 366 this.type = new CodeableConcept(); 367 return this.type; 368 } 369 else if (name.equals("reference")) { 370 return addReference(); 371 } 372 else 373 return super.addChild(name); 374 } 375 376 public MedicationKnowledgeRelatedMedicationKnowledgeComponent copy() { 377 MedicationKnowledgeRelatedMedicationKnowledgeComponent dst = new MedicationKnowledgeRelatedMedicationKnowledgeComponent(); 378 copyValues(dst); 379 return dst; 380 } 381 382 public void copyValues(MedicationKnowledgeRelatedMedicationKnowledgeComponent dst) { 383 super.copyValues(dst); 384 dst.type = type == null ? null : type.copy(); 385 if (reference != null) { 386 dst.reference = new ArrayList<Reference>(); 387 for (Reference i : reference) 388 dst.reference.add(i.copy()); 389 }; 390 } 391 392 @Override 393 public boolean equalsDeep(Base other_) { 394 if (!super.equalsDeep(other_)) 395 return false; 396 if (!(other_ instanceof MedicationKnowledgeRelatedMedicationKnowledgeComponent)) 397 return false; 398 MedicationKnowledgeRelatedMedicationKnowledgeComponent o = (MedicationKnowledgeRelatedMedicationKnowledgeComponent) other_; 399 return compareDeep(type, o.type, true) && compareDeep(reference, o.reference, true); 400 } 401 402 @Override 403 public boolean equalsShallow(Base other_) { 404 if (!super.equalsShallow(other_)) 405 return false; 406 if (!(other_ instanceof MedicationKnowledgeRelatedMedicationKnowledgeComponent)) 407 return false; 408 MedicationKnowledgeRelatedMedicationKnowledgeComponent o = (MedicationKnowledgeRelatedMedicationKnowledgeComponent) other_; 409 return true; 410 } 411 412 public boolean isEmpty() { 413 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, reference); 414 } 415 416 public String fhirType() { 417 return "MedicationKnowledge.relatedMedicationKnowledge"; 418 419 } 420 421 } 422 423 @Block() 424 public static class MedicationKnowledgeMonographComponent extends BackboneElement implements IBaseBackboneElement { 425 /** 426 * The category of documentation about the medication. (e.g. professional monograph, patient education monograph). 427 */ 428 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 429 @Description(shortDefinition="The category of medication document", formalDefinition="The category of documentation about the medication. (e.g. professional monograph, patient education monograph)." ) 430 protected CodeableConcept type; 431 432 /** 433 * Associated documentation about the medication. 434 */ 435 @Child(name = "source", type = {DocumentReference.class}, order=2, min=0, max=1, modifier=false, summary=false) 436 @Description(shortDefinition="Associated documentation about the medication", formalDefinition="Associated documentation about the medication." ) 437 protected Reference source; 438 439 private static final long serialVersionUID = -197893751L; 440 441 /** 442 * Constructor 443 */ 444 public MedicationKnowledgeMonographComponent() { 445 super(); 446 } 447 448 /** 449 * @return {@link #type} (The category of documentation about the medication. (e.g. professional monograph, patient education monograph).) 450 */ 451 public CodeableConcept getType() { 452 if (this.type == null) 453 if (Configuration.errorOnAutoCreate()) 454 throw new Error("Attempt to auto-create MedicationKnowledgeMonographComponent.type"); 455 else if (Configuration.doAutoCreate()) 456 this.type = new CodeableConcept(); // cc 457 return this.type; 458 } 459 460 public boolean hasType() { 461 return this.type != null && !this.type.isEmpty(); 462 } 463 464 /** 465 * @param value {@link #type} (The category of documentation about the medication. (e.g. professional monograph, patient education monograph).) 466 */ 467 public MedicationKnowledgeMonographComponent setType(CodeableConcept value) { 468 this.type = value; 469 return this; 470 } 471 472 /** 473 * @return {@link #source} (Associated documentation about the medication.) 474 */ 475 public Reference getSource() { 476 if (this.source == null) 477 if (Configuration.errorOnAutoCreate()) 478 throw new Error("Attempt to auto-create MedicationKnowledgeMonographComponent.source"); 479 else if (Configuration.doAutoCreate()) 480 this.source = new Reference(); // cc 481 return this.source; 482 } 483 484 public boolean hasSource() { 485 return this.source != null && !this.source.isEmpty(); 486 } 487 488 /** 489 * @param value {@link #source} (Associated documentation about the medication.) 490 */ 491 public MedicationKnowledgeMonographComponent setSource(Reference value) { 492 this.source = value; 493 return this; 494 } 495 496 protected void listChildren(List<Property> children) { 497 super.listChildren(children); 498 children.add(new Property("type", "CodeableConcept", "The category of documentation about the medication. (e.g. professional monograph, patient education monograph).", 0, 1, type)); 499 children.add(new Property("source", "Reference(DocumentReference)", "Associated documentation about the medication.", 0, 1, source)); 500 } 501 502 @Override 503 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 504 switch (_hash) { 505 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The category of documentation about the medication. (e.g. professional monograph, patient education monograph).", 0, 1, type); 506 case -896505829: /*source*/ return new Property("source", "Reference(DocumentReference)", "Associated documentation about the medication.", 0, 1, source); 507 default: return super.getNamedProperty(_hash, _name, _checkValid); 508 } 509 510 } 511 512 @Override 513 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 514 switch (hash) { 515 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 516 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // Reference 517 default: return super.getProperty(hash, name, checkValid); 518 } 519 520 } 521 522 @Override 523 public Base setProperty(int hash, String name, Base value) throws FHIRException { 524 switch (hash) { 525 case 3575610: // type 526 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 527 return value; 528 case -896505829: // source 529 this.source = TypeConvertor.castToReference(value); // Reference 530 return value; 531 default: return super.setProperty(hash, name, value); 532 } 533 534 } 535 536 @Override 537 public Base setProperty(String name, Base value) throws FHIRException { 538 if (name.equals("type")) { 539 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 540 } else if (name.equals("source")) { 541 this.source = TypeConvertor.castToReference(value); // Reference 542 } else 543 return super.setProperty(name, value); 544 return value; 545 } 546 547 @Override 548 public void removeChild(String name, Base value) throws FHIRException { 549 if (name.equals("type")) { 550 this.type = null; 551 } else if (name.equals("source")) { 552 this.source = null; 553 } else 554 super.removeChild(name, value); 555 556 } 557 558 @Override 559 public Base makeProperty(int hash, String name) throws FHIRException { 560 switch (hash) { 561 case 3575610: return getType(); 562 case -896505829: return getSource(); 563 default: return super.makeProperty(hash, name); 564 } 565 566 } 567 568 @Override 569 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 570 switch (hash) { 571 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 572 case -896505829: /*source*/ return new String[] {"Reference"}; 573 default: return super.getTypesForProperty(hash, name); 574 } 575 576 } 577 578 @Override 579 public Base addChild(String name) throws FHIRException { 580 if (name.equals("type")) { 581 this.type = new CodeableConcept(); 582 return this.type; 583 } 584 else if (name.equals("source")) { 585 this.source = new Reference(); 586 return this.source; 587 } 588 else 589 return super.addChild(name); 590 } 591 592 public MedicationKnowledgeMonographComponent copy() { 593 MedicationKnowledgeMonographComponent dst = new MedicationKnowledgeMonographComponent(); 594 copyValues(dst); 595 return dst; 596 } 597 598 public void copyValues(MedicationKnowledgeMonographComponent dst) { 599 super.copyValues(dst); 600 dst.type = type == null ? null : type.copy(); 601 dst.source = source == null ? null : source.copy(); 602 } 603 604 @Override 605 public boolean equalsDeep(Base other_) { 606 if (!super.equalsDeep(other_)) 607 return false; 608 if (!(other_ instanceof MedicationKnowledgeMonographComponent)) 609 return false; 610 MedicationKnowledgeMonographComponent o = (MedicationKnowledgeMonographComponent) other_; 611 return compareDeep(type, o.type, true) && compareDeep(source, o.source, true); 612 } 613 614 @Override 615 public boolean equalsShallow(Base other_) { 616 if (!super.equalsShallow(other_)) 617 return false; 618 if (!(other_ instanceof MedicationKnowledgeMonographComponent)) 619 return false; 620 MedicationKnowledgeMonographComponent o = (MedicationKnowledgeMonographComponent) other_; 621 return true; 622 } 623 624 public boolean isEmpty() { 625 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, source); 626 } 627 628 public String fhirType() { 629 return "MedicationKnowledge.monograph"; 630 631 } 632 633 } 634 635 @Block() 636 public static class MedicationKnowledgeCostComponent extends BackboneElement implements IBaseBackboneElement { 637 /** 638 * The date range for which the cost information of the medication is effective. 639 */ 640 @Child(name = "effectiveDate", type = {Period.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 641 @Description(shortDefinition="The date range for which the cost is effective", formalDefinition="The date range for which the cost information of the medication is effective." ) 642 protected List<Period> effectiveDate; 643 644 /** 645 * The category of the cost information. For example, manufacturers' cost, patient cost, claim reimbursement cost, actual acquisition cost. 646 */ 647 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 648 @Description(shortDefinition="The category of the cost information", formalDefinition="The category of the cost information. For example, manufacturers' cost, patient cost, claim reimbursement cost, actual acquisition cost." ) 649 protected CodeableConcept type; 650 651 /** 652 * The source or owner that assigns the price to the medication. 653 */ 654 @Child(name = "source", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 655 @Description(shortDefinition="The source or owner for the price information", formalDefinition="The source or owner that assigns the price to the medication." ) 656 protected StringType source; 657 658 /** 659 * The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication. 660 */ 661 @Child(name = "cost", type = {Money.class, CodeableConcept.class}, order=4, min=1, max=1, modifier=false, summary=false) 662 @Description(shortDefinition="The price or category of the cost of the medication", formalDefinition="The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication." ) 663 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-cost-category") 664 protected DataType cost; 665 666 private static final long serialVersionUID = 747402134L; 667 668 /** 669 * Constructor 670 */ 671 public MedicationKnowledgeCostComponent() { 672 super(); 673 } 674 675 /** 676 * Constructor 677 */ 678 public MedicationKnowledgeCostComponent(CodeableConcept type, DataType cost) { 679 super(); 680 this.setType(type); 681 this.setCost(cost); 682 } 683 684 /** 685 * @return {@link #effectiveDate} (The date range for which the cost information of the medication is effective.) 686 */ 687 public List<Period> getEffectiveDate() { 688 if (this.effectiveDate == null) 689 this.effectiveDate = new ArrayList<Period>(); 690 return this.effectiveDate; 691 } 692 693 /** 694 * @return Returns a reference to <code>this</code> for easy method chaining 695 */ 696 public MedicationKnowledgeCostComponent setEffectiveDate(List<Period> theEffectiveDate) { 697 this.effectiveDate = theEffectiveDate; 698 return this; 699 } 700 701 public boolean hasEffectiveDate() { 702 if (this.effectiveDate == null) 703 return false; 704 for (Period item : this.effectiveDate) 705 if (!item.isEmpty()) 706 return true; 707 return false; 708 } 709 710 public Period addEffectiveDate() { //3 711 Period t = new Period(); 712 if (this.effectiveDate == null) 713 this.effectiveDate = new ArrayList<Period>(); 714 this.effectiveDate.add(t); 715 return t; 716 } 717 718 public MedicationKnowledgeCostComponent addEffectiveDate(Period t) { //3 719 if (t == null) 720 return this; 721 if (this.effectiveDate == null) 722 this.effectiveDate = new ArrayList<Period>(); 723 this.effectiveDate.add(t); 724 return this; 725 } 726 727 /** 728 * @return The first repetition of repeating field {@link #effectiveDate}, creating it if it does not already exist {3} 729 */ 730 public Period getEffectiveDateFirstRep() { 731 if (getEffectiveDate().isEmpty()) { 732 addEffectiveDate(); 733 } 734 return getEffectiveDate().get(0); 735 } 736 737 /** 738 * @return {@link #type} (The category of the cost information. For example, manufacturers' cost, patient cost, claim reimbursement cost, actual acquisition cost.) 739 */ 740 public CodeableConcept getType() { 741 if (this.type == null) 742 if (Configuration.errorOnAutoCreate()) 743 throw new Error("Attempt to auto-create MedicationKnowledgeCostComponent.type"); 744 else if (Configuration.doAutoCreate()) 745 this.type = new CodeableConcept(); // cc 746 return this.type; 747 } 748 749 public boolean hasType() { 750 return this.type != null && !this.type.isEmpty(); 751 } 752 753 /** 754 * @param value {@link #type} (The category of the cost information. For example, manufacturers' cost, patient cost, claim reimbursement cost, actual acquisition cost.) 755 */ 756 public MedicationKnowledgeCostComponent setType(CodeableConcept value) { 757 this.type = value; 758 return this; 759 } 760 761 /** 762 * @return {@link #source} (The source or owner that assigns the price to the medication.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 763 */ 764 public StringType getSourceElement() { 765 if (this.source == null) 766 if (Configuration.errorOnAutoCreate()) 767 throw new Error("Attempt to auto-create MedicationKnowledgeCostComponent.source"); 768 else if (Configuration.doAutoCreate()) 769 this.source = new StringType(); // bb 770 return this.source; 771 } 772 773 public boolean hasSourceElement() { 774 return this.source != null && !this.source.isEmpty(); 775 } 776 777 public boolean hasSource() { 778 return this.source != null && !this.source.isEmpty(); 779 } 780 781 /** 782 * @param value {@link #source} (The source or owner that assigns the price to the medication.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 783 */ 784 public MedicationKnowledgeCostComponent setSourceElement(StringType value) { 785 this.source = value; 786 return this; 787 } 788 789 /** 790 * @return The source or owner that assigns the price to the medication. 791 */ 792 public String getSource() { 793 return this.source == null ? null : this.source.getValue(); 794 } 795 796 /** 797 * @param value The source or owner that assigns the price to the medication. 798 */ 799 public MedicationKnowledgeCostComponent setSource(String value) { 800 if (Utilities.noString(value)) 801 this.source = null; 802 else { 803 if (this.source == null) 804 this.source = new StringType(); 805 this.source.setValue(value); 806 } 807 return this; 808 } 809 810 /** 811 * @return {@link #cost} (The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.) 812 */ 813 public DataType getCost() { 814 return this.cost; 815 } 816 817 /** 818 * @return {@link #cost} (The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.) 819 */ 820 public Money getCostMoney() throws FHIRException { 821 if (this.cost == null) 822 this.cost = new Money(); 823 if (!(this.cost instanceof Money)) 824 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.cost.getClass().getName()+" was encountered"); 825 return (Money) this.cost; 826 } 827 828 public boolean hasCostMoney() { 829 return this.cost instanceof Money; 830 } 831 832 /** 833 * @return {@link #cost} (The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.) 834 */ 835 public CodeableConcept getCostCodeableConcept() throws FHIRException { 836 if (this.cost == null) 837 this.cost = new CodeableConcept(); 838 if (!(this.cost instanceof CodeableConcept)) 839 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.cost.getClass().getName()+" was encountered"); 840 return (CodeableConcept) this.cost; 841 } 842 843 public boolean hasCostCodeableConcept() { 844 return this.cost instanceof CodeableConcept; 845 } 846 847 public boolean hasCost() { 848 return this.cost != null && !this.cost.isEmpty(); 849 } 850 851 /** 852 * @param value {@link #cost} (The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.) 853 */ 854 public MedicationKnowledgeCostComponent setCost(DataType value) { 855 if (value != null && !(value instanceof Money || value instanceof CodeableConcept)) 856 throw new FHIRException("Not the right type for MedicationKnowledge.cost.cost[x]: "+value.fhirType()); 857 this.cost = value; 858 return this; 859 } 860 861 protected void listChildren(List<Property> children) { 862 super.listChildren(children); 863 children.add(new Property("effectiveDate", "Period", "The date range for which the cost information of the medication is effective.", 0, java.lang.Integer.MAX_VALUE, effectiveDate)); 864 children.add(new Property("type", "CodeableConcept", "The category of the cost information. For example, manufacturers' cost, patient cost, claim reimbursement cost, actual acquisition cost.", 0, 1, type)); 865 children.add(new Property("source", "string", "The source or owner that assigns the price to the medication.", 0, 1, source)); 866 children.add(new Property("cost[x]", "Money|CodeableConcept", "The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.", 0, 1, cost)); 867 } 868 869 @Override 870 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 871 switch (_hash) { 872 case -930389515: /*effectiveDate*/ return new Property("effectiveDate", "Period", "The date range for which the cost information of the medication is effective.", 0, java.lang.Integer.MAX_VALUE, effectiveDate); 873 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The category of the cost information. For example, manufacturers' cost, patient cost, claim reimbursement cost, actual acquisition cost.", 0, 1, type); 874 case -896505829: /*source*/ return new Property("source", "string", "The source or owner that assigns the price to the medication.", 0, 1, source); 875 case 956138899: /*cost[x]*/ return new Property("cost[x]", "Money|CodeableConcept", "The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.", 0, 1, cost); 876 case 3059661: /*cost*/ return new Property("cost[x]", "Money|CodeableConcept", "The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.", 0, 1, cost); 877 case -286697229: /*costMoney*/ return new Property("cost[x]", "Money", "The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.", 0, 1, cost); 878 case -238369772: /*costCodeableConcept*/ return new Property("cost[x]", "CodeableConcept", "The price or representation of the cost (for example, Band A, Band B or $, $$) of the medication.", 0, 1, cost); 879 default: return super.getNamedProperty(_hash, _name, _checkValid); 880 } 881 882 } 883 884 @Override 885 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 886 switch (hash) { 887 case -930389515: /*effectiveDate*/ return this.effectiveDate == null ? new Base[0] : this.effectiveDate.toArray(new Base[this.effectiveDate.size()]); // Period 888 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 889 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // StringType 890 case 3059661: /*cost*/ return this.cost == null ? new Base[0] : new Base[] {this.cost}; // DataType 891 default: return super.getProperty(hash, name, checkValid); 892 } 893 894 } 895 896 @Override 897 public Base setProperty(int hash, String name, Base value) throws FHIRException { 898 switch (hash) { 899 case -930389515: // effectiveDate 900 this.getEffectiveDate().add(TypeConvertor.castToPeriod(value)); // Period 901 return value; 902 case 3575610: // type 903 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 904 return value; 905 case -896505829: // source 906 this.source = TypeConvertor.castToString(value); // StringType 907 return value; 908 case 3059661: // cost 909 this.cost = TypeConvertor.castToType(value); // DataType 910 return value; 911 default: return super.setProperty(hash, name, value); 912 } 913 914 } 915 916 @Override 917 public Base setProperty(String name, Base value) throws FHIRException { 918 if (name.equals("effectiveDate")) { 919 this.getEffectiveDate().add(TypeConvertor.castToPeriod(value)); 920 } else if (name.equals("type")) { 921 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 922 } else if (name.equals("source")) { 923 this.source = TypeConvertor.castToString(value); // StringType 924 } else if (name.equals("cost[x]")) { 925 this.cost = TypeConvertor.castToType(value); // DataType 926 } else 927 return super.setProperty(name, value); 928 return value; 929 } 930 931 @Override 932 public void removeChild(String name, Base value) throws FHIRException { 933 if (name.equals("effectiveDate")) { 934 this.getEffectiveDate().remove(value); 935 } else if (name.equals("type")) { 936 this.type = null; 937 } else if (name.equals("source")) { 938 this.source = null; 939 } else if (name.equals("cost[x]")) { 940 this.cost = null; 941 } else 942 super.removeChild(name, value); 943 944 } 945 946 @Override 947 public Base makeProperty(int hash, String name) throws FHIRException { 948 switch (hash) { 949 case -930389515: return addEffectiveDate(); 950 case 3575610: return getType(); 951 case -896505829: return getSourceElement(); 952 case 956138899: return getCost(); 953 case 3059661: return getCost(); 954 default: return super.makeProperty(hash, name); 955 } 956 957 } 958 959 @Override 960 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 961 switch (hash) { 962 case -930389515: /*effectiveDate*/ return new String[] {"Period"}; 963 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 964 case -896505829: /*source*/ return new String[] {"string"}; 965 case 3059661: /*cost*/ return new String[] {"Money", "CodeableConcept"}; 966 default: return super.getTypesForProperty(hash, name); 967 } 968 969 } 970 971 @Override 972 public Base addChild(String name) throws FHIRException { 973 if (name.equals("effectiveDate")) { 974 return addEffectiveDate(); 975 } 976 else if (name.equals("type")) { 977 this.type = new CodeableConcept(); 978 return this.type; 979 } 980 else if (name.equals("source")) { 981 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.cost.source"); 982 } 983 else if (name.equals("costMoney")) { 984 this.cost = new Money(); 985 return this.cost; 986 } 987 else if (name.equals("costCodeableConcept")) { 988 this.cost = new CodeableConcept(); 989 return this.cost; 990 } 991 else 992 return super.addChild(name); 993 } 994 995 public MedicationKnowledgeCostComponent copy() { 996 MedicationKnowledgeCostComponent dst = new MedicationKnowledgeCostComponent(); 997 copyValues(dst); 998 return dst; 999 } 1000 1001 public void copyValues(MedicationKnowledgeCostComponent dst) { 1002 super.copyValues(dst); 1003 if (effectiveDate != null) { 1004 dst.effectiveDate = new ArrayList<Period>(); 1005 for (Period i : effectiveDate) 1006 dst.effectiveDate.add(i.copy()); 1007 }; 1008 dst.type = type == null ? null : type.copy(); 1009 dst.source = source == null ? null : source.copy(); 1010 dst.cost = cost == null ? null : cost.copy(); 1011 } 1012 1013 @Override 1014 public boolean equalsDeep(Base other_) { 1015 if (!super.equalsDeep(other_)) 1016 return false; 1017 if (!(other_ instanceof MedicationKnowledgeCostComponent)) 1018 return false; 1019 MedicationKnowledgeCostComponent o = (MedicationKnowledgeCostComponent) other_; 1020 return compareDeep(effectiveDate, o.effectiveDate, true) && compareDeep(type, o.type, true) && compareDeep(source, o.source, true) 1021 && compareDeep(cost, o.cost, true); 1022 } 1023 1024 @Override 1025 public boolean equalsShallow(Base other_) { 1026 if (!super.equalsShallow(other_)) 1027 return false; 1028 if (!(other_ instanceof MedicationKnowledgeCostComponent)) 1029 return false; 1030 MedicationKnowledgeCostComponent o = (MedicationKnowledgeCostComponent) other_; 1031 return compareValues(source, o.source, true); 1032 } 1033 1034 public boolean isEmpty() { 1035 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(effectiveDate, type, source 1036 , cost); 1037 } 1038 1039 public String fhirType() { 1040 return "MedicationKnowledge.cost"; 1041 1042 } 1043 1044 } 1045 1046 @Block() 1047 public static class MedicationKnowledgeMonitoringProgramComponent extends BackboneElement implements IBaseBackboneElement { 1048 /** 1049 * Type of program under which the medication is monitored. 1050 */ 1051 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1052 @Description(shortDefinition="Type of program under which the medication is monitored", formalDefinition="Type of program under which the medication is monitored." ) 1053 protected CodeableConcept type; 1054 1055 /** 1056 * Name of the reviewing program. 1057 */ 1058 @Child(name = "name", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1059 @Description(shortDefinition="Name of the reviewing program", formalDefinition="Name of the reviewing program." ) 1060 protected StringType name; 1061 1062 private static final long serialVersionUID = -280346281L; 1063 1064 /** 1065 * Constructor 1066 */ 1067 public MedicationKnowledgeMonitoringProgramComponent() { 1068 super(); 1069 } 1070 1071 /** 1072 * @return {@link #type} (Type of program under which the medication is monitored.) 1073 */ 1074 public CodeableConcept getType() { 1075 if (this.type == null) 1076 if (Configuration.errorOnAutoCreate()) 1077 throw new Error("Attempt to auto-create MedicationKnowledgeMonitoringProgramComponent.type"); 1078 else if (Configuration.doAutoCreate()) 1079 this.type = new CodeableConcept(); // cc 1080 return this.type; 1081 } 1082 1083 public boolean hasType() { 1084 return this.type != null && !this.type.isEmpty(); 1085 } 1086 1087 /** 1088 * @param value {@link #type} (Type of program under which the medication is monitored.) 1089 */ 1090 public MedicationKnowledgeMonitoringProgramComponent setType(CodeableConcept value) { 1091 this.type = value; 1092 return this; 1093 } 1094 1095 /** 1096 * @return {@link #name} (Name of the reviewing program.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1097 */ 1098 public StringType getNameElement() { 1099 if (this.name == null) 1100 if (Configuration.errorOnAutoCreate()) 1101 throw new Error("Attempt to auto-create MedicationKnowledgeMonitoringProgramComponent.name"); 1102 else if (Configuration.doAutoCreate()) 1103 this.name = new StringType(); // bb 1104 return this.name; 1105 } 1106 1107 public boolean hasNameElement() { 1108 return this.name != null && !this.name.isEmpty(); 1109 } 1110 1111 public boolean hasName() { 1112 return this.name != null && !this.name.isEmpty(); 1113 } 1114 1115 /** 1116 * @param value {@link #name} (Name of the reviewing program.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1117 */ 1118 public MedicationKnowledgeMonitoringProgramComponent setNameElement(StringType value) { 1119 this.name = value; 1120 return this; 1121 } 1122 1123 /** 1124 * @return Name of the reviewing program. 1125 */ 1126 public String getName() { 1127 return this.name == null ? null : this.name.getValue(); 1128 } 1129 1130 /** 1131 * @param value Name of the reviewing program. 1132 */ 1133 public MedicationKnowledgeMonitoringProgramComponent setName(String value) { 1134 if (Utilities.noString(value)) 1135 this.name = null; 1136 else { 1137 if (this.name == null) 1138 this.name = new StringType(); 1139 this.name.setValue(value); 1140 } 1141 return this; 1142 } 1143 1144 protected void listChildren(List<Property> children) { 1145 super.listChildren(children); 1146 children.add(new Property("type", "CodeableConcept", "Type of program under which the medication is monitored.", 0, 1, type)); 1147 children.add(new Property("name", "string", "Name of the reviewing program.", 0, 1, name)); 1148 } 1149 1150 @Override 1151 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1152 switch (_hash) { 1153 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of program under which the medication is monitored.", 0, 1, type); 1154 case 3373707: /*name*/ return new Property("name", "string", "Name of the reviewing program.", 0, 1, name); 1155 default: return super.getNamedProperty(_hash, _name, _checkValid); 1156 } 1157 1158 } 1159 1160 @Override 1161 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1162 switch (hash) { 1163 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1164 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1165 default: return super.getProperty(hash, name, checkValid); 1166 } 1167 1168 } 1169 1170 @Override 1171 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1172 switch (hash) { 1173 case 3575610: // type 1174 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1175 return value; 1176 case 3373707: // name 1177 this.name = TypeConvertor.castToString(value); // StringType 1178 return value; 1179 default: return super.setProperty(hash, name, value); 1180 } 1181 1182 } 1183 1184 @Override 1185 public Base setProperty(String name, Base value) throws FHIRException { 1186 if (name.equals("type")) { 1187 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1188 } else if (name.equals("name")) { 1189 this.name = TypeConvertor.castToString(value); // StringType 1190 } else 1191 return super.setProperty(name, value); 1192 return value; 1193 } 1194 1195 @Override 1196 public void removeChild(String name, Base value) throws FHIRException { 1197 if (name.equals("type")) { 1198 this.type = null; 1199 } else if (name.equals("name")) { 1200 this.name = null; 1201 } else 1202 super.removeChild(name, value); 1203 1204 } 1205 1206 @Override 1207 public Base makeProperty(int hash, String name) throws FHIRException { 1208 switch (hash) { 1209 case 3575610: return getType(); 1210 case 3373707: return getNameElement(); 1211 default: return super.makeProperty(hash, name); 1212 } 1213 1214 } 1215 1216 @Override 1217 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1218 switch (hash) { 1219 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1220 case 3373707: /*name*/ return new String[] {"string"}; 1221 default: return super.getTypesForProperty(hash, name); 1222 } 1223 1224 } 1225 1226 @Override 1227 public Base addChild(String name) throws FHIRException { 1228 if (name.equals("type")) { 1229 this.type = new CodeableConcept(); 1230 return this.type; 1231 } 1232 else if (name.equals("name")) { 1233 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.monitoringProgram.name"); 1234 } 1235 else 1236 return super.addChild(name); 1237 } 1238 1239 public MedicationKnowledgeMonitoringProgramComponent copy() { 1240 MedicationKnowledgeMonitoringProgramComponent dst = new MedicationKnowledgeMonitoringProgramComponent(); 1241 copyValues(dst); 1242 return dst; 1243 } 1244 1245 public void copyValues(MedicationKnowledgeMonitoringProgramComponent dst) { 1246 super.copyValues(dst); 1247 dst.type = type == null ? null : type.copy(); 1248 dst.name = name == null ? null : name.copy(); 1249 } 1250 1251 @Override 1252 public boolean equalsDeep(Base other_) { 1253 if (!super.equalsDeep(other_)) 1254 return false; 1255 if (!(other_ instanceof MedicationKnowledgeMonitoringProgramComponent)) 1256 return false; 1257 MedicationKnowledgeMonitoringProgramComponent o = (MedicationKnowledgeMonitoringProgramComponent) other_; 1258 return compareDeep(type, o.type, true) && compareDeep(name, o.name, true); 1259 } 1260 1261 @Override 1262 public boolean equalsShallow(Base other_) { 1263 if (!super.equalsShallow(other_)) 1264 return false; 1265 if (!(other_ instanceof MedicationKnowledgeMonitoringProgramComponent)) 1266 return false; 1267 MedicationKnowledgeMonitoringProgramComponent o = (MedicationKnowledgeMonitoringProgramComponent) other_; 1268 return compareValues(name, o.name, true); 1269 } 1270 1271 public boolean isEmpty() { 1272 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, name); 1273 } 1274 1275 public String fhirType() { 1276 return "MedicationKnowledge.monitoringProgram"; 1277 1278 } 1279 1280 } 1281 1282 @Block() 1283 public static class MedicationKnowledgeIndicationGuidelineComponent extends BackboneElement implements IBaseBackboneElement { 1284 /** 1285 * Indication or reason for use of the medication that applies to the specific administration guideline. 1286 */ 1287 @Child(name = "indication", type = {CodeableReference.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1288 @Description(shortDefinition="Indication for use that applies to the specific administration guideline", formalDefinition="Indication or reason for use of the medication that applies to the specific administration guideline." ) 1289 protected List<CodeableReference> indication; 1290 1291 /** 1292 * The guidelines for the dosage of the medication for the indication. 1293 */ 1294 @Child(name = "dosingGuideline", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1295 @Description(shortDefinition="Guidelines for dosage of the medication", formalDefinition="The guidelines for the dosage of the medication for the indication." ) 1296 protected List<MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent> dosingGuideline; 1297 1298 private static final long serialVersionUID = 256409722L; 1299 1300 /** 1301 * Constructor 1302 */ 1303 public MedicationKnowledgeIndicationGuidelineComponent() { 1304 super(); 1305 } 1306 1307 /** 1308 * @return {@link #indication} (Indication or reason for use of the medication that applies to the specific administration guideline.) 1309 */ 1310 public List<CodeableReference> getIndication() { 1311 if (this.indication == null) 1312 this.indication = new ArrayList<CodeableReference>(); 1313 return this.indication; 1314 } 1315 1316 /** 1317 * @return Returns a reference to <code>this</code> for easy method chaining 1318 */ 1319 public MedicationKnowledgeIndicationGuidelineComponent setIndication(List<CodeableReference> theIndication) { 1320 this.indication = theIndication; 1321 return this; 1322 } 1323 1324 public boolean hasIndication() { 1325 if (this.indication == null) 1326 return false; 1327 for (CodeableReference item : this.indication) 1328 if (!item.isEmpty()) 1329 return true; 1330 return false; 1331 } 1332 1333 public CodeableReference addIndication() { //3 1334 CodeableReference t = new CodeableReference(); 1335 if (this.indication == null) 1336 this.indication = new ArrayList<CodeableReference>(); 1337 this.indication.add(t); 1338 return t; 1339 } 1340 1341 public MedicationKnowledgeIndicationGuidelineComponent addIndication(CodeableReference t) { //3 1342 if (t == null) 1343 return this; 1344 if (this.indication == null) 1345 this.indication = new ArrayList<CodeableReference>(); 1346 this.indication.add(t); 1347 return this; 1348 } 1349 1350 /** 1351 * @return The first repetition of repeating field {@link #indication}, creating it if it does not already exist {3} 1352 */ 1353 public CodeableReference getIndicationFirstRep() { 1354 if (getIndication().isEmpty()) { 1355 addIndication(); 1356 } 1357 return getIndication().get(0); 1358 } 1359 1360 /** 1361 * @return {@link #dosingGuideline} (The guidelines for the dosage of the medication for the indication.) 1362 */ 1363 public List<MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent> getDosingGuideline() { 1364 if (this.dosingGuideline == null) 1365 this.dosingGuideline = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent>(); 1366 return this.dosingGuideline; 1367 } 1368 1369 /** 1370 * @return Returns a reference to <code>this</code> for easy method chaining 1371 */ 1372 public MedicationKnowledgeIndicationGuidelineComponent setDosingGuideline(List<MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent> theDosingGuideline) { 1373 this.dosingGuideline = theDosingGuideline; 1374 return this; 1375 } 1376 1377 public boolean hasDosingGuideline() { 1378 if (this.dosingGuideline == null) 1379 return false; 1380 for (MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent item : this.dosingGuideline) 1381 if (!item.isEmpty()) 1382 return true; 1383 return false; 1384 } 1385 1386 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent addDosingGuideline() { //3 1387 MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent t = new MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent(); 1388 if (this.dosingGuideline == null) 1389 this.dosingGuideline = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent>(); 1390 this.dosingGuideline.add(t); 1391 return t; 1392 } 1393 1394 public MedicationKnowledgeIndicationGuidelineComponent addDosingGuideline(MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent t) { //3 1395 if (t == null) 1396 return this; 1397 if (this.dosingGuideline == null) 1398 this.dosingGuideline = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent>(); 1399 this.dosingGuideline.add(t); 1400 return this; 1401 } 1402 1403 /** 1404 * @return The first repetition of repeating field {@link #dosingGuideline}, creating it if it does not already exist {3} 1405 */ 1406 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent getDosingGuidelineFirstRep() { 1407 if (getDosingGuideline().isEmpty()) { 1408 addDosingGuideline(); 1409 } 1410 return getDosingGuideline().get(0); 1411 } 1412 1413 protected void listChildren(List<Property> children) { 1414 super.listChildren(children); 1415 children.add(new Property("indication", "CodeableReference(ClinicalUseDefinition)", "Indication or reason for use of the medication that applies to the specific administration guideline.", 0, java.lang.Integer.MAX_VALUE, indication)); 1416 children.add(new Property("dosingGuideline", "", "The guidelines for the dosage of the medication for the indication.", 0, java.lang.Integer.MAX_VALUE, dosingGuideline)); 1417 } 1418 1419 @Override 1420 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1421 switch (_hash) { 1422 case -597168804: /*indication*/ return new Property("indication", "CodeableReference(ClinicalUseDefinition)", "Indication or reason for use of the medication that applies to the specific administration guideline.", 0, java.lang.Integer.MAX_VALUE, indication); 1423 case -1792856970: /*dosingGuideline*/ return new Property("dosingGuideline", "", "The guidelines for the dosage of the medication for the indication.", 0, java.lang.Integer.MAX_VALUE, dosingGuideline); 1424 default: return super.getNamedProperty(_hash, _name, _checkValid); 1425 } 1426 1427 } 1428 1429 @Override 1430 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1431 switch (hash) { 1432 case -597168804: /*indication*/ return this.indication == null ? new Base[0] : this.indication.toArray(new Base[this.indication.size()]); // CodeableReference 1433 case -1792856970: /*dosingGuideline*/ return this.dosingGuideline == null ? new Base[0] : this.dosingGuideline.toArray(new Base[this.dosingGuideline.size()]); // MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent 1434 default: return super.getProperty(hash, name, checkValid); 1435 } 1436 1437 } 1438 1439 @Override 1440 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1441 switch (hash) { 1442 case -597168804: // indication 1443 this.getIndication().add(TypeConvertor.castToCodeableReference(value)); // CodeableReference 1444 return value; 1445 case -1792856970: // dosingGuideline 1446 this.getDosingGuideline().add((MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent) value); // MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent 1447 return value; 1448 default: return super.setProperty(hash, name, value); 1449 } 1450 1451 } 1452 1453 @Override 1454 public Base setProperty(String name, Base value) throws FHIRException { 1455 if (name.equals("indication")) { 1456 this.getIndication().add(TypeConvertor.castToCodeableReference(value)); 1457 } else if (name.equals("dosingGuideline")) { 1458 this.getDosingGuideline().add((MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent) value); 1459 } else 1460 return super.setProperty(name, value); 1461 return value; 1462 } 1463 1464 @Override 1465 public void removeChild(String name, Base value) throws FHIRException { 1466 if (name.equals("indication")) { 1467 this.getIndication().remove(value); 1468 } else if (name.equals("dosingGuideline")) { 1469 this.getDosingGuideline().remove((MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent) value); 1470 } else 1471 super.removeChild(name, value); 1472 1473 } 1474 1475 @Override 1476 public Base makeProperty(int hash, String name) throws FHIRException { 1477 switch (hash) { 1478 case -597168804: return addIndication(); 1479 case -1792856970: return addDosingGuideline(); 1480 default: return super.makeProperty(hash, name); 1481 } 1482 1483 } 1484 1485 @Override 1486 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1487 switch (hash) { 1488 case -597168804: /*indication*/ return new String[] {"CodeableReference"}; 1489 case -1792856970: /*dosingGuideline*/ return new String[] {}; 1490 default: return super.getTypesForProperty(hash, name); 1491 } 1492 1493 } 1494 1495 @Override 1496 public Base addChild(String name) throws FHIRException { 1497 if (name.equals("indication")) { 1498 return addIndication(); 1499 } 1500 else if (name.equals("dosingGuideline")) { 1501 return addDosingGuideline(); 1502 } 1503 else 1504 return super.addChild(name); 1505 } 1506 1507 public MedicationKnowledgeIndicationGuidelineComponent copy() { 1508 MedicationKnowledgeIndicationGuidelineComponent dst = new MedicationKnowledgeIndicationGuidelineComponent(); 1509 copyValues(dst); 1510 return dst; 1511 } 1512 1513 public void copyValues(MedicationKnowledgeIndicationGuidelineComponent dst) { 1514 super.copyValues(dst); 1515 if (indication != null) { 1516 dst.indication = new ArrayList<CodeableReference>(); 1517 for (CodeableReference i : indication) 1518 dst.indication.add(i.copy()); 1519 }; 1520 if (dosingGuideline != null) { 1521 dst.dosingGuideline = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent>(); 1522 for (MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent i : dosingGuideline) 1523 dst.dosingGuideline.add(i.copy()); 1524 }; 1525 } 1526 1527 @Override 1528 public boolean equalsDeep(Base other_) { 1529 if (!super.equalsDeep(other_)) 1530 return false; 1531 if (!(other_ instanceof MedicationKnowledgeIndicationGuidelineComponent)) 1532 return false; 1533 MedicationKnowledgeIndicationGuidelineComponent o = (MedicationKnowledgeIndicationGuidelineComponent) other_; 1534 return compareDeep(indication, o.indication, true) && compareDeep(dosingGuideline, o.dosingGuideline, true) 1535 ; 1536 } 1537 1538 @Override 1539 public boolean equalsShallow(Base other_) { 1540 if (!super.equalsShallow(other_)) 1541 return false; 1542 if (!(other_ instanceof MedicationKnowledgeIndicationGuidelineComponent)) 1543 return false; 1544 MedicationKnowledgeIndicationGuidelineComponent o = (MedicationKnowledgeIndicationGuidelineComponent) other_; 1545 return true; 1546 } 1547 1548 public boolean isEmpty() { 1549 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(indication, dosingGuideline 1550 ); 1551 } 1552 1553 public String fhirType() { 1554 return "MedicationKnowledge.indicationGuideline"; 1555 1556 } 1557 1558 } 1559 1560 @Block() 1561 public static class MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent extends BackboneElement implements IBaseBackboneElement { 1562 /** 1563 * The overall intention of the treatment, for example, prophylactic, supporative, curative, etc. 1564 */ 1565 @Child(name = "treatmentIntent", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 1566 @Description(shortDefinition="Intention of the treatment", formalDefinition="The overall intention of the treatment, for example, prophylactic, supporative, curative, etc." ) 1567 protected CodeableConcept treatmentIntent; 1568 1569 /** 1570 * Dosage for the medication for the specific guidelines. 1571 */ 1572 @Child(name = "dosage", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1573 @Description(shortDefinition="Dosage for the medication for the specific guidelines", formalDefinition="Dosage for the medication for the specific guidelines." ) 1574 protected List<MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent> dosage; 1575 1576 /** 1577 * The type of the treatment that the guideline applies to, for example, long term therapy, first line treatment, etc. 1578 */ 1579 @Child(name = "administrationTreatment", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1580 @Description(shortDefinition="Type of treatment the guideline applies to", formalDefinition="The type of the treatment that the guideline applies to, for example, long term therapy, first line treatment, etc." ) 1581 protected CodeableConcept administrationTreatment; 1582 1583 /** 1584 * Characteristics of the patient that are relevant to the administration guidelines (for example, height, weight, gender, etc.). 1585 */ 1586 @Child(name = "patientCharacteristic", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1587 @Description(shortDefinition="Characteristics of the patient that are relevant to the administration guidelines", formalDefinition="Characteristics of the patient that are relevant to the administration guidelines (for example, height, weight, gender, etc.)." ) 1588 protected List<MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent> patientCharacteristic; 1589 1590 private static final long serialVersionUID = 882198366L; 1591 1592 /** 1593 * Constructor 1594 */ 1595 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent() { 1596 super(); 1597 } 1598 1599 /** 1600 * @return {@link #treatmentIntent} (The overall intention of the treatment, for example, prophylactic, supporative, curative, etc.) 1601 */ 1602 public CodeableConcept getTreatmentIntent() { 1603 if (this.treatmentIntent == null) 1604 if (Configuration.errorOnAutoCreate()) 1605 throw new Error("Attempt to auto-create MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent.treatmentIntent"); 1606 else if (Configuration.doAutoCreate()) 1607 this.treatmentIntent = new CodeableConcept(); // cc 1608 return this.treatmentIntent; 1609 } 1610 1611 public boolean hasTreatmentIntent() { 1612 return this.treatmentIntent != null && !this.treatmentIntent.isEmpty(); 1613 } 1614 1615 /** 1616 * @param value {@link #treatmentIntent} (The overall intention of the treatment, for example, prophylactic, supporative, curative, etc.) 1617 */ 1618 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent setTreatmentIntent(CodeableConcept value) { 1619 this.treatmentIntent = value; 1620 return this; 1621 } 1622 1623 /** 1624 * @return {@link #dosage} (Dosage for the medication for the specific guidelines.) 1625 */ 1626 public List<MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent> getDosage() { 1627 if (this.dosage == null) 1628 this.dosage = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent>(); 1629 return this.dosage; 1630 } 1631 1632 /** 1633 * @return Returns a reference to <code>this</code> for easy method chaining 1634 */ 1635 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent setDosage(List<MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent> theDosage) { 1636 this.dosage = theDosage; 1637 return this; 1638 } 1639 1640 public boolean hasDosage() { 1641 if (this.dosage == null) 1642 return false; 1643 for (MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent item : this.dosage) 1644 if (!item.isEmpty()) 1645 return true; 1646 return false; 1647 } 1648 1649 public MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent addDosage() { //3 1650 MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent t = new MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent(); 1651 if (this.dosage == null) 1652 this.dosage = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent>(); 1653 this.dosage.add(t); 1654 return t; 1655 } 1656 1657 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent addDosage(MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent t) { //3 1658 if (t == null) 1659 return this; 1660 if (this.dosage == null) 1661 this.dosage = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent>(); 1662 this.dosage.add(t); 1663 return this; 1664 } 1665 1666 /** 1667 * @return The first repetition of repeating field {@link #dosage}, creating it if it does not already exist {3} 1668 */ 1669 public MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent getDosageFirstRep() { 1670 if (getDosage().isEmpty()) { 1671 addDosage(); 1672 } 1673 return getDosage().get(0); 1674 } 1675 1676 /** 1677 * @return {@link #administrationTreatment} (The type of the treatment that the guideline applies to, for example, long term therapy, first line treatment, etc.) 1678 */ 1679 public CodeableConcept getAdministrationTreatment() { 1680 if (this.administrationTreatment == null) 1681 if (Configuration.errorOnAutoCreate()) 1682 throw new Error("Attempt to auto-create MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent.administrationTreatment"); 1683 else if (Configuration.doAutoCreate()) 1684 this.administrationTreatment = new CodeableConcept(); // cc 1685 return this.administrationTreatment; 1686 } 1687 1688 public boolean hasAdministrationTreatment() { 1689 return this.administrationTreatment != null && !this.administrationTreatment.isEmpty(); 1690 } 1691 1692 /** 1693 * @param value {@link #administrationTreatment} (The type of the treatment that the guideline applies to, for example, long term therapy, first line treatment, etc.) 1694 */ 1695 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent setAdministrationTreatment(CodeableConcept value) { 1696 this.administrationTreatment = value; 1697 return this; 1698 } 1699 1700 /** 1701 * @return {@link #patientCharacteristic} (Characteristics of the patient that are relevant to the administration guidelines (for example, height, weight, gender, etc.).) 1702 */ 1703 public List<MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent> getPatientCharacteristic() { 1704 if (this.patientCharacteristic == null) 1705 this.patientCharacteristic = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent>(); 1706 return this.patientCharacteristic; 1707 } 1708 1709 /** 1710 * @return Returns a reference to <code>this</code> for easy method chaining 1711 */ 1712 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent setPatientCharacteristic(List<MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent> thePatientCharacteristic) { 1713 this.patientCharacteristic = thePatientCharacteristic; 1714 return this; 1715 } 1716 1717 public boolean hasPatientCharacteristic() { 1718 if (this.patientCharacteristic == null) 1719 return false; 1720 for (MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent item : this.patientCharacteristic) 1721 if (!item.isEmpty()) 1722 return true; 1723 return false; 1724 } 1725 1726 public MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent addPatientCharacteristic() { //3 1727 MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent t = new MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent(); 1728 if (this.patientCharacteristic == null) 1729 this.patientCharacteristic = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent>(); 1730 this.patientCharacteristic.add(t); 1731 return t; 1732 } 1733 1734 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent addPatientCharacteristic(MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent t) { //3 1735 if (t == null) 1736 return this; 1737 if (this.patientCharacteristic == null) 1738 this.patientCharacteristic = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent>(); 1739 this.patientCharacteristic.add(t); 1740 return this; 1741 } 1742 1743 /** 1744 * @return The first repetition of repeating field {@link #patientCharacteristic}, creating it if it does not already exist {3} 1745 */ 1746 public MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent getPatientCharacteristicFirstRep() { 1747 if (getPatientCharacteristic().isEmpty()) { 1748 addPatientCharacteristic(); 1749 } 1750 return getPatientCharacteristic().get(0); 1751 } 1752 1753 protected void listChildren(List<Property> children) { 1754 super.listChildren(children); 1755 children.add(new Property("treatmentIntent", "CodeableConcept", "The overall intention of the treatment, for example, prophylactic, supporative, curative, etc.", 0, 1, treatmentIntent)); 1756 children.add(new Property("dosage", "", "Dosage for the medication for the specific guidelines.", 0, java.lang.Integer.MAX_VALUE, dosage)); 1757 children.add(new Property("administrationTreatment", "CodeableConcept", "The type of the treatment that the guideline applies to, for example, long term therapy, first line treatment, etc.", 0, 1, administrationTreatment)); 1758 children.add(new Property("patientCharacteristic", "", "Characteristics of the patient that are relevant to the administration guidelines (for example, height, weight, gender, etc.).", 0, java.lang.Integer.MAX_VALUE, patientCharacteristic)); 1759 } 1760 1761 @Override 1762 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1763 switch (_hash) { 1764 case 1744920884: /*treatmentIntent*/ return new Property("treatmentIntent", "CodeableConcept", "The overall intention of the treatment, for example, prophylactic, supporative, curative, etc.", 0, 1, treatmentIntent); 1765 case -1326018889: /*dosage*/ return new Property("dosage", "", "Dosage for the medication for the specific guidelines.", 0, java.lang.Integer.MAX_VALUE, dosage); 1766 case 1197121978: /*administrationTreatment*/ return new Property("administrationTreatment", "CodeableConcept", "The type of the treatment that the guideline applies to, for example, long term therapy, first line treatment, etc.", 0, 1, administrationTreatment); 1767 case 1770130432: /*patientCharacteristic*/ return new Property("patientCharacteristic", "", "Characteristics of the patient that are relevant to the administration guidelines (for example, height, weight, gender, etc.).", 0, java.lang.Integer.MAX_VALUE, patientCharacteristic); 1768 default: return super.getNamedProperty(_hash, _name, _checkValid); 1769 } 1770 1771 } 1772 1773 @Override 1774 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1775 switch (hash) { 1776 case 1744920884: /*treatmentIntent*/ return this.treatmentIntent == null ? new Base[0] : new Base[] {this.treatmentIntent}; // CodeableConcept 1777 case -1326018889: /*dosage*/ return this.dosage == null ? new Base[0] : this.dosage.toArray(new Base[this.dosage.size()]); // MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent 1778 case 1197121978: /*administrationTreatment*/ return this.administrationTreatment == null ? new Base[0] : new Base[] {this.administrationTreatment}; // CodeableConcept 1779 case 1770130432: /*patientCharacteristic*/ return this.patientCharacteristic == null ? new Base[0] : this.patientCharacteristic.toArray(new Base[this.patientCharacteristic.size()]); // MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent 1780 default: return super.getProperty(hash, name, checkValid); 1781 } 1782 1783 } 1784 1785 @Override 1786 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1787 switch (hash) { 1788 case 1744920884: // treatmentIntent 1789 this.treatmentIntent = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1790 return value; 1791 case -1326018889: // dosage 1792 this.getDosage().add((MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent) value); // MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent 1793 return value; 1794 case 1197121978: // administrationTreatment 1795 this.administrationTreatment = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1796 return value; 1797 case 1770130432: // patientCharacteristic 1798 this.getPatientCharacteristic().add((MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent) value); // MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent 1799 return value; 1800 default: return super.setProperty(hash, name, value); 1801 } 1802 1803 } 1804 1805 @Override 1806 public Base setProperty(String name, Base value) throws FHIRException { 1807 if (name.equals("treatmentIntent")) { 1808 this.treatmentIntent = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1809 } else if (name.equals("dosage")) { 1810 this.getDosage().add((MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent) value); 1811 } else if (name.equals("administrationTreatment")) { 1812 this.administrationTreatment = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 1813 } else if (name.equals("patientCharacteristic")) { 1814 this.getPatientCharacteristic().add((MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent) value); 1815 } else 1816 return super.setProperty(name, value); 1817 return value; 1818 } 1819 1820 @Override 1821 public void removeChild(String name, Base value) throws FHIRException { 1822 if (name.equals("treatmentIntent")) { 1823 this.treatmentIntent = null; 1824 } else if (name.equals("dosage")) { 1825 this.getDosage().remove((MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent) value); 1826 } else if (name.equals("administrationTreatment")) { 1827 this.administrationTreatment = null; 1828 } else if (name.equals("patientCharacteristic")) { 1829 this.getPatientCharacteristic().remove((MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent) value); 1830 } else 1831 super.removeChild(name, value); 1832 1833 } 1834 1835 @Override 1836 public Base makeProperty(int hash, String name) throws FHIRException { 1837 switch (hash) { 1838 case 1744920884: return getTreatmentIntent(); 1839 case -1326018889: return addDosage(); 1840 case 1197121978: return getAdministrationTreatment(); 1841 case 1770130432: return addPatientCharacteristic(); 1842 default: return super.makeProperty(hash, name); 1843 } 1844 1845 } 1846 1847 @Override 1848 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1849 switch (hash) { 1850 case 1744920884: /*treatmentIntent*/ return new String[] {"CodeableConcept"}; 1851 case -1326018889: /*dosage*/ return new String[] {}; 1852 case 1197121978: /*administrationTreatment*/ return new String[] {"CodeableConcept"}; 1853 case 1770130432: /*patientCharacteristic*/ return new String[] {}; 1854 default: return super.getTypesForProperty(hash, name); 1855 } 1856 1857 } 1858 1859 @Override 1860 public Base addChild(String name) throws FHIRException { 1861 if (name.equals("treatmentIntent")) { 1862 this.treatmentIntent = new CodeableConcept(); 1863 return this.treatmentIntent; 1864 } 1865 else if (name.equals("dosage")) { 1866 return addDosage(); 1867 } 1868 else if (name.equals("administrationTreatment")) { 1869 this.administrationTreatment = new CodeableConcept(); 1870 return this.administrationTreatment; 1871 } 1872 else if (name.equals("patientCharacteristic")) { 1873 return addPatientCharacteristic(); 1874 } 1875 else 1876 return super.addChild(name); 1877 } 1878 1879 public MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent copy() { 1880 MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent dst = new MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent(); 1881 copyValues(dst); 1882 return dst; 1883 } 1884 1885 public void copyValues(MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent dst) { 1886 super.copyValues(dst); 1887 dst.treatmentIntent = treatmentIntent == null ? null : treatmentIntent.copy(); 1888 if (dosage != null) { 1889 dst.dosage = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent>(); 1890 for (MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent i : dosage) 1891 dst.dosage.add(i.copy()); 1892 }; 1893 dst.administrationTreatment = administrationTreatment == null ? null : administrationTreatment.copy(); 1894 if (patientCharacteristic != null) { 1895 dst.patientCharacteristic = new ArrayList<MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent>(); 1896 for (MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent i : patientCharacteristic) 1897 dst.patientCharacteristic.add(i.copy()); 1898 }; 1899 } 1900 1901 @Override 1902 public boolean equalsDeep(Base other_) { 1903 if (!super.equalsDeep(other_)) 1904 return false; 1905 if (!(other_ instanceof MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent)) 1906 return false; 1907 MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent o = (MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent) other_; 1908 return compareDeep(treatmentIntent, o.treatmentIntent, true) && compareDeep(dosage, o.dosage, true) 1909 && compareDeep(administrationTreatment, o.administrationTreatment, true) && compareDeep(patientCharacteristic, o.patientCharacteristic, true) 1910 ; 1911 } 1912 1913 @Override 1914 public boolean equalsShallow(Base other_) { 1915 if (!super.equalsShallow(other_)) 1916 return false; 1917 if (!(other_ instanceof MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent)) 1918 return false; 1919 MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent o = (MedicationKnowledgeIndicationGuidelineDosingGuidelineComponent) other_; 1920 return true; 1921 } 1922 1923 public boolean isEmpty() { 1924 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(treatmentIntent, dosage, administrationTreatment 1925 , patientCharacteristic); 1926 } 1927 1928 public String fhirType() { 1929 return "MedicationKnowledge.indicationGuideline.dosingGuideline"; 1930 1931 } 1932 1933 } 1934 1935 @Block() 1936 public static class MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent extends BackboneElement implements IBaseBackboneElement { 1937 /** 1938 * The type or category of dosage for a given medication (for example, prophylaxis, maintenance, therapeutic, etc.). 1939 */ 1940 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1941 @Description(shortDefinition="Category of dosage for a medication", formalDefinition="The type or category of dosage for a given medication (for example, prophylaxis, maintenance, therapeutic, etc.)." ) 1942 protected CodeableConcept type; 1943 1944 /** 1945 * Dosage for the medication for the specific guidelines. 1946 */ 1947 @Child(name = "dosage", type = {Dosage.class}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1948 @Description(shortDefinition="Dosage for the medication for the specific guidelines", formalDefinition="Dosage for the medication for the specific guidelines." ) 1949 protected List<Dosage> dosage; 1950 1951 private static final long serialVersionUID = 1578257961L; 1952 1953 /** 1954 * Constructor 1955 */ 1956 public MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent() { 1957 super(); 1958 } 1959 1960 /** 1961 * Constructor 1962 */ 1963 public MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent(CodeableConcept type, Dosage dosage) { 1964 super(); 1965 this.setType(type); 1966 this.addDosage(dosage); 1967 } 1968 1969 /** 1970 * @return {@link #type} (The type or category of dosage for a given medication (for example, prophylaxis, maintenance, therapeutic, etc.).) 1971 */ 1972 public CodeableConcept getType() { 1973 if (this.type == null) 1974 if (Configuration.errorOnAutoCreate()) 1975 throw new Error("Attempt to auto-create MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent.type"); 1976 else if (Configuration.doAutoCreate()) 1977 this.type = new CodeableConcept(); // cc 1978 return this.type; 1979 } 1980 1981 public boolean hasType() { 1982 return this.type != null && !this.type.isEmpty(); 1983 } 1984 1985 /** 1986 * @param value {@link #type} (The type or category of dosage for a given medication (for example, prophylaxis, maintenance, therapeutic, etc.).) 1987 */ 1988 public MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent setType(CodeableConcept value) { 1989 this.type = value; 1990 return this; 1991 } 1992 1993 /** 1994 * @return {@link #dosage} (Dosage for the medication for the specific guidelines.) 1995 */ 1996 public List<Dosage> getDosage() { 1997 if (this.dosage == null) 1998 this.dosage = new ArrayList<Dosage>(); 1999 return this.dosage; 2000 } 2001 2002 /** 2003 * @return Returns a reference to <code>this</code> for easy method chaining 2004 */ 2005 public MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent setDosage(List<Dosage> theDosage) { 2006 this.dosage = theDosage; 2007 return this; 2008 } 2009 2010 public boolean hasDosage() { 2011 if (this.dosage == null) 2012 return false; 2013 for (Dosage item : this.dosage) 2014 if (!item.isEmpty()) 2015 return true; 2016 return false; 2017 } 2018 2019 public Dosage addDosage() { //3 2020 Dosage t = new Dosage(); 2021 if (this.dosage == null) 2022 this.dosage = new ArrayList<Dosage>(); 2023 this.dosage.add(t); 2024 return t; 2025 } 2026 2027 public MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent addDosage(Dosage t) { //3 2028 if (t == null) 2029 return this; 2030 if (this.dosage == null) 2031 this.dosage = new ArrayList<Dosage>(); 2032 this.dosage.add(t); 2033 return this; 2034 } 2035 2036 /** 2037 * @return The first repetition of repeating field {@link #dosage}, creating it if it does not already exist {3} 2038 */ 2039 public Dosage getDosageFirstRep() { 2040 if (getDosage().isEmpty()) { 2041 addDosage(); 2042 } 2043 return getDosage().get(0); 2044 } 2045 2046 protected void listChildren(List<Property> children) { 2047 super.listChildren(children); 2048 children.add(new Property("type", "CodeableConcept", "The type or category of dosage for a given medication (for example, prophylaxis, maintenance, therapeutic, etc.).", 0, 1, type)); 2049 children.add(new Property("dosage", "Dosage", "Dosage for the medication for the specific guidelines.", 0, java.lang.Integer.MAX_VALUE, dosage)); 2050 } 2051 2052 @Override 2053 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2054 switch (_hash) { 2055 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type or category of dosage for a given medication (for example, prophylaxis, maintenance, therapeutic, etc.).", 0, 1, type); 2056 case -1326018889: /*dosage*/ return new Property("dosage", "Dosage", "Dosage for the medication for the specific guidelines.", 0, java.lang.Integer.MAX_VALUE, dosage); 2057 default: return super.getNamedProperty(_hash, _name, _checkValid); 2058 } 2059 2060 } 2061 2062 @Override 2063 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2064 switch (hash) { 2065 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2066 case -1326018889: /*dosage*/ return this.dosage == null ? new Base[0] : this.dosage.toArray(new Base[this.dosage.size()]); // Dosage 2067 default: return super.getProperty(hash, name, checkValid); 2068 } 2069 2070 } 2071 2072 @Override 2073 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2074 switch (hash) { 2075 case 3575610: // type 2076 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2077 return value; 2078 case -1326018889: // dosage 2079 this.getDosage().add(TypeConvertor.castToDosage(value)); // Dosage 2080 return value; 2081 default: return super.setProperty(hash, name, value); 2082 } 2083 2084 } 2085 2086 @Override 2087 public Base setProperty(String name, Base value) throws FHIRException { 2088 if (name.equals("type")) { 2089 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2090 } else if (name.equals("dosage")) { 2091 this.getDosage().add(TypeConvertor.castToDosage(value)); 2092 } else 2093 return super.setProperty(name, value); 2094 return value; 2095 } 2096 2097 @Override 2098 public void removeChild(String name, Base value) throws FHIRException { 2099 if (name.equals("type")) { 2100 this.type = null; 2101 } else if (name.equals("dosage")) { 2102 this.getDosage().remove(value); 2103 } else 2104 super.removeChild(name, value); 2105 2106 } 2107 2108 @Override 2109 public Base makeProperty(int hash, String name) throws FHIRException { 2110 switch (hash) { 2111 case 3575610: return getType(); 2112 case -1326018889: return addDosage(); 2113 default: return super.makeProperty(hash, name); 2114 } 2115 2116 } 2117 2118 @Override 2119 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2120 switch (hash) { 2121 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2122 case -1326018889: /*dosage*/ return new String[] {"Dosage"}; 2123 default: return super.getTypesForProperty(hash, name); 2124 } 2125 2126 } 2127 2128 @Override 2129 public Base addChild(String name) throws FHIRException { 2130 if (name.equals("type")) { 2131 this.type = new CodeableConcept(); 2132 return this.type; 2133 } 2134 else if (name.equals("dosage")) { 2135 return addDosage(); 2136 } 2137 else 2138 return super.addChild(name); 2139 } 2140 2141 public MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent copy() { 2142 MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent dst = new MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent(); 2143 copyValues(dst); 2144 return dst; 2145 } 2146 2147 public void copyValues(MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent dst) { 2148 super.copyValues(dst); 2149 dst.type = type == null ? null : type.copy(); 2150 if (dosage != null) { 2151 dst.dosage = new ArrayList<Dosage>(); 2152 for (Dosage i : dosage) 2153 dst.dosage.add(i.copy()); 2154 }; 2155 } 2156 2157 @Override 2158 public boolean equalsDeep(Base other_) { 2159 if (!super.equalsDeep(other_)) 2160 return false; 2161 if (!(other_ instanceof MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent)) 2162 return false; 2163 MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent o = (MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent) other_; 2164 return compareDeep(type, o.type, true) && compareDeep(dosage, o.dosage, true); 2165 } 2166 2167 @Override 2168 public boolean equalsShallow(Base other_) { 2169 if (!super.equalsShallow(other_)) 2170 return false; 2171 if (!(other_ instanceof MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent)) 2172 return false; 2173 MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent o = (MedicationKnowledgeIndicationGuidelineDosingGuidelineDosageComponent) other_; 2174 return true; 2175 } 2176 2177 public boolean isEmpty() { 2178 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, dosage); 2179 } 2180 2181 public String fhirType() { 2182 return "MedicationKnowledge.indicationGuideline.dosingGuideline.dosage"; 2183 2184 } 2185 2186 } 2187 2188 @Block() 2189 public static class MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 2190 /** 2191 * The categorization of the specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender). 2192 */ 2193 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 2194 @Description(shortDefinition="Categorization of specific characteristic that is relevant to the administration guideline", formalDefinition="The categorization of the specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender)." ) 2195 protected CodeableConcept type; 2196 2197 /** 2198 * The specific characteristic (e.g. height, weight, gender, etc.). 2199 */ 2200 @Child(name = "value", type = {CodeableConcept.class, Quantity.class, Range.class}, order=2, min=0, max=1, modifier=false, summary=false) 2201 @Description(shortDefinition="The specific characteristic", formalDefinition="The specific characteristic (e.g. height, weight, gender, etc.)." ) 2202 protected DataType value; 2203 2204 private static final long serialVersionUID = -1659186716L; 2205 2206 /** 2207 * Constructor 2208 */ 2209 public MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent() { 2210 super(); 2211 } 2212 2213 /** 2214 * Constructor 2215 */ 2216 public MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent(CodeableConcept type) { 2217 super(); 2218 this.setType(type); 2219 } 2220 2221 /** 2222 * @return {@link #type} (The categorization of the specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender).) 2223 */ 2224 public CodeableConcept getType() { 2225 if (this.type == null) 2226 if (Configuration.errorOnAutoCreate()) 2227 throw new Error("Attempt to auto-create MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent.type"); 2228 else if (Configuration.doAutoCreate()) 2229 this.type = new CodeableConcept(); // cc 2230 return this.type; 2231 } 2232 2233 public boolean hasType() { 2234 return this.type != null && !this.type.isEmpty(); 2235 } 2236 2237 /** 2238 * @param value {@link #type} (The categorization of the specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender).) 2239 */ 2240 public MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent setType(CodeableConcept value) { 2241 this.type = value; 2242 return this; 2243 } 2244 2245 /** 2246 * @return {@link #value} (The specific characteristic (e.g. height, weight, gender, etc.).) 2247 */ 2248 public DataType getValue() { 2249 return this.value; 2250 } 2251 2252 /** 2253 * @return {@link #value} (The specific characteristic (e.g. height, weight, gender, etc.).) 2254 */ 2255 public CodeableConcept getValueCodeableConcept() throws FHIRException { 2256 if (this.value == null) 2257 this.value = new CodeableConcept(); 2258 if (!(this.value instanceof CodeableConcept)) 2259 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 2260 return (CodeableConcept) this.value; 2261 } 2262 2263 public boolean hasValueCodeableConcept() { 2264 return this.value instanceof CodeableConcept; 2265 } 2266 2267 /** 2268 * @return {@link #value} (The specific characteristic (e.g. height, weight, gender, etc.).) 2269 */ 2270 public Quantity getValueQuantity() throws FHIRException { 2271 if (this.value == null) 2272 this.value = new Quantity(); 2273 if (!(this.value instanceof Quantity)) 2274 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 2275 return (Quantity) this.value; 2276 } 2277 2278 public boolean hasValueQuantity() { 2279 return this.value instanceof Quantity; 2280 } 2281 2282 /** 2283 * @return {@link #value} (The specific characteristic (e.g. height, weight, gender, etc.).) 2284 */ 2285 public Range getValueRange() throws FHIRException { 2286 if (this.value == null) 2287 this.value = new Range(); 2288 if (!(this.value instanceof Range)) 2289 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 2290 return (Range) this.value; 2291 } 2292 2293 public boolean hasValueRange() { 2294 return this.value instanceof Range; 2295 } 2296 2297 public boolean hasValue() { 2298 return this.value != null && !this.value.isEmpty(); 2299 } 2300 2301 /** 2302 * @param value {@link #value} (The specific characteristic (e.g. height, weight, gender, etc.).) 2303 */ 2304 public MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent setValue(DataType value) { 2305 if (value != null && !(value instanceof CodeableConcept || value instanceof Quantity || value instanceof Range)) 2306 throw new FHIRException("Not the right type for MedicationKnowledge.indicationGuideline.dosingGuideline.patientCharacteristic.value[x]: "+value.fhirType()); 2307 this.value = value; 2308 return this; 2309 } 2310 2311 protected void listChildren(List<Property> children) { 2312 super.listChildren(children); 2313 children.add(new Property("type", "CodeableConcept", "The categorization of the specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender).", 0, 1, type)); 2314 children.add(new Property("value[x]", "CodeableConcept|Quantity|Range", "The specific characteristic (e.g. height, weight, gender, etc.).", 0, 1, value)); 2315 } 2316 2317 @Override 2318 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2319 switch (_hash) { 2320 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The categorization of the specific characteristic that is relevant to the administration guideline (e.g. height, weight, gender).", 0, 1, type); 2321 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|Quantity|Range", "The specific characteristic (e.g. height, weight, gender, etc.).", 0, 1, value); 2322 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|Quantity|Range", "The specific characteristic (e.g. height, weight, gender, etc.).", 0, 1, value); 2323 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "The specific characteristic (e.g. height, weight, gender, etc.).", 0, 1, value); 2324 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "The specific characteristic (e.g. height, weight, gender, etc.).", 0, 1, value); 2325 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "The specific characteristic (e.g. height, weight, gender, etc.).", 0, 1, value); 2326 default: return super.getNamedProperty(_hash, _name, _checkValid); 2327 } 2328 2329 } 2330 2331 @Override 2332 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2333 switch (hash) { 2334 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2335 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 2336 default: return super.getProperty(hash, name, checkValid); 2337 } 2338 2339 } 2340 2341 @Override 2342 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2343 switch (hash) { 2344 case 3575610: // type 2345 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2346 return value; 2347 case 111972721: // value 2348 this.value = TypeConvertor.castToType(value); // DataType 2349 return value; 2350 default: return super.setProperty(hash, name, value); 2351 } 2352 2353 } 2354 2355 @Override 2356 public Base setProperty(String name, Base value) throws FHIRException { 2357 if (name.equals("type")) { 2358 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2359 } else if (name.equals("value[x]")) { 2360 this.value = TypeConvertor.castToType(value); // DataType 2361 } else 2362 return super.setProperty(name, value); 2363 return value; 2364 } 2365 2366 @Override 2367 public void removeChild(String name, Base value) throws FHIRException { 2368 if (name.equals("type")) { 2369 this.type = null; 2370 } else if (name.equals("value[x]")) { 2371 this.value = null; 2372 } else 2373 super.removeChild(name, value); 2374 2375 } 2376 2377 @Override 2378 public Base makeProperty(int hash, String name) throws FHIRException { 2379 switch (hash) { 2380 case 3575610: return getType(); 2381 case -1410166417: return getValue(); 2382 case 111972721: return getValue(); 2383 default: return super.makeProperty(hash, name); 2384 } 2385 2386 } 2387 2388 @Override 2389 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2390 switch (hash) { 2391 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2392 case 111972721: /*value*/ return new String[] {"CodeableConcept", "Quantity", "Range"}; 2393 default: return super.getTypesForProperty(hash, name); 2394 } 2395 2396 } 2397 2398 @Override 2399 public Base addChild(String name) throws FHIRException { 2400 if (name.equals("type")) { 2401 this.type = new CodeableConcept(); 2402 return this.type; 2403 } 2404 else if (name.equals("valueCodeableConcept")) { 2405 this.value = new CodeableConcept(); 2406 return this.value; 2407 } 2408 else if (name.equals("valueQuantity")) { 2409 this.value = new Quantity(); 2410 return this.value; 2411 } 2412 else if (name.equals("valueRange")) { 2413 this.value = new Range(); 2414 return this.value; 2415 } 2416 else 2417 return super.addChild(name); 2418 } 2419 2420 public MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent copy() { 2421 MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent dst = new MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent(); 2422 copyValues(dst); 2423 return dst; 2424 } 2425 2426 public void copyValues(MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent dst) { 2427 super.copyValues(dst); 2428 dst.type = type == null ? null : type.copy(); 2429 dst.value = value == null ? null : value.copy(); 2430 } 2431 2432 @Override 2433 public boolean equalsDeep(Base other_) { 2434 if (!super.equalsDeep(other_)) 2435 return false; 2436 if (!(other_ instanceof MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent)) 2437 return false; 2438 MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent o = (MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent) other_; 2439 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 2440 } 2441 2442 @Override 2443 public boolean equalsShallow(Base other_) { 2444 if (!super.equalsShallow(other_)) 2445 return false; 2446 if (!(other_ instanceof MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent)) 2447 return false; 2448 MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent o = (MedicationKnowledgeIndicationGuidelineDosingGuidelinePatientCharacteristicComponent) other_; 2449 return true; 2450 } 2451 2452 public boolean isEmpty() { 2453 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 2454 } 2455 2456 public String fhirType() { 2457 return "MedicationKnowledge.indicationGuideline.dosingGuideline.patientCharacteristic"; 2458 2459 } 2460 2461 } 2462 2463 @Block() 2464 public static class MedicationKnowledgeMedicineClassificationComponent extends BackboneElement implements IBaseBackboneElement { 2465 /** 2466 * The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification). 2467 */ 2468 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 2469 @Description(shortDefinition="The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification)", formalDefinition="The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification)." ) 2470 protected CodeableConcept type; 2471 2472 /** 2473 * Either a textual source of the classification or a reference to an online source. 2474 */ 2475 @Child(name = "source", type = {StringType.class, UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2476 @Description(shortDefinition="The source of the classification", formalDefinition="Either a textual source of the classification or a reference to an online source." ) 2477 protected DataType source; 2478 2479 /** 2480 * Specific category assigned to the medication (e.g. anti-infective, anti-hypertensive, antibiotic, etc.). 2481 */ 2482 @Child(name = "classification", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2483 @Description(shortDefinition="Specific category assigned to the medication", formalDefinition="Specific category assigned to the medication (e.g. anti-infective, anti-hypertensive, antibiotic, etc.)." ) 2484 protected List<CodeableConcept> classification; 2485 2486 private static final long serialVersionUID = 1598220123L; 2487 2488 /** 2489 * Constructor 2490 */ 2491 public MedicationKnowledgeMedicineClassificationComponent() { 2492 super(); 2493 } 2494 2495 /** 2496 * Constructor 2497 */ 2498 public MedicationKnowledgeMedicineClassificationComponent(CodeableConcept type) { 2499 super(); 2500 this.setType(type); 2501 } 2502 2503 /** 2504 * @return {@link #type} (The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification).) 2505 */ 2506 public CodeableConcept getType() { 2507 if (this.type == null) 2508 if (Configuration.errorOnAutoCreate()) 2509 throw new Error("Attempt to auto-create MedicationKnowledgeMedicineClassificationComponent.type"); 2510 else if (Configuration.doAutoCreate()) 2511 this.type = new CodeableConcept(); // cc 2512 return this.type; 2513 } 2514 2515 public boolean hasType() { 2516 return this.type != null && !this.type.isEmpty(); 2517 } 2518 2519 /** 2520 * @param value {@link #type} (The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification).) 2521 */ 2522 public MedicationKnowledgeMedicineClassificationComponent setType(CodeableConcept value) { 2523 this.type = value; 2524 return this; 2525 } 2526 2527 /** 2528 * @return {@link #source} (Either a textual source of the classification or a reference to an online source.) 2529 */ 2530 public DataType getSource() { 2531 return this.source; 2532 } 2533 2534 /** 2535 * @return {@link #source} (Either a textual source of the classification or a reference to an online source.) 2536 */ 2537 public StringType getSourceStringType() throws FHIRException { 2538 if (this.source == null) 2539 this.source = new StringType(); 2540 if (!(this.source instanceof StringType)) 2541 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.source.getClass().getName()+" was encountered"); 2542 return (StringType) this.source; 2543 } 2544 2545 public boolean hasSourceStringType() { 2546 return this.source instanceof StringType; 2547 } 2548 2549 /** 2550 * @return {@link #source} (Either a textual source of the classification or a reference to an online source.) 2551 */ 2552 public UriType getSourceUriType() throws FHIRException { 2553 if (this.source == null) 2554 this.source = new UriType(); 2555 if (!(this.source instanceof UriType)) 2556 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.source.getClass().getName()+" was encountered"); 2557 return (UriType) this.source; 2558 } 2559 2560 public boolean hasSourceUriType() { 2561 return this.source instanceof UriType; 2562 } 2563 2564 public boolean hasSource() { 2565 return this.source != null && !this.source.isEmpty(); 2566 } 2567 2568 /** 2569 * @param value {@link #source} (Either a textual source of the classification or a reference to an online source.) 2570 */ 2571 public MedicationKnowledgeMedicineClassificationComponent setSource(DataType value) { 2572 if (value != null && !(value instanceof StringType || value instanceof UriType)) 2573 throw new FHIRException("Not the right type for MedicationKnowledge.medicineClassification.source[x]: "+value.fhirType()); 2574 this.source = value; 2575 return this; 2576 } 2577 2578 /** 2579 * @return {@link #classification} (Specific category assigned to the medication (e.g. anti-infective, anti-hypertensive, antibiotic, etc.).) 2580 */ 2581 public List<CodeableConcept> getClassification() { 2582 if (this.classification == null) 2583 this.classification = new ArrayList<CodeableConcept>(); 2584 return this.classification; 2585 } 2586 2587 /** 2588 * @return Returns a reference to <code>this</code> for easy method chaining 2589 */ 2590 public MedicationKnowledgeMedicineClassificationComponent setClassification(List<CodeableConcept> theClassification) { 2591 this.classification = theClassification; 2592 return this; 2593 } 2594 2595 public boolean hasClassification() { 2596 if (this.classification == null) 2597 return false; 2598 for (CodeableConcept item : this.classification) 2599 if (!item.isEmpty()) 2600 return true; 2601 return false; 2602 } 2603 2604 public CodeableConcept addClassification() { //3 2605 CodeableConcept t = new CodeableConcept(); 2606 if (this.classification == null) 2607 this.classification = new ArrayList<CodeableConcept>(); 2608 this.classification.add(t); 2609 return t; 2610 } 2611 2612 public MedicationKnowledgeMedicineClassificationComponent addClassification(CodeableConcept t) { //3 2613 if (t == null) 2614 return this; 2615 if (this.classification == null) 2616 this.classification = new ArrayList<CodeableConcept>(); 2617 this.classification.add(t); 2618 return this; 2619 } 2620 2621 /** 2622 * @return The first repetition of repeating field {@link #classification}, creating it if it does not already exist {3} 2623 */ 2624 public CodeableConcept getClassificationFirstRep() { 2625 if (getClassification().isEmpty()) { 2626 addClassification(); 2627 } 2628 return getClassification().get(0); 2629 } 2630 2631 protected void listChildren(List<Property> children) { 2632 super.listChildren(children); 2633 children.add(new Property("type", "CodeableConcept", "The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification).", 0, 1, type)); 2634 children.add(new Property("source[x]", "string|uri", "Either a textual source of the classification or a reference to an online source.", 0, 1, source)); 2635 children.add(new Property("classification", "CodeableConcept", "Specific category assigned to the medication (e.g. anti-infective, anti-hypertensive, antibiotic, etc.).", 0, java.lang.Integer.MAX_VALUE, classification)); 2636 } 2637 2638 @Override 2639 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2640 switch (_hash) { 2641 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification).", 0, 1, type); 2642 case -1698413947: /*source[x]*/ return new Property("source[x]", "string|uri", "Either a textual source of the classification or a reference to an online source.", 0, 1, source); 2643 case -896505829: /*source*/ return new Property("source[x]", "string|uri", "Either a textual source of the classification or a reference to an online source.", 0, 1, source); 2644 case 1327821836: /*sourceString*/ return new Property("source[x]", "string", "Either a textual source of the classification or a reference to an online source.", 0, 1, source); 2645 case -1698419887: /*sourceUri*/ return new Property("source[x]", "uri", "Either a textual source of the classification or a reference to an online source.", 0, 1, source); 2646 case 382350310: /*classification*/ return new Property("classification", "CodeableConcept", "Specific category assigned to the medication (e.g. anti-infective, anti-hypertensive, antibiotic, etc.).", 0, java.lang.Integer.MAX_VALUE, classification); 2647 default: return super.getNamedProperty(_hash, _name, _checkValid); 2648 } 2649 2650 } 2651 2652 @Override 2653 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2654 switch (hash) { 2655 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2656 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // DataType 2657 case 382350310: /*classification*/ return this.classification == null ? new Base[0] : this.classification.toArray(new Base[this.classification.size()]); // CodeableConcept 2658 default: return super.getProperty(hash, name, checkValid); 2659 } 2660 2661 } 2662 2663 @Override 2664 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2665 switch (hash) { 2666 case 3575610: // type 2667 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2668 return value; 2669 case -896505829: // source 2670 this.source = TypeConvertor.castToType(value); // DataType 2671 return value; 2672 case 382350310: // classification 2673 this.getClassification().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 2674 return value; 2675 default: return super.setProperty(hash, name, value); 2676 } 2677 2678 } 2679 2680 @Override 2681 public Base setProperty(String name, Base value) throws FHIRException { 2682 if (name.equals("type")) { 2683 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 2684 } else if (name.equals("source[x]")) { 2685 this.source = TypeConvertor.castToType(value); // DataType 2686 } else if (name.equals("classification")) { 2687 this.getClassification().add(TypeConvertor.castToCodeableConcept(value)); 2688 } else 2689 return super.setProperty(name, value); 2690 return value; 2691 } 2692 2693 @Override 2694 public void removeChild(String name, Base value) throws FHIRException { 2695 if (name.equals("type")) { 2696 this.type = null; 2697 } else if (name.equals("source[x]")) { 2698 this.source = null; 2699 } else if (name.equals("classification")) { 2700 this.getClassification().remove(value); 2701 } else 2702 super.removeChild(name, value); 2703 2704 } 2705 2706 @Override 2707 public Base makeProperty(int hash, String name) throws FHIRException { 2708 switch (hash) { 2709 case 3575610: return getType(); 2710 case -1698413947: return getSource(); 2711 case -896505829: return getSource(); 2712 case 382350310: return addClassification(); 2713 default: return super.makeProperty(hash, name); 2714 } 2715 2716 } 2717 2718 @Override 2719 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2720 switch (hash) { 2721 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2722 case -896505829: /*source*/ return new String[] {"string", "uri"}; 2723 case 382350310: /*classification*/ return new String[] {"CodeableConcept"}; 2724 default: return super.getTypesForProperty(hash, name); 2725 } 2726 2727 } 2728 2729 @Override 2730 public Base addChild(String name) throws FHIRException { 2731 if (name.equals("type")) { 2732 this.type = new CodeableConcept(); 2733 return this.type; 2734 } 2735 else if (name.equals("sourceString")) { 2736 this.source = new StringType(); 2737 return this.source; 2738 } 2739 else if (name.equals("sourceUri")) { 2740 this.source = new UriType(); 2741 return this.source; 2742 } 2743 else if (name.equals("classification")) { 2744 return addClassification(); 2745 } 2746 else 2747 return super.addChild(name); 2748 } 2749 2750 public MedicationKnowledgeMedicineClassificationComponent copy() { 2751 MedicationKnowledgeMedicineClassificationComponent dst = new MedicationKnowledgeMedicineClassificationComponent(); 2752 copyValues(dst); 2753 return dst; 2754 } 2755 2756 public void copyValues(MedicationKnowledgeMedicineClassificationComponent dst) { 2757 super.copyValues(dst); 2758 dst.type = type == null ? null : type.copy(); 2759 dst.source = source == null ? null : source.copy(); 2760 if (classification != null) { 2761 dst.classification = new ArrayList<CodeableConcept>(); 2762 for (CodeableConcept i : classification) 2763 dst.classification.add(i.copy()); 2764 }; 2765 } 2766 2767 @Override 2768 public boolean equalsDeep(Base other_) { 2769 if (!super.equalsDeep(other_)) 2770 return false; 2771 if (!(other_ instanceof MedicationKnowledgeMedicineClassificationComponent)) 2772 return false; 2773 MedicationKnowledgeMedicineClassificationComponent o = (MedicationKnowledgeMedicineClassificationComponent) other_; 2774 return compareDeep(type, o.type, true) && compareDeep(source, o.source, true) && compareDeep(classification, o.classification, true) 2775 ; 2776 } 2777 2778 @Override 2779 public boolean equalsShallow(Base other_) { 2780 if (!super.equalsShallow(other_)) 2781 return false; 2782 if (!(other_ instanceof MedicationKnowledgeMedicineClassificationComponent)) 2783 return false; 2784 MedicationKnowledgeMedicineClassificationComponent o = (MedicationKnowledgeMedicineClassificationComponent) other_; 2785 return true; 2786 } 2787 2788 public boolean isEmpty() { 2789 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, source, classification 2790 ); 2791 } 2792 2793 public String fhirType() { 2794 return "MedicationKnowledge.medicineClassification"; 2795 2796 } 2797 2798 } 2799 2800 @Block() 2801 public static class MedicationKnowledgePackagingComponent extends BackboneElement implements IBaseBackboneElement { 2802 /** 2803 * The cost of the packaged medication. 2804 */ 2805 @Child(name = "cost", type = {MedicationKnowledgeCostComponent.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2806 @Description(shortDefinition="Cost of the packaged medication", formalDefinition="The cost of the packaged medication." ) 2807 protected List<MedicationKnowledgeCostComponent> cost; 2808 2809 /** 2810 * A reference to a PackagedProductDefinition that provides the details of the product that is in the packaging and is being priced. 2811 */ 2812 @Child(name = "packagedProduct", type = {PackagedProductDefinition.class}, order=2, min=0, max=1, modifier=false, summary=false) 2813 @Description(shortDefinition="The packaged medication that is being priced", formalDefinition="A reference to a PackagedProductDefinition that provides the details of the product that is in the packaging and is being priced." ) 2814 protected Reference packagedProduct; 2815 2816 private static final long serialVersionUID = -337249398L; 2817 2818 /** 2819 * Constructor 2820 */ 2821 public MedicationKnowledgePackagingComponent() { 2822 super(); 2823 } 2824 2825 /** 2826 * @return {@link #cost} (The cost of the packaged medication.) 2827 */ 2828 public List<MedicationKnowledgeCostComponent> getCost() { 2829 if (this.cost == null) 2830 this.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 2831 return this.cost; 2832 } 2833 2834 /** 2835 * @return Returns a reference to <code>this</code> for easy method chaining 2836 */ 2837 public MedicationKnowledgePackagingComponent setCost(List<MedicationKnowledgeCostComponent> theCost) { 2838 this.cost = theCost; 2839 return this; 2840 } 2841 2842 public boolean hasCost() { 2843 if (this.cost == null) 2844 return false; 2845 for (MedicationKnowledgeCostComponent item : this.cost) 2846 if (!item.isEmpty()) 2847 return true; 2848 return false; 2849 } 2850 2851 public MedicationKnowledgeCostComponent addCost() { //3 2852 MedicationKnowledgeCostComponent t = new MedicationKnowledgeCostComponent(); 2853 if (this.cost == null) 2854 this.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 2855 this.cost.add(t); 2856 return t; 2857 } 2858 2859 public MedicationKnowledgePackagingComponent addCost(MedicationKnowledgeCostComponent t) { //3 2860 if (t == null) 2861 return this; 2862 if (this.cost == null) 2863 this.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 2864 this.cost.add(t); 2865 return this; 2866 } 2867 2868 /** 2869 * @return The first repetition of repeating field {@link #cost}, creating it if it does not already exist {3} 2870 */ 2871 public MedicationKnowledgeCostComponent getCostFirstRep() { 2872 if (getCost().isEmpty()) { 2873 addCost(); 2874 } 2875 return getCost().get(0); 2876 } 2877 2878 /** 2879 * @return {@link #packagedProduct} (A reference to a PackagedProductDefinition that provides the details of the product that is in the packaging and is being priced.) 2880 */ 2881 public Reference getPackagedProduct() { 2882 if (this.packagedProduct == null) 2883 if (Configuration.errorOnAutoCreate()) 2884 throw new Error("Attempt to auto-create MedicationKnowledgePackagingComponent.packagedProduct"); 2885 else if (Configuration.doAutoCreate()) 2886 this.packagedProduct = new Reference(); // cc 2887 return this.packagedProduct; 2888 } 2889 2890 public boolean hasPackagedProduct() { 2891 return this.packagedProduct != null && !this.packagedProduct.isEmpty(); 2892 } 2893 2894 /** 2895 * @param value {@link #packagedProduct} (A reference to a PackagedProductDefinition that provides the details of the product that is in the packaging and is being priced.) 2896 */ 2897 public MedicationKnowledgePackagingComponent setPackagedProduct(Reference value) { 2898 this.packagedProduct = value; 2899 return this; 2900 } 2901 2902 protected void listChildren(List<Property> children) { 2903 super.listChildren(children); 2904 children.add(new Property("cost", "@MedicationKnowledge.cost", "The cost of the packaged medication.", 0, java.lang.Integer.MAX_VALUE, cost)); 2905 children.add(new Property("packagedProduct", "Reference(PackagedProductDefinition)", "A reference to a PackagedProductDefinition that provides the details of the product that is in the packaging and is being priced.", 0, 1, packagedProduct)); 2906 } 2907 2908 @Override 2909 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2910 switch (_hash) { 2911 case 3059661: /*cost*/ return new Property("cost", "@MedicationKnowledge.cost", "The cost of the packaged medication.", 0, java.lang.Integer.MAX_VALUE, cost); 2912 case 1893956145: /*packagedProduct*/ return new Property("packagedProduct", "Reference(PackagedProductDefinition)", "A reference to a PackagedProductDefinition that provides the details of the product that is in the packaging and is being priced.", 0, 1, packagedProduct); 2913 default: return super.getNamedProperty(_hash, _name, _checkValid); 2914 } 2915 2916 } 2917 2918 @Override 2919 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2920 switch (hash) { 2921 case 3059661: /*cost*/ return this.cost == null ? new Base[0] : this.cost.toArray(new Base[this.cost.size()]); // MedicationKnowledgeCostComponent 2922 case 1893956145: /*packagedProduct*/ return this.packagedProduct == null ? new Base[0] : new Base[] {this.packagedProduct}; // Reference 2923 default: return super.getProperty(hash, name, checkValid); 2924 } 2925 2926 } 2927 2928 @Override 2929 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2930 switch (hash) { 2931 case 3059661: // cost 2932 this.getCost().add((MedicationKnowledgeCostComponent) value); // MedicationKnowledgeCostComponent 2933 return value; 2934 case 1893956145: // packagedProduct 2935 this.packagedProduct = TypeConvertor.castToReference(value); // Reference 2936 return value; 2937 default: return super.setProperty(hash, name, value); 2938 } 2939 2940 } 2941 2942 @Override 2943 public Base setProperty(String name, Base value) throws FHIRException { 2944 if (name.equals("cost")) { 2945 this.getCost().add((MedicationKnowledgeCostComponent) value); 2946 } else if (name.equals("packagedProduct")) { 2947 this.packagedProduct = TypeConvertor.castToReference(value); // Reference 2948 } else 2949 return super.setProperty(name, value); 2950 return value; 2951 } 2952 2953 @Override 2954 public void removeChild(String name, Base value) throws FHIRException { 2955 if (name.equals("cost")) { 2956 this.getCost().remove((MedicationKnowledgeCostComponent) value); 2957 } else if (name.equals("packagedProduct")) { 2958 this.packagedProduct = null; 2959 } else 2960 super.removeChild(name, value); 2961 2962 } 2963 2964 @Override 2965 public Base makeProperty(int hash, String name) throws FHIRException { 2966 switch (hash) { 2967 case 3059661: return addCost(); 2968 case 1893956145: return getPackagedProduct(); 2969 default: return super.makeProperty(hash, name); 2970 } 2971 2972 } 2973 2974 @Override 2975 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2976 switch (hash) { 2977 case 3059661: /*cost*/ return new String[] {"@MedicationKnowledge.cost"}; 2978 case 1893956145: /*packagedProduct*/ return new String[] {"Reference"}; 2979 default: return super.getTypesForProperty(hash, name); 2980 } 2981 2982 } 2983 2984 @Override 2985 public Base addChild(String name) throws FHIRException { 2986 if (name.equals("cost")) { 2987 return addCost(); 2988 } 2989 else if (name.equals("packagedProduct")) { 2990 this.packagedProduct = new Reference(); 2991 return this.packagedProduct; 2992 } 2993 else 2994 return super.addChild(name); 2995 } 2996 2997 public MedicationKnowledgePackagingComponent copy() { 2998 MedicationKnowledgePackagingComponent dst = new MedicationKnowledgePackagingComponent(); 2999 copyValues(dst); 3000 return dst; 3001 } 3002 3003 public void copyValues(MedicationKnowledgePackagingComponent dst) { 3004 super.copyValues(dst); 3005 if (cost != null) { 3006 dst.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 3007 for (MedicationKnowledgeCostComponent i : cost) 3008 dst.cost.add(i.copy()); 3009 }; 3010 dst.packagedProduct = packagedProduct == null ? null : packagedProduct.copy(); 3011 } 3012 3013 @Override 3014 public boolean equalsDeep(Base other_) { 3015 if (!super.equalsDeep(other_)) 3016 return false; 3017 if (!(other_ instanceof MedicationKnowledgePackagingComponent)) 3018 return false; 3019 MedicationKnowledgePackagingComponent o = (MedicationKnowledgePackagingComponent) other_; 3020 return compareDeep(cost, o.cost, true) && compareDeep(packagedProduct, o.packagedProduct, true) 3021 ; 3022 } 3023 3024 @Override 3025 public boolean equalsShallow(Base other_) { 3026 if (!super.equalsShallow(other_)) 3027 return false; 3028 if (!(other_ instanceof MedicationKnowledgePackagingComponent)) 3029 return false; 3030 MedicationKnowledgePackagingComponent o = (MedicationKnowledgePackagingComponent) other_; 3031 return true; 3032 } 3033 3034 public boolean isEmpty() { 3035 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(cost, packagedProduct); 3036 } 3037 3038 public String fhirType() { 3039 return "MedicationKnowledge.packaging"; 3040 3041 } 3042 3043 } 3044 3045 @Block() 3046 public static class MedicationKnowledgeStorageGuidelineComponent extends BackboneElement implements IBaseBackboneElement { 3047 /** 3048 * Reference to additional information about the storage guidelines. 3049 */ 3050 @Child(name = "reference", type = {UriType.class}, order=1, min=0, max=1, modifier=false, summary=false) 3051 @Description(shortDefinition="Reference to additional information", formalDefinition="Reference to additional information about the storage guidelines." ) 3052 protected UriType reference; 3053 3054 /** 3055 * Additional notes about the storage. 3056 */ 3057 @Child(name = "note", type = {Annotation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3058 @Description(shortDefinition="Additional storage notes", formalDefinition="Additional notes about the storage." ) 3059 protected List<Annotation> note; 3060 3061 /** 3062 * Duration that the medication remains stable if the environmentalSetting is respected. 3063 */ 3064 @Child(name = "stabilityDuration", type = {Duration.class}, order=3, min=0, max=1, modifier=false, summary=false) 3065 @Description(shortDefinition="Duration remains stable", formalDefinition="Duration that the medication remains stable if the environmentalSetting is respected." ) 3066 protected Duration stabilityDuration; 3067 3068 /** 3069 * Describes a setting/value on the environment for the adequate storage of the medication and other substances. Environment settings may involve temperature, humidity, or exposure to light. 3070 */ 3071 @Child(name = "environmentalSetting", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3072 @Description(shortDefinition="Setting or value of environment for adequate storage", formalDefinition="Describes a setting/value on the environment for the adequate storage of the medication and other substances. Environment settings may involve temperature, humidity, or exposure to light." ) 3073 protected List<MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent> environmentalSetting; 3074 3075 private static final long serialVersionUID = -304442588L; 3076 3077 /** 3078 * Constructor 3079 */ 3080 public MedicationKnowledgeStorageGuidelineComponent() { 3081 super(); 3082 } 3083 3084 /** 3085 * @return {@link #reference} (Reference to additional information about the storage guidelines.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 3086 */ 3087 public UriType getReferenceElement() { 3088 if (this.reference == null) 3089 if (Configuration.errorOnAutoCreate()) 3090 throw new Error("Attempt to auto-create MedicationKnowledgeStorageGuidelineComponent.reference"); 3091 else if (Configuration.doAutoCreate()) 3092 this.reference = new UriType(); // bb 3093 return this.reference; 3094 } 3095 3096 public boolean hasReferenceElement() { 3097 return this.reference != null && !this.reference.isEmpty(); 3098 } 3099 3100 public boolean hasReference() { 3101 return this.reference != null && !this.reference.isEmpty(); 3102 } 3103 3104 /** 3105 * @param value {@link #reference} (Reference to additional information about the storage guidelines.). This is the underlying object with id, value and extensions. The accessor "getReference" gives direct access to the value 3106 */ 3107 public MedicationKnowledgeStorageGuidelineComponent setReferenceElement(UriType value) { 3108 this.reference = value; 3109 return this; 3110 } 3111 3112 /** 3113 * @return Reference to additional information about the storage guidelines. 3114 */ 3115 public String getReference() { 3116 return this.reference == null ? null : this.reference.getValue(); 3117 } 3118 3119 /** 3120 * @param value Reference to additional information about the storage guidelines. 3121 */ 3122 public MedicationKnowledgeStorageGuidelineComponent setReference(String value) { 3123 if (Utilities.noString(value)) 3124 this.reference = null; 3125 else { 3126 if (this.reference == null) 3127 this.reference = new UriType(); 3128 this.reference.setValue(value); 3129 } 3130 return this; 3131 } 3132 3133 /** 3134 * @return {@link #note} (Additional notes about the storage.) 3135 */ 3136 public List<Annotation> getNote() { 3137 if (this.note == null) 3138 this.note = new ArrayList<Annotation>(); 3139 return this.note; 3140 } 3141 3142 /** 3143 * @return Returns a reference to <code>this</code> for easy method chaining 3144 */ 3145 public MedicationKnowledgeStorageGuidelineComponent setNote(List<Annotation> theNote) { 3146 this.note = theNote; 3147 return this; 3148 } 3149 3150 public boolean hasNote() { 3151 if (this.note == null) 3152 return false; 3153 for (Annotation item : this.note) 3154 if (!item.isEmpty()) 3155 return true; 3156 return false; 3157 } 3158 3159 public Annotation addNote() { //3 3160 Annotation t = new Annotation(); 3161 if (this.note == null) 3162 this.note = new ArrayList<Annotation>(); 3163 this.note.add(t); 3164 return t; 3165 } 3166 3167 public MedicationKnowledgeStorageGuidelineComponent addNote(Annotation t) { //3 3168 if (t == null) 3169 return this; 3170 if (this.note == null) 3171 this.note = new ArrayList<Annotation>(); 3172 this.note.add(t); 3173 return this; 3174 } 3175 3176 /** 3177 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist {3} 3178 */ 3179 public Annotation getNoteFirstRep() { 3180 if (getNote().isEmpty()) { 3181 addNote(); 3182 } 3183 return getNote().get(0); 3184 } 3185 3186 /** 3187 * @return {@link #stabilityDuration} (Duration that the medication remains stable if the environmentalSetting is respected.) 3188 */ 3189 public Duration getStabilityDuration() { 3190 if (this.stabilityDuration == null) 3191 if (Configuration.errorOnAutoCreate()) 3192 throw new Error("Attempt to auto-create MedicationKnowledgeStorageGuidelineComponent.stabilityDuration"); 3193 else if (Configuration.doAutoCreate()) 3194 this.stabilityDuration = new Duration(); // cc 3195 return this.stabilityDuration; 3196 } 3197 3198 public boolean hasStabilityDuration() { 3199 return this.stabilityDuration != null && !this.stabilityDuration.isEmpty(); 3200 } 3201 3202 /** 3203 * @param value {@link #stabilityDuration} (Duration that the medication remains stable if the environmentalSetting is respected.) 3204 */ 3205 public MedicationKnowledgeStorageGuidelineComponent setStabilityDuration(Duration value) { 3206 this.stabilityDuration = value; 3207 return this; 3208 } 3209 3210 /** 3211 * @return {@link #environmentalSetting} (Describes a setting/value on the environment for the adequate storage of the medication and other substances. Environment settings may involve temperature, humidity, or exposure to light.) 3212 */ 3213 public List<MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent> getEnvironmentalSetting() { 3214 if (this.environmentalSetting == null) 3215 this.environmentalSetting = new ArrayList<MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent>(); 3216 return this.environmentalSetting; 3217 } 3218 3219 /** 3220 * @return Returns a reference to <code>this</code> for easy method chaining 3221 */ 3222 public MedicationKnowledgeStorageGuidelineComponent setEnvironmentalSetting(List<MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent> theEnvironmentalSetting) { 3223 this.environmentalSetting = theEnvironmentalSetting; 3224 return this; 3225 } 3226 3227 public boolean hasEnvironmentalSetting() { 3228 if (this.environmentalSetting == null) 3229 return false; 3230 for (MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent item : this.environmentalSetting) 3231 if (!item.isEmpty()) 3232 return true; 3233 return false; 3234 } 3235 3236 public MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent addEnvironmentalSetting() { //3 3237 MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent t = new MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent(); 3238 if (this.environmentalSetting == null) 3239 this.environmentalSetting = new ArrayList<MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent>(); 3240 this.environmentalSetting.add(t); 3241 return t; 3242 } 3243 3244 public MedicationKnowledgeStorageGuidelineComponent addEnvironmentalSetting(MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent t) { //3 3245 if (t == null) 3246 return this; 3247 if (this.environmentalSetting == null) 3248 this.environmentalSetting = new ArrayList<MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent>(); 3249 this.environmentalSetting.add(t); 3250 return this; 3251 } 3252 3253 /** 3254 * @return The first repetition of repeating field {@link #environmentalSetting}, creating it if it does not already exist {3} 3255 */ 3256 public MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent getEnvironmentalSettingFirstRep() { 3257 if (getEnvironmentalSetting().isEmpty()) { 3258 addEnvironmentalSetting(); 3259 } 3260 return getEnvironmentalSetting().get(0); 3261 } 3262 3263 protected void listChildren(List<Property> children) { 3264 super.listChildren(children); 3265 children.add(new Property("reference", "uri", "Reference to additional information about the storage guidelines.", 0, 1, reference)); 3266 children.add(new Property("note", "Annotation", "Additional notes about the storage.", 0, java.lang.Integer.MAX_VALUE, note)); 3267 children.add(new Property("stabilityDuration", "Duration", "Duration that the medication remains stable if the environmentalSetting is respected.", 0, 1, stabilityDuration)); 3268 children.add(new Property("environmentalSetting", "", "Describes a setting/value on the environment for the adequate storage of the medication and other substances. Environment settings may involve temperature, humidity, or exposure to light.", 0, java.lang.Integer.MAX_VALUE, environmentalSetting)); 3269 } 3270 3271 @Override 3272 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3273 switch (_hash) { 3274 case -925155509: /*reference*/ return new Property("reference", "uri", "Reference to additional information about the storage guidelines.", 0, 1, reference); 3275 case 3387378: /*note*/ return new Property("note", "Annotation", "Additional notes about the storage.", 0, java.lang.Integer.MAX_VALUE, note); 3276 case 1823268957: /*stabilityDuration*/ return new Property("stabilityDuration", "Duration", "Duration that the medication remains stable if the environmentalSetting is respected.", 0, 1, stabilityDuration); 3277 case 105846514: /*environmentalSetting*/ return new Property("environmentalSetting", "", "Describes a setting/value on the environment for the adequate storage of the medication and other substances. Environment settings may involve temperature, humidity, or exposure to light.", 0, java.lang.Integer.MAX_VALUE, environmentalSetting); 3278 default: return super.getNamedProperty(_hash, _name, _checkValid); 3279 } 3280 3281 } 3282 3283 @Override 3284 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3285 switch (hash) { 3286 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // UriType 3287 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 3288 case 1823268957: /*stabilityDuration*/ return this.stabilityDuration == null ? new Base[0] : new Base[] {this.stabilityDuration}; // Duration 3289 case 105846514: /*environmentalSetting*/ return this.environmentalSetting == null ? new Base[0] : this.environmentalSetting.toArray(new Base[this.environmentalSetting.size()]); // MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent 3290 default: return super.getProperty(hash, name, checkValid); 3291 } 3292 3293 } 3294 3295 @Override 3296 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3297 switch (hash) { 3298 case -925155509: // reference 3299 this.reference = TypeConvertor.castToUri(value); // UriType 3300 return value; 3301 case 3387378: // note 3302 this.getNote().add(TypeConvertor.castToAnnotation(value)); // Annotation 3303 return value; 3304 case 1823268957: // stabilityDuration 3305 this.stabilityDuration = TypeConvertor.castToDuration(value); // Duration 3306 return value; 3307 case 105846514: // environmentalSetting 3308 this.getEnvironmentalSetting().add((MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent) value); // MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent 3309 return value; 3310 default: return super.setProperty(hash, name, value); 3311 } 3312 3313 } 3314 3315 @Override 3316 public Base setProperty(String name, Base value) throws FHIRException { 3317 if (name.equals("reference")) { 3318 this.reference = TypeConvertor.castToUri(value); // UriType 3319 } else if (name.equals("note")) { 3320 this.getNote().add(TypeConvertor.castToAnnotation(value)); 3321 } else if (name.equals("stabilityDuration")) { 3322 this.stabilityDuration = TypeConvertor.castToDuration(value); // Duration 3323 } else if (name.equals("environmentalSetting")) { 3324 this.getEnvironmentalSetting().add((MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent) value); 3325 } else 3326 return super.setProperty(name, value); 3327 return value; 3328 } 3329 3330 @Override 3331 public void removeChild(String name, Base value) throws FHIRException { 3332 if (name.equals("reference")) { 3333 this.reference = null; 3334 } else if (name.equals("note")) { 3335 this.getNote().remove(value); 3336 } else if (name.equals("stabilityDuration")) { 3337 this.stabilityDuration = null; 3338 } else if (name.equals("environmentalSetting")) { 3339 this.getEnvironmentalSetting().remove((MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent) value); 3340 } else 3341 super.removeChild(name, value); 3342 3343 } 3344 3345 @Override 3346 public Base makeProperty(int hash, String name) throws FHIRException { 3347 switch (hash) { 3348 case -925155509: return getReferenceElement(); 3349 case 3387378: return addNote(); 3350 case 1823268957: return getStabilityDuration(); 3351 case 105846514: return addEnvironmentalSetting(); 3352 default: return super.makeProperty(hash, name); 3353 } 3354 3355 } 3356 3357 @Override 3358 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3359 switch (hash) { 3360 case -925155509: /*reference*/ return new String[] {"uri"}; 3361 case 3387378: /*note*/ return new String[] {"Annotation"}; 3362 case 1823268957: /*stabilityDuration*/ return new String[] {"Duration"}; 3363 case 105846514: /*environmentalSetting*/ return new String[] {}; 3364 default: return super.getTypesForProperty(hash, name); 3365 } 3366 3367 } 3368 3369 @Override 3370 public Base addChild(String name) throws FHIRException { 3371 if (name.equals("reference")) { 3372 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.storageGuideline.reference"); 3373 } 3374 else if (name.equals("note")) { 3375 return addNote(); 3376 } 3377 else if (name.equals("stabilityDuration")) { 3378 this.stabilityDuration = new Duration(); 3379 return this.stabilityDuration; 3380 } 3381 else if (name.equals("environmentalSetting")) { 3382 return addEnvironmentalSetting(); 3383 } 3384 else 3385 return super.addChild(name); 3386 } 3387 3388 public MedicationKnowledgeStorageGuidelineComponent copy() { 3389 MedicationKnowledgeStorageGuidelineComponent dst = new MedicationKnowledgeStorageGuidelineComponent(); 3390 copyValues(dst); 3391 return dst; 3392 } 3393 3394 public void copyValues(MedicationKnowledgeStorageGuidelineComponent dst) { 3395 super.copyValues(dst); 3396 dst.reference = reference == null ? null : reference.copy(); 3397 if (note != null) { 3398 dst.note = new ArrayList<Annotation>(); 3399 for (Annotation i : note) 3400 dst.note.add(i.copy()); 3401 }; 3402 dst.stabilityDuration = stabilityDuration == null ? null : stabilityDuration.copy(); 3403 if (environmentalSetting != null) { 3404 dst.environmentalSetting = new ArrayList<MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent>(); 3405 for (MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent i : environmentalSetting) 3406 dst.environmentalSetting.add(i.copy()); 3407 }; 3408 } 3409 3410 @Override 3411 public boolean equalsDeep(Base other_) { 3412 if (!super.equalsDeep(other_)) 3413 return false; 3414 if (!(other_ instanceof MedicationKnowledgeStorageGuidelineComponent)) 3415 return false; 3416 MedicationKnowledgeStorageGuidelineComponent o = (MedicationKnowledgeStorageGuidelineComponent) other_; 3417 return compareDeep(reference, o.reference, true) && compareDeep(note, o.note, true) && compareDeep(stabilityDuration, o.stabilityDuration, true) 3418 && compareDeep(environmentalSetting, o.environmentalSetting, true); 3419 } 3420 3421 @Override 3422 public boolean equalsShallow(Base other_) { 3423 if (!super.equalsShallow(other_)) 3424 return false; 3425 if (!(other_ instanceof MedicationKnowledgeStorageGuidelineComponent)) 3426 return false; 3427 MedicationKnowledgeStorageGuidelineComponent o = (MedicationKnowledgeStorageGuidelineComponent) other_; 3428 return compareValues(reference, o.reference, true); 3429 } 3430 3431 public boolean isEmpty() { 3432 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reference, note, stabilityDuration 3433 , environmentalSetting); 3434 } 3435 3436 public String fhirType() { 3437 return "MedicationKnowledge.storageGuideline"; 3438 3439 } 3440 3441 } 3442 3443 @Block() 3444 public static class MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent extends BackboneElement implements IBaseBackboneElement { 3445 /** 3446 * Identifies the category or type of setting (e.g., type of location, temperature, humidity). 3447 */ 3448 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 3449 @Description(shortDefinition="Categorization of the setting", formalDefinition="Identifies the category or type of setting (e.g., type of location, temperature, humidity)." ) 3450 protected CodeableConcept type; 3451 3452 /** 3453 * Value associated to the setting. E.g., 40° ? 50°F for temperature. 3454 */ 3455 @Child(name = "value", type = {Quantity.class, Range.class, CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 3456 @Description(shortDefinition="Value of the setting", formalDefinition="Value associated to the setting. E.g., 40° ? 50°F for temperature." ) 3457 protected DataType value; 3458 3459 private static final long serialVersionUID = -1659186716L; 3460 3461 /** 3462 * Constructor 3463 */ 3464 public MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent() { 3465 super(); 3466 } 3467 3468 /** 3469 * Constructor 3470 */ 3471 public MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent(CodeableConcept type, DataType value) { 3472 super(); 3473 this.setType(type); 3474 this.setValue(value); 3475 } 3476 3477 /** 3478 * @return {@link #type} (Identifies the category or type of setting (e.g., type of location, temperature, humidity).) 3479 */ 3480 public CodeableConcept getType() { 3481 if (this.type == null) 3482 if (Configuration.errorOnAutoCreate()) 3483 throw new Error("Attempt to auto-create MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent.type"); 3484 else if (Configuration.doAutoCreate()) 3485 this.type = new CodeableConcept(); // cc 3486 return this.type; 3487 } 3488 3489 public boolean hasType() { 3490 return this.type != null && !this.type.isEmpty(); 3491 } 3492 3493 /** 3494 * @param value {@link #type} (Identifies the category or type of setting (e.g., type of location, temperature, humidity).) 3495 */ 3496 public MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent setType(CodeableConcept value) { 3497 this.type = value; 3498 return this; 3499 } 3500 3501 /** 3502 * @return {@link #value} (Value associated to the setting. E.g., 40° ? 50°F for temperature.) 3503 */ 3504 public DataType getValue() { 3505 return this.value; 3506 } 3507 3508 /** 3509 * @return {@link #value} (Value associated to the setting. E.g., 40° ? 50°F for temperature.) 3510 */ 3511 public Quantity getValueQuantity() throws FHIRException { 3512 if (this.value == null) 3513 this.value = new Quantity(); 3514 if (!(this.value instanceof Quantity)) 3515 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 3516 return (Quantity) this.value; 3517 } 3518 3519 public boolean hasValueQuantity() { 3520 return this.value instanceof Quantity; 3521 } 3522 3523 /** 3524 * @return {@link #value} (Value associated to the setting. E.g., 40° ? 50°F for temperature.) 3525 */ 3526 public Range getValueRange() throws FHIRException { 3527 if (this.value == null) 3528 this.value = new Range(); 3529 if (!(this.value instanceof Range)) 3530 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 3531 return (Range) this.value; 3532 } 3533 3534 public boolean hasValueRange() { 3535 return this.value instanceof Range; 3536 } 3537 3538 /** 3539 * @return {@link #value} (Value associated to the setting. E.g., 40° ? 50°F for temperature.) 3540 */ 3541 public CodeableConcept getValueCodeableConcept() throws FHIRException { 3542 if (this.value == null) 3543 this.value = new CodeableConcept(); 3544 if (!(this.value instanceof CodeableConcept)) 3545 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 3546 return (CodeableConcept) this.value; 3547 } 3548 3549 public boolean hasValueCodeableConcept() { 3550 return this.value instanceof CodeableConcept; 3551 } 3552 3553 public boolean hasValue() { 3554 return this.value != null && !this.value.isEmpty(); 3555 } 3556 3557 /** 3558 * @param value {@link #value} (Value associated to the setting. E.g., 40° ? 50°F for temperature.) 3559 */ 3560 public MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent setValue(DataType value) { 3561 if (value != null && !(value instanceof Quantity || value instanceof Range || value instanceof CodeableConcept)) 3562 throw new FHIRException("Not the right type for MedicationKnowledge.storageGuideline.environmentalSetting.value[x]: "+value.fhirType()); 3563 this.value = value; 3564 return this; 3565 } 3566 3567 protected void listChildren(List<Property> children) { 3568 super.listChildren(children); 3569 children.add(new Property("type", "CodeableConcept", "Identifies the category or type of setting (e.g., type of location, temperature, humidity).", 0, 1, type)); 3570 children.add(new Property("value[x]", "Quantity|Range|CodeableConcept", "Value associated to the setting. E.g., 40° ? 50°F for temperature.", 0, 1, value)); 3571 } 3572 3573 @Override 3574 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3575 switch (_hash) { 3576 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Identifies the category or type of setting (e.g., type of location, temperature, humidity).", 0, 1, type); 3577 case -1410166417: /*value[x]*/ return new Property("value[x]", "Quantity|Range|CodeableConcept", "Value associated to the setting. E.g., 40° ? 50°F for temperature.", 0, 1, value); 3578 case 111972721: /*value*/ return new Property("value[x]", "Quantity|Range|CodeableConcept", "Value associated to the setting. E.g., 40° ? 50°F for temperature.", 0, 1, value); 3579 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Value associated to the setting. E.g., 40° ? 50°F for temperature.", 0, 1, value); 3580 case 2030761548: /*valueRange*/ return new Property("value[x]", "Range", "Value associated to the setting. E.g., 40° ? 50°F for temperature.", 0, 1, value); 3581 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "Value associated to the setting. E.g., 40° ? 50°F for temperature.", 0, 1, value); 3582 default: return super.getNamedProperty(_hash, _name, _checkValid); 3583 } 3584 3585 } 3586 3587 @Override 3588 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3589 switch (hash) { 3590 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 3591 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 3592 default: return super.getProperty(hash, name, checkValid); 3593 } 3594 3595 } 3596 3597 @Override 3598 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3599 switch (hash) { 3600 case 3575610: // type 3601 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3602 return value; 3603 case 111972721: // value 3604 this.value = TypeConvertor.castToType(value); // DataType 3605 return value; 3606 default: return super.setProperty(hash, name, value); 3607 } 3608 3609 } 3610 3611 @Override 3612 public Base setProperty(String name, Base value) throws FHIRException { 3613 if (name.equals("type")) { 3614 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 3615 } else if (name.equals("value[x]")) { 3616 this.value = TypeConvertor.castToType(value); // DataType 3617 } else 3618 return super.setProperty(name, value); 3619 return value; 3620 } 3621 3622 @Override 3623 public void removeChild(String name, Base value) throws FHIRException { 3624 if (name.equals("type")) { 3625 this.type = null; 3626 } else if (name.equals("value[x]")) { 3627 this.value = null; 3628 } else 3629 super.removeChild(name, value); 3630 3631 } 3632 3633 @Override 3634 public Base makeProperty(int hash, String name) throws FHIRException { 3635 switch (hash) { 3636 case 3575610: return getType(); 3637 case -1410166417: return getValue(); 3638 case 111972721: return getValue(); 3639 default: return super.makeProperty(hash, name); 3640 } 3641 3642 } 3643 3644 @Override 3645 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3646 switch (hash) { 3647 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3648 case 111972721: /*value*/ return new String[] {"Quantity", "Range", "CodeableConcept"}; 3649 default: return super.getTypesForProperty(hash, name); 3650 } 3651 3652 } 3653 3654 @Override 3655 public Base addChild(String name) throws FHIRException { 3656 if (name.equals("type")) { 3657 this.type = new CodeableConcept(); 3658 return this.type; 3659 } 3660 else if (name.equals("valueQuantity")) { 3661 this.value = new Quantity(); 3662 return this.value; 3663 } 3664 else if (name.equals("valueRange")) { 3665 this.value = new Range(); 3666 return this.value; 3667 } 3668 else if (name.equals("valueCodeableConcept")) { 3669 this.value = new CodeableConcept(); 3670 return this.value; 3671 } 3672 else 3673 return super.addChild(name); 3674 } 3675 3676 public MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent copy() { 3677 MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent dst = new MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent(); 3678 copyValues(dst); 3679 return dst; 3680 } 3681 3682 public void copyValues(MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent dst) { 3683 super.copyValues(dst); 3684 dst.type = type == null ? null : type.copy(); 3685 dst.value = value == null ? null : value.copy(); 3686 } 3687 3688 @Override 3689 public boolean equalsDeep(Base other_) { 3690 if (!super.equalsDeep(other_)) 3691 return false; 3692 if (!(other_ instanceof MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent)) 3693 return false; 3694 MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent o = (MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent) other_; 3695 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 3696 } 3697 3698 @Override 3699 public boolean equalsShallow(Base other_) { 3700 if (!super.equalsShallow(other_)) 3701 return false; 3702 if (!(other_ instanceof MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent)) 3703 return false; 3704 MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent o = (MedicationKnowledgeStorageGuidelineEnvironmentalSettingComponent) other_; 3705 return true; 3706 } 3707 3708 public boolean isEmpty() { 3709 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 3710 } 3711 3712 public String fhirType() { 3713 return "MedicationKnowledge.storageGuideline.environmentalSetting"; 3714 3715 } 3716 3717 } 3718 3719 @Block() 3720 public static class MedicationKnowledgeRegulatoryComponent extends BackboneElement implements IBaseBackboneElement { 3721 /** 3722 * The authority that is specifying the regulations. 3723 */ 3724 @Child(name = "regulatoryAuthority", type = {Organization.class}, order=1, min=1, max=1, modifier=false, summary=false) 3725 @Description(shortDefinition="Specifies the authority of the regulation", formalDefinition="The authority that is specifying the regulations." ) 3726 protected Reference regulatoryAuthority; 3727 3728 /** 3729 * Specifies if changes are allowed when dispensing a medication from a regulatory perspective. 3730 */ 3731 @Child(name = "substitution", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3732 @Description(shortDefinition="Specifies if changes are allowed when dispensing a medication from a regulatory perspective", formalDefinition="Specifies if changes are allowed when dispensing a medication from a regulatory perspective." ) 3733 protected List<MedicationKnowledgeRegulatorySubstitutionComponent> substitution; 3734 3735 /** 3736 * Specifies the schedule of a medication in jurisdiction. 3737 */ 3738 @Child(name = "schedule", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3739 @Description(shortDefinition="Specifies the schedule of a medication in jurisdiction", formalDefinition="Specifies the schedule of a medication in jurisdiction." ) 3740 protected List<CodeableConcept> schedule; 3741 3742 /** 3743 * The maximum number of units of the medication that can be dispensed in a period. 3744 */ 3745 @Child(name = "maxDispense", type = {}, order=4, min=0, max=1, modifier=false, summary=false) 3746 @Description(shortDefinition="The maximum number of units of the medication that can be dispensed in a period", formalDefinition="The maximum number of units of the medication that can be dispensed in a period." ) 3747 protected MedicationKnowledgeRegulatoryMaxDispenseComponent maxDispense; 3748 3749 private static final long serialVersionUID = -2005823416L; 3750 3751 /** 3752 * Constructor 3753 */ 3754 public MedicationKnowledgeRegulatoryComponent() { 3755 super(); 3756 } 3757 3758 /** 3759 * Constructor 3760 */ 3761 public MedicationKnowledgeRegulatoryComponent(Reference regulatoryAuthority) { 3762 super(); 3763 this.setRegulatoryAuthority(regulatoryAuthority); 3764 } 3765 3766 /** 3767 * @return {@link #regulatoryAuthority} (The authority that is specifying the regulations.) 3768 */ 3769 public Reference getRegulatoryAuthority() { 3770 if (this.regulatoryAuthority == null) 3771 if (Configuration.errorOnAutoCreate()) 3772 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatoryComponent.regulatoryAuthority"); 3773 else if (Configuration.doAutoCreate()) 3774 this.regulatoryAuthority = new Reference(); // cc 3775 return this.regulatoryAuthority; 3776 } 3777 3778 public boolean hasRegulatoryAuthority() { 3779 return this.regulatoryAuthority != null && !this.regulatoryAuthority.isEmpty(); 3780 } 3781 3782 /** 3783 * @param value {@link #regulatoryAuthority} (The authority that is specifying the regulations.) 3784 */ 3785 public MedicationKnowledgeRegulatoryComponent setRegulatoryAuthority(Reference value) { 3786 this.regulatoryAuthority = value; 3787 return this; 3788 } 3789 3790 /** 3791 * @return {@link #substitution} (Specifies if changes are allowed when dispensing a medication from a regulatory perspective.) 3792 */ 3793 public List<MedicationKnowledgeRegulatorySubstitutionComponent> getSubstitution() { 3794 if (this.substitution == null) 3795 this.substitution = new ArrayList<MedicationKnowledgeRegulatorySubstitutionComponent>(); 3796 return this.substitution; 3797 } 3798 3799 /** 3800 * @return Returns a reference to <code>this</code> for easy method chaining 3801 */ 3802 public MedicationKnowledgeRegulatoryComponent setSubstitution(List<MedicationKnowledgeRegulatorySubstitutionComponent> theSubstitution) { 3803 this.substitution = theSubstitution; 3804 return this; 3805 } 3806 3807 public boolean hasSubstitution() { 3808 if (this.substitution == null) 3809 return false; 3810 for (MedicationKnowledgeRegulatorySubstitutionComponent item : this.substitution) 3811 if (!item.isEmpty()) 3812 return true; 3813 return false; 3814 } 3815 3816 public MedicationKnowledgeRegulatorySubstitutionComponent addSubstitution() { //3 3817 MedicationKnowledgeRegulatorySubstitutionComponent t = new MedicationKnowledgeRegulatorySubstitutionComponent(); 3818 if (this.substitution == null) 3819 this.substitution = new ArrayList<MedicationKnowledgeRegulatorySubstitutionComponent>(); 3820 this.substitution.add(t); 3821 return t; 3822 } 3823 3824 public MedicationKnowledgeRegulatoryComponent addSubstitution(MedicationKnowledgeRegulatorySubstitutionComponent t) { //3 3825 if (t == null) 3826 return this; 3827 if (this.substitution == null) 3828 this.substitution = new ArrayList<MedicationKnowledgeRegulatorySubstitutionComponent>(); 3829 this.substitution.add(t); 3830 return this; 3831 } 3832 3833 /** 3834 * @return The first repetition of repeating field {@link #substitution}, creating it if it does not already exist {3} 3835 */ 3836 public MedicationKnowledgeRegulatorySubstitutionComponent getSubstitutionFirstRep() { 3837 if (getSubstitution().isEmpty()) { 3838 addSubstitution(); 3839 } 3840 return getSubstitution().get(0); 3841 } 3842 3843 /** 3844 * @return {@link #schedule} (Specifies the schedule of a medication in jurisdiction.) 3845 */ 3846 public List<CodeableConcept> getSchedule() { 3847 if (this.schedule == null) 3848 this.schedule = new ArrayList<CodeableConcept>(); 3849 return this.schedule; 3850 } 3851 3852 /** 3853 * @return Returns a reference to <code>this</code> for easy method chaining 3854 */ 3855 public MedicationKnowledgeRegulatoryComponent setSchedule(List<CodeableConcept> theSchedule) { 3856 this.schedule = theSchedule; 3857 return this; 3858 } 3859 3860 public boolean hasSchedule() { 3861 if (this.schedule == null) 3862 return false; 3863 for (CodeableConcept item : this.schedule) 3864 if (!item.isEmpty()) 3865 return true; 3866 return false; 3867 } 3868 3869 public CodeableConcept addSchedule() { //3 3870 CodeableConcept t = new CodeableConcept(); 3871 if (this.schedule == null) 3872 this.schedule = new ArrayList<CodeableConcept>(); 3873 this.schedule.add(t); 3874 return t; 3875 } 3876 3877 public MedicationKnowledgeRegulatoryComponent addSchedule(CodeableConcept t) { //3 3878 if (t == null) 3879 return this; 3880 if (this.schedule == null) 3881 this.schedule = new ArrayList<CodeableConcept>(); 3882 this.schedule.add(t); 3883 return this; 3884 } 3885 3886 /** 3887 * @return The first repetition of repeating field {@link #schedule}, creating it if it does not already exist {3} 3888 */ 3889 public CodeableConcept getScheduleFirstRep() { 3890 if (getSchedule().isEmpty()) { 3891 addSchedule(); 3892 } 3893 return getSchedule().get(0); 3894 } 3895 3896 /** 3897 * @return {@link #maxDispense} (The maximum number of units of the medication that can be dispensed in a period.) 3898 */ 3899 public MedicationKnowledgeRegulatoryMaxDispenseComponent getMaxDispense() { 3900 if (this.maxDispense == null) 3901 if (Configuration.errorOnAutoCreate()) 3902 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatoryComponent.maxDispense"); 3903 else if (Configuration.doAutoCreate()) 3904 this.maxDispense = new MedicationKnowledgeRegulatoryMaxDispenseComponent(); // cc 3905 return this.maxDispense; 3906 } 3907 3908 public boolean hasMaxDispense() { 3909 return this.maxDispense != null && !this.maxDispense.isEmpty(); 3910 } 3911 3912 /** 3913 * @param value {@link #maxDispense} (The maximum number of units of the medication that can be dispensed in a period.) 3914 */ 3915 public MedicationKnowledgeRegulatoryComponent setMaxDispense(MedicationKnowledgeRegulatoryMaxDispenseComponent value) { 3916 this.maxDispense = value; 3917 return this; 3918 } 3919 3920 protected void listChildren(List<Property> children) { 3921 super.listChildren(children); 3922 children.add(new Property("regulatoryAuthority", "Reference(Organization)", "The authority that is specifying the regulations.", 0, 1, regulatoryAuthority)); 3923 children.add(new Property("substitution", "", "Specifies if changes are allowed when dispensing a medication from a regulatory perspective.", 0, java.lang.Integer.MAX_VALUE, substitution)); 3924 children.add(new Property("schedule", "CodeableConcept", "Specifies the schedule of a medication in jurisdiction.", 0, java.lang.Integer.MAX_VALUE, schedule)); 3925 children.add(new Property("maxDispense", "", "The maximum number of units of the medication that can be dispensed in a period.", 0, 1, maxDispense)); 3926 } 3927 3928 @Override 3929 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3930 switch (_hash) { 3931 case 711233419: /*regulatoryAuthority*/ return new Property("regulatoryAuthority", "Reference(Organization)", "The authority that is specifying the regulations.", 0, 1, regulatoryAuthority); 3932 case 826147581: /*substitution*/ return new Property("substitution", "", "Specifies if changes are allowed when dispensing a medication from a regulatory perspective.", 0, java.lang.Integer.MAX_VALUE, substitution); 3933 case -697920873: /*schedule*/ return new Property("schedule", "CodeableConcept", "Specifies the schedule of a medication in jurisdiction.", 0, java.lang.Integer.MAX_VALUE, schedule); 3934 case -1977784607: /*maxDispense*/ return new Property("maxDispense", "", "The maximum number of units of the medication that can be dispensed in a period.", 0, 1, maxDispense); 3935 default: return super.getNamedProperty(_hash, _name, _checkValid); 3936 } 3937 3938 } 3939 3940 @Override 3941 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3942 switch (hash) { 3943 case 711233419: /*regulatoryAuthority*/ return this.regulatoryAuthority == null ? new Base[0] : new Base[] {this.regulatoryAuthority}; // Reference 3944 case 826147581: /*substitution*/ return this.substitution == null ? new Base[0] : this.substitution.toArray(new Base[this.substitution.size()]); // MedicationKnowledgeRegulatorySubstitutionComponent 3945 case -697920873: /*schedule*/ return this.schedule == null ? new Base[0] : this.schedule.toArray(new Base[this.schedule.size()]); // CodeableConcept 3946 case -1977784607: /*maxDispense*/ return this.maxDispense == null ? new Base[0] : new Base[] {this.maxDispense}; // MedicationKnowledgeRegulatoryMaxDispenseComponent 3947 default: return super.getProperty(hash, name, checkValid); 3948 } 3949 3950 } 3951 3952 @Override 3953 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3954 switch (hash) { 3955 case 711233419: // regulatoryAuthority 3956 this.regulatoryAuthority = TypeConvertor.castToReference(value); // Reference 3957 return value; 3958 case 826147581: // substitution 3959 this.getSubstitution().add((MedicationKnowledgeRegulatorySubstitutionComponent) value); // MedicationKnowledgeRegulatorySubstitutionComponent 3960 return value; 3961 case -697920873: // schedule 3962 this.getSchedule().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 3963 return value; 3964 case -1977784607: // maxDispense 3965 this.maxDispense = (MedicationKnowledgeRegulatoryMaxDispenseComponent) value; // MedicationKnowledgeRegulatoryMaxDispenseComponent 3966 return value; 3967 default: return super.setProperty(hash, name, value); 3968 } 3969 3970 } 3971 3972 @Override 3973 public Base setProperty(String name, Base value) throws FHIRException { 3974 if (name.equals("regulatoryAuthority")) { 3975 this.regulatoryAuthority = TypeConvertor.castToReference(value); // Reference 3976 } else if (name.equals("substitution")) { 3977 this.getSubstitution().add((MedicationKnowledgeRegulatorySubstitutionComponent) value); 3978 } else if (name.equals("schedule")) { 3979 this.getSchedule().add(TypeConvertor.castToCodeableConcept(value)); 3980 } else if (name.equals("maxDispense")) { 3981 this.maxDispense = (MedicationKnowledgeRegulatoryMaxDispenseComponent) value; // MedicationKnowledgeRegulatoryMaxDispenseComponent 3982 } else 3983 return super.setProperty(name, value); 3984 return value; 3985 } 3986 3987 @Override 3988 public void removeChild(String name, Base value) throws FHIRException { 3989 if (name.equals("regulatoryAuthority")) { 3990 this.regulatoryAuthority = null; 3991 } else if (name.equals("substitution")) { 3992 this.getSubstitution().remove((MedicationKnowledgeRegulatorySubstitutionComponent) value); 3993 } else if (name.equals("schedule")) { 3994 this.getSchedule().remove(value); 3995 } else if (name.equals("maxDispense")) { 3996 this.maxDispense = (MedicationKnowledgeRegulatoryMaxDispenseComponent) value; // MedicationKnowledgeRegulatoryMaxDispenseComponent 3997 } else 3998 super.removeChild(name, value); 3999 4000 } 4001 4002 @Override 4003 public Base makeProperty(int hash, String name) throws FHIRException { 4004 switch (hash) { 4005 case 711233419: return getRegulatoryAuthority(); 4006 case 826147581: return addSubstitution(); 4007 case -697920873: return addSchedule(); 4008 case -1977784607: return getMaxDispense(); 4009 default: return super.makeProperty(hash, name); 4010 } 4011 4012 } 4013 4014 @Override 4015 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4016 switch (hash) { 4017 case 711233419: /*regulatoryAuthority*/ return new String[] {"Reference"}; 4018 case 826147581: /*substitution*/ return new String[] {}; 4019 case -697920873: /*schedule*/ return new String[] {"CodeableConcept"}; 4020 case -1977784607: /*maxDispense*/ return new String[] {}; 4021 default: return super.getTypesForProperty(hash, name); 4022 } 4023 4024 } 4025 4026 @Override 4027 public Base addChild(String name) throws FHIRException { 4028 if (name.equals("regulatoryAuthority")) { 4029 this.regulatoryAuthority = new Reference(); 4030 return this.regulatoryAuthority; 4031 } 4032 else if (name.equals("substitution")) { 4033 return addSubstitution(); 4034 } 4035 else if (name.equals("schedule")) { 4036 return addSchedule(); 4037 } 4038 else if (name.equals("maxDispense")) { 4039 this.maxDispense = new MedicationKnowledgeRegulatoryMaxDispenseComponent(); 4040 return this.maxDispense; 4041 } 4042 else 4043 return super.addChild(name); 4044 } 4045 4046 public MedicationKnowledgeRegulatoryComponent copy() { 4047 MedicationKnowledgeRegulatoryComponent dst = new MedicationKnowledgeRegulatoryComponent(); 4048 copyValues(dst); 4049 return dst; 4050 } 4051 4052 public void copyValues(MedicationKnowledgeRegulatoryComponent dst) { 4053 super.copyValues(dst); 4054 dst.regulatoryAuthority = regulatoryAuthority == null ? null : regulatoryAuthority.copy(); 4055 if (substitution != null) { 4056 dst.substitution = new ArrayList<MedicationKnowledgeRegulatorySubstitutionComponent>(); 4057 for (MedicationKnowledgeRegulatorySubstitutionComponent i : substitution) 4058 dst.substitution.add(i.copy()); 4059 }; 4060 if (schedule != null) { 4061 dst.schedule = new ArrayList<CodeableConcept>(); 4062 for (CodeableConcept i : schedule) 4063 dst.schedule.add(i.copy()); 4064 }; 4065 dst.maxDispense = maxDispense == null ? null : maxDispense.copy(); 4066 } 4067 4068 @Override 4069 public boolean equalsDeep(Base other_) { 4070 if (!super.equalsDeep(other_)) 4071 return false; 4072 if (!(other_ instanceof MedicationKnowledgeRegulatoryComponent)) 4073 return false; 4074 MedicationKnowledgeRegulatoryComponent o = (MedicationKnowledgeRegulatoryComponent) other_; 4075 return compareDeep(regulatoryAuthority, o.regulatoryAuthority, true) && compareDeep(substitution, o.substitution, true) 4076 && compareDeep(schedule, o.schedule, true) && compareDeep(maxDispense, o.maxDispense, true); 4077 } 4078 4079 @Override 4080 public boolean equalsShallow(Base other_) { 4081 if (!super.equalsShallow(other_)) 4082 return false; 4083 if (!(other_ instanceof MedicationKnowledgeRegulatoryComponent)) 4084 return false; 4085 MedicationKnowledgeRegulatoryComponent o = (MedicationKnowledgeRegulatoryComponent) other_; 4086 return true; 4087 } 4088 4089 public boolean isEmpty() { 4090 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(regulatoryAuthority, substitution 4091 , schedule, maxDispense); 4092 } 4093 4094 public String fhirType() { 4095 return "MedicationKnowledge.regulatory"; 4096 4097 } 4098 4099 } 4100 4101 @Block() 4102 public static class MedicationKnowledgeRegulatorySubstitutionComponent extends BackboneElement implements IBaseBackboneElement { 4103 /** 4104 * Specifies the type of substitution allowed. 4105 */ 4106 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 4107 @Description(shortDefinition="Specifies the type of substitution allowed", formalDefinition="Specifies the type of substitution allowed." ) 4108 protected CodeableConcept type; 4109 4110 /** 4111 * Specifies if regulation allows for changes in the medication when dispensing. 4112 */ 4113 @Child(name = "allowed", type = {BooleanType.class}, order=2, min=1, max=1, modifier=false, summary=false) 4114 @Description(shortDefinition="Specifies if regulation allows for changes in the medication when dispensing", formalDefinition="Specifies if regulation allows for changes in the medication when dispensing." ) 4115 protected BooleanType allowed; 4116 4117 private static final long serialVersionUID = 396354861L; 4118 4119 /** 4120 * Constructor 4121 */ 4122 public MedicationKnowledgeRegulatorySubstitutionComponent() { 4123 super(); 4124 } 4125 4126 /** 4127 * Constructor 4128 */ 4129 public MedicationKnowledgeRegulatorySubstitutionComponent(CodeableConcept type, boolean allowed) { 4130 super(); 4131 this.setType(type); 4132 this.setAllowed(allowed); 4133 } 4134 4135 /** 4136 * @return {@link #type} (Specifies the type of substitution allowed.) 4137 */ 4138 public CodeableConcept getType() { 4139 if (this.type == null) 4140 if (Configuration.errorOnAutoCreate()) 4141 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatorySubstitutionComponent.type"); 4142 else if (Configuration.doAutoCreate()) 4143 this.type = new CodeableConcept(); // cc 4144 return this.type; 4145 } 4146 4147 public boolean hasType() { 4148 return this.type != null && !this.type.isEmpty(); 4149 } 4150 4151 /** 4152 * @param value {@link #type} (Specifies the type of substitution allowed.) 4153 */ 4154 public MedicationKnowledgeRegulatorySubstitutionComponent setType(CodeableConcept value) { 4155 this.type = value; 4156 return this; 4157 } 4158 4159 /** 4160 * @return {@link #allowed} (Specifies if regulation allows for changes in the medication when dispensing.). This is the underlying object with id, value and extensions. The accessor "getAllowed" gives direct access to the value 4161 */ 4162 public BooleanType getAllowedElement() { 4163 if (this.allowed == null) 4164 if (Configuration.errorOnAutoCreate()) 4165 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatorySubstitutionComponent.allowed"); 4166 else if (Configuration.doAutoCreate()) 4167 this.allowed = new BooleanType(); // bb 4168 return this.allowed; 4169 } 4170 4171 public boolean hasAllowedElement() { 4172 return this.allowed != null && !this.allowed.isEmpty(); 4173 } 4174 4175 public boolean hasAllowed() { 4176 return this.allowed != null && !this.allowed.isEmpty(); 4177 } 4178 4179 /** 4180 * @param value {@link #allowed} (Specifies if regulation allows for changes in the medication when dispensing.). This is the underlying object with id, value and extensions. The accessor "getAllowed" gives direct access to the value 4181 */ 4182 public MedicationKnowledgeRegulatorySubstitutionComponent setAllowedElement(BooleanType value) { 4183 this.allowed = value; 4184 return this; 4185 } 4186 4187 /** 4188 * @return Specifies if regulation allows for changes in the medication when dispensing. 4189 */ 4190 public boolean getAllowed() { 4191 return this.allowed == null || this.allowed.isEmpty() ? false : this.allowed.getValue(); 4192 } 4193 4194 /** 4195 * @param value Specifies if regulation allows for changes in the medication when dispensing. 4196 */ 4197 public MedicationKnowledgeRegulatorySubstitutionComponent setAllowed(boolean value) { 4198 if (this.allowed == null) 4199 this.allowed = new BooleanType(); 4200 this.allowed.setValue(value); 4201 return this; 4202 } 4203 4204 protected void listChildren(List<Property> children) { 4205 super.listChildren(children); 4206 children.add(new Property("type", "CodeableConcept", "Specifies the type of substitution allowed.", 0, 1, type)); 4207 children.add(new Property("allowed", "boolean", "Specifies if regulation allows for changes in the medication when dispensing.", 0, 1, allowed)); 4208 } 4209 4210 @Override 4211 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4212 switch (_hash) { 4213 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Specifies the type of substitution allowed.", 0, 1, type); 4214 case -911343192: /*allowed*/ return new Property("allowed", "boolean", "Specifies if regulation allows for changes in the medication when dispensing.", 0, 1, allowed); 4215 default: return super.getNamedProperty(_hash, _name, _checkValid); 4216 } 4217 4218 } 4219 4220 @Override 4221 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4222 switch (hash) { 4223 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 4224 case -911343192: /*allowed*/ return this.allowed == null ? new Base[0] : new Base[] {this.allowed}; // BooleanType 4225 default: return super.getProperty(hash, name, checkValid); 4226 } 4227 4228 } 4229 4230 @Override 4231 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4232 switch (hash) { 4233 case 3575610: // type 4234 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4235 return value; 4236 case -911343192: // allowed 4237 this.allowed = TypeConvertor.castToBoolean(value); // BooleanType 4238 return value; 4239 default: return super.setProperty(hash, name, value); 4240 } 4241 4242 } 4243 4244 @Override 4245 public Base setProperty(String name, Base value) throws FHIRException { 4246 if (name.equals("type")) { 4247 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4248 } else if (name.equals("allowed")) { 4249 this.allowed = TypeConvertor.castToBoolean(value); // BooleanType 4250 } else 4251 return super.setProperty(name, value); 4252 return value; 4253 } 4254 4255 @Override 4256 public void removeChild(String name, Base value) throws FHIRException { 4257 if (name.equals("type")) { 4258 this.type = null; 4259 } else if (name.equals("allowed")) { 4260 this.allowed = null; 4261 } else 4262 super.removeChild(name, value); 4263 4264 } 4265 4266 @Override 4267 public Base makeProperty(int hash, String name) throws FHIRException { 4268 switch (hash) { 4269 case 3575610: return getType(); 4270 case -911343192: return getAllowedElement(); 4271 default: return super.makeProperty(hash, name); 4272 } 4273 4274 } 4275 4276 @Override 4277 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4278 switch (hash) { 4279 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 4280 case -911343192: /*allowed*/ return new String[] {"boolean"}; 4281 default: return super.getTypesForProperty(hash, name); 4282 } 4283 4284 } 4285 4286 @Override 4287 public Base addChild(String name) throws FHIRException { 4288 if (name.equals("type")) { 4289 this.type = new CodeableConcept(); 4290 return this.type; 4291 } 4292 else if (name.equals("allowed")) { 4293 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.regulatory.substitution.allowed"); 4294 } 4295 else 4296 return super.addChild(name); 4297 } 4298 4299 public MedicationKnowledgeRegulatorySubstitutionComponent copy() { 4300 MedicationKnowledgeRegulatorySubstitutionComponent dst = new MedicationKnowledgeRegulatorySubstitutionComponent(); 4301 copyValues(dst); 4302 return dst; 4303 } 4304 4305 public void copyValues(MedicationKnowledgeRegulatorySubstitutionComponent dst) { 4306 super.copyValues(dst); 4307 dst.type = type == null ? null : type.copy(); 4308 dst.allowed = allowed == null ? null : allowed.copy(); 4309 } 4310 4311 @Override 4312 public boolean equalsDeep(Base other_) { 4313 if (!super.equalsDeep(other_)) 4314 return false; 4315 if (!(other_ instanceof MedicationKnowledgeRegulatorySubstitutionComponent)) 4316 return false; 4317 MedicationKnowledgeRegulatorySubstitutionComponent o = (MedicationKnowledgeRegulatorySubstitutionComponent) other_; 4318 return compareDeep(type, o.type, true) && compareDeep(allowed, o.allowed, true); 4319 } 4320 4321 @Override 4322 public boolean equalsShallow(Base other_) { 4323 if (!super.equalsShallow(other_)) 4324 return false; 4325 if (!(other_ instanceof MedicationKnowledgeRegulatorySubstitutionComponent)) 4326 return false; 4327 MedicationKnowledgeRegulatorySubstitutionComponent o = (MedicationKnowledgeRegulatorySubstitutionComponent) other_; 4328 return compareValues(allowed, o.allowed, true); 4329 } 4330 4331 public boolean isEmpty() { 4332 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, allowed); 4333 } 4334 4335 public String fhirType() { 4336 return "MedicationKnowledge.regulatory.substitution"; 4337 4338 } 4339 4340 } 4341 4342 @Block() 4343 public static class MedicationKnowledgeRegulatoryMaxDispenseComponent extends BackboneElement implements IBaseBackboneElement { 4344 /** 4345 * The maximum number of units of the medication that can be dispensed. 4346 */ 4347 @Child(name = "quantity", type = {Quantity.class}, order=1, min=1, max=1, modifier=false, summary=false) 4348 @Description(shortDefinition="The maximum number of units of the medication that can be dispensed", formalDefinition="The maximum number of units of the medication that can be dispensed." ) 4349 protected Quantity quantity; 4350 4351 /** 4352 * The period that applies to the maximum number of units. 4353 */ 4354 @Child(name = "period", type = {Duration.class}, order=2, min=0, max=1, modifier=false, summary=false) 4355 @Description(shortDefinition="The period that applies to the maximum number of units", formalDefinition="The period that applies to the maximum number of units." ) 4356 protected Duration period; 4357 4358 private static final long serialVersionUID = -441724185L; 4359 4360 /** 4361 * Constructor 4362 */ 4363 public MedicationKnowledgeRegulatoryMaxDispenseComponent() { 4364 super(); 4365 } 4366 4367 /** 4368 * Constructor 4369 */ 4370 public MedicationKnowledgeRegulatoryMaxDispenseComponent(Quantity quantity) { 4371 super(); 4372 this.setQuantity(quantity); 4373 } 4374 4375 /** 4376 * @return {@link #quantity} (The maximum number of units of the medication that can be dispensed.) 4377 */ 4378 public Quantity getQuantity() { 4379 if (this.quantity == null) 4380 if (Configuration.errorOnAutoCreate()) 4381 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatoryMaxDispenseComponent.quantity"); 4382 else if (Configuration.doAutoCreate()) 4383 this.quantity = new Quantity(); // cc 4384 return this.quantity; 4385 } 4386 4387 public boolean hasQuantity() { 4388 return this.quantity != null && !this.quantity.isEmpty(); 4389 } 4390 4391 /** 4392 * @param value {@link #quantity} (The maximum number of units of the medication that can be dispensed.) 4393 */ 4394 public MedicationKnowledgeRegulatoryMaxDispenseComponent setQuantity(Quantity value) { 4395 this.quantity = value; 4396 return this; 4397 } 4398 4399 /** 4400 * @return {@link #period} (The period that applies to the maximum number of units.) 4401 */ 4402 public Duration getPeriod() { 4403 if (this.period == null) 4404 if (Configuration.errorOnAutoCreate()) 4405 throw new Error("Attempt to auto-create MedicationKnowledgeRegulatoryMaxDispenseComponent.period"); 4406 else if (Configuration.doAutoCreate()) 4407 this.period = new Duration(); // cc 4408 return this.period; 4409 } 4410 4411 public boolean hasPeriod() { 4412 return this.period != null && !this.period.isEmpty(); 4413 } 4414 4415 /** 4416 * @param value {@link #period} (The period that applies to the maximum number of units.) 4417 */ 4418 public MedicationKnowledgeRegulatoryMaxDispenseComponent setPeriod(Duration value) { 4419 this.period = value; 4420 return this; 4421 } 4422 4423 protected void listChildren(List<Property> children) { 4424 super.listChildren(children); 4425 children.add(new Property("quantity", "Quantity", "The maximum number of units of the medication that can be dispensed.", 0, 1, quantity)); 4426 children.add(new Property("period", "Duration", "The period that applies to the maximum number of units.", 0, 1, period)); 4427 } 4428 4429 @Override 4430 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4431 switch (_hash) { 4432 case -1285004149: /*quantity*/ return new Property("quantity", "Quantity", "The maximum number of units of the medication that can be dispensed.", 0, 1, quantity); 4433 case -991726143: /*period*/ return new Property("period", "Duration", "The period that applies to the maximum number of units.", 0, 1, period); 4434 default: return super.getNamedProperty(_hash, _name, _checkValid); 4435 } 4436 4437 } 4438 4439 @Override 4440 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4441 switch (hash) { 4442 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // Quantity 4443 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Duration 4444 default: return super.getProperty(hash, name, checkValid); 4445 } 4446 4447 } 4448 4449 @Override 4450 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4451 switch (hash) { 4452 case -1285004149: // quantity 4453 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 4454 return value; 4455 case -991726143: // period 4456 this.period = TypeConvertor.castToDuration(value); // Duration 4457 return value; 4458 default: return super.setProperty(hash, name, value); 4459 } 4460 4461 } 4462 4463 @Override 4464 public Base setProperty(String name, Base value) throws FHIRException { 4465 if (name.equals("quantity")) { 4466 this.quantity = TypeConvertor.castToQuantity(value); // Quantity 4467 } else if (name.equals("period")) { 4468 this.period = TypeConvertor.castToDuration(value); // Duration 4469 } else 4470 return super.setProperty(name, value); 4471 return value; 4472 } 4473 4474 @Override 4475 public void removeChild(String name, Base value) throws FHIRException { 4476 if (name.equals("quantity")) { 4477 this.quantity = null; 4478 } else if (name.equals("period")) { 4479 this.period = null; 4480 } else 4481 super.removeChild(name, value); 4482 4483 } 4484 4485 @Override 4486 public Base makeProperty(int hash, String name) throws FHIRException { 4487 switch (hash) { 4488 case -1285004149: return getQuantity(); 4489 case -991726143: return getPeriod(); 4490 default: return super.makeProperty(hash, name); 4491 } 4492 4493 } 4494 4495 @Override 4496 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4497 switch (hash) { 4498 case -1285004149: /*quantity*/ return new String[] {"Quantity"}; 4499 case -991726143: /*period*/ return new String[] {"Duration"}; 4500 default: return super.getTypesForProperty(hash, name); 4501 } 4502 4503 } 4504 4505 @Override 4506 public Base addChild(String name) throws FHIRException { 4507 if (name.equals("quantity")) { 4508 this.quantity = new Quantity(); 4509 return this.quantity; 4510 } 4511 else if (name.equals("period")) { 4512 this.period = new Duration(); 4513 return this.period; 4514 } 4515 else 4516 return super.addChild(name); 4517 } 4518 4519 public MedicationKnowledgeRegulatoryMaxDispenseComponent copy() { 4520 MedicationKnowledgeRegulatoryMaxDispenseComponent dst = new MedicationKnowledgeRegulatoryMaxDispenseComponent(); 4521 copyValues(dst); 4522 return dst; 4523 } 4524 4525 public void copyValues(MedicationKnowledgeRegulatoryMaxDispenseComponent dst) { 4526 super.copyValues(dst); 4527 dst.quantity = quantity == null ? null : quantity.copy(); 4528 dst.period = period == null ? null : period.copy(); 4529 } 4530 4531 @Override 4532 public boolean equalsDeep(Base other_) { 4533 if (!super.equalsDeep(other_)) 4534 return false; 4535 if (!(other_ instanceof MedicationKnowledgeRegulatoryMaxDispenseComponent)) 4536 return false; 4537 MedicationKnowledgeRegulatoryMaxDispenseComponent o = (MedicationKnowledgeRegulatoryMaxDispenseComponent) other_; 4538 return compareDeep(quantity, o.quantity, true) && compareDeep(period, o.period, true); 4539 } 4540 4541 @Override 4542 public boolean equalsShallow(Base other_) { 4543 if (!super.equalsShallow(other_)) 4544 return false; 4545 if (!(other_ instanceof MedicationKnowledgeRegulatoryMaxDispenseComponent)) 4546 return false; 4547 MedicationKnowledgeRegulatoryMaxDispenseComponent o = (MedicationKnowledgeRegulatoryMaxDispenseComponent) other_; 4548 return true; 4549 } 4550 4551 public boolean isEmpty() { 4552 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(quantity, period); 4553 } 4554 4555 public String fhirType() { 4556 return "MedicationKnowledge.regulatory.maxDispense"; 4557 4558 } 4559 4560 } 4561 4562 @Block() 4563 public static class MedicationKnowledgeDefinitionalComponent extends BackboneElement implements IBaseBackboneElement { 4564 /** 4565 * Associated definitions for this medication. 4566 */ 4567 @Child(name = "definition", type = {MedicinalProductDefinition.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4568 @Description(shortDefinition="Definitional resources that provide more information about this medication", formalDefinition="Associated definitions for this medication." ) 4569 protected List<Reference> definition; 4570 4571 /** 4572 * Describes the form of the item. Powder; tablets; capsule. 4573 */ 4574 @Child(name = "doseForm", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 4575 @Description(shortDefinition="powder | tablets | capsule +", formalDefinition="Describes the form of the item. Powder; tablets; capsule." ) 4576 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-form-codes") 4577 protected CodeableConcept doseForm; 4578 4579 /** 4580 * The intended or approved route of administration. 4581 */ 4582 @Child(name = "intendedRoute", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4583 @Description(shortDefinition="The intended or approved route of administration", formalDefinition="The intended or approved route of administration." ) 4584 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/route-codes") 4585 protected List<CodeableConcept> intendedRoute; 4586 4587 /** 4588 * Identifies a particular constituent of interest in the product. 4589 */ 4590 @Child(name = "ingredient", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4591 @Description(shortDefinition="Active or inactive ingredient", formalDefinition="Identifies a particular constituent of interest in the product." ) 4592 protected List<MedicationKnowledgeDefinitionalIngredientComponent> ingredient; 4593 4594 /** 4595 * Specifies descriptive properties of the medicine, such as color, shape, imprints, etc. 4596 */ 4597 @Child(name = "drugCharacteristic", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 4598 @Description(shortDefinition="Specifies descriptive properties of the medicine", formalDefinition="Specifies descriptive properties of the medicine, such as color, shape, imprints, etc." ) 4599 protected List<MedicationKnowledgeDefinitionalDrugCharacteristicComponent> drugCharacteristic; 4600 4601 private static final long serialVersionUID = 2050532775L; 4602 4603 /** 4604 * Constructor 4605 */ 4606 public MedicationKnowledgeDefinitionalComponent() { 4607 super(); 4608 } 4609 4610 /** 4611 * @return {@link #definition} (Associated definitions for this medication.) 4612 */ 4613 public List<Reference> getDefinition() { 4614 if (this.definition == null) 4615 this.definition = new ArrayList<Reference>(); 4616 return this.definition; 4617 } 4618 4619 /** 4620 * @return Returns a reference to <code>this</code> for easy method chaining 4621 */ 4622 public MedicationKnowledgeDefinitionalComponent setDefinition(List<Reference> theDefinition) { 4623 this.definition = theDefinition; 4624 return this; 4625 } 4626 4627 public boolean hasDefinition() { 4628 if (this.definition == null) 4629 return false; 4630 for (Reference item : this.definition) 4631 if (!item.isEmpty()) 4632 return true; 4633 return false; 4634 } 4635 4636 public Reference addDefinition() { //3 4637 Reference t = new Reference(); 4638 if (this.definition == null) 4639 this.definition = new ArrayList<Reference>(); 4640 this.definition.add(t); 4641 return t; 4642 } 4643 4644 public MedicationKnowledgeDefinitionalComponent addDefinition(Reference t) { //3 4645 if (t == null) 4646 return this; 4647 if (this.definition == null) 4648 this.definition = new ArrayList<Reference>(); 4649 this.definition.add(t); 4650 return this; 4651 } 4652 4653 /** 4654 * @return The first repetition of repeating field {@link #definition}, creating it if it does not already exist {3} 4655 */ 4656 public Reference getDefinitionFirstRep() { 4657 if (getDefinition().isEmpty()) { 4658 addDefinition(); 4659 } 4660 return getDefinition().get(0); 4661 } 4662 4663 /** 4664 * @return {@link #doseForm} (Describes the form of the item. Powder; tablets; capsule.) 4665 */ 4666 public CodeableConcept getDoseForm() { 4667 if (this.doseForm == null) 4668 if (Configuration.errorOnAutoCreate()) 4669 throw new Error("Attempt to auto-create MedicationKnowledgeDefinitionalComponent.doseForm"); 4670 else if (Configuration.doAutoCreate()) 4671 this.doseForm = new CodeableConcept(); // cc 4672 return this.doseForm; 4673 } 4674 4675 public boolean hasDoseForm() { 4676 return this.doseForm != null && !this.doseForm.isEmpty(); 4677 } 4678 4679 /** 4680 * @param value {@link #doseForm} (Describes the form of the item. Powder; tablets; capsule.) 4681 */ 4682 public MedicationKnowledgeDefinitionalComponent setDoseForm(CodeableConcept value) { 4683 this.doseForm = value; 4684 return this; 4685 } 4686 4687 /** 4688 * @return {@link #intendedRoute} (The intended or approved route of administration.) 4689 */ 4690 public List<CodeableConcept> getIntendedRoute() { 4691 if (this.intendedRoute == null) 4692 this.intendedRoute = new ArrayList<CodeableConcept>(); 4693 return this.intendedRoute; 4694 } 4695 4696 /** 4697 * @return Returns a reference to <code>this</code> for easy method chaining 4698 */ 4699 public MedicationKnowledgeDefinitionalComponent setIntendedRoute(List<CodeableConcept> theIntendedRoute) { 4700 this.intendedRoute = theIntendedRoute; 4701 return this; 4702 } 4703 4704 public boolean hasIntendedRoute() { 4705 if (this.intendedRoute == null) 4706 return false; 4707 for (CodeableConcept item : this.intendedRoute) 4708 if (!item.isEmpty()) 4709 return true; 4710 return false; 4711 } 4712 4713 public CodeableConcept addIntendedRoute() { //3 4714 CodeableConcept t = new CodeableConcept(); 4715 if (this.intendedRoute == null) 4716 this.intendedRoute = new ArrayList<CodeableConcept>(); 4717 this.intendedRoute.add(t); 4718 return t; 4719 } 4720 4721 public MedicationKnowledgeDefinitionalComponent addIntendedRoute(CodeableConcept t) { //3 4722 if (t == null) 4723 return this; 4724 if (this.intendedRoute == null) 4725 this.intendedRoute = new ArrayList<CodeableConcept>(); 4726 this.intendedRoute.add(t); 4727 return this; 4728 } 4729 4730 /** 4731 * @return The first repetition of repeating field {@link #intendedRoute}, creating it if it does not already exist {3} 4732 */ 4733 public CodeableConcept getIntendedRouteFirstRep() { 4734 if (getIntendedRoute().isEmpty()) { 4735 addIntendedRoute(); 4736 } 4737 return getIntendedRoute().get(0); 4738 } 4739 4740 /** 4741 * @return {@link #ingredient} (Identifies a particular constituent of interest in the product.) 4742 */ 4743 public List<MedicationKnowledgeDefinitionalIngredientComponent> getIngredient() { 4744 if (this.ingredient == null) 4745 this.ingredient = new ArrayList<MedicationKnowledgeDefinitionalIngredientComponent>(); 4746 return this.ingredient; 4747 } 4748 4749 /** 4750 * @return Returns a reference to <code>this</code> for easy method chaining 4751 */ 4752 public MedicationKnowledgeDefinitionalComponent setIngredient(List<MedicationKnowledgeDefinitionalIngredientComponent> theIngredient) { 4753 this.ingredient = theIngredient; 4754 return this; 4755 } 4756 4757 public boolean hasIngredient() { 4758 if (this.ingredient == null) 4759 return false; 4760 for (MedicationKnowledgeDefinitionalIngredientComponent item : this.ingredient) 4761 if (!item.isEmpty()) 4762 return true; 4763 return false; 4764 } 4765 4766 public MedicationKnowledgeDefinitionalIngredientComponent addIngredient() { //3 4767 MedicationKnowledgeDefinitionalIngredientComponent t = new MedicationKnowledgeDefinitionalIngredientComponent(); 4768 if (this.ingredient == null) 4769 this.ingredient = new ArrayList<MedicationKnowledgeDefinitionalIngredientComponent>(); 4770 this.ingredient.add(t); 4771 return t; 4772 } 4773 4774 public MedicationKnowledgeDefinitionalComponent addIngredient(MedicationKnowledgeDefinitionalIngredientComponent t) { //3 4775 if (t == null) 4776 return this; 4777 if (this.ingredient == null) 4778 this.ingredient = new ArrayList<MedicationKnowledgeDefinitionalIngredientComponent>(); 4779 this.ingredient.add(t); 4780 return this; 4781 } 4782 4783 /** 4784 * @return The first repetition of repeating field {@link #ingredient}, creating it if it does not already exist {3} 4785 */ 4786 public MedicationKnowledgeDefinitionalIngredientComponent getIngredientFirstRep() { 4787 if (getIngredient().isEmpty()) { 4788 addIngredient(); 4789 } 4790 return getIngredient().get(0); 4791 } 4792 4793 /** 4794 * @return {@link #drugCharacteristic} (Specifies descriptive properties of the medicine, such as color, shape, imprints, etc.) 4795 */ 4796 public List<MedicationKnowledgeDefinitionalDrugCharacteristicComponent> getDrugCharacteristic() { 4797 if (this.drugCharacteristic == null) 4798 this.drugCharacteristic = new ArrayList<MedicationKnowledgeDefinitionalDrugCharacteristicComponent>(); 4799 return this.drugCharacteristic; 4800 } 4801 4802 /** 4803 * @return Returns a reference to <code>this</code> for easy method chaining 4804 */ 4805 public MedicationKnowledgeDefinitionalComponent setDrugCharacteristic(List<MedicationKnowledgeDefinitionalDrugCharacteristicComponent> theDrugCharacteristic) { 4806 this.drugCharacteristic = theDrugCharacteristic; 4807 return this; 4808 } 4809 4810 public boolean hasDrugCharacteristic() { 4811 if (this.drugCharacteristic == null) 4812 return false; 4813 for (MedicationKnowledgeDefinitionalDrugCharacteristicComponent item : this.drugCharacteristic) 4814 if (!item.isEmpty()) 4815 return true; 4816 return false; 4817 } 4818 4819 public MedicationKnowledgeDefinitionalDrugCharacteristicComponent addDrugCharacteristic() { //3 4820 MedicationKnowledgeDefinitionalDrugCharacteristicComponent t = new MedicationKnowledgeDefinitionalDrugCharacteristicComponent(); 4821 if (this.drugCharacteristic == null) 4822 this.drugCharacteristic = new ArrayList<MedicationKnowledgeDefinitionalDrugCharacteristicComponent>(); 4823 this.drugCharacteristic.add(t); 4824 return t; 4825 } 4826 4827 public MedicationKnowledgeDefinitionalComponent addDrugCharacteristic(MedicationKnowledgeDefinitionalDrugCharacteristicComponent t) { //3 4828 if (t == null) 4829 return this; 4830 if (this.drugCharacteristic == null) 4831 this.drugCharacteristic = new ArrayList<MedicationKnowledgeDefinitionalDrugCharacteristicComponent>(); 4832 this.drugCharacteristic.add(t); 4833 return this; 4834 } 4835 4836 /** 4837 * @return The first repetition of repeating field {@link #drugCharacteristic}, creating it if it does not already exist {3} 4838 */ 4839 public MedicationKnowledgeDefinitionalDrugCharacteristicComponent getDrugCharacteristicFirstRep() { 4840 if (getDrugCharacteristic().isEmpty()) { 4841 addDrugCharacteristic(); 4842 } 4843 return getDrugCharacteristic().get(0); 4844 } 4845 4846 protected void listChildren(List<Property> children) { 4847 super.listChildren(children); 4848 children.add(new Property("definition", "Reference(MedicinalProductDefinition)", "Associated definitions for this medication.", 0, java.lang.Integer.MAX_VALUE, definition)); 4849 children.add(new Property("doseForm", "CodeableConcept", "Describes the form of the item. Powder; tablets; capsule.", 0, 1, doseForm)); 4850 children.add(new Property("intendedRoute", "CodeableConcept", "The intended or approved route of administration.", 0, java.lang.Integer.MAX_VALUE, intendedRoute)); 4851 children.add(new Property("ingredient", "", "Identifies a particular constituent of interest in the product.", 0, java.lang.Integer.MAX_VALUE, ingredient)); 4852 children.add(new Property("drugCharacteristic", "", "Specifies descriptive properties of the medicine, such as color, shape, imprints, etc.", 0, java.lang.Integer.MAX_VALUE, drugCharacteristic)); 4853 } 4854 4855 @Override 4856 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4857 switch (_hash) { 4858 case -1014418093: /*definition*/ return new Property("definition", "Reference(MedicinalProductDefinition)", "Associated definitions for this medication.", 0, java.lang.Integer.MAX_VALUE, definition); 4859 case 1303858817: /*doseForm*/ return new Property("doseForm", "CodeableConcept", "Describes the form of the item. Powder; tablets; capsule.", 0, 1, doseForm); 4860 case -767798050: /*intendedRoute*/ return new Property("intendedRoute", "CodeableConcept", "The intended or approved route of administration.", 0, java.lang.Integer.MAX_VALUE, intendedRoute); 4861 case -206409263: /*ingredient*/ return new Property("ingredient", "", "Identifies a particular constituent of interest in the product.", 0, java.lang.Integer.MAX_VALUE, ingredient); 4862 case -844126885: /*drugCharacteristic*/ return new Property("drugCharacteristic", "", "Specifies descriptive properties of the medicine, such as color, shape, imprints, etc.", 0, java.lang.Integer.MAX_VALUE, drugCharacteristic); 4863 default: return super.getNamedProperty(_hash, _name, _checkValid); 4864 } 4865 4866 } 4867 4868 @Override 4869 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4870 switch (hash) { 4871 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // Reference 4872 case 1303858817: /*doseForm*/ return this.doseForm == null ? new Base[0] : new Base[] {this.doseForm}; // CodeableConcept 4873 case -767798050: /*intendedRoute*/ return this.intendedRoute == null ? new Base[0] : this.intendedRoute.toArray(new Base[this.intendedRoute.size()]); // CodeableConcept 4874 case -206409263: /*ingredient*/ return this.ingredient == null ? new Base[0] : this.ingredient.toArray(new Base[this.ingredient.size()]); // MedicationKnowledgeDefinitionalIngredientComponent 4875 case -844126885: /*drugCharacteristic*/ return this.drugCharacteristic == null ? new Base[0] : this.drugCharacteristic.toArray(new Base[this.drugCharacteristic.size()]); // MedicationKnowledgeDefinitionalDrugCharacteristicComponent 4876 default: return super.getProperty(hash, name, checkValid); 4877 } 4878 4879 } 4880 4881 @Override 4882 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4883 switch (hash) { 4884 case -1014418093: // definition 4885 this.getDefinition().add(TypeConvertor.castToReference(value)); // Reference 4886 return value; 4887 case 1303858817: // doseForm 4888 this.doseForm = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4889 return value; 4890 case -767798050: // intendedRoute 4891 this.getIntendedRoute().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 4892 return value; 4893 case -206409263: // ingredient 4894 this.getIngredient().add((MedicationKnowledgeDefinitionalIngredientComponent) value); // MedicationKnowledgeDefinitionalIngredientComponent 4895 return value; 4896 case -844126885: // drugCharacteristic 4897 this.getDrugCharacteristic().add((MedicationKnowledgeDefinitionalDrugCharacteristicComponent) value); // MedicationKnowledgeDefinitionalDrugCharacteristicComponent 4898 return value; 4899 default: return super.setProperty(hash, name, value); 4900 } 4901 4902 } 4903 4904 @Override 4905 public Base setProperty(String name, Base value) throws FHIRException { 4906 if (name.equals("definition")) { 4907 this.getDefinition().add(TypeConvertor.castToReference(value)); 4908 } else if (name.equals("doseForm")) { 4909 this.doseForm = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 4910 } else if (name.equals("intendedRoute")) { 4911 this.getIntendedRoute().add(TypeConvertor.castToCodeableConcept(value)); 4912 } else if (name.equals("ingredient")) { 4913 this.getIngredient().add((MedicationKnowledgeDefinitionalIngredientComponent) value); 4914 } else if (name.equals("drugCharacteristic")) { 4915 this.getDrugCharacteristic().add((MedicationKnowledgeDefinitionalDrugCharacteristicComponent) value); 4916 } else 4917 return super.setProperty(name, value); 4918 return value; 4919 } 4920 4921 @Override 4922 public void removeChild(String name, Base value) throws FHIRException { 4923 if (name.equals("definition")) { 4924 this.getDefinition().remove(value); 4925 } else if (name.equals("doseForm")) { 4926 this.doseForm = null; 4927 } else if (name.equals("intendedRoute")) { 4928 this.getIntendedRoute().remove(value); 4929 } else if (name.equals("ingredient")) { 4930 this.getIngredient().remove((MedicationKnowledgeDefinitionalIngredientComponent) value); 4931 } else if (name.equals("drugCharacteristic")) { 4932 this.getDrugCharacteristic().remove((MedicationKnowledgeDefinitionalDrugCharacteristicComponent) value); 4933 } else 4934 super.removeChild(name, value); 4935 4936 } 4937 4938 @Override 4939 public Base makeProperty(int hash, String name) throws FHIRException { 4940 switch (hash) { 4941 case -1014418093: return addDefinition(); 4942 case 1303858817: return getDoseForm(); 4943 case -767798050: return addIntendedRoute(); 4944 case -206409263: return addIngredient(); 4945 case -844126885: return addDrugCharacteristic(); 4946 default: return super.makeProperty(hash, name); 4947 } 4948 4949 } 4950 4951 @Override 4952 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4953 switch (hash) { 4954 case -1014418093: /*definition*/ return new String[] {"Reference"}; 4955 case 1303858817: /*doseForm*/ return new String[] {"CodeableConcept"}; 4956 case -767798050: /*intendedRoute*/ return new String[] {"CodeableConcept"}; 4957 case -206409263: /*ingredient*/ return new String[] {}; 4958 case -844126885: /*drugCharacteristic*/ return new String[] {}; 4959 default: return super.getTypesForProperty(hash, name); 4960 } 4961 4962 } 4963 4964 @Override 4965 public Base addChild(String name) throws FHIRException { 4966 if (name.equals("definition")) { 4967 return addDefinition(); 4968 } 4969 else if (name.equals("doseForm")) { 4970 this.doseForm = new CodeableConcept(); 4971 return this.doseForm; 4972 } 4973 else if (name.equals("intendedRoute")) { 4974 return addIntendedRoute(); 4975 } 4976 else if (name.equals("ingredient")) { 4977 return addIngredient(); 4978 } 4979 else if (name.equals("drugCharacteristic")) { 4980 return addDrugCharacteristic(); 4981 } 4982 else 4983 return super.addChild(name); 4984 } 4985 4986 public MedicationKnowledgeDefinitionalComponent copy() { 4987 MedicationKnowledgeDefinitionalComponent dst = new MedicationKnowledgeDefinitionalComponent(); 4988 copyValues(dst); 4989 return dst; 4990 } 4991 4992 public void copyValues(MedicationKnowledgeDefinitionalComponent dst) { 4993 super.copyValues(dst); 4994 if (definition != null) { 4995 dst.definition = new ArrayList<Reference>(); 4996 for (Reference i : definition) 4997 dst.definition.add(i.copy()); 4998 }; 4999 dst.doseForm = doseForm == null ? null : doseForm.copy(); 5000 if (intendedRoute != null) { 5001 dst.intendedRoute = new ArrayList<CodeableConcept>(); 5002 for (CodeableConcept i : intendedRoute) 5003 dst.intendedRoute.add(i.copy()); 5004 }; 5005 if (ingredient != null) { 5006 dst.ingredient = new ArrayList<MedicationKnowledgeDefinitionalIngredientComponent>(); 5007 for (MedicationKnowledgeDefinitionalIngredientComponent i : ingredient) 5008 dst.ingredient.add(i.copy()); 5009 }; 5010 if (drugCharacteristic != null) { 5011 dst.drugCharacteristic = new ArrayList<MedicationKnowledgeDefinitionalDrugCharacteristicComponent>(); 5012 for (MedicationKnowledgeDefinitionalDrugCharacteristicComponent i : drugCharacteristic) 5013 dst.drugCharacteristic.add(i.copy()); 5014 }; 5015 } 5016 5017 @Override 5018 public boolean equalsDeep(Base other_) { 5019 if (!super.equalsDeep(other_)) 5020 return false; 5021 if (!(other_ instanceof MedicationKnowledgeDefinitionalComponent)) 5022 return false; 5023 MedicationKnowledgeDefinitionalComponent o = (MedicationKnowledgeDefinitionalComponent) other_; 5024 return compareDeep(definition, o.definition, true) && compareDeep(doseForm, o.doseForm, true) && compareDeep(intendedRoute, o.intendedRoute, true) 5025 && compareDeep(ingredient, o.ingredient, true) && compareDeep(drugCharacteristic, o.drugCharacteristic, true) 5026 ; 5027 } 5028 5029 @Override 5030 public boolean equalsShallow(Base other_) { 5031 if (!super.equalsShallow(other_)) 5032 return false; 5033 if (!(other_ instanceof MedicationKnowledgeDefinitionalComponent)) 5034 return false; 5035 MedicationKnowledgeDefinitionalComponent o = (MedicationKnowledgeDefinitionalComponent) other_; 5036 return true; 5037 } 5038 5039 public boolean isEmpty() { 5040 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(definition, doseForm, intendedRoute 5041 , ingredient, drugCharacteristic); 5042 } 5043 5044 public String fhirType() { 5045 return "MedicationKnowledge.definitional"; 5046 5047 } 5048 5049 } 5050 5051 @Block() 5052 public static class MedicationKnowledgeDefinitionalIngredientComponent extends BackboneElement implements IBaseBackboneElement { 5053 /** 5054 * A reference to the resource that provides information about the ingredient. 5055 */ 5056 @Child(name = "item", type = {CodeableReference.class}, order=1, min=1, max=1, modifier=false, summary=true) 5057 @Description(shortDefinition="Substances contained in the medication", formalDefinition="A reference to the resource that provides information about the ingredient." ) 5058 protected CodeableReference item; 5059 5060 /** 5061 * Indication of whether this ingredient affects the therapeutic action of the drug. 5062 */ 5063 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 5064 @Description(shortDefinition="A code that defines the type of ingredient, active, base, etc", formalDefinition="Indication of whether this ingredient affects the therapeutic action of the drug." ) 5065 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://terminology.hl7.org/ValueSet/v3-RoleClassIngredientEntity") 5066 protected CodeableConcept type; 5067 5068 /** 5069 * Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet. 5070 */ 5071 @Child(name = "strength", type = {Ratio.class, CodeableConcept.class, Quantity.class}, order=3, min=0, max=1, modifier=false, summary=false) 5072 @Description(shortDefinition="Quantity of ingredient present", formalDefinition="Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet." ) 5073 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-ingredientstrength") 5074 protected DataType strength; 5075 5076 private static final long serialVersionUID = 1772676131L; 5077 5078 /** 5079 * Constructor 5080 */ 5081 public MedicationKnowledgeDefinitionalIngredientComponent() { 5082 super(); 5083 } 5084 5085 /** 5086 * Constructor 5087 */ 5088 public MedicationKnowledgeDefinitionalIngredientComponent(CodeableReference item) { 5089 super(); 5090 this.setItem(item); 5091 } 5092 5093 /** 5094 * @return {@link #item} (A reference to the resource that provides information about the ingredient.) 5095 */ 5096 public CodeableReference getItem() { 5097 if (this.item == null) 5098 if (Configuration.errorOnAutoCreate()) 5099 throw new Error("Attempt to auto-create MedicationKnowledgeDefinitionalIngredientComponent.item"); 5100 else if (Configuration.doAutoCreate()) 5101 this.item = new CodeableReference(); // cc 5102 return this.item; 5103 } 5104 5105 public boolean hasItem() { 5106 return this.item != null && !this.item.isEmpty(); 5107 } 5108 5109 /** 5110 * @param value {@link #item} (A reference to the resource that provides information about the ingredient.) 5111 */ 5112 public MedicationKnowledgeDefinitionalIngredientComponent setItem(CodeableReference value) { 5113 this.item = value; 5114 return this; 5115 } 5116 5117 /** 5118 * @return {@link #type} (Indication of whether this ingredient affects the therapeutic action of the drug.) 5119 */ 5120 public CodeableConcept getType() { 5121 if (this.type == null) 5122 if (Configuration.errorOnAutoCreate()) 5123 throw new Error("Attempt to auto-create MedicationKnowledgeDefinitionalIngredientComponent.type"); 5124 else if (Configuration.doAutoCreate()) 5125 this.type = new CodeableConcept(); // cc 5126 return this.type; 5127 } 5128 5129 public boolean hasType() { 5130 return this.type != null && !this.type.isEmpty(); 5131 } 5132 5133 /** 5134 * @param value {@link #type} (Indication of whether this ingredient affects the therapeutic action of the drug.) 5135 */ 5136 public MedicationKnowledgeDefinitionalIngredientComponent setType(CodeableConcept value) { 5137 this.type = value; 5138 return this; 5139 } 5140 5141 /** 5142 * @return {@link #strength} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.) 5143 */ 5144 public DataType getStrength() { 5145 return this.strength; 5146 } 5147 5148 /** 5149 * @return {@link #strength} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.) 5150 */ 5151 public Ratio getStrengthRatio() throws FHIRException { 5152 if (this.strength == null) 5153 this.strength = new Ratio(); 5154 if (!(this.strength instanceof Ratio)) 5155 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.strength.getClass().getName()+" was encountered"); 5156 return (Ratio) this.strength; 5157 } 5158 5159 public boolean hasStrengthRatio() { 5160 return this.strength instanceof Ratio; 5161 } 5162 5163 /** 5164 * @return {@link #strength} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.) 5165 */ 5166 public CodeableConcept getStrengthCodeableConcept() throws FHIRException { 5167 if (this.strength == null) 5168 this.strength = new CodeableConcept(); 5169 if (!(this.strength instanceof CodeableConcept)) 5170 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.strength.getClass().getName()+" was encountered"); 5171 return (CodeableConcept) this.strength; 5172 } 5173 5174 public boolean hasStrengthCodeableConcept() { 5175 return this.strength instanceof CodeableConcept; 5176 } 5177 5178 /** 5179 * @return {@link #strength} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.) 5180 */ 5181 public Quantity getStrengthQuantity() throws FHIRException { 5182 if (this.strength == null) 5183 this.strength = new Quantity(); 5184 if (!(this.strength instanceof Quantity)) 5185 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.strength.getClass().getName()+" was encountered"); 5186 return (Quantity) this.strength; 5187 } 5188 5189 public boolean hasStrengthQuantity() { 5190 return this.strength instanceof Quantity; 5191 } 5192 5193 public boolean hasStrength() { 5194 return this.strength != null && !this.strength.isEmpty(); 5195 } 5196 5197 /** 5198 * @param value {@link #strength} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.) 5199 */ 5200 public MedicationKnowledgeDefinitionalIngredientComponent setStrength(DataType value) { 5201 if (value != null && !(value instanceof Ratio || value instanceof CodeableConcept || value instanceof Quantity)) 5202 throw new FHIRException("Not the right type for MedicationKnowledge.definitional.ingredient.strength[x]: "+value.fhirType()); 5203 this.strength = value; 5204 return this; 5205 } 5206 5207 protected void listChildren(List<Property> children) { 5208 super.listChildren(children); 5209 children.add(new Property("item", "CodeableReference(Substance)", "A reference to the resource that provides information about the ingredient.", 0, 1, item)); 5210 children.add(new Property("type", "CodeableConcept", "Indication of whether this ingredient affects the therapeutic action of the drug.", 0, 1, type)); 5211 children.add(new Property("strength[x]", "Ratio|CodeableConcept|Quantity", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength)); 5212 } 5213 5214 @Override 5215 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5216 switch (_hash) { 5217 case 3242771: /*item*/ return new Property("item", "CodeableReference(Substance)", "A reference to the resource that provides information about the ingredient.", 0, 1, item); 5218 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Indication of whether this ingredient affects the therapeutic action of the drug.", 0, 1, type); 5219 case 127377567: /*strength[x]*/ return new Property("strength[x]", "Ratio|CodeableConcept|Quantity", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength); 5220 case 1791316033: /*strength*/ return new Property("strength[x]", "Ratio|CodeableConcept|Quantity", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength); 5221 case 2141786186: /*strengthRatio*/ return new Property("strength[x]", "Ratio", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength); 5222 case -1455903456: /*strengthCodeableConcept*/ return new Property("strength[x]", "CodeableConcept", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength); 5223 case -1793570836: /*strengthQuantity*/ return new Property("strength[x]", "Quantity", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet but can also be expressed a quantity when the denominator is assumed to be 1 tablet.", 0, 1, strength); 5224 default: return super.getNamedProperty(_hash, _name, _checkValid); 5225 } 5226 5227 } 5228 5229 @Override 5230 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5231 switch (hash) { 5232 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // CodeableReference 5233 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 5234 case 1791316033: /*strength*/ return this.strength == null ? new Base[0] : new Base[] {this.strength}; // DataType 5235 default: return super.getProperty(hash, name, checkValid); 5236 } 5237 5238 } 5239 5240 @Override 5241 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5242 switch (hash) { 5243 case 3242771: // item 5244 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 5245 return value; 5246 case 3575610: // type 5247 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5248 return value; 5249 case 1791316033: // strength 5250 this.strength = TypeConvertor.castToType(value); // DataType 5251 return value; 5252 default: return super.setProperty(hash, name, value); 5253 } 5254 5255 } 5256 5257 @Override 5258 public Base setProperty(String name, Base value) throws FHIRException { 5259 if (name.equals("item")) { 5260 this.item = TypeConvertor.castToCodeableReference(value); // CodeableReference 5261 } else if (name.equals("type")) { 5262 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5263 } else if (name.equals("strength[x]")) { 5264 this.strength = TypeConvertor.castToType(value); // DataType 5265 } else 5266 return super.setProperty(name, value); 5267 return value; 5268 } 5269 5270 @Override 5271 public void removeChild(String name, Base value) throws FHIRException { 5272 if (name.equals("item")) { 5273 this.item = null; 5274 } else if (name.equals("type")) { 5275 this.type = null; 5276 } else if (name.equals("strength[x]")) { 5277 this.strength = null; 5278 } else 5279 super.removeChild(name, value); 5280 5281 } 5282 5283 @Override 5284 public Base makeProperty(int hash, String name) throws FHIRException { 5285 switch (hash) { 5286 case 3242771: return getItem(); 5287 case 3575610: return getType(); 5288 case 127377567: return getStrength(); 5289 case 1791316033: return getStrength(); 5290 default: return super.makeProperty(hash, name); 5291 } 5292 5293 } 5294 5295 @Override 5296 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5297 switch (hash) { 5298 case 3242771: /*item*/ return new String[] {"CodeableReference"}; 5299 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 5300 case 1791316033: /*strength*/ return new String[] {"Ratio", "CodeableConcept", "Quantity"}; 5301 default: return super.getTypesForProperty(hash, name); 5302 } 5303 5304 } 5305 5306 @Override 5307 public Base addChild(String name) throws FHIRException { 5308 if (name.equals("item")) { 5309 this.item = new CodeableReference(); 5310 return this.item; 5311 } 5312 else if (name.equals("type")) { 5313 this.type = new CodeableConcept(); 5314 return this.type; 5315 } 5316 else if (name.equals("strengthRatio")) { 5317 this.strength = new Ratio(); 5318 return this.strength; 5319 } 5320 else if (name.equals("strengthCodeableConcept")) { 5321 this.strength = new CodeableConcept(); 5322 return this.strength; 5323 } 5324 else if (name.equals("strengthQuantity")) { 5325 this.strength = new Quantity(); 5326 return this.strength; 5327 } 5328 else 5329 return super.addChild(name); 5330 } 5331 5332 public MedicationKnowledgeDefinitionalIngredientComponent copy() { 5333 MedicationKnowledgeDefinitionalIngredientComponent dst = new MedicationKnowledgeDefinitionalIngredientComponent(); 5334 copyValues(dst); 5335 return dst; 5336 } 5337 5338 public void copyValues(MedicationKnowledgeDefinitionalIngredientComponent dst) { 5339 super.copyValues(dst); 5340 dst.item = item == null ? null : item.copy(); 5341 dst.type = type == null ? null : type.copy(); 5342 dst.strength = strength == null ? null : strength.copy(); 5343 } 5344 5345 @Override 5346 public boolean equalsDeep(Base other_) { 5347 if (!super.equalsDeep(other_)) 5348 return false; 5349 if (!(other_ instanceof MedicationKnowledgeDefinitionalIngredientComponent)) 5350 return false; 5351 MedicationKnowledgeDefinitionalIngredientComponent o = (MedicationKnowledgeDefinitionalIngredientComponent) other_; 5352 return compareDeep(item, o.item, true) && compareDeep(type, o.type, true) && compareDeep(strength, o.strength, true) 5353 ; 5354 } 5355 5356 @Override 5357 public boolean equalsShallow(Base other_) { 5358 if (!super.equalsShallow(other_)) 5359 return false; 5360 if (!(other_ instanceof MedicationKnowledgeDefinitionalIngredientComponent)) 5361 return false; 5362 MedicationKnowledgeDefinitionalIngredientComponent o = (MedicationKnowledgeDefinitionalIngredientComponent) other_; 5363 return true; 5364 } 5365 5366 public boolean isEmpty() { 5367 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item, type, strength); 5368 } 5369 5370 public String fhirType() { 5371 return "MedicationKnowledge.definitional.ingredient"; 5372 5373 } 5374 5375 } 5376 5377 @Block() 5378 public static class MedicationKnowledgeDefinitionalDrugCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 5379 /** 5380 * A code specifying which characteristic of the medicine is being described (for example, colour, shape, imprint). 5381 */ 5382 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 5383 @Description(shortDefinition="Code specifying the type of characteristic of medication", formalDefinition="A code specifying which characteristic of the medicine is being described (for example, colour, shape, imprint)." ) 5384 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationknowledge-characteristic") 5385 protected CodeableConcept type; 5386 5387 /** 5388 * Description of the characteristic. 5389 */ 5390 @Child(name = "value", type = {CodeableConcept.class, StringType.class, Quantity.class, Base64BinaryType.class, Attachment.class}, order=2, min=0, max=1, modifier=false, summary=false) 5391 @Description(shortDefinition="Description of the characteristic", formalDefinition="Description of the characteristic." ) 5392 protected DataType value; 5393 5394 private static final long serialVersionUID = -1659186716L; 5395 5396 /** 5397 * Constructor 5398 */ 5399 public MedicationKnowledgeDefinitionalDrugCharacteristicComponent() { 5400 super(); 5401 } 5402 5403 /** 5404 * @return {@link #type} (A code specifying which characteristic of the medicine is being described (for example, colour, shape, imprint).) 5405 */ 5406 public CodeableConcept getType() { 5407 if (this.type == null) 5408 if (Configuration.errorOnAutoCreate()) 5409 throw new Error("Attempt to auto-create MedicationKnowledgeDefinitionalDrugCharacteristicComponent.type"); 5410 else if (Configuration.doAutoCreate()) 5411 this.type = new CodeableConcept(); // cc 5412 return this.type; 5413 } 5414 5415 public boolean hasType() { 5416 return this.type != null && !this.type.isEmpty(); 5417 } 5418 5419 /** 5420 * @param value {@link #type} (A code specifying which characteristic of the medicine is being described (for example, colour, shape, imprint).) 5421 */ 5422 public MedicationKnowledgeDefinitionalDrugCharacteristicComponent setType(CodeableConcept value) { 5423 this.type = value; 5424 return this; 5425 } 5426 5427 /** 5428 * @return {@link #value} (Description of the characteristic.) 5429 */ 5430 public DataType getValue() { 5431 return this.value; 5432 } 5433 5434 /** 5435 * @return {@link #value} (Description of the characteristic.) 5436 */ 5437 public CodeableConcept getValueCodeableConcept() throws FHIRException { 5438 if (this.value == null) 5439 this.value = new CodeableConcept(); 5440 if (!(this.value instanceof CodeableConcept)) 5441 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 5442 return (CodeableConcept) this.value; 5443 } 5444 5445 public boolean hasValueCodeableConcept() { 5446 return this.value instanceof CodeableConcept; 5447 } 5448 5449 /** 5450 * @return {@link #value} (Description of the characteristic.) 5451 */ 5452 public StringType getValueStringType() throws FHIRException { 5453 if (this.value == null) 5454 this.value = new StringType(); 5455 if (!(this.value instanceof StringType)) 5456 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 5457 return (StringType) this.value; 5458 } 5459 5460 public boolean hasValueStringType() { 5461 return this.value instanceof StringType; 5462 } 5463 5464 /** 5465 * @return {@link #value} (Description of the characteristic.) 5466 */ 5467 public Quantity getValueQuantity() throws FHIRException { 5468 if (this.value == null) 5469 this.value = new Quantity(); 5470 if (!(this.value instanceof Quantity)) 5471 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 5472 return (Quantity) this.value; 5473 } 5474 5475 public boolean hasValueQuantity() { 5476 return this.value instanceof Quantity; 5477 } 5478 5479 /** 5480 * @return {@link #value} (Description of the characteristic.) 5481 */ 5482 public Base64BinaryType getValueBase64BinaryType() throws FHIRException { 5483 if (this.value == null) 5484 this.value = new Base64BinaryType(); 5485 if (!(this.value instanceof Base64BinaryType)) 5486 throw new FHIRException("Type mismatch: the type Base64BinaryType was expected, but "+this.value.getClass().getName()+" was encountered"); 5487 return (Base64BinaryType) this.value; 5488 } 5489 5490 public boolean hasValueBase64BinaryType() { 5491 return this.value instanceof Base64BinaryType; 5492 } 5493 5494 /** 5495 * @return {@link #value} (Description of the characteristic.) 5496 */ 5497 public Attachment getValueAttachment() throws FHIRException { 5498 if (this.value == null) 5499 this.value = new Attachment(); 5500 if (!(this.value instanceof Attachment)) 5501 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 5502 return (Attachment) this.value; 5503 } 5504 5505 public boolean hasValueAttachment() { 5506 return this.value instanceof Attachment; 5507 } 5508 5509 public boolean hasValue() { 5510 return this.value != null && !this.value.isEmpty(); 5511 } 5512 5513 /** 5514 * @param value {@link #value} (Description of the characteristic.) 5515 */ 5516 public MedicationKnowledgeDefinitionalDrugCharacteristicComponent setValue(DataType value) { 5517 if (value != null && !(value instanceof CodeableConcept || value instanceof StringType || value instanceof Quantity || value instanceof Base64BinaryType || value instanceof Attachment)) 5518 throw new FHIRException("Not the right type for MedicationKnowledge.definitional.drugCharacteristic.value[x]: "+value.fhirType()); 5519 this.value = value; 5520 return this; 5521 } 5522 5523 protected void listChildren(List<Property> children) { 5524 super.listChildren(children); 5525 children.add(new Property("type", "CodeableConcept", "A code specifying which characteristic of the medicine is being described (for example, colour, shape, imprint).", 0, 1, type)); 5526 children.add(new Property("value[x]", "CodeableConcept|string|Quantity|base64Binary|Attachment", "Description of the characteristic.", 0, 1, value)); 5527 } 5528 5529 @Override 5530 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5531 switch (_hash) { 5532 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A code specifying which characteristic of the medicine is being described (for example, colour, shape, imprint).", 0, 1, type); 5533 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|string|Quantity|base64Binary|Attachment", "Description of the characteristic.", 0, 1, value); 5534 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|string|Quantity|base64Binary|Attachment", "Description of the characteristic.", 0, 1, value); 5535 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept", "Description of the characteristic.", 0, 1, value); 5536 case -1424603934: /*valueString*/ return new Property("value[x]", "string", "Description of the characteristic.", 0, 1, value); 5537 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "Quantity", "Description of the characteristic.", 0, 1, value); 5538 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "base64Binary", "Description of the characteristic.", 0, 1, value); 5539 case -475566732: /*valueAttachment*/ return new Property("value[x]", "Attachment", "Description of the characteristic.", 0, 1, value); 5540 default: return super.getNamedProperty(_hash, _name, _checkValid); 5541 } 5542 5543 } 5544 5545 @Override 5546 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5547 switch (hash) { 5548 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 5549 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DataType 5550 default: return super.getProperty(hash, name, checkValid); 5551 } 5552 5553 } 5554 5555 @Override 5556 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5557 switch (hash) { 5558 case 3575610: // type 5559 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5560 return value; 5561 case 111972721: // value 5562 this.value = TypeConvertor.castToType(value); // DataType 5563 return value; 5564 default: return super.setProperty(hash, name, value); 5565 } 5566 5567 } 5568 5569 @Override 5570 public Base setProperty(String name, Base value) throws FHIRException { 5571 if (name.equals("type")) { 5572 this.type = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 5573 } else if (name.equals("value[x]")) { 5574 this.value = TypeConvertor.castToType(value); // DataType 5575 } else 5576 return super.setProperty(name, value); 5577 return value; 5578 } 5579 5580 @Override 5581 public void removeChild(String name, Base value) throws FHIRException { 5582 if (name.equals("type")) { 5583 this.type = null; 5584 } else if (name.equals("value[x]")) { 5585 this.value = null; 5586 } else 5587 super.removeChild(name, value); 5588 5589 } 5590 5591 @Override 5592 public Base makeProperty(int hash, String name) throws FHIRException { 5593 switch (hash) { 5594 case 3575610: return getType(); 5595 case -1410166417: return getValue(); 5596 case 111972721: return getValue(); 5597 default: return super.makeProperty(hash, name); 5598 } 5599 5600 } 5601 5602 @Override 5603 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5604 switch (hash) { 5605 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 5606 case 111972721: /*value*/ return new String[] {"CodeableConcept", "string", "Quantity", "base64Binary", "Attachment"}; 5607 default: return super.getTypesForProperty(hash, name); 5608 } 5609 5610 } 5611 5612 @Override 5613 public Base addChild(String name) throws FHIRException { 5614 if (name.equals("type")) { 5615 this.type = new CodeableConcept(); 5616 return this.type; 5617 } 5618 else if (name.equals("valueCodeableConcept")) { 5619 this.value = new CodeableConcept(); 5620 return this.value; 5621 } 5622 else if (name.equals("valueString")) { 5623 this.value = new StringType(); 5624 return this.value; 5625 } 5626 else if (name.equals("valueQuantity")) { 5627 this.value = new Quantity(); 5628 return this.value; 5629 } 5630 else if (name.equals("valueBase64Binary")) { 5631 this.value = new Base64BinaryType(); 5632 return this.value; 5633 } 5634 else if (name.equals("valueAttachment")) { 5635 this.value = new Attachment(); 5636 return this.value; 5637 } 5638 else 5639 return super.addChild(name); 5640 } 5641 5642 public MedicationKnowledgeDefinitionalDrugCharacteristicComponent copy() { 5643 MedicationKnowledgeDefinitionalDrugCharacteristicComponent dst = new MedicationKnowledgeDefinitionalDrugCharacteristicComponent(); 5644 copyValues(dst); 5645 return dst; 5646 } 5647 5648 public void copyValues(MedicationKnowledgeDefinitionalDrugCharacteristicComponent dst) { 5649 super.copyValues(dst); 5650 dst.type = type == null ? null : type.copy(); 5651 dst.value = value == null ? null : value.copy(); 5652 } 5653 5654 @Override 5655 public boolean equalsDeep(Base other_) { 5656 if (!super.equalsDeep(other_)) 5657 return false; 5658 if (!(other_ instanceof MedicationKnowledgeDefinitionalDrugCharacteristicComponent)) 5659 return false; 5660 MedicationKnowledgeDefinitionalDrugCharacteristicComponent o = (MedicationKnowledgeDefinitionalDrugCharacteristicComponent) other_; 5661 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 5662 } 5663 5664 @Override 5665 public boolean equalsShallow(Base other_) { 5666 if (!super.equalsShallow(other_)) 5667 return false; 5668 if (!(other_ instanceof MedicationKnowledgeDefinitionalDrugCharacteristicComponent)) 5669 return false; 5670 MedicationKnowledgeDefinitionalDrugCharacteristicComponent o = (MedicationKnowledgeDefinitionalDrugCharacteristicComponent) other_; 5671 return true; 5672 } 5673 5674 public boolean isEmpty() { 5675 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, value); 5676 } 5677 5678 public String fhirType() { 5679 return "MedicationKnowledge.definitional.drugCharacteristic"; 5680 5681 } 5682 5683 } 5684 5685 /** 5686 * Business identifier for this medication. 5687 */ 5688 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5689 @Description(shortDefinition="Business identifier for this medication", formalDefinition="Business identifier for this medication." ) 5690 protected List<Identifier> identifier; 5691 5692 /** 5693 * A code that specifies this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems. 5694 */ 5695 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 5696 @Description(shortDefinition="Code that identifies this medication", formalDefinition="A code that specifies this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems." ) 5697 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 5698 protected CodeableConcept code; 5699 5700 /** 5701 * A code to indicate if the medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. The status refers to the validity about the information of the medication and not to its medicinal properties. 5702 */ 5703 @Child(name = "status", type = {CodeType.class}, order=2, min=0, max=1, modifier=true, summary=true) 5704 @Description(shortDefinition="active | entered-in-error | inactive", formalDefinition="A code to indicate if the medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. The status refers to the validity about the information of the medication and not to its medicinal properties." ) 5705 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medicationknowledge-status") 5706 protected Enumeration<MedicationKnowledgeStatusCodes> status; 5707 5708 /** 5709 * The creator or owner of the knowledge or information about the medication. 5710 */ 5711 @Child(name = "author", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=false) 5712 @Description(shortDefinition="Creator or owner of the knowledge or information about the medication", formalDefinition="The creator or owner of the knowledge or information about the medication." ) 5713 protected Reference author; 5714 5715 /** 5716 * Lists the jurisdictions that this medication knowledge was written for. 5717 */ 5718 @Child(name = "intendedJurisdiction", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5719 @Description(shortDefinition="Codes that identify the different jurisdictions for which the information of this resource was created", formalDefinition="Lists the jurisdictions that this medication knowledge was written for." ) 5720 protected List<CodeableConcept> intendedJurisdiction; 5721 5722 /** 5723 * All of the names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol. 5724 */ 5725 @Child(name = "name", type = {StringType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5726 @Description(shortDefinition="A name associated with the medication being described", formalDefinition="All of the names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol." ) 5727 protected List<StringType> name; 5728 5729 /** 5730 * Associated or related medications. For example, if the medication is a branded product (e.g. Crestor), this is the Therapeutic Moeity (e.g. Rosuvastatin) or if this is a generic medication (e.g. Rosuvastatin), this would link to a branded product (e.g. Crestor. 5731 */ 5732 @Child(name = "relatedMedicationKnowledge", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5733 @Description(shortDefinition="Associated or related medication information", formalDefinition="Associated or related medications. For example, if the medication is a branded product (e.g. Crestor), this is the Therapeutic Moeity (e.g. Rosuvastatin) or if this is a generic medication (e.g. Rosuvastatin), this would link to a branded product (e.g. Crestor." ) 5734 protected List<MedicationKnowledgeRelatedMedicationKnowledgeComponent> relatedMedicationKnowledge; 5735 5736 /** 5737 * Links to associated medications that could be prescribed, dispensed or administered. 5738 */ 5739 @Child(name = "associatedMedication", type = {Medication.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5740 @Description(shortDefinition="The set of medication resources that are associated with this medication", formalDefinition="Links to associated medications that could be prescribed, dispensed or administered." ) 5741 protected List<Reference> associatedMedication; 5742 5743 /** 5744 * Category of the medication or product (e.g. branded product, therapeutic moeity, generic product, innovator product, etc.). 5745 */ 5746 @Child(name = "productType", type = {CodeableConcept.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5747 @Description(shortDefinition="Category of the medication or product", formalDefinition="Category of the medication or product (e.g. branded product, therapeutic moeity, generic product, innovator product, etc.)." ) 5748 protected List<CodeableConcept> productType; 5749 5750 /** 5751 * Associated documentation about the medication. 5752 */ 5753 @Child(name = "monograph", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5754 @Description(shortDefinition="Associated documentation about the medication", formalDefinition="Associated documentation about the medication." ) 5755 protected List<MedicationKnowledgeMonographComponent> monograph; 5756 5757 /** 5758 * The instructions for preparing the medication. 5759 */ 5760 @Child(name = "preparationInstruction", type = {MarkdownType.class}, order=10, min=0, max=1, modifier=false, summary=false) 5761 @Description(shortDefinition="The instructions for preparing the medication", formalDefinition="The instructions for preparing the medication." ) 5762 protected MarkdownType preparationInstruction; 5763 5764 /** 5765 * The price of the medication. 5766 */ 5767 @Child(name = "cost", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5768 @Description(shortDefinition="The pricing of the medication", formalDefinition="The price of the medication." ) 5769 protected List<MedicationKnowledgeCostComponent> cost; 5770 5771 /** 5772 * The program under which the medication is reviewed. 5773 */ 5774 @Child(name = "monitoringProgram", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 5775 @Description(shortDefinition="Program under which a medication is reviewed", formalDefinition="The program under which the medication is reviewed." ) 5776 protected List<MedicationKnowledgeMonitoringProgramComponent> monitoringProgram; 5777 5778 /** 5779 * Guidelines or protocols that are applicable for the administration of the medication based on indication. 5780 */ 5781 @Child(name = "indicationGuideline", type = {}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5782 @Description(shortDefinition="Guidelines or protocols for administration of the medication for an indication", formalDefinition="Guidelines or protocols that are applicable for the administration of the medication based on indication." ) 5783 protected List<MedicationKnowledgeIndicationGuidelineComponent> indicationGuideline; 5784 5785 /** 5786 * Categorization of the medication within a formulary or classification system. 5787 */ 5788 @Child(name = "medicineClassification", type = {}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5789 @Description(shortDefinition="Categorization of the medication within a formulary or classification system", formalDefinition="Categorization of the medication within a formulary or classification system." ) 5790 protected List<MedicationKnowledgeMedicineClassificationComponent> medicineClassification; 5791 5792 /** 5793 * Information that only applies to packages (not products). 5794 */ 5795 @Child(name = "packaging", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5796 @Description(shortDefinition="Details about packaged medications", formalDefinition="Information that only applies to packages (not products)." ) 5797 protected List<MedicationKnowledgePackagingComponent> packaging; 5798 5799 /** 5800 * Potential clinical issue with or between medication(s) (for example, drug-drug interaction, drug-disease contraindication, drug-allergy interaction, etc.). 5801 */ 5802 @Child(name = "clinicalUseIssue", type = {ClinicalUseDefinition.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5803 @Description(shortDefinition="Potential clinical issue with or between medication(s)", formalDefinition="Potential clinical issue with or between medication(s) (for example, drug-drug interaction, drug-disease contraindication, drug-allergy interaction, etc.)." ) 5804 protected List<Reference> clinicalUseIssue; 5805 5806 /** 5807 * Information on how the medication should be stored, for example, refrigeration temperatures and length of stability at a given temperature. 5808 */ 5809 @Child(name = "storageGuideline", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5810 @Description(shortDefinition="How the medication should be stored", formalDefinition="Information on how the medication should be stored, for example, refrigeration temperatures and length of stability at a given temperature." ) 5811 protected List<MedicationKnowledgeStorageGuidelineComponent> storageGuideline; 5812 5813 /** 5814 * Regulatory information about a medication. 5815 */ 5816 @Child(name = "regulatory", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5817 @Description(shortDefinition="Regulatory information about a medication", formalDefinition="Regulatory information about a medication." ) 5818 protected List<MedicationKnowledgeRegulatoryComponent> regulatory; 5819 5820 /** 5821 * Along with the link to a Medicinal Product Definition resource, this information provides common definitional elements that are needed to understand the specific medication that is being described. 5822 */ 5823 @Child(name = "definitional", type = {}, order=19, min=0, max=1, modifier=false, summary=false) 5824 @Description(shortDefinition="Minimal definition information about the medication", formalDefinition="Along with the link to a Medicinal Product Definition resource, this information provides common definitional elements that are needed to understand the specific medication that is being described." ) 5825 protected MedicationKnowledgeDefinitionalComponent definitional; 5826 5827 private static final long serialVersionUID = -814493741L; 5828 5829 /** 5830 * Constructor 5831 */ 5832 public MedicationKnowledge() { 5833 super(); 5834 } 5835 5836 /** 5837 * @return {@link #identifier} (Business identifier for this medication.) 5838 */ 5839 public List<Identifier> getIdentifier() { 5840 if (this.identifier == null) 5841 this.identifier = new ArrayList<Identifier>(); 5842 return this.identifier; 5843 } 5844 5845 /** 5846 * @return Returns a reference to <code>this</code> for easy method chaining 5847 */ 5848 public MedicationKnowledge setIdentifier(List<Identifier> theIdentifier) { 5849 this.identifier = theIdentifier; 5850 return this; 5851 } 5852 5853 public boolean hasIdentifier() { 5854 if (this.identifier == null) 5855 return false; 5856 for (Identifier item : this.identifier) 5857 if (!item.isEmpty()) 5858 return true; 5859 return false; 5860 } 5861 5862 public Identifier addIdentifier() { //3 5863 Identifier t = new Identifier(); 5864 if (this.identifier == null) 5865 this.identifier = new ArrayList<Identifier>(); 5866 this.identifier.add(t); 5867 return t; 5868 } 5869 5870 public MedicationKnowledge addIdentifier(Identifier t) { //3 5871 if (t == null) 5872 return this; 5873 if (this.identifier == null) 5874 this.identifier = new ArrayList<Identifier>(); 5875 this.identifier.add(t); 5876 return this; 5877 } 5878 5879 /** 5880 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 5881 */ 5882 public Identifier getIdentifierFirstRep() { 5883 if (getIdentifier().isEmpty()) { 5884 addIdentifier(); 5885 } 5886 return getIdentifier().get(0); 5887 } 5888 5889 /** 5890 * @return {@link #code} (A code that specifies this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.) 5891 */ 5892 public CodeableConcept getCode() { 5893 if (this.code == null) 5894 if (Configuration.errorOnAutoCreate()) 5895 throw new Error("Attempt to auto-create MedicationKnowledge.code"); 5896 else if (Configuration.doAutoCreate()) 5897 this.code = new CodeableConcept(); // cc 5898 return this.code; 5899 } 5900 5901 public boolean hasCode() { 5902 return this.code != null && !this.code.isEmpty(); 5903 } 5904 5905 /** 5906 * @param value {@link #code} (A code that specifies this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.) 5907 */ 5908 public MedicationKnowledge setCode(CodeableConcept value) { 5909 this.code = value; 5910 return this; 5911 } 5912 5913 /** 5914 * @return {@link #status} (A code to indicate if the medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. The status refers to the validity about the information of the medication and not to its medicinal properties.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 5915 */ 5916 public Enumeration<MedicationKnowledgeStatusCodes> getStatusElement() { 5917 if (this.status == null) 5918 if (Configuration.errorOnAutoCreate()) 5919 throw new Error("Attempt to auto-create MedicationKnowledge.status"); 5920 else if (Configuration.doAutoCreate()) 5921 this.status = new Enumeration<MedicationKnowledgeStatusCodes>(new MedicationKnowledgeStatusCodesEnumFactory()); // bb 5922 return this.status; 5923 } 5924 5925 public boolean hasStatusElement() { 5926 return this.status != null && !this.status.isEmpty(); 5927 } 5928 5929 public boolean hasStatus() { 5930 return this.status != null && !this.status.isEmpty(); 5931 } 5932 5933 /** 5934 * @param value {@link #status} (A code to indicate if the medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. The status refers to the validity about the information of the medication and not to its medicinal properties.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 5935 */ 5936 public MedicationKnowledge setStatusElement(Enumeration<MedicationKnowledgeStatusCodes> value) { 5937 this.status = value; 5938 return this; 5939 } 5940 5941 /** 5942 * @return A code to indicate if the medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. The status refers to the validity about the information of the medication and not to its medicinal properties. 5943 */ 5944 public MedicationKnowledgeStatusCodes getStatus() { 5945 return this.status == null ? null : this.status.getValue(); 5946 } 5947 5948 /** 5949 * @param value A code to indicate if the medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. The status refers to the validity about the information of the medication and not to its medicinal properties. 5950 */ 5951 public MedicationKnowledge setStatus(MedicationKnowledgeStatusCodes value) { 5952 if (value == null) 5953 this.status = null; 5954 else { 5955 if (this.status == null) 5956 this.status = new Enumeration<MedicationKnowledgeStatusCodes>(new MedicationKnowledgeStatusCodesEnumFactory()); 5957 this.status.setValue(value); 5958 } 5959 return this; 5960 } 5961 5962 /** 5963 * @return {@link #author} (The creator or owner of the knowledge or information about the medication.) 5964 */ 5965 public Reference getAuthor() { 5966 if (this.author == null) 5967 if (Configuration.errorOnAutoCreate()) 5968 throw new Error("Attempt to auto-create MedicationKnowledge.author"); 5969 else if (Configuration.doAutoCreate()) 5970 this.author = new Reference(); // cc 5971 return this.author; 5972 } 5973 5974 public boolean hasAuthor() { 5975 return this.author != null && !this.author.isEmpty(); 5976 } 5977 5978 /** 5979 * @param value {@link #author} (The creator or owner of the knowledge or information about the medication.) 5980 */ 5981 public MedicationKnowledge setAuthor(Reference value) { 5982 this.author = value; 5983 return this; 5984 } 5985 5986 /** 5987 * @return {@link #intendedJurisdiction} (Lists the jurisdictions that this medication knowledge was written for.) 5988 */ 5989 public List<CodeableConcept> getIntendedJurisdiction() { 5990 if (this.intendedJurisdiction == null) 5991 this.intendedJurisdiction = new ArrayList<CodeableConcept>(); 5992 return this.intendedJurisdiction; 5993 } 5994 5995 /** 5996 * @return Returns a reference to <code>this</code> for easy method chaining 5997 */ 5998 public MedicationKnowledge setIntendedJurisdiction(List<CodeableConcept> theIntendedJurisdiction) { 5999 this.intendedJurisdiction = theIntendedJurisdiction; 6000 return this; 6001 } 6002 6003 public boolean hasIntendedJurisdiction() { 6004 if (this.intendedJurisdiction == null) 6005 return false; 6006 for (CodeableConcept item : this.intendedJurisdiction) 6007 if (!item.isEmpty()) 6008 return true; 6009 return false; 6010 } 6011 6012 public CodeableConcept addIntendedJurisdiction() { //3 6013 CodeableConcept t = new CodeableConcept(); 6014 if (this.intendedJurisdiction == null) 6015 this.intendedJurisdiction = new ArrayList<CodeableConcept>(); 6016 this.intendedJurisdiction.add(t); 6017 return t; 6018 } 6019 6020 public MedicationKnowledge addIntendedJurisdiction(CodeableConcept t) { //3 6021 if (t == null) 6022 return this; 6023 if (this.intendedJurisdiction == null) 6024 this.intendedJurisdiction = new ArrayList<CodeableConcept>(); 6025 this.intendedJurisdiction.add(t); 6026 return this; 6027 } 6028 6029 /** 6030 * @return The first repetition of repeating field {@link #intendedJurisdiction}, creating it if it does not already exist {3} 6031 */ 6032 public CodeableConcept getIntendedJurisdictionFirstRep() { 6033 if (getIntendedJurisdiction().isEmpty()) { 6034 addIntendedJurisdiction(); 6035 } 6036 return getIntendedJurisdiction().get(0); 6037 } 6038 6039 /** 6040 * @return {@link #name} (All of the names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol.) 6041 */ 6042 public List<StringType> getName() { 6043 if (this.name == null) 6044 this.name = new ArrayList<StringType>(); 6045 return this.name; 6046 } 6047 6048 /** 6049 * @return Returns a reference to <code>this</code> for easy method chaining 6050 */ 6051 public MedicationKnowledge setName(List<StringType> theName) { 6052 this.name = theName; 6053 return this; 6054 } 6055 6056 public boolean hasName() { 6057 if (this.name == null) 6058 return false; 6059 for (StringType item : this.name) 6060 if (!item.isEmpty()) 6061 return true; 6062 return false; 6063 } 6064 6065 /** 6066 * @return {@link #name} (All of the names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol.) 6067 */ 6068 public StringType addNameElement() {//2 6069 StringType t = new StringType(); 6070 if (this.name == null) 6071 this.name = new ArrayList<StringType>(); 6072 this.name.add(t); 6073 return t; 6074 } 6075 6076 /** 6077 * @param value {@link #name} (All of the names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol.) 6078 */ 6079 public MedicationKnowledge addName(String value) { //1 6080 StringType t = new StringType(); 6081 t.setValue(value); 6082 if (this.name == null) 6083 this.name = new ArrayList<StringType>(); 6084 this.name.add(t); 6085 return this; 6086 } 6087 6088 /** 6089 * @param value {@link #name} (All of the names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol.) 6090 */ 6091 public boolean hasName(String value) { 6092 if (this.name == null) 6093 return false; 6094 for (StringType v : this.name) 6095 if (v.getValue().equals(value)) // string 6096 return true; 6097 return false; 6098 } 6099 6100 /** 6101 * @return {@link #relatedMedicationKnowledge} (Associated or related medications. For example, if the medication is a branded product (e.g. Crestor), this is the Therapeutic Moeity (e.g. Rosuvastatin) or if this is a generic medication (e.g. Rosuvastatin), this would link to a branded product (e.g. Crestor.) 6102 */ 6103 public List<MedicationKnowledgeRelatedMedicationKnowledgeComponent> getRelatedMedicationKnowledge() { 6104 if (this.relatedMedicationKnowledge == null) 6105 this.relatedMedicationKnowledge = new ArrayList<MedicationKnowledgeRelatedMedicationKnowledgeComponent>(); 6106 return this.relatedMedicationKnowledge; 6107 } 6108 6109 /** 6110 * @return Returns a reference to <code>this</code> for easy method chaining 6111 */ 6112 public MedicationKnowledge setRelatedMedicationKnowledge(List<MedicationKnowledgeRelatedMedicationKnowledgeComponent> theRelatedMedicationKnowledge) { 6113 this.relatedMedicationKnowledge = theRelatedMedicationKnowledge; 6114 return this; 6115 } 6116 6117 public boolean hasRelatedMedicationKnowledge() { 6118 if (this.relatedMedicationKnowledge == null) 6119 return false; 6120 for (MedicationKnowledgeRelatedMedicationKnowledgeComponent item : this.relatedMedicationKnowledge) 6121 if (!item.isEmpty()) 6122 return true; 6123 return false; 6124 } 6125 6126 public MedicationKnowledgeRelatedMedicationKnowledgeComponent addRelatedMedicationKnowledge() { //3 6127 MedicationKnowledgeRelatedMedicationKnowledgeComponent t = new MedicationKnowledgeRelatedMedicationKnowledgeComponent(); 6128 if (this.relatedMedicationKnowledge == null) 6129 this.relatedMedicationKnowledge = new ArrayList<MedicationKnowledgeRelatedMedicationKnowledgeComponent>(); 6130 this.relatedMedicationKnowledge.add(t); 6131 return t; 6132 } 6133 6134 public MedicationKnowledge addRelatedMedicationKnowledge(MedicationKnowledgeRelatedMedicationKnowledgeComponent t) { //3 6135 if (t == null) 6136 return this; 6137 if (this.relatedMedicationKnowledge == null) 6138 this.relatedMedicationKnowledge = new ArrayList<MedicationKnowledgeRelatedMedicationKnowledgeComponent>(); 6139 this.relatedMedicationKnowledge.add(t); 6140 return this; 6141 } 6142 6143 /** 6144 * @return The first repetition of repeating field {@link #relatedMedicationKnowledge}, creating it if it does not already exist {3} 6145 */ 6146 public MedicationKnowledgeRelatedMedicationKnowledgeComponent getRelatedMedicationKnowledgeFirstRep() { 6147 if (getRelatedMedicationKnowledge().isEmpty()) { 6148 addRelatedMedicationKnowledge(); 6149 } 6150 return getRelatedMedicationKnowledge().get(0); 6151 } 6152 6153 /** 6154 * @return {@link #associatedMedication} (Links to associated medications that could be prescribed, dispensed or administered.) 6155 */ 6156 public List<Reference> getAssociatedMedication() { 6157 if (this.associatedMedication == null) 6158 this.associatedMedication = new ArrayList<Reference>(); 6159 return this.associatedMedication; 6160 } 6161 6162 /** 6163 * @return Returns a reference to <code>this</code> for easy method chaining 6164 */ 6165 public MedicationKnowledge setAssociatedMedication(List<Reference> theAssociatedMedication) { 6166 this.associatedMedication = theAssociatedMedication; 6167 return this; 6168 } 6169 6170 public boolean hasAssociatedMedication() { 6171 if (this.associatedMedication == null) 6172 return false; 6173 for (Reference item : this.associatedMedication) 6174 if (!item.isEmpty()) 6175 return true; 6176 return false; 6177 } 6178 6179 public Reference addAssociatedMedication() { //3 6180 Reference t = new Reference(); 6181 if (this.associatedMedication == null) 6182 this.associatedMedication = new ArrayList<Reference>(); 6183 this.associatedMedication.add(t); 6184 return t; 6185 } 6186 6187 public MedicationKnowledge addAssociatedMedication(Reference t) { //3 6188 if (t == null) 6189 return this; 6190 if (this.associatedMedication == null) 6191 this.associatedMedication = new ArrayList<Reference>(); 6192 this.associatedMedication.add(t); 6193 return this; 6194 } 6195 6196 /** 6197 * @return The first repetition of repeating field {@link #associatedMedication}, creating it if it does not already exist {3} 6198 */ 6199 public Reference getAssociatedMedicationFirstRep() { 6200 if (getAssociatedMedication().isEmpty()) { 6201 addAssociatedMedication(); 6202 } 6203 return getAssociatedMedication().get(0); 6204 } 6205 6206 /** 6207 * @return {@link #productType} (Category of the medication or product (e.g. branded product, therapeutic moeity, generic product, innovator product, etc.).) 6208 */ 6209 public List<CodeableConcept> getProductType() { 6210 if (this.productType == null) 6211 this.productType = new ArrayList<CodeableConcept>(); 6212 return this.productType; 6213 } 6214 6215 /** 6216 * @return Returns a reference to <code>this</code> for easy method chaining 6217 */ 6218 public MedicationKnowledge setProductType(List<CodeableConcept> theProductType) { 6219 this.productType = theProductType; 6220 return this; 6221 } 6222 6223 public boolean hasProductType() { 6224 if (this.productType == null) 6225 return false; 6226 for (CodeableConcept item : this.productType) 6227 if (!item.isEmpty()) 6228 return true; 6229 return false; 6230 } 6231 6232 public CodeableConcept addProductType() { //3 6233 CodeableConcept t = new CodeableConcept(); 6234 if (this.productType == null) 6235 this.productType = new ArrayList<CodeableConcept>(); 6236 this.productType.add(t); 6237 return t; 6238 } 6239 6240 public MedicationKnowledge addProductType(CodeableConcept t) { //3 6241 if (t == null) 6242 return this; 6243 if (this.productType == null) 6244 this.productType = new ArrayList<CodeableConcept>(); 6245 this.productType.add(t); 6246 return this; 6247 } 6248 6249 /** 6250 * @return The first repetition of repeating field {@link #productType}, creating it if it does not already exist {3} 6251 */ 6252 public CodeableConcept getProductTypeFirstRep() { 6253 if (getProductType().isEmpty()) { 6254 addProductType(); 6255 } 6256 return getProductType().get(0); 6257 } 6258 6259 /** 6260 * @return {@link #monograph} (Associated documentation about the medication.) 6261 */ 6262 public List<MedicationKnowledgeMonographComponent> getMonograph() { 6263 if (this.monograph == null) 6264 this.monograph = new ArrayList<MedicationKnowledgeMonographComponent>(); 6265 return this.monograph; 6266 } 6267 6268 /** 6269 * @return Returns a reference to <code>this</code> for easy method chaining 6270 */ 6271 public MedicationKnowledge setMonograph(List<MedicationKnowledgeMonographComponent> theMonograph) { 6272 this.monograph = theMonograph; 6273 return this; 6274 } 6275 6276 public boolean hasMonograph() { 6277 if (this.monograph == null) 6278 return false; 6279 for (MedicationKnowledgeMonographComponent item : this.monograph) 6280 if (!item.isEmpty()) 6281 return true; 6282 return false; 6283 } 6284 6285 public MedicationKnowledgeMonographComponent addMonograph() { //3 6286 MedicationKnowledgeMonographComponent t = new MedicationKnowledgeMonographComponent(); 6287 if (this.monograph == null) 6288 this.monograph = new ArrayList<MedicationKnowledgeMonographComponent>(); 6289 this.monograph.add(t); 6290 return t; 6291 } 6292 6293 public MedicationKnowledge addMonograph(MedicationKnowledgeMonographComponent t) { //3 6294 if (t == null) 6295 return this; 6296 if (this.monograph == null) 6297 this.monograph = new ArrayList<MedicationKnowledgeMonographComponent>(); 6298 this.monograph.add(t); 6299 return this; 6300 } 6301 6302 /** 6303 * @return The first repetition of repeating field {@link #monograph}, creating it if it does not already exist {3} 6304 */ 6305 public MedicationKnowledgeMonographComponent getMonographFirstRep() { 6306 if (getMonograph().isEmpty()) { 6307 addMonograph(); 6308 } 6309 return getMonograph().get(0); 6310 } 6311 6312 /** 6313 * @return {@link #preparationInstruction} (The instructions for preparing the medication.). This is the underlying object with id, value and extensions. The accessor "getPreparationInstruction" gives direct access to the value 6314 */ 6315 public MarkdownType getPreparationInstructionElement() { 6316 if (this.preparationInstruction == null) 6317 if (Configuration.errorOnAutoCreate()) 6318 throw new Error("Attempt to auto-create MedicationKnowledge.preparationInstruction"); 6319 else if (Configuration.doAutoCreate()) 6320 this.preparationInstruction = new MarkdownType(); // bb 6321 return this.preparationInstruction; 6322 } 6323 6324 public boolean hasPreparationInstructionElement() { 6325 return this.preparationInstruction != null && !this.preparationInstruction.isEmpty(); 6326 } 6327 6328 public boolean hasPreparationInstruction() { 6329 return this.preparationInstruction != null && !this.preparationInstruction.isEmpty(); 6330 } 6331 6332 /** 6333 * @param value {@link #preparationInstruction} (The instructions for preparing the medication.). This is the underlying object with id, value and extensions. The accessor "getPreparationInstruction" gives direct access to the value 6334 */ 6335 public MedicationKnowledge setPreparationInstructionElement(MarkdownType value) { 6336 this.preparationInstruction = value; 6337 return this; 6338 } 6339 6340 /** 6341 * @return The instructions for preparing the medication. 6342 */ 6343 public String getPreparationInstruction() { 6344 return this.preparationInstruction == null ? null : this.preparationInstruction.getValue(); 6345 } 6346 6347 /** 6348 * @param value The instructions for preparing the medication. 6349 */ 6350 public MedicationKnowledge setPreparationInstruction(String value) { 6351 if (Utilities.noString(value)) 6352 this.preparationInstruction = null; 6353 else { 6354 if (this.preparationInstruction == null) 6355 this.preparationInstruction = new MarkdownType(); 6356 this.preparationInstruction.setValue(value); 6357 } 6358 return this; 6359 } 6360 6361 /** 6362 * @return {@link #cost} (The price of the medication.) 6363 */ 6364 public List<MedicationKnowledgeCostComponent> getCost() { 6365 if (this.cost == null) 6366 this.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 6367 return this.cost; 6368 } 6369 6370 /** 6371 * @return Returns a reference to <code>this</code> for easy method chaining 6372 */ 6373 public MedicationKnowledge setCost(List<MedicationKnowledgeCostComponent> theCost) { 6374 this.cost = theCost; 6375 return this; 6376 } 6377 6378 public boolean hasCost() { 6379 if (this.cost == null) 6380 return false; 6381 for (MedicationKnowledgeCostComponent item : this.cost) 6382 if (!item.isEmpty()) 6383 return true; 6384 return false; 6385 } 6386 6387 public MedicationKnowledgeCostComponent addCost() { //3 6388 MedicationKnowledgeCostComponent t = new MedicationKnowledgeCostComponent(); 6389 if (this.cost == null) 6390 this.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 6391 this.cost.add(t); 6392 return t; 6393 } 6394 6395 public MedicationKnowledge addCost(MedicationKnowledgeCostComponent t) { //3 6396 if (t == null) 6397 return this; 6398 if (this.cost == null) 6399 this.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 6400 this.cost.add(t); 6401 return this; 6402 } 6403 6404 /** 6405 * @return The first repetition of repeating field {@link #cost}, creating it if it does not already exist {3} 6406 */ 6407 public MedicationKnowledgeCostComponent getCostFirstRep() { 6408 if (getCost().isEmpty()) { 6409 addCost(); 6410 } 6411 return getCost().get(0); 6412 } 6413 6414 /** 6415 * @return {@link #monitoringProgram} (The program under which the medication is reviewed.) 6416 */ 6417 public List<MedicationKnowledgeMonitoringProgramComponent> getMonitoringProgram() { 6418 if (this.monitoringProgram == null) 6419 this.monitoringProgram = new ArrayList<MedicationKnowledgeMonitoringProgramComponent>(); 6420 return this.monitoringProgram; 6421 } 6422 6423 /** 6424 * @return Returns a reference to <code>this</code> for easy method chaining 6425 */ 6426 public MedicationKnowledge setMonitoringProgram(List<MedicationKnowledgeMonitoringProgramComponent> theMonitoringProgram) { 6427 this.monitoringProgram = theMonitoringProgram; 6428 return this; 6429 } 6430 6431 public boolean hasMonitoringProgram() { 6432 if (this.monitoringProgram == null) 6433 return false; 6434 for (MedicationKnowledgeMonitoringProgramComponent item : this.monitoringProgram) 6435 if (!item.isEmpty()) 6436 return true; 6437 return false; 6438 } 6439 6440 public MedicationKnowledgeMonitoringProgramComponent addMonitoringProgram() { //3 6441 MedicationKnowledgeMonitoringProgramComponent t = new MedicationKnowledgeMonitoringProgramComponent(); 6442 if (this.monitoringProgram == null) 6443 this.monitoringProgram = new ArrayList<MedicationKnowledgeMonitoringProgramComponent>(); 6444 this.monitoringProgram.add(t); 6445 return t; 6446 } 6447 6448 public MedicationKnowledge addMonitoringProgram(MedicationKnowledgeMonitoringProgramComponent t) { //3 6449 if (t == null) 6450 return this; 6451 if (this.monitoringProgram == null) 6452 this.monitoringProgram = new ArrayList<MedicationKnowledgeMonitoringProgramComponent>(); 6453 this.monitoringProgram.add(t); 6454 return this; 6455 } 6456 6457 /** 6458 * @return The first repetition of repeating field {@link #monitoringProgram}, creating it if it does not already exist {3} 6459 */ 6460 public MedicationKnowledgeMonitoringProgramComponent getMonitoringProgramFirstRep() { 6461 if (getMonitoringProgram().isEmpty()) { 6462 addMonitoringProgram(); 6463 } 6464 return getMonitoringProgram().get(0); 6465 } 6466 6467 /** 6468 * @return {@link #indicationGuideline} (Guidelines or protocols that are applicable for the administration of the medication based on indication.) 6469 */ 6470 public List<MedicationKnowledgeIndicationGuidelineComponent> getIndicationGuideline() { 6471 if (this.indicationGuideline == null) 6472 this.indicationGuideline = new ArrayList<MedicationKnowledgeIndicationGuidelineComponent>(); 6473 return this.indicationGuideline; 6474 } 6475 6476 /** 6477 * @return Returns a reference to <code>this</code> for easy method chaining 6478 */ 6479 public MedicationKnowledge setIndicationGuideline(List<MedicationKnowledgeIndicationGuidelineComponent> theIndicationGuideline) { 6480 this.indicationGuideline = theIndicationGuideline; 6481 return this; 6482 } 6483 6484 public boolean hasIndicationGuideline() { 6485 if (this.indicationGuideline == null) 6486 return false; 6487 for (MedicationKnowledgeIndicationGuidelineComponent item : this.indicationGuideline) 6488 if (!item.isEmpty()) 6489 return true; 6490 return false; 6491 } 6492 6493 public MedicationKnowledgeIndicationGuidelineComponent addIndicationGuideline() { //3 6494 MedicationKnowledgeIndicationGuidelineComponent t = new MedicationKnowledgeIndicationGuidelineComponent(); 6495 if (this.indicationGuideline == null) 6496 this.indicationGuideline = new ArrayList<MedicationKnowledgeIndicationGuidelineComponent>(); 6497 this.indicationGuideline.add(t); 6498 return t; 6499 } 6500 6501 public MedicationKnowledge addIndicationGuideline(MedicationKnowledgeIndicationGuidelineComponent t) { //3 6502 if (t == null) 6503 return this; 6504 if (this.indicationGuideline == null) 6505 this.indicationGuideline = new ArrayList<MedicationKnowledgeIndicationGuidelineComponent>(); 6506 this.indicationGuideline.add(t); 6507 return this; 6508 } 6509 6510 /** 6511 * @return The first repetition of repeating field {@link #indicationGuideline}, creating it if it does not already exist {3} 6512 */ 6513 public MedicationKnowledgeIndicationGuidelineComponent getIndicationGuidelineFirstRep() { 6514 if (getIndicationGuideline().isEmpty()) { 6515 addIndicationGuideline(); 6516 } 6517 return getIndicationGuideline().get(0); 6518 } 6519 6520 /** 6521 * @return {@link #medicineClassification} (Categorization of the medication within a formulary or classification system.) 6522 */ 6523 public List<MedicationKnowledgeMedicineClassificationComponent> getMedicineClassification() { 6524 if (this.medicineClassification == null) 6525 this.medicineClassification = new ArrayList<MedicationKnowledgeMedicineClassificationComponent>(); 6526 return this.medicineClassification; 6527 } 6528 6529 /** 6530 * @return Returns a reference to <code>this</code> for easy method chaining 6531 */ 6532 public MedicationKnowledge setMedicineClassification(List<MedicationKnowledgeMedicineClassificationComponent> theMedicineClassification) { 6533 this.medicineClassification = theMedicineClassification; 6534 return this; 6535 } 6536 6537 public boolean hasMedicineClassification() { 6538 if (this.medicineClassification == null) 6539 return false; 6540 for (MedicationKnowledgeMedicineClassificationComponent item : this.medicineClassification) 6541 if (!item.isEmpty()) 6542 return true; 6543 return false; 6544 } 6545 6546 public MedicationKnowledgeMedicineClassificationComponent addMedicineClassification() { //3 6547 MedicationKnowledgeMedicineClassificationComponent t = new MedicationKnowledgeMedicineClassificationComponent(); 6548 if (this.medicineClassification == null) 6549 this.medicineClassification = new ArrayList<MedicationKnowledgeMedicineClassificationComponent>(); 6550 this.medicineClassification.add(t); 6551 return t; 6552 } 6553 6554 public MedicationKnowledge addMedicineClassification(MedicationKnowledgeMedicineClassificationComponent t) { //3 6555 if (t == null) 6556 return this; 6557 if (this.medicineClassification == null) 6558 this.medicineClassification = new ArrayList<MedicationKnowledgeMedicineClassificationComponent>(); 6559 this.medicineClassification.add(t); 6560 return this; 6561 } 6562 6563 /** 6564 * @return The first repetition of repeating field {@link #medicineClassification}, creating it if it does not already exist {3} 6565 */ 6566 public MedicationKnowledgeMedicineClassificationComponent getMedicineClassificationFirstRep() { 6567 if (getMedicineClassification().isEmpty()) { 6568 addMedicineClassification(); 6569 } 6570 return getMedicineClassification().get(0); 6571 } 6572 6573 /** 6574 * @return {@link #packaging} (Information that only applies to packages (not products).) 6575 */ 6576 public List<MedicationKnowledgePackagingComponent> getPackaging() { 6577 if (this.packaging == null) 6578 this.packaging = new ArrayList<MedicationKnowledgePackagingComponent>(); 6579 return this.packaging; 6580 } 6581 6582 /** 6583 * @return Returns a reference to <code>this</code> for easy method chaining 6584 */ 6585 public MedicationKnowledge setPackaging(List<MedicationKnowledgePackagingComponent> thePackaging) { 6586 this.packaging = thePackaging; 6587 return this; 6588 } 6589 6590 public boolean hasPackaging() { 6591 if (this.packaging == null) 6592 return false; 6593 for (MedicationKnowledgePackagingComponent item : this.packaging) 6594 if (!item.isEmpty()) 6595 return true; 6596 return false; 6597 } 6598 6599 public MedicationKnowledgePackagingComponent addPackaging() { //3 6600 MedicationKnowledgePackagingComponent t = new MedicationKnowledgePackagingComponent(); 6601 if (this.packaging == null) 6602 this.packaging = new ArrayList<MedicationKnowledgePackagingComponent>(); 6603 this.packaging.add(t); 6604 return t; 6605 } 6606 6607 public MedicationKnowledge addPackaging(MedicationKnowledgePackagingComponent t) { //3 6608 if (t == null) 6609 return this; 6610 if (this.packaging == null) 6611 this.packaging = new ArrayList<MedicationKnowledgePackagingComponent>(); 6612 this.packaging.add(t); 6613 return this; 6614 } 6615 6616 /** 6617 * @return The first repetition of repeating field {@link #packaging}, creating it if it does not already exist {3} 6618 */ 6619 public MedicationKnowledgePackagingComponent getPackagingFirstRep() { 6620 if (getPackaging().isEmpty()) { 6621 addPackaging(); 6622 } 6623 return getPackaging().get(0); 6624 } 6625 6626 /** 6627 * @return {@link #clinicalUseIssue} (Potential clinical issue with or between medication(s) (for example, drug-drug interaction, drug-disease contraindication, drug-allergy interaction, etc.).) 6628 */ 6629 public List<Reference> getClinicalUseIssue() { 6630 if (this.clinicalUseIssue == null) 6631 this.clinicalUseIssue = new ArrayList<Reference>(); 6632 return this.clinicalUseIssue; 6633 } 6634 6635 /** 6636 * @return Returns a reference to <code>this</code> for easy method chaining 6637 */ 6638 public MedicationKnowledge setClinicalUseIssue(List<Reference> theClinicalUseIssue) { 6639 this.clinicalUseIssue = theClinicalUseIssue; 6640 return this; 6641 } 6642 6643 public boolean hasClinicalUseIssue() { 6644 if (this.clinicalUseIssue == null) 6645 return false; 6646 for (Reference item : this.clinicalUseIssue) 6647 if (!item.isEmpty()) 6648 return true; 6649 return false; 6650 } 6651 6652 public Reference addClinicalUseIssue() { //3 6653 Reference t = new Reference(); 6654 if (this.clinicalUseIssue == null) 6655 this.clinicalUseIssue = new ArrayList<Reference>(); 6656 this.clinicalUseIssue.add(t); 6657 return t; 6658 } 6659 6660 public MedicationKnowledge addClinicalUseIssue(Reference t) { //3 6661 if (t == null) 6662 return this; 6663 if (this.clinicalUseIssue == null) 6664 this.clinicalUseIssue = new ArrayList<Reference>(); 6665 this.clinicalUseIssue.add(t); 6666 return this; 6667 } 6668 6669 /** 6670 * @return The first repetition of repeating field {@link #clinicalUseIssue}, creating it if it does not already exist {3} 6671 */ 6672 public Reference getClinicalUseIssueFirstRep() { 6673 if (getClinicalUseIssue().isEmpty()) { 6674 addClinicalUseIssue(); 6675 } 6676 return getClinicalUseIssue().get(0); 6677 } 6678 6679 /** 6680 * @return {@link #storageGuideline} (Information on how the medication should be stored, for example, refrigeration temperatures and length of stability at a given temperature.) 6681 */ 6682 public List<MedicationKnowledgeStorageGuidelineComponent> getStorageGuideline() { 6683 if (this.storageGuideline == null) 6684 this.storageGuideline = new ArrayList<MedicationKnowledgeStorageGuidelineComponent>(); 6685 return this.storageGuideline; 6686 } 6687 6688 /** 6689 * @return Returns a reference to <code>this</code> for easy method chaining 6690 */ 6691 public MedicationKnowledge setStorageGuideline(List<MedicationKnowledgeStorageGuidelineComponent> theStorageGuideline) { 6692 this.storageGuideline = theStorageGuideline; 6693 return this; 6694 } 6695 6696 public boolean hasStorageGuideline() { 6697 if (this.storageGuideline == null) 6698 return false; 6699 for (MedicationKnowledgeStorageGuidelineComponent item : this.storageGuideline) 6700 if (!item.isEmpty()) 6701 return true; 6702 return false; 6703 } 6704 6705 public MedicationKnowledgeStorageGuidelineComponent addStorageGuideline() { //3 6706 MedicationKnowledgeStorageGuidelineComponent t = new MedicationKnowledgeStorageGuidelineComponent(); 6707 if (this.storageGuideline == null) 6708 this.storageGuideline = new ArrayList<MedicationKnowledgeStorageGuidelineComponent>(); 6709 this.storageGuideline.add(t); 6710 return t; 6711 } 6712 6713 public MedicationKnowledge addStorageGuideline(MedicationKnowledgeStorageGuidelineComponent t) { //3 6714 if (t == null) 6715 return this; 6716 if (this.storageGuideline == null) 6717 this.storageGuideline = new ArrayList<MedicationKnowledgeStorageGuidelineComponent>(); 6718 this.storageGuideline.add(t); 6719 return this; 6720 } 6721 6722 /** 6723 * @return The first repetition of repeating field {@link #storageGuideline}, creating it if it does not already exist {3} 6724 */ 6725 public MedicationKnowledgeStorageGuidelineComponent getStorageGuidelineFirstRep() { 6726 if (getStorageGuideline().isEmpty()) { 6727 addStorageGuideline(); 6728 } 6729 return getStorageGuideline().get(0); 6730 } 6731 6732 /** 6733 * @return {@link #regulatory} (Regulatory information about a medication.) 6734 */ 6735 public List<MedicationKnowledgeRegulatoryComponent> getRegulatory() { 6736 if (this.regulatory == null) 6737 this.regulatory = new ArrayList<MedicationKnowledgeRegulatoryComponent>(); 6738 return this.regulatory; 6739 } 6740 6741 /** 6742 * @return Returns a reference to <code>this</code> for easy method chaining 6743 */ 6744 public MedicationKnowledge setRegulatory(List<MedicationKnowledgeRegulatoryComponent> theRegulatory) { 6745 this.regulatory = theRegulatory; 6746 return this; 6747 } 6748 6749 public boolean hasRegulatory() { 6750 if (this.regulatory == null) 6751 return false; 6752 for (MedicationKnowledgeRegulatoryComponent item : this.regulatory) 6753 if (!item.isEmpty()) 6754 return true; 6755 return false; 6756 } 6757 6758 public MedicationKnowledgeRegulatoryComponent addRegulatory() { //3 6759 MedicationKnowledgeRegulatoryComponent t = new MedicationKnowledgeRegulatoryComponent(); 6760 if (this.regulatory == null) 6761 this.regulatory = new ArrayList<MedicationKnowledgeRegulatoryComponent>(); 6762 this.regulatory.add(t); 6763 return t; 6764 } 6765 6766 public MedicationKnowledge addRegulatory(MedicationKnowledgeRegulatoryComponent t) { //3 6767 if (t == null) 6768 return this; 6769 if (this.regulatory == null) 6770 this.regulatory = new ArrayList<MedicationKnowledgeRegulatoryComponent>(); 6771 this.regulatory.add(t); 6772 return this; 6773 } 6774 6775 /** 6776 * @return The first repetition of repeating field {@link #regulatory}, creating it if it does not already exist {3} 6777 */ 6778 public MedicationKnowledgeRegulatoryComponent getRegulatoryFirstRep() { 6779 if (getRegulatory().isEmpty()) { 6780 addRegulatory(); 6781 } 6782 return getRegulatory().get(0); 6783 } 6784 6785 /** 6786 * @return {@link #definitional} (Along with the link to a Medicinal Product Definition resource, this information provides common definitional elements that are needed to understand the specific medication that is being described.) 6787 */ 6788 public MedicationKnowledgeDefinitionalComponent getDefinitional() { 6789 if (this.definitional == null) 6790 if (Configuration.errorOnAutoCreate()) 6791 throw new Error("Attempt to auto-create MedicationKnowledge.definitional"); 6792 else if (Configuration.doAutoCreate()) 6793 this.definitional = new MedicationKnowledgeDefinitionalComponent(); // cc 6794 return this.definitional; 6795 } 6796 6797 public boolean hasDefinitional() { 6798 return this.definitional != null && !this.definitional.isEmpty(); 6799 } 6800 6801 /** 6802 * @param value {@link #definitional} (Along with the link to a Medicinal Product Definition resource, this information provides common definitional elements that are needed to understand the specific medication that is being described.) 6803 */ 6804 public MedicationKnowledge setDefinitional(MedicationKnowledgeDefinitionalComponent value) { 6805 this.definitional = value; 6806 return this; 6807 } 6808 6809 protected void listChildren(List<Property> children) { 6810 super.listChildren(children); 6811 children.add(new Property("identifier", "Identifier", "Business identifier for this medication.", 0, java.lang.Integer.MAX_VALUE, identifier)); 6812 children.add(new Property("code", "CodeableConcept", "A code that specifies this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.", 0, 1, code)); 6813 children.add(new Property("status", "code", "A code to indicate if the medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. The status refers to the validity about the information of the medication and not to its medicinal properties.", 0, 1, status)); 6814 children.add(new Property("author", "Reference(Organization)", "The creator or owner of the knowledge or information about the medication.", 0, 1, author)); 6815 children.add(new Property("intendedJurisdiction", "CodeableConcept", "Lists the jurisdictions that this medication knowledge was written for.", 0, java.lang.Integer.MAX_VALUE, intendedJurisdiction)); 6816 children.add(new Property("name", "string", "All of the names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol.", 0, java.lang.Integer.MAX_VALUE, name)); 6817 children.add(new Property("relatedMedicationKnowledge", "", "Associated or related medications. For example, if the medication is a branded product (e.g. Crestor), this is the Therapeutic Moeity (e.g. Rosuvastatin) or if this is a generic medication (e.g. Rosuvastatin), this would link to a branded product (e.g. Crestor.", 0, java.lang.Integer.MAX_VALUE, relatedMedicationKnowledge)); 6818 children.add(new Property("associatedMedication", "Reference(Medication)", "Links to associated medications that could be prescribed, dispensed or administered.", 0, java.lang.Integer.MAX_VALUE, associatedMedication)); 6819 children.add(new Property("productType", "CodeableConcept", "Category of the medication or product (e.g. branded product, therapeutic moeity, generic product, innovator product, etc.).", 0, java.lang.Integer.MAX_VALUE, productType)); 6820 children.add(new Property("monograph", "", "Associated documentation about the medication.", 0, java.lang.Integer.MAX_VALUE, monograph)); 6821 children.add(new Property("preparationInstruction", "markdown", "The instructions for preparing the medication.", 0, 1, preparationInstruction)); 6822 children.add(new Property("cost", "", "The price of the medication.", 0, java.lang.Integer.MAX_VALUE, cost)); 6823 children.add(new Property("monitoringProgram", "", "The program under which the medication is reviewed.", 0, java.lang.Integer.MAX_VALUE, monitoringProgram)); 6824 children.add(new Property("indicationGuideline", "", "Guidelines or protocols that are applicable for the administration of the medication based on indication.", 0, java.lang.Integer.MAX_VALUE, indicationGuideline)); 6825 children.add(new Property("medicineClassification", "", "Categorization of the medication within a formulary or classification system.", 0, java.lang.Integer.MAX_VALUE, medicineClassification)); 6826 children.add(new Property("packaging", "", "Information that only applies to packages (not products).", 0, java.lang.Integer.MAX_VALUE, packaging)); 6827 children.add(new Property("clinicalUseIssue", "Reference(ClinicalUseDefinition)", "Potential clinical issue with or between medication(s) (for example, drug-drug interaction, drug-disease contraindication, drug-allergy interaction, etc.).", 0, java.lang.Integer.MAX_VALUE, clinicalUseIssue)); 6828 children.add(new Property("storageGuideline", "", "Information on how the medication should be stored, for example, refrigeration temperatures and length of stability at a given temperature.", 0, java.lang.Integer.MAX_VALUE, storageGuideline)); 6829 children.add(new Property("regulatory", "", "Regulatory information about a medication.", 0, java.lang.Integer.MAX_VALUE, regulatory)); 6830 children.add(new Property("definitional", "", "Along with the link to a Medicinal Product Definition resource, this information provides common definitional elements that are needed to understand the specific medication that is being described.", 0, 1, definitional)); 6831 } 6832 6833 @Override 6834 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6835 switch (_hash) { 6836 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Business identifier for this medication.", 0, java.lang.Integer.MAX_VALUE, identifier); 6837 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code that specifies this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.", 0, 1, code); 6838 case -892481550: /*status*/ return new Property("status", "code", "A code to indicate if the medication referred to by this MedicationKnowledge is in active use within the drug database or inventory system. The status refers to the validity about the information of the medication and not to its medicinal properties.", 0, 1, status); 6839 case -1406328437: /*author*/ return new Property("author", "Reference(Organization)", "The creator or owner of the knowledge or information about the medication.", 0, 1, author); 6840 case 2136596300: /*intendedJurisdiction*/ return new Property("intendedJurisdiction", "CodeableConcept", "Lists the jurisdictions that this medication knowledge was written for.", 0, java.lang.Integer.MAX_VALUE, intendedJurisdiction); 6841 case 3373707: /*name*/ return new Property("name", "string", "All of the names for a medication, for example, the name(s) given to a medication in different countries. For example, acetaminophen and paracetamol or salbutamol and albuterol.", 0, java.lang.Integer.MAX_VALUE, name); 6842 case 723067972: /*relatedMedicationKnowledge*/ return new Property("relatedMedicationKnowledge", "", "Associated or related medications. For example, if the medication is a branded product (e.g. Crestor), this is the Therapeutic Moeity (e.g. Rosuvastatin) or if this is a generic medication (e.g. Rosuvastatin), this would link to a branded product (e.g. Crestor.", 0, java.lang.Integer.MAX_VALUE, relatedMedicationKnowledge); 6843 case 1312779381: /*associatedMedication*/ return new Property("associatedMedication", "Reference(Medication)", "Links to associated medications that could be prescribed, dispensed or administered.", 0, java.lang.Integer.MAX_VALUE, associatedMedication); 6844 case -1491615543: /*productType*/ return new Property("productType", "CodeableConcept", "Category of the medication or product (e.g. branded product, therapeutic moeity, generic product, innovator product, etc.).", 0, java.lang.Integer.MAX_VALUE, productType); 6845 case -1442980789: /*monograph*/ return new Property("monograph", "", "Associated documentation about the medication.", 0, java.lang.Integer.MAX_VALUE, monograph); 6846 case 1025456503: /*preparationInstruction*/ return new Property("preparationInstruction", "markdown", "The instructions for preparing the medication.", 0, 1, preparationInstruction); 6847 case 3059661: /*cost*/ return new Property("cost", "", "The price of the medication.", 0, java.lang.Integer.MAX_VALUE, cost); 6848 case 569848092: /*monitoringProgram*/ return new Property("monitoringProgram", "", "The program under which the medication is reviewed.", 0, java.lang.Integer.MAX_VALUE, monitoringProgram); 6849 case -347044108: /*indicationGuideline*/ return new Property("indicationGuideline", "", "Guidelines or protocols that are applicable for the administration of the medication based on indication.", 0, java.lang.Integer.MAX_VALUE, indicationGuideline); 6850 case 1791551680: /*medicineClassification*/ return new Property("medicineClassification", "", "Categorization of the medication within a formulary or classification system.", 0, java.lang.Integer.MAX_VALUE, medicineClassification); 6851 case 1802065795: /*packaging*/ return new Property("packaging", "", "Information that only applies to packages (not products).", 0, java.lang.Integer.MAX_VALUE, packaging); 6852 case 251885509: /*clinicalUseIssue*/ return new Property("clinicalUseIssue", "Reference(ClinicalUseDefinition)", "Potential clinical issue with or between medication(s) (for example, drug-drug interaction, drug-disease contraindication, drug-allergy interaction, etc.).", 0, java.lang.Integer.MAX_VALUE, clinicalUseIssue); 6853 case 1618773173: /*storageGuideline*/ return new Property("storageGuideline", "", "Information on how the medication should be stored, for example, refrigeration temperatures and length of stability at a given temperature.", 0, java.lang.Integer.MAX_VALUE, storageGuideline); 6854 case -27327848: /*regulatory*/ return new Property("regulatory", "", "Regulatory information about a medication.", 0, java.lang.Integer.MAX_VALUE, regulatory); 6855 case 101791934: /*definitional*/ return new Property("definitional", "", "Along with the link to a Medicinal Product Definition resource, this information provides common definitional elements that are needed to understand the specific medication that is being described.", 0, 1, definitional); 6856 default: return super.getNamedProperty(_hash, _name, _checkValid); 6857 } 6858 6859 } 6860 6861 @Override 6862 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6863 switch (hash) { 6864 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 6865 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 6866 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MedicationKnowledgeStatusCodes> 6867 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 6868 case 2136596300: /*intendedJurisdiction*/ return this.intendedJurisdiction == null ? new Base[0] : this.intendedJurisdiction.toArray(new Base[this.intendedJurisdiction.size()]); // CodeableConcept 6869 case 3373707: /*name*/ return this.name == null ? new Base[0] : this.name.toArray(new Base[this.name.size()]); // StringType 6870 case 723067972: /*relatedMedicationKnowledge*/ return this.relatedMedicationKnowledge == null ? new Base[0] : this.relatedMedicationKnowledge.toArray(new Base[this.relatedMedicationKnowledge.size()]); // MedicationKnowledgeRelatedMedicationKnowledgeComponent 6871 case 1312779381: /*associatedMedication*/ return this.associatedMedication == null ? new Base[0] : this.associatedMedication.toArray(new Base[this.associatedMedication.size()]); // Reference 6872 case -1491615543: /*productType*/ return this.productType == null ? new Base[0] : this.productType.toArray(new Base[this.productType.size()]); // CodeableConcept 6873 case -1442980789: /*monograph*/ return this.monograph == null ? new Base[0] : this.monograph.toArray(new Base[this.monograph.size()]); // MedicationKnowledgeMonographComponent 6874 case 1025456503: /*preparationInstruction*/ return this.preparationInstruction == null ? new Base[0] : new Base[] {this.preparationInstruction}; // MarkdownType 6875 case 3059661: /*cost*/ return this.cost == null ? new Base[0] : this.cost.toArray(new Base[this.cost.size()]); // MedicationKnowledgeCostComponent 6876 case 569848092: /*monitoringProgram*/ return this.monitoringProgram == null ? new Base[0] : this.monitoringProgram.toArray(new Base[this.monitoringProgram.size()]); // MedicationKnowledgeMonitoringProgramComponent 6877 case -347044108: /*indicationGuideline*/ return this.indicationGuideline == null ? new Base[0] : this.indicationGuideline.toArray(new Base[this.indicationGuideline.size()]); // MedicationKnowledgeIndicationGuidelineComponent 6878 case 1791551680: /*medicineClassification*/ return this.medicineClassification == null ? new Base[0] : this.medicineClassification.toArray(new Base[this.medicineClassification.size()]); // MedicationKnowledgeMedicineClassificationComponent 6879 case 1802065795: /*packaging*/ return this.packaging == null ? new Base[0] : this.packaging.toArray(new Base[this.packaging.size()]); // MedicationKnowledgePackagingComponent 6880 case 251885509: /*clinicalUseIssue*/ return this.clinicalUseIssue == null ? new Base[0] : this.clinicalUseIssue.toArray(new Base[this.clinicalUseIssue.size()]); // Reference 6881 case 1618773173: /*storageGuideline*/ return this.storageGuideline == null ? new Base[0] : this.storageGuideline.toArray(new Base[this.storageGuideline.size()]); // MedicationKnowledgeStorageGuidelineComponent 6882 case -27327848: /*regulatory*/ return this.regulatory == null ? new Base[0] : this.regulatory.toArray(new Base[this.regulatory.size()]); // MedicationKnowledgeRegulatoryComponent 6883 case 101791934: /*definitional*/ return this.definitional == null ? new Base[0] : new Base[] {this.definitional}; // MedicationKnowledgeDefinitionalComponent 6884 default: return super.getProperty(hash, name, checkValid); 6885 } 6886 6887 } 6888 6889 @Override 6890 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6891 switch (hash) { 6892 case -1618432855: // identifier 6893 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 6894 return value; 6895 case 3059181: // code 6896 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6897 return value; 6898 case -892481550: // status 6899 value = new MedicationKnowledgeStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 6900 this.status = (Enumeration) value; // Enumeration<MedicationKnowledgeStatusCodes> 6901 return value; 6902 case -1406328437: // author 6903 this.author = TypeConvertor.castToReference(value); // Reference 6904 return value; 6905 case 2136596300: // intendedJurisdiction 6906 this.getIntendedJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6907 return value; 6908 case 3373707: // name 6909 this.getName().add(TypeConvertor.castToString(value)); // StringType 6910 return value; 6911 case 723067972: // relatedMedicationKnowledge 6912 this.getRelatedMedicationKnowledge().add((MedicationKnowledgeRelatedMedicationKnowledgeComponent) value); // MedicationKnowledgeRelatedMedicationKnowledgeComponent 6913 return value; 6914 case 1312779381: // associatedMedication 6915 this.getAssociatedMedication().add(TypeConvertor.castToReference(value)); // Reference 6916 return value; 6917 case -1491615543: // productType 6918 this.getProductType().add(TypeConvertor.castToCodeableConcept(value)); // CodeableConcept 6919 return value; 6920 case -1442980789: // monograph 6921 this.getMonograph().add((MedicationKnowledgeMonographComponent) value); // MedicationKnowledgeMonographComponent 6922 return value; 6923 case 1025456503: // preparationInstruction 6924 this.preparationInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 6925 return value; 6926 case 3059661: // cost 6927 this.getCost().add((MedicationKnowledgeCostComponent) value); // MedicationKnowledgeCostComponent 6928 return value; 6929 case 569848092: // monitoringProgram 6930 this.getMonitoringProgram().add((MedicationKnowledgeMonitoringProgramComponent) value); // MedicationKnowledgeMonitoringProgramComponent 6931 return value; 6932 case -347044108: // indicationGuideline 6933 this.getIndicationGuideline().add((MedicationKnowledgeIndicationGuidelineComponent) value); // MedicationKnowledgeIndicationGuidelineComponent 6934 return value; 6935 case 1791551680: // medicineClassification 6936 this.getMedicineClassification().add((MedicationKnowledgeMedicineClassificationComponent) value); // MedicationKnowledgeMedicineClassificationComponent 6937 return value; 6938 case 1802065795: // packaging 6939 this.getPackaging().add((MedicationKnowledgePackagingComponent) value); // MedicationKnowledgePackagingComponent 6940 return value; 6941 case 251885509: // clinicalUseIssue 6942 this.getClinicalUseIssue().add(TypeConvertor.castToReference(value)); // Reference 6943 return value; 6944 case 1618773173: // storageGuideline 6945 this.getStorageGuideline().add((MedicationKnowledgeStorageGuidelineComponent) value); // MedicationKnowledgeStorageGuidelineComponent 6946 return value; 6947 case -27327848: // regulatory 6948 this.getRegulatory().add((MedicationKnowledgeRegulatoryComponent) value); // MedicationKnowledgeRegulatoryComponent 6949 return value; 6950 case 101791934: // definitional 6951 this.definitional = (MedicationKnowledgeDefinitionalComponent) value; // MedicationKnowledgeDefinitionalComponent 6952 return value; 6953 default: return super.setProperty(hash, name, value); 6954 } 6955 6956 } 6957 6958 @Override 6959 public Base setProperty(String name, Base value) throws FHIRException { 6960 if (name.equals("identifier")) { 6961 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 6962 } else if (name.equals("code")) { 6963 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 6964 } else if (name.equals("status")) { 6965 value = new MedicationKnowledgeStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 6966 this.status = (Enumeration) value; // Enumeration<MedicationKnowledgeStatusCodes> 6967 } else if (name.equals("author")) { 6968 this.author = TypeConvertor.castToReference(value); // Reference 6969 } else if (name.equals("intendedJurisdiction")) { 6970 this.getIntendedJurisdiction().add(TypeConvertor.castToCodeableConcept(value)); 6971 } else if (name.equals("name")) { 6972 this.getName().add(TypeConvertor.castToString(value)); 6973 } else if (name.equals("relatedMedicationKnowledge")) { 6974 this.getRelatedMedicationKnowledge().add((MedicationKnowledgeRelatedMedicationKnowledgeComponent) value); 6975 } else if (name.equals("associatedMedication")) { 6976 this.getAssociatedMedication().add(TypeConvertor.castToReference(value)); 6977 } else if (name.equals("productType")) { 6978 this.getProductType().add(TypeConvertor.castToCodeableConcept(value)); 6979 } else if (name.equals("monograph")) { 6980 this.getMonograph().add((MedicationKnowledgeMonographComponent) value); 6981 } else if (name.equals("preparationInstruction")) { 6982 this.preparationInstruction = TypeConvertor.castToMarkdown(value); // MarkdownType 6983 } else if (name.equals("cost")) { 6984 this.getCost().add((MedicationKnowledgeCostComponent) value); 6985 } else if (name.equals("monitoringProgram")) { 6986 this.getMonitoringProgram().add((MedicationKnowledgeMonitoringProgramComponent) value); 6987 } else if (name.equals("indicationGuideline")) { 6988 this.getIndicationGuideline().add((MedicationKnowledgeIndicationGuidelineComponent) value); 6989 } else if (name.equals("medicineClassification")) { 6990 this.getMedicineClassification().add((MedicationKnowledgeMedicineClassificationComponent) value); 6991 } else if (name.equals("packaging")) { 6992 this.getPackaging().add((MedicationKnowledgePackagingComponent) value); 6993 } else if (name.equals("clinicalUseIssue")) { 6994 this.getClinicalUseIssue().add(TypeConvertor.castToReference(value)); 6995 } else if (name.equals("storageGuideline")) { 6996 this.getStorageGuideline().add((MedicationKnowledgeStorageGuidelineComponent) value); 6997 } else if (name.equals("regulatory")) { 6998 this.getRegulatory().add((MedicationKnowledgeRegulatoryComponent) value); 6999 } else if (name.equals("definitional")) { 7000 this.definitional = (MedicationKnowledgeDefinitionalComponent) value; // MedicationKnowledgeDefinitionalComponent 7001 } else 7002 return super.setProperty(name, value); 7003 return value; 7004 } 7005 7006 @Override 7007 public void removeChild(String name, Base value) throws FHIRException { 7008 if (name.equals("identifier")) { 7009 this.getIdentifier().remove(value); 7010 } else if (name.equals("code")) { 7011 this.code = null; 7012 } else if (name.equals("status")) { 7013 value = new MedicationKnowledgeStatusCodesEnumFactory().fromType(TypeConvertor.castToCode(value)); 7014 this.status = (Enumeration) value; // Enumeration<MedicationKnowledgeStatusCodes> 7015 } else if (name.equals("author")) { 7016 this.author = null; 7017 } else if (name.equals("intendedJurisdiction")) { 7018 this.getIntendedJurisdiction().remove(value); 7019 } else if (name.equals("name")) { 7020 this.getName().remove(value); 7021 } else if (name.equals("relatedMedicationKnowledge")) { 7022 this.getRelatedMedicationKnowledge().remove((MedicationKnowledgeRelatedMedicationKnowledgeComponent) value); 7023 } else if (name.equals("associatedMedication")) { 7024 this.getAssociatedMedication().remove(value); 7025 } else if (name.equals("productType")) { 7026 this.getProductType().remove(value); 7027 } else if (name.equals("monograph")) { 7028 this.getMonograph().remove((MedicationKnowledgeMonographComponent) value); 7029 } else if (name.equals("preparationInstruction")) { 7030 this.preparationInstruction = null; 7031 } else if (name.equals("cost")) { 7032 this.getCost().remove((MedicationKnowledgeCostComponent) value); 7033 } else if (name.equals("monitoringProgram")) { 7034 this.getMonitoringProgram().remove((MedicationKnowledgeMonitoringProgramComponent) value); 7035 } else if (name.equals("indicationGuideline")) { 7036 this.getIndicationGuideline().remove((MedicationKnowledgeIndicationGuidelineComponent) value); 7037 } else if (name.equals("medicineClassification")) { 7038 this.getMedicineClassification().remove((MedicationKnowledgeMedicineClassificationComponent) value); 7039 } else if (name.equals("packaging")) { 7040 this.getPackaging().remove((MedicationKnowledgePackagingComponent) value); 7041 } else if (name.equals("clinicalUseIssue")) { 7042 this.getClinicalUseIssue().remove(value); 7043 } else if (name.equals("storageGuideline")) { 7044 this.getStorageGuideline().remove((MedicationKnowledgeStorageGuidelineComponent) value); 7045 } else if (name.equals("regulatory")) { 7046 this.getRegulatory().remove((MedicationKnowledgeRegulatoryComponent) value); 7047 } else if (name.equals("definitional")) { 7048 this.definitional = (MedicationKnowledgeDefinitionalComponent) value; // MedicationKnowledgeDefinitionalComponent 7049 } else 7050 super.removeChild(name, value); 7051 7052 } 7053 7054 @Override 7055 public Base makeProperty(int hash, String name) throws FHIRException { 7056 switch (hash) { 7057 case -1618432855: return addIdentifier(); 7058 case 3059181: return getCode(); 7059 case -892481550: return getStatusElement(); 7060 case -1406328437: return getAuthor(); 7061 case 2136596300: return addIntendedJurisdiction(); 7062 case 3373707: return addNameElement(); 7063 case 723067972: return addRelatedMedicationKnowledge(); 7064 case 1312779381: return addAssociatedMedication(); 7065 case -1491615543: return addProductType(); 7066 case -1442980789: return addMonograph(); 7067 case 1025456503: return getPreparationInstructionElement(); 7068 case 3059661: return addCost(); 7069 case 569848092: return addMonitoringProgram(); 7070 case -347044108: return addIndicationGuideline(); 7071 case 1791551680: return addMedicineClassification(); 7072 case 1802065795: return addPackaging(); 7073 case 251885509: return addClinicalUseIssue(); 7074 case 1618773173: return addStorageGuideline(); 7075 case -27327848: return addRegulatory(); 7076 case 101791934: return getDefinitional(); 7077 default: return super.makeProperty(hash, name); 7078 } 7079 7080 } 7081 7082 @Override 7083 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7084 switch (hash) { 7085 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 7086 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 7087 case -892481550: /*status*/ return new String[] {"code"}; 7088 case -1406328437: /*author*/ return new String[] {"Reference"}; 7089 case 2136596300: /*intendedJurisdiction*/ return new String[] {"CodeableConcept"}; 7090 case 3373707: /*name*/ return new String[] {"string"}; 7091 case 723067972: /*relatedMedicationKnowledge*/ return new String[] {}; 7092 case 1312779381: /*associatedMedication*/ return new String[] {"Reference"}; 7093 case -1491615543: /*productType*/ return new String[] {"CodeableConcept"}; 7094 case -1442980789: /*monograph*/ return new String[] {}; 7095 case 1025456503: /*preparationInstruction*/ return new String[] {"markdown"}; 7096 case 3059661: /*cost*/ return new String[] {}; 7097 case 569848092: /*monitoringProgram*/ return new String[] {}; 7098 case -347044108: /*indicationGuideline*/ return new String[] {}; 7099 case 1791551680: /*medicineClassification*/ return new String[] {}; 7100 case 1802065795: /*packaging*/ return new String[] {}; 7101 case 251885509: /*clinicalUseIssue*/ return new String[] {"Reference"}; 7102 case 1618773173: /*storageGuideline*/ return new String[] {}; 7103 case -27327848: /*regulatory*/ return new String[] {}; 7104 case 101791934: /*definitional*/ return new String[] {}; 7105 default: return super.getTypesForProperty(hash, name); 7106 } 7107 7108 } 7109 7110 @Override 7111 public Base addChild(String name) throws FHIRException { 7112 if (name.equals("identifier")) { 7113 return addIdentifier(); 7114 } 7115 else if (name.equals("code")) { 7116 this.code = new CodeableConcept(); 7117 return this.code; 7118 } 7119 else if (name.equals("status")) { 7120 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.status"); 7121 } 7122 else if (name.equals("author")) { 7123 this.author = new Reference(); 7124 return this.author; 7125 } 7126 else if (name.equals("intendedJurisdiction")) { 7127 return addIntendedJurisdiction(); 7128 } 7129 else if (name.equals("name")) { 7130 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.name"); 7131 } 7132 else if (name.equals("relatedMedicationKnowledge")) { 7133 return addRelatedMedicationKnowledge(); 7134 } 7135 else if (name.equals("associatedMedication")) { 7136 return addAssociatedMedication(); 7137 } 7138 else if (name.equals("productType")) { 7139 return addProductType(); 7140 } 7141 else if (name.equals("monograph")) { 7142 return addMonograph(); 7143 } 7144 else if (name.equals("preparationInstruction")) { 7145 throw new FHIRException("Cannot call addChild on a singleton property MedicationKnowledge.preparationInstruction"); 7146 } 7147 else if (name.equals("cost")) { 7148 return addCost(); 7149 } 7150 else if (name.equals("monitoringProgram")) { 7151 return addMonitoringProgram(); 7152 } 7153 else if (name.equals("indicationGuideline")) { 7154 return addIndicationGuideline(); 7155 } 7156 else if (name.equals("medicineClassification")) { 7157 return addMedicineClassification(); 7158 } 7159 else if (name.equals("packaging")) { 7160 return addPackaging(); 7161 } 7162 else if (name.equals("clinicalUseIssue")) { 7163 return addClinicalUseIssue(); 7164 } 7165 else if (name.equals("storageGuideline")) { 7166 return addStorageGuideline(); 7167 } 7168 else if (name.equals("regulatory")) { 7169 return addRegulatory(); 7170 } 7171 else if (name.equals("definitional")) { 7172 this.definitional = new MedicationKnowledgeDefinitionalComponent(); 7173 return this.definitional; 7174 } 7175 else 7176 return super.addChild(name); 7177 } 7178 7179 public String fhirType() { 7180 return "MedicationKnowledge"; 7181 7182 } 7183 7184 public MedicationKnowledge copy() { 7185 MedicationKnowledge dst = new MedicationKnowledge(); 7186 copyValues(dst); 7187 return dst; 7188 } 7189 7190 public void copyValues(MedicationKnowledge dst) { 7191 super.copyValues(dst); 7192 if (identifier != null) { 7193 dst.identifier = new ArrayList<Identifier>(); 7194 for (Identifier i : identifier) 7195 dst.identifier.add(i.copy()); 7196 }; 7197 dst.code = code == null ? null : code.copy(); 7198 dst.status = status == null ? null : status.copy(); 7199 dst.author = author == null ? null : author.copy(); 7200 if (intendedJurisdiction != null) { 7201 dst.intendedJurisdiction = new ArrayList<CodeableConcept>(); 7202 for (CodeableConcept i : intendedJurisdiction) 7203 dst.intendedJurisdiction.add(i.copy()); 7204 }; 7205 if (name != null) { 7206 dst.name = new ArrayList<StringType>(); 7207 for (StringType i : name) 7208 dst.name.add(i.copy()); 7209 }; 7210 if (relatedMedicationKnowledge != null) { 7211 dst.relatedMedicationKnowledge = new ArrayList<MedicationKnowledgeRelatedMedicationKnowledgeComponent>(); 7212 for (MedicationKnowledgeRelatedMedicationKnowledgeComponent i : relatedMedicationKnowledge) 7213 dst.relatedMedicationKnowledge.add(i.copy()); 7214 }; 7215 if (associatedMedication != null) { 7216 dst.associatedMedication = new ArrayList<Reference>(); 7217 for (Reference i : associatedMedication) 7218 dst.associatedMedication.add(i.copy()); 7219 }; 7220 if (productType != null) { 7221 dst.productType = new ArrayList<CodeableConcept>(); 7222 for (CodeableConcept i : productType) 7223 dst.productType.add(i.copy()); 7224 }; 7225 if (monograph != null) { 7226 dst.monograph = new ArrayList<MedicationKnowledgeMonographComponent>(); 7227 for (MedicationKnowledgeMonographComponent i : monograph) 7228 dst.monograph.add(i.copy()); 7229 }; 7230 dst.preparationInstruction = preparationInstruction == null ? null : preparationInstruction.copy(); 7231 if (cost != null) { 7232 dst.cost = new ArrayList<MedicationKnowledgeCostComponent>(); 7233 for (MedicationKnowledgeCostComponent i : cost) 7234 dst.cost.add(i.copy()); 7235 }; 7236 if (monitoringProgram != null) { 7237 dst.monitoringProgram = new ArrayList<MedicationKnowledgeMonitoringProgramComponent>(); 7238 for (MedicationKnowledgeMonitoringProgramComponent i : monitoringProgram) 7239 dst.monitoringProgram.add(i.copy()); 7240 }; 7241 if (indicationGuideline != null) { 7242 dst.indicationGuideline = new ArrayList<MedicationKnowledgeIndicationGuidelineComponent>(); 7243 for (MedicationKnowledgeIndicationGuidelineComponent i : indicationGuideline) 7244 dst.indicationGuideline.add(i.copy()); 7245 }; 7246 if (medicineClassification != null) { 7247 dst.medicineClassification = new ArrayList<MedicationKnowledgeMedicineClassificationComponent>(); 7248 for (MedicationKnowledgeMedicineClassificationComponent i : medicineClassification) 7249 dst.medicineClassification.add(i.copy()); 7250 }; 7251 if (packaging != null) { 7252 dst.packaging = new ArrayList<MedicationKnowledgePackagingComponent>(); 7253 for (MedicationKnowledgePackagingComponent i : packaging) 7254 dst.packaging.add(i.copy()); 7255 }; 7256 if (clinicalUseIssue != null) { 7257 dst.clinicalUseIssue = new ArrayList<Reference>(); 7258 for (Reference i : clinicalUseIssue) 7259 dst.clinicalUseIssue.add(i.copy()); 7260 }; 7261 if (storageGuideline != null) { 7262 dst.storageGuideline = new ArrayList<MedicationKnowledgeStorageGuidelineComponent>(); 7263 for (MedicationKnowledgeStorageGuidelineComponent i : storageGuideline) 7264 dst.storageGuideline.add(i.copy()); 7265 }; 7266 if (regulatory != null) { 7267 dst.regulatory = new ArrayList<MedicationKnowledgeRegulatoryComponent>(); 7268 for (MedicationKnowledgeRegulatoryComponent i : regulatory) 7269 dst.regulatory.add(i.copy()); 7270 }; 7271 dst.definitional = definitional == null ? null : definitional.copy(); 7272 } 7273 7274 protected MedicationKnowledge typedCopy() { 7275 return copy(); 7276 } 7277 7278 @Override 7279 public boolean equalsDeep(Base other_) { 7280 if (!super.equalsDeep(other_)) 7281 return false; 7282 if (!(other_ instanceof MedicationKnowledge)) 7283 return false; 7284 MedicationKnowledge o = (MedicationKnowledge) other_; 7285 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) && compareDeep(status, o.status, true) 7286 && compareDeep(author, o.author, true) && compareDeep(intendedJurisdiction, o.intendedJurisdiction, true) 7287 && compareDeep(name, o.name, true) && compareDeep(relatedMedicationKnowledge, o.relatedMedicationKnowledge, true) 7288 && compareDeep(associatedMedication, o.associatedMedication, true) && compareDeep(productType, o.productType, true) 7289 && compareDeep(monograph, o.monograph, true) && compareDeep(preparationInstruction, o.preparationInstruction, true) 7290 && compareDeep(cost, o.cost, true) && compareDeep(monitoringProgram, o.monitoringProgram, true) 7291 && compareDeep(indicationGuideline, o.indicationGuideline, true) && compareDeep(medicineClassification, o.medicineClassification, true) 7292 && compareDeep(packaging, o.packaging, true) && compareDeep(clinicalUseIssue, o.clinicalUseIssue, true) 7293 && compareDeep(storageGuideline, o.storageGuideline, true) && compareDeep(regulatory, o.regulatory, true) 7294 && compareDeep(definitional, o.definitional, true); 7295 } 7296 7297 @Override 7298 public boolean equalsShallow(Base other_) { 7299 if (!super.equalsShallow(other_)) 7300 return false; 7301 if (!(other_ instanceof MedicationKnowledge)) 7302 return false; 7303 MedicationKnowledge o = (MedicationKnowledge) other_; 7304 return compareValues(status, o.status, true) && compareValues(name, o.name, true) && compareValues(preparationInstruction, o.preparationInstruction, true) 7305 ; 7306 } 7307 7308 public boolean isEmpty() { 7309 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, status 7310 , author, intendedJurisdiction, name, relatedMedicationKnowledge, associatedMedication 7311 , productType, monograph, preparationInstruction, cost, monitoringProgram, indicationGuideline 7312 , medicineClassification, packaging, clinicalUseIssue, storageGuideline, regulatory 7313 , definitional); 7314 } 7315 7316 @Override 7317 public ResourceType getResourceType() { 7318 return ResourceType.MedicationKnowledge; 7319 } 7320 7321 /** 7322 * Search parameter: <b>identifier</b> 7323 * <p> 7324 * Description: <b>Multiple Resources: 7325 7326* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 7327* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 7328* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 7329* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 7330* [Citation](citation.html): External identifier for the citation 7331* [CodeSystem](codesystem.html): External identifier for the code system 7332* [ConceptMap](conceptmap.html): External identifier for the concept map 7333* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 7334* [EventDefinition](eventdefinition.html): External identifier for the event definition 7335* [Evidence](evidence.html): External identifier for the evidence 7336* [EvidenceReport](evidencereport.html): External identifier for the evidence report 7337* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 7338* [ExampleScenario](examplescenario.html): External identifier for the example scenario 7339* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 7340* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 7341* [Library](library.html): External identifier for the library 7342* [Measure](measure.html): External identifier for the measure 7343* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 7344* [MessageDefinition](messagedefinition.html): External identifier for the message definition 7345* [NamingSystem](namingsystem.html): External identifier for the naming system 7346* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 7347* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 7348* [PlanDefinition](plandefinition.html): External identifier for the plan definition 7349* [Questionnaire](questionnaire.html): External identifier for the questionnaire 7350* [Requirements](requirements.html): External identifier for the requirements 7351* [SearchParameter](searchparameter.html): External identifier for the search parameter 7352* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 7353* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 7354* [StructureMap](structuremap.html): External identifier for the structure map 7355* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 7356* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 7357* [TestPlan](testplan.html): An identifier for the test plan 7358* [TestScript](testscript.html): External identifier for the test script 7359* [ValueSet](valueset.html): External identifier for the value set 7360</b><br> 7361 * Type: <b>token</b><br> 7362 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 7363 * </p> 7364 */ 7365 @SearchParamDefinition(name="identifier", path="ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition\r\n* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition\r\n* [Citation](citation.html): External identifier for the citation\r\n* [CodeSystem](codesystem.html): External identifier for the code system\r\n* [ConceptMap](conceptmap.html): External identifier for the concept map\r\n* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition\r\n* [EventDefinition](eventdefinition.html): External identifier for the event definition\r\n* [Evidence](evidence.html): External identifier for the evidence\r\n* [EvidenceReport](evidencereport.html): External identifier for the evidence report\r\n* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable\r\n* [ExampleScenario](examplescenario.html): External identifier for the example scenario\r\n* [GraphDefinition](graphdefinition.html): External identifier for the graph definition\r\n* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide\r\n* [Library](library.html): External identifier for the library\r\n* [Measure](measure.html): External identifier for the measure\r\n* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication\r\n* [MessageDefinition](messagedefinition.html): External identifier for the message definition\r\n* [NamingSystem](namingsystem.html): External identifier for the naming system\r\n* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition\r\n* [OperationDefinition](operationdefinition.html): External identifier for the search parameter\r\n* [PlanDefinition](plandefinition.html): External identifier for the plan definition\r\n* [Questionnaire](questionnaire.html): External identifier for the questionnaire\r\n* [Requirements](requirements.html): External identifier for the requirements\r\n* [SearchParameter](searchparameter.html): External identifier for the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition\r\n* [StructureDefinition](structuredefinition.html): External identifier for the structure definition\r\n* [StructureMap](structuremap.html): External identifier for the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic\r\n* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities\r\n* [TestPlan](testplan.html): An identifier for the test plan\r\n* [TestScript](testscript.html): External identifier for the test script\r\n* [ValueSet](valueset.html): External identifier for the value set\r\n", type="token" ) 7366 public static final String SP_IDENTIFIER = "identifier"; 7367 /** 7368 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 7369 * <p> 7370 * Description: <b>Multiple Resources: 7371 7372* [ActivityDefinition](activitydefinition.html): External identifier for the activity definition 7373* [ActorDefinition](actordefinition.html): External identifier for the Actor Definition 7374* [CapabilityStatement](capabilitystatement.html): External identifier for the capability statement 7375* [ChargeItemDefinition](chargeitemdefinition.html): External identifier for the charge item definition 7376* [Citation](citation.html): External identifier for the citation 7377* [CodeSystem](codesystem.html): External identifier for the code system 7378* [ConceptMap](conceptmap.html): External identifier for the concept map 7379* [ConditionDefinition](conditiondefinition.html): External identifier for the condition definition 7380* [EventDefinition](eventdefinition.html): External identifier for the event definition 7381* [Evidence](evidence.html): External identifier for the evidence 7382* [EvidenceReport](evidencereport.html): External identifier for the evidence report 7383* [EvidenceVariable](evidencevariable.html): External identifier for the evidence variable 7384* [ExampleScenario](examplescenario.html): External identifier for the example scenario 7385* [GraphDefinition](graphdefinition.html): External identifier for the graph definition 7386* [ImplementationGuide](implementationguide.html): External identifier for the implementation guide 7387* [Library](library.html): External identifier for the library 7388* [Measure](measure.html): External identifier for the measure 7389* [MedicationKnowledge](medicationknowledge.html): Business identifier for this medication 7390* [MessageDefinition](messagedefinition.html): External identifier for the message definition 7391* [NamingSystem](namingsystem.html): External identifier for the naming system 7392* [ObservationDefinition](observationdefinition.html): The unique identifier associated with the specimen definition 7393* [OperationDefinition](operationdefinition.html): External identifier for the search parameter 7394* [PlanDefinition](plandefinition.html): External identifier for the plan definition 7395* [Questionnaire](questionnaire.html): External identifier for the questionnaire 7396* [Requirements](requirements.html): External identifier for the requirements 7397* [SearchParameter](searchparameter.html): External identifier for the search parameter 7398* [SpecimenDefinition](specimendefinition.html): The unique identifier associated with the SpecimenDefinition 7399* [StructureDefinition](structuredefinition.html): External identifier for the structure definition 7400* [StructureMap](structuremap.html): External identifier for the structure map 7401* [SubscriptionTopic](subscriptiontopic.html): Business Identifier for SubscriptionTopic 7402* [TerminologyCapabilities](terminologycapabilities.html): External identifier for the terminology capabilities 7403* [TestPlan](testplan.html): An identifier for the test plan 7404* [TestScript](testscript.html): External identifier for the test script 7405* [ValueSet](valueset.html): External identifier for the value set 7406</b><br> 7407 * Type: <b>token</b><br> 7408 * Path: <b>ActivityDefinition.identifier | ActorDefinition.identifier | CapabilityStatement.identifier | ChargeItemDefinition.identifier | Citation.identifier | CodeSystem.identifier | ConceptMap.identifier | ConditionDefinition.identifier | EventDefinition.identifier | Evidence.identifier | EvidenceReport.identifier | EvidenceVariable.identifier | ExampleScenario.identifier | GraphDefinition.identifier | ImplementationGuide.identifier | Library.identifier | Measure.identifier | MedicationKnowledge.identifier | MessageDefinition.identifier | NamingSystem.identifier | ObservationDefinition.identifier | OperationDefinition.identifier | PlanDefinition.identifier | Questionnaire.identifier | Requirements.identifier | SearchParameter.identifier | SpecimenDefinition.identifier | StructureDefinition.identifier | StructureMap.identifier | SubscriptionTopic.identifier | TerminologyCapabilities.identifier | TestPlan.identifier | TestScript.identifier | ValueSet.identifier</b><br> 7409 * </p> 7410 */ 7411 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 7412 7413 /** 7414 * Search parameter: <b>status</b> 7415 * <p> 7416 * Description: <b>Multiple Resources: 7417 7418* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 7419* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 7420* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 7421* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 7422* [Citation](citation.html): The current status of the citation 7423* [CodeSystem](codesystem.html): The current status of the code system 7424* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 7425* [ConceptMap](conceptmap.html): The current status of the concept map 7426* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 7427* [EventDefinition](eventdefinition.html): The current status of the event definition 7428* [Evidence](evidence.html): The current status of the evidence 7429* [EvidenceReport](evidencereport.html): The current status of the evidence report 7430* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 7431* [ExampleScenario](examplescenario.html): The current status of the example scenario 7432* [GraphDefinition](graphdefinition.html): The current status of the graph definition 7433* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 7434* [Library](library.html): The current status of the library 7435* [Measure](measure.html): The current status of the measure 7436* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 7437* [MessageDefinition](messagedefinition.html): The current status of the message definition 7438* [NamingSystem](namingsystem.html): The current status of the naming system 7439* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 7440* [OperationDefinition](operationdefinition.html): The current status of the operation definition 7441* [PlanDefinition](plandefinition.html): The current status of the plan definition 7442* [Questionnaire](questionnaire.html): The current status of the questionnaire 7443* [Requirements](requirements.html): The current status of the requirements 7444* [SearchParameter](searchparameter.html): The current status of the search parameter 7445* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 7446* [StructureDefinition](structuredefinition.html): The current status of the structure definition 7447* [StructureMap](structuremap.html): The current status of the structure map 7448* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 7449* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 7450* [TestPlan](testplan.html): The current status of the test plan 7451* [TestScript](testscript.html): The current status of the test script 7452* [ValueSet](valueset.html): The current status of the value set 7453</b><br> 7454 * Type: <b>token</b><br> 7455 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 7456 * </p> 7457 */ 7458 @SearchParamDefinition(name="status", path="ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status", description="Multiple Resources: \r\n\r\n* [ActivityDefinition](activitydefinition.html): The current status of the activity definition\r\n* [ActorDefinition](actordefinition.html): The current status of the Actor Definition\r\n* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement\r\n* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition\r\n* [Citation](citation.html): The current status of the citation\r\n* [CodeSystem](codesystem.html): The current status of the code system\r\n* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition\r\n* [ConceptMap](conceptmap.html): The current status of the concept map\r\n* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition\r\n* [EventDefinition](eventdefinition.html): The current status of the event definition\r\n* [Evidence](evidence.html): The current status of the evidence\r\n* [EvidenceReport](evidencereport.html): The current status of the evidence report\r\n* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable\r\n* [ExampleScenario](examplescenario.html): The current status of the example scenario\r\n* [GraphDefinition](graphdefinition.html): The current status of the graph definition\r\n* [ImplementationGuide](implementationguide.html): The current status of the implementation guide\r\n* [Library](library.html): The current status of the library\r\n* [Measure](measure.html): The current status of the measure\r\n* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error\r\n* [MessageDefinition](messagedefinition.html): The current status of the message definition\r\n* [NamingSystem](namingsystem.html): The current status of the naming system\r\n* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown\r\n* [OperationDefinition](operationdefinition.html): The current status of the operation definition\r\n* [PlanDefinition](plandefinition.html): The current status of the plan definition\r\n* [Questionnaire](questionnaire.html): The current status of the questionnaire\r\n* [Requirements](requirements.html): The current status of the requirements\r\n* [SearchParameter](searchparameter.html): The current status of the search parameter\r\n* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown\r\n* [StructureDefinition](structuredefinition.html): The current status of the structure definition\r\n* [StructureMap](structuremap.html): The current status of the structure map\r\n* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown\r\n* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities\r\n* [TestPlan](testplan.html): The current status of the test plan\r\n* [TestScript](testscript.html): The current status of the test script\r\n* [ValueSet](valueset.html): The current status of the value set\r\n", type="token" ) 7459 public static final String SP_STATUS = "status"; 7460 /** 7461 * <b>Fluent Client</b> search parameter constant for <b>status</b> 7462 * <p> 7463 * Description: <b>Multiple Resources: 7464 7465* [ActivityDefinition](activitydefinition.html): The current status of the activity definition 7466* [ActorDefinition](actordefinition.html): The current status of the Actor Definition 7467* [CapabilityStatement](capabilitystatement.html): The current status of the capability statement 7468* [ChargeItemDefinition](chargeitemdefinition.html): The current status of the charge item definition 7469* [Citation](citation.html): The current status of the citation 7470* [CodeSystem](codesystem.html): The current status of the code system 7471* [CompartmentDefinition](compartmentdefinition.html): The current status of the compartment definition 7472* [ConceptMap](conceptmap.html): The current status of the concept map 7473* [ConditionDefinition](conditiondefinition.html): The current status of the condition definition 7474* [EventDefinition](eventdefinition.html): The current status of the event definition 7475* [Evidence](evidence.html): The current status of the evidence 7476* [EvidenceReport](evidencereport.html): The current status of the evidence report 7477* [EvidenceVariable](evidencevariable.html): The current status of the evidence variable 7478* [ExampleScenario](examplescenario.html): The current status of the example scenario 7479* [GraphDefinition](graphdefinition.html): The current status of the graph definition 7480* [ImplementationGuide](implementationguide.html): The current status of the implementation guide 7481* [Library](library.html): The current status of the library 7482* [Measure](measure.html): The current status of the measure 7483* [MedicationKnowledge](medicationknowledge.html): active | inactive | entered-in-error 7484* [MessageDefinition](messagedefinition.html): The current status of the message definition 7485* [NamingSystem](namingsystem.html): The current status of the naming system 7486* [ObservationDefinition](observationdefinition.html): Publication status of the ObservationDefinition: draft, active, retired, unknown 7487* [OperationDefinition](operationdefinition.html): The current status of the operation definition 7488* [PlanDefinition](plandefinition.html): The current status of the plan definition 7489* [Questionnaire](questionnaire.html): The current status of the questionnaire 7490* [Requirements](requirements.html): The current status of the requirements 7491* [SearchParameter](searchparameter.html): The current status of the search parameter 7492* [SpecimenDefinition](specimendefinition.html): Publication status of the SpecimenDefinition: draft, active, retired, unknown 7493* [StructureDefinition](structuredefinition.html): The current status of the structure definition 7494* [StructureMap](structuremap.html): The current status of the structure map 7495* [SubscriptionTopic](subscriptiontopic.html): draft | active | retired | unknown 7496* [TerminologyCapabilities](terminologycapabilities.html): The current status of the terminology capabilities 7497* [TestPlan](testplan.html): The current status of the test plan 7498* [TestScript](testscript.html): The current status of the test script 7499* [ValueSet](valueset.html): The current status of the value set 7500</b><br> 7501 * Type: <b>token</b><br> 7502 * Path: <b>ActivityDefinition.status | ActorDefinition.status | CapabilityStatement.status | ChargeItemDefinition.status | Citation.status | CodeSystem.status | CompartmentDefinition.status | ConceptMap.status | ConditionDefinition.status | EventDefinition.status | Evidence.status | EvidenceReport.status | EvidenceVariable.status | ExampleScenario.status | GraphDefinition.status | ImplementationGuide.status | Library.status | Measure.status | MedicationKnowledge.status | MessageDefinition.status | NamingSystem.status | ObservationDefinition.status | OperationDefinition.status | PlanDefinition.status | Questionnaire.status | Requirements.status | SearchParameter.status | SpecimenDefinition.status | StructureDefinition.status | StructureMap.status | SubscriptionTopic.status | TerminologyCapabilities.status | TestPlan.status | TestScript.status | ValueSet.status</b><br> 7503 * </p> 7504 */ 7505 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 7506 7507 /** 7508 * Search parameter: <b>classification-type</b> 7509 * <p> 7510 * Description: <b>The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification)</b><br> 7511 * Type: <b>token</b><br> 7512 * Path: <b>MedicationKnowledge.medicineClassification.type</b><br> 7513 * </p> 7514 */ 7515 @SearchParamDefinition(name="classification-type", path="MedicationKnowledge.medicineClassification.type", description="The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification)", type="token" ) 7516 public static final String SP_CLASSIFICATION_TYPE = "classification-type"; 7517 /** 7518 * <b>Fluent Client</b> search parameter constant for <b>classification-type</b> 7519 * <p> 7520 * Description: <b>The type of category for the medication (for example, therapeutic classification, therapeutic sub-classification)</b><br> 7521 * Type: <b>token</b><br> 7522 * Path: <b>MedicationKnowledge.medicineClassification.type</b><br> 7523 * </p> 7524 */ 7525 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASSIFICATION_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLASSIFICATION_TYPE); 7526 7527 /** 7528 * Search parameter: <b>classification</b> 7529 * <p> 7530 * Description: <b>Specific category assigned to the medication</b><br> 7531 * Type: <b>token</b><br> 7532 * Path: <b>MedicationKnowledge.medicineClassification.classification</b><br> 7533 * </p> 7534 */ 7535 @SearchParamDefinition(name="classification", path="MedicationKnowledge.medicineClassification.classification", description="Specific category assigned to the medication", type="token" ) 7536 public static final String SP_CLASSIFICATION = "classification"; 7537 /** 7538 * <b>Fluent Client</b> search parameter constant for <b>classification</b> 7539 * <p> 7540 * Description: <b>Specific category assigned to the medication</b><br> 7541 * Type: <b>token</b><br> 7542 * Path: <b>MedicationKnowledge.medicineClassification.classification</b><br> 7543 * </p> 7544 */ 7545 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASSIFICATION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLASSIFICATION); 7546 7547 /** 7548 * Search parameter: <b>code</b> 7549 * <p> 7550 * Description: <b>Code that identifies this medication</b><br> 7551 * Type: <b>token</b><br> 7552 * Path: <b>MedicationKnowledge.code</b><br> 7553 * </p> 7554 */ 7555 @SearchParamDefinition(name="code", path="MedicationKnowledge.code", description="Code that identifies this medication", type="token" ) 7556 public static final String SP_CODE = "code"; 7557 /** 7558 * <b>Fluent Client</b> search parameter constant for <b>code</b> 7559 * <p> 7560 * Description: <b>Code that identifies this medication</b><br> 7561 * Type: <b>token</b><br> 7562 * Path: <b>MedicationKnowledge.code</b><br> 7563 * </p> 7564 */ 7565 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 7566 7567 /** 7568 * Search parameter: <b>doseform</b> 7569 * <p> 7570 * Description: <b>powder | tablets | capsule +</b><br> 7571 * Type: <b>token</b><br> 7572 * Path: <b>MedicationKnowledge.definitional.doseForm</b><br> 7573 * </p> 7574 */ 7575 @SearchParamDefinition(name="doseform", path="MedicationKnowledge.definitional.doseForm", description="powder | tablets | capsule +", type="token" ) 7576 public static final String SP_DOSEFORM = "doseform"; 7577 /** 7578 * <b>Fluent Client</b> search parameter constant for <b>doseform</b> 7579 * <p> 7580 * Description: <b>powder | tablets | capsule +</b><br> 7581 * Type: <b>token</b><br> 7582 * Path: <b>MedicationKnowledge.definitional.doseForm</b><br> 7583 * </p> 7584 */ 7585 public static final ca.uhn.fhir.rest.gclient.TokenClientParam DOSEFORM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_DOSEFORM); 7586 7587 /** 7588 * Search parameter: <b>ingredient-code</b> 7589 * <p> 7590 * Description: <b>Reference to a concept (by class)</b><br> 7591 * Type: <b>token</b><br> 7592 * Path: <b>MedicationKnowledge.definitional.ingredient.item.concept</b><br> 7593 * </p> 7594 */ 7595 @SearchParamDefinition(name="ingredient-code", path="MedicationKnowledge.definitional.ingredient.item.concept", description="Reference to a concept (by class)", type="token" ) 7596 public static final String SP_INGREDIENT_CODE = "ingredient-code"; 7597 /** 7598 * <b>Fluent Client</b> search parameter constant for <b>ingredient-code</b> 7599 * <p> 7600 * Description: <b>Reference to a concept (by class)</b><br> 7601 * Type: <b>token</b><br> 7602 * Path: <b>MedicationKnowledge.definitional.ingredient.item.concept</b><br> 7603 * </p> 7604 */ 7605 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INGREDIENT_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INGREDIENT_CODE); 7606 7607 /** 7608 * Search parameter: <b>ingredient</b> 7609 * <p> 7610 * Description: <b>Reference to a resource (by instance)</b><br> 7611 * Type: <b>reference</b><br> 7612 * Path: <b>MedicationKnowledge.definitional.ingredient.item.reference</b><br> 7613 * </p> 7614 */ 7615 @SearchParamDefinition(name="ingredient", path="MedicationKnowledge.definitional.ingredient.item.reference", description="Reference to a resource (by instance)", type="reference", target={Substance.class } ) 7616 public static final String SP_INGREDIENT = "ingredient"; 7617 /** 7618 * <b>Fluent Client</b> search parameter constant for <b>ingredient</b> 7619 * <p> 7620 * Description: <b>Reference to a resource (by instance)</b><br> 7621 * Type: <b>reference</b><br> 7622 * Path: <b>MedicationKnowledge.definitional.ingredient.item.reference</b><br> 7623 * </p> 7624 */ 7625 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INGREDIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INGREDIENT); 7626 7627/** 7628 * Constant for fluent queries to be used to add include statements. Specifies 7629 * the path value of "<b>MedicationKnowledge:ingredient</b>". 7630 */ 7631 public static final ca.uhn.fhir.model.api.Include INCLUDE_INGREDIENT = new ca.uhn.fhir.model.api.Include("MedicationKnowledge:ingredient").toLocked(); 7632 7633 /** 7634 * Search parameter: <b>monitoring-program-name</b> 7635 * <p> 7636 * Description: <b>Name of the reviewing program</b><br> 7637 * Type: <b>token</b><br> 7638 * Path: <b>MedicationKnowledge.monitoringProgram.name</b><br> 7639 * </p> 7640 */ 7641 @SearchParamDefinition(name="monitoring-program-name", path="MedicationKnowledge.monitoringProgram.name", description="Name of the reviewing program", type="token" ) 7642 public static final String SP_MONITORING_PROGRAM_NAME = "monitoring-program-name"; 7643 /** 7644 * <b>Fluent Client</b> search parameter constant for <b>monitoring-program-name</b> 7645 * <p> 7646 * Description: <b>Name of the reviewing program</b><br> 7647 * Type: <b>token</b><br> 7648 * Path: <b>MedicationKnowledge.monitoringProgram.name</b><br> 7649 * </p> 7650 */ 7651 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MONITORING_PROGRAM_NAME = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MONITORING_PROGRAM_NAME); 7652 7653 /** 7654 * Search parameter: <b>monitoring-program-type</b> 7655 * <p> 7656 * Description: <b>Type of program under which the medication is monitored</b><br> 7657 * Type: <b>token</b><br> 7658 * Path: <b>MedicationKnowledge.monitoringProgram.type</b><br> 7659 * </p> 7660 */ 7661 @SearchParamDefinition(name="monitoring-program-type", path="MedicationKnowledge.monitoringProgram.type", description="Type of program under which the medication is monitored", type="token" ) 7662 public static final String SP_MONITORING_PROGRAM_TYPE = "monitoring-program-type"; 7663 /** 7664 * <b>Fluent Client</b> search parameter constant for <b>monitoring-program-type</b> 7665 * <p> 7666 * Description: <b>Type of program under which the medication is monitored</b><br> 7667 * Type: <b>token</b><br> 7668 * Path: <b>MedicationKnowledge.monitoringProgram.type</b><br> 7669 * </p> 7670 */ 7671 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MONITORING_PROGRAM_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MONITORING_PROGRAM_TYPE); 7672 7673 /** 7674 * Search parameter: <b>monograph-type</b> 7675 * <p> 7676 * Description: <b>The category of medication document</b><br> 7677 * Type: <b>token</b><br> 7678 * Path: <b>MedicationKnowledge.monograph.type</b><br> 7679 * </p> 7680 */ 7681 @SearchParamDefinition(name="monograph-type", path="MedicationKnowledge.monograph.type", description="The category of medication document", type="token" ) 7682 public static final String SP_MONOGRAPH_TYPE = "monograph-type"; 7683 /** 7684 * <b>Fluent Client</b> search parameter constant for <b>monograph-type</b> 7685 * <p> 7686 * Description: <b>The category of medication document</b><br> 7687 * Type: <b>token</b><br> 7688 * Path: <b>MedicationKnowledge.monograph.type</b><br> 7689 * </p> 7690 */ 7691 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MONOGRAPH_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MONOGRAPH_TYPE); 7692 7693 /** 7694 * Search parameter: <b>monograph</b> 7695 * <p> 7696 * Description: <b>Associated documentation about the medication</b><br> 7697 * Type: <b>reference</b><br> 7698 * Path: <b>MedicationKnowledge.monograph.source</b><br> 7699 * </p> 7700 */ 7701 @SearchParamDefinition(name="monograph", path="MedicationKnowledge.monograph.source", description="Associated documentation about the medication", type="reference", target={DocumentReference.class } ) 7702 public static final String SP_MONOGRAPH = "monograph"; 7703 /** 7704 * <b>Fluent Client</b> search parameter constant for <b>monograph</b> 7705 * <p> 7706 * Description: <b>Associated documentation about the medication</b><br> 7707 * Type: <b>reference</b><br> 7708 * Path: <b>MedicationKnowledge.monograph.source</b><br> 7709 * </p> 7710 */ 7711 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MONOGRAPH = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MONOGRAPH); 7712 7713/** 7714 * Constant for fluent queries to be used to add include statements. Specifies 7715 * the path value of "<b>MedicationKnowledge:monograph</b>". 7716 */ 7717 public static final ca.uhn.fhir.model.api.Include INCLUDE_MONOGRAPH = new ca.uhn.fhir.model.api.Include("MedicationKnowledge:monograph").toLocked(); 7718 7719 /** 7720 * Search parameter: <b>packaging-cost-concept</b> 7721 * <p> 7722 * Description: <b>The cost of the packaged medication, if the cost is a CodeableConcept</b><br> 7723 * Type: <b>token</b><br> 7724 * Path: <b>null</b><br> 7725 * </p> 7726 */ 7727 @SearchParamDefinition(name="packaging-cost-concept", path="", description="The cost of the packaged medication, if the cost is a CodeableConcept", type="token" ) 7728 public static final String SP_PACKAGING_COST_CONCEPT = "packaging-cost-concept"; 7729 /** 7730 * <b>Fluent Client</b> search parameter constant for <b>packaging-cost-concept</b> 7731 * <p> 7732 * Description: <b>The cost of the packaged medication, if the cost is a CodeableConcept</b><br> 7733 * Type: <b>token</b><br> 7734 * Path: <b>null</b><br> 7735 * </p> 7736 */ 7737 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PACKAGING_COST_CONCEPT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PACKAGING_COST_CONCEPT); 7738 7739 /** 7740 * Search parameter: <b>packaging-cost</b> 7741 * <p> 7742 * Description: <b>The cost of the packaged medication, if the cost is Money</b><br> 7743 * Type: <b>quantity</b><br> 7744 * Path: <b>null</b><br> 7745 * </p> 7746 */ 7747 @SearchParamDefinition(name="packaging-cost", path="", description="The cost of the packaged medication, if the cost is Money", type="quantity" ) 7748 public static final String SP_PACKAGING_COST = "packaging-cost"; 7749 /** 7750 * <b>Fluent Client</b> search parameter constant for <b>packaging-cost</b> 7751 * <p> 7752 * Description: <b>The cost of the packaged medication, if the cost is Money</b><br> 7753 * Type: <b>quantity</b><br> 7754 * Path: <b>null</b><br> 7755 * </p> 7756 */ 7757 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam PACKAGING_COST = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_PACKAGING_COST); 7758 7759 /** 7760 * Search parameter: <b>product-type</b> 7761 * <p> 7762 * Description: <b>Category of the medication or product</b><br> 7763 * Type: <b>token</b><br> 7764 * Path: <b>MedicationKnowledge.productType</b><br> 7765 * </p> 7766 */ 7767 @SearchParamDefinition(name="product-type", path="MedicationKnowledge.productType", description="Category of the medication or product", type="token" ) 7768 public static final String SP_PRODUCT_TYPE = "product-type"; 7769 /** 7770 * <b>Fluent Client</b> search parameter constant for <b>product-type</b> 7771 * <p> 7772 * Description: <b>Category of the medication or product</b><br> 7773 * Type: <b>token</b><br> 7774 * Path: <b>MedicationKnowledge.productType</b><br> 7775 * </p> 7776 */ 7777 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRODUCT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRODUCT_TYPE); 7778 7779 /** 7780 * Search parameter: <b>source-cost</b> 7781 * <p> 7782 * Description: <b>The source or owner for the price information</b><br> 7783 * Type: <b>token</b><br> 7784 * Path: <b>MedicationKnowledge.cost.source</b><br> 7785 * </p> 7786 */ 7787 @SearchParamDefinition(name="source-cost", path="MedicationKnowledge.cost.source", description="The source or owner for the price information", type="token" ) 7788 public static final String SP_SOURCE_COST = "source-cost"; 7789 /** 7790 * <b>Fluent Client</b> search parameter constant for <b>source-cost</b> 7791 * <p> 7792 * Description: <b>The source or owner for the price information</b><br> 7793 * Type: <b>token</b><br> 7794 * Path: <b>MedicationKnowledge.cost.source</b><br> 7795 * </p> 7796 */ 7797 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SOURCE_COST = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SOURCE_COST); 7798 7799 7800} 7801